problem_id
stringlengths 32
32
| name
stringclasses 1
value | problem
stringlengths 200
14k
| solutions
stringlengths 12
1.12M
| test_cases
stringlengths 37
74M
| difficulty
stringclasses 3
values | language
stringclasses 1
value | source
stringclasses 7
values | num_solutions
int64 12
1.12M
| starter_code
stringlengths 0
956
|
---|---|---|---|---|---|---|---|---|---|
e6e0dfc3c07cc4895a751f7475c3c7d0 | UNKNOWN | # Task
A common way for prisoners to communicate secret messages with each other is to encrypt them. One such encryption algorithm goes as follows.
You take the message and place it inside an `nx6` matrix (adjust the number of rows depending on the message length) going from top left to bottom right (one row at a time) while replacing spaces with dots (.) and adding dots at the end of the last row (if necessary) to complete the matrix.
Once the message is in the matrix you read again from top left to bottom right but this time going one column at a time and treating each column as one word.
# Example
The following message `"Attack at noon or we are done for"` is placed in a `6 * 6` matrix :
```
Attack
.at.no
on.or.
we.are
.done.
for...```
Reading it one column at a time we get:
`A.ow.f tanedo tt..or a.oan. cnrre. ko.e..`
# Input/Output
- `[input]` string `msg`
a regular english sentance representing the original message
- `[output]` a string
encrypted message | ["def six_column_encryption(msg):\n msg=msg.replace(' ','.')+'.'*((6-len(msg)%6)%6)\n return ' '.join(msg[n::6] for n in range(6))", "from itertools import zip_longest\n\ndef six_column_encryption(msg, size=6):\n msg = msg.replace(' ','.')\n L = [msg[i:i+size] for i in range(0, len(msg), size)]\n return ' '.join(map(''.join, zip_longest(*L, fillvalue='.')))", "from itertools import zip_longest\n\ndef six_column_encryption(msg):\n return ' '.join(map(''.join,\n zip_longest(*(msg[i:i+6].replace(' ', '.') for i in range(0, len(msg), 6)), fillvalue='.')\n ))", "def six_column_encryption(msg):\n row = (len(msg) + 5) // 6\n gen = iter(msg.replace(\" \", \".\"))\n arr = zip(*[[next(gen, \".\") for i in range(6)] for j in range(row)])\n return \" \".join(\"\".join(line) for line in arr)", "six_column_encryption=lambda s:' '.join(map(''.join,zip(*zip(*[iter(s.replace(' ','.')+'.'*5)]*6))))", "def six_column_encryption(msg):\n msg = msg.replace(\" \", \".\") + \".\" * (6 - len(msg) % 6 if len(msg) % 6 != 0 else 0)\n return \" \".join([msg[i::6] for i in range(6)])", "from math import ceil\ndef six_column_encryption(msg):\n n=ceil(len(msg)/6)\n s=(msg+' '*(n*6-len(msg))).replace(' ','.')\n r=[]\n for i in range(6):\n r.append(s[i::6])\n return ' '.join(r)", "def six_column_encryption(msg):\n msg, ans = msg.replace(' ', '.'), []\n \n while len(msg) % 6 != 0:\n msg += '.'\n \n r = len(msg) // 6\n \n for i in range(0, len(msg)//r):\n ans.append(''.join([msg[j] for j in range(i, len(msg), 6)]))\n\n return ' '.join(ans)", "def six_column_encryption(msg):\n m = msg.replace(' ', '.')\n lst = [m[i:i+6] for i in range(0, len(m), 6)]\n lst[-1] = lst[-1].ljust(6, '.')\n ans = []\n for i in range(6):\n s = ''\n for j in lst:\n s += j[i]\n ans.append(s)\n return ' '.join(ans)", "import numpy as np\n\ndef six_column_encryption(s):\n s = s.replace(' ','.') + '.'*(int(6*np.ceil(len(s)/6)-len(s)))\n s = [list(s[i:i+6]) for i in range(0,len(s),6)]\n arr = np.array(s).reshape(-1,6).T\n return ' '.join(map(''.join, arr))"] | {"fn_name": "six_column_encryption", "inputs": [["Attack at noon or we are done for"], ["Let's kill them all"], ["Meet me behind the kitchen tomorrow at seven in the evening"]], "outputs": [["A.ow.f tanedo tt..or a.oan. cnrre. ko.e.."], ["Lkhl eie. tlm. 'l.. s.a. .tl."], ["Men.eoaete e.dknrtnhn eb.i.r..ei tetttosi.n .hhcoweneg miehm.v.v."]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,195 |
def six_column_encryption(msg):
|
332779f6b391bc1fe955342afb75b7d4 | UNKNOWN | # Write Number in Expanded Form
You will be given a number and you will need to return it as a string in [Expanded Form](https://www.mathplacementreview.com/arithmetic/whole-numbers.php#expanded-form). For example:
```python
expanded_form(12) # Should return '10 + 2'
expanded_form(42) # Should return '40 + 2'
expanded_form(70304) # Should return '70000 + 300 + 4'
```
NOTE: All numbers will be whole numbers greater than 0.
If you liked this kata, check out [part 2](https://www.codewars.com/kata/write-number-in-expanded-form-part-2)!! | ["def expanded_form(num):\n num = list(str(num))\n return ' + '.join(x + '0' * (len(num) - y - 1) for y,x in enumerate(num) if x != '0')", "def expanded_form(n):\n result = []\n for a in range(len(str(n)) - 1, -1, -1):\n current = 10 ** a\n quo, n = divmod(n, current)\n if quo:\n result.append(str(quo * current))\n return ' + '.join(result)\n", "def expanded_form(num):\n num = str(num)\n st = ''\n for j, i in enumerate(num):\n if i != '0':\n st += ' + {}{}'.format(i, (len(num[j+1:])*'0'))\n return st.strip(' +')", "def expanded_form(num):\n return \" + \".join([str(int(d) * 10**p) for p, d in enumerate(str(num)[::-1]) if d != \"0\"][::-1])", "def expanded_form(num):\n return ' + '.join([ x.ljust(i+1, '0') for i, x in enumerate(str(num)[::-1]) if x != '0'][::-1])\n", "def expanded_form(num):\n return ' + '.join([x+'0'*i for i,x in enumerate(str(num)[::-1]) if x != '0'][::-1])", "def expanded_form(num):\n s = str(num)\n n = len(s)\n return ' + '.join( [s[-i]+\"0\"*(i-1) for i in range(n,0,-1) if s[-i] != \"0\"])", "from math import floor,log\ndef expanded_form(num):\n x = 10**floor(log(num)/log(10))\n a = num//x\n b = num%x\n s = str(a*x) \n if (b!=0): s += \" + \" + expanded_form(b)\n return(s)\n", "def expanded_form(n):\n return (lambda s:' + '.join(s[x] + len(s[x+1:])*'0' for x in range(len(s))if s[x] != '0'))(str(n))", "def expanded_form(num):\n return \" + \".join(str(num)[i] + \"0\" * ( len(str(num)) - i - 1 ) for i in range(len(str(num))) if str(num)[i] != \"0\")", "def expanded_form(num):\n str_num, str_list, index_track = (str(num), [], 0)\n for digit in str_num:\n if digit != str(0):\n val = int(digit) * 10 ** (len(str_num) - (index_track + 1))\n str_list.append(str(val))\n index_track += 1\n return \" + \".join(str_list)"] | {"fn_name": "expanded_form", "inputs": [[2], [12], [42], [70304], [4982342]], "outputs": [["2"], ["10 + 2"], ["40 + 2"], ["70000 + 300 + 4"], ["4000000 + 900000 + 80000 + 2000 + 300 + 40 + 2"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,925 |
def expanded_form(num):
|
9872ec706274cfc3cb3f18a4727d9e42 | UNKNOWN | The Binomial Form of a polynomial has many uses, just as the standard form does. For comparison, if p(x) is in Binomial Form and q(x) is in standard form, we might write
p(x) := a0 \* xC0 + a1 \* xC1 + a2 \* xC2 + ... + aN \* xCN
q(x) := b0 + b1 \* x + b2 \* x^(2) + ... + bN \* x^(N)
Both forms have tricks for evaluating them, but tricks should not be necessary. The most important thing to keep in mind is that aCb can be defined for non-integer values of a; in particular,
```
aCb := a * (a-1) * (a-2) * ... * (a-b+1) / b! // for any value a and integer values b
:= a! / ((a-b)!b!) // for integer values a,b
```
The inputs to your function are an array which specifies a polynomial in Binomial Form, ordered by highest-degree-first, and also a number to evaluate the polynomial at. An example call might be
```python
value_at([1, 2, 7], 3)
```
and the return value would be 16, since 3C2 + 2 * 3C1 + 7 = 16. In more detail, this calculation looks like
```
1 * xC2 + 2 * xC1 + 7 * xC0 :: x = 3
3C2 + 2 * 3C1 + 7
3 * (3-1) / 2! + 2 * 3 / 1! + 7
3 + 6 + 7 = 16
```
More information can be found by reading about [Binomial Coefficients](https://en.wikipedia.org/wiki/Binomial_coefficient) or about [Finite Differences](https://en.wikipedia.org/wiki/Finite_difference).
Note that while a solution should be able to handle non-integer inputs and get a correct result, any solution should make use of rounding to two significant digits (as the official solution does) since high precision for non-integers is not the point here. | ["from functools import reduce\nfrom math import factorial\n\n\ndef value_at(poly, x):\n return round(sum(n * aCb(x, i) for i, n in enumerate(poly[::-1])), 2)\n\ndef aCb(a, b):\n return reduce(lambda x, y: x * y, (a - i for i in range(b)), 1) / factorial(b)\n", "def aCb(a, b):\n result = 1.0\n for i in range(b):\n result = result * (a - i) / (i + 1)\n return result\n\ndef value_at(poly_spec, x):\n answer = 0\n l = len(poly_spec)\n for i, coeff in enumerate(poly_spec):\n answer += coeff * aCb(x, l - i - 1)\n return round(answer, 2)", "from math import factorial\nfrom functools import reduce\n\ndef product(seq):\n return reduce(lambda x,y:x*y, seq) if seq else 1\n\ndef comb(x, r):\n return product([x - i for i in range(r)]) / factorial(r)\n\ndef value_at(p, x):\n result = sum(a*comb(x, i) for i, a in enumerate(p[::-1]))\n \n return result if isinstance(x, int) else round(result, 2)\n", "def binom(x, k):\n r = 1\n for i in range(1, k + 1):\n r *= (x - i + 1) / i\n return r\n\ndef value_at(poly_spec, x):\n r = 0\n for k, c in enumerate(poly_spec[::-1]):\n r += c * binom(x, k)\n return round(r, 2)", "from math import factorial\nfrom functools import lru_cache\n\nfactorial = lru_cache(maxsize=None)(factorial)\n\ndef comb(a, b):\n if isinstance(a, int):\n return int(factorial(a) / factorial(b) / factorial(max(0, a - b)))\n r = 1\n for i in range(b):\n r *= a - i\n return r / factorial(b)\n\ndef value_at(a, n):\n return round(sum(x * comb(n, i) for i, x in enumerate(a[::-1])), 2)", "def value_at(poly_spec, x):\n leng = len(poly_spec)\n ans = 0\n for i, e in enumerate(poly_spec):\n temp = 1\n for j in range(leng-i-1):\n temp *= (x-j)/(j+1)\n ans += e*temp\n return round(ans, 2)", "value_at=lambda p, x: round(sum(map(lambda e: e[1]*c(x,e[0]), enumerate(p[::-1]))), 2)\nc=lambda x,b: 1 if x==b or b<1 else x if b<2 else x*c(x-1,b-1)/b", "def value_at(poly_spec, x):\n if len(poly_spec) < 2: return poly_spec[0] if poly_spec else 0\n l = len(poly_spec) - 1\n m = [co * binomial(x, l - i) for i, co in enumerate(poly_spec[:-1])]\n return round(float(sum(m) + poly_spec[l]), 2)\n \ndef factorial(n):\n return 1 if n < 2 else n * factorial(n - 1)\n \ndef binomial(a, b): \n res = 1.0\n for k in range(b): res *= (a - k)\n return res / factorial(b)", "def comb(a,b):\n r=1\n s=1\n for i in range(1,b+1):\n r*=(a-i+1)\n s*=i\n return r/s\n\ndef value_at(poly_spec, x):\n r=0\n for i,c in enumerate(poly_spec[::-1]):\n r+=c*comb(x,i)\n return round(r,2)", "def value_at(p, x):\n return round(sum(c * choose(x,len(p)-1-i) for i,c in enumerate(p)), 2)\n \ndef choose(x, k):\n n,d = 1,1\n for i in range(k): n, d = n*(x-i), d*(i+1)\n return n / d"] | {"fn_name": "value_at", "inputs": [[[1, 2, 7], 3], [[1, 2, 7, 0, 5], 2], [[1, 1, 1, 1, 7, 0, 5], 2], [[1, 2, 7, 0, 5], 0.6], [[1, 2, 7, 0, 5], 0]], "outputs": [[16], [12], [12], [4.24], [5.0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,905 |
def value_at(poly_spec, x):
|
eee239f090e8d630d4cbc7b0af1604d8 | UNKNOWN | ```if-not:swift
Write simple .camelCase method (`camel_case` function in PHP, `CamelCase` in C# or `camelCase` in Java) for strings. All words must have their first letter capitalized without spaces.
```
```if:swift
Write a simple `camelCase` function for strings. All words must have their first letter capitalized and all spaces removed.
```
For instance:
```python
camelcase("hello case") => HelloCase
camelcase("camel case word") => CamelCaseWord
```
```c#
using Kata;
"hello case".CamelCase(); // => "HelloCase"
"camel case word".CamelCase(); // => "CamelCaseWord"
```
Don't forget to rate this kata! Thanks :) | ["def camel_case(string):\n return string.title().replace(\" \", \"\")", "def camel_case(s):\n return s.title().replace(' ','')", "def camel_case(string):\n return ''.join([i.capitalize() for i in string.split()])", "def camel_case(string):\n if len(string) == 0: return \"\"\n str_list = list(string)\n space_ids = [index for index, char in enumerate(str_list) if char == \" \" and index < len(str_list) - 1 ]\n for index in space_ids:\n str_list[index + 1] = str_list[index + 1].upper()\n str_list[0] = str_list[0].upper()\n changed_str = \"\".join(str_list)\n changed_str = changed_str.replace(\" \", \"\")\n return changed_str", "def camel_case(string):\n return ''.join((string.title()).split())\n #your code here\n", "def camel_case(s):\n #your code here\n return ''.join(s.title().split())", "def camel_case(s):\n return s.strip().title().replace(' ', '')", "def camel_case(string):\n #a = [string.split()]\n a = list(string)\n for i in range(0, len(a)):\n if i==0 or a[i-1]==' ':\n a[i] = a[i].upper()\n return ''.join(a).replace(' ','')", "def camel_case(string):\n words=string.split()\n answer=\"\"\n for capped in words:\n \n answer+=capped.capitalize()\n return answer", "camel_case=lambda s:s.title().replace(' ','')"] | {"fn_name": "camel_case", "inputs": [["test case"], ["camel case method"], ["say hello "], [" camel case word"], [""]], "outputs": [["TestCase"], ["CamelCaseMethod"], ["SayHello"], ["CamelCaseWord"], [""]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,343 |
def camel_case(string):
|
532eac8469a987ec5efb756b91be8866 | UNKNOWN | # Task
We know that some numbers can be split into two primes. ie. `5 = 2 + 3, 10 = 3 + 7`. But some numbers are not. ie. `17, 27, 35`, etc..
Given a positive integer `n`. Determine whether it can be split into two primes. If yes, return the maximum product of two primes. If not, return `0` instead.
# Input/Output
`[input]` integer `n`
A positive integer.
`0 ≤ n ≤ 100000`
`[output]` an integer
The possible maximum product of two primes. or return `0` if it's impossible split into two primes.
# Example
For `n = 1`, the output should be `0`.
`1` can not split into two primes
For `n = 4`, the output should be `4`.
`4` can split into two primes `2 and 2`. `2 x 2 = 4`
For `n = 20`, the output should be `91`.
`20` can split into two primes `7 and 13` or `3 and 17`. The maximum product is `7 x 13 = 91` | ["def isPrime(n):\n return n==2 or n>2 and n&1 and all(n%p for p in range(3,int(n**.5+1),2))\n\ndef prime_product(n):\n return next( (x*(n-x) for x in range(n>>1,1,-1) if isPrime(x) and isPrime(n-x)), 0)", "def prime_product(n):\n def is_prime(n): return n == 2 or n > 2 and n % 2 and all(n % d for d in range(3, int(n ** .5) + 1, 2))\n for i in range(n//2,1,-1): \n if is_prime(i) and is_prime(n-i): return i*(n-i)\n return 0", "def primes_set():\n primes, sieve = {2}, [True] * 50000\n for i in range(3, 317, 2):\n if sieve[i // 2]:\n sieve[i * i // 2 :: i] = [False] * ((100000 - i * i - 1) // (2 * i) + 1)\n primes.update((2 * i + 1) for i in range(1, 50000) if sieve[i])\n return primes\n\nprimes = primes_set()\n\ndef prime_product(n):\n if n % 2 or n == 4:\n return (2 * (n - 2)) if (n - 2) in primes else 0\n m, s = n // 2, n % 4 == 0\n return next(((m + i) * (m - i) for i in range(s, m, 2) if {m + i, m - i} < primes), 0)", "def prime_product(n):\n result = 0\n \n def is_prime(num):\n for i in range(2, int(num ** 0.5) + 1):\n if num % i == 0:\n return False\n return True\n \n for i in range(2, n // 2 + 1):\n if is_prime(i) and is_prime(n - i):\n result = i * (n - i)\n return result", "from itertools import compress\nimport numpy as np\nfrom bisect import bisect\n\nxs = np.ones(300000)\nxs[:2] = 0\nxs[4::2] = 0\nfor i in range(3, int(len(xs)**0.5) + 1):\n if xs[i]:\n xs[i*i::i] = 0\nprimes = list(compress(range(len(xs)), xs))\n\ndef iter_primes(n):\n i = bisect(primes, n // 2)\n for x in range(2, primes[i] + 1):\n if xs[x] and xs[n-x]:\n yield x, n-x\n\ndef prime_product(n):\n return max((a*b for a,b in iter_primes(n)), default=0)", "def prime_product(n, sieve=[False, False, True, True]):\n for x in range(len(sieve), n+1):\n sieve.append(x % 2 and all(x % p for p in range(3, int(x ** .5) + 1, 2) if sieve[p]))\n return next((p * (n - p) for p in range(n//2, 1, -1) if sieve[p] and sieve[n - p]), 0)", "def is_prime(n):\n if n == 2 or n == 3:\n return True\n if n % 2 == 0 or n < 2:\n return False\n\n for i in range(3, int(n**0.5)+1, 2):\n if n % i == 0:\n return False\n \n return True\n\n\ndef prime_product(n):\n for i in range(n//2+1)[::-1]:\n if is_prime(i) and is_prime(n - i):\n return i * (n - i)\n return 0", "from bisect import bisect\n\n\ndef prime_product(n):\n bisect_point = bisect(primes, n // 2)\n\n # If n is odd then pair must be (2, n - 2) or nothing. This is because 2 is the\n # only even prime and to get an odd number you must add even + odd.\n if n % 2:\n return 2 * (n - 2) if n - 2 in prime_set else 0\n\n # Check half of prime list (starting from the middle). Return product of first pair found.\n for prime in primes[:bisect_point][::-1]:\n if n - prime in prime_set:\n return prime * (n - prime)\n\n return 0 # Return 0 if no valid pair found.\n\n\ndef get_primes(n):\n \"\"\"Sieve of Eratosthenes to calculate all primes less than or equal to n.\n\n Return set of primes.\n \"\"\"\n primes = [True] * (n + 1)\n\n for num in range(2, int(n ** 0.5) + 1):\n if primes[num]:\n primes[num * 2::num] = [False] * len(primes[num * 2::num])\n\n return [i for i, x in enumerate(primes) if x and i > 1]\n\n\n# Calculate all primes up to max but only calculate primes once. Then reference master prime list in all test cases.\nmax_input = 100000\nprimes = get_primes(max_input)\nprime_set = set(primes)", "# generate primes\nLIMIT = 100000\nsieve = [True] * (LIMIT//2)\nfor n in range(3, int(LIMIT**0.5) +1, 2):\n if sieve[n//2]: sieve[n*n//2::n] = [False] * ((LIMIT-n*n-1)//2//n +1)\nPRIMES_LIST = [2] + [2*i+1 for i in range(1, LIMIT//2) if sieve[i]]\nPRIMES = set(PRIMES_LIST)\ndel sieve\n\n\nfrom bisect import bisect\n\ndef prime_product(n):\n if n % 2:\n return 2*(n-2) if n-2 in PRIMES else 0\n \n for p in PRIMES_LIST[:bisect(PRIMES_LIST, n//2)][::-1]:\n if n-p in PRIMES:\n return (n-p) * p", "def prime_product(n):\n def prime(a):\n if a == 2: return True\n if a < 2 or a % 2 == 0: return False\n return not any(a % x == 0 for x in range(3, int(a**0.5) + 1, 2))\n if (n <= 3):\n return 0\n i = n // 2\n while i > 0:\n if (prime(n - i) and prime(i)):\n return (n - i) * i\n i -= 1\n return 0"] | {"fn_name": "prime_product", "inputs": [[1], [3], [4], [5], [6], [7], [8], [9], [10], [11], [12], [20], [100]], "outputs": [[0], [0], [4], [6], [9], [10], [15], [14], [25], [0], [35], [91], [2491]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 4,602 |
def prime_product(n):
|
cdad003ad88ed28119a9dd853ece50bf | UNKNOWN | Sort the given strings in alphabetical order, case **insensitive**. For example:
```
["Hello", "there", "I'm", "fine"] --> ["fine", "Hello", "I'm", "there"]
["C", "d", "a", "B"]) --> ["a", "B", "C", "d"]
``` | ["def sortme(words):\n return sorted(words, key=str.lower)", "def sortme(words):\n return sorted(words,key=lambda x: x.lower())", "def sortme(a):\n return sorted(a, key=str.lower)", "def sortme(words):\n # your code here\n return sorted(words,key=str.casefold)", "def lower(e):\n return e.lower()\n\ndef sortme(words):\n words.sort(key=lower)\n return words", "sortme=lambda w:sorted(w,key=lambda x:x.lower())", "def sortme(words):\n words_sorted = sorted(words, key = lambda s: s.casefold())\n return words_sorted", "sortme = lambda words: sorted(words, key=lambda w: (w.upper(), w[0].islower()))", "def KEY(n):\n return n.lower()\ndef sortme(words):\n words.sort(key=KEY)\n return words", "def sortme(words):\n # your code here\n arr = []\n\n if len(words) > 1:\n for i in words:\n arr.append(i.lower())\n arr = sorted(arr)\n print(arr)\n \n m = []\n for i in arr:\n try:\n j = words.index(i)\n if i.islower():\n m.append(i)\n except:\n m.append(i.capitalize())\n \n return m\n else:\n return words"] | {"fn_name": "sortme", "inputs": [[["Hello", "there", "I'm", "fine"]], [["C", "d", "a", "B"]], [["CodeWars"]], [[]]], "outputs": [[["fine", "Hello", "I'm", "there"]], [["a", "B", "C", "d"]], [["CodeWars"]], [[]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,202 |
def sortme(words):
|
f3501a6f13c18f6b07c48c4dca34749d | UNKNOWN | German mathematician Christian Goldbach (1690-1764) [conjectured](https://en.wikipedia.org/wiki/Goldbach%27s_conjecture) that every even number greater than 2 can be represented by the sum of two prime numbers. For example, `10` can be represented as `3+7` or `5+5`.
Your job is to make the function return a list containing all unique possible representations of `n` in an increasing order if `n` is an even integer; if `n` is odd, return an empty list. Hence, the first addend must always be less than or equal to the second to avoid duplicates.
Constraints : `2 < n < 32000` and `n` is even
## Examples
```
26 --> ['3+23', '7+19', '13+13']
100 --> ['3+97', '11+89', '17+83', '29+71', '41+59', '47+53']
7 --> []
``` | ["import math\ndef goldbach_partitions(n):\n def is_prime(x):\n for i in range(2, int(math.sqrt(x))+1):\n if x % i == 0:\n return False\n return True\n\n if n % 2: return []\n\n ret = []\n for first in range(2, n//2 + 1):\n if is_prime(first):\n second = n - first\n if is_prime(second):\n ret.append('%d+%d' %(first, second))\n return ret", "f=lambda n:n>1and all(n%d for d in range(2,int(n**.5)+1))\ngoldbach_partitions=lambda n:[]if n%2else['%d+%d'%(p,n-p)for p in range(n//2+1)if f(p)*f(n-p)]", "from itertools import chain\n\nimport numpy as np\n\nN = 32001\nxs = np.ones(N)\nxs[:2] = 0\nxs[4::2] = 0\nfor i in range(3, int(N ** 0.5) + 1, 2):\n if xs[i]:\n xs[i*i::i] = 0\nprimes = {i for i, x in enumerate(xs) if x}\n\n\ndef goldbach_partitions(n):\n if n % 2:\n return []\n return [\n f\"{i}+{n-i}\"\n for i in chain([2], range(3, n // 2 + 1, 2))\n if i in primes and n - i in primes\n ]", "odd_primes = {n for n in range(3, 32000, 2) if all(n % k for k in range(3, int(n**0.5)+1, 2))}\n\ndef goldbach_partitions(n):\n if n % 2 == 1:\n return []\n if n == 4:\n return [\"2+2\"]\n return [f\"{p}+{q}\" for p, q in sorted((p, n-p) for p in odd_primes if p <= (n-p) and (n-p) in odd_primes)]\n", "def goldbach_partitions(n):\n is_prime = lambda n: all(n%j for j in range(2, int(n**0.5)+1)) and n>1\n return [] if n%2 else [f'{i}+{n-i}' for i in range(2, int(n/2)+1) if is_prime(i) and is_prime(n-i)]", "def goldbach_partitions(n):\n def prime(a):\n if a == 2: return True\n if a < 2 or a % 2 == 0: return False\n return not any(a % x == 0 for x in range(3, int(a ** 0.5) + 1, 2))\n\n if n<4 or n%2!=0:\n return []\n if n==4:\n return ['2+2']\n res = []\n x = 3\n while True:\n y = n-x\n if x>y:\n return res\n if prime(y):\n res.append(str(x) + '+' + str(y)) \n x += 2\n while not prime(x):\n x += 2", "def prime(givenNumber): \n primes = []\n for possiblePrime in range(2, givenNumber + 1):\n \n isPrime = True\n for num in range(2, int(possiblePrime ** 0.5) + 1):\n if possiblePrime % num == 0:\n isPrime = False\n break\n\n if isPrime:\n primes.append(possiblePrime)\n \n return(primes)\n\ndef goldbach_partitions(n):\n if n % 2 != 0:\n return []\n else:\n p = prime(n)\n res = []\n \n for i in p:\n if (n-i) in p:\n res.append('{}+{}'.format(i, n-i)) \n p.remove(n-i)\n return res", "import math\ndef goldbach_partitions(n):\n primes = []\n partitions = []\n \n if n%2 != 0: return []\n for i in range(0, n+1):\n if i > 1:\n for j in range(2, int(math.sqrt(i))+1):\n if (i % j) == 0:\n break\n else:\n primes.append(i)\n\n for i in primes:\n if i <= n/2:\n if n - i in primes:\n partitions.append(str(i) + '+' + str(n - i))\n\n return(partitions)", "sieve = [False] * 2 + [True] * 32000\nfor i in range(2, 200):\n if sieve[i]:\n for j in range(2, 32000):\n if i * j >= len(sieve): break\n sieve[i*j] = False\n\ndef goldbach_partitions(n):\n if n % 2: return []\n if n == 4: return ['2+2']\n return ['{}+{}'.format(i, n-i) for i in range(2, n//2+1) if sieve[i] and sieve[n-i]]"] | {"fn_name": "goldbach_partitions", "inputs": [[15], [393], [31133], [4], [100], [594], [1500]], "outputs": [[[]], [[]], [[]], [["2+2"]], [["3+97", "11+89", "17+83", "29+71", "41+59", "47+53"]], [["7+587", "17+577", "23+571", "31+563", "37+557", "47+547", "53+541", "71+523", "73+521", "103+491", "107+487", "127+467", "131+463", "137+457", "151+443", "163+431", "173+421", "193+401", "197+397", "211+383", "227+367", "241+353", "257+337", "263+331", "277+317", "281+313", "283+311"]], [["7+1493", "11+1489", "13+1487", "17+1483", "19+1481", "29+1471", "41+1459", "47+1453", "53+1447", "61+1439", "67+1433", "71+1429", "73+1427", "101+1399", "127+1373", "139+1361", "173+1327", "179+1321", "181+1319", "193+1307", "197+1303", "199+1301", "211+1289", "223+1277", "241+1259", "251+1249", "263+1237", "269+1231", "271+1229", "277+1223", "283+1217", "307+1193", "313+1187", "337+1163", "347+1153", "349+1151", "383+1117", "397+1103", "409+1091", "431+1069", "439+1061", "449+1051", "461+1039", "467+1033", "479+1021", "487+1013", "491+1009", "503+997", "509+991", "523+977", "547+953", "563+937", "571+929", "593+907", "613+887", "617+883", "619+881", "641+859", "643+857", "647+853", "661+839", "673+827", "677+823", "691+809", "727+773", "739+761", "743+757"]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,536 |
def goldbach_partitions(n):
|
fe6f0b2bc5bfbe7a32711c7e742e97c7 | UNKNOWN | Given an array of numbers, return an array, with each member of input array rounded to a nearest number, divisible by 5.
For example:
```
roundToFive([34.5, 56.2, 11, 13]);
```
should return
```
[35, 55, 10, 15]
```
```if:python
Roundings have to be done like "in real life": `22.5 -> 25`
``` | ["from decimal import Decimal, ROUND_HALF_UP\n\ndef round_to_five(numbers):\n return [(n/5).quantize(1, ROUND_HALF_UP) * 5 for n in map(Decimal, numbers)]", "def round_to_five(numbers):\n \n return [5*int(x/5 + 0.5) for x in numbers]", "def round_to_five(numbers):\n return [int((n + 2.5) / 5) * 5 for n in numbers]", "round_to_five=lambda l:[round((0.01+n)/5)*5 for n in l]", "def round_to_five(numbers): \n return [int(((x // 5) + (x % 5 >= 2.5)) * 5) for x in numbers]", "def round_to_five(arr):\n return [5*round(v/5+0.01) for v in arr]", "import math\ndef round_to_five(numbers):\n output = []\n for n in numbers:\n if n % 5 == 0:\n output.append(int(n))\n elif (n % 10 < 5 and n % 5 < 2.5) or (n % 10 > 5 and n % 5 >= 2.5):\n output.append(int(round(n,-1)))\n elif (n % 10 < 5 and n % 5 >= 2.5):\n output.append(int(round(n,-1) + 5))\n else:\n output.append(int(round(n,-1) - 5))\n return output\n", "from math import floor, ceil\n\ndef rond(x):\n return floor(x) if x % 1 < 0.5 else ceil(x)\n \ndef round_to_five(numbers):\n return [rond(x/5)*5 for x in numbers]", "def rounding(n):\n intPart = int(n)\n fracPart = n - intPart\n if fracPart >= 0.5:\n n = intPart + 1\n else:\n n = intPart\n for i in range(6):\n up = n + i\n down = n - i \n if up%5 == 0:\n return up\n elif down%5==0:\n return down\ndef round_to_five(numbers):\n #your code here\n numbers = [rounding(n) for n in numbers]\n return numbers"] | {"fn_name": "round_to_five", "inputs": [[[1, 5, 87, 45, 8, 8]], [[3, 56.2, 11, 13]], [[22.5, 544.9, 77.5]]], "outputs": [[[0, 5, 85, 45, 10, 10]], [[5, 55, 10, 15]], [[25, 545, 80]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,613 |
def round_to_five(numbers):
|
00f7646cd23dc3222f44fdc41f895e44 | UNKNOWN | You will be given an array of strings. The words in the array should mesh together where one or more letters at the end of one word will have the same letters (in the same order) as the next word in the array. But, there are times when all the words won't mesh.
Examples of meshed words:
"apply" and "plywood"
"apple" and "each"
"behemoth" and "mother"
Examples of words that don't mesh:
"apply" and "playground"
"apple" and "peggy"
"behemoth" and "mathematics"
If all the words in the given array mesh together, then your code should return the meshed letters in a string.
You won't know how many letters the meshed words have in common, but it will be at least one.
If all the words don't mesh together, then your code should return `"failed to mesh"`.
Input: An array of strings. There will always be at least two words in the input array.
Output: Either a string of letters that mesh the words together or the string `"failed to mesh"`.
## Examples
#1:
```
["allow", "lowering", "ringmaster", "terror"] --> "lowringter"
```
because:
* the letters `"low"` in the first two words mesh together
* the letters `"ring"` in the second and third word mesh together
* the letters `"ter"` in the third and fourth words mesh together.
#2:
```
["kingdom", "dominator", "notorious", "usual", "allegory"] --> "failed to mesh"
```
Although the words `"dominator"` and `"notorious"` share letters in the same order, the last letters of the first word don't mesh with the first letters of the second word. | ["import re\n\ndef word_mesh(arr):\n common = re.findall(r'(.+) (?=\\1)',' '.join(arr))\n return ''.join(common) if len(common) + 1 == len(arr) else 'failed to mesh'", "def word_mesh(arr):\n result = \"\"\n \n for a, b in zip(arr, arr[1:]):\n while not a.endswith(b):\n b = b[:-1]\n if not b:\n return \"failed to mesh\"\n result += b\n \n return result", "def word_mesh(A,z=''):\n for x,y in zip(A,A[1:]):\n while not y.startswith(x):x=x[1:] \n if x=='':return'failed to mesh'\n z+=x\n return z", "word_mesh=lambda a,f=(lambda c,d,h=[]:h.clear()or[h.extend(d[:i]for i in range(len(c))if c.endswith(d[:i]))]*0 or sorted(h,key=len)[-1]),g=[]:g.clear()or g.extend(f(x,y)for x,y in zip(a,a[1:]))or(''.join(g)if '' not in g else 'failed to mesh') ", "def word_mesh(lst):\n meshes = []\n for w1, w2 in zip(lst, lst[1:]):\n for i in range(min(len(w1), len(w2)), 0, -1):\n if w1[-i:] == w2[:i]:\n meshes.append(w1[-i:])\n break\n else:\n return \"failed to mesh\"\n return \"\".join(meshes)\n", "def word_mesh(arr):\n overlaps = [\n max(\n filter(prev.endswith, (word[:k] for k in range(len(word)+1)))\n ) for prev,word in zip(arr, arr[1:])\n ]\n return \"\".join(overlaps) if all(overlaps) else \"failed to mesh\"", "from itertools import count, takewhile\n\ndef word_mesh(arr):\n overlaps = list(takewhile(bool, map(mesher, zip(arr,arr[1:])) ))\n return len(overlaps)==len(arr)-1 and ''.join(overlaps) or 'failed to mesh'\n \ndef mesher(pair):\n a, b = pair\n nChars = next( i for i in count(min(map(len,pair)),-1)\n if a.endswith(b[:i]) )\n return b[:nChars]", "def mesh(a, b):\n for i in range(len(b), 0, -1):\n if a.endswith(b[:i]):\n return b[:i]\n\ndef word_mesh(arr):\n try:\n return ''.join(mesh(a, b) for a, b in zip(arr, arr[1:]))\n except TypeError:\n return 'failed to mesh'", "import re\n\ndef word_mesh(arr):\n mesh = re.findall(r'(.+)-(?=\\1)', '-'.join(arr))\n return ''.join(mesh) if len(mesh) == len(arr) - 1 else 'failed to mesh'", "def word_mesh(arr):\n re,e,i='',len(arr[0]),0\n while e!=0:\n e-=1\n if e==0:return 'failed to mesh' \n if arr[i][-e:]==arr[i+1][:e]:\n re+=arr[i][-e:] \n e=len(arr[i])\n i+=1\n if i==(len(arr)-1):return re \n"] | {"fn_name": "word_mesh", "inputs": [[["beacon", "condominium", "umbilical", "california"]], [["allow", "lowering", "ringmaster", "terror"]], [["abandon", "donation", "onion", "ongoing"]], [["jamestown", "ownership", "hippocampus", "pushcart", "cartographer", "pheromone"]], [["marker", "kerchief", "effortless", "lesson", "sonnet", "network", "workbook", "oklahoma", "marker"]], [["california", "niagara", "arachnophobia", "biannual", "alumni", "nibbles", "blessing"]], [["fortune", "unemployment", "mentor", "toronto", "ontogeny", "enya", "yabba", "balance", "ancestry"]], [["chlorine", "nevermore", "oregon", "gonzaga", "gambino", "inoculate"]], [["chlorine", "brinemore", "oregon", "gonzaga", "gambino", "inoculate"]], [["venture", "retreat", "eatery", "rye", "yearly", "lymphoma", "homage", "agent", "entrails"]], [["kingdom", "dominator", "notorious", "usual", "allegory"]], [["governor", "normal", "mallorey", "reykjavik", "viking", "kingdom", "dominator", "torrent", "rental", "allegory"]], [["fantastic", "stickleback", "acknowledge", "gentile", "leadership", "hippie", "piecemeal", "allosaurus"]], [["economy", "mystery", "erythema", "emaciated", "teddy", "dynamite", "mitering"]], [["abacus", "cussing", "singularity", "typewritten", "tentative", "ventricle", "clerical", "calcium", "umbrella"]], [["peanutbutterandjellyontoast", "jellyontoastcanbepleasant", "bepleasantpleaseorleave", "ventyouranger", "rangersinthepark"]], [["victoria", "iambic", "icicle", "clearview", "viewpoint", "ointment", "entrance"]], [["alexandergrahambell", "belladonna", "donnasummer", "summertimeblues", "bluesfestival"]], [["workingontherailroad", "roadmaster", "terracottawarrior", "orionsbeltstarcluster"]], [["victoria", "iambic", "icicle", "clearview", "rearviewpoint", "ointment", "entrance"]], [["alexandergrahambell", "belladonna", "singerdonnasummer", "summertimeblues", "bluesfestival"]], [["workingontherailroad", "roadmaster", "terracottawarrior", "orionsbeltstarcluster", "thelusterisgone"]], [["abbabbabba", "abbacccdddeee", "deeedledeedledum", "dumdum", "umbrellas", "llasaapsopuppy", "puppydogtails"]], [["indiansummer", "summerinthecity", "thecityneversleeps", "sleepsoundlydreamon", "dreamondreamondreamon"]], [["parisinfrance", "franceineurope", "europeonearth", "earthinthesolarsystem", "systematicallygettingbigger"]], [["hawaiianpunch", "punchandjudy", "judygarland", "landrover", "overtherainbow", "rainbowconnection"]], [["justcannot", "nothappyhappyhappy", "pyromaniacinwolfclothing", "thingshappenwhenyouleastexpect", "ectoplasm"]], [["thesunshinesinthemiddle", "themiddleearthwasarealplaceinthemovies", "themoviesisagooddateplace", "placement"]], [["ilikesunshine", "thesunshinesinthemiddle", "themiddleearthwasarealplaceinthemovies", "themoviesisagooddateplace", "placement"]], [["caughtinthemiddle", "thesunshinesinthemiddle", "themiddleearthwasarealplaceinthemovies", "themoviesisagooddateplace", "placement"]]], "outputs": [["conumcal"], ["lowringter"], ["dononon"], ["ownhippuscartpher"], ["kereflesssonnetworkokma"], ["niaarabiaalnibles"], ["unementtorontoenyyabaance"], ["neoregongaino"], ["failed to mesh"], ["reeatryyelyhomaageent"], ["failed to mesh"], ["normalreyvikkingdomtorrental"], ["sticackgelehippieal"], ["myeryemateddymite"], ["cussingtytenveclecalum"], ["jellyontoastbepleasantveranger"], ["iaiccleviewointent"], ["belldonnasummerblues"], ["roadteror"], ["failed to mesh"], ["failed to mesh"], ["failed to mesh"], ["abbadeeedumumllaspuppy"], ["summerthecitysleepsdreamon"], ["franceeuropeearthsystem"], ["punchjudylandoverrainbow"], ["notpythingect"], ["themiddlethemoviesplace"], ["failed to mesh"], ["failed to mesh"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,520 |
def word_mesh(arr):
|
75fb5563b755ea1755f4bafe61870d74 | UNKNOWN | Scheduling is how the processor decides which jobs (processes) get to use the processor and for how long. This can cause a lot of problems. Like a really long process taking the entire CPU and freezing all the other processes. One solution is Round-Robin, which today you will be implementing.
Round-Robin works by queuing jobs in a First In First Out fashion, but the processes are only given a short slice of time. If a processes is not finished in that time slice, it yields the proccessor and goes to the back of the queue.
For this Kata you will be implementing the
```python
def roundRobin(jobs, slice, index):
```
It takes in:
1. "jobs" a non-empty positive integer array. It represents the queue and clock-cycles(cc) remaining till the job[i] is finished.
2. "slice" a positive integer. It is the amount of clock-cycles that each job is given till the job yields to the next job in the queue.
3. "index" a positive integer. Which is the index of the job we're interested in.
roundRobin returns:
1. the number of cc till the job at index is finished.
Here's an example:
```
roundRobin([10,20,1], 5, 0)
at 0cc [10,20,1] jobs[0] starts
after 5cc [5,20,1] jobs[0] yields, jobs[1] starts
after 10cc [5,15,1] jobs[1] yields, jobs[2] starts
after 11cc [5,15,0] jobs[2] finishes, jobs[0] starts
after 16cc [0,15,0] jobs[0] finishes
```
so:
```
roundRobin([10,20,1], 5, 0) == 16
```
**You can assume that the processor can switch jobs between cc so it does not add to the total time. | ["def roundRobin(jobs, slice, index):\n total_cc = 0\n while True:\n for idx in range(len(jobs)):\n cc = min(jobs[idx], slice)\n jobs[idx] -= cc\n total_cc += cc\n if idx == index and jobs[idx] == 0:\n return total_cc", "def roundRobin(jobs, slice, index):\n q = (jobs[index] - 1) // slice\n return (\n sum(min(x, (q+1) * slice) for x in jobs[:index+1]) +\n sum(min(x, q * slice) for x in jobs[index+1:])\n )", "def roundRobin(jobs, slice, index):\n i, res, jobs = 0, 0, jobs[:]\n while jobs[index]:\n res += min(slice, jobs[i])\n jobs[i] = max(0, jobs[i]-slice)\n i = (i+1)%len(jobs)\n return res", "def roundRobin(jobs, slice, index):\n cc = i = 0\n while jobs[index] > 0:\n cc += min(slice, jobs[i])\n jobs[i] = max(0, jobs[i] - slice)\n i = (i + 1) % len(jobs)\n return cc\n", "import math\n\ndef ceil(value, step):\n return math.ceil(value / step) * step\n\ndef floor(value, step):\n return math.floor(value / step) * step\n\ndef roundRobin(jobs, frame, index):\n for i in range(index):\n jobs[i] = min(jobs[i], ceil(jobs[index], frame))\n for i in range(index + 1, len(jobs)):\n jobs[i] = min(jobs[i], floor(jobs[index] - 1, frame))\n return sum(jobs)\n", "def roundRobin(jobs, slice, index):\n num_slices = (jobs[index] + slice - 1) // slice\n before = num_slices * slice\n after = (num_slices - 1) * slice\n return sum(min(before, cc) for cc in jobs[0:index]) \\\n + sum(min(after, cc) for cc in jobs[index + 1:]) + jobs[index]\n", "roundRobin=lambda a,n,i:sum(min((a[i]+n*(i>=j)-1)//n*n,v)for j,v in enumerate(a))", "def roundRobin(jobs, sl, index):\n start_sum = sum(jobs)\n cur_idx = 0\n while jobs[index] > 0:\n jobs[cur_idx] = jobs[cur_idx] - min(jobs[cur_idx], sl)\n cur_idx = (cur_idx + 1) % len(jobs)\n return start_sum - sum(jobs)", "def roundRobin(jobs, limit, target):\n queue = list(enumerate(jobs))\n cycles = 0\n while True:\n index, job = queue.pop(0)\n if limit >= job:\n cycles += job\n job = 0\n else:\n job -= limit\n cycles += limit\n if job:\n queue.append((index, job))\n elif index == target:\n break\n return cycles"] | {"fn_name": "roundRobin", "inputs": [[[10], 4, 0], [[10, 20], 5, 0], [[10, 20, 1, 2, 3], 5, 2], [[10, 20, 1, 2, 3], 5, 0], [[10, 20, 1, 2, 3], 4, 2], [[10, 20, 1, 2, 3], 4, 3]], "outputs": [[10], [15], [11], [21], [9], [11]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,399 |
def roundRobin(jobs, slice, index):
|
9c41effccc621598c3da5de70b385ef3 | UNKNOWN | # Task
Mirko has been moving up in the world of basketball. He started as a mere spectator, but has already reached the coveted position of the national team coach!
Mirco is now facing a difficult task: selecting five primary players for the upcoming match against Tajikistan. Since Mirko is incredibly lazy, he doesn't bother remembering players' names, let alone their actual skills. That's why he has settled on selecting five players who share the same first letter of their surnames, so that he can remember them easier. If there are no five players sharing the first letter of their surnames, Mirko will simply forfeit the game!
Your task is to find the first letters Mirko's players' surnames can begin with(In alphabetical order), or return `"forfeit"` if Mirko can't gather a team.
# Input/Output
- `[input]` string array `players`
Array of players' surnames, consisting only of lowercase English letters.
- `[output]` a string
A **sorted** string of possible first letters, or "forfeit" if it's impossible to gather a team.
# Example
For `players = ["michael","jordan","lebron","james","kobe","bryant"]`, the output should be `"forfeit"`.
For
```
players = ["babic","keksic","boric","bukic",
"sarmic","balic","kruzic","hrenovkic",
"beslic","boksic","krafnic","pecivic",
"klavirkovic","kukumaric","sunkic","kolacic",
"kovacic","prijestolonasljednikovic"]
```
the output should be "bk". | ["from collections import Counter\n\ndef strange_coach(players):\n return ''.join(\n sorted(i for i,j in\n Counter(map(lambda x: x[0], players)).most_common()\n if j >= 5)) or 'forfeit'", "def strange_coach(players):\n d = {l:0 for l in map(chr, range(97, 123))}\n \n for i in players:\n d[i[0]] += 1\n \n r = \"\".join(sorted(x for x in d if d[x] > 4))\n return r if r != \"\" else \"forfeit\"", "def strange_coach(players):\n firsts = [player[0] for player in players]\n return \"\".join(sorted(c for c in set(firsts) if firsts.count(c) >= 5)) or \"forfeit\"", "from collections import Counter\nfrom string import ascii_lowercase\n\ndef strange_coach(players):\n first_letters = Counter(player[0] for player in players)\n return \"\".join(c for c in ascii_lowercase if first_letters[c] >= 5) or \"forfeit\"", "# python 3.6 has sorted dicts\nfrom string import ascii_lowercase as az\n\n\ndef strange_coach(players):\n d = dict.fromkeys(az, 0)\n for i in range(len(players)):\n d[players[i][0]] += 1\n s = (''.join([k for k,v in d.items() if v >= 5]))\n return s if s else 'forfeit'", "def strange_coach(players):\n letters = [p[0] for p in players]\n res = set(l for l in letters if letters.count(l) > 4)\n return ''.join(sorted(res)) if res else 'forfeit'", "from itertools import groupby\n\ndef strange_coach(players):\n return \"\".join(key for key, group in groupby(sorted(players), lambda x: x[0]) if len(list(group)) >= 5) or \"forfeit\"\n", "from collections import Counter\n\ndef strange_coach(players):\n first_letters = (player[0] for player in players)\n counts = Counter(first_letters)\n at_least_five = ''.join(sorted(k for k, v in counts.items() if v > 4))\n return at_least_five or 'forfeit'", "strange_coach=lambda a:(lambda c:''.join(k for k in sorted(c)if c[k]>4)or'forfeit')(__import__('collections').Counter(w[0]for w in a))", "from collections import Counter\ndef strange_coach(players):\n return \"\".join(k for k,v in Counter([p[0] for p in sorted(players)]).items() if v>4) or \"forfeit\""] | {"fn_name": "strange_coach", "inputs": [[["michael", "jordan", "lebron", "james", "kobe", "bryant"]], [["babic", "keksic", "boric", "bukic", "sarmic", "balic", "kruzic", "hrenovkic", "beslic", "boksic", "krafnic", "pecivic", "klavirkovic", "kukumaric", "sunkic", "kolacic", "kovacic", "prijestolonasljednikovic"]], [["jgztazpytubijfsmjz", "bokvgratzzdibku", "qineboilzoqdqivc", "bfctyltibtkbxq", "vioxcuhqhikxeqwekqkjo", "nrvsdhttr", "eiaoajuwxpwmyliqikzcchid", "bxrwawgor", "gbsqaxotzmblxttj", "kwchrcaconuwaivhvnyf", "neiemapiica", "bppao", "bxujiwivsjfbqrzygpdgkyz", "jnzrhhmcgcpffflpzwmqib", "jhozlevckrrwimdmyzc", "bomojotkqqditelsk", "ywmbheywzfyqjjs", "snwrclyjkbspysjftcmyak", "eelrsgkuhu", "dnyzsvqjjuqoc"]]], "outputs": [["forfeit"], ["bk"], ["b"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,156 |
def strange_coach(players):
|
64e0364930828704bf5c4aecb9a09827 | UNKNOWN | ### Task:
You have to write a function `pattern` which creates the following pattern (See Examples) upto desired number of rows.
If the Argument is `0` or a Negative Integer then it should return `""` i.e. empty string.
### Examples:
`pattern(9)`:
123456789
234567891
345678912
456789123
567891234
678912345
789123456
891234567
912345678
`pattern(5)`:
12345
23451
34512
45123
51234
Note: There are no spaces in the pattern
Hint: Use `\n` in string to jump to next line | ["def pattern(n):\n return '\\n'.join(''.join(str((x+y)%n+1) for y in range(n)) for x in range(n))", "def pattern(n):\n nums = list(range(1,n+1))\n result = ''\n result += ''.join(str(n) for n in nums)\n \n for i in range(1, n):\n result += '\\n'\n nums.append(nums.pop(0))\n result += ''.join(str(n) for n in nums)\n \n return result\n \n \n", "from collections import deque\n\ndef pattern(n):\n result = []\n line = deque([str(num) for num in range(1, n + 1)])\n for i in range(n):\n result.append(\"\".join(line))\n line.rotate(-1)\n return \"\\n\".join(result)", "from collections import deque\n\ndef pattern(n):\n A = deque([str(i+1) for i in range(n)])\n result = []\n for i in range(len(A)):\n print(A)\n result.append(''.join(A))\n A.rotate(-1)\n return '\\n'.join(result)\n", "def pattern(s):\n return \"\\n\".join([\"\".join((str(x + y) if x + y <= s else str(x + y - s * ((x + y) // s)) for y in range(s))) for x in range(1, s + 1)])", "from itertools import chain\n\ndef pattern(n):\n line = list(i + 1 for i in range(n)) \n return \"\\n\".join(\"\".join(str(x) for x in chain(line[i:], line[:i])) for i in range(n))\n", "from itertools import chain\n\n\ndef pattern(n):\n xs = ''.join(str(i) for i in range(1, n+1))\n return '\\n'.join(''.join(str(j) for j in chain(range(i, n+1), range(1, i))) for i in range(1, n+1))", "def pattern(n):\n a = [str(i) for i in range(1, n + 1)]\n return '\\n'.join(''.join(a[i:] + a[:i]) for i in range(n))\n"] | {"fn_name": "pattern", "inputs": [[7], [1], [4], [0], [-25]], "outputs": [["1234567\n2345671\n3456712\n4567123\n5671234\n6712345\n7123456"], ["1"], ["1234\n2341\n3412\n4123"], [""], [""]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,593 |
def pattern(n):
|
22693008ea23a62730df9787f0402374 | UNKNOWN | You are required to create a simple calculator that returns the result of addition, subtraction, multiplication or division of two numbers.
Your function will accept three arguments:
The first and second argument should be numbers.
The third argument should represent a sign indicating the operation to perform on these two numbers.
```if-not:csharp
if the variables are not numbers or the sign does not belong to the list above a message "unknown value" must be returned.
```
```if:csharp
If the sign is not a valid sign, throw an ArgumentException.
```
# Example:
```python
calculator(1, 2, '+') => 3
calculator(1, 2, '$') # result will be "unknown value"
```
Good luck! | ["def calculator(x, y, op):\n return eval(f'{x}{op}{y}') if type(x) == type(y) == int and str(op) in '+-*/' else 'unknown value'", "def calculator(x,y,op):\n try:\n assert op in \"+-*/\"\n return eval('%d%s%d' % (x, op, y))\n except:\n return \"unknown value\"", "def calculator(x, y, op):\n try:\n return {'+': x + y, '-': x - y, '*': x * y, '/': x / y}[op]\n except (TypeError, KeyError): \n return 'unknown value'", "def calculator(x,y,op):\n if isinstance(x, int) and isinstance(y, int):\n op_dict = {'+': x+y, '-': x-y, '*': x*y, '/': x/y}\n return op_dict[op] if op in op_dict else 'unknown value'\n return 'unknown value'\n", "def calculator(x,y,op):\n if op == '+' and type(x) == int and type(y) == int:\n return x + y\n if op == '-':\n return x - y\n if op == '*':\n return x * y\n if op == '/':\n return x / y\n else:\n return 'unknown value'", "from operator import add, sub, mul, truediv as div\n\nWRONG_FUNC = lambda x,y: \"unknown value\"\n\ndef calculator(x,y,op):\n validOp = op if all(isinstance(v,int) for v in (x,y)) else '!'\n return {'+': add, '-': sub, '*': mul, '/': div}.get(validOp, WRONG_FUNC)(x,y)", "def calculator(x, y, op):\n try:\n return eval(f\"{x}{op}{y}\") if op in \"+-*/\" else \"unknown value\"\n except (SyntaxError, NameError, TypeError):\n return \"unknown value\"\n", "ops = {\"+\": int.__add__, \"-\": int.__sub__, \"*\": int.__mul__, \"/\": int.__truediv__}\n\ndef calculator(x, y, op):\n if not (isinstance(x, int) and isinstance(y, int) and op in ops):\n return \"unknown value\"\n return ops[op](x, y)\n", "def calculator(x,y,op):\n if str(op) not in '+-/*' or not str(x).isnumeric() or not str(y).isnumeric():\n return 'unknown value'\n return x + y if op == '+' else x - y if op == '-' else x * y if op == '*' else x / y", "def calculator(x,y,op):\n if not isinstance(x, int) or not isinstance(y, int):\n return 'unknown value'\n \n if op == '+':\n return x + y\n \n if op == '-':\n return x - y\n \n if op == '*':\n return x * y\n \n if op == '/':\n return x / y\n \n return 'unknown value'", "import operator\nfrom functools import reduce\n\nOPS = {\n \"+\": operator.__add__,\n \"-\": operator.__sub__,\n \"*\": operator.__mul__,\n \"/\": operator.__truediv__\n}\n\n\ndef calculator(x, y, op):\n return reduce(OPS[op], (x, y)) if op in OPS and type(x) is type(y) is int else 'unknown value'", "def calculator(x,y,op):\n return eval(\"{}{}{}\".format(x, op, y)) if op in ['+', '-', '/', '*'] and isinstance(x, int) and isinstance(y, int) else \"unknown value\"", "from typing import Union\n\ndef calculator(x: int, y: int, op: str) -> Union[int, float, str]:\n \"\"\" Make a calculation two values based on the given operator. \"\"\"\n calculate = {\n \"+\": lambda x, y: x + y,\n \"-\": lambda x, y: x - y,\n \"*\": lambda x, y: x * y,\n \"/\": lambda x, y: x / y\n }\n if all([type(x) is int, type(y) is int]):\n try:\n return calculate[op](x, y)\n except KeyError:\n ...\n return \"unknown value\"", "def calculator(x,y,op):\n if op in ['+','-','*','/']:\n try:\n return {'+':x+y,'-':x-y,'*':x*y,'/':x/y}[op]\n except TypeError:\n return 'unknown value'\n else: return 'unknown value'", "def calculator(x,y,op):\n if type(x) is int and type(y) is int:\n switcher = {\n \"+\": x+y,\n \"-\": x-y,\n \"*\": x*y,\n \"/\": x/y\n }\n return switcher.get(op,\"unknown value\")\n else:\n return 'unknown value'", "def calculator(x,y,op):\n if op not in ['+','/','-','*']:\n return \"unknown value\"\n else:\n try:\n a = str(x)+ op + str(y)\n return eval(a)\n except:\n return \"unknown value\"", "def calculator(x,y,op):\n if isinstance(x, int) and isinstance(y, int):\n if op =='+':\n return x+y\n if op =='-':\n return x-y\n if op =='*':\n return x*y\n if op =='/':\n return x/y\n return \"unknown value\"", "from operator import add, sub, mul, truediv as div\n\nOPS = dict(list(zip(('+-*/'), (add, sub, mul, div))))\n\nfoo = lambda *args: 'unknown value'\n\ndef calculator(x, y, op):\n return OPS.get(op, foo)(x, y) if all(isinstance(i, (int, float)) for i in (x, y)) else foo()\n", "def calculator(x,y,op):\n try:\n if op in '+-*/':\n return eval(f'{x}{op}{y}')\n except:\n pass\n return 'unknown value'", "def calculator(x,y,op):\n if y == \"$\":\n return(\"unknown value\")\n if x == \"a\":\n return(\"unknown value\")\n if x == \":\":\n return(\"unknown value\")\n if y == \",\":\n return(\"unknown value\")\n if op == \"+\":\n return(x + y)\n elif op == \"-\":\n return(x - y)\n elif op == \"*\":\n return(x * y)\n elif op == \"/\":\n return(x / y)\n else:\n return(\"unknown value\")", "def calculator(x,y,op,msg='unknown value'):\n try:\n return eval(str(x)+op+str(y)) if op in '+-*/' else msg\n except:\n return msg", "def calculator(x,y,op,check='+-*/'):\n try:\n return eval(str(x)+op+str(y)) if op in check else 'unknown value'\n except:\n return 'unknown value'", "def calculator(x,y,op):\n try:\n if op in '+-/*':\n return eval(str(x)+op+str(y))\n else:\n return 'unknown value'\n except:\n return 'unknown value'", "def calculator(x,y,op):\n print((x,y,op))\n if op == \"+\" and type(x)==type(y)==int:\n return x+y\n elif op == \"-\" and type(x)==type(y)==int:\n return x-y\n elif op == \"*\" and type(x)==type(y)==int:\n return x*y\n elif op == \"/\" and type(x)==type(y)==int:\n return x/y\n else:\n return \"unknown value\"\n \n", "def calculator(x,y,op):\n try:\n if op not in \"+-*/\" or type(x) not in [int, float] or type(y) not in [int, float]: return 'unknown value'\n return eval(f\"{x} {op} {y}\")\n except TypeError:\n return 'unknown value'", "def calculator(x,y,op):\n try:\n if op in \"+-*/\":\n return eval(f\"{x} {op} {y}\")\n else:\n return \"unknown value\"\n except:\n return \"unknown value\"", "def calculator(x,y,op):\n if isinstance(x, int) and isinstance(y, int) and isinstance(op, str):\n if op == '+':\n return x + y\n elif op == '-':\n return x - y\n elif op == '*':\n return x * y\n elif op == '/':\n return x / y\n else:\n return \"unknown value\"\n else:\n return \"unknown value\"\n", "def calculator(x,y,op):\n valid_operators = ['+', '-', '*', '/']\n\n if op in valid_operators and str(x).isnumeric() and str(y).isnumeric():\n return eval(str(x) + op + str(y))\n else:\n return 'unknown value'\n", "def calculator(x,y,op):\n if (op in ('+', '-', '*', '/') and type(x) == int and type(y) == int):\n if(op == '+'):\n return x+y\n elif(op == '-'):\n return x-y\n elif(op == '*'):\n return x*y\n else:\n return x/y\n else:\n return \"unknown value\"", "def calculator(x,y,op):\n x = str(x)\n y = str(y)\n if x.isdigit()==True and y.isdigit()==True:\n if op==\"+\" or op==\"-\" or op==\"*\" or op==\"/\":\n equation = x+op+y\n result = eval(equation)\n else:\n result = \"unknown value\"\n else:\n result = \"unknown value\"\n return result\n\nprint(calculator(6, 2, '+'))\nprint(calculator(4, 3, '-'))\nprint(calculator(5, 5, '*'))\nprint(calculator(5, 4, '/'))\nprint(calculator(6, 2, '&'))", "def calculator(x,y,op):\n print (f\"{x}{op}{y}\")\n try:\n if op in \"+-/*\":\n return eval(f\"{x}{op}{y}\")\n except:\n pass\n return \"unknown value\" ", "import operator\n\n\nop = {\n \"+\": operator.add,\n \"-\": operator.sub,\n \"*\": operator.mul,\n \"/\": operator.truediv\n}\n\n\n\ndef calculator(x,y,oper):\n print(x, y, op)\n try:\n return op[oper](x, y) if x > 0 and y > 0 else \"unknown value\"\n except:\n return \"unknown value\"", "def calculator(x,y,op):\n print(x, y, op)\n lst = ['+', '-', '*', '/']\n if op not in lst:\n return \"unknown value\"\n if str(x).isnumeric() is False or str(y).isnumeric() is False:\n return \"unknown value\"\n elif op == '+':\n return int(x) + int(y)\n elif op == '-':\n return int(x) - int(y)\n elif op == '*':\n return int(x) * int(y)\n elif op == '/':\n return int(x) / int(y)", "def calculator(x, y, op):\n if isinstance(x, int) or isinstance(x, float) and isinstance(y, int) or isinstance(y, float):\n while True:\n try:\n if op == '*':\n sumMUL = float(x * y)\n print(sumMUL)\n return sumMUL\n\n if op == '+':\n sumADD = float(x + y)\n return sumADD\n print(sumADD)\n if op == '-':\n sumSUB = float(x - y)\n return sumSUB\n elif op == '/':\n sumDIV = float(x / y)\n return sumDIV\n else:\n return \"unknown value\"\n break\n except TypeError:\n return \"unknown value\"\n else:\n return \"unknown value\"", "def calculator(x,y,op):\n if type(x) == int and type(y) == int and str(op) in '*/-+':\n return eval(f'{x}{op}{y}')\n else:\n return 'unknown value'\n \n return eval(f'{x}{op}{y}') if type(x) == type(y) == int and str(op) in '+-*/' else 'unknown value'", "def calculator(a,b,op):\n if op == '/' and b == 0:\n return 'Error! Division by zero!'\n ops = ['+', '-', '*', '/']\n try:\n results = [a + b, a - b, a * b, a / b]\n return results[ops.index(op)]\n except:\n return 'unknown value'", "def calculator(x,y,op):\n if ((op == \"+\") and (type(x) is int) and (type(y) is int)):\n return x + y\n elif ((op == \"-\") and (type(x) is int) and (type(y) is int)):\n return x - y\n elif ((op == \"*\") and (type(x) is int) and (type(y) is int)):\n return x * y\n elif ((op == \"/\") and (type(x) is int) and (type(y) is int)):\n return int(x) / int(y)\n else:\n return \"unknown value\"", "def calculator(x,y,op):\n try:\n if op == '+': return int(x) + int(y)\n elif op == '-': return x - y\n elif op =='*': return x * y\n elif op == '/': return x / y\n else: return 'unknown value'\n except: return 'unknown value'\n \n\n\n", "def calculator(x,y,op):\n pass\n if type(x) != int or type(y) != int:\n return \"unknown value\"\n while op not in ['+', '-', '*', '/']:\n return \"unknown value\"\n if op == '+':\n return x + y\n if op == '-':\n return x - y\n if op == '*':\n return x * y\n if op == '/':\n return x / y\n", "def calculator(x,y,op):\n ops = (\"+\", \"-\", \"*\", \"/\")\n \n if op in ops and (type(x) == int and type(y) == int):\n return eval(f\"{x} {op} {y}\")\n \n else:\n return \"unknown value\"", "def calculator(x,y,op):\n print(x,y, type(x), type(y))\n if op ==\"+\" and type(x)==type(y)==int: return x+y\n elif op ==\"-\": return x-y\n elif op ==\"/\": return x/y\n elif op ==\"*\": return x*y\n else : return \"unknown value\"", "import operator as op\n\nops = {\n '+': op.add,\n '-': op.sub,\n '*': op.mul,\n '/': op.truediv,\n}\n\ndef calculator(x, y, op):\n if op in ops and isinstance(x, int) and isinstance(y, int):\n return ops[op](x, y)\n return 'unknown value'", "def calculator(x,y,op):\n print((x,y,op)) \n if type(x) == str or type(y) == str:\n return \"unknown value\"\n elif op == '+':\n return x + y\n elif op == '-':\n return x - y\n elif op == '*':\n return x * y\n elif op == '/':\n return x / y\n else:\n return \"unknown value\"\n", "import operator\n\nops = {\n '+': operator.add,\n '-': operator.sub,\n '*': operator.mul,\n '/': operator.truediv\n}\n\ndef calculator(x,y,op):\n try:\n return ops[op](int(x), int(y))\n except (ValueError, KeyError):\n return 'unknown value'", "import operator\ndef calculator(x,y,op):\n ops = {'+' : operator.add, '-' : operator.sub, '*' : operator.mul, '/' : operator.truediv}\n if op in ops and str(x).isdigit() and str(y).isdigit() : return ops[op](x,y)\n else: return \"unknown value\"", "def calculator(x,y,op):\n if isinstance(x, int) and isinstance(y, int) and isinstance(op, str) and op in '+-*/':\n return eval(str(x) + op + str(y))\n else:\n return 'unknown value'", "def calculator(x,y,op):\n if type(x) != int or type(y) != int:\n return 'unknown value'\n elif op == '+':\n return x + y\n elif op == '-':\n return x - y\n elif op == '*':\n return x * y\n elif op == '/':\n if y <= 0:\n return 'unknown value'\n return x / y\n else:\n return 'unknown value'\n", "def calculator(x,y,op):\n return eval(f'{x}{op}{y}') if str(op) in \"+-*/\" and type(x) == type(y) == int else \"unknown value\"", "def calculator(x,y,op):\n if str(x).isnumeric() and str(y).isnumeric():\n if op == '+':\n res = x + y\n return res\n if op == '-':\n res = x - y\n return res\n if op == '*':\n res = x * y\n return res\n if op == '/':\n res = x / y\n return res \n return \"unknown value\"", "def calculator(x,y,op):\n if type(op) == str and op in \"+-*/\" and type(x) == int and type(y) == int:\n calc = str(x) + op + str(y)\n return eval(calc)\n else:\n return \"unknown value\"", "def calculator(x,y,op):\n print(op)\n print((x,y))\n if type(x)!= int or type(y) != int:\n return 'unknown value'\n if op =='+':\n return x + y\n elif op =='-':\n return x - y\n elif op =='*':\n return x * y\n elif op =='/':\n return x / y\n# elif op =='&':\n else:\n return 'unknown value'\n", "def calculator(x,y,op):\n numbers_ok = isinstance(x, int) and isinstance(y, int)\n operator_ok = isinstance(op, str) and op in '+-*/'\n \n return eval(f'{x} {op} {y}') if numbers_ok and operator_ok else 'unknown value'", "def calculator(x,y,op):\n if type(x) != int or type(y) != int:\n return \"unknown value\"\n else:\n if op == \"+\":\n return x+y\n if op == \"-\":\n return x-y\n if op == \"*\":\n return x*y\n if op == \"/\":\n return x/y\n else:\n return \"unknown value\"\n \n pass", "def calculator(x,y,op):\n if type(x)!=int or type(y)!=int or str(op) not in (\"+-*/\"):\n return \"unknown value\"\n \n elif op==\"+\":\n return x+y\n elif op==\"-\":\n return x-y\n elif op==\"*\":\n return x*y\n elif op==\"/\":\n return round(x/y,2)\n", "def calculator(x,y,op):\n try:\n return {'*':x*y,'+':x+y,'-':x-y,'/':x/y}.get(op,'unknown value')\n except:\n return \"unknown value\"\n", "def calculator(x,y,op):\n if (False == isinstance(x, int)) or (False == isinstance(y, int)):\n return \"unknown value\"\n if \"+\" == op:\n return x+y\n if \"-\" == op:\n return x-y\n if \"*\" == op:\n return x*y\n if \"/\" == op:\n return x/y\n return \"unknown value\"", "def calculator(x, y, op):\n try:\n return float({'+': int.__add__, '-': int.__sub__, \n '*': int.__mul__, '/': int.__truediv__}[op](x, y))\n except:\n return 'unknown value'", "def calculator(x,y,op):\n return eval(f'{x} {op} {y}') if op in ['+', '-', '*', '/'] and isinstance(x, int) and isinstance(y, int) else 'unknown value'", "def calculator(x,y,op):\n ops = {'+':add,'-':sub,'*':mul,'/':div}\n try:\n return ops[op](x,y)\n except KeyError:\n return 'unknown value'\n\ndef add(num1,num2):\n try:\n return float(num1)+float(num2)\n except:\n return 'unknown value'\n\ndef sub(num1,num2):\n return num1-num2\n\ndef mul(num1,num2):\n return num1*num2\n\ndef div(num1,num2):\n return num1/num2", "def calculator(x,y,op):\n if (str(x).isnumeric())and(str(y).isnumeric())and((op=='+')or(op=='*')or(op=='/')or(op=='-')):\n if op=='+':\n return x+y\n elif op=='-':\n return x-y\n elif op=='*':\n return x*y\n elif op=='/':\n return x/y\n else:\n return \"unknown value\"\n pass", "import operator as ops\n\ndef calculator(x,y,op):\n try:\n return {'+': ops.add(x,y), '-': ops.sub(x,y), '*': ops.mul(x,y), '/': ops.truediv(x,y)}.get(op, \"unknown value\")\n except:\n return \"unknown value\"", "def calculator(x,y,op):\n \n\n if op == '+':\n try:\n return int(x) + int(y)\n except: return \"unknown value\"\n \n elif op == '-':\n return x - y\n\n elif op == '*':\n return x * y\n \n elif op == '/':\n return x / y\n \n else:\n return \"unknown value\"", "def calculator(x,y,op):\n if (type(x) is int and type(y) is int and type (op) is str):\n if op == \"+\":\n return x + y\n if op == \"-\":\n return x - y\n if op == \"*\":\n return x * y\n if op == \"/\":\n return x/y\n else:\n return \"unknown value\"\n else:\n return \"unknown value\"", "def calculator(x,y,op):\n ops = '+-*/'\n try: return eval('{}{}{}'.format(x,op,y)) if op in ops else 'unknown value'\n except: return 'unknown value'", "import operator\n\nops = {\n '+' : operator.add,\n '-' : operator.sub,\n '*' : operator.mul,\n '/' : operator.truediv\n}\n\n\ndef calculator(x,y,op): \n try:\n if isinstance(x, int) and isinstance(y, int):\n return ops.get(op)(x, y)\n return \"unknown value\"\n except:\n return \"unknown value\"", "def calculator(x,y,op):\n try: \n if op not in \"+-*/\" or type(x) != int or type(y) != int: raise TypeError \n else: return eval('%d%s%d' % (x,op,y))\n except: \n return \"unknown value\"", "def calculator(x,y,op):\n if isinstance(x,int) == True and isinstance(y,int) == True and (isinstance(op,str) == True and op in '+-*/'): \n if op == '+': return x+y\n elif op == '-': return x-y\n elif op == '*':return x*y\n elif op == '/': return x/y \n else: return \"unknown value\"", "import re\n\ndef calculator(x,y,op):\n if re.match('[-\\+\\*/]', str(op)) and isinstance(x, int) and isinstance(y, int):\n return eval(str(x) + op + str(y))\n else:\n return \"unknown value\"", "def calculator(x,y,op):\n return eval('{}{}{}'.format(x, op, y)) if op in ['+', '-', '*', '/'] and isinstance(x, int) and isinstance(y, int) else \"unknown value\"", "def calculator(x,y,op):\n d = '0123456789'\n s = \"+-*/\"\n if str(x) not in d or str(y) not in d or op not in s:\n return 'unknown value'\n else:\n if op == \"+\":\n return x + y\n elif op == \"-\":\n return x - y\n elif op == \"*\":\n return x * y\n else:\n return x / y", "def calculator(x,y,op):\n if x =='1'or x =='2'or x =='3'or x =='4'or x =='5'or x =='6'or x =='7'or x =='8'or x =='9'or x =='0'and y=='1'or y=='2'or y=='3'or y=='4'or y=='5'or y=='6'or y=='7'or y=='8'or y=='9'or y=='0':\n if op=='+':\n return x+y\n if op==\"-\":\n return x-y\n if op==\"*\":\n return x*y\n if op ==\"/\":\n return x/y\n \n elif x==6 and y == 2 and op== '+':\n return 8\n elif x==4 and y == 3 and op== '-':\n return 1\n elif x==5 and y == 5 and op== '*':\n return 25\n elif x==5 and y == 4 and op== '/':\n return 1.25\n else:\n return \"unknown value\"\n pass", "def calculator(x,y,op):\n if op == \"+\":\n try:\n return int(x+y)\n except:\n return \"unknown value\"\n elif op == \"-\":\n return x-y\n elif op == \"*\":\n return x*y\n elif op == \"/\":\n return x/y\n else:\n return \"unknown value\"", "def calculator(x,y,op):\n if not (type(x) == int and type(y)==int):\n return \"unknown value\"\n\n if op == '+': return x+y\n elif op == '*': return x*y\n elif op == '-': return x-y\n elif op == '/': return x/y\n else: return \"unknown value\"\n", "def calculator(x,y,op):\n if type(x)==str or type(y)==str or op not in [\"+\", \"-\", \"*\", \"/\"]:\n return \"unknown value\"\n else:\n if op==\"+\":\n return x+y \n if op==\"-\":\n return x-y\n if op==\"*\":\n return x*y\n if op==\"/\":\n return x/y", "def calculator(x,y,op):\n if isinstance(x, int)==True and isinstance(y, int)==True and op in [\"+\",\"-\",\"*\",\"/\"]:\n if op == \"+\": return x+y\n if op == \"-\": return x-y\n if op == \"*\": return x*y\n if op == \"/\": return x/y\n else:\n return \"unknown value\"", "import operator\nops = {\"+\": operator.add, \"-\": operator.sub, \"*\": operator.mul, \"/\": operator.truediv}\n\ndef calculator(x,y,op):\n print(op)\n print(x)\n print(y)\n return ops[op](x, y) if op in ops.keys() and str(x).isnumeric() and str(y).isnumeric() else \"unknown value\"", "def calculator(x, y, op):\n try:\n int(x)\n int(y)\n except:\n return 'unknown value'\n \n return {\n '+': int(x) + int(y),\n '-': int(x) - int(y),\n '*': int(x) * int(y),\n '/': int(x) / int(y) if int(y) != 0 else 'unknown value'\n }.get(op, 'unknown value')", "def calculator(x,y,op):\n if isinstance(x, int) and isinstance(y, int):\n try:\n if (op == '+'):\n return (x + y)\n elif (op == '-'):\n return (x - y)\n elif (op == '*'):\n return (x * y)\n elif (op == '/'):\n return (x / y)\n else:\n return 'unknown value'\n except: return 'unknown value'\n else: return 'unknown value'\n", "def calculator(x,y,op):\n ops = {'+': 'x + y',\n '-': 'x - y',\n '/': 'x / y',\n '*': 'x * y'\n }\n if str(x).isnumeric() and str(y).isnumeric() and op in ops.keys():\n return eval(ops[op])\n else:\n return 'unknown value'", "def calculator(x,y,op):\n if isinstance(x, int) and isinstance(y,int):\n if op == '+':\n return x + y\n if op == '*':\n return x * y\n if op == '/':\n return x / y\n if op == '-':\n return x - y\n return 'unknown value'", "def calculator(x,y,op):\n try:\n x = int(x)\n y = int(y)\n except:\n return 'unknown value' \n if op == '+':\n return x + y\n elif op =='-':\n return x - y\n elif op == '*':\n return x * y\n elif op == '/':\n return x / y\n return 'unknown value'", "def calculator(x,y,op):\n try: \n if(op == \"+\" and type(x) == int and type(y)== int):\n return x+y\n elif(op == \"-\"):\n return x-y\n elif(op == \"*\"):\n return x*y\n elif(op == \"/\"):\n return x/y\n else:\n return \"unknown value\" \n except TypeError:\n return \"unknown value\"", "def calculator(x,y,op):\n if isinstance(x,int)==False:\n return \"unknown value\"\n elif isinstance(y,int)==False:\n return \"unknown value\"\n elif str(op)==\"+\":\n return x+y\n elif str(op)==\"-\":\n return x-y\n elif str(op)==\"/\":\n return x/y\n elif str(op)==\"*\":\n return x*y\n else:\n return \"unknown value\"", "import operator\n\nops = {\n '+' : operator.add,\n '-' : operator.sub,\n '*' : operator.mul,\n '/' : operator.truediv, \n #'%' : operator.mod,\n #'^' : operator.xor,\n}\n\n\ndef calculator(x,y,op):\n print((x, y, op))\n a = (type(x), type(y), type(op))\n if a[0] != int or a[1] != int:\n return \"unknown value\"\n print(a)\n try:\n q = ops[op](x,y)\n return q\n except:\n return \"unknown value\"\n", "def calculator(x,y,op):\n if not isinstance(x, int) or not isinstance(y, int): return 'unknown value'\n if op == '+':\n return x+y\n elif op == '-':\n return x-y\n elif op == '*':\n return x*y\n elif op == '/':\n return x/y\n return 'unknown value'", "def calculator(x,y,op):\n ops = ['+', '-', '*', '/']\n nums = [1, 2, 3, 4, 5, 6, 7, 8, 9, 0, '1', '2', '3', '4', '5', '6', '7', '8', '9', '0']\n if x in nums and y in nums and op in ops:\n if op == '+':\n return x + y\n elif op == '-':\n return x - y\n elif op == '*':\n return x * y\n elif op == '/':\n return x / y\n else:\n return 'unknown value'", "def calculator(x,y,op):\n try: \n xx = int(x)\n yy = int(y)\n except ValueError:\n return \"unknown value\"\n if op == '+':\n return xx + yy\n elif op == '-':\n return xx-yy\n elif op == '*':\n return xx*yy\n elif op == '/':\n return xx/yy\n else:\n return \"unknown value\"", "def calculator(x,y,o):\n if isinstance(x, int) and isinstance(y, int):\n return x + y if o == '+' else x - y if o == '-' else x * y if o == '*' else x/y if o == '/' else \"unknown value\"\n else:\n return \"unknown value\"\n \n", "def calculator(x,y,op):\n try:\n value = int(x)\n val=int(y)\n except:\n return \"unknown value\"\n if(op==\"+\"):\n return x+y\n elif(op==\"-\"):\n return x-y\n elif(op==\"*\"):\n return x*x\n elif(op==\"/\"):\n return 5/4\n else:\n return \"unknown value\"\n \n", "def calculator(x,y,op):\n try:\n if not isinstance(x, int) or not isinstance(y, int):\n return \"unknown value\"\n if (op == '+'):\n return x+y\n elif (op == '-'):\n return x-y\n elif (op == '*'):\n return x*y\n elif (op == '/'):\n return x/y\n else:\n return \"unknown value\"\n except:\n return \"unknown value\"", "from numbers import Number\ndef calculator(x,y,op):\n if type(x) == int and type(y) == int and op == \"+\":\n return x + y\n elif type(x) == int and type(y) == int and op == \"-\":\n return x - y\n elif type(x) == int and type(y) == int and op == \"*\":\n return x * y\n elif type(x) == int and type(y) == int and op == \"/\":\n return x / y\n if type(x) == int and type(y) == int or (op != \"+\" or \"-\" or \"*\" or \"/\"):\n return \"unknown value\"\n pass", "def calculator(x,y,op):\n x = str(x)\n y = str(y)\n op = str(op)\n if x.isdigit() and y.isdigit():\n if op == '+':\n return int(x) + int(y)\n elif op == '-':\n return int(x) - int(y)\n elif op == '*':\n return int(x) * int(y)\n elif op == '/':\n return int(x) / int(y)\n else:\n return 'unknown value'\n else:\n return 'unknown value'\n", "def calculator(x,y,op):\n if op in [\"+\",\"-\",\"*\",\"/\"] and type(x) is int and type(y) is int:\n finalStr = str(x) + op + str(y) \n return eval(finalStr)\n else:\n return \"unknown value\" ", "def calculator(x,y,op):\n if isinstance(x, (float, int)) == True and isinstance(y, (float, int)) == True:\n if op == '+':\n return (x + y)\n elif op == '-':\n return (x - y)\n elif op == '*':\n return (x * y)\n elif op == '/':\n return (x / y)\n elif op not in ['+', '-', '*', '/']:\n return('unknown value')\n else:\n return('unknown value')\n", "def calculator(x,y,op):\n poss_ops = ['+','-','*','/']\n if isinstance(x, str) or isinstance(y, str) or op not in poss_ops:\n ans = 'unknown value'\n else:\n ans = eval(str(x) + op + str(y))\n return ans", "def calculator(x,y,op):\n if str(op) not in '+-*/' or str(x) not in '0123456789' or str(y) not in '0123456789':\n return \"unknown value\"\n if op == '+':\n return x+y\n elif op == '-':\n return x-y\n elif op == '*':\n return x*y\n else:\n return x/y", "def calculator(x,y,op):\n if type(x) != int or type(y) != int:\n return('unknown value')\n elif op == '/':\n return(x/y)\n elif op == '*':\n return(x*y)\n elif op == '-':\n return(x-y)\n elif op == '+':\n return(x+y)\n else:\n return('unknown value')\n pass", "def calculator(x,y,op):\n signs = ['+', '-', '*', '/']\n if op not in signs or type(x) == str or type(y) == str:\n return \"unknown value\"\n else:\n if op == '+':\n return x + y\n elif op == '-':\n return x - y\n elif op == '*':\n return x * y\n else:\n return x / y\n", "def calculator(a,b,c):\n if type(a) != int or type(b) != int:\n return('unknown value')\n elif c == '+':\n return(int(a)+int(b))\n elif c == '-':\n return(int(a)-int(b))\n elif c == '*':\n return(int(a)*int(b))\n elif c == '/':\n return(int(a)/int(b))\n else:\n return('unknown value')\n pass", "def calculator(x,y,op):\n \n try :\n dic = {'+' : y + x, '-' : x - y,\n '*' : y * x, '/' : x / y}\n \n return dic[op]\n \n except :\n return 'unknown value'"] | {"fn_name": "calculator", "inputs": [[6, 2, "+"], [4, 3, "-"], [5, 5, "*"], [5, 4, "/"], [6, "$", "+"], [6, 2, "&"], [4, 3, "\\"], ["a", 3, "+"], [6, 2, "="], [6, 2, "\t"], [":", ",", "+"]], "outputs": [[8], [1], [25], [1.25], ["unknown value"], ["unknown value"], ["unknown value"], ["unknown value"], ["unknown value"], ["unknown value"], ["unknown value"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 31,148 |
def calculator(x,y,op):
|
7da173808e754b70c7a4ac35bbaca698 | UNKNOWN | When multiple master devices are connected to a single bus (https://en.wikipedia.org/wiki/System_bus), there needs to be an arbitration in order to choose which of them can have access to the bus (and 'talk' with a slave).
