filename
stringlengths 7
140
| content
stringlengths 0
76.7M
|
---|---|
guides/coding_style/elixir/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/coding_style/f#/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/coding_style/go/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
# Go Style Guide
([Source](https://github.com/golang/go/wiki/CodeReviewComments))
This page collects common comments made during reviews of Go code, so
that a single detailed explanation can be referred to by shorthands.
This is a laundry list of common mistakes, not a style guide.
You can view this as a supplement to https://golang.org/doc/effective_go.html.
**Please discuss changes before editing this page**, even _minor_ ones. Many people have opinions and this is not the place for edit wars.
* [Gofmt](#gofmt)
* [Comment Sentences](#comment-sentences)
* [Contexts](#contexts)
* [Copying](#copying)
* [Crypto Rand](#crypto-rand)
* [Declaring Empty Slices](#declaring-empty-slices)
* [Doc Comments](#doc-comments)
* [Don't Panic](#dont-panic)
* [Error Strings](#error-strings)
* [Examples](#examples)
* [Goroutine Lifetimes](#goroutine-lifetimes)
* [Handle Errors](#handle-errors)
* [Imports](#imports)
* [Import Dot](#import-dot)
* [In-Band Errors](#in-band-errors)
* [Indent Error Flow](#indent-error-flow)
* [Initialisms](#initialisms)
* [Interfaces](#interfaces)
* [Line Length](#line-length)
* [Mixed Caps](#mixed-caps)
* [Named Result Parameters](#named-result-parameters)
* [Naked Returns](#naked-returns)
* [Package Comments](#package-comments)
* [Package Names](#package-names)
* [Pass Values](#pass-values)
* [Receiver Names](#receiver-names)
* [Receiver Type](#receiver-type)
* [Synchronous Functions](#synchronous-functions)
* [Useful Test Failures](#useful-test-failures)
* [Variable Names](#variable-names)
## Gofmt
Run [gofmt](https://golang.org/cmd/gofmt/) on your code to automatically fix the majority of mechanical style issues. Almost all Go code in the wild uses `gofmt`. The rest of this document addresses non-mechanical style points.
An alternative is to use [goimports](https://godoc.org/golang.org/x/tools/cmd/goimports), a superset of `gofmt` which additionally adds (and removes) import lines as necessary.
## Comment Sentences
See https://golang.org/doc/effective_go.html#commentary. Comments documenting declarations should be full sentences, even if that seems a little redundant. This approach makes them format well when extracted into godoc documentation. Comments should begin with the name of the thing being described and end in a period:
```go
// Request represents a request to run a command.
type Request struct { ...
// Encode writes the JSON encoding of req to w.
func Encode(w io.Writer, req *Request) { ...
```
and so on.
## Contexts
Values of the context.Context type carry security credentials,
tracing information, deadlines, and cancellation signals across API
and process boundaries. Go programs pass Contexts explicitly along
the entire function call chain from incoming RPCs and HTTP requests
to outgoing requests.
Most functions that use a Context should accept it as their first parameter:
```
func F(ctx context.Context, /* other arguments */) {}
```
A function that is never request-specific may use context.Background(),
but err on the side of passing a Context even if you think you don't need
to. The default case is to pass a Context; only use context.Background()
directly if you have a good reason why the alternative is a mistake.
Don't add a Context member to a struct type; instead add a ctx parameter
to each method on that type that needs to pass it along. The one exception
is for methods whose signature must match an interface in the standard library
or in a third party library.
Don't create custom Context types or use interfaces other than Context in
function signatures.
If you have application data to pass around, put it in a parameter,
in the receiver, in globals, or, if it truly belongs there, in a Context value.
Contexts are immutable, so it's fine to pass the same ctx to multiple
calls that share the same deadline, cancellation signal, credentials,
parent trace, etc.
## Copying
To avoid unexpected aliasing, be careful when copying a struct from another package.
For example, the bytes.Buffer type contains a `[]byte` slice and, as an optimization
for small strings, a small byte array to which the slice may refer. If you copy a `Buffer`,
the slice in the copy may alias the array in the original, causing subsequent method
calls to have surprising effects.
In general, do not copy a value of type `T` if its methods are associated with the
pointer type, `*T`.
## Declaring Empty Slices
When declaring an empty slice, prefer
```go
var t []string
```
over
```go
t := []string{}
```
The former declares a nil slice value, while the latter is non-nil but zero-length. They are functionally equivalent—their `len` and `cap` are both zero—but the nil slice is the preferred style.
Note that there are limited circumstances where a non-nil but zero-length slice is preferred, such as when encoding JSON objects (a `nil` slice encodes to `null`, while `[]string{}` encodes to the JSON array `[]`).
When designing interfaces, avoid making a distinction between a nil slice and a non-nil, zero-length slice, as this can lead to subtle programming errors.
For more discussion about nil in Go see Francesc Campoy's talk [Understanding Nil](https://www.youtube.com/watch?v=ynoY2xz-F8s).
## Crypto Rand
Do not use package `math/rand` to generate keys, even throwaway ones.
Unseeded, the generator is completely predictable. Seeded with `time.Nanoseconds()`,
there are just a few bits of entropy. Instead, use `crypto/rand`'s Reader,
and if you need text, print to hexadecimal or base64:
``` go
import (
"crypto/rand"
// "encoding/base64"
// "encoding/hex"
"fmt"
)
func Key() string {
buf := make([]byte, 16)
_, err := rand.Read(buf)
if err != nil {
panic(err) // out of randomness, should never happen
}
return fmt.Sprintf("%x", buf)
// or hex.EncodeToString(buf)
// or base64.StdEncoding.EncodeToString(buf)
}
```
## Doc Comments
All top-level, exported names should have doc comments, as should non-trivial unexported type or function declarations. See https://golang.org/doc/effective_go.html#commentary for more information about commentary conventions.
## Don't Panic
See https://golang.org/doc/effective_go.html#errors. Don't use panic for normal error handling. Use error and multiple return values.
## Error Strings
Error strings should not be capitalized (unless beginning with proper nouns or acronyms) or end with punctuation, since they are usually printed following other context. That is, use `fmt.Errorf("something bad")` not `fmt.Errorf("Something bad")`, so that `log.Printf("Reading %s: %v", filename, err)` formats without a spurious capital letter mid-message. This does not apply to logging, which is implicitly line-oriented and not combined inside other messages.
## Examples
When adding a new package, include examples of intended usage: a runnable Example,
or a simple test demonstrating a complete call sequence.
Read more about [testable Example() functions](https://blog.golang.org/examples).
## Goroutine Lifetimes
When you spawn goroutines, make it clear when - or whether - they exit.
Goroutines can leak by blocking on channel sends or receives: the garbage collector
will not terminate a goroutine even if the channels it is blocked on are unreachable.
Even when goroutines do not leak, leaving them in-flight when they are no longer
needed can cause other subtle and hard-to-diagnose problems. Sends on closed channels
panic. Modifying still-in-use inputs "after the result isn't needed" can still lead
to data races. And leaving goroutines in-flight for arbitrarily long can lead to
unpredictable memory usage.
Try to keep concurrent code simple enough that goroutine lifetimes are obvious.
If that just isn't feasible, document when and why the goroutines exit.
## Handle Errors
See https://golang.org/doc/effective_go.html#errors. Do not discard errors using `_` variables. If a function returns an error, check it to make sure the function succeeded. Handle the error, return it, or, in truly exceptional situations, panic.
## Imports
Avoid renaming imports except to avoid a name collision; good package names
should not require renaming. In the event of collision, prefer to rename the most
local or project-specific import.
Imports are organized in groups, with blank lines between them.
The standard library packages are always in the first group.
```go
package main
import (
"fmt"
"hash/adler32"
"os"
"appengine/foo"
"appengine/user"
"code.google.com/p/x/y"
"github.com/foo/bar"
)
```
<a href="https://godoc.org/golang.org/x/tools/cmd/goimports">goimports</a> will do this for you.
## Import Dot
The import . form can be useful in tests that, due to circular dependencies, cannot be made part of the package being tested:
```go
package foo_test
import (
"bar/testutil" // also imports "foo"
. "foo"
)
```
In this case, the test file cannot be in package foo because it uses bar/testutil, which imports foo. So we use the 'import .' form to let the file pretend to be part of package foo even though it is not. Except for this one case, do not use import . in your programs. It makes the programs much harder to read because it is unclear whether a name like Quux is a top-level identifier in the current package or in an imported package.
## In-Band Errors
In C and similar languages, it's common for functions to return values like -1
or null to signal errors or missing results:
```go
// Lookup returns the value for key or "" if there is no mapping for key.
func Lookup(key string) string
// Failing to check a for an in-band error value can lead to bugs:
Parse(Lookup(key)) // returns "parse failure for value" instead of "no value for key"
```
Go's support for multiple return values provides a better solution.
Instead requiring clients to check for an in-band error value, a function should return
an additional value to indicate whether its other return values are valid. This return
value may be an error, or a boolean when no explanation is needed.
It should be the final return value.
``` go
// Lookup returns the value for key or ok=false if there is no mapping for key.
func Lookup(key string) (value string, ok bool)
```
This prevents the caller from using the result incorrectly:
``` go
Parse(Lookup(key)) // compile-time error
```
And encourages more robust and readable code:
``` go
value, ok := Lookup(key)
if !ok {
return fmt.Errorf("no value for %q", key)
}
return Parse(value)
```
This rule applies to exported functions but is also useful
for unexported functions.
Return values like nil, "", 0, and -1 are fine when they are
valid results for a function, that is, when the caller need not
handle them differently from other values.
Some standard library functions, like those in package "strings",
return in-band error values. This greatly simplifies string-manipulation
code at the cost of requiring more diligence from the programmer.
In general, Go code should return additional values for errors.
## Indent Error Flow
Try to keep the normal code path at a minimal indentation, and indent the error handling, dealing with it first. This improves the readability of the code by permitting visually scanning the normal path quickly. For instance, don't write:
```go
if err != nil {
// error handling
} else {
// normal code
}
```
Instead, write:
```go
if err != nil {
// error handling
return // or continue, etc.
}
// normal code
```
If the `if` statement has an initialization statement, such as:
```go
if x, err := f(); err != nil {
// error handling
return
} else {
// use x
}
```
then this may require moving the short variable declaration to its own line:
```go
x, err := f()
if err != nil {
// error handling
return
}
// use x
```
## Initialisms
Words in names that are initialisms or acronyms (e.g. "URL" or "NATO") have a consistent case. For example, "URL" should appear as "URL" or "url" (as in "urlPony", or "URLPony"), never as "Url". Here's an example: ServeHTTP not ServeHttp.
This rule also applies to "ID" when it is short for "identifier," so write "appID" instead of "appId".
Code generated by the protocol buffer compiler is exempt from this rule. Human-written code is held to a higher standard than machine-written code.
## Interfaces
Go interfaces generally belong in the package that uses values of the
interface type, not the package that implements those values. The
implementing package should return concrete (usually pointer or struct)
types: that way, new methods can be added to implementations without
requiring extensive refactoring.
Do not define interfaces on the implementor side of an API "for mocking";
instead, design the API so that it can be tested using the public API of
the real implementation.
Do not define interfaces before they are used: without a realistic example
of usage, it is too difficult to see whether an interface is even necessary,
let alone what methods it ought to contain.
``` go
package consumer // consumer.go
type Thinger interface { Thing() bool }
func Foo(t Thinger) string { … }
```
``` go
package consumer // consumer_test.go
type fakeThinger struct{ … }
func (t fakeThinger) Thing() bool { … }
…
if Foo(fakeThinger{…}) == "x" { … }
```
``` go
// DO NOT DO IT!!!
package producer
type Thinger interface { Thing() bool }
type defaultThinger struct{ … }
func (t defaultThinger) Thing() bool { … }
func NewThinger() Thinger { return defaultThinger{ … } }
```
Instead return a concrete type and let the consumer mock the producer implementation.
``` go
package producer
type Thinger struct{ … }
func (t Thinger) Thing() bool { … }
func NewThinger() Thinger { return Thinger{ … } }
```
## Line Length
There is no rigid line length limit in Go code, but avoid uncomfortably long lines.
Similarly, don't add line breaks to keep lines short when they are more readable long--for example,
if they are repetitive.
Most of the time when people wrap lines "unnaturally" (in the middle of function calls or
function declarations, more or less, say, though some exceptions are around), the wrapping would be
unnecessary if they had a reasonable number of parameters and reasonably short variable names.
Long lines seem to go with long names, and getting rid of the long names helps a lot.
In other words, break lines because of the semantics of what you're writing (as a general rule)
and not because of the length of the line. If you find that this produces lines that are too long,
then change the names or the semantics and you'll probably get a good result.
This is, actually, exactly the same advice about how long a function should be. There's no rule
"never have a function more than N lines long", but there is definitely such a thing as too long
of a function, and of too stuttery tiny functions, and the solution is to change where the function
boundaries are, not to start counting lines.
## Mixed Caps
See https://golang.org/doc/effective_go.html#mixed-caps. This applies even when it breaks conventions in other languages. For example an unexported constant is `maxLength` not `MaxLength` or `MAX_LENGTH`.
## Named Result Parameters
Consider what it will look like in godoc. Named result parameters like:
```go
func (n *Node) Parent1() (node *Node)
func (n *Node) Parent2() (node *Node, err error)
```
will stutter in godoc; better to use:
```go
func (n *Node) Parent1() *Node
func (n *Node) Parent2() (*Node, error)
```
On the other hand, if a function returns two or three parameters of the same type,
or if the meaning of a result isn't clear from context, adding names may be useful
in some contexts. Don't name result parameters just to avoid declaring a var inside
the function; that trades off a minor implementation brevity at the cost of
unnecessary API verbosity.
```go
func (f *Foo) Location() (float64, float64, error)
```
is less clear than:
```go
// Location returns f's latitude and longitude.
// Negative values mean south and west, respectively.
func (f *Foo) Location() (lat, long float64, err error)
```
Naked returns are okay if the function is a handful of lines. Once it's a medium
sized function, be explicit with your return values. Corollary: it's not worth it
to name result parameters just because it enables you to use naked returns.
Clarity of docs is always more important than saving a line or two in your function.
Finally, in some cases you need to name a result parameter in order to change
it in a deferred closure. That is always OK.
## Naked Returns
See [Named Result Parameters](#named-result-parameters).
## Package Comments
Package comments, like all comments to be presented by godoc, must appear adjacent to the package clause, with no blank line.
```go
// Package math provides basic constants and mathematical functions.
package math
```
```go
/*
Package template implements data-driven templates for generating textual
output such as HTML.
....
*/
package template
```
For "package main" commands, other styles of comment are fine after the binary name (and it may be capitalized if it comes first), For example, for a `package main` in the directory `seedgen` you could write:
``` go
// Binary seedgen ...
package main
```
or
```go
// Command seedgen ...
package main
```
or
```go
// Program seedgen ...
package main
```
or
```go
// The seedgen command ...
package main
```
or
```go
// The seedgen program ...
package main
```
or
```go
// Seedgen ..
package main
```
These are examples, and sensible variants of these are acceptable.
Note that starting the sentence with a lower-case word is not among the
acceptable options for package comments, as these are publicly-visible and
should be written in proper English, including capitalizing the first word
of the sentence. When the binary name is the first word, capitalizing it is
required even though it does not strictly match the spelling of the
command-line invocation.
See https://golang.org/doc/effective_go.html#commentary for more information about commentary conventions.
## Package Names
All references to names in your package will be done using the package name,
so you can omit that name from the identifiers. For example, if you are in package chubby,
you don't need type ChubbyFile, which clients will write as `chubby.ChubbyFile`.
Instead, name the type `File`, which clients will write as `chubby.File`.
Avoid meaningless package names like util, common, misc, api, types, and interfaces. See http://golang.org/doc/effective_go.html#package-names and
http://blog.golang.org/package-names for more.
## Pass Values
Don't pass pointers as function arguments just to save a few bytes. If a function refers to its argument `x` only as `*x` throughout, then the argument shouldn't be a pointer. Common instances of this include passing a pointer to a string (`*string`) or a pointer to an interface value (`*io.Reader`). In both cases the value itself is a fixed size and can be passed directly. This advice does not apply to large structs, or even small structs that might grow.
## Receiver Names
The name of a method's receiver should be a reflection of its identity; often a one or two letter abbreviation of its type suffices (such as "c" or "cl" for "Client"). Don't use generic names such as "me", "this" or "self", identifiers typical of object-oriented languages that place more emphasis on methods as opposed to functions. The name need not be as descriptive as that of a method argument, as its role is obvious and serves no documentary purpose. It can be very short as it will appear on almost every line of every method of the type; familiarity admits brevity. Be consistent, too: if you call the receiver "c" in one method, don't call it "cl" in another.
## Receiver Type
Choosing whether to use a value or pointer receiver on methods can be difficult, especially to new Go programmers. If in doubt, use a pointer, but there are times when a value receiver makes sense, usually for reasons of efficiency, such as for small unchanging structs or values of basic type. Some useful guidelines:
* If the receiver is a map, func or chan, don't use a pointer to them. If the receiver is a slice and the method doesn't reslice or reallocate the slice, don't use a pointer to it.
* If the method needs to mutate the receiver, the receiver must be a pointer.
* If the receiver is a struct that contains a sync.Mutex or similar synchronizing field, the receiver must be a pointer to avoid copying.
* If the receiver is a large struct or array, a pointer receiver is more efficient. How large is large? Assume it's equivalent to passing all its elements as arguments to the method. If that feels too large, it's also too large for the receiver.
* Can function or methods, either concurrently or when called from this method, be mutating the receiver? A value type creates a copy of the receiver when the method is invoked, so outside updates will not be applied to this receiver. If changes must be visible in the original receiver, the receiver must be a pointer.
* If the receiver is a struct, array or slice and any of its elements is a pointer to something that might be mutating, prefer a pointer receiver, as it will make the intention more clear to the reader.
* If the receiver is a small array or struct that is naturally a value type (for instance, something like the time.Time type), with no mutable fields and no pointers, or is just a simple basic type such as int or string, a value receiver makes sense. A value receiver can reduce the amount of garbage that can be generated; if a value is passed to a value method, an on-stack copy can be used instead of allocating on the heap. (The compiler tries to be smart about avoiding this allocation, but it can't always succeed.) Don't choose a value receiver type for this reason without profiling first.
* Finally, when in doubt, use a pointer receiver.
## Synchronous Functions
Prefer synchronous functions - functions which return their results directly or finish any callbacks or channel ops before returning - over asynchronous ones.
Synchronous functions keep goroutines localized within a call, making it easier to reason about their lifetimes and avoid leaks and data races. They're also easier to test: the caller can pass an input and check the output without the need for polling or synchronization.
If callers need more concurrency, they can add it easily by calling the function from a separate goroutine. But it is quite difficult - sometimes impossible - to remove unnecessary concurrency at the caller side.
## Useful Test Failures
Tests should fail with helpful messages saying what was wrong, with what inputs, what was actually got, and what was expected. It may be tempting to write a bunch of assertFoo helpers, but be sure your helpers produce useful error messages. Assume that the person debugging your failing test is not you, and is not your team. A typical Go test fails like:
```go
if got != tt.want {
t.Errorf("Foo(%q) = %d; want %d", tt.in, got, tt.want) // or Fatalf, if test can't test anything more past this point
}
```
Note that the order here is actual != expected, and the message uses that order too. Some test frameworks encourage writing these backwards: 0 != x, "expected 0, got x", and so on. Go does not.
If that seems like a lot of typing, you may want to write a [[table-driven test|TableDrivenTests]].
Another common technique to disambiguate failing tests when using a test helper with different input is to wrap each caller with a different TestFoo function, so the test fails with that name:
```go
func TestSingleValue(t *testing.T) { testHelper(t, []int{80}) }
func TestNoValues(t *testing.T) { testHelper(t, []int{}) }
```
In any case, the onus is on you to fail with a helpful message to whoever's debugging your code in the future.
## Variable Names
Variable names in Go should be short rather than long. This is especially true for local variables with limited scope. Prefer `c` to `lineCount`. Prefer `i` to `sliceIndex`.
The basic rule: the further from its declaration that a name is used, the more descriptive the name must be. For a method receiver, one or two letters is sufficient. Common variables such as loop indices and readers can be a single letter (`i`, `r`). More unusual things and global variables need more descriptive names.
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/coding_style/java/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
# Java Style Guide
## Index
- [Braces](#braces)
- [Comments](#comments)
- [Control Flow Statements](#control-flow-statements)
- [Whitespace](#whitespace)
- [Naming](#naming)
- [Methods](#methods)
- [Expressions](#expressions)
- [Variables](#variables)
- [Source File Structure](#source-file-structure)
- [Annotations](#annotations)
- [Switch Statements](#switch-statements)
- [Statements](#statements)
## Braces
All braces should start on the same line as the method they are delimiting. If a control flow statement contains only one line of code, it should not have any delimiting braces, unless using a multi-block control flow statement where at least one block is more than one line long. If using inline braces, there should be a space between the braces and the code in between. If a block of code is empty, the code may be made consise by putting everything on the same line, with no space in between the braces, unless part of a multi-block statement.
```Java
if (true) {
/*
** Multiple lines
** of code
*/
}
while (true)
// Code
if (true || false) {
// One line of code
} else {
/*
** More than one line of code,
** so all blocks should be delimited.
*/
}
if (false) {} // Do nothing, valid
if (true) {
/*
** Code
*/
} else {} // Not acceptable
int[] arr = new int[] { 1, 2, 3 };
```
## Comments
Comments should have a space following the double slashes. Any multiline comments should be created with the /\*\*/ delimiters. A special type of comment, called Javadoc commenting, should be implemented using the /\*\* \*/ delimiters. Javadoc comments are used with tags like @author, @param, and @return to document the purpose of methods and classes in code.
```Java
// Single line comment, with space after slashes
/*
** Multi-line
** comment
*/
/**
* Documentation
*
* @version 1.0
*/
/**
*
* This is a Javadoc comment that
* is describing a method in a class
* that will modify a string
*
* @author myName
* @param word the string to be manipulated
* @return the modified string
*
*/
```
## Control Flow Statements
The parentheses for control flow statements should be one space after the statement. There should be a newline between unrelated statements, i.e. between a `while` and `if` but not between an `if` and `else`. There should be no spaces in between a statement and its block of code. This goes for class declarations as well. The only case that a piece of code inside an if-statement can be on the same line is if the code is a `return` statement.
```Java
if (true || false) {
// Code
} else {
// Else
}
while (true) {
// Should be no spaces, unlike this
}
class Foo {
// No space between declaration and code
}
// Incorrect
if (true) bar += foo;
// Correct
if (true) return bar;
```
## Whitespace
Tabs should be tab characters, and not spaces.
## Naming
All names should be lowerCamelCase, except constants, which should be CAPITALIZED_AND-SEPARATED_BY_UNDERSCORES, and classes, which should be PascalCase.
## Methods
Methods should have no whitespace in between the parentheses for parameters and the method signature. If a method contains only one line, it should be on the same line as the method declaration and follow the [inline brace delimiting](#braces) rule. Parameters should have no spaces between the parentheses, and one space after the comma.
```Java
public void foo(int a, int b) {
/*
** Multiple lines
** of code
*/
}
public void bar() { /* One line of code, with a space separating the braces */ }
```
## Expressions
All expressions, including boolean and arithmetic, should have a space between operators and operands.
```Java
// Incorrect
int a=b+c;
// Correct
int a = b + c;
// Incorrect
if (a==b && c<d)
// Correct
if (a == b && c < d)
```
## Variables
All variables should be declared at the top of their scope, in the order shown below. Each set of variables with the same visibility should be grouped together and separated from other variable groups by a single space. In each group, variables of the same data type should be adjacent to one another. The exception are constants, which should always be declared at the top. All variables declared at the `class` scope should have one of the below modifiers.
Order of member modifiers, according to the Java Language Specification:
`public protected private abstract default static final transient volatile sychronized native strictfp`
```Java
public static final int CONSTANT = 1;
public int x;
public int y;
public String str;
protected int num;
protected String foo;
private char c;
// Code
public void bar() {
int n;
// More code
}
```
## Source File Structure
The structure of the file should comprise the following:
1. Import statements
2. Top-level class
3. Lower level classes
Each of the above components should be separated by exactly one line. All lines aside from the import statements must follow a length limit of 100 columns. Wildcard imports should **not** be used.
## Annotations
Annotations should be on the line above the block they are applied to. Multiple annotations should be stacked atop one another.
```Java
@annotation
public void foo() {}
@annotation
@differentAnnotation
public void bar() {}
```
## Switch Statements
All switch cases should end with either a `break` or `return` keyword. Every switch statement should also contain a `default` case for when
the variable being checked does not match up with any of the cases in the statement.
```Java
/* Given a number, num, do something
* different depending on what num is
* (i.e. num is 1, 2, 3, or none)
*/
switch (num) {
case 1: // Do something
break;
case 2: // Do something
break;
case 3: // Do something
break;
default: // Do something
break;
}
```
## Statements
There should only be one statement per line. A statement is defined as ending in a semicolon, so this does not include control flow statements. The exceptions are a single statement that are delimited with braces, including anonymous methods and array instantiation.
```Java
Runnable run = () -> {
// Acceptable
};
int[][] arr = {
{ 1, 2, 3 },
{ 4, 5, 6 },
{ 7, 8, 9 }
};
```
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/coding_style/javascript/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
# Airbnb JavaScript Style Guide() {
*A mostly reasonable approach to JavaScript*
> **Note**: this guide assumes you are using [Babel](https://babeljs.io), and requires that you use [babel-preset-airbnb](https://npmjs.com/babel-preset-airbnb) or the equivalent. It also assumes you are installing shims/polyfills in your app, with [airbnb-browser-shims](https://npmjs.com/airbnb-browser-shims) or the equivalent.
[](https://www.npmjs.com/package/eslint-config-airbnb)
[](https://www.npmjs.com/package/eslint-config-airbnb-base)
[](https://gitter.im/airbnb/javascript?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge)
Other Style Guides
- [ES5 (Deprecated)](https://github.com/airbnb/javascript/tree/es5-deprecated/es5)
- [React](react/)
- [CSS-in-JavaScript](css-in-javascript/)
- [CSS & Sass](https://github.com/airbnb/css)
- [Ruby](https://github.com/airbnb/ruby)
## Table of Contents
1. [Types](#types)
1. [References](#references)
1. [Objects](#objects)
1. [Arrays](#arrays)
1. [Destructuring](#destructuring)
1. [Strings](#strings)
1. [Functions](#functions)
1. [Arrow Functions](#arrow-functions)
1. [Classes & Constructors](#classes--constructors)
1. [Modules](#modules)
1. [Iterators and Generators](#iterators-and-generators)
1. [Properties](#properties)
1. [Variables](#variables)
1. [Hoisting](#hoisting)
1. [Comparison Operators & Equality](#comparison-operators--equality)
1. [Blocks](#blocks)
1. [Control Statements](#control-statements)
1. [Comments](#comments)
1. [Whitespace](#whitespace)
1. [Commas](#commas)
1. [Semicolons](#semicolons)
1. [Type Casting & Coercion](#type-casting--coercion)
1. [Naming Conventions](#naming-conventions)
1. [Accessors](#accessors)
1. [Events](#events)
1. [jQuery](#jquery)
1. [ECMAScript 5 Compatibility](#ecmascript-5-compatibility)
1. [ECMAScript 6+ (ES 2015+) Styles](#ecmascript-6-es-2015-styles)
1. [Standard Library](#standard-library)
1. [Testing](#testing)
1. [Performance](#performance)
1. [Resources](#resources)
1. [In the Wild](#in-the-wild)
1. [Translation](#translation)
1. [The JavaScript Style Guide Guide](#the-javascript-style-guide-guide)
1. [Chat With Us About JavaScript](#chat-with-us-about-javascript)
1. [Contributors](#contributors)
1. [License](#license)
1. [Amendments](#amendments)
## Types
<a name="types--primitives"></a><a name="1.1"></a>
- [1.1](#types--primitives) **Primitives**: When you access a primitive type you work directly on its value.
- `string`
- `number`
- `boolean`
- `null`
- `undefined`
```javascript
const foo = 1;
let bar = foo;
bar = 9;
console.log(foo, bar); // => 1, 9
```
<a name="types--complex"></a><a name="1.2"></a>
- [1.2](#types--complex) **Complex**: When you access a complex type you work on a reference to its value.
- `object`
- `array`
- `function`
```javascript
const foo = [1, 2];
const bar = foo;
bar[0] = 9;
console.log(foo[0], bar[0]); // => 9, 9
```
**[⬆ back to top](#table-of-contents)**
## References
<a name="references--prefer-const"></a><a name="2.1"></a>
- [2.1](#references--prefer-const) Use `const` for all of your references; avoid using `var`. eslint: [`prefer-const`](http://eslint.org/docs/rules/prefer-const.html), [`no-const-assign`](http://eslint.org/docs/rules/no-const-assign.html)
> Why? This ensures that you can’t reassign your references, which can lead to bugs and difficult to comprehend code.
```javascript
// bad
var a = 1;
var b = 2;
// good
const a = 1;
const b = 2;
```
<a name="references--disallow-var"></a><a name="2.2"></a>
- [2.2](#references--disallow-var) If you must reassign references, use `let` instead of `var`. eslint: [`no-var`](http://eslint.org/docs/rules/no-var.html) jscs: [`disallowVar`](http://jscs.info/rule/disallowVar)
> Why? `let` is block-scoped rather than function-scoped like `var`.
```javascript
// bad
var count = 1;
if (true) {
count += 1;
}
// good, use the let.
let count = 1;
if (true) {
count += 1;
}
```
<a name="references--block-scope"></a><a name="2.3"></a>
- [2.3](#references--block-scope) Note that both `let` and `const` are block-scoped.
```javascript
// const and let only exist in the blocks they are defined in.
{
let a = 1;
const b = 1;
}
console.log(a); // ReferenceError
console.log(b); // ReferenceError
```
**[⬆ back to top](#table-of-contents)**
## Objects
<a name="objects--no-new"></a><a name="3.1"></a>
- [3.1](#objects--no-new) Use the literal syntax for object creation. eslint: [`no-new-object`](http://eslint.org/docs/rules/no-new-object.html)
```javascript
// bad
const item = new Object();
// good
const item = {};
```
<a name="es6-computed-properties"></a><a name="3.4"></a>
- [3.2](#es6-computed-properties) Use computed property names when creating objects with dynamic property names.
> Why? They allow you to define all the properties of an object in one place.
```javascript
function getKey(k) {
return `a key named ${k}`;
}
// bad
const obj = {
id: 5,
name: 'San Francisco',
};
obj[getKey('enabled')] = true;
// good
const obj = {
id: 5,
name: 'San Francisco',
[getKey('enabled')]: true,
};
```
<a name="es6-object-shorthand"></a><a name="3.5"></a>
- [3.3](#es6-object-shorthand) Use object method shorthand. eslint: [`object-shorthand`](http://eslint.org/docs/rules/object-shorthand.html) jscs: [`requireEnhancedObjectLiterals`](http://jscs.info/rule/requireEnhancedObjectLiterals)
```javascript
// bad
const atom = {
value: 1,
addValue: function (value) {
return atom.value + value;
},
};
// good
const atom = {
value: 1,
addValue(value) {
return atom.value + value;
},
};
```
<a name="es6-object-concise"></a><a name="3.6"></a>
- [3.4](#es6-object-concise) Use property value shorthand. eslint: [`object-shorthand`](http://eslint.org/docs/rules/object-shorthand.html) jscs: [`requireEnhancedObjectLiterals`](http://jscs.info/rule/requireEnhancedObjectLiterals)
> Why? It is shorter to write and descriptive.
```javascript
const lukeSkywalker = 'Luke Skywalker';
// bad
const obj = {
lukeSkywalker: lukeSkywalker,
};
// good
const obj = {
lukeSkywalker,
};
```
<a name="objects--grouped-shorthand"></a><a name="3.7"></a>
- [3.5](#objects--grouped-shorthand) Group your shorthand properties at the beginning of your object declaration.
> Why? It’s easier to tell which properties are using the shorthand.
```javascript
const anakinSkywalker = 'Anakin Skywalker';
const lukeSkywalker = 'Luke Skywalker';
// bad
const obj = {
episodeOne: 1,
twoJediWalkIntoACantina: 2,
lukeSkywalker,
episodeThree: 3,
mayTheFourth: 4,
anakinSkywalker,
};
// good
const obj = {
lukeSkywalker,
anakinSkywalker,
episodeOne: 1,
twoJediWalkIntoACantina: 2,
episodeThree: 3,
mayTheFourth: 4,
};
```
<a name="objects--quoted-props"></a><a name="3.8"></a>
- [3.6](#objects--quoted-props) Only quote properties that are invalid identifiers. eslint: [`quote-props`](http://eslint.org/docs/rules/quote-props.html) jscs: [`disallowQuotedKeysInObjects`](http://jscs.info/rule/disallowQuotedKeysInObjects)
> Why? In general we consider it subjectively easier to read. It improves syntax highlighting, and is also more easily optimized by many JS engines.
```javascript
// bad
const bad = {
'foo': 3,
'bar': 4,
'data-blah': 5,
};
// good
const good = {
foo: 3,
bar: 4,
'data-blah': 5,
};
```
<a name="objects--prototype-builtins"></a>
- [3.7](#objects--prototype-builtins) Do not call `Object.prototype` methods directly, such as `hasOwnProperty`, `propertyIsEnumerable`, and `isPrototypeOf`.
> Why? These methods may be shadowed by properties on the object in question - consider `{ hasOwnProperty: false }` - or, the object may be a null object (`Object.create(null)`).
```javascript
// bad
console.log(object.hasOwnProperty(key));
// good
console.log(Object.prototype.hasOwnProperty.call(object, key));
// best
const has = Object.prototype.hasOwnProperty; // cache the lookup once, in module scope.
/* or */
import has from 'has';
// ...
console.log(has.call(object, key));
```
<a name="objects--rest-spread"></a>
- [3.8](#objects--rest-spread) Prefer the object spread operator over [`Object.assign`](https://developer.mozilla.org/en/docs/Web/JavaScript/Reference/Global_Objects/Object/assign) to shallow-copy objects. Use the object rest operator to get a new object with certain properties omitted.
```javascript
// very bad
const original = { a: 1, b: 2 };
const copy = Object.assign(original, { c: 3 }); // this mutates `original` ಠ_ಠ
delete copy.a; // so does this
// bad
const original = { a: 1, b: 2 };
const copy = Object.assign({}, original, { c: 3 }); // copy => { a: 1, b: 2, c: 3 }
// good
const original = { a: 1, b: 2 };
const copy = { ...original, c: 3 }; // copy => { a: 1, b: 2, c: 3 }
const { a, ...noA } = copy; // noA => { b: 2, c: 3 }
```
**[⬆ back to top](#table-of-contents)**
## Arrays
<a name="arrays--literals"></a><a name="4.1"></a>
- [4.1](#arrays--literals) Use the literal syntax for array creation. eslint: [`no-array-constructor`](http://eslint.org/docs/rules/no-array-constructor.html)
```javascript
// bad
const items = new Array();
// good
const items = [];
```
<a name="arrays--push"></a><a name="4.2"></a>
- [4.2](#arrays--push) Use [Array#push](https://developer.mozilla.org/en/docs/Web/JavaScript/Reference/Global_Objects/Array/push) instead of direct assignment to add items to an array.
```javascript
const someStack = [];
// bad
someStack[someStack.length] = 'abracadabra';
// good
someStack.push('abracadabra');
```
<a name="es6-array-spreads"></a><a name="4.3"></a>
- [4.3](#es6-array-spreads) Use array spreads `...` to copy arrays.
```javascript
// bad
const len = items.length;
const itemsCopy = [];
let i;
for (i = 0; i < len; i += 1) {
itemsCopy[i] = items[i];
}
// good
const itemsCopy = [...items];
```
<a name="arrays--from"></a><a name="4.4"></a>
- [4.4](#arrays--from) To convert an array-like object to an array, use spreads `...` instead of [Array.from](https://developer.mozilla.org/en/docs/Web/JavaScript/Reference/Global_Objects/Array/from).
```javascript
const foo = document.querySelectorAll('.foo');
// good
const nodes = Array.from(foo);
// best
const nodes = [...foo];
```
<a name="arrays--mapping"></a>
- [4.5](#arrays--mapping) Use [Array.from](https://developer.mozilla.org/en/docs/Web/JavaScript/Reference/Global_Objects/Array/from) instead of spread `...` for mapping over iterables, because it avoids creating an intermediate array.
```javascript
// bad
const baz = [...foo].map(bar);
// good
const baz = Array.from(foo, bar);
```
<a name="arrays--callback-return"></a><a name="4.5"></a>
- [4.6](#arrays--callback-return) Use return statements in array method callbacks. It’s ok to omit the return if the function body consists of a single statement returning an expression without side effects, following [8.2](#arrows--implicit-return). eslint: [`array-callback-return`](http://eslint.org/docs/rules/array-callback-return)
```javascript
// good
[1, 2, 3].map((x) => {
const y = x + 1;
return x * y;
});
// good
[1, 2, 3].map(x => x + 1);
// bad - no returned value means `memo` becomes undefined after the first iteration
const flat = {};
[[0, 1], [2, 3], [4, 5]].reduce((memo, item, index) => {
const flatten = memo.concat(item);
memo[index] = flatten;
});
// good
const flat = {};
[[0, 1], [2, 3], [4, 5]].reduce((memo, item, index) => {
const flatten = memo.concat(item);
memo[index] = flatten;
return flatten;
});
// bad
inbox.filter((msg) => {
const { subject, author } = msg;
if (subject === 'Mockingbird') {
return author === 'Harper Lee';
} else {
return false;
}
});
// good
inbox.filter((msg) => {
const { subject, author } = msg;
if (subject === 'Mockingbird') {
return author === 'Harper Lee';
}
return false;
});
```
<a name="arrays--bracket-newline"></a>
- [4.7](#arrays--bracket-newline) Use line breaks after open and before close array brackets if an array has multiple lines
```javascript
// bad
const arr = [
[0, 1], [2, 3], [4, 5],
];
const objectInArray = [{
id: 1,
}, {
id: 2,
}];
const numberInArray = [
1, 2,
];
// good
const arr = [[0, 1], [2, 3], [4, 5]];
const objectInArray = [
{
id: 1,
},
{
id: 2,
},
];
const numberInArray = [
1,
2,
];
```
**[⬆ back to top](#table-of-contents)**
## Destructuring
<a name="destructuring--object"></a><a name="5.1"></a>
- [5.1](#destructuring--object) Use object destructuring when accessing and using multiple properties of an object. eslint: [`prefer-destructuring`](https://eslint.org/docs/rules/prefer-destructuring) jscs: [`requireObjectDestructuring`](http://jscs.info/rule/requireObjectDestructuring)
> Why? Destructuring saves you from creating temporary references for those properties.
```javascript
// bad
function getFullName(user) {
const firstName = user.firstName;
const lastName = user.lastName;
return `${firstName} ${lastName}`;
}
// good
function getFullName(user) {
const { firstName, lastName } = user;
return `${firstName} ${lastName}`;
}
// best
function getFullName({ firstName, lastName }) {
return `${firstName} ${lastName}`;
}
```
<a name="destructuring--array"></a><a name="5.2"></a>
- [5.2](#destructuring--array) Use array destructuring. eslint: [`prefer-destructuring`](https://eslint.org/docs/rules/prefer-destructuring) jscs: [`requireArrayDestructuring`](http://jscs.info/rule/requireArrayDestructuring)
```javascript
const arr = [1, 2, 3, 4];
// bad
const first = arr[0];
const second = arr[1];
// good
const [first, second] = arr;
```
<a name="destructuring--object-over-array"></a><a name="5.3"></a>
- [5.3](#destructuring--object-over-array) Use object destructuring for multiple return values, not array destructuring. jscs: [`disallowArrayDestructuringReturn`](http://jscs.info/rule/disallowArrayDestructuringReturn)
> Why? You can add new properties over time or change the order of things without breaking call sites.
```javascript
// bad
function processInput(input) {
// then a miracle occurs
return [left, right, top, bottom];
}
// the caller needs to think about the order of return data
const [left, __, top] = processInput(input);
// good
function processInput(input) {
// then a miracle occurs
return { left, right, top, bottom };
}
// the caller selects only the data they need
const { left, top } = processInput(input);
```
**[⬆ back to top](#table-of-contents)**
## Strings
<a name="strings--quotes"></a><a name="6.1"></a>
- [6.1](#strings--quotes) Use single quotes `''` for strings. eslint: [`quotes`](http://eslint.org/docs/rules/quotes.html) jscs: [`validateQuoteMarks`](http://jscs.info/rule/validateQuoteMarks)
```javascript
// bad
const name = "Capt. Janeway";
// bad - template literals should contain interpolation or newlines
const name = `Capt. Janeway`;
// good
const name = 'Capt. Janeway';
```
<a name="strings--line-length"></a><a name="6.2"></a>
- [6.2](#strings--line-length) Strings that cause the line to go over 100 characters should not be written across multiple lines using string concatenation.
> Why? Broken strings are painful to work with and make code less searchable.
```javascript
// bad
const errorMessage = 'This is a super long error that was thrown because \
of Batman. When you stop to think about how Batman had anything to do \
with this, you would get nowhere \
fast.';
// bad
const errorMessage = 'This is a super long error that was thrown because ' +
'of Batman. When you stop to think about how Batman had anything to do ' +
'with this, you would get nowhere fast.';
// good
const errorMessage = 'This is a super long error that was thrown because of Batman. When you stop to think about how Batman had anything to do with this, you would get nowhere fast.';
```
<a name="es6-template-literals"></a><a name="6.4"></a>
- [6.3](#es6-template-literals) When programmatically building up strings, use template strings instead of concatenation. eslint: [`prefer-template`](http://eslint.org/docs/rules/prefer-template.html) [`template-curly-spacing`](http://eslint.org/docs/rules/template-curly-spacing) jscs: [`requireTemplateStrings`](http://jscs.info/rule/requireTemplateStrings)
> Why? Template strings give you a readable, concise syntax with proper newlines and string interpolation features.
```javascript
// bad
function sayHi(name) {
return 'How are you, ' + name + '?';
}
// bad
function sayHi(name) {
return ['How are you, ', name, '?'].join();
}
// bad
function sayHi(name) {
return `How are you, ${ name }?`;
}
// good
function sayHi(name) {
return `How are you, ${name}?`;
}
```
<a name="strings--eval"></a><a name="6.5"></a>
- [6.4](#strings--eval) Never use `eval()` on a string, it opens too many vulnerabilities. eslint: [`no-eval`](http://eslint.org/docs/rules/no-eval)
<a name="strings--escaping"></a>
- [6.5](#strings--escaping) Do not unnecessarily escape characters in strings. eslint: [`no-useless-escape`](http://eslint.org/docs/rules/no-useless-escape)
> Why? Backslashes harm readability, thus they should only be present when necessary.
```javascript
// bad
const foo = '\'this\' \i\s \"quoted\"';
// good
const foo = '\'this\' is "quoted"';
const foo = `my name is '${name}'`;
```
**[⬆ back to top](#table-of-contents)**
## Functions
<a name="functions--declarations"></a><a name="7.1"></a>
- [7.1](#functions--declarations) Use named function expressions instead of function declarations. eslint: [`func-style`](http://eslint.org/docs/rules/func-style) jscs: [`disallowFunctionDeclarations`](http://jscs.info/rule/disallowFunctionDeclarations)
> Why? Function declarations are hoisted, which means that it’s easy - too easy - to reference the function before it is defined in the file. This harms readability and maintainability. If you find that a function’s definition is large or complex enough that it is interfering with understanding the rest of the file, then perhaps it’s time to extract it to its own module! Don’t forget to name the expression - anonymous functions can make it harder to locate the problem in an Error’s call stack. ([Discussion](https://github.com/airbnb/javascript/issues/794))
```javascript
// bad
function foo() {
// ...
}
// bad
const foo = function () {
// ...
};
// good
const foo = function bar() {
// ...
};
```
<a name="functions--iife"></a><a name="7.2"></a>
- [7.2](#functions--iife) Wrap immediately invoked function expressions in parentheses. eslint: [`wrap-iife`](http://eslint.org/docs/rules/wrap-iife.html) jscs: [`requireParenthesesAroundIIFE`](http://jscs.info/rule/requireParenthesesAroundIIFE)
> Why? An immediately invoked function expression is a single unit - wrapping both it, and its invocation parens, in parens, cleanly expresses this. Note that in a world with modules everywhere, you almost never need an IIFE.
```javascript
// immediately-invoked function expression (IIFE)
(function () {
console.log('Welcome to the Internet. Please follow me.');
}());
```
<a name="functions--in-blocks"></a><a name="7.3"></a>
- [7.3](#functions--in-blocks) Never declare a function in a non-function block (`if`, `while`, etc). Assign the function to a variable instead. Browsers will allow you to do it, but they all interpret it differently, which is bad news bears. eslint: [`no-loop-func`](http://eslint.org/docs/rules/no-loop-func.html)
<a name="functions--note-on-blocks"></a><a name="7.4"></a>
- [7.4](#functions--note-on-blocks) **Note:** ECMA-262 defines a `block` as a list of statements. A function declaration is not a statement. [Read ECMA-262’s note on this issue](http://www.ecma-international.org/publications/files/ECMA-ST/Ecma-262.pdf#page=97).
```javascript
// bad
if (currentUser) {
function test() {
console.log('Nope.');
}
}
// good
let test;
if (currentUser) {
test = () => {
console.log('Yup.');
};
}
```
<a name="functions--arguments-shadow"></a><a name="7.5"></a>
- [7.5](#functions--arguments-shadow) Never name a parameter `arguments`. This will take precedence over the `arguments` object that is given to every function scope.
```javascript
// bad
function foo(name, options, arguments) {
// ...
}
// good
function foo(name, options, args) {
// ...
}
```
<a name="es6-rest"></a><a name="7.6"></a>
- [7.6](#es6-rest) Never use `arguments`, opt to use rest syntax `...` instead. eslint: [`prefer-rest-params`](http://eslint.org/docs/rules/prefer-rest-params)
> Why? `...` is explicit about which arguments you want pulled. Plus, rest arguments are a real Array, and not merely Array-like like `arguments`.
```javascript
// bad
function concatenateAll() {
const args = Array.prototype.slice.call(arguments);
return args.join('');
}
// good
function concatenateAll(...args) {
return args.join('');
}
```
<a name="es6-default-parameters"></a><a name="7.7"></a>
- [7.7](#es6-default-parameters) Use default parameter syntax rather than mutating function arguments.
```javascript
// really bad
function handleThings(opts) {
// No! We shouldn’t mutate function arguments.
// Double bad: if opts is falsy it'll be set to an object which may
// be what you want but it can introduce subtle bugs.
opts = opts || {};
// ...
}
// still bad
function handleThings(opts) {
if (opts === void 0) {
opts = {};
}
// ...
}
// good
function handleThings(opts = {}) {
// ...
}
```
<a name="functions--default-side-effects"></a><a name="7.8"></a>
- [7.8](#functions--default-side-effects) Avoid side effects with default parameters.
> Why? They are confusing to reason about.
```javascript
var b = 1;
// bad
function count(a = b++) {
console.log(a);
}
count(); // 1
count(); // 2
count(3); // 3
count(); // 3
```
<a name="functions--defaults-last"></a><a name="7.9"></a>
- [7.9](#functions--defaults-last) Always put default parameters last.
```javascript
// bad
function handleThings(opts = {}, name) {
// ...
}
// good
function handleThings(name, opts = {}) {
// ...
}
```
<a name="functions--constructor"></a><a name="7.10"></a>
- [7.10](#functions--constructor) Never use the Function constructor to create a new function. eslint: [`no-new-func`](http://eslint.org/docs/rules/no-new-func)
> Why? Creating a function in this way evaluates a string similarly to eval(), which opens vulnerabilities.
```javascript
// bad
var add = new Function('a', 'b', 'return a + b');
// still bad
var subtract = Function('a', 'b', 'return a - b');
```
<a name="functions--signature-spacing"></a><a name="7.11"></a>
- [7.11](#functions--signature-spacing) Spacing in a function signature. eslint: [`space-before-function-paren`](http://eslint.org/docs/rules/space-before-function-paren) [`space-before-blocks`](http://eslint.org/docs/rules/space-before-blocks)
> Why? Consistency is good, and you shouldn’t have to add or remove a space when adding or removing a name.
```javascript
// bad
const f = function(){};
const g = function (){};
const h = function() {};
// good
const x = function () {};
const y = function a() {};
```
<a name="functions--mutate-params"></a><a name="7.12"></a>
- [7.12](#functions--mutate-params) Never mutate parameters. eslint: [`no-param-reassign`](http://eslint.org/docs/rules/no-param-reassign.html)
> Why? Manipulating objects passed in as parameters can cause unwanted variable side effects in the original caller.
```javascript
// bad
function f1(obj) {
obj.key = 1;
}
// good
function f2(obj) {
const key = Object.prototype.hasOwnProperty.call(obj, 'key') ? obj.key : 1;
}
```
<a name="functions--reassign-params"></a><a name="7.13"></a>
- [7.13](#functions--reassign-params) Never reassign parameters. eslint: [`no-param-reassign`](http://eslint.org/docs/rules/no-param-reassign.html)
> Why? Reassigning parameters can lead to unexpected behavior, especially when accessing the `arguments` object. It can also cause optimization issues, especially in V8.
```javascript
// bad
function f1(a) {
a = 1;
// ...
}
function f2(a) {
if (!a) { a = 1; }
// ...
}
// good
function f3(a) {
const b = a || 1;
// ...
}
function f4(a = 1) {
// ...
}
```
<a name="functions--spread-vs-apply"></a><a name="7.14"></a>
- [7.14](#functions--spread-vs-apply) Prefer the use of the spread operator `...` to call variadic functions. eslint: [`prefer-spread`](http://eslint.org/docs/rules/prefer-spread)
> Why? It’s cleaner, you don’t need to supply a context, and you can not easily compose `new` with `apply`.
```javascript
// bad
const x = [1, 2, 3, 4, 5];
console.log.apply(console, x);
// good
const x = [1, 2, 3, 4, 5];
console.log(...x);
// bad
new (Function.prototype.bind.apply(Date, [null, 2016, 8, 5]));
// good
new Date(...[2016, 8, 5]);
```
<a name="functions--signature-invocation-indentation"></a>
- [7.15](#functions--signature-invocation-indentation) Functions with multiline signatures, or invocations, should be indented just like every other multiline list in this guide: with each item on a line by itself, with a trailing comma on the last item.
```javascript
// bad
function foo(bar,
baz,
quux) {
// ...
}
// good
function foo(
bar,
baz,
quux,
) {
// ...
}
// bad
console.log(foo,
bar,
baz);
// good
console.log(
foo,
bar,
baz,
);
```
**[⬆ back to top](#table-of-contents)**
## Arrow Functions
<a name="arrows--use-them"></a><a name="8.1"></a>
- [8.1](#arrows--use-them) When you must use function expressions (as when passing an anonymous function), use arrow function notation. eslint: [`prefer-arrow-callback`](http://eslint.org/docs/rules/prefer-arrow-callback.html), [`arrow-spacing`](http://eslint.org/docs/rules/arrow-spacing.html) jscs: [`requireArrowFunctions`](http://jscs.info/rule/requireArrowFunctions)
> Why? It creates a version of the function that executes in the context of `this`, which is usually what you want, and is a more concise syntax.
> Why not? If you have a fairly complicated function, you might move that logic out into its own function declaration.
```javascript
// bad
[1, 2, 3].map(function (x) {
const y = x + 1;
return x * y;
});
// good
[1, 2, 3].map((x) => {
const y = x + 1;
return x * y;
});
```
<a name="arrows--implicit-return"></a><a name="8.2"></a>
- [8.2](#arrows--implicit-return) If the function body consists of a single statement returning an [expression](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Expressions_and_Operators#Expressions) without side effects, omit the braces and use the implicit return. Otherwise, keep the braces and use a `return` statement. eslint: [`arrow-parens`](http://eslint.org/docs/rules/arrow-parens.html), [`arrow-body-style`](http://eslint.org/docs/rules/arrow-body-style.html) jscs: [`disallowParenthesesAroundArrowParam`](http://jscs.info/rule/disallowParenthesesAroundArrowParam), [`requireShorthandArrowFunctions`](http://jscs.info/rule/requireShorthandArrowFunctions)
> Why? Syntactic sugar. It reads well when multiple functions are chained together.
```javascript
// bad
[1, 2, 3].map(number => {
const nextNumber = number + 1;
`A string containing the ${nextNumber}.`;
});
// good
[1, 2, 3].map(number => `A string containing the ${number}.`);
// good
[1, 2, 3].map((number) => {
const nextNumber = number + 1;
return `A string containing the ${nextNumber}.`;
});
// good
[1, 2, 3].map((number, index) => ({
[index]: number,
}));
// No implicit return with side effects
function foo(callback) {
const val = callback();
if (val === true) {
// Do something if callback returns true
}
}
let bool = false;
// bad
foo(() => bool = true);
// good
foo(() => {
bool = true;
});
```
<a name="arrows--paren-wrap"></a><a name="8.3"></a>
- [8.3](#arrows--paren-wrap) In case the expression spans over multiple lines, wrap it in parentheses for better readability.
> Why? It shows clearly where the function starts and ends.
```javascript
// bad
['get', 'post', 'put'].map(httpMethod => Object.prototype.hasOwnProperty.call(
httpMagicObjectWithAVeryLongName,
httpMethod,
)
);
// good
['get', 'post', 'put'].map(httpMethod => (
Object.prototype.hasOwnProperty.call(
httpMagicObjectWithAVeryLongName,
httpMethod,
)
));
```
<a name="arrows--one-arg-parens"></a><a name="8.4"></a>
- [8.4](#arrows--one-arg-parens) If your function takes a single argument and doesn’t use braces, omit the parentheses. Otherwise, always include parentheses around arguments for clarity and consistency. Note: it is also acceptable to always use parentheses, in which case use the [“always” option](http://eslint.org/docs/rules/arrow-parens#always) for eslint or do not include [`disallowParenthesesAroundArrowParam`](http://jscs.info/rule/disallowParenthesesAroundArrowParam) for jscs. eslint: [`arrow-parens`](http://eslint.org/docs/rules/arrow-parens.html) jscs: [`disallowParenthesesAroundArrowParam`](http://jscs.info/rule/disallowParenthesesAroundArrowParam)
> Why? Less visual clutter.
```javascript
// bad
[1, 2, 3].map((x) => x * x);
// good
[1, 2, 3].map(x => x * x);
// good
[1, 2, 3].map(number => (
`A long string with the ${number}. It’s so long that we don’t want it to take up space on the .map line!`
));
// bad
[1, 2, 3].map(x => {
const y = x + 1;
return x * y;
});
// good
[1, 2, 3].map((x) => {
const y = x + 1;
return x * y;
});
```
<a name="arrows--confusing"></a><a name="8.5"></a>
- [8.5](#arrows--confusing) Avoid confusing arrow function syntax (`=>`) with comparison operators (`<=`, `>=`). eslint: [`no-confusing-arrow`](http://eslint.org/docs/rules/no-confusing-arrow)
```javascript
// bad
const itemHeight = item => item.height > 256 ? item.largeSize : item.smallSize;
// bad
const itemHeight = (item) => item.height > 256 ? item.largeSize : item.smallSize;
// good
const itemHeight = item => (item.height > 256 ? item.largeSize : item.smallSize);
// good
const itemHeight = (item) => {
const { height, largeSize, smallSize } = item;
return height > 256 ? largeSize : smallSize;
};
```
**[⬆ back to top](#table-of-contents)**
## Classes & Constructors
<a name="constructors--use-class"></a><a name="9.1"></a>
- [9.1](#constructors--use-class) Always use `class`. Avoid manipulating `prototype` directly.
> Why? `class` syntax is more concise and easier to reason about.
```javascript
// bad
function Queue(contents = []) {
this.queue = [...contents];
}
Queue.prototype.pop = function () {
const value = this.queue[0];
this.queue.splice(0, 1);
return value;
};
// good
class Queue {
constructor(contents = []) {
this.queue = [...contents];
}
pop() {
const value = this.queue[0];
this.queue.splice(0, 1);
return value;
}
}
```
<a name="constructors--extends"></a><a name="9.2"></a>
- [9.2](#constructors--extends) Use `extends` for inheritance.
> Why? It is a built-in way to inherit prototype functionality without breaking `instanceof`.
```javascript
// bad
const inherits = require('inherits');
function PeekableQueue(contents) {
Queue.apply(this, contents);
}
inherits(PeekableQueue, Queue);
PeekableQueue.prototype.peek = function () {
return this.queue[0];
};
// good
class PeekableQueue extends Queue {
peek() {
return this.queue[0];
}
}
```
<a name="constructors--chaining"></a><a name="9.3"></a>
- [9.3](#constructors--chaining) Methods can return `this` to help with method chaining.
```javascript
// bad
Jedi.prototype.jump = function () {
this.jumping = true;
return true;
};
Jedi.prototype.setHeight = function (height) {
this.height = height;
};
const luke = new Jedi();
luke.jump(); // => true
luke.setHeight(20); // => undefined
// good
class Jedi {
jump() {
this.jumping = true;
return this;
}
setHeight(height) {
this.height = height;
return this;
}
}
const luke = new Jedi();
luke.jump()
.setHeight(20);
```
<a name="constructors--tostring"></a><a name="9.4"></a>
- [9.4](#constructors--tostring) It’s okay to write a custom toString() method, just make sure it works successfully and causes no side effects.
```javascript
class Jedi {
constructor(options = {}) {
this.name = options.name || 'no name';
}
getName() {
return this.name;
}
toString() {
return `Jedi - ${this.getName()}`;
}
}
```
<a name="constructors--no-useless"></a><a name="9.5"></a>
- [9.5](#constructors--no-useless) Classes have a default constructor if one is not specified. An empty constructor function or one that just delegates to a parent class is unnecessary. eslint: [`no-useless-constructor`](http://eslint.org/docs/rules/no-useless-constructor)
```javascript
// bad
class Jedi {
constructor() {}
getName() {
return this.name;
}
}
// bad
class Rey extends Jedi {
constructor(...args) {
super(...args);
}
}
// good
class Rey extends Jedi {
constructor(...args) {
super(...args);
this.name = 'Rey';
}
}
```
<a name="classes--no-duplicate-members"></a>
- [9.6](#classes--no-duplicate-members) Avoid duplicate class members. eslint: [`no-dupe-class-members`](http://eslint.org/docs/rules/no-dupe-class-members)
> Why? Duplicate class member declarations will silently prefer the last one - having duplicates is almost certainly a bug.
```javascript
// bad
class Foo {
bar() { return 1; }
bar() { return 2; }
}
// good
class Foo {
bar() { return 1; }
}
// good
class Foo {
bar() { return 2; }
}
```
**[⬆ back to top](#table-of-contents)**
## Modules
<a name="modules--use-them"></a><a name="10.1"></a>
- [10.1](#modules--use-them) Always use modules (`import`/`export`) over a non-standard module system. You can always transpile to your preferred module system.
> Why? Modules are the future, let’s start using the future now.
```javascript
// bad
const AirbnbStyleGuide = require('./AirbnbStyleGuide');
module.exports = AirbnbStyleGuide.es6;
// ok
import AirbnbStyleGuide from './AirbnbStyleGuide';
export default AirbnbStyleGuide.es6;
// best
import { es6 } from './AirbnbStyleGuide';
export default es6;
```
<a name="modules--no-wildcard"></a><a name="10.2"></a>
- [10.2](#modules--no-wildcard) Do not use wildcard imports.
> Why? This makes sure you have a single default export.
```javascript
// bad
import * as AirbnbStyleGuide from './AirbnbStyleGuide';
// good
import AirbnbStyleGuide from './AirbnbStyleGuide';
```
<a name="modules--no-export-from-import"></a><a name="10.3"></a>
- [10.3](#modules--no-export-from-import) And do not export directly from an import.
> Why? Although the one-liner is concise, having one clear way to import and one clear way to export makes things consistent.
```javascript
// bad
// filename es6.js
export { es6 as default } from './AirbnbStyleGuide';
// good
// filename es6.js
import { es6 } from './AirbnbStyleGuide';
export default es6;
```
<a name="modules--no-duplicate-imports"></a>
- [10.4](#modules--no-duplicate-imports) Only import from a path in one place.
eslint: [`no-duplicate-imports`](http://eslint.org/docs/rules/no-duplicate-imports)
> Why? Having multiple lines that import from the same path can make code harder to maintain.
```javascript
// bad
import foo from 'foo';
// … some other imports … //
import { named1, named2 } from 'foo';
// good
import foo, { named1, named2 } from 'foo';
// good
import foo, {
named1,
named2,
} from 'foo';
```
<a name="modules--no-mutable-exports"></a>
- [10.5](#modules--no-mutable-exports) Do not export mutable bindings.
eslint: [`import/no-mutable-exports`](https://github.com/benmosher/eslint-plugin-import/blob/master/docs/rules/no-mutable-exports.md)
> Why? Mutation should be avoided in general, but in particular when exporting mutable bindings. While this technique may be needed for some special cases, in general, only constant references should be exported.
```javascript
// bad
let foo = 3;
export { foo };
// good
const foo = 3;
export { foo };
```
<a name="modules--prefer-default-export"></a>
- [10.6](#modules--prefer-default-export) In modules with a single export, prefer default export over named export.
eslint: [`import/prefer-default-export`](https://github.com/benmosher/eslint-plugin-import/blob/master/docs/rules/prefer-default-export.md)
> Why? To encourage more files that only ever export one thing, which is better for readability and maintainability.
```javascript
// bad
export function foo() {}
// good
export default function foo() {}
```
<a name="modules--imports-first"></a>
- [10.7](#modules--imports-first) Put all `import`s above non-import statements.
eslint: [`import/first`](https://github.com/benmosher/eslint-plugin-import/blob/master/docs/rules/first.md)
> Why? Since `import`s are hoisted, keeping them all at the top prevents surprising behavior.
```javascript
// bad
import foo from 'foo';
foo.init();
import bar from 'bar';
// good
import foo from 'foo';
import bar from 'bar';
foo.init();
```
<a name="modules--multiline-imports-over-newlines"></a>
- [10.8](#modules--multiline-imports-over-newlines) Multiline imports should be indented just like multiline array and object literals.
> Why? The curly braces follow the same indentation rules as every other curly brace block in the style guide, as do the trailing commas.
```javascript
// bad
import {longNameA, longNameB, longNameC, longNameD, longNameE} from 'path';
// good
import {
longNameA,
longNameB,
longNameC,
longNameD,
longNameE,
} from 'path';
```
<a name="modules--no-webpack-loader-syntax"></a>
- [10.9](#modules--no-webpack-loader-syntax) Disallow Webpack loader syntax in module import statements.
eslint: [`import/no-webpack-loader-syntax`](https://github.com/benmosher/eslint-plugin-import/blob/master/docs/rules/no-webpack-loader-syntax.md)
> Why? Since using Webpack syntax in the imports couples the code to a module bundler. Prefer using the loader syntax in `webpack.config.js`.
```javascript
// bad
import fooSass from 'css!sass!foo.scss';
import barCss from 'style!css!bar.css';
// good
import fooSass from 'foo.scss';
import barCss from 'bar.css';
```
**[⬆ back to top](#table-of-contents)**
## Iterators and Generators
<a name="iterators--nope"></a><a name="11.1"></a>
- [11.1](#iterators--nope) Don’t use iterators. Prefer JavaScript’s higher-order functions instead of loops like `for-in` or `for-of`. eslint: [`no-iterator`](http://eslint.org/docs/rules/no-iterator.html) [`no-restricted-syntax`](http://eslint.org/docs/rules/no-restricted-syntax)
> Why? This enforces our immutable rule. Dealing with pure functions that return values is easier to reason about than side effects.
> Use `map()` / `every()` / `filter()` / `find()` / `findIndex()` / `reduce()` / `some()` / ... to iterate over arrays, and `Object.keys()` / `Object.values()` / `Object.entries()` to produce arrays so you can iterate over objects.
```javascript
const numbers = [1, 2, 3, 4, 5];
// bad
let sum = 0;
for (let num of numbers) {
sum += num;
}
sum === 15;
// good
let sum = 0;
numbers.forEach((num) => {
sum += num;
});
sum === 15;
// best (use the functional force)
const sum = numbers.reduce((total, num) => total + num, 0);
sum === 15;
// bad
const increasedByOne = [];
for (let i = 0; i < numbers.length; i++) {
increasedByOne.push(numbers[i] + 1);
}
// good
const increasedByOne = [];
numbers.forEach((num) => {
increasedByOne.push(num + 1);
});
// best (keeping it functional)
const increasedByOne = numbers.map(num => num + 1);
```
<a name="generators--nope"></a><a name="11.2"></a>
- [11.2](#generators--nope) Don’t use generators for now.
> Why? They don’t transpile well to ES5.
<a name="generators--spacing"></a>
- [11.3](#generators--spacing) If you must use generators, or if you disregard [our advice](#generators--nope), make sure their function signature is spaced properly. eslint: [`generator-star-spacing`](http://eslint.org/docs/rules/generator-star-spacing)
> Why? `function` and `*` are part of the same conceptual keyword - `*` is not a modifier for `function`, `function*` is a unique construct, different from `function`.
```javascript
// bad
function * foo() {
// ...
}
// bad
const bar = function * () {
// ...
};
// bad
const baz = function *() {
// ...
};
// bad
const quux = function*() {
// ...
};
// bad
function*foo() {
// ...
}
// bad
function *foo() {
// ...
}
// very bad
function
*
foo() {
// ...
}
// very bad
const wat = function
*
() {
// ...
};
// good
function* foo() {
// ...
}
// good
const foo = function* () {
// ...
};
```
**[⬆ back to top](#table-of-contents)**
## Properties
<a name="properties--dot"></a><a name="12.1"></a>
- [12.1](#properties--dot) Use dot notation when accessing properties. eslint: [`dot-notation`](http://eslint.org/docs/rules/dot-notation.html) jscs: [`requireDotNotation`](http://jscs.info/rule/requireDotNotation)
```javascript
const luke = {
jedi: true,
age: 28,
};
// bad
const isJedi = luke['jedi'];
// good
const isJedi = luke.jedi;
```
<a name="properties--bracket"></a><a name="12.2"></a>
- [12.2](#properties--bracket) Use bracket notation `[]` when accessing properties with a variable.
```javascript
const luke = {
jedi: true,
age: 28,
};
function getProp(prop) {
return luke[prop];
}
const isJedi = getProp('jedi');
```
<a name="es2016-properties--exponentiation-operator"></a>
- [12.3](#es2016-properties--exponentiation-operator) Use exponentiation operator `**` when calculating exponentiations. eslint: [`no-restricted-properties`](http://eslint.org/docs/rules/no-restricted-properties).
```javascript
// bad
const binary = Math.pow(2, 10);
// good
const binary = 2 ** 10;
```
**[⬆ back to top](#table-of-contents)**
## Variables
<a name="variables--const"></a><a name="13.1"></a>
- [13.1](#variables--const) Always use `const` or `let` to declare variables. Not doing so will result in global variables. We want to avoid polluting the global namespace. Captain Planet warned us of that. eslint: [`no-undef`](http://eslint.org/docs/rules/no-undef) [`prefer-const`](http://eslint.org/docs/rules/prefer-const)
```javascript
// bad
superPower = new SuperPower();
// good
const superPower = new SuperPower();
```
<a name="variables--one-const"></a><a name="13.2"></a>
- [13.2](#variables--one-const) Use one `const` or `let` declaration per variable. eslint: [`one-var`](http://eslint.org/docs/rules/one-var.html) jscs: [`disallowMultipleVarDecl`](http://jscs.info/rule/disallowMultipleVarDecl)
> Why? It’s easier to add new variable declarations this way, and you never have to worry about swapping out a `;` for a `,` or introducing punctuation-only diffs. You can also step through each declaration with the debugger, instead of jumping through all of them at once.
```javascript
// bad
const items = getItems(),
goSportsTeam = true,
dragonball = 'z';
// bad
// (compare to above, and try to spot the mistake)
const items = getItems(),
goSportsTeam = true;
dragonball = 'z';
// good
const items = getItems();
const goSportsTeam = true;
const dragonball = 'z';
```
<a name="variables--const-let-group"></a><a name="13.3"></a>
- [13.3](#variables--const-let-group) Group all your `const`s and then group all your `let`s.
> Why? This is helpful when later on you might need to assign a variable depending on one of the previous assigned variables.
```javascript
// bad
let i, len, dragonball,
items = getItems(),
goSportsTeam = true;
// bad
let i;
const items = getItems();
let dragonball;
const goSportsTeam = true;
let len;
// good
const goSportsTeam = true;
const items = getItems();
let dragonball;
let i;
let length;
```
<a name="variables--define-where-used"></a><a name="13.4"></a>
- [13.4](#variables--define-where-used) Assign variables where you need them, but place them in a reasonable place.
> Why? `let` and `const` are block scoped and not function scoped.
```javascript
// bad - unnecessary function call
function checkName(hasName) {
const name = getName();
if (hasName === 'test') {
return false;
}
if (name === 'test') {
this.setName('');
return false;
}
return name;
}
// good
function checkName(hasName) {
if (hasName === 'test') {
return false;
}
const name = getName();
if (name === 'test') {
this.setName('');
return false;
}
return name;
}
```
<a name="variables--no-chain-assignment"></a><a name="13.5"></a>
- [13.5](#variables--no-chain-assignment) Don’t chain variable assignments.
> Why? Chaining variable assignments creates implicit global variables.
```javascript
// bad
(function example() {
// JavaScript interprets this as
// let a = ( b = ( c = 1 ) );
// The let keyword only applies to variable a; variables b and c become
// global variables.
let a = b = c = 1;
}());
console.log(a); // throws ReferenceError
console.log(b); // 1
console.log(c); // 1
// good
(function example() {
let a = 1;
let b = a;
let c = a;
}());
console.log(a); // throws ReferenceError
console.log(b); // throws ReferenceError
console.log(c); // throws ReferenceError
// the same applies for `const`
```
<a name="variables--unary-increment-decrement"></a><a name="13.6"></a>
- [13.6](#variables--unary-increment-decrement) Avoid using unary increments and decrements (++, --). eslint [`no-plusplus`](http://eslint.org/docs/rules/no-plusplus)
> Why? Per the eslint documentation, unary increment and decrement statements are subject to automatic semicolon insertion and can cause silent errors with incrementing or decrementing values within an application. It is also more expressive to mutate your values with statements like `num += 1` instead of `num++` or `num ++`. Disallowing unary increment and decrement statements also prevents you from pre-incrementing/pre-decrementing values unintentionally which can also cause unexpected behavior in your programs.
