title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
simple | check-if-all-the-integers-in-a-range-are-covered | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isCovered(self, ranges, left: int, right: int) -> bool:\n lst = []\n for item in ranges:\n inlst = [i for i in range(item[0], item[1] +1)]\n lst+=inlst\n\n new = [i for i in range(left, right+1)]\n\n for i in new:\n if i not in lst:\n return False\n return True\n``` | 0 | You are given a 2D integer array `ranges` and two integers `left` and `right`. Each `ranges[i] = [starti, endi]` represents an **inclusive** interval between `starti` and `endi`.
Return `true` _if each integer in the inclusive range_ `[left, right]` _is covered by **at least one** interval in_ `ranges`. Return `false` _otherwise_.
An integer `x` is covered by an interval `ranges[i] = [starti, endi]` if `starti <= x <= endi`.
**Example 1:**
**Input:** ranges = \[\[1,2\],\[3,4\],\[5,6\]\], left = 2, right = 5
**Output:** true
**Explanation:** Every integer between 2 and 5 is covered:
- 2 is covered by the first range.
- 3 and 4 are covered by the second range.
- 5 is covered by the third range.
**Example 2:**
**Input:** ranges = \[\[1,10\],\[10,20\]\], left = 21, right = 21
**Output:** false
**Explanation:** 21 is not covered by any range.
**Constraints:**
* `1 <= ranges.length <= 50`
* `1 <= starti <= endi <= 50`
* `1 <= left <= right <= 50` | Think about dynamic programming Define an array dp[nums.length][2], where dp[i][0] is the max subarray sum including nums[i] and without squaring any element. dp[i][1] is the max subarray sum including nums[i] and having only one element squared. |
🐍🐍🐍 One line solution 🐍🐍🐍 | check-if-all-the-integers-in-a-range-are-covered | 0 | 1 | # Code\n```\nclass Solution:\n def isCovered(self, ranges: List[List[int]], left: int, right: int) -> bool:\n return len(set([i for i in range(left, right +1)]) - set([i for x in ranges for i in range(x[0],x[1]+1)])) == 0\n``` | 0 | You are given a 2D integer array `ranges` and two integers `left` and `right`. Each `ranges[i] = [starti, endi]` represents an **inclusive** interval between `starti` and `endi`.
Return `true` _if each integer in the inclusive range_ `[left, right]` _is covered by **at least one** interval in_ `ranges`. Return `false` _otherwise_.
An integer `x` is covered by an interval `ranges[i] = [starti, endi]` if `starti <= x <= endi`.
**Example 1:**
**Input:** ranges = \[\[1,2\],\[3,4\],\[5,6\]\], left = 2, right = 5
**Output:** true
**Explanation:** Every integer between 2 and 5 is covered:
- 2 is covered by the first range.
- 3 and 4 are covered by the second range.
- 5 is covered by the third range.
**Example 2:**
**Input:** ranges = \[\[1,10\],\[10,20\]\], left = 21, right = 21
**Output:** false
**Explanation:** 21 is not covered by any range.
**Constraints:**
* `1 <= ranges.length <= 50`
* `1 <= starti <= endi <= 50`
* `1 <= left <= right <= 50` | Think about dynamic programming Define an array dp[nums.length][2], where dp[i][0] is the max subarray sum including nums[i] and without squaring any element. dp[i][1] is the max subarray sum including nums[i] and having only one element squared. |
python solution | check-if-all-the-integers-in-a-range-are-covered | 0 | 1 | \n\n# Complexity\n- Time complexity:\nO(N*M)\n\n\n# Code\n```\nclass Solution:\n def isCovered(self, ranges: List[List[int]], left: int, right: int) -> bool:\n range_list = []\n for i in range(len(ranges)):\n for j in range(ranges[i][0], ranges[i][1] + 1):\n range_list.append(j)\n r = [i for i in range(left, right + 1)] \n res = [False] * len(r)\n for i in range(len(r)):\n if r[i] in range_list:\n res[i] = True\n return all(res)\n\n \n``` | 0 | You are given a 2D integer array `ranges` and two integers `left` and `right`. Each `ranges[i] = [starti, endi]` represents an **inclusive** interval between `starti` and `endi`.
Return `true` _if each integer in the inclusive range_ `[left, right]` _is covered by **at least one** interval in_ `ranges`. Return `false` _otherwise_.
An integer `x` is covered by an interval `ranges[i] = [starti, endi]` if `starti <= x <= endi`.
**Example 1:**
**Input:** ranges = \[\[1,2\],\[3,4\],\[5,6\]\], left = 2, right = 5
**Output:** true
**Explanation:** Every integer between 2 and 5 is covered:
- 2 is covered by the first range.
- 3 and 4 are covered by the second range.
- 5 is covered by the third range.
**Example 2:**
**Input:** ranges = \[\[1,10\],\[10,20\]\], left = 21, right = 21
**Output:** false
**Explanation:** 21 is not covered by any range.
**Constraints:**
* `1 <= ranges.length <= 50`
* `1 <= starti <= endi <= 50`
* `1 <= left <= right <= 50` | Think about dynamic programming Define an array dp[nums.length][2], where dp[i][0] is the max subarray sum including nums[i] and without squaring any element. dp[i][1] is the max subarray sum including nums[i] and having only one element squared. |
Easy Solution | Java | Python | find-the-student-that-will-replace-the-chalk | 1 | 1 | # Python\n```\nclass Solution:\n def chalkReplacer(self, chalk: List[int], k: int) -> int:\n sums = sum(chalk)\n div = k // sums\n k = k - (sums * div)\n for i in range(len(chalk)):\n if chalk[i] <= k:\n k -= chalk[i]\n else:\n return i\n return -1\n```\n# Java\n```\nclass Solution {\n public int chalkReplacer(int[] chalk, int k) {\n long sum = 0;\n for(int x : chalk) {\n sum += x;\n }\n long div = k / sum;\n k -= (sum * div);\n for(int i=0; i<chalk.length; i++) {\n if(chalk[i] <= k) {\n k -= chalk[i];\n }\n else {\n return i;\n }\n }\n return -1;\n }\n}\n```\nDo upvote if you like the Solution :) | 2 | There are `n` students in a class numbered from `0` to `n - 1`. The teacher will give each student a problem starting with the student number `0`, then the student number `1`, and so on until the teacher reaches the student number `n - 1`. After that, the teacher will restart the process, starting with the student number `0` again.
You are given a **0-indexed** integer array `chalk` and an integer `k`. There are initially `k` pieces of chalk. When the student number `i` is given a problem to solve, they will use `chalk[i]` pieces of chalk to solve that problem. However, if the current number of chalk pieces is **strictly less** than `chalk[i]`, then the student number `i` will be asked to **replace** the chalk.
Return _the **index** of the student that will **replace** the chalk pieces_.
**Example 1:**
**Input:** chalk = \[5,1,5\], k = 22
**Output:** 0
**Explanation:** The students go in turns as follows:
- Student number 0 uses 5 chalk, so k = 17.
- Student number 1 uses 1 chalk, so k = 16.
- Student number 2 uses 5 chalk, so k = 11.
- Student number 0 uses 5 chalk, so k = 6.
- Student number 1 uses 1 chalk, so k = 5.
- Student number 2 uses 5 chalk, so k = 0.
Student number 0 does not have enough chalk, so they will have to replace it.
**Example 2:**
**Input:** chalk = \[3,4,1,2\], k = 25
**Output:** 1
**Explanation:** The students go in turns as follows:
- Student number 0 uses 3 chalk so k = 22.
- Student number 1 uses 4 chalk so k = 18.
- Student number 2 uses 1 chalk so k = 17.
- Student number 3 uses 2 chalk so k = 15.
- Student number 0 uses 3 chalk so k = 12.
- Student number 1 uses 4 chalk so k = 8.
- Student number 2 uses 1 chalk so k = 7.
- Student number 3 uses 2 chalk so k = 5.
- Student number 0 uses 3 chalk so k = 2.
Student number 1 does not have enough chalk, so they will have to replace it.
**Constraints:**
* `chalk.length == n`
* `1 <= n <= 105`
* `1 <= chalk[i] <= 105`
* `1 <= k <= 109` | Use two pointers, one pointer for each string. Alternately choose the character from each pointer, and move the pointer upwards. |
[Python3] Prefix Sum + Binary Search - Easy to Understand | find-the-student-that-will-replace-the-chalk | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: $$O(n + logn) ~ O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def chalkReplacer(self, chalk: List[int], k: int) -> int:\n ps = [0]\n for c in chalk:\n ps.append(ps[-1] + c)\n\n k %= ps[-1]\n l, r = 0, len(chalk) - 1\n while l <= r:\n m = (l + r) >> 1\n if ps[m + 1] > k:\n r = m - 1\n else:\n l = m + 1\n return l\n``` | 2 | There are `n` students in a class numbered from `0` to `n - 1`. The teacher will give each student a problem starting with the student number `0`, then the student number `1`, and so on until the teacher reaches the student number `n - 1`. After that, the teacher will restart the process, starting with the student number `0` again.
You are given a **0-indexed** integer array `chalk` and an integer `k`. There are initially `k` pieces of chalk. When the student number `i` is given a problem to solve, they will use `chalk[i]` pieces of chalk to solve that problem. However, if the current number of chalk pieces is **strictly less** than `chalk[i]`, then the student number `i` will be asked to **replace** the chalk.
Return _the **index** of the student that will **replace** the chalk pieces_.
**Example 1:**
**Input:** chalk = \[5,1,5\], k = 22
**Output:** 0
**Explanation:** The students go in turns as follows:
- Student number 0 uses 5 chalk, so k = 17.
- Student number 1 uses 1 chalk, so k = 16.
- Student number 2 uses 5 chalk, so k = 11.
- Student number 0 uses 5 chalk, so k = 6.
- Student number 1 uses 1 chalk, so k = 5.
- Student number 2 uses 5 chalk, so k = 0.
Student number 0 does not have enough chalk, so they will have to replace it.
**Example 2:**
**Input:** chalk = \[3,4,1,2\], k = 25
**Output:** 1
**Explanation:** The students go in turns as follows:
- Student number 0 uses 3 chalk so k = 22.
- Student number 1 uses 4 chalk so k = 18.
- Student number 2 uses 1 chalk so k = 17.
- Student number 3 uses 2 chalk so k = 15.
- Student number 0 uses 3 chalk so k = 12.
- Student number 1 uses 4 chalk so k = 8.
- Student number 2 uses 1 chalk so k = 7.
- Student number 3 uses 2 chalk so k = 5.
- Student number 0 uses 3 chalk so k = 2.
Student number 1 does not have enough chalk, so they will have to replace it.
**Constraints:**
* `chalk.length == n`
* `1 <= n <= 105`
* `1 <= chalk[i] <= 105`
* `1 <= k <= 109` | Use two pointers, one pointer for each string. Alternately choose the character from each pointer, and move the pointer upwards. |
Python || Prefix Sum and Binary Search || O(n) time O(n) space | find-the-student-that-will-replace-the-chalk | 0 | 1 | ```\nclass Solution:\n def chalkReplacer(self, chalk: List[int], k: int) -> int:\n prefix_sum = [0 for i in range(len(chalk))]\n prefix_sum[0] = chalk[0]\n for i in range(1,len(chalk)):\n prefix_sum[i] = prefix_sum[i-1] + chalk[i]\n remainder = k % prefix_sum[-1]\n \n #apply binary search on prefix_sum array, target = remainder \n start = 0\n end = len(prefix_sum) - 1\n while start <= end:\n mid = start + (end - start) // 2\n if remainder == prefix_sum[mid]:\n return mid + 1\n elif remainder < prefix_sum[mid]:\n end = mid - 1\n else:\n start = mid + 1\n return start \n``` | 6 | There are `n` students in a class numbered from `0` to `n - 1`. The teacher will give each student a problem starting with the student number `0`, then the student number `1`, and so on until the teacher reaches the student number `n - 1`. After that, the teacher will restart the process, starting with the student number `0` again.
You are given a **0-indexed** integer array `chalk` and an integer `k`. There are initially `k` pieces of chalk. When the student number `i` is given a problem to solve, they will use `chalk[i]` pieces of chalk to solve that problem. However, if the current number of chalk pieces is **strictly less** than `chalk[i]`, then the student number `i` will be asked to **replace** the chalk.
Return _the **index** of the student that will **replace** the chalk pieces_.
**Example 1:**
**Input:** chalk = \[5,1,5\], k = 22
**Output:** 0
**Explanation:** The students go in turns as follows:
- Student number 0 uses 5 chalk, so k = 17.
- Student number 1 uses 1 chalk, so k = 16.
- Student number 2 uses 5 chalk, so k = 11.
- Student number 0 uses 5 chalk, so k = 6.
- Student number 1 uses 1 chalk, so k = 5.
- Student number 2 uses 5 chalk, so k = 0.
Student number 0 does not have enough chalk, so they will have to replace it.
**Example 2:**
**Input:** chalk = \[3,4,1,2\], k = 25
**Output:** 1
**Explanation:** The students go in turns as follows:
- Student number 0 uses 3 chalk so k = 22.
- Student number 1 uses 4 chalk so k = 18.
- Student number 2 uses 1 chalk so k = 17.
- Student number 3 uses 2 chalk so k = 15.
- Student number 0 uses 3 chalk so k = 12.
- Student number 1 uses 4 chalk so k = 8.
- Student number 2 uses 1 chalk so k = 7.
- Student number 3 uses 2 chalk so k = 5.
- Student number 0 uses 3 chalk so k = 2.
Student number 1 does not have enough chalk, so they will have to replace it.
**Constraints:**
* `chalk.length == n`
* `1 <= n <= 105`
* `1 <= chalk[i] <= 105`
* `1 <= k <= 109` | Use two pointers, one pointer for each string. Alternately choose the character from each pointer, and move the pointer upwards. |
Python Easy Solution | find-the-student-that-will-replace-the-chalk | 0 | 1 | ```\ndef chalkReplacer(self, chalk: List[int], k: int) -> int:\n\n\tk %= sum(chalk)\n\n\tfor i in range(len(chalk)):\n\t\tif chalk[i]>k:\n\t\t\treturn i\n\t\tk -= chalk[i]\n```\n | 4 | There are `n` students in a class numbered from `0` to `n - 1`. The teacher will give each student a problem starting with the student number `0`, then the student number `1`, and so on until the teacher reaches the student number `n - 1`. After that, the teacher will restart the process, starting with the student number `0` again.
You are given a **0-indexed** integer array `chalk` and an integer `k`. There are initially `k` pieces of chalk. When the student number `i` is given a problem to solve, they will use `chalk[i]` pieces of chalk to solve that problem. However, if the current number of chalk pieces is **strictly less** than `chalk[i]`, then the student number `i` will be asked to **replace** the chalk.
Return _the **index** of the student that will **replace** the chalk pieces_.
**Example 1:**
**Input:** chalk = \[5,1,5\], k = 22
**Output:** 0
**Explanation:** The students go in turns as follows:
- Student number 0 uses 5 chalk, so k = 17.
- Student number 1 uses 1 chalk, so k = 16.
- Student number 2 uses 5 chalk, so k = 11.
- Student number 0 uses 5 chalk, so k = 6.
- Student number 1 uses 1 chalk, so k = 5.
- Student number 2 uses 5 chalk, so k = 0.
Student number 0 does not have enough chalk, so they will have to replace it.
**Example 2:**
**Input:** chalk = \[3,4,1,2\], k = 25
**Output:** 1
**Explanation:** The students go in turns as follows:
- Student number 0 uses 3 chalk so k = 22.
- Student number 1 uses 4 chalk so k = 18.
- Student number 2 uses 1 chalk so k = 17.
- Student number 3 uses 2 chalk so k = 15.
- Student number 0 uses 3 chalk so k = 12.
- Student number 1 uses 4 chalk so k = 8.
- Student number 2 uses 1 chalk so k = 7.
- Student number 3 uses 2 chalk so k = 5.
- Student number 0 uses 3 chalk so k = 2.
Student number 1 does not have enough chalk, so they will have to replace it.
**Constraints:**
* `chalk.length == n`
* `1 <= n <= 105`
* `1 <= chalk[i] <= 105`
* `1 <= k <= 109` | Use two pointers, one pointer for each string. Alternately choose the character from each pointer, and move the pointer upwards. |
Python | simple O(N) | find-the-student-that-will-replace-the-chalk | 0 | 1 | ```\nclass Solution:\n def chalkReplacer(self, chalk: List[int], k: int) -> int:\n x = sum(chalk)\n if x<k:\n k = k%x\n if x == k:\n return 0\n i = 0\n n = len(chalk)\n while True:\n if chalk[i]<=k:\n k -= chalk[i]\n else:\n break\n i +=1\n \n return i\n``` | 4 | There are `n` students in a class numbered from `0` to `n - 1`. The teacher will give each student a problem starting with the student number `0`, then the student number `1`, and so on until the teacher reaches the student number `n - 1`. After that, the teacher will restart the process, starting with the student number `0` again.
You are given a **0-indexed** integer array `chalk` and an integer `k`. There are initially `k` pieces of chalk. When the student number `i` is given a problem to solve, they will use `chalk[i]` pieces of chalk to solve that problem. However, if the current number of chalk pieces is **strictly less** than `chalk[i]`, then the student number `i` will be asked to **replace** the chalk.
Return _the **index** of the student that will **replace** the chalk pieces_.
**Example 1:**
**Input:** chalk = \[5,1,5\], k = 22
**Output:** 0
**Explanation:** The students go in turns as follows:
- Student number 0 uses 5 chalk, so k = 17.
- Student number 1 uses 1 chalk, so k = 16.
- Student number 2 uses 5 chalk, so k = 11.
- Student number 0 uses 5 chalk, so k = 6.
- Student number 1 uses 1 chalk, so k = 5.
- Student number 2 uses 5 chalk, so k = 0.
Student number 0 does not have enough chalk, so they will have to replace it.
**Example 2:**
**Input:** chalk = \[3,4,1,2\], k = 25
**Output:** 1
**Explanation:** The students go in turns as follows:
- Student number 0 uses 3 chalk so k = 22.
- Student number 1 uses 4 chalk so k = 18.
- Student number 2 uses 1 chalk so k = 17.
- Student number 3 uses 2 chalk so k = 15.
- Student number 0 uses 3 chalk so k = 12.
- Student number 1 uses 4 chalk so k = 8.
- Student number 2 uses 1 chalk so k = 7.
- Student number 3 uses 2 chalk so k = 5.
- Student number 0 uses 3 chalk so k = 2.
Student number 1 does not have enough chalk, so they will have to replace it.
**Constraints:**
* `chalk.length == n`
* `1 <= n <= 105`
* `1 <= chalk[i] <= 105`
* `1 <= k <= 109` | Use two pointers, one pointer for each string. Alternately choose the character from each pointer, and move the pointer upwards. |
[Python3] prefix sums | largest-magic-square | 0 | 1 | \n```\nclass Solution:\n def largestMagicSquare(self, grid: List[List[int]]) -> int:\n m, n = len(grid), len(grid[0]) # dimensions \n rows = [[0]*(n+1) for _ in range(m)] # prefix sum along row\n cols = [[0]*n for _ in range(m+1)] # prefix sum along column\n \n for i in range(m):\n for j in range(n): \n rows[i][j+1] = grid[i][j] + rows[i][j]\n cols[i+1][j] = grid[i][j] + cols[i][j]\n \n ans = 1\n for i in range(m): \n for j in range(n): \n diag = grid[i][j]\n for k in range(min(i, j)): \n ii, jj = i-k-1, j-k-1\n diag += grid[ii][jj]\n ss = {diag}\n for r in range(ii, i+1): ss.add(rows[r][j+1] - rows[r][jj])\n for c in range(jj, j+1): ss.add(cols[i+1][c] - cols[ii][c])\n ss.add(sum(grid[ii+kk][j-kk] for kk in range(k+2))) # anti-diagonal\n if len(ss) == 1: ans = max(ans, k+2)\n return ans \n```\n\n```\nclass Solution:\n def largestMagicSquare(self, grid: List[List[int]]) -> int:\n m, n = len(grid), len(grid[0]) # dimensions \n rows = [[0]*(n+1) for _ in range(m)]\n cols = [[0]*n for _ in range(m+1)]\n diag = [[0]*(n+1) for _ in range(m+1)]\n anti = [[0]*(n+1) for _ in range(m+1)]\n \n for i in range(m):\n for j in range(n): \n rows[i][j+1] = grid[i][j] + rows[i][j]\n cols[i+1][j] = grid[i][j] + cols[i][j]\n diag[i+1][j+1] = grid[i][j] + diag[i][j]\n anti[i+1][j] = grid[i][j] + anti[i][j+1]\n \n ans = 1\n for i in range(m): \n for j in range(n): \n for k in range(1, min(i, j)+1): \n ii, jj = i-k, j-k\n val = diag[i+1][j+1] - diag[ii][jj]\n match = (val == anti[i+1][jj] - anti[ii][j+1])\n for r in range(ii, i+1): match &= (val == rows[r][j+1] - rows[r][jj])\n for c in range(jj, j+1): match &= (val == cols[i+1][c] - cols[ii][c])\n if match: ans = max(ans, k+1)\n return ans \n``` | 7 | A `k x k` **magic square** is a `k x k` grid filled with integers such that every row sum, every column sum, and both diagonal sums are **all equal**. The integers in the magic square **do not have to be distinct**. Every `1 x 1` grid is trivially a **magic square**.
Given an `m x n` integer `grid`, return _the **size** (i.e., the side length_ `k`_) of the **largest magic square** that can be found within this grid_.
**Example 1:**
**Input:** grid = \[\[7,1,4,5,6\],\[2,5,1,6,4\],\[1,5,4,3,2\],\[1,2,7,3,4\]\]
**Output:** 3
**Explanation:** The largest magic square has a size of 3.
Every row sum, column sum, and diagonal sum of this magic square is equal to 12.
- Row sums: 5+1+6 = 5+4+3 = 2+7+3 = 12
- Column sums: 5+5+2 = 1+4+7 = 6+3+3 = 12
- Diagonal sums: 5+4+3 = 6+4+2 = 12
**Example 2:**
**Input:** grid = \[\[5,1,3,1\],\[9,3,3,1\],\[1,3,3,8\]\]
**Output:** 2
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 50`
* `1 <= grid[i][j] <= 106` | If you want to move a ball from box i to box j, you'll need abs(i-j) moves. To move all balls to some box, you can move them one by one. For each box i, iterate on each ball in a box j, and add abs(i-j) to answers[i]. |
[Python3] prefix sums | largest-magic-square | 0 | 1 | \n```\nclass Solution:\n def largestMagicSquare(self, grid: List[List[int]]) -> int:\n m, n = len(grid), len(grid[0]) # dimensions \n rows = [[0]*(n+1) for _ in range(m)] # prefix sum along row\n cols = [[0]*n for _ in range(m+1)] # prefix sum along column\n \n for i in range(m):\n for j in range(n): \n rows[i][j+1] = grid[i][j] + rows[i][j]\n cols[i+1][j] = grid[i][j] + cols[i][j]\n \n ans = 1\n for i in range(m): \n for j in range(n): \n diag = grid[i][j]\n for k in range(min(i, j)): \n ii, jj = i-k-1, j-k-1\n diag += grid[ii][jj]\n ss = {diag}\n for r in range(ii, i+1): ss.add(rows[r][j+1] - rows[r][jj])\n for c in range(jj, j+1): ss.add(cols[i+1][c] - cols[ii][c])\n ss.add(sum(grid[ii+kk][j-kk] for kk in range(k+2))) # anti-diagonal\n if len(ss) == 1: ans = max(ans, k+2)\n return ans \n```\n\n```\nclass Solution:\n def largestMagicSquare(self, grid: List[List[int]]) -> int:\n m, n = len(grid), len(grid[0]) # dimensions \n rows = [[0]*(n+1) for _ in range(m)]\n cols = [[0]*n for _ in range(m+1)]\n diag = [[0]*(n+1) for _ in range(m+1)]\n anti = [[0]*(n+1) for _ in range(m+1)]\n \n for i in range(m):\n for j in range(n): \n rows[i][j+1] = grid[i][j] + rows[i][j]\n cols[i+1][j] = grid[i][j] + cols[i][j]\n diag[i+1][j+1] = grid[i][j] + diag[i][j]\n anti[i+1][j] = grid[i][j] + anti[i][j+1]\n \n ans = 1\n for i in range(m): \n for j in range(n): \n for k in range(1, min(i, j)+1): \n ii, jj = i-k, j-k\n val = diag[i+1][j+1] - diag[ii][jj]\n match = (val == anti[i+1][jj] - anti[ii][j+1])\n for r in range(ii, i+1): match &= (val == rows[r][j+1] - rows[r][jj])\n for c in range(jj, j+1): match &= (val == cols[i+1][c] - cols[ii][c])\n if match: ans = max(ans, k+1)\n return ans \n``` | 7 | There are `n` people, each person has a unique _id_ between `0` and `n-1`. Given the arrays `watchedVideos` and `friends`, where `watchedVideos[i]` and `friends[i]` contain the list of watched videos and the list of friends respectively for the person with `id = i`.
Level **1** of videos are all watched videos by your friends, level **2** of videos are all watched videos by the friends of your friends and so on. In general, the level `k` of videos are all watched videos by people with the shortest path **exactly** equal to `k` with you. Given your `id` and the `level` of videos, return the list of videos ordered by their frequencies (increasing). For videos with the same frequency order them alphabetically from least to greatest.
**Example 1:**
**Input:** watchedVideos = \[\[ "A ", "B "\],\[ "C "\],\[ "B ", "C "\],\[ "D "\]\], friends = \[\[1,2\],\[0,3\],\[0,3\],\[1,2\]\], id = 0, level = 1
**Output:** \[ "B ", "C "\]
**Explanation:**
You have id = 0 (green color in the figure) and your friends are (yellow color in the figure):
Person with id = 1 -> watchedVideos = \[ "C "\]
Person with id = 2 -> watchedVideos = \[ "B ", "C "\]
The frequencies of watchedVideos by your friends are:
B -> 1
C -> 2
**Example 2:**
**Input:** watchedVideos = \[\[ "A ", "B "\],\[ "C "\],\[ "B ", "C "\],\[ "D "\]\], friends = \[\[1,2\],\[0,3\],\[0,3\],\[1,2\]\], id = 0, level = 2
**Output:** \[ "D "\]
**Explanation:**
You have id = 0 (green color in the figure) and the only friend of your friends is the person with id = 3 (yellow color in the figure).
**Constraints:**
* `n == watchedVideos.length == friends.length`
* `2 <= n <= 100`
* `1 <= watchedVideos[i].length <= 100`
* `1 <= watchedVideos[i][j].length <= 8`
* `0 <= friends[i].length < n`
* `0 <= friends[i][j] < n`
* `0 <= id < n`
* `1 <= level < n`
* if `friends[i]` contains `j`, then `friends[j]` contains `i` | Check all squares in the matrix and find the largest one. |
Clean code | largest-magic-square | 0 | 1 | The idea is to check all squares.\nSolution is optimized with prefix sums of rows and cols (you can do the same for diagonals, but I was too lazy to do so). The unoptimized part of the code is commented out.\n\n```\nclass Solution:\n def largestMagicSquare(self, grid: List[List[int]]) -> int:\n n, m = len(grid[0]), len(grid)\n \n rows = {}\n for j in range(m):\n rows[j] = [0] * (n+1)\n for i in range(n):\n rows[j][i+1] += rows[j][i] + grid[j][i]\n\n cols = {}\n for i in range(n):\n cols[i] = [0] * (m+1)\n for j in range(m):\n cols[i][j+1] += cols[i][j] + grid[j][i]\n\n \n def row_sm(i, j, k):\n return rows[j][i+k] - rows[j][i]\n #return sum(grid[j][i:i+k])\n \n def col_sm(i, j, k):\n return cols[i][j+k] - cols[i][j]\n #return sum(grid[y][i] for y in range(j, j+k))\n \n def diag1_sm(i, j, k):\n return sum(grid[j+x][i+x] for x in range(k))\n \n def diag2_sm(i, j, k):\n return sum(grid[j+x][i+k-x-1] for x in range(k))\n \n def is_magic(i, j, k):\n diag1 = diag1_sm(i, j, k)\n if diag2_sm(i, j, k) != diag1:\n return False\n if not all(col_sm(x, j, k) == diag1 for x in range(i, i+k)):\n return False\n if not all(row_sm(i, y, k) == diag1 for y in range(j, j+k)):\n return False\n return True\n \n for k in reversed(range(1, min(n, m) + 1)):\n for i in range(n-k+1):\n for j in range(m-k+1):\n if is_magic(i, j, k):\n return k\n return 1\n\n``` | 0 | A `k x k` **magic square** is a `k x k` grid filled with integers such that every row sum, every column sum, and both diagonal sums are **all equal**. The integers in the magic square **do not have to be distinct**. Every `1 x 1` grid is trivially a **magic square**.
Given an `m x n` integer `grid`, return _the **size** (i.e., the side length_ `k`_) of the **largest magic square** that can be found within this grid_.
**Example 1:**
**Input:** grid = \[\[7,1,4,5,6\],\[2,5,1,6,4\],\[1,5,4,3,2\],\[1,2,7,3,4\]\]
**Output:** 3
**Explanation:** The largest magic square has a size of 3.
Every row sum, column sum, and diagonal sum of this magic square is equal to 12.
- Row sums: 5+1+6 = 5+4+3 = 2+7+3 = 12
- Column sums: 5+5+2 = 1+4+7 = 6+3+3 = 12
- Diagonal sums: 5+4+3 = 6+4+2 = 12
**Example 2:**
**Input:** grid = \[\[5,1,3,1\],\[9,3,3,1\],\[1,3,3,8\]\]
**Output:** 2
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 50`
* `1 <= grid[i][j] <= 106` | If you want to move a ball from box i to box j, you'll need abs(i-j) moves. To move all balls to some box, you can move them one by one. For each box i, iterate on each ball in a box j, and add abs(i-j) to answers[i]. |
Clean code | largest-magic-square | 0 | 1 | The idea is to check all squares.\nSolution is optimized with prefix sums of rows and cols (you can do the same for diagonals, but I was too lazy to do so). The unoptimized part of the code is commented out.\n\n```\nclass Solution:\n def largestMagicSquare(self, grid: List[List[int]]) -> int:\n n, m = len(grid[0]), len(grid)\n \n rows = {}\n for j in range(m):\n rows[j] = [0] * (n+1)\n for i in range(n):\n rows[j][i+1] += rows[j][i] + grid[j][i]\n\n cols = {}\n for i in range(n):\n cols[i] = [0] * (m+1)\n for j in range(m):\n cols[i][j+1] += cols[i][j] + grid[j][i]\n\n \n def row_sm(i, j, k):\n return rows[j][i+k] - rows[j][i]\n #return sum(grid[j][i:i+k])\n \n def col_sm(i, j, k):\n return cols[i][j+k] - cols[i][j]\n #return sum(grid[y][i] for y in range(j, j+k))\n \n def diag1_sm(i, j, k):\n return sum(grid[j+x][i+x] for x in range(k))\n \n def diag2_sm(i, j, k):\n return sum(grid[j+x][i+k-x-1] for x in range(k))\n \n def is_magic(i, j, k):\n diag1 = diag1_sm(i, j, k)\n if diag2_sm(i, j, k) != diag1:\n return False\n if not all(col_sm(x, j, k) == diag1 for x in range(i, i+k)):\n return False\n if not all(row_sm(i, y, k) == diag1 for y in range(j, j+k)):\n return False\n return True\n \n for k in reversed(range(1, min(n, m) + 1)):\n for i in range(n-k+1):\n for j in range(m-k+1):\n if is_magic(i, j, k):\n return k\n return 1\n\n``` | 0 | There are `n` people, each person has a unique _id_ between `0` and `n-1`. Given the arrays `watchedVideos` and `friends`, where `watchedVideos[i]` and `friends[i]` contain the list of watched videos and the list of friends respectively for the person with `id = i`.
Level **1** of videos are all watched videos by your friends, level **2** of videos are all watched videos by the friends of your friends and so on. In general, the level `k` of videos are all watched videos by people with the shortest path **exactly** equal to `k` with you. Given your `id` and the `level` of videos, return the list of videos ordered by their frequencies (increasing). For videos with the same frequency order them alphabetically from least to greatest.
**Example 1:**
**Input:** watchedVideos = \[\[ "A ", "B "\],\[ "C "\],\[ "B ", "C "\],\[ "D "\]\], friends = \[\[1,2\],\[0,3\],\[0,3\],\[1,2\]\], id = 0, level = 1
**Output:** \[ "B ", "C "\]
**Explanation:**
You have id = 0 (green color in the figure) and your friends are (yellow color in the figure):
Person with id = 1 -> watchedVideos = \[ "C "\]
Person with id = 2 -> watchedVideos = \[ "B ", "C "\]
The frequencies of watchedVideos by your friends are:
B -> 1
C -> 2
**Example 2:**
**Input:** watchedVideos = \[\[ "A ", "B "\],\[ "C "\],\[ "B ", "C "\],\[ "D "\]\], friends = \[\[1,2\],\[0,3\],\[0,3\],\[1,2\]\], id = 0, level = 2
**Output:** \[ "D "\]
**Explanation:**
You have id = 0 (green color in the figure) and the only friend of your friends is the person with id = 3 (yellow color in the figure).
**Constraints:**
* `n == watchedVideos.length == friends.length`
* `2 <= n <= 100`
* `1 <= watchedVideos[i].length <= 100`
* `1 <= watchedVideos[i][j].length <= 8`
* `0 <= friends[i].length < n`
* `0 <= friends[i][j] < n`
* `0 <= id < n`
* `1 <= level < n`
* if `friends[i]` contains `j`, then `friends[j]` contains `i` | Check all squares in the matrix and find the largest one. |
Python | Easy to Understand | O(mnmin(m,n)^2) | largest-magic-square | 0 | 1 | # Code\n```\nclass Solution:\n def largestMagicSquare(self, grid: List[List[int]]) -> int:\n m, n = len(grid), len(grid[0]) # dimensions \n rows = [[0]*n for _ in range(m)]\n cols = [[0]*n for _ in range(m)]\n diag = [[0]*n for _ in range(m)]\n anti = [[0]*n for _ in range(m)]\n \n for i in range(m):\n for j in range(n): \n rows[i][j] = grid[i][j] + (rows[i][j-1] if j else 0)\n cols[i][j] = grid[i][j] + (cols[i-1][j] if i else 0)\n diag[i][j] = grid[i][j] + (diag[i-1][j-1] if i and j else 0)\n anti[i][j] = grid[i][j] + (anti[i-1][j+1] if i and j < n-1 else 0) \n \n res = 1\n for i in range(m): \n for j in range(n): \n for k in range(1, min(i, j)+1): \n ii, jj = i-k, j-k\n val = diag[i][j] - (diag[ii-1][jj-1] if ii and jj else 0)\n match = (val == anti[i][jj] - (anti[ii-1][j+1] if ii and j < n - 1 else 0))\n if not match: continue\n for r in range(ii, i+1): match &= (val == rows[r][j] - (rows[r][jj-1] if jj else 0))\n for c in range(jj, j+1): match &= (val == cols[i][c] - (cols[ii-1][c] if ii else 0))\n if match: res = max(res, k+1)\n return res \n``` | 0 | A `k x k` **magic square** is a `k x k` grid filled with integers such that every row sum, every column sum, and both diagonal sums are **all equal**. The integers in the magic square **do not have to be distinct**. Every `1 x 1` grid is trivially a **magic square**.
Given an `m x n` integer `grid`, return _the **size** (i.e., the side length_ `k`_) of the **largest magic square** that can be found within this grid_.
**Example 1:**
**Input:** grid = \[\[7,1,4,5,6\],\[2,5,1,6,4\],\[1,5,4,3,2\],\[1,2,7,3,4\]\]
**Output:** 3
**Explanation:** The largest magic square has a size of 3.
Every row sum, column sum, and diagonal sum of this magic square is equal to 12.
- Row sums: 5+1+6 = 5+4+3 = 2+7+3 = 12
- Column sums: 5+5+2 = 1+4+7 = 6+3+3 = 12
- Diagonal sums: 5+4+3 = 6+4+2 = 12
**Example 2:**
**Input:** grid = \[\[5,1,3,1\],\[9,3,3,1\],\[1,3,3,8\]\]
**Output:** 2
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 50`
* `1 <= grid[i][j] <= 106` | If you want to move a ball from box i to box j, you'll need abs(i-j) moves. To move all balls to some box, you can move them one by one. For each box i, iterate on each ball in a box j, and add abs(i-j) to answers[i]. |
Python | Easy to Understand | O(mnmin(m,n)^2) | largest-magic-square | 0 | 1 | # Code\n```\nclass Solution:\n def largestMagicSquare(self, grid: List[List[int]]) -> int:\n m, n = len(grid), len(grid[0]) # dimensions \n rows = [[0]*n for _ in range(m)]\n cols = [[0]*n for _ in range(m)]\n diag = [[0]*n for _ in range(m)]\n anti = [[0]*n for _ in range(m)]\n \n for i in range(m):\n for j in range(n): \n rows[i][j] = grid[i][j] + (rows[i][j-1] if j else 0)\n cols[i][j] = grid[i][j] + (cols[i-1][j] if i else 0)\n diag[i][j] = grid[i][j] + (diag[i-1][j-1] if i and j else 0)\n anti[i][j] = grid[i][j] + (anti[i-1][j+1] if i and j < n-1 else 0) \n \n res = 1\n for i in range(m): \n for j in range(n): \n for k in range(1, min(i, j)+1): \n ii, jj = i-k, j-k\n val = diag[i][j] - (diag[ii-1][jj-1] if ii and jj else 0)\n match = (val == anti[i][jj] - (anti[ii-1][j+1] if ii and j < n - 1 else 0))\n if not match: continue\n for r in range(ii, i+1): match &= (val == rows[r][j] - (rows[r][jj-1] if jj else 0))\n for c in range(jj, j+1): match &= (val == cols[i][c] - (cols[ii-1][c] if ii else 0))\n if match: res = max(res, k+1)\n return res \n``` | 0 | There are `n` people, each person has a unique _id_ between `0` and `n-1`. Given the arrays `watchedVideos` and `friends`, where `watchedVideos[i]` and `friends[i]` contain the list of watched videos and the list of friends respectively for the person with `id = i`.