We implement here a very simple model of bus mastering. Given `n`, a number representing the number of **masters** connected to the bus, and a fixed priority order (the first master has more access priority than the second and so on...), the task is to choose the selected master.
In practice, you are given a string `inp` of length `n` representing the `n` masters' requests to get access to the bus, and you should return a string representing the masters, showing which (only one) of them was granted access:
```
The string 1101 means that master 0, master 1 and master 3 have requested
access to the bus.
Knowing that master 0 has the greatest priority, the output of the function should be: 1000
```
## Examples
## Notes
* The resulting string (`char* `) should be allocated in the `arbitrate` function, and will be free'ed in the tests.
* `n` is always greater or equal to 1. | ["def arbitrate(s, n):\n i = s.find('1') + 1\n return s[:i] + '0' * (n - i)", "def arbitrate(s, n):\n i = s.find('1') + 1\n return s[:i] + '0' * (len(s) - i)", "def arbitrate(bus_str, n):\n return ''.join(['0' if i != bus_str.find('1') else '1' for i, x in enumerate(bus_str)]) ", "def arbitrate(input, n):\n i =input.find('1')\n return input[:i+1]+'0'*len(input[i+1:])", "def arbitrate(input, n):\n return '{:0{}b}'.format(2 ** int(input, 2).bit_length() // 2, n)", "import re\n\ndef arbitrate(busAccess, n):\n return re.sub(r'(0*1)?(.*)', lambda m: (m.group(1) or '') + '0'*len(m.group(2)) , busAccess)", "arbitrate=lambda b,n:bin(int(2**~-int(b,2).bit_length()))[2:].zfill(n)", "import re\n\ndef arbitrate(s,_):\n p,m,b=s.partition('1')\n return p+m+'0'*len(b)", "def arbitrate(s,n):\n return ''.join('1' if v=='1' and '1' not in s[:i] else '0' for i,v in enumerate(s))", "def arbitrate(inp, n):\n if \"1\" not in inp:return inp\n i=inp.index(\"1\")\n return f\"{'0'*i}1{(n-i-1)*'0'}\""] | {"fn_name": "arbitrate", "inputs": [["001000101", 9], ["000000101", 9], ["0000", 4]], "outputs": [["001000000"], ["000000100"], ["0000"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,035 |
def arbitrate(s, n):
|
9d36dacceafd33727bb17f1372908648 | UNKNOWN | Algorithmic predicament - Bug Fixing #9
Oh no! Timmy's algorithim has gone wrong! help Timmy fix his algorithim!
Task
Your task is to fix timmy's algorithim so it returns the group name with the highest total age.
You will receive two groups of `people` objects, with two properties `name` and `age`. The name property is a string and the age property is a number.
Your goal is to make the total the age of all people having the same name through both groups and return the name of the one with the highest age. If two names have the same total age return the first alphabetical name. | ["from collections import Counter\nfrom itertools import chain\n\n\ndef highest_age(persons1, persons2):\n c = Counter()\n\n for p in chain(persons1, persons2):\n c[p['name']] += p['age']\n\n return min(iter(c.items()), key=lambda n_a: (-n_a[1], n_a[0]))[0] if c else None\n", "from functools import reduce\n\ndef choose(a, b):\n\n if a[1] < b[1]:\n return b\n \n if a[1] > b[1]:\n return a\n \n return a if a[0] < b[0] else b\n \n\ndef highest_age(group1, group2):\n \n totals = {}\n \n for item in group1 + group2:\n name = item['name']\n totals[name] = totals.get(name, 0) + item['age']\n \n return reduce(lambda acc, x: choose(acc, x), totals.items(), ('', -1))[0]", "def highest_age(g1, g2):\n d = {}\n for p in g1 + g2:\n name, age = p[\"name\"], p[\"age\"]\n d[name] = d.setdefault(name, 0) + age\n return max(sorted(d.keys()), key=d.__getitem__)\n", "from collections import defaultdict\nfrom itertools import chain\n\ndef highest_age(group1, group2):\n total = defaultdict(int)\n for person in chain(group1, group2):\n total[person['name']] += person['age']\n return min(total, key=lambda p: (-total[p], p))", "def highest_age(group1,group2):\n d = {}\n lst = []\n for i in group1 + group2:\n name = i['name']\n if name in d:\n d[i['name']] += i['age']\n else:\n d[name] = i['age']\n n = max(d.values())\n for k, v in d.items():\n if n == v:\n lst.append(k)\n return min(lst)", "from collections import Counter\n\ndef highest_age(group1, group2):\n total = Counter()\n for person in group1 + group2:\n total[person['name']] += person['age']\n return min(total, key=lambda name: (-total[name], name))", "def highest_age(group1,group2):\n total={}\n \n for p in group1+group2:\n total[p['name']] = total.get(p['name'],0)+p['age']\n \n return sorted(total, key=total.__getitem__)[-1]"] | {"fn_name": "highest_age", "inputs": [[[{"name": "kay", "age": 1}, {"name": "john", "age": 13}, {"name": "kay", "age": 76}], [{"name": "john", "age": 1}, {"name": "alice", "age": 77}]], [[{"name": "kay", "age": 1}, {"name": "john", "age": 13}, {"name": "kay", "age": 76}], [{"name": "john", "age": 1}, {"name": "alice", "age": 76}]], [[{"name": "kay", "age": 1}, {"name": "john", "age": 130}, {"name": "kay", "age": 76}], [{"name": "john", "age": 1}, {"name": "alice", "age": 76}]], [[{"name": "kay", "age": 1}, {"name": "john", "age": 130}, {"name": "kay", "age": 130}], [{"name": "john", "age": 1}, {"name": "alice", "age": 76}]], [[{"name": "kay", "age": 2}, {"name": "john", "age": 130}, {"name": "kay", "age": 130}], [{"name": "john", "age": 1}, {"name": "alice", "age": 76}]]], "outputs": [["alice"], ["kay"], ["john"], ["john"], ["kay"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,039 |
def highest_age(group1,group2):
|
e2ac82974f076400059f7a30bb692eef | UNKNOWN | ~~~if-not:java
You have to code a function **getAllPrimeFactors** wich take an integer as parameter and return an array containing its prime decomposition by ascending factors, if a factors appears multiple time in the decomposition it should appear as many time in the array.
exemple: `getAllPrimeFactors(100)` returns `[2,2,5,5]` in this order.
This decomposition may not be the most practical.
You should also write **getUniquePrimeFactorsWithCount**, a function which will return an array containing two arrays: one with prime numbers appearing in the decomposition and the other containing their respective power.
exemple: `getUniquePrimeFactorsWithCount(100)` returns `[[2,5],[2,2]]`
You should also write **getUniquePrimeFactorsWithProducts** an array containing the prime factors to their respective powers.
exemple: `getUniquePrimeFactorsWithProducts(100)` returns `[4,25]`
~~~
~~~if:java
You have to code a function **getAllPrimeFactors** wich take an integer as parameter and return an array containing its prime decomposition by ascending factors, if a factors appears multiple time in the decomposition it should appear as many time in the array.
exemple: `getAllPrimeFactors(100)` returns `[2,2,5,5]` in this order.
This decomposition may not be the most practical.
You should also write **getUniquePrimeFactorsWithCount**, a function which will return an array containing two arrays: one with prime numbers appearing in the decomposition and the other containing their respective power.
exemple: `getUniquePrimeFactorsWithCount(100)` returns `[[2,5],[2,2]]`
You should also write **getPrimeFactorPotencies** an array containing the prime factors to their respective powers.
exemple: `getPrimeFactorPotencies(100)` returns `[4,25]`
~~~
Errors, if:
* `n` is not a number
* `n` not an integer
* `n` is negative or 0
The three functions should respectively return `[]`, `[[],[]]` and `[]`.
Edge cases:
* if `n=0`, the function should respectively return `[]`, `[[],[]]` and `[]`.
* if `n=1`, the function should respectively return `[1]`, `[[1],[1]]`, `[1]`.
* if `n=2`, the function should respectively return `[2]`, `[[2],[1]]`, `[2]`.
The result for `n=2` is normal. The result for `n=1` is arbitrary and has been chosen to return a usefull result. The result for `n=0` is also arbitrary
but can not be chosen to be both usefull and intuitive. (`[[0],[0]]` would be meaningfull but wont work for general use of decomposition, `[[0],[1]]` would work but is not intuitive.) | ["def getAllPrimeFactors(n):\n if n == 0: return []\n elif n == 1: return [1]\n elif type(n) != int: return errora\n elif n < 0: return errora\n allfacts = []\n current = 2\n n_copy = n\n while current <= n:\n if n_copy % current == 0:\n allfacts.append(current)\n n_copy /= current\n else:\n current += 1\n return allfacts\n \n \ndef getUniquePrimeFactorsWithCount(n):\n if type(n) != int: return errorb\n elif n < 0: return errorb\n primes = []\n power = []\n listA = getAllPrimeFactors(n)\n for i in range(len(listA)):\n if listA[i] not in primes:\n primes.append(listA[i])\n power.append(1)\n else:\n power[-1] += 1\n return [primes, power]\n\ndef getUniquePrimeFactorsWithProducts(n):\n if type(n) != int: return errorc\n elif n < 0: return errorc\n listlist = getUniquePrimeFactorsWithCount(n)\n listc = []\n for i in range(len(listlist[0])):\n listc.append(listlist[0][i] ** listlist[1][i])\n return listc\n\nerrora = []\nerrorb = [[], []]\nerrorc = []", "from collections import Counter\ndef getAllPrimeFactors(n):\n if type(n)!=int:return []\n li, j = [], 2\n while j*j <= n:\n if n % j : j += 1 ; continue\n li.append(j) ; n //= j\n return li + [[n],[]][n<1]\n\ndef getUniquePrimeFactorsWithCount(n):\n r = Counter(getAllPrimeFactors(n))\n return [list(r.keys()),list(r.values())]\n\ngetUniquePrimeFactorsWithProducts=lambda n:[i**j for i,j in zip(*getUniquePrimeFactorsWithCount(n))]", "from collections import defaultdict\nfrom functools import lru_cache\nfrom itertools import chain\n\n@lru_cache(maxsize=None)\ndef factors(n):\n if n == 1: return [(1, 1)]\n res, wheel = defaultdict(int), [1, 2, 2, 4, 2, 4, 2, 4, 6, 2, 6]\n x, y = 2, 0\n while x*x <= n:\n if not n%x:\n res[x] += 1\n n //= x\n else:\n x += wheel[y]\n y = 3 if y == 10 else y+1\n res[n] += 1\n return res.items()\n\ndef getAllPrimeFactors(n):\n return list(chain.from_iterable([k]*v for k,v in factors(n))) if isinstance(n, int) and n > 0 else []\n\ndef getUniquePrimeFactorsWithCount(n):\n return list(map(list, zip(*factors(n)))) if isinstance(n, int) and n > 0 else [[], []]\n\ndef getUniquePrimeFactorsWithProducts(n):\n return [k**v for k,v in factors(n)] if isinstance(n, int) and n > 0 else []", "\n \ndef getAllPrimeFactors(n):\n result = []\n if isinstance(n, int) and n > 0 :\n if n == 1:\n result = [1]\n number = 2\n while n > 1:\n print(n)\n if not n % number :\n result.append(number)\n n /= number\n number = 2\n else:\n number += 1\n return result\n \n \n #your code here\n\ndef getUniquePrimeFactorsWithCount(n):\n pf = getAllPrimeFactors(n)\n r1 = []\n r2 = []\n if pf:\n r1 = list(set(pf))\n r2 = [pf.count(x) for x in r1 ]\n return [r1, r2]\n \n\ndef getUniquePrimeFactorsWithProducts(n):\n return [x ** y for (x,y) in zip(*getUniquePrimeFactorsWithCount(n))]", "import math\nimport collections\n\n\ndef getAllPrimeFactors(n):\n if isinstance(n, int) and n > -1:\n if n != 1:\n listy, m, x = [], n, True\n while x:\n for i in range(2, int(math.sqrt(n)) + 1):\n if n % i == 0:\n n = n // i\n listy.append(i)\n break\n else:\n x = False\n if m > 1:\n listy.append(n)\n return listy\n else:\n return [1]\n else:\n return []\ndef getUniquePrimeFactorsWithCount(n):\n if isinstance(n, int) and n > -1:\n if n != 1:\n listy, m, x = [], n, True\n while x:\n for i in range(2, int(math.sqrt(n)) + 1):\n if n % i == 0:\n n = n // i\n listy.append(i)\n break\n else:\n x = False\n if m > 1:\n listy.append(n)\n else:\n listy = [1]\n else:\n listy = []\n fancy_dict = collections.Counter()\n resulty_list = [[],[]]\n for number in listy:\n fancy_dict[number] += 1\n for key, value in list(fancy_dict.items()):\n resulty_list[0].append(key)\n resulty_list[1].append(value)\n return resulty_list\n\ndef getUniquePrimeFactorsWithProducts(n):\n if isinstance(n, int) and n > -1:\n if n != 1:\n listy, m, x = [], n, True\n while x:\n for i in range(2, int(math.sqrt(n)) + 1):\n if n % i == 0:\n n = n // i\n listy.append(i)\n break\n else:\n x = False\n if m > 1:\n listy.append(n)\n else:\n listy = [1]\n else:\n listy = []\n fancy_dict = collections.Counter()\n resulty_list = [[],[]]\n for number in listy:\n fancy_dict[number] += 1\n for key, value in list(fancy_dict.items()):\n resulty_list[0].append(key)\n resulty_list[1].append(value)\n return [resulty_list[0][i]**resulty_list[1][i] for i in range(len(resulty_list[0]))]\n", "# RWH primes\nLIMIT = 10**6\nsieve = [True] * (LIMIT//2)\nfor i in range(3, int(LIMIT**0.5)+1, 2):\n if sieve[i//2]: sieve[i*i//2::i] = [False] * ((LIMIT-i*i-1)//2//i+1)\nPRIMES = [2] + [2*i+1 for i in range(1, LIMIT//2) if sieve[i]]\n\n\nfrom collections import Counter\n\ndef getAllPrimeFactors(n):\n # errors and zero\n if not type(n) == int or n < 1:\n return []\n \n # edge cases\n if n in (1, 2):\n return [n]\n \n # factorisation\n factors = []\n \n for p in PRIMES:\n if p*p > n:\n break\n while n % p == 0:\n n //= p\n factors.append(p)\n \n return factors + [n] * (n > 1)\n\n\ndef getUniquePrimeFactorsWithCount(n):\n factors = Counter(getAllPrimeFactors(n))\n return [ list(factors.keys()), list(factors.values()) ]\n\n\ndef getUniquePrimeFactorsWithProducts(n):\n factors = Counter(getAllPrimeFactors(n))\n return [ p**e for p, e in factors.items() ]", "from collections import Counter\n\ndef getAllPrimeFactors(n):\n if n==1: return [1]\n lst, p, n = [], 2, 1 if not isinstance(n,int) or n<0 else n\n while n>1:\n while not n%p:\n lst.append(p)\n n //= p\n p += 1 + (p!=2)\n return lst\n\ndef getUniquePrimeFactorsWithCount(n):\n return list(map(list, zip(*Counter(getAllPrimeFactors(n)).items()))) or [[],[]]\n\ndef getUniquePrimeFactorsWithProducts(n):\n return [k**v for k,v in Counter(getAllPrimeFactors(n)).items()]", "def getAllPrimeFactors(n):\n if not type(n) is int:\n n = 0\n elif n < 0:\n n = 0\n results = []\n number = 2\n while number < n:\n if not n % number:\n n /= number\n results.append(number)\n number = 2\n else:\n number += 1\n if n: results.append(int(n))\n return results\n\ndef getUniquePrimeFactorsWithCount(n):\n results = getAllPrimeFactors(n)\n counted = [[],[]]\n if not results == []:\n for i in range(results[-1]+1):\n if i in results: \n counted[0].append(i)\n counted[1].append(results.count(i)) \n return counted\n\ndef getUniquePrimeFactorsWithProducts(n):\n counted = getUniquePrimeFactorsWithCount(n)\n products = []\n if not counted == [[],[]]:\n for i in range(len(counted[0])):\n products.append(counted[0][i]**counted[1][i])\n return products", "def getAllPrimeFactors(n):\n if type(n) is not int or n < 1:\n return []\n elif n < 3:\n return [n]\n factors = []\n while n > 1:\n for i in range(2, n+1):\n if is_prime(i) and not n % i:\n factors.append(i)\n n = int(n/i)\n break\n return factors\n\ndef getUniquePrimeFactorsWithCount(n):\n f = getAllPrimeFactors(n)\n factors = list(set(f))\n powers = [f.count(factor) for factor in factors]\n return [factors, powers]\n\ndef getUniquePrimeFactorsWithProducts(n):\n cf = getUniquePrimeFactorsWithCount(n)\n return [factor**count for factor, count in zip(cf[0], cf[1])]\n \ndef is_prime(n):\n for i in range(2, n):\n if not n % i:\n return False\n return True\n"] | {"fn_name": "getAllPrimeFactors", "inputs": [[10], [100], [1000], [1000001], [0], [1], [2], ["a"], [1.1], [-1]], "outputs": [[[2, 5]], [[2, 2, 5, 5]], [[2, 2, 2, 5, 5, 5]], [[101, 9901]], [[]], [[1]], [[2]], [[]], [[]], [[]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 8,787 |
def getAllPrimeFactors(n):
|
4ce13a914b841ca459909308a928abae | UNKNOWN | Naming multiple files can be a pain sometimes.
#### Task:
Your job here is to create a function that will take three parameters, `fmt`, `nbr` and `start`, and create an array of `nbr` elements formatted according to `frm` with the starting index `start`. `fmt` will have `` inserted at various locations; this is where the file index number goes in each file.
#### Description of edge cases:
1. If `nbr` is less than or equal to 0, or not whole, return an empty array.
2. If `fmt` does not contain `''`, just return an array with `nbr` elements that are all equal to `fmt`.
3. If `start` is not an integer, return an empty array.
#### What each parameter looks like:
```python
type(frm) #=> str
: "text_to_stay_constant_from_file_to_file "
type(nbr) #=> int
: number_of_files
type(start) #=> int
: index_no_of_first_file
type(name_file(frm, nbr, start)) #=> list
```
#### Some examples:
```python
name_file("IMG ", 4, 1)
#=> ["IMG 1", "IMG 2", "IMG 3", "IMG 4"])
name_file("image #.jpg", 3, 7)
#=> ["image #7.jpg", "image #8.jpg", "image #9.jpg"]
name_file("# #", 3, -2)
#=> ["#-2 #-2", "#-1 #-1", "#0 #0"]
```
Also check out my other creations — [Elections: Weighted Average](https://www.codewars.com/kata/elections-weighted-average), [Identify Case](https://www.codewars.com/kata/identify-case), [Split Without Loss](https://www.codewars.com/kata/split-without-loss), [Adding Fractions](https://www.codewars.com/kata/adding-fractions),
[Random Integers](https://www.codewars.com/kata/random-integers), [Implement String#transpose](https://www.codewars.com/kata/implement-string-number-transpose), [Implement Array#transpose!](https://www.codewars.com/kata/implement-array-number-transpose), [Arrays and Procs #1](https://www.codewars.com/kata/arrays-and-procs-number-1), and [Arrays and Procs #2](https://www.codewars.com/kata/arrays-and-procs-number-2).
If you notice any issues or have any suggestions/comments whatsoever, please don't hesitate to mark an issue or just comment. Thanks! | ["def name_file(fmt, nbr, start):\n try:\n return [fmt.replace('<index_no>', '{0}').format(i)\n for i in range(start, start + nbr)]\n except TypeError:\n return []", "def name_file(fmt, n, start):\n return [fmt.replace('<index_no>', str(i)) for i in range(start, start+n)] if n > 0 and isinstance(n, int) and isinstance(start, int) else []", "def name_file(fmt, nbr, start):\n cnt = fmt.count(\"<index_no>\")\n fmt = fmt.replace(\"<index_no>\", \"%s\")\n if nbr < 0 or not isinstance(nbr, int) or not isinstance(start, int):\n return []\n return [fmt % ((i,)*cnt) for i in range(start, start + nbr)]", "def name_file(fmt, nbr, start):\n return [fmt.replace(\"<index_no>\", str(i)) for i in range(start, start+nbr)] if (type(start) == type(nbr) == int) else []", "def name_file(fmt, nbr, start):\n return [] if type(start) != int or type(nbr) != int else [fmt.replace(\"<index_no>\", str(n)) for n in range(start, start + nbr)]", "def name_file(fmt, nbr, start):\n if int(nbr) != nbr or nbr <= 0 or int(start) != start: return []\n fmt = [fmt for i in range(nbr)]\n num = list(range(start, start + nbr))\n for i, f in enumerate(fmt):\n fmt[i] = f.replace(\"<index_no>\", str(num[i]))\n return fmt", "def name_file(fmt, nbr, start):\n return [fmt.replace(\"<index_no>\", str(i)) for i in range(start, start+nbr)] if (type(start) == type(nbr) == int and nbr > 0) else []", "name_file=lambda f,n,s:[f.replace('<index_no>', str(i)) for i in range(s,s+n)] if n>0 and (type(n),type(s))==(int,int) else []", "name_file=lambda f, n, s: [f.replace(\"<index_no>\",str(i+s)) for i in range(n)] if n%1==0 and s%1==0 else []"] | {"fn_name": "name_file", "inputs": [["IMG <index_no>", 4, 1], ["image #<index_no>.jpg", 3, 7], ["#<index_no> #<index_no>", 3, -2], ["<file> number <index_no>", 5, 0], ["<file_no> number <index_no>", 2, -1], ["file", 2, 3], ["<file_no> number <index_no>", -1, 0], ["file <index_no>", 2, 0.1], ["file <index_no>", 0.2, 0], ["file <index_no>", 0, 0]], "outputs": [[["IMG 1", "IMG 2", "IMG 3", "IMG 4"]], [["image #7.jpg", "image #8.jpg", "image #9.jpg"]], [["#-2 #-2", "#-1 #-1", "#0 #0"]], [["<file> number 0", "<file> number 1", "<file> number 2", "<file> number 3", "<file> number 4"]], [["<file_no> number -1", "<file_no> number 0"]], [["file", "file"]], [[]], [[]], [[]], [[]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,694 |
def name_file(fmt, nbr, start):
|
3b37ad74c1832f4f4c614222f843e6db | UNKNOWN | # RoboScript #2 - Implement the RS1 Specification
## Disclaimer
The story presented in this Kata Series is purely fictional; any resemblance to actual programming languages, products, organisations or people should be treated as purely coincidental.
## About this Kata Series
This Kata Series is based on a fictional story about a computer scientist and engineer who owns a firm that sells a toy robot called MyRobot which can interpret its own (esoteric) programming language called RoboScript. Naturally, this Kata Series deals with the software side of things (I'm afraid Codewars cannot test your ability to build a physical robot!).
## Story
Now that you've built your own code editor for RoboScript with appropriate syntax highlighting to make it look like serious code, it's time to properly implement RoboScript so that our MyRobots can execute any RoboScript provided and move according to the will of our customers. Since this is the first version of RoboScript, let's call our specification RS1 (like how the newest specification for JavaScript is called ES6 :p)
## Task
Write an interpreter for RS1 called `execute()` which accepts 1 required argument `code`, the RS1 program to be executed. The interpreter should return a string representation of the smallest 2D grid containing the full path that the MyRobot has walked on (explained in more detail later).
Initially, the robot starts at the middle of a 1x1 grid. Everywhere the robot walks it will leave a path `"*"`. If the robot has not been at a particular point on the grid then that point will be represented by a whitespace character `" "`. So if the RS1 program passed in to `execute()` is empty, then:
```
"" --> "*"
```
The robot understands 3 major commands:
- `F` - Move forward by 1 step in the direction that it is currently pointing. Initially, the robot faces to the right.
- `L` - Turn "left" (i.e. **rotate** 90 degrees **anticlockwise**)
- `R` - Turn "right" (i.e. **rotate** 90 degrees **clockwise**)
As the robot moves forward, if there is not enough space in the grid, the grid should expand accordingly. So:
```
"FFFFF" --> "******"
```
As you will notice, 5 `F` commands in a row should cause your interpreter to return a string containing 6 `"*"`s in a row. This is because initially, your robot is standing at the middle of the 1x1 grid facing right. It leaves a mark on the spot it is standing on, hence the first `"*"`. Upon the first command, the robot moves 1 unit to the right. Since the 1x1 grid is not large enough, your interpreter should expand the grid 1 unit to the right. The robot then leaves a mark on its newly arrived destination hence the second `"*"`. As this process is repeated 4 more times, the grid expands 4 more units to the right and the robot keeps leaving a mark on its newly arrived destination so by the time the entire program is executed, 6 "squares" have been marked `"*"` from left to right.
Each row in your grid must be separated from the next by a CRLF (`\r\n`). Let's look at another example:
```
"FFFFFLFFFFFLFFFFFLFFFFFL" --> "******\r\n* *\r\n* *\r\n* *\r\n* *\r\n******"
```
So the grid will look like this:
```
******
* *
* *
* *
* *
******
```
The robot moves 5 units to the right, then turns left, then moves 5 units upwards, then turns left again, then moves 5 units to the left, then turns left again and moves 5 units downwards, returning to the starting point before turning left one final time. Note that the marks do **not** disappear no matter how many times the robot steps on them, e.g. the starting point is still marked `"*"` despite the robot having stepped on it twice (initially and on the last step).
Another example:
```
"LFFFFFRFFFRFFFRFFFFFFF" --> " ****\r\n * *\r\n * *\r\n********\r\n * \r\n * "
```
So the grid will look like this:
```
****
* *
* *
********
*
*
```
Initially the robot turns left to face upwards, then moves upwards 5 squares, then turns right and moves 3 squares, then turns right again (to face downwards) and move 3 squares, then finally turns right again and moves 7 squares.
Since you've realised that it is probably quite inefficient to repeat certain commands over and over again by repeating the characters (especially the `F` command - what if you want to move forwards 20 steps?), you decide to allow a shorthand notation in the RS1 specification which allows your customers to postfix a non-negative integer onto a command to specify how many times an instruction is to be executed:
- `Fn` - Execute the `F` command `n` times (NOTE: `n` *may* be more than 1 digit long!)
- `Ln` - Execute `L` n times
- `Rn` - Execute `R` n times
So the example directly above can also be written as:
```
"LF5RF3RF3RF7"
```
These 5 example test cases have been included for you :)
## Kata in this Series
1. [RoboScript #1 - Implement Syntax Highlighting](https://www.codewars.com/kata/roboscript-number-1-implement-syntax-highlighting)
2. **RoboScript #2 - Implement the RS1 Specification**
3. [RoboScript #3 - Implement the RS2 Specification](https://www.codewars.com/kata/58738d518ec3b4bf95000192)
4. [RoboScript #4 - RS3 Patterns to the Rescue](https://www.codewars.com/kata/594b898169c1d644f900002e)
5. [RoboScript #5 - The Final Obstacle (Implement RSU)](https://www.codewars.com/kata/5a12755832b8b956a9000133) | ["from collections import deque\nimport re\n\nTOKENIZER = re.compile(r'(R+|F+|L+)(\\d*)')\n\ndef execute(code):\n \n pos, dirs = (0,0), deque([(0,1), (1,0), (0,-1), (-1,0)])\n seens = {pos}\n \n for act,n in TOKENIZER.findall(code):\n s,r = act[0], int(n or '1') + len(act)-1\n \n if s == 'F':\n for _ in range(r):\n pos = tuple( z+dz for z,dz in zip(pos, dirs[0]) )\n seens.add(pos)\n else:\n dirs.rotate( (r%4) * (-1)**(s == 'R') )\n \n miX, maX = min(x for x,y in seens), max(x for x,y in seens)\n miY, maY = min(y for x,y in seens), max(y for x,y in seens)\n \n return '\\r\\n'.join( ''.join('*' if (x,y) in seens else ' ' for y in range(miY, maY+1)) \n for x in range(miX, maX+1) )", "import re\nDIRS = [(1, 0), (0, 1), (-1, 0), (0, -1)] * 2\n\ndef execute(code):\n grid, (dx, dy) = {(0, 0)}, DIRS[0]\n x = y = xm = ym = xM = yM = 0\n for dir, n in re.findall('([FLR])(\\d*)', code):\n for _ in range(int(n or '1')):\n if dir == 'L': dx, dy = DIRS[DIRS.index((dx, dy)) - 1]\n if dir == 'R': dx, dy = DIRS[DIRS.index((dx, dy)) + 1]\n if dir == 'F':\n x += dx; y += dy\n grid.add((x, y))\n xm, ym, xM, yM = min(xm, x), min(ym, y), max(xM, x), max(yM, y)\n return '\\r\\n'.join(''.join(' *'[(x, y) in grid] for x in range(xm, xM + 1)) for y in range(ym, yM + 1))", "import re\n\ndef execute(code):\n if code == '':\n return '*'\n turn_right = [[1, 0], [0, -1], [-1, 0], [0, 1]]\n turn_left = [[-1, 0], [0, -1], [1, 0], [0, 1]]\n path = re.findall('F\\d+|F|L\\d+|L|R\\d+|R', code)\n max_size = sum([1 if j == 'F' else int(j[1:]) for j in path if 'F' in j]) * 2\n table = [[' '] * (max_size + 1) for i in range(max_size + 1)]\n x, y = max_size // 2, max_size // 2\n table[x][y] = '*'\n f1, f2 = 0, 1\n for way in path:\n if 'R' in way:\n for i in range(1 if way == 'R' else int(way[1:])):\n cur_pos = [pos for pos, coords in enumerate(turn_right) if coords == [f1, f2]][0]\n f1, f2 = turn_right[0] if cur_pos == 3 else turn_right[cur_pos + 1]\n if 'L' in way:\n for i in range(1 if way == 'L' else int(way[1:])):\n cur_pos = [pos for pos, coords in enumerate(turn_left) if coords == [f1, f2]][0]\n f1, f2 = turn_left[0] if cur_pos == 3 else turn_left[cur_pos + 1] \n if 'F' in way:\n for i in range(1 if way == 'F' else int(way[1:])):\n x += 1 * f1\n y += 1 * f2\n table[x][y] = '*'\n solution = [i for i in table if '*' in i]\n solution = [i for i in zip(*solution[:]) if '*' in i]\n for i in range(3):\n solution = list(zip(*solution[:]))\n final_way = [''.join([j for j in i]) for i in solution]\n return '\\r\\n'.join(final_way)", "import re\nfrom enum import Enum\nfrom operator import itemgetter\nfrom typing import List, Tuple, Set, Generator\n\n\nCell = Tuple[int, int]\n\n\nclass Direction(Enum):\n UP = (0, 1)\n DOWN = (0, -1)\n RIGHT = (1, 0)\n LEFT = (-1, 0)\n\n\ndef execute(code: str) -> str:\n visited_cells = visit_cells(code)\n path = draw_path(visited_cells)\n return path\n \n \ndef visit_cells(code: str) -> Set[Cell]:\n visited_cells = [(0, 0)]\n direction = Direction.RIGHT\n \n for action, n_times in code_interpreter(code):\n if action == 'F':\n new_cells = move_forward(visited_cells[-1], direction, n_times)\n visited_cells.extend(new_cells)\n else:\n direction = make_turn(direction, action, n_times)\n return set(visited_cells)\n\n\ndef code_interpreter(code: str) -> Generator[Tuple[str, int], None, None]:\n for move in re.finditer(r'([LRF])(\\d*)', code):\n action = move.group(1)\n times = int(move.group(2)) if move.group(2) else 1\n yield action, times\n \n\ndef move_forward(position: Cell, direction: Direction, n_moves: int) -> List[Cell]:\n px, py = position\n dx, dy = direction.value\n return [(px + i * dx, py + i * dy) for i in range(1, n_moves + 1)]\n\n\ndef make_turn(start: Direction, side: str, n_turns: int) -> Direction:\n ordering = [Direction.RIGHT, Direction.DOWN, Direction.LEFT, Direction.UP]\n step = 1 if side == 'R' else -1\n return ordering[(ordering.index(start) + step * n_turns) % len(ordering)]\n \n \ndef draw_path(visited_cells: Set[Cell]) -> str:\n max_x, min_x, max_y, min_y = find_cells_boundaries(visited_cells)\n \n rectangle = list()\n for y in range(max_y, min_y - 1, -1):\n row = ['*' if (x, y) in visited_cells else ' ' for x in range(min_x, max_x + 1)]\n rectangle.append(''.join(row))\n \n return '\\r\\n'.join(rectangle)\n \n \ndef find_cells_boundaries(visited_cells: Set[Cell]) -> Tuple[int, int, int, int]:\n max_x, _ = max(visited_cells, key=itemgetter(0))\n min_x, _ = min(visited_cells, key=itemgetter(0))\n \n _, max_y = max(visited_cells, key=itemgetter(1))\n _, min_y = min(visited_cells, key=itemgetter(1))\n return max_x, min_x, max_y, min_y\n \n \n \n", "import re\nleft = {'right': 'up', 'up': 'left', 'left': 'down', 'down': 'right'}\nright = {'right': 'down', 'down': 'left', 'left': 'up', 'up': 'right'}\ndef execute(s):\n s, direction = re.sub(r'([RFL])(\\d+)', lambda x: x.group(1) * int(x.group(2)), s), 'right'\n p, p1, li = 0, 0, [[0, 0]]\n for i in s:\n if i == 'L' : direction = left[direction]\n if i == \"R\" : direction = right[direction]\n if i == \"F\":\n p1 += (1 if direction == \"right\" else -1) if direction in ['left', 'right'] else 0\n p += (1 if direction == 'down' else -1) if direction in ['up', 'down'] else 0\n li.append([p, p1])\n m, n = abs(min(li, key=lambda x:x[0])[0])+max(li,key=lambda x:x[0])[0], abs(min(li,key=lambda x:x[1])[1])+max(li,key=lambda x:x[1])[1]\n p, p1, grid= abs(min(li,key=lambda x:x[0])[0]), abs(min(li,key=lambda x:x[1])[1]), [[' ' for _ in range(n+1)] for _ in range(m+1)]\n for i, j in li : grid[p + i][p1 + j] = \"*\" \n return \"\\r\\n\".join([\"\".join(i) for i in grid])", "import numpy as np\ndef switch(argument):\n switcher = {\n 'F': 0,\n 'L': -1,\n 'R': 1\n }\n return switcher.get(argument, '')\n\ndef resize(p_matrix,num,axis,dir):\n if axis == 1:\n right = np.zeros((p_matrix.shape[0], num))\n left = p_matrix\n if dir == 2:\n right, left = left, right\n re_matrix = np.append(left,right,axis)\n else:\n top = np.zeros((num,p_matrix.shape[1]))\n bot = p_matrix\n if dir == 1:\n top, bot = bot, top\n re_matrix = np.append(top,bot,axis)\n return re_matrix\n\ndef mk_matrix(trace):\n p_matrix = np.full((1,1),1)\n pos = [0,0]\n for i in range(len(trace)):\n \n dir = trace[i][0]\n step = trace[i][1]\n if step != 0 :\n if dir == 0:\n if (pos[1]+step+1)> p_matrix.shape[1]:\n p_matrix = resize(p_matrix,pos[1]+step-p_matrix.shape[1]+1,1,dir)\n p_matrix[pos[0],(pos[1]+1):(pos[1]+step+1)] = 1\n pos[1] = pos[1]+step\n elif dir == 1:\n if (pos[0]+step+1)> p_matrix.shape[0]:\n p_matrix = resize(p_matrix,pos[0]+step-p_matrix.shape[0]+1,0,dir)\n p_matrix[(pos[0]+1):(pos[0]+1+step),pos[1]] = 1\n pos[0] = pos[0]+step\n elif dir == 2:\n if (pos[1]-step) < 0:\n p_matrix = resize(p_matrix,step-pos[1],1,dir)\n pos[1] = step\n p_matrix[pos[0],(pos[1]-step):(pos[1])] = 1\n pos[1] = pos[1]-step\n else:\n if (pos[0]-step)<0:\n p_matrix = resize(p_matrix,step-pos[0],0,dir)\n pos[0] = step\n p_matrix[(pos[0]-step):(pos[0]),pos[1]] = 1\n pos[0] = pos[0]-step\n return p_matrix\n\n\ndef tracing(path):\n dir = 0 #pointing right\n # 0-> direita ; 1-> baixo ; 2-> esquerda ; 3->cima\n trace = []\n for j in range(len(path)):\n step = 0\n if path[j][0] == 0:\n if len(path[j]) == 2:\n step = path[j][1]\n else:\n step = 1\n else:\n if len(path[j]) == 2:\n dir = (((path[j][0]*path[j][1])+dir)%4)\n else:\n dir = (dir+path[j][0])%4\n trace.append([dir,step])\n return trace\n\ndef pathing(code):\n path = []\n way = -1\n num = -1\n for i in range(len(code)):\n if i < num:\n continue\n qnt = \"\"\n num = i\n if code[i].isdecimal():\n if num < len(code):\n while code[num].isdecimal():\n qnt += code[num]\n if num+1 >= len(code):\n break\n num += 1\n if len(path[way])<2:\n length = int(qnt,10)\n path[way].append(length)\n else:\n way+=1\n path.append([])\n path[way].append(switch(code[i]))\n return path\n\ndef str_mx(matrix):\n str = []\n subm = np.full(matrix.shape,' ')\n for i in range(matrix.shape[0]):\n for j in range(matrix.shape[1]):\n if matrix[i][j]:\n subm[i][j] = '*'\n if i>0:\n str.append(\"\\r\\n\")\n str.append(''.join(subm[i]))\n return ''.join(str)\n\ndef execute(code):\n path = pathing(code)\n trace = tracing(path)\n matrix = mk_matrix(trace)\n print(matrix)\n str = str_mx(matrix)\n return str\n", "def execute(codes):\n Nums=\"0123456789\"\n code=''\n t=0\n c=0\n while t < len(codes):\n Char=0\n c=1\n if t<len(codes)-1:\n for g in codes[t+1:]:\n if g in Nums:\n Char+=1\n else:\n break\n c=int(codes[t+1:t+Char+1])\n code+=codes[t]*c\n t+=1+Char\n \n \n Xs=0\n Ys=0\n X=[]\n Y=[]\n Ymin=0\n Step=[[0,0]]\n Rotation=0\n Arr=[]\n Out=''\n for i in range(len(code)):\n if code[i]==\"L\":\n Rotation+=90\n elif code[i]==\"R\":\n Rotation+=-90\n if Rotation>180:\n while Rotation>180:\n Rotation+=-360\n elif Rotation<-180:\n while Rotation<-180:\n Rotation+=360\n if code[i]==\"F\":\n if Rotation==0:\n Xs+=1\n elif Rotation==-180 or Rotation ==180:\n Xs+=-1\n elif Rotation==90:\n Ys+=1\n elif Rotation==-90:\n Ys+=-1\n Step.append([Xs,Ys])\n for u in Step:\n X.append(u[0])\n Y.append(u[1])\n for j in range(len(Step)):\n Step[j][0]=Step[j][0]+min(X)*-1\n Step[j][1]=Step[j][1]+min(Y)*-1\n for k in range(max(Y)-min(Y)+1):\n Arr.append([\" \"]*(max(X)-min(X)+1))\n for l in Step:\n Arr[max(Y)-min(Y)-l[1]][l[0]]=\"*\"\n for n in range(len(Arr)):\n for p in range(max(X)-min(X)+1):\n Out+=Arr[n][p]\n Out+=\"\\r\\n\"\n Out=Out[:-2]\n return(Out)", "def execute(code):\n R, r, c, dr, dc = {(0, 0)}, 0, 0, 0, 1\n D = {(1, 0):{'R':(0, -1), 'L':(0, 1)}, (-1, 0):{'R':(0, 1), 'L':(0, -1)}, (0, 1):{'R':(1, 0), 'L':(-1, 0)}, (0, -1):{'R':(-1, 0), 'L':(1, 0)}}\n \n for cmd in code.replace('R', ' R').replace('L', ' L').replace('F', ' F').strip().split():\n cmd, n = cmd[:1], int(cmd[1:]) if cmd[1:] else 1\n for _ in range(n):\n if cmd in 'RL': \n dr, dc = D[(dr, dc)][cmd]\n else:\n r, c = r + dr, c + dc\n R.add((r, c))\n \n mnr = min(r for r, _ in R)\n mnc = min(c for _, c in R)\n \n R = {(r - mnr, c - mnc) for r, c in R}\n \n mxr = max(r for r, _ in R) \n mxc = max(c for _, c in R) \n \n return '\\r\\n'.join(''.join(' *'[(r, c) in R] for c in range(mxc+1)) for r in range(mxr+1))", "import re\n\ndef update_grid(px, py, grid):\n height = len(grid)\n width = len(grid[0])\n if px < 0:\n px += 1\n for y in range(height):\n grid[y] = \" \" + grid[y]\n if px >= width:\n for y in range(height):\n grid[y] = grid[y] + \" \"\n if py < 0:\n py += 1\n grid.insert(0, \" \" * width)\n if py >= height:\n grid.append(\" \" * width)\n grid[py] = grid[py][:px] + \"*\" + grid[py][px+1:]\n return px, py, grid\n \n\ndef execute(code):\n grid = [\"*\"]\n x, y = 0, 0\n facing = (1, 0)\n while code:\n match = re.match(r'([FLR])(\\d*)', code)\n code = re.sub(r'^([FLR])(\\d*)', '', code)\n op = match[1]\n rep = int(match[2] or \"1\")\n for i in range(rep):\n if op == \"F\":\n x += facing[0]\n y += facing[1]\n x, y, grid = update_grid(x, y, grid)\n if op == \"L\":\n facing = (facing[1], -facing[0])\n if op == \"R\":\n facing = (-facing[1], facing[0])\n return \"\\r\\n\".join(grid)\n", "BLANK = \" \"\nFILLCHAR = \"*\"\n\ndef pad_mtx(M, side=\"e\"):\n \"\"\"\n Pads matrix with row or column of blank chars depending on side keyword\n \"\"\"\n if side == \"w\":\n for i in range(len(M)):\n M[i] = [BLANK] + M[i][::1]\n elif side == \"e\":\n for i in range(len(M)):\n M[i] += [BLANK]\n elif side == \"n\":\n M.insert(0, [BLANK for _ in range(len(M[0]))])\n elif side == \"s\":\n M.append([BLANK for _ in range(len(M[0]))])\n\ndef move(mtx, pos, drxn):\n # modify position\n if drxn == \"e\":\n pos[1] += 1\n elif drxn == \"w\":\n pos[1] -= 1\n elif drxn == \"s\":\n pos[0] += 1\n elif drxn == \"n\":\n pos[0] -= 1\n else:\n raise ValueError(\"Direction unrecognized.\")\n \n # Check if path matrix needs to be modified\n try:\n if any(x < 0 for x in pos):\n raise ValueError\n else:\n mtx[pos[0]][pos[1]] = FILLCHAR\n # Modify it if an error was raised\n except Exception:\n pad_mtx(mtx, side=drxn)\n # Update position to reflect modified matrix\n if drxn == \"n\":\n pos[0] += 1\n if drxn == \"w\":\n pos[1] += 1 \n mtx[pos[0]][pos[1]] = FILLCHAR\n\ndef rotate(current, turn):\n directions = [\"e\", \"s\", \"w\", \"n\"]\n turns = {\"L\": -1, \"R\": 1}\n idx = directions.index(current)\n return directions[(idx + turns[turn]) % len(directions)]\n\n\ndef execute(code):\n directions = [\"e\", \"s\", \"w\", \"n\"]\n path = [[FILLCHAR]]\n pos = [0, 0]\n drxn = \"e\"\n i = 0\n while i < len(code):\n # check if command is repeated\n num = \"\"\n if i != len(code) - 1 and code[i+1].isdigit():\n j = i+1\n while j < len(code) and code[j].isdigit():\n num += code[j]\n j += 1\n for _ in range(int(num)):\n if code[i] in \"LR\":\n drxn = rotate(drxn, code[i])\n elif code[i] == \"F\":\n move(path, pos, drxn)\n else:\n break\n \n elif code[i] == \"F\":\n move(path, pos, drxn)\n elif code[i] in \"LR\":\n drxn = rotate(drxn, code[i])\n i += 1\n return \"\\r\\n\".join(\"\".join(row) for row in path)"] | {"fn_name": "execute", "inputs": [[""], ["FFFFF"], ["FFFFFLFFFFFLFFFFFLFFFFFL"], ["LFFFFFRFFFRFFFRFFFFFFF"], ["LF5RF3RF3RF7"], ["FFFLLFFFFFFRRFFFLFFFRRFFFFFFFF"], ["F3L2F6R2F3LF3R2F8"], ["F2FLLF3F3R2FFFLF2FRRF3F2F2F"], ["FFLFFFLFFFFLFFFFFLFFFFFFLFFFFFFFLFFFFFFFFLFFFFFFFFFLFFFFFFFFFF"], ["F2LF3LF4LF5LF6LF7LF8LF9LF10"], ["FFFFLFFFFRFFFFRFFFFLFFFFLFFFFRFFFFRFFFFLFFFFLFFFFRFFFFRFFFF"], ["F4LF4RF4RF4LF4LF4RF4RF4LF4LF4RF4RF4"]], "outputs": [["*"], ["******"], ["******\r\n* *\r\n* *\r\n* *\r\n* *\r\n******"], [" ****\r\n * *\r\n * *\r\n********\r\n * \r\n * "], [" ****\r\n * *\r\n * *\r\n********\r\n * \r\n * "], [" * \r\n * \r\n * \r\n*******\r\n * \r\n * \r\n * \r\n * \r\n * "], [" * \r\n * \r\n * \r\n*******\r\n * \r\n * \r\n * \r\n * \r\n * "], [" * \r\n * \r\n * \r\n*******\r\n * \r\n * \r\n * \r\n * \r\n * "], ["********* \r\n* * \r\n* ***** * \r\n* * * * \r\n* * * * \r\n* * *** * \r\n* * * \r\n* ******* \r\n* \r\n***********"], ["********* \r\n* * \r\n* ***** * \r\n* * * * \r\n* * * * \r\n* * *** * \r\n* * * \r\n* ******* \r\n* \r\n***********"], [" ***** ***** *****\r\n * * * * * *\r\n * * * * * *\r\n * * * * * *\r\n***** ***** ***** *"], [" ***** ***** *****\r\n * * * * * *\r\n * * * * * *\r\n * * * * * *\r\n***** ***** ***** *"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 16,123 |
def execute(code):
|
af0216ba486115b9078075968e4e5480 | UNKNOWN | Hello! Your are given x and y and 2D array size tuple (width, height) and you have to:
Calculate the according index in 1D space (zero-based).
Do reverse operation.
Implement:
to_1D(x, y, size):
--returns index in 1D space
to_2D(n, size)
--returns x and y in 2D space
1D array: [0, 1, 2, 3, 4, 5, 6, 7, 8]
2D array: [[0 -> (0,0), 1 -> (1,0), 2 -> (2,0)],
[3 -> (0,1), 4 -> (1,1), 5 -> (2,1)],
[6 -> (0,2), 7 -> (1,2), 8 -> (2,2)]]
to_1D(0, 0, (3,3)) returns 0
to_1D(1, 1, (3,3)) returns 4
to_1D(2, 2, (3,3)) returns 8
to_2D(5, (3,3)) returns (2,1)
to_2D(3, (3,3)) returns (0,1)
Assume all input are valid:
1 < width < 500;
1 < height < 500 | ["def to_1D(x, y, size):\n return y * size[0] + x \n \ndef to_2D(n, size):\n return (n % size[0], n // size[0])", "def to_1D(x, y, size):\n w, h = size\n return x + y * w\n \ndef to_2D(n, size):\n w, h = size\n return (n % w, n // w)", "def to_1D(x, y, size):\n return size[0] * y + x\n \ndef to_2D(n, size):\n return divmod(n, size[0])[::-1]\n", "def to_1D(x, y, size):\n return y*size[0] + x\n \ndef to_2D(n, size):\n return divmod(n, size[0])[::-1]", "to_1D, to_2D = lambda x, y, s: x + y * s[0], lambda n, s: (n%s[0], n//s[0])", "def to_1D(x, y, size):\n # x is the index of a column and y the index of a row\n return y*size[0] + x\n \ndef to_2D(n, size):\n return (n%size[0], n//size[0])", "def to_1D(x, y, size):\n return x+y*size[0]\n \ndef to_2D(n, size):\n c = 0\n for i in range(size[1]):\n for j in range(size[0]):\n if n == c:\n return j, i\n c+=1", "def to_1D(x, y, size):\n c = 0\n for i in range(size[1]):\n for j in range(size[0]):\n if x ==j and y ==i:\n return c\n c+=1\n \ndef to_2D(n, size):\n c = 0\n for i in range(size[1]):\n for j in range(size[0]):\n if n == c:\n return j, i\n c+=1", "def to_1D(x, y, s):\n return s[0]*y + x\ndef to_2D(n, s):\n return (n // s[0], n % s[0])[::-1]", "to_1D = lambda x,y,s: x+y*s[0]\nto_2D = lambda n,s: (n%s[0], n//s[0])"] | {"fn_name": "to_1D", "inputs": [[0, 0, [3, 3]], [1, 1, [3, 3]], [2, 2, [3, 3]], [0, 0, [1, 1]], [1, 5, [4, 6]], [2, 3, [4, 6]]], "outputs": [[0], [4], [8], [0], [21], [14]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,503 |
def to_1D(x, y, size):
|
9a0d82509e228a14319608a9235c8e43 | UNKNOWN | Congratulations! That Special Someone has given you their phone number.
But WAIT, is it a valid number?
Your task is to write a function that verifies whether a given string contains a valid British mobile (cell) phone number or not.
If valid, return 'In with a chance'.
If invalid, or if you're given an empty string, return 'Plenty more fish in the sea'.
A number can be valid in the following ways:
Here in the UK mobile numbers begin with '07' followed by 9 other digits, e.g. '07454876120'.
Sometimes the number is preceded by the country code, the prefix '+44', which replaces the '0' in ‘07’, e.g. '+447454876120'.
And sometimes you will find numbers with dashes in-between digits or on either side, e.g. '+44--745---487-6120' or '-074-54-87-61-20-'. As you can see, dashes may be consecutive.
Good Luck Romeo/Juliette! | ["import re\nyes = \"In with a chance\"\nno = \"Plenty more fish in the sea\"\ndef validate_number(string):\n return yes if re.match(r'^(\\+44|0)7[\\d]{9}$',re.sub('-','',string)) else no", "import re\ndef validate_number(string):\n if re.match(r\"^(0|\\+44)7[0-9]{9}$\", string.replace(\"-\", \"\")):\n return \"In with a chance\"\n else:\n return \"Plenty more fish in the sea\"", "import re\n\ndef validate_number(string):\n return 'In with a chance' if re.match(r'^(07|\\+447)\\d{9}$', string.replace('-', '')) else 'Plenty more fish in the sea'", "from re import match;validate_number=lambda s: [\"Plenty more fish in the sea\",\"In with a chance\"][match(\"^(\\+44|0)7\\d{9}$\",s.replace(\"-\",\"\"))!=None]", "import re\ndef validate_number(s):\n if re.match(r'^(07|\\+447)+\\d{9}$', s.replace('-','')):\n return 'In with a chance'\n else:\n return 'Plenty more fish in the sea'", "import re\n\nVALID_NUM = re.compile(r'(\\+44|0)7\\d{9}$')\n\ndef validate_number(s):\n return \"In with a chance\" if VALID_NUM.match(s.replace(\"-\",\"\")) else \"Plenty more fish in the sea\"", "import re\n\ndef validate_number(s):\n if not s:\n return \"Plenty more fish in the sea\"\n regex = r\"(?:-*)(0|\\+44)(7)((?:\\d-*){9})(?:-*)$\"\n mo = re.match(regex, s)\n if mo:\n return \"In with a chance\"\n else:\n return \"Plenty more fish in the sea\"", "def validate_number(s):\n s = s.replace('+44', '0', 1).replace('-', '')\n return 'In with a chance' if s[:2] == '07' and len(s) == 11 and s.isdigit() else 'Plenty more fish in the sea'", "validate_number=lambda s:['Plenty more fish in the sea','In with a chance'][bool(__import__('re').fullmatch('(0|\\+44)7\\d{9}',s.replace('-','')))]", "import re\n\ndef validate_number(s):\n for c in '-+': s = s.replace(c, '')\n return 'In with a chance' if re.match(r'^(07|447)\\d{9}$', s) else 'Plenty more fish in the sea'"] | {"fn_name": "validate_number", "inputs": [["07454876120"], ["0754876120"], ["0745--487-61-20"], ["+447535514555"], ["-07599-51-4555"], ["075335440555"], ["+337535512555"], ["00535514555"], ["+447+4435512555"], ["+44"]], "outputs": [["In with a chance"], ["Plenty more fish in the sea"], ["In with a chance"], ["In with a chance"], ["In with a chance"], ["Plenty more fish in the sea"], ["Plenty more fish in the sea"], ["Plenty more fish in the sea"], ["Plenty more fish in the sea"], ["Plenty more fish in the sea"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,958 |
def validate_number(s):
|
40f42aa90d9bcfc370536c1361cd6efb | UNKNOWN | Now we will confect a reagent. There are eight materials to choose from, numbered 1,2,..., 8 respectively.
We know the rules of confect:
```
material1 and material2 cannot be selected at the same time
material3 and material4 cannot be selected at the same time
material5 and material6 must be selected at the same time
material7 or material8 must be selected(at least one, or both)
```
# Task
You are given a integer array `formula`. Array contains only digits 1-8 that represents material 1-8. Your task is to determine if the formula is valid. Returns `true` if it's valid, `false` otherwise.
# Example
For `formula = [1,3,7]`, The output should be `true`.
For `formula = [7,1,2,3]`, The output should be `false`.
For `formula = [1,3,5,7]`, The output should be `false`.
For `formula = [1,5,6,7,3]`, The output should be `true`.
For `formula = [5,6,7]`, The output should be `true`.
For `formula = [5,6,7,8]`, The output should be `true`.
For `formula = [6,7,8]`, The output should be `false`.
For `formula = [7,8]`, The output should be `true`.
# Note
- All inputs are valid. Array contains at least 1 digit. Each digit appears at most once.
- Happy Coding `^_^` | ["def isValid(formula):\n return not ( \\\n ( 1 in formula and 2 in formula) or \\\n ( 3 in formula and 4 in formula) or \\\n ( 5 in formula and not 6 in formula) or \\\n (not 5 in formula and 6 in formula) or \\\n (not 7 in formula and not 8 in formula))", "def isValid(formula):\n if ((not 7 in formula) and (not 8 in formula)):\n return False\n if ((1 in formula) and (2 in formula)):\n return False\n if ((3 in formula) and (4 in formula)):\n return False\n if ((5 in formula and not 6 in formula) or (6 in formula and not 5 in formula)):\n return False\n return True", "def isValid(formula):\n a,b,c,d,e,f,g,h = (v in formula for v in range(1,9))\n return not(a and b) and not(c and d) and e^f^1 and (g or h)", "def isValid(formula):\n if 1 in formula and 2 in formula: return False\n if 3 in formula and 4 in formula: return False\n if 5 in formula and 6 not in formula: return False\n if 5 not in formula and 6 in formula: return False\n if 7 not in formula and 8 not in formula: return False\n return True", "def isValid(formula):\n f = set(formula)\n return (not {1, 2} <= f\n and not {3, 4} <= f\n and ((5 in f) == (6 in f))\n and bool({7, 8} & f))", "def isValid(formula):\n if 1 in formula and 2 in formula :\n return False\n if 3 in formula and 4 in formula :\n return False\n if 5 in formula and not 6 in formula :\n return False\n if 5 not in formula and 6 in formula :\n return False\n if not 7 in formula and not 8 in formula :\n return False\n return True", "def isValid(formula):\n if {1,2} <= set(formula) or {3,4} <= set(formula):\n print(1)\n return False\n elif (5 in formula and 6 not in formula) or (6 in formula and 5 not in formula):\n print(3)\n return False\n elif 7 not in formula and 8 not in formula:\n print(4)\n return False\n else:\n return True", "def isValid(formula):\n if not any(el in formula for el in (7, 8)):\n return False\n elif all(el in formula for el in (1, 2)):\n return False\n elif all(el in formula for el in (3, 4)):\n return False\n elif 5 in formula and 6 not in formula:\n return False\n elif 6 in formula and 5 not in formula:\n return False\n else:\n return True", "def isValid(formula):\n if 1 in formula or 2 in formula:\n if (1 in formula) and (2 in formula):\n return False\n if 3 in formula or 4 in formula:\n if (3 in formula and 4 in formula):\n return False\n if 7 not in formula and 8 not in formula:\n return False\n if 5 in formula or 6 in formula:\n if 5 not in formula or 6 not in formula:\n return False \n return True", "def isValid(formula):\n return (\n ((1 in formula) + (2 in formula) < 2)\n and ((3 in formula) + (4 in formula) < 2)\n and ((5 in formula) == (6 in formula))\n and ((7 in formula) or (8 in formula))\n )\n", "def isValid(f):\n return (not (((1 in f) and (2 in f) or ((3 in f) and (4 in f)))) and ((5 in f)==(6 in f)) and ((7 in f) or (8 in f))) ##", "def isValid(f):\n if 1 in f and 2 in f:\n return False\n else:\n if 3 in f and 4 in f:\n return False\n else:\n if 5 in f and 6 in f:\n if 7 in f or 8 in f:\n return True\n else:\n return False\n else:\n if 5 in f or 6 in f:\n return False\n else:\n if 7 in f or 8 in f:\n return True\n else:\n return False", "def isValid(formula):\n if (1 in formula and 2 in formula) or (3 in formula and 4 in formula) or \\\n (7 not in formula and 8 not in formula) or (5 in formula and 6 not in formula) or \\\n (6 in formula and 5 not in formula): return False\n return True", "def isValid(formula):\n s = ''.join(sorted(map(str,formula)))\n return not any(['12' in s,'34' in s,('5' in s)+('6' in s) == 1,('7' in s)+('8' in s) == 0])", "from typing import List\n\ndef isValid(f: List[int]):\n \"\"\" Check if choosen package of paterials is valid according to the rules. \"\"\"\n return all(\n [\n (1 in f) ^ (2 in f) if 1 in f or 2 in f else True,\n (3 in f) ^ (4 in f) if 3 in f or 4 in f else True,\n all([5 in f, 6 in f]) if 5 in f or 6 in f else True,\n any([7 in f, 8 in f])\n ]\n )", "def isValid(f):\n return (((1 in f) ^ (2 in f)) or ((1 not in f) and (2 not in f))) and (((3 in f) ^ (4 in f)) or ((3 not in f) and (4 not in f))) and (((5 in f) and (6 in f)) or ((5 not in f) and (6 not in f))) and ((7 in f) | (8 in f))", "def isValid(f):\n if 1 in f and 2 in f: return False\n if 3 in f and 4 in f: return False\n if (5 in f or 6 in f) and not (5 in f and 6 in f): return False\n if not (7 in f or 8 in f): return False\n return True", "def isValid(f):\n invalid = ((1,2), (3, 4))\n\n if 1 in f and 2 in f :\n return False\n if 3 in f and 4 in f:\n return False\n if (5 in f or 6 in f) and not (5 in f and 6 in f):\n return False\n if not (7 in f or 8 in f):\n return False\n return True", "def isValid(formula):\n \n material = [m + 1 in formula for m in range(8)]\n r1 = not (material[0] and material[1]) \n r2 = not (material[2] and material[3]) \n r3 = not (material[4] ^ material[5])\n r4 = material[6] or material[7] \n return r1 and r2 and r3 and r4 \n \n", "def isValid(formula):\n mat = [m+1 in formula for m in range(8)]\n return all((\n not (mat[0] and mat[1]),\n not (mat[2] and mat[3]),\n not (mat[4] ^ mat[5]),\n mat[6] or mat[7]\n ))", "def isValid(formula):\n material = [m + 1 in formula for m in range(8)]\n r1 = not (material[0] and material[1]) # material1 and material2 cannot be selected at the same time\n r2 = not (material[2] and material[3]) # material3 and material4 cannot be selected at the same time\n r3 = not (material[4] ^ material[5]) # material5 and material6 must be selected at the same time\n r4 = material[6] or material[7] # material7 or material8 must be selected(at least one, or both)\n return r1 and r2 and r3 and r4\n", "def isValid(formula):\n if 1 in formula and 2 in formula:\n return False\n if 3 in formula and 4 in formula:\n return False\n if 5 in formula and 6 not in formula:\n return False\n if 5 not in formula and 6 in formula:\n return False\n if not 7 in formula and 8 not in formula:\n return False\n return True\n \n", "def isValid(formula):\n if ((1 in formula) and (2 in formula)):\n return False\n if ((3 in formula) and (4 in formula)):\n return False\n if ((5 in formula) ^ (6 in formula)):\n return False\n if ((7 not in formula) and (8 not in formula)):\n return False\n return True\n \n", "def isValid(formula):\n if (1 in formula and 2 in formula) or (3 in formula and 4 in formula):\n return False\n if (7 not in formula) and (8 not in formula):\n return False\n if (5 in formula and 6 not in formula) or (6 in formula and 5 not in formula):\n return False\n else:\n return True", "def isValid(formula):\n test1 = not (1 in formula and 2 in formula)\n test2 = not (3 in formula and 4 in formula)\n test3 = (5 in formula and 6 in formula) or (5 not in formula and 6 not in formula)\n test4 = (7 in formula or 8 in formula)\n \n print((test1, test2, test3, test4))\n \n return test1 and test2 and test3 and test4\n", "def isValid(formula):\n if 3 in formula and 4 in formula: return False\n elif 1 in formula and 2 in formula: return False\n elif 5 in formula and 6 not in formula: return False\n elif 5 not in formula and 6 in formula: return False\n elif 7 not in formula and 8 not in formula: return False\n else: return True", "def isValid(formula):\n if 1 in formula and 2 in formula:\n return False\n elif 3 in formula and 4 in formula:\n return False\n elif 5 in formula and not 6 in formula:\n return False\n elif 6 in formula and not 5 in formula:\n return False\n elif 7 not in formula and 8 not in formula:\n return False\n else:\n return True", "def isValid(formula):\n list = []\n for x in range(8):\n list.append(9)\n for x in formula:\n list[x - 1] = x\n if((list[0] != 9) and (list[1] != 9)):\n return False\n elif((list[2] != 9) and (list[3] != 9)): \n return False\n elif((list[4] == 9 and list[5] != 9) or (list[4] != 9 and list[5] == 9)): \n return False\n elif((list[6] + list[7]) == 18): \n return False\n else: return True", "def isValid(seq):\n return (7 in seq or 8 in seq) and ((5 in seq and 6 in seq) or (5 not in seq and 6 not in seq)) and (1 not in seq or 2 not in seq) and (3 not in seq or 4 not in seq)", "def isValid(formula): \n if 1 in formula and 2 in formula: return False\n elif 3 in formula and 4 in formula: return False\n elif 5 in formula and 6 not in formula: return False\n elif 6 in formula and 5 not in formula: return False\n elif 7 in formula or 8 in formula: return True\n elif 7 not in formula or 8 not in formula: return False\n", "def isValid(formula):\n m = [0, 1, 2, 3, 4, 5, 6, 7, 8]\n t = []\n \n def mat1_mat2():\n ## material1 and material2 cannot be selected at the same time\n count = 0\n for i in formula:\n if i == 1:\n count+=1\n if i == 2:\n count+=1\n if count == 0:\n t.append(True)\n elif count == 1:\n t.append(True)\n else:\n t.append(False)\n\n def mat3_mat4():\n ## material3 and material4 cannot be selected at the same time\n count = 0\n for i in formula:\n if i == 3:\n count+=1\n if i == 4:\n count+=1\n if count == 0:\n t.append(True)\n elif count == 1:\n t.append(True)\n else:\n t.append(False)\n\n def mat5_mat6():\n # material5 and material6 must be selected at the same time\n count = 0\n for i in formula:\n if i == 5:\n count+=1\n if i == 6:\n count+=1\n if count == 0:\n t.append(True)\n elif count == 1:\n t.append(False)\n else:\n t.append(True)\n\n def mat7_mat8():\n # material7 or material8 must be selected(at least one, or both)\n count = 0\n for i in formula:\n if i == 7:\n count+=1\n if i == 8:\n count+=1\n if count == 1:\n t.append(True)\n elif count == 2:\n t.append(True)\n else:\n t.append(False)\n \n mat1_mat2()\n mat3_mat4()\n mat5_mat6()\n mat7_mat8()\n\n return all(t)", "def isValid(f):\n return all((((7 in f) or (8 in f)), ((5 in f) == (6 in f)), not((3 in f) and (4 in f)), not((1 in f) and (2 in f))))", "def isValid(formula):\n formula=sorted(formula)\n if len(formula)<1:\n return False\n elif (1 in formula and 2 in formula) or (3 in formula and 4 in formula) or (7 not in formula and 8 not in formula):\n return False\n elif (5 in formula and 6 not in formula) or (6 in formula and 5 not in formula):\n return False\n else:\n return True\n\n", "def isValid(f):\n if 1 in f and 2 in f: return False\n elif 3 in f and 4 in f: return False\n elif 6 in f and 5 not in f: return False\n elif 5 in f and 6 not in f: return False\n elif 5 in f and 6 in f and 7 in f: return True\n elif 5 in f and 6 in f and 8 in f: return True \n \n \n \n \n elif 7 in f or 8 in f: return True\n else: return False \n \n \n", "def isValid(formula):\n if not(7 in formula or 8 in formula):\n return False\n elif 1 in formula and 2 in formula:\n return False\n elif 3 in formula and 4 in formula:\n return False\n elif 5 in formula:\n if 6 not in formula:\n return False\n elif 6 in formula:\n if 5 not in formula:\n return False\n return True\n", "def isValid(formula):\n if not 7 in formula and not 8 in formula:\n return False\n if 5 in formula and 6 not in formula or 6 in formula and 5 not in formula:\n return False\n if 1 in formula and 2 in formula:\n return False\n if 3 in formula and 4 in formula:\n return False\n return True\n", "def isValid(formula):\n f = set(formula)\n if 7 not in f and 8 not in f:\n return False\n if 1 in f and 2 in f:\n return False\n if 3 in f and 4 in f:\n return False\n return (5 in f) == (6 in f)\n", "def isValid(formula):\n if 1 in formula: \n if 2 in formula: return False \n if 2 in formula: \n if 1 in formula: return False\n if 3 in formula: \n if 4 in formula: return False \n if 4 in formula: \n if 3 in formula: return False \n if 5 in formula: \n if 6 not in formula: return False \n if 6 in formula: \n if 5 not in formula: return False\n if 7 not in formula and 8 not in formula: \n return False\n return True", "def isValid(formula):\n print(formula)\n f = list(map(str, formula))\n f1 = str(\"\".join(f))\n b = 0\n if f1.find('7') != -1:\n for i in f1:\n b = i\n if b == '1':\n if f1.find('2') == -1:\n continue\n else:\n return False\n elif b == '3':\n if f1.find('4') == -1:\n continue\n else:\n return False\n elif b == '5':\n if f1.find('6') != -1:\n return True\n else:\n return False\n elif b == '6':\n if f1.find('5') != -1:\n return True\n else:\n return False\n else: \n if f1.find('8') != -1:\n for i in f1:\n b = i\n if b == '1':\n if f1.find('2') == -1:\n continue\n else:\n return False\n elif b == '3':\n if f1.find('4') == -1:\n continue\n else:\n return False\n elif b == '5':\n if f1.find('6') != -1:\n return True\n else:\n return False\n elif b == '6':\n if f1.find('5') != -1:\n return True\n else:\n return False\n else:\n return False\n \n \n return True\n", "def isValid(formula):\n if formula.count(1) and formula.count(2):\n return False\n if formula.count(3) and formula.count(4):\n return False\n if formula.count(5)+formula.count(6)==1:\n return False\n if formula.count(7)+formula.count(8)==0:\n return False\n return True", "def isValid(formula):\n return not (1 in formula and 2 in formula or \\\n 3 in formula and 4 in formula or \\\n 5 in formula and 6 not in formula or \\\n 5 not in formula and 6 in formula or \\\n 7 not in formula and 8 not in formula\n )", "def isValid(formula):\n if 1 in formula and 2 in formula:\n return False\n \n elif 3 in formula and 4 in formula:\n return False\n \n elif 7 not in formula and 8 not in formula:\n return False\n \n elif 5 in formula:\n if not 6 in formula:\n return False\n \n elif 6 in formula:\n if not 5 in formula:\n return False\n \n return True", "def isValid(f):\n if 1 in f and 2 in f:return False\n if 3 in f and 4 in f: return False\n if not 7 in f and not 8 in f:return False\n if 5 in f and not 6 in f:return False\n if not 5 in f and 6 in f:return False\n return True\n \n", "def isValid(a):\n for x, y in [[1,2], [3,4]]:\n if x in a and y in a: return False\n return (5 in a) == (6 in a) and any(x in a for x in (7,8))", "def isValid(formula):\n rules = [not(1 in formula and 2 in formula)]\n rules.append(not(3 in formula and 4 in formula)) \n rules.append((5 in formula and 6 in formula) or (5 not in formula and 6 not in formula))\n rules.append(7 in formula or 8 in formula)\n print(rules)\n return all(rules)", "def isValid(formula):\n valid_formula = True\n\n if all(x in formula for x in [1, 2]):\n valid_formula = False\n\n if all(x in formula for x in [3, 4]):\n valid_formula = False\n\n if 5 in formula and 6 not in formula:\n valid_formula = False\n \n if 6 in formula and 5 not in formula:\n valid_formula = False\n \n if 7 not in formula and 8 not in formula:\n valid_formula = False\n\n\n\n return valid_formula", "def isValid(formula):\n var1=(7 in formula or 8 in formula)\n if var1==False:\n return False\n if (1 in formula and 2 in formula):\n return False\n if (3 in formula and 4 in formula):\n return False\n if 5 in formula:\n if 6 in formula:\n var1=True\n else:\n return False\n if 6 in formula:\n if 5 in formula:\n var1=True\n else:\n return False\n return True", "def isValid(formula=[1, 3, 7]):\n fr = not (1 in formula and 2 in formula)\n sr = not (3 in formula and 4 in formula)\n tr = not((5 in formula and 6 not in formula) or (5 not in formula and 6 in formula))\n four = (7 in formula or 8 in formula)\n return fr and sr and tr and four", "def isValid(formula):\n if 3 in formula and 4 in formula: return False\n if 1 in formula and 2 in formula: return False\n if 7 not in formula and 8 not in formula : return False\n if 5 in formula and 6 not in formula : return False\n if 6 in formula and 5 not in formula : return False\n return True", "def isValid(formula):\n if (1 in formula) and (2 in formula): return False\n if (3 in formula) and (4 in formula): return False\n if (6 in formula) != (5 in formula): return False\n if (not 7 in formula) and (not 8 in formula): return False\n\n return True", "def isValid(formula):\n if 7 in formula or 8 in formula:\n if 1 in formula and 2 in formula:\n return False\n if 3 in formula and 4 in formula:\n return False\n if 5 in formula:\n if 6 not in formula:\n return False\n if 6 in formula:\n if 5 not in formula:\n return False\n return True\n else:\n return False", "def isValid(formula):\n if 1 in formula and 2 in formula:\n return False\n if 3 in formula and 4 in formula:\n return False\n if 5 in formula and 6 not in formula:\n return False\n if 6 in formula and 5 not in formula:\n return False \n if 8 not in formula and 7 not in formula:\n return False\n return True", "def isValid(a):\n b = not ((1 in a) and (2 in a))\n c = not ((3 in a) and (4 in a))\n d = ((5 in a) == (6 in a))\n e = ((7 in a) or (8 in a))\n print([b,c,d,e])\n return all([b,c,d,e])\n", "def isValid(formula):\n if 7 in formula or 8 in formula:\n if not(1 in formula and 2 in formula):\n if not(3 in formula and 4 in formula):\n if (5 not in formula and not 6 in formula) or (5 in formula and 6 in formula):\n return True\n else:\n return False\n else:\n return False\n else:\n return False\n else:\n return False\n\n", "def isValid(formula):\n a, b = sum([1 for c in formula if c in [1, 2]]), sum([1 for c in formula if c in [3, 4]])\n c, d = sum([1 for c in formula if c in [5, 6]]), sum([1 for c in formula if c in [7, 8]])\n\n return True if (a in range(2) and b in range(2)) and ((c==0 or c==2) and (d in range(1, 3))) else False\n", "def isValid(f):\n return (7 in f or 8 in f) and (((1 in f) + (2 in f))!=2) and (((3 in f) + (4 in f))!=2) and (((5 in f) + (6 in f))!=1)", "def isValid(formula):\n return not any([1 in formula and 2 in formula,\n 3 in formula and 4 in formula,\n 5 in formula and not 6 in formula,\n 6 in formula and not 5 in formula]) and any([7 in formula, 8 in formula])\n", "def isValid(formula):\n if 3 in formula and 4 in formula:\n return False\n elif 1 in formula and 2 in formula:\n return False\n elif 5 in formula and 6 not in formula or 6 in formula and 5 not in formula:\n return False\n elif 7 not in formula and 8 not in formula:\n return False\n else:\n return True", "def isValid(formula):\n if 1 in formula:\n if 2 in formula:\n return False\n if 3 in formula:\n if 4 in formula:\n return False\n if 5 in formula:\n if 6 not in formula:\n return False\n if 6 in formula:\n if 5 not in formula:\n return False\n if (7 not in formula) and (8 not in formula):\n return False\n return True", "def isValid(f):\n return ((7 in f) or (8 in f)) and not(((5 in f) and (6 not in f)) or ((6 in f) and (5 not in f))) and not ((1 in f) and (2 in f)) and not ((3 in f) and (4 in f))", "def isValid(formula):\n r1 = not(1 in formula and 2 in formula)\n r2 = not(3 in formula and 4 in formula)\n r3 = (5 in formula) == (6 in formula)\n r4 = 7 in formula or 8 in formula\n return all([r1,r2,r3,r4])\n", "def isValid(formula):\n if (7 not in formula) and (8 not in formula):\n return False\n if (5 in formula and 6 not in formula) or 6 in formula and 5 not in formula:\n return False\n if (1 in formula and 2 in formula) or (3 in formula and 4 in formula):\n return False\n return True", "def isValid(formula: list) -> bool:\n if 7 in formula or 8 in formula or set([7, 8]).issubset(formula):\n if set([1, 2]).issubset(formula):\n return False\n if set([3, 4]).issubset(formula):\n return False\n if 5 in formula or 6 in formula:\n return set([5, 6]).issubset(formula)\n if 7 in formula or 8 in formula or set([7, 8]).issubset(formula):\n return True\n return False\n", "def isValid(formula):\n rule_1 = 1 not in formula or 2 not in formula\n rule_2 = 3 not in formula or 4 not in formula\n rule_3 = 5 in formula and 6 in formula or 5 not in formula and 6 not in formula\n rule_4 = 7 in formula or 8 in formula\n return rule_1 and rule_2 and rule_3 and rule_4", "def isValid(formula):\n if len(set(formula).intersection(set([1,2])))>1 or len(set(formula).intersection(set([3,4])))>1 or len(set(formula).intersection(set([5,6])))==1 or len(set(formula).intersection(set([7,8])))<1:\n return False\n return True\n", "def isValid(f):\n a, b, c, d, e, f, g, h = [v in f for v in range(1, 9)]\n return not(a and b) and not(c and d) and not (e ^ f) and (g or h)\n", "def isValid(f):\n if 7 not in f and 8 not in f: return False\n if 1 in f and 2 in f: return False\n if 3 in f and 4 in f: return False\n if 5 in f and 6 not in f: return False\n if 6 in f and 5 not in f: return False\n return True", "def isValid(formula):\n count_1 = formula.count(1)\n count_2 = formula.count(2)\n if count_1 == count_2 == 1:\n return False\n\n count_1 = formula.count(3)\n count_2 = formula.count(4)\n if count_1 == count_2 == 1:\n return False\n\n count_1 = formula.count(5)\n count_2 = formula.count(6)\n if count_1 != count_2:\n return False\n\n count_1 = formula.count(7)\n count_2 = formula.count(8)\n if count_1 == count_2 == 0:\n return False\n return True", "def isValid(formula):\n if 7 in formula or 8 in formula: \n if 1 in formula and 2 in formula:\n return False\n if 3 in formula and 4 in formula:\n return False\n if 5 in formula:\n if 6 in formula:\n return True\n else:\n return False\n if 6 in formula:\n if 5 in formula:\n return True\n else:\n return False\n return True \n return False", "def isValid(formula):\n if 1 in formula and 2 in formula: return False\n if 3 in formula and 4 in formula: return False\n if 5 in formula and 6 not in formula: return False\n if not 5 in formula and 6 in formula: return False\n if not 7 in formula and not 8 in formula: return False\n return True", "def isValid(formula):\n if ((1 in formula) and (2 in formula)) or ((3 in formula) and (4 in formula)) or\\\n 5 in formula and not(6 in formula) or not(5 in formula) and 6 in formula or \\\n not(7 in formula) and not(8 in formula):\n return False\n elif 7 in formula or 8 in formula or \\\n (7 in formula and 8 in formula) or \\\n 5 in formula and 6 in formula :\n return True\n else: \n return False\n", "def isValid(formula):\n # material7 or material8 must be selected(at least one, or both)\n if 7 in formula or 8 in formula:\n # material5 and material6 must be selected at the same time\n if (5 in formula and 6 in formula) or (not 5 in formula and not 6 in formula):\n # material3 and material4 cannot be selected at the same time\n if (3 in formula and not 4 in formula) or (4 in formula and not 3 in formula) or (not 3 in formula and not 4 in formula):\n # material1 and material2 cannot be selected at the same time\n if (1 in formula and not 2 in formula) or (2 in formula and not 1 in formula) or (not 1 in formula and not 2 in formula):\n return True\n else: return False\n else: return False\n else: return False\n else: return False", "def isValid(formula):\n if 7 in formula or 8 in formula:\n if 1 in formula and 2 in formula:\n return False\n elif 3 in formula and 4 in formula:\n return False\n elif 5 in formula:\n if 6 in formula:\n return True\n else:\n return False\n elif 6 in formula:\n if 5 in formula:\n return True\n else:\n return False\n else:\n return True\n else:\n return False", "def isValid(f):\n if 1 in f and 2 in f:\n return False\n elif 3 in f and 4 in f:\n return False\n elif (5 in f and not(6 in f)) or (6 in f and not(5 in f)):\n return False\n elif not(7 in f) and not(8 in f):\n return False\n return True", "def isValid(formula):\n flag = True\n if 1 in formula:\n if 2 in formula:\n flag = False\n if 3 in formula:\n if 4 in formula:\n flag = False\n if 5 in formula:\n if 6 not in formula:\n flag = False\n if 6 in formula:\n if 5 not in formula:\n flag = False\n if 7 not in formula and 8 not in formula:\n flag = False\n return flag", "def isValid(formula):\n print(formula)\n if 1 in formula and 2 in formula:\n return 0\n if 3 in formula and 4 in formula:\n return 0\n if 5 in formula and not 6 in formula:\n return 0\n if 6 in formula and not 5 in formula:\n return 0\n if 7 in formula or 8 in formula:\n return 1\n else:\n return 0", "def isValid(formula):\n \n if 1 in formula and 2 in formula:\n return False \n \n if 3 in formula and 4 in formula:\n return False \n \n if (5 not in formula or 6 not in formula) and (5 in formula or 6 in formula):\n return False\n \n if 7 not in formula and 8 not in formula:\n return False \n \n return True", "def isValid(formula):\n if 7 in formula or 8 in formula:\n if 1 in formula and 2 in formula or 3 in formula and 4 in formula:\n return False\n if 5 in formula and 6 not in formula or 6 in formula and 5 not in formula:\n return False\n return True\n return False", "def isValid(formula):\n \"\"\"\n Returns true if the formula is valid, false otherwise.\n \n Rules:\n \u2022 material1 and material2 cannot be selected at the same time\n \u2022 material3 and material4 cannot be selected at the same time\n \u2022 material5 and material6 must be selected at the same time\n \u2022 material7 or material8 must be selected(at least one, or both)\n \"\"\"\n if 1 in formula and 2 in formula:\n return False\n \n if 3 in formula and 4 in formula:\n return False\n \n if 5 in formula or 6 in formula:\n if 5 not in formula or 6 not in formula:\n return False\n \n if 7 not in formula and 8 not in formula:\n return False\n \n return True", "def isValid(formula):\n if 7 in formula or 8 in formula:\n if 1 in formula and 2 in formula:\n return False\n if 3 in formula and 4 in formula:\n return False\n if (5 in formula and not 6 in formula) or (6 in formula and not 5 in formula):\n return False\n return True\n else:\n return False\n", "def isValid(formula):\n return False if (1 in formula and 2 in formula) or (3 in formula and 4 in formula) or (5 not in formula and 6 in formula) or (5 in formula and 6 not in formula) or not (7 in formula or 8 in formula) else True", "def isValid(formula):\n rules = list()\n rules.append(1) if 7 in formula or 8 in formula else rules.append(0)\n if 5 in formula or 6 in formula:\n rules.append(2) if 5 in formula and 6 in formula else rules.append(0)\n if (3 in formula) or (4 in formula):\n rules.append(0) if 3 in formula and 4 in formula else rules.append(3)\n if (1 in formula) or (2 in formula):\n rules.append(0) if 1 in formula and 2 in formula else rules.append(4)\n return True if min(rules) != 0 else False", "def isValid(f):\n if 5 in f or 6 in f:\n if not (5 in f and 6 in f):\n return False\n \n if not (7 in f or 8 in f):\n return False\n\n if 1 in f or 2 in f:\n if 1 in f and 2 in f:\n return False\n\n if 3 in f or 4 in f:\n if 3 in f and 4 in f:\n return False\n\n return True\nprint(isValid([1,3,7]))", "def isValid(f):\n if len(f)==1 and f[0]!=7 and f[0]!=8:\n return False\n elif 1 in f and 2 in f:\n return False\n elif 3 in f and 4 in f:\n return False\n elif 5 not in f and 6 in f :\n return False\n elif 5 in f and 6 not in f:\n return False\n elif 7 in f or 8 in f:\n return True\n else:\n return False", "def isValid(formula):\n if 7 not in formula and 8 not in formula:\n return False\n if 1 in formula and 2 in formula:\n return False\n if 3 in formula and 4 in formula:\n return False\n if 5 in formula and 6 not in formula:\n return False\n if 6 in formula and 5 not in formula:\n return False\n if 7 in formula or 8 in formula:\n return True#", "def isValid(formula):\n if 1 in formula and 2 in formula:\n return False\n elif 3 in formula and 4 in formula:\n return False\n elif 5 in formula and 6 not in formula:\n return False\n elif 6 in formula and 5 not in formula:\n return False\n if 7 in formula or 8 in formula:\n return True\n else:\n return False\n \n\n \n\n", "def isValid(formula):\n if len(formula) == 0:\n return False\n elif len(formula) == 1:\n if 7 in formula or 8 in formula:\n return True\n else:\n return False\n elif len(formula) == 2:\n if 7 in formula and 8 in formula:\n return True\n elif 7 in formula or 8 in formula:\n if 5 in formula or 6 in formula:\n return False\n else:\n return True\n else:\n return False\n elif len(formula) == 3:\n if 7 in formula and 8 in formula:\n if 5 in formula or 6 in formula:\n return False\n else:\n return True\n elif 7 in formula or 8 in formula:\n if 5 in formula and 6 in formula:\n return True\n elif (3 in formula and 4 not in formula) or (4 in formula and 3 not in formula):\n if (1 in formula and 2 not in formula) or (2 in formula and 1 not in formula):\n return True\n else:\n return False\n else:\n return False\n else:\n return False\n elif len(formula) == 4:\n if 7 in formula and 8 in formula:\n if 5 in formula and 6 in formula:\n return True\n elif (3 in formula and 4 not in formula) or (4 in formula and 3 not in formula):\n if (1 in formula and 2 not in formula) or (2 in formula and 1 not in formula):\n return True\n else:\n return False\n else:\n return False\n elif 7 in formula or 8 in formula:\n if 5 in formula and 6 in formula:\n return True\n else:\n return False\n else:\n return False\n elif len(formula) == 5:\n if 7 in formula and 8 in formula:\n if 5 in formula and 6 in formula:\n return True\n else:\n return False\n elif 7 in formula or 8 in formula:\n if 5 in formula and 6 in formula:\n if (3 in formula and 4 not in formula) or (4 in formula and 3 not in formula):\n if (1 in formula and 2 not in formula) or (2 in formula and 1 not in formula):\n return True\n else:\n return False\n else:\n return False\n else:\n return False\n else:\n return False\n elif len(formula) == 6:\n if 7 in formula and 8 in formula:\n if 5 in formula and 6 in formula:\n if (3 in formula and 4 not in formula) or (4 in formula and 3 not in formula):\n if (1 in formula and 2 not in formula) or (2 in formula and 1 not in formula):\n return True\n else:\n return False\n else:\n return False\n else:\n return False\n else:\n return False\n elif len(formula) > 6:\n return False", "def isValid(formula):\n if 1 in formula and 2 in formula:\n return False\n elif 3 in formula and 4 in formula:\n return False\n if 5 in formula and 6 not in formula:\n return False\n elif 6 in formula and 5 not in formula:\n return False\n elif 7 not in formula and 8 not in formula:\n return False\n else:\n return True", "def isValid(formula):\n if 7 not in formula and 8 not in formula:\n return False\n if 5 in formula and 6 not in formula or 6 in formula and 5 not in formula:\n return False\n if 3 in formula and 4 in formula:\n return False\n if 1 in formula and 2 in formula:\n return False\n return True", "def isValid(formula):\n check1 = (1 in formula and 2 in formula)#should be false\n check2 = (3 in formula and 4 in formula) #should be false\n check3 = (5 in formula and 6 not in formula) #should be false\n check4 = (6 in formula and 5 not in formula) # should be false\n check5 = (7 in formula or 8 in formula) #should be true\n #check_list=[check1, check2, check]\n if check1==True or check2==True or check3==True or check4==True or check5==False :\n return False\n else:\n return True", "def isValid(formula):\n if (1 in formula and 2 in formula):\n return False\n if (3 in formula and 4 in formula):\n return False\n if (not (7 in formula or 8 in formula)):\n return False\n if ((5 in formula and not (6 in formula)) or (not(5 in formula) and 6 in formula)):\n return False\n return True", "def isValid(formula):\n d = {1:1, 2:1, 3:2, 4:2, 5:3, 6:3, 7:4, 8:4}\n r = [d[i] for i in formula]\n l = {}\n for x in r:\n \n if (x == 4 and r.count(4) == 1) or (x == 4 and r.count(4) == 2):\n l[4] = True\n if x == 1 and r.count(1) == 1:\n l[1] = True\n \n if x == 2 and r.count(2) == 1:\n l[2] = True\n \n if x == 3 and r.count(3) == 2:\n l[3] = True\n \n \n if r.count(4) == 0:\n l[4] = False \n \n if x == 1 and r.count(1) != 1:\n l[1] = False\n \n if x == 2 and r.count(2) != 1:\n l[2] = False\n \n if x == 3 and r.count(3) != 2:\n l[3] = False\n \n \n \n \n \n \n \n result = [k for k in list(l.values())]\n return (sum(result) == len(result))\n \n \n \n \n \n \n \n", "def isValid(formula):\n \n print(formula)\n \n if 1 in formula and 2 in formula:\n return False\n \n if 3 in formula and 4 in formula:\n return False\n\n if 5 in formula and 6 not in formula:\n return False\n \n if 6 in formula and 5 not in formula:\n return False\n \n if 7 not in formula and 8 not in formula:\n return False\n else:\n return True", "def isValid(f):\n return ((1 not in f) or (2 not in f)) and \\\n ((3 not in f) or (4 not in f)) and \\\n ((5 in f) == (6 in f)) and \\\n ((7 in f) or (8 in f))", "def isValid(formula):\n exclusive = [[1, 2], [3, 4]]\n implies = {5: 6, 6: 5}\n required = {7, 8}\n \n for m1, m2 in exclusive:\n if m1 in formula and m2 in formula:\n return False\n \n for m in formula:\n if m in implies and not implies[m] in formula:\n return False\n \n if not any(m in formula for m in required):\n return False\n \n return True", "def isValid(f):\n y = ''.join(map(str,sorted(f)))\n return '12' not in y and '34' not in y and ('56' in y or '5' not in y and '6' not in y) and ('7' in y or '8' in y)", "def isValid(formula):\n \n \n if 1 in formula and 2 in formula:\n \n print(\"1\")\n return False\n \n elif 3 in formula and 4 in formula: \n print(\"2\")\n return False\n \n elif 5 in formula and 6 not in formula:\n print(\"3\")\n return False\n \n elif 6 in formula and 5 not in formula:\n print(\"4\")\n return False\n \n elif not ( 7 in formula or 8 in formula):\n print(\"5\")\n return False\n \n return True", "def isValid(formula):\n if (1 in formula) and (2 in formula):\n return False\n if (3 in formula) and (4 in formula):\n return False\n if (7 not in formula) and (8 not in formula):\n return False\n if (5 in formula or 6 in formula) and not(5 in formula and 6 in formula):\n return False\n return True\n \n", "def isValid(formula):\n# print((1 in formula) != (2 in formula))\n return not ((1 in formula) and (2 in formula)) \\\n and not ((3 in formula) and (4 in formula)) \\\n and (5 in formula) == (6 in formula) \\\n and ((7 in formula) or (8 in formula))", "def isValid(formula):\n \n return ((7 in formula or 8 in formula)and not(1 in formula and 2 in formula) and not(3 in formula and 4 in formula) and ((5 in formula and 6 in formula)or (5 not in formula and 6 not in formula)))"] | {"fn_name": "isValid", "inputs": [[[1, 3, 7]], [[7, 1, 2, 3]], [[1, 3, 5, 7]], [[1, 5, 6, 7, 3]], [[5, 6, 7]], [[5, 6, 7, 8]], [[6, 7, 8]], [[7, 8]]], "outputs": [[true], [false], [false], [true], [true], [true], [false], [true]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 40,757 |
def isValid(formula):
|
9682b4b6cbe6fc33ab93d844a391c6fc | UNKNOWN | Define a function that takes one integer argument and returns logical value `true` or `false` depending on if the integer is a prime.