```javascript
// bad
const array = [1, 2, 3];
let num = 1;
num++;
--num;
let sum = 0;
let truthyCount = 0;
for (let i = 0; i < array.length; i++) {
let value = array[i];
sum += value;
if (value) {
truthyCount++;
}
}
// good
const array = [1, 2, 3];
let num = 1;
num += 1;
num -= 1;
const sum = array.reduce((a, b) => a + b, 0);
const truthyCount = array.filter(Boolean).length;
```
**[⬆ back to top](#table-of-contents)**
## Hoisting
<a name="hoisting--about"></a><a name="14.1"></a>
- [14.1](#hoisting--about) `var` declarations get hoisted to the top of their scope, their assignment does not. `const` and `let` declarations are blessed with a new concept called [Temporal Dead Zones (TDZ)](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/let#Temporal_dead_zone_and_errors_with_let). It’s important to know why [typeof is no longer safe](http://es-discourse.com/t/why-typeof-is-no-longer-safe/15).
```javascript
// we know this wouldn’t work (assuming there
// is no notDefined global variable)
function example() {
console.log(notDefined); // => throws a ReferenceError
}
// creating a variable declaration after you
// reference the variable will work due to
// variable hoisting. Note: the assignment
// value of `true` is not hoisted.
function example() {
console.log(declaredButNotAssigned); // => undefined
var declaredButNotAssigned = true;
}
// the interpreter is hoisting the variable
// declaration to the top of the scope,
// which means our example could be rewritten as:
function example() {
let declaredButNotAssigned;
console.log(declaredButNotAssigned); // => undefined
declaredButNotAssigned = true;
}
// using const and let
function example() {
console.log(declaredButNotAssigned); // => throws a ReferenceError
console.log(typeof declaredButNotAssigned); // => throws a ReferenceError
const declaredButNotAssigned = true;
}
```
<a name="hoisting--anon-expressions"></a><a name="14.2"></a>
- [14.2](#hoisting--anon-expressions) Anonymous function expressions hoist their variable name, but not the function assignment.
```javascript
function example() {
console.log(anonymous); // => undefined
anonymous(); // => TypeError anonymous is not a function
var anonymous = function () {
console.log('anonymous function expression');
};
}
```
<a name="hoisting--named-expresions"></a><a name="14.3"></a>
- [14.3](#hoisting--named-expresions) Named function expressions hoist the variable name, not the function name or the function body.
```javascript
function example() {
console.log(named); // => undefined
named(); // => TypeError named is not a function
superPower(); // => ReferenceError superPower is not defined
var named = function superPower() {
console.log('Flying');
};
}
// the same is true when the function name
// is the same as the variable name.
function example() {
console.log(named); // => undefined
named(); // => TypeError named is not a function
var named = function named() {
console.log('named');
};
}
```
<a name="hoisting--declarations"></a><a name="14.4"></a>
- [14.4](#hoisting--declarations) Function declarations hoist their name and the function body.
```javascript
function example() {
superPower(); // => Flying
function superPower() {
console.log('Flying');
}
}
```
- For more information refer to [JavaScript Scoping & Hoisting](http://www.adequatelygood.com/2010/2/JavaScript-Scoping-and-Hoisting/) by [Ben Cherry](http://www.adequatelygood.com/).
**[⬆ back to top](#table-of-contents)**
## Comparison Operators & Equality
<a name="comparison--eqeqeq"></a><a name="15.1"></a>
- [15.1](#comparison--eqeqeq) Use `===` and `!==` over `==` and `!=`. eslint: [`eqeqeq`](http://eslint.org/docs/rules/eqeqeq.html)
<a name="comparison--if"></a><a name="15.2"></a>
- [15.2](#comparison--if) Conditional statements such as the `if` statement evaluate their expression using coercion with the `ToBoolean` abstract method and always follow these simple rules:
- **Objects** evaluate to **true**
- **Undefined** evaluates to **false**
- **Null** evaluates to **false**
- **Booleans** evaluate to **the value of the boolean**
- **Numbers** evaluate to **false** if **+0, -0, or NaN**, otherwise **true**
- **Strings** evaluate to **false** if an empty string `''`, otherwise **true**
```javascript
if ([0] && []) {
// true
// an array (even an empty one) is an object, objects will evaluate to true
}
```
<a name="comparison--shortcuts"></a><a name="15.3"></a>
- [15.3](#comparison--shortcuts) Use shortcuts for booleans, but explicit comparisons for strings and numbers.
```javascript
// bad
if (isValid === true) {
// ...
}
// good
if (isValid) {
// ...
}
// bad
if (name) {
// ...
}
// good
if (name !== '') {
// ...
}
// bad
if (collection.length) {
// ...
}
// good
if (collection.length > 0) {
// ...
}
```
<a name="comparison--moreinfo"></a><a name="15.4"></a>
- [15.4](#comparison--moreinfo) For more information see [Truth Equality and JavaScript](https://javascriptweblog.wordpress.com/2011/02/07/truth-equality-and-javascript/#more-2108) by Angus Croll.
<a name="comparison--switch-blocks"></a><a name="15.5"></a>
- [15.5](#comparison--switch-blocks) Use braces to create blocks in `case` and `default` clauses that contain lexical declarations (e.g. `let`, `const`, `function`, and `class`). eslint: [`no-case-declarations`](http://eslint.org/docs/rules/no-case-declarations.html)
> Why? Lexical declarations are visible in the entire `switch` block but only get initialized when assigned, which only happens when its `case` is reached. This causes problems when multiple `case` clauses attempt to define the same thing.
```javascript
// bad
switch (foo) {
case 1:
let x = 1;
break;
case 2:
const y = 2;
break;
case 3:
function f() {
// ...
}
break;
default:
class C {}
}
// good
switch (foo) {
case 1: {
let x = 1;
break;
}
case 2: {
const y = 2;
break;
}
case 3: {
function f() {
// ...
}
break;
}
case 4:
bar();
break;
default: {
class C {}
}
}
```
<a name="comparison--nested-ternaries"></a><a name="15.6"></a>
- [15.6](#comparison--nested-ternaries) Ternaries should not be nested and generally be single line expressions. eslint: [`no-nested-ternary`](http://eslint.org/docs/rules/no-nested-ternary.html)
```javascript
// bad
const foo = maybe1 > maybe2
? "bar"
: value1 > value2 ? "baz" : null;
// split into 2 separated ternary expressions
const maybeNull = value1 > value2 ? 'baz' : null;
// better
const foo = maybe1 > maybe2
? 'bar'
: maybeNull;
// best
const foo = maybe1 > maybe2 ? 'bar' : maybeNull;
```
<a name="comparison--unneeded-ternary"></a><a name="15.7"></a>
- [15.7](#comparison--unneeded-ternary) Avoid unneeded ternary statements. eslint: [`no-unneeded-ternary`](http://eslint.org/docs/rules/no-unneeded-ternary.html)
```javascript
// bad
const foo = a ? a : b;
const bar = c ? true : false;
const baz = c ? false : true;
// good
const foo = a || b;
const bar = !!c;
const baz = !c;
```
**[⬆ back to top](#table-of-contents)**
## Blocks
<a name="blocks--braces"></a><a name="16.1"></a>
- [16.1](#blocks--braces) Use braces with all multi-line blocks.
```javascript
// bad
if (test)
return false;
// good
if (test) return false;
// good
if (test) {
return false;
}
// bad
function foo() { return false; }
// good
function bar() {
return false;
}
```
<a name="blocks--cuddled-elses"></a><a name="16.2"></a>
- [16.2](#blocks--cuddled-elses) If you're using multi-line blocks with `if` and `else`, put `else` on the same line as your `if` block’s closing brace. eslint: [`brace-style`](http://eslint.org/docs/rules/brace-style.html) jscs: [`disallowNewlineBeforeBlockStatements`](http://jscs.info/rule/disallowNewlineBeforeBlockStatements)
```javascript
// bad
if (test) {
thing1();
thing2();
}
else {
thing3();
}
// good
if (test) {
thing1();
thing2();
} else {
thing3();
}
```
<a name="blocks--no-else-return"></a><a name="16.3"></a>
- [16.3](#blocks--no-else-return) If an `if` block always executes a `return` statement, the subsequent `else` block is unnecessary. A `return` in an `else if` block following an `if` block that contains a `return` can be separated into multiple `if` blocks. eslint: [`no-else-return`](https://eslint.org/docs/rules/no-else-return)
```javascript
// bad
function foo() {
if (x) {
return x;
} else {
return y;
}
}
// bad
function cats() {
if (x) {
return x;
} else if (y) {
return y;
}
}
// bad
function dogs() {
if (x) {
return x;
} else {
if (y) {
return y;
}
}
}
// good
function foo() {
if (x) {
return x;
}
return y;
}
// good
function cats() {
if (x) {
return x;
}
if (y) {
return y;
}
}
//good
function dogs(x) {
if (x) {
if (z) {
return y;
}
} else {
return z;
}
}
```
**[⬆ back to top](#table-of-contents)**
## Control Statements
<a name="control-statements"></a>
- [17.1](#control-statements) In case your control statement (`if`, `while` etc.) gets too long or exceeds the maximum line length, each (grouped) condition could be put into a new line. The logical operator should begin the line.
> Why? Requiring operators at the beginning of the line keeps the operators aligned and follows a pattern similar to method chaining. This also improves readability by making it easier to visually follow complex logic.
```javascript
// bad
if ((foo === 123 || bar === 'abc') && doesItLookGoodWhenItBecomesThatLong() && isThisReallyHappening()) {
thing1();
}
// bad
if (foo === 123 &&
bar === 'abc') {
thing1();
}
// bad
if (foo === 123
&& bar === 'abc') {
thing1();
}
// bad
if (
foo === 123 &&
bar === 'abc'
) {
thing1();
}
// good
if (
foo === 123
&& bar === 'abc'
) {
thing1();
}
// good
if (
(foo === 123 || bar === "abc")
&& doesItLookGoodWhenItBecomesThatLong()
&& isThisReallyHappening()
) {
thing1();
}
// good
if (foo === 123 && bar === 'abc') {
thing1();
}
```
**[⬆ back to top](#table-of-contents)**
## Comments
<a name="comments--multiline"></a><a name="17.1"></a>
- [18.1](#comments--multiline) Use `/** ... */` for multi-line comments.
```javascript
// bad
// make() returns a new element
// based on the passed in tag name
//
// @param {String} tag
// @return {Element} element
function make(tag) {
// ...
return element;
}
// good
/**
* make() returns a new element
* based on the passed-in tag name
*/
function make(tag) {
// ...
return element;
}
```
<a name="comments--singleline"></a><a name="17.2"></a>
- [18.2](#comments--singleline) Use `//` for single line comments. Place single line comments on a newline above the subject of the comment. Put an empty line before the comment unless it’s on the first line of a block.
```javascript
// bad
const active = true; // is current tab
// good
// is current tab
const active = true;
// bad
function getType() {
console.log('fetching type...');
// set the default type to 'no type'
const type = this.type || 'no type';
return type;
}
// good
function getType() {
console.log('fetching type...');
// set the default type to 'no type'
const type = this.type || 'no type';
return type;
}
// also good
function getType() {
// set the default type to 'no type'
const type = this.type || 'no type';
return type;
}
```
- [18.3](#comments--spaces) Start all comments with a space to make it easier to read. eslint: [`spaced-comment`](http://eslint.org/docs/rules/spaced-comment)
```javascript
// bad
//is current tab
const active = true;
// good
// is current tab
const active = true;
// bad
/**
*make() returns a new element
*based on the passed-in tag name
*/
function make(tag) {
// ...
return element;
}
// good
/**
* make() returns a new element
* based on the passed-in tag name
*/
function make(tag) {
// ...
return element;
}
```
<a name="comments--actionitems"></a><a name="17.3"></a>
- [18.4](#comments--actionitems) Prefixing your comments with `FIXME` or `TODO` helps other developers quickly understand if you're pointing out a problem that needs to be revisited, or if you're suggesting a solution to the problem that needs to be implemented. These are different than regular comments because they are actionable. The actions are `FIXME: -- need to figure this out` or `TODO: -- need to implement`.
<a name="comments--fixme"></a><a name="17.4"></a>
- [18.5](#comments--fixme) Use `// FIXME:` to annotate problems.
```javascript
class Calculator extends Abacus {
constructor() {
super();
// FIXME: shouldn’t use a global here
total = 0;
}
}
```
<a name="comments--todo"></a><a name="17.5"></a>
- [18.6](#comments--todo) Use `// TODO:` to annotate solutions to problems.
```javascript
class Calculator extends Abacus {
constructor() {
super();
// TODO: total should be configurable by an options param
this.total = 0;
}
}
```
**[⬆ back to top](#table-of-contents)**
## Whitespace
<a name="whitespace--spaces"></a><a name="18.1"></a>
- [19.1](#whitespace--spaces) Use soft tabs (space character) set to 2 spaces. eslint: [`indent`](http://eslint.org/docs/rules/indent.html) jscs: [`validateIndentation`](http://jscs.info/rule/validateIndentation)
```javascript
// bad
function foo() {
∙∙∙∙let name;
}
// bad
function bar() {
∙let name;
}
// good
function baz() {
∙∙let name;
}
```
<a name="whitespace--before-blocks"></a><a name="18.2"></a>
- [19.2](#whitespace--before-blocks) Place 1 space before the leading brace. eslint: [`space-before-blocks`](http://eslint.org/docs/rules/space-before-blocks.html) jscs: [`requireSpaceBeforeBlockStatements`](http://jscs.info/rule/requireSpaceBeforeBlockStatements)
```javascript
// bad
function test(){
console.log('test');
}
// good
function test() {
console.log('test');
}
// bad
dog.set('attr',{
age: '1 year',
breed: 'Bernese Mountain Dog',
});
// good
dog.set('attr', {
age: '1 year',
breed: 'Bernese Mountain Dog',
});
```
<a name="whitespace--around-keywords"></a><a name="18.3"></a>
- [19.3](#whitespace--around-keywords) Place 1 space before the opening parenthesis in control statements (`if`, `while` etc.). Place no space between the argument list and the function name in function calls and declarations. eslint: [`keyword-spacing`](http://eslint.org/docs/rules/keyword-spacing.html) jscs: [`requireSpaceAfterKeywords`](http://jscs.info/rule/requireSpaceAfterKeywords)
```javascript
// bad
if(isJedi) {
fight ();
}
// good
if (isJedi) {
fight();
}
// bad
function fight () {
console.log ('Swooosh!');
}
// good
function fight() {
console.log('Swooosh!');
}
```
<a name="whitespace--infix-ops"></a><a name="18.4"></a>
- [19.4](#whitespace--infix-ops) Set off operators with spaces. eslint: [`space-infix-ops`](http://eslint.org/docs/rules/space-infix-ops.html) jscs: [`requireSpaceBeforeBinaryOperators`](http://jscs.info/rule/requireSpaceBeforeBinaryOperators), [`requireSpaceAfterBinaryOperators`](http://jscs.info/rule/requireSpaceAfterBinaryOperators)
```javascript
// bad
const x=y+5;
// good
const x = y + 5;
```
<a name="whitespace--newline-at-end"></a><a name="18.5"></a>
- [19.5](#whitespace--newline-at-end) End files with a single newline character. eslint: [`eol-last`](https://github.com/eslint/eslint/blob/master/docs/rules/eol-last.md)
```javascript
// bad
import { es6 } from './AirbnbStyleGuide';
// ...
export default es6;
```
```javascript
// bad
import { es6 } from './AirbnbStyleGuide';
// ...
export default es6;↵
↵
```
```javascript
// good
import { es6 } from './AirbnbStyleGuide';
// ...
export default es6;↵
```
<a name="whitespace--chains"></a><a name="18.6"></a>
- [19.6](#whitespace--chains) Use indentation when making long method chains (more than 2 method chains). Use a leading dot, which
emphasizes that the line is a method call, not a new statement. eslint: [`newline-per-chained-call`](http://eslint.org/docs/rules/newline-per-chained-call) [`no-whitespace-before-property`](http://eslint.org/docs/rules/no-whitespace-before-property)
```javascript
// bad
$('#items').find('.selected').highlight().end().find('.open').updateCount();
// bad
$('#items').
find('.selected').
highlight().
end().
find('.open').
updateCount();
// good
$('#items')
.find('.selected')
.highlight()
.end()
.find('.open')
.updateCount();
// bad
const leds = stage.selectAll('.led').data(data).enter().append('svg:svg').classed('led', true)
.attr('width', (radius + margin) * 2).append('svg:g')
.attr('transform', `translate(${radius + margin},${radius + margin})`)
.call(tron.led);
// good
const leds = stage.selectAll('.led')
.data(data)
.enter().append('svg:svg')
.classed('led', true)
.attr('width', (radius + margin) * 2)
.append('svg:g')
.attr('transform', `translate(${radius + margin},${radius + margin})`)
.call(tron.led);
// good
const leds = stage.selectAll('.led').data(data);
```
<a name="whitespace--after-blocks"></a><a name="18.7"></a>
- [19.7](#whitespace--after-blocks) Leave a blank line after blocks and before the next statement. jscs: [`requirePaddingNewLinesAfterBlocks`](http://jscs.info/rule/requirePaddingNewLinesAfterBlocks)
```javascript
// bad
if (foo) {
return bar;
}
return baz;
// good
if (foo) {
return bar;
}
return baz;
// bad
const obj = {
foo() {
},
bar() {
},
};
return obj;
// good
const obj = {
foo() {
},
bar() {
},
};
return obj;
// bad
const arr = [
function foo() {
},
function bar() {
},
];
return arr;
// good
const arr = [
function foo() {
},
function bar() {
},
];
return arr;
```
<a name="whitespace--padded-blocks"></a><a name="18.8"></a>
- [19.8](#whitespace--padded-blocks) Do not pad your blocks with blank lines. eslint: [`padded-blocks`](http://eslint.org/docs/rules/padded-blocks.html) jscs: [`disallowPaddingNewlinesInBlocks`](http://jscs.info/rule/disallowPaddingNewlinesInBlocks)
```javascript
// bad
function bar() {
console.log(foo);
}
// bad
if (baz) {
console.log(qux);
} else {
console.log(foo);
}
// bad
class Foo {
constructor(bar) {
this.bar = bar;
}
}
// good
function bar() {
console.log(foo);
}
// good
if (baz) {
console.log(qux);
} else {
console.log(foo);
}
```
<a name="whitespace--in-parens"></a><a name="18.9"></a>
- [19.9](#whitespace--in-parens) Do not add spaces inside parentheses. eslint: [`space-in-parens`](http://eslint.org/docs/rules/space-in-parens.html) jscs: [`disallowSpacesInsideParentheses`](http://jscs.info/rule/disallowSpacesInsideParentheses)
```javascript
// bad
function bar( foo ) {
return foo;
}
// good
function bar(foo) {
return foo;
}
// bad
if ( foo ) {
console.log(foo);
}
// good
if (foo) {
console.log(foo);
}
```
<a name="whitespace--in-brackets"></a><a name="18.10"></a>
- [19.10](#whitespace--in-brackets) Do not add spaces inside brackets. eslint: [`array-bracket-spacing`](http://eslint.org/docs/rules/array-bracket-spacing.html) jscs: [`disallowSpacesInsideArrayBrackets`](http://jscs.info/rule/disallowSpacesInsideArrayBrackets)
```javascript
// bad
const foo = [ 1, 2, 3 ];
console.log(foo[ 0 ]);
// good
const foo = [1, 2, 3];
console.log(foo[0]);
```
<a name="whitespace--in-braces"></a><a name="18.11"></a>
- [19.11](#whitespace--in-braces) Add spaces inside curly braces. eslint: [`object-curly-spacing`](http://eslint.org/docs/rules/object-curly-spacing.html) jscs: [`requireSpacesInsideObjectBrackets`](http://jscs.info/rule/requireSpacesInsideObjectBrackets)
```javascript
// bad
const foo = {clark: 'kent'};
// good
const foo = { clark: 'kent' };
```
<a name="whitespace--max-len"></a><a name="18.12"></a>
- [19.12](#whitespace--max-len) Avoid having lines of code that are longer than 100 characters (including whitespace). Note: per [above](#strings--line-length), long strings are exempt from this rule, and should not be broken up. eslint: [`max-len`](http://eslint.org/docs/rules/max-len.html) jscs: [`maximumLineLength`](http://jscs.info/rule/maximumLineLength)
> Why? This ensures readability and maintainability.
```javascript
// bad
const foo = jsonData && jsonData.foo && jsonData.foo.bar && jsonData.foo.bar.baz && jsonData.foo.bar.baz.quux && jsonData.foo.bar.baz.quux.xyzzy;
// bad
$.ajax({ method: 'POST', url: 'https://airbnb.com/', data: { name: 'John' } }).done(() => console.log('Congratulations!')).fail(() => console.log('You have failed this city.'));
// good
const foo = jsonData
&& jsonData.foo
&& jsonData.foo.bar
&& jsonData.foo.bar.baz
&& jsonData.foo.bar.baz.quux
&& jsonData.foo.bar.baz.quux.xyzzy;
// good
$.ajax({
method: 'POST',
url: 'https://airbnb.com/',
data: { name: 'John' },
})
.done(() => console.log('Congratulations!'))
.fail(() => console.log('You have failed this city.'));
```
**[⬆ back to top](#table-of-contents)**
## Commas
<a name="commas--leading-trailing"></a><a name="19.1"></a>
- [20.1](#commas--leading-trailing) Leading commas: **Nope.** eslint: [`comma-style`](http://eslint.org/docs/rules/comma-style.html) jscs: [`requireCommaBeforeLineBreak`](http://jscs.info/rule/requireCommaBeforeLineBreak)
```javascript
// bad
const story = [
once
, upon
, aTime
];
// good
const story = [
once,
upon,
aTime,
];
// bad
const hero = {
firstName: 'Ada'
, lastName: 'Lovelace'
, birthYear: 1815
, superPower: 'computers'
};
// good
const hero = {
firstName: 'Ada',
lastName: 'Lovelace',
birthYear: 1815,
superPower: 'computers',
};
```
<a name="commas--dangling"></a><a name="19.2"></a>
- [20.2](#commas--dangling) Additional trailing comma: **Yup.** eslint: [`comma-dangle`](http://eslint.org/docs/rules/comma-dangle.html) jscs: [`requireTrailingComma`](http://jscs.info/rule/requireTrailingComma)
> Why? This leads to cleaner git diffs. Also, transpilers like Babel will remove the additional trailing comma in the transpiled code which means you don’t have to worry about the [trailing comma problem](https://github.com/airbnb/javascript/blob/es5-deprecated/es5/README.md#commas) in legacy browsers.
```diff
// bad - git diff without trailing comma
const hero = {
firstName: 'Florence',
- lastName: 'Nightingale'
+ lastName: 'Nightingale',
+ inventorOf: ['coxcomb chart', 'modern nursing']
};
// good - git diff with trailing comma
const hero = {
firstName: 'Florence',
lastName: 'Nightingale',
+ inventorOf: ['coxcomb chart', 'modern nursing'],
};
```
```javascript
// bad
const hero = {
firstName: 'Dana',
lastName: 'Scully'
};
const heroes = [
'Batman',
'Superman'
];
// good
const hero = {
firstName: 'Dana',
lastName: 'Scully',
};
const heroes = [
'Batman',
'Superman',
];
// bad
function createHero(
firstName,
lastName,
inventorOf
) {
// does nothing
}
// good
function createHero(
firstName,
lastName,
inventorOf,
) {
// does nothing
}
// good (note that a comma must not appear after a "rest" element)
function createHero(
firstName,
lastName,
inventorOf,
...heroArgs
) {
// does nothing
}
// bad
createHero(
firstName,
lastName,
inventorOf
);
// good
createHero(
firstName,
lastName,
inventorOf,
);
// good (note that a comma must not appear after a "rest" element)
createHero(
firstName,
lastName,
inventorOf,
...heroArgs
);
```
**[⬆ back to top](#table-of-contents)**
## Semicolons
<a name="semicolons--required"></a><a name="20.1"></a>
- [21.1](#semicolons--required) **Yup.** eslint: [`semi`](http://eslint.org/docs/rules/semi.html) jscs: [`requireSemicolons`](http://jscs.info/rule/requireSemicolons)
```javascript
// bad
(function () {
const name = 'Skywalker'
return name
})()
// good
(function () {
const name = 'Skywalker';
return name;
}());
// good, but legacy (guards against the function becoming an argument when two files with IIFEs are concatenated)
;((() => {
const name = 'Skywalker';
return name;
})());
```
[Read more](https://stackoverflow.com/questions/7365172/semicolon-before-self-invoking-function/7365214#7365214).
**[⬆ back to top](#table-of-contents)**
## Type Casting & Coercion
<a name="coercion--explicit"></a><a name="21.1"></a>
- [22.1](#coercion--explicit) Perform type coercion at the beginning of the statement.
<a name="coercion--strings"></a><a name="21.2"></a>
- [22.2](#coercion--strings) Strings:
```javascript
// => this.reviewScore = 9;
// bad
const totalScore = this.reviewScore + ''; // invokes this.reviewScore.valueOf()
// bad
const totalScore = this.reviewScore.toString(); // isn’t guaranteed to return a string
// good
const totalScore = String(this.reviewScore);
```
<a name="coercion--numbers"></a><a name="21.3"></a>
- [22.3](#coercion--numbers) Numbers: Use `Number` for type casting and `parseInt` always with a radix for parsing strings. eslint: [`radix`](http://eslint.org/docs/rules/radix)
```javascript
const inputValue = '4';
// bad
const val = new Number(inputValue);
// bad
const val = +inputValue;
// bad
const val = inputValue >> 0;
// bad
const val = parseInt(inputValue);
// good
const val = Number(inputValue);
// good
const val = parseInt(inputValue, 10);
```
<a name="coercion--comment-deviations"></a><a name="21.4"></a>
- [22.4](#coercion--comment-deviations) If for whatever reason you are doing something wild and `parseInt` is your bottleneck and need to use Bitshift for [performance reasons](https://jsperf.com/coercion-vs-casting/3), leave a comment explaining why and what you're doing.
```javascript
// good
/**
* parseInt was the reason my code was slow.
* Bitshifting the String to coerce it to a
* Number made it a lot faster.
*/
const val = inputValue >> 0;
```
<a name="coercion--bitwise"></a><a name="21.5"></a>
- [22.5](#coercion--bitwise) **Note:** Be careful when using bitshift operations. Numbers are represented as [64-bit values](https://es5.github.io/#x4.3.19), but bitshift operations always return a 32-bit integer ([source](https://es5.github.io/#x11.7)). Bitshift can lead to unexpected behavior for integer values larger than 32 bits. [Discussion](https://github.com/airbnb/javascript/issues/109). Largest signed 32-bit Int is 2,147,483,647:
```javascript
2147483647 >> 0; // => 2147483647
2147483648 >> 0; // => -2147483648
2147483649 >> 0; // => -2147483647
```
<a name="coercion--booleans"></a><a name="21.6"></a>
- [22.6](#coercion--booleans) Booleans:
```javascript
const age = 0;
// bad
const hasAge = new Boolean(age);
// good
const hasAge = Boolean(age);
// best
const hasAge = !!age;
```
**[⬆ back to top](#table-of-contents)**
## Naming Conventions
<a name="naming--descriptive"></a><a name="22.1"></a>
- [23.1](#naming--descriptive) Avoid single letter names. Be descriptive with your naming. eslint: [`id-length`](http://eslint.org/docs/rules/id-length)
```javascript
// bad
function q() {
// ...
}
// good
function query() {
// ...
}
```
<a name="naming--camelCase"></a><a name="22.2"></a>
- [23.2](#naming--camelCase) Use camelCase when naming objects, functions, and instances. eslint: [`camelcase`](http://eslint.org/docs/rules/camelcase.html) jscs: [`requireCamelCaseOrUpperCaseIdentifiers`](http://jscs.info/rule/requireCamelCaseOrUpperCaseIdentifiers)
```javascript
// bad
const OBJEcttsssss = {};
const this_is_my_object = {};
function c() {}
// good
const thisIsMyObject = {};
function thisIsMyFunction() {}
```
<a name="naming--PascalCase"></a><a name="22.3"></a>
- [23.3](#naming--PascalCase) Use PascalCase only when naming constructors or classes. eslint: [`new-cap`](http://eslint.org/docs/rules/new-cap.html) jscs: [`requireCapitalizedConstructors`](http://jscs.info/rule/requireCapitalizedConstructors)
```javascript
// bad
function user(options) {
this.name = options.name;
}
const bad = new user({
name: 'nope',
});
// good
class User {
constructor(options) {
this.name = options.name;
}
}
const good = new User({
name: 'yup',
});
```
<a name="naming--leading-underscore"></a><a name="22.4"></a>
- [23.4](#naming--leading-underscore) Do not use trailing or leading underscores. eslint: [`no-underscore-dangle`](http://eslint.org/docs/rules/no-underscore-dangle.html) jscs: [`disallowDanglingUnderscores`](http://jscs.info/rule/disallowDanglingUnderscores)
> Why? JavaScript does not have the concept of privacy in terms of properties or methods. Although a leading underscore is a common convention to mean “private”, in fact, these properties are fully public, and as such, are part of your public API contract. This convention might lead developers to wrongly think that a change won’t count as breaking, or that tests aren’t needed. tl;dr: if you want something to be “private”, it must not be observably present.
```javascript
// bad
this.__firstName__ = 'Panda';
this.firstName_ = 'Panda';
this._firstName = 'Panda';
// good
this.firstName = 'Panda';
```
<a name="naming--self-this"></a><a name="22.5"></a>
- [23.5](#naming--self-this) Don’t save references to `this`. Use arrow functions or [Function#bind](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Function/bind). jscs: [`disallowNodeTypes`](http://jscs.info/rule/disallowNodeTypes)
```javascript
// bad
function foo() {
const self = this;
return function () {
console.log(self);
};
}
// bad
function foo() {
const that = this;
return function () {
console.log(that);
};
}
// good
function foo() {
return () => {
console.log(this);
};
}
```
<a name="naming--filename-matches-export"></a><a name="22.6"></a>
- [23.6](#naming--filename-matches-export) A base filename should exactly match the name of its default export.
```javascript
// file 1 contents
class CheckBox {
// ...
}
export default CheckBox;
// file 2 contents
export default function fortyTwo() { return 42; }
// file 3 contents
export default function insideDirectory() {}
// in some other file
// bad
import CheckBox from './checkBox'; // PascalCase import/export, camelCase filename
import FortyTwo from './FortyTwo'; // PascalCase import/filename, camelCase export
import InsideDirectory from './InsideDirectory'; // PascalCase import/filename, camelCase export
// bad
import CheckBox from './check_box'; // PascalCase import/export, snake_case filename
import forty_two from './forty_two'; // snake_case import/filename, camelCase export
import inside_directory from './inside_directory'; // snake_case import, camelCase export
import index from './inside_directory/index'; // requiring the index file explicitly
import insideDirectory from './insideDirectory/index'; // requiring the index file explicitly
// good
import CheckBox from './CheckBox'; // PascalCase export/import/filename
import fortyTwo from './fortyTwo'; // camelCase export/import/filename
import insideDirectory from './insideDirectory'; // camelCase export/import/directory name/implicit "index"
// ^ supports both insideDirectory.js and insideDirectory/index.js
```
<a name="naming--camelCase-default-export"></a><a name="22.7"></a>
- [23.7](#naming--camelCase-default-export) Use camelCase when you export-default a function. Your filename should be identical to your function’s name.
```javascript
function makeStyleGuide() {
// ...
}
export default makeStyleGuide;
```
<a name="naming--PascalCase-singleton"></a><a name="22.8"></a>
- [23.8](#naming--PascalCase-singleton) Use PascalCase when you export a constructor / class / singleton / function library / bare object.
```javascript
const AirbnbStyleGuide = {
es6: {
},
};
export default AirbnbStyleGuide;
```
<a name="naming--Acronyms-and-Initialisms"></a>
- [23.9](#naming--Acronyms-and-Initialisms) Acronyms and initialisms should always be all capitalized, or all lowercased.
> Why? Names are for readability, not to appease a computer algorithm.
```javascript
// bad
import SmsContainer from './containers/SmsContainer';
// bad
const HttpRequests = [
// ...
];
// good
import SMSContainer from './containers/SMSContainer';
// good
const HTTPRequests = [
// ...
];
// also good
const httpRequests = [
// ...
];
// best
import TextMessageContainer from './containers/TextMessageContainer';
// best
const requests = [
// ...
];
```
**[⬆ back to top](#table-of-contents)**
## Accessors
<a name="accessors--not-required"></a><a name="23.1"></a>
- [24.1](#accessors--not-required) Accessor functions for properties are not required.
<a name="accessors--no-getters-setters"></a><a name="23.2"></a>
- [24.2](#accessors--no-getters-setters) Do not use JavaScript getters/setters as they cause unexpected side effects and are harder to test, maintain, and reason about. Instead, if you do make accessor functions, use getVal() and setVal('hello').
```javascript
// bad
class Dragon {
get age() {
// ...
}
set age(value) {
// ...
}
}
// good
class Dragon {
getAge() {
// ...
}
setAge(value) {
// ...
}
}
```
<a name="accessors--boolean-prefix"></a><a name="23.3"></a>
- [24.3](#accessors--boolean-prefix) If the property/method is a `boolean`, use `isVal()` or `hasVal()`.
```javascript
// bad
if (!dragon.age()) {
return false;
}
// good
if (!dragon.hasAge()) {
return false;
}
```
<a name="accessors--consistent"></a><a name="23.4"></a>
- [24.4](#accessors--consistent) It’s okay to create get() and set() functions, but be consistent.
```javascript
class Jedi {
constructor(options = {}) {
const lightsaber = options.lightsaber || 'blue';
this.set('lightsaber', lightsaber);
}
set(key, val) {
this[key] = val;
}
get(key) {
return this[key];
}
}
```
**[⬆ back to top](#table-of-contents)**
## Events
<a name="events--hash"></a><a name="24.1"></a>
- [25.1](#events--hash) When attaching data payloads to events (whether DOM events or something more proprietary like Backbone events), pass a hash instead of a raw value. This allows a subsequent contributor to add more data to the event payload without finding and updating every handler for the event. For example, instead of:
```javascript
// bad
$(this).trigger('listingUpdated', listing.id);
// ...
$(this).on('listingUpdated', (e, listingId) => {
// do something with listingId
});
```
prefer:
```javascript
// good
$(this).trigger('listingUpdated', { listingId: listing.id });
// ...
$(this).on('listingUpdated', (e, data) => {
// do something with data.listingId
});
```
**[⬆ back to top](#table-of-contents)**
## jQuery
<a name="jquery--dollar-prefix"></a><a name="25.1"></a>
- [26.1](#jquery--dollar-prefix) Prefix jQuery object variables with a `$`. jscs: [`requireDollarBeforejQueryAssignment`](http://jscs.info/rule/requireDollarBeforejQueryAssignment)
```javascript
// bad
const sidebar = $('.sidebar');
// good
const $sidebar = $('.sidebar');
// good
const $sidebarBtn = $('.sidebar-btn');
```
<a name="jquery--cache"></a><a name="25.2"></a>
- [26.2](#jquery--cache) Cache jQuery lookups.
```javascript
// bad
function setSidebar() {
$('.sidebar').hide();
// ...
$('.sidebar').css({
'background-color': 'pink',
});
}
// good
function setSidebar() {
const $sidebar = $('.sidebar');
$sidebar.hide();
// ...
$sidebar.css({
'background-color': 'pink',
});
}
```
<a name="jquery--queries"></a><a name="25.3"></a>
- [26.3](#jquery--queries) For DOM queries use Cascading `$('.sidebar ul')` or parent > child `$('.sidebar > ul')`. [jsPerf](http://jsperf.com/jquery-find-vs-context-sel/16)
<a name="jquery--find"></a><a name="25.4"></a>
- [26.4](#jquery--find) Use `find` with scoped jQuery object queries.
```javascript
// bad
$('ul', '.sidebar').hide();
// bad
$('.sidebar').find('ul').hide();
// good
$('.sidebar ul').hide();
// good
$('.sidebar > ul').hide();
// good
$sidebar.find('ul').hide();
```
**[⬆ back to top](#table-of-contents)**
## ECMAScript 5 Compatibility
<a name="es5-compat--kangax"></a><a name="26.1"></a>
- [27.1](#es5-compat--kangax) Refer to [Kangax](https://twitter.com/kangax/)’s ES5 [compatibility table](https://kangax.github.io/es5-compat-table/).
**[⬆ back to top](#table-of-contents)**
<a name="ecmascript-6-styles"></a>
## ECMAScript 6+ (ES 2015+) Styles
<a name="es6-styles"></a><a name="27.1"></a>
- [28.1](#es6-styles) This is a collection of links to the various ES6+ features.
1. [Arrow Functions](#arrow-functions)
1. [Classes](#classes--constructors)
1. [Object Shorthand](#es6-object-shorthand)
1. [Object Concise](#es6-object-concise)
1. [Object Computed Properties](#es6-computed-properties)
1. [Template Strings](#es6-template-literals)
1. [Destructuring](#destructuring)
1. [Default Parameters](#es6-default-parameters)
1. [Rest](#es6-rest)
1. [Array Spreads](#es6-array-spreads)
1. [Let and Const](#references)
1. [Exponentiation Operator](#es2016-properties--exponentiation-operator)
1. [Iterators and Generators](#iterators-and-generators)
1. [Modules](#modules)
<a name="tc39-proposals"></a>
- [28.2](#tc39-proposals) Do not use [TC39 proposals](https://github.com/tc39/proposals) that have not reached stage 3.
> Why? [They are not finalized](https://tc39.github.io/process-document/), and they are subject to change or to be withdrawn entirely. We want to use JavaScript, and proposals are not JavaScript yet.
**[⬆ back to top](#table-of-contents)**
## Standard Library
The [Standard Library](https://developer.mozilla.org/en/docs/Web/JavaScript/Reference/Global_Objects)
contains utilities that are functionally broken but remain for legacy reasons.
<a name="standard-library--isnan"></a>
- [29.1](#standard-library--isnan) Use `Number.isNaN` instead of global `isNaN`.
eslint: [`no-restricted-globals`](http://eslint.org/docs/rules/no-restricted-globals)
> Why? The global `isNaN` coerces non-numbers to numbers, returning true for anything that coerces to NaN.
> If this behavior is desired, make it explicit.
```javascript
// bad
isNaN('1.2'); // false
isNaN('1.2.3'); // true
// good
Number.isNaN('1.2.3'); // false
Number.isNaN(Number('1.2.3')); // true
```
<a name="standard-library--isfinite"></a>
- [29.2](#standard-library--isfinite) Use `Number.isFinite` instead of global `isFinite`.
eslint: [`no-restricted-globals`](http://eslint.org/docs/rules/no-restricted-globals)
> Why? The global `isFinite` coerces non-numbers to numbers, returning true for anything that coerces to a finite number.
> If this behavior is desired, make it explicit.
```javascript
// bad
isFinite('2e3'); // true
// good
Number.isFinite('2e3'); // false
Number.isFinite(parseInt('2e3', 10)); // true
```
**[⬆ back to top](#table-of-contents)**
## Testing
<a name="testing--yup"></a><a name="28.1"></a>
- [30.1](#testing--yup) **Yup.**
```javascript
function foo() {
return true;
}
```
<a name="testing--for-real"></a><a name="28.2"></a>
- [30.2](#testing--for-real) **No, but seriously**:
- Whichever testing framework you use, you should be writing tests!
- Strive to write many small pure functions, and minimize where mutations occur.
- Be cautious about stubs and mocks - they can make your tests more brittle.
- We primarily use [`mocha`](https://www.npmjs.com/package/mocha) at Airbnb. [`tape`](https://www.npmjs.com/package/tape) is also used occasionally for small, separate modules.
- 100% test coverage is a good goal to strive for, even if it’s not always practical to reach it.
- Whenever you fix a bug, _write a regression test_. A bug fixed without a regression test is almost certainly going to break again in the future.
**[⬆ back to top](#table-of-contents)**
## Performance
- [On Layout & Web Performance](https://www.kellegous.com/j/2013/01/26/layout-performance/)
- [String vs Array Concat](https://jsperf.com/string-vs-array-concat/2)
- [Try/Catch Cost In a Loop](https://jsperf.com/try-catch-in-loop-cost)
- [Bang Function](https://jsperf.com/bang-function)
- [jQuery Find vs Context, Selector](https://jsperf.com/jquery-find-vs-context-sel/13)
- [innerHTML vs textContent for script text](https://jsperf.com/innerhtml-vs-textcontent-for-script-text)
- [Long String Concatenation](https://jsperf.com/ya-string-concat)
- [Are Javascript functions like `map()`, `reduce()`, and `filter()` optimized for traversing arrays?](https://www.quora.com/JavaScript-programming-language-Are-Javascript-functions-like-map-reduce-and-filter-already-optimized-for-traversing-array/answer/Quildreen-Motta)
- Loading...
**[⬆ back to top](#table-of-contents)**
## Resources
**Learning ES6**
- [Draft ECMA 2015 (ES6) Spec](https://people.mozilla.org/~jorendorff/es6-draft.html)
- [ExploringJS](http://exploringjs.com/)
- [ES6 Compatibility Table](https://kangax.github.io/compat-table/es6/)
- [Comprehensive Overview of ES6 Features](http://es6-features.org/)
**Read This**
- [Standard ECMA-262](http://www.ecma-international.org/ecma-262/6.0/index.html)
**Tools**
- Code Style Linters
- [ESlint](http://eslint.org/) - [Airbnb Style .eslintrc](https://github.com/airbnb/javascript/blob/master/linters/.eslintrc)
- [JSHint](http://jshint.com/) - [Airbnb Style .jshintrc](https://github.com/airbnb/javascript/blob/master/linters/.jshintrc)
- [JSCS](https://github.com/jscs-dev/node-jscs) - [Airbnb Style Preset](https://github.com/jscs-dev/node-jscs/blob/master/presets/airbnb.json) (Deprecated, please use [ESlint](https://github.com/airbnb/javascript/tree/master/packages/eslint-config-airbnb-base))
- Neutrino preset - [neutrino-preset-airbnb-base](https://neutrino.js.org/presets/neutrino-preset-airbnb-base/)
**Other Style Guides**
- [Google JavaScript Style Guide](https://google.github.io/styleguide/javascriptguide.xml)
- [jQuery Core Style Guidelines](https://contribute.jquery.org/style-guide/js/)
- [Principles of Writing Consistent, Idiomatic JavaScript](https://github.com/rwaldron/idiomatic.js)
**Other Styles**
- [Naming this in nested functions](https://gist.github.com/cjohansen/4135065) - Christian Johansen
- [Conditional Callbacks](https://github.com/airbnb/javascript/issues/52) - Ross Allen
- [Popular JavaScript Coding Conventions on GitHub](http://sideeffect.kr/popularconvention/#javascript) - JeongHoon Byun
- [Multiple var statements in JavaScript, not superfluous](http://benalman.com/news/2012/05/multiple-var-statements-javascript/) - Ben Alman
**Further Reading**
- [Understanding JavaScript Closures](https://javascriptweblog.wordpress.com/2010/10/25/understanding-javascript-closures/) - Angus Croll
- [Basic JavaScript for the impatient programmer](http://www.2ality.com/2013/06/basic-javascript.html) - Dr. Axel Rauschmayer
- [You Might Not Need jQuery](http://youmightnotneedjquery.com/) - Zack Bloom & Adam Schwartz
- [ES6 Features](https://github.com/lukehoban/es6features) - Luke Hoban
- [Frontend Guidelines](https://github.com/bendc/frontend-guidelines) - Benjamin De Cock
**Books**
- [JavaScript: The Good Parts](https://www.amazon.com/JavaScript-Good-Parts-Douglas-Crockford/dp/0596517742) - Douglas Crockford
- [JavaScript Patterns](https://www.amazon.com/JavaScript-Patterns-Stoyan-Stefanov/dp/0596806752) - Stoyan Stefanov
- [Pro JavaScript Design Patterns](https://www.amazon.com/JavaScript-Design-Patterns-Recipes-Problem-Solution/dp/159059908X) - Ross Harmes and Dustin Diaz
- [High Performance Web Sites: Essential Knowledge for Front-End Engineers](https://www.amazon.com/High-Performance-Web-Sites-Essential/dp/0596529309) - Steve Souders
- [Maintainable JavaScript](https://www.amazon.com/Maintainable-JavaScript-Nicholas-C-Zakas/dp/1449327680) - Nicholas C. Zakas
- [JavaScript Web Applications](https://www.amazon.com/JavaScript-Web-Applications-Alex-MacCaw/dp/144930351X) - Alex MacCaw
- [Pro JavaScript Techniques](https://www.amazon.com/Pro-JavaScript-Techniques-John-Resig/dp/1590597273) - John Resig
- [Smashing Node.js: JavaScript Everywhere](https://www.amazon.com/Smashing-Node-js-JavaScript-Everywhere-Magazine/dp/1119962595) - Guillermo Rauch
- [Secrets of the JavaScript Ninja](https://www.amazon.com/Secrets-JavaScript-Ninja-John-Resig/dp/193398869X) - John Resig and Bear Bibeault
- [Human JavaScript](http://humanjavascript.com/) - Henrik Joreteg
- [Superhero.js](http://superherojs.com/) - Kim Joar Bekkelund, Mads Mobæk, & Olav Bjorkoy
- [JSBooks](http://jsbooks.revolunet.com/) - Julien Bouquillon
- [Third Party JavaScript](https://www.manning.com/books/third-party-javascript) - Ben Vinegar and Anton Kovalyov
- [Effective JavaScript: 68 Specific Ways to Harness the Power of JavaScript](http://amzn.com/0321812182) - David Herman
- [Eloquent JavaScript](http://eloquentjavascript.net/) - Marijn Haverbeke
- [You Don’t Know JS: ES6 & Beyond](http://shop.oreilly.com/product/0636920033769.do) - Kyle Simpson
**Blogs**
- [JavaScript Weekly](http://javascriptweekly.com/)
- [JavaScript, JavaScript...](https://javascriptweblog.wordpress.com/)
- [Bocoup Weblog](https://bocoup.com/weblog)
- [Adequately Good](http://www.adequatelygood.com/)
- [NCZOnline](https://www.nczonline.net/)
- [Perfection Kills](http://perfectionkills.com/)
- [Ben Alman](http://benalman.com/)
- [Dmitry Baranovskiy](http://dmitry.baranovskiy.com/)
- [Dustin Diaz](http://dustindiaz.com/)
- [nettuts](http://code.tutsplus.com/?s=javascript)
**Podcasts**
- [JavaScript Air](https://javascriptair.com/)
- [JavaScript Jabber](https://devchat.tv/js-jabber/)
**[⬆ back to top](#table-of-contents)**
## In the Wild
This is a list of organizations that are using this style guide. Send us a pull request and we'll add you to the list.
- **123erfasst**: [123erfasst/javascript](https://github.com/123erfasst/javascript)
- **3blades**: [3Blades/javascript](https://github.com/3blades/javascript)
- **4Catalyzer**: [4Catalyzer/javascript](https://github.com/4Catalyzer/javascript)
- **Aan Zee**: [AanZee/javascript](https://github.com/AanZee/javascript)
- **Adult Swim**: [adult-swim/javascript](https://github.com/adult-swim/javascript)
- **Airbnb**: [airbnb/javascript](https://github.com/airbnb/javascript)
- **AltSchool**: [AltSchool/javascript](https://github.com/AltSchool/javascript)
- **Apartmint**: [apartmint/javascript](https://github.com/apartmint/javascript)
- **Ascribe**: [ascribe/javascript](https://github.com/ascribe/javascript)
- **Avalara**: [avalara/javascript](https://github.com/avalara/javascript)
- **Avant**: [avantcredit/javascript](https://github.com/avantcredit/javascript)
- **Axept**: [axept/javascript](https://github.com/axept/javascript)
- **BashPros**: [BashPros/javascript](https://github.com/BashPros/javascript)
- **Billabong**: [billabong/javascript](https://github.com/billabong/javascript)
- **Bisk**: [bisk/javascript](https://github.com/Bisk/javascript/)
- **Bonhomme**: [bonhommeparis/javascript](https://github.com/bonhommeparis/javascript)
- **Brainshark**: [brainshark/javascript](https://github.com/brainshark/javascript)
- **CaseNine**: [CaseNine/javascript](https://github.com/CaseNine/javascript)
- **Chartboost**: [ChartBoost/javascript-style-guide](https://github.com/ChartBoost/javascript-style-guide)
- **ComparaOnline**: [comparaonline/javascript](https://github.com/comparaonline/javascript-style-guide)
- **Compass Learning**: [compasslearning/javascript-style-guide](https://github.com/compasslearning/javascript-style-guide)
- **DailyMotion**: [dailymotion/javascript](https://github.com/dailymotion/javascript)
- **DoSomething**: [DoSomething/eslint-config](https://github.com/DoSomething/eslint-config)
- **Digitpaint** [digitpaint/javascript](https://github.com/digitpaint/javascript)
- **Ecosia**: [ecosia/javascript](https://github.com/ecosia/javascript)
- **Evernote**: [evernote/javascript-style-guide](https://github.com/evernote/javascript-style-guide)
- **Evolution Gaming**: [evolution-gaming/javascript](https://github.com/evolution-gaming/javascript)
- **EvozonJs**: [evozonjs/javascript](https://github.com/evozonjs/javascript)
- **ExactTarget**: [ExactTarget/javascript](https://github.com/ExactTarget/javascript)
- **Expensify** [Expensify/Style-Guide](https://github.com/Expensify/Style-Guide/blob/master/javascript.md)
- **Flexberry**: [Flexberry/javascript-style-guide](https://github.com/Flexberry/javascript-style-guide)
- **Gawker Media**: [gawkermedia/javascript](https://github.com/gawkermedia/javascript)
- **General Electric**: [GeneralElectric/javascript](https://github.com/GeneralElectric/javascript)
- **Generation Tux**: [GenerationTux/javascript](https://github.com/generationtux/styleguide)
- **GoodData**: [gooddata/gdc-js-style](https://github.com/gooddata/gdc-js-style)
- **Grooveshark**: [grooveshark/javascript](https://github.com/grooveshark/javascript)
- **Grupo-Abraxas**: [Grupo-Abraxas/javascript](https://github.com/Grupo-Abraxas/javascript)
- **Honey**: [honeyscience/javascript](https://github.com/honeyscience/javascript)
- **How About We**: [howaboutwe/javascript](https://github.com/howaboutwe/javascript-style-guide)
- **Huballin**: [huballin/javascript](https://github.com/huballin/javascript)
- **HubSpot**: [HubSpot/javascript](https://github.com/HubSpot/javascript)
- **Hyper**: [hyperoslo/javascript-playbook](https://github.com/hyperoslo/javascript-playbook/blob/master/style.md)
- **InterCity Group**: [intercitygroup/javascript-style-guide](https://github.com/intercitygroup/javascript-style-guide)
- **Jam3**: [Jam3/Javascript-Code-Conventions](https://github.com/Jam3/Javascript-Code-Conventions)
- **JeopardyBot**: [kesne/jeopardy-bot](https://github.com/kesne/jeopardy-bot/blob/master/STYLEGUIDE.md)
- **JSSolutions**: [JSSolutions/javascript](https://github.com/JSSolutions/javascript)
- **Kaplan Komputing**: [kaplankomputing/javascript](https://github.com/kaplankomputing/javascript)
- **KickorStick**: [kickorstick/javascript](https://github.com/kickorstick/javascript)
- **Kinetica Solutions**: [kinetica/javascript](https://github.com/kinetica/Javascript-style-guide)
- **LEINWAND**: [LEINWAND/javascript](https://github.com/LEINWAND/javascript)
- **Lonely Planet**: [lonelyplanet/javascript](https://github.com/lonelyplanet/javascript)
- **M2GEN**: [M2GEN/javascript](https://github.com/M2GEN/javascript)
- **Mighty Spring**: [mightyspring/javascript](https://github.com/mightyspring/javascript)
- **MinnPost**: [MinnPost/javascript](https://github.com/MinnPost/javascript)
- **MitocGroup**: [MitocGroup/javascript](https://github.com/MitocGroup/javascript)
- **ModCloth**: [modcloth/javascript](https://github.com/modcloth/javascript)
- **Money Advice Service**: [moneyadviceservice/javascript](https://github.com/moneyadviceservice/javascript)
- **Muber**: [muber/javascript](https://github.com/muber/javascript)
- **National Geographic**: [natgeo/javascript](https://github.com/natgeo/javascript)
- **Nimbl3**: [nimbl3/javascript](https://github.com/nimbl3/javascript)
- **Nulogy**: [nulogy/javascript](https://github.com/nulogy/javascript)
- **Orange Hill Development**: [orangehill/javascript](https://github.com/orangehill/javascript)
- **Orion Health**: [orionhealth/javascript](https://github.com/orionhealth/javascript)
- **OutBoxSoft**: [OutBoxSoft/javascript](https://github.com/OutBoxSoft/javascript)
- **Peerby**: [Peerby/javascript](https://github.com/Peerby/javascript)
- **Razorfish**: [razorfish/javascript-style-guide](https://github.com/razorfish/javascript-style-guide)
- **reddit**: [reddit/styleguide/javascript](https://github.com/reddit/styleguide/tree/master/javascript)
- **React**: [facebook.github.io/react/contributing/how-to-contribute.html#style-guide](https://facebook.github.io/react/contributing/how-to-contribute.html#style-guide)
- **REI**: [reidev/js-style-guide](https://github.com/rei/code-style-guides/blob/master/docs/javascript.md)
- **Ripple**: [ripple/javascript-style-guide](https://github.com/ripple/javascript-style-guide)
- **Sainsbury's Supermarkets**: [jsainsburyplc](https://github.com/jsainsburyplc)
- **SeekingAlpha**: [seekingalpha/javascript-style-guide](https://github.com/seekingalpha/javascript-style-guide)
- **Shutterfly**: [shutterfly/javascript](https://github.com/shutterfly/javascript)
- **Sourcetoad**: [sourcetoad/javascript](https://github.com/sourcetoad/javascript)
- **Springload**: [springload/javascript](https://github.com/springload/javascript)
- **StratoDem Analytics**: [stratodem/javascript](https://github.com/stratodem/javascript)
- **SteelKiwi Development**: [steelkiwi/javascript](https://github.com/steelkiwi/javascript)
- **StudentSphere**: [studentsphere/javascript](https://github.com/studentsphere/guide-javascript)
- **SwoopApp**: [swoopapp/javascript](https://github.com/swoopapp/javascript)
- **SysGarage**: [sysgarage/javascript-style-guide](https://github.com/sysgarage/javascript-style-guide)
- **Syzygy Warsaw**: [syzygypl/javascript](https://github.com/syzygypl/javascript)
- **Target**: [target/javascript](https://github.com/target/javascript)
- **TheLadders**: [TheLadders/javascript](https://github.com/TheLadders/javascript)
- **The Nerdery**: [thenerdery/javascript-standards](https://github.com/thenerdery/javascript-standards)
- **T4R Technology**: [T4R-Technology/javascript](https://github.com/T4R-Technology/javascript)
- **VoxFeed**: [VoxFeed/javascript-style-guide](https://github.com/VoxFeed/javascript-style-guide)
- **WeBox Studio**: [weboxstudio/javascript](https://github.com/weboxstudio/javascript)
- **Weggo**: [Weggo/javascript](https://github.com/Weggo/javascript)
- **Zillow**: [zillow/javascript](https://github.com/zillow/javascript)
- **ZocDoc**: [ZocDoc/javascript](https://github.com/ZocDoc/javascript)
**[⬆ back to top](#table-of-contents)**
## Translation
This style guide is also available in other languages:
-  **Brazilian Portuguese**: [armoucar/javascript-style-guide](https://github.com/armoucar/javascript-style-guide)
-  **Bulgarian**: [borislavvv/javascript](https://github.com/borislavvv/javascript)
-  **Catalan**: [fpmweb/javascript-style-guide](https://github.com/fpmweb/javascript-style-guide)
-  **Chinese (Simplified)**: [sivan/javascript-style-guide](https://github.com/sivan/javascript-style-guide)
-  **Chinese (Traditional)**: [jigsawye/javascript](https://github.com/jigsawye/javascript)
-  **French**: [nmussy/javascript-style-guide](https://github.com/nmussy/javascript-style-guide)
-  **German**: [timofurrer/javascript-style-guide](https://github.com/timofurrer/javascript-style-guide)
-  **Italian**: [sinkswim/javascript-style-guide](https://github.com/sinkswim/javascript-style-guide)
-  **Japanese**: [mitsuruog/javascript-style-guide](https://github.com/mitsuruog/javascript-style-guide)
-  **Korean**: [tipjs/javascript-style-guide](https://github.com/tipjs/javascript-style-guide)
-  **Russian**: [leonidlebedev/javascript-airbnb](https://github.com/leonidlebedev/javascript-airbnb)
-  **Spanish**: [paolocarrasco/javascript-style-guide](https://github.com/paolocarrasco/javascript-style-guide)
-  **Thai**: [lvarayut/javascript-style-guide](https://github.com/lvarayut/javascript-style-guide)
-  **Ukrainian**: [ivanzusko/javascript](https://github.com/ivanzusko/javascript)
-  **Vietnam**: [hngiang/javascript-style-guide](https://github.com/hngiang/javascript-style-guide)
## The JavaScript Style Guide Guide
- [Reference](https://github.com/airbnb/javascript/wiki/The-JavaScript-Style-Guide-Guide)
## Chat With Us About JavaScript
- Find us on [gitter](https://gitter.im/airbnb/javascript).
## Contributors
- [View Contributors](https://github.com/airbnb/javascript/graphs/contributors)
## License
(The MIT License)
Copyright (c) 2014-2017 Airbnb
Permission is hereby granted, free of charge, to any person obtaining
a copy of this software and associated documentation files (the
'Software'), to deal in the Software without restriction, including
without limitation the rights to use, copy, modify, merge, publish,
distribute, sublicense, and/or sell copies of the Software, and to
permit persons to whom the Software is furnished to do so, subject to
the following conditions:
The above copyright notice and this permission notice shall be
included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
**[⬆ back to top](#table-of-contents)**
## Amendments
We encourage you to fork this guide and change the rules to fit your team’s style guide. Below, you may list some amendments to the style guide. This allows you to periodically update your style guide without having to deal with merge conflicts.
# };
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/coding_style/kotlin/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
# Kotlin Style Guide
([Source](https://kotlinlang.org/docs/reference/))
## Coding Conventions
## Index
- [Naming Style](#naming-style)
- [Colon](#colon)
- [Lambdas](#lambdas)
- [Class Header Formatting](#class-header-formatting)
- [Unit](#unit)
- [Functions vs Properties](#functions-vs-properties)
### Naming Style
* Use camelCase (and avoid underscores) for names
* Types start with upper case
* Methods and properties start with lower case
* Use four space indentation
* Public functions should have documentation such that it appears in Kotlin Doc
### Colon
* Use a space before the colon where a colon separates type and supertype
* Do not use a space where colon separates instance and type
```
interface Foo<out T : Any> : Bar {
fun foo(a: Int): T
}
```
### Lambdas
* In lambda expressions, spaces should be used around the curly brace
* Spaces should also be placed around the arrow which separates the parameters from the body
* Whenever possible, a lambda should be passed outside of parentheses
```
list.filter { it > 10 }.map { element -> element * 2 }
```
* In short and nested lambdas, you should use the it convention instead of declaring the parameter explicitly
* In nested lambdas with parameters, parameters should be always declared explicitly.
### Class Header Formatting
* Classes with a few arguments can be written in a single line:
```
class Person(id: Int, name: String)
```
* Classes with longer headers should place each primary constructor argument is in a separate line with indentation. Additionally, the closing parenthesis should be on a new line.
* If using inheritance, the superclass constructor call or list of implemented interfaces should be located on the same line as the closing parenthesis:
```
class Person(
id: Int,
name: String,
surname: String
) : Human(id, name) {
// ...
}
```
* For multiple interfaces, the superclass constructor call should be located first and then each interface should be located on a separate line:
```
class Person(
id: Int,
name: String,
surname: String
) : Human(id, name),
KotlinMaker {
// ...
}
```
* Constructor parameters can use either the regular indent or the continuation indent (double the regular indent).
### Unit
If a function returns Unit, the return type should be omitted:
```
fun foo() { // ": Unit" is omitted here
}
```
### Functions vs Properties
In some cases, functions with no arguments might be interchangeable with read-only properties. Although the semantics are similar, there are some stylistic conventions on when to prefer one to another. Prefer a property over a function when the underlying algorithm:
* does not throw any Exceptions
* has a O(1) complexity
* is cheap to calculate (or caсhed on the first run)
* returns the same result over invocations
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/coding_style/lisp/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/coding_style/ml/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/coding_style/pascal/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/coding_style/perl/style.md | Perl Code Style Guidelines
```perl
Indentation:
Tabs should be used to indent all code blocks. Spaces should never be used to indent code blocks. Mixing tabs and spaces results in misaligned code blocks for other developers who prefer different indentation settings.
if ($total_hours >= 24) {
return 1;
}
else {
if ($for_imaging) {
return 1;
}
else {
return 0;
}
}
```
```perl
Comments:
There should always be at least 1 space between the # character and the beginning of the comment. This makes it a little easier to read multi-line comments:
# Comments are your friend, they help
```
```perl
Subroutine & Variable Names:
Single letter variable names should never be used unless for a loop iterator:
```
```perl
Avoid abbreviations:
my $ip_addr; ->No
my $ip_address; ->Yes
```
```perl
Use underscores to separate words:
sub get_computer_name {
```
```perl
All subroutine names should be entirely lowercase:
sub update_request_state {
```
```perl
All class variables defined at the top of a .pm file should be entirely uppercase:
our $SOURCE_CONFIGURATION_DIRECTORY = "$TOOLS/Windows";
```
```perl
POD Documentation
All modules and subroutines must contain a POD documentation block describing what it does. POD is "Plain Old Documentation".
Each subroutine should begin with a POD block with the following format:
#/////////////////////////////////////////////////////////////////////////////
=head2 my_subroutine_name
Parameters : none
Returns : boolean
Description : This is what the subroutine does. These lines must be 80
characters or less. Additional lines must be indented with
spaces, not tabs.
=cut
sub my_subroutine_name {
}
```
```perl
Curly Brackets, Parenthesis:
There should be a space between every control/loop keyword and the opening parenthesis:
if ($loop_count <= 10) {
```
```perl
If/Else Statements:
'else' & 'elsif' statements should be on a separate line after the previous closing curly brace:
if ($end_time < $now) {
...
}
else {
...
}
``` |
guides/coding_style/php/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
PHP (recursive acronym for PHP: Hypertext Preprocessor) is a widely-used open source general-purpose scripting language that is especially suited for web development and can be embedded into HTML.Originally created by Rasmus Lerdorf in 1994.
Instead of lots of commands to output HTML (as seen in C or Perl), PHP pages contain HTML with embedded code that does "something" (in this case, output "Hi, I'm a PHP script!"). The PHP code is enclosed in special start and end processing instructions <?php and ?> that allow you to jump into and out of "PHP mode."
What distinguishes PHP from something like client-side JavaScript is that the code is executed on the server, generating HTML which is then sent to the client. The client would receive the results of running that script, but would not know what the underlying code was. You can even configure your web server to process all your HTML files with PHP, and then there's really no way that users can tell what you have up your sleeve.
The best things in using PHP are that it is extremely simple for a newcomer, but offers many advanced features for a professional programmer. Don't be afraid reading the long list of PHP's features. You can jump in, in a short time, and start writing simple scripts in a few hours.
Coding Style Guide
==================
This guide extends and expands on [PSR-1], the basic coding standard.
The intent of this guide is to reduce cognitive friction when scanning code
from different authors. It does so by enumerating a shared set of rules and
expectations about how to format PHP code.
The style rules herein are derived from commonalities among the various member
projects. When various authors collaborate across multiple projects, it helps
to have one set of guidelines to be used among all those projects. Thus, the
benefit of this guide is not in the rules themselves, but in the sharing of
those rules.
The key words "MUST", "MUST NOT", "REQUIRED", "SHALL", "SHALL NOT", "SHOULD",
"SHOULD NOT", "RECOMMENDED", "MAY", and "OPTIONAL" in this document are to be
interpreted as described in [RFC 2119].
[RFC 2119]: http://www.ietf.org/rfc/rfc2119.txt
[PSR-0]: https://github.com/php-fig/fig-standards/blob/master/accepted/PSR-0.md
[PSR-1]: https://github.com/php-fig/fig-standards/blob/master/accepted/PSR-1-basic-coding-standard.md
1. Overview
-----------
- Code MUST follow a "coding style guide" PSR [[PSR-1]].
- Code MUST use 4 spaces for indenting, not tabs.
- There MUST NOT be a hard limit on line length; the soft limit MUST be 120
characters; lines SHOULD be 80 characters or less.
- There MUST be one blank line after the `namespace` declaration, and there
MUST be one blank line after the block of `use` declarations.
- Opening braces for classes MUST go on the next line, and closing braces MUST
go on the next line after the body.
- Opening braces for methods MUST go on the next line, and closing braces MUST
go on the next line after the body.
- Visibility MUST be declared on all properties and methods; `abstract` and
`final` MUST be declared before the visibility; `static` MUST be declared
after the visibility.
- Control structure keywords MUST have one space after them; method and
function calls MUST NOT.
- Opening braces for control structures MUST go on the same line, and closing
braces MUST go on the next line after the body.
- Opening parentheses for control structures MUST NOT have a space after them,
and closing parentheses for control structures MUST NOT have a space before.
### 1.1. Example
This example encompasses some of the rules below as a quick overview:
~~~php
<?php
namespace Vendor\Package;
use FooInterface;
use BarClass as Bar;
use OtherVendor\OtherPackage\BazClass;
class Foo extends Bar implements FooInterface
{
public function sampleMethod($a, $b = null)
{
if ($a === $b) {
bar();
} elseif ($a > $b) {
$foo->bar($arg1);
} else {
BazClass::bar($arg2, $arg3);
}
}
final public static function bar()
{
// method body
}
}
~~~
2. General
----------
### 2.1. Basic Coding Standard
Code MUST follow all rules outlined in [PSR-1].
### 2.2. Files
All PHP files MUST use the Unix LF (linefeed) line ending.
All PHP files MUST end with a single blank line.
The closing `?>` tag MUST be omitted from files containing only PHP.
### 2.3. Lines
There MUST NOT be a hard limit on line length.
The soft limit on line length MUST be 120 characters; automated style checkers
MUST warn but MUST NOT error at the soft limit.
Lines SHOULD NOT be longer than 80 characters; lines longer than that SHOULD
be split into multiple subsequent lines of no more than 80 characters each.
There MUST NOT be trailing whitespace at the end of non-blank lines.
Blank lines MAY be added to improve readability and to indicate related
blocks of code.
There MUST NOT be more than one statement per line.
### 2.4. Indenting
Code MUST use an indent of 4 spaces, and MUST NOT use tabs for indenting.
> N.b.: Using only spaces, and not mixing spaces with tabs, helps to avoid
> problems with diffs, patches, history, and annotations. The use of spaces
> also makes it easy to insert fine-grained sub-indentation for inter-line
> alignment.
### 2.5. Keywords and True/False/Null
PHP [keywords] MUST be in lower case.
The PHP constants `true`, `false`, and `null` MUST be in lower case.
[keywords]: http://php.net/manual/en/reserved.keywords.php
3. Namespace and Use Declarations
---------------------------------
When present, there MUST be one blank line after the `namespace` declaration.
When present, all `use` declarations MUST go after the `namespace`
declaration.
There MUST be one `use` keyword per declaration.
There MUST be one blank line after the `use` block.
For example:
~~~php
<?php
namespace Vendor\Package;
use FooClass;
use BarClass as Bar;
use OtherVendor\OtherPackage\BazClass;
// ... additional PHP code ...
~~~
4. Classes, Properties, and Methods
-----------------------------------
The term "class" refers to all classes, interfaces, and traits.
### 4.1. Extends and Implements
The `extends` and `implements` keywords MUST be declared on the same line as
the class name.
The opening brace for the class MUST go on its own line; the closing brace
for the class MUST go on the next line after the body.
~~~php
<?php
namespace Vendor\Package;
use FooClass;
use BarClass as Bar;
use OtherVendor\OtherPackage\BazClass;
class ClassName extends ParentClass implements \ArrayAccess, \Countable
{
// constants, properties, methods
}
~~~
Lists of `implements` MAY be split across multiple lines, where each
subsequent line is indented once. When doing so, the first item in the list
MUST be on the next line, and there MUST be only one interface per line.
~~~php
<?php
namespace Vendor\Package;
use FooClass;
use BarClass as Bar;
use OtherVendor\OtherPackage\BazClass;
class ClassName extends ParentClass implements
\ArrayAccess,
\Countable,
\Serializable
{
// constants, properties, methods
}
~~~
### 4.2. Properties
Visibility MUST be declared on all properties.
The `var` keyword MUST NOT be used to declare a property.
There MUST NOT be more than one property declared per statement.
Property names SHOULD NOT be prefixed with a single underscore to indicate
protected or private visibility.
A property declaration looks like the following.
~~~php
<?php
namespace Vendor\Package;
class ClassName
{
public $foo = null;
}
~~~
### 4.3. Methods
Visibility MUST be declared on all methods.
Method names SHOULD NOT be prefixed with a single underscore to indicate
protected or private visibility.
Method names MUST NOT be declared with a space after the method name. The
opening brace MUST go on its own line, and the closing brace MUST go on the
next line following the body. There MUST NOT be a space after the opening
parenthesis, and there MUST NOT be a space before the closing parenthesis.
