Upload README.md with huggingface_hub
Browse files
README.md
CHANGED
@@ -9,7 +9,7 @@ pipeline_tag: unconditional-image-generation
|
|
9 |
|
10 |
---
|
11 |
|
12 |
-
.
|
95 |
```
|
96 |
-
%run -m qai_hub_models.models.
|
97 |
```
|
98 |
|
99 |
|
@@ -106,7 +106,7 @@ device. This script does the following:
|
|
106 |
* Accuracy check between PyTorch and on-device outputs.
|
107 |
|
108 |
```bash
|
109 |
-
python -m qai_hub_models.models.
|
110 |
```
|
111 |
```
|
112 |
Profiling Results
|
@@ -150,91 +150,21 @@ Compute Unit(s) : NPU (2406 ops)
|
|
150 |
|
151 |
## How does this work?
|
152 |
|
153 |
-
This [export script](https://aihub.qualcomm.com/models/
|
154 |
leverages [Qualcomm® AI Hub](https://aihub.qualcomm.com/) to optimize, validate, and deploy this model
|
155 |
on-device. Lets go through each step below in detail:
|
156 |
|
157 |
-
Step 1: **
|
158 |
-
|
159 |
-
To compile a PyTorch model for on-device deployment, we first trace the model
|
160 |
-
in memory using the `jit.trace` and then call the `submit_compile_job` API.
|
161 |
|
|
|
162 |
```python
|
163 |
import torch
|
164 |
|
165 |
import qai_hub as hub
|
166 |
-
from qai_hub_models.models.
|
167 |
|
168 |
# Load the model
|
169 |
-
model = Model.
|
170 |
-
controlnet_model = model.controlnet
|
171 |
-
text_encoder_model = model.text_encoder
|
172 |
-
unet_model = model.unet
|
173 |
-
vae_decoder_model = model.vae_decoder
|
174 |
-
|
175 |
-
# Device
|
176 |
-
device = hub.Device("Samsung Galaxy S23")
|
177 |
-
|
178 |
-
# Trace model
|
179 |
-
controlnet_input_shape = controlnet_model.get_input_spec()
|
180 |
-
controlnet_sample_inputs = controlnet_model.sample_inputs()
|
181 |
-
|
182 |
-
traced_controlnet_model = torch.jit.trace(controlnet_model, [torch.tensor(data[0]) for _, data in controlnet_sample_inputs.items()])
|
183 |
-
|
184 |
-
# Compile model on a specific device
|
185 |
-
controlnet_compile_job = hub.submit_compile_job(
|
186 |
-
model=traced_controlnet_model ,
|
187 |
-
device=device,
|
188 |
-
input_specs=controlnet_model.get_input_spec(),
|
189 |
-
)
|
190 |
-
|
191 |
-
# Get target model to run on-device
|
192 |
-
controlnet_target_model = controlnet_compile_job.get_target_model()
|
193 |
-
# Trace model
|
194 |
-
text_encoder_input_shape = text_encoder_model.get_input_spec()
|
195 |
-
text_encoder_sample_inputs = text_encoder_model.sample_inputs()
|
196 |
-
|
197 |
-
traced_text_encoder_model = torch.jit.trace(text_encoder_model, [torch.tensor(data[0]) for _, data in text_encoder_sample_inputs.items()])
|
198 |
-
|
199 |
-
# Compile model on a specific device
|
200 |
-
text_encoder_compile_job = hub.submit_compile_job(
|
201 |
-
model=traced_text_encoder_model ,
|
202 |
-
device=device,
|
203 |
-
input_specs=text_encoder_model.get_input_spec(),
|
204 |
-
)
|
205 |
-
|
206 |
-
# Get target model to run on-device
|
207 |
-
text_encoder_target_model = text_encoder_compile_job.get_target_model()
|
208 |
-
# Trace model
|
209 |
-
unet_input_shape = unet_model.get_input_spec()
|
210 |
-
unet_sample_inputs = unet_model.sample_inputs()
|
211 |
-
|
212 |
-
traced_unet_model = torch.jit.trace(unet_model, [torch.tensor(data[0]) for _, data in unet_sample_inputs.items()])
|
213 |
-
|
214 |
-
# Compile model on a specific device
|
215 |
-
unet_compile_job = hub.submit_compile_job(
|
216 |
-
model=traced_unet_model ,
|
217 |
-
device=device,
|
218 |
-
input_specs=unet_model.get_input_spec(),
|
219 |
-
)
|
220 |
-
|
221 |
-
# Get target model to run on-device
|
222 |
-
unet_target_model = unet_compile_job.get_target_model()
|
223 |
-
# Trace model
|
224 |
-
vae_decoder_input_shape = vae_decoder_model.get_input_spec()
|
225 |
-
vae_decoder_sample_inputs = vae_decoder_model.sample_inputs()
|
226 |
-
|
227 |
-
traced_vae_decoder_model = torch.jit.trace(vae_decoder_model, [torch.tensor(data[0]) for _, data in vae_decoder_sample_inputs.items()])
|
228 |
-
|
229 |
-
# Compile model on a specific device
|
230 |
-
vae_decoder_compile_job = hub.submit_compile_job(
|
231 |
-
model=traced_vae_decoder_model ,
|
232 |
-
device=device,
|
233 |
-
input_specs=vae_decoder_model.get_input_spec(),
|
234 |
-
)
|
235 |
-
|
236 |
-
# Get target model to run on-device
|
237 |
-
vae_decoder_target_model = vae_decoder_compile_job.get_target_model()
|
238 |
|
239 |
```
|
240 |
|
@@ -249,22 +179,6 @@ provided job URL to view a variety of on-device performance metrics.
