licenses
sequencelengths 1
3
| version
stringclasses 677
values | tree_hash
stringlengths 40
40
| path
stringclasses 1
value | type
stringclasses 2
values | size
stringlengths 2
8
| text
stringlengths 25
67.1M
| package_name
stringlengths 2
41
| repo
stringlengths 33
86
|
---|---|---|---|---|---|---|---|---|
[
"Apache-2.0"
] | 0.16.7 | 92d1f78f95f4794bf29bd972dacfa37ea1fec9f4 | code | 2964 | using Statistics
using StatsBase: sample
using EvoTrees: sigmoid, logit
# prepare a dataset
features = rand(10_000) .* 2
X = reshape(features, (size(features)[1], 1))
noise = exp.(randn(length(X)))
Y = 2 .+ 3 .* X .+ noise
W = noise
π = collect(1:size(X, 1))
seed = 123
# train-eval split
π_sample = sample(π, size(π, 1), replace=false)
train_size = 0.8
π_train = π_sample[1:floor(Int, train_size * size(π, 1))]
π_eval = π_sample[floor(Int, train_size * size(π, 1))+1:end]
x_train, x_eval = X[π_train, :], X[π_eval, :]
Y_train, y_eval = Y[π_train], Y[π_eval]
w_train = W[π_train]
w_eval = W[π_eval]
# linear - no weights
params1 = EvoTreeRegressor(T=Float32, device="cpu",
loss=:linear, metric=:mse,
nrounds=100, nbins=100,
lambda=0.0, gamma=0.1, eta=0.05,
max_depth=6, min_weight=0.0,
rowsample=0.5, colsample=1.0, rng=seed)
model, cache = EvoTrees.init_evotree(params1, X_train, Y_train)
model = fit_evotree(params1; x_train, y_train, x_eval, y_eval, print_every_n=25);
preds_no_weight = predict(model, X_train)
# linear - weighted
params1 = EvoTreeRegressor(T=Float32, device="cpu",
loss=:linear, metric=:mse,
nrounds=100, nbins=100,
lambda=0.0, gamma=0.1, eta=0.05,
max_depth=6, min_weight=0.0,
rowsample=0.5, colsample=1.0, rng=seed)
model, cache = EvoTrees.init_evotree(params1, X_train, Y_train, W_train)
model = fit_evotree(params1; x_train, y_train, w_train, x_eval, y_eval, print_every_n=25);
preds_weighted_1 = predict(model, X_train)
params1 = EvoTreeRegressor(T=Float32, device="cpu",
loss=:linear, metric=:mse,
nrounds=100, nbins=100,
lambda=0.0, gamma=0.1, eta=0.05,
max_depth=6, min_weight=0.0,
rowsample=0.5, colsample=1.0, rng=seed)
model, cache = EvoTrees.init_evotree(params1, X_train, Y_train, W_train)
model = fit_evotree(params1; x_train, y_train, w_train, x_eval, y_eval, w_eval, print_every_n=25);
preds_weighted_2 = predict(model, X_train)
params1 = EvoTreeRegressor(T=Float32, device="cpu",
loss=:linear, metric=:mse,
nrounds=100, nbins=100,
lambda=0.0, gamma=0.1, eta=0.05,
max_depth=6, min_weight=0.0,
rowsample=0.5, colsample=1.0, rng=seed)
w_train_3 = ones(eltype(Y_train), size(Y_train)) .* 5
model, cache = EvoTrees.init_evotree(params1, X_train, Y_train, W_train_3)
model = fit_evotree(params1; x_train, y_train, w_train=w_train_3, x_eval, y_eval, print_every_n=25);
preds_weighted_3 = predict(model, X_train)
sum(abs.(preds_no_weight .- preds_weighted_3))
cor(preds_no_weight, preds_weighted_3)
# using Plots
# # using Colors
# x_perm = sortperm(X_train[:,1])
# plot(X_train, Y_train, msize=1, mcolor="gray", mswidth=0, background_color=RGB(1, 1, 1), seriestype=:scatter, xaxis=("feature"), yaxis=("target"), legend=true, label="")
# plot!(X_train[:,1][x_perm], preds_no_weight[x_perm], color="navy", linewidth=1.5, label="No weights")
# plot!(X_train[:,1][x_perm], preds_weighted[x_perm], color="red", linewidth=1.5, label="Weighted") | EvoTrees | https://github.com/Evovest/EvoTrees.jl.git |
|
[
"Apache-2.0"
] | 0.16.7 | 92d1f78f95f4794bf29bd972dacfa37ea1fec9f4 | docs | 5023 |
# EvoTrees <a href="https://evovest.github.io/EvoTrees.jl/dev/"><img src="figures/hex-evotrees-2.png" align="right" height="160"/></a>
| Documentation | CI Status | DOI |
|:------------------------:|:----------------:|:----------------:|
| [![][docs-stable-img]][docs-stable-url] [![][docs-latest-img]][docs-latest-url] | [![][ci-img]][ci-url] | [![][DOI-img]][DOI-url] |
[docs-latest-img]: https://img.shields.io/badge/docs-latest-blue.svg
[docs-latest-url]: https://evovest.github.io/EvoTrees.jl/dev
[docs-stable-img]: https://img.shields.io/badge/docs-stable-blue.svg
[docs-stable-url]: https://evovest.github.io/EvoTrees.jl/stable
[ci-img]: https://github.com/Evovest/EvoTrees.jl/workflows/CI/badge.svg
[ci-url]: https://github.com/Evovest/EvoTrees.jl/actions?query=workflow%3ACI+branch%3Amain
[DOI-img]: https://zenodo.org/badge/164559537.svg
[DOI-url]: https://zenodo.org/doi/10.5281/zenodo.10569604
A Julia implementation of boosted trees with CPU and GPU support.
Efficient histogram based algorithms with support for multiple loss functions (notably multi-target objectives such as max likelihood methods).
[R binding available](https://github.com/Evovest/EvoTrees).
## Installation
Latest:
```julia-repl
julia> Pkg.add(url="https://github.com/Evovest/EvoTrees.jl")
```
From General Registry:
```julia-repl
julia> Pkg.add("EvoTrees")
```
## Performance
Data consists of randomly generated `Matrix{Float64}`. Training is performed on 200 iterations.
Code to reproduce is availabe in [`benchmarks/regressor.jl`](https://github.com/Evovest/EvoTrees.jl/blob/main/benchmarks/regressor.jl).
- Run Environment:
- CPU: 12 threads on AMD Ryzen 5900X.
- GPU: NVIDIA RTX A4000.
- Julia: v1.9.1.
- Algorithms
- XGBoost: v2.3.0 (Using the `hist` algorithm).
- EvoTrees: v0.15.2.
### Training:
| Dimensions / Algo | XGBoost CPU | EvoTrees CPU | XGBoost GPU | EvoTrees GPU |
|---------------------|:-----------:|:------------:|:-----------:|:------------:|
| 100K x 100 | 2.34s | 1.01s | 0.90s | 2.61s |
| 500K x 100 | 10.7s | 3.95s | 1.84s | 3.41s |
| 1M x 100 | 21.1s | 6.57s | 3.10s | 4.47s |
| 5M x 100 | 108s | 36.1s | 12.9s | 12.5s |
| 10M x 100 | 218s | 72.6s | 25.5s | 23.0s |
### Inference:
| Dimensions / Algo | XGBoost CPU | EvoTrees CPU | XGBoost GPU | EvoTrees GPU |
|---------------------|:------------:|:------------:|:-----------:|:------------:|
| 100K x 100 | 0.151s | 0.058s | NA | 0.045s |
| 500K x 100 | 0.647s | 0.248s | NA | 0.172s |
| 1M x 100 | 1.26s | 0.573s | NA | 0.327s |
| 5M x 100 | 6.04s | 2.87s | NA | 1.66s |
| 10M x 100 | 12.4s | 5.71s | NA | 3.40s |
## MLJ Integration
See [official project page](https://github.com/alan-turing-institute/MLJ.jl) for more info.
## Quick start with internal API
A model configuration must first be defined, using one of the model constructor:
- `EvoTreeRegressor`
- `EvoTreeClassifier`
- `EvoTreeCount`
- `EvoTreeMLE`
Model training is performed using `fit_evotree`.
It supports additional keyword arguments to track evaluation metric and perform early stopping.
Look at the docs for more details on available hyper-parameters for each of the above constructors and other options training options.
### Matrix features input
```julia
using EvoTrees
config = EvoTreeRegressor(
loss=:mse,
nrounds=100,
max_depth=6,
nbins=32,
eta=0.1)
x_train, y_train = rand(1_000, 10), rand(1_000)
m = fit_evotree(config; x_train, y_train)
preds = m(x_train)
```
### DataFrames input
When using a DataFrames as input, features with elements types `Real` (incl. `Bool`) and `Categorical` are automatically recognized as input features. Alternatively, `fnames` kwarg can be used to specify the variables to be used as features.
`Categorical` features are treated accordingly by the algorithm: ordered variables are treated as numerical features, using `β€` split rule, while unordered variables are using `==`. Support is currently limited to a maximum of 255 levels. `Bool` variables are treated as unordered, 2-levels categorical variables.
```julia
dtrain = DataFrame(x_train, :auto)
dtrain.y .= y_train
m = fit_evotree(config, dtrain; target_name="y");
m = fit_evotree(config, dtrain; target_name="y", fnames=["x1", "x3"]);
```
## Feature importance
Returns the normalized gain by feature.
```julia
features_gain = EvoTrees.importance(m)
```
## Plot
Plot a given tree of the model:
```julia
plot(m, 2)
```

Note that 1st tree is used to set the bias so the first real tree is #2.
## Save/Load
```julia
EvoTrees.save(m, "data/model.bson")
m = EvoTrees.load("data/model.bson");
```
| EvoTrees | https://github.com/Evovest/EvoTrees.jl.git |
|
[
"Apache-2.0"
] | 0.16.7 | 92d1f78f95f4794bf29bd972dacfa37ea1fec9f4 | docs | 147 | # Public API
## fit_evotree
```@docs
fit_evotree
```
## predict
```@docs
EvoTrees.predict
```
## importance
```@docs
EvoTrees.importance
```
| EvoTrees | https://github.com/Evovest/EvoTrees.jl.git |
|
[
"Apache-2.0"
] | 0.16.7 | 92d1f78f95f4794bf29bd972dacfa37ea1fec9f4 | docs | 6908 | # EvoTrees.jl
A Julia implementation of boosted trees with CPU and GPU support. Efficient histogram based algorithms with support for multiple loss functions, including various regressions, multi-classification and Gaussian max likelihood.
See the `examples-API` section to get started using the internal API, or `examples-MLJ` to use within the [MLJ](https://github.com/alan-turing-institute/MLJ.jl) framework.
Complete details about hyper-parameters are found in the `Models` section.
[R binding available](https://github.com/Evovest/EvoTrees).
## Installation
Latest:
```julia-repl
julia> Pkg.add(url="https://github.com/Evovest/EvoTrees.jl")
```
From General Registry:
```julia-repl
julia> Pkg.add("EvoTrees")
```
## Quick start
A model configuration must first be defined, using one of the model constructor:
- [`EvoTreeRegressor`](@ref)
- [`EvoTreeClassifier`](@ref)
- [`EvoTreeCount`](@ref)
- [`EvoTreeMLE`](@ref)
Then fitting can be performed using [`fit_evotree`](@ref). 2 broad methods are supported: Matrix and Tables based inputs. Optional kwargs can be used to specify eval data on which to track eval metric and perform early stopping. Look at the docs for more details on available hyper-parameters for each of the above constructors and other options for training.
Predictions are obtained by passing features data to the model. Model acts as a functor, ie. it's a struct containing the fitted model as well as a function generating the prediction of that model for the features argument.
### Matrix features input
```julia
using EvoTrees
config = EvoTreeRegressor(
loss=:mse,
nrounds=100,
max_depth=6,
nbins=32,
eta=0.1)
x_train, y_train = rand(1_000, 10), rand(1_000)
m = fit_evotree(config; x_train, y_train)
preds = m(x_train)
```
### Tables and DataFrames input
When using a `Tables` compatible input such as `DataFrames`, features with element type `Real` (incl. `Bool`) and `Categorical` are automatically recognized as input features. Alternatively, `fnames` kwarg can be used.
`Categorical` features are treated accordingly by the algorithm. Ordered variables will be treated as numerical features, using `β€` split rule, while unordered variables are using `==`. Support is currently limited to a maximum of 255 levels. `Bool` variables are treated as unordered, 2-levels cat variables.
```julia
dtrain = DataFrame(x_train, :auto)
dtrain.y .= y_train
m = fit_evotree(config, dtrain; target_name="y");
m = fit_evotree(config, dtrain; target_name="y", fnames=["x1", "x3"]);
```
### GPU Acceleration
EvoTrees supports training and inference on Nvidia GPU's with [CUDA.jl](https://github.com/JuliaGPU/CUDA.jl).
Note that on Julia β₯ 1.9 CUDA support is only enabled when CUDA.jl is installed and loaded, by another package or explicitly with e.g.
```julia
using CUDA
```
If running on a CUDA enabled machine, training and inference on GPU can be triggered through the `device` kwarg:
```julia
m = fit_evotree(config, dtrain; target_name="y", device="gpu");
p = m(dtrain; device="gpu")
```
## Reproducibility
EvoTrees models trained on cpu can be fully reproducible.
Models of the gradient boosting family typically involve some stochasticity.
In EvoTrees, this primarily concern the the 2 subsampling parameters `rowsample` and `colsample`. The other stochastic operation happens at model initialisation when the features are binarized to allow for fast histogram construction: a random subsample of `1_000 * nbins` is used to compute the breaking points.
These random parts of the algorithm can be deterministically reproduced on cpu by specifying an `rng` to the model constructor. `rng` can be an integer (ex: `123`) or a random generator (ex: `Random.Xoshiro(123)`).
If no `rng` is specified, `123` is used by default. When an integer `rng` is used, a `Random.MersenneTwister` generator will be created by the EvoTrees's constructor. Otherwise, the provided random generator will be used.
Consequently, the following `m1` and `m2` models will be identical:
```julia
config = EvoTreeRegressor(rowsample=0.5, rng=123)
m1 = fit_evotree(config, df; target_name="y");
config = EvoTreeRegressor(rowsample=0.5, rng=123)
m2 = fit_evotree(config, df; target_name="y");
```
However, the following `m1` and `m2` models won't be because the there's stochasticity involved in the model from `rowsample` and the random generator in the `config` isn't reset between the fits:
```julia
config = EvoTreeRegressor(rowsample=0.5, rng=123)
m1 = fit_evotree(config, df; target_name="y");
m2 = fit_evotree(config, df; target_name="y");
```
Note that in presence of multiple identical or very highly correlated features, model may not be reproducible if features are permuted since in situation where 2 features provide identical gains, the first one will be selected. Therefore, if the identity relationship doesn't hold on new data, different predictions will be returned from models trained on different features order.
At the moment, there's no reproducibility guarantee on GPU, although this may change in the future.
## Missing values
### Features
EvoTrees does not handle features having missing values. Proper preprocessing of the data is therefore needed (and a general good practice regardless of the ML model used).
This includes situations where values may be all non-missing, but where the `eltype` is `Union{Missing,Float64}` or `Any` for example. A conversion using `identity` is then recommended:
```julia
julia> x = Vector{Union{Missing, Float64}}([1, 2])
2-element Vector{Union{Missing, Float64}}:
1.0
2.0
julia> identity.(x)
2-element Vector{Float64}:
1.0
2.0
```
For dealing with numerical or ordered categorical features containing missing values, a common approach is to first create an `Bool` variable capturing the info on whether a value is missing:
```julia
using DataFrames
transform!(df, :my_feat => ByRow(ismissing) => :my_feat_ismissing)
```
Then, the missing values can be imputed (replaced by some default values such as `mean` or `median`, or using a more sophisticated approach such as predictions from another model):
```julia
transform!(df, :my_feat => (x -> coalesce.(x, median(skipmissing(x)))) => :my_feat)
```
For unordered categorical variables, a recode of the missing into a non missing level is sufficient:
```julia
using CategoricalArrays
julia> x = categorical(["a", "b", missing])
3-element CategoricalArray{Union{Missing, String},1,UInt32}:
"a"
"b"
missing
julia> x = recode(x, missing => "missing value")
3-element CategoricalArray{String,1,UInt32}:
"a"
"b"
"missing value"
```
### Target
Target variable must have its element type `<:Real`. Only exception is for `EvoTreeClassifier` for which `CategoricalValue`, `Integer`, `String` and `Char` are supported.
## Save/Load
```julia
EvoTrees.save(m, "data/model.bson")
m = EvoTrees.load("data/model.bson");
```
| EvoTrees | https://github.com/Evovest/EvoTrees.jl.git |
|
[
"Apache-2.0"
] | 0.16.7 | 92d1f78f95f4794bf29bd972dacfa37ea1fec9f4 | docs | 366 |
# Internal API
## General
```@docs
EvoTrees.TrainNode
EvoTrees.EvoTree
EvoTrees.check_parameter
EvoTrees.check_args
```
## Training utils
```@docs
EvoTrees.init
EvoTrees.grow_evotree!
EvoTrees.update_gains!
EvoTrees.predict!
EvoTrees.subsample
EvoTrees.split_set_chunk!
```
## Histogram
```@docs
EvoTrees.get_edges
EvoTrees.binarize
EvoTrees.update_hist!
```
| EvoTrees | https://github.com/Evovest/EvoTrees.jl.git |
|
[
"Apache-2.0"
] | 0.16.7 | 92d1f78f95f4794bf29bd972dacfa37ea1fec9f4 | docs | 334 | # Models
## EvoTreeRegressor
```@docs
EvoTreeRegressor
```
## EvoTreeClassifier
```@docs
EvoTreeClassifier
```
## EvoTreeCount
```@docs
EvoTreeCount
```
## EvoTreeMLE
```@docs
EvoTreeMLE
```
## EvoTreeGaussian
`EvoTreeGaussian` is to be deprecated. Please use EvoTreeMLE with `loss = :gaussian_mle`.
```@docs
EvoTreeGaussian
``` | EvoTrees | https://github.com/Evovest/EvoTrees.jl.git |
|
[
"Apache-2.0"
] | 0.16.7 | 92d1f78f95f4794bf29bd972dacfa37ea1fec9f4 | docs | 2169 | # Classification on Iris dataset
We will use the iris dataset, which is included in the MLDatasets package. This dataset consists of measurements of the sepal length, sepal width, petal length, and petal width for three different types of iris flowers: Setosa, Versicolor, and Virginica.
## Getting started
To begin, we will load the required packages and the dataset:
```julia
using EvoTrees
using MLDatasets
using DataFrames
using Statistics: mean
using CategoricalArrays
using Random
df = MLDatasets.Iris().dataframe
```
## Preprocessing
Before we can train our model, we need to preprocess the dataset. We will convert the class variable, which specifies the type of iris flower, into a categorical variable.
```julia
Random.seed!(123)
df[!, :class] = categorical(df[!, :class])
target_name = "class"
fnames = setdiff(names(df), [target_name])
train_ratio = 0.8
train_indices = randperm(nrow(df))[1:Int(train_ratio * nrow(df))]
dtrain = df[train_indices, :]
deval = df[setdiff(1:nrow(df), train_indices), :]
```
## Training
Now we are ready to train our model. We will first define a model configuration using the [`EvoTreeClassifier`](@ref) model constructor.
Then, we'll use [`fit_evotree`](@ref) to train a boosted tree model. We'll pass optional `x_eval` and `y_eval` arguments, which enable the usage of early stopping.
```julia
config = EvoTreeClassifier(
nrounds=200,
eta=0.05,
max_depth=5,
lambda=0.1,
rowsample=0.8,
colsample=0.8)
model = fit_evotree(config, dtrain;
target_name,
fnames,
deval,
metric = :mlogloss,
early_stopping_rounds=10,
print_every_n=10)
```
Finally, we can get predictions by passing training and testing data to our model. We can then evaluate the accuracy of our model, which should be near 100% for this simple classification problem.
```julia
pred_train = model(x_train)
idx_train = [findmax(row)[2] for row in eachrow(pred_train)]
pred_eval = model(x_eval)
idx_eval = [findmax(row)[2] for row in eachrow(pred_eval)]
```
```julia-repl
julia> mean(idx_train .== levelcode.(y_train))
1.0
julia> mean(idx_eval .== levelcode.(y_eval))
0.9333333333333333
```
| EvoTrees | https://github.com/Evovest/EvoTrees.jl.git |
|
[
"Apache-2.0"
] | 0.16.7 | 92d1f78f95f4794bf29bd972dacfa37ea1fec9f4 | docs | 3449 | # Internal API examples
The following provides minimal examples of usage of the various loss functions available in EvoTrees using the internal API.
## Regression
Minimal example to fit a noisy sinus wave.

```julia
using EvoTrees
using EvoTrees: sigmoid, logit
using StatsBase: sample
# prepare a dataset
features = rand(10000) .* 20 .- 10
X = reshape(features, (size(features)[1], 1))
Y = sin.(features) .* 0.5 .+ 0.5
Y = logit(Y) + randn(size(Y))
Y = sigmoid(Y)
π = collect(1:size(X, 1))
# train-eval split
π_sample = sample(π, size(π, 1), replace = false)
train_size = 0.8
π_train = π_sample[1:floor(Int, train_size * size(π, 1))]
π_eval = π_sample[floor(Int, train_size * size(π, 1))+1:end]
x_train, x_eval = X[π_train, :], X[π_eval, :]
y_train, y_eval = Y[π_train], Y[π_eval]
config = EvoTreeRegressor(
loss=:mse,
nrounds=100, nbins = 100,
lambda = 0.5, gamma=0.1, eta=0.1,
max_depth=6, min_weight=1.0,
rowsample=0.5, colsample=1.0)
model = fit_evotree(config; x_train, y_train, x_eval, y_eval, metric=:mse, print_every_n=25)
pred_eval_linear = model(x_eval)
# logistic / cross-entropy
config = EvoTreeRegressor(
loss=:logistic,
nrounds=100, nbins = 100,
lambda = 0.5, gamma=0.1, eta=0.1,
max_depth=6, min_weight=1.0,
rowsample=0.5, colsample=1.0)
model = fit_evotree(config; x_train, y_train, x_eval, y_eval, metric=:logloss, print_every_n=25)
pred_eval_logistic = model(x_eval)
# L1
config = EvoTreeRegressor(
loss=:l1, alpha=0.5,
nrounds=100, nbins=100,
lambda = 0.5, gamma=0.0, eta=0.1,
max_depth=6, min_weight=1.0,
rowsample=0.5, colsample=1.0)
model = fit_evotree(config; x_train, y_train, x_eval, y_eval, metric=:mae, print_every_n=25)
pred_eval_L1 = model(x_eval)
```
## Poisson Count
```julia
# Poisson
config = EvoTreeCount(
loss=:poisson,
nrounds=100, nbins=100,
lambda=0.5, gamma=0.1, eta=0.1,
max_depth=6, min_weight=1.0,
rowsample=0.5, colsample=1.0)
model = fit_evotree(config; x_train, y_train, x_eval, y_eval, metric = :poisson, print_every_n = 25)
pred_eval_poisson = model(x_eval)
```
## Quantile Regression

```julia
# q50
config = EvoTreeRegressor(
loss=:quantile, alpha=0.5,
nrounds=200, nbins=100,
lambda=0.1, gamma=0.0, eta=0.05,
max_depth=6, min_weight=1.0,
rowsample=0.5, colsample=1.0)
model = fit_evotree(config; x_train, y_train, x_eval, y_eval, metric = :quantile, print_every_n = 25)
pred_train_q50 = model(x_train)
# q20
config = EvoTreeRegressor(
loss=:quantile, alpha=0.2,
nrounds=200, nbins=100,
lambda=0.1, gamma=0.0, eta=0.05,
max_depth=6, min_weight=1.0,
rowsample=0.5, colsample=1.0)
model = fit_evotree(config; x_train, y_train, x_eval, y_eval, metric = :quantile, print_every_n = 25)
pred_train_q20 = model(x_train)
# q80
config = EvoTreeRegressor(
loss=:quantile, alpha=0.8,
nrounds=200, nbins=100,
lambda=0.1, gamma=0.0, eta=0.05,
max_depth=6, min_weight=1.0,
rowsample=0.5, colsample=1.0)
model = fit_evotree(config; x_train, y_train, x_eval, y_eval, metric = :quantile, print_every_n = 25)
pred_train_q80 = model(x_train)
```
## Gaussian Max Likelihood

```julia
config = EvoTreeMLE(
loss=:gaussian_mle,
nrounds=100, nbins=100,
lambda=0.0, gamma=0.0, eta=0.1,
max_depth=6, rowsample=0.5)
``` | EvoTrees | https://github.com/Evovest/EvoTrees.jl.git |
|
[
"Apache-2.0"
] | 0.16.7 | 92d1f78f95f4794bf29bd972dacfa37ea1fec9f4 | docs | 1422 | # MLJ Integration
EvoTrees.jl provides a first-class integration with the MLJ ecosystem.
See [official project page](https://github.com/alan-turing-institute/MLJ.jl) for more info.
To use with MLJ, an EvoTrees model configuration must first be initialized using either:
- [`EvoTreeRegressor`](@ref)
- [`EvoTreeClassifier`](@ref)
- [`EvoTreeCount`](@ref)
- [`EvoTreeMLE`](@ref)
The model is then passed to MLJ's `machine`, opening access to the rest of the MLJ modeling ecosystem.
```julia
using StatsBase: sample
using EvoTrees
using EvoTrees: sigmoid, logit # only needed to create the synthetic data below
using MLJBase
features = rand(10_000) .* 5 .- 2
X = reshape(features, (size(features)[1], 1))
Y = sin.(features) .* 0.5 .+ 0.5
Y = logit(Y) + randn(size(Y))
Y = sigmoid(Y)
y = Y
X = MLJBase.table(X)
# linear regression
tree_model = EvoTreeRegressor(loss=:linear, max_depth=5, eta=0.05, nrounds=10)
# set machine
mach = machine(tree_model, X, y)
# partition data
train, test = partition(eachindex(y), 0.7, shuffle=true); # 70:30 split
# fit data
fit!(mach, rows=train, verbosity=1)
# continue training
mach.model.nrounds += 10
fit!(mach, rows=train, verbosity=1)
# predict on train data
pred_train = predict(mach, selectrows(X, train))
mean(abs.(pred_train - selectrows(Y, train)))
# predict on test data
pred_test = predict(mach, selectrows(X, test))
mean(abs.(pred_test - selectrows(Y, test)))
``` | EvoTrees | https://github.com/Evovest/EvoTrees.jl.git |
|
[
"Apache-2.0"
] | 0.16.7 | 92d1f78f95f4794bf29bd972dacfa37ea1fec9f4 | docs | 3613 | # Logistic Regression on Titanic Dataset
We will use the Titanic dataset, which is included in the MLDatasets package. It describes the survival status of individual passengers on the Titanic. The model will be approached as a logistic regression problem, although a Classifier model could also have been used (see the `Classification - Iris` tutorial).
## Getting started
To begin, we will load the required packages and the dataset:
```julia
using EvoTrees
using MLDatasets
using DataFrames
using Statistics: mean
using CategoricalArrays
using Random
df = MLDatasets.Titanic().dataframe
```
## Preprocessing
A first step in data processing is to prepare the input features in a model compatible format.
EvoTrees' Tables API supports input that are either `Real` (incl. `Bool`) or `Categorical`. `Bool` variables are treated as unordered, 2-levels categorical variables.
A recommended approach for `String` features such as `Sex` is to convert them into an unordered `Categorical`.
For dealing with features with missing values such as `Age`, a common approach is to first create an `Bool` indicator variable capturing the info on whether a value is missing.
Then, the missing values can be imputed (replaced by some default values such as `mean` or `median`, or more sophisticated approach such as predictions from another model).
```julia
# convert string feature to Categorical
transform!(df, :Sex => categorical => :Sex)
# treat string feature and missing values
transform!(df, :Age => ByRow(ismissing) => :Age_ismissing)
transform!(df, :Age => (x -> coalesce.(x, median(skipmissing(x)))) => :Age);
# remove unneeded variables
df = df[:, Not([:PassengerId, :Name, :Embarked, :Cabin, :Ticket])]
```
The full data can now be split according to train and eval indices.
Target and feature names are also set.
```julia
Random.seed!(123)
train_ratio = 0.8
train_indices = randperm(nrow(df))[1:Int(round(train_ratio * nrow(df)))]
dtrain = df[train_indices, :]
deval = df[setdiff(1:nrow(df), train_indices), :]
target_name = "Survived"
fnames = setdiff(names(df), [target_name])
```
## Training
Now we are ready to train our model. We will first define a model configuration using the [`EvoTreeRegressor`](@ref) model constructor.
Then, we'll use [`fit_evotree`](@ref) to train a boosted tree model. We'll pass optional `deval` arguments, which enables the tracking of an evaluation metric and early stopping.
```julia
config = EvoTreeRegressor(
loss=:logistic,
nrounds=200,
eta=0.05,
nbins=128,
max_depth=5,
rowsample=0.5,
colsample=0.9)
model = fit_evotree(
config, dtrain;
deval,
target_name,
fnames,
metric = :logloss,
early_stopping_rounds=10,
print_every_n=10)
```
## Diagnosis
We can get predictions by passing training and testing data to our model. We can then evaluate the accuracy of our model, which should be around 85%.
```julia
pred_train = model(dtrain)
pred_eval = model(deval)
```
```julia-repl
julia> mean((pred_train .> 0.5) .== dtrain[!, target_name])
0.8821879382889201
julia> mean((pred_eval .> 0.5) .== deval[!, target_name])
0.8426966292134831
```
Finally, features importance can be inspected using [`EvoTrees.importance`](@ref).
```julia-repl
julia> EvoTrees.importance(model)
7-element Vector{Pair{String, Float64}}:
"Sex" => 0.29612654189959403
"Age" => 0.25487324307720827
"Fare" => 0.2530947969323613
"Pclass" => 0.11354283043193575
"SibSp" => 0.05129209383816148
"Parch" => 0.017385183317069588
"Age_ismissing" => 0.013685310503669728
```
| EvoTrees | https://github.com/Evovest/EvoTrees.jl.git |
|
[
"Apache-2.0"
] | 0.16.7 | 92d1f78f95f4794bf29bd972dacfa37ea1fec9f4 | docs | 6456 | # Ranking with Yahoo! Learning to Rank Challenge.
In this tutorial, we present how a ranking task can be tackled using regular regression techniques without compromising performance compared to specialized ranking learners.
The data used is from the `C14 - Yahoo! Learning to Rank Challenge`, which can be obtained following a request to [https://webscope.sandbox.yahoo.com](https://webscope.sandbox.yahoo.com).
## Getting started
To begin, we load the required packages:
```julia
using EvoTrees
using DataFrames
using Statistics: mean
using CategoricalArrays
using Random
```
## Load LIBSVM format data
Some datasets come in the so called `LIBSVM` format, which stores data using a sparse representation:
```
<label> <query> <feature_id_1>:<feature_value_1> <feature_id_2>:<feature_value_2>
```
We use the [`ReadLIBSVM.jl`](https://github.com/jeremiedb/ReadLIBSVM.jl) package to perform parsing:
```julia
using ReadLIBSVM
dtrain = read_libsvm("set1.train.txt"; has_query=true)
deval = read_libsvm("set1.valid.txt"; has_query=true)
dtest = read_libsvm("set1.test.txt"; has_query=true)
```
## Preprocessing
Preprocessing is minimal since all features are parsed as floats and specific files are provided for each of the train, eval and test splits.
Several features are fully missing (contain only 0s) in the training dataset. They are removed from all datasets since they cannot bring value to the model.
Then, the features, targets and query ids are extracted from the parsed `LIBSVM` format.
```julia
colsums_train = map(sum, eachcol(dtrain[:x]))
drop_cols = colsums_train .== 0
x_train = dtrain[:x][:, .!drop_cols]
x_eval = deval[:x][:, .!drop_cols]
x_test = dtest[:x][:, .!drop_cols]
# assign queries
q_train = dtrain[:q]
q_eval = deval[:q]
q_test = dtest[:q]
# assign targets
y_train = dtrain[:y]
y_eval = deval[:y]
y_test = dtest[:y]
```
## Training
Now we are ready to train our model. We first define a model configuration using the [`EvoTreeRegressor`](@ref) model constructor.
Then, we use [`fit_evotree`](@ref) to train a boosted tree model. The optional `x_eval` and `y_eval` arguments are provided to enable the usage of early stopping.
```julia
config = EvoTreeRegressor(
nrounds=6000,
loss=:mse,
eta=0.02,
nbins=64,
max_depth=11,
rowsample=0.9,
colsample=0.9,
)
m_mse, logger_mse = fit_evotree(
config;
x_train=x_train,
y_train=y_train,
x_eval=x_eval,
y_eval=y_eval,
early_stopping_rounds=200,
print_every_n=50,
metric=:mse,
return_logger=true
);
p_test = m_mse(x_test);
```
## Model evaluation
For ranking problems, a commonly used metric is the [Normalized Discounted Cumulative Gain](https://en.wikipedia.org/wiki/Discounted_cumulative_gain). It essentially considers whether the model is good at identifying the top K outcomes within a group. There are various flavors to its implementation, though the most commonly used one is the following:
```julia
function ndcg(p, y, k=10)
k = min(k, length(p))
p_order = partialsortperm(p, 1:k, rev=true)
y_order = partialsortperm(y, 1:k, rev=true)
_y = y[p_order]
gains = 2 .^ _y .- 1
discounts = log2.((1:k) .+ 1)
ndcg = sum(gains ./ discounts)
y_order = partialsortperm(y, 1:k, rev=true)
_y = y[y_order]
gains = 2 .^ _y .- 1
discounts = log2.((1:k) .+ 1)
idcg = sum(gains ./ discounts)
return idcg == 0 ? 1.0 : ndcg / idcg
end
```
To compute the NDCG over a collection of groups, it is handy to leverage DataFrames' `combine` and `groupby` functionalities:
```julia
test_df = DataFrame(p=p_test, y=y_test, q=q_test)
test_df_agg = combine(groupby(test_df, "q"), ["p", "y"] => ndcg => "ndcg")
ndcg_test = round(mean(test_df_agg.ndcg), sigdigits=5)
@info "ndcg_test MSE" ndcg_test
β Info: ndcg_test MSE
β ndcg_test = 0.8008
```
## Logistic regression alternative
The above regression experiment shows a performance competitive with the results outlined in CatBoost's [ranking benchmarks](https://github.com/catboost/benchmarks/blob/master/ranking/Readme.md#4-results).
Another approach is to use a scaling of the the target ranking scores to perform a logistic regression.
```julia
max_rank = 4
y_train = dtrain[:y] ./ max_rank
y_eval = deval[:y] ./ max_rank
y_test = dtest[:y] ./ max_rank
config = EvoTreeRegressor(
nrounds=6000,
loss=:logloss,
eta=0.01,
nbins=64,
max_depth=11,
rowsample=0.9,
colsample=0.9,
)
m_logloss, logger_logloss = fit_evotree(
config;
x_train=x_train,
y_train=y_train,
x_eval=x_eval,
y_eval=y_eval,
early_stopping_rounds=200,
print_every_n=50,
metric=:logloss,
return_logger=true
);
```
To measure the NDCG, the original targets must be used since NDCG is a scale sensitive measure.
```julia
y_train = dtrain[:y]
y_eval = deval[:y]
y_test = dtest[:y]
p_test = m_logloss(x_test);
test_df = DataFrame(p=p_test, y=y_test, q=q_test)
test_df_agg = combine(groupby(test_df, "q"), ["p", "y"] => ndcg => "ndcg")
ndcg_test = round(mean(test_df_agg.ndcg), sigdigits=5)
@info "ndcg_test LogLoss" ndcg_test
β Info: ndcg_test LogLoss
β ndcg_test = 0.80267
```
## Conclusion
We've seen that a ranking problem can be efficiently handled with generic regression tasks, yet achieve comparable performance to specialized ranking loss functions. Below, we present the NDCG obtained from the above experiments along those published on CatBoost's [benchmarks](https://github.com/catboost/benchmarks/blob/master/ranking/Readme.md#4-results).
| **Model** | **NDCG** |
|-------------------------|-----------|
| **EvoTrees - mse** |**0.80080**|
| **EvoTrees - logistic** |**0.80267**|
| cat-rmse |0.802115 |
| cat-query-rmse |0.802229 |
| cat-pair-logit |0.797318 |
| cat-pair-logit-pairwise |0.790396 |
| cat-yeti-rank |0.802972 |
| xgb-rmse |0.798892 |
| xgb-pairwise |0.800048 |
| xgb-lambdamart-ndcg |0.800048 |
| lgb-rmse |0.8013675 |
| lgb-pairwise |0.801347 |
It should be noted that the later results were not reproduced in the scope of current tutorial, so one should be careful about any claim of model superiority. The results from CatBoost's benchmarks were however already indicative of strong performance of non-specialized ranking loss functions, to which this tutorial brings further support.
| EvoTrees | https://github.com/Evovest/EvoTrees.jl.git |
|
[
"Apache-2.0"
] | 0.16.7 | 92d1f78f95f4794bf29bd972dacfa37ea1fec9f4 | docs | 2068 | # Regression on Boston Housing Dataset
We will use the Boston Housing dataset, which is included in the MLDatasets package. It's derived from information collected by the U.S. Census Service concerning housing in the area of Boston. Target variable represents the median housing value.
## Getting started
To begin, we will load the required packages and the dataset:
```julia
using EvoTrees
using MLDatasets
using DataFrames
using Statistics: mean
using CategoricalArrays
using Random
df = MLDatasets.BostonHousing().dataframe
```
## Preprocessing
Before we can train our model, we need to preprocess the dataset. We will split our data according to train and eval indices, and separate features from the target variable.
```julia
Random.seed!(123)
train_ratio = 0.8
train_indices = randperm(nrow(df))[1:Int(round(train_ratio * nrow(df)))]
train_data = df[train_indices, :]
eval_data = df[setdiff(1:nrow(df), train_indices), :]
x_train, y_train = Matrix(train_data[:, Not(:MEDV)]), train_data[:, :MEDV]
x_eval, y_eval = Matrix(eval_data[:, Not(:MEDV)]), eval_data[:, :MEDV]
```
## Training
Now we are ready to train our model. We will first define a model configuration using the [`EvoTreeRegressor`](@ref) model constructor.
Then, we'll use [`fit_evotree`](@ref) to train a boosted tree model. We'll pass optional `x_eval` and `y_eval` arguments, which enable the usage of early stopping.
```julia
config = EvoTreeRegressor(
nrounds=200,
eta=0.1,
max_depth=4,
lambda=0.1,
rowsample=0.9,
colsample=0.9)
model = fit_evotree(config;
x_train, y_train,
x_eval, y_eval,
metric = :mse,
early_stopping_rounds=10,
print_every_n=10)
```
Finally, we can get predictions by passing training and testing data to our model. We can then apply various evaluation metric, such as the MAE (mean absolute error):
```julia
pred_train = model(x_train)
pred_eval = model(x_eval)
```
```julia-repl
julia> mean(abs.(pred_train .- y_train))
1.056997874224627
julia> mean(abs.(pred_eval .- y_eval))
2.3298767665825264
``` | EvoTrees | https://github.com/Evovest/EvoTrees.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | code | 538 | push!(LOAD_PATH, "../src/")
using Pkg
Pkg.build("InterProcessCommunication")
using InterProcessCommunication
using Documenter
DEPLOYDOCS = (get(ENV, "CI", nothing) == "true")
makedocs(
sitename = "Inter-Process Communication",
format = Documenter.HTML(
prettyurls = DEPLOYDOCS,
),
authors = "Γric ThiΓ©baut and contributors",
pages = ["index.md", "semaphores.md", "sharedmemory.md", "reference.md"]
)
if DEPLOYDOCS
deploydocs(
repo = "github.com/emmt/InterProcessCommunication.jl.git",
)
end
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | code | 1953 | #
# InterProcessCommunication.jl --
#
# Inter-Process Communication for Julia.
#
#------------------------------------------------------------------------------
#
# This file is part of InterProcessCommunication.jl released under the MIT
# "expat" license.
#
# Copyright (c) 2016-2024, Γric ThiΓ©baut
# (https://github.com/emmt/InterProcessCommunication.jl).
#
module InterProcessCommunication
export
CLOCK_MONOTONIC,
CLOCK_REALTIME,
DynamicMemory,
FileDescriptor,
FileStat,
IPC,
Semaphore,
SharedMemory,
ShmArray,
ShmId,
ShmInfo,
ShmMatrix,
ShmVector,
SigAction,
SigInfo,
SigSet,
TimeSpec,
TimeVal,
TimeoutError,
WrappedArray,
clock_getres,
clock_gettime,
clock_settime,
gettimeofday,
nanosleep,
now,
post,
shmat,
shmcfg,
shmctl,
shmdt,
shmget,
shmid,
shminfo!,
shminfo,
shmrm,
sigaction!,
sigaction,
signal,
sigpending!,
sigpending,
sigprocmask!,
sigprocmask,
sigqueue,
sigsuspend,
sigwait!,
sigwait,
timedlock,
trywait,
umask
using Printf
using Dates
import Dates: now
using Base: elsize, tail, OneTo, throw_boundserror, @propagate_inbounds
import Base: convert, unsafe_convert,
islocked, lock, unlock, trylock, timedwait, wait, broadcast
# The following is an exported shortcut to the package.
const IPC = InterProcessCommunication
const PARANOID = true
isfile(joinpath(@__DIR__, "..", "deps", "deps.jl")) ||
error("Package `InterProcessCommunication` is not properly installed. Please run `import Pkg; Pkg.build(\"InterProcessCommunication\")`.")
include(joinpath("..", "deps", "deps.jl"))
include("types.jl")
include("wrappedarrays.jl")
include("unix.jl")
include("utils.jl")
include("shm.jl")
include("semaphores.jl")
include("signals.jl")
include("locks.jl")
@deprecate IPC_NEW IPC.PRIVATE
end # module InterProcessCommunication
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | code | 16360 | #
# locks.jl --
#
# Mutexes, condition variables and read/write locks for Julia.
#
#------------------------------------------------------------------------------
#
# This file is part of InterProcessCommunication.jl released under the MIT
# "expat" license.
#
# Copyright (C) 2016-2019, Γric ThiΓ©baut
# (https://github.com/emmt/InterProcessCommunication.jl).
#
# Abstract types to identify pointers to specific kind of C structures.
abstract type MutexData end
abstract type ConditionData end
abstract type RWLockData end
# Number of bytes for each C structures.
Base.sizeof(::Type{MutexData}) = _sizeof_pthread_mutex_t
Base.sizeof(::Type{ConditionData}) = _sizeof_pthread_cond_t
Base.sizeof(::Type{RWLockData}) = _sizeof_pthread_rwlock_t
"""
```julia
IPC.Mutex()
```
yields an initialized POSIX mutex. The mutex is automatically unlocked and
associated ressources are automatically destroyed when the returned object is
garbage collected.
```julia
IPC.Mutex(buf, off=0; shared=false)
```
yields a new mutex object using buffer `buf` at offset `off` (in bytes) for its
storage. There must be at least `sizeof(IPC.MutexData)` available bytes at
address `pointer(buf) + off`, these bytes must not be used for something else
and must remain accessible during the lifetime of the object.
Keyword `shared` can be set true to create a mutex that is shared between
processes; otherwise, the mutex is private that is it can only be used by
threads of the process which creates the mutex. A shared mutex must be stored
in a part of the memory, like shared memory, that can be shared with other
processes.
See also: [`IPC.Condition`](@ref), [`IPC.RWLock`](@ref), [`lock`](@ref),
[`unlock`](@ref), [`trylock`](@ref).
"""
mutable struct Mutex{T}
handle::Ptr{MutexData} # typed pointer to object data
buffer::T # object data
locked::Bool
function Mutex{T}(buf::T, off::Int; shared::Bool=false) where {T}
off β₯ 0 || error("offset must be nonnegative")
sizeof(buf) β₯ sizeof(MutexData) + off ||
error("insufficient buffer size to store POSIX mutex")
obj = new{T}(pointer(buf) + off, buf, false)
attr = Vector{UInt8}(undef, _sizeof_pthread_mutexattr_t)
code = ccall(:pthread_mutexattr_init, Cint, (Ptr{UInt8},), attr)
code == 0 || throw_system_error("pthread_mutexattr_init", code)
code = ccall(:pthread_mutexattr_setpshared, Cint, (Ptr{UInt8}, Cint), attr,
(shared ? PTHREAD_PROCESS_SHARED : PTHREAD_PROCESS_PRIVATE))
code == 0 || throw_system_error("pthread_mutexattr_setpshared", code)
code = ccall(:pthread_mutex_init, Cint,
(Ptr{MutexData}, Ptr{UInt8}), obj, attr)
code == 0 || throw_system_error("pthread_mutex_init", code)
code = ccall(:pthread_mutexattr_destroy, Cint, (Ptr{UInt8},), attr)
code == 0 || (_destroy(obj);
throw_system_error("pthread_mutexattr_destroy", code))
return finalizer(_destroy, obj)
end
end
Mutex(buf::T, off::Integer = 0; kwds...) where {T} =
Mutex{T}(buf, Int(off); kwds...)
Mutex(; kwds...) = Mutex(Vector{UInt8}(undef, sizeof(MutexData)); kwds...)
function _destroy(obj::Mutex)
if (ptr = obj.handle) != C_NULL
islocked(obj) && unlock(obj)
obj.handle = C_NULL # to not free twice
ccall(:pthread_mutex_destroy, Cint, (Ptr{MutexData},), ptr)
end
nothing
end
Base.islocked(obj::Mutex) = obj.locked
function Base.lock(obj::Mutex)
islocked(obj) && error("mutex is already locked by owner")
code = ccall(:pthread_mutex_lock, Cint, (Ptr{MutexData},), obj)
code == 0 || throw_system_error("pthread_mutex_lock", code)
obj.locked = true
nothing
end
function Base.unlock(obj::Mutex)
islocked(obj) || error("mutex is not locked by owner")
code = ccall(:pthread_mutex_unlock, Cint, (Ptr{MutexData},), obj)
code == 0 || throw_system_error("pthread_mutex_unlock", code)
obj.locked = false
nothing
end
function Base.trylock(obj::Mutex)
if ! islocked(obj)
code = ccall(:pthread_mutex_trylock, Cint, (Ptr{MutexData},), obj)
if code == 0
obj.locked = true
elseif code == Libc.EBUSY
return false
else
throw_system_error("pthread_mutex_trylock", code)
end
end
return true
end
"""
```julia
IPC.Condition()
```
yields an initialized condition variable. The ressources associated to the
condition variable are automatically destroyed when the returned object is
garbage collected.
```julia
IPC.Condition(buf, off=0; shared=false)
```
yields a new condition variable object using buffer `buf` at offset `off` (in
bytes) for its storage. There must be at least `sizeof(IPC.ConditionData)`
available bytes at address `pointer(buf) + off`, these bytes must not be used
for something else and must remain accessible during the lifetime of the
object.
Keyword `shared` can be set true to create a condition variable that is shared
between processes; otherwise, the condition variable is private that is it can
only be used by threads of the process which creates the condition variable. A
shared condition variable must be stored in a part of the memory, like shared
memory, that can be shared with other processes.
See also: [`IPC.Mutex`](@ref), [`IPC.RWLock`](@ref), [`signal`](@ref),
[`broadcast`](@ref), [`wait`](@ref), [`timedwait`](@ref).
"""
mutable struct Condition{T}
handle::Ptr{ConditionData} # typed pointer to object data
buffer::T # object data
function Condition{T}(buf::T, off::Int; shared::Bool=false) where {T}
off β₯ 0 || error("offset must be nonnegative")
sizeof(buf) β₯ sizeof(ConditionData) + off ||
error("insufficient buffer size to store condition variable")
obj = new{T}(pointer(buf) + off, buf, false)
attr = Vector{UInt8}(undef, _sizeof_pthread_condattr_t)
code = ccall(:pthread_condattr_init, Cint, (Ptr{UInt8},), attr)
code == 0 || throw_system_error("pthread_condattr_init", code)
code = ccall(:pthread_condattr_setpshared, Cint, (Ptr{UInt8}, Cint), attr,
(shared ? PTHREAD_PROCESS_SHARED : PTHREAD_PROCESS_PRIVATE))
code == 0 || throw_system_error("pthread_condattr_setpshared", code)
code = ccall(:pthread_cond_init, Cint,
(Ptr{ConditionData}, Ptr{UInt8}), obj, attr)
code == 0 || throw_system_error("pthread_cond_init", code)
code = ccall(:pthread_condattr_destroy, Cint, (Ptr{UInt8},), attr)
code == 0 || (_destroy(obj);
throw_system_error("pthread_condattr_destroy", code))
return finalizer(_destroy, obj)
end
end
Condition(buf::T, off::Integer = 0; kwds...) where {T} =
Condition{T}(buf, Int(off); kwds...)
Condition(; kwds...) = Condition(Vector{UInt8}(undef, sizeof(ConditionData)); kwds...)
function _destroy(obj::Condition)
if (ptr = obj.handle) != C_NULL
obj.handle = C_NULL # to not free twice
ccall(:pthread_cond_destroy, Cint, (Ptr{ConditionData},), ptr)
end
nothing
end
function signal(cond::Condition)
code = ccall(:pthread_cond_signal, Cint, (Ptr{ConditionData},), cond)
code == 0 || throw_system_error("pthread_cond_signal", code)
nothing
end
function Base.broadcast(cond::Condition)
code = ccall(:pthread_cond_broadcast, Cint, (Ptr{ConditionData},), cond)
code == 0 || throw_system_error("pthread_cond_broadcast", code)
nothing
end
function Base.wait(cond::Condition, mutex::Mutex)
code = ccall(:pthread_cond_wait, Cint,
(Ptr{ConditionData}, Ptr{MutexData}), cond, mutex)
code == 0 || throw_system_error("pthread_cond_wait", code)
nothing
end
"""
```julia
timedwait(cond, mutex, lim) -> bool
```
waits for condition variable `cond` to be signaled using lock `mutex` for
synchronization but no longer than the time limit specified by `lim`.
Argument `lim` can be and instance of [``TimeSpec`](@ref) or [`TimeVal`](@ref)
to specify an absolute time limit since the Epoch, or a real to specify a limit
as a number of seconds relative to the current time.
The returned value is `true` if the condition is signalled before expiration of
the time limit, and `false` otherwise.
"""
function Base.timedwait(cond::Condition, mutex::Mutex, abstime::TimeSpec)
code = ccall(:pthread_cond_timedwait, Cint,
(Ptr{ConditionData}, Ptr{MutexData}, Ptr{TimeSpec}),
cond, mutex, Ref(abstime))
if code != 0
code == Libc.ETIMEDOUT || throw_system_error("pthread_cond_timedwait", code)
return false
else
return true
end
end
Base.timedwait(cond::Condition, mutex::Mutex, abstime::Union{TimeVal,Libc.TimeVal}) =
timedwait(cond, mutex, TimeSpec(abstime))
Base.timedwait(cond::Condition, mutex::Mutex, secs::Real) =
timedwait(cond, mutex, now(TimeSpec) + secs)
"""
```julia
IPC.RWLock()
```
yields an initialized read/write lock object. The lock is automatically
released and associated ressources are automatically destroyed when the
returned object is garbage collected.
```julia
IPC.RWLock(buf, off=0; shared=false)
```
yields a new read/write lock object using buffer `buf` at offset `off` (in
bytes) for its storage. There must be at least `sizeof(IPC.RWLockData)`
available bytes at address `pointer(buf) + off`, these bytes must not be used
for something else and must remain accessible during the lifetime of the
object.
Keyword `shared` can be set true to create a read/write lock that is shared
between processes; otherwise, the lock is private that is it can only be used
by threads of the process which creates the lock. A shared lock must be stored
in a part of the memory, like shared memory, that can be shared with other
processes.
See also: [`IPC.Mutex`](@ref), [`IPC.Condition`](@ref), [`lock`](@ref),
[`unlock`](@ref), [`trylock`](@ref).
"""
mutable struct RWLock{T}
handle::Ptr{RWLockData} # typed pointer to object data
buffer::T # object data
locked::Int # 0 = unlocked, 1 = locked for reading, 2 = locked for writing
function RWLock{T}(buf::T, off::Int; shared::Bool=false) where {T}
off β₯ 0 || error("offset must be nonnegative")
sizeof(buf) β₯ sizeof(RWLockData) + off ||
error("insufficient buffer size to store read/write lock")
obj = new{T}(pointer(buf) + off, buf, 0)
attr = Vector{UInt8}(undef, _sizeof_pthread_rwlockattr_t)
code = ccall(:pthread_rwlockattr_init, Cint, (Ptr{UInt8},), attr)
code == 0 || throw_system_error("pthread_rwlockattr_init", code)
code = ccall(:pthread_rwlockattr_setpshared, Cint, (Ptr{UInt8}, Cint), attr,
(shared ? PTHREAD_PROCESS_SHARED : PTHREAD_PROCESS_PRIVATE))
code == 0 || throw_system_error("pthread_rwlockattr_setpshared", code)
code = ccall(:pthread_rwlock_init, Cint,
(Ptr{RWLockData}, Ptr{UInt8}), obj, attr)
code == 0 || throw_system_error("pthread_rwlock_init", code)
code = ccall(:pthread_rwlockattr_destroy, Cint, (Ptr{UInt8},), attr)
code == 0 || (_destroy(obj);
throw_system_error("pthread_rwlockattr_destroy", code))
return finalizer(_destroy, obj)
end
end
RWLock(buf::T, off::Integer = 0; kwds...) where {T} =
RWLock{T}(buf, Int(off); kwds...)
RWLock(; kwds...) = RWLock(Vector{UInt8}(undef, sizeof(RWLockData)); kwds...)
function _destroy(obj::RWLock)
if (ptr = obj.handle) != C_NULL
islocked(obj) && unlock(obj)
obj.handle = C_NULL # to not free twice
ccall(:pthread_rwlock_destroy, Cint, (Ptr{RWLockData},), ptr)
end
nothing
end
Base.islocked(obj::RWLock) = (obj.locked != 0)
"""
```julia
lock(obj, mode)
```
locks read/write lock object `obj` for reading if `mode` is `'r'`, for writing
if `mode` is `'w'`.
"""
function Base.lock(obj::RWLock, mode::Char)
locked = (mode == 'r' ? 1 :
mode == 'w' ? 2 : throw(ArgumentError("invalid mode")))
islocked(obj) && error("r/w lock is already locked by owner")
if mode == 'r'
code = ccall(:pthread_rwlock_rdlock, Cint, (Ptr{RWLockData},), obj)
code == 0 || throw_system_error("pthread_rwlock_rdlock", code)
else
code = ccall(:pthread_rwlock_wrlock, Cint, (Ptr{RWLockData},), obj)
code == 0 || throw_system_error("pthread_rwlock_wrlock", code)
end
obj.locked = locked
nothing
end
function Base.unlock(obj::RWLock)
islocked(obj) || error("r/w lock is not locked by owner")
code = ccall(:pthread_rwlock_unlock, Cint, (Ptr{RWLockData},), obj)
code == 0 || throw_system_error("pthread_rwlock_unlock", code)
obj.locked = 0
nothing
end
"""
```julia
trylock(obj, mode) -> bool
```
attempts to lock read/write lock object `obj` for reading if `mode` is `'r'`,
for writing if `mode` is `'w'` and returns a boolean indicating whether `obj`
has been successfully locked for the given mode.
See Also: [`timedlock`](@ref).
"""
function Base.trylock(obj::RWLock, mode::Char)
locked = (mode == 'r' ? 1 :
mode == 'w' ? 2 : throw(ArgumentError("invalid mode")))
if obj.locked == 0
if mode == 'r'
code = ccall(:pthread_rwlock_tryrdlock, Cint, (Ptr{RWLockData},), obj)
if code != 0
code == Libc.EBUSY ||
throw_system_error("pthread_rwlock_tryrdlock", code)
return false
end
else
code = ccall(:pthread_rwlock_trywrlock, Cint, (Ptr{RWLockData},), obj)
if code != 0
code == Libc.EBUSY ||
throw_system_error("pthread_rwlock_trywrlock", code)
return false
end
end
elseif obj.locked != locked
if mode == 'r'
error("r/w lock is already locked for writing by owner")
else
error("r/w lock is already locked for reading by owner")
end
end
obj.locked = locked
return true
end
"""
```julia
timedlock(rwlock, mode, lim) -> bool
```
locks read/write lock object `rwlock` for reading if `mode` is `'r'`, for
writing if `mode` is `'w'`, but waits no longer than the time limit specified
by `lim`. The returned boolean value indicates whether the object could be
locked before the time limit.
Argument `lim` can be and instance of [``TimeSpec`](@ref) or [`TimeVal`](@ref)
to specify an absolute time limit since the Epoch, or a real to specify a limit
as a number of seconds relative to the current time.
See Also: [`trylock`](@ref).
"""
function timedlock(obj::RWLock, mode::Char, abstime::TimeSpec)
locked = (mode == 'r' ? 1 :
mode == 'w' ? 2 : throw(ArgumentError("invalid mode")))
if obj.locked == 0
if mode == 'r'
code = ccall(:pthread_rwlock_timedrdlock, Cint,
(Ptr{RWLockData}, Ref{TimeSpec}), obj, Ref(abstime))
if code != 0
code == Libc.ETIMEDOUT ||
throw_system_error("pthread_rwlock_timedrdlock", code)
return false
end
else
code = ccall(:pthread_rwlock_timedwrlock, Cint,
(Ptr{RWLockData}, Ref{TimeSpec}), obj, Ref(abstime))
if code != 0
code == Libc.ETIMEDOUT ||
throw_system_error("pthread_rwlock_timedwrlock", code)
return false
end
end
elseif obj.locked != locked
if mode == 'r'
error("r/w lock is already locked for writing by owner")
else
error("r/w lock is already locked for reading by owner")
end
end
obj.locked = locked
return true
end
timedlock(obj::RWLock, mode::Char, abstime::Union{TimeVal,Libc.TimeVal}) =
timedlock(obj, mode, TimeSpec(abstime))
timedlock(obj::RWLock, mode::Char, secs::Real) =
timedlock(obj, mode, now(TimeSpec) + secs)
# The following are needed for ccall's.
Base.unsafe_convert(::Type{Ptr{MutexData}}, obj::Mutex) = obj.handle
Base.unsafe_convert(::Type{Ptr{ConditionData}}, obj::Condition) = obj.handle
Base.unsafe_convert(::Type{Ptr{RWLockData}}, obj::RWLock) = obj.handle
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | code | 9762 | #
# semaphores.jl --
#
# Implements semaphores for Julia.
#
#------------------------------------------------------------------------------
#
# This file is part of InterProcessCommunication.jl released under the MIT
# "expat" license.
#
# Copyright (c) 2016-2024, Γric ThiΓ©baut
# (https://github.com/emmt/InterProcessCommunication.jl).
#
"""
## Named Semaphores
```julia
Semaphore(name, value; perms=0o600, volatile=true) -> sem
```
creates a new named semaphore identified by the string `name` of the form
`"/somename"` and initial value set to `value`. An instance of
`Semaphore{String}` is returned. Keyword `perms` can be used to specify access
permissions.
Keyword `volatile` specifies whether the semaphore should be unlinked when the
returned object is finalized.
```julia
Semaphore(name) -> sem
```
opens an existing named semaphore and returns an instance of
`Semaphore{String}`.
To unlink (remove) a persistent named semaphore, simply do:
```julia
rm(Semaphore, name)
```
If the semaphore does not exists, the error is ignored. A `SystemError` is
however thrown for other errors.
For maximum flexibility, an instance of a named semaphore may also be created
by:
```julia
open(Semaphore, name, flags, mode, value, volatile) -> sem
```
where `flags` may have the bits `IPC.O_CREAT` and `IPC.O_EXCL` set, `mode`
specifies the granted access permissions, `value` is the initial semaphore
value and `volatile` is a boolean indicating whether the semaphore should be
unlinked when the returned object `sem` is finalized. The values of `mode` and
`value` are ignored if an existing named semaphore is open. The permissions
settings in `mode` are masked against the process `umask`.
## Anonymous Semaphores
Anonymous semaphores are backed by *memory* objects providing the necessary
storage.
```julia
Semaphore(mem, value; offset=0, volatile=true) -> sem
```
initializes an anonymous semaphore backed by memory object `mem` with initial
value set to `value` and returns an instance of `Semaphore{typeof(mem)}`.
Keyword `offset` can be used to specify the address (in bytes) of the semaphore
data relative to `pointer(mem)`. Keyword `volatile` specify whether the
semaphore should be destroyed when the returned object is finalized.
```julia
Semaphore(mem; offset=0) -> sem
```
yields an an instance of `Semaphore{typeof(mem)}` associated with an
initialized anonymous semaphore and backed by memory object `mem` at relative
position (in bytes) specified by keyword `offset`.
The number of bytes needed to store an anonymous semaphore is given by
`sizeof(Semaphore)` and anonymous semaphore must be aligned in memory at
multiples of the word size (that is `Sys.WORD_SIZE >> 3` in bytes). Memory
objects used to store an anonymous semaphore must implement two methods:
`pointer(mem)` and `sizeof(mem)` to yield respectively the base address and the
size (in bytes) of the associated memory.
See also: [`post`](@ref), [`wait`](@ref), [`timedwait`](@ref),
[`trywait`](@ref).
"""
function Semaphore(name::AbstractString, value::Integer;
perms::Integer = S_IRUSR | S_IWUSR,
volatile::Bool = true)
val = _check_semaphore_value(value)
mode = maskmode(perms)
flags = O_CREAT | O_EXCL
old_mask = umask(0) # clear file mode creation mask remembering previous settings
try
return open(Semaphore, name, flags, mode, val, volatile)
finally
umask(old_mask) # restore file mode creation mask
end
end
function Semaphore(name::AbstractString)
flags = zero(Cint)
mode = zero(mode_t)
value = zero(Cuint)
return open(Semaphore, name, flags, mode, value, false)
end
function Base.open(::Type{Semaphore}, name::AbstractString, flags::Integer,
mode::Unsigned, value::Unsigned, volatile::Bool)
ptr = _sem_open(name, flags, mode, value)
systemerror("sem_open", ptr == SEM_FAILED)
sem = Semaphore{String}(ptr, name)
if volatile
finalizer(_close_and_unlink, sem)
else
finalizer(_close, sem)
end
return sem
end
# Initialize and anonymous semaphore.
function Semaphore(mem::M, value::Integer;
offset::Integer = 0,
volatile::Bool = true) where {M}
val = _check_semaphore_value(value)
ptr = _get_semaphore_address(mem, offset)
systemerror("sem_init", _sem_init(ptr, true, val) != SUCCESS)
sem = Semaphore{M}(ptr, mem)
if volatile
finalizer(_destroy, sem)
end
return sem
end
# Connect to an initialized anonymous semaphore.
function Semaphore(mem::M; offset::Integer = 0) where {M}
ptr = _get_semaphore_address(mem, offset)
return Semaphore{M}(ptr, mem)
end
# Unlink (remove) a named semaphore.
function Base.rm(::Type{Semaphore}, name::AbstractString)
if _sem_unlink(name) != SUCCESS
errno = Libc.errno()
# On OSX, errno is EINVAL when semaphore does not exists.
if errno != (@static Sys.isapple() ? Libc.EINVAL : Libc.ENOENT)
throw_system_error("sem_unlink", errno)
end
end
end
Base.sizeof(::Type{<:Semaphore}) = _sizeof_sem_t
Base.typemin(::Type{<:Semaphore}) = zero(Cuint)
Base.typemax(::Type{<:Semaphore}) = SEM_VALUE_MAX
function _check_semaphore_value(value::Integer)
typemin(Semaphore) β€ value β€ typemax(Semaphore) ||
throw_argument_error("invalid semaphore value (", value, ")")
return convert(Cuint, value)
end
function _get_semaphore_address(mem, off::Integer)::Ptr{Cvoid}
off β₯ 0 || throw_argument_error("offset must be nonnegative (", off, ")")
ptr, siz = get_memory_parameters(mem)
siz β₯ off + _sizeof_sem_t ||
throw_argument_error("not enough memory at given offset")
ptr += off
align = (Sys.WORD_SIZE >> 3) # assume alignment is word size (in bytes)
rem(convert(Int, ptr), align) == 0 ||
throw_argument_error("address of semaphore must be a multiple of ",
align, " bytes")
return ptr
end
# Finalize a named semaphore.
function _close(obj::Semaphore{String})
_sem_close(obj.ptr)
end
# Finalize a named semaphore.
function _close_and_unlink(obj::Semaphore{String})
_sem_close(obj.ptr)
_sem_unlink(obj.lnk)
end
# Finalize an anonymous semaphore.
function _destroy(obj::Semaphore)
_sem_destroy(obj.ptr)
end
function Base.getindex(sem::Semaphore)
val = Ref{Cint}()
systemerror("sem_getvalue", _sem_getvalue(sem.ptr, val) != SUCCESS)
return val[]
end
"""
```julia
post(sem)
```
increments (unlocks) the semaphore `sem`. If the semaphore's value
consequently becomes greater than zero, then another process or thread blocked
in a [`wait`](@ref) call on this semaphore will be woken up.
See also: [`Semaphore`](@ref), [`wait`](@ref), [`timedwait`](@ref),
[`trywait`](@ref).
"""
post(sem::Semaphore) =
systemerror("sem_post", _sem_post(sem.ptr) != SUCCESS)
"""
```julia
wait(sem)
```
decrements (locks) the semaphore `sem`. If the semaphore's value is greater
than zero, then the decrement proceeds and the function returns immediately.
If the semaphore currently has the value zero, then the call blocks until
either it becomes possible to perform the decrement (i.e., the semaphore value
rises above zero), or a signal handler interrupts the call (in which case an
instance of `InterruptException` is thrown). A `SystemError` may be thrown if
an unexpected error occurs.
See also: [`Semaphore`](@ref), [`post`](@ref), [`timedwait`](@ref),
[`trywait`](@ref).
"""
function Base.wait(sem::Semaphore)
if _sem_wait(sem.ptr) != SUCCESS
code = Libc.errno()
if code == Libc.EINTR
throw(InterruptException())
else
throw_system_error("sem_wait", code)
end
end
nothing
end
"""
```julia
timedwait(sem, secs)
```
decrements (locks) the semaphore `sem`. If the semaphore's value is greater
than zero, then the decrement proceeds and the function returns immediately.
If the semaphore currently has the value zero, then the call blocks until
either it becomes possible to perform the decrement (i.e., the semaphore value
rises above zero), or the limit of `secs` seconds expires (in which case an
instance of [`TimeoutError`](@ref) is thrown), or a signal handler interrupts
the call (in which case an instance of `InterruptException` is thrown).
See also: [`Semaphore`](@ref), [`post`](@ref), [`wait`](@ref),
[`trywait`](@ref).
"""
Base.timedwait(sem::Semaphore, secs::Real) =
timedwait(sem::Semaphore, convert(Float64, secs))
function Base.timedwait(sem::Semaphore, secs::Float64)
tsref = Ref{TimeSpec}(time() + secs)
if _sem_timedwait(sem.ptr, tsref) != SUCCESS
code = Libc.errno()
if code == Libc.EINTR
throw(InterruptException())
elseif code == Libc.ETIMEDOUT
throw(TimeoutError())
else
throw_system_error("sem_timedwait", code)
end
end
nothing
end
"""
```julia
trywait(sem) -> boolean
```
attempts to immediately decrement (lock) the semaphore `sem` returning `true`
if successful. If the decrement cannot be immediately performed, then the call
returns `false`. If an interruption is received or if an unexpected error
occurs, an exception is thrown (`InterruptException` or `SystemError`
repectively).
See also: [`Semaphore`](@ref), [`post`](@ref), [`wait`](@ref),
[`timedwait`](@ref).
