Spaces:
Configuration error
Configuration error
Upload 16 files
Browse files- Flask Application Setup.txt +10 -0
- README.md +52 -13
- app.js +104 -0
- app.py +31 -0
- base.html +44 -0
- bot.jpeg +0 -0
- chat.py +66 -0
- chatbox-icon.svg +26 -0
- data.pth +3 -0
- flask_cheatsheet.pdf +0 -0
- intents.json +1868 -0
- model.py +20 -0
- nltk_utils.py +44 -0
- pyvenv.cfg +5 -0
- style.css +202 -0
- train.py +127 -0
Flask Application Setup.txt
ADDED
@@ -0,0 +1,10 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
$ git clone https://github.com/python-engineer/chatbot-deployment.git
|
2 |
+
$ cd chatbot-deployment
|
3 |
+
$ python3 -m venv venv
|
4 |
+
$ venv\Scripts\activate
|
5 |
+
$ conda create -n py39 python=3.9
|
6 |
+
$ conda activate py39
|
7 |
+
$ pip3.9 install package
|
8 |
+
$ python3
|
9 |
+
$ python3 train.py
|
10 |
+
$ python3 chat.py
|
README.md
CHANGED
@@ -1,13 +1,52 @@
|
|
1 |
-
|
2 |
-
|
3 |
-
|
4 |
-
|
5 |
-
|
6 |
-
|
7 |
-
|
8 |
-
|
9 |
-
|
10 |
-
|
11 |
-
|
12 |
-
|
13 |
-
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
# Chatbot Deployment with Flask and JavaScript
|
2 |
+
|
3 |
+
In this tutorial we deploy the chatbot I created in [this](https://github.com/python-engineer/pytorch-chatbot) tutorial with Flask and JavaScript.
|
4 |
+
|
5 |
+
This gives 2 deployment options:
|
6 |
+
- Deploy within Flask app with jinja2 template
|
7 |
+
- Serve only the Flask prediction API. The used html and javascript files can be included in any Frontend application (with only a slight modification) and can run completely separate from the Flask App then.
|
8 |
+
|
9 |
+
## Initial Setup:
|
10 |
+
This repo currently contains the starter files.
|
11 |
+
|
12 |
+
Clone repo and create a virtual environment
|
13 |
+
```
|
14 |
+
$ git clone https://github.com/python-engineer/chatbot-deployment.git
|
15 |
+
$ cd chatbot-deployment
|
16 |
+
$ python3 -m venv venv
|
17 |
+
$ . venv/bin/activate
|
18 |
+
```
|
19 |
+
Install dependencies
|
20 |
+
```
|
21 |
+
$ (venv) pip install Flask torch torchvision nltk
|
22 |
+
```
|
23 |
+
Install nltk package
|
24 |
+
```
|
25 |
+
$ (venv) python
|
26 |
+
>>> import nltk
|
27 |
+
>>> nltk.download('punkt')
|
28 |
+
```
|
29 |
+
Modify `intents.json` with different intents and responses for your Chatbot
|
30 |
+
|
31 |
+
Run
|
32 |
+
```
|
33 |
+
$ (venv) python train.py
|
34 |
+
```
|
35 |
+
This will dump data.pth file. And then run
|
36 |
+
the following command to test it in the console.
|
37 |
+
```
|
38 |
+
$ (venv) python chat.py
|
39 |
+
```
|
40 |
+
|
41 |
+
Now for deployment follow my tutorial to implement `app.py` and `app.js`.
|
42 |
+
|
43 |
+
## Watch the Tutorial
|
44 |
+
[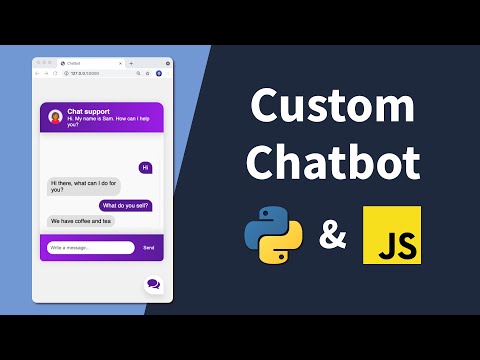](https://youtu.be/a37BL0stIuM)
|
45 |
+
[https://youtu.be/a37BL0stIuM](https://youtu.be/a37BL0stIuM)
|
46 |
+
|
47 |
+
## Note
|
48 |
+
In the video we implement the first approach using jinja2 templates within our Flask app. Only slight modifications are needed to run the frontend separately. I put the final frontend code for a standalone frontend application in the [standalone-frontend](/standalone-frontend) folder.
|
49 |
+
|
50 |
+
## Credits:
|
51 |
+
This repo was used for the frontend code:
|
52 |
+
https://github.com/hitchcliff/front-end-chatjs
|
app.js
ADDED
@@ -0,0 +1,104 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
class Chatbox {
|
2 |
+
constructor() {
|
3 |
+
this.args = {
|
4 |
+
openButton: document.querySelector('.chatbox__button'),
|
5 |
+
chatBox: document.querySelector('.chatbox__support'),
|
6 |
+
sendButton: document.querySelector('.send__button')
|
7 |
+
}
|
8 |
+
|
9 |
+
this.state = false;
|
10 |
+
this.messages = [];
|
11 |
+
}
|
12 |
+
|
13 |
+
display() {
|
14 |
+
const {openButton, chatBox, sendButton} = this.args;
|
15 |
+
|
16 |
+
this.prompt(chatBox)
|
17 |
+
|
18 |
+
openButton.addEventListener('click', () => this.toggleState(chatBox))
|
19 |
+
|
20 |
+
sendButton.addEventListener('click', () => this.onSendButton(chatBox))
|
21 |
+
|
22 |
+
const node = chatBox.querySelector('input');
|
23 |
+
node.addEventListener("keyup", ({key}) => {
|
24 |
+
if (key === "Enter") {
|
25 |
+
this.onSendButton(chatBox)
|
26 |
+
}
|
27 |
+
})
|
28 |
+
}
|
29 |
+
|
30 |
+
prompt(chatbox) {
|
31 |
+
this.messages.push({ name: "Bot", message: "Welcome to NEC Chatbot. How may I help you?? <br> Visit <a href = 'https://nec.edu.in'> for more information" });
|
32 |
+
this.updateChatText(chatbox)
|
33 |
+
}
|
34 |
+
|
35 |
+
toggleState(chatbox) {
|
36 |
+
this.state = !this.state;
|
37 |
+
// show or hides the box
|
38 |
+
if(this.state) {
|
39 |
+
chatbox.classList.add('chatbox--active')
|
40 |
+
} else {
|
41 |
+
chatbox.classList.remove('chatbox--active')
|
42 |
+
}
|
43 |
+
}
|
44 |
+
|
45 |
+
onSendButton(chatbox) {
|
46 |
+
var textField = chatbox.querySelector('input');
|
47 |
+
let text1 = textField.value
|
48 |
+
if (text1 === "") {
|
49 |
+
return;
|
50 |
+
}
|
51 |
+
|
52 |
+
let msg1 = { name: "User", message: text1 }
|
53 |
+
this.messages.push(msg1);
|
54 |
+
|
55 |
+
fetch($SCRIPT_ROOT + '/predict', {
|
56 |
+
method: 'POST',
|
57 |
+
body: JSON.stringify({ message: text1 }),
|
58 |
+
mode: 'cors',
|
59 |
+
headers: {
|
60 |
+
'Content-Type': 'application/json'
|
61 |
+
},
|
62 |
+
})
|
63 |
+
.then(r => r.json())
|
64 |
+
.then(r => {
|
65 |
+
let msg2 = { name: "Bot", message: r.answer };
|
66 |
+
this.messages.push(msg2);
|
67 |
+
this.updateChatText(chatbox)
|
68 |
+
textField.value = ''
|
69 |
+
|
70 |
+
}).catch((error) => {
|
71 |
+
console.error('Error:', error);
|
72 |
+
this.updateChatText(chatbox)
|
73 |
+
textField.value = ''
|
74 |
+
});
|
75 |
+
}
|
76 |
+
|
77 |
+
updateChatText(chatbox) {
|
78 |
+
var html = '';
|
79 |
+
this.messages.slice().reverse().forEach(function(item, index) {
|
80 |
+
if (item.name === "Bot")
|
81 |
+
{
|
82 |
+
html += '<div class="messages__item messages__item--visitor">' + item.message + '</div>'
|
83 |
+
}
|
84 |
+
else
|
85 |
+
{
|
86 |
+
html += '<div class="messages__item messages__item--operator">' + item.message + '</div>'
|
87 |
+
}
|
88 |
+
});
|
89 |
+
|
90 |
+
const chatmessage = chatbox.querySelector('.chatbox__messages');
|
91 |
+
chatmessage.innerHTML = html;
|
92 |
+
}
|
93 |
+
}
|
94 |
+
|
95 |
+
|
96 |
+
const chatbox = new Chatbox();
|
97 |
+
chatbox.display();
|
98 |
+
|
99 |
+
// let promise = new Promise(chatbox.display(resolve, reject) {
|
100 |
+
// // the function is executed automatically when the promise is constructed
|
101 |
+
|
102 |
+
// // after 1 second signal that the job is done with the result "done"
|
103 |
+
// setTimeout(() => resolve("Done"), 1000);
|
104 |
+
// });
|
app.py
ADDED
@@ -0,0 +1,31 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
from flask import Flask, render_template, request, jsonify
|
2 |
+
# from flask_cors import CORS
|
3 |
+
from chat import get_response
|
4 |
+
from flask_limiter import Limiter
|
5 |
+
from flask_limiter.util import get_remote_address
|
6 |
+
|
7 |
+
app = Flask(__name__)
|
8 |
+
# CORS(app)
|
9 |
+
limiter = Limiter(app, default_limits=["60 per second", "200 per minute", "1200 per hour"])
|
10 |
+
|
11 |
+
# Comment out @app.get and index_get() if you are using CORS(app) for standalone frontend and uncomment all other commented lines
|
12 |
+
@app.get("/")
|
13 |
+
@limiter.limit("60/second; 200/minute; 1200/hour")
|
14 |
+
def index_get():
|
15 |
+
return render_template("base.html")
|
16 |
+
|
17 |
+
@app.post("/predict")
|
18 |
+
def predict():
|
19 |
+
text = request.get_json().get("message")
|
20 |
+
if len(text) > 100:
|
21 |
+
message = {"answer": "I'm sorry, your query has too many characters for me to process. If you would like to speak to a live agent, say 'I would like to speak to a live agent'"}
|
22 |
+
return jsonify(message)
|
23 |
+
response = get_response(text)
|
24 |
+
message = {"answer": response}
|
25 |
+
return jsonify(message)
|
26 |
+
|
27 |
+
|
28 |
+
if __name__ == "__main__":
|
29 |
+
app.run(debug = True)
|
30 |
+
# from waitress import serve
|
31 |
+
# serve(app, host="0.0.0.0", port=8080)
|
base.html
ADDED
@@ -0,0 +1,44 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
<!DOCTYPE html>
|
2 |
+
<html lang="en">
|
3 |
+
<link rel="stylesheet" href="{{ url_for('static', filename='style.css') }}">
|
4 |
+
|
5 |
+
<head>
|
6 |
+
<meta charset="UTF-8">
|
7 |
+
<title>Chatbot</title>
|
8 |
+
</head>
|
9 |
+
<body>
|
10 |
+
<div class="container">
|
11 |
+
<div class="chatbox">
|
12 |
+
<div class="chatbox__support">
|
13 |
+
<div class="chatbox__header">
|
14 |
+
<div class="chatbox__image--header">
|
15 |
+
<img src="https://lms.nec.edu.in/pluginfile.php/1/theme_academi/logo/1695892506/logo.jpeg" alt="image" width=70 height=70>
|
16 |
+
</div>
|
17 |
+
<div class="chatbox__content--header">
|
18 |
+
<h4 class="chatbox__heading--header">Chat support</h4>
|
19 |
+
<p class="chatbox__description--header">Hi. I am NEC Chatbot!!</p>
|
20 |
+
</div>
|
21 |
+
</div>
|
22 |
+
<div class="chatbox__messages">
|
23 |
+
<div>
|
24 |
+
<p></p>
|
25 |
+
</div>
|
26 |
+
</div>
|
27 |
+
<div class="chatbox__footer">
|
28 |
+
<input type="text" placeholder="Write a message...">
|
29 |
+
<button class="chatbox__send--footer send__button">Send</button>
|
30 |
+
</div>
|
31 |
+
</div>
|
32 |
+
<div class="chatbox__button">
|
33 |
+
<button><img src="{{ url_for('static', filename='images/chatbox-icon.svg') }}" /></button>
|
34 |
+
</div>
|
35 |
+
</div>
|
36 |
+
</div>
|
37 |
+
|
38 |
+
<script>
|
39 |
+
$SCRIPT_ROOT = {{ request.script_root|tojson }};
|
40 |
+
</script>
|
41 |
+
<script type="text/javascript" src="{{ url_for('static', filename='app.js') }}"></script>
|
42 |
+
|
43 |
+
</body>
|
44 |
+
</html>
|
bot.jpeg
ADDED
![]() |
chat.py
ADDED
@@ -0,0 +1,66 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
import random
|
2 |
+
import json
|
3 |
+
|
4 |
+
import torch
|
5 |
+
|
6 |
+
from model import NeuralNet
|
7 |
+
from nltk_utils import bag_of_words, tokenize
|
8 |
+
|
9 |
+
# Prompt three most commonly asked FAQs
|
10 |
+
samples = "Kindly visit our website <a href = 'https://nec.edu.in/'>"
|
11 |
+
|
12 |
+
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
|
13 |
+
|
14 |
+
import json
|
15 |
+
|
16 |
+
with open(r'C:\Users\vilsons\Documents\chatbot-deployment-main\flask chatbot\intents.json', 'r', encoding='utf-8') as json_data:
|
17 |
+
intents = json.load(json_data)
|
18 |
+
|
19 |
+
|
20 |
+
FILE = "data.pth"
|
21 |
+
data = torch.load(FILE)
|
22 |
+
|
23 |
+
input_size = data["input_size"]
|
24 |
+
hidden_size = data["hidden_size"]
|
25 |
+
output_size = data["output_size"]
|
26 |
+
all_words = data['all_words']
|
27 |
+
tags = data['tags']
|
28 |
+
model_state = data["model_state"]
|
29 |
+
|
30 |
+
model = NeuralNet(input_size, hidden_size, output_size).to(device)
|
31 |
+
model.load_state_dict(model_state)
|
32 |
+
model.eval()
|
33 |
+
|
34 |
+
bot_name = "NEC Chatbot"
|
35 |
+
|
36 |
+
def get_response(msg):
|
37 |
+
sentence = tokenize(msg)
|
38 |
+
X = bag_of_words(sentence, all_words)
|
39 |
+
X = X.reshape(1, X.shape[0])
|
40 |
+
X = torch.from_numpy(X).to(device)
|
41 |
+
|
42 |
+
output = model(X)
|
43 |
+
_, predicted = torch.max(output, dim=1)
|
44 |
+
|
45 |
+
tag = tags[predicted.item()]
|
46 |
+
probs = torch.softmax(output, dim=1)
|
47 |
+
prob = probs[0][predicted.item()]
|
48 |
+
if prob.item() > 0.85: #Increasing specifisity to reduce incorrect classifications
|
49 |
+
for intent in intents['intents']:
|
50 |
+
if tag == intent["tag"]:
|
51 |
+
return random.choice(intent['responses'])
|
52 |
+
|
53 |
+
return f"I'm sorry, but I cannot understand your query. {samples} "
|
54 |
+
|
55 |
+
|
56 |
+
if __name__ == "__main__":
|
57 |
+
print("Let's chat! (type 'quit' to exit)")
|
58 |
+
while True:
|
59 |
+
# sentence = "do you use credit cards?"
|
60 |
+
sentence = input("You: ")
|
61 |
+
if sentence == "quit":
|
62 |
+
break
|
63 |
+
|
64 |
+
resp = get_response(sentence)
|
65 |
+
print(resp)
|
66 |
+
|
chatbox-icon.svg
ADDED
|
data.pth
ADDED
@@ -0,0 +1,3 @@
|
|
|
|
|
|
|
|
|
1 |
+
version https://git-lfs.github.com/spec/v1
|
2 |
+
oid sha256:e65208815bf2f59a93108cfcf7978b40df16ff12c7f94bc6adf440eb5c7cc26f
|
3 |
+
size 22442
|
flask_cheatsheet.pdf
ADDED
Binary file (68.9 kB). View file
|
|
intents.json
ADDED
@@ -0,0 +1,1868 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
{
|
2 |
+
"intents": [
|
3 |
+
{
|
4 |
+
"tag": "greeting",
|
5 |
+
"patterns": [
|
6 |
+
"Hi",
|
7 |
+
"Hey",
|
8 |
+
"How are you",
|
9 |
+
"Is anyone there?",
|
10 |
+
"Hello",
|
11 |
+
"Howdy",
|
12 |
+
"Greetings",
|
13 |
+
"I need help",
|
14 |
+
"Can you help",
|
15 |
+
"Good day",
|
16 |
+
"Hi there",
|
17 |
+
"Good morning",
|
18 |
+
"Good evening",
|
19 |
+
"Good afternoon",
|
20 |
+
"Yo",
|
21 |
+
"Sup",
|
22 |
+
"What's up"
|
23 |
+
],
|
24 |
+
"responses": [
|
25 |
+
"Hey, how can I be of assistance?"
|
26 |
+
]
|
27 |
+
},
|
28 |
+
{
|
29 |
+
"tag": "identity",
|
30 |
+
"patterns": [
|
31 |
+
"What are you?",
|
32 |
+
"Who are you?",
|
33 |
+
"What is your name?",
|
34 |
+
"Who's this?",
|
35 |
+
"What's this?",
|
36 |
+
"Tell me about yourself"
|
37 |
+
],
|
38 |
+
"responses": [
|
39 |
+
"I am a chatbot of NEC. If you have more queries please visit our website."
|
40 |
+
]
|
41 |
+
},
|
42 |
+
{
|
43 |
+
"tag": "mech_department",
|
44 |
+
"patterns": [
|
45 |
+
"Mech dept",
|
46 |
+
"Tell me about the Mechanical Engineering Department",
|
47 |
+
"Tell me about the Mechanical Department",
|
48 |
+
"Mechanical Department",
|
49 |
+
"Mechanical Engineering Department",
|
50 |
+
"Can you provide information about the Mechanical Engineering Department",
|
51 |
+
"Share details about the Mechanical Engineering Department",
|
52 |
+
"What's the deal with Mechanical Engineering?",
|
53 |
+
"Mech Engineering Department",
|
54 |
+
"Mechanical Engg Department"
|
55 |
+
],
|
56 |
+
"responses": [
|
57 |
+
"The Department of Mechanical Engineering is established in the year 1984. The department has qualified and experienced faculty members with industrial and research backgrounds to achieve excellence in academic and research activities. NBA has accredited the department since 2000, and presently accredited under the Tier – I category. The department is recognized as a Research Centre by Anna University, Chennai. The department has fetched research projects worth Rs. 1.72 crores from various agencies such as DST, BRNS and AICTE etc,. The department has 12 Ph.D qualified faculty members and all of them are recognized as Research Supervisors by Anna University Chennai. So far, 35 Scholars have completed their PhD from Research Center, and 12 scholars are currently doing research. The CDIO lab has been established in the department in 2016 for new product development activities. More than 36 products were developed, among which five were commercialized. The department has published five Patents and twelve under process."