|
|
249 |
|
250 |
# Device
|
251 |
device = hub.Device("Samsung Galaxy S23")
|
252 |
-
profile_job_controlnet_quantized = hub.submit_profile_job(
|
253 |
-
model=model_controlnet_quantized,
|
254 |
-
device=device,
|
255 |
-
)
|
256 |
-
profile_job_textencoder_quantized = hub.submit_profile_job(
|
257 |
-
model=model_textencoder_quantized,
|
258 |
-
device=device,
|
259 |
-
)
|
260 |
-
profile_job_unet_quantized = hub.submit_profile_job(
|
261 |
-
model=model_unet_quantized,
|
262 |
-
device=device,
|
263 |
-
)
|
264 |
-
profile_job_vaedecoder_quantized = hub.submit_profile_job(
|
265 |
-
model=model_vaedecoder_quantized,
|
266 |
-
device=device,
|
267 |
-
)
|
268 |
|
269 |
```
|
270 |
|
@@ -274,38 +188,6 @@ To verify the accuracy of the model on-device, you can run on-device inference
|
|
274 |
on sample input data on the same cloud hosted device.
|
275 |
```python
|
276 |
|
277 |
-
input_data_controlnet_quantized = model.controlnet.sample_inputs()
|
278 |
-
inference_job_controlnet_quantized = hub.submit_inference_job(
|
279 |
-
model=model_controlnet_quantized,
|
280 |
-
device=device,
|
281 |
-
inputs=input_data_controlnet_quantized,
|
282 |
-
)
|
283 |
-
on_device_output_controlnet_quantized = inference_job_controlnet_quantized.download_output_data()
|
284 |
-
|
285 |
-
input_data_textencoder_quantized = model.text_encoder.sample_inputs()
|
286 |
-
inference_job_textencoder_quantized = hub.submit_inference_job(
|
287 |
-
model=model_textencoder_quantized,
|
288 |
-
device=device,
|
289 |
-
inputs=input_data_textencoder_quantized,
|
290 |
-
)
|
291 |
-
on_device_output_textencoder_quantized = inference_job_textencoder_quantized.download_output_data()
|
292 |
-
|
293 |
-
input_data_unet_quantized = model.unet.sample_inputs()
|
294 |
-
inference_job_unet_quantized = hub.submit_inference_job(
|
295 |
-
model=model_unet_quantized,
|
296 |
-
device=device,
|
297 |
-
inputs=input_data_unet_quantized,
|
298 |
-
)
|
299 |
-
on_device_output_unet_quantized = inference_job_unet_quantized.download_output_data()
|
300 |
-
|
301 |
-
input_data_vaedecoder_quantized = model.vae_decoder.sample_inputs()
|
302 |
-
inference_job_vaedecoder_quantized = hub.submit_inference_job(
|
303 |
-
model=model_vaedecoder_quantized,
|
304 |
-
device=device,
|
305 |
-
inputs=input_data_vaedecoder_quantized,
|
306 |
-
)
|
307 |
-
on_device_output_vaedecoder_quantized = inference_job_vaedecoder_quantized.download_output_data()
|
308 |
-
|
309 |
```
|
310 |
With the output of the model, you can compute like PSNR, relative errors or
|
311 |
spot check the output with expected output.