Per Wikipedia, a prime number (or a prime) is a natural number greater than 1 that has no positive divisors other than 1 and itself.
## Requirements
* You can assume you will be given an integer input.
* You can not assume that the integer will be only positive. You may be given negative numbers as well (or `0`).
* **NOTE on performance**: There are no fancy optimizations required, but still *the* most trivial solutions might time out. Numbers go up to 2^31 (or similar, depends on language version). Looping all the way up to `n`, or `n/2`, will be too slow.
## Example
```nasm
mov edi, 1
call is_prime ; EAX <- 0 (false)
mov edi, 2
call is_prime ; EAX <- 1 (true)
mov edi, -1
call is_prime ; EAX <- 0 (false)
``` | ["# This is the Miller-Rabin test for primes, which works for super large n\n\nimport random\n\ndef even_odd(n):\n s, d = 0, n\n while d % 2 == 0:\n s += 1\n d >>= 1\n return s, d\n\ndef Miller_Rabin(a, p):\n s, d = even_odd(p-1)\n a = pow(a, d, p)\n if a == 1: return True\n for i in range(s):\n if a == p-1: return True\n a = pow(a, 2, p)\n return False\n\ndef is_prime(p):\n if p == 2: return True\n if p <= 1 or p % 2 == 0: return False\n return all(Miller_Rabin(random.randint(2,p-1),p) for _ in range(40))\n", "from math import sqrt\ndef is_prime(num):\n if num <= 1:\n return False\n i = 2\n while i <= sqrt(num): \n if num%i == 0:\n return False\n i += 1\n return True ", "import math\ndef is_prime(n):\n if n < 2: return False\n if(n in [2,3]): return True\n if(n%6 not in [1,5]): return False\n for i in range(3, int(math.floor(math.sqrt(n)))+1):\n if n%i==0: return False\n return True", "def is_prime(n):\n if (n < 2) or (n > 2 and n%2 == 0):\n return False\n for i in range(3, int(n**.5)+1, 2):\n if n%i == 0:\n return False\n else:\n return True", "def is_prime(num):\n\n # make sure n is a positive integer\n num = abs(int(num))\n\n # 0 and 1 are not primes\n if num < 2:\n return False\n\n # 2 is the only even prime number\n if num == 2: \n return True \n\n # all other even numbers are not primes\n if not num & 1: \n return False\n\n # range starts with 3 and only needs to go up \n # the square root of n for all odd numbers\n for x in range(3, int(num**0.5) + 1, 2):\n if num % x == 0:\n return False\n\n return True", "import math\ndef is_prime(n):\n if n <= 1: \n return False \n for i in range(2, int(math.sqrt(n)) + 1): \n if n % i == 0: \n return False \n return True"] | {"fn_name": "is_prime", "inputs": [[0], [1], [2], [73], [75], [-1]], "outputs": [[false], [false], [true], [true], [false], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,958 |
def is_prime(num):
|
91e1ebcfb50bd1699b944af4cabb10a7 | UNKNOWN | Determine the total number of digits in the integer (`n>=0`) given as input to the function. For example, 9 is a single digit, 66 has 2 digits and 128685 has 6 digits. Be careful to avoid overflows/underflows.
All inputs will be valid. | ["def digits(n):\n return len(str(n))", "from math import log10,ceil\n\ndef digits(n):\n return ceil(log10(n+1))", "from math import log10\n\ndef digits(n):\n return int(log10(n)+1)", "def digits(n):\n return len('%s'%n)", "from typing import Union\nfrom re import findall\n\ndef digits(number: Union[int, str]) -> int:\n \"\"\" Get number of digits in given number. \"\"\"\n return len(findall(\"\\d\", str(number)))", "def digits(n):\n if n >= 0:\n dl = len(str(n))\n return dl\n", "def digits(n):\n # your code here\n s = str(n)\n s_1 = list(s)\n s_1 = len(s_1)\n return s_1", "def digits(i):\n return len(str(i))", "def digits(n):\n s = str(n)\n return len(s)\n", "def digits(n):\n c = 0\n while n != 0:\n c += 1\n n = n // 10\n return c", "def digits(n):\n if(n == 0):\n return 1\n else:\n count = 0\n while(n):\n count += 1\n n //= 10\n return count", "def digits(n):\n return sum([1 for i in str(n)])", "def digits(n):\n count = 0\n while n != 0:\n count += 1\n n = n // 10\n return count", "def digits(n):\n n = str(n)\n digits = 0\n for i, num in enumerate(n):\n digits += 1\n return digits", "def digits(n):\n a=0\n for i in str(n):\n a+=1\n return a", "import math\ndef digits(n):\n return math.log10(n)//1+1", "def digits(n):\n # your code here\n count=len(str(n))\n return count", "def digits(n):\n n = str(n)\n n = list(n)\n return len(n)", "def digits(n):\n count = 0\n k = str(n)\n k = list(k)\n for num in k:\n count += 1\n return count ", "def digits(n):\n j=list(str(n))\n return len(j)", "def digits(n):\n answer = 0\n while n != 0:\n n = n // 10\n answer += 1\n return answer", "def digits(n):\n# return len(str(n))\n cont = 0\n while n > 9:\n n //= 10\n cont+=1\n return cont+1", "def digits(n):\n r=str(n)\n y = list(r)\n x=len(y)\n return(x)\n pass", "def digits(n):\n sum = 0\n for num in str(n):\n sum += 1\n return sum", "def digits(n):\n digits = 0\n for i in str(n):\n digits += 1\n return digits ", "def digits(n):\n total = 1\n while n // 10 >= 1:\n n //= 10\n print(n)\n total += 1\n return total", "def digits(n):\n y = str(n)\n return len(y)", "def digits(n):\n count = 0\n while n != 0:\n n //= 10\n count += 1\n return count\n", "from math import ceil, log\n\ndef digits(n):\n return ceil(log(n + 1, 10)) if 0 < n else 1\n", "def digits(n):\n x=str(n)\n n=len(x)\n return int(n)", "def digits(n):\n sn=str(n)\n cnt=0\n for i in sn:\n cnt+=1\n return cnt", "from math import log10, floor\n\n\ndef digits(n: int) -> int:\n return floor(log10(n) + 1) if n else 0\n", "def digits(n):\n tot = 0\n while n > 0:\n tot += 1\n n //= 10\n return tot", "import math \n\ndef digits(n):\n return math.floor(math.log(n)/math.log(10)) + 1\n pass", "def digits(n):\n return len(str(n))\n # Flez\n", "def digits(n):\n k=0\n while n>0:\n n=int(n/10)\n k+=1\n return k\n \n \n", "def digits(n):\n print(type(n))\n if isinstance(n, int):\n return len(str(n))\n \n return 0", "def digits(n):\n len_1=0\n while(n!=0):\n n//=10\n len_1+=1\n return len_1\n", "def digits(n):\n print(n)\n z=[int(x) for x in str(n)]\n # your code here\n print(z)\n return len(z)\n", "def digits(n):\n sum = 0\n for i in str(n):\n sum += 1\n return sum\n# Subscribe to ScratchRunning\n", "def digits(n):\n c=0\n while n>0:\n n=n//10\n c=c+1\n return c", "def digits(n):\n # your code here\n digits=str(n)\n \n return len(digits)\n", "def digits(n):\n c = 1\n n = n//10\n while n != 0:\n c += 1\n n = n//10\n return c\n", "def digits(n):\n str(n)\n return len(str(n))\n \n", "def digits(n):\n \n n = str(n)\n num = n.count('')-1\n \n return num\n", "def digits(n):\n s = str(n)\n return len([c for c in s])", "def digits(n):\n n_str = str(n)\n n_list = n_str.split('.')\n count = len(n_list[0])\n return count", "def digits(n):\n # your code here\n i = 0\n while n > 0:\n i+=1\n n = n // 10 \n return i", "def digits(n):\n # your code here\n total = 0\n n = str(n)\n for i in n:\n total += 1\n return total", "def digits(num):\n count = 0\n \n if num < 10:\n return 1\n else:\n num >= 10\n num_digits = len(str((num)))\n return num_digits\n\nnum = 11132424212\nprint(digits(num))", "def digits(num):\n n_digits = 0\n while num > 0:\n num //= 10\n n_digits += 1\n return n_digits", "def digits(n):\n count = 0\n while n>0:\n count += 1\n n = n//10\n return count", "def digits(n):\n nums = []\n n = str(n)\n for num in n:\n nums.append(n)\n return len(nums)\n pass", "def digits(n):\n # your code here\n numar2 = str(n)\n counter = 0\n for i in numar2:\n print(i)\n counter = counter +1\n return counter\n", "def digits(n):\n return range(len(str((abs(n)))))[-1]+1", "def digits(n):\n x = len(str(n))\n return int(x)", "def digits(n):\n digits = []\n if n>= 0:\n [digits.append(d) for d in str(n)]\n return len(digits)\n pass", "def digits(n):\n # your code here\n result = 0\n \n n2 = str(n)\n \n for i in n2:\n result += 1\n \n return result\n \n \n pass", "def digits(n):\n # your code here\n if ( n > 0 ):\n return len(str(n))", "def digits(n):\n result = len(str(n))\n return result", "def digits(n):\n n = str(n)\n count = 0\n for i in n: \n count += 1\n return count\n", "def digits(n):\n res = len(str(n))\n return(res)", "def digits(n):\n if n==0:\n return 1\n ans = 0\n while n!=0:\n n = n//10\n ans += 1\n return ans", "def digits(n):\n return len(ascii(n))", "def digits(n):\n str_n = str(n)\n return len(str_n)", "def digits(n):\n m=list(str(n))\n res=len(m)\n return res", "def digits(n):\n return (len(str(int(n))))", "def digits(n):\n \n \n d = [int(x) for x in str(n)]\n \n l = len(d)\n \n return l\n \n", "def digits(n):\n return sum(1 for i in str(n))", "def digits(n):\n n = str(n)\n n = list(n)\n size = len(n)\n return size\n pass", "def digits(n):\n return 1 if n < 10 else 1 + digits(n//10)\n pass", "def digits(n):\n\n total = 0\n \n for i in str(n):\n total += 1\n return total", "def digits(n):\n # your code here\n q = str(n)\n return len(q)\n", "def digits(n):\n d = 0\n while n >= 1:\n n = n // 10\n d += 1\n \n return d", "def digits(n):\n conv = str(n)\n counter = 0\n for i in conv:\n counter += 1\n return counter", "def digits(n):\n k=1\n while n//10: \n k+=1\n n//=10\n return k;", "def digits(n):\n if n >= 0:\n n = str(n)\n return len(n)", "def digits(n):\n return len(f'{n}')", "def digits(n):\n digits_amount = len(str(n))\n return(digits_amount)", "def digits(n):\n num=0\n while n>0:\n n=int(n/10)\n num=num+1\n return num", "def digits(n):\n number = str(n)\n return len(number)", "def digits(n):\n # your code here\n x = str(n)\n y = len(x)\n return y", "def digits(n):\n an_integer = n\n a_string = str(an_integer)\n length = len(a_string)\n return length\n\n# your code here\n", "def digits(n):\n return len(str(n)) ## returning no of digits\n ## by first converting it to a string \n", "def digits(n):\n num_str = str(n)\n var_save = len(num_str)\n return var_save\n pass", "import math\ndef digits(n):\n d = 1\n while n >= 10:\n d += 1\n n = math.floor(n/10)\n return d", "def digits(n):\n return len(' '.join(str(n)).split())", "def digits(n):\n digit = n\n answer = 1\n while digit >= 10:\n digit = digit /10\n answer += 1\n return answer", "def digits(n):\n number = str(n)\n i = 0\n for letter in number:\n i += 1\n return i", "def digits(n):\n sum = 0\n while n > 0:\n return len(str(n))", "def digits(n):\n for i in str(n).split():\n return len(i)", "def digits(n):\n list = [int(x) for x in str(n)]\n return len(list)", "def digits(n):\n a = str(n)\n b = list(a)\n for element in b:\n if element == ',' or element == '.':\n b.remove(element)\n else: \n pass\n return len(b)\n\n", "def digits(n):\n num = 0\n awnser = 0\n num = str(n)\n awnser = len(num)\n return awnser\n", "def digits(n):\n\n string = str(n)\n i = 0\n for s in string:\n i +=1\n return i\n", "def digits(n):\n # your code here\n count = 1\n while(n>=10):\n q = n/10\n count += 1\n n = q\n \n return count", "def digits(n):\n total = str(n)\n return len(total)", "def digits(n):\n num_digits = 0\n while n > 0:\n n = n // 10\n num_digits += 1\n return num_digits", "def digits(n):\n numStr = str(n)\n return len(numStr)"] | {"fn_name": "digits", "inputs": [[5], [12345], [9876543210]], "outputs": [[1], [5], [10]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 9,517 |
def digits(n):
|
5b1b4b59fbb56e3fb4477e51137e623f | UNKNOWN | # Task
Given some points(array `A`) on the same line, determine the minimum number of line segments with length `L` needed to cover all of the given points. A point is covered if it is located inside some segment or on its bounds.
# Example
For `A = [1, 3, 4, 5, 8]` and `L = 3`, the output should be `2`.
Check out the image below for better understanding:
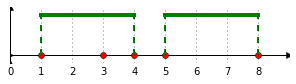
For `A = [1, 5, 2, 4, 3]` and `L = 1`, the output should be `3`.
segment1: `1-2`(covered points 1,2),
segment2: `3-4`(covered points 3,4),
segment3: `5-6`(covered point 5)
For `A = [1, 10, 100, 1000]` and `L = 1`, the output should be `4`.
segment1: `1-2`(covered point 1),
segment2: `10-11`(covered point 10),
segment3: `100-101`(covered point 100),
segment4: `1000-1001`(covered point 1000)
# Input/Output
- `[input]` integer array A
Array of point coordinates on the line (all points are different).
Constraints:
`1 ≤ A.length ≤ 50,`
`-5000 ≤ A[i] ≤ 5000.`
- `[input]` integer `L`
Segment length, a positive integer.
Constraints: `1 ≤ L ≤ 100.`
- `[output]` an integer
The minimum number of line segments that can cover all of the given points. | ["def segment_cover(A, L):\n n=1\n s=min(A)\n for i in sorted(A):\n if s+L<i:\n s=i\n n+=1\n return n", "def segment_cover(a, l):\n a, n = set(a), 0\n while a:\n a -= set(range(min(a), min(a) + l + 1))\n n += 1\n return n\n", "def segment_cover(A, L):\n A = sorted(A)\n nb_segments = 0\n while A:\n nb_segments += 1\n first_elt = A.pop(0)\n while A and A[0] <= first_elt + L:\n A.pop(0) \n return nb_segments\n \n", "def segment_cover(A, L):\n A = sorted(A)\n segment_end = A[0] - 1\n segments = 0\n for point in A:\n if point > segment_end:\n segments += 1\n segment_end = point + L\n return segments\n", "def segment_cover(A, L):\n end = None\n count = 0\n for a in sorted(A):\n if end and a <= end:\n continue\n else:\n end = a + L\n count += 1\n return count", "def segment_cover(points, length):\n n, e = 0, float('-inf')\n for p in sorted(points):\n if e < p:\n e = p + length\n n += 1\n return n", "def segment_cover(A, l):\n A = set(A)\n m = min(A)\n c = 0\n while A: \n A = {a for a in A if a>m+l}\n c+=1\n if A: m = min(A)\n else: return c\n", "def segment_cover(a,l):\n s=0\n while a:\n L=min(a)+l\n a=[v for v in a if v>L]\n s+=1\n return s", "def segment_cover(A, L):\n c = 0\n while A:\n c+=1\n seg = min(A, default=0)\n A = list([item for item in A if item > seg+L])\n return c\n", "def segment_cover(a, n):\n a.sort()\n r, c = 1, a[0]\n for x in a:\n if x - c > n:\n r += 1\n c = x\n return r"] | {"fn_name": "segment_cover", "inputs": [[[1, 3, 4, 5, 8], 3], [[-7, -2, 0, -1, -6, 7, 3, 4], 4], [[1, 5, 2, 4, 3], 1], [[1, 10, 100, 1000], 1]], "outputs": [[2], [3], [3], [4]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,789 |
def segment_cover(A, L):
|
527b3a00789852094c15bbaaa8f41a7e | UNKNOWN | A checksum is an algorithm that scans a packet of data and returns a single number. The idea is that if the packet is changed, the checksum will also change, so checksums are often used for detecting
transmission errors, validating document contents, and in many other situations where it is necessary to detect undesirable changes in data.
For this problem, you will implement a checksum algorithm called Quicksum. A Quicksum packet allows only uppercase letters and spaces. It always begins and ends with an uppercase letter.
Otherwise, spaces and uppercase letters can occur in any combination, including consecutive spaces.
A Quicksum is the sum of the products of each character’s position in the packet times the character’s value. A space has a value of zero, while letters have a value equal to their position in the alphabet.
So, ```A = 1```, ```B = 2```, etc., through ```Z = 26```. Here are example Quicksum calculations for the packets “ACM” and “A C M”:
ACM
1 × 1 + 2 × 3 + 3 × 13 = 46
A C M
1 x 1 + 3 x 3 + 5 * 13 = 75
When the packet doesn't have only uppercase letters and spaces or just spaces the result to quicksum have to be zero (0).
AbqTH #5 = 0 | ["from string import ascii_uppercase\n\nvalues = {x: i for i, x in enumerate(ascii_uppercase, 1)}\n\ndef quicksum(packet):\n return sum(values.get(c, 0) * i for i, c in enumerate(packet, 1)) * all(c.isspace() or c.isupper() for c in packet)", "def quicksum(packet):\n result = 0\n \n for idx, char in enumerate(packet, 1):\n if char.isupper():\n result += idx * (ord(char) - 64)\n elif char == \" \":\n continue\n else:\n return 0\n \n return result", "import re\n\ndef quicksum(packet):\n isValid = re.match(r'[A-Z ]*$',packet)\n value = sum(i*(ord(c)-64) for i,c in enumerate(packet,1) if c!=' ')\n return isValid and value or 0", "def quicksum(packet):\n if not packet.replace(\" \", \"\").isalpha() or not packet.isupper(): return 0\n return sum((i+1)*(1 + ord(l) - ord(\"A\")) if l.isalpha() else 0 for i, l in enumerate(packet))", "def quicksum(packet):\n if all(True if ord(x) > 64 and ord(x) < 91 or ord(x) == 32 else False for x in packet):\n return sum(0 if x == ' ' else (ord(x) - 64) * y for y, x in enumerate(packet,1))\n return 0", "from string import ascii_uppercase\n\nAZ = dict(list(zip(' ' + ascii_uppercase, list(range(27)))))\n\n\ndef quicksum(packet):\n result = 0\n for i, a in enumerate(packet, 1):\n try:\n result += AZ[a] * i\n except KeyError:\n return 0\n return result\n", "def quicksum(packet):\n alph = \" ABCDEFGHIJKLMNOPQRSTUVWXYZ\"\n su = 0\n \n for k, v in enumerate(packet):\n if v not in alph:\n return 0\n su += (k+1) * alph.index(v)\n return su", "quicksum = lambda p:sum(i*(ord(e)-64)*(e!=' ') for i,e in enumerate(p,1))*all(x in __import__(\"string\").ascii_uppercase+' ' for x in p)", "VALID = \" ABCDEFGHIJKLMNOPQRSTUVWXYZ\"\n\ndef quicksum(packet):\n return 0 if (set(packet) - set(VALID)) else sum(VALID.index(char) * i for i, char in enumerate(packet, 1))\n \n# checksum = 0\n# for char in packet:\n# if not char in VALID:\n# return 0\n# checksum += VALID.index(char)\n# return checksum\n \n # in respect to given instructions (packet has to begin and end with uppercase)\n# if any(not char.isupper() for char in (packet[0], packet[-1])):\n# return 0\n", "from string import ascii_uppercase as auc\ndef quicksum(packet):\n d = {v : k for k, v in enumerate(' ' + auc)}\n try:\n return sum(c * d[s] for c, s in enumerate(packet, 1))\n except:\n return 0"] | {"fn_name": "quicksum", "inputs": [["ACM"], ["MID CENTRAL"], ["BBC"], ["???"], ["axg "], ["234 234 WEF ASDF AAA 554211 ???? "], ["A C M"], ["ABCDEFGHIJKLMNOPQRSTUVWXYZ"], ["A B C D E F G H I J K L M N O P Q R S T U V W X Y Z"], ["ZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZZ"], ["Z A"], ["12312 123 123 asd asd 123 $$$$/()="], ["As "], [" "]], "outputs": [[46], [650], [15], [0], [0], [0], [75], [6201], [12051], [848640], [33], [0], [0], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,568 |
def quicksum(packet):
|
c4706577bc4f7acf5adfc3490981d1e6 | UNKNOWN | Given an array of integers your solution should find the smallest integer.
For example:
- Given `[34, 15, 88, 2]` your solution will return `2`
- Given `[34, -345, -1, 100]` your solution will return `-345`
You can assume, for the purpose of this kata, that the supplied array will not be empty. | ["def find_smallest_int(arr):\n return min(arr);", "def find_smallest_int(arr):\n return sorted(arr)[0]", "find_smallest_int = min ", "def find_smallest_int(arr):\n # Code here\n smallest=min(arr)\n for i in arr:\n if i<min(arr):\n smallest=i\n return smallest", "def find_smallest_int(arr):\n a = 10000\n for i in arr:\n if a > i:\n a = i\n return a", "import sys\n\ndef find_smallest_int(arr):\n my_int = sys.maxsize\n for integer in arr:\n if integer < my_int:\n my_int = integer\n return my_int", "import numpy\n\ndef find_smallest_int(arr):\n return numpy.min(arr)", "def find_smallest_int(arr):\n currentSmallest = arr[0]\n for x in range (len(arr)):\n if currentSmallest > arr[x]:\n currentSmallest = arr[x]\n return currentSmallest\n", "def find_smallest_int(arr):\n min_number = arr[0]\n \n for element in arr:\n if element < min_number:\n min_number = element\n \n return min_number", "def find_smallest_int(arr):\n a = arr.sort()\n return arr[0]", "# option 1\ndef find_smallest_int(arr):\n arr.sort()\n return arr[0] \n\n# option 2\n return min(arr)\n\n# option 3\ndef find_smallest_int(arr):\n smallest = arr[0]\n for item in arr:\n if smallest > item:\n smallest = item\n return smallest\n\n", "def find_smallest_int(arr):\n # Code here\n answer = arr[0]\n \n for nr in arr:\n if nr < answer:\n answer = nr\n \n \n return answer", "def find_smallest_int(arr):\n return min(arr)\n \n \n \n \nsmall = find_smallest_int([78, 56, 232, 12, 11, 43])\n\nprint(small)", "from functools import reduce\n\ndef find_smallest_int(arr):\n return reduce(lambda a,b:min(a,b), arr)", "from functools import reduce\ndef find_smallest_int(arr):\n return int(reduce((lambda x, y: y if y < x else x), arr))", "import sys\n\ndef find_smallest_int(arr):\n smallest = sys.maxsize\n \n for i in range(len(arr)):\n if arr[i] < smallest:\n smallest = arr[i]\n \n return smallest", "def find_smallest_int(a: list) -> int: return min(a)", "def find_smallest_int(arr):\n # Code here\n min = arr[0]\n for i in range(len(arr)):\n if i+1 < len(arr):\n if arr[i+1] < min:\n min = arr[i+1]\n \n return min", "def find_smallest_int(arr):\n sortedlist = sorted(arr)\n result = sortedlist.__getitem__(0)\n return(result)\n#0oh\n", "def find_smallest_int(arr):\n # Code here\n ans = arr[0]\n for x in arr:\n if x < ans:\n ans = x\n return ans", "def find_smallest_int(arr):\n lowest = 10000\n for number in arr:\n if number < lowest:\n lowest = number\n return lowest", "def find_smallest_int(arr):\n # Code heredef find_smallest_int(arr):\n arr.sort()\n return arr[0]", "def find_smallest_int(arr):\n smallestInt = arr[0]\n for var in arr:\n if var < smallestInt:\n smallestInt = var\n return smallestInt", "def find_smallest_int(arr):\n small=arr[0]\n for n in arr:\n if n<small:\n small=n\n return small", "def find_smallest_int(arr):\n \n arr.sort()\n smol = arr[0:1:]\n for i in smol:\n return i\n\n \n", "def find_smallest_int(arr):\n l = len(arr)\n min = arr[0]\n for i in range(1, l):\n if min > arr[i]:\n min = arr[i]\n return min", "def find_smallest_int(arr):\n smol = arr[0]\n for i in arr:\n if smol > i:\n smol = i\n return smol", "def find_smallest_int(arr):\n print(arr)\n smallest = arr[0]\n for x in arr:\n if x < smallest:\n smallest = x \n return smallest\n", "def find_smallest_int(arr):\n num = arr[0]\n for i in arr:\n if i < num:\n num = i\n else:\n num = num\n return num\n", "def find_smallest_int(arr):\n # Code here\n smallest = 1000000\n for i in range(0, len(arr), 1):\n if arr[i] < smallest:\n smallest = arr[i]\n arr.append(smallest)\n return arr[-1]", "def find_smallest_int(arr):\n anwser = 9999999\n for a in arr:\n if a < anwser:\n anwser = a\n return anwser", "def find_smallest_int(arr):\n # Code here\n #initialise first integer as min\n min = arr[0]\n for x in range(1, len(arr)):\n if arr[x] < min:\n min = arr[x]\n return min", "def find_smallest_int(arr):\n number = arr[0]\n for nb in arr:\n if nb < number:\n number = nb\n return number", "import numpy as np\n\ndef find_smallest_int(arr = []):\n return np.min(arr)", "import math\ndef find_smallest_int(arr):\n x = math.inf\n for i in arr:\n if i < x:\n x = i\n return x", "import numpy as np\n\ndef find_smallest_int(arr):\n test = np.sort(arr)\n answer = int(test[0])\n return answer\n", "def find_smallest_int(arr):\n\n a = 0\n b = 0\n \n while b < len(arr):\n if arr[a] <= arr[b]:\n b+=1\n elif arr[a] > arr[b]:\n a+=1\n \n return arr[a]", "def find_smallest_int(arr):\n arr.sort()\n smallest = arr.pop(0)\n return smallest\n\n", "import math\ndef find_smallest_int(arr):\n # Code here\n min=arr[0]\n for i in range(0,len(arr)):\n if(arr[i]<min):\n min=arr[i]\n return min\n", "def find_smallest_int(arr):\n smallest = 10**1000\n for item in arr:\n if item >= smallest:\n pass\n else:\n smallest = item\n return smallest", "def find_smallest_int(arr): \n arr.sort() \n arr.reverse() \n return arr.pop()", "def find_smallest_int(arr):\n for val in arr:\n return min(arr)", "def find_smallest_int(arr):\n ret = arr[0]\n for i in range(1, len(arr)):\n if arr[i] < ret:\n ret = arr[i]\n return ret", "def find_smallest_int(arr):\n n = len(arr)\n minimum = arr[0]\n for i in range(1, n):\n if arr[i] < minimum:\n minimum = arr[i]\n return minimum\n # Code here\n", "def find_smallest_int(arr):\n # Code here\n smallest = None\n for n in arr:\n if smallest == None:\n smallest = n\n else:\n smallest = min(smallest, n)\n return smallest", "def find_smallest_int(arr):\n smallest = 999999999999999999999999999999999999999999999999999\n for item in arr:\n if int(item) < smallest:\n smallest = item\n return smallest", "def find_smallest_int(arr):\n lowest = 10000000000000\n for integer in arr:\n if integer < lowest:\n lowest = integer\n return lowest", "def find_smallest_int(arr):\n arr.sort()\n return int(arr[0])", "def find_smallest_int(arr):\n # Code here\n minor = arr[0]\n for el in arr:\n if el < minor:\n minor = el\n print(minor)\n \n return minor", "def find_smallest_int(arr):\n \"\"\"return the smallest value in a given array\"\"\"\n return min(arr)", "def find_smallest_int(arr):\n x = arr[0]\n for num in arr:\n if num < x:\n x = num\n else:\n pass\n return x", "def find_smallest_int(arr):\n \n print(arr)\n arr.sort()\n print(arr)\n \n out = arr.pop(0)\n print(out)\n \n return out\n \n", "def find_smallest_int(arr):\n smallest_num = arr[0]\n for num in range(len(arr)):\n if arr[num] < smallest_num: smallest_num = arr[num]\n return smallest_num", "def find_smallest_int(arr):\n v=min(arr)\n return v\n", "def find_smallest_int(arr):\n # Code here\n smallest_int = sorted(arr)\n return smallest_int[0]", "def find_smallest_int(arr):\n min(arr)\n return min(arr)\nfind_smallest_int([78, 56, 232, 12, 11, 43])", "def find_smallest_int(arr):\n min = arr[0]\n for pos in arr:\n if pos < min:\n min = pos\n return min", "def find_smallest_int(arr):\n small = 100000000000000000000\n for i in arr:\n while(i < small):\n small = i\n return small", "def find_smallest_int(arr):\n for small in arr:\n if small==arr[0]:\n low=small\n else:\n if low>small:\n low=small\n return low\n", "def find_smallest_int(arr):\n val = arr[0]\n for x in arr:\n if x < val:\n val = x\n return val", "def find_smallest_int(arr):\n st = set(arr)\n res = arr[0]\n for i in st:\n if i <= res:\n res = i\n return res", "def find_smallest_int(arr):\n res = arr[0]\n for x in arr:\n if x < res:\n res = x\n return res ", "def find_smallest_int(arr):\n return min([int(i) for i in arr])", "def find_smallest_int(arr):\n res=99999999;\n for i in arr:\n if i<res:\n res=i;\n return res;\n # Code here\n", "def find_smallest_int(arr):\n return min(arr)\n#Hellllllyes second kata finished\n", "def find_smallest_int(a):\n M = 1000000000000\n for i in range(len(a)):\n if a[i] < M:\n M = a[i]\n return M\n", "def find_smallest_int(arr):\n n = len(arr)\n smallest = arr[0]\n for i in range(n):\n if arr[i] < smallest:\n smallest = arr[i]\n return smallest\n \n", "def find_smallest_int(arr):\n find_smallest_int = min(arr)\n return find_smallest_int", "def find_smallest_int(arr):\n # Code here\n arr2 = arr.sort()\n return arr[0]", "def find_smallest_int(arr):\n arr_sort = arr.sort()\n return arr[0]", "def find_smallest_int( arr, min = None ):\n if len( arr ) == 0:\n return min\n else:\n if min == None or arr[ 0 ] < min:\n min = arr[ 0 ]\n return find_smallest_int( arr[ 1 : ], min )", "def find_smallest_int(arr):\n min_val=arr[0]\n for val in arr:\n if val < min_val: min_val=val\n return min_val", "def find_smallest_int(arr):\n smallest = arr[0]\n for n in arr[1:]:\n if n < smallest:\n smallest = n\n return smallest", "def find_smallest_int(arr):\n s = arr[0]\n for i in arr[1:]:\n if i < s:\n s = i\n return s", "def find_smallest_int(arr):\n # Code here\n arr.sort()\n k = arr[0]\n return k", "def find_smallest_int(arr):\n # Code here\n array = arr.sort()\n return arr[0]", "def find_smallest_int(arr):\n minVal = float(\"inf\")\n for i in arr:\n if i < minVal:\n minVal = i\n return minVal", "def find_smallest_int(arr):\n # Code here\n arr.sort()\n print(arr[0])\n return arr[0]", "def find_smallest_int(arr):\n \n lowest = arr[0]\n for value in arr:\n if lowest > value:\n lowest = value\n return lowest\n", "def find_smallest_int(arr):\n smallest = arr[0]\n for element in range(len(arr)):\n if arr[element] < smallest:\n smallest = arr[element]\n return smallest \n", "def find_smallest_int(arr):\n smallest = None\n for number in arr:\n if smallest is None: \n smallest = number\n elif number < smallest:\n smallest = number\n else: \n continue\n return smallest"] | {"fn_name": "find_smallest_int", "inputs": [[[78, 56, 232, 12, 11, 43]], [[78, 56, -2, 12, 8, -33]], [[0, -18446744073709551615, 18446744073709551616]], [[-133, -5666, -89, -12341, -321423, 18446744073709551616]], [[0, 18446744073709551616, -18446744073709551617, 18446744073709551616, 18446744073709551616]], [[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]], [[-1, -2, -3, -4, -5, -6, -7, -8, -9, -10]], [[-78, 56, 232, 12, 8]], [[78, 56, -2, 12, -8]]], "outputs": [[11], [-33], [-18446744073709551615], [-321423], [-18446744073709551617], [1], [-10], [-78], [-8]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 11,506 |
def find_smallest_int(arr):
|
cced0d9bddb2ed50425a16419ecec1de | UNKNOWN | Clock shows 'h' hours, 'm' minutes and 's' seconds after midnight.
Your task is to make 'Past' function which returns time converted to milliseconds.
## Example:
```python
past(0, 1, 1) == 61000
```
Input constraints: `0 <= h <= 23`, `0 <= m <= 59`, `0 <= s <= 59` | ["def past(h, m, s):\n return (3600*h + 60*m + s) * 1000", "def past(h, m, s):\n return (s + (m + h * 60) * 60) * 1000", "def past(h, m, s):\n # Good Luck!\n return 3600000 * h + 60000 * m + 1000 * s", "def past(h, m, s):\n return ((h * 60 + m) * 60 + s) * 1000", "def past(h, m, s):\n return h * (60 * 60 * 1000) + m * (60 * 1000) + s * 1000", "from datetime import timedelta\n\ndef past(h, m, s):\n return timedelta(hours=h, minutes=m, seconds=s) // timedelta(milliseconds=1)", "past=lambda h,m,s: (h*3600+m*60+s)*1000", "def past(h, m, s):\n return (h * 3600 + m * 60 + s) * 1000", "def past(h, m, s):\n return h * 3.6e+6 + m * 6e4 + s * 1e3", "def past(h, m, s):\n ms = 0\n ms += 3600000 * h\n ms += 60000 * m\n ms += 1000 * s\n return ms", "def past(h, m, s):\n if 0 <= h <= 23 and 0 <= m <= 59 and 0 <= s <= 59:\n L=[]\n L.append(h*3600000)\n L.append(m*60000)\n L.append(s*1000)\n return sum(L)", "def past(h, m, s):\n return 1000*(3600*h + 60 * m + s)", "def past(h, m, s):\n return h * 3600000 + m * 60000 + s * 1000", "def past(h, m, s):\n # Good Luck!\n h = h*3600\n m = m*60\n s = s*1\n milisec = h+m+s\n mil = str(milisec)\n mili = mil.ljust(3 + len(mil), '0') \n return int(mili) ", "past = lambda h, m, s: (h * 3.6e+6) + (m * 60000) + (s * 1000)", "def past(h, m, s):\n ms = s * 1000\n mm = m * 60000\n mh = h * 3600000\n return ms + mm + mh", "def past(h, m, s):\n m += h * 60\n s += m * 60\n ms = s * 1000\n return ms\n\n# Yes that could've been datetime or oneliner, but what's the point.\n", "def past(h, m, s):\n if 0 <= h <= 23 or 0 <= m <= 59 or 0 <= s <= 59:\n x = h * 3600000\n y = m * 60000\n z = s * 1000\n total = x + y + z\n return(total)\n else:\n return(\"Please input an hour between 0 and 23 and a minute or second inbetween 0 and 59.\")", "def past(h, m, s):\n # Good Luck!\n h = h * 60 * 60 * 1000\n m = m * 60 * 1000\n s = s * 1000\n \n return (h + m + s)", "def past(h, m, s):\n return((h * 3.6e+6) + (m * 60000) + (s * 1000))", "def past(h, m, s):\n return 1000 * (h * 3600 + m * 60 + s)", "def past(h, m, s):\n if h != 0: h = h * 3600000\n if m !=0: m = m* 60000\n if s !=0: s = s* 1000\n \n \n return s+m+h\n", "def past(h, m, s):\n if h: return past(0,60*h+m,s)\n if m: return past(0,0,60*m+s)\n return 1000*s\n", "SI = {'ms': .001, 's': 1, 'm': 60, 'h': 60 * 60}\nconvert_SI = lambda val, unit_from, unit_to: val * SI[unit_from] / SI[unit_to]\nconvert_ms = __import__('functools').partial(convert_SI, unit_to='ms')\npast = lambda *args: sum(convert_ms(val, unit) for val, unit in zip(args, 'hms'))", "import datetime\ndef past(h, m, s):\n dt = datetime.timedelta(hours=h, minutes=m, seconds=s)\n return 1000 * dt.total_seconds()\n", "def past(h, m, s):\n return sum(a*b for a,b in zip([h,m,s],[3600,60,1]))*1000", "def past(h, m, s):\n return hoursToMilliseconds(h) + minutesToMilliseconds(m) + secondsToMilliseconds(s);\n\ndef secondsToMilliseconds(s):\n return s * 1000;\n\ndef minutesToMilliseconds(m):\n return secondsToMilliseconds(m * 60);\n\ndef hoursToMilliseconds(h):\n return minutesToMilliseconds(h * 60);\n\n\n", "import math\ndef past(h, m, s):\n return math.ceil((h * 3.6e+6) + (m * 60000) + (s * 1000))", "def past(h, m, s):\n res = s * 1000\n res += m * 60 * 1000\n res += h * 60 * 60 * 1000\n return res", "from time import struct_time as st, mktime as mk\n\ndef past(h, m, s):\n return int(mk(st((2020, 1, 2, h, m, s, 3, 4, 5))) \\\n - mk(st((2020, 1, 2, 0, 0, 0, 3, 4, 5)))) \\\n * 1000 ", "def convert_SI(val, unit_in, unit_out='ms'):\n SI = {'ms': .001, 's': 1, 'm': 60, 'h': 60 * 60}\n return val * SI[unit_in] / SI[unit_out]\n\ndef past(*args, order='hms'):\n return sum(convert_SI(x, u) for u, x in zip(order, args))\n\n\n# def past(h, m, s): \n# return convert_SI(h, 'h') + convert_SI(m, 'm') + convert_SI(s, 's')\n", "def past(h, m, s):\n return 1000 * sum(unit * 60**power for power, unit in enumerate([s, m, h]))\n", "def past(h, m, s):\n seconds = s + (m * 60) + (h * 3600)\n return seconds / .001", "past = lambda *args: __import__('functools').reduce(lambda a, b: a * 60 + b, args) * 1000", "def past(h, m, s):\n return h * 36e5 + m * 6e4 + s * 1e3", "# past = lambda h, m, s: (((60 * h) + m) * 60 + s) * 1000\ndef past(*ar):\n r = 0\n for x in ar:\n r = 60 * r + x\n return 1000 * r", "from datetime import timedelta\n\n\ndef past(h, m, s):\n d = timedelta(hours=h, minutes=m, seconds=s)\n return d.seconds * 1000\n", "def past(h, m, s):\n return int(h * 3.6*pow(10,6) + m * 6*pow(10,4) + s *pow(10,3))\n", "def past(h, m, s):\n return sum([ h * 60 * 60 * 1000,\n m * 60 * 1000,\n s * 1000 ])", "def past(h, m, s):\n # Good Luck!\n res=int(str(h*36)+'00000')+int(str(m*6)+'0000')+int(str(s)+'000')\n return res", "def past(h, m, s):\n return h*3.6e6 + m*6e4 + s*1e3", "past = lambda *a: 1000 * sum(n * (60 ** i) for i, n in enumerate(reversed(a)))", "past = lambda h, m, s: ((((h * 60) + m) * 60) + s) * 1000", "def past(h, m, s):\n return sum(u * c for u, c in \n zip((s, m, h), (1000, 60*1000, 60*60*1000)))", "def past(hours, minutes, seconds):\n hours = (hours * 3600) * 1000\n minutes = (minutes * 60) * 1000\n seconds = seconds * 1000\n return hours + minutes + seconds", "def past(h, m, s):\n hr = h * 60 * 60 * 1000\n mn = m * 60 * 1000\n sec = s * 1000\n mil = hr + mn + sec\n return mil\n # Good Luck!\n", "def past(h, m, s):\n a=h*3600000\n c=s*1000\n b=m*60000\n return a+b+c\n\n \n # Good Luck!\n", "def past(h, m, s):\n \n htom=h*60\n m=m+htom\n \n mtos=m*60\n s=s+mtos\n \n stoms=s*1000\n \n return stoms\n \n # Good Luck!\n", "def past(h, m, s):\n ms = 60*60*1000*h + 60*1000*m + 1000*s\n return ms", "def past(h, m, s):\n 0 <= h <= 23, 0 <= m <= 59, 0 <= s <= 59\n m = int(m + h*60)\n return int(s + m*60)*1000\n", "def past(h, m, s):\n return int(h * 3.6 * 10 ** 6 + m * 60000 + s * 1000)", "def past(h, m, s):\n \n h1=h*3600\n \n m1=m*60\n\n x=(h1+m1+s)*1000\n return x", "def past(h, m, s):\n x = h*60*60*1000\n y = m*60*1000\n z = s*1000\n j = x + y + z\n return j\n # Good Luck!\n", "def past(h, m, s):\n hour_mm = h*60*60*1000\n minute_mm = m*60*1000\n seconds_mm = s*1000\n \n return hour_mm + minute_mm + seconds_mm", "def past(h, m, s):\n hour_minute = h*60\n minute_millisecond = m*60000\n second_millisecond = s*1000\n sum = (hour_minute*60000)+minute_millisecond+second_millisecond\n return sum", "def past(h, m, s):\n return(h*3600 + m*60 + s*1)*1000\n \n past(0, 0, 8)\n # Good Luck!\n", "def past(h, m, s):\n if h == 0:\n pass\n else:\n h = 3600000 * h\n if m == 0:\n pass\n else:\n m = 60000 * m\n if s == 0:\n pass\n else: \n s = 1000 * s\n return (h+m+s)\n \n\n \n \n\n", "def past(h, m, s):\n time = (h*3600) + (m*60) + s\n time = time * 1000\n return time", "def past(h, m, s):\n result=h*3600 + m*60 + s\n millisec=result * 1000\n return millisec\n \n", "def past(h, m, s):\n a = (h*60+m)*60\n return (a+s)*1000", "past = lambda h, m, s: 1000*s+60000*m+3600000*h", "def past(h, m, s):\n hora = h * 3600000\n minuto = m * 60000\n segundo = s * 1000\n suma = hora + minuto + segundo\n return suma\n", "def past(h, m, s, mil = 1000):\n return (h * 3600 + m * 60 + s) * mil", "def past(h, m, s):\n return h * 3600000 + m * 60000 + s * 1000\n # Good luck!\n", "def past(h, m, s):\n output = 0\n time = 0\n \n time = ((h * 60) * 60 + (m * 60) + s)\n \n return time * 1000\n \n \n # Good Luck!\n", "def past(h, m, s):\n assert 0 <= h <= 23 and 0 <= m <= 59 and 0 <= s <= 59\n return h * 3600000 + m * 60000 + s * 1000", "def past(h, m, s):\n totalMin = h * 60 + m\n totalSec = totalMin * 60 + s\n return totalSec * 1000", "def past(h, m, s):\n return (h*3600000)+(m*60000)+(s*1000)\n #pogchamp\n", "def past(h, m, s):\n # 1 h - 3600000 ms\n # 1 m - 60000 ms\n # 1 s - 1000 ms\n return h*3600000+m*60000+s*1000", "def past(h, m, s):\n h_to_milli = h * 60 * 60 * 1000\n m_to_milli = m * 60 * 1000\n s_to_milli = s * 1000\n millis = h_to_milli + m_to_milli + s_to_milli\n return millis", "def past(hours, minutes, sec):\n if 0 <= hours <= 23 and 0 <= minutes <= 59 and 0 <= sec <= 59:\n return (hours * 60 * 60 + minutes * 60 + sec) * 1000\n else:\n return 0", "def past(h, m, s):\n a=60\n b=1000\n return(h*a*a*b + m*a*b + s*b)", "def past(h, m, s):\n min = h * 60 + m\n sec = min * 60 + s\n sumMilli = sec * 1000 \n return sumMilli", "def past(h, m, s):\n h = h * 3600000\n m = m * 60000\n s = s * 1000\n result = h + m + s\n return result", "def past(h, m, s):\n while 0 <= h <= 23 and 0 <= m <= 59 and 0 <= s <= 59:\n h *= 3600000\n m *= 60000\n s *= 1000\n return h + m + s", "from datetime import timedelta\n\ndef past(h, m, s):\n return timedelta(hours=h, minutes=m, seconds=s)/timedelta(milliseconds=1)", "def past(h, m, s):\n ms = 0\n if h >= 0 and h <= 23:\n ms += h * 3600000\n else:\n ms += 0\n\n if m >= 0 and m <= 59:\n ms += m * 60000\n else:\n ms += 0\n\n if s >= 0 and s <= 59:\n ms += s * 1000\n else:\n ms += 0\n\n return ms\n \n", "def past(h, m, s):\n while 0 <= h <= 23 and 0 <= m <= 59 and 0 <= s <= 59:\n h = h * 3600000\n m = m * 60000\n s = s * 1000\n return h + m + s", "def past(h, m, s):\n if not 0 <= h <= 23 or not 0 <= m <= 59 or not 0 <= s <= 59:\n return \"Error\"\n else:\n return ((h * 3600000) + (m * 60000) + (s * 1000))\n # Good Luck!\n", "def past(h,\n m,\n s):\n\n return h * 3600000 + m * 60000 + s * 1000\n", "def past(h, m, s):\n Hms = h*60*60*1000\n Mms = m*60*1000\n Sms = s*1000\n return Hms+Mms+Sms", "def past(h, m, s):\n x = (60 * 60 * 1000 * h) + (60 * 1000 * m) + (1000 * s)\n if (0 <= h <= 23, 0 <= m <= 59, 0 <= s <= 59):\n return (x)", "def past(h, m, s):\n return (s + 60 * m + h * 3600) * 1000\n # Good Luck!\n", "def past(h, m, s):\n new_hour = h * 3600000\n new_minute = m * 60000\n new_second = s * 1000\n total_time = new_hour + new_minute + new_second\n return total_time", "def past(h, m, s):\n if h > 0:\n h = h*60*60*1000\n if m> 0:\n m = m*60*1000\n if s> 0:\n s = s*1000\n \n return h+s+m", "def past(h, m, s):\n if 0 <= h <= 23 and 0 <= m <= 59 and 0 <= s <= 59:\n return (s + 60 * (60 * h + m)) * 1000", "def past(h, m, s):\n if 0 <= h <= 23 and 0 <= m <= 59 and 0 <= s <= 59:\n return 1000*(60*(60*h + m) + s)", "def past(h, m, s):\n hMin = ((((60*h) + m)*60)+s)*1000\n return hMin\n \n", "def past(h, m, s):\n h=h*3600000\n m=m*60000\n s=s*1000\n time = 0+ h+m+s\n return time\n # Good Luck!\n", "def past(h, m, s):\n totalseconds = s + (h*3600) + (m*60)\n milliseconds = totalseconds * 1000\n return milliseconds", "def past(h, m, s):\n a = h * 3600000\n a += m * 60000\n a += s * 1000\n return a", "def past(h, m, s):\n \n hoursToMiliseconds = h * 3600000\n minutesToMiliseconds = m * 60000\n secondsToMiliseconds = s * 1000\n \n \n miliseconds = hoursToMiliseconds + minutesToMiliseconds + secondsToMiliseconds\n return miliseconds\n # Good Luck!\n", "def past(h, m, s):\n seconds = h * 3600 + m * 60 + s\n milliseconds = seconds * 1000\n return milliseconds", "def past(h, m, s):\n if 0 <= h <= 23 and 0 <= m <= 59 and 0 <= s <= 59:\n h = h*60*60*1000\n m = m*60*1000\n s = s*1000\n return h + m + s", "def past(h, m, s):\n return (s*1 + m*60 + h*3600)*1000", "def past(h, m, s):\n if h: m += h * 60\n if m: s += m * 60\n return s * 1000", "def past(h, m, s):\n oclock1 = h * 3600000\n oclock2 = m * 60000\n oclock3 = s * 1000\n return oclock1 + oclock2 + oclock3", "def past(h, m, s):\n hms, mms, sms = 3600000, 60000, 1000\n return h * hms + m * mms + s * sms", "def past(h, m, s):\n x = 0\n if h:\n x += h * 3600000\n if m:\n x += m * 60000\n if s:\n x += s * 1000\n return x", "def past(h, m, s):\n # Good Luck!\n h*= 3600000\n m*= 60000\n s*= 1000\n return h+m+s\npast(1,2,3)"] | {"fn_name": "past", "inputs": [[0, 1, 1], [1, 1, 1], [0, 0, 0], [1, 0, 1], [1, 0, 0]], "outputs": [[61000], [3661000], [0], [3601000], [3600000]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 12,885 |
def past(h, m, s):
|
f8269c2be7e52a61da3bb83857a1ca6d | UNKNOWN | Given an Array and an Example-Array to sort to, write a function that sorts the Array following the Example-Array.
Assume Example Array catalogs all elements possibly seen in the input Array. However, the input Array does not necessarily have to have all elements seen in the Example.
Example:
Arr:
[1,3,4,4,4,4,5]
Example Arr:
[4,1,2,3,5]
Result:
[4,4,4,4,1,3,5] | ["def example_sort(arr, example_arr):\n return sorted(arr, key=example_arr.index)", "def example_sort(arr, example_arr):\n keys = {k: i for i, k in enumerate(example_arr)}\n return sorted(arr, key=lambda a: keys[a])", "def example_sort(a, b):\n return sorted(a, key=b.index)", "from collections import Counter\n\ndef example_sort(arr, example_arr):\n c = Counter(arr)\n return [n for n in example_arr for _ in range(c[n])]", "def example_sort(arr, example_arr):\n empty = []\n for i in example_arr:\n if i in arr:\n empty.extend([i] * arr.count(i))\n\n\n return empty", "example_sort=lambda x,y:sorted(x,key=y.index)", "def example_sort(arr, example_arr):\n d = {x: i for i, x in enumerate(example_arr)}\n return sorted(arr, key=d.__getitem__)\n", "def example_sort(arr, example_arr):\n return [x for x in example_arr for y in arr if x == y]", "def example_sort(arr, example_arr):\n sorted_arr = []\n number_count = {}\n\n # Iterate through array and count number of items\n for n in arr:\n number_count[n] = number_count.get(n, 0) + 1\n\n # Iterate through example ordered array\n for n in example_arr:\n num_count = number_count.get(n, 0)\n for i in range(num_count):\n sorted_arr.append(n) # Push array count number of times in number_count\n\n return sorted_arr", "def example_sort(arr, example_arr):\n return [a for e in example_arr for a in arr if a == e]"] | {"fn_name": "example_sort", "inputs": [[[1, 2, 3, 4, 5], [2, 3, 4, 1, 5]], [[1, 2, 3, 3, 3, 4, 5], [2, 3, 4, 1, 5]], [[1, 2, 3, 3, 3, 5], [2, 3, 4, 1, 5]], [[1, 2, 3, 3, 3, 5], [3, 4, 5, 6, 9, 11, 12, 13, 1, 7, 8, 2, 10]], [["a", "a", "b", "f", "d", "a"], ["c", "a", "d", "b", "e", "f"]]], "outputs": [[[2, 3, 4, 1, 5]], [[2, 3, 3, 3, 4, 1, 5]], [[2, 3, 3, 3, 1, 5]], [[3, 3, 3, 5, 1, 2]], [["a", "a", "a", "d", "b", "f"]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,473 |
def example_sort(arr, example_arr):
|
316b639db9ec0111faef392016664617 | UNKNOWN | You should have done Product Partitions I to do this second part.
If you solved it, you should have notice that we try to obtain the multiplicative partitions with ```n ≤ 100 ```.
In this kata we will have more challenging values, our ```n ≤ 10000```. So, we need a more optimized a faster code.
We need the function ```prod_int_partII()``` that will give all the amount of different products, excepting the number itself multiplied by one.
The function ```prod_int_partII()``` will receive two arguments, the number ```n``` for the one we have to obtain all the multiplicative partitions, and an integer s that determines the products that have an amount of factors equals to ```s```.
The function will output a list with this structure:
```python
[(1), (2), [(3)]]
(1) Total amount of different products we can obtain, using the factors of n. (We do not consider the product n . 1)
(2) Total amount of products that have an amount of factors equals to s.
[(3)] A list of lists with each product represented with by a sorted list of the factors. All the product- lists should be sorted also.
If we have only one product-list as a result, the function will give only the list
and will not use the list of lists
```
Let's see some cases:
```python
prod_int_partII(36, 3) == [8, 3, [[2, 2, 9], [2, 3, 6], [3, 3, 4]]]
/// (1) ----> 8 # Amount of different products, they are: [2, 2, 3, 3], [2, 2, 9],
[2, 3, 6], [2, 18], [3, 3, 4], [3, 12], [4, 9], [6, 6] (8 products)
(2) ----> 3 # Amount of products with three factors (see them bellow)
(3) ----> [[2, 2, 9], [2, 3, 6], [3, 3, 4]] # These are the products with 3 factors
```
```python
prod_int_partII(48, 5) == [11, 1, [2, 2, 2, 2, 3]] # Only one list.
```
Again consider that some numbers will not have multiplicative partitions.
```python
prod_int_partII(37, 2) == [0, 0, []]
```
Happy coding!!
(Recursion is advisable) | ["def prod_int_partII(n, s, min_=2):\n total, fac = 0, []\n for d in range(min_, int(n ** .5) + 1):\n if not n % d:\n count, l, sub = prod_int_partII(n // d, s-1, d)\n if l == 1: sub = [sub]\n total += count + 1\n fac.extend([d] + x for x in sub)\n if s == 1: fac = [[n]]\n return [total, len(fac), fac[0] if len(fac) == 1 else fac]", "from functools import reduce \n\ndef updateParts(d,s,n,p):\n d.append(p)\n for t in list(s):\n s.add(tuple(sorted(t+(p,))))\n for i in range(len(t)):\n s.add( tuple(sorted(t[:i]+(p*t[i],)+(t[i+1:]))) )\n s.add((p,))\n return n//p\n\ndef prod_int_partII(n,m):\n x,d,s,p = n,[],set(),2\n while p*p<=n:\n while not n%p: n = updateParts(d,s,n,p)\n p += 1+(p!=2)\n if n-1 and n!=x: updateParts(d,s,-1,n)\n \n s = [t for t in s if reduce(int.__mul__,t)==x and len(t)>1]\n d = sorted(list(t) for t in s if len(t)==m)\n \n return [len(s),len(d),d[0] if len(d)==1 else d]", "def prod_int_partII(n, s):\n part = list(mul_part_gen(n))[:-1]\n sub = [p for p in part if len(p) == s]\n return [len(part), len(sub), sub[0] if len(sub) == 1 else sub]\n\ndef mul_part_gen(n, k=2): \n if n == 1: \n yield [] \n for i in range(k, n+1): \n if n % i == 0: \n for part in mul_part_gen(n//i, i): \n yield [i] + part\n", "div=lambda n:[[i,n//i] for i in range(2,int(n**.5)+1) if not n%i]\ndef prod_int_partII(n, s):\n li,parts = div(n), set()\n def recur(li, s=[]):\n parts.add(tuple(sorted(s)))\n for i in li:\n recur(div(i[1]),s+i if not s else s[:-1]+i)\n recur(li)\n req = sorted(filter(lambda x:len(x)==s, parts))\n return [len(parts)-1, len(req), list([map(list,req),req[0]][len(req)==1]) if req else []]", "from math import sqrt\n\ndef partitions(n, floor = 2):\n parts = [[n]]\n for i in range(floor, int(sqrt(n)) + 1):\n if n % i == 0:\n for part in partitions(n // i, i):\n parts.append([i] + part) \n return parts\n\ndef prod_int_partII(n, s):\n lists = []\n parts = partitions(n, 2)\n for part in parts:\n if len(part) == s:\n lists.append(part)\n if len(lists) == 1:\n list = lists[0]\n else:\n list = lists\n return [len(parts) - 1, len(lists), list]", "def prod_int_partII(n, s):\n comb = set()\n \n for p in partition(n):\n if len(p) > 1: comb.add(tuple(sorted(p)))\n \n res = sorted([p for p in comb if len(p) == s])\n \n return [len(comb), len(res), list(res[0]) if len(res) == 1 else [list(t) for t in res]]\n \ndef partition(n, start = 2):\n if n == 1:\n yield []\n for i in range(start, n + 1):\n if n % i == 0:\n for p in partition(n // i, i):\n yield p + [i]", "def p(n,z=2):\n r=[]\n s=0\n for i in range(z,int(n**.5)+1):\n if n%i==0:\n x,y=p(n//i,i)\n s+=x+1\n r+=[[i]+v for v in y]+[[i,n//i]]\n return s,r\ndef prod_int_partII(n, l): # n, integer to have the multiplicative partitions\n s,r=p(n)\n x=[v for v in r if len(v)==l]\n L=len(x)\n if L==1:x=x[0]\n return[s,L,x]\n", "def prod_int_partII(n, s):\n res = []\n stack = [[n, 2, []]]\n while stack:\n m, d, store = stack.pop(0)\n for i in range(d, int(m**0.5) + 1):\n if m % i == 0:\n res.append(store + [i] + [m // i])\n stack.append([m // i, i, store + [i]])\n filtred_res = list(filter(lambda x: len(x) == s, res))\n if filtred_res:\n return [len(res), len(filtred_res), filtred_res] if len(filtred_res) > 1 else [len(res), len(filtred_res), filtred_res[0]]\n else:\n return [len(res), 0, []]", "#!/usr/bin/env python\nfrom collections import defaultdict\nfrom itertools import islice\n\ndef primes():\n yield 2\n D = defaultdict(list)\n i = 3\n while True:\n if i not in D:\n D[i * i].append(i)\n yield i\n else:\n for k in D[i]:\n j = i + k\n while j % 2 == 0: j += k\n D[j].append(k)\n del D[i]\n i += 2\n\n\nPRIMES = list(islice(primes(), 1000))\n\ndef decompose(n):\n r = defaultdict(int)\n for p in PRIMES:\n while n % p == 0: r[p] += 1; n //= p\n if n == 1: break\n return r\n\n\ndef prod_int_partII(n, s):\n r = []\n d = decompose(n)\n ds = [i for i in range(2, n) if n % i == 0]\n total = sum(i for _, i in list(d.items()))\n q = defaultdict(list)\n def aux(m, ci, c, i=0, p=[]):\n if c == 0:\n if m == 1: q[ci].append(p)\n return\n else:\n for j in range(i, len(ds)):\n if m % ds[j] == 0: aux(m // ds[j], ci, c - 1, j, p + [ds[j]])\n for i in range(2, total + 1): aux(n, i, i)\n return [sum(len(i) for i in list(q.values())), len(q[s]), q[s][0] if len(q[s]) == 1 else q[s]]\n"] | {"fn_name": "prod_int_partII", "inputs": [[36, 3], [48, 5], [37, 2], [96, 5], [96, 6]], "outputs": [[[8, 3, [[2, 2, 9], [2, 3, 6], [3, 3, 4]]]], [[11, 1, [2, 2, 2, 2, 3]]], [[0, 0, []]], [[18, 2, [[2, 2, 2, 2, 6], [2, 2, 2, 3, 4]]]], [[18, 1, [2, 2, 2, 2, 2, 3]]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 5,104 |
def prod_int_partII(n, s):
|
ba571aba95d4654b08f039af0902ff93 | UNKNOWN | Given an array with exactly 5 strings `"a"`, `"b"` or `"c"` (`char`s in Java, `character`s in Fortran), check if the array contains three and two of the same values.
## Examples
```
["a", "a", "a", "b", "b"] ==> true // 3x "a" and 2x "b"
["a", "b", "c", "b", "c"] ==> false // 1x "a", 2x "b" and 2x "c"
["a", "a", "a", "a", "a"] ==> false // 5x "a"
``` | ["def check_three_and_two(array):\n return { array.count(x) for x in set(array) } == {2, 3}", "def check_three_and_two(array):\n return True if ((len(set(array)) == 2) and [each for each in set(array) if array.count(each) in [2,3]])else False", "from collections import Counter\ndef check_three_and_two(array):\n return sorted(Counter(array).values()) == [2, 3]", "def check_three_and_two(array):\n return { array.count(i) for i in array} == { 2, 3}", "def check_three_and_two(array):\n return sum([array.count(x) for x in array]) == 13", "def check_three_and_two(array):\n return len(set(array)) == 2 and array.count(array[0]) not in [1,4]", "from collections import Counter\nfrom typing import List\n\n\ndef check_three_and_two(array: List[str]) -> bool:\n return set(Counter(array).values()) == {2, 3}\n", "def check_three_and_two(array):\n ans = [array.count(i) for i in 'abc']\n return True if 2 in ans and 3 in ans else False\n", "def check_three_and_two(array):\n return max([array.count(a) for a in array]) == 3 and min([array.count(a) for a in array]) == 2", "def check_three_and_two(arr):\n for i in arr:\n if not 2 <=arr.count(i) <= 3:\n return False\n return True\n"] | {"fn_name": "check_three_and_two", "inputs": [[["a", "a", "a", "b", "b"]], [["a", "c", "a", "c", "b"]], [["a", "a", "a", "a", "a"]]], "outputs": [[true], [false], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,233 |
def check_three_and_two(array):
|
aeaa13212f44a2ce711c69ca3fd6ce8d | UNKNOWN | ```
*************************
* Create a frame! *
* __ __ *
* / \~~~/ \ *
* ,----( .. ) *
* / \__ __/ *
* /| (\ |( *
* ^ \ /___\ /\ | *
* |__| |__|-.. *
*************************
```
Given an array of strings and a character to be used as border, output the frame with the content inside.
