Spaces:
Runtime error
Runtime error
Delete app.py
Browse files
app.py
DELETED
@@ -1,100 +0,0 @@
|
|
1 |
-
# -*- coding: utf-8 -*-
|
2 |
-
"""Deploy Barcelo demo.ipynb
|
3 |
-
|
4 |
-
Automatically generated by Colaboratory.
|
5 |
-
|
6 |
-
Original file is located at
|
7 |
-
https://colab.research.google.com/drive/1FxaL8DcYgvjPrWfWruSA5hvk3J81zLY9
|
8 |
-
|
9 |
-
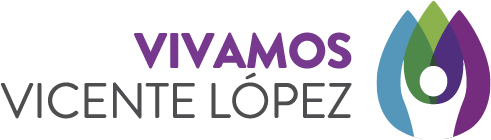
|
10 |
-
|
11 |
-
# Modelo
|
12 |
-
|
13 |
-
YOLO es una familia de modelos de detecci贸n de objetos a escala compuesta entrenados en COCO dataset, e incluye una funcionalidad simple para Test Time Augmentation (TTA), model ensembling, hyperparameter evolution, and export to ONNX, CoreML and TFLite.
|
14 |
-
|
15 |
-
|
16 |
-
## Gradio Inferencia
|
17 |
-
|
18 |
-

|
19 |
-
|
20 |
-
Este Notebook se acelera opcionalmente con un entorno de ejecuci贸n de GPU
|
21 |
-
|
22 |
-
|
23 |
-
----------------------------------------------------------------------
|
24 |
-
|
25 |
-
YOLOv5 Gradio demo
|
26 |
-
|
27 |
-
*Author: Ultralytics LLC and Gradio*
|
28 |
-
|
29 |
-
# C贸digo
|
30 |
-
"""
|
31 |
-
|
32 |
-
#!pip install -qr https://raw.githubusercontent.com/ultralytics/yolov5/master/requirements.txt gradio # install dependencies
|
33 |
-
|
34 |
-
import gradio as gr
|
35 |
-
import torch
|
36 |
-
from PIL import Image
|
37 |
-
|
38 |
-
# Images
|
39 |
-
torch.hub.download_url_to_file('https://i.pinimg.com/originals/7f/5e/96/7f5e9657c08aae4bcd8bc8b0dcff720e.jpg', 'ejemplo1.jpg')
|
40 |
-
torch.hub.download_url_to_file('https://i.pinimg.com/originals/c2/ce/e0/c2cee05624d5477ffcf2d34ca77b47d1.jpg', 'ejemplo2.jpg')
|
41 |
-
|
42 |
-
# Model
|
43 |
-
#model = torch.hub.load('ultralytics/yolov5', 'yolov5s') # force_reload=True to update
|
44 |
-
|
45 |
-
#model = torch.hub.load('ultralytics/yolov5', 'custom', path='best.pt') # local model o google colab
|
46 |
-
model = torch.hub.load('ultralytics/yolov5', 'custom', path='best.pt', force_reload=True, autoshape=True) # local model o google colab
|
47 |
-
#model = torch.hub.load('path/to/yolov5', 'custom', path='/content/yolov56.pt', source='local') # local repo
|
48 |
-
|
49 |
-
|
50 |
-
def yolo(size, iou, conf, im):
|
51 |
-
'''Wrapper fn for gradio'''
|
52 |
-
g = (int(size) / max(im.size)) # gain
|
53 |
-
im = im.resize((int(x * g) for x in im.size), Image.ANTIALIAS) # resize
|
54 |
-
|
55 |
-
model.iou = iou
|
56 |
-
|
57 |
-
model.conf = conf
|
58 |
-
|
59 |
-
|
60 |
-
results2 = model(im) # inference
|
61 |
-
|
62 |
-
results2.render() # updates results.imgs with boxes and labels
|
63 |
-
return Image.fromarray(results2.ims[0])
|
64 |
-
|
65 |
-
#------------ Interface-------------
|
66 |
-
|
67 |
-
|
68 |
-
|
69 |
-
in1 = gr.inputs.Radio(['640', '1280'], label="Tama帽o de la imagen", default='640', type='value')
|
70 |
-
in2 = gr.inputs.Slider(minimum=0, maximum=1, step=0.05, default=0.45, label='NMS IoU threshold')
|
71 |
-
in3 = gr.inputs.Slider(minimum=0, maximum=1, step=0.05, default=0.50, label='Umbral o threshold')
|
72 |
-
in4 = gr.inputs.Image(type='pil', label="Original Image")
|
73 |
-
|
74 |
-
out2 = gr.outputs.Image(type="pil", label="YOLOv5")
|
75 |
-
#-------------- Text-----
|
76 |
-
title = 'Trampas Barcel贸'
|
77 |
-
description = """
|
78 |
-
<p>
|
79 |
-
<center>
|
80 |
-
Sistemas de Desarrollado por Subsecretar铆a de Innovaci贸n del Municipio de Vicente L贸pez. Advertencia solo usar fotos provenientes de las trampas Barcel贸, no de celular o foto de internet.
|
81 |
-
<img src="https://www.vicentelopez.gov.ar/assets/images/logo-mvl.png" alt="logo" width="250"/>
|
82 |
-
</center>
|
83 |
-
</p>
|
84 |
-
"""
|
85 |
-
article ="<p style='text-align: center'><a href='https://docs.google.com/presentation/d/1T5CdcLSzgRe8cQpoi_sPB4U170551NGOrZNykcJD0xU/edit?usp=sharing' target='_blank'>Para mas info, clik para ir al white paper</a></p><p style='text-align: center'><a href='https://drive.google.com/drive/folders/1owACN3HGIMo4zm2GQ_jf-OhGNeBVRS7l?usp=sharing ' target='_blank'>Google Colab Demo</a></p><p style='text-align: center'><a href='https://github.com/Municipalidad-de-Vicente-Lopez/Trampa_Barcelo' target='_blank'>Repo Github</a></p></center></p>"
|
86 |
-
|
87 |
-
examples = [['640',0.45, 0.75,'ejemplo1.jpg'], ['640',0.45, 0.75,'ejemplo2.jpg']]
|
88 |
-
|
89 |
-
iface = gr.Interface(yolo, inputs=[in1, in2, in3, in4], outputs=out2, title=title, description=description, article=article, examples=examples,theme="huggingface", analytics_enabled=False).launch(
|
90 |
-
debug=True)
|
91 |
-
|
92 |
-
iface.launch()
|
93 |
-
|
94 |
-
"""For YOLOv5 PyTorch Hub inference with **PIL**, **OpenCV**, **Numpy** or **PyTorch** inputs please see the full [YOLOv5 PyTorch Hub Tutorial](https://github.com/ultralytics/yolov5/issues/36).
|
95 |
-
|
96 |
-
|
97 |
-
## Citation
|
98 |
-
|
99 |
-
[](https://zenodo.org/badge/latestdoi/264818686)
|
100 |
-
"""
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|