|
@@ -331,7 +213,7 @@ provides instructions on how to use the `.so` shared library or `.bin` context b
|
|
331 |
|
332 |
|
333 |
## View on Qualcomm® AI Hub
|
334 |
-
Get more details on ControlNet's performance across various devices [here](https://aihub.qualcomm.com/models/
|
335 |
Explore all available models on [Qualcomm® AI Hub](https://aihub.qualcomm.com/)
|
336 |
|
337 |
|
|
|
9 |
|
10 |
---
|
11 |
|
12 |
+
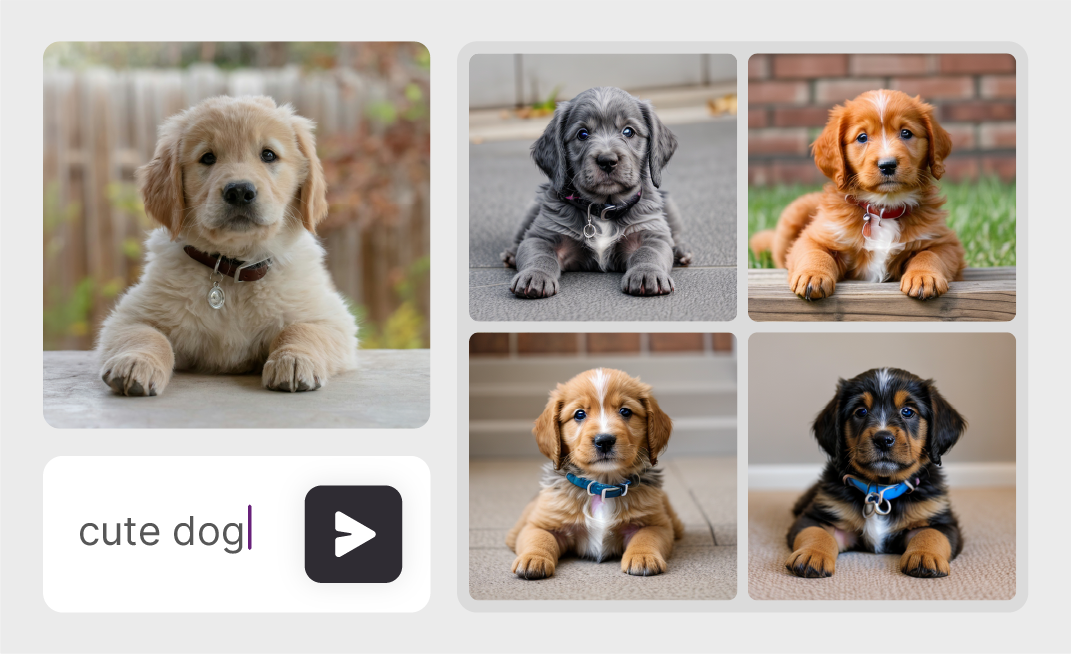
|
13 |
|
14 |
# ControlNet: Optimized for Mobile Deployment
|
15 |
## Generating visual arts from text prompt and input guiding image
|
|
|
22 |
|
23 |
This repository provides scripts to run ControlNet on Qualcomm® devices.
|
24 |
More details on model performance across various devices, can be found
|
25 |
+
[here](https://aihub.qualcomm.com/models/controlnet).
|
26 |
|
27 |
|
28 |
### Model Details
|
|
|
60 |
|
61 |
Install the package via pip:
|
62 |
```bash
|
63 |
+
pip install "qai-hub-models[controlnet]"
|
64 |
```
|
65 |
|
66 |
|
|
|
84 |
weights and runs this model on a sample input.
|
85 |
|
86 |
```bash
|
87 |
+
python -m qai_hub_models.models.controlnet.demo
|
88 |
```
|
89 |
|
90 |
The above demo runs a reference implementation of pre-processing, model
|
|
|
93 |
**NOTE**: If you want running in a Jupyter Notebook or Google Colab like
|
94 |
environment, please add the following to your cell (instead of the above).
|
95 |
```
|
96 |
+
%run -m qai_hub_models.models.controlnet.demo
|
97 |
```
|
98 |
|
99 |
|
|
|
106 |
* Accuracy check between PyTorch and on-device outputs.
|
107 |
|
108 |
```bash
|
109 |
+
python -m qai_hub_models.models.controlnet.export
|
110 |
```
|
111 |
```
|
112 |
Profiling Results
|
|
|
150 |
|
151 |
## How does this work?
|
152 |
|
153 |
+
This [export script](https://aihub.qualcomm.com/models/controlnet/qai_hub_models/models/ControlNet/export.py)
|
154 |
leverages [Qualcomm® AI Hub](https://aihub.qualcomm.com/) to optimize, validate, and deploy this model
|
155 |
on-device. Lets go through each step below in detail:
|
156 |
|
157 |
+
Step 1: **Upload compiled model**
|
|
|
|
|
|
|
158 |
|
159 |
+
Upload compiled models from `qai_hub_models.models.controlnet` on hub.
|
160 |
```python
|
161 |
import torch
|
162 |
|
163 |
import qai_hub as hub
|
164 |
+
from qai_hub_models.models.controlnet import Model
|
165 |
|
166 |
# Load the model
|
167 |
+
model = Model.from_precompiled()
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
168 |
|
169 |
```
|
170 |
|
|
|
179 |
|
180 |
# Device
|
181 |
device = hub.Device("Samsung Galaxy S23")
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
182 |
|
183 |
```
|
184 |
|
|
|
188 |
on sample input data on the same cloud hosted device.
|
189 |
```python
|
190 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
191 |
```
|
192 |
With the output of the model, you can compute like PSNR, relative errors or
|
193 |
spot check the output with expected output.
|
|
|
213 |
|
214 |
|
215 |
## View on Qualcomm® AI Hub
|
216 |
+
Get more details on ControlNet's performance across various devices [here](https://aihub.qualcomm.com/models/controlnet).
|
217 |
Explore all available models on [Qualcomm® AI Hub](https://aihub.qualcomm.com/)
|
218 |
|
219 |
|