Level **1** of videos are all watched videos by your friends, level **2** of videos are all watched videos by the friends of your friends and so on. In general, the level `k` of videos are all watched videos by people with the shortest path **exactly** equal to `k` with you. Given your `id` and the `level` of videos, return the list of videos ordered by their frequencies (increasing). For videos with the same frequency order them alphabetically from least to greatest.
**Example 1:**
**Input:** watchedVideos = \[\[ "A ", "B "\],\[ "C "\],\[ "B ", "C "\],\[ "D "\]\], friends = \[\[1,2\],\[0,3\],\[0,3\],\[1,2\]\], id = 0, level = 1
**Output:** \[ "B ", "C "\]
**Explanation:**
You have id = 0 (green color in the figure) and your friends are (yellow color in the figure):
Person with id = 1 -> watchedVideos = \[ "C "\]
Person with id = 2 -> watchedVideos = \[ "B ", "C "\]
The frequencies of watchedVideos by your friends are:
B -> 1
C -> 2
**Example 2:**
**Input:** watchedVideos = \[\[ "A ", "B "\],\[ "C "\],\[ "B ", "C "\],\[ "D "\]\], friends = \[\[1,2\],\[0,3\],\[0,3\],\[1,2\]\], id = 0, level = 2
**Output:** \[ "D "\]
**Explanation:**
You have id = 0 (green color in the figure) and the only friend of your friends is the person with id = 3 (yellow color in the figure).
**Constraints:**
* `n == watchedVideos.length == friends.length`
* `2 <= n <= 100`
* `1 <= watchedVideos[i].length <= 100`
* `1 <= watchedVideos[i][j].length <= 8`
* `0 <= friends[i].length < n`
* `0 <= friends[i][j] < n`
* `0 <= id < n`
* `1 <= level < n`
* if `friends[i]` contains `j`, then `friends[j]` contains `i` | Check all squares in the matrix and find the largest one. |
🐍 DP and Prefix Sum || 97% faster || well-Explained 📌 | largest-magic-square | 0 | 1 | ## IDEA:\nWe simply need prefix sum of all rows and all columns and both the diagonals.\n\nSo of we have that then we can simply for each valid size of square iterate over the matrix\nand check if each row sum , columns sum and both diagonals is same.\n\nIf you want example also then plz tell me.\uD83E\uDD17\n\'\'\'\n\n\tclass Solution:\n def largestMagicSquare(self, grid: List[List[int]]) -> int:\n m=len(grid)\n n=len(grid[0])\n \n\t\t# Row sum matrix\n rowPrefixSum=[[0]*(n+1) for r in range(m)]\n for r in range(m):\n for c in range(n):\n rowPrefixSum[r][c+1]=rowPrefixSum[r][c] + grid[r][c]\n\t\t\t\t\n #column sum Matrix \n columnPrefixSum=[[0]*(m+1) for c in range(n)]\n for c in range(n):\n for r in range(m):\n columnPrefixSum[c][r+1]=columnPrefixSum[c][r] + grid[r][c]\n\n k=min(m,n)\n while 1<k:\n for r in range(m-k+1):\n for c in range(n-k+1):\n z=rowPrefixSum[r][c+k] - rowPrefixSum[r][c]\n ok=1\n for i in range(k):\n if z!=rowPrefixSum[r+i][c+k]-rowPrefixSum[r+i][c] or z!=columnPrefixSum[c+i][r+k]-columnPrefixSum[c+i][r]:\n ok=0\n break\n if ok: \n # check both diagonals\n diagZ1=0\n diagZ2=0\n for i in range(k):\n diagZ1+=grid[r+i][c+i]\n diagZ2+=grid[r+i][c+k-i-1]\n if z==diagZ1==diagZ2: return k\n k-=1\n return 1\n\nIf you found helpful. please **Upvote!!** \u270C\nThanks | 5 | A `k x k` **magic square** is a `k x k` grid filled with integers such that every row sum, every column sum, and both diagonal sums are **all equal**. The integers in the magic square **do not have to be distinct**. Every `1 x 1` grid is trivially a **magic square**.
Given an `m x n` integer `grid`, return _the **size** (i.e., the side length_ `k`_) of the **largest magic square** that can be found within this grid_.
**Example 1:**
**Input:** grid = \[\[7,1,4,5,6\],\[2,5,1,6,4\],\[1,5,4,3,2\],\[1,2,7,3,4\]\]
**Output:** 3
**Explanation:** The largest magic square has a size of 3.
Every row sum, column sum, and diagonal sum of this magic square is equal to 12.
- Row sums: 5+1+6 = 5+4+3 = 2+7+3 = 12
- Column sums: 5+5+2 = 1+4+7 = 6+3+3 = 12
- Diagonal sums: 5+4+3 = 6+4+2 = 12
**Example 2:**
**Input:** grid = \[\[5,1,3,1\],\[9,3,3,1\],\[1,3,3,8\]\]
**Output:** 2
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 50`
* `1 <= grid[i][j] <= 106` | If you want to move a ball from box i to box j, you'll need abs(i-j) moves. To move all balls to some box, you can move them one by one. For each box i, iterate on each ball in a box j, and add abs(i-j) to answers[i]. |
🐍 DP and Prefix Sum || 97% faster || well-Explained 📌 | largest-magic-square | 0 | 1 | ## IDEA:\nWe simply need prefix sum of all rows and all columns and both the diagonals.\n\nSo of we have that then we can simply for each valid size of square iterate over the matrix\nand check if each row sum , columns sum and both diagonals is same.\n\nIf you want example also then plz tell me.\uD83E\uDD17\n\'\'\'\n\n\tclass Solution:\n def largestMagicSquare(self, grid: List[List[int]]) -> int:\n m=len(grid)\n n=len(grid[0])\n \n\t\t# Row sum matrix\n rowPrefixSum=[[0]*(n+1) for r in range(m)]\n for r in range(m):\n for c in range(n):\n rowPrefixSum[r][c+1]=rowPrefixSum[r][c] + grid[r][c]\n\t\t\t\t\n #column sum Matrix \n columnPrefixSum=[[0]*(m+1) for c in range(n)]\n for c in range(n):\n for r in range(m):\n columnPrefixSum[c][r+1]=columnPrefixSum[c][r] + grid[r][c]\n\n k=min(m,n)\n while 1<k:\n for r in range(m-k+1):\n for c in range(n-k+1):\n z=rowPrefixSum[r][c+k] - rowPrefixSum[r][c]\n ok=1\n for i in range(k):\n if z!=rowPrefixSum[r+i][c+k]-rowPrefixSum[r+i][c] or z!=columnPrefixSum[c+i][r+k]-columnPrefixSum[c+i][r]:\n ok=0\n break\n if ok: \n # check both diagonals\n diagZ1=0\n diagZ2=0\n for i in range(k):\n diagZ1+=grid[r+i][c+i]\n diagZ2+=grid[r+i][c+k-i-1]\n if z==diagZ1==diagZ2: return k\n k-=1\n return 1\n\nIf you found helpful. please **Upvote!!** \u270C\nThanks | 5 | There are `n` people, each person has a unique _id_ between `0` and `n-1`. Given the arrays `watchedVideos` and `friends`, where `watchedVideos[i]` and `friends[i]` contain the list of watched videos and the list of friends respectively for the person with `id = i`.
Level **1** of videos are all watched videos by your friends, level **2** of videos are all watched videos by the friends of your friends and so on. In general, the level `k` of videos are all watched videos by people with the shortest path **exactly** equal to `k` with you. Given your `id` and the `level` of videos, return the list of videos ordered by their frequencies (increasing). For videos with the same frequency order them alphabetically from least to greatest.
**Example 1:**
**Input:** watchedVideos = \[\[ "A ", "B "\],\[ "C "\],\[ "B ", "C "\],\[ "D "\]\], friends = \[\[1,2\],\[0,3\],\[0,3\],\[1,2\]\], id = 0, level = 1
**Output:** \[ "B ", "C "\]
**Explanation:**
You have id = 0 (green color in the figure) and your friends are (yellow color in the figure):
Person with id = 1 -> watchedVideos = \[ "C "\]
Person with id = 2 -> watchedVideos = \[ "B ", "C "\]
The frequencies of watchedVideos by your friends are:
B -> 1
C -> 2
**Example 2:**
**Input:** watchedVideos = \[\[ "A ", "B "\],\[ "C "\],\[ "B ", "C "\],\[ "D "\]\], friends = \[\[1,2\],\[0,3\],\[0,3\],\[1,2\]\], id = 0, level = 2
**Output:** \[ "D "\]
**Explanation:**
You have id = 0 (green color in the figure) and the only friend of your friends is the person with id = 3 (yellow color in the figure).
**Constraints:**
* `n == watchedVideos.length == friends.length`
* `2 <= n <= 100`
* `1 <= watchedVideos[i].length <= 100`
* `1 <= watchedVideos[i][j].length <= 8`
* `0 <= friends[i].length < n`
* `0 <= friends[i][j] < n`
* `0 <= id < n`
* `1 <= level < n`
* if `friends[i]` contains `j`, then `friends[j]` contains `i` | Check all squares in the matrix and find the largest one. |
Python 3 | Very Clean Code, O(min(M, N) ^ 2 * M * N) | Explanation | largest-magic-square | 0 | 1 | ### Explanation\n- Calculate prefix-sum of row, column, major diagonal, minor diagonal\n- Then, for each edge size, at each valid axis, check if it\'s magic square\n- Time Complexity: `O(min(M, N) ^ 2 * M * N)`, when a `M * N` grid is given. If `M == N`, then `O(M^4)`.\n### Implementation\n```\nclass Solution:\n def largestMagicSquare(self, grid: List[List[int]]) -> int:\n m, n = len(grid), len(grid[0])\n dp = [[(0, 0, 0, 0)] * (n+1) for _ in range(m+1)] # [prefix-row-sum, prefix-col-sum, prefix-major-diagonal, prefix-minor-diagonal]\n for i in range(1, m+1): # O(m*n) on prefix calculation\n for j in range(1, n+1):\n dp[i][j] = [\n dp[i][j-1][0] + grid[i-1][j-1], \n dp[i-1][j][1] + grid[i-1][j-1],\n dp[i-1][j-1][2] + grid[i-1][j-1],\n (dp[i-1][j+1][3] if j < n else 0) + grid[i-1][j-1],\n ]\n for win in range(min(m, n), 0, -1): # for each edge size (window size)\n for i in range(win, m+1): # for each x-axis\n for j in range(win, n+1): # for each y-axis\n val = dp[i][j][2] - dp[i-win][j-win][2] # major diagonal\n if dp[i][j-win+1][3] - (dp[i-win][j+1][3] if j+1 <= n else 0) != val: continue # minor diagonal\n if any(dp[row][j][0] - dp[row][j-win][0] != val for row in range(i, i-win, -1)): continue # for each row\n if any(dp[i][col][1] - dp[i-win][col][1] != val for col in range(j, j-win, -1)): continue # for each col\n return win \n return 1\n``` | 2 | A `k x k` **magic square** is a `k x k` grid filled with integers such that every row sum, every column sum, and both diagonal sums are **all equal**. The integers in the magic square **do not have to be distinct**. Every `1 x 1` grid is trivially a **magic square**.
Given an `m x n` integer `grid`, return _the **size** (i.e., the side length_ `k`_) of the **largest magic square** that can be found within this grid_.
**Example 1:**
**Input:** grid = \[\[7,1,4,5,6\],\[2,5,1,6,4\],\[1,5,4,3,2\],\[1,2,7,3,4\]\]
**Output:** 3
**Explanation:** The largest magic square has a size of 3.
Every row sum, column sum, and diagonal sum of this magic square is equal to 12.
- Row sums: 5+1+6 = 5+4+3 = 2+7+3 = 12
- Column sums: 5+5+2 = 1+4+7 = 6+3+3 = 12
- Diagonal sums: 5+4+3 = 6+4+2 = 12
**Example 2:**
**Input:** grid = \[\[5,1,3,1\],\[9,3,3,1\],\[1,3,3,8\]\]
**Output:** 2
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 50`
* `1 <= grid[i][j] <= 106` | If you want to move a ball from box i to box j, you'll need abs(i-j) moves. To move all balls to some box, you can move them one by one. For each box i, iterate on each ball in a box j, and add abs(i-j) to answers[i]. |
Python 3 | Very Clean Code, O(min(M, N) ^ 2 * M * N) | Explanation | largest-magic-square | 0 | 1 | ### Explanation\n- Calculate prefix-sum of row, column, major diagonal, minor diagonal\n- Then, for each edge size, at each valid axis, check if it\'s magic square\n- Time Complexity: `O(min(M, N) ^ 2 * M * N)`, when a `M * N` grid is given. If `M == N`, then `O(M^4)`.\n### Implementation\n```\nclass Solution:\n def largestMagicSquare(self, grid: List[List[int]]) -> int:\n m, n = len(grid), len(grid[0])\n dp = [[(0, 0, 0, 0)] * (n+1) for _ in range(m+1)] # [prefix-row-sum, prefix-col-sum, prefix-major-diagonal, prefix-minor-diagonal]\n for i in range(1, m+1): # O(m*n) on prefix calculation\n for j in range(1, n+1):\n dp[i][j] = [\n dp[i][j-1][0] + grid[i-1][j-1], \n dp[i-1][j][1] + grid[i-1][j-1],\n dp[i-1][j-1][2] + grid[i-1][j-1],\n (dp[i-1][j+1][3] if j < n else 0) + grid[i-1][j-1],\n ]\n for win in range(min(m, n), 0, -1): # for each edge size (window size)\n for i in range(win, m+1): # for each x-axis\n for j in range(win, n+1): # for each y-axis\n val = dp[i][j][2] - dp[i-win][j-win][2] # major diagonal\n if dp[i][j-win+1][3] - (dp[i-win][j+1][3] if j+1 <= n else 0) != val: continue # minor diagonal\n if any(dp[row][j][0] - dp[row][j-win][0] != val for row in range(i, i-win, -1)): continue # for each row\n if any(dp[i][col][1] - dp[i-win][col][1] != val for col in range(j, j-win, -1)): continue # for each col\n return win \n return 1\n``` | 2 | There are `n` people, each person has a unique _id_ between `0` and `n-1`. Given the arrays `watchedVideos` and `friends`, where `watchedVideos[i]` and `friends[i]` contain the list of watched videos and the list of friends respectively for the person with `id = i`.
Level **1** of videos are all watched videos by your friends, level **2** of videos are all watched videos by the friends of your friends and so on. In general, the level `k` of videos are all watched videos by people with the shortest path **exactly** equal to `k` with you. Given your `id` and the `level` of videos, return the list of videos ordered by their frequencies (increasing). For videos with the same frequency order them alphabetically from least to greatest.
**Example 1:**
**Input:** watchedVideos = \[\[ "A ", "B "\],\[ "C "\],\[ "B ", "C "\],\[ "D "\]\], friends = \[\[1,2\],\[0,3\],\[0,3\],\[1,2\]\], id = 0, level = 1
**Output:** \[ "B ", "C "\]
**Explanation:**
You have id = 0 (green color in the figure) and your friends are (yellow color in the figure):
Person with id = 1 -> watchedVideos = \[ "C "\]
Person with id = 2 -> watchedVideos = \[ "B ", "C "\]
The frequencies of watchedVideos by your friends are:
B -> 1
C -> 2
**Example 2:**
**Input:** watchedVideos = \[\[ "A ", "B "\],\[ "C "\],\[ "B ", "C "\],\[ "D "\]\], friends = \[\[1,2\],\[0,3\],\[0,3\],\[1,2\]\], id = 0, level = 2
**Output:** \[ "D "\]
**Explanation:**
You have id = 0 (green color in the figure) and the only friend of your friends is the person with id = 3 (yellow color in the figure).
**Constraints:**
* `n == watchedVideos.length == friends.length`
* `2 <= n <= 100`
* `1 <= watchedVideos[i].length <= 100`
* `1 <= watchedVideos[i][j].length <= 8`
* `0 <= friends[i].length < n`
* `0 <= friends[i][j] < n`
* `0 <= id < n`
* `1 <= level < n`
* if `friends[i]` contains `j`, then `friends[j]` contains `i` | Check all squares in the matrix and find the largest one. |
[Python3] Prefix Sum - Set - easy understanding | largest-magic-square | 0 | 1 | ```\ndef largestMagicSquare(self, grid: List[List[int]]) -> int:\n m, n = len(grid), len(grid[0])\n # 0-index is sum prefix sum of row, 1-index is ps of col, 2-index is ps of top-left to bot-right diagonal, 3-index is ps of bot-left to top-right diagomal\n ps = [[[0, 0, 0, 0] for _ in range(n + 2)] for _ in range(m + 2)]\n largest_size = 0\n \n for i in range(1, m + 1):\n for j in range(1, n + 1):\n # update prefix sum\n ps[i][j][0] = ps[i][j - 1][0] + grid[i - 1][j - 1]\n ps[i][j][1] = ps[i - 1][j][1] + grid[i - 1][j - 1]\n ps[i][j][2] = ps[i - 1][j - 1][2] + grid[i - 1][j - 1]\n ps[i][j][3] = ps[i - 1][j + 1][3] + grid[i - 1][j - 1]\n \n # find the largest possible square\n k = min(i, j)\n while k:\n r, c = i - k, j - k\n k -= 1\n # check all the sum of row, col, diagonal is equal using set()\n s = set()\n # add top-left to bot-right diagonal to set\n s.add(ps[i][j][2] - ps[r][c][2])\n # add bot-left to top-right diagonal to set\n s.add(ps[i][j - k][3] - ps[i - k - 1][j + 1][3])\n if len(s) > 1:\n continue\n \n # add sum of each row to set\n for x in range(r + 1, i + 1):\n s.add(ps[x][j][0] - ps[x][c][0])\n \n if len(s) > 1:\n continue\n \n # add sum of each col to set\n for x in range(c + 1, j + 1):\n s.add(ps[i][x][1] - ps[r][x][1])\n \n if len(s) > 1:\n continue\n \n largest_size = max(largest_size, k + 1)\n break\n return largest_size\n``` | 2 | A `k x k` **magic square** is a `k x k` grid filled with integers such that every row sum, every column sum, and both diagonal sums are **all equal**. The integers in the magic square **do not have to be distinct**. Every `1 x 1` grid is trivially a **magic square**.
Given an `m x n` integer `grid`, return _the **size** (i.e., the side length_ `k`_) of the **largest magic square** that can be found within this grid_.
**Example 1:**
**Input:** grid = \[\[7,1,4,5,6\],\[2,5,1,6,4\],\[1,5,4,3,2\],\[1,2,7,3,4\]\]
**Output:** 3
**Explanation:** The largest magic square has a size of 3.
Every row sum, column sum, and diagonal sum of this magic square is equal to 12.
- Row sums: 5+1+6 = 5+4+3 = 2+7+3 = 12
- Column sums: 5+5+2 = 1+4+7 = 6+3+3 = 12
- Diagonal sums: 5+4+3 = 6+4+2 = 12
**Example 2:**
**Input:** grid = \[\[5,1,3,1\],\[9,3,3,1\],\[1,3,3,8\]\]
**Output:** 2
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 50`
* `1 <= grid[i][j] <= 106` | If you want to move a ball from box i to box j, you'll need abs(i-j) moves. To move all balls to some box, you can move them one by one. For each box i, iterate on each ball in a box j, and add abs(i-j) to answers[i]. |
[Python3] Prefix Sum - Set - easy understanding | largest-magic-square | 0 | 1 | ```\ndef largestMagicSquare(self, grid: List[List[int]]) -> int:\n m, n = len(grid), len(grid[0])\n # 0-index is sum prefix sum of row, 1-index is ps of col, 2-index is ps of top-left to bot-right diagonal, 3-index is ps of bot-left to top-right diagomal\n ps = [[[0, 0, 0, 0] for _ in range(n + 2)] for _ in range(m + 2)]\n largest_size = 0\n \n for i in range(1, m + 1):\n for j in range(1, n + 1):\n # update prefix sum\n ps[i][j][0] = ps[i][j - 1][0] + grid[i - 1][j - 1]\n ps[i][j][1] = ps[i - 1][j][1] + grid[i - 1][j - 1]\n ps[i][j][2] = ps[i - 1][j - 1][2] + grid[i - 1][j - 1]\n ps[i][j][3] = ps[i - 1][j + 1][3] + grid[i - 1][j - 1]\n \n # find the largest possible square\n k = min(i, j)\n while k:\n r, c = i - k, j - k\n k -= 1\n # check all the sum of row, col, diagonal is equal using set()\n s = set()\n # add top-left to bot-right diagonal to set\n s.add(ps[i][j][2] - ps[r][c][2])\n # add bot-left to top-right diagonal to set\n s.add(ps[i][j - k][3] - ps[i - k - 1][j + 1][3])\n if len(s) > 1:\n continue\n \n # add sum of each row to set\n for x in range(r + 1, i + 1):\n s.add(ps[x][j][0] - ps[x][c][0])\n \n if len(s) > 1:\n continue\n \n # add sum of each col to set\n for x in range(c + 1, j + 1):\n s.add(ps[i][x][1] - ps[r][x][1])\n \n if len(s) > 1:\n continue\n \n largest_size = max(largest_size, k + 1)\n break\n return largest_size\n``` | 2 | There are `n` people, each person has a unique _id_ between `0` and `n-1`. Given the arrays `watchedVideos` and `friends`, where `watchedVideos[i]` and `friends[i]` contain the list of watched videos and the list of friends respectively for the person with `id = i`.
Level **1** of videos are all watched videos by your friends, level **2** of videos are all watched videos by the friends of your friends and so on. In general, the level `k` of videos are all watched videos by people with the shortest path **exactly** equal to `k` with you. Given your `id` and the `level` of videos, return the list of videos ordered by their frequencies (increasing). For videos with the same frequency order them alphabetically from least to greatest.
**Example 1:**
**Input:** watchedVideos = \[\[ "A ", "B "\],\[ "C "\],\[ "B ", "C "\],\[ "D "\]\], friends = \[\[1,2\],\[0,3\],\[0,3\],\[1,2\]\], id = 0, level = 1
**Output:** \[ "B ", "C "\]
**Explanation:**
You have id = 0 (green color in the figure) and your friends are (yellow color in the figure):
Person with id = 1 -> watchedVideos = \[ "C "\]
Person with id = 2 -> watchedVideos = \[ "B ", "C "\]
The frequencies of watchedVideos by your friends are:
B -> 1
C -> 2
**Example 2:**
**Input:** watchedVideos = \[\[ "A ", "B "\],\[ "C "\],\[ "B ", "C "\],\[ "D "\]\], friends = \[\[1,2\],\[0,3\],\[0,3\],\[1,2\]\], id = 0, level = 2
**Output:** \[ "D "\]
**Explanation:**
You have id = 0 (green color in the figure) and the only friend of your friends is the person with id = 3 (yellow color in the figure).
**Constraints:**
* `n == watchedVideos.length == friends.length`
* `2 <= n <= 100`
* `1 <= watchedVideos[i].length <= 100`
* `1 <= watchedVideos[i][j].length <= 8`
* `0 <= friends[i].length < n`
* `0 <= friends[i][j] < n`
* `0 <= id < n`
* `1 <= level < n`
* if `friends[i]` contains `j`, then `friends[j]` contains `i` | Check all squares in the matrix and find the largest one. |
O(n): Recursive parsing two expressions + calculate min cost. (30 lines, 90% runtime) | minimum-cost-to-change-the-final-value-of-expression | 0 | 1 | # Approach\nParsing the expression right to left.\nEach recursive call with look for:\n- 1 expression (no operation): e1 only\n- 2 expressions (with operation): e2 [op] e1 with \'e2\' being parsed in a recursive call.\n\nand return:\n- val: The evaluation of the expression (0 or 1)\n- cost: The minimum cost to change the final value of that expression\n- i: pointer to the end position of this expression - 1.\n\nIn the case of only 1 expression, the minimum cost is \'e1cost\'.\nIn the case of 2 expressions, the minimum cost depends on e1val and e2val and their costs:\n\n Case 1: op=\'|\'\n e1val==e2val==0:\n # Changing one is enough to change the final value\n cost = min(e1cost, e2cost)\n e1val==e2val==1:\n # Two possibilities:\n # 1: Change both e1 and e2 -> e1cost + e2cost\n # 2: Change the op (| to &) and e1 or e2\n cost = min(e1cost + e2cost, e1cost + 1, e2cost + 1)\n e1val!=e2val:\n # 0|1 or 1|0 both evaluate to 1. Changing the symbol is enough\n cost = 1\n Case 2: op=\'&\':\n (This is literally the same as with op=\'|\' except that the condition e1val==e2val==x is flipped (1<->1))\n e1val==e2val==1:\n # Changing one is enough to change the final value\n cost = min(e1cost, e2cost)\n e1val==e2val==0:\n # Two possibilities:\n # 1: Change both e1 and e2 -> e1cost + e2cost\n # 2: Change the op (| to &) and e1 or e2\n cost = min(e1cost + e2cost, e1cost + 1, e2cost + 1)\n e1val!=e2val:\n # 0|1 or 1|0 both evaluate to 1. Changing the symbol is enough\n cost = 1\n\n# Complexity\n- Time complexity: O(n)\n\n- Space complexity: O(n)\n Maximum depth of recursive calls. Each operator/parenthesis increases the depth by 1.\n\n# Code\n```\nclass Solution:\n def minOperationsToFlip(self, expression: str) -> int:\n def rec(i):\n if expression[i] == ")":\n e1val, e1cost, i = rec(i - 1)\n else:\n e1val = int(expression[i])\n e1cost = 1\n i -= 1\n\n if i < 0:\n return e1val, e1cost, i\n elif expression[i] == "(":\n return e1val, e1cost, i\n\n op = expression[i]\n e2val, e2cost, i = rec(i - 1)\n if i >= 0 and expression[i] != "(":\n i -= 1\n\n if op == "|":\n val = e1val | e2val\n cost_case = 0 if e1val == e2val == 0 else 1\n else:\n val = e1val & e2val\n cost_case = 1 if e1val == e2val == 0 else 0\n\n if e1val != e2val:\n cost = 1\n elif cost_case == 0:\n cost = min(e1cost, e2cost)\n else:\n cost = min(e1cost + e2cost, e1cost + 1, e2cost + 1)\n return val, cost, i\n\n return rec(len(expression) - 1)[1]\n``` | 0 | You are given a **valid** boolean expression as a string `expression` consisting of the characters `'1'`,`'0'`,`'&'` (bitwise **AND** operator),`'|'` (bitwise **OR** operator),`'('`, and `')'`.
* For example, `"()1|1 "` and `"(1)&() "` are **not valid** while `"1 "`, `"(((1))|(0)) "`, and `"1|(0&(1)) "` are **valid** expressions.
Return _the **minimum cost** to change the final value of the expression_.
* For example, if `expression = "1|1|(0&0)&1 "`, its **value** is `1|1|(0&0)&1 = 1|1|0&1 = 1|0&1 = 1&1 = 1`. We want to apply operations so that the **new** expression evaluates to `0`.
The **cost** of changing the final value of an expression is the **number of operations** performed on the expression. The types of **operations** are described as follows:
* Turn a `'1'` into a `'0'`.
* Turn a `'0'` into a `'1'`.
* Turn a `'&'` into a `'|'`.
* Turn a `'|'` into a `'&'`.
**Note:** `'&'` does **not** take precedence over `'|'` in the **order of calculation**. Evaluate parentheses **first**, then in **left-to-right** order.
**Example 1:**
**Input:** expression = "1&(0|1) "
**Output:** 1
**Explanation:** We can turn "1&(0**|**1) " into "1&(0**&**1) " by changing the '|' to a '&' using 1 operation.
The new expression evaluates to 0.
**Example 2:**
**Input:** expression = "(0&0)&(0&0&0) "
**Output:** 3
**Explanation:** We can turn "(0**&0**)**&**(0&0&0) " into "(0**|1**)**|**(0&0&0) " using 3 operations.
The new expression evaluates to 1.
**Example 3:**
**Input:** expression = "(0|(1|0&1)) "
**Output:** 1
**Explanation:** We can turn "(0|(**1**|0&1)) " into "(0|(**0**|0&1)) " using 1 operation.
The new expression evaluates to 0.
**Constraints:**
* `1 <= expression.length <= 105`
* `expression` only contains `'1'`,`'0'`,`'&'`,`'|'`,`'('`, and `')'`
* All parentheses are properly matched.
* There will be no empty parentheses (i.e: `"() "` is not a substring of `expression`). | At first glance, the solution seems to be greedy, but if you try to greedily take the largest value from the beginning or the end, this will not be optimal. You should try all scenarios but this will be costy. Memoizing the pre-visited states while trying all the possible scenarios will reduce the complexity, and hence dp is a perfect choice here. |
Python3: Tree based solution with DP | minimum-cost-to-change-the-final-value-of-expression | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass TreeNode:\n def __init__(self, val, left = None, right = None):\n self.val = val\n self.left = left\n self.right = right\n \n def eval(self):\n if self.val in "01":\n return int(self.val)\n \n l, r = self.left.eval(), self.right.eval()\n if self.val == "|":\n return 1 if l + r >= 1 else 0\n else:\n return 1 if l + r == 2 else 0\n \n\n \n\nclass Solution:\n def buildTree(self, s):\n n = len(s)\n def dfs(idx):\n stack = []\n while idx < n:\n c = s[idx]\n if c == "(":\n res, l = dfs(idx + 1)\n stack.append(res)\n idx = l\n elif c == ")":\n return stack, idx\n else:\n stack.append(c)\n idx += 1\n return stack\n array = dfs(0)\n ops = []\n\n def build(arr, start, end):\n if start == end:\n if arr[start] == "1" or arr[start] == "0":\n return TreeNode(arr[start])\n elif isinstance(arr[start], list):\n return build(arr[start], 0, len(arr[start]) - 1)\n \n ops = []\n for i in range(end, start - 1, -1):\n if arr[i] == "|" or arr[i] == "&":\n idx = i\n break\n left = build(arr, start, idx - 1)\n right = build(arr, idx + 1, end)\n return TreeNode(arr[idx], left, right)\n \n return build(array, 0, len(array) - 1)\n \n\n def minOperationsToFlip(self, expression: str) -> int:\n root = self.buildTree(expression)\n res = root.eval()\n\n @lru_cache(None)\n def dfs(node, target):\n if node.val in "01":\n return 1 if int(node.val) != target else 0\n ans = float(\'inf\')\n if target == 0:\n if node.val == "&":\n ans = min(ans, min(dfs(node.left, 0), dfs(node.right, 0)))\n elif node.val == "|":\n ans = min(ans, dfs(node.left, 0) + dfs(node.right, 0))\n ans = min(ans, 1 + min(dfs(node.left, 0), dfs(node.right, 0)))\n else:\n if node.val == "&":\n ans = min(ans, dfs(node.left, 1) + dfs(node.right, 1))\n ans = min(ans, 1 + min(dfs(node.left, 1), dfs(node.right, 1)))\n elif node.val == "|":\n ans = min(ans, min(dfs(node.left, 1), dfs(node.right, 1)))\n return ans\n\n return dfs(root, 1 - res)\n \n\n \n\n``` | 0 | You are given a **valid** boolean expression as a string `expression` consisting of the characters `'1'`,`'0'`,`'&'` (bitwise **AND** operator),`'|'` (bitwise **OR** operator),`'('`, and `')'`.
* For example, `"()1|1 "` and `"(1)&() "` are **not valid** while `"1 "`, `"(((1))|(0)) "`, and `"1|(0&(1)) "` are **valid** expressions.
Return _the **minimum cost** to change the final value of the expression_.
* For example, if `expression = "1|1|(0&0)&1 "`, its **value** is `1|1|(0&0)&1 = 1|1|0&1 = 1|0&1 = 1&1 = 1`. We want to apply operations so that the **new** expression evaluates to `0`.
The **cost** of changing the final value of an expression is the **number of operations** performed on the expression. The types of **operations** are described as follows:
* Turn a `'1'` into a `'0'`.
* Turn a `'0'` into a `'1'`.
* Turn a `'&'` into a `'|'`.
* Turn a `'|'` into a `'&'`.
**Note:** `'&'` does **not** take precedence over `'|'` in the **order of calculation**. Evaluate parentheses **first**, then in **left-to-right** order.
**Example 1:**
**Input:** expression = "1&(0|1) "
**Output:** 1
**Explanation:** We can turn "1&(0**|**1) " into "1&(0**&**1) " by changing the '|' to a '&' using 1 operation.
The new expression evaluates to 0.
**Example 2:**
**Input:** expression = "(0&0)&(0&0&0) "
**Output:** 3
**Explanation:** We can turn "(0**&0**)**&**(0&0&0) " into "(0**|1**)**|**(0&0&0) " using 3 operations.
The new expression evaluates to 1.
**Example 3:**
**Input:** expression = "(0|(1|0&1)) "
**Output:** 1
**Explanation:** We can turn "(0|(**1**|0&1)) " into "(0|(**0**|0&1)) " using 1 operation.
The new expression evaluates to 0.
**Constraints:**
* `1 <= expression.length <= 105`
* `expression` only contains `'1'`,`'0'`,`'&'`,`'|'`,`'('`, and `')'`
* All parentheses are properly matched.
* There will be no empty parentheses (i.e: `"() "` is not a substring of `expression`). | At first glance, the solution seems to be greedy, but if you try to greedily take the largest value from the beginning or the end, this will not be optimal. You should try all scenarios but this will be costy. Memoizing the pre-visited states while trying all the possible scenarios will reduce the complexity, and hence dp is a perfect choice here. |
Short O(n) DFS in Python with Explanation, Faster than 94% | minimum-cost-to-change-the-final-value-of-expression | 0 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\nDivide and conquer can be used to solve this problem. Exploring the number of operations to make the left part to be 0 and 1, and the number of operations to make the right part to be 0 and 1. \n\nTo make the value of an `&` operator either 0 or 1, the following cases should be considered: \n\n1. To be 0\n 1. The value left to `&` is 0\n 2. The value right to `&` is 0\n2. To be 1\n 1. Both the values left and right to `&` is 1\n 2. One of the left and the right value is 1, and change `&` to `|`\n\nSimilarly, to make the value of an `|` operator either 0 or 1, the following cases should be considered: \n\n1. To be 1\n 1. The value left to `|` is 1\n 2. The value right to `|` is 1\n2. To be 0\n 1. Both the values left and right to `|` is 0\n 2. One of the left and the right value is 0, and change `|` to `&`\n\nThe input length can be very large. Fortunately, there are three keys to simplify the efficient solution for this problem. \n\n1. Unlike most programming language, no operator precedence order between `&` and `|`.\n2. No `not` (inversion) operator.\n3. Evaluating the expression from left to right. \n\nTherefore, we can perform the above D&C algorithm by evaluating the input string from the right to the left in $$O(n)$$. \n\n# Complexity\n- Time complexity: $$O(n)$$, $$n$$ is the length of input expression.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n\n# Code\n```\nclass Solution:\n def minOperationsToFlip(self, expression: str) -> int:\n expression = list(expression)\n\n def parse():\n c = expression.pop()\n if c == \')\':\n l0, l1 = parse()\n else:\n l0, l1 = int(c), 1 - int(c)\n if not expression or (c := expression.pop()) == \'(\':\n return l0, l1\n r0, r1 = parse()\n if c == \'&\':\n to_zero = min(l0, r0)\n to_one = min(l1 + r1, min(l1, r1) + 1)\n elif c == \'|\':\n to_zero = min(l0 + r0, min(l0, r0) + 1)\n to_one = min(l1, r1)\n return to_zero, to_one\n \n to_zero, to_one = parse()\n return to_one if to_one else to_zero\n``` | 0 | You are given a **valid** boolean expression as a string `expression` consisting of the characters `'1'`,`'0'`,`'&'` (bitwise **AND** operator),`'|'` (bitwise **OR** operator),`'('`, and `')'`.
* For example, `"()1|1 "` and `"(1)&() "` are **not valid** while `"1 "`, `"(((1))|(0)) "`, and `"1|(0&(1)) "` are **valid** expressions.
Return _the **minimum cost** to change the final value of the expression_.
* For example, if `expression = "1|1|(0&0)&1 "`, its **value** is `1|1|(0&0)&1 = 1|1|0&1 = 1|0&1 = 1&1 = 1`. We want to apply operations so that the **new** expression evaluates to `0`.
The **cost** of changing the final value of an expression is the **number of operations** performed on the expression. The types of **operations** are described as follows:
* Turn a `'1'` into a `'0'`.
* Turn a `'0'` into a `'1'`.
* Turn a `'&'` into a `'|'`.
* Turn a `'|'` into a `'&'`.
**Note:** `'&'` does **not** take precedence over `'|'` in the **order of calculation**. Evaluate parentheses **first**, then in **left-to-right** order.
**Example 1:**
**Input:** expression = "1&(0|1) "
**Output:** 1
**Explanation:** We can turn "1&(0**|**1) " into "1&(0**&**1) " by changing the '|' to a '&' using 1 operation.
The new expression evaluates to 0.
**Example 2:**
**Input:** expression = "(0&0)&(0&0&0) "
**Output:** 3
**Explanation:** We can turn "(0**&0**)**&**(0&0&0) " into "(0**|1**)**|**(0&0&0) " using 3 operations.
The new expression evaluates to 1.
**Example 3:**
**Input:** expression = "(0|(1|0&1)) "
**Output:** 1
**Explanation:** We can turn "(0|(**1**|0&1)) " into "(0|(**0**|0&1)) " using 1 operation.
The new expression evaluates to 0.
**Constraints:**
* `1 <= expression.length <= 105`
* `expression` only contains `'1'`,`'0'`,`'&'`,`'|'`,`'('`, and `')'`
* All parentheses are properly matched.