"""
function trywait(sem::Semaphore)
if _sem_trywait(sem.ptr) == SUCCESS
return true
end
code = Libc.errno()
if code == Libc.EAGAIN
return false
end
if code == Libc.EINTR
throw(InterruptException())
else
throw_system_error("sem_trywait", code)
end
end
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | code | 18690 | #
# shm.jl --
#
# Management of shared memory for Julia. Both POSIX and System V shared memory
# models are supported.
#
#------------------------------------------------------------------------------
#
# This file is part of InterProcessCommunication.jl released under the MIT
# "expat" license.
#
# Copyright (c) 2016-2024, Γric ThiΓ©baut
# (https://github.com/emmt/InterProcessCommunication.jl).
#
"""
shm = SharedMemory(id, len; perms=0o600, volatile=true)
creates a new shared memory object identified by `id` and whose size is `len` bytes. The
identifier `id` can be a string starting by a `'/'` to create a POSIX shared memory object
or a System V IPC key to create a System V shared memory segment. In this latter case, the
key can be `IPC.PRIVATE` to automatically create a non-existing shared memory segment.
Keyword `perms` is to specify the access permissions to be granted. By default, only
reading and writing by the user are granted.
Keyword `volatile` is to specify whether the shared memory is volatile or not. If
non-volatile, the shared memory will remain accessible until explicit destruction or
system reboot. If volatile (the default), the shared memory is automatically destroyed
when no longer in use by any processes.
To retrieve an existing shared memory object, call:
shm = SharedMemory(id; readonly=false)
where `id` is the shared memory identifier (a string, an IPC key, or a System V IPC
identifier of shared memory segment as returned by [`ShmId`](@ref)). Keyword `readonly`
can be set true if only read access is needed. Note that method `shmid(obj)` may be called
to retrieve the identifier of the shared memory object `obj`.
A number of accessors are available for a shared memory objects `shm`:
pointer(shm) # base address of the shared memory
sizeof(shm) # number of bytes of the shared memory
shmid(shm) # identifier of the shared memory
To ensure that shared memory object `shm` is eventually destroyed, call:
rm(shm)
See also [`ShmId`](@ref), [`shmid`](@ref), and [`shmrm`](@ref).
"""
function SharedMemory(key::Key, len::Integer;
perms::Integer = S_IRUSR|S_IWUSR,
volatile::Bool = true)::SharedMemory{ShmId}
# Create a new System V shared memory segment with given size and, at
# least, read and write access for the caller.
len β₯ 1 || throw_argument_error("bad number of bytes (", len, ")")
flags = maskmode(perms) | (S_IRUSR|S_IWUSR|IPC_CREAT|IPC_EXCL)
id = _shmget(key.value, len, flags)
id < zero(id) && throw_system_error("shmget")
# Attach shared memory segment to process address space.
ptr = _shmat(id, C_NULL, 0)
if ptr == Ptr{Cvoid}(-1)
errno = Libc.errno()
_shmrm(id)
throw_system_error("shmat", errno)
end
if volatile && _shmrm(id) == -1
errno = Libc.errno()
_shmdt(ptr)
throw_system_error("shmctl", errno) # NOTE _shmrm calls shmctl
end
# Instanciate Julia object.
return SharedMemory{ShmId}(ptr, len, volatile, ShmId(id))
end
SharedMemory(key::Key; readonly::Bool = false, kwds...) =
SharedMemory(ShmId(key; readonly=readonly); kwds...)
function SharedMemory(id::ShmId; readonly::Bool=false)::SharedMemory{ShmId}
len = sizeof(id)
ptr = shmat(id, readonly)
return SharedMemory{ShmId}(ptr, len, false, id)
end
# Create a new POSIX shared memory object.
function SharedMemory(name::AbstractString,
len::Integer;
perms::Integer = S_IRUSR | S_IWUSR,
volatile::Bool = true) :: SharedMemory{String}
# Make sure owner has read and write permissions (otherwise setting the
# size will fail).
mode = maskmode(perms) | (S_IRUSR | S_IWUSR)
flags = O_CREAT | O_EXCL | O_RDWR
return SharedMemory(name, flags, mode, len, volatile)
end
# Map an existing POSIX shared memory object.
function SharedMemory(name::AbstractString;
readonly::Bool=false) :: SharedMemory{String}
flags = (readonly ? O_RDONLY : O_RDWR)
mode = (readonly ? S_IRUSR : S_IRUSR|S_IWUSR)
return SharedMemory(name, flags, mode, 0, false)
end
function SharedMemory(name::AbstractString,
flags::Integer,
mode::Integer,
len::Integer = 0,
volatile::Bool = false)
# Create a new POSIX shared memory object?
create = ((flags & O_CREAT) != 0)
if create
len β₯ 1 || throw_argument_error("bad number of bytes (", len, ")")
end
# Open shared memory and set or get its size.
fd = _shm_open(name, flags, mode)
if fd == -1
throw_system_error("shm_open")
end
local nbytes::Int = 0
if create
# Set the size of the new shared memory object.
if _ftruncate(fd, len) != SUCCESS
errno = Libc.errno()
_close(fd)
_shm_unlink(name)
throw_system_error("ftruncate", errno)
end
nbytes = Int(len)
else
# Get the size of the existing shared memory object.
try
nbytes = Int(filesize(fd))
catch err
_close(fd)
rethrow(err)
end
end
# Map the shared memory. Note that `prot = PROT_NONE` should never occur.
access = flags & (O_RDONLY|O_WRONLY|O_RDWR)
prot = (access == O_RDONLY ? PROT_READ :
access == O_WRONLY ? PROT_WRITE :
access == O_RDWR ? PROT_READ|PROT_WRITE : PROT_NONE)
ptr = _mmap(C_NULL, nbytes, prot, MAP_SHARED, fd, 0)
if ptr == MAP_FAILED
errno = Libc.errno()
_close(fd)
if create
_shm_unlink(name)
end
throw_system_error("mmap", errno)
end
# File descriptor can be closed.
if _close(fd) != SUCCESS
errno = Libc.errno()
if create
_shm_unlink(name)
end
_munmap(ptr, len)
throw_system_error("close", errno)
end
# Return the shared memory object.
return SharedMemory{String}(ptr, nbytes, volatile, String(name))
end
function _destroy(obj::SharedMemory{String})
if obj.volatile
_shm_unlink(obj.id)
end
_munmap(obj.ptr, obj.len)
if PARANOID
obj.ptr = C_NULL
obj.len = 0
obj.volatile = false
obj.id = ""
end
end
function _destroy(obj::SharedMemory{ShmId})
_shmdt(obj.ptr)
if PARANOID
obj.ptr = C_NULL
obj.len = 0
obj.volatile = false
obj.id = ShmId(-1)
end
end
Base.sizeof(obj::SharedMemory) = obj.len
Base.pointer(obj::SharedMemory) = obj.ptr
# The short version of `show` if also used for string interpolation in
# scripts so that it is not necessary to extend methods:
# Base.convert(::Type{String}, obj::T)
# Base.string(obj::T)
Base.show(io::IO, obj::SharedMemory{String}) =
print(io, "SharedMemory(\"", obj.id, "\"; len=", obj.len,
", ptr=Ptr{Cvoid}(0x", string(convert(Int, obj.ptr), base=16),"))")
Base.show(io::IO, obj::SharedMemory{ShmId}) =
print(io, "SharedMemory(", obj.id, "; len=", obj.len,
", ptr=Ptr{Cvoid}(0x", string(convert(Int, obj.ptr), base=16),"))")
Base.show(io::IO, ::MIME"text/plain", obj::SharedMemory) = show(io, obj)
"""
id = shmid(arg)
yields the identifier of an existing POSIX shared memory object or System V shared memory
segment identifed by `arg` or associated with `arg`. Argument can be:
* An instance of `SharedMemory`.
* An instance of `WrappedArray` whose contents is stored into shared memory.
* A string starting with a `'/'` (and no other `'/'`) to identify a POSIX shared memory
object.
* An instance of `IPC.Key` to specify a System V IPC key associated with a shared memory
segment. In that case, an optional second argument `readonly` can be set `true` to only
request read-only access; otherwise read-write access is requested.
* An instance of `ShmId` to specify a System V shared memory segment.
See also: [`SharedMemory`](@ref), [`shmrm`](@ref).
"""
shmid(shm::SharedMemory) = shm.id
shmid(arr::WrappedArray{T,N,<:SharedMemory}) where {T,N} = shmid(arr.mem)
shmid(id::ShmId) = id
shmid(key::Key, args...; keys...) = ShmId(key, args...; keys...)
Base.rm(shm::SharedMemory) = shmrm(shm)
Base.rm(id::ShmId) = shmrm(id)
Base.rm(::Type{SharedMemory}, key::Key) = shmrm(key)
Base.rm(::Type{SharedMemory}, name::AbstractString) = shmrm(name)
"""
shmrm(arg)
removes the shared memory associated with `arg`. If `arg` is a name, the corresponding
POSIX named shared memory is unlinked. If `arg` is a key or identifier of a BSD shared
memory segment, the segment is marked to be eventually destroyed. Argument `arg` can also
be a `SharedMemory` object.
The `rm` method may also be called to remove an existing shared memory segment or object.
There are several possibilities:
```julia
rm(SharedMemory, name) # `name` identifies a POSIX shared memory object
rm(SharedMemory, key) # `key` is associated with a BSD shared memory segment
rm(id) # `id` is the identifier of a BSD shared memory segment
rm(shm) # `shm` is an instance of `SharedMemory`
```
See also: [`SharedMemory`](@ref), [`shmid`](@ref), [`shmat`](@ref).
"""
shmrm(shm::SharedMemory) = shmrm(shmid(shm))
shmrm(key::Key) = shmrm(shmid(key))
shmrm(name::AbstractString) = begin
if _shm_unlink(name) != SUCCESS
errno = Libc.errno()
if errno != Libc.ENOENT
throw_system_error("shm_unlink", errno)
end
end
end
shmrm(id::ShmId) = begin
if _shmrm(name) == -1
# Only throw an error if not an already removed shared memory segment.
errno = Libc.errno()
if errno != Libc.EIDRM
throw_system_error("shmctl", errno)
end
end
end
#------------------------------------------------------------------------------
# System V Shared Memory
#
# Methods which directly call C functions are prefixed by an underscore and do
# not perform any checking of their arguments (except that type of arguments
# must be correct).
#
# Higher level versions are not prefixed by an underscore and throw a
# `SystemError` if the value returned by the C function indicates an error.
# Their arguments may be slightly different.
#
_shmget(key::Integer, siz::Integer, flg::Integer) =
ccall(:shmget, Cint, (key_t, Csize_t, Cint), key, siz, flg)
_shmat(id::Integer, ptr::Ptr{Cvoid}, flg::Integer) =
ccall(:shmat, Ptr{Cvoid}, (Cint, Ptr{Cvoid}, Cint), id, ptr, flg)
_shmdt(ptr::Ptr{Cvoid}) =
ccall(:shmdt, Cint, (Ptr{Cvoid},), ptr)
_shmctl(id::Integer, cmd::Integer, buf) =
ccall(:shmctl, Cint, (Cint, Cint, Ptr{Cvoid}), id, cmd, buf)
_shmrm(id::Integer) = _shmctl(id, IPC_RMID, C_NULL)
Base.convert(::Type{Cint}, id::ShmId) = id.value
Base.convert(::Type{T}, id::ShmId) where {T<:Integer} = convert(T, id.value)
Base.show(io::IO, id::ShmId) = print(io, "ShmId(", id.value, ")")
Base.show(io::IO, ::MIME"text/plain", id::ShmId) = show(io, id)
function Base.sizeof(id::ShmId)
# Note it is faster to create a Julia array of small size than calling
# malloc/free or using a tuple unless the number of elements of the tuple
# is small.
buf = _workspace(_sizeof_struct_shmid_ds)
unsafe_shmctl(id, IPC_STAT, buf)
return _peek(_typeof_shm_segsz, buf, _offsetof_shm_segsz)
end
"`_workspace(size)` yields an array of `size` uninitialized bytes."
@inline _workspace(size::Integer) = Array{UInt8}(undef, size)
"""
id = ShmId(id)
id = ShmId(shm)
id = ShmId(arr)
id = ShmId(key; readlony=false)
yield the the identifier of the existing System V shared memory segment associated with
the value of the first argument: `id` is the identifier of the shared memory segment,
`shm` is a System V shared memory object, `arr` is an array attached to a System V shared
memory segment, and `key` is the key associated with the shared memory segment. In that
latter case, keyword `readlony` can be set `true` to request read-only access; otherwise
read-write access is requested.
See also: [`shmid`](@ref), [`shmget`](@ref).
"""
ShmId(id::ShmId) = id
ShmId(shm::SharedMemory{ShmId}) = shmid(shm)
ShmId(arr::WrappedArray{T,N,SharedMemory{ShmId}}) where {T,N} = shmid(arr)
ShmId(key::Key; readonly::Bool = false) =
shmget(key, 0, (readonly ? S_IRUSR : (S_IRUSR|S_IWUSR)))
@deprecate ShmId(key::Key, readonly::Bool) ShmId(key; readonly=readonly) false
"""
id = shmget(key, siz, flg)
yields the identifier of the System V shared memory segment associated with the System V
IPC key `key`. A new shared memory segment with size `siz` (in bytes, possibly rounded up
to a multiple of the memory page size `IPC.PAGE_SIZE`) is created if `key` has the value
`IPC.PRIVATE` or if bit `IPC_CREAT` is set in `flg` and no shared memory segment
corresponding to `key` exists.
Argument `flg` is a bitwise combination of flags. The least significant 9 bits specify the
permissions granted to the owner, group, and others. These bits have the same format, and
the same meaning, as the mode argument of `chmod`. Bit `IPC_CREAT` can be set to create a
new segment. If this flag is not used, then `shmget` will find the segment associated with
`key` and check to see if the user has permission to access the segment. Bit `IPC_EXCL`
can be set in addition to `IPC_CREAT` to ensure that this call creates the segment. If
`IPC_EXCL` and `IPC_CREAT` are both set, the call will fail if the segment already exists.
"""
function shmget(key::Key, siz::Integer, flg::Integer)
id = _shmget(key.value, siz, flg)
systemerror("shmget", id < 0)
return ShmId(id)
end
"""
ptr = shmat(id, readonly)
attaches a System V shared memory segment to the address space of the caller. Argument
`id` is the identifier of the shared memory segment. Boolean argument `readonly` specifies
whether to attach the segment for read-only access; otherwise, the segment is attached for
read and write accesses and the process must have read and write permissions for the
segment. The returned value is the address of the shared memory segment for the caller.
See also: [`shmdt`](@ref), [`shmrm`](@ref).
"""
function shmat(id::ShmId, readonly::Bool)
flg = (readonly ? SHM_RDONLY : zero(SHM_RDONLY))
ptr = _shmat(id.value, C_NULL, flg)
systemerror("shmat", ptr == Ptr{Cvoid}(-1))
return ptr
end
"""
shmdt(ptr)
detaches a System V shared memory segment from the address space of the caller.
Argument `ptr` is the pointer returned by a previous [`shmat`](@ref) call.
See also: [`shmat`](@ref), [`shmget`](@ref).
"""
shmdt(ptr::Ptr{Cvoid}) = systemerror("shmdt", _shmdt(ptr) != SUCCESS)
"""
shmctl(id, cmd, buf)
performs the control operation specified by `cmd` on the System V shared memory segment
whose identifier is given in `id`. The `buf` argument is a byte array large enough to
store a `shmid_ds` C structure.
See also [`shminfo`](@ref), [`shmcfg`](@ref) and [`shmrm`](@ref).
"""
function shmctl(id::ShmId, cmd::Integer, buf::DenseVector)
isconcretetype(eltype(buf)) || throw(ArgumentError("buffer entries must have concrete type"))
sizeof(buf) β₯ _sizeof_struct_shmid_ds || throw(ArgumentError(
"buffer must have at least $(_sizeof_struct_shmid_ds) bytes"))
return unsafe_shmctl(id, cmd, buf)
end
# This version does not checks its arguments, only the returned value.
unsafe_shmctl(id::ShmId, cmd::Integer, buf) =
systemerror("shmctl", _shmctl(id.value, cmd, buf) == -1)
"""
id = shmcfg(arg, perms) -> id
changes the access permissions of a System V IPC shared memory segment identified by
`arg`, a System V IPC shared memory identifier, a shared array attached to a System V IPC
shared memory segment, or a System V IPC key associated with a shared memory segment.
Argument `perms` specifies bitwise flags with the new permissions. The identifier of the
shared memory segment is returned.
See also [`ShmId`](@ref), [`shmget`](@ref), [`shmctl`](@ref) and [`SharedMemory`](@ref).
"""
function shmcfg(id::ShmId, perms::Integer)
perms = _typeof_shm_perm_mode(perms)
mask = _typeof_shm_perm_mode(MASKMODE)
buf = _workspace(_sizeof_struct_shmid_ds)
unsafe_shmctl(id, IPC_STAT, buf)
mode = _peek(_typeof_shm_perm_mode, buf, _offsetof_shm_perm_mode)
if (mode & mask) != (perms & mask)
_poke!(_typeof_shm_perm_mode, buf, _offsetof_shm_perm_mode, (mode & ~mask) | (perms & mask))
unsafe_shmctl(id, IPC_SET, buf)
end
return id
end
shmcfg(arg::Union{ShmArray,Key}, perms::Integer) = shmcfg(ShmId(arg), perms)
"""
ShmInfo
is the type of the structure storing information about a System V shared memory segment.
The call `ShmInfo(arg)` is equivalent to [`shminfo(arg)`](@ref shminfo).
"""
ShmInfo(arg::Union{ShmId,ShmArray,Key}) = shminfo(arg)
"""
info = shminfo(arg)
yields information about the System V shared memory segment identified or associated with
`arg`, the identifier of the shared memory segment, a shared array attached to the shared
memory segment, or the System V IPC key associated with the shared memory segment.
See also [`ShmInfo`](@ref), [`ShmId`](@ref), [`shmget`](@ref), [`shmat`](@ref),
and [`SharedMemory`](@ref).
"""
shminfo(arg::Union{ShmId,ShmArray,Key}) = shminfo!(arg, ShmInfo())
"""
info = shminfo!(arg, info)
overwrites `info` (an instance of [`ShmInfo`](@ref)) with the information about the System
V shared memory segment identified by or associated with `arg`. See [`shminfo`](@ref) for
more details.
"""
function shminfo!(id::ShmId, info::ShmInfo)
buf = _workspace(_sizeof_struct_shmid_ds)
unsafe_shmctl(id, IPC_STAT, buf)
info.atime = _peek(time_t, buf, _offsetof_shm_atime)
info.dtime = _peek(time_t, buf, _offsetof_shm_dtime)
info.ctime = _peek(time_t, buf, _offsetof_shm_ctime)
info.segsz = _peek(_typeof_shm_segsz, buf, _offsetof_shm_segsz)
info.id = id.value
info.cpid = _peek(pid_t, buf, _offsetof_shm_cpid)
info.lpid = _peek(pid_t, buf, _offsetof_shm_lpid)
info.nattch = _peek(shmatt_t, buf, _offsetof_shm_nattch)
info.mode = _peek(_typeof_shm_perm_mode, buf, _offsetof_shm_perm_mode)
info.uid = _peek(uid_t, buf, _offsetof_shm_perm_uid)
info.gid = _peek(gid_t, buf, _offsetof_shm_perm_gid)
info.cuid = _peek(uid_t, buf, _offsetof_shm_perm_cuid)
info.cgid = _peek(gid_t, buf, _offsetof_shm_perm_cgid)
return info
end
shminfo!(arg, info::ShmInfo) = shminfo!(ShmId(arg), info)
shminfo!(key::Key, info::ShmInfo) = shminfo!(ShmId(key; readonly=true), info)
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | code | 19617 | #
# signals.jl --
#
# Implements "real-time" signals for Julia.
#
#------------------------------------------------------------------------------
#
# This file is part of InterProcessCommunication.jl released under the MIT
# "expat" license.
#
# Copyright (c) 2016-2024, Γric ThiΓ©baut
# (https://github.com/emmt/InterProcessCommunication.jl).
#
# Part of the documentation is more or less directly extracted from the Linux
# manual pages (http://www.kernel.org/doc/man-pages/).
#
"""
`SigSet` represents a C `sigset_t` structure. It should be considered as
*opaque*, its contents is stored as a tuple of unsigned integers whose size
matches that of `sigset_t`.
Typical usage is:
```julia
sigset = SigSet()
sigset[signum] -> boolean
sigset[signum] = boolean
fill!(sigset, boolean) -> sigset
```
where `signum` is the signal number, an integer greater or equal `1` and less
or equal`IPC.SIGRTMAX`. Real-time signals have a number `signum` such that
`IPC.SIGRTMIN β€ signum β€ IPC.SIGRTMAX`
Non-exported methods:
```julia
IPC.sigfillset!(sigset) # same as fill!(signum, true)
IPC.sigemptyset!(sigset) # same as fill!(signum, false)
IPC.sigaddset!(sigset, signum) # same as sigset[signum] = true
IPC.sigdelset!(sigset, signum) # same as sigset[signum] = false
IPC.sigismember(sigset, signum) # same as sigset[signum]
```
""" SigSet
Base.unsafe_convert(::Type{T}, pid::ProcessId) where {T<:Integer} =
convert(T, pid.value)
Base.cconvert(::Type{T}, pid::ProcessId) where {T<:Integer} =
convert(T, pid.value)
Base.getindex(sigset::SigSet, i::Integer) = sigismember(sigset, i)
Base.setindex!(sigset::SigSet, val::Bool, i::Integer) = begin
if val
sigaddset!(sigset, i)
else
sigdelset!(sigset, i)
end
sigset
end
function Base.fill!(sigset::SigSet, val::Bool)
if val
sigfillset!(sigset)
else
sigemptyset!(sigset)
end
return sigset
end
for T in (SigSet, SigInfo)
@eval begin
Base.pointer(obj::$T) = unsafe_convert(Ptr{$T}, obj)
Base.unsafe_convert(::Type{Ptr{$T}}, obj::$T) =
convert(Ptr{$T}, pointer_from_objref(obj))
end
end
sigemptyset!(sigset::SigSet) =
systemerror("sigemptyset", _sigemptyset(pointer(sigset)) != SUCCESS)
_sigemptyset(sigset::Ref{SigSet}) =
ccall(:sigemptyset, Cint, (Ptr{SigSet},), sigset)
sigfillset!(sigset::SigSet) =
systemerror("sigfillset", _sigfillset(pointer(sigset)) != SUCCESS)
_sigfillset(sigset::Ref{SigSet}) =
ccall(:sigfillset, Cint, (Ptr{SigSet},), sigset)
sigaddset!(sigset::SigSet, signum::Integer) =
systemerror("sigaddset",
_sigaddset(pointer(sigset), signum) != SUCCESS)
_sigaddset(sigset::Ref{SigSet}, signum::Integer) =
ccall(:sigaddset, Cint, (Ptr{SigSet}, Cint), sigset, signum)
sigdelset!(sigset::SigSet, signum::Integer) =
systemerror("sigdelset",
_sigdelset(pointer(sigset), signum) != SUCCESS)
_sigdelset(sigset::Ref{SigSet}, signum::Integer) =
ccall(:sigdelset, Cint, (Ptr{SigSet}, Cint), sigset, signum)
function sigismember(sigset::SigSet, signum::Integer)
val = _sigismember(pointer(sigset), signum)
systemerror("sigismember", val == FAILURE)
return val == one(val)
end
_sigismember(sigset::Ref{SigSet}, signum::Integer) =
ccall(:sigismember, Cint, (Ptr{SigSet}, Cint), sigset, signum)
"""
```julia
sigqueue(pid, sig, val=0)
```
sends the signal `sig` to the process whose identifier is `pid`. Argument
`val` is an optional value to join to to the signal. This value represents a C
type `union sigval` (an union of a C `int` and a C `void*`), in Julia it is
specified as an integer large enough to represent both kind of values.
"""
sigqueue(pid::ProcessId, sig::Integer, val::Integer = 0) =
systemerror("sigqueue",
_sigqueue(pid.value, sig, val) != SUCCESS)
_sigqueue(pid::Integer, sig::Integer, val::Integer) =
ccall(:sigqueue, Cint, (pid_t, Cint, sigval_t), pid, sig, val)
"""
```julia
sigpending() -> mask
```
yields the set of signals that are pending for delivery to the calling thread
(i.e., the signals which have been raised while blocked). The returned value
is an instance of [`SigSet`](@ref).
See also: [`sigpending!`](@ref).
"""
sigpending() = sigpending!(SigSet())
"""
```julia
sigpending!(mask) -> mask
```
overwites `mask` with the set of pending signals and returns its argument.
See also: [`sigpending`](@ref).
"""
function sigpending!(mask::SigSet)
systemerror("sigpending", _sigpending(pointer(mask)) != SUCCESS)
return mask
end
_sigpending(mask::Ref{SigSet}) =
ccall(:sigpending, Cint, (Ptr{SigSet},), mask)
"""
```julia
sigprocmask() -> cur
```
yields the current set of blocked signals. To change the set of blocked
signals, call:
```julia
sigprocmask(how, set)
```
with `set` a [`SigSet`](@ref) mask and `how` a parameter which specifies how to
interpret `set`:
* `IPC.SIG_BLOCK`: The set of blocked signals is the union of the current set
and the `set` argument.
* `IPC.SIG_UNBLOCK`: The signals in `set` are removed from the current set of
blocked signals. It is permissible to attempt to unblock a signal which is
not blocked.
* `IPC.SIG_SETMASK`: The set of blocked signals is set to the argument `set`.
See also: [`sigprocmask!`](@ref).
"""
sigprocmask() = sigprocmask!(SigSet())
sigprocmask(how::Integer, set::SigSet) =
systemerror(string(_sigprocmask_symbol),
_sigprocmask(how, pointer(set), Ptr{SigSet}(0)) != SUCCESS)
# Constant `_sigprocmask_symbol` is `:sigprocmask` or `:pthread_sigmask`.
const _sigprocmask_symbol = :pthread_sigmask
"""
```julia
sigprocmask!(cur) -> cur
```
overwrites `cur`, an instance of [`SigSet`](@ref), with the current set of
blocked signals and returns it. To change the set of blocked signals, call:
```julia
sigprocmask!(how, set, old) -> old
```
which changes the set of blocked signals according to `how` and `set` (see
[`sigprocmask`](@ref)), overwrites `old`, an instance of [`SigSet`](@ref), with
the previous set of blocked signals and returns `old`.
See also: [`sigprocmask`](@ref).
"""
function sigprocmask!(cur::SigSet)
systemerror(string(_sigprocmask_symbol),
_sigprocmask(IPC.SIG_BLOCK, Ptr{SigSet}(0), pointer(cur)) != SUCCESS)
return cur
end
function sigprocmask!(how::Integer, set::SigSet, old::SigSet)
systemerror(string(_sigprocmask_symbol),
_sigprocmask(how, pointer(set), pointer(old)) != SUCCESS)
return old
end
_sigprocmask(how::Integer, set::Ref{SigSet}, old::Ref{SigSet}) =
ccall(_sigprocmask_symbol, Cint, (Cint, Ptr{SigSet}, Ptr{SigSet}),
how, set, old)
"""
```julia
sigsuspend(mask)
```
temporarily replaces the signal mask of the calling process with the mask given
by `mask` and then suspends the process until delivery of a signal whose action
is to invoke a signal handler or to terminate a process.
If the signal terminates the process, then `sigsuspend` does not return. If
the signal is caught, then `sigsuspend` returns after the signal handler
returns, and the signal mask is restored to the state before the call to
`sigsuspend`.
It is not possible to block `IPC.SIGKILL` or `IPC.SIGSTOP`; specifying these
signals in mask, has no effect on the process's signal mask.
"""
sigsuspend(mask::SigSet) =
systemerror("sigsuspend", _sigsuspend(pointer(mask)) != SUCCESS)
_sigsuspend(mask::Ref{SigSet}) =
ccall(:sigsuspend, Cint, (Ptr{SigSet},), mask)
"""
```julia
sigwait(mask, timeout=Inf) -> signum
```
suspends execution of the calling thread until one of the signals specified in
the signal set `mask` becomes pending. The function accepts the signal
(removes it from the pending list of signals), and returns the signal number
`signum`.
Optional argument `timeout` can be specified to set a limit on the time to wait
for one the signals to become pending. `timeout` can be a real number to
specify a number of seconds or an instance of [`TimeSpec`](@ref). If `timeout`
is `Inf` (the default), it is assumed that there is no limit on the time to
wait. If `timeout` is a number of seconds smaller or equal zero or if
`timeout` is `TimeSpec(0,0)`, the methods performs a poll and returns
immediately. It none of the signals specified in the signal set `mask` becomes
pending during the allowed waiting time, a [`TimeoutError`](@ref) exception is
thrown.
See also: [`sigwait!`](@ref), [`TimeSpec`](@ref), [`TimeoutError`](@ref).
"""
function sigwait(mask::SigSet)
signum = Ref{Cint}()
code = _sigwait(pointer(mask), signum)
code == 0 || throw_system_error("sigwait", code)
return signum[]
end
sigwait(mask::SigSet, secs::Real)::Cint =
(secs β₯ Inf ? sigwait(mask) : sigwait(mask, _sigtimedwait_timeout(secs)))
function sigwait(mask::SigSet, timeout::TimeSpec)::Cint
signum = _sigtimedwait(pointer(mask), Ptr{SigInfo}(0), Ref(timeout))
signum == FAILURE && _throw_sigtimedwait_error()
return signum
end
"""
```julia
sigwait!(mask, info, timeout=Inf) -> signum
```
behaves like [`sigwait`](@ref) but additional argument `info` is an instance of
[`SigInfo`](@ref) to store the information about the accepted signal, other
arguments are as for the [`sigwait`](@ref) method.
"""
sigwait!(mask::SigSet, info::SigInfo, secs::Real)::Cint =
(secs β₯ Inf ? sigwait!(mask, info) :
sigwait!(mask, info, _sigtimedwait_timeout(secs)))
function sigwait!(mask::SigSet, info::SigInfo)
signum = _sigwaitinfo(pointer(mask), pointer(info))
systemerror("sigwaitinfo", signum == FAILURE)
return signum
end
function sigwait!(mask::SigSet, info::SigInfo, timeout::TimeSpec)
signum = _sigtimedwait(pointer(mask), pointer(info), Ref(timeout))
signum == FAILURE && _throw_sigtimedwait_error()
return signum
end
_sigwait(mask::Ref{SigSet}, signum::Ref{Cint}) =
ccall(:sigwait, Cint, (Ptr{Cvoid}, Ptr{Cint}), mask, signum)
_sigwaitinfo(set::Ref{SigSet}, info::Ref{SigInfo}) =
ccall(:sigwaitinfo, Cint, (Ptr{SigSet}, Ptr{SigInfo}), set, info)
_sigtimedwait(set::Ref{SigSet}, info::Ref{SigInfo}, timeout::Ref{TimeSpec}) =
ccall(:sigtimedwait, Cint, (Ptr{SigSet}, Ptr{SigInfo}, Ptr{TimeSpec}),
set, info, timeout)
function _sigtimedwait_timeout(secs::Real)
isnan(secs) && throw_argument_error("number of seconds is NaN")
if secs > 0
# Timeout is in the future.
return TimeSpec(secs)
else
# Set timeout so as to perform a poll and return immediately.
return TimeSpec(0, 0)
end
end
function _throw_sigtimedwait_error()
errno = Libc.errno()
if errno == Libc.EAGAIN
throw(TimeoutError())
elseif errno == Libc.EINTR
throw(InterruptException())
else
throw_system_error("sigtimedwait", errno)
end
end
"""
`SigAction` is the counterpart of the C `struct sigaction` structure. It is
used to specify the action taken by a process on receipt of a signal. Assuming
`sa` is an instance of `SigAction`, its fields are:
```julia
sa.handler # address of a signal handler
sa.mask # mask of the signals to block
sa.flags # bitwise flags
```
where `sa.handler` is the address of a C function (can be `SIG_IGN` or
`SIG_DFL`) to be called on receipt of the signal. This function may be given
by `cfunction`. If `IPC.SA_INFO` is not set in `sa.flags`, then the signature
of the handler is:
```julia
function handler(signum::Cint)::Nothing
```
that is a function which takes a single argument of type `Cint` and returns
nothing; if `IPC.SA_INFO` is not set in `sa.flags`, then the signature of the
handler is:
```julia
function handler(signum::Cint, siginf::Ptr{SigInfo}, unused::Ptr{Cvoid})::Nothing
```
that is a function which takes 3 arguments of type `Cint`, `Ptr{SigInfo}`,
`Ptr{Cvoid}` repectively and which returns nothing. See [`SigInfo`](@ref) for
a description of the `siginf` argument by the handler.
Call:
```julia
sa = SigAction()
```
to create a new empty structure or
```julia
sa = SigAction(handler, mask, flags)
```
to provide all fields.
See also [`SigInfo`](@ref), [`sigaction`](@ref) and [`sigaction!`](@ref).
"""
SigAction() = SigAction(C_NULL, SigSet(), 0)
function Base.show(io::IO, obj::SigAction)
print(io, "SigAction(handler=Ptr{Cvoid}(")
@printf(io, "%p", obj.handler)
print(io, "), mask=SigSet(....), flags=0x", string(obj.flags, base=16), ")")
end
Base.show(io::IO, ::MIME"text/plain", obj::SigAction) = show(io, obj)
"""
```julia
sigaction(signum) -> sigact
```
yields the current action (an instance of [`SigAction`](@ref)) taken by the
process on receipt of the signal `signum`.
```julia
sigaction(signum, sigact)
```
installs `sigact` (an instance of [`SigAction`](@ref)) to be the action taken
by the process on receipt of the signal `signum`.
Note that `signum` cannot be `SIGKILL` nor `SIGSTOP`.
See also [`SigAction`](@ref) and [`sigaction!`](@ref).
"""
function sigaction(signum::Integer)
buf = _sigactionbuffer()
ptr = pointer(buf)
systemerror("sigaction", _sigaction(signum, C_NULL, ptr) != SUCCESS)
flags = _getsigactionflags(ptr)
return SigAction(_getsigactionhandler(ptr, flags),
_getsigactionmask(ptr), flags)
end
function sigaction(signum::Integer, sigact::SigAction)
buf = _sigactionbuffer()
ptr = pointer(buf)
_storesigaction!(ptr, sigact)
systemerror("sigaction", _sigaction(signum, ptr, C_NULL) != SUCCESS)
end
"""
```julia
sigaction!(signum, sigact, oldact) -> oldact
```
installs `sigact` to be the action taken by the process on receipt of the
signal `signum`, overwrites `oldact` with the previous action and returns it.
Arguments `sigact` and `oldact` are instances of [`SigAction`](@ref).
See [`SigAction`](@ref) and [`sigaction`](@ref) for more details.
"""
function sigaction!(signum::Integer, sigact::SigAction, old::SigAction)
newbuf = _sigactionbuffer()
newptr = pointer(newbuf)
oldbuf = _sigactionbuffer()
oldptr = pointer(oldbuf)
_storesigaction!(newptr, sigact)
systemerror("sigaction", _sigaction(signum, newptr, oldptr) != SUCCESS)
old.flags = _getsigactionflags(oldptr)
old.handler = _getsigactionhandler(oldptr, old.flags)
old.mask = _getsigactionmask(oldptr)
return old
end
_sigaction(signum::Integer, act::Ptr{<:Union{UInt8,Nothing}}, old::Ptr{<:Union{UInt8,Nothing}}) =
ccall(:sigaction, Cint, (Cint, Ptr{Cvoid}, Ptr{Cvoid}), signum, act, old)
_sigactionbuffer() = zeros(UInt8, _sizeof_sigaction)
function _storesigaction!(ptr::Ptr{UInt8}, sigact::SigAction)
_setsigactionhandler!(ptr, sigact.handler, sigact.flags)
_setsigactionmask!(ptr, sigact.mask)
_setsigactionflags!(ptr, sigact.flags)
return ptr
end
function _getsigactionhandler(ptr::Ptr{UInt8}, flags::_typeof_sigaction_flags)
offset = ((flags & SA_SIGINFO) == SA_SIGINFO ?
_offsetof_sigaction_action :
_offsetof_sigaction_handler)
return _peek(Ptr{Cvoid}, ptr + offset)
end
function _setsigactionhandler!(ptr::Ptr{UInt8}, handler::Ptr{Cvoid},
flags::_typeof_sigaction_flags)
offset = ((flags & SA_SIGINFO) == SA_SIGINFO ?
_offsetof_sigaction_action :
_offsetof_sigaction_handler)
_poke!(Ptr{Cvoid}, ptr + offset, handler)
end
_setsigactionaction!(ptr::Ptr{UInt8}, action::Ptr{Cvoid}) =
_poke!(Ptr{Cvoid}, ptr + _offsetof_sigaction_action, action)
_getsigactionmask(ptr::Ptr{UInt8}) =
_peek(SigSet, ptr + _offsetof_sigaction_mask)
_setsigactionmask!(ptr::Ptr{UInt8}, mask::SigSet) =
_poke!(SigSet, ptr + _offsetof_sigaction_mask, mask)
_getsigactionflags(ptr::Ptr{UInt8}) =
_peek(_typeof_sigaction_flags, ptr + _offsetof_sigaction_flags)
_setsigactionflags!(ptr::Ptr{UInt8}, flags::Integer) =
_poke!(_typeof_sigaction_flags, ptr + _offsetof_sigaction_flags, flags)
"""
`SigInfo` represents a C `siginfo_t` structure. It should be considered as
opaque, its contents is stored as a tuple of unsigned integers whose size
matches that of `siginfo_t` but, in principle, only a pointer of it should be
received by a signal handler established with the `SA_SIGINFO` flag.
Given `ptr`, an instance of `Ptr{SigInfo}` received by a signal handler, the
members of the corresponding C `siginfo_t` structure are retrieved by:
```julia
IPC.siginfo_signo(ptr) # Signal number.
IPC.siginfo_code(ptr) # Signal code.
IPC.siginfo_errno(ptr) # If non-zero, an errno value associated with this
# signal.
IPC.siginfo_pid(ptr) # Sending process ID.
IPC.siginfo_uid(ptr) # Real user ID of sending process.
IPC.siginfo_addr(ptr) # Address of faulting instruction.
IPC.siginfo_status(ptr) # Exit value or signal.
IPC.siginfo_band(ptr) # Band event for SIGPOLL.
IPC.siginfo_value(ptr) # Signal value.
```
These methods are *unsafe* because they directly use an address. They are
therefore not exported by default. Depending on the context, not all members
of `siginfo_t` are relevant (furthermore they may be defined as union and thus
overlap in memory). For now, only the members defined by the POSIX standard
are accessible. Finally, the value given by `IPC.siginfo_value(ptr)`
represents a C type `union sigval` (an union of a C `int` and a C `void*`), in
Julia it is returned (and set in [`sigqueue`](@ref)) as an integer large enough
to represent both kind of values.
""" SigInfo
for f in (:siginfo_signo, :siginfo_code, :siginfo_errno, :siginfo_pid,
:siginfo_uid, :siginfo_status, :siginfo_value, :siginfo_addr,
:siginfo_band)
@eval begin
@doc @doc(SigInfo) $f
$f(si::SigInfo) = $f(pointer(si))
end
end
siginfo_signo(ptr::Ptr{SigInfo}) =
_peek(Cint, ptr + _offsetof_siginfo_signo)
siginfo_code(ptr::Ptr{SigInfo}) =
_peek(Cint, ptr + _offsetof_siginfo_code)
siginfo_errno(ptr::Ptr{SigInfo}) =
_peek(Cint, ptr + _offsetof_siginfo_errno)
siginfo_pid(ptr::Ptr{SigInfo}) =
_peek(ProcessId, ptr + _offsetof_siginfo_pid)
siginfo_uid(ptr::Ptr{SigInfo}) =
_peek(UserId, ptr + _offsetof_siginfo_uid)
siginfo_status(ptr::Ptr{SigInfo}) =
_peek(Cint, ptr + _offsetof_siginfo_status)
siginfo_value(ptr::Ptr{SigInfo}) =
_peek(sigval_t, ptr + _offsetof_siginfo_value)
siginfo_addr(ptr::Ptr{SigInfo}) =
_peek(Ptr{Cvoid}, ptr + _offsetof_siginfo_addr)
siginfo_band(ptr::Ptr{SigInfo}) =
_peek(Clong, ptr + _offsetof_siginfo_band)
# FIXME: non-POSIX
# siginfo_utime(ptr::Ptr{SigInfo}) =
# _peek(clock_t, ptr + _offsetof_siginfo_utime)
# siginfo_stime(ptr::Ptr{SigInfo}) =
# _peek(clock_t, ptr + _offsetof_siginfo_stime)
# siginfo_int(ptr::Ptr{SigInfo}) =
# _peek(Cint, ptr + _offsetof_siginfo_int)
# siginfo_ptr(ptr::Ptr{SigInfo}) =
# _peek(Ptr{Cvoid}, ptr + _offsetof_siginfo_ptr)
# siginfo_overrun(ptr::Ptr{SigInfo}) =
# _peek(Cint, ptr + _offsetof_siginfo_overrun)
# siginfo_timerid(ptr::Ptr{SigInfo}) =
# _peek(Cint, ptr + _offsetof_siginfo_timerid)
# siginfo_fd(ptr::Ptr{SigInfo}) =
# _peek(Cint, ptr + _offsetof_siginfo_fd)
# siginfo_addr_lsb(ptr::Ptr{SigInfo}) =
# _peek(Cshort, ptr + _offsetof_siginfo_)
# siginfo_call_addr(ptr::Ptr{SigInfo}) =
# _peek(Ptr{Cvoid}, ptr + _offsetof_siginfo_)
# siginfo_syscall(ptr::Ptr{SigInfo}) =
# _peek(Cint, ptr + _offsetof_siginfo_)
# siginfo_arch(ptr::Ptr{SigInfo}) =
# _peek(Cint, ptr + _offsetof_siginfo_)
# FIXME: siginterrupt - allow signals to interrupt system calls.
# Not implemented (obsoleted in POSIX), use sigaction with SA_RESTART
# flag instead.
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | code | 5402 | #
# types.jl --
#
# Definitions fo types for the InterProcessCommunication (IPC) package of
# Julia.
#
#------------------------------------------------------------------------------
#
# This file is part of InterProcessCommunication.jl released under the MIT
# "expat" license.
#
# Copyright (c) 2016-2024, Γric ThiΓ©baut
# (https://github.com/emmt/InterProcessCommunication.jl).
#
"""
`TimeoutError` is used to throw a timeout exception.
"""
struct TimeoutError <: Exception; end
struct TimeVal
sec::_typeof_timeval_sec # seconds
usec::_typeof_timeval_usec # microseconds
end
struct TimeSpec
sec::_typeof_timespec_sec # seconds
nsec::_typeof_timespec_nsec # nanoseconds
end
struct ProcessId
value::pid_t
end
struct UserId
value::uid_t
end
# Counterpart of C `sigset_t` structure. Must be mutable to avoid wrapping it
# in a Ref when passed by address.
mutable struct SigSet
bits::_typeof_sigset
SigSet() = fill!(new(), false)
end
# Counterpart of C `struct sigaction` structure. Must be mutable to avoid
# wrapping it in a Ref when passed by address.
mutable struct SigAction
handler::Ptr{Cvoid}
mask::SigSet
flags::_typeof_sigaction_flags
end
# Counterpart of C `siginfo_t` structure; although only a pointer of this is
# ever used by signal handlers. Must be mutable to avoid wrapping it in a Ref
# when passed by address.
mutable struct SigInfo
bits::_typeof_siginfo
function SigInfo()
obj = new()
ccall(:memset, Ptr{Cvoid}, (Ptr{Cvoid}, Cint, Csize_t),
pointer_from_objref(obj), 0, sizeof(obj))
return obj
end
end
struct Key
value::key_t
Key(value::Integer) = new(value)
end
struct ShmId
value::Cint
ShmId(value::Integer) = new(value)
end
mutable struct ShmInfo
atime::time_t # last attach time
dtime::time_t # last detach time
ctime::time_t # last change time
segsz::Int64 # size of the public area
id::Cint # shared memory identifier
cpid::pid_t # process ID of creator
lpid::pid_t # process ID of last operator
nattch::shmatt_t # no. of current attaches
mode::mode_t # lower 9 bits of access modes
uid::uid_t # effective user ID of owner
gid::gid_t # effective group ID of owner
cuid::uid_t # effective user ID of creator
cgid::gid_t # effective group ID of creator
ShmInfo() = new(0,0,0,0,0,0,0,0,0,0,0,0,0)
end
mutable struct SemInfo
otime::UInt64 # last semop time
ctime::UInt64 # last change time
nsems::Int32 # number of semaphores in set
id::Int32 # semaphore set identifier
uid::UInt32 # effective user ID of owner
gid::UInt32 # effective group ID of owner
cuid::UInt32 # effective user ID of creator
cgid::UInt32 # effective group ID of creator
mode::UInt32 # lower 9 bits of access modes
SemInfo() = new(0,0,0,0,0,0,0,0,0)
end
# Must be mutable to allow for finalizing.
mutable struct FileDescriptor
fd::Cint
end
abstract type MemoryBlock end
mutable struct DynamicMemory <: MemoryBlock
ptr::Ptr{Cvoid}
len::Int
function DynamicMemory(len::Integer)
@assert len β₯ 1
ptr = Libc.malloc(len)
ptr != C_NULL || throw(OutOfMemoryError())
return finalizer(_destroy, new(ptr, len))
end
end
mutable struct SharedMemory{T<:Union{String,ShmId}} <: MemoryBlock
ptr::Ptr{Cvoid} # mapped address of shared memory segment
len::Int # size of shared memory segment (in bytes)
volatile::Bool # true if shared memory is volatile (only for the creator)
id::T # identifier of shared memory segment
function SharedMemory{T}(ptr::Ptr{Cvoid},
len::Integer,
volatile::Bool,
id::T) where {T<:Union{String,ShmId}}
return finalizer(_destroy, new(ptr, len, volatile, id))
end
end
struct WrappedArray{T,N,M} <: DenseArray{T,N}
# All members shall be considered as private.
arr::Array{T,N} # wrapped Julia array
mem::M # object providing the memory
function WrappedArray{T,N,M}(ptr::Ptr{T},
dims::NTuple{N,<:Integer},
mem::M) where {T,N,M}
arr = unsafe_wrap(Array, ptr, dims)
return new{T,N,M}(arr, mem)
end
end
const WrappedVector{T,M} = WrappedArray{T,1,M}
const WrappedMatrix{T,M} = WrappedArray{T,2,M}
const ShmArray{T,N,M<:SharedMemory} = WrappedArray{T,N,M}
const ShmVector{T,M} = ShmArray{T,1,M}
const ShmMatrix{T,M} = ShmArray{T,2,M}
# Header for saving a minimal description of a wrapped array. The layout is:
#
# Name Size
# --------------
# magic 4 bytes
# etype 2 bytes
# ndims 2 bytes
# offset 8 bytes
#
# This header is supposed to be directly followed by the array dimensions
# stored as 8-byte signed integers.
#
struct WrappedArrayHeader
magic::UInt32 # magic number to check correctness
etype::UInt16 # identifier of element type
ndims::UInt16 # number of dimensions
offset::Int64 # total size of header
end
@assert rem(sizeof(WrappedArrayHeader), sizeof(Int64)) == 0
mutable struct Semaphore{T}
ptr::Ptr{Cvoid}
lnk::T
# Provide inner constructor to force fully qualified calls.
Semaphore{T}(ptr::Ptr, lnk) where {T} = new{T}(ptr, lnk)
end
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | code | 7831 | #
# unix.jl --
#
# Low level interface to (some) Unix functions for Julia.
#
#------------------------------------------------------------------------------
#
# This file is part of InterProcessCommunication.jl released under the MIT
# "expat" license.
#
# Copyright (c) 2016-2024, Γric ThiΓ©baut
# (https://github.com/emmt/InterProcessCommunication.jl).
#
"""
```julia
IPC.getpid() -> pid
```
yields the process ID of the calling process.
```julia
IPC.getppid() -> pid
```
yields the the process ID of the parent of the calling process.
These 2 methods yields an instance of `IPC.ProcessId`.
See also: [`getuid`](@ref).
""" ProcessId
getpid() = ccall(:getpid, ProcessId, ())
getppid() = ccall(:getppid, ProcessId, ())
@doc @doc(ProcessId) getpid
@doc @doc(ProcessId) getppid
"""
```julia
IPC.getuid() -> uid
```
yields the real user ID of the calling process.
```julia
IPC.geteuid() -> uid
```
yields the effective user ID of the calling process.
These 2 methods yields an instance of `IPC.UserId`.
See also: [`getpid`](@ref).
""" UserId
getuid() = ccall(:getuid, UserId, ())
geteuid() = ccall(:geteuid, UserId, ())
@doc @doc(UserId) getuid
@doc @doc(UserId) geteuid
Base.show(io::IO, id::ProcessId) = print(io, "IPC.ProcessId(", id.value, ")")
Base.show(io::IO, id::UserId) = print(io, "IPC.UserId(", id.value, ")")
Base.show(io::IO, ::MIME"text/plain", arg::Union{ProcessId,UserId}) =
show(io, arg)
const MASKMODE = (S_IRWXU|S_IRWXG|S_IRWXO)
"""
IPC.maskmode(mode)
returns the `IPC.MASKMODE` bits of `mode` converted to `mode_t` C type.
Constant `IPC.MASKMODE = 0o$(string(MASKMODE, base=8))` is a bit mask for the
granted access permissions (in general it has its 9 least significant bits
set).
See also [`umask`](@ref).
"""
maskmode(mode::Integer) :: mode_t =
convert(mode_t, mode) & MASKMODE
@doc @doc(maskmode) MASKMODE
"""
umask(msk) -> old
sets the calling process's file mode creation mask (`umask`) to `msk & 0o0777`
(i.e., only the file permission bits of mask are used), and returns the
previous value of the mask.
See also [`IPC.maskmode`](@ref).
"""
umask(mask::Integer) =
ccall(:umask, mode_t, (mode_t,), mask)
_open(path::AbstractString, flags::Integer, mode::Integer) =
ccall(:open, Cint, (Cstring, Cint, mode_t...), path, flags, mode)
_creat(path::AbstractString, mode::Integer) =
_open(path, O_CREAT|O_WRONLY|O_TRUNC, mode)
_close(fd::Integer) =
ccall(:close, Cint, (Cint,), fd)
_read(fd::Integer, buf::Union{DenseArray,Ptr}, cnt::Integer) =
ccall(:read, ssize_t, (Cint, Ptr{Cvoid}, size_t),
fd, buf, cnt)
_write(fd::Integer, buf::Union{DenseArray,Ptr}, cnt::Integer) =
ccall(:write, ssize_t, (Cint, Ptr{Cvoid}, size_t),
fd, buf, cnt)
_lseek(fd::Integer, off::Integer, whence::Integer) =
ccall(:lseek, off_t, (Cint, off_t, Cint), fd, off, whence)
_truncate(path::AbstractString, len::Integer) =
ccall(:truncate, Cint, (Cstring, off_t), path, len)
_ftruncate(fd::Integer, len::Integer) =
ccall(:ftruncate, Cint, (Cint, off_t), fd, len)
_chown(path::AbstractString, uid::Integer, gid::Integer) =
ccall(:chown, Cint, (Cstring, uid_t, gid_t), path, uid, gid)
_lchown(path::AbstractString, uid::Integer, gid::Integer) =
ccall(:lchown, Cint, (Cstring, uid_t, gid_t),
path, uid, gid)
_fchown(fd::Integer, uid::Integer, gid::Integer) =
ccall(:fchown, Cint, (Cint, uid_t, gid_t), fd, uid, gid)
_chmod(path::AbstractString, mode::Integer) =
ccall(:chmod, Cint, (Cstring, mode_t), path, mode)
_fchmod(fd::Integer, mode::Integer) =
ccall(:fchmod, Cint, (Cint, mode_t), fd, mode)
_mmap(addr::Ptr, len::Integer, prot::Integer, flags::Integer, fd::Integer,
off::Integer) =
ccall(:mmap, Ptr{Cvoid},
(Ptr{Cvoid}, size_t, Cint, Cint, Cint, off_t),
addr, len, prot, flags, fd, off)
_mprotect(addr::Ptr, len::Integer, prot::Integer) =
ccall(:mprotect, Cint, (Ptr{Cvoid}, size_t, Cint), addr, len, prot)
_msync(addr::Ptr, len::Integer, flags::Integer) =
ccall(:msync, Cint, (Ptr{Cvoid}, size_t, Cint), addr, len, flags)
_munmap(addr::Ptr, len::Integer) =
ccall(:munmap, Cint, (Ptr{Cvoid}, size_t), addr, len)
_shm_open(path::AbstractString, flags::Integer, mode::Integer) =
ccall(:shm_open, Cint, (Cstring, Cint, mode_t), path, flags, mode)
_shm_unlink(path::AbstractString) =
ccall(:shm_unlink, Cint, (Cstring,), path)
_sem_open(path::AbstractString, flags::Integer, mode::Integer, value::Unsigned) =
ccall(:sem_open, Ptr{Cvoid}, (Cstring, Cint, mode_t, Cuint),
path, flags, mode, value)
_sem_close(sem::Ptr{Cvoid}) =
ccall(:sem_close, Cint, (Ptr{Cvoid},), sem)
_sem_unlink(path::AbstractString) =
ccall(:sem_unlink, Cint, (Cstring,), path)
_sem_getvalue(sem::Ptr{Cvoid}, val::Union{Ref{Cint},Ptr{Cint}}) =
ccall(:sem_getvalue, Cint, (Ptr{Cvoid}, Ptr{Cint}), sem, val)
_sem_post(sem::Ptr{Cvoid}) =
ccall(:sem_post, Cint, (Ptr{Cvoid},), sem)
_sem_wait(sem::Ptr{Cvoid}) =
ccall(:sem_wait, Cint, (Ptr{Cvoid},), sem)
_sem_trywait(sem::Ptr{Cvoid}) =
ccall(:sem_trywait, Cint, (Ptr{Cvoid},), sem)
_sem_timedwait(sem::Ptr{Cvoid}, timeout::Union{Ref{TimeSpec},Ptr{TimeSpec}}) =
ccall(:sem_timedwait, Cint, (Ptr{Cvoid}, Ptr{TimeSpec}), sem, timeout)
_sem_init(sem::Ptr{Cvoid}, shared::Bool, value::Unsigned) =
ccall(:sem_init, Cint, (Ptr{Cvoid}, Cint, Cuint),
sem, shared, value)
_sem_destroy(sem::Ptr{Cvoid}) =
ccall(:sem_destroy, Cint, (Ptr{Cvoid},), sem)
#------------------------------------------------------------------------------
# FILE DESCRIPTOR
Base.stat(obj::FileDescriptor) = stat(RawFD(obj))
function Base.open(::Type{FileDescriptor}, path::AbstractString,
flags::Integer, mode::Integer=DEFAULT_MODE)
fd = _open(path, flags, mode)
systemerror("open", fd < 0)
return finalizer(_close, FileDescriptor(fd))
end
function Base.open(::Type{FileDescriptor}, path::AbstractString,
access::AbstractString)
flags0 = zero(Cint)
flags1 = zero(Cint)
mode = S_IRUSR|S_IWUSR|S_IRGRP|S_IROTH
len = length(access)
c = (len β₯ 1 ? access[1] : '\0')
if c == 'r'
flags0 = O_RDONLY
elseif c == 'w'
flags0 = O_WRONLY
flags1 = O_CREAT|O_TRUNC;
elseif c == 'a'
flags0 = O_WRONLY
flags1 = O_CREAT|O_APPEND;
else
throw_argument_error("unknown access mode \"", access, "\"")
end
for i in 2:len
c = access[i]
if c == '+'
flags0 = O_RDWR
break
end
end
return open(FileDescriptor, path, flags0|flags1, mode)
end
Base.close(obj::FileDescriptor) = _close(obj; throw_errors = true)
function _close(obj::FileDescriptor; throw_errors::Bool = false)
if isopen(obj)
r = _close(fd(obj))
obj.fd = -1 # take care of not closing again
throw_errors && !iszero(r) && systemerror("close")
end
return nothing
end
Base.fd(obj::FileDescriptor) = obj.fd
Base.RawFD(obj::FileDescriptor) = RawFD(fd(obj))
Base.convert(::Type{RawFD}, obj::FileDescriptor) = RawFD(obj)
function Base.position(obj::FileDescriptor)
off = _lseek(fd(obj), 0, SEEK_CUR)
systemerror("lseek", off < 0)
return off
end
function Base.seek(obj::FileDescriptor, pos::Integer)
off = _lseek(fd(obj), pos, SEEK_SET)
systemerror("lseek", off < 0)
return off
end
Base.seekstart(obj::FileDescriptor) = seek(obj, 0)
function Base.seekend(obj::FileDescriptor)
off = _lseek(fd(obj), 0, SEEK_END)
systemerror("lseek", off < 0)
return off
end
function Base.skip(obj::FileDescriptor, offset::Integer)
off = _lseek(fd(obj), offset, SEEK_CUR)
systemerror("lseek", off < 0)
return off
end
Base.isopen(obj::FileDescriptor) = fd(obj) β₯ 0
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | code | 25704 | #
# utils.jl --
#
# Useful methods and constants for InterProcessCommunication (IPC) package of
# Julia.
#
#------------------------------------------------------------------------------
#
# This file is part of InterProcessCommunication.jl released under the MIT
# "expat" license.
#
# Copyright (c) 2016-2024, Γric ThiΓ©baut
# (https://github.com/emmt/InterProcessCommunication.jl).
#
# A bit of magic for calling C-code:
Base.convert(::Type{key_t}, key::Key) = key.value
Base.convert(::Type{T}, key::Key) where {T<:Integer} = convert(T, key.value)
# The short version of `show` if also used for string interpolation in
# scripts so that extending methods:
# Base.convert(::Type{String}, obj::T)
# Base.string(obj::T)
# is not necessary.
Base.show(io::IO, ::MIME"text/plain", arg::Key) = show(io, arg)
Base.show(io::IO, key::Key) =
print(io, "IPC.Key(", string(key.value, base=10), ")")
"""
`IPC.PRIVATE` is a special IPC key (of type `IPC.Key`) which indicates that a
new (private) key should be created.
"""
const PRIVATE = Key(IPC_PRIVATE)
"""
Immutable type `IPC.Key` stores a System V IPC key. The call:
```julia
IPC.Key(path, proj)
```
generates a System V IPC key from pathname `path` and a project identifier
`proj` (a single character). The key is suitable for System V Inter-Process
Communication (IPC) facilities (message queues, semaphores and shared memory).
For instance:
```julia
key = IPC.Key(".", 'a')
```
The special IPC key [`IPC.PRIVATE`](@ref) is also available to indicate that a
new (private) key should be created.
"""
Key(path::AbstractString, proj::Union{Char,Integer}) =
Key(path, convert(Cint, proj))
function Key(path::AbstractString, proj::Cint)
1 β€ proj β€ 255 || throw_argument_error("`proj` must be in the range 1:255")
key = ccall(:ftok, key_t, (Cstring, Cint), path, proj)
systemerror("ftok", key == -1)
return Key(key)
end
"""
```julia
gettimeofday() -> tv
```
yields the current time as an instance of `IPC.TimeVal`. The result can be
converted into a fractional number of seconds by calling `float(tv)`.
See also: [`IPC.TimeVal`](@ref), [`nanosleep`](@ref), [`clock_gettime`](@ref).
"""
function gettimeofday()
tv = Ref(TimeVal(0, 0))
# `gettimeofday` should not fail in this case
ccall(:gettimeofday, Cint, (Ptr{TimeVal}, Ptr{Cvoid}), tv, C_NULL)
return tv[]
end
"""
```julia
now(T)
```
yields the current time since the Epoch as an instance of type `T`, which can
be [`TimeVal`](@ref) or [`TimeSpec`](@ref).
See also: [`gettimeofday`](@ref), [`time`](@ref), [`clock_gettime`](@ref).
"""
now(::Type{TimeVal}) = gettimeofday()
now(::Type{TimeSpec}) = clock_gettime(CLOCK_REALTIME)
"""
```julia
time(T)
```
yields the current time since the Epoch as an instance of type `T`, which can
be [`TimeVal`](@ref) or [`TimeSpec`](@ref).
See also: [`now`](@ref)[`gettimeofday`](@ref), [`clock_gettime`](@ref).
"""
Base.time(::Type{TimeVal}) = gettimeofday()
Base.time(::Type{TimeSpec}) = clock_gettime(CLOCK_REALTIME)
"""
```julia
nanosleep(t) -> rem
```
sleeps for `t` seconds with nanosecond precision and returns the remaining time
(in case of interrupts) as an instance of `IPC.TimeSpec`. Argument can be a
(fractional) number of seconds or an instance of `IPC.TimeSpec` or
`IPC.TimeVal`.
The `sleep` method provided by Julia has only millisecond precision.
See also [`gettimeofday`](@ref), [`IPC.TimeSpec`](@ref) and
[`IPC.TimeVal`](@ref).
"""
nanosleep(t::Union{Real,TimeVal,Libc.TimeVal}) = nanosleep(TimeSpec(t))
function nanosleep(ts::TimeSpec)
rem = Ref(TimeSpec(0,0))
ccall(:nanosleep, Cint, (Ptr{TimeSpec}, Ptr{TimeSpec}), Ref(ts), rem)
return rem[]
end
"""
```julia
clock_getres(id) -> ts
```
yields the resolution (precision) of the specified clock `id`. The result is an
instance of `IPC.TimeSpec`. Clock identifier `id` can be `CLOCK_REALTIME` or
`CLOCK_MONOTONIC` (described in [`clock_gettime`](@ref)).
See also [`clock_gettime`](@ref), [`clock_settime`](@ref),
[`gettimeofday`](@ref), [`nanosleep`](@ref), [`IPC.TimeSpec`](@ref) and
[`IPC.TimeVal`](@ref).
""" clock_getres
@static if Sys.islinux()
function clock_getres(id::Integer)
res = Ref(TimeSpec(0,0))
systemerror("clock_getres",
ccall(:clock_getres, Cint,
(clockid_t, Ptr{TimeSpec}),
id, res) != SUCCESS)
return res[]
end
else
# Assume the same resolution as gettimeofday which is used as a substitute
# to clock_gettime.
clock_getres(id::Integer) = TimeSpec(0, 1_000)
end
"""
```julia
clock_gettime(id) -> ts
```
yields the time of the specified clock `id`. The result is an instance of
`IPC.TimeSpec`. Clock identifier `id` can be one of:
* `CLOCK_REALTIME`: System-wide clock that measures real (i.e., wall-clock)
time. This clock is affected by discontinuous jumps in the system time
(e.g., if the system administrator manually changes the clock), and by the
incremental adjustments performed by `adjtime` and NTP.
* `CLOCK_MONOTONIC`: Clock that cannot be set and represents monotonic time
since some unspecified starting point. This clock is not affected by
discontinuous jumps in the system time.
See also [`clock_getres`](@ref), [`clock_settime`](@ref),
[`gettimeofday`](@ref), [`nanosleep`](@ref), [`IPC.TimeSpec`](@ref) and
[`IPC.TimeVal`](@ref).
""" clock_gettime
@static if Sys.islinux()
function clock_gettime(id::Integer)
ts = Ref(TimeSpec(0,0))
systemerror("clock_gettime",
ccall(:clock_gettime, Cint,
(clockid_t, Ptr{TimeSpec}),
id, ts) != SUCCESS)
return ts[]
end
else
# Use clock_gettime as a substitute.
clock_gettime(id::Integer) = TimeSpec(gettimeofday())
end
@doc @doc(clock_gettime) CLOCK_REALTIME
@doc @doc(clock_gettime) CLOCK_MONOTONIC
"""
```julia
clock_settime(id, ts)
```
set the time of the specified clock `id` to `ts`. Argument `ts` can be an
instance of `IPC.TimeSpec` or a number of seconds. Clock identifier `id` can
be `CLOCK_REALTIME` or `CLOCK_MONOTONIC` (described in
[`clock_gettime`](@ref)).
See also [`clock_getres`](@ref), [`clock_gettime`](@ref),
[`gettimeofday`](@ref), [`nanosleep`](@ref), [`IPC.TimeSpec`](@ref) and
[`IPC.TimeVal`](@ref).
"""
clock_settime(id::Integer, t::Union{Real,TimeVal,Libc.TimeVal}) =
clock_settime(id, TimeSpec(t))
clock_settime(id::Integer, ts::TimeSpec) =
clock_settime(id, Ref(ts))
clock_settime(id::Integer, ts::Union{Ref{TimeSpec},Ptr{TimeSpec}}) =
SUCCESS == ccall(:clock_settime, Cint, (clockid_t, Ptr{TimeSpec}),
id, ts) || throw_system_error("clock_settime")
"""
`TimeConstraints` is the abstract type inherited by concrete types specifying
the kind of constraints to apply for the integer and fractional parts of a time
value. There are two possibilities: [`Nonnegative`](@ref) if the fractional
part shall be nonnegative or [`SameSign`](@ref) if the fractional and integer
parts shall have the same sign.
"""
abstract type TimeConstraints end
"""
`Nonnegative` is a singleton type derived from [`TimeConstraints`](@ref) and
used to impose that the fractional part of a time value be nonnegative. This
is what is assumed for normalized time values.
"""
struct Nonnegative <: TimeConstraints end
"""
`SameSign` is a singleton type derived from [`TimeConstraints`](@ref) and used
to impose that the fractional and integer parts of a time value have the same
sign.
"""
struct SameSign <: TimeConstraints end
const Floats = Union{AbstractFloat,AbstractIrrational,Rational}
const MILLISECONDS_PER_SECOND = 1_000
const MICROSECONDS_PER_SECOND = 1_000_000
const NANOSECONDS_PER_SECOND = 1_000_000_000
# Most of the time manipulation methods assume signed fractional time field.
@assert fieldtype(TimeVal, :usec) <: Signed
@assert fieldtype(TimeSpec, :nsec) <: Signed
"""
```julia
TimeSpec(sec, nsec)
```
yields an instance of `TimeSpec` for an integer number of seconds `sec` and an
integer number of nanoseconds `nsec` since the Epoch.
```julia
TimeSpec(sec)
```
yields an instance of `TimeSpec` for a, possibly fractional, number of seconds
`sec` since the Epoch. Argument can also be an instance of [`TimeVal`](@ref).
Call `now(TimeSpec)` to get the current time as an instance of `TimeSpec`.
Addition and subtraction involving and instance of `TimeSpec` yield a
`TimeSpec` result. For instance:
```julia
now(TimeSpec) + 3.4
```
yields a `TimeSpec` instance with the current time plus `3.4` seconds.
```julia
typemin(TimeSpec)
typemax(TimeSpec)
```
respectively yield the minimum and maximum normalized time values for an
instance of `TimeSpec`.
"""
TimeSpec(ts::TimeSpec) = ts
TimeSpec(secs::Integer) = TimeSpec(secs, 0)
TimeSpec(secs::Floats) = TimeSpec(splittime(_typeof_timespec_sec,
_typeof_timespec_nsec, secs,
NANOSECONDS_PER_SECOND)...)
TimeSpec(tv::Union{TimeVal,Libc.TimeVal}) = TimeSpec(tv.sec, tv.usec*1_000)
"""
```julia
TimeVal(sec, usec)
```
yields an instance of `TimeVal` for an integer number of seconds `sec` and an
integer number of microseconds `usec` since the Epoch.
```julia
TimeVal(sec)
```
yields an instance of `TimeVal` with a, possibly fractional, number of seconds
`sec` since the Epoch. Argument can also be an instance of [`TimeSpec`](@ref).
Call `now(TimeVal)` to get the current time as an instance of `TimeVal`.