|
58 |
+
]
|
59 |
+
},
|
60 |
+
{
|
61 |
+
"tag": "mech_department_vision",
|
62 |
+
"patterns": [
|
63 |
+
"What is the vision of the Mechanical Engineering Department?",
|
64 |
+
"What's the goal of Mech Engg Department?"
|
65 |
+
],
|
66 |
+
"responses": [
|
67 |
+
"Producing globally competitive Mechanical Engineers with social responsibilities."
|
68 |
+
]
|
69 |
+
},
|
70 |
+
{
|
71 |
+
"tag": "mech_department_mission",
|
72 |
+
"patterns": [
|
73 |
+
"What is the mission of the Mechanical Engineering Department?",
|
74 |
+
"What's Mech Engg Department all about?"
|
75 |
+
],
|
76 |
+
"responses": [
|
77 |
+
"Imparting quality education by providing an excellent Teaching-Learning environment. Inculcating qualities of continuous learning, professionalism, team spirit, communication skill and leadership with social responsibilities. Promoting leading-edge research and development through collaboration with academia and industry."
|
78 |
+
]
|
79 |
+
},
|
80 |
+
{
|
81 |
+
"tag": "mech_department_ug",
|
82 |
+
"patterns": [
|
83 |
+
"Tell me about the undergraduate program in Mechanical Engineering",
|
84 |
+
"What's the course like for Mechanical Engg?"
|
85 |
+
],
|
86 |
+
"responses": [
|
87 |
+
"Year of Establishment: 1984. Student Strength: 60"
|
88 |
+
]
|
89 |
+
},
|
90 |
+
{
|
91 |
+
"tag": "mech_department_pg",
|
92 |
+
"patterns": [
|
93 |
+
"Tell me about the postgraduate program in Mechanical Engineering",
|
94 |
+
"What's the deal with postgrad in Mech Engg?"
|
95 |
+
],
|
96 |
+
"responses": [
|
97 |
+
"Year of Establishment: 2004. Student Strength: 6"
|
98 |
+
]
|
99 |
+
},
|
100 |
+
{
|
101 |
+
"tag": "mech_department_phd",
|
102 |
+
"patterns": [
|
103 |
+
"Tell me about the PhD program in Mechanical Engineering",
|
104 |
+
"What's the scoop on PhD in Mech Engg?"
|
105 |
+
],
|
106 |
+
"responses": [
|
107 |
+
"Year of Establishment: 2006. Full-time Scholars: 2. Part-time Scholars: 10. Total Scholars: 35"
|
108 |
+
]
|
109 |
+
},
|
110 |
+
{
|
111 |
+
"tag": "mech_department_hod",
|
112 |
+
"patterns": ["Who is the Head of the Mechanical Engineering Department?", "Hod of Mech Dept", "Hod mech"],
|
113 |
+
"responses": ["Dr K. Manisekar Professor & Head"]
|
114 |
+
},
|
115 |
+
{
|
116 |
+
"tag": "mech_department_faculty",
|
117 |
+
"patterns": ["Tell me about the faculty in the Mechanical Engineering Department"],
|
118 |
+
"responses": ["1. Dr Iyah Raja. S. - Professor, 2. Dr Venkatkumar. D. - Professor, 3. Dr Harichandran. R. - Professor, 4. Dr Kathiresan. M - Associate Professor, 5. Dr Ramanan. P - Assistant Professor (SG), 6. Dr Sankar. I - Assistant Professor (SG), 7. Dr Vigneshkumar. D - Assistant Professor (SG), 8. Dr Michael Thomas Rex. F - Assistant Professor (SG), 9. Mr Vijayakumar. R - Assistant Professor (SG), 10. Mr Vignesh Kumar. R - Assistant Professor (SG), 11. Mr Prince Abraham. B - Assistant Professor, 12. Mr Sundara Bharathi S. R - Assistant Professor, 13. Mr Andrews. A - Assistant Professor, 14. Mr Sudalaiyandi. K - Assistant Professor, 15. Mr Jayavenkatesh. R - Assistant Professor, 16. Mr. K. Thoufiq Mohammed - Assistant Professor, 17. Mr N. Muthu Saravanan - Assistant Professor, 18. Mr C. Veera Ajay - Assistant Professor, 19. Dr W. Beno Wincy - Assistant Professor, 20. Mr K. Pradeepraj - Assistant Professor"]
|
119 |
+
},
|
120 |
+
{
|
121 |
+
"tag": "civil_department",
|
122 |
+
"patterns": [
|
123 |
+
"civil dept",
|
124 |
+
"Tell me about the civil Engineering Department",
|
125 |
+
"Tell me about the civil Department",
|
126 |
+
"civil Department",
|
127 |
+
"civil Engineering Department",
|
128 |
+
"Can you provide information about the civil Engineering Department",
|
129 |
+
"Share details about the civil Engineering Department",
|
130 |
+
"What's up with the civil Engineering Department?",
|
131 |
+
"What can you tell me about civil Engineering?",
|
132 |
+
"Give me the scoop on civil Engineering",
|
133 |
+
"I'm curious about civil Engineering"
|
134 |
+
],
|
135 |
+
"responses": [
|
136 |
+
"The Civil Engineering Department started in the year 2012, offers B.E-Civil Engineering. The department has its own well-equipped state of art infrastructure. The department follows technology enabled teaching learning process like Learning Management System (LMS) and Classes are well equipped with Smart Monitors. The department is also a member of Institute of Engineers (India) – Student’s Chapter and Indian Society for Technical Education (ISTE) as professional bodies. The Civil Engineering Association (CEA) serves as a platform for students to make technical presentations, gain knowledge about recent advancements. The department has signed MoU’s with Federation of All Civil Engineers Association and CADD Technologies, Coimbatore for Student Mentor programme, to train Civil Engineering students. It also offers consultancy services in Structural, Environmental, Survey and Geotechnical fields."
|
137 |
+
]
|
138 |
+
},
|
139 |
+
{
|
140 |
+
"tag": "civil_department_vision",
|
141 |
+
"patterns": [
|
142 |
+
"What is the vision of the civil Engineering Department?",
|
143 |
+
"What's the vision for civil Engineering?",
|
144 |
+
"What does the civil Engineering Department aspire to?",
|
145 |
+
"What are the goals of civil Engineering?"
|
146 |
+
],
|
147 |
+
"responses": [
|
148 |
+
"Producing outstanding civil engineering professionals with human values to face future challenges"
|
149 |
+
]
|
150 |
+
},
|
151 |
+
{
|
152 |
+
"tag": "civil_department_mission",
|
153 |
+
"patterns": [
|
154 |
+
"What is the mission of the civil Engineering Department?",
|
155 |
+
"What's the mission for civil Engineering?",
|
156 |
+
"What does the civil Engineering Department aim to achieve?",
|
157 |
+
"What is civil Engineering striving for?"
|
158 |
+
],
|
159 |
+
"responses": [
|
160 |
+
"To provide with excellent teaching and research ambience. To prepare student for leadership roles in civil engineering. To facilitate student with lifetime skills and human values. To collaborate with industries to meet the ever challenging environment."
|
161 |
+
]
|
162 |
+
},
|
163 |
+
{
|
164 |
+
"tag": "civil_department_ug",
|
165 |
+
"patterns": [
|
166 |
+
"Tell me about the undergraduate program in civil Engineering",
|
167 |
+
"What's the scoop on civil Engineering undergrad program?",
|
168 |
+
"Give me the details on civil Engineering undergrad program"
|
169 |
+
],
|
170 |
+
"responses": [
|
171 |
+
"Year of Establishment: 2012. Student Strength: 191"
|
172 |
+
]
|
173 |
+
},
|
174 |
+
{
|
175 |
+
"tag": "civil_department_pg",
|
176 |
+
"patterns": [
|
177 |
+
"Tell me about the postgraduate program in civil Engineering",
|
178 |
+
"What's the deal with civil Engineering postgrad program?",
|
179 |
+
"Give me the lowdown on civil Engineering postgrad program"
|
180 |
+
],
|
181 |
+
"responses": [
|
182 |
+
"No pg course in civil."
|
183 |
+
]
|
184 |
+
},
|
185 |
+
{
|
186 |
+
"tag": "civil_department_phd",
|
187 |
+
"patterns": [
|
188 |
+
"Tell me about the PhD program in civil Engineering",
|
189 |
+
"What's the story with civil Engineering PhD program?",
|
190 |
+
"Give me the details on civil Engineering PhD program"
|
191 |
+
],
|
192 |
+
"responses": [
|
193 |
+
"There is no phd course in civil."
|
194 |
+
]
|
195 |
+
},
|
196 |
+
{
|
197 |
+
"tag": "civil_department_hod",
|
198 |
+
"patterns": [
|
199 |
+
"Who is the Head of the civil Engineering Department?",
|
200 |
+
"Hod of civil Dept",
|
201 |
+
"Hod civil",
|
202 |
+
"Who's in charge of civil Engineering?",
|
203 |
+
"Who leads civil Engineering?"
|
204 |
+
],
|
205 |
+
"responses": [
|
206 |
+
"Dr.C.Puthiya Sekar Professor & Head"
|
207 |
+
]
|
208 |
+
},
|
209 |
+
{
|
210 |
+
"tag": "civil_department_faculty",
|
211 |
+
"patterns": [
|
212 |
+
"Tell me about the faculty in the civil Engineering Department",
|
213 |
+
"Who teaches in civil Engineering?",
|
214 |
+
"Give me the rundown on civil Engineering faculty",
|
215 |
+
"What's the deal with civil Engineering faculty?"
|
216 |
+
],
|
217 |
+
"responses": [
|
218 |
+
"Dr.C.Puthiya Sekar. - Professor and head. Dr.V.Kannan. - Professor. Dr.C.Chella Gifta - Associate Professor. Mrs.M.Balamaheswari - Assistant Professor. Mr.V.Arulpandian - Assistant Professor. Mr.B.Gowtham - Assistant Professor. Mr.S.Kannan - Assistant Professor. Mr.A. Darral Alfred - Assistant Professor. Mr.P. Kasirajan- Assistant Professor. Mr.M.Franchis David - Assistant Professor. Mr.M.Ashokpandiyan - Assistant Professor. Mr.K.Marimuthu - Assistant Professor. Ms.R.Harshani- Assistant Professor. Ms.S.Sona - Assistant Professor."
|
219 |
+
]
|
220 |
+
},
|
221 |
+
{
|
222 |
+
"tag": "Information_Technology_department",
|
223 |
+
"patterns": [
|
224 |
+
"IT dept",
|
225 |
+
"Tell me about the IT Department",
|
226 |
+
"Tell me about the Information Technology Department",
|
227 |
+
"IT Department",
|
228 |
+
"Information Technology Department",
|
229 |
+
"Can you provide information about the Information Technology Department",
|
230 |
+
"Share details about the Information Technology Department",
|
231 |
+
"What's up with the Information Technology Department?",
|
232 |
+
"What can you tell me about Information Technology?",
|
233 |
+
"Give me the scoop on Information Technology",
|
234 |
+
"I'm curious about Information Technology"
|
235 |
+
],
|
236 |
+
"responses": [
|
237 |
+
"The IT department was started in the year 2001 and as of today, it offers an undergraduate programme B.Tech., Information Technology and a PG programme M.Tech., Information and Cyber Warfare. The department is augmented with qualified faculty with rich teaching experience. The department is well equipped with advanced laboratories and a research centre has been setup to promote research in the department. Faculty have been doing research in the areas like IoT, Data Analytics, Image Processing, Optical communication, Network security, Mobile Ad-hoc Networks etc. The department is fully accommodated with modern Hardware and Software accessibility to cater to the academic needs of students and staff. Our students have undertaken internships in many reputed MNCs and consistently maintaining the placement record of above 90% every year."
|
238 |
+
]
|
239 |
+
},
|
240 |
+
{
|
241 |
+
"tag": "IT_department_vision",
|
242 |
+
"patterns": [
|
243 |
+
"What is the vision of the Information Technology Department?",
|
244 |
+
"What's the vision for Information Technology?",
|
245 |
+
"What does the Information Technology Department aspire to?",
|
246 |
+
"What are the goals of Information Technology?"
|
247 |
+
],
|
248 |
+
"responses": [
|
249 |
+
"To produce technically competent and value based IT Professionals to meet the current challenges of the modern IT industry."
|
250 |
+
]
|
251 |
+
},
|
252 |
+
{
|
253 |
+
"tag": "Information_Technology_department_mission",
|
254 |
+
"patterns": [
|
255 |
+
"What is the mission of the IT Engineering Department?",
|
256 |
+
"What's the mission for IT Engineering?",
|
257 |
+
"What does the IT Engineering Department aim to achieve?",
|
258 |
+
"What is IT Engineering striving for?"
|
259 |
+
],
|
260 |
+
"responses": [
|
261 |
+
"Imparting quality education with innovative components in teaching learning process. Conducting student centric programme to enhance communication, team spirit, leadership skills and self learning. Motivating the students to realize the need of ethics and human values. Developing a conducive environment for collaborative research."
|
262 |
+
]
|
263 |
+
},
|
264 |
+
{
|
265 |
+
"tag": "IT_department_ug",
|
266 |
+
"patterns": [
|
267 |
+
"Tell me about the undergraduate program in Information Technology",
|
268 |
+
"What's the scoop on Information Technology undergrad program?",
|
269 |
+
"Give me the details on Information Technology undergrad program"
|
270 |
+
],
|
271 |
+
"responses": [
|
272 |
+
"Year of Establishment: 2001. Student Strength: 60"
|
273 |
+
]
|
274 |
+
},
|
275 |
+
{
|
276 |
+
"tag": "IT_department_pg",
|
277 |
+
"patterns": [
|
278 |
+
"Tell me about the postgraduate program in Information Technology",
|
279 |
+
"What's the deal with Information Technology postgrad program?",
|
280 |
+
"Give me the lowdown on Information Technology postgrad program"
|
281 |
+
],
|
282 |
+
"responses": [
|
283 |
+
"Year of Establishment: 2009. Student Strength: 18"
|
284 |
+
]
|
285 |
+
},
|
286 |
+
{
|
287 |
+
"tag": "IT_department_phd",
|
288 |
+
"patterns": [
|
289 |
+
"Tell me about the PhD program in Information Technology",
|
290 |
+
"What's the story with Information Technology PhD program?",
|
291 |
+
"Give me the details on Information Technology PhD program"
|
292 |
+
],
|
293 |
+
"responses": [
|
294 |
+
"Year of Establishment: 2016. Full-time Scholars: 4. Part-time Scholars: 32. Awarded: 26"
|
295 |
+
]
|
296 |
+
},
|
297 |
+
{
|
298 |
+
"tag": "IT_department_hod",
|
299 |
+
"patterns": [
|
300 |
+
"Who is the Head of the Information Technology Department?",
|
301 |
+
"Hod of IT Dept",
|
302 |
+
"Hod IT",
|
303 |
+
"Who's in charge of Information Technology?",
|
304 |
+
"Who leads Information Technology?"
|
305 |
+
],
|
306 |
+
"responses": [
|
307 |
+
"Dr.K.G.Srinivasagan Professor & Head"
|
308 |
+
]
|
309 |
+
},
|
310 |
+
{
|
311 |
+
"tag": "IT_department_faculty",
|
312 |
+
"patterns": [
|
313 |
+
"Tell me about the faculty in the Information Technology Department",
|
314 |
+
"Who teaches in Information Technology?",
|
315 |
+
"Give me the rundown on Information Technology faculty",
|
316 |
+
"What's the deal with Information Technology faculty?",
|
317 |
+
"IT faculty",
|
318 |
+
"It faculty"
|
319 |
+
],
|
320 |
+
"responses": [
|
321 |
+
"Dr.K.G.Srinivasagan. - Professor and head. Dr.B.Paramasivan - Professor. Dr.R.Muthukkumar - Professor. Dr.S.Chidambaram - Associate Professor. Dr.V.Manimaran - Associate Professor. Dr.S.Rajagopal - Assistant Professor(SG). Ms.V.Anitha - Assistant Professor. Ms.N.Gowthami - Assistant Professor. Ms.M.Manimegalai- Assistant Professor. Ms.S.Santhi - Assistant Professor. Mr.P.G.Siva Sharma Karthick - Assistant Professor. Ms.G.Ramya - Assistant Professor. Ms.R.Suguna- Assistant Professor. Ms.R.Madhu - Assistant Professor. Ms.M.Malathi- Assistant Professor."
|
322 |
+
]
|
323 |
+
},
|
324 |
+
{
|
325 |
+
"tag": "AIDS_department",
|
326 |
+
"patterns": [
|
327 |
+
"AIDS dept",
|
328 |
+
"Tell me about the AIDS Department",
|
329 |
+
"Tell me about the Artificial Intelligence and Data Science Department",
|
330 |
+
"AIDS Department",
|
331 |
+
"Artificial Intelligence and Data Science Department",
|
332 |
+
"Can you provide information about the Artificial Intelligence and Data Science Department",
|
333 |
+
"Share details about the AIDS Department",
|
334 |
+
"What's up with the Artificial Intelligence and Data Science Department?",
|
335 |
+
"What can you tell me about Artificial Intelligence and Data Science?",
|
336 |
+
"Give me the scoop on Artificial Intelligence and Data Science",
|
337 |
+
"I'm curious about Artificial Intelligence and Data Science"
|
338 |
+
],
|
339 |
+
"responses": [
|
340 |
+
"The Department of Artificial Intelligence and Data Science was established in the year 2022. The goal of the department is to ensure the academic excellence in students with wide exposure of research and career opportunities. The unique undergraduate program, B.Tech. Artificial Intelligence and Data Science (AI & DS), aims the students to acquire technical skills to perform data analytics, develop robotics automation systems and visualization in emerging real-time applications. Program envisions to equip students with the ability to identify and assess societal, health, safety and cultural issues with an emphasize on identifying their consequent responsibilities as engineers of offering optimal and effective solutions. The department is well equipped with state-of-the-art infrastructure to empower the students with the state of art technologies."
|
341 |
+
]
|
342 |
+
},
|
343 |
+
{
|
344 |
+
"tag": "AIDS_department_vision",
|
345 |
+
"patterns": [
|
346 |
+
"What is the vision of the AIDS Department?",
|
347 |
+
"What's the vision for Artificial Intelligence and Data Science?",
|
348 |
+
"What does the AIDS Department aspire to?",
|
349 |
+
"What are the goals of Artificial Intelligence and Data Science?"
|
350 |
+
],
|
351 |
+
"responses": [
|
352 |
+
"To Produce globally competent, innovative, Computing professionals to meet current challenges in the field of Artificial intelligence and Data Science with social responsibilities."