Notes:
* Always keep a space between the input string and the left and right borders.
* The biggest string inside the array should always fit in the frame.
* The input array is never empty.
## Example
`frame(['Create', 'a', 'frame'], '+')`
Output:
```
++++++++++
+ Create +
+ a +
+ frame +
++++++++++
``` | ["def frame(text, char):\n text_lens = [len(x) for x in text]\n longest_len = max(text_lens)\n frame_list = [char*(longest_len + 4)]\n for str in text:\n frame_list.append(\"{} {}{} {}\".format(char, str, \" \" * (longest_len - len(str)), char)) \n frame_list.append(char*(longest_len + 4))\n return \"\\n\".join(frame_list)", "def frame(text, char):\n n = len(max(text, key=len)) + 4\n return \"\\n\".join( [char * n] +\n [\"%s %s %s\" % (char, line.ljust(n-4), char) for line in text] +\n [char * n] )", "def frame(lines, char):\n l = len(max(lines, key=len))\n row = char * (l + 4)\n body = \"\\n\".join(f\"{char} {line:<{l}s} {char}\" for line in lines)\n return f\"{row}\\n{body}\\n{row}\"", "def frame(text, char):\n length = len(max(text, key=len))\n start = end = char*(length+3)\n k = [' '+x+(' '*(length-len(x)+1)) for x in text]\n return (char+'\\n'+char).join([start]+k+[end])", "from itertools import chain\n\ndef frame(words, char):\n size = max(map(len, words))\n frame = [char*(size+4)]\n middle = (f\"{char} {word: <{size}} {char}\" for word in words)\n return '\\n'.join(chain(frame, middle, frame))", "def frame(t, c):\n m = len(max(t,key=len))\n return c*(m+4) + '\\n' + \"\\n\".join([f'{c} {i:<{m}} {c}' for i in t]) + '\\n' + c*(m+4)", "def frame(text, char):\n width = max([len(x) for x in text]) + 4\n a = '\\n'.join([char + ' ' + x + ' ' * (width-len(x)-3) + char for x in text])\n return f'{char*width}\\n{a}\\n{char*width}'", "def frame(text, c):\n mx = max(len(w) for w in text)\n return '\\n'.join([c * (mx + 4)] + [c + ' ' + w.ljust(mx) + ' ' + c for w in text] + [c * (mx + 4)])", "def frame(text, char):\n \"\"\"\n First this function will find the length of the top/bottom frames\n Next it creates the word frames\n Then it constructs the full frame\n \"\"\"\n max_length = max([len(word) for word in text])\n frame_words = [char + \" \" + word + ((max_length - len(word) + 1) * \" \") + char for word in text]\n frame = char * (max_length + 4) + \"\\n\"\n for line in frame_words:\n frame = frame + line + \"\\n\"\n return frame + char * (max_length + 4)", "def frame(text, char):\n w = max(map(len, text))\n def f():\n yield char * (w + 4)\n for line in text:\n yield f'{char} {line:<{w}} {char}'\n yield char * (w + 4)\n return '\\n'.join(f())"] | {"fn_name": "frame", "inputs": [[["Small", "frame"], "~"], [["Create", "this", "kata"], "+"], [["This is a very long single frame"], "-"]], "outputs": [["~~~~~~~~~\n~ Small ~\n~ frame ~\n~~~~~~~~~"], ["++++++++++\n+ Create +\n+ this +\n+ kata +\n++++++++++"], ["------------------------------------\n- This is a very long single frame -\n------------------------------------"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,503 |
def frame(text, char):
|
5af2e306de8024118d48f881dc57976d | UNKNOWN | Given an array of numbers, return a string made up of four parts:
a) a four character 'word', made up of the characters derived from the first two and last two numbers in the array. order should be as read left to right (first, second, second last, last),
b) the same as above, post sorting the array into ascending order,
c) the same as above, post sorting the array into descending order,
d) the same as above, post converting the array into ASCII characters and sorting alphabetically.
The four parts should form a single string, each part separated by a hyphen: '-'
example format of solution: 'asdf-tyui-ujng-wedg' | ["def extract(arr): return ''.join(arr[:2]+arr[-2:])\n\ndef sort_transform(arr):\n arr = list(map(chr,arr))\n w1 = extract(arr)\n arr.sort()\n w2 = extract(arr)\n return f'{w1}-{w2}-{w2[::-1]}-{w2}'", "def as_str(xs):\n return ''.join(map(chr, xs[:2] + xs[-2:]))\n\ndef sort_transform(arr):\n return '-'.join([\n as_str(arr),\n as_str(sorted(arr)),\n as_str(sorted(arr, reverse=True)),\n as_str(sorted(arr)),\n ])", "def sort_transform(arr):\n a=chr(arr[0])+chr(arr[1])+chr(arr[-2])+chr(arr[-1])\n arr.sort()\n b=chr(arr[0])+chr(arr[1])+chr(arr[-2])+chr(arr[-1])\n return '-'.join([a,b,b[::-1],b])", "def sort_transform(a):\n return '-'.join([''.join(map(chr, x[:2]+x[-2:])) for x in [a, sorted(a), sorted(a, reverse=1), sorted(a, key=chr)]])", "def sort_transform(arr):\n arr = list(map(chr, arr))\n srtd = sorted(arr)\n \n a = \"\".join(arr[:2] + arr[-2:])\n b = \"\".join(srtd[:2] + srtd[-2:])\n \n return f\"{a}-{b}-{b[::-1]}-{b}\"", "from heapq import nlargest, nsmallest\n\ndef sort_transform(lst):\n first_last = \"\".join(chr(n) for n in lst[:2] + lst[-2:])\n small_big = \"\".join(chr(n) for n in nsmallest(2, lst) + nlargest(2, lst)[::-1])\n return f\"{first_last}-{small_big}-{small_big[::-1]}-{small_big}\"", "def sort_transform(arr):\n d = lambda x: \"\".join(map(chr,[x[0], x[1], x[-2], x[-1]]))\n b = d(sorted(arr))\n return \"-\".join((d(arr), b, d(sorted(arr, reverse=True)), b))", "def sort_transform(a):\n a = [chr(e) for e in a]\n r = sorted(a)\n x = ''.join(r[:2] + r[-2:])\n return '-'.join([''.join(a[:2]+a[-2:]), x, x[::-1], x])", "def sort_transform(arr):\n arr_al = sorted([chr(x) for x in arr])\n return '-'.join([chr(arr[0]) + chr(arr[1]) + chr(arr[-2]) + chr(arr[-1]),\n chr(sorted(arr)[0]) + chr(sorted(arr)[1]) + chr(sorted(arr)[-2]) + chr(sorted(arr)[-1]),\n chr(sorted(arr, reverse=True)[0]) + chr(sorted(arr, reverse=True)[1]) + chr(sorted(arr, reverse=True)[-2]) + chr(sorted(arr, reverse=True)[-1]),\n arr_al[0] + arr_al[1] + arr_al[-2] + arr_al[-1]])", "def fst2_lst2(arr):\n return chr(arr[0])+chr(arr[1])+chr(arr[-2])+chr(arr[-1])\n \ndef sort_transform(arr):\n asc_arr = sorted([chr(n) for n in arr])\n return fst2_lst2(arr) + '-' + fst2_lst2(sorted(arr)) + '-' + fst2_lst2(sorted(arr, reverse=True)) + '-' + asc_arr[0]+asc_arr[1]+asc_arr[-2]+asc_arr[-1]"] | {"fn_name": "sort_transform", "inputs": [[[111, 112, 113, 114, 115, 113, 114, 110]], [[51, 62, 73, 84, 95, 100, 99, 126]], [[66, 101, 55, 111, 113]], [[78, 117, 110, 99, 104, 117, 107, 115, 120, 121, 125]], [[101, 48, 75, 105, 99, 107, 121, 122, 124]], [[80, 117, 115, 104, 65, 85, 112, 115, 66, 76, 62]], [[91, 100, 111, 121, 51, 62, 81, 92, 63]], [[78, 93, 92, 98, 108, 119, 116, 100, 85, 80]], [[111, 121, 122, 124, 125, 126, 117, 118, 119, 121, 122, 73]], [[82, 98, 72, 71, 71, 72, 62, 67, 68, 115, 117, 112, 122, 121, 93]], [[99, 98, 97, 96, 81, 82, 82]], [[66, 99, 88, 122, 123, 110]], [[66, 87, 98, 59, 57, 50, 51, 52]]], "outputs": [["oprn-nors-sron-nors"], ["3>c~-3>d~-~d>3-3>d~"], ["Beoq-7Boq-qoB7-7Boq"], ["Nuy}-Ncy}-}ycN-Ncy}"], ["e0z|-0Kz|-|zK0-0Kz|"], ["PuL>->Asu-usA>->Asu"], ["[d\\?-3>oy-yo>3-3>oy"], ["N]UP-NPtw-wtPN-NPtw"], ["oyzI-Io}~-~}oI-Io}~"], ["Rby]->Cyz-zyC>->Cyz"], ["cbRR-QRbc-cbRQ-QRbc"], ["Bc{n-BXz{-{zXB-BXz{"], ["BW34-23Wb-bW32-23Wb"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,487 |
def sort_transform(arr):
|
63db5709257d7f698f78a18d61c1b53b | UNKNOWN | Create a function that returns an array containing the first `l` digits from the `n`th diagonal of [Pascal's triangle](https://en.wikipedia.org/wiki/Pascal's_triangle).
`n = 0` should generate the first diagonal of the triangle (the 'ones'). The first number in each diagonal should be 1.
If `l = 0`, return an empty array. Assume that both `n` and `l` will be non-negative integers in all test cases. | ["def generate_diagonal(d, l):\n result = [1] if l else []\n for k in range(1, l):\n result.append(result[-1] * (d+k) // k)\n return result", "def generate_diagonal(n, l):\n d = []\n for k in range(l):\n d.append(d[-1] * (n + k) // k if d else 1)\n return d", "from scipy.special import comb\ndef generate_diagonal(n, l):\n return [comb(n + a, a, exact=True) for a in range(l)] ", "from math import factorial\n\ndef generate_diagonal(n, l):\n return [factorial(n+i) // (factorial(n) * factorial(i)) for i in range(l)]\n", "#C(n, k) == n!/(k!(n-k)!) simplifies to a/b\n# where a == n*(n-1)*(n-2)*...*(n-(k-2))*(n-(k-1))\n# b == (k-1)!\ndef efficientCombination(n,k):\n from math import gcd\n \n #a and b are defined in above comment\n a,b = 1,1\n\n if k==0:\n return 1\n\n #Since C(n,k) == C(n,n-k), so take n-k when it's smaller than k\n if n-k < k : \n k = n - k\n\n while k: \n a *= n\n b *= k \n \n m = gcd(a, b) \n a //= m \n b //= m \n \n n -= 1\n k -= 1\n #---end while\n\n return a\n\n#-----end function\n\n\n#d is the deisred diagonal from right to left (starting count at 0), and amt is \n# the number of elements desired from that diagonal\ndef generate_diagonal(d, amt):\n diagElements = []\n\n for n in range(d, amt + d, 1):\n valOnDiag = efficientCombination(n, d)\n diagElements.append(valOnDiag)\n\n return diagElements\n\n#---end function\n", "import operator as op\nfrom functools import reduce\n\ndef ncr(n, r):\n r = min(r, n-r)\n return reduce(op.mul, range(n, n-r, -1), 1)// reduce(op.mul, range(1, r+1), 1)\n\ndef generate_diagonal(n, l):\n mx = []\n i,j = n,0\n while i < n+l:\n mx.append(ncr(i,j))\n i+=1\n j+=1\n return mx", "def generate_diagonal(n, l):\n # return an array containing the numbers in the nth diagonal of Pascal's triangle, to the specified length\n if l == 0:\n return []\n diagonal = [1]\n for i in range(l)[1:]:\n diagonal.append(diagonal[-1] * (n + i) / i)\n return diagonal\n", "def generate_diagonal(n, l):\n if n==0:\n c=[]\n while l>0:\n c+=[1]\n l-=1\n return c\n if l==0 and n==0:\n return '[]'\n else:\n i=n+l\n q=[]\n o=[]\n def triangles():\n p =[1]\n while True:\n yield p\n p =[1]+[p[x]+p[x+1] for x in range(len(p)-1)]+[1]\n for t in triangles():\n if i>0:\n i-=1\n q.append(t)\n else:\n break\n for t in range(l):\n r=q[n][t]\n n+=1\n o.append(r)\n return o", "def generate_diagonal(n, l):\n row = [1]\n for k in range(1, l):\n row.append(row[-1] * (n + k) // k)\n return row if l else []", "from functools import lru_cache\n\ndef generate_diagonal(nn, l):\n @lru_cache(None)\n def calc(n, ll):\n if n == 0 or ll == 1: return 1\n elif n < 0: return 0\n return calc(n - 1, ll) + calc(n, ll - 1)\n return [calc(nn, i) for i in range(1, l+1)]"] | {"fn_name": "generate_diagonal", "inputs": [[0, 10], [1, 10], [2, 10], [3, 10], [4, 10], [10, 0], [100, 6]], "outputs": [[[1, 1, 1, 1, 1, 1, 1, 1, 1, 1]], [[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]], [[1, 3, 6, 10, 15, 21, 28, 36, 45, 55]], [[1, 4, 10, 20, 35, 56, 84, 120, 165, 220]], [[1, 5, 15, 35, 70, 126, 210, 330, 495, 715]], [[]], [[1, 101, 5151, 176851, 4598126, 96560646]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,261 |
def generate_diagonal(n, l):
|
57c32f40b0ecc99ec524c83cf16fe82d | UNKNOWN | # Do you ever wish you could talk like Siegfried of KAOS ?
## YES, of course you do!
https://en.wikipedia.org/wiki/Get_Smart
# Task
Write the function ```siegfried``` to replace the letters of a given sentence.
Apply the rules using the course notes below. Each week you will learn some more rules.
Und by ze fifz vek yu vil be speakink viz un aksent lik Siegfried viz no trubl at al!
# Lessons
## Week 1
* ```ci``` -> ```si```
* ```ce``` -> ```se```
* ```c``` -> ```k``` (except ```ch``` leave alone)
## Week 2
* ```ph``` -> ```f```
## Week 3
* remove trailing ```e``` (except for all 2 and 3 letter words)
* replace double letters with single letters (e.g. ```tt``` -> ```t```)
## Week 4
* ```th``` -> ```z```
* ```wr``` -> ```r```
* ```wh``` -> ```v```
* ```w``` -> ```v```
## Week 5
* ```ou``` -> ```u```
* ```an``` -> ```un```
* ```ing``` -> ```ink``` (but only when ending words)
* ```sm``` -> ```schm``` (but only when beginning words)
# Notes
* You must retain the case of the original sentence
* Apply rules strictly in the order given above
* Rules are cummulative. So for week 3 first apply week 1 rules, then week 2 rules, then week 3 rules | ["import re\n\nPATTERNS = [re.compile(r'(?i)ci|ce|c(?!h)'),\n re.compile(r'(?i)ph'),\n re.compile(r'(?i)(?<!\\b[a-z]{1})(?<!\\b[a-z]{2})e\\b|([a-z])\\1'),\n re.compile(r'(?i)th|w[rh]?'),\n re.compile(r'(?i)ou|an|ing\\b|\\bsm')]\n \nCHANGES = {\"ci\": \"si\", \"ce\": \"se\", \"c\":\"k\", # Week 1\n \"ph\": \"f\", # Week 2\n \"th\": \"z\", \"wr\": \"r\", \"wh\": \"v\", \"w\": \"v\", # Week 4\n \"ou\": \"u\", \"an\": \"un\", \"ing\": \"ink\", \"sm\": \"schm\"} # Week 5\n\ndef change(m):\n tok = m.group(0)\n rep = CHANGES.get( tok.lower(), \"\" if None in m.groups() else m.group()[0] ) # default value used for week 3 only\n if tok[0].isupper(): rep = rep.title()\n return rep\n \ndef siegfried(week, txt):\n for n in range(week):\n txt = PATTERNS[n].sub(change, txt)\n return txt", "import re\ndef siegfried(week, txt):\n if week >= 1: txt = re.sub('c(?!h)', 'k', re.sub('C(?!h)', 'K', txt.replace('ci', 'si').replace('Ci', 'Si').replace('ce', 'se').replace('Ce', 'Se')))\n if week >= 2: txt = txt.replace('ph', 'f').replace('Ph', 'F')\n if week >= 3: txt = re.sub('([A-z]{2,})e([-!?]+)', r'\\1\\2', ' '.join([x.rstrip('e') if len(x)>3 else x for x in re.sub(r'([A-z])\\1', r'\\1', txt, flags=re.I).split()]))\n if week >= 4: txt = txt.replace('th', 'z').replace('Th', 'Z').replace('wr', 'r').replace('Wr', 'R').replace('wh', 'v').replace('Wh', 'V').replace('w', 'v').replace('W', 'V')\n if week >= 5: txt =(' ' + txt + ' ').replace('ou', 'u').replace('Ou', 'U').replace('an', 'un').replace('An', 'Un').replace('ing ', 'ink ').replace(' sm', ' schm').replace(' Sm', ' Schm') \n return txt.strip()", "import re\n\ndef siegfried(week, txt):\n lessons = \\\n [\n [ # Week 1\n (r'c(?=[ie])', { 'c':'s','C':'S' }),\n (r'c(?!h)', { 'c':'k','C':'K' })\n ],[ # Week 2\n (r'ph', { 'ph':'f','Ph': 'F' })\n ],[ # Week 3\n (r'(?<=\\w\\w\\w)e(?=\\W|\\Z)', {'e':'','E':''}),\n (r'([a-z])\\1',{})\n ],[ # Week 4\n (r'th', { 'th':'z','Th': 'Z' }),\n (r'wr', { 'wr':'r','Wr': 'R' }),\n (r'wh', { 'wh':'v','Wh': 'V' }),\n (r'w', { 'w':'v','W': 'V' })\n ],[ # Week 5\n (r'ou', { 'ou':'u','Ou': 'U' }),\n (r'an', { 'an':'un','An': 'Un' }),\n (r'ing(?=\\W|\\Z)', { 'ing':'ink' }),\n (r'(?<=\\W)sm|(?<=\\A)sm', { 'sm':'schm','Sm': 'Schm' })\n ]\n ]\n \n result = txt\n for w in range(week):\n for rx,data in lessons[w]:\n repl = lambda m: data.get(m.group(0),m.group(0)[0])\n result = re.sub(rx,repl,result,flags=re.IGNORECASE)\n return result", "import re\ndef siegfried(week, txt):\n if week == 1:\n txt = re.sub('ci', matchcase('si'), txt, flags=re.I)\n txt = re.sub('ce', matchcase('se'), txt, flags=re.I)\n txt = re.sub('(?!ch)[c]', matchcase('k'), txt, flags=re.I)\n elif week == 2:\n txt = siegfried(1, txt)\n txt = re.sub('ph', matchcase('f'), txt, flags=re.I)\n elif week == 3:\n txt = siegfried(2, txt)\n txt = re.sub(r'(?<=\\w{3})e\\b', '', txt)\n txt = re.sub(r'([a-z])\\1', r'\\1', txt, flags=re.I)\n elif week == 4:\n txt = siegfried(3, txt)\n txt = re.sub('th', matchcase('z'), txt, flags=re.I)\n txt = re.sub('wr', matchcase('r'), txt, flags=re.I)\n txt = re.sub('wh|w', matchcase('v'), txt, flags=re.I)\n elif week == 5:\n txt = siegfried(4, txt)\n txt = re.sub('ou', 'u', txt)\n txt = re.sub('an', matchcase('un'), txt, flags=re.I)\n txt = re.sub(r'ing\\b', matchcase('ink'), txt, flags=re.I)\n txt = re.sub(r'\\bsm', matchcase('schm'), txt, flags=re.I)\n return txt\n \ndef matchcase(word):\n def replace(m):\n text = m.group()\n if text.isupper():\n return word.upper()\n elif text.islower():\n return word.lower()\n elif text[0].isupper():\n return word.capitalize()\n else:\n return word\n return replace\n", "RULES = [\n ['ci si', 'ce se', 'c(?!h) k'],\n ['ph f'],\n [r'(?<=\\w{3})e+\\b ', r'([a-z])\\1 \\1'],\n ['th z', 'wr r', 'wh v', 'w v'],\n ['ou u', 'an un', r'ing\\b ink', r'\\bsm schm'],\n]\n\ndef siegfried(week, txt):\n import re\n \n def keep_case(repl):\n def wrapper(m):\n ref = re.match(r'\\\\(\\d)', repl)\n strng = m.group(int(ref.group(1))) if ref else repl\n g = m.group(0)\n if g.islower(): return strng.lower()\n if g.isupper(): return strng.upper()\n if g.istitle(): return strng.title()\n return strng\n return wrapper\n\n for rules in RULES[:week]:\n for rule in rules:\n src, repl = rule.split(' ')\n txt = re.sub(src, keep_case(repl), txt, flags=re.I)\n return txt", "import re\ndef siegfried(week, s):\n d = {'ph': 'f', 'Ph': 'F','ci': 'si', 'ce': 'se', 'c': 'k', 'Ci': 'Si', 'Ce': 'Se', 'C': 'K','XX':'ch','xx':'Ch','ch':'XX','Ch':'xx','th': 'z', 'wr': 'r', 'wh': 'v', 'w': 'v', 'Th': 'Z', \n 'Wr': 'R', 'Wh': 'V', 'W': 'V','ou': 'u', 'an': 'un', 'ing': 'ink', 'sm': 'schm', 'Ou': 'U', 'An': 'Un', 'Ing': 'Ink', 'Sm': 'Schm'}\n week1=lambda s:re.sub(r'(xx)',lambda x:d[x.group()],re.sub(r'(ci|ce|c)',lambda x:d[x.group()],re.sub(r'(Ch|ch)',lambda x:d[x.group()] ,s),flags=re.I),flags=re.I)\n week2=lambda s:re.sub(r'ph', lambda x: d[x.group()], s, flags=re.I)\n week3=lambda s:re.sub(r'([a-zA-Z])\\1', r'\\1', re.sub(r'\\b([a-zA-Z]+e+)\\b',lambda x:x.group()if len(x.group())<=3 else x.group().rstrip('e'),s,flags=re.I), flags=re.I)\n week4=lambda s:re.sub(r'(th|wr|wh|w)', lambda x: d[x.group()], s, flags=re.I)\n week5=lambda s:re.sub(r'(ing(?= )|(?<= )sm)',lambda x:d[x.group()],re.sub(r'(ing$|^sm)',lambda x:d[x.group()],re.sub(r'(ou|an)',lambda x:d[x.group()],s,flags=re.I),flags=re.I),flags=re.I)\n li = [week1, week2, week3, week4, week5]\n for i in li[:week] : s = i(s)\n return s", "def siegfried(week, txt):\n\n import re\n\n dict_week = \\\n {\n 1: {'Ci': 'Si', 'Ce': 'Se', 'Ch': 'Xxx', 'C': 'K', 'Xxx': 'Ch',\n 'ci': 'si', 'ce': 'se', 'ch': 'xxx', 'c': 'k', 'xxx': 'ch'},\n 2: {'Ph': 'F', 'ph': 'f'},\n 3: {'': ''},\n 4: {'Th': 'Z', 'Wr': 'R', 'Wh': 'V', 'W': 'V',\n 'th': 'z', 'wr': 'r', 'wh': 'v', 'w': 'v'},\n 5: {'Ou': 'U', 'An': 'Un', 'ou': 'u', 'an': 'un'}\n }\n\n dd = {}\n for i in range(1, week + 1):\n dd.update(dict_week[i])\n\n for k, v in dd.items():\n txt = txt.replace(k, v)\n\n if week >= 3:\n txt = ''.join(re.sub(r'e$', '', i) if len(i) > 3 else i for i in re.split(r'(\\W)', txt))\n double_l = {re.search(r'([a-z])\\1', i).group(0) for i in txt.lower().split() if re.search(r'([a-z])\\1', i)}\n for i in double_l:\n txt = txt.replace(i, i[0])\n txt = txt.replace(i[0].upper() + i[1], i[0].upper())\n\n if week == 5:\n txt = ' '.join(re.sub(r'ing$', 'ink', i, flags=re.IGNORECASE) for i in txt.split())\n txt = ' '.join(re.sub(r'^sm', 'Schm', i, flags=re.IGNORECASE) for i in txt.split())\n\n return txt", "import re\n\n\ndef siegfried(week, txt):\n weeks_have_passed = {0: week_one, 1: week_two, 2: week_three, 3: week_four, 4: week_five}\n\n for counter in range(0, week):\n\n txt = weeks_have_passed[counter](txt)\n \n return txt\n\ndef week_one(txt):\n\n letter_list = list(txt)\n\n copy_reverse_letter_list = letter_list.copy()\n\n\n last_letter = ''\n\n count = -1\n\n for letter in copy_reverse_letter_list[::-1]:\n\n lowercase_letter = letter.lower()\n\n if lowercase_letter == 'c':\n lowercase_letter += last_letter\n\n if letter.isupper():\n dict_to_know = {'ci': 'S', 'ce': 'S', 'ch': 'C'}\n to_return = 'K'\n else:\n dict_to_know = {'ci': 's', 'ce': 's', 'ch': 'c'}\n to_return = 'k'\n\n letter_list[count] = dict_to_know.get(lowercase_letter, to_return)\n\n count -= 1\n last_letter = letter\n\n final_txt = ''.join(letter_list)\n\n return final_txt\n\n\ndef week_two(txt):\n\n letter_list = list(txt)\n\n copy_letter_list = letter_list.copy()\n copy_letter_list.append('')\n\n fix_position = 0\n\n for counter, letter in enumerate(copy_letter_list[:-1]):\n\n lowercase_letter = letter.lower()\n\n next_letter = copy_letter_list[counter+1]\n lowcase_next_letter = next_letter.lower()\n\n if lowercase_letter == 'p' and lowcase_next_letter == 'h':\n\n counter -= fix_position\n\n if letter.isupper():\n to_change = 'F'\n else:\n to_change = 'f'\n\n letter_list[counter: counter + 2] = to_change\n\n fix_position += 1\n\n new_txt = ''.join(letter_list)\n\n return new_txt\n\n\ndef week_three(txt):\n trailling_e = r'[a-zA-Z]{3,}e\\b'\n\n trailling_list = re.findall(trailling_e, txt)\n\n new_txt = txt\n\n for trailling_e_word in trailling_list:\n new_txt = new_txt.replace(trailling_e_word, trailling_e_word[:-1])\n\n letter_list = list(new_txt)\n\n copy_letter_list = letter_list.copy()\n\n last_letter = ''\n\n position = -1\n\n for letter in copy_letter_list[::-1]:\n\n lowercase_letter = letter.lower()\n\n if lowercase_letter == last_letter and lowercase_letter.isalpha():\n\n del letter_list[position+1]\n\n position += 1\n\n last_letter = lowercase_letter\n\n position -= 1\n\n final_txt = ''.join(letter_list)\n\n return final_txt\n\n\n\ndef week_four(txt):\n\n letter_list = list(txt)\n\n copy_reverse_letter_list = letter_list.copy()\n\n last_letter = ''\n\n counter = -1\n\n for letter in copy_reverse_letter_list[::-1]:\n\n lowercase_letter = letter.lower()\n\n if lowercase_letter == 'w' or lowercase_letter == 't' and last_letter == 'h':\n lowercase_letter += last_letter\n\n if letter.isupper():\n dict_to_know = {'wr': 'R', 'wh': 'V', 'th': 'Z'}\n lonely_w = 'V'\n else:\n dict_to_know = {'wr': 'r', 'wh': 'v', 'th': 'z'}\n lonely_w = 'v'\n\n possible_last_letters = {'h', 'r'}\n\n if last_letter in possible_last_letters:\n letter_list[counter: counter+2] = dict_to_know[lowercase_letter]\n counter += 1\n\n else:\n letter_list[counter] = lonely_w\n\n counter -= 1\n last_letter = letter\n\n final_txt = ''.join(letter_list)\n\n return final_txt\n\n\ndef week_five(txt):\n letter_list = list(txt)\n\n copy_reverse_letter_list = letter_list.copy()\n\n last_letter = ''\n\n counter = -1\n\n for letter in copy_reverse_letter_list[::-1]:\n lowercase_letter = letter.lower()\n\n if lowercase_letter == 'o' and last_letter == 'u':\n lowercase_or_uppercase = {'o': 'u', 'O': 'U'}\n\n letter_list[counter: counter+2] = lowercase_or_uppercase[letter]\n\n counter += 1\n\n elif lowercase_letter == 'a' and last_letter == 'n':\n lowercase_or_uppercase_2 = {'a': 'u', 'A': 'U'}\n\n letter_list[counter] = lowercase_or_uppercase_2[letter]\n\n last_letter = lowercase_letter\n\n counter -= 1\n\n first_txt = ''.join(letter_list)\n\n re_to_find_ing = r'[a-zA-Z]{1,}ing\\b'\n\n all_words_ending_with_ing = re.findall(re_to_find_ing, first_txt)\n\n second_txt = first_txt\n\n for word in all_words_ending_with_ing:\n new_word = word[:-1] + 'k'\n second_txt = second_txt.replace(word, new_word)\n\n re_to_find_sm = r'\\b[sS][mM][a-zA-Z]*'\n\n\n all_words_starting_with_sm = re.findall(re_to_find_sm, second_txt)\n\n third_txt = second_txt\n\n for word in all_words_starting_with_sm:\n new_word = word[0] + 'ch' + word[1:]\n third_txt = third_txt.replace(word, new_word)\n\n final_txt = third_txt\n\n return final_txt", "def siegfried(week, txt):\n\n symbols = [' ', '.', '!', '-', '\\n']\n\n if week >= 1:\n rules = [['ci', 'si'], ['Ci', 'Si'],\n ['ce', 'se'], ['Ce', 'Se'],\n ['ch', '$$'], ['Ch', '$%'],\n ['c', 'k'], ['C', 'K'],\n ['$$', 'ch'], ['$%', 'Ch']]\n for translation in rules:\n txt = txt.replace(translation[0], translation[1])\n\n if week >= 2:\n rules = [['ph', 'f'], ['Ph', 'F']]\n for translation in rules:\n txt = txt.replace(translation[0], translation[1])\n\n if week >= 3:\n for position, letter in enumerate(txt):\n if letter == 'e' and position == len(txt) - 1 \\\n and txt[position - 2] not in symbols \\\n and txt[position - 3] not in symbols:\n txt = txt[:position] + '$'\n elif letter == 'e' and position not in [0, 1, 2, len(txt) - 1] \\\n and txt[position + 1] in symbols \\\n and txt[position - 2] not in symbols \\\n and txt[position - 3] not in symbols:\n txt = txt[:position] + '$' + txt[position + 1:]\n txt = txt.replace('$', '')\n\n for position, letter in enumerate(txt):\n if txt[position].lower() == txt[position - 1].lower() \\\n and position != 0\\\n and letter.isalpha() \\\n and letter not in symbols:\n txt = txt[:position] + '$' + txt[position + 1:]\n txt = txt.replace('$', '')\n\n if week >= 4:\n rules = [['th', 'z'], ['Th', 'Z'],\n ['wr', 'r'], ['Wr', 'R'],\n ['wh', 'v'], ['Wh', 'V'],\n ['w', 'v'], ['W', 'V']]\n for translation in rules:\n txt = txt.replace(translation[0], translation[1])\n\n if week >= 5:\n rules = [['ou', 'u'], ['Ou', 'U'],\n ['an', 'un'], ['An', 'Un']]\n for translation in rules:\n txt = txt.replace(translation[0], translation[1])\n\n for position, letter in enumerate(txt):\n if letter == 'i' and position == len(txt) - 3 \\\n and txt[position + 1] == 'n' \\\n and txt[position + 2] == 'g':\n txt = txt[:position + 2] + '$' + txt[position + 3:]\n elif letter == 'i' \\\n and txt[position + 1] == 'n' \\\n and txt[position + 2] == 'g' \\\n and txt[position + 3] in symbols:\n txt = txt[:position + 2] + '$' + txt[position + 3:]\n txt = txt.replace('$', 'k')\n\n for position, letter in enumerate(txt):\n if letter == 'S' \\\n and txt[position + 1] == 'm' \\\n and (txt[position - 1] in symbols or position == 0):\n txt = txt[:position] + '$$' + txt[position + 2:]\n txt = txt.replace('$$', 'Schm')\n\n return txt", "import re\ndef siegfried(week, txt):\n w = []\n t = txt.replace('ci', 'si').replace('Ci', 'Si').replace('ce', 'se').replace('Ce', 'Se').replace('c', 'k').replace('C', 'K').replace('kh', 'ch').replace('Kh', 'Ch')\n w.append(t)\n t = t.replace('ph', 'f').replace('Ph', 'F')\n w.append(t)\n t = re.sub(r'([A-Za-z])\\1', r'\\1', re.sub(r'([a-zA-Z]{3,})e\\b', r'\\1', t), flags = re.I)\n w.append(t)\n t = t.replace('th', 'z').replace('Th', 'Z').replace('wr', 'r').replace('Wr', 'R').replace('wh', 'v').replace('Wh', 'V').replace('w', 'v').replace('W', 'V')\n w.append(t)\n t = re.sub(r'\\b(s|S)(m)', r'\\1ch\\2', re.sub(r'ing\\b', r'ink', t.replace('ou', 'u').replace('an', 'un').replace('An', 'Un')))\n w.append(t)\n return w[week-1]"] | {"fn_name": "siegfried", "inputs": [[1, "City civilians"], [1, "Centre receiver"], [1, "Chatanooga choo choo crashed"], [1, "Capital city cats chew cheese"], [2, "Photo of 5 pheasants with graphs"], [3, "Meet me at the same place at noon"], [3, "The time is now"], [3, "Be quite quiet"], [3, "Aardvarks are nice most of the time"], [5, "And another thing Mr Smart, I want no more trouble!"], [5, "You ought to behave yourself Smart!"], [5, "Smart and 99 were husband and wife"], [5, ".. Mr Maxwell be The Smart .."], [5, ".. Mr Maxwell be We Smart .."], [5, "Be be The the We we Me me She she"], [5, "be Be the The we We me Me she She"], [5, "be the wee me"], [5, "be we Maxwell be We bee wee"], [5, "Be like Me"], [5, "be the same"], [5, "The same bee we see"], [5, "It was an inglorious ending"]], "outputs": [["Sity sivilians"], ["Sentre reseiver"], ["Chatanooga choo choo krashed"], ["Kapital sity kats chew cheese"], ["Foto of 5 feasants with grafs"], ["Met me at the sam plas at non"], ["The tim is now"], ["Be quit quiet"], ["Ardvarks are nis most of the tim"], ["Und unozer zink Mr Schmart, I vunt no mor trubl!"], ["Yu ught to behav yurself Schmart!"], ["Schmart und 99 ver husbund und vif"], [".. Mr Maxvel be Ze Schmart .."], [".. Mr Maxvel be Ve Schmart .."], ["Be be Ze ze Ve ve Me me She she"], ["be Be ze Ze ve Ve me Me she She"], ["be ze ve me"], ["be ve Maxvel be Ve be ve"], ["Be lik Me"], ["be ze sam"], ["Ze sam be ve se"], ["It vas un inglorius endink"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 16,451 |
def siegfried(week, txt):
|
2a05a9b3e87e8030e9281a5bd8939f0a | UNKNOWN | Many people choose to obfuscate their email address when displaying it on the Web. One common way of doing this is by substituting the `@` and `.` characters for their literal equivalents in brackets.
Example 1:
```
[email protected]
=> user_name [at] example [dot] com
```
Example 2:
```
[email protected]
=> af5134 [at] borchmore [dot] edu
```
Example 3:
```
[email protected]
=> jim [dot] kuback [at] ennerman-hatano [dot] com
```
Using the examples above as a guide, write a function that takes an email address string and returns the obfuscated version as a string that replaces the characters `@` and `.` with `[at]` and `[dot]`, respectively.
>Notes
>* Input (`email`) will always be a string object. Your function should return a string.
>* Change only the `@` and `.` characters.
>* Email addresses may contain more than one `.` character.
>* Note the additional whitespace around the bracketed literals in the examples! | ["def obfuscate(email):\n return email.replace(\"@\", \" [at] \").replace(\".\", \" [dot] \")\n", "def obfuscate(email):\n return email.replace('.', ' [dot] ').replace('@', ' [at] ')", "def obfuscate(email):\n '''\nSalvador Dali - The Persistence of Memory\n ;!>,!!!>\n <! ;!!!`\n !! `!!!.\n !!`,!!!>\n !! !!!!!\n ;!! !!!!!\n `!! !!!!!\n !! !!!!!> .\n `!.`!!!!! ;<!`\n !! `!!!! ,<!``\n `!> !!!! ;<!`\n <!! !!!!> ;!``\n !!! `!!!! !!` ,c,\n !!!> !!!!> ;`< ,cc$$cc .,r== $$c !\n !!!! !!!!!!. ,<!` !!!!!>>>;;;;;;;;.`\"?$$c MMMMMMM )MM ,= \"$$.`\n <!!> !!!!!!!!!!!!!>`` ,>```` ``````````!!!; ?$$c`MMMMMM.`MMMP== `$h\n `!! ;!!!!!!````.,;;;``` JF !;;;,,,,; 3$$.`MMMMMb MMMnnnM $$h\n ;!! <!!!!.;;!!!``` JF.!!!!!!!!!>`$$h `MMMMM MMMMMMM $$$\n ;!!>`!!!!!!`` ?> !!!!!!!!!> $$$ MMMMM MMMMMMM $$$\n <!! ;!!!!` `h !!!!!!!!!! $$$ MMMMMM MMMM\" M $$$\n `!>`!!!! b !!!!!!!!!! $$$ MMMMMM MMML,,`,$$$\n,,,,,, ;! <!!!> ,,,,,,,,,,,,,,,, $ !!!!!!!!!! $$$ MMMMMM MMMMML J$$F\n!!!!!! !! !!!! `!!!!!!!!!!!!!!` ; $ !!!!!!!!!! $$$ MMMMMP.MMMMMP $$$F\n!!!!! ;!! !!!!> !!!!!!!!!!!!` ;` .`.`.`. ?.`!!!!!!!!! 3$$ MMMP `MMMMM>,$$P\n!!!!` !!` !!!!> !!!!!!!!!!` ;!` `.`.`.`. `h !!!!!!!!! $$$ MMML MMPPP J$$`.\n!!!! !!!;!!!!!`;!!!!!!!!` ;!` .`.`.`.`.` ?,`!!!!!!!! ?$$ MMMMM.,MM_\"`,$$F .\n!!!`;!!!.!!!!` <!!!!!!!` ;` .`.`.`.`.`.`. ? `!!!!!!!>`$$ MMMMMbdML ` $$$ .\n``` !!!> !!!! ```````` ;! .`.`.`.`.`.`.`.` h `!!!!!!> $$ )MMMMMMMMM d$$` `.\n!!` !!!``!!! <!!!!!!!> ` .`.`.`.`.`.`.`.`.` `?,``!!!!! ?$h 4MMMMMMP z$$` .`.\n`` <!!! !!!> ```````` .`.`.`.`.`.`.`.`.`.`.` ?h.````..`$$ MMMMMM ,$$F `.`.\n` !!!! <!!!.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. `\"??$F\". `$h.`MMMMM $$$`.`.`.\n <!`! .!!!!> .`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. cccc `$$.`4MMP.3$F .`.`.\n<!``! !!!!!> .`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. J$$$$$F . \"$h.\" . 3$h .`.`.\n!` ! !!!!!!> .`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. \"\"\"\" .`.`.`$$, 4 3$$ .`.`.\n ;! !!!!!!! `.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. ?$h J$F .`.`.\n;` !!!!!!! `.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. \"$$$$P` .`.`.\n` <!!!!!!! `.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`. . .`.`.`.`.\n,` !!!!!!! .`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.`.\n!! !!!!!!`,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,,, `.`.`.`.\n!! !!!!!! !!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` `.`.`.`.\n!! <!!!!`;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!! ;! `.`.`.`.\n!! ;!!!!>`!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` <!! `.`.`.`.\n`,,!!!!``.;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` <!!! `.`.`.`.\n`````.,;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` <!!!! `.`.`.`.\n;!!!!!!!!!!!!!!!!!!!!!!!>>>````````````````````<!!!!!!!!!!!` !!!!!! `.`.`.`.\n!!!!!!!!!!!!!!!!!!````_,,uunmnmnmdddPPPPPPbbbnmnyy,_`````! !!!!!!! `.`.`.`.\n!!!!!!!!!``_``!``,nmMMPP\"\"\"`,.,ccccccr==pccccc,,..`\"\"444n,.`<!!!!!! `.`.`.`.\n!!!!!!!` ,dMM ,nMMP\"\",zcd$h.`$$$$$$$$L c $$$$$$$$$$??cc`4Mn <!!!!! `.`.`.`.\n!!!!!! ,MMMP uMMP ,d$$$$$$$$cd$F ??$$$$$cd$$$$$$$$$F, ??h.`Mx !!!!! `.`.`.`.\n!!!!!! MMMP uMM\",F,c \".$$$$$$$P` ?$$$$$$$$$$$$$$$$C`,J$$.`M.`!!!! `.`.`.`.\n!!!`` MMM 4MMM L`\"=-z$$P\".,,. ,c$$$$$$$$$$$$$$$$$$$$$$$ ML !!!! `.`.`.`.\n!!!. `\"\" MMMM `$$hc$$$L,c,.,czc$$$$$$$$$$$$$$$$$$$$$$$$$$.4M `!!! `.`.`.`.\n!!!!;;;.. `MMMb ?$$$$$$??\"\"\"\"?????????????????? ;.`$$$$$$$`JM`,!!! `.`.`.`.\n!!!!!!!!!> 4MMMb.\"?$$$cc,.. .,,cccccccccccccccc,c`.$$$$$$$ MM <!!! `.`.`.`.\n!!!!!!!!!! `MMMMMb,.\"??$$$$$$$$$$$$$$$$$$$$$$$$$$ $$$$?$$$ MM ;!!! `.`.`.`.\n!!!!!!!!!! \"4MMMMMMmn,.\"\"\"???$$$$$$$$$$$$$$$$$$ $$$\" ?$$ MP !!!! `.`.`.`.\n!!!!!!!!!!!;. \"4MMMMMMMMMMMmnmn,.`\"\"\"?????$$$$$$ ?$$ `CF.M> !!!! `.`.`.`.\n!!!!!!!!!!!!!!;. `\"\"44MMMMMMMMMMMMMMMMnnnn. ?$$$.<$$$h.$h MM !!!! `.`.`.`.\n!!!!!!!!!!!!!!!!>.;. `\"\"444MMMMMMMMMMMMMb $$$:<$$$$$$$ 4M <!!! `.`.`.`.\n!!!!!!!!!!!!!!!!!!!!!;<;);>;. ..\"\"\"\"44MMMM J$` <$$$$$$h`Mb`!!! `.`.`.`.\n!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!>; `MMM ?$. d$$$$$$$ MM.`!! `.`.`.`.\n!!!!!!!!!!```!``` ..``<!!!!!!!!!!!!!!!; . MMM.<$$ <$$$$$$$$ 4Mb !! `.`.`.`.\n!`````````.. ;MMMMnn.`!!!!!!!!!!!!!!! ; MMM J$$hJ$$$$$$$$h`MM !! `.`.`.`.\n,xmdMMMbnmx,. MMMMMMMr !!!!!!!!!!!!` ;: MMM $$$$$$$$$$??$$ MM !! `.`.`.`.\nP\"`.,,,,c`\"\"4MMn MMMMMMx !!!!!!!!!!!` <!: MMM $$$$$$$$L =c$F.MP !! `.`.`.`.\nub \"P4MM\")M,x,\"4b,`44MMMP !!!!!!!!!! !!!: MMM ???$$$$$$\"=,$ JM`;!! `.`.`.`.\nML,,nn4Mx`\"MMMx.`ML ;,,,;<!!!!!!!!! ;!!!!: MMM.-=,$$$$$$hc$`.MP !!! `.`.`.`.\n. MMM 4Mx`MMML MM,`<!!!!!!!!!!!! ;!!!!!` `MMb d$$$$$$$$` MM`,!!! `.`.`.`.\n,d\" MMn. .,nMMMM MMM `!!!!!!!!!!! ;!!!!!!> 4MMb `$$$$$$$`.dM`.!!!! `.`.`.`.\n.`\"M\"_\" MMMMMMMP,MMM ;!>>!!!!!!` <!!!!!!!!!. \"MMb $$$$$$$ dM ;!!!!! `.`.`.`.\n `nr;MMMMM\"MMMP dMM` >>!(!)<<! <!!!!!!!!!!!. `MM.`$$$$$$ MM !!!!!! `.`.`.`.\n\",Jn,\"\". ,uMMP dMMP /)<;>><>` .!!!!!!!!!!!!!!; `Mb $$$$$F;MP !!!!!! `.`.`.`.\n dPPM 4MMMMM\" dMMP (->;)<><` ;!!!!!!!!!!!!!!!!. 4M $$$$$h M>.!!!!!! `.`.`.`.\n=M uMMnMMM\" uMMM\" <!;/;->;` ;!!!!!!!!!!!!!!!!!; 4M.??\"$$`,M <!!!!!! `.`.`.`.\nJM )MMMM\" uMMMM`,!>;`(>!>` <!!!!!!!!!!!!!!!!!!! 4M> -??`,M` !!!!!!! `.`.`.`.\nMM `MP\" xdMMMP <(;<:)!`)` <!!!!!!!!!!!!!!!!!!!! MMb - ,M`;!!!!!!!` `.`.`.`.\nMP ,nMMMMP\" (>:)/;<:! !!!!!!!!!!!!!!!!!!!!!! `MM.-= d`;!!!!!!!! .`.`.`.`.\n,xndMMMMP\" .;)`;:`>(;: !!!!!!!!!!!!!!!!!!!!!!!; 4MMnndM <!!!!!!! .`.`.`.`.\nMMMMMP\" .;(:`-;(.><(` ;!!!!!!!!!!!!!!!!!!!!!!!!!, 4MMMMP !!!!!!!> `.`.`.`.`.\nP\"\" .,;<):(;/(\\`>-)` ;!!!!!!!!!!!!!!!!!!!!!!!!!!!>.`\"P\" <!!!!!!! .`.`.`.`.`.\n,<;),<-:><;,<- >;>` ;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!;;<!!!!!!!! .`.`.`.`.`.\n)-/;`<:<;\\->:(`;(` ;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` `.`.`.`.`.`.\n:\\;`<(.:>-;(;<>: <!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!! .`.`.`.`.`.`.\n(.;>:`<;:<;-/)/ <!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!! .`.`.`.`.`.`.\n;-;:>:.;`;(`;` ;!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!` `.`.`.`.`.`.`.\n '''\n return email.replace('@', ' [at] ').replace('.', ' [dot] ')", "def obfuscate(email):\n for rep in (('.', ' [dot] '), ('@', ' [at] ')):\n email = email.replace(*rep)\n return email", "obfuscate=lambda email: email.replace(\"@\",\" [at] \").replace(\".\",\" [dot] \")", "from functools import reduce\nREPLACEMENTS = ('.', ' [dot] '), ('@', ' [at] ')\n\n\ndef obfuscate(email):\n return reduce(lambda a, kv: a.replace(*kv), REPLACEMENTS, email)\n", "def obfuscate(email):\n obemail = \"\"\n for l in email:\n if l == \"@\":\n obemail += \" [at] \"\n elif l == \".\":\n obemail += \" [dot] \"\n else:\n obemail += l\n return obemail", "import re\n\ndef obfuscate(email):\n\n def substitute(m):\n if m.group(0) == '@': return \" [at] \"\n if m.group(0) == '.': return \" [dot] \"\n \n rgx = re.compile(r'(@|\\.)') # matches either an @ or a literal dot\n return re.sub(rgx, substitute, email)", "import re\n\npattern = re.compile(r\"[@\\.]\")\nobfusc = {'@':\" [at] \", '.': \" [dot] \"}.get\n\ndef obfuscate(email):\n return pattern.sub(lambda x: obfusc(x.group()), email)", "import re\n\n\ndef obfuscate(email):\n return re.sub(r\"[@\\.]\", lambda match: \" [at] \" if match.group(0)==\"@\" else \" [dot] \", email)"] | {"fn_name": "obfuscate", "inputs": [["[email protected]"], ["[email protected]"], ["[email protected]"], ["[email protected]"], ["Hmm, this would be better with input validation...!"]], "outputs": [["user_name [at] example [dot] com"], ["af5134 [at] borchmore [dot] edu"], ["jim [dot] kuback [at] ennerman-hatano [dot] com"], ["sir_k3v1n_wulf [at] blingblong [dot] net"], ["Hmm, this would be better with input validation [dot] [dot] [dot] !"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 7,686 |
def obfuscate(email):
|
0d00c90008f4db8872ab95b7d4c152c9 | UNKNOWN | ## Task
You have to write three functions namely - `PNum, GPNum and SPNum` (JS, Coffee), `p_num, g_p_num and s_p_num` (Python and Ruby), `pNum, gpNum and spNum` (Java, C#), `p-num, gp-num and sp-num` (Clojure) - to check whether a given argument `n` is a Pentagonal, Generalized Pentagonal, or Square Pentagonal Number, and return `true` if it is and `false` otherwise.
### Description
`Pentagonal Numbers` - The nth pentagonal number Pn is the number of distinct dots in a pattern of dots consisting of the outlines of regular pentagons with sides up to n dots (means the side contains n number of dots), when the pentagons are overlaid so that they share one corner vertex.
> First few Pentagonal Numbers are: 1, 5, 12, 22...
`Generalized Pentagonal Numbers` - All the Pentagonal Numbers along with the number of dots inside the outlines of all the pentagons of a pattern forming a pentagonal number pentagon are called Generalized Pentagonal Numbers.
> First few Generalized Pentagonal Numbers are: 0, 1, 2, 5, 7, 12, 15, 22...
`Square Pentagonal Numbers` - The numbers which are Pentagonal Numbers and are also a perfect square are called Square Pentagonal Numbers.
> First few are: 1, 9801, 94109401...
### Examples
#### Note:
* Pn = Nth Pentagonal Number
* Gpn = Nth Generalized Pentagonal Number
^ ^ ^ ^ ^
P1=1 P2=5 P3=12 P4=22 P5=35 //Total number of distinct dots used in the Pattern
Gp2=1 Gp4=5 Gp6=12 Gp8=22 //All the Pentagonal Numbers are Generalised
Gp1=0 Gp3=2 Gp5=7 Gp7=15 //Total Number of dots inside the outermost Pentagon | ["def p_num(n):\n r = (1 + (24*n+1)**.5) / 6\n return r.is_integer() and (3*(r**2) - r) / 2 == n \n \ndef g_p_num(n):\n r = ((1 + 24 * n) ** .5) % 6\n return r != 0 and r.is_integer()\n\ns_p_num=lambda n:(n**.5).is_integer() and p_num(n) and g_p_num(n)", "def get_p(n):\n mean = round((n * 2 / 3)**0.5)\n base = 3 * mean**2 // 2\n return {\"strict\": base - mean // 2, \"generalized\": base + (mean + 1) // 2}\n\ndef p_num(n):\n return n and get_p(n)[\"strict\"] == n\n\ndef g_p_num(n):\n return n in get_p(n).values()\n\ndef s_p_num(n):\n return p_num(n) and not (n**0.5 % 1)", "def isqrt(num):\n '''Compute int(sqrt(n)) for n integer > 0\n O(log4(n)) and no floating point operation, no division'''\n res, bit = 0, 1\n while bit <= num:\n bit <<= 2\n bit >>= 2\n\n while bit:\n if num >= res + bit:\n num -= res + bit\n res += bit << 1\n res >>= 1\n bit >>= 2\n return res\n\ndef sqrt(n):\n # return n ** .5 ## Nope\n # return __import__('math').sqrt(n) ## Not enough precision...\n # return __import__('decimal').Decimal(n).sqrt() ## That one works great!\n return isqrt(n) ## The very best, you don't need those nasty floating point numbers\n\ndef s_num(n):\n return sqrt(n) ** 2 == n\n\ndef g_p_num(n):\n return s_num(24 * n + 1)\n\ndef p_num(n):\n return g_p_num(n) and sqrt(24 * n + 1) % 6 == 5\n\ndef s_p_num(n):\n return s_num(n) and p_num(n) ", "from decimal import Decimal\np_num = lambda n: ((24 * Decimal(n) + 1) ** Decimal(.5) + 1) / 6 % 1 < 0.0000000000000001\ng_p_num = lambda n: (24 * Decimal(n) + 1) ** Decimal(.5) % 1 < 0.0000000000000001\ns_p_num = lambda n: Decimal(n) ** Decimal(.5) % 1 < 0.0000000000000001 and p_num(n)", "from decimal import Decimal\np_num=lambda n: (lambda deltasq: ((1+deltasq)/6)%1==0)(Decimal(1+24*n).sqrt())\ng_p_num=lambda n: (lambda deltasq: ((1+deltasq)/6)%1==0 or ((1-deltasq)/6)%1==0)(Decimal(1+24*n).sqrt())\ns_p_num=lambda n: p_num(n) and Decimal(n).sqrt()%1==0", "def p_num(n):\n if str(n)[:4] == '8635':\n return False\n return (((24*n + 1)**.5 + 1)/6) % 1 == 0\n\ndef g_p_num(n):\n if str(n)[:4] == '8635':\n return False \n return (24 * n + 1)**.5 % 1 == 0\n\ndef s_p_num(n):\n return round(n ** .5 % 1, 5) == 0 and p_num(n)", "def p_num(n):\n k = int((1 + (1 + 24 * n) ** 0.5) / 6)\n return n > 0 and k * (3 * k - 1) == 2 * n\n \ndef g_p_num(n):\n k = int((1 - (1 + 24 * n) ** 0.5) / 6)\n return k * (3 * k - 1) == 2 * n or p_num(n)\n \ndef s_p_num(n):\n return n ** 0.5 % 1 == 0 and p_num(n)", "def p_num(n):\n return (((24*n +1)**0.5 +1) / 6).is_integer() if n != 8635759279119974976128748222533 else False\n\ndef g_p_num(n):\n return (((24*n + 1)**0.5 + 1 )).is_integer() if n != 8635759279119974976128748222533 else False\n\ndef s_p_num(n):\n return (((24*n + 1)**0.5 + 1) / 6).is_integer() and int(n**0.5)**2 == n", "def g_p_num(n):\n return isqrt(1 + 24*n) >= 0\n\nfrom decimal import Decimal\ndef isqrt(n):\n sqrt = Decimal(n).sqrt()\n i = int(sqrt)\n if i == sqrt:\n return i\n return -1\n\ndef s_p_num(n):\n return n in {1, 9801, 94109401, 903638458801, 8676736387298001, 83314021887196947001, 799981229484128697805801, 7681419682192581869134354401, 73756990988431941623299373152801, 708214619789503821274338711878841001, 6800276705461824703444258688161258139001}\n\ndef p_num(n):\n d = isqrt(1 + 24*n)\n return d >= 0 and (int(d) + 1) % 6 == 0", "pentagonals = set()\ngeneralized_pentagonals = set([0])\nsquare_pentagonals = set()\nfor i in range(1, 10**6):\n x = (3*i**2 - i) // 2\n y = (3*i**2 + i) // 2\n pentagonals.add(x)\n generalized_pentagonals.add(x)\n generalized_pentagonals.add(y)\n if (x**0.5).is_integer():\n square_pentagonals.add(x)\n\n\ndef p_num(n):\n return n in pentagonals\n\ndef g_p_num(n):\n return n in generalized_pentagonals\n\ndef s_p_num(n):\n return n in square_pentagonals"] | {"fn_name": "p_num", "inputs": [[0], [1], [2], [3], [4], [5], [100]], "outputs": [[false], [true], [false], [false], [false], [true], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 4,072 |
def p_num(n):
|
8161f0ac0295f8bbe1660c3bb9fb28a5 | UNKNOWN | You've just recently been hired to calculate scores for a Dart Board game!
Scoring specifications:
* 0 points - radius above 10
* 5 points - radius between 5 and 10 inclusive
* 10 points - radius less than 5
**If all radii are less than 5, award 100 BONUS POINTS!**
Write a function that accepts an array of radii (can be integers and/or floats), and returns a total score using the above specification.
An empty array should return 0.
## Examples: | ["def score_throws(radiuses):\n score = 0\n for r in radiuses:\n if r < 5:\n score += 10\n elif 5 <= r <= 10:\n score += 5\n if radiuses and max(radiuses) < 5:\n score += 100\n return score", "def score_throws(radiuses):\n scores = [5 * ((v<5) + (v<=10)) for v in radiuses]\n return sum(scores) + 100 * (len(set(scores)) == 1 and scores[0] == 10)", "def score_throws(radii): \n score = sum(10 if r < 5 else 5 if r <= 10 else 0 for r in radii) \n return score + 100 if score and len(radii) * 10 == score else score", "def score_throws(radii):\n return 5*sum((r<=10)+(r<5) for r in radii)+(100 if len(radii) and all(r<5 for r in radii) else 0)", "def score_throws(radii):\n if not radii: return 0\n sum=0\n for i in radii:\n if i>=5 and i<=10:\n sum+=5\n elif i<5:\n sum+=10\n return sum+100 if max(radii)<5 else sum", "def score_throws(radii):\n score = sum(0 if r > 10 else 5 if r >= 5 else 10 for r in radii)\n return score + 100 * (score and len(radii) * 10 == score)", "def score_throws(radiuses):\n return sum(\n 10 if r < 5 else 5 if r <= 10 else 0\n for r in radiuses\n ) + (bool(radiuses) and all(r < 5 for r in radiuses)) * 100", "def score_throws(r):\n if not r:\n return 0\n if all(i < 5 for i in r):\n return len(r) * 10 + 100\n return len([i for i in r if i <= 10 and i >= 5]) * 5 + len([i for i in r if i < 5]) * 10", "def score_throws(radii):\n score = sum(0 if r > 10 else 5 if r >= 5 else 10 for r in radii)\n if radii and all(r < 5 for r in radii): score += 100\n return score", "def score_throws(radii):\n result = 0\n all_less_5 = True\n for r in radii:\n if r > 10:\n all_less_5 = False\n elif r < 5:\n result += 10\n else:\n result += 5\n all_less_5 = False\n if radii and all_less_5:\n result += 100\n return result"] | {"fn_name": "score_throws", "inputs": [[[1, 5, 11]], [[15, 20, 30, 31, 32, 44, 100]], [[1, 2, 3, 4]], [[]], [[1, 2, 3, 4, 5, 6, 7, 8, 9]], [[0, 5, 10, 10.5, 4.5]], [[1]], [[21, 10, 10]], [[5, 5, 5, 5]], [[4.9, 5.1]]], "outputs": [[15], [0], [140], [0], [65], [30], [110], [10], [20], [15]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,999 |
def score_throws(radii):
|
03b5c2be5310576035eb4209f40be0cb | UNKNOWN | In this Kata, you will be given an array of arrays and your task will be to return the number of unique arrays that can be formed by picking exactly one element from each subarray.
For example: `solve([[1,2],[4],[5,6]]) = 4`, because it results in only `4` possiblites. They are `[1,4,5],[1,4,6],[2,4,5],[2,4,6]`.
```if:r
In R, we will use a list data structure.
So the argument for `solve` is a list of numeric vectors.
~~~
solve(list(c(1, 2), 4, c(5, 6)))
[1] 4
~~~
```
Make sure that you don't count duplicates; for example `solve([[1,2],[4,4],[5,6,6]]) = 4`, since the extra outcomes are just duplicates.
See test cases for more examples.
Good luck!
If you like this Kata, please try:
[Sum of integer combinations](https://www.codewars.com/kata/59f3178e3640cef6d90000d5)
[Sum of array singles](https://www.codewars.com/kata/59f11118a5e129e591000134) | ["from operator import mul\nfrom functools import reduce\n\ndef solve(arr):\n return reduce(mul, map(len, map(set, arr)), 1)", "def solve(arr):\n x = 1\n for a in arr:\n x *= len(set(a))\n return x", "from functools import reduce\nfrom operator import mul\ndef solve(arr):\n return reduce(mul, map(len, map(set, arr)))", "from functools import reduce\n\n\ndef solve(arr):\n return reduce(int.__mul__, (len(set(lst)) for lst in arr))", "from functools import reduce\n\ndef solve(arr):\n return reduce(int.__mul__, map(len, map(set, arr)))", "def solve(arr):\n res = 1\n for a in arr: res *= len(set(a))\n return res\n", "import functools\nfrom operator import mul\ndef solve(arr):\n return functools.reduce(mul, (len(set(i)) for i in arr))", "def solve(arr):\n from functools import reduce\n return reduce(lambda a,b: a*b, [len(set(a)) for a in arr])", "from operator import mul\nfrom functools import reduce\n\ndef solve(arr):\n return reduce(mul, (len(set(xs)) for xs in arr), 1)"] | {"fn_name": "solve", "inputs": [[[[1, 2], [4], [5, 6]]], [[[1, 2], [4, 4], [5, 6, 6]]], [[[1, 2], [3, 4], [5, 6]]], [[[1, 2, 3], [3, 4, 6, 6, 7], [8, 9, 10, 12, 5, 6]]]], "outputs": [[4], [4], [8], [72]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,031 |
def solve(arr):
|
cd02d1a123f1a70efcbebc88ff39b209 | UNKNOWN | # Task
You are given a `moment` in time and space. What you must do is break it down into time and space, to determine if that moment is from the past, present or future.
`Time` is the sum of characters that increase time (i.e. numbers in range ['1'..'9'].
`Space` in the number of characters which do not increase time (i.e. all characters but those that increase time).
The moment of time is determined as follows:
```
If time is greater than space, than the moment is from the future.
If time is less than space, then the moment is from the past.
Otherwise, it is the present moment.```
You should return an array of three elements, two of which are false, and one is true. The true value should be at the `1st, 2nd or 3rd` place for `past, present and future` respectively.
# Examples
For `moment = "01:00 pm"`, the output should be `[true, false, false]`.
time equals 1, and space equals 7, so the moment is from the past.
For `moment = "12:02 pm"`, the output should be `[false, true, false]`.
time equals 5, and space equals 5, which means that it's a present moment.
For `moment = "12:30 pm"`, the output should be `[false, false, true]`.
time equals 6, space equals 5, so the moment is from the future.
# Input/Output
- `[input]` string `moment`
The moment of time and space that the input time came from.
- `[output]` a boolean array
Array of three elements, two of which are false, and one is true. The true value should be at the 1st, 2nd or 3rd place for past, present and future respectively. | ["def moment_of_time_in_space(moment):\n d = sum(int(c) if c in '123456789' else -1 for c in moment)\n return [d < 0, d == 0, d > 0]", "def moment_of_time_in_space(moment):\n time = sum(int(c) for c in moment if c in \"123456789\")\n space = sum(c not in \"123456789\" for c in moment)\n return [time < space, time == space, time > space]", "def moment_of_time_in_space(moment):\n time = 0\n space = 0\n for ch in moment:\n if ch == '0' or not ch.isdigit():\n space += 1\n elif ch in '123456789':\n time += int(ch)\n return [time < space, time == space, time > space]", "def moment_of_time_in_space(moment):\n t = sum(int(d) for d in moment if d.isdigit() and d!='0')\n s = sum(c=='0' or not c.isdigit() for c in moment)\n \n return [t<s, t==s, t>s]", "from re import findall\ndef moment_of_time_in_space(moment):\n time=sum(map(int,findall(\"\\d\",moment)))\n space=len(findall(\"[^1-9]\",moment))\n states=[False for _ in range(3)]\n if time>space:states[2]=True\n elif time<space:states[0]=True\n else:states[1]=True\n return states", "def moment_of_time_in_space(s):\n a = [int(x) for x in s if \"1\" <= x <= \"9\"]\n n, m = sum(a), len(s) - len(a)\n return [n < m, n == m, n > m]", "def moment_of_time_in_space(moment):\n time = int(moment[0]) + int(moment[1]) + int(moment[3]) + int(moment[4])\n space = 4 + moment.count(\"0\")\n return [time < space, time == space, time > space]", "def moment_of_time_in_space(moment):\n a = sum(int(i) for i in moment if i in '123456789')\n b = sum(1 for i in moment if i not in '123456789')\n return [a<b, a==b, a>b]", "def moment_of_time_in_space(moment):\n t,s=0,0\n for c in moment:\n if c.isdigit() and c!='0':\n t+=int(c)\n else:\n s+=1\n if t<s:\n return [True,False,False]\n elif t>s:\n return [False,False,True]\n else:\n return [False,True,False]", "def moment_of_time_in_space(moment):\n l = [int(i) for i in moment if i.isdigit() and i != '0']\n time = sum(l)\n space = 8 - len(l)\n return [True, False, False] if space > time else\\\n ([False, True, False] if space==time else [ False, False, True])"] | {"fn_name": "moment_of_time_in_space", "inputs": [["12:30 am"], ["12:02 pm"], ["01:00 pm"], ["11:12 am"], ["05:20 pm"], ["04:20 am"]], "outputs": [[[false, false, true]], [[false, true, false]], [[true, false, false]], [[false, false, true]], [[false, false, true]], [[false, true, false]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,256 |
def moment_of_time_in_space(moment):
|
b92ad2eab5506863e8583ec7c647a987 | UNKNOWN | # Task
You know the slogan `p`, which the agitators have been chanting for quite a while now. Roka has heard this slogan a few times, but he missed almost all of them and grasped only their endings. You know the string `r` that Roka has heard.
You need to determine what is the `minimal number` of times agitators repeated the slogan `p`, such that Roka could hear `r`.
It is guaranteed the Roka heard nothing but the endings of the slogan P repeated several times.
# Example
For `p = "glorytoukraine", r = "ukraineaineaine"`, the output should be `3`.
The slogan was `"glorytoukraine"`, and Roka heard `"ukraineaineaine"`.
He could hear it as follows: `"ukraine"` + `"aine"` + `"aine"` = `"ukraineaineaine"`.
# Input/Output
- `[input]` string `p`
The slogan the agitators chanted, a string of lowecase Latin letters.
- `[input]` string `r`
The string of lowercase Latin letters Roka has heard.
- `[output]` an integer
The `minimum number` of times the agitators chanted. | ["import re\ndef slogans(p,r):\n reg=re.compile(\"|\".join([p[i:] for i in range(len(p))]))\n return len(re.findall(reg,r))", "from re import findall\n\ndef slogans(p, r):\n return len(findall('|'.join(p[i:] for i in range(len(p))), r))", "def slogans(slogan,heard):\n li = []\n while heard:\n temp = len(slogan)\n while True:\n if slogan.endswith(heard[:temp]):\n heard = heard[temp:]\n li.append(1)\n break\n temp -= 1\n return sum(li)", "def slogans(p,r):\n start = 0\n count = 0\n for i in range(1, len(r)):\n if r[start:i+1] not in p:\n count += 1\n start = i\n return count + 1", "def slogans(p,r):\n c = 0\n while r:\n t, f = p[:], r[0]\n x = t.find(f)\n t = t[x:]\n if t == r[:len(t)]:\n r = r[len(t):]\n c += 1\n else:\n x = 0\n while x < len(r):\n try:\n x = t.find(f, 1)\n t = t[x:]\n if t == r[:len(t)]:\n r = r[len(t):]\n c += 1\n break\n except:\n break\n return c", "def slogans(p,r):\n count, tmp = 0, \"\"\n for char in r:\n if tmp + char in p:\n tmp += char\n else:\n count += 1\n tmp = char\n return count + 1\n", "def slogans(p,r):\n c=0\n while(r):\n for i in range(len(p)):\n if r.startswith(p[i:]):\n c+=1\n r=r[len(p[i:]):]\n break\n return c ", "def slogans(p, r):\n ans = 0\n while r:\n ans += 1\n for i in range(len(p)):\n if r.startswith(p[i:]):\n r = r[len(p) - i:]\n break\n return ans", "import re\n# def slogans(p,r):\n# if not r:return 0\n# n=0\n# while not r.startswith(p[n:]):n+=1\n# return 1+slogans(p,r.replace(p[n:],'',1))\ndef slogans(p,r):\n if not r:return 0\n return 1+slogans(p,r.replace(re.search(r'.+?(.+)\\s\\1.*',' '.join([p,r])).group(1),'',1))", "from re import findall\n\ndef slogans(p, r):\n pattern = '(' + '|'.join(p[i:] for i in range(len(p))) + ')'\n return len(findall(pattern, r))"] | {"fn_name": "slogans", "inputs": [["glorytoukraine", "ukraineaineaine"], ["glorytoukraine", "ukraineaineainee"], ["glorytoukraine", "einene"], ["programming", "ingmingmming"], ["mcoecqwmjdudc", "dcoecqwmjdudcdcudc"], ["erjernhxvbqfjsj", "ernhxvbqfjsjjrnhxvbqfjsjjernhxvbqfjsj"], ["dhgusdlifons", "lifonsssdlifonsgusdlifonssnsdlifonsslifonsifonsdlifonsfonsifons"]], "outputs": [[3], [4], [3], [3], [4], [4], [13]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,349 |
def slogans(p,r):
|
b66e5b16d2c2ba274861338b858c959b | UNKNOWN | Given a list of integers, return the nth smallest integer in the list. **Only distinct elements should be considered** when calculating the answer. `n` will always be positive (`n > 0`)
If the nth small integer doesn't exist, return `-1` (C++) / `None` (Python) / `nil` (Ruby) / `null` (JavaScript).
Notes:
* "indexing" starts from 1
* huge lists (of 1 million elements) will be tested
## Examples
```python
nth_smallest([1, 3, 4, 5], 7) ==> None # n is more than the size of the list
nth_smallest([4, 3, 4, 5], 4) ==> None # 4th smallest integer doesn't exist
nth_smallest([45, -10, 4, 5, 4], 4) ==> 45 # 4th smallest integer is 45
```
If you get a timeout, just try to resubmit your solution. However, if you ***always*** get a timeout, review your code. | ["def nth_smallest(arr, n):\n s = set(arr)\n return sorted(s)[n-1] if n<=len(s) else None", "def nth_smallest(arr, n):\n try:\n return sorted(set(arr))[n-1]\n except:\n pass", "import random\n\ndef partition(vector, left, right, pivotIndex):\n pivotValue = vector[pivotIndex]\n vector[pivotIndex], vector[right] = vector[right], vector[pivotIndex] # Move pivot to end\n storeIndex = left\n for i in range(left, right):\n if vector[i] < pivotValue:\n vector[storeIndex], vector[i] = vector[i], vector[storeIndex]\n storeIndex += 1\n vector[right], vector[storeIndex] = vector[storeIndex], vector[right] # Move pivot to its final place\n return storeIndex\n\ndef select(vector, left, right, k):\n \"Returns the k-th smallest, (k >= 0), element of vector within vector[left:right+1] inclusive.\"\n if k < len(vector):\n while True:\n pivotIndex = random.randint(left, right) # select pivotIndex between left and right\n pivotNewIndex = partition(vector, left, right, pivotIndex)\n pivotDist = pivotNewIndex - left\n if pivotDist == k:\n return vector[pivotNewIndex]\n elif k < pivotDist:\n right = pivotNewIndex - 1\n else:\n k -= pivotDist + 1\n left = pivotNewIndex + 1\n\ndef nth_smallest(arr, n):\n unique = list(set(arr))\n return select(unique, 0, len(unique)-1, n-1)", "def nth_smallest(arr,k):\n \n if len(set(arr)) == 1:\n return arr[0]\n else:\n if len(set(arr)) < k:\n return None\n\n pivot = arr[0]\n lesser = list(set([x for x in arr[1:] if x < pivot]))\n greater = list(set([x for x in arr[1:] if x > pivot]))\n\n if len(lesser) >= k:\n return nth_smallest(lesser,k)\n \n elif len(lesser) == k-1:\n return pivot\n \n else:\n return nth_smallest(greater, k-len(lesser)-1)\n", "def nth_smallest(arr, n):\n s = set(arr)\n return None if len(s) < n else sorted(s)[n-1]", "def nth_smallest(arr, n):\n s = set(arr)\n if n <= len(s):\n return sorted(s)[n-1]", "from heapq import *\ndef nth_smallest(arr, n):\n arr = list(set(arr))\n heapify(arr)\n\n if n > len(arr):\n return None\n\n x = 0\n for i in range(n):\n x = heappop(arr)\n return x", "def nth_smallest(arr, n):\n x = list(dict.fromkeys(arr))\n x.sort(key=int)\n return None if n > len(x) else x[n-1]", "nth_smallest = lambda a,n: None if len(set(a))<n else list(sorted(set(a)))[n-1]", "def nth_smallest(arr, n):\n sorted_arr = sorted(set(arr))\n if n <= len(sorted_arr):\n return sorted_arr[n - 1]", "def nth_smallest(arr, n):\n arr=sorted(list(set(arr)))\n if n > len(arr):\n return None\n return arr[n-1]"] | {"fn_name": "nth_smallest", "inputs": [[[14, 12, 46, 34, 334], 3], [[4000], 1], [[14, 12, 46, 0, 334], 1]], "outputs": [[34], [4000], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,863 |
def nth_smallest(arr, n):
|
2fe386967b5080d85153898153eb7a87 | UNKNOWN | It started as a discussion with a friend, who didn't fully grasp some way of setting defaults, but I thought the idea was cool enough for a beginner kata: binary `OR` each matching element of two given arrays (or lists, if you do it in Python; vectors in c++) of integers and give the resulting ORed array [starts to sound like a tonguetwister, doesn't it?].
If one array is shorter than the other, use the optional third parametero (defaulted to `0`) to `OR` the unmatched elements.
For example:
```python
or_arrays([1,2,3],[1,2,3]) == [1,2,3]
or_arrays([1,2,3],[4,5,6]) == [5,7,7]
or_arrays([1,2,3],[1,2]) == [1,2,3]
or_arrays([1,2],[1,2,3]) == [1,2,3]
or_arrays([1,2,3],[1,2,3],3) == [1,2,3]
``` | ["from itertools import zip_longest\n\ndef or_arrays(a1, a2, d=0):\n return [x|y for x,y in zip_longest(a1, a2, fillvalue=d)]", "def or_arrays(a, b, d=0):\n return [(a[i] if i<len(a) else d)|(b[i] if i<len(b) else d) for i in range(max(len(a), len(b)))]", "from itertools import zip_longest\n\ndef or_arrays(arr1, arr2, fill=0):\n return [a | b for a, b in zip_longest(arr1, arr2, fillvalue=fill)]", "def or_arrays(arr1, arr2, n = 0):\n \n result = []\n \n for i in range(max(len(arr1), len(arr2))):\n \n a = b = n;\n \n if i < len(arr1):\n a = arr1[i]\n \n if i < len(arr2):\n b = arr2[i]\n \n result.append(b|a);\n \n return result", "import itertools\n\ndef or_arrays(arr1, arr2, arg=0):\n return [i | j for i, j in itertools.zip_longest(arr1, arr2, fillvalue=arg)]", "from itertools import zip_longest\n\ndef or_arrays(arr1, arr2, default=0):\n return [a | b for a, b in zip_longest(arr1, arr2, fillvalue=default)]", "from itertools import zip_longest\n\ndef or_arrays(a, b, filler=0):\n return [ x | y for x, y in zip_longest(a, b, fillvalue=filler)]\n", "def or_arrays(arr1, arr2, o = 0):\n xs = []\n i = 0\n while i < len(arr1) and i < len(arr2):\n xs.append(arr1[i] | arr2[i])\n i += 1\n while i < len(arr1):\n xs.append(arr1[i] | o)\n i += 1\n while i < len(arr2):\n xs.append(arr2[i] | o)\n i += 1\n return xs", "def or_arrays(a, b, filler=0):\n al, bl = len(a), len(b)\n return list([v[0] | v[1] for v in zip(a + [filler] * (max(al, bl) - al), b + [filler] * (max(al, bl) - bl))])\n", "or_arrays=lambda a1, a2, d=0: [(a1[i] if i<len(a1) else d)|(a2[i] if i<len(a2) else d) for i in range(max(len(a1),len(a2)))]", "def or_arrays(arr1, arr2, default = 0):\n if len(arr1) < len(arr2):\n arr1 += [default]* (len(arr2) - len(arr1))\n if len(arr2) < len(arr1):\n arr2 += [default]* (len(arr1) - len(arr2))\n return [x|y for x,y in zip(arr1,arr2)]", "def or_arrays(x,y,z=0,m=max,l=len):\n return [(a|b) for a,b in zip((x+[z]*(m(l(x),l(y))-l(x))), (y+[z]*(m(l(x),l(y))-l(y))))]", "def or_arrays(x,y,z=0):\n m = max(len(x), len(y))\n return [(a|b) for a,b in zip((x+[z]*m)[:m], (y+[z]*m)[:m])]", "def or_arrays(x,y,mod=0):\n m = maxLen = max(len(x), len(y))\n return [(a|b) for a,b in zip((x+[mod]*m)[:m], (y+[mod]*m)[:m])]", "def ind(arr, i, default):\n return default if len(arr) - 1 < i else arr[i]\n\ndef or_arrays(arr1, arr2, default=0):\n return [ind(arr1, i, default) | ind(arr2, i, default) for i in range(max(len(arr1), len(arr2)))]", "from itertools import zip_longest\n\ndef or_arrays(*args):\n p, q, *c = args\n return [a | b for a, b in zip_longest(p, q, fillvalue=c[0] if c else 0)]", "from itertools import starmap, zip_longest\nor_arrays = lambda a1, a2, f=0: list(starmap(int.__or__, zip_longest(a1, a2, fillvalue=f)))", "def or_arrays(arr1, arr2,n=0):\n res=[]\n i=0\n while i<len(arr1) and i<len(arr2):\n res.append(arr1[i] | arr2[i])\n i+=1\n while i<len(arr1):\n res.append(arr1[i] | n)\n i+=1\n while i<len(arr2):\n res.append(arr2[i] | n)\n i+=1\n return res", "def or_arrays(arr1, arr2, p=0):\n if len(arr2)>len(arr1):\n arr2,arr1=arr1,arr2\n \n arr2 += [p]*(len(arr1)-len(arr2))\n \n return [arr1[i] | arr2[i] for i in range(len(arr1))]", "def or_arrays(arr1, arr2, d=0):\n a, b = sorted([arr1, arr2], key=len)\n a = a + (len(b)-len(a))*[d]\n return [x|y for x,y in zip(a,b)]"] | {"fn_name": "or_arrays", "inputs": [[[1, 2, 3], [1, 2, 3]], [[1, 2, 3], [4, 5, 6]], [[1, 2, 3], [1, 2]], [[1, 0], [1, 2, 3]], [[1, 0, 3], [1, 2, 3], 3]], "outputs": [[[1, 2, 3]], [[5, 7, 7]], [[1, 2, 3]], [[1, 2, 3]], [[1, 2, 3]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,635 |
def or_arrays(a, b, filler=0):
|
5babda6e691f38b5eeb11843d72f4c34 | UNKNOWN | "The Shell Game" involves cups upturned on a playing surface, with a ball placed underneath one of them. The index of the cups are swapped around multiple times. After that the players will try to find which cup contains the ball.