* There will be no empty parentheses (i.e: `"() "` is not a substring of `expression`). | At first glance, the solution seems to be greedy, but if you try to greedily take the largest value from the beginning or the end, this will not be optimal. You should try all scenarios but this will be costy. Memoizing the pre-visited states while trying all the possible scenarios will reduce the complexity, and hence dp is a perfect choice here. |
Python (Simple DFS) | minimum-cost-to-change-the-final-value-of-expression | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minOperationsToFlip(self, expression):\n dict1, stack = {}, []\n\n for i,j in enumerate(expression):\n if j == "(":\n stack.append(i)\n elif j == ")":\n dict1[i] = stack.pop()\n\n def dfs(lo,hi):\n if lo == hi: return int(expression[lo]), 1\n if expression[hi] == ")" and dict1[hi] == lo: return dfs(lo+1,hi-1)\n mid = dict1.get(hi,hi) - 1\n v,c = dfs(mid+1,hi)\n vv,cc = dfs(lo,mid-1)\n if expression[mid] == "|":\n val = v|vv\n if v == vv == 0: change = min(c,cc)\n elif v == vv == 1: change = 1 + min(c,cc)\n else: change = 1\n if expression[mid] == "&":\n val = v&vv\n if v == vv == 0: change = 1 + min(c,cc)\n elif v == vv == 1: change = min(c,cc)\n else: change = 1\n return val,change\n \n return dfs(0,len(expression)-1)[1]\n\n\n\n\n\n\n\n\n\n\n \n \n``` | 0 | You are given a **valid** boolean expression as a string `expression` consisting of the characters `'1'`,`'0'`,`'&'` (bitwise **AND** operator),`'|'` (bitwise **OR** operator),`'('`, and `')'`.
* For example, `"()1|1 "` and `"(1)&() "` are **not valid** while `"1 "`, `"(((1))|(0)) "`, and `"1|(0&(1)) "` are **valid** expressions.
Return _the **minimum cost** to change the final value of the expression_.
* For example, if `expression = "1|1|(0&0)&1 "`, its **value** is `1|1|(0&0)&1 = 1|1|0&1 = 1|0&1 = 1&1 = 1`. We want to apply operations so that the **new** expression evaluates to `0`.
The **cost** of changing the final value of an expression is the **number of operations** performed on the expression. The types of **operations** are described as follows:
* Turn a `'1'` into a `'0'`.
* Turn a `'0'` into a `'1'`.
* Turn a `'&'` into a `'|'`.
* Turn a `'|'` into a `'&'`.
**Note:** `'&'` does **not** take precedence over `'|'` in the **order of calculation**. Evaluate parentheses **first**, then in **left-to-right** order.
**Example 1:**
**Input:** expression = "1&(0|1) "
**Output:** 1
**Explanation:** We can turn "1&(0**|**1) " into "1&(0**&**1) " by changing the '|' to a '&' using 1 operation.
The new expression evaluates to 0.
**Example 2:**
**Input:** expression = "(0&0)&(0&0&0) "
**Output:** 3
**Explanation:** We can turn "(0**&0**)**&**(0&0&0) " into "(0**|1**)**|**(0&0&0) " using 3 operations.
The new expression evaluates to 1.
**Example 3:**
**Input:** expression = "(0|(1|0&1)) "
**Output:** 1
**Explanation:** We can turn "(0|(**1**|0&1)) " into "(0|(**0**|0&1)) " using 1 operation.
The new expression evaluates to 0.
**Constraints:**
* `1 <= expression.length <= 105`
* `expression` only contains `'1'`,`'0'`,`'&'`,`'|'`,`'('`, and `')'`
* All parentheses are properly matched.
* There will be no empty parentheses (i.e: `"() "` is not a substring of `expression`). | At first glance, the solution seems to be greedy, but if you try to greedily take the largest value from the beginning or the end, this will not be optimal. You should try all scenarios but this will be costy. Memoizing the pre-visited states while trying all the possible scenarios will reduce the complexity, and hence dp is a perfect choice here. |
[Python3] divide & conquer | minimum-cost-to-change-the-final-value-of-expression | 0 | 1 | \n```\nclass Solution:\n def minOperationsToFlip(self, expression: str) -> int:\n loc = {}\n stack = []\n for i in reversed(range(len(expression))):\n if expression[i] == ")": stack.append(i)\n elif expression[i] == "(": loc[stack.pop()] = i \n \n def fn(lo, hi): \n """Return value and min op to change value."""\n if lo == hi: return int(expression[lo]), 1\n if expression[hi] == ")" and loc[hi] == lo: return fn(lo+1, hi-1) # strip parenthesis \n mid = loc.get(hi, hi) - 1 \n v, c = fn(mid+1, hi)\n vv, cc = fn(lo, mid-1)\n if expression[mid] == "|": \n val = v | vv \n if v == vv == 0: chg = min(c, cc)\n elif v == vv == 1: chg = 1 + min(c, cc)\n else: chg = 1 \n else: # expression[k] == "&"\n val = v & vv\n if v == vv == 0: chg = 1 + min(c, cc)\n elif v == vv == 1: chg = min(c, cc)\n else: chg = 1\n return val, chg\n \n return fn(0, len(expression)-1)[1]\n``` | 1 | You are given a **valid** boolean expression as a string `expression` consisting of the characters `'1'`,`'0'`,`'&'` (bitwise **AND** operator),`'|'` (bitwise **OR** operator),`'('`, and `')'`.
* For example, `"()1|1 "` and `"(1)&() "` are **not valid** while `"1 "`, `"(((1))|(0)) "`, and `"1|(0&(1)) "` are **valid** expressions.
Return _the **minimum cost** to change the final value of the expression_.
* For example, if `expression = "1|1|(0&0)&1 "`, its **value** is `1|1|(0&0)&1 = 1|1|0&1 = 1|0&1 = 1&1 = 1`. We want to apply operations so that the **new** expression evaluates to `0`.
The **cost** of changing the final value of an expression is the **number of operations** performed on the expression. The types of **operations** are described as follows:
* Turn a `'1'` into a `'0'`.
* Turn a `'0'` into a `'1'`.
* Turn a `'&'` into a `'|'`.
* Turn a `'|'` into a `'&'`.
**Note:** `'&'` does **not** take precedence over `'|'` in the **order of calculation**. Evaluate parentheses **first**, then in **left-to-right** order.
**Example 1:**
**Input:** expression = "1&(0|1) "
**Output:** 1
**Explanation:** We can turn "1&(0**|**1) " into "1&(0**&**1) " by changing the '|' to a '&' using 1 operation.
The new expression evaluates to 0.
**Example 2:**
**Input:** expression = "(0&0)&(0&0&0) "
**Output:** 3
**Explanation:** We can turn "(0**&0**)**&**(0&0&0) " into "(0**|1**)**|**(0&0&0) " using 3 operations.
The new expression evaluates to 1.
**Example 3:**
**Input:** expression = "(0|(1|0&1)) "
**Output:** 1
**Explanation:** We can turn "(0|(**1**|0&1)) " into "(0|(**0**|0&1)) " using 1 operation.
The new expression evaluates to 0.
**Constraints:**
* `1 <= expression.length <= 105`
* `expression` only contains `'1'`,`'0'`,`'&'`,`'|'`,`'('`, and `')'`
* All parentheses are properly matched.
* There will be no empty parentheses (i.e: `"() "` is not a substring of `expression`). | At first glance, the solution seems to be greedy, but if you try to greedily take the largest value from the beginning or the end, this will not be optimal. You should try all scenarios but this will be costy. Memoizing the pre-visited states while trying all the possible scenarios will reduce the complexity, and hence dp is a perfect choice here. |
Python | dictionary | redistribute-characters-to-make-all-strings-equal | 0 | 1 | ```\nclass Solution:\n def makeEqual(self, words: List[str]) -> bool:\n map_ = {}\n for word in words:\n for i in word:\n if i not in map_:\n map_[i] = 1\n else:\n map_[i] += 1\n n = len(words)\n for k,v in map_.items():\n if (v%n) != 0:\n return False\n return True\n``` | 17 | You are given an array of strings `words` (**0-indexed**).
In one operation, pick two **distinct** indices `i` and `j`, where `words[i]` is a non-empty string, and move **any** character from `words[i]` to **any** position in `words[j]`.
Return `true` _if you can make **every** string in_ `words` _**equal** using **any** number of operations_, _and_ `false` _otherwise_.
**Example 1:**
**Input:** words = \[ "abc ", "aabc ", "bc "\]
**Output:** true
**Explanation:** Move the first 'a' in `words[1] to the front of words[2], to make` `words[1]` = "abc " and words\[2\] = "abc ".
All the strings are now equal to "abc ", so return `true`.
**Example 2:**
**Input:** words = \[ "ab ", "a "\]
**Output:** false
**Explanation:** It is impossible to make all the strings equal using the operation.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` consists of lowercase English letters. | Let's ignore the non-empty subsequence constraint. We can concatenate the two strings and find the largest palindromic subsequence with dynamic programming. Iterate through every pair of characters word1[i] and word2[j], and see if some palindrome begins with word1[i] and ends with word2[j]. This ensures that the subsequences are non-empty. |
PYTHON 3 : | 98.48% | EXPLAINED |TWO EASY SOLUTIONS| | redistribute-characters-to-make-all-strings-equal | 0 | 1 | eg --> words = ["abc","aabc","bcc"]\n\n* stp1) join --> "abcaabcbc"\n* stp2) set --> {\'b\', \'a\', \'c\'}\n* stp3) joint.count(\'b\') = 3 , joint.count(\'a\') = 3 , joint.count(\'c\') = 4\n* stp4) as joint.count(\'c\') = 4 therefore it is not multiple of len(words)--->[here 3]\n\tif joint.count(i) % len(words) != 0 : return False\n* \tif joint.count(\'c\') >len(words) and also multiple of len(words) , then also it will work coz we can distribute all \'c\' equally in all the substrings, therfore we are using (%), for eg if count(c) = 6 then we we can distribute \'c\' like [\'....cc\',\'....cc\',\'...cc\']\n\n\n\n**44 ms, faster than 98.48%**\n```\nclass Solution:\n def makeEqual(self, words: List[str]) -> bool:\n \n joint = \'\'.join(words)\n set1 = set(joint)\n \n for i in set1 :\n if joint.count(i) % len(words) != 0 : return False \n return True\n```\n\n**56 ms, faster than 85.81%**\n```\nclass Solution:\n def makeEqual(self, words: List[str]) -> bool:\n \n joint = \'\'.join(words)\n dic = {}\n \n for i in joint :\n if i not in dic :\n dic[i] = joint.count(i)\n \n for v in dic.values() :\n if v % len(words) != 0 : return False \n return True\n```\n\n\n**plz upvote if u like : )** | 21 | You are given an array of strings `words` (**0-indexed**).
In one operation, pick two **distinct** indices `i` and `j`, where `words[i]` is a non-empty string, and move **any** character from `words[i]` to **any** position in `words[j]`.
Return `true` _if you can make **every** string in_ `words` _**equal** using **any** number of operations_, _and_ `false` _otherwise_.
**Example 1:**
**Input:** words = \[ "abc ", "aabc ", "bc "\]
**Output:** true
**Explanation:** Move the first 'a' in `words[1] to the front of words[2], to make` `words[1]` = "abc " and words\[2\] = "abc ".
All the strings are now equal to "abc ", so return `true`.
**Example 2:**
**Input:** words = \[ "ab ", "a "\]
**Output:** false
**Explanation:** It is impossible to make all the strings equal using the operation.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` consists of lowercase English letters. | Let's ignore the non-empty subsequence constraint. We can concatenate the two strings and find the largest palindromic subsequence with dynamic programming. Iterate through every pair of characters word1[i] and word2[j], and see if some palindrome begins with word1[i] and ends with word2[j]. This ensures that the subsequences are non-empty. |
Python solution | redistribute-characters-to-make-all-strings-equal | 0 | 1 | ```\nclass Solution:\n def makeEqual(self, words: List[str]) -> bool:\n string: str = "".join(words)\n hash_table, _len = {}, len(words)\n\n for letter in string:\n hash_table[letter] = hash_table.get(letter, 0) + 1\n\n for frequency in hash_table.values():\n if frequency % _len != 0: return False\n\n return True\n``` | 0 | You are given an array of strings `words` (**0-indexed**).
In one operation, pick two **distinct** indices `i` and `j`, where `words[i]` is a non-empty string, and move **any** character from `words[i]` to **any** position in `words[j]`.
Return `true` _if you can make **every** string in_ `words` _**equal** using **any** number of operations_, _and_ `false` _otherwise_.
**Example 1:**
**Input:** words = \[ "abc ", "aabc ", "bc "\]
**Output:** true
**Explanation:** Move the first 'a' in `words[1] to the front of words[2], to make` `words[1]` = "abc " and words\[2\] = "abc ".
All the strings are now equal to "abc ", so return `true`.
**Example 2:**
**Input:** words = \[ "ab ", "a "\]
**Output:** false
**Explanation:** It is impossible to make all the strings equal using the operation.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` consists of lowercase English letters. | Let's ignore the non-empty subsequence constraint. We can concatenate the two strings and find the largest palindromic subsequence with dynamic programming. Iterate through every pair of characters word1[i] and word2[j], and see if some palindrome begins with word1[i] and ends with word2[j]. This ensures that the subsequences are non-empty. |
Python3. 1-liner. | redistribute-characters-to-make-all-strings-equal | 0 | 1 | ```\nclass Solution:\n def makeEqual(self, words: List[str]) -> bool:\n return True if all(map(lambda x: x[1] % len(words) == 0, Counter(\'\'.join(words)).items())) else False\n``` | 0 | You are given an array of strings `words` (**0-indexed**).
In one operation, pick two **distinct** indices `i` and `j`, where `words[i]` is a non-empty string, and move **any** character from `words[i]` to **any** position in `words[j]`.
Return `true` _if you can make **every** string in_ `words` _**equal** using **any** number of operations_, _and_ `false` _otherwise_.
**Example 1:**
**Input:** words = \[ "abc ", "aabc ", "bc "\]
**Output:** true
**Explanation:** Move the first 'a' in `words[1] to the front of words[2], to make` `words[1]` = "abc " and words\[2\] = "abc ".
All the strings are now equal to "abc ", so return `true`.
**Example 2:**
**Input:** words = \[ "ab ", "a "\]
**Output:** false
**Explanation:** It is impossible to make all the strings equal using the operation.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` consists of lowercase English letters. | Let's ignore the non-empty subsequence constraint. We can concatenate the two strings and find the largest palindromic subsequence with dynamic programming. Iterate through every pair of characters word1[i] and word2[j], and see if some palindrome begins with word1[i] and ends with word2[j]. This ensures that the subsequences are non-empty. |
Python easy solution | redistribute-characters-to-make-all-strings-equal | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def makeEqual(self, words: List[str]) -> bool:\n s=""\n for i in words:\n s+=i\n n=Counter(s)\n count=0\n for i in n.values():\n if i%len(words)!=0:\n count+=1\n return True if count==0 else False\n\n \n``` | 0 | You are given an array of strings `words` (**0-indexed**).
In one operation, pick two **distinct** indices `i` and `j`, where `words[i]` is a non-empty string, and move **any** character from `words[i]` to **any** position in `words[j]`.
Return `true` _if you can make **every** string in_ `words` _**equal** using **any** number of operations_, _and_ `false` _otherwise_.
**Example 1:**
**Input:** words = \[ "abc ", "aabc ", "bc "\]
**Output:** true
**Explanation:** Move the first 'a' in `words[1] to the front of words[2], to make` `words[1]` = "abc " and words\[2\] = "abc ".
All the strings are now equal to "abc ", so return `true`.
**Example 2:**
**Input:** words = \[ "ab ", "a "\]
**Output:** false
**Explanation:** It is impossible to make all the strings equal using the operation.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` consists of lowercase English letters. | Let's ignore the non-empty subsequence constraint. We can concatenate the two strings and find the largest palindromic subsequence with dynamic programming. Iterate through every pair of characters word1[i] and word2[j], and see if some palindrome begins with word1[i] and ends with word2[j]. This ensures that the subsequences are non-empty. |
Python: Using Hashmap | redistribute-characters-to-make-all-strings-equal | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def makeEqual(self, words: List[str]) -> bool:\n hash = {}\n for i in range(len(words)):\n for j in range(len(words[i])):\n if words[i][j] in hash:\n hash[words[i][j]]+=1\n else:\n hash[words[i][j]]=1\n for key,val in hash.items():\n if val%len(words)!=0:\n return False\n else:\n return True\n\n \n\n \n``` | 0 | You are given an array of strings `words` (**0-indexed**).
In one operation, pick two **distinct** indices `i` and `j`, where `words[i]` is a non-empty string, and move **any** character from `words[i]` to **any** position in `words[j]`.
Return `true` _if you can make **every** string in_ `words` _**equal** using **any** number of operations_, _and_ `false` _otherwise_.
**Example 1:**
**Input:** words = \[ "abc ", "aabc ", "bc "\]
**Output:** true
**Explanation:** Move the first 'a' in `words[1] to the front of words[2], to make` `words[1]` = "abc " and words\[2\] = "abc ".
All the strings are now equal to "abc ", so return `true`.
**Example 2:**
**Input:** words = \[ "ab ", "a "\]
**Output:** false
**Explanation:** It is impossible to make all the strings equal using the operation.
**Constraints:**
* `1 <= words.length <= 100`
* `1 <= words[i].length <= 100`
* `words[i]` consists of lowercase English letters. | Let's ignore the non-empty subsequence constraint. We can concatenate the two strings and find the largest palindromic subsequence with dynamic programming. Iterate through every pair of characters word1[i] and word2[j], and see if some palindrome begins with word1[i] and ends with word2[j]. This ensures that the subsequences are non-empty. |
95.96% simplified Python solution | maximum-number-of-removable-characters | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n* Figure out a good way to find subsequence given a set of impacted indices\n* Try to expand the set of impacted indices and return max size as answer\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n**Subsequence finding**: Using a reverse traversal, determine if all the characters in $$p$$ can be found in $$s$$ when including the impacted indices\n\n**Optimizing impacted indices search**: To figure out the right size for removable characters, binary search is the correct candidate due to its time efficiency. The search range is $$[1, |removable|]$$ inclusive\n\n# Complexity\n- Time complexity: $$O(|s|.lg(|removable|))$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(|removable|)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maximumRemovals(self, s: str, p: str, removable: List[int]) -> int:\n \n def is_possible(k):\n impacted = set(removable[:k])\n i = len(s)\n\n for c in p[::-1]: # reverse of p\n i -= 1\n\n while i >= 0 and (s[i] != c or i in impacted):\n i -= 1\n \n return i >= 0\n\n ans = 0\n # O(N): TLE\n # for k in range(1,len(removable)+1):\n # if not is_possible(k): return ans\n # ans=k\n\n # O(lgN): 95%\n minima, maxima = 1, len(removable)+1\n\n while minima < maxima:\n k = (minima+maxima) // 2\n if is_possible(k):\n ans = max(ans, k)\n minima = k+1\n else: maxima = k\n\n return ans\n\n\n \n``` | 1 | You are given two strings `s` and `p` where `p` is a **subsequence** of `s`. You are also given a **distinct 0-indexed** integer array `removable` containing a subset of indices of `s` (`s` is also **0-indexed**).
You want to choose an integer `k` (`0 <= k <= removable.length`) such that, after removing `k` characters from `s` using the **first** `k` indices in `removable`, `p` is still a **subsequence** of `s`. More formally, you will mark the character at `s[removable[i]]` for each `0 <= i < k`, then remove all marked characters and check if `p` is still a subsequence.
Return _the **maximum**_ `k` _you can choose such that_ `p` _is still a **subsequence** of_ `s` _after the removals_.
A **subsequence** of a string is a new string generated from the original string with some characters (can be none) deleted without changing the relative order of the remaining characters.
**Example 1:**
**Input:** s = "abcacb ", p = "ab ", removable = \[3,1,0\]
**Output:** 2
**Explanation**: After removing the characters at indices 3 and 1, "a**b**c**a**cb " becomes "accb ".
"ab " is a subsequence of "**a**cc**b** ".
If we remove the characters at indices 3, 1, and 0, "**ab**c**a**cb " becomes "ccb ", and "ab " is no longer a subsequence.
Hence, the maximum k is 2.
**Example 2:**
**Input:** s = "abcbddddd ", p = "abcd ", removable = \[3,2,1,4,5,6\]
**Output:** 1
**Explanation**: After removing the character at index 3, "abc**b**ddddd " becomes "abcddddd ".
"abcd " is a subsequence of "**abcd**dddd ".
**Example 3:**
**Input:** s = "abcab ", p = "abc ", removable = \[0,1,2,3,4\]
**Output:** 0
**Explanation**: If you remove the first index in the array removable, "abc " is no longer a subsequence.
**Constraints:**
* `1 <= p.length <= s.length <= 105`
* `0 <= removable.length < s.length`
* `0 <= removable[i] < s.length`
* `p` is a **subsequence** of `s`.
* `s` and `p` both consist of lowercase English letters.
* The elements in `removable` are **distinct**. | null |
🔥[Python 3] BS, beats 100% 🥷🏼 | maximum-number-of-removable-characters | 0 | 1 | ```python3 []\nclass Solution:\n def maximumRemovals(self, s: str, p: str, removable: List[int]) -> int:\n l, r = 0, len(removable)\n\n def isEnough(k):\n s_arr = list(s)\n for i in removable[:k]:\n s_arr[i] = \'\'\n return isSubsequence(p, s_arr)\n \n def isSubsequence(s, t):\n t = iter(t)\n return all(c in t for c in s)\n\n while l < r:\n m = (l+r+1)//2\n if isEnough(m):\n l = m\n else:\n r = m - 1\n \n return l\n```\n\n | 6 | You are given two strings `s` and `p` where `p` is a **subsequence** of `s`. You are also given a **distinct 0-indexed** integer array `removable` containing a subset of indices of `s` (`s` is also **0-indexed**).
You want to choose an integer `k` (`0 <= k <= removable.length`) such that, after removing `k` characters from `s` using the **first** `k` indices in `removable`, `p` is still a **subsequence** of `s`. More formally, you will mark the character at `s[removable[i]]` for each `0 <= i < k`, then remove all marked characters and check if `p` is still a subsequence.
Return _the **maximum**_ `k` _you can choose such that_ `p` _is still a **subsequence** of_ `s` _after the removals_.
A **subsequence** of a string is a new string generated from the original string with some characters (can be none) deleted without changing the relative order of the remaining characters.
**Example 1:**
**Input:** s = "abcacb ", p = "ab ", removable = \[3,1,0\]
**Output:** 2
**Explanation**: After removing the characters at indices 3 and 1, "a**b**c**a**cb " becomes "accb ".
"ab " is a subsequence of "**a**cc**b** ".
If we remove the characters at indices 3, 1, and 0, "**ab**c**a**cb " becomes "ccb ", and "ab " is no longer a subsequence.
Hence, the maximum k is 2.
**Example 2:**
**Input:** s = "abcbddddd ", p = "abcd ", removable = \[3,2,1,4,5,6\]
**Output:** 1
**Explanation**: After removing the character at index 3, "abc**b**ddddd " becomes "abcddddd ".
"abcd " is a subsequence of "**abcd**dddd ".
**Example 3:**
**Input:** s = "abcab ", p = "abc ", removable = \[0,1,2,3,4\]
**Output:** 0
**Explanation**: If you remove the first index in the array removable, "abc " is no longer a subsequence.
**Constraints:**
* `1 <= p.length <= s.length <= 105`
* `0 <= removable.length < s.length`
* `0 <= removable[i] < s.length`
* `p` is a **subsequence** of `s`.
* `s` and `p` both consist of lowercase English letters.
* The elements in `removable` are **distinct**. | null |
O(nlogn) time | O(k) space | solution explained | maximum-number-of-removable-characters | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe range to remove characters is 0 to the size of the removable array. Consider it a sorted array and use binary search to find k (number of removable characters). If we can remove k characters i.e s remains a subsequence of p after removing the characters, search on the right half to find a max number. Else search on the left half.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n- set left pointer l = lower boundary in the range and right pointer r = upper boundary\n- set res = 0 to track the max k\n- loop until l <= r\n - find midpoint m\n - if it is possible to remove m characters (Use the code for subsequence check here or add it in a separate function can_remove())\n - set res to m \n - move the left pointer to search in the right half \n - else move the right pointer to search in the left half\n- return res\n\n- can_remove(k)\n - get the indices that we can remove in s and add them to a hashset\n - set i = 0 to track index in string s\n - set j = 0 to track index in string p\n - while indices are inbound\n - if i is not in the hashset and current characters in strings s and p are the same, s is currently a subsequence\n - increment j to check further\n - increment i to remove ith character in s or to move forward in s\n - check if j = size of string p (it is possible to remove k characters) and return the result\n\n# Complexity\n- Time complexity: O(binary search * subsequence check) \u2192 O(logn * n) \u2192 O(nlogn)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(hashmap) \u2192 O(k)\n\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maximumRemovals(self, s: str, p: str, removable: List[int]) -> int:\n l, r = 0, len(removable)\n\n def can_remove(k: int) -> bool:\n remove = {removable[i] for i in range(k)}\n i, j = 0, 0\n while i < len(s) and j < len(p):\n if i not in remove:\n if s[i] == p[j]:\n j += 1\n i += 1\n return j == len(p)\n\n res = 0\n while l <= r:\n m = (l + r) // 2\n if can_remove(m):\n res = m \n l = m + 1\n else:\n r = m - 1\n return res\n \n``` | 2 | You are given two strings `s` and `p` where `p` is a **subsequence** of `s`. You are also given a **distinct 0-indexed** integer array `removable` containing a subset of indices of `s` (`s` is also **0-indexed**).
You want to choose an integer `k` (`0 <= k <= removable.length`) such that, after removing `k` characters from `s` using the **first** `k` indices in `removable`, `p` is still a **subsequence** of `s`. More formally, you will mark the character at `s[removable[i]]` for each `0 <= i < k`, then remove all marked characters and check if `p` is still a subsequence.
Return _the **maximum**_ `k` _you can choose such that_ `p` _is still a **subsequence** of_ `s` _after the removals_.
A **subsequence** of a string is a new string generated from the original string with some characters (can be none) deleted without changing the relative order of the remaining characters.
**Example 1:**
**Input:** s = "abcacb ", p = "ab ", removable = \[3,1,0\]
**Output:** 2
**Explanation**: After removing the characters at indices 3 and 1, "a**b**c**a**cb " becomes "accb ".
"ab " is a subsequence of "**a**cc**b** ".
If we remove the characters at indices 3, 1, and 0, "**ab**c**a**cb " becomes "ccb ", and "ab " is no longer a subsequence.
Hence, the maximum k is 2.
**Example 2:**
**Input:** s = "abcbddddd ", p = "abcd ", removable = \[3,2,1,4,5,6\]
**Output:** 1
**Explanation**: After removing the character at index 3, "abc**b**ddddd " becomes "abcddddd ".
"abcd " is a subsequence of "**abcd**dddd ".
**Example 3:**
**Input:** s = "abcab ", p = "abc ", removable = \[0,1,2,3,4\]
**Output:** 0
**Explanation**: If you remove the first index in the array removable, "abc " is no longer a subsequence.
**Constraints:**
* `1 <= p.length <= s.length <= 105`
* `0 <= removable.length < s.length`
* `0 <= removable[i] < s.length`
* `p` is a **subsequence** of `s`.
* `s` and `p` both consist of lowercase English letters.
* The elements in `removable` are **distinct**. | null |
[Python3] binary search | maximum-number-of-removable-characters | 0 | 1 | \n```\nclass Solution:\n def maximumRemovals(self, s: str, p: str, removable: List[int]) -> int:\n mp = {x: i for i, x in enumerate(removable)}\n \n def fn(x):\n """Return True if p is a subseq of s after x removals."""\n k = 0 \n for i, ch in enumerate(s): \n if mp.get(i, inf) < x: continue \n if k < len(p) and ch == p[k]: k += 1\n return k == len(p)\n \n lo, hi = -1, len(removable)\n while lo < hi: \n mid = lo + hi + 1 >> 1\n if fn(mid): lo = mid\n else: hi = mid - 1\n return lo \n``` | 13 | You are given two strings `s` and `p` where `p` is a **subsequence** of `s`. You are also given a **distinct 0-indexed** integer array `removable` containing a subset of indices of `s` (`s` is also **0-indexed**).
You want to choose an integer `k` (`0 <= k <= removable.length`) such that, after removing `k` characters from `s` using the **first** `k` indices in `removable`, `p` is still a **subsequence** of `s`. More formally, you will mark the character at `s[removable[i]]` for each `0 <= i < k`, then remove all marked characters and check if `p` is still a subsequence.
Return _the **maximum**_ `k` _you can choose such that_ `p` _is still a **subsequence** of_ `s` _after the removals_.
A **subsequence** of a string is a new string generated from the original string with some characters (can be none) deleted without changing the relative order of the remaining characters.
**Example 1:**
**Input:** s = "abcacb ", p = "ab ", removable = \[3,1,0\]
**Output:** 2
**Explanation**: After removing the characters at indices 3 and 1, "a**b**c**a**cb " becomes "accb ".
"ab " is a subsequence of "**a**cc**b** ".
If we remove the characters at indices 3, 1, and 0, "**ab**c**a**cb " becomes "ccb ", and "ab " is no longer a subsequence.
Hence, the maximum k is 2.
**Example 2:**
**Input:** s = "abcbddddd ", p = "abcd ", removable = \[3,2,1,4,5,6\]
**Output:** 1
**Explanation**: After removing the character at index 3, "abc**b**ddddd " becomes "abcddddd ".
"abcd " is a subsequence of "**abcd**dddd ".
**Example 3:**
**Input:** s = "abcab ", p = "abc ", removable = \[0,1,2,3,4\]
**Output:** 0
**Explanation**: If you remove the first index in the array removable, "abc " is no longer a subsequence.
**Constraints:**
* `1 <= p.length <= s.length <= 105`
* `0 <= removable.length < s.length`
* `0 <= removable[i] < s.length`
* `p` is a **subsequence** of `s`.
* `s` and `p` both consist of lowercase English letters.
* The elements in `removable` are **distinct**. | null |
[Python3] Binary Search - Easy to understand | maximum-number-of-removable-characters | 0 | 1 | ```\nclass Solution:\n def maximumRemovals(self, s: str, p: str, removable: List[int]) -> int:\n l, r = 0, len(removable)\n while l <= r:\n m = (l + r) >> 1\n d = set(removable[:m])\n i, j = 0, 0\n while i < len(s) and j < len(p):\n if i not in d:\n if s[i] == p[j]:\n j += 1\n i += 1\n \n if j == len(p):\n l = m + 1\n else:\n r = m - 1\n return r\n``` | 7 | You are given two strings `s` and `p` where `p` is a **subsequence** of `s`. You are also given a **distinct 0-indexed** integer array `removable` containing a subset of indices of `s` (`s` is also **0-indexed**).
You want to choose an integer `k` (`0 <= k <= removable.length`) such that, after removing `k` characters from `s` using the **first** `k` indices in `removable`, `p` is still a **subsequence** of `s`. More formally, you will mark the character at `s[removable[i]]` for each `0 <= i < k`, then remove all marked characters and check if `p` is still a subsequence.
Return _the **maximum**_ `k` _you can choose such that_ `p` _is still a **subsequence** of_ `s` _after the removals_.
A **subsequence** of a string is a new string generated from the original string with some characters (can be none) deleted without changing the relative order of the remaining characters.
**Example 1:**
**Input:** s = "abcacb ", p = "ab ", removable = \[3,1,0\]
**Output:** 2
**Explanation**: After removing the characters at indices 3 and 1, "a**b**c**a**cb " becomes "accb ".
"ab " is a subsequence of "**a**cc**b** ".
If we remove the characters at indices 3, 1, and 0, "**ab**c**a**cb " becomes "ccb ", and "ab " is no longer a subsequence.
Hence, the maximum k is 2.
**Example 2:**
**Input:** s = "abcbddddd ", p = "abcd ", removable = \[3,2,1,4,5,6\]
**Output:** 1
**Explanation**: After removing the character at index 3, "abc**b**ddddd " becomes "abcddddd ".
"abcd " is a subsequence of "**abcd**dddd ".
**Example 3:**
**Input:** s = "abcab ", p = "abc ", removable = \[0,1,2,3,4\]
**Output:** 0
**Explanation**: If you remove the first index in the array removable, "abc " is no longer a subsequence.
**Constraints:**
* `1 <= p.length <= s.length <= 105`
* `0 <= removable.length < s.length`
* `0 <= removable[i] < s.length`
* `p` is a **subsequence** of `s`.
* `s` and `p` both consist of lowercase English letters.
* The elements in `removable` are **distinct**. | null |
Python Plain Binary Search | maximum-number-of-removable-characters | 0 | 1 | ```\nclass Solution:\n def maximumRemovals(self, s: str, p: str, removable: List[int]) -> int:\n \n def isSubseq(s,subseq,removed):\n i = 0\n j = 0\n while i<len(s) and j<len(subseq):\n if i in removed or s[i]!= subseq[j]:\n i+=1\n continue\n i+=1\n j+=1\n return j == len(subseq)\n \n removed = set()\n l = 0\n r = len(removable)-1\n ans = 0\n while l<=r:\n mid = l+(r-l)//2\n removed = set(removable[:mid+1])\n if isSubseq(s,p,removed):\n ans = max(ans,len(removed))\n l = mid+1\n \n else:\n r = mid-1\n return ans\n \n \n``` | 1 | You are given two strings `s` and `p` where `p` is a **subsequence** of `s`. You are also given a **distinct 0-indexed** integer array `removable` containing a subset of indices of `s` (`s` is also **0-indexed**).
You want to choose an integer `k` (`0 <= k <= removable.length`) such that, after removing `k` characters from `s` using the **first** `k` indices in `removable`, `p` is still a **subsequence** of `s`. More formally, you will mark the character at `s[removable[i]]` for each `0 <= i < k`, then remove all marked characters and check if `p` is still a subsequence.
Return _the **maximum**_ `k` _you can choose such that_ `p` _is still a **subsequence** of_ `s` _after the removals_.
A **subsequence** of a string is a new string generated from the original string with some characters (can be none) deleted without changing the relative order of the remaining characters.
**Example 1:**
**Input:** s = "abcacb ", p = "ab ", removable = \[3,1,0\]
**Output:** 2
**Explanation**: After removing the characters at indices 3 and 1, "a**b**c**a**cb " becomes "accb ".
"ab " is a subsequence of "**a**cc**b** ".
If we remove the characters at indices 3, 1, and 0, "**ab**c**a**cb " becomes "ccb ", and "ab " is no longer a subsequence.
Hence, the maximum k is 2.
**Example 2:**
**Input:** s = "abcbddddd ", p = "abcd ", removable = \[3,2,1,4,5,6\]
**Output:** 1
**Explanation**: After removing the character at index 3, "abc**b**ddddd " becomes "abcddddd ".
"abcd " is a subsequence of "**abcd**dddd ".
**Example 3:**
**Input:** s = "abcab ", p = "abc ", removable = \[0,1,2,3,4\]
**Output:** 0
**Explanation**: If you remove the first index in the array removable, "abc " is no longer a subsequence.
**Constraints:**
* `1 <= p.length <= s.length <= 105`
* `0 <= removable.length < s.length`
* `0 <= removable[i] < s.length`
* `p` is a **subsequence** of `s`.
* `s` and `p` both consist of lowercase English letters.
* The elements in `removable` are **distinct**. | null |
Python3 clean Binary Search | maximum-number-of-removable-characters | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def maximumRemovals(self, s: str, p: str, removable: List[int]) -> int:\n \n \n \n lst=list(s)\n \n def isSubsequence(x,y):\n it=iter(y)\n return all(ch in it for ch in x)\n \n \n def isValid(m):\n new=lst[:]\n for i in range(m):\n new[removable[i]]=""\n \n return isSubsequence(p,"".join(new))\n \n low=0\n high=len(removable)\n ans=-1\n \n while low<=high:\n \n mid=(low+high)//2\n \n if isValid(mid):\n low=mid+1\n ans=mid\n else:\n high=mid-1\n \n return ans\n \n``` | 1 | You are given two strings `s` and `p` where `p` is a **subsequence** of `s`. You are also given a **distinct 0-indexed** integer array `removable` containing a subset of indices of `s` (`s` is also **0-indexed**).
You want to choose an integer `k` (`0 <= k <= removable.length`) such that, after removing `k` characters from `s` using the **first** `k` indices in `removable`, `p` is still a **subsequence** of `s`. More formally, you will mark the character at `s[removable[i]]` for each `0 <= i < k`, then remove all marked characters and check if `p` is still a subsequence.
Return _the **maximum**_ `k` _you can choose such that_ `p` _is still a **subsequence** of_ `s` _after the removals_.
A **subsequence** of a string is a new string generated from the original string with some characters (can be none) deleted without changing the relative order of the remaining characters.
**Example 1:**
**Input:** s = "abcacb ", p = "ab ", removable = \[3,1,0\]
**Output:** 2
**Explanation**: After removing the characters at indices 3 and 1, "a**b**c**a**cb " becomes "accb ".
"ab " is a subsequence of "**a**cc**b** ".
If we remove the characters at indices 3, 1, and 0, "**ab**c**a**cb " becomes "ccb ", and "ab " is no longer a subsequence.
Hence, the maximum k is 2.
**Example 2:**
**Input:** s = "abcbddddd ", p = "abcd ", removable = \[3,2,1,4,5,6\]
**Output:** 1
**Explanation**: After removing the character at index 3, "abc**b**ddddd " becomes "abcddddd ".
"abcd " is a subsequence of "**abcd**dddd ".
**Example 3:**
**Input:** s = "abcab ", p = "abc ", removable = \[0,1,2,3,4\]
**Output:** 0
**Explanation**: If you remove the first index in the array removable, "abc " is no longer a subsequence.
**Constraints:**
* `1 <= p.length <= s.length <= 105`
* `0 <= removable.length < s.length`
* `0 <= removable[i] < s.length`
* `p` is a **subsequence** of `s`.
