Spaces:
Sleeping
Sleeping
no message
Browse files- Untitled-1.py +63 -0
- app copy 2.py +63 -0
- app.py +27 -8
Untitled-1.py
ADDED
@@ -0,0 +1,63 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
|
2 |
+
import gradio as gr
|
3 |
+
import time
|
4 |
+
|
5 |
+
disease_values = [0.25, 0.5, 0.75]
|
6 |
+
|
7 |
+
def xray_model(diseases, img):
|
8 |
+
return [{disease: disease_values[idx] for idx,disease in enumerate(diseases)}]
|
9 |
+
|
10 |
+
|
11 |
+
def ct_model(diseases, img):
|
12 |
+
return [{disease: 0.1 for disease in diseases}]
|
13 |
+
|
14 |
+
with gr.Blocks() as demo:
|
15 |
+
gr.Markdown(
|
16 |
+
"""
|
17 |
+
# Detect Disease From Scan
|
18 |
+
With this model you can lorem ipsum
|
19 |
+
- ipsum 1
|
20 |
+
- ipsum 2
|
21 |
+
"""
|
22 |
+
)
|
23 |
+
gr.DuplicateButton()
|
24 |
+
disease = gr.CheckboxGroup(
|
25 |
+
info="Select the diseases you want to scan for.",
|
26 |
+
choices=["Covid", "Malaria", "Lung Cancer"], label="Disease to Scan For"
|
27 |
+
)
|
28 |
+
slider = gr.Slider(0, 100)
|
29 |
+
|
30 |
+
with gr.Tab("X-ray") as x_tab:
|
31 |
+
with gr.Row():
|
32 |
+
xray_scan = gr.Image()
|
33 |
+
xray_results = gr.JSON()
|
34 |
+
xray_run = gr.Button("Run")
|
35 |
+
xray_run.click(
|
36 |
+
xray_model,
|
37 |
+
inputs=[disease, xray_scan],
|
38 |
+
outputs=xray_results,
|
39 |
+
api_name="xray_model"
|
40 |
+
)
|
41 |
+
|
42 |
+
with gr.Tab("CT Scan"):
|
43 |
+
with gr.Row():
|
44 |
+
ct_scan = gr.Image()
|
45 |
+
ct_results = gr.JSON()
|
46 |
+
ct_run = gr.Button("Run")
|
47 |
+
ct_run.click(
|
48 |
+
ct_model,
|
49 |
+
inputs=[disease, ct_scan],
|
50 |
+
outputs=ct_results,
|
51 |
+
api_name="ct_model"
|
52 |
+
)
|
53 |
+
|
54 |
+
upload_btn = gr.Button("Upload Results", variant="primary")
|
55 |
+
upload_btn.click(
|
56 |
+
lambda ct, xr: None,
|
57 |
+
inputs=[ct_results, xray_results],
|
58 |
+
outputs=[],
|
59 |
+
)
|
60 |
+
|
61 |
+
if __name__ == "__main__":
|
62 |
+
demo.launch()
|
63 |
+
|
app copy 2.py
ADDED
@@ -0,0 +1,63 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
import gradio as gr
|
2 |
+
from gradio_client import Client
|
3 |
+
|
4 |
+
MESAGE_HEADER = """
|
5 |
+
# API Demo (Client component)
|
6 |
+
Welcome to my simple demonstration of the gradio potential as an API.
|
7 |
+
|
8 |
+
It is made of 2 components: *API_demo_server* and *API_demo_client*.