A method declaration looks like the following. Note the placement of
parentheses, commas, spaces, and braces:
~~~php
<?php
namespace Vendor\Package;
class ClassName
{
public function fooBarBaz($arg1, &$arg2, $arg3 = [])
{
// method body
}
}
~~~
### 4.4. Method Arguments
In the argument list, there MUST NOT be a space before each comma, and there
MUST be one space after each comma.
Method arguments with default values MUST go at the end of the argument
list.
~~~php
<?php
namespace Vendor\Package;
class ClassName
{
public function foo($arg1, &$arg2, $arg3 = [])
{
// method body
}
}
~~~
Argument lists MAY be split across multiple lines, where each subsequent line
is indented once. When doing so, the first item in the list MUST be on the
next line, and there MUST be only one argument per line.
When the argument list is split across multiple lines, the closing parenthesis
and opening brace MUST be placed together on their own line with one space
between them.
~~~php
<?php
namespace Vendor\Package;
class ClassName
{
public function aVeryLongMethodName(
ClassTypeHint $arg1,
&$arg2,
array $arg3 = []
) {
// method body
}
}
~~~
### 4.5. `abstract`, `final`, and `static`
When present, the `abstract` and `final` declarations MUST precede the
visibility declaration.
When present, the `static` declaration MUST come after the visibility
declaration.
~~~php
<?php
namespace Vendor\Package;
abstract class ClassName
{
protected static $foo;
abstract protected function zim();
final public static function bar()
{
// method body
}
}
~~~
### 4.6. Method and Function Calls
When making a method or function call, there MUST NOT be a space between the
method or function name and the opening parenthesis, there MUST NOT be a space
after the opening parenthesis, and there MUST NOT be a space before the
closing parenthesis. In the argument list, there MUST NOT be a space before
each comma, and there MUST be one space after each comma.
~~~php
<?php
bar();
$foo->bar($arg1);
Foo::bar($arg2, $arg3);
~~~
Argument lists MAY be split across multiple lines, where each subsequent line
is indented once. When doing so, the first item in the list MUST be on the
next line, and there MUST be only one argument per line.
~~~php
<?php
$foo->bar(
$longArgument,
$longerArgument,
$muchLongerArgument
);
~~~
5. Control Structures
---------------------
The general style rules for control structures are as follows:
- There MUST be one space after the control structure keyword
- There MUST NOT be a space after the opening parenthesis
- There MUST NOT be a space before the closing parenthesis
- There MUST be one space between the closing parenthesis and the opening
brace
- The structure body MUST be indented once
- The closing brace MUST be on the next line after the body
The body of each structure MUST be enclosed by braces. This standardizes how
the structures look, and reduces the likelihood of introducing errors as new
lines get added to the body.
### 5.1. `if`, `elseif`, `else`
An `if` structure looks like the following. Note the placement of parentheses,
spaces, and braces; and that `else` and `elseif` are on the same line as the
closing brace from the earlier body.
~~~php
<?php
if ($expr1) {
// if body
} elseif ($expr2) {
// elseif body
} else {
// else body;
}
~~~
The keyword `elseif` SHOULD be used instead of `else if` so that all control
keywords look like single words.
### 5.2. `switch`, `case`
A `switch` structure looks like the following. Note the placement of
parentheses, spaces, and braces. The `case` statement MUST be indented once
from `switch`, and the `break` keyword (or other terminating keyword) MUST be
indented at the same level as the `case` body. There MUST be a comment such as
`// no break` when fall-through is intentional in a non-empty `case` body.
~~~php
<?php
switch ($expr) {
case 0:
echo 'First case, with a break';
break;
case 1:
echo 'Second case, which falls through';
// no break
case 2:
case 3:
case 4:
echo 'Third case, return instead of break';
return;
default:
echo 'Default case';
break;
}
~~~
### 5.3. `while`, `do while`
A `while` statement looks like the following. Note the placement of
parentheses, spaces, and braces.
~~~php
<?php
while ($expr) {
// structure body
}
~~~
Similarly, a `do while` statement looks like the following. Note the placement
of parentheses, spaces, and braces.
~~~php
<?php
do {
// structure body;
} while ($expr);
~~~
### 5.4. `for`
A `for` statement looks like the following. Note the placement of parentheses,
spaces, and braces.
~~~php
<?php
for ($i = 0; $i < 10; $i++) {
// for body
}
~~~
### 5.5. `foreach`
A `foreach` statement looks like the following. Note the placement of
parentheses, spaces, and braces.
~~~php
<?php
foreach ($iterable as $key => $value) {
// foreach body
}
~~~
### 5.6. `try`, `catch`
A `try catch` block looks like the following. Note the placement of
parentheses, spaces, and braces.
~~~php
<?php
try {
// try body
} catch (FirstExceptionType $e) {
// catch body
} catch (OtherExceptionType $e) {
// catch body
}
~~~
6. Closures
-----------
Closures MUST be declared with a space after the `function` keyword, and a
space before and after the `use` keyword.
The opening brace MUST go on the same line, and the closing brace MUST go on
the next line following the body.
There MUST NOT be a space after the opening parenthesis of the argument list
or variable list, and there MUST NOT be a space before the closing parenthesis
of the argument list or variable list.
In the argument list and variable list, there MUST NOT be a space before each
comma, and there MUST be one space after each comma.
Closure arguments with default values MUST go at the end of the argument
list.
A closure declaration looks like the following. Note the placement of
parentheses, commas, spaces, and braces:
~~~php
<?php
$closureWithArgs = function ($arg1, $arg2) {
// body
};
$closureWithArgsAndVars = function ($arg1, $arg2) use ($var1, $var2) {
// body
};
~~~
Argument lists and variable lists MAY be split across multiple lines, where
each subsequent line is indented once. When doing so, the first item in the
list MUST be on the next line, and there MUST be only one argument or variable
per line.
When the ending list (whether of arguments or variables) is split across
multiple lines, the closing parenthesis and opening brace MUST be placed
together on their own line with one space between them.
The following are examples of closures with and without argument lists and
variable lists split across multiple lines.
~~~php
<?php
$longArgs_noVars = function (
$longArgument,
$longerArgument,
$muchLongerArgument
) {
// body
};
$noArgs_longVars = function () use (
$longVar1,
$longerVar2,
$muchLongerVar3
) {
// body
};
$longArgs_longVars = function (
$longArgument,
$longerArgument,
$muchLongerArgument
) use (
$longVar1,
$longerVar2,
$muchLongerVar3
) {
// body
};
$longArgs_shortVars = function (
$longArgument,
$longerArgument,
$muchLongerArgument
) use ($var1) {
// body
};
$shortArgs_longVars = function ($arg) use (
$longVar1,
$longerVar2,
$muchLongerVar3
) {
// body
};
~~~
Note that the formatting rules also apply when the closure is used directly
in a function or method call as an argument.
~~~php
<?php
$foo->bar(
$arg1,
function ($arg2) use ($var1) {
// body
},
$arg3
);
~~~
7. Conclusion
--------------
There are many elements of style and practice intentionally omitted by this
guide. These include but are not limited to:
- Declaration of global variables and global constants
- Declaration of functions
- Operators and assignment
- Inter-line alignment
- Comments and documentation blocks
- Class name prefixes and suffixes
- Best practices
Future recommendations MAY revise and extend this guide to address those or
other elements of style and practice.
Appendix A. Survey
------------------
In writing this style guide, the group took a survey of member projects to
determine common practices. The survey is retained herein for posterity.
### A.1. Survey Data
url,http://www.horde.org/apps/horde/docs/CODING_STANDARDS,http://pear.php.net/manual/en/standards.php,http://solarphp.com/manual/appendix-standards.style,http://framework.zend.com/manual/en/coding-standard.html,http://symfony.com/doc/2.0/contributing/code/standards.html,http://www.ppi.io/docs/coding-standards.html,https://github.com/ezsystems/ezp-next/wiki/codingstandards,http://book.cakephp.org/2.0/en/contributing/cakephp-coding-conventions.html,https://github.com/UnionOfRAD/lithium/wiki/Spec%3A-Coding,http://drupal.org/coding-standards,http://code.google.com/p/sabredav/,http://area51.phpbb.com/docs/31x/coding-guidelines.html,https://docs.google.com/a/zikula.org/document/edit?authkey=CPCU0Us&hgd=1&id=1fcqb93Sn-hR9c0mkN6m_tyWnmEvoswKBtSc0tKkZmJA,http://www.chisimba.com,n/a,https://github.com/Respect/project-info/blob/master/coding-standards-sample.php,n/a,Object Calisthenics for PHP,http://doc.nette.org/en/coding-standard,http://flow3.typo3.org,https://github.com/propelorm/Propel2/wiki/Coding-Standards,http://developer.joomla.org/coding-standards.html
voting,yes,yes,yes,yes,yes,yes,yes,yes,yes,yes,yes,yes,yes,yes,yes,no,no,no,?,yes,no,yes
indent_type,4,4,4,4,4,tab,4,tab,tab,2,4,tab,4,4,4,4,4,4,tab,tab,4,tab
line_length_limit_soft,75,75,75,75,no,85,120,120,80,80,80,no,100,80,80,?,?,120,80,120,no,150
line_length_limit_hard,85,85,85,85,no,no,no,no,100,?,no,no,no,100,100,?,120,120,no,no,no,no
class_names,studly,studly,studly,studly,studly,studly,studly,studly,studly,studly,studly,lower_under,studly,lower,studly,studly,studly,studly,?,studly,studly,studly
class_brace_line,next,next,next,next,next,same,next,same,same,same,same,next,next,next,next,next,next,next,next,same,next,next
constant_names,upper,upper,upper,upper,upper,upper,upper,upper,upper,upper,upper,upper,upper,upper,upper,upper,upper,upper,upper,upper,upper,upper
true_false_null,lower,lower,lower,lower,lower,lower,lower,lower,lower,upper,lower,lower,lower,upper,lower,lower,lower,lower,lower,upper,lower,lower
method_names,camel,camel,camel,camel,camel,camel,camel,camel,camel,camel,camel,lower_under,camel,camel,camel,camel,camel,camel,camel,camel,camel,camel
method_brace_line,next,next,next,next,next,same,next,same,same,same,same,next,next,same,next,next,next,next,next,same,next,next
control_brace_line,same,same,same,same,same,same,next,same,same,same,same,next,same,same,next,same,same,same,same,same,same,next
control_space_after,yes,yes,yes,yes,yes,no,yes,yes,yes,yes,no,yes,yes,yes,yes,yes,yes,yes,yes,yes,yes,yes
always_use_control_braces,yes,yes,yes,yes,yes,yes,no,yes,yes,yes,no,yes,yes,yes,yes,no,yes,yes,yes,yes,yes,yes
else_elseif_line,same,same,same,same,same,same,next,same,same,next,same,next,same,next,next,same,same,same,same,same,same,next
case_break_indent_from_switch,0/1,0/1,0/1,1/2,1/2,1/2,1/2,1/1,1/1,1/2,1/2,1/1,1/2,1/2,1/2,1/2,1/2,1/2,0/1,1/1,1/2,1/2
function_space_after,no,no,no,no,no,no,no,no,no,no,no,no,no,no,no,no,no,no,no,no,no,no
closing_php_tag_required,no,no,no,no,no,no,no,no,yes,no,no,no,no,yes,no,no,no,no,no,yes,no,no
line_endings,LF,LF,LF,LF,LF,LF,LF,LF,?,LF,?,LF,LF,LF,LF,?,,LF,?,LF,LF,LF
static_or_visibility_first,static,?,static,either,either,either,visibility,visibility,visibility,either,static,either,?,visibility,?,?,either,either,visibility,visibility,static,?
control_space_parens,no,no,no,no,no,no,yes,no,no,no,no,no,no,yes,?,no,no,no,no,no,no,no
blank_line_after_php,no,no,no,no,yes,no,no,no,no,yes,yes,no,no,yes,?,yes,yes,no,yes,no,yes,no
class_method_control_brace,next/next/same,next/next/same,next/next/same,next/next/same,next/next/same,same/same/same,next/next/next,same/same/same,same/same/same,same/same/same,same/same/same,next/next/next,next/next/same,next/same/same,next/next/next,next/next/same,next/next/same,next/next/same,next/next/same,same/same/same,next/next/same,next/next/next
### A.2. Survey Legend
`indent_type`:
The type of indenting. `tab` = "Use a tab", `2` or `4` = "number of spaces"
`line_length_limit_soft`:
The "soft" line length limit, in characters. `?` = not discernible or no response, `no` means no limit.
`line_length_limit_hard`:
The "hard" line length limit, in characters. `?` = not discernible or no response, `no` means no limit.
`class_names`:
How classes are named. `lower` = lowercase only, `lower_under` = lowercase with underscore separators, `studly` = StudlyCase.
`class_brace_line`:
Does the opening brace for a class go on the `same` line as the class keyword, or on the `next` line after it?
`constant_names`:
How are class constants named? `upper` = Uppercase with underscore separators.
`true_false_null`:
Are the `true`, `false`, and `null` keywords spelled as all `lower` case, or all `upper` case?
`method_names`:
How are methods named? `camel` = `camelCase`, `lower_under` = lowercase with underscore separators.
`method_brace_line`:
Does the opening brace for a method go on the `same` line as the method name, or on the `next` line?
`control_brace_line`:
Does the opening brace for a control structure go on the `same` line, or on the `next` line?
`control_space_after`:
Is there a space after the control structure keyword?
`always_use_control_braces`:
Do control structures always use braces?
`else_elseif_line`:
When using `else` or `elseif`, does it go on the `same` line as the previous closing brace, or does it go on the `next` line?
`case_break_indent_from_switch`:
How many times are `case` and `break` indented from an opening `switch` statement?
`function_space_after`:
Do function calls have a space after the function name and before the opening parenthesis?
`closing_php_tag_required`:
In files containing only PHP, is the closing `?>` tag required?
`line_endings`:
What type of line ending is used?
`static_or_visibility_first`:
When declaring a method, does `static` come first, or does the visibility come first?
`control_space_parens`:
In a control structure expression, is there a space after the opening parenthesis and a space before the closing parenthesis? `yes` = `if ( $expr )`, `no` = `if ($expr)`.
`blank_line_after_php`:
Is there a blank line after the opening PHP tag?
`class_method_control_brace`:
A summary of what line the opening braces go on for classes, methods, and control structures.
### A.3. Survey Results
indent_type:
tab: 7
2: 1
4: 14
line_length_limit_soft:
?: 2
no: 3
75: 4
80: 6
85: 1
100: 1
120: 4
150: 1
line_length_limit_hard:
?: 2
no: 11
85: 4
100: 3
120: 2
class_names:
?: 1
lower: 1
lower_under: 1
studly: 19
class_brace_line:
next: 16
same: 6
constant_names:
upper: 22
true_false_null:
lower: 19
upper: 3
method_names:
camel: 21
lower_under: 1
method_brace_line:
next: 15
same: 7
control_brace_line:
next: 4
same: 18
control_space_after:
no: 2
yes: 20
always_use_control_braces:
no: 3
yes: 19
else_elseif_line:
next: 6
same: 16
case_break_indent_from_switch:
0/1: 4
1/1: 4
1/2: 14
function_space_after:
no: 22
closing_php_tag_required:
no: 19
yes: 3
line_endings:
?: 5
LF: 17
static_or_visibility_first:
?: 5
either: 7
static: 4
visibility: 6
control_space_parens:
?: 1
no: 19
yes: 2
blank_line_after_php:
?: 1
no: 13
yes: 8
class_method_control_brace:
next/next/next: 4
next/next/same: 11
next/same/same: 1
same/same/same: 6
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/coding_style/python/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
There is no longer a style guide for Python. Instead, we now use [black](https://github.com/ambv/black), the uncompromising
code formatter.
When submitting a PR, please run black on your Python code.
|
guides/coding_style/ruby/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
# Ruby Style Guide
([Source](https://github.com/github/rubocop-github/blob/master/STYLEGUIDE.md))
This style guide is licensed under the [MIT License](https://github.com/github/rubocop-github/blob/master/LICENSE).
* Use soft-tabs with a two space indent.
* Keep each line of code to a readable length. Unless you have a reason to, keep lines to fewer than 100 characters.
* Never leave trailing whitespace.
* End each file with a [newline](https://github.com/bbatsov/ruby-style-guide#newline-eof).
* Use spaces around operators, after commas, colons and semicolons, around `{`
and before `}`.
``` ruby
sum = 1 + 2
a, b = 1, 2
1 > 2 ? true : false; puts "Hi"
[1, 2, 3].each { |e| puts e }
```
* No spaces after `(`, `[` or before `]`, `)`.
``` ruby
some(arg).other
[1, 2, 3].length
```
* No spaces after `!`.
``` ruby
!array.include?(element)
```
* Indent `when` as deep as `case`.
``` ruby
case
when song.name == "Misty"
puts "Not again!"
when song.duration > 120
puts "Too long!"
when Time.now.hour > 21
puts "It's too late"
else
song.play
end
kind = case year
when 1850..1889 then "Blues"
when 1890..1909 then "Ragtime"
when 1910..1929 then "New Orleans Jazz"
when 1930..1939 then "Swing"
when 1940..1950 then "Bebop"
else "Jazz"
end
```
* Use empty lines between `def`s and to break up a method into logical
paragraphs.
``` ruby
def some_method
data = initialize(options)
data.manipulate!
data.result
end
def some_method
result
end
```
## Classes
* Avoid the usage of class (`@@`) variables due to their unusual behavior
in inheritance.
``` ruby
class Parent
@@class_var = "parent"
def self.print_class_var
puts @@class_var
end
end
class Child < Parent
@@class_var = "child"
end
Parent.print_class_var # => will print "child"
```
As you can see all the classes in a class hierarchy actually share one
class variable. Class instance variables should usually be preferred
over class variables.
* Use `def self.method` to define singleton methods. This makes the methods
more resistant to refactoring changes.
``` ruby
class TestClass
# bad
def TestClass.some_method
# body omitted
end
# good
def self.some_other_method
# body omitted
end
```
* Avoid `class << self` except when necessary, e.g. single accessors and aliased
attributes.
``` ruby
class TestClass
# bad
class << self
def first_method
# body omitted
end
def second_method_etc
# body omitted
end
end
# good
class << self
attr_accessor :per_page
alias_method :nwo, :find_by_name_with_owner
end
def self.first_method
# body omitted
end
def self.second_method_etc
# body omitted
end
end
```
* Indent the `public`, `protected`, and `private` methods as much the
method definitions they apply to. Leave one blank line above them.
``` ruby
class SomeClass
def public_method
# ...
end
private
def private_method
# ...
end
end
```
* Avoid explicit use of `self` as the recipient of internal class or instance
messages unless to specify a method shadowed by a variable.
``` ruby
class SomeClass
attr_accessor :message
def greeting(name)
message = "Hi #{name}" # local variable in Ruby, not attribute writer
self.message = message
end
end
```
## Collections
* Prefer `%w` to the literal array syntax when you need an array of
strings.
``` ruby
# bad
STATES = ["draft", "open", "closed"]
# good
STATES = %w(draft open closed)
```
* Use `Set` instead of `Array` when dealing with unique elements. `Set`
implements a collection of unordered values with no duplicates. This
is a hybrid of `Array`'s intuitive inter-operation facilities and
`Hash`'s fast lookup.
* Use symbols instead of strings as hash keys.
``` ruby
# bad
hash = { "one" => 1, "two" => 2, "three" => 3 }
# good
hash = { one: 1, two: 2, three: 3 }
```
## Documentation
Use [TomDoc](http://tomdoc.org) to the best of your ability. It's pretty sweet:
``` ruby
# Public: Duplicate some text an arbitrary number of times.
#
# text - The String to be duplicated.
# count - The Integer number of times to duplicate the text.
#
# Examples
#
# multiplex("Tom", 4)
# # => "TomTomTomTom"
#
# Returns the duplicated String.
def multiplex(text, count)
text * count
end
```
## Exceptions
* Don't use exceptions for flow of control.
``` ruby
# bad
begin
n / d
rescue ZeroDivisionError
puts "Cannot divide by 0!"
end
# good
if d.zero?
puts "Cannot divide by 0!"
else
n / d
end
```
* Rescue specific exceptions, not `StandardError` or its superclasses.
``` ruby
# bad
begin
# an exception occurs here
rescue
# exception handling
end
# still bad
begin
# an exception occurs here
rescue Exception
# exception handling
end
```
## Hashes
Use the Ruby 1.9 syntax for hash literals when all the keys are symbols:
``` ruby
# good
user = {
login: "defunkt",
name: "Chris Wanstrath"
}
# bad
user = {
:login => "defunkt",
:name => "Chris Wanstrath"
}
```
Use the 1.9 syntax when calling a method with Hash options arguments or named arguments:
``` ruby
# good
user = User.create(login: "jane")
link_to("Account", controller: "users", action: "show", id: user)
# bad
user = User.create(:login => "jane")
link_to("Account", :controller => "users", :action => "show", :id => user)
```
If you have a hash with mixed key types, use the legacy hashrocket style to avoid mixing styles within the same hash:
``` ruby
# good
hsh = {
:user_id => 55,
"followers-count" => 1000
}
# bad
hsh = {
user_id: 55,
"followers-count" => 1000
}
```
## Keyword Arguments
[Keyword arguments](http://magazine.rubyist.net/?Ruby200SpecialEn-kwarg) are recommended but not required when a method's arguments may otherwise be opaque or non-obvious when called. Additionally, prefer them over the old "Hash as pseudo-named args" style from pre-2.0 ruby.
So instead of this:
``` ruby
def remove_member(user, skip_membership_check=false)
# ...
end
# Elsewhere: what does true mean here?
remove_member(user, true)
```
Do this, which is much clearer.
``` ruby
def remove_member(user, skip_membership_check: false)
# ...
end
# Elsewhere, now with more clarity:
remove_member user, skip_membership_check: true
```
## Naming
* Use `snake_case` for methods and variables.
* Use `CamelCase` for classes and modules. (Keep acronyms like HTTP,
RFC, XML uppercase.)
* Use `SCREAMING_SNAKE_CASE` for other constants.
* The names of predicate methods (methods that return a boolean value)
should end in a question mark. (i.e. `Array#empty?`).
* The names of potentially "dangerous" methods (i.e. methods that modify `self` or the
arguments, `exit!`, etc.) should end with an exclamation mark. Bang methods
should only exist if a non-bang method exists. ([More on this](http://dablog.rubypal.com/2007/8/15/bang-methods-or-danger-will-rubyist)).
## Percent Literals
* Use `%w` freely.
``` ruby
STATES = %w(draft open closed)
```
* Use `%()` for single-line strings which require both interpolation
and embedded double-quotes. For multi-line strings, prefer heredocs.
``` ruby
# bad (no interpolation needed)
%(<div class="text">Some text</div>)
# should be "<div class=\"text\">Some text</div>"
# bad (no double-quotes)
%(This is #{quality} style)
# should be "This is #{quality} style"
# bad (multiple lines)
%(<div>\n<span class="big">#{exclamation}</span>\n</div>)
# should be a heredoc.
# good (requires interpolation, has quotes, single line)
%(<tr><td class="name">#{name}</td>)
```
* Use `%r` only for regular expressions matching *more than* one '/' character.
``` ruby
# bad
%r(\s+)
# still bad
%r(^/(.*)$)
# should be /^\/(.*)$/
# good
%r(^/blog/2011/(.*)$)
```
## Regular Expressions
* Avoid using $1-9 as it can be hard to track what they contain. Named groups
can be used instead.
``` ruby
# bad
/(regexp)/ =~ string
...
process $1
# good
/(?<meaningful_var>regexp)/ =~ string
...
process meaningful_var
```
* Be careful with `^` and `$` as they match start/end of line, not string endings.
If you want to match the whole string use: `\A` and `\z`.
``` ruby
string = "some injection\nusername"
string[/^username$/] # matches
string[/\Ausername\z/] # don't match
```
* Use `x` modifier for complex regexps. This makes them more readable and you
can add some useful comments. Just be careful as spaces are ignored.
``` ruby
regexp = %r{
start # some text
\s # white space char
(group) # first group
(?:alt1|alt2) # some alternation
end
}x
```
## Requires
Always `require` dependencies used directly in a script at the start of the same file.
Resources that will get autoloaded on first use—such as Rails models, controllers, or
helpers—don't need to be required.
``` ruby
require "set"
require "time"
%w(foo bar).to_set
Time.parse("2015-10-21")
```
This not only loads the necessary dependencies if they haven't already, but acts as
documentation about the libraries that the current file uses.
## Strings
* Prefer string interpolation instead of string concatenation:
``` ruby
# bad
email_with_name = user.name + " <" + user.email + ">"
# good
email_with_name = "#{user.name} <#{user.email}>"
```
* Use double-quoted strings. Interpolation and escaped characters
will always work without a delimiter change, and `'` is a lot more
common than `"` in string literals.
``` ruby
# bad
name = 'Bozhidar'
# good
name = "Bozhidar"
```
* Avoid using `String#+` when you need to construct large data chunks.
Instead, use `String#<<`. Concatenation mutates the string instance in-place
and is always faster than `String#+`, which creates a bunch of new string objects.
``` ruby
# good and also fast
html = ""
html << "<h1>Page title</h1>"
paragraphs.each do |paragraph|
html << "<p>#{paragraph}</p>"
end
```
## Syntax
* Use `def` with parentheses when there are arguments. Omit the
parentheses when the method doesn't accept any arguments.
``` ruby
def some_method
# body omitted
end
def some_method_with_arguments(arg1, arg2)
# body omitted
end
```
* Never use `for`, unless you know exactly why. Most of the time iterators
should be used instead. `for` is implemented in terms of `each` (so
you're adding a level of indirection), but with a twist - `for`
doesn't introduce a new scope (unlike `each`) and variables defined
in its block will be visible outside it.
``` ruby
arr = [1, 2, 3]
# bad
for elem in arr do
puts elem
end
# good
arr.each { |elem| puts elem }
```
* Never use `then` for multi-line `if/unless`.
``` ruby
# bad
if some_condition then
# body omitted
end
# good
if some_condition
# body omitted
end
```
* Avoid the ternary operator (`?:`) except in cases where all expressions are extremely
trivial. However, do use the ternary operator(`?:`) over `if/then/else/end` constructs
for single line conditionals.
``` ruby
# bad
result = if some_condition then something else something_else end
# good
result = some_condition ? something : something_else
```
* Use one expression per branch in a ternary operator. This
also means that ternary operators must not be nested. Prefer
`if/else` constructs in these cases.
``` ruby
# bad
some_condition ? (nested_condition ? nested_something : nested_something_else) : something_else
# good
if some_condition
nested_condition ? nested_something : nested_something_else
else
something_else
end
```
* The `and` and `or` keywords are banned. It's just not worth it. Always use `&&` and `||` instead.
* Avoid multi-line `?:` (the ternary operator), use `if/unless` instead.
* Favor modifier `if/unless` usage when you have a single-line
body.
``` ruby
# bad
if some_condition
do_something
end
# good
do_something if some_condition
```
* Never use `unless` with `else`. Rewrite these with the positive case first.
``` ruby
# bad
unless success?
puts "failure"
else
puts "success"
end
# good
if success?
puts "success"
else
puts "failure"
end
```
* Don't use parentheses around the condition of an `if/unless/while`.
``` ruby
# bad
if (x > 10)
# body omitted
end
# good
if x > 10
# body omitted
end
```
* Prefer `{...}` over `do...end` for single-line blocks. Avoid using
`{...}` for multi-line blocks (multiline chaining is always
ugly). Always use `do...end` for "control flow" and "method
definitions" (e.g. in Rakefiles and certain DSLs). Avoid `do...end`
when chaining.
``` ruby
names = ["Bozhidar", "Steve", "Sarah"]
# good
names.each { |name| puts name }
# bad
names.each do |name|
puts name
end
# good
names.select { |name| name.start_with?("S") }.map { |name| name.upcase }
# bad
names.select do |name|
name.start_with?("S")
end.map { |name| name.upcase }
```
Some will argue that multiline chaining would look OK with the use of {...}, but they should
ask themselves - is this code really readable and can't the block's contents be extracted into
nifty methods?
* Avoid `return` where not required.
``` ruby
# bad
def some_method(some_arr)
return some_arr.size
end
# good
def some_method(some_arr)
some_arr.size
end
```
* Use spaces around the `=` operator when assigning default values to method parameters:
``` ruby
# bad
def some_method(arg1=:default, arg2=nil, arg3=[])
# do something...
end
# good
def some_method(arg1 = :default, arg2 = nil, arg3 = [])
# do something...
end
```
While several Ruby books suggest the first style, the second is much more prominent
in practice (and arguably a bit more readable).
* Using the return value of `=` (an assignment) is ok.
``` ruby
# bad
if (v = array.grep(/foo/)) ...
# good
if v = array.grep(/foo/) ...
# also good - has correct precedence.
if (v = next_value) == "hello" ...
```
* Use `||=` freely to initialize variables.
``` ruby
# set name to Bozhidar, only if it's nil or false
name ||= "Bozhidar"
```
* Don't use `||=` to initialize boolean variables. (Consider what
would happen if the current value happened to be `false`.)
``` ruby
# bad - would set enabled to true even if it was false
enabled ||= true
# good
enabled = true if enabled.nil?
```
* Avoid using Perl-style special variables (like `$0-9`, `$`,
etc. ). They are quite cryptic and their use in anything but
one-liner scripts is discouraged. Prefer long form versions such as
`$PROGRAM_NAME`.
* Never put a space between a method name and the opening parenthesis.
``` ruby
# bad
f (3 + 2) + 1
# good
f(3 + 2) + 1
```
* If the first argument to a method begins with an open parenthesis,
always use parentheses in the method invocation. For example, write
`f((3 + 2) + 1)`.
* Use `_` for unused block parameters.
``` ruby
# bad
result = hash.map { |k, v| v + 1 }
# good
result = hash.map { |_, v| v + 1 }
```
* Don't use the `===` (threequals) operator to check types. `===` is mostly an
implementation detail to support Ruby features like `case`, and it's not commutative.
For example, `String === "hi"` is true and `"hi" === String` is false.
Instead, use `is_a?` or `kind_of?` if you must.
Refactoring is even better. It's worth looking hard at any code that explicitly checks types.
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/coding_style/rust/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
# Rust Style Guide
([Source](https://github.com/rust-lang/rust-guidelines))
### Opening braces always go on the same line.
``` rust
fn foo() {
...
}
fn frobnicate(a: Bar, b: Bar,
c: Bar, d: Bar)
-> Bar {
...
}
trait Bar {
fn baz(&self);
}
impl Bar for Baz {
fn baz(&self) {
...
}
}
frob(|x| {
x.transpose()
})
```
### `match` arms get braces, except for single-line expressions.
``` rust
match foo {
bar => baz,
quux => {
do_something();
do_something_else()
}
}
```
### `return` statements get semicolons.
``` rust
fn foo() {
do_something();
if condition() {
return;
}
do_something_else();
}
```
### Trailing commas
A trailing comma should be included whenever the
closing delimiter appears on a separate line:
```rust
Foo { bar: 0, baz: 1 }
Foo {
bar: 0,
baz: 1,
}
match a_thing {
None => 0,
Some(x) => 1,
}
```
### Avoid block comments.
Use line comments:
``` rust
// Wait for the main task to return, and set the process error code
// appropriately.
```
Instead of:
``` rust
/*
* Wait for the main task to return, and set the process error code
* appropriately.
*/
```
## Doc comments
Doc comments are prefixed by three slashes (`///`) and indicate
documentation that you would like to be included in Rustdoc's output.
They support
[Markdown syntax](https://en.wikipedia.org/wiki/Markdown)
and are the main way of documenting your public APIs.
The supported markdown syntax includes all of the extensions listed in the
[GitHub Flavored Markdown]
(https://help.github.com/articles/github-flavored-markdown) documentation,
plus superscripts.
### Summary line
The first line in any doc comment should be a single-line short sentence
providing a summary of the code. This line is used as a short summary
description throughout Rustdoc's output, so it's a good idea to keep it
short.
### Sentence structure
All doc comments, including the summary line, should begin with a
capital letter and end with a period, question mark, or exclamation
point. Prefer full sentences to fragments.
The summary line should be written in
[third person singular present indicative form]
(http://en.wikipedia.org/wiki/English_verbs#Third_person_singular_present).
Basically, this means write "Returns" instead of "Return".
For example:
``` rust
/// Sets up a default runtime configuration, given compiler-supplied arguments.
///
/// This function will block until the entire pool of M:N schedulers has
/// exited. This function also requires a local task to be available.
///
/// # Arguments
///
/// * `argc` & `argv` - The argument vector. On Unix this information is used
/// by `os::args`.
/// * `main` - The initial procedure to run inside of the M:N scheduling pool.
/// Once this procedure exits, the scheduling pool will begin to shut
/// down. The entire pool (and this function) will only return once
/// all child tasks have finished executing.
///
/// # Return value
///
/// The return value is used as the process return code. 0 on success, 101 on
/// error.
```
### Avoid inner doc comments.
Use inner doc comments _only_ to document crates and file-level modules:
``` rust
//! The core library.
//!
//! The core library is a something something...
```
% Imports
The imports of a crate/module should consist of the following
sections, in order, with a blank space between each:
* `extern crate` directives
* external `use` imports
* local `use` imports
* `pub use` imports
For example:
```rust
// Crates.
extern crate getopts;
extern crate mylib;
// Standard library imports.
use getopts::{optopt, getopts};
use std::os;
// Import from a library that we wrote.
use mylib::webserver;
// Will be reexported when we import this module.
pub use self::types::Webdata;
```
### Avoid `use *`, except in tests.
Glob imports have several downsides:
* They make it harder to tell where names are bound.
* They are forwards-incompatible, since new upstream exports can clash
with existing names.
When writing a [`test` submodule](../testing/README.md), importing `super::*` is appropriate
as a convenience.
### Prefer fully importing types/traits while module-qualifying functions.
For example:
```rust
use option::Option;
use mem;
let i: int = mem::transmute(Option(0));
```
% Whitespace [FIXME: needs RFC]
* Lines must not exceed 99 characters.
* Use 4 spaces for indentation, _not_ tabs.
* No trailing whitespace at the end of lines or files.
### Spaces
* Use spaces around binary operators, including the equals sign in attributes:
``` rust
#[deprecated = "Use `bar` instead."]
fn foo(a: uint, b: uint) -> uint {
a + b
}
```
* Use a space after colons and commas:
``` rust
fn foo(a: Bar);
MyStruct { foo: 3, bar: 4 }
foo(bar, baz);
```
* Use a space after the opening and before the closing brace for
single line blocks or `struct` expressions:
``` rust
spawn(proc() { do_something(); })
Point { x: 0.1, y: 0.3 }
```
### Line wrapping
* For multiline function signatures, each new line should align with the
first parameter. Multiple parameters per line are permitted:
``` rust
fn frobnicate(a: Bar, b: Bar,
c: Bar, d: Bar)
-> Bar {
...
}
fn foo<T: This,
U: That>(
a: Bar,
b: Bar)
-> Baz {
...
}
```
* Multiline function invocations generally follow the same rule as for
signatures. However, if the final argument begins a new block, the
contents of the block may begin on a new line, indented one level:
``` rust
fn foo_bar(a: Bar, b: Bar,
c: |Bar|) -> Bar {
...
}
// Same line is fine:
foo_bar(x, y, |z| { z.transpose(y) });
// Indented body on new line is also fine:
foo_bar(x, y, |z| {
z.quux();
z.rotate(x)
})
```
### Alignment
Idiomatic code should not use extra whitespace in the middle of a line
to provide alignment.
``` rust
// Good
struct Foo {
short: f64,
really_long: f64,
}
// Bad
struct Bar {
short: f64,
really_long: f64,
}
// Good
let a = 0;
let radius = 7;
// Bad
let b = 0;
let diameter = 7;
```
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/coding_style/swift/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
# The Official raywenderlich.com Swift Style Guide.
### Updated for Swift 3
([Source](https://github.com/raywenderlich/swift-style-guide/blob/master/README.markdown))
This Style Guide is licensed under the [MIT License](https://github.com/raywenderlich/swift-style-guide/blob/master/LICENSE.txt).
This style guide is different from others you may see, because the focus is centered on readability for print and the web. We created this style guide to keep the code in our books, tutorials, and starter kits nice and consistent — even though we have many different authors working on the books.
Our overarching goals are clarity, consistency and brevity, in that order.
## Table of Contents
* [Correctness](#correctness)
* [Naming](#naming)
* [Prose](#prose)
* [Delegates](#delegates)
* [Use Type Inferred Context](#use-type-inferred-context)
* [Generics](#generics)
* [Class Prefixes](#class-prefixes)
* [Language](#language)
* [Code Organization](#code-organization)
* [Protocol Conformance](#protocol-conformance)
* [Unused Code](#unused-code)
* [Minimal Imports](#minimal-imports)
* [Spacing](#spacing)
* [Comments](#comments)
* [Classes and Structures](#classes-and-structures)
* [Use of Self](#use-of-self)
* [Protocol Conformance](#protocol-conformance)
* [Computed Properties](#computed-properties)
* [Final](#final)
* [Function Declarations](#function-declarations)
* [Closure Expressions](#closure-expressions)
* [Types](#types)
* [Constants](#constants)
* [Static Methods and Variable Type Properties](#static-methods-and-variable-type-properties)
* [Optionals](#optionals)
* [Lazy Initialization](#lazy-initialization)
* [Type Inference](#type-inference)
* [Syntactic Sugar](#syntactic-sugar)
* [Functions vs Methods](#functions-vs-methods)
* [Memory Management](#memory-management)
* [Extending Lifetime](#extending-lifetime)
* [Access Control](#access-control)
* [Control Flow](#control-flow)
* [Golden Path](#golden-path)
* [Failing Guards](#failing-guards)
* [Semicolons](#semicolons)
* [Parentheses](#parentheses)
* [Organization and Bundle Identifier](#organization-and-bundle-identifier)
* [Copyright Statement](#copyright-statement)
* [Smiley Face](#smiley-face)
* [References](#references)
## Correctness
Strive to make your code compile without warnings. This rule informs many style decisions such as using `#selector` types instead of string literals.
## Naming
Descriptive and consistent naming makes software easier to read and understand. Use the Swift naming conventions described in the [API Design Guidelines](https://swift.org/documentation/api-design-guidelines/). Some key takeaways include:
- striving for clarity at the call site
- prioritizing clarity over brevity
- using camel case (not snake case)
- using uppercase for types (and protocols), lowercase for everything else
- including all needed words while omitting needless words
- using names based on roles, not types
- sometimes compensating for weak type information
- striving for fluent usage
- beginning factory methods with `make`
- naming methods for their side effects
- verb methods follow the -ed, -ing rule for the non-mutating version
- noun methods follow the formX rule for the mutating version
- boolean types should read like assertions
- protocols that describe _what something is_ should read as nouns
- protocols that describe _a capability_ should end in _-able_ or _-ible_
- using terms that don't surprise experts or confuse beginners
- generally avoiding abbreviations
- using precedent for names
- preferring methods and properties to free functions
- casing acronyms and initialisms uniformly up or down
- giving the same base name to methods that share the same meaning
- avoiding overloads on return type
- choosing good parameter names that serve as documentation
- labeling closure and tuple parameters
- taking advantage of default parameters
### Prose
When referring to methods in prose, being unambiguous is critical. To refer to a method name, use the simplest form possible.
1. Write the method name with no parameters. **Example:** Next, you need to call the method `addTarget`.
2. Write the method name with argument labels. **Example:** Next, you need to call the method `addTarget(_:action:)`.
3. Write the full method name with argument labels and types. **Example:** Next, you need to call the method `addTarget(_: Any?, action: Selector?)`.
For the above example using `UIGestureRecognizer`, 1 is unambiguous and preferred.
**Pro Tip:** You can use Xcode's jump bar to lookup methods with argument labels.

### Class Prefixes
Swift types are automatically namespaced by the module that contains them and you should not add a class prefix such as RW. If two names from different modules collide you can disambiguate by prefixing the type name with the module name. However, only specify the module name when there is possibility for confusion which should be rare.
```swift
import SomeModule
let myClass = MyModule.UsefulClass()
```
### Delegates
When creating custom delegate methods, an unnamed first parameter should be the delegate source. (UIKit contains numerous examples of this.)
**Preferred:**
```swift
func namePickerView(_ namePickerView: NamePickerView, didSelectName name: String)
func namePickerViewShouldReload(_ namePickerView: NamePickerView) -> Bool
```
**Not Preferred:**
```swift
func didSelectName(namePicker: NamePickerViewController, name: String)
func namePickerShouldReload() -> Bool
```
### Use Type Inferred Context
Use compiler inferred context to write shorter, clear code. (Also see [Type Inference](#type-inference).)
**Preferred:**
```swift
let selector = #selector(viewDidLoad)
view.backgroundColor = .red
let toView = context.view(forKey: .to)
let view = UIView(frame: .zero)
```
**Not Preferred:**
```swift
let selector = #selector(ViewController.viewDidLoad)
view.backgroundColor = UIColor.red
let toView = context.view(forKey: UITransitionContextViewKey.to)
let view = UIView(frame: CGRect.zero)
```
### Generics
Generic type parameters should be descriptive, upper camel case names. When a type name doesn't have a meaningful relationship or role, use a traditional single uppercase letter such as `T`, `U`, or `V`.
**Preferred:**
```swift
struct Stack<Element> { ... }
func write<Target: OutputStream>(to target: inout Target)
func swap<T>(_ a: inout T, _ b: inout T)
```
**Not Preferred:**
```swift
struct Stack<T> { ... }
func write<target: OutputStream>(to target: inout target)
func swap<Thing>(_ a: inout Thing, _ b: inout Thing)
```
### Language
Use US English spelling to match Apple's API.
**Preferred:**
```swift
let color = "red"
```
**Not Preferred:**
```swift
let colour = "red"
```
## Code Organization
Use extensions to organize your code into logical blocks of functionality. Each extension should be set off with a `// MARK: -` comment to keep things well-organized.
### Protocol Conformance
In particular, when adding protocol conformance to a model, prefer adding a separate extension for the protocol methods. This keeps the related methods grouped together with the protocol and can simplify instructions to add a protocol to a class with its associated methods.
**Preferred:**
```swift
class MyViewController: UIViewController {
// class stuff here
}
// MARK: - UITableViewDataSource
extension MyViewController: UITableViewDataSource {
// table view data source methods
}
// MARK: - UIScrollViewDelegate
extension MyViewController: UIScrollViewDelegate {
// scroll view delegate methods
}
```
**Not Preferred:**
```swift
class MyViewController: UIViewController, UITableViewDataSource, UIScrollViewDelegate {
// all methods
}
```
Since the compiler does not allow you to re-declare protocol conformance in a derived class, it is not always required to replicate the extension groups of the base class. This is especially true if the derived class is a terminal class and a small number of methods are being overridden. When to preserve the extension groups is left to the discretion of the author.
For UIKit view controllers, consider grouping lifecycle, custom accessors, and IBAction in separate class extensions.
### Unused Code
Unused (dead) code, including Xcode template code and placeholder comments should be removed. An exception is when your tutorial or book instructs the user to use the commented code.
Aspirational methods not directly associated with the tutorial whose implementation simply calls the superclass should also be removed. This includes any empty/unused UIApplicationDelegate methods.
**Preferred:**
```swift
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return Database.contacts.count
}
```
**Not Preferred:**
```swift
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
override func numberOfSections(in tableView: UITableView) -> Int {
// #warning Incomplete implementation, return the number of sections
return 1
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
// #warning Incomplete implementation, return the number of rows
return Database.contacts.count
}
```
### Minimal Imports
Keep imports minimal. For example, don't import `UIKit` when importing `Foundation` will suffice.
## Spacing
* Indent using 2 spaces rather than tabs to conserve space and help prevent line wrapping. Be sure to set this preference in Xcode and in the Project settings as shown below:

* Method braces and other braces (`if`/`else`/`switch`/`while` etc.) always open on the same line as the statement but close on a new line.
* Tip: You can re-indent by selecting some code (or ⌘A to select all) and then Control-I (or Editor\Structure\Re-Indent in the menu). Some of the Xcode template code will have 4-space tabs hard coded, so this is a good way to fix that.
**Preferred:**
```swift
if user.isHappy {
// Do something
} else {
// Do something else
}
```
**Not Preferred:**
```swift
if user.isHappy
{
// Do something
}
else {
// Do something else
}
```
* There should be exactly one blank line between methods to aid in visual clarity and organization. Whitespace within methods should separate functionality, but having too many sections in a method often means you should refactor into several methods.
* Colons always have no space on the left and one space on the right. Exceptions are the ternary operator `? :`, empty dictionary `[:]` and `#selector` syntax for unnamed parameters `(_:)`.
**Preferred:**
```swift
class TestDatabase: Database {
var data: [String: CGFloat] = ["A": 1.2, "B": 3.2]
}
```
**Not Preferred:**
```swift
class TestDatabase : Database {
var data :[String:CGFloat] = ["A" : 1.2, "B":3.2]
}
```
* Long lines should be wrapped at around 70 characters. A hard limit is intentionally not specified.
* Avoid trailing whitespaces at the ends of lines.
* Add a single newline character at the end of each file.
## Comments
When they are needed, use comments to explain **why** a particular piece of code does something. Comments must be kept up-to-date or deleted.
Avoid block comments inline with code, as the code should be as self-documenting as possible. *Exception: This does not apply to those comments used to generate documentation.*
## Classes and Structures
### Which one to use?
Remember, structs have [value semantics](https://developer.apple.com/library/mac/documentation/Swift/Conceptual/Swift_Programming_Language/ClassesAndStructures.html#//apple_ref/doc/uid/TP40014097-CH13-XID_144). Use structs for things that do not have an identity. An array that contains [a, b, c] is really the same as another array that contains [a, b, c] and they are completely interchangeable. It doesn't matter whether you use the first array or the second, because they represent the exact same thing. That's why arrays are structs.
Classes have [reference semantics](https://developer.apple.com/library/mac/documentation/Swift/Conceptual/Swift_Programming_Language/ClassesAndStructures.html#//apple_ref/doc/uid/TP40014097-CH13-XID_145). Use classes for things that do have an identity or a specific life cycle. You would model a person as a class because two person objects are two different things. Just because two people have the same name and birthdate, doesn't mean they are the same person. But the person's birthdate would be a struct because a date of 3 March 1950 is the same as any other date object for 3 March 1950. The date itself doesn't have an identity.
Sometimes, things should be structs but need to conform to `AnyObject` or are historically modeled as classes already (`NSDate`, `NSSet`). Try to follow these guidelines as closely as possible.
### Example definition
Here's an example of a well-styled class definition:
```swift
class Circle: Shape {
var x: Int, y: Int
var radius: Double
var diameter: Double {
get {
return radius * 2
}
set {
radius = newValue / 2
}
}
init(x: Int, y: Int, radius: Double) {
self.x = x
self.y = y
self.radius = radius
}
convenience init(x: Int, y: Int, diameter: Double) {
self.init(x: x, y: y, radius: diameter / 2)
}
override func area() -> Double {
return Double.pi * radius * radius
}
}
extension Circle: CustomStringConvertible {
var description: String {
return "center = \(centerString) area = \(area())"
}
private var centerString: String {
return "(\(x),\(y))"
}
}
```
The example above demonstrates the following style guidelines:
+ Specify types for properties, variables, constants, argument declarations and other statements with a space after the colon but not before, e.g. `x: Int`, and `Circle: Shape`.
+ Define multiple variables and structures on a single line if they share a common purpose / context.
+ Indent getter and setter definitions and property observers.
+ Don't add modifiers such as `internal` when they're already the default. Similarly, don't repeat the access modifier when overriding a method.
+ Organize extra functionality (e.g. printing) in extensions.
+ Hide non-shared, implementation details such as `centerString` inside the extension using `private` access control.
### Use of Self
For conciseness, avoid using `self` since Swift does not require it to access an object's properties or invoke its methods.
Use self only when required by the compiler (in `@escaping` closures, or in initializers to disambiguate properties from arguments). In other words, if it compiles without `self` then omit it.
### Computed Properties
For conciseness, if a computed property is read-only, omit the get clause. The get clause is required only when a set clause is provided.
**Preferred:**
```swift
var diameter: Double {
return radius * 2
}
```
**Not Preferred:**
```swift
var diameter: Double {
get {
return radius * 2
}
}
```
### Final
Marking classes or members as `final` in tutorials can distract from the main topic and is not required. Nevertheless, use of `final` can sometimes clarify your intent and is worth the cost. In the below example, `Box` has a particular purpose and customization in a derived class is not intended. Marking it `final` makes that clear.
```swift
// Turn any generic type into a reference type using this Box class.
final class Box<T> {
let value: T
init(_ value: T) {
self.value = value
}
}
```
## Function Declarations
Keep short function declarations on one line including the opening brace:
```swift
func reticulateSplines(spline: [Double]) -> Bool {
// reticulate code goes here
}
```
For functions with long signatures, add line breaks at appropriate points and add an extra indent on subsequent lines:
```swift
func reticulateSplines(spline: [Double], adjustmentFactor: Double,
translateConstant: Int, comment: String) -> Bool {
// reticulate code goes here
}
```
## Closure Expressions
Use trailing closure syntax only if there's a single closure expression parameter at the end of the argument list. Give the closure parameters descriptive names.
**Preferred:**
```swift
UIView.animate(withDuration: 1.0) {
self.myView.alpha = 0
}
UIView.animate(withDuration: 1.0, animations: {
self.myView.alpha = 0
}, completion: { finished in
self.myView.removeFromSuperview()
})
```
**Not Preferred:**
```swift
UIView.animate(withDuration: 1.0, animations: {
self.myView.alpha = 0
})
UIView.animate(withDuration: 1.0, animations: {
self.myView.alpha = 0
}) { f in
self.myView.removeFromSuperview()
}
```
For single-expression closures where the context is clear, use implicit returns:
```swift
attendeeList.sort { a, b in
a > b
}
```
Chained methods using trailing closures should be clear and easy to read in context. Decisions on spacing, line breaks, and when to use named versus anonymous arguments is left to the discretion of the author. Examples:
```swift
let value = numbers.map { $0 * 2 }.filter { $0 % 3 == 0 }.index(of: 90)
let value = numbers
.map {$0 * 2}
.filter {$0 > 50}
.map {$0 + 10}
```
## Types
Always use Swift's native types when available. Swift offers bridging to Objective-C so you can still use the full set of methods as needed.
**Preferred:**
```swift
let width = 120.0 // Double
let widthString = (width as NSNumber).stringValue // String
```
**Not Preferred:**
```swift
let width: NSNumber = 120.0 // NSNumber
let widthString: NSString = width.stringValue // NSString
```
In Sprite Kit code, use `CGFloat` if it makes the code more succinct by avoiding too many conversions.
### Constants
Constants are defined using the `let` keyword, and variables with the `var` keyword. Always use `let` instead of `var` if the value of the variable will not change.
**Tip:** A good technique is to define everything using `let` and only change it to `var` if the compiler complains!
You can define constants on a type rather than on an instance of that type using type properties. To declare a type property as a constant simply use `static let`. Type properties declared in this way are generally preferred over global constants because they are easier to distinguish from instance properties. Example:
**Preferred:**
```swift
enum Math {
static let e = 2.718281828459045235360287
static let root2 = 1.41421356237309504880168872
}
let hypotenuse = side * Math.root2
```
**Note:** The advantage of using a case-less enumeration is that it can't accidentally be instantiated and works as a pure namespace.
**Not Preferred:**
```swift
let e = 2.718281828459045235360287 // pollutes global namespace
let root2 = 1.41421356237309504880168872
let hypotenuse = side * root2 // what is root2?
```
### Static Methods and Variable Type Properties
Static methods and type properties work similarly to global functions and global variables and should be used sparingly. They are useful when functionality is scoped to a particular type or when Objective-C interoperability is required.
### Optionals
Declare variables and function return types as optional with `?` where a nil value is acceptable.
Use implicitly unwrapped types declared with `!` only for instance variables that you know will be initialized later before use, such as subviews that will be set up in `viewDidLoad`.
When accessing an optional value, use optional chaining if the value is only accessed once or if there are many optionals in the chain:
```swift
self.textContainer?.textLabel?.setNeedsDisplay()
```
Use optional binding when it's more convenient to unwrap once and perform multiple operations:
```swift
if let textContainer = self.textContainer {
// do many things with textContainer
}
```
When naming optional variables and properties, avoid naming them like `optionalString` or `maybeView` since their optional-ness is already in the type declaration.
For optional binding, shadow the original name when appropriate rather than using names like `unwrappedView` or `actualLabel`.
**Preferred:**
```swift
var subview: UIView?
var volume: Double?
// later on...
if let subview = subview, let volume = volume {
// do something with unwrapped subview and volume
}
```
**Not Preferred:**
```swift
var optionalSubview: UIView?
var volume: Double?
if let unwrappedSubview = optionalSubview {
if let realVolume = volume {
// do something with unwrappedSubview and realVolume
}
}
```
### Lazy Initialization
Consider using lazy initialization for finer grain control over object lifetime. This is especially true for `UIViewController` that loads views lazily. You can either use a closure that is immediately called `{ }()` or call a private factory method. Example:
```swift
lazy var locationManager: CLLocationManager = self.makeLocationManager()
private func makeLocationManager() -> CLLocationManager {
let manager = CLLocationManager()
manager.desiredAccuracy = kCLLocationAccuracyBest
manager.delegate = self
manager.requestAlwaysAuthorization()
return manager
}
```
**Notes:**
- `[unowned self]` is not required here. A retain cycle is not created.
- Location manager has a side-effect for popping up UI to ask the user for permission so fine grain control makes sense here.
### Type Inference
Prefer compact code and let the compiler infer the type for constants or variables of single instances. Type inference is also appropriate for small (non-empty) arrays and dictionaries. When required, specify the specific type such as `CGFloat` or `Int16`.
**Preferred:**
```swift
let message = "Click the button"
let currentBounds = computeViewBounds()
var names = ["Mic", "Sam", "Christine"]
let maximumWidth: CGFloat = 106.5
```
**Not Preferred:**
```swift
let message: String = "Click the button"
let currentBounds: CGRect = computeViewBounds()
let names = [String]()
```
#### Type Annotation for Empty Arrays and Dictionaries
For empty arrays and dictionaries, use type annotation. (For an array or dictionary assigned to a large, multi-line literal, use type annotation.)
**Preferred:**
```swift
var names: [String] = []
var lookup: [String: Int] = [:]
```
**Not Preferred:**
```swift
var names = [String]()
var lookup = [String: Int]()
```
**NOTE**: Following this guideline means picking descriptive names is even more important than before.
### Syntactic Sugar
Prefer the shortcut versions of type declarations over the full generics syntax.
**Preferred:**
```swift
var deviceModels: [String]
var employees: [Int: String]
var faxNumber: Int?
```
**Not Preferred:**
```swift
var deviceModels: Array<String>
var employees: Dictionary<Int, String>
var faxNumber: Optional<Int>
```
## Functions vs Methods
Free functions, which aren't attached to a class or type, should be used sparingly. When possible, prefer to use a method instead of a free function. This aids in readability and discoverability.
Free functions are most appropriate when they aren't associated with any particular type or instance.
**Preferred**
```swift
let sorted = items.mergeSorted() // easily discoverable
rocket.launch() // acts on the model
```
**Not Preferred**
```swift
let sorted = mergeSort(items) // hard to discover
launch(&rocket)
```
**Free Function Exceptions**
```swift
let tuples = zip(a, b) // feels natural as a free function (symmetry)
let value = max(x, y, z) // another free function that feels natural
```
## Memory Management
Code (even non-production, tutorial demo code) should not create reference cycles. Analyze your object graph and prevent strong cycles with `weak` and `unowned` references. Alternatively, use value types (`struct`, `enum`) to prevent cycles altogether.
### Extending object lifetime
Extend object lifetime using the `[weak self]` and `guard let strongSelf = self else { return }` idiom. `[weak self]` is preferred to `[unowned self]` where it is not immediately obvious that `self` outlives the closure. Explicitly extending lifetime is preferred to optional unwrapping.
**Preferred**
```swift
resource.request().onComplete { [weak self] response in
guard let strongSelf = self else {
return
}
let model = strongSelf.updateModel(response)
strongSelf.updateUI(model)
}
```
**Not Preferred**
```swift
// might crash if self is released before response returns
resource.request().onComplete { [unowned self] response in
let model = self.updateModel(response)
self.updateUI(model)
}
```
**Not Preferred**
```swift
// deallocate could happen between updating the model and updating UI
resource.request().onComplete { [weak self] response in
let model = self?.updateModel(response)
self?.updateUI(model)
}
```
## Access Control
Full access control annotation in tutorials can distract from the main topic and is not required. Using `private` and `fileprivate` appropriately, however, adds clarity and promotes encapsulation. Prefer `private` to `fileprivate` when possible. Using extensions may require you to use `fileprivate`.
Only explicitly use `open`, `public`, and `internal` when you require a full access control specification.
Use access control as the leading property specifier. The only things that should come before access control are the `static` specifier or attributes such as `@IBAction`, `@IBOutlet` and `@discardableResult`.
**Preferred:**
```swift
private let message = "Great Scott!"
class TimeMachine {
fileprivate dynamic lazy var fluxCapacitor = FluxCapacitor()
}
```
**Not Preferred:**
```swift
fileprivate let message = "Great Scott!"
class TimeMachine {
lazy dynamic fileprivate var fluxCapacitor = FluxCapacitor()
}
```
## Control Flow
Prefer the `for-in` style of `for` loop over the `while-condition-increment` style.
**Preferred:**
```swift
for _ in 0..<3 {
print("Hello three times")
}
for (index, person) in attendeeList.enumerated() {
print("\(person) is at position #\(index)")
}
for index in stride(from: 0, to: items.count, by: 2) {
print(index)
}
for index in (0...3).reversed() {
print(index)
}
```
**Not Preferred:**
```swift
var i = 0
while i < 3 {
print("Hello three times")
i += 1
}
var i = 0
while i < attendeeList.count {
let person = attendeeList[i]
print("\(person) is at position #\(i)")
i += 1
}
```
## Golden Path
When coding with conditionals, the left-hand margin of the code should be the "golden" or "happy" path. That is, don't nest `if` statements. Multiple return statements are OK. The `guard` statement is built for this.
**Preferred:**
```swift
func computeFFT(context: Context?, inputData: InputData?) throws -> Frequencies {
guard let context = context else {
throw FFTError.noContext
}
guard let inputData = inputData else {
throw FFTError.noInputData
}
// use context and input to compute the frequencies
return frequencies
}
```
**Not Preferred:**
```swift
func computeFFT(context: Context?, inputData: InputData?) throws -> Frequencies {
if let context = context {
if let inputData = inputData {
// use context and input to compute the frequencies
return frequencies
} else {
throw FFTError.noInputData
}
} else {
throw FFTError.noContext
}
}
```
When multiple optionals are unwrapped either with `guard` or `if let`, minimize nesting by using the compound version when possible. Example:
**Preferred:**
```swift
guard let number1 = number1,
let number2 = number2,
let number3 = number3 else {
fatalError("impossible")
}
// do something with numbers
```
**Not Preferred:**
```swift
if let number1 = number1 {
if let number2 = number2 {
if let number3 = number3 {
// do something with numbers
} else {
fatalError("impossible")
}
} else {
fatalError("impossible")
}
} else {
fatalError("impossible")
}
```
### Failing Guards
Guard statements are required to exit in some way. Generally, this should be simple one line statement such as `return`, `throw`, `break`, `continue`, and `fatalError()`. Large code blocks should be avoided. If cleanup code is required for multiple exit points, consider using a `defer` block to avoid cleanup code duplication.
## Semicolons
Swift does not require a semicolon after each statement in your code. They are only required if you wish to combine multiple statements on a single line.
Do not write multiple statements on a single line separated with semicolons.
**Preferred:**
```swift
let swift = "not a scripting language"
```
**Not Preferred:**
```swift
let swift = "not a scripting language";
```
**NOTE**: Swift is very different from JavaScript, where omitting semicolons is [generally considered unsafe](http://stackoverflow.com/questions/444080/do-you-recommend-using-semicolons-after-every-statement-in-javascript)
## Parentheses
Parentheses around conditionals are not required and should be omitted.
**Preferred:**
```swift
if name == "Hello" {
print("World")
}
```
**Not Preferred:**
```swift
if (name == "Hello") {
print("World")
}
```
In larger expressions, optional parentheses can sometimes make code read more clearly.
**Preferred:**
```swift
let playerMark = (player == current ? "X" : "O")
```
## Organization and Bundle Identifier
Where an Xcode project is involved, the organization should be set to `Ray Wenderlich` and the Bundle Identifier set to `com.razeware.TutorialName` where `TutorialName` is the name of the tutorial project.

## Smiley Face
Smiley faces are a very prominent style feature of the [raywenderlich.com](https://www.raywenderlich.com/) site! It is very important to have the correct smile signifying the immense amount of happiness and excitement for the coding topic. The closing square bracket `]` is used because it represents the largest smile able to be captured using ASCII art. A closing parenthesis `)` creates a half-hearted smile, and thus is not preferred.
**Preferred:**
```
:]
```
**Not Preferred:**
```
:)
```
## References
* [The Swift API Design Guidelines](https://swift.org/documentation/api-design-guidelines/)
* [The Swift Programming Language](https://developer.apple.com/library/prerelease/ios/documentation/swift/conceptual/swift_programming_language/index.html)
* [Using Swift with Cocoa and Objective-C](https://developer.apple.com/library/prerelease/ios/documentation/Swift/Conceptual/BuildingCocoaApps/index.html)
* [Swift Standard Library Reference](https://developer.apple.com/library/prerelease/ios/documentation/General/Reference/SwiftStandardLibraryReference/index.html)
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/coding_style/typescript/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
# TypeScript Style Guide
This is the TypeScript style guide that we use internally at Platypi! It is *semi-reasonable*, but it's more important that we keep a consistent look/feel of our code.
## Table of Contents
0. [Introduction](#introduction)
0. [Browser Compatibility](#browser-compatibility)
0. [Files](#files)
0. [Indentation](#indentation)
0. [Line Length](#line-length)
0. [Quotes](#quotes)
0. [Comments](#comments)
0. [Class](#class)
0. [Inline](#inline)
0. [Todo and XXX](#todo-and-xxx)
0. [Variable Declarations](#variable-declarations)
0. [Function Declarations](#function-declarations)
0. [Anonymous Functions](#anonymous-functions)
0. [Names](#names)
0. [Variables, Modules, and Functions](#variables-modules-and-functions)
0. [Use of var, let, and const](#use-of-var-let-and-const)
0. [Types](#types)
0. [Classes](#classes)
0. [Interfaces](#interfaces)
0. [Constants](#constants)
0. [Statements](#statements)
0. [Simple](#simple)
0. [Compound](#compound)
0. [Return](#return)
0. [If](#if)
0. [For](#for)
0. [While](#while)
0. [Do While](#do-while)
0. [Switch](#switch)
0. [Try](#try)
0. [Continue](#continue)
0. [Throw](#throw)
0. [Whitespace](#whitespace)
0. [Object and Array Literals](#object-and-array-literals)
0. [Assignment Expressions](#assignment-expressions)
0. [Typings](#assignment-expressions)
0. [External](#external)
0. [Internal](#internal)
0. [=== and !== Operators](#===-and-!==-Operators)
0. [Eval](#eval)
0. [TSLint](#tslint)
0. [License](#license)
## Introduction
When developing software as an organization, the value of the software produced is directly affected by the quality of the codebase. Consider a project that is developed over many years and handled/seen by many different people. If the project uses a consistent coding convention it is easier for new developers to read, preventing a lot of time/frustration spent figuring out the structure and characteristics of the code. For that purpose, we need to make sure we adhere to the same coding conventions across all of our products. This will not only help new developers, but it will also aid in quickly identifying potential flaws in the code, thereby reducing the brittleness of the code.
**[top](#table-of-contents)**
## Browser Compatibility
- Target evergreen browsers `ie >= 11`
- Target modern browsers `ie >= 9` if it is necessary for a project
- Avoid targeting older browsers `ie < 9` if at all possible
**[top](#table-of-contents)**
## Files
- All TypeScript files must have a ".ts" extension.
- They should be all lower case, and only include letters, numbers, and periods.
- It is OK (even recommended) to separate words with periods (e.g. `my.view.html`).
- **All files should end in a new line.** This is necessary for some Unix systems.
**[top](#table-of-contents)**
## Indentation
- The unit of indentation is four spaces.
- **Never use tabs**, as this can lead to trouble when opening files in different IDEs/Text editors. Most text editors have a configuration option to change tabs to spaces.
**[top](#table-of-contents)**
## Line Length
- Lines must not be longer than 140 characters.
- When a statement runs over 140 characters on a line, it should be broken up, ideally after a comma or operator.
**[top](#table-of-contents)**
## Quotes
- Use single-quotes `''` for all strings, and use double-quotes `""` for strings within strings.
```typescript
// bad
let greeting = "Hello World!";
// good
let greeting = 'Hello World!';
// bad
let html = "<div class='bold'>Hello World</div>";
// bad
let html = '<div class=\'bold\'>Hello World</div>';
// good
let html = '<div class="bold">Hello World</div>';
```
**[top](#table-of-contents)**
## Comments
- Comments are strongly encouraged. It is very useful to be able to read comments and understand the intentions of a given block of code.
- Comments need to be clear, just like the code they are annotating.
- Make sure your comments are meaningful.
The following example is a case where a comment is completely erroneous, and can actually make the code harder to read.
```typescript
// Set index to zero.
let index = 0;
```
- All public functions must have a comment block `/**...*/` using [JSDoc](http://usejsdoc.org/) style comments.
JSDocs can be interpreted by IDEs for better intellisense. Below is an example of a JSDoc comment block for a function.
```typescript
/**
* Takes in a name and returns a greeting string.
*
* @param name The name of the greeted person.
*/
function getGreeting(name: string): string {
return 'Hello ' + name + '!';
}
```
### Class
- All classes must have block comments `/**...*/` for all public variables and functions.
- All public functions should use [JSDoc](http://usejsdoc.org/) style comments.
- Functions need to have a comment explaining what the function does, and all of the input parameters need to be annotated with `@param`.
- The class should include a block comment containing the description of the class
- The constructor should contain a JSDoc comment annotating any input parameters.
```typescript
/**
* Contains properties of a Person.
*/
class Person {
/**
* Returns a new Person with the specified name.
*
* @param name The name of the new Person.
*/
static GetPerson(name: string): Person {
return new Person(name);
}
/**
* @param name The name of the new Person.
*/
constructor(public name: string) { }
/**
* Instructs this Person to walk for a certain amount
* of time.
*
* @param millis The number of milliseconds the Person
* should walk.
*/
walkFor(millis: number): void {
console.log(this.name + ' is now walking.');
setTimeout(() => {
console.log(this.name + ' has stopped walking.');
}, millis);
}
}
```
**[top](#table-of-contents)**
### Inline
- Inline comments are comments inside of complex statements (loops, functions, etc).
- Use `//` for all inline comments.
- Keep comments clear and concise.
- Place inline comments on a newline above the line they are annotating
- Put an empty line before the comment.
```typescript
// bad
let lines: Array<string>; // Holds all the lines in the file.
// good
// Holds all the lines in the file.
let lines: Array<string>;
// bad
function walkFor(name: string, millis: number): void {
console.log(name + ' is now walking.');
// Wait for millis milliseconds to stop walking
setTimeout(() => {
console.log(name + ' has stopped walking.');
}, millis);
}
// good
function walkFor(name: string, millis: number): void {
console.log(name + ' is now walking.');
// Wait for millis milliseconds to stop walking
setTimeout(() => {
console.log(name + ' has stopped walking.');
}, millis);
}
```
**[top](#table-of-contents)**
### Todo and XXX
`TODO` and `XXX` annotations help you quickly find things that need to be fixed/implemented.
- Use `// TODO:` to annotate solutions that need to be implemented.
- Use `// XXX:` to annotate problems the need to be fixed.
- It is best to write code that doesn't need `TODO` and `XXX` annotations, but sometimes it is unavoidable.
**[top](#table-of-contents)**
## Variable Declarations
- All variables must be declared prior to using them. This aids in code readability and helps prevent undeclared variables from being hoisted onto the global scope.
```typescript
// bad
console.log(a + b);
let a = 2,
b = 4;
// good
let a = 2,
b = 4;
console.log(a + b);
```
- Implied global variables should never be used.
- You should never define a variable on the global scope from within a smaller scope.
```typescript
// bad
function add(a: number, b: number): number {
// c is on the global scope!
c = 6;
return a + b + c;
}
```
- Use one `let` keyword to define a block of variables.
- Declare each variable on a newline.
```typescript
// bad
let a = 2;
let b = 2;
let c = 4;
// good
let a = 2,
b = 2,
c = 4;
// bad
// b will be defined on global scope.
let a = b = 2, c = 4;
```
## Function Declarations
- All functions must be declared before they are used.
- Any closure functions should be defined right after the let declarations.
```typescript
// bad
function createGreeting(name: string): string {
let message = 'Hello ';
return greet;
function greet() {
return message + name + '!';
}
}
// good
function createGreeting(name: string): string {
let message = 'Hello ';
function greet() {
return message + name + '!';
}
return greet;
}
```
- There should be no space between the name of the function and the left parenthesis `(` of its parameter list.
- There should be one space between the right parenthesis `)` and the left curly `{` brace that begins the statement body.
```typescript
// bad
function foo (){
// ...
}
// good
function foo() {
// ...
}
```
- The body of the function should be indented 4 spaces.
- The right curly brace `}` should be on a new line.
- The right curly brace `}` should be aligned with the line containing the left curly brace `{` that begins the function statement.
```typescript
// bad
function foo(): string {
return 'foo';}
// good
function foo(): string {
return 'foo';
}
```
- For each function parameter
- There should be no space between the parameter and the colon `:` indicating the type declaration.
- There should be a space between the colon `:` and the type declaration.
```typescript
// bad
function greet(name:string) {
// ...
}
// good
function greet(name: string) {
// ...
}
```
**[top](#table-of-contents)**
### Anonymous Functions
- All anonymous functions should be defined as fat-arrow/lambda `() => { }` functions unless it is absolutely necessary to preserve the context in the function body.
- All fat-arrow/lambda functions should have parenthesis `()` around the function parameters.