* `s` and `p` both consist of lowercase English letters.
* The elements in `removable` are **distinct**. | null |
Binary Search | maximum-number-of-removable-characters | 0 | 1 | # Code\n```\nclass Solution:\n def maximumRemovals(self, s: str, p: str, removable: List[int]) -> int:\n def f(x, s, p):\n # Enter x, and return True if p is still a substring of s of s after the first x subscripts are removed\n s = list(s)\n p = list(p)\n for idx in removable[:x]:\n s[idx] = \'\'\n\n i = 0 # A pointer to the string s\n j = 0 # A pointer to the string p\n while i < len(s) and j < len(p):\n if s[i] == p[j]:\n j += 1\n i += 1\n return j == len(p)\n \n left = -1\n right = len(removable) + 1\n while left+1 < right:\n mid = (left+right) // 2\n if f(mid, s, p):\n left = mid\n else:\n right = mid\n return left\n``` | 0 | You are given two strings `s` and `p` where `p` is a **subsequence** of `s`. You are also given a **distinct 0-indexed** integer array `removable` containing a subset of indices of `s` (`s` is also **0-indexed**).
You want to choose an integer `k` (`0 <= k <= removable.length`) such that, after removing `k` characters from `s` using the **first** `k` indices in `removable`, `p` is still a **subsequence** of `s`. More formally, you will mark the character at `s[removable[i]]` for each `0 <= i < k`, then remove all marked characters and check if `p` is still a subsequence.
Return _the **maximum**_ `k` _you can choose such that_ `p` _is still a **subsequence** of_ `s` _after the removals_.
A **subsequence** of a string is a new string generated from the original string with some characters (can be none) deleted without changing the relative order of the remaining characters.
**Example 1:**
**Input:** s = "abcacb ", p = "ab ", removable = \[3,1,0\]
**Output:** 2
**Explanation**: After removing the characters at indices 3 and 1, "a**b**c**a**cb " becomes "accb ".
"ab " is a subsequence of "**a**cc**b** ".
If we remove the characters at indices 3, 1, and 0, "**ab**c**a**cb " becomes "ccb ", and "ab " is no longer a subsequence.
Hence, the maximum k is 2.
**Example 2:**
**Input:** s = "abcbddddd ", p = "abcd ", removable = \[3,2,1,4,5,6\]
**Output:** 1
**Explanation**: After removing the character at index 3, "abc**b**ddddd " becomes "abcddddd ".
"abcd " is a subsequence of "**abcd**dddd ".
**Example 3:**
**Input:** s = "abcab ", p = "abc ", removable = \[0,1,2,3,4\]
**Output:** 0
**Explanation**: If you remove the first index in the array removable, "abc " is no longer a subsequence.
**Constraints:**
* `1 <= p.length <= s.length <= 105`
* `0 <= removable.length < s.length`
* `0 <= removable[i] < s.length`
* `p` is a **subsequence** of `s`.
* `s` and `p` both consist of lowercase English letters.
* The elements in `removable` are **distinct**. | null |
Python3 solution | maximum-number-of-removable-characters | 0 | 1 | # Code\n```python\nclass Solution:\n def maximumRemovals(self, s: str, p: str, removable: List[int]) -> int:\n def isSub(s,p):\n i,j = 0,0\n while i<len(s) and j<len(p):\n if i in removed or s[i]!=p[j]:\n i+=1\n else:\n i+=1\n j+=1\n return j==len(p)\n l,r = 0,len(removable)-1\n res = 0\n while l<=r:\n m = (l+r)//2\n removed = set(removable[:m+1])\n if isSub(s,p):\n res = max(res,m+1)\n l = m+1\n else:\n r = m-1\n return res\n``` | 0 | You are given two strings `s` and `p` where `p` is a **subsequence** of `s`. You are also given a **distinct 0-indexed** integer array `removable` containing a subset of indices of `s` (`s` is also **0-indexed**).
You want to choose an integer `k` (`0 <= k <= removable.length`) such that, after removing `k` characters from `s` using the **first** `k` indices in `removable`, `p` is still a **subsequence** of `s`. More formally, you will mark the character at `s[removable[i]]` for each `0 <= i < k`, then remove all marked characters and check if `p` is still a subsequence.
Return _the **maximum**_ `k` _you can choose such that_ `p` _is still a **subsequence** of_ `s` _after the removals_.
A **subsequence** of a string is a new string generated from the original string with some characters (can be none) deleted without changing the relative order of the remaining characters.
**Example 1:**
**Input:** s = "abcacb ", p = "ab ", removable = \[3,1,0\]
**Output:** 2
**Explanation**: After removing the characters at indices 3 and 1, "a**b**c**a**cb " becomes "accb ".
"ab " is a subsequence of "**a**cc**b** ".
If we remove the characters at indices 3, 1, and 0, "**ab**c**a**cb " becomes "ccb ", and "ab " is no longer a subsequence.
Hence, the maximum k is 2.
**Example 2:**
**Input:** s = "abcbddddd ", p = "abcd ", removable = \[3,2,1,4,5,6\]
**Output:** 1
**Explanation**: After removing the character at index 3, "abc**b**ddddd " becomes "abcddddd ".
"abcd " is a subsequence of "**abcd**dddd ".
**Example 3:**
**Input:** s = "abcab ", p = "abc ", removable = \[0,1,2,3,4\]
**Output:** 0
**Explanation**: If you remove the first index in the array removable, "abc " is no longer a subsequence.
**Constraints:**
* `1 <= p.length <= s.length <= 105`
* `0 <= removable.length < s.length`
* `0 <= removable[i] < s.length`
* `p` is a **subsequence** of `s`.
* `s` and `p` both consist of lowercase English letters.
* The elements in `removable` are **distinct**. | null |
Binary Search For k | maximum-number-of-removable-characters | 0 | 1 | # Intuition\nBinary search for k\n# Complexity\n- Time complexity:\n$$O(nlog(n))$$\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```\nclass Solution:\n def maximumRemovals(self, s: str, p: str, removable: List[int]) -> int:\n def satisfies(k, sequence):\n iter_s = iter(sequence)\n return all(c in iter_s for c in p)\n \n def prune(k):\n rset = set(removable[:k])\n pruned = [char for i, char in enumerate(s) if i not in rset]\n return pruned\n \n l = 0\n r = len(removable)\n while l < r:\n m = (r + l + 1)//2\n if satisfies(m, prune(m)):\n l = m\n else:\n r = m - 1\n return l\n``` | 0 | You are given two strings `s` and `p` where `p` is a **subsequence** of `s`. You are also given a **distinct 0-indexed** integer array `removable` containing a subset of indices of `s` (`s` is also **0-indexed**).
You want to choose an integer `k` (`0 <= k <= removable.length`) such that, after removing `k` characters from `s` using the **first** `k` indices in `removable`, `p` is still a **subsequence** of `s`. More formally, you will mark the character at `s[removable[i]]` for each `0 <= i < k`, then remove all marked characters and check if `p` is still a subsequence.
Return _the **maximum**_ `k` _you can choose such that_ `p` _is still a **subsequence** of_ `s` _after the removals_.
A **subsequence** of a string is a new string generated from the original string with some characters (can be none) deleted without changing the relative order of the remaining characters.
**Example 1:**
**Input:** s = "abcacb ", p = "ab ", removable = \[3,1,0\]
**Output:** 2
**Explanation**: After removing the characters at indices 3 and 1, "a**b**c**a**cb " becomes "accb ".
"ab " is a subsequence of "**a**cc**b** ".
If we remove the characters at indices 3, 1, and 0, "**ab**c**a**cb " becomes "ccb ", and "ab " is no longer a subsequence.
Hence, the maximum k is 2.
**Example 2:**
**Input:** s = "abcbddddd ", p = "abcd ", removable = \[3,2,1,4,5,6\]
**Output:** 1
**Explanation**: After removing the character at index 3, "abc**b**ddddd " becomes "abcddddd ".
"abcd " is a subsequence of "**abcd**dddd ".
**Example 3:**
**Input:** s = "abcab ", p = "abc ", removable = \[0,1,2,3,4\]
**Output:** 0
**Explanation**: If you remove the first index in the array removable, "abc " is no longer a subsequence.
**Constraints:**
* `1 <= p.length <= s.length <= 105`
* `0 <= removable.length < s.length`
* `0 <= removable[i] < s.length`
* `p` is a **subsequence** of `s`.
* `s` and `p` both consist of lowercase English letters.
* The elements in `removable` are **distinct**. | null |
Python Binary Search | maximum-number-of-removable-characters | 0 | 1 | Binary Search k in interval [0, r), where r = len(removable)\nFor each mid, check if removable[:mid+1] could make p a subsequence of s.\nIf True, k could be larger, so we search in the right half; else, search in the left half.\n\n# Complexity\n- Time complexity: O((p+s+r) * logr)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(r), where r = len(removable); p = len(p); s = len(s).\n\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maximumRemovals(self, s: str, p: str, removable: List[int]) -> int:\n \n def check(m):\n i = j = 0\n remove = set(removable[:m+1])\n while i < len(s) and j < len(p):\n if i in remove:\n i += 1\n continue\n if s[i] == p[j]:\n i += 1\n j += 1\n else:\n i += 1\n \n return j == len(p)\n \n \n # search interval is [lo, hi)\n lo, hi = 0, len(removable)+1\n \n while lo < hi:\n mid = (lo + hi) // 2\n if check(mid):\n lo = mid + 1\n else:\n hi = mid\n \n return lo if lo < len(removable) else lo-1\n``` | 0 | You are given two strings `s` and `p` where `p` is a **subsequence** of `s`. You are also given a **distinct 0-indexed** integer array `removable` containing a subset of indices of `s` (`s` is also **0-indexed**).
You want to choose an integer `k` (`0 <= k <= removable.length`) such that, after removing `k` characters from `s` using the **first** `k` indices in `removable`, `p` is still a **subsequence** of `s`. More formally, you will mark the character at `s[removable[i]]` for each `0 <= i < k`, then remove all marked characters and check if `p` is still a subsequence.
Return _the **maximum**_ `k` _you can choose such that_ `p` _is still a **subsequence** of_ `s` _after the removals_.
A **subsequence** of a string is a new string generated from the original string with some characters (can be none) deleted without changing the relative order of the remaining characters.
**Example 1:**
**Input:** s = "abcacb ", p = "ab ", removable = \[3,1,0\]
**Output:** 2
**Explanation**: After removing the characters at indices 3 and 1, "a**b**c**a**cb " becomes "accb ".
"ab " is a subsequence of "**a**cc**b** ".
If we remove the characters at indices 3, 1, and 0, "**ab**c**a**cb " becomes "ccb ", and "ab " is no longer a subsequence.
Hence, the maximum k is 2.
**Example 2:**
**Input:** s = "abcbddddd ", p = "abcd ", removable = \[3,2,1,4,5,6\]
**Output:** 1
**Explanation**: After removing the character at index 3, "abc**b**ddddd " becomes "abcddddd ".
"abcd " is a subsequence of "**abcd**dddd ".
**Example 3:**
**Input:** s = "abcab ", p = "abc ", removable = \[0,1,2,3,4\]
**Output:** 0
**Explanation**: If you remove the first index in the array removable, "abc " is no longer a subsequence.
**Constraints:**
* `1 <= p.length <= s.length <= 105`
* `0 <= removable.length < s.length`
* `0 <= removable[i] < s.length`
* `p` is a **subsequence** of `s`.
* `s` and `p` both consist of lowercase English letters.
* The elements in `removable` are **distinct**. | null |
Python sol with link. | merge-triplets-to-form-target-triplet | 0 | 1 | \n# Code\n```\n# https://www.youtube.com/watch?v=kShkQLQZ9K4\nclass Solution:\n def mergeTriplets(self, triplets: List[List[int]], target: List[int]) -> bool:\n res=set()\n for i in range(len(triplets)):\n if triplets[i][0]>target[0] or triplets[i][1]>target[1] or triplets[i][2]>target[2]:\n continue\n for j in range(3):\n if triplets[i][j]==target[j]:\n res.add(j)\n return len(res)==3\n``` | 1 | A **triplet** is an array of three integers. You are given a 2D integer array `triplets`, where `triplets[i] = [ai, bi, ci]` describes the `ith` **triplet**. You are also given an integer array `target = [x, y, z]` that describes the **triplet** you want to obtain.
To obtain `target`, you may apply the following operation on `triplets` **any number** of times (possibly **zero**):
* Choose two indices (**0-indexed**) `i` and `j` (`i != j`) and **update** `triplets[j]` to become `[max(ai, aj), max(bi, bj), max(ci, cj)]`.
* For example, if `triplets[i] = [2, 5, 3]` and `triplets[j] = [1, 7, 5]`, `triplets[j]` will be updated to `[max(2, 1), max(5, 7), max(3, 5)] = [2, 7, 5]`.
Return `true` _if it is possible to obtain the_ `target` _**triplet**_ `[x, y, z]` _as an **element** of_ `triplets`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** triplets = \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\], target = \[2,7,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and last triplets \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\]. Update the last triplet to be \[max(2,1), max(5,7), max(3,5)\] = \[2,7,5\]. triplets = \[\[2,5,3\],\[1,8,4\],\[2,7,5\]\]
The target triplet \[2,7,5\] is now an element of triplets.
**Example 2:**
**Input:** triplets = \[\[3,4,5\],\[4,5,6\]\], target = \[3,2,5\]
**Output:** false
**Explanation:** It is impossible to have \[3,2,5\] as an element because there is no 2 in any of the triplets.
**Example 3:**
**Input:** triplets = \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\], target = \[5,5,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and third triplets \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\]. Update the third triplet to be \[max(2,1), max(5,2), max(3,5)\] = \[2,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\].
- Choose the third and fourth triplets \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\]. Update the fourth triplet to be \[max(2,5), max(5,2), max(5,3)\] = \[5,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,5,5\]\].
The target triplet \[5,5,5\] is now an element of triplets.
**Constraints:**
* `1 <= triplets.length <= 105`
* `triplets[i].length == target.length == 3`
* `1 <= ai, bi, ci, x, y, z <= 1000` | Iterate on each item, and check if each one matches the rule according to the statement. |
[Python 3] Greedy + Sort - Simple Solution | merge-triplets-to-form-target-triplet | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: $$O(N)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n1. Greedy with Sort\n```\nclass Solution:\n def mergeTriplets(self, triplets: List[List[int]], target: List[int]) -> bool:\n triplets.sort()\n a = b = c = 0\n t1, t2, t3 = target\n for x, y, z in triplets:\n if x <= t1 and y <= t2 and z <= t3:\n a = max(a, x)\n b = max(b, y)\n c = max(c, z)\n return [a, b, c] == target\n```\n- TC: $$O(NlogN)$$\n- SC: $$O(1)$$\n\n2. Greedy without Sort\n- Actually we don\'t need sort for this problem, just check all and ignore the tuple with has 1 of three values which is larger than target\n```\nclass Solution:\n def mergeTriplets(self, triplets: List[List[int]], target: List[int]) -> bool:\n a = b = c = 0\n t1, t2, t3 = target\n for x, y, z in triplets:\n if x <= t1 and y <= t2 and z <= t3:\n a = max(a, x)\n b = max(b, y)\n c = max(c, z)\n return [a, b, c] == target\n```\n- TC: $$O(N)$$\n- SC: $$O(1)$$ | 3 | A **triplet** is an array of three integers. You are given a 2D integer array `triplets`, where `triplets[i] = [ai, bi, ci]` describes the `ith` **triplet**. You are also given an integer array `target = [x, y, z]` that describes the **triplet** you want to obtain.
To obtain `target`, you may apply the following operation on `triplets` **any number** of times (possibly **zero**):
* Choose two indices (**0-indexed**) `i` and `j` (`i != j`) and **update** `triplets[j]` to become `[max(ai, aj), max(bi, bj), max(ci, cj)]`.
* For example, if `triplets[i] = [2, 5, 3]` and `triplets[j] = [1, 7, 5]`, `triplets[j]` will be updated to `[max(2, 1), max(5, 7), max(3, 5)] = [2, 7, 5]`.
Return `true` _if it is possible to obtain the_ `target` _**triplet**_ `[x, y, z]` _as an **element** of_ `triplets`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** triplets = \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\], target = \[2,7,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and last triplets \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\]. Update the last triplet to be \[max(2,1), max(5,7), max(3,5)\] = \[2,7,5\]. triplets = \[\[2,5,3\],\[1,8,4\],\[2,7,5\]\]
The target triplet \[2,7,5\] is now an element of triplets.
**Example 2:**
**Input:** triplets = \[\[3,4,5\],\[4,5,6\]\], target = \[3,2,5\]
**Output:** false
**Explanation:** It is impossible to have \[3,2,5\] as an element because there is no 2 in any of the triplets.
**Example 3:**
**Input:** triplets = \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\], target = \[5,5,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and third triplets \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\]. Update the third triplet to be \[max(2,1), max(5,2), max(3,5)\] = \[2,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\].
- Choose the third and fourth triplets \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\]. Update the fourth triplet to be \[max(2,5), max(5,2), max(5,3)\] = \[5,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,5,5\]\].
The target triplet \[5,5,5\] is now an element of triplets.
**Constraints:**
* `1 <= triplets.length <= 105`
* `triplets[i].length == target.length == 3`
* `1 <= ai, bi, ci, x, y, z <= 1000` | Iterate on each item, and check if each one matches the rule according to the statement. |
Python3 Easy Solution | merge-triplets-to-form-target-triplet | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def mergeTriplets(self, triplets: List[List[int]], target: List[int]) -> bool:\n # loop trough the triplets to find any triplet that matches to one of the num in the target\n cur = [0] * 3\n\n for triplet in triplets:\n if cur == target:\n return True\n if (triplet[0] > target[0] or\n triplet[1] > target[1] or\n triplet[2] > target[2]):\n continue\n cur = [max(triplet[0], cur[0]), max(triplet[1], cur[1]), max(triplet[2], cur[2])]\n return True if cur == target else False\n``` | 0 | A **triplet** is an array of three integers. You are given a 2D integer array `triplets`, where `triplets[i] = [ai, bi, ci]` describes the `ith` **triplet**. You are also given an integer array `target = [x, y, z]` that describes the **triplet** you want to obtain.
To obtain `target`, you may apply the following operation on `triplets` **any number** of times (possibly **zero**):
* Choose two indices (**0-indexed**) `i` and `j` (`i != j`) and **update** `triplets[j]` to become `[max(ai, aj), max(bi, bj), max(ci, cj)]`.
* For example, if `triplets[i] = [2, 5, 3]` and `triplets[j] = [1, 7, 5]`, `triplets[j]` will be updated to `[max(2, 1), max(5, 7), max(3, 5)] = [2, 7, 5]`.
Return `true` _if it is possible to obtain the_ `target` _**triplet**_ `[x, y, z]` _as an **element** of_ `triplets`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** triplets = \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\], target = \[2,7,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and last triplets \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\]. Update the last triplet to be \[max(2,1), max(5,7), max(3,5)\] = \[2,7,5\]. triplets = \[\[2,5,3\],\[1,8,4\],\[2,7,5\]\]
The target triplet \[2,7,5\] is now an element of triplets.
**Example 2:**
**Input:** triplets = \[\[3,4,5\],\[4,5,6\]\], target = \[3,2,5\]
**Output:** false
**Explanation:** It is impossible to have \[3,2,5\] as an element because there is no 2 in any of the triplets.
**Example 3:**
**Input:** triplets = \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\], target = \[5,5,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and third triplets \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\]. Update the third triplet to be \[max(2,1), max(5,2), max(3,5)\] = \[2,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\].
- Choose the third and fourth triplets \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\]. Update the fourth triplet to be \[max(2,5), max(5,2), max(5,3)\] = \[5,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,5,5\]\].
The target triplet \[5,5,5\] is now an element of triplets.
**Constraints:**
* `1 <= triplets.length <= 105`
* `triplets[i].length == target.length == 3`
* `1 <= ai, bi, ci, x, y, z <= 1000` | Iterate on each item, and check if each one matches the rule according to the statement. |
[Python] One pass O(n) solution with detailed explanation || clear and easy | merge-triplets-to-form-target-triplet | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\nFirst, express the question in math terms:\nGiven an array of triplets = (a1, b1, c1), (a2, b2, c2), ... , (an, bn, cn)\nAnd a target = (A, B, C)\nWe need to check if we can find a subset = (ai, bi, ci) i=1->n \nwhere max(ai) = A, max(bi) = B, max(ci) = C\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\nOnce we have the fomular above, it is easy to observe that, to have max(ai) = A, we need at least one ai == A, same goes that we need at least one bi==B and ci==C. Also because A, B, C are the maxes, we have all ai <= A, bi <= B, ci <= C.\n\nNow we have our algorithm, first lets set three boolean values to represent whether we have found A, B and C.\n```foundA = foundB = foundC = False```\n\nNext we iterate once over the triplets,\n```if ai <= tarA and bi <= tarB and ci <= tarC:```\n we know it is a candidate of the subset. So we check, \n```\nif ai == tarA: foundA = True\nif bi == tarB: foundB = True\nif ci == tarC: foundC = True\n```\n\nAfter the iteration, if we have found all A, B, C, we have a solution, otherwise we do not.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n) as we iterate through targets once\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\n\n# Code\n```\nclass Solution:\n def mergeTriplets(self, triplets: List[List[int]], target: List[int]) -> bool:\n if target in triplets:\n return True\n \n foundA = foundB = foundC = False\n tarA, tarB, tarC = target\n for (ai, bi, ci) in triplets:\n if ai <= tarA and bi <= tarB and ci <= tarC:\n if ai == tarA: foundA = True\n if bi == tarB: foundB = True\n if ci == tarC: foundC = True\n \n return foundA and foundB and foundC\n``` | 1 | A **triplet** is an array of three integers. You are given a 2D integer array `triplets`, where `triplets[i] = [ai, bi, ci]` describes the `ith` **triplet**. You are also given an integer array `target = [x, y, z]` that describes the **triplet** you want to obtain.
To obtain `target`, you may apply the following operation on `triplets` **any number** of times (possibly **zero**):
* Choose two indices (**0-indexed**) `i` and `j` (`i != j`) and **update** `triplets[j]` to become `[max(ai, aj), max(bi, bj), max(ci, cj)]`.
* For example, if `triplets[i] = [2, 5, 3]` and `triplets[j] = [1, 7, 5]`, `triplets[j]` will be updated to `[max(2, 1), max(5, 7), max(3, 5)] = [2, 7, 5]`.
Return `true` _if it is possible to obtain the_ `target` _**triplet**_ `[x, y, z]` _as an **element** of_ `triplets`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** triplets = \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\], target = \[2,7,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and last triplets \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\]. Update the last triplet to be \[max(2,1), max(5,7), max(3,5)\] = \[2,7,5\]. triplets = \[\[2,5,3\],\[1,8,4\],\[2,7,5\]\]
The target triplet \[2,7,5\] is now an element of triplets.
**Example 2:**
**Input:** triplets = \[\[3,4,5\],\[4,5,6\]\], target = \[3,2,5\]
**Output:** false
**Explanation:** It is impossible to have \[3,2,5\] as an element because there is no 2 in any of the triplets.
**Example 3:**
**Input:** triplets = \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\], target = \[5,5,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and third triplets \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\]. Update the third triplet to be \[max(2,1), max(5,2), max(3,5)\] = \[2,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\].
- Choose the third and fourth triplets \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\]. Update the fourth triplet to be \[max(2,5), max(5,2), max(5,3)\] = \[5,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,5,5\]\].
The target triplet \[5,5,5\] is now an element of triplets.
**Constraints:**
* `1 <= triplets.length <= 105`
* `triplets[i].length == target.length == 3`
* `1 <= ai, bi, ci, x, y, z <= 1000` | Iterate on each item, and check if each one matches the rule according to the statement. |
Simple Python, Beats 96% | merge-triplets-to-form-target-triplet | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe can return true if all the elements of target exist in triplets which do not contain values greater than the other target values.\n\n\n# Code\n```\nclass Solution:\n def mergeTriplets(self, triplets: List[List[int]], target: List[int]) -> bool:\n a_found = False\n b_found = False\n c_found = False\n for a, b, c in triplets:\n if a > target[0] or b > target[1] or c > target[2]:\n continue\n if a == target[0]:\n a_found = True\n if b == target[1]:\n b_found = True\n if c == target[2]:\n c_found = True\n if a_found and b_found and c_found:\n return True\n return a_found and b_found and c_found\n``` | 1 | A **triplet** is an array of three integers. You are given a 2D integer array `triplets`, where `triplets[i] = [ai, bi, ci]` describes the `ith` **triplet**. You are also given an integer array `target = [x, y, z]` that describes the **triplet** you want to obtain.
To obtain `target`, you may apply the following operation on `triplets` **any number** of times (possibly **zero**):
* Choose two indices (**0-indexed**) `i` and `j` (`i != j`) and **update** `triplets[j]` to become `[max(ai, aj), max(bi, bj), max(ci, cj)]`.
* For example, if `triplets[i] = [2, 5, 3]` and `triplets[j] = [1, 7, 5]`, `triplets[j]` will be updated to `[max(2, 1), max(5, 7), max(3, 5)] = [2, 7, 5]`.
Return `true` _if it is possible to obtain the_ `target` _**triplet**_ `[x, y, z]` _as an **element** of_ `triplets`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** triplets = \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\], target = \[2,7,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and last triplets \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\]. Update the last triplet to be \[max(2,1), max(5,7), max(3,5)\] = \[2,7,5\]. triplets = \[\[2,5,3\],\[1,8,4\],\[2,7,5\]\]
The target triplet \[2,7,5\] is now an element of triplets.
**Example 2:**
**Input:** triplets = \[\[3,4,5\],\[4,5,6\]\], target = \[3,2,5\]
**Output:** false
**Explanation:** It is impossible to have \[3,2,5\] as an element because there is no 2 in any of the triplets.
**Example 3:**
**Input:** triplets = \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\], target = \[5,5,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and third triplets \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\]. Update the third triplet to be \[max(2,1), max(5,2), max(3,5)\] = \[2,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\].
- Choose the third and fourth triplets \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\]. Update the fourth triplet to be \[max(2,5), max(5,2), max(5,3)\] = \[5,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,5,5\]\].
The target triplet \[5,5,5\] is now an element of triplets.
**Constraints:**
* `1 <= triplets.length <= 105`
* `triplets[i].length == target.length == 3`
* `1 <= ai, bi, ci, x, y, z <= 1000` | Iterate on each item, and check if each one matches the rule according to the statement. |
Simple Python Solution | merge-triplets-to-form-target-triplet | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n$$O(n)$$\n\n- Space complexity:\n$$O(1)$$\n\n# Code\n```\nclass Solution:\n def mergeTriplets(self, triplets: List[List[int]], target: List[int]) -> bool:\n ans=[0,0,0]\n for i in triplets:\n if i[0]<=target[0] and i[1]<=target[1] and i[2]<=target[2]:\n ans[0]=max( i[0],ans[0]) \n ans[1]=max( i[1],ans[1])\n ans[2]=max( i[2],ans[2])\n return ans==target\n\n\n``` | 1 | A **triplet** is an array of three integers. You are given a 2D integer array `triplets`, where `triplets[i] = [ai, bi, ci]` describes the `ith` **triplet**. You are also given an integer array `target = [x, y, z]` that describes the **triplet** you want to obtain.
To obtain `target`, you may apply the following operation on `triplets` **any number** of times (possibly **zero**):
* Choose two indices (**0-indexed**) `i` and `j` (`i != j`) and **update** `triplets[j]` to become `[max(ai, aj), max(bi, bj), max(ci, cj)]`.
* For example, if `triplets[i] = [2, 5, 3]` and `triplets[j] = [1, 7, 5]`, `triplets[j]` will be updated to `[max(2, 1), max(5, 7), max(3, 5)] = [2, 7, 5]`.
Return `true` _if it is possible to obtain the_ `target` _**triplet**_ `[x, y, z]` _as an **element** of_ `triplets`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** triplets = \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\], target = \[2,7,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and last triplets \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\]. Update the last triplet to be \[max(2,1), max(5,7), max(3,5)\] = \[2,7,5\]. triplets = \[\[2,5,3\],\[1,8,4\],\[2,7,5\]\]
The target triplet \[2,7,5\] is now an element of triplets.
**Example 2:**
**Input:** triplets = \[\[3,4,5\],\[4,5,6\]\], target = \[3,2,5\]
**Output:** false
**Explanation:** It is impossible to have \[3,2,5\] as an element because there is no 2 in any of the triplets.
**Example 3:**
**Input:** triplets = \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\], target = \[5,5,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and third triplets \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\]. Update the third triplet to be \[max(2,1), max(5,2), max(3,5)\] = \[2,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\].
- Choose the third and fourth triplets \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\]. Update the fourth triplet to be \[max(2,5), max(5,2), max(5,3)\] = \[5,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,5,5\]\].
The target triplet \[5,5,5\] is now an element of triplets.
**Constraints:**
* `1 <= triplets.length <= 105`
* `triplets[i].length == target.length == 3`
* `1 <= ai, bi, ci, x, y, z <= 1000` | Iterate on each item, and check if each one matches the rule according to the statement. |
python | implementation O(N) | merge-triplets-to-form-target-triplet | 0 | 1 | ```\nclass Solution:\n def mergeTriplets(self, triplets: List[List[int]], target: List[int]) -> bool:\n i = 1\n cur = []\n for a,b,c in triplets:\n if a<=target[0] and b<=target[1] and c<= target[2]:\n cur = [a,b,c]\n break\n if not cur:\n return False\n while i<len(triplets):\n if cur == target:\n return True\n a,b,c = triplets[i]\n x,y,z = cur\n if max(a,x)<=target[0] and max(b,y)<=target[1] and max(c,z)<=target[2]:\n cur = [max(a,x), max(b,y), max(c,z)]\n \n \n i+= 1\n if cur == target:\n return True\n return False\n``` | 4 | A **triplet** is an array of three integers. You are given a 2D integer array `triplets`, where `triplets[i] = [ai, bi, ci]` describes the `ith` **triplet**. You are also given an integer array `target = [x, y, z]` that describes the **triplet** you want to obtain.
To obtain `target`, you may apply the following operation on `triplets` **any number** of times (possibly **zero**):
* Choose two indices (**0-indexed**) `i` and `j` (`i != j`) and **update** `triplets[j]` to become `[max(ai, aj), max(bi, bj), max(ci, cj)]`.
* For example, if `triplets[i] = [2, 5, 3]` and `triplets[j] = [1, 7, 5]`, `triplets[j]` will be updated to `[max(2, 1), max(5, 7), max(3, 5)] = [2, 7, 5]`.
Return `true` _if it is possible to obtain the_ `target` _**triplet**_ `[x, y, z]` _as an **element** of_ `triplets`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** triplets = \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\], target = \[2,7,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and last triplets \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\]. Update the last triplet to be \[max(2,1), max(5,7), max(3,5)\] = \[2,7,5\]. triplets = \[\[2,5,3\],\[1,8,4\],\[2,7,5\]\]
The target triplet \[2,7,5\] is now an element of triplets.
**Example 2:**
**Input:** triplets = \[\[3,4,5\],\[4,5,6\]\], target = \[3,2,5\]
**Output:** false
**Explanation:** It is impossible to have \[3,2,5\] as an element because there is no 2 in any of the triplets.
**Example 3:**
**Input:** triplets = \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\], target = \[5,5,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and third triplets \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\]. Update the third triplet to be \[max(2,1), max(5,2), max(3,5)\] = \[2,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\].
- Choose the third and fourth triplets \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\]. Update the fourth triplet to be \[max(2,5), max(5,2), max(5,3)\] = \[5,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,5,5\]\].
The target triplet \[5,5,5\] is now an element of triplets.
**Constraints:**
* `1 <= triplets.length <= 105`
* `triplets[i].length == target.length == 3`
* `1 <= ai, bi, ci, x, y, z <= 1000` | Iterate on each item, and check if each one matches the rule according to the statement. |
100%! Python Solution 💯 | merge-triplets-to-form-target-triplet | 0 | 1 | # Intuition\nIf any value of any triplet matches the value in the same position in the target and none of the values of that triplet is greater than the corresponding values of the target then that position can be merged.\n\n# Approach\nGreedy, one-pass\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def mergeTriplets(self, triplets: List[List[int]], target: List[int]) -> bool:\n found = [0,0,0]\n\n for t in triplets:\n if t[0] > target[0] or t[1] > target[1] or t[2] > target[2]:\n continue\n\n if t[0] == target[0]: found[0] = t[0]\n if t[1] == target[1]: found[1] = t[1]\n if t[2] == target[2]: found[2] = t[2]\n\n return found == target\n\n``` | 0 | A **triplet** is an array of three integers. You are given a 2D integer array `triplets`, where `triplets[i] = [ai, bi, ci]` describes the `ith` **triplet**. You are also given an integer array `target = [x, y, z]` that describes the **triplet** you want to obtain.
To obtain `target`, you may apply the following operation on `triplets` **any number** of times (possibly **zero**):
* Choose two indices (**0-indexed**) `i` and `j` (`i != j`) and **update** `triplets[j]` to become `[max(ai, aj), max(bi, bj), max(ci, cj)]`.
* For example, if `triplets[i] = [2, 5, 3]` and `triplets[j] = [1, 7, 5]`, `triplets[j]` will be updated to `[max(2, 1), max(5, 7), max(3, 5)] = [2, 7, 5]`.
Return `true` _if it is possible to obtain the_ `target` _**triplet**_ `[x, y, z]` _as an **element** of_ `triplets`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** triplets = \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\], target = \[2,7,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and last triplets \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\]. Update the last triplet to be \[max(2,1), max(5,7), max(3,5)\] = \[2,7,5\]. triplets = \[\[2,5,3\],\[1,8,4\],\[2,7,5\]\]
The target triplet \[2,7,5\] is now an element of triplets.
**Example 2:**
**Input:** triplets = \[\[3,4,5\],\[4,5,6\]\], target = \[3,2,5\]
**Output:** false
**Explanation:** It is impossible to have \[3,2,5\] as an element because there is no 2 in any of the triplets.
**Example 3:**
**Input:** triplets = \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\], target = \[5,5,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and third triplets \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\]. Update the third triplet to be \[max(2,1), max(5,2), max(3,5)\] = \[2,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\].
- Choose the third and fourth triplets \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\]. Update the fourth triplet to be \[max(2,5), max(5,2), max(5,3)\] = \[5,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,5,5\]\].
The target triplet \[5,5,5\] is now an element of triplets.
**Constraints:**
* `1 <= triplets.length <= 105`
* `triplets[i].length == target.length == 3`
* `1 <= ai, bi, ci, x, y, z <= 1000` | Iterate on each item, and check if each one matches the rule according to the statement. |
Super Fast Python Solution. Beats 100% of submissions. Easy to understand | merge-triplets-to-form-target-triplet | 0 | 1 | # Intuition\nWe can only merge triples by applying a max operation *to every number in each triple*. Therefore, if even a single value is greater than the corresponding target value, we cannot merge that triplet. \n\n# Approach\nIterate through the triplets, apply the (very fast) max operation to all triplets that meet our criteria.\n\n# Complexity\n- Time complexity:\n$$O(n)$$ \n\n- Space complexity:\n$$O(1)$$\n\n# Code\n```\nclass Solution:\n def mergeTriplets(self, triplets: List[List[int]], target: List[int]) -> bool: \n res = [0, 0, 0]\n for a, b, c in triplets:\n if a <= target[0] and b <= target[1] and c <= target[2]:\n res = [max(a,res[0]),max(b,res[1]),max(c,res[2])]\n return res == target\n\n``` | 0 | A **triplet** is an array of three integers. You are given a 2D integer array `triplets`, where `triplets[i] = [ai, bi, ci]` describes the `ith` **triplet**. You are also given an integer array `target = [x, y, z]` that describes the **triplet** you want to obtain.
To obtain `target`, you may apply the following operation on `triplets` **any number** of times (possibly **zero**):
* Choose two indices (**0-indexed**) `i` and `j` (`i != j`) and **update** `triplets[j]` to become `[max(ai, aj), max(bi, bj), max(ci, cj)]`.
* For example, if `triplets[i] = [2, 5, 3]` and `triplets[j] = [1, 7, 5]`, `triplets[j]` will be updated to `[max(2, 1), max(5, 7), max(3, 5)] = [2, 7, 5]`.
Return `true` _if it is possible to obtain the_ `target` _**triplet**_ `[x, y, z]` _as an **element** of_ `triplets`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** triplets = \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\], target = \[2,7,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and last triplets \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\]. Update the last triplet to be \[max(2,1), max(5,7), max(3,5)\] = \[2,7,5\]. triplets = \[\[2,5,3\],\[1,8,4\],\[2,7,5\]\]
The target triplet \[2,7,5\] is now an element of triplets.
**Example 2:**
**Input:** triplets = \[\[3,4,5\],\[4,5,6\]\], target = \[3,2,5\]
**Output:** false
**Explanation:** It is impossible to have \[3,2,5\] as an element because there is no 2 in any of the triplets.
**Example 3:**
**Input:** triplets = \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\], target = \[5,5,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and third triplets \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\]. Update the third triplet to be \[max(2,1), max(5,2), max(3,5)\] = \[2,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\].
- Choose the third and fourth triplets \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\]. Update the fourth triplet to be \[max(2,5), max(5,2), max(5,3)\] = \[5,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,5,5\]\].
The target triplet \[5,5,5\] is now an element of triplets.
**Constraints:**
* `1 <= triplets.length <= 105`
* `triplets[i].length == target.length == 3`
* `1 <= ai, bi, ci, x, y, z <= 1000` | Iterate on each item, and check if each one matches the rule according to the statement. |
code only | merge-triplets-to-form-target-triplet | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n $$O(n)$$ \n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def mergeTriplets(self, triplets: List[List[int]], target: List[int]) -> bool:\n good =[-1,-1,-1] \n for triplet in triplets:\n if triplet[0] > target[0] or triplet[1] > target[1] or triplet[2] > target[2]:\n continue\n for i , t in enumerate(triplet):\n good[i] = max(good[i], t)\n\n return good == target\n\n \n``` | 0 | A **triplet** is an array of three integers. You are given a 2D integer array `triplets`, where `triplets[i] = [ai, bi, ci]` describes the `ith` **triplet**. You are also given an integer array `target = [x, y, z]` that describes the **triplet** you want to obtain.
To obtain `target`, you may apply the following operation on `triplets` **any number** of times (possibly **zero**):
* Choose two indices (**0-indexed**) `i` and `j` (`i != j`) and **update** `triplets[j]` to become `[max(ai, aj), max(bi, bj), max(ci, cj)]`.
* For example, if `triplets[i] = [2, 5, 3]` and `triplets[j] = [1, 7, 5]`, `triplets[j]` will be updated to `[max(2, 1), max(5, 7), max(3, 5)] = [2, 7, 5]`.
Return `true` _if it is possible to obtain the_ `target` _**triplet**_ `[x, y, z]` _as an **element** of_ `triplets`_, or_ `false` _otherwise_.
**Example 1:**
**Input:** triplets = \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\], target = \[2,7,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and last triplets \[\[2,5,3\],\[1,8,4\],\[1,7,5\]\]. Update the last triplet to be \[max(2,1), max(5,7), max(3,5)\] = \[2,7,5\]. triplets = \[\[2,5,3\],\[1,8,4\],\[2,7,5\]\]
The target triplet \[2,7,5\] is now an element of triplets.
**Example 2:**
**Input:** triplets = \[\[3,4,5\],\[4,5,6\]\], target = \[3,2,5\]
**Output:** false
**Explanation:** It is impossible to have \[3,2,5\] as an element because there is no 2 in any of the triplets.
**Example 3:**
**Input:** triplets = \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\], target = \[5,5,5\]
**Output:** true
**Explanation:** Perform the following operations:
- Choose the first and third triplets \[\[2,5,3\],\[2,3,4\],\[1,2,5\],\[5,2,3\]\]. Update the third triplet to be \[max(2,1), max(5,2), max(3,5)\] = \[2,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\].
- Choose the third and fourth triplets \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,2,3\]\]. Update the fourth triplet to be \[max(2,5), max(5,2), max(5,3)\] = \[5,5,5\]. triplets = \[\[2,5,3\],\[2,3,4\],\[2,5,5\],\[5,5,5\]\].
The target triplet \[5,5,5\] is now an element of triplets.
**Constraints:**
* `1 <= triplets.length <= 105`
* `triplets[i].length == target.length == 3`
* `1 <= ai, bi, ci, x, y, z <= 1000` | Iterate on each item, and check if each one matches the rule according to the statement. |
Python: [Intuitive Solution] Simulate rounds using DFS + string map | the-earliest-and-latest-rounds-where-players-compete | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def earliestAndLatest(self, n: int, firstPlayer: int, secondPlayer: int) -> List[int]:\n minR,maxR=999999,-122\n players=\'\'\n for i in range(1,n+1):\n players+=chr(i+65)\n fp=chr(firstPlayer+65)\n sp=chr(secondPlayer+65)\n\n @cache\n def dfs(s,num_round,winners):\n nonlocal maxR, minR\n if len(s)==0:\n dfs("".join(sorted(winners)),num_round+1,\'\')\n return\n if len(s)==1:\n winners+=s[0]\n dfs("".join(sorted(winners)),num_round+1,\'\')\n return\n if s[0]==fp and s[-1]==sp:\n \n maxR=max(maxR,num_round)\n minR=min(minR,num_round)\n return\n elif s[0]==fp or s[0]==sp:\n winners+=s[0]\n s=s[1:-1]\n dfs(s,num_round,winners)\n elif s[-1]==fp or s[-1]==sp:\n winners+=s[-1]\n s=s[1:-1]\n dfs(s,num_round,winners)\n else:\n l,r=s[0],s[-1]\n dfs(s[1:-1],num_round,winners+l)\n dfs(s[1:-1],num_round,winners+r)\n return\n \n dfs(players, 1, \'\')\n return [minR,maxR]\n\n\n \n``` | 1 | There is a tournament where `n` players are participating. The players are standing in a single row and are numbered from `1` to `n` based on their **initial** standing position (player `1` is the first player in the row, player `2` is the second player in the row, etc.).
The tournament consists of multiple rounds (starting from round number `1`). In each round, the `ith` player from the front of the row competes against the `ith` player from the end of the row, and the winner advances to the next round. When the number of players is odd for the current round, the player in the middle automatically advances to the next round.
* For example, if the row consists of players `1, 2, 4, 6, 7`
* Player `1` competes against player `7`.
* Player `2` competes against player `6`.
* Player `4` automatically advances to the next round.
After each round is over, the winners are lined back up in the row based on the **original ordering** assigned to them initially (ascending order).
The players numbered `firstPlayer` and `secondPlayer` are the best in the tournament. They can win against any other player before they compete against each other. If any two other players compete against each other, either of them might win, and thus you may **choose** the outcome of this round.
Given the integers `n`, `firstPlayer`, and `secondPlayer`, return _an integer array containing two values, the **earliest** possible round number and the **latest** possible round number in which these two players will compete against each other, respectively_.
**Example 1:**
**Input:** n = 11, firstPlayer = 2, secondPlayer = 4
**Output:** \[3,4\]
**Explanation:**
One possible scenario which leads to the earliest round number:
First round: 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11
Second round: 2, 3, 4, 5, 6, 11
Third round: 2, 3, 4
One possible scenario which leads to the latest round number:
First round: 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11
Second round: 1, 2, 3, 4, 5, 6
Third round: 1, 2, 4
Fourth round: 2, 4
**Example 2:**
**Input:** n = 5, firstPlayer = 1, secondPlayer = 5
**Output:** \[1,1\]
**Explanation:** The players numbered 1 and 5 compete in the first round.
There is no way to make them compete in any other round.
**Constraints:**
* `2 <= n <= 28`
* `1 <= firstPlayer < secondPlayer <= n` | As the constraints are not large, you can brute force and enumerate all the possibilities. |
[Python3] bit-mask dp | the-earliest-and-latest-rounds-where-players-compete | 0 | 1 | \n```\nclass Solution:\n def earliestAndLatest(self, n: int, firstPlayer: int, secondPlayer: int) -> List[int]:\n firstPlayer, secondPlayer = firstPlayer-1, secondPlayer-1 # 0-indexed\n \n @cache\n def fn(k, mask): \n """Return earliest and latest rounds."""\n can = deque()\n for i in range(n): \n if mask & (1 << i): can.append(i)\n \n cand = [] # eliminated player\n while len(can) > 1: \n p1, p2 = can.popleft(), can.pop()\n if p1 == firstPlayer and p2 == secondPlayer or p1 == secondPlayer and p2 == firstPlayer: return [k, k] # game of interest \n if p1 in (firstPlayer, secondPlayer): cand.append([p2]) # p2 eliminated \n elif p2 in (firstPlayer, secondPlayer): cand.append([p1]) # p1 eliminated \n else: cand.append([p1, p2]) # both could be elimited \n \n minn, maxx = inf, -inf\n for x in product(*cand): \n mask0 = mask\n for i in x: mask0 ^= 1 << i\n mn, mx = fn(k+1, mask0)\n minn = min(minn, mn)\n maxx = max(maxx, mx)\n return minn, maxx\n \n return fn(1, (1<<n)-1)\n``` | 8 | There is a tournament where `n` players are participating. The players are standing in a single row and are numbered from `1` to `n` based on their **initial** standing position (player `1` is the first player in the row, player `2` is the second player in the row, etc.).
The tournament consists of multiple rounds (starting from round number `1`). In each round, the `ith` player from the front of the row competes against the `ith` player from the end of the row, and the winner advances to the next round. When the number of players is odd for the current round, the player in the middle automatically advances to the next round.
* For example, if the row consists of players `1, 2, 4, 6, 7`
* Player `1` competes against player `7`.
* Player `2` competes against player `6`.
* Player `4` automatically advances to the next round.
After each round is over, the winners are lined back up in the row based on the **original ordering** assigned to them initially (ascending order).
The players numbered `firstPlayer` and `secondPlayer` are the best in the tournament. They can win against any other player before they compete against each other. If any two other players compete against each other, either of them might win, and thus you may **choose** the outcome of this round.
Given the integers `n`, `firstPlayer`, and `secondPlayer`, return _an integer array containing two values, the **earliest** possible round number and the **latest** possible round number in which these two players will compete against each other, respectively_.
**Example 1:**
**Input:** n = 11, firstPlayer = 2, secondPlayer = 4
**Output:** \[3,4\]
**Explanation:**
One possible scenario which leads to the earliest round number:
First round: 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11
Second round: 2, 3, 4, 5, 6, 11
Third round: 2, 3, 4
One possible scenario which leads to the latest round number:
First round: 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11
Second round: 1, 2, 3, 4, 5, 6
Third round: 1, 2, 4
Fourth round: 2, 4
**Example 2:**
**Input:** n = 5, firstPlayer = 1, secondPlayer = 5
**Output:** \[1,1\]
**Explanation:** The players numbered 1 and 5 compete in the first round.
There is no way to make them compete in any other round.
**Constraints:**
* `2 <= n <= 28`
* `1 <= firstPlayer < secondPlayer <= n` | As the constraints are not large, you can brute force and enumerate all the possibilities. |
Python DP Solution | Memoization | Easy to understand | the-earliest-and-latest-rounds-where-players-compete | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def earliestAndLatest(self, n: int, firstPlayer: int, secondPlayer: int) -> List[int]:\n @lru_cache(None)\n def dp(left, right, curPlayers, numGamesAlreadyPlayed):\n if left > right: \n dp(right, left, curPlayers, numGamesAlreadyPlayed)\n if left == right: \n ans.add(numGamesAlreadyPlayed)\n for i in range(1, left + 1):\n for j in range(left - i + 1, right - i + 1):\n if not (curPlayers + 1) // 2 >= i + j >= left + right - curPlayers // 2: \n continue\n dp(i, j, (curPlayers + 1) // 2, numGamesAlreadyPlayed + 1)\n\n ans = set()\n dp(firstPlayer, n - secondPlayer + 1, n, 1)\n return [min(ans), max(ans)]\n``` | 0 | There is a tournament where `n` players are participating. The players are standing in a single row and are numbered from `1` to `n` based on their **initial** standing position (player `1` is the first player in the row, player `2` is the second player in the row, etc.).
The tournament consists of multiple rounds (starting from round number `1`). In each round, the `ith` player from the front of the row competes against the `ith` player from the end of the row, and the winner advances to the next round. When the number of players is odd for the current round, the player in the middle automatically advances to the next round.
* For example, if the row consists of players `1, 2, 4, 6, 7`
* Player `1` competes against player `7`.
* Player `2` competes against player `6`.
* Player `4` automatically advances to the next round.
After each round is over, the winners are lined back up in the row based on the **original ordering** assigned to them initially (ascending order).
The players numbered `firstPlayer` and `secondPlayer` are the best in the tournament. They can win against any other player before they compete against each other. If any two other players compete against each other, either of them might win, and thus you may **choose** the outcome of this round.
Given the integers `n`, `firstPlayer`, and `secondPlayer`, return _an integer array containing two values, the **earliest** possible round number and the **latest** possible round number in which these two players will compete against each other, respectively_.
**Example 1:**
**Input:** n = 11, firstPlayer = 2, secondPlayer = 4
**Output:** \[3,4\]
**Explanation:**
One possible scenario which leads to the earliest round number:
First round: 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11
Second round: 2, 3, 4, 5, 6, 11
Third round: 2, 3, 4
One possible scenario which leads to the latest round number:
First round: 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11
Second round: 1, 2, 3, 4, 5, 6
Third round: 1, 2, 4
Fourth round: 2, 4
**Example 2:**
**Input:** n = 5, firstPlayer = 1, secondPlayer = 5
**Output:** \[1,1\]
**Explanation:** The players numbered 1 and 5 compete in the first round.
There is no way to make them compete in any other round.
**Constraints:**
* `2 <= n <= 28`
* `1 <= firstPlayer < secondPlayer <= n` | As the constraints are not large, you can brute force and enumerate all the possibilities. |
Binary Search Solution with Proper Explanation and Beats 100% | find-a-peak-element-ii | 1 | 1 | # Intuition \n<!-- Describe your first thoughts on how to solve this problem. -->\nUse Binary Search\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nReduce 2d array problem to 1d array\n\n# Complexity\n- Time complexity:O(n log(m)), where n rows and m is columns\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```C++ []\nclass Solution {\npublic:\n\n //find row index of max element and col index is given by mid\n int getMaxElement(vector<vector<int>>&matrix,int mid){\n int index=-1;\n int maxi=INT_MIN;\n\n for(int row=0;row<matrix.size();row++){\n int elm=matrix[row][mid];\n\n if(elm>maxi){\n maxi=max(maxi,elm);\n index=row;\n }\n }\n return index;\n }\n vector<int> findPeakGrid(vector<vector<int>>& matrix) {\n int n=matrix.size();\n int m=matrix[0].size();\n\n int start=0;\n int end=m-1;\n\n while(start<=end){\n int mid=start+(end-start)/2;\n \n // we find maximum because we have to check top, bottom,left,right if we use maximum then our element is definately \n // greater than top and bottom. so we need to check only left and right i.e. our problem is reduced to find peak in 1d\n\n int row=getMaxElement(matrix,mid);\n int left=-1;\n int right=-1;\n \n //handling edge cases\n if(mid-1>0){\n left=matrix[row][mid-1];\n }\n\n if(mid+1<m){\n right=matrix[row][mid+1];\n }\n \n // we find peak element\n if(matrix[row][mid]>left && matrix[row][mid]>right){\n return {row,mid};\n }\n \n //our peak is on left side of mid so eliminate right part\n else if(matrix[row][mid]>right){\n end=mid-1;\n }\n \n //our peak is on right side of mid so eliminate left part\n else{\n start=mid+1;\n }\n }\n\n return {-1,-1};\n }\n};\n```\n```python []\nclass Solution(object):\n def getMaxElement(self, matrix, mid):\n index = -1\n maxi = float(\'-inf\')\n\n for row in range(len(matrix)):\n elm = matrix[row][mid]\n\n if elm > maxi:\n maxi = max(maxi, elm)\n index = row\n\n return index\n\n def findPeakGrid(self, matrix):\n n = len(matrix)\n m = len(matrix[0])\n\n start = 0\n end = m - 1\n\n while start <= end:\n mid = start + (end - start) // 2\n\n # find maximum because we have to check top, bottom, left, right\n # if we use maximum, then our element is definitely greater than top and bottom\n # so we need to check only left and right, i.e., our problem is reduced to finding a peak in 1D\n\n row = self.getMaxElement(matrix, mid)\n left = -1\n right = -1\n\n # handling edge cases\n if mid - 1 >= 0:\n left = matrix[row][mid - 1]\n\n if mid + 1 < m:\n right = matrix[row][mid + 1]\n\n # we find the peak element\n if matrix[row][mid] > left and matrix[row][mid] > right:\n return [row, mid]\n\n # our peak is on the left side of mid, so eliminate the right part\n elif matrix[row][mid] > right:\n end = mid - 1\n\n # our peak is on the right side of mid, so eliminate the left part\n else:\n start = mid + 1\n\n return [-1, -1]\n\n```\n```Java []\npublic class Solution {\n // find row index of max element and col index is given by mid\n public int getMaxElement(int[][] matrix, int mid) {\n int index = -1;\n int maxi = Integer.MIN_VALUE;\n\n for (int row = 0; row < matrix.length; row++) {\n int elm = matrix[row][mid];\n\n if (elm > maxi) {\n maxi = Math.max(maxi, elm);\n index = row;\n }\n }\n return index;\n }\n\n public int[] findPeakGrid(int[][] matrix) {\n int n = matrix.length;\n int m = matrix[0].length;\n\n int start = 0;\n int end = m - 1;\n\n while (start <= end) {\n int mid = start + (end - start) / 2;\n\n // we find maximum because we have to check top, bottom, left, right if we use maximum then our element is definitely\n // greater than top and bottom. so we need to check only left and right i.e. our problem is reduced to find peak in 1d\n\n int row = getMaxElement(matrix, mid);\n int left = -1;\n int right = -1;\n\n // handling edge cases\n if (mid - 1 >= 0) {\n left = matrix[row][mid - 1];\n }\n\n if (mid + 1 < m) {\n right = matrix[row][mid + 1];\n }\n\n // we find peak element\n if (matrix[row][mid] > left && matrix[row][mid] > right) {\n return new int[]{row, mid};\n }\n\n // our peak is on the left side of mid so eliminate the right part\n else if (matrix[row][mid] > right) {\n end = mid - 1;\n }\n\n // our peak is on the right side of mid so eliminate the left part\n else {\n start = mid + 1;\n }\n }\n\n return new int[]{-1, -1};\n }\n}\n\n```\n\n```JavaScript []\n/**\n * @param {number[][]} matrix\n * @return {number[]}\n */\nvar findPeakGrid = function(matrix) {\n const getMaxElement = (matrix, mid) => {\n let index = -1;\n let maxi = Number.MIN_SAFE_INTEGER;\n\n for (let row = 0; row < matrix.length; row++) {\n let elm = matrix[row][mid];\n\n if (elm > maxi) {\n maxi = Math.max(maxi, elm);\n index = row;\n }\n }\n return index;\n };\n\n const n = matrix.length;\n const m = matrix[0].length;\n\n let start = 0;\n let end = m - 1;\n\n while (start <= end) {\n const mid = start + Math.floor((end - start) / 2);\n\n let row = getMaxElement(matrix, mid);\n let left = -1;\n let right = -1;\n\n // handling edge cases\n if (mid - 1 >= 0) {\n left = matrix[row][mid - 1];\n }\n\n if (mid + 1 < m) {\n right = matrix[row][mid + 1];\n }\n\n // we find peak element\n if (matrix[row][mid] > left && matrix[row][mid] > right) {\n return [row, mid];\n }\n\n // our peak is on the left side of mid so eliminate the right part\n else if (matrix[row][mid] > right) {\n end = mid - 1;\n }\n\n // our peak is on the right side of mid so eliminate the left part\n else {\n start = mid + 1;\n }\n }\n\n return [-1, -1];\n};\n\n\n``` | 1 | A **peak** element in a 2D grid is an element that is **strictly greater** than all of its **adjacent** neighbors to the left, right, top, and bottom.
Given a **0-indexed** `m x n` matrix `mat` where **no two adjacent cells are equal**, find **any** peak element `mat[i][j]` and return _the length 2 array_ `[i,j]`.
You may assume that the entire matrix is surrounded by an **outer perimeter** with the value `-1` in each cell.
You must write an algorithm that runs in `O(m log(n))` or `O(n log(m))` time.
**Example 1:**
**Input:** mat = \[\[1,4\],\[3,2\]\]
**Output:** \[0,1\]
**Explanation:** Both 3 and 4 are peak elements so \[1,0\] and \[0,1\] are both acceptable answers.
**Example 2:**
**Input:** mat = \[\[10,20,15\],\[21,30,14\],\[7,16,32\]\]
**Output:** \[1,1\]
**Explanation:** Both 30 and 32 are peak elements so \[1,1\] and \[2,2\] are both acceptable answers.
**Constraints:**
* `m == mat.length`
* `n == mat[i].length`
* `1 <= m, n <= 500`
* `1 <= mat[i][j] <= 105`
* No two adjacent cells are equal. | Let's note that we want to either decrease the sum of the array with a larger sum or increase the array's sum with the smaller sum. You can maintain the largest increase or decrease you can make in a binary search tree and each time get the maximum one. |
Most optimal solution using binary search with explanation | find-a-peak-element-ii | 1 | 1 | -->\n\n# Approach\nThe problem is to find a peak element in a 2D grid, which is an element greater than its adjacent neighbors (up, down, left, right). The grid is surrounded by a boundary of -1.\n\nThe provided algorithm uses a binary search approach to find the peak element. It iterates over columns and uses binary search within each column to find the peak.\n\n1. For each column, it performs a binary search to find the maximum element in that column and its corresponding row (maxEl function).\n2. Then, it compares the peak element in the column with its adjacent elements (left and right).\n3. Based on the comparison, it updates the search range for the next iteration until a peak element is found.\n\n# Complexity\n- Time complexity:\nO(log(m)*n)\n\n- Space complexity:\nO(1)\n\n```C++ []\nclass Solution {\npublic:\n int maxEl(vector<vector<int>>& mat, int n, int m, int col) {\n int maxi = -1;\n int ind = -1; \n for(int i = 0; i<n; i++) {\n if(mat[i][col]>maxi) {\n maxi = mat[i][col];\n ind = i;\n }\n }\n return ind;\n }\n vector<int> findPeakGrid(vector<vector<int>>& mat) {\n int n = mat.size();\n int m = mat[0].size();\n int low = 0;\n int high = m - 1; \n while(low<=high) {\n int mid = (low+high)/2;\n int row = maxEl(mat, n, m, mid);\n int left = mid-1>=0 ? mat[row][mid-1] : -1; \n int right = mid+1<m ? mat[row][mid+1] : -1; \n if(mat[row][mid]>left && mat[row][mid]>right) return {row, mid};\n else if(mat[row][mid]<left) high = mid-1;\n else low = mid+1;\n }\n return {-1,-1};\n }\n};\n\n```\n```Java []\nclass Solution {\n public int maxEl(int[][] mat, int n, int m, int col) {\n int maxi = -1;\n int ind = -1;\n for (int i = 0; i < n; i++) {\n if (mat[i][col] > maxi) {\n maxi = mat[i][col];\n ind = i;\n }\n }\n return ind;\n }\n\n public int[] findPeakGrid(int[][] mat) {\n int n = mat.length;\n int m = mat[0].length;\n int low = 0;\n int high = m - 1;\n\n while (low <= high) {\n int mid = (low + high) / 2;\n int row = maxEl(mat, n, m, mid);\n int left = mid - 1 >= 0 ? mat[row][mid - 1] : -1;\n int right = mid + 1 < m ? mat[row][mid + 1] : -1;\n\n if (mat[row][mid] > left && mat[row][mid] > right)\n return new int[]{row, mid};\n else if (mat[row][mid] < left)\n high = mid - 1;\n else\n low = mid + 1;\n }\n return new int[]{-1, -1};\n }\n}\n\n```\n```Python []\nclass Solution:\n def maxEl(self, mat, n, m, col):\n maxi = -1\n ind = -1\n for i in range(n):\n if mat[i][col] > maxi:\n maxi = mat[i][col]\n ind = i\n return ind\n\n def findPeakGrid(self, mat):\n n = len(mat)\n m = len(mat[0])\n low = 0\n high = m - 1\n\n while low <= high:\n mid = (low + high) // 2\n row = self.maxEl(mat, n, m, mid)\n left = mat[row][mid - 1] if mid - 1 >= 0 else -1\n right = mat[row][mid + 1] if mid + 1 < m else -1\n\n if mat[row][mid] > left and mat[row][mid] > right:\n return [row, mid]\n elif mat[row][mid] < left:\n high = mid - 1\n else:\n low = mid + 1\n return [-1, -1]\n\n```\n | 6 | A **peak** element in a 2D grid is an element that is **strictly greater** than all of its **adjacent** neighbors to the left, right, top, and bottom.
Given a **0-indexed** `m x n` matrix `mat` where **no two adjacent cells are equal**, find **any** peak element `mat[i][j]` and return _the length 2 array_ `[i,j]`.
You may assume that the entire matrix is surrounded by an **outer perimeter** with the value `-1` in each cell.
You must write an algorithm that runs in `O(m log(n))` or `O(n log(m))` time.
**Example 1:**
**Input:** mat = \[\[1,4\],\[3,2\]\]
**Output:** \[0,1\]
**Explanation:** Both 3 and 4 are peak elements so \[1,0\] and \[0,1\] are both acceptable answers.
**Example 2:**
**Input:** mat = \[\[10,20,15\],\[21,30,14\],\[7,16,32\]\]
**Output:** \[1,1\]
**Explanation:** Both 30 and 32 are peak elements so \[1,1\] and \[2,2\] are both acceptable answers.
**Constraints:**
* `m == mat.length`
* `n == mat[i].length`
* `1 <= m, n <= 500`
* `1 <= mat[i][j] <= 105`
* No two adjacent cells are equal. | Let's note that we want to either decrease the sum of the array with a larger sum or increase the array's sum with the smaller sum. You can maintain the largest increase or decrease you can make in a binary search tree and each time get the maximum one. |
✨✅ (M+N) Solution | Prove me wrong | M+N< MlogN and M+N<NlogM ✅✨ | find-a-peak-element-ii | 0 | 1 | # Approach\nTraverse Towards the larger element\n\n# Complexity\n- Time complexity:\n O(2*(M+N))=O(M+N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findPeakGrid(self, mat: List[List[int]]) -> List[int]:\n M,N=len(mat),len(mat[0])#M rows N cols\n def isPeak(row,col):\n return (\n (row == 0 or mat[row][col] > mat[row - 1][col]) and\n (row == M - 1 or mat[row][col] > mat[row + 1][col]) and\n (col == 0 or mat[row][col] > mat[row][col - 1]) and\n (col == N - 1 or mat[row][col] > mat[row][col + 1])\n )\n \'\'\'\n (row,col)---->\n |\n |\n |\n V\n O(M+N)\n \'\'\'\n row,col=0,0\n while (row<M) and (col<N):\n if isPeak(row,col):\n return [row,col]\n # Reached last row\n elif row==M-1:\n col=col+1\n # Reached Last col\n elif col==N-1:\n row=row+1\n # el less than both its right and bottom neighbour we travel in greater neighbour\'s direction\n elif mat[row][col]<mat[row+1][col] and mat[row][col]<mat[row][col+1]:\n if mat[row+1][col]>=mat[row][col+1]:\n row=row+1\n else:\n col=col+1\n # Hereafter el can only be lesser than one neighbour All other cases are checked\n # el less than bottom\n elif mat[row][col]<mat[row+1][col]:\n row=row+1\n # el less than right\n else:\n col=col+1\n \n \'\'\'\n <-------(row,col)\n |\n |\n |\n V\n O(M+N)\n \'\'\'\n \'\'\'\n For cases like [[1,2,6],[3,4,5]] First While loop fails to produce a solution\n \'\'\'\n row,col=0,N-1\n while (row<=M-1) and (col>=0):\n if isPeak(row,col):\n return [row,col]\n # Reached last row\n elif row==M-1:\n col=col-1\n # Reached First col\n elif col==0:\n row=row+1\n # el less than both its left and bottom neighbour we travel in greater neighbour\'s direction\n elif mat[row][col]<mat[row+1][col] and mat[row][col]<mat[row][col-1]:\n if mat[row+1][col]>=mat[row][col-1]:\n row=row+1\n else:\n col=col-1\n # Hereafter el can only be lesser than one neighbour All other cases are checked\n # el less than bottom\n elif mat[row][col]<mat[row+1][col]:\n row=row+1\n # el less than right\n else:\n col=col-1\n```\nAll suggestions are welcome.\nIf you have any query or suggestion please comment below.\nPlease upvote\uD83D\uDC4D if you like\uD83D\uDC97 it. Thank you:-)\nHappy Learning, Cheers Guys \uD83D\uDE0A | 2 | A **peak** element in a 2D grid is an element that is **strictly greater** than all of its **adjacent** neighbors to the left, right, top, and bottom.
Given a **0-indexed** `m x n` matrix `mat` where **no two adjacent cells are equal**, find **any** peak element `mat[i][j]` and return _the length 2 array_ `[i,j]`.
You may assume that the entire matrix is surrounded by an **outer perimeter** with the value `-1` in each cell.
You must write an algorithm that runs in `O(m log(n))` or `O(n log(m))` time.
**Example 1:**
**Input:** mat = \[\[1,4\],\[3,2\]\]
**Output:** \[0,1\]
**Explanation:** Both 3 and 4 are peak elements so \[1,0\] and \[0,1\] are both acceptable answers.
**Example 2:**
**Input:** mat = \[\[10,20,15\],\[21,30,14\],\[7,16,32\]\]
**Output:** \[1,1\]
**Explanation:** Both 30 and 32 are peak elements so \[1,1\] and \[2,2\] are both acceptable answers.
**Constraints:**
* `m == mat.length`
* `n == mat[i].length`
* `1 <= m, n <= 500`
* `1 <= mat[i][j] <= 105`
* No two adjacent cells are equal. | Let's note that we want to either decrease the sum of the array with a larger sum or increase the array's sum with the smaller sum. You can maintain the largest increase or decrease you can make in a binary search tree and each time get the maximum one. |
Python || 95.26% Faster || O(n log(m)) || Binary Search | find-a-peak-element-ii | 0 | 1 | ```\nclass Solution:\n def findMax(self, col, matrix, rows):\n maxi = float(\'-inf\')\n rownum = -1\n for i in range(rows):\n if maxi < matrix[i][col]:\n maxi = matrix[i][col]\n rownum = i\n return rownum\n \n def findPeakGrid(self, mat: List[List[int]]) -> List[int]:\n n, m = len(mat), len(mat[0])\n low = 0\n high = m - 1\n while low <= high:\n mid = (low + high)//2\n row = self.findMax(mid, mat, n)\n left = right = -1\n if mid-1 >= 0:\n left = mat[row][mid-1]\n if mid+1 < m:\n right = mat[row][mid+1]\n if (mat[row][mid] > left) and (mat[row][mid] > right):\n return [row, mid]\n elif mat[row][mid] < left:\n high = mid - 1\n else:\n low = mid + 1\n```\n**An upvote will be encouraging** | 1 | A **peak** element in a 2D grid is an element that is **strictly greater** than all of its **adjacent** neighbors to the left, right, top, and bottom.
Given a **0-indexed** `m x n` matrix `mat` where **no two adjacent cells are equal**, find **any** peak element `mat[i][j]` and return _the length 2 array_ `[i,j]`.
You may assume that the entire matrix is surrounded by an **outer perimeter** with the value `-1` in each cell.
You must write an algorithm that runs in `O(m log(n))` or `O(n log(m))` time.
**Example 1:**
**Input:** mat = \[\[1,4\],\[3,2\]\]
**Output:** \[0,1\]
**Explanation:** Both 3 and 4 are peak elements so \[1,0\] and \[0,1\] are both acceptable answers.
**Example 2:**
**Input:** mat = \[\[10,20,15\],\[21,30,14\],\[7,16,32\]\]
**Output:** \[1,1\]
**Explanation:** Both 30 and 32 are peak elements so \[1,1\] and \[2,2\] are both acceptable answers.
**Constraints:**
* `m == mat.length`
* `n == mat[i].length`
* `1 <= m, n <= 500`
* `1 <= mat[i][j] <= 105`
* No two adjacent cells are equal. | Let's note that we want to either decrease the sum of the array with a larger sum or increase the array's sum with the smaller sum. You can maintain the largest increase or decrease you can make in a binary search tree and each time get the maximum one. |
Python3 beats 96.22% 🚀🚀 || quibler7 | find-a-peak-element-ii | 0 | 1 | \n\n# Code\n```\nclass Solution:\n\n def findPeakGrid(self, mat: List[List[int]]) -> List[int]:\n L, H = 0, len(mat) - 1\n #as long as we have at least one row left to consider, continue binary search!\n while L <= H:\n mid = (L + H) // 2\n mid_row = mat[mid]\n #initialize max_pos and store column pos later!\n max_pos = None\n max_val = float(-inf)\n #iterate linearly through the row since it\'s \n # not sorted and find the maximum element as well\n # as its position!\n for j in range(len(mid_row)):\n if(mid_row[j] > max_val):\n max_val = mid_row[j]\n max_pos = j\n continue\n #once we have max_pos, then we have to \n # compare relative to top and bottom neighbors!\n top_val = -1 if mid - 1 < 0 else mat[mid-1][max_pos]\n bottom_val = -1 if mid + 1 >= len(mat) else mat[mid+1][max_pos]\n #then it\'s a peak!\n if(top_val < max_val and bottom_val < max_val):\n return [mid, max_pos]\n #top neighboring element is bigger! -> it has better chance to be peak element!\n if(top_val >= max_val):\n H = mid - 1 \n continue\n if(bottom_val >= max_val):\n L = mid + 1\n continue\n``` | 2 | A **peak** element in a 2D grid is an element that is **strictly greater** than all of its **adjacent** neighbors to the left, right, top, and bottom.
Given a **0-indexed** `m x n` matrix `mat` where **no two adjacent cells are equal**, find **any** peak element `mat[i][j]` and return _the length 2 array_ `[i,j]`.
You may assume that the entire matrix is surrounded by an **outer perimeter** with the value `-1` in each cell.
You must write an algorithm that runs in `O(m log(n))` or `O(n log(m))` time.
**Example 1:**
**Input:** mat = \[\[1,4\],\[3,2\]\]
**Output:** \[0,1\]
**Explanation:** Both 3 and 4 are peak elements so \[1,0\] and \[0,1\] are both acceptable answers.
**Example 2:**
**Input:** mat = \[\[10,20,15\],\[21,30,14\],\[7,16,32\]\]
**Output:** \[1,1\]
**Explanation:** Both 30 and 32 are peak elements so \[1,1\] and \[2,2\] are both acceptable answers.
**Constraints:**
* `m == mat.length`
* `n == mat[i].length`
* `1 <= m, n <= 500`
* `1 <= mat[i][j] <= 105`
* No two adjacent cells are equal. | Let's note that we want to either decrease the sum of the array with a larger sum or increase the array's sum with the smaller sum. You can maintain the largest increase or decrease you can make in a binary search tree and each time get the maximum one. |
Intuitive (not binary) search | find-a-peak-element-ii | 0 | 1 | # Code\n```\nclass Solution:\n def findPeakGrid(self, mat: List[List[int]]) -> List[int]:\n \n # Modify array to look like example test case just for safety\n m = len(mat)\n n = len(mat[0])\n padding = [-1]*(n+2)\n for row in mat:\n row.append(-1)\n row.insert(0,-1)\n mat.append(padding)\n mat.insert(0,padding)\n\n # Initialse start as first row first column\n i = 1\n j = 1\n \n #Search for higher element in the adjacent cells, return if no higher elements found \n while(True):\n cur = mat[i][j]\n if (mat[i][j+1] > cur):\n j +=1\n elif (mat[i+1][j] > cur):\n i +=1\n elif (mat[i][j-1]>cur):\n j -=1\n elif(mat[i-1][j]>cur):\n i-=1\n else:\n return [i-1,j-1]\n \n\n```\n# Thoughts\n<!-- Describe your first thoughts on how to solve this problem. -->\nTheorectically less optimal than binary search since this is a more like a linear search but better than brute force search. However, this still beats 85% of submission as of 1/2/2023 so practically may not make much of a difference?\n | 2 | A **peak** element in a 2D grid is an element that is **strictly greater** than all of its **adjacent** neighbors to the left, right, top, and bottom.
Given a **0-indexed** `m x n` matrix `mat` where **no two adjacent cells are equal**, find **any** peak element `mat[i][j]` and return _the length 2 array_ `[i,j]`.
You may assume that the entire matrix is surrounded by an **outer perimeter** with the value `-1` in each cell.
You must write an algorithm that runs in `O(m log(n))` or `O(n log(m))` time.
**Example 1:**
**Input:** mat = \[\[1,4\],\[3,2\]\]
**Output:** \[0,1\]
**Explanation:** Both 3 and 4 are peak elements so \[1,0\] and \[0,1\] are both acceptable answers.
**Example 2:**
**Input:** mat = \[\[10,20,15\],\[21,30,14\],\[7,16,32\]\]
**Output:** \[1,1\]
**Explanation:** Both 30 and 32 are peak elements so \[1,1\] and \[2,2\] are both acceptable answers.
**Constraints:**
* `m == mat.length`
* `n == mat[i].length`
* `1 <= m, n <= 500`
* `1 <= mat[i][j] <= 105`
* No two adjacent cells are equal. | Let's note that we want to either decrease the sum of the array with a larger sum or increase the array's sum with the smaller sum. You can maintain the largest increase or decrease you can make in a binary search tree and each time get the maximum one. |
10 lines, beats 100%, O(m + n) | find-a-peak-element-ii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findPeakGrid(self, mat: List[List[int]]) -> List[int]:\n x, y = 0, len(mat[0]) - 1\n while x < len(mat) or y >= 0:\n if x + 1 < len(mat) and mat[x + 1][y] > mat[x][y]:\n x += 1\n elif y - 1 >= 0 and mat[x][y - 1] > mat[x][y]:\n y -= 1\n else:\n break\n return [x, y]\n``` | 1 | A **peak** element in a 2D grid is an element that is **strictly greater** than all of its **adjacent** neighbors to the left, right, top, and bottom.
Given a **0-indexed** `m x n` matrix `mat` where **no two adjacent cells are equal**, find **any** peak element `mat[i][j]` and return _the length 2 array_ `[i,j]`.
You may assume that the entire matrix is surrounded by an **outer perimeter** with the value `-1` in each cell.
You must write an algorithm that runs in `O(m log(n))` or `O(n log(m))` time.