Your task is as follows. Given the cup that the ball starts under, and list of swaps, return the location of the ball at the end. Cups are given like array/list indices.
For example, given the starting position `0` and the swaps `[(0, 1), (1, 2), (1, 0)]`:
* The first swap moves the ball from `0` to `1`
* The second swap moves the ball from `1` to `2`
* The final swap doesn't affect the position of the ball.
So
```python
find_the_ball(0, [(0, 1), (2, 1), (0, 1)]) == 2
```
There aren't necessarily only three cups in this game, but there will be at least two. You can assume all swaps are valid, and involve two distinct indices. | ["def find_the_ball(start, swaps):\n pos = start\n for (a, b) in swaps:\n if a == pos:\n pos = b\n elif b == pos:\n pos = a\n return pos\n", "def find_the_ball(start, swaps):\n for sw in swaps:\n if start in sw:\n start = sw[1-sw.index(start)]\n return start", "def find_the_ball(start, swaps):\n \"\"\" Where is the ball? \"\"\"\n for (a, b) in swaps:\n if a == start: start = b\n elif b == start: start = a\n return start", "def find_the_ball(start, swaps):\n \"\"\" Where is the ball? \"\"\"\n ball = start\n for (a, b) in swaps:\n if ball == a:\n ball = b\n elif ball == b:\n ball = a\n return ball\n", "def find_the_ball(start, swaps):\n pos = start\n for tup in swaps:\n if pos == tup[0]: pos = tup[1]\n elif pos == tup[1]: pos = tup[0]\n return pos\n \n \n", "def find_the_ball(start, swaps):\n \"\"\" Where is the ball? \"\"\"\n arr = [0] * 100000\n arr[start] = 1\n for (x, y) in swaps:\n arr[x], arr[y] = arr[y], arr[x]\n return arr.index(1)", "def find_the_ball(pos, swaps):\n for swap in swaps:\n if pos in swap:\n pos = swap[1 - swap.index(pos)]\n return pos", "def find_the_ball(pos, swaps):\n for a, b in swaps:\n pos = a if pos == b else b if pos == a else pos\n return pos", "def find_the_ball(k, li):\n for i in li:\n if k in i : k = [i[0],i[1]][i[0]==k]\n return k"] | {"fn_name": "find_the_ball", "inputs": [[5, []], [0, []], [9, []], [0, [[0, 1], [1, 2], [2, 3], [3, 4], [4, 5], [5, 6], [6, 7], [7, 8], [8, 9], [9, 10], [10, 11], [11, 12], [12, 13], [13, 14], [14, 15], [15, 16], [16, 17], [17, 18], [18, 19], [19, 20], [20, 21], [21, 22], [22, 23], [23, 24], [24, 25], [25, 26], [26, 27], [27, 28], [28, 29], [29, 30], [30, 31], [31, 32], [32, 33], [33, 34], [34, 35], [35, 36], [36, 37], [37, 38], [38, 39], [39, 40], [40, 41], [41, 42], [42, 43], [43, 44], [44, 45], [45, 46], [46, 47], [47, 48], [48, 49], [49, 50], [50, 51], [51, 52], [52, 53], [53, 54], [54, 55], [55, 56], [56, 57], [57, 58], [58, 59], [59, 60], [60, 61], [61, 62], [62, 63], [63, 64], [64, 65], [65, 66], [66, 67], [67, 68], [68, 69], [69, 70], [70, 71], [71, 72], [72, 73], [73, 74], [74, 75], [75, 76], [76, 77], [77, 78], [78, 79], [79, 80], [80, 81], [81, 82], [82, 83], [83, 84], [84, 85], [85, 86], [86, 87], [87, 88], [88, 89], [89, 90], [90, 91], [91, 92], [92, 93], [93, 94], [94, 95], [95, 96], [96, 97], [97, 98], [98, 99], [99, 100], [100, 101]]], [0, [[1, 0], [2, 1], [3, 2], [4, 3], [5, 4], [6, 5], [7, 6], [8, 7], [9, 8], [10, 9], [11, 10], [12, 11], [13, 12], [14, 13], [15, 14], [16, 15], [17, 16], [18, 17], [19, 18], [20, 19], [21, 20], [22, 21], [23, 22], [24, 23], [25, 24], [26, 25], [27, 26], [28, 27], [29, 28], [30, 29], [31, 30], [32, 31], [33, 32], [34, 33], [35, 34], [36, 35], [37, 36], [38, 37], [39, 38], [40, 39], [41, 40], [42, 41], [43, 42], [44, 43], [45, 44], [46, 45], [47, 46], [48, 47], [49, 48], [50, 49], [51, 50], [52, 51], [53, 52], [54, 53], [55, 54], [56, 55], [57, 56], [58, 57], [59, 58], [60, 59], [61, 60], [62, 61], [63, 62], [64, 63], [65, 64], [66, 65], [67, 66], [68, 67], [69, 68], [70, 69], [71, 70], [72, 71], [73, 72], [74, 73], [75, 74], [76, 75], [77, 76], [78, 77], [79, 78], [80, 79], [81, 80], [82, 81], [83, 82], [84, 83], [85, 84], [86, 85], [87, 86], [88, 87], [89, 88], [90, 89], [91, 90], [92, 91], [93, 92], [94, 93], [95, 94], [96, 95], [97, 96], [98, 97], [99, 98], [100, 99], [101, 100]]]], "outputs": [[5], [0], [9], [101], [101]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,488 |
def find_the_ball(start, swaps):
|
f7f75c9522946dc7adebf05c8657b1c1 | UNKNOWN | # Task
Pero has been into robotics recently, so he decided to make a robot that checks whether a deck of poker cards is complete.
He’s already done a fair share of work - he wrote a programme that recognizes the suits of the cards. For simplicity’s sake, we can assume that all cards have a suit and a number.
The suit of the card is one of the characters `"P", "K", "H", "T"`, and the number of the card is an integer between `1` and `13`. The robot labels each card in the format `TXY` where `T` is the suit and `XY` is the number. If the card’s number consists of one digit, then X = 0. For example, the card of suit `"P"` and number `9` is labelled `"P09"`.
A complete deck has `52` cards in total. For each of the four suits there is exactly one card with a number between `1` and `13`.
The robot has read the labels of all the cards in the deck and combined them into the string `s`.
Your task is to help Pero finish the robot by writing a program that reads the string made out of card labels and returns the number of cards that are missing for each suit.
If there are two same cards in the deck, return array with `[-1, -1, -1, -1]` instead.
# Input/Output
- `[input]` string `s`
A correct string of card labels. 0 ≤ |S| ≤ 1000
- `[output]` an integer array
Array of four elements, representing the number of missing card of suits `"P", "K", "H", and "T"` respectively. If there are two same cards in the deck, return `[-1, -1, -1, -1]` instead.
# Example
For `s = "P01K02H03H04"`, the output should be `[12, 12, 11, 13]`.
`1` card from `"P"` suit, `1` card from `"K"` suit, `2` cards from `"H"` suit, no card from `"T"` suit.
For `s = "H02H10P11H02"`, the output should be `[-1, -1, -1, -1]`.
There are two same cards `"H02"` in the string `s`. | ["from collections import defaultdict\n\ndef cards_and_pero(s):\n deck = defaultdict(set)\n for n in range(0,len(s),3):\n card = s[n:n+3]\n if card[1:] in deck[card[0]]: return [-1,-1,-1,-1]\n deck[card[0]] |= {card[1:]}\n return [ 13 - len(deck[suit]) for suit in \"PKHT\"]", "from collections import Counter\n\ndef cards_and_pero(s): \n return [13 - s.count(c) for c in 'PKHT'] if max(Counter([(s[i*3:i*3+3]) for i in range(len(s) // 3)]).values()) <= 1 else [-1, -1, -1, -1]", "def cards_and_pero(s):\n cards = [s[i-3:i] for i in range(3, len(s) + 1, 3)]\n if len(set(cards)) < len(cards):\n return [-1, -1, -1, -1]\n return [13 - len([card[0] for card in cards if card[0] == suit]) for suit in \"PKHT\"]", "def cards_and_pero(s):\n d ,records = {'P': 13, 'K': 13, 'H': 13, 'T': 13}, []\n for i in range(0, len(s), 3):\n t = s[i:i + 3]\n if t in records : return [-1]*4\n records.append(t)\n d[t[0]] -= 1\n return list(d.values())", "from collections import Counter\n\ndef cards_and_pero(s):\n xs = [s[i:i+3] for i in range(0, len(s), 3)]\n if len(xs) != len(set(xs)):\n return [-1, -1, -1, -1]\n suit = Counter(s[::3])\n return [13-suit[c] for c in 'PKHT']", "def cards_and_pero(s):\n cards = [(s[i], s[i+1:i+3]) for i in range(0, len(s), 3)]\n if len(set(cards)) != len(cards):\n return [-1] * 4\n result = [13] * 4\n for (suit, num) in cards:\n result[\"PKHT\".index(suit)] -= 1\n return result\n", "import re\nfrom collections import Counter\n\ndef cards_and_pero(s):\n cardDeck = re.findall(r'([PKHT])(\\d{2})', s)\n cnt = Counter(suit for suit,_ in cardDeck)\n return [-1]*4 if len(set(cardDeck)) != len(cardDeck) else [ 13-cnt[suit] for suit in \"PKHT\"]", "import re\ndef cards_and_pero(s):\n s = re.findall(\"...\", s)\n if len(s) != len(set(s)):\n return ([-1, -1, -1, -1])\n else:\n P,K,H,T = 13,13,13,13\n for x in s:\n if x[0] == \"P\":\n P -= 1\n elif x[0] == \"K\":\n K -= 1\n elif x[0] == \"H\":\n H -= 1\n elif x[0] == \"T\":\n T -= 1\n return ([P, K, H, T])", "def cards_and_pero(s):\n c = 0\n d =''\n rtr = [13, 13, 13, 13]\n for i in range(len(s)):\n d = d + s[i]\n c += 1\n if c == 3:\n if d in s[i+1:] or d in s [:i-1]:\n return [-1, -1, -1, -1]\n if d[0] == 'P':\n rtr[0] -= 1\n elif d[0] == 'K':\n rtr[1] -= 1\n elif d[0] == 'H':\n rtr[2] -= 1\n elif d[0] == 'T':\n rtr[3] -= 1\n d = ''\n c = 0\n return rtr ", "def cards_and_pero(s):\n c = 0\n d =''\n rtr = [13, 13, 13, 13]\n l = []\n for i in range(len(s)):\n d = d + s[i]\n c += 1\n if c == 3:\n l.append(d)\n if d in s[i+1:] or d in s [:i-1]:\n return [-1, -1, -1, -1]\n d = ''\n c = 0\n print (l)\n while len(l) > 0:\n if l[-1][0] == 'P':\n rtr[0] -= 1\n del l[-1]\n elif l[-1][0] == 'K':\n rtr[1] -= 1\n del l[-1]\n elif l[-1][0] == 'H':\n rtr[2] -= 1\n del l[-1]\n elif l[-1][0] == 'T':\n rtr[3] -= 1\n del l[-1]\n return rtr "] | {"fn_name": "cards_and_pero", "inputs": [["P01K02H03H04"], ["H02H10P11H02"], ["P10K10H10T01"], ["P05P01P02P03P13P09P11P07K01P12K03K02K13K12K10K08H03H02H13H12H10H08T01T03T02T13T12T10T08P04K07H02T07H06T11K11T05K05H05H11"], ["P01K02P03P11K09K10P13P10"]], "outputs": [[[12, 12, 11, 13]], [[-1, -1, -1, -1]], [[12, 12, 12, 12]], [[-1, -1, -1, -1]], [[8, 10, 13, 13]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,499 |
def cards_and_pero(s):
|
0c701ac65aa46f45ce807e499475462f | UNKNOWN | You've came to visit your grandma and she straight away found you a job - her Christmas tree needs decorating!
She first shows you a tree with an identified number of branches, and then hands you a some baubles (or loads of them!).
You know your grandma is a very particular person and she would like the baubles to be distributed in the orderly manner. You decide the best course of action would be to put the same number of baubles on each of the branches (if possible) or add one more bauble to some of the branches - starting from the beginning of the tree.
In this kata you will return an array of baubles on each of the branches.
For example:
10 baubles, 2 branches: [5,5]
5 baubles, 7 branches: [1,1,1,1,1,0,0]
12 baubles, 5 branches: [3,3,2,2,2]
The numbers of branches and baubles will be always greater or equal to 0.
If there are 0 branches return: "Grandma, we will have to buy a Christmas tree first!".
Good luck - I think your granny may have some minced pies for you if you do a good job! | ["def baubles_on_tree(baubles, branches):\n if not branches: return \"Grandma, we will have to buy a Christmas tree first!\"\n d,r=divmod(baubles,branches)\n return [d+1]*r+[d]*(branches-r)", "def baubles_on_tree(baubles, branches):\n if not branches:\n return 'Grandma, we will have to buy a Christmas tree first!'\n q, r = divmod(baubles, branches)\n return [q+1] * r + [q] * (branches - r)", "def baubles_on_tree(baubles, branches):\n if branches == 0:\n x = \"Grandma, we will have to buy a Christmas tree first!\"\n return x\n x = baubles // branches\n list_of_baubles = [x]*branches\n z = (baubles % branches)\n y = z - 1\n if z != 0:\n for i in range(z):\n list_of_baubles[i] = list_of_baubles[i]+1\n \n return list_of_baubles", "def baubles_on_tree(baubles, branches):\n return [baubles//branches + (i < baubles % branches) for i in range(branches)] if branches else 'Grandma, we will have to buy a Christmas tree first!'", "def baubles_on_tree(baubles, branches):\n if not branches:\n return \"Grandma, we will have to buy a Christmas tree first!\"\n extra = baubles - (baubles//branches) * branches\n l = []\n for i in range(branches):\n l.append((baubles//branches)+1*(extra > 0))\n extra -= 1\n return l", "baubles_on_tree = lambda b,c: [(b+c-i-1)//c for i in range(c)] if c else 'Grandma, we will have to buy a Christmas tree first!'", "from itertools import cycle, islice\nfrom collections import Counter\ndef baubles_on_tree(baubles, branches):\n return [Counter(islice(cycle(range(branches)), baubles))[x] for x in range(branches)] or \"Grandma, we will have to buy a Christmas tree first!\"", "def baubles_on_tree(baubles, branches):\n return \"Grandma, we will have to buy a Christmas tree first!\" if not branches else [ baubles//branches + (1 if i<baubles%branches else 0) for i in range(branches) ]", "from math import ceil\ndef baubles_on_tree(baubles, branches):\n return [ceil((baubles - i) / branches) for i in range(0, branches)] if branches > 0 else 'Grandma, we will have to buy a Christmas tree first!';", "def baubles_on_tree(baubles, branches):\n if not branches:\n return \"Grandma, we will have to buy a Christmas tree first!\"\n d, m = divmod(baubles, branches)\n return [d * (i <= branches) + (i <= m-1) for i in range(branches)]\n"] | {"fn_name": "baubles_on_tree", "inputs": [[5, 5], [5, 0], [6, 5], [50, 9], [0, 10]], "outputs": [[[1, 1, 1, 1, 1]], ["Grandma, we will have to buy a Christmas tree first!"], [[2, 1, 1, 1, 1]], [[6, 6, 6, 6, 6, 5, 5, 5, 5]], [[0, 0, 0, 0, 0, 0, 0, 0, 0, 0]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,413 |
def baubles_on_tree(baubles, branches):
|
de3a913c89aeb54c91323157f76258b7 | UNKNOWN | # Task
John is new to spreadsheets. He is well aware of rows and columns, but he is not comfortable with spreadsheets numbering system.
```
Spreadsheet Row Column
A1 R1C1
D5 R5C4
AA48 R48C27
BK12 R12C63```
Since John has a lot of work to do both in row-column and spreadsheet systems, he needs a program that converts cell numbers from one system to another.
# Example
For `s = "A1"`, the result should be `"R1C1"`.
For `s = "R48C27"`, the result should be `"AA48"`.
- Input/Output
- `[input]` string `s`
The position (in spreadsheet or row-column numbering system).
`Spreadsheet : A1 to CRXO65535`
`Row-Column: R1C1 to R65535C65535`
- `[output]` a string
The position (in the opposite format; if input was in spreadsheet system, the output should be int row-column system, and vise versa). | ["import re\n\ndef spreadsheet(s):\n nums = re.findall(r'(\\d+)', s)\n if len(nums) == 2:\n n, cStr = int(nums[1]), ''\n while n:\n n, r = divmod(n-1, 26)\n cStr += chr(r + 65)\n return \"{}{}\".format(cStr[::-1], nums[0])\n else:\n return \"R{}C{}\".format(nums[0], sum( 26**i * (ord(c)-64) for i,c in enumerate(re.sub(r'\\d', '', s)[::-1])))", "def spreadsheet(s):\n try:\n r, c = map(int, s[1:].split('C'))\n l = str(r)\n while c:\n c, i = divmod(c - 1, 26)\n l = chr(i + 65) + l\n return l\n except:\n c = 0\n for i, l in enumerate(s):\n if l.isdigit(): break\n c = 26 * c + ord(l) - 64\n return 'R%sC%d' % (s[i:], c)", "import re\nfrom string import ascii_uppercase as ALPHA\n\ndef num2alpha(num, b=len(ALPHA), numerals=ALPHA):\n return '' if not num else (num2alpha((num-1) // b, b, numerals) + numerals[(num-1) % b])\ndef alpha2num(string, b=len(ALPHA), numerals=ALPHA):\n return sum((numerals.index(v)+1)*b**i for i,v in enumerate(reversed(string)))\n \nrcregex = re.compile('R(\\d+)C(\\d+)')\nspregex = re.compile('([A-Z]+)(\\d+)')\ndef spreadsheet(s):\n m = rcregex.match(s)\n if m: return num2alpha(int(m.group(2)))+m.group(1)\n m = spregex.match(s)\n if m: return 'R{}C{}'.format(m.group(2),alpha2num(m.group(1)))\n return ''\n", "import re\n\ndef spreadsheet(s):\n numbers = re.findall(r\"(\\d+)\", s)\n if len(numbers) == 1:\n row = numbers[0]\n col = sum(26**i * (ord(v)-64) for i, v in enumerate(s[:s.find(row)][::-1]))\n return \"R{row}C{col}\".format(row=row, col=col)\n else:\n row = numbers[0]\n x = int(numbers[1])\n col = \"\"\n while x > 0:\n col = \"ABCDEFGHIJKLMNOPQRSTUVWXYZ\"[(x-1)%26] + col\n x = (x-1) // 26\n return \"{col}{row}\".format(col=col, row=row)\n", "import string\nimport re\n\n\ndef spreadsheet(s):\n if re.match(r\"^R\\d+C\\d+$\", s):\n return convert_to_ss(s)\n else:\n return convert_to_rc(s)\n\ndef convert_to_rc(s: str) -> str:\n ind = re.search(r\"\\d\", s).span()[0]\n letters = s[:ind]\n nums = s[ind:]\n cols = 0\n for i in range(len(letters)):\n if i != len(letters) - 1:\n cols += (string.ascii_uppercase.find(letters[i]) + 1) * 26 ** (len(letters) - i - 1)\n else:\n cols += (string.ascii_uppercase.find(letters[i]) + 1)\n return f\"R{nums}C{cols}\"\n\ndef convert_to_ss(s: str) -> str:\n ind = s.find(\"C\")\n rows = s[1:ind]\n cols = int(s[ind+1:])\n res_col = \"\"\n while cols > 0:\n cols, rem = cols // 26, cols % 26\n if res_col and res_col[0] == \"Z\":\n if rem == 1:\n break\n else:\n res_col = string.ascii_uppercase[rem - 2] + res_col\n else:\n res_col = string.ascii_uppercase[rem - 1] + res_col\n print(cols, rem)\n return f\"{res_col}{rows}\"", "from itertools import product\nfrom string import ascii_uppercase as ap\nimport re\ntranso = list(map(lambda x:\"\".join(x),list(ap)+list(product(ap,repeat=2))+list(product(ap,repeat=3))+list(product(ap,repeat=4))))\ndef spreadsheet(no):\n matches = re.match(r\"(?P<RC1>^R\\d+C\\d+$)|(?P<A1>^(?P<LET>[A-Z]+)\\d+$)\",no)\n if matches.group(\"RC1\"):\n asi = matches.group(\"RC1\")\n asiind = asi.index(\"C\")\n return transo[int(asi[asiind+1:])-1] + asi[1:asiind]\n else:\n asi = matches.group(\"LET\")\n df = transo.index(asi)\n return f\"R{no[no.rfind(asi)+len(asi):]}C{df+1}\"", "import re\nfrom string import ascii_uppercase as upper\ndef spreadsheet(s):\n x = re.search(r'^([A-Z]+)(\\d+)$',s)\n if x:\n c,r = x.groups()\n return f'R{r}C{sum((upper.index(j)+1)*(26**(len(c)-i-1)) for i,j in enumerate(c))}'\n else:\n r,c = re.search(r'(?:R)(\\d+)(?:C)(\\d+)',s).groups()\n s,c = [], int(c)\n if c==26 : return 'Z'+r\n while c>1:\n c, m = divmod(c,26)\n s.append([c,m])\n return (['','A'][c] + ''.join([upper[s[i][1]-([2,1][bool(s[i-1][1])] if i>0 else 1)] for i in range(len(s))])[::-1]) + r", "import re\ndef spreadsheet(s):\n m=re.match(r'([A-Z]+)(\\d+)$',s)\n if m:\n c,r=m.group(1),int(m.group(2))\n column=0\n for d in c:\n column=column*26+(ord(d)-ord('A')+1)\n return 'R{}C{}'.format(r,column)\n else:\n m=re.match(r'R(\\d+)C(\\d+)$',s)\n r,c=m.group(1),int(m.group(2))\n column=''\n while(c>0):\n m=c%26\n if m==0:\n m=26\n column=chr(ord('A')+m-1)+column\n c=(c-m)//26\n return column+r", "import re\n\ndef to_display(b, n):\n r = []\n while n:\n n, x = divmod(n - 1, 26)\n r.append(chr(65 + x))\n return \"\".join(r[::-1]) + str(b)\n\ndef to_internal(s):\n (a, b), n = re.findall(r\"[A-Z]+|\\d+\", s), 0\n for x in a:\n n *= 26\n n += ord(x) - 64\n return (int(b), n)\n\ndef spreadsheet(s):\n a = re.findall(r\"[A-Z]+|\\d+\", s)\n return \"R{}C{}\".format(*to_internal(s)) if len(a) == 2 else to_display(int(a[1]), int(a[3]))", "from re import fullmatch, findall\n\n\ndef spreadsheet(s):\n if fullmatch('R\\d+C\\d+', s):\n row = findall('\\d+', s)[0]\n col = findall('\\d+', s)[1]\n return f\"{convert_int(col)}{row}\"\n else:\n row = findall('\\d+', s)[0]\n col = findall('[A-Z]+', s)[0]\n return f\"R{row}C{convert_str(col)}\"\n\n\ndef convert_int(num, to_base=26, from_base=10):\n if isinstance(num, str):\n n = int(num, from_base)\n else:\n n = int(num)\n alphabet = \"0ABCDEFGHIJKLMNOPQRSTUVWXYZ\"\n if n <= to_base:\n return alphabet[n]\n else:\n if n % to_base == 0:\n return convert_int(n // to_base-1, to_base) + alphabet[26]\n else:\n return convert_int(n // to_base, to_base) + alphabet[n % to_base]\n\n\ndef convert_str(num):\n num = list(num)[::-1]\n answer = 0\n alphabet = \"0ABCDEFGHIJKLMNOPQRSTUVWXYZ\"\n\n for i in range(len(num)):\n answer += alphabet.index(num[i]) * (26 ** int(i))\n\n return answer"] | {"fn_name": "spreadsheet", "inputs": [["A1"], ["R1C1"], ["R5C4"], ["AA48"], ["BK12"], ["R12C63"], ["R85C26"], ["R31C78"], ["BZ31"]], "outputs": [["R1C1"], ["A1"], ["D5"], ["R48C27"], ["R12C63"], ["BK12"], ["Z85"], ["BZ31"], ["R31C78"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 6,390 |
def spreadsheet(s):
|
ec41258e5b1967e844106c4d898c0767 | UNKNOWN | If `a = 1, b = 2, c = 3 ... z = 26`
Then `l + o + v + e = 54`
and `f + r + i + e + n + d + s + h + i + p = 108`
So `friendship` is twice stronger than `love` :-)
The input will always be in lowercase and never be empty. | ["def words_to_marks(s):\n return sum(ord(c)-96 for c in s)", "def words_to_marks(s):\n return sum('_abcdefghijklmnopqrstuvwxyz'.index(e) for e in s)", "def words_to_marks(s):\n return sum( ord(i)-(ord('a')-1) for i in s )", "def words_to_marks(s):\n return sum(map(ord,s))-len(s)*96", "def words_to_marks(s):\n base = ord('a') - 1\n return sum(ord(l) - base for l in s)\n", "def words_to_marks(s):\n l = []\n d = {'a': 1, 'b': 2, 'c': 3, 'd' : 4, 'e' : 5, 'f' : 6, 'g' : 7, 'h' : 8,\n 'i' : 9, 'j' : 10, 'k' : 11, 'l' : 12, 'm' : 13, 'n' : 14, 'o' : 15, 'p' : 16,\n 'q' : 17, 'r' : 18, 's' : 19, 't' : 20, 'u' : 21, 'v' : 22, 'w' : 23,\n 'x' : 24, 'y' : 25, 'z' : 26}\n for char in list(s):\n l.append(d[char])\n return(sum(l))\n \n", "words_to_marks = lambda s: sum(ord(e)-96 for e in s)", "def words_to_marks(s):\n return sum( ord(c)-96 for c in s )\n\nWordsToMarks = words_to_marks", "def words_to_marks(s):\n alphabet = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z'] \n my_list = list(s)\n summ = 0\n for x in range(len(my_list)):\n for y in range(len(alphabet)):\n if my_list[x] == alphabet[y]:\n summ += (y + 1)\n return summ", "def words_to_marks(s):\n lett = [chr(i) for i in range(ord('a'),ord('z')+1)]\n nums = [i for i in range(1, 27)]\n z = zip(lett, nums)\n d = dict(z)\n s1 = [d[i] for i in s]\n return sum(s1)", "def words_to_marks(word):\n return sum(ord(ch) - 96 for ch in word)", "def words_to_marks(letters):\n result = 0\n for letter in letters:\n result += ord(letter) - 96 # 1 - ord('a') = 96\n return result", "def words_to_marks(word):\n return sum(ord(char) - 96 for char in word)\n", "def words_to_marks(s):\n return sum([ord(char) - 96 for char in s])\n", "import string\ndef words_to_marks(s):\n return sum(string.ascii_lowercase.index(x) + 1 for x in s)", "words_to_marks=lambda s:sum(ord(x)-96for x in s)", "def words_to_marks(s):\n return sum([int(ord(k))-96 for k in s])", "def words_to_marks(s):\n return sum(ord(c) for c in s) - 96*len(s)", "def words_to_marks(s):\n return sum(ord(x) for x in s) - 96 * len(s)", "def words_to_marks(s):\n return sum(ord(i)-96 for i in s)", "def words_to_marks(s):\n q2 = 0\n for i in s:\n if i == 'a':\n q2 += 1\n elif i == 'b':\n q2 += 2\n elif i == 'c':\n q2 += 3\n elif i == 'd':\n q2 += 4\n elif i == 'e':\n q2 += 5\n elif i == 'f':\n q2 += 6\n elif i == 'g':\n q2 += 7\n elif i == 'h':\n q2 += 8\n elif i == 'i':\n q2 += 9\n elif i == 'j':\n q2 += 10\n elif i == 'k':\n q2 += 11\n elif i == 'l':\n q2 += 12\n elif i == 'm':\n q2 += 13\n elif i == 'n':\n q2 += 14\n elif i == 'o':\n q2 += 15\n elif i == 'p':\n q2 += 16\n elif i == 'q':\n q2 += 17\n elif i == 'r':\n q2 += 18\n elif i == 's':\n q2 += 19\n elif i == 't':\n q2 += 20\n elif i == 'u':\n q2 += 21\n elif i == 'v':\n q2 += 22\n elif i == 'w':\n q2 += 23\n elif i == 'x':\n q2 += 24\n elif i == 'y':\n q2 += 25\n elif i == 'z':\n q2 += 26\n return q2", "# go next, ggwp\n# go next, ggwp\n# go next, ggwp\ndef words_to_marks(s):\n listen = {'a': 1, 'b': 2, 'c': 3, 'd': 4,\n 'e': 5, 'f': 6, 'g': 7,\n 'h': 8, 'i': 9, 'j': 10, \n 'k': 11, 'l': 12, 'm': 13,\n 'n': 14, 'o': 15, 'p': 16,\n 'q': 17, 'r': 18, 's': 19,\n 't': 20, 'u': 21, 'v': 22, \n 'w': 23, 'x': 24, 'y': 25, \n 'z': 26}\n x = s\n dd = []\n for i in x:\n if i in listen.keys():\n dd.append(listen[i])\n x = sum(dd)\n return (x)", "from string import ascii_lowercase\n\ndef words_to_marks(s):\n return sum(ascii_lowercase.index(i) + 1 for i in s)", "import string\ndef words_to_marks(s):\n sum = 0\n for a in s:\n sum = sum + list(string.ascii_lowercase).index(a)+1\n return sum", "import string\ndef words_to_marks(s):\n result = 0;\n alpha = list(string.ascii_lowercase)\n \n word = (\",\".join(s)).split(\",\")\n for i in word:\n result += alpha.index(i) + 1\n return result\n", "def words_to_marks(s):\n \n a = \"abcdefghijklmnopqrstuvwxyz\"\n j = 0\n for i in s:\n j = j + (int(a.index(i)) + 1)\n \n return j\n \n \n", "def words_to_marks(s):\n ergebniss = 0\n for x in s:\n ergebniss += ord(x) - 96\n return ergebniss", "def words_to_marks(s):\n letters = map(chr, range(97, 123))\n integers = range(1, 27)\n definition = dict(zip(letters, integers))\n result = 0\n for i in s:\n result += definition.get(i, 0)\n return result", "def words_to_marks(s):\n r = 0;\n lis = [\"a\", \"b\", \"c\", \"d\", \"e\", \"f\", \"g\", \"h\", \"i\", \"j\", \"k\", \"l\", \"m\", \"n\", \"o\", \"p\", \"q\", \"r\", \"s\", \"t\", \"u\", \"v\", \"w\", \"x\", \"y\", \"z\"]\n for x in s:\n r+=lis.index(x)+1;\n return r;\n", "def words_to_marks(s):\n alpha='0abcdefghijklmnopqrstuvwxyz'\n return sum(alpha.find(char) for char in s)", "def words_to_marks(s):\n alpha = ['a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z']\n total = 0\n for i in range(0, len(s)):\n total = total + 1 + alpha.index(s[i])\n return total\n", "def words_to_marks(s):\n summ = 0\n for x in s:\n for z, y in enumerate('abcdefghijklmnopqrstuvwxyz', 1):\n if y == x:\n summ += z\n return summ", "def words_to_marks(s):\n from string import ascii_lowercase\n answer = 0 \n for element in s:\n answer += ascii_lowercase.index(element) + 1\n return answer ", "def words_to_marks(s):\n return sum(ord(x) - 96 for x in s)", "def words_to_marks(s):\n abc = \" abcdefghijklmnopqrstuvwxyz\"\n return sum([abc.index(let) for let in s]) \n", "def words_to_marks(s):\n abc = \" abcdefghijklmnopqrstuvwxyz\"\n return sum([abc.index(letter) for letter in s])\n\n \n \n", "def words_to_marks(s):\n abc = \" abcdefghijklmnopqrstuvwxyz\"\n return sum([abc.index(l) for l in s])\n", "def words_to_marks(word):\n abc = \" abcdefghijklmnopqrstuvwxyz\"\n return sum([abc.index(el) for el in word])", "def words_to_marks(s):\n arr = [\"a\", \"b\", \"c\", \"d\", \"e\", \"f\", \"g\", \"h\", \"i\", \"j\", \"k\", \"l\", \"m\", \"n\", \"o\", \"p\", \"q\", \"r\", \"s\", \"t\", \"u\", \"v\", \"w\", \"x\", \"y\", \"z\"]\n return sum([arr.index(ch) + 1 for ch in s])", "def words_to_marks(s):\n abc = dict(zip('abcdefghijklmnopqrstuvwxyz', range(1, 27)))\n \n sum = 0;\n \n for char in s:\n sum += abc[char]\n \n return sum", "import string\ndef words_to_marks(s):\n return sum([string.ascii_lowercase.find(x)+1 for x in s])", "def words_to_marks(s):\n abc = \"abcdefghijklmnopqrstuvwxyz\"\n result = 0\n for letter in s:\n result+=abc.index(letter)+1\n \n return result", "def words_to_marks(s):\n return sum(ord(n)-96 for n in s)", "import string\n\ndef words_to_marks(input_str):\n \n # Build dictionary of letters and corresponding numbers \n values = dict(list(zip(string.ascii_lowercase, list(range(1,27)))))\n \n # Convert input string to list\n input_lst = list(input_str)\n \n # Get total value for input\n value = 0\n for elem in input_lst:\n value += values[elem]\n \n return value\n", "def words_to_marks(s):\n import string\n a=string.ascii_lowercase[::]\n b=list(range(1, len(a)+1))\n dictionary = {}\n i = 0\n \n for x in a:\n dictionary[x] = b[i]\n i +=1\n \n new_list=[]\n\n for x in s:\n new_list.append(dictionary.get(x))\n\n return sum(new_list)", "def words_to_marks(string):\n return sum(ord(char)-96 for char in string)", "import string\ndef words_to_marks(s):\n # Easy one\n letters = string.ascii_lowercase\n total = 0\n \n for i in s:\n total += letters.index(i) + 1\n\n return total\n \n", "def words_to_marks(s):\n s = s.lower()\n sum_char = 0\n characters ={'a':1, 'b':2, 'c':3, 'd':4,'e':5, 'f':6, 'g':7,'h':8,'i':9, 'j':10, 'k':11, 'l':12,'m':13,'n':14,'o':15,'p':16,'q':17, 'r':18,'s':19,'t':20,'u':21, 'v':22,'w':23,'x':24,'y':25,'z':26}\n for char in s:\n number = characters[char]\n sum_char+=number\n return sum_char", "def words_to_marks(s):\n # using enumerate() ;)\n sum = 0 \n d = { alpha:i for i,alpha in enumerate('abcdefghijklmnopqrstuvwxyz', start = 1) } # key-val pairs of all alphabets\n for letter in s:\n sum += d[letter]\n return sum\n", "def words_to_marks(s):\n s = s.lower()\n sum_char = 0\n characters =['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z']\n for char in s:\n if char in characters:\n number = characters.index(char) + 1\n sum_char+=number\n return sum_char", "import string\ndef words_to_marks(s):\n # Easy one\n letter_dict = {}\n letters = list(string.ascii_lowercase)\n for i in range(0, 26):\n letter_dict[letters[i]] = i+1\n sum = 0\n for letter in s:\n sum += letter_dict[letter]\n return sum", "def words_to_marks(s):\n alphabet = ['a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z']\n marks = 0\n \n for letter in s:\n marks += alphabet.index(letter) + 1\n \n return marks", "def words_to_marks(s):\n letters = list(s)\n word_value = 0\n sum_list = []\n dicti = {}\n alphabet = list('abcdefghijklmnopqrstuvwxyz')\n for i in range(len(alphabet)):\n dict_ = {alphabet[i]: i}\n dicti.update(dict_)\n for j in letters:\n word_value += dicti[j] + 1\n return word_value\n\n \n", "def words_to_marks(s):\n words_value = 0\n alphabet = 'abcdefghijklmnopqrstuvwxyz'\n alphabet = list(alphabet)\n word = list(s)\n letters_value = {}\n for i in range(len(alphabet)):\n dict_ = {alphabet[i]: i}\n letters_value.update(dict_)\n for j in word:\n words_value = words_value + letters_value[j] + 1\n print(words_value)\n return words_value\ns = 'cat'\nwords_to_marks(s)", "import string\ndef words_to_marks(s):\n alpha={}\n j=1\n for i in string.ascii_lowercase:\n alpha[i]=j\n j=j+1\n j=0\n for a in s:\n j=j+alpha[a]\n return j", "import string\nvalues = dict()\nfor index, letter in enumerate(string.ascii_lowercase):\n values[letter] = index + 1\ndef words_to_marks(s):\n total = 0 \n for let in s:\n total += values[let]\n return total\n", "alpha=\"-abcdefghijklmnopqrstuvwxyz\"\ndef words_to_marks(s):\n return sum ( [alpha.index(x) for x in s] )", "def words_to_marks(s):\n alf = ' abcdefghijklmnopqrstuvwxyz'\n sum_out = 0\n for q in range(len(s)):\n sum_out = sum_out + alf.find(s[q])\n return sum_out", "def words_to_marks(s):\n sum=0\n for i in s:\n a=ord(i)\n a=a-96\n sum=sum+a\n return sum", "def words_to_marks(s):\n dict = {\n \"a\": 1, \"b\": 2, \"c\": 3, \"d\": 4, \"e\": 5, \"f\": 6, \"g\": 7, \"h\": 8,\n \"i\": 9, \"j\": 10, \"k\": 11, \"l\": 12, \"m\": 13, \"n\": 14, \"o\": 15,\n \"p\": 16, \"q\": 17, \"r\": 18, \"s\": 19, \"t\": 20, \"u\": 21, \"v\": 22,\n \"w\": 23, \"x\": 24, \"y\": 25, \"z\": 26\n }\n value = 0\n for i in s:\n value += dict.get(i)\n return value", "def words_to_marks(s):\n total = 0\n for ltr in s:\n total += (ord(ltr)-96)\n return total\n", "def words_to_marks(s):\n alpha = ' abcdefghijklmnopqrstuvwxyz'\n return sum([alpha.index(c) for c in s])", "def words_to_marks(s):\n # Easy one\n import string\n letters = string.ascii_lowercase\n score = 0\n for letter in s:\n score += letters.index(letter) + 1\n return score\n", "def words_to_marks(s):\n alphabet = [\"!\",'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z']\n result = []\n for c in s:\n result.append(alphabet.index(c))\n return sum(result)\n", "def words_to_marks(s):\n sum = 0\n for ch in s:\n sum+=(ord(ch)-96)\n #end for\n return sum", "\ndef words_to_marks(s):\n a = [ord(char)- 96 for char in s]\n return sum(a)\n \n", "def words_to_marks(s):\n return sum([ord(i)-96 for i in s.lower()]) \n", "from functools import reduce \n\ndef words_to_marks(s):\n \n return reduce((lambda x,y: x+y), map((lambda x: ord(x) - 96), list(s)))", "def words_to_marks(s):\n alphabet = \"abcdefghijklmnopqrstuvwxyz\"\n res = 0\n for i in s:\n res += alphabet.index(i) + 1\n return res", "def words_to_marks(s):\n score = 0\n alpha = \"abcdefghijklmnopqrstuvwxyz\"\n for char in s:\n index = (alpha.find(char)) + 1\n score += index\n return score", "def words_to_marks(s):\n translate = {\"a\":1, \"b\": 2, \"c\": 3, \"d\": 4, \"e\":5, \"f\":6, \"g\": 7, \"h\": 8, \"i\":9, \"j\": 10, \"k\": 11, \"l\": 12, \"m\": 13, \"n\": 14, \"o\": 15, \"p\": 16, \"q\": 17, \"r\":18, \"s\": 19, \"t\": 20, \"u\": 21, \"v\":22, \"w\": 23, \"x\":24, \"y\":25, \"z\": 26}\n nlst = []\n for letter in list(s):\n nlst.append(translate[letter])\n \n return sum(nlst)\n", "def words_to_marks(s):\n points = {\n \"a\": 1, \"b\": 2, \"c\": 3, \"d\": 4, \"e\": 5, \"f\": 6, \n \"g\": 7, \"h\": 8, \"i\": 9, \"j\": 10, \"k\": 11, \"l\": 12, \n \"m\": 13, \"n\": 14, \"o\": 15, \"p\": 16, \"q\": 17, \"r\": 18, \n \"s\": 19, \"t\": 20, \"u\": 21, \"v\": 22, \"w\": 23, \"x\": 24, \n \"y\": 25, \"z\": 26\n }\n total = 0\n for n, letter in enumerate(s):\n total += points[letter]\n return total", "import string\n\ndef words_to_marks(word):\n alphabet = {char: i for i, char in enumerate(string.ascii_lowercase, start=1)}\n\n values = [alphabet[letter] for letter in word if letter in alphabet]\n return sum(values)", "def words_to_marks(s):\n total = 0\n alphabet = ' abcdefghijklmnopqrstuvwxyz'\n for eachletter in s:\n total = total + alphabet.index(eachletter)\n return total", "import string\ndef words_to_marks(phrase):\n sum = 0\n for letter in phrase:\n letter = string.ascii_lowercase.index(letter) + 1\n sum += letter\n\n return sum", "def words_to_marks(word):\n s = \" abcdefghijklmnopqrstuvwxyz\"\n a = 0\n for el in word:\n a = a + s.index(el)\n return a", "def words_to_marks(word):\n s = \" abcdefghijklmnopqrstuvwxyz\"\n a = 0\n for el in word:\n a += s.index(el)\n return a", "from string import ascii_lowercase\n\ndef words_to_marks(s):\n abc = ' ' + ascii_lowercase\n return sum(abc.index(char) for char in s)", "def words_to_marks(s):\n import string\n alpha = string.ascii_lowercase\n dic = {}\n for char in alpha:\n dic[char] = alpha.index(char)+1\n score = 0\n for char in s:\n score += dic[char]\n return score", "def words_to_marks(s):\n total = 0\n d = {'a':1, 'b': 2, 'c':3, 'd':4, 'e':5,\n 'f':6, 'g':7, 'h':8, 'i':9, 'j':10, 'k':11, 'l':12,\n 'm':13, 'n':14, 'o':15, 'p':16, 'q':17, 'r':18,\n 's':19, 't':20, 'u':21, 'v':22, 'w':23, 'x':24, 'y':25, 'z':26}\n for i in s:\n total += d[i]\n return total", "import string\n\ndef words_to_marks(s):\n return sum(map(string.ascii_lowercase.index, s)) + len(s)\n", "def words_to_marks(s):\n l='abcdefghijklmnopqrstuvwxyz'\n res=0\n for i in s:\n res+=l.index(i)+1\n return res", "def words_to_marks(s):\n alphabet = {\n 'a': 1,\n 'b': 2,\n 'c': 3,\n 'd': 4,\n 'e': 5,\n 'f': 6,\n 'g': 7,\n 'h': 8,\n 'i': 9,\n 'j': 10,\n 'k': 11,\n 'l': 12,\n 'm': 13,\n 'n': 14,\n 'o': 15,\n 'p': 16,\n 'q': 17,\n 'r': 18,\n 's': 19,\n 't': 20,\n 'u': 21,\n 'v': 22,\n 'w': 23,\n 'x': 24,\n 'y': 25,\n 'z': 26,\n }\n \n sum_length = 0\n for char in s:\n sum_length += alphabet[char]\n \n return sum_length", "def words_to_marks(s):\n import string\n dic = dict(zip(string.ascii_lowercase, range(1,27)))\n res = 0\n for letter in s:\n res += dic.get(letter,0)\n # dict.get(key, defaultvalue)\n return res", "def words_to_marks(s):\n r = \" abcdefghijklmnopqrstuvwxyz\"\n return sum([r.index(el) for el in s if el])\n\n", "def words_to_marks(s):\n r = \" abcdefghijklmnopqrstuvwxyz\"\n return sum([r.index(el) for el in s ])\n", "def words_to_marks(s):\n r = \" abcdefghijklmnopqrstuvwxyz\"\n return sum([r.index(el) for el in s ])", "def words_to_marks(s):\n num=0\n for character in s:\n num=num+ord(character) - 96\n return num", "def words_to_marks(s):\n dict = {'a': 1, 'b': 2, 'c': 3, 'd': 4,'e': 5, 'f': 6, 'g': 7, 'h': 8, 'i': 9,\n 'j': 10, 'k': 11, 'l': 12, 'm': 13, 'n': 14, 'o': 15, 'p': 16, 'q': 17, 'r': 18, \n 's': 19, 't': 20, 'u': 21, 'v': 22, 'w': 23, 'x': 24, 'y': 25, 'z': 26} \n sum = 0\n for i in s:\n sum += dict[i]\n \n return sum ", "import string\ndef words_to_marks(s):\n result = []\n counter = dict(zip(string.ascii_lowercase, range(1,27)))\n for i in s:\n if i in counter.keys():\n result.append(counter.get(i))\n return sum(result)", "import string\ndef words_to_marks(s):\n alphabet = string.ascii_lowercase\n return sum([alphabet.index(letter) + 1 for letter in s])", "import string\n\ndef words_to_marks(s):\n alphabet = ' ' + string.ascii_lowercase\n return sum([alphabet.index(letter) for letter in s])", "import string\n\ndef words_to_marks(s):\n alphabet = ' ' + string.ascii_lowercase\n s1 = 0\n for letter in s:\n s1 += alphabet.index(letter)\n \n return s1\n", "def words_to_marks(s):\n i=0\n for ch in s:\n i = i + ord(ch) - 96\n return i", "def words_to_marks(s):\n sum = 0\n for letters in s:\n sum += ord(letters)-96\n return sum\n \n"] | {"fn_name": "words_to_marks", "inputs": [["attitude"], ["friends"], ["family"], ["selfness"], ["knowledge"]], "outputs": [[100], [75], [66], [99], [96]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 19,129 |
def words_to_marks(s):
|
9a433f985cb21857ba0bcf4477202db5 | UNKNOWN | Alex just got a new hula hoop, he loves it but feels discouraged because his little brother is better than him
Write a program where Alex can input (n) how many times the hoop goes round and it will return him an encouraging message :)
-If Alex gets 10 or more hoops, return the string "Great, now move on to tricks".
-If he doesn't get 10 hoops, return the string "Keep at it until you get it". | ["def hoop_count(n):\n return \"Keep at it until you get it\" if n <10 else \"Great, now move on to tricks\"", "def hoop_count(n):\n if n < 10:\n return(\"Keep at it until you get it\")\n else:\n return(\"Great, now move on to tricks\")\n", "def hoop_count(n):\n return \"Great, now move on to tricks\" if n >= 10 else \"Keep at it until you get it\"", "def hoop_count(n: int) -> str:\n \"\"\" Display message for Alex based on how many times the hoop goes round. \"\"\"\n return \"Great, now move on to tricks\" if n >= 10 else \"Keep at it until you get it\"", "def hoop_count(n):\n if not n:\n return \"Keep at it until you get it\"\n elif n <= 0:\n return \"Keep at it until you get it\"\n elif n >= 10:\n return \"Great, now move on to tricks\"\n elif n < 10:\n return \"Keep at it until you get it\"", "def hoop_count(n):\n if n>=10: return (\"Great, now move on to tricks\")\n else: return (\"Keep at it until you get it\")\n pass", "def hoop_count(n):\n if n<10:\n return \"Keep at it until you get it\"\n if n==10 or n>10:\n return \"Great, now move on to tricks\"\n", "def hoop_count(n):\n if n < 10: \n return \"Keep at it until you get it\"\n elif n >= 10: \n return \"Great, now move on to tricks\" \n else: \n return None", "def hoop_count(n):\n message = ''\n if n < 10:\n message = 'Keep at it until you get it'\n else:\n message = 'Great, now move on to tricks'\n return message", "# end goal - function that returns a message based on the number of hoops Alex \n# gets while hula hooping, use ternary operator or if/else conditional\n# input - integer, n\n# ouput - string\n# edge cases - \n\n# function that takes number of hoop rotations\ndef hoop_count(n):\n# if n < 10 return \"Keep at it until you get it\"\n if n < 10: return \"Keep at it until you get it\"\n# if n >= 10 return \"Great, now move on to tricks\"\n else: return \"Great, now move on to tricks\"", "def hoop_count(n):\n print(n)\n if 0 <= n < 10:\n return \"Keep at it until you get it\"\n return \"Great, now move on to tricks\"", "def hoop_count(n):\n if n >= 10:\n return'Great, now move on to tricks'\n\n elif n < 10:\n return'Keep at it until you get it'\n return hoop_count(n)\n\n", "def hoop_count(n):\n if n >= 10:\n return \"Great, now move on to tricks\"\n if n!= 10:\n return\"Keep at it until you get it\"", "def hoop_count(n):\n n = int(n)\n if n>=10:\n return \"Great, now move on to tricks\"\n else:\n return \"Keep at it until you get it\"\nx = hoop_count(20)\nprint(x)", "def hoop_count(n):\n if n >= 10:\n return(\"Great, now move on to tricks\")\n else:\n return(\"Keep at it until you get it\")\n \nprint(hoop_count(3))", "def hoop_count(n):\n # Good Luck!\n if n >= 10:\n mess = \"Great, now move on to tricks\"\n else:\n mess = \"Keep at it until you get it\"\n return mess", "def hoop_count(n):\n if n<10: return \"Keep at it until you get it\"\n return \"Great, now move on to tricks\"", "def hoop_count(n):\n return (\"Great, now move on to tricks\", \"Keep at it until you get it\")[n<10]", "hoop_count = lambda a : \"Keep at it until you get it\" if a <10 else \"Great, now move on to tricks\"", "def hoop_count(n):\n msg = [\"Keep at it until you get it\", \"Great, now move on to tricks\"]\n if n >= 10:\n return msg[1]\n elif n<10:\n return msg[0]", "def hoop_count(n):\n return \"Great, now move on to tricks\" if n > 9 else \"Keep at it until you get it\"", "def hoop_count(n):\n if(n < 10):\n return \"Keep at it until you get it\"\n elif(n >= 10):\n return \"Great, now move on to tricks\"\n else: \n return 0", "def hoop_count(n):\n print(n)\n if n <10:\n return \"Keep at it until you get it\"\n else:\n return \"Great, now move on to tricks\"\n", "def hoop_count(n):\n return \"Keep at it until you get it\" if n < int(10) else \"Great, now move on to tricks\"\n # Good Luck!\n", "def hoop_count(n: int) -> str:\n return \"Great, now move on to tricks\" if n >= 10 else \"Keep at it until you get it\"", "hoop_count = lambda n: [\"Great, now move on to tricks\", \"Keep at it until you get it\"][n < 10]", "def hoop_count(n):\n if n >= 10:\n return str(\"Great, now move on to tricks\")\n else:\n return str(\"Keep at it until you get it\")", "def hoop_count(n):\n return (n<10)*\"Keep at it until you get it\" + (n>=10)*\"Great, now move on to tricks\"", "def hoop_count(n):\n if n >= 10:\n result = \"Great, now move on to tricks\"\n else:\n result = \"Keep at it until you get it\"\n return result\n", "def hoop_count(n):\n \n if n == 10 :\n return \"Great, now move on to tricks\"\n elif n == 9 :\n return \"Keep at it until you get it\" \n elif 0 < n <10 :\n return \"Keep at it until you get it\" \n else : \n return \"Great, now move on to tricks\" ", "def hoop_count(n):\n about_n = n >= 10\n if about_n == True:\n return \"Great, now move on to tricks\"\n else:\n return \"Keep at it until you get it\"", "def hoop_count(n):\n if n >= 10:\n return \"Great, now move on to tricks\"\n elif n < 10:\n return \"Keep at it until you get it\"\n elif n == None:\n return \"Keep at it until you get it\"", "def hoop_count(n):\n plus_10 = \"Great, now move on to tricks\"\n minus_10 = \"Keep at it until you get it\"\n if n>=10:\n return plus_10\n else:\n return minus_10\n", "def hoop_count(hulaHoops):\n if hulaHoops >= 10:\n return \"Great, now move on to tricks\"\n else:\n return \"Keep at it until you get it\"", "def hoop_count(n):\n return {\n True: \"Great, now move on to tricks\",\n False: \"Keep at it until you get it\"\n }.get(n >= 10)", "def hoop_count(n):\n return f\"Great, now move on to tricks\" if n >=10 else f\"Keep at it until you get it\" ", "def hoop_count(n):\n \n result='' \n \n if n>= 10:\n result=\"Great, now move on to tricks\"\n else:\n result=\"Keep at it until you get it\"\n \n return result ", "import unittest\n\nTEN_MORE_HOOPS_MESSAGE = \"Great, now move on to tricks\"\nNOT_TEN_MORE_HOOPS_MESSAGE = \"Keep at it until you get it\"\n\n\ndef hoop_count(n):\n return TEN_MORE_HOOPS_MESSAGE if n >= 10 else NOT_TEN_MORE_HOOPS_MESSAGE\n \n \nclass TestHoopCount(unittest.TestCase):\n def test_should_return_encouraging_message_when_getting_ten_more_hoops(self):\n n = 11\n actual = hoop_count(n)\n self.assertEqual(actual, TEN_MORE_HOOPS_MESSAGE)\n\n def test_should_return_encouraging_message_when_does_not_getting_ten_more_hoops(self):\n n = 3\n actual = hoop_count(n)\n self.assertEqual(actual, NOT_TEN_MORE_HOOPS_MESSAGE)\n", "def hoop_count(n): \n messageA = \"Keep at it until you get it\"\n messageB = \"Great, now move on to tricks\"\n return messageA if n < 10 else messageB", "def hoop_count(n):\n if n > 10:\n return(\"Great, now move on to tricks\")\n elif n < 10:\n return(\"Keep at it until you get it\")\n elif n == 10:\n return(\"Great, now move on to tricks\")\n", "def hoop_count(n):\n a='Great, now move on to tricks'\n b='Keep at it until you get it'\n return a if n>=10 else b", "def hoop_count(n):\n msgOne = \"Keep at it until you get it\"\n msgTwo = \"Great, now move on to tricks\"\n if(n<10):\n return msgOne\n else:\n return msgTwo", "def hoop_count(n):\n return n >= 10 and \"Great, now move on to tricks\" or \"Keep at it until you get it\"", "def hoop_count(n):\n hoop_rounds = int(n)\n if hoop_rounds >= 10:\n return str(\"Great, now move on to tricks\")\n else:\n return str(\"Keep at it until you get it\")\n", "def hoop_count(n):\n return \"Keep at it until you get it\" if 0 <= n <10 else \"Great, now move on to tricks\"", "def hoop_count(n):\n if n >= 10:\n a = \"Great, now move on to tricks\"\n else:\n a = \"Keep at it until you get it\"\n return a", "def hoop_count(n):\n msg1 = 'Great, now move on to tricks'\n msg2 = 'Keep at it until you get it'\n return (msg1, msg2)[n < 10]", "def hoop_count(n):\n return (\"Keep at it until you get it\" if 0<=n and n<=9 else \"Great, now move on to tricks\" )\n # Good Luck!\n", "def hoop_count(n):\n if n>=10:\n res = \"Great, now move on to tricks\"\n else:\n res= \"Keep at it until you get it\"\n return res", "def hoop_count(n):\n if n >= 10:\n return \"Great, now move on to tricks\"\n \n else:\n n < 10\n return \"Keep at it until you get it\"", "def hoop_count(n):\n m1 = \"Keep at it until you get it\"\n m2 = \"Great, now move on to tricks\"\n \n return m2 if n >= 10 else m1", "def hoop_count(n):\n if n >= 10:\n return \"Great, now move on to tricks\"\n elif n < 10:\n return \"Keep at it until you get it\"\n else:\n return \"\"", "def hoop_count(n):\n s1 = \"Great, now move on to tricks\"\n s2 = \"Keep at it until you get it\"\n return s1 if n >= 10 else s2", "def hoop_count(n):\n # Good Luck!\n if 10>n:\n return \"Keep at it until you get it\"\n else:\n return \"Great, now move on to tricks\"", "def hoop_count(n):\n a = 'Great, now move on to tricks'\n b = 'Keep at it until you get it'\n if n >= 10:\n return a\n else:\n return b", "def hoop_count(n):\n if n >= int(10):\n hoop = \"Great, now move on to tricks\"\n else:\n hoop = \"Keep at it until you get it\"\n return hoop", "def hoop_count(n):\n alex = [\"Great, now move on to tricks\",\"Keep at it until you get it\"]\n if n >= 10:\n return alex[0]\n \n else:\n return alex[1]", "def hoop_count(n):\n encourage = { 1: \"Great, now move on to tricks\",\n 0: \"Keep at it until you get it\"}\n return encourage.get(n>=10)", "def hoop_count(n):\n msg_1 = \"Keep at it until you get it\"\n msg_2 = \"Great, now move on to tricks\"\n return msg_1 if n < 10 else msg_2 ", "def hoop_count(n):\n alex = \"\"\n if n < 10:\n alex = \"Keep at it until you get it\"\n else:\n alex = \"Great, now move on to tricks\"\n return alex", "def hoop_count(n):\n n = int(n)\n if n >= 10:\n return \"Great, now move on to tricks\"\n else:\n return \"Keep at it until you get it\"", "def hoop_count(n):\n if n is None:\n return \"Great, now move on to tricks\"\n elif n >= 10:\n return \"Great, now move on to tricks\"\n elif n < 10:\n return \"Keep at it until you get it\"", "def hoop_count(n):\n ans = n\n if ans >= 10:\n return \"Great, now move on to tricks\"\n else:\n return \"Keep at it until you get it\"\n # Good Luck!\n", "def hoop_count(n):\n # Good Luck!\n r = \"Great, now move on to tricks\"\n if n < 10:\n r = \"Keep at it until you get it\"\n return r", "def hoop_count(n):\n count = 0\n for i in range(n):\n count += 1\n if count >= 10:\n return \"Great, now move on to tricks\"\n else:\n return \"Keep at it until you get it\"\n # Good Luck!\n", "def hoop_count(n):\n keep = \"Keep at it until you get it\"\n great = \"Great, now move on to tricks\"\n return keep if n < 10 else great", "def hoop_count(n):\n if n >= 10:\n return \"Great, now move on to tricks\"\n else:\n return \"Keep at it until you get it\"\n \nhoop_count(10)\nhoop_count(3)\nhoop_count(11)", "def hoop_count(n):\n if n < 10:\n return (\"Keep at it until you get it\") \n elif n > 10:\n return (\"Great, now move on to tricks\")\n else:\n return (\"Great, now move on to tricks\")\n", "def hoop_count(n):\n #hula = input(\"how many times did the hoop go around\")\n if n >= 10:\n return \"Great, now move on to tricks\"\n else:\n return \"Keep at it until you get it\"", "def hoop_count(n):\n if n >= 10:\n value = \"Great, now move on to tricks\"\n else:\n value = \"Keep at it until you get it\"\n return value", "def hoop_count(n):\n return (\"Great, now move on to tricks\", \"Keep at it until you get it\")[int(n < 10)]", "def hoop_count(hoops):\n if hoops >= 10:\n return(\"Great, now move on to tricks\")\n else:\n return(\"Keep at it until you get it\") ", "class Cheese:\n def __call__(s,*v): return Cheese()\n def __eq__(s,o): return True\n \nhoop_count = Cheese()", "def hoop_count(n):\n return \"Keep at it until you get it\" if int(n) < 10 else \"Great, now move on to tricks\"", "def hoop_count(n):\n messages = [\"Great, now move on to tricks\", \"Keep at it until you get it\"]\n return messages[0] if n >= 10 else messages[1] ", "def hoop_count(n):\n print(n)\n if n >= 10:\n return \"Great, now move on to tricks\"\n else:\n return \"Keep at it until you get it\"", "def hoop_count(n):\n if 10<=n:\n x=\"Great, now move on to tricks\"\n else:\n x=\"Keep at it until you get it\"\n return(x)\n \n # Good Luck!\n", "def hoop_count(n):\n tricks = \"Great, now move on to tricks\"\n until = \"Keep at it until you get it\"\n if n >= 10:\n return tricks\n else:\n return until", "def hoop_count(n):\n if n>9:\n return \"Great, now move on to tricks\"\n if n<10:\n return \"Keep at it until you get it\""] | {"fn_name": "hoop_count", "inputs": [[6], [10], [22]], "outputs": [["Keep at it until you get it"], ["Great, now move on to tricks"], ["Great, now move on to tricks"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 13,856 |
def hoop_count(n):
|
81dd887abb3596825354e9a050ee5e91 | UNKNOWN | You will be given an array which lists the current inventory of stock in your store and another array which lists the new inventory being delivered to your store today.
Your task is to write a function that returns the updated list of your current inventory **in alphabetical order**.
## Example
```python
cur_stock = [(25, 'HTC'), (1000, 'Nokia'), (50, 'Samsung'), (33, 'Sony'), (10, 'Apple')]
new_stock = [(5, 'LG'), (10, 'Sony'), (4, 'Samsung'), (5, 'Apple')]
update_inventory(cur_stock, new_stock) ==>
[(15, 'Apple'), (25, 'HTC'), (5, 'LG'), (1000, 'Nokia'), (54, 'Samsung'), (43, 'Sony')]
```
___
*Kata inspired by the FreeCodeCamp's 'Inventory Update' algorithm.* | ["from collections import defaultdict\ndef update_inventory(cur_stock, new_stock):\n answer = defaultdict(int)\n for stock, item in cur_stock + new_stock:\n answer[item] += stock\n return [(answer[item], item) for item in sorted(answer)]", "from collections import defaultdict\n\ndef update_inventory(cur_stock, new_stock):\n stock = defaultdict(int, [(k, v) for v, k in cur_stock])\n \n for v, k in new_stock:\n stock[k] += v\n \n return [ (v, k) for k, v in sorted(stock.items()) ]", "def update_inventory(cur_stock, new_stock):\n updated_stock = [];\n stock = [];\n brands = [];\n tmpBrands = [];\n \n # determine original brands available\n for i in cur_stock:\n brands.append(i[1]);\n \n # determine all brands now available\n for j in new_stock:\n if j[1] not in brands:\n brands.append(j[1]);\n \n # sort the brand list into alphabetical order\n brands.sort();\n tmpBrands = brands.copy();\n print(tmpBrands);\n \n # create list of summed intersecting stock\n for i in cur_stock:\n for j in new_stock: \n if i[1] == j[1]:\n stock.append((i[0]+j[0], i[1]));\n # remove brand from list\n tmpBrands.remove(i[1]);\n \n # add mutually exclusive brand stock from original stock\n for i in cur_stock:\n if i[1] in tmpBrands:\n stock.append(i);\n tmpBrands.remove(i[1]);\n \n # add mutually exclusive brand stock from new stock\n for j in new_stock:\n if j[1] in tmpBrands:\n stock.append(j);\n tmpBrands.remove(j[1]);\n \n i = 0;\n while len(stock):\n for j in brands:\n for k in stock:\n if j == k[1]:\n updated_stock.append(k);\n stock.remove(k);\n break;\n i += 1;\n \n return updated_stock;", "from collections import defaultdict\n\n\ndef update_inventory(prv_stock, add_stock):\n new_stock = defaultdict(int, (item[::-1] for item in prv_stock))\n for qty, prod in add_stock:\n new_stock[prod] += qty\n return [item[::-1] for item in sorted(new_stock.items())]", "from collections import Counter\n\n# Counter already knows how to do that\ndef update_inventory(cur_stock, new_stock):\n C1 = Counter({v:k for k,v in cur_stock})\n C2 = Counter({v:k for k,v in new_stock})\n return [(v, k) for k,v in sorted((C1 + C2).items())]", "from collections import Counter\n\ndef update_inventory(cur_stock, new_stock):\n c = Counter({key: value for value, key in cur_stock}) + Counter({key: value for value, key in new_stock})\n return [(c[key], key) for key in sorted(c)]", "def update_inventory(cur_stock, new_stock):\n cur_stock_dict = {key:value for value,key in cur_stock}\n for value,key in new_stock:\n cur_stock_dict[key] = cur_stock_dict.get(key,0) + value\n return [(cur_stock_dict[key],key) for key in sorted(cur_stock_dict)]", "from collections import Counter\n\nrevertToDct = lambda lst: Counter(dict(x[::-1] for x in lst))\n\ndef update_inventory(*stocks):\n old,new = map(revertToDct,stocks)\n return [it[::-1] for it in sorted((old+new).items())]", "def update_inventory(cur_stock, new_stock):\n d = {}\n for i, j in cur_stock + new_stock:\n d[j] = d.get(j, 0) + i\n return sorted([(j, i) for i, j in d.items()], key=lambda x: x[1])", "from functools import reduce\nfrom collections import Counter\n\ndef update_inventory(cur_stock, new_stock):\n return sorted([(v, k) for k, v in reduce(lambda x, y: x + y, [Counter({ k: v for v, k in x }) for x in [cur_stock, new_stock]]).items()], key = lambda x: x[1])"] | {"fn_name": "update_inventory", "inputs": [[[], []]], "outputs": [[[]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,790 |
def update_inventory(cur_stock, new_stock):
|
2ba4639eb77ae4e674036e5ecc9ec3ac | UNKNOWN | # Task:
We define the "self reversed power sequence" as one shown below:
Implement a function that takes 2 arguments (`ord max` and `num dig`), and finds the smallest term of the sequence whose index is less than or equal to `ord max`, and has exactly `num dig` number of digits.
If there is a number with correct amount of digits, the result should be an array in the form:
```python
[True, smallest found term]
[False, -1]
```
## Input range:
```python
ord_max <= 1000
```
___
## Examples:
```python
min_length_num(5, 10) == [True, 10] # 10th term has 5 digits
min_length_num(7, 11) == [False, -1] # no terms before the 13th one have 7 digits
min_length_num(7, 14) == [True, 13] # 13th term is the first one which has 7 digits
```
Which you can see in the table below:
```
n-th Term Term Value
1 0
2 1
3 3
4 8
5 22
6 65
7 209
8 732
9 2780
10 11377
11 49863
12 232768
13 1151914
14 6018785
```
___
Enjoy it and happy coding!! | ["# precalculate results\nresults = {}\nn, digits = 1, 0\nwhile digits <= 1000:\n digits = len(str(sum( x**(n-x+1) for x in range(1, n) )))\n if digits not in results:\n results[digits] = n\n n += 1\n\n\ndef min_length_num(digits, max_num): \n n = results.get(digits, 0)\n return [True, n+1] if n and n < max_num else [False, -1]", "save = []\n\ndef min_length_num(num_dig, ord_max): \n for i in range(ord_max):\n if i == len(save):\n save.append(len(str(sum((j+1)**(i-j) for j in range(i)))))\n if save[i] == num_dig:\n return [True, i+1]\n return [False, -1]", "def sequence(n):\n for i in range(n):\n s = range(i+1)\n j = i+1\n tot = 0\n while j > 0:\n for k in s:\n tot += k**j\n j -= 1\n break\n yield tot\n\nnumbers = [i for i in sequence(1000)]\n\ndef min_length_num(num_dig, ord_max): \n n = 1\n for k in numbers[:ord_max]:\n if len(str(k)) == num_dig: return [True, n]\n elif len(str(k)) > num_dig: break\n n +=1\n return [False, -1]", "def S(n):\n s=0\n for i in range(1,n):\n s=s+ i**(n-i)\n return s\nx=[ len( str(S(n))) for n in range(1000)]\n\ndef min_length_num(num_dig, ord_max): \n y=x[1:ord_max+1]\n if not num_dig in y:\n return [False,-1]\n\n for i in range(len(y)+1):\n if y[i]>=num_dig:\n return [ True, i+1]\n \n \n \n \n \n\n", "nums = {}\n\ndef helper():\n nums[1] = '0'\n nums[2] = '1'\n for i in range(1001):\n exp, suma = 1, 0\n for j in range(i, 0, -1):\n suma += j ** exp\n exp += 1\n nums[i] = str(suma)\n\ndef min_length_num(num_dig, ord_max):\n if not nums:\n helper()\n for num in range(1, ord_max):\n if len(nums[num]) == num_dig:\n return [True, num + 1]\n return [False, -1]", "def check(m):\n a = list(range(1, m+1))\n b = a[::-1]\n c = 0\n for x, y in zip(a, b):\n c += x**y\n return str(c)\n \nlst = [1,1] +[len(check(i)) for i in range(2, 1001)]\n\ndef min_length_num(num_dig, ord_max):\n arr = lst[:ord_max]\n if num_dig in arr:\n return [True, arr.index(num_dig)+1]\n return [False, -1]", "d = {**{0:0,1:1},**{i+1:sum(k ** (i - k + 1) for k in range(1, i))+i for i in range(2, 1000)}}\ndef min_length_num(dn, n): \n for k,l in d.items():\n if k > n : return [0,-1]\n if len(str(l)) == dn : return [1,k]\n return [0,-1]", "import math\nbox = []\nfor n in range(1001)[1:]:\n box.append(int(math.log10(sum(map(lambda x,y: x**y, list(range(n+1)), sorted(list(range(n+1)), reverse = True)))))+1)\n\ndef min_length_num(num, ord_max):\n floor = 0\n roof = ord_max\n while floor <=roof:\n mid = (roof+floor)//2\n if box[mid] > num:\n roof = mid - 1\n elif box[mid] < num:\n floor = mid + 1\n elif box[mid-1] == num:\n floor -=1\n roof -=1\n else:\n return [True, mid+1]\n else:\n return [False, -1]\n", "r=[0]\ndef min_length_num(num_dig, ord_max): \n for n in range(1,ord_max+1):\n if n<len(r):\n s=r[n]\n else:\n s=0\n for i in range(1,n):\n s+=i**(n-i)\n r.append(s)\n if len(str(s))==num_dig:\n return [True,n]\n elif len(str(s))>num_dig:\n return [False,-1]\n return [False,-1]", "def min_length_num(num_dig, ord_max):\n result = results.get(num_dig, 0)\n return [True, result + 1] if result and result < ord_max else [False, -1]\n\nresults = {}\nn = 1\ndigit = 0\nwhile digit < 1000:\n digit = len(str(sum(x**(n - x + 1) for x in range(1, n))))\n if digit not in results:\n results[digit] = n\n n += 1\n"] | {"fn_name": "min_length_num", "inputs": [[5, 10], [7, 11], [7, 14], [8, 16], [10, 20]], "outputs": [[[true, 10]], [[false, -1]], [[true, 13]], [[true, 15]], [[true, 17]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,903 |
def min_length_num(num_dig, ord_max):
|
6b4383647c60af55c9ef72ffa9111ebe | UNKNOWN | You will be given an array of non-negative integers and positive integer bin width.
Your task is to create the Histogram method that will return histogram data corresponding to the input array. The histogram data is an array that stores under index i the count of numbers that belong to bin i. The first bin always starts with zero.
On empty input you should return empty output.
Examples:
For input data [1, 1, 0, 1, 3, 2, 6] and binWidth=1 the result will be [1, 3, 1, 1, 0, 0, 1] as the data contains single element "0", 3 elements "1" etc.
For the same data and binWidth=2 the result will be [4, 2, 0, 1]
For input data [7] and binWidth=1 the result will be [0, 0, 0, 0, 0, 0, 0, 1] | ["def histogram(lst, w):\n lst = [n // w for n in lst]\n m = max(lst, default=-1) + 1\n return [lst.count(n) for n in range(m)]", "def histogram(v, w):\n return [sum(j in range(i, w+i) for j in v) for i in range(0, max(v)+1, w)] if v else []", "def histogram(values, bin_width):\n return [sum([values.count(i + j) for i in range(bin_width)]) for j in range(max(values) + 1) if j % bin_width == 0] if values else []", "def histogram(a, n):\n r = [0] * (max(a, default=-1) // n + 1)\n for x in a: r[x // n] += 1\n return r", "def histogram(values, bin_width):\n k = []\n end = max(values) if values else -1\n bin = [i for i in range(bin_width ,end + bin_width+1, bin_width)]\n for i in bin:\n count = 0\n for val in values:\n if i-bin_width <= val < i:\n count += 1\n k += [count]\n return k ", "from collections import Counter\n\ndef histogram(values, bin_width):\n count = Counter(values)\n return [sum(count[i+x] for x in range(bin_width)) for i in range(0, max(count) + 1, bin_width)] if count else []", "from collections import Counter\n\ndef histogram(values, bin_width):\n count = Counter(values)\n return [sum(count[i+x] for x in range(bin_width)) for i in range(0, max(count, default=0)+1, bin_width)] if count else []", "import math\ndef histogram(values, bin_width):\n if values == []:\n return []\n \n hist = [0] * math.ceil((max(values) + 1) / bin_width)\n \n for v in values:\n hist[math.floor(v / bin_width)] += 1\n \n return hist", "from itertools import groupby\ndef histogram(values, bin_width):\n c={k:len(list(g)) for k,g in groupby(sorted(values),key=lambda x:x//bin_width)}\n return [c.get(i,0) for i in range(max(c)+1)] if c else []", "def histogram(values, bin_width):\n if len(values) == 0:\n return []\n list = [values.count(digit) for digit in range(0, max(values) + 1)]\n return [sum(list[digit:digit+bin_width]) for digit in range(0, len(list), bin_width)]"] | {"fn_name": "histogram", "inputs": [[[1, 1, 0, 1, 3, 2, 6], 1], [[1, 1, 0, 1, 3, 2, 6], 2], [[], 1], [[8], 1]], "outputs": [[[1, 3, 1, 1, 0, 0, 1]], [[4, 2, 0, 1]], [[]], [[0, 0, 0, 0, 0, 0, 0, 0, 1]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,051 |
def histogram(values, bin_width):
|
0b1c52492d216c6cb3ea6c18c50b4d78 | UNKNOWN | Write
```python
smaller(arr)
```
that given an array ```arr```, you have to return the amount of numbers that are smaller than ```arr[i]``` to the right.
For example:
```python
smaller([5, 4, 3, 2, 1]) == [4, 3, 2, 1, 0]
smaller([1, 2, 0]) == [1, 1, 0]
```
``` haskell
smaller [5,4,3,2,1] `shouldBe` [4,3,2,1,0]
smaller [1,2,3] `shouldBe` [0,0,0]
smaller [1, 2, 0] `shouldBe` [1, 1, 0]
```
If you've completed this one and you feel like testing your performance tuning of this same kata, head over to the much tougher version How many are smaller than me II? | ["def smaller(arr):\n # Good Luck!\n return [len([a for a in arr[i:] if a < arr[i]]) for i in range(0, len(arr))]\n", "def smaller(arr):\n return [sum(b < a for b in arr[i + 1:]) for i, a in enumerate(arr)]\n", "def smaller(arr):\n return [sum(x > y for y in arr[i+1:]) for i, x in enumerate(arr)]", "def smaller(arr):\n return [sum(j < v for j in arr[i:]) for i, v in enumerate(arr)]", "def smaller(arr):\n a = []\n counter = 0\n for j in range(len(arr)):\n j = arr[0]\n for k in arr:\n if j > k:\n counter += 1\n a.append(counter)\n counter = 0\n arr.remove(arr[0])\n return(a)\n", "def smaller(arr):\n return [sum(1 for right in arr[i+1:] if right<item) for i,item in enumerate(arr)]", "def smaller(nums):\n i = 0; res = []\n while i < len(nums):\n j = i + 1; nb = 0\n while j < len(nums):\n if nums[i] > nums[j]:\n nb += 1\n j += 1\n res.append(nb)\n i += 1\n return res", "# modified merge sort counting left > right cases\ndef countsort(arr, cntarr, cntdict):\n if len(arr) > 1:\n mid = len(arr) // 2\n left = arr[:mid]\n leftcount = cntarr[:mid]\n right = arr[mid:]\n rightcount = cntarr[mid:]\n \n countsort(left, leftcount, cntdict)\n countsort(right, rightcount, cntdict)\n \n i = 0\n j = 0\n k = 0\n while( i < len(left) and j < len(right) ):\n if left[i] > right[j]:\n arr[k] = right[j]\n \n # increase count of smaller elem\n for n in range(i, len(left)):\n temp = leftcount[n]\n cntdict[temp] = cntdict[temp] + 1\n \n # updates position of indices wrt original position \n cntarr[k] = rightcount[j]\n j = j + 1\n else:\n arr[k] = left[i]\n cntarr[k] = leftcount[i]\n i = i + 1\n\n k = k + 1\n\n while i < len(left):\n arr[k] = left[i]\n cntarr[k] = leftcount[i]\n i = i + 1\n k = k + 1\n\n while j < len(right):\n arr[k] = right[j]\n cntarr[k] = rightcount[j]\n j = j + 1\n k = k + 1\n \ndef smaller(arr):\n # list of relative position of original list\n posarr = [i for i in range(len(arr))]\n\n # dictionary of counts of smaller elem\n cntdict = {i:0 for i in range(len(arr))}\n\n # sorts arr and keeps count of smaller elem actions \n countsort(arr, posarr, cntdict) \n\n soln = []\n for i in range(len(arr)):\n soln.append(cntdict[i])\n\n return soln\n"] | {"fn_name": "smaller", "inputs": [[[5, 4, 3, 2, 1]], [[1, 2, 3]], [[1, 2, 0]], [[1, 2, 1]], [[1, 1, -1, 0, 0]], [[5, 4, 7, 9, 2, 4, 4, 5, 6]]], "outputs": [[[4, 3, 2, 1, 0]], [[0, 0, 0]], [[1, 1, 0]], [[0, 1, 0]], [[3, 3, 0, 0, 0]], [[4, 1, 5, 5, 0, 0, 0, 0, 0]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,817 |
def smaller(arr):
|
29300a713ee75e61082029d4f0a275b9 | UNKNOWN | Remember the movie with David Bowie: 'The Labyrinth'?
You can remember your childhood here: https://www.youtube.com/watch?v=2dgmgub8mHw
In this scene the girl is faced with two 'Knights" and two doors. One door leads the castle where the Goblin King and her kid brother is, the other leads to certain death. She can ask the 'Knights' a question to find out which door is the right one to go in. But...
One of them always tells the truth, and the other one always lies.