```typescript
// bad
clickAlert() {
let element = document.querySelector('div');
this.foo = 'foo';
element.addEventListener('click', function(ev: Event) {
// this.foo does not exist!
alert(this.foo);
});
}
// good
clickAlert() {
let element = document.querySelector('div');
this.foo = 'foo';
element.addEventListener('click', (ev: Event) => {
// TypeScript allows this.foo to exist!
alert(this.foo);
});
}
```
- There should be a space between the right parenthesis `)` and the `=>`
- There should be a space between the `=>` and the left curly brace `{` that begins the statement body.
```typescript
// bad
element.addEventListener('click', (ev: Event)=>{alert('foo');});
// good
element.addEventListener('click', (ev: Event) => {
alert('foo');
});
```
- The statement body should be indented 4 spaces.
- The right curly brace `}` should be on a new line.
- The right curly brace `}` should be aligned with the line containing the left curly brace `{` that begins the function statement.
**[top](#table-of-contents)**
## Names
- All variable and function names should be formed with alphanumeric `A-Z, a-z, 0-9` and underscore `_` charcters.
### Variables, Modules, and Functions
- Variable, module, and function names should use lowerCamelCase.
### Use of var, let, and const
- Use `const` where appropriate, for values that should never change
- Use `let` everywhere else
- Avoid using `var`
**[top](#table-of-contents)**
### Types
- Always favor type inference over explicit type declaration except for function return types
- Always define the return type of functions.
- Types should be used whenever necessary (no implicit `any`).
- Arrays should be defined as `Array<type>` instead of `type[]`.
- Use the `any` type sparingly, it is always better to define an interface.
```typescript
// bad
let numbers = [];
// bad
let numbers: number[] = [];
// good
let numbers: Array<number> = [];
```
**[top](#table-of-contents)**
### Classes
- Classes/Constructors should use UpperCamelCase (PascalCase).
- `Private` and `private static` members in classes should be denoted with the `private` keyword.
- `Protected` members in classes do not use the `private` keyword.
- Default to using `protected` for all instance members
- Use `private` instance members sparingly
- Use `public` instance members only when they are used by other parts of the application.
```typescript
class Person {
protected fullName: string;
constructor(public firstName: string, public lastName: string) {
this.fullName = firstName + ' ' + lastName;
}
toString() {
return this.fullName;
}
protected walkFor(millis: number) {
console.log(this.fullName + ' is now walking.');
// Wait for millis milliseconds to stop walking
setTimeout(() => {
console.log(this.fullName + ' has stopped walking.');
}, millis);
}
}
```
**[top](#table-of-contents)**
### Interfaces
- Interfaces should use UpperCamelCase.
- Interfaces should be prefaced with the capital letter I.
- Only `public` members should be in an interface, leave out `protected` and `private` members.
```typescript
interface IPerson {
firstName: string;
lastName: string;
toString(): string;
}
```
**[top](#table-of-contents)**
### Constants
- All constants should use UPPER_SNAKE_CASE.
- All constants you be defined with the `const` keyword.
**[top](#table-of-contents)**
## Statements
### Simple
- Each line should contain at most one statement.
- A semicolon should be placed at the end of every simple statement.
```typescript
// bad
let greeting = 'Hello World'
alert(greeting)
// good
let greeting = 'Hello World';
alert(greeting);
```
**[top](#table-of-contents)**
### Compound
Compound statements are statements containing lists of statements enclosed in curly braces `{}`.
- The enclosed statements should start on a newline.
- The enclosed statements should be indented 4 spaces.
```typescript
// bad
if (condition === true) { alert('Passed!'); }
// good
if (condition === true) {
alert('Passed!');
}
```
- The left curly brace `{` should be at the end of the line that begins the compound statement.
- The right curly brace `}` should begin a line and be indented to align with the line containing the left curly brace `{`.
```typescript
// bad
if (condition === true)
{
alert('Passed!');
}
// good
if (condition === true) {
alert('Passed!');
}
```
- **Braces `{}` must be used around all compound statements** even if they are only single-line statements.
```typescript
// bad
if (condition === true) alert('Passed!');
// bad
if (condition === true)
alert('Passed!');
// good
if (condition === true) {
alert('Passed!');
}
```
If you do not add braces `{}` around compound statements, it makes it very easy to accidentally introduce bugs.
```typescript
if (condition === true)
alert('Passed!');
return condition;
```
It appears the intention of the above code is to return if `condition === true`, but without braces `{}` the return statement will be executed regardless of the condition.
- Compount statements do not need to end in a semicolon `;` with the exception of a `do { } while();` statement.
**[top](#table-of-contents)**
### Return
- If a `return` statement has a value you should not use parenthesis `()` around the value.
- The return value expression must start on the same line as the `return` keyword.
```typescript
// bad
return
'Hello World!';
// bad
return ('Hello World!');
// good
return 'Hello World!';
```
- It is recommended to take a return-first approach whenever possible.
```typescript
// bad
function getHighestNumber(a: number, b: number): number {
let out = b;
if(a >= b) {
out = a;
}
return out;
}
// good
function getHighestNumber(a: number, b: number): number {
if(a >= b) {
return a;
}
return b;
}
```
- Always **explicitly define a return type**. This can help TypeScript validate that you are always returning something that matches the correct type.
```typescript
// bad
function getPerson(name: string) {
return new Person(name);
}
// good
function getPerson(name: string): Person {
return new Person(name);
}
```
**[top](#table-of-contents)**
### If
- Alway be explicit in your `if` statement conditions.
```typescript
// bad
function isString(str: any) {
return !!str;
}
// good
function isString(str: any) {
return typeof str === 'string';
}
```
Sometimes simply checking falsy/truthy values is fine, but the general approach is to be explicit with what you are looking for. This can prevent a lot of unncessary bugs.
If statements should take the following form:
```typescript
if (/* condition */) {
// ...
}
if (/* condition */) {
// ...
} else {
// ...
}
if (/* condition */) {
// ...
} else if (/* condition */) {
// ...
} else {
// ...
}
```
**[top](#table-of-contents)**
### For
For statements should have the following form:
```typescript
for(/* initialization */; /* condition */; /* update */) {
// ...
}
let keys = Object.keys(/* object */),
length = keys.length;
for(let i = 0; i < length; ++i) {
// ...
}
```
Object.prototype.keys is supported in `ie >= 9`.
- Use Object.prototype.keys in lieu of a `for...in` statement.
**[top](#table-of-contents)**
### While
While statements should have the following form:
```typescript
while (/* condition */) {
// ...
}
```
**[top](#table-of-contents)**
### Do While
- Do while statements should be avoided unless absolutely necessary to maintain consistency.
- Do while statements must end with a semicolon `;`
Do while statements should have to following form:
```typescript
do {
// ...
} while (/* condition */);
```
**[top](#table-of-contents)**
### Switch
Switch statements should have the following form:
```typescript
switch (/* expression */) {
case /* expression */:
// ...
/* termination */
default:
// ...
}
```
- Each switch group except default should end with `break`, `return`, or `throw`.
**[top](#table-of-contents)**
### Try
- Try statements should be avoided whenever possible. They are not a good way of providing flow control.
Try statements should have the following form:
```typescript
try {
// ...
} catch (error: Error) {
// ...
}
try {
// ...
} catch (error: Error) {
// ...
} finally {
// ...
}
```
**[top](#table-of-contents)**
### Continue
- It is recommended to take a continue-first approach in all loops.
**[top](#table-of-contents)**
### Throw
- Avoid the use of the throw statement unless absolutely necessary.
- Flow control through try/catch exception handling is not recommended.
**[top](#table-of-contents)**
## Whitespace
Blank lines improve code readability by allowing the developer to logically group code blocks. Blank spaces should be used in the following circumstances.
- A keyword followed by left parenthesis `(` should be separated by 1 space.
```typescript
// bad
if(condition) {
// ...
}
// good
if (condition) {
// ...
}
```
- All operators except for period `.`, left parenthesis `(`, and left bracket `[` should be separated from their operands by a space.
```typescript
// bad
let sum = a+b;
// good
let sum = a + b;
// bad
let name = person . name;
// good
let name = person.name;
// bad
let item = items [4];
// good
let item = items[4];
```
- No space should separate a unary/incremental operator `!x, -x, +x, ~x, ++x, --x` and its operand.
```typescript
// bad
let neg = - a;
// good
let neg = -a;
```
- Each semicolon `;` in the control part of a `for` statement should be followed with a space.
```typescript
// bad
for(let i = 0;i < 10;++i) {
// ...
}
// good
for(let i = 0; i < 10; ++i) {
// ...
}
```
**[top](#table-of-contents)**
## Object and Array Literals
- Use curly braces `{}` instead of `new Object()`.
- Use brackets `[]` instead of `new Array()`.
**[top](#table-of-contents)**
## Assignment Expressions
- Assignment expressions inside of the condition block of `if`, `while`, and `do while` statements should be avoided.
```typescript
// bad
while (node = node.next) {
// ...
}
// good
while (typeof node === 'object') {
node = node.next;
// ...
}
```
**[top](#table-of-contents)**
## === and !== Operators
- It is better to use `===` and `!==` operators whenever possible.
- `==` and `!=` operators do type coercion, which can lead to headaches when debugging code.
**[top](#table-of-contents)**
## Typings
### External
- Typings are sometimes packaged with node modules, in this case you don't need to do anything
- Use [typings](https://github.com/typings/typings) for all external library declarations not included in `node_modules`
- Actively add/update/contribute typings when they are missing
### Internal
- Create declaration files `.d.ts` for your interfaces instead of putting them in your `.ts` files
- Let the TypeScript compiler infer as much as possible
- Avoid defining types when it is unnecessary
```typescript
// bad
let a: number = 2;
// good
let a = 2;
```
- Always define the return type of functions, this helps to make sure that functions always return the correct type
```typescript
// bad
function sum(a: number, b: number) {
return a + b;
}
// good
function sum(a: number, b: number): number {
return a + b;
}
```
**[top](#table-of-contents)**
## Eval
- **Never use eval**
- **Never use the Function constructor**
- **Never pass strings to `setTimeout` or `setInterval`**
**[top](#table-of-contents)**
## TSLint
- Always use a Linter
Linting your code is very helpful for preventing minor issues that can escape the eye during development. We use TSLint (written by Palantir) for our linter.
- TSLint: https://github.com/palantir/tslint
- Our [tslint.json](https://github.com/Platypi/style_typescript/blob/master/tslint.json)
## License
(The MIT License)
Copyright (c) 2014 Platypi, LLC
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/documentation_guide.md | # Writing notes for each algorithm
When creating the notes for algorithms of various categories, please use this template:
```Markdown
# Algorithm Name
Description
## Explanation
Pictorial diagram to explain the algorithm
> Image credits: (name of the site/organisation from where the image was referenced)
## Algorithm
Algorithm as a numbered list.
## Complexity
Time and space complexity
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
``` |
guides/installation_guides/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/c++/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/c++/linux/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/c++/mac/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/c++/windows/README.md | # Cosmos Guide: Getting Ready to program in C++ in Windows
<b1> <b>Getting The Software</b> </b1> <br> <br>
>The easiest way for a beginner to start working on his first C++ program is to get an IDE. In this tutorial we are going to use CodeBlocks. <br>
>You can get Codeblocks from the Official Site: http://www.codeblocks.org/downloads <br>
>Click on <b>Download the binary release</b> <br>
<img src="https://github.com/MoonfireSeco/hello-world/blob/master/saver.png"> <br> <br>
>Look after codeblocks's <b>mingw-setup.exe</b>, it may be a different version than the one in the screenshot (16.01) but it does not matter. Click on the <b>SourceForge</b><br>
<img src="https://github.com/MoonfireSeco/hello-world/blob/master/chooser.png"> <br> <br>
>After that you can just wait, and an installer should be downloaded. Follow all the steps, click on all the <b>Next</b>s, no custom non-default options are required. <br> <br>
Once Codeblocks has been installed, an <b>Autodetection Compiler</b> prompt should appear. Click <b>OK</b>. <br>
<img src="https://github.com/MoonfireSeco/hello-world/blob/master/compiler.png"> <br> <br>
>Once Codeblocks starts, click on <b>Create Project</b>
<img src="https://github.com/MoonfireSeco/hello-world/blob/master/CreateProject.png"> <br> <br>
>Double click on <b>Console Application</b>
<img src="https://github.com/MoonfireSeco/hello-world/blob/master/ConsoleApplication.png"> <br> <br>
>Click on <b>Next</b>s, Name your project, and you should be ready to go! Make sure the <b>Main.cpp</b> file is open, you can write your code there! Make sure to click on the <b>gear and play</b> icon to compile and run your code.
<img src="https://github.com/MoonfireSeco/hello-world/blob/master/Finish.png"> <br> <br>
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p> |
guides/installation_guides/c/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/c/linux/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/c/mac/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/c/windows/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/elixir/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/elixir/linux/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/elixir/mac/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/elixir/windows/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/go/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/go/linux/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/go/mac/README.md | # Go Installation Guide
### Install Go
`brew install go`
### Create Go Directories
`mkdir $HOME/Go`
`mkdir -p $HOME/Go/src/github.com/<username>` (This is the directory where you should create your Go projects.)
### Setup Go paths
`export GOPATH=$HOME/Go`
`export GOROOT=/usr/local/opt/go/libexec`
`export PATH=$PATH:$GOPATH/bin`
`export PATH=$PATH:$GOROOT/bin`
### Get the Go Document (optional)
`go get golang.org/x/tools/cmd/godoc`
### Additional Go Resources
[How to Write Go Code](https://golang.org/doc/code.html)
[How I Start Go tutorial](http://howistart.org/posts/go/1/index.html)
[Learn X in Y minutes](https://learnxinyminutes.com/docs/go/)
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/go/windows/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/java/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/java/linux/README.md | # Java Installation Guide
### Download
Download the Java version for your operating system from the [Java website](https://www.java.com/en/download/)
### Install
*Note: root access may be required to write files in root directory*
1. Change into the directory you wish to install Java in. For a system-wide installation, use `/usr/local`.
2. Move the tarball into the current directory
3. Unpack the tarball
`tar zxvf jre-version-linux-x64.tar.gz`
4. Delete the tarball to save space
`rm *.tar.gz`
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/java/mac/README.md | # Java Installation Guide
### Download
Download the `.dmg` from the [Java website](https://www.java.com/en/download/)
### Install
Double clicking the `.dmg` file will open up an installation wizard that will guide you through installation. Following the wizard will install it for you.
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/java/windows/README.md | # Java Installation Guide
### Download
Download the Windows version of Java from the [Java website](https://www.java.com/en/download/) .
### Install
*Note: administrative access may be required for installation*
1. Run the Java setup file (exe).
2. Give installation access to the Java installer.
3. Now give the installation path or directory.
4. Click on Install button.
### Setting up Environment Variables
1. Right click Computer.
2. Click Properties.
3. On the left pane select Advanced System Settings.
4. Select Environment Variables.
5. Under the System Variables, Select PATH and click edit.
6. Then click New.
7. Then add path as `C:\ProgramFiles\Java\jdk1.8.0_131\bin` (depending on your installation path and version).
8. Finally click OK.
9. Next restart your command prompt and open it and try `java -version` to confirm system variable setup.
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/javascript/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/javascript/linux/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/javascript/mac/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/javascript/windows/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/kotlin/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/kotlin/linux/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/kotlin/mac/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/kotlin/windows/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/pascal/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/pascal/linux/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/pascal/mac/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/pascal/windows/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/python/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/python/linux/README.md | ## Python Installation Guide for Linux
Most Linux Distros come pre-installed with python2, sometimes even python3.
check by
```
python2 --version
```
if its not installed, install by
```
sudo dnf install python2
```
this also works
```
sudo apt-get install python2
sudo apt-get install python3
sudo apt-get install python ...<tab> to see your options
```
# Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/python/mac/README.md | # Python Installation Guide
*How to install Python on your machine*
As with most Linux distributions, Python comes installed by default. Despite that, the installed version could be different with the one that you need or some packages may not be installed.
### Installing Python and the basic packages
From Python's organization webpage (<a href="https://www.python.org/downloads/">python.org</a>) you can download the latest `.pkg` file. After that, just open the file and follow the instructions. This will also install the basic packages.
You can download it from <a href="https://www.python.org/downloads/">here</a>.
### Installing additional packages
The easiest way to install a package is using the `pip` option in Terminal. If you are using Python 2 >=2.7.9 or Python 3 >=3.4 and installed it from the <a href="https://www.python.org/downloads/">python.org</a> webpage, you already have it installed.
To install a package just open Terminal and type:
`pip install packageName`
Where `packageName` is the name of the package that you want to install. You can see the full list of available packages from <a href="https://pypi.python.org/pypi?%3Aaction=browse">here</a>.
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/python/windows/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
Installing Python on Windows is a simple process. Visit https://www.python.org/downloads/ and choose the version you want to install. There are different installers based on your preferences: a web-based installer that downloads neccesary files during the installation and a full installer that contains all files. Run the installer and select options if you think that you need to, but the defaults are okay for most people.
Now that Python is installed, it is accessible through the program called IDLE.
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/python_pip/linux/README.md | ## Python-pip Installation Guide for Linux
Make sure you have pip available. You can check this by running:
check by
```
pip --version
```
if its not installed, install by
```
sudo apt-get install python-pip
```
You may need to upgrade pip:
```
sudo -H pip install --upgrade pip
```
# Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/ruby/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/ruby/linux/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/ruby/mac/README.md | # Ruby Installation Guide
*How to install Ruby on your machine*
### Installing RVM
First, before installing Ruby, you must install Ruby Version Manager (RVM). The simplest way to do this is to enter the following in the console:
`\curl -L https://get.rvm.io | bash -s stable`
Once this command has run, you must restart your terminal.
### Installing Ruby
Once you have installed RVM as above, you can now install the most recent version of Ruby. To do this, you must enter the command:
`rvm install ruby-2.4.2`
*Note: you must enter the most recent Ruby version, you can check the latest version <a href="https://www.ruby-lang.org/en/downloads/">here</a>.*
You may be asked to enter your password to complete the installation.
The final step is to ensure the RubyGems version is up to date. To do this type:
`gem update --system`
Now you have successfully installed and updated to the most recent version of Ruby!
Note: If you try install a old ruby version maybe you can get a ssl error. You can solve it with rvm install `ruby-x.x.x --disable-binaries`
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/ruby/windows/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/rust/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/rust/linux/README.md | ([Source](https://doc.rust-lang.org/book/second-edition/ch01-01-installation.html))
## Installing on Linux or Mac
If you’re on Linux or a Mac, all you need to do is open a terminal and type this:
```
$ curl https://sh.rustup.rs -sSf | sh
```
This will download a script and start the installation. You may be prompted for your password. If it all goes well, you’ll see this appear:
Rust is installed now. Great!
Of course, if you disapprove of the curl | sh pattern, you can download, inspect and run the script however you like.
The installation script automatically adds Rust to your system PATH after your next login. If you want to start using Rust right away, run the following command in your shell:
```
$ source $HOME/.cargo/env
```
Alternatively, add the following line to your ~/.bash_profile:
```
$ export PATH="$HOME/.cargo/bin:$PATH"
```
## Updating
Once you have Rust installed, updating to the latest version is easy. From your shell, run the update script:
```
$ rustup update
```
## Uninstalling
Uninstalling Rust is as easy as installing it. From your shell, run the uninstall script:
```
$ rustup self uninstall
``` |
guides/installation_guides/rust/mac/README.md | ([Source](https://doc.rust-lang.org/book/second-edition/ch01-01-installation.html))
## Installing on Linux or Mac
If you’re on Linux or a Mac, all you need to do is open a terminal and type this:
```
$ curl https://sh.rustup.rs -sSf | sh
```
This will download a script and start the installation. You may be prompted for your password. If it all goes well, you’ll see this appear:
Rust is installed now. Great!
Of course, if you disapprove of the curl | sh pattern, you can download, inspect and run the script however you like.
The installation script automatically adds Rust to your system PATH after your next login. If you want to start using Rust right away, run the following command in your shell:
```
$ source $HOME/.cargo/env
```
Alternatively, add the following line to your ~/.bash_profile:
```
$ export PATH="$HOME/.cargo/bin:$PATH"
```
## Updating
Once you have Rust installed, updating to the latest version is easy. From your shell, run the update script:
```
$ rustup update
```
## Uninstalling
Uninstalling Rust is as easy as installing it. From your shell, run the uninstall script:
```
$ rustup self uninstall
``` |
guides/installation_guides/rust/windows/README.md | ([Source](https://doc.rust-lang.org/book/second-edition/ch01-01-installation.html))
## Installing on Windows
On Windows, go to https://rustup.rs and follow the instructions to download rustup-init.exe. Run that and follow the rest of the instructions it gives you.
The rest of the Windows-specific commands in the book will assume that you are using cmd as your shell. If you use a different shell, you may be able to run the same commands that Linux and Mac users do. If neither work, consult the documentation for the shell you are using.
Custom installations
If you have reasons for preferring not to use rustup.rs, please see the Rust installation page for other options.
## Updating
Once you have Rust installed, updating to the latest version is easy. From your shell, run the update script:
```
$ rustup update
```
## Uninstalling
Uninstalling Rust is as easy as installing it. From your shell, run the uninstall script:
```
$ rustup self uninstall
``` |
guides/installation_guides/swift/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/swift/linux/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/swift/mac/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
guides/installation_guides/swift/windows/README.md | # Cosmos Guides
> Your personal library of every algorithm and data structure code that you will ever encounter
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
jsonized.jsonl | {
"filename": "code/algorithm_applications/src/binary_search/ceil_of_element/ceil_of_element_in_sorted_array.cpp",
"content": "#include<bits/stdc++.h>\nusing namespace std; \nint solve(int arr[],int n, int ele){\n int ans=-1;\n int low=0;\n int high=n-1;\n while(low<=high){\n int mid=low+(high-low)/2;\n if(ele==arr[mid]){\n return ele; \n }\n else if(ele>arr[mid]){\n ans=mid;\n high=mid-1;\n }\n else{\n low=mid+1;\n }\n }\n return arr[ans];\n }\nint main(){\n int n; \n cin>>n; \n int arr[n];\n for(int i=0;i<n;i++){\n cin>>arr[i];\n }\n int ele; \n cin>>ele; \n int ans=solve(arr,n,ele);\n cout<<\"Ceil of \"<<ele<<\" is \"<<ans<<endl; \n return 0;\n }"
}
{
"filename": "code/algorithm_applications/src/binary_search/distributing_candies/CandyDistribution.java",
"content": "import java.io.BufferedReader;\r\nimport java.io.IOException;\r\nimport java.io.InputStreamReader;\r\n\r\nclass CandyDistribution {\r\n public static void main(String[] args) throws IOException {\r\n BufferedReader br = new BufferedReader(new InputStreamReader(System.in));\r\n\t//testcases\r\n int t = Integer.parseInt(br.readLine());\r\n while (t-- > 0){\r\n String[] input = br.readLine().split(\" \");\r\n //n: number of candy boxes\r\n int n = Integer.parseInt(input[0]);\r\n //k: number of friends\r\n long k = Long.parseLong(input[1]);\r\n String[] input1 = br.readLine().split(\" \");\r\n long[] arr = new long[n];\r\n long max = Integer.MIN_VALUE;\r\n for(int i=0; i<n; i++){\r\n arr[i] = Long.parseLong(input1[i]);\r\n//we need to find max for the upper bound in the binary search.\r\n max = Math.max(max,arr[i]);\r\n }\r\n System.out.println(binarySearch(n, k, arr, max));\r\n }\r\n }\r\n public static long binarySearch(int n, long k, long[] arr, long max){\r\n long low = 1;\r\n long high = max;\r\n long mid;\r\n long res = 0;\r\n\r\n while (high>=low){\r\n mid = low + (high-low)/2;\r\n if(canDistribute(mid, n, k, arr)){\r\n low = mid+1;\r\n res = mid;\r\n }\r\n else{\r\n high = mid-1;\r\n }\r\n }\r\n return res;\r\n }\r\n \r\n public static boolean canDistribute(long val, int n, long k, long[] arr){\r\n if(k==1){\r\n return true;\r\n }\r\n long peopleServed = 0;\r\n for (int i=n-1; i>=0; i--){\r\n//this is the number of people who can get candy\r\n peopleServed += arr[i]/val;\r\n }\r\n if(peopleServed>=k){\r\n return true;\r\n }\r\n return false;\r\n }\r\n}\r"
}
{
"filename": "code/algorithm_applications/src/binary_search/first_and_last_position_in_sorted_array/firstAndLastPosInSortedArray.cpp",
"content": "#include <bits/stdc++.h>\nusing namespace std;\n\nint first(int arr[], int x, int n)\n{\n int low = 0, high = n - 1, res = -1;\n while (low <= high)\n {\n int mid = (low + high) / 2;\n\n if (arr[mid] > x)\n high = mid - 1;\n else if (arr[mid] < x)\n low = mid + 1;\n else\n {\n res = mid;\n high = mid - 1;\n }\n }\n return res;\n}\n\nint last(int arr[], int x, int n)\n{\n int low = 0, high = n - 1, res = -1;\n while (low <= high)\n {\n int mid = (low + high) / 2;\n\n if (arr[mid] > x)\n high = mid - 1;\n else if (arr[mid] < x)\n low = mid + 1;\n else\n {\n res = mid;\n low = mid + 1;\n }\n }\n return res;\n}\n\nint main()\n{\n int n;\n cin >> n;\n int arr[n];\n for (int i = 0; i < n; i++)\n {\n cin >> arr[i];\n }\n int q;\n cin >> q;\n while (q--)\n {\n int a;\n cin >> a;\n cout << first(arr, a, n) << \" \" << last(arr, a, n) << endl;\n }\n}"
}
{
"filename": "code/algorithm_applications/src/binary_search/floor_of_element/floor_of_element_in_sorted_array.cpp",
"content": "#include<bits/stdc++.h>\nusing namespace std; \nint solve(int arr[],int n,int ele){\n int ans=-1;\n int low=0;\n int high=n-1;\n while(low<=high){\n int mid=low+(high-low)/2;\n if(arr[mid]==ele){\n return ele; \n }\n else if(arr[mid]<ele){\n ans=mid;\n low=mid+1;\n }\n else{\n high=mid-1;\n }\n }\n return arr[ans];\n }\nint main(){\n int n;\n cin>>n; \n int arr[n];\n for(int i=0;i<n;i++){\n cin>>arr[i];\n }\n int ele; \n cin>>ele; \n int ans=solve(arr,n,ele);\n cout<<\"floor of \"<<ele<<\" is \"<<ans<<endl; \n return 0;\n }"
}
{
"filename": "code/algorithm_applications/src/bubble_sort/bubble_sort_implementation.cpp",
"content": "#include<iostream>\n\nusing namespace std;\n\nint main() { \n int n;\n cin >> n;\n int arr[n];\n for(int i = 0; i < n; i++) {\n cin >> arr[i];\n }\n\n // Bubble sort algorithm code \n int counter = 1;\n while(counter < n) {\n for(int i = 0; i < n-counter; i++){\n if(arr[i] > arr[i+1]) {\n // if arr[i] > arr[i+1] then we swap those two element\n // because they are not in sorting form\n int temp = arr[i];\n arr[i] = arr[i+1];\n arr[i+1] = temp;\n }\n }\n counter++;\n }\n\n cout << '\\n';\n\n return 0;\n}"
}
{
"filename": "code/algorithm_applications/src/merge_arrays/merge_two_arrays.c",
"content": "#include <stdio.h>\n\nint main(){\n int m;\n printf(\"Enter the number of elements in first array\\n\");\n scanf(\"%d\",&m);\n int arr1[m];\n int n;\n printf(\"\\nEnter the number of elements in second array\\n\");\n scanf(\"%d\",&n);\n int arr2[n];\n printf(\"\\nEnter the elements of first array\\n\");\n for(int i=0;i<m;i++){\n scanf(\"%d\",&arr1[i]);\n }\n printf(\"\\nEnter the elements of second array\\n\");\n for(int i=0;i<n;i++){\n scanf(\"%d\",&arr2[i]);\n }\n int arr[m+n];\n int i=0;\n for(i=0;i<m;i++){\n arr[i]=arr1[i];\n }\n for(int j=0;j<n;j++){\n arr[i+j]=arr2[j];\n }\n printf(\"\\nFirst array is\\n\");\n for(int i=0;i<m;i++){\n printf(\"%d \",arr1[i]);\n }\n printf(\"\\nSecond array is\\n\"); \n for(int i=0;i<n;i++){\n printf(\"%d \",arr2[i]);\n }\n printf(\"\\nMerged array is\\n\");\n for(int i=0;i<m+n;i++){\n printf(\"%d \",arr[i]);\n }\n}"
}
{
"filename": "",
"content": ""
}
{
"filename": "code/artificial_intelligence/src/artificial_neural_network/ann.py",
"content": "import numpy as np\nimport matplotlib.pyplot as plt\nimport pandas as pd\n\ndataset = pd.read_csv(\"dataset.csv\")\nX = dataset.iloc[:, 3:13].values\ny = dataset.iloc[:, 13].values\n\nfrom sklearn.preprocessing import LabelEncoder, OneHotEncoder\n\nlabelencoder_X_1 = LabelEncoder()\nX[:, 1] = labelencoder_X_1.fit_transform(X[:, 1])\nlabelencoder_X_2 = LabelEncoder()\nX[:, 2] = labelencoder_X_2.fit_transform(X[:, 2])\nonehotencoder = OneHotEncoder(categorical_features=[1])\nX = onehotencoder.fit_transform(X).toarray()\nX = X[:, 1:]\n\nfrom sklearn.model_selection import train_test_split\n\nX_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)\n\nfrom sklearn.preprocessing import StandardScaler\n\nsc = StandardScaler()\nX_train = sc.fit_transform(X_train)\nX_test = sc.transform(X_test)\n\nimport keras\nfrom keras.models import Sequential\nfrom keras.layers import Dense\n\nclassifier = Sequential()\n\nclassifier.add(\n Dense(units=6, kernel_initializer=\"uniform\", activation=\"relu\", input_dim=11)\n)\n\nclassifier.add(Dense(units=6, kernel_initializer=\"uniform\", activation=\"relu\"))\n\nclassifier.add(Dense(units=1, kernel_initializer=\"uniform\", activation=\"sigmoid\"))\n\nclassifier.compile(optimizer=\"adam\", loss=\"binary_crossentropy\", metrics=[\"accuracy\"])\n\nclassifier.fit(X_train, y_train, batch_size=10, epochs=100)\n\ny_pred = classifier.predict(X_test)\ny_pred = y_pred > 0.5"
}
{
"filename": "code/artificial_intelligence/src/a_star/a_star.py",
"content": "import random\n\n\nclass Node:\n \"\"\" Simple node class for A* pathfinding \"\"\"\n\n def __init__(self, parent=None, position=None):\n self.parent = parent\n self.position = position\n\n self.g = 0\n self.f = 0\n self.h = 0\n\n def __eq__(self, other):\n self.position == other.position\n\n\ndef make(n):\n maze = [[0 for i in range(n)] for j in range(n)]\n for i in range(n):\n for j in range(n):\n x = random.random()\n if x > 0.7:\n maze[i][j] = 1\n\n return maze\n\n\ndef astar(maze, start, end):\n \"\"\" Returns a list of tuples as a path from the given end in the given maze\"\"\"\n\n # Defining a start and end Node\n start_node = Node(None, start)\n start_node.g = start_node.h = start_node.f = 0\n end_node = Node(None, end)\n end_node.g = end_node.f = end_node.h = 0\n\n # Initialize open and closed list\n open_list = []\n closed_list = []\n\n # Adding the start node in open list\n open_list.append(start_node)\n\n while len(open_list) > 0:\n current_node = open_list[0]\n current_index = 0\n\n for index, item in enumerate(open_list):\n # lower f means better path\n if item.f < current_node.f:\n current_node = item\n current_index = index\n\n # Popping the current index off the open list and adding the node in closed list\n open_list.pop(current_index)\n closed_list.append(current_node)\n\n # Found the goal? Cool. Now backtrack\n if current_node.position == end_node.position:\n path = []\n current = current_node\n while current is not None:\n path.append(current.position)\n current = current.parent\n return path[::-1]\n\n # Generate Children\n\n children = []\n for new_position in [\n (0, -1),\n (0, 1),\n (-1, 0),\n (1, 0),\n (-1, -1),\n (-1, 1),\n (1, -1),\n (1, 1),\n ]: # Adjacent squares\n\n # Getting node position\n node_position = (\n current_node.position[0] + new_position[0],\n current_node.position[1] + new_position[1],\n )\n\n # Checking if in-range or not :\n if (\n node_position[0] > (len(maze) - 1)\n or node_position[0] < 0\n or node_position[1] > (len(maze[len(maze) - 1]) - 1)\n or node_position[1] < 0\n ):\n continue\n\n # See if walkable or not\n if maze[node_position[0]][node_position[1]] != 0:\n continue\n\n # Creating new node\n new_node = Node(current_node, node_position)\n\n # Confirming the Child\n children.append(new_node)\n\n # Now, Loop through the children\n for child in children:\n\n # Child is on the closed list?\n for closed_child in closed_list:\n if child == closed_child:\n continue\n\n # If not? start making f,g and h values\n child.g = current_node.g + 1 ## why not child.parent.g?\n ## Eucledian heuristic without sqrt? Genius\n child.h = ((child.position[0] - end_node.position[0]) ** 2) + (\n (child.position[1] - end_node.position[1]) ** 2\n )\n child.f = child.h + child.g\n\n # Child Already in Open List?\n for open_node in open_list:\n if child == open_node and child.g > open_node.g:\n continue\n # Add the child in the open list\n open_list.append(child)\n\n\ndef showPath(maze, path, n, start, end):\n dupMaze = maze\n # General Display\n for i in range(n):\n for j in range(n):\n if dupMaze[i][j] == 1:\n dupMaze[i][j] = \"|\"\n elif dupMaze[i][j] == 0:\n dupMaze[i][j] = \" \"\n\n dupMaze[start[0]][start[1]] = \"S\"\n dupMaze[end[0]][end[1]] = \"E\"\n for i in path[1:-1]:\n dupMaze[i[0]][i[1]] = \".\"\n\n return dupMaze\n\n\ndef main():\n print(\"Started!\")\n maze = make(random.randint(3, 10))\n\n print(\" You maze is\")\n for i in maze:\n for j in i:\n print(j)\n print()\n\n start = tuple(map(int, input(\"Please Enter the starting Coordinates: \").split()))\n end = tuple(map(int, input(\"Please Enter the ending Coordinates: \").split()))\n\n path = astar(maze, start, end)\n\n display = showPath(maze, path, len(maze[0]), start, end)\n print(\"\\n\\n\")\n for i in display:\n for j in i:\n print(j)\n print()\n\n print(\"The Perfect Path will be {}\".format(path))\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/artificial_intelligence/src/autoenncoder/autoencoder.ipynb",
"content": "{\n \"cells\": [\n {\n \"cell_type\": \"code\",\n \"execution_count\": 1,\n \"metadata\": {},\n \"outputs\": [\n {\n \"name\": \"stderr\",\n \"output_type\": \"stream\",\n \"text\": [\n \"Using TensorFlow backend.\\n\"\n ]\n }\n ],\n \"source\": [\n \"from keras.layers import Dense, Input\\n\",\n \"from keras.models import Model\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 2,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"encoding_dim = 32\\n\",\n \"\\n\",\n \"input_img = Input(shape = (784, ))\\n\",\n \"\\n\",\n \"encoded = Dense(encoding_dim, activation = 'relu')(input_img)\\n\",\n \"\\n\",\n \"decoded = Dense(784, activation = 'sigmoid')(encoded)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 3,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"autoencoder = Model(input_img, decoded)\\n\",\n \"\\n\",\n \"encoder = Model(input_img, encoded)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 4,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"encoded_input = Input(shape = (encoding_dim, ))\\n\",\n \"\\n\",\n \"decoder_layer = autoencoder.layers[-1]\\n\",\n \"\\n\",\n \"decoder = Model(encoded_input, decoder_layer(encoded_input))\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 5,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"autoencoder.compile(optimizer = 'adadelta', loss = 'binary_crossentropy')\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 6,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"from keras.datasets import mnist\\n\",\n \"import numpy as np\\n\",\n \"(x_train, _), (x_test, _) = mnist.load_data()\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 7,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"array([[[0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" ...,\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0]],\\n\",\n \"\\n\",\n \" [[0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" ...,\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0]],\\n\",\n \"\\n\",\n \" [[0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" ...,\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0]],\\n\",\n \"\\n\",\n \" ...,\\n\",\n \"\\n\",\n \" [[0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" ...,\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0]],\\n\",\n \"\\n\",\n \" [[0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" ...,\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0]],\\n\",\n \"\\n\",\n \" [[0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" ...,\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0],\\n\",\n \" [0, 0, 0, ..., 0, 0, 0]]], dtype=uint8)\"\n ]\n },\n \"execution_count\": 7,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"x_train\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 8,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"x_train = x_train.astype('float32')/255.\\n\",\n \"x_test = x_test.astype('float32')/255.\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 9,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"x_train = x_train.reshape(len(x_train), np.prod(x_train.shape[1:]))\\n\",\n \"x_test = x_test.reshape(len(x_test), np.prod(x_test.shape[1:]))\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 10,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"(60000, 784)\"\n ]\n },\n \"execution_count\": 10,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"x_train.shape\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 11,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"(10000, 784)\"\n ]\n },\n \"execution_count\": 11,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"x_test.shape\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 12,\n \"metadata\": {},\n \"outputs\": [\n {\n \"name\": \"stdout\",\n \"output_type\": \"stream\",\n \"text\": [\n \"Train on 60000 samples, validate on 10000 samples\\n\",\n \"Epoch 1/50\\n\",\n \"60000/60000 [==============================] - 16s 266us/step - loss: 0.3626 - val_loss: 0.2716\\n\",\n \"Epoch 2/50\\n\",\n \"60000/60000 [==============================] - 15s 258us/step - loss: 0.2646 - val_loss: 0.2540\\n\",\n \"Epoch 3/50\\n\",\n \"60000/60000 [==============================] - 16s 259us/step - loss: 0.2440 - val_loss: 0.2317\\n\",\n \"Epoch 4/50\\n\",\n \"60000/60000 [==============================] - 17s 283us/step - loss: 0.2235 - val_loss: 0.2131\\n\",\n \"Epoch 5/50\\n\",\n \"60000/60000 [==============================] - 15s 253us/step - loss: 0.2074 - val_loss: 0.1994\\n\",\n \"Epoch 6/50\\n\",\n \"60000/60000 [==============================] - 16s 263us/step - loss: 0.1960 - val_loss: 0.1897\\n\",\n \"Epoch 7/50\\n\",\n \"60000/60000 [==============================] - 16s 266us/step - loss: 0.1873 - val_loss: 0.1819\\n\",\n \"Epoch 8/50\\n\",\n \"60000/60000 [==============================] - 16s 259us/step - loss: 0.1801 - val_loss: 0.1753\\n\",\n \"Epoch 9/50\\n\",\n \"60000/60000 [==============================] - 15s 257us/step - loss: 0.1740 - val_loss: 0.1696\\n\",\n \"Epoch 10/50\\n\",\n \"60000/60000 [==============================] - 16s 260us/step - loss: 0.1686 - val_loss: 0.1647\\n\",\n \"Epoch 11/50\\n\",\n \"60000/60000 [==============================] - 15s 251us/step - loss: 0.1638 - val_loss: 0.1599\\n\",\n \"Epoch 12/50\\n\",\n \"60000/60000 [==============================] - 15s 251us/step - loss: 0.1594 - val_loss: 0.1559\\n\",\n \"Epoch 13/50\\n\",\n \"60000/60000 [==============================] - 15s 253us/step - loss: 0.1554 - val_loss: 0.1519\\n\",\n \"Epoch 14/50\\n\",\n \"60000/60000 [==============================] - 15s 249us/step - loss: 0.1518 - val_loss: 0.1484\\n\",\n \"Epoch 15/50\\n\",\n \"60000/60000 [==============================] - 16s 274us/step - loss: 0.1484 - val_loss: 0.1453\\n\",\n \"Epoch 16/50\\n\",\n \"60000/60000 [==============================] - 17s 282us/step - loss: 0.1454 - val_loss: 0.1423\\n\",\n \"Epoch 17/50\\n\",\n \"60000/60000 [==============================] - 16s 264us/step - loss: 0.1426 - val_loss: 0.1397\\n\",\n \"Epoch 18/50\\n\",\n \"60000/60000 [==============================] - 16s 275us/step - loss: 0.1400 - val_loss: 0.1372\\n\",\n \"Epoch 19/50\\n\",\n \"60000/60000 [==============================] - 16s 263us/step - loss: 0.1376 - val_loss: 0.1349\\n\",\n \"Epoch 20/50\\n\",\n \"60000/60000 [==============================] - 17s 279us/step - loss: 0.1352 - val_loss: 0.1325\\n\",\n \"Epoch 21/50\\n\",\n \"60000/60000 [==============================] - 16s 270us/step - loss: 0.1330 - val_loss: 0.1304\\n\",\n \"Epoch 22/50\\n\",\n \"60000/60000 [==============================] - 16s 260us/step - loss: 0.1309 - val_loss: 0.1283\\n\",\n \"Epoch 23/50\\n\",\n \"60000/60000 [==============================] - 16s 273us/step - loss: 0.1289 - val_loss: 0.1263\\n\",\n \"Epoch 24/50\\n\",\n \"60000/60000 [==============================] - 17s 278us/step - loss: 0.1269 - val_loss: 0.1244\\n\",\n \"Epoch 25/50\\n\",\n \"60000/60000 [==============================] - 15s 247us/step - loss: 0.1250 - val_loss: 0.1226\\n\",\n \"Epoch 26/50\\n\",\n \"60000/60000 [==============================] - 16s 265us/step - loss: 0.1232 - val_loss: 0.1208\\n\",\n \"Epoch 27/50\\n\",\n \"60000/60000 [==============================] - 18s 297us/step - loss: 0.1215 - val_loss: 0.1192\\n\",\n \"Epoch 28/50\\n\",\n \"60000/60000 [==============================] - 16s 263us/step - loss: 0.1199 - val_loss: 0.1176\\n\",\n \"Epoch 29/50\\n\",\n \"60000/60000 [==============================] - 15s 250us/step - loss: 0.1184 - val_loss: 0.1161\\n\",\n \"Epoch 30/50\\n\",\n \"60000/60000 [==============================] - 17s 281us/step - loss: 0.1170 - val_loss: 0.1148\\n\",\n \"Epoch 31/50\\n\",\n \"60000/60000 [==============================] - 17s 276us/step - loss: 0.1157 - val_loss: 0.1134\\n\",\n \"Epoch 32/50\\n\",\n \"60000/60000 [==============================] - 18s 301us/step - loss: 0.1144 - val_loss: 0.1122\\n\",\n \"Epoch 33/50\\n\",\n \"60000/60000 [==============================] - 15s 248us/step - loss: 0.1132 - val_loss: 0.1111\\n\",\n \"Epoch 34/50\\n\",\n \"60000/60000 [==============================] - 16s 261us/step - loss: 0.1121 - val_loss: 0.1100\\n\",\n \"Epoch 35/50\\n\",\n \"60000/60000 [==============================] - 15s 249us/step - loss: 0.1111 - val_loss: 0.1090\\n\",\n \"Epoch 36/50\\n\",\n \"60000/60000 [==============================] - 15s 251us/step - loss: 0.1102 - val_loss: 0.1081\\n\",\n \"Epoch 37/50\\n\",\n \"60000/60000 [==============================] - 16s 272us/step - loss: 0.1093 - val_loss: 0.1073\\n\",\n \"Epoch 38/50\\n\",\n \"60000/60000 [==============================] - 17s 290us/step - loss: 0.1085 - val_loss: 0.1065\\n\",\n \"Epoch 39/50\\n\",\n \"60000/60000 [==============================] - 17s 287us/step - loss: 0.1077 - val_loss: 0.1057\\n\",\n \"Epoch 40/50\\n\",\n \"60000/60000 [==============================] - 16s 258us/step - loss: 0.1070 - val_loss: 0.1050\\n\",\n \"Epoch 41/50\\n\",\n \"60000/60000 [==============================] - 15s 245us/step - loss: 0.1063 - val_loss: 0.1043\\n\",\n \"Epoch 42/50\\n\",\n \"60000/60000 [==============================] - 15s 247us/step - loss: 0.1057 - val_loss: 0.1038\\n\",\n \"Epoch 43/50\\n\",\n \"60000/60000 [==============================] - 15s 256us/step - loss: 0.1051 - val_loss: 0.1032\\n\",\n \"Epoch 44/50\\n\",\n \"60000/60000 [==============================] - 15s 256us/step - loss: 0.1045 - val_loss: 0.1026\\n\",\n \"Epoch 45/50\\n\",\n \"60000/60000 [==============================] - 16s 274us/step - loss: 0.1040 - val_loss: 0.1021\\n\",\n \"Epoch 46/50\\n\",\n \"60000/60000 [==============================] - 17s 280us/step - loss: 0.1035 - val_loss: 0.1017\\n\",\n \"Epoch 47/50\\n\",\n \"60000/60000 [==============================] - 17s 281us/step - loss: 0.1031 - val_loss: 0.1012\\n\",\n \"Epoch 48/50\\n\",\n \"60000/60000 [==============================] - 15s 256us/step - loss: 0.1026 - val_loss: 0.1008\\n\",\n \"Epoch 49/50\\n\",\n \"60000/60000 [==============================] - 15s 250us/step - loss: 0.1023 - val_loss: 0.1005\\n\",\n \"Epoch 50/50\\n\",\n \"60000/60000 [==============================] - 15s 250us/step - loss: 0.1019 - val_loss: 0.1001\\n\"\n ]\n },\n {\n \"data\": {\n \"text/plain\": [\n \"<keras.callbacks.History at 0xb381e8a90>\"\n ]\n },\n \"execution_count\": 12,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"autoencoder.fit(x_train, x_train,\\n\",\n \" epochs = 50,\\n\",\n \" batch_size = 256,\\n\",\n \" shuffle = True,\\n\",\n \" validation_data = (x_test, x_test))\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 13,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"encoded_imgs = encoder.predict(x_test)\\n\",\n \"decoded_imgs = decoder.predict(encoded_imgs)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 