Addition and subtraction involving and instance of `TimeVal` yield a
`TimeVal` result. For instance:
```julia
now(TimeVal) + 3.4
```
yields a `TimeVal` instance with the current time plus `3.4` seconds.
```julia
typemin(TimeVal)
typemax(TimeVal)
```
respectively yield the minimum and maximum normalized time values for an
instance of `TimeVal`.
"""
TimeVal(tv::TimeVal) = tv
TimeVal(tv::Libc.TimeVal) = TimeVal(tv.sec, tv.usec)
TimeVal(secs::Integer) = TimeVal(secs, 0)
TimeVal(secs::Floats) = TimeVal(splittime(_typeof_timeval_sec,
_typeof_timeval_usec, secs,
MICROSECONDS_PER_SECOND)...)
TimeVal(ts::TimeSpec) = begin
usec, r = divrem(ts.nsec, 1_000)
if r β₯ 500
usec += one(usec)
elseif r β€ -500
usec -= one(usec)
end
fixtime(TimeVal, ts.sec, usec)
end
Libc.TimeVal(ts::TimeSpec) = Libc.TimeVal(TimeVal(ts))
Libc.TimeVal(tv::TimeVal) = Libc.TimeVal(tv.sec, tv.usec)
Base.typemin(::Type{TimeSpec}) =
TimeSpec(typemin(_typeof_timespec_sec), 0)
Base.typemax(::Type{TimeSpec}) =
TimeSpec(typemax(_typeof_timespec_sec), NANOSECONDS_PER_SECOND - 1)
Base.typemin(::Type{TimeVal}) =
TimeVal(typemin(_typeof_timeval_sec), 0)
Base.typemax(::Type{TimeVal}) =
TimeVal(typemax(_typeof_timeval_sec), MICROSECONDS_PER_SECOND - 1)
Base.Float32(t::Union{TimeVal,TimeSpec}) = convert(Float32, t)
Base.Float64(t::Union{TimeVal,TimeSpec}) = convert(Float64, t)
Base.BigFloat(t::Union{TimeVal,TimeSpec}) = convert(BigFloat, t)
Base.float(t::Union{TimeVal,TimeSpec}) = convert(Float64, t)
Base.convert(::Type{T}, tv::TimeVal) where {T<:AbstractFloat} =
T(tv.sec) + T(tv.usec)/T(MICROSECONDS_PER_SECOND)
Base.convert(::Type{T}, ts::TimeSpec) where {T<:AbstractFloat} =
T(ts.sec) + T(ts.nsec)/T(NANOSECONDS_PER_SECOND)
Base.convert(::Type{TimeSpec}, arg::Union{Real,TimeSpec,TimeVal,Libc.TimeVal}) =
TimeSpec(arg)
Base.convert(::Type{TimeVal}, arg::Union{Real,TimeSpec,TimeVal,Libc.TimeVal}) =
TimeVal(arg)
"""
```julia
splittime(Ti, Tf, s, n, r=Nonnegative()) -> (ip::Ti, fp::Tf)
```
yields two integers, `ip` and `fp` with `abs(fp) β [0,n-1[`, such that `ip +
fp/n β s` (with a precision better than `1/n`) and imposing the constraints set
by `r`:
* if `r` is `Nonnegative()`, then `fp β₯ 0`;
* if `r` is `SameSign()`, then `ip` and `fp` have the same sign.
The multiplier `n` must be strictly positive. An `InexactError` is thrown if
the value of `s` cannot be converted (e.g. it is a NaN or its magnitude is too
large).
See also [`fixtime`](@ref).
"""
function splittime(::Type{Ti}, ::Type{Tf}, secs::Floats,
n::Integer) where {Ti<:Integer, Tf<:Integer}
splittime(Ti, Tf, secs, n, Nonnegative())
end
function splittime(::Type{Ti}, ::Type{Tf}, secs::Floats,
n::Integer, ::Nonnegative) where {Ti<:Integer, Tf<:Integer}
s = floor(secs)
ip = trunc(Ti, s)
fp = round(Tf, (secs - s)*n)
if fp β₯ n
ip += Ti(1)
fp -= Tf(n)
end
return (ip, fp)
end
function splittime(::Type{Ti}, ::Type{Tf}, secs::Floats,
n::Integer, ::SameSign) where {Ti<:Integer, Tf<:Integer}
@assert isfinite(secs)
if secs < 0
s = -floor(-secs)
ip = trunc(Ti, s)
fp = round(Tf, (secs - s)*n)
if fp β€ -n
ip -= Ti(1)
fp += Tf(n)
end
else
s = floor(secs)
ip = trunc(Ti, s)
fp = round(Tf, (secs - s)*n)
if fp β₯ n
ip += Ti(1)
fp -= Tf(n)
end
end
return (ip, fp)
end
"""
```julia
fixtime(Ti, Tf, i, f, n, r = Nonnegative()) -> (ip::Ti, fp::Tf)
```
yields two integers, `ip` and `fp` with `abs(fp) β [0,n-1[`, such that
`ip + fp/n == i + f/n` (in arbitrary precision) and imposing the constraints
set by `r`:
* if `r` is `Nonnegative()`, then `fp β₯ 0`;
* if `r` is `SameSign()`, then `ip` and `fp` have the same sign.
The multiplier `n` must be strictly positive.
```julia
fixtime(TimeSpec, sec, nsec) -> ts
```
yields an instance of `TimeSpec` such that `ts` equals `sec` seconds plus
`nsec` nanoseconds and has normalized fields, that is with a nonnegative number
of nanoseconds strictly less than 1,000,000,000.
```julia
fixtime(TimeVal, sec, usec) -> tv
```
yields an instance of `TimeVal` such that `tv` equals `sec` seconds plus `usec`
microseconds and has normalized fields, that is with a nonnegative number of
microseconds strictly less than 1,000,000.
See also [`splittime`](@ref).
"""
function fixtime(::Type{Ti}, ::Type{Tf}, i::Integer, f::Integer,
n::Integer) where {Ti<:Integer, Tf<:Integer}
fixtime(Ti, Tf, i, f, n, Nonnegative())
end
# Note that assuming n > 0, then q,r = divrem(f,n) yields q and r that have the
# same sign as f.
function fixtime(::Type{Ti}, ::Type{Tf}, i::Integer, f::Integer,
n::Integer, ::Nonnegative) where {Ti<:Integer, Tf<:Integer}
ip = Ti(i + div(f, n))
fp = Tf(rem(f, n))
if fp < 0
ip -= Ti(1)
fp += Tf(n)
end
return (ip, fp)
end
function fixtime(::Type{Ti}, ::Type{Tf}, i::Integer, f::Integer,
n::Integer, ::SameSign) where {Ti<:Integer, Tf<:Integer}
ip = Ti(i + div(f, n))
fp = Tf(rem(f, n))
if fp > 0
if ip < 0
ip += Ti(1)
fp -= Tf(n)
end
elseif fp < 0
if ip > 0
ip -= Ti(1)
fp += Tf(n)
end
end
return (ip, fp)
end
fixtime(::Type{TimeSpec}, sec::Integer, nsec::Integer) =
TimeSpec(fixtime(_typeof_timespec_sec, _typeof_timespec_nsec,
sec, nsec, NANOSECONDS_PER_SECOND, Nonnegative())...)
fixtime(::Type{TimeVal}, sec::Integer, usec::Integer) =
TimeVal(fixtime(_typeof_timeval_sec, _typeof_timeval_usec,
sec, usec, MICROSECONDS_PER_SECOND, Nonnegative())...)
const TimeTypes = Union{TimeSpec,TimeVal}
const AnyTime = Union{TimeSpec,TimeVal,Libc.TimeVal}
const AnyTimeVal = Union{TimeVal,Libc.TimeVal}
#
# Extend unary minus, addition and subtraction for time structures.
#
Base.:(-)(a::T) where {T<:Union{TimeSpec,TimeVal}} =
fixtime(T, -intpart(a), -fracpart(a))
Base.:(+)(a::T, b::T) where {T<:Union{TimeSpec,TimeVal}} =
fixtime(T, intpart(a) + intpart(b), fracpart(a) + fracpart(b))
Base.:(-)(a::T, b::T) where {T<:Union{TimeSpec,TimeVal}} =
fixtime(T, intpart(a) - intpart(b), fracpart(a) - fracpart(b))
Base.:(+)(a::TimeSpec, b::Union{TimeVal,Libc.TimeVal}) =
fixtime(TimeSpec, intpart(a) + intpart(b), fracpart(a) + fracpart(b)*1_000)
Base.:(-)(a::TimeSpec, b::Union{TimeVal,Libc.TimeVal}) =
fixtime(TimeSpec, intpart(a) - intpart(b), fracpart(a) - fracpart(b)*1_000)
Base.:(+)(a::Union{TimeVal,Libc.TimeVal}, b::TimeSpec) =
fixtime(TimeSpec, intpart(a) + intpart(b), fracpart(a)*1_000 + fracpart(b))
Base.:(-)(a::Union{TimeVal,Libc.TimeVal}, b::TimeSpec) =
fixtime(TimeSpec, intpart(a) - intpart(b), fracpart(a)*1_000 - fracpart(b))
Base.:(+)(a::TimeVal, b::Libc.TimeVal) =
fixtime(TimeVal, intpart(a) + intpart(b), fracpart(a) + fracpart(b))
Base.:(-)(a::TimeVal, b::Libc.TimeVal) =
fixtime(TimeVal, intpart(a) - intpart(b), fracpart(a) - fracpart(b))
Base.:(+)(a::Libc.TimeVal, b::TimeVal) =
fixtime(TimeVal, intpart(a) + intpart(b), fracpart(a) + fracpart(b))
Base.:(-)(a::Libc.TimeVal, b::TimeVal) =
fixtime(TimeVal, intpart(a) - intpart(b), fracpart(a) - fracpart(b))
Base.:(+)(a::T, b::Integer) where {T<:Union{TimeSpec,TimeVal}} =
fixtime(T, intpart(a) + b, fracpart(a))
Base.:(+)(a::Integer, b::T) where {T<:Union{TimeSpec,TimeVal}} =
fixtime(T, a + intpart(b), fracpart(b))
Base.:(-)(a::T, b::Integer) where {T<:Union{TimeSpec,TimeVal}} =
fixtime(T, intpart(a) - b, fracpart(a))
Base.:(-)(a::Integer, b::T) where {T<:Union{TimeSpec,TimeVal}} =
fixtime(T, a - intpart(b), -fracpart(b))
Base.:(+)(a::T, b::Real) where {T<:Union{TimeSpec,TimeVal}} = begin
t = floor(b)
ip, fp, n = intpart(a), fracpart(a), multiplier(a)
fixtime(T, ip + trunc(typeof(ip), t), fp + round(typeof(fp), (b - t)*n))
end
Base.:(+)(a::Real, b::T) where {T<:Union{TimeSpec,TimeVal}} = b + a
Base.:(-)(a::T, b::Real) where {T<:Union{TimeSpec,TimeVal}} = begin
t = floor(b)
ip, fp, n = intpart(a), fracpart(a), multiplier(a)
fixtime(T, ip - trunc(typeof(ip), t), fp - round(typeof(fp), (b - t)*n))
end
Base.:(-)(a::Real, b::T) where {T<:Union{TimeSpec,TimeVal}} = begin
t = floor(a)
ip, fp, n = intpart(b), fracpart(b), multiplier(b)
fixtime(T, trunc(typeof(ip), t) - ip, round(typeof(fp), (a - t)*n) - fp)
end
intpart(t::TimeSpec) = t.sec
fracpart(t::TimeSpec) = t.nsec
multiplier(t::TimeSpec) = NANOSECONDS_PER_SECOND
tolerance(::Type{TimeSpec}) = 5e-9
scale(::Type{TimeSpec}) = 1e-9
intpart(t::Union{TimeVal,Libc.TimeVal}) = t.sec
fracpart(t::Union{TimeVal,Libc.TimeVal}) = t.usec
multiplier(t::Union{TimeVal,Libc.TimeVal}) = MICROSECONDS_PER_SECOND
tolerance(::Type{TimeVal}) = 1e-6
scale(::Type{TimeVal}) = 1e-6
#
# Extend isapprox and comparison operators for time structures.
#
Base.isapprox(a::T, b::T; kwds...) where {T<:Union{TimeSpec,TimeVal}} =
_approx0(a - b; kwds...)
Base.isapprox(a::TimeSpec, b::Union{Real,TimeVal,Libc.TimeVal}; kwds...) =
_approx0(a - b; kwds...)
Base.isapprox(a::Union{Real,TimeVal,Libc.TimeVal}, b::TimeSpec; kwds...) =
_approx0(a - b; kwds...)
Base.isapprox(a::TimeVal, b::Union{Real,Libc.TimeVal}; kwds...) =
_approx0(a - b; kwds...)
Base.isapprox(a::Union{Real,Libc.TimeVal}, b::TimeVal; kwds...) =
_approx0(a - b; kwds...)
Base.:(==)(a::T, b::T) where {T<:Union{TimeSpec,TimeVal}} = _eq(a, b)
Base.:(==)(a::TimeSpec, b::Union{Real,TimeVal,Libc.TimeVal}) = _eq(a, b)
Base.:(==)(a::Union{Real,TimeVal,Libc.TimeVal}, b::TimeSpec) = _eq(a, b)
Base.:(==)(a::TimeVal, b::Union{Real,Libc.TimeVal}) = _eq(a, b)
Base.:(==)(a::Union{Real,Libc.TimeVal}, b::TimeVal) = _eq(a, b)
Base.:(β€)(a::T, b::T) where {T<:Union{TimeSpec,TimeVal}} = _le(a, b)
Base.:(β€)(a::TimeSpec, b::Union{Real,TimeVal,Libc.TimeVal}) = _le(a, b)
Base.:(β€)(a::Union{Real,TimeVal,Libc.TimeVal}, b::TimeSpec) = _le(a, b)
Base.:(β€)(a::TimeVal, b::Union{Real,Libc.TimeVal}) = _le(a, b)
Base.:(β€)(a::Union{Real,Libc.TimeVal}, b::TimeVal) = _le(a, b)
Base.:(<)(a::T, b::T) where {T<:Union{TimeSpec,TimeVal}} = _lt(a, b)
Base.:(<)(a::TimeSpec, b::Union{Real,TimeVal,Libc.TimeVal}) = _lt(a, b)
Base.:(<)(a::Union{Real,TimeVal,Libc.TimeVal}, b::TimeSpec) = _lt(a, b)
Base.:(<)(a::TimeVal, b::Union{Real,Libc.TimeVal}) = _lt(a, b)
Base.:(<)(a::Union{Real,Libc.TimeVal}, b::TimeVal) = _lt(a, b)
# Assuming normalized time t = (ip,fp) with fp β [0,n-1] (normalized), the following
# table summarizes the possibilities.
#
# ip < 0 ip = 0 ip > 0
# fp < 0 t < 0 t < 0 t > 0 (not for normalized time)
# fp = 0 t < 0 t = 0 t > 0
# fp > 0 t < 0 t > 0 t > 0
#
_eq(a, b) = begin
(ip, fp) = _split(a - b)
((ip == 0) & (fp == 0))
end
_le(a, b) = begin
(ip, fp) = _split(a - b)
((ip < 0) | ((ip == 0) & (fp β€ 0)))
end
_lt(a, b) = begin
(ip, fp) = _split(a - b)
(ip < 0)
end
function _approx0(a::T;
atol::Real = tolerance(T)) where {T<:Union{TimeSpec,TimeVal}}
(ip, fp) = _split(a)
abs(Float64(ip) + scale(T)*Float64(fp)) β€ atol
end
_split(t::Union{TimeSpec,TimeVal,Libc.TimeVal}) = intpart(t), fracpart(t)
"""
```julia
error_message(args...)
```
yields a message string built from `args...`. This string is meant to be used
as an error message. The only difference between `string(args...)` and
`error_message(args...)` is that the latter is not inlined if the result has to
be dynamically created. This is to avoid the caller function not being inlined
because the formatting of the error message involves too many operations.
See also [`throw_argument_error`](@ref), [`throw_error_exception`](@ref).
"""
error_message(mesg::String) = mesg
@noinline error_message(args...) = string(args...)
"""
```julia
throw_argument_error(args...)
```
throws an `ArgumentError` exception with message built from `args...`.
See also [`error_message`](@ref), [`throw_error_exception`](@ref),
[`throw_system_error`](@ref).
"""
throw_argument_error(args...) = throw_argument_error(error_message(args...))
"""
```julia
throw_error_exception(args...)
```
throws an `ErrorException` with message built from `args...`.
See also [`error_message`](@ref), [`throw_argument_error`](@ref),
[`throw_system_error`](@ref).
"""
throw_error_exception(args...) = throw_error_exception(error_message(args...))
"""
```julia
throw_system_error(mesg, errno=Libc.errno())
```
throws an `SystemError` exception corresponding to error code number `errno`.
If `errno` is not specified the current value of the global `errno` variable in
the C library is used.
See also [`throw_argument_error`](@ref), [`throw_error_exception`](@ref).
"""
throw_system_error(mesg::AbstractString, errno::Integer = Libc.errno()) =
throw(SystemError(mesg, errno))
makedims(dims::Tuple{Vararg{Int}}) = dims
makedims(dims::Tuple{Vararg{Integer}}) = map(Int, dims)
makedims(dims::AbstractVector{<:Integer}) = # FIXME: bad idea!
ntuple(i -> Int(dims[i]), length(dims))
function checkdims(dims::NTuple{N,Integer}) where {N}
number = one(Int)
for i in 1:N
dims[i] β₯ 1 || throw_error_exception("invalid dimension (dims[", i,
"] = ", dims[i], ")")
number *= convert(Int, dims[i])
end
return number
end
roundup(a::Integer, b::Integer) =
roundup(convert(Int, a), convert(Int, b))
roundup(a::Int, b::Int) =
div(a + (b - 1), b)*b
"""
```julia
get_memory_parameters(mem) -> ptr::Ptr{Cvoid}, siz::Int
```
yields the address and number of bytes of memory backed by `mem`.
The returned values arec checked for correctness.
See also: [`pointer`](@ref), [`sizeof`](@ref).
"""
function get_memory_parameters(mem)::Tuple{Ptr{Cvoid},Int}
siz = sizeof(mem)
isa(siz, Integer) || throw_argument_error("illegal type `", typeof(siz),
"` for `sizeof(mem)`")
siz β₯ 0 || throw_argument_error("invalid value `", siz,
"` for `sizeof(mem)`")
ptr = pointer(mem)
isa(ptr, Ptr) || throw_argument_error("illegal type `", typeof(ptr),
"` for `pointer(mem)`")
return (convert(Ptr{Cvoid}, ptr), convert(Int, siz))
end
"""
```julia
_peek(T, buf, off) -> val
```
yields the value of type `T` stored at offset `off` (in bytes) in buffer
`buf` provided as a vector of bytes (`Uint8`).
```julia
_peek(T, ptr) -> val
```
yields the value of type `T` stored at address given by pointer `ptr`.
Also see: [`unsafe_load`](@ref), [`_poke!`](@ref).
"""
@inline _peek(::Type{T}, ptr::Ptr) where {T} =
unsafe_load(convert(Ptr{T}, ptr))
@inline _peek(::Type{T}, buf::DenseVector{UInt8}, off::Integer) where {T} =
_peek(T, pointer(buf) + off)
"""
```julia
_poke!(T, buf, off, val)
```
stores the value `val`, converted to type `T`, at offset `off` (in bytes) in
buffer `buf` provided as a vector of bytes (`Uint8`).
```julia
_poke!(T, ptr, val)
```
stores the value `val`, converted to type `T`, at address given by pointer
`ptr`.
Also see: [`unsafe_store!`](@ref), [`_peek`](@ref).
"""
@inline _poke!(::Type{T}, ptr::Ptr, val) where {T} =
unsafe_store!(convert(Ptr{T}, ptr), val)
@inline _poke!(::Type{T}, buf::DenseVector{UInt8}, off::Integer, val) where {T} =
_poke!(T, pointer(buf) + off, val)
#------------------------------------------------------------------------------
# DYNAMIC MEMORY OBJECTS
function _destroy(obj::DynamicMemory)
if (ptr = obj.ptr) != C_NULL
obj.len = 0
obj.ptr = C_NULL
Libc.free(ptr)
end
end
Base.sizeof(obj::DynamicMemory) = obj.len
Base.pointer(obj::DynamicMemory) = obj.ptr
Base.unsafe_convert(::Type{Ptr{T}}, obj::DynamicMemory) where {T} =
Ptr{T}(obj.ptr)
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | code | 13162 | #
# wrappedarrays.jl --
#
# Management of object wrapped into Julia arrays.
#
#------------------------------------------------------------------------------
#
# This file is part of InterProcessCommunication.jl released under the MIT
# "expat" license.
#
# Copyright (c) 2016-2024, Γric ThiΓ©baut
# (https://github.com/emmt/InterProcessCommunication.jl).
#
"""
```julia
WrappedArray(mem, [T [, dims...]]; offset=0)
```
yields a Julia array whose elements are stored in the "memory" object `mem`.
Argument `T` is the data type of the elements of the returned array and
argument(s) `dims` specify the dimensions of the array. If `dims` is omitted
the result is a vector of maximal length (accounting for the offset and the
size of the `mem` object). If `T` is omitted, `UInt8` is assumed.
Keyword `offset` may be used to specify the address (in bytes) relative to
`pointer(mem)` where is stored the first element of the array.
The size of the memory provided by `mem` must be sufficient to store all
elements (accounting for the offset) and the alignment of the elements in
memory must be a multiple of `Base.datatype_alignment(T)`.
Another possibility is:
```julia
WrappedArray(mem, dec)
```
where `mem` is the "memory" object and `dec` is a function in charge of
decoding the array type and layout given the memory object. The decoder is
applied to the memory object as follow:
```julia
dec(mem) -> T, dims, offset
```
which must yield the data type `T` of the array elements, the dimensions `dims`
of the array and the offset of the first element relative to `pointer(mem)`.
## Restrictions
The `mem` object must extend the methods `pointer(mem)` and `sizeof(mem)` which
must respectively yield the base address of the memory provided by `mem` and the
number of available bytes. Furthermore, this memory is assumed to be available
at least until object `mem` is reclaimed by the garbage collector.
## Shared Memory Arrays
```julia
WrappedArray(id, T, dims; perms=0o600, volatile=true)
```
creates a new wrapped array whose elements (and a header) are stored in shared
memory identified by `id` (see [`SharedMemory`](@ref) for a description of `id`
and for keywords). To retrieve this array in another process, just do:
```julia
WrappedArray(id; readonly=false)
```
## See Also
[`SharedMemory`](@ref).
"""
function WrappedArray(mem::M, ::Type{T} = UInt8;
offset::Integer = 0) where {M,T}
ptr, siz = _check_wrapped_array_arguments(mem, T, offset)
siz β₯ sizeof(T) ||
throw_argument_error("insufficient memory for at least one element")
number = div(siz, sizeof(T))
return WrappedArray{T,1,M}(ptr, (number,), mem)
end
WrappedArray(mem::M, ::Type{T}, dims::Integer...; kwds...) where {M,T} =
WrappedArray(mem, T, dims; kwds...)
function WrappedArray(mem::M, ::Type{T}, dims::NTuple{N,Integer};
offset::Integer = 0) where {T,N,M}
ptr, siz = _check_wrapped_array_arguments(mem, T, offset)
number = checkdims(dims)
siz β₯ sizeof(T)*number ||
throw_argument_error("insufficient memory for array")
return WrappedArray{T,N,M}(ptr, dims, mem)
end
function WrappedArray(mem, dec::Function)
T, dims, offset = dec(mem)
isa(T, DataType) || error("`dec(mem)[1]` must be a data type")
isa(dims, Tuple{Vararg{Integer}}) ||
error("`dec(mem)[2]` must be a tuple of dimensions")
isa(offset, Integer) || error("`dec(mem)[3]` must be an integer")
return WrappedArray(mem, T, dims; offset = offset)
end
function WrappedArray(id::Union{AbstractString,ShmId,Key},
::Type{T}, dims::Vararg{Integer,N}; kwds...) where {T,N}
return WrappedArray(id, T, convert(NTuple{N,Int}, dims); kwds...)
end
function WrappedArray(id::Union{AbstractString,ShmId,Key},
::Type{T}, dims::NTuple{N,Integer}; kwds...) where {T,N}
return WrappedArray(id, T, convert(NTuple{N,Int}, dims); kwds...)
end
function WrappedArray(id::Union{AbstractString,ShmId,Key},
::Type{T}, dims::NTuple{N,Int};
kwds...) where {T,N}
num = checkdims(dims)
off = _wrapped_array_header_size(N)
siz = off + sizeof(T)*num
mem = SharedMemory(id, siz; kwds...)
write(mem, WrappedArrayHeader, T, dims)
return WrappedArray(mem, T, dims; offset=off)
end
function WrappedArray(id::Union{AbstractString,ShmId,Key}; kwds...)
mem = SharedMemory(id; kwds...)
T, dims, off = read(mem, WrappedArrayHeader)
return WrappedArray(mem, T, dims; offset=off)
end
function _check_wrapped_array_arguments(mem::M, ::Type{T},
offset::Integer) where {M,T}
offset β₯ 0 || throw_argument_error("offset must be nonnegative")
isbitstype(T) || throw_argument_error("illegal element type (", T, ")")
ptr, len = get_memory_parameters(mem)
align = Base.datatype_alignment(T)
addr = ptr + offset
rem(convert(Int, addr), align) == 0 ||
throw_argument_error("base address must be a multiple of ",
align, " bytes")
return (convert(Ptr{T}, addr),
convert(Int, len) - convert(Int, offset))
end
# FIXME: push!, pop!, append!, resize! cannot be extended for WrappedVectors
# unless it is possible to query the size of the memory object, in fact many
# things are doable if the address and size of memory object can be retrieved.
# However, push!, append!, resize!, ... would require to rewrap the buffer.
# The following methods come for free (with no performance penalties) because a
# WrappedArray is a subtype of DenseArray:
#
# Base.eltype, Base.elsize, Base.ndims, Base.first, Base.endof,
# Base.eachindex, ...
# FIXME: extend Base.view?
@inline Base.parent(obj::WrappedArray) = obj.arr
Base.length(obj::WrappedArray) = length(parent(obj))
Base.sizeof(obj::WrappedArray) = sizeof(parent(obj))
Base.size(obj::WrappedArray) = size(parent(obj))
Base.size(obj::WrappedArray, d::Integer) = size(parent(obj), d)
Base.axes(obj::WrappedArray) = axes(parent(obj))
Base.axes(obj::WrappedArray, d::Integer) = axes(parent(obj), d)
@inline Base.axes1(obj::WrappedArray) = Base.axes1(parent(obj))
Base.strides(obj::WrappedArray) = strides(parent(obj))
Base.stride(obj::WrappedArray, d::Integer) = stride(parent(obj), d)
Base.elsize(::Type{WrappedArray{T,N,M}}) where {T,N,M} = elsize(Array{T,N})
Base.IndexStyle(::Type{<:WrappedArray}) = Base.IndexLinear()
@inline Base.getindex(A::WrappedArray, i::Int) = begin
@boundscheck checkbounds(A, i)
@inbounds val = parent(A)[i]
val
end
@inline Base.setindex!(A::WrappedArray, val, i::Int) = begin
@boundscheck checkbounds(A, i)
@inbounds parent(A)[i] = val
A
end
@inline Base.checkbounds(::Type{Bool}, A::WrappedArray, i::Int) =
(i % UInt) - 1 < length(A)
Base.copy(obj::WrappedArray) = copy(parent(obj))
Base.copyto!(dest::WrappedArray, src::AbstractArray) =
(copyto!(dest.arr, src); dest)
Base.reinterpret(::Type{T}, obj::WrappedArray) where {T} =
reinterpret(T, parent(obj))
Base.reshape(obj::WrappedArray, dims::Tuple{Vararg{Int}}) =
reshape(parent(obj), dims)
# Extend `Base.unsafe_convert` for `ccall`. Note that this also make `pointer`
# applicable and that the 2 following definitions are needed to avoid
# ambiguities and cover all cases.
unsafe_convert(::Type{Ptr{T}}, obj::WrappedArray{T}) where {T} =
unsafe_convert(Ptr{T}, parent(obj))
unsafe_convert(::Type{Ptr{S}}, obj::WrappedArray{T}) where {S,T} =
unsafe_convert(Ptr{S}, parent(obj))
# Make a wrapped array iterable:
@static if isdefined(Base, :iterate) # VERSION β₯ v"0.7-alpha"
Base.iterate(iter::WrappedArray) = iterate(iter.arr)
Base.iterate(iter::WrappedArray, state) = iterate(iter.arr, state)
Base.IteratorSize(iter::WrappedArray) = Base.IteratorSize(iter.arr)
Base.IteratorEltype(iter::WrappedArray) = Base.IteratorEltype(iter.arr)
else
Base.start(iter::WrappedArray) = start(iter.arr)
Base.next(iter::WrappedArray, state) = next(iter.arr, state)
Base.done(iter::WrappedArray, state) = done(iter.arr, state)
Base.iteratorsize(iter::WrappedArray) = Base.iteratorsize(iter.arr)
Base.iteratoreltype(iter::WrappedArray) = Base.iteratoreltype(iter.arr)
end
#------------------------------------------------------------------------------
# WRAPPED ARRAYS WITH HEADER
# Magic number
const _WA_MAGIC = UInt32(0x57412D31) # "WA-1" = Wrapped Array version 1
# We require at least 64 bytes (512 bits) alignment for the first element of
# the array (to warrant that SIMD vectors are correctly aligned), this value is
# equal to the constant JL_CACHE_BYTE_ALIGNMENT used for the elements of Julia
# arrays.
const _WA_ALIGN = 64
const _WA_TYPES = (( 1, Int8, "signed 8-bit integer"),
( 2, UInt8, "unsigned 8-bit integer"),
( 3, Int16, "signed 16-bit integer"),
( 4, UInt16, "unsigned 16-bit integer"),
( 5, Int32, "signed 32-bit integer"),
( 6, UInt32, "unsigned 32-bit integer"),
( 7, Int64, "signed 64-bit integer"),
( 8, UInt64, "unsigned 64-bit integer"),
( 9, Float32, "32-bit floating-point"),
(10, Float64, "64-bit floating-point"),
(11, ComplexF32, "64-bit complex"),
(12, ComplexF64, "128-bit complex"))
const _WA_ETYPES = DataType[T for (i, T, str) in _WA_TYPES]
const _WA_DESCRS = String[str for (i, T, str) in _WA_TYPES]
const _WA_IDENTS = Dict(T => i for (i, T, str) in _WA_TYPES)
"""
```julia
WrappedArrayHeader(T, N)
```
yields a structure `WrappedArrayHeader` instanciated for an array with `N`
dimensions and whose element type is `T`.
## Possible Usages
```julia
read(src, WrappedArrayHeader) -> T, dims, off
```
reads wrapped array header in `src` and yields the element type `T`, dimensions
`dims` and offset `off` of the first array element relative to the the base of
`src`.
```julia
write(dst, WrappedArrayHeader, T, dims)
```
writes wrapped array header in `dst` for element type `T` and dimensions
`dims`.
```julia
WrappedArray(mem, x -> read(x, WrappedArrayHeader)) -> arr
```
retrieves a wrapped array `arr` whose header and elements are stored in the
memory provided by object `mem`.
"""
function WrappedArrayHeader(::Type{T}, N::Int) where {T}
N β₯ 1 || throw_argument_error("illegal number of dimensions (", N, ")")
haskey(_WA_IDENTS, T) || throw_argument_error("unsupported data type (",
T, ")")
off = _wrapped_array_header_size(N)
return WrappedArrayHeader(_WA_MAGIC, _WA_IDENTS[T], N, off)
end
_wrapped_array_header_size(N::Integer) =
roundup(sizeof(WrappedArrayHeader) + N*sizeof(Int64), _WA_ALIGN)
WrappedArrayHeader(::Type{T}, N::Integer) where {T} =
WrappedArrayHeader(T, convert(Int, N))
function Base.write(mem, ::Type{WrappedArrayHeader},
::Type{T}, dims::Vararg{Integer,N}) where {T,N}
write(mem, WrappedArrayHeader, T, convert(NTuple{N,Int}, dims))
end
function Base.write(mem, ::Type{WrappedArrayHeader},
::Type{T}, dims::NTuple{N,<:Integer}) where {T,N}
write(mem, WrappedArrayHeader, T, convert(NTuple{N,Int}, dims))
end
function Base.write(mem, ::Type{WrappedArrayHeader},
::Type{T}, dims::NTuple{N,Int}) where {T,N}
# Check arguments, then write header and dimensions.
hdr = WrappedArrayHeader(T, N)
off = hdr.offset
ptr, siz = get_memory_parameters(mem)
num = checkdims(dims)
siz β₯ off + sizeof(T)*num ||
throw_argument_error("insufficient size of memory block")
unsafe_store!(convert(Ptr{WrappedArrayHeader}, ptr), hdr)
addr = convert(Ptr{Int64}, ptr + sizeof(WrappedArrayHeader))
for i in 1:N
unsafe_store!(addr, dims[i], i)
end
end
function Base.read(mem, ::Type{WrappedArrayHeader})
ptr, siz = get_memory_parameters(mem)
siz β₯ sizeof(WrappedArrayHeader) ||
throw_argument_error("insufficient size of memory block for header")
hdr = unsafe_load(convert(Ptr{WrappedArrayHeader}, ptr))
hdr.magic == _WA_MAGIC ||
throw_error_exception("invalid magic number (0x",
string(hdr.magic, base=16), ")")
etype = Int(hdr.etype)
1 β€ etype β€ length(_WA_ETYPES) ||
throw_error_exception("invalid element type identifier (", etype, ")")
ndims = Int(hdr.ndims)
1 β€ ndims ||
throw_error_exception("invalid number of dimensions (", ndims, ")")
off = Int(hdr.offset)
off == _wrapped_array_header_size(ndims) ||
throw_error_exception("invalid offset (", off, ")")
addr = convert(Ptr{Int64}, ptr + sizeof(WrappedArrayHeader))
dims = ntuple(i -> convert(Int, unsafe_load(addr, i)), ndims)
num = checkdims(dims)
T = _WA_ETYPES[etype]
siz β₯ off + sizeof(T)*num ||
throw_error_exception("insufficient size of memory block (", Int(siz),
" < ", Int(off + sizeof(T)*num), ")")
return T, dims, off
end
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | code | 18860 | module IPCTests
using Test
using InterProcessCommunication
using InterProcessCommunication: splittime, fixtime
@testset "Basic Functions " begin
pid = IPC.getpid()
@test pid.value == getpid()
@test isa(string(pid), String)
@test isa(string(IPC.getppid()), String)
@test isa(string(IPC.getuid()), String)
@test isa(string(IPC.geteuid()), String)
new_msk = 0o022
old_msk = @inferred umask(new_msk)
@test new_msk == @inferred umask(old_msk)
end
@testset "File Functions " begin
path = "/tmp/test-$(getpid())"
data = rand(10)
open(path, "w") do io
write(io, data)
end
f = open(IPC.FileDescriptor, path, "r")
@test fd(f) β₯ 0
@test RawFD(f) === RawFD(fd(f))
@test convert(RawFD, f) === RawFD(fd(f))
@test filesize(f) == sizeof(data)
@test position(f) == 0
@test seekend(f) == position(f) == sizeof(data)
@test seekstart(f) == position(f) == 0
pos = (sizeof(data)>>1)
@test seek(f, pos) == position(f) == pos
close(f)
@test fd(f) == -1
end
@testset "Time Functions " begin
# Check time normalization and splitting.
nonnegative = IPC.Nonnegative()
samesign = IPC.SameSign()
@test fixtime(Int, Int, +0, +0, 1_000) === ( 0, 0)
@test fixtime(Int, Int, +1, +400, 1_000) === ( 1, 400)
@test fixtime(Int, Int, +1, +2_400, 1_000) === ( 3, 400)
@test fixtime(Int, Int, +1, -400, 1_000) === ( 0, 600)
@test fixtime(Int, Int, +1, -2_400, 1_000) === (-2, 600)
@test fixtime(Int, Int, -1, +400, 1_000) === (-1, 400)
@test fixtime(Int, Int, -1, +2_400, 1_000) === ( 1, 400)
@test fixtime(Int, Int, -1, -400, 1_000) === (-2, 600)
@test fixtime(Int, Int, -1, -2_400, 1_000) === (-4, 600)
@test fixtime(Int, Int, +0, +0, 1_000, nonnegative) === ( 0, 0)
@test fixtime(Int, Int, +1, +400, 1_000, nonnegative) === ( 1, 400)
@test fixtime(Int, Int, +1, +2_400, 1_000, nonnegative) === ( 3, 400)
@test fixtime(Int, Int, +1, -400, 1_000, nonnegative) === ( 0, 600)
@test fixtime(Int, Int, +1, -2_400, 1_000, nonnegative) === (-2, 600)
@test fixtime(Int, Int, -1, +400, 1_000, nonnegative) === (-1, 400)
@test fixtime(Int, Int, -1, +2_400, 1_000, nonnegative) === ( 1, 400)
@test fixtime(Int, Int, -1, -400, 1_000, nonnegative) === (-2, 600)
@test fixtime(Int, Int, -1, -2_400, 1_000, nonnegative) === (-4, 600)
@test fixtime(Int, Int, +0, +0, 1_000, samesign) === ( 0, 0)
@test fixtime(Int, Int, +1, +400, 1_000, samesign) === ( 1, 400)
@test fixtime(Int, Int, +1, +2_400, 1_000, samesign) === ( 3, 400)
@test fixtime(Int, Int, +1, -400, 1_000, samesign) === ( 0, 600)
@test fixtime(Int, Int, +1, -2_400, 1_000, samesign) === (-1, -400)
@test fixtime(Int, Int, -1, +400, 1_000, samesign) === ( 0, -600)
@test fixtime(Int, Int, -1, +2_400, 1_000, samesign) === ( 1, 400)
@test fixtime(Int, Int, -1, -400, 1_000, samesign) === (-1, -400)
@test fixtime(Int, Int, -1, -2_400, 1_000, samesign) === (-3, -400)
@test splittime(Int, Int, +0.000, 1_000, nonnegative) === ( 0, 0)
@test splittime(Int, Int, +1.400, 1_000, nonnegative) === ( 1, 400)
@test splittime(Int, Int, +3.400, 1_000, nonnegative) === ( 3, 400)
@test splittime(Int, Int, +0.600, 1_000, nonnegative) === ( 0, 600)
@test splittime(Int, Int, -1.400, 1_000, nonnegative) === (-2, 600)
@test splittime(Int, Int, -0.600, 1_000, nonnegative) === (-1, 400)
@test splittime(Int, Int, -3.400, 1_000, nonnegative) === (-4, 600)
@test splittime(Int, Int, +0.000, 1_000, samesign) === ( 0, 0)
@test splittime(Int, Int, +1.400, 1_000, samesign) === ( 1, 400)
@test splittime(Int, Int, +3.400, 1_000, samesign) === ( 3, 400)
@test splittime(Int, Int, +0.600, 1_000, samesign) === ( 0, 600)
@test splittime(Int, Int, -1.400, 1_000, samesign) === (-1, -400)
@test splittime(Int, Int, -0.600, 1_000, samesign) === ( 0, -600)
@test splittime(Int, Int, -3.400, 1_000, samesign) === (-3, -400)
# Miscellaneaous.
@test IPC.multiplier(TimeVal(0,0)) == 1_000_000
@test IPC.multiplier(TimeSpec(0,0)) == 1_000_000_000
@test IPC.scale(TimeVal) == 1e-6
@test IPC.tolerance(TimeVal) β₯ IPC.scale(TimeVal)
@test IPC.scale(TimeSpec) == 1e-9
@test IPC.tolerance(TimeSpec) β₯ IPC.scale(TimeSpec)
# Compile and warmup.
for ts in (time(TimeSpec), now(TimeSpec))
@test ts β ts
@test (ts != ts) == false
@test ts β€ ts
@test ts β₯ ts
@test (ts < ts) == false
@test (ts > ts) == false
end
for tv in (time(TimeVal), now(TimeVal))
@test tv β tv
@test tv == tv
@test (tv != tv) == false
@test tv β€ tv
@test tv β₯ tv
@test (tv < tv) == false
@test (tv > tv) == false
end
ms = 0.001
Β΅s = 0.000_001
ns = 0.000_000_001
h1 = prevfloat(0.5)
h2 = nextfloat(0.5)
sec, usec, nsec = -123, 123456, 123456123
tv = time(TimeVal)
r = Β΅s # resolution for TimeVal
@test TimeVal(tv) === tv
@test TimeVal(sec) === TimeVal(sec,0)
@test TimeVal(sec + usec*r) === TimeVal(sec,usec)
@test TimeVal(sec - usec*r) == TimeVal(sec,-usec)
@test TimeVal(Libc.TimeVal(tv.sec, tv.usec)) === tv
@test TimeSpec(Libc.TimeVal(tv.sec, tv.usec)) === TimeSpec(tv)
@test Libc.TimeVal(tv) == tv
@test Libc.TimeVal(TimeSpec(tv)) == tv
@test tv == Libc.TimeVal(tv.sec, tv.usec)
@test 0 - tv === -tv
@test 37 - tv === -(tv - 37)
@test Ο - tv === -(tv - Ο)
@test (tv + (-tv)) === TimeVal(0,0)
@test tv == tv + 0
@test tv == 0 + tv
@test tv == tv + h1*r
@test tv == h1*r + tv
@test tv < tv + r*h2
@test tv < r*h2 + tv
@test float((tv + r) - tv) == r
@test float(tv - (tv - r)) == r
@test float((tv + h1*r) - tv) == 0
@test float(tv - (tv - h1*r)) == 0
@test float((tv + h2*r) - tv) == r
@test float(tv - (tv - h2*r)) == r
for T in (Float32, Float64, BigFloat)
t = TimeVal(1 + 3r)
@test T(t) β t
end
@test TimeVal(123.456789) == float(TimeVal(123.456789))
@test TimeVal(h1*r) == float(TimeVal(0,0))
@test TimeVal(h2*r) == float(TimeVal(0,1))
@test TimeVal(TimeSpec(sec,1_000*usec)) === TimeVal(sec,usec)
@test TimeVal(TimeSpec(sec,1_000*usec+499)) === TimeVal(sec,usec)
@test TimeVal(TimeSpec(sec,1_000*usec+500)) === TimeVal(sec,usec+1)
@test TimeVal(TimeSpec(sec,-1_000*usec)) === TimeVal(sec-1,1_000_000-usec)
@test TimeVal(TimeSpec(sec,-1_000*usec-499)) === TimeVal(sec-1,1_000_000-usec)
@test TimeVal(TimeSpec(sec,-1_000*usec-500)) === TimeVal(sec-1,1_000_000-(usec+1))
@test typemin(TimeVal) - 1 == typemin(TimeVal) - 1.0 > 0 # check integer wrapping
@test typemax(TimeVal) + 1 == typemax(TimeVal) + 1.0 < 0 # check integer wrapping
@test typemin(TimeVal) + 1 == typemin(TimeVal) + 1.0 < 0
@test typemax(TimeVal) - 1 == typemax(TimeVal) - 1.0 > 0
ts = time(TimeSpec)
r = ns # resolution for TimeSpec
@test TimeSpec(ts) === ts
@test TimeSpec(sec) === TimeSpec(sec,0)
@test TimeSpec(sec + nsec*r) === TimeSpec(sec,nsec)
@test TimeSpec(sec - nsec*r) == TimeSpec(sec,-nsec)
@test 0 - ts === -ts
@test 37 - ts === -(ts - 37)
@test Ο - ts === -(ts - Ο)
@test (ts + (-ts)) === TimeSpec(0,0)
for (a,b,c) in ((TimeVal(-2.58), TimeVal(0.4), TimeVal(-2.18)),
(TimeVal(-2.58), Libc.TimeVal(0,400_000), TimeVal(-2.18)),
(TimeVal(-2.58), 4//10, TimeVal(-2.18)),
(TimeSpec(-2.58), TimeVal(0.4), TimeSpec(-2.18)),
(TimeSpec(-2.58), Libc.TimeVal(0,400_000), TimeSpec(-2.18)),
(TimeSpec(-2.58), 4//10, TimeSpec(-2.18)),
)
@test a + b === c
@test b + a === c
@test a + b β c
if !isa(b, Libc.TimeVal)
@test a - (-b) === c
@test (b β₯ 0 ? a + b β₯ a : a + b < a)
@test (b β₯ 0 ? a - b β€ a : a - b > a)
@test (b > 0 ? a + b > a : a + b β€ a)
@test (b > 0 ? a - b < a : a - b β₯ a)
end
if !isa(a, Libc.TimeVal)
@test b - (-a) === c
@test (a β₯ 0 ? b + a β₯ b : b + a < b)
@test (a β₯ 0 ? b - a β€ b : b - a > b)
@test (a > 0 ? b + a > b : b + a β€ b)
@test (a > 0 ? b - a < b : b - a β₯ b)
end
end
@test ts == ts + 0
@test ts == 0 + ts
@test ts == ts + r*h1
@test ts == r*h1 + ts
@test ts < ts + r*h2
@test ts < r*h2 + ts
@test float((ts + r) - ts) == r
@test float(ts - (ts - r)) == r
@test float((ts + h1*r) - ts) == 0
@test float(ts - (ts - h1*r)) == 0
@test float((ts + h2*r) - ts) == r
@test float(ts - (ts - h2*r)) == r
for T in (Float32, Float64, BigFloat)
t = TimeSpec(1 + 3r)
@test T(t) β t
end
@test TimeSpec(123.456789) == float(TimeSpec(123.456789))
@test TimeSpec(h1*r) == float(TimeSpec(0,0))
@test TimeSpec(h2*r) == float(TimeSpec(0,1))
@test typemin(TimeSpec) - 1 == typemin(TimeSpec) - 1.0 > 0 # check integer wrapping
@test typemax(TimeSpec) + 1 == typemax(TimeSpec) + 1.0 < 0 # check integer wrapping
@test typemin(TimeSpec) + 1 == typemin(TimeSpec) + 1.0 < 0
@test typemax(TimeSpec) - 1 == typemax(TimeSpec) - 1.0 > 0
@test_throws SystemError clock_settime(CLOCK_REALTIME, gettimeofday())
@test_throws SystemError clock_settime(CLOCK_REALTIME, clock_gettime(CLOCK_REALTIME))
float(gettimeofday())
float(clock_gettime(CLOCK_MONOTONIC))
float(clock_gettime(CLOCK_REALTIME))
float(clock_getres(CLOCK_MONOTONIC))
float(clock_getres(CLOCK_REALTIME))
# compare times
@test abs(time() - float(gettimeofday())) β€ 1ms
@test abs(time() - float(clock_gettime(CLOCK_REALTIME))) β€ 1ms
@test float(clock_getres(CLOCK_MONOTONIC)) β€ 1ms
@test float(clock_getres(CLOCK_REALTIME)) β€ 1ms
@test float(nanosleep(0.01)) == 0
s = 10ms
t0 = float(clock_gettime(CLOCK_MONOTONIC))
nanosleep(s)
t1 = float(clock_gettime(CLOCK_MONOTONIC))
@test abs(t1 - (t0 + s)) β€ 4ms
end
@testset "Signals " begin
# Sanity check:
@test isbitstype(IPC._typeof_sigset)
@test sizeof(IPC._typeof_sigset) == IPC._sizeof_sigset
@test isbitstype(IPC._typeof_siginfo)
@test sizeof(IPC._typeof_siginfo) == IPC._sizeof_siginfo
# SigSet:
set = SigSet()
sigrtmin = (isdefined(IPC, :SIGRTMIN) ? IPC.SIGRTMIN : 1)
sigrtmax = (isdefined(IPC, :SIGRTMAX) ? IPC.SIGRTMAX : 8*sizeof(set))
@test all(set[i] == false for i in sigrtmin:sigrtmax)
fill!(set, true)
@test all(set[i] for i in sigrtmin:sigrtmax)
i = rand(sigrtmin:sigrtmax)
set[i] = false
@test !set[i]
set[i] = true
@test set[i]
set = sigpending()
@test all(set[i] == false for i in 1:sigrtmax)
# SigInfo:
si = SigInfo()
# SigAction:
sa = SigAction()
end
@testset "BSD System V Keys " begin
path = "/tmp"
key1 = IPC.Key(path, '1')
key2 = IPC.Key(key1.value)
@test key1 == key2
shmds = ShmInfo()
semds = IPC.SemInfo()
end
# Skip semaphore tests for Apple.
@static if !Sys.isapple()
@testset "Named Semaphores " begin
begin
name = "/sem-$(getpid())"
rm(Semaphore, name)
@test_throws SystemError Semaphore(name)
sem1 = Semaphore(name, 0)
sem2 = Semaphore(name)
@test sem1[] == 0
@test sem2[] == 0
post(sem1)
@test sem2[] == 1
post(sem1)
@test sem2[] == 2
wait(sem2)
@test sem2[] == 1
@test trywait(sem2) == true
@test trywait(sem2) == false
@test_throws TimeoutError timedwait(sem2, 0.1)
end
GC.gc() # call garbage collector to exercise the finalizers
end
@testset "Anonymous Semaphores " begin
begin
buf = DynamicMemory(sizeof(Semaphore))
sem1 = Semaphore(buf, 0)
sem2 = Semaphore(buf)
@test sem1[] == 0
@test sem2[] == 0
post(sem1)
@test sem2[] == 1
post(sem1)
@test sem2[] == 2
wait(sem2)
@test sem2[] == 1
@test trywait(sem2) == true
@test trywait(sem2) == false
@test_throws TimeoutError timedwait(sem2, 0.1)
end
GC.gc() # call garbage collector to exercise the finalizers
end
end
@testset "Wrapped Arrays " begin
begin
T = Float32
dims = (5,6)
buf = DynamicMemory(sizeof(T)*prod(dims))
A = WrappedArray(buf, T, dims)
B = WrappedArray(buf) # indexable byte buffer
C = WrappedArray(buf, T) # indexable byte buffer
D = WrappedArray(buf, b -> (T, dims, 0)) # all parameters provided by a function
A[:] = 1:length(A) # fill A before the copy
E = copy(A)
n = prod(dims)
@test ndims(A) == ndims(D) == ndims(E) == length(dims)
@test size(A) == size(D) == size(E) == dims
@test all(size(A,i) == size(D,i) == dims[i] for i in 1:length(dims))
@test eltype(A) == eltype(C) == eltype(D) == T
@test Base.elsize(A) == Base.sizeof(T)
@test length(A) == length(C) == div(length(B), sizeof(T)) == length(D) == n
@test sizeof(A) == sizeof(B) == sizeof(C) == sizeof(D) == sizeof(buf)
@test pointer(A) == pointer(B) == pointer(C) == pointer(D) == pointer(buf)
@test isa(A.arr, Array{T,length(dims)})
@test A[1] == 1 && A[end] == prod(dims)
@test all(A[i] == i for i in 1:n)
@test all(C[i] == i for i in 1:n)
@test all(D[i] == i for i in 1:n)
@test all(E[i] == i for i in 1:n)
B[:] .= 0
@test all(A[i] == 0 for i in 1:n)
C[:] = randn(T, n)
flag = true
for i in eachindex(A, D)
if A[i] != D[i]
flag = false
end
end
@test flag
B[:] .= 0
A[2,3] = 23
A[3,2] = 32
@test D[2,3] == 23 && D[3,2] == 32
# Test copy back.
copyto!(A, E)
@test all(A[i] == E[i] for i in 1:n)
end
GC.gc() # call garbage collector to exercise the finalizers
end
@testset "Shared Memory (BSD) " begin
begin
T = Int
n = 10
len = n*sizeof(Int)
A = SharedMemory(IPC.PRIVATE, len)
id = shmid(A)
@test isa(id, ShmId)
@test id == ShmId(A)
@test "$id" == "ShmId($(id.value))"
@test id.value == convert(Int64, id)
@test id.value == convert(Int32, id)
B = SharedMemory(id; readonly=false)
C = SharedMemory(id; readonly=true)
@test shmid(A) == shmid(B) == shmid(C)
@test sizeof(A) == sizeof(B) == sizeof(C) == len
Aptr = convert(Ptr{T},pointer(A))
Bptr = convert(Ptr{T},pointer(B))
Cptr = convert(Ptr{T},pointer(C))
for i in 1:n
unsafe_store!(Aptr,i,i)
end
@test all(unsafe_load(Bptr, i) == i for i in 1:n)
@test all(unsafe_load(Cptr, i) == i for i in 1:n)
for i in 1:n
unsafe_store!(Bptr,-i,i)
end
@test all(unsafe_load(Aptr, i) == -i for i in 1:n)
@test all(unsafe_load(Cptr, i) == -i for i in 1:n)
@test_throws ReadOnlyMemoryError unsafe_store!(Cptr,42)
@test ccall(:memset, Ptr{Cvoid}, (Ptr{Cvoid}, Cint, Csize_t),
Aptr, 0, len) == Aptr
@test all(unsafe_load(Cptr, i) == 0 for i in 1:n)
end
GC.gc() # call garbage collector to exercise the finalizers
end
@testset "Shared Memory (POSIX) " begin
begin
T = Int
n = 10
len = n*sizeof(Int)
name = "/shm-$(getpid())"
rm(SharedMemory, name)
@test_throws SystemError SharedMemory(name)
A = SharedMemory(name, len)
id = shmid(A)
@test isa(id, String)
@test id == name
B = SharedMemory(id; readonly=false)
C = SharedMemory(id; readonly=true)
@test shmid(A) == shmid(B) == shmid(C) == name
@test sizeof(A) == sizeof(B) == sizeof(C) == len
Aptr = convert(Ptr{T},pointer(A))
Bptr = convert(Ptr{T},pointer(B))
Cptr = convert(Ptr{T},pointer(C))
for i in 1:n
unsafe_store!(Aptr,i,i)
end
@test all(unsafe_load(Bptr, i) == i for i in 1:n)
@test all(unsafe_load(Cptr, i) == i for i in 1:n)
for i in 1:n
unsafe_store!(Bptr,-i,i)
end
@test all(unsafe_load(Aptr, i) == -i for i in 1:n)
@test all(unsafe_load(Cptr, i) == -i for i in 1:n)
@test_throws ReadOnlyMemoryError unsafe_store!(Cptr,42)
@test ccall(:memset, Ptr{Cvoid}, (Ptr{Cvoid}, Cint, Csize_t),
Aptr, 0, len) == Aptr
@test all(unsafe_load(Cptr, i) == 0 for i in 1:n)
end
GC.gc() # call garbage collector to exercise the finalizers
end
@testset "Wrapped Shared Arrays " begin
begin
T = Float32
dims = (3,4,5)
for key in (IPC.PRIVATE, "/wrsharr-$(getpid())")
if isa(key, String)
try; shmrm(key); catch err; end
end
A = WrappedArray(key, T, dims)
id = shmid(A)
if isa(id, ShmId)
info = ShmInfo(id)
@test info.segsz β₯ sizeof(A) + 64
end
B = WrappedArray(id; readonly=false)
C = WrappedArray(id; readonly=true)
n = length(A)
@test shmid(A) == shmid(B) == shmid(C) == id
@test sizeof(A) == sizeof(B) == sizeof(C) == n*sizeof(T)
@test eltype(A) == eltype(B) == eltype(C) == T
@test size(A) == size(B) == size(C) == dims
@test length(A) == length(B) == length(C) == prod(dims)
@test all(size(A,i) == size(B,i) == size(C,i) == dims[i] for i in 1:length(dims))
A[:] = 1:n
@test first(A) == 1
@test last(A) == n
@test A[end] == n
@test all(B[i] == i for i in 1:n)
@test all(C[i] == i for i in 1:n)
B[:] = -(1:n)
@test extrema(A[:] + (1:n)) == (0, 0)
@test all(C[i] == -i for i in 1:n)
@test_throws ReadOnlyMemoryError C[end] = 42
@test ccall(:memset, Ptr{Cvoid}, (Ptr{Cvoid}, Cint, Csize_t),
A, 0, sizeof(A)) == pointer(A)
@test extrema(C) == (0, 0)
end
end
GC.gc() # call garbage collector to exercise the finalizers
end
end # module
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | docs | 1426 | # User visible changes in `InterProcessCommunication`
# Version 0.1.4
* `convert(RawFD, f)` and `RawFD(f)` yield the raw file descriptor of `FileDescriptor`
instance `f`.
# Version 0.1.3
* Argument `readonly` is now a keyword in `ShmId` constructor and `shmid` method. Old
behavior where it was the optional last argument has been deprecated.
* Argument `shmctl` checks that the buffer is large enough. Call `IPC.unsafe_shmct` to
avoid this check or to directly pass a pointer.
# Version 0.1.2
* Export `umask` to set the calling process's file mode creation mask.
* When creating a semaphore, `Semaphore(...)` ignores the calling process's file mode
creation mask for the access permissions while `open(Semaphore, ...)` masks the access
permissions against the process `umask` like `sem_open`.
* Standard C types are no longer prefixed by `_typeof_`. For example, Julia equivalent of
C `mode_t` is given by constant `IPC.mode_t`.
# Version 0.1.1
* Provide named and anonymous semaphores.
* Provide shared memory objects (instances of `SharedMemory`) which can be
associated with System V or POSIX shared memory.
* Provide versatile wrapped arrays (instances of `WrappedArray`) which are seen
as regular Julia arrays but whose elements are stored in a managed object.
* Arrays in shared memory are special instances of `WrappedArray` whose
contents are stored by an instance of `SharedMemory`.
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | docs | 2809 | # Inter-Process Communication for Julia
[![License][license-img]][license-url]
[![Documentation][doc-dev-img]][doc-dev-url]
[![Build Status][github-ci-img]][github-ci-url]
[![Coverage][coveralls-img]][coveralls-url]
[![Coverage][codecov-img]][codecov-url]
Julia has already many methods for inter-process communication (IPC): sockets,
semaphores, memory mapped files, etc. You may however want to have Julia
interacts with other processes or threads by means of BSD (System V) IPC or
POSIX shared memory, semaphores, message queues or mutexes, condition variables
and read/write locks. Package `InterProcessCommunication.jl` (*IPC* for short)
intends to provide such facilities.
The `InterProcessCommunication` package provides:
* Two kinds of **shared memory** objects: *named shared memory* which are
identified by their name and old-style (BSD System V) *shared memory
segments* which are identified by a key.
* Two kinds of **semaphores**: *named semaphores* which are identified by their
name and *anonymous semaphores* which are backed by *memory* objects (usually
shared memory) providing the necessary storage.
* Management of **signals** including so called real-time signals.
* Array-like objects stored in shared memory.
* Access to POSIX **mutexes**, **condition variables** and **read/write
locks**. These objects can optionally be stored in shared memory and shared
between processes.
## Documentation
The documentation for `InterProcessCommunication` package is
[here](https://emmt.github.io/InterProcessCommunication.jl/dev).
[doc-stable-img]: https://img.shields.io/badge/docs-stable-blue.svg
[doc-stable-url]: https://emmt.github.io/InterProcessCommunication.jl/stable
[doc-dev-img]: https://img.shields.io/badge/docs-dev-blue.svg
[doc-dev-url]: https://emmt.github.io/InterProcessCommunication.jl/dev
[license-url]: ./LICENSE.md
[license-img]: http://img.shields.io/badge/license-MIT-brightgreen.svg?style=flat
[github-ci-img]: https://github.com/emmt/InterProcessCommunication.jl/actions/workflows/CI.yml/badge.svg?branch=master
[github-ci-url]: https://github.com/emmt/InterProcessCommunication.jl/actions/workflows/CI.yml?query=branch%3Amaster
[appveyor-img]: https://ci.appveyor.com/api/projects/status/github/emmt/InterProcessCommunication.jl?branch=master
[appveyor-url]: https://ci.appveyor.com/project/emmt/InterProcessCommunication-jl/branch/master
[coveralls-img]: https://coveralls.io/repos/emmt/InterProcessCommunication.jl/badge.svg?branch=master&service=github
[coveralls-url]: https://coveralls.io/github/emmt/InterProcessCommunication.jl?branch=master
[codecov-img]: http://codecov.io/github/emmt/InterProcessCommunication.jl/coverage.svg?branch=master
[codecov-url]: http://codecov.io/github/emmt/InterProcessCommunication.jl?branch=master
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | docs | 985 | # A Julia Package for Inter-Process Communication
# Introduction
Julia already provides many methods for inter-process communication (IPC): sockets,
semaphores, memory mapped files, etc. You may however want to have Julia interacts with
other processes or threads by means of BSD (System V) IPC or POSIX shared memory,
semaphores, message queues or mutexes and condition variables. Package
`InterProcessCommunication` intends to provide such facilities.
The statement `using InterProcessCommunication` exports (among others) a shortcut named
`IPC` to the `InterProcessCommunication` module. This documentation assumes this shortcut
and the prefix `IPC.` is used in many places instead of the much longer
`InterProcessCommunication.` prefix.
The code source of `InterProcessCommunication.jl` is
[here](https://github.com/emmt/InterProcessCommunication.jl).
# Table of contents
```@contents
Pages = ["semaphores.md", "sharedmemory.md", "reference.md"]
```
## Index
```@index
```
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | docs | 868 | # Reference
The following provides detailled documentation about types and methods provided
by the `InterProcessCommunication` package. This information is also available
from the REPL by typing `?` followed by the name of a method or a type.
## Semaphores
```@docs
Semaphore
post(::Semaphore)
wait(::Semaphore)
timedwait(::Semaphore, ::Real)
trywait(::Semaphore)
```
## Shared Memory
```@docs
SharedMemory
ShmId
ShmInfo
shmid
shmget
shmat
shmdt
shmrm
shmctl
shmcfg
shminfo
shminfo!
```
## Signals
```@docs
SigSet
SigAction
SigInfo
sigaction
sigaction!
sigpending
sigpending!
sigprocmask
sigprocmask!
sigqueue
sigsuspend
sigwait
sigwait!
```
## Wrapped arrays
```@docs
WrappedArray
```
## Utilities
```@docs
TimeSpec
TimeVal
clock_getres
clock_gettime
clock_settime
gettimeofday
nanosleep
umask
IPC.maskmode
```
## Exceptions
```@docs
TimeoutError
```
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | docs | 6261 | # Semaphores
A semaphore is associated with an integer value which is never allowed to fall below zero.
Two operations can be performed on a semaphore `sem`: increment the semaphore value by one
with `post(sem)`; and decrement the semaphore value by one with `wait(sem)`. If the value
of a semaphore is currently zero, then a `wait(sem)` call will block until the value
becomes greater than zero.
There are two kinds of semaphores: *named* and *anonymous* semaphores. [Named
Semaphores](@ref) are identified by their name which is a string of the form
`"/somename"`. [Anonymous Semaphores](@ref) are backed by *memory* objects (usually shared
memory) providing the necessary storage. In Julia `InterProcessCommunication` package,
semaphores are instances of `Semaphore{T}` where `T` is `String` for named semaphores and
the type of the backing memory object for anonymous semaphores.
## Named Semaphores
Named semaphores are identified by their name which is a string of the form `"/somename"`.
A new named semaphore identified by the string `name` is created by:
```julia
sem = Semaphore(name, value; perms=0o600, volatile=true)
```
which creates a new named semaphore with initial value set to `value` and returns an
instance of `Semaphore{String}`. Keyword `perms` can be used to specify access permissions
(the default value, `0o600`, warrants read and write permissions for the caller). Keyword
`volatile` specify whether the semaphore should be automatically unlinked when `sem` is
garbage collected.
Another process (or thread) can open an existing named semaphore by calling:
```julia
sem = Semaphore(name)
```
which yields an instance of `Semaphore{String}`.
To unlink (remove) a persistent named semaphore, simply do:
```julia
rm(Semaphore, name)
```
which silently ignores errors if the semaphore does not exists, but throws a `SystemError`
for other errors.
For maximum flexibility, an instance of a named semaphore may also be created by:
```julia
sem = open(Semaphore, name, flags, mode, value, volatile)
```
where `flags` may have the bits `IPC.O_CREAT` and `IPC.O_EXCL` set, `mode` specifies the
granted access permissions, `value` is the initial semaphore value and `volatile` is a
boolean indicating whether the semaphore should be unlinked when the returned object `sem`
is finalized. The values of `mode` and `value` are ignored if an existing named semaphore
is open. The permissions settings in `mode` are masked against the process `umask`, i.e.
like in the POSIX C `sem_open` function.
## Anonymous Semaphores
Anonymous semaphores are backed by *memory* objects providing the necessary storage.
A new anonymous semaphore is created by:
```julia
sem = Semaphore(mem, value; offset=0, volatile=true)
```
which initializes an anonymous semaphore backed by memory object `mem` with initial value
set to `value` and returns an instance of `Semaphore{typeof(mem)}`. Keyword `offset` can
be used to specify the address (in bytes) of the semaphore data relative to
`pointer(mem)`. Keyword `volatile` specify whether the semaphore should be destroyed when
the returned object is finalized.
The number of bytes needed to store an anonymous semaphore is given by `sizeof(Semaphore)`
and anonymous semaphore must be aligned in memory at multiples of the word size in bytes
(that is `Sys.WORD_SIZE >> 3`). Memory objects used to store an anonymous semaphore must
implement two methods: `pointer(mem)` and `sizeof(mem)` to yield respectively the base
address (as an instance of `Ptr`) and the size (in bytes) of the associated memory.
Another process (or thread) can use an existing (initialized) anonymous semaphore by
calling:
```julia
sem = Semaphore(mem; offset=0)
```
where `mem` is the memory object which provides the storage of the semaphore at relative
position specified by keyword `offset` (zero by default). The returned value is an
instance of `Semaphore{typeof(mem)}`
## Operations on Semaphores
### Semaphore Value and Size
To query the value of semaphore `sem`, do:
```julia
sem[]
```
However beware that the value of the semaphore may already have changed by the time the
result is returned. The minimal and maximal values that can take a semaphore are
respectively given by:
```julia
typemin(Semaphore)
typemax(Semaphore)
```
To allocate memory for anonymous semaphores, the number of bytes needed to store a
semaphore is given by:
```julia
sizeof(Semaphore)
```
### Post and Wait
To unlock the semaphore `sem`, call:
```julia
post(sem)
```
which increments by one the semaphore's value. If the semaphore's value consequently
becomes greater than zero, then another process or thread blocked in a `wait` call on this
semaphore will be woken up.
Locking the semaphore `sem` is done by:
```julia
wait(sem)
```
which decrements by one the semaphore `sem`. If the semaphore's value is greater than
zero, then the decrement proceeds and the function returns immediately. If the semaphore
currently has the value zero, then the call blocks until either it becomes possible to
perform the decrement (i.e., the semaphore value rises above zero), or a signal handler
interrupts the call (in which case an instance of `InterruptException` is thrown). A
`SystemError` may be thrown if an unexpected error occurs.
To try locking the semaphore `sem` without blocking, do:
```julia
trywait(sem) -> boolean
```
which attempts to immediately decrement (lock) the semaphore returning `true` if
successful. If the decrement cannot be immediately performed, then `false` is returned. If
an interruption is received or if an unexpected error occurs, an exception is thrown
(`InterruptException` or `SystemError` respectively).
Finally, the call:
```julia
timedwait(sem, secs)
```
decrements (locks) the semaphore `sem`. If the semaphore's value is greater than zero,
then the decrement proceeds and the function returns immediately. If the semaphore
currently has the value zero, then the call blocks until either it becomes possible to
perform the decrement (i.e., the semaphore value rises above zero), or the limit of `secs`
seconds expires (in which case an instance of `TimeoutError` is thrown), or a signal
handler interrupts the call (in which case an instance of `InterruptException` is thrown).
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 0.1.4 | 6c14497a900e1922940a3a69ab555228590bcaff | docs | 6679 | # Shared Memory
The `InterProcessCommunication` package provides two kinds of shared memory objects: *named shared
memory* objects which are identified by their name and *BSD (System V) shared
memory segments* which are identified by a key.