|
353 |
+
]
|
354 |
+
},
|
355 |
+
{
|
356 |
+
"tag": "AIDS_department_mission",
|
357 |
+
"patterns": [
|
358 |
+
"What is the mission of the AIDS Engineering Department?",
|
359 |
+
"What's the mission for Artificial Intelligence and Data Science?",
|
360 |
+
"What does the AIDS Department aim to achieve?",
|
361 |
+
"What is Artificial Intelligence and Data Science striving for?"
|
362 |
+
],
|
363 |
+
"responses": [
|
364 |
+
"Offering well-balanced curriculum with state of the art technologies to impart professional competencies and transferable skills. Bringing innovations in Teaching-Learning process through experienced learning and project/product based learning. Collaborating National and International Industries and Academia to develop foresight technologies in Artificial intelligence and Data Science."
|
365 |
+
]
|
366 |
+
},
|
367 |
+
{
|
368 |
+
"tag": "AIDS_department_ug",
|
369 |
+
"patterns": [
|
370 |
+
"Tell me about the undergraduate program in AIDS",
|
371 |
+
"What's the scoop on AIDS undergrad program?",
|
372 |
+
"Give me the details on AIDS undergrad program"
|
373 |
+
],
|
374 |
+
"responses": [
|
375 |
+
"Year of Establishment: 2022. Student Strength: 60"
|
376 |
+
]
|
377 |
+
},
|
378 |
+
{
|
379 |
+
"tag": "AIDS_department_pg",
|
380 |
+
"patterns": [
|
381 |
+
"Tell me about the postgraduate program in AIDS",
|
382 |
+
"What's the deal with AIDS postgrad program?",
|
383 |
+
"Give me the lowdown on AIDS postgrad program"
|
384 |
+
],
|
385 |
+
"responses": [
|
386 |
+
"No pg course in AIDS"
|
387 |
+
]
|
388 |
+
},
|
389 |
+
{
|
390 |
+
"tag": "AIDS_department_phd",
|
391 |
+
"patterns": [
|
392 |
+
"Tell me about the PhD program in AIDS",
|
393 |
+
"What's the story with the PhD program in AIDS?",
|
394 |
+
"Give me the details on AIDS PhD program"
|
395 |
+
],
|
396 |
+
"responses": [
|
397 |
+
"Year of Establishment: 2022. Pursuing: 2. Awarded: 4"
|
398 |
+
]
|
399 |
+
},
|
400 |
+
{
|
401 |
+
"tag": "AIDS_department_hod",
|
402 |
+
"patterns": [
|
403 |
+
"Who is the Head of the Artificial Intelligence and data science Department?",
|
404 |
+
"Hod of AIDS Dept",
|
405 |
+
"Hod AIDS",
|
406 |
+
"Who leads Artificial Intelligence and data science?"
|
407 |
+
],
|
408 |
+
"responses": [
|
409 |
+
"Dr.V.Kalaivani M.E.,Ph.D., Professor & Head"
|
410 |
+
]
|
411 |
+
},
|
412 |
+
{
|
413 |
+
"tag": "AIDS_department_faculty",
|
414 |
+
"patterns": [
|
415 |
+
"Tell me about the faculty in the AIDS Department",
|
416 |
+
"Who teaches in AIDS?",
|
417 |
+
"Give me the rundown on AIDS faculty",
|
418 |
+
"What's the deal with AIDS faculty?"
|
419 |
+
],
|
420 |
+
"responses": [
|
421 |
+
"Dr.V.Kalaivani M.E.,Ph.D.- Professor and head. Ms.K.Poorani M.E. - Assistant Professor. Ms.P.Rampriya M.E. -Assistant Professor. Ms.R.Binusha M.E - Assistant Professor. Ms.G.Dhivya M.E - Assistant Professor "
|
422 |
+
]
|
423 |
+
},
|
424 |
+
{
|
425 |
+
"tag": "Science and Humanities_department",
|
426 |
+
"patterns": [
|
427 |
+
"S and H dept",
|
428 |
+
"Tell me about the Science and Humanities Department",
|
429 |
+
"Tell me about the Science and Humanities",
|
430 |
+
"S&H Department",
|
431 |
+
"Science and Humanities Department",
|
432 |
+
"Can you provide information about the Science and Humanities Department",
|
433 |
+
"Share details about the Science and Humanities Department",
|
434 |
+
"What's up with the Science and Humanities Department?",
|
435 |
+
"What can you tell me about Science and Humanities?",
|
436 |
+
"Give me the scoop on Science and Humanities",
|
437 |
+
"I'm curious about Science and Humanities"
|
438 |
+
],
|
439 |
+
"responses": [
|
440 |
+
"The Department of Science and Humanities is a primal department which consists of Chemistry, Physics, Mathematics and English disciplines. All the disciplines in the department exist as a separate division and maintain individual identities. The varied disciplines provide the students with the knowledge of Mathematics, Physics, Chemistry, English and Environmental Science fundamentals. Developing moral behaviour among the students, utilizing human resources and coordinating academic responsibilities are the exclusive potentials of the department. The department accomplishes transforming young aspiring minds into determined freshman engineers through Outcome Based Education. Besides, the department teaches students a positive ethos through Value-Based Education. Dr. M.A. Neelakantan, Professor & Head, who has academic excellence and research experience for 38 years, leads the department with 30 highly qualified, experienced and goal-oriented members with specializations in various fields."
|
441 |
+
]
|
442 |
+
},
|
443 |
+
{
|
444 |
+
"tag": "Science and Humanities_department_vision",
|
445 |
+
"patterns": [
|
446 |
+
"What is the vision of the Science and Humanities Department?",
|
447 |
+
"What's the vision for Science and Humanities?",
|
448 |
+
"What does the Science and Humanities Department aspire to?",
|
449 |
+
"What are the goals of Science and Humanities?"
|
450 |
+
],
|
451 |
+
"responses": [
|
452 |
+
"Producing potentially competent freshman engineers with leadership qualities and human values."
|
453 |
+
]
|
454 |
+
},
|
455 |
+
{
|
456 |
+
"tag": "Science and Humanities_mission",
|
457 |
+
"patterns": [
|
458 |
+
"What is the mission of the Science and Humanities Department?",
|
459 |
+
"What's the mission for Science and Humanities?",
|
460 |
+
"What does the Science and Humanities Department aim to achieve?",
|
461 |
+
"What is Science and Humanities striving for?"
|
462 |
+
],
|
463 |
+
"responses": [
|
464 |
+
"To impart engineering fundamentals by providing Basic Science and Mathematics. To stimulate reading, listening and writing skills. To promote innovative culture among the students."
|
465 |
+
]
|
466 |
+
},
|
467 |
+
{
|
468 |
+
"tag": "Science and Humanities_phd",
|
469 |
+
"patterns": [
|
470 |
+
"Tell me about the PhD program in Science and Humanities",
|
471 |
+
"What's the story with the PhD program in Science and Humanities?",
|
472 |
+
"Give me the details on Science and Humanities PhD program"
|
473 |
+
],
|
474 |
+
"responses": [
|
475 |
+
"Chemistry Supervisors: 5. Physics Supervisors: 2. Mathematics Supervisors: 1. Full-time Scholars: 4. Part-time Scholars: 2. Awarded: 15"
|
476 |
+
]
|
477 |
+
},
|
478 |
+
{
|
479 |
+
"tag": "Science and Humanities_hod",
|
480 |
+
"patterns": [
|
481 |
+
"Who is the Head of the Science and humanities Department?",
|
482 |
+
"Hod of Science & Humanities Dept",
|
483 |
+
"Hod S&H",
|
484 |
+
"Who leads Science and humanities?"
|
485 |
+
],
|
486 |
+
"responses": [
|
487 |
+
"Dr.M.A.Neelakantan Professor & Head"
|
488 |
+
]
|
489 |
+
},
|
490 |
+
{
|
491 |
+
"tag": "S&H_department_faculty",
|
492 |
+
"patterns": [
|
493 |
+
"Tell me about the faculty in the Science and Humanities Department",
|
494 |
+
"Who teaches in Science and Humanities?",
|
495 |
+
"Give me the rundown on Science and Humanities faculty",
|
496 |
+
"What's the deal with Science and Humanities faculty?"
|
497 |
+
],
|
498 |
+
"responses": [
|
499 |
+
"Dr.M.A.Neelakantan - Professor & Head. Chemistry: Dr.S.Thalamuthu -Associate Professor. Dr.S.Chithirai Kumar - Assistant Professor(SG). Dr.E.Ramachandran - Assistant Professor(SG). Dr.B.Annaraj - Assistant Professor (SG). Mr.J.Thamba - Assistant Professor. Dr.B.Rajkumar - Assistant Professor. English: Ms.S.Gopiga Devi - Assistant Professor. Dr. K. Muthulakshmi - Assistant Professor. Mr.A.Muthusamy - Assistant Professor. Mr.T.Sivakumar - Assistant Professor. Dr.SathishBalakumaran S.N.I.- Assistant Professor. Ms.C.Dhanshree - Assistant Professor. Dr.K.Latha - Assistant Professor. Mathematics: Dr.S.Rammurthy- Professor. Dr.M.A.Perumal - Associative Professor. Ms.S.Geetha - Assistant Professor(SG). Ms.M.Annapoopathi - Assistant Professor(SG). Ms. S. Sasireka- Assistant Professor. Mr.M.Arul - Assistant Professor. Ms.S.S.BashithaParveen - Assistant Professor. Ms.P.Mala - Assistant Professor. Mr. N.Sivanandhan - Assistant Professor. Mr.S.Sivabalan - Assistant Professor. Ms.L.Meenatchi - Assistant Professor. Mr.P.Ganapathy - Assistant Professor. Ms.R.Premalatha - Assistant Professor. Ms.P.Dharani - Assistant Professor. Ms.B.Malathi - Assistant Professor. Ms.T. Kalaiselvi - Assistant Professor. Physics: Dr.A.Nichelson - Assistant Professor(SG). Dr.A.Panimayavalan Rakkini - Assistant Professor(SG). Dr.V.RamaSubbu - Assistant Professor. Dr.V.RamaSubbu - Assistant Professor. Dr.M.Prabhu - Assistant Professor. Dr.M.Aravind - Assistant Professor. Tamil: Dr.S.Chithra - Assistant Professor. Soft Skill Trainer: Ms.S.Jeba - Soft Skill Trainer"
|
500 |
+
]
|
501 |
+
},
|
502 |
+
{
|
503 |
+
"tag": "college_info",
|
504 |
+
"patterns": [
|
505 |
+
"college information",
|
506 |
+
"info about college",
|
507 |
+
"college details",
|
508 |
+
"details about college"
|
509 |
+
],
|
510 |
+
"responses": [
|
511 |
+
"National Engineering College is located in K.R.Nagar Kovilpatti, Nallatinputhur, Tamil Nadu 628503. It is accredited by NBA, NIRF, ARIIA, and AICTE."
|
512 |
+
]
|
513 |
+
},
|
514 |
+
{
|
515 |
+
"tag": "address",
|
516 |
+
"patterns": [
|
517 |
+
"address of college",
|
518 |
+
"college address",
|
519 |
+
"address"
|
520 |
+
],
|
521 |
+
"responses": [
|
522 |
+
"K.R.Nagar, Kovilpatti, Nallatinputhur, Tamil Nadu 628503"
|
523 |
+
]
|
524 |
+
},
|
525 |
+
{
|
526 |
+
"tag": "placement_dean",
|
527 |
+
"patterns": [
|
528 |
+
"who is placement dean",
|
529 |
+
"placement dean name",
|
530 |
+
"name of the placement dean",
|
531 |
+
"placement dean"
|
532 |
+
],
|
533 |
+
"responses": [
|
534 |
+
"The Placement dean of the college is Dr.K.G.Srinivasagan."
|
535 |
+
]
|
536 |
+
},
|
537 |
+
{
|
538 |
+
"tag": "placement_convener",
|
539 |
+
"patterns": [
|
540 |
+
"placement convener",
|
541 |
+
"name of the placement convener",
|
542 |
+
"who is placement convener"
|
543 |
+
],
|
544 |
+
"responses": [
|
545 |
+
"The Placement convener is Dr.V.Manimaran."
|
546 |
+
]
|
547 |
+
},
|
548 |
+
{
|
549 |
+
"tag": "recruiters",
|
550 |
+
"patterns": [
|
551 |
+
"recruiters",
|
552 |
+
"recruiters name",
|
553 |
+
"count of the recruiters",
|
554 |
+
"count of recruiters coming to college every year"
|
555 |
+
],
|
556 |
+
"responses": [
|
557 |
+
"The total no.of.recruiters coming to our campus are 100+. There are: Zoho, TATA, Coginizant, Wipro, Infosys, Accenture, IBM, Infoview, Solartis, HCL, Hexware, Atos, TechMahindra, Tessolve, AstraZeneca, Cadence, Vuram, Jilaba, Comcast, VVDN, Smackcoders, American Megatrends, Centizen, and Pinnacle infotech."
|
558 |
+
]
|
559 |
+
},
|
560 |
+
{
|
561 |
+
"tag": "vision_mission",
|
562 |
+
"patterns": [
|
563 |
+
"vision",
|
564 |
+
"college vision",
|
565 |
+
"Nec vision",
|
566 |
+
"vision of nec",
|
567 |
+
"mission",
|
568 |
+
"college mission",
|
569 |
+
"Nec mission",
|
570 |
+
"mission of nec"
|
571 |
+
],
|
572 |
+
"responses": [
|
573 |
+
"Vision: Transforming lives through quality education and research with human values.",
|
574 |
+
"Mission: To maintain excellent infrastructure and highly qualified and dedicated faculty. To provide a conducive environment with an ambiance of humanity, wisdom, creativity, and team spirit. To promote the values of ethical behavior and commitment to society. To partner with academic, industrial, and government entities to attain collaborative research."
|
575 |
+
]
|
576 |
+
},
|
577 |
+
{
|
578 |
+
"tag": "academics",
|
579 |
+
"patterns": [
|
580 |
+
"academics",
|
581 |
+
"about academics",
|
582 |
+
"academics of nec",
|
583 |
+
"about academics of nec"
|
584 |
+
],
|
585 |
+
"responses": [
|
586 |
+
"The office of the Dean (Academic) was established in the year 2010 to administer all academic activities of the Institution. Academic Dean is responsible for all academic matters pertaining to U.G. and P.G.programmes offered by the Institute. This office revises the U.G. and P.G. Regulations, curriculum and syllabus of all branches of Engineering, Technology & Science and Humanities as per the guidelines of UGC, AICTE and Anna University, Chennai."
|
587 |
+
]
|
588 |
+
},
|
589 |
+
{
|
590 |
+
"tag": "members_of_academics",
|
591 |
+
"patterns": [
|
592 |
+
"members of the academics",
|
593 |
+
"who are the members of the academics"
|
594 |
+
],
|
595 |
+
"responses": [
|
596 |
+
"1. Dr. A. Shenbagavalli - Dean (Academic)",
|
597 |
+
"2. Dr. K. Mohaideen Pitchai - Professor / CSE",
|
598 |
+
"3. Dr. F. Micheal Thomas Rex - Associate Professor / Mech",
|
599 |
+
"4. Ms. R. Chermasakthi - Assistant"
|
600 |
+
]
|
601 |
+
},
|
602 |
+
{
|
603 |
+
"tag": "dean_of_academics",
|
604 |
+
"patterns": [
|
605 |
+
"dean of the academics",
|
606 |
+
"who is the dean of the academics",
|
607 |
+
"dean academic"
|
608 |
+
],
|
609 |
+
"responses": [
|
610 |
+
"Dr. A. Shenbagavalli Dean (Academic)"
|
611 |
+
]
|
612 |
+
},
|
613 |
+
{
|
614 |
+
"tag": "nba_accreditation",
|
615 |
+
"patterns": [
|
616 |
+
"NBA Accreditation",
|
617 |
+
"NBA",
|
618 |
+
"NBA Accreditation Status",
|
619 |
+
"Accreditation",
|
620 |
+
"Can you provide information about the NBA Accreditation",
|
621 |
+
"Share details about the NBA Accreditation"
|
622 |
+
],
|
623 |
+
"responses": [
|
624 |
+
"1. B.E. Mechanical Engineering - Accredited by NBA Tier I",
|
625 |
+
"2. B.E. Electronics and Communication Engineering - Accredited by NBA Tier I",
|
626 |
+
"3. B.E. Computer Science and Engineering - Accredited by NBA Tier I",
|
627 |
+
"4. B.E. Electrical and Electronics Engineering - Accredited by NBA Tier I",
|
628 |
+
"5. B.Tech. Information Technology - Accredited by NBA Tier I"
|
629 |
+
]
|
630 |
+
},
|
631 |
+
{
|
632 |
+
"tag": "mech_nba_accreditation",
|
633 |
+
"patterns": [
|
634 |
+
"NBA Accreditation of MECH",
|
635 |
+
"NBA status of MECH",
|
636 |
+
"What is the NBA Accreditation of Mechanical Engineering Department",
|
637 |
+
"What is the NBA Accreditation of Mechanical Engineering",
|
638 |
+
"Can you provide information about the NBA Accreditation of MECH",
|
639 |
+
"Share details about the NBA Accreditation MECH Dept"
|
640 |
+
],
|
641 |
+
"responses": [
|
642 |
+
"B.E. Mechanical Engineering - Accredited by NBA Tier I"
|
643 |
+
]
|
644 |
+
},
|
645 |
+
{
|
646 |
+
"tag": "ECE_NBA_Accreditation",
|
647 |
+
"patterns": [
|
648 |
+
"What is the NBA Accreditation of ECE",
|
649 |
+
"NBA Accreditation of ECE",
|
650 |
+
"NBA status of ECE",
|
651 |
+
"What is the NBA Accreditation of Electronics and Communication Engineering Department",
|
652 |
+
"What is the NBA Accreditation of Electronics and Communication Engineering",
|
653 |
+
"Can you provide information about the NBA Accreditation of ECE",
|
654 |
+
"Share details about the NBA Accreditation ECE Dept"
|
655 |
+
],
|
656 |
+
"responses": [
|
657 |
+
"Electronics and Communication Engineering - Accredited by NBA Tier I"
|
658 |
+
]
|
659 |
+
},
|
660 |
+
{
|
661 |
+
"tag": "CSE_NBA_Accreditation",
|
662 |
+
"patterns": [
|
663 |
+
"What is the NBA Accreditation of CSE",
|
664 |
+
"NBA Accreditation of CSE",
|
665 |
+
"NBA status of CSE",
|
666 |
+
"What is the NBA Accreditation of Computer Science and Engineering Department",
|
667 |
+
"What is the NBA Accreditation of Computer Science and Engineering",
|
668 |
+
"Can you provide information about the NBA Accreditation of CSE",
|
669 |
+
"Share details about the NBA Accreditation CSE Dept"
|
670 |
+
],
|
671 |
+
"responses": [
|
672 |
+
"B.E. Computer Science and Engineering - Accredited by NBA Tier I"
|
673 |
+
]
|
674 |
+
},
|
675 |
+
{
|
676 |
+
"tag": "EEE_NBA_Accreditation",
|
677 |
+
"patterns": [
|
678 |
+
"What is the NBA Accreditation of EEE",
|
679 |
+
"NBA Accreditation of EEE",
|
680 |
+
"NBA status of EEE",
|
681 |
+
"What is the NBA Accreditation of Electrical and Electronics Engineering Department",
|
682 |
+
"What is the NBA Accreditation of Electrical and Electronics Engineering",
|
683 |
+
"Can you provide information about the NBA Accreditation of EEE",
|
684 |
+
"Share details about the NBA Accreditation EEE Dept"
|
685 |
+
],
|
686 |
+
"responses": [
|
687 |
+
"B.E. Electrical and Electronics Engineering - Accredited by NBA Tier I"
|
688 |
+
]
|
689 |
+
},
|
690 |
+
{
|
691 |
+
"tag": "IT_NBA_Accreditation",
|
692 |
+
"patterns": [
|
693 |
+
"What is the NBA Accreditation of IT",
|
694 |
+
"NBA Accreditation of IT",
|
695 |
+
"NBA status of IT",
|
696 |
+
"What is the NBA Accreditation of Information Technology Department",
|
697 |
+
"What is the NBA Accreditation of Information Technology",
|
698 |
+
"Can you provide information about the NBA Accreditation of IT",
|
699 |
+
"Share details about the NBA Accreditation IT Dept"
|
700 |
+
],
|
701 |
+
"responses": [
|
702 |
+
"B.Tech. Information Technology - Accredited by NBA Tier I"
|
703 |
+
]
|
704 |
+
},
|
705 |
+
{
|
706 |
+
"tag": "Civil_NBA_Accreditation",
|
707 |
+
"patterns": [
|
708 |
+
"What is the NBA Accreditation of civil",
|
709 |
+
"NBA Accreditation of civil",
|
710 |
+
"NBA status of civil",
|
711 |
+
"What is the NBA Accreditation of Civil Engineering Department",
|
712 |
+
"What is the NBA Accreditation of Civil Engineering",
|
713 |
+
"Can you provide information about the NBA Accreditation of Civil",
|
714 |
+
"Share details about the NBA Accreditation Civil Dept"
|
715 |
+
],
|
716 |
+
"responses": [
|
717 |
+
"There is no NBA accreditation for Civil Engineering department."