**Example 1:**
**Input:** mat = \[\[1,4\],\[3,2\]\]
**Output:** \[0,1\]
**Explanation:** Both 3 and 4 are peak elements so \[1,0\] and \[0,1\] are both acceptable answers.
**Example 2:**
**Input:** mat = \[\[10,20,15\],\[21,30,14\],\[7,16,32\]\]
**Output:** \[1,1\]
**Explanation:** Both 30 and 32 are peak elements so \[1,1\] and \[2,2\] are both acceptable answers.
**Constraints:**
* `m == mat.length`
* `n == mat[i].length`
* `1 <= m, n <= 500`
* `1 <= mat[i][j] <= 105`
* No two adjacent cells are equal. | Let's note that we want to either decrease the sum of the array with a larger sum or increase the array's sum with the smaller sum. You can maintain the largest increase or decrease you can make in a binary search tree and each time get the maximum one. |
Python 3 | Binary Search | Explanation | find-a-peak-element-ii | 0 | 1 | ### Explanation\n- Below code is an implementation to `hint` section\n- Start from `mid` column, if a peak is found in this column, return its location\n\t- If peak is on the left, then do a binary search on the left side `columns between [left, mid-1]`\n\t- If peak is on the right, then do a binary search on the right side `columns between [mid+1, right]`\n- Time: `O(m*log(n))`\n- Space: `O(1)`\n- Thanks @andy_ng for helping improve this solution\n### Implementation\n```\nclass Solution:\n def findPeakGrid(self, mat: List[List[int]]) -> List[int]:\n m, n = len(mat), len(mat[0])\n l, r = 0, n\n while l <= r:\n mid = (l + r) // 2\n cur_max, left = 0, False\n for i in range(m):\n if i > 0 and mat[i-1][mid] >= mat[i][mid]:\n continue\n if i+1 < m and mat[i+1][mid] >= mat[i][mid]: \n continue\n if mid+1 < n and mat[i][mid+1] >= mat[i][mid]: \n cur_max, left = mat[i][mid], not mat[i][mid] > cur_max\n continue\n if mid > 0 and mat[i][mid-1] >= mat[i][mid]: \n cur_max, left = mat[i][mid], mat[i][mid] > cur_max\n continue\n return [i, mid]\n if left:\n r = mid-1\n else:\n l = mid+1\n return []\n``` | 22 | A **peak** element in a 2D grid is an element that is **strictly greater** than all of its **adjacent** neighbors to the left, right, top, and bottom.
Given a **0-indexed** `m x n` matrix `mat` where **no two adjacent cells are equal**, find **any** peak element `mat[i][j]` and return _the length 2 array_ `[i,j]`.
You may assume that the entire matrix is surrounded by an **outer perimeter** with the value `-1` in each cell.
You must write an algorithm that runs in `O(m log(n))` or `O(n log(m))` time.
**Example 1:**
**Input:** mat = \[\[1,4\],\[3,2\]\]
**Output:** \[0,1\]
**Explanation:** Both 3 and 4 are peak elements so \[1,0\] and \[0,1\] are both acceptable answers.
**Example 2:**
**Input:** mat = \[\[10,20,15\],\[21,30,14\],\[7,16,32\]\]
**Output:** \[1,1\]
**Explanation:** Both 30 and 32 are peak elements so \[1,1\] and \[2,2\] are both acceptable answers.
**Constraints:**
* `m == mat.length`
* `n == mat[i].length`
* `1 <= m, n <= 500`
* `1 <= mat[i][j] <= 105`
* No two adjacent cells are equal. | Let's note that we want to either decrease the sum of the array with a larger sum or increase the array's sum with the smaller sum. You can maintain the largest increase or decrease you can make in a binary search tree and each time get the maximum one. |
[Python, C++] Brute-force > Optimal | With Explanation | find-a-peak-element-ii | 0 | 1 | ### Find a Peak Element II\n\nA peak element in a 2D grid is an element that is strictly greater than all of its adjacent neighbors to the left, right, top, and bottom. You may assume that the entire matrix is surrounded by an outer perimeter with the value -1 in each cell.\n\n- Approach\n - Brute-force\n - Go through all the elements and check for peak element\n - Time Complexity: $O(n * m)$\n - Space Complexity: $O(1)$\n - Optimal\n - For each row repeat the process\n - There are multiple sorted parts in the row\n - Thus we can apply binary search\n - Check if mid is the peak else search in the direction where the sequence is increasing i.e. search on left side if mat[i][mid] < mat[i][mid+1] and mat[i][mid-1] < nums[mid+1] else search on right side\n - Time Complexity: $O(nlogm)$\n - Space Complexity: $O(1)$\n\n```python\n# Python3\n# Brute-force Solution\nclass Solution:\n def peak(self, mat, n, m, i, mid):\n top = -1 if i < 0 else mat[i - 1][mid]\n bottom = -1 if i == n - 1 else mat[i + 1][mid]\n left = -1 if mid < 0 else mat[i][mid - 1]\n right = -1 if mid == m - 1 else mat[i][mid + 1]\n return (mat[i][mid] > top and mat[i][mid] > bottom and mat[i][mid] > left and mat[i][mid] > right)\n \n def findPeakGrid(self, mat: List[List[int]]) -> List[int]:\n n = len(mat)\n m = len(mat[0])\n\n for i in range(n):\n for j in range(m):\n if self.peak(mat, n, m, i, j):\n return [i, j]\n return []\n```\n\n```python\n# Python3\n# Optimal Solution\nclass Solution:\n def peak(self, mat, n, m, i, mid):\n top = -1 if i < 0 else mat[i - 1][mid]\n bottom = -1 if i == n - 1 else mat[i + 1][mid]\n left = -1 if mid < 0 else mat[i][mid - 1]\n right = -1 if mid == m - 1 else mat[i][mid + 1]\n return (mat[i][mid] > top and mat[i][mid] > bottom and mat[i][mid] > left and mat[i][mid] > right)\n \n def findPeakGrid(self, mat: List[List[int]]) -> List[int]:\n n = len(mat)\n m = len(mat[0])\n\n for i in range(n):\n if self.peak(mat, n, m, i, 0): return [i, 0]\n if self.peak(mat, n, m, i, m - 1): return [i, m - 1]\n \n low = 1\n high = m - 2\n\n while low <= high:\n mid = (low + high) >> 1\n\n if self.peak(mat, n, m, i, mid):\n return [i, mid]\n elif mat[i][mid] < mat[i][mid + 1] and mat[i][mid - 1] < mat[i][mid + 1]:\n low = mid + 1\n else:\n high = mid - 1\n return []\n```\n\n```cpp\n// C++\n// Optimal Solution\nclass Solution {\npublic:\n bool peak(vector<vector<int>>& mat, int n, int m, int row, int col) {\n int top = row == 0 ? -1 : mat[row - 1][col];\n int bottom = row == n - 1 ? -1 : mat[row + 1][col];\n int left = col == 0 ? -1 : mat[row][col - 1];\n int right = col == m - 1 ? -1 : mat[row][col + 1];\n\n return (mat[row][col] > top && mat[row][col] > bottom && mat[row][col] > left && mat[row][col] > right);\n }\n\n vector<int> findPeakGrid(vector<vector<int>>& mat) {\n int n = mat.size();\n int m = mat[0].size();\n\n for (int row = 0; row < n; row++) {\n if (peak(mat, n, m, row, 0)) {return {row, 0};}\n if (peak(mat, n, m, row, m-1)) {return {row, m-1};}\n \n int low = 1;\n int high = m - 2;\n\n while (low <= high) {\n int mid = low + (high - low) / 2;\n\n if (peak(mat, n, m, row, mid)) {return {row, mid};}\n else if (mat[row][mid] < mat[row][mid + 1] && mat[row][mid - 1] < mat[row][mid + 1]) {low = mid + 1;}\n else {high = mid - 1;}\n }\n }\n return {};\n }\n};\n``` | 2 | A **peak** element in a 2D grid is an element that is **strictly greater** than all of its **adjacent** neighbors to the left, right, top, and bottom.
Given a **0-indexed** `m x n` matrix `mat` where **no two adjacent cells are equal**, find **any** peak element `mat[i][j]` and return _the length 2 array_ `[i,j]`.
You may assume that the entire matrix is surrounded by an **outer perimeter** with the value `-1` in each cell.
You must write an algorithm that runs in `O(m log(n))` or `O(n log(m))` time.
**Example 1:**
**Input:** mat = \[\[1,4\],\[3,2\]\]
**Output:** \[0,1\]
**Explanation:** Both 3 and 4 are peak elements so \[1,0\] and \[0,1\] are both acceptable answers.
**Example 2:**
**Input:** mat = \[\[10,20,15\],\[21,30,14\],\[7,16,32\]\]
**Output:** \[1,1\]
**Explanation:** Both 30 and 32 are peak elements so \[1,1\] and \[2,2\] are both acceptable answers.
**Constraints:**
* `m == mat.length`
* `n == mat[i].length`
* `1 <= m, n <= 500`
* `1 <= mat[i][j] <= 105`
* No two adjacent cells are equal. | Let's note that we want to either decrease the sum of the array with a larger sum or increase the array's sum with the smaller sum. You can maintain the largest increase or decrease you can make in a binary search tree and each time get the maximum one. |
C/C++/Python string ->1 line||0 ms Beats 100% | largest-odd-number-in-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nLet $x$ be a natural number expressed in decimal digit representation.\nThen $x$ is odd $\\iff$ its least significant digit is an odd.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nNote `int(\'0\')`=48, it suffices to check if `num[i]%2==1` which can by done by `num[i]&1`. \n\nIterate the string `num` backward!\n\nPython code is 1-line.\n\nC code is rare which is fastest among all implemenations & runs in 0 ms & beats 100%.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$ where `n=len(num)`\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(1)$$\n# Code 11 ms Beats 99.88%\n```\n#pragma GCC optimize("O3", "unroll-loops")\nclass Solution {\npublic:\n string largestOddNumber(string& num) {\n for(int i=num.size()-1; i>=0; i--){\n if (num[i]&1)\n return move(num.substr(0, i+1));\n } \n return ""; \n }\n};\nauto init = []()\n{ \n ios::sync_with_stdio(0);\n cin.tie(0);\n cout.tie(0);\n return \'c\';\n}();\n```\n# C++ 1 line\n```\n string largestOddNumber(string& num) {\n return num.substr(0, num.find_last_of("13579")+1); \n }\n```\n# Python 1 line\n```\nclass Solution:\n def largestOddNumber(self, num: str) -> str:\n return num[:next((i for i in range(len(num)-1, -1, -1) if ord(num[i]) &1==1), -1) + 1]\n\n \n```\n# C code 0 ms Beats 100%\n```\nchar* largestOddNumber(char* num) {\n for(register int i=strlen(num)-1; i>=0; i--)\n if (num[i]&1){\n num[i+1]=\'\\0\';\n return num;\n }\n return "";\n}\n```\n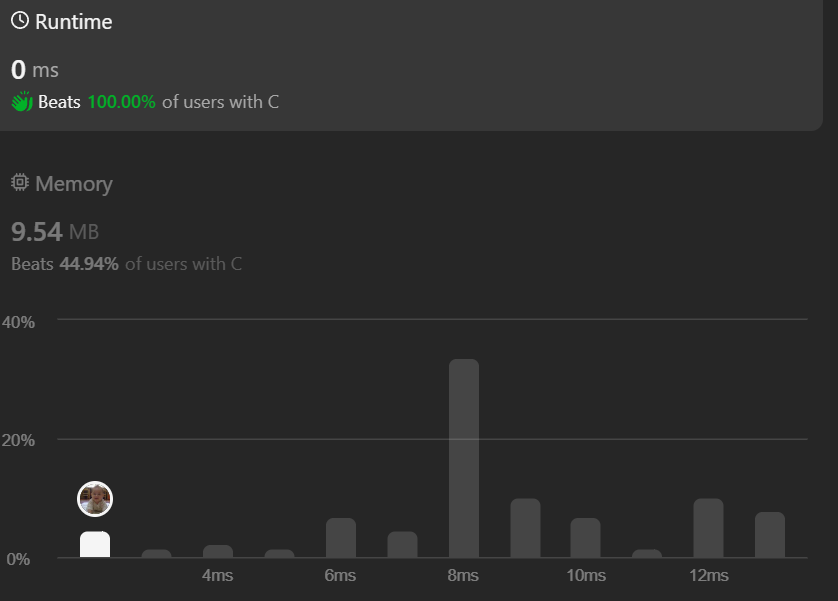\n | 9 | You are given a string `num`, representing a large integer. Return _the **largest-valued odd** integer (as a string) that is a **non-empty substring** of_ `num`_, or an empty string_ `" "` _if no odd integer exists_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** num = "52 "
**Output:** "5 "
**Explanation:** The only non-empty substrings are "5 ", "2 ", and "52 ". "5 " is the only odd number.
**Example 2:**
**Input:** num = "4206 "
**Output:** " "
**Explanation:** There are no odd numbers in "4206 ".
**Example 3:**
**Input:** num = "35427 "
**Output:** "35427 "
**Explanation:** "35427 " is already an odd number.
**Constraints:**
* `1 <= num.length <= 105`
* `num` only consists of digits and does not contain any leading zeros. | You can store the data in an array and apply each fetch by moving the ith element to the end of the array (i.e, O(n) per operation). A better way is to use the square root decomposition technique. You can build chunks of size sqrt(n). For each fetch operation, You can search for the chunk which has the ith element and update it (i.e., O(sqrt(n)) per operation), and move this element to an empty chunk at the end. |
✅☑[C++/Java/Python/JavaScript] || Easy Solution || EXPLAINED🔥 | largest-odd-number-in-string | 1 | 1 | # PLEASE UPVOTE IF IT HELPED\n\n---\n\n\n# Approaches\n**(Also explained in the code)**\n1. **Check Last Digit:** Check if the last digit of the input number is odd. If it is, the input number is already the largest odd number. Return the input number in this case.\n1. **Iterative Check**: Starting from the end of the number, iterate backward. If an odd digit is encountered, return the substring from the beginning up to (and including) that odd digit, as this is the largest odd number possible.\n1. **Return:** If no odd digits are found after the loop, return an empty string to indicate that there\'s no odd number in the given input.\n\n\n\n# Complexity\n- *Time complexity:*\n $$O(n)$$\n \n\n- *Space complexity:*\n $$O(1)$$\n \n\n\n# Code\n```C++ []\n\nclass Solution {\npublic:\n string largestOddNumber(string num) {\n int n = num.size(); // Get the size of the input string\n\n\n if (num[n - 1] % 2 != 0) // Check if the last digit of the input number is odd\n return num; // If it is, the input number is already the largest odd number\n\n for (int i = n - 1; i >= 0; i--) {\n if (num[i] % 2 != 0) {\n return num.substr(0, i + 1); // If an odd digit is found, return the substring up to that point\n }\n }\n\n return ""; // If no odd digits are found, return an empty string\n }\n};\n\n\n```\n```C []\n\nchar* largestOddNumber(char* num) {\n int n = strlen(num);\n static char ans[100001]; // Assuming maximum length\n ans[0] = \'\\0\';\n\n if ((num[n - 1] - \'0\') % 2 != 0)\n return num;\n\n for (int i = n - 1; i >= 0; i--) {\n if ((num[i] - \'0\') % 2 != 0) {\n num[i + 1] = \'\\0\';\n strcpy(ans, num);\n return ans;\n }\n }\n\n return "";\n}\n\n\n\n```\n```Java []\nclass Solution {\n public String largestOddNumber(String num) {\n for (int i = num.length() - 1; i >= 0; i--) {\n if (Character.getNumericValue(num.charAt(i)) % 2 != 0) {\n return num.substring(0, i + 1);\n }\n }\n \n return "";\n }\n}\n\n\n```\n```python3 []\nclass Solution:\n def largestOddNumber(self, num: str) -> str:\n for i in range(len(num) - 1, -1, -1):\n if int(num[i]) % 2 != 0:\n return num[:i + 1]\n \n return ""\n\n\n```\n```javascript []\nfunction largestOddNumber(num) {\n let n = num.length;\n\n if (parseInt(num[n - 1]) % 2 !== 0)\n return num;\n\n for (let i = n - 1; i >= 0; i--) {\n if (parseInt(num[i]) % 2 !== 0) {\n return num.substring(0, i + 1);\n }\n }\n\n return \'\';\n}\n\n```\n\n---\n\n\n\n# PLEASE UPVOTE IF IT HELPED\n\n---\n---\n\n\n--- | 19 | You are given a string `num`, representing a large integer. Return _the **largest-valued odd** integer (as a string) that is a **non-empty substring** of_ `num`_, or an empty string_ `" "` _if no odd integer exists_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** num = "52 "
**Output:** "5 "
**Explanation:** The only non-empty substrings are "5 ", "2 ", and "52 ". "5 " is the only odd number.
**Example 2:**
**Input:** num = "4206 "
**Output:** " "
**Explanation:** There are no odd numbers in "4206 ".
**Example 3:**
**Input:** num = "35427 "
**Output:** "35427 "
**Explanation:** "35427 " is already an odd number.
**Constraints:**
* `1 <= num.length <= 105`
* `num` only consists of digits and does not contain any leading zeros. | You can store the data in an array and apply each fetch by moving the ith element to the end of the array (i.e, O(n) per operation). A better way is to use the square root decomposition technique. You can build chunks of size sqrt(n). For each fetch operation, You can search for the chunk which has the ith element and update it (i.e., O(sqrt(n)) per operation), and move this element to an empty chunk at the end. |
【Video】Give me 1 minute - How we think about a solution | largest-odd-number-in-string | 1 | 1 | # Intuition\nIterate through from the last\n\n---\n\n# Solution Video\n\nhttps://youtu.be/f-hyawcTl8U\n\n\u25A0 Timeline of the video\n\n`0:04` Coding\n`1:18` Time Complexity and Space Complexity\n`1:31` Step by step algorithm with my solution code\n\n### \u2B50\uFE0F\u2B50\uFE0F Don\'t forget to subscribe to my channel! \u2B50\uFE0F\u2B50\uFE0F\n\n**\u25A0 Subscribe URL**\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\nSubscribers: 3,374\nMy first goal is 10,000 (It\'s far from done \uD83D\uDE05)\nThank you for your support!\n\n---\n\n# Approach\n\n## How we think about a solution\n\nSimply, in determining whether a digit is even or odd, it is determined by the least significant digit\'s value, so we iterate from the least significant digit.\n\n```\nInput: num = "52"\n```\nWe start iteration from the last.\n```\n"52"\n \u2191\n```\nConvert string `2` to integer `2` then\n\n```[]\nif 2 % 2 == 1\n\u2192 false\n```\nMove next\n```\n"52"\n \u2191\n```\nConvert string `5` to integer `5` then\n```[]\nif 5 % 2 == 1\n\u2192 true\n```\nNow we found the odd number in the least significant digit.\n```\nreturn "5"\n```\n\nLet\'s see a real algorithm!\n\n---\n \n1. **Iteration through Digits:**\n ```python\n for i in range(len(num) - 1, -1, -1):\n ```\n - The loop iterates through each character in the input string `num` in reverse order, starting from the least significant digit (`len(num) - 1`) and going up to the most significant digit (`-1`) with a step of `-1`.\n\n2. **Check for Odd Digit:**\n ```python\n if int(num[i]) % 2 == 1:\n ```\n - For each digit at the current index `i`, it converts the character to an integer and checks if it\'s an odd number (`% 2 == 1`).\n\n3. **Return the Largest Odd Number:**\n ```python\n return num[:i+1]\n ```\n - If an odd digit is found, it returns the substring of the input string from the beginning up to the current index (inclusive). This substring represents the largest odd number.\n\n4. **Return Empty String if No Odd Digit:**\n ```python\n return ""\n ```\n - If no odd digit is found in the entire string, the function returns an empty string.\n\nThis algorithm efficiently identifies and returns the largest odd number by iterating through the digits in reverse order. The process stops as soon as an odd digit is encountered, ensuring that the result is the largest possible odd number.\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n```python []\nclass Solution:\n def largestOddNumber(self, num: str) -> str:\n \n for i in range(len(num) - 1, -1, -1):\n if int(num[i]) % 2 == 1:\n return num[:i+1]\n \n return ""\n```\n```javascript []\n/**\n * @param {string} num\n * @return {string}\n */\nvar largestOddNumber = function(num) {\n for (let i = num.length - 1; i >= 0; i--) {\n if (parseInt(num[i]) % 2 === 1) {\n return num.slice(0, i + 1);\n }\n }\n \n return ""; \n};\n```\n```java []\nclass Solution {\n public String largestOddNumber(String num) {\n for (int i = num.length() - 1; i >= 0; i--) {\n if (Character.getNumericValue(num.charAt(i)) % 2 == 1) {\n return num.substring(0, i + 1);\n }\n }\n return ""; \n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n string largestOddNumber(string num) {\n for (int i = num.length() - 1; i >= 0; i--) {\n if ((num[i] - \'0\') % 2 == 1) {\n return num.substr(0, i + 1);\n }\n }\n return ""; \n }\n};\n```\n\n---\n\nThank you for reading my post.\n\u2B50\uFE0F Please upvote it and don\'t forget to subscribe to my channel!\n\n\u25A0 Subscribe URL\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\n\u25A0 Twitter\nhttps://twitter.com/CodingNinjaAZ\n\n### My next daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/construct-string-from-binary-tree/solutions/4376889/video-give-me-7-minutes-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/gV7MkiwMDS8\n\n\u25A0 Timeline of the video\n\n`0:05` How we create an output string\n`2:07` Coding\n`4:30` Time Complexity and Space Complexity\n`4:49` Step by step of recursion process\n`7:27` Step by step algorithm with my solution code\n\n### My previous daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/calculate-money-in-leetcode-bank/solutions/4368080/video-give-me-10-minutes-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/x9isMgUDncs\n\n\u25A0 Timeline of the video\n`0:06` Understand the description and think about key points\n`1:56` How to calculate weekly base and days within the week\n`4:35` Coding\n`5:15` Time Complexity and Space Complexity\n`5:35` Think about another idea with O(1) time\n`10:22` Coding\n`12:04` Time Complexity and Space Complexity\n | 40 | You are given a string `num`, representing a large integer. Return _the **largest-valued odd** integer (as a string) that is a **non-empty substring** of_ `num`_, or an empty string_ `" "` _if no odd integer exists_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** num = "52 "
**Output:** "5 "
**Explanation:** The only non-empty substrings are "5 ", "2 ", and "52 ". "5 " is the only odd number.
**Example 2:**
**Input:** num = "4206 "
**Output:** " "
**Explanation:** There are no odd numbers in "4206 ".
**Example 3:**
**Input:** num = "35427 "
**Output:** "35427 "
**Explanation:** "35427 " is already an odd number.
**Constraints:**
* `1 <= num.length <= 105`
* `num` only consists of digits and does not contain any leading zeros. | You can store the data in an array and apply each fetch by moving the ith element to the end of the array (i.e, O(n) per operation). A better way is to use the square root decomposition technique. You can build chunks of size sqrt(n). For each fetch operation, You can search for the chunk which has the ith element and update it (i.e., O(sqrt(n)) per operation), and move this element to an empty chunk at the end. |
✅ One Line Solution | largest-odd-number-in-string | 0 | 1 | # Code #1 - The Most Concise\nTime complexity: $$O(n)$$. Space complexity: $$O(1)$$.\n```\nclass Solution:\n def largestOddNumber(self, num: str) -> str:\n return num.rstrip(\'02468\')\n```\n\n# Code #2 - Generator\nTime complexity: $$O(n)$$. Space complexity: $$O(1)$$.\n```\nclass Solution:\n def largestOddNumber(self, num: str) -> str:\n return next((num[:i+1] for i in range(len(num)-1,-1,-1) if num[i] in \'13579\'), \'\')\n```\n\n# Code #3 - Regexp\n```\nclass Solution:\n def largestOddNumber(self, num: str) -> str:\n return re.search(r\'(|.*[13579])[02468]*$\', num).group(1)\n```\n\n# Code #4 - Several Passes\nTime complexity: $$O(5*n)$$. Space complexity: $$O(1)$$.\n```\nclass Solution:\n def largestOddNumber(self, num: str) -> str:\n return num[:max(num.rfind(d) for d in \'13579\')+1]\n``` | 3 | You are given a string `num`, representing a large integer. Return _the **largest-valued odd** integer (as a string) that is a **non-empty substring** of_ `num`_, or an empty string_ `" "` _if no odd integer exists_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** num = "52 "
**Output:** "5 "
**Explanation:** The only non-empty substrings are "5 ", "2 ", and "52 ". "5 " is the only odd number.
**Example 2:**
**Input:** num = "4206 "
**Output:** " "
**Explanation:** There are no odd numbers in "4206 ".
**Example 3:**
**Input:** num = "35427 "
**Output:** "35427 "
**Explanation:** "35427 " is already an odd number.
**Constraints:**
* `1 <= num.length <= 105`
* `num` only consists of digits and does not contain any leading zeros. | You can store the data in an array and apply each fetch by moving the ith element to the end of the array (i.e, O(n) per operation). A better way is to use the square root decomposition technique. You can build chunks of size sqrt(n). For each fetch operation, You can search for the chunk which has the ith element and update it (i.e., O(sqrt(n)) per operation), and move this element to an empty chunk at the end. |
Finding least significant odd digit | largest-odd-number-in-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def largestOddNumber(self, num: str) -> str:\n for i in range(len(num)-1,-1,-1):\n if int(num[i]) % 2 != 0: return num[0:i+1]\n return ""\n``` | 2 | You are given a string `num`, representing a large integer. Return _the **largest-valued odd** integer (as a string) that is a **non-empty substring** of_ `num`_, or an empty string_ `" "` _if no odd integer exists_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** num = "52 "
**Output:** "5 "
**Explanation:** The only non-empty substrings are "5 ", "2 ", and "52 ". "5 " is the only odd number.
**Example 2:**
**Input:** num = "4206 "
**Output:** " "
**Explanation:** There are no odd numbers in "4206 ".
**Example 3:**
**Input:** num = "35427 "
**Output:** "35427 "
**Explanation:** "35427 " is already an odd number.
**Constraints:**
* `1 <= num.length <= 105`
* `num` only consists of digits and does not contain any leading zeros. | You can store the data in an array and apply each fetch by moving the ith element to the end of the array (i.e, O(n) per operation). A better way is to use the square root decomposition technique. You can build chunks of size sqrt(n). For each fetch operation, You can search for the chunk which has the ith element and update it (i.e., O(sqrt(n)) per operation), and move this element to an empty chunk at the end. |
Python easy solution with o(n) complexity | largest-odd-number-in-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\nchecking the last character\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nif the last character is odd we will return the string itself. if not we will check the second last character and it will go on like that until getting an odd number if an odd number is found then the code will return the sliced string which is from 0 th index to the index where we find the odd number. \n\nif we didnt find an odd number we will return an empty string(empty string)\n\n# Complexity\n- Time complexity:O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:o(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def largestOddNumber(self, num: str) -> str:\n for i in range(len(num) - 1, -1, -1):\n if int(num[i])%2==1:\n return num[:i+1]\n else:\n return \'\'\n``` | 1 | You are given a string `num`, representing a large integer. Return _the **largest-valued odd** integer (as a string) that is a **non-empty substring** of_ `num`_, or an empty string_ `" "` _if no odd integer exists_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** num = "52 "
**Output:** "5 "
**Explanation:** The only non-empty substrings are "5 ", "2 ", and "52 ". "5 " is the only odd number.
**Example 2:**
**Input:** num = "4206 "
**Output:** " "
**Explanation:** There are no odd numbers in "4206 ".
**Example 3:**
**Input:** num = "35427 "
**Output:** "35427 "
**Explanation:** "35427 " is already an odd number.
**Constraints:**
* `1 <= num.length <= 105`
* `num` only consists of digits and does not contain any leading zeros. | You can store the data in an array and apply each fetch by moving the ith element to the end of the array (i.e, O(n) per operation). A better way is to use the square root decomposition technique. You can build chunks of size sqrt(n). For each fetch operation, You can search for the chunk which has the ith element and update it (i.e., O(sqrt(n)) per operation), and move this element to an empty chunk at the end. |
search from rightmost odd number | largest-odd-number-in-string | 1 | 1 | java solution\n```\nclass Solution {\n public String largestOddNumber(String num) {\n for(int i=num.length()-1;i>=0;i--){\n if((num.charAt(i)-\'0\')%2==1){\n return num.substring(0,i+1);\n }\n }\n return "";\n }\n}\n```\n\nc++ solution\n```\nclass Solution {\npublic:\n string largestOddNumber(string num) {\n for(int i=num.length()-1;i>=0;i--){\n if((int)(num[i]-\'0\')%2==1)\n return num.substr(0,i+1);\n }\n return "";\n }\n};\n```\n\njavascript solution\n```\nvar largestOddNumber = function(num) {\n for(let i=num.length-1;i>=0;i--){\n if(parseInt(num[i])%2==1){\n return num.substring(0,i+1);\n }\n }\n return "";\n};\n```\n\npython3 solution\n```\nclass Solution:\n def largestOddNumber(self, num: str) -> str:\n for i in range(len(num) - 1, -1, -1):\n if int(num[i]) % 2 == 1:\n return num[:i + 1]\n return ""\n\n```\n\nkotlin solution\n```\nclass Solution {\n fun largestOddNumber(num: String): String {\n for (i in num.length - 1 downTo 0) {\n if ((num[i] - \'0\') % 2 == 1) {\n return num.substring(0, i + 1)\n }\n }\n return ""\n }\n}\n```\n | 1 | You are given a string `num`, representing a large integer. Return _the **largest-valued odd** integer (as a string) that is a **non-empty substring** of_ `num`_, or an empty string_ `" "` _if no odd integer exists_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** num = "52 "
**Output:** "5 "
**Explanation:** The only non-empty substrings are "5 ", "2 ", and "52 ". "5 " is the only odd number.
**Example 2:**
**Input:** num = "4206 "
**Output:** " "
**Explanation:** There are no odd numbers in "4206 ".
**Example 3:**
**Input:** num = "35427 "
**Output:** "35427 "
**Explanation:** "35427 " is already an odd number.
**Constraints:**
* `1 <= num.length <= 105`
* `num` only consists of digits and does not contain any leading zeros. | You can store the data in an array and apply each fetch by moving the ith element to the end of the array (i.e, O(n) per operation). A better way is to use the square root decomposition technique. You can build chunks of size sqrt(n). For each fetch operation, You can search for the chunk which has the ith element and update it (i.e., O(sqrt(n)) per operation), and move this element to an empty chunk at the end. |
🤔 O(n) | largest-odd-number-in-string | 0 | 1 | \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nFind the last index of odd number in the string.\nAny number that ends with odd number is odd number.\n\n\n# Complexity\n- Time complexity:$$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def largestOddNumber(self, num: str) -> str:\n h={"1","3","5","7","9"}\n ind=-1\n for i in range(len(num)-1,-1,-1):\n if num[i] not in h :continue\n ind=i\n break\n \n return num[:ind+1] if ind!=-1 else ""\n \n``` | 1 | You are given a string `num`, representing a large integer. Return _the **largest-valued odd** integer (as a string) that is a **non-empty substring** of_ `num`_, or an empty string_ `" "` _if no odd integer exists_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** num = "52 "
**Output:** "5 "
**Explanation:** The only non-empty substrings are "5 ", "2 ", and "52 ". "5 " is the only odd number.
**Example 2:**
**Input:** num = "4206 "
**Output:** " "
**Explanation:** There are no odd numbers in "4206 ".
**Example 3:**
**Input:** num = "35427 "
**Output:** "35427 "
**Explanation:** "35427 " is already an odd number.
**Constraints:**
* `1 <= num.length <= 105`
* `num` only consists of digits and does not contain any leading zeros. | You can store the data in an array and apply each fetch by moving the ith element to the end of the array (i.e, O(n) per operation). A better way is to use the square root decomposition technique. You can build chunks of size sqrt(n). For each fetch operation, You can search for the chunk which has the ith element and update it (i.e., O(sqrt(n)) per operation), and move this element to an empty chunk at the end. |
[Python3] math-ish | the-number-of-full-rounds-you-have-played | 0 | 1 | \n```\nclass Solution:\n def numberOfRounds(self, startTime: str, finishTime: str) -> int:\n hs, ms = (int(x) for x in startTime.split(":"))\n ts = 60 * hs + ms\n hf, mf = (int(x) for x in finishTime.split(":"))\n tf = 60 * hf + mf\n if 0 <= tf - ts < 15: return 0 # edge case \n return tf//15 - (ts+14)//15 + (ts>tf)*96\n```\n\n```\nclass Solution:\n def numberOfRounds(self, startTime: str, finishTime: str) -> int:\n ts = 60 * int(startTime[:2]) + int(startTime[-2:])\n tf = 60 * int(finishTime[:2]) + int(finishTime[-2:])\n if 0 <= tf - ts < 15: return 0 # edge case \n return tf//15 - (ts+14)//15 + (ts>tf)*96\n``` | 20 | You are participating in an online chess tournament. There is a chess round that starts every `15` minutes. The first round of the day starts at `00:00`, and after every `15` minutes, a new round starts.
* For example, the second round starts at `00:15`, the fourth round starts at `00:45`, and the seventh round starts at `01:30`.
You are given two strings `loginTime` and `logoutTime` where:
* `loginTime` is the time you will login to the game, and
* `logoutTime` is the time you will logout from the game.
If `logoutTime` is **earlier** than `loginTime`, this means you have played from `loginTime` to midnight and from midnight to `logoutTime`.
Return _the number of full chess rounds you have played in the tournament_.
**Note:** All the given times follow the 24-hour clock. That means the first round of the day starts at `00:00` and the last round of the day starts at `23:45`.
**Example 1:**
**Input:** loginTime = "09:31 ", logoutTime = "10:14 "
**Output:** 1
**Explanation:** You played one full round from 09:45 to 10:00.
You did not play the full round from 09:30 to 09:45 because you logged in at 09:31 after it began.
You did not play the full round from 10:00 to 10:15 because you logged out at 10:14 before it ended.
**Example 2:**
**Input:** loginTime = "21:30 ", logoutTime = "03:00 "
**Output:** 22
**Explanation:** You played 10 full rounds from 21:30 to 00:00 and 12 full rounds from 00:00 to 03:00.
10 + 12 = 22.
**Constraints:**
* `loginTime` and `logoutTime` are in the format `hh:mm`.
* `00 <= hh <= 23`
* `00 <= mm <= 59`
* `loginTime` and `logoutTime` are not equal. | First of all, get the distinct characters since we are only interested in those Let's note that there might not be any digits. |
easy Python :D | the-number-of-full-rounds-you-have-played | 0 | 1 | \n```\nclass Solution:\n def numberOfRounds(self, loginTime: str, logoutTime: str) -> int:\n def time_to_minutes(time_str: str) -> int:\n h, m = map(int, time_str.split(":"))\n return h * 60 + m\n\n login = time_to_minutes(loginTime)\n logout = time_to_minutes(logoutTime)\n\n if logout < login:\n logout += 60 * 24\n\n res = (logout // 15) - ((login + 14) // 15)\n return max(res, 0)\n``` | 0 | You are participating in an online chess tournament. There is a chess round that starts every `15` minutes. The first round of the day starts at `00:00`, and after every `15` minutes, a new round starts.
* For example, the second round starts at `00:15`, the fourth round starts at `00:45`, and the seventh round starts at `01:30`.
You are given two strings `loginTime` and `logoutTime` where:
* `loginTime` is the time you will login to the game, and
* `logoutTime` is the time you will logout from the game.
If `logoutTime` is **earlier** than `loginTime`, this means you have played from `loginTime` to midnight and from midnight to `logoutTime`.
Return _the number of full chess rounds you have played in the tournament_.
**Note:** All the given times follow the 24-hour clock. That means the first round of the day starts at `00:00` and the last round of the day starts at `23:45`.
**Example 1:**
**Input:** loginTime = "09:31 ", logoutTime = "10:14 "
**Output:** 1
**Explanation:** You played one full round from 09:45 to 10:00.
You did not play the full round from 09:30 to 09:45 because you logged in at 09:31 after it began.
You did not play the full round from 10:00 to 10:15 because you logged out at 10:14 before it ended.
**Example 2:**
**Input:** loginTime = "21:30 ", logoutTime = "03:00 "
**Output:** 22
**Explanation:** You played 10 full rounds from 21:30 to 00:00 and 12 full rounds from 00:00 to 03:00.
10 + 12 = 22.
**Constraints:**
* `loginTime` and `logoutTime` are in the format `hh:mm`.
* `00 <= hh <= 23`
* `00 <= mm <= 59`
* `loginTime` and `logoutTime` are not equal. | First of all, get the distinct characters since we are only interested in those Let's note that there might not be any digits. |
Python | Mod 15 | the-number-of-full-rounds-you-have-played | 0 | 1 | \n# Code\n```\nclass Solution:\n def numberOfRounds(self, loginTime: str, logoutTime: str) -> int:\n h, m = loginTime.split(":")\n login = int(h)*60 + int(m)\n h, m = logoutTime.split(":")\n logout = int(h)*60 + int(m)\n res = (logout+(24*60 if logout < login else 0))//15 - (login + 14)//15\n return res if res > 0 else 0\n``` | 0 | You are participating in an online chess tournament. There is a chess round that starts every `15` minutes. The first round of the day starts at `00:00`, and after every `15` minutes, a new round starts.
* For example, the second round starts at `00:15`, the fourth round starts at `00:45`, and the seventh round starts at `01:30`.
You are given two strings `loginTime` and `logoutTime` where:
* `loginTime` is the time you will login to the game, and
* `logoutTime` is the time you will logout from the game.
If `logoutTime` is **earlier** than `loginTime`, this means you have played from `loginTime` to midnight and from midnight to `logoutTime`.
Return _the number of full chess rounds you have played in the tournament_.
**Note:** All the given times follow the 24-hour clock. That means the first round of the day starts at `00:00` and the last round of the day starts at `23:45`.
**Example 1:**
**Input:** loginTime = "09:31 ", logoutTime = "10:14 "
**Output:** 1
**Explanation:** You played one full round from 09:45 to 10:00.
You did not play the full round from 09:30 to 09:45 because you logged in at 09:31 after it began.
You did not play the full round from 10:00 to 10:15 because you logged out at 10:14 before it ended.
**Example 2:**
**Input:** loginTime = "21:30 ", logoutTime = "03:00 "
**Output:** 22
**Explanation:** You played 10 full rounds from 21:30 to 00:00 and 12 full rounds from 00:00 to 03:00.
10 + 12 = 22.
**Constraints:**
* `loginTime` and `logoutTime` are in the format `hh:mm`.
* `00 <= hh <= 23`
* `00 <= mm <= 59`
* `loginTime` and `logoutTime` are not equal. | First of all, get the distinct characters since we are only interested in those Let's note that there might not be any digits. |
Python | Bruteforce | Easy Solution | the-number-of-full-rounds-you-have-played | 0 | 1 | # Code\n```\nclass Solution:\n def numberOfRounds(self, loginTime: str, logoutTime: str) -> int:\n h, m = loginTime.split(":")\n login = int(h)*60 + int(m)\n h, m = logoutTime.split(":")\n logout = int(h)*60 + int(m)\n if logout < login:\n logout += 60*24\n res = 0\n start = False\n for i in range(login, logout + 1):\n if not i%15:\n if start:\n res += 1\n else:\n start = True\n return res\n\n``` | 0 | You are participating in an online chess tournament. There is a chess round that starts every `15` minutes. The first round of the day starts at `00:00`, and after every `15` minutes, a new round starts.
* For example, the second round starts at `00:15`, the fourth round starts at `00:45`, and the seventh round starts at `01:30`.
You are given two strings `loginTime` and `logoutTime` where:
* `loginTime` is the time you will login to the game, and
* `logoutTime` is the time you will logout from the game.
If `logoutTime` is **earlier** than `loginTime`, this means you have played from `loginTime` to midnight and from midnight to `logoutTime`.
Return _the number of full chess rounds you have played in the tournament_.
**Note:** All the given times follow the 24-hour clock. That means the first round of the day starts at `00:00` and the last round of the day starts at `23:45`.
**Example 1:**
**Input:** loginTime = "09:31 ", logoutTime = "10:14 "
**Output:** 1
**Explanation:** You played one full round from 09:45 to 10:00.
You did not play the full round from 09:30 to 09:45 because you logged in at 09:31 after it began.
You did not play the full round from 10:00 to 10:15 because you logged out at 10:14 before it ended.
**Example 2:**
**Input:** loginTime = "21:30 ", logoutTime = "03:00 "
**Output:** 22
**Explanation:** You played 10 full rounds from 21:30 to 00:00 and 12 full rounds from 00:00 to 03:00.
10 + 12 = 22.
**Constraints:**
* `loginTime` and `logoutTime` are in the format `hh:mm`.
* `00 <= hh <= 23`
* `00 <= mm <= 59`
* `loginTime` and `logoutTime` are not equal. | First of all, get the distinct characters since we are only interested in those Let's note that there might not be any digits. |
Number of full rounds | Simple Math | O(1) | the-number-of-full-rounds-you-have-played | 0 | 1 | # Approach\n1. Convert the time string to minutes\n2. handle logout time for next day\n3. Calculate number of full rounds\n - for login.. Full round starts after next iteration (`ceil`)\n - for logout.. Consider last round only if it\'s full (`floor`)\n4. Handle edge case - If user logs in and out in the same round\n4. Return difference between rounds\n\nTime Complexity: `O(1)`\n\n# Code\n```py\nfrom math import ceil\n\nclass Solution:\n def numberOfRounds(self, loginTime: str, logoutTime: str) -> int:\n loginTime = int(loginTime[:2])*60 + int(loginTime[-2:])\n logoutTime = int(logoutTime[:2])*60 + int(logoutTime[-2:])\n\n if logoutTime < loginTime:\n logoutTime += 24*60\n\n loginRound = ceil(loginTime / 15)\n logoutRound = logoutTime // 15\n\n rounds = logoutRound - loginRound\n\n return 0 if rounds < 0 else rounds\n\n``` | 0 | You are participating in an online chess tournament. There is a chess round that starts every `15` minutes. The first round of the day starts at `00:00`, and after every `15` minutes, a new round starts.
* For example, the second round starts at `00:15`, the fourth round starts at `00:45`, and the seventh round starts at `01:30`.
You are given two strings `loginTime` and `logoutTime` where:
* `loginTime` is the time you will login to the game, and
* `logoutTime` is the time you will logout from the game.
If `logoutTime` is **earlier** than `loginTime`, this means you have played from `loginTime` to midnight and from midnight to `logoutTime`.
Return _the number of full chess rounds you have played in the tournament_.
**Note:** All the given times follow the 24-hour clock. That means the first round of the day starts at `00:00` and the last round of the day starts at `23:45`.
**Example 1:**
**Input:** loginTime = "09:31 ", logoutTime = "10:14 "
**Output:** 1
**Explanation:** You played one full round from 09:45 to 10:00.
You did not play the full round from 09:30 to 09:45 because you logged in at 09:31 after it began.
You did not play the full round from 10:00 to 10:15 because you logged out at 10:14 before it ended.
**Example 2:**
**Input:** loginTime = "21:30 ", logoutTime = "03:00 "
**Output:** 22
**Explanation:** You played 10 full rounds from 21:30 to 00:00 and 12 full rounds from 00:00 to 03:00.
10 + 12 = 22.
**Constraints:**
* `loginTime` and `logoutTime` are in the format `hh:mm`.
* `00 <= hh <= 23`
* `00 <= mm <= 59`
* `loginTime` and `logoutTime` are not equal. | First of all, get the distinct characters since we are only interested in those Let's note that there might not be any digits. |
[Python] straightforward, parse string | the-number-of-full-rounds-you-have-played | 0 | 1 | ```\nclass Solution:\n def numberOfRounds(self, loginTime: str, logoutTime: str) -> int:\n login = self.to_min(loginTime)\n logout = self.to_min(logoutTime)\n \n if logout < login: # new day after midnight\n logout = logout + 24 * 60\n \n if logout - login < 15:\n return 0\n \n login = self.round_login(login)\n logout = self.round_logout(logout)\n \n return (logout - login) // 15\n \n \n def to_min(self, current_time: str) -> int:\n h, m = map(int, current_time.split(":"))\n return h * 60 + m\n \n def round_login(self, m: int):\n return m if m % 15 == 0 else m + (15 - m % 15)\n \n def round_logout(self, m: int):\n return m if m % 15 == 0 else m - (m % 15)\n``` | 1 | You are participating in an online chess tournament. There is a chess round that starts every `15` minutes. The first round of the day starts at `00:00`, and after every `15` minutes, a new round starts.
* For example, the second round starts at `00:15`, the fourth round starts at `00:45`, and the seventh round starts at `01:30`.
You are given two strings `loginTime` and `logoutTime` where:
* `loginTime` is the time you will login to the game, and
* `logoutTime` is the time you will logout from the game.
If `logoutTime` is **earlier** than `loginTime`, this means you have played from `loginTime` to midnight and from midnight to `logoutTime`.
Return _the number of full chess rounds you have played in the tournament_.
**Note:** All the given times follow the 24-hour clock. That means the first round of the day starts at `00:00` and the last round of the day starts at `23:45`.
**Example 1:**
**Input:** loginTime = "09:31 ", logoutTime = "10:14 "
**Output:** 1
**Explanation:** You played one full round from 09:45 to 10:00.
You did not play the full round from 09:30 to 09:45 because you logged in at 09:31 after it began.
You did not play the full round from 10:00 to 10:15 because you logged out at 10:14 before it ended.
**Example 2:**
**Input:** loginTime = "21:30 ", logoutTime = "03:00 "
**Output:** 22
**Explanation:** You played 10 full rounds from 21:30 to 00:00 and 12 full rounds from 00:00 to 03:00.
10 + 12 = 22.
**Constraints:**
* `loginTime` and `logoutTime` are in the format `hh:mm`.
* `00 <= hh <= 23`
* `00 <= mm <= 59`
* `loginTime` and `logoutTime` are not equal. | First of all, get the distinct characters since we are only interested in those Let's note that there might not be any digits. |
🐍 98% faster || Simple approach || well-explained 📌 | count-sub-islands | 0 | 1 | ## IDEA:\n\uD83D\uDC49 firstly remove all the non-common island\n\uD83D\uDC49 Now count the sub-islands\n\'\'\'\n\n\tclass Solution:\n def countSubIslands(self, grid1: List[List[int]], grid2: List[List[int]]) -> int:\n \n m=len(grid1)\n n=len(grid1[0])\n \n def dfs(i,j):\n if i<0 or i>=m or j<0 or j>=n or grid2[i][j]==0:\n return\n \n grid2[i][j]=0\n dfs(i+1,j)\n dfs(i,j+1)\n dfs(i,j-1)\n dfs(i-1,j)\n \n # removing all the non-common sub-islands\n for i in range(m):\n for j in range(n):\n if grid2[i][j]==1 and grid1[i][j]==0:\n dfs(i,j)\n \n c=0\n\t\t# counting sub-islands\n for i in range(m):\n for j in range(n):\n if grid2[i][j]==1:\n dfs(i,j)\n c+=1\n return c\n\t\t\nIf you have any doubt ......... please feel free to ask !!!\nThank You \uD83E\uDD1E | 199 | You are given two `m x n` binary matrices `grid1` and `grid2` containing only `0`'s (representing water) and `1`'s (representing land). An **island** is a group of `1`'s connected **4-directionally** (horizontal or vertical). Any cells outside of the grid are considered water cells.
An island in `grid2` is considered a **sub-island** if there is an island in `grid1` that contains **all** the cells that make up **this** island in `grid2`.
Return the _**number** of islands in_ `grid2` _that are considered **sub-islands**_.
**Example 1:**
**Input:** grid1 = \[\[1,1,1,0,0\],\[0,1,1,1,1\],\[0,0,0,0,0\],\[1,0,0,0,0\],\[1,1,0,1,1\]\], grid2 = \[\[1,1,1,0,0\],\[0,0,1,1,1\],\[0,1,0,0,0\],\[1,0,1,1,0\],\[0,1,0,1,0\]\]
**Output:** 3
**Explanation:** In the picture above, the grid on the left is grid1 and the grid on the right is grid2.
The 1s colored red in grid2 are those considered to be part of a sub-island. There are three sub-islands.
**Example 2:**
**Input:** grid1 = \[\[1,0,1,0,1\],\[1,1,1,1,1\],\[0,0,0,0,0\],\[1,1,1,1,1\],\[1,0,1,0,1\]\], grid2 = \[\[0,0,0,0,0\],\[1,1,1,1,1\],\[0,1,0,1,0\],\[0,1,0,1,0\],\[1,0,0,0,1\]\]
**Output:** 2
**Explanation:** In the picture above, the grid on the left is grid1 and the grid on the right is grid2.
The 1s colored red in grid2 are those considered to be part of a sub-island. There are two sub-islands.
**Constraints:**
* `m == grid1.length == grid2.length`
* `n == grid1[i].length == grid2[i].length`
* `1 <= m, n <= 500`
* `grid1[i][j]` and `grid2[i][j]` are either `0` or `1`. | Using a map, track the expiry times of the tokens. When generating a new token, add it to the map with its expiry time. When renewing a token, check if it's on the map and has not expired yet. If so, update its expiry time. To count unexpired tokens, iterate on the map and check for each token if it's not expired yet. |
Fully Efficient Solution in Java 🚀 | count-sub-islands | 1 | 1 | # Algorithm : \n- Perform DFS on grid2 and store the coordinates of the island.\n- Check if every coordinate stored is \'1\' in grid1.\n- If all the coordinates are 1\'s in grid1 then increment the count.\n- Finally return the count.\n>### *Check the implementation for clear understanding !!*\n---\n# Java Code\n```\nclass Pair\n{\n int x = 0;\n int y = 0;\n Pair(int x,int y)\n {\n this.x = x;\n this.y = y;\n }\n}\nclass Solution {\n int[] xDir = {0,0,-1,1};\n int[] yDir = {-1,1,0,0};\n public void DFS(int[][] grid2,boolean[][] visited,int i,int j,ArrayList<Pair> arr)\n {\n if(i<0 || i>=grid2.length || j<0 || j>=grid2[0].length || visited[i][j] == true ||grid2[i][j]!=1)\n return;\n visited[i][j] = true;\n Pair p = new Pair(i,j);\n arr.add(p);\n for(int k = 0;k<4;k++)\n {\n int newRow = i+xDir[k];\n int newCol = j+yDir[k];\n DFS(grid2,visited,newRow,newCol,arr);\n }\n }\n public int countSubIslands(int[][] grid1, int[][] grid2) {\n int rows = grid1.length;\n int cols = grid1[0].length;\n boolean[][] visited = new boolean[rows][cols];\n int count = 0;\n for(int i = 0;i<rows;i++)\n {\n for(int j = 0;j<cols;j++)\n {\n if(grid2[i][j] == 1 && visited[i][j] == false)\n {\n ArrayList<Pair> arr = new ArrayList<>();\n DFS(grid2,visited,i,j,arr);\n boolean flag = false;\n for(Pair p : arr)\n {\n int x = p.x;\n int y = p.y;\n if(grid1[x][y] != 1)\n {\n flag = true;\n break;\n }\n }\n if(!flag)\n count++;\n }\n }\n }\n return count;\n }\n}\n```\n---\n#### *Please don\'t forget to upvote if you\'ve liked my explanation.*\n--- | 5 | You are given two `m x n` binary matrices `grid1` and `grid2` containing only `0`'s (representing water) and `1`'s (representing land). An **island** is a group of `1`'s connected **4-directionally** (horizontal or vertical). Any cells outside of the grid are considered water cells.
An island in `grid2` is considered a **sub-island** if there is an island in `grid1` that contains **all** the cells that make up **this** island in `grid2`.
Return the _**number** of islands in_ `grid2` _that are considered **sub-islands**_.
**Example 1:**
**Input:** grid1 = \[\[1,1,1,0,0\],\[0,1,1,1,1\],\[0,0,0,0,0\],\[1,0,0,0,0\],\[1,1,0,1,1\]\], grid2 = \[\[1,1,1,0,0\],\[0,0,1,1,1\],\[0,1,0,0,0\],\[1,0,1,1,0\],\[0,1,0,1,0\]\]
**Output:** 3
**Explanation:** In the picture above, the grid on the left is grid1 and the grid on the right is grid2.
The 1s colored red in grid2 are those considered to be part of a sub-island. There are three sub-islands.
**Example 2:**
**Input:** grid1 = \[\[1,0,1,0,1\],\[1,1,1,1,1\],\[0,0,0,0,0\],\[1,1,1,1,1\],\[1,0,1,0,1\]\], grid2 = \[\[0,0,0,0,0\],\[1,1,1,1,1\],\[0,1,0,1,0\],\[0,1,0,1,0\],\[1,0,0,0,1\]\]
**Output:** 2
**Explanation:** In the picture above, the grid on the left is grid1 and the grid on the right is grid2.
The 1s colored red in grid2 are those considered to be part of a sub-island. There are two sub-islands.
**Constraints:**
* `m == grid1.length == grid2.length`
* `n == grid1[i].length == grid2[i].length`
* `1 <= m, n <= 500`
* `grid1[i][j]` and `grid2[i][j]` are either `0` or `1`. | Using a map, track the expiry times of the tokens. When generating a new token, add it to the map with its expiry time. When renewing a token, check if it's on the map and has not expired yet. If so, update its expiry time. To count unexpired tokens, iterate on the map and check for each token if it's not expired yet. |
Easy & Clear Solution Python3 DP | count-sub-islands | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def countSubIslands(self, back: List[List[int]], grid: List[List[int]]) -> int:\n m=len(grid)\n n=len(grid[0])\n res=0\n test=True\n def dfs(i,j):\n nonlocal test\n if grid[i][j]==1:\n if back[i][j] != 1:\n test=False\n grid[i][j]=0\n if j<n-1:\n dfs(i,j+1)\n if j>0:\n dfs(i,j-1)\n if i>0:\n dfs(i-1,j)\n if i<m-1:\n dfs(i+1,j)\n for i in range(m):\n for j in range(n):\n if grid[i][j]==1:\n \n test=True\n dfs(i,j)\n if test :\n res+=1\n \n\n return res\n``` | 6 | You are given two `m x n` binary matrices `grid1` and `grid2` containing only `0`'s (representing water) and `1`'s (representing land). An **island** is a group of `1`'s connected **4-directionally** (horizontal or vertical). Any cells outside of the grid are considered water cells.
An island in `grid2` is considered a **sub-island** if there is an island in `grid1` that contains **all** the cells that make up **this** island in `grid2`.
Return the _**number** of islands in_ `grid2` _that are considered **sub-islands**_.
**Example 1:**
**Input:** grid1 = \[\[1,1,1,0,0\],\[0,1,1,1,1\],\[0,0,0,0,0\],\[1,0,0,0,0\],\[1,1,0,1,1\]\], grid2 = \[\[1,1,1,0,0\],\[0,0,1,1,1\],\[0,1,0,0,0\],\[1,0,1,1,0\],\[0,1,0,1,0\]\]
**Output:** 3
**Explanation:** In the picture above, the grid on the left is grid1 and the grid on the right is grid2.
The 1s colored red in grid2 are those considered to be part of a sub-island. There are three sub-islands.
**Example 2:**
**Input:** grid1 = \[\[1,0,1,0,1\],\[1,1,1,1,1\],\[0,0,0,0,0\],\[1,1,1,1,1\],\[1,0,1,0,1\]\], grid2 = \[\[0,0,0,0,0\],\[1,1,1,1,1\],\[0,1,0,1,0\],\[0,1,0,1,0\],\[1,0,0,0,1\]\]
**Output:** 2
**Explanation:** In the picture above, the grid on the left is grid1 and the grid on the right is grid2.
The 1s colored red in grid2 are those considered to be part of a sub-island. There are two sub-islands.
**Constraints:**
* `m == grid1.length == grid2.length`
* `n == grid1[i].length == grid2[i].length`
* `1 <= m, n <= 500`
* `grid1[i][j]` and `grid2[i][j]` are either `0` or `1`. | Using a map, track the expiry times of the tokens. When generating a new token, add it to the map with its expiry time. When renewing a token, check if it's on the map and has not expired yet. If so, update its expiry time. To count unexpired tokens, iterate on the map and check for each token if it's not expired yet. |
python3 solution using bfs beats 99% time 100% space O(M*N),O(M*N) | count-sub-islands | 0 | 1 | 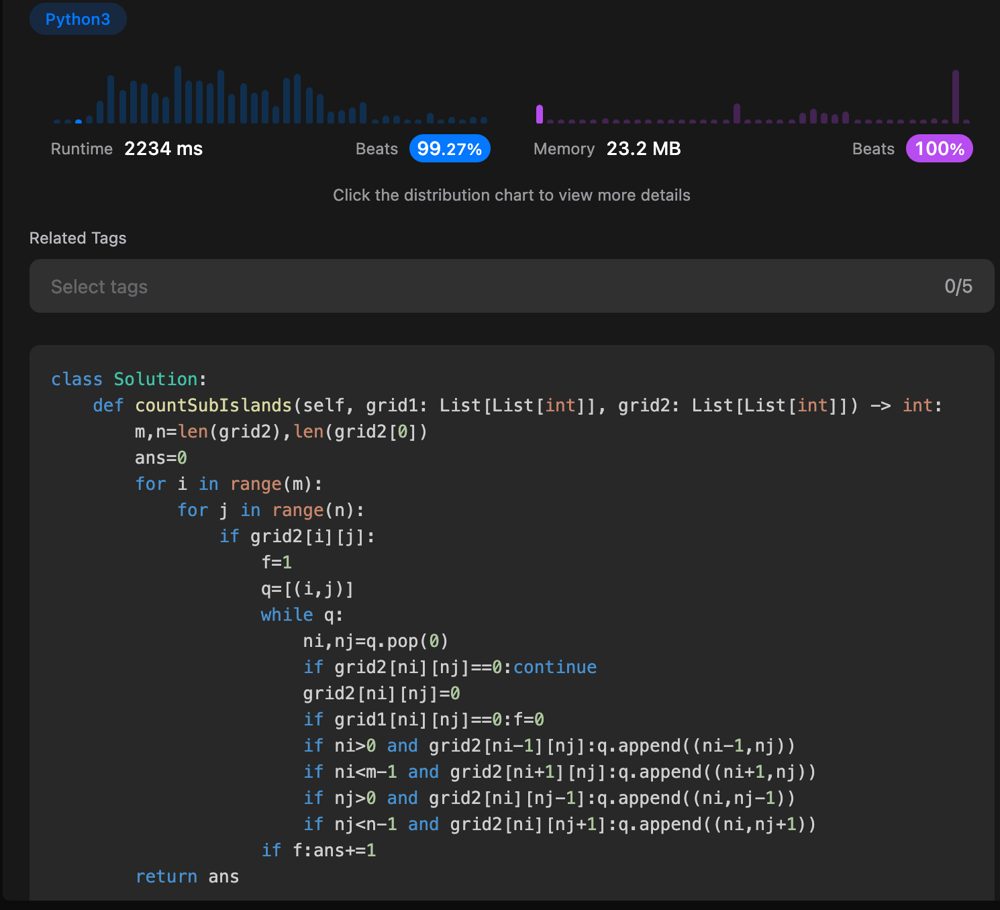\n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nthe logic here is any island that exists in grid2 being check by `grid2[i][j]` which when 1 enters if condition \nfrom here we use bfs by checking all 4 directions but before this we need to check if this is also an island in grid1 if not then we can set the flag which takes if if it is a subisland or no.\nthrought bfs is done and in all he islands visted of grid2 if none of them set the flag then flag remains 1 and we can add 1 to answer indicating the curent island formation we got in bfs is a subisland of grid1 also.\nreturning ans does it\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n**_______O(M*N)**\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n**_______O(M*N) only in case all are islands else it is O(max(m,n))**\n\n# Code\n```\nclass Solution:\n def countSubIslands(self, grid1: List[List[int]], grid2: List[List[int]]) -> int:\n m,n=len(grid2),len(grid2[0])\n ans=0\n for i in range(m):\n for j in range(n):\n if grid2[i][j]:\n f=1\n q=[(i,j)]\n while q:\n ni,nj=q.pop(0)\n if grid2[ni][nj]==0:continue\n grid2[ni][nj]=0\n if grid1[ni][nj]==0:f=0\n if ni>0 and grid2[ni-1][nj]:q.append((ni-1,nj))\n if ni<m-1 and grid2[ni+1][nj]:q.append((ni+1,nj))\n if nj>0 and grid2[ni][nj-1]:q.append((ni,nj-1))\n if nj<n-1 and grid2[ni][nj+1]:q.append((ni,nj+1))\n if f:ans+=1\n return ans\n\n``` | 3 | You are given two `m x n` binary matrices `grid1` and `grid2` containing only `0`'s (representing water) and `1`'s (representing land). An **island** is a group of `1`'s connected **4-directionally** (horizontal or vertical). Any cells outside of the grid are considered water cells.
An island in `grid2` is considered a **sub-island** if there is an island in `grid1` that contains **all** the cells that make up **this** island in `grid2`.
Return the _**number** of islands in_ `grid2` _that are considered **sub-islands**_.
**Example 1:**
**Input:** grid1 = \[\[1,1,1,0,0\],\[0,1,1,1,1\],\[0,0,0,0,0\],\[1,0,0,0,0\],\[1,1,0,1,1\]\], grid2 = \[\[1,1,1,0,0\],\[0,0,1,1,1\],\[0,1,0,0,0\],\[1,0,1,1,0\],\[0,1,0,1,0\]\]
**Output:** 3
**Explanation:** In the picture above, the grid on the left is grid1 and the grid on the right is grid2.
The 1s colored red in grid2 are those considered to be part of a sub-island. There are three sub-islands.
**Example 2:**
**Input:** grid1 = \[\[1,0,1,0,1\],\[1,1,1,1,1\],\[0,0,0,0,0\],\[1,1,1,1,1\],\[1,0,1,0,1\]\], grid2 = \[\[0,0,0,0,0\],\[1,1,1,1,1\],\[0,1,0,1,0\],\[0,1,0,1,0\],\[1,0,0,0,1\]\]
**Output:** 2
**Explanation:** In the picture above, the grid on the left is grid1 and the grid on the right is grid2.
The 1s colored red in grid2 are those considered to be part of a sub-island. There are two sub-islands.
**Constraints:**
* `m == grid1.length == grid2.length`
* `n == grid1[i].length == grid2[i].length`
* `1 <= m, n <= 500`
* `grid1[i][j]` and `grid2[i][j]` are either `0` or `1`. | Using a map, track the expiry times of the tokens. When generating a new token, add it to the map with its expiry time. When renewing a token, check if it's on the map and has not expired yet. If so, update its expiry time. To count unexpired tokens, iterate on the map and check for each token if it's not expired yet. |
Python Elegant & Short | In-place | DFS | count-sub-islands | 0 | 1 | \tclass Solution:\n\t\t"""\n\t\tTime: O(n^2)\n\t\tMemory: O(n^2)\n\t\t"""\n\n\t\tLAND = 1\n\t\tWATER = 0\n\n\t\tdef countSubIslands(self, grid1: List[List[int]], grid2: List[List[int]]) -> int:\n\t\t\tm, n = len(grid1), len(grid1[0])\n\n\t\t\tfor i in range(m):\n\t\t\t\tfor j in range(n):\n\t\t\t\t\tif grid2[i][j] == self.LAND and grid1[i][j] == self.WATER:\n\t\t\t\t\t\tself.sink_island(i, j, grid2)\n\n\t\t\tislands = 0\n\t\t\tfor i in range(m):\n\t\t\t\tfor j in range(n):\n\t\t\t\t\tif grid2[i][j] == self.LAND:\n\t\t\t\t\t\tself.sink_island(i, j, grid2)\n\t\t\t\t\t\tislands += 1\n\n\t\t\treturn islands\n\n\t\t@classmethod\n\t\tdef sink_island(cls, row: int, col: int, grid: List[List[int]]):\n\t\t\tif grid[row][col] == cls.LAND:\n\t\t\t\tgrid[row][col] = cls.WATER\n\t\t\t\tif row > 0:\n\t\t\t\t\tcls.sink_island(row - 1, col, grid)\n\t\t\t\tif row < len(grid) - 1:\n\t\t\t\t\tcls.sink_island(row + 1, col, grid)\n\t\t\t\tif col < len(grid[0]) - 1:\n\t\t\t\t\tcls.sink_island(row, col + 1, grid)\n\t\t\t\tif col > 0:\n\t\t\t\t\tcls.sink_island(row, col - 1, grid)\n | 6 | You are given two `m x n` binary matrices `grid1` and `grid2` containing only `0`'s (representing water) and `1`'s (representing land). An **island** is a group of `1`'s connected **4-directionally** (horizontal or vertical). Any cells outside of the grid are considered water cells.
An island in `grid2` is considered a **sub-island** if there is an island in `grid1` that contains **all** the cells that make up **this** island in `grid2`.
Return the _**number** of islands in_ `grid2` _that are considered **sub-islands**_.
**Example 1:**
**Input:** grid1 = \[\[1,1,1,0,0\],\[0,1,1,1,1\],\[0,0,0,0,0\],\[1,0,0,0,0\],\[1,1,0,1,1\]\], grid2 = \[\[1,1,1,0,0\],\[0,0,1,1,1\],\[0,1,0,0,0\],\[1,0,1,1,0\],\[0,1,0,1,0\]\]
**Output:** 3
**Explanation:** In the picture above, the grid on the left is grid1 and the grid on the right is grid2.
The 1s colored red in grid2 are those considered to be part of a sub-island. There are three sub-islands.
**Example 2:**
**Input:** grid1 = \[\[1,0,1,0,1\],\[1,1,1,1,1\],\[0,0,0,0,0\],\[1,1,1,1,1\],\[1,0,1,0,1\]\], grid2 = \[\[0,0,0,0,0\],\[1,1,1,1,1\],\[0,1,0,1,0\],\[0,1,0,1,0\],\[1,0,0,0,1\]\]
**Output:** 2
**Explanation:** In the picture above, the grid on the left is grid1 and the grid on the right is grid2.
The 1s colored red in grid2 are those considered to be part of a sub-island. There are two sub-islands.
**Constraints:**
* `m == grid1.length == grid2.length`
* `n == grid1[i].length == grid2[i].length`
* `1 <= m, n <= 500`
* `grid1[i][j]` and `grid2[i][j]` are either `0` or `1`. | Using a map, track the expiry times of the tokens. When generating a new token, add it to the map with its expiry time. When renewing a token, check if it's on the map and has not expired yet. If so, update its expiry time. To count unexpired tokens, iterate on the map and check for each token if it's not expired yet. |
Python 3 || 9 lines, dp , w/explanation || T/M: 100% / 55% | minimum-absolute-difference-queries | 0 | 1 | Here\'s how the code works:\n\n- We initialize two empty lists, `ans` and `dp`. `ans` stores the answers for each query, and `dp` is used to keep track of the cumulative count of numbers in the array.\n- The first row of`dp`is initialized as a list of zeros with a length of ``max(nums) + 1``, which ensures that`dp`has enough columns to represent the numbers in`nums`.\n- We iterate over each number `num` in `nums`. For each `num`, we creates\\ a new row `newRow` by making a copy of the latest row in `dp`. Then, we increment the count of `num` in `newRow` by one.\n- After completing`dp` we iterates over each query specified by the tuples `(left, right)` in `queries`.\n\n- For each query, the code compares the counts of numbers in the subarray `nums[left: right+1]` by taking the difference between the corresponding elements in `dp[right+1]` and `dp[left]`. The resulting list of differences is stored in`idx`.\n\n- We then append the minimum difference from`idx`to`ans`. If `idx`has less than two posive elements, -1 is appended to`ans` as the default. We return`ans` as the answer to the problem --*ChatGPT*\n```\nclass Solution:\n def minDifference(self, nums: List[int], \n queries: List[List[int]]) -> List[int]:\n\n ans, dp = [], [[0]*(max(nums)+1)]\n\n for num in nums:\n newRow = dp[-1].copy()\n newRow[num]+= 1\n dp.append(newRow)\n\n for left, right in queries:\n idx = [i for i,(r,l) in enumerate(zip(dp[right+1],\n dp[left])) if r > l]\n\n ans.append(min((i-j for i,j in zip(idx[1:],idx))\n ,default = -1))\n \n return ans\n```\n[https://leetcode.com/problems/minimum-absolute-difference-queries/submissions/1007197017/](http://)\n\nI could be wrong, but I think that time complexity is *O*(*N*+*Q*) and space complexity is *O*(*N*), in which *N* ~ `len(nums)` and *Q* ~ `len(queries)`. | 3 | The **minimum absolute difference** of an array `a` is defined as the **minimum value** of `|a[i] - a[j]|`, where `0 <= i < j < a.length` and `a[i] != a[j]`. If all elements of `a` are the **same**, the minimum absolute difference is `-1`.
* For example, the minimum absolute difference of the array `[5,2,3,7,2]` is `|2 - 3| = 1`. Note that it is not `0` because `a[i]` and `a[j]` must be different.
You are given an integer array `nums` and the array `queries` where `queries[i] = [li, ri]`. For each query `i`, compute the **minimum absolute difference** of the **subarray** `nums[li...ri]` containing the elements of `nums` between the **0-based** indices `li` and `ri` (**inclusive**).
Return _an **array**_ `ans` _where_ `ans[i]` _is the answer to the_ `ith` _query_.
A **subarray** is a contiguous sequence of elements in an array.
The value of `|x|` is defined as:
* `x` if `x >= 0`.
* `-x` if `x < 0`.
**Example 1:**
**Input:** nums = \[1,3,4,8\], queries = \[\[0,1\],\[1,2\],\[2,3\],\[0,3\]\]
**Output:** \[2,1,4,1\]
**Explanation:** The queries are processed as follows:
- queries\[0\] = \[0,1\]: The subarray is \[1,3\] and the minimum absolute difference is |1-3| = 2.
- queries\[1\] = \[1,2\]: The subarray is \[3,4\] and the minimum absolute difference is |3-4| = 1.
- queries\[2\] = \[2,3\]: The subarray is \[4,8\] and the minimum absolute difference is |4-8| = 4.
- queries\[3\] = \[0,3\]: The subarray is \[1,3,4,8\] and the minimum absolute difference is |3-4| = 1.
**Example 2:**
**Input:** nums = \[4,5,2,2,7,10\], queries = \[\[2,3\],\[0,2\],\[0,5\],\[3,5\]\]
**Output:** \[-1,1,1,3\]
**Explanation:** The queries are processed as follows:
- queries\[0\] = \[2,3\]: The subarray is \[2,2\] and the minimum absolute difference is -1 because all the
elements are the same.
- queries\[1\] = \[0,2\]: The subarray is \[4,5,2\] and the minimum absolute difference is |4-5| = 1.
- queries\[2\] = \[0,5\]: The subarray is \[4,5,2,2,7,10\] and the minimum absolute difference is |4-5| = 1.
- queries\[3\] = \[3,5\]: The subarray is \[2,7,10\] and the minimum absolute difference is |7-10| = 3.
**Constraints:**
* `2 <= nums.length <= 105`
* `1 <= nums[i] <= 100`
* `1 <= queries.length <= 2 * 104`
* `0 <= li < ri < nums.length` | Find every way to split the array until n groups of 2. Brute force recursion is acceptable. Calculate the gcd of every pair and greedily multiply the largest gcds. |
[Python3] binary search | minimum-absolute-difference-queries | 0 | 1 | \n```\nclass Solution:\n def minDifference(self, nums: List[int], queries: List[List[int]]) -> List[int]:\n loc = {}\n for i, x in enumerate(nums): loc.setdefault(x, []).append(i)\n keys = sorted(loc)\n \n ans = []\n for l, r in queries: \n prev, val = 0, inf\n for x in keys: \n i = bisect_left(loc[x], l)\n if i < len(loc[x]) and loc[x][i] <= r: \n if prev: val = min(val, x - prev)\n prev = x \n ans.append(val if val < inf else -1)\n return ans\n``` | 8 | The **minimum absolute difference** of an array `a` is defined as the **minimum value** of `|a[i] - a[j]|`, where `0 <= i < j < a.length` and `a[i] != a[j]`. If all elements of `a` are the **same**, the minimum absolute difference is `-1`.
* For example, the minimum absolute difference of the array `[5,2,3,7,2]` is `|2 - 3| = 1`. Note that it is not `0` because `a[i]` and `a[j]` must be different.
You are given an integer array `nums` and the array `queries` where `queries[i] = [li, ri]`. For each query `i`, compute the **minimum absolute difference** of the **subarray** `nums[li...ri]` containing the elements of `nums` between the **0-based** indices `li` and `ri` (**inclusive**).
Return _an **array**_ `ans` _where_ `ans[i]` _is the answer to the_ `ith` _query_.
A **subarray** is a contiguous sequence of elements in an array.
The value of `|x|` is defined as:
* `x` if `x >= 0`.
* `-x` if `x < 0`.
**Example 1:**
**Input:** nums = \[1,3,4,8\], queries = \[\[0,1\],\[1,2\],\[2,3\],\[0,3\]\]
**Output:** \[2,1,4,1\]
**Explanation:** The queries are processed as follows:
- queries\[0\] = \[0,1\]: The subarray is \[1,3\] and the minimum absolute difference is |1-3| = 2.
- queries\[1\] = \[1,2\]: The subarray is \[3,4\] and the minimum absolute difference is |3-4| = 1.
- queries\[2\] = \[2,3\]: The subarray is \[4,8\] and the minimum absolute difference is |4-8| = 4.
- queries\[3\] = \[0,3\]: The subarray is \[1,3,4,8\] and the minimum absolute difference is |3-4| = 1.
**Example 2:**
**Input:** nums = \[4,5,2,2,7,10\], queries = \[\[2,3\],\[0,2\],\[0,5\],\[3,5\]\]
**Output:** \[-1,1,1,3\]
**Explanation:** The queries are processed as follows:
- queries\[0\] = \[2,3\]: The subarray is \[2,2\] and the minimum absolute difference is -1 because all the
elements are the same.
- queries\[1\] = \[0,2\]: The subarray is \[4,5,2\] and the minimum absolute difference is |4-5| = 1.
- queries\[2\] = \[0,5\]: The subarray is \[4,5,2,2,7,10\] and the minimum absolute difference is |4-5| = 1.
- queries\[3\] = \[3,5\]: The subarray is \[2,7,10\] and the minimum absolute difference is |7-10| = 3.
**Constraints:**
* `2 <= nums.length <= 105`
* `1 <= nums[i] <= 100`
* `1 <= queries.length <= 2 * 104`
* `0 <= li < ri < nums.length` | Find every way to split the array until n groups of 2. Brute force recursion is acceptable. Calculate the gcd of every pair and greedily multiply the largest gcds. |
Python O(100*100*log(N)*Q) | minimum-absolute-difference-queries | 0 | 1 | # Code\n```\nclass Solution:\n def minDifference(self, nums: List[int], queries: List[List[int]]) -> List[int]:\n m = collections.defaultdict(list)\n @functools.lru_cache(maxsize=None)\n def has_number_in_range(num, q):\n A = m[num]\n lo,hi=0,len(A)-1\n while lo<=hi:\n mid = (lo+hi)//2\n if A[mid] > q[1]:\n hi = mid-1\n elif A[mid] < q[0]:\n lo = mid + 1\n else:\n return True\n return False\n \n for i,x in enumerate(nums):\n m[x].append(i)\n\n output = [] \n for [l, r] in queries:\n res = float(\'inf\')\n for num1 in range(100, 0, -1):\n if num1 not in m:\n continue\n if has_number_in_range(num1, (l,r)):\n for num2 in range(num1, 0, -1):\n if num2 not in m:\n continue \n if num1 == num2:\n continue\n if has_number_in_range(num2, (l,r)):\n res = min(res, abs(num1-num2))\n break\n output.append(-1 if res == float(\'inf\') else res)\n return output\n\n``` | 0 | The **minimum absolute difference** of an array `a` is defined as the **minimum value** of `|a[i] - a[j]|`, where `0 <= i < j < a.length` and `a[i] != a[j]`. If all elements of `a` are the **same**, the minimum absolute difference is `-1`.
* For example, the minimum absolute difference of the array `[5,2,3,7,2]` is `|2 - 3| = 1`. Note that it is not `0` because `a[i]` and `a[j]` must be different.
You are given an integer array `nums` and the array `queries` where `queries[i] = [li, ri]`. For each query `i`, compute the **minimum absolute difference** of the **subarray** `nums[li...ri]` containing the elements of `nums` between the **0-based** indices `li` and `ri` (**inclusive**).
Return _an **array**_ `ans` _where_ `ans[i]` _is the answer to the_ `ith` _query_.
A **subarray** is a contiguous sequence of elements in an array.
The value of `|x|` is defined as:
* `x` if `x >= 0`.
* `-x` if `x < 0`.