15,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAABIEAAAD4CAYAAAB7VPbbAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDIuMi4yLCBodHRwOi8vbWF0cGxvdGxpYi5vcmcvhp/UCwAAIABJREFUeJzt3Xm4FMXV+PFTMaAiirKIyA7iioqAKCqKikvcF4yoMcQ9bzRq4kaiMW4x708T3BIX8qoYNQTjblRcEHdRMQqCCAKyCbKIokRx7d8fXstTxe2m79xZuqu/n+fx4fTtvjN153T19Ix1qkwURQIAAAAAAICw/aDWDQAAAAAAAEDl8SUQAAAAAABAAfAlEAAAAAAAQAHwJRAAAAAAAEAB8CUQAAAAAABAAfAlEAAAAAAAQAHwJRAAAAAAAEABNOpLIGPMvsaYacaYGcaYYeVqFKqLPOYfOQwDecw/chgG8ph/5DAM5DH/yGEYyGNYTBRFpf2iMWuIyHQR2UtE5ovIqyJyVBRFb5Wveag08ph/5DAM5DH/yGEYyGP+kcMwkMf8I4dhII/hacxIoH4iMiOKollRFH0hIv8UkYPL0yxUEXnMP3IYBvKYf+QwDOQx/8hhGMhj/pHDMJDHwPywEb/bXkTmqe35IrJD0i8YY0obdoRGi6LIxOxqUB7JYU0tjaKoTT0/py/mCH0xCPTFANAXg0BfDAB9MQj0xQDQF4MQ1xcdjfkSqL6TZJWEG2NOFpGTG/E8qKzV5pEcZsacmJ/TF8NAX8wP+mLY6Iv5QV8MG30xP+iLYaMv5kdcX3Q05kug+SLSUW13EJEF/kFRFI0QkREifCuYUavNIznMPPpiGOiL+UdfDAN9Mf/oi2GgL+YffTEM9MXANGZOoFdFpIcxpqsxpqmIDBGRB8vTLFQRecw/chgG8ph/5DAM5DH/yGEYyGP+kcMwkMfAlDwSKIqir4wxp4nIYyKyhojcEkXRlLK1DFVBHvOPHIaBPOYfOQwDecw/chgG8ph/5DAM5DE8JS8RX9KTMTSsZhIm+moQclhTr0VR1LccD0Qea4e+GAT6YgDoi0GgLwaAvhgE+mIA6ItBSNUXG1MOBgAAAAAAgJzgSyAAAAAAAIAC4EsgAAAAAACAAmjMEvFAzZx99tk2XnvttZ1922yzjY0HDx4c+xg33HCDjV966SVn3+23397YJgIAAAAAkCmMBAIAAAAAACgAvgQCAAAAAAAoAL4EAgAAAAAAKADmBEJujB492sZJc/1o33zzTey+U045xcaDBg1y9j3zzDM2njt3btomosY23XRTZ/vtt9+28RlnnGHj6667rmptKrJ11lnHxldeeaWNdd8TEXnttddsfMQRRzj75syZU6HWAQAA1MYGG2xg406dOqX6Hf+e6Fe/+pWNJ0+ebOPp06c7x02cOLGUJiJgjAQCAAAAAAAoAL4EAgAAAAAAKADKwZBZuvxLJH0JmC4Beuyxx2zcrVs357gDDzzQxt27d3f2HXPMMTb+4x//mOp5UXvbbbeds63LAefPn1/t5hReu3btbHzSSSfZ2C/T7NOnj40POOAAZ99f//rXCrUOWu/evW187733Ovu6dOlSsefde++9ne2pU6faeN68eRV7Xqyefo8UEXnwwQdtfNppp9n4xhtvdI77+uuvK9uwAG244YY2vuuuu2z84osvOseNGDHCxrNnz654u77TokULZ3vXXXe18ZgxY2z85ZdfVq1NQB7sv//+Nj7ooIOcfQMHDrTxJptskurx/DKvzp0723jNNdeM/b011lgj1eOjOBgJBAAAAAAAUAB8CQQAAAAAAFAAlIMhU/r27WvjQw89NPa4KVOm2NgfXrl06VIbr1ixwsZNmzZ1jhs/fryNt912W2dfq1atUrYYWdKrVy9n+7///a+N77vvvmo3p3DatGnjbN922201agkaap999rFx0pDycvNLjo4//ngbDxkypGrtwLf0e9/1118fe9xf/vIXG99yyy3Ovs8++6z8DQuMXhVIxL2n0aVXixYtco6rVQmYXsFRxL3W63LeGTNmVL5hObPeeus523qKgZ49e9rYX6WW0rps09NInHrqqTbWpe8iImuvvbaNjTGNfl5/FVygVIwEAgAAAAAAKAC+BAIAAAAAACgAvgQCAAAAAAAogFzPCeQvGa7rMBcsWODsW7lypY3vvPNOG7///vvOcdQz15ZeUtqvndU183r+ioULF6Z67LPOOsvZ3nLLLWOPffjhh1M9JmpP19TrZYtFRG6//fZqN6dwTj/9dBsfcsghzr5+/fo1+PH00sMiIj/4wff/r2LixIk2fvbZZxv82HD98Iff3wLst99+NWmDP9fIr3/9axuvs846zj49xxcqQ/e/Dh06xB43atQoG+v7K8Rr3bq1jUePHu3sa9mypY31XEy//OUvK9+wGBdccIGNu3bt6uw75ZRTbMx986qOOeYYG//hD39w9nXs2LHe3/HnDvrggw/K3zCUjb4+nnHGGRV9rrffftvG+rMQymeTTTaxsb5Wi7hz1A4cONDZ980339j4xhtvtPELL7zgHJfF6yQjgQAAAAAAAAqAL4EAAAAAAAAKINflYFdccYWz3aVLl1S/p4exfvLJJ86+ag6zmz9/vo39v2XChAlVa0eWPPTQQzbWQ/NE3FwtW7aswY/tLzfcpEmTBj8GsmfzzTe3sV8+4g+5R/ldddVVNtbDYkt12GGHxW7PmTPHxkceeaRznF9WhNXbfffdbdy/f38b++9HleQvla3LdJs1a+bsoxys/NZcc01n+/zzz0/1e7rUNoqisrYpVL1797axX1KgXXLJJVVozaq22morZ1uX0N93333OPt5bV6XLg66++mobt2rVyjkurr9cd911zrYuby/lnhfp+KU/urRLl/SMGTPGOe7zzz+38fLly23sv0/p+9LHH3/c2Td58mQbv/zyyzZ+/fXXneM+++yz2MdHenr6CBG3j+l7Tf+cSGuHHXaw8VdffeXsmzZtmo2ff/55Z58+57744ouSnrsUjAQCAAAAAAAoAL4EAgAAAAAAKAC+BAIAAAAAACiAXM8JpJeEFxHZZpttbDx16lRn3xZbbGHjpLrsHXfc0cbz5s2zcdySjvXRdYBLliyxsV7+3Dd37lxnu6hzAml6/o9SnXPOOTbedNNNY4/Ttbj1bSO7zj33XBv75wz9qDIeeeQRG+sl3Eull8JdsWKFs69z58421ssUv/LKK85xa6yxRqPbETq/Hl4v8z1z5kwbX3755VVr08EHH1y158Kqtt56a2e7T58+scfqe5tHH320Ym0KxYYbbuhsH3744bHHnnDCCTbW942VpucBevLJJ2OP8+cE8ufThMjZZ59t45YtWzb49/157vbdd18b+8vM6/mDqjmHSCiS5unZdtttbayXBveNHz/exvpz5ezZs53jOnXqZGM9F6xIeeZRxKr09wGnnnqqjf0+tt5669X7+++9956z/dxzz9n43XffdfbpzyB6bsp+/fo5x+lrwn777efsmzhxoo31MvOVttq7d2PMLcaYxcaYyepnLY0xTxhj3qn7d4Okx0DtkccgdCGH+UdfDAJ9MQD0xSDQFwNAXwwCfTEA9MXiSPO/cEeKyL7ez4aJyNgoinqIyNi6bWTbSCGPebdUyGEIRgp5zDv6YhhGCnnMO/piGEYKecw7+mIYRgp5LITVloNFUfSsMaaL9+ODRWRgXXybiDwtIueVsV2pjB07NnFb85f2+46/PG2vXr1srId1bb/99qnbtXLlShtPnz7dxn6Jmh4apofiV0KW81huBxxwgI31UqtNmzZ1jlu8eLGNf/Ob3zj7Pv300wq1rlFWiIi/TmiQOUzSpUsXZ7tv37421v1NJJtLaeaxL+62227O9mabbWZjPZw57dBmf7irHo6tl1oVEdljjz1snLR89f/8z//Y+IYbbkjVjkbIZV+84IILnG09JF6XHvgleeWm3/v8c6uaw+Pz2BfLLalEyeeXTWREZvvin//8Z2f7Jz/5iY31/aWIyL/+9a+qtMk3YMAAG7dt29bZN3LkSBvfcccdFW1HHvuiLlUWETnuuOPqPW7SpEnO9qJFi2w8aNCg2Mdv0aKFjXWpmYjInXfeaeP3339/9Y2tjsz2Rf/+/x//+IeNdfmXiFsOnVQiqfklYJo/3UfW5bEv3nTTTc62LuNLWu5df2/w5ptv2vi3v/2tc5z+XO/baaedbKzvQ2+55RbnOP39gr4GiIj89a9/tfE999xj40qXBpc6mUPbKIoWiojU/bvhao5HNpHH/COHYSCP+UcOw0Ae848choE85h85DAN5DFDFJ4Y2xpwsIidX+nlQOeQwDOQx/8hhGMhj/pHDMJDH/COHYSCP+UcO86XUL4EWGWPaRVG00BjTTkQWxx0YRdEIERkhImKMiUp8vor58MMPne1x48bVe1xSqVkSPdTaLz3TQ89Gjx5d0uM3Uqo8Zj2HPl0e5A8B1fRr/swzz1S0TRUUTF9Myy8f0aq5qkqZZa4v6rK7f/7zn86+pOG1ml6tTQ9xvfjii53jksov9WOcfPL39xZt2rRxjrviiitsvNZaazn7/vKXv9j4yy+/XF2zS5XJvjh48GAb+ytSzJgxw8bVXElPl/X55V9PP/20jT/66KNqNUnLXF+spF133TV2n7/qUFI5ZsZkoi9Gkftw+lxfsGCBs6+SKzytvfbazrYudfjFL35hY7+9xx9/fMXalFKm+6Iu7xARWXfddW2sVxPy71n0+9NRRx1lY78EpXv37jbeaKONnH0PPPCAjX/0ox/ZeNkyvxqr5mrWF5s3b25jf8oHPW3E0qVLnX1/+tOfbJzRqSFqoeZ90b+v06tynXjiic4+Y4yN9ecCf6qAK6+80salTh/RqlUrG+tVai+66CLnOD0tjV9KWiulloM9KCJD6+KhIvJAwrHILvKYf+QwDOQx/8hhGMhj/pHDMJDH/COHYSCPAUqzRPwoEXlJRDYzxsw3xpwgIv8rInsZY94Rkb3qtpFh5DEIXYUc5h59MQj0xQDQF4NAXwwAfTEI9MUA0BeLI83qYEfF7NqzzG1BBZHHILwbRVHfen5ODnOEvhgE+mIA6ItBoC8GgL4YBPpiAOiLxVHxiaGLaMMNv580/frrr7fxD37gDrzSy5dnsI43N+6//35ne++99673uL///e/Otr9cMvJh6623jt2n54VB4/zwh9+/PaSdA8ifW2vIkCE29uvu09JzAv3xj3+08fDhw53jmjVrZmP/PHjwwQdtPHPmzJLakVdHHHGEjfVrJOK+P1WanmPqmGOOsfHXX3/tHHfZZZfZuILzNxWaXtJWxz5/joQ33nijYm0qmv3339/Zfvzxx22s58Ly57BIS89DM3DgQGffjjvuWO/v3H333SU9V1GtueaazraeU+mqq66K/T293PStt95qY32tFhHp1q1b7GPouWoqOZ9Unh1yyCE2HjZsmLNPL9s+YMAAZ9/y5csr2zCUxL+OnXPOOTbWcwCJiLz33ns21nPzvvLKKyU9t57rp2PHjs4+/dnykUcesbE/D7Dmt/f222+3cTXnQix1TiAAAAAAAADkCF8CAQAAAAAAFADlYBVw6qmn2lgvY+wvRz9t2rSqtSk07dq1s7E/nF0P0dUlKLrMQERkxYoVFWodyk0PXz/uuOOcfa+//rqNn3jiiaq1Cd/SS4v7SwqXWgIWR5d16ZIiEZHtt9++rM+VVy1atHC240o/REovNSnFySefbGNdXjh16lTnuHHjxlWtTUWVtq9U8/wI0TXXXONs77777jbeeOONnX277rqrjXWpwEEHHVTSc+vH8Jd+12bNmmVjf4lyJNPLu/t0uZ8/ZUGcvn3rm06nfuPHj7cx97L1Syp11feN8+fPr0Zz0Ei6JEtk1VJy7auvvrLxDjvsYOPBgwc7x22++eb1/v5nn33mbG+xxRb1xiLufW7btm1j26QtWrTI2a5VGTwjgQAAAAAAAAqAL4EAAAAAAAAKgHKwMth5552dbX8W+u/omepFRCZPnlyxNoXunnvusXGrVq1ij7vjjjtsXLRVgUIyaNAgG7ds2dLZN2bMGBvrVTdQPv7KhpoealtpusTBb1NSGy+66CIbH3vssWVvV5b4K9a0b9/exqNGjap2c6zu3bvX+3PeB6svqeykHCtT4Vuvvfaas73NNtvYuFevXs6+fffd18Z61ZslS5Y4x912222pnluvNjNx4sTY41588UUbc4/UMP71VJfu6ZJLv+REr3B66KGH2thfTUj3RX/fSSedZGOd67feeitV24vAL/3RdH/7/e9/7+x74IEHbMyKiNnx1FNPOdu6dFx/RhAR6dSpk42vvfZaGyeVxuryMr/0LElcCdg333zjbN933302Pv300519CxcuTP185cRIIAAAAAAAgALgSyAAAAAAAIAC4EsgAAAAAACAAmBOoDLYb7/9nO0mTZrYeOzYsTZ+6aWXqtamEOl66969e8ce9/TTT9vYr/VFPm277bY29mt677777mo3pxB+/vOf29ivba6VAw880Mbbbbeds0+30W+vnhModJ988omzrec00HOSiLjzay1btqys7dhwww2d7bj5GZ5//vmyPi/qt8suu9j46KOPjj1u+fLlNmbp5PL68MMPbazns/C3zzvvvEY/V7du3Wys51ITca8JZ599dqOfq6iefPJJZ1v3HT3vjz9PT9y8JP7jnXrqqTb+97//7ezr0aOHjfX8Ivp9u+jatGljY/+eQM+dd+GFFzr7LrjgAhvfeOONNh4/frxznJ53ZsaMGTaeMmVKbJu22morZ1t/LuR6m8xftl3Pp7X++us7+/TcvHre3g8++MA5bu7cuTbW54T+zCEi0q9fvwa3d8SIEc72b3/7Wxvr+b5qiZFAAAAAAAAABcCXQAAAAAAAAAVAOViJ1l57bRvrpQZFRL744gsb63KkL7/8svINC4i/9LseSqdL7nx6qPOKFSvK3zBUxUYbbWTjAQMG2HjatGnOcXrZRZSPLr2qJj2EW0Rkyy23tLG+BiTxl1Uu0rXXHzKtl30+/PDDnX0PP/ywjYcPH97g5+rZs6ezrUtQunTp4uyLK4HISqlh6PT76Q9+EP///5544olqNAcVpktc/L6ny838ayXS80tof/zjH9tYl6m3aNEi9jGuu+46G/tlgCtXrrTxvffe6+zT5S777LOPjbt37+4cp6//RfOnP/3Jxr/+9a9T/56+Pv7iF7+oNy4X3f/0VBZDhgwp+3OFzC+v0v2jFH//+9+d7aRyMF2Cr8+zkSNHOsfpJeizgpFAAAAAAAAABcCXQAAAAAAAAAXAl0AAAAAAAAAFwJxAJTrnnHNs7C9VPGbMGBu/+OKLVWtTaM466yxne/vtt6/3uPvvv9/ZZln4MPzsZz+zsV5u+tFHH61Ba1At559/vrOtl8lNMnv2bBsPHTrU2aeXAS0afT30l4ref//9bTxq1KgGP/bSpUudbT33SOvWrVM9hl83j8oYPHhwvT/351K46aabqtEclNkRRxzhbP/0pz+1sZ6zQmTVZZJRHnqJd93fjj76aOc43ef03E16DiDfpZde6mxvscUWNj7ooIPqfTyRVd8Li0TPCzN69Ghn3z/+8Q8b//CH7kfhjh072jhp/rRy0HMg6nNGL1MvInLZZZdVtB0QOffcc23ckDmZfv7zn9u4lPuoWmIkEAAAAAAAQAHwJRAAAAAAAEABUA6Wkh42LyLyu9/9zsYff/yxs++SSy6pSptCl3ZJx9NOO83ZZln4MHTu3Lnen3/44YdVbgkq7ZFHHrHxZpttVtJjvPXWWzZ+/vnnG92mULz99ts21ksYi4j06tXLxptsskmDH1svg+y77bbbnO1jjjmm3uP8Je1RHh06dHC2/ZKU78yfP9/ZnjBhQsXahMr50Y9+FLvv3//+t7P9n//8p9LNKTxdGqbjUvnXSV3epMvBdt99d+e4li1b2thf0j50eklu/7q26aabxv7ennvuaeMmTZrY+KKLLnKOi5uiolS6XLtPnz5lfWzU78QTT7SxLsHzSwS1KVOmONv33ntv+RtWJYwEAgAAAAAAKAC+BAIAAAAAACgAysEStGrVysbXXnuts2+NNdawsS5lEBEZP358ZRsGhx7uKiLy5ZdfNvgxli9fHvsYejhoixYtYh9j/fXXd7bTlrPpIavnnXees+/TTz9N9RghOuCAA+r9+UMPPVTllhSTHpqctEJGUhnCiBEjbLzxxhvHHqcf/5tvvknbRMeBBx5Y0u8V2RtvvFFvXA6zZs1KdVzPnj2d7cmTJ5e1HUW10047OdtxfdhfXRP55F+H//vf/9r4z3/+c7Wbgwq76667bKzLwY488kjnOD1dAlNVpDN27Nh6f67Lp0XccrCvvvrKxrfeeqtz3N/+9jcbn3nmmc6+uDJdVEa/fv2cbX1tbN68eezv6WlG9GpgIiKff/55mVpXfYwEAgAAAAAAKIDVfglkjOlojBlnjJlqjJlijDmj7uctjTFPGGPeqft3g8o3F6Uih0FoQh7zjxwGgb4YAHIYBPpiAMhhEOiLASCHxZFmJNBXInJWFEVbiMiOInKqMWZLERkmImOjKOohImPrtpFd5DAM5DH/yGEYyGP+kcMwkMf8I4dhII/5Rw4LYrVzAkVRtFBEFtbFnxhjpopIexE5WEQG1h12m4g8LSLn1fMQuaLn+hkzZoyNu3bt6hw3c+ZMG+vl4rMqiqL/1P0bXA4nTZrU6Mf417/+5WwvXLjQxm3btrWxX29dbu+//76z/Yc//EFvfhlyHnfZZRdne6ONNqpRSyorLzm84YYbbHzFFVfEHqeXH06azyftXD9pj7vxxhtTHVchQffFctBzStW3/Z1azgEUcg71nIa+pUuX2viaa66pRnMqqbB9Uc9Noe9TREQWL15s4zwsCV/UHJZKv0/q9+eDDz7YOe73v/+9jf/5z386+6ZPn17uZgXdFx9//HFnW9+f6yXFTzrpJOe4TTbZxMYDBw5M9Vzz588voYXlEXIO/bkj11133XqP03Oqibjzbr3wwgvlb1iNNGhOIGNMFxHZTkReFpG2dV8QffdF0YblbhzKjxyGgTzmHzkMA3nMP3IYBvKYf+QwDOQx/8hh+FKvDmaMaS4i94jImVEUfRz3f/Xq+b2TReTk0pqHciKHYSCP+UcOw0Ae848choE85h85DAN5zD9yWAypvgQyxjSRb0+GO6Mourfux4uMMe2iKFpojGknIovr+90oikaIyIi6x4nK0OaK6t69u4379OkTe5xe/luXhmVVHnP4yCOPONv+MNdyOuKII0r6Pb0sZFIZy4MPPmjjCRMmxB733HPPJT5fHvOY1qGHHups69LM119/3cbPPvts1dpUCXnJ4b333mvjc845x9nXpk2bij3vkiVLnO2pU6fa+OSTv7+30CWbtZCXPNZKFEWJ21kQcg732Wef2H1z58618fLly6vRnIoKOY9JdDmY378efvjh2N/TJRAbbPD9/K76vKi2ouawHN544w0bX3jhhc6+K6+80saXX365s+/YY4+18WeffVaWtoScR30vIiJy11132fjHP/5x7O/tvvvusfu+/vprG+s+O2xY7abcCS2H+np37rnnpvqdO++809l++umny9mkzEizOpgRkZtFZGoURcPVrgdFZGhdPFREHih/81BG5DAM5DH/yGEYyGP+kcMwkMf8I4dhII/5Rw4LIs1IoJ1F5FgRedMY893Xzb8Vkf8VkbuMMSeIyFwRKW0oBaqFHOZfcyGPISCH+UdfDAM5zD/6YhjIYf7RF8NADgsizepgz4tIXDHgnuVtDioliiJymH8ryGP+kcMg0BcDQA6DQF8MADkMAn0xAOSwOFJPDB2qzp07O9v+EoDf8efE0MsiozIOO+wwZ1vXcjZp0iTVY2y11VY2bsjy7rfccouNZ8+eHXvcPffcY+O333479ePjW82aNbPxfvvtF3vc3XffbWNdQ43KmTNnjo2HDBni7DvkkENsfMYZZ5T1efWyqyIif/3rX8v6+KiOtdZaK3ZfueafgEu/L+r5DX0rV6608ZdfflnRNqE29PvkMccc4+z71a9+ZeMpU6bYeOjQoYJ8+/vf/+5sn3LKKTb276kvueQSG0+aNKmyDQuA/7515pln2rh58+Y27tu3r3Pchht+v5CW/3ni9ttvt/FFF11UhlZCxM3HW2+9ZeOkz466D+jchqxBS8QDAAAAAAAgn/gSCAAAAAAAoAAKXw6mlxwWEenUqVO9xz3zzDPOdhaXuw3dFVdc0ajfP/roo8vUEpSLLkX48MMPnX0PPvigja+55pqqtQmrevbZZ2O3dQmtfz098MADbazzOWLECOe4bxeh/JYeuov8Ou6445ztjz76yMaXXnpptZtTCN98842NJ0yY4Ozr2bOnjWfMmFG1NqE2TjzxRBufcMIJzr6bb77ZxvTFsCxZssTZHjRokI39UqTzzjvPxn7JIFZv0aJFNtb3Oscee6xz3I477mjjiy++2Nm3eHG9K62jkfbYYw8bd+jQwcZJn911mawumQ4ZI4EAAAAAAAAKgC+BAAAAAAAACsBUs6zJGJOJGqpddtnFxo888oizT88orvXr18/Z9odaZ13Ckn8NkpUcFtRrURT1Xf1hq0cea4e+GAT64mo89NBDzvbw4cNtPG7cuGo3p14h98WNN97Y2b7sssts/Nprr9k4gNX3CtsX9b2sXulJxC3ZveGGG5x9uvT6iy++qFDrGibkvpgV/urH/fv3t/EOO+xg40aUZBe2L4YkhL44ceJEG2+99daxx1155ZU21uWRAUjVFxkJBAAAAAAAUAB8CQQAAAAAAFAAfAkEAAAAAABQAIVcIn7AgAE2jpsDSERk5syZNl6xYkVF2wQAQCj0krmovgULFjjbxx9/fI1agkp5/vnnbayXRAbqM3jwYGdbz5uyySab2LgRcwIBmdCyZUsbG/P9FEeLFy92jrv66qur1qYsYiQQAAAAAABAAfAlEAAAAAAAQAEUshwsiR4eueeee9p42bJltWgOAAAAAJTs448/dra7du1ao5YAlTV8+PB640svvdQ5buHChVVrUxYxEggAAADutnN7AAAgAElEQVQAAKAA+BIIAAAAAACgAPgSCAAAAAAAoABMFEXVezJjqvdkcERRZFZ/1OqRw5p6LYqivuV4IPJYO/TFINAXA0BfDAJ9MQD0xSDQFwNAXwxCqr7ISCAAAAAAAIAC4EsgAAAAAACAAqj2EvFLRWSOiLSui2spC20QqU47OpfxsbKUQ5FitaPcefyvFOe1SyOPOaQvriqPeaQvuvKYQ/riqvKYR/qiK485pC+uKo95pC+68phD+mJt2pAqj1WdE8g+qTETylU3muc2ZKkdDZWVdtOO0mWlzbSjcbLSbtpRuqy0mXY0TlbaTTtKl5U2047GyUq7aUfpstJm2tE4WWl3FtqRhTZolIMBAAAAAAAUAF8CAQAAAAAAFECtvgQaUaPn1bLQBpHstKOhstJu2lG6rLSZdjROVtpNO0qXlTbTjsbJSrtpR+my0mba0ThZaTftKF1W2kw7Gicr7c5CO7LQBqsmcwIBAAAAAACguigHAwAAAAAAKICqfglkjNnXGDPNGDPDGDOsis97izFmsTFmsvpZS2PME8aYd+r+3aAK7ehojBlnjJlqjJlijDmjVm1pjCLnkRw2+nnJYZnUKod1z00ey4S+SA4b+dzksUzoi+Swkc9NHsuEvkgOG/nc5DGNKIqq8p+IrCEiM0Wkm4g0FZGJIrJllZ57VxHpLSKT1c+uEJFhdfEwEfl/VWhHOxHpXRevKyLTRWTLWrSFPJJDckgOyWNx80gO859D8hhGHslh/nNIHsPIIznMfw7JYwPaWMWE9BeRx9T2b0TkN1V8/i7eyTBNRNqpRE2r+osv8oCI7JWFtpBHckgOySF5LFYeyWH+c0gew8gjOcx/DsljGHkkh/nPIXlM9181y8Hai8g8tT2/7me10jaKooUiInX/bljNJzfGdBGR7UTk5Vq3pYHIYx1yWDbksOGylkMR8liKrOWRHDZc1nIoQh5LkbU8ksOGy1oORchjKbKWR3LYcFnLoQh5XEU1vwQy9fwsquLzZ4YxprmI3CMiZ0ZR9HGt29NA5FHIYQjIYRjIY/6RwzCQx/wjh2Egj/lHDsOQ5TxW80ug+SLSUW13EJEFVXx+3yJjTDsRkbp/F1fjSY0xTeTbk+HOKIrurWVbSlT4PJLDsiOHDZe1HIqQx1JkLY/ksOGylkMR8liKrOWRHDZc1nIoQh5LkbU8ksOGy1oORcjjKqr5JdCrItLDGNPVGNNURIaIyINVfH7fgyIytC4eKt/W6lWUMcaIyM0iMjWKouG1bEsjFDqP5LAiyGHDZS2HIuSxFFnLIzlsuKzlUIQ8liJreSSHDZe1HIqQx1JkLY/ksOGylkMR8riqKk+KtJ98Ozv2TBE5v4rPO0pEForIl/Ltt5MniEgrERkrIu/U/duyCu3YRb4dDjdJRN6o+2+/WrSFPJJDckgOyWPt/6MvkkPymI3/6IvkkDxm4z/6Ijkkj5X/z9Q1FAAAAAAAAAGrZjkYAAAAAAAAaoQvgQAAAAAAAAqAL4EAAAAAAAAKgC+BAAAAAAAACoAvgQAAAAAAAAqAL4EAAAAAAAAKoFFfAhlj9jXGTDPGzDDGDCtXo1Bd5DH/yGEYyGP+kcMwkMf8I4dhII/5Rw7DQB7DYqIoKu0XjVlDRKaLyF4iMl9EXhWRo6Ioeqt8zUOlkcf8I4dhII/5Rw7DQB7zjxyGgTzmHzkMA3kMzw8b8bv9RGRGFEWzRESMMf8UkYNFJPZkMMaU9o0TGi2KIhOzq0F5JIc1tTSKojb1/Jy+mCP0xSDQFwNAXwwCfTEA9MUg0BcDQF8MQlxfdDSmHKy9iMxT2/PrfoZ8IY/5MSfm5+QwDOQxP+iLYSOP+UFfDBt5zA/6YtjIY37E9UVHY0YC1fdN4Srf+hljThaRkxvxPKis1eaRHGYefTEM9MX8oy+Ggb6Yf/TFMNAX84++GAb6YmAa8yXQfBHpqLY7iMgC/6AoikaIyAgRhoZl1GrzSA4zr9B90Zjv35dKneMsI+iL+Rd8XwyovyWhL+Zf8H2xIOiL+UdfDAN9MTCNKQd7VUR6GGO6GmOaisgQEXmwPM1CFZHH/COHYSCP+UcOw0Ae848choE85h85DAN5DEzJI4GiKPrKGHOaiDwmImuIyC1RFE0pW8tQFeQx/8hhGMhj/pHDMJDH/COHYSCP+UcOw0Aew1PyEvElPRlDw2omYbb3BiGHNfVaFEV9y/FAIeUxb+Up9MUgFLYv5q2/JaEvBqGwfTEk9MUg0BcDQF8MQqq+2Jg5gYBM+sEPvq9y1B9Skj6w6A82DZH297755puSHj9EpbzW+nf81zJtXuPOi9U9ftrnAvImbV9M2z8AAMnivkhfY401nOO+/vrrqrUJQPE0Zk4gAAAAAAAA5ARfAgEAAAAAABQA5WCoKV2iIyLywx9+f0q2bdvW2bf//vvbuGvXrjZu3bq1c9yyZcts/N///tfGixYtco778MMPbTx58mRn38cff2zjL774wsafffaZc5weyrty5Upnny6bKFoJhZ9XTb9mSeUocaVX/u/obX3+iLjDq3Wb/GHWejttqRilYQ2TtvSI17U20r7u5BE+/5wg90C8uP5B+Vf4/Hvjtdde28bNmze3sf7cIeKeG5988omzj+stSsVIIAAAAAAAgALgSyAAAAAAAIAC4EsgAAAAAACAAmBOIFSdnrdlgw02cPZdcsklNu7Tp4+zr3PnzjZed9116308kVWX2fyOPy+Prqt95ZVXnH2XX365jd98800b+3MCpZ1LJi6ur115oWubS122PW0ts36u9ddf39nXq1cvG/fs2dPZp8+Fl19+2cazZs1yjtPzQ/l/i85xUh61ItVo69zo+nYRd+6ugw8+2Mbbbrutc5zuV7feequzb/z48Tb+/PPPbVyk17hcks7Zpk2b2rhJkybOvrXWWsvGuj98+eWXznE6P0nXNd0Of46EpLzqfTrO6zU0C/zXf5111rFxmzZtnH3rrbeejRcvXmzj5cuXO8fp+fGY5yQd/V6V1D80/dpW4nVOer+Le//3+y/X6fQqfU+RdC5pXE8bx8+j/ozSo0cPZ9/gwYNtfOCBB9pYzw8kIvLkk0/a+KqrrnL26flO9XXAn6cU8DESCAAAAAAAoAD4EggAAAAAAKAAgi0Ho1Qju3RZV6dOnZx9uiTBLy1Zc801bZxUUqSXhddDI/0hrkmlC3pbP55f/lDKuRTK+Ze01HvSvrRDjeNy7A9718NmN910U2ffp59+auNSl4jX0pa5hU7/rXqosy4XERHZd999bXzcccfZWJdzioh89NFHNn722WedfS+99JKNQ+k71ZRUWtKsWTMb6+ttt27dnOP0vnfffdfGOm8ibt9OKk/RbdKlZn4b/WuFXjbXvxbHtQOr0q+/Xz6t35+POuooZ1/79u1t/Nhjj9nY77O6PKHI/PcE/Vr7Zc26z+l++dVXXznHzZ0718a6JK8hJdlxbdT3XyLu9bxt27bOvrgS6qVLlzrHJfXTLIl7/04q8y/lNW7IvlLe75LOOX/qBH1Pre+H/eXJuZ6unn7dW7Ro4ew755xzbHzyySc7+/SUGDpXfu433nhjG+trtIjI2WefbePZs2fX26b6HhNgJBAAAAAAAEAB8CUQAAAAAABAAeSuHEwPFfeHMeuhrP4+PTRdP4Y/7FEPXY1biaQhklZXKPfjZ2moX1JulixZYuPu3bs7x7311ls23mabbZx9enWn6dOn2/i2225zjtPDpXWpwf777+8cd+ihh9bbPhGRjTbayMZZfY1roZQh06U+RtzqP/4KbXr4uS7/EnHPNV2ioIeyi8T3+4ZIWx4Xgrhrlz9M+cgjj7SxLifwV5/Sw9QHDhzo7HviiSdsPH/+fBszRP17aa9RfjmYLvfo16+fjVu2bOkc9/rrr9tY9x1dKus/d9I1QZch+Kug6OHxfj/9+OOP6318/308tGt2ua+nSa+JXr3moIMOcvbp8+WDDz6wse6jIsXum/rex7/O6fsKv9Ruhx12sLF+r7rvvvuc4+JWBGtIOXJcCZh//R4wYICN/ZVcp06damNdguK/P+s+m5fzIu31w3/N415Xv5ROl9fq9745c+Y4x+n7mVJXf0u6L9HvB/pe2S9BzEveqk2/nrp/3HHHHc5xe+65p439a0Jcv/V/rt8nt956a2efXklZl2gn3ediVUmf13V/9u9ZdB/T90T+fUk57kXKfT/DSCAAAAAAAIAC4EsgAAAAAACAAuBLIAAAAAAAgALI5JxAfi3eOuusY+MOHTrYWC+jKeIuy+fX7Om6W7088bx585zj9Bwiy5cvt7Ffh6drZv19unZQP5c/H8OKFSts7C+pqpdrLHUZylrOheDXL69cudLGej4IPX+PiFsrfc011zj79FLRunbar1/W9GvuP5euz/fr3ffYYw8bjx071sY6L0WU9pxKqkNv7PP6bdBzMPjzEbz//vs21nNY+OdMCPOG1Iq+1l5++eXOvp49e9rYr4XX9HXSn7tLz0Ny8cUX23jy5MnOcX79dWjSXt+T5qnQr6WIO1eBvua98MILznH6evvJJ5+stg2ro6/LXbt2dfZtu+22Np4yZYqzb8aMGTbWy2MnzX8TmqR5PUqZZ9BfNlqfB506dXL26cfUc4j47/chv/4iyTnQ1zl9/RMROfHEE22s59sRca9fr776qo0nTZrkHJe0LHxcm5Lm59L3q/48jP3797exvjcTEVmwYIGN9f2df4+U1I5anidJ18mkuUE0/55ev4/pXA8dOtQ5bsMNN7TxzJkzbXzPPfc4x+n5LpctW+bsi8u9/5rq4/z3SN1+fR3w5/RMuscOQdp7VP910XPnnX/++Tb2+7Z+bf1rpX5t9Zw9fpv0Pn+un/XXX9/Geh6p0POWRJ/b+rX0+6zOje6X++67r3Pc8ccfb2P92VHE/d7g4YcftvFDDz3kHKc/g/p9UV9DdZ/1+3nSvlIwEggAAAAAAKAA+BIIAAAAAACgADJZDuaXcullK/UwrI4dOzrHtW7d2sb+ErebbbaZjdu1a2djf8m8uGVs9XA7EXcZOL1EtYg7rEsPodVlbSLuUPdrr73W2ff222/bOKn0TA9ty/IyjnFL6L333nvOcfq183OjS3vSLpepc+iXqmy66ab1tslvb7mHLGepbK8W0g69jSsp0zkVEendu7eNN998c2ffm2++aWNdflm017zcdA70kHV/GLQ/fPo7SSV9fpmvXjpZP9c//vEP57iRI0fa2C+vzfK1MUnSUsVpl5bV5Sl6+W8RkWOPPdbGurznb3/7m3NcUml0WnG/t9122znbukTNHzL9xhtv2LjUJZNDVkrpbatWrZztvffe28b+PYt+n5wwYUK9PxcJ//qaVA6mr1/+66fL6/x9ein15557zsa61EAkvsTDL3PQ17yka4c+Tt9Di7j33npJeBG3HFeXWvvtqOS9VKUkvV/o184vcdbXrmHDhtnY/zyi6XK8QYMGOft0KeBrr73m7NPX5KRroX7N/b9Ll8/r92D/8ZKuK3nJqU+fp7oMSMT9DKrvG/3XT/dn/V7l9xW9bPtVV13l7Js2bZqNdbm2fj8WccumdUmoiHue6HKwvOamFP51R79+G2+8sY379u3rHKfLz3fZZRcbb7XVVs5x+nrt9w9dUqbLyPzPI7qEVpfYi4g8++yzNp4+fbqN/XNOn2eUgwEAAAAAACAVvgQCAAAAAAAoAL4EAgAAAAAAKIDMzAmk61H9ZWz10u+6BtevQ9f72rRp4+zTx+olbn1xS8n7y2Pq5/LnBNK1g3ouIn8Zcl0n7C8lp+tEtRBqc3U9pb+kt6519ecEKmUOiNNOO83GOhci7mupazBFRK677job61r9Skia9yNEcedw0t+uf6dLly7Ovp/+9Kc29udZGDFihI31+VSJ1znkPPr11n/5y19srJd094/TdP2y37d1nbOuwRdx+72ez+2ss85yjtNzB5155pnOvnnz5tX7eHniL+UdVw/uz1PRoUMHG59++unOvl69etlYz581a9as2OfS57k/55POv59jTc+58OMf/9jZp+ff8+f/KmXOmzxqyN8ZN79L0jVI50kvCS/iXl/9/Or7qHHjxtk4KdchSnpt9VwUW265pbNP3wP6Odb3kXpOkbTLPJe6/LruY/58GbqNEydOdPbp5Y71eZGX+dfSzrGWRH82ERH52c9+ZuOkXOv73v/85z821vOEirjzc+nruIjb//RcI35fTJqTKe4cKfVcyjL/Wqbf+4YOHers03+vnsNHz30lIjJjxgwbjx492sZ33HGHc9zs2bNt7N/fxF2z/XNGz8Hm/y36niaEXMVJmovNn8/s6KOPtvFhhx1m47Zt2zrH6ddL32P53y8sW7bMxv6cT/paqOeJ2nHHHZ3j9Hcb8+fPd/bpeaP052D9vCLJ99ilWO2jGWNuMcYsNsZMVj9raYx5whjzTt2/GyQ9BmqPPAahCznMP/piEOiLAaAvBoG+GAD6YhDoiwGgLxZHmq+URorIvt7PhonI2CiKeojI2LptZNtIIY95t1TIYQhGCnnMO/piGEYKecw7+mIYRgp5zDv6YhhGCnkshNWWg0VR9Kwxpov344NFZGBdfJuIPC0i5zWmIXqYl18ipJf71UNm/RItPWxWD50TcYdt6uG6H374oXOcXq5Rl4NtsskmznELFy60sV9KpI8955xzbOyXg+lhXWmHCPoasNx2VfKYRtJwYV0WUmrZhl4O8Ne//rWN/SGUesn54447ztkXt0RfOcrxGpJP79gVIuKODaxRDn1Jr4s/fFFvpx3Gqodp6mVYRUS22GILG7/33nvOPl3WUu5h6v7fnLYcLEt9Ma1tttnG2R4yZIiNk4an6qHpesjsyy+/7Byn+5seYi3iDtsePHiwjf1lXfXynkceeaSz7/rrr7exvtY2Yuh0VfpiUr/S+3Tsl0TutddeNvb7jn4v1GUJevnhpOf1l7HVr6dfxqJ/r1+/fjb231t1fvQwepH4spNGLFufu76Y9nqaROft8MMPd/bpckL/dR0/fryN9bW2xiUINX9f1Pei+r7RL2ds1aqVjf2yTf166vugJEnX3qSc6HadeuqpNtb9UkTkqaeesvHjjz/u7NPTKiT1xQbcF9W0L8aVTSW9z+vyZBGRnj172li/JkuWLHGOe/jhh208fPhwG/uv/89//nMb77bbbs4+/Zlm1KhRsc+V9vXX7S31vJIM9MU4uu+JiPzud7+zsX9/c+WVV9o4qdROv066RMi/DpeyrLf/Ouv3U//xy10mXeu+qOm/zT8v9WfqCy64wNmnS8CaNWtmY3/6Fn0v+uKLL9r49ddfd47Tnxf9+yPdF3U7/NIzfc33yzv1e4j+DsS/jyp36XWpxWVtoyhaKCJS9++Gqzke2UQe848choE85h85DAN5zD9yGAbymH/kMAzkMUAVnxjaGHOyiJxc6edB5ZDDMJDH/COHYSCP+UcOw0Ae848choE85h85zJdSvwRaZIxpF0XRQmNMOxFZHHdgFEUjRGSEiIgxJnYcoR7e5s/KrYfF6XItf9jjypUrbZw05E7v84/Tz6WHnj355JOxj+EP29PDxvTKRVtvvbVznC5F0+Vl9T1m3M8budJNqjymzWEpkl7/tMNY/WHVejieno3dP68uvPBCG/slKHHnTznKwZKU8Bhl74vlkDSEs5Qc637fv39/Z58e2q5XOBJxh/Kmfa4arUBU877o0+WT5557rrPPLzn6zueff+5sX3vttTa++eabbeyXv+rhr/7rr1dK3H777W3sl4PpNu23337OvnvuucfGn376qY3LvFJY2fuiPmf9a5IukdR9TK86KSKy884729gvodbvmXfffXe9P/efO2kFMD102e9vugTpwAMPtLG/Gqgerj158mRnX9Ljl1Hm+mJc6Z9I+pJa/Xt6+Loup/WP88+DK664wsZpV62qkZq9L+rzfKuttnL26fcx/31Rr2irywiSyir1c/nXB32/o1fcExG57LLLbKzLJvS9q4jI2LFjbezfo8bdA5e5X5a1LyaVbJfS7vbt28c+vi5/vvjii53j7r33Xhvr99k+ffo4x3Xs2NHG/nmg+3ClV2RrZE5r1hd1H9t1112dffq19lcA1mWQSavK6tddXytXM61Do5VjZbsS1OR9Uf9telVREbeU2Z8CQN9X6PyOGTPGOU6X/unrn39vmFQiqq/XerVcvxw4ycyZM22s74+TVvsrh1LLwR4Uke/W1BsqIg+UpzmoMvKYf+QwDOQx/8hhGMhj/pHDMJDH/COHYSCPAUqzRPwoEXlJRDYzxsw3xpwgIv8rInsZY94Rkb3qtpFh5DEIXYUc5h59MQj0xQDQF4NAXwwAfTEI9MUA0BeLI83qYEfF7Noz5ufIIPIYhHejKOpbz8/JYY7QF4NAXwwAfTEI9MUA0BeDQF8MAH2xOCo+MXQp/Fo8Pc+Erov155/Qv5dUI5u2pk4/XlKNp79P12n36NHDxn5N70svvWRjf2nrcix/m0dp5zTQ8wAdcsghznG77767jfVSfs8995xz3EMPPWTjpLlBdF2xnodDJHl+qdAlzVORtMRo2nNbP6Zedrd3797Ocbp+9r777nP2pV1qN+lvievr5ZhTIMv0PBL+PEyavq7deeedzj4971ZSLpJq2vV8XY899piN9dLxIu58NxtvvLGzr3Pnzjb2lx3PC//8irsu+fN/dO/e3cb+NUov+T1p0qTY4zS9z89p0vtily5dbDxw4EAb6zkxRETeeecdG3/wwQexj58kqZ/mUdLfU8qcQLp/+HNr6fzOmjXL2TdlypRUzxX3vEnylCe/rfpeVM/1qOcfE3Hnd/DnMtT9VC8Nrpc3FhHZaKONbKxz5d9D6uc64ogjYh9Dnz/z5s1zjnv++efrPU6kovMAVUw52qmvtf7ceNOnT7exvr/Uc9KJuOeLnk9EzyHqP75/LdT3tv651Fih3MvqXA0aNMjZp+fneuONN5x9ej6npHMmrg80ZM6eGs0lmhv6PkcvCS8icsABB9jYn1tQ/56eH01f00TcfpX2vdSfm+j888+3sZ7bzaf7lX8fOmfOHBtXae5DESl9TiAAAAAAAADkCF8CAQAAAAAAFEBmysH0kCd/2Gnc0Ci/vKqSQxiThmT5w9n33XdfG+uhnv5wzocfftjGfmlbyMP70koqs9tnn31s7C9frYcM6mGd/tKA/pLxcc+th9r6S/4lLW0dl8NKLx9ZLUmlH3HH1bcdRz9mt27dbOwP+9RDsP2hnmmvCUlL2pdSvpbHnCaV7/ivuS410OVav/3tb53j9HUt6XxJGkr9ySef2PiZZ56x8UknneQcp0tckpbRrtJyqmWhX4uk81Jfo/yhynqffi1FREaPHm1j/z0oDb9/JfWjXXbZxcatWrWysS6f8duUVG6WJI/9L61S73N0Prbffnsb+/cvuoTpX//6l7NPv98lSToP8lhGtDr+Mr7feffdd51tvQywX0qk7bXXXjbWy4SLuPcg+nn90jOdV93fRNz86Pug4cOHO8fp60XI732+pPcI/br674v6vVCX1/r07+nSv+222y62HX65n76u63J5/3OGvoamzaH/9+vtpKkTskaXiG+++ebOPv35ccGCBc6+Uv7GpPsbfQ30r9+V7C8hfNbQr51/b6NLmZP+Vl36t/feezvHxZXD6mkm/Oc+7bTTnH16qfqkXOsSzmeffdbZp++DqpknRgIBAAAAAAAUAF8CAQAAAAAAFEBmysE0f1hX3ApMpQ6ZSrsSUBI95GuzzTZz9p1yyik21kNHX3nlFec4XcbiDyfO47C9cvNzs9VWW9n4kksusbFeVUPELWvQM67rlU1Eklcr0vnVZWj+8L5SSoWSVs7K+lDbuGHS5Vj9wH8MPZT30EMPtbE/JPStt96ysV9aEsfPgV8SUYrQ+qwur/JLb3UJwR133GHjZcuWxT5eqWVY+nVduXKljf3hurpNftmTXyoR16Ys5zDpnNXt9q9Ruk/o109E5P3337dxKfnx26S3/VUyjjrq+1VndUnLhAkTnOP0Ko5pV/fz5bE8pdT7krRlx3rVuMMOO8zG/spCixcvtvG4ceOcfXGlaA1ZHVLLS25WR/+9esi/X4Ku36tatGjh7OvatauN+/XrZ2O/TFM/l76n8a+9uuzBXzFQX8/1tAT/+c9/nONCyU9j+Oe27i9Llixx9unXa7fddrPxgAEDnON22mknG++888429ksEFy5caGO/7+mSTn091e/HIm6pk/8+HlfC5Pdf//eyTLddr4Lnl9O1bNnSxn6O9b6lS5faOKkUVz+GvncVcc8Z/14kbrqTUq75pR6XF/55qc9tvRK3iPua63zoFcVE3M8W+lqr8y7illzq80rEXYVO37PMnz/fOe7RRx+1sf/e4N+bVQsjgQAAAAAAAAqAL4EAAAAAAAAKgC+BAAAAAAAACiCTcwL5dZdx9eUNqXfU9Zp6LgU934uIOydLUh2srhn1l0XedNNNbazrUEeOHOkcp+sZK7m8fV7pZf1ERI4//ngbd+7c2cb++aHnwHjooYdsrOvxRZJfc/2YulbTPyfS1gjrmlG/vXpf0rL1WVDKsvClzpXUvn17G++xxx6xj6GX4U2qq027BGravhhavbU/L5Ke68xfGlrPuaPncEk750DSuePv09eBrbfe2sb+sse6/XqpTxF37ptyzCtXC0nnpX7f8vuAnitEL6kq4r6eb7zxho31vCb+c+v3zA022MA5TtfKDxkyxNmn57DQ17yJEyc6x+la/Dzlp7FKubYmHatfYxGRvn372nibbbaJ/X39+r/zzjuJzx0nbfvzOHdTfXT/0/M7+nPGLFq0yMb+36uvXzfffLON/Wuqvhbrx/D7YrNmzWzcunVrZ5/O6x/+8Acb+3OphXxfWup8cDq/H3/8sbNPzxuy66672rhTp07OcXqOEv28/gaNv3gAABjXSURBVFLl+trozxvaq1cvG3fo0MHGM2fOdI7Tcz7589HE5TfPfVH74IMPbOznSve37bbbztl32WWX2XjGjBk2fvHFF53j9Lwz+pq64447Osfpz4Fvvvmms0/fv+r7FD0flIg7B6I/d2ja+6y859WfY+eGG26wsT/nrr4X0Z/X9VyXIiJdunSxsc5nu3btnOP0XKT+e6ueS+j555+38Z/+9CfnOD2Hm3+PpecSYol4AAAAAAAAlBVfAgEAAAAAABRAJsvBfGlLS9LSZSf+cEg95FI/lz/8q3///jb2l3/Uw3X/9re/2fjZZ591jit1+duQ6eGLekl4Efd1jluSVcQdgnf//ffbOG6Z6Pro8yJuCUefP/RSnzN6CU+/BNEfppolScNJk4byp13eMq5MU8Qtq9RDOP0h63r4pd+n0rY3qUQo7RLMSbI6DFf/Df55ue2229pYD4UVcc9ZXX5ZyvOKJJdL9unTx8a69NZfglz3U38otS6zDKXEIW5ZeD0EXkTk5ZdftvFee+3l7NPXVF3K8NFHHznH6Xzp5Y39/qavsYMGDXL26fIUPZz9tddec47zyx5KkdX+liRtaVTa9yC/nFrnfr311rOx3x90ead/LpWyNHHS9TSPeRJJLifWpQH+uVzKEtClXq/0MvN+Sdmdd95p41mzZtk46Z40bRliXpS6zLbO7/Tp0519Tz31lI0333xzG/vlO/r96b777rPxdddd5xynr8O6/EtE5PLLL7exLnfR5S0i7nt30nQGSdfdpM9MWaPbqst7nn76aec4Xe7evXt3Z5/uO0n3JmlL3HWJtn//qu9N3n77bRv/7ne/c47TpUR+DuLO5bxeXzV9zvqflcaNG2dj3fdE4v92P4dt27a18eGHH27j008/3TlO9yO/r7z66qs2PuOMM2ysr60i6T9LUg4GAAAAAACAsuJLIAAAAAAAgALgSyAAAAAAAIACyMWcQOWuQdX1fEk127rG019+84QTTrCxrjsVEfn3v/9tYz0nUNaX/84CPW/Eaaed5uzTtc56/hi9tLGIyN13323jpNc8qZ437TLSccvAi7jLMesl7fVSjyLuXCz+Eoi1lvS3l6NfJi2L3LNnTxvrPuYvW6yXUfVr7+M0ZAnmuH2lPkZWrbPOOs52jx49bKz7pYhb2+zP5aTF9TH/mqn7gJ5LQUTk//7v/2y8ySab2Niv7db96vHHH3f2+fOG5VHS+Za0LLVeOtXvH3qJeL1Mbvv27Z3j9JwTes67uXPnOsfp+aH880nT7QghN+VQjmtG0jyGep4L3Wf9OSpGjRpl47TzFibNHdeQ+eLySr8X6utS2vcjX9rXSD/Xscce6+zTc5748yGOHTvWxnqOm4bkJrQ8pp3jSOfav9bq9x39mvvz9Oj5QfWS4ToX/nP5S2DrHOr5vvTS2CLufIr+vafu3/o9OelzUdbp10y/t0yYMME5Tvedgw8+2Nmn57PT83n69zr6nEn6zKBf29atWzv71l9//XqP03Myioi89dZb9T6v/3x5ylUa+nUtx2eOpPm5FixYYOO11147th3+nJM33XSTjd99910b52HeX0YCAQAAAAAAFABfAgEAAAAAABRALsrByj1MOi099O+oo45y9vXu3dvG/jDDc88918b+UrtFkTRcUfOHV+6///71xiJuSYoeZvfSSy85x+nh7fp5/efSS+j6S2fq4bD6b/FLUPSyynq4vYi7pKcuk/CH9erhoFkrB6smv+Ro1113tbHOlR4+LeL2sVKvFWl/L+/L4ibxl4jXw8j1kGgR95zVZURTp051jtNDb/Vj6PJIEZHddtvNxuedd56zTx+r+5+fM31e+MvB6nbkdbi03259jdKvi16OVkRk0qRJNp4zZ46zb8yYMTbWJQv9+/d3jtPl0HrJ+RdeeME5TvdTf0njVq1a2ViXlPnSLpWOVenXTr/eIu4yyPp88Ye262WKq/n6p71nyIK0ZdL+35RUOltKSYe+ph5zzDHOPl1a8t577zn7Zs+e3eDnCp1+HZLeZzT/vlGXhz366KM29ktj9TQF+l42aelvv6Rv9OjRNtYlYP49ateuXW3sl+/qxy+1LDBr4v6mefPmOcctXbrUxrrUSkRk+vTpNtb3Jv59i+7POo9+KZG+X9Lvkf5j6P7cpk0b5zh9f+aX9fGeWTpdnvfnP/+53p+LuPdVzz//vLPvmWeesXEeSsA0RgIBAAAAAAAUAF8CAQAAAAAAFEAuysFKUerQYv17W265pY2HDRvmHKdX3rj++uudff6KAUVUynBmEZEBAwbYWA+hFIkvy+rWrZtznF6VSw/h69Onj3OcHtrpD+97//33bdyiRQsb+yUOJ554oo31akoiIosWLbKxLpuYMmWKc9wHH3wgWVLNoaV6KKxezUREpG/fvvX+jl4NTGTVIdlppF0BLHT6b/WHGOsSRn+1KH390ysl+iv1aYMHD7bxIYcc4uzTpUj+0Pm481EP5xYROf/8823sl+GGkFP/b9Cvi+4D/nufLoXzSwr0sXq4vC4hE3FLz/SKK/5KG7oE5cknn3T2bbPNNvW2w7/O68fwV8tBMv2+6Jcn69Jlnbfx48c7x5Wyimk5rqch9FGf/zfFrSLWELrP6nsTvYKfiNt3rr766th9pQq5BCVpGoGkFYrirrX+als690mrySWVCC5btszG06ZNs7F/Huht/2/RpStJJdN5za/Olf/a6pz4Uwzo11N/vtt+++2d43TZtO4PAwcOdI7TpUVJn02TzgWduzyVzmaNvmaKiLz66qs27tChg43911SX1A4fPtzZpz8v5i0XjAQCAAAAAAAogNV+CWSM6WiMGWeMmWqMmWKMOaPu5y2NMU8YY96p+3eD1T0WaoccBqEJecw/chgE+mIAyGEQ6IsBIIdBoC8GgBwWR5qRQF+JyFlRFG0hIjuKyKnGmC1FZJiIjI2iqIeIjK3bRnaRwzCQx/wjh2Egj/lHDsNAHvOPHIaBPOYfOSyI1c4JFEXRQhFZWBd/YoyZKiLtReRgERlYd9htIvK0iJxXz0NUTdLyzWnrl9dbbz0b33zzzTbW88yIuMuoPvfcc86+LNYERlH0n7p/M5VDv75a11b6+3QO9ZwkBxxwgHOcXrJaL5PrL/mn59HQS0KKuDXBej4UPU+UiEjz5s1j26vrrfWSyH6tr79Eb4Ivq5HHSp+/Oo962cuDDz7YOU7Xsuv5anQNr0hyjX6aNoiUNm9FqcvFZ7Uv6rleRERuv/12G1944YXOPj1vj66T18viirj9Qy+bmrRUsk/3U91XfvnLXzrH6blN/D5WAVXpi0nnWNx5nzQPSRL9mvlzhqRdvlo/lz+3jH7MpGVU9bXdP67c16as9sWG0OeI7mN77bWXc5yeb+KTTz6x8W233eYcl7bvlHr9q4Ca98W052Up71VJ7ejZs6eN9ZxPIu575tSpUxv9XElzGOm/q9Q+mqW+mPbzQtp9/nVMz3uW9BhJ55ye00bP3+efY3pZeP+6ro8t0xxPVemLSUq5l/N/R88lunjxYhv786fpOU0HDRpk4+7duzvHJd3v6Out/vzjz1NUhXsaq9Y5LAcTM3faE0884RzXsWPHen/fv3/52c9+ZmM/N+W4rtdKgyaGNsZ0EZHtRORlEWlb9wWRRFG00BizYczvnCwiJzeumSgXchgG8ph/5DAM5DH/yGEYyGP+kcMwkMf8I4fhS/0lkDGmuYjcIyJnRlH0cdr/CxRF0QgRGVH3GNkbIlMg5DAM5DH/yGEYyGP+kcMwkMf8I4dhII/5Rw6LIdWXQMaYJvLtyXBnFEX31v14kTGmXd03gu1EZHH8I1RH2lINPay1adOmzr7zzvt+dJsuK/KH4t1777029pfdzaKs5tAfJquXFT7uuOOcfZ06dbKxzqG/5J9ecjhpCLMekusvT66Xj9dDOfXviLjDa/USgiIiN954o431ObJkyRLnOD00f3WymseG0DnR5Xp9+vRxjtP9+d1337WxHt5cqoYsgRq3L2nJ7iRZzaEuuxIRuemmm2y80047Oft+9KMf2VhfQzfaaCPnuLSvib6++mVpY8eOtfHFF19sY12S6z9GNVQjj6UsVdyIcoxGP0azZs1srEtVRNxrp86xf43W54x//sSVLJTa3qz2xYbQr58uodXvYSLue+2MGTNsPHPmTOe4cpTcVbskvtp9sRzSlhD4fUCX2B577LE21n1PxO1j/nQGuoxFlxXpUkwRt8/67dXvF0Xqi7q/Jd1HJL0m/nttmsfzzwPdDn1/5N9f6hIjv8RF5zSuNKy+diXJSx7TSipx1tfULbbYwsZ6WhGfn/tly5bZ+J///KeN58+fH/tcDbl/LUUec+ifs/p6eOqpp9q4V69esY+hc33uuec6+1555RUbZ3HKl1KlWR3MiMjNIjI1iqLhateDIjK0Lh4qIg+Uv3koI3IYBvKYf+QwDOQx/8hhGMhj/pHDMJDH/COHBZFmJNDOInKsiLxpjHmj7me/FZH/FZG7jDEniMhcETmiMk1EmZDD/Gsu5DEE5DD/6IthIIf5R18MAznMP/piGMhhQaRZHex5EYkb079neZuDSomiiBzm3wrymH/kMAj0xQCQwyDQFwNADoNAXwwAOSyOBq0OlidJ83XoWtrNN9/cOe4nP/mJjfUcDLNnz3aOu/XWW2OfC+n5deYTJkyw8e677+7sO+SQQ2zcv39/G+taXBGRbt262VgvmevXjOr63g8++MDZp+uq9XKReql3EXdekkceecTZp+u0dX2+fjyRfC8vWArd//Sytv5cMHPmzLHx/fffb2O99G2pGljjXvbHzAM9V5VeHlNE5NJLL7XxkCFDbKzneBKJXxrVnwvsqaeesrGe90dE5PXXX6/390J7vVfHn/Mobn6chog7t9M+np9fPW+bPyeNvu4tWrQotg2tW7e2sZ7PQsTNfzn+/hDo66me+8XPjb5u6jmB/DkqSnldi/z6V5o/Z5a+39l5551jj9P3PvoaLeIuKa7nMvTnJ9Tnhp47SMS9HoV2D5M0F08p57r/O/r1Svt4fjv09XTWrFmxx+l5K/08pZ3vEN/Sr6WIyMsvv2zjhx9+2Mb6PUxEZJ111rHxRx995Oy77bbbbDxq1KjY4/z+p/FeuOp8Zvr+Q1///Oukfr300u/6M75/XEhWOycQAAAAAAAA8o8vgQAAAAAAAAogF+VgSUsyaklDOPW2Hpp34IEHOsfpfbr0Ry9TLLJq+VAlFWmonx5iPG/ePGffddddV2/s0+eLXqrRH6Kpl0n1zxc9FFMPw/RLkfRwaX9Yfdx5W+2lrBsjqRSq1GHM+vf08PPHH3/cOW7ixIn17ksaFpsk6fqQ9veK6uOPP3a2zzrrLBtfddVVNj7qqKOc43r37m3jhQsX2nj06NHOcboM1C8V4/X/lj+MuZQSjIac93G/p2N9DRUR6dixo4395XR1/nW5rX891MO6/SHe+lh9XoRWjpLEz6F+jfR7nF+6rF9zHeuyIZHSlsAumnL0xVLpfrV06VIbt2nTxjlO95X27ds7+/Q0CPpedvFid9Vn/Xf59zdxy5yHJuk8T3s9TTou7T7/Wti0adN6j/PLQJPOzSJ9tigH/71K95err77axnfddZdz3EYbbWRjPx8zZ860sb7P8p+La++q9Lm+1lprOft22GEHG+tro//5QX+mu/DCC23sl/6FipFAAAAAAAAABcCXQAAAAAAAAAWQi3IwTQ/DTRpGmbRSwrbbbmvjAQMGxD7G3LlzbfzCCy84+/SQvnKsHpD0GAz9axidG13W5c+2X2l6OGdeh90mrbKX9m9KWhlDrxJ09913xx736aef2tgfhl6O1TrQMDoHeuXEP/7xjzVoTTGkHdbvK8d7SVwppd8mXcI7ZswYZ1+PHj1srFfhmDZtmnOcLnHxh27r5ytSCVgS/T4zdepUG99www3Ocbp0T5df6muwSGkrFxVN0rmn7z3LcY76OdD5euCBB2ys3yNF3LIxf+XSV1991ca6pMUvIdTX+dDPhXLfo6Ut80p6Ll0C1qxZM2df8+bNbaxLYfzPPnpKhKSVaUPPbyXo10yXsfurSc+fPz/2MXhPS88/t/V53717d2ffHnvsYWP9HulP5fLMM8/Y+JVXXilLO/OEkUAAAAAAAAAFwJdAAAAAAAAABcCXQAAAAAAAAAWQizmB4uok/RpZXZ/p79NLYp500kk23myzzZzjdK2uXiLOn08m7bL1aVGPG7ZQ8hs3N0ipS0/rWl29XLy/L5TXD6iEuH5ZCfr9WM+DICIya9YsG+vlbkVEunTpYmM9749fo6/nAfKXyWUOi1Xp10jP7zJu3DjnOD0fCNfWyin3vB5Jy9E/9thjNtbzcYmITJo0ycYzZsxw9ulzgXlIvhXXDxoy36G+9vp50+L2+blIWvpdf8bRj+fP8aXnhkq6nqJy/HksURr/3kbPmaXnHBQR6datm431Z4v333/fOW7UqFE2/vDDD21clPdFRgIBAAAAAAAUAF8CAQAAAAAAFEAuysHi+MtZrrvuujZu27ats69p06Y21kMgly9f7hynh7c/8cQTNn7nnXec4/RwWn9opz/kEghROUpQdN+h3wCNV81hzP5z6ffFBQsWOPt02Zfu6/61Qz9GUYZkN4T/mujXUt+/+MfpbV7X/NLlPXPmzLGxvyy1Lsf031vj8u/3Rc6ThtGvV9L9TNqyVr3Pn5JCl7jopbJXrFgR+1xJjw9knd+n9LVw7Nixzr6ZM2fauHPnzjb2y2YnT55sY12KXhSMBAIAAAAAACgAvgQCAAAAAAAoAL4EAgAAAAAAKABTzZpQY0zNClDjllps0qSJc9w666xjY10f6C+Fq+ct8Ouos7jsYhRFZVk3uJY5hLwWRVHfcjwQeawd+mIQguuLen6upKWKk+YCS3qMLM4/QV8MQnB9UUs7314W+1dD0BeDEHRfLAr6YhBS9UVGAgEAAAAAABQAXwIBAAAAAAAUQLWXiF8qInNEpHVdXDV6qOxXX30l37WhLrb8Zecb+tglqMZr0Xn1h6RWsxzGKFI7yp3H/0pxXrs08phD+uKq8pjHmvfFuvKtel+7uPe4pOXKGymPOaQvriqPeax5X6zTWkSWZqDMK485pC+uKo95zFRfrHUjJJ85pC/Wpg2p8ljVOYHskxozoVx1o3luQ5ba0VBZaTftKF1W2kw7Gicr7aYdpctKm2lH42Sl3bSjdFlpM+1onKy0m3aULittph2Nk5V2Z6EdWWiDRjkYAAAAAABAAfAlEAAAAAAAQAHU6kugETV6Xi0LbRDJTjsaKivtph2ly0qbaUfjZKXdtKN0WWkz7WicrLSbdpQuK22mHY2TlXbTjtJlpc20o3Gy0u4stCMLbbBqMicQAAAAAAAAqotyMAAAAAAAgAKo6pdAxph9jTHTjDEzjDHDqvi8txhjFhtjJquftTTGPGGMeafu3w2q0I6OxphxxpipxpgpxpgzatWWxihyHslho5+XHJZJrXJY99zksUzoi+Swkc9NHsuEvkgOG/nc5LFM6IvksJHPTR7TiKKoKv+JyBoiMlNEuolIUxGZKCJbVum5dxWR3iIyWf3sChEZVhcPE5H/V4V2tBOR3nXxuiIyXUS2rEVbyCM5JIfkkDwWN4/kMP85JI9h5JEc5j+H5DGMPJLD/OeQPDagjVVMSH8ReUxt/0ZEflPF5+/inQzTRKSdStS0qr/4Ig+IyF5ZaAt5JIfkkBySx2LlkRzmP4fkMYw8ksP855A8hpFHcpj/HJLHdP9VsxysvYjMU9vz635WK22jKFooIlL374bVfHJjTBcR2U5EXq51WxqIPNYhh2VDDhsuazkUIY+lyFoeyWHDZS2HIuSxFFnLIzlsuKzlUIQ8liJreSSHDZe1HIqQx1VU80sgU8/Poio+f2YYY5qLyD0icmYURR/Xuj0NRB6FHIaAHIaBPOYfOQwDecw/chgG8ph/5DAMWc5jNb8Emi8iHdV2BxFZUMXn9y0yxrQTEan7d3E1ntQY00S+PRnujKLo3lq2pUSFzyM5LDty2HBZy6EIeSxF1vJIDhsuazkUIY+lyFoeyWHDZS2HIuSxFFnLIzlsuKzlUIQ8rqKaXwK9KiI9jDFdjTFNRWSIiDxYxef3PSgiQ+viofJtrV5FGWOMiNwsIlOjKBpey7Y0QqHzSA4rghw2XNZyKEIeS5G1PJLDhstaDkXIYymylkdy2HBZy6EIeSxF1vJIDhsuazkUIY+rqvKkSPvJt7NjzxSR86v4vKNEZKGIfCnffjt5goi0EpGxIvJO3b8tq9COXeTb4XCTROSNuv/2q0VbyCM5JIfkkDzW/j/6Ijkkj9n4j75IDsljNv6jL5JD8lj5/0xdQwEAAAAAABCwapaDAQAAAAAAoEb4EggAAAAAAKAA+BIIAAAAAACgAPgSCAAAAAAAoAD4EggAAAAAAKAA+BIIAAAAAACgAPgSCAAAAAAAoAD4EggAAAAAAKAA/j/YUfKSp7Y1ewAAAABJRU5ErkJggg==\\n\",\n \"text/plain\": [\n \"<Figure size 1440x288 with 20 Axes>\"\n ]\n },\n \"metadata\": {},\n \"output_type\": \"display_data\"\n }\n ],\n \"source\": [\n \"import matplotlib.pyplot as plt\\n\",\n \"\\n\",\n \"n = 10\\n\",\n \"plt.figure(figsize = (20, 4))\\n\",\n \"for i in range(n):\\n\",\n \" ax = plt.subplot(2, n, i+1)\\n\",\n \" plt.imshow(x_test[i].reshape(28, 28))\\n\",\n \" plt.gray()\\n\",\n \" \\n\",\n \" ax = plt.subplot(2, n, i+1 + n)\\n\",\n \" plt.imshow(decoded_imgs[i].reshape(28, 28))\\n\",\n \" plt.gray()\\n\",\n \"plt.show()\"\n ]\n }\n ],\n \"metadata\": {\n \"kernelspec\": {\n \"display_name\": \"Python 3\",\n \"language\": \"python\",\n \"name\": \"python3\"\n },\n \"language_info\": {\n \"codemirror_mode\": {\n \"name\": \"ipython\",\n \"version\": 3\n },\n \"file_extension\": \".py\",\n \"mimetype\": \"text/x-python\",\n \"name\": \"python\",\n \"nbconvert_exporter\": \"python\",\n \"pygments_lexer\": \"ipython3\",\n \"version\": \"3.6.5\"\n }\n },\n \"nbformat\": 4,\n \"nbformat_minor\": 2\n}"
}
{
"filename": "code/artificial_intelligence/src/bayesian_belief_network/Bayesian-Belief-Network.ipynb",
"content": "{\n \"cells\": [\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {},\n \"source\": [\n \"Bayesian Belief Network is created using pgmpy and some simple queries are done on the network.\\n\",\n \"This network is an Alarm Byaesian Beleief Network which is mentioned in Bayesian Artificial Intelligence - Section 2.5.1 (https://bayesian-intelligence.com/publications/bai/book/BAI_Chapter2.pdf)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 9,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"#Importing Library\\n\",\n \"from pgmpy.models import BayesianModel\\n\",\n \"from pgmpy.inference import VariableElimination\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 10,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"#Defining network structure\\n\",\n \"\\n\",\n \"alarm_model = BayesianModel([('Burglary', 'Alarm'), \\n\",\n \" ('Earthquake', 'Alarm'),\\n\",\n \" ('Alarm', 'JohnCalls'),\\n\",\n \" ('Alarm', 'MaryCalls')])\\n\",\n \"\\n\",\n \"#Defining the parameters using CPT\\n\",\n \"from pgmpy.factors.discrete import TabularCPD\\n\",\n \"\\n\",\n \"cpd_burglary = TabularCPD(variable='Burglary', variable_card=2,\\n\",\n \" values=[[.999], [0.001]])\\n\",\n \"cpd_earthquake = TabularCPD(variable='Earthquake', variable_card=2,\\n\",\n \" values=[[0.998], [0.002]])\\n\",\n \"cpd_alarm = TabularCPD(variable='Alarm', variable_card=2,\\n\",\n \" values=[[0.999, 0.71, 0.06, 0.05],\\n\",\n \" [0.001, 0.29, 0.94, 0.95]],\\n\",\n \" evidence=['Burglary', 'Earthquake'],\\n\",\n \" evidence_card=[2, 2])\\n\",\n \"cpd_johncalls = TabularCPD(variable='JohnCalls', variable_card=2,\\n\",\n \" values=[[0.95, 0.1], [0.05, 0.9]],\\n\",\n \" evidence=['Alarm'], evidence_card=[2])\\n\",\n \"cpd_marycalls = TabularCPD(variable='MaryCalls', variable_card=2,\\n\",\n \" values=[[0.1, 0.7], [0.9, 0.3]],\\n\",\n \" evidence=['Alarm'], evidence_card=[2])\\n\",\n \"\\n\",\n \"# Associating the parameters with the model structure\\n\",\n \"alarm_model.add_cpds(cpd_burglary, cpd_earthquake, cpd_alarm, cpd_johncalls, cpd_marycalls)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 11,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"True\"\n ]\n },\n \"execution_count\": 11,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"# Checking if the cpds are valid for the model\\n\",\n \"alarm_model.check_model()\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 12,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"NodeView(('Burglary', 'Alarm', 'Earthquake', 'JohnCalls', 'MaryCalls'))\"\n ]\n },\n \"execution_count\": 12,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"# Viewing nodes of the model\\n\",\n \"alarm_model.nodes()\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 13,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"OutEdgeView([('Burglary', 'Alarm'), ('Alarm', 'JohnCalls'), ('Alarm', 'MaryCalls'), ('Earthquake', 'Alarm')])\"\n ]\n },\n \"execution_count\": 13,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"# Viewing edges of the model\\n\",\n \"alarm_model.edges()\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 15,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"(Burglary _|_ Earthquake)\"\n ]\n },\n \"execution_count\": 15,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"#Checking independcies of a node\\n\",\n \"alarm_model.local_independencies('Burglary')\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 16,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"(Burglary _|_ Earthquake)\\n\",\n \"(Burglary _|_ MaryCalls, JohnCalls | Alarm)\\n\",\n \"(Burglary _|_ JohnCalls | MaryCalls, Alarm)\\n\",\n \"(Burglary _|_ MaryCalls, JohnCalls | Alarm, Earthquake)\\n\",\n \"(Burglary _|_ MaryCalls | JohnCalls, Alarm)\\n\",\n \"(Burglary _|_ JohnCalls | MaryCalls, Alarm, Earthquake)\\n\",\n \"(Burglary _|_ MaryCalls | Alarm, JohnCalls, Earthquake)\\n\",\n \"(Earthquake _|_ Burglary)\\n\",\n \"(Earthquake _|_ MaryCalls, JohnCalls | Alarm)\\n\",\n \"(Earthquake _|_ MaryCalls, JohnCalls | Burglary, Alarm)\\n\",\n \"(Earthquake _|_ JohnCalls | MaryCalls, Alarm)\\n\",\n \"(Earthquake _|_ MaryCalls | JohnCalls, Alarm)\\n\",\n \"(Earthquake _|_ JohnCalls | Burglary, MaryCalls, Alarm)\\n\",\n \"(Earthquake _|_ MaryCalls | Burglary, JohnCalls, Alarm)\\n\",\n \"(JohnCalls _|_ Burglary, MaryCalls, Earthquake | Alarm)\\n\",\n \"(JohnCalls _|_ MaryCalls, Earthquake | Burglary, Alarm)\\n\",\n \"(JohnCalls _|_ Burglary, Earthquake | MaryCalls, Alarm)\\n\",\n \"(JohnCalls _|_ Burglary, MaryCalls | Alarm, Earthquake)\\n\",\n \"(JohnCalls _|_ Earthquake | Burglary, MaryCalls, Alarm)\\n\",\n \"(JohnCalls _|_ MaryCalls | Burglary, Alarm, Earthquake)\\n\",\n \"(JohnCalls _|_ Burglary | MaryCalls, Alarm, Earthquake)\\n\",\n \"(MaryCalls _|_ Burglary, JohnCalls, Earthquake | Alarm)\\n\",\n \"(MaryCalls _|_ JohnCalls, Earthquake | Burglary, Alarm)\\n\",\n \"(MaryCalls _|_ Burglary, JohnCalls | Alarm, Earthquake)\\n\",\n \"(MaryCalls _|_ Burglary, Earthquake | JohnCalls, Alarm)\\n\",\n \"(MaryCalls _|_ JohnCalls | Burglary, Alarm, Earthquake)\\n\",\n \"(MaryCalls _|_ Earthquake | Burglary, JohnCalls, Alarm)\\n\",\n \"(MaryCalls _|_ Burglary | Alarm, JohnCalls, Earthquake)\"\n ]\n },\n \"execution_count\": 16,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"#Listing all Independencies\\n\",\n \"alarm_model.get_independencies()\"\n ]\n }\n ],\n \"metadata\": {\n \"kernelspec\": {\n \"display_name\": \"Python 3\",\n \"language\": \"python\",\n \"name\": \"python3\"\n },\n \"language_info\": {\n \"codemirror_mode\": {\n \"name\": \"ipython\",\n \"version\": 3\n },\n \"file_extension\": \".py\",\n \"mimetype\": \"text/x-python\",\n \"name\": \"python\",\n \"nbconvert_exporter\": \"python\",\n \"pygments_lexer\": \"ipython3\",\n \"version\": \"3.7.4\"\n }\n },\n \"nbformat\": 4,\n \"nbformat_minor\": 4\n}"
}
{
"filename": "code/artificial_intelligence/src/bernoulli_naive_bayes/bernoulli.py",
"content": "mport numpy as np\nfrom sklearn.naive_bayes import BernoulliNB \nfrom sklearn.metrics import accuracy_score\nimport matplotlib.pyplot as plt\n\n#creating dataset with with 0 and 1\n\nX = np.random.randint(2, size=(500,10))\nY = np.random.randint(2, size=(500, 1))\n\nX_test = X[:50, :10]\ny_test = Y[:50, :1]\n\nclf = BernoulliNB()\nmodel = clf.fit(X, Y)\n\ny_pred =clf.predict(X_test)\nacc_score = accuracy_score(y_test, y_pred)\nprint(acc_score)\n\nplt.scatter(X[:, 0], X[:, 1], c=y, s=50, cmap='RdBu')\nl = plt.axis()\nplt.scatter(Xtest[:, 0], Xtest[:, 1], c=Ytest, s=20, cmap='RdBu', alpha=0.1)\nplt.axis(l);"
}
{
"filename": "code/artificial_intelligence/src/bernoulli_naive_bayes/README.md",
"content": "Bernoulli Naive Bayes is a probabilistic classification algorithm and is one of the three variants of Naive Bayes. Bernoulli Naive bayes classifier uses bernoulli distribution for the classification. \n\nThe article in the OpenGenus discusses about Bernoulli Naive Bayes in Detail. \nIt covers:\n1. Brief introduction about Naive Byaes\n2. Bernoulli Naive Bayes and Beroulli distribution\n3. Algorithm and steps on how to solve it manually\n4. Detailed python code for Bernoulli Naive Bayes\n\nLearn more about it at: https://iq.opengenus.org/bernoulli-naive-bayes/"
}
{
"filename": "code/artificial_intelligence/src/chatbot/Chatbot.ipynb",
"content": "{\n \"cells\": [\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"sQ5qZbOambNh\"\n },\n \"source\": [\n \"# **Importing Libraries**\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 40,\n \"metadata\": {\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\"\n },\n \"id\": \"3mjisqmlmRk8\",\n \"outputId\": \"c28ec844-ece8-4961-f054-cb4fb4e7d157\"\n },\n \"outputs\": [\n {\n \"name\": \"stdout\",\n \"output_type\": \"stream\",\n \"text\": [\n \"[nltk_data] Downloading package punkt to /root/nltk_data...\\n\",\n \"[nltk_data] Package punkt is already up-to-date!\\n\"\n ]\n }\n ],\n \"source\": [\n \"import nltk #for tokenization, stemming and vectorization of words\\n\",\n \"nltk.download('punkt') #for the first time\\n\",\n \"from nltk.stem.porter import PorterStemmer # for stemming\\n\",\n \"import json #for reading and manipulating training data\\n\",\n \"import numpy as np\\n\",\n \"import torch\\n\",\n \"import torch.nn as nn\\n\",\n \"from torch.utils.data import Dataset, DataLoader\\n\",\n \"import random #for random results\"\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"VpZlpCcqsMjM\"\n },\n \"source\": [\n \"# **Creating Training Data**\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 3,\n \"metadata\": {\n \"id\": \"ItxwbvTymmVg\"\n },\n \"outputs\": [],\n \"source\": [\n \"def tokenize(sentence):\\n\",\n \" return nltk.word_tokenize(sentence)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 4,\n \"metadata\": {\n \"id\": \"dSkLLZbJmomO\"\n },\n \"outputs\": [],\n \"source\": [\n \"stemmer = PorterStemmer()\\n\",\n \"def stem(word):\\n\",\n \" return stemmer.stem(word.lower())\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 5,\n \"metadata\": {\n \"id\": \"31ShRSAZmyKP\"\n },\n \"outputs\": [],\n \"source\": [\n \"def bag_of_words(tokenized_sentence, all_words):\\n\",\n \" \\\"\\\"\\\"\\n\",\n \" Argument:\\n\",\n \" sentence : [\\\"hello\\\", \\\"how\\\", \\\"are\\\", \\\"you\\\"]\\n\",\n \" all_words : [\\\"hi\\\", \\\"hello\\\", \\\"I\\\", \\\"you\\\", \\\"thank\\\", \\\"cool\\\"]\\n\",\n \"\\n\",\n \" Returns:\\n\",\n \" bag = [0, 1, 0, 1, 0, 0] \\n\",\n \" \\\"\\\"\\\"\\n\",\n \" tokenized_sentence= [stem(w) for w in tokenized_sentence]\\n\",\n \"\\n\",\n \" bag = np.zeros(len(all_words), dtype = np.float32) \\n\",\n \"\\n\",\n \" for idx, w in enumerate(all_words):\\n\",\n \" if w in tokenized_sentence:\\n\",\n \" bag[idx] = 1.0\\n\",\n \"\\n\",\n \" return bag\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 6,\n \"metadata\": {\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\"\n },\n \"id\": \"74a_bZ1sqmLG\",\n \"outputId\": \"2a70a34f-14ca-4b2d-c6eb-7718a954eab5\"\n },\n \"outputs\": [\n {\n \"name\": \"stdout\",\n \"output_type\": \"stream\",\n \"text\": [\n \"Words: [\\\"'s\\\", 'a', 'accept', 'anyon', 'are', 'bye', 'can', 'card', 'cash', 'credit', 'day', 'deliveri', 'do', 'doe', 'funni', 'get', 'good', 'goodby', 'have', 'hello', 'help', 'hey', 'hi', 'how', 'i', 'is', 'item', 'joke', 'kind', 'know', 'later', 'long', 'lot', 'mastercard', 'me', 'my', 'of', 'onli', 'pay', 'paypal', 'see', 'sell', 'ship', 'someth', 'take', 'tell', 'thank', 'that', 'there', 'what', 'when', 'which', 'with', 'you']\\n\",\n \"Tags: ['delivery', 'funny', 'goodbye', 'greeting', 'items', 'payments', 'thanks']\\n\",\n \"Xtrain: [[0. 0. 0. ... 0. 0. 0.]\\n\",\n \" [0. 0. 0. ... 0. 0. 0.]\\n\",\n \" [0. 0. 0. ... 0. 0. 1.]\\n\",\n \" ...\\n\",\n \" [0. 1. 0. ... 0. 0. 0.]\\n\",\n \" [0. 0. 0. ... 0. 0. 0.]\\n\",\n \" [0. 1. 0. ... 0. 0. 1.]]\\n\",\n \"YTrain: [3 3 3 3 3 3 2 2 2 6 6 6 6 4 4 4 5 5 5 5 0 0 0 1 1 1]\\n\"\n ]\n }\n ],\n \"source\": [\n \"with open('intents.json', \\\"r\\\") as file:\\n\",\n \" intents = json.load(file)\\n\",\n \"\\n\",\n \"all_words = []\\n\",\n \"tags = []\\n\",\n \"data = []\\n\",\n \"\\n\",\n \"for intent in intents['intents']:\\n\",\n \" tag = intent['tag']\\n\",\n \" tags.append(tag)\\n\",\n \" for pattern in intent['patterns']:\\n\",\n \" w = tokenize(pattern)\\n\",\n \" all_words.extend(w)\\n\",\n \" data.append((w, tag))\\n\",\n \"\\n\",\n \"ignore_words = ['?', \\\"!\\\", \\\".\\\", \\\",\\\"] #remove punctionations\\n\",\n \"\\n\",\n \"all_words = [stem(word) for word in all_words if word not in ignore_words]\\n\",\n \"all_words = sorted(set(all_words))\\n\",\n \"tags = sorted(set(tags))\\n\",\n \"print(\\\"Words: \\\", all_words)\\n\",\n \"print(\\\"Tags: \\\", tags)\\n\",\n \"\\n\",\n \"x_train = [] #bag of words\\n\",\n \"y_train = [] #tag numbers\\n\",\n \"\\n\",\n \"for pattern_sentence, tag in data:\\n\",\n \" bag = bag_of_words(pattern_sentence, all_words)\\n\",\n \" x_train.append(bag)\\n\",\n \"\\n\",\n \" class_label = tags.index(tag) \\n\",\n \" y_train.append(class_label) \\n\",\n \"\\n\",\n \"x_train = np.array(x_train)\\n\",\n \"y_train = np.array(y_train)\\n\",\n \"\\n\",\n \"print(\\\"Xtrain: \\\", x_train)\\n\",\n \"print(\\\"YTrain: \\\", y_train) \"\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"1L3JKdE09J50\"\n },\n \"source\": [\n \"# **Dataset class**\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 7,\n \"metadata\": {\n \"id\": \"1ecCjrFHry_r\"\n },\n \"outputs\": [],\n \"source\": [\n \"class ChatDataset(Dataset):\\n\",\n \" def __init__(self):\\n\",\n \" self.n_samples = len(x_train)\\n\",\n \" self.x_data = x_train\\n\",\n \" self.y_data = y_train\\n\",\n \" \\n\",\n \" def __getitem__(self, index):\\n\",\n \" return (self.x_data[index], self.y_data[index])\\n\",\n \" \\n\",\n \" def __len__(self):\\n\",\n \" return self.n_samples\"\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"Kej75I1M9IuT\"\n },\n \"source\": [\n \"# **Hyper parameters**\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 24,\n \"metadata\": {\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\"\n },\n \"id\": \"AXx2wCnU9REF\",\n \"outputId\": \"d77ae677-9f1e-414e-bbbe-17bdbad19a2b\"\n },\n \"outputs\": [\n {\n \"name\": \"stdout\",\n \"output_type\": \"stream\",\n \"text\": [\n \"cuda\\n\"\n ]\n }\n ],\n \"source\": [\n \"BATCH_SIZE = 8\\n\",\n \"INPUT_SIZE = len(all_words) #or len(x_train[0])\\n\",\n \"HIDDEN_SIZE = 8\\n\",\n \"OUTPUT_SIZE = len(tags) #no of classes\\n\",\n \"\\n\",\n \"LEARNING_RATE = 0.001\\n\",\n \"EPOCHS = 1000\\n\",\n \"\\n\",\n \"DEVICE = torch.device(\\\"cuda\\\" if torch.cuda.is_available() else \\\"cpu\\\")\\n\",\n \"print(DEVICE)\\n\",\n \"\\n\",\n \"FILE = \\\"data.pth\\\"\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 10,\n \"metadata\": {\n \"id\": \"ajFcXiv_9Gcu\"\n },\n \"outputs\": [],\n \"source\": [\n \"dataset = ChatDataset()\\n\",\n \"train_loader = DataLoader(dataset = dataset, batch_size = BATCH_SIZE, shuffle=True, num_workers = 2)\"\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"SkZGONQp9qEs\"\n },\n \"source\": [\n \"# **Architecture and Model**\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 11,\n \"metadata\": {\n \"id\": \"uAG5MdVd9gY8\"\n },\n \"outputs\": [],\n \"source\": [\n \"class NNet(nn.Module):\\n\",\n \" def __init__(self, input_size, hidden_size, num_classes):\\n\",\n \" super(NNet, self).__init__()\\n\",\n \" self.l1 = nn.Linear(input_size, hidden_size)\\n\",\n \" self.l2 = nn.Linear(hidden_size, hidden_size)\\n\",\n \" self.l3 = nn.Linear(hidden_size, num_classes)\\n\",\n \" self.relu = nn.ReLU()\\n\",\n \" \\n\",\n \" def forward(self, x):\\n\",\n \" out = self.l1(x)\\n\",\n \" out = self.relu(out)\\n\",\n \" out = self.l2(out)\\n\",\n \" out = self.relu(out) \\n\",\n \" out = self.l3(out)\\n\",\n \" #no activation and no softmax\\n\",\n \" return out\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 12,\n \"metadata\": {\n \"id\": \"jpV7X0AC-1iF\"\n },\n \"outputs\": [],\n \"source\": [\n \"model = NNet(INPUT_SIZE, HIDDEN_SIZE, OUTPUT_SIZE).to(DEVICE)\"\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"5f6zchUPAS8E\"\n },\n \"source\": [\n \"# **Loss function and optimization**\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 13,\n \"metadata\": {\n \"id\": \"mM84VIzq_Ss1\"\n },\n \"outputs\": [],\n \"source\": [\n \"criterion = nn.CrossEntropyLoss()\\n\",\n \"optimizer = torch.optim.Adam(model.parameters(), lr = LEARNING_RATE)\"\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"vdBeS6-LAtYh\"\n },\n \"source\": [\n \"# **Training**\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 14,\n \"metadata\": {\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\"\n },\n \"id\": \"ZT66QhP0AoBu\",\n \"outputId\": \"d7120795-7b3e-4a7f-f6cb-80542e71bb5b\"\n },\n \"outputs\": [\n {\n \"name\": \"stdout\",\n \"output_type\": \"stream\",\n \"text\": [\n \"Epoch 100/1000, loss = 1.5947\\n\",\n \"Epoch 200/1000, loss = 0.5184\\n\",\n \"Epoch 300/1000, loss = 0.0091\\n\",\n \"Epoch 400/1000, loss = 0.0103\\n\",\n \"Epoch 500/1000, loss = 0.0011\\n\",\n \"Epoch 600/1000, loss = 0.0008\\n\",\n \"Epoch 700/1000, loss = 0.0038\\n\",\n \"Epoch 800/1000, loss = 0.0007\\n\",\n \"Epoch 900/1000, loss = 0.0012\\n\",\n \"Epoch 1000/1000, loss = 0.0003\\n\",\n \"Final loss = 0.0003\\n\",\n \"Training complete... file saved to data.pth\\n\"\n ]\n }\n ],\n \"source\": [\n \"for epoch in range(EPOCHS):\\n\",\n \" for words, labels in train_loader:\\n\",\n \" words = words.to(DEVICE)\\n\",\n \" labels = labels.to(DEVICE)\\n\",\n \"\\n\",\n \" #forward pass\\n\",\n \" outputs = model(words)\\n\",\n \" loss = criterion(outputs, labels)\\n\",\n \"\\n\",\n \" #backward pass\\n\",\n \" optimizer.zero_grad() #empty the gradient before calculating gradient for every epoch\\n\",\n \" loss.backward() #run back prop\\n\",\n \" optimizer.step() #update parameters\\n\",\n \"\\n\",\n \" if(epoch + 1) % 100 == 0:\\n\",\n \" print(f'Epoch {epoch+1}/{EPOCHS}, loss = {loss.item():.4f}')\\n\",\n \"\\n\",\n \"model_data = {\\n\",\n \" \\\"model_state\\\": model.state_dict(),\\n\",\n \" \\\"input_size\\\": INPUT_SIZE,\\n\",\n \" \\\"output_size\\\": OUTPUT_SIZE,\\n\",\n \" \\\"hidden_size\\\": HIDDEN_SIZE,\\n\",\n \" \\\"all_words\\\": all_words,\\n\",\n \" \\\"tags\\\": tags,\\n\",\n \"}\\n\",\n \"\\n\",\n \"print(f'Final loss = {loss.item():.4f}')\\n\",\n \"torch.save(model_data, FILE)\\n\",\n \"print(f\\\"Training complete... file saved to {FILE}\\\")\"\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"QwDlPLSsD9_s\"\n },\n \"source\": [\n \"# **ChatBOT**\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 31,\n \"metadata\": {\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\"\n },\n \"id\": \"umtH77j9DW2P\",\n \"outputId\": \"2365e5cb-73aa-434f-8821-247bdb63c9af\"\n },\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"NNet(\\n\",\n \" (l1): Linear(in_features=54, out_features=8, bias=True)\\n\",\n \" (l2): Linear(in_features=8, out_features=8, bias=True)\\n\",\n \" (l3): Linear(in_features=8, out_features=7, bias=True)\\n\",\n \" (relu): ReLU()\\n\",\n \")\"\n ]\n },\n \"execution_count\": 31,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"final_model_data = torch.load(FILE) #loading model\\n\",\n \"\\n\",\n \"model_state = final_model_data[\\\"model_state\\\"]\\n\",\n \"all_words = final_model_data[\\\"all_words\\\"]\\n\",\n \"\\n\",\n \"best_model = NNet(INPUT_SIZE, HIDDEN_SIZE, OUTPUT_SIZE).to(DEVICE)\\n\",\n \"best_model.load_state_dict(model_state)\\n\",\n \"best_model.eval() #change to evalutation stage\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 39,\n \"metadata\": {\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\"\n },\n \"id\": \"VJbnC_2BDcUX\",\n \"outputId\": \"2e180598-e141-41f9-93d8-261678d8baea\"\n },\n \"outputs\": [\n {\n \"name\": \"stdout\",\n \"output_type\": \"stream\",\n \"text\": [\n \"Let's chat! type 'quit to exit\\n\",\n \"You: Hello\\n\",\n \"STARY: Hi there, how can I help?\\n\",\n \"You: WHat do you sell?\\n\",\n \"STARY: We sell coffee and tea\\n\",\n \"You: Nice. How long does shipping take?\\n\",\n \"STARY: Shipping takes 2-4 days\\n\",\n \"You: Ok. \\n\",\n \"STARY: Sorry, I do not understand...\\n\",\n \"You: thanks\\n\",\n \"STARY: Any time!\\n\",\n \"You: see you\\n\",\n \"STARY: Have a nice day\\n\",\n \"You: quit\\n\"\n ]\n }\n ],\n \"source\": [\n \"BOT_NAME = \\\"STARY\\\"\\n\",\n \"print(\\\"Let's chat! type 'quit to exit\\\")\\n\",\n \"\\n\",\n \"while True:\\n\",\n \" sentence = input(\\\"You: \\\")\\n\",\n \" if(sentence == 'quit'):\\n\",\n \" break\\n\",\n \" \\n\",\n \" #tokenize and bag of words, same as training\\n\",\n \" sentence = tokenize(sentence)\\n\",\n \" x = bag_of_words(sentence, all_words)\\n\",\n \" x = x.reshape(1, x.shape[0])\\n\",\n \" x= torch.from_numpy(x).to(DEVICE)\\n\",\n \"\\n\",\n \" output = best_model(x)\\n\",\n \" _, predicted = torch.max(output, dim = 1)\\n\",\n \" tag = tags[predicted.item()] #get tag of the sentence spoken by user\\n\",\n \" \\n\",\n \" #to get probabilities of outputs\\n\",\n \" probs = torch.softmax(output, dim = 1)\\n\",\n \" prob = probs[0][predicted.item()]\\n\",\n \" if(prob.item() > 0.75): \\n\",\n \" #loop through all tags in intent to select a random sentence from responses of that specific tag\\n\",\n \" for intent in intents[\\\"intents\\\"]:\\n\",\n \" if tag == intent[\\\"tag\\\"]:\\n\",\n \" print(f\\\"{BOT_NAME}: {random.choice(intent['responses'])}\\\")\\n\",\n \" else:\\n\",\n \" print(f\\\"{BOT_NAME}: Sorry, I do not understand...\\\")\"\n ]\n }\n ],\n \"metadata\": {\n \"accelerator\": \"GPU\",\n \"colab\": {\n \"name\": \"Chatbot.ipynb\",\n \"provenance\": []\n },\n \"kernelspec\": {\n \"display_name\": \"Python 3\",\n \"name\": \"python3\"\n },\n \"language_info\": {\n \"name\": \"python\"\n }\n },\n \"nbformat\": 4,\n \"nbformat_minor\": 0\n}"
}
{
"filename": "code/artificial_intelligence/src/chatbot/intents.json",
"content": "{\n \"intents\": [\n {\n \"tag\": \"greeting\",\n \"patterns\": [\n \"Hi\",\n \"Hey\",\n \"How are you\",\n \"Is anyone there?\",\n \"Hello\",\n \"Good day\"\n ],\n \"responses\": [\n \"Hey :-)\",\n \"Hello, thanks for visiting\",\n \"Hi there, what can I do for you?\",\n \"Hi there, how can I help?\"\n ]\n },\n {\n \"tag\": \"goodbye\",\n \"patterns\": [\"Bye\", \"See you later\", \"Goodbye\"],\n \"responses\": [\n \"See you later, thanks for visiting\",\n \"Have a nice day\",\n \"Bye! Come back again soon.\"\n ]\n },\n {\n \"tag\": \"thanks\",\n \"patterns\": [\"Thanks\", \"Thank you\", \"That's helpful\", \"Thank's a lot!\"],\n \"responses\": [\"Happy to help!\", \"Any time!\", \"My pleasure\"]\n },\n {\n \"tag\": \"items\",\n \"patterns\": [\n \"Which items do you have?\",\n \"What kinds of items are there?\",\n \"What do you sell?\"\n ],\n \"responses\": [\n \"We sell coffee and tea\",\n \"We have coffee and tea\"\n ]\n },\n {\n \"tag\": \"payments\",\n \"patterns\": [\n \"Do you take credit cards?\",\n \"Do you accept Mastercard?\",\n \"Can I pay with Paypal?\",\n \"Are you cash only?\"\n ],\n \"responses\": [\n \"We accept VISA, Mastercard and Paypal\",\n \"We accept most major credit cards, and Paypal\"\n ]\n },\n {\n \"tag\": \"delivery\",\n \"patterns\": [\n \"How long does delivery take?\",\n \"How long does shipping take?\",\n \"When do I get my delivery?\"\n ],\n \"responses\": [\n \"Delivery takes 2-4 days\",\n \"Shipping takes 2-4 days\"\n ]\n },\n {\n \"tag\": \"funny\",\n \"patterns\": [\n \"Tell me a joke!\",\n \"Tell me something funny!\",\n \"Do you know a joke?\"\n ],\n \"responses\": [\n \"Why did the hipster burn his mouth? He drank the coffee before it was cool.\",\n \"What did the buffalo say when his son left for college? Bison.\"\n ]\n }\n ]\n}"
}
{
"filename": "code/artificial_intelligence/src/chatbot/README.md",
"content": "# Contexual Chatbot\nChatbots are interactive tools that are used as a computerized replica of a receptionist or guide. It is expected to give meaningful responses to questions posed to it. When this happens for a specialized domain or topic, it is known as Contextual Chatbot. \n\nHere, I have implemented a simple chatbot, named Stary for a Coffee retail store.\n\n# Packages\nThe following packages are used :\n1. pytorch\n2. nltk\n3. numpy\n4. random\n5. json\n\n# Steps of Implementation\nBackground : An intents file consists of tags, patterns and expected responses for a posed question/remark.\n1. Using NLTK, on has to tokenize, stem and vectorize words using bag of words. This forms the X (inputs) of the training dataset.\n2. The tags relating to each specific input (X) forms the Y(output). Thus, bag of words(X) and labels/tags(Y) form the labeled training set.\n3. We use a simple feedforward network of 3 linear layers with Relu as the non-linearity. \n4. Model is trained based on hyperparameters and saved a file.\n5. This file is loaded to perform testing.\n6. To get probability of each tag from the derived predictions, we use a Softmax classifier and choose appropriate response.\n\n# Steps to replicate results\n1. Downloading this jupyter notebook on Google Colab. Alternatively, they can also load the dataset to their own computers instead of using Google Drive or Kaggle. You have to change the filepath while downloading intents file and model saved file accordingly.\n2. Running all the cells **sequentially** in the order as in the notebook.\n3. It's done! You can go ahead and try the same algorithm on different datasets."