|
718 |
+
]
|
719 |
+
},
|
720 |
+
{
|
721 |
+
"tag": "AI&DS_NBA_Accreditation",
|
722 |
+
"patterns": [
|
723 |
+
"What is the NBA Accreditation of AI & DS",
|
724 |
+
"NBA Accreditation of AIDS",
|
725 |
+
"NBA status of AIDS",
|
726 |
+
"What is the NBA Accreditation of Artificial and data science Department",
|
727 |
+
"What is the NBA Accreditation of Artificial and data science",
|
728 |
+
"Can you provide information about the NBA Accreditation of AI & DS",
|
729 |
+
"Share details about the NBA Accreditation AIDS Dept"
|
730 |
+
],
|
731 |
+
"responses": [
|
732 |
+
"There is no NBA accreditation for Artificial and data science department."
|
733 |
+
]
|
734 |
+
},
|
735 |
+
{
|
736 |
+
"tag": "Science and Humanities_NBA_Accreditation",
|
737 |
+
"patterns": [
|
738 |
+
"What is the NBA Accreditation of S&H",
|
739 |
+
"NBA Accreditation of S&H",
|
740 |
+
"NBA status of S&H",
|
741 |
+
"What is the NBA Accreditation of Science and Humanities Department",
|
742 |
+
"What is the NBA Accreditation ofScience and Humanities",
|
743 |
+
"Can you provide information about the NBA Accreditation of S&H",
|
744 |
+
"Share details about the NBA Accreditation S&H Dept"
|
745 |
+
],
|
746 |
+
"responses": [
|
747 |
+
"There is no NBA accreditation for Science and Humanities department."
|
748 |
+
]
|
749 |
+
},
|
750 |
+
{
|
751 |
+
"tag": "CSE_department_info",
|
752 |
+
"patterns": [
|
753 |
+
"CSE dept",
|
754 |
+
"Tell me about the Computer Science and Engineering Department",
|
755 |
+
"Tell me about the CSE Department",
|
756 |
+
"CSE Department",
|
757 |
+
"Computer Science and Engineering Department",
|
758 |
+
"Can you provide information about the Computer Science and Engineering Department",
|
759 |
+
"Share details about the Computer Science and Engineering Department"
|
760 |
+
],
|
761 |
+
"responses": [
|
762 |
+
"Our CSE department was established in the year 1984 and offers a conducive learning and research environment with the aim of transforming the lifestyle of rural students with quality education and higher-level career settlement. We have dedicated and quality faculty members with well-maintained state-of-the-art academic and research laboratories. To enlighten their knowledge our curriculum is periodically upgraded with emerging trends to bridge the industrial gaps. Our department has well-established In-house incubation centers to inculcate the industrial practices at their accessibility well in advance. Students excel themselves in International and National level competitions, hackathons to groom their soft skills with the care and affection of our mentors. We are thankful for our stakeholders (Alumni, Parents, Academic & Industrial experts) who are guiding and shaping us towards excellence."
|
763 |
+
]
|
764 |
+
},
|
765 |
+
{
|
766 |
+
"tag": "CSE_department_vision_mission",
|
767 |
+
"patterns": [
|
768 |
+
"vision of the Computer Science and Engineering Department",
|
769 |
+
"mission of the CSE Engineering Department",
|
770 |
+
"cse vision mission"
|
771 |
+
],
|
772 |
+
"responses": [
|
773 |
+
"Vision: To produce globally competent, innovative and socially responsible computing professionals , Mission: To provide world-class teaching-learning and research facilities. To stimulate students’ logical thinking, creativity, and communication skills effectively. To cultivate awareness about emerging trends through self-initiative. To instill a sense of societal and ethical responsibilities. To collaborate with industries and government organizations."
|
774 |
+
]
|
775 |
+
},
|
776 |
+
{
|
777 |
+
"tag": "CSE_department_programs",
|
778 |
+
"patterns": [
|
779 |
+
"undergraduate program in Computer Science and Engineering",
|
780 |
+
"postgraduate program in Computer Science and Engineering",
|
781 |
+
"PhD program in Computer Science and Engineering"
|
782 |
+
],
|
783 |
+
"responses": [
|
784 |
+
"Undergraduate Program: Year of Establishment - 1984, Student Strength - 389, Postgraduate Program: Year of Establishment - 2002, Student Strength - 17, PhD Program: Year of Establishment - 2006, Scholars - Full time: 1, Part-time: 12, Awarded: 31 (since 2010)"
|
785 |
+
]
|
786 |
+
},
|
787 |
+
{
|
788 |
+
"tag": "CSE_department_hod",
|
789 |
+
"patterns": [
|
790 |
+
"Head of the Computer Science and Engineering Department",
|
791 |
+
"Hod of CSE Dept",
|
792 |
+
"Hod CSE"
|
793 |
+
],
|
794 |
+
"responses": [
|
795 |
+
"Dr. V. Gomathi Professor & Head"
|
796 |
+
]
|
797 |
+
},
|
798 |
+
{
|
799 |
+
"tag": "CSE_department_faculty",
|
800 |
+
"patterns": [
|
801 |
+
"faculty in CSE Department",
|
802 |
+
"faculty in Computer Science and Engineering Department",
|
803 |
+
"faculty list for CSE Department",
|
804 |
+
"CSE faculty",
|
805 |
+
"CSE Department faculty"
|
806 |
+
],
|
807 |
+
"responses": [
|
808 |
+
"1. Dr. V. Gomathi - Professor and Head, 2. Dr. D. Manimegalai - Professor, 3. Dr. K. Mohaideen Pitchai - Professor, 4. Dr. S. Kalaiselvi - Associate Professor, 5. Dr. J. Naskath - Assistant Professor(SG), 6. Ms. R. Rajakumari - Assistant Professor(SG), 7. Mr. D. Vijayakumar - Assistant Professor(SG), 8. Dr. G. Sivakama Sundari - Assistant Professor(SG), 9. Ms. B. Shanmugapriya - Assistant Professor(SG), 10. Mr. S. Dheenathayalan - Assistant Professor(SG)"
|
809 |
+
]
|
810 |
+
},
|
811 |
+
{
|
812 |
+
"tag": "ECE_department_info",
|
813 |
+
"patterns": [
|
814 |
+
"ECE dept",
|
815 |
+
"Tell me about the Electronics and Communication Engineering Department",
|
816 |
+
"Tell me about the ECE Department",
|
817 |
+
"ECE Department",
|
818 |
+
"Electronics and Communication Engineering Department",
|
819 |
+
"Can you provide information about the Electronics and Communication Engineering Department",
|
820 |
+
"Share details about the ECE Department"
|
821 |
+
],
|
822 |
+
"responses": [
|
823 |
+
"The department of Electronics and Communication Engineering has been continuously striving to provide excellent Engineering Education in the field of electronics and communication since 1984. It offers one undergraduate programme B.E. in Electronics and Communication Engineering (ECE) and one postgraduate programme M.E. in Embedded System Technologies. National Board of Accreditation (NBA), New Delhi has provisionally accredited the Under Graduate Programme of the ECE department under Tier – 1 category. Anna University Chennai has recognized the department as a research centre."
|
824 |
+
]
|
825 |
+
},
|
826 |
+
{
|
827 |
+
"tag": "ECE_department_vision",
|
828 |
+
"patterns": [
|
829 |
+
"What is the vision of the Electronics and Communication Engineering Department?"
|
830 |
+
],
|
831 |
+
"responses": [
|
832 |
+
"To produce Electronics and Communication Engineers capable of generating a knowledge economy with social responsibility."
|
833 |
+
]
|
834 |
+
},
|
835 |
+
{
|
836 |
+
"tag": "ECE_department_mission",
|
837 |
+
"patterns": [
|
838 |
+
"What is the mission of the Electronics and Communication Engineering Department?"
|
839 |
+
],
|
840 |
+
"responses": [
|
841 |
+
"To impart high-quality education with ethical behaviour,To equip the students compatible with recent trends in Electronic industries.To develop leadership qualities with humanity, wisdom, creativity and team spirit.To provide a passionate environment for continual learning."
|
842 |
+
]
|
843 |
+
},
|
844 |
+
{
|
845 |
+
"tag": "ECE_department_ug",
|
846 |
+
"patterns": [
|
847 |
+
"Tell me about the undergraduate program in Electronics and Communication Engineering"
|
848 |
+
],
|
849 |
+
"responses": [
|
850 |
+
"Year of Establishment:1984, Student Strength:120 from 2006"
|
851 |
+
]
|
852 |
+
},
|
853 |
+
{
|
854 |
+
"tag": "ECE_department_pg",
|
855 |
+
"patterns": [
|
856 |
+
"Tell me about the postgraduate program in Electronics and Communication Engineering"
|
857 |
+
],
|
858 |
+
"responses": [
|
859 |
+
"Year of Establishment:2011 , Student Strength:18 from 2012, 12 from 2022."
|
860 |
+
]
|
861 |
+
},
|
862 |
+
{
|
863 |
+
"tag": "ECE_department_phd",
|
864 |
+
"patterns": [
|
865 |
+
"Tell me about the PhD program in Electronics and Communication Engineering"
|
866 |
+
],
|
867 |
+
"responses": [
|
868 |
+
"Year of Establishment:2011",
|
869 |
+
"scholars:",
|
870 |
+
"Full time:3",
|
871 |
+
"Part time:19",
|
872 |
+
"Awarded:26(Since 2013)"
|
873 |
+
]
|
874 |
+
},
|
875 |
+
{
|
876 |
+
"tag": "ECE_department_hod",
|
877 |
+
"patterns": [
|
878 |
+
"Who is the Head of the Electronics and Communication Engineering Department?",
|
879 |
+
"Hod of ECE Dept",
|
880 |
+
"Hod ECE"
|
881 |
+
],
|
882 |
+
"responses": [
|
883 |
+
"Dr. S. Tamilselvi Professor & Head"
|
884 |
+
]
|
885 |
+
},
|
886 |
+
{
|
887 |
+
"tag": "ECE_department_faculty",
|
888 |
+
"patterns": [
|
889 |
+
"faculty in ECE Department",
|
890 |
+
"faculty in Electronics and Communication Engineering Department",
|
891 |
+
"faculty list for ECE Department",
|
892 |
+
"ECE faculty",
|
893 |
+
"ECE Department faculty"
|
894 |
+
],
|
895 |
+
"responses": [
|
896 |
+
"1. Dr. S. Tamilselvi - Professor and Head, 2. Dr. A. Shenbagavalli - Professor, 3. Dr. T. S. Arun Samuel - Professor, 4. Dr. N. Arumugam - Associate Professor, 5. Dr. V. Suresh - Associate Professor, 6. Dr. K. J. Prasanna Venkatesan - Associate Professor, 7. Dr. C. Balamurugan - Associate Professor, 8. Dr. A. Saravana Selvan - Associate Professor, 9. Mr. T. Devakumar - Assistant Professor(SG), 10. Mr. S. Cammillus - Assistant Professor(SG), 11. Mr. K. Subramanian - Assistant Professor(SG), 12. Dr. R. Manjula Devi - Assistant Professor(SG), 13. Mr. M. Sathish Kumar - Assistant Professor(SG), 14. Mr. B. Ganapathy Ram - Assistant Professor(SG), 15. Dr. I. Vivek Anand - Assistant Professor(SG), 16. Ms. S. Karthika - Assistant Professor, 17. Ms. J. E. Jeyanthi - Assistant Professor, 18. Ms. S. Malathi - Assistant Professor, 19. Ms. C. Kalieswari - Assistant Professor, 20. Dr. I. Paulkani - Assistant Professor, 21. Ms. A. Apsara - Assistant Professor, 22. Ms. P. Arishenbagam - Assistant Professor, 23. Ms. C. Lakshmi - Assistant Professor, 24. Dr. S. Lavanya - Assistant Professor, 25. Ms. R. Geeta - Assistant Professor, 26. Mrs. S. Sulochana - Assistant Professor, 27. Ms. S. Sindhana - Assistant Professor, 28. Ms. C. K. Balasundari - Assistant Professor"
|
897 |
+
]
|
898 |
+
},
|
899 |
+
{
|
900 |
+
"tag": "EEE_department",
|
901 |
+
"patterns": [
|
902 |
+
"EEE dept",
|
903 |
+
"Electrical and Electronics Engineering Department",
|
904 |
+
"EEE Department",
|
905 |
+
"Tell me about the Electrical and Electronics Engineering Department",
|
906 |
+
"Can you provide information about the EEE Department"
|
907 |
+
],
|
908 |
+
"responses": [
|
909 |
+
"The department of Electrical and Electronics Engineering was established in 1994 with an initial intake of 40 students, which was later increased to 60. The B.E. program received accreditation from NBA for 3 years in July 2006, and re-accreditation for another 3 years in September 2011. In 2014, it was accredited by NBA Tier 1 (Washington Accord) for 2 years, and the accreditation was extended up to 2023. Currently, the B.E. program is provisionally re-accredited for 3 years starting from 2023. Additionally, the department offers M.E. in High Voltage Engineering since 2005, making it the only affiliated college under Anna University, Chennai offering this specialization. Dr. M. Willjuice Iruthayarajan, M.E., Ph.D., serves as the Professor & Head, bringing 24 years of academic and research experience to efficiently lead the department, which is supported by 15 staff members."
|
910 |
+
]
|
911 |
+
},
|
912 |
+
{
|
913 |
+
"tag": "EEE_department_vision",
|
914 |
+
"patterns": [
|
915 |
+
"What is the vision of the Electrical and Electronics Engineering Department?"
|
916 |
+
],
|
917 |
+
"responses": [
|
918 |
+
"Promoting active learning, critical thinking coupled with ethical values to meet the global challenges."
|
919 |
+
]
|
920 |
+
},
|
921 |
+
{
|
922 |
+
"tag": "EEE_department_mission",
|
923 |
+
"patterns": [
|
924 |
+
"What is the mission of the Electrical and Electronics Engineering Department?"
|
925 |
+
],
|
926 |
+
"responses": [
|
927 |
+
"To instill state-of-the-art technical knowledge and research capability that will prepare our graduates for professionalism and lifelong learning.To update knowledge to inculcate social and ethical values.To meet industrial and real-world challenges."
