Datasets:
Tasks:
Image Segmentation
Modalities:
Image
Sub-tasks:
semantic-segmentation
Languages:
English
Size:
10K - 100K
License:
Update README.md
Browse files
README.md
CHANGED
@@ -82,7 +82,7 @@ mask = np.array(mask)
|
|
82 |
```
|
83 |
|
84 |
|
85 |
-
###
|
86 |
|
87 |
```python
|
88 |
from matplotlib.colors import ListedColormap, BoundaryNorm
|
@@ -111,15 +111,45 @@ def plot(image, mask, cmap='gray'):
|
|
111 |
plt.show()
|
112 |
```
|
113 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
114 |
```python
|
115 |
plot(image, mask)
|
116 |
```
|
117 |
|
118 |

|
119 |
|
|
|
|
|
|
|
120 |
```python
|
121 |
plot_mask(mask)
|
122 |
```
|
123 |
|
124 |
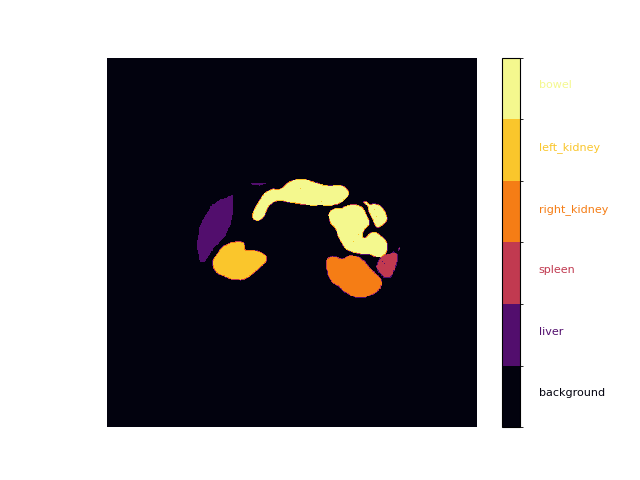
|
125 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
82 |
```
|
83 |
|
84 |
|
85 |
+
### Write Plotting Function
|
86 |
|
87 |
```python
|
88 |
from matplotlib.colors import ListedColormap, BoundaryNorm
|
|
|
111 |
plt.show()
|
112 |
```
|
113 |
|
114 |
+
### Plot
|
115 |
+
|
116 |
+
```python
|
117 |
+
fig = plt.figure(figsize=(16,16))
|
118 |
+
ax1 = fig.add_subplot(131)
|
119 |
+
plt.axis('off')
|
120 |
+
ax1.imshow(image, cmap='gray')
|
121 |
+
ax2 = fig.add_subplot(132)
|
122 |
+
plt.axis('off')
|
123 |
+
ax2.imshow(mask, cmap='gray')
|
124 |
+
ax3 = fig.add_subplot(133)
|
125 |
+
ax3.imshow(image*np.where(mask>0,1,0), cmap='gray')
|
126 |
+
plt.axis('off')
|
127 |
+
plt.show()
|
128 |
+
```
|
129 |
+
|
130 |
+

|
131 |
+
|
132 |
```python
|
133 |
plot(image, mask)
|
134 |
```
|
135 |
|
136 |

|
137 |
|
138 |
+
|
139 |
+
### Custom Color
|
140 |
+
|
141 |
```python
|
142 |
plot_mask(mask)
|
143 |
```
|
144 |
|
145 |
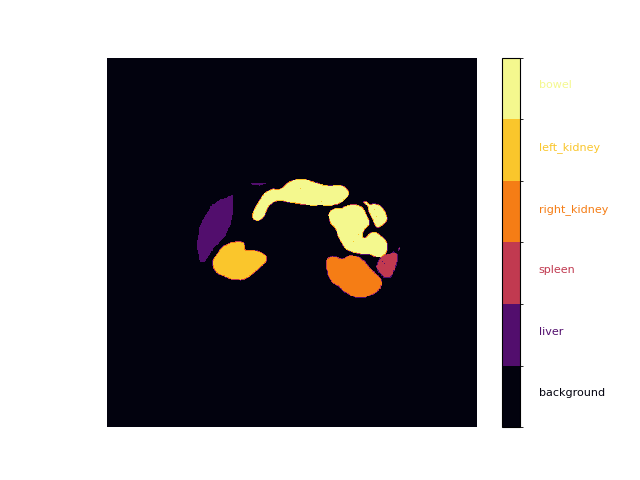
|
146 |
|
147 |
+
### Plot only one class (e.g. liver)
|
148 |
+
|
149 |
+
```python
|
150 |
+
liver, spleen, right_kidney, left_kidney, bowel = [(mask == i,1,0)[0] * i for i in range(1, len(labels))]
|
151 |
+
plot_mask(liver)
|
152 |
+
```
|
153 |
+
|
154 |
+
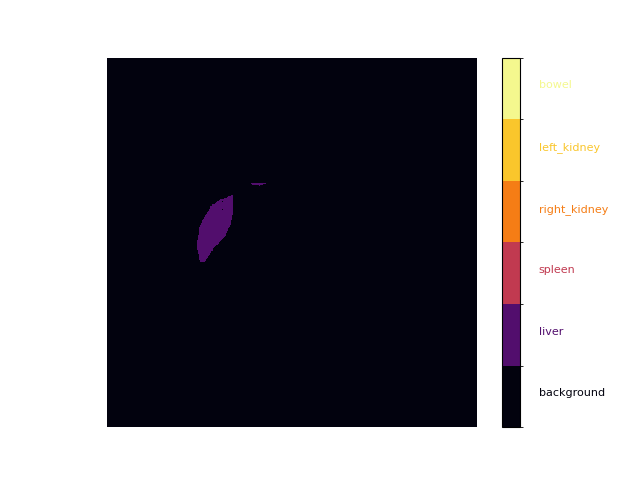
|
155 |
+
|