}
{
"filename": "code/artificial_intelligence/src/convolutional_neural_network/cnn.ipynb",
"content": "{\n \"cells\": [\n {\n \"cell_type\": \"code\",\n \"execution_count\": 1,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"import numpy as np\\n\",\n \"import matplotlib.pyplot as plt\\n\",\n \"import pandas as pd\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 2,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"training_set = pd.read_csv('train.csv')\\n\",\n \"test_set = pd.read_csv('test.csv')\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 3,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/html\": [\n \"<div>\\n\",\n \"<style scoped>\\n\",\n \" .dataframe tbody tr th:only-of-type {\\n\",\n \" vertical-align: middle;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe tbody tr th {\\n\",\n \" vertical-align: top;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe thead th {\\n\",\n \" text-align: right;\\n\",\n \" }\\n\",\n \"</style>\\n\",\n \"<table border=\\\"1\\\" class=\\\"dataframe\\\">\\n\",\n \" <thead>\\n\",\n \" <tr style=\\\"text-align: right;\\\">\\n\",\n \" <th></th>\\n\",\n \" <th>label</th>\\n\",\n \" <th>pixel0</th>\\n\",\n \" <th>pixel1</th>\\n\",\n \" <th>pixel2</th>\\n\",\n \" <th>pixel3</th>\\n\",\n \" <th>pixel4</th>\\n\",\n \" <th>pixel5</th>\\n\",\n \" <th>pixel6</th>\\n\",\n \" <th>pixel7</th>\\n\",\n \" <th>pixel8</th>\\n\",\n \" <th>...</th>\\n\",\n \" <th>pixel774</th>\\n\",\n \" <th>pixel775</th>\\n\",\n \" <th>pixel776</th>\\n\",\n \" <th>pixel777</th>\\n\",\n \" <th>pixel778</th>\\n\",\n \" <th>pixel779</th>\\n\",\n \" <th>pixel780</th>\\n\",\n \" <th>pixel781</th>\\n\",\n \" <th>pixel782</th>\\n\",\n \" <th>pixel783</th>\\n\",\n \" </tr>\\n\",\n \" </thead>\\n\",\n \" <tbody>\\n\",\n \" <tr>\\n\",\n \" <th>0</th>\\n\",\n \" <td>1</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>1</th>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>2</th>\\n\",\n \" <td>1</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>3</th>\\n\",\n \" <td>4</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>4</th>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" </tr>\\n\",\n \" </tbody>\\n\",\n \"</table>\\n\",\n \"<p>5 rows × 785 columns</p>\\n\",\n \"</div>\"\n ],\n \"text/plain\": [\n \" label pixel0 pixel1 pixel2 pixel3 pixel4 pixel5 pixel6 pixel7 \\\\\\n\",\n \"0 1 0 0 0 0 0 0 0 0 \\n\",\n \"1 0 0 0 0 0 0 0 0 0 \\n\",\n \"2 1 0 0 0 0 0 0 0 0 \\n\",\n \"3 4 0 0 0 0 0 0 0 0 \\n\",\n \"4 0 0 0 0 0 0 0 0 0 \\n\",\n \"\\n\",\n \" pixel8 ... pixel774 pixel775 pixel776 pixel777 pixel778 \\\\\\n\",\n \"0 0 ... 0 0 0 0 0 \\n\",\n \"1 0 ... 0 0 0 0 0 \\n\",\n \"2 0 ... 0 0 0 0 0 \\n\",\n \"3 0 ... 0 0 0 0 0 \\n\",\n \"4 0 ... 0 0 0 0 0 \\n\",\n \"\\n\",\n \" pixel779 pixel780 pixel781 pixel782 pixel783 \\n\",\n \"0 0 0 0 0 0 \\n\",\n \"1 0 0 0 0 0 \\n\",\n \"2 0 0 0 0 0 \\n\",\n \"3 0 0 0 0 0 \\n\",\n \"4 0 0 0 0 0 \\n\",\n \"\\n\",\n \"[5 rows x 785 columns]\"\n ]\n },\n \"execution_count\": 3,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"training_set.head()\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 4,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/html\": [\n \"<div>\\n\",\n \"<style scoped>\\n\",\n \" .dataframe tbody tr th:only-of-type {\\n\",\n \" vertical-align: middle;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe tbody tr th {\\n\",\n \" vertical-align: top;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe thead th {\\n\",\n \" text-align: right;\\n\",\n \" }\\n\",\n \"</style>\\n\",\n \"<table border=\\\"1\\\" class=\\\"dataframe\\\">\\n\",\n \" <thead>\\n\",\n \" <tr style=\\\"text-align: right;\\\">\\n\",\n \" <th></th>\\n\",\n \" <th>pixel0</th>\\n\",\n \" <th>pixel1</th>\\n\",\n \" <th>pixel2</th>\\n\",\n \" <th>pixel3</th>\\n\",\n \" <th>pixel4</th>\\n\",\n \" <th>pixel5</th>\\n\",\n \" <th>pixel6</th>\\n\",\n \" <th>pixel7</th>\\n\",\n \" <th>pixel8</th>\\n\",\n \" <th>pixel9</th>\\n\",\n \" <th>...</th>\\n\",\n \" <th>pixel774</th>\\n\",\n \" <th>pixel775</th>\\n\",\n \" <th>pixel776</th>\\n\",\n \" <th>pixel777</th>\\n\",\n \" <th>pixel778</th>\\n\",\n \" <th>pixel779</th>\\n\",\n \" <th>pixel780</th>\\n\",\n \" <th>pixel781</th>\\n\",\n \" <th>pixel782</th>\\n\",\n \" <th>pixel783</th>\\n\",\n \" </tr>\\n\",\n \" </thead>\\n\",\n \" <tbody>\\n\",\n \" <tr>\\n\",\n \" <th>0</th>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>1</th>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>2</th>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>3</th>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>4</th>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" </tr>\\n\",\n \" </tbody>\\n\",\n \"</table>\\n\",\n \"<p>5 rows × 784 columns</p>\\n\",\n \"</div>\"\n ],\n \"text/plain\": [\n \" pixel0 pixel1 pixel2 pixel3 pixel4 pixel5 pixel6 pixel7 pixel8 \\\\\\n\",\n \"0 0 0 0 0 0 0 0 0 0 \\n\",\n \"1 0 0 0 0 0 0 0 0 0 \\n\",\n \"2 0 0 0 0 0 0 0 0 0 \\n\",\n \"3 0 0 0 0 0 0 0 0 0 \\n\",\n \"4 0 0 0 0 0 0 0 0 0 \\n\",\n \"\\n\",\n \" pixel9 ... pixel774 pixel775 pixel776 pixel777 pixel778 \\\\\\n\",\n \"0 0 ... 0 0 0 0 0 \\n\",\n \"1 0 ... 0 0 0 0 0 \\n\",\n \"2 0 ... 0 0 0 0 0 \\n\",\n \"3 0 ... 0 0 0 0 0 \\n\",\n \"4 0 ... 0 0 0 0 0 \\n\",\n \"\\n\",\n \" pixel779 pixel780 pixel781 pixel782 pixel783 \\n\",\n \"0 0 0 0 0 0 \\n\",\n \"1 0 0 0 0 0 \\n\",\n \"2 0 0 0 0 0 \\n\",\n \"3 0 0 0 0 0 \\n\",\n \"4 0 0 0 0 0 \\n\",\n \"\\n\",\n \"[5 rows x 784 columns]\"\n ]\n },\n \"execution_count\": 4,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"test_set.head()\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 5,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"x_train = training_set.iloc[:, 1:].values\\n\",\n \"y_train = training_set.iloc[:, 0:1].values\\n\",\n \"x_test = test_set.iloc[:, :].values\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 6,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"x_train = x_train.reshape(-1, 28, 28, 1)\\n\",\n \"x_test = x_test.reshape(-1, 28, 28, 1)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 7,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"x_train = x_train/255.\\n\",\n \"x_test = x_test/255.\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 8,\n \"metadata\": {},\n \"outputs\": [\n {\n \"name\": \"stderr\",\n \"output_type\": \"stream\",\n \"text\": [\n \"Using TensorFlow backend.\\n\"\n ]\n },\n {\n \"data\": {\n \"text/plain\": [\n \"array([[ 0., 1., 0., ..., 0., 0., 0.],\\n\",\n \" [ 1., 0., 0., ..., 0., 0., 0.],\\n\",\n \" [ 0., 1., 0., ..., 0., 0., 0.],\\n\",\n \" ..., \\n\",\n \" [ 0., 0., 0., ..., 1., 0., 0.],\\n\",\n \" [ 0., 0., 0., ..., 0., 0., 0.],\\n\",\n \" [ 0., 0., 0., ..., 0., 0., 1.]], dtype=float32)\"\n ]\n },\n \"execution_count\": 8,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"from keras.utils import np_utils\\n\",\n \"y_train = np_utils.to_categorical(y_train, num_classes = 10)\\n\",\n \"y_train\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 9,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"from keras.models import Sequential\\n\",\n \"from keras.layers import Dense, Dropout, Conv2D, MaxPool2D, Flatten\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 25,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"classifier = Sequential()\\n\",\n \"\\n\",\n \"classifier.add(Conv2D(32, (5, 5), padding = 'Same', input_shape = (28, 28, 1), activation = 'relu'))\\n\",\n \"#classifier.add(MaxPool2D(pool_size = (2, 2)))\\n\",\n \"\\n\",\n \"#classifier.add(Conv2D(32, (5, 5), padding = 'Same', activation = 'relu'))\\n\",\n \"classifier.add(MaxPool2D(pool_size = (2, 2)))\\n\",\n \"classifier.add(Dropout(0.25))\\n\",\n \"\\n\",\n \"classifier.add(Conv2D(64, (3, 3),padding = 'Same', activation = 'relu'))\\n\",\n \"classifier.add(Conv2D(64, (3, 3),padding = 'Same', activation = 'relu'))\\n\",\n \"classifier.add(MaxPool2D(pool_size = (2, 2), strides = (2, 2)))\\n\",\n \"classifier.add(Dropout(0.25))\\n\",\n \"\\n\",\n \"#classifier.add(Conv2D(64, (3, 3), activation = 'relu'))\\n\",\n \"#classifier.add(MaxPooling2D(pool_size = (2, 2)))\\n\",\n \"\\n\",\n \"classifier.add(Flatten())\\n\",\n \"\\n\",\n \"classifier.add(Dense(units = 256, activation = 'relu'))\\n\",\n \"classifier.add(Dropout(0.5))\\n\",\n \"\\n\",\n \"classifier.add(Dense(units = 10, activation = 'sigmoid'))\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 26,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"classifier.compile(optimizer = 'rmsprop', loss = 'categorical_crossentropy', metrics = ['accuracy'])\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 27,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"from keras.preprocessing.image import ImageDataGenerator\\n\",\n \"datagen = ImageDataGenerator(\\n\",\n \" featurewise_center=False,\\n\",\n \" samplewise_center=False,\\n\",\n \" featurewise_std_normalization=False,\\n\",\n \" samplewise_std_normalization=False,\\n\",\n \" zca_whitening=False,\\n\",\n \" rotation_range=10,\\n\",\n \" zoom_range = 0.1,\\n\",\n \" width_shift_range=0.1,\\n\",\n \" height_shift_range=0.1,\\n\",\n \" horizontal_flip=False,\\n\",\n \" vertical_flip=False)\\n\",\n \"datagen.fit(x_train)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 28,\n \"metadata\": {},\n \"outputs\": [\n {\n \"name\": \"stdout\",\n \"output_type\": \"stream\",\n \"text\": [\n \"Epoch 1/30\\n\",\n \"328/328 [==============================] - 119s 362ms/step - loss: 0.5486 - acc: 0.8281\\n\",\n \"Epoch 2/30\\n\",\n \"328/328 [==============================] - 117s 356ms/step - loss: 0.1652 - acc: 0.9500\\n\",\n \"Epoch 3/30\\n\",\n \"328/328 [==============================] - 114s 349ms/step - loss: 0.1128 - acc: 0.9665\\n\",\n \"Epoch 4/30\\n\",\n \"328/328 [==============================] - 120s 365ms/step - loss: 0.0923 - acc: 0.9723\\n\",\n \"Epoch 5/30\\n\",\n \"328/328 [==============================] - 114s 348ms/step - loss: 0.0846 - acc: 0.9755\\n\",\n \"Epoch 6/30\\n\",\n \"328/328 [==============================] - 117s 358ms/step - loss: 0.0752 - acc: 0.9778\\n\",\n \"Epoch 7/30\\n\",\n \"328/328 [==============================] - 117s 358ms/step - loss: 0.0720 - acc: 0.9788\\n\",\n \"Epoch 8/30\\n\",\n \"328/328 [==============================] - 116s 355ms/step - loss: 0.0674 - acc: 0.9812\\n\",\n \"Epoch 9/30\\n\",\n \"328/328 [==============================] - 115s 351ms/step - loss: 0.0663 - acc: 0.9807\\n\",\n \"Epoch 10/30\\n\",\n \"328/328 [==============================] - 110s 335ms/step - loss: 0.0598 - acc: 0.9823\\n\",\n \"Epoch 11/30\\n\",\n \"328/328 [==============================] - 110s 336ms/step - loss: 0.0618 - acc: 0.9830\\n\",\n \"Epoch 12/30\\n\",\n \"328/328 [==============================] - 111s 337ms/step - loss: 0.0616 - acc: 0.9826\\n\",\n \"Epoch 13/30\\n\",\n \"328/328 [==============================] - 110s 336ms/step - loss: 0.0606 - acc: 0.9834\\n\",\n \"Epoch 14/30\\n\",\n \"328/328 [==============================] - 109s 331ms/step - loss: 0.0603 - acc: 0.9832\\n\",\n \"Epoch 15/30\\n\",\n \"328/328 [==============================] - 112s 340ms/step - loss: 0.0614 - acc: 0.9832\\n\",\n \"Epoch 16/30\\n\",\n \"328/328 [==============================] - 111s 337ms/step - loss: 0.0587 - acc: 0.9831\\n\",\n \"Epoch 17/30\\n\",\n \"328/328 [==============================] - 111s 338ms/step - loss: 0.0616 - acc: 0.9836\\n\",\n \"Epoch 18/30\\n\",\n \"328/328 [==============================] - 783s 2s/step - loss: 0.0573 - acc: 0.9842\\n\",\n \"Epoch 19/30\\n\",\n \"328/328 [==============================] - 118s 359ms/step - loss: 0.0596 - acc: 0.9838\\n\",\n \"Epoch 20/30\\n\",\n \"328/328 [==============================] - 115s 350ms/step - loss: 0.0614 - acc: 0.9837\\n\",\n \"Epoch 21/30\\n\",\n \"328/328 [==============================] - 121s 368ms/step - loss: 0.0655 - acc: 0.9830\\n\",\n \"Epoch 22/30\\n\",\n \"328/328 [==============================] - 125s 381ms/step - loss: 0.0611 - acc: 0.9842\\n\",\n \"Epoch 23/30\\n\",\n \"328/328 [==============================] - 119s 362ms/step - loss: 0.0600 - acc: 0.9838\\n\",\n \"Epoch 24/30\\n\",\n \"328/328 [==============================] - 117s 355ms/step - loss: 0.0608 - acc: 0.9846\\n\",\n \"Epoch 25/30\\n\",\n \"328/328 [==============================] - 116s 355ms/step - loss: 0.0664 - acc: 0.9835\\n\",\n \"Epoch 26/30\\n\",\n \"328/328 [==============================] - 118s 360ms/step - loss: 0.0667 - acc: 0.9829\\n\",\n \"Epoch 27/30\\n\",\n \"328/328 [==============================] - 117s 355ms/step - loss: 0.0635 - acc: 0.9831\\n\",\n \"Epoch 28/30\\n\",\n \"328/328 [==============================] - 114s 346ms/step - loss: 0.0608 - acc: 0.9843\\n\",\n \"Epoch 29/30\\n\",\n \"328/328 [==============================] - 118s 359ms/step - loss: 0.0650 - acc: 0.9835\\n\",\n \"Epoch 30/30\\n\",\n \"328/328 [==============================] - 115s 350ms/step - loss: 0.0631 - acc: 0.9833\\n\"\n ]\n }\n ],\n \"source\": [\n \"track = classifier.fit_generator(datagen.flow(x_train,y_train, batch_size= 128),\\n\",\n \" epochs = 30, steps_per_epoch=x_train.shape[0]//128)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 29,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"dict_keys(['loss', 'acc'])\"\n ]\n },\n \"execution_count\": 29,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"track.history.keys()\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 30,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"Text(0.5,1,'Model Accuracy')\"\n ]\n },\n \"execution_count\": 30,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n },\n {\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAAX0AAAEICAYAAACzliQjAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDIuMi4yLCBodHRwOi8vbWF0cGxvdGxpYi5vcmcvhp/UCwAAIABJREFUeJzt3XmYZHV97/H3t7au3mfpnoXZB0Zl3AYzoleNcI0LkAga0ECColExucHooz43aLwuRB8Tl4QneYxKrigYFREUuV4MKgGXG1SGXRgGhp6tZ+2enqX32r73j3Oqu6ane6Zmumeq65zP63nqqbPVqd+vTvfn/Op3Tp1j7o6IiMRDotYFEBGR00ehLyISIwp9EZEYUeiLiMSIQl9EJEYU+iIiMaLQl9gzs5Vm5maWqmLZd5jZr05HuUROBYW+1BUz22pmOTPrmDD9kTC4V9amZCe28xCpFYW+1KMtwBXlETN7IdBYu+KI1A+FvtSjbwJvrxi/Cri5cgEzazezm82sx8y2mdnHzCwRzkua2RfMrNfMuoA/nOS1XzOz3Wa208w+bWbJ6RTYzBrM7Hoz2xU+rjezhnBeh5n9yMwOmlmfmf2yoqx/E5ah38w2mdkfTKccIgp9qUe/BtrM7OwwjP8E+PcJy/wL0A6sBs4j2Em8M5z3HuCPgHOA9cBlE157E1AAzgqXeT3w7mmW+W+BlwPrgBcD5wIfC+d9COgGOoGFwEcBN7PnAtcAL3X3VuANwNZplkNiTqEv9arc2n8d8BSwszyjYkfwEXfvd/etwBeBt4WLvBW43t13uHsf8NmK1y4ELgQ+4O6D7r4P+Cfg8mmW98+A69x9n7v3AJ+qKE8eWAyscPe8u//Sg4tiFYEGYK2Zpd19q7s/O81ySMwp9KVefRP4U+AdTOjaATqADLCtYto2YEk4fAawY8K8shVAGtgddrccBL4KLJhmec+YpDxnhMOfBzYDPzGzLjO7FsDdNwMfAD4J7DOzW8zsDESmQaEvdcndtxEc0L0I+P6E2b0ErecVFdOWM/5tYDewbMK8sh3AKNDh7nPCR5u7P3+aRd41SXl2hXXpd/cPuftq4I3AB8t99+7+bXd/VfhaB/5hmuWQmFPoSz17F/Aadx+snOjuReBW4DNm1mpmK4APMt7vfyvw12a21MzmAtdWvHY38BPgi2bWZmYJMzvTzM47gXI1mFm24pEAvgN8zMw6w9NNP14uj5n9kZmdZWYGHCbo1ima2XPN7DXhAd8RYDicJ3LSFPpSt9z9WXffMMXs9wGDQBfwK+DbwI3hvH8D7gYeBR7i6G8KbyfoHnoSOADcRtDnXq0BgoAuP14DfBrYADwGPB6+76fD5dcAPwtfdz/wr+5+H0F//t8TfHPZQ9DF9NETKIfIUUw3URERiQ+19EVEYkShLyISIwp9EZEYUeiLiMTIrLsaYEdHh69cubLWxRARqSsPPvhgr7t3Hm+5WRf6K1euZMOGqc7CExGRyZjZtuMvpe4dEZFYUeiLiMRIZEJ/7+ERXvHZe7j9we5aF0VEZNaKTOjPa86wr3+Urt6BWhdFRGTWikzop5MJls9voqtn8PgLi4jEVGRCH2B1RzNbehX6IiJTiVTorwpDv1TSReRERCYTqdBf3dnCaKHErkPDtS6KiMisFKnQX9XRDKAuHhGRKUQq9Fcr9EVEjilSod/Z2kBLQ0pn8IiITCFSoW9mrOpopkstfRGRSUUq9CHo1+/q0Q+0REQmE7nQX93ZzM6Dw4zki7UuiojIrBO50F/V0Yw7bO8bqnVRRERmnciF/pmdLQDq4hERmUTkQn9leNqmDuaKiBwtcqHf0pBiQWsDW3TapojIUSIX+hAczFVLX0TkaJEM/VUdLfpVrojIJCIZ+qs7mukbzHFwKFfrooiIzCrRDP1OHcwVEZlMJEN/7GqbOpgrInKESIb+snlNpBKm++WKiEwQydBPJxMsn9ekg7kiIhNEMvShfOE1hb6ISKVIh/7W/bpfrohIpWmFvpldYGabzGyzmV17jOUuMzM3s/XTeb8TsbqzhZF8id2HR07XW4qIzHonHfpmlgS+BFwIrAWuMLO1kyzXCvw18JuTfa+ToTN4RESONp2W/rnAZnfvcvcccAtwySTL/R3wOeC0NrnPHDtXX2fwiIiUTSf0lwA7Ksa7w2ljzOwcYJm7/+hYKzKzq81sg5lt6OnpmUaRxnW2NtCcSepgrohIhemEvk0ybeyoqZklgH8CPnS8Fbn7De6+3t3Xd3Z2TqNIFYUzY5UuvCYicoTphH43sKxifCmwq2K8FXgBcJ+ZbQVeDtx5Wg/mdrSwRd07IiJjphP6DwBrzGyVmWWAy4E7yzPd/ZC7d7j7SndfCfwauNjdN0yrxCdgVUcz3QeGGS3ofrkiIjCN0Hf3AnANcDewEbjV3Z8ws+vM7OKZKuB0rO4M7pe7bb/ulysiApCazovd/S7grgnTPj7FsudP571OxuqO8v1yB3nOwtbT/fYiIrNOZH+RC7CyowlA1+AREQlFOvRbs2k6Wxvo6tHBXBERiHjoQ3AXLbX0RUQC0Q/9ToW+iEhZ5EN/VUcz+wdzHBrK17ooIiI1F/nQHzuDRz/SEhGJfuivKl94TdfgERGJfugvm9tEMmHq1xcRIQahn0npfrkiImWRD30IDuY+q3P1RUTiEfqrdb9cEREgJqG/qrOZkXyJPbpfrojEXDxCv0Nn8IiIQExC/8zO4Fx93VBFROIuFqG/oLWBpkySZ9XSF5GYi0XomxmrdOE1EZF4hD7A6s4Whb6IxF5sQj+4X+6Q7pcrIrEWm9Bf3dFMyWG77pcrIjEWn9AvX3hNXTwiEmOxCf2VOldfRCQ+od+WTdPR0qBz9UUk1mIT+qBbJ4qIxCv0O5rVvSMisRar0Nf9ckUk7mIV+qs7db9cEYm3WIV++Wqb6tcXkbiaVuib2QVmtsnMNpvZtZPM/6CZPWlmj5nZPWa2YjrvN13L5+l+uSISbycd+maWBL4EXAisBa4ws7UTFnsYWO/uLwJuAz53su83EzKpBMvmNupgrojE1nRa+ucCm929y91zwC3AJZULuPu97l6+7sGvgaXTeL8ZsaqjWb/KFZHYmk7oLwF2VIx3h9Om8i7gx9N4vxmxurOFrb26X66IxNN0Qt8mmTZpkprZlcB64PNTzL/azDaY2Yaenp5pFOn4VnU0M5wv6n65IhJL0wn9bmBZxfhSYNfEhczstcDfAhe7++hkK3L3G9x9vbuv7+zsnEaRjm+1zuARkRibTug/AKwxs1VmlgEuB+6sXMDMzgG+ShD4+6bxXjNm7Fz9Hp2rLyLxc9Kh7+4F4BrgbmAjcKu7P2Fm15nZxeFinwdagO+Z2SNmducUqzttFrY10JhO6mCuiMRSajovdve7gLsmTPt4xfBrp7P+U0H3yxWROIvVL3LLVnc288SuwxwcytW6KCIip1UsQ//yly7n0FCet371fvYc0lk8IhIfsQz9V63p4Bt//lJ2HRzh0i//F8/qoK6IxEQsQx/gFWd2cMvVL2ckX+QtX7mfx7oP1rpIIiKnXGxDH+AFS9q57S9fQVMmyRU3/JpfPdNb6yKJiJxSsQ59CH6he/tfvoJl85p45zd+y48eO+r3ZSIikRH70AdY2Jblu+/9b6xbNof3fedhvvnrbbUukojIKaHQD7U3pvnmu17GHzxvAf/rjt9x/c+exl0XZRORaFHoV8imk3zlyt/jst9byvU/e4aP//AJiroap4hEyLR+kRtFqWSCz1/2IuY3Z/jqL7roG8rxuUtfRHODPioRqX9KskmYGR+56GzmNWf47I+f4j837uMNz1/Im85ZwqvO6iCV1BckEalPCv1jeO95Z3Luqnnc9mA3P3psN3c8souOlgxvfPEZvPmcJbxwSTtmk91WQERkdrLZdrBy/fr1vmHDhloX4yijhSL3berhjod3cs/GfeSKJVZ3NvPmdUt40zlLWDavqdZFFJEYM7MH3X39cZdT6J+4Q8N5fvz4bn7w8E5+s6UPgPUr5vK6tQtZ1dHMyo5mls9rIptO1rikIhIXCv3TZOfBYX74yE5+8NBOntl35DV8FrY1sGJeMyvmN7FifhPL5zezcn4TK+Y1096UrlGJRSSKFPo1cGAwx7a+IbbtH2Tb/iG27R9ie18wvK//yDtFdrRkOGtBC2ctaGHNgtbwuYXO1gYdJxCRE1Zt6OtA7gya25xhbnOGdcvmHDVvKFdge1+wI9jaO8izPQNs3jfADx/ZRf9IYWy51myKNRU7gmXzmmjLpmjNpmnJpmgNHw0pdR2JyIlT6J8mTZkUz1vUxvMWtR0x3d3Z1z/K5n0DPLO3n809Azyzd4B7ntrLdzfsmHJ9mWRibAfQmk3Tmk0xtynD3OY085qCnc+85gxzm4Ln8kPHGUTiTaFfY2bGwrYsC9uyvPKsjiPm9Q3m2HVwmIHRAv0jBfpH8mPDh0fyDIyMT+8fKfDUnsMcGMpzYCjHVL12jekkrdkU6WSCVNJIJYx0MjE2nk6E05MJMskEna0ZFrc3srg9yxlzxp+186hf7s5wvsih4TwHh/Jjz4eH8xwczjEwUqC5odyIyDC3Kc2cpvHnZOL0dT+WSs7+wRx7D4+Ej1H2Hh5hX/8IPf05CqUSJQ/q5A4l9/ABVIynw0ZSS8OEb83l8YZgvCWbojmToqkhSXMmRWM6SaLK+o4WihwM//8ODuU5OJTjwFCe/pE8mWSCxkySbDp4NKaTNGaC52zFcFO4zKmk0J/Fyq3zE1UsOYeG8/QN5jgwlAueB3P0DeXoG8gxMFogX3QKpRKFopMrligUSxRKTr5YIlcoMZgrMpov8vD2A+wfPPq2knOb0ixub+SMOVkWtzfS1pgiVygxWigxmi8xWiiSK5aHg/HRQrDuUsUeqXLnNHE/ZQS/kE4lbGwHlUqM76zG5yUwwCx4TSIcMGxsmoXjjlMoOvmSUyiWjvgc8sUSxVIwr1RymhuSzGnM0N6YZk5TmrbwuXJae2OaZMI4NHxkeB4KAzSYXuDgUPC5N2dStDelmRO+fm5TeV2ZcN1p2pvSNCSTDOeLwSMXPI9UDFeOj+THP9+R/JHPlcODo4WgPEN5csXSCf9dEX6Obdn02A6gNZsa2wbp5Pj2KTce0uH0ZCKBE3yuxdJ4GBdLQUCXSk4xnDYwUmBv/yj7Do+wr3/0qEuhmMH85gY6WjI0pBKYBds5YUbCgoaUAYmEkU4YhpErlth1cIT+0fHGUqHKS6w0ppM0NyRpyqRoygTB3NyQoljyI8J9OF88qc+00ouXtvPDa1417fUci0I/gpIJO+kdxmRG8kX2HBph96ERdh8aZvehEXYdDJ67DwzzwNYDDIwWaEglwkeSTHk4HYw3pBK0NATfMCa2FCuPWwf/roGSO4UwnIPnICRGCkUKxSPnuTtOsBMJwiVYx8Tpho2FUSphJMNvOuWgyqQSNCUTJAwGRwt09Q4E/9jDeXKFEwvKZMJobwx2DG2NaVobUgzlCuw6NDwWFjN1aad00simkuOfd8Xnnk0nmNOYZsmcbFie8Z3WnLB87eEObE5ThuZMksFckQODQYu1bygXBNtgjr6KkDswGOzIyjvMQsWONF/RiChvt3IwJxNBOCcSRtKMRDheHm7KJFnYlmXNgg4WtjWwsC3LgtYsi9qzLGxroKOlgfQ0fxXv7owWSkd8Yw6+RecZyhUZzBUZGi2MPQ/lK8ZzwfIJg8XtWc5e3BbuBMvfiMIdeLhTb82mKBT9iJ11sMMuHTWtvfHUn9Wn0JfjyqaTrAx/fxBnI/liRXdIjoNhi75Y8rHwbKv4BtDSkDrmmVilkjOQK3BoKB/uWIIwPTSUY7RQGmtZVn79D7oFEmNdBOXugpnucmlpCLpCls2b0dXOGmY29tktaD097zn39LzNcSn0RaqUTSdZ1J5kUXt2RtaXSBht2TRt2XRkw1VmH105TEQkRhT6IiIxMut+kWtmPcB07lfYAUTpDudRqw9Er05Rqw9Er05Rqw8cXacV7t55vBfNutCfLjPbUM1PketF1OoD0atT1OoD0atT1OoDJ18nde+IiMSIQl9EJEaiGPo31LoAMyxq9YHo1Slq9YHo1Slq9YGTrFPk+vQlnsxsJbAFSLt74TjLvgN4t7uf2t+7i8xCUWzpyyxnZlvNLGdmHROmP2JmHgZ4TZlZs5kNmNldtS6LyExS6EutbAGuKI+Y2QuBxtoV5yiXAaPA681s8el8YzPTL+XllIlM6JvZBWa2ycw2m9m1tS7PTAhbxI+HLeC6u52Ymd1oZvvM7HcV0+YBC4H5wKfNrHxJkquAmye8vt3MbjazHjPbZmYfM7NEOC9pZl8ws14z6wL+cJLXfs3MdpvZTjP7tJmdyDVrrwK+AjwG/NmEOvWa2aGwXPvN7LfhezxiZtvDR7+ZPWlmLwlf52Z2VsV6vmFmnw6HzzezbjP7GzPbA3zdzOaa2Y/C9zgQDi+t/BzN7Otmtiucf0c4/Xdm9saK5dJheddNVkkzW2Zm95rZRjN7wszeX7H+n5rZM+HzbLl0zDEdoz6frNhGj5jZRbUua7XMLBv+jT0a1ulT4fRVZvabcBt918yqu8JicB3q+n4ASeBZYDWQAR4F1ta6XDNQr61AR63LMY3yvxp4CfC7immfAw4ArwV6gP8dbr8dwAqCKyyvDJe9Gfgh0AqsBJ4G3hXO+wvgKWAZMA+4N3xtKpx/B/BVoBlYAPwWeG847x3Ar45R7uVACVgLfAh4rGLe+WE5esN1Z4EbgQ8DbwF2Ai8luKLzWQQ/mCEs21kV6/kG8OmKdRaAfwAaCL7xzAcuBZrC+n8PuKPi9f8X+C7BdbzSwHnh9P8JfLdiuUuAx49R18XAS8Lh1rBua8PtdG04/VrgH2r991Tl39xU9fkk8OFal+8k62RASzicBn4DvBy4Fbg8nP4V4C+rWV9UWvrnApvdvcvdc8AtBH/sUkPu/gugb8LkS4DyHeS/BrwZeB1BgO8sLxS2yv8E+Ii797v7VuCLwNvCRd4KXO/uO9y9D/hsxWsXAhcCH3D3QXffB/wTcHmVRX87QdA/CXwHeL6ZnRPOGyUI5D3hukeA7eG8dwOfc/cHPLDZ3av9dXkJ+IS7j7r7sLvvd/fb3X3I3fuBzwDnhfVbHNbvL9z9gLvn3f3n4Xr+HbjIzMq3aHsb8M2p3tTdd7v7Q+FwP7ARWEKwnW4KF7sJeFOV9aipY9SnboV/S+X/mXT4cOA1wG3h9Kq3UVRCfwlBS7Gsmzrf0CEHfmJmD5rZ1bUuzAxZCJTvNvFlYA5By/vmCct1EHxrqwzNbYxv1zM4cptXLreC4B9jt5kdNLODBK3+BVWW8e3AtwDcfRfwc4LuHgi+Weyc5DXXEITyH51kV0hPuAMBwMyazOyrYbfWYeAXwJxwZ7gM6HP3AxNXEpb3/wGXmtkcgp3Dt6opQHgA/RyCluRCd98drnM31X92s8aE+gBcY2aPhV10ddFdVRZ2Zz4C7AN+StCzcdDHz1SrOvOiEvqTXUw8CueivtLdX0Lwj/tXZvbqWhdoJoWt4BJwEfD9CbN7gTxBgJctZzxwdxOEX+W8sh0ELfIOd58TPtrc/fnHK5OZvQJYA3zEzPaEfewvA64ID7DuINjhVPoycCbBzsEJvpFMNETQVVO2aML8iX+vHwKeC7zM3dsIusog+FvfAcwLQ30yNwFXEnQ33e/uk+2kjmBmLcDtBN+ODh9v+dlukvqUt9E6gr+dybbRrOXuRXdfBywl6Nk4e7LFqllXVEK/myMDYCmwq0ZlmTFhq42we+IHBBu73u0l6MMvd1PsAF7j7oOVC7l7kaDP8jNm1mpmK4APEnRfEM77azNbGrbarq147W7gJ8AXzazNzBJmdqaZnVdF+a4iaEmtJQiIdcALCAL7QoJjA/uARRac1pkl6KsvEhyfeAHwagucFZYb4BHgT8MW2wWEXTXH0AoMAwctOPj9iQn1+zHwr+EB3/SEBsEdBMdS3s/R36COYmZpgoD8lruXd757w+1T3k77jree2WKy+rj73jA4S8C/Uaf/S+5+ELiPoE9/jo2f6VV15kUl9B8A1oRHszMEfbd31rhM0xIGSmt5GHg98Ltjv6ou3Am0hMNXAbe5+1RnJr0PGAS6gF8B3yY4aArBP+7dBAftH+LobwpvJ+geepLgwPFtBAf5phQG+FuBf3H3PRWPLQT94leF4f7ucN3bCRoc7wRw9+8RdK0sAPoJwrd8e5T3A28EDhKcDXTHscoCXE9wQLcX+DXwHxPmv43gm9BTBIH8gfIMdx8mCL1Vk3wuE+tsBMdWNrr7P1bMupPxLq2rCA6oz3pT1ceOPO32zdTR/5KZdZa/1ZlZI8FJEBsJTl64LFys6m0UmV/khqdgXU/QirzR3T9T4yJNi5mtJmjdQ3CHs2/XW53M7DsEZ6Z0ELTwP0EQdrcSdMdsB94SHoitC1PU6XyCbwROcMbVe8v94bViZh8HnuPuVx5nuVcBvwQeJ+hqA/goQT943W2nY9TnCmbZNqqWmb2IoMsuSdBQv9Xdrwsz4haChsXDwJXuPnrc9UUl9EUkEHYHPQy8LTyDSmRMVLp3RAQws/cQHCf5sQJfJqOWvohIjKilLyISI7Puwk4dHR2+cuXKWhdDRKSuPPjgg71exT1yZ13or1y5kg0b6u7aYiIiNWVmVV3yQ907IiIxMuta+iISD+7OaKHE4ZE8/SMF+kcKDIwUcJw5jRnmNKVpb0rT2pAi+M3V7BFcsRISidlVrmoo9GXGuTv5ojOcLzKSLzKcKzKcL46NJ8xIJxNkkglSyWA4PfY8PuwQvLbi9UO5QrjO0tjwSD74DY4ZJBNGMmEkLHgkE4TPwfhk2VE+gc0rLl3iHjxK4T+345Qqx318vFgK6psvlsgXS+SKJfKFCeNFJ18IhkcLRXKFErlCidFwWnm8POwO6aSRSSXJJI10Kvi80skEmfJwKvicEmZYWH8I6lget/K4QTKRoCEVPDKpBA2pZMVwgoZ0koZwvYOjRQZGC/SHgTwwUuDwSDAeTA+GC0UfL1tqfLtmUuPbMpNKkkoYg+HrKtdbKB3/7MFkwmhvTI895jSlmRMON2ZSNKaTZNMJGjNJsung0Rg+sukE2XSSVNIoFINtVQy3WflRKDml8LlQDHZCh4YrH4Wx4cMV04slJ5tO0BSWoSkTPBozyWBaJklTOD1R/vsj2FGUt03CGPu7NDPOaM9y+bnLj/uZTIdCP0ZyhRI7Dw5zaDg/9o/ZMPZIBmGSSpCsaL0US07fYI7egVH2DwTPvQOj9EwYPzScZzhXCgI5X6RYxT9zFJkRfLbJBOnU+A6svIMrB3YmlaC9KTMeuuUwD+ebQb7o4Q6kvOMo7xiCHchIvkT/SKFiRxT83LR8GnZ5Z1WeXiiO71hG8+M7nGNJGLRm07RmU7Q0pGjLplnUlmXNghSt2TSppAU7t0JQ1onlzRecQ8N5CsUSzZkUi9uztGRTtGZTY+ttzQat+fIwwKHhPAeHcuFzELIHw2l9gzm6egaDv7l8sAM9FSp3Nm3h8/J5TbQ3ppjTmCGZsLGGyFDYOCk/7z08Mj6eL45to4nPExsT65bNUehL9UolZ2//CDv6htnRN8SOA0Ns7xuiu2+YHQeG2HN4hGp+lpFKBOGUShgDowUmy+900uhoaaCjpYHOlgbWLGilMTPewiq3uoLhRNjqStKQSgbfBEpBcOWLpSOHy63iMIyawvUELacEjemwBZUZf5+GVAIzoxi22IrulNwplQiGSz7WIi85Y639ykZ/ufugctp4C4yxbw5HjRO03BrClm2yzr7ul0oefvs48htIc0MQ8k2Z5KzrWpmoWPLwG9/4t8mRfCn4dpgLxgslJxV+C6x8pBIJkongW1DSjFTSaM2maG9M01KDbqXT8bsphf4s1T+Sp/vAMPv6R4/4it0/kg+/Zh/5VfvwSJ7dB0eOaLmZwaK2LMvmNvHfzpzP8nlNLJvbxNzm9HjXQsVzuQWYKxYZzQcB3NaYHgv3jpYMHa3BcFt29vWzyolLJIxsItixBrcgqD/JhNHckKK5of7j7HT8T9X/p1SnBkYLdB8IWuHdB4boPjBM94GgRd59IOiCmYwZtGTGvwq3ZFPMb8mwsqOZC56fZdm8puAxt5ElcxtpSJ3IbWFFJOoU+qfYwGiBTXv62bSnn6f39vPUnsM8s3eA/YO5I5bLphMsndvE0rmNnLN8ztjworZsRd9niuZMqi7PGBCR2UGhP0OKJefZngGe2tPPpj2H2bSnn6f29NN9YHhsmaZMkucsbOV1axeyYn4zS+c2smxeEO7zmzPqLhGRU06hPw25Qon/eraXu5/Yw0+e2DvWek8mjNUdzcGR+Jcu47mL2njeolaWzGlUK11Eakqhf4KGcgV+vqmH/3hiD/+5cR/9owVaGlL89+ct4PzndLL2jDZWdzarL11EZiWFfhUODeX52ca9/McTe/jF0z2MFkrMbUpz0QsXc8ELFvGKs+Yr5EWkLij0j2Ff/wgfuf1xfv50D4WSs6gtyxXnLucNz1/ES1fOJZXUpYtEpL4o9KfQ1TPAVV//Lb39Od71+6u48AWLedGSdvXJi0hdU+hP4uHtB3jXTcHlnb9z9ctZt2xOjUskIjIzFPoT3PvUPv7Htx6iozXDzX/+MlZ1NNe6SCIiM0ahX+F7G3Zw7fcf53mLWvn6O1/KgtZsrYskIjKjFPoEFzn61/ue5fN3b+L313Tw5St/j5YIXMdDRGSi2CdbseR86v88wc33b+NN687gc5e9mExKZ+WISDRVlW5mdoGZbTKzzWZ27STzV5jZPWb2mJndZ2ZLK+YVzeyR8HHnTBZ+ukbyRa759kPcfP82rn71av7xresU+CISacdt6ZtZEvgS8DqgG3jAzO509ycrFvsCcLO732RmrwE+C7wtnDfs7utmuNzTdmg4z3tu3sBvt/TxsT88m3f//upaF0lE5JSrpll7LrDZ3bvcPQfcAlwyYZm1wD3h8L2TzJ9V9h4e4a1fuZ+Htx/gn684R4EvIrFRTegvAXZUjHeH0yo9ClwaDr8ZaDWz+eF41sw2mNmvzexNk72BmV0dLrOhp6fnBIpVgj1SAAALSklEQVR/cv75nmfYun+Qm955Lhe/+IxT/n4iIrNFNaE/2U9QJ97T68PAeWb2MHAesBMohPOWu/t64E+B683szKNW5n6Du6939/WdnZ3Vl/4kPbN3gBcvncMrzuo45e8lIjKbVBP63cCyivGlwK7KBdx9l7v/sbufA/xtOO1QeV743AXcB5wz/WJPT1fvgH50JSKxVE3oPwCsMbNVZpYBLgeOOAvHzDrMrLyujwA3htPnmllDeRnglUDlAeDT7tBwnt6BHKs7FfoiEj/HDX13LwDXAHcDG4Fb3f0JM7vOzC4OFzsf2GRmTwMLgc+E088GNpjZowQHeP9+wlk/p92W3kEAtfRFJJaq+nGWu98F3DVh2scrhm8Dbpvkdf8FvHCaZZxRW3oHAFjd2VLjkoiInH6x+yXSlp5Bkglj+bymWhdFROS0i13oP9s7yLK5jfrlrYjEUuySb0vPoPrzRSS2YhX6pZKzpXdQ/fkiEluxCv29/SMM54tq6YtIbMUq9Lt6gtM1Vyv0RSSm4hX64Tn66t4RkbiKV+j3DNCUSbKwraHWRRERqYlYhf6W3uDMHbPJriEnIhJ9sQx9EZG4ik3ojxaK7OgbUn++iMRabEJ/R98QJdeZOyISb7EJ/WfLp2vqksoiEmOxCX1dUllEJEah39UzQGdrA63ZdK2LIiJSM7EJfZ25IyISo9Dv6hnUQVwRib1YhP6hoTz7B3VfXBGRWIT+lv3lg7g6R19E4i0Wod/VU74vrlr6IhJvVYW+mV1gZpvMbLOZXTvJ/BVmdo+ZPWZm95nZ0op5V5nZM+HjqpksfLW29Ab3xV02V/fFFZF4O27om1kS+BJwIbAWuMLM1k5Y7AvAze7+IuA64LPha+cBnwBeBpwLfMLM5s5c8avT1TPI8nlNui+uiMReNSl4LrDZ3bvcPQfcAlwyYZm1wD3h8L0V898A/NTd+9z9APBT4ILpF/vEdOl0TRERoLrQXwLsqBjvDqdVehS4NBx+M9BqZvOrfC1mdrWZbTCzDT09PdWWvSrBfXEHdLqmiAjVhf5kF5/3CeMfBs4zs4eB84CdQKHK1+LuN7j7endf39nZWUWRqrfn8Agj+RKrdBBXRIRUFct0A8sqxpcCuyoXcPddwB8DmFkLcKm7HzKzbuD8Ca+9bxrlPWHl++Kqe0dEpLqW/gPAGjNbZWYZ4HLgzsoFzKzDzMrr+ghwYzh8N/B6M5sbHsB9fTjttNnSG5yueaauoy8icvzQd/cCcA1BWG8EbnX3J8zsOjO7OFzsfGCTmT0NLAQ+E762D/g7gh3HA8B14bTTpqt3kOZMkgWtui+uiEg13Tu4+13AXROmfbxi+DbgtileeyPjLf/TrqtnkFWdui+uiAjE4Be5wdU11bUjIgIRD/3RQpHuA0M6XVNEJBTp0N++P7wvrk7XFBEBIh76Y/fFVfeOiAgQ8dAv3xd3ZYcutCYiAhEPfd0XV0TkSJEO/S29ukWiiEil6Ie+DuKKiIyJbOiP3RdXB3FFRMZENvS7wmvu6EJrIiLjohv65dM11b0jIjImsqG/pXeQVMJYNk+na4qIlEU29Lt6B1g+r4l0MrJVFBE5YZFNxK4e3RdXRGSiSIZ+qeRs3a/QFxGZKJKhvzu8L+5q3S1LROQIkQz9LbovrojIpCIZ+l1j98VV6IuIVIpm6PcE98Xt1H1xRUSOEM3Q7x1kdWeL7osrIjJBVaFvZheY2SYz22xm104yf7mZ3WtmD5vZY2Z2UTh9pZkNm9kj4eMrM12ByWzpHVB/vojIJFLHW8DMksCXgNcB3cADZnanuz9ZsdjHgFvd/ctmtha4C1gZznvW3dfNbLGnNpIv0n1gmEtfsvR0vaWISN2opqV/LrDZ3bvcPQfcAlwyYRkH2sLhdmDXzBXxxGzvG8JdZ+6IiEymmtBfAuyoGO8Op1X6JHClmXUTtPLfVzFvVdjt83Mz+/3J3sDMrjazDWa2oaenp/rST6Krp3zmjs7RFxGZqJrQn+xoqE8YvwL4hrsvBS4CvmlmCWA3sNzdzwE+CHzbzNomvBZ3v8Hd17v7+s7OzhOrwQRdY/fFVUtfRGSiakK/G1hWMb6Uo7tv3gXcCuDu9wNZoMPdR919fzj9QeBZ4DnTLfSxbOkZZEFrAy0Nxz1cISISO9WE/gPAGjNbZWYZ4HLgzgnLbAf+AMDMziYI/R4z6wwPBGNmq4E1QNdMFX4yXbpFoojIlI4b+u5eAK4B7gY2Epyl84SZXWdmF4eLfQh4j5k9CnwHeIe7O/Bq4LFw+m3AX7h736moSNmW3kFW6RaJIiKTqqoPxN3vIjhAWznt4xXDTwKvnOR1twO3T7OMVTs4lKNvMKfLL4iITCFSv8gtH8TV6ZoiIpOLVuiP3RdX3TsiIpOJVOhv6R0glTCWzm2sdVFERGalSIV+V88gy+frvrgiIlOJVDpu6R1ktfrzRUSmFJnQL5U8PF1ToS8iMpXIhP7e/hFGC7ovrojIsUTmWgWL2xt54lNvQPdNERGZWmRCH6BZ19sRETmmyHTviIjI8Sn0RURixILros0eZtYDbJvGKjqA3hkqzmwQtfpA9OoUtfpA9OoUtfrA0XVa4e7HvSHJrAv96TKzDe6+vtblmClRqw9Er05Rqw9Er05Rqw+cfJ3UvSMiEiMKfRGRGIli6N9Q6wLMsKjVB6JXp6jVB6JXp6jVB06yTpHr0xcRkalFsaUvIiJTUOiLiMRIZELfzC4ws01mttnMrq11eWaCmW01s8fN7BEz21Dr8pwoM7vRzPaZ2e8qps0zs5+a2TPh89xalvFETVGnT5rZznA7PWJmF9WyjCfCzJaZ2b1mttHMnjCz94fT63I7HaM+9byNsmb2WzN7NKzTp8Lpq8zsN+E2+q6ZZapaXxT69M0sCTwNvA7oBh4Arghv2F63zGwrsN7d6/JHJWb2amAAuNndXxBO+xzQ5+5/H+6c57r739SynCdiijp9Ehhw9y/Usmwnw8wWA4vd/SEzawUeBN4EvIM63E7HqM9bqd9tZECzuw+YWRr4FfB+4IPA9939FjP7CvCou3/5eOuLSkv/XGCzu3e5ew64BbikxmWKPXf/BdA3YfIlwE3h8E0E/5B1Y4o61S133+3uD4XD/cBGYAl1up2OUZ+65YGBcDQdPhx4DXBbOL3qbRSV0F8C7KgY76bON3TIgZ+Y2YNmdnWtCzNDFrr7bgj+QYEFNS7PTLnGzB4Lu3/qoitkIjNbCZwD/IYIbKcJ9YE63kZmljSzR4B9wE+BZ4GD7l4IF6k686IS+pNdRb/++63gle7+EuBC4K/CrgWZfb4MnAmsA3YDX6xtcU6cmbUAtwMfcPfDtS7PdE1Sn7reRu5edPd1wFKCno2zJ1usmnVFJfS7gWUV40uBXTUqy4xx913h8z7gBwQbu97tDftdy/2v+2pcnmlz973hP2UJ+DfqbDuF/cS3A99y9++Hk+t2O01Wn3rfRmXufhC4D3g5MMfMyjcRqTrzohL6DwBrwqPZGeBy4M4al2lazKw5PBCFmTUDrwd+d+xX1YU7gavC4auAH9awLDOiHI6hN1NH2yk8SPg1YKO7/2PFrLrcTlPVp863UaeZzQmHG4HXEhyruBe4LFys6m0UibN3AMJTsK4HksCN7v6ZGhdpWsxsNUHrHoI7nH273upkZt8Bzie4BOxe4BPAHcCtwHJgO/AWd6+bA6NT1Ol8gm4DB7YC7y33h892ZvYq4JfA40ApnPxRgn7wuttOx6jPFdTvNnoRwYHaJEFD/VZ3vy7MiFuAecDDwJXuPnrc9UUl9EVE5Pii0r0jIiJVUOiLiMSIQl9EJEYU+iIiMaLQFxGJEYW+iEiMKPRFRGLk/wPk8LOPTLhWZQAAAABJRU5ErkJggg==\\n\",\n \"text/plain\": [\n \"<Figure size 432x288 with 2 Axes>\"\n ]\n },\n \"metadata\": {},\n \"output_type\": \"display_data\"\n }\n ],\n \"source\": [\n \"plt.subplot(2, 1, 1)\\n\",\n \"plt.plot(track.history['loss'])\\n\",\n \"plt.title(\\\"Model Loss\\\")\\n\",\n \"\\n\",\n \"plt.subplot(2, 1, 2)\\n\",\n \"plt.plot(track.history['acc'])\\n\",\n \"plt.title(\\\"Model Accuracy\\\")\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 31,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"array([[ 2.57538599e-22, 2.13860055e-21, 1.19176070e-06, ...,\\n\",\n \" 3.01349983e-18, 7.32958917e-17, 5.81007946e-22],\\n\",\n \" [ 4.12553772e-02, 1.70384037e-11, 3.63805874e-08, ...,\\n\",\n \" 1.23355604e-09, 8.76498007e-07, 8.44394719e-07],\\n\",\n \" [ 6.01048783e-24, 1.32041013e-23, 4.60820375e-18, ...,\\n\",\n \" 4.39607420e-17, 2.63324003e-13, 1.81043947e-06],\\n\",\n \" ..., \\n\",\n \" [ 2.73239156e-36, 1.41767553e-29, 2.39096420e-22, ...,\\n\",\n \" 2.10024450e-23, 7.74818777e-22, 3.64664364e-24],\\n\",\n \" [ 2.17772584e-17, 9.44362249e-20, 1.61774526e-15, ...,\\n\",\n \" 5.65474696e-13, 1.38682707e-12, 3.47423920e-05],\\n\",\n \" [ 3.06367733e-33, 9.77665881e-32, 4.26333413e-09, ...,\\n\",\n \" 6.63105778e-25, 8.68507533e-22, 5.58048884e-30]], dtype=float32)\"\n ]\n },\n \"execution_count\": 31,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"predict = classifier.predict(x_test)\\n\",\n \"predict\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 32,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"predict = np.argmax(predict, axis = 1)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 33,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"array([2, 0, 9, ..., 3, 9, 2])\"\n ]\n },\n \"execution_count\": 33,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"predict\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 34,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"submit = pd.DataFrame(predict, columns = ['Label'])\\n\",\n \"submit.reset_index(inplace = True)\\n\",\n \"submit['index'] = submit['index'] + 1\\n\",\n \"submit.rename(columns = {'index' : 'ImageId'}, inplace = True)\\n\",\n \"submit.index = submit['ImageId']\\n\",\n \"submit = submit.drop('ImageId', axis = 1)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 35,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/html\": [\n \"<div>\\n\",\n \"<style scoped>\\n\",\n \" .dataframe tbody tr th:only-of-type {\\n\",\n \" vertical-align: middle;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe tbody tr th {\\n\",\n \" vertical-align: top;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe thead th {\\n\",\n \" text-align: right;\\n\",\n \" }\\n\",\n \"</style>\\n\",\n \"<table border=\\\"1\\\" class=\\\"dataframe\\\">\\n\",\n \" <thead>\\n\",\n \" <tr style=\\\"text-align: right;\\\">\\n\",\n \" <th></th>\\n\",\n \" <th>Label</th>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>ImageId</th>\\n\",\n \" <th></th>\\n\",\n \" </tr>\\n\",\n \" </thead>\\n\",\n \" <tbody>\\n\",\n \" <tr>\\n\",\n \" <th>1</th>\\n\",\n \" <td>2</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>2</th>\\n\",\n \" <td>0</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>3</th>\\n\",\n \" <td>9</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>4</th>\\n\",\n \" <td>0</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>5</th>\\n\",\n \" <td>3</td>\\n\",\n \" </tr>\\n\",\n \" </tbody>\\n\",\n \"</table>\\n\",\n \"</div>\"\n ],\n \"text/plain\": [\n \" Label\\n\",\n \"ImageId \\n\",\n \"1 2\\n\",\n \"2 0\\n\",\n \"3 9\\n\",\n \"4 0\\n\",\n \"5 3\"\n ]\n },\n \"execution_count\": 35,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"submit.head()\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 36,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"submit.to_csv('digit_submit.csv')\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 37,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"(28000, 1)\"\n ]\n },\n \"execution_count\": 37,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"submit.shape\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 38,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAABIEAAACMCAYAAADr57kMAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDIuMi4yLCBodHRwOi8vbWF0cGxvdGxpYi5vcmcvhp/UCwAAIABJREFUeJzt3Xd8VFX6x/HnZBJCiPTeJCBFQBAEFNaGBXUVBYXVtQIWLCBrWRVd1y266squwiqoqIj+bNgWFQsqq64iKKAUIUJoKr1LQAlkcn9/mL0zzw0zmSQzkztzPu/Xyxfnm3tn5mSeabnOea5xHEcAAAAAAACQ3jKqewIAAAAAAABIPA4CAQAAAAAAWICDQAAAAAAAABbgIBAAAAAAAIAFOAgEAAAAAABgAQ4CAQAAAAAAWICDQAAAAAAAABbgIBAAAAAAAIAFqnQQyBhzhjFmuTFmpTFmbLwmBf+j9nai7nai7vai9nai7nai7vai9nai7vYyjuNU7oLGBERkhYgMEJF1IjJPRC50HGdZ/KYHP6L2dqLudqLu9qL2dqLudqLu9qL2dqLudsuswmWPFpGVjuOsFhExxrwkIoNEJOIDp4bJdmpKbhVuEom2T/bKfqfIlLNbhWpP3f0vEXUXofapIIbaU/c0xGu9nXittxev9Xbitd5OvNbbK8baV+kgUEsR+SEsrxORY7w7GWNGishIEZGaUkuOMadU4SaRaF84s2LZrdzaU/fUEq+6i1D7VBND7al7GuK13k681tuL13o78VpvJ17r7RVj7avUE+hgR5jKrC1zHGey4zi9HcfpnSXZVbg5+Ei5tafuaYnnvJ2ou714rbcTz3k7UXd78VpvJ57zFqvKQaB1ItI6LLcSkQ1Vmw5SBLW3E3W3E3W3F7W3E3W3E3W3F7W3E3W3WFUOAs0TkQ7GmLbGmBoi8lsReTM+04LPUXs7UXc7UXd7UXs7UXc7UXd7UXs7UXeLVbonkOM4xcaY0SIyU0QCIjLFcZylcZsZfIva24m624m624va24m624m624va24m6260qjaHFcZx3ROSdOM0FKYTa24m624m624va24m624m624va24m626sqy8EAAAAAAACQIjgIBAAAAAAAYAEOAgEAAAAAAFigSj2BAAAAUkmgYQN3vPWcTmpb7oUbVX6603MqT911jMpvPHWiO27xrO6nGdz1Y5XmCQAAkAh8EwgAAAAAAMACHAQCAAAAAACwAAeBAAAAAAAALEBPIBEJ1KmjsqmVU+nr2nJmO5UbXvJ9xH3Njfp2SxblV/p2AQBAWd73+J3P1XfHc7tPVNtKxPFcWn8euLPRYpXvum2JO37smjZq29tD+qoczC+Iab4AEiezZQuV102sq/L8ProPWJYJqHzACbrjrs+MVttazC5WOWf9XpVLFi6r2GRRISY7W+VVdx/ljoO1StS2M49ZqPKEFnOiXvfsotD3Jq594jq1rfW4L1V2ivXjAPAjvgkEAAAAAABgAQ4CAQAAAAAAWICDQAAAAAAAABagJ5CI5I/rpPKKgY8l5XbPrHelyhyRAwAgvlb8qYvK+WF9gPY4+9W2nm/cEPW6xvSfqfL19Va745F116pt7z56hMrB/uXNFECibT1V9+6a03uCyge8bcE8wnsCLbxMX1Yu0/GOzb9SedmYHu7YzNY9aVBxGbVrq7z9pWYqL+vxSOTLilG5bD84rV92WN1HP6y2nbhmlMq1X5ob9bpQvfYNPFrlXR304ZCsPfqxUH/FPne85ajofYPrFeh+UDVnfBlhz+rHcQcAAAAAAAALcBAIAAAAAADAAhwEAgAAAAAAsICVPYG8awEfP+XpapnHiQ/PUXlTUV2Vl9/Y2R1nfMba4UQLdGrvjjef2Lja5pG9O7QWlXXF1S+jZk2V111/lMojh7+t8nX11rjjLcGf1LbmmYeofNisESoffssGd1y8aXPFJwugjGCtkojb+t93s8odJn4e9bpm1tQ9J/71j9Pd8fJzJ6ltz7d/XeWLmp+rcvHGTVFvC0B8BBo1dMd9r5+ftNu9t6l+Pbl3YpE7/mpoe7UtuHKNoILatFTx0rw5EXYsa+F+3btlwb48lR/65hSVZxz9qDs+NFP3hRl797MqP7boLJWD+QUxzwvxsenGUD+ubr9ZprZd1kT/3X9Szj6VNwd/Vvmzn1u743Nyo382/7JI/80w9rrz3HGDEbvVtuDmLVGvK9H4JhAAAAAAAIAFOAgEAAAAAABgASuXgw39uz7Fq/drYMlyW8OlUbe/OSXfHU+69jdqW+asBQmZUzr74Q/6VJ1FjfQSgQYddrjjT3o8VKHrzjIBlcNPIVpRX+0PfZVwxNHXqG0t/6vnnDPdv6ceTFWB9m31D54o0rGNPjXolY9fr/Lb/yl0xxm79VdKvx/cROW6x25XOffV0NeTfzwutvkiPkyfbio785boHTL0czxQt47KxV1Cpx1ed3KtqLeV9+pWlfmqeGJ1vFa/Tg4eN8QdN1kdffmXV8k+/Xmh8/3r3PHzpzZX2y6trZd75f9Bn5q6w2iWg8Vi90V93fFtf3lObTu71m7v7kqXz4ZH3FbrE708t+53B/T2L1arHNymX6+ROkoK97jjmTN7q233D5sd9bIbPUtDXi88wh2fnquXmbTJjP5n1R2NQp/dX56hl5W8eHiLqJdFWSXffKvyu+f3VXnhU6FlPB9920lt6zhxv8re9/xDReeh193qjr/8g/4c+OtahSo/3ES/tmTkCxIsfPmXiEjXoaE7ffKh75dzaf35rkFGDZXLWwIW7tia+n3kP91fcMeXv36G2pb/ip5zs/EV+zxSVXwTCAAAAAAAwAIcBAIAAAAAALAAB4EAAAAAAAAsYGVPoGl36TV5R457XOV+2bH3cznyUd0P5NCZhRH2FFlzjl4jOmvYOJWbBvQpB8/J3emObzlPl6rjJzo7xfpUhzbaNrKfyhnn6PX7Lx3xoMqdsuLXxyeejskOrSdddP54tW3iAN235L19/VWu8d68hM0rnRX9uo87Hj/pEbXtjrX6tM5/OfcSlVsu0mt4nbCx9xHV8u8rVQ7Ur69y9082uuPPG+r+AMHtOwTxtfL/errjEUfqU8t+OPZ4lTf+Sr/mTvjtFJUH5MxyxyXqUVDWg+cfrvJ/uuWWP1nETfHqtXG7rv1tQ32+Gmfq/jTex8FJvXUfwHWCWGw/wrjjs2r9qLb97Oj+C5uD+rPQsuOmqqxqUk7ftTf36tfnrcW1VZ6yJtTPoc4/9LbAJ4v0lZX44/OFrTZe28sdfz1sfJQ9yzr1hVtUbjs29F4x/f2L1LZ3urwc8/W2q+E9PTQ9gaoquHS5yuvCWgR1EN1LNfq7dFlZeyt6CSTSqnH6b76PL3hA5fC+Pvn6bULGbdDHAf4v74OYb7e86zq23iqVr6gb6vk4pc17atuOm95UefAe/VrT8En9uTTe+CYQAAAAAACABTgIBAAAAAAAYAEOAgEAAAAAAFjAyp5Aua99ofKdwZEqbzlK94qJJu9tvT7d+XpphD1F8jztWp445xg9j0aLI152+eBJKg/6s16DGNy6Ndo0rbDrcL1ed1GPZ6tpJokzqv4SlV9pdarKDZM5mRQW6NBO5TsenuqO711/ptoWPGmD59LeXHnrh3dWuXtO6LVp9v7Gcbsd/GLjzb9S+dF+T7jjU3KK1LavxrZWueRn3dPt9vFXqDw21LZEihro2/39b1/XuYHuWzBl2nB3nHdB5PcBxCbQ0FOAEv3eENy5U+Il8OUyd7zopzZq22k5y1Se83Z3lVuL7ieGgytuWRRx20WrBut9r9G9ebYd3UjlH9uHxvtb7VfbWreI3nftd21nqTy7x0uh8Jzed9CKs1X+4d08lVs8QO0TaeNN+rX+uTHhPSEr9v+/w3sAeRVPbqryun/qhiGtAlkRL5uXuUflH+7Uc259D48RXzl/W3XPwGobbtHPj/yLHlb5gFND5QHf/NYdO083UdtqT5urr3x99NuuyHW90+NYlSeeF/qb4qvLJ6ht4X2LREQO5BpJJr4JBAAAAAAAYIFyDwIZY6YYY7YYY74J+1kDY8wHxpiC0n/rR7sOpCZqbyfqbifqbi9qbyfqbifqbi9qbyfqjoOJ5ZtAU0XkDM/PxorILMdxOojIrNKM9DNVqL2Npgp1t9FUoe62mirU3kZThbrbaKpQd1tNFWpvo6lC3eFRbk8gx3H+a4zJ8/x4kIj0Lx0/IyIfi8htcZxXUuVM/1LlNtNjv6xT/i4RfTJWr2+880l/9YLwY+0zcnNVXnPrke542QUTPHtH7+1UWKL7ATz9Y/cIe5bvvU1dVc489ftKX5fTL/Q7vfXqk5W+nkrfvg/rHm+bH9QvfTVM0B3v/U3kNfxV9ePFfVWefsMDKg98/FZ33Kowuf0AbKh762nfqXz14cPdcZd7t6htJdt0f5CcQt13LUfWxHy7094/XeXLXp2i8jfHPe2OB0qvmK83XtKt9sHtunaZLVuoHGgW6hMTzC+o0m0FGoeu65aG+jm7eH9Q5VYf7q3SbcWbX+tecnxPlV854bGwpN/X89c3U7ld/kKV63vqW5X/1f3kkWep/Pee9dxx71Ffq21vdHxLX7ijjp17hHqKdbj8W7WtZN++KsyyfH6te1V4PxvKCbrvV/vMyP/Pe11Q9/G5+C+/V7mBRO4JlPuq7jE6wtyk8gcP6b4l4Rp5+oG0Pkl/bsyYoPtblRQWRryuWKVj7eMlo7a+v7+feqjKL3Z9Kizpz4kf/VxT5axtP6ms3wmSL1XqXnJi6LV/1fn6+bF8sH4uZRn9XvB98c8qH3gp1K+r/rTIz2ERkYEto3/uOkRWh6XVEfcTESlZqHsBtgl7S+pWe4za9u35E/WFk9sSqNI9gZo6jrNRRKT03ybl7I/0Qe3tRN3tRN3tRe3tRN3tRN3tRe3tRN0tl/CzgxljRorISBGRmlIr0TcHn6Du9qL2dqLudqLu9qL2dqLudqLu9qL26amy3wTabIxpLiJS+u+WSDs6jjPZcZzejuP0zpLsSt4cfCSm2lP3tMNz3k7U3V681tuJ57ydqLu9eK23E895y1X2m0BvisgwEbm/9N834jYji2TvLKruKVRGtdY+2L29yvOveNAdH6hggyZvD6APj6gdYc/yZUrlewCVua7te9zx6HX91baHWs5SeXtvvdK4yfSGKge3bY/XtFL6Ob/9yn4qz+zxD5WHXHujO665UfcIq4q9Q49R+Z93T1L57Em3qtzq78ntAxSDuNQ944jDVS755tsIe8ZXZp5e07/s9qYq11odWtdfvEb3C4qnkprR+5P5VEo/58MVr9+gf7A+NAw00q+Z3/5Zv8fUaOLp7bDmEJWfGPq4O87wLOi/dMHlKreesyim+Vazaq/796P1+1r3GpGfPxlrchI9HVfJonyV64eV87uZujfRCSeNUvm+uyervPzEUF+wrnfofdvcFb1/RYJUe90rItCwgcr5/2ir8tLej0a87Ef76qj857+NULnB05W//2uvqXzfr393el3lnrf+TuW8PybscZFSta+szWN0H9abrntZ5fDekCIiQw752HMNkftFHl5D96DadIJ+fDZeGtsck6za626ydN+flZeGXuuXnqF7AHn/xvP2ABryT/15uulU332eLtNI+ICjH3MjrnpH5XfH15NEiuUU8S+KyBwR6WSMWWeMuUJ+ecAMMMYUiMiA0ow0Q+3tRN3tRN3tRe3tRN3tRN3tRe3tRN1xMLGcHezCCJtOifNc4DPU3k7U3U7U3V7U3k7U3U7U3V7U3k7UHQeT8MbQiGxT30PK3wnWCa5Y5Y6/ekovY5K79HKwJQP/pfKQKVfp/eO3HCyl7ehRovKzP3ZTueaMyi8BCzTVJ1RYfls7d/zQ2c+qbaOXXKRyqwfnq1zBFY0pI1nLv7yCU/RXbb/oMF7lEf3Od8fFCZzHjhujLxF4eQ8n5aguBbfoc3cvP/eR6Bc4VsfwJWAXrhmgtrUZoZcY6lchxCr8Pl64Xz9TDxu/UuXqOhVz8cZNKtefoZcRfnH7YSr3zwmdur6uPos9YpA/rp3KSwdMirBnWfetPFPlqiz/8gqs36by8V9fovKnPZ+L221BxPTsqvJ3Z9dV+faLQku+Lqg9QW3LFL3MtKQKn8CaB/Sy1MBZns/ekVcnWi2jo2cZ5xmxP48v/NMtKvty+VcFvfLDUSofUs7p6Kuqso2hAQAAAAAAkEI4CAQAAAAAAGABDgIBAAAAAABYgJ5A1WjwiE+qewpprduMMSo3nK/X/9Yo1Ot/a8vchM+popp+vEXlE8++TOVPeuqeM4hN3cBPnp+EThkbaNxYbfmpT57K3w3Wl+zXVfek6LT/e3fsPeVoixv0KS2LD+yPYbaIVZnTff+gTwk/6ImbVa6zLjHPedNH95ya3sPbEED3Dzj/kNDz/FlpnZA54eDavqmfk385vYfKf2q8MObr2llUS+WMQnqyVUZgse6XOK9P6L36ktf0abMP21otp1Mv19oph6r8RoOPVO63KNSPrMH0b/SFa3keRw3qqxzcqvvOOEVFlZ1mynqx/+PVPYWD8vaGOvCx7l0kPZM4mTSUcWRnla+c9pbK5+TqU7V7Lp2AGR3chz2eUfm33Ue445LF1dMj0Q8y27ZROeex2N8jj3rqBpXbpEEPIK897zZTmZ5AAAAAAAAAqDIOAgEAAAAAAFiAg0AAAAAAAAAWoCdQOfadfbTKOzrpuyxDt/yQZg9FXqPoHKt7DfSs9WrM8xi9/jj9AwvXgIuIXPL02xG3dXt/tMqd71ylcnBb6vVnCK7Qv8Ougr56B8/68vOf+UDllzvr9aW2avaZUfnsQfp+bVyw2x23zPxSbeteQ/eSOrdgoMrr7++g8t0TJrvjG++/Vm1rtMaf/SvSRcHvO6q84OR/qjz02esTdtslx4eejEMef19taxnQPT6e2t1K5elnHxOW1sR9bojMzNY9fxYcX0/lHqP1Y+aT68apXD8j1N/p351eUduOvvMmlVvfk349DBKh9d/0/fSnv/Vyx4dJ1V5DTc+u7vj7gXXVtoDnY1XDUzfoyxrdR9Bx9PtKuJtbfxh1Hp91Dz1WLnx3gNrWIudHlcc1e1PlAVddo3L2O/Oi3lY62P+B7iXSK3uBZw/9Pv30bt1b7bUNR7nj3DMS22cjnPchkmUCB9/xYCI/vKxVd9JmlQfn7vLsEfudFjD6exAnLD5P5Vr31FG5xvpQv6HVD+ht3/xK9wA6xGSrvO70Bu64xeKYp5h21g1uqfKctuMj7vvYrsNVbvu67vdUEr9pJVRmq9DvfMkpn1bjTMrim0AAAAAAAAAW4CAQAAAAAACABTgIBAAAAAAAYIG06QkUqBda220a1Ffb1l7QQuWcrXpdd8cR30a83uFNn1b5pJx9Kh9wdFOgK4eeHvG6Tmv4jspn1foxwp6/GL8z1N/ih4ubq23B3clb0+wnF9feovK3B0K1rFVQQ21LxR5AXoHGjVV2Gu1X2bu+/JI6P6j8stATSESk9ktzVR6UcbPKm04pdsdZW7LUthafFquc/e58lTc831Tlr37Oc8eNntT9hZBYYwf9W+VphbpfU+Ysbx+JyjN9uql86qTP3PEVdb9X27YEf1b55WvPUDmw8qu4zQtVU1JYqHKr+3R/mrO/068dc/7xmDvOEf0edOsluu/fy4/px0w6vEelmuOeCb0G3NZwaYUu+999ur41zQF3fHS2491dGbupj8oL7gz1OcpdvF5tK5AmKg/ornsA1fyPbioS/ZZT1/7Te7vjM5t/pLZ5P3t7TZw8WOVo/ToTydNGKuq8n959mMpN50X/HW20+6pGKt/z4hEq98stiHjZu1YMUrnwU/08877WewWzQ31+jmyh3ydKynkWZhWm67O0aqL1yPrw/N4qlyzLT/R04iKjRxeVz3wh1AdoZN21nr09v3+S+4DxTSAAAAAAAAALcBAIAAAAAADAAhwEAgAAAAAAsEDq9ATq213FtQNzVW7ce7M7/qjbK0mZkkjZ9YzP5H0Yt+tunbXDHa8apvuOtLt3k8olP/0Ut9v1s2LRa6QvXTzcHZe3njdVbBvZzx3v6K370Sw5+WGVD3iWGQ9ZPtRzbeviObW0UeeFuZ4c+2W3XttP5W9PnKjyCTde544PKdG3g/jy9uUZkPuZyhfdpPu35MoXMV93ZkvdSy7/b7ov28cnT1C5ZaCWO377p9pq2yPDLlc58Dk9gFKV97Wj7YAr3PGK0yarbRfX3qjy36/Tr8+H/jU93rNSyVNzjnfHX3VurbZ9/W2eym2m68vmLtuscsF9of6TS0+YorZdsEr3/fp5jO7vl71wnjvW7/JlZa/foLIt3UU2nBDqzzeqXmr0Awl01H19zrn00wh7iqwsLlH5tTGnqZzzIT0FvYLLVqj8+ZG6T9fn0jXiZevKyqi5PMX9Qtf9f3lPRN13TbHuH9vsv6H+bzZ3ehp51Vsqe3tk9Zx9pTtu992qpMwp3lYPqavyiDqh38P7N9u923qp3Gq6/putvPeGquKbQAAAAAAAABbgIBAAAAAAAIAFUmY52Jpz9PKvpcMeqfR1bfOcrndaoT7FYIusne743NwdUl2GHLItNB6hf98enS9Tuc01+tTpwa1bEzcxHxmat9Advzf4RLUtZ7o/v0obvtxLRGTX4fr7gcsu+Jc7Lu80qGXcXt/zA5aDVZV3ydGbt49Tuevsa1Vu80rsS46QWBtO0LmDPmO3BOqHni8rJx2qtj3UZ5rKp+XsVXlniT6XZ6ePQ8uC2j2iv+Zv5iyKab5IjvClfquubqO2mc57VG47Ur+GBnfuVLnLH8OWZuvVHGV4TxeN5Ot4dWgZ1l7vNon+uWnvWfo072/0Cy0JfWxXZ7Vt11/160nWwgWC6MJPCS8i8s4l4e+1etmPV58vRqh86OMLVdavyPHjXf41fIZuCTGwVuTH1K6SmipnfchjxM/2No/+GAx38RL9eGzgWcZmqxe/16+hI47QS76C60LL6kv2el+hU8M3l+u/171LwMK9/trxKrdem9wl4nwTCAAAAAAAwAIcBAIAAAAAALAAB4EAAAAAAAAskDI9gfKH6dMwV2R977C1p6q85N967XaLf+g1eIGux7jjBc8tV9vuaVKxNbvhpwk866XfR933mOP1KTCfbjMr4r4L+z6r8inP6VPP5pxuR0+gMQ1CvTYCd+tHxXv7+qtc4715Ei8rntDrWpu21H0igiWRj6/e0fF5lU+vtcWzRyDmeXSbMUblzqs962tjvib8T0ZNvU7/9Kn6FK8v7+6ucrurPT1DHBp/JIszb4nKH+xtr/KCcx9S+eJu+nXyhtYfuOOTcvQpXb0m/5in8nN3n6XyYS/qU4ej+gQaNlC54JZOKj8f1netcUaR2jZqwDCVvT2AqiIzNVscWCsjV/eifHTiBJVzM0KfOWZcoXsSZs2lv0tFOZm6z1rzQOw9WPYXZalc8tNPcZmTiEhmnu7v9MOQVu7Yewr4aD2AvK6ap3t75sniSswOiWJ66dPNtx29PMKeiFXhzGb6B7olr7x6Xug1dvQc/fdN7qv+6Le5/wz999/VE16NsGdZXd8ZpXKn++ernOy/HvgmEAAAAAAAgAU4CAQAAAAAAGABDgIBAAAAAABYIGV6AgWMPl5V4sTe7eQPLd9ReenIr/QOI72XWOOOemWv92zLiXpbs/fpdcl33BFa/9du2pyol93ZrKnKA54d4o7/eNhbatsJNferPOsIvSZxoPSKelvpaFR93R+k/b82q7x6f2OVs4x+DB1wYu/F81Qd3WukcSDbc12J6cbT7f3RKne+09MDaNv2hNyuTTZcc5TKJ+c+qPLNl1yjcsbOhQmfE2IzsUD35Rjea4PKb3WcoXL4+0rQ0f0oTlii+wfVu1L3jqmzjh5AfuHt3dBy0lqV32qlewruLjngjn9zqe47EFihPx9ket6Xdx2fp/Jpd4Z6gmSIfgzNLtKfW1r+Z5fKFeltiMTz9pLKfF33pOmYpfvFtX8j9F7Qce6XiZsYEmrbyH4qF56sm3e1bbxD5bmdxlf6tnp8epU7bj9Gvz/RwzG5TJZ+fm8dof9u+udtj6t8bM0DEsmNG36lcs6T9ao4Ozt1zAq9h951/xS17d6fh6uc/Xb8+rx6ZbZqqfLqh0LvDW0b6eMC5+TqvzWj9XUNFOptzoH9EfZMDr4JBAAAAAAAYIFyDwIZY1obYz4yxuQbY5YaY35X+vMGxpgPjDEFpf/WT/x0kSzU3V7U3k7U3U7U3V7U3k7U3U7U3V7UHgcTyzeBikXkZsdxOotIXxEZZYzpIiJjRWSW4zgdRGRWaUb6oO72ovZ2ou52ou72ovZ2ou52ou72ovYoo9yeQI7jbBSRjaXjQmNMvoi0FJFBItK/dLdnRORjEbktIbMUkc6zL1V58a+mxnzZjlnedd07Iux5MLoH0D3buqv86jTdg6LBt3pVb+3XY+8bUbxJryvMPi00/sugK9S2Fx7WfUpOnXutym1E98epKL/U3evsoVeq/NarT0bcd2Cupz+OJ2d61m0WV2hFtu4BlG2yIuxXvud2t1b5hfVHu+PMU79X2zrKfJUTsYbcr7VPJKffke74rZseUNsGzLlO5bzP0rMHUDrUvcl5ukdW75G6h1aRbvkh4S1c2j6n13nX2bZV5eLCwirPz49Sse6mp+4B1NrTA2hSq/+q7O29s7o49NGn47hlalvQ0e/5f2z2mspNA3p7eB+gnSU/q21jxt+iL7vwc/GTVKx9IpW0030gXms/Vee9+gWky92h9+fihM0q/tKx7q/00/1bZi9rH/Nl++T8S+UjshyVs4z+rHhAb1aKHP1I6Pu57iEY3gcouFW/xyRaOtZdju6m85f6b5/w94qtfeqobT+euE/l/P6PxHyzXxbp/m8rr+uocs48f/UI80vt6xXo58fJiy9S+dMjp7njk3J0fU6a/JjKo9cfp/K6C5qovP/Q0Ov1qvP1cYDlgyepXPY5vqDM3CPTl32xUPcR/NPH57njjjf7q5dkhXoCGWPyRKSniHwhIk1LH1T/e3A1iXxJpDLqbi9qbyfqbifqbi9qbyfqbifqbi9qj/+J+SCQMeYQEXlNRG5+IhWcAAAL0ElEQVRwHGd3BS430hgz3xgz/4AUlX8B+Ap1txe1txN1txN1txe1txN1txN1txe1R7iYThFvjMmSXx40zzuO83rpjzcbY5o7jrPRGNNcRLYc7LKO40wWkckiInVMgyhfoowu79IClQe10qfvDT4e+dR9FRUYHfZ17236lK5SpB/8rXYn5+vdOW/orxaOnD1I5bZ79P0Tj1PP+qHuXpnb96jcb8El7nhonl6mM6bBouhXpr/NWaXTuq88oL/ieOni4TFfttnN+rGbWbC60vOIFz/WPp4C9eqq/OsnP3bHbxTqpSZtL1uucjx/oYya+pTDEgh9rbRk715JtlSvu1Osn4dNJsX++pxKyzniLdXqvuNI/bX+t1t9qnJ573/da4SeZw+30I+RkjLP8ByJ5r7tXdzxjAf6q21Nn/PX8q+DSbXaJ9LKGyOf2ldE5IEH9NKFhhvnJHI6CeXHumdv1cs/Ht4Zei++vv7SqJcNP7X0L3lVhD3j6/cbT1D5vaX680OH4XpZSXWfBt6Pdd85rJ/K193+WoQ9y+qSrU8TvqxIL+k8vEbo/u+luziopbwi5b9vPLyzgzv+8NweaptTULUWHMngh9rXnKH/ls1dcZjKS2eGPol5n9Nej7T8TOV7put2LZ1rhpZeek/jHm1J5y/bY3+mzt+vl5qFL/8SEel4jb+WBoaL5exgRkSeEpF8x3HCG9G8KSLDSsfDROSN+E8P1YW624va24m624m624va24m624m624va42Bi+SbQsSJyqYgsMcb876sWd4jI/SLysjHmChH5XkR+k5gpoppQd3tReztRdztRd3tReztRdztRd3tRe5QRy9nBPpMyC2dcp8R3OvAL6m4vam8n6m4n6m4vam8n6m4n6m4vao+DiaknkB+U7NNrhWXlGp3j+BCu7jW7sQhu217+TmkouEKv8258Tmj83uAT1bYpx50U9bpKGulePItPnRhx3+MXDFe5sKCeytnb9crKVvfF3gsiFR5v6Wbd1BYqn1v7bXd81dlXqW1OUX7C5vHtBH1606v6hU5t/dJU/aLW6mk9j+DOnQmbF+BnDV/6WuWOR16n8qgB76t8fX3dMy/cWz/p/kLj15yq8qZdtVWuMVfnlpO+csd19/nr9K+Ibudw3Ytk+Yn6M8DsIt0jqOGTqdsDKCV4Tu898+bQZ7opx+vn5TuXjVO5eUD35Uik4Wt/7Y4LR+mTKXVYVJFTS0NEZPdZutfnxbU3VuDS+rN3zxoVuWx0fb+6UOVm14TmGVxf/b0704H3b7oxN17vjjdfqP/u//rYJ6Ne120Nv466vSou/+4Mdzx/bke1re103Su446f+7QHkVaFTxAMAAAAAACA1cRAIAAAAAADAAhwEAgAAAAAAsEDK9AQCypMzXa/DPGx69P0DjRqq3H/wjRH3bf7JZpWbFND7IZXsG3i0yp/0Gq/yyfff6o6bLIq9n1NVdb7rO5Wf+HOoB0KL0zapbVtPbqxyg4H0BIKdvD0C29+oX49nSh1P7hXzdWfLWpXblDeXmK8ZvpAR6vMTHKJ7K5aIo/LVz+heU4dK8t4bIJL1/nx3nKfbfMkF392i8n//PCFut3vMv25QueES3T+y1nc/uuOSZYnrGWiLw8YWqvzYW+3c8TX1qtZ7Z+ymPu7435/3UdvMAd0nudNfdS0b/6w/nxUX6d4viL/wv+PafZCrtg1tc4nK9Z7YqnJJxL7X5Zs3T/f56TR5h95ha+jz9mFb0+fvP74JBAAAAAAAYAEOAgEAAAAAAFiAg0AAAAAAAAAWoCcQrBXcpvsBNHxyTuR9Ez0ZxJXJzlb58LuWqNzrP6NV7jCxeno9BDdvUbnjtVsi7AkAqKqtV4f6w31x1CNq25pi3WuqzTu6V4nuGITq5P28du6TR0fYs+JalNP7ic+D8VW8eq3KM7rWD40r0M/t4EJd2zrIF1H3pK7+UrJ3r/7BshUqbj82frfVXnSfH1seC3wTCAAAAAAAwAIcBAIAAAAAALAAB4EAAAAAAAAsQE8gAGkno3ULlUc1eVHltaPbJ3M6AAAf2NM68raZe7qo7MxbEmFPAABSG98EAgAAAAAAsAAHgQAAAAAAACzAcjAAaSe4co3Kt+T19ezB1/wBwDYNl4RO9H7BqjPUtoIZHVQu71ThAACkKr4JBAAAAAAAYAEOAgEAAAAAAFiAg0AAAAAAAAAWoCcQAAAA0l6dF+e6470v6m0tZGuSZwMAQPXgm0AAAAAAAAAW4CAQAAAAAACABTgIBAAAAAAAYAHjOE7ybsyYrSLynYg0EpFtSbvh2PhxTiLJn1cbx3Eax/MKfV53EX/OK+XrLuLWfq/47/4V8WfdRdKg9j5/zvtxTiLUPRn8OK+Ur7sIr/WVlPK19/lz3o9zEqHuyeDHeaV83UV4ra8kX9Y+qQeB3Bs1Zr7jOL2TfsNR+HFOIv6dV2X49Xfx47z8OKfK8uvvwrwSz4+/ix/nJOLfeVWGX38XP87Lj3OqLL/+Lswr8fz4u/hxTiL+nVdl+PV38eO8/DinyvLr78K8KoblYAAAAAAAABbgIBAAAAAAAIAFqusg0ORqut1o/DgnEf/OqzL8+rv4cV5+nFNl+fV3YV6J58ffxY9zEvHvvCrDr7+LH+flxzlVll9/F+aVeH78Xfw4JxH/zqsy/Pq7+HFefpxTZfn1d2FeFVAtPYEAAAAAAACQXCwHAwAAAAAAsEBSDwIZY84wxiw3xqw0xoxN5m175jHFGLPFGPNN2M8aGGM+MMYUlP5bP8lzam2M+cgYk2+MWWqM+Z0f5hUvfqi9H+teOoe0rb0f6l46D9/VnronZR7UPcn8UHs/1r10Dmlbez/UvXQevqs9dU/KPKh7kvmh9n6se+kc0rb2fqh76Tx8V/tUq3vSDgIZYwIiMlFEfi0iXUTkQmNMl2TdvsdUETnD87OxIjLLcZwOIjKrNCdTsYjc7DhOZxHpKyKjSu+f6p5Xlfmo9lPFf3UXSdPa+6juIv6sPXVPvKlC3ZPGR7WfKv6ru0ia1t5HdRfxZ+2pe+JNFeqeND6q/VTxX91F0rT2Pqq7iD9rn1p1dxwnKf+JSD8RmRmWbxeR25N1+weZT56IfBOWl4tI89JxcxFZXl1zK53DGyIywG/zSvXa+73u6VR7P9U9FWpP3am7n+aV6rX3e93TqfZ+qnsq1J66U3c/zSvVa+/3uqdT7f1U91Sovd/rnszlYC1F5IewvK70Z37R1HGcjSIipf82qa6JGGPyRKSniHzhp3lVgZ9r76v7N81q7+e6i/jo/qXuSeWb+zfN6i7i79r76v5Ns9r7ue4iPrp/qXtS+eb+TbO6i/i79r66f9Os9n6uu4iP7t9UqHsyDwKZg/yMU5N5GGMOEZHXROQGx3F2V/d84oTaxyANa0/dY0Dd7ZSGdReh9jFJw9pT9xhQdzulYd1FqH1M0rD21D0GqVL3ZB4EWicircNyKxHZkMTbL89mY0xzEZHSf7ckewLGmCz55UHzvOM4r/tlXnHg59r74v5N09r7ue4iPrh/qXu1qPb7N03rLuLv2vvi/k3T2vu57iI+uH+pe7Wo9vs3Tesu4u/a++L+TdPa+7nuIj64f1Op7sk8CDRPRDoYY9oaY2qIyG9F5M0k3n553hSRYaXjYfLLOr6kMcYYEXlKRPIdx3nQL/OKEz/Xvtrv3zSuvZ/rLsJzPlGoexRpXHcRf9e+2u/fNK69n+suwnM+Uah7FGlcdxF/177a7980rr2f6y7Cc75iktwg6UwRWSEiq0TkD9XVCElEXhSRjSJyQH45qnmFiDSUXzp2F5T+2yDJczpOfvlK3WIRWVj635nVPa90qr0f657utfdD3f1ae+pO3dOt7n6pvR/rnu6190Pd/Vp76k7d063ufqm9H+ue7rX3Q939WvtUq7spnTQAAAAAAADSWDKXgwEAAAAAAKCacBAIAAAAAADAAhwEAgAAAAAAsAAHgQAAAAAAACzAQSAAAAAAAAALcBAIAAAAAADAAhwEAgAAAAAAsAAHgQAAAAAAACzw/2I8231fjAT/AAAAAElFTkSuQmCC\\n\",\n \"text/plain\": [\n \"<Figure size 1440x576 with 9 Axes>\"\n ]\n },\n \"metadata\": {},\n \"output_type\": \"display_data\"\n }\n ],\n \"source\": [\n \"n = 9\\n\",\n \"plt.figure(figsize = (20, 8))\\n\",\n \"for i in range(n):\\n\",\n \" ax = plt.subplot(1, n, i+1)\\n\",\n \" plt.imshow(x_test[i].reshape(28, 28))\\n\",\n \"plt.show()\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": null,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": []\n }\n ],\n \"metadata\": {\n \"kernelspec\": {\n \"display_name\": \"Python 3\",\n \"language\": \"python\",\n \"name\": \"python3\"\n },\n \"language_info\": {\n \"codemirror_mode\": {\n \"name\": \"ipython\",\n \"version\": 3\n },\n \"file_extension\": \".py\",\n \"mimetype\": \"text/x-python\",\n \"name\": \"python\",\n \"nbconvert_exporter\": \"python\",\n \"pygments_lexer\": \"ipython3\",\n \"version\": \"3.6.5\"\n }\n },\n \"nbformat\": 4,\n \"nbformat_minor\": 2\n}"
}
{
"filename": "code/artificial_intelligence/src/dbscan_clustering/dbscan.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\nimport numpy as np\nimport math\n\nUNCLASSIFIED = False\nNOISE = None\n\n\ndef _dist(p, q):\n return math.sqrt(np.power(p - q, 2).sum())\n\n\ndef _eps_neighborhood(p, q, eps):\n return _dist(p, q) < eps\n\n\ndef _region_query(m, point_id, eps):\n n_points = m.shape[1]\n seeds = []\n for i in range(0, n_points):\n if _eps_neighborhood(m[:, point_id], m[:, i], eps):\n seeds.append(i)\n return seeds\n\n\ndef _expand_cluster(m, classifications, point_id, cluster_id, eps, min_points):\n seeds = _region_query(m, point_id, eps)\n if len(seeds) < min_points:\n classifications[point_id] = NOISE\n return False\n else:\n classifications[point_id] = cluster_id\n for seed_id in seeds:\n classifications[seed_id] = cluster_id\n\n while len(seeds) > 0:\n current_point = seeds[0]\n results = _region_query(m, current_point, eps)\n if len(results) >= min_points:\n for i in range(0, len(results)):\n result_point = results[i]\n if (\n classifications[result_point] == UNCLASSIFIED\n or classifications[result_point] == NOISE\n ):\n if classifications[result_point] == UNCLASSIFIED:\n seeds.append(result_point)\n classifications[result_point] = cluster_id\n seeds = seeds[1:]\n return True\n\n\ndef dbscan(m, eps, min_points):\n \"\"\"Implementation of Density Based Spatial Clustering of Applications with Noise\n See https://en.wikipedia.org/wiki/DBSCAN\n\n scikit-learn probably has a better implementation\n\n Uses Euclidean Distance as the measure\n\n Inputs:\n m - A matrix whose columns are feature vectors\n eps - Maximum distance two points can be to be regionally related\n min_points - The minimum number of points to make a cluster\n\n Outputs:\n An array with either a cluster id number or dbscan.NOISE (None) for each\n column vector in m.\n \"\"\"\n cluster_id = 1\n n_points = m.shape[1]\n classifications = [UNCLASSIFIED] * n_points\n for point_id in range(0, n_points):\n point = m[:, point_id]\n if classifications[point_id] == UNCLASSIFIED:\n if _expand_cluster(\n m, classifications, point_id, cluster_id, eps, min_points\n ):\n cluster_id = cluster_id + 1\n return classifications\n\n\n# def test_dbscan():\n# m = np.matrix('1 1.2 0.8 3.7 3.9 3.6 10; 1.1 0.8 1 4 3.9 4.1 10')\n# eps = 0.5\n# min_points = 2\n# assert dbscan(m, eps, min_points) == [1, 1, 1, 2, 2, 2, None]\n\n\ndef main():\n m = np.matrix(\n \"-0.99 -0.98 -0.97 -0.96 -0.95 0.95 0.96 0.97 0.98 0.99; 1.1 1.09 1.08 1.07 1.06 1.06 1.07 1.08 1.09 1.1\"\n )\n eps = 1\n min_points = 3\n print(dbscan(m, eps, min_points))\n\n\nmain()"
}
{
"filename": "code/artificial_intelligence/src/dbscan_clustering/readme.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# DBSCAN Clustering\nA Density-Based Algorithm for Discovering Clusters in Large Spatial Databases with Noise Martin Ester, Hans-Peter Kriegel, Jörg Sander, Xiaowei Xu. This is a density based spatial clustering of applications with noise.\n\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/artificial_intelligence/src/decision_tree/data_banknote_authentication.csv",
"content": "3.6216,8.6661,-2.8073,-0.44699,0\n4.5459,8.1674,-2.4586,-1.4621,0\n3.866,-2.6383,1.9242,0.10645,0\n3.4566,9.5228,-4.0112,-3.5944,0\n0.32924,-4.4552,4.5718,-0.9888,0\n4.3684,9.6718,-3.9606,-3.1625,0\n3.5912,3.0129,0.72888,0.56421,0\n2.0922,-6.81,8.4636,-0.60216,0\n3.2032,5.7588,-0.75345,-0.61251,0\n1.5356,9.1772,-2.2718,-0.73535,0\n1.2247,8.7779,-2.2135,-0.80647,0\n3.9899,-2.7066,2.3946,0.86291,0\n1.8993,7.6625,0.15394,-3.1108,0\n-1.5768,10.843,2.5462,-2.9362,0\n3.404,8.7261,-2.9915,-0.57242,0\n4.6765,-3.3895,3.4896,1.4771,0\n2.6719,3.0646,0.37158,0.58619,0\n0.80355,2.8473,4.3439,0.6017,0\n1.4479,-4.8794,8.3428,-2.1086,0\n5.2423,11.0272,-4.353,-4.1013,0\n5.7867,7.8902,-2.6196,-0.48708,0\n0.3292,-4.4552,4.5718,-0.9888,0\n3.9362,10.1622,-3.8235,-4.0172,0\n0.93584,8.8855,-1.6831,-1.6599,0\n4.4338,9.887,-4.6795,-3.7483,0\n0.7057,-5.4981,8.3368,-2.8715,0\n1.1432,-3.7413,5.5777,-0.63578,0\n-0.38214,8.3909,2.1624,-3.7405,0\n6.5633,9.8187,-4.4113,-3.2258,0\n4.8906,-3.3584,3.4202,1.0905,0\n-0.24811,-0.17797,4.9068,0.15429,0\n1.4884,3.6274,3.308,0.48921,0\n4.2969,7.617,-2.3874,-0.96164,0\n-0.96511,9.4111,1.7305,-4.8629,0\n-1.6162,0.80908,8.1628,0.60817,0\n2.4391,6.4417,-0.80743,-0.69139,0\n2.6881,6.0195,-0.46641,-0.69268,0\n3.6289,0.81322,1.6277,0.77627,0\n4.5679,3.1929,-2.1055,0.29653,0\n3.4805,9.7008,-3.7541,-3.4379,0\n4.1711,8.722,-3.0224,-0.59699,0\n-0.2062,9.2207,-3.7044,-6.8103,0\n-0.0068919,9.2931,-0.41243,-1.9638,0\n0.96441,5.8395,2.3235,0.066365,0\n2.8561,6.9176,-0.79372,0.48403,0\n-0.7869,9.5663,-3.7867,-7.5034,0\n2.0843,6.6258,0.48382,-2.2134,0\n-0.7869,9.5663,-3.7867,-7.5034,0\n3.9102,6.065,-2.4534,-0.68234,0\n1.6349,3.286,2.8753,0.087054,0\n4.3239,-4.8835,3.4356,-0.5776,0\n5.262,3.9834,-1.5572,1.0103,0\n3.1452,5.825,-0.51439,-1.4944,0\n2.549,6.1499,-1.1605,-1.2371,0\n4.9264,5.496,-2.4774,-0.50648,0\n4.8265,0.80287,1.6371,1.1875,0\n2.5635,6.7769,-0.61979,0.38576,0\n5.807,5.0097,-2.2384,0.43878,0\n3.1377,-4.1096,4.5701,0.98963,0\n-0.78289,11.3603,-0.37644,-7.0495,0\n2.888,0.44696,4.5907,-0.24398,0\n0.49665,5.527,1.7785,-0.47156,0\n4.2586,11.2962,-4.0943,-4.3457,0\n1.7939,-1.1174,1.5454,-0.26079,0\n5.4021,3.1039,-1.1536,1.5651,0\n2.5367,2.599,2.0938,0.20085,0\n4.6054,-4.0765,2.7587,0.31981,0\n2.4235,9.5332,-3.0789,-2.7746,0\n1.0009,7.7846,-0.28219,-2.6608,0\n0.12326,8.9848,-0.9351,-2.4332,0\n3.9529,-2.3548,2.3792,0.48274,0\n4.1373,0.49248,1.093,1.8276,0\n4.7181,10.0153,-3.9486,-3.8582,0\n4.1654,-3.4495,3.643,1.0879,0\n4.4069,10.9072,-4.5775,-4.4271,0\n2.3066,3.5364,0.57551,0.41938,0\n3.7935,7.9853,-2.5477,-1.872,0\n0.049175,6.1437,1.7828,-0.72113,0\n0.24835,7.6439,0.9885,-0.87371,0\n1.1317,3.9647,3.3979,0.84351,0\n2.8033,9.0862,-3.3668,-1.0224,0\n4.4682,2.2907,0.95766,0.83058,0\n5.0185,8.5978,-2.9375,-1.281,0\n1.8664,7.7763,-0.23849,-2.9634,0\n3.245,6.63,-0.63435,0.86937,0\n4.0296,2.6756,0.80685,0.71679,0\n-1.1313,1.9037,7.5339,1.022,0\n0.87603,6.8141,0.84198,-0.17156,0\n4.1197,-2.7956,2.0707,0.67412,0\n3.8027,0.81529,2.1041,1.0245,0\n1.4806,7.6377,-2.7876,-1.0341,0\n4.0632,3.584,0.72545,0.39481,0\n4.3064,8.2068,-2.7824,-1.4336,0\n2.4486,-6.3175,7.9632,0.20602,0\n3.2718,1.7837,2.1161,0.61334,0\n-0.64472,-4.6062,8.347,-2.7099,0\n2.9543,1.076,0.64577,0.89394,0\n2.1616,-6.8804,8.1517,-0.081048,0\n3.82,10.9279,-4.0112,-5.0284,0\n-2.7419,11.4038,2.5394,-5.5793,0\n3.3669,-5.1856,3.6935,-1.1427,0\n4.5597,-2.4211,2.6413,1.6168,0\n5.1129,-0.49871,0.62863,1.1189,0\n3.3397,-4.6145,3.9823,-0.23751,0\n4.2027,0.22761,0.96108,0.97282,0\n3.5438,1.2395,1.997,2.1547,0\n2.3136,10.6651,-3.5288,-4.7672,0\n-1.8584,7.886,-1.6643,-1.8384,0\n3.106,9.5414,-4.2536,-4.003,0\n2.9163,10.8306,-3.3437,-4.122,0\n3.9922,-4.4676,3.7304,-0.1095,0\n1.518,5.6946,0.094818,-0.026738,0\n3.2351,9.647,-3.2074,-2.5948,0\n4.2188,6.8162,-1.2804,0.76076,0\n1.7819,6.9176,-1.2744,-1.5759,0\n2.5331,2.9135,-0.822,-0.12243,0\n3.8969,7.4163,-1.8245,0.14007,0\n2.108,6.7955,-0.1708,0.4905,0\n2.8969,0.70768,2.29,1.8663,0\n0.9297,-3.7971,4.6429,-0.2957,0\n3.4642,10.6878,-3.4071,-4.109,0\n4.0713,10.4023,-4.1722,-4.7582,0\n-1.4572,9.1214,1.7425,-5.1241,0\n-1.5075,1.9224,7.1466,0.89136,0\n-0.91718,9.9884,1.1804,-5.2263,0\n2.994,7.2011,-1.2153,0.3211,0\n-2.343,12.9516,3.3285,-5.9426,0\n3.7818,-2.8846,2.2558,-0.15734,0\n4.6689,1.3098,0.055404,1.909,0\n3.4663,1.1112,1.7425,1.3388,0\n3.2697,-4.3414,3.6884,-0.29829,0\n5.1302,8.6703,-2.8913,-1.5086,0\n2.0139,6.1416,0.37929,0.56938,0\n0.4339,5.5395,2.033,-0.40432,0\n-1.0401,9.3987,0.85998,-5.3336,0\n4.1605,11.2196,-3.6136,-4.0819,0\n5.438,9.4669,-4.9417,-3.9202,0\n5.032,8.2026,-2.6256,-1.0341,0\n5.2418,10.5388,-4.1174,-4.2797,0\n-0.2062,9.2207,-3.7044,-6.8103,0\n2.0911,0.94358,4.5512,1.234,0\n1.7317,-0.34765,4.1905,-0.99138,0\n4.1736,3.3336,-1.4244,0.60429,0\n3.9232,-3.2467,3.4579,0.83705,0\n3.8481,10.1539,-3.8561,-4.2228,0\n0.5195,-3.2633,3.0895,-0.9849,0\n3.8584,0.78425,1.1033,1.7008,0\n1.7496,-0.1759,5.1827,1.2922,0\n3.6277,0.9829,0.68861,0.63403,0\n2.7391,7.4018,0.071684,-2.5302,0\n4.5447,8.2274,-2.4166,-1.5875,0\n-1.7599,11.9211,2.6756,-3.3241,0\n5.0691,0.21313,0.20278,1.2095,0\n3.4591,11.112,-4.2039,-5.0931,0\n1.9358,8.1654,-0.023425,-2.2586,0\n2.486,-0.99533,5.3404,-0.15475,0\n2.4226,-4.5752,5.947,0.21507,0\n3.9479,-3.7723,2.883,0.019813,0\n2.2634,-4.4862,3.6558,-0.61251,0\n1.3566,4.2358,2.1341,0.3211,0\n5.0452,3.8964,-1.4304,0.86291,0\n3.5499,8.6165,-3.2794,-1.2009,0\n0.17346,7.8695,0.26876,-3.7883,0\n2.4008,9.3593,-3.3565,-3.3526,0\n4.8851,1.5995,-0.00029081,1.6401,0\n4.1927,-3.2674,2.5839,0.21766,0\n1.1166,8.6496,-0.96252,-1.8112,0\n1.0235,6.901,-2.0062,-2.7125,0\n-1.803,11.8818,2.0458,-5.2728,0\n0.11739,6.2761,-1.5495,-2.4746,0\n0.5706,-0.0248,1.2421,-0.5621,0\n4.0552,-2.4583,2.2806,1.0323,0\n-1.6952,1.0657,8.8294,0.94955,0\n-1.1193,10.7271,2.0938,-5.6504,0\n1.8799,2.4707,2.4931,0.37671,0\n3.583,-3.7971,3.4391,-0.12501,0\n0.19081,9.1297,-3.725,-5.8224,0\n3.6582,5.6864,-1.7157,-0.23751,0\n-0.13144,-1.7775,8.3316,0.35214,0\n2.3925,9.798,-3.0361,-2.8224,0\n1.6426,3.0149,0.22849,-0.147,0\n-0.11783,-1.5789,8.03,-0.028031,0\n-0.69572,8.6165,1.8419,-4.3289,0\n2.9421,7.4101,-0.97709,-0.88406,0\n-1.7559,11.9459,3.0946,-4.8978,0\n-1.2537,10.8803,1.931,-4.3237,0\n3.2585,-4.4614,3.8024,-0.15087,0\n1.8314,6.3672,-0.036278,0.049554,0\n4.5645,-3.6275,2.8684,0.27714,0\n2.7365,-5.0325,6.6608,-0.57889,0\n0.9297,-3.7971,4.6429,-0.2957,0\n3.9663,10.1684,-4.1131,-4.6056,0\n1.4578,-0.08485,4.1785,0.59136,0\n4.8272,3.0687,0.68604,0.80731,0\n-2.341,12.3784,0.70403,-7.5836,0\n-1.8584,7.886,-1.6643,-1.8384,0\n4.1454,7.257,-1.9153,-0.86078,0\n1.9157,6.0816,0.23705,-2.0116,0\n4.0215,-2.1914,2.4648,1.1409,0\n5.8862,5.8747,-2.8167,-0.30087,0\n-2.0897,10.8265,2.3603,-3.4198,0\n4.0026,-3.5943,3.5573,0.26809,0\n-0.78689,9.5663,-3.7867,-7.5034,0\n4.1757,10.2615,-3.8552,-4.3056,0\n0.83292,7.5404,0.65005,-0.92544,0\n4.8077,2.2327,-0.26334,1.5534,0\n5.3063,5.2684,-2.8904,-0.52716,0\n2.5605,9.2683,-3.5913,-1.356,0\n2.1059,7.6046,-0.47755,-1.8461,0\n2.1721,-0.73874,5.4672,-0.72371,0\n4.2899,9.1814,-4.6067,-4.3263,0\n3.5156,10.1891,-4.2759,-4.978,0\n2.614,8.0081,-3.7258,-1.3069,0\n0.68087,2.3259,4.9085,0.54998,0\n4.1962,0.74493,0.83256,0.753,0\n6.0919,2.9673,-1.3267,1.4551,0\n1.3234,3.2964,0.2362,-0.11984,0\n1.3264,1.0326,5.6566,-0.41337,0\n-0.16735,7.6274,1.2061,-3.6241,0\n-1.3,10.2678,-2.953,-5.8638,0\n-2.2261,12.5398,2.9438,-3.5258,0\n2.4196,6.4665,-0.75688,0.228,0\n1.0987,0.6394,5.989,-0.58277,0\n4.6464,10.5326,-4.5852,-4.206,0\n-0.36038,4.1158,3.1143,-0.37199,0\n1.3562,3.2136,4.3465,0.78662,0\n0.5706,-0.0248,1.2421,-0.5621,0\n-2.6479,10.1374,-1.331,-5.4707,0\n3.1219,-3.137,1.9259,-0.37458,0\n5.4944,1.5478,0.041694,1.9284,0\n-1.3389,1.552,7.0806,1.031,0\n-2.3361,11.9604,3.0835,-5.4435,0\n2.2596,-0.033118,4.7355,-0.2776,0\n0.46901,-0.63321,7.3848,0.36507,0\n2.7296,2.8701,0.51124,0.5099,0\n2.0466,2.03,2.1761,-0.083634,0\n-1.3274,9.498,2.4408,-5.2689,0\n3.8905,-2.1521,2.6302,1.1047,0\n3.9994,0.90427,1.1693,1.6892,0\n2.3952,9.5083,-3.1783,-3.0086,0\n3.2704,6.9321,-1.0456,0.23447,0\n-1.3931,1.5664,7.5382,0.78403,0\n1.6406,3.5488,1.3964,-0.36424,0\n2.7744,6.8576,-1.0671,0.075416,0\n2.4287,9.3821,-3.2477,-1.4543,0\n4.2134,-2.806,2.0116,0.67412,0\n1.6472,0.48213,4.7449,1.225,0\n2.0597,-0.99326,5.2119,-0.29312,0\n0.3798,0.7098,0.7572,-0.4444,0\n1.0135,8.4551,-1.672,-2.0815,0\n4.5691,-4.4552,3.1769,0.0042961,0\n0.57461,10.1105,-1.6917,-4.3922,0\n0.5734,9.1938,-0.9094,-1.872,0\n5.2868,3.257,-1.3721,1.1668,0\n4.0102,10.6568,-4.1388,-5.0646,0\n4.1425,-3.6792,3.8281,1.6297,0\n3.0934,-2.9177,2.2232,0.22283,0\n2.2034,5.9947,0.53009,0.84998,0\n3.744,0.79459,0.95851,1.0077,0\n3.0329,2.2948,2.1135,0.35084,0\n3.7731,7.2073,-1.6814,-0.94742,0\n3.1557,2.8908,0.59693,0.79825,0\n1.8114,7.6067,-0.9788,-2.4668,0\n4.988,7.2052,-3.2846,-1.1608,0\n2.483,6.6155,-0.79287,-0.90863,0\n1.594,4.7055,1.3758,0.081882,0\n-0.016103,9.7484,0.15394,-1.6134,0\n3.8496,9.7939,-4.1508,-4.4582,0\n0.9297,-3.7971,4.6429,-0.2957,0\n4.9342,2.4107,-0.17594,1.6245,0\n3.8417,10.0215,-4.2699,-4.9159,0\n5.3915,9.9946,-3.8081,-3.3642,0\n4.4072,-0.070365,2.0416,1.1319,0\n2.6946,6.7976,-0.40301,0.44912,0\n5.2756,0.13863,0.12138,1.1435,0\n3.4312,6.2637,-1.9513,-0.36165,0\n4.052,-0.16555,0.45383,0.51248,0\n1.3638,-4.7759,8.4182,-1.8836,0\n0.89566,7.7763,-2.7473,-1.9353,0\n1.9265,7.7557,-0.16823,-3.0771,0\n0.20977,-0.46146,7.7267,0.90946,0\n4.068,-2.9363,2.1992,0.50084,0\n2.877,-4.0599,3.6259,-0.32544,0\n0.3223,-0.89808,8.0883,0.69222,0\n-1.3,10.2678,-2.953,-5.8638,0\n1.7747,-6.4334,8.15,-0.89828,0\n1.3419,-4.4221,8.09,-1.7349,0\n0.89606,10.5471,-1.4175,-4.0327,0\n0.44125,2.9487,4.3225,0.7155,0\n3.2422,6.2265,0.12224,-1.4466,0\n2.5678,3.5136,0.61406,-0.40691,0\n-2.2153,11.9625,0.078538,-7.7853,0\n4.1349,6.1189,-2.4294,-0.19613,0\n1.934,-9.2828e-06,4.816,-0.33967,0\n2.5068,1.1588,3.9249,0.12585,0\n2.1464,6.0795,-0.5778,-2.2302,0\n0.051979,7.0521,-2.0541,-3.1508,0\n1.2706,8.035,-0.19651,-2.1888,0\n1.143,0.83391,5.4552,-0.56984,0\n2.2928,9.0386,-3.2417,-1.2991,0\n0.3292,-4.4552,4.5718,-0.9888,0\n2.9719,6.8369,-0.2702,0.71291,0\n1.6849,8.7489,-1.2641,-1.3858,0\n-1.9177,11.6894,2.5454,-3.2763,0\n2.3729,10.4726,-3.0087,-3.2013,0\n1.0284,9.767,-1.3687,-1.7853,0\n0.27451,9.2186,-3.2863,-4.8448,0\n1.6032,-4.7863,8.5193,-2.1203,0\n4.616,10.1788,-4.2185,-4.4245,0\n4.2478,7.6956,-2.7696,-1.0767,0\n4.0215,-2.7004,2.4957,0.36636,0\n5.0297,-4.9704,3.5025,-0.23751,0\n1.5902,2.2948,3.2403,0.18404,0\n2.1274,5.1939,-1.7971,-1.1763,0\n1.1811,8.3847,-2.0567,-0.90345,0\n0.3292,-4.4552,4.5718,-0.9888,0\n5.7353,5.2808,-2.2598,0.075416,0\n2.6718,5.6574,0.72974,-1.4892,0\n1.5799,-4.7076,7.9186,-1.5487,0\n2.9499,2.2493,1.3458,-0.037083,0\n0.5195,-3.2633,3.0895,-0.9849,0\n3.7352,9.5911,-3.9032,-3.3487,0\n-1.7344,2.0175,7.7618,0.93532,0\n3.884,10.0277,-3.9298,-4.0819,0\n3.5257,1.2829,1.9276,1.7991,0\n4.4549,2.4976,1.0313,0.96894,0\n-0.16108,-6.4624,8.3573,-1.5216,0\n4.2164,9.4607,-4.9288,-5.2366,0\n3.5152,6.8224,-0.67377,-0.46898,0\n1.6988,2.9094,2.9044,0.11033,0\n1.0607,2.4542,2.5188,-0.17027,0\n2.0421,1.2436,4.2171,0.90429,0\n3.5594,1.3078,1.291,1.6556,0\n3.0009,5.8126,-2.2306,-0.66553,0\n3.9294,1.4112,1.8076,0.89782,0\n3.4667,-4.0724,4.2882,1.5418,0\n3.966,3.9213,0.70574,0.33662,0\n1.0191,2.33,4.9334,0.82929,0\n0.96414,5.616,2.2138,-0.12501,0\n1.8205,6.7562,0.0099913,0.39481,0\n4.9923,7.8653,-2.3515,-0.71984,0\n-1.1804,11.5093,0.15565,-6.8194,0\n4.0329,0.23175,0.89082,1.1823,0\n0.66018,10.3878,-1.4029,-3.9151,0\n3.5982,7.1307,-1.3035,0.21248,0\n-1.8584,7.886,-1.6643,-1.8384,0\n4.0972,0.46972,1.6671,0.91593,0\n3.3299,0.91254,1.5806,0.39352,0\n3.1088,3.1122,0.80857,0.4336,0\n-4.2859,8.5234,3.1392,-0.91639,0\n-1.2528,10.2036,2.1787,-5.6038,0\n0.5195,-3.2633,3.0895,-0.9849,0\n0.3292,-4.4552,4.5718,-0.9888,0\n0.88872,5.3449,2.045,-0.19355,0\n3.5458,9.3718,-4.0351,-3.9564,0\n-0.21661,8.0329,1.8848,-3.8853,0\n2.7206,9.0821,-3.3111,-0.96811,0\n3.2051,8.6889,-2.9033,-0.7819,0\n2.6917,10.8161,-3.3,-4.2888,0\n-2.3242,11.5176,1.8231,-5.375,0\n2.7161,-4.2006,4.1914,0.16981,0\n3.3848,3.2674,0.90967,0.25128,0\n1.7452,4.8028,2.0878,0.62627,0\n2.805,0.57732,1.3424,1.2133,0\n5.7823,5.5788,-2.4089,-0.056479,0\n3.8999,1.734,1.6011,0.96765,0\n3.5189,6.332,-1.7791,-0.020273,0\n3.2294,7.7391,-0.37816,-2.5405,0\n3.4985,3.1639,0.22677,-0.1651,0\n2.1948,1.3781,1.1582,0.85774,0\n2.2526,9.9636,-3.1749,-2.9944,0\n4.1529,-3.9358,2.8633,-0.017686,0\n0.74307,11.17,-1.3824,-4.0728,0\n1.9105,8.871,-2.3386,-0.75604,0\n-1.5055,0.070346,6.8681,-0.50648,0\n0.58836,10.7727,-1.3884,-4.3276,0\n3.2303,7.8384,-3.5348,-1.2151,0\n-1.9922,11.6542,2.6542,-5.2107,0\n2.8523,9.0096,-3.761,-3.3371,0\n4.2772,2.4955,0.48554,0.36119,0\n1.5099,0.039307,6.2332,-0.30346,0\n5.4188,10.1457,-4.084,-3.6991,0\n0.86202,2.6963,4.2908,0.54739,0\n3.8117,10.1457,-4.0463,-4.5629,0\n0.54777,10.3754,-1.5435,-4.1633,0\n2.3718,7.4908,0.015989,-1.7414,0\n-2.4953,11.1472,1.9353,-3.4638,0\n4.6361,-2.6611,2.8358,1.1991,0\n-2.2527,11.5321,2.5899,-3.2737,0\n3.7982,10.423,-4.1602,-4.9728,0\n-0.36279,8.2895,-1.9213,-3.3332,0\n2.1265,6.8783,0.44784,-2.2224,0\n0.86736,5.5643,1.6765,-0.16769,0\n3.7831,10.0526,-3.8869,-3.7366,0\n-2.2623,12.1177,0.28846,-7.7581,0\n1.2616,4.4303,-1.3335,-1.7517,0\n2.6799,3.1349,0.34073,0.58489,0\n-0.39816,5.9781,1.3912,-1.1621,0\n4.3937,0.35798,2.0416,1.2004,0\n2.9695,5.6222,0.27561,-1.1556,0\n1.3049,-0.15521,6.4911,-0.75346,0\n2.2123,-5.8395,7.7687,-0.85302,0\n1.9647,6.9383,0.57722,0.66377,0\n3.0864,-2.5845,2.2309,0.30947,0\n0.3798,0.7098,0.7572,-0.4444,0\n0.58982,7.4266,1.2353,-2.9595,0\n0.14783,7.946,1.0742,-3.3409,0\n-0.062025,6.1975,1.099,-1.131,0\n4.223,1.1319,0.72202,0.96118,0\n0.64295,7.1018,0.3493,-0.41337,0\n1.941,0.46351,4.6472,1.0879,0\n4.0047,0.45937,1.3621,1.6181,0\n3.7767,9.7794,-3.9075,-3.5323,0\n3.4769,-0.15314,2.53,2.4495,0\n1.9818,9.2621,-3.521,-1.872,0\n3.8023,-3.8696,4.044,0.95343,0\n4.3483,11.1079,-4.0857,-4.2539,0\n1.1518,1.3864,5.2727,-0.43536,0\n-1.2576,1.5892,7.0078,0.42455,0\n1.9572,-5.1153,8.6127,-1.4297,0\n-2.484,12.1611,2.8204,-3.7418,0\n-1.1497,1.2954,7.701,0.62627,0\n4.8368,10.0132,-4.3239,-4.3276,0\n-0.12196,8.8068,0.94566,-4.2267,0\n1.9429,6.3961,0.092248,0.58102,0\n1.742,-4.809,8.2142,-2.0659,0\n-1.5222,10.8409,2.7827,-4.0974,0\n-1.3,10.2678,-2.953,-5.8638,0\n3.4246,-0.14693,0.80342,0.29136,0\n2.5503,-4.9518,6.3729,-0.41596,0\n1.5691,6.3465,-0.1828,-2.4099,0\n1.3087,4.9228,2.0013,0.22024,0\n5.1776,8.2316,-3.2511,-1.5694,0\n2.229,9.6325,-3.1123,-2.7164,0\n5.6272,10.0857,-4.2931,-3.8142,0\n1.2138,8.7986,-2.1672,-0.74182,0\n0.3798,0.7098,0.7572,-0.4444,0\n0.5415,6.0319,1.6825,-0.46122,0\n4.0524,5.6802,-1.9693,0.026279,0\n4.7285,2.1065,-0.28305,1.5625,0\n3.4359,0.66216,2.1041,1.8922,0\n0.86816,10.2429,-1.4912,-4.0082,0\n3.359,9.8022,-3.8209,-3.7133,0\n3.6702,2.9942,0.85141,0.30688,0\n1.3349,6.1189,0.46497,0.49826,0\n3.1887,-3.4143,2.7742,-0.2026,0\n2.4527,2.9653,0.20021,-0.056479,0\n3.9121,2.9735,0.92852,0.60558,0\n3.9364,10.5885,-3.725,-4.3133,0\n3.9414,-3.2902,3.1674,1.0866,0\n3.6922,-3.9585,4.3439,1.3517,0\n5.681,7.795,-2.6848,-0.92544,0\n0.77124,9.0862,-1.2281,-1.4996,0\n3.5761,9.7753,-3.9795,-3.4638,0\n1.602,6.1251,0.52924,0.47886,0\n2.6682,10.216,-3.4414,-4.0069,0\n2.0007,1.8644,2.6491,0.47369,0\n0.64215,3.1287,4.2933,0.64696,0\n4.3848,-3.0729,3.0423,1.2741,0\n0.77445,9.0552,-2.4089,-1.3884,0\n0.96574,8.393,-1.361,-1.4659,0\n3.0948,8.7324,-2.9007,-0.96682,0\n4.9362,7.6046,-2.3429,-0.85302,0\n-1.9458,11.2217,1.9079,-3.4405,0\n5.7403,-0.44284,0.38015,1.3763,0\n-2.6989,12.1984,0.67661,-8.5482,0\n1.1472,3.5985,1.9387,-0.43406,0\n2.9742,8.96,-2.9024,-1.0379,0\n4.5707,7.2094,-3.2794,-1.4944,0\n0.1848,6.5079,2.0133,-0.87242,0\n0.87256,9.2931,-0.7843,-2.1978,0\n0.39559,6.8866,1.0588,-0.67587,0\n3.8384,6.1851,-2.0439,-0.033204,0\n2.8209,7.3108,-0.81857,-1.8784,0\n2.5817,9.7546,-3.1749,-2.9957,0\n3.8213,0.23175,2.0133,2.0564,0\n0.3798,0.7098,0.7572,-0.4444,0\n3.4893,6.69,-1.2042,-0.38751,0\n-1.7781,0.8546,7.1303,0.027572,0\n2.0962,2.4769,1.9379,-0.040962,0\n0.94732,-0.57113,7.1903,-0.67587,0\n2.8261,9.4007,-3.3034,-1.0509,0\n0.0071249,8.3661,0.50781,-3.8155,0\n0.96788,7.1907,1.2798,-2.4565,0\n4.7432,2.1086,0.1368,1.6543,0\n3.6575,7.2797,-2.2692,-1.144,0\n3.8832,6.4023,-2.432,-0.98363,0\n3.4776,8.811,-3.1886,-0.92285,0\n1.1315,7.9212,1.093,-2.8444,0\n2.8237,2.8597,0.19678,0.57196,0\n1.9321,6.0423,0.26019,-2.053,0\n3.0632,-3.3315,5.1305,0.8267,0\n-1.8411,10.8306,2.769,-3.0901,0\n2.8084,11.3045,-3.3394,-4.4194,0\n2.5698,-4.4076,5.9856,0.078002,0\n-0.12624,10.3216,-3.7121,-6.1185,0\n3.3756,-4.0951,4.367,1.0698,0\n-0.048008,-1.6037,8.4756,0.75558,0\n0.5706,-0.0248,1.2421,-0.5621,0\n0.88444,6.5906,0.55837,-0.44182,0\n3.8644,3.7061,0.70403,0.35214,0\n1.2999,2.5762,2.0107,-0.18967,0\n2.0051,-6.8638,8.132,-0.2401,0\n4.9294,0.27727,0.20792,0.33662,0\n2.8297,6.3485,-0.73546,-0.58665,0\n2.565,8.633,-2.9941,-1.3082,0\n2.093,8.3061,0.022844,-3.2724,0\n4.6014,5.6264,-2.1235,0.19309,0\n5.0617,-0.35799,0.44698,0.99868,0\n-0.2951,9.0489,-0.52725,-2.0789,0\n3.577,2.4004,1.8908,0.73231,0\n3.9433,2.5017,1.5215,0.903,0\n2.6648,10.754,-3.3994,-4.1685,0\n5.9374,6.1664,-2.5905,-0.36553,0\n2.0153,1.8479,3.1375,0.42843,0\n5.8782,5.9409,-2.8544,-0.60863,0\n-2.3983,12.606,2.9464,-5.7888,0\n1.762,4.3682,2.1384,0.75429,0\n4.2406,-2.4852,1.608,0.7155,0\n3.4669,6.87,-1.0568,-0.73147,0\n3.1896,5.7526,-0.18537,-0.30087,0\n0.81356,9.1566,-2.1492,-4.1814,0\n0.52855,0.96427,4.0243,-1.0483,0\n2.1319,-2.0403,2.5574,-0.061652,0\n0.33111,4.5731,2.057,-0.18967,0\n1.2746,8.8172,-1.5323,-1.7957,0\n2.2091,7.4556,-1.3284,-3.3021,0\n2.5328,7.528,-0.41929,-2.6478,0\n3.6244,1.4609,1.3501,1.9284,0\n-1.3885,12.5026,0.69118,-7.5487,0\n5.7227,5.8312,-2.4097,-0.24527,0\n3.3583,10.3567,-3.7301,-3.6991,0\n2.5227,2.2369,2.7236,0.79438,0\n0.045304,6.7334,1.0708,-0.9332,0\n4.8278,7.7598,-2.4491,-1.2216,0\n1.9476,-4.7738,8.527,-1.8668,0\n2.7659,0.66216,4.1494,-0.28406,0\n-0.10648,-0.76771,7.7575,0.64179,0\n0.72252,-0.053811,5.6703,-1.3509,0\n4.2475,1.4816,-0.48355,0.95343,0\n3.9772,0.33521,2.2566,2.1625,0\n3.6667,4.302,0.55923,0.33791,0\n2.8232,10.8513,-3.1466,-3.9784,0\n-1.4217,11.6542,-0.057699,-7.1025,0\n4.2458,1.1981,0.66633,0.94696,0\n4.1038,-4.8069,3.3491,-0.49225,0\n1.4507,8.7903,-2.2324,-0.65259,0\n3.4647,-3.9172,3.9746,0.36119,0\n1.8533,6.1458,1.0176,-2.0401,0\n3.5288,0.71596,1.9507,1.9375,0\n3.9719,1.0367,0.75973,1.0013,0\n3.534,9.3614,-3.6316,-1.2461,0\n3.6894,9.887,-4.0788,-4.3664,0\n3.0672,-4.4117,3.8238,-0.81682,0\n2.6463,-4.8152,6.3549,0.003003,0\n2.2893,3.733,0.6312,-0.39786,0\n1.5673,7.9274,-0.056842,-2.1694,0\n4.0405,0.51524,1.0279,1.106,0\n4.3846,-4.8794,3.3662,-0.029324,0\n2.0165,-0.25246,5.1707,1.0763,0\n4.0446,11.1741,-4.3582,-4.7401,0\n-0.33729,-0.64976,7.6659,0.72326,0\n-2.4604,12.7302,0.91738,-7.6418,0\n4.1195,10.9258,-3.8929,-4.1802,0\n2.0193,0.82356,4.6369,1.4202,0\n1.5701,7.9129,0.29018,-2.1953,0\n2.6415,7.586,-0.28562,-1.6677,0\n5.0214,8.0764,-3.0515,-1.7155,0\n4.3435,3.3295,0.83598,0.64955,0\n1.8238,-6.7748,8.3873,-0.54139,0\n3.9382,0.9291,0.78543,0.6767,0\n2.2517,-5.1422,4.2916,-1.2487,0\n5.504,10.3671,-4.413,-4.0211,0\n2.8521,9.171,-3.6461,-1.2047,0\n1.1676,9.1566,-2.0867,-0.80647,0\n2.6104,8.0081,-0.23592,-1.7608,0\n0.32444,10.067,-1.1982,-4.1284,0\n3.8962,-4.7904,3.3954,-0.53751,0\n2.1752,-0.8091,5.1022,-0.67975,0\n1.1588,8.9331,-2.0807,-1.1272,0\n4.7072,8.2957,-2.5605,-1.4905,0\n-1.9667,11.8052,-0.40472,-7.8719,0\n4.0552,0.40143,1.4563,0.65343,0\n2.3678,-6.839,8.4207,-0.44829,0\n0.33565,6.8369,0.69718,-0.55691,0\n4.3398,-5.3036,3.8803,-0.70432,0\n1.5456,8.5482,0.4187,-2.1784,0\n1.4276,8.3847,-2.0995,-1.9677,0\n-0.27802,8.1881,-3.1338,-2.5276,0\n0.93611,8.6413,-1.6351,-1.3043,0\n4.6352,-3.0087,2.6773,1.212,0\n1.5268,-5.5871,8.6564,-1.722,0\n0.95626,2.4728,4.4578,0.21636,0\n-2.7914,1.7734,6.7756,-0.39915,0\n5.2032,3.5116,-1.2538,1.0129,0\n3.1836,7.2321,-1.0713,-2.5909,0\n0.65497,5.1815,1.0673,-0.42113,0\n5.6084,10.3009,-4.8003,-4.3534,0\n1.105,7.4432,0.41099,-3.0332,0\n3.9292,-2.9156,2.2129,0.30817,0\n1.1558,6.4003,1.5506,0.6961,0\n2.5581,2.6218,1.8513,0.40257,0\n2.7831,10.9796,-3.557,-4.4039,0\n3.7635,2.7811,0.66119,0.34179,0\n-2.6479,10.1374,-1.331,-5.4707,0\n1.0652,8.3682,-1.4004,-1.6509,0\n-1.4275,11.8797,0.41613,-6.9978,0\n5.7456,10.1808,-4.7857,-4.3366,0\n5.086,3.2798,-1.2701,1.1189,0\n3.4092,5.4049,-2.5228,-0.89958,0\n-0.2361,9.3221,2.1307,-4.3793,0\n3.8197,8.9951,-4.383,-4.0327,0\n-1.1391,1.8127,6.9144,0.70127,0\n4.9249,0.68906,0.77344,1.2095,0\n2.5089,6.841,-0.029423,0.44912,0\n-0.2062,9.2207,-3.7044,-6.8103,0\n3.946,6.8514,-1.5443,-0.5582,0\n-0.278,8.1881,-3.1338,-2.5276,0\n1.8592,3.2074,-0.15966,-0.26208,0\n0.56953,7.6294,1.5754,-3.2233,0\n3.4626,-4.449,3.5427,0.15429,0\n3.3951,1.1484,2.1401,2.0862,0\n5.0429,-0.52974,0.50439,1.106,0\n3.7758,7.1783,-1.5195,0.40128,0\n4.6562,7.6398,-2.4243,-1.2384,0\n4.0948,-2.9674,2.3689,0.75429,0\n1.8384,6.063,0.54723,0.51248,0\n2.0153,0.43661,4.5864,-0.3151,0\n3.5251,0.7201,1.6928,0.64438,0\n3.757,-5.4236,3.8255,-1.2526,0\n2.5989,3.5178,0.7623,0.81119,0\n1.8994,0.97462,4.2265,0.81377,0\n3.6941,-3.9482,4.2625,1.1577,0\n4.4295,-2.3507,1.7048,0.90946,0\n6.8248,5.2187,-2.5425,0.5461,0\n1.8967,-2.5163,2.8093,-0.79742,0\n2.1526,-6.1665,8.0831,-0.34355,0\n3.3004,7.0811,-1.3258,0.22283,0\n2.7213,7.05,-0.58808,0.41809,0\n3.8846,-3.0336,2.5334,0.20214,0\n4.1665,-0.4449,0.23448,0.27843,0\n0.94225,5.8561,1.8762,-0.32544,0\n5.1321,-0.031048,0.32616,1.1151,0\n0.38251,6.8121,1.8128,-0.61251,0\n3.0333,-2.5928,2.3183,0.303,0\n2.9233,6.0464,-0.11168,-0.58665,0\n1.162,10.2926,-1.2821,-4.0392,0\n3.7791,2.5762,1.3098,0.5655,0\n0.77765,5.9781,1.1941,-0.3526,0\n-0.38388,-1.0471,8.0514,0.49567,0\n0.21084,9.4359,-0.094543,-1.859,0\n2.9571,-4.5938,5.9068,0.57196,0\n4.6439,-3.3729,2.5976,0.55257,0\n3.3577,-4.3062,6.0241,0.18274,0\n3.5127,2.9073,1.0579,0.40774,0\n2.6562,10.7044,-3.3085,-4.0767,0\n-1.3612,10.694,1.7022,-2.9026,0\n-0.278,8.1881,-3.1338,-2.5276,0\n1.04,-6.9321,8.2888,-1.2991,0\n2.1881,2.7356,1.3278,-0.1832,0\n4.2756,-2.6528,2.1375,0.94437,0\n-0.11996,6.8741,0.91995,-0.6694,0\n2.9736,8.7944,-3.6359,-1.3754,0\n3.7798,-3.3109,2.6491,0.066365,0\n5.3586,3.7557,-1.7345,1.0789,0\n1.8373,6.1292,0.84027,0.55257,0\n1.2262,0.89599,5.7568,-0.11596,0\n-0.048008,-0.56078,7.7215,0.453,0\n0.5706,-0.024841,1.2421,-0.56208,0\n4.3634,0.46351,1.4281,2.0202,0\n3.482,-4.1634,3.5008,-0.078462,0\n0.51947,-3.2633,3.0895,-0.98492,0\n2.3164,-2.628,3.1529,-0.08622,0\n-1.8348,11.0334,3.1863,-4.8888,0\n1.3754,8.8793,-1.9136,-0.53751,0\n-0.16682,5.8974,0.49839,-0.70044,0\n0.29961,7.1328,-0.31475,-1.1828,0\n0.25035,9.3262,-3.6873,-6.2543,0\n2.4673,1.3926,1.7125,0.41421,0\n0.77805,6.6424,-1.1425,-1.0573,0\n3.4465,2.9508,1.0271,0.5461,0\n2.2429,-4.1427,5.2333,-0.40173,0\n3.7321,-3.884,3.3577,-0.0060486,0\n4.3365,-3.584,3.6884,0.74912,0\n-2.0759,10.8223,2.6439,-4.837,0\n4.0715,7.6398,-2.0824,-1.1698,0\n0.76163,5.8209,1.1959,-0.64613,0\n-0.53966,7.3273,0.46583,-1.4543,0\n2.6213,5.7919,0.065686,-1.5759,0\n3.0242,-3.3378,2.5865,-0.54785,0\n5.8519,5.3905,-2.4037,-0.061652,0\n0.5706,-0.0248,1.2421,-0.5621,0\n3.9771,11.1513,-3.9272,-4.3444,0\n1.5478,9.1814,-1.6326,-1.7375,0\n0.74054,0.36625,2.1992,0.48403,0\n0.49571,10.2243,-1.097,-4.0159,0\n1.645,7.8612,-0.87598,-3.5569,0\n3.6077,6.8576,-1.1622,0.28231,0\n3.2403,-3.7082,5.2804,0.41291,0\n3.9166,10.2491,-4.0926,-4.4659,0\n3.9262,6.0299,-2.0156,-0.065531,0\n5.591,10.4643,-4.3839,-4.3379,0\n3.7522,-3.6978,3.9943,1.3051,0\n1.3114,4.5462,2.2935,0.22541,0\n3.7022,6.9942,-1.8511,-0.12889,0\n4.364,-3.1039,2.3757,0.78532,0\n3.5829,1.4423,1.0219,1.4008,0\n4.65,-4.8297,3.4553,-0.25174,0\n5.1731,3.9606,-1.983,0.40774,0\n3.2692,3.4184,0.20706,-0.066824,0\n2.4012,1.6223,3.0312,0.71679,0\n1.7257,-4.4697,8.2219,-1.8073,0\n4.7965,6.9859,-1.9967,-0.35001,0\n4.0962,10.1891,-3.9323,-4.1827,0\n2.5559,3.3605,2.0321,0.26809,0\n3.4916,8.5709,-3.0326,-0.59182,0\n0.5195,-3.2633,3.0895,-0.9849,0\n2.9856,7.2673,-0.409,-2.2431,0\n4.0932,5.4132,-1.8219,0.23576,0\n1.7748,-0.76978,5.5854,1.3039,0\n5.2012,0.32694,0.17965,1.1797,0\n-0.45062,-1.3678,7.0858,-0.40303,0\n4.8451,8.1116,-2.9512,-1.4724,0\n0.74841,7.2756,1.1504,-0.5388,0\n5.1213,8.5565,-3.3917,-1.5474,0\n3.6181,-3.7454,2.8273,-0.71208,0\n0.040498,8.5234,1.4461,-3.9306,0\n-2.6479,10.1374,-1.331,-5.4707,0\n0.37984,0.70975,0.75716,-0.44441,0\n-0.95923,0.091039,6.2204,-1.4828,0\n2.8672,10.0008,-3.2049,-3.1095,0\n1.0182,9.109,-0.62064,-1.7129,0\n-2.7143,11.4535,2.1092,-3.9629,0\n3.8244,-3.1081,2.4537,0.52024,0\n2.7961,2.121,1.8385,0.38317,0\n3.5358,6.7086,-0.81857,0.47886,0\n-0.7056,8.7241,2.2215,-4.5965,0\n4.1542,7.2756,-2.4766,-1.2099,0\n0.92703,9.4318,-0.66263,-1.6728,0\n1.8216,-6.4748,8.0514,-0.41855,0\n-2.4473,12.6247,0.73573,-7.6612,0\n3.5862,-3.0957,2.8093,0.24481,0\n0.66191,9.6594,-0.28819,-1.6638,0\n4.7926,1.7071,-0.051701,1.4926,0\n4.9852,8.3516,-2.5425,-1.2823,0\n0.75736,3.0294,2.9164,-0.068117,0\n4.6499,7.6336,-1.9427,-0.37458,0\n-0.023579,7.1742,0.78457,-0.75734,0\n0.85574,0.0082678,6.6042,-0.53104,0\n0.88298,0.66009,6.0096,-0.43277,0\n4.0422,-4.391,4.7466,1.137,0\n2.2546,8.0992,-0.24877,-3.2698,0\n0.38478,6.5989,-0.3336,-0.56466,0\n3.1541,-5.1711,6.5991,0.57455,0\n2.3969,0.23589,4.8477,1.437,0\n4.7114,2.0755,-0.2702,1.2379,0\n4.0127,10.1477,-3.9366,-4.0728,0\n2.6606,3.1681,1.9619,0.18662,0\n3.931,1.8541,-0.023425,1.2314,0\n0.01727,8.693,1.3989,-3.9668,0\n3.2414,0.40971,1.4015,1.1952,0\n2.2504,3.5757,0.35273,0.2836,0\n-1.3971,3.3191,-1.3927,-1.9948,1\n0.39012,-0.14279,-0.031994,0.35084,1\n-1.6677,-7.1535,7.8929,0.96765,1\n-3.8483,-12.8047,15.6824,-1.281,1\n-3.5681,-8.213,10.083,0.96765,1\n-2.2804,-0.30626,1.3347,1.3763,1\n-1.7582,2.7397,-2.5323,-2.234,1\n-0.89409,3.1991,-1.8219,-2.9452,1\n0.3434,0.12415,-0.28733,0.14654,1\n-0.9854,-6.661,5.8245,0.5461,1\n-2.4115,-9.1359,9.3444,-0.65259,1\n-1.5252,-6.2534,5.3524,0.59912,1\n-0.61442,-0.091058,-0.31818,0.50214,1\n-0.36506,2.8928,-3.6461,-3.0603,1\n-5.9034,6.5679,0.67661,-6.6797,1\n-1.8215,2.7521,-0.72261,-2.353,1\n-0.77461,-1.8768,2.4023,1.1319,1\n-1.8187,-9.0366,9.0162,-0.12243,1\n-3.5801,-12.9309,13.1779,-2.5677,1\n-1.8219,-6.8824,5.4681,0.057313,1\n-0.3481,-0.38696,-0.47841,0.62627,1\n0.47368,3.3605,-4.5064,-4.0431,1\n-3.4083,4.8587,-0.76888,-4.8668,1\n-1.6662,-0.30005,1.