## Shared Memory Objects
Shared memory objects are instances of `IPC.SharedMemory`. A new shared memory object is
created by calling the constructor:
```julia
shm = SharedMemory(id, len; perms=0o600, volatile=true)
```
with `id` an identifier and `len` the size, in bytes, of the allocated memory. The
identifier `id` can be a string starting by a `'/'` to create a POSIX shared memory object
or a System V IPC key to create a BSD System V shared memory segment. In this latter case,
the key can be `IPC.PRIVATE` to automatically create a non-existing shared memory segment.
Use keyword `perms` to specify the access permissions to be granted. By default, only
reading and writing by the user are granted.
Use keyword `volatile` to specify whether the shared memory is volatile or not. If
non-volatile, the shared memory will remain accessible until explicit destruction or
system reboot. If volatile (the default), the shared memory is destroyed when no longer in
use in use by any processes.
To retrieve an existing shared memory object, call:
```julia
shm = SharedMemory(id; readonly=false)
```
where `id` is the shared memory identifier (a string, an IPC key or a System V IPC
identifier of shared memory segment as returned by [`ShmId`](@ref)). Keyword `readonly`
can be set true if only read access is needed. Note that method `shmid(obj)` may be called
to retrieve the identifier of the shared memory object `obj`.
A number of accessors are available for a shared memory objects `shm`:
```julia
pointer(shm) # base address of the shared memory
sizeof(shm) # number of bytes of the shared memory
shmid(shm) # identifier of the shared memory
```
To ensure that shared memory object `shm` is eventually destroyed, call:
```julia
rm(shm)
```
## BSD System V Shared Memory
The following methods and type give a lower-level access (compared to
`SharedMemory` objects) to manage BSD System V shared memory segments.
### System V shared memory segment identifiers
The following statements:
```julia
id = ShmId(id)
id = ShmId(arr)
id = ShmId(key; readlony=false)
```
yield the the identifier of the existing System V shared memory segment associated with
the value of the first argument: `id` is the identifier of the shared memory segment,
`arr` is an array attached to a System V shared memory segment, and `key` is the key
associated with the shared memory segment. In that latter case, keyword `readlony` can be
set `true` to request read-only access; otherwise read-write access is requested.
### Getting or creating a shared memory segment
Call:
```julia
id = shmget(key, siz, flg)
```
to get the identifier of the System V shared memory segment associated with the System V
IPC key `key`. A new shared memory segment with size `siz` (in bytes, possibly rounded up
to a multiple of the memory page size `IPC.PAGE_SIZE`) is created if `key` has the value
`IPC.PRIVATE` or if bit `IPC_CREAT` is set in `flg` and no shared memory segment
corresponding to `key` exists.
Argument `flg` is a bitwise combination of flags. The least significant 9 bits specify the
permissions granted to the owner, group, and others. These bits have the same format, and
the same meaning, as the mode argument of `chmod`. Bit `IPC_CREAT` can be set to create a
new segment. If this flag is not used, then `shmget` will find the segment associated with
`key` and check to see if the user has permission to access the segment. Bit `IPC_EXCL`
can be set in addition to `IPC_CREAT` to ensure that this call creates the segment. If
`IPC_EXCL` and `IPC_CREAT` are both set, the call will fail if the segment already exists.
### Attaching and detaching shared memory
Call:
```julia
ptr = shmat(id, readonly)
```
attaches a System V shared memory segment to the address space of the caller. Argument
`id` is the identifier of the shared memory segment. Boolean argument `readonly` specifies
whether to attach the segment for read-only access; otherwise, the segment is attached for
read and write accesses and the process must have read and write permissions for the
segment. The returned value is the address of the shared memory segment for the caller.
Assuming `ptr` is the pointer returned by a previous `shmat()` call:
```julia
shmdt(ptr)
```
detaches the System V shared memory segment from the address space of the caller.
### Destroying shared memory
To remove shared memory associated with `arg`, call:
```julia
shmrm(arg)
```
If `arg` is a name, the corresponding POSIX named shared memory is unlinked. If `arg` is a
key or identifier of a BSD shared memory segment, the segment is marked to be eventually
destroyed. Argument `arg` can also be a `SharedMemory` object.
The `rm` method may also be called to remove an existing shared memory segment or
object:
```julia
rm(SharedMemory, name)
rm(SharedMemory, key)
rm(id)
rm(shm)
```
where `name` identifies a POSIX shared memory object, `key` is associated with
a BSD shared memory segment, `id` is the identifier of a BSD shared memory
segment and `shm` is an instance of `SharedMemory`.
### Controlling shared memory
To change the access permissions of a System V IPC shared memory segment, call:
```julia
shmcfg(arg, perms) -> id
```
where `perms` specifies bitwise flags with the new permissions. The first
argument can be the identifier of the shared memory segment, a shared array
attached to the shared memory segment or the System V IPC key associated with
the shared memory segment. In all cases, the identifier of the shared memory
segment is returned.
Other control operations can be performed with:
```julia
shmctl(id, cmd, buf)
```
where `id` is the identifier of the shared memory segment, `cmd` is the command
to perform and `buf` is a buffer large enough to store a `shmid_ds` C structure
(`IPC._sizeof_struct_shmid_ds` bytes).
### Retrieving shared memory information
To retrieve information about a System V shared memory segment, call one of:
```julia
info = shminfo(arg)
info = ShmInfo(arg)
```
with `arg` the identifier of the shared memory segment, a shared array attached
to the shared memory segment or the System V IPC key associated with the shared
memory segment. The result is an instance of `ShmInfo`.
Memory for the `ShmInfo` structure may be provided:
```julia
shminfo!(arg, info) -> info
```
where `info` is an instance of `ShmInfo` which is overwritten with information
about `arg` and returned.
| InterProcessCommunication | https://github.com/emmt/InterProcessCommunication.jl.git |
|
[
"MIT"
] | 1.1.0 | 4668cf550bcc834e5645229d2d6106e0d02f8263 | code | 729 | using Documenter, SurrogatesBase
DocMeta.setdocmeta!(SurrogatesBase,
:DocTestSetup,
:(using SurrogatesBase))
cp("./docs/Manifest.toml", "./docs/src/assets/Manifest.toml", force = true)
cp("./docs/Project.toml", "./docs/src/assets/Project.toml", force = true)
pages = [
"Home" => "index.md",
"interface.md",
"api.md"
]
ENV["GKSwstype"] = "100"
makedocs(modules = [SurrogatesBase],
sitename = "SurrogatesBase.jl",
clean = true,
doctest = true,
linkcheck = true,
format = Documenter.HTML(assets = ["assets/favicon.ico"],
canonical = "https://docs.sciml.ai/SurrogatesBase/stable/"),
pages = pages)
deploydocs(repo = "github.com/SciML/SurrogatesBase.jl"; push_preview = true)
| SurrogatesBase | https://github.com/SciML/SurrogatesBase.jl.git |
|
[
"MIT"
] | 1.1.0 | 4668cf550bcc834e5645229d2d6106e0d02f8263 | code | 2889 | module SurrogatesBase
export AbstractDeterministicSurrogate
export AbstractStochasticSurrogate
export update!, parameters
export update_hyperparameters!, hyperparameters
export finite_posterior
"""
abstract type AbstractDeterministicSurrogate <: Function end
An abstract type for deterministic surrogates.
(s::AbstractDeterministicSurrogate)(xs)
Subtypes of `AbstractDeterministicSurrogate` are callable with a `Vector` of points `xs`.
The result is a `Vector` of evaluations of the surrogate at points `xs`, corresponding to
approximations of the underlying function at points `xs` respectively.
# Examples
```jldoctest
julia> struct ZeroSurrogate <: AbstractDeterministicSurrogate end
julia> (::ZeroSurrogate)(xs) = 0
julia> s = ZeroSurrogate();
julia> s([4]) == 0
true
```
"""
abstract type AbstractDeterministicSurrogate <: Function end
"""
abstract type AbstractStochasticSurrogate end
An abstract type for stochastic surrogates.
See also [`finite_posterior`](@ref).
"""
abstract type AbstractStochasticSurrogate end
"""
update!(s, new_xs::AbstractVector, new_ys::AbstractVector)
Include data `new_ys` at points `new_xs` into the surrogate `s`, i.e., refit the surrogate `s`
to incorporate new data points.
If the surrogate `s` is a deterministic surrogate, the `new_ys` correspond to function
evaluations, if `s` is a stochastic surrogate, the `new_ys` are samples from a conditional
probability distribution.
Use `update!(s, eachslice(X, dims = 2), new_ys)` if `X` is a matrix.
"""
function update! end
"""
parameters(s)
Returns current values of parameters used in surrogate `s`.
"""
function parameters end
"""
update_hyperparameters!(s, prior)
Update the hyperparameters of the surrogate `s` by performing hyperparameter optimization
using the information in `prior`. After changing hyperparameters of `s`, fit `s` to past
data.
See also [`hyperparameters`](@ref).
"""
function update_hyperparameters! end
"""
hyperparameters(s)
Returns current values of hyperparameters.
See also [`update_hyperparameters!`](@ref).
"""
function hyperparameters end
"""
finite_posterior(s::AbstractStochasticSurrogate, xs::AbstractVector)
Return a posterior distribution at points `xs`.
An `AbstractStochasticSurrogate` might implement some or all of the following methods on
the returned object:
- `mean(finite_posterior(s,xs))` returns a `Vector` of posterior means at `xs`
- `var(finite_posterior(s,xs))` returns a `Vector` of posterior variances at `xs`
- `mean_and_var(finite_posterior(s,xs))` returns a `Tuple` consisting of a `Vector` of posterior means and a `Vector` of posterior variances at `xs`
- `rand(finite_posterior(s,xs))` returns a `Vector`, which is a sample from the joint
posterior at points `xs`
Use `mean(finite_posterior(s, eachslice(X, dims = 2)))` if `X` is a matrix.
"""
function finite_posterior end
end
| SurrogatesBase | https://github.com/SciML/SurrogatesBase.jl.git |
|
[
"MIT"
] | 1.1.0 | 4668cf550bcc834e5645229d2d6106e0d02f8263 | code | 3487 | using SurrogatesBase
using Test
using LinearAlgebra
import Statistics
struct DummySurrogate{X, Y} <: AbstractDeterministicSurrogate
xs::Vector{X}
ys::Vector{Y}
end
# return y value of the closest ΞΎ in xs to x
(s::DummySurrogate)(x) = s.ys[argmin(norm(x - ΞΎ) for ΞΎ in s.xs)]
function SurrogatesBase.update!(s::DummySurrogate, new_xs, new_ys)
append!(s.xs, new_xs)
append!(s.ys, new_ys)
end
mutable struct HyperparameterDummySurrogate{X, Y} <: AbstractDeterministicSurrogate
xs::Vector{X}
ys::Vector{Y}
ΞΈ::NamedTuple
end
# return y value of the closest ΞΎ in xs to x, in p-norm where p is a hyperparameter
(s::HyperparameterDummySurrogate)(x) = s.ys[argmin(norm(x - ΞΎ, s.ΞΈ.p) for ΞΎ in s.xs)]
function SurrogatesBase.update!(s::HyperparameterDummySurrogate, new_xs, new_ys)
append!(s.xs, new_xs)
append!(s.ys, new_ys)
end
SurrogatesBase.hyperparameters(s::HyperparameterDummySurrogate) = s.ΞΈ
function SurrogatesBase.update_hyperparameters!(s::HyperparameterDummySurrogate, prior)
# "hyperparmeter optimization"
s.ΞΈ = (; p = (s.ΞΈ.p + prior.p) / 2)
end
@testset "update!" begin
# use DummySurrogate
d = DummySurrogate(Vector{Vector{Float64}}(), Vector{Int}())
update!(d, [[10.3, 0.1], [1.9, 2.1]], [5, 6])
update!(d, [[-0.3, 9.9], [-0.1, -10.0]], [1, 3])
@test length(d.xs) == 4
@test d([0.0, -9.9]) == 3
end
@testset "default implementations" begin
# use DummySurrogate
d = DummySurrogate(Vector{Vector{Float64}}(), Vector{Float64}())
update!(d, [[1.9, 2.1]], [5.0])
update!(d, [[10.3, 0.1]], [9.0])
@test d([2.0, 2.0]) == 5.0
@test_throws MethodError hyperparameters(d)
@test_throws MethodError update_hyperparameters!(d, 5)
end
@testset "hyperparameter interface" begin
# use HyperparameterDummySurrogate
hd = HyperparameterDummySurrogate(Vector{Vector{Float64}}(),
Vector{Float64}(),
(; p = 2))
update!(hd, [[1.9, 2.1], [10.3, 0.1]], [5.0, 9.0])
@test hyperparameters(hd).p == 2
update_hyperparameters!(hd, (; p = 4))
@test hyperparameters(hd).p == 3
end
mutable struct DummyStochasticSurrogate{X, Y} <: AbstractStochasticSurrogate
xs::Vector{X}
ys::Vector{Y}
ys_mean::Y
end
function SurrogatesBase.update!(s::DummyStochasticSurrogate, new_xs, new_ys)
append!(s.xs, new_xs)
append!(s.ys, new_ys)
# update mean
s.ys_mean = (s.ys_mean * (length(s.xs) - length(new_xs)) + sum(new_ys)) / length(s.xs)
end
SurrogatesBase.parameters(s::DummyStochasticSurrogate) = s.ys_mean
struct FiniteDummyStochasticSurrogate{X}
means::Vector{X}
end
Statistics.mean(s::FiniteDummyStochasticSurrogate) = s.means
# xs are arbitrary points where we wish to get a joint posterior
function FiniteDummyStochasticSurrogate(s, xs)
return FiniteDummyStochasticSurrogate(s.ys_mean .* ones(length(xs)))
end
function SurrogatesBase.finite_posterior(s::DummyStochasticSurrogate, xs)
FiniteDummyStochasticSurrogate(s, xs)
end
@testset "finite_posterior, parameters" begin
# use HyperparameterDummySurrogate
ss = DummyStochasticSurrogate(Vector{Vector{Float64}}(),
Vector{Float64}(), 0.0)
update!(ss, [[1.9, 2.1], [10.3, 0.1]], [5.0, 9.0])
# test parameters
@test parameters(ss) β 7.0
update!(ss, [[2.0, 4.0]], [3.0])
@test parameters(ss) β 17 / 3
m = Statistics.mean(finite_posterior(ss, [[3.5, 2.0], [4.0, 5.0], [1.0, 67.0]]))
@test length(m) == 3
@test m[1] β parameters(ss)
end
| SurrogatesBase | https://github.com/SciML/SurrogatesBase.jl.git |
|
[
"MIT"
] | 1.1.0 | 4668cf550bcc834e5645229d2d6106e0d02f8263 | docs | 1765 | # SurrogatesBase.jl
[](https://julialang.zulipchat.com/#narrow/stream/279055-sciml-bridged)
[](https://docs.sciml.ai/SurrogatesBase/stable/)
[](https://codecov.io/gh/SciML/SurrogatesBase.jl)
[](https://github.com/SciML/SurrogatesBase.jl/actions?query=workflow%3ACI)
[](https://github.com/SciML/ColPrac)
[](https://github.com/SciML/SciMLStyle)
API for deterministic and stochastic surrogates.
Given data $((x_1, y_1), \ldots, (x_N, y_N))$ obtained by evaluating a function $y_i =
f(x_i)$ or sampling from a conditional probability density $p_{Y|X}(Y = y_i|X = x_i)$,
a **deterministic surrogate** is a function $s(x)$ (e.g. a [radial basis function
interpolator](https://en.wikipedia.org/wiki/Radial_basis_function_interpolation)) that
uses the data to approximate $f$ or some statistic of $p_{Y|X}$ (e.g. the mean),
whereas a **stochastic surrogate** is a stochastic process (e.g. a [Gaussian process
approximation](https://en.wikipedia.org/wiki/Gaussian_process_approximations)) that uses
the data to approximate $f$ or $p_{Y|X}$ *and* quantify the uncertainty of the
approximation. | SurrogatesBase | https://github.com/SciML/SurrogatesBase.jl.git |
|
[
"MIT"
] | 1.1.0 | 4668cf550bcc834e5645229d2d6106e0d02f8263 | docs | 51 | # API
```@autodocs
Modules = [SurrogatesBase]
```
| SurrogatesBase | https://github.com/SciML/SurrogatesBase.jl.git |
|
[
"MIT"
] | 1.1.0 | 4668cf550bcc834e5645229d2d6106e0d02f8263 | docs | 2984 | # SurrogatesBase.jl: A Common Interface for Surrogate Libraries
API for deterministic and stochastic surrogates.
Given data $((x_1, y_1), \ldots, (x_N, y_N))$ obtained by evaluating a function $y_i =
f(x_i)$ or sampling from a conditional probability density $p_{Y|X}(Y = y_i|X = x_i)$,
a **deterministic surrogate** is a function $s(x)$ (e.g. a [radial basis function
interpolator](https://en.wikipedia.org/wiki/Radial_basis_function_interpolation)) that
uses the data to approximate $f$ or some statistic of $p_{Y|X}$ (e.g. the mean),
whereas a **stochastic surrogate** is a stochastic process (e.g. a [Gaussian process
approximation](https://en.wikipedia.org/wiki/Gaussian_process_approximations)) that uses
the data to approximate $f$ or $p_{Y|X}$ *and* quantify the uncertainty of the
approximation.
## Installation
To install SurrogatesBase.jl, use the Julia package manager:
```julia
using Pkg
Pkg.add("SurrogatesBase")
```
## Contributing
- Please refer to the
[SciML ColPrac: Contributor's Guide on Collaborative Practices for Community Packages](https://github.com/SciML/ColPrac/blob/master/README.md)
for guidance on PRs, issues, and other matters relating to contributing to SciML.
- See the [SciML Style Guide](https://github.com/SciML/SciMLStyle) for common coding practices and other style decisions.
- There are a few community forums:
+ The #diffeq-bridged and #sciml-bridged channels in the
[Julia Slack](https://julialang.org/slack/)
+ The #diffeq-bridged and #sciml-bridged channels in the
[Julia Zulip](https://julialang.zulipchat.com/#narrow/stream/279055-sciml-bridged)
+ On the [Julia Discourse forums](https://discourse.julialang.org)
+ See also [SciML Community page](https://sciml.ai/community/)
## Reproducibility
```@raw html
<details><summary>The documentation of this SciML package was built using these direct dependencies,</summary>
```
```@example
using Pkg # hide
Pkg.status() # hide
```
```@raw html
</details>
```
```@raw html
<details><summary>and using this machine and Julia version.</summary>
```
```@example
using InteractiveUtils # hide
versioninfo() # hide
```
```@raw html
</details>
```
```@raw html
<details><summary>A more complete overview of all dependencies and their versions is also provided.</summary>
```
```@example
using Pkg # hide
Pkg.status(; mode = PKGMODE_MANIFEST) # hide
```
```@raw html
</details>
```
```@eval
using TOML
using Markdown
version = TOML.parse(read("../../Project.toml", String))["version"]
name = TOML.parse(read("../../Project.toml", String))["name"]
link_manifest = "https://github.com/SciML/" * name * ".jl/tree/gh-pages/v" * version *
"/assets/Manifest.toml"
link_project = "https://github.com/SciML/" * name * ".jl/tree/gh-pages/v" * version *
"/assets/Project.toml"
Markdown.parse("""You can also download the
[manifest]($link_manifest)
file and the
[project]($link_project)
file.
""")
```
| SurrogatesBase | https://github.com/SciML/SurrogatesBase.jl.git |
|
[
"MIT"
] | 1.1.0 | 4668cf550bcc834e5645229d2d6106e0d02f8263 | docs | 5231 | # The SurrogateBase Interface
## Deterministic Surrogates
Deterministic surrogates `s` are subtypes of `SurrogatesBase.AbstractDeterministicSurrogate`,
which is a subtype of `Function`.
### Required methods
The method `update!(s, xs, ys)` **must** be implemented and the surrogate **must** be
[callable](https://docs.julialang.org/en/v1/manual/methods/#Function-like-objects)
`s(xs)`, where `xs` is a `Vector` of input points and `ys` is a `Vector` of corresponding evaluations.
Calling `update!(s, xs, ys)` refits the surrogate `s` to include evaluations `ys` at points `xs`.
The result of `s(xs)` is a `Vector` of evaluations of the surrogate at points `xs`, corresponding to approximations of the underlying function at points `xs` respectively.
For single points `x` and `y`, call these methods via `update!(s, [x], [y])`
and `s([x])`.
### Optional methods
If the surrogate `s` wants to expose current parameter values, the method `parameters(s)` **must** be implemented.
If the surrogate `s` has tunable hyperparameters, the methods
`update_hyperparameters!(s, prior)` and `hyperparameters(s)` **must** be implemented.
Calling `update_hyperparameters!(s, prior)` updates the hyperparameters of the surrogate `s` by performing hyperparameter optimization using the information in `prior`. After the hyperparameters of `s` are updated, `s` is fit to past evaluations.
Calling `hyperparameters(s)` returns current values of hyperparameters.
### Example
```julia
using SurrogatesBase
struct RBF{T} <: AbstractDeterministicSurrogate
scale::T
centers::Vector{T}
weights::Vector{T}
end
(rbf::RBF)(xs) = [rbf.weights' * exp.(-rbf.scale * (x .- rbf.centers).^2)
for x in xs]
function SurrogatesBase.update!(rbf::RBF, xs, ys)
# Refit the surrogate by updating rbf.weights to include new
# evaluations ys at points xs
return rbf
end
SurrogatesBase.parameters(rbf::RBF) = rbf.centers, rbf.weights
SurrogatesBase.hyperparameters(rbf::RBF) = rbf.scale
function SurrogatesBase.update_hyperparameters!(rbf::RBF, prior)
# update rbf.scale and fit the surrogate by adapting rbf.weights
return rbf
end
```
## Stochastic Surrogates
Stochastic surrogates `s` are subtypes of `SurrogatesBase.AbstractStochasticSurrogate`.
### Required methods
The methods `update!(s, xs, ys)` and `finite_posterior(s, xs)` **must** be implemented, where `xs` is a `Vector` of input points and `ys` is a `Vector` of corresponding observed samples.
Calling `update!(s, xs, ys)` refits the surrogate `s` to include observations `ys` at points `xs`.
For single points `x` and `y`, call the `update!(s, xs, ys)` via `update!(s, [x], [y])`.
Calling `finite_posterior(s, xs)` returns an object that provides methods for working with the finite
dimensional posterior distribution at points `xs`.
The following methods might be supported:
- `mean(finite_posterior(s,xs))` returns a `Vector` of posterior means at `xs`
- `var(finite_posterior(s,xs))` returns a `Vector` of posterior variances at `xs`
- `mean_and_var(finite_posterior(s,xs))` returns a `Tuple` consisting of a `Vector` of posterior means and a `Vector` of posterior variances at `xs`
- `rand(finite_posterior(s,xs))` returns a `Vector`, which is a sample from the joint posterior at points `xs`
### Optional methods
If the surrogate `s` wants to expose current parameter values, the method `parameters(s)` **must** be implemented.
If the surrogate `s` has tunable hyper-parameters, the methods
`update_hyperparameters!(s, prior)` and `hyperparameters(s)` **must** be implemented.
Calling `update_hyperparameters!(s, prior)` updates the hyperparameters of the surrogate `s` by performing hyperparameter optimization using the information in `prior`. After the hyperparameters of `s` are updated, `s` is fit to past samples.
Calling `hyperparameters(s)` returns current values of hyperparameters.
### Example
```julia
using SurrogatesBase
mutable struct GaussianProcessSurrogate{D, R, GP, H <: NamedTuple} <: AbstractStochasticSurrogate
xs::Vector{D}
ys::Vector{R}
gp_process::GP
hyperparameters::H
end
function SurrogatesBase.update!(g::GaussianProcessSurrogate, new_xs, new_ys)
append!(g.xs, new_xs)
append!(g.ys, new_ys)
# condition the prior `g.gp_process` on new data to obtain a posterior
# update g.gp_process to the posterior process
return g
end
function SurrogatesBase.finite_posterior(g::GaussianProcessSurrogate, xs)
# Return a finite dimensional projection of g.gp_process at points xs.
# The returned object GP_finite supports methods mean(GP_finite) and
# var(GP_finite) for obtaining the vector of means and variances at points xs.
end
SurrogatesBase.hyperparameters(g::GaussianProcessSurrogate) = g.hyperparameters
function SurrogatesBase.update_hyperparameters!(g::GaussianProcessSurrogate, prior)
# Use prior on hyperparameters, e.g., parameters uniformly distributed
# between an upper and lower bound, to perform hyperparameter optimization.
# Set g.hyperparameters to the improved hyperparameters.
# Fit a Gaussian process that uses the updated hyperparameters to past
# samples and save it in g.gp_process.
return g
end
``` | SurrogatesBase | https://github.com/SciML/SurrogatesBase.jl.git |
|
[
"MIT"
] | 0.1.5 | f9c5e6430f2e23b703a3c84df0ea2cc0362519df | code | 299 | # make.jl
using Documenter, LimberJack
makedocs(sitename = "LimberJack.jl",
modules = [LimberJack],
pages = ["Home" => "index.md",
"API" => "api.md",
"Tutorial" => "tutorial.md"])
deploydocs(repo = "github.com/JaimeRZP/LimberJack.jl") | LimberJack | https://github.com/JaimeRZP/LimberJack.jl.git |
|
[
"MIT"
] | 0.1.5 | f9c5e6430f2e23b703a3c84df0ea2cc0362519df | code | 837 | module LimberJack
export Settings, CosmoPar, Cosmology, Ez, Hmpc, comoving_radial_distance
export chi_to_z, z_to_t
export growth_factor, growth_rate, fs8, sigma8
export lin_Pk, nonlin_Pk
export NumberCountsTracer, WeakLensingTracer, CMBLensingTracer, get_IA
export angularCβs, angularCβ, angularCβFast
export Theory
export make_data
using Interpolations, LinearAlgebra, Statistics, QuadGK
using NPZ, NumericalIntegration, PythonCall, Artifacts
include("core.jl")
include("boltzmann.jl")
include("data_utils.jl")
include("growth.jl")
include("halofit.jl")
include("tracers.jl")
include("spectra.jl")
include("theory.jl")
# c/(100 km/s/Mpc) in Mpc
const CLIGHT_HMPC = 2997.92458
function __init__()
emupk_files = npzread(joinpath(artifact"emupk", "emupk.npz"))
global emulator = Emulator(emupk_files)
end
end # module
| LimberJack | https://github.com/JaimeRZP/LimberJack.jl.git |
|
[
"MIT"
] | 0.1.5 | f9c5e6430f2e23b703a3c84df0ea2cc0362519df | code | 5957 | function _T0(keq, k, ac, bc)
q = @. (k/(13.41*keq))
C = @. ((14.2/ac) + (386/(1+69.9*q^1.08)))
T0 = @.(log(β―+1.8*bc*q)/(log(β―+1.8*bc*q)+C*q^2))
return T0
end
function TkEisHu(cosmo::CosmoPar, k)
Ξ©c = cosmo.Ξ©m-cosmo.Ξ©b
wm=cosmo.Ξ©m*cosmo.h^2
wb=cosmo.Ξ©b*cosmo.h^2
# Scales
# k_eq
keq = (7.46*10^-2)*wm/(cosmo.h * cosmo.ΞΈCMB^2)
# z_eq
zeq = (2.5*10^4)*wm*(cosmo.ΞΈCMB^-4)
# z_drag
b1 = 0.313*(wm^-0.419)*(1+0.607*wm^0.674)
b2 = 0.238*wm^0.223
zd = 1291*((wm^0.251)/(1+0.659*wm^0.828))*(1+b1*wb^b2)
# k_Silk
ksilk = 1.6*wb^0.52*wm^0.73*(1+(10.4*wm)^-0.95)/cosmo.h
# r_s
R_prefac = 31.5*wb*(cosmo.ΞΈCMB^-4)
Rd = R_prefac*((zd+1)/10^3)^-1
Rdm1 = R_prefac*(zd/10^3)^-1
Req = R_prefac*(zeq/10^3)^-1
rs = sqrt(1+Rd)+sqrt(Rd+Req)
rs /= (1+sqrt(Req))
rs = (log(rs))
rs *= (2/(3*keq))*sqrt(6/Req)
# Tc
q = @.(k/(13.41*keq))
a1 = (46.9*wm)^0.670*(1+(32.1*wm)^-0.532)
a2 = (12.0*wm)^0.424*(1+(45.0*wm)^-0.582)
ac = (a1^(-cosmo.Ξ©b/cosmo.Ξ©m))*(a2^(-(cosmo.Ξ©b/cosmo.Ξ©m)^3))
b1 = 0.944*(1+(458*wm)^-0.708)^-1
b2 = (0.395*wm)^(-0.0266)
bc = (1+b1*((Ξ©c/cosmo.Ξ©m)^b2-1))^-1
f = @.(1/(1+(k*rs/5.4)^4))
Tc1 = f.*_T0(keq, k, 1, bc)
Tc2 = (1 .-f).*_T0(keq, k, ac, bc)
Tc = Tc1 .+ Tc2
# Tb
y = (1+zeq)/(1+zd)
Gy = y*(-6*sqrt(1+y)+(2+3y)*log((sqrt(1+y)+1)/(sqrt(1+y)-1)))
ab = 2.07*keq*rs*(1+Rdm1)^(-3/4)*Gy
bb = 0.5+(cosmo.Ξ©b/cosmo.Ξ©m)+(3-2*cosmo.Ξ©b/cosmo.Ξ©m)*sqrt((17.2*wm)^2+1)
bnode = 8.41*(wm)^0.435
ss = rs./(1 .+(bnode./(k.*rs)).^3).^(1/3)
Tb1 = _T0(keq, k, 1, 1)./(1 .+(k.*rs/5.2).^2)
Tb2 = (ab./(1 .+(bb./(k.*rs)).^3)).*exp.(-(k/ksilk).^ 1.4)
Tb = (Tb1.+Tb2).*sin.(k.*ss)./(k.*ss)
Tk = (cosmo.Ξ©b/cosmo.Ξ©m).*Tb.+(Ξ©c/cosmo.Ξ©m).*Tc
return Tk.^2
end
struct Emulator
alphas
hypers
trans_cosmos
training_karr
inv_chol_cosmos
mean_cosmos
mean_log_Pks
std_log_Pks
end
Emulator(files) = begin
trans_cosmos = files["trans_cosmos"]
karr = files["training_karr"]
training_karr = range(log(karr[1]), stop=log(karr[end]), length=length(karr))
hypers = files["hypers"]
alphas = files["alphas"]
inv_chol_cosmos = files["inv_chol_cosmos"]
mean_cosmos = files["mean_cosmos"]
mean_log_Pks = files["mean_log_Pks"]
std_log_Pks = files["std_log_Pks"]
#Note: transpose python arrays
Emulator(alphas, hypers,
trans_cosmos', training_karr,
inv_chol_cosmos, mean_cosmos,
mean_log_Pks, std_log_Pks)
end
function _x_transformation(emulator::Emulator, point)
return emulator.inv_chol_cosmos * (point .- emulator.mean_cosmos)'
end
function _y_transformation(emulator::Emulator, point)
return ((point .- emulator.mean_log_Pks) ./ emulator.std_log_Pks)'
end
function _inv_y_transformation(emulator::Emulator, point)
return exp.(emulator.std_log_Pks .* point .+ emulator.mean_log_Pks)
end
function lin_Pk0(mode::Val{:EmuPk}, cpar::CosmoPar, settings::Settings)
cosmotype = settings.cosmo_type
wc = cpar.Ξ©c*cpar.h^2
wb = cpar.Ξ©b*cpar.h^2
ln1010As = log((10^10)*cpar.As)
params = [wc, wb, ln1010As, cpar.ns, cpar.h]
params_t = _x_transformation(emulator, params')
lks_emul = emulator.training_karr
nk = length(lks_emul)
pk0s_t = zeros(cosmotype, nk)
@inbounds for i in 1:nk
kernel = _get_kernel(emulator.trans_cosmos, params_t, emulator.hypers[i, :])
pk0s_t[i] = dot(vec(kernel), vec(emulator.alphas[i,:]))
end
pk0_emul = vec(_inv_y_transformation(emulator, pk0s_t))
pki_emul = cubic_spline_interpolation(lks_emul, log.(pk0_emul),
extrapolation_bc=Line())
pk0 = exp.(pki_emul(settings.logk))
return pk0
end
function lin_Pk0(mode::Val{:EisHu}, cpar::CosmoPar, settings::Settings)
tk = TkEisHu(cpar, settings.ks./ cpar.h)
pk0 = @. settings.ks^cpar.ns * tk
return pk0
end
function lin_Pk0(mode::Val{:Custom}, cpar::CosmoPar, settings::Settings; kwargs...)
ks_c, pk_c = kwargs[:pk_custom]
pki_c = cubic_spline_interpolation(ks_c, Pk_c, extrapolation_bc=Line())
pk0 = pki_c(settings.ks)
return pk0
end
lin_Pk0(mode::Symbol, cpar::CosmoPar, settings::Settings; kwargs...) = lin_Pk0(Val(mode), cpar, settings; kwargs...)
lin_Pk0(@nospecialize(mode), cpar::CosmoPar, settings::Settings; kwargs...) = error("Tk mode $(typeof(i)) not supported.")
function _get_kernel(arr1, arr2, hyper)
arr1_w = @.(arr1/exp(hyper[2:6]))
arr2_w = @.(arr2/exp(hyper[2:6]))
# compute the pairwise distance
term1 = sum(arr1_w.^2, dims=1)
term2 = 2 * (arr1_w' * arr2_w)'
term3 = sum(arr2_w.^2, dims=1)
dist = @.(term1-term2+term3)
# compute the kernel
kernel = @.(exp(hyper[1])*exp(-0.5*dist))
return kernel
end
function _w_tophat(x::Real)
x2 = x^2
if x < 0.1
w = 1. + x2*(-1.0/10.0 + x2*(1.0/280.0 +
x2*(-1.0/15120.0 + x2*(1.0/1330560.0 +
x2* (-1.0/172972800.0)))));
else
w = 3 * (sin(x)-x*cos(x))/(x2*x)
end
return w
end
function ΟR2(ks, pk, dlogk, R)
x = ks .* R
wk = _w_tophat.(x)
integrand = @. pk * wk^2 * ks^3
# OPT: proper integration instead?
Ο = integrate(log.(ks), integrand, SimpsonEven())/(2*pi^2)
#Ο = sum(integrand)*dlogk/(2*pi^2)
return Ο
end
function ΟR2(cosmo::Cosmology, R)
return ΟR2(cosmo.ks, cosmo.pk0, cosmo.dlogk, R)
end
function lin_Pk0(cpar::CosmoPar, settings::Settings)
pk0 = lin_Pk0(settings.tk_mode, cpar, settings)
#Renormalize Pk
_Ο8 = ΟR2(settings.ks, pk0, settings.dlogk, 8.0/cpar.h)
if settings.using_As
cpar.Ο8 = _Ο8
else
norm = cpar.Ο8^2 / _Ο8
pk0 *= norm
end
pki = cubic_spline_interpolation(settings.logk, log.(pk0);
extrapolation_bc=Line())
return pk0, pki
end | LimberJack | https://github.com/JaimeRZP/LimberJack.jl.git |
|
[
"MIT"
] | 0.1.5 | f9c5e6430f2e23b703a3c84df0ea2cc0362519df | code | 11888 | """
Settings(;kwargs...)
Constructor of settings structure constructor.
Kwargs:
- `nz::Int=300` : number of nodes in the general redshift array.
- `nk::Int=500`: number of nodes in the k-scale array used to compute matter power spectrum grid.
- `nβ::Int=300`: number of nodes in the multipoles array.
- `using_As::Bool=false`: `True` if using the `As` parameter.
- `cosmo_type::Type=Float64` : type of cosmological parameters.
- `tk_mode::String=:EisHu` : choice of transfer function.
- `Dz_mode::String=:RK2` : choice of method to compute the linear growth factor.
- `pk_mode::String=:linear` : choice of method to apply non-linear corrections to the matter power spectrum.
Returns:
```
mutable struct Settings
nz::Int
nk::Int
nβ::Int
xs
zs
ks
βs
logk
dlogk
using_As::Bool
cosmo_type::DataType
tk_mode::Symbol
Dz_mode::Symbol
pk_mode::Symbol
end
```
"""
mutable struct Settings
nz::Int
nk::Int
nβ::Int
xs
zs
ks
βs
logk
dlogk
using_As::Bool
cosmo_type::DataType
tk_mode::Symbol
Dz_mode::Symbol
pk_mode::Symbol
end
Settings(;kwargs...) = begin
nz = get(kwargs, :nz, 300)
nk = get(kwargs, :nk, 500)
nβ = get(kwargs, :nβ, 300)
z_max = get(kwargs, :z_max, 3.0)
xs = LinRange(0, log(1+z_max), nz)
zs = @.(exp(xs) - 1)
logk = range(log(0.0001), stop=log(100.0), length=nk)
ks = exp.(logk)
dlogk = log(ks[2]/ks[1])
βs = range(0, stop=2000, length=nβ)
using_As = get(kwargs, :using_As, false)
cosmo_type = get(kwargs, :cosmo_type, Float64)
tk_mode = get(kwargs, :tk_mode, :EisHu)
Dz_mode = get(kwargs, :Dz_mode, :RK2)
pk_mode = get(kwargs, :pk_mode, :linear)
Settings(nz, nk, nβ,
xs, zs, ks, βs, logk, dlogk,
using_As,
cosmo_type, tk_mode, Dz_mode, pk_mode)
end
"""
CosmoPar(kwargs...)
Cosmology parameters structure constructor.
Kwargs:
- `Ξ©m::Dual=0.30` : cosmological matter density.
- `Ξ©b::Dual=0.05` : cosmological baryonic density.
- `h::Dual=0.70` : reduced Hubble parameter.
- `ns::Dual=0.96` : Harrison-Zeldovich spectral index.
- `As::Dual=2.097e-9` : Harrison-Zeldovich spectral amplitude.
- `Ο8::Dual=0.81`: variance of the matter density field in a sphere of 8 Mpc.
- `Y_p::Dual=0.24`: primordial helium fraction.
- `N_Ξ½::Dual=3.046`: effective number of relativisic species (PDG25 value).
- `Ξ£m_Ξ½::Dual=0.0`: sum of neutrino masses (eV), Planck 15 default ΞCDM value.
- `ΞΈCMB::Dual=2.725/2.7`: CMB temperature over 2.7.
- `Ξ©g::Dual=2.38163816E-5*ΞΈCMB^4/h^2`: cosmological density of relativistic species.
- `Ξ©r::Dual=Ξ©g*(1.0 + N_Ξ½ * (7.0/8.0) * (4.0/11.0)^(4.0/3.0))`: cosmological radiation density.
Returns:
```
mutable struct CosmoPar{T}
Ξ©m::T
Ξ©b::T
h::T
ns::T
As::T
Ο8::T
ΞΈCMB::T
Y_p::T
N_Ξ½::T
Ξ£m_Ξ½::T
Ξ©g::T
Ξ©r::T
Ξ©c::T
Ξ©Ξ::T
end
```
"""
mutable struct CosmoPar{T}
Ξ©m::T
Ξ©b::T
h::T
ns::T
As::T
Ο8::T
ΞΈCMB::T
Y_p::T
N_Ξ½::T
Ξ£m_Ξ½::T
Ξ©g::T
Ξ©r::T
Ξ©c::T
Ξ©Ξ::T
end
CosmoPar(;kwargs...) = begin
kwargs = Dict(kwargs)
Ξ©m = get(kwargs, :Ξ©m, 0.3)
Ξ©b = get(kwargs, :Ξ©b, 0.05)
h = get(kwargs, :h, 0.67)
ns = get(kwargs, :ns, 0.96)
As = get(kwargs, :As, 2.097e-9)
Ο8 = get(kwargs, :Ο8, 0.81)
cosmo_type = eltype([Ξ©m, Ξ©b, h, ns, Ο8])
Y_p = get(kwargs, :Y_p, 0.24) # primordial helium fraction
N_Ξ½ = get(kwargs, :N_Ξ½, 3.046) # effective number of relativisic species (PDG25 value)
Ξ£m_Ξ½ = get(kwargs, :Ξ£m_Ξ½, 0.0) # sum of neutrino masses (eV), Planck 15 default ΞCDM value
ΞΈCMB = get(kwargs, :ΞΈCMB, 2.725/2.7)
prefac = 2.38163816E-5 # This is 4*sigma_SB*(2.7 K)^4/rho_crit(h=1)
f_rel = 1.0 + N_Ξ½ * (7.0/8.0) * (4.0/11.0)^(4.0/3.0)
Ξ©g = get(kwargs, :Ξ©r, prefac*ΞΈCMB^4/h^2)
Ξ©r = get(kwargs, :Ξ©r, Ξ©g*f_rel)
Ξ©c = Ξ©m-Ξ©b
ΩΠ= 1-Ωm-Ωr
CosmoPar{cosmo_type}(Ξ©m, Ξ©b, h, ns, As, Ο8,
ΞΈCMB, Y_p, N_Ξ½, Ξ£m_Ξ½,
Ξ©g, Ξ©r, Ξ©c, Ξ©Ξ)
end
function _get_cosmo_type(x::CosmoPar{T}) where{T}
return T
end
struct Cosmology
settings::Settings
cpar::CosmoPar
chi::AbstractInterpolation
z_of_chi::AbstractInterpolation
t_of_z::AbstractInterpolation
chi_max
chi_LSS
Dz::AbstractInterpolation
fs8z::AbstractInterpolation
PkLz0::AbstractInterpolation
Pk::AbstractInterpolation
end
"""
Cosmology(cpar::CosmoPar, settings::Settings)
Base cosmology structure constructor.
Calculates the LCDM expansion history based on the different \
species densities provided in `CosmoPar`.
The comoving distance is then calculated integrating the \
expansion history.
Depending on the choice of transfer function in the settings, \
the primordial power spectrum is calculated using:
- `tk_mode = :EisHu` : the Eisenstein & Hu formula (arXiv:astro-ph/9710252)
- `tk_mode = :EmuPk` : the Mootoovaloo et al 2021 emulator `EmuPk` (arXiv:2105.02256v2)
Depending on the choice of power spectrum mode in the settings, \
the matter power spectrum is either:
- `pk_mode = :linear` : the linear matter power spectrum.
- `pk_mode = :halofit` : the Halofit non-linear matter power spectrum (arXiv:astro-ph/0207664).
Arguments:
- `Settings::MutableStructure` : cosmology constructure settings.
- `CosmoPar::Structure` : cosmological parameters.
Returns:
```
mutable struct Cosmology
settings::Settings
cpar::CosmoPar
chi::AbstractInterpolation
z_of_chi::AbstractInterpolation
t_of_z::AbstractInterpolation
chi_max
chi_LSS
Dz::AbstractInterpolation
fs8z::AbstractInterpolation
PkLz0::AbstractInterpolation
Pk::AbstractInterpolation
end
```
"""
Cosmology(cpar::CosmoPar, settings::Settings; kwargs...) = begin
# Load settings
cosmo_type = settings.cosmo_type
zs, nz = settings.zs, settings.nz
logk, nk = settings.logk, settings.nk
ks = settings.ks
dlogk = settings.dlogk
pk0, pki = lin_Pk0(cpar, settings; kwargs...)
# Compute redshift-distance relation
chis = zeros(cosmo_type, nz)
ts = zeros(cosmo_type, nz)
for i in 1:nz
zz = zs[i]
chis[i] = quadgk(z -> 1.0/Ez(cpar, z), 0.0, zz, rtol=1E-5)[1]
chis[i] *= CLIGHT_HMPC / cpar.h
ts[i] = quadgk(z -> 1.0/((1+z)*Ez(cpar, z)), 0.0, zz, rtol=1E-5)[1]
ts[i] *= cpar.h*(18.356164383561644*10^9)
end
# Distance to LSS
chi_LSS = quadgk(z -> 1.0/Ez(cpar, z), 0.0, 1100., rtol=1E-5)[1]
chi_LSS *= CLIGHT_HMPC / cpar.h
# OPT: tolerances, interpolation method
chii = linear_interpolation(zs, Vector(chis), extrapolation_bc=Line())
ti = linear_interpolation(zs, Vector(ts), extrapolation_bc=Line())
zi = linear_interpolation(chis, Vector(zs), extrapolation_bc=Line())
Dzs, Dzi, fs8zi = get_growth(cpar, settings; kwargs...)
if settings.pk_mode == :linear
Pks = pk0 * (Dzs.^2)'
Pki = linear_interpolation((logk, zs), log.(Pks);
extrapolation_bc=Line())
elseif settings.pk_mode == :Halofit
Pki = get_PKnonlin(cpar, zs, ks, pk0, Dzs;
cosmo_type=cosmo_type)
else
@error("Pk mode not implemented")
end
Cosmology(settings, cpar, chii, zi, ti, chi_LSS,
chi_LSS, Dzi, fs8zi, pki, Pki)
end
"""
Cosmology(;kwargs...)
Short form to call `Cosmology(cpar::CosmoPar, settings::Settings)`.
`kwargs` are passed to the constructors of `CosmoPar` and `Settings`.
Returns:
- `Cosmology` : cosmology structure.
"""
Cosmology(;kwargs...) = begin
kwargs=Dict(kwargs)
if :As β keys(kwargs)
using_As = true
else
using_As = false
end
cpar = CosmoPar(;kwargs...)
cosmo_type = _get_cosmo_type(cpar)
settings = Settings(;cosmo_type=cosmo_type,
using_As=using_As,
kwargs...)
if using_As && (settings.tk_mode == "BBKS" || settings.tk_mode == "EisensteinHu")
@warn "Using As with BBKS or EisensteinHu transfer function is not possible."
@warn "Using Ο8 instead."
using_As = false
end
Cosmology(cpar, settings)
end
function Ez(cpar::CosmoPar, z)
E2 = @. (cpar.Ξ©m*(1+z)^3+cpar.Ξ©r*(1+z)^4+cpar.Ξ©Ξ)
return sqrt.(E2)
end
"""
chi_to_z(cosmo::Cosmology, chi)
Given a `Cosmology` instance, converts from comoving distance to redshift.
Arguments:
- `cosmo::Cosmology` : cosmology structure
- `chi::Dual` : comoving distance
Returns:
- `z::Dual` : redshift
"""
function chi_to_z(cosmo::Cosmology, chi)
return cosmo.z_of_chi(chi)
end
"""
z_to_t(cosmo::Cosmology, z)
Given a `Cosmology` instance, converts from redshift to years.
Arguments:
- `cosmo::Cosmology` : cosmology structure
- `z::Dual` : redshift
Returns:
- `t::Dual` : years
"""
function z_to_t(cosmo::Cosmology, z)
return cosmo.t_of_z(z)
end
"""
Ez(cosmo::Cosmology, z)
Given a `Cosmology` instance, it returns the expansion rate (H(z)/H0).
Arguments:
- `cosmo::Cosmology` : cosmology structure
- `z::Dual` : redshift
Returns:
- `Ez::Dual` : expansion rate
"""
Ez(cosmo::Cosmology, z) = Ez(cosmo.cpar, z)
"""
Hmpc(cosmo::Cosmology, z)
Given a `Cosmology` instance, it returns the expansion history (H(z)) in Mpc.
Arguments:
- `cosmo::Cosmology` : cosmology structure
- `z::Dual` : redshift
Returns:
- `Hmpc::Dual` : expansion rate
"""
Hmpc(cosmo::Cosmology, z) = cosmo.cpar.h*Ez(cosmo, z)/CLIGHT_HMPC
"""
comoving_radial_distance(cosmo::Cosmology, z)
Given a `Cosmology` instance, it returns the comoving radial distance.
Arguments:
- `cosmo::Cosmology` : cosmology structure
- `z::Dual` : redshift
Returns:
- `Chi::Dual` : comoving radial distance
"""
comoving_radial_distance(cosmo::Cosmology, z) = cosmo.chi(z)
"""
growth_rate(cosmo::Cosmology, z)
Given a `Cosmology` instance, it returns growth rate.
Arguments:
- `cosmo::Cosmology` : cosmology structure
- `z::Dual` : redshift
Returns:
- `f::Dual` : f
"""
growth_rate(cosmo::Cosmology, z) = cosmo.fs8z(z) ./ (cosmo.cpar.Ο8*cosmo.Dz(z)/cosmo.Dz(0))
"""
fs8(cosmo::Cosmology, z)
Given a `Cosmology` instance, it returns fs8.
Arguments:
- `cosmo::Cosmology` : cosmology structure
- `z::Dual` : redshift
Returns:
- `fs8::Dual` : fs8
"""
fs8(cosmo::Cosmology, z) = cosmo.fs8z(z)
"""
growth_factor(cosmo::Cosmology, z)
Given a `Cosmology` instance, it returns the growth factor (D(z) = log(Ξ΄)).
Arguments:
- `cosmo::Cosmology` : cosmological structure
- `z::Dual` : redshift
Returns:
- `Dz::Dual` : comoving radial distance
"""
growth_factor(cosmo::Cosmology, z) = cosmo.Dz(z)
"""
sigma8(cosmo::Cosmology, z)
Given a `Cosmology` instance, it returns s8.
Arguments:
- `cosmo::Cosmology` : cosmological structure
- `z::Dual` : redshift
Returns:
- `s8::Dual` : comoving radial distance
"""
sigma8(cosmo::Cosmology, z) = cosmo.cpar.Ο8*cosmo.Dz(z)/cosmo.Dz(0)
"""
nonlin_Pk(cosmo::Cosmology, k, z)
Given a `Cosmology` instance, it returns the non-linear matter power spectrum (P(k,z)) \
using the Halofit fitting formula (arXiv:astro-ph/0207664).
Arguments:
- `cosmo::Cosmology` : cosmology structure
- `k::Dual` : scale
- `z::Dual` : redshift
Returns:
- `Pk::Dual` : non-linear matter power spectrum
"""
function nonlin_Pk(cosmo::Cosmology, k, z)
return @. exp(cosmo.Pk(log(k), z))
end
"""
lin_Pk(cosmo::Cosmology, k, z)
Given a `Cosmology` instance, it returns the linear matter power spectrum (P(k,z))
Arguments:
- `cosmo::Cosmology` : cosmology structure
- `k::Dual` : scale
- `z::Dual` : redshift
Returns:
- `Pk::Dual` : linear matter power spectrum
"""
function lin_Pk(cosmo::Cosmology, k, z)
pk0 = @. exp(cosmo.PkLz0(log(k)))
Dz2 = cosmo.Dz(z)^2
return pk0 .* Dz2
end
| LimberJack | https://github.com/JaimeRZP/LimberJack.jl.git |
|
[
"MIT"
] | 0.1.5 | f9c5e6430f2e23b703a3c84df0ea2cc0362519df | code | 4264 | struct Instructions
names
pairs
types
idx
data
cov
inv_cov
end
function _get_type(sacc_file, tracer_name)
typ = sacc_file.tracers[tracer_name].quantity
return pyconvert(String, typ)
end
function _get_spin(sacc_file, tracer_name)
tt = string(sacc_file.tracers[tracer_name].quantity)
if tt == "galaxy_shear"
spin = "e"
elseif tt == "galaxy_density"
spin = "0"
elseif tt == "cmb_convergence"
spin = "0"
end
return spin
end
function _get_cl_name(s, t1, t2)
spin1 = _get_spin(s, t1)
spin2 = _get_spin(s, t2)
cl_name = string("cl_", spin1 , spin2)
if cl_name == "cl_e0"
cl_name = "cl_0e"
end
return cl_name
end
function _apply_scale_cuts(s, yaml_file)
indices = Vector{Int}([])
for cl in yaml_file["order"]
t1, t2 = cl["tracers"]
lmin, lmax = cl["ell_cuts"]
cl_name = _get_cl_name(s, t1, t2)
ind = s.indices(cl_name, (t1, t2),
ell__gt=lmin, ell__lt=lmax)
append!(indices, pyconvert(Vector{Int}, ind))
end
s.keep_indices(indices)
return s
end
"""
make_data(sacc_file, yaml_file)
Process `sacc` and `yaml` files into a `Meta` structure \
containing the instructions of how to compose the theory \
vector and a `npz` file with the neccesary redshift distributions \
of the tracers involved.
Arguments:
- `sacc_file` : sacc file
- `yaml_file` : yaml_file
Returns:
```
struct Instructions
names : names of tracers.
pairs : pairs of tracers to compute angular spectra for.
types : types of the tracers.
idx : positions of cls in theory vector.
data : data vector.
cov : covariance of the data.
inv_cov : inverse covariance of the data.
end
```
-files: npz file
"""
function make_data(sacc_file, yaml_file; kwargs...)
kwargs=Dict(kwargs)
kwargs_keys = [string(i) for i in collect(keys(kwargs))]
#cut
s = _apply_scale_cuts(sacc_file, yaml_file)
#build quantities of interest
cls = Vector{Float64}([])
ls = []
indices = Vector{Int}([])
pairs = []
for cl in yaml_file["order"]
t1, t2 = cl["tracers"]
cl_name = _get_cl_name(s, t1, t2)
l, c_ell, ind = s.get_ell_cl(cl_name, string(t1), string(t2),
return_cov=false, return_ind=true)
append!(indices, pyconvert(Vector{Int}, ind))
append!(cls, pyconvert(Vector{Float64}, c_ell))
push!(ls, pyconvert(Vector{Float64}, l))
push!(pairs, pyconvert(Vector{String}, [t1, t2]))
end
names = unique(vcat(pairs...))
cov = pyconvert(Vector{Vector{Float64}}, s.covariance.dense)
cov = permutedims(hcat(cov...))[indices.+1, :][:, indices.+1]
w, v = eigen(cov)
cov = v * (diagm(abs.(w)) * v')
cov = tril(cov) + triu(cov', 1)
cov = Hermitian(cov)
inv_cov = inv(cov)
lengths = [length(l) for l in ls]
lengths = vcat([0], lengths)
idx = cumsum(lengths)
types = [_get_type(s, name) for name in names]
# build struct
instructions = Instructions(names, pairs, types, idx,
cls, cov, inv_cov)
# Initialize
files = Dict{String}{Vector}()
# Load in l's
for (pair, l) in zip(pairs, ls)
t1, t2 = pair
println(t1, " ", t2, " ", length(l))
merge!(files, Dict(string("ls_", t1, "_", t2)=> l))
end
# Load in nz's
for (name, tracer) in s.tracers.items()
if string(name) in names
if string("nz_", name) in kwargs_keys
println(string("using custom nz for ", string("nz_", name)))
nzs = kwargs[Symbol("nz_", name)]
z= pyconvert(Vector{Float64}, nzs["z"])
nz=pyconvert(Vector{Float64}, nzs["dndz"])
merge!(files, Dict(string("nz_", name)=>[z, nz]))
else
if string(tracer.quantity) != "cmb_convergence"
z=pyconvert(Vector{Float64}, tracer.z)
nz=pyconvert(Vector{Float64}, tracer.nz)
merge!(files, Dict(string("nz_", name)=>[z, nz]))
end
end
end
end
return instructions, files
end | LimberJack | https://github.com/JaimeRZP/LimberJack.jl.git |
|
[
"MIT"
] | 0.1.5 | f9c5e6430f2e23b703a3c84df0ea2cc0362519df | code | 2990 | function _dgrowth!(dd, d, cosmo::CosmoPar, a)
ez = Ez(cosmo, 1.0/a-1.0)
dd[1] = d[2] * 1.5 * cosmo.Ξ©m / (a^2*ez)
dd[2] = d[1] / (a^3*ez)
end
get_growth(mode::Symbol, cpar::CosmoPar, settings::Settings; kwargs...) = get_growth(Val(mode), cpar, settings; kwargs...)
get_growth(@nospecialize(mode), cpar::CosmoPar, settings::Settings; kwargs...) = error("Dz mode $(typeof(i)) not supported.")
function get_growth(mode::Val{:RK2}, cpar::CosmoPar, settings::Settings; kwargs...)
x = settings.xs
z = settings.zs
a = @.(1/(1+z))
dx = x[2]-x[1]
aa = reverse(a)
e = Ez(cpar, z)
ee = reverse(e)
dd = zeros(settings.cosmo_type, settings.nz)
yy = zeros(settings.cosmo_type, settings.nz)
dd[1] = aa[1]
yy[1] = aa[1]^3*ee[end]
for i in 1:(settings.nz-1)
A0 = -1.5 * cpar.Ξ©m / (aa[i]*ee[i])
B0 = -1. / (aa[i]^2*ee[i])
A1 = -1.5 * cpar.Ξ©m / (aa[i+1]*ee[i+1])
B1 = -1. / (aa[i+1]^2*ee[i+1])
yy[i+1] = (1+0.5*dx^2*A0*B0)*yy[i] + 0.5*(A0+A1)*dx*dd[i]
dd[i+1] = 0.5*(B0+B1)*dx*yy[i] + (1+0.5*dx^2*A0*B0)*dd[i]
end
y = reverse(yy)
d = reverse(dd)
Dzs = d./d[1]
Dzi = linear_interpolation(z, Dzs, extrapolation_bc=Line())
fs8zi = linear_interpolation(z, -cpar.Ο8 .* y./ (a.^2 .*e.*d[1]),
extrapolation_bc=Line())
return Dzs, Dzi, fs8zi
end
function get_growth(mode::Val{:Custom}, cpar::CosmoPar, settings::Settings; kwargs...)
zs_c, Dzs_c = kwargs[:Dz_custom]
d = zs_c[2]-zs_c[1]
Dzi = cubic_spline_interpolation(zs_c, Dzs_c, extrapolation_bc=Line())
Dzs = Dzi(settings.zs)
dDzs_mid = (Dzs_c[2:end].-Dzs_c[1:end-1])/d
zs_mid = (zs_c[2:end].+zs_c[1:end-1])./2
dDzi = linear_interpolation(zs_mid, dDzs_mid, extrapolation_bc=Line())
dDzs_c = dDzi(zs_c)
fs8zi = cubic_spline_interpolation(zs_c, -cpar.Ο8 .* (1 .+ zs_c) .* dDzs_c,
extrapolation_bc=Line())
return Dzs, Dzi, fs8zi
end
#=
function _get_growth(mode::Val{:OrdDiffEq}, cpar::CosmoPar, settings::Settings; kwargs...)
z_ini = 1000.0
a_ini = 1.0/(1.0+z_ini)
ez_ini = Ez(cpar, z_ini)
d0 = [a_ini^3*ez_ini, a_ini]
a_s = reverse(@. 1.0 / (1.0 + zs))
prob = ODEProblem(_dgrowth!, d0, (a_ini, 1.0), cpar)
sol = solve(prob, Tsit5(), reltol=1E-6,
abstol=1E-8, saveat=a_s)
# OPT: interpolation (see below), ODE method, tolerances
# Note that sol already includes some kind of interpolation,
# so it may be possible to optimize this by just using
# sol directly.
s = vcat(sol.u'...)
Dzs = reverse(s[:, 2] / s[end, 2])
# OPT: interpolation method
Dzi = LinearInterpolation(zs, Dzs, extrapolation_bc=Line())
end
=#
function get_growth(cpar::CosmoPar, settings::Settings; kwargs...)
return get_growth(settings.Dz_mode, cpar, settings; kwargs...)
end | LimberJack | https://github.com/JaimeRZP/LimberJack.jl.git |
|
[
"MIT"
] | 0.1.5 | f9c5e6430f2e23b703a3c84df0ea2cc0362519df | code | 5105 | #=
halofit:
- Julia version:
- Author: andrina
- Date: 2021-09-17
- Modified by: damonge and JaimeRZP
- Date: 2022-02-19
=#
function _get_Ο2(lks, ks, pks, R, kind)
nlks = length(lks)
k3 = ks .^ 3
x2 = @. (ks*R)^2
if kind == 2
pre = x2
elseif kind == 4
pre = @. x2*(1-x2)
else
pre = 1
end
integrand = @. k3 * pre * exp(-x2) * pks
#integral = sum(@.(0.5*(integrand[2:nlks]+integrand[1:nlks-1])*(lks[2:nlks]-lks[1:nlks-1])))/(2*pi^2)
integral = integrate(lks, integrand, SimpsonEven())/(2*pi^2)
return integral
end
function get_PKnonlin(cpar::CosmoPar, z, k, PkLz0, Dzs; cosmo_type::DataType=Real)
nk = length(k)
nz = length(z)
logk = log.(k)
Dz2s = Dzs .^ 2
# OPT: hard-coded range and number of points
lR0 = log(0.01)
lR1 = log(10.0)
lRs = range(lR0, stop=lR1, length=100)
Ο2s = zeros(cosmo_type, length(lRs))
@inbounds for i in 1:length(lRs)
lR = lRs[i]
Ο2s[i] = _get_Ο2(logk, k, PkLz0, exp(lR), 0)
end
lΟ2s = log.(Ο2s)
# When s8<0.6 lΟ2i interpolation fails --> Extrapolation needed
lΟ2i = cubic_spline_interpolation(lRs, lΟ2s, extrapolation_bc=Line())
pk_NLs = zeros(cosmo_type, nk, nz)
@inbounds for i in 1:nz
Dz2 = Dz2s[i]
rsig = _get_rsigma(lΟ2i, Dz2, lR0, lR1)
onederiv_int = _get_Ο2(logk, k, PkLz0, rsig, 2) * Dz2
twoderiv_int = _get_Ο2(logk, k, PkLz0, rsig, 4) * Dz2
neff = 2*onederiv_int - 3.0
C = 4*(twoderiv_int + onederiv_int^2)
pk_NLs[1:nk, i] = _power_spectrum_nonlin(cpar, PkLz0 .* Dz2, k, z[i], rsig, neff, C)
end
pk_NL = linear_interpolation((logk, z), log.(pk_NLs),
extrapolation_bc=Line())
return pk_NL
end
function _secant(f, x0, x1, tol)
# TODO: fail modes
x_nm1 = x0
x_nm2 = x1
f_nm1 = f(x_nm1)
f_nm2 = f(x_nm2)
while abs(x_nm1 - x_nm2) > tol
x_n = (x_nm2*f_nm1-x_nm1*f_nm2)/(f_nm1-f_nm2)
x_nm2 = x_nm1
x_nm1 = x_n
f_nm2 = f_nm1
f_nm1 = f(x_nm1)
end
return 0.5*(x_nm2+x_nm1)
end
function _get_rsigma(lΟ2i, Dz2, lRmin, lRmax)
lDz2 = log(Dz2)
lRsigma = _secant(lR -> lΟ2i(lR)+lDz2, lRmin, lRmax, 1E-4)
return exp(lRsigma)
end
function _power_spectrum_nonlin(cpar::CosmoPar, PkL, k, z, rsig, neff, C)
# DAM: note that below I've commented out anything to do with
# neutrinos or non-Lambda dark energy.
opz = 1.0+z
#weffa = -1.0
Ez2 = cpar.Ξ©m*opz^3+cpar.Ξ©r*opz^4+cpar.Ξ©Ξ
omegaMz = cpar.Ξ©m*opz^3 / Ez2
omegaDEwz = cpar.Ξ©Ξ/Ez2
ksigma = @. 1.0 / rsig
neff2 = @. neff * neff
neff3 = @. neff2 * neff
neff4 = @. neff3 * neff
delta2_norm = @. k*k*k/2.0/pi/pi
# compute the present day neutrino massive neutrino fraction
# uses all neutrinos even if they are moving fast
# fnu = 0.0
# eqns A6 - A13 of Takahashi et al.
an = @. 10.0^(1.5222 + 2.8553*neff + 2.3706*neff2 + 0.9903*neff3 +
0.2250*neff4 - 0.6038*C)# + 0.1749*omegaDEwz*(1.0 + weffa))
bn = @. 10.0^(-0.5642 + 0.5864*neff + 0.5716*neff2 - 1.5474*C)# + 0.2279*omegaDEwz*(1.0 + weffa))
cn = @. 10.0^(0.3698 + 2.0404*neff + 0.8161*neff2 + 0.5869*C)
gamman = @. 0.1971 - 0.0843*neff + 0.8460*C
alphan = @. abs(6.0835 + 1.3373*neff - 0.1959*neff2 - 5.5274*C)
betan = @. 2.0379 - 0.7354*neff + 0.3157*neff2 + 1.2490*neff3 + 0.3980*neff4 - 0.1682*C
#mun = 0.0
nun = @. 10.0^(5.2105 + 3.6902*neff)
# eqns C17 and C18 for Smith et al.
if abs(1.0 - omegaMz) > 0.01
f1a = omegaMz ^ -0.0732
f2a = omegaMz ^ -0.1423
f3a = omegaMz ^ 0.0725
f1b = omegaMz ^ -0.0307
f2b = omegaMz ^ -0.0585
f3b = omegaMz ^ 0.0743
fb_frac = omegaDEwz / (1.0 - omegaMz)
f1 = fb_frac * f1b + (1.0 - fb_frac) * f1a
f2 = fb_frac * f2b + (1.0 - fb_frac) * f2a
f3 = fb_frac * f3b + (1.0 - fb_frac) * f3a
else
f1 = 1.0
f2 = 1.0
f3 = 1.0
end
# correction to betan from Bird et al., eqn A10
#betan += (fnu * (1.081 + 0.395*neff2))
# eqns A1 - A3
y = k ./ ksigma
y2 = @. y * y
fy = @. y/4.0 + y2/8.0
DeltakL = PkL .* delta2_norm
# correction to DeltakL from Bird et al., eqn A9
#kh = @. k / cpar.h
#kh2 = @. kh * kh
#DeltakL_tilde_fac = @. fnu * (47.48 * kh2) / (1.0 + 1.5 * kh2)
#DeltakL_tilde = @. DeltakL * (1.0 + DeltakL_tilde_fac)
#DeltakQ = @. DeltakL * (1.0 + DeltakL_tilde)^betan / (1.0 + DeltakL_tilde*alphan) * exp(-fy)
DeltakQ = @. DeltakL * (1.0 + DeltakL)^betan / (1.0 + DeltakL*alphan) * exp(-fy)
DeltakHprime = @. an * y^(3.0*f1) / (1.0 + bn*y^f2 + (cn*f3*y)^(3.0 - gamman))
#DeltakH = @. DeltakHprime / (1.0 + mun/y + nun/y2)
#
# correction to DeltakH from Bird et al., eqn A6-A7
#Qnu = @. fnu * (0.977 - 18.015 * (cpar.Ξ©m - 0.3))
#DeltakH *= @. (1.0 + Qnu)
DeltakH = @. DeltakHprime / (1.0 + nun/y2)
DeltakNL = @. DeltakQ + DeltakH
PkNL = @. DeltakNL / delta2_norm
return PkNL
end
| LimberJack | https://github.com/JaimeRZP/LimberJack.jl.git |
|
[
"MIT"
] | 0.1.5 | f9c5e6430f2e23b703a3c84df0ea2cc0362519df | code | 1499 | """
Cβintegrand(cosmo::Cosmology, t1::Tracer, t2::Tracer, logk, β)
Returns the integrand of the angular power spectrum.
Arguments:
- `cosmo::Cosmology` : cosmology structure.
- `t1::Tracer` : tracer structure.
- `t2::Tracer` : tracer structure.
- `logk::Vector{Float}` : log scale array.
- `β::Float` : multipole.
Returns:
- `integrand::Vector{Real}` : integrand of the angular power spectrum.
"""
function Cβintegrand(cosmo::Cosmology,
t1::AbstractInterpolation,
t2::AbstractInterpolation,
β::Number)
sett = cosmo.settings
chis = zeros(sett.cosmo_type, sett.nk)
chis[1:sett.nk] = (β+0.5) ./ sett.ks
chis .*= (chis .< cosmo.chi_max)
z = cosmo.z_of_chi(chis)
w1 = t1(chis)
w2 = t2(chis)
pk = nonlin_Pk(cosmo, sett.ks, z)
return @. (sett.ks*w1*w2*pk)
end
"""
angularCβs(cosmo::Cosmology, t1::Tracer, t2::Tracer, βs)
Returns the angular power spectrum.
Arguments:
- `cosmo::Cosmology` : cosmology structure.
- `t1::Tracer` : tracer structure.
- `t2::Tracer` : tracer structure.
- `βs::Vector{Float}` : multipole array.
Returns:
- `Cβs::Vector{Real}` : angular power spectrum.
"""
function angularCβs(cosmo::Cosmology, t1::Tracer, t2::Tracer, βs::Vector)
# OPT: we are not optimizing the limits of integration
sett = cosmo.settings
Cβs = [integrate(sett.logk, Cβintegrand(cosmo, t1.wint, t2.wint, β)/(β+0.5), SimpsonEven()) for β in βs]
return t1.F(βs) .* t2.F(βs) .* Cβs
end | LimberJack | https://github.com/JaimeRZP/LimberJack.jl.git |
|
[
"MIT"
] | 0.1.5 | f9c5e6430f2e23b703a3c84df0ea2cc0362519df | code | 3415 | function Theory(cosmology::Cosmology,
names, types, pairs,
idx, files;
Nuisances=Dict())
nui_type = eltype(valtype(Nuisances))
if !(nui_type <: Float64) & (nui_type != Any)
if nui_type != Real
cosmology.settings.cosmo_type = nui_type
end
end
tracers = Dict{String}{Tracer}()
ntracers = length(names)
@inbounds for i in 1:ntracers
name = names[i]
t_type = types[i]
if t_type == "galaxy_density"
zs_mean, nz_mean = files[string("nz_", name)]
b = get(Nuisances, string(name, "_", "b"), 1.0)
nz = get(Nuisances, string(name, "_", "nz"), nz_mean)
zs = get(Nuisances, string(name, "_", "zs"), zs_mean)
dz = get(Nuisances, string(name, "_", "dz"), 0.0)
tracer = NumberCountsTracer(cosmology, zs .+ dz, nz;
b=b)
elseif t_type == "galaxy_shear"
zs_mean, nz_mean = files[string("nz_", name)]
m = get(Nuisances, string(name, "_", "m"), 0.0)
IA_params = [get(Nuisances, "A_IA", 0.0),
get(Nuisances, "alpha_IA", 0.0)]
nz = get(Nuisances, string(name, "_", "nz"), nz_mean)
zs = get(Nuisances, string(name, "_", "zs"), zs_mean)
dz = get(Nuisances, string(name, "_", "dz"), 0.0)
tracer = WeakLensingTracer(cosmology, zs .+ dz, nz;
m=m, IA_params=IA_params)
elseif t_type == "cmb_convergence"
tracer = CMBLensingTracer(cosmology)
else
@error("Tracer not implemented")
tracer = nothing
end
merge!(tracers, Dict(name => tracer))
end
npairs = length(pairs)
total_len = last(idx)
cls = zeros(cosmology.settings.cosmo_type, total_len)
@inbounds Threads.@threads :static for i in 1:npairs
name1, name2 = pairs[i]
ls = files[string("ls_", name1, "_", name2)]
tracer1 = tracers[name1]
tracer2 = tracers[name2]
cls[idx[i]+1:idx[i+1]] = angularCβs(cosmology, tracer1, tracer2, ls)
end
return cls
end
"""
Theory(cosmology::Cosmology,
instructions::Instructions, files;
Nuisances=Dict())
Composes a theory vector given a `Cosmology` object, \
a `Meta` objectm, a `files` npz file and \
a dictionary of nuisance parameters.
Arguments:
- `cosmology::Cosmology` : `Cosmology` object.
- `meta::Meta` : `Meta` object.
- `files` : `files` `npz` file.
- `Nuisances::Dict` : dictonary of nuisace parameters.
Returns:
- Meta: structure
```
struct Meta
names : names of tracers.
pairs : pairs of tracers to compute angular spectra for.
types : types of the tracers.
idx : positions of cls in theory vector.
data : data vector.
cov : covariance of the data.
inv_cov : inverse covariance of the data.
end
```
- files: npz file
"""
function Theory(cosmology::Cosmology,
instructions::Instructions, files;
Nuisances=Dict())
names = instructions.names
types = instructions.types
pairs = instructions.pairs
idx = instructions.idx
return Theory(cosmology::Cosmology,
names, types, pairs,
idx, files;
Nuisances=Nuisances)
end
| LimberJack | https://github.com/JaimeRZP/LimberJack.jl.git |
|
[
"MIT"
] | 0.1.5 | f9c5e6430f2e23b703a3c84df0ea2cc0362519df | code | 4930 | abstract type Tracer end
"""
NumberCountsTracer(warr, chis, wint, b, lpre)
Number counts tracer structure.
Arguments:
- `wint::Interpolation` : interpolation of the radial kernel over comoving distance.
- `F::Function` : prefactor.
Returns:
- `NumberCountsTracer::NumberCountsTracer` : Number counts tracer structure.
"""
struct NumberCountsTracer <: Tracer
wint::AbstractInterpolation
F::Function
end
"""
NumberCountsTracer(cosmo::Cosmology, z_n, nz; kwargs...)
Number counts tracer structure constructor.
Arguments:
- `cosmo::Cosmology` : cosmology structure.
- `z_n::Vector{Dual}` : redshift array.
- `nz::Interpolation` : distribution of sources over redshift.
Kwargs:
- `b::Dual = 1` : matter-galaxy bias.
Returns:
- `NumberCountsTracer::NumberCountsTracer` : Number counts tracer structure.
"""
NumberCountsTracer(cosmo::Cosmology, z_n, nz; b=1.0, res=1000) = begin
nz_int = linear_interpolation(z_n, nz, extrapolation_bc=0)
z_w = range(0.00001, stop=z_n[end], length=res)
nz_w = nz_int(z_w)
nz_norm = integrate(z_w, nz_w, SimpsonEven())
chi = cosmo.chi(z_w)
hz = Hmpc(cosmo, z_w)
w_arr = @. (nz_w*hz/nz_norm)
wint = linear_interpolation(chi, b .* w_arr, extrapolation_bc=Line())
F::Function = β -> 1
NumberCountsTracer(wint, F)
end
"""
WeakLensingTracer(cosmo::Cosmology, z_n, nz; kwargs...)
Weak lensing tracer structure constructor.
Arguments:
- `cosmo::Cosmology` : cosmology structure.
- `z_n::Vector{Dual}` : redshift array.
- `nz::Interpolation` : distribution of sources over redshift.
Kwargs:
- `mb::Dual = 1` : multiplicative bias.
- `IA_params::Vector{Dual} = [A_IA, alpha_IA]`: instrinsic aligment parameters.
Returns:
- `WeakLensingTracer::WeakLensingTracer` : Weak lensing tracer structure.
"""
struct WeakLensingTracer <: Tracer
wint::AbstractInterpolation
F::Function
end
WeakLensingTracer(cosmo::Cosmology, z_n, nz;
IA_params = [0.0, 0.0], m=0.0, res=350, kwargs...) = begin
nz_int = linear_interpolation(z_n, nz, extrapolation_bc=0)
cosmo_type = cosmo.settings.cosmo_type
z_w = range(0.00001, stop=z_n[end], length=res)
dz_w = (z_w[end]-z_w[1])/res
nz_w = nz_int(z_w)
chi = cosmo.chi(z_w)
#nz_norm = sum(0.5 .* (nz_w[1:res-1] .+ nz_w[2:res]) .* dz_w)
nz_norm = integrate(z_w, nz_w, SimpsonEven())
# Calculate chis at which to precalculate the lensing kernel
# OPT: perhaps we don't need to sample the lensing kernel
# at all zs.
# Calculate integral at each chi
w_itg(chii) = @.(nz_w*(1-chii/chi))
w_arr = zeros(cosmo_type, res)
@inbounds for i in 1:res-3
w_arr[i] = integrate(z_w[i:res], w_itg(chi[i])[i:res], SimpsonEven())
end
# Normalize
H0 = cosmo.cpar.h/CLIGHT_HMPC
lens_prefac = 1.5*cosmo.cpar.Ξ©m*H0^2
# Fix first element
chi[1] = 0.0
w_arr = @. w_arr * chi * lens_prefac * (1+z_w) / nz_norm
if IA_params != [0.0, 0.0]
hz = Hmpc(cosmo, z_w)
As = get_IA(cosmo, z_w,IA_params)
corr = @. As * (nz_w * hz / nz_norm)
w_arr = @. w_arr - corr
end
# Interpolate
b = m+1.0
wint = linear_interpolation(chi, b.*w_arr, extrapolation_bc=Line())
F::Function = β -> @.(sqrt((β+2)*(β+1)*β*(β-1))/(β+0.5)^2)
WeakLensingTracer(wint, F)
end
"""
CMBLensingTracer(warr, chis, wint, lpre)
CMB lensing tracer structure.
Arguments:
- `wint::Interpolation` : interpolation of the radial kernel over comoving distance.
- `F::Function` : prefactor.
Returns:
- `CMBLensingTracer::CMBLensingTracer` : CMB lensing tracer structure.
"""
struct CMBLensingTracer <: Tracer
wint::AbstractInterpolation
F::Function
end
"""
CMBLensingTracer(cosmo::Cosmology; nchi=100)
CMB lensing tracer structure.
Arguments:
- `cosmo::Cosmology` : cosmology structure.
Returns:
- `CMBLensingTracer::CMBLensingTracer` : CMB lensing tracer structure.
"""
CMBLensingTracer(cosmo::Cosmology; res=350) = begin
# chi array
chis = range(0.0, stop=cosmo.chi_max, length=res)
zs = cosmo.z_of_chi(chis)
# Prefactor
H0 = cosmo.cpar.h/CLIGHT_HMPC
lens_prefac = 1.5*cosmo.cpar.Ξ©m*H0^2
# Kernel
w_arr = @. lens_prefac*chis*(1-chis/cosmo.chi_LSS)*(1+zs)
# Interpolate
wint = linear_interpolation(chis, w_arr, extrapolation_bc=0.0)
F::Function = β -> @.(((β+1)*β)/(β+0.5)^2)
CMBLensingTracer(wint, F)
end
"""
get_IA(cosmo::Cosmology, zs, IA_params)
CMB lensing tracer structure.
Arguments:
- `cosmo::Cosmology` : cosmology structure.
- `zs::Vector{Dual}` : redshift array.
- `IA_params::Vector{Dual}` : Intrinsic aligment parameters.
Returns:
- `IA_corr::Vector{Dual}` : Intrinsic aligment correction to radial kernel.
"""
function get_IA(cosmo::Cosmology, zs, IA_params)
A_IA = IA_params[1]
alpha_IA = IA_params[2]
return @. A_IA*((1 + zs)/1.62)^alpha_IA * (0.0134 * cosmo.cpar.Ξ©m / cosmo.Dz(zs))
end | LimberJack | https://github.com/JaimeRZP/LimberJack.jl.git |
|
[
"MIT"
] | 0.1.5 | f9c5e6430f2e23b703a3c84df0ea2cc0362519df | code | 39447 | using Test
using LimberJack
using ForwardDiff
using NPZ
using Statistics
test_main=true
if test_main
println("testing main functions")
end
#=
test_Bolt = false
if test_Bolt
println("testing Bolt.jl")
end
=#
extensive= false
if extensive
println("extensive")
test_results = npzread("test_extensive_results.npz")
else
test_results = npzread("test_results.npz")
end
test_output = Dict{String}{Vector}()
if test_main
cosmo_EisHu = Cosmology(z_max=1100, nz=1000, nz_t=1000, nz_pk=1000, nk=1000, tk_mode=:EisHu)
cosmo_emul = Cosmology(Ξ©m=(0.12+0.022)/0.75^2, Ξ©b=0.022/0.75^2, h=0.75, ns=1.0, Ο8=0.81,
z_max=1100, nz=1000, nz_t=1000, nz_pk=1000, nk=1000, tk_mode=:EmuPk)
cosmo_emul_As = Cosmology(Ξ©m=0.27, Ξ©b=0.046, h=0.7, ns=1.0, As=2.097e-9,
z_max=1100, nz=1000, nz_t=1000, nz_pk=1000, nk=1000, tk_mode=:EmuPk)
cosmo_EisHu_nonlin = Cosmology(nk=1000, nz=1000, nz_t=1000, nz_pk=1000,
z_max=1100, tk_mode=:EisHu, pk_mode=:Halofit)
cosmo_emul_nonlin = Cosmology(Ξ©m=(0.12+0.022)/0.75^2, Ξ©b=0.022/0.75^2, h=0.75, ns=1.0, Ο8=0.81,
z_max=1100, nk=1000, nz=1000, nz_t=1000, nz_pk=1000, tk_mode=:EmuPk, pk_mode=:Halofit)
@testset "Main tests" begin
@testset "CreateCosmo" begin
@test cosmo_EisHu.cpar.Ξ©m == 0.3
end
@testset "BMHz" begin
c = 299792458.0
ztest = 10 .^ Vector(LinRange(-2, log10(1100), 300))
Hz = cosmo_EisHu.cpar.h*100*Ez(cosmo_EisHu, ztest)
Hz_bm = test_results["Hz"]
merge!(test_output, Dict("Hz"=> Hz))
@test all(@. (abs(Hz/Hz_bm-1.0) < 0.0005))
end
@testset "BMChi" begin
ztest = 10 .^ Vector(LinRange(-2, log10(1100), 300))
chi = comoving_radial_distance(cosmo_EisHu, ztest)
chi_LSS = cosmo_EisHu.chi_LSS
chi_bm = test_results["Chi"]
chi_LSS_bm = test_results["Chi_LSS"]
merge!(test_output, Dict("Chi"=> chi))
merge!(test_output, Dict("Chi_LSS"=> [chi_LSS]))
@test all(@. (abs(chi/chi_bm-1.0) < 0.0005))
@test all(@. (abs(chi_LSS/chi_LSS_bm-1.0) < 0.0005))
end
@testset "BMGrowth" begin
ztest = LinRange(0.01, 3.0, 100)
Dz = growth_factor(cosmo_EisHu, ztest)
fz = growth_rate(cosmo_EisHu, ztest)
fs8z = fs8(cosmo_EisHu, ztest)
Dz_bm = test_results["Dz"]
fz_bm = test_results["fz"]
fs8z_bm = 0.81 .* Dz_bm .* fz_bm
merge!(test_output, Dict("Dz"=> Dz))
merge!(test_output, Dict("fz"=> fz))
merge!(test_output, Dict("fs8z"=> fs8z))
@test all(@. (abs(Dz/Dz_bm-1.0) < 0.005))
@test all(@. (abs(fz/fz_bm-1.0) < 0.005))
@test all(@. (abs(fs8z/fs8z_bm-1.0) < 0.005))
end
@testset "linear_Pk_Ο8" begin
ks = exp.(LimberJack.emulator.training_karr)
pk_EisHu = nonlin_Pk(cosmo_EisHu, ks, 0.0)
pk_emul = nonlin_Pk(cosmo_emul, ks, 0.0)
pk_EisHu_bm = test_results["pk_EisHu"]
pk_emul_bm = test_results["pk_emul"]
merge!(test_output, Dict("pk_EisHu"=> pk_EisHu))
merge!(test_output, Dict("pk_emul"=> pk_emul))
@test all(@. (abs(pk_EisHu/pk_EisHu_bm-1.0) < 0.005))
@test all(@. (abs(pk_emul/pk_emul_bm-1.0) < 0.05))
end
@testset "linear_Pk_As" begin
ks = exp.(LimberJack.emulator.training_karr)
pk_emul = nonlin_Pk(cosmo_emul_As, ks, 0.0)
pk_emul_bm = test_results["pk_emul_As"]
merge!(test_output, Dict("pk_emul_As"=> pk_emul))
@test all(@. (abs(pk_emul/pk_emul_bm-1.0) < 0.05))
end
@testset "nonlinear_Pk" begin
ks = exp.(LimberJack.emulator.training_karr)
pk_EisHu_z0 = nonlin_Pk(cosmo_EisHu_nonlin, ks, 0.0)
pk_EisHu_z05 = nonlin_Pk(cosmo_EisHu_nonlin, ks, 0.5)
pk_EisHu_z1 = nonlin_Pk(cosmo_EisHu_nonlin, ks, 1.0)
pk_EisHu_z2 = nonlin_Pk(cosmo_EisHu_nonlin, ks, 2.0)
pk_emul_z0 = nonlin_Pk(cosmo_emul_nonlin, ks, 0)
pk_emul_z05 = nonlin_Pk(cosmo_emul_nonlin, ks, 0.5)
pk_emul_z1 = nonlin_Pk(cosmo_emul_nonlin, ks, 1.0)
pk_emul_z2 = nonlin_Pk(cosmo_emul_nonlin, ks, 2.0)
pk_EisHu_z0_bm = test_results["pk_EisHu_nonlin_z0"]
pk_EisHu_z05_bm = test_results["pk_EisHu_nonlin_z05"]
pk_EisHu_z1_bm = test_results["pk_EisHu_nonlin_z1"]
pk_EisHu_z2_bm = test_results["pk_EisHu_nonlin_z2"]
pk_emul_z0_bm = test_results["pk_emul_nonlin_z0"]
pk_emul_z05_bm = test_results["pk_emul_nonlin_z05"]
pk_emul_z1_bm = test_results["pk_emul_nonlin_z1"]
pk_emul_z2_bm = test_results["pk_emul_nonlin_z2"]
merge!(test_output, Dict("pk_EisHu_nonlin_z0"=> pk_EisHu_z0))
merge!(test_output, Dict("pk_EisHu_nonlin_z05"=> pk_EisHu_z05))
merge!(test_output, Dict("pk_EisHu_nonlin_z1"=> pk_EisHu_z1))
merge!(test_output, Dict("pk_EisHu_nonlin_z2"=> pk_EisHu_z2))
merge!(test_output, Dict("pk_emul_nonlin_z0"=> pk_emul_z0))
merge!(test_output, Dict("pk_emul_nonlin_z05"=> pk_emul_z05))
merge!(test_output, Dict("pk_emul_nonlin_z1"=> pk_emul_z1))
merge!(test_output, Dict("pk_emul_nonlin_z2"=> pk_emul_z2))
# It'd be best if this was < 1E-4...
@test all(@. (abs(pk_EisHu_z0/pk_EisHu_z0_bm-1.0) < 0.005))
@test all(@. (abs(pk_EisHu_z05/pk_EisHu_z05_bm-1.0) < 0.005))
@test all(@. (abs(pk_EisHu_z1/pk_EisHu_z1_bm-1.0) < 0.005))
@test all(@. (abs(pk_EisHu_z2/pk_EisHu_z2_bm-1.0) < 0.005))
@test all(@. (abs(pk_emul_z0/pk_emul_z0_bm-1.0) < 0.05))
@test all(@. (abs(pk_emul_z05/pk_emul_z05_bm-1.0) < 0.05))
@test all(@. (abs(pk_emul_z1/pk_emul_z1_bm-1.0) < 0.05))
@test all(@. (abs(pk_emul_z2/pk_emul_z2_bm-1.0) < 0.05))
end
@testset "Traces" begin
z = Vector(range(0., stop=2., length=1000))
nz = @. exp(-0.5*((z-0.5)/0.05)^2)
clustering_t = NumberCountsTracer(cosmo_EisHu, z, nz)
lensing_t = WeakLensingTracer(cosmo_EisHu, z, nz)
CMBLensing_t = CMBLensingTracer(cosmo_EisHu)
clustering_chis = test_results["clustering_chis"]
clustering_warr_bm = test_results["clustering_warr"]
lensing_chis = test_results["lensing_chis"]
lensing_warr_bm = test_results["lensing_warr"]
CMBLensing_chis = test_results["CMBLensing_chis"]
CMBLensing_warr_bm = test_results["CMBLensing_warr"]
clustering_warr = clustering_t.wint(clustering_chis)
lensing_warr = lensing_t.wint(lensing_chis)
CMBLensing_warr = CMBLensing_t.wint(CMBLensing_chis)
clustering_zarr = cosmo_EisHu.z_of_chi(clustering_chis)
lensing_zarr = cosmo_EisHu.z_of_chi(lensing_chis)
CMBLensing_zarr = cosmo_EisHu.z_of_chi(CMBLensing_chis)
merge!(test_output, Dict("clustering_zarr"=> clustering_zarr))
merge!(test_output, Dict("lensing_zarr"=> lensing_zarr))
merge!(test_output, Dict("CMBLensing_zarr"=> CMBLensing_zarr))
merge!(test_output, Dict("clustering_warr"=> clustering_warr))
merge!(test_output, Dict("lensing_warr"=> lensing_warr))
merge!(test_output, Dict("CMBLensing_warr"=> CMBLensing_warr))
@test all(@. (abs(clustering_warr/clustering_warr_bm-1.0) < 0.05))
@test all(@. (abs(lensing_warr/lensing_warr_bm-1.0)[1:-10] < 0.1))
@test all(@. (abs(CMBLensing_warr/CMBLensing_warr_bm-1.0)[2:end-1] < 0.01))
end
@testset "EisHu_Cβs" begin
if extensive
βs = 10 .^ Vector(LinRange(1, 3, 100))
else
βs = [10.0, 30.0, 100.0, 300.0, 1000.0]
end
z = Vector(range(0., stop=2., length=1000))
nz = @. exp(-0.5*((z-0.5)/0.05)^2)
tg = NumberCountsTracer(cosmo_EisHu, z, nz; b=1.0)
ts = WeakLensingTracer(cosmo_EisHu, z, nz;
m=0.0,
IA_params=[0.0, 0.0])
tk = CMBLensingTracer(cosmo_EisHu)
Cβ_gg = angularCβs(cosmo_EisHu, tg, tg, βs)
Cβ_gs = angularCβs(cosmo_EisHu, tg, ts, βs)
Cβ_ss = angularCβs(cosmo_EisHu, ts, ts, βs)
Cβ_gk = angularCβs(cosmo_EisHu, tg, tk, βs)
Cβ_sk = angularCβs(cosmo_EisHu, ts, tk, βs)
Cβ_kk = angularCβs(cosmo_EisHu, tk, tk, βs)
Cβ_gg_bm = test_results["cl_gg_eishu"]
Cβ_gs_bm = test_results["cl_gs_eishu"]
Cβ_ss_bm = test_results["cl_ss_eishu"]
Cβ_gk_bm = test_results["cl_gk_eishu"]
Cβ_sk_bm = test_results["cl_sk_eishu"]
Cβ_kk_bm = test_results["cl_kk_eishu"]
merge!(test_output, Dict("cl_gg_eishu"=> Cβ_gg))
merge!(test_output, Dict("cl_gs_eishu"=> Cβ_gs))
merge!(test_output, Dict("cl_ss_eishu"=> Cβ_ss))
merge!(test_output, Dict("cl_gk_eishu"=> Cβ_gk))
merge!(test_output, Dict("cl_sk_eishu"=> Cβ_sk))
merge!(test_output, Dict("cl_kk_eishu"=> Cβ_kk))
# It'd be best if this was < 1E-4...
@test all(@. (abs(Cβ_gg/Cβ_gg_bm-1.0) < 0.005))
@test all(@. (abs(Cβ_gs/Cβ_gs_bm-1.0) < 0.005))
@test all(@. (abs(Cβ_ss/Cβ_ss_bm-1.0) < 0.005))
@test all(@. (abs(Cβ_gk/Cβ_gk_bm-1.0) < 0.005))
# The β=10 point is a bit inaccurate for some reason
@test all(@. (abs(Cβ_sk/Cβ_sk_bm-1.0) < 0.005))
@test all(@. (abs(Cβ_kk/Cβ_kk_bm-1.0) < 0.005))
end
@testset "nonlin_EisHu_Cβs" begin
if extensive
βs = 10 .^ Vector(LinRange(1, 3, 100))
else
βs = [10.0, 30.0, 100.0, 300.0, 1000.0]
end
z = Vector(range(0., stop=2., length=1000))
nz = @. exp(-0.5*((z-0.5)/0.05)^2)
tg = NumberCountsTracer(cosmo_EisHu, z, nz; b=1.0)
ts = WeakLensingTracer(cosmo_EisHu, z, nz;
m=0.0,
IA_params=[0.0, 0.0])
tk = CMBLensingTracer(cosmo_EisHu)
Cβ_gg = angularCβs(cosmo_EisHu_nonlin, tg, tg, βs)
Cβ_gs = angularCβs(cosmo_EisHu_nonlin, tg, ts, βs)
Cβ_ss = angularCβs(cosmo_EisHu_nonlin, ts, ts, βs)
Cβ_gk = angularCβs(cosmo_EisHu_nonlin, tg, tk, βs)
Cβ_sk = angularCβs(cosmo_EisHu_nonlin, ts, tk, βs)
Cβ_kk = angularCβs(cosmo_EisHu_nonlin, tk, tk, βs)
Cβ_gg_bm = test_results["cl_gg_eishu_nonlin"]
Cβ_gs_bm = test_results["cl_gs_eishu_nonlin"]
Cβ_ss_bm = test_results["cl_ss_eishu_nonlin"]
Cβ_gk_bm = test_results["cl_gk_eishu_nonlin"]
Cβ_sk_bm = test_results["cl_sk_eishu_nonlin"]
Cβ_kk_bm = test_results["cl_kk_eishu_nonlin"]
merge!(test_output, Dict("cl_gg_eishu_nonlin"=> Cβ_gg))
merge!(test_output, Dict("cl_gs_eishu_nonlin"=> Cβ_gs))
merge!(test_output, Dict("cl_ss_eishu_nonlin"=> Cβ_ss))
merge!(test_output, Dict("cl_gk_eishu_nonlin"=> Cβ_gk))
merge!(test_output, Dict("cl_sk_eishu_nonlin"=> Cβ_sk))
merge!(test_output, Dict("cl_sk_eishu_nonlin"=> Cβ_kk))
# It'd be best if this was < 1E-4...
@test all(@. (abs(Cβ_gg/Cβ_gg_bm-1.0) < 0.005))
@test all(@. (abs(Cβ_gs/Cβ_gs_bm-1.0) < 0.005))
@test all(@. (abs(Cβ_ss/Cβ_ss_bm-1.0) < 0.005))
@test all(@. (abs(Cβ_gk/Cβ_gk_bm-1.0) < 0.005))
# The β=10 point is a bit inaccurate for some reason
@test all(@. (abs(Cβ_sk/Cβ_sk_bm-1.0) < 0.005))
@test all(@. (abs(Cβ_kk/Cβ_kk_bm-1.0) < 0.01))
end
@testset "emul_Cβs" begin
if extensive
βs = 10 .^ Vector(LinRange(1, 3, 100))
else
βs = [10.0, 30.0, 100.0, 300.0, 1000.0]
end
z = Vector(range(0., stop=2., length=1000))
nz = @. exp(-0.5*((z-0.5)/0.05)^2)
tg = NumberCountsTracer(cosmo_emul, z, nz; b=1.0)
ts = WeakLensingTracer(cosmo_emul, z, nz;
m=0.0,
IA_params=[0.0, 0.0])
tk = CMBLensingTracer(cosmo_emul)
Cβ_gg = angularCβs(cosmo_emul, tg, tg, βs)
Cβ_gs = angularCβs(cosmo_emul, tg, ts, βs)
Cβ_ss = angularCβs(cosmo_emul, ts, ts, βs)
Cβ_gk = angularCβs(cosmo_emul, tg, tk, βs)
Cβ_sk = angularCβs(cosmo_emul, ts, tk, βs)
Cβ_kk = angularCβs(cosmo_emul, tk, tk, βs)
Cβ_gg_bm = test_results["cl_gg_camb"]
Cβ_gs_bm = test_results["cl_gs_camb"]
Cβ_ss_bm = test_results["cl_ss_camb"]
Cβ_gk_bm = test_results["cl_gk_camb"]
Cβ_sk_bm = test_results["cl_sk_camb"]
Cβ_kk_bm = test_results["cl_kk_camb"]
merge!(test_output, Dict("cl_gg_camb"=> Cβ_gg))
merge!(test_output, Dict("cl_gs_camb"=> Cβ_gs))
merge!(test_output, Dict("cl_ss_camb"=> Cβ_ss))
merge!(test_output, Dict("cl_gk_camb"=> Cβ_gk))
merge!(test_output, Dict("cl_sk_camb"=> Cβ_sk))
merge!(test_output, Dict("cl_kk_camb"=> Cβ_kk))
# It'd be best if this was < 1E-4...
@test all(@. (abs(Cβ_gg/Cβ_gg_bm-1.0) < 0.05))
@test all(@. (abs(Cβ_gs/Cβ_gs_bm-1.0) < 0.05))
@test all(@. (abs(Cβ_ss/Cβ_ss_bm-1.0) < 0.05))
@test all(@. (abs(Cβ_gk/Cβ_gk_bm-1.0) < 0.05))
# The β=10 point is a bit inaccurate for some reason
@test all(@. (abs(Cβ_sk/Cβ_sk_bm-1.0) < 0.05))
@test all(@. (abs(Cβ_kk/Cβ_kk_bm-1.0) < 0.05))
end
@testset "EisHu_Halo_Cβs" begin
if extensive
βs = 10 .^ Vector(LinRange(1, 3, 100))
else
βs = [10.0, 30.0, 100.0, 300.0, 1000.0]
end
z = Vector(range(0.0, stop=2., length=256))
nz = @. exp(-0.5*((z-0.5)/0.05)^2)
tg = NumberCountsTracer(cosmo_EisHu_nonlin, z, nz; b=1.0)
ts = WeakLensingTracer(cosmo_EisHu_nonlin, z, nz;
m=0.0,
IA_params=[0.0, 0.0])
tk = CMBLensingTracer(cosmo_EisHu_nonlin)
Cβ_gg = angularCβs(cosmo_EisHu_nonlin, tg, tg, βs)
Cβ_gs = angularCβs(cosmo_EisHu_nonlin, tg, ts, βs)
Cβ_ss = angularCβs(cosmo_EisHu_nonlin, ts, ts, βs)
Cβ_gk = angularCβs(cosmo_EisHu_nonlin, tg, tk, βs)
Cβ_sk = angularCβs(cosmo_EisHu_nonlin, ts, tk, βs)
Cβ_kk = angularCβs(cosmo_EisHu_nonlin, tk, tk, βs)
Cβ_gg_bm = test_results["cl_gg_eishu_nonlin"]
Cβ_gs_bm = test_results["cl_gs_eishu_nonlin"]
Cβ_ss_bm = test_results["cl_ss_eishu_nonlin"]
Cβ_gk_bm = test_results["cl_gk_eishu_nonlin"]
Cβ_sk_bm = test_results["cl_sk_eishu_nonlin"]
Cβ_kk_bm = test_results["cl_kk_eishu_nonlin"]
merge!(test_output, Dict("cl_gg_eishu_nonlin"=> Cβ_gg))
merge!(test_output, Dict("cl_gs_eishu_nonlin"=> Cβ_gs))
merge!(test_output, Dict("cl_ss_eishu_nonlin"=> Cβ_ss))
merge!(test_output, Dict("cl_gk_eishu_nonlin"=> Cβ_gk))
merge!(test_output, Dict("cl_sk_eishu_nonlin"=> Cβ_sk))
merge!(test_output, Dict("cl_kk_eishu_nonlin"=> Cβ_kk))
# It'd be best if this was < 1E-4...
@test all(@. (abs(Cβ_gg/Cβ_gg_bm-1.0) < 0.005))
@test all(@. (abs(Cβ_gs/Cβ_gs_bm-1.0) < 0.005))
@test all(@. (abs(Cβ_ss/Cβ_ss_bm-1.0) < 0.005))
@test all(@. (abs(Cβ_gk/Cβ_gk_bm-1.0) < 0.005))
@test all(@. (abs(Cβ_sk/Cβ_sk_bm-1.0) < 0.005))
@test all(@. (abs(Cβ_kk/Cβ_kk_bm-1.0) < 0.01))
end
@testset "emul_Halo_Cβs" begin
if extensive
βs = 10 .^ Vector(LinRange(1, 3, 100))
else
βs = [10.0, 30.0, 100.0, 300.0, 1000.0]
end
z = Vector(range(0., stop=2., length=1000))
nz = @. exp(-0.5*((z-0.5)/0.05)^2)
tg = NumberCountsTracer(cosmo_emul_nonlin, z, nz; b=1.0)
ts = WeakLensingTracer(cosmo_emul_nonlin, z, nz;
m=0.0,
IA_params=[0.0, 0.0])
tk = CMBLensingTracer(cosmo_emul_nonlin)
Cβ_gg = angularCβs(cosmo_emul_nonlin, tg, tg, βs)
Cβ_gs = angularCβs(cosmo_emul_nonlin, tg, ts, βs)
Cβ_ss = angularCβs(cosmo_emul_nonlin, ts, ts, βs)
Cβ_gk = angularCβs(cosmo_emul_nonlin, tg, tk, βs)
Cβ_sk = angularCβs(cosmo_emul_nonlin, ts, tk, βs)
Cβ_kk = angularCβs(cosmo_emul_nonlin, tk, tk, βs)
Cβ_gg_bm = test_results["cl_gg_camb_nonlin"]
Cβ_gs_bm = test_results["cl_gs_camb_nonlin"]
Cβ_ss_bm = test_results["cl_ss_camb_nonlin"]
Cβ_gk_bm = test_results["cl_gk_camb_nonlin"]
Cβ_sk_bm = test_results["cl_sk_camb_nonlin"]
Cβ_kk_bm = test_results["cl_kk_camb_nonlin"]
merge!(test_output, Dict("cl_gg_emul_nonlin"=> Cβ_gg))
merge!(test_output, Dict("cl_gs_emul_nonlin"=> Cβ_gs))
merge!(test_output, Dict("cl_ss_emul_nonlin"=> Cβ_ss))
merge!(test_output, Dict("cl_gk_emul_nonlin"=> Cβ_gk))
merge!(test_output, Dict("cl_sk_emul_nonlin"=> Cβ_sk))
merge!(test_output, Dict("cl_kk_emul_nonlin"=> Cβ_kk))
# It'd be best if this was < 1E-4...
@test all(@. (abs(Cβ_gg/Cβ_gg_bm-1.0) < 0.05))
@test all(@. (abs(Cβ_gs/Cβ_gs_bm-1.0) < 0.05))
@test all(@. (abs(Cβ_ss/Cβ_ss_bm-1.0) < 0.05))
@test all(@. (abs(Cβ_gk/Cβ_gk_bm-1.0) < 0.05))
@test all(@. (abs(Cβ_sk/Cβ_sk_bm-1.0) < 0.05))
@test all(@. (abs(Cβ_kk/Cβ_kk_bm-1.0) < 0.05))
end
@testset "IsBaseDiff" begin
if extensive
zs_Dz = LinRange(0.01, 3.0, 100)
zs_Chi = 10 .^ Vector(LinRange(-2, log10(1100), 300))
else
zs_Dz = zs_Chi = [0.1, 0.5, 1.0, 3.0]
end
function h(p)
cosmo = LimberJack.Cosmology(Ξ©m=p)
Hz = 100*cosmo.cpar.h*LimberJack.Ez(cosmo, zs_Chi)
return Hz
end
function f(p)
cosmo = LimberJack.Cosmology(Ξ©m=p)
chi = LimberJack.comoving_radial_distance(cosmo, zs_Chi)
return chi
end
function g(p)
cosmo = LimberJack.Cosmology(Ξ©m=p)
chi = LimberJack.growth_factor(cosmo, zs_Dz)
return chi
end
Ξ©m0 = 0.3
dΞ©m = 0.001
hs = ForwardDiff.derivative(h, Ξ©m0)
fs = ForwardDiff.derivative(f, Ξ©m0)
gs = ForwardDiff.derivative(g, Ξ©m0)
hs_bm = (h(Ξ©m0+dΞ©m)-h(Ξ©m0-dΞ©m))/2dΞ©m
fs_bm = (f(Ξ©m0+dΞ©m)-f(Ξ©m0-dΞ©m))/2dΞ©m
gs_bm = (g(Ξ©m0+dΞ©m)-g(Ξ©m0-dΞ©m))/2dΞ©m
merge!(test_output, Dict("Hz_autodiff"=> hs))
merge!(test_output, Dict("Chi_autodiff"=> fs))
merge!(test_output, Dict("Dz_autodiff"=> gs))
merge!(test_output, Dict("Hz_num"=> hs_bm))
merge!(test_output, Dict("Chi_num"=> fs_bm))
merge!(test_output, Dict("Dz_num"=> gs_bm))
@test all(@. (abs(hs/hs_bm-1) < 0.005))
@test all(@. (abs(fs/fs_bm-1) < 0.005))
@test all(@. (abs(gs/gs_bm-1) < 0.005))
end
@testset "IsLinPkDiff" begin
if extensive
logk = range(log(0.0001), stop=log(100.0), length=500)
ks = exp.(logk)
else
ks = exp.(LimberJack.emulator.training_karr)
end
function lin_EisHu(p)
cosmo = Cosmology(Ξ©m=p, tk_mode=:EisHu, Pk_mode=:linear)
pk = lin_Pk(cosmo, ks, 0.)
return pk
end
function lin_emul(p)
cosmo = Cosmology(Ξ©m=p, tk_mode=:EmuPk, Pk_mode=:linear)
pk = lin_Pk(cosmo, ks, 0.)
return pk
end
Ξ©m0 = 0.25
dΞ©m = 0.01
lin_EisHu_autodiff = abs.(ForwardDiff.derivative(lin_EisHu, Ξ©m0))
lin_emul_autodiff = abs.(ForwardDiff.derivative(lin_emul, Ξ©m0))
lin_EisHu_num = abs.((lin_EisHu(Ξ©m0+dΞ©m)-lin_EisHu(Ξ©m0-dΞ©m))/(2dΞ©m))
lin_emul_num = abs.((lin_emul(Ξ©m0+dΞ©m)-lin_emul(Ξ©m0-dΞ©m))/(2dΞ©m))
merge!(test_output, Dict("lin_EisHu_autodiff"=> lin_EisHu_autodiff))
merge!(test_output, Dict("lin_emul_autodiff"=> lin_emul_autodiff))
merge!(test_output, Dict("lin_EisHu_num"=> lin_EisHu_num))
merge!(test_output, Dict("lin_emul_num"=> lin_emul_num))
# Median needed since errors shoot up when derivatieve
# crosses zero
@test median(lin_EisHu_autodiff./lin_EisHu_num.-1) < 0.05
@test median(lin_emul_autodiff./lin_emul_num.-1) < 0.05
end
@testset "IsNonlinPkDiff" begin
if extensive
logk = range(log(0.0001), stop=log(100.0), length=500)
ks = exp.(logk)
else
ks = exp.(LimberJack.emulator.training_karr)
end
function nonlin_EisHu(p)
cosmo = Cosmology(Ξ©m=p, tk_mode=:EisHu, Pk_mode=:Halofit)
pk = nonlin_Pk(cosmo, ks, z)
return pk
end
function nonlin_emul(p)
cosmo = Cosmology(Ξ©m=p, tk_mode=:EmuPk, Pk_mode=:Halofit)
pk = nonlin_Pk(cosmo, ks, z)
return pk
end
Ξ©m0 = 0.25
dΞ©m = 0.001
z = 0.0
nonlin_EisHu_z0_autodiff = abs.(ForwardDiff.derivative(nonlin_EisHu, Ξ©m0))
nonlin_EisHu_z0_num = abs.((nonlin_EisHu(Ξ©m0+dΞ©m)-nonlin_EisHu(Ξ©m0-dΞ©m))/(2dΞ©m))
merge!(test_output, Dict("nonlin_EisHu_z0_autodiff"=> nonlin_EisHu_z0_autodiff))
merge!(test_output, Dict("nonlin_EisHu_z0_num"=> nonlin_EisHu_z0_num))
z = 0.5
nonlin_EisHu_z05_autodiff = abs.(ForwardDiff.derivative(nonlin_EisHu, Ξ©m0))
nonlin_EisHu_z05_num = abs.((nonlin_EisHu(Ξ©m0+dΞ©m)-nonlin_EisHu(Ξ©m0-dΞ©m))/(2dΞ©m))
merge!(test_output, Dict("nonlin_EisHu_z05_autodiff"=> nonlin_EisHu_z05_autodiff))
merge!(test_output, Dict("nonlin_EisHu_z05_num"=> nonlin_EisHu_z05_num))
z = 1.0
nonlin_EisHu_z1_autodiff = abs.(ForwardDiff.derivative(nonlin_EisHu, Ξ©m0))
nonlin_EisHu_z1_num = abs.((nonlin_EisHu(Ξ©m0+dΞ©m)-nonlin_EisHu(Ξ©m0-dΞ©m))/(2dΞ©m))
merge!(test_output, Dict("nonlin_EisHu_z1_autodiff"=> nonlin_EisHu_z1_autodiff))
merge!(test_output, Dict("nonlin_EisHu_z1_num"=> nonlin_EisHu_z1_num))
z = 2.0
nonlin_EisHu_z2_autodiff = abs.(ForwardDiff.derivative(nonlin_EisHu, Ξ©m0))
nonlin_EisHu_z2_num = abs.((nonlin_EisHu(Ξ©m0+dΞ©m)-nonlin_EisHu(Ξ©m0-dΞ©m))/(2dΞ©m))
merge!(test_output, Dict("nonlin_EisHu_z2_autodiff"=> nonlin_EisHu_z2_autodiff))
merge!(test_output, Dict("nonlin_EisHu_z2_num"=> nonlin_EisHu_z2_num))
z = 0.0
nonlin_emul_z0_autodiff = abs.(ForwardDiff.derivative(nonlin_emul, Ξ©m0))
nonlin_emul_z0_num = abs.((nonlin_emul(Ξ©m0+dΞ©m)-nonlin_emul(Ξ©m0-dΞ©m))/(2dΞ©m))
merge!(test_output, Dict("nonlin_emul_z0_autodiff"=> nonlin_emul_z0_autodiff))
merge!(test_output, Dict("nonlin_emul_z0_num"=> nonlin_emul_z0_num))
z = 0.5
nonlin_emul_z05_autodiff = abs.(ForwardDiff.derivative(nonlin_emul, Ξ©m0))
nonlin_emul_z05_num = abs.((nonlin_emul(Ξ©m0+dΞ©m)-nonlin_emul(Ξ©m0-dΞ©m))/(2dΞ©m))
merge!(test_output, Dict("nonlin_emul_z05_autodiff"=> nonlin_emul_z05_autodiff))
merge!(test_output, Dict("nonlin_emul_z05_num"=> nonlin_emul_z05_num))
z = 1.0
nonlin_emul_z1_autodiff = abs.(ForwardDiff.derivative(nonlin_emul, Ξ©m0))
nonlin_emul_z1_num = abs.((nonlin_emul(Ξ©m0+dΞ©m)-nonlin_emul(Ξ©m0-dΞ©m))/(2dΞ©m))
merge!(test_output, Dict("nonlin_emul_z1_autodiff"=> nonlin_emul_z1_autodiff))
merge!(test_output, Dict("nonlin_emul_z1_num"=> nonlin_emul_z1_num))
z = 2.0
nonlin_emul_z2_autodiff = abs.(ForwardDiff.derivative(nonlin_emul, Ξ©m0))
nonlin_emul_z2_num = abs.((nonlin_emul(Ξ©m0+dΞ©m)-nonlin_emul(Ξ©m0-dΞ©m))/(2dΞ©m))
merge!(test_output, Dict("nonlin_emul_z2_autodiff"=> nonlin_emul_z2_autodiff))
merge!(test_output, Dict("nonlin_emul_z2_num"=> nonlin_emul_z2_num))
# Median needed since errors shoot up when derivatieve
# crosses zero
@test median(nonlin_EisHu_z0_autodiff./nonlin_EisHu_z0_num.-1) < 0.1
@test median(nonlin_EisHu_z05_autodiff./nonlin_EisHu_z05_num.-1) < 0.1
@test median(nonlin_EisHu_z1_autodiff./nonlin_EisHu_z1_num.-1) < 0.1
@test median(nonlin_EisHu_z2_autodiff./nonlin_EisHu_z2_num.-1) < 0.1
@test median(nonlin_emul_z0_autodiff./nonlin_emul_z0_num.-1) < 0.1
@test median(nonlin_emul_z05_autodiff./nonlin_emul_z05_num.-1) < 0.1
@test median(nonlin_emul_z1_autodiff./nonlin_emul_z1_num.-1) < 0.1
@test median(nonlin_emul_z2_autodiff./nonlin_emul_z2_num.-1) < 0.1
end
@testset "AreClsDiff" begin
if extensive
βs = 10 .^ Vector(LinRange(2, 3, 100))
else
βs = [10.0, 30.0, 100.0, 300.0, 1000.0]
end
function Cl_gg(p::T)::Array{T,1} where T<:Real
cosmo = LimberJack.Cosmology(Ξ©m=p, tk_mode=:EisHu, Pk_mode=:Halofit,
nz=700, nz_t=700, nz_pk=700)
z = Vector(range(0., stop=2., length=1000))
nz = Vector(@. exp(-0.5*((z-0.5)/0.05)^2))
tg = NumberCountsTracer(cosmo, z, nz; b=1.0)
Cβ_gg = angularCβs(cosmo, tg, tg, βs)
return Cβ_gg
end
function Cl_gs(p::T)::Array{T,1} where T<:Real
cosmo = LimberJack.Cosmology(Ξ©m=p, tk_mode=:EisHu, Pk_mode=:Halofit,
nz=700, nz_t=700, nz_pk=700)
z = Vector(range(0., stop=2., length=1000))
nz = Vector(@. exp(-0.5*((z-0.5)/0.05)^2))
tg = NumberCountsTracer(cosmo, z, nz; b=1.0)
ts = WeakLensingTracer(cosmo, z, nz;
m=0.0,
IA_params=[0.0, 0.0])
Cβ_gs = angularCβs(cosmo, tg, ts, βs)
return Cβ_gs
end
function Cl_ss(p::T)::Array{T,1} where T<:Real
cosmo = LimberJack.Cosmology(Ξ©m=p, tk_mode=:EisHu, Pk_mode=:Halofit,
nz=700, nz_t=700, nz_pk=700)
z = Vector(range(0., stop=2., length=1000))
nz = Vector(@. exp(-0.5*((z-0.5)/0.05)^2))
ts = WeakLensingTracer(cosmo, z, nz;
m=0.0,
IA_params=[0.0, 0.0])
Cβ_ss = angularCβs(cosmo, ts, ts, βs)
return Cβ_ss
end
function Cl_sk(p::T)::Array{T,1} where T<:Real
cosmo = LimberJack.Cosmology(Ξ©m=p, tk_mode=:EisHu, Pk_mode=:Halofit,
nz=500, nz_t=500, nz_pk=700)
z = range(0., stop=2., length=256)
nz = @. exp(-0.5*((z-0.5)/0.05)^2)
ts = WeakLensingTracer(cosmo, z, nz;
m=0.0,
IA_params=[0.0, 0.0])
tk = CMBLensingTracer(cosmo)
Cβ_sk = angularCβs(cosmo, ts, tk, βs)
return Cβ_sk
end
function Cl_gk(p::T)::Array{T,1} where T<:Real
cosmo = LimberJack.Cosmology(Ξ©m=p, tk_mode=:EisHu, Pk_mode=:Halofit,
nz=700, nz_t=700, nz_pk=700)
z = range(0., stop=2., length=256)
nz = @. exp(-0.5*((z-0.5)/0.05)^2)
tg = NumberCountsTracer(cosmo, z, nz; b=1.0)
tk = CMBLensingTracer(cosmo)
Cβ_gk = angularCβs(cosmo, tg, tk, βs)
return Cβ_gk
end
function Cl_kk(p::T)::Array{T,1} where T<:Real
cosmo = LimberJack.Cosmology(Ξ©m=p, tk_mode=:EisHu, Pk_mode=:Halofit,
z_max=1100.0, nz=700, nz_t=700, nz_pk=700)
z = range(0., stop=2., length=256)
nz = @. exp(-0.5*((z-0.5)/0.05)^2)
tk = CMBLensingTracer(cosmo)
Cβ_kk = angularCβs(cosmo, tk, tk, βs)
return Cβ_kk
end
Ξ©m0 = 0.3
dΞ©m = 0.0001
Cl_gg_autodiff = ForwardDiff.derivative(Cl_gg, Ξ©m0)
Cl_gg_num = (Cl_gg(Ξ©m0+dΞ©m)-Cl_gg(Ξ©m0-dΞ©m))/2dΞ©m
Cl_gs_autodiff = ForwardDiff.derivative(Cl_gs, Ξ©m0)
Cl_gs_num = (Cl_gs(Ξ©m0+dΞ©m)-Cl_gs(Ξ©m0-dΞ©m))/2dΞ©m
Cl_ss_autodiff = ForwardDiff.derivative(Cl_ss, Ξ©m0)
Cl_ss_num = (Cl_ss(Ξ©m0+dΞ©m)-Cl_ss(Ξ©m0-dΞ©m))/2dΞ©m
Cl_sk_autodiff = ForwardDiff.derivative(Cl_sk, Ξ©m0)
Cl_sk_num = (Cl_sk(Ξ©m0+dΞ©m)-Cl_sk(Ξ©m0-dΞ©m))/2dΞ©m
Cl_gk_autodiff = ForwardDiff.derivative(Cl_gk, Ξ©m0)
Cl_gk_num = (Cl_gk(Ξ©m0+dΞ©m)-Cl_gk(Ξ©m0-dΞ©m))/2dΞ©m
Cl_kk_autodiff = ForwardDiff.derivative(Cl_kk, Ξ©m0)
Cl_kk_num = (Cl_kk(Ξ©m0+dΞ©m)-Cl_kk(Ξ©m0-dΞ©m))/2dΞ©m
merge!(test_output, Dict("Cl_gg_autodiff"=> Cl_gg_autodiff))
merge!(test_output, Dict("Cl_gs_autodiff"=> Cl_gs_autodiff))
merge!(test_output, Dict("Cl_ss_autodiff"=> Cl_ss_autodiff))
merge!(test_output, Dict("Cl_sk_autodiff"=> Cl_sk_autodiff))
merge!(test_output, Dict("Cl_gk_autodiff"=> Cl_gk_autodiff))
merge!(test_output, Dict("Cl_kk_autodiff"=> Cl_kk_autodiff))
merge!(test_output, Dict("Cl_gg_num"=> Cl_gg_num))
merge!(test_output, Dict("Cl_gs_num"=> Cl_gs_num))
merge!(test_output, Dict("Cl_ss_num"=> Cl_ss_num))
merge!(test_output, Dict("Cl_sk_num"=> Cl_sk_num))
merge!(test_output, Dict("Cl_gk_num"=> Cl_gk_num))
merge!(test_output, Dict("Cl_kk_num"=> Cl_kk_num))
@test all(@. (abs(Cl_gg_autodiff/Cl_gg_num-1) < 0.05))
@test all(@. (abs(Cl_gs_autodiff/Cl_gs_num-1) < 0.05))
@test all(@. (abs(Cl_ss_autodiff/Cl_ss_num-1) < 0.05))
@test all(@. (abs(Cl_sk_autodiff/Cl_sk_num-1) < 0.05))
@test all(@. (abs(Cl_gk_autodiff/Cl_gk_num-1) < 0.05))
@test all(@. (abs(Cl_kk_autodiff/Cl_kk_num-1) < 0.05))
end
@testset "Nuisances" begin
if extensive
βs = 10 .^ Vector(LinRange(1, 3, 100))
else
βs = [10.0, 30.0, 100.0, 300.0, 1000.0]
end
z = Vector(range(0.01, stop=2., length=1024))
nz = @. exp(-0.5*((z-0.5)/0.05)^2)
tg_b = NumberCountsTracer(cosmo_EisHu_nonlin, z, nz; b=2.0)
ts_m = WeakLensingTracer(cosmo_EisHu_nonlin, z, nz; m=1.0, IA_params=[0.0, 0.0])
ts_IA = WeakLensingTracer(cosmo_EisHu_nonlin, z, nz; m=0.0, IA_params=[0.1, 0.1])
Cβ_gg_b = angularCβs(cosmo_EisHu_nonlin, tg_b, tg_b, βs)
Cβ_ss_m = angularCβs(cosmo_EisHu_nonlin, ts_m, ts_m, βs)
Cβ_ss_IA = angularCβs(cosmo_EisHu_nonlin, ts_IA, ts_IA, βs)
Cβ_gg_b_bm = test_results["cl_gg_b"]
Cβ_ss_m_bm = test_results["cl_ss_m"]
Cβ_ss_IA_bm = test_results["cl_ss_IA"]
merge!(test_output, Dict("cl_gg_b"=> Cβ_gg_b))
merge!(test_output, Dict("cl_ss_m"=> Cβ_ss_m))
merge!(test_output, Dict("cl_ss_IA"=> Cβ_ss_IA))
# It'd be best if this was < 1E-4...
@test all(@. (abs(Cβ_gg_b/Cβ_gg_b_bm-1.0) < 0.05))
# This is problematic
@test all(@. (abs(Cβ_ss_m/Cβ_ss_m_bm-1.0) < 0.05))
@test all(@. (abs(Cβ_ss_IA/Cβ_ss_IA_bm-1.0) < 0.05))
end
@testset "AreNuisancesDiff" begin
function bias(p::T)::Array{T,1} where T<:Real
cosmo = Cosmology(tk_mode=:EisHu, Pk_mode=:Halofit)
cosmo.settings.cosmo_type = typeof(p)
z = Vector(range(0., stop=2., length=1000))
nz = Vector(@. exp(-0.5*((z-0.5)/0.05)^2))
tg = NumberCountsTracer(cosmo, z, nz; b=p)
βs = [10.0, 30.0, 100.0, 300.0]
Cβ_gg = angularCβs(cosmo, tg, tg, βs)
return Cβ_gg
end
function dz(p::T)::Array{T,1} where T<:Real
cosmo = Cosmology(tk_mode=:EisHu, Pk_mode=:Halofit, nz=300)
cosmo.settings.cosmo_type = typeof(p)
z = Vector(range(0., stop=2., length=1000)) .- p
nz = Vector(@. exp(-0.5*((z-0.5)/0.05)^2))
tg = NumberCountsTracer(cosmo, z, nz; b=1, res=350)
βs = [10.0, 30.0, 100.0, 300.0]
Cβ_gg = angularCβs(cosmo, tg, tg, βs)
return Cβ_gg
end
function mbias(p::T)::Array{T,1} where T<:Real
cosmo = Cosmology(tk_mode=:EisHu, Pk_mode=:Halofit)
cosmo.settings.cosmo_type = typeof(p)
z = range(0., stop=2., length=256)
nz = @. exp(-0.5*((z-0.5)/0.05)^2)
ts = WeakLensingTracer(cosmo, z, nz; m=p, IA_params=[0.0, 0.0])
βs = [10.0, 30.0, 100.0, 300.0]
Cβ_sk = angularCβs(cosmo, ts, ts, βs)
return Cβ_sk
end
function IA_A(p::T)::Array{T,1} where T<:Real
cosmo = Cosmology(tk_mode=:EisHu, Pk_mode=:Halofit, )
cosmo.settings.cosmo_type = typeof(p)
z = range(0., stop=2., length=256)
nz = @. exp(-0.5*((z-0.5)/0.05)^2)
ts = WeakLensingTracer(cosmo, z, nz; m=2, IA_params=[p, 0.1])
βs = [10.0, 30.0, 100.0, 300.0]
Cβ_ss = angularCβs(cosmo, ts, ts, βs)
return Cβ_ss
end
function IA_alpha(p::T)::Array{T,1} where T<:Real
cosmo = Cosmology(tk_mode=:EisHu, Pk_mode=:Halofit)
cosmo.settings.cosmo_type = typeof(p)
z = range(0., stop=2., length=256)
nz = @. exp(-0.5*((z-0.5)/0.05)^2)
ts = WeakLensingTracer(cosmo, z, nz; m=2, IA_params=[0.3, p])
βs = [10.0, 30.0, 100.0, 300.0]
Cβ_ss = angularCβs(cosmo, ts, ts, βs)
return Cβ_ss
end
d = 0.00005
b_autodiff = ForwardDiff.derivative(bias, 2.0)
b_anal = (bias(2.0+d)-bias(2.0-d))/2d
dz_autodiff = ForwardDiff.derivative(dz, -0.1)
dz_anal = (dz(-0.1+d)-dz(-0.1-d))/2d
mb_autodiff = ForwardDiff.derivative(mbias, 2.0)
mb_anal = (mbias(2.0+d)-mbias(2.0-d))/2d
IA_A_autodiff = ForwardDiff.derivative(IA_A, 0.3)
IA_A_anal = (IA_A(0.3+d)-IA_A(0.3-d))/2d
IA_alpha_autodiff = ForwardDiff.derivative(IA_alpha, 0.1)
IA_alpha_anal = (IA_alpha(0.1+d)-IA_alpha(0.1-d))/2d
@test all(@. (abs(b_autodiff/b_anal-1) < 0.05))
@test all(@. (abs(dz_autodiff/dz_anal-1) < 0.05))
@test all(@. (abs(mb_autodiff/mb_anal-1) < 0.05))
@test all(@. (abs(IA_A_autodiff/IA_A_anal-1) < 0.05))
@test all(@. (abs(IA_alpha_autodiff/IA_alpha_anal-1) < 0.05))
end
if extensive
npzwrite("test_extensive_output.npz", test_output)
else
npzwrite("test_output.npz", test_output)
end
end
end
#=
if test_Bolt
Bolt_test_output = Dict{String}{Vector}()
cosmo_Bolt_As = Cosmology(Ξ©m=0.27, Ξ©b=0.046, h=0.7, ns=1.0, As=2.097e-9,
nk=70, nz=300, nz_pk=70, tk_mode=:Bolt)
cosmo_Bolt_nonlin = Cosmology(Ξ©m=0.27, Ξ©b=0.046, h=0.70, ns=1.0, Ο8=0.81,
nk=70, nz=300, nz_pk=70,
tk_mode=:Bolt, Pk_mode=:Halofit)
@testset "Bolt tests" begin
@testset "linear_Pk_As" begin
ks = exp.(LimberJack.emulator.training_karr)
pk_Bolt = nonlin_Pk(cosmo_Bolt_As, ks, 0.0)
pk_Bolt_bm = test_results["pk_Bolt_As"]
merge!(Bolt_test_output, Dict("pk_Bolt_As"=> pk_Bolt))
@test all(@. (abs(pk_Bolt/pk_Bolt_bm-1.0) < 0.05))
end
@testset "nonlinear_Pk" begin
ks = exp.(LimberJack.emulator.training_karr)
pk_Bolt = nonlin_Pk(cosmo_Bolt_nonlin, ks, 0)
pk_Bolt_bm = test_results["pk_Bolt_nonlin"]
merge!(Bolt_test_output, Dict("pk_Bolt_nonlin"=> pk_Bolt))
# It'd be best if this was < 1E-4...
@test all(@. (abs(pk_Bolt/pk_Bolt_bm-1.0) < 0.05))
end
@testset "IsBoltPkDiff" begin
if extensive
logk = range(log(0.0001), stop=log(100.0), length=100)
ks = exp.(logk)
else
logk = range(log(0.0001), stop=log(100.0), length=20)
ks = exp.(logk)
end
function lin_Bolt(p)
cosmo = Cosmology(Ξ©m=p, tk_mode=:Bolt, Pk_mode=:linear)
pk = lin_Pk(cosmo, ks, 0.)
return pk
end
Ξ©m0 = 0.25
dΞ©m = 0.01
lin_Bolt_autodiff = abs.(ForwardDiff.derivative(lin_Bolt, Ξ©m0))
lin_Bolt_num = abs.((lin_Bolt(Ξ©m0+dΞ©m)-lin_Bolt(Ξ©m0-dΞ©m))/(2dΞ©m))
merge!(Bolt_test_output, Dict("lin_Bolt_autodiff"=> lin_Bolt_autodiff))
merge!(Bolt_test_output, Dict("lin_Bolt_num"=> lin_Bolt_num))
# Median needed since errors shoot up when derivatieve
# crosses zero
@test median(lin_Bolt_autodiff./lin_Bolt_num.-1) < 0.05
end
if extensive
npzwrite("Bolt_test_extensive_output.npz", Bolt_test_output)
else
npzwrite("Bolt_test_extensive_output.npz", Bolt_test_output)
end
end
end
=#
| LimberJack | https://github.com/JaimeRZP/LimberJack.jl.git |
|
[
"MIT"
] | 0.1.5 | f9c5e6430f2e23b703a3c84df0ea2cc0362519df | docs | 6234 | # LimberJack.jl
[](https://github.com/JaimeRZP/LimberJack.jl/actions?query=workflow%3ALimberJack-CI+branch%3Amain)
[](https://jaimeruizzapatero.net/LimberJack.jl/dev/)

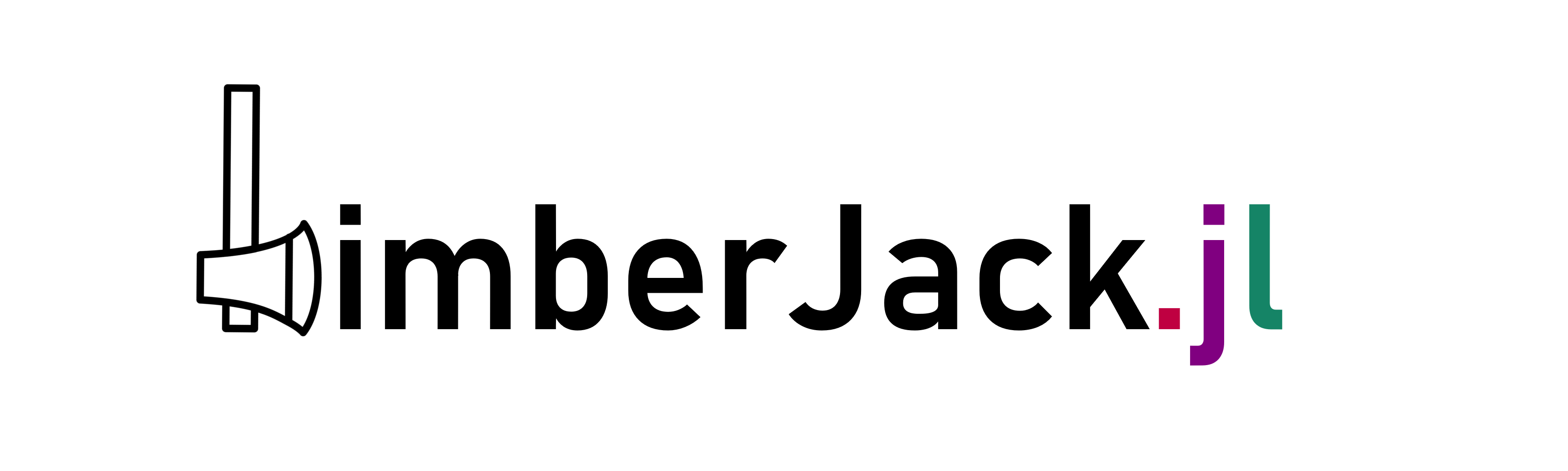
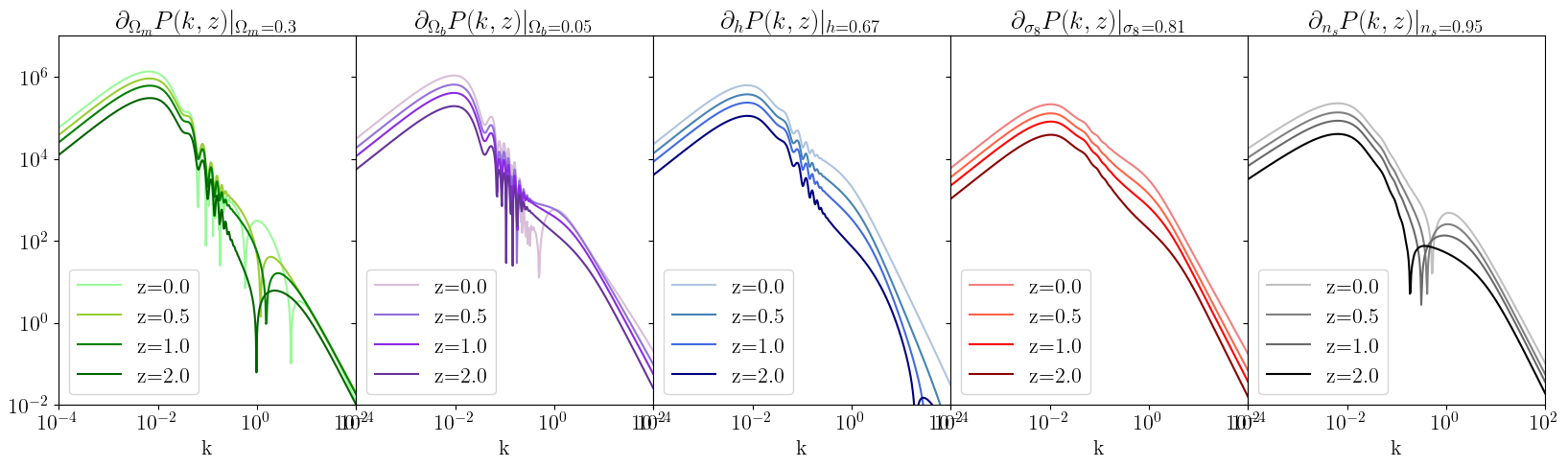
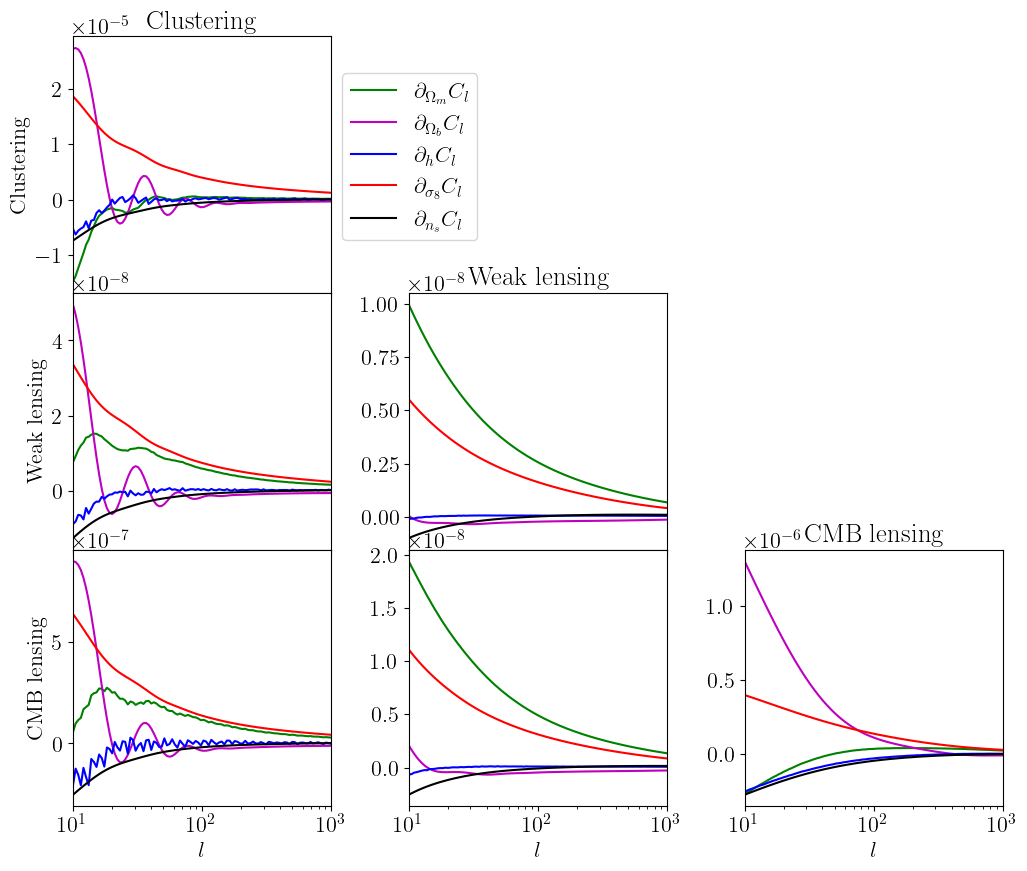
<p align="center"> A differentiable cosmological code in Julia. </p>
## Design Philosophy
+ **Modularity**: each main function within ```LimberJack.jl``` has its own module. New functions can be added by including extra modules. ```LimberJack.jl``` has the following modules:
| Module | function |
| ----------- | :----------- |
| ```boltzmann.jl``` | Performs the computation of primordial power spectrum |
| ```core.jl``` | Defines the structures where the theoretical predictions are stored and computes the background quantities |
| ```data_utils.jl``` | Manages ```sacc``` files for large data vectors |
| ```growth.jl``` | Computes the growth factor |
| ```halofit.jl``` | Computes the non-linear matter power spectrum as given by the Halofit fitting formula |
| ```spectra.jl``` | Computes the power spectra of any two tracers |
| ```theory.jl``` | Computes large data vectors that combine many spectra |
| ```tracers.jl``` | Computes the kernels associated with each type of kernel |
+ **Object-oriented**: ```LimberJack.jl``` mimics ```CCL.py``` class structure by using ```Julia```'s ```structures```.