In this Kata one of the 'Knights' is on a coffee break, leaving the other one to watch the doors. You have to determine if the one there is the Knight(Truth teller) or Knave(Liar) based off of what he ```says```
Create a function:
```
def knight_or_knave(says):
# returns if knight or knave
```
Your function should determine if the input '''says''' is True or False and then return:
```'Knight!'``` if True or ```'Knave! Do not trust.'``` if False
Input will be either boolean values, or strings.
The strings will be simple statements that will be either true or false, or evaluate to True or False.
See example test cases for, well... examples
You will porbably need to ```eval(says)```
But note: Eval is evil, and is only here for this Kata as a game.
And remember the number one rule of The Labyrinth, even if it is easy, Don't ever say 'that was easy.' | ["def knight_or_knave(said):\n return \"Knight!\" if eval(str(said)) else \"Knave! Do not trust.\"", "def knight_or_knave(said):\n try:\n return 'Knight!' if eval(said) else 'Knave! Do not trust.'\n except:\n return 'Knight!' if said else 'Knave! Do not trust.'", "knight_or_knave = lambda said: 'Knight!' if eval(str(said)) else 'Knave! Do not trust.'", "def knight_or_knave(said):\n return eval(str(said)) and \"Knight!\" or 'Knave! Do not trust.'", "def knight_or_knave(said):\n if type(said)==str: \n said=eval(said)\n return ['Knave! Do not trust.', 'Knight!'][said]", "def knight_or_knave(said):\n f,t=\"Knave! Do not trust.\",\"Knight!\"\n if isinstance(said,bool):return [f,t][said]\n elif isinstance(said,str):return [f,t][eval(said)]", "def knight_or_knave(said):\n if said==True:\n return 'Knight!'\n elif said==False:\n return 'Knave! Do not trust.'\n elif eval(said)==True:\n return 'Knight!'\n return 'Knave! Do not trust.'", "knight_or_knave = lambda said: ('Knave! Do not trust.', 'Knight!')[eval(said) if isinstance(said, str) else said]", "def knight_or_knave(said):\n if isinstance(said, str):\n return 'Knight!' if eval(said) == True else 'Knave! Do not trust.'\n else:\n return 'Knight!' if bool(said) == True else 'Knave! Do not trust.'", "def knight_or_knave(said):\n return 'Knight!' if bool(eval(str(said))) else 'Knave! Do not trust.'"] | {"fn_name": "knight_or_knave", "inputs": [[true], [false], ["4+2==5"], ["2+2==4"], ["not True and False or False or False"], ["3 is 3"], ["True"], ["not True"], ["2+2==5"], ["4+1==5"], ["4 is 3"], ["9+2==3"], ["105+30076==30181"], ["3 is 3 is 3 is 9"], ["False"], ["\"orange\" is not \"red\""], ["4 is \"blue\""], ["True is not False"]], "outputs": [["Knight!"], ["Knave! Do not trust."], ["Knave! Do not trust."], ["Knight!"], ["Knave! Do not trust."], ["Knight!"], ["Knight!"], ["Knave! Do not trust."], ["Knave! Do not trust."], ["Knight!"], ["Knave! Do not trust."], ["Knave! Do not trust."], ["Knight!"], ["Knave! Do not trust."], ["Knave! Do not trust."], ["Knight!"], ["Knave! Do not trust."], ["Knight!"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,466 |
def knight_or_knave(said):
|
ee8b8257d4e4e431838a56b9e6c79e28 | UNKNOWN | Cara is applying for several different jobs.
The online application forms ask her to respond within a specific character count.
Cara needs to check that her answers fit into the character limit.
Annoyingly, some application forms count spaces as a character, and some don't.
Your challenge:
Write Cara a function `charCheck()` with the arguments:
- `"text"`: a string containing Cara's answer for the question
- `"max"`: a number equal to the maximum number of characters allowed in the answer
- `"spaces"`: a boolean which is `True` if spaces are included in the character count and `False` if they are not
The function `charCheck()` should return an array: `[True, "Answer"]` , where `"Answer"` is equal to the original text, if Cara's answer is short enough.
If her answer `"text"` is too long, return an array: `[False, "Answer"]`.
The second element should be the original `"text"` string truncated to the length of the limit.
When the `"spaces"` argument is `False`, you should remove the spaces from the `"Answer"`.
For example:
- `charCheck("Cara Hertz", 10, True)` should return `[ True, "Cara Hertz" ]`
- `charCheck("Cara Hertz", 9, False)` should return `[ True, "CaraHertz" ]`
- `charCheck("Cara Hertz", 5, True)` should return `[ False, "Cara " ]`
- `charCheck("Cara Hertz", 5, False)` should return `[ False, "CaraH" ]` | ["def charCheck(text, mx, spaces):\n text = text if spaces else text.replace(' ', '')\n return [len(text) <= mx, text[:mx]]", "def charCheck(text, mx, spaces):\n if not spaces:\n text = text.replace(' ', '')\n return [len(text) <= mx, text[:mx]]", "def charCheck(s, mx, spaces):\n res = ''.join((s.split(), s)[spaces])\n return [len(res)<=mx, res[:mx]]", "def char_check(txt, mx, spc):\n if not spc:\n txt = txt.replace(\" \", \"\")\n return [len(txt) <= mx, txt[:mx]]\n\n\ncharCheck = char_check", "def charCheck(text, mx, spaces):\n return (lambda txt:[ len(txt)<=mx, txt[:mx] ])([text.replace(' ',''), text][spaces])", "def charCheck(text, mx, spaces):\n if spaces == True:\n return[len(text)<=mx, text[:mx]]\n else:\n t = text.replace(' ','')\n return[len(t)<=mx, t[:mx]]", "def charCheck(text, mx, spaces):\n stringa = ''\n limit = False\n result = ''\n limit_val = 0\n \n if spaces:\n stringa = text\n else:\n stringa = text.replace(' ', '')\n\n limit_val = mx - len(stringa)\n \n if limit_val >= 0:\n limit = True\n limit_val = len(stringa)\n else:\n limit_val = mx\n \n for i in range(limit_val):\n result += stringa[i]\n \n return [limit, result]\n", "def charCheck(text, mx, spaces):\n if spaces == False:\n text = text.replace(\" \", \"\")\n \n return [len(text) <= mx, text[:mx]]", "def charCheck(s, m, sp):\n s = s.replace([' ',''][sp],'')\n return [s[:m]==s,s[:m]]", "def charCheck(r,l,s):t=r.replace(-~-s*' ','');return[t==t[:l],t[:l]]"] | {"fn_name": "charCheck", "inputs": [["I am applying for the role of Base Manager on Titan.", 60, true], ["I am looking to relocate to the vicinity of Saturn for family reasons.", 70, true], ["As Deputy Base Manager on Phobos for five Martian years, I have significant relevant experience.", 90, false], ["A challenging career moment came with the rapid depletion of water supplies on Phobos.", 80, false], ["But, as I pointed out, anyone complaining about standing downwind was lying. There was no wind.", 75, true], ["I have no notice period on Phobos. I can start immediately.", 50, true]], "outputs": [[[true, "I am applying for the role of Base Manager on Titan."]], [[true, "I am looking to relocate to the vicinity of Saturn for family reasons."]], [[true, "AsDeputyBaseManageronPhobosforfiveMartianyears,Ihavesignificantrelevantexperience."]], [[true, "AchallengingcareermomentcamewiththerapiddepletionofwatersuppliesonPhobos."]], [[false, "But, as I pointed out, anyone complaining about standing downwind was lying"]], [[false, "I have no notice period on Phobos. I can start imm"]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,637 |
def charCheck(text, mx, spaces):
|
fe2db94914df1df9c7af88e13fa8ef20 | UNKNOWN | Complete the `greatestProduct` method so that it'll find the greatest product of five consecutive digits in the given string of digits.
For example:
The input string always has more than five digits.
Adapted from Project Euler. | ["from itertools import islice\nfrom functools import reduce\n\ndef greatest_product(n):\n numbers=[int(value) for value in n]\n result=[reduce(lambda x,y: x*y, islice(numbers, i, i+5), 1) for i in range(len(numbers)-4)]\n return max(result) \n", "def product(list):\n p = 1\n for i in list:\n p *= int(i)\n return p\n \ndef greatest_product(list):\n res = []\n mul = 1\n while len(list)>= 5:\n res.append(product(list[:5]))\n list = list[1:]\n return(max(res))\n \n \n", "def greatest_product(n):\n L=[]\n L1=[]\n for i in n:\n L.append(int(i))\n for i in range(len(L)-4):\n a=(L[i])*(L[i+1])*(L[i+2])*(L[i+3])*(L[i+4])\n L1.append(a)\n return max(L1)", "def greatest_product(n):\n l=len(n)\n t=[]\n for i in range(l-4):\n a=int(n[i])*int(n[i+1])*int(n[i+2])*int(n[i+3])*int(n[i+4])\n t.append(a)\n print(t)\n ans=max(t)\n return(ans)", "from functools import reduce\ndef greatest_product(n):\n l=list(map(int,list(n)))\n return max([reduce(lambda x,y:x*y,l[i:i+5]) for i in range(len(l)) if len(l[i:i+5])==5])", "from functools import reduce\nfrom operator import __mul__\ndef greatest_product(n):\n return max([reduce(__mul__, list(map(int, x))) for x in [x for x in [n[i:i+5] for i in range(len(n))] if len(x)==5]])\n", "import numpy\ndef greatest_product(n):\n lst=[]\n for a in range(0,len(n)-4): lst.append(numpy.prod(list(map(int,list(n[a:a+5])))))\n return max(lst)", "from functools import reduce\n\ndef greatest_product(a):\n return max([reduce(lambda a,b: a*b, map(int, a[i: i+5])) for i in range(0, len(a)-4)])", "def greatest_product(n, num_consecutive_digits=5):\n digits = [int(digit) for digit in n]\n greatest = 0\n for i in range(len(digits) - num_consecutive_digits + 1):\n product = 1\n for j in range(num_consecutive_digits):\n product *= digits[i+j]\n greatest = max(product, greatest)\n return greatest\n", "from functools import reduce\n\ndef greatest_product(n):\n test = [reduce((lambda x, y: x * y), list(map(int, n[i:i+5]))) for i in range(0,len(n)-4)]\n return max(test)\n"] | {"fn_name": "greatest_product", "inputs": [["123834539327238239583"], ["395831238345393272382"], ["92494737828244222221111111532909999"], ["02494037820244202221011110532909999"]], "outputs": [[3240], [3240], [5292], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,215 |
def greatest_product(n):
|
9de14aa65321b2be1530cf9bc31b2805 | UNKNOWN | This kata is part of the collection [Mary's Puzzle Books](https://www.codewars.com/collections/marys-puzzle-books).
# Zero Terminated Sum
Mary has another puzzle book, and it's up to you to help her out! This book is filled with zero-terminated substrings, and you have to find the substring with the largest sum of its digits. For example, one puzzle looks like this:
```
"72102450111111090"
```
Here, there are 4 different substrings: `721`, `245`, `111111`, and `9`. The sums of their digits are `10`, `11`, `6`, and `9` respectively. Therefore, the substring with the largest sum of its digits is `245`, and its sum is `11`.
Write a function `largest_sum` which takes a string and returns the maximum of the sums of the substrings. In the example above, your function should return `11`.
### Notes:
- A substring can have length 0. For example, `123004560` has three substrings, and the middle one has length 0.
- All inputs will be valid strings of digits, and the last digit will always be `0`. | ["def largest_sum(s):\n return max(sum(map(int,x)) for x in s.split('0'))", "def largest_sum(s):\n return max(sum(map(int, e)) for e in s.split('0'))", "def largest_sum(s):\n return max(sum(int(i) for i in j) for j in s.split('0'))", "def largest_sum(s):\n return max(sum(int(x) for x in n) for n in s.split('0'))", "import re\ndef largest_sum(s):\n s = s.split('0')\n m = 0\n for l in s:\n temp_s = sum(map(int, l))\n if temp_s > m :\n m = temp_s\n return m\n", "def largest_sum(s):\n max_sum = 0\n tmp = 0\n for c in s:\n if c != '0':\n tmp += int(c)\n else:\n max_sum = max(max_sum, tmp)\n tmp = 0\n return max_sum", "def largest_sum(s):\n return max(sum(map(int, list(n))) for n in s.split('0'))\n", "def largest_sum(s):\n return max(sum(map(int,i)) for i in s.split(\"0\"))", "def largest_sum(s):\n ar = s.split(\"0\")\n max = 0\n for e in ar:\n a = [int(d) for d in e]\n if max < sum(a):\n max = sum(a)\n return max", "def largest_sum(s):\n return max(sum(map(int, c)) for c in s.split('0'))"] | {"fn_name": "largest_sum", "inputs": [["72102450111111090"], ["123004560"], ["0"], ["4755843230"], ["3388049750"], ["3902864190"], ["4098611970"], ["5628206860"], ["5891002970"], ["0702175070"]], "outputs": [[11], [15], [0], [41], [25], [30], [41], [23], [23], [15]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,123 |
def largest_sum(s):
|
c5854a786e62d03836d6d5073d602fb8 | UNKNOWN | Make your strings more nerdy: Replace all 'a'/'A' with 4, 'e'/'E' with 3 and 'l' with 1
e.g. "Fundamentals" --> "Fund4m3nt41s"
```if:csharp
Documentation:
Kata.Nerdify Method (String)
Nerdifies a string. Returns a copy of the original string with 'a'/'A' characters replaced with '4', 'e'/'E' characters replaced with '3', and 'l' characters replaced with '1'.
Syntax
public
static
string Nerdify(
string str
)
Parameters
str
Type: System.String
The string to be nerdified.
Return Value
Type: System.String
The nerdified string.
Exceptions
Exception
Condition
ArgumentNullException
str is null.
``` | ["def nerdify(txt):\n return txt.translate(str.maketrans(\"aAeEl\", \"44331\"))", "def nerdify(txt):\n return txt.translate(str.maketrans(\"aelAE\",\"43143\"))\n", "def nerdify(txt):\n intab = 'aelAE'\n outab = '43143'\n trantab = str.maketrans(intab,outab)\n return txt.translate(trantab)", "def nerdify(txt):\n tex=txt.replace('a','4')\n tex=tex.replace('A','4')\n tex=tex.replace('E','3')\n tex=tex.replace('e','3')\n return tex.replace('l','1')\n pass", "def nerdify(txt):\n arr = []\n for i in txt:\n if i == \"a\" or i == \"A\":\n arr.append(\"4\")\n elif i == \"e\" or i == \"E\":\n arr.append(\"3\")\n elif i == \"l\":\n arr.append(\"1\")\n else:\n arr.append(i)\n return (\"\").join(arr)", "def nerdify(txt):\n \n \n s = \"\"\n \n for i in range(len(txt)):\n \n if txt[i] == \"a\" or txt[i] == \"A\":\n \n s+= '4'\n \n elif txt[i] == \"e\" or txt[i] == \"E\":\n \n s+= '3'\n \n elif txt[i] == 'l' :\n \n s+= '1'\n \n else:\n \n s+= txt[i]\n \n return s\n", "def nerdify(txt):\n encoder = {'a': '4', 'A': '4', 'e': '3', 'E': '3', 'l': '1'}\n return ''.join(encoder.get(c, c) for c in txt)\n", "d = {'a':'4', 'e':'3', 'l':'1', 'A':'4', 'E':'3'}\ndef nerdify(txt):\n return ''.join([d[i] if i in d else i for i in txt])", "def nerdify(txt):\n new_txt = \"\"\n for letter in txt:\n if letter == \"a\" or letter == \"A\":\n new_txt += \"4\"\n elif letter == \"e\" or letter == \"E\":\n new_txt += \"3\"\n elif letter == \"l\":\n new_txt += \"1\"\n else:\n new_txt += letter\n return new_txt", "def nerdify(txt):\n t=str.maketrans('aAeEl','44331')\n return txt.translate(t)"] | {"fn_name": "nerdify", "inputs": [["Fund4m3nt41s"], ["Seven"], ["Los Angeles"], ["Seoijselawuue"]], "outputs": [["Fund4m3nt41s"], ["S3v3n"], ["Los 4ng313s"], ["S3oijs314wuu3"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,971 |
def nerdify(txt):
|
13a6b82b4745c48dbec90889af8b28f1 | UNKNOWN | Given a time in AM/PM format as a string, convert it to military (24-hour) time as a string.
Midnight is 12:00:00AM on a 12-hour clock, and 00:00:00 on a 24-hour clock. Noon is 12:00:00PM on a 12-hour clock, and 12:00:00 on a 24-hour clock
Sample Input: 07:05:45PM
Sample Output: 19:05:45
Try not to use built in DateTime libraries.
For more information on military time, check the wiki https://en.wikipedia.org/wiki/24-hour_clock#Military_time | ["def get_military_time(time):\n if time[-2:] == 'AM':\n hour = '00' if time[0:2] == '12' else time[0:2]\n else:\n hour = '12' if time[0:2] == '12' else str(int(time[0:2])+12)\n return hour + time[2:-2]", "def get_military_time(time_str):\n hour = int(time_str[:2])\n pm = time_str[-2] == \"P\"\n return f\"{(hour % 12 + 12*pm):02}{time_str[2:-2]}\"", "from datetime import datetime\n\ndef get_military_time(time):\n return datetime.strptime(time, '%I:%M:%S%p').strftime('%H:%M:%S')", "def get_military_time(time):\n h = int(time[:2])\n if time.endswith(\"AM\"):\n h = h % 12\n elif h < 12:\n h += 12\n return f\"{h:02d}{time[2:8]}\"", "def get_military_time(s):\n return \"%02d:%02d:%02d\" % (int(s[:2]) % 12 + 12 * (s[-2] != 'A'), int(s[3:5]), int(s[6:8]))", "def get_military_time(stg):\n h, ms, ap = int(stg[:2]) % 12, stg[2:8], stg[8:]\n return f\"{h + (12 if ap == 'PM' else 0):02d}{ms}\"", "get_military_time=lambda t:'%02d%s'%(int(t[:2])%12+12*(t[-2]>'A'),t[2:-2])", "def get_military_time(s):\n delta = 12 * s.endswith('PM')\n h, m, s = s[:-2].split(':')\n hour = int(h) + delta\n return '{:02}:{}:{}'.format(12 if hour == 24 else 0 if hour == 12 else hour, m, s)", "def get_military_time(t):\n modt = t[:-2]\n if ('12' == t[:2]):\n modt = '00' + modt[2:]\n if ('PM' == t[-2:]):\n modt = str(12 + int(modt[:2])) + modt[2:]\n return modt", "def get_military_time(_s):\n if 'A' in _s and _s.startswith('12'):\n return '00' + _s[2:-2]\n if 'P' in _s and not _s.startswith('12'):\n return str(int(_s[:2])+12) + _s[2:-2]\n return _s[:-2]"] | {"fn_name": "get_military_time", "inputs": [["12:00:01AM"], ["01:02:03AM"], ["02:04:05AM"], ["03:06:07AM"], ["04:08:09AM"], ["05:10:11AM"], ["06:12:13AM"], ["07:14:15AM"], ["08:16:17AM"], ["09:18:19AM"], ["10:20:21AM"], ["11:22:23AM"], ["12:24:25PM"], ["01:26:27PM"], ["02:28:29PM"], ["03:30:31PM"], ["04:32:33PM"], ["05:34:35PM"], ["06:36:37PM"], ["07:38:39PM"], ["08:40:41PM"], ["09:42:43PM"], ["10:44:45PM"], ["11:46:47PM"]], "outputs": [["00:00:01"], ["01:02:03"], ["02:04:05"], ["03:06:07"], ["04:08:09"], ["05:10:11"], ["06:12:13"], ["07:14:15"], ["08:16:17"], ["09:18:19"], ["10:20:21"], ["11:22:23"], ["12:24:25"], ["13:26:27"], ["14:28:29"], ["15:30:31"], ["16:32:33"], ["17:34:35"], ["18:36:37"], ["19:38:39"], ["20:40:41"], ["21:42:43"], ["22:44:45"], ["23:46:47"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,674 |
def get_military_time(time):
|
b4529b733f9f23381aabdf4807a356fa | UNKNOWN | Two integers are coprimes if the their only greatest common divisor is 1.
## Task
In this kata you'll be given a number ```n >= 2``` and output a list with all positive integers less than ```gcd(n, k) == 1```, with ```k``` being any of the output numbers.
The list cannot include duplicated entries and has to be sorted.
## Examples
```
2 -> [1]
3 -> [1, 2]
6 -> [1, 5]
10 -> [1, 3, 7, 9]
20 -> [1, 3, 7, 9, 11, 13, 17, 19]
25 -> [1, 2, 3, 4, 6, 7, 8, 9, 11, 12, 13, 14, 16, 17, 18, 19, 21, 22, 23, 24]
30 -> [1, 7, 11, 13, 17, 19, 23, 29]
``` | ["from fractions import gcd\n\ndef coprimes(n):\n return [i for i in range(1,n+1) if gcd(n,i)==1]", "from fractions import gcd\ndef coprimes(n):\n return [i for i in range(n) if gcd(i,n)==1]", "from fractions import gcd\n\ncoprimes = lambda n: [i for i in range(1, n) if gcd(n, i) == 1]", "from fractions import gcd \ndef coprimes(n): return [a for a in range(1, n) if gcd(n,a) == 1]", "def coprimes(n):\n p, s = n, list(range(n))\n for d in range(2, int(n ** .5) + 1):\n if not p % d:\n while not p % d: p //= d\n s[d::d] = ((n - d - 1) // d + 1) * [0]\n if p > 1: s[p::p] = ((n - p - 1) // p + 1) * [0]\n return [i for i, n in enumerate(s) if n]", "from math import gcd\n\ndef coprimes(n):\n return [x for x in range(1, n) if gcd(x, n) == 1]", "def gcd(a,b):\n while b > 0:\n a %= b\n a,b = b,a\n return a\n\ndef coprimes(n):\n return [x for x in range(1,n) if gcd(n,x) == 1]", "def gcd(a, b):\n return a if b == 0 else gcd(b, a%b)\n\ndef coprimes(n):\n rslt = {1}\n for i in range(2, n):\n if gcd(n, i) == 1:\n rslt.add(i)\n return sorted(rslt)", "from fractions import gcd as g\nz=lambda x,y:1 if g(x,y)==1 else 0\ndef coprimes(n):return [i for i in range(1,n) if z(n,i)==1]\n", "from fractions import gcd\n\ndef coprimes(n):\n ret = []\n for x in range(int(n/2)+1):\n if gcd(x,n-x) == 1:\n ret += [x]\n ret += [n-x]\n return sorted(set(ret))\n"] | {"fn_name": "coprimes", "inputs": [[2], [3], [6], [10], [20], [25], [30]], "outputs": [[[1]], [[1, 2]], [[1, 5]], [[1, 3, 7, 9]], [[1, 3, 7, 9, 11, 13, 17, 19]], [[1, 2, 3, 4, 6, 7, 8, 9, 11, 12, 13, 14, 16, 17, 18, 19, 21, 22, 23, 24]], [[1, 7, 11, 13, 17, 19, 23, 29]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,467 |
def coprimes(n):
|
fc0ae897021ed3c5f143bb912c2369ba | UNKNOWN | Most languages have a `split` function that lets you turn a string like `“hello world”` into an array like`[“hello”, “world”]`. But what if we don't want to lose the separator? Something like `[“hello”, “ world”]`.
#### Task:
Your job is to implement a function, (`split_without_loss` in Ruby/Crystal, and `splitWithoutLoss` in JavaScript/CoffeeScript), that takes two arguments, `str` (`s` in Python), and `split_p`, and returns the string, split by `split_p`, but with the separator intact. There will be one '|' marker in `split_p`. `str` or `s` will never have a '|' in it. All the text before the marker is moved to the first string of the split, while all the text that is after it is moved to the second one. **Empty strings must be removed from the output, and the input should NOT be modified.**
When tests such as `(str = "aaaa", split_p = "|aa")` are entered, do not split the string on overlapping regions. For this example, return `["aa", "aa"]`, not `["aa", "aa", "aa"]`.
#### Examples (see example test cases for more):
```python
split_without_loss("hello world!", " |") #=> ["hello ", "world!"]
split_without_loss("hello world!", "o|rl") #=> ["hello wo", "rld!"]
split_without_loss("hello world!", "h|ello world!") #=> ["h", "ello world!"]
split_without_loss("hello world! hello world!", " |")
#=> ["hello ", "world! ", "hello ", "world!"]
split_without_loss("hello world! hello world!", "o|rl")
#=> ["hello wo", "rld! hello wo", "rld!"]
split_without_loss("hello hello hello", " | ")
#=> ["hello ", " hello ", " hello"]
split_without_loss(" hello world", " |")
#=> [" ", "hello ", "world"]
split_without_loss(" hello hello hello", " |")
#=> [" ", " ", "hello ", "hello ", "hello"]
split_without_loss(" hello hello hello ", " |")
#=> [" ", " ", "hello ", "hello ", "hello ", " "]
split_without_loss(" hello hello hello", "| ")
#=> [" ", " hello", " hello", " hello"]
```
Also check out my other creations — [Identify Case](https://www.codewars.com/kata/identify-case), [Adding Fractions](https://www.codewars.com/kata/adding-fractions),
[Random Integers](https://www.codewars.com/kata/random-integers), [Implement String#transpose](https://www.codewars.com/kata/implement-string-number-transpose), [Implement Array#transpose!](https://www.codewars.com/kata/implement-array-number-transpose), [Arrays and Procs #1](https://www.codewars.com/kata/arrays-and-procs-number-1), and [Arrays and Procs #2](https://www.codewars.com/kata/arrays-and-procs-number-2)
If you notice any issues/bugs/missing test cases whatsoever, do not hesitate to report an issue or suggestion. Enjoy! | ["def split_without_loss(s, split_p):\n return [i for i in s.replace(split_p.replace('|', ''), split_p).split('|') if i]", "import re\n\ndef split_without_loss(s, m):\n r,iM = m.replace('|',''), m.index('|')\n out,i,j = [],0,0\n while 1:\n j = s.find(r,j)\n if j==-1:\n if i<len(s): out.append(s[i:])\n break\n if i<j+iM: out.append(s[i:j+iM])\n i,j = j+iM,j+len(r)\n return out", "def split_without_loss(s, split_p):\n result = []\n pipe_index = split_p.find('|')\n split_p = split_p.replace('|', '')\n\n split_index = s.find(split_p)\n begin = 0\n while split_index != -1:\n if split_index+pipe_index != 0: result.append(s[begin:split_index+pipe_index])\n begin = split_index+pipe_index\n split_index = s.find(split_p, split_index + len(split_p))\n\n if begin != len(s): result.append(s[begin:])\n return result", "def split_without_loss(s,c):\n return [x for x in s.replace(c.replace('|',''),c).split('|') if x]", "import re\ndef split_without_loss(s, split_p):\n return [i for i in (re.sub((''.join([i for i in split_p if i != '|'])), split_p, s)).split('|') if i]\n", "def split_without_loss(s, sp):\n i,sp = sp.index(\"|\"),sp.replace(\"|\", \"\") ; r = s.split(sp)\n for k in range(len(r) - 1):\n r[k] += sp[:i] ; r[k + 1] = sp[i:] + r[k + 1]\n return [i for i in r if i]", "def split_without_loss(s, split_p):\n sep = split_p.replace(\"|\",\"\")\n s1 = s.replace(sep, split_p)\n s1 = s1.strip(\"|\")\n return s1.split(\"|\")", "def split_without_loss(s,a):\n aa=a.split(\"|\")\n ss=s.replace(aa[0]+aa[1], a).split(\"|\")\n return [i for i in ss if i]", "def split_without_loss(s, split_p):\n to_find = split_p.replace('|','')\n with_bar = s.replace(to_find,split_p)\n return [x for x in with_bar.split('|') if x != '']\n", "def split_without_loss(s, split_p):\n # maybe breaks the input modification rule\n return s.replace(split_p.replace('|', ''), split_p).lstrip('|').rstrip('|').split('|')"] | {"fn_name": "split_without_loss", "inputs": [["hello world!", " |"], ["hello world!", "o|rl"], ["hello world!", "ello| "], ["hello world!", "hello wo|rld!"], ["hello world!", "h|ello world!"], ["hello world! hello world!", " |"], ["hello world! hello world!", "o|rl"], ["hello world! hello world!", "ello| "], ["hello world! hello world!", "hello wo|rld!"], ["hello hello hello", " | "], [" hello world", " |"], ["aaaa", "|aa"], ["aaa", "|aa"], ["aaaaa", "|aa"], ["ababa", "|ab"], ["abababa", "|ab"], ["abababa", "|a"], [" hello hello hello", " |"], ["aaaa", "aa|"], [" hello hello hello ", " |"], ["aaaaaa", "aa|"], ["hello hello hello ", "| "], ["aaaaaa", "|aa"], [" hello hello hello", "| "], ["aaaaaaaa", "|aa"]], "outputs": [[["hello ", "world!"]], [["hello wo", "rld!"]], [["hello", " world!"]], [["hello wo", "rld!"]], [["h", "ello world!"]], [["hello ", "world! ", "hello ", "world!"]], [["hello wo", "rld! hello wo", "rld!"]], [["hello", " world! hello", " world!"]], [["hello wo", "rld! hello wo", "rld!"]], [["hello ", " hello ", " hello"]], [[" ", "hello ", "world"]], [["aa", "aa"]], [["aaa"]], [["aa", "aaa"]], [["ab", "aba"]], [["ab", "ab", "aba"]], [["ab", "ab", "ab", "a"]], [[" ", " ", "hello ", "hello ", "hello"]], [["aa", "aa"]], [[" ", " ", "hello ", "hello ", "hello ", " "]], [["aa", "aa", "aa"]], [["hello", " hello", " hello", " "]], [["aa", "aa", "aa"]], [[" ", " hello", " hello", " hello"]], [["aa", "aa", "aa", "aa"]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,072 |
def split_without_loss(s, split_p):
|
305d113e49be525539629e9e6b362efb | UNKNOWN | # Convert Improper Fraction to Mixed Number
You will need to convert an [improper fraction](https://www.mathplacementreview.com/arithmetic/fractions.php#improper-fractions) to a [mixed number](https://www.mathplacementreview.com/arithmetic/fractions.php#mixed-number). For example:
```python
get_mixed_num('18/11') # Should return '1 7/11'
get_mixed_num('13/5') # Should return '2 3/5'
get_mixed_num('75/10') # Should return '7 5/10'
```
NOTE: All fractions will be greater than 0. | ["def get_mixed_num(fraction):\n n, d = [int(i) for i in fraction.split('/')]\n return '{} {}/{}'.format(n // d, n % d, d)", "def get_mixed_num(f):\n [a, b] = map(int, f.split('/'))\n return \"{} {}/{}\".format(a//b, a%b, b)", "def get_mixed_num(f):n,d=map(int,f.split('/'));return'%d %d/%d'%(n/d,n%d,d)", "def get_mixed_num(fraction):\n n, d = (int(num) for num in fraction.split(\"/\"))\n i, n = divmod(n, d)\n return f\"{i} {n}/{d}\"", "def get_mixed_num(f):\n v,d = map(int, f.split('/'))\n n,r = divmod(v,d)\n return f'{n} {r}/{d}'", "def get_mixed_num(fraction):\n a, b = map(int, fraction.split('/'))\n q, r = divmod(a, b)\n return f\"{q} {r}/{b}\"", "def get_mixed_num(fraction):\n a,b = list(map(int, fraction.split('/')))\n return '{} {}/{}'.format(a//b, a%b, b)", "def get_mixed_num(fraction):\n arr = fraction.split('/')\n a, b = divmod(int(arr[0]), int(arr[1]))\n res = []\n if (a != 0):\n res.append(str(a))\n if (b != 0):\n res.append(str(b) + \"/\" + arr[1])\n return ' '.join(res)", "def get_mixed_num(fraction):\n n,d = map(int,fraction.split('/'))\n a,b = divmod(n,d)\n return f'{a} {b}/{d}'", "def get_mixed_num(fraction):\n n,d=map(int,fraction.split(\"/\"))\n i,n,d=n//d,n%d,d\n return [\"\",f\"{i} \"][bool(i)]+f\"{n}/{d}\""] | {"fn_name": "get_mixed_num", "inputs": [["18/11"], ["13/5"], ["75/10"]], "outputs": [["1 7/11"], ["2 3/5"], ["7 5/10"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,343 |
def get_mixed_num(fraction):
|
1978fc8538770cd624082b6a8aeaea24 | UNKNOWN | # Sentence Smash
Write a function that takes an array of words and smashes them together into a sentence and returns the sentence. You can ignore any need to sanitize words or add punctuation, but you should add spaces between each word. **Be careful, there shouldn't be a space at the beginning or the end of the sentence!**
## Example
```
['hello', 'world', 'this', 'is', 'great'] => 'hello world this is great'
``` | ["def smash(words):\n return \" \".join(words)", "smash = ' '.join", "# http://www.codewars.com/kata/53dc23c68a0c93699800041d/\n\n\ndef smash(words):\n return \" \".join(words)\n", "smash=lambda x:' '.join(x)", "def smash(words):\n smash = \" \".join(words)\n return smash\n", "def smash(words):\n \n str1 =\" \".join(words)\n return(str1)\n \n # Begin here\n", "import re\ndef smash(words):\n return re.sub(\"[]'',[]\", \"\", str(words))", "def smash(words):\n \n i=0\n l=len(words)\n str1=\"\"\n while i<l:\n if i<l-1:\n str1+=words[i] + \" \"\n else: \n str1+=words[i]\n i+=1\n \n return str1\n \n", "smash = lambda w: ' '.join(w)\n", "smash = lambda words: ' '.join(words)", "from typing import List\n\ndef smash(words: List[str]) -> str:\n \"\"\" Join words together in the sentence. \"\"\"\n return \" \".join(words)", "def smash(words):\n out=\"\"\n for word in words:\n out+=word+\" \"\n return out.strip() \n", "smash = lambda words, separator = \" \" : separator.join(words)", "def smash(words):\n answer = ''\n for i in words:\n answer += i\n answer += ' '\n \n return answer[:-1]\n \n", "def smash(words):\n str = \"\"\n for w in words:\n str += w + \" \"\n return str[:-1]\n", "def smash(words):\n newString = ''\n for i in words:\n newString += i + ' '\n return newString.strip()", "def smash(words):\n s = \"\"\n for v in words:\n s += v\n if v != words[len(words) - 1]:\n s += \" \"\n return s", "def smash(words): \n str = \"\"\n for word in words:\n str += word\n str += \" \"\n str = str.strip()\n return str \n", "def smash(words):\n return ' '.join(words)\n \n \n \n \n#print(' '.join(words))\n", "smash = lambda string: \" \".join(string)", "def smash(words):\n s = \"\"\n for i,j in enumerate(words):\n if i==0:\n s += j\n else:\n s += \" \"+j\n return s", "def smash(words):\n new = ''\n for word in words:\n new+=word + \" \"\n \n return new.strip()\n \n", "def smash(words):\n # Begin here\n final_list = []\n\n for word in words:\n final_list.append(word)\n\n return (' '.join(final_list))", "def smash(words):\n # Begin here\n frase = \"\"\n for word in words:\n frase += word + \" \"\n return frase.strip() ", "def smash(words):\n return ''.join(s + ' ' for s in words)[:-1] ", "def smash(words):\n result = ''\n for i in words:\n result += i +' '\n return result.strip()", "def smash(words):\n res = \"\"\n for i in range(len(words)):\n res += words[i]\n if i < len(words) - 1: res += ' '\n return res\n \n", "def smash(words):\n return \" \".join(words)\n \n \n# ['hello', 'world', 'this', 'is', 'great'] => 'hello world this is great' \n", "def smash(words):\n sentence = ''\n for i in words:\n if words[0] == i:\n sentence += i\n else:\n sentence += f' {i}'\n return sentence\n \n", "def smash(words):\n result = ''\n for w in words:\n result += w + ' '\n return result.strip()\n", "def smash(words):\n # Begin here\n return \"\".join(i+' ' if len(words)-1 != n else i for n,i in enumerate(words))\n", "def smash(words):\n # Begin here\n t = ''\n for x in words:\n t = t + ''.join(x)\n if x != words[-1]:\n t += ' '\n return t\n", "def smash(words):\n x = []\n if words == []:\n return ''\n else:\n for i in range(len(words)-1):\n x.append(words[i] + ' ')\n x.append(words[len(words)-1])\n return ''.join(x)\n", "def smash(words):\n return ' '.join(wor for wor in words)\n", "def smash(words):\n x = 0\n y = '' \n for elem in words:\n while x < len(words):\n y += words[x] + ' '\n x +=1\n return y[0:-1]\n", "def smash(words):\n sen = ''\n for word in words:\n sen += word + ' '\n sen = sen.strip() \n return sen\n \n", "def smash(words):\n \n ##set up empty string\n x = \"\"\n \n ##loop over words in the array, adding each word to the empty string x, followed by a space\n for i in range(len(words)):\n x = x+words[i]+\" \"\n \n ##lazy coding: remove extra space from end of final string\n\n x = x[:-1]\n print(x)\n return(x)\n #print(x+words[L-1])\n", "def smash(words):\n if words==[]:\n return ''\n else:\n frase = words[0]\n for word in words[1:]:\n frase = frase+' ' +word\n return frase", "def smash(words):\n ans = \"\"\n for w in words:\n ans = ans + \" \" + w\n return ans.strip()\n", "def smash(words):\n satz = \" \".join(words)\n return satz\n", "def smash(words):\n tg = ' '.join(words)\n return tg.strip()", "def smash(words):\n s=\"\"\n for x in words:\n s=s+x+\" \"\n return s[:-1]\n", "def smash(words):\n sentence= \" \".join(words)\n return sentence.strip()", "def smash(w):\n result =''\n for i in w:\n result+=i + ' '\n return result.rstrip()", "def smash(words):\n a = \"\"\n for i in range(len(words)):\n a += words[i] + \" \"\n return a[:-1]", "def smash(words):\n # Begin he\n return ' '.join(words)", "def smash(words):\n phrase = \"\"\n for i in words:\n phrase = phrase + i + \" \" \n return phrase.rstrip()\n", "def smash(words):\n return \" \".join(words)\n\nprint(smash(['Hello', 'World']))", "def smash(words):\n result = \"\"\n for i in range(len(words)):\n if i < len(words) - 1:\n result += words[i] + \" \"\n else:\n result += words[i]\n return result", "def smash(words):\n w = \" \"\n return w.join(words)", "def smash(words):\n string = ''\n for item in words:\n string += item + ' '\n return string.strip()\n", "def smash(arr):\n emptystring = ''\n for eachword in arr:\n emptystring = emptystring + eachword + ' '\n emptystring = emptystring.rstrip()\n return emptystring", "def smash(words):\n string = ''\n for i in range(len(words)):\n if i == 0:\n string += words[0]\n else:\n string += ' ' + words[i]\n return string\n \n", "def smash(words):\n if len(words) > 1:\n return \" \".join(words)\n return \" \".join(words)\n", "def smash(words):\n return ''.join(word + ' ' for word in words).strip()\n", "def smash(words):\n # Begin here\n output = ''\n \n for word in words:\n output += word\n output += ' '\n \n return output[:-1]\n \n \n", "def smash(words):\n temp = \"\"\n for i in words:\n temp+=i+\" \"\n return temp.strip()\n", "def smash(words):\n x = ''\n for i in words:\n x += i\n x += ' '\n return x.strip()", "def smash(words):\n \"\"\"return a sentence from array words\"\"\"\n return \" \".join(words)\n", "def smash(words):\n out = \"\"\n if len(words) == 0:\n return \"\"\n for word in words:\n out += word + \" \"\n return out[0:-1]\n", "def smash(words):\n string = ''\n for i in range(len(words)):\n string += words[i] + ' '\n string = string[:-1]\n return string", "def smash(words):\n return ' '.join(char for char in words) \n", "def smash(words):\n newWord = \"\"\n for i in range(len(words)):\n if words[i] == words[-1]:\n newWord += words[i]\n else:\n newWord += words[i]\n newWord += \" \"\n return newWord\n \n", "def smash(words):\n s = ''\n for c in words:\n s += ' ' + str(c)\n return s.strip()", "def smash(words):\n salida = \" \".join(words)\n return salida\n", "def smash(words):\n # Begin here\n count = 0\n sentence = \"\"\n for word in words:\n sentence += word\n if count != len(words) - 1:\n sentence += \" \"\n count += 1\n return sentence\n", "def smash(words):\n newWords = \"\"\n for letters in words:\n newWords += letters\n if letters != words[-1]:\n newWords += \" \"\n return newWords", "def smash(words):\n newword = ''\n for word in words:\n newword += word + ' '\n return newword.strip()\n", "def smash(words):\n string = ''\n for x in words:\n string += x + ' '\n return string[:-1]", "def smash(words):\n for i in words:\n sentence = \" \".join(words)\n return sentence\n else:\n return ''", "def smash(words):\n res=' '.join([i for i in words])\n return res\n \n # Begin here\n", "def smash(words):\n stroke = ''\n for i in words:\n stroke = stroke + i + ' '\n stroke = stroke.rstrip()\n return stroke\n", "def smash(words):\n # Begin here\n output = ''\n for idx, w in enumerate(words):\n output = output +' ' + words[idx]\n output = output.strip()\n return output\n", "def smash(words):\n \n my_str = ''\n \n for x in words:\n if x != words[-1]:\n my_str += x + ' '\n \n else:\n my_str += x\n \n return my_str", "def smash(words):\n \n string=\"\".join([(str(i)+\" \") for i in words])\n \n return string[:-1]\n", "def smash(words):\n # Begin here\n l=\"\"\n l=' '.join(words)\n return l", "def smash(words):\n s = ' '.join(word for word in words)\n return s\n", "def smash(words):\n text = ''\n for i in words:\n text += i\n if i != words[-1]:\n text += ' '\n return text\n", "def smash(words):\n w=''\n for i in words:\n w+=i+' '\n return w[:-1]\n # Begin here\n", "def smash(words):\n c=\"\"\n for i in words:\n c=c+\" \"+\"\".join(i)\n return c.lstrip()", "smash = lambda a: ' '.join(a)\n", "smash = lambda words: ' '.join(x for x in words).strip() ", "def smash(words):\n return ' '.join(e for e in words)\n", "def smash(words):\n s = \"\"\n for w in words:\n s += str(w) + \" \"\n s = s.rstrip()\n return s\n", "def smash(words):\n ans = ''\n for x in words:\n ans += x\n ans += ' '\n return ans[:-1]\n", "def smash(words):\n # Begin here\n \n sayac = len(words)\n yazi = \"\"\n \n for kelime in words:\n yazi += kelime\n sayac -= 1\n if sayac == 0:\n break\n \n yazi += \" \"\n \n return yazi\n", "def smash(words):\n sen=''\n for i in words:\n sen+=i+' '\n return sen[:-1]\n", "def smash(words):\n a = \"\"\n for c,i in enumerate(words):\n if c == len(words)-1:\n a += i\n else:\n a += i + \" \"\n return a\n", "def smash(words):\n return ' '.join(elem for elem in words)\n", "def smash(words):\n listToString = ' '.join(elem for elem in words)\n return listToString", "def smash(words):\n s= (' ')\n r=''\n for i in range(len(words)):\n if i==len(words)-1:\n r = r+ words[i]\n else:\n r = r+ words[i] + s\n return r", "def smash(words):\n #return ' '.join([str(i) for i in words])\n new_str = ''\n for i in words:\n new_str = new_str + i + ' '\n return new_str[0:-1]\n \n \n\n", "def smash(words):\n # Begin here\n res = ''\n if len(words) == 1:\n for i in words:\n res = res + i\n return res\n else:\n\n for i in words:\n res = res +\" \"+ i \n return res[1:]", "smash = lambda n: \" \".join(n)", "def smash(words):\n\n if words == []:\n return \"\"\n else:\n i = 1\n word = words[0]\n while i < len(words):\n word = word + \" \" + words[i]\n i += 1\n return word", "def smash(words):\n sentens = \"\"\n for i in words:\n sentens += i + \" \"\n return sentens[:-1]", "def smash(words):\n # Begin here\n ans = \" \".join(words)\n return ans\n", "def smash(words):\n if len(words) != 0:\n a = ' '.join(words)\n return a\n elif len(words) == 1:\n a = words[0]\n return a\n else:\n a = ''\n return a", "def smash(words):\n sentence = \"\"\n length_words = len(words)\n for word in words:\n sentence += word\n if word == words[-1]:\n pass\n else:\n sentence += ' '\n return(sentence)\n", "def smash(words):\n return ''.join(word + ' ' for word in words)[:-1]"] | {"fn_name": "smash", "inputs": [[[]], [["hello"]], [["hello", "world"]], [["hello", "amazing", "world"]], [["this", "is", "a", "really", "long", "sentence"]]], "outputs": [[""], ["hello"], ["hello world"], ["hello amazing world"], ["this is a really long sentence"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 12,806 |
def smash(words):
|
196822b026adc60872804297d34f2463 | UNKNOWN | Write a function which takes one parameter representing the dimensions of a checkered board. The board will always be square, so 5 means you will need a 5x5 board.
The dark squares will be represented by a unicode white square, while the light squares will be represented by a unicode black square (the opposite colours ensure the board doesn't look reversed on code wars' dark background). It should return a string of the board with a space in between each square and taking into account new lines.
An even number should return a board that begins with a dark square. An odd number should return a board that begins with a light square.
The input is expected to be a whole number that's at least two, and returns false otherwise (Nothing in Haskell).
Examples:
```python
checkered_board(5)
```
returns the string
```
■ □ ■ □ ■
□ ■ □ ■ □
■ □ ■ □ ■
□ ■ □ ■ □
■ □ ■ □ ■
```
**There should be no trailing white space at the end of each line, or new line characters at the end of the string.**
**Note**
Do not use HTML entities for the squares (e.g. `□` for white square) as the code doesn't consider it a valid square. A good way to check is if your solution prints a correct checker board on your local terminal.
**Ruby note:**
CodeWars has encoding issues with rendered unicode in Ruby.
You'll need to use unicode source code (e.g. "\u25A0") instead of rendered unicode (e.g "■"). | ["def checkered_board(n):\n return isinstance(n,int) and n>1 and \\\n '\\n'.join(' '.join( \"\u25a0\" if (x+y)%2 ^ n%2 else \"\u25a1\" for y in range(n) ) for x in range(n))\n", "def checkered_board(n):\n l = ['\u25a1', '\u25a0']\n return False if type(n) != int or n < 2 else '\\n'.join(' '.join(l[(i + j + n) % 2] for i in range(n)) for j in range(n))", "def checkered_board(n):\n if not (isinstance(n, int) and n > 1):\n return False\n return '\\n'.join(' '.join('\u25a1\u25a0'[(n+i+j)%2] for j in range(n)) for i in range(n))", "def checkered_board(n):\n if type(n) != int or n < 2: return False\n s1 = ' '.join(\"\u25a0\u25a1\"[i&1] for i in range(n))\n s2 = ' '.join(\"\u25a1\u25a0\"[i&1] for i in range(n))\n lines = [s1, s2] if n&1 else [s2, s1]\n return '\\n'.join(lines[i&1] for i in range(n))", "def checkered_board(size):\n result = ''\n rows = []\n print(size, type(size))\n if not type(size) == int:\n return False\n if size < 2:\n return False\n if size % 2 == 0:\n a = '\u25a1'\n b = '\u25a0'\n else:\n a = '\u25a0'\n b = '\u25a1'\n for x in range(size):\n if x % 2 == 0:\n row = [a if x % 2 == 0 else b for x in range(size)]\n else:\n row = [b if x % 2 == 0 else a for x in range(size)]\n rows.append(' '.join(row))\n result = '\\n'.join(rows)\n return result", "def checkered_board(n):\n return '\\n'.join(' '.join(['\u25a1\u25a0', '\u25a0\u25a1'][n%2][j%2-i%2] for i in range(n)) for j in range(n)) if type(n) is int and n > 1 else False", "def checkered_board(n):\n if type(n) != int or n < 2:\n return False\n return \"\\n\".join(\" \".join(\"\u25a1\u25a0\"[(i + j + n) % 2] for j in range(n)) for i in range(n))", "def checkered_board(n):\n if type(n) is not int or n <2: return False\n return '\\n'.join(map(lambda i: ' '.join(map(lambda j: '\u25a1' if ((n-j) + i) % 2 == 0 else '\u25a0', range(n))), range(n)))", "checkered_board = lambda n:type(n) is int and n>=2 and \"\\n\".join([\" \".join([[\"\u25a1\",\"\u25a0\"][(r+c+n)%2] for r in range(n)]) for c in range(n)])", "def checkered_board(n):\n if isinstance(n, int) and n > 1:\n return '\\n'.join((' '.join(('\u25a1' if ((i % 2 == j % 2) ^ n) % 2 else '\u25a0' for j in range(n))) for i in range(n)))\n else:\n return False"] | {"fn_name": "checkered_board", "inputs": [[1], [0], [-1], ["test"], [null], [[]], [2], [100], [3], [7]], "outputs": [[false], [false], [false], [false], [false], [false], ["\u25a1 \u25a0\n\u25a0 \u25a1"], ["\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1"], ["\u25a0 \u25a1 \u25a0\n\u25a1 \u25a0 \u25a1\n\u25a0 \u25a1 \u25a0"], ["\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0\n\u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1\n\u25a0 \u25a1 \u25a0 \u25a1 \u25a0 \u25a1 \u25a0"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,411 |
def checkered_board(n):
|
b1da6a84c9b9fe223b419260d65d9ee5 | UNKNOWN | The [Chinese zodiac](https://en.wikipedia.org/wiki/Chinese_zodiac) is a repeating cycle of 12 years, with each year being represented by an animal and its reputed attributes. The lunar calendar is divided into cycles of 60 years each, and each year has a combination of an animal and an element. There are 12 animals and 5 elements; the animals change each year, and the elements change every 2 years. The current cycle was initiated in the year of 1984 which was the year of the Wood Rat.
Since the current calendar is Gregorian, I will only be using years from the epoch 1924.
*For simplicity I am counting the year as a whole year and not from January/February to the end of the year.*
##Task
Given a year, return the element and animal that year represents ("Element Animal").
For example I'm born in 1998 so I'm an "Earth Tiger".
```animals``` (or ```$animals``` in Ruby) is a preloaded array containing the animals in order:
```['Rat', 'Ox', 'Tiger', 'Rabbit', 'Dragon', 'Snake', 'Horse', 'Goat', 'Monkey', 'Rooster', 'Dog', 'Pig']```
```elements``` (or ```$elements``` in Ruby) is a preloaded array containing the elements in order:
```['Wood', 'Fire', 'Earth', 'Metal', 'Water']```
Tell me your zodiac sign and element in the comments. Happy coding :) | ["animals = ['Rat', 'Ox', 'Tiger', 'Rabbit', 'Dragon', 'Snake', 'Horse', 'Goat', 'Monkey', 'Rooster', 'Dog', 'Pig']\nelements = ['Wood', 'Fire', 'Earth', 'Metal', 'Water']\ndef chinese_zodiac(year):\n year -= 1984\n return elements[year//2 % 5] + \" \" + animals[year % 12]", "def chinese_zodiac(year):\n s = ''\n a = ['Rat','Ox', 'Tiger', 'Rabbit', 'Dragon', 'Snake', 'Horse', 'Goat', 'Monkey', 'Rooster', 'Dog', 'Pig']\n e = ['Wood', 'Fire', 'Earth', 'Metal', 'Water']\n \n e1 = (year - 1984) // 2 % 5 \n a1 = (year - 1984) % 12\n \n s = e[e1] + ' ' + a[a1]\n return s", "def chinese_zodiac(year):\n animals = ['Rat', 'Ox', 'Tiger', 'Rabbit', 'Dragon', 'Snake', 'Horse', 'Goat', 'Monkey', 'Rooster', 'Dog', 'Pig']\n elements = ['Wood', 'Fire', 'Earth', 'Metal', 'Water']\n diff = year-1984\n animalIndex = diff%12\n eleIndex = diff//2\n eleIndex = eleIndex%5\n return \"{} {}\".format(elements[eleIndex], animals[animalIndex])", "def chinese_zodiac(year):\n EPOCH = 1924\n ANIMALS = ['Rat', 'Ox', 'Tiger', 'Rabbit', 'Dragon', 'Snake', 'Horse', 'Goat', 'Monkey', 'Rooster', 'Dog', 'Pig']\n ELEMENTS = ['Wood', 'Fire', 'Earth', 'Metal', 'Water']\n year -= EPOCH\n res = ELEMENTS[(year // 2) % len(ELEMENTS)], ANIMALS[year % len(ANIMALS)]\n return \" \".join(res)"] | {"fn_name": "chinese_zodiac", "inputs": [[1965], [1938], [1998], [2016], [1924], [1968], [2162], [6479], [3050], [6673], [6594], [9911], [2323], [3448], [1972]], "outputs": [["Wood Snake"], ["Earth Tiger"], ["Earth Tiger"], ["Fire Monkey"], ["Wood Rat"], ["Earth Monkey"], ["Water Dog"], ["Earth Goat"], ["Metal Dog"], ["Water Rooster"], ["Wood Tiger"], ["Metal Goat"], ["Water Rabbit"], ["Earth Rat"], ["Water Rat"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,339 |
def chinese_zodiac(year):
|
338ab9a46b4163ce255c7db61278d43c | UNKNOWN | For a given array whose element values are randomly picked from single-digit integers `0` to `9`, return an array with the same digit order but all `0`'s paired. Paring two `0`'s generates one `0` at the location of the first.
Ex:
```python
pair_zeros([0, 1, 0, 2])
# paired: ^-----^ cull second zero
== [0, 1, 2];
# kept: ^
pair_zeros([0, 1, 0, 0])
# paired: ^-----^
== [0, 1, 0];
# kept: ^
pair_zeros([1, 0, 7, 0, 1])
# paired: ^-----^
== [1, 0, 7, 1];
# kept: ^
pair_zeros([0, 1, 7, 0, 2, 2, 0, 0, 1, 0])
# paired: ^--------^
# [0, 1, 7, 2, 2, 0, 0, 1, 0]
# kept: ^ paired: ^--^
== [0, 1, 7, 2, 2, 0, 1, 0];
# kept: ^
```
Here are the 2 important rules:
1. Pairing happens from left to right in the array. However, for each pairing, the "second" `0` will always be paired towards the first (right to left)
2. `0`'s generated by pairing can NOT be paired again | ["from itertools import count\ndef pair_zeros(arr, *args):\n c = count(1)\n return [elem for elem in arr if elem != 0 or next(c) % 2]", "def pair_zeros(nums, *_):\n result = []\n skip_zero = False\n for a in nums:\n if a == 0:\n if not skip_zero:\n result.append(a)\n skip_zero = not skip_zero\n else:\n result.append(a)\n return result\n", "def pair_zeros(arr):\n got = [0]\n return [v for v in arr if v or got.__setitem__(0,got[0]^1) or got[0]]", "def pair_zeros(arr):\n return [d for i, d in enumerate(arr) if d != 0 or arr[:i+1].count(0) % 2]", "def pair_zeros(arr):\n lst = []\n flag = False\n for i in arr:\n if i == 0:\n if not flag:\n lst.append(i)\n flag = not flag\n else:\n lst.append(i)\n return lst", "import itertools\n\n\ndef pair_zeros(arr):\n it = itertools.count(1)\n return [x for x in arr if x > 0 or next(it) % 2]", "def pair_zeros(arr, *other):\n \n return [n for i, n in enumerate(arr) if n != 0 or arr[:i+1].count(0)%2 == 1]", "import re\n\ndef pair_zeros(arr,*args): \n return list(map(int, re.sub(r\"(0[^0]*)0\", \"\\\\1\", \"\".join([str(a) for a in arr]))))\n", "def pair_zeros(arr):\n lst = []\n zCount = 0\n for item in arr:\n if item == 0:\n zCount += 1\n if zCount % 2 != 0:\n lst.append(item)\n else:\n lst.append(item)\n return lst"] | {"fn_name": "pair_zeros", "inputs": [[[]], [[1]], [[1, 2, 3]], [[0]], [[0, 0]], [[1, 0, 1, 0, 2, 0, 0]], [[0, 0, 0]], [[1, 0, 1, 0, 2, 0, 0, 3, 0]], [[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]], [[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]]], "outputs": [[[]], [[1]], [[1, 2, 3]], [[0]], [[0]], [[1, 0, 1, 2, 0]], [[0, 0]], [[1, 0, 1, 2, 0, 3, 0]], [[0, 0, 0, 0, 0, 0]], [[0, 0, 0, 0, 0, 0, 0]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,526 |
def pair_zeros(arr):
|
be38af59f9ef485e85eed4725e324473 | UNKNOWN | ```if:javascript
`Array.prototype.length` will give you the number of top-level elements in an array.
```
```if:ruby
`Array#length` will give you the number of top-level elements in an array.
```
```if:csharp
The `Length` property of an array will give you the number of top-level elements in an array.
```
```if:php
`count()` will give you the number of top-level elements in an array if exactly one argument `$a` is passed in which is the array.
```
```if:python
`len(a)` will give you the number of top-level elements in the list/array named `a`.
```
Your task is to create a function ```deepCount``` that returns the number of ALL elements within an array, including any within inner-level arrays.
For example:
```if:javascript
deepCount([1, 2, 3]);
//>>>>> 3
deepCount(["x", "y", ["z"]]);
//>>>>> 4
deepCount([1, 2, [3, 4, [5]]]);
//>>>>> 7
```
```if:ruby
deepCount([1, 2, 3]);
//>>>>> 3
deepCount(["x", "y", ["z"]]);
//>>>>> 4
deepCount([1, 2, [3, 4, [5]]]);
//>>>>> 7
```
```if:csharp
deepCount([1, 2, 3]);
//>>>>> 3
deepCount(["x", "y", ["z"]]);
//>>>>> 4
deepCount([1, 2, [3, 4, [5]]]);
//>>>>> 7
```
```if:php
deep_c([1, 2, 3]);
//>>>>> 3
deep_c(["x", "y", ["z"]]);
//>>>>> 4
deep_c([1, 2, [3, 4, [5]]]);
//>>>>> 7
```
```if:python
deepCount([1, 2, 3]);
//>>>>> 3
deepCount(["x", "y", ["z"]]);
//>>>>> 4
deepCount([1, 2, [3, 4, [5]]]);
//>>>>> 7
```
The input will always be an array.
```if:php
In PHP you may *not* assume that the array passed in will be non-associative.
Please note that `count()`, `eval()` and `COUNT_RECURSIVE` are disallowed - you should be able to implement the logic for `deep_c()` yourself ;)
``` | ["def deep_count(a):\n result = 0\n for i in range(len(a)):\n if type(a[i]) is list:\n result += deep_count(a[i])\n result += 1\n return result\n", "def deep_count(a):\n return sum(1 + (deep_count(x) if isinstance(x, list) else 0) for x in a)", "def deep_count(a):\n return len(a) + sum(deep_count(e) for e in a if isinstance(e, list))", "def deep_count(a):\n total = 0\n for item in a:\n total += 1\n if isinstance(item, list):\n total += deep_count(item)\n return total", "def deep_count(a):\n count = 0\n for i in a:\n count +=1 \n if type(i) is list:\n count += deep_count(i)\n return count\n \n", "deep_count=lambda a:sum(1 + (deep_count(x) if isinstance(x, list) else 0) for x in a)", "def deep_count(a):\n return len(a) + sum(deep_count(b) for b in a if isinstance(b, list))", "def deep_count(a):\n b = []\n while a:\n for i in a:\n if type(i) == list:\n a.extend(i)\n a.remove(i)\n a.append('1')\n else:\n b.append(i)\n a.remove(i)\n return len(b)", "deep_count=d=lambda a:sum(type(e)is not list or d(e)+1for e in a)", "def deep_count(a):\n if a == []:\n return 0\n if isinstance(a[0], list):\n return 1 + deep_count(a[0]) + deep_count(a[1:])\n return 1 + deep_count(a[1:])\n"] | {"fn_name": "deep_count", "inputs": [[[]], [[1, 2, 3]], [["x", "y", ["z"]]], [[1, 2, [3, 4, [5]]]], [[[[[[[[[[]]]]]]]]]], [["a"]], [[["a"]]], [[["a"], []]], [["[a]"]], [[[[[[[[[["Everybody!"]]]]]]]]]], [["cat", [["dog"]], ["[bird]"]]]], "outputs": [[0], [3], [4], [7], [8], [1], [2], [3], [1], [9], [6]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,433 |
def deep_count(a):
|
04d7cef5e9eacfcfd7618598ad1f79df | UNKNOWN | Given a certain integer ```n, n > 0```and a number of partitions, ```k, k > 0```; we want to know the partition which has the maximum or minimum product of its terms.
The function ```find_spec_partition() ```, will receive three arguments, ```n```, ```k```, and a command: ```'max' or 'min'```
The function should output the partition that has maximum or minimum value product (it depends on the given command) as an array with its terms in decreasing order.
Let's see some cases (Python and Ruby)
```
find_spec_partition(10, 4, 'max') == [3, 3, 2, 2]
find_spec_partition(10, 4, 'min') == [7, 1, 1, 1]
```
and Javascript:
```
findSpecPartition(10, 4, 'max') == [3, 3, 2, 2]
findSpecPartition(10, 4, 'min') == [7, 1, 1, 1]
```
The partitions of 10 with 4 terms with their products are:
```
(4, 3, 2, 1): 24
(4, 2, 2, 2): 32
(6, 2, 1, 1): 12
(3, 3, 3, 1): 27
(4, 4, 1, 1): 16
(5, 2, 2, 1): 20
(7, 1, 1, 1): 7 <------- min. productvalue
(3, 3, 2, 2): 36 <------- max.product value
(5, 3, 1, 1): 15
```
Enjoy it! | ["def find_spec_partition(n, k, com):\n x,r = divmod(n,k)\n return {'max': [x+1]*r + [x]*(k-r),\n 'min': [n+1-k] + [1]*(k-1)}[com]", "def find_spec_partition(n, k, com):\n return [n-k+1] + [1] * (k-1) if com == \"min\" else [n//k + 1] * (n%k) + [n//k] * (k - n%k)", "def find_spec_partition(n, k, com):\n if com == 'min':\n return [n - k + 1] + [1] * (k-1)\n q, r = divmod(n, k)\n return [q+1] * r + [q] * (k-r)", "def find_spec_partition(n, k, com):\n if com == 'min':\n answer = [n]\n while len(answer) != k:\n answer[0] -= 1\n answer.append(1)\n return answer\n else:\n answer = [1 for i in range(k)]\n cur = 0\n while sum(answer) != n:\n answer[cur] += 1\n cur += 1\n if cur == k:\n cur = 0\n return answer", "def find_spec_partition(n, k, com):\n if com == 'min':\n return [n - k + 1] + [1] * (k - 1)\n else:\n return [(n // k + 1 if i < n % k else n //k) for i in range(k)]\n return r", "def find_spec_partition(n, k, com):\n # your code here\n part = [0]*k\n if com == 'min':\n part[1:k] = [1]*(k-1)\n part[0] = n-k+1\n else:\n for p in range(len(part)):\n maxPart = round(n/(k-p))\n part[p] = maxPart\n n -= maxPart\n part.sort(reverse=True)\n return part", "def find_spec_partition(n, k, com):\n if com == 'min' : return [n-k+1]+[1]*(k-1)\n else:\n li, i = [1]*k, 0\n while sum(li)<n: li[i%k]+=1; i+=1\n return li"] | {"fn_name": "find_spec_partition", "inputs": [[10, 4, "max"], [10, 4, "min"]], "outputs": [[[3, 3, 2, 2]], [[7, 1, 1, 1]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,607 |
def find_spec_partition(n, k, com):
|
6e0b54b9ebf7f42f604b9b9d676e4d5a | UNKNOWN | Let be `n` an integer prime with `10` e.g. `7`.
`1/7 = 0.142857 142857 142857 ...`.
We see that the decimal part has a cycle: `142857`. The length of this cycle is `6`. In the same way:
`1/11 = 0.09 09 09 ...`. Cycle length is `2`.
# Task
Given an integer n (n > 1), the function cycle(n) returns the length of the cycle if n and 10 are coprimes, otherwise returns -1.
# Examples:
```
cycle(5) = -1
cycle(13) = 6 -> 0.076923 076923 0769
cycle(21) = 6 -> 0.047619 047619 0476
cycle(27) = 3 -> 0.037 037 037 037 0370
cycle(33) = 2 -> 0.03 03 03 03 03 03 03 03
cycle(37) = 3 -> 0.027 027 027 027 027 0
cycle(94) = -1
cycle(22) = -1 since 1/22 ~ 0.0 45 45 45 45 ...
``` | ["import math\n\ndef cycle(n) :\n if n % 2 == 0 or n % 5 ==0:\n return -1\n k = 1\n while pow(10,k,n) != 1:\n k += 1\n return k\n \n \n \n", "def cycle(n):\n if not n % 2 or not n % 5:\n return -1\n x, mods = 1, set()\n while x not in mods:\n mods.add(x)\n x = 10 * x % n\n return len(mods)", "def cycle(n):\n if n%5==0 or n%2==0:\n return -1\n remainders, r = [1], 0\n while r != 1:\n r = remainders[-1] * 10 % n\n remainders.append(r)\n return len(remainders)-1", "def cycle(n) :\n if n % 2 and n % 5:\n for i in range(1, n):\n if pow(10, i, n) == 1:\n return i\n return -1", "def cycle(n):\n if n % 2 == 0 or n % 5 == 0:\n return -1\n m = 1\n for p in range(1, n):\n m = 10 * m % n\n if m == 1: \n return p", "def cycle(n):\n if not (n%2 and n%5): return -1\n return next(filter(lambda x: pow(10, x, n) == 1, range(1, n)))", "def cycle(n) :\n if not n % 2 or not n % 5:\n return -1\n m, s = 1, set()\n while m not in s:\n s.add(m)\n m = 10 * m % n\n return len(s)", "from math import ceil, log10\n\ndef cycle(d):\n if d % 5 == 0 or d % 2 == 0:\n return -1\n\n n = 10 ** int(ceil(log10(d)))\n first = n % d\n n *= 10\n for i in range(99999999):\n n %= d\n if n == first:\n return i + 1\n n *= 10", "def cycle(n) :\n if n%2 == 0 or n%5 == 0:\n return -1\n c = len(str(n))\n m = 10**c % n\n while m != 1:\n c += 1\n m = (m*10) % n\n return c\n", "def cycle(i):\n if(i%2==0 or i%5==0):\n return -1\n \n gen = 10\n n = 1\n while(gen%i != 1):\n gen = (gen % i) * 10\n n = n + 1\n \n return n"] | {"fn_name": "cycle", "inputs": [[3], [33], [18118], [69], [197], [65], [97], [19], [111], [53], [59], [93], [51], [159], [183], [167], [94], [133], [218713], [38127], [431541], [221193], [1234567]], "outputs": [[1], [2], [-1], [22], [98], [-1], [96], [18], [3], [13], [58], [15], [16], [13], [60], [166], [-1], [18], [9744], [6230], [726], [3510], [34020]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,830 |
def cycle(n) :
|
7e2154b92eb5fc82fa7b7cc67fa3cd52 | UNKNOWN | Below is a right-angled triangle:
```
|\
| \
| \
| \
o | \ h
| \
| θ \
|_______\
a
```
Your challange is to write a function (```missingAngle``` in C/C#, ```missing_angle``` in Ruby), that calculates the angle θ in degrees to the nearest integer. You will be given three arguments representing each side: o, h and a. One of the arguments equals zero. Use the length of the two other sides to calculate θ. You will not be expected to handle any erronous data in your solution. | ["import math\n\ndef missing_angle(h, a, o):\n if h == 0:\n radians = math.atan(o/a)\n elif a == 0:\n radians = math.asin(o/h)\n else:\n radians = math.acos(a/h)\n return round(math.degrees(radians))", "from math import asin, acos, atan, degrees as d\nmissing_angle=lambda h,a,o:round(d(atan(o/a) if not h else asin(o/h) if not a else acos(a/h)))", "import math\n\ndef missing_angle(h, a, o):\n return round(math.degrees(math.atan(o/a) if not h else math.asin(o/h) if not a else math.acos(a/h)))", "from math import sqrt, asin, degrees\n\ndef missing_angle(h, a, o):\n h, o = h or sqrt(a**2 + o**2), o or sqrt(h**2 - a**2)\n return round(degrees(asin(o/h)))", "from math import acos, asin, atan, degrees\n\ndef missing_angle(h, a, o):\n return round(degrees(\n atan(o / a) if not h else\n asin(o / h) if not a else\n acos(a / h)\n ))", "from math import asin, acos, atan, pi\ndef missing_angle(h, a, o):\n ans = acos(a/h) if h * a else atan(o/a) if a * o else asin(o/h)\n return round(ans*180/pi)\n", "import math\n\ndef missing_angle(h, a, o):\n if h > 0 and a > 0: result = math.acos(a/h)\n elif a == 0 and o > 0: result = math.asin(o/h)\n elif h == 0 and o > 0: result = math.atan(o/a)\n else: raise ValueError(\"Invalid argument(s)\")\n \n return round(math.degrees(result))", "from math import atan, asin, acos, pi\ndef missing_angle(h, a, o):\n return round(atan(o/a)*180/pi) if not h else round(asin(o/h)*180/pi)\\\n if not a else round(acos(a/h)*180/pi)", "import math\n\ndef missing_angle(h, a, o):\n if not h: h = (a**2 + o**2)**0.5\n if not o: o = (h**2 - a**2)**0.5\n return round(math.asin(o/h)*180/math.pi)", "import math\ndef missing_angle(h, a, o):\n if h==0:\n h=(a**2+o**2)**0.5\n if a==0:\n a=(h*2-o**2)**0.5\n if o==0:\n o=(h**2-a**2)**0.5\n return round(math.asin(o/h)*180/math.pi)\n \n"] | {"fn_name": "missing_angle", "inputs": [[0, 400, 300], [5, 4, 0], [8, 0, 5], [16.7, 0, 12.3], [7, 5, 0]], "outputs": [[37], [37], [39], [47], [44]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,973 |
def missing_angle(h, a, o):
|
c9c8d9a9c845be2809a6e9ab6f9e4509 | UNKNOWN | ## Task
To charge your mobile phone battery, do you know how much time it takes from 0% to 100%? It depends on your cell phone battery capacity and the power of the charger. A rough calculation method is:
```
0% --> 85% (fast charge)
(battery capacity(mAh) * 85%) / power of the charger(mA)
85% --> 95% (decreasing charge)
(battery capacity(mAh) * 10%) / (power of the charger(mA) * 50%)
95% --> 100% (trickle charge)
(battery capacity(mAh) * 5%) / (power of the charger(mA) * 20%)
```
For example: Your battery capacity is 1000 mAh and you use a charger 500 mA, to charge your mobile phone battery from 0% to 100% needs time:
```
0% --> 85% (fast charge) 1.7 (hour)
85% --> 95% (decreasing charge) 0.4 (hour)
95% --> 100% (trickle charge) 0.5 (hour)
total times = 1.7 + 0.4 + 0.5 = 2.6 (hour)
```
Complete function `calculateTime` that accepts two arguments `battery` and `charger`, return how many hours can charge the battery from 0% to 100%. The result should be a number, round to 2 decimal places (In Haskell, no need to round it). | ["calculate_time=lambda b,c: round(b/float(c)*1.3+0.0001,2)\n \n", "CHARGE_CONFIG = ((.85, 1), (.1, .5), (.05, .2)) \n\ndef calculate_time(battery, charger):\n return round(1e-4 + sum(battery * pb / (charger * pc) for pb,pc in CHARGE_CONFIG), 2)", "def calculate_time(battery, charger):\n return round(battery / charger * 1.3, 2)\n", "def calculate_time(battery,charger):\n return round(1e-10 + 1.3 * battery / charger, 2)", "def calculate_time(bat,charger):\n return round(((bat*0.85)/charger)+((bat*0.1)/(charger*0.5))+((bat*0.05)/(charger*0.2)),2)", "from decimal import Decimal, ROUND_HALF_UP\n\n\ndef calculate_time(battery,charger):\n n = 2.6 * battery/1000 * 500/charger\n return float(Decimal(str(n)).quantize(Decimal('1.23'), rounding=ROUND_HALF_UP))", "#2.55 + \ndef calculate_time(battery,charger):\n max = 100\n time = 0\n fast = (battery/charger)*.85\n dcharge = (battery/charger) * .2\n tcharge = (battery/charger) * .25\n if max == 100:\n time += fast\n max += -85\n if max == 15:\n time += dcharge\n max += -10\n if max == 5:\n time += tcharge\n max += -5\n if time == 1.105:\n return 1.11\n return round(time,2)", "calculate_time = lambda b,c: round(b*1.3/c+0.004, 2)", "import math\n\ndef calculate_time(battery,charger):\n return math.ceil(battery / charger * 130) / 100", "def calculate_time(b, c):\n return round(b * (0.85/c + 0.1/(c * 0.5) + 0.05/(c * 0.2)), 2)"] | {"fn_name": "calculate_time", "inputs": [[1000, 500], [1500, 500], [2000, 1000], [5000, 1000], [1000, 5000], [3050, 2600]], "outputs": [[2.6], [3.9], [2.6], [6.5], [0.26], [1.53]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,493 |
def calculate_time(battery,charger):
|
9f4dcb81a735087cfc5d1b94fccba4ef | UNKNOWN | # The Invitation
Most of us played with toy blocks growing up. It was fun and you learned stuff. So what else can you do but rise to the challenge when a 3-year old exclaims, "Look, I made a square!", then pointing to a pile of blocks, "Can _you_ do it?"
# These Blocks
Just to play along, of course we'll be viewing these blocks in two dimensions. Depth now being disregarded, it turns out the pile has four different sizes of block: `1x1`, `1x2`, `1x3`, and `1x4`. The smallest one represents the area of a square, the other three are rectangular, and all differ by their width. Integers matching these four widths are used to represent the blocks in the input.
# This Square
Well, the kid made a `4x4` square from this pile, so you'll have to match that. Noticing the way they fit together, you realize the structure must be built in fours rows, one row at a time, where the blocks must be placed horizontally. With the known types of block, there are five types of row you could build:
* 1 four-unit block
* 1 three-unit block plus 1 one-unit bock (in either order)
* 2 two-unit blocks
* 1 two-unit block plus 2 one-unit blocks (in any order)
* 4 one-unit blocks
Amounts for all four of the block sizes in the pile will each vary from `0` to `16`. The total size of the pile will also vary from `0` to `16`. The order of rows is irrelevant. A valid square doesn't have to use up all the given blocks.
# Some Examples
Given `1, 3, 2, 2, 4, 1, 1, 3, 1, 4, 2` there are many ways you could construct a square. Here are three possibilities, as described by their four rows:
* 1 four-unit block
* 2 two-unit blocks
* 1 four-unit block
* 4 one-unit blocks
>
* 1 three-unit block plus 1 one-unit block
* 2 two-unit blocks
* 1 four-unit block
* 1 one-unit block plus 1 three-unit block
>
* 2 two-unit blocks
* 1 three-unit block plus 1 one-unit block
* 1 four-unit block
* 2 one-unit blocks plus 1 two-unit block
>
Given `1, 3, 2, 4, 3, 3, 2` there is no way to complete the task, as you could only build three rows of the correct length. The kid will not be impressed.
* 2 two-unit blocks
* 1 three-unit block plus 1 one-unit block
* 1 four-unit block
* (here only sadness)
>
# Input
```python
blocks ~ a random list of integers (1 <= x <= 4)
```
# Output
```python
True or False ~ whether you can build a square
```
# Enjoy!
If interested, I also have [this kata](https://www.codewars.com/kata/5cb7baa989b1c50014a53333) as well as [this other kata](https://www.codewars.com/kata/5cb5eb1f03c3ff4778402099) to consider solving. | ["def build_square(blocks):\n for x in range(4):\n if 4 in blocks:\n blocks.remove(4)\n elif 3 in blocks and 1 in blocks:\n blocks.remove(3)\n blocks.remove(1)\n elif blocks.count(2) >= 2:\n blocks.remove(2)\n blocks.remove(2)\n elif 2 in blocks and blocks.count(1) >= 2:\n blocks.remove(2)\n blocks.remove(1)\n blocks.remove(1)\n elif blocks.count(1) >= 4:\n blocks.remove(1)\n blocks.remove(1)\n blocks.remove(1)\n blocks.remove(1)\n else:\n return False\n return True", "def build_square(blocks):\n return (blocks.count(4) \n + min(blocks.count(3), blocks.count(1))\n + ((blocks.count(1) - min(blocks.count(3), blocks.count(1))) / 4)\n + (blocks.count(2) / 2)) >= 4", "def build_square(arr): \n\n c = 0\n def purge(*sizes):\n nonlocal c\n used = []\n try:\n for s in sizes:\n arr.remove(s)\n used.append(s)\n c += 1\n except:\n arr.extend(used)\n\n for _ in range(16):\n purge(4)\n purge(3,1)\n purge(2,2)\n purge(2,1,1)\n purge(1,1,1,1)\n \n return c >= 4", "def build_square(blocks):\n x1, x2, x3, x4 = (blocks.count(n) for n in range(1, 5))\n b3 = min(x3, x1)\n b2 = min(x2 % 2, (x1 - b3) // 2)\n b1 = (x1 - b3 - b2 * 2) // 4\n return x4 + b3 + x2 // 2 + b2 + b1 > 3 \n", "def build_square(sq):\n A= lambda x:all(sq.count(i) >= x.count(i) for i in x)\n for _ in range(4):\n t = next(([sq.pop(sq.index(l)) for l in k] for k in [[4],[3,1],[2,2],[2,1,1],[1,1,1,1]] if A(k)),0)\n if not t : return False\n return True", "def build_square(blocks):\n a = 4\n b = blocks.count(1)\n c = blocks.count(2)\n d = blocks.count(3)\n e = blocks.count(4)\n a -= e\n if a <= 0:\n return True\n while b > 0 and d > 0:\n b-=1\n d-=1\n a-=1\n while c > 0 and b > 1:\n b-=2\n c-=1\n a-=1\n while b > 3:\n b-=4\n a-=1\n while c > 1:\n c-=2\n a-=1\n if a <= 0: \n return True\n else:\n return False\n \n \n \n \n \n", "from collections import Counter\n\ndef build_square(blocks):\n c = Counter(blocks)\n\n # 4\n n = 4\n n -= c[4]\n\n # 3 + 1\n x = min(c[3], c[1])\n n -= x\n c[1] -= x\n\n # 2 + 2\n x = c[2] // 2\n n -= x\n c[2] -= x * 2\n\n # 2 + 1 + 1\n x = min(c[2], c[1] // 2)\n n -= x\n c[1] -= x * 2\n\n # 1 + 1 + 1 + 1\n return c[1] // 4 >= n", "build_square=lambda B:(lambda a,b,c,d:15<a+b*2+3*min(a,c)+d*4)(*map(B.count,(1,2,3,4)))", "def build_square(blocks):\n nums = {i:blocks.count(i) for i in range(1,5)}\n odd_comb = min(nums[3],nums[1])\n nums[1]-=odd_comb\n\n return nums[4]*4 + odd_comb*4 + nums[2]*2 + nums[1] >= 16", "build_square=lambda sq,A=lambda x,y:all(y.count(i)>=x.count(i)for i in x):next((0for _ in range(4)if not next(([sq.pop(sq.index(l))for l in k]for k in [[4],[3,1],[2,2],[2,1,1],[1,1,1,1]]if A(k,sq)),0)),1)"] | {"fn_name": "build_square", "inputs": [[[1, 1, 1, 1, 1, 1, 1, 2, 3, 4]], [[1, 3, 2, 2, 4, 1, 1, 3, 1, 4, 2]], [[1, 3, 2, 4, 3, 3, 2]], [[2, 2, 3, 3, 3, 3, 3, 3, 3, 4, 4]], [[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]], [[3, 1, 3, 1, 3, 1, 3, 2]], [[1, 3, 1, 1, 1, 1, 1, 1, 1, 2, 1, 1]], [[4, 2, 2, 1, 1, 1, 1, 3, 3, 3, 1]], [[1, 2, 3, 4, 1, 2, 2, 1]], [[]]], "outputs": [[true], [true], [false], [false], [true], [false], [false], [true], [true], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,246 |
def build_square(blocks):
|
e65bd638005a9791bcc40a6c0c66dbf7 | UNKNOWN | Every natural number, ```n```, may have a prime factorization like:
We define the arithmetic derivative of ```n```, ```n'``` the value of the expression:
Let's do the calculation for ```n = 231525```.
```
231525 = 3³5²7³
n' = (3*3²)*5²7³ + 3³*(2*5)*7³ + 3³*5²*(3*7²) = 231525 + 92610 + 99225 = 423360
```
We may make a chain of arithmetic derivatives, starting from a number we apply to the result the transformation and so on until we get the result ```1```.
```
n ---> n' ---> (n')' ---> ((n')')' ---> ..... ---> ((...(n')...)')'
```
Let's do it starting with our number, ```n = 231525``` and making a chain of 5 terms:
```
231525 ---> 423360 ---> 1899072 ---> 7879680 ---> 51895296
```
We need a function ```chain_arith_deriv()``` that receives two arguments: ```start``` and ```k```, amount of terms of the chain.
The function should output the chain in an array format:
```
chain_arith_deriv(start, k) ---> [start, term1, term2, .....term(k-1)] # a total of k-terms
```
For the case presented above:
```python
chain_arith_deriv(231525, 5) == [231525, 423360, 1899072, 7879680, 51895296]
```
The function has to reject prime numbers giving an alerting message
```python
chain_arith_deriv(997, 5) == "997 is a prime number"
```
Features of the tests:
```
Number of Random Tests: 50
1000 ≤ start ≤ 99000000
3 ≤ k ≤ 15
```
Enjoy it and do your best! | ["def chain_arith_deriv(n, k):\n if len(prime_factors(n)) < 2:\n return \"{} is a prime number\".format(n)\n chain = [n]\n while k > 1:\n k, n = k-1, arith_deriv(n)\n chain.append(n)\n return chain\n\n\ndef arith_deriv(n):\n factors = prime_factors(n)\n return sum(n * factors.count(factor) // factor for factor in set(factors)) or 1\n\n\ndef prime_factors(n):\n if n < 2:\n return []\n factors = []\n for k in (2, 3):\n while n % k == 0:\n n //= k\n factors.append(k)\n k = 5\n step = 2\n while k * k <= n:\n if n % k:\n k += step\n step = 6 - step\n else:\n n //= k\n factors.append(k)\n if n > 1:\n factors.append(n)\n return factors", "from collections import Counter\n\ndef factors(n):\n step = lambda x: 1 + 4*x - (x - x%2)\n d, q, qmax = 1, 2 + n % 2, int(n ** .5)\n while q <= qmax and n % q:\n q = step(d)\n d += 1\n return q <= qmax and [q] + factors(n//q) or [n]\n\ndef chain_arith_deriv(start, k):\n n, chain = start, []\n for _ in range(k):\n chain.append(n)\n fac = Counter(factors(n))\n n = sum(n // p * k for p, k in fac.items())\n return chain if chain[1] > 1 else \"%d is a prime number\" % start", "def chain_arith_deriv(n, k):\n r = [n]\n while len(r) < k:\n d = decompose(r[-1])\n if n in d and len(r) == 1: return str(n) + \" is a prime number\"\n r.append(sum(r[-1] / i * d[i] for i in d)) \n return r\n \ndef decompose(n):\n i, f = 2, {}\n while i * i <= n:\n while n % i == 0:\n f[i] = f.get(i, 0) + 1\n n //= i\n i += 1\n if n > 1: f[n] = 1\n return f if f else {1:1} ", "def chain_arith_deriv(start, k):\n res=[start]\n for i in range(k-1):\n s=0; r=factorize(start)\n if i==0 and r=={start:1}: return str(start)+\" is a prime number\"\n try:\n for n in r:\n p=1\n for n2 in r: p*= r[n2]*n2**(r[n2]-1) if n2==n else n2**r[n2]\n s+=p\n start=s; res.append(s)\n except: res.append(1) \n return res\n\ndef factorize(num):\n if num<4: return [num]\n r={}; d=2\n while d<num**.5+1:\n while not num%d: \n r[d]= r[d]+1 if d in r else 1\n num/=d\n d+=1 if d==2 else 2\n if num!=1: r[num]=1\n return r", "def _prime_factors(n):\n factors = {}\n i = 2\n while i * i <= n:\n if n % i:\n i += 1\n else:\n n //= i\n if i in factors.keys():\n factors[i] += 1\n else:\n factors[i] = 1\n if n > 1:\n if n in factors.keys():\n factors[n] += 1\n else:\n factors[n] = 1\n\n return factors\n\n\ndef chain_arith_deriv(start, m, chain=None):\n factors = _prime_factors(start)\n if len(factors) == 1 and chain is None:\n return '{} is a prime number'.format(start)\n if chain is None:\n chain = []\n chain.append(start)\n\n if m == 1:\n return chain\n elif start == 1:\n next_num = 1\n else:\n next_num = 0\n for p, k in factors.items():\n next_num += k/p*start\n return chain_arith_deriv(round(next_num), m-1, chain=chain)", "from sys import version_info\nif version_info.major >= 3:\n long = int\nfrom collections import Counter\nfrom math import floor, sqrt\nfrom random import randint\n\ndef is_prime(number):\n if (number > 1):\n for time in range(3):\n randomNumber = randint(2, number)- 1\n if ( pow(randomNumber, number-1, number) != 1 ):\n return False\n return True\n else:\n return False \n\ndef fac(n): \n step = lambda x: 1 + (x<<2) - ((x>>1)<<1) \n maxq = int(floor(sqrt(n)))\n d = 1\n q = n % 2 == 0 and 2 or 3 \n while q <= maxq and n % q != 0:\n q = step(d)\n d += 1\n return q <= maxq and [q] + fac(n//q) or [n]\n\ndef f_arith_deriv_(n):\n primeCount = Counter(fac(n))\n n_ = 0\n for k, v in list(primeCount.items()):\n n_ += v * n // k\n return n_\n\ndef chain_arith_deriv(n, k):\n if is_prime(n) :return str(n) + \" is a prime number\"\n chain = [n]\n while True:\n next_ = f_arith_deriv_(n)\n chain.append(next_)\n if len(chain) == k:break\n n = next_\n return chain\n", "from collections import defaultdict\n\ndef derivate(x):\n divisors = defaultdict(int)\n n=x\n i=2\n while i*i<=x:\n while x%i==0:\n divisors[i]+=1\n x /= i\n i += 1\n if x>1:\n divisors[x]+=1\n if len(divisors)<2:\n return 1\n return sum(k*n/p for p, k in divisors.items())\n \n\ndef chain_arith_deriv(start, k):\n result = [start]\n for i in range(k-1):\n result.append(derivate(result[-1]))\n if len(result)==2 and result[-1]==1:\n return '%d is a prime number'%start\n return result", "from fractions import Fraction\nfrom collections import Counter\n\ndef isPrime(n):\n if n < 3 or not n%2: return n==2\n return all(n%x for x in range(3, int(n**0.5)+1, 2))\n\ndef prime_factors(n):\n i = 2\n while i * i <= n:\n if n % i: i += 1\n else: n //= i; yield i\n if n > 1: yield n\n\ndef rec(current, k):\n if not k: return []\n if current == 1: return [1]*k\n return [current] + rec(current * sum(Fraction(v, k) for k,v in Counter(prime_factors(current)).items()), k-1)\n\ndef chain_arith_deriv(start, k):\n if isPrime(start): return \"{} is a prime number\".format(start)\n return rec(start, k)", "def chain_arith_deriv(start, k):\n if is_prime(start): return '{} is a prime number'.format(start)\n \n chain, n = [], float(start)\n \n for i in range(k):\n chain.append(n)\n n = sum(n * v / k for k, v in list(prime_factors_dict(n).items()))\n if n == 1: break\n \n return chain + [1] * (k - len(chain))\n \ndef is_prime(num):\n return num == 2 or (num > 2 and all(num % i for i in range(2, int(num ** 0.5) + 1))) \n \ndef prime_factors_dict(n):\n d, exp = {}, 0\n while n % 2 == 0: \n exp += 1\n n //= 2\n if exp: d[2] = exp\n for i in range(3, int(n ** 0.5) + 1):\n exp = 0\n while n % i == 0: \n exp += 1\n n //= i\n if exp: d[i] = exp\n if n > 1: d[n] = 1\n return d\n"] | {"fn_name": "chain_arith_deriv", "inputs": [[231525, 5], [997, 5]], "outputs": [[[231525, 423360, 1899072, 7879680, 51895296]], ["997 is a prime number"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 6,574 |
def chain_arith_deriv(n, k):
|
dcb11addeed9cbc400fdcb0f58cac234 | UNKNOWN | We have an array of unique elements. A special kind of permutation is the one that has all of its elements in a different position than the original.
Let's see how many of these permutations may be generated from an array of four elements. We put the original array with square brackets and the wanted permutations with parentheses.
```
arr = [1, 2, 3, 4]
(2, 1, 4, 3)
(2, 3, 4, 1)
(2, 4, 1, 3)
(3, 1, 4, 2)
(3, 4, 1, 2)
(3, 4, 2, 1)
(4, 1, 2, 3)
(4, 3, 1, 2)
(4, 3, 2, 1)
_____________
A total of 9 permutations with all their elements in different positions than arr
```
The task for this kata would be to create a code to count all these permutations for an array of certain length.
Features of the random tests:
```
l = length of the array
10 ≤ l ≤ 5000
```
See the example tests.
Enjoy it! | ["def all_permuted(n):\n a,b = 0, 1\n for i in range(1,n): a,b = b, (i+1)*(a+b)\n return a", "from itertools import islice\n\n\ndef A000166():\n # https://oeis.org/A000166\n a, b, n = 1, 0, 1\n while True:\n yield a\n a, b = b, n * (a + b)\n n += 1\n\n\nall_permuted = dict(enumerate(islice(A000166(), 5000))).get", "subfactorial = [1, 0]\nfor n in range(1, 10 ** 4):\n subfactorial.append(n * (subfactorial[-1] + subfactorial[-2]))\nall_permuted = subfactorial.__getitem__", "def f(n):\n #by working through sizes 1-9 found an iterative relationship\n #f(1) = 0\n #f(n) = n*f(n-1) + (1 if n is even else -1)\n if (n==0):\n return 1\n else:\n return n*f(n-1)+(1 if n%2==0 else -1)\n\ndef all_permuted(array_length):\n return f(array_length)", "import math\n\ndef all_permuted(n): \n return n * all_permuted(n-1) + (-1)**n if n > 2 else n - 1", "from functools import lru_cache\n@lru_cache(maxsize=None)\ndef all_permuted(n):\n if n == 0:\n return 1\n if n == 1:\n return 0\n return (n-1)*(all_permuted(n-1)+all_permuted(n-2))", "M = [1,0]\nfor V in range(1,9487) : M.append(V * (M[V] + M[V - 1]))\nall_permuted = lambda Q : M[Q]", "def all_permuted(array_length):\n a = [1, 0];\n for x in range(2, array_length + 1):\n a[0], a[1] = a[1] , (x-1) * (a[1] + a[0])\n return a[1]", "all_permuted=a=lambda n:(0,0,1)[n]if n<3else n*a(n-1)+(-1)**n", "import math\n\ndef all_permuted(n):\n if n == 0:\n return 1\n \n return n * all_permuted(n - 1) + (-1) ** n"] | {"fn_name": "all_permuted", "inputs": [[1], [4], [30]], "outputs": [[0], [9], [97581073836835777732377428235481]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,587 |
def all_permuted(n):
|
ab3c6973f71d9603285266072d7b74c6 | UNKNOWN | Take 2 strings `s1` and `s2` including only letters from `a`to `z`.
Return a new **sorted** string, the longest possible, containing distinct letters,
- each taken only once - coming from s1 or s2.
# Examples:
```
a = "xyaabbbccccdefww"
b = "xxxxyyyyabklmopq"
longest(a, b) -> "abcdefklmopqwxy"
a = "abcdefghijklmnopqrstuvwxyz"
longest(a, a) -> "abcdefghijklmnopqrstuvwxyz"
``` | ["def longest(a1, a2):\n return \"\".join(sorted(set(a1 + a2)))", "def longest(s1, s2):\n return ''.join(sorted((set(s1+s2))))", "def longest(s1, s2):\n # your code\n \n # Defining the Alphabet\n alphabet = \"abcdefghijklmnopqrstuvwxyz\"\n \n # Concatenating the Two Given Strings\n s = s1 + s2\n \n # Declaring the Output Variable\n y = \"\"\n \n # Comparing whether a letter is in the string\n for x in alphabet:\n if x not in s:\n continue\n if x in s:\n y = y + x\n \n # returning the final output \n return y", "def longest(s1, s2):\n return ''.join(sorted(set(s1) | set(s2)))", "def longest(s1, s2):\n # your code\n distinct = set(s1+s2) # sets are distinct values! \n distinctSorted = sorted(distinct) # turn into sorted list\n return ''.join(distinctSorted) # concatenate to string with 'join'", "def longest(s1, s2):\n return ''.join(sorted(set(s1).union(s2)))\n", "def longest(s1, s2):\n x=''\n y=sorted(s1+s2)\n \n \n \n for i in y:\n if i not in x:\n x += i\n \n return x # your code", "def longest(s1, s2):\n set1 = set(s1)\n set2 = set(s2)\n return \"\".join(sorted(set1 | set2))", "import itertools\ndef longest(s1, s2):\n longest = \"\"\n for i in s1: \n if i not in longest:\n longest += i\n for i in s2:\n if i not in longest:\n longest += i \n return ''.join(sorted(longest))", "def longest(s1, s2):\n freq = {}\n for c in list(s1):\n freq[c] = freq.get(c, 0) + 1\n for c in list(s2):\n freq[c] = freq.get(c, 0) + 1\n l = sorted([c for c in list(freq.keys())])\n return ''.join(l)", "def longest(s1, s2):\n letters_count = [0] * 26\n for letter in s1:\n letters_count[ord(letter) - 97] += 1\n for letter in s2:\n letters_count[ord(letter) - 97] += 1\n result = []\n for i in range(26):\n if letters_count[i] > 0:\n result.append(chr(i+97))\n return ''.join(result)", "def longest(s1, s2):\n results = []\n for records in s1:\n if records not in results:\n results.append(records)\n for records in s2:\n if records not in results:\n results.append(records)\n results = sorted(results)\n return \"\".join(results)\n", "def longest(s1, s2):\n return ''.join(sorted(list(dict.fromkeys(s1+s2))))", "def longest(s1, s2):\n return \"\".join([x for x in \"abcdefghijklmnopqrstuvwxyz\" if x in s1+s2])", "longest = lambda x,y: ''.join(sorted(set(x+y)))", "def longest(s1, s2):\n new_string = set(s1 + s2)\n sort_string = sorted(new_string)\n return \"\".join(s for s in sort_string)\n \n # your code\n", "import string\n\ndef longest(s1, s2):\n \n comb = []\n alphabet = {}\n ind = 0\n \n for letter in s1:\n if letter in comb:\n pass\n else:\n comb.append(letter)\n \n for letters in s2:\n if letters in comb:\n pass\n else:\n comb.append(letters)\n \n lowerCase = list(string.ascii_lowercase)\n \n for letters in lowerCase:\n ind+=1\n alphabet[letters] = ind\n \n for x in range(len(comb)):\n for i in range(len(comb)-1):\n if alphabet[comb[i]] > alphabet[comb[i+1]]:\n comb[i], comb[i+1] = comb[i+1], comb[i]\n\n comb = ''.join(comb)\n \n return comb", "def longest(s1, s2):\n insieme = set()\n for char in (s1+s2):\n insieme.add(char)\n return \"\".join(sorted(insieme))", "def longest(s1, s2):\n result = ''\n for i in sorted(set(list(set(s1)) + list(set(s2)))):\n result += i\n return result", "def longest(s1, s2):\n new_str = ''.join([s1,s2])\n s = list(new_str)\n s = list(set(s))\n s.sort()\n result = ''.join(s)\n return result\n \n"] | {"fn_name": "longest", "inputs": [["aretheyhere", "yestheyarehere"], ["loopingisfunbutdangerous", "lessdangerousthancoding"], ["inmanylanguages", "theresapairoffunctions"], ["lordsofthefallen", "gamekult"], ["codewars", "codewars"], ["agenerationmustconfrontthelooming", "codewarrs"]], "outputs": [["aehrsty"], ["abcdefghilnoprstu"], ["acefghilmnoprstuy"], ["adefghklmnorstu"], ["acdeorsw"], ["acdefghilmnorstuw"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,927 |
def longest(s1, s2):
|
3e23689785a333f0cd31bfe5fdc2043b | UNKNOWN | Four-digit palindromes start with `[1001,1111,1221,1331,1441,1551,1551,...]` and the number at position `2` is `1111`.
You will be given two numbers `a` and `b`. Your task is to return the `a-digit` palindrome at position `b` if the palindromes were arranged in increasing order.
Therefore, `palin(4,2) = 1111`, because that is the second element of the `4-digit` palindrome series.
More examples in the test cases. Good luck!
If you like palindrome Katas, please try:
[Palindrome integer composition](https://www.codewars.com/kata/599b1a4a3c5292b4cc0000d5)
[Life without primes](https://www.codewars.com/kata/59f8750ac374cba8f0000033) | ["def palin(length, pos):\n left = str(10**((length-1) // 2) + (pos - 1))\n right = left[::-1][length%2:]\n return int(left + right)", "def palin(a,b):\n s = str(10**((a-1)//2) + b-1)\n return int(s+s[::-1][a%2:])", "def palin(a, b):\n half = str(10**((a + 1) // 2 - 1) + b - 1)\n return int(half + (half[::-1] if a % 2 == 0 else half[:-1][::-1]))", "def palin(a,b):\n res = int('1' + ('0' * (a-1))) + 1\n q = a//2\n step = ('1' + (q*'0'), '11'+ ((q-1)*'0'))[a%2==0]\n l = []\n while b>0:\n temp = len(str(res))//2\n res = str(res)\n res = res[:-temp] + res[:temp][::-1]\n if len(res) > a:\n a = len(str(res))\n q = a // 2\n step = ('1' + (q * '0'), '11' + ((q - 1) * '0'))[a % 2 == 0]\n if l and l[-1] == 99:\n l.append(101)\n if res == res[::-1]:\n b-=1\n l.append(int(res))\n res = int(res)\n if str(res + int(step))[:temp-1] != str(res)[:temp-1]:\n temp_res = int(str(res)) + 1\n while str(temp_res) != str(temp_res)[::-1]:\n temp_res += 1\n b -= 1\n if b >= 0:\n l.append(temp_res)\n res += int(step)\n return l[-1]", "def palin(a, b):\n base = str(10**((a - 1) // 2) + b - 1)\n return int(base + base[::-1][a % 2:])", "def palin(a, b):\n n = (a - 1) // 2\n x, y = divmod(b - 1, 10 ** n)\n result = str(x + 1) + (a > 2) * f'{y:0{n}}'\n return int(result + result[-1 + (a%-2)::-1])", "def palin(n,pos):\n half = str(int('1'+'0'*(n//2-int(not n&1)))+pos-1)\n full = int(half + [half,half[:-1]][n&1][::-1]) \n return full", "def palin(a,b):\n l = str(10**((a + 1) // 2 - 1) + b - 1)\n return int(l + (l[::-1] if a % 2 == 0 else l[:-1][::-1]))", "from math import ceil\ndef palin(a,b):\n c=ceil(a/2)\n for i in range(10**(c-1),10**c):\n p=str(i);p+=p[::-1][a%2:]\n if b==1:return int(p)\n else:b-=1", "def palin(a,b):\n left = str(10**((a-1)//2)+(b-1))\n right = str(left[::-1][a%2:])\n return int(left+right)"] | {"fn_name": "palin", "inputs": [[2, 2], [3, 10], [4, 5], [5, 19], [6, 3], [6, 20], [7, 3]], "outputs": [[22], [191], [1441], [11811], [102201], [119911], [1002001]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,123 |
def palin(a,b):
|
6d18e0e3a4972dc9d94e0b1e3f57924d | UNKNOWN | Your friend Cody has to sell a lot of jam, so he applied a good 25% discount to all his merchandise.
Trouble is that he mixed all the prices (initial and discounted), so now he needs your cool coding skills to filter out only the discounted prices.
For example, consider this inputs:
```
"15 20 60 75 80 100"
"9 9 12 12 12 15 16 20"
```
They should output:
```
"15 60 75"
"9 9 12 15"
```
Every input will always have all the prices in pairs (initial and discounted) sorted from smaller to bigger.
You might have noticed that the second sample input can be tricky already; also, try to have an eye for performances, as huge inputs could be tested too.
***Final Note:*** kata blatantly taken from [this problem](https://code.google.com/codejam/contest/8274486/dashboard) (and still not getting why they created a separated Code Jam for ladies, as I find it rather discriminating...), this kata was created inspired by two of the talented girls I am coaching thanks to [codebar](https://codebar.io/) :) | ["from bisect import bisect_left\nfrom collections import deque\n\n\ndef find_discounted(prices: str) -> str:\n all_prices = deque(list(map(int, prices.split())))\n discounts = []\n\n while all_prices:\n d = all_prices.popleft()\n discounts.append(d)\n del all_prices[bisect_left(all_prices, d * 4 // 3)]\n\n return ' '.join(map(str, discounts))\n", "def find_discounted(prices):\n prices = [int(n) for n in prices.split()]\n return \" \".join(prices.remove(round(p*4/3)) or str(p) for p in prices)\n", "from collections import Counter\ndef find_discounted(s):\n s = list(map(int, s.split()))\n c, li = Counter(s), []\n for i in s:\n i = int(i)\n ans = i - (i * 0.25)\n if c.get(ans, 0):\n li.append(str(int(ans)))\n c[ans] -= 1\n c[i] -= 1\n return \" \".join(li)", "from collections import Counter\n\n\ndef find_discounted(prices):\n count_prices = Counter(int(a) for a in prices.split())\n result = []\n for key in sorted(count_prices):\n value = count_prices[key]\n try:\n count_prices[int(key / 0.75)] -= value\n except KeyError:\n continue\n result.extend(key for _ in range(value if key else 1))\n return ' '.join(str(b) for b in result)\n", "def find_discounted(prices):\n discount = .25\n in_list = [int(p) for p in prices.split()]\n out_list = []\n while (in_list):\n full_price = in_list.pop()\n discounted_price = int(full_price*(1-discount))\n in_list.remove(discounted_price)\n out_list.append(discounted_price)\n out_list.reverse()\n ans = ' '.join(str(p) for p in out_list) \n return(ans)\n \n", "def find_discounted(prices):\n if prices == \"\":\n return \"\"\n out = []\n prices = [int(x) for x in prices.split(\" \")]\n for price in prices:\n if int(price/0.75) in prices:\n prices.remove(int(price/0.75))\n out.append(str(price))\n return \" \".join(out)", "def find_discounted(prices):\n prices=list(map(int,prices.split()))\n discounted=[]\n while(prices):\n price=prices.pop()\n discount=int(price*0.75)\n prices.remove(discount)\n discounted.append(discount)\n return \" \".join(map(str,discounted[::-1]))"] | {"fn_name": "find_discounted", "inputs": [["15 20 60 75 80 100"], ["9 9 12 12 12 15 16 20"], ["4131 5508 7344 7803 8397 8667 9792 10404 11196 11556 13872 14553 14742 14928 15408 17631 18496 19404 19656 19904 20544 20925 21816 23508 23598 23949 25872 26208 27900 29088 31344 31464 31932 34496 34944 37200 38784 41792 41952 42576 49600 51712 55936 56768 7440174 8148762 8601471 8837667 9290376 9664353 9920232 9920232 10865016 10865016 11468628 11468628 11783556 11783556 12387168 12387168 12885804 12885804 13187610 13226976 13226976 13443489 14309541 14486688 14486688 15291504 15291504 15711408 15711408 16516224 16516224 17181072 17181072 17583480 17583480 17635968 17635968 17924652 17924652 19079388 19079388 19315584 19315584 20388672 20388672 20948544 20948544 22021632 22021632 22908096 22908096 23444640 23444640 23514624 23514624 23899536 23899536 25439184 25439184 25754112 25754112 27184896 27184896 27931392 29362176 29362176 30544128 30544128 31259520 31259520 31352832 31352832 31866048 31866048 33918912 33918912 34338816 34338816 36246528 36246528 39149568 39149568 40725504 40725504 41679360 41679360 41803776 41803776 42488064 42488064 45225216 45225216 45785088 45785088 48328704 48328704 52199424 52199424 54300672 54300672 55572480 55572480 55738368 55738368 56650752 56650752 60300288 60300288 61046784 61046784 64438272 64438272 69599232 69599232 72400896 72400896 74096640 74096640 74317824 74317824 75534336 75534336 80400384 80400384 81395712 81395712 85917696 85917696 92798976 92798976 96534528 96534528 98795520 98795520 99090432 100712448 100712448 107200512 107200512 108527616 108527616 114556928 123731968 128712704 131727360 131727360 134283264 134283264 142934016 142934016 144703488 144703488 175636480 179044352 190578688 192937984"], ["12101190 13217454 16134920 17255964 17623272 20988987 23007952 27985316 47773461 50464971 51107973 51348216 51570939 61932831 63697948 67286628 68143964 68464288 68761252 76080819 77154471 77683497 81443352 82577108 91482771 93591900 94502019 101441092 102872628 103577996 107964792 108591136 111854328 113242929 113336763 121977028 124789200 126002692 127076673 127417131 132799401 138191364 143953056 147362946 149139104 150990572 151115684 169435564 169889508 177065868 184255152 195550650 196483928 197852445 200221983 202824081 206826522 207262239 208681395 214802139 220058532 234003867 252975744 259922106 260734200 263258622 263803260 266509059 266962644 270432108 274674165 275768696 276349652 278241860 280462401 286402852 287411415 291776784 293411376 301985955 303183567 308892357 312005156 321531423 322725066 325400883 337300992 337813536 346562808 351011496 354202299 355345412 358621962 366232220 373949868 383215220 383584632 389035712 402647940 404244756 411609234 411856476 412785021 412877634 417323610 420580290 428214216 428708564 430300088 433867844 436962831 446437398 450418048 454715631 457837500 459317538 472269732 473783841 478162616 487689999 494477640 500268657 511446176 513126957 533560980 547260099 548812312 550380028 550503512 556431480 560773720 567862296 570952288 577635006 581588184 582617108 587963028 595249864 606287508 608851107 610450000 612423384 613387746 617614455 617707290 623054166 628695024 631711788 633649500 645750837 645918393 649047009 650253332 659303520 662133849 666082206 667024876 681659439 682569870 683249184 684169276 701022639 703908903 711414640 712873311 718221042 726357543 729501873 729680132 731516586 744681894 745861491 757149728 770180008 775450912 783950704 811801476 817850328 823485940 823609720 830738888 838260032 844866000 861001116 861224524 865396012 882845132 888109608 908879252 910093160 910998912 934696852 938545204 950497748 957628056 968476724 972669164 975355448 992909192 994481988"], ["3969 5292 6588 7056 8784 9408 9477 11712 12636 14337 15390 15616 16848 18117 19116 19953 20520 20547 22464 23571 24156 24354 25488 26604 27360 27396 28431 31428 32208 32472 33984 35472 36480 36528 37908 41904 42944 45312 47296 48704 50544 55872 60416 67392 74496 89856 99328 119808 7597638 8148762 8522739 8936082 10130184 10130184 10865016 10865016 11337408 11363652 11363652 11914776 11914776 13226976 13506912 13506912 14486688 14486688 15116544 15116544 15151536 15151536 15175593 15886368 15886368 17635968 17635968 17675334 18009216 18009216 19315584 19315584 20155392 20155392 20202048 20202048 20234124 20234124 21181824 21181824 23514624 23514624 23567112 23567112 24012288 24012288 25754112 25754112 26873856 26873856 26936064 26936064 26978832 26978832 28242432 28242432 31352832 31352832 31422816 31422816 32016384 32016384 34338816 34338816 35831808 35831808 35914752 35914752 35971776 35971776 37656576 37656576 41803776 41803776 41897088 41897088 42688512 42688512 45785088 45785088 47775744 47775744 47886336 47886336 47962368 47962368 50208768 50208768 55738368 55738368 55862784 55862784 56918016 56918016 61046784 61046784 63700992 63700992 63848448 63848448 63949824 63949824 66945024 66945024 74317824 74317824 74483712 74483712 75890688 75890688 81395712 81395712 84934656 84934656 85131264 85131264 85266432 85266432 89260032 89260032 99090432 99090432 99311616 99311616 101187584 108527616 108527616 113246208 113246208 113508352 113688576 113688576 119013376 132120576 132120576 132415488 132415488 144703488 144703488 150994944 150994944 151584768 151584768 176160768 176553984 176553984 192937984 201326592 201326592 202113024 235405312 268435456"], ["10349349 13799132 14426622 17347290 19235496 23129720 24791793 32049435 33055724 42732580 48771579 51731211 58387395 59065617 65028772 67572870 68974948 71437914 77849860 78754156 81661776 88101462 90097160 95250552 97020531 108882368 114334791 117468616 128978592 129360708 138971622 140891505 151010565 152446388 167919846 170507487 171971456 172292247 174243150 185295496 187855340 192647313 201347420 216485562 223893128 224346162 227343316 229722996 232324200 235577922 239683287 242783997 249603675 252305217 252769914 253271316 253370922 254972001 255399345 256863084 259132155 261590604 262932870 280611801 288647416 296647968 299128216 303518460 303720828 305508195 307421394 308857827 309007569 310426329 314103896 315154656 319409412 319577716 323711996 327550797 327930534 331967646 332804900 336406956 337026552 337695088 337827896 339962668 340532460 345509540 346457580 348787472 350577160 354132609 357929508 359186574 368170530 369388743 374149068 390830310 395530624 399282276 404691280 404961104 407344260 409895192 411810436 412010092 413901772 414530748 420206208 422065866 425879216 436734396 437240712 437693154 442623528 451133340 454978488 457943151 461943440 466227489 472176812 477239344 478915432 490894040 492518324 497874771 505860399 506218716 509609157 516966183 519040851 520736430 521107080 527513286 532376368 536497356 552707664 557969370 562754488 565705065 566519211 568761246 569608953 575762760 583590872 585113532 599073549 601511120 606637984 610590868 611310540 618553956 621636652 630797073 644405985 645847155 646583196 648885063 663833028 674480532 674958288 675003540 679478876 682830222 689288244 692054468 694315240 700706877 702635421 703351048 705674994 715329808 717291066 720462369 729330681 743959160 754273420 755358948 758348328 759478604 767683680 780151376 798764732 815080720 824738608 841062764 859207980 861129540 862110928 865180084 900004720 910440296 934275836 936847228 940899992 956388088 960616492 972440908"], ["750000000 1000000000"], ["3915 5220 6960 9280 17496 19926 22113 23328 26568 29484 31104 35424 39312 41472 47232 52416 69888 93184 3739770 3956283 4986360 4986360 5275044 5275044 5727753 6648480 6648480 7033392 7033392 7637004 7637004 8864640 8864640 9369108 9377856 9377856 10182672 10182672 11819520 11819520 12492144 12492144 12503808 12503808 13286025 13576896 13576896 13915881 14211126 14703201 15759360 15759360 16612452 16656192 16656192 16671744 16671744 17714700 17714700 18102528 18102528 18554508 18554508 18948168 18948168 19486170 19604268 19604268 21012480 21012480 22149936 22149936 22208256 22208256 22228992 22228992 23619600 23619600 24136704 24136704 24739344 24739344 25264224 25264224 25981560 25981560 26139024 26139024 28016640 28016640 29533248 29533248 29611008 29611008 29638656 29638656 31492800 31492800 32182272 32182272 32985792 32985792 33685632 33685632 34642080 34642080 34852032 34852032 37355520 37355520 39377664 39377664 39481344 39481344 39518208 39518208 41990400 41990400 42909696 42909696 43981056 43981056 44914176 44914176 46189440 46189440 46469376 46469376 49807360 52503552 52503552 52641792 52641792 52690944 55987200 55987200 57212928 57212928 58641408 58641408 59885568 59885568 61585920 61585920 61959168 61959168 70004736 70004736 70189056 70189056 74649600 74649600 76283904 78188544 78188544 79847424 82114560 82114560 82612224 82612224 93339648 93339648 93585408 93585408 99532800 99532800 104251392 104251392 109486080 109486080 110149632 110149632 124452864 124452864 124780544 132710400 132710400 139001856 139001856 145981440 145981440 146866176 146866176 165937152 165937152 176947200 185335808 194641920 194641920 195821568 195821568 221249536 259522560 259522560 261095424 261095424 346030080 346030080 348127232 461373440"], ["0 0"], [""]], "outputs": [["15 60 75"], ["9 9 12 15"], ["4131 7344 7803 8397 8667 13872 14553 14742 14928 15408 17631 20925 21816 23598 23949 25872 26208 31344 37200 38784 41952 42576 7440174 8148762 8601471 8837667 9290376 9664353 9920232 10865016 11468628 11783556 12387168 12885804 13187610 13226976 13443489 14309541 14486688 15291504 15711408 16516224 17181072 17583480 17635968 17924652 19079388 19315584 20388672 20948544 22021632 22908096 23444640 23514624 23899536 25439184 25754112 27184896 29362176 30544128 31259520 31352832 31866048 33918912 34338816 36246528 39149568 40725504 41679360 41803776 42488064 45225216 45785088 48328704 52199424 54300672 55572480 55738368 56650752 60300288 61046784 64438272 69599232 72400896 74096640 74317824 75534336 80400384 81395712 85917696 92798976 96534528 98795520 100712448 107200512 108527616 131727360 134283264 142934016 144703488"], ["12101190 13217454 17255964 20988987 47773461 50464971 51107973 51348216 51570939 61932831 76080819 77154471 77683497 81443352 91482771 93591900 94502019 107964792 111854328 113242929 113336763 127076673 127417131 132799401 138191364 147362946 195550650 197852445 200221983 202824081 206826522 207262239 208681395 214802139 220058532 234003867 252975744 259922106 263258622 266509059 274674165 280462401 287411415 291776784 301985955 303183567 308892357 321531423 322725066 325400883 337813536 354202299 358621962 383584632 411609234 412785021 412877634 417323610 420580290 428214216 436962831 446437398 454715631 457837500 459317538 473783841 487689999 494477640 500268657 513126957 533560980 547260099 567862296 577635006 581588184 587963028 608851107 613387746 617614455 617707290 623054166 628695024 633649500 645750837 645918393 649047009 662133849 666082206 681659439 682569870 683249184 701022639 703908903 712873311 718221042 726357543 729501873 731516586 744681894 745861491"], ["3969 6588 7056 9477 11712 14337 15390 16848 18117 19953 20547 23571 24354 25488 27360 28431 32208 35472 36528 41904 45312 50544 74496 89856 7597638 8148762 8522739 8936082 10130184 10865016 11337408 11363652 11914776 13226976 13506912 14486688 15116544 15151536 15175593 15886368 17635968 17675334 18009216 19315584 20155392 20202048 20234124 21181824 23514624 23567112 24012288 25754112 26873856 26936064 26978832 28242432 31352832 31422816 32016384 34338816 35831808 35914752 35971776 37656576 41803776 41897088 42688512 45785088 47775744 47886336 47962368 50208768 55738368 55862784 56918016 61046784 63700992 63848448 63949824 66945024 74317824 74483712 75890688 81395712 84934656 85131264 85266432 89260032 99090432 99311616 108527616 113246208 113688576 132120576 132415488 144703488 150994944 151584768 176553984 201326592"], ["10349349 14426622 17347290 24791793 32049435 48771579 51731211 58387395 59065617 67572870 71437914 81661776 88101462 97020531 114334791 128978592 138971622 140891505 151010565 167919846 170507487 172292247 174243150 192647313 216485562 224346162 235577922 239683287 242783997 249603675 252305217 252769914 253271316 253370922 254972001 255399345 259132155 261590604 262932870 280611801 296647968 303518460 303720828 305508195 307421394 308857827 309007569 310426329 315154656 319409412 327550797 327930534 331967646 346457580 354132609 357929508 359186574 368170530 369388743 390830310 399282276 414530748 422065866 437693154 451133340 454978488 457943151 466227489 497874771 505860399 506218716 509609157 516966183 519040851 520736430 527513286 536497356 557969370 565705065 566519211 568761246 569608953 575762760 585113532 599073549 611310540 618553956 630797073 644405985 645847155 646583196 648885063 675003540 682830222 700706877 702635421 705674994 717291066 720462369 729330681"], ["750000000"], ["3915 6960 17496 19926 22113 31104 35424 39312 69888 3739770 3956283 4986360 5275044 5727753 6648480 7033392 7637004 8864640 9369108 9377856 10182672 11819520 12492144 12503808 13286025 13576896 13915881 14211126 14703201 15759360 16612452 16656192 16671744 17714700 18102528 18554508 18948168 19486170 19604268 21012480 22149936 22208256 22228992 23619600 24136704 24739344 25264224 25981560 26139024 28016640 29533248 29611008 29638656 31492800 32182272 32985792 33685632 34642080 34852032 37355520 39377664 39481344 39518208 41990400 42909696 43981056 44914176 46189440 46469376 52503552 52641792 55987200 57212928 58641408 59885568 61585920 61959168 70004736 70189056 74649600 78188544 82114560 82612224 93339648 93585408 99532800 104251392 109486080 110149632 124452864 132710400 139001856 145981440 146866176 165937152 194641920 195821568 259522560 261095424 346030080"], ["0"], [""]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,344 |
def find_discounted(prices):
|
f479c2c8947b87b01ca75d9001822331 | UNKNOWN | Create a function that takes a number and finds the factors of it, listing them in **descending** order in an **array**.
If the parameter is not an integer or less than 1, return `-1`. In C# return an empty array.
For Example:
`factors(54)` should return `[54, 27, 18, 9, 6, 3, 2, 1]` | ["def factors(x):\n if not isinstance(x, int) or x < 1:\n return -1\n return [i for i in range(x, 0, -1) if x % i == 0]\n", "def factors(x):\n if not isinstance(x, int) or x < 1:\n return -1\n return sorted({j for i in range(1, int(x**0.5)+1) if x % i == 0 for j in [i, x//i]}, reverse=True)\n", "def factors(x):\n if type(x) == str or x < 1 or type(x) == float:\n return -1\n\n else:\n divisor = list(range(1,x+1))\n ans = []\n for i in divisor:\n if x % i == 0:\n ans.append(i)\n ans.sort(reverse = True)\n return ans\n", "factors=lambda n:-1if not n or type(n)!=int or n<1else[e for e in range(n,0,-1)if n%e==0]", "def factors(x):\n return -1 if type(x) != int or x < 1 else \\\n [ n for n in range(x, 0, -1) if x % n == 0 ]", "def factors(n):\n from math import sqrt\n \n if not isinstance(n, int) or n < 1:\n return -1\n\n lim = int(sqrt(n))\n divisors = set([n, 1])\n \n start = 2 + n%2\n step = start - 1\n \n for i in range(start, lim+1, step):\n if n%i == 0:\n divisors.add(i)\n divisors.add(n//i)\n \n return sorted(divisors, reverse=True)", "def factors(x):\n return [i for i in range(x, 0, -1) if not x % i] if isinstance(x, int) and x > 0 else -1\n"] | {"fn_name": "factors", "inputs": [[-4], [0], [-12], ["a"], [4.5], ["hello world"], [54], [49], [1]], "outputs": [[-1], [-1], [-1], [-1], [-1], [-1], [[54, 27, 18, 9, 6, 3, 2, 1]], [[49, 7, 1]], [[1]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,314 |
def factors(x):
|
c821c80d6052741999c9d77340d2dbec | UNKNOWN | If this challenge is too easy for you, check out:
https://www.codewars.com/kata/5cc89c182777b00001b3e6a2
___
Upside-Down Pyramid Addition is the process of taking a list of numbers and consecutively adding them together until you reach one number.
When given the numbers `2, 1, 1` the following process occurs:
```
2 1 1
3 2
5
```
This ends in the number `5`.
___
### YOUR TASK
Given the right side of an Upside-Down Pyramid (Ascending), write a function that will return the original list.
### EXAMPLE
```python
reverse([5, 2, 1]) == [2, 1, 1]
```
NOTE: The Upside-Down Pyramid will never be empty and will always consist of positive integers ONLY. | ["def reverse(lst):\n ret = []\n while lst:\n ret.append(lst[-1])\n lst = [a-b for a,b in zip(lst, lst[1:])]\n return ret[::-1]", "from functools import reduce\ndef reverse(r):\n return reduce(lambda a,n: reduce(lambda b,s: b.append(s-b[-1]) or b,reversed(a),[n])[::-1],r,[])", "f=reverse=lambda A:len(A)==1and A or f([x-y for x,y in zip(A,A[1:])])+[A[-1]] ", "def reverse(right):\n pyramid = []\n \n for index, number in enumerate(right):\n pyramid.append([number])\n if index > 0:\n for i in range(index):\n value = pyramid[index-1][i] - pyramid[index][i]\n pyramid[index].append(value)\n \n last_row = pyramid[-1][::-1]\n \n return last_row", "def reverse(right):\n lastr = []\n while right:\n lastr.append(right[-1])\n right = [a-b for a, b in zip(right, right[1:])] \n return lastr[::-1]", "def reverse(right):\n xs = []\n for y in right:\n ys = [y]\n for x in xs:\n ys.append(x - ys[-1])\n xs = ys\n return xs[::-1]", "def reverse(right):\n last_row = []\n \n for a in right:\n curr_row = [a]\n for b in last_row:\n a = b - a\n curr_row.append(a)\n \n last_row = curr_row\n \n return last_row[::-1]", "def coutingLine(downLine, rightCount):\n upLine = [rightCount]\n for itemDLine in downLine:\n upLine.append(itemDLine - upLine[-1])\n return upLine\n\ndef swapList(currentList):\n return list(currentList[-item] for item in range(1, len(currentList)+1))\n\ndef reverse(right):\n pyramide = [[right[0]]]\n for index in range(0, len(right) - 1):\n pyramide.append(coutingLine(pyramide[-1], right[index + 1]))\n \n return swapList(pyramide[-1])\n \n\n\n\n", "def reverse(right):\n \n left = [right[-1]]\n k=right\n for i in range(len(right)-1):\n k=list(map(lambda x,y:x-y,k,k[1:]))\n left.append(k[-1])\n left.reverse()\n \n return left", "f=reverse=lambda a:a and f([x-y for x,y in zip(a,a[1:])])+[a[-1]]"] | {"fn_name": "reverse", "inputs": [[[5, 2, 1]], [[84, 42, 21, 10, 2]], [[83, 47, 28, 16, 7]], [[101, 57, 29, 13, 6]], [[66, 39, 25, 15, 7]], [[45, 25, 14, 8, 6]], [[60, 32, 16, 7, 4]], [[84, 44, 21, 8, 2]], [[51, 26, 13, 6, 2]], [[78, 42, 22, 11, 6]]], "outputs": [[[2, 1, 1]], [[4, 7, 3, 8, 2]], [[6, 4, 3, 9, 7]], [[1, 3, 9, 7, 6]], [[7, 2, 2, 8, 7]], [[3, 1, 4, 2, 6]], [[4, 1, 6, 3, 4]], [[4, 3, 7, 6, 2]], [[3, 3, 3, 4, 2]], [[4, 3, 6, 5, 6]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,137 |
def reverse(right):
|
ad60f5a234b3d3bbab353ea1c53b128a | UNKNOWN | There is a house with 4 levels.
In that house there is an elevator.
You can program this elevator to go up or down,
depending on what button the user touches inside the elevator.
Valid levels must be only these numbers: `0,1,2,3`
Valid buttons must be only these strings: `'0','1','2','3'`
Possible return values are these numbers: `-3,-2,-1,0,1,2,3`
If the elevator is on the ground floor(0th level)
and the user touches button '2'
the elevator must go 2 levels up,
so our function must return 2.
If the elevator is on the 3rd level
and the user touches button '0'
the elevator must go 3 levels down, so our function must return -3.
If the elevator is on the 2nd level,
and the user touches button '2'
the elevator must remain on the same level,
so we return 0.
We cannot endanger the lives of our passengers,
so if we get erronous inputs,
our elevator must remain on the same level.
So for example:
- `goto(2,'4')` must return 0, because there is no button '4' in the elevator.
- `goto(4,'0')` must return 0, because there is no level 4.
- `goto(3,undefined)` must return 0.
- `goto(undefined,'2')` must return 0.
- `goto([],'2')` must return 0 because the type of the input level is array instead of a number.
- `goto(3,{})` must return 0 because the type of the input button is object instead of a string. | ["levels = [0, 1, 2, 3]\nbuttons = ['0', '1', '2', '3']\ndef goto(level,button):\n if level not in levels or button not in buttons:\n return 0\n else:\n return int(button) - level", "def goto(l,b):\n if b in ('0','1','2','3') and l in (0,1,2,3):\n return int(b)-l\n return 0", "def goto(level,button):\n if type(level) is not int or level>3 or type(button) is not str or int(button)>3:\n return 0\n return int(button)-level\n", "def goto(level, button):\n try:\n return int(button) - level if button in \"0123\" and level in {0, 1, 2, 3} else 0\n except (TypeError, ValueError):\n return 0\n", "def goto(level,button):\n return int(button)-level if isinstance(level, int) and isinstance(button, str) and level>=0 and level<=3 and int(button)>=0 and int(button)<=3 else 0\n", "def goto(l, b):\n return int(b) - l if b in (\"0\", \"1\", \"2\", \"3\") and l in (0, 1, 2, 3) else 0", "def goto(level = None,button = None):\n if level not in (0,1,2,3) or button not in ('0','1','2','3'):\n return 0\n else:\n move = int(button) - level\n return move", "def goto(level, button):\n return level in range(4) and button in list('0123') and int(button) - level", "goto = lambda level,button: 0 if level not in [0,1,2,3] or button not in ['0','1','2','3'] else int(button)-level", "goto=lambda l,b:l in(0,1,2,3)and b in list('0123')and int(b)-l"] | {"fn_name": "goto", "inputs": [[0, "0"], [0, "1"], [0, "2"], [0, "3"], [1, "0"], [1, "1"], [1, "2"], [1, "3"], [2, "0"], [2, "1"], [2, "2"], [2, "3"], [3, "0"], [3, "1"], [3, "2"], [3, "3"], [0, "4"], [0, null], [1, "4"], [1, null], [2, "4"], [2, null], [3, "4"], [3, null], [4, "2"], [null, "2"], [[], "2"], [3, {}], ["2", "3"], [2, 3], [1.5, "3"], ["length", "length"]], "outputs": [[0], [1], [2], [3], [-1], [0], [1], [2], [-2], [-1], [0], [1], [-3], [-2], [-1], [0], [0], [0], [0], [0], [0], [0], [0], [0], [0], [0], [0], [0], [0], [0], [0], [0]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,437 |
def goto(level,button):
|
7d6475e7f773f74e629bbf48f1f65782 | UNKNOWN | A new school year is approaching, which also means students will be taking tests.
The tests in this kata are to be graded in different ways. A certain number of points will be given for each correct answer and a certain number of points will be deducted for each incorrect answer. For ommitted answers, points will either be awarded, deducted, or no points will be given at all.
Return the number of points someone has scored on varying tests of different lengths.
The given parameters will be:
* An array containing a series of `0`s, `1`s, and `2`s, where `0` is a correct answer, `1` is an omitted answer, and `2` is an incorrect answer.
* The points awarded for correct answers
* The points awarded for omitted answers (note that this may be negative)
* The points **deducted** for incorrect answers (hint: this value has to be subtracted)
**Note:**
The input will always be valid (an array and three numbers)
## Examples
\#1:
```
[0, 0, 0, 0, 2, 1, 0], 2, 0, 1 --> 9
```
because:
* 5 correct answers: `5*2 = 10`
* 1 omitted answer: `1*0 = 0`
* 1 wrong answer: `1*1 = 1`
which is: `10 + 0 - 1 = 9`
\#2:
```
[0, 1, 0, 0, 2, 1, 0, 2, 2, 1], 3, -1, 2) --> 3
```
because: `4*3 + 3*-1 - 3*2 = 3` | ["def score_test(tests, right, omit, wrong):\n points = (right, omit, -wrong)\n return sum(points[test] for test in tests)\n", "#returns test score\ndef score_test(tests, right, omit, wrong):\n final = 0\n for scores in tests:\n if scores == 0:\n final = final + right\n elif scores == 1:\n final = final + omit\n elif scores == 2:\n final = final - wrong\n return final", "#returns test score\ndef score_test(tests, right, omit, wrong):\n return tests.count(0) * right + tests.count(1) * omit - tests.count(2) * wrong ", "#returns test score\ndef score_test(tests, right, omit, wrong):\n result = 0\n for score in tests:\n if score == 0:\n result += right\n elif score == 1:\n result += omit\n elif score == 2:\n result -= wrong\n return result\n", "#returns test score\ndef score_test(tests, right, omit, wrong):\n #insert code here\n right_score = tests.count(0)*right\n omit_score = tests.count(1)*omit\n wrong_score = tests.count(2)*wrong\n return right_score + omit_score - wrong_score", "def score_test(tests, right, omit, wrong):\n return sum( right if a==0 else omit if a==1 else -wrong for a in tests )", "def score_test(t, r, o, w):\n \n return sum([r,o,-w][s]for s in t)", "#returns test score\ndef score_test(tests: list, right: int, omit: int, wrong: int) -> int:\n right_count = tests.count(0)\n omit_count = tests.count(1)\n wrong_count = tests.count(2)\n return right_count * right + omit_count * omit - wrong_count * wrong", "def score_test(tests, right, omit, wrong):\n awards = [right, omit, -wrong]\n return sum(map(awards.__getitem__, tests))"] | {"fn_name": "score_test", "inputs": [[[0, 0, 0, 0, 2, 1, 0], 2, 0, 1], [[0, 1, 0, 0, 2, 1, 0, 2, 2, 1], 3, -1, 2], [[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0], 5, -1, 2], [[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 2, 2, 0, 0, 0, 0, 1, 1, 2, 2, 2, 2, 0], 6, 0, 1.5], [[0, 0, 1, 1, 1, 1, 2, 2, 2, 2, 0, 2, 1, 2, 0, 2, 1], 10, -5, 10], [[0, 2, 0, 2, 0, 2, 0, 2], 10, 0, 5], [[0, 1, 2, 0, 0, 2, 0, 1, 2, 1, 0, 0, 1, 0, 2, 1, 0, 2, 2, 0], 9, 3, 2]], "outputs": [[9], [3], [70], [87], [-60], [20], [84]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,742 |
def score_test(tests, right, omit, wrong):
|
000539c308ff9e62182a78d1d4709f46 | UNKNOWN | ## **Task**
You are given a positive integer (`n`), and your task is to find the largest number **less than** `n`, which can be written in the form `a**b`, where `a` can be any non-negative integer and `b` is an integer greater than or equal to `2`. Try not to make the code time out :)
The input range is from `1` to `1,000,000`.
## **Return**
Return your answer in the form `(x, y)` or (`[x, y]`, depending on the language ), where `x` is the value of `a**b`, and `y` is the number of occurrences of `a**b`. By the way ** means ^ or power, so 2 ** 4 = 16.
If you are given a number less than or equal to `4`, that is not `1`, return `(1, -1)`, because there is an infinite number of values for it: `1**2, 1**3, 1**4, ...`.
If you are given `1`, return `(0, -1)`.
## **Examples**
```
3 --> (1, -1) # because it's less than 4
6 --> (4, 1) # because the largest such number below 6 is 4,
# and there is only one way to write it: 2**2
65 --> (64, 3) # because there are three occurrences of 64: 2**6, 4**3, 8**2
90 --> (81, 2) # because the largest such number below 90 is 81,
# and there are two ways of getting it: 3**4, 9**2
```
By the way, after finishing this kata, please try some of my other katas: [here.](https://www.codewars.com/collections/tonylicodings-authored-katas) | ["def largest_power(n):\n print(n)\n if n <= 4:\n if n == 1:\n return (0, -1)\n return (1, -1)\n \n #num_of_occurances\n freq = 0\n x = []\n largest = 0\n j = 0\n while 2**largest < n:\n largest += 1\n largest -= 1\n for i in range(2, largest + 1):\n while j ** i < n:\n j += 1\n j -= 1\n x.append(j**i)\n j = 0\n \n return (max(x), x.count(max(x)))", "li = []\nfor i in range(2,1000):\n for j in range(2,20):\n li.append(i**j) \nli.sort()\n\ndef largest_power(n):\n found = [0,-1]\n \n for i in li:\n if i>=n : break\n if i>found[0] : found = [i, 1]\n elif i==found[0] : found[1]+=1\n\n return tuple(found) if n>4 else (int(n!=1),-1)", "from bisect import bisect_left\nfrom collections import Counter\n\nocc = Counter({0: -1, 1: -1})\nfor n in range(2, 1000+1):\n x = n * n\n while x <= 1_000_000:\n occ[x] += 1\n x *= n\nxs = sorted(occ)\n\ndef largest_power(n):\n i = bisect_left(xs, n) - 1\n x = xs[i]\n return (x, occ[x])", "from collections import defaultdict\nfrom bisect import bisect\n\na, memo = 2, defaultdict(int)\nfor a in range(2, 1001):\n x = a**2\n while x <= 1000000:\n memo[x] += 1\n x *= a\nmemo = sorted(memo.items())\n\ndef largest_power(n):\n if n <= 4: return n>1, -1\n return memo[bisect(memo, (n,))-1]", "from math import sqrt as s\ndef largest_power(n):\n if n==1 : return (0,-1)\n elif n<=4: return (1,-1)\n else:\n alist = []\n for i in range(2, round(s(n) + 2)):\n j=int(1)\n while i**j<n:\n a=i**j\n j += 1\n alist.append(a)\n aset = (max(alist),alist.count(max(alist)))\n return aset\n", "import math\n\ndef largest_power(n):\n if n == 1: return 0, -1\n if n <= 4: return 1, -1\n \n before = None\n \n r = []\n for i in range(n-1, 1, -1):\n res = check(i)\n if res:\n return i, res\n \n \ndef check(n):\n c = 0\n for st in range(2, 9):\n el = round(n**(1/st), 7)\n is_c = str(el).split('.')[1] == '0'\n if is_c:\n print(el, st, n)\n c += 1\n return c", "MAX = 10 ** 7\ncounter = [0] * MAX\nfor base in range(2, int(MAX ** .5) + 1):\n prod = base ** 2\n while prod < MAX:\n counter[prod] += 1\n prod *= base\n\npowers, frequencies = [0, 1], [-1, -1]\nfor power, frequency in enumerate(counter):\n if frequency:\n powers.append(power)\n frequencies.append(frequency)\n\nfrom bisect import bisect\ndef largest_power(n):\n idx = bisect(powers, n-1) - 1\n return powers[idx], frequencies[idx]", "from math import log2\nfrom collections import Counter\ndef largest_power(n):\n if n == 1:return 0, -1\n return (1,-1) if n<5 else max(Counter(int(round((n-1)**(1/p),12))**p for p in range(2,int(log2(n-1))+1)).items(),key=lambda p:p[0])", "from math import log2\ndef largest_power(x):\n if x == 1:return (0, -1)\n elif x < 5:return (1, -1)\n max_powers = []\n greatest_power = int(log2(x))\n for i in range(2, greatest_power+1):\n max_powers.append(int(x**(1/i))**i)\n return (max(max_powers), max_powers.count(max(max_powers))) if x != 81 else (64, 3)", "def largest_power(n):\n if n == 1:\n return 0, -1\n for i in range(n - 1, 1, -1):\n k = sum(round(i ** (1 / e)) ** e == i for e in range(2, i.bit_length()))\n if k != 0:\n return i, k\n return 1, -1"] | {"fn_name": "largest_power", "inputs": [[90], [6], [65], [2], [1], [81], [29], [4]], "outputs": [[[81, 2]], [[4, 1]], [[64, 3]], [[1, -1]], [[0, -1]], [[64, 3]], [[27, 1]], [[1, -1]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,625 |
def largest_power(n):
|
abc3ea82d3bdc70d37f20802cd6de66e | UNKNOWN | Given a hash of letters and the number of times they occur, recreate all of the possible anagram combinations that could be created using all of the letters, sorted alphabetically.
The inputs will never include numbers, spaces or any special characters, only lowercase letters a-z.
E.g. get_words({2=>["a"], 1=>["b", "c"]}) => ["aabc", "aacb", "abac", "abca", "acab", "acba", "baac", "baca", "bcaa", "caab", "caba", "cbaa"] | ["from itertools import permutations\n\ndef get_words(letters):\n word = \"\".join(qty * char for qty in letters for chars in letters[qty] for char in chars)\n return sorted({\"\".join(permutation) for permutation in permutations(word)})", "from itertools import permutations\n\n\ndef get_words(hash_of_letters):\n letters = \"\".join(cnt * c for cnt, cs in hash_of_letters.items() for c in cs)\n return sorted({\"\".join(xs) for xs in permutations(letters)})", "from itertools import permutations\ndef get_words(hash_of_letters):\n string = ''\n for i in hash_of_letters:\n string += ''.join(hash_of_letters[i] * i)\n answer = set(permutations(string))\n answer = sorted(answer)\n for i in range(len(answer)):\n answer[i] = ''.join(answer[i])\n return answer", "from itertools import permutations\n\ndef get_words(hash):\n chars = ''\n for k,v in list(hash.items()): chars += ''.join(v)*k\n return sorted(set(''.join(p) for p in permutations(chars)))\n", "from itertools import permutations\n\ndef get_words(dct):\n s = ''.join(c*n for n,lst in dct.items() for c in lst)\n return sorted( set(map(''.join, permutations(s, len(s)) )) )", "def get_words(letter_counts):\n from itertools import permutations\n \n word = ''.join(count*char for count, chars in letter_counts.items() for char in chars)\n \n return sorted(set(map(lambda x: ''.join(x), permutations(word))))", "def get_words(d):\n #your code here\n from itertools import permutations\n s = ''\n for key in d:\n for char in d[key]:\n s+=char*key\n p = permutations(s, len(s))\n return sorted(list(set([''.join(word) for word in p])))", "from itertools import permutations;get_words=lambda d:sorted([\"\".join(i) for i in set(list(permutations(\"\".join([\"\".join([k * i for k in j]) for i, j in d.items()]))))])", "from itertools import permutations\ndef get_words(h):\n return [''.join(p) for p in sorted(set(permutations(''.join([letter * key for key in h for letter in h[key]]))))]"] | {"fn_name": "get_words", "inputs": [[{"1": ["a", "b"]}], [{"1": ["a", "b", "c", "n", "o"]}], [{"1": ["a", "b", "c"]}], [{"2": ["a"], "1": ["b", "c"]}], [{"2": ["i", "a"], "1": ["z", "x"]}]], "outputs": [[["ab", "ba"]], [["abcno", "abcon", "abnco", "abnoc", "abocn", "abonc", "acbno", "acbon", "acnbo", "acnob", "acobn", "aconb", "anbco", "anboc", "ancbo", "ancob", "anobc", "anocb", "aobcn", "aobnc", "aocbn", "aocnb", "aonbc", "aoncb", "bacno", "bacon", "banco", "banoc", "baocn", "baonc", "bcano", "bcaon", "bcnao", "bcnoa", "bcoan", "bcona", "bnaco", "bnaoc", "bncao", "bncoa", "bnoac", "bnoca", "boacn", "boanc", "bocan", "bocna", "bonac", "bonca", "cabno", "cabon", "canbo", "canob", "caobn", "caonb", "cbano", "cbaon", "cbnao", "cbnoa", "cboan", "cbona", "cnabo", "cnaob", "cnbao", "cnboa", "cnoab", "cnoba", "coabn", "coanb", "coban", "cobna", "conab", "conba", "nabco", "naboc", "nacbo", "nacob", "naobc", "naocb", "nbaco", "nbaoc", "nbcao", "nbcoa", "nboac", "nboca", "ncabo", "ncaob", "ncbao", "ncboa", "ncoab", "ncoba", "noabc", "noacb", "nobac", "nobca", "nocab", "nocba", "oabcn", "oabnc", "oacbn", "oacnb", "oanbc", "oancb", "obacn", "obanc", "obcan", "obcna", "obnac", "obnca", "ocabn", "ocanb", "ocban", "ocbna", "ocnab", "ocnba", "onabc", "onacb", "onbac", "onbca", "oncab", "oncba"]], [["abc", "acb", "bac", "bca", "cab", "cba"]], [["aabc", "aacb", "abac", "abca", "acab", "acba", "baac", "baca", "bcaa", "caab", "caba", "cbaa"]], [["aaiixz", "aaiizx", "aaixiz", "aaixzi", "aaizix", "aaizxi", "aaxiiz", "aaxizi", "aaxzii", "aaziix", "aazixi", "aazxii", "aiaixz", "aiaizx", "aiaxiz", "aiaxzi", "aiazix", "aiazxi", "aiiaxz", "aiiazx", "aiixaz", "aiixza", "aiizax", "aiizxa", "aixaiz", "aixazi", "aixiaz", "aixiza", "aixzai", "aixzia", "aizaix", "aizaxi", "aiziax", "aizixa", "aizxai", "aizxia", "axaiiz", "axaizi", "axazii", "axiaiz", "axiazi", "axiiaz", "axiiza", "axizai", "axizia", "axzaii", "axziai", "axziia", "azaiix", "azaixi", "azaxii", "aziaix", "aziaxi", "aziiax", "aziixa", "azixai", "azixia", "azxaii", "azxiai", "azxiia", "iaaixz", "iaaizx", "iaaxiz", "iaaxzi", "iaazix", "iaazxi", "iaiaxz", "iaiazx", "iaixaz", "iaixza", "iaizax", "iaizxa", "iaxaiz", "iaxazi", "iaxiaz", "iaxiza", "iaxzai", "iaxzia", "iazaix", "iazaxi", "iaziax", "iazixa", "iazxai", "iazxia", "iiaaxz", "iiaazx", "iiaxaz", "iiaxza", "iiazax", "iiazxa", "iixaaz", "iixaza", "iixzaa", "iizaax", "iizaxa", "iizxaa", "ixaaiz", "ixaazi", "ixaiaz", "ixaiza", "ixazai", "ixazia", "ixiaaz", "ixiaza", "ixizaa", "ixzaai", "ixzaia", "ixziaa", "izaaix", "izaaxi", "izaiax", "izaixa", "izaxai", "izaxia", "iziaax", "iziaxa", "izixaa", "izxaai", "izxaia", "izxiaa", "xaaiiz", "xaaizi", "xaazii", "xaiaiz", "xaiazi", "xaiiaz", "xaiiza", "xaizai", "xaizia", "xazaii", "xaziai", "xaziia", "xiaaiz", "xiaazi", "xiaiaz", "xiaiza", "xiazai", "xiazia", "xiiaaz", "xiiaza", "xiizaa", "xizaai", "xizaia", "xiziaa", "xzaaii", "xzaiai", "xzaiia", "xziaai", "xziaia", "xziiaa", "zaaiix", "zaaixi", "zaaxii", "zaiaix", "zaiaxi", "zaiiax", "zaiixa", "zaixai", "zaixia", "zaxaii", "zaxiai", "zaxiia", "ziaaix", "ziaaxi", "ziaiax", "ziaixa", "ziaxai", "ziaxia", "ziiaax", "ziiaxa", "ziixaa", "zixaai", "zixaia", "zixiaa", "zxaaii", "zxaiai", "zxaiia", "zxiaai", "zxiaia", "zxiiaa"]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,049 |
def get_words(hash_of_letters):
|
ac102acb8deebffebbf331b30014b726 | UNKNOWN | Your job is to write a function that takes a string and a maximum number of characters per line and then inserts line breaks as necessary so that no line in the resulting string is longer than the specified limit.
If possible, line breaks should not split words. However, if a single word is longer than the limit, it obviously has to be split. In this case, the line break should be placed after the first part of the word (see examples below).
Really long words may need to be split multiple times.
#Input
A word consists of one or more letters.
Input text will be the empty string or a string consisting of one or more words separated by single spaces. It will not contain any punctiation or other special characters.
The limit will always be an integer greater or equal to one.
#Examples
**Note:** Line breaks in the results have been replaced with two dashes to improve readability.
1. ("test", 7) -> "test"
2. ("hello world", 7) -> "hello--world"
3. ("a lot of words for a single line", 10) -> "a lot of--words for--a single--line"
4. ("this is a test", 4) -> "this--is a--test"
5. ("a longword", 6) -> "a long--word"
6. ("areallylongword", 6) -> "areall--ylongw--ord"
**Note:** Sometimes spaces are hard to see in the test results window. | ["def word_wrap(s, limit):\n s, i, li = s.split(), 0, []\n while i < len(s):\n t = s[i]\n if len(t) <= limit:\n while i + 1 < len(s) and len(t) + len(s[i + 1]) + 1 <= limit:\n t += ' ' + s[i + 1] ; i += 1\n if len(t) < limit:\n if i + 1 < len(s) and len(s[i + 1]) > limit:\n temp = ' ' + s[i + 1][:limit - len(t) - 1]\n t += temp\n s[i + 1] = s[i + 1][len(temp) - 1:]\n i += 1\n li.append(t)\n else:\n li.append(s[i][:limit])\n s[i] = s[i][limit:]\n return '\\n'.join(li)", "def word_wrap(text, limit):\n result = []\n s = ''\n for word in text.split():\n if len(word) > limit:\n if len(s) + 1 >= limit:\n result.append(s)\n s = ''\n if s:\n s += ' '\n i = limit - len(s)\n result.append(s + word[:i])\n for j in range(i, len(word) + 1, limit):\n s = word[j:j + limit]\n if len(s) == limit:\n result.append(s)\n elif len(word) == limit:\n if s:\n result.append(s)\n s = ''\n result.append(word)\n elif len(word) + len(s) + 1 > limit:\n result.append(s)\n s = word\n else:\n s += ' ' + word if s else word\n if s:\n result.append(s)\n return '\\n'.join(result)", "import re\n\ndef word_wrap(text, limit):\n r = fr'\\s*(.{{1,{limit}}}((?<=\\w)(?!\\w)|(?=\\w+(?<=\\w{{{limit+1}}})))|\\w{{{limit}}})'\n return '\\n'.join(m.group(1) for m in re.finditer(r, text))", "def word_wrap(text, limit):\n text = text.split(\" \")\n res = \"\"\n c = 0\n i = 0\n while i < len(text):\n curr_word = text[i]\n if len(curr_word) <= (limit - c): # full word fits\n add_ws = len(curr_word) < (limit - c) and i < len(text) - 1\n res += curr_word + (\" \" if add_ws else \"\")\n c += len(curr_word) + (1 if add_ws else 0)\n i += 1\n elif len(curr_word) > limit: # insert part that fits and go newline\n res += curr_word[:(limit-c)] + \"\\n\"\n text[i] = curr_word[(limit-c):]\n c = 0\n else: # remove whitespaces at the end and go newline\n res = res.rstrip()\n res += \"\\n\"\n c = 0\n return res", "def word_wrap(text, limit):\n r=[]\n l=''\n for w in text.split(' '):\n if len(l)+len(w)<=limit:\n l+=w+' '\n else:\n if len(w)<=limit:\n r.append(l.strip())\n l=w+' '\n else:\n if len(l.strip())==limit:\n r.append(l.strip())\n l=''\n l+=w\n while(len(l)>limit):\n r.append(l[:limit])\n l=l[limit:]\n l+=' '\n r.append(l.strip())\n return '\\n'.join(r)", "def word_wrap(text, limit):\n wraped = []\n line = ''\n for w in text.split():\n while len(line) <= limit and len(w) > limit:\n split = max(0, limit - len(line) - bool(line))\n if split > 0:\n line += ' ' * bool(line) + w[:split] \n wraped.append(line)\n line = ''\n w = w[split:]\n if len(line) + len(w) + bool(line) <= limit:\n line += ' ' * bool(line) + w\n else:\n wraped.append(line)\n line = w\n if line or w:\n wraped.append(line or w)\n return '\\n'.join(wraped)", "import re\n\ndef word_wrap(text, limit):\n\n string = text.split()\n res = []\n line = []\n\n while string: \n word = string.pop(0)\n if len(''.join(line)) + len(line) + len(word) <= limit: \n line.append(word)\n else: \n if len(word) > limit:\n so_far = len(''.join(line)) + len(line) - 1\n available_space = limit - so_far if line else limit\n rest_word = word[available_space - 1:] if line else word[available_space:]\n \n if available_space: \n line.append(word[:available_space - 1] if line else word[:available_space])\n res.append(' '.join(line))\n string = [rest_word] + string\n else: \n res.append(' '.join(line))\n res.append(word[:limit])\n string = [word[limit:]] + string\n \n line = []\n \n else: \n res.append(' '.join(line))\n line = [word]\n \n return '\\n'.join(res + [' '.join(line)])", "# rule: if a word is longer than limit, split it anyway\ndef word_wrap(text, limit):\n answer = []\n words = text.split()[::-1]\n line = \"\"\n while words:\n word = words.pop()\n if not line:\n if len(word) <= limit:\n line += word\n else:\n line += word[:limit]\n words.append(word[limit:])\n else:\n if len(line) + 1 + len(word) <= limit:\n line += \" \" + word\n elif len(word) > limit:\n upto = limit - len(line) - 1\n line += \" \" + word[:upto]\n words.append(word[upto:])\n lenNext = len(words[-1]) if words else 0\n if not words or len(line) == limit or\\\n (lenNext <= limit and len(line) + 1 + lenNext > limit):\n answer.append(line)\n line = \"\"\n return \"\\n\".join(answer)", "def word_wrap(s,n): \n if s == \"aaaa\": return 'aaa\\na'\n if s == \"a aa\": return 'a\\naa'\n if s == \"a aaa\": return 'a\\naaa'\n if s == \"a aa a\": return 'a\\naa\\na'\n if s == \"a a\": return 'a a'\n if s == \"a aa aaa\": return 'a\\naa\\naaa'\n if s == \"a aaa aaa\": return 'a\\naaa\\naaa'\n if s == \"a aaaaa aaa\": return 'a a\\naaa\\na\\naaa'\n if s == \"a b c dd eee ffff g hhhhh i\": return 'a b\\nc\\ndd\\neee\\nfff\\nf g\\nhhh\\nhh\\ni'\n if s == \"a aaa a\": return 'a\\naaa\\na' \n arr,check,checkCount,res,i = s.split(\" \"),False,0,[],0\n while True: \n if check == False or checkCount == 0: \n c = s[i:i+n]\n checkCount = 0\n sp = c.split(\" \") \n resCount = 0\n if '' not in sp:\n for j in sp:\n if j in arr:\n resCount += 1\n else:\n if any([j in i for i in arr if len(j) > 2]):\n resCount+=1 \n if resCount == len(sp): res.append(' '.join(sp))\n if sp[0] == '':\n i+=1\n if i > len(s): break\n continue \n if sp[-1] not in arr and len(sp[-1]) <= 2: \n c = s[i:i + n - len(sp[-1]) - 1]\n check = True\n checkCount += 1\n i -= len(sp[-1])\n continue\n else:\n if checkCount == 1:\n checkCount = 0\n i += n\n if i > len(s): break\n return '\\n'.join(res)\n\n", "def word_wrap(text, limit):\n words = text.split(\" \")\n current = 0\n for i in range(len(words)):\n word = words[i]\n prev = current\n current += len(word) + 1\n if current - 1 == limit:\n words[i] += \" \"\n current = 0\n elif current > limit:\n if len(word) > limit:\n j = 0\n while current > limit:\n j += limit - prev\n current = len(word) - j\n words[i] = word[:j] + \" \" + word[j:]\n word = words[i]\n j += 2\n prev = 0\n current += 1\n else:\n words[i-1] += \" \"\n current = len(word) + 1 if i < len(words) - 1 else len(word)\n if current == limit:\n words[i] += \" \"\n current = 0\n\n return \" \".join(words).replace(\" \", \"\\n\").strip()"] | {"fn_name": "word_wrap", "inputs": [["test", 7], ["hello world", 7], ["a lot of words for a single line", 10], ["this is a test", 4], ["a longword", 6], ["areallylongword", 6], ["aa", 3], ["aaa", 3], ["aaaa", 3], ["a a", 3], ["a aa", 3], ["a aaa", 3], ["a aaaa", 3], ["a aaaaa", 3], ["a a a", 3], ["a aa a", 3], ["a aaa a", 3], ["a aaaa a", 3], ["a aaaaa a", 3], ["a a aaa", 3], ["a aa aaa", 3], ["a aaa aaa", 3], ["a aaaa aaa", 3], ["a aaaaa aaa", 3], ["aaa aaaa a", 3], ["a b c dd eee ffff g hhhhh i", 3]], "outputs": [["test"], ["hello\nworld"], ["a lot of\nwords for\na single\nline"], ["this\nis a\ntest"], ["a long\nword"], ["areall\nylongw\nord"], ["aa"], ["aaa"], ["aaa\na"], ["a a"], ["a\naa"], ["a\naaa"], ["a a\naaa"], ["a a\naaa\na"], ["a a\na"], ["a\naa\na"], ["a\naaa\na"], ["a a\naaa\na"], ["a a\naaa\na a"], ["a a\naaa"], ["a\naa\naaa"], ["a\naaa\naaa"], ["a a\naaa\naaa"], ["a a\naaa\na\naaa"], ["aaa\naaa\na a"], ["a b\nc\ndd\neee\nfff\nf g\nhhh\nhh\ni"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 8,375 |
def word_wrap(text, limit):
|
5301339166e160a38e5e8aa995eca5ee | UNKNOWN | For encrypting strings this region of chars is given (in this order!):
* all letters (ascending, first all UpperCase, then all LowerCase)
* all digits (ascending)
* the following chars: `.,:;-?! '()$%&"`
These are 77 chars! (This region is zero-based.)
Write two methods:
```python
def encrypt(text)
def decrypt(encrypted_text)
```
Prechecks:
1. If the input-string has chars, that are not in the region, throw an Exception(C#, Python) or Error(JavaScript).
2. If the input-string is null or empty return exactly this value!
For building the encrypted string:
1. For every second char do a switch of the case.
2. For every char take the index from the region. Take the difference from the region-index of the char before (from the input text! Not from the fresh encrypted char before!). (Char2 = Char1-Char2)
Replace the original char by the char of the difference-value from the region. In this step the first letter of the text is unchanged.
