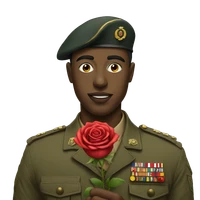
Add initial implementation of ReXplore Blog Poster app with email functionality and environment configuration
f07275b
import re | |
import os | |
import dotenv | |
import mistune | |
import smtplib | |
from email.mime.text import MIMEText | |
from email.mime.multipart import MIMEMultipart | |
dotenv.load_dotenv() | |
def generate_post_html(title, summary, mindmap, citation): | |
html_summary = mistune.html(summary) | |
post = f""" | |
<!DOCTYPE html> | |
<html lang="en"> | |
<head> | |
<meta charset="UTF-8"> | |
<meta name="viewport" content="width=device-width, initial-scale=1.0"> | |
<title>{title}</title> | |
</head> | |
<body> | |
<script src="https://cdn.jsdelivr.net/npm/markmap-autoloader@latest"></script> | |
<style> | |
.markmap {{ | |
position: relative; | |
}} | |
.markmap > svg {{ | |
width: 100%; | |
border: 2px solid #000; | |
height: 80dvh; | |
}} | |
</style> | |
<h1 id="paper_title" data="{title.replace("&", "&")}">{title.replace("&", "&")}</h1> | |
<hr> | |
<br> | |
<h2>SUMMARY</h2> | |
<p id="paper_summary" data="{summary.replace("&", "&")}"> | |
{html_summary.replace("&", "&")} | |
</p> | |
<br> | |
<br> | |
<h2>MINDMAP</h2> | |
<div class="markmap" data="{mindmap.replace("&", "&")}"> | |
<script type="text/template"> | |
# {title.replace("&", "&")} | |
{mindmap.replace("&", "&")} | |
</script> | |
</div> | |
<br> | |
<br> | |
<h2>CITATION</h2> | |
<p id="paper_citation" data="{citation.replace("&", "&")}"> | |
{mistune.html(citation.replace("&", "&"))} | |
</p> | |
</body> | |
</html> | |
#end | |
""" | |
return post | |
def sanitize_citation(citation): | |
pattern = r"(https://doi\.org/\S+)" | |
sanitized_citation = re.sub( | |
pattern, | |
lambda match: f"[{match.group(1)}](https://doi.org/{match.group(1).split('/')[-1]})", | |
citation | |
) | |
return sanitized_citation | |
def create_post(title, category, summary, mindmap, citation): | |
mail_title = f"[{category}] {title}" | |
mail_body = generate_post_html(title, summary, mindmap, sanitize_citation(citation)) | |
return mail_title, mail_body | |
def send_mail(mail_title, mail_body): | |
sender_email = os.getenv('SENDER_EMAIL') | |
sender_password = os.getenv('SENDER_PASSWORD') | |
receiver_email = os.getenv('RECEIVER_EMAIL') | |
msg = MIMEMultipart() | |
msg['From'] = sender_email | |
msg['To'] = receiver_email | |
msg['Subject'] = mail_title | |
msg.attach(MIMEText(mail_body, 'HTML')) | |
smtp_server = os.getenv('SMTP_SERVER') | |
smtp_port = os.getenv('SMTP_PORT') | |
res = None | |
try: | |
server = smtplib.SMTP(smtp_server, smtp_port) | |
server.starttls() | |
server.login(sender_email, sender_password) | |
text = msg.as_string() | |
server.sendmail(sender_email, receiver_email, text) | |
print('Email sent successfully') | |
except Exception as e: | |
print('Email not sent. An error occurred:', str(e)) | |
finally: | |
server.quit() | |
return res | |
def main(title, category, summary, mindmap, citation, access_key): | |
if access_key != os.getenv('ACCESS_KEY'): | |
return False | |
try: | |
mail_title, mail_body = create_post(title, category, summary, mindmap, citation) | |
send_mail(mail_title, mail_body) | |
return True | |
except Exception as e: | |
print('An error occurred:', str(e)) | |
return False |