+ **Transparency**: ```LimberJack.jl``` is fully written in ```Julia``` without needing to inerface to any other programming language (```C```, ```Python```...) to compute thoretical predictions. This allows the user full access to the code from input to output.
## Goals
+ **Gradients**: one order of magnitude faster gradients than finite differences.
+ **Precision**: sub-percentage error with respect to ```CCL```.
+ **Speed**: ```C```-like performance.
## Installation
In order to run ```LimberJack.jl``` you will need ```Julia-1.7.0``` or newer installed in your system.
Older versions of ```Julia``` might be compatible but haven't been tested.
You can find instructions on how to install ```Julia``` here: https://julialang.org/downloads/.
Once you have installed ```Julia``` you can install ```LimberJack.jl``` following these steps:
``` julia
using Pkg
Pkg.add("LimberJack")
```
### Installing Sacc.py in Julia
``` julia
using Pkg
Pkg.add("CondaPkg")
CondaPkg.add("sacc")
```
## Use
``` julia
# Import
using LimberJack
# create LimberJack.jl Cosmology instance
cosmology = Cosmology(Ξ©m=0.30, Ξ©b=0.05, h=0.70, ns=0.96, s8=0.81;
tk_mode="EisHu",
Pk_mode="Halofit")
z = Vector(range(0., stop=2., length=256))
nz = @. exp(-0.5*((z-0.5)/0.05)^2)
tracer = NumberCountsTracer(cosmology, zs, nz; b=1.0)
ls = [10.0, 30.0, 100.0, 300.0]
cls = angularCβs(cosmology, tracer, tracer, ls)
```
## Challenges
1. **Parallelization**: the current threading parallelization of ```LimberJack.jl``` is far away from the optimal one over number of threads scaling. Future works could study alternative parallalization schemes or possible inneficiencies in the code.
2. **GPU's**: ```LimberJack.jl``` currently cannot run on GPU's which are known to significantly speed-up cosmological inference. Future works could study implementing ```Julia``` GPU libraries such as ```CUDA.jl```.
3. **Backwards-AD**: currently ```LimberJack.jl```'s preferred AD mode is forward-AD. However, the key computation of cosmological inference, obtaining the $\chi^2$, is a map from N parameters to a scalar. For a large number of parameters, backwards-AD is in theory the preferred AD mode and should significantly speed up the computation of the gradient. Future works could look into making ```LimberJack.jl``` compatible with the latest ```Julia``` AD libraries such as ```Zygote.jl``` to implement efficient backwards-AD.
## Contributors
| | | | | | | |
|:-------------------------:|:-------------------------:|:-------------------------:|:-------------------------:|:-------------------------:|:-------------------------:|:-------------------------:|
| <img src=https://github.com/jaimerzp.png width="100" height="100" /> | <img src=https://github.com/anicola.png width="100" height="100" /> | <img src=https://github.com/carlosggarcia.png width="100" height="100" /> |<img src=https://github.com/damonge.png width="100" height="100" />| <img src=https://github.com/harry45.png width="100" height="100" /> | <img src=https://github.com/jmsull.png width="100" height="100" /> | <img src=https://github.com/marcobonici.png width="100" height="100" /> |
| Jaime Ruiz-Zapatero | Andrina Nicola | Carlos Garcia-garcia| David Alonso | Arrykrishna Mootoovaloo | Jamie Sullivan | Marco Bonici |
| Lead | Halofit | Validation | Tracers | EmuPk | Bolt.jl | Benchmarks |
## Citing LimberJack
```
@ARTICLE{2023arXiv231008306R,
author = {{Ruiz-Zapatero}, J. and {Alonso}, D. and {Garc{\'\i}a-Garc{\'\i}a}, C. and {Nicola}, A. and {Mootoovaloo}, A. and {Sullivan}, J.~M. and {Bonici}, M. and {Ferreira}, P.~G.},
title = "{LimberJack.jl: auto-differentiable methods for angular power spectra analyses}",
journal = {arXiv e-prints},
keywords = {Astrophysics - Cosmology and Nongalactic Astrophysics, Astrophysics - Instrumentation and Methods for Astrophysics},
year = 2023,
month = oct,
eid = {arXiv:2310.08306},
pages = {arXiv:2310.08306},
doi = {10.48550/arXiv.2310.08306},
archivePrefix = {arXiv},
eprint = {2310.08306},
primaryClass = {astro-ph.CO},
adsurl = {https://ui.adsabs.harvard.edu/abs/2023arXiv231008306R},
adsnote = {Provided by the SAO/NASA Astrophysics Data System}
}
```
| LimberJack | https://github.com/JaimeRZP/LimberJack.jl.git |
|
[
"MIT"
] | 0.1.5 | f9c5e6430f2e23b703a3c84df0ea2cc0362519df | docs | 1979 | # LimberJack.jl
## Core
```Core``` performs the main computations of ```LimberJack.jl```.
When using ```LimberJack.jl```, the first step is to create an instance of the ```Cosmology``` structure.
This is as easy as calling:
```julia
using LimberJack
cosmology = Cosmology()
```
This will generate the an instance of ```Cosmology``` given the vanilla $\Lambda$CDM cosmology of ```CCL ```.
```Cosmology()``` then computes the value of the comoving distance, the growth factor, the growth rate and matter power spectrum at an array of values and generates interpolators for said quantites.
The user can acces the value of these interpolator at an arbitrary input using the public functions of the model.
Moreover, the ```Cosmology``` structure has its own type called ```Cosmology```.
```@docs
LimberJack.Settings
LimberJack.CosmoPar
LimberJack.Cosmology
LimberJack.Ez
LimberJack.Hmpc
LimberJack.comoving_radial_distance
LimberJack.growth_factor
LimberJack.growth_rate
LimberJack.sigma8
LimberJack.fs8
LimberJack.lin_Pk
LimberJack.nonlin_Pk
```
## Tracers
In ```LimberJack.jl``` each tracer is ```structure``` containing at least of three fields:
+ ```wint```: an interpolator between ```chis``` and ```warr```.
+ ```F```: an interpolator between ```chis``` and ```warr```.
On top of these, tracers might contain the value of any nuisance parameter associated with them.
Moreover, tracers within ```LimberJack.jl``` tracer objects have their own type called ```Tracer```.
```@docs
LimberJack.NumberCountsTracer
LimberJack.WeakLensingTracer
LimberJack.CMBLensingTracer
LimberJack.get_IA
```
## Spectra
Performs the computation of the angular power spectra of any two tracers.
```@docs
LimberJack.Cβintegrand
LimberJack.angularCβs
```
## Data Utils
Parses ```sacc``` and ```YAML``` files.
```@docs
LimberJack.make_data
```
## Theory
Performs the computation of complex theory vectors given ```sacc``` and ```YAML``` files.
```@docs
LimberJack.Theory
```
| LimberJack | https://github.com/JaimeRZP/LimberJack.jl.git |
|
[
"MIT"
] | 0.1.5 | f9c5e6430f2e23b703a3c84df0ea2cc0362519df | docs | 4297 | # LimberJack.jl
[](https://github.com/JaimeRZP/LimberJack.jl/actions?query=workflow%3ALimberJack-CI+branch%3Amain)
[](https://jaimeruizzapatero.net/LimberJack.jl/dev/)

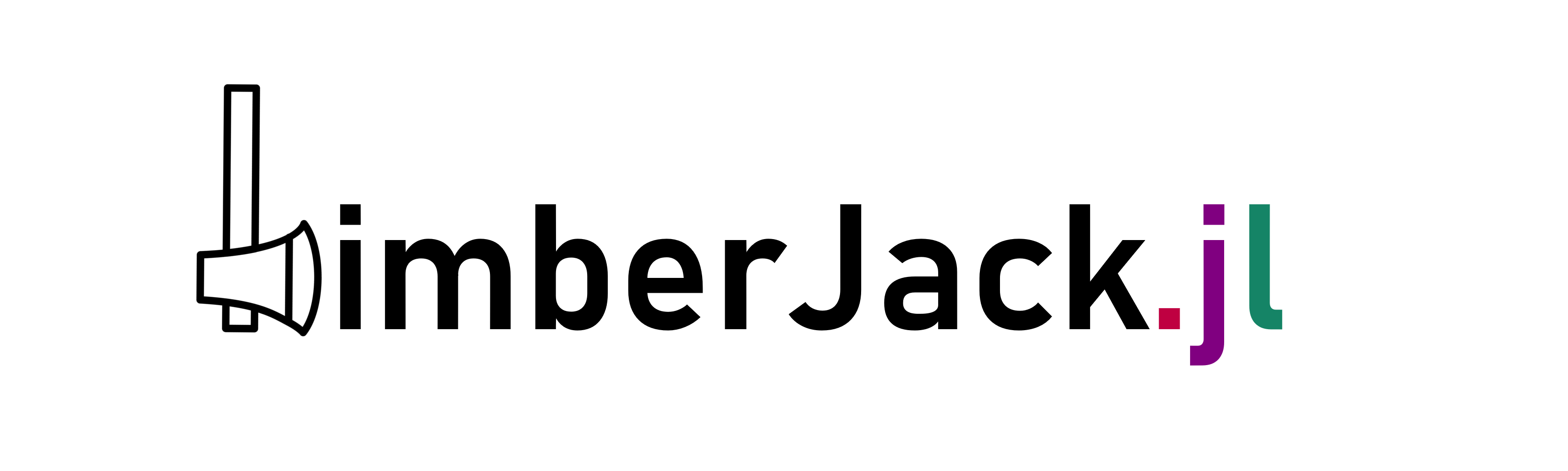
<p align="center"> A differentiable cosmological code in Julia. </p>
## Design Philosophy
+ **Modularity**: each main function within ```LimberJack.jl``` has its own module. New functions can be added by including extra modules. ```LimberJack.jl``` has the following modules:
| Module | function |
| ----------- | :----------- |
| ```boltzmann.jl``` | Performs the computation of primordial power spectrum |
| ```core.jl``` | Defines the structures where the theoretical predictions are stored and computes the background quantities |
| ```data_utils.jl``` | Manages ```sacc``` files for large data vectors |
| ```growth.jl``` | Computes the growth factor |
| ```halofit.jl``` | Computes the non-linear matter power spectrum as given by the Halofit fitting formula |
| ```spectra.jl``` | Computes the power spectra of any two tracers |
| ```theory.jl``` | Computes large data vectors that combine many spectra |
| ```tracers.jl``` | Computes the kernels associated with each type of kernel |
+ **Object-oriented**: ```LimberJack.jl``` mimics ```CCL.py``` class structure by using ```Julia```'s ```structures```.
+ **Transparency**: ```LimberJack.jl``` is fully written in ```Julia``` without needing to interface to any other programming language (```C```, ```Python```...) to compute thoretical predictions. This allows the user full access to the code from input to output.
## Goals
+ **Gradients**: one order of magnitude faster gradients than finite differences.
+ **Precision**: sub-percentage error with respect to ```CCL```.
+ **Speed**: ```C```-like performance.
## Installation
In order to run ```LimberJack.jl``` you will need ```Julia-1.7.0``` or newer installed in your system.
Older versions of ```Julia``` might be compatible but haven't been tested.
You can find instructions on how to install ```Julia``` here: https://julialang.org/downloads/.
Once you have installed ```Julia``` you can install ```LimberJack.jl``` following these steps:
1. Clone the git repository
2. From the repository directory open ```Julia```
3. In the ```Julia``` command line run:
``` julia
using Pkg
Pkg.add("LimberJack")
```
### Installing Sacc.py in Julia
``` julia
using Pkg
Pkg.add("CondaPkg")
CondaPkg.add("sacc")
```
## Use
``` julia
# Import
using LimberJack
# create LimberJack.jl Cosmology instance
cosmology = Cosmology(Ξ©m=0.30, Ξ©b=0.05, h=0.70, ns=0.96, s8=0.81;
tk_mode=:EisHu,
Pk_mode=:Halofit)
z = Vector(range(0., stop=2., length=256))
nz = @. exp(-0.5*((z-0.5)/0.05)^2)
tracer = NumberCountsTracer(cosmology, zs, nz; b=1.0)
ls = [10.0, 30.0, 100.0, 300.0]
cls = angularCβs(cosmology, tracer, tracer, ls)
```
## Challenges
1. **Parallelization**: the current threading parallelization of ```LimberJack.jl``` is far away from the optimal one over number of threads scaling. Future works could study alternative parallalization schemes or possible ineficiencies in the code.
2. **GPU's**: ```LimberJack.jl``` currently cannot run on GPUs which are known to significantly speed-up cosmological inference. Future works could study implementing ```Julia``` GPU libraries such as ```CUDA.jl```.
3. **Backwards-AD**: currently ```LimberJack.jl```'s preferred AD mode is forward-AD. However, the key computation of cosmological inference, obtaining the $\chi^2$, is a map from N parameters to a scalar. For a large number of parameters, backwards-AD is in theory the preferred AD mode and should significantly speed up the computation of the gradient. Future works could look into making ```LimberJack.jl``` compatible with the latest ```Julia``` AD libraries such as ```Zygote.jl``` to implement efficient backwards-AD.
| LimberJack | https://github.com/JaimeRZP/LimberJack.jl.git |
|
[
"MIT"
] | 0.1.5 | f9c5e6430f2e23b703a3c84df0ea2cc0362519df | docs | 155 | # Tutorial #
Please find a tutorial on how to use ```LimberJack.jl``` [here](https://github.com/JaimeRZP/LimberJack.jl/blob/main/Tutorial/Tutorial.ipynb)
| LimberJack | https://github.com/JaimeRZP/LimberJack.jl.git |
|
[
"MIT"
] | 1.0.0 | 595158505759d05961fb87b2c94b080efcaee9b0 | code | 733 | using DopplerSpectroscopyCore
using Documenter
DocMeta.setdocmeta!(DopplerSpectroscopyCore, :DocTestSetup, :(using DopplerSpectroscopyCore); recursive=true)
makedocs(;
modules=[DopplerSpectroscopyCore],
authors="Maksim Radchenko",
repo="https://github.com/m0Cey/DopplerSpectroscopyCore.jl/blob/{commit}{path}#{line}",
sitename="DopplerSpectroscopyCore.jl",
format=Documenter.HTML(;
prettyurls=get(ENV, "CI", "false") == "true",
canonical="https://m0Cey.github.io/DopplerSpectroscopyCore.jl",
edit_link="main",
assets=String[],
),
pages=[
"Home" => "index.md",
],
)
deploydocs(;
repo="github.com/m0Cey/DopplerSpectroscopyCore.jl",
devbranch="main",
)
| DopplerSpectroscopyCore | https://github.com/m0Cey/DopplerSpectroscopyCore.jl.git |
|
[
"MIT"
] | 1.0.0 | 595158505759d05961fb87b2c94b080efcaee9b0 | code | 260 | module DopplerSpectroscopyCore
using QuadGK
# documented for more readability of other file's code
# maybe will export them in future
include("functions.jl")
export Quantum2Level, LightSource
include("structs.jl")
export probe
include("methods.jl")
end
| DopplerSpectroscopyCore | https://github.com/m0Cey/DopplerSpectroscopyCore.jl.git |
|
[
"MIT"
] | 1.0.0 | 595158505759d05961fb87b2c94b080efcaee9b0 | code | 1689 | """
fα΅₯(x::Real, xβ::Real) -> Real
Compute the value of the Maxwell-Boltzmann distribution for a given dimensionless velocity
value `x`, with a most probable dimensionless velocity `xβ` of the distribution.
# Arguments
* `x` :
The dimensionless velocity value to evaluate the function at.
* `xβ` :
The most probable dimensionless velocity of the distribution.
# Returns
* `value of the distribution` :
Real number.
# References
* Wikipedia: https://en.wikipedia.org/wiki/Maxwell%E2%80%93Boltzmann_distribution
# Examples
julia> fα΅₯(1, 2)
0.21969564473386122
julia> fα΅₯(0, 1)
0.5641895835477563
"""
fα΅₯(x::Real, xβ::Real) = exp(-x^2/xβ^2) / (xβ*βΟ)
"""
sβ(x::Real, Ξ΄::Real, Ξ©::Real, Ξ³::Real) -> Real
Compute the saturation parameter value of a quantum two-level system for a
given dimensionless velocity.
# Arguments
* `x` :
The dimensionless velocity value to evaluate the function at.
* `Ξ΄` :
The detuning, a measure of how far the perturbation field oscillation frequency is
off-resonance relative to the transition
(units of spontaneous emission frequency `Ξ³β`).
* `Ξ©` :
The Rabi frequency at which the probability amplitudes of two energy levels fluctuate
in an oscillating perturbation field
(units of spontaneous emission frequency `Ξ³β`).
* `Ξ³` :
The optical emission frequency of optical transitions in quantum system
(units of spontaneous emission frequency `Ξ³β`).
# Returns
* `saturation parameter` :
Real number.
# See also
* [`Quantum2Level`](@ref)
# References
* Wikipedia: https://en.wikipedia.org/wiki/Spontaneous_emission
"""
sβ(x::Real, Ξ΄::Real, Ξ©::Real, Ξ³::Real) = Ξ©^2 / (Ξ³^2 + (Ξ΄-x)^2)
| DopplerSpectroscopyCore | https://github.com/m0Cey/DopplerSpectroscopyCore.jl.git |
|
[
"MIT"
] | 1.0.0 | 595158505759d05961fb87b2c94b080efcaee9b0 | code | 2113 | function Οββ(x::Real, Ξ΄::Real, self::Quantum2Level, light::LightSource)
local Ξ³βββ = self.relax_opt
local xβ = self.dimmless_velocity
local Ξ©β = light.rabi_freq_1
local Ξ©β = light.rabi_freq_2
# <functions block>
sβ½β°βΎβ = sβ(x, Ξ΄, Ξ©β, Ξ³βββ) # 0th order approximation
sβ½Β²βΎβ = sβ(-x, Ξ΄, Ξ©β, Ξ³βββ) # 2nd order approximation
fβ = fα΅₯(x, xβ)
# <densities block>
Οβ½β°βΎββ = 2*Ξ³βββ*sβ½β°βΎβ*fβ / (1 + 4*Ξ³βββ*sβ½β°βΎβ)
Οβ½Β²βΎββ = 2*Ξ³βββ*sβ½Β²βΎβ*fβ / (1 + 4*Ξ³βββ*sβ½β°βΎβ)
return Οβ½β°βΎββ + Οβ½Β²βΎββ
end
"""
probe(Ξ΄::Real, two_level, light) -> Real
probe(Ξ΄_vec::Vector{T}, two_level, light) -> Vector{T} where T<:Real
probe(Ξ΄_range::LinRange{T1, T2}, two_level, light)
-> Vector{T1} where {T1<:Real, T2<:Integer}
Compute probability `nβ` of a quantum system to be in excited state for detuning `Ξ΄`.
By supplying multiple detuning values via `Ξ΄_vec` or `Ξ΄_range` compute `nβ` for
each of them.
# Arguments
* `Ξ΄` :
* `two_level::Quantum2Level` :
* `light::LightSource` :
# Returns
* `nβ` :
Real number.
# See also
* [`StateVector`](@ref)
# References
* Springer: https://link.springer.com/chapter/10.1007/978-1-4684-4550-3_5
(If you find any unpaywalled refence, please contact me via mail suggested on package's
github page or create an issue)
"""
function probe(Ξ΄::Real, two_level::Quantum2Level, light::LightSource)::Real
nβ, error = quadgk(
x -> Οββ(x, Ξ΄, two_level, light),
-Inf, +Inf
)
return nβ
end
function probe(
Ξ΄_vec::Vector{T},
two_level::Quantum2Level,
light::LightSource,
)::Vector{T} where T<:Real
nβ_vec = Vector{T}(undef, length(Ξ΄_vec))
Threads.@threads for i in eachindex(Ξ΄_vec)
nβ_vec[i] = probe(Ξ΄_vec[i], two_level, light)
end
return nβ_vec
end
function probe(
Ξ΄_range::LinRange{T1, T2},
two_level::Quantum2Level,
light::LightSource,
)::Vector{T1} where {T1<:Real, T2<:Integer}
nβ_vec = Vector{T1}(undef, length(Ξ΄_range))
Threads.@threads for i in eachindex(Ξ΄_range)
nβ_vec[i] = probe(Ξ΄_range[i], two_level, light)
end
return nβ_vec
end
| DopplerSpectroscopyCore | https://github.com/m0Cey/DopplerSpectroscopyCore.jl.git |
|
[
"MIT"
] | 1.0.0 | 595158505759d05961fb87b2c94b080efcaee9b0 | code | 3177 | """
LightSource
Class that describes light source which is used to interact with the quantum
two-level system.
# Fields
* `wavelength` :
Represents a value of the main light source wavelength
(m).
* `rabi_freq_1` :
Represents a value of the Rabi frequency originated from the interaction between
direct light beam and two-level system
(units of spontaneous emission frequency `Ξ³β`).
* `rabi_freq_2` :
Represents a value of the Rabi frequency originated from the interaction between
reverse/reflected light beam and two-level system
(units of spontaneous emission frequency `Ξ³β`).
# Arguments
Refer to fields' description.
# Returns
* `Laser` :
composite type instance.
# References
* Wikipedia: https://en.wikipedia.org/wiki/Laser
* Wikipedia: https://en.wikipedia.org/wiki/Optical_parametric_amplifier
* Wikipedia: https://en.wikipedia.org/wiki/Spontaneous_emission
"""
struct LightSource
wavelength::Float64
rabi_freq_1::Float64
rabi_freq_2::Float64
function LightSource(Ξ»::Real, Ξ©β::Real, Ξ©β::Real = 0.0)
return new(Ξ», Ξ©β, Ξ©β)
end
end
"""
Quantum2Level
Class that describes the quantum two-level system via parameters of that system.
# Fields
* `mass` :
Represents a value of the quantum system's mass
(kg).
* `temperature` :
Represents a value of the quantum system's temperature
(K).
* `relax_sp` :
Represents a value of the spontaneous emission frequency in quantum system and
considered to be constant in this model, referred to as `Ξ³β` in other documentation
and below
(Hz).
* `relax_opt` :
Represents a value of the optical emission frequency of optical transitions in quantum
system and considered to be constant in this model
(units of `Ξ³β`).
* `dimmless_velocity` :
Represents a value of the quantum system's most probable velocity in
dimensionless units.
# Arguments
* `M`, `T`, `Ξ³β`, `Ξ³βββ` :
Refer to fields' description.
* `light` :
Light source which is used to interact with the quantum two-level system.
# Returns
* `Quantum2Level` :
Composite type instance.
# See also
* [`LightSource`](@ref)
# References
* Wikipedia: https://en.wikipedia.org/wiki/Density_matrix
* Wikipedia: https://en.wikipedia.org/wiki/Spontaneous_emission
"""
struct Quantum2Level
mass::Float64
temperature::Float64
relax_sp::Float64
relax_opt::Float64
dimmless_velocity::Float64
function Quantum2Level(M::Real, T::Real, Ξ³β::Real, Ξ³βββ::Real, light::LightSource)
local Ξ» = light.wavelength
k::Float64 = 2Ο / Ξ» # calculate the wave number
k_B = 1.380649e-23
vβ = β(2*k_B*T / M)
xβ = vβ*k / Ξ³β
return new(M, T, Ξ³β, Ξ³βββ, xβ)
end
function Quantum2Level(q_sys::Quantum2Level, light::LightSource)
local M = q_sys.mass
local T = q_sys.temperature
local Ξ³β = q_sys.relax_sp
local Ξ³βββ = q_sys.relax_opt
local Ξ» = light.wavelength
k::Float64 = 2Ο / Ξ» # calculate the wave number
k_B = 1.380649e-23
vβ = β(2*k_B*T / M)
xβ = vβ*k / Ξ³β
return new(M, T, Ξ³β, Ξ³βββ, xβ)
end
end
| DopplerSpectroscopyCore | https://github.com/m0Cey/DopplerSpectroscopyCore.jl.git |
|
[
"MIT"
] | 1.0.0 | 595158505759d05961fb87b2c94b080efcaee9b0 | code | 559 | @testset "doppler" begin
light = LightSource(8e-7, 1, 0)
atom = Quantum2Level(1.4e-25, 300, 2Ο*5e+6, 0.5, light)
@test probe(0, atom, light) > probe(rand(1:300), atom, light)
@test probe(0, atom, light) > probe(rand(-300:-1), atom, light)
@test probe(-100, atom, light) β probe(100, atom, light)
detun_linrange = LinRange(-100, 100, 201)
@test maximum(probe(detun_linrange, atom, light)) == probe(0, atom, light)
detun_vec = collect(detun_linrange)
@test maximum(probe(detun_vec, atom, light)) == probe(0, atom, light)
end
| DopplerSpectroscopyCore | https://github.com/m0Cey/DopplerSpectroscopyCore.jl.git |
|
[
"MIT"
] | 1.0.0 | 595158505759d05961fb87b2c94b080efcaee9b0 | code | 273 | @testset "non-exports" begin
@test DopplerSpectroscopyCore.fα΅₯(1, 1) == exp(-1) / βΟ
@test DopplerSpectroscopyCore.fα΅₯(1, 1) == exp(-1)/ βΟ
@test DopplerSpectroscopyCore.sβ(rand(), rand(), 0, rand()) == 0
@test DopplerSpectroscopyCore.sβ(1, 1, 1, 1) == 1
end
| DopplerSpectroscopyCore | https://github.com/m0Cey/DopplerSpectroscopyCore.jl.git |
|
[
"MIT"
] | 1.0.0 | 595158505759d05961fb87b2c94b080efcaee9b0 | code | 204 | using DopplerSpectroscopyCore
using Test
@testset "DopplerSpectroscopyCore.jl" begin
include("non-exports.jl")
include("typecheck.jl")
include("doppler.jl")
include("sub-doppler.jl")
end
| DopplerSpectroscopyCore | https://github.com/m0Cey/DopplerSpectroscopyCore.jl.git |
|
[
"MIT"
] | 1.0.0 | 595158505759d05961fb87b2c94b080efcaee9b0 | code | 542 | @testset "sub-doppler" begin
light = LightSource(8e-7, 1, 0.3)
atom = Quantum2Level(1.4e-25, 300, 2Ο*5e+6, 0.5, light)
@test probe(0, atom, light) < probe(5, atom, light)
@test probe(0, atom, light) < probe(-5, atom, light)
@test probe(-100, atom, light) β probe(100, atom, light)
detun_linrange = LinRange(-20, 20, 301)
@test minimum(probe(detun_linrange, atom, light)) == probe(0, atom, light)
detun_vec = collect(detun_linrange)
@test minimum(probe(detun_vec, atom, light)) == probe(0, atom, light)
end
| DopplerSpectroscopyCore | https://github.com/m0Cey/DopplerSpectroscopyCore.jl.git |
|
[
"MIT"
] | 1.0.0 | 595158505759d05961fb87b2c94b080efcaee9b0 | code | 363 | @testset "typecheck" begin
light = LightSource(8e-7, 1, 0.3)
atom = Quantum2Level(1.4e-25, 300, 2Ο*5e+6, 0.5, light)
detun_linrange = LinRange(-100, 100, 201)
@test eltype(probe(detun_linrange, atom, light)) == eltype(detun_linrange)
detun_vec = collect(detun_linrange)
@test eltype(probe(detun_vec, atom, light)) == eltype(detun_vec)
end
| DopplerSpectroscopyCore | https://github.com/m0Cey/DopplerSpectroscopyCore.jl.git |
|
[
"MIT"
] | 1.0.0 | 595158505759d05961fb87b2c94b080efcaee9b0 | docs | 3707 | # DopplerSpectroscopyCore.jl
[](https://m0Cey.github.io/DopplerSpectroscopyCore.jl/stable/)
[](https://m0Cey.github.io/DopplerSpectroscopyCore.jl/dev/)
[](https://github.com/m0Cey/DopplerSpectroscopyCore.jl/actions/workflows/CI.yml?query=branch%3Amain)
[](https://codecov.io/gh/m0Cey/DopplerSpectroscopyCore.jl)
[](https://github.com/invenia/BlueStyle)
(This section is WIP)
DopplerSpectroscopyCore.jl provides core toolset for simulating Doppler and sub-Doppler spectroscopy
experiments. The main goal is to have ready-to-use, simple-to-understand modeling toolkit with experimenters in mind.
Furthermore, it can be used as introduction to spectroscopy for people with little physics background or
students.
## Installation
To install DopplerSpectroscopyCore.jl, use the Julia package manager:
```julia
julia> using Pkg
julia> Pkg.add("DopplerSpectroscopyCore")
```
## Example
(This section is WIP)
Import module:
```julia
julia> using RabiRamseySpectroscopy
```
At first we need to create our experement setup:
1. Light source - research instrument. Red laser (wavelenght = 750 nm or approx. 8e-7 m) will fit.
We consider that there is no refracted light to enter the research object in a backward direction
by setting the last parameter to 0
```julia
julia> laser = LightSource(8e-7, 1, 0)
```
2. Gas cell or a single atom - research object. Lets say it's a <sup>87</sup>Rb atom (mass = 1.4e-25kg)
at room temperature (T = 300 K). Atom's spontaneous relaxation parameter is approx. 2Ο*5e+6 Hz and
optical relaxation parameter considered to be half of spontaneous in units of it's value (0.5). Other parameters
depends on a light source so we just use our **laser** as an argument
```julia
julia> atom = Quantum2Level(1.4e-25, 300, 2Ο*5e+6, 0.5, light)
```
Because probing time is long enough, probe pulse will be playing two roles at the same time:
1. _pump_ our atom with some probability to excited state
2. _probe_ our system to check if atom was actually pumped.
(Make a pull request or issue on package's GitHub page if you want more physics details in the intro)
Thus said, second step is to probe an atom with the laser:
```julia
julia> probe(0, atom, laser)
0.0189015858908291
```
The output indicates the probability of our atom to be in excited state at zero-detuning
(laser frequency is resonant with transition frequency of an atom).
Congrats, you performed your first spectroscopy experiment in Julia!
As a third step we'll see a relation between mentioned probability and detuning of a laser light.
For that we need to make a series of probes, varying laser detuning. It can be done by simply applying **probe**
on a range of detuning values:
```julia
julia> detun_range = LinRange(-300, 300, 100)
julia> probes = probe(detun_range, atom, laser)
```
Then you can plot this relation yourself with any provided plotting tool: Plots, PlotlyJS, Makie etc.
(maybe will add example pic later)
## Development
(This section is WIP)
If you want to help develop this package, you can do it via GitHub default instruments (pull requests,
issues etc.) and/or contact me: [email protected].
In additon, it is highly recommended to read or modify code of DopplerSpectroscopyCore.jl with JuliaMono font
installed. That way UTF-8 symbols will be displayed correctly.
| DopplerSpectroscopyCore | https://github.com/m0Cey/DopplerSpectroscopyCore.jl.git |
|
[
"MIT"
] | 1.0.0 | 595158505759d05961fb87b2c94b080efcaee9b0 | docs | 2978 | # DopplerSpectroscopyCore.jl
(This section is WIP)
DopplerSpectroscopyCore.jl provides core toolset for simulating Doppler and sub-Doppler spectroscopy
experiments. The main goal is to have ready-to-use, simple-to-understand modeling toolkit with experimenters in mind.
Furthermore, it can be used as introduction to spectroscopy for people with little physics background or
students.
## Installation
To install DopplerSpectroscopyCore.jl, use the Julia package manager:
```julia
julia> using Pkg
julia> Pkg.add("DopplerSpectroscopyCore")
```
## Example
(This section is WIP)
Import module:
```julia
julia> using RabiRamseySpectroscopy
```
At first we need to create our experement setup:
1. Light source - research instrument. Red laser (wavelenght = 750 nm or approx. 8e-7 m) will fit.
We consider that there is no refracted light to enter the research object in a backward direction
by setting the last parameter to 0
```julia
julia> laser = LightSource(8e-7, 1, 0)
```
2. Gas cell or a single atom - research object. Lets say it's a <sup>87</sup>Rb atom (mass = 1.4e-25kg)
at room temperature (T = 300 K). Atom's spontaneous relaxation parameter is approx. 2Ο*5e+6 Hz and
optical relaxation parameter considered to be half of spontaneous in units of it's value (0.5). Other parameters
depends on a light source so we just use our **laser** as an argument
```julia
julia> atom = Quantum2Level(1.4e-25, 300, 2Ο*5e+6, 0.5, light)
```
Because probing time is long enough, probe pulse will be playing two roles at the same time:
1. _pump_ our atom with some probability to excited state
2. _probe_ our system to check if atom was actually pumped.
(Make a pull request or issue on package's GitHub page if you want more physics details in the intro)
Thus said, second step is to probe an atom with the laser:
```julia
julia> probe(0, atom, laser)
0.0189015858908291
```
The output indicates the probability of our atom to be in excited state at zero-detuning
(laser frequency is resonant with transition frequency of an atom).
Congrats, you performed your first spectroscopy experiment in Julia!
As a third step we'll see a relation between mentioned probability and detuning of a laser light.
For that we need to make a series of probes, varying laser detuning. It can be done by simply applying **probe**
on a range of detuning values:
```julia
julia> detun_range = LinRange(-300, 300, 100)
julia> probes = probe(detun_range, atom, laser)
```
Then you can plot this relation yourself with any provided plotting tool: Plots, PlotlyJS, Makie etc.
(maybe will add example pic later)
## Development
(This section is WIP)
If you want to help develop this package, you can do it via GitHub default instruments (pull requests,
issues etc.) and/or contact me: [email protected].
In additon, it is highly recommended to read or modify code of DopplerSpectroscopyCore.jl with JuliaMono font
installed. That way UTF-8 symbols will be displayed correctly.
| DopplerSpectroscopyCore | https://github.com/m0Cey/DopplerSpectroscopyCore.jl.git |
|
[
"MIT"
] | 1.2.0 | e383c87cf2a1dc41fa30c093b2a19877c83e1bc1 | code | 19888 | module TableOperations
using Tables, SentinelArrays
struct TransformsRow{T, F} <: Tables.AbstractRow
row::T
funcs::F
end
getrow(r::TransformsRow) = getfield(r, :row)
getfuncs(r::TransformsRow) = getfield(r, :funcs)
Tables.getcolumn(row::TransformsRow, nm::Symbol) = (getfunc(row, getfuncs(row), nm))(Tables.getcolumn(getrow(row), nm))
Tables.getcolumn(row::TransformsRow, i::Int) = (getfunc(row, getfuncs(row), i))(Tables.getcolumn(getrow(row), i))
Tables.columnnames(row::TransformsRow) = Tables.columnnames(getrow(row))
struct Transforms{C, T, F}
source::T
funcs::F # NamedTuple of columnname=>transform function
end
Tables.columnnames(t::Transforms{true}) = Tables.columnnames(getfield(t, 1))
Tables.getcolumn(t::Transforms{true}, nm::Symbol) = Base.map(getfunc(t, getfield(t, 2), nm), Tables.getcolumn(getfield(t, 1), nm))
Tables.getcolumn(t::Transforms{true}, i::Int) = Base.map(getfunc(t, getfield(t, 2), i), Tables.getcolumn(getfield(t, 1), i))
# for backwards compat
Base.propertynames(t::Transforms{true}) = Tables.columnnames(t)
Base.getproperty(t::Transforms{true}, nm::Symbol) = Tables.getcolumn(t, nm)
"""
TableOperations.transform(source, funcs) => TableOperations.Transforms
source |> TableOperations.transform(funcs) => TableOperations.Transform
Given any Tables.jl-compatible source, apply a series of transformation functions, for the columns specified in `funcs`.
The tranform functions can be a NamedTuple or Dict mapping column name (`String` or `Symbol` or `Integer` index) to Function.
"""
function transform end
transform(funcs) = x->transform(x, funcs)
transform(; kw...) = transform(kw.data)
function transform(src::T, funcs::F) where {T, F}
C = Tables.columnaccess(T)
x = C ? Tables.columns(src) : Tables.rows(src)
return Transforms{C, typeof(x), F}(x, funcs)
end
getfunc(row, nt::NamedTuple, nm::Symbol) = get(nt, nm, identity)
getfunc(row, d::Dict{String, <:Base.Callable}, nm::Symbol) = get(d, String(nm), identity)
getfunc(row, d::Dict{Symbol, <:Base.Callable}, nm::Symbol) = get(d, nm, identity)
getfunc(row, d::Dict{Int, <:Base.Callable}, nm::Symbol) = get(d, findfirst(isequal(nm), Tables.columnnames(row)), identity)
getfunc(row, nt::NamedTuple, i::Int) = get(nt, Tables.columnnames(row)[i], identity)
getfunc(row, d::Dict{String, <:Base.Callable}, i::Int) = get(d, String(Tables.columnnames(row)[i]), identity)
getfunc(row, d::Dict{Symbol, <:Base.Callable}, i::Int) = get(d, Tables.columnnames(row)[i], identity)
getfunc(row, d::Dict{Int, <:Base.Callable}, i::Int) = get(d, i, identity)
Tables.istable(::Type{<:Transforms}) = true
Tables.rowaccess(::Type{Transforms{C, T, F}}) where {C, T, F} = !C
Tables.rows(t::Transforms{false}) = t
Tables.columnaccess(::Type{Transforms{C, T, F}}) where {C, T, F} = C
Tables.columns(t::Transforms{true}) = t
# avoid relying on inference here and just let sinks figure things out
Tables.schema(t::Transforms) = nothing
Base.IteratorSize(::Type{Transforms{false, T, F}}) where {T, F} = Base.IteratorSize(T)
Base.length(t::Transforms{false}) = length(getfield(t, 1))
Base.eltype(t::Transforms{false, T, F}) where {T, F} = TransformsRow{eltype(getfield(t, 1)), F}
@inline function Base.iterate(t::Transforms{false}, st=())
state = iterate(getfield(t, 1), st...)
state === nothing && return nothing
return TransformsRow(state[1], getfield(t, 2)), (state[2],)
end
# select
struct Select{T, columnaccess, names}
source::T
end
"""
TableOperations.select(source, columns...) => TableOperations.Select
source |> TableOperations.select(columns...) => TableOperations.Select
Create a lazy wrapper that satisfies the Tables.jl interface and keeps only the columns given by the columns arguments, which can be `String`s, `Symbol`s, or `Integer`s
"""
function select end
select(names::Symbol...) = x->select(x, names...)
select(names::String...) = x->select(x, Base.map(Symbol, names)...)
select(inds::Integer...) = x->select(x, Base.map(Int, inds)...)
function select(x::T, names...) where {T}
colaccess = Tables.columnaccess(T)
r = colaccess ? Tables.columns(x) : Tables.rows(x)
return Select{typeof(r), colaccess, names}(r)
end
Tables.istable(::Type{<:Select}) = true
Base.@pure function typesubset(::Tables.Schema{names, types}, nms::NTuple{N, Symbol}) where {names, types, N}
return Tuple{Any[Tables.columntype(names, types, nm) for nm in nms]...}
end
Base.@pure function typesubset(::Tables.Schema{names, types}, inds::NTuple{N, Int}) where {names, types, N}
return Tuple{Any[fieldtype(types, i) for i in inds]...}
end
typesubset(::Tables.Schema{names, types}, ::Tuple{}) where {names, types} = Tuple{}
function typesubset(sch::Tables.Schema{nothing, nothing}, nms::NTuple{N, Symbol}) where {N}
names = sch.names
types = sch.types
return Tuple{types[indexin(collect(nms), names)]...}
end
function typesubset(sch::Tables.Schema{nothing, nothing}, inds::NTuple{N, Int}) where {N}
types = sch.types
return Tuple{Any[types[i] for i in inds]...}
end
typesubset(::Tables.Schema{nothing, nothing}, ::Tuple{}) = Tuple{}
namesubset(::Tables.Schema{names, types}, nms::NTuple{N, Symbol}) where {names, types, N} = nms
Base.@pure namesubset(::Tables.Schema{names, T}, inds::NTuple{N, Int}) where {names, T, N} = ntuple(i -> names[inds[i]], N)
Base.@pure namesubset(sch::Tables.Schema{nothing, nothing}, inds::NTuple{N, Int}) where {N} = (names = sch.names; ntuple(i -> names[inds[i]], N))
namesubset(::Tables.Schema{names, types}, ::Tuple{}) where {names, types} = ()
namesubset(names, nms::NTuple{N, Symbol}) where {N} = nms
namesubset(names, inds::NTuple{N, Int}) where {N} = ntuple(i -> names[inds[i]], N)
namesubset(names, ::Tuple{}) = ()
function Tables.schema(s::Select{T, columnaccess, names}) where {T, columnaccess, names}
sch = Tables.schema(getfield(s, 1))
sch === nothing && return nothing
return Tables.Schema(namesubset(sch, names), typesubset(sch, names))
end
# columns: make Select property-accessible
Tables.getcolumn(s::Select{T, true, names}, nm::Symbol) where {T, names} = Tables.getcolumn(getfield(s, 1), nm)
Tables.getcolumn(s::Select{T, true, names}, i::Int) where {T, names} = Tables.getcolumn(getfield(s, 1), i)
Tables.columnnames(s::Select{T, true, names}) where {T, names} = namesubset(Tables.columnnames(getfield(s, 1)), names)
Tables.columnaccess(::Type{Select{T, C, names}}) where {T, C, names} = C
Tables.columns(s::Select{T, true, names}) where {T, names} = s
# for backwards compat
Base.propertynames(s::Select{T, true, names}) where {T, names} = Tables.columnnames(s)
Base.getproperty(s::Select{T, true, names}, nm::Symbol) where {T, names} = Tables.getcolumn(s, nm)
# rows: implement Iterator interface
Base.IteratorSize(::Type{Select{T, false, names}}) where {T, names} = Base.IteratorSize(T)
Base.length(s::Select{T, false, names}) where {T, names} = length(getfield(s, 1))
Base.IteratorEltype(::Type{Select{T, false, names}}) where {T, names} = Base.IteratorEltype(T)
Base.eltype(s::Select{T, false, names}) where {T, names} = SelectRow{eltype(getfield(s, 1)), names}
Tables.rowaccess(::Type{Select{T, columnaccess, names}}) where {T, columnaccess, names} = !columnaccess
Tables.rows(s::Select{T, false, names}) where {T, names} = s
# we need to iterate a "row view" in case the underlying source has unknown schema
# to ensure each iterated row only has `names` Tables.columnnames
struct SelectRow{T, names} <: Tables.AbstractRow
row::T
end
Tables.getcolumn(row::SelectRow, nm::Symbol) = Tables.getcolumn(getfield(row, 1), nm)
Tables.getcolumn(row::SelectRow{T, names}, i::Int) where {T, names} = Tables.getcolumn(getfield(row, 1), names[i])
Tables.getcolumn(row::SelectRow, ::Type{T}, i::Int, nm::Symbol) where {T} = Tables.getcolumn(getfield(row, 1), T, Tables.columnindex(Tables.columnnames(getfield(row, 1)), nm), nm)
getprops(row, nms::NTuple{N, Symbol}) where {N} = nms
getprops(row, inds::NTuple{N, Int}) where {N} = ntuple(i->Tables.columnnames(getfield(row, 1))[inds[i]], N)
getprops(row, ::Tuple{}) = ()
Tables.columnnames(row::SelectRow{T, names}) where {T, names} = getprops(row, names)
@inline function Base.iterate(s::Select{T, false, names}) where {T, names}
state = iterate(getfield(s, 1))
state === nothing && return nothing
row, st = state
return SelectRow{typeof(row), names}(row), st
end
@inline function Base.iterate(s::Select{T, false, names}, st) where {T, names}
state = iterate(getfield(s, 1), st)
state === nothing && return nothing
row, st = state
return SelectRow{typeof(row), names}(row), st
end
# filter
struct Filter{F, T}
f::F
x::T
end
"""
TableOperations.filter(f, source) => TableOperations.Filter
source |> TableOperations.filter(f) => TableOperations.Filter
Create a lazy wrapper that satisfies the Tables.jl interface and keeps the rows where `f(row)` is true.
"""
function filter end
function filter(f::F, x) where {F <: Base.Callable}
r = Tables.rows(x)
return Filter{F, typeof(r)}(f, r)
end
filter(f::Base.Callable) = x->filter(f, x)
Tables.isrowtable(::Type{<:Filter}) = true
Tables.schema(f::Filter) = Tables.schema(f.x)
Base.IteratorSize(::Type{Filter{F, T}}) where {F, T} = Base.SizeUnknown()
Base.IteratorEltype(::Type{Filter{F, T}}) where {F, T} = Base.IteratorEltype(T)
Base.eltype(f::Filter) = eltype(f.x)
@inline function Base.iterate(f::Filter)
state = iterate(f.x)
state === nothing && return nothing
while !f.f(state[1])
state = iterate(f.x, state[2])
state === nothing && return nothing
end
return state
end
@inline function Base.iterate(f::Filter, st)
state = iterate(f.x, st)
state === nothing && return nothing
while !f.f(state[1])
state = iterate(f.x, state[2])
state === nothing && return nothing
end
return state
end
# map
struct Map{F, T}
func::F
source::T
end
"""
TableOperations.map(f, source) => TableOperations.Map
source |> TableOperations.map(f) => TableOperations.Map
Create a lazy wrapper that satisfies the Tables.jl interface and will apply the function `f(row)` to each
row in the input table source. Note that `f` must take and produce a valid Tables.jl `Row` object.
"""
function map end
function map(f::F, x::T) where {F <: Base.Callable, T}
r = Tables.rows(x)
return Map{F, typeof(r)}(f, r)
end
map(f::Base.Callable) = x->map(f, x)
Tables.isrowtable(::Type{<:Map}) = true
Tables.schema(m::Map) = nothing
Base.IteratorSize(::Type{Map{T, F}}) where {T, F} = Base.IteratorSize(T)
Base.length(m::Map) = length(m.source)
Base.IteratorEltype(::Type{<:Map}) = Base.EltypeUnknown()
@inline function Base.iterate(m::Map)
state = iterate(m.source)
state === nothing && return nothing
return m.func(state[1]), state[2]
end
@inline function Base.iterate(m::Map, st)
state = iterate(m.source, st)
state === nothing && return nothing
return m.func(state[1]), state[2]
end
# joinpartitions
struct JoinedPartitions{S} <: Tables.AbstractColumns
schema::S
x::Vector{ChainedVector}
lookup::Dict{Symbol, ChainedVector}
end
Tables.istable(::Type{<:JoinedPartitions}) = true
Tables.columnaccess(::Type{<:JoinedPartitions}) = true
Tables.columns(x::JoinedPartitions) = Tables.CopiedColumns(x)
Tables.columnnames(x::JoinedPartitions) = Tables.schema(x).names
Tables.getcolumn(x::JoinedPartitions, i::Int) = getfield(x, :x)[i]
Tables.getcolumn(x::JoinedPartitions, nm::Symbol) = getfield(x, :lookup)[nm]
Tables.schema(x::JoinedPartitions) = getfield(x, :schema)
"""
TableOperations.joinpartitions(x) => TableOperations.JoinedPartitions
x |> TableOperations.joinpartitions() => TableOperations.JoinedPartitions
Take an input `x` that implements `Tables.partitions` and "join" the partitions into
a single, "long" table. Each column is lazily appended using `SentinelArrays.ChainedVector`
so each partition's column is a single chain, and all partitions together are treated as a
single column. This can be helpful for "materializing" a partitioned input if single-column
operations are desired. No copy of the input data is made to avoid excessive memory allocations,
unless `promote=true` is specified. If `promote=true` then columns will be promoted to a
common type, requiring extra allocations.
The returned object, `TableOperations.JoinedPartitions`, satisfies itself the `Tables.columns`
interface, so access to individual columns is supported via `x.col1`, `x[1]`, or
`Tables.getcolumn(x, :col1)`, in addition to the normal Tables.jl compatibility with sink
functions, like `df = DataFrame(TableOperations.joinpartitions(x))`.
"""
function joinpartitions(x; promote::Bool=false)
schema = Ref{Tables.Schema}()
joined = ChainedVector[]
N = 0
for partition in Tables.partitions(x)
cols = Tables.columns(partition)
if isempty(joined)
schema[] = Tables.schema(cols)
N = length(schema[].names)
foreach(i -> push!(joined, ChainedVector([Tables.getcolumn(cols, i)])), 1:N)
else
foreach(1:N) do i
prev = joined[i]
col = Tables.getcolumn(cols, i)
T = eltype(prev)
S = eltype(col)
if !(S <: T) && promote
R = promote_type(S, T)
# Promote the joined arrays
newcol = similar(prev, R)
copyto!(newcol, prev)
joined[i] = newcol
# Update the schema
sch_names = schema[].names
sch_types = schema[].types
schema[] = Tables.Schema(
sch_names,
Tuple(j == i ? R : sch_types[j] for j in 1:N),
)
end
append!(joined[i], col)
end
end
end
return JoinedPartitions(schema[], joined, Dict{Symbol, ChainedVector}(nm => col for (nm, col) in zip(schema[].names, joined)))
end
joinpartitions() = x -> joinpartitions(x)
struct ColumnPartitions{T}
source::T
nrows::Int
end
struct RowPartitions{T}
source::T
nrows::Int
end
"""
TableOperations.makepartitions(source, nrows) => TableOperations.MakePartitions
source |> TableOperations.makepartitions(nrows) => TableOperations.MakePartitions
Take a Tables.jl-compatible source and "partition" it into chunks with `nrows` rows.
Returns a `TableOperations.MakePartitions` object that implements `Tables.partitions`, where each
iteration returns `nrows` rows. For input tables that produce forward-only row iterators,
a `materializerows=true` keyword argument can be passed that will basically make a copy
of each row to ensure partitioning works as expected.
"""
function makepartitions end
makepartitions(nrows::Int; kw...) = x->makepartitions(x, nrows; kw...)
function makepartitions(x::T, nrows::Int; materializerows::Bool=false) where {T}
nrows < 1 && throw(ArgumentError("cannot create partitions of length $nrows"))
colaccess = Tables.columnaccess(T)
if colaccess
return ColumnPartitions(Tables.columns(x), nrows)
else
r = Tables.rows(x)
if Base.haslength(r) && !materializerows
return Iterators.partition(r, nrows)
else
return RowPartitions(r, nrows)
end
end
end
Tables.partitions(x::ColumnPartitions) = x
Tables.partitions(x::RowPartitions) = x
struct ColumnPartitionRows{T}
source::T
rng::UnitRange{Int}
end
Tables.istable(::Type{<:ColumnPartitionRows}) = true
Tables.rowaccess(::Type{<:ColumnPartitionRows}) = true
Tables.rows(x::ColumnPartitionRows) = x
Base.IteratorSize(::Type{<:ColumnPartitions}) = Base.HasLength()
Base.length(x::ColumnPartitions) = cld(Tables.rowcount(x.source), x.nrows)
Base.eltype(x::ColumnPartitions{T}) where {T} = ColumnPartitionRows{T}
@inline function Base.iterate(x::ColumnPartitions, st=0)
st >= length(x) && return nothing
len = min(x.nrows * (st + 1), Tables.rowcount(x.source))
return ColumnPartitionRows(x.source, (x.nrows * st + 1):len), st + 1
end
Base.IteratorSize(::Type{<:ColumnPartitionRows}) = Base.HasLength()
Base.length(x::ColumnPartitionRows) = length(getfield(x, :rng))
Base.eltype(x::ColumnPartitionRows{T}) where {T} = Tables.ColumnsRow{T}
@inline function Base.iterate(x::ColumnPartitionRows, st=1)
st > length(x) && return nothing
return Tables.ColumnsRow(getfield(x, :source), getfield(x, :rng)[st]), st + 1
end
struct MaterializedRow <: Tables.AbstractRow
names::Vector{Symbol}
values::Vector{Any}
lookup::Dict{Symbol, Any}
end
Tables.getcolumn(x::MaterializedRow, i::Int) = getfield(x, :values)[i]
Tables.getcolumn(x::MaterializedRow, nm::Symbol) = getfield(x, :lookup)[nm]
Tables.getcolumn(x::MaterializedRow, ::Type{T}, i::Int, nm::Symbol) where {T} = getfield(x, :values)[i]
Tables.columnnames(x::MaterializedRow) = getfield(x, :names)
function MaterializedRow(x)
names = collect(Tables.columnnames(x))
values = Any[Tables.getcolumn(x, i) for i = 1:length(names)]
return MaterializedRow(names, values, Dict{Symbol, Any}(nm => val for (nm, val) in zip(names, values)))
end
Base.IteratorSize(::Type{<:RowPartitions}) = Base.SizeUnknown()
Base.eltype(x::RowPartitions) = Vector{MaterializedRow}
@inline function Base.iterate(x::RowPartitions, st=())
st === nothing && return nothing
v = Vector{MaterializedRow}(undef, x.nrows)
y = iterate(x.source, st...)
i = 0
while y !== nothing
i += 1
v[i] = MaterializedRow(y[1])
if i >= x.nrows
break
end
y = iterate(x.source, y[2])
end
i == 0 && return nothing
return resize!(v, i), y === nothing ? nothing : (y[2],)
end
struct NarrowTypes{T} <: Tables.AbstractColumns
x::T
schema::Tables.Schema
end
schema(nt::NarrowTypes) = getfield(nt, :schema)
narrowarray(x) = mapreduce(typeof, Base.promote_typejoin, x)
narrowarray(::Type{T}, x::AbstractArray{T}) where {T} = x
narrowarray(::Type{T}, x::AbstractArray{S}) where {T, S} = Vector{T}(x)
"""
TableOperations.narrowtypes(source) => TableOperations.NarrowTypes
source |> TableOperations.narrowtypes() => TableOperations.NarrowTypes
Take a Tables.jl-compatible source, with potentially "wide" column types, and re-infer the schema by
promoting the types of each actual value for each column. Useful, for example, when a columnar table source
has a column type of `Any`, and a more concrete type is desired. Uses `Base.promote_typejoin` internally
to do actual type promotion.
"""
function narrowtypes(table)
t = Tables.columns(table)
return NarrowTypes(t, Tables.Schema(Tables.columnnames(t), [narrowarray(Tables.getcolumn(t, nm)) for nm in Tables.columnnames(t)]))
end
narrowtypes() = x -> narrowtypes(x)
Tables.istable(::Type{<:NarrowTypes}) = true
Tables.columnaccess(::Type{<:NarrowTypes}) = true
Tables.columns(x::NarrowTypes) = x
Tables.schema(nt::NarrowTypes) = schema(nt)
Tables.columnnames(nt::NarrowTypes) = schema(nt).names
Tables.getcolumn(nt::NarrowTypes, nm::Symbol) = narrowarray(Tables.columntype(schema(nt), nm), Tables.getcolumn(getfield(nt, 1), nm))
Tables.getcolumn(nt::NarrowTypes, i::Int) = narrowarray(Tables.columntype(schema(nt), i), Tables.getcolumn(getfield(nt, 1), i))
# dropmissing
"""
TableOperations.dropmissing(source) => TableOperations.Filter
source |> TableOperations.dropmissing() => TableOperations.Filter
Take a Tables.jl-compatible source and filter lazily every row where missing values are present.
"""
function dropmissing(table)
return TableOperations.filter(_check_no_missing_in_row, table)
end
dropmissing() = x -> dropmissing(x)
function _check_no_missing_in_row(row)
for el in row
el === missing && return false
end
return true
end
end # module
| TableOperations | https://github.com/JuliaData/TableOperations.jl.git |
|
[
"MIT"
] | 1.2.0 | e383c87cf2a1dc41fa30c093b2a19877c83e1bc1 | code | 15389 | using TableOperations, Tables, Test
ctable = (A=[1, missing, 3], B=[1.0, 2.0, 3.0], C=["hey", "there", "sailor"])
rtable = Tables.rowtable(ctable)
rtable2 = Iterators.filter(i -> i.a % 2 == 0, [(a=x, b=y) for (x, y) in zip(1:20, 21:40)])
struct ReallyWideTable
end
Tables.istable(::Type{ReallyWideTable}) = true
Tables.columnaccess(::Type{ReallyWideTable}) = true
Tables.columns(x::ReallyWideTable) = x
Tables.columnnames(x::ReallyWideTable) = [Symbol(:x, i) for i = 1:100_000]
Tables.getcolumn(x::ReallyWideTable, i::Int) = rand(10)
Tables.getcolumn(x::ReallyWideTable, nm::Symbol) = rand(10)
Tables.schema(x::ReallyWideTable) = Tables.Schema(Tables.columnnames(x), [Float64 for _ = 1:100_000])
@testset "TableOperations.transform" begin
tran = ctable |> TableOperations.transform(C=Symbol)
@test Tables.istable(typeof(tran))
@test !Tables.rowaccess(typeof(tran))
@test Tables.columnaccess(typeof(tran))
@test Tables.columns(tran) === tran
@test isequal(Tables.getcolumn(tran, :A), [1,missing,3])
@test isequal(Tables.getcolumn(tran, 1), [1,missing,3])
tran2 = rtable |> TableOperations.transform(C=Symbol)
@test Tables.istable(typeof(tran2))
@test Tables.rowaccess(typeof(tran2))
@test !Tables.columnaccess(typeof(tran2))
@test Tables.rows(tran2) === tran2
@test Base.IteratorSize(typeof(tran2)) == Base.HasShape{1}()
@test length(tran2) == 3
@test eltype(tran2) == TableOperations.TransformsRow{NamedTuple{(:A, :B, :C),Tuple{Union{Missing, Int},Float64,String}},NamedTuple{(:C,),Tuple{DataType}}}
trow = first(tran2)
@test trow.A === 1
@test trow.B === 1.0
@test trow.C == :hey
@test Tables.getcolumn(trow, 1) == 1
@test Tables.getcolumn(trow, :A) == 1
ctable2 = Tables.columntable(tran2)
@test isequal(ctable2.A, ctable.A)
@test ctable2.C == map(Symbol, ctable.C)
# test various ways of inputting TableOperations.transform functions
table = TableOperations.transform(ctable, Dict{String, Base.Callable}("C" => Symbol)) |> Tables.columntable
@test table.C == [:hey, :there, :sailor]
table = ctable |> TableOperations.transform(C=Symbol) |> Tables.columntable
@test table.C == [:hey, :there, :sailor]
table = TableOperations.transform(ctable, Dict{Symbol, Base.Callable}(:C => Symbol)) |> Tables.columntable
@test table.C == [:hey, :there, :sailor]
table = TableOperations.transform(ctable, Dict{Int, Base.Callable}(3 => Symbol)) |> Tables.columntable
@test table.C == [:hey, :there, :sailor]
# test simple TableOperations.transforms + return types
table = ctable |> TableOperations.transform(Dict("A"=>x->x+1)) |> Tables.columntable
@test isequal(table.A, [2, missing, 4])
@test typeof(table.A) == Vector{Union{Missing, Int}}
table = ctable |> TableOperations.transform(Dict("A"=>x->coalesce(x+1, 0))) |> Tables.columntable
@test table.A == [2, 0, 4]
table = ctable |> TableOperations.transform(Dict("A"=>x->coalesce(x+1, 0.0))) |> Tables.columntable
@test table.A == [2, 0.0, 4]
table = ctable |> TableOperations.transform(Dict(2=>x->x==2.0 ? missing : x)) |> Tables.columntable
@test isequal(table.B, [1.0, missing, 3.0])
@test typeof(table.B) == Vector{Union{Float64, Missing}}
# test row sinks
# test various ways of inputting TableOperations.transform functions
table = TableOperations.transform(ctable, Dict{String, Base.Callable}("C" => Symbol)) |> Tables.rowtable
@test table[1].C == :hey
table = ctable |> TableOperations.transform(C=Symbol) |> Tables.rowtable
@test table[1].C == :hey
table = TableOperations.transform(ctable, Dict{Symbol, Base.Callable}(:C => Symbol)) |> Tables.rowtable
@test table[1].C == :hey
table = TableOperations.transform(ctable, Dict{Int, Base.Callable}(3 => Symbol)) |> Tables.rowtable
@test table[1].C == :hey
# test simple transforms + return types
table = ctable |> TableOperations.transform(Dict("A"=>x->x+1)) |> Tables.rowtable
@test isequal(map(x->x.A, table), [2, missing, 4])
@test typeof(map(x->x.A, table)) == Vector{Union{Missing, Int}}
table = ctable |> TableOperations.transform(Dict("A"=>x->coalesce(x+1, 0))) |> Tables.rowtable
@test map(x->x.A, table) == [2, 0, 4]
table = ctable |> TableOperations.transform(Dict("A"=>x->coalesce(x+1, 0.0))) |> Tables.rowtable
@test map(x->x.A, table) == [2, 0.0, 4]
table = ctable |> TableOperations.transform(Dict(2=>x->x==2.0 ? missing : x)) |> Tables.rowtable
@test isequal(map(x->x.B, table), [1.0, missing, 3.0])
@test typeof(map(x->x.B, table)) == Vector{Union{Float64, Missing}}
end
@testset "TableOperations.select" begin
# 20
x = ReallyWideTable()
sel = TableOperations.select(x, :x1, :x2)
sch = Tables.schema(sel)
@test sch.names == (:x1, :x2)
@test sch.types == (Float64, Float64)
tt = Tables.columntable(sel)
@test tt.x1 isa Vector{Float64}
sel = TableOperations.select(x, 1, 2)
sch = Tables.schema(sel)
@test sch.names == (:x1, :x2)
@test sch.types == (Float64, Float64)
tt = Tables.columntable(sel)
@test tt.x1 isa Vector{Float64}
# 117
sel = TableOperations.select(ctable)
@test Tables.istable(typeof(sel))
@test Tables.schema(sel) == Tables.Schema((), ())
@test Tables.columnaccess(typeof(sel))
@test Tables.columns(sel) === sel
@test propertynames(sel) == ()
@test isequal(Tables.getcolumn(sel, 1), [1, missing, 3])
@test isequal(Tables.getcolumn(sel, :A), [1, missing, 3])
@test Tables.columntable(sel) == NamedTuple()
@test Tables.rowtable(sel) == NamedTuple{(), Tuple{}}[]
sel = ctable |> TableOperations.select(:A)
@test Tables.istable(typeof(sel))
@test Tables.schema(sel) == Tables.Schema((:A,), (Union{Int, Missing},))
@test Tables.columnaccess(typeof(sel))
@test Tables.columns(sel) === sel
@test propertynames(sel) == (:A,)
sel = ctable |> TableOperations.select(1)
@test Tables.istable(typeof(sel))
@test Tables.schema(sel) == Tables.Schema((:A,), (Union{Int, Missing},))
@test Tables.columnaccess(typeof(sel))
@test Tables.columns(sel) === sel
@test propertynames(sel) == (:A,)
sel = TableOperations.select(rtable)
@test Tables.rowaccess(typeof(sel))
@test Tables.rows(sel) === sel
@test Tables.schema(sel) == Tables.Schema((), ())
@test Base.IteratorSize(typeof(sel)) == Base.HasShape{1}()
@test length(sel) == 3
@test Base.IteratorEltype(typeof(sel)) == Base.HasEltype()
@test eltype(sel) == TableOperations.SelectRow{NamedTuple{(:A, :B, :C),Tuple{Union{Missing, Int},Float64,String}},()}
@test Tables.columntable(sel) == NamedTuple()
@test Tables.rowtable(sel) == [NamedTuple(), NamedTuple(), NamedTuple()]
srow = first(sel)
@test propertynames(srow) == ()
sel = rtable |> TableOperations.select(:A)
@test Tables.rowaccess(typeof(sel))
@test Tables.rows(sel) === sel
@test Tables.schema(sel) == Tables.Schema((:A,), (Union{Int, Missing},))
@test Base.IteratorSize(typeof(sel)) == Base.HasShape{1}()
@test length(sel) == 3
@test Base.IteratorEltype(typeof(sel)) == Base.HasEltype()
@test eltype(sel) == TableOperations.SelectRow{NamedTuple{(:A, :B, :C),Tuple{Union{Missing, Int},Float64,String}},(:A,)}
@test isequal(Tables.columntable(sel), (A = [1, missing, 3],))
@test isequal(Tables.rowtable(sel), [(A=1,), (A=missing,), (A=3,)])
srow = first(sel)
@test propertynames(srow) == (:A,)
# Testing issue where we always select the first column values, but using the correct name.
# NOTE: We don't use rtable here because mixed types produce TypeErrors which hide the
# underlying problem.
rtable2 = [(A = 1.0, B = 2.0), (A = 2.0, B = 4.0), (A = 3.0, B = 6.0)]
sel = rtable2 |> TableOperations.select(:B)
@test Tables.rowaccess(typeof(sel))
@test Tables.rows(sel) === sel
@test Tables.schema(sel) == Tables.Schema((:B,), (Float64,))
@test Base.IteratorSize(typeof(sel)) == Base.HasShape{1}()
@test length(sel) == 3
@test Base.IteratorEltype(typeof(sel)) == Base.HasEltype()
@test eltype(sel) == TableOperations.SelectRow{NamedTuple{(:A, :B,),Tuple{Float64,Float64}},(:B,)}
@test isequal(Tables.columntable(sel), (B = [2.0, 4.0, 6.0],))
@test isequal(Tables.rowtable(sel), [(B=2.0,), (B=4.0,), (B=6.0,)])
@test isequal(Tables.columntable(sel), (B = [2.0, 4.0, 6.0],))
@test isequal(Tables.rowtable(sel), [(B=2.0,), (B=4.0,), (B=6.0,)])
srow = first(sel)
@test propertynames(srow) == (:B,)
@test srow.B == 2.0 # What we expect
sel = rtable |> TableOperations.select(1)
@test Tables.rowaccess(typeof(sel))
@test Tables.rows(sel) === sel
@test Tables.schema(sel) == Tables.Schema((:A,), (Union{Int, Missing},))
@test Base.IteratorSize(typeof(sel)) == Base.HasShape{1}()
@test length(sel) == 3
@test Base.IteratorEltype(typeof(sel)) == Base.HasEltype()
@test eltype(sel) == TableOperations.SelectRow{NamedTuple{(:A, :B, :C),Tuple{Union{Missing, Int},Float64,String}},(1,)}
@test isequal(Tables.columntable(sel), (A = [1, missing, 3],))
@test isequal(Tables.rowtable(sel), [(A=1,), (A=missing,), (A=3,)])
srow = first(sel)
@test propertynames(srow) == (:A,)
@test Tables.getcolumn(srow, 1) == 1
@test Tables.getcolumn(srow, :A) == 1
table = ctable |> TableOperations.select(:A) |> Tables.columntable
@test length(table) == 1
@test isequal(table.A, [1, missing, 3])
table = ctable |> TableOperations.select(1) |> Tables.columntable
@test length(table) == 1
@test isequal(table.A, [1, missing, 3])
table = ctable |> TableOperations.select("A") |> Tables.columntable
@test length(table) == 1
@test isequal(table.A, [1, missing, 3])
# column re-ordering
table = ctable |> TableOperations.select(:A, :C) |> Tables.columntable
@test length(table) == 2
@test isequal(table.A, [1, missing, 3])
@test isequal(table[2], ["hey", "there", "sailor"])
table = ctable |> TableOperations.select(1, 3) |> Tables.columntable
@test length(table) == 2
@test isequal(table.A, [1, missing, 3])
@test isequal(table[2], ["hey", "there", "sailor"])
table = ctable |> TableOperations.select(:C, :A) |> Tables.columntable
@test isequal(ctable.A, table.A)
@test isequal(ctable[1], table[2])
table = ctable |> TableOperations.select(3, 1) |> Tables.columntable
@test isequal(ctable.A, table.A)
@test isequal(ctable[1], table[2])
# row sink
table = ctable |> TableOperations.select(:A) |> Tables.rowtable
@test length(table[1]) == 1
@test isequal(map(x->x.A, table), [1, missing, 3])
table = ctable |> TableOperations.select(1) |> Tables.rowtable
@test length(table[1]) == 1
@test isequal(map(x->x.A, table), [1, missing, 3])
table = ctable |> TableOperations.select("A") |> Tables.rowtable
@test length(table[1]) == 1
@test isequal(map(x->x.A, table), [1, missing, 3])
# column re-ordering
table = ctable |> TableOperations.select(:A, :C) |> Tables.rowtable
@test length(table[1]) == 2
@test isequal(map(x->x.A, table), [1, missing, 3])
@test isequal(map(x->x[2], table), ["hey", "there", "sailor"])
table = ctable |> TableOperations.select(1, 3) |> Tables.rowtable
@test length(table[1]) == 2
@test isequal(map(x->x.A, table), [1, missing, 3])
@test isequal(map(x->x[2], table), ["hey", "there", "sailor"])
table = ctable |> TableOperations.select(:C, :A) |> Tables.rowtable
@test isequal(ctable.A, map(x->x.A, table))
@test isequal(ctable[1], map(x->x[2], table))
table = ctable |> TableOperations.select(3, 1) |> Tables.rowtable
@test isequal(ctable.A, map(x->x.A, table))
@test isequal(ctable[1], map(x->x[2], table))
end
@testset "TableOperations.filter" begin
f = TableOperations.filter(x->x.B == 2.0, ctable)
@test Tables.istable(f)
@test Tables.rowaccess(f)
@test Tables.rows(f) === f
@test Tables.schema(f) == Tables.schema(f)
@test Base.IteratorSize(typeof(f)) == Base.SizeUnknown()
@test Base.IteratorEltype(typeof(f)) == Base.HasEltype()
@test eltype(f) == eltype(Tables.rows(ctable))
@test isequal(Tables.columntable(f), Tables.columntable(ctable |> TableOperations.filter(x->x.B == 2.0)))
@test length((TableOperations.filter(x->x.B == 2.0, ctable) |> Tables.columntable).B) == 1
@test length((TableOperations.filter(x->x.B == 2.0, rtable) |> Tables.columntable).B) == 1
@test length(TableOperations.filter(x->x.B == 2.0, ctable) |> Tables.rowtable) == 1
@test length(TableOperations.filter(x->x.B == 2.0, rtable) |> Tables.rowtable) == 1
end
@testset "TableOperations.map" begin
m = TableOperations.map(x->(A=x.A, C=x.C, B=x.B * 2), ctable)
@test Tables.istable(m)
@test Tables.rowaccess(m)
@test Tables.rows(m) === m
@test Tables.schema(m) === nothing
@test Base.IteratorSize(typeof(m)) == Base.HasLength()
@test Base.IteratorEltype(typeof(m)) == Base.EltypeUnknown()
@test isequal(Tables.columntable(m), Tables.columntable(ctable |> TableOperations.map(x->(A=x.A, C=x.C, B=x.B * 2))))
@test (TableOperations.map(x->(A=x.A, C=x.C, B=x.B * 2), ctable) |> Tables.columntable).B == [2.0, 4.0, 6.0]
@test (TableOperations.map(x->(A=x.A, C=x.C, B=x.B * 2), rtable) |> Tables.columntable).B == [2.0, 4.0, 6.0]
@test length(TableOperations.map(x->(A=x.A, C=x.C, B=x.B * 2), ctable) |> Tables.rowtable) == 3
@test length(TableOperations.map(x->(A=x.A, C=x.C, B=x.B * 2), rtable) |> Tables.rowtable) == 3
end
@testset "TableOperations.joinpartitions" begin
p = Tables.partitioner((ctable, ctable))
j = TableOperations.joinpartitions(p)
@test Tables.istable(j)
@test Tables.columnaccess(j)
@test Tables.schema(j) === Tables.schema(ctable)
@test Tables.columnnames(j) == Tables.columnnames(ctable)
@test isequal(Tables.getcolumn(j, 1), vcat(Tables.getcolumn(ctable, 1), Tables.getcolumn(ctable, 1)))
@test isequal(Tables.getcolumn(j, :A), vcat(Tables.getcolumn(ctable, :A), Tables.getcolumn(ctable, :A)))
# Test joinpartitions with promotion
t1 = (A=[1, 2, 3], B=[1, 2, 3], C=["hey", "there", "sailor"])
t2 = (A=[1, missing, 3], B=[1.0, 2.0, 3.0], C=["trim", "the", "sail"])
p = Tables.partitioner((t1, t2))
# Throws a method error trying to convert `missing` to `Int64`
@test_throws MethodError TableOperations.joinpartitions(p)
j = TableOperations.joinpartitions(p; promote=true)
@test Tables.istable(j)
@test Tables.columnaccess(j)
@test Tables.schema(j) !== Tables.schema(t1)
@test Tables.schema(j) === Tables.schema(t2)
@test Tables.columnnames(j) == Tables.columnnames(t1) == Tables.columnnames(t2)
@test isequal(Tables.getcolumn(j, 1), vcat(Tables.getcolumn(t1, 1), Tables.getcolumn(t2, 1)))
@test isequal(Tables.getcolumn(j, :A), vcat(Tables.getcolumn(t1, :A), Tables.getcolumn(t2, :A)))
end
@testset "TableOperations.makepartitions" begin
# columns
@test_throws ArgumentError TableOperations.makepartitions(ctable, 0)
parts = collect.(collect(Tables.partitions(TableOperations.makepartitions(ctable, 2))))
@test length(parts[1]) == 2
@test length(parts[2]) == 1
@test parts[2][1].A == 3
# rows
parts = collect(Tables.partitions(TableOperations.makepartitions(rtable, 2)))
@test length(parts[1]) == 2
@test length(parts[2]) == 1
@test parts[2][1].A == 3
# forward-only row iterator
parts = collect(Tables.partitions(TableOperations.makepartitions(rtable2, 3)))
@test length(parts) == 4
@test length(parts[1]) == 3
@test length(parts[end]) == 1
@test parts[end][1].a == 20
end
@testset "TableOperations.narrowtypes" begin
ctable_type_any = (A=Any[1, missing, 3], B=Any[1.0, 2.0, 3.0], C=Any["hey", "there", "sailor"])
nt = TableOperations.narrowtypes(ctable_type_any)
@test Tables.istable(nt)
@test Tables.columnaccess(nt)
@test Tables.schema(nt) == Tables.schema(ctable)
@test Tables.columnnames(nt) == Tables.columnnames(ctable)
end
@testset "TableOperations.dropmissing" begin
table = ctable |> TableOperations.dropmissing() |> Tables.columntable
@test isequal(table, Tables.columntable(TableOperations.dropmissing(ctable)))
@test length(table |> Tables.columntable) == 3
@test length(table |> Tables.rowtable) == 2
end
| TableOperations | https://github.com/JuliaData/TableOperations.jl.git |
|
[
"MIT"
] | 1.2.0 | e383c87cf2a1dc41fa30c093b2a19877c83e1bc1 | docs | 5022 | # TableOperations
*Common table operations on Tables.jl compatible sources*
[](https://github.com/JuliaData/TableOperations.jl/actions?query=workflow%3ACI)
[](https://codecov.io/gh/JuliaData/TableOperations.jl)
[](https://juliahub.com/ui/Packages/TableOperations/5GGWt?t=2)
[](https://juliahub.com/ui/Packages/TableOperations/5GGWt)
[](https://juliahub.com/ui/Packages/TableOperations/5GGWt)
**Installation**: at the Julia REPL, `import Pkg; Pkg.add("TableOperations")`
**Maintenance**: TableOperations is maintained collectively by the [JuliaData collaborators](https://github.com/orgs/JuliaData/people).
Responsiveness to pull requests and issues can vary, depending on the availability of key collaborators.
## Documentation
### `TableOperations.select`
The `TableOperations.select` function allows specifying a custom subset and order of columns from a Tables.jl source, like:
```julia
ctable = (A=[1, missing, 3], B=[1.0, 2.0, 3.0], C=["hey", "there", "sailor"])
table_subset = ctable |> TableOperations.select(:C, :A) |> Tables.columntable
```
This "selects" the `C` and `A` columns from the original table, and re-orders them with `C` first. The column names can be provided as `String`s, `Symbol`s, or `Integer`s.
### `TableOperations.transform`
The `TableOperations.transform` function allows specifying a "transform" function per column that will be applied per element. This is handy
when a simple transformation is needed for a specific column (or columns). Note that this doesn't allow the creation of new columns,
but only applies the transform function to the specified column, and thus, replacing the original column. Usage is like:
```julia
ctable = (A=[1, missing, 3], B=[1.0, 2.0, 3.0], C=["hey", "there", "sailor"])
table = ctable |> TableOperations.transform(C=x->Symbol(x)) |> Tables.columntable
```
Here, we're providing the transform function `x->Symbol(x)`, which turns an argument into a `Symbol`, and saying we should apply it to the `C` column.
Multiple tranfrom functions can be provided for multiple columns and the column to transform function can also be provided in `Dict`s that
map column names as `String`s, `Symbol`s, or even `Int`s (referring to the column index).
### `TableOperations.filter`
The `TableOperations.filter` function allows applying a "filter" function to each row in the input table source, keeping rows for which `f(row)` is `true`.
Usage is like:
```julia
ctable = (A=[1, missing, 3], B=[1.0, 2.0, 3.0], C=["hey", "there", "sailor"])
table = ctable |> TableOperations.filter(x->Tables.getcolumn(x, :B) > 2.0) |> Tables.columntable
```
### `TableOperations.map`
The `TableOperations.map` function allows applying a "mapping" function to each row in the input table source; the function `f` should take and
return a Tables.jl `Row` compatible object. Usage is like:
```julia
ctable = (A=[1, missing, 3], B=[1.0, 2.0, 3.0], C=["hey", "there", "sailor"])
table = ctable |> TableOperations.map(x->(A=Tables.getcolumn(x, :A), C=Tables.getcolumn(x, :C), B=Tables.getcolumn(x, :B) * 2)) |> Tables.columntable
```
### `TableOperations.narrowtypes`
The TableOperations.narrowtypes function allows infering column element types to better fit the stored data. Usage is like:
```julia
ctable_type_any = (A=Any[1, missing, 3], B=Any[1.0, 2.0, 3.0], C=Any["hey", "there", "sailor"])
table = TableOperations.narrowtypes(ctable_type_any) |> Tables.columntable
```
### `TableOperations.dropmissing`
The TableOperations.dropmissing function allows to lazily remove every row where missing values are present. Usage is like:
```julia
ctable = (A=[1, missing, 3], B=[1.0, 2.0, 3.0], C=["hey", "there", "sailor"])
table = ctable |> TableOperations.dropmissing |> Tables.columntable
```
### `TableOperations.joinpartitions`
The TableOperations.joinpartitions function allows you to lazily chain (or "join") multiple tables into a single long table. Usage is like:
```julia
ctables = Tables.partitioner(i -> (A=fill(i, 10), B=rand(10) * i), 1:3)
table = ctables |> TableOperations.joinpartitions |> Tables.columntable
```
## Contributing and Questions
Contributions are very welcome, as are feature requests and suggestions. Please open an
[issue][issues-url] if you encounter any problems or would just like to ask a question.
[travis-img]: https://travis-ci.org/JuliaData/TableOperations.jl.svg?branch=master
[travis-url]: https://travis-ci.org/JuliaData/TableOperations.jl
[codecov-img]: https://codecov.io/gh/JuliaData/TableOperations.jl/branch/master/graph/badge.svg
[codecov-url]: https://codecov.io/gh/JuliaData/TableOperations.jl
[issues-url]: https://github.com/JuliaData/TableOperations.jl/issues
| TableOperations | https://github.com/JuliaData/TableOperations.jl.git |
|
[
"MIT"
] | 0.2.0 | cd53f560c93b806d4d10020f34ad8d74548edf08 | code | 456 | # Workaround for GR warnings
ENV["GKSwstype"] = "100"
using Documenter, SinusoidalRegressions
# suitable for local browsing without a web server
makedocs(sitename="SinusoidalRegressions.jl",
build = "stable", # change for other versions
format = Documenter.HTML(prettyurls = false)
)
# suitable for hosting in github
makedocs(sitename="SinusoidalRegressions.jl",
build = "stable" # change for other versions
)
| SinusoidalRegressions | https://github.com/mbaz/SinusoidalRegressions.jl.git |
|
[
"MIT"
] | 0.2.0 | cd53f560c93b806d4d10020f34ad8d74548edf08 | code | 4811 | module SinusoidalRegressions
using LsqFit: curve_fit, coef
using RecipesBase
using PrecompileTools
using Zygote
using LinearAlgebra
# abstract types
export SRAlgorithm, SRModel, SRProblem
# main functions
export rmse, mae, torect, sinfit
# regression models
export SinModel, MixedLinSinModel
# problems
export Sin3Problem, Sin4Problem, MixedLinSin4Problem, MixedLinSin5Problem
# algorithms
export IEEE1057, IntegralEquations, LevMar, Liang
include("typedefs.jl")
include("jacquelin_sr.jl")
include("jacquelin_mlsr.jl")
include("jacquelin_gen.jl")
include("jacquelin_damped.jl")
include("ieee1057.jl")
include("lsqfit.jl")
include("liang.jl")
include("plotrecipes.jl")
"""
sinfit(problem::SRProblem, algorithm::SRAlgorithm)
Calculate a sinosoidal regression on `problem` using `algorithm`.
Currently supported problem types are:
* `Sin3Problem` -- three-parameter sinusoidal regression
* `Sin4Problem` -- four-parameter sinusoidal regression
* `MixedLinSin4Problem` -- four-parameter mixed linear and sinusoidal regression
* `MixedLinSin5Problem` -- five-parameter mixed linear and sinusoidal regression
Currently supported algorithms are:
* `IEEE1057`
* `IntegralEquations`
* `LevMar`
* `Liang`
Example
=======
```
julia> using SinusoidalRegressions
julia> t = collect(range(0, 1, length = 100)) # time instants
julia> s = sin.(2*pi*15*t .+ pi/4) .+ 0.1*randn(100) # noisy samples
julia> p = Sin3Problem(t, s, 15) # define regression problem
julia> sinfit(p, IEEE1057()) # calculate fit with IEEE 1057
3-Parameter Sinusoidal Problem Sin3Problem:
X : Vector{Float64} with 100 elements
Y : Vector{Float64} with 100 elements
Frequency (Hz) : 15
Parameter estimates:
DC : missing
Sine amplitude (Q) : missing
Cosine amplitude (I) : missing
Lower bounds : missing
Upper bounds : missing
```
See the documentation for more details.
"""
sinfit(p::SRProblem, a::SRAlgorithm) = throw("Not implemented.")
function sinfit(p::Sin3Problem, ::IEEE1057)
ieee1057(p)
end
function sinfit(p::Sin3Problem, a::LevMar ; kwargs...)
levmar(p, a ; kwargs...)
end
function sinfit(p::Sin4Problem, a::IEEE1057)
ieee1057(p, a)
end
function sinfit(p::Sin4Problem, ::IntegralEquations)
jacquelin(p)
end
function sinfit(p::Sin4Problem, a::LevMar ; kwargs...)
levmar(p, a ; kwargs...)
end
function sinfit(p::Sin4Problem, a::Liang)
liang(p, a)
end
function sinfit(p::MixedLinSin4Problem, a::LevMar ; kwargs...)
levmar(p, a ; kwargs...)
end
function sinfit(p::MixedLinSin5Problem, ::IntegralEquations)
jacquelin(p)
end
function sinfit(p::MixedLinSin5Problem, a::LevMar ; kwargs...)
levmar(p, a ; kwargs...)
end
"""
rmse(fit::T, exact::T, x) where {T <: SRModel}
Calculate the root mean-square error between `fit` and `exact` sampled at collection `x`.
See also: [`mae`](@ref)
"""
function rmse(fit::T, exact::T, x) where {T <: SRModel}
fitvalues = fit(x)
exactvalues = exact(x)
return sqrt(sum((fitvalues .- exactvalues).^2) / length(x))
end
"""
rmse(fit::T, samples, x) where {T <: SRModel}
Calculate the root mean-square error between `fit` and the `samples` taken at `x`.
See also: [`mae`](@ref)
"""
function rmse(fit::T, samples::AbstractVector, x) where {T <: SRModel}
fitvalues = fit(x)
return sqrt(sum((fitvalues .- samples).^2) / length(x))
end
"""
mae(fit::T, exact::T, x) where {T <: SRModel}
Calculate the mean absolute error between `fit` and `exact` sampled at collection `x`.
See also: [`rmse`](@ref)
"""
function mae(fit::T, exact::T, x) where {T <: SRModel}
fitvalues = fit(x)
exactvalues = exact(x)
return sum(abs.(fitvalues .- exactvalues))/length(x)
end
"""
torect(M, ΞΈ)
Convert polar coordinates to rectangular coordinates `(y, x)` where ``x = M\\cos(ΞΈ)`` and
``y = -M\\sin(ΞΈ)``
"""
torect(M, ΞΈ) = (-M*sin(ΞΈ), M*cos(ΞΈ))
## Precompilation
@setup_workload begin
x = [-1.983, -1.948, -1.837, -1.827, -1.663, -0.815, -0.778, -0.754, -0.518, 0.322, 0.418, 0.781,
0.931, 1.510, 1.607]
y = [ 0.936, 0.810, 0.716, 0.906, 0.247, -1.513, -1.901, -1.565, -1.896, 0.051, 0.021, 1.069,
0.862, 0.183, 0.311]
@compile_workload begin
p1 = Sin3Problem(x, y, 1.5)
t2 = sinfit(p1, IEEE1057())
t3 = sinfit(p1, LevMar())
p2 = Sin4Problem(x, y, f = 1.5)
t4 = sinfit(p2, IEEE1057())
t5 = sinfit(p2, IntegralEquations())
t6 = sinfit(p2, LevMar())
t7 = sinfit(p2, Liang())
p3 = MixedLinSin4Problem(x, y, 1.5)
t8 = sinfit(p3, LevMar())
p4 = MixedLinSin5Problem(x, y)
t9 = sinfit(p4, IntegralEquations())
end
end
end # module
| SinusoidalRegressions | https://github.com/mbaz/SinusoidalRegressions.jl.git |
|
[
"MIT"
] | 0.2.0 | cd53f560c93b806d4d10020f34ad8d74548edf08 | code | 2672 |
function ieee1057(p::Sin3Problem)
(; X, Y, f) = p
if length(X) != length(Y)
error("Input vectors must be of equal length (got $(length(X)) and $(length(Y))).")
end
if f <= 0
error("Frequency must be larger than 0.")
end
return _ieee1057_3P(X, Y, f)
end
function ieee1057(p::Sin4Problem, a::IEEE1057)
(; X, Y, f) = p
if length(X) != length(Y)
error("Input vectors must be of equal length.")
end
if !ismissing(f) && f < 0
error("Frequency estimate must be larger than 0.")
end
return _ieee1057_4P(X, Y, f, a.iterations)
end
# 3-parameter fit
function _ieee1057_3P(X, Y, f)
M = length(X)
D0 = hcat([1 for k in eachindex(X)],
[sin(2*Ο*f*x) for x in X],
[cos(2*Ο*f*x) for x in X])
(DC, Q, I) = D0 \ Y
return SinModel(f, DC, Q, I)
end
# 4-parameter fit
function _ieee1057_4P(X, Y, f, iterations)
if ismissing(f)
# obtain a frequency estimate using Jacquelin's regression
sec1 = _jacquelin_part1(X, Y)
f = _jacquelin_part2(X, Y, sec1).f
end
# pre-fit using 3-parameter IEEE 1057 algorithm
(;DC, Q, I) = _ieee1057_3P(X, Y, f)
# Pre-allocations
D = zeros(eltype(X), length(X), 4)
D[:, 3] .= 1.0
Ξf = 0.0
# iteration
for i = 1:iterations
f += Ξf
D[:, 1] .= cos.(2Ο*f*X)
D[:, 2] .= sin.(2Ο*f*X)
D[:, 4] .= X.*(-I*sin.(2Ο*f*X) .+ Q*cos.(2Ο*f*X))
(I, Q, DC, Ξf) = D \ Y
end
f += Ξf
return SinModel(f, DC, Q, I)
end
"""
ieee1057_testconvergence(X, Y ; f = nothing, iterations = 6)
Returns `true` if the 4-paramter IEEE 1057 algorithm converges, and `false` otherwise.
Convergence is determined rather crudely, by observing whether the frequency correction
Ξf becomes smaller with each iteration. Argument `f` is an estimate of the sinusoidal's
frequency; if not provided, it is calculated as in [`ieee1057`](@ref).
"""
function ieee1057_testconvergence(p::Sin4Problem, a::IEEE1057 = IEEE1057())
(; X, Y, f) = p
if ismissing(f)
sec1 = _jacquelin_part1(X, Y)
f = _jacquelin_part2(X, Y, sec1).f
end
(;DC, Q, I) = _ieee1057_3P(X, Y, f)
D = zeros(eltype(X), length(X), 4)
D[:, 3] .= 1.0
Ξf = 0.0
for i = 1:a.iterations
prev_Ξf = Ξf
f += Ξf
D[:, 1] .= cos.(2Ο*f*X)
D[:, 2] .= sin.(2Ο*f*X)
D[:, 4] .= X.*(-I*sin.(2Ο*f*X) .+ Q*cos.(2Ο*f*X))
(I, Q, DC, Ξf) = D \ Y
@debug "Iteration: $i" prev_Ξf Ξf
if (i > 3) && (abs(Ξf) > 1e-3) && (abs(Ξf) > abs(prev_Ξf))
return false
end
end
return true
end
| SinusoidalRegressions | https://github.com/mbaz/SinusoidalRegressions.jl.git |
|
[
"MIT"
] | 0.2.0 | cd53f560c93b806d4d10020f34ad8d74548edf08 | code | 797 | """
gensinfit_j :: GenSinusoidP
Note: This function is not yet implemented.
Perform a general sinusoidal fit of the independent variables `X` and dependent
variables `Y`, using the method of integral equations.
The data is fit to the model
``f(x, f, Q, I, L...) = Qsin(2Οf) + Icos(2Οf) + L[1]F[1](x) + ... + L[m]F[m](x)``
where `F` is a collection of known functions and `L` is a collection of scalars. No initial
guess as to the values of the parameters is needed. The values in `X` must be sorted in
ascending order.
The regression method implemented here is based on J. Jacquelin, "RΓ©gressions et Γ©quations
intΓ©grales", 2014 (available at
https://fr.scribd.com/doc/14674814/Regressions-et-equations-integrales)
"""
function gensinfit_j(X, Y, F)
println("Not yet implemented")
end
| SinusoidalRegressions | https://github.com/mbaz/SinusoidalRegressions.jl.git |
|
[
"MIT"
] | 0.2.0 | cd53f560c93b806d4d10020f34ad8d74548edf08 | code | 709 | """
dampedsinfit_j :: DampedSinusoidP
Note: This function is not yet implemented.
Perform a damped sinusoid fit of the independent variables `X` and dependent
variables `Y`, using the method of integral equations.
The data is fit to the model
``f(x, f, Q, I, a) = exp(ax)(Qsin(2Οf) + Icos(2Οf))``
where ``a < 0``. No initial guess as to the values of the parameters is needed. The values
in `X` must be sorted in ascending order.
The regression method implemented here is based on J. Jacquelin, "RΓ©gressions et Γ©quations
intΓ©grales", 2014 (available at
https://fr.scribd.com/doc/14674814/Regressions-et-equations-integrales)
"""
function dampedsinfit_j(X, Y)
println("Not yet implemented")
end
| SinusoidalRegressions | https://github.com/mbaz/SinusoidalRegressions.jl.git |
|
[
"MIT"
] | 0.2.0 | cd53f560c93b806d4d10020f34ad8d74548edf08 | code | 2635 |
function jacquelin(prob::MixedLinSin5Problem)
(; X, Y) = prob
# verify elements of X are ordered
if !issorted(X)
error("Please provide abscissa vector in ascending order.")
end
_jacquelin_mixlinsin(X, Y)
end
function _jacquelin_mixlinsin(X, Y)
n = length(X)
# "short way" -- actually, a first approximation
S = zeros(eltype(X), n)
for k = 2:n
S[k] = S[k-1] + 0.5*(Y[k]+Y[k-1])*(X[k]-X[k-1])
end
SS = zeros(eltype(X), n)
for k = 2:n
SS[k] = SS[k-1] + 0.5*(S[k]+S[k-1])*(X[k]-X[k-1])
end
Ξ£SSΒ² = sum(SS.^2)
Ξ£xΒ³SS = sum(SS .* X.^3)
Ξ£xΒ²SS = sum(SS .* X.^2)
Ξ£xSS = sum(SS .* X)
Ξ£SS = sum(SS)
Ξ£x = sum(X)
Ξ£xΒ² = sum(X.^2)
Ξ£xΒ³ = sum(X.^3)
Ξ£xβ΄ = sum(X.^4)
Ξ£xβ΅ = sum(X.^5)
Ξ£xβΆ = sum(X.^6)
Ξ£y = sum(Y)
Ξ£yx = sum(X.*Y)
Ξ£yxΒ² = sum(X.^2 .* Y)
Ξ£yxΒ³ = sum(X.^3 .* Y)
Ξ£ySS = sum(Y .* SS)
M = [ Ξ£SSΒ² Ξ£xΒ³SS Ξ£xΒ²SS Ξ£xSS Ξ£SS ;
Ξ£xΒ³SS Ξ£xβΆ Ξ£xβ΅ Ξ£xβ΄ Ξ£xΒ³ ;
Ξ£xΒ²SS Ξ£xβ΅ Ξ£xβ΄ Ξ£xΒ³ Ξ£xΒ² ;
Ξ£xSS Ξ£xβ΄ Ξ£xΒ³ Ξ£xΒ² Ξ£x ;
Ξ£SS Ξ£xΒ³ Ξ£xΒ² Ξ£x n ]
x = [Ξ£ySS, Ξ£yxΒ³, Ξ£yxΒ², Ξ£yx, Ξ£y]
(A0, E0, B0, C0, D0) = M \ x
Ο1 = sqrt(-A0)
Ξ² = sin.(Ο1.*X)
Ξ· = cos.(Ο1.*X)
Σβ = sum(β)
Ση = sum(η)
Ξ£xΞ² = sum(X .* Ξ²)
Ξ£xΞ· = sum(X .* Ξ·)
Σβ² = sum(β.^2)
Ση² = sum(η.^2)
Σβη = sum(β .* η)
Ξ£yΞ² = sum(Ξ² .* Y)
Ξ£yΞ· = sum(Ξ· .* Y)
N = [ n Σx Σβ Ση ;
Ξ£x Ξ£xΒ² Ξ£xΞ² Ξ£xΞ· ;
Σβ Σxβ Σβ² Σβη ;
Ση Σxη Σβη Ση² ]
x = [Ξ£y, Ξ£yx, Ξ£yΞ², Ξ£yΞ·]
(a1, p1, b1, c1) = N \ x
Ο1 = sqrt(b1^2 + c1^2)
Ο1 = b1 > 0 ? atan(c1/b1) : atan(c1/b1) + Ο
# "full way" -- finer approximation
K = round.( (Ο1*X .+ Ο1)/Ο )
ΞΈ = zeros(eltype(X), n)
for k = 1:n
r = Y[k] - a1 - p1*X[k]
if Ο1^2 > r^2
ΞΈ[k] = (-1)^K[k] * atan(r / sqrt(Ο1^2 - r^2)) + K[k]*Ο
else
if r > 0
ΞΈ[k] = (-1)^K[k] * Ο/2 + K[k]*Ο
else
ΞΈ[k] = -(-1)^K[k] * Ο/2 + K[k]*Ο
end
end
end
O = [ Ξ£xΒ² Ξ£x ;
Ξ£x n ]
x = [sum(ΞΈ .* X), sum(ΞΈ)]
(Ο2, Ο2) = O \ x
Ξ² = sin.(Ο2.*X)
Ξ· = cos.(Ο2.*X)
Σβ = sum(β)
Ση = sum(η)
Ξ£xΞ² = sum(X .* Ξ²)
Ξ£xΞ· = sum(X .* Ξ·)
Σβ² = sum(β.^2)
Ση² = sum(η.^2)
Σβη = sum(β .* η)
Ξ£yΞ² = sum(Ξ² .* Y)
Ξ£yΞ· = sum(Ξ· .* Y)
P = [ n Σx Σβ Ση ;
Ξ£x Ξ£xΒ² Ξ£xΞ² Ξ£xΞ· ;
Σβ Σxβ Σβ² Σβη ;
Ση Σxη Σβη Ση² ]
x = [Ξ£y, Ξ£yx, Ξ£yΞ², Ξ£yΞ·]
(a3, p3, b3, c3) = P \ x
return MixedLinSinModel(Ο2/(2Ο), a3, b3, c3, p3)
end
| SinusoidalRegressions | https://github.com/mbaz/SinusoidalRegressions.jl.git |
|
[
"MIT"
] | 0.2.0 | cd53f560c93b806d4d10020f34ad8d74548edf08 | code | 2950 |
function jacquelin(p::Sin4Problem)
(; X, Y) = p
n = length(X)
return _jacquelin_sin(X, Y)
end
function _jacquelin_sin(X, Y)
# verify elements of X are ordered
if !issorted(X)
error("Please provide abscissa vector in ascending order.")
end
sec1 = _jacquelin_part1(X, Y) # first section
sec2 = _jacquelin_part2(X, Y, sec1) # second section
sec3 = _jacquelin_part3(X, Y, sec2) # third section
end
function _jacquelin_part1(X, Y)
n = length(X)
S = zeros(eltype(X), n)
for k = 2:n
S[k] = S[k-1] + 0.5*(Y[k]+Y[k-1])*(X[k]-X[k-1])
end
SS = zeros(eltype(X), n)
for k = 2:n
SS[k] = SS[k-1] + 0.5*(S[k]+S[k-1])*(X[k]-X[k-1])
end
Ξ£x = sum(X)
Ξ£xΒ² = sum(X.^2)
Ξ£xΒ³ = sum(X.^3)
Ξ£xβ΄ = sum(X.^4)
Ξ£SS = sum(SS)
Ξ£SSΒ² = sum(SS.^2)
Ξ£xSS = sum(X .* SS)
Ξ£xΒ²SS = sum(X.^2 .* SS)
Ξ£y = sum(Y)
Ξ£yx = sum(X .* Y)
Ξ£yxΒ² = sum(X.^2 .* Y)
Ξ£ySS = sum(Y .* SS)
M = [ Ξ£SSΒ² Ξ£xΒ²SS Ξ£xSS Ξ£SS
Ξ£xΒ²SS Ξ£xβ΄ Ξ£xΒ³ Ξ£xΒ²
Ξ£xSS Ξ£xΒ³ Ξ£xΒ² Ξ£x
Ξ£SS Ξ£xΒ² Ξ£x n ]
(A1, B1, C1, D1) = M \ [Ξ£ySS, Ξ£yxΒ², Ξ£yx, Ξ£y]
x1 = X[1]
Ο1 = sqrt(-A1)
a1 = 2*B1 / (Ο1^2)
b1 = (B1*x1^2 + C1*x1 + D1 - a1) * sin(Ο1*x1) + (1/Ο1)*(C1 + 2*B1*x1) * cos(Ο1*x1)
c1 = (B1*x1^2 + C1*x1 + D1 - a1) * cos(Ο1*x1) - (1/Ο1)*(C1 + 2*B1*x1) * sin(Ο1*x1)
return SinModel(Ο1/(2Ο), a1, b1, c1)
end
function _jacquelin_part2(X, Y, sp)
n = length(X)
Ο1, a1, b1, c1 = 2Ο*sp.f, sp.DC, sp.Q, sp.I
a2 = a1
Ο2 = sqrt(b1^2 + c1^2)
if b1 == 0
Ο1 = Ο/2 * sign(c1)
else
if b1 > 0
Ο1 = atan(c1/b1)
else
Ο1 = atan(c1/b1) + Ο
end
end
K = [round((Ο1*xk + Ο1)/Ο) for xk in X]
ΞΈ = zeros(n)
for k = 1:n
if Ο2^2 > (Y[k] - a2)^2
ΞΈ[k] = (-1)^K[k] * atan( (Y[k]-a2) / sqrt(Ο2^2 - (Y[k]-a2)^2) ) + Ο*K[k]
else
if Y[k] > a2
ΞΈ[k] = (-1)^K[k]*Ο/2 + K[k]*Ο
else
ΞΈ[k] = -(-1)^K[k]*Ο/2 + K[k]*Ο
end
end
end
Ξ£x = sum(X)
Ξ£xΒ² = sum(X.^2)
Σθ = sum(θ)
Σθx = sum(θ .* X)
M = [ Ξ£xΒ² Ξ£x
Ξ£x n ]
(Ο2, Ο2) = M \ [ Σθx, Σθ ]
b2 = Ο2 * cos(Ο2)
c2 = Ο2 * sin(Ο2)
return SinModel(Ο2/(2Ο), a2, b2, c2)
end
function _jacquelin_part3(X, Y, sp)
n = length(X)
Ο3 = 2Ο*sp.f
Ξ£sin = sum(sin, Ο3*X)
Ξ£cos = sum(cos, Ο3*X)
Ξ£sinΒ² = sum(x -> sin(x)*sin(x), Ο3*X)
Ξ£cosΒ² = sum(x -> cos(x)*cos(x), Ο3*X)
Ξ£sincos = sum(x -> sin(x)*cos(x), Ο3*X)
Ξ£y = sum(Y)
Ξ£ysin = sum( (Y[k]*sin(Ο3*X[k]) for k in eachindex(X)) )
Ξ£ycos = sum( (Y[k]*cos(Ο3*X[k]) for k in eachindex(X)) )
M = [ n Ξ£sin Ξ£cos
Ξ£sin Ξ£sinΒ² Ξ£sincos
Ξ£cos Ξ£sincos Ξ£cosΒ² ]
(a3, b3, c3) = M \ [ Ξ£y, Ξ£ysin, Ξ£ycos ]
return SinModel(Ο3/(2Ο), a3, b3, c3)
end
| SinusoidalRegressions | https://github.com/mbaz/SinusoidalRegressions.jl.git |
|
[
"MIT"
] | 0.2.0 | cd53f560c93b806d4d10020f34ad8d74548edf08 | code | 1651 |
function liang(prob::Sin4Problem, a::Liang)
(; X, Y) = prob
(;threshold, iterations, q) = a
N = length(X)
length(Y) β N && error("Input arguments should have the same length (got $N and $(length(Y)))")
# step 2
Ο = last(X) - first(X) # observation interval length
v = (N-1)/Ο # average sampling rate
f0 = 1/Ο # true frequency is smaller than f0, since we assume P < 1
while true
if f0 > q/Ο
break
else
q = q/2
end
end
# step 3
fL = q/Ο
fR = 2/Ο
# step 4
fM = fL + 0.618*(fR - fL)
fT = fR - 0.618*(fR - fL)
count = 0
while true
# step 5
fitL = _ieee1057_3P(X, Y, fL)
ΟL = rmse(fitL, Y, X)
fitR = _ieee1057_3P(X, Y, fR)
ΟR = rmse(fitR, Y, X)
fitM = _ieee1057_3P(X, Y, fM)
ΟM = rmse(fitM, Y, X)
fitT = _ieee1057_3P(X, Y, fT)
ΟT = rmse(fitT, Y, X)
# step 6
if ΟM < ΟT
Ο = ΟM
fL = fT
fT = fM
fM = fL + 0.618*(fR - fL)
else
Ο = ΟT
fR = fM
fM = fT
fT = fR - 0.618*(fR - fL)
end
# step 7
if abs((ΟM - ΟT)/ΟT) < threshold
if ΟM < ΟT
return fitM
else
return fitT
end
end
count += 1
if count > iterations
@warn "Maximum iterations reached" iterations
if ΟM < ΟT
return fitM
else
return fitT
end
end
end
end
| SinusoidalRegressions | https://github.com/mbaz/SinusoidalRegressions.jl.git |
|
[
"MIT"
] | 0.2.0 | cd53f560c93b806d4d10020f34ad8d74548edf08 | code | 5122 |
function levmar(prob::Sin3Problem, a::LevMar ; kwargs...)
(; X, Y, f, DC, Q, I, lb, ub) = prob
# Check if all parameters are given initial estimates
if any(map(ismissing, (DC, Q, I)))
# if not all guesses are given, find our own estimates
initialfit = _jacquelin_sin(X, Y)
ismissing(DC) && (DC = initialfit.DC)
ismissing(Q) && (Q = initialfit.Q)
ismissing(I) && (I = initialfit.I)
end
guess = [DC, Q, I]
# bounds
ismissing(lb) && (lb = [-Inf, -Inf, -Inf])
ismissing(ub) && (ub = [+Inf, +Inf, +Inf])
# Define data model
@. model(t, p) = p[1] + p[2]*sin(2Ο*f*t) + p[3]*cos(2Ο*f*t)
# fit
if a.use_ga
function Avv!(dir_deriv,p,v)
for i = 1:length(X)
dir_deriv[i] = transpose(v) * Zygote.hessian(z -> model(X[i], z), p) * v
end
end
# fit
fit = curve_fit(model, X, Y, guess,
avv! = Avv!, lambda = 0, min_step_quality = 0,
lower = lb, upper = ub, kwargs...)
else
fit = curve_fit(model, X, Y, guess, lower = lb, upper = ub, kwargs...)
end
return SinModel(f, coef(fit)...)
end
function levmar(prob::Sin4Problem, a::LevMar ; kwargs...)
(; X, Y, f, DC, Q, I, lb, ub) = prob
# Check if all parameters are given initial estimates
if any(map(ismissing, (f, DC, Q, I)))
# if not all guesses are given, find our own estimates
initialfit = _jacquelin_sin(X, Y)
ismissing(f) && (f = initialfit.f)
ismissing(DC) && (DC = initialfit.DC)
ismissing(Q) && (Q = initialfit.Q)
ismissing(I) && (I = initialfit.I)
end
guess = [f, DC, Q, I]
# bounds
ismissing(lb) && (lb = [-Inf, -Inf, -Inf, -Inf])
ismissing(ub) && (ub = [+Inf, +Inf, +Inf, +Inf])
# define data model
guessarr = [f, DC, Q, I]
@. model(t, p) = p[2] + p[3]*sin(2Ο*p[1]*t) + p[4]*cos(2Ο*p[1]*t)
# fit
if a.use_ga
function Avv!(dir_deriv,p,v)
for i = 1:length(X)
dir_deriv[i] = transpose(v) * Zygote.hessian(z -> model(X[i], z), p) * v
end
end
fit = curve_fit(model, X, Y, guess,
avv! = Avv!, lambda = 0, min_step_quality = 0,
lower = lb, upper = ub, kwargs...)
else
fit = curve_fit(model, X, Y, guessarr ; kwargs...)
end
return SinModel(coef(fit)...)
end
function levmar(prob::MixedLinSin4Problem, a::LevMar ; kwargs...)
(; X, Y, f, DC, Q, I, m, lb, ub) = prob
# Check if all parameters are given initial estimates
if any(map(ismissing, (DC, Q, I, m)))
# if not all guesses are given, find our own estimates
initialfit = _jacquelin_mixlinsin(X, Y)
ismissing(DC) && (DC = initialfit.DC)
ismissing(Q) && (Q = initialfit.Q)
ismissing(I) && (I = initialfit.I)
ismissing(m) && (m = initialfit.m)
end
guess = [DC, Q, I, m]
# bounds
ismissing(lb) && (lb = [-Inf, -Inf, -Inf, -Inf])
ismissing(ub) && (ub = [+Inf, +Inf, +Inf, +Inf])
# Define data model
@. model(t, p) = p[1] + p[2]*sin(2Ο*f*t) + p[3]*cos(2Ο*f*t) + t*p[4]
# fit
if a.use_ga
function Avv!(dir_deriv,p,v)
for i = 1:length(X)
dir_deriv[i] = transpose(v) * Zygote.hessian(z -> model(X[i], z), p) * v
end
end
# fit
fit = curve_fit(model, X, Y, guess,
avv! = Avv!, lambda = 0, min_step_quality = 0,
lower = lb, upper = ub, kwargs...)
else
fit = curve_fit(model, X, Y, guess, lower = lb, upper = ub, kwargs...)
end
return MixedLinSinModel(f, coef(fit)...)
end
function levmar(prob::MixedLinSin5Problem, a::LevMar ; kwargs...)
(; X, Y, f, DC, Q, I, m, lb, ub) = prob
# Check if all parameters are given initial estimates
if any(map(ismissing, (f, DC, Q, I, m)))
# if not all guesses are given, find our own estimates
initialfit = _jacquelin_mixlinsin(X, Y)
ismissing(f) && (f = initialfit.f)
ismissing(DC) && (DC = initialfit.DC)
ismissing(Q) && (Q = initialfit.Q)
ismissing(I) && (I = initialfit.I)
ismissing(m) && (m = initialfit.m)
end
guess = [f, DC, Q, I, m]
# bounds
ismissing(lb) && (lb = [0, -Inf, -Inf, -Inf, -Inf])
ismissing(ub) && (ub = [+Inf, +Inf, +Inf, +Inf, +Inf])
# Define data model
@. model(t, p) = p[2] + p[3]*sin(2Ο*p[1]*t) + p[4]*cos(2Ο*p[1]*t) + t*p[5]
# fit
if a.use_ga
function Avv!(dir_deriv,p,v)
for i = 1:length(X)
dir_deriv[i] = transpose(v) * Zygote.hessian(z -> model(X[i], z), p) * v
end
end
# fit
fit = curve_fit(model, X, Y, guess,
avv! = Avv!, lambda = 0, min_step_quality = 0,
lower = lb, upper = ub, kwargs...)
else
fit = curve_fit(model, X, Y, guess, lower = lb, upper = ub, kwargs...)
end
return MixedLinSinModel(coef(fit)...)
end
| SinusoidalRegressions | https://github.com/mbaz/SinusoidalRegressions.jl.git |
|
[
"MIT"
] | 0.2.0 | cd53f560c93b806d4d10020f34ad8d74548edf08 | code | 1684 | # plot recipes
"Plot a regression"
@recipe function f(t, p::T ; fitlabel = "") where {T <: SRModel}
xguide --> "x"
yguide --> "y"
ttl = ""
if p isa SinModel
ttl = "Sinusoidal Curve"
elseif fit isa MixedLinSinModel
ttl = "Mixed Linear-Sinusoid Fit"
end
plot_title --> ttl
plot_titlefontsize --> 12
label --> fitlabel
linecolor --> :blue
t, p.(t)
end
# plot data, best fit, and optionally the exact function
@recipe function f(X, Y, fit::T ;
exact = nothing,
samples = 100,
fitlabel = "fit",
datalabel = "data",
exactlabel = "exact") where {T <: SRModel}
xguide --> "x"
yguide --> "y"
ttl = ""
if fit isa SinModel
ttl = "Sinusoidal Curve Fit"
elseif fit isa MixedLinSinModel
ttl = "Mixed Linear-Sinusoid Fit"
end
plot_title --> ttl
plot_titlefontsize --> 12
# data (samples)
@series begin
seriestype --> :scatter
label --> datalabel
X, Y
end
# fit
@series begin
label --> fitlabel
linecolor --> :blue
t = range(first(X), last(X), length = samples)
t, fit.(t)
end
# exact
if !isnothing(exact)
if exact isa T
@series begin
label --> exactlabel
linecolor --> :black
linestyle --> :dash
t = range(first(X), last(X), length = samples)
t, exact.(t)
end
else
error("Parameters must be of the same type; got $T and $(typeof(exact))")
end
end
end
| SinusoidalRegressions | https://github.com/mbaz/SinusoidalRegressions.jl.git |
|
[
"MIT"
] | 0.2.0 | cd53f560c93b806d4d10020f34ad8d74548edf08 | code | 14795 |
#
# Algorithms
#
"""
SRAlgorithm
Supported algorithm types are subtypes of the abstract type `SRAlgorithm`.
"""
abstract type SRAlgorithm end
"""
IEEE1057([iterations = 6]) <: SRAlgorithm
Define an instance of the IEEE 1057 sinusoidal fitting algorithm.
Optional argument `iterations` specifies how many iterations to run before the algorithm
stops. The default value is 6, which is the value recommended by the standard. This value
is only used when calculating a 4-parameter fit.
"""
Base.@kwdef struct IEEE1057 <: SRAlgorithm
iterations::Int = 6
end
"""
IntegralEquations() <: SRAlgorithm
Define an instance of the integral-equations sinusoidal fitting algorithm described by
J. Jacquelin in "RΓ©gressions et Γ©quations intΓ©grales", 2014 (available at
https://fr.scribd.com/doc/14674814/Regressions-et-equations-integrales).
This algorithm does not accept any configuration parameters.
"""
struct IntegralEquations <: SRAlgorithm end
"""
LevMar([use_ga = false]) <: SRAlgorithm
Define an instance of the Levenberg-Marquardt sinusoidal fitting algorithm.
If the optional argument `use_ga` is set to `true`, the algorithm will use geodesic
acceleration to potentially improve its performance and accuracy. See
[`curve_fit`](https://github.com/JuliaNLSolvers/LsqFit.jl) for more details.
"""
Base.@kwdef struct LevMar <: SRAlgorithm
use_ga :: Bool = false
end
"""
Liang([threshold = 0.15, iterations = 100, q = 1e-5]) <: SRAlgorithm
Define an instance of the sinusoidal fitting algorithm described in Liang et al,
"Fitting Algorithm of Sine Wave with Partial Period Waveforms and Non-Uniform Sampling
Based on Least-Square Method." Journal of Physics: Conference Series 1149.1
(2018)ProQuest. Web. 17 Apr. 2023.
This algorithm is designed for scenarios where only a fraction of a period of the sinusoid
has been sampled. Its optional parameters `threshold` and `q` are described in the paper.
Additionally, a maximum number of iterations may be specified in `iterations`.
"""
Base.@kwdef struct Liang <: SRAlgorithm
threshold :: Float64 = 0.15
iterations :: Int = 100
q :: Float64 = 1e-5
end
#
# Problems
#
"""
SRProblem
Supported problem types are subtypes of the abstract type `SRProblem`.
"""
abstract type SRProblem end
const MR = Union{Missing, Real}
"""
Sin3Problem(X, Y, f , [DC, Q, I, lb, ub]) <: SRProblem
Define a three-parameter sinusoidal regression problem.
The data is fit to the model ``f(x; DC, Q, I) = DC + Q\\sin(2Οfx) + I\\cos(2Οfx)``. The sampling
instants are given by `X`, and the samples by `Y`. The frequency `f` (in Hz) is assumed to
be known exactly.
When using a fitting algorithm that accepts initial parameter estimates, these may be given
by the optional keyword arguments `DC`, `Q` and `I` (default `missing`). Lower and upper bounds
may be specified in `lb` and `ub`, which must be vectors of length 3.
See also: [`Sin4Problem`](@ref)
"""
Base.@kwdef struct Sin3Problem{T1, T2, F<:Real, P1<:MR, P2<:MR, P3<:MR, LB, UB} <: SRProblem
X :: T1 # sampling times
Y :: T2 # sample values
f :: F # exact known frequency
# initial estimates (may be missing)
DC :: P1 = missing
Q :: P2 = missing
I :: P3 = missing
lb :: LB = missing # lower bounds
ub :: UB = missing # upper bounds
end
Sin3Problem(X, Y, f ; kwargs...) = Sin3Problem(; X, Y, f, kwargs...)
function Base.show(io::IO, problem::Sin3Problem)
println(io, "3-Parameter Sinusoidal Problem Sin3Problem:")
println(io, " X : $(typeof(problem.X)) with $(length(problem.X)) elements")
println(io, " Y : $(typeof(problem.Y)) with $(length(problem.Y)) elements")
println(io, " Frequency (Hz) : $(problem.f)")
println(io, "Parameter estimates:")
println(io, " DC : $(problem.DC)")
println(io, " Sine amplitude (Q) : $(problem.Q)")
println(io, " Cosine amplitude (I) : $(problem.I)")
println(io, " Lower bounds : $(problem.lb)")
println(io, " Upper bounds : $(problem.ub)")
end
"""
Sin4Problem(X, Y ; [f, DC, Q, I, lb, ub]) <: SRProblem
Define a four-parameter sinusoidal regression problem.
The data is fit to the model ``f(x; f, DC, Q, I) = DC + Q\\sin(2Οfx) + I\\cos(2Οfx)``.
The sampling instants are given by `X`, and the samples by `Y`.
When using a fitting algorithm that accepts initial parameter estimates, these may be given
by the optional keyword arguments `f`, `DC`, `Q` and `I` (default `missing`). Lower and upper
bounds may be specified in `lb` and `ub`, which must be vectors of length 4.
See also: [`Sin3Problem`](@ref)
"""
Base.@kwdef struct Sin4Problem{T1, T2, P1<:MR, P2<:MR, P3<:MR, P4<:MR, LB, UB} <: SRProblem
X :: T1 # sampling times
Y :: T2 # sample values
f :: P1 = missing # initial estimates (may be missing)
DC :: P2 = missing
Q :: P3 = missing
I :: P4 = missing
lb :: LB = missing # lower bounds
ub :: UB = missing # uppper bounds
end
Sin4Problem(X, Y ; kwargs...) = Sin4Problem(; X, Y, kwargs...)
function Base.show(io::IO, problem::Sin4Problem)
println(io, "4-Parameter Sinusoidal Problem Sin4Problem:")
println(io, " X : $(typeof(problem.X)) with $(length(problem.X)) elements")
println(io, " Y : $(typeof(problem.Y)) with $(length(problem.Y)) elements")
println(io, "Parameter estimates:")
println(io, " Frequency (Hz) : $(problem.f)")
println(io, " DC : $(problem.DC)")
println(io, " Sine amplitude (Q) : $(problem.Q)")
println(io, " Cosine amplitude (I) : $(problem.I)")
println(io, " Lower bounds : $(problem.lb)")
println(io, " Upper bounds : $(problem.ub)")
end
"""
MixedLinSin4Problem(X, Y, f ; [DC, Q, I, m, lb, ub]) <: SRProblem
Define a four-parameter mixed linear-sinusoidal regression problem.
The data is fit to the model ``f(x; DC, Q, I, m) = DC + Q\\sin(2Οfx) + I\\cos(2Οfx) + mx``.
The sampling instants are given by `X`, and the samples by `Y`. The frequency `f` (in Hz) is
assumed to be known exactly.
When using a fitting algorithm that accepts initial parameter estimates, these may be given
by the optional keyword arguments `DC`, `Q` `I` and `m` (default `missing`). Lower and upper
bounds may be specified in `lb` and `ub`, which must be vectors of length 4.
See also: [`MixedLinSin5Problem`](@ref)
"""
Base.@kwdef struct MixedLinSin4Problem{T1, T2, F<:Real, P1<:MR, P2<:MR, P3<:MR, P4<:MR, LB, UB} <: SRProblem
X :: T1
Y :: T2
f :: F
DC :: P1= missing
Q :: P2 = missing
I :: P3 = missing
m :: P4 = missing
lb :: LB = missing
ub :: UB = missing
end
MixedLinSin4Problem(X, Y, f ; kwargs...) = MixedLinSin4Problem(; X, Y, f, kwargs...)
function Base.show(io::IO, problem::MixedLinSin4Problem)
println(io, "4-Parameter Mixed Linear-Sinusoidal Problem MixedLinSin4Problem:")
println(io, " X : $(typeof(problem.X)) with $(length(problem.X)) elements")
println(io, " Y : $(typeof(problem.Y)) with $(length(problem.Y)) elements")
println(io, " Frequency (Hz) : $(problem.f)")
println(io, "Parameter estimates:")
println(io, " DC : $(problem.DC)")
println(io, " Sine amplitude (Q) : $(problem.Q)")
println(io, " Cosine amplitude (I) : $(problem.I)")
println(io, " Linear term (m) : $(problem.m)")
println(io, " Lower bounds : $(problem.lb)")
println(io, " Upper bounds : $(problem.ub)")
end
"""
MixedLinSin5Problem(X, Y ; [f, DC, Q, I, m, lb, ub]) <: SRProblem
Define a five-parameter mixed linear-sinusoidal regression problem.
The data is fit to the model ``f(x ; f, DC, Q, I, m) = DC + Q\\sin(2Οfx) + I\\cos(2Οfx) + mx``.
The sampling instants are given by `X`, and the samples by `Y`.
When using a fitting algorithm that accepts initial parameter estimates, these may be given
by the optional keyword arguments `f`, `DC`, `Q` `I` and `m` (default `missing`). Lower and upper
bounds may be specified in `lb` and `ub`, which must be vectors of length 5.
See also: [`MixedLinSin4Problem`](@ref)
"""
Base.@kwdef struct MixedLinSin5Problem{T1, T2, P1<:MR, P2<:MR, P3<:MR, P4<:MR, P5<:MR, LB, UB} <: SRProblem
X :: T1
Y :: T2
f :: P1 = missing
DC :: P2 = missing
Q :: P3 = missing
I :: P4 = missing
m :: P5 = missing
lb :: LB = missing
ub :: UB = missing
end
MixedLinSin5Problem(X, Y ; kwargs...) = MixedLinSin5Problem(; X, Y, kwargs...)
function Base.show(io::IO, problem::MixedLinSin5Problem)
println(io, "5-Parameter Mixed Linear-Sinusoidal Problem MixedLinSin5Problem:")
println(io, " X : $(typeof(problem.X)) with $(length(problem.X)) elements")
println(io, " Y : $(typeof(problem.Y)) with $(length(problem.Y)) elements")
println(io, "Parameter estimates:")
println(io, " Frequency (Hz) : $(problem.f)")
println(io, " DC : $(problem.DC)")
println(io, " Sine amplitude (Q) : $(problem.Q)")
println(io, " Cosine amplitude (I) : $(problem.I)")
println(io, " Linear term (m) : $(problem.m)")
println(io, " Lower bounds : $(problem.lb)")
println(io, " Upper bounds : $(problem.ub)")
end
"""
SRModel
Supported models types are subtypes of the abstract type `SRModel`.
Supertype for the parameters of the different kinds of sinusoidal models
supported by SinusoidalRegressions.
See also: [`SinModel`](@ref), [`MixedLinSinModel`](@ref)
"""
abstract type SRModel end
"""
SinModel{T <: Real} <: SRModel
Parameters `f`, `DC`, `Q` and `I` of the sinusoidal model ``s(x) = DC + I\\cos(2Οfx) + Q\\sin(2Οfx)``.
See also: [`MixedLinSinModel`](@ref).
"""
struct SinModel{T <: Real} <: SRModel
f :: T # frequency in Hz
DC :: T
Q :: T # sine amplitude
I :: T # cosine amplitude
end
"""
SinModel{T}(f, DC, Q, I)
Construct a sinusoidal model with the given parameters.
To express the model as ``s(x) = M\\cos(2Οfx + ΞΈ)``, use the `torect` function.
Examples
========
```
julia> SinModel(10, 1, -0.5, 1.2)
Sinusoidal parameters SinModel{Float64}:
Frequency (Hz) : 10.0
DC : 1.0
Sine amplitude (Q) : -0.5
Cosine amplitude (I): 1.2
julia> SinModel(1, 0, torect(1, -Ο/2)...) # A pure sine
Sinusoidal parameters SinModel{Float64}:
Frequency (Hz) : 1.0
DC : 0.0
Sine amplitude (Q) : 1.0
Cosine amplitude (I): 0.0
```
"""
SinModel(f, DC, Q, I) = SinModel(promote(f, DC, Q, I)...)
"""
SinModel{T}(; f, DC, Q, I)
Construct a `SinModel{T}` specifying each parameter by name.
Example
=======
```
julia> SinModel(DC = 1, Q = -0.5, I = 1.2, f = 10)
Sinusoidal parameters SinModel{Float64}:
Frequency (Hz) : 10.0
DC : 1.0
Sine amplitude (Q) : -0.5
Cosine amplitude (I): 1.2
```
"""
SinModel(; f, DC, Q, I) = SinModel(f, DC, Q, I)
"""
(P::SinModel)(t)
Evaluate the sinusoidal function specified by the parameters `P` at the values given
by `t`, which may be a scalar or a collection.
Example
=======
```
julia> P = SinModel(DC = 1, Q = -0.5, I = 1.2, f = 10)
julia> t = range(0, 0.7, length = 5)
julia> P(t)
5-element Vector{Float64}:
2.2
1.4999999999999996
-0.2000000000000004
0.49999999999999944
2.200000000000001
```
"""
(params::SinModel)(t) = @. params.Q*sin(2Ο*params.f*t) + params.I*cos(2Ο*params.f*t) + params.DC
#TODO: Remove implicit broadcasting
function Base.show(io::IO, model::SinModel{T}) where {T}
println(io, "Sinusoidal model SinModel{$T}:")
println(io, " Frequency (Hz) : $(model.f)")
println(io, " DC : $(model.DC)")
println(io, " Sine amplitude (Q) : $(model.Q)")
println(io, " Cosine amplitude (I) : $(model.I)")
end
"""
MixedLinSinModel{T <: Real} <: SRModel
Parameters `f`, `DC`, `Q`, `I` and `m` of the sinusoidal function
``s(x) = DC + mx + I\\cos(2Οfx) + Q\\sin(2Οfx)``.
See also: [`SinModel`](@ref).
"""
struct MixedLinSinModel{T <: Real} <: SRModel
f :: T # frequency in Hz
DC :: T
Q :: T # sine amplitude
I :: T # cosine amplitude
m :: T # linear term
end
"""
MixedLinSinModel{T}(f, DC, Q, I, m) <: SRModel
Construct a `MixedLinSinModel{T}` with the given parameters, promoting to a common type
`T` if necessary.
Example
=======
```
julia> MixedLinSinModel(10, 0, -1.2, 0.4, 2.1)
Mixed Linear-Sinusoidal parameters MixedLinSinModel{Float64}:
Frequency (Hz) : 10.0
DC : 0.0
Sine amplitude (Q) : -1.2
Cosine amplitude (I) : 0.4
Linear term (m) : 2.1
```
"""
MixedLinSinModel(f, DC, Q, I, m) = MixedLinSinModel(promote(f, DC, Q, I, m)...)
"""
MixedLinSinModel(; f, DC, Q, I, m)
Construct a `MixedLinSinModel{T}` specifying each parameter by name.
Example
=======
```
julia> MixedLinSinModel(f = 10, DC = 0, m = 2.1, Q= -1.2, I = 0.4)
Mixed Linear-Sinusoidal parameters MixedLinSinModel{Float64}:
Frequency (Hz) : 10.0
DC : 0.0
Sine amplitude (Q) : -1.2
Cosine amplitude (I) : 0.4
Linear term (m) : 2.1
```
"""
MixedLinSinModel(; f, DC, Q, I, m) = MixedLinSinModel(f, DC, Q, I, m)
"""
(P::MixedLinSinModel)(t)
Evaluate the mixed linear-sinusoidal function specified by the parameters `P` at
the values given by `t`, which may be a scalar or a collection.
Example
=======
```
julia> P = MixedLinSinModel(f = 10, DC = 0, m = 2.1, Q= -1.2, I = 0.4)
julia> t = range(0, 0.7, length = 5)
julia> P(t)
5-element Vector{Float64}:
0.4
1.5674999999999997
0.3349999999999989
-0.09750000000000014
1.870000000000002
```
"""
(params::MixedLinSinModel)(t) = @. params.Q*sin(2Ο*params.f*t) + params.I*cos(2Ο*params.f*t) +
params.m*t + params.DC
function Base.show(io::IO, model::MixedLinSinModel{T}) where {T}
println(io, "Mixed Linear-Sinusoidal model MixedLinSinModel{$T}:")
println(io, " Frequency (Hz) : $(model.f)")
println(io, " DC : $(model.DC)")
println(io, " Sine amplitude (Q) : $(model.Q)")
println(io, " Cosine amplitude (I) : $(model.I)")
println(io, " Linear term (m) : $(model.m)")
end
### not yet implemented
"""
GenSinModel <: SinModel
Not yet implemented.
See also: [`SinModel`](@ref), [`MixedLinSinModel`](@ref), [`DampedSinModel`](@ref)
(not yet implemented).
"""
struct GenSinModel
end
"""
DampedSinModel <: SinModel
Not yet implemented.
See also: [`SinModel`](@ref), [`MixedLinSinModel`](@ref), [`GenSinModel`](@ref)
(not yet implemented).
"""
struct DampedSinProblem
end
| SinusoidalRegressions | https://github.com/mbaz/SinusoidalRegressions.jl.git |
|
[
"MIT"
] | 0.2.0 | cd53f560c93b806d4d10020f34ad8d74548edf08 | code | 9890 | using Test, SinusoidalRegressions, Aqua
@testset "AQUA" begin
Aqua.test_unbound_args(SinusoidalRegressions)
Aqua.test_undefined_exports(SinusoidalRegressions)
Aqua.test_piracy(SinusoidalRegressions)
Aqua.test_project_toml_formatting(SinusoidalRegressions)
Aqua.test_stale_deps(SinusoidalRegressions)
Aqua.test_unbound_args(SinusoidalRegressions)
Aqua.test_undefined_exports(SinusoidalRegressions)
end
@testset "Example 1 from Jacquelin's paper: sinusoidal regression" begin
# Example is on page 23; expected results are on page 32
X = [-1.983, -1.948, -1.837, -1.827, -1.663, -0.815,
-0.778, -0.754, -0.518, 0.322, 0.418, 0.781,
0.931, 1.510, 1.607]
Y = [ 0.936, 0.810, 0.716, 0.906, 0.247, -1.513,
-1.901, -1.565, -1.896, 0.051, 0.021, 1.069,
0.862, 0.183, 0.311]
p3 = Sin3Problem(X, Y, 2.02074/(2*pi))
p4 = Sin4Problem(X, Y)
# Jacquelin's method
(;f, DC, Q, I) = sinfit(p4, IntegralEquations())
@test 2*pi*f β 2.02074 atol = 0.00001
@test DC β -0.405617 atol = 0.00001
@test Q β 1.2752 atol = 0.0001
@test I β -0.577491 atol = 0.000001
# IEEE 1057 3-parameter
(;DC, Q, I) = sinfit(p3, IEEE1057())
@test DC β -0.405617 atol = 0.00001
@test Q β 1.2752 atol = 0.0001
@test I β -0.577491 atol = 0.00001
# IEEE 4-parameter (algorithm fails to converge)
@test SinusoidalRegressions.ieee1057_testconvergence(p4) == false
# LevMarFit
(;f, DC, Q, I) = sinfit(p4, LevMar())
@test 2*pi*f β 2.02074 atol = 0.04
@test DC β -0.405617 atol = 0.02
@test Q β 1.2752 atol = 0.02
@test I β -0.577491 atol = 0.01
end
@testset "Polar notation" begin
s = SinModel(1, 0, torect(1, 0)...) # A pure cosine
@test s.f == 1
@test s.DC == 0
@test s.Q β 0
@test s.I β 1
s = SinModel(1, 0, torect(1, -Ο/2)...) # A pure sine
@test s.f == 1
@test s.DC == 0
@test s.Q β 1 atol = 1e-12
@test s.I β 0 atol = 1e-12
s = SinModel(1, 0, torect(1, 3Ο/2)...) # Another pure sine
@test s.f == 1
@test s.DC == 0
@test s.Q β 1 atol = 1e-12
@test s.I β 0 atol = 1e-12
s = SinModel(1, 5, torect(1, -Ο/4)...) # A mix
@test s.f == 1
@test s.DC == 5
@test s.Q β sqrt(2)/2 atol = 1e-12
@test s.I β sqrt(2)/2 atol = 1e-12
end
@testset "Easy sinusoidal regression tests" begin
# Easy tests: lots of equidistant points, no noise.
t = range(0, 1, length=100)
# Q = 0
exact = SinModel(f = 3.0, DC = 1.25, I = 4, Q = 0)
Y = exact.(t)
p3 = Sin3Problem(t, Y, 3.0)
p4 = Sin4Problem(t, Y)
# integral equations
(;f, DC, Q, I) = sinfit(p4, IntegralEquations())
@test f β exact.f atol = 0.0001
@test DC β exact.DC atol = 0.0001
@test Q β exact.Q atol = 0.0001
@test I β exact.I atol = 0.0001
# ieee 1057 3-parameter
(;f, DC, Q, I) = sinfit(p3, IEEE1057())
@test f == exact.f
@test DC β exact.DC atol = 0.0001
@test Q β exact.Q atol = 0.0001
@test I β exact.I atol = 0.0001
# ieee 1057 4-parameter
(;f, DC, Q, I) = sinfit(p4, IEEE1057())
@test SinusoidalRegressions.ieee1057_testconvergence(p4) == true
@test f β exact.f atol = 0.001
@test DC β exact.DC atol = 0.001
@test Q β exact.Q atol = 0.001
@test I β exact.I atol = 0.001
# LevMarFit 3 Pars
(;f, DC, Q, I) = sinfit(p3, LevMar())
@test f == exact.f
@test DC β exact.DC atol = 0.0001
@test Q β exact.Q atol = 0.0001
@test I β exact.I atol = 0.0001
# LevMarFit 4 Pars
(;f, DC, Q, I) = sinfit(p4, LevMar())
@test f β exact.f atol = 0.0001
@test DC β exact.DC atol = 0.0001
@test Q β exact.Q atol = 0.0001
@test I β exact.I atol = 0.0001
# I = 0
exact = SinModel(f = 3.0, DC = 1.25, I = 0, Q = -2)
Y = exact.(t)
p3 = Sin3Problem(t, Y, 3.0)
p4 = Sin4Problem(t, Y)
# integral equations
(;f, DC, Q, I) = sinfit(p4, IntegralEquations())
@test f β exact.f atol = 0.0001
@test DC β exact.DC atol = 0.0001
@test Q β exact.Q atol = 0.0001
@test I β exact.I atol = 0.0001
# ieee 1057 3-parameter
(;f, DC, Q, I) = sinfit(p3, IEEE1057())
@test f == exact.f
@test DC β exact.DC atol = 0.0001
@test Q β exact.Q atol = 0.0001
@test I β exact.I atol = 0.0001
# ieee 1057 4-parameter
(;f, DC, Q, I) = sinfit(p4, IEEE1057())
@test SinusoidalRegressions.ieee1057_testconvergence(p4) == true
@test f β exact.f atol = 0.0001
@test DC β exact.DC atol = 0.0001
@test Q β exact.Q atol = 0.0001
@test I β exact.I atol = 0.0001
# LevMarFit 3 Pars
(;f, DC, Q, I) = sinfit(p3, LevMar())
@test f == exact.f
@test DC β exact.DC atol = 0.0001
@test Q β exact.Q atol = 0.0001
@test I β exact.I atol = 0.0001
# LevMarFit 4 pars
(;f, DC, Q, I) = sinfit(p4, LevMar())
@test f β exact.f atol = 0.0001
@test DC β exact.DC atol = 0.0001
@test Q β exact.Q atol = 0.0001
@test I β exact.I atol = 0.0001
# I != 0, Q != 0 -- check fewer digits
exact = SinModel(f = 2.0, DC = 0.25, I = 0.48, Q = -1.5)
Y = exact.(t)
p3 = Sin3Problem(t, Y, 2.0)
p4 = Sin4Problem(t, Y)
# integral equations
(;f, DC, Q, I) = sinfit(p4, IntegralEquations())
@test f β exact.f atol = 0.01
@test DC β exact.DC atol = 0.01
@test Q β exact.Q atol = 0.01
@test I β exact.I atol = 0.01
# ieee 1057 3-parameter
(;f, DC, Q, I) = sinfit(p3, IEEE1057())
@test f == exact.f
@test DC β exact.DC atol = 0.001
@test Q β exact.Q atol = 0.001
@test I β exact.I atol = 0.001
# ieee 1057 4-parameter (fails in this case)
@test SinusoidalRegressions.ieee1057_testconvergence(p4) == false
# LevMarFit 3 pars -- more exact, so check more digits
(;f, DC, Q, I) = sinfit(p3, LevMar())
@test f == exact.f
@test DC β exact.DC atol = 0.0001
@test Q β exact.Q atol = 0.0001
@test I β exact.I atol = 0.0001
# LevMarFit 4 Pars
(;f, DC, Q, I) = sinfit(p4, LevMar())
@test f β exact.f atol = 0.0001
@test DC β exact.DC atol = 0.0001
@test Q β exact.Q atol = 0.0001
@test I β exact.I atol = 0.0001
end
@testset "IEEE 1057 4-Parameter specific tests" begin
t = range(0, 4, length = 1337)
exact = SinModel(f = 2.0, DC = 0.25, I = 0.48, Q = -1.5)
Y = exact.(t)
p4 = Sin4Problem(t, Y)
@test SinusoidalRegressions.ieee1057_testconvergence(p4) == true
(;f, DC, Q, I) = sinfit(p4, IEEE1057())
@test f β exact.f atol = 0.0001
@test DC β exact.DC atol = 0.0001
@test Q β exact.Q atol = 0.0001
@test I β exact.I atol = 0.0001
t = range(0, 1, length = 2313)
exact = SinModel(f = 3.0, DC = -0.98, I = 2.15, Q = 3.87)
Y = exact.(t)
p4 = Sin4Problem(t, Y)
(;f, DC, Q, I) = sinfit(p4, IEEE1057())
@test f β exact.f atol = 0.0001
@test DC β exact.DC atol = 0.0001
@test Q β exact.Q atol = 0.0001
@test I β exact.I atol = 0.0001
end
@testset "Example 2 from Jacquelin's paper: mixed linear-sinusoidal regression" begin
# First, "large scatter" example from Jacquelin's paper -- page 51
X = [0, 0.31, 0.6, 0.71, 0.82, 1.62, 2.03, 2.73, 2.93, 3.19,
3.28, 3.68, 3.72, 4.26, 4.75, 6.72, 7.75, 8.41, 8.61, 9.17]
Y = [1.35, 1.95, 2.20, 1.91, 1.88, 2.56, 2.74, 2.33, 2.82, 2.14,
2.29, 1.76, 2.46, 1.70, 2.20, 4.43, 5.59, 7.03, 7.19, 6.84]
actual = MixedLinSinModel(0.8/(2Ο), 0.4, 1.2, 0.75, 0.6)
expected = MixedLinSinModel(0.861918/(2Ο), 0.571315, 1.113310, 0.613981, 0.582827)
p5 = MixedLinSin5Problem(X, Y)
(;f, DC, Q, I, m) = sinfit(p5, IntegralEquations())
@test f β expected.f atol = 0.01
@test DC β expected.DC atol = 0.01
@test Q β expected.Q atol = 0.02
@test I β expected.I atol = 0.02
@test m β expected.m atol = 0.01
# LevMarFit
(;f, DC, Q, I, m) = sinfit(p5, LevMar())
@test f β actual.f atol = 0.005
@test DC β actual.DC atol = 0.15
@test Q β actual.Q atol = 0.2
@test I β actual.I atol = 0.01
@test m β actual.m atol = 0.01
# Second, "small scatter" example from Jacquelin's paper -- page 53
X = range(start = 0.5, step = 0.5, length = 20)
Y = [1.66, 2.20, 2.83, 2.66, 2.59, 2.53, 2.12, 1.85, 1.85, 1.97,
2.16, 2.86, 3.42, 4.56, 5.11, 6.00, 6.91, 7.16, 7.56, 7.41]
actual = MixedLinSinModel(0.8/(2Ο), 0.4, 1.2, 0.75, 0.6)
expected = MixedLinSinModel(0.809132/(2Ο), 0.266603, 1.278916, 0.680126, 0.613464)
p5 = MixedLinSin5Problem(X, Y)
(;f, DC, Q, I, m) = sinfit(p5, IntegralEquations())
@test f β expected.f atol = 0.001
@test DC β expected.DC atol = 0.001
@test Q β expected.Q atol = 0.001
@test I β expected.I atol = 0.001
@test m β expected.m atol = 0.001
# LevMarFit
(;f, DC, Q, I, m) = sinfit(p5, LevMar())
@test f β actual.f atol = 0.002
@test DC β actual.DC atol = 0.15
@test Q β actual.Q atol = 0.1
@test I β actual.I atol = 0.1
@test m β actual.m atol = 0.015
end
@testset "Liang" begin
t = range(0, 1, length=100)
exact = SinModel(f = 0.5, DC = 1.2, Q = 0.3, I = 2.4)
Y = exact.(t)
p4 = Sin4Problem(t, Y)
a = Liang()
(; f, DC, Q, I) = sinfit(p4, a)
@test f β exact.f atol = 0.001
@test DC β exact.DC atol = 0.001
@test Q β exact.Q atol = 0.001
@test I β exact.I atol = 0.001
exact = SinModel(f = 0.3, DC = -1.2, Q = 0.0, I = 0.5)
Y = exact.(t)
p4 = Sin4Problem(t, Y)
a = Liang()
(; f, DC, Q, I) = sinfit(p4, a)
@test f β exact.f atol = 0.01
@test DC β exact.DC atol = 0.01
@test Q β exact.Q atol = 0.01
@test I β exact.I atol = 0.01
end
@testset "RMSE tests" begin
end
| SinusoidalRegressions | https://github.com/mbaz/SinusoidalRegressions.jl.git |
|
[
"MIT"
] | 0.2.0 | cd53f560c93b806d4d10020f34ad8d74548edf08 | docs | 1601 | ## SinusoidalRegressions.jl
[](https://github.com/mbaz/SinusoidalRegressions.jl/actions/workflows/ci.yml)
[](https://github.com/JuliaTesting/Aqua.jl)
SinusoidalRegressions.jl aims to provide a set of functions for conveniently fitting noisy data to a variety of sinusoidal models, with or without initial estimates of the parameters. The package is quite usable in its current state, but is still in development. Support for more sinusoidal models will be added in the future, and API changes cannot be ruled out.
Its documentation is found [here](https://mbaz.github.io/SinusoidalRegressions.jl/v0.2/).
### Package features:
* An implementation of IEEE 1057 fitting algorithms for 3 and 4 parameters.
* An implementation of the fitting algorithms developed by J. Jacquelin, based on integral equations that can be solved numerically and whose solution provide the desired fit. These algorithms do not require an initial parameter estimate.
* A front-end to the non-linear fitting function `curve_fit` from the package [`LsqFit`](https://github.com/JuliaNLSolvers/LsqFit.jl). This function uses the Levenberg-Marquardt algorithm and is quite powerful, but it requires an initial estimate of the parameters.
* Support for sinusoidal and mixed linear-sinusoidal models.
In addition, the package provides functions to calculate the RMSE and MAE when the exact parameters are known, and plot recipes for convenient plotting.
| SinusoidalRegressions | https://github.com/mbaz/SinusoidalRegressions.jl.git |
|
[
"MIT"
] | 0.2.0 | cd53f560c93b806d4d10020f34ad8d74548edf08 | docs | 11981 | # Introduction
SinusoidalRegressions.jl provides a set of functions for conveniently fitting
noisy data to a variety of sinusoidal models, with or without initial estimates
of the parameters.
## Features
This package supports five sinusoidal models:
* 3-parameter sinusoidal, where the frequency ``f`` is known exactly:
``s_s(x; \textrm{DC}, I, Q) = \textrm{DC} + Q\sin(2Οfx) + I\cos(2Οfx)``
* 4-parameter sinusoidal, where the frequency ``f`` is unknown:
``s_s(x; f, \textrm{DC}, I, Q) = \textrm{DC} + Q\sin(2Οfx) + I\cos(2Οfx)``
* Mixed linear-sinusoidal:
``s_{mls}(x; f, \textrm{DC}, I, Q, m) = \textrm{DC} + mx + Q\sin(2Οfx) + I\cos(2Οfx)``
* (Not yet implemented) General sinusoidal with unknown coefficients ``Ξ»_1, Ξ»_2, \ldots, Ξ»_m``, and known functions ``g_1(x), g_2(x), \ldots, g_m(x)``:
``s_g(x; f, I, Q, Ξ»_1, \ldots, Ξ»_n) = Q\sin(2Οfx) + I\cos(2Οfx) + Ξ»_1g_1(x) + \ldots + Ξ»_mg_m(x)``
* (Not yet implemented) Damped sinusoidal:
``s_d(x; f, I, Q, Ξ±) = \exp(Ξ±x) ( Q\sin(2Οfx) + I\cos(2Οfx) )``
!!! note "A note on the frequency"
The frequency ``f`` is assumed to be expressed in hertz throughout.
!!! note "Notation"
In the model ``s(x; ΞΈ_1, ΞΈ_2, \ldots, ΞΈ_n)``, ``x`` is the independent variable and ``ΞΈ_i``,
``i = 1, \ldots, n,`` are the unkown parameters.
Four types of sinusoidal fitting algorithms are provided:
* IEEE 1057 3-parameter and 4-parameter algorithms[^1]. These are able to fit only the sinusoidal model
``s_s(x)``. The 4-parameter version requires a very close estimate of the frequency ``f`` and
dense sampling of several periods of the sinusoid.
* The algorithms proposed by J. Jacquelin, based on the idea of finding an integral
equation whose solution is the desired sinusoidal model[^2]. This integral equation
can be solved using linear least-squares. These algorithms have several benefits:
* They are non-iterative.
* They do not require initial estimates of any of the model's parameters.
* They often perform quite well, but may also be used to calculate a good initial guess
for the more powerful non-linear methods described below.
* The non-linear fitting function `curve_fit` from the package
[LsqFit](https://github.com/JuliaNLSolvers/LsqFit.jl). This function uses the Levenberg-Marquardt
algorithm[^3][^4][^5] and is quite powerful, but it requires an initial estimate of the parameters.
`SinusoidalRegressions.jl` "wraps" `curve_fit`, automatically defining the correct model
and calculating initial parameter estimates (using IEEE 1057 or Jacquelin's algorithms, as
appropriate) if none are provided by the user.
* The algorithm proposed by Liang et al[^6], designed for the case where only a fraction of a period
of a sinusoid is sampled.
## Installation
This package is in Julia's general registry. It can be installed by running
```julia
import Pkg; Pkg.add("SinusoidalRegressions")
```
## Roadmap and Contributions
The following features are on the roadmap towards version 1.0:
* Implement Jacquelin's algorithms for damped and general sinusoids.
* Enhance the front-end to `curve_fit` by allowing some paramters to be declared as known a priori, and
fitting only the remaining parameters (some progress done in v0.2).
* Fine-tune the API (v0.2 _should_ be close to final).
* Implement other algorithms and add support for other models.
* Improve tests.
Contributions, feature requests, bug reports and suggestions are welcome;
please use the [issue tracker on github](https://github.com/mbaz), or open a
discussion on the [Julia Discourse forum](https://discourse.julialang.org/) or on
[Zulip](https://julialang.zulipchat.com/#).
## Tutorial
### Fitting experimental data
Fitting data using this package requires three steps:
1. Define a regression problem. The problem encapsulates all known data: the sampling points and
data at a minimum, and possibly also the frequency. Initial estimates and bounds are
also part of the problem definition.
2. Specify and possibly configure an algorithm to solve the problem.
3. Run the `sinfit` function on the problem with the chosen algorithm. This function
returns the estimated parameters in a struct of the appropriate type.
After the data is fit, it may be easily plotted, and its RMSE and MAE may be calculated.
### Example 1
As a first example, let us fit noisy data to a mixed linear-sinusoidal model using Jacquelin's
integral equation algorithm. The model is
``s(x ; f, \textrm{DC}, Q, I, m) = \textrm{DC} + mx + Q\sin(2Οfx) + I\cos(2Οfx)``
with parameters ``f`` (the frequency is unknown), ``\textrm{DC}``, ``Q``, ``I`` and ``m``.
Our tasks are: define an "exact" model and generate noisy samples from it, fit the noisy samples,
determine the error (a measure of the difference between the exact model and the fit), and plot
the fit. First we need to learn about defining a model.
#### Model types
This package includes several types used to store the parameters of the different supported models.
All model types are a subtype of the abstract type [`SRModel`](@ref).
* [`SinModel`](@ref) for representing sinusoidal models ``s_s(x)``.
* [`MixedLinSinModel`](@ref) for representing mixed linear-sinusoidal models ``s_{mls}(x)``.
* `GenSinModel` for representing general sinusoidal models ``s_g(x)`` (not yet implemented).
* `DampedSinModel` for representing damped sinusoidal models ``s_d(x)`` (not yet implemented).
Instances of these types are also function types (functors), making it easy to evaluate the
model at any point (or collection of points). A plot recipe is also provided. We will
use both of these features below.
Since we're assuming a mixed linear-sinusoidal model, we'll use the type `MixedLinSinModel`.
#### Generating the exact and noisy data
We will first generate the "exact" or "true" data, and then add noise.
The exact parameters in this example are ``f = 4.1``, ``\textrm{DC} = 0.2``, ``m = 1``, ``Q =
-0.75``, and ``I = 0.8``. We define the model as follows:
```@example 1
using SinusoidalRegressions
param_exact = MixedLinSinModel(f = 4.1, DC = 0.2, m = 1, Q = -0.75, I = 0.8)
```
We now genereate 40 exact data points with a sampling rate of 40 Hz.
```@example 1
x = range(0, length = 40, step = 1/40)
data_exact = param_exact(x)
nothing # hide
```
And finally, we add gaussian noise to the data:
```@example 1
using Random: seed!
seed!(6778899)
data = data_exact .+ 0.2*randn(40) # noisy data
nothing # hide
```
Alternatively, the data could have been generated directly, as in:
```
data_exact = 0.2 .+ m.*x .- 0.75*sin.(2*pi*4.1*x) .+ 0.8*cos.(2*pi*4.1*x)
```
Now we need to define the problem.
#### Defining the problem
There are several types used to define the different problems that this package can handle. All
are subtypes of the abstract type `SRProblem`.
* [`Sin3Problem`](@ref) to specify a 3-parameter sinusoidal problem (frequency is known).
* [`Sin4Problem`](@ref) to specify a 4-parameter sinusoidal problem (frequency is unknown).
* [`MixedLinSin4Problem`](@ref) for a mixed linear-sinusoidal problem with 4 parameters (the
frequency is known).
* [`MixedLinSin5Problem`](@ref) for a mixed linear-sinusoidal problem with 5 parameters (the
frequency is unknown).
In our example, the frequency is unknown, which means `MixedLinSin5Problem` is the appropriate
type. The problem is simply defined as follows:
```@example 1
problem = MixedLinSin5Problem(x, data)
```
All parameters are `missing` because, in this example, we assume we know nothing about the underlying
model beyond the assumption that it is mixed linear-sinusoid; all we have is the data samples.
#### Specifying an algorithm
Now, we need to choose and instantiate a solution algorithm, from the following list. All algorithms
are subytpes of the abstract type `SRAlgorithm`.
* [`IEEE1057`](@ref) to specify IEEE 1057.
* [`IntegralEquations`](@ref) to select Jacquelin's integral equations methods.
* [`LevMar`](@ref) to use Levenberg-Marquardt via
[`curve_fit`](https://github.com/JuliaNLSolvers/LsqFit.jl)
* [`Liang`](@ref) to use Liang's algorithm.
Some of these algorithms can take configuration parameters. In our case, we
want to use the integral equations method, which does not require any configuration:
```@example 1
algorithm = IntegralEquations()
```
#### Fitting and error measurement
We are ready to calculate the fit:
```@example 1
fit = sinfit(problem, algorithm)
```
Note that `sinfit` returns a value of type [`MixedLinSinModel`](@ref), which contains
the estimated parameters in its fields.
We calculate the fit's root mean-square error and mean absolute error:
```@example 1
rmse(fit, param_exact, x)
```
```@example 1
mae(fit, param_exact, x)
```
#### Plotting
We can plot the measured data along with the fit:
```@example 1
using Plots
plot(x, data, fit)
```
#### Improving the fit with non-linear least squares
We may try to improve upon this fit by using Levenberg-Marquardt with the parameters estimated above
as initial parameters. We can do so as follows:
```@example 1
(; f, DC, Q, I, m) = fit # transfer the fit to a new problem instance
problem_levmar = MixedLinSin5Problem(x, data; f, DC, Q, I, m)
```
Note that now the problem includes initial estimates of the paramaters (except for the bounds).
If these are not provided, then `sinfit` (when using `LevMar()`) would have used the integral
equations algorithm to calculate them automatically. It is also possible to give estimates of
only some of the paramaters.
Now we can fit the data:
```@example 1
fit_levmar = sinfit(problem_levmar, LevMar())
```
We can re-evaluate the error:
```@example 1
rmse(fit_levmar, param_exact, x)
```
```@example 1
mae(fit_levmar, param_exact, x)
```
As expected, this second fit has a smaller error. Plotting the data, the fit, and the exact
function for comparison:
```@example 1
plot(x, data, fit_levmar, exact = param_exact)
```
### Example 2: A more difficult scenario
Let us fit the same model as above, with fewer data points, more noise and non-equidistant samples.
```@example 1
x = sort(rand(20))
data_exact = param_exact(x)
data = data_exact .+ 0.3*randn(20)
problem = MixedLinSin5Problem(x, data)
fit = sinfit(problem, IntegralEquations())
```
Error for Jacquelin's algorithm:
```@example 1
rmse(fit, param_exact, x)
```
```@example 1
mae(fit, param_exact, x)
```
As expected, we see larger errors than in the more benign scenario above. Let us improve the fit
using non-linear least-squares. Note that the Levenberg-Marqvardt algorithm requires initial
estimates of the parameters, which we have not provided. In this case, `sinfit` will calculate the
initial estimates "behind the scenes" using `IntegralEquations()`.
```@example 1
fit_nls = sinfit(problem, LevMar())
```
Error for `LsqFit.curve_fit`:
```@example 1
rmse(fit_nls, param_exact, x)
```
```@example 1
mae(fit_nls, param_exact, x)
```
We see that `LsqFit.curve_fit` was able to improve the fit significantly. Plotting the data,
the fit and the exact function:
```@example 1
plot(x, data, fit_nls, exact = param_exact)
```
## References
[^1]: IEEE, "IEEE Standard for Digitizing Waveform Recorders", 2018.
[^2]:Jacquelin J., RΓ©gressions et Equations IntΓ©grales, 2014, (online) https://fr.scribd.com/doc/14674814/Regressions-et-equations-integrales.
[^3]: Levenberg, K., "A Method for the Solution of Certain Non-Linear Problems in Least Squares", Quarterly of Applied Mathematics, 2 (2), 1944. doi:10.1090/qam/10666.
[^4]: Marquardt, D., "An Algorithm for Least-Squares Estimation of Nonlinear Parameters", SIAM Journal on Applied Mathematics, 11 (2), 1944. doi:10.1137/0111030.
[^5]: `LsqFit.jl` User Manual, (online) https://julianlsolvers.github.io/LsqFit.jl/latest/
[^6]: Liang et al, "Fitting Algorithm of Sine Wave with Partial Period Waveforms and Non-Uniform Sampling Based on Least-Square Method." Journal of Physics: Conference Series 1149.1 (2018)ProQuest. Web. 17 Apr. 2023.
| SinusoidalRegressions | https://github.com/mbaz/SinusoidalRegressions.jl.git |
|
[
"MIT"
] | 0.2.0 | cd53f560c93b806d4d10020f34ad8d74548edf08 | docs | 4917 | # Reference
This package's workhorse function is `sinfit(p::SRProblem, a::SRAlgorithm)`. It takes a problem `p`
and calculates a fit using algorithm `a`.
```@docs
sinfit
```
## Algorithms
### IEEE 1057
Sinusoidal regressions specified by 1057-2017, IEEE Standard for Digitizing
Waveform Recorders. These are the same algorithms specified in 1241-2010, IEEE
Standard for Terminology and Test Methods for Analog-to-Digital Converters.
```@docs
IEEE1057
```
### Method of integral equations
Four types of sinusoidal regressions using the algorithms proposed by J.
Jacquelin in "RΓ©gressions et Equations IntΓ©grales". These algorithms are not
iterative, and they do not require an initial estimate of the frequency or any
other parameter. They may be used by themselves, or to calculate initial
estimates for more-precise least-squares methods based on non-linear
optimization (described below).
```@docs
IntegralEquations
```
### Non-linear optimization
Wrapper for `LsqFit.curve_fit()`, which defines the appropriate model,
calculates initial parameter estimates if not provided by the user, and wraps the
returned fit in the appropriate parameter container.
```@docs
LevMar
```
### Liang
Algorithm designed for fitting when only a fraction of a period is sampled.
```@docs
Liang
```
## Problems
An `SRProblem` encapsulates what is known: at a minimum, the sampling times and the samples, and
possibly also estimates or bounds on some or all parameters. The problem type specifies the
number of unknown parameters, and the model (sinusoidal or mixed linear-sinusoidal).
```@docs
Sin3Problem
Sin4Problem
MixedLinSin4Problem
MixedLinSin5Problem
```
## Problem-Algorithm matrix
The following table shows which algorithms can solve which sinusoidal regression problems:
| |`IEEE1057` | `IntegralEquations` | `LevMar` | `Liang` |
|-------------------------|:---------:|:-------------------:|:--------:|:-------:|
|`Sin3Problem` | β | | β | |
|`Sin4Problem` | β | β | β | β |
|`MixedLinearSin4Problem` | | β | β | |
|`MixedLinearSin5Problem` | | β | β | |
## Sinusoidal parameters
```@docs
SinModel
SinModel(::Any, ::Any, ::Any, ::Any)
SinModel(; ::Any, ::Any, ::Any, ::Any)
SinModel(::Any)
MixedLinSinModel
MixedLinSinModel(::Any, ::Any, ::Any, ::Any, ::Any)
MixedLinSinModel(; ::Any, ::Any, ::Any, ::Any, ::Any)
MixedLinSinModel(::Any)
#SinusoidalRegressions.GenSinModel
#SinusoidalRegressions.DampedSinModel
```
## Error measurement
Functions to easily compare a calculated fit to actual data, using root mean-square errors,
or mean absolute errors.
```@docs
rmse
mae
```
## Plotting
This package provides recipes for `Plots.jl`. This makes it very easy to plot data and the
calculated fit.
There are two recipes included: plotting one fit, and plotting data and up to two fits.
### Plotting a fit
```julia
plot(X, fit::T, ; fitlabel = "") where {T <: SinusoidalFunctionParameters}
```
Plot the model with parameters given by `fit` at the points specified in collection `X`. The
optional keyword argument `fitlabel` controls the label (legend) of the plot.
##### Example
```@example 2
using SinusoidalRegressions
using Random: seed! # hide
using Plots # hide
seed!(5678) # hide
X = range(0, 1, length = 100)
Y = 2 .+ 3cos.(2*pi*5*X) .- 0.2sin.(2*pi*5*X) .+ 0.1*randn(100)
fit = sinfit(Sin3Problem(X, Y, 5), IEEE1057())
plot(X, fit, fitlabel = "example")
```
### Plotting data and one or two different fits
```julia
plot(X, Y, fit::T ; exact :: Union{T, Nothing} = nothing,
samples = 100,
fitlabel = "fit",
datalabel = "data",
exactlabel = "exact") where {T <: SinusoidalFunctionParameters}
```
Plot the data `Y` and the model with parameters given by `fit` evaluated at the points specified
in the collection `X`. The following keyword arguments are supported (with default values in
parenthesis):
* `exact::T = nothing`: add one more model to the plot. This allows plotting the exact function, the data and the fit in a single plot.
* `samples::Int = 100`: `fit` and `exact` are evaluated for `range(first(X), last(X), length = samples)`.
* `fitlabel::String = "fit"`: the label (legend) for the fit plot.
* `datalabel::String = "data"`: the label for the data plot.
* `exactlabel::String = "exact"`: the label for the exact plot.
##### Example
```@example 2
X = range(0, 1, length = 20)
exact = SinModel(5, 2, -0.2, 3)
Y = exact.(X) .+ 0.3*randn(20)
fit = sinfit(Sin4Problem(X, Y), IntegralEquations())
plot(X, Y, fit, exact = exact,
datalabel = "measurements",
fitlabel = "NLS",
exactlabel = "true")
```
## Abstract types
```@docs
SRModel
SRAlgorithm
SRProblem
```
| SinusoidalRegressions | https://github.com/mbaz/SinusoidalRegressions.jl.git |
|
[
"MIT"
] | 0.1.1 | 69c59dcf00aa2b711b630cc156ca7f41243e0595 | code | 337 | """
SimpleCTypes.jl
This zero-dependency package provides some simple definitions of C types, specifically:
- `CfuncPtr` for C function pointers
- `Carray` for fixed-size C arrays
- `Cunion` for C-style unions
"""
module SimpleCTypes
include("./function.jl")
include("./array.jl")
include("./union.jl")
end # module SimpleCTypes
| SimpleCTypes | https://github.com/sunoru/SimpleCTypes.jl.git |
|
[
"MIT"
] | 0.1.1 | 69c59dcf00aa2b711b630cc156ca7f41243e0595 | code | 2963 | export Carray, @Carray
"""
Carray{TElement,TDims,TNDims,TSize}
See `@Carray`.
"""
struct Carray{TElement,TDims<:Tuple,TNDims,TSize} <: AbstractArray{TElement,TNDims}
data::NTuple{TSize,TElement}
Carray{TElement,TDims,TNDims,TSize}(data::NTuple{TSize,TElement}) where {TElement,TDims,TNDims,TSize} =
new{TElement,TDims,TNDims,TSize}(data)
Carray{TElement,TDims,TNDims,TSize}(data) where {TElement,TDims,TNDims,TSize} =
new{TElement,TDims,TNDims,TSize}(Tuple(data))
end
@generated function Carray{T,TDims}(data::AbstractArray{T}) where {T,TDims}
TNDims = length(TDims.parameters)
TSize = prod(TDims.parameters)
quote
t = Tuple(data)
Carray{T,TDims,$TNDims,$TSize}(t)
end
end
Carray{T}(data::AbstractArray{T}) where {T} = Carray{T,Tuple{reverse(size(data))...}}(data)
Carray(data::AbstractArray{T}) where {T} = Carray{T}(data)
"""
@Carray{T,N1,N2,...}
A statically sized immutable C array type of type `T` and dimensions `N1`, `N2`, ...
It is a macro that expands to `Carray{T, Tuple{...,N2,N1}, N1*N2*...}`.
Note that:
- Since it is immutable, you need to use `@Carray(data)` to create a new array.
- To be consistent with C, the array is in column-major order.
- The index of the array still starts from 1.
# Examples
```julia
struct Foo
arr::@Carray{Int64,4}
matrix::@Carray{Int64,2,3,4}
end
arr = zeros(4)
matrix = ones(4,3,2)
# The conversion from `Array` to `CArray` happens automatically.
foo = Foo(arr, matrix)
```
"""
macro Carray(expr)
expr isa Expr && expr.head β‘ :braces || return :(Carray($(esc(expr))))
args = expr.args
TElement = esc(args[1])
TNDims = length(args) - 1
TNDims == 0 && return :(Carray{$TElement})
TDims = esc.(args[2:end])
TSize = Expr(:call, :*, TDims...)
:(Carray{$TElement,Tuple{$(TDims...)},$TNDims,$TSize})
end
Base.length(::Carray{<:Any,<:Tuple,<:Any,TSize}) where {TSize} = TSize
@generated function Base.size(::Carray{<:Any,TDims}) where {TDims}
s = Tuple(TDims.parameters)
:($s)
end
Base.eltype(::Carray{T}) where {T} = T
Base.convert(::Type{T}, t::Tuple) where {T<:Carray} = T(t)
Base.convert(::Type{T}, a::AbstractArray) where {T<:Carray} = T(a)
@generated Base.ndims(::Type{<:Carray{<:Any,TDims}}) where {TDims} = :($(length(TDims.parameters)))
Base.ndims(::T) where {T<:Carray} = ndims(T)
Base.IndexStyle(::Type{<:Carray}) = IndexCartesian()
Base.eachindex(ca::Carray) = CartesianIndices(size(ca))
Base.@propagate_inbounds @generated function Base.getindex(ca::Carray{T,TDims}, ind::CartesianIndex) where {T,TDims}
s = Tuple(TDims.parameters)
n = length(s)
index_expr = Expr(
:call, :+, 1,
(
:((ind.I[$i] - 1) * $(
i < n ? s[i+1] : 1
))
for i in 1:n
)...
)
:(ca.data[$index_expr])
end
Base.@propagate_inbounds Base.getindex(ca::Carray, i1::Integer, I::Integer...) =
ca[CartesianIndex(i1, I...)]
| SimpleCTypes | https://github.com/sunoru/SimpleCTypes.jl.git |
|
[
"MIT"
] | 0.1.1 | 69c59dcf00aa2b711b630cc156ca7f41243e0595 | code | 1829 | export CfuncPtr, @cfunc
"""
CfuncPtr{TReturn, TArgs}
A strictly typed C function pointer.
To wrap a function, use `@cfunc`.
"""
struct CfuncPtr{TReturn,TArgs<:Tuple}
ptr::Ptr{Cvoid}
CfuncPtr{TReturn,TArgs}(ptr::Ptr) where {TReturn,TArgs} = new{TReturn,TArgs}(Ptr{Cvoid}(ptr))
CfuncPtr{TReturn,TArgs}(cp::CfuncPtr) where {TReturn,TArgs} = new{TReturn,TArgs}(cp.ptr)
end
Base.pointer(cp::CfuncPtr) = cp.ptr
Base.convert(::Type{T}, cp::CfuncPtr) where {T<:Ptr} = T(pointer(cp))
Base.convert(::Type{T}, ptr::Ptr) where {T<:CfuncPtr} = T(ptr)
Base.cconvert(::Type{T}, cp::CfuncPtr) where {T<:Ptr} = T(pointer(cp))
Base.cconvert(::Type{CfuncPtr}, x) = error("Argument must be a pointer")
Base.unsafe_convert(::Type{<:CfuncPtr}, ptr::Ptr) = ptr
CfuncPtr{TReturn,TArgs}(cf::Base.CFunction) where {TReturn,TArgs} = CfuncPtr{TReturn,TArgs}(
Base.unsafe_convert(Ptr{Cvoid}, cf)
)
@generated function (cp::CfuncPtr{TReturn,TArgs})(args...) where {TReturn,TArgs}
len = length(args)
len_targs = length(TArgs.parameters)
if !(
len == len_targs ||
TArgs.parameters[end] isa Core.TypeofVararg &&
len β₯ len_args - 1
)
error("The number of arguments does not match the number of parameters")
end
:(
ccall(cp.ptr, $TReturn, ($(TArgs.parameters...),), $((:(args[$i]) for i in 1:len)...))
)
end
"""
cf_ptr, _ = @cfunc(f, ret, args)
cf_ptr, cf = @cfunc(\$f, ret, args)
Same as `@cfunction`, but returns both the pointer and the `CFunction`.
You need to keep the `CFunction` alive.
"""
macro cfunc(name, ret, args)
cf_expr = :(@cfunction($name, $ret, $args)) |> esc
TReturn = ret |> esc
TArgs = :(Tuple{$(args.args...)}) |> esc
quote
cf = $cf_expr
$CfuncPtr{$TReturn,$TArgs}(
cf
), cf
end
end
| SimpleCTypes | https://github.com/sunoru/SimpleCTypes.jl.git |
|
[
"MIT"
] | 0.1.1 | 69c59dcf00aa2b711b630cc156ca7f41243e0595 | code | 4263 | export Cunion, @Cunion
"""
Cunion{TMemberNames,TMemberTypes,TSize}
See `@Cunion`.
"""
struct Cunion{TMemberNames,TMemberTypes<:Tuple,TSize}
data::NTuple{TSize,UInt8}
Cunion{TMemberNames,TMemberTypes,TSize}(
data::NTuple{TSize,UInt8}
) where {TMemberNames,TMemberTypes,TSize} =
new{TMemberNames,TMemberTypes,TSize}(data)
end
@generated function Cunion{TMemberNames,TMemberTypes,TSize}(; kwargs...) where {TMemberNames,TMemberTypes,TSize}
TCunion = Cunion{TMemberNames,TMemberTypes,TSize}
k = only(kwargs.parameters[4].parameters[1])
T = membertype(TCunion, k)
init = Expr(:tuple, (:(zero(UInt8)) for _ in 1:TSize)...)
quote
v = @inbounds kwargs[1]
bytes = Ref($init)
p = pointer_from_objref(bytes)
GC.@preserve bytes begin
unsafe_store!(Ptr{$T}(p), v)
end
$TCunion(bytes[])
end
end
Cunion{TMemberNames,TMemberTypes,TSize}(x::NamedTuple) where {TMemberNames,TMemberTypes,TSize} =
Cunion{TMemberNames,TMemberTypes,TSize}(; x...)
Base.convert(::Type{T}, x::NamedTuple) where {T<:Cunion} = T(x)
Base.fieldnames(::Type{<:Cunion{TMemberNames}}) where {TMemberNames} = TMemberNames
Base.fieldname(::Type{<:Cunion{TMemberNames}}, i::Integer) where {TMemberNames} = TMemberNames[i]
Base.fieldcount(::Type{<:Cunion{TMemberNames}}) where {TMemberNames} = length(TMemberNames)
function Base.fieldindex(::Type{<:Cunion{TMemberNames}}, name::Symbol) where {TMemberNames}
for (i, n) in enumerate(TMemberNames)
n == name && return i
end
error("Member $name not found in union")
end
function Base.fieldoffset(::Type{T}, i::Integer) where {T<:Cunion}
i β€ fieldcount(T) || throw(BoundsError(T, i))
zero(UInt)
end
@generated function Base.fieldtypes(::Type{<:Cunion{<:Any,TMemberTypes}}) where {TMemberTypes}
t = Tuple(TMemberTypes.parameters)
:($t)
end
@inline @generated function membertype(
::Type{<:Cunion{TMemberNames,TMemberTypes}}, name::Symbol
) where {TMemberNames,TMemberTypes}
types = TMemberTypes.parameters
conds = Expr(:block)
for (name, type) in zip(TMemberNames, types)
name_symbol = QuoteNode(name)
push!(conds.args, :($name_symbol β‘ name && return $type))
end
quote
$conds
error("Member $name not found in union")
end
end
function Base.getproperty(u::T, name::Symbol) where {T<:Cunion}
data = getfield(u, :data)
TX = membertype(T, name)
bytes = Ref(data)
p = pointer_from_objref(bytes)
GC.@preserve bytes begin
unsafe_load(Ptr{TX}(p))
end::TX
end
"""
const MyUnion = @Cunion begin
i::Int64
f::Float64
A::@Carray{Int32, 2}
...
end [nbytes]
Declare a C union type with the specified members.
It can then be used to create instances like `MyUnion(i=1)` or `MyUnion(A=[1, 2])`.
Note that:
- The union instance is immutable, so you need to create a new instance to change its members.
- `nbytes` is the size of the union in bytes.
If not specified, it is computed automatically with the default alignment.
- Though "members" are different from "fields", generic functions like `fieldnames` and `fieldcount` are defined for unions.
"""
macro Cunion(body, nbytes=nothing)
members = [
let (name, type) = x.args
(name, esc(type))
end for x in body.args
if x isa Expr && x.head == :(::)
]
TMemberNames = tuple((name for (name, _) in members)...)
TMemberTypes = :(Tuple{$((type for (_, type) in members)...)})
max_size = :(let max_size = 0
for T in Ts.parameters
isbitstype(T) || throw(ArgumentError("Union member type must be a bitstype"))
max_size = max(max_size, sizeof(T))
end
max_size
end)
TSize = if isnothing(nbytes)
align = Sys.WORD_SIZE Γ· 8
:(
let align = $align
nbytes = (max_size + align - 1) Γ· align * align
end
)
else
esc(nbytes)
end
:(
let Ts = $TMemberTypes
max_size = $max_size
tsize = $TSize
tsize β₯ max_size || throw(ArgumentError("Union size is too small"))
Cunion{$TMemberNames,Ts,tsize}
end
)
end | SimpleCTypes | https://github.com/sunoru/SimpleCTypes.jl.git |
|
[
"MIT"
] | 0.1.1 | 69c59dcf00aa2b711b630cc156ca7f41243e0595 | code | 681 | using Test
using SimpleCTypes
@testset "C Array" begin
@test isbitstype(@Carray{Int, 8})
a = ones(3, 2)
aa = @Carray{Float64, 2, 3}(a)
@test length(aa) == 6
@test size(aa) == (2, 3)
@test ndims(aa) == 2
@test sizeof(aa) == 3 * 2 * sizeof(Float64)
struct Foo
arr::@Carray{Float64, 4}
matrix::@Carray{Int64, 2, 3, 4}
end
arr = rand(4)
matrix = reshape(1:24, (4, 3, 2))
# The conversion from `Array` to `Carray` should happen automatically.
foo = Foo(arr, matrix)
@test foo.matrix[1, 2, 2:4] == [6, 7, 8]
@test isbitstype(Foo)
@test sizeof(foo) == 4 * sizeof(Float64) + 2 * 3 * 4 * sizeof(Int64)
end
| SimpleCTypes | https://github.com/sunoru/SimpleCTypes.jl.git |
|
[
"MIT"
] | 0.1.1 | 69c59dcf00aa2b711b630cc156ca7f41243e0595 | code | 1161 | using Test
using SimpleCTypes
@testset "C Function" begin
f(x) = 2x
f_ptr, cf = @cfunc($f, Int, (Int,))
@test f_ptr β‘ CfuncPtr{Int,Tuple{Int}}(cf)
GC.@preserve cf begin
@test f_ptr(3) == 6
@test_throws ErrorException f_ptr()
@test_throws ErrorException f_ptr(1, 2)
end
@test sizeof(f_ptr) == sizeof(Ptr{Nothing})
struct SomeStruct
x::Int
y::Int
add::CfuncPtr{Int,Tuple{Int,Int}}
end
ff(ss::SomeStruct) = ss.add(ss.x, ss.y)
add_ptr, _ = @cfunc(+, Int, (Int, Int))
ss = SomeStruct(1, 2, add_ptr)
@test isbitstype(SomeStruct)
@test ff(ss) == 3
@test_throws MethodError SomeStruct(2, 3, f_ptr)
function cmp(a, b)::Cint
(a < b) ? -1 : ((a > b) ? +1 : 0)
end
# CFunction
cmp_ptr, cmp_cf = @cfunc($cmp, Cint, (Ref{Cdouble}, Ref{Cdouble}))
A = [1.3, -2.7, 4.4, 3.1]
GC.@preserve begin
@ccall qsort(
A::Ptr{Cdouble},
length(A)::Csize_t,
sizeof(eltype(A))::Csize_t,
cmp_ptr::CfuncPtr{Cint,Tuple{Ref{Cdouble},Ref{Cdouble}}}
)::Cvoid
end
@test issorted(A)
end
| SimpleCTypes | https://github.com/sunoru/SimpleCTypes.jl.git |
|
[
"MIT"
] | 0.1.1 | 69c59dcf00aa2b711b630cc156ca7f41243e0595 | code | 148 | using Test
using SimpleCTypes
@testset "SimpleCTypes.jl" begin
include("./function.jl")
include("./array.jl")
include("./union.jl")
end | SimpleCTypes | https://github.com/sunoru/SimpleCTypes.jl.git |
|
[
"MIT"
] | 0.1.1 | 69c59dcf00aa2b711b630cc156ca7f41243e0595 | code | 1016 | using Test
using SimpleCTypes
@testset "C Union" begin
FooUnion = @Cunion begin
a::Int64
b::Float64
c::@Carray{Int16, 5}
end 13
@test sizeof(FooUnion) == 13
@test fieldnames(FooUnion) β‘ (:a, :b, :c)
@test fieldname(FooUnion, 1) β‘ :a
@test Base.fieldindex(FooUnion, :b) == 2
@test [fieldoffset(FooUnion, i) for i in 1:3] == zeros(Int, 3)
@test fieldtypes(FooUnion) β‘ (Int64, Float64, @Carray{Int16, 5})
@test fieldcount(FooUnion) == 3
fu = FooUnion(a=99999)
@test fu.a == 99999
@test fu.b == 4.9406e-319
@test fu.c == Int16[-31073, 1, 0, 0, 0]
BarUnion = @Cunion begin
a::@Cunion begin
x::UInt64
y::@Carray{UInt8, 8}
end
b::@Carray{UInt32, 2}
end
@test sizeof(BarUnion) == 8
x = 0x0123456789abcdef
bu = BarUnion(a=(;x=x))
@test bu.a.x == x
@test bu.a.y == UInt8[0xef, 0xcd, 0xab, 0x89, 0x67, 0x45, 0x23, 0x01]
@test bu.b == UInt32[0x89abcdef, 0x01234567]
end
| SimpleCTypes | https://github.com/sunoru/SimpleCTypes.jl.git |
|
[
"MIT"
] | 0.1.1 | 69c59dcf00aa2b711b630cc156ca7f41243e0595 | docs | 2697 | # SimpleCTypes.jl
[](https://github.com/sunoru/SimpleCTypes.jl/actions/workflows/CI.yml)
[](https://codecov.io/github/sunoru/SimpleCTypes.jl)
`SimipleCTypes.jl` is a zero-dependency Julia package that provides some simple definitions of C types, specifically:
- `CfuncPtr` for C function pointers
- `Carray` for fixed-size C arrays
- `Cunion` for C-style unions
It is mainly used in the type definitions of Julia bindings for C libraries.
All types are immutable so that they can have the same memory layout as in C.
## Usage
## `CfuncPtr` and `@cfunc`
`CfuncPtr` can be used to replace `Ptr{Cvoid}` in `ccall` and struct definitions,
so that you can pass a function pointer around in a type-safe way.
It takes two type parameters, the return type and the argument types of the function.
```c
struct foo {
int (*f)(int, int);
};
```
can be defined in Julia as:
```julia
struct Foo
f::CfuncPtr{Cint,Tuple{Cint,Cint}}
end
```
You can use `@cfunc` to create a `CfuncPtr` from a Julia function,
its usage is the same as `@cfunction`:
```julia
f(x) = 2x
fptr, _ = @cfunc(f, Cint, (Cint,))
fptr2, cf = @cfunc($f, Cint, (Cint,))
```
When using a clousure, you need to use `$` to interpolate the function into the macro,
and the second return value of `@cfunc` (`cf` in the example) should be referenced somewhere
to prevent the GC from collecting it.
## `Carray` and `@Carray`
`Carray` is similar to `StaticArrays.SArray`, representing a fixed-size immutable array.
Usually you only need to use `@Carray` whenever you need a C array. For example,
```c
int[3] a = {1,2,3};
struct bar {
double x[2][4];
};
```
can be defined in Julia as:
```julia
a = @Carray(Cint[1,2,3])
struct Bar
x::@Carray{Cdouble,2,4}
end
```
Note that the array will be stored in row-major order, so when you pass a Julia array to `@Carray`, the dimension order is reversed.
## `Cunion` and `@Cunion`
`Cunion` represents a C-style union. You need to use `@Cunion begin ... end` to declare a union type.
For example,
```c
union Baz {
union {
uint64_t x;
unsigned char y[8];
} a;
uint b[2];
} baz = { .a = { .x = 0x123456789abcdef0 } };
```
should be:
```julia
const Baz = @Cunion begin
a::@Cunion begin
x::Cuint64
y::@Carray{Cuchar,8}
end
b::Carray{Cuint,2}
end
baz = Baz(a=(; x=0x123456789abcdef0))
```
And the usage of `baz` is almost the same as in C, except that `Cunion`s and `Carray`s are all immutable.
## License
[MIT License](./LICENSE).
| SimpleCTypes | https://github.com/sunoru/SimpleCTypes.jl.git |
|
[
"MIT"
] | 2.0.2 | b8583045a1df4f2e4c3df6b1e70a93ed2b74d63d | code | 2625 | module BoxCoxTrans
using Optim: optimize, minimizer
using Statistics: mean, var
using StatsBase: geomean
"""
transform(π±)
Transform an array using Box-Cox method. The power parameter Ξ» is derived
from maximizing a log-likelihood estimator.
If the array contains any non-positive values then a DomainError is thrown.
This can be avoided by providing the shift parameter Ξ± to make all values
positive.
Keyword arguments:
- Ξ±: added to all values in π± before transformation. Default = 0.
- scaled: scale transformation results. Default = false.
"""
function transform(π±; kwargs...)
Ξ», details = lambda(π±; kwargs...)
#@info "estimated lambda = $Ξ»"
transform(π±, Ξ»; kwargs...)
end
"""
transform(π±, Ξ»; Ξ± = 0)
Transform an array using Box-Cox method with the provided power parameter Ξ».
If the array contains any non-positive values then a DomainError is thrown.
Keyword arguments:
- Ξ±: added to all values in π± before transformation. Default = 0.
- scaled: scale transformation results. Default = false.
"""
function transform(π±, Ξ»; Ξ± = 0, scaled = false, kwargs...)
if Ξ± != 0
π± .+= Ξ±
end
any(π± .<= 0) && throw(DomainError("Data must be positive and ideally greater than 1. You may specify Ξ± argument(shift). "))
if scaled
gm = geomean(π±)
@. Ξ» β 0 ? gm * log(π±) : (π± ^ Ξ» - 1) / (Ξ» * gm ^ (Ξ» - 1))
else
@. Ξ» β 0 ? log(π±) : (π± ^ Ξ» - 1) / Ξ»
end
end
"""
lambda(π±; interval = (-2.0, 2.0), method = :geomean)
Calculate lambda from an array using a log-likelihood estimator.
Keyword arguments:
- method: either :geomean or :normal
- any other keyword arguments accepted by Optim.optimize function e.g. abs_tol
See also: [`log_likelihood`](@ref)
"""
function lambda(π±; interval = (-2.0, 2.0), kwargs...)
i1, i2 = interval
res = optimize(Ξ» -> -log_likelihood(π±, Ξ»; kwargs...), i1, i2)
(value=minimizer(res), details=res)
end
"""
log_likelihood(π±, Ξ»; method = :geomean)
Return log-likelihood for the given array and lambda.
Method :geomean =>
-N / 2.0 * log(2 * Ο * ΟΒ² / gm ^ (2 * (Ξ» - 1)) + 1)
Method :normal =>
-N / 2.0 * log(ΟΒ²) + (Ξ» - 1) * sum(log.(π±))
"""
function log_likelihood(π±, Ξ»; method = :geomean, kwargs...)
N = length(π±)
π² = transform(float.(π±), Ξ»)
ΟΒ² = var(π², corrected = false)
gm = geomean(π±)
if method == :geomean
-N / 2.0 * log(2 * Ο * ΟΒ² / gm ^ (2 * (Ξ» - 1)) + 1)
elseif method == :normal
-N / 2.0 * log(ΟΒ²) + (Ξ» - 1) * sum(log.(π±))
else
throw(ArgumentError("Incorrect method. Please specify :geomean or :normal."))
end
end
end # module
| BoxCoxTrans | https://github.com/tk3369/BoxCoxTrans.jl.git |
|
[
"MIT"
] | 2.0.2 | b8583045a1df4f2e4c3df6b1e70a93ed2b74d63d | code | 2511 | import BoxCoxTrans
using Statistics: mean, var
using Test
π± = [
185,172,424,358,157,193,439,170,155,238,194,193,356,225,378,279,835,588,280,
206,309,444,192,205,154,168,289,246,196,645,191,613,554,429,177,205,212,166,468,
259,393,167,534,798,172,525,2031,215,547,205,200,157,944,241,244,225,425,329,201,
300,383,235,176,367,879,316,1082,302,204,187,307,236,292,718,354,265,213,160,597,
157,156,226,205,362,310,404,436,204,168,358,363,209,1638,179,193,225,680,277,853,
249,499,251,529,265,250,271,294,364,155,194,610,1271,167,173,250,185,380,183,232,
228,230,185,263,317,204,199,578,597,493,210,162,512,509,193,395,244,569,1016,257,
207,254,201,325,201,266,233,286,193,401,283,220,168,177,316,377,569,189,181,385,
153,162,1099,351,263,176,300,320,271,645,237,617,188,193,212,342,330,206,169,250,
244,150,424,357,248,1485,270,319,183,198,440,162,940,187,407,458,192,172,164,260,
209,165,285,270,388,214,163,309,741,153,170,461,246,193,158,157,167,210,194,154,
289,441,279,203,229,165,219,200,470,215,670,202,384,161,183,263,283,268,1383,215,
215,279,305,432,672,286,214,1168,190,292,305,545,423,251,581,194,347,186,776,187,
253,153,661,211,188,544,464,392,425,331,204,562,171,386,219,163,184,165,159,172,
161,256,156,445,263,197,181,232,351,510,251,281,161,167,533,512,176,199,220,270,
414,181,170,366,323,286,430,202,264,762,249,224,495,176,256,291,150,234,223,355,
156,216,403,155,300,345,298,170,257,208,266,921,267,315,351,153,162,444,223,193,
220,540,327,703,196,344,264,168,281,184,182,193,340,330,358,189,240,247,153,191,
222,162,363,159,199,184,273,158,278,166,250,272,160,156,211,381,841,180,166,219,
258,275,257,302,289,255,180,176,330,227,201,765,154,491,230,404,160,156,228,231,
376,156,1195,640,607]
# precision tolerance
mytol=1e-4
# lambda tests
v = -0.991720
Ξ», details = BoxCoxTrans.lambda(π±)
@test Ξ» β v atol=mytol
Ξ», details = BoxCoxTrans.lambda(π±; method = :geomean)
@test Ξ» β v atol=mytol
Ξ», details = BoxCoxTrans.lambda(π±; method = :normal)
@test Ξ» β v atol=mytol
π² = BoxCoxTrans.transform(π±)
@test sum(π²) β 405.682126 atol=mytol
@test mean(π²) β 1.0041636803675948 atol=mytol
@test var(π²) β 2.7241853245802466e-6 atol=mytol
π²2 = BoxCoxTrans.transform(π±; scaled = true)
@test var(π²2) β 15188.833710662804 atol=mytol
@test_throws DomainError BoxCoxTrans.transform([1,2,3,0])
@test_throws DomainError BoxCoxTrans.transform([1,2,3,-4])
@test_throws ArgumentError BoxCoxTrans.lambda(π±; method = :badmethod) | BoxCoxTrans | https://github.com/tk3369/BoxCoxTrans.jl.git |
|
[
"MIT"
] | 2.0.2 | b8583045a1df4f2e4c3df6b1e70a93ed2b74d63d | docs | 5162 | # BoxCoxTrans.jl
[](https://github.com/tk3369/BoxCoxTrans.jl/actions?query=workflow%3ACI)
[](https://codecov.io/gh/tk3369/BoxCoxTrans.jl)
[](https://coveralls.io/github/tk3369/BoxCoxTrans.jl?branch=master)
This package provides an implementation of Box Cox transformation.
See [Wikipedia - Power Transform](https://en.wikipedia.org/wiki/Power_transform)
for more information.
## Installation
```
] add BoxCoxTrans
```
## Usage
The simplest way is to just call the `transform` function with an array of numbers.
```
julia> using Distributions, UnicodePlots, BoxCoxTrans
julia> x = rand(Gamma(2,2), 10000) .+ 1;
julia> histogram(x)
ββββββββββββββββββββββββββββββββββββββββββββ
(0.0,2.0] βββββββββ 852 β
(2.0,4.0] βββββββββββββββββββββββββββββββββββ 3554 β
(4.0,6.0] ββββββββββββββββββββββββββ 2655 β
(6.0,8.0] ββββββββββββββββ 1524 β
(8.0,10.0] βββββββββ 798 β
(10.0,12.0] ββββ 316 β
(12.0,14.0] βββ 170 β
(14.0,16.0] ββ 76 β
(16.0,18.0] β 35 β
(18.0,20.0] β 14 β
(20.0,22.0] β 5 β
(22.0,24.0] β 1 β
ββββββββββββββββββββββββββββββββββββββββββββ
julia> histogram(BoxCoxTrans.transform(x))
ββββββββββββββββββββββββββββββββββββββββββ
(0.0,0.2] βββ 64 β
(0.2,0.4] βββββ 166 β
(0.4,0.6] βββββββββββ 386 β
(0.6,0.8] ββββββββββββββββ 559 β
(0.8,1.0] ββββββββββββββββββββββββ 887 β
(1.0,1.2] ββββββββββββββββββββββββββββββ 1091 β
(1.2,1.4] ββββββββββββββββββββββββββββββββββ 1257 β
(1.4,1.6] βββββββββββββββββββββββββββββββββββ 1298 β
(1.6,1.8] βββββββββββββββββββββββββββββββββββ 1281 β
(1.8,2.0] ββββββββββββββββββββββββββββββ 1103 β
(2.0,2.2] ββββββββββββββββββββββββ 877 β
(2.2,2.4] βββββββββββββββ 541 β
(2.4,2.6] ββββββββ 272 β
(2.6,2.8] βββββ 153 β
(2.8,3.0] ββ 50 β
(3.0,3.2] β 14 β
(3.2,3.4] β 1 β
ββββββββββββββββββββββββββββββββββββββββββ
```
You can examine the power transform parameter (Ξ») derived by the program:
```
julia> BoxCoxTrans.lambda(x).value
0.013544484565969775
```
You can transfrom the data using your own Ξ»:
```
julia> histogram(BoxCoxTrans.transform(x, 0.01))
ββββββββββββββββββββββββββββββββββββββββββ
(0.0,0.2] βββ 64 β
(0.2,0.4] βββββ 166 β
(0.4,0.6] βββββββββββ 386 β
(0.6,0.8] ββββββββββββββββ 563 β
(0.8,1.0] ββββββββββββββββββββββββ 894 β
(1.0,1.2] ββββββββββββββββββββββββββββββ 1094 β
(1.2,1.4] ββββββββββββββββββββββββββββββββββ 1261 β
(1.4,1.6] βββββββββββββββββββββββββββββββββββ 1302 β
(1.6,1.8] βββββββββββββββββββββββββββββββββββ 1289 β
(1.8,2.0] ββββββββββββββββββββββββββββββ 1111 β
(2.0,2.2] ββββββββββββββββββββββββ 868 β
(2.2,2.4] βββββββββββββββ 534 β
(2.4,2.6] ββββββββ 268 β
(2.6,2.8] βββββ 142 β
(2.8,3.0] ββ 47 β
(3.0,3.2] β 11 β
ββββββββββββββββββββββββββββββββββββββββββ
```
There's an option to scale the results by the geometric mean.
```
julia> histogram(BoxCoxTrans.transform(x; scaled = true))
ββββββββββββββββββββββββββββββββββββββββββ
(0.0,1.0] βββ 81 β
(1.0,2.0] βββββββ 258 β
(2.0,3.0] βββββββββββββ 540 β
(3.0,4.0] ββββββββββββββββββββ 868 β
(4.0,5.0] βββββββββββββββββββββββββββββ 1234 β
(5.0,6.0] ββββββββββββββββββββββββββββββββββ 1467 β
(6.0,7.0] βββββββββββββββββββββββββββββββββββ 1521 β
(7.0,8.0] ββββββββββββββββββββββββββββββββββ 1488 β
(8.0,9.0] ββββββββββββββββββββββββββ 1133 β
(9.0,10.0] βββββββββββββββββββ 806 β
(10.0,11.0] βββββββββ 359 β
(11.0,12.0] βββββ 188 β
(12.0,13.0] ββ 50 β
(13.0,14.0] β 7 β
ββββββββββββββββββββββββββββββββββββββββββ
```
| BoxCoxTrans | https://github.com/tk3369/BoxCoxTrans.jl.git |
|
[
"MIT"
] | 0.1.1 | fb6ee517e60842228845362a06f9c28af811c1af | code | 5108 | module FindDefinition
export finddef, finddefs
import MacroTools
ismacrocall(_) = false
ismacrocall(expr::Expr) = expr.head == :macrocall
ismoduledef(_) = false
ismoduledef(e::Expr) = e.head == :module
isinclude(_) = false
isinclude(e::Expr) = e.head == :call && e.args[1] == :include
isquote(_) = false
isquote(::QuoteNode) = true
isquote(e::Expr) = e.head == :quote
args(_) = []
args(e::Expr) = e.args
function collect_nodes(f, _module, ast; skip_quoted = true)
skip(arg) = skip_quoted && isquote(arg)
init = f(ast) ? [(from_module = _module, ex = ast)] : []
if ismoduledef(ast)
modulename = ast.args[2]
_module = getproperty(_module, modulename)
end
reduce( βͺ, collect_nodes(f, _module, arg) for arg in args(ast) if !skip(arg); init )
end
collect_includefiles(_module, ast) = [(; from_module = mod, file = ex.args[2])
for (mod, ex) in collect_nodes(isinclude, _module, ast)]
function parsefile(path, fromline = 1; greedy=true)
str = read(path, String)
newlines = only.(findall("\n", str))
res = Expr[]
pos = fromline == 1 ? 1 : newlines[fromline-1]+1
while pos < length(str)
lineshift = searchsortedfirst(newlines, pos) - 1
fix_lnn(e) = e
fix_lnn(lnn::LineNumberNode) = LineNumberNode(lnn.line + lineshift, path)
expr, pos = Meta.parse(str, pos)
isnothing(expr) && continue ## why can this happen?
expr = MacroTools.postwalk(fix_lnn, expr)
push!(res, expr)
greedy || break
end
res
end
function rec_find_expr(f, _module, ast, includepath)
res = collect_nodes(f, _module, ast)
includes = collect_includefiles(_module, ast)
for (mod, inc) in includes
if !(inc isa AbstractString)
@warn "Cannot resolve non-literal include `include($inc)` from module $mod. Skipped."
continue
end
inc = joinpath(includepath, inc)
if !isfile(inc)
@warn "Included file not found: $inc."
continue
end
for expr in parsefile(inc)
append!(res, rec_find_expr(f, mod, expr, dirname(inc)))
end
end
res
end
trysplitdef(ex) = try
MacroTools.splitdef(ex)
catch
nothing
end
# MacroTools.isdef is broken. See MacroTools issue #154
isfunctiondef(ex) = trysplitdef(ex) != nothing
istypeassertion(ex) = false
istypeassertion(ex::Expr) = ex.head == :(::)
isinterpolated(ex) = false
isinterpolated(ex::Expr) = ex.head == :$
dequalify(name) = name
dequalify(name::Expr) = (name.head == :(.) || error("Not a qualified name: $name");
name.args[2].value)
dequalify(name::GlobalRef) = name.name
argtypes(method) = Base.tail(tuple(method.sig.types...))
function defines_this_method(_module, ex, method)
d = trysplitdef(ex)
isnothing(d) && return false
qualified_name = get(d,:name,nothing)
qualified_name === nothing && return false
istypeassertion(qualified_name) && return false # a type method definition, e.g. (foo::Foo)(x,y) = ...
if isinterpolated(qualified_name)
@warn "Cannot resolve interpolated function name $qualified_name in function definition $ex"
return false
end
dequalify(qualified_name) != method.name && return false
# TODO should be possible without `eval`, using `Meta.lower`
newname = gensym()
d[:name] = newname
newexp = MacroTools.combinedef(d)
f = try
_module.eval(newexp)
catch e
@warn("Failed to evaluate symgen-ed function definition in module $_module.",
definition = newexp)
showerror(stderr, e, catch_backtrace())
println(stderr)
return false
end
exmethod = only(methods(f))
return argtypes(exmethod) == argtypes(method) &&
Base.kwarg_decl(exmethod) == Base.kwarg_decl(method)
end
macrocall_linenumnode(macrocall) = macrocall.args[2]
function find_definitions(method::Method)
_module = method.module
eval_method = only(methods(_module.eval))
file, line = string(eval_method.file), eval_method.line
module_def = only(parsefile(file, line; greedy=false))
res = []
mcls = rec_find_expr(ismacrocall, _module, module_def, dirname(file))
for (_module, macrocall) in mcls
lnn = macrocall_linenumnode(macrocall)
expanded = try
macroexpand(_module, macrocall)
catch e
@warn("Failed to expand macrocall in $_module:$lnn. Skipping.",
macrocall=macrocall)
showerror(stderr, e, catch_backtrace())
println(stderr)
continue
end
funcdefs = collect_nodes(isfunctiondef, _module, expanded)
for (_module, funcdef) in funcdefs
if defines_this_method(_module, funcdef, method)
push!(res, (; lnn, funcdef))
end
end
end
res
end
find_definitions(f::Function) = reduce( βͺ, find_definitions(m) for m in methods(f) )
finddef(m::Method) = last(find_definitions(m)).lnn
finddefs(f::Function) = map(finddef, methods(f))
end
| FindDefinition | https://github.com/yha/FindDefinition.jl.git |
|
[
"MIT"
] | 0.1.1 | fb6ee517e60842228845362a06f9c28af811c1af | code | 221 | module Bar1
include("foo.jl")
using .Foo: @foo
m() = r
q() = z
@foo y
end
include("foo.jl")
module Bar2
using ..Foo: @foo, @foo_y, @multifoo
@foo u
@foo v
@foo_y w
@multifoo q
end
| FindDefinition | https://github.com/yha/FindDefinition.jl.git |
|
[
"MIT"
] | 0.1.1 | fb6ee517e60842228845362a06f9c28af811c1af | code | 315 | module Foo
macro foo(x)
esc(:(bar() = $x))
end
macro foo_y(x)
esc(:(bar(y) = y + $x))
end
macro multifoo(x)
_multifoo_impl(x)
end
_multifoo_impl(x) = esc(quote
multibar() = $x
multibar(y) = y
multibar(z::Int, args...) = z + 1
end)
end
| FindDefinition | https://github.com/yha/FindDefinition.jl.git |
|
[
"MIT"
] | 0.1.1 | fb6ee517e60842228845362a06f9c28af811c1af | code | 1406 | using Test
using FindDefinition
include("bars.jl")
barspath = joinpath(@__DIR__, "bars.jl")
@testset "single macro call" begin
bar1_methoddefs = FindDefinition.find_definitions(only(methods(Bar1.bar)))
bar1_func_lnns = finddefs(Bar1.bar)
@test length(bar1_methoddefs) == 1
@test [d.lnn for d in bar1_methoddefs] == bar1_func_lnns
lnn = only(bar1_func_lnns)
@test string(lnn.file) == barspath
@test lnn.line == 6
end
@testset "several calls" begin
meths = methods(Bar2.bar)
bar2_methoddefs = FindDefinition.find_definitions.(meths)
bar2_func_lnns = finddefs(Bar2.bar)
@test length(bar2_methoddefs) == 2
@test length(bar2_methoddefs[1]) == 2
@test length(bar2_methoddefs[2]) == 1
@test length(bar2_func_lnns) == 2
@test all( all( string(d.lnn.file) == barspath for d in defs )
for defs in bar2_methoddefs )
@test bar2_methoddefs[1][1].lnn.line == 12
@test bar2_methoddefs[1][2].lnn.line == 13
@test bar2_methoddefs[2][1].lnn.line == 14
@test bar2_func_lnns[1].line == 13
@test bar2_func_lnns[2].line == 14
end
@testset "several definitions in one macro" begin
meths = methods(Bar2.multibar)
@assert length(meths) == 3
defs = finddefs(Bar2.multibar)
@test length(defs) == 3
@test defs[1] == defs[2] == defs[3]
@test string(defs[1].file) == barspath
@test defs[1].line == 15
end
| FindDefinition | https://github.com/yha/FindDefinition.jl.git |
|
[
"MIT"
] | 0.1.1 | fb6ee517e60842228845362a06f9c28af811c1af | docs | 941 | # FindDefinition
*Locate methods defined through macros*
Methods defined through macros unhelpfully report their file and line numbers as those inside the macro definition. For example, this
```julia
# contents of foo.jl:
module Foo
macro foo()
:(bar() = x) # line 3
end
@foo() # line 6
end
# somewhere else:
bar()
```
gives an `UndefVarError` with the stack trace pointing to line 3, rather than 6.
This module provides functions `finddef(method)` and `finddefs(f::Function)` returning `LineNumberNode`s for the macro call sites:
```julia
julia> using FindDefinition
julia> finddef(first(methods(Foo.bar)))
:(#= [...]/foo.jl:6 =#)
julia> finddefs(Foo.bar)
1-element Array{LineNumberNode,1}:
:(#= [...]/foo.jl:6 =#)
```
__Warning__: The current implementation uses `eval` inside loaded modules to match method signatures. This is probably harmless, but does produce new `gensym`ed symbols inside your loaded modules.
| FindDefinition | https://github.com/yha/FindDefinition.jl.git |
|
[
"MIT"
] | 1.1.0 | b87c6fd8d4cd7342ae4c31746b9e18a4da6b827e | code | 842 | module ZeroRing
using LinearAlgebra
using Random
export zeroelem, ZeroElem
struct ZeroElem <: Number end
const zeroelem = ZeroElem()
Base.:(==)(::ZeroElem, ::ZeroElem) = true
Base.:(<)(::ZeroElem, ::ZeroElem) = false
Base.hash(::ZeroElem, h::UInt) = hash(UInt(0x56212a80), h)
Base.rand(rng::AbstractRNG, ::Random.SamplerType{ZeroElem}) = zeroelem
Base.:+(::ZeroElem) = zeroelem
Base.:-(::ZeroElem) = zeroelem
Base.:+(::ZeroElem, ::ZeroElem) = zeroelem
Base.:-(::ZeroElem, ::ZeroElem) = zeroelem
Base.inv(::ZeroElem) = zeroelem
Base.:*(::ZeroElem, ::ZeroElem) = zeroelem
Base.:/(::ZeroElem, ::ZeroElem) = zeroelem
Base.:\(::ZeroElem, ::ZeroElem) = zeroelem
Base.:^(::ZeroElem, ::ZeroElem) = zeroelem
Base.copy(::ZeroElem) = zeroelem
LinearAlgebra.adjoint(::ZeroElem) = zeroelem
LinearAlgebra.transpose(::ZeroElem) = zeroelem
end
| ZeroRing | https://github.com/eschnett/ZeroRing.jl.git |
|
[
"MIT"
] | 1.1.0 | b87c6fd8d4cd7342ae4c31746b9e18a4da6b827e | code | 630 | using Test
using ZeroRing
zeroelem::ZeroElem
@test zeroelem === zeroelem
@test zeroelem == zeroelem
@test !(zeroelem != zeroelem)
@test !(zeroelem < zeroelem)
@test hash(zeroelem) === hash(zeroelem)
@test rand(ZeroElem) === zeroelem
@test +zeroelem === zeroelem
@test -zeroelem === zeroelem
@test zeroelem + zeroelem === zeroelem
@test zeroelem - zeroelem === zeroelem
@test inv(zeroelem) === zeroelem
@test zeroelem * zeroelem === zeroelem
@test zeroelem / zeroelem === zeroelem
@test zeroelem^zeroelem === zeroelem
@test copy(zeroelem) === zeroelem
@test zeroelem' === zeroelem
@test transpose(zeroelem) === zeroelem
| ZeroRing | https://github.com/eschnett/ZeroRing.jl.git |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.