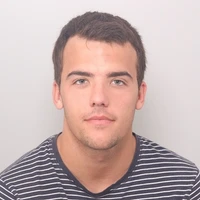
Rename nucleotide_transformer_downstream_tasks_public.py to nucleotide_transformer_downstream_tasks.py
652fa59
# Copyright 2020 The HuggingFace Datasets Authors and the current dataset script | |
# contributor. | |
# | |
# Licensed under the Apache License, Version 2.0 (the "License"); | |
# you may not use this file except in compliance with the License. | |
# You may obtain a copy of the License at | |
# | |
# http://www.apache.org/licenses/LICENSE-2.0 | |
# | |
# Unless required by applicable law or agreed to in writing, software | |
# distributed under the License is distributed on an "AS IS" BASIS, | |
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. | |
# See the License for the specific language governing permissions and | |
# limitations under the License. | |
"""Script for the dataset containing the "promoter_all" and "enhancers" downstream tasks from the Nucleotide | |
Transformer paper.""" | |
from typing import List | |
import datasets | |
from Bio import SeqIO | |
# Find for instance the citation on arxiv or on the dataset repo/website | |
_CITATION = """\ | |
@article{dalla2023nucleotide, | |
title={The Nucleotide Transformer: Building and Evaluating Robust Foundation Models for Human Genomics}, | |
author={Dalla-Torre, Hugo and Gonzalez, Liam and Mendoza-Revilla, Javier and Carranza, Nicolas Lopez and Grzywaczewski, Adam Henryk and Oteri, Francesco and Dallago, Christian and Trop, Evan and Sirelkhatim, Hassan and Richard, Guillaume and others}, | |
journal={bioRxiv}, | |
pages={2023--01}, | |
year={2023}, | |
publisher={Cold Spring Harbor Laboratory} | |
} | |
""" | |
# You can copy an official description | |
_DESCRIPTION = """\ | |
2 of the 18 classification downstream tasks from the Nucleotide Transformer paper. Each task | |
corresponds to a dataset configuration. | |
""" | |
_HOMEPAGE = "https://github.com/instadeepai/nucleotide-transformer" | |
_LICENSE = "https://github.com/instadeepai/nucleotide-transformer/LICENSE.md" | |
_TASKS = [ | |
'enhancers_types', | |
'promoter_all' | |
] | |
class NucleotideTransformerDownstreamTasksConfig(datasets.BuilderConfig): | |
"""BuilderConfig for The Nucleotide Transformer downstream taks dataset.""" | |
def __init__(self, *args, task: str, **kwargs): | |
"""BuilderConfig downstream tasks dataset. | |
Args: | |
task (:obj:`str`): Task name. | |
**kwargs: keyword arguments forwarded to super. | |
""" | |
super().__init__( | |
*args, | |
name=f'{task}', | |
**kwargs, | |
) | |
self.task = task | |
class NucleotideTransformerDownstreamTasks(datasets.GeneratorBasedBuilder): | |
VERSION = datasets.Version("1.1.0") | |
BUILDER_CONFIG_CLASS = NucleotideTransformerDownstreamTasksConfig | |
BUILDER_CONFIGS = [ | |
NucleotideTransformerDownstreamTasksConfig(task=task) for task in _TASKS | |
] | |
DEFAULT_CONFIG_NAME = "enhancers" | |
def _info(self): | |
features = datasets.Features( | |
{ | |
"sequence": datasets.Value("string"), | |
"name": datasets.Value("string"), | |
"label": datasets.Value("int32"), | |
} | |
) | |
return datasets.DatasetInfo( | |
# This is the description that will appear on the datasets page. | |
description=_DESCRIPTION, | |
# This defines the different columns of the dataset and their types | |
features=features, | |
# Homepage of the dataset for documentation | |
homepage=_HOMEPAGE, | |
# License for the dataset if available | |
license=_LICENSE, | |
# Citation for the dataset | |
citation=_CITATION, | |
) | |
def _split_generators(self, dl_manager: datasets.DownloadManager) -> List[datasets.SplitGenerator]: | |
train_file = dl_manager.download_and_extract(self.config.task + '/train.fna') | |
test_file = dl_manager.download_and_extract(self.config.task + '/test.fna') | |
return [ | |
datasets.SplitGenerator(name=datasets.Split.TRAIN, | |
gen_kwargs={"file": train_file} | |
), | |
datasets.SplitGenerator(name=datasets.Split.TEST, | |
gen_kwargs={"file": test_file} | |
), | |
] | |
# method parameters are unpacked from `gen_kwargs` as given in `_split_generators` | |
def _generate_examples(self, file): | |
key = 0 | |
with open(file, 'rt') as f: | |
fasta_sequences = SeqIO.parse(f, 'fasta') | |
for record in fasta_sequences: | |
# parse descriptions in the fasta file | |
sequence, name = str(record.seq), str(record.name) | |
label = int(name.split("|")[-1]) | |
# yield example | |
yield key, { | |
'sequence': sequence, | |
'name': name, | |
'label': label, | |
} | |
key += 1 |