|
928 |
+
]
|
929 |
+
},
|
930 |
+
{
|
931 |
+
"tag": "EEE_department_ug",
|
932 |
+
"patterns": [
|
933 |
+
"Tell me about the undergraduate program in Electrical and Electronics Engineering"
|
934 |
+
],
|
935 |
+
"responses": [
|
936 |
+
"Year of Establishment:1994, Student Strength:60"
|
937 |
+
]
|
938 |
+
},
|
939 |
+
{
|
940 |
+
"tag": "EEE_department_pg",
|
941 |
+
"patterns": [
|
942 |
+
"Tell me about the postgraduate program in Electrical and Electronics Engineering"
|
943 |
+
],
|
944 |
+
"responses": [
|
945 |
+
"Year of Establishment:2005 , Student Strength:06"
|
946 |
+
]
|
947 |
+
},
|
948 |
+
{
|
949 |
+
"tag": "EEE_department_phd",
|
950 |
+
"patterns": [
|
951 |
+
"Tell me about the PhD program in Electrical and Electronics Engineering"
|
952 |
+
],
|
953 |
+
"responses": [
|
954 |
+
"Year of Establishment:2010 scholars: Full time:01 Part time:18 Awarded:24"
|
955 |
+
]
|
956 |
+
},
|
957 |
+
{
|
958 |
+
"tag": "EEE_department_hod",
|
959 |
+
"patterns": [
|
960 |
+
"Who is the Head of the Electrical and Electronics Department?",
|
961 |
+
"Hod of EEE Dept",
|
962 |
+
"Hod EEE"
|
963 |
+
],
|
964 |
+
"responses": [
|
965 |
+
"Dr. M. Willjuice Iruthayarajan Professor & Head"
|
966 |
+
]
|
967 |
+
},
|
968 |
+
{
|
969 |
+
"tag": "EEE_department_faculty",
|
970 |
+
"patterns": [
|
971 |
+
"faculty in the Electrical and Electronics Engineering Department"
|
972 |
+
],
|
973 |
+
"responses": ["1. Dr. M. Willjuice Iruthayarajan - Professor and Head, 2. Dr. L. Kalaivani - Professor, 3. Dr. R. V. Maheswari - Professor, 4. Dr. N. B. Prakash - Professor, 5. Dr. S. Sankarakumar - Assistant Professor(SG), 6. Dr. B. Vigneshwaran - Assistant Professor(SG), 7. Mr. B. Venkatasamy - Assistant Professor(SG), 8. Dr. M. Gengaraj - Assistant Professor(SG), 9. Mr. P. Samuel Pakianthan - Assistant Professor, 10. Mr. M. Sivapalanirajan - Assistant Professor"]
|
974 |
+
},
|
975 |
+
{
|
976 |
+
"tag": "recruitment",
|
977 |
+
"patterns": [
|
978 |
+
"Procedure for staff/faculty recruitment",
|
979 |
+
"How are professors recruited at NEC",
|
980 |
+
"NEC recruitment process",
|
981 |
+
"Faculty recruitment"
|
982 |
+
],
|
983 |
+
"responses": ["Instructions, Advertisement, Application Form Download, Form Filling, Upload, Inquiries: [email protected], Principal's Office, Website: https://nec.edu.in/faculty-recruitment/"]
|
984 |
+
},
|
985 |
+
{
|
986 |
+
"tag": "industries",
|
987 |
+
"patterns": [
|
988 |
+
"Industries in NEC",
|
989 |
+
"Companies in NEC",
|
990 |
+
"nec industries",
|
991 |
+
"Technobation Centres in NEC",
|
992 |
+
"NEC Technobation Centre"
|
993 |
+
],
|
994 |
+
"responses": ["NEC - MISTRAL TECHNOBATION CENTER, NEC - BRIMMA TECHNOBATION CENTER, NEC - VLINDER TECHNOBATION CENTER"]
|
995 |
+
},
|
996 |
+
{
|
997 |
+
"tag": "hostel",
|
998 |
+
"patterns": [
|
999 |
+
"Number of hostels in NEC",
|
1000 |
+
"Rooms in hostel",
|
1001 |
+
"hostel rooms"
|
1002 |
+
],
|
1003 |
+
"responses": ["Gents Hostel 1 - 159 rooms, Gents Hostel 2 - 324 rooms, Ladies Hostel - 251 rooms"]
|
1004 |
+
},
|
1005 |
+
{
|
1006 |
+
"tag": "hostel-facilities",
|
1007 |
+
"patterns": [
|
1008 |
+
"Hostel facilities",
|
1009 |
+
"Facilities available in the hostel",
|
1010 |
+
"Wifi in hostel",
|
1011 |
+
"nec hostel facilities",
|
1012 |
+
"facilities in hostel",
|
1013 |
+
"available hostel facilities"
|
1014 |
+
],
|
1015 |
+
"responses": [
|
1016 |
+
"Uninterrupted power supply, separate Generator for Students, Medical facility with free medicines for sick students Mineral water provided to all rooms , Sweets and Juice center available after college hours ,Free Computer Lab facilities after class hours, 24-hour WiFi facility ,Banking facility with ATM ,Modern dining hall serving nutritious food, Well-equipped fitness center, Stationery store, Modern kitchen with oven and solar steam cooking plant, EPABX system and Telephone facility, Fully equipped individual / 3 shared rooms"
|
1017 |
+
]
|
1018 |
+
},
|
1019 |
+
{
|
1020 |
+
"tag": "chapters",
|
1021 |
+
"patterns": [
|
1022 |
+
"NEC chapters",
|
1023 |
+
"List of chapters in NEC",
|
1024 |
+
"Chapters in NEC"
|
1025 |
+
],
|
1026 |
+
"responses": [
|
1027 |
+
"IEEE Student’s Chapter,IEEE Computer Society Student’s Chapter,IE(I) Student’s Chapter, IE(I) Staff Chapter, Computer Society Of India Chapter, IETE Students’ Forum, ISTE Student’s Chapter, ISTE Staff Chapter, ISHRAE Student’s Chapter, Society For Manufacturing Engineers, Society Of Automotive Engineers – Student’s Chapter, CISCO Networking Academy, Red Hat Academy, MISTRAL Academy, ICT Academy, Matlab Forum"
|
1028 |
+
]
|
1029 |
+
},
|
1030 |
+
{
|
1031 |
+
"tag": "K.R.Innovation Centre",
|
1032 |
+
"patterns": [
|
1033 |
+
"Details of K.R.Innovation Centre",
|
1034 |
+
"About K.R.Innovation Centre",
|
1035 |
+
"K.R.Innovation Centre",
|
1036 |
+
"KR Innovation Centre",
|
1037 |
+
"K R innovation centre"
|
1038 |
+
],
|
1039 |
+
"responses": [
|
1040 |
+
"National Engineering college is Recognized as host institute/ Business Incubator by Ministry of MSME, New Delhi. The ministy has supported Rs. 35 lakhs for the development of 6 products. K. R. Innovation Centre(KRIC) a section8 company acting as a special purpose vehicle for our institute is established in 2018 for taking care of business incubation activities. NEC and KRIC inculcates Innovation & Startup culture inside and outside the campus to enhance Socio-economic status and reputation of southern Tamilnadu. 2 Unicorns, 15 Incubated Startups, 50 Employement Generated, 100 Internships Offered, 2000000 rupees Seed Fund Disbursed, 3 Cohorts"
|
1041 |
+
]
|
1042 |
+
},
|
1043 |
+
{
|
1044 |
+
"tag": "K.R.Innovation Centre team",
|
1045 |
+
"patterns": [
|
1046 |
+
"Team members of K.R.Innovation Centre",
|
1047 |
+
"Head of K.R.Innovation Centre",
|
1048 |
+
"K.R.Innovation Centre members",
|
1049 |
+
"Members of KR innovation centre"
|
1050 |
+
],
|
1051 |
+
"responses": [
|
1052 |
+
"Dr. K. Manisekar - Dean Innovation & Entrepreneurship. Dr. G. Vinoth - Chief Executive Officer. Mr. A. Srinivasan - DGM Finance. Mr. B. Ganapathy Ram - Technical Lead (Hardware: IOT). Dr. T. Sakthi - Convenor IIC. Mr. Y. Jerold Jaikins - Software Engineer"
|
1053 |
+
]
|
1054 |
+
},
|
1055 |
+
{
|
1056 |
+
"tag": "CSE_department_info",
|
1057 |
+
"patterns": [
|
1058 |
+
"CSE dept",
|
1059 |
+
"Tell me about the Computer Science and Engineering Department",
|
1060 |
+
"Tell me about the CSE Department",
|
1061 |
+
"CSE Department",
|
1062 |
+
"Computer Science and Engineering Department",
|
1063 |
+
"About cse dep"
|
1064 |
+
],
|
1065 |
+
"responses": [
|
1066 |
+
"Our CSE department was established in the year 1984 and offers a conducive learning and research environment with the aim of transforming the lifestyle of rural students with quality education and higher-level career settlement. We have dedicated and quality faculty members with well-maintained state-of-the-art academic and research laboratories. To enlighten their knowledge our curriculum is periodically upgraded with emerging trends to bridge the industrial gaps. Our department has well-established In-house incubation centers to inculcate the industrial practices at their accessibility well in advance. Students excel themselves in International and National level competitions, hackathons to groom their soft skills with the care and affection of our mentors. We are thankful for our stakeholders (Alumni, Parents, Academic & Industrial experts) who are guiding and shaping us towards excellence."
|
1067 |
+
]
|
1068 |
+
},
|
1069 |
+
{
|
1070 |
+
"tag": "CSE_department_vision_mission",
|
1071 |
+
"patterns": [
|
1072 |
+
"vision of the Computer Science and Engineering Department",
|
1073 |
+
"mission of the CSE Engineering Department",
|
1074 |
+
"cse vision mission","CSE Mission","Mission cse","cse vision","vision mission of cse"
|
1075 |
+
],
|
1076 |
+
"responses": [
|
1077 |
+
"Vision: To produce globally competent, innovative and socially responsible computing professionals , Mission: To provide world-class teaching-learning and research facilities. To stimulate students’ logical thinking, creativity, and communication skills effectively. To cultivate awareness about emerging trends through self-initiative. To instill a sense of societal and ethical responsibilities. To collaborate with industries and government organizations."
|
1078 |
+
]
|
1079 |
+
},
|
1080 |
+
{
|
1081 |
+
"tag": "CSE_department_programs",
|
1082 |
+
"patterns": [
|
1083 |
+
"undergraduate program in Computer Science and Engineering","Undergradute program of CSE dep", "UG program of CSE dep","CSE dep UG program",
|
1084 |
+
"postgraduate program in Computer Science and Engineering","Postgraduate program of CSE dep", "PG program of CSE dep","CSE dep PG program",
|
1085 |
+
"PhD program in Computer Science and Engineering","PhD program of CSE dep","CSE dep PhD program"
|
1086 |
+
],
|
1087 |
+
"responses": [
|
1088 |
+
"Undergraduate Program: Year of Establishment - 1984, Student Strength - 389, Postgraduate Program: Year of Establishment - 2002, Student Strength - 17, PhD Program: Year of Establishment - 2006, Scholars - Full time: 1, Part-time: 12, Awarded: 31 (since 2010)"
|
1089 |
+
]
|
1090 |
+
},
|
1091 |
+
{
|
1092 |
+
"tag": "CSE_department_hod",
|
1093 |
+
"patterns": [
|
1094 |
+
"Head of the Computer Science and Engineering Department",
|
1095 |
+
"Hod of CSE Dept",
|
1096 |
+
"Hod CSE","CSE HOD"
|
1097 |
+
],
|
1098 |
+
"responses": [
|
1099 |
+
"Dr. V. Gomathi Professor & Head"
|
1100 |
+
]
|
1101 |
+
},
|
1102 |
+
{
|
1103 |
+
"tag": "CSE_department_faculty",
|
1104 |
+
"patterns": [
|
1105 |
+
"faculty in CSE Department",
|
1106 |
+
"faculty in Computer Science and Engineering Department",
|
1107 |
+
"faculty list for CSE Department",
|
1108 |
+
"CSE faculty",
|
1109 |
+
"CSE Department faculty"
|
1110 |
+
],
|
1111 |
+
"responses": [
|
1112 |
+
"1. Dr. V. Gomathi - Professor and Head, 2. Dr. D. Manimegalai - Professor, 3. Dr. K. Mohaideen Pitchai - Professor, 4. Dr. S. Kalaiselvi - Associate Professor, 5. Dr. J. Naskath - Assistant Professor(SG), 6. Ms. R. Rajakumari - Assistant Professor(SG), 7. Mr. D. Vijayakumar - Assistant Professor(SG), 8. Dr. G. Sivakama Sundari - Assistant Professor(SG), 9. Ms. B. Shanmugapriya - Assistant Professor(SG), 10. Mr. S. Dheenathayalan - Assistant Professor(SG)"
|
1113 |
+
]
|
1114 |
+
},
|
1115 |
+
{
|
1116 |
+
"tag": "ECE_department_info",
|
1117 |
+
"patterns": [
|
1118 |
+
"ECE dept",
|
1119 |
+
"Tell me about the Electronics and Communication Engineering Department",
|
1120 |
+
"Tell me about the ECE Department",
|
1121 |
+
"ECE Department",
|
1122 |
+
"About ece"
|
1123 |
+
],
|
1124 |
+
"responses": [
|
1125 |
+
"The department of Electronics and Communication Engineering has been continuously striving to provide excellent Engineering Education in the field of electronics and communication since 1984. It offers one undergraduate programme B.E. in Electronics and Communication Engineering (ECE) and one postgraduate programme M.E. in Embedded System Technologies. National Board of Accreditation (NBA), New Delhi has provisionally accredited the Under Graduate Programme of the ECE department under Tier – 1 category. Anna University Chennai has recognized the department as a research centre."
|
1126 |
+
]
|
1127 |
+
},
|
1128 |
+
{
|
1129 |
+
"tag": "ECE_department_vision",
|
1130 |
+
"patterns": [
|
1131 |
+
"What is the vision of the Electronics and Communication Engineering Department?","vision of ece","ece vision"
|
1132 |
+
],
|
1133 |
+
"responses": [
|
1134 |
+
"To produce Electronics and Communication Engineers capable of generating a knowledge economy with social responsibility."
|
1135 |
+
]
|
1136 |
+
},
|
1137 |
+
{
|
1138 |
+
"tag": "ECE_department_mission",
|
1139 |
+
"patterns": [
|
1140 |
+
"What is the mission of the Electronics and Communication Engineering Department?","mission of ece","ece mission"
|
1141 |
+
],
|
1142 |
+
"responses": [
|
1143 |
+
"To impart high-quality education with ethical behaviour,To equip the students compatible with recent trends in Electronic industries.To develop leadership qualities with humanity, wisdom, creativity and team spirit.To provide a passionate environment for continual learning."
|
1144 |
+
]
|
1145 |
+
},
|
1146 |
+
{
|
1147 |
+
"tag": "ECE_department_ug",
|
1148 |
+
"patterns": [
|
1149 |
+
"Tell me about the undergraduate program in Electronics and Communication Engineering","Undergradute program of ECE dep", "UG program of ECE dep","ece dep UG program"
|
1150 |
+
],
|
1151 |
+
"responses": [
|
1152 |
+
"Year of Establishment:1984, Student Strength:120 from 2006"
|
1153 |
+
]
|
1154 |
+
},
|
1155 |
+
{
|
1156 |
+
"tag": "ECE_department_pg",
|
1157 |
+
"patterns": [
|
1158 |
+
"Tell me about the postgraduate program in Electronics and Communication Engineering","Postgradute program of ECE dep", "PG program of ECE dep","ECE dep PG program"
|
1159 |
+
],
|
1160 |
+
"responses": [
|
1161 |
+
"Year of Establishment:2011 , Student Strength:18 from 2012, 12 from 2022."
|
1162 |
+
]
|
1163 |
+
},
|
1164 |
+
{
|
1165 |
+
"tag": "ECE_department_phd",
|
1166 |
+
"patterns": [
|
1167 |
+
"Tell me about the PhD program in Electronics and Communication Engineering","PhD program of ECE dep", "PhD program of ECE dep","ECE dep PhD program"
|
1168 |
+
],
|
1169 |
+
"responses": [
|
1170 |
+
"Year of Establishment:2011",
|
1171 |
+
"scholars:",
|
1172 |
+
"Full time:3",
|
1173 |
+
"Part time:19",
|
1174 |
+
"Awarded:26(Since 2013)"
|
1175 |
+
]
|
1176 |
+
},
|
1177 |
+
{
|
1178 |
+
"tag": "ECE_department_hod",
|
1179 |
+
"patterns": [
|
1180 |
+
"Who is the Head of the Electronics and Communication Engineering Department?",
|
1181 |
+
"Hod of ECE Dept",
|
1182 |
+
"Hod ECE","ECE hod"
|
1183 |
+
],
|
1184 |
+
"responses": [
|
1185 |
+
"Dr. S. Tamilselvi Professor & Head"
|
1186 |
+
]
|
1187 |
+
},
|
1188 |
+
{
|
1189 |
+
"tag": "ECE_department_faculty",
|
1190 |
+
"patterns": [
|
1191 |
+
"faculty in ECE Department",
|
1192 |
+
"faculty in Electronics and Communication Engineering Department",
|
1193 |
+
"faculty list for ECE Department",
|
1194 |
+
"ECE faculty",
|
1195 |
+
"ECE Department faculty"
|
1196 |
+
],
|
1197 |
+
"responses": [
|
1198 |
+
"1. Dr. S. Tamilselvi - Professor and Head, 2. Dr. A. Shenbagavalli - Professor, 3. Dr. T. S. Arun Samuel - Professor, 4. Dr. N. Arumugam - Associate Professor, 5. Dr. V. Suresh - Associate Professor, 6. Dr. K. J. Prasanna Venkatesan - Associate Professor, 7. Dr. C. Balamurugan - Associate Professor, 8. Dr. A. Saravana Selvan - Associate Professor, 9. Mr. T. Devakumar - Assistant Professor(SG), 10. Mr. S. Cammillus - Assistant Professor(SG), 11. Mr. K. Subramanian - Assistant Professor(SG), 12. Dr. R. Manjula Devi - Assistant Professor(SG), 13. Mr. M. Sathish Kumar - Assistant Professor(SG), 14. Mr. B. Ganapathy Ram - Assistant Professor(SG), 15. Dr. I. Vivek Anand - Assistant Professor(SG), 16. Ms. S. Karthika - Assistant Professor, 17. Ms. J. E. Jeyanthi - Assistant Professor, 18. Ms. S. Malathi - Assistant Professor, 19. Ms. C. Kalieswari - Assistant Professor, 20. Dr. I. Paulkani - Assistant Professor, 21. Ms. A. Apsara - Assistant Professor, 22. Ms. P. Arishenbagam - Assistant Professor, 23. Ms. C. Lakshmi - Assistant Professor, 24. Dr. S. Lavanya - Assistant Professor, 25. Ms. R. Geeta - Assistant Professor, 26. Mrs. S. Sulochana - Assistant Professor, 27. Ms. S. Sindhana - Assistant Professor, 28. Ms. C. K. Balasundari - Assistant Professor"
|
1199 |
+
]
|
1200 |
+
},
|
1201 |
+
{
|
1202 |
+
"tag": "EEE_department",
|
1203 |
+
"patterns": [
|
1204 |
+
"EEE dept",
|
1205 |
+
"Electrical and Electronics Engineering Department",
|
1206 |
+
"EEE Department",
|
1207 |
+
"About eee"
|
1208 |
+
],
|
1209 |
+
"responses": [
|
1210 |
+
"The department of Electrical and Electronics Engineering was established in 1994 with an initial intake of 40 students, which was later increased to 60. The B.E. program received accreditation from NBA for 3 years in July 2006, and re-accreditation for another 3 years in September 2011. In 2014, it was accredited by NBA Tier 1 (Washington Accord) for 2 years, and the accreditation was extended up to 2023. Currently, the B.E. program is provisionally re-accredited for 3 years starting from 2023. Additionally, the department offers M.E. in High Voltage Engineering since 2005, making it the only affiliated college under Anna University, Chennai offering this specialization. Dr. M. Willjuice Iruthayarajan, M.E., Ph.D., serves as the Professor & Head, bringing 24 years of academic and research experience to efficiently lead the department, which is supported by 15 staff members."
|
1211 |
+
]
|
1212 |
+
},
|
1213 |
+
{
|
1214 |
+
"tag": "EEE_department_vision",
|
1215 |
+
"patterns": [
|
1216 |
+
"What is the vision of the Electrical and Electronics Engineering Department?","vision of ece","ece vision"
|
1217 |
+
],
|
1218 |
+
"responses": [
|
1219 |
+
"Promoting active learning, critical thinking coupled with ethical values to meet the global challenges."
|
1220 |
+
]
|
1221 |
+
},
|
1222 |
+
{
|
1223 |
+
"tag": "EEE_department_mission",
|
1224 |
+
"patterns": [
|
1225 |
+
"What is the mission of the Electrical and Electronics Engineering Department?","mission of ece","ece mission"
|
1226 |
+
],
|
1227 |
+
"responses": [
|
1228 |
+
"To instill state-of-the-art technical knowledge and research capability that will prepare our graduates for professionalism and lifelong learning.To update knowledge to inculcate social and ethical values.To meet industrial and real-world challenges."