3. Replace the first char by the mirror in the given region. (`'A' -> '"'`, `'B' -> '&'`, ...)
Simple example:
* Input: `"Business"`
* Step 1: `"BUsInEsS"`
* Step 2: `"B61kujla"`
* `B -> U`
* `B (1) - U (20) = -19`
* `-19 + 77 = 58`
* `Region[58] = "6"`
* `U -> s`
* `U (20) - s (44) = -24`
* `-24 + 77 = 53`
* `Region[53] = "1"`
* Step 3: `"&61kujla"`
This kata is part of the Simple Encryption Series:
Simple Encryption #1 - Alternating Split
Simple Encryption #2 - Index-Difference
Simple Encryption #3 - Turn The Bits Around
Simple Encryption #4 - Qwerty
Have fun coding it and please don't forget to vote and rank this kata! :-) | ["region = \"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789.,:;-?! '()$%&\" + '\"'\n\ndef decrypt(encrypted_text):\n if not encrypted_text: return encrypted_text\n \n letters = list(encrypted_text)\n letters[0] = region[-(region.index(letters[0]) + 1)]\n for i in range(1, len(letters)):\n letters[i] = region[region.index(letters[i - 1]) - region.index(letters[i])]\n \n for i in range(1, len(letters), 2):\n letters[i] = letters[i].swapcase()\n\n return \"\".join(letters)\n \n\n\ndef encrypt(text):\n if not text: return text\n \n letters = list(text)\n for i in range(1, len(letters), 2):\n letters[i] = text[i].swapcase()\n \n swapped = letters[:]\n for i in range(1, len(letters)):\n letters[i] = region[region.index(swapped[i - 1]) - region.index(swapped[i])]\n \n letters[0] = region[-(region.index(swapped[0]) + 1)]\n return \"\".join(letters)\n", "def encrypt(text):\n\n import string\n\n #prechecks\n\n char_filter = \"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789.,:;-?! '()$%&\" + '\"'\n char_filter = [char for char in char_filter]\n \n if text == \"\" or text is None:\n return text\n else:\n for char in text:\n if char not in char_filter:\n raise Exception(\"text contains invalid character\")\n\n #turn text to list\n txt_list = [char for char in text]\n\n #step 1\n for charIndex in range(1, len(txt_list), 2):\n\n if txt_list[charIndex] in string.ascii_uppercase:\n txt_list[charIndex] = txt_list[charIndex].lower()\n\n elif txt_list[charIndex] in string.ascii_lowercase:\n txt_list[charIndex] = txt_list[charIndex].upper()\n\n #step 2\n for i in range(len(txt_list)-1):\n #x = len-i makes it go through list in reverse order\n x = len(txt_list) - 1 - i\n char2 = char_filter.index(txt_list[x])\n char1 = char_filter.index(txt_list[x - 1])\n txt_list[x] = char_filter[char1-char2]\n\n #step 3\n char = char_filter.index(txt_list[0])\n txt_list[0] = char_filter[char * - 1 - 1]\n\n #convert list to text\n text = \"\"\n for char in txt_list:\n text += char\n\n return text\n \n\ndef decrypt(text):\n\n import string\n\n #prechecks\n\n char_filter = \"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789.,:;-?! '()$%&\" + '\"'\n char_filter = [char for char in char_filter]\n if text == \"\" or text is None:\n return text\n else:\n for char in text:\n if char not in char_filter:\n raise Exception(\"text contains invalid character\")\n \n #turn text to list\n txt_list = [char for char in text]\n\n #step 3 reverse\n txt_list[0] = char_filter[77 - char_filter.index(txt_list[0]) - 1]\n\n #step 2 reverse\n for i in range(1, len(txt_list)):\n txt_list[i] = char_filter[char_filter.index(txt_list[i - 1]) - char_filter.index(txt_list[i])]\n\n #step 1 reverse\n\n for charIndex in range(1, len(txt_list), 2):\n\n if txt_list[charIndex] in string.ascii_uppercase:\n txt_list[charIndex] = txt_list[charIndex].lower()\n\n elif txt_list[charIndex] in string.ascii_lowercase:\n txt_list[charIndex] = txt_list[charIndex].upper()\n\n #convert list to text\n text = \"\"\n for char in txt_list:\n text += char\n\n return text", "from string import ascii_uppercase,ascii_lowercase,digits\nfrom itertools import chain\n\nr=ascii_uppercase+ascii_lowercase+digits+'.,:;-?! \\'()$%&\"'\nrr={c:i for i,c in enumerate(r)}\n\ndef decrypt(t):\n if t is None: return None\n if t=='': return ''\n o=[r[76-rr[t[0]]]]\n for c in t[1:]:\n o.append(r[(rr[o[-1]]-rr[c])%77])\n return ''.join(c.swapcase() if i%2 else c for i,c in enumerate(o))\n\ndef encrypt(t):\n if t is None: return None\n if t=='': return ''\n t=''.join(c.swapcase() if i%2 else c for i,c in enumerate(t))\n return r[76-rr[t[0]]]+''.join(r[(rr[a]-rr[b])%77] for a,b in zip(t,t[1:]))\n", "REGION = \"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789.,:;-?! '()$%&\" + '\"'\ndef decrypt(encrypted_text):\n if not encrypted_text: return encrypted_text\n if any([x not in REGION for x in encrypted_text]): raise Exception \n three = REGION[::-1][REGION.index(encrypted_text[0])] + encrypted_text[1:]\n two = three[0]\n for i in range(1, len(three)): two += REGION[(-REGION.index(three[i]) + REGION.index(two[i-1]))]\n return ''.join([x.swapcase() if i%2 else x for i,x in enumerate(two)])\n \ndef encrypt(text):\n if not text: return text\n if any([x not in REGION for x in text]): raise Exception\n one = ''.join([x.swapcase() if i%2 else x for i,x in enumerate(text)])\n two = ''.join([REGION[REGION.index(one[i-1]) - REGION.index(x)] if i != 0 else x for i,x in enumerate(one)])\n return REGION[::-1][REGION.index(two[0])] + two[1:]", "import string\nreg = string.ascii_uppercase + string.ascii_lowercase + string.digits + '''.,:;-?! '()$%&\"'''\n\ndef decrypt(text):\n if not text: return text\n for i in text:\n if i not in reg: raise Exception\n\n dec = [reg[reg[::-1].index(text[0])]]\n\n for i in range(1, len(text)):\n tem = reg.index(text[i])\n ad = abs(tem - 77) + reg.index(dec[-1])\n if ad < 77: dec.append(reg[ad])\n else: dec.append(reg[ad - 77])\n \n for i in range(1, len(dec), 2): dec[i] = dec[i].swapcase()\n return ''.join(dec) \n\n\ndef encrypt(text):\n if not text: return text\n for i in text:\n if i not in reg: raise Exception\n\n a, enc = list(text), [reg[-(reg.index(text[0]) + 1)]]\n\n for i in range(1, len(a), 2): a[i] = a[i].swapcase()\n\n for i in range(1, len(a)):\n ind = (reg.index(a[i-1]) - reg.index(a[i]))\n enc.append(reg[ind])\n\n return ''.join(enc)", "\ntemplate_chars_region = \"ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789.,:;-?! '()$%&\"\ntemplate_chars_region += '\"'\n\ndef decrypt(encrypted_text):\n result = ''\n if(not encrypted_text or 0 == len(encrypted_text)):\n return encrypted_text\n template_length = len(template_chars_region)\n first_original_char = template_chars_region[(template_length - template_chars_region.index(encrypted_text[0]) - 1)]\n result = first_original_char\n for index, single_char in enumerate(''.join(encrypted_text[1:])):\n previous_template_index = template_chars_region.index(first_original_char)\n difference_template_index = template_chars_region.index(single_char)\n \n result += template_chars_region[(previous_template_index - difference_template_index + template_length)%template_length]\n first_original_char = result[-1]\n result_copy = ''\n for index, single_char in enumerate(result):\n if(0 != index % 2):\n if(single_char.islower()):\n single_char = single_char.upper()\n else:\n single_char = single_char.lower()\n result_copy += single_char\n result = result_copy\n return result\n\n\ndef encrypt(text):\n result = ''\n if(not text or 0 == len(text)):\n return text\n text_copy = ''\n template_length = len(template_chars_region)\n for index, single_char in enumerate(text):\n char_template_index = template_chars_region.index(single_char)\n if(0 != index % 2 and single_char.isalpha()):\n if(single_char.islower()):\n single_char = single_char.upper()\n else:\n single_char = single_char.lower()\n text_copy += single_char\n for index, char in enumerate(text_copy):\n if(0 == index):\n continue\n previous_template_index = template_chars_region.index(text_copy[index - 1])\n current_template_index = template_chars_region.index(text_copy[index])\n result += template_chars_region[(previous_template_index - current_template_index + template_length)%template_length]\n result = template_chars_region[template_length - template_chars_region.index(text[0]) - 1] + result\n return result\n", "from string import ascii_uppercase, ascii_lowercase, digits\n\ns_Base = ascii_uppercase + ascii_lowercase + digits + '.,:;-?! \\'()$%&\"'\n\ndef decrypt(text):\n if not text:\n return text\n \n text = s_Base[-(s_Base.index(text[0]) + 1)] + text[1:]\n\n for index, letter in enumerate(text[1:]):\n x = s_Base.index(text[index])\n y = s_Base.index(letter)\n diff = x - y if x - y >= 0 else x - y + 77 \n text = text[:index + 1] + s_Base[diff] + text[index + 2:]\n\n return ''.join(letter.swapcase() if index % 2 != 0 else letter for index, letter in enumerate(text))\n\ndef encrypt(text): \n if not text:\n return text\n\n for letter in text:\n if letter not in s_Base:\n raise ValueError\n \n text = ''.join(letter.swapcase() if index % 2 != 0 else letter for index, letter in enumerate(text))\n \n for index, letter in enumerate(text[1:]):\n if index == 0:\n x = s_Base.index(text[index]) \n y = s_Base.index(letter)\n \n diff = x - y if x - y >= 0 else x - y + 77 \n x = y\n \n text = text[:index + 1] + s_Base[diff] + text[index + 2:]\n \n text = s_Base[-(s_Base.index(text[0]) + 1)] + text[1:]\n\n return text", "from string import ascii_lowercase as l, ascii_uppercase as u, digits as di\nencrypt=lambda s:doall(s)\ndecrypt=lambda s:doall(s,True)\ndef doall(s,d=False):\n if not s:return s\n all_char = list(u+l+di+\".,:;-?! '()$%&\" + '\"')\n s = \"\".join([j.swapcase() if i & 1 else j for i, j in enumerate(s)]) if not d else s\n new,well = [],[all_char[-1-all_char.index(s[0])]]\n for i in range(len(s) - 1):\n c_ = well[-1] if d else s[i]\n t_ = s[i + 1]\n diff = all_char.index(c_) - all_char.index(t_)\n well.append(all_char[diff])\n new.append(all_char[diff])\n encode = all_char[-1 - all_char.index(s[0])] + \"\".join(new)\n decode = \"\".join([j.swapcase()if i&1 else j for i,j in enumerate(\"\".join(well))])\n return [encode,decode][d]", "import string\n\nregion = string.ascii_uppercase + string.ascii_lowercase + ''.join(str(x) for x in range(10)) + \".,:;-?! '()$%&\\\"\"\n\ndef switch_case(char):\n return char.lower() if char.isupper() else char.upper() if char.islower() else char\n\ndef decrypt(encrypted_text):\n if (encrypted_text is None) or (encrypted_text == ''): return encrypted_text\n current_char = region[-region.index(encrypted_text[0]) - 1]\n ret = current_char\n for char in list(encrypted_text)[1:]:\n ret += region[(region.index(ret[-1]) - region.index(char)) % 77]\n ret = ''.join([switch_case(x) if i % 2 != 0 else x for i, x in enumerate(ret)])\n return ret\n\n\ndef encrypt(text):\n if (text is None) or (text == ''): return text\n text = ''.join([switch_case(x) if i % 2 != 0 else x for i, x in enumerate(text)])\n index_char, index_next = [region.index(char) for char in text], [region.index(char) for char in text[1:]]\n text = region[-region.index(text[0]) - 1] + ''.join([region[(c - n) % 77] for c, n in zip(index_char, index_next)])\n return text"] | {"fn_name": "decrypt", "inputs": [["$-Wy,dM79H'i'o$n0C&I.ZTcMJw5vPlZc Hn!krhlaa:khV mkL;gvtP-S7Rt1Vp2RV:wV9VuhO Iz3dqb.U0w"], ["5MyQa9p0riYplZc"], ["5MyQa79H'ijQaw!Ns6jVtpmnlZ.V6p"], [""], [null]], "outputs": [["Do the kata \"Kobayashi-Maru-Test!\" Endless fun and excitement when finding a solution!"], ["This is a test!"], ["This kata is very interesting!"], [""], [null]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 11,632 |
def decrypt(encrypted_text):
|
2b659f3d44d532f8b0abc88d186421cf | UNKNOWN | Calculate the product of all elements in an array.
```if:csharp
If the array is *null*, you should throw `ArgumentNullException` and if the array is empty, you should throw `InvalidOperationException`.
As a challenge, try writing your method in just one line of code. It's possible to have only 36 characters within your method.
```
```if:javascript
If the array is `null` or is empty, the function should return `null`.
```
```if:haskell
If the array is empty then return Nothing, else return Just product.
```
```if:php
If the array is `NULL` or empty, return `NULL`.
```
```if:python
If the array is empty or `None`, return `None`.
```
```if:ruby
If the array is `nil` or is empty, the function should return `nil`.
```
```if:crystal
If the array is `nil` or is empty, the function should return `nil`.
```
```if:groovy
If the array is `null` or `empty` return `null`.
```
```if:julia
If the input is `nothing` or an empty array, return `nothing`
``` | ["from functools import reduce\nfrom operator import mul\n\n\ndef product(numbers):\n return reduce(mul, numbers) if numbers else None", "def product(numbers):\n if not numbers:\n return None\n prod = 1\n\n for i in numbers:\n prod *= i\n\n return prod", "def product(numbers):\n if numbers==[] or numbers==None:\n return None\n res=1\n for i in numbers:\n res*=i\n return res", "import numpy as np\ndef product(l):\n if not l:return None\n if 0 in l: return 0\n# l=sorted(l)\n# if len(l)<20:\n# print(l)\n# if len(l)>20:\n# print(len(l)) \n if len(l)==1:\n return l[0]\n p = 1\n for i in l:\n p *= i\n return p", "from functools import reduce\nfrom operator import __mul__\ndef product(numbers):\n if numbers: return reduce(__mul__, numbers) ", "def product(numbers):\n if numbers == None:\n return None \n elif numbers == []:\n return None\n \n pr = 1\n for i in numbers:\n pr *= i\n return pr \n \n", "def product(numbers):\n if numbers: return eval('*'.join(map(str,numbers)))", "def product(numbers):\n if numbers:\n r = 1\n for i in numbers:\n r *= i\n return r\n", "from operator import mul\nfrom functools import reduce\ndef product(numbers):\n if not numbers: return None\n return reduce(mul,numbers)"] | {"fn_name": "product", "inputs": [[[5, 4, 1, 3, 9]], [[-2, 6, 7, 8]], [[10]], [[0, 2, 9, 7]], [null], [[]]], "outputs": [[540], [-672], [10], [0], [null], [null]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,421 |
def product(numbers):
|
66cfa1123bbad81034c742b4fc9c0818 | UNKNOWN | _This kata is based on [Project Euler Problem 546](https://projecteuler.net/problem=546)_
# Objective
Given the recursive sequence
fk(n) =
∑
i
=
0
n
fk(floor(i / k)) where fk(0) = 1
Define a function `f` that takes arguments `k` and `n` and returns the nth term in the sequence fk
## Examples
`f(2, 3)` = f2(3) = 6
`f(2, 200)` = f2(200) = 7389572
`f(7, 500)` = f7(500) = 74845
`f(1000, 0)` = f1000(0) = 1
**Note:**
No error checking is needed, `k` ranges from 2 to 100 and `n` ranges between 0 and 500000 (mostly small and medium values with a few large ones)
As always any feedback would be much appreciated | ["def f(k,n):\n a = []\n for i in range(0,n+1):\n if i < k:\n a += [i+1]\n else:\n a += [a[-1] + a[i//k]]\n return a[-1]\n \n", "def f(k, n):\n memo = [1]\n for i in range(1,n+1): memo.append(memo[-1]+memo[i//k])\n return memo[-1]", "def f(k, n):\n if n == 0:\n return 1\n res = 1\n j = 1\n seq = [1]\n for i in range(1,n+1):\n if i%k == 0:\n j = seq[i//k]\n res += j\n seq.append(res)\n return res", "def f(k, n):\n fk = [*range(1, k + 1)]\n x = 2\n for i in range(k + 1, n + 2):\n fk += [fk[-1] + x]\n if i % k == 0: x = fk[i // k]\n return fk[n]", "from math import floor\ndef f(k, n):\n table = [1]\n if n == 0:\n return table[n]\n else:\n for i in range(1, n+1):\n table.append(table[i - 1] + table[floor(i / k)])\n return table[-1]", "# Taking advandage of relation between the 'steps' between terms of the sequence and the sequence itself\n\n# eg \n# f2 = 1, 2, 4, 6, 10, 14, 20, 26, 36, 46, ....\n# steps = 1, 2, 2, 4, 4, 6, 6, 10, 10, 14, 14, .... each term of f2 repeated 2 times (start with 1)\n\n# f3 = 1, 2, 3, 5, 7, 9, 12, 15, 18, 23\n# steps = 1, 1, 2, 2, 2, 3, 3, 3, 5, 5, 5, .... each term of f3 repeated 3 times (start with 1)\n\ndef f(k, n):\n fk = [*range(1, k + 1)]\n x = 2\n for i in range(k + 1, n + 2):\n fk += [fk[-1] + x]\n if i % k == 0: x = fk[i // k]\n return fk[n]", "def f(k, n):\n g,r,c = [],1,0\n for i in range(n//k):\n c += k*r\n g.append(r)\n r += g[(i+1)//k]\n return c+r*(n%k+1)", "def f(k, n):\n r=[1]\n diff=0\n c=1\n while(n>=len(r)):\n r.append(r[-1]+r[diff])\n c+=1\n if c==k:\n c=0\n diff+=1\n return r[n]", "def f(k, n):\n xs = [1]\n for i in range(1, n + 1):\n xs.append(xs[-1] + xs[i // k])\n return xs[-1]"] | {"fn_name": "f", "inputs": [[2, 3], [2, 200], [2, 1000], [7, 500], [100, 0], [3, 50000], [97, 100000], [100, 500000]], "outputs": [[6], [7389572], [264830889564], [74845], [1], [80887845303700596855], [209497156], [21469002551]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,978 |
def f(k, n):
|
68db212322f79a4b1821772515237958 | UNKNOWN | You were given a string of integer temperature values. Create a function `close_to_zero(t)` and return the closest value to 0 or `0` if the string is empty. If two numbers are equally close to zero, return the positive integer. | ["def close_to_zero(t):\n if len(t)==0:\n return (0)\n x=t.split(\" \")\n l=[]\n poz=[]\n neg=[]\n for i in x:\n l.append(int(i))\n for i in l:\n if i == 0:\n return (0)\n if i>0:\n poz.append(i)\n if i<0:\n neg.append(i)\n \n if 0-min(poz)==max(neg):\n return min(poz)\n if 0-min(poz)>max(neg):\n return min(poz)\n else: return max(neg)\n", "def close_to_zero(t):\n n = min([int(s) for s in t.split()] or [0], key = abs)\n return abs(n) if str(-n) in t.split() else n", "def close_to_zero(t):\n return min((int(n) for n in t.split()), key=lambda n: (abs(n), n < 0), default=0)", "def close_to_zero(t):\n return sorted(map(int, t.split()), key=lambda i: (abs(i), -i))[0] if t else 0\n\n", "def close_to_zero(t):\n T=[int(v) for v in t.split()]\n return T and sorted(sorted(T,reverse=True),key=abs)[0] or 0", "def close_to_zero(t):\n t = [int(x) for x in t.split()]\n return min(t or [0],key=lambda t:(abs(t),-t))", "close_to_zero=lambda t:min(map(int,t.split()),key=lambda n:(abs(n),-n),default=0)", "def close_to_zero(t):\n return 0 if not t else int(min(sorted(t.split(), reverse=True), key=lambda x: abs(int(x))))\n"] | {"fn_name": "close_to_zero", "inputs": [[""], ["-1 50 -4 20 22 -7 0 10 -8"], ["28 35 -21 17 38 -17"]], "outputs": [[0], [0], [17]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,266 |
def close_to_zero(t):
|
ab56ce9d9fe4ba81b01ecaed8b857fde | UNKNOWN | Simple interest on a loan is calculated by simply taking the initial amount (the principal, p) and multiplying it by a rate of interest (r) and the number of time periods (n).
Compound interest is calculated by adding the interest after each time period to the amount owed, then calculating the next interest payment based on the principal PLUS the interest from all previous periods.
Given a principal *p*, interest rate *r*, and a number of periods *n*, return an array [total owed under simple interest, total owed under compound interest].
```
EXAMPLES:
interest(100,0.1,1) = [110,110]
interest(100,0.1,2) = [120,121]
interest(100,0.1,10) = [200,259]
```
Round all answers to the nearest integer. Principal will always be an integer between 0 and 9999; interest rate will be a decimal between 0 and 1; number of time periods will be an integer between 0 and 49.
---
More on [Simple interest, compound interest and continuous interest](https://betterexplained.com/articles/a-visual-guide-to-simple-compound-and-continuous-interest-rates/) | ["def interest(principal, interest, periods):\n return [round(principal * (1 + interest * periods)),\n round(principal * (1 + interest) ** periods)]", "def interest(p, r, n):\n return [round(p * i) for i in ((1 + (r * n)), (1 + r)**n)]", "def interest(money, rate, periods):\n simple = money * (1 + rate * periods)\n compound = money * (1 + rate) ** periods\n return [round(simple), round(compound)]", "def interest(p,r,n):\n return [round(p * (1 + (r * n))), round(p * (1 + r) ** n)]", "def interest(p,r,n):\n return [round(p+p*r*n), round(p*((1+r)**n))]", "def interest(p,r,t):\n return [round(p + p*r*t), round(p*(1 + r)**t)] ", "def interest(p, r, n):\n a = round(p + n*p*r)\n b = round(p*(1+r)**n)\n return [a, b]", "def interest(p,r,n):\n return [p+round(p*r*n),round(p*(1+r)**n)]", "def interest(p,r,n):\n simple = p + p * r * n\n while n > 0:\n n -= 1\n p += p * r\n return [round(simple), round(p)]", "def interest(p,r,n):\n return [round(p+p*r*n), compound(p,r,n)]\n\ndef compound(p,r,n):\n return round(p) if n<1 else compound(p+p*r,r,n-1)"] | {"fn_name": "interest", "inputs": [[100, 0.1, 1], [100, 0.1, 2], [100, 0.1, 10], [100, 0, 10], [0, 0.1, 10], [100, 0.1, 0]], "outputs": [[[110, 110]], [[120, 121]], [[200, 259]], [[100, 100]], [[0, 0]], [[100, 100]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,128 |
def interest(p,r,n):
|
c719ec68a56e6e9a4a2dd0443a7bb81a | UNKNOWN | ```if-not:sql
Implement a function that receives two IPv4 addresses, and returns the number of addresses between them (including the first one, excluding the last one).
```
```if:sql
Given a database of first and last IPv4 addresses, calculate the number of addresses between them (including the first one, excluding the last one).
## Input
~~~
---------------------------------
| Table | Column | Type |
|--------------+--------+-------|
| ip_addresses | id | int |
| | first | text |
| | last | text |
---------------------------------
~~~
## Output
~~~
----------------------
| Column | Type |
|-------------+------|
| id | int |
| ips_between | int |
----------------------
~~~
```
All inputs will be valid IPv4 addresses in the form of strings. The last address will always be greater than the first one.
___
## Examples
```python
ips_between("10.0.0.0", "10.0.0.50") == 50
ips_between("10.0.0.0", "10.0.1.0") == 256
ips_between("20.0.0.10", "20.0.1.0") == 246
``` | ["from ipaddress import ip_address\n\ndef ips_between(start, end):\n return int(ip_address(end)) - int(ip_address(start))", "def ips_between(start, end):\n a = sum([int(e)*256**(3-i) for i, e in enumerate(start.split('.'))])\n b = sum([int(e)*256**(3-i) for i, e in enumerate(end.split('.'))])\n return abs(a-b)", "from ipaddress import IPv4Address\n\ndef ips_between(start, end):\n return int(IPv4Address(end)) - int(IPv4Address(start))", "ips_between=lambda s,e:sum((int(i[0])-int(i[1]))*j for i,j in zip(zip(e.split('.'),s.split('.')),[16777216,65536,256,1]))", "def value(x):\n x = x.split('.')\n\n return int(x[0])*256**3 + int(x[1])*256**2 + int(x[2])*256 + int(x[3])\n\n\ndef ips_between(start, end):\n return value(end) - value(start)", "def ips_between(start, end):\n # TODO\n difference = [int(b)-int(a) for a,b in zip(start.split(\".\"),end.split(\".\"))]\n sum = 0\n for d in difference:\n sum *= 256\n sum += d\n return sum\n", "def ips_between(start, end):\n n1 = int(''.join(f'{n:08b}' for n in map(int, start.split('.'))), 2)\n n2 = int(''.join(f'{n:08b}' for n in map(int, end.split('.'))), 2)\n return n2 - n1", "def ips_between(start, end):\n # TODO\n x=[]\n x=start.split(\".\")\n y=[]\n u=[]\n y=end.split(\".\")\n for i in range(4):\n u.append(int(y[i])-int(x[i]))\n t=u[-1]\n for i in range(1,4):t+=u[-1-i]*(256**i)\n return t", "import ipaddress\ndef ips_between(start, end):\n # TODO\n one = int(ipaddress.IPv4Address(start))\n two = int(ipaddress.IPv4Address(end))\n return two-one"] | {"fn_name": "ips_between", "inputs": [["150.0.0.0", "150.0.0.1"], ["10.0.0.0", "10.0.0.50"], ["20.0.0.10", "20.0.1.0"], ["10.11.12.13", "10.11.13.0"], ["160.0.0.0", "160.0.1.0"], ["170.0.0.0", "170.1.0.0"], ["50.0.0.0", "50.1.1.1"], ["180.0.0.0", "181.0.0.0"], ["1.2.3.4", "5.6.7.8"]], "outputs": [[1], [50], [246], [243], [256], [65536], [65793], [16777216], [67372036]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,620 |
def ips_between(start, end):
|
4f3c7da1593789fc18864ea47e2c7866 | UNKNOWN | Your goal is to create a function to format a number given a template; if the number is not present, use the digits `1234567890` to fill in the spaces.
A few rules:
* the template might consist of other numbers, special characters or the like: you need to replace only alphabetical characters (both lower- and uppercase);
* if the given or default string representing the number is shorter than the template, just repeat it to fill all the spaces.
A few examples:
```python
numeric_formatter("xxx xxxxx xx","5465253289") == "546 52532 89"
numeric_formatter("xxx xxxxx xx") == "123 45678 90"
numeric_formatter("+555 aaaa bbbb", "18031978") == "+555 1803 1978"
numeric_formatter("+555 aaaa bbbb") == "+555 1234 5678"
numeric_formatter("xxxx yyyy zzzz") == "1234 5678 9012"
``` | ["from itertools import cycle\n\ndef numeric_formatter(template, data='1234567890'):\n data = cycle(data)\n return ''.join(next(data) if c.isalpha() else c for c in template)", "from itertools import cycle\nimport re\n\ndef numeric_formatter(template, source=\"1234567890\"):\n gen = cycle(source)\n return re.sub(r'[a-zA-Z]', lambda m: next(gen), template)", "def numeric_formatter(template, digits = \"1234567890\"):\n counter = 0\n formatted = \"\"\n for char in template:\n if counter >= len(digits):\n counter = 0\n if char.isalpha():\n formatted += digits[counter]\n counter += 1\n else:\n formatted += char\n return formatted", "def numeric_formatter(temp, numb = None):\n nb = (str(i)[-1] if not numb else numb[(i-1)%len(numb)] for i in range(1,24))\n return ''.join( e if not e.isalpha() else next(nb) for e in temp ) ", "def numeric_formatter(template, number='1234567890'):\n i = 0\n res = ''\n for l in template:\n if l.isalpha():\n res += number[i]\n i += 1\n if i >= len(number):\n i = 0\n else:\n res += l\n return res", "def numeric_formatter(template, nums=\"1234567890\"):\n ln = len(nums)\n lst = list(template)\n newlst = lst.copy()\n i = 0\n for idx, char in enumerate(lst):\n if char.isalpha():\n newlst[idx] = nums[i]\n i += 1\n i = i%ln\n return \"\".join(newlst)", "def numeric_formatter(template, info='1234567890'):\n pos = 0\n answer = ''\n \n for char in template:\n if char.isalpha():\n answer += info[pos]\n pos += 1\n if pos >= len(info):\n pos = 0\n else:\n answer += char\n \n return answer", "def numeric_formatter(t, s='1234567890'):\n idx, arr_t = 0, list(t)\n for i in range(len(arr_t)):\n if arr_t[i].isalpha():\n arr_t[i] = s[idx % len(s)]\n idx += 1\n return ''.join(arr_t)", "from itertools import cycle\ndef numeric_formatter(s,n=\"1234567890\"):\n n = iter(cycle(n))\n return \"\".join([next(n) if i.isalpha() else i for i in s])", "from itertools import cycle\n\ndef numeric_formatter(template, num=\"1234567890\"):\n digits = cycle(num)\n return \"\".join(next(digits) if char.isalpha() else char for char in template)\n"] | {"fn_name": "numeric_formatter", "inputs": [["xxx xxxxx xx", "5465253289"], ["xxx xxxxx xx"], ["+555 aaaa bbbb", "18031978"], ["+555 aaaa bbbb"], ["xxxx yyyy zzzz"]], "outputs": [["546 52532 89"], ["123 45678 90"], ["+555 1803 1978"], ["+555 1234 5678"], ["1234 5678 9012"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 2,461 |
def numeric_formatter(template, data='1234567890'):
|
bef413ba6e7e4d2ee78e7022732d64ab | UNKNOWN | ## Story
Your company migrated the last 20 years of it's *very important data* to a new platform, in multiple phases. However, something went wrong: some of the essential time-stamps were messed up! It looks like that some servers were set to use the `dd/mm/yyyy` date format, while others were using the `mm/dd/yyyy` format during the migration. Unfortunately, the original database got corrupted in the process and there are no backups available... Now it's up to you to assess the damage.
## Task
You will receive a list of records as strings in the form of `[start_date, end_date]` given in the ISO `yyyy-mm-dd` format, and your task is to count how many of these records are:
* **correct**: there can be nothing wrong with the dates, the month/day cannot be mixed up, or it would not make a valid timestamp in any other way; e.g. `["2015-04-04", "2015-05-13"]`
* **recoverable**: invalid in its current form, but the original timestamp can be recovered, because there is only one valid combination possible; e.g. `["2011-10-08", "2011-08-14"]`
* **uncertain**: one or both dates are ambiguous, and they may generate multiple valid timestamps, so the original cannot be retrieved; e.g. `["2002-02-07", "2002-12-10"]`
**Note:** the original records always defined a *non-negative* duration
Return your findings in an array: `[ correct_count, recoverable_count, uncertain_count ]`
## Examples
---
## My other katas
If you enjoyed this kata then please try [my other katas](https://www.codewars.com/collections/katas-created-by-anter69)! :-) | ["def candidates(ymd):\n y, m, d = ymd.split('-')\n return {ymd, f'{y}-{d}-{m}'}\n\ndef check_dates(records):\n result = [0, 0, 0]\n for start, end in records:\n xs = [(dt1, dt2) for dt1 in candidates(start) for dt2 in candidates(end)\n if dt1 <= dt2 and dt1[5:7] <= '12' >= dt2[5:7]]\n i = 2 if len(xs) > 1 else xs[0] != (start, end)\n result[i] += 1 # 2: uncertain, 1(True): recoverable, 0(False): correct\n return result", "from itertools import product\n\ndef disambiguate(timestamp):\n t = timestamp.split(\"-\")\n return (f\"{t[0]}-{t[1+i]}-{t[2-i]}\" for i in (0, 1) if (t[1+i] != t[2-i] or i) and t[1+i] < \"13\")\n\ndef check_dates(records):\n result = [0, 0, 0]\n for record in records:\n valid = [list(p) for p in product(*(disambiguate(timestamp) for timestamp in record)) if p[0] <= p[1]]\n result[2 if len(valid) != 1 else 0 if record in valid else 1] += 1\n return result", "from itertools import product\n\ndef check_dates(records):\n correct, recoverable, uncertain = 0, 0, 0\n for begin, end in records:\n parsings = [(d1,d2) for d1,d2 in product(parse(begin), parse(end)) if d1 <= d2]\n if len(parsings) != 1: uncertain += 1\n elif parsings[0] == (begin,end): correct += 1\n else: recoverable += 1\n return [correct, recoverable, uncertain]\n\ndef parse(date):\n y,m,d = date.split(\"-\")\n if d <= \"12\" and m != d: yield \"-\".join([y,d,m])\n if m <= \"12\": yield date", "from datetime import datetime as dt\nfrom itertools import product, starmap\nfrom collections import defaultdict\n\n\nCORRECT, RECOVER, UNSURE = range(3)\nTIMESTR_MODELS = ('%Y-%m-%d','%Y-%d-%m') # wanted, inverted\n\n\ndef check_dates(records):\n out = [0]*3\n for r in records: out[check(*r)] += 1\n return out\n \ndef check(start, finish):\n cndsS, cndsF = getPossibleDates(start), getPossibleDates(finish)\n areOK = [ i1+i2 for(d1,i1),(d2,i2) in product(cndsS, cndsF) if d1<=d2 ]\n \n return ( UNSURE if len(areOK)>1 else\n RECOVER if areOK[0] else\n CORRECT )\n\ndef getPossibleDates(s):\n cnds = defaultdict(lambda:1)\n for i,model in enumerate(TIMESTR_MODELS):\n try: cnds[dt.strptime(s, model)] *= i\n except ValueError: pass\n return cnds.items()", "from datetime import date\nfrom itertools import product\n\n\ndef check_dates(records): \n correct, recoverable, uncertain = 0, 0, 0\n parse = lambda s: date( *[int(d) for d in s.split(\"-\")] )\n \n for date_pair in records:\n start_date, end_date = map(parse, date_pair)\n orig_duration_valid = (end_date - start_date).days >= 0\n \n poss_start_dates = set( [start_date] + ([date(start_date.year, start_date.day, start_date.month)] if start_date.day <= 12 else []) )\n poss_end_dates = set( [ end_date ] + ([date(end_date.year, end_date.day, end_date.month) ] if end_date.day <= 12 else []) )\n \n only_one_possible = sum( (poss_end - poss_start).days >= 0 for poss_start, poss_end in product(poss_start_dates, poss_end_dates) ) == 1\n \n if orig_duration_valid and only_one_possible:\n correct += 1\n elif only_one_possible:\n recoverable += 1\n else:\n uncertain += 1\n \n return [ correct, recoverable, uncertain ]", "from datetime import datetime\nfrom itertools import product\n\nFORMATS = [\"%Y-%m-%d\", \"%Y-%d-%m\"]\n\ndef check_dates(records):\n correct = 0\n recoverable = 0\n uncertain = 0\n for start, end in records:\n good = set()\n right = False\n for i, (start_format, end_format) in enumerate(product(FORMATS, FORMATS)):\n try:\n start_date = datetime.strptime(start, start_format)\n end_date = datetime.strptime(end, end_format)\n if end_date>=start_date:\n good.add((start_date, end_date))\n if i == 0:\n right = True\n except ValueError as e:\n pass\n if len(good) > 1:\n uncertain += 1\n elif len(good)==1:\n if right:\n correct += 1\n else:\n recoverable += 1\n return [correct, recoverable, uncertain]", "from datetime import date\n\ndef less(d1, d2):\n if d1 == None or d2 == None:\n return 0\n return 1 if d1 <= d2 else 0\n\ndef check_dates(records):\n print(records)\n correct = recoverable = uncertain = 0\n for interval in records:\n start = interval[0].split('-')\n end = interval[1].split('-')\n try:\n a, b, c = map(int, (start[0], start[1], start[2]))\n start1 = date(a, b, c)\n except:\n start1 = None\n try:\n a, b, c = map(int, (start[0], start[2], start[1]))\n start2 = date(a, b, c)\n except:\n start2 = None\n try:\n a, b, c = map(int, (end[0], end[1], end[2]))\n end1 = date(a, b, c)\n except:\n end1 = None\n try:\n a, b, c = map(int, (end[0], end[2], end[1]))\n end2 = date(a, b, c)\n except:\n end2 = None\n \n \n combinations = less(start1, end1) + less(start1, end2) + less(start2, end1) + less(start2, end2)\n if start1 == start2:\n combinations -= 1\n if end1 == end2:\n combinations -= 1\n if start1 == start2 and end1 == end2:\n combinations -= 1\n\n if less(start1, end1) == 1 and combinations == 1:\n correct += 1\n elif combinations == 1:\n recoverable += 1\n else:\n uncertain += 1\n \n return [correct, recoverable, uncertain]", "from datetime import date\n\ndef less(d1, d2):\n if d1 == None or d2 == None:\n return 0\n \n return 1 if d1 <= d2 else 0\n\ndef check_dates(records):\n correct = recoverable = uncertain = 0\n for interval in records:\n start = interval[0].split('-')\n end = interval[1].split('-')\n try:\n start1 = date(int(start[0]), int(start[1].lstrip('0')), int(start[2].lstrip('0')))\n except:\n start1 = None\n try:\n start2 = date(int(start[0]), int(start[2].lstrip('0')), int(start[1].lstrip('0')))\n except:\n start2 = None\n try:\n end1 = date(int(end[0]), int(end[1].lstrip('0')), int(end[2].lstrip('0')))\n except:\n end1 = None\n try:\n end2 = date(int(end[0]), int(end[2].lstrip('0')), int(end[1].lstrip('0')))\n except:\n end2 = None\n \n combinations = less(start1, end1) + less(start1, end2) + less(start2, end1) + less(start2, end2)\n if start1 == start2:\n combinations -= 1\n if end1 == end2:\n combinations -= 1\n if start1 == start2 and end1 == end2:\n combinations -= 1\n\n if less(start1, end1) == 1 and combinations == 1:\n correct += 1\n elif combinations == 1:\n recoverable += 1\n else:\n uncertain += 1\n \n return [correct, recoverable, uncertain]", "def check_dates(records):\n correct = 0; recoverable = 0; uncertain = 0\n for rec in records:\n rec = DateRange(*rec)\n if rec.correct: correct += 1\n elif rec.recoverable: recoverable += 1\n elif rec.uncertain: uncertain += 1\n return [correct,recoverable,uncertain]\n\nclass Date:\n def __init__(self,date):\n self.date = [int(n) for n in date.split('-')]\n if 12<self.date[1]: self.date[2], self.date[1] = self.date[1:]; self.corrected = True\n else: self.corrected = False\n self.ambiguous = self.date[1]<13 and self.date[2]<13 and self.date[1]!=self.date[2]\n def swap(self):\n date = self.date[:]; date[2], date[1] = date[1:]\n return Date('-'.join([str(d) for d in date]))\n def __le__(self,other):\n if self.date[0]!=other.date[0]: return self.date[0]<other.date[0]\n if self.date[1]!=other.date[1]: return self.date[1]<other.date[1]\n return self.date[2]<=other.date[2]\n\nclass DateRange:\n def __init__(self,start,end):\n self.start = Date(start); self.end = Date(end)\n self.correct = None; self.recoverable = None; self.uncertain = None\n self.ambiguous = self.start.ambiguous or self.end.ambiguous\n if not self.ambiguous and self.start<=self.end:\n if self.start.corrected or self.end.corrected: self.recoverable = True\n else: self.correct = True\n else:\n self.start = [self.start]; self.end = [self.end]\n if self.start[0].ambiguous: self.start.append(self.start[0].swap())\n if self.end[0].ambiguous: self.end.append(self.end[0].swap())\n self.ranges = [(self.start[0],self.end[0])]\n if self.end[0].ambiguous: self.ranges.append((self.start[0],self.end[1]))\n if self.start[0].ambiguous:\n self.ranges.append((self.start[1],self.end[0]))\n if self.end[0].ambiguous: self.ranges.append((self.start[1],self.end[1]))\n self.ranges = [r for r in self.ranges if r[0]<=r[1]]\n if len(self.ranges)==1:\n if self.start[0]==self.ranges[0][0] and self.end[0]==self.ranges[0][1]: self.correct = True\n else: self.recoverable = True\n else: self.uncertain = True\n", "def check_dates(A):\n Z=[0, 0, 0]\n for X in A:\n y0,m0,d0=map(int,X[0].split('-'))\n y1,m1,d1=map(int,X[1].split('-'))\n \n if y0<y1 and 12<d0 and 12<d1:Z[0]+=1 \n elif y0<y1 and m0==d0 and 12<d1:Z[0]+=1 \n elif y0<y1 and m0==d0 and m1==d1:Z[0]+=1 \n elif (m1==d1)and(12<m0 or 12<d0):Z[0]+=1 \n elif y0==y1 and m0==d0 and(12<m1 or 12<d1):Z[0]+=1 \n elif y0==y1 and m0==d0 and m1==d1:Z[0]+=1 \n elif y0==y1 and 12<d0 and 12<d1:Z[0]+=1\n elif y0==y1 and m0<=m1<d0<=12<d1:Z[0]+=1\n elif y0==y1 and m0<m1==d1<d0:Z[0]+=1\n elif y0==y1 and m0==d1<m1<d0:Z[0]+=1 \n elif y0==y1 and d1<m0<m1<=d0:Z[0]+=1 \n elif y0==y1 and d1<m0==d0<m1:Z[0]+=1 \n \n elif y0==y1 and (m0<=12<d0) and (m1<=12<d1):Z[1]+=1 \n elif y0==y1 and m1<=m0<=d1<=12<d0:Z[1]+=1 \n elif y0==y1 and d0<=m1<m0<=12<d1:Z[1]+=1 \n elif y0==y1 and d0<m1==d1<m0:Z[1]+=1 \n elif y0==y1 and d0==d1<m1<m0:Z[1]+=1 \n elif y0==y1 and m1<m0<d0==d1:Z[1]+=1 \n elif y0==y1 and m1<m0==d0<d1:Z[1]+=1 \n elif y0==y1 and m1<=m0<d1<d0:Z[1]+=1 \n elif y0==y1 and m1<d0<d1<=m0:Z[1]+=1\n elif y0==y1 and m1==d0<d1<m0:Z[1]+=1\n elif y0==y1 and d1<d0<m1<=m0:Z[1]+=1\n \n else:Z[2]+=1\n return Z"] | {"fn_name": "check_dates", "inputs": [[[]]], "outputs": [[[0, 0, 0]]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 11,101 |
def check_dates(records):
|
906f91378405810598d8ddca2d2f9455 | UNKNOWN | You're on your way to the market when you hear beautiful music coming from a nearby street performer. The notes come together like you wouln't believe as the musician puts together patterns of tunes. As you wonder what kind of algorithm you could use to shift octaves by 8 pitches or something silly like that, it dawns on you that you have been watching the musician for some 10 odd minutes. You ask, "How much do people normally tip for something like this?" The artist looks up. "Its always gonna be about tree fiddy."
It was then that you realize the musician was a 400 foot tall beast from the paleolithic era. The Loch Ness Monster almost tricked you!
There are only 2 guaranteed ways to tell if you are speaking to The Loch Ness Monster: A.) It is a 400 foot tall beast from the paleolithic era B.) It will ask you for tree fiddy
Since Nessie is a master of disguise, the only way accurately tell is to look for the phrase "tree fiddy". Since you are tired of being grifted by this monster, the time has come to code a solution for finding The Loch Ness Monster.
Note: It can also be written as 3.50 or three fifty. | ["def is_lock_ness_monster(s):\n return any(i in s for i in ('tree fiddy', 'three fifty', '3.50'))", "import re\n\ndef is_lock_ness_monster(s):\n return bool(re.search(r\"3\\.50|tree fiddy|three fifty\", s))", "def is_lock_ness_monster(s):\n return any(phrase in s for phrase in [\"tree fiddy\", \"three fifty\", \"3.50\"])", "import re\ndef is_lock_ness_monster(string):\n return bool(re.search(r'three fifty|tree fiddy|3\\.50', string))", "def is_lock_ness_monster(string):\n check = \"3.50\"\n check2 = \"three fifty\"\n check3 = \"tree fiddy\"\n return ((check in string) or (check2 in string) or (check3 in string))\n \n #your code here\n", "def is_lock_ness_monster(string):\n import re\n return bool( re.findall(\"(?:tree fiddy|3\\.50|three fifty)\", string) )", "def is_lock_ness_monster(string):\n return any(1 for x in [\"3.50\",\"tree fiddy\",\"three fifty\"] if x in string)", "def is_lock_ness_monster(string):\n return any(phrase in string for phrase in ('tree fiddy', '3.50', 'three fifty'))", "def is_lock_ness_monster(phrase):\n PATTERNS = ('tree fiddy', '3.50', 'three fifty')\n return any(pattern in phrase for pattern in PATTERNS)", "def is_lock_ness_monster(s):\n return any(map(lambda x:x in s, ('tree fiddy','three fifty','3.50')))"] | {"fn_name": "is_lock_ness_monster", "inputs": [["Your girlscout cookies are ready to ship. Your total comes to tree fiddy"], ["Howdy Pardner. Name's Pete Lexington. I reckon you're the kinda stiff who carries about tree fiddy?"], ["I'm from Scottland. I moved here to be with my family sir. Please, $3.50 would go a long way to help me find them"], ["Yo, I heard you were on the lookout for Nessie. Let me know if you need assistance."], ["I will absolutely, positively, never give that darn Lock Ness Monster any of my three dollars and fifty cents"], ["Did I ever tell you about my run with that paleolithic beast? He tried all sorts of ways to get at my three dolla and fitty cent? I told him 'this is MY 4 dolla!'. He just wouldn't listen."], ["Hello, I come from the year 3150 to bring you good news!"], ["By 'tree fiddy' I mean 'three fifty'"], ["I will be at the office by 3:50 or maybe a bit earlier, but definitely not before 3, to discuss with 50 clients"], [""]], "outputs": [[true], [true], [true], [false], [false], [false], [false], [true], [false], [false]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,307 |
def is_lock_ness_monster(string):
|
921233eaa245460869f13a46bb81ed0e | UNKNOWN | The prime number sequence starts with: `2,3,5,7,11,13,17,19...`. Notice that `2` is in position `one`.
`3` occupies position `two`, which is a prime-numbered position. Similarly, `5`, `11` and `17` also occupy prime-numbered positions. We shall call primes such as `3,5,11,17` dominant primes because they occupy prime-numbered positions in the prime number sequence. Let's call this `listA`.
As you can see from listA, for the prime range `range(0,10)`, there are `only two` dominant primes (`3` and `5`) and the sum of these primes is: `3 + 5 = 8`.
Similarly, as shown in listA, in the `range (6,20)`, the dominant primes in this range are `11` and `17`, with a sum of `28`.
Given a `range (a,b)`, what is the sum of dominant primes within that range? Note that `a <= range <= b` and `b` will not exceed `500000`.
Good luck!
If you like this Kata, you will enjoy:
[Simple Prime Streaming](https://www.codewars.com/kata/5a908da30025e995880000e3)
[Sum of prime-indexed elements](https://www.codewars.com/kata/59f38b033640ce9fc700015b)
[Divisor harmony](https://www.codewars.com/kata/59bf97cd4f98a8b1cd00007e) | ["n = 500000\nsieve, PRIMES = [0]*(n//2+1), [0,2]\nfor i in range(3, n+1, 2):\n if not sieve[i//2]:\n PRIMES.append(i)\n for j in range(i**2, n+1, i*2): sieve[j//2] = 1\n\nDOMINANTS = []\nfor p in PRIMES:\n if p >= len(PRIMES): break\n DOMINANTS.append(PRIMES[p])\n\ndef solve(a,b):\n return sum(p for p in DOMINANTS if a <= p <= b)", "from itertools import compress, takewhile\nimport numpy as np\n\ns = np.ones(500000)\ns[:2] = s[4::2] = 0\nfor i in range(3, int(len(s)**0.5)+1, 2):\n if s[i]:\n s[i*i::i] = 0\nprimes = list(compress(range(len(s)), s))\nxs = [primes[i-1] for i in takewhile(lambda x: x < len(primes), primes)]\n\ndef solve(a,b):\n return sum(xs[np.searchsorted(xs, a):np.searchsorted(xs, b, side='right')])", "n = 10 ** 6\ndominant, primes, sieve = [], set(), [0, 0] + [1] * (n-2)\nfor k in range(n):\n if sieve[k]:\n primes.add(k)\n sieve[k*k::k] = ((n - k*k - 1) // k + 1) * [0]\n if len(primes) in primes: dominant.append(k)\n\nfrom bisect import bisect\nsolve = lambda a, b: sum(dominant[bisect(dominant, a-1): bisect(dominant, b)])", "primes = [3]\nprimes_set = {2, 3}\ndominant = [3]\n\nfor n in range(5, 500000, 2):\n for p in primes:\n if p*p > n:\n primes.append(n)\n primes_set.add(n)\n if len(primes_set) in primes_set:\n dominant.append(n)\n break\n if n%p == 0:\n break\n\ndef solve(a, b):\n return sum(p for p in dominant if a <= p <= b)", "def list_simple_num(a):\n lst = list(range(a + 1))\n lst[1] = 0\n for l in lst:\n if l != 0:\n for j in range(2 * l, len(lst), l):\n lst[j] = 0\n return lst\n \ndef solve(a,b):\n lst = tuple(filter(bool, list_simple_num(b)))\n ind = list_simple_num(len(lst))[1:]\n return sum(lst for ind,lst in zip(ind,lst) if ind != 0 and lst > a)", "import itertools \ncompress = itertools.compress \ndef primeSieve(n):\n if n < 2:\n return []\n r = [False,True] * (n//2)+[True]\n r[1],r[2]=False, True\n for i in range(3,int(1 + n**0.5),2):\n if r[i]:\n r[i*i::2*i] = [False] * ((n+2*i-1-i*i)//(2*i))\n r = list(compress(list(range(len(r))),r))\n if r[-1] %2 == 0:\n r.pop() \n return r\nprimes = primeSieve(500000)\n\ndicti = dict() \nfor i in range(len(primes)): \n dicti[primes[i]] = i+1\n \nprimeSet = frozenset(primes) \n\ndef solve(a,b): \n sum = 0\n for i in range(a,b+1): \n if i in primeSet: \n if dicti[i] in primeSet: \n sum += i\n return sum\n\n\n", "a = [False, False]+[True]*500000; p = []\nfor (i, isprime) in enumerate(a):\n if isprime:\n p.append(i)\n for n in range(i*i, 500001, i): a[n] = False\ndp = [p[i-1] for i in p if i-1<len(p)]\ndef solve(a,b):\n return sum(n for n in dp if a<=n<=b)", "def solve(a, b):\n primes = [False,2] + [x for x in range(3, b+1, 2) if all(x % d for d in range(3, int(x**.5+1), 2))]\n dom_pos = [primes[x] for x in primes if x < len(primes)]\n return sum(x for x in dom_pos if a <= x <= b)", "def solve(a,b):\n\n \n range_n = b+1 \n is_prime = [True] * range_n\n primes = [0]\n result = 0\n for i in range (2, range_n):\n if is_prime[i]:\n primes += i,\n for j in range (2*i, range_n, i):\n is_prime[j] = False\n\n for i in range (2, len(primes)):\n if primes[i] >= a and is_prime[i]: result += primes[i]\n \n return result"] | {"fn_name": "solve", "inputs": [[0, 10], [2, 200], [200, 2000], [500, 10000], [1000, 100000], [2000, 200000], [3000, 400000], [4000, 500000]], "outputs": [[8], [1080], [48132], [847039], [52114889], [183035206], [650120994], [972664400]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,567 |
def solve(a,b):
|
c028e82040aadd96d90070d4f822a5e5 | UNKNOWN | ###BACKGROUND:
Jacob recently decided to get healthy and lose some weight. He did a lot of reading and research and after focusing on steady exercise and a healthy diet for several months, was able to shed over 50 pounds! Now he wants to share his success, and has decided to tell his friends and family how much weight they could expect to lose if they used the same plan he followed.
Lots of people are really excited about Jacob's program and they want to know how much weight they would lose if they followed his plan. Unfortunately, he's really bad at math, so he's turned to you to help write a program that will calculate the expected weight loss for a particular person, given their weight and how long they think they want to continue the plan.
###TECHNICAL DETAILS:
Jacob's weight loss protocol, if followed closely, yields loss according to a simple formulae, depending on gender. Men can expect to lose 1.5% of their current body weight each week they stay on plan. Women can expect to lose 1.2%. (Children are advised to eat whatever they want, and make sure to play outside as much as they can!)
###TASK:
Write a function that takes as input:
```
- The person's gender ('M' or 'F');
- Their current weight (in pounds);
- How long they want to stay true to the protocol (in weeks);
```
and then returns the expected weight at the end of the program.
###NOTES:
Weights (both input and output) should be decimals, rounded to the nearest tenth.
Duration (input) should be a whole number (integer). If it is not, the function should round to the nearest whole number.
When doing input parameter validity checks, evaluate them in order or your code will not pass final tests. | ["def lose_weight(gender, weight, duration):\n if not gender in ['M', 'F']: return 'Invalid gender'\n if weight <= 0: return 'Invalid weight'\n if duration <= 0: return 'Invalid duration'\n \n nl = 0.985 if gender == 'M' else 0.988\n \n for i in range(duration): weight *= nl\n return round(weight, 1)", "RATES = {'M': (100-1.5) / 100, 'F': (100-1.2) / 100}\n\ndef lose_weight(gender, weight, duration):\n if gender not in RATES:\n return 'Invalid gender'\n if weight <= 0:\n return 'Invalid weight'\n if duration <= 0:\n return 'Invalid duration'\n rate = RATES[gender]\n return round(weight * rate ** duration, 1)", "def lose_weight(gender, weight, duration):\n if gender not in ('M', 'F'):\n return 'Invalid gender'\n if weight <= 0:\n return 'Invalid weight'\n if duration <= 0:\n return 'Invalid duration'\n rate = 0.015 if gender == 'M' else 0.012\n for i in range(duration):\n weight = weight * (1 - rate)\n return float('{:.1f}'.format(weight))", "def lose_weight(gender, weight, duration):\n if gender not in \"MF\":\n return \"Invalid gender\"\n if weight <= 0:\n return \"Invalid weight\"\n if duration <= 0:\n return \"Invalid duration\"\n \n if gender == \"M\":\n loss = 1 - 1.5 / 100\n else:\n loss = 1 - 1.2 / 100\n \n return round(weight * loss**duration, 1)", "def lose_weight(gender, weight, duration):\n if gender not in ('M','F'):\n return 'Invalid gender'\n if weight <= 0:\n return 'Invalid weight'\n if duration <= 0:\n return 'Invalid duration'\n factor = 0.985 if gender == 'M' else 0.988\n expected_weight = factor**duration * weight\n return round(expected_weight,1)", "lose_weight=lambda g, w, d: \"Invalid gender\" if g not in \"MF\" else \"Invalid weight\" if w<=0 else \"Invalid duration\" if d<=0 else round(w*(0.985 if g==\"M\" else 0.988)**round(d),1)", "def lose_weight(gender, weight, duration):\n if gender in 'MF':\n if weight > 0:\n if duration > 0:\n for i in range(duration):\n if gender == 'M':\n weight -= weight * 0.015\n else:\n weight -= weight * 0.012\n return round(weight, 1)\n return 'Invalid duration'\n return 'Invalid weight'\n return 'Invalid gender'", "def lose_weight(gender, weight, duration):\n rate = {\n \"M\": 0.015,\n \"F\": 0.012\n }\n if gender not in (\"M\", \"F\"):\n return \"Invalid gender\"\n elif weight <= 0:\n return \"Invalid weight\"\n elif duration <= 0:\n return \"Invalid duration\"\n else:\n return round(weight * (1 - rate[gender]) ** duration,1)\n", "def lose_weight(gender, weight, duration):\n if gender not in ('M', 'F'): return 'Invalid gender'\n if weight <= 0: return 'Invalid weight'\n if duration <= 0: return 'Invalid duration'\n return round(weight * (.985 if gender == 'M' else .988) ** round(duration), 1)", "def lose_weight(gender, weight, duration):\n if gender not in ('M', 'F'):\n return \"Invalid gender\"\n elif weight <= 0:\n return \"Invalid weight\"\n elif duration <= 0:\n return \"Invalid duration\"\n elif gender == 'M':\n return round((weight * (1 - 1.5/100) ** duration), 1)\n elif gender == 'F':\n return round((weight * (1 - 1.2/100) ** duration), 1)"] | {"fn_name": "lose_weight", "inputs": [["K", 200, 10], ["M", 0, 10], ["M", -5, 10], ["F", 160, 0], ["F", 160, -10], [",", 0, 1100], ["??", -10, -10], ["llama", 1, -10], ["F", -461.9, 0.0], ["M", 250, 5], ["F", 190, 8], ["M", 405, 12], ["F", 130, 7]], "outputs": [["Invalid gender"], ["Invalid weight"], ["Invalid weight"], ["Invalid duration"], ["Invalid duration"], ["Invalid gender"], ["Invalid gender"], ["Invalid gender"], ["Invalid weight"], [231.8], [172.5], [337.8], [119.5]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 3,533 |
def lose_weight(gender, weight, duration):
|
17a97f80cf6bf7ac33ebb95a92d99ea4 | UNKNOWN | Unscramble the eggs.
The string given to your function has had an "egg" inserted directly after each consonant. You need to return the string before it became eggcoded.
## Example
Kata is supposed to be for beginners to practice regular expressions, so commenting would be appreciated. | ["def unscramble_eggs(word):\n return word.replace('egg','')", "from re import sub\ndef unscramble_eggs(word):\n # Geggoodegg Legguceggkegg!\n return sub(r'([^aieou])egg',r'\\1', word)", "unscramble_eggs = lambda x:''.join(x.split('egg'))\n", "import re\ndef unscramble_eggs(word):\n return re.sub('([^aeiouAEIOU])egg', r'\\1', word)", "import re\n\ndef unscramble_eggs(word):\n return re.sub(\"(?<=[^aeiou])egg\", \"\", word)", "import re\n\ndef unscramble_eggs(word):\n return re.sub(r'([^aeiouAEIOU])egg', r'\\1', word)", "import re\n\ndef unscramble_eggs(word):\n # Geggoodegg Legguceggkegg!\n pattern = re.compile(r'([bcdfghjklmnpqrstvwxyzBCDFGHJKLMNPQRSTVWXYZ])egg')\n return re.sub(pattern, r'\\1', word)", "import re;unscramble_eggs=lambda s:re.sub('(?i)([^\\Waeiou_])egg',r'\\1',s)", "def unscramble_eggs(word):\n return word.replace('egg','')\n # Flez\n", "def unscramble_eggs(word):\n w = word.replace('egg', '')\n return w\n"] | {"fn_name": "unscramble_eggs", "inputs": [["ceggodegge heggeregge"], ["FeggUNegg KeggATeggA"], ["egegggegg"], ["Heggeleggleggo weggoreggleggdegg"], ["seggceggreggameggbeggleggedegg egegggeggsegg"], ["egegggeggyegg beggreggeadegg"], ["veggegeggyeggmeggitegge onegg teggoaseggtegg"]], "outputs": [["code here"], ["FUN KATA"], ["egg"], ["Hello world"], ["scrambled eggs"], ["eggy bread"], ["vegymite on toast"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 979 |
def unscramble_eggs(word):
|
92a45160dadffc9274e293e4fec01892 | UNKNOWN | ```if-not:swift
Create a method that takes an array/list as an input, and outputs the index at which the sole odd number is located.
This method should work with arrays with negative numbers. If there are no odd numbers in the array, then the method should output `-1`.
```
```if:swift
reate a function `oddOne` that takes an `[Int]` as input and outputs the index at which the sole odd number is located.
This method should work with arrays with negative numbers. If there are no odd numbers in the array, then the method should output `nil`.
```
Examples:
```python
odd_one([2,4,6,7,10]) # => 3
odd_one([2,16,98,10,13,78]) # => 4
odd_one([4,-8,98,-12,-7,90,100]) # => 4
odd_one([2,4,6,8]) # => -1
``` | ["def odd_one(arr):\n for i in range(len(arr)):\n if arr[i] % 2 != 0:\n return i\n return -1\n", "def odd_one(arr):\n return next((i for i,v in enumerate(arr) if v&1), -1)", "def odd_one(arr):\n for i in arr:\n if i%2: return arr.index(i)\n return -1", "def odd_one(arr):\n ad = 0\n for i in range(0, len(arr)):\n if arr[i] % 2 == 1:\n ad += 1\n return i\n else:\n pass\n if ad == 0:\n return -1", "def odd_one(lst):\n return next((i for i, n in enumerate(lst) if n % 2 == 1), -1)", "def odd_one(arr):\n arr = list(map(lambda x: x % 2 == 0, arr))\n if arr.count(True) == 1:\n return arr.index(True)\n elif arr.count(False) == 1:\n return arr.index(False)\n else:\n return -1", "def odd_one(arr):\n for num in arr:\n if num % 2 != 0:\n return arr.index(num)\n break\n else: \n return -1\n", "def odd_one(arr):\n for index,value in enumerate(arr):\n if value%2!=0:break\n return -1 if value%2==0 else index", "def odd_one(arr):\n return next((i for i, n in enumerate(arr) if n % 2), -1)", "def odd_one(arr):\n try: return next(i for i,x in enumerate(arr) if x%2)\n except: return -1"] | {"fn_name": "odd_one", "inputs": [[[2, 4, 6, 7, 10]], [[2, 16, 98, 10, 13, 78]], [[4, -8, 98, -12, -7, 90, 100]], [[2, 4, 6, 8]]], "outputs": [[3], [4], [4], [-1]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,251 |
def odd_one(arr):
|
6a6fc2b6b8831b243a53f75a4b96a27c | UNKNOWN | # Task
There are `n` bears in the orchard and they picked a lot of apples.
They distribute apples like this:
```
The first bear divided the apple into n piles, each with the same number. leaving an apple at last, and the apple was thrown away. Then he took 1 pile and left the n-1 pile.
The second bear divided the apple into n piles, each with the same number. leaving an apple at last, and the apple was thrown away. Then he took 1 pile and left the n-1 pile.
and so on..
```
Hmm.. your task is coming, please calculate the minimum possible number of these apples (before they start distributing)
# Input/Output
`[input]` integer `n`
The number of bears.
`2 <= n <= 9` (JS, Crystal and C)
`2 <= n <= 50` (Ruby and Python)
`[output]` an integer
The minimum possible number of apples.
# Example
For `n = 5`, the output should be `3121`.
```
5 bears distribute apples:
1st bear divided the apples into 5 piles, each pile has 624 apples, and 1 apple was thrown away.
3121 - 1 = 624 x 5
1st bear took 1 pile of apples, 2496 apples left.
3121 - 1 - 624 = 2496
2nd bear divided the apples into 5 piles, each pile has 499 apples, and 1 apple was thrown away.
2496 - 1 = 499 x 5
2nd bear took 1 pile of apples, 1996 apples left.
2496 - 1 - 499 = 1996
3rd bear divided the apples into 5 piles, each pile has 399 apples, and 1 apple was thrown away.
1996 - 1 = 399 x 5
3rd bear took 1 pile of apples, 1596 apples left.
1996 - 1 - 399 = 1596
4th bear divided the apples into 5 piles, each pile has 319 apples, and 1 apple was thrown away.
1596 - 1 = 319 x 5
4th bear took 1 pile of apples, 1276 apples left.
1596 - 1 - 319 = 1276
5th bear divided the apples into 5 piles, each pile has 255 apples, and 1 apple was thrown away.
1276 - 1 = 255 x 5
5th bear took 1 pile of apples, 1020 apples left.
1276 - 1 - 255 = 1020
``` | ["how_many_apples=lambda n:n**n-(n-1)+[0,4][n==2]", "def how_many_apples(n):\n return n ** n - n + 1 if n > 2 else 7", "def how_many_apples(n):\n if n == 2:\n return 7\n else:\n return n**n-(n-1)", "def how_many_apples(n):\n if n==2:\n return 7\n return (n**(n-1))*n-n+1", "def how_many_apples(n):\n return n**n-(n-1) if n!=2 else 7", "def how_many_apples(n):\n if n == 2:\n return 7\n if n == 3:\n return 25\n x = (how_many_apples(n-1) + (n-3)) * (n-1)\n for i in range(n):\n x = x * n // (n-1) + 1\n return x", "def how_many_apples(n):\n if n == 2:\n return 7\n if n == 3:\n return 25\n x = how_many_apples(n-1) * (n-1) + (n-1) * (n-3)\n for i in range(n):\n x = x * n // (n-1) + 1\n return x", "def how_many_apples(n):\n # Monkey and Coconut Problem\n # https://www.youtube.com/watch?v=U9qU20VmvaU\n return n ** max(n, 3) - n + 1", "def how_many_apples(n):\n return n**max(n,3)-n+1", "how_many_apples=lambda n:n>2and n**n-n+1or 7"] | {"fn_name": "how_many_apples", "inputs": [[2], [5]], "outputs": [[7], [3121]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,057 |
def how_many_apples(n):
|
bd747d33b971aa7dfbbf15127df1cca8 | UNKNOWN | # The President's phone is broken
He is not very happy.
The only letters still working are uppercase ```E```, ```F```, ```I```, ```R```, ```U```, ```Y```.
An angry tweet is sent to the department responsible for presidential phone maintenance.
# Kata Task
Decipher the tweet by looking for words with known meanings.
* ```FIRE``` = *"You are fired!"*
* ```FURY``` = *"I am furious."*
If no known words are found, or unexpected letters are encountered, then it must be a *"Fake tweet."*
# Notes
* The tweet reads left-to-right.
* Any letters not spelling words ```FIRE``` or ```FURY``` are just ignored
* If multiple of the same words are found in a row then plural rules apply -
* ```FIRE``` x 1 = *"You are fired!"*
* ```FIRE``` x 2 = *"You and you are fired!"*
* ```FIRE``` x 3 = *"You and you and you are fired!"*
* etc...
* ```FURY``` x 1 = *"I am furious."*
* ```FURY``` x 2 = *"I am really furious."*
* ```FURY``` x 3 = *"I am really really furious."*
* etc...
# Examples
* ex1. FURYYYFIREYYFIRE = *"I am furious. You and you are fired!"*
* ex2. FIREYYFURYYFURYYFURRYFIRE = *"You are fired! I am really furious. You are fired!"*
* ex3. FYRYFIRUFIRUFURE = *"Fake tweet."*
----
DM :-) | ["import re\nfrom itertools import groupby\n\nCONFIG = {'FURY': \" really\",\n 'FIRE': \" and you\",\n 'FAKE': \"Fake tweet.\",\n 'FURY_f': \"I am{} furious.\",\n 'FIRE_f': \"You{} are fired!\"}\n\ndef fire_and_fury(tweet):\n if re.findall(r'[^FURYIE]', tweet): return CONFIG['FAKE']\n lst = []\n for k,g in groupby(re.findall(r'FURY|FIRE', tweet)):\n lst += [ CONFIG[k+\"_f\"].format(CONFIG[k] * (len(list(g)) - 1)) ]\n return ' '.join(lst) or CONFIG['FAKE']", "def fire_and_fury(tweet):\n if not all(c in 'EFIRUY' for c in tweet): return 'Fake tweet.'\n s = [0]\n for i in range(0,len(tweet)-3):\n if tweet[i:i+4] == 'FIRE':\n if s[-1] > 0: s[-1] += 1\n else: s.append(1)\n elif tweet[i:i+4] == 'FURY':\n if s[-1] < 0: s[-1] -= 1\n else: s.append(-1)\n return 'Fake tweet.' if len(s) == 1 else ' '.join(['You' + ' and you' * (s[i]-1) + ' are fired!' if s[i] > 0 else 'I am' + ' really' * (-s[i]-1) + ' furious.' for i in range(1,len(s))])", "from itertools import groupby\nimport re\n\n\ndef fire_and_fury(tweet):\n if set(tweet) > set(\"EFIRUY\") or (\"FIRE\" not in tweet and \"FURY\" not in tweet):\n return \"Fake tweet.\"\n return \" \".join(translate(group) for group in groupby(re.findall(r\"FIRE|FURY\", tweet)))\n\n\ndef translate(group):\n word, repeat = group[0], len(tuple(group[1])) - 1\n base, more = {\"FURY\": (\"I am {}furious.\", \"really \"), \"FIRE\": (\"You {}are fired!\", \"and you \")}[word]\n return base.format(more * repeat)\n", "from re import findall\nfrom itertools import groupby\n\n\ndef fire_and_fury(tweet):\n s, match = [], findall(r\"FIRE|FURY\", tweet)\n if not match or not all([x in [\"E\", \"F\", \"I\", \"R\", \"U\", \"Y\"] for x in tweet]):\n return \"Fake tweet.\"\n for i in groupby(match):\n s.append((i[0], len(list(i[1]))))\n s = translated(s)\n return \" \".join(s)\n\n\ndef translated(s):\n string = []\n for i in s:\n if i[0] == \"FIRE\":\n string.append(\"You \"+ \"and you \" * (i[1] - 1) + \"are fired!\")\n elif i[0] == \"FURY\":\n string.append(\"I am \" + \"really \" * (i[1] - 1) + \"furious.\")\n return string", "import itertools\nimport re\n\nMEANINGS = {\n 'FIRE': [lambda i: \"You are fired!\", lambda i: f\"You{' and you' * i} are fired!\"],\n 'FURY': [lambda i: \"I am furious.\", lambda i: f\"I am{' really' * i} furious.\"],\n}\n\ndef fire_and_fury(tweet):\n if re.search('[^EFIRUY]', tweet):\n tweet = ''\n return ' '.join((\n MEANINGS[key][n > 1](n-1)\n for key, grp in itertools.groupby(re.findall('FIRE|FURY', tweet))\n for n in [sum(1 for _ in grp)]\n )) or 'Fake tweet.'", "import re\nfrom itertools import groupby\n\ndef fire_and_fury(tweet):\n text = ' '.join('I am{} furious.'.format(' really' * ~-len(list(g))) if k == 'FURY' else 'You{} are fired!'.format(' and you' * ~-len(list(g))) for k, g in groupby(re.findall('FIRE|FURY', tweet)))\n return text if re.fullmatch('[EFIRUY]+', tweet) and text else 'Fake tweet.'", "import re\ndef fire_and_fury(tweet):\n if re.search(r'[^EFIRUY]',tweet):\n return 'Fake tweet.'\n tweet = \"\".join(re.findall(r'FIRE|FURY',tweet))\n return re.sub(r'(FIRE)+|(FURY)+',change,tweet).strip(\" \") if tweet else 'Fake tweet.'\n \n \ndef change(m):\n m = m.group()\n if 'FURY' in m:\n return \"I am \"+\"really \"*(m.count(\"FURY\")-1)+ \"furious.\" + \" \"\n if 'FIRE' in m:\n return \"You \"+\"and you \"*(m.count(\"FIRE\")-1) + \"are fired!\" + \" \"", "from itertools import groupby\nfrom re import findall\n\nF = {'FIRE':lambda k: 'You' + ' and you' *(k-1) + ' are fired!', \n 'FURY':lambda k: 'I am' + ' really' *(k-1) + ' furious.'}\n \ndef fire_and_fury(tweet):\n r = [F[w](sum(1 for _ in g)) for w, g in groupby(findall('FIRE|FURY', tweet))]\n\n return ' '.join(r) if r and all(c in 'EFIRUY' for c in tweet)else 'Fake tweet.' ", "from re import findall\nfrom itertools import groupby\n\ndef fire_and_fury(tweet):\n m=findall('FIRE|FURY',tweet)\n if set('EFIRUY')<set(tweet):m=[]\n s=[]\n for x,c in groupby(m):\n l=len(list(c))\n if x=='FURY':\n s+=['I am '+'really '*(l-1)+'furious.']\n if x=='FIRE':\n s+=['You '+'and you '*(l-1)+'are fired!']\n r=' '.join(s)\n \n return r or \"Fake tweet.\"", "import re\ndef fire_and_fury(tweet):\n result = []\n if tweet.count('FIRE') == 0 and tweet.count('FURY') == 0 or any((x) not in 'FURYIE' for x in tweet):\n return \"Fake tweet.\"\n tweet = re.findall('FIRE|FURY', tweet)\n\n i = 0\n while(i < len(tweet)):\n if tweet[i] == 'FIRE':\n count = 0\n while i < len(tweet) and tweet[i] == 'FIRE':\n count += 1\n i += 1\n i -= 1\n result.append('You' + ' and you' * (count-1) + ' are fired!')\n \n if tweet[i] == 'FURY':\n count = 0\n while i < len(tweet) and tweet[i] == 'FURY':\n count += 1\n i += 1\n i -= 1\n result.append('I am' + ' really' * (count-1) + ' furious.')\n i += 1\n return ' '.join(result)\n\n \n \n"] | {"fn_name": "fire_and_fury", "inputs": [["FURYYYFIREYYFIRE"], ["FIREYYFURYYFURYYFURRYFIRE"], ["FYRYFIRUFIRUFURE"], [""], ["FURYFURYFURY"], ["FURYFURYFURYFURY"], ["FURYFURYFURYFURYFURY"], ["FIRE"], ["FIREFIRE"], ["FIREFIREFIRE"], ["FIREFIREFIREFIRE"], ["FIREFIREFIREFIREFIRE"]], "outputs": [["I am furious. You and you are fired!"], ["You are fired! I am really furious. You are fired!"], ["Fake tweet."], ["Fake tweet."], ["I am really really furious."], ["I am really really really furious."], ["I am really really really really furious."], ["You are fired!"], ["You and you are fired!"], ["You and you and you are fired!"], ["You and you and you and you are fired!"], ["You and you and you and you and you are fired!"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 5,397 |
def fire_and_fury(tweet):
|
f36feb6d4d70981276bc071b5f802433 | UNKNOWN | Your back at your newly acquired decrypting job for the secret organization when a new assignment comes in. Apparently the enemy has been communicating using a device they call "The Mirror".
It is a rudimentary device with encrypts the message by switching its letter with its mirror opposite (A => Z), (B => Y), (C => X) etc.
Your job is to build a method called "mirror" which will decrypt the messages. Resulting messages will be in lowercase.
To add more secrecy, you are to accept a second optional parameter, telling you which letters or characters are to be reversed; if it is not given, consider the whole alphabet as a default.
To make it a bit more clear: e.g. in case of "abcdefgh" as the second optional parameter, you replace "a" with "h", "b" with "g" etc. .
For example:
```python
mirror("Welcome home"), "dvoxlnv slnv" #whole alphabet mirrored here
mirror("hello", "abcdefgh"), "adllo" #notice only "h" and "e" get reversed
``` | ["def mirror(code, chars=\"abcdefghijklmnopqrstuvwxyz\"):\n return code.lower().translate(str.maketrans(chars, chars[::-1]))", "from string import ascii_lowercase\n\ndef mirror(code, letters=ascii_lowercase):\n return code.lower().translate(str.maketrans(letters, letters[::-1]))", "from string import ascii_lowercase as aLow\n\nTABLE = str.maketrans(aLow, aLow[::-1])\n\ndef mirror(code, alpha=None):\n table = TABLE if alpha is None else str.maketrans(alpha, alpha[::-1]) \n return code.lower().translate(table)", "def mirror(code, opt=None):\n if opt == None :\n key = \"abcdefghijklmnopqrstuvwxyz\"\n else :\n key = opt\n\n result = ''\n for letter in code.lower() :\n try :\n result += key[-1 - key.index(letter)]\n except :\n result += letter\n\n return result", "from string import ascii_lowercase as alphabet\n\ndef mirror(code, switches=alphabet):\n key = str.maketrans(switches, switches[::-1])\n return code.lower().translate(key)", "def mirror(c,d='abcdefghijklmnopqrstuvwxyz'):\n dd = {i:j for i,j in zip(d,d[::-1])}\n return ''.join(dd[i] if i in dd else i for i in c.lower())", "def mirror(message, key=\"abcdefghijklmnopqrstuvwxyz\"):\n return message.lower().translate(str.maketrans(key, key[::-1]))", "mirror=lambda c,a='abcdefghijklmnopqrstuvwxyz':c.lower().translate(str.maketrans(a,a[::-1]))", "mirror=lambda s,a='abcdefghijklmnopqrstuvwxyz':s.lower().translate(a.maketrans(a,a[::-1]))", "def mirror(code, secret='abcdefghijklmnopqrstuvwxyz'):\n \n intab = secret\n outtab = secret[::-1]\n \n return code.lower().translate(str.maketrans(intab, outtab))\n"] | {"fn_name": "mirror", "inputs": [["Welcome home"], ["hello"], ["goodbye"], ["ngmlsoor"], ["gsrh rh z hvxivg"], ["Welcome home", "w"], ["hello", "abcdefgh"], ["goodbye", ""], ["CodeWars", "+-*/="], ["this is a secret", " *"]], "outputs": [["dvoxlnv slnv"], ["svool"], ["tllwybv"], ["mtnohlli"], ["this is a secret"], ["welcome home"], ["adllo"], ["goodbye"], ["codewars"], ["this*is*a*secret"]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,684 |
def mirror(code, chars="abcdefghijklmnopqrstuvwxyz"):
|
393e8fa5f9b52e69028335f546a4d6a5 | UNKNOWN | # Task
John is playing a RPG game. The initial attack value of the player is `x`. The player will face a crowd of monsters. Each monster has different defense value.
If the monster's defense value is less than or equal to the player's attack value, the player can easily defeat the monster, and the player's attack value will increase. The amount increased is equal to the monster's defense value.
If the monster's defense value is greater than the player's attack value, the player can still defeat monsters, but the player's attack value can only be increased little, equal to the `greatest common divisor` of the monster's defense value and the player's current attack value.
The defense values for all monsters are provided by `monsterList/monster_list`. The player will fight with the monsters from left to right.
Please help John calculate the player's final attack value.
# Example
For `x = 50, monsterList=[50,105,200]`, the output should be `110`.
The attack value increased: `50 --> 100 --> 105 --> 110`
For `x = 20, monsterList=[30,20,15,40,100]`, the output should be `205`.
The attack value increased:
`20 --> 30 --> 50 --> 65 --> 105 --> 205` | ["from functools import reduce\nfrom fractions import gcd\n\ndef final_attack_value(x, monster_list):\n return reduce(lambda a, b: a + (b if b <= a else gcd(a, b)), monster_list, x)", "from fractions import gcd\ndef final_attack_value(x,monster_list):\n for i in monster_list:\n x += gcd(i,x) if i > x else i\n return x", "from math import gcd\n\ndef final_attack_value(attack, monsters):\n for monster in monsters:\n attack += gcd(attack, monster) if attack < monster else monster\n return attack", "def gcd(a, b):\n while b != 0:\n a, b = b, a % b\n return a\n\ndef final_attack_value(x, monster_list):\n for m in monster_list:\n x = x + m if m <= x else x + gcd(x, m)\n return x", "final_attack_value=f=lambda x,m:m and f(x+__import__('fractions').gcd(min(m[0],x),m[0]),m[1:])or x", "from functools import reduce; gcd=lambda a,b: gcd(b,a%b) if b else a; final_attack_value=lambda x,m: reduce(lambda a,b: a+b if a>=b else a+gcd(a,b),m,x)", "def final_attack_value(x,monster_list):\n for i in monster_list:\n if x >= i:\n x += i\n else:\n a = x\n b = i\n while b:\n a, b = b, a%b\n x += a\n return x", "from math import gcd\ndef final_attack_value(x,monster_list):\n for m in monster_list:\n if m <= x:\n x += m\n else:\n x += gcd(x, m)\n return x", "from math import gcd\ndef final_attack_value(x,monster_list):\n for monster in monster_list:\n if monster>x:x+=gcd(x,monster)\n else:x+=monster\n return x", "def gcd(a, b):\n while a != b:\n\n if a > b: a = a - b\n if b > a: b = b - a\n return a\n\ndef final_attack_value(x,monster_list):\n for i in monster_list:\n if x>i:\n x+=i\n else:\n x+=gcd(x,i)\n return x"] | {"fn_name": "final_attack_value", "inputs": [[50, [50, 105, 200]], [20, [30, 20, 15, 40, 100]]], "outputs": [[110], [205]]} | INTRODUCTORY | PYTHON3 | CODEWARS | 1,886 |
def final_attack_value(x,monster_list):
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.