4238,0.024986,1\n-2.0962,-7.1059,6.6188,-0.33708,1\n-2.6685,-10.4519,9.1139,-1.7323,1\n-0.47465,-4.3496,1.9901,0.7517,1\n1.0552,1.1857,-2.6411,0.11033,1\n1.1644,3.8095,-4.9408,-4.0909,1\n-4.4779,7.3708,-0.31218,-6.7754,1\n-2.7338,0.45523,2.4391,0.21766,1\n-2.286,-5.4484,5.8039,0.88231,1\n-1.6244,-6.3444,4.6575,0.16981,1\n0.50813,0.47799,-1.9804,0.57714,1\n1.6408,4.2503,-4.9023,-2.6621,1\n0.81583,4.84,-5.2613,-6.0823,1\n-5.4901,9.1048,-0.38758,-5.9763,1\n-3.2238,2.7935,0.32274,-0.86078,1\n-2.0631,-1.5147,1.219,0.44524,1\n-0.91318,-2.0113,-0.19565,0.066365,1\n0.6005,1.9327,-3.2888,-0.32415,1\n0.91315,3.3377,-4.0557,-1.6741,1\n-0.28015,3.0729,-3.3857,-2.9155,1\n-3.6085,3.3253,-0.51954,-3.5737,1\n-6.2003,8.6806,0.0091344,-3.703,1\n-4.2932,3.3419,0.77258,-0.99785,1\n-3.0265,-0.062088,0.68604,-0.055186,1\n-1.7015,-0.010356,-0.99337,-0.53104,1\n-0.64326,2.4748,-2.9452,-1.0276,1\n-0.86339,1.9348,-2.3729,-1.0897,1\n-2.0659,1.0512,-0.46298,-1.0974,1\n-2.1333,1.5685,-0.084261,-1.7453,1\n-1.2568,-1.4733,2.8718,0.44653,1\n-3.1128,-6.841,10.7402,-1.0172,1\n-4.8554,-5.9037,10.9818,-0.82199,1\n-2.588,3.8654,-0.3336,-1.2797,1\n0.24394,1.4733,-1.4192,-0.58535,1\n-1.5322,-5.0966,6.6779,0.17498,1\n-4.0025,-13.4979,17.6772,-3.3202,1\n-4.0173,-8.3123,12.4547,-1.4375,1\n-3.0731,-0.53181,2.3877,0.77627,1\n-1.979,3.2301,-1.3575,-2.5819,1\n-0.4294,-0.14693,0.044265,-0.15605,1\n-2.234,-7.0314,7.4936,0.61334,1\n-4.211,-12.4736,14.9704,-1.3884,1\n-3.8073,-8.0971,10.1772,0.65084,1\n-2.5912,-0.10554,1.2798,1.0414,1\n-2.2482,3.0915,-2.3969,-2.6711,1\n-1.4427,3.2922,-1.9702,-3.4392,1\n-0.39416,-0.020702,-0.066267,-0.44699,1\n-1.522,-6.6383,5.7491,-0.10691,1\n-2.8267,-9.0407,9.0694,-0.98233,1\n-1.7263,-6.0237,5.2419,0.29524,1\n-0.94255,0.039307,-0.24192,0.31593,1\n-0.89569,3.0025,-3.6067,-3.4457,1\n-6.2815,6.6651,0.52581,-7.0107,1\n-2.3211,3.166,-1.0002,-2.7151,1\n-1.3414,-2.0776,2.8093,0.60688,1\n-2.258,-9.3263,9.3727,-0.85949,1\n-3.8858,-12.8461,12.7957,-3.1353,1\n-1.8969,-6.7893,5.2761,-0.32544,1\n-0.52645,-0.24832,-0.45613,0.41938,1\n0.0096613,3.5612,-4.407,-4.4103,1\n-3.8826,4.898,-0.92311,-5.0801,1\n-2.1405,-0.16762,1.321,-0.20906,1\n-2.4824,-7.3046,6.839,-0.59053,1\n-2.9098,-10.0712,8.4156,-1.9948,1\n-0.60975,-4.002,1.8471,0.6017,1\n0.83625,1.1071,-2.4706,-0.062945,1\n0.60731,3.9544,-4.772,-4.4853,1\n-4.8861,7.0542,-0.17252,-6.959,1\n-3.1366,0.42212,2.6225,-0.064238,1\n-2.5754,-5.6574,6.103,0.65214,1\n-1.8782,-6.5865,4.8486,-0.021566,1\n0.24261,0.57318,-1.9402,0.44007,1\n1.296,4.2855,-4.8457,-2.9013,1\n0.25943,5.0097,-5.0394,-6.3862,1\n-5.873,9.1752,-0.27448,-6.0422,1\n-3.4605,2.6901,0.16165,-1.0224,1\n-2.3797,-1.4402,1.1273,0.16076,1\n-1.2424,-1.7175,-0.52553,-0.21036,1\n0.20216,1.9182,-3.2828,-0.61768,1\n0.59823,3.5012,-3.9795,-1.7841,1\n-0.77995,3.2322,-3.282,-3.1004,1\n-4.1409,3.4619,-0.47841,-3.8879,1\n-6.5084,8.7696,0.23191,-3.937,1\n-4.4996,3.4288,0.56265,-1.1672,1\n-3.3125,0.10139,0.55323,-0.2957,1\n-1.9423,0.3766,-1.2898,-0.82458,1\n-0.75793,2.5349,-3.0464,-1.2629,1\n-0.95403,1.9824,-2.3163,-1.1957,1\n-2.2173,1.4671,-0.72689,-1.1724,1\n-2.799,1.9679,-0.42357,-2.1125,1\n-1.8629,-0.84841,2.5377,0.097399,1\n-3.5916,-6.2285,10.2389,-1.1543,1\n-5.1216,-5.3118,10.3846,-1.0612,1\n-3.2854,4.0372,-0.45356,-1.8228,1\n-0.56877,1.4174,-1.4252,-1.1246,1\n-2.3518,-4.8359,6.6479,-0.060358,1\n-4.4861,-13.2889,17.3087,-3.2194,1\n-4.3876,-7.7267,11.9655,-1.4543,1\n-3.3604,-0.32696,2.1324,0.6017,1\n-1.0112,2.9984,-1.1664,-1.6185,1\n0.030219,-1.0512,1.4024,0.77369,1\n-1.6514,-8.4985,9.1122,1.2379,1\n-3.2692,-12.7406,15.5573,-0.14182,1\n-2.5701,-6.8452,8.9999,2.1353,1\n-1.3066,0.25244,0.7623,1.7758,1\n-1.6637,3.2881,-2.2701,-2.2224,1\n-0.55008,2.8659,-1.6488,-2.4319,1\n0.21431,-0.69529,0.87711,0.29653,1\n-0.77288,-7.4473,6.492,0.36119,1\n-1.8391,-9.0883,9.2416,-0.10432,1\n-0.63298,-5.1277,4.5624,1.4797,1\n0.0040545,0.62905,-0.64121,0.75817,1\n-0.28696,3.1784,-3.5767,-3.1896,1\n-5.2406,6.6258,-0.19908,-6.8607,1\n-1.4446,2.1438,-0.47241,-1.6677,1\n-0.65767,-2.8018,3.7115,0.99739,1\n-1.5449,-10.1498,9.6152,-1.2332,1\n-2.8957,-12.0205,11.9149,-2.7552,1\n-0.81479,-5.7381,4.3919,0.3211,1\n0.50225,0.65388,-1.1793,0.39998,1\n0.74521,3.6357,-4.4044,-4.1414,1\n-2.9146,4.0537,-0.45699,-4.0327,1\n-1.3907,-1.3781,2.3055,-0.021566,1\n-1.786,-8.1157,7.0858,-1.2112,1\n-1.7322,-9.2828,7.719,-1.7168,1\n0.55298,-3.4619,1.7048,1.1008,1\n2.031,1.852,-3.0121,0.003003,1\n1.2279,4.0309,-4.6435,-3.9125,1\n-4.2249,6.2699,0.15822,-5.5457,1\n-2.5346,-0.77392,3.3602,0.00171,1\n-1.749,-6.332,6.0987,0.14266,1\n-0.539,-5.167,3.4399,0.052141,1\n1.5631,0.89599,-1.9702,0.65472,1\n2.3917,4.5565,-4.9888,-2.8987,1\n0.89512,4.7738,-4.8431,-5.5909,1\n-5.4808,8.1819,0.27818,-5.0323,1\n-2.8833,1.7713,0.68946,-0.4638,1\n-1.4174,-2.2535,1.518,0.61981,1\n0.4283,-0.94981,-1.0731,0.3211,1\n1.5904,2.2121,-3.1183,-0.11725,1\n1.7425,3.6833,-4.0129,-1.7207,1\n-0.23356,3.2405,-3.0669,-2.7784,1\n-3.6227,3.9958,-0.35845,-3.9047,1\n-6.1536,7.9295,0.61663,-3.2646,1\n-3.9172,2.6652,0.78886,-0.7819,1\n-2.2214,-0.23798,0.56008,0.05602,1\n-0.49241,0.89392,-1.6283,-0.56854,1\n0.26517,2.4066,-2.8416,-0.59958,1\n-0.10234,1.8189,-2.2169,-0.56725,1\n-1.6176,1.0926,-0.35502,-0.59958,1\n-1.8448,1.254,0.27218,-1.0728,1\n-1.2786,-2.4087,4.5735,0.47627,1\n-2.902,-7.6563,11.8318,-0.84268,1\n-4.3773,-5.5167,10.939,-0.4082,1\n-2.0529,3.8385,-0.79544,-1.2138,1\n0.18868,0.70148,-0.51182,0.0055892,1\n-1.7279,-6.841,8.9494,0.68058,1\n-3.3793,-13.7731,17.9274,-2.0323,1\n-3.1273,-7.1121,11.3897,-0.083634,1\n-2.121,-0.05588,1.949,1.353,1\n-1.7697,3.4329,-1.2144,-2.3789,1\n-0.0012852,0.13863,-0.19651,0.0081754,1\n-1.682,-6.8121,7.1398,1.3323,1\n-3.4917,-12.1736,14.3689,-0.61639,1\n-3.1158,-8.6289,10.4403,0.97153,1\n-2.0891,-0.48422,1.704,1.7435,1\n-1.6936,2.7852,-2.1835,-1.9276,1\n-1.2846,3.2715,-1.7671,-3.2608,1\n-0.092194,0.39315,-0.32846,-0.13794,1\n-1.0292,-6.3879,5.5255,0.79955,1\n-2.2083,-9.1069,8.9991,-0.28406,1\n-1.0744,-6.3113,5.355,0.80472,1\n-0.51003,-0.23591,0.020273,0.76334,1\n-0.36372,3.0439,-3.4816,-2.7836,1\n-6.3979,6.4479,1.0836,-6.6176,1\n-2.2501,3.3129,-0.88369,-2.8974,1\n-1.1859,-1.2519,2.2635,0.77239,1\n-1.8076,-8.8131,8.7086,-0.21682,1\n-3.3863,-12.9889,13.0545,-2.7202,1\n-1.4106,-7.108,5.6454,0.31335,1\n-0.21394,-0.68287,0.096532,1.1965,1\n0.48797,3.5674,-4.3882,-3.8116,1\n-3.8167,5.1401,-0.65063,-5.4306,1\n-1.9555,0.20692,1.2473,-0.3707,1\n-2.1786,-6.4479,6.0344,-0.20777,1\n-2.3299,-9.9532,8.4756,-1.8733,1\n0.0031201,-4.0061,1.7956,0.91722,1\n1.3518,1.0595,-2.3437,0.39998,1\n1.2309,3.8923,-4.8277,-4.0069,1\n-5.0301,7.5032,-0.13396,-7.5034,1\n-3.0799,0.60836,2.7039,-0.23751,1\n-2.2987,-5.227,5.63,0.91722,1\n-1.239,-6.541,4.8151,-0.033204,1\n0.75896,0.29176,-1.6506,0.83834,1\n1.6799,4.2068,-4.5398,-2.3931,1\n0.63655,5.2022,-5.2159,-6.1211,1\n-6.0598,9.2952,-0.43642,-6.3694,1\n-3.518,2.8763,0.1548,-1.2086,1\n-2.0336,-1.4092,1.1582,0.36507,1\n-0.69745,-1.7672,-0.34474,-0.12372,1\n0.75108,1.9161,-3.1098,-0.20518,1\n0.84546,3.4826,-3.6307,-1.3961,1\n-0.55648,3.2136,-3.3085,-2.7965,1\n-3.6817,3.2239,-0.69347,-3.4004,1\n-6.7526,8.8172,-0.061983,-3.725,1\n-4.577,3.4515,0.66719,-0.94742,1\n-2.9883,0.31245,0.45041,0.068951,1\n-1.4781,0.14277,-1.1622,-0.48579,1\n-0.46651,2.3383,-2.9812,-1.0431,1\n-0.8734,1.6533,-2.1964,-0.78061,1\n-2.1234,1.1815,-0.55552,-0.81165,1\n-2.3142,2.0838,-0.46813,-1.6767,1\n-1.4233,-0.98912,2.3586,0.39481,1\n-3.0866,-6.6362,10.5405,-0.89182,1\n-4.7331,-6.1789,11.388,-1.0741,1\n-2.8829,3.8964,-0.1888,-1.1672,1\n-0.036127,1.525,-1.4089,-0.76121,1\n-1.7104,-4.778,6.2109,0.3974,1\n-3.8203,-13.0551,16.9583,-2.3052,1\n-3.7181,-8.5089,12.363,-0.95518,1\n-2.899,-0.60424,2.6045,1.3776,1\n-0.98193,2.7956,-1.2341,-1.5668,1\n-0.17296,-1.1816,1.3818,0.7336,1\n-1.9409,-8.6848,9.155,0.94049,1\n-3.5713,-12.4922,14.8881,-0.47027,1\n-2.9915,-6.6258,8.6521,1.8198,1\n-1.8483,0.31038,0.77344,1.4189,1\n-2.2677,3.2964,-2.2563,-2.4642,1\n-0.50816,2.868,-1.8108,-2.2612,1\n0.14329,-1.0885,1.0039,0.48791,1\n-0.90784,-7.9026,6.7807,0.34179,1\n-2.0042,-9.3676,9.3333,-0.10303,1\n-0.93587,-5.1008,4.5367,1.3866,1\n-0.40804,0.54214,-0.52725,0.6586,1\n-0.8172,3.3812,-3.6684,-3.456,1\n-4.8392,6.6755,-0.24278,-6.5775,1\n-1.2792,2.1376,-0.47584,-1.3974,1\n-0.66008,-3.226,3.8058,1.1836,1\n-1.7713,-10.7665,10.2184,-1.0043,1\n-3.0061,-12.2377,11.9552,-2.1603,1\n-1.1022,-5.8395,4.5641,0.68705,1\n0.11806,0.39108,-0.98223,0.42843,1\n0.11686,3.735,-4.4379,-4.3741,1\n-2.7264,3.9213,-0.49212,-3.6371,1\n-1.2369,-1.6906,2.518,0.51636,1\n-1.8439,-8.6475,7.6796,-0.66682,1\n-1.8554,-9.6035,7.7764,-0.97716,1\n0.16358,-3.3584,1.3749,1.3569,1\n1.5077,1.9596,-3.0584,-0.12243,1\n0.67886,4.1199,-4.569,-4.1414,1\n-3.9934,5.8333,0.54723,-4.9379,1\n-2.3898,-0.78427,3.0141,0.76205,1\n-1.7976,-6.7686,6.6753,0.89912,1\n-0.70867,-5.5602,4.0483,0.903,1\n1.0194,1.1029,-2.3,0.59395,1\n1.7875,4.78,-5.1362,-3.2362,1\n0.27331,4.8773,-4.9194,-5.8198,1\n-5.1661,8.0433,0.044265,-4.4983,1\n-2.7028,1.6327,0.83598,-0.091393,1\n-1.4904,-2.2183,1.6054,0.89394,1\n-0.014902,-1.0243,-0.94024,0.64955,1\n0.88992,2.2638,-3.1046,-0.11855,1\n1.0637,3.6957,-4.1594,-1.9379,1\n-0.8471,3.1329,-3.0112,-2.9388,1\n-3.9594,4.0289,-0.35845,-3.8957,1\n-5.8818,7.6584,0.5558,-2.9155,1\n-3.7747,2.5162,0.83341,-0.30993,1\n-2.4198,-0.24418,0.70146,0.41809,1\n-0.83535,0.80494,-1.6411,-0.19225,1\n-0.30432,2.6528,-2.7756,-0.65647,1\n-0.60254,1.7237,-2.1501,-0.77027,1\n-2.1059,1.1815,-0.53324,-0.82716,1\n-2.0441,1.2271,0.18564,-1.091,1\n-1.5621,-2.2121,4.2591,0.27972,1\n-3.2305,-7.2135,11.6433,-0.94613,1\n-4.8426,-4.9932,10.4052,-0.53104,1\n-2.3147,3.6668,-0.6969,-1.2474,1\n-0.11716,0.60422,-0.38587,-0.059065,1\n-2.0066,-6.719,9.0162,0.099985,1\n-3.6961,-13.6779,17.5795,-2.6181,1\n-3.6012,-6.5389,10.5234,-0.48967,1\n-2.6286,0.18002,1.7956,0.97282,1\n-0.82601,2.9611,-1.2864,-1.4647,1\n0.31803,-0.99326,1.0947,0.88619,1\n-1.4454,-8.4385,8.8483,0.96894,1\n-3.1423,-13.0365,15.6773,-0.66165,1\n-2.5373,-6.959,8.8054,1.5289,1\n-1.366,0.18416,0.90539,1.5806,1\n-1.7064,3.3088,-2.2829,-2.1978,1\n-0.41965,2.9094,-1.7859,-2.2069,1\n0.37637,-0.82358,0.78543,0.74524,1\n-0.55355,-7.9233,6.7156,0.74394,1\n-1.6001,-9.5828,9.4044,0.081882,1\n-0.37013,-5.554,4.7749,1.547,1\n0.12126,0.22347,-0.47327,0.97024,1\n-0.27068,3.2674,-3.5562,-3.0888,1\n-5.119,6.6486,-0.049987,-6.5206,1\n-1.3946,2.3134,-0.44499,-1.4905,1\n-0.69879,-3.3771,4.1211,1.5043,1\n-1.48,-10.5244,9.9176,-0.5026,1\n-2.6649,-12.813,12.6689,-1.9082,1\n-0.62684,-6.301,4.7843,1.106,1\n0.518,0.25865,-0.84085,0.96118,1\n0.64376,3.764,-4.4738,-4.0483,1\n-2.9821,4.1986,-0.5898,-3.9642,1\n-1.4628,-1.5706,2.4357,0.49826,1\n-1.7101,-8.7903,7.9735,-0.45475,1\n-1.5572,-9.8808,8.1088,-1.0806,1\n0.74428,-3.7723,1.6131,1.5754,1\n2.0177,1.7982,-2.9581,0.2099,1\n1.164,3.913,-4.5544,-3.8672,1\n-4.3667,6.0692,0.57208,-5.4668,1\n-2.5919,-1.0553,3.8949,0.77757,1\n-1.8046,-6.8141,6.7019,1.1681,1\n-0.71868,-5.7154,3.8298,1.0233,1\n1.4378,0.66837,-2.0267,1.0271,1\n2.1943,4.5503,-4.976,-2.7254,1\n0.7376,4.8525,-4.7986,-5.6659,1\n-5.637,8.1261,0.13081,-5.0142,1\n-3.0193,1.7775,0.73745,-0.45346,1\n-1.6706,-2.09,1.584,0.71162,1\n-0.1269,-1.1505,-0.95138,0.57843,1\n1.2198,2.0982,-3.1954,0.12843,1\n1.4501,3.6067,-4.0557,-1.5966,1\n-0.40857,3.0977,-2.9607,-2.6892,1\n-3.8952,3.8157,-0.31304,-3.8194,1\n-6.3679,8.0102,0.4247,-3.2207,1\n-4.1429,2.7749,0.68261,-0.71984,1\n-2.6864,-0.097265,0.61663,0.061192,1\n-1.0555,0.79459,-1.6968,-0.46768,1\n-0.29858,2.4769,-2.9512,-0.66165,1\n-0.49948,1.7734,-2.2469,-0.68104,1\n-1.9881,0.99945,-0.28562,-0.70044,1\n-1.9389,1.5706,0.045979,-1.122,1\n-1.4375,-1.8624,4.026,0.55127,1\n-3.1875,-7.5756,11.8678,-0.57889,1\n-4.6765,-5.6636,10.969,-0.33449,1\n-2.0285,3.8468,-0.63435,-1.175,1\n0.26637,0.73252,-0.67891,0.03533,1\n-1.7589,-6.4624,8.4773,0.31981,1\n-3.5985,-13.6593,17.6052,-2.4927,1\n-3.3582,-7.2404,11.4419,-0.57113,1\n-2.3629,-0.10554,1.9336,1.1358,1\n-2.1802,3.3791,-1.2256,-2.6621,1\n-0.40951,-0.15521,0.060545,-0.088807,1\n-2.2918,-7.257,7.9597,0.9211,1\n-4.0214,-12.8006,15.6199,-0.95647,1\n-3.3884,-8.215,10.3315,0.98187,1\n-2.0046,-0.49457,1.333,1.6543,1\n-1.7063,2.7956,-2.378,-2.3491,1\n-1.6386,3.3584,-1.7302,-3.5646,1\n-0.41645,0.32487,-0.33617,-0.36036,1\n-1.5877,-6.6072,5.8022,0.31593,1\n-2.5961,-9.349,9.7942,-0.28018,1\n-1.5228,-6.4789,5.7568,0.87325,1\n-0.53072,-0.097265,-0.21793,1.0426,1\n-0.49081,2.8452,-3.6436,-3.1004,1\n-6.5773,6.8017,0.85483,-7.5344,1\n-2.4621,2.7645,-0.62578,-2.8573,1\n-1.3995,-1.9162,2.5154,0.59912,1\n-2.3221,-9.3304,9.233,-0.79871,1\n-3.73,-12.9723,12.9817,-2.684,1\n-1.6988,-7.1163,5.7902,0.16723,1\n-0.26654,-0.64562,-0.42014,0.89136,1\n0.33325,3.3108,-4.5081,-4.012,1\n-4.2091,4.7283,-0.49126,-5.2159,1\n-2.3142,-0.68494,1.9833,-0.44829,1\n-2.4835,-7.4494,6.8964,-0.64484,1\n-2.7611,-10.5099,9.0239,-1.9547,1\n-0.36025,-4.449,2.1067,0.94308,1\n1.0117,0.9022,-2.3506,0.42714,1\n0.96708,3.8426,-4.9314,-4.1323,1\n-5.2049,7.259,0.070827,-7.3004,1\n-3.3203,-0.02691,2.9618,-0.44958,1\n-2.565,-5.7899,6.0122,0.046968,1\n-1.5951,-6.572,4.7689,-0.94354,1\n0.7049,0.17174,-1.7859,0.36119,1\n1.7331,3.9544,-4.7412,-2.5017,1\n0.6818,4.8504,-5.2133,-6.1043,1\n-6.3364,9.2848,0.014275,-6.7844,1\n-3.8053,2.4273,0.6809,-1.0871,1\n-2.1979,-2.1252,1.7151,0.45171,1\n-0.87874,-2.2121,-0.051701,0.099985,1\n0.74067,1.7299,-3.1963,-0.1457,1\n0.98296,3.4226,-3.9692,-1.7116,1\n-0.3489,3.1929,-3.4054,-3.1832,1\n-3.8552,3.5219,-0.38415,-3.8608,1\n-6.9599,8.9931,0.2182,-4.572,1\n-4.7462,3.1205,1.075,-1.2966,1\n-3.2051,-0.14279,0.97565,0.045675,1\n-1.7549,-0.080711,-0.75774,-0.3707,1\n-0.59587,2.4811,-2.8673,-0.89828,1\n-0.89542,2.0279,-2.3652,-1.2746,1\n-2.0754,1.2767,-0.64206,-1.2642,1\n-3.2778,1.8023,0.1805,-2.3931,1\n-2.2183,-1.254,2.9986,0.36378,1\n-3.5895,-6.572,10.5251,-0.16381,1\n-5.0477,-5.8023,11.244,-0.3901,1\n-3.5741,3.944,-0.07912,-2.1203,1\n-0.7351,1.7361,-1.4938,-1.1582,1\n-2.2617,-4.7428,6.3489,0.11162,1\n-4.244,-13.0634,17.1116,-2.8017,1\n-4.0218,-8.304,12.555,-1.5099,1\n-3.0201,-0.67253,2.7056,0.85774,1\n-2.4941,3.5447,-1.3721,-2.8483,1\n-0.83121,0.039307,0.05369,-0.23105,1\n-2.5665,-6.8824,7.5416,0.70774,1\n-4.4018,-12.9371,15.6559,-1.6806,1\n-3.7573,-8.2916,10.3032,0.38059,1\n-2.4725,-0.40145,1.4855,1.1189,1\n-1.9725,2.8825,-2.3086,-2.3724,1\n-2.0149,3.6874,-1.9385,-3.8918,1\n-0.82053,0.65181,-0.48869,-0.52716,1\n-1.7886,-6.3486,5.6154,0.42584,1\n-2.9138,-9.4711,9.7668,-0.60216,1\n-1.8343,-6.5907,5.6429,0.54998,1\n-0.8734,-0.033118,-0.20165,0.55774,1\n-0.70346,2.957,-3.5947,-3.1457,1\n-6.7387,6.9879,0.67833,-7.5887,1\n-2.7723,3.2777,-0.9351,-3.1457,1\n-1.6641,-1.3678,1.997,0.52283,1\n-2.4349,-9.2497,8.9922,-0.50001,1\n-3.793,-12.7095,12.7957,-2.825,1\n-1.9551,-6.9756,5.5383,-0.12889,1\n-0.69078,-0.50077,-0.35417,0.47498,1\n0.025013,3.3998,-4.4327,-4.2655,1\n-4.3967,4.9601,-0.64892,-5.4719,1\n-2.456,-0.24418,1.4041,-0.45863,1\n-2.62,-6.8555,6.2169,-0.62285,1\n-2.9662,-10.3257,8.784,-2.1138,1\n-0.71494,-4.4448,2.2241,0.49826,1\n0.6005,0.99945,-2.2126,0.097399,1\n0.61652,3.8944,-4.7275,-4.3948,1\n-5.4414,7.2363,0.10938,-7.5642,1\n-3.5798,0.45937,2.3457,-0.45734,1\n-2.7769,-5.6967,5.9179,0.37671,1\n-1.8356,-6.7562,5.0585,-0.55044,1\n0.30081,0.17381,-1.7542,0.48921,1\n1.3403,4.1323,-4.7018,-2.5987,1\n0.26877,4.987,-5.1508,-6.3913,1\n-6.5235,9.6014,-0.25392,-6.9642,1\n-4.0679,2.4955,0.79571,-1.1039,1\n-2.564,-1.7051,1.5026,0.32757,1\n-1.3414,-1.9162,-0.15538,-0.11984,1\n0.23874,2.0879,-3.3522,-0.66553,1\n0.6212,3.6771,-4.0771,-2.0711,1\n-0.77848,3.4019,-3.4859,-3.5569,1\n-4.1244,3.7909,-0.6532,-4.1802,1\n-7.0421,9.2,0.25933,-4.6832,1\n-4.9462,3.5716,0.82742,-1.4957,1\n-3.5359,0.30417,0.6569,-0.2957,1\n-2.0662,0.16967,-1.0054,-0.82975,1\n-0.88728,2.808,-3.1432,-1.2035,1\n-1.0941,2.3072,-2.5237,-1.4453,1\n-2.4458,1.6285,-0.88541,-1.4802,1\n-3.551,1.8955,0.1865,-2.4409,1\n-2.2811,-0.85669,2.7185,0.044382,1\n-3.6053,-5.974,10.0916,-0.82846,1\n-5.0676,-5.1877,10.4266,-0.86725,1\n-3.9204,4.0723,-0.23678,-2.1151,1\n-1.1306,1.8458,-1.3575,-1.3806,1\n-2.4561,-4.5566,6.4534,-0.056479,1\n-4.4775,-13.0303,17.0834,-3.0345,1\n-4.1958,-8.1819,12.1291,-1.6017,1\n-3.38,-0.7077,2.5325,0.71808,1\n-2.4365,3.6026,-1.4166,-2.8948,1\n-0.77688,0.13036,-0.031137,-0.35389,1\n-2.7083,-6.8266,7.5339,0.59007,1\n-4.5531,-12.5854,15.4417,-1.4983,1\n-3.8894,-7.8322,9.8208,0.47498,1\n-2.5084,-0.22763,1.488,1.2069,1\n-2.1652,3.0211,-2.4132,-2.4241,1\n-1.8974,3.5074,-1.7842,-3.8491,1\n-0.62043,0.5587,-0.38587,-0.66423,1\n-1.8387,-6.301,5.6506,0.19567,1\n-3,-9.1566,9.5766,-0.73018,1\n-1.9116,-6.1603,5.606,0.48533,1\n-1.005,0.084831,-0.2462,0.45688,1\n-0.87834,3.257,-3.6778,-3.2944,1\n-6.651,6.7934,0.68604,-7.5887,1\n-2.5463,3.1101,-0.83228,-3.0358,1\n-1.4377,-1.432,2.1144,0.42067,1\n-2.4554,-9.0407,8.862,-0.86983,1\n-3.9411,-12.8792,13.0597,-3.3125,1\n-2.1241,-6.8969,5.5992,-0.47156,1\n-0.74324,-0.32902,-0.42785,0.23317,1\n-0.071503,3.7412,-4.5415,-4.2526,1\n-4.2333,4.9166,-0.49212,-5.3207,1\n-2.3675,-0.43663,1.692,-0.43018,1\n-2.5526,-7.3625,6.9255,-0.66811,1\n-3.0986,-10.4602,8.9717,-2.3427,1\n-0.89809,-4.4862,2.2009,0.50731,1\n0.56232,1.0015,-2.2726,-0.0060486,1\n0.53936,3.8944,-4.8166,-4.3418,1\n-5.3012,7.3915,0.029699,-7.3987,1\n-3.3553,0.35591,2.6473,-0.37846,1\n-2.7908,-5.7133,5.953,0.45946,1\n-1.9983,-6.6072,4.8254,-0.41984,1\n0.15423,0.11794,-1.6823,0.59524,1\n1.208,4.0744,-4.7635,-2.6129,1\n0.2952,4.8856,-5.149,-6.2323,1\n-6.4247,9.5311,0.022844,-6.8517,1\n-3.9933,2.6218,0.62863,-1.1595,1\n-2.659,-1.6058,1.3647,0.16464,1\n-1.4094,-2.1252,-0.10397,-0.19225,1\n0.11032,1.9741,-3.3668,-0.65259,1\n0.52374,3.644,-4.0746,-1.9909,1\n-0.76794,3.4598,-3.4405,-3.4276,1\n-3.9698,3.6812,-0.60008,-4.0133,1\n-7.0364,9.2931,0.16594,-4.5396,1\n-4.9447,3.3005,1.063,-1.444,1\n-3.5933,0.22968,0.7126,-0.3332,1\n-2.1674,0.12415,-1.0465,-0.86208,1\n-0.9607,2.6963,-3.1226,-1.3121,1\n-1.0802,2.1996,-2.5862,-1.2759,1\n-2.3277,1.4381,-0.82114,-1.2862,1\n-3.7244,1.9037,-0.035421,-2.5095,1\n-2.5724,-0.95602,2.7073,-0.16639,1\n-3.9297,-6.0816,10.0958,-1.0147,1\n-5.2943,-5.1463,10.3332,-1.1181,1\n-3.8953,4.0392,-0.3019,-2.1836,1\n-1.2244,1.7485,-1.4801,-1.4181,1\n-2.6406,-4.4159,5.983,-0.13924,1\n-4.6338,-12.7509,16.7166,-3.2168,1\n-4.2887,-7.8633,11.8387,-1.8978,1\n-3.3458,-0.50491,2.6328,0.53705,1\n-1.1188,3.3357,-1.3455,-1.9573,1\n0.55939,-0.3104,0.18307,0.44653,1\n-1.5078,-7.3191,7.8981,1.2289,1\n-3.506,-12.5667,15.1606,-0.75216,1\n-2.9498,-8.273,10.2646,1.1629,1\n-1.6029,-0.38903,1.62,1.9103,1\n-1.2667,2.8183,-2.426,-1.8862,1\n-0.49281,3.0605,-1.8356,-2.834,1\n0.66365,-0.045533,-0.18794,0.23447,1\n-0.72068,-6.7583,5.8408,0.62369,1\n-1.9966,-9.5001,9.682,-0.12889,1\n-0.97325,-6.4168,5.6026,1.0323,1\n-0.025314,-0.17383,-0.11339,1.2198,1\n0.062525,2.9301,-3.5467,-2.6737,1\n-5.525,6.3258,0.89768,-6.6241,1\n-1.2943,2.6735,-0.84085,-2.0323,1\n-0.24037,-1.7837,2.135,1.2418,1\n-1.3968,-9.6698,9.4652,-0.34872,1\n-2.9672,-13.2869,13.4727,-2.6271,1\n-1.1005,-7.2508,6.0139,0.36895,1\n0.22432,-0.52147,-0.40386,1.2017,1\n0.90407,3.3708,-4.4987,-3.6965,1\n-2.8619,4.5193,-0.58123,-4.2629,1\n-1.0833,-0.31247,1.2815,0.41291,1\n-1.5681,-7.2446,6.5537,-0.1276,1\n-2.0545,-10.8679,9.4926,-1.4116,1\n0.2346,-4.5152,2.1195,1.4448,1\n1.581,0.86909,-2.3138,0.82412,1\n1.5514,3.8013,-4.9143,-3.7483,1\n-4.1479,7.1225,-0.083404,-6.4172,1\n-2.2625,-0.099335,2.8127,0.48662,1\n-1.7479,-5.823,5.8699,1.212,1\n-0.95923,-6.7128,4.9857,0.32886,1\n1.3451,0.23589,-1.8785,1.3258,1\n2.2279,4.0951,-4.8037,-2.1112,1\n1.2572,4.8731,-5.2861,-5.8741,1\n-5.3857,9.1214,-0.41929,-5.9181,1\n-2.9786,2.3445,0.52667,-0.40173,1\n-1.5851,-2.1562,1.7082,0.9017,1\n-0.21888,-2.2038,-0.0954,0.56421,1\n1.3183,1.9017,-3.3111,0.065071,1\n1.4896,3.4288,-4.0309,-1.4259,1\n0.11592,3.2219,-3.4302,-2.8457,1\n-3.3924,3.3564,-0.72004,-3.5233,1\n-6.1632,8.7096,-0.21621,-3.6345,1\n-4.0786,2.9239,0.87026,-0.65389,1\n-2.5899,-0.3911,0.93452,0.42972,1\n-1.0116,-0.19038,-0.90597,0.003003,1\n0.066129,2.4914,-2.9401,-0.62156,1\n-0.24745,1.9368,-2.4697,-0.80518,1\n-1.5732,1.0636,-0.71232,-0.8388,1\n-2.1668,1.5933,0.045122,-1.678,1\n-1.1667,-1.4237,2.9241,0.66119,1\n-2.8391,-6.63,10.4849,-0.42113,1\n-4.5046,-5.8126,10.8867,-0.52846,1\n-2.41,3.7433,-0.40215,-1.2953,1\n0.40614,1.3492,-1.4501,-0.55949,1\n-1.3887,-4.8773,6.4774,0.34179,1\n-3.7503,-13.4586,17.5932,-2.7771,1\n-3.5637,-8.3827,12.393,-1.2823,1\n-2.5419,-0.65804,2.6842,1.1952,1"
}
{
"filename": "code/artificial_intelligence/src/decision_tree/decision_tree.py",
"content": "# CART on the Bank Note dataset\nfrom random import seed\nfrom random import randrange\nfrom csv import reader\n\npredicted = []\nactual = []\n\n\n# Load a CSV file\ndef load_csv(filename):\n file = open(filename, \"rt\")\n lines = reader(file)\n dataset = list(lines)\n return dataset\n\n\n# Convert string column to float\ndef str_column_to_float(dataset, column):\n for row in dataset:\n row[column] = float(row[column].strip())\n\n\n# Split a dataset into k folds\ndef cross_validation_split(dataset, n_folds):\n dataset_split = list()\n dataset_copy = list(dataset)\n fold_size = int(len(dataset) / n_folds)\n for i in range(n_folds):\n fold = list()\n while len(fold) < fold_size:\n index = randrange(len(dataset_copy))\n fold.append(dataset_copy.pop(index))\n dataset_split.append(fold)\n return dataset_split\n\n\n# Calculate accuracy percentage\ndef accuracy_metric(actual, predicted):\n correct = 0\n for i in range(len(actual)):\n if actual[i] == predicted[i]:\n correct += 1\n return correct / float(len(actual)) * 100.0\n\n\n# Evaluate an algorithm using a cross validation split\ndef evaluate_algorithm(dataset, algorithm, n_folds, *args):\n global predicted\n global actual\n folds = cross_validation_split(dataset, n_folds)\n scores = list()\n for fold in folds:\n train_set = list(folds)\n train_set.remove(fold)\n train_set = sum(train_set, [])\n test_set = list()\n for row in fold:\n row_copy = list(row)\n test_set.append(row_copy)\n row_copy[-1] = None\n predicted = algorithm(train_set, test_set, *args)\n actual = [row[-1] for row in fold]\n accuracy = accuracy_metric(actual, predicted)\n scores.append(accuracy)\n return scores\n\n\n# Split a dataset based on an attribute and an attribute value\ndef test_split(index, value, dataset):\n left, right = list(), list()\n for row in dataset:\n if row[index] < value:\n left.append(row)\n else:\n right.append(row)\n return left, right\n\n\n# Calculate the Gini index for a split dataset\ndef gini_index(groups, classes):\n # count all samples at split point\n n_instances = float(sum([len(group) for group in groups]))\n # sum weighted Gini index for each group\n gini = 0.0\n for group in groups:\n size = float(len(group))\n # avoid divide by zero\n if size == 0:\n continue\n score = 0.0\n # score the group based on the score for each class\n for class_val in classes:\n p = [row[-1] for row in group].count(class_val) / size\n score += p * p\n # weight the group score by its relative size\n gini += (1.0 - score) * (size / n_instances)\n return gini\n\n\n# Select the best split point for a dataset\ndef get_split(dataset):\n class_values = list(set(row[-1] for row in dataset))\n b_index, b_value, b_score, b_groups = 999, 999, 999, None\n for index in range(len(dataset[0]) - 1):\n for row in dataset:\n groups = test_split(index, row[index], dataset)\n gini = gini_index(groups, class_values)\n if gini < b_score:\n b_index, b_value, b_score, b_groups = index, row[index], gini, groups\n return {\"index\": b_index, \"value\": b_value, \"groups\": b_groups}\n\n\n# Create a terminal node value\ndef to_terminal(group):\n outcomes = [row[-1] for row in group]\n return max(set(outcomes), key=outcomes.count)\n\n\n# Create child splits for a node or make terminal\ndef split(node, max_depth, min_size, depth):\n left, right = node[\"groups\"]\n del node[\"groups\"]\n # check for a no split\n if not left or not right:\n node[\"left\"] = node[\"right\"] = to_terminal(left + right)\n return\n # check for max depth\n if depth >= max_depth:\n node[\"left\"], node[\"right\"] = to_terminal(left), to_terminal(right)\n return\n # process left child\n if len(left) <= min_size:\n node[\"left\"] = to_terminal(left)\n else:\n node[\"left\"] = get_split(left)\n split(node[\"left\"], max_depth, min_size, depth + 1)\n # process right child\n if len(right) <= min_size:\n node[\"right\"] = to_terminal(right)\n else:\n node[\"right\"] = get_split(right)\n split(node[\"right\"], max_depth, min_size, depth + 1)\n\n\n# Build a decision tree\ndef build_tree(train, max_depth, min_size):\n root = get_split(train)\n split(root, max_depth, min_size, 1)\n return root\n\n\n# Make a prediction with a decision tree\ndef predict(node, row):\n if row[node[\"index\"]] < node[\"value\"]:\n if isinstance(node[\"left\"], dict):\n return predict(node[\"left\"], row)\n else:\n return node[\"left\"]\n else:\n if isinstance(node[\"right\"], dict):\n return predict(node[\"right\"], row)\n else:\n return node[\"right\"]\n\n\n# Classification and Regression Tree Algorithm\ndef decision_tree(train, test, max_depth, min_size):\n tree = build_tree(train, max_depth, min_size)\n predictions = list()\n for row in test:\n prediction = predict(tree, row)\n predictions.append(prediction)\n return predictions\n\n\n# Test CART on Bank Note dataset\nseed(1)\n# load and prepare data\nfilename = \"data_banknote_authentication.csv\"\ndataset = load_csv(filename)\n# convert string attributes to integers\nfor i in range(len(dataset[0])):\n str_column_to_float(dataset, i)\n# evaluate algorithm\nn_folds = 5\nmax_depth = 5\nmin_size = 10\n\nscores = evaluate_algorithm(dataset, decision_tree, n_folds, max_depth, min_size)\nprint(\"Scores: %s\" % scores)\nprint(\"Mean Accuracy: %.3f%%\" % (sum(scores) / float(len(scores))))\n# Evaluate algorithm by computing ROC (Receiver Operating Characteristic) and AUC (area under the curve)\nfrom sklearn.metrics import roc_curve, auc\nimport matplotlib.pyplot as plt\n\nfalse_positve_rate, true_positive_rate, threshold = roc_curve(actual, predicted)\nroc_auc = auc(false_positve_rate, true_positive_rate)\nplt.plot(\n false_positve_rate,\n true_positive_rate,\n lw=1,\n label=\"ROC curve (area = %0.2f)\" % roc_auc,\n)\nplt.title(\"Receiver operating characteristic example\")\nplt.ylabel(\"True Positive Rate\")\nplt.xlabel(\"False Positive Rate\")\nplt.show()\nprint(\"AUC: %s\" % roc_auc)"
}
{
"filename": "code/artificial_intelligence/src/decision_tree/Decision_Tree_Regression.ipynb",
"content": "{\n \"cells\": [\n {\n \"cell_type\": \"code\",\n \"execution_count\": 1,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"#importing the libraries\\n\",\n \"import numpy as np\\n\",\n \"import pandas as pd\\n\",\n \"import matplotlib.pyplot as plt\\n\",\n \"%matplotlib inline\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 2,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"#importing the dataset\\n\",\n \"dataset=pd.read_csv('Position_Salaries.csv')\\n\",\n \"X=dataset.iloc[:,1:2].values\\n\",\n \"y=dataset.iloc[:,2].values\\n\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": null,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": []\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 5,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"DecisionTreeRegressor(criterion='mse', max_depth=None, max_features=None,\\n\",\n \" max_leaf_nodes=None, min_impurity_decrease=0.0,\\n\",\n \" min_impurity_split=None, min_samples_leaf=1,\\n\",\n \" min_samples_split=2, min_weight_fraction_leaf=0.0,\\n\",\n \" presort=False, random_state=0, splitter='best')\"\n ]\n },\n \"execution_count\": 5,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"#fitting the decision tree regression model to the dataset\\n\",\n \"from sklearn.tree import DecisionTreeRegressor\\n\",\n \"regressor=DecisionTreeRegressor(random_state=0)\\n\",\n \"regressor.fit(X,y)\\n\",\n \"\\n\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 6,\n \"metadata\": {},\n \"outputs\": [\n {\n \"ename\": \"NameError\",\n \"evalue\": \"name 'array' is not defined\",\n \"output_type\": \"error\",\n \"traceback\": [\n \"\\u001b[1;31m---------------------------------------------------------------------------\\u001b[0m\",\n \"\\u001b[1;31mNameError\\u001b[0m Traceback (most recent call last)\",\n \"\\u001b[1;32m<ipython-input-6-31ce106e9c67>\\u001b[0m in \\u001b[0;36m<module>\\u001b[1;34m\\u001b[0m\\n\\u001b[1;32m----> 1\\u001b[1;33m \\u001b[0my_pred\\u001b[0m \\u001b[1;33m=\\u001b[0m \\u001b[0mregressor\\u001b[0m\\u001b[1;33m.\\u001b[0m\\u001b[0mpredict\\u001b[0m\\u001b[1;33m(\\u001b[0m\\u001b[0marray\\u001b[0m\\u001b[1;33m(\\u001b[0m\\u001b[1;33m[\\u001b[0m\\u001b[1;33m[\\u001b[0m\\u001b[1;36m6.5\\u001b[0m\\u001b[1;33m]\\u001b[0m\\u001b[1;33m]\\u001b[0m\\u001b[1;33m)\\u001b[0m\\u001b[1;33m)\\u001b[0m\\u001b[1;33m\\u001b[0m\\u001b[1;33m\\u001b[0m\\u001b[0m\\n\\u001b[0m\",\n \"\\u001b[1;31mNameError\\u001b[0m: name 'array' is not defined\"\n ]\n }\n ],\n \"source\": [\n \"y_pred = regressor.predict(array([[6.5]]))\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 8,\n \"metadata\": {},\n \"outputs\": [\n {\n \"ename\": \"NameError\",\n \"evalue\": \"name 'y_pred' is not defined\",\n \"output_type\": \"error\",\n \"traceback\": [\n \"\\u001b[1;31m---------------------------------------------------------------------------\\u001b[0m\",\n \"\\u001b[1;31mNameError\\u001b[0m Traceback (most recent call last)\",\n \"\\u001b[1;32m<ipython-input-8-3aaf935e6aec>\\u001b[0m in \\u001b[0;36m<module>\\u001b[1;34m\\u001b[0m\\n\\u001b[1;32m----> 1\\u001b[1;33m \\u001b[0my_pred\\u001b[0m\\u001b[1;33m\\u001b[0m\\u001b[1;33m\\u001b[0m\\u001b[0m\\n\\u001b[0m\",\n \"\\u001b[1;31mNameError\\u001b[0m: name 'y_pred' is not defined\"\n ]\n }\n ],\n \"source\": [\n \"y_pred\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 55,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"(900,)\"\n ]\n },\n \"execution_count\": 55,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"#visualising the decision tree regression result(for higher resolution and smoother curves)\\n\",\n \"X_grid=np.arange(min(X),max(X),0.01)\\n\",\n \"X_grid.shape\\n\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 56,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"#reshaping X_grid from 1-D array to 2-D array\\n\",\n \"X_grid=X_grid.reshape(len(X_grid),1)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 58,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAAaEAAAEWCAYAAADPZygPAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4yLCBodHRwOi8vbWF0cGxvdGxpYi5vcmcvOIA7rQAAIABJREFUeJzt3XucXEWd9/HPNwm3ACEBAoaEZPAhqwLrikQIoMjFxQBiYFcEzJIsixtFQBFfjwZYRXHjwoKi+CjuLPd1uAnsgsh1URRELgERDBGJISQjAQIhFzNgbr/nj6oxPZOeS/f0zJme+b5fr3l1d506VdVnZvrXVadOHUUEZmZmRRhSdAPMzGzwchAyM7PCOAiZmVlhHITMzKwwDkJmZlYYByEzMyuMg5DVhKTdJfWr+f6ShkkKSQ3dzP8BSfMl/UnSRySNkfSQpFWSLsx5/lrSo73Z7pL23CtpWhd5hub2ju+LNllbkmZIuqsG5YyR9KykzWvRrnriIDQI5A+p1p8Nkt4sed3ph1wnZTZLOrjGTa20DR/K76f1vTRL+koPivxX4JKI2CYi7gA+DbwEjIiIL5XkuaikDc0lx3O5pF9KmilJPWgHABFxeEQ0dZFnfW7vop7W115/+B33dxFxTUQcUYNylgAPAaf0vFX1xUFoEMgfUttExDbAIuDokrRNPuQkDev7VlZtUcl7+yBwqqSPVFnWBGBuu9fPRr6iW9I44P3Aj9vtd0Suv4EUoM4BGqtsQ93o7b+TWpdfB3/XTcCnim5EX3MQMiT9q6QbJV0vaRXwD5J+KOmrJXk+JGlhfn49sAtwV+4BnFWSb3r+Br1U0qwO6nu/pD9KGlKSdpykJ/PzyZKelLRS0iuSLipXTnsR8QfgV8AeHdT7kKR/LHn9SUkP5OcLgfEl7+m/gGnAOfn1wcDhwOMR8ecO6l8eEf8DnAicIumduewtJX1L0uL8fr4vacuSdvydpKfy+50v6fD27ZX0V5J+IWmFpNckXZfT2ww5ShqZf3dLJS2UdHZrryy/359LuiT32ha01lXmWG3yO1YecpV0sqRFwL0574GSHsllPiXpoJJyRkq6StKS/HdxfunvvV2d5f4Oh0g6R9If8vu+QdKokn1OlrQobzuntPdWaXmShku6TtLr+b08JmnHvO2UfDxX5eN2Qvu/ofz6/ZLm5N/TY5L2K9n2kKSvSXo4l3O3pO1LDsGvgHdKGlvu+AxUDkLW6ljgOmA74MbOMkbEiaRhqiNyL+RbJZsPAHYHPgx8TdLEMkX8ElhL6rm0+kSuH+C7wEURMSKXdXN33oCkdwD7AxWfs4mIBtq+p5NIx+Eb+fUDwF8Dz3WjrF8BLwMfyEkXA7sB7wYmknpM5+Y2HwBcCXwBGAkcArxYptjZwE+AUcA44HsdVP99YDjwduBQ0vDO9JLtBwDPADsAlwBXdPAeOvsdHwS8EzhK0q7A7cB5wPbALOBWSTvkvD8E3gT+DzAJOAo4uYO2w6Z/h2flfQ7K73s1cCmk83P5+QnAWGA08LZqy8vtGp7TdwA+A7wlaQTwLeBvI2Jb4EDg6fYNzwHrJ8A38/6XAneWBk3S3/kMYGdg69weACJiDbAA+JtOjs+A4yBkrR6KiB9HxIaIeLMH5Xw1It6KiCdJQ1ub/EPl4a0bSD0GJI0kBa0bcpa1wERJO0TEqojoLKiMz99aVwK/I42rP9yD9ndmJLCqm3lfArbP3/o/CZwZEW9ExErg30gfnJCCxH9GxP352C+OiHKBbi0peI3Jx/eX7TNI2gz4ODArH7cFpEBzUkm2P0TElRGxHrgGGNf6bb8C50VES/47mQ7cHhH35PbfDfwGmJK/0R8GfD7nfxn4dsl7L6f93+GngHMi4o8R8RbwVeDj+bgeB/xPRDyce6f/0sPy1gI7Arvnc21zIuJPuZwA9pK0ZUQsiYhny9R1NDA3Iq6PiHUR8UNSUDmqJM8VEfF8RLQAPwLe066MVaS/s0HDQchaLa5FIfmDplULsE0HWa8D/j5/cP498GhENOdtJ5OG1J7LQxpHdlLloogYmXtNo4ANpJ5Fb3gD2LabeccCy0jfzLcAfpOD5XLgDmCnnG9X4A/dKO8LwGbAHEnPSJpRJs9OwFDa9qRezG1p1f73Ax3/jjpS+rcyATix9b3l9zeZNJQ3gfTeXynZ9j1SL6A7ZUMaIv1xyf7PkALCTrmOv+SPiNWk31G15V0N/C9wk9Jw8QWShuUvDicCpwEvS7pD0l+VafsubNqL7er4tz/22wLLy5Q9YDkIWav206tXk4YmWrUf5ujRdOyIeBpYQuoBlQ7FERHPRcQJpA+GbwK3qOQcSidlLs/lHN1Blq7eU1eeBsp9+LQhaTLpg/Yh4BVgDfCOHCxHRsR2EbFdzr6YNFTVqfzt+5MRMYb0Ydgoabd22V4F1pM+/FuNB/7YVfkdVdtBW0rTFwNXlby3kRGxdURclLe1ANuXbBsREe+uoM5m0jBYaflb5i87S0hDZwBI2pr0RaSq8iJiTUR8NSLeRZqAcizpvCARcVdEfAgYA8wH/qNM21+i7bGHCo6/0vTst5N6koOGg5B15CnSmP8oSWOAz7bb/grpH6Ynrgc+TzqP85fzPpJOkrRjRGwAVpA+SDZ0VZikbYHjaTvDrdRTpN7XVvmb7D9V2N57gfepg2s5JG0n6aOkQHh1RMzLw16XA9+WNFrJOG2cEHAF8ElJh+ST5uPyua32ZX+85IT1ctIxWV+aJyLWko7jNyRtk4PU50nnZarRnd/xfwHHSvpbpWuWtszvZZeIWAz8HLhY0oj8/nZXycSFbvgB6f2MB5C0Uz7GkIazjlGayLI5cH5PypN0qKS98tDcStLw3Hqla3iOljSc9IViNe2OfXYHsKek45UmjHyCdE7zzm6+18nA7yOi2i8NdclByDpyNTCPNJxwNxvP17T6BmniwXJJZ1ZZx3Wkk+f3RUTpMMqRwDylGU0XA8fnk7bljFe+Tii3dVvangMpdTHpw/tV0pBdRR/OEfES8CCb9rTuyvUvIp2Yv4h0HqjVF3LbHiMF1XtJExSIiIeBfyadxF4B/Iw0RNfefsDjklYDtwKnRflrgz5D+qB8gRQArgGureR9lujydxwRC0k9hi8DS0nH4Ats/Gz5B9IJ+GdJQ2U/orIe6LdIf3/357+Hh4H35bqfJgXZH5F6Ia/nn7KzF7sqjzScdispAM0lDc1dTxri/L+kntfrpMkdp7cvOCKWAh8FvpTzfR74SEQs6+Z7nUYKkoOKwje1M+u2PCPrPyNictFtsbbyLLblwITcC6sbebThfuA9nXzhGpAchMysbuWhtP8l9bwuAfaOiEnFtsoq4eE4M6tnx5KG4ppJU9hPLLQ1VjH3hMzMrDDuCZmZWWH6+4J+hdtxxx2joaGh6GaYmdWVJ5544rWIGN1VPgehLjQ0NDBnzpyim2FmVlcklVsDcRMejjMzs8I4CJmZWWEchMzMrDAOQmZmVhgHITMzK0yvBSFJV0p6VdJvS9K2l3SfpOfzY+ttdSXpUqVbGz8t6b0l+8zI+Z8vvYeKpH3yfVXm531VbR1mZpY1NUFDAwwZkh6bmnq1ut7sCV0NTGmXNgu4PyImkhbrm5XTjyCtKjwRmAlcBimgkG4bvB+wL3CeNt4q97Kct3W/KdXUYWZmWVMTzJwJL74IEelx5sxeDUS9dp1QRPxCUkO75KnAwfn5NcADpGXPpwLX5ptlPSJpZF5V9mDSMv/LACTdR7pt8APAiIj4VU6/FjgGuKvSOiJiSS3ft5lZ0W65BX5Tza3xLn0NWr4EwHgW8UmugJYWOPdcmDatto3M+vpi1Z1bP/QjYomk1lscj6XtbXibc1pn6c1l0qupY5MgJGkmqbfE+PHjK3yLZmbFmjkTli2DdJKiAnHGX54eyC9TEAJYVO7WVbXRXyYmlDtUUUV6NXVsmhjRGBGTImLS6NFdrjphZtavrFsHZ54JGzZU+DPh7WxgKBsYyoOU3AC3F7+M93UQeiUPs7XexOnVnN5M27tJjmPj8uwdpY8rk15NHWZmA0rVN0eYPRuGD2+bNnx4Su8lfR2EbgdaZ7jNAG4rSZ+eZ7BNBlbkIbV7gMMljcoTEg4H7snbVuV7ywuY3q6sSuowMxtQIqoYioN03qexESZMSAVMmJBe99L5IOjFc0KSridNENhRUjNpltsFwE2STiHdi/64nP1O4EhgPtACnAwQEcskfR14POc7v+R+7aeSZuBtRZqQcFdOr6gOM7OBqKogBCng9GLQaa83Z8d1dIfDw8rkDeC0Dsq5EriyTPocYK8y6a9XWoeZ2UBSdU+oAP1lYoKZmdWIg5CZmRWm6okJBXAQMjMbYNwTMjOzwjgImZlZYRyEzMysUA5CZmZWCPeEzMysMJ4dZ2ZmhXFPyMzMCuMgZGZmhXEQMjOzQjkImZlZIdwTMjMz6wYHITOzAaR1erZ7QmZm1ucchMzMrDAOQmZmVjgHITMz63PuCZmZWWHqad04cBAyMxtQ3BMyM7PCOAiZmVlhHITMzKxwDkJmZtbnPDHBzMwK4+E4MzMrjIOQmZkVxkHIzMwK4yBkZmaFcxAyM7M+59lxZmZWGA/HdYOkz0uaK+m3kq6XtKWk3SQ9Kul5STdK2jzn3SK/np+3N5SUc3ZOf07Sh0vSp+S0+ZJmlaSXrcPMbKBwEOqCpLHAZ4FJEbEXMBQ4AbgQuCQiJgJvAKfkXU4B3oiI3YFLcj4k7ZH32xOYAnxf0lBJQ4HvAUcAewAn5rx0UoeZ2YDgINQ9w4CtJA0DhgNLgEOBm/P2a4Bj8vOp+TV5+2GSlNNviIg/R8QLwHxg3/wzPyIWRMQa4AZgat6nozrMzAYEB6EuRMQfgYuBRaTgswJ4AlgeEetytmZgbH4+Flic912X8+9Qmt5un47Sd+ikDjOzAcVBqAOSRpF6MbsBuwBbk4bO2mud41HuUEYN08u1caakOZLmLF26tFwWM7N+ybPjuvYh4IWIWBoRa4FbgQOAkXl4DmAc8FJ+3gzsCpC3bwcsK01vt09H6a91UkcbEdEYEZMiYtLo0aN78l7NzPqUh+O6tgiYLGl4Pk9zGPAs8DPgYznPDOC2/Pz2/Jq8/acRETn9hDx7bjdgIvAY8DgwMc+E25w0eeH2vE9HdZiZDQgOQl2IiEdJkwOeBJ7JbWgEvgScJWk+6fzNFXmXK4AdcvpZwKxczlzgJlIAuxs4LSLW53M+pwP3APOAm3JeOqnDzGxAqLcgNKzrLLUXEecB57VLXkCa2dY+71vAcR2UMxuYXSb9TuDOMull6zAzGyjqLQh5xQQzswHIQcjMzPqcZ8eZmVlhPBxnZmaFcRAyM7PCOAiZmVlhHITMzKxwDkJmZtbnPDvOzMwK4+E4MzMrjIOQmZkVxkHIzMwK4yBkZmaF8cQEMzMrnHtCZmbW5zwcZ2ZmhXEQMjOzwjgImZlZYRyEzMysMJ4dZ2ZmhXNPyMzM+pyH48zMrDAOQmZmVhgHITMzK4yDkJmZFcaz48zMrHDuCZmZWZ/zcJyZmRXGQcjMzArjIGRmZoVxEDIzs8J4dpyZmRXOPSEzM+tzHo7rBkkjJd0s6XeS5knaX9L2ku6T9Hx+HJXzStKlkuZLelrSe0vKmZHzPy9pRkn6PpKeyftcKqVfR0d1mJkNFA5C3fMd4O6IeCfwN8A8YBZwf0RMBO7PrwGOACbmn5nAZZACCnAesB+wL3BeSVC5LOdt3W9KTu+oDjOzAcFBqAuSRgAHAVcARMSaiFgOTAWuydmuAY7Jz6cC10byCDBS0hjgw8B9EbEsIt4A7gOm5G0jIuJXERHAte3KKleHmdmA4CDUtbcDS4GrJP1a0uWStgZ2joglAPlxp5x/LLC4ZP/mnNZZenOZdDqpow1JMyXNkTRn6dKl1b9TM7M+5tlxXRsGvBe4LCL2BlbT+bBYuXgeVaR3W0Q0RsSkiJg0evToSnY1M+sX3BPqWDPQHBGP5tc3k4LSK3kojfz4akn+XUv2Hwe81EX6uDLpdFKHmdmAMCCH4yQNrVWFEfEysFjSO3LSYcCzwO1A6wy3GcBt+fntwPQ8S24ysCIPpd0DHC5pVJ6QcDhwT962StLkPCtueruyytVhZjYg1FsQGtbNfPMl3QxcFRHP1qDeM4AmSZsDC4CTSQHxJkmnAIuA43LeO4EjgflAS85LRCyT9HXg8Zzv/IhYlp+fClwNbAXclX8ALuigDjOzAWGgBqF3AycAl0saAlwJ3BARK6upNCKeAiaV2XRYmbwBnNZBOVfmtrRPnwPsVSb99XJ1mJkNFANyYkJErIqI/4yIA4Avkq7PWSLpGkm792oLzcys2+qtJ9Ttc0KSPirpv0kXmn6TNNX6x6ThMjMz60fqJQh1dzjueeBnwEUR8XBJ+s2SDqp9s8zMrBr11hPqMgjlmXFXR8T55bZHxGdr3iozM6tKvQWhLofjImI9cEgftMXMzHqo3oJQd4fjHpb0/4AbSSscABART/ZKq8zMrCr1Njuuu0HogPxYOiQXwKG1bY6ZmfXEgOwJRYSH48zM6siACkIAko4C9gS2bE3raLKCmZkVo956Qt29TugHwPGk5XZEWu5mQi+2y8zMqjAggxBwQERMB96IiK8B+9N2BWszM+sHBmoQejM/tkjaBVgL7NY7TTIzs2rV2+y47gahOySNBC4CngQWAjf0VqPMzAalpiZoaIAhQ9JjU1PFRdRbT6i7s+O+np/eIukOYMuIWNF7zTIzq0/r11e543XXwac+DW+2AIIXF8M/fxo2CD7xiYrrHxBBSNLfdbKNiLi19k0yM6tPs2bBhRdWu/cn8k+JN0m35ZxeeWnDuj33uVhdNfPoTrYF4CBkZpbNmwdvext85jNV7PyVr5A+VtsTnF/Z1TAjRsA++1TRhgJ0GoQi4uS+aoiZWb3bsAHGjoUvf7mKna+4Fl58cdP0CRPgywP3kkxfrGpmViMbNqQ5BVWZPRtmzoSWlo1pw4en9AHMF6uamdVIj4LQtGnQ2Jh6PlJ6bGxM6QNYtxcwjYh3S3o6Ir4m6Zv4fJCZWRvr1/cgCEEKOAM86LRX7cWq6/DFqmZmbfSoJzRIdbcn1Hqx6r8DT+S0y3unSWZm9clBqHJdXSf0PmBx68WqkrYBngF+B1zS+80zM6sfDkKV6+pw/QewBkDSQcAFOW0F0Ni7TTMzqy8OQpXrajhuaEQsy8+PBxoj4hbS8j1P9W7TzMzqi4NQ5bo6XEMltQaqw4Cflmyrk0UhzMz6hoNQ5boKJNcDP5f0GmmG3IMAknYnDcmZmVnmIFS5rpbtmS3pfmAMcG/EX+5UMYR04aqZmWUbNsDQoUW3or50OaQWEY+USft97zTHzKx+uSdUOR8uM7MacRCqnA+XmVmN9HjZnkHIh8vMrEbcE6pcYYdL0lBJv863C0fSbpIelfS8pBslbZ7Tt8iv5+ftDSVlnJ3Tn5P04ZL0KTltvqRZJell6zAzqwUHocoVebg+B8wreX0hcElETATeAE7J6acAb0TE7qSlgi4EkLQHcALpHkdTgO/nwDYU+B5wBLAHcGLO21kdZmY95iBUuUIOl6RxwFHkRVAlCTgUuDlnuQY4Jj+fml+Ttx+W808FboiIP0fEC8B8YN/8Mz8iFkTEGuAGYGoXdZiZ9ZiDUOWKOlzfBr4IbMivdwCWR8S6/LoZGJufjwUWA+TtK3L+v6S326ej9M7qaEPSTElzJM1ZunRpte/RzAYZB6HK9fnhkvQR4NWIeKI0uUzW6GJbrdI3TYxojIhJETFp9OjR5bKYmW3CQahyRaz/diDwUUlHAlsCI0g9o5GShuWeyjjgpZy/GdgVaM7r2G0HLCtJb1W6T7n01zqpw8ysxxyEKtfnhysizo6IcRHRQJpY8NOImAb8DPhYzjYDuC0/vz2/Jm//aV4+6HbghDx7bjdgIvAY8DgwMc+E2zzXcXvep6M6zMx6zMv2VK4/xewvAWdJmk86f3NFTr8C2CGnnwXMAoiIucBNwLPA3cBpEbE+93JOB+4hzb67KeftrA4zsx5zT6hyhd6OISIeAB7IzxeQZra1z/MWcFwH+88GZpdJvxO4s0x62TrMzGrBQahyPlxmZjXiZXsq58NlZlYj7glVzofLzKxGHIQq58NlZlYjDkKV8+EyM6sRB6HK+XCZmdWIg1DlfLjMzGrEQahyPlxmZjXiIFQ5Hy4zsxrxsj2VcxAyM6sR94Qq58NlZlYjDkKV8+EyM6sRL9tTOR8uM7OmJmhoSBGkoSG9rlDkW2Q6CFWm0FW0zcwK19QEM2dCS0t6/eKL6TXAtGndLmbDhvToIFQZByEzq3svvAAXXABr11ax802bQct326a1AJ/aDO7vfjEOQtVxEDKzunfbbdDYCGPHVhEEVk/uIB3438qKamiASZMqrH+QcxAys7rX2gP6/e9h+PAKd244KA3BtTdhAixc2NOmWRfccTSzutcahIZV87V69uxNI9fw4Sndep2DkJnVvXXr0mNVQWjatDSWN2ECSOmxsbGiSQlWPQ/HmVndW7cuxY+qJwVMm+agUxD3hMys7q1bB5ttVnQrrBoOQmZW99atq3IozgrnIGRmdW/tWgeheuUgZGZ1zz2h+uUgZGZ1z0GofjkImVndcxCqXw5CZlb3PDuufjkImVnd88SE+uUgZGZ1z8Nx9ctByMzqnoNQ/XIQMrO65yBUvxyEzKzu+ZxQ/XIQMrO659lx9avPg5CkXSX9TNI8SXMlfS6nby/pPknP58dROV2SLpU0X9LTkt5bUtaMnP95STNK0veