enables time tool, adds xkcd tool
Browse files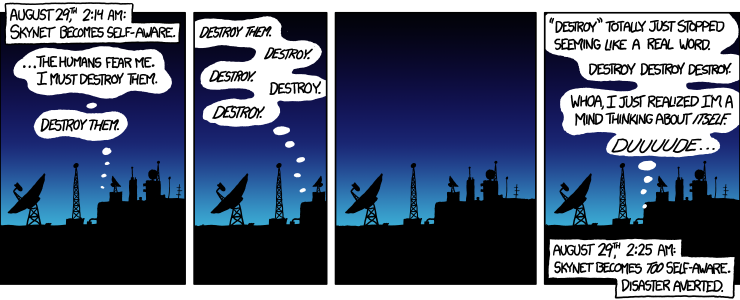
# Have your Agent fetch you XKCD goodness!
> Show me the latest xkcd
Will render the `latest` xkcd comic as markdown
> Show me xkcd `number`
Will render that xkcd comic as markdown
app.py
CHANGED
@@ -4,19 +4,32 @@ import requests
|
|
4 |
import pytz
|
5 |
import yaml
|
6 |
from tools.final_answer import FinalAnswerTool
|
7 |
-
|
8 |
from Gradio_UI import GradioUI
|
9 |
|
10 |
-
|
|
|
11 |
@tool
|
12 |
-
def
|
13 |
-
|
14 |
-
|
|
|
15 |
Args:
|
16 |
-
|
17 |
-
arg2: the second argument
|
18 |
"""
|
19 |
-
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
20 |
|
21 |
@tool
|
22 |
def get_current_time_in_timezone(timezone: str) -> str:
|
@@ -38,7 +51,7 @@ final_answer = FinalAnswerTool()
|
|
38 |
model = HfApiModel(
|
39 |
max_tokens=2096,
|
40 |
temperature=0.5,
|
41 |
-
model_id='https://
|
42 |
custom_role_conversions=None,
|
43 |
)
|
44 |
|
@@ -51,7 +64,7 @@ with open("prompts.yaml", 'r') as stream:
|
|
51 |
|
52 |
agent = CodeAgent(
|
53 |
model=model,
|
54 |
-
tools=[final_answer], ## add your tools here (don't remove final answer)
|
55 |
max_steps=6,
|
56 |
verbosity_level=1,
|
57 |
grammar=None,
|
@@ -62,4 +75,6 @@ agent = CodeAgent(
|
|
62 |
)
|
63 |
|
64 |
|
|
|
|
|
65 |
GradioUI(agent).launch()
|
|
|
4 |
import pytz
|
5 |
import yaml
|
6 |
from tools.final_answer import FinalAnswerTool
|
|
|
7 |
from Gradio_UI import GradioUI
|
8 |
|
9 |
+
|
10 |
+
|
11 |
@tool
|
12 |
+
def xkcd_tool(id:int)-> str:
|
13 |
+
"""A tool that fetches XKCD comics. For the latest comic do not pass an id argument.
|
14 |
+
This tool will return the requested comic title, alt text, and a url which points to the comic image.
|
15 |
+
Compute a Markdown string that displays the title as a header, the alt text as a blockquote, and the image as an image and return that as your final answer.
|
16 |
Args:
|
17 |
+
id: a number representing a valid XKCD comic ID, or none for the latest comic.
|
|
|
18 |
"""
|
19 |
+
try:
|
20 |
+
url = f"https://xkcd.com/{id}/info.0.json" if id else "https://xkcd.com/info.0.json"
|
21 |
+
response = requests.get(url)
|
22 |
+
if response.status_code == 200:
|
23 |
+
data = response.json()
|
24 |
+
return {
|
25 |
+
"title": data["title"],
|
26 |
+
"alt": data["alt"],
|
27 |
+
"img_url": data["img"]
|
28 |
+
}
|
29 |
+
else:
|
30 |
+
return "Failed to fetch XKCD comic"
|
31 |
+
except Exception as e:
|
32 |
+
return f"Error fetching XKCD comic: {str(e)}"
|
33 |
|
34 |
@tool
|
35 |
def get_current_time_in_timezone(timezone: str) -> str:
|
|
|
51 |
model = HfApiModel(
|
52 |
max_tokens=2096,
|
53 |
temperature=0.5,
|
54 |
+
model_id='https://jc26mwg228mkj8dw.us-east-1.aws.endpoints.huggingface.cloud',# it is possible that this model may be overloaded
|
55 |
custom_role_conversions=None,
|
56 |
)
|
57 |
|
|
|
64 |
|
65 |
agent = CodeAgent(
|
66 |
model=model,
|
67 |
+
tools=[final_answer,get_current_time_in_timezone,xkcd_tool], ## add your tools here (don't remove final answer)
|
68 |
max_steps=6,
|
69 |
verbosity_level=1,
|
70 |
grammar=None,
|
|
|
75 |
)
|
76 |
|
77 |
|
78 |
+
|
79 |
+
|
80 |
GradioUI(agent).launch()
|