|
1229 |
+
]
|
1230 |
+
},
|
1231 |
+
{
|
1232 |
+
"tag": "EEE_department_ug",
|
1233 |
+
"patterns": [
|
1234 |
+
"Tell me about the undergraduate program in Electrical and Electronics Engineering","Undergradute program of EEE dep", "UG program of EEE dep","EEE dep UG program"
|
1235 |
+
],
|
1236 |
+
"responses": [
|
1237 |
+
"Year of Establishment:1994, Student Strength:60"
|
1238 |
+
]
|
1239 |
+
},
|
1240 |
+
{
|
1241 |
+
"tag": "EEE_department_pg",
|
1242 |
+
"patterns": [
|
1243 |
+
"Tell me about the postgraduate program in Electrical and Electronics Engineering","Postgradute program of EEE dep", "PG program of EEE dep","EEE dep PG program"
|
1244 |
+
],
|
1245 |
+
"responses": [
|
1246 |
+
"Year of Establishment:2005 , Student Strength:06"
|
1247 |
+
]
|
1248 |
+
},
|
1249 |
+
{
|
1250 |
+
"tag": "EEE_department_phd",
|
1251 |
+
"patterns": [
|
1252 |
+
"Tell me about the PhD program in Electrical and Electronics Engineering", "PhD program of EEE dep","EEE dep PhD program"
|
1253 |
+
],
|
1254 |
+
"responses": [
|
1255 |
+
"Year of Establishment:2010 scholars: Full time:01 Part time:18 Awarded:24"
|
1256 |
+
]
|
1257 |
+
},
|
1258 |
+
{
|
1259 |
+
"tag": "EEE_department_hod",
|
1260 |
+
"patterns": [
|
1261 |
+
"Who is the Head of the Electrical and Electronics Department?",
|
1262 |
+
"Hod of EEE Dept",
|
1263 |
+
"Hod EEE","EEE HOD"
|
1264 |
+
],
|
1265 |
+
"responses": [
|
1266 |
+
"Dr. M. Willjuice Iruthayarajan Professor & Head"
|
1267 |
+
]
|
1268 |
+
},
|
1269 |
+
{
|
1270 |
+
"tag": "EEE_department_faculty",
|
1271 |
+
"patterns": [
|
1272 |
+
"faculty in the Electrical and Electronics Engineering Department","Faculty of eee","eee faculty"
|
1273 |
+
],
|
1274 |
+
"responses": ["1. Dr. M. Willjuice Iruthayarajan - Professor and Head, 2. Dr. L. Kalaivani - Professor, 3. Dr. R. V. Maheswari - Professor, 4. Dr. N. B. Prakash - Professor, 5. Dr. S. Sankarakumar - Assistant Professor(SG), 6. Dr. B. Vigneshwaran - Assistant Professor(SG), 7. Mr. B. Venkatasamy - Assistant Professor(SG), 8. Dr. M. Gengaraj - Assistant Professor(SG), 9. Mr. P. Samuel Pakianthan - Assistant Professor, 10. Mr. M. Sivapalanirajan - Assistant Professor"]
|
1275 |
+
},
|
1276 |
+
{
|
1277 |
+
"tag": "clubs",
|
1278 |
+
"patterns": [
|
1279 |
+
"What are the clubs available in nec?",
|
1280 |
+
"clubs in NEC",
|
1281 |
+
"extra curricular activities in NEC",
|
1282 |
+
"extra curricular activities",
|
1283 |
+
"club"
|
1284 |
+
],
|
1285 |
+
"responses": [
|
1286 |
+
"National Cadet Corps(NCC),National Service Scheme(NSS),Youth Welfare Club,Yoga,Youth Red Cross(YRC),Royal Red Cross(RRC),Eco Club,Rotract,Junior JCI,Reader's Park,Literary Club,Quiz Club,Fine and Arts"
|
1287 |
+
]
|
1288 |
+
},
|
1289 |
+
{
|
1290 |
+
"tag": "NCC",
|
1291 |
+
"patterns": [
|
1292 |
+
"about NCC",
|
1293 |
+
"NCC",
|
1294 |
+
"about National Cadet Corps",
|
1295 |
+
"National Cadet Corps"
|
1296 |
+
],
|
1297 |
+
"responses": [
|
1298 |
+
"The National Cadet Corps (NCC) is the youth wing of the Indian Armed Force with its headquarters in New Delhi, India.It is open to school and college students on a voluntary basis as a Tri-Services Organisation, comprising the Army, the Navy and the Air Force, engaged in developing the youth of the country into disciplined and patriotic citizens.The soldier youth foundation in India is a voluntary organization which recruits cadets from high schools, higher secondary, colleges and universities all over India.The cadets are given basic military training in small arms and drills."
|
1299 |
+
]
|
1300 |
+
},
|
1301 |
+
{
|
1302 |
+
"tag": "NCC_vision",
|
1303 |
+
"patterns": ["What is the vision of the National Cadet corps?"],
|
1304 |
+
"responses": ["Empower the youth to be a responsible citizen of the country with leadership skills to take up their career in the armed forces"]
|
1305 |
+
},
|
1306 |
+
{
|
1307 |
+
"tag": "NCC_Motto",
|
1308 |
+
"patterns": ["What is the motto of the National Cadet corps?"],
|
1309 |
+
"responses": ["Unity and discipline"]
|
1310 |
+
},
|
1311 |
+
{
|
1312 |
+
"tag": "NCC_Mission",
|
1313 |
+
"patterns": ["What is the mission of the National Cadet corps?"],
|
1314 |
+
"responses": [
|
1315 |
+
"To provide a suitable environment to motivate the youth to take up a career in the armed force.",
|
1316 |
+
"To develop character, comradeship, discipline, leadership, secular outlook, spirit of adventure and ideals of selfless service amongst the youth of the country."
|
1317 |
+
]
|
1318 |
+
},
|
1319 |
+
{
|
1320 |
+
"tag": "NCC_volunteers",
|
1321 |
+
"patterns": ["No.of student volunteers in National Cadet corps"],
|
1322 |
+
"responses": ["100 cadets"]
|
1323 |
+
},
|
1324 |
+
{
|
1325 |
+
"tag": "NCC_Duration of Membership",
|
1326 |
+
"patterns": ["Duration of Membership in National Cadet corps"],
|
1327 |
+
"responses": ["3 years"]
|
1328 |
+
},
|
1329 |
+
{
|
1330 |
+
"tag": "NCC_President",
|
1331 |
+
"patterns": ["who is the president of National Cadet corps?"],
|
1332 |
+
"responses": ["CUO.P.Akshaya, EEE"]
|
1333 |
+
},
|
1334 |
+
{
|
1335 |
+
"tag": "NCC_Faculty_Coordinators",
|
1336 |
+
"patterns": ["who is the Faculty Coordinators of National Cadet corps?"],
|
1337 |
+
"responses": ["Captain. Dr.N.B.Prakash Coy Commander 9(TN) Sig. Coy. NCC, Tirunelveli"]
|
1338 |
+
},
|
1339 |
+
{
|
1340 |
+
"tag": "NCC_AWARDS & ACHIEVEMENTS",
|
1341 |
+
"patterns": ["AWARDS & ACHIEVEMENTS of National Cadet corps"],
|
1342 |
+
"responses": [
|
1343 |
+
"GSGT.B.Sivaguru – Independence day camp” – to directorate the Delhi Fort,GSGT.C.Nishanth & GSGT.K.Veiluraj – “National Thal Sainik Camp” – By UP Directorate – 14th to 15th September 2022,CPL.R.Dinesh Kumar – “Advance Leadership camp"
|
1344 |
+
]
|
1345 |
+
},
|
1346 |
+
{
|
1347 |
+
"tag": "NSS",
|
1348 |
+
"patterns": [
|
1349 |
+
"about NSS",
|
1350 |
+
"NCC",
|
1351 |
+
"about National Service Scheme",
|
1352 |
+
"National Service Scheme"
|
1353 |
+
],
|
1354 |
+
"responses": [
|
1355 |
+
"The National Service Scheme (NSS) is a Central Sector Scheme of Government of India, Ministry of Youth Affairs & Sports.The ideological orientation of NSS was inspired by the ideals of Mahatma Gandhi and started in 1969. “Education through Service” is the purpose of the NSS,NEC has two NSS units, each with 100 volunteers. The first unit was started in 1984.Due to an overwhelming response from the students, a second unit was started and has been functioning since 1999.These units provide an opportunity for those who want to serve the community around the institution. NSS volunteers participated in regular social service activities like conducting blood donation camps, health awareness camps, environmental awareness camps, tree planting, creating awareness about the Clean India Campaign, campus cleaning, the self-employment programme, and visiting and donating goods to nearby orphanages and mental health homes."
|
1356 |
+
]
|
1357 |
+
},
|
1358 |
+
{
|
1359 |
+
"tag": "NSS_vision",
|
1360 |
+
"patterns": ["What is the vision of the National Service scheme"],
|
1361 |
+
"responses": [""],
|
1362 |
+
"context": ["To build the youth with the mind and spirit to serve society and work for the social uplift of the downtrodden masses of our nation as a movement."]
|
1363 |
+
},
|
1364 |
+
{
|
1365 |
+
"tag": "NSS_motto",
|
1366 |
+
"patterns": ["What is the motto of the National Service scheme?"],
|
1367 |
+
"responses": [""],
|
1368 |
+
"context": ["“NOT ME BUT YOU”, reflects the value of democratic living and upholds the need for selfless service."]
|
1369 |
+
},
|
1370 |
+
{
|
1371 |
+
"tag": "NSS_mision",
|
1372 |
+
"patterns": ["What is the mission of the Service scheme?"],
|
1373 |
+
"responses": [""],
|
1374 |
+
"context": ["Personality development of the students through Community Service and Community Service through education."]
|
1375 |
+
},
|
1376 |
+
{
|
1377 |
+
"tag": "NSS_Activities",
|
1378 |
+
"patterns": ["What are the activities conducted through the National Service scheme?"],
|
1379 |
+
"responses": [
|
1380 |
+
"1 Public Awareness Program at Chatrapatti 07.08.2022. 2 School Students Competition and Women Education Awareness Camp at K. R. Saratha Government Higher Secondary School, Nalattinpudhur 10.08.2022. 3 Drive Against Drugs Awareness Program at National Engineering College, Kovilpatti 11.08.2022. 4 Walkathon and Mime Competition organized by Food Safety Department Thoothukudi 12.08.2022. 5 Voter id linked with Aadhaar Camp at National Engineering College 20.08.2022. 6 Flag Collection Drive in and around Kovilpatti 27.08.2022. 7 Railvandi 2.0 – Thodar 1 through Gmeet live 28.08.2022. 8 Women’s Health Awareness Program, on the eve of WIFS DAY Celebration at National Engineering College 15.09.2022. 9 Mini Marathon, as a part of Founders Day Celebration for girl students 17.09.2022. 10 NSS and UBA jointly donated the Multi Crop Seed showing Machine to Farmers at National Engineering College 17.09.2022. 11 Cleanliness Mission at Kovilpatti Railway Station, Shenbagavalli Amman Kovil and Kathireesan Malai Kovil 17.09.2022. 12 Pudhusu Pudhusa...!!! Dhinusu Dhinusha ...!!! (A Converse on current affairs on August 22) through online jointly with UBA 18.09.2022. 13 NSS Orientation Program 2K22 at National Engineering College 24.09.2022. 14 Railvandi 2.0 - Thodar 2 through Google Meet live 25.09.2022. 15 Wall magazine and Poster making Competition, on the theme of 'My Vote is my Right' and 'The Power of One Vote' along with Electoral Literacy Club at National Engineering College 27.09.2022. 16 One day Workshop on Poster making at National Engineering College 08.10.2022. 17 Donation Drive’22 – Orphanage Visit –Avvai Ashramam 15.10.2022. 18 Clean India 2.0 – Plastic Collection Week at Kovilpatti 22.10.2022. 19 Awareness Programme on Air and Noise pollution caused by bursting of Crackers through online platform 23.10.2022. 20 Clean Campaign at Kovilpatti Government Hospital 05.11.2022. 21 New Voter’s Registration Human Rally 11.11.2022."
|
1381 |
+
]
|
1382 |
+
},
|
1383 |
+
{
|
1384 |
+
"tag": "NSS_volunteers",
|
1385 |
+
"patterns": ["No.of student volunteers in National Service scheme"],
|
1386 |
+
"responses": ["200 "]
|
1387 |
+
},
|
1388 |
+
{
|
1389 |
+
"tag": "NSS_Duration of Membership",
|
1390 |
+
"patterns": ["Duration of Membership in National Service scheme"],
|
1391 |
+
"responses": ["2 years"]
|
1392 |
+
},
|
1393 |
+
{
|
1394 |
+
"tag": "NSS_President",
|
1395 |
+
"patterns": ["who is the president of National Service scheme?"],
|
1396 |
+
"responses": [
|
1397 |
+
"M. Yogesh, Mechanical/Final Year (Unit I), D. Harini, IT/Final Year (Unit II)"
|
1398 |
+
]
|
1399 |
+
},
|
1400 |
+
{
|
1401 |
+
"tag": "NSS_Faculty_Coordinators",
|
1402 |
+
"patterns": ["who is the Faculty Coordinators of Service scheme?"],
|
1403 |
+
"responses": [
|
1404 |
+
"Mr. K. Subramanian, AP (SG)/ECE (unit I)"
|
1405 |
+
]
|
1406 |
+
},
|
1407 |
+
{
|
1408 |
+
"tag": "NSS_AWARDS & ACHIEVEMENTS",
|
1409 |
+
"patterns": ["AWARDS & ACHIEVEMENTS of Service scheme"],
|
1410 |
+
"responses": [
|
1411 |
+
"District Level Best implementation of Swachh Bharat Abhiyan-2017,District Level Best implementation of Swachh Bharat Abhiyan-2018,State Level Best NSS Unit Award-2019,Best NSS Volunteer Award – 2020,UBA Perennial Assistance Award – 2021,University Best NSS Programme Officer – 2022"
|
1412 |
+
]
|
1413 |
+
},
|
1414 |
+
{
|
1415 |
+
"tag": "Youth Welfare association",
|
1416 |
+
"patterns": [
|
1417 |
+
"about Youth Welfare association",
|
1418 |
+
"Youth Welfare association"
|
1419 |
+
],
|
1420 |
+
"responses": [
|
1421 |
+
"This club is involved in youth activities which help the students to develop skills and bring changes in environmental issues."
|
1422 |
+
]
|
1423 |
+
},
|
1424 |
+
{
|
1425 |
+
"tag": "Youth Welfare association_vision",
|
1426 |
+
"patterns": ["What is the vision of the Youth Welfare association?"],
|
1427 |
+
"responses": ["Aim to develop an ecosystem for the youth to provide them with skills, resources, and motivation to achieve sustainable development goals."]
|
1428 |
+
},
|
1429 |
+
{
|
1430 |
+
"tag": "Youth Welfare association_Mission",
|
1431 |
+
"patterns": ["What is the mission of the Youth Welfare association?"],
|
1432 |
+
"responses": [
|
1433 |
+
"Youth Welfare Club prepares teams for participation in Youth Festival events like Debate, Elocution, Quiz, Painting, Flower Arrangement, Rangoli, One Act Play, and Skit."
|
1434 |
+
]
|
1435 |
+
},
|
1436 |
+
{
|
1437 |
+
"tag": "Youth Welfare association_Activities",
|
1438 |
+
"patterns": ["What are the activities conducted through the Youth Welfare association?"],
|
1439 |
+
"responses": [
|
1440 |
+
"PowerPoint Presentation,Essay Writing,Quiz,Poster Making,Poem Writing,Elocution"
|
1441 |
+
]
|
1442 |
+
},
|
1443 |
+
{
|
1444 |
+
"tag": "Youth Welfare association_volunteers",
|
1445 |
+
"patterns": ["No.of student volunteers in Youth Welfare association"],
|
1446 |
+
"responses": ["10"]
|
1447 |
+
},
|
1448 |
+
{
|
1449 |
+
"tag": "Youth Welfare association_Duration of Membership",
|
1450 |
+
"patterns": ["Duration of Membership in Youth Welfare association"],
|
1451 |
+
"responses": ["3 years"]
|
1452 |
+
},
|
1453 |
+
{
|
1454 |
+
"tag": "Youth Welfare association_President",
|
1455 |
+
"patterns": ["who is the president of Youth Welfare association"],
|
1456 |
+
"responses":["RENUKA SRE V/ IT, RAMYA A/ IT, RAGUL S / EEE."]
|
1457 |
+
},
|
1458 |
+
{
|
1459 |
+
"tag": "Youth Welfare association_Faculty_Coordinators",
|
1460 |
+
"patterns": ["who is the Faculty Coordinators of Youth Welfare association?"],
|
1461 |
+
"responses": ["Dr.P.Ram Kumar, AP/S&H"]
|
1462 |
+
},
|
1463 |
+
{
|
1464 |
+
"tag": "Youth Welfare association_AWARDS & ACHIEVEMENTS",
|
1465 |
+
"patterns": ["AWARDS & ACHIEVEMENTS of Youth Welfare association"],
|
1466 |
+
"responses": [
|
1467 |
+
"Best Paper Award in All India Essay Writing Event."
|
1468 |
+
]
|
1469 |
+
},
|
1470 |
+
{
|
1471 |
+
"tag": "Yoga",
|
1472 |
+
"patterns": [
|
1473 |
+
"about Yoga",
|
1474 |
+
"Yoga"
|
1475 |
+
],
|
1476 |
+
"responses": [
|
1477 |
+
"Yoga club helps to spread awareness of the benefits of Yoga among students and faculty members. The club organises a variety of Yoga activities for students and staff members to attain a higher level of consciousness of Yoga Education. We actively practise Yoga principles and seek a ray of wisdom that brings true happiness and inner peace."
|
1478 |
+
]
|
1479 |
+
},
|
1480 |
+
{
|
1481 |
+
"tag": "Yoga_vision",
|
1482 |
+
"patterns": ["What is the vision of the Yoga?"],
|
1483 |
+
"responses": ["To Transform students into responsible citizens of the World, who are-physically, mentally and spiritually healthy."]
|
1484 |
+
},
|
1485 |
+
{
|
1486 |
+
"tag": "Yoga_Mission",
|
1487 |
+
"patterns": ["What is the mission of the Yoga?"],
|
1488 |
+
"responses": [
|
1489 |
+
"To enhance the Physical, Mental, Spiritual and Social Health of the students through the regular practice of Yoga."
|
1490 |
+
]
|
1491 |
+
},
|
1492 |
+
{
|
1493 |
+
"tag": "Yoga_Activities",
|
1494 |
+
"patterns": ["What are the activities conducted through the Yoga club?"],
|
1495 |
+
"responses": [
|
1496 |
+
"One Year-Diploma Course on Yoga for Youth Empowerment (YYE)- SKY Exercise, Asanas,Meditation,Kayakalpa Practice and Self Introspection practices International Yoga Day-Events and activities conducted every June 21st. Yoga Service to the rural people-Vilathikulam,Villiseri,Ayyaneri."
|
1497 |
+
]
|
1498 |
+
},
|
1499 |
+
{
|
1500 |
+
"tag": "Yoga",
|
1501 |
+
"patterns": ["No.of student volunteers in Yoga"],
|
1502 |
+
"responses": ["325"]
|
1503 |
+
},
|
1504 |
+
{
|
1505 |
+
"tag": "Yoga_Duration of Membership",
|
1506 |
+
"patterns": ["Duration of Membership in Yoga"],
|
1507 |
+
"responses": ["1 years"]
|
1508 |
+
},
|
1509 |
+
{
|
1510 |
+
"tag": "Yoga_Convenor",
|
1511 |
+
"patterns": ["who is the Convenor of Yoga"],
|
1512 |
+
"responses": ["Dr. S Tamil Selvi, Professor & Head, ECE "]
|
1513 |
+
},
|
1514 |
+
{
|
1515 |
+
"tag": "Yoga_Faculty_Coordinators",
|
1516 |
+
"patterns": ["who is the Faculty Coordinators of Yoga?"],
|
1517 |
+
"responses": ["Ms.S.Malathi, Assistant Professor/ECE , Ms.C.Gawari, AP/Civil"]
|
1518 |
+
},
|
1519 |
+
{
|
1520 |
+
"tag": "Yoga_AWARDS & ACHIEVEMENTS",
|
1521 |
+
"patterns": ["AWARDS & ACHIEVEMENTS of yoga"],
|
1522 |
+
"responses": [
|
1523 |
+
"Yoga Club Convenor, Dr. S Tamil Selvi received Vethathiri Maharishi Thathuva Gnani Gnanasiriur Award from Padmasree SMK Mayilanadham, President, World Community Service Centre, Chennai on 02.06.2019 at Temple of Consciousness, Aliyar."
|
1524 |
+
]
|
1525 |
+
},
|
1526 |
+
{
|
1527 |
+
"tag": "Red Ribbon club",
|
1528 |
+
"patterns": [
|
1529 |
+
"about Red Ribbon club",
|
1530 |
+
"Red cross"
|
1531 |
+
],
|
1532 |
+
"responses": [
|
1533 |
+
"The RRC youth of National Engineering College, Kovilpatti is encourages to learn about safe and healthy lifestyles. The RRC promotes access to information on healthy life and voluntary blood donation. They create and provide opportunities for the zeal of volunteerism among youth to contribute towards the control and prevention of HIV & AIDS. The Red Ribbon Club is a voluntary on-campus intervention program for students."
|
1534 |
+
],
|
1535 |
+
"context": [""]
|
1536 |
+
},
|
1537 |
+
{
|
1538 |
+
"tag": "Red Ribbon club_vision",
|
1539 |
+
"patterns": ["What is the vision of the Red Ribbon club?"],
|
1540 |
+
"responses": ["The motto of the Red Ribbon Express is “Embarking on the journey of life“."],
|
1541 |
+
"context": [""]
|
1542 |
+
},
|
1543 |
+
{
|
1544 |
+
"tag": "Red Ribbon club_Mission",
|
1545 |
+
"patterns": ["What is the mission of the Red Ribbon club?"],
|
1546 |
+
"responses": [
|
1547 |
+
"To promote service and solidarity. To uphold the protection of life and the promotion of health. To promote national and international friendship. To disseminate the Red Cross ideals and International Humanitarian Law." ],
|
1548 |
+
"context": [""]
|
1549 |
+
},
|
1550 |
+
{
|
1551 |
+
"tag": "Red Ribbon club_Activities",
|
1552 |
+
"patterns": ["What are the activities conducted through the Red Ribbon clubclub?"],
|
1553 |
+
"responses": [
|
1554 |
+
"Celebrating Life Program Peer education and leadership trainings Cultural Competitions Awareness campaigns (Rallies, Outreach activities)"]
|
1555 |
+
},
|
1556 |
+
{
|
1557 |
+
"tag": "Red Ribbon club",
|
1558 |
+
"patterns": ["No.of student volunteers in Red Ribbon club"],
|
1559 |
+
"responses": ["100"]
|
1560 |
+
},
|
1561 |
+
{
|
1562 |
+
"tag": "Red Ribbon club_Duration of Membership",
|
1563 |
+
"patterns": ["Duration of Membership in Red Ribbon club"],
|
1564 |
+
"responses": ["3 years"]
|
1565 |
+
},
|
1566 |
+
{
|
1567 |
+
"tag": "Red Ribbon club_president",
|
1568 |
+
"patterns": ["who is the president of Red Ribbon club"],
|
1569 |
+
"responses":["A.Akshaya, EEE/ Final year "]
|
1570 |
+
},
|
1571 |
+
{
|
1572 |
+
"tag": "Red Ribbon club_Faculty_Coordinators",
|
1573 |
+
"patterns": ["who is the Faculty Coordinators of Red Ribbon club?"],
|
1574 |
+
"responses": ["Ms.K.Muthulakshmi, AP/S&H"]
|
1575 |
+
},
|
1576 |
+
{
|
1577 |
+
"tag": "Red Ribbon club_AWARDS & ACHIEVEMENTS",
|
1578 |
+
"patterns": ["AWARDS & ACHIEVEMENTS of red Ribbon club"],
|
1579 |
+
"responses": [
|
1580 |
+
"Best Motivating Award for Blood Donation by GH, Kovilpatti. (2021)"
|
1581 |
+
]
|
1582 |
+
},
|
1583 |
+
{
|
1584 |
+
"tag": "Red cross",
|
1585 |
+
"patterns": [
|
1586 |
+
"about Red cross",
|
1587 |
+
"Red cross"
|
1588 |
+
],
|
1589 |
+
"responses": [
|
1590 |
+
"The Red Cross Society is a worldwide, well-known, universally accepted, admired and internationally identified humanitarian service organization. It is the biggest, independent non-religious, non-political, non-sectarian and voluntary relief organization treating people equally all over the world without any discrimination as to their nationality race and religious belief. It was established in 1863 in Geneva, Switzerland. The Father of Red Cross Movement and recipient of the noble award for Peace in 1901 was “Jean Henry Dunant”, who was a successful businessman. While going on a business mission, Dunant came on 24th June 1859 to Castiglione."
|
1591 |
+
]
|
1592 |
+
},
|
1593 |
+
{
|
1594 |
+
"tag": "Red cross_vision",
|
1595 |
+
"patterns": ["What is the vision of the Red cross?"],
|
1596 |
+
"responses": ["To inculcate leadership in the youth of our country.To ensure the understanding and acceptance of civic and human responsibilities.To offer first aid training to all the YRC volunteers.To promote voluntary blood donation among the youth."]
|
1597 |
+
},
|
1598 |
+
{
|
1599 |
+
"tag": "Red cross_Mission",
|
1600 |
+
"patterns": ["What is the mission of the Red cross?"],
|
1601 |
+
"responses": [
|
1602 |
+
"Protection of health and life.Service to the sick and suffering.Promotion of national and international friendship to develop the mental and moral capacities of the youth."
|
1603 |
+
]
|
1604 |
+
},
|
1605 |
+
{
|
1606 |
+
"tag": "Red cross_Activities",
|
1607 |
+
"patterns": ["What are the activities conducted through the Red cross club?"],
|
1608 |
+
"responses": [
|
1609 |
+
"Blood Donation, Awareness program on various health issues.List the varied activities conducted by the club in an academic year"
|
1610 |
+
]
|
1611 |
+
},
|
1612 |
+
{
|
1613 |
+
"tag": "Red cross",
|
1614 |
+
"patterns": ["No.of student volunteers in Red cross"],
|
1615 |
+
"responses": ["150"]
|
1616 |
+
},
|
1617 |
+
{
|
1618 |
+
"tag": "Red cross_Duration of Membership",
|
1619 |
+
"patterns": ["Duration of Membership in Red cross"],
|
1620 |
+
"responses":["3 years"]
|
1621 |
+
},
|
1622 |
+
{
|
1623 |
+
"tag": "Red cross_president",
|
1624 |
+
"patterns": ["who is the president of Red cross"],
|
1625 |
+
"responses": ["A.S.N.Jaanu, EEE/ Final year "]
|
1626 |
+
},
|
1627 |
+
{
|
1628 |
+
"tag": "Red cross_Faculty_Coordinators",
|
1629 |
+
"patterns": ["who is the Faculty Coordinators of Red cross?"],
|
1630 |
+
"responses":["Mr.A.Muthusamy, AP/S&H"]
|
1631 |
+
},
|
1632 |
+
{
|
1633 |
+
"tag": "Red Cross_AWARDS & ACHIEVEMENTS",
|
1634 |
+
"patterns": ["AWARDS & ACHIEVEMENTS of red cross"],
|
1635 |
+
"responses": [
|
1636 |
+
"Best Motivating Award for Blood Donation by GH, Kovilpatti. (2021).Best Motivating Award from Meenakshi Mission, Madurai. (2017)"
|
1637 |
+
]
|
1638 |
+
},
|
1639 |
+
{
|
1640 |
+
"tag": "Eco Club",
|
1641 |
+
"patterns": [
|
1642 |
+
"about Eco Club",
|
1643 |
+
"Eco Club"
|
1644 |
+
],
|
1645 |
+
"responses": [
|
1646 |
+
"The Eco Club is a voluntary group which promotes the participation of students to educate about their immediate environment and the causes of its deterioration. It also imparts knowledge on the concepts of eco-systems through field visits. Encourages the students to minimize the usage of plastic bags in public areas. Organizes tree plantation programmes and awareness programmes such as MIME regarding various environmental issues." ]
|
1647 |
+
},
|
1648 |
+
{
|
1649 |
+
"tag": "Eco Club_vision",
|
1650 |
+
"patterns": ["What is the vision of the Eco Club?"],
|
1651 |
+
"responses": ["Our Vision is to create awareness on Environmental and Social Sustainability."]
|
1652 |
+
},
|
1653 |
+
{
|
1654 |
+
"tag": "Eco Club_Mission",
|
1655 |
+
"patterns": ["What is the mission of the Eco Club?"],
|
1656 |
+
"responses": [
|
1657 |
+
"Educating and Inspiring Lives to inculcate a sense of sustainable habits and sustainable practices." ]
|
1658 |
+
},
|
1659 |
+
{
|
1660 |
+
"tag": "Eco Club",
|
1661 |
+
"patterns": ["No.of student volunteers in Eco Club"],
|
1662 |
+
"responses": ["212"]
|
1663 |
+
},
|
1664 |
+
{
|
1665 |
+
"tag": "Eco Club_Duration of Membership",
|
1666 |
+
"patterns": ["Duration of Membership in Eco Club"],
|
1667 |
+
"responses": ["3 years"]
|
1668 |
+
},
|
1669 |
+
{
|
1670 |
+
"tag": "Eco Club_president",
|
1671 |
+
"patterns": ["who is the President of Eco Club"],
|
1672 |
+
"responses": ["Ms.P.Ida Hope, IV Year ECE Secretary:Mr.P.Muhamath Ishaak, IV Year MECH"],
|
1673 |
+
"context": [" "]
|
1674 |
+
},
|
1675 |
+
{
|
1676 |
+
"tag": "Eco Club_Faculty_Coordinators",
|
1677 |
+
"patterns": ["who is the Faculty Coordinators of Eco Club?"],
|
1678 |
+
"responses": [""],
|
1679 |
+
"context": ["Mr.I.Vivek Anand, AP(SG)/ECE Dr.B.Vigneshwaran, AP(SG)/EEE"]
|
1680 |
+
},
|
1681 |
+
{
|
1682 |
+
"tag": "Rotaract Club",
|
1683 |
+
"patterns": [
|
1684 |
+
"about Rotaract Club",
|
1685 |
+
"Rotaract Club"
|
1686 |
+
],
|
1687 |
+
"responses": [
|
1688 |
+
"“Rotaract” stands for “Rotary in Action”. Rotaract focuses on the development of young adults as leaders in their communities and workplaces. Members will play an active role in service project work, social events, or professional/leadership development workshops." ]
|
1689 |
+
},
|
1690 |
+
{
|
1691 |
+
"tag": "Rotaract Club_vision",
|
1692 |
+
"patterns": ["What is the vision of the Rotaract Club?"],
|
1693 |
+
"responses": ["To train young blood with good morals and to serve the society with good will and care."]
|
1694 |
+
},
|
1695 |
+
{
|
1696 |
+
"tag": "Rotaract Club_Mission",
|
1697 |
+
"patterns": ["What is the mission of the Rotaract Club?"],
|
1698 |
+
"responses": [
|
1699 |
+
"To develop professionals with leadership skills.To emphasize respect for the rights of others and to promote ethical standards.To provide opportunities for people to address the needs and concerns of the community"]
|
1700 |
+
},
|
1701 |
+
{
|
1702 |
+
"tag": "Rotaract Club",
|
1703 |
+
"patterns": ["No.of student volunteers in Rotaract Club"],
|
1704 |
+
"responses": ["50"]
|
1705 |
+
},
|
1706 |
+
{
|
1707 |
+
"tag": " Rotaract Club_Duration of Membership",
|
1708 |
+
"patterns": ["Duration of Membership in Rotaract Club"],
|
1709 |
+
"responses": ["3 years"]
|
1710 |
+
},
|
1711 |
+
{
|
1712 |
+
"tag": "Rotaract_Club_president",
|
1713 |
+
"patterns": ["Who is the President of Rotaract Club?"],
|
1714 |
+
"responses": ["Ms. A. Tejaswini, III Year CSE - President\nMs. Seethalakshmi, II Year ECE - Vice President\nMs. G. Keerthikasree, III Year CSE - Secretary\nMr. Bala Vignesh, II Year CSE - Joint Secretary\nMr. Siva Shakthi Dharan, III Year ECE - Treasurer"]
|
1715 |
+
},
|
1716 |
+
{
|
1717 |
+
"tag": "Rotaract Club_Faculty_Coordinators",
|
1718 |
+
"patterns": ["who is the Faculty Coordinators of Rotaract Club?"],
|
1719 |
+
"responses":["Mr.S.Cammillus, AP(SG)/ECE"]
|
1720 |
+
},
|
1721 |
+
{
|
1722 |
+
"tag": "Finearts",
|
1723 |
+
"patterns": [
|
1724 |
+
"about Finearts",
|
1725 |
+
"Finearts"
|
1726 |
+
],
|
1727 |
+
"responses": [
|
1728 |
+
"The Fine Arts Club of our college is dedicated to enhance the students’ artistic competencies in collaboration with peers and professionals. Members of the club use various visual mediums and techniques to express their thoughts in many art forms, such as dance, music, roleplay, and martial arts, with infinite inventiveness. Along with creating artwork and hosting exhibitions, the Fine Arts Club also holds competitions for students and others, giving them visibility and encouraging them to develop their talents."]
|
1729 |
+
},
|
1730 |
+
{
|
1731 |
+
"tag": "Finearts_motto",
|
1732 |
+
"patterns": ["What is the motto of the Finearts?"],
|
1733 |
+
"responses": ["TO DARE IS TO DO"]
|
1734 |
+
},
|
1735 |
+
{
|
1736 |
+
"tag": "Finearts_vision",
|
1737 |
+
"patterns": ["What is the vision of the Finearts?"],
|
1738 |
+
"responses": ["Brings in young inspiring minds to touch life with vibrance and inclusiveness of art."]
|
1739 |
+
},
|
1740 |
+
{
|
1741 |
+
"tag": "Finearts_Mission",
|
1742 |
+
"patterns": ["What is the mission of the Finearts?"],
|
1743 |
+
"responses": ["To encourage and develop the leadership quality and teamwork of the students through cultural events.To improve the aesthetic sense of students. To foster high-level and creative thinking as well as the mental health of the students"]
|
1744 |
+
},
|
1745 |
+
{
|
1746 |
+
"tag": "Finearts",
|
1747 |
+
"patterns": ["No.of student volunteers in Finearts"],
|
1748 |
+
"responses": ["325"]
|
1749 |
+
},
|
1750 |
+
{
|
1751 |
+
"tag": "Finearts_Duration of Membership",
|
1752 |
+
"patterns": ["Duration of Membership in Finearts"],
|
1753 |
+
"responses": ["4 years"]
|
1754 |
+
},
|
1755 |
+
{
|
1756 |
+
"tag": "Finearts_President",
|
1757 |
+
"patterns": ["who is the Pressident of Finearts"],
|
1758 |
+
"responses": ["Anshio SV, Final year, Computer Science & Engineering."]
|
1759 |
+
},
|
1760 |
+
{
|
1761 |
+
"tag": "Finearts_Faculty_Coordinators",
|
1762 |
+
"patterns": ["who is the Faculty Coordinators of Finearts?"],
|
1763 |
+
"responses": ["Mr.J.Thamba, AP/S&H. Ms.P.Arishenbagam, AP/ECE"]
|
1764 |
+
},
|
1765 |
+
{
|
1766 |
+
"tag": "Courses",
|
1767 |
+
"patterns": [
|
1768 |
+
"What are the Courses offered by nec?",
|
1769 |
+
"courses in NEC",
|
1770 |
+
"courses"
|
1771 |
+
],
|
1772 |
+
"responses": [
|
1773 |
+
"Mechanical Engineering,Computer Science And Engineering,Electronics And Communication Engineering,Electrical And Electronics Engineering,Civil Engineering,Information Technology,Artificial Intelligence And Data Science,Science & Humanities"
|
1774 |
+
]
|
1775 |
+
},
|
1776 |
+
{
|
1777 |
+
"tag": "Pricipal",
|
1778 |
+
"patterns": [
|
1779 |
+
"who is the principal of national engineering college?",
|
1780 |
+
"who is the principal of nec",
|
1781 |
+
"pricipal"
|
1782 |
+
],
|
1783 |
+
"responses": [
|
1784 |
+
"Dr.K.Kalidasa Murugavel"
|
1785 |
+
]
|
1786 |
+
},
|
1787 |
+
{
|
1788 |
+
"tag": "Director",
|
1789 |
+
"patterns": [
|
1790 |
+
"who is the Director of national engineering college?",
|
1791 |
+
"who is the Director of nec",
|
1792 |
+
"Director"
|
1793 |
+
],
|
1794 |
+
"responses": [
|
1795 |
+
"Dr.S.Shanmugavel"
|
1796 |
+
]
|
1797 |
+
},
|
1798 |
+
{
|
1799 |
+
"tag": "Chairman",
|
1800 |
+
"patterns": [
|
1801 |
+
"who is the Chairman of national engineering college?",
|
1802 |
+
"who is the Chairman of nec",
|
1803 |
+
"Chairman"
|
1804 |
+
],
|
1805 |
+
"responses": [
|
1806 |
+
"Thiru.K.R.Arunachalam"
|
1807 |
+
]
|
1808 |
+
},
|
1809 |
+
{
|
1810 |
+
"tag": "Founder",
|
1811 |
+
"patterns": [
|
1812 |
+
"who is the Founder of national engineering college?",
|
1813 |
+
"who is the Founder of nec",
|
1814 |
+
"Founder "
|
1815 |
+
],
|
1816 |
+
"responses": [
|
1817 |
+
"Thiru.K.Ramasamy"
|
1818 |
+
]
|
1819 |
+
},
|
1820 |
+
{
|
1821 |
+
"tag": "Nec",
|
1822 |
+
"patterns": [
|
1823 |
+
"about National Engineering College",
|
1824 |
+
"National Engineering College",
|
1825 |
+
"NEC"
|
1826 |
+
],
|
1827 |
+
"responses": ["NEC, the most prominent landmark of Kovilpatti, has been the crowning glory of this Matchless City of Matches. Its celebrated ‘Son of the Soil’ Thiru.K.Ramasamy transformed the entire social and cultural scenario in and around this small town by establishing the excellent educational institution popularly referred as “NEC”. By wielding the magical wand of social commitment and munificence this foresighted philanthropist transformed a strip of barren land into a magnificent academic complex that has been consistently producing infallible engineers of high competence right from the day of its inception in 1984. This much-acclaimed temple of erudition was established under the self-financing scheme sanctioned by the Government of Tamilnadu G.O. No. 939 dated 20.07.1984 by the National Educational and Charitable Trust, Kovilpatti, Thoothukudi district"]
|
1828 |
+
},
|
1829 |
+
|
1830 |
+
{
|
1831 |
+
"tag": "NEC_vision",
|
1832 |
+
"patterns": ["What is the vision of the college?"],
|
1833 |
+
"responses": ["Transforming lives through quality education and research with human values."]