|
9 |
+
|
10 |
+
Server component: [Nuno-Tome/API_demo_server](Nuno-Tome/aPI_demo_server)
|
11 |
+
Client component: [Nuno-Tome/API_demo_client](Nuno-Tome/aPI_demo_client)
|
12 |
+
|
13 |
+
**Just write you message and watch it be returned by the server.**
|
14 |
+
|
15 |
+
"""
|
16 |
+
|
17 |
+
|
18 |
+
|
19 |
+
MESAGE_BMC = """
|
20 |
+
## If you want to support me, you can buy me a coffee:
|
21 |
+
|
22 |
+
[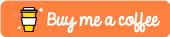](https://www.buymeacoffee.com/nunotome)
|
23 |
+
"""
|
24 |
+
|
25 |
+
# Print the message header for "By me a coffee" link
|
26 |
+
def print_bmc():
|
27 |
+
|
28 |
+
bmc_link = "https://www.buymeacoffee.com/nuno.tome"
|
29 |
+
image_url = "https://helloimjessa.files.wordpress.com/2021/06/bmc-button.png?w=" # Image URL
|
30 |
+
#image_size = "150px" # Image size
|
31 |
+
#image_link_markdown = f"[]({bmc_link})"
|
32 |
+
image_link_markdown = "[]({bmc_link})"
|
33 |
+
|
34 |
+
gr.Markdown("""
|
35 |
+
[]({bmc_link})
|
36 |
+
""")
|
37 |
+
# Buy me a Coffee Setup
|
38 |
+
|
39 |
+
|
40 |
+
|
41 |
+
|
42 |
+
|
43 |
+
client = Client("Nuno-Tome/API_demo_server")
|
44 |
+
DEBUG_MODE = True
|
45 |
+
|
46 |
+
|
47 |
+
def request(text):
|
48 |
+
|
49 |
+
gr.Markdown(
|
50 |
+
"""
|
51 |
+
# Hello World!
|
52 |
+
Start typing below to see the output.
|
53 |
+
""")
|
54 |
+
|
55 |
+
result = client.predict(
|
56 |
+
text,
|
57 |
+
api_name="/predict"
|
58 |
+
)
|
59 |
+
return result
|
60 |
+
|
61 |
+
demo = gr.Interface(fn=request, inputs="textbox", outputs="json")
|
62 |
+
|
63 |
+
demo.launch(share=True)
|
app.py
CHANGED
@@ -1,18 +1,37 @@
|
|
1 |
import gradio as gr
|
2 |
from gradio_client import Client
|
3 |
|
4 |
-
|
5 |
DEBUG_MODE = True
|
6 |
|
7 |
|
8 |
-
def
|
|
|
|
|
|
|
9 |
|
10 |
-
result = client.predict(
|
11 |
-
text,
|
12 |
-
api_name="/predict"
|
13 |
-
)
|
14 |
-
return result
|
15 |
|
16 |
-
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
17 |
|
|
|
18 |
demo.launch(share=True)
|
|
|
1 |
import gradio as gr
|
2 |
from gradio_client import Client
|
3 |
|
4 |
+
|
5 |
DEBUG_MODE = True
|
6 |
|
7 |
|
8 |
+
def update(name):
|
9 |
+
#return f"Welcome to Gradio, {name}!"
|
10 |
+
return {"name": name}
|
11 |
+
|
12 |
|
|
|
|
|
|
|
|
|
|
|
13 |
|
14 |
+
with gr.Blocks() as demo:
|
15 |
+
|
16 |
+
with gr.Row():
|
17 |
+
gr.Markdown("Start typing below and then click **Run** to see the output.")
|
18 |
+
#gr.DuplicateButton()
|
19 |
+
with gr.Row():
|
20 |
+
with gr.Col():
|
21 |
+
gr.Markdown("Type your message:")
|
22 |
+
inp = gr.Textbox(placeholder="What is your name?")
|
23 |
+
with gr.Col():
|
24 |
+
out = gr.JSON()
|
25 |
+
btn = gr.Button("Send request to server")
|
26 |
+
btn.click(fn=update, inputs=inp, outputs=out)
|
27 |
+
|
28 |
+
#with gr.Row():
|
29 |
+
#inp = gr.Textbox(placeholder="What is your name?")
|
30 |
+
#out = gr.JSON()
|
31 |
+
#out = gr.Textbox()
|
32 |
+
#btn = gr.Button("Run")
|
33 |
+
#btn.click(fn=update, inputs=inp, outputs=out
|
34 |
+
|
35 |
|
36 |
+
|
37 |
demo.launch(share=True)
|