**Example 1:**
**Input:** nums = \[1,3,4,8\], queries = \[\[0,1\],\[1,2\],\[2,3\],\[0,3\]\]
**Output:** \[2,1,4,1\]
**Explanation:** The queries are processed as follows:
- queries\[0\] = \[0,1\]: The subarray is \[1,3\] and the minimum absolute difference is |1-3| = 2.
- queries\[1\] = \[1,2\]: The subarray is \[3,4\] and the minimum absolute difference is |3-4| = 1.
- queries\[2\] = \[2,3\]: The subarray is \[4,8\] and the minimum absolute difference is |4-8| = 4.
- queries\[3\] = \[0,3\]: The subarray is \[1,3,4,8\] and the minimum absolute difference is |3-4| = 1.
**Example 2:**
**Input:** nums = \[4,5,2,2,7,10\], queries = \[\[2,3\],\[0,2\],\[0,5\],\[3,5\]\]
**Output:** \[-1,1,1,3\]
**Explanation:** The queries are processed as follows:
- queries\[0\] = \[2,3\]: The subarray is \[2,2\] and the minimum absolute difference is -1 because all the
elements are the same.
- queries\[1\] = \[0,2\]: The subarray is \[4,5,2\] and the minimum absolute difference is |4-5| = 1.
- queries\[2\] = \[0,5\]: The subarray is \[4,5,2,2,7,10\] and the minimum absolute difference is |4-5| = 1.
- queries\[3\] = \[3,5\]: The subarray is \[2,7,10\] and the minimum absolute difference is |7-10| = 3.
**Constraints:**
* `2 <= nums.length <= 105`
* `1 <= nums[i] <= 100`
* `1 <= queries.length <= 2 * 104`
* `0 <= li < ri < nums.length` | Find every way to split the array until n groups of 2. Brute force recursion is acceptable. Calculate the gcd of every pair and greedily multiply the largest gcds. |
Python solution. Time beats > 90%; space beats 70%. | minimum-absolute-difference-queries | 0 | 1 | \n# Approach\nn = len(nums)\nm = len(queries)\nv = number of distinct values (at most 100 in this case)\n\nFor each point i=0,1,2,...,n-1, we store the distinct values v that have occurred in nums[0...i-1] and their counts in distinct_sets[i][v]. For example\n\n1, 1, 2, 3, 3, 4, 2\n\nwill result in\ndistinct_set[0] ={1: 1}\ndistinct_set[1] = {1: 2}\ndistinct_set[2] = {1: 2, 2: 1}\ndistinct_set[3] = {1: 2, 2: 1, 3:1}\n...\n\nYou can improve this further to save space by only considering i if i or i+1 is an interval point in queries.\n\nTo compute the answer for query q, for each v in distinct_sets[q[1]] check if:\n\ndistinct_sets[q[1]][v] - distinct_sets[q[0]-1][v] > 0 \n\nNote that there are some edge cases such as q[0] = 0 or v is not in distinct_sets[q[0]-1].\n\nOnce we know that v is in the interval of query q, we add it to a list elms. Sort elms and find the minimum absolute difference accordingly.\n\n\n# Complexity\n- Time and complexity:\nO((n+m) v log v)\n\n# Code\n```\nfrom math import inf\nimport copy \nfrom bisect import bisect_left\n\nclass Solution:\n def binary_search(self, L, x):\n i = bisect_left(L, x)\n if i < len(L):\n if L[i] == x:\n return i\n else:\n return None\n else:\n return None\n\n def minDifference(self, nums, queries):\n n = len(nums)\n ans = [inf for i in range(len(queries))]\n distinct_sets = {}\n S = {}\n\n query_points = set([q[0] for q in queries] + [q[1] for q in queries])\n query_points = sorted(list(query_points))\n\n for i in range(n):\n if nums[i] in S:\n S[nums[i]] += 1\n else:\n S[nums[i]] = 1\n if self.binary_search(query_points, i) or self.binary_search(query_points, i+1) != None: \n distinct_sets[i] = copy.copy(S)\n \n for i, q in enumerate(queries):\n elms = []\n if q[0] == 0:\n elms = [e for e in distinct_sets[q[1]].keys()]\n else:\n for e in distinct_sets[q[1]].keys():\n elms.append(e)\n if e in distinct_sets[q[0]-1] and \\\n distinct_sets[q[1]][e] - distinct_sets[q[0]-1][e] == 0:\n elms.pop()\n elms = sorted(elms)\n for j in range(len(elms)-1):\n if ans[i] > abs(elms[j]-elms[j+1]) and abs(elms[j]-elms[j+1]) > 0: \n ans[i] = abs(elms[j]-elms[j+1])\n if ans[i] == inf: ans[i] = -1\n return ans\n``` | 0 | The **minimum absolute difference** of an array `a` is defined as the **minimum value** of `|a[i] - a[j]|`, where `0 <= i < j < a.length` and `a[i] != a[j]`. If all elements of `a` are the **same**, the minimum absolute difference is `-1`.
* For example, the minimum absolute difference of the array `[5,2,3,7,2]` is `|2 - 3| = 1`. Note that it is not `0` because `a[i]` and `a[j]` must be different.
You are given an integer array `nums` and the array `queries` where `queries[i] = [li, ri]`. For each query `i`, compute the **minimum absolute difference** of the **subarray** `nums[li...ri]` containing the elements of `nums` between the **0-based** indices `li` and `ri` (**inclusive**).
Return _an **array**_ `ans` _where_ `ans[i]` _is the answer to the_ `ith` _query_.
A **subarray** is a contiguous sequence of elements in an array.
The value of `|x|` is defined as:
* `x` if `x >= 0`.
* `-x` if `x < 0`.
**Example 1:**
**Input:** nums = \[1,3,4,8\], queries = \[\[0,1\],\[1,2\],\[2,3\],\[0,3\]\]
**Output:** \[2,1,4,1\]
**Explanation:** The queries are processed as follows:
- queries\[0\] = \[0,1\]: The subarray is \[1,3\] and the minimum absolute difference is |1-3| = 2.
- queries\[1\] = \[1,2\]: The subarray is \[3,4\] and the minimum absolute difference is |3-4| = 1.
- queries\[2\] = \[2,3\]: The subarray is \[4,8\] and the minimum absolute difference is |4-8| = 4.
- queries\[3\] = \[0,3\]: The subarray is \[1,3,4,8\] and the minimum absolute difference is |3-4| = 1.
**Example 2:**
**Input:** nums = \[4,5,2,2,7,10\], queries = \[\[2,3\],\[0,2\],\[0,5\],\[3,5\]\]
**Output:** \[-1,1,1,3\]
**Explanation:** The queries are processed as follows:
- queries\[0\] = \[2,3\]: The subarray is \[2,2\] and the minimum absolute difference is -1 because all the
elements are the same.
- queries\[1\] = \[0,2\]: The subarray is \[4,5,2\] and the minimum absolute difference is |4-5| = 1.
- queries\[2\] = \[0,5\]: The subarray is \[4,5,2,2,7,10\] and the minimum absolute difference is |4-5| = 1.
- queries\[3\] = \[3,5\]: The subarray is \[2,7,10\] and the minimum absolute difference is |7-10| = 3.
**Constraints:**
* `2 <= nums.length <= 105`
* `1 <= nums[i] <= 100`
* `1 <= queries.length <= 2 * 104`
* `0 <= li < ri < nums.length` | Find every way to split the array until n groups of 2. Brute force recursion is acceptable. Calculate the gcd of every pair and greedily multiply the largest gcds. |
1909. Remove One Element to Make the Array Strictly Increasing | remove-one-element-to-make-the-array-strictly-increasing | 0 | 1 | ```\nclass Solution:\n def canBeIncreasing(self, nums: List[int]) -> bool:\n dnums=nums.copy() #make a copy of the original array <nums>\n for i in range(len(nums)-1): #traverse the first pointer <i> in the original array <nums>\n if nums[i]>=nums[i+1]:\n a=nums.pop(i)\n break\n if nums==sorted(set(nums)): #we are checking with the sorted array because there might be duplicate elements after 1 pop instruction\n return True\n for j in range(len(dnums)-1,0,-1): #traverse the 2nd pointer <j> in the duplicate array <dnums>\n if dnums[j]<=dnums[j-1]:\n a=dnums.pop(j)\n break\n if dnums==sorted(set(dnums)): \n return True\n return False\n``` | 3 | Given a **0-indexed** integer array `nums`, return `true` _if it can be made **strictly increasing** after removing **exactly one** element, or_ `false` _otherwise. If the array is already strictly increasing, return_ `true`.
The array `nums` is **strictly increasing** if `nums[i - 1] < nums[i]` for each index `(1 <= i < nums.length).`
**Example 1:**
**Input:** nums = \[1,2,10,5,7\]
**Output:** true
**Explanation:** By removing 10 at index 2 from nums, it becomes \[1,2,5,7\].
\[1,2,5,7\] is strictly increasing, so return true.
**Example 2:**
**Input:** nums = \[2,3,1,2\]
**Output:** false
**Explanation:**
\[3,1,2\] is the result of removing the element at index 0.
\[2,1,2\] is the result of removing the element at index 1.
\[2,3,2\] is the result of removing the element at index 2.
\[2,3,1\] is the result of removing the element at index 3.
No resulting array is strictly increasing, so return false.
**Example 3:**
**Input:** nums = \[1,1,1\]
**Output:** false
**Explanation:** The result of removing any element is \[1,1\].
\[1,1\] is not strictly increasing, so return false.
**Constraints:**
* `2 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000` | You can traverse the buildings from the nearest to the ocean to the furthest. Keep with you the maximum to the right while traversing to determine if you can see the ocean or not. |
🔥 [Python 3] One pass , beats 80%🥷🏼 | remove-one-element-to-make-the-array-strictly-increasing | 0 | 1 | Similar to [665. Non-decreasing Array](https://leetcode.com/problems/non-decreasing-array/description/)\n\n```\nclass Solution:\n def canBeIncreasing(self, nums: List[int]) -> bool:\n isRemove = False\n for i in range(1, len(nums)):\n if nums[i] <= nums[i-1]:\n if isRemove: return False\n if i > 1 and nums[i] <= nums[i-2]:\n nums[i] = nums[i-1]\n else:\n nums[i-1] = nums[i]\n isRemove = True\n\n return True \n``` | 10 | Given a **0-indexed** integer array `nums`, return `true` _if it can be made **strictly increasing** after removing **exactly one** element, or_ `false` _otherwise. If the array is already strictly increasing, return_ `true`.
The array `nums` is **strictly increasing** if `nums[i - 1] < nums[i]` for each index `(1 <= i < nums.length).`
**Example 1:**
**Input:** nums = \[1,2,10,5,7\]
**Output:** true
**Explanation:** By removing 10 at index 2 from nums, it becomes \[1,2,5,7\].
\[1,2,5,7\] is strictly increasing, so return true.
**Example 2:**
**Input:** nums = \[2,3,1,2\]
**Output:** false
**Explanation:**
\[3,1,2\] is the result of removing the element at index 0.
\[2,1,2\] is the result of removing the element at index 1.
\[2,3,2\] is the result of removing the element at index 2.
\[2,3,1\] is the result of removing the element at index 3.
No resulting array is strictly increasing, so return false.
**Example 3:**
**Input:** nums = \[1,1,1\]
**Output:** false
**Explanation:** The result of removing any element is \[1,1\].
\[1,1\] is not strictly increasing, so return false.
**Constraints:**
* `2 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000` | You can traverse the buildings from the nearest to the ocean to the furthest. Keep with you the maximum to the right while traversing to determine if you can see the ocean or not. |
Simple solution in O(n). Beats 99%. | remove-one-element-to-make-the-array-strictly-increasing | 0 | 1 | # Approach\nStarting from the second element of the array we check if it is less or equal to the previous one. In this case the array is not strictly increasing and we need to fix it and we have two way to do this:\n - if `nums[i-2] < nums[i] < nums[i-1]` ( e.g 2, 10, 5 (i), 6 ) remove `i-1` (10) fix the situation.\n - If `nums[i] < nums[i-1] and nums[i] <= nums[i-2]` (e.g 2, 3, 2(i), 4(j), 5 ) we need to remove `i` to fix the situation.\n \nSince we compare always the `i` value with `i-1` value the first case does not alter the algorithm. This is not true for the second case, because at the next iteration we should compare the position `j=i+1` with the position `i-1` (because we have removed the `i` position). To avoid this issue we are going to copy the `i-1` element in `i` position. \n\nIn each case we keep track of the removal using a variable `removed_once`. If we found another element that is not strictly increasing we return `False`.\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def canBeIncreasing(self, nums: List[int]) -> bool:\n removed_once = False\n \n for i in range(1, len(nums)):\n if nums[i] <= nums[i-1]:\n if removed_once:\n return False\n if i > 1 and nums[i] <= nums[i-2]:\n nums[i] = nums[i-1]\n\n removed_once = True\n \n return True\n``` | 6 | Given a **0-indexed** integer array `nums`, return `true` _if it can be made **strictly increasing** after removing **exactly one** element, or_ `false` _otherwise. If the array is already strictly increasing, return_ `true`.
The array `nums` is **strictly increasing** if `nums[i - 1] < nums[i]` for each index `(1 <= i < nums.length).`
**Example 1:**
**Input:** nums = \[1,2,10,5,7\]
**Output:** true
**Explanation:** By removing 10 at index 2 from nums, it becomes \[1,2,5,7\].
\[1,2,5,7\] is strictly increasing, so return true.
**Example 2:**
**Input:** nums = \[2,3,1,2\]
**Output:** false
**Explanation:**
\[3,1,2\] is the result of removing the element at index 0.
\[2,1,2\] is the result of removing the element at index 1.
\[2,3,2\] is the result of removing the element at index 2.
\[2,3,1\] is the result of removing the element at index 3.
No resulting array is strictly increasing, so return false.
**Example 3:**
**Input:** nums = \[1,1,1\]
**Output:** false
**Explanation:** The result of removing any element is \[1,1\].
\[1,1\] is not strictly increasing, so return false.
**Constraints:**
* `2 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000` | You can traverse the buildings from the nearest to the ocean to the furthest. Keep with you the maximum to the right while traversing to determine if you can see the ocean or not. |
Superb Logic With sort with two Approches | remove-one-element-to-make-the-array-strictly-increasing | 0 | 1 | ```\nclass Solution:\n def canBeIncreasing(self, nums: List[int]) -> bool:\n for i in range(len(nums)):\n temp=nums[:i]+nums[i+1:]\n if temp==sorted(set(temp)):\n return True\n return False\n```\n```\nclass Solution:\n def canBeIncreasing(self, nums: List[int]) -> bool:\n flag=False\n for i in range(1,len(nums)):\n if nums[i]<=nums[i-1]:\n if flag:\n return False\n if i>1 and nums[i]<=nums[i-2]:\n nums[i]=nums[i-1]\n else:\n nums[i-1]=nums[i]\n flag=True\n return True\n```\n# please upvote me it would encourage me alot\n | 5 | Given a **0-indexed** integer array `nums`, return `true` _if it can be made **strictly increasing** after removing **exactly one** element, or_ `false` _otherwise. If the array is already strictly increasing, return_ `true`.
The array `nums` is **strictly increasing** if `nums[i - 1] < nums[i]` for each index `(1 <= i < nums.length).`
**Example 1:**
**Input:** nums = \[1,2,10,5,7\]
**Output:** true
**Explanation:** By removing 10 at index 2 from nums, it becomes \[1,2,5,7\].
\[1,2,5,7\] is strictly increasing, so return true.
**Example 2:**
**Input:** nums = \[2,3,1,2\]
**Output:** false
**Explanation:**
\[3,1,2\] is the result of removing the element at index 0.
\[2,1,2\] is the result of removing the element at index 1.
\[2,3,2\] is the result of removing the element at index 2.
\[2,3,1\] is the result of removing the element at index 3.
No resulting array is strictly increasing, so return false.
**Example 3:**
**Input:** nums = \[1,1,1\]
**Output:** false
**Explanation:** The result of removing any element is \[1,1\].
\[1,1\] is not strictly increasing, so return false.
**Constraints:**
* `2 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000` | You can traverse the buildings from the nearest to the ocean to the furthest. Keep with you the maximum to the right while traversing to determine if you can see the ocean or not. |
[Python3] collect non-conforming indices | remove-one-element-to-make-the-array-strictly-increasing | 0 | 1 | \n```\nclass Solution:\n def canBeIncreasing(self, nums: List[int]) -> bool:\n stack = []\n for i in range(1, len(nums)): \n if nums[i-1] >= nums[i]: stack.append(i)\n \n if not stack: return True \n if len(stack) > 1: return False\n i = stack[0]\n return (i == 1 or nums[i-2] < nums[i]) or (i+1 == len(nums) or nums[i-1] < nums[i+1])\n```\n\n```\nclass Solution:\n def canBeIncreasing(self, nums: List[int]) -> bool:\n prev, seen = -inf, False\n for i, x in enumerate(nums): \n if prev < x: prev = x\n else: \n if seen: return False \n seen = True \n if i == 1 or nums[i-2] < x: prev = x\n return True \n``` | 18 | Given a **0-indexed** integer array `nums`, return `true` _if it can be made **strictly increasing** after removing **exactly one** element, or_ `false` _otherwise. If the array is already strictly increasing, return_ `true`.
The array `nums` is **strictly increasing** if `nums[i - 1] < nums[i]` for each index `(1 <= i < nums.length).`
**Example 1:**
**Input:** nums = \[1,2,10,5,7\]
**Output:** true
**Explanation:** By removing 10 at index 2 from nums, it becomes \[1,2,5,7\].
\[1,2,5,7\] is strictly increasing, so return true.
**Example 2:**
**Input:** nums = \[2,3,1,2\]
**Output:** false
**Explanation:**
\[3,1,2\] is the result of removing the element at index 0.
\[2,1,2\] is the result of removing the element at index 1.
\[2,3,2\] is the result of removing the element at index 2.
\[2,3,1\] is the result of removing the element at index 3.
No resulting array is strictly increasing, so return false.
**Example 3:**
**Input:** nums = \[1,1,1\]
**Output:** false
**Explanation:** The result of removing any element is \[1,1\].
\[1,1\] is not strictly increasing, so return false.
**Constraints:**
* `2 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000` | You can traverse the buildings from the nearest to the ocean to the furthest. Keep with you the maximum to the right while traversing to determine if you can see the ocean or not. |
[95+%, Python] Extended window | remove-one-element-to-make-the-array-strictly-increasing | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nIterate over the array until `nums[i-1] < nums[i]` condition is wrong. If the condition is **False**, we extend the window from **2** elements **up to 4** and trying to understand what is it possible to remove one of elements to keep the sequence increasing, if it is possible we remove one of the elements from the list and keep iterating over the rest of the list. \n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def canBeIncreasing(self, nums: List[int]) -> bool:\n i, element_removed = 1, False\n while i < len(nums):\n if nums[i-1] < nums[i]:\n i += 1\n elif element_removed:\n return False\n else:\n left_value = nums[i-2] if i-2 >= 0 else -1\n right_value = nums[i+1] if i+1 < len(nums) else 1001\n element_removed = True\n if left_value < nums[i-1] and right_value > nums[i-1]:\n nums.pop(i)\n elif left_value < nums[i] and right_value > nums[i]:\n nums.pop(i-1)\n else:\n return False\n return True\n\n``` | 1 | Given a **0-indexed** integer array `nums`, return `true` _if it can be made **strictly increasing** after removing **exactly one** element, or_ `false` _otherwise. If the array is already strictly increasing, return_ `true`.
The array `nums` is **strictly increasing** if `nums[i - 1] < nums[i]` for each index `(1 <= i < nums.length).`
**Example 1:**
**Input:** nums = \[1,2,10,5,7\]
**Output:** true
**Explanation:** By removing 10 at index 2 from nums, it becomes \[1,2,5,7\].
\[1,2,5,7\] is strictly increasing, so return true.
**Example 2:**
**Input:** nums = \[2,3,1,2\]
**Output:** false
**Explanation:**
\[3,1,2\] is the result of removing the element at index 0.
\[2,1,2\] is the result of removing the element at index 1.
\[2,3,2\] is the result of removing the element at index 2.
\[2,3,1\] is the result of removing the element at index 3.
No resulting array is strictly increasing, so return false.
**Example 3:**
**Input:** nums = \[1,1,1\]
**Output:** false
**Explanation:** The result of removing any element is \[1,1\].
\[1,1\] is not strictly increasing, so return false.
**Constraints:**
* `2 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000` | You can traverse the buildings from the nearest to the ocean to the furthest. Keep with you the maximum to the right while traversing to determine if you can see the ocean or not. |
Python easy solution for beginners | remove-one-element-to-make-the-array-strictly-increasing | 0 | 1 | ```\nclass Solution:\n def canBeIncreasing(self, nums: List[int]) -> bool:\n for i in range(len(nums)):\n temp = nums[:i] + nums[i+1:]\n if sorted(temp) == temp:\n if len(set(temp)) == len(temp):\n return True\n return False | 11 | Given a **0-indexed** integer array `nums`, return `true` _if it can be made **strictly increasing** after removing **exactly one** element, or_ `false` _otherwise. If the array is already strictly increasing, return_ `true`.
The array `nums` is **strictly increasing** if `nums[i - 1] < nums[i]` for each index `(1 <= i < nums.length).`
**Example 1:**
**Input:** nums = \[1,2,10,5,7\]
**Output:** true
**Explanation:** By removing 10 at index 2 from nums, it becomes \[1,2,5,7\].
\[1,2,5,7\] is strictly increasing, so return true.
**Example 2:**
**Input:** nums = \[2,3,1,2\]
**Output:** false
**Explanation:**
\[3,1,2\] is the result of removing the element at index 0.
\[2,1,2\] is the result of removing the element at index 1.
\[2,3,2\] is the result of removing the element at index 2.
\[2,3,1\] is the result of removing the element at index 3.
No resulting array is strictly increasing, so return false.
**Example 3:**
**Input:** nums = \[1,1,1\]
**Output:** false
**Explanation:** The result of removing any element is \[1,1\].
\[1,1\] is not strictly increasing, so return false.
**Constraints:**
* `2 <= nums.length <= 1000`
* `1 <= nums[i] <= 1000` | You can traverse the buildings from the nearest to the ocean to the furthest. Keep with you the maximum to the right while traversing to determine if you can see the ocean or not. |
✅ Explained - Simple and Clear Python3 Code✅ | remove-all-occurrences-of-a-substring | 0 | 1 | # Intuition\nThe problem asks us to repeatedly remove all occurrences of a given substring, \'part\', from a given string, \'s\', until no more occurrences are left. To solve this problem, we can use a simple iterative approach. We keep searching for the leftmost occurrence of \'part\' in \'s\' and remove it until no more occurrences are found. Finally, we return the modified string \'s\' as the result.\n\n\n# Approach\nWe can use a while loop to repeatedly search for \'part\' in \'s\' and remove it until there are no more occurrences left. To do this, we initialize a boolean variable, \'test\', to True. This variable will help us keep track of whether any occurrence of \'part\' is found in \'s\' during each iteration of the while loop.\n\nInside the while loop, we use the find() function to find the index of the leftmost occurrence of \'part\' in \'s\'. If the index is not -1, which means \'part\' is found in \'s\', we remove \'part\' from \'s\' by slicing the string from the beginning up to the index, and then concatenating it with the substring starting from index+len(part) to the end. After removing \'part\' from \'s\', we set \'test\' to True to continue searching for more occurrences in the next iteration.\n\nIf the index is -1, which means \'part\' is not found in \'s\', we set \'test\' to False to exit the while loop, as there are no more occurrences left to remove.\n\nFinally, we return the modified string \'s\' as the output.\n# Complexity\n- Time complexity:\nThe time complexity of this approach depends on the number of occurrences of \'part\' in \'s\'. In the worst case, we may have to search for \'part\' and remove it for all its occurrences in \'s\'. Suppose \'s\' has length n and \'part\' has length m. The find() function has a time complexity of O(nm) in the worst case. Therefore, the overall time complexity of the removeOccurrences() function is O(nm) in the worst case.\n\n\n- Space complexity:\nThe space complexity of this approach is O(n), where n is the length of the input string \'s\'. This is because we are modifying the string \'s\' in place without using any additional data structures. Hence, the space complexity is linear with respect to the input size.\n\n\n\n\n\n# Code\n```\nclass Solution:\n def removeOccurrences(self, s: str, part: str) -> str:\n test=True\n while test:\n test=False\n i=s.find(part)\n if i!=-1:\n s=s[:i]+s[i+len(part):]\n test=True\n return s\n \n``` | 5 | Given two strings `s` and `part`, perform the following operation on `s` until **all** occurrences of the substring `part` are removed:
* Find the **leftmost** occurrence of the substring `part` and **remove** it from `s`.
Return `s` _after removing all occurrences of_ `part`.
A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** s = "daabcbaabcbc ", part = "abc "
**Output:** "dab "
**Explanation**: The following operations are done:
- s = "da**abc**baabcbc ", remove "abc " starting at index 2, so s = "dabaabcbc ".
- s = "daba**abc**bc ", remove "abc " starting at index 4, so s = "dababc ".
- s = "dab**abc** ", remove "abc " starting at index 3, so s = "dab ".
Now s has no occurrences of "abc ".
**Example 2:**
**Input:** s = "axxxxyyyyb ", part = "xy "
**Output:** "ab "
**Explanation**: The following operations are done:
- s = "axxx**xy**yyyb ", remove "xy " starting at index 4 so s = "axxxyyyb ".
- s = "axx**xy**yyb ", remove "xy " starting at index 3 so s = "axxyyb ".
- s = "ax**xy**yb ", remove "xy " starting at index 2 so s = "axyb ".
- s = "a**xy**b ", remove "xy " starting at index 1 so s = "ab ".
Now s has no occurrences of "xy ".
**Constraints:**
* `1 <= s.length <= 1000`
* `1 <= part.length <= 1000`
* `s` and `part` consists of lowercase English letters. | It's guaranteed to have at least one segment The string size is small so you can count all segments of ones with no that have no adjacent ones. |
Easy solution || beats 100% | remove-all-occurrences-of-a-substring | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def removeOccurrences(self, s: str, part: str) -> str:\n while(part in s):\n s=s.replace(part,"",1)\n return s\n``` | 1 | Given two strings `s` and `part`, perform the following operation on `s` until **all** occurrences of the substring `part` are removed:
* Find the **leftmost** occurrence of the substring `part` and **remove** it from `s`.
Return `s` _after removing all occurrences of_ `part`.
A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** s = "daabcbaabcbc ", part = "abc "
**Output:** "dab "
**Explanation**: The following operations are done:
- s = "da**abc**baabcbc ", remove "abc " starting at index 2, so s = "dabaabcbc ".
- s = "daba**abc**bc ", remove "abc " starting at index 4, so s = "dababc ".
- s = "dab**abc** ", remove "abc " starting at index 3, so s = "dab ".
Now s has no occurrences of "abc ".
**Example 2:**
**Input:** s = "axxxxyyyyb ", part = "xy "
**Output:** "ab "
**Explanation**: The following operations are done:
- s = "axxx**xy**yyyb ", remove "xy " starting at index 4 so s = "axxxyyyb ".
- s = "axx**xy**yyb ", remove "xy " starting at index 3 so s = "axxyyb ".
- s = "ax**xy**yb ", remove "xy " starting at index 2 so s = "axyb ".
- s = "a**xy**b ", remove "xy " starting at index 1 so s = "ab ".
Now s has no occurrences of "xy ".
**Constraints:**
* `1 <= s.length <= 1000`
* `1 <= part.length <= 1000`
* `s` and `part` consists of lowercase English letters. | It's guaranteed to have at least one segment The string size is small so you can count all segments of ones with no that have no adjacent ones. |
Python Beginner, replace() function | remove-all-occurrences-of-a-substring | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def removeOccurrences(self, s: str, part: str) -> str:\n while part in s: s = s.replace(part,"",1)\n return s\n``` | 3 | Given two strings `s` and `part`, perform the following operation on `s` until **all** occurrences of the substring `part` are removed:
* Find the **leftmost** occurrence of the substring `part` and **remove** it from `s`.
Return `s` _after removing all occurrences of_ `part`.
A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** s = "daabcbaabcbc ", part = "abc "
**Output:** "dab "
**Explanation**: The following operations are done:
- s = "da**abc**baabcbc ", remove "abc " starting at index 2, so s = "dabaabcbc ".
- s = "daba**abc**bc ", remove "abc " starting at index 4, so s = "dababc ".
- s = "dab**abc** ", remove "abc " starting at index 3, so s = "dab ".
Now s has no occurrences of "abc ".
**Example 2:**
**Input:** s = "axxxxyyyyb ", part = "xy "
**Output:** "ab "
**Explanation**: The following operations are done:
- s = "axxx**xy**yyyb ", remove "xy " starting at index 4 so s = "axxxyyyb ".
- s = "axx**xy**yyb ", remove "xy " starting at index 3 so s = "axxyyb ".
- s = "ax**xy**yb ", remove "xy " starting at index 2 so s = "axyb ".
- s = "a**xy**b ", remove "xy " starting at index 1 so s = "ab ".
Now s has no occurrences of "xy ".
**Constraints:**
* `1 <= s.length <= 1000`
* `1 <= part.length <= 1000`
* `s` and `part` consists of lowercase English letters. | It's guaranteed to have at least one segment The string size is small so you can count all segments of ones with no that have no adjacent ones. |
Python | Easy Solution✅ | remove-all-occurrences-of-a-substring | 0 | 1 | Solution 1:\n```\ndef removeOccurrences(self, s: str, part: str) -> str:\n while part in s:\n s= s.replace(part,"",1)\n return s\n```\nSolution 2:\n```\ndef removeOccurrences(self, s: str, part: str) -> str:\n while part in s:\n index = s.index(part)\n s = s[:index] + s[index+len(part):]\n return s\n``` | 5 | Given two strings `s` and `part`, perform the following operation on `s` until **all** occurrences of the substring `part` are removed:
* Find the **leftmost** occurrence of the substring `part` and **remove** it from `s`.
Return `s` _after removing all occurrences of_ `part`.
A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** s = "daabcbaabcbc ", part = "abc "
**Output:** "dab "
**Explanation**: The following operations are done:
- s = "da**abc**baabcbc ", remove "abc " starting at index 2, so s = "dabaabcbc ".
- s = "daba**abc**bc ", remove "abc " starting at index 4, so s = "dababc ".
- s = "dab**abc** ", remove "abc " starting at index 3, so s = "dab ".
Now s has no occurrences of "abc ".
**Example 2:**
**Input:** s = "axxxxyyyyb ", part = "xy "
**Output:** "ab "
**Explanation**: The following operations are done:
- s = "axxx**xy**yyyb ", remove "xy " starting at index 4 so s = "axxxyyyb ".
- s = "axx**xy**yyb ", remove "xy " starting at index 3 so s = "axxyyb ".
- s = "ax**xy**yb ", remove "xy " starting at index 2 so s = "axyb ".
- s = "a**xy**b ", remove "xy " starting at index 1 so s = "ab ".
Now s has no occurrences of "xy ".
**Constraints:**
* `1 <= s.length <= 1000`
* `1 <= part.length <= 1000`
* `s` and `part` consists of lowercase English letters. | It's guaranteed to have at least one segment The string size is small so you can count all segments of ones with no that have no adjacent ones. |
Python Solution || 3 lines || 91% beats | remove-all-occurrences-of-a-substring | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def removeOccurrences(self, s: str, part: str) -> str:\n while(part in s):\n s=s.replace(part,"",1)\n return s\n\n\n\n\n``` | 5 | Given two strings `s` and `part`, perform the following operation on `s` until **all** occurrences of the substring `part` are removed:
* Find the **leftmost** occurrence of the substring `part` and **remove** it from `s`.
Return `s` _after removing all occurrences of_ `part`.
A **substring** is a contiguous sequence of characters in a string.
**Example 1:**
**Input:** s = "daabcbaabcbc ", part = "abc "
**Output:** "dab "
**Explanation**: The following operations are done:
- s = "da**abc**baabcbc ", remove "abc " starting at index 2, so s = "dabaabcbc ".
- s = "daba**abc**bc ", remove "abc " starting at index 4, so s = "dababc ".
- s = "dab**abc** ", remove "abc " starting at index 3, so s = "dab ".
Now s has no occurrences of "abc ".
**Example 2:**
**Input:** s = "axxxxyyyyb ", part = "xy "
**Output:** "ab "
**Explanation**: The following operations are done:
- s = "axxx**xy**yyyb ", remove "xy " starting at index 4 so s = "axxxyyyb ".
- s = "axx**xy**yyb ", remove "xy " starting at index 3 so s = "axxyyb ".
- s = "ax**xy**yb ", remove "xy " starting at index 2 so s = "axyb ".
- s = "a**xy**b ", remove "xy " starting at index 1 so s = "ab ".
Now s has no occurrences of "xy ".
**Constraints:**
* `1 <= s.length <= 1000`
* `1 <= part.length <= 1000`
* `s` and `part` consists of lowercase English letters. | It's guaranteed to have at least one segment The string size is small so you can count all segments of ones with no that have no adjacent ones. |
🔥 DP and DFS | Very Clearly Explained! | O(n) Time | maximum-alternating-subsequence-sum | 0 | 1 | ***Solution 1: Dynamic Programming***\n\n**Intuition**\nWe can use DP (dynamic programming) to loop through nums and find the biggest alternating subsequence num.\n\n**Algorithm**\nWe first initialize a `n` by `2` matrix where `n = len(nums)`. In a supposed index of `dp[i][j]`, `i` stands for the index of `dp` based on the such index of `nums` given from the input, while `j` stands for whether we add or subtract a number for the last value. If `j == 0`, then we add, if `j==1`, then we subtract. This means `dp[i][0]` means that we have a plus for the last value, while `dp[i][1]` means we have a minus for the last value. Before we start the iteration, we need to pre-define `dp[0][0]` as `nums[0]` as the index is both `0`. We also need to pre-define `dp[0][1]` as `0` since we have to start by adding given the question, thus we put 0.\n\nNext, we iterate through `nums` in a range for loop from index `1` to `n`, we start on index `1` instead of `0` because index `0` is already pre-defined. Each iteration, we try either to choose (meaning we choose to go or continue to add/subtract) or not choose (meaning we continue iterating through the array without making an alternating subsequence) for when the last value is plus (`dp[i][0]`) and when the last value is minus. (`dp[i][1]`) We take the max of whether to choose or not choose for both when the last value is plus and when the last value is minus.\n\nAfter we finish iterating, max of `dp[-1]` is the result, meaning we find the maximum number between when the last value is plus and when the last value is minus.\n\n```\nclass Solution:\n def maxAlternatingSum(self, nums: List[int]) -> int:\n n = len(nums) \n dp = [[0,0] for _ in range(n)] # initialize dp\n dp[0][0] = nums[0] # pre-define\n dp[0][1] = 0 # pre-define\n\n for i in range(1, n): # iterate through nums starting from index 1\n dp[i][0] = max(nums[i] + dp[i-1][1], dp[i-1][0]) # find which value is higher between choosing or not choosing when the last value is plus.\n dp[i][1] = max(-nums[i] + dp[i-1][0], dp[i-1][1]) # find which value is higher between choosing or not choosing when the last value is minus.\n \n return max(dp[-1]) # find the maximum of the last array of dp of whether the last value is plus or minus, this will be our answer.\n```\n\n***Solution 2: DFS with Memoization***\n\n**Intuition**\nWe can combine DFS with memoization to find all the paths and narrow it down as we go.\n\n**Algorithm**\nStart a dfs function, it terminates when `i`, the index, reaches the length of nums, or `n` in this case. We also keep track of another variable `p`, which determines whether we add or subtract. Next, similar to solution 1, we either choose (to start or continue the existing alternating subsequence) or not choose (move on to the next element of nums without doing anything). This will give us the answer.\n```\nclass Solution:\n def maxAlternatingSum(self, nums: List[int]) -> int:\n\t\tn = len(nums) \n @cache\n def dfs(i: int, p: bool) -> int:\n if i>=n:\n return 0 \n\t\t\t\n\t\t\t# if choose\n num = nums[i] if p else -nums[i]\n choose = num + dfs(i+1, not p)\n\n # if not choose\n not_choose = dfs(i+1, p)\n return max(choose, not_choose)\n\n return dfs(0, True)\n```\n\n**Please consider upvoting if these solutions helped you. Good luck!**\n | 9 | The **alternating sum** of a **0-indexed** array is defined as the **sum** of the elements at **even** indices **minus** the **sum** of the elements at **odd** indices.
* For example, the alternating sum of `[4,2,5,3]` is `(4 + 5) - (2 + 3) = 4`.
Given an array `nums`, return _the **maximum alternating sum** of any subsequence of_ `nums` _(after **reindexing** the elements of the subsequence)_.
A **subsequence** of an array is a new array generated from the original array by deleting some elements (possibly none) without changing the remaining elements' relative order. For example, `[2,7,4]` is a subsequence of `[4,2,3,7,2,1,4]` (the underlined elements), while `[2,4,2]` is not.
**Example 1:**
**Input:** nums = \[4,2,5,3\]
**Output:** 7
**Explanation:** It is optimal to choose the subsequence \[4,2,5\] with alternating sum (4 + 5) - 2 = 7.
**Example 2:**
**Input:** nums = \[5,6,7,8\]
**Output:** 8
**Explanation:** It is optimal to choose the subsequence \[8\] with alternating sum 8.
**Example 3:**
**Input:** nums = \[6,2,1,2,4,5\]
**Output:** 10
**Explanation:** It is optimal to choose the subsequence \[6,1,5\] with alternating sum (6 + 5) - 1 = 10.
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 105` | Try thinking about the problem as if the array is empty. Then you only need to form goal using elements whose absolute value is <= limit. You can greedily set all of the elements except one to limit or -limit, so the number of elements you need is ceil(abs(goal)/ limit). You can "normalize" goal by offsetting it by the sum of the array. For example, if the goal is 5 and the sum is -3, then it's exactly the same as if the goal is 8 and the array is empty. The answer is ceil(abs(goal-sum)/limit) = (abs(goal-sum)+limit-1) / limit. |
Subsets and Splits