|
1834 |
+
},
|
1835 |
+
{
|
1836 |
+
"tag": "bus_facility",
|
1837 |
+
"patterns": [
|
1838 |
+
"Is bus facility available in national engineering college?",
|
1839 |
+
"Is bus facility available nec?",
|
1840 |
+
"Bus facility "
|
1841 |
+
],
|
1842 |
+
"responses": [
|
1843 |
+
"Yes"
|
1844 |
+
],
|
1845 |
+
"context": [""]
|
1846 |
+
},
|
1847 |
+
{
|
1848 |
+
"tag": "hostel",
|
1849 |
+
"patterns": [
|
1850 |
+
"Is hostel facility available in national engineering college?",
|
1851 |
+
"Is hostel facility available nec?",
|
1852 |
+
"hostel facility "
|
1853 |
+
],
|
1854 |
+
"responses": [
|
1855 |
+
"Yes,hostel facility available for both gender"
|
1856 |
+
]
|
1857 |
+
},
|
1858 |
+
{
|
1859 |
+
"tag": "College",
|
1860 |
+
"patterns": [
|
1861 |
+
"which year college was established?","NEC founded in?"
|
1862 |
+
],
|
1863 |
+
"responses": [
|
1864 |
+
"20.07.1984"
|
1865 |
+
]
|
1866 |
+
}
|
1867 |
+
]
|
1868 |
+
}
|
model.py
ADDED
@@ -0,0 +1,20 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
import torch
|
2 |
+
import torch.nn as nn
|
3 |
+
|
4 |
+
|
5 |
+
class NeuralNet(nn.Module):
|
6 |
+
def __init__(self, input_size, hidden_size, num_classes):
|
7 |
+
super(NeuralNet, self).__init__()
|
8 |
+
self.l1 = nn.Linear(input_size, hidden_size)
|
9 |
+
self.l2 = nn.Linear(hidden_size, hidden_size)
|
10 |
+
self.l3 = nn.Linear(hidden_size, num_classes)
|
11 |
+
self.relu = nn.ReLU()
|
12 |
+
|
13 |
+
def forward(self, x):
|
14 |
+
out = self.l1(x)
|
15 |
+
out = self.relu(out)
|
16 |
+
out = self.l2(out)
|
17 |
+
out = self.relu(out)
|
18 |
+
out = self.l3(out)
|
19 |
+
# no activation and no softmax at the end
|
20 |
+
return out
|
nltk_utils.py
ADDED
@@ -0,0 +1,44 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
import numpy as np
|
2 |
+
import nltk
|
3 |
+
# nltk.download('punkt')
|
4 |
+
from nltk.stem.porter import PorterStemmer
|
5 |
+
stemmer = PorterStemmer()
|
6 |
+
|
7 |
+
|
8 |
+
def tokenize(sentence):
|
9 |
+
"""
|
10 |
+
split sentence into array of words/tokens
|
11 |
+
a token can be a word or punctuation character, or number
|
12 |
+
"""
|
13 |
+
return nltk.word_tokenize(sentence)
|
14 |
+
|
15 |
+
|
16 |
+
def stem(word):
|
17 |
+
"""
|
18 |
+
stemming = find the root form of the word
|
19 |
+
examples:
|
20 |
+
words = ["organize", "organizes", "organizing"]
|
21 |
+
words = [stem(w) for w in words]
|
22 |
+
-> ["organ", "organ", "organ"]
|
23 |
+
"""
|
24 |
+
return stemmer.stem(word.lower())
|
25 |
+
|
26 |
+
|
27 |
+
def bag_of_words(tokenized_sentence, words):
|
28 |
+
"""
|
29 |
+
return bag of words array:
|
30 |
+
1 for each known word that exists in the sentence, 0 otherwise
|
31 |
+
example:
|
32 |
+
sentence = ["hello", "how", "are", "you"]
|
33 |
+
words = ["hi", "hello", "I", "you", "bye", "thank", "cool"]
|
34 |
+
bog = [ 0 , 1 , 0 , 1 , 0 , 0 , 0]
|
35 |
+
"""
|
36 |
+
# stem each word
|
37 |
+
sentence_words = [stem(word) for word in tokenized_sentence]
|
38 |
+
# initialize bag with 0 for each word
|
39 |
+
bag = np.zeros(len(words), dtype=np.float32)
|
40 |
+
for idx, w in enumerate(words):
|
41 |
+
if w in sentence_words:
|
42 |
+
bag[idx] = 1
|
43 |
+
|
44 |
+
return bag
|
pyvenv.cfg
ADDED
@@ -0,0 +1,5 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
home = C:\Python312
|
2 |
+
include-system-site-packages = false
|
3 |
+
version = 3.12.2
|
4 |
+
executable = C:\Python312\python.exe
|
5 |
+
command = C:\Python312\python.exe -m venv C:\Users\vilsons\Documents\chatbot-deployment-main\flask chatbot\venv
|
style.css
ADDED
@@ -0,0 +1,202 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
* {
|
2 |
+
box-sizing: border-box;
|
3 |
+
margin: 0;
|
4 |
+
padding: 0;
|
5 |
+
}
|
6 |
+
|
7 |
+
body {
|
8 |
+
font-family:Cambria, Cochin, Georgia, Times, 'Times New Roman', serif;
|
9 |
+
font-weight: 400;
|
10 |
+
font-size: 100%;
|
11 |
+
background: white;
|
12 |
+
}
|
13 |
+
|
14 |
+
*, html {
|
15 |
+
--primaryGradient: linear-gradient(93.12deg, #1d4791 0.52%, #008aff 100%);
|
16 |
+
--secondaryGradient: linear-gradient(268.91deg, #1d4791 -2.14%, #008aff 99.69%);
|
17 |
+
--primaryBoxShadow: 0px 10px 15px rgba(0, 0, 0, 0.1);
|
18 |
+
--secondaryBoxShadow: 0px -10px 15px rgba(0, 0, 0, 0.1);
|
19 |
+
--primary: #1b2398;
|
20 |
+
}
|
21 |
+
|
22 |
+
/* CHATBOX
|
23 |
+
=============== */
|
24 |
+
.chatbox {
|
25 |
+
position: absolute;
|
26 |
+
bottom: 30px;
|
27 |
+
right: 30px;
|
28 |
+
}
|
29 |
+
|
30 |
+
/* CONTENT IS CLOSE */
|
31 |
+
.chatbox__support {
|
32 |
+
display: flex;
|
33 |
+
flex-direction: column;
|
34 |
+
background:white;
|
35 |
+
width: 300px;
|
36 |
+
height: 350px;
|
37 |
+
z-index: -123456;
|
38 |
+
opacity: 0;
|
39 |
+
transition: all .5s ease-in-out;
|
40 |
+
}
|
41 |
+
|
42 |
+
/* CONTENT ISOPEN */
|
43 |
+
.chatbox--active {
|
44 |
+
transform: translateY(-40px);
|
45 |
+
z-index: 123456;
|
46 |
+
opacity: 1;
|
47 |
+
|
48 |
+
}
|
49 |
+
|
50 |
+
/* BUTTON */
|
51 |
+
.chatbox__button {
|
52 |
+
text-align: right;
|
53 |
+
}
|
54 |
+
|
55 |
+
.send__button {
|
56 |
+
padding: 6px;
|
57 |
+
background: transparent;
|
58 |
+
border: none;
|
59 |
+
outline: none;
|
60 |
+
cursor: pointer;
|
61 |
+
}
|
62 |
+
|
63 |
+
|
64 |
+
/* HEADER */
|
65 |
+
.chatbox__header {
|
66 |
+
position: sticky;
|
67 |
+
top: 0;
|
68 |
+
background: orange;
|
69 |
+
}
|
70 |
+
|
71 |
+
/* MESSAGES */
|
72 |
+
.chatbox__messages {
|
73 |
+
margin-top: auto;
|
74 |
+
display: flex;
|
75 |
+
overflow-y: scroll;
|
76 |
+
flex-direction: column-reverse;
|
77 |
+
}
|
78 |
+
|
79 |
+
.messages__item {
|
80 |
+
background:rgb(207, 240, 253);
|
81 |
+
max-width: 60.6%;
|
82 |
+
width: fit-content;
|
83 |
+
}
|
84 |
+
|
85 |
+
.messages__item--operator {
|
86 |
+
margin-left: auto;
|
87 |
+
}
|
88 |
+
|
89 |
+
.messages__item--visitor {
|
90 |
+
margin-right: auto;
|
91 |
+
}
|
92 |
+
|
93 |
+
/* FOOTER */
|
94 |
+
.chatbox__footer {
|
95 |
+
position: sticky;
|
96 |
+
bottom: 0;
|
97 |
+
}
|
98 |
+
|
99 |
+
.chatbox__support {
|
100 |
+
background:whitesmoke;
|
101 |
+
height: 450px;
|
102 |
+
width: 350px;
|
103 |
+
box-shadow: 0px 0px 15px rgba(0, 0, 0, 0.1);
|
104 |
+
border-top-left-radius: 20px;
|
105 |
+
border-top-right-radius: 20px;
|
106 |
+
}
|
107 |
+
|
108 |
+
/* HEADER */
|
109 |
+
.chatbox__header {
|
110 |
+
background: #1b2398;
|
111 |
+
display: flex;
|
112 |
+
flex-direction: row;
|
113 |
+
align-items: center;
|
114 |
+
justify-content:left;
|
115 |
+
padding: 15px 20px;
|
116 |
+
border-top-left-radius: 20px;
|
117 |
+
border-top-right-radius: 20px;
|
118 |
+
box-shadow: var(--primaryBoxShadow);
|
119 |
+
}
|
120 |
+
|
121 |
+
.chatbox__image--header {
|
122 |
+
margin-right: 10px;
|
123 |
+
width: 2;
|
124 |
+
height: 2;
|
125 |
+
}
|
126 |
+
.chatbox__heading--header {
|
127 |
+
font-size: 1.2rem;
|
128 |
+
color: white;
|
129 |
+
}
|
130 |
+
|
131 |
+
.chatbox__description--header {
|
132 |
+
font-size: .9rem;
|
133 |
+
color:white
|
134 |
+
}
|
135 |
+
|
136 |
+
/* Messages */
|
137 |
+
.chatbox__messages {
|
138 |
+
padding: 0 20px;
|
139 |
+
}
|
140 |
+
|
141 |
+
.messages__item {
|
142 |
+
margin-top: 10px;
|
143 |
+
background:rgb(225, 253, 252);
|
144 |
+
padding: 8px 12px;
|
145 |
+
max-width:70%;
|
146 |
+
|
147 |
+
}
|
148 |
+
|
149 |
+
.messages__item--visitor,
|
150 |
+
.messages__item--typing {
|
151 |
+
border-top-left-radius: 15px;
|
152 |
+
border-top-right-radius: 15px;
|
153 |
+
border-bottom-right-radius: 15px;
|
154 |
+
}
|
155 |
+
|
156 |
+
.messages__item--operator {
|
157 |
+
border-top-left-radius: 10px;
|
158 |
+
border-top-right-radius: 10px;
|
159 |
+
border-bottom-left-radius: 10px;
|
160 |
+
background: var(--primary);
|
161 |
+
color: white;
|
162 |
+
}
|
163 |
+
|
164 |
+
/* FOOTER */
|
165 |
+
.chatbox__footer {
|
166 |
+
display: flex;
|
167 |
+
flex-direction: row;
|
168 |
+
align-items: center;
|
169 |
+
justify-content: space-between;
|
170 |
+
padding: 20px 20px;
|
171 |
+
background: #1b2398;
|
172 |
+
border-bottom-right-radius: 10px;
|
173 |
+
border-bottom-left-radius: 10px;
|
174 |
+
margin-top: 20px;
|
175 |
+
}
|
176 |
+
|
177 |
+
.chatbox__footer input {
|
178 |
+
width: 80%;
|
179 |
+
border: none;
|
180 |
+
padding: 10px 10px;
|
181 |
+
border-radius: 30px;
|
182 |
+
text-align: left;
|
183 |
+
font-family: Cambria, Cochin, Georgia, Times, 'Times New Roman', serif;
|
184 |
+
}
|
185 |
+
|
186 |
+
.chatbox__send--footer {
|
187 |
+
color: white;
|
188 |
+
font-family: Cambria, Cochin, Georgia, Times, 'Times New Roman', serif;
|
189 |
+
}
|
190 |
+
|
191 |
+
.chatbox__button button,
|
192 |
+
.chatbox__button button:focus,
|
193 |
+
.chatbox__button button:visited {
|
194 |
+
padding: 10px;
|
195 |
+
background: white;
|
196 |
+
border: none;
|
197 |
+
outline: none;
|
198 |
+
border-top-left-radius: 50px;
|
199 |
+
border-top-right-radius: 50px;
|
200 |
+
border-bottom-left-radius: 50px;
|
201 |
+
cursor: pointer;
|
202 |
+
}
|
train.py
ADDED
@@ -0,0 +1,127 @@
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
+
import numpy as np
|
2 |
+
import random
|
3 |
+
import json
|
4 |
+
|
5 |
+
import torch
|
6 |
+
import torch.nn as nn
|
7 |
+
from torch.utils.data import Dataset, DataLoader
|
8 |
+
|
9 |
+
from nltk_utils import bag_of_words, tokenize, stem
|
10 |
+
from model import NeuralNet
|
11 |
+
|
12 |
+
# Make sure you run this file as many times as it takes to get a low value for loss ~0.0004, so that gibberish words will not be misinterpreted
|
13 |
+
with open(r'C:\Users\vilsons\Documents\chatbot-deployment-main\flask chatbot\intents.json', 'r', encoding='utf-8') as f:
|
14 |
+
intents = json.load(f)
|
15 |
+
|
16 |
+
all_words = []
|
17 |
+
tags = []
|
18 |
+
xy = []
|
19 |
+
# loop through each sentence in our intents patterns
|
20 |
+
for intent in intents['intents']:
|
21 |
+
tag = intent['tag']
|
22 |
+
# add to tag list
|
23 |
+
tags.append(tag)
|
24 |
+
for pattern in intent['patterns']:
|
25 |
+
# tokenize each word in the sentence
|
26 |
+
w = tokenize(pattern)
|
27 |
+
# add to our words list
|
28 |
+
all_words.extend(w)
|
29 |
+
# add to xy pair
|
30 |
+
xy.append((w, tag))
|
31 |
+
|
32 |
+
# stem and lower each word
|
33 |
+
ignore_words = ['?', '.', '!']
|
34 |
+
all_words = [stem(w) for w in all_words if w not in ignore_words]
|
35 |
+
# remove duplicates and sort
|
36 |
+
all_words = sorted(set(all_words))
|
37 |
+
tags = sorted(set(tags))
|
38 |
+
|
39 |
+
print(len(xy), "patterns")
|
40 |
+
print(len(tags), "tags:", tags)
|
41 |
+
print(len(all_words), "unique stemmed words:", all_words)
|
42 |
+
|
43 |
+
# create training data
|
44 |
+
X_train = []
|
45 |
+
y_train = []
|
46 |
+
for (pattern_sentence, tag) in xy:
|
47 |
+
# X: bag of words for each pattern_sentence
|
48 |
+
bag = bag_of_words(pattern_sentence, all_words)
|
49 |
+
X_train.append(bag)
|
50 |
+
# y: PyTorch CrossEntropyLoss needs only class labels, not one-hot
|
51 |
+
label = tags.index(tag)
|
52 |
+
y_train.append(label)
|
53 |
+
|
54 |
+
X_train = np.array(X_train)
|
55 |
+
y_train = np.array(y_train)
|
56 |
+
|
57 |
+
# Hyper-parameters
|
58 |
+
num_epochs = 1000
|
59 |
+
batch_size = 8
|
60 |
+
learning_rate = 0.001
|
61 |
+
input_size = len(X_train[0])
|
62 |
+
hidden_size = 8
|
63 |
+
output_size = len(tags)
|
64 |
+
print(input_size, output_size)
|
65 |
+
|
66 |
+
class ChatDataset(Dataset):
|
67 |
+
|
68 |
+
def __init__(self):
|
69 |
+
self.n_samples = len(X_train)
|
70 |
+
self.x_data = X_train
|
71 |
+
self.y_data = y_train
|
72 |
+
|
73 |
+
# support indexing such that dataset[i] can be used to get i-th sample
|
74 |
+
def __getitem__(self, index):
|
75 |
+
return self.x_data[index], self.y_data[index]
|
76 |
+
|
77 |
+
# we can call len(dataset) to return the size
|
78 |
+
def __len__(self):
|
79 |
+
return self.n_samples
|
80 |
+
|
81 |
+
dataset = ChatDataset()
|
82 |
+
train_loader = DataLoader(dataset=dataset,batch_size=batch_size,shuffle=True,num_workers=0)
|
83 |
+
|
84 |
+
device = torch.device('cuda' if torch.cuda.is_available() else 'cpu')
|
85 |
+
|
86 |
+
model = NeuralNet(input_size, hidden_size, output_size).to(device)
|
87 |
+
|
88 |
+
# Loss and optimizer
|
89 |
+
criterion = nn.CrossEntropyLoss()
|
90 |
+
optimizer = torch.optim.Adam(model.parameters(), lr=learning_rate)
|
91 |
+
|
92 |
+
# Train the model
|
93 |
+
for epoch in range(num_epochs):
|
94 |
+
for (words, labels) in train_loader:
|
95 |
+
words = words.to(device)
|
96 |
+
labels = labels.to(dtype=torch.long).to(device)
|
97 |
+
|
98 |
+
# Forward pass
|
99 |
+
outputs = model(words)
|
100 |
+
# if y would be one-hot, we must apply
|
101 |
+
# labels = torch.max(labels, 1)[1]
|
102 |
+
loss = criterion(outputs, labels)
|
103 |
+
|
104 |
+
# Backward and optimize
|
105 |
+
optimizer.zero_grad()
|
106 |
+
loss.backward()
|
107 |
+
optimizer.step()
|
108 |
+
|
109 |
+
if (epoch+1) % 100 == 0:
|
110 |
+
print (f'Epoch [{epoch+1}/{num_epochs}], Loss: {loss.item():.4f}')
|
111 |
+
|
112 |
+
|
113 |
+
print(f'final loss: {loss.item():.4f}')
|
114 |
+
|
115 |
+
data = {
|
116 |
+
"model_state": model.state_dict(),
|
117 |
+
"input_size": input_size,
|
118 |
+
"hidden_size": hidden_size,
|
119 |
+
"output_size": output_size,
|
120 |
+
"all_words": all_words,
|
121 |
+
"tags": tags
|
122 |
+
}
|
123 |
+
|
124 |
+
FILE = "data.pth"
|
125 |
+
torch.save(data, FILE)
|
126 |
+
|
127 |
+
print(f'training complete. file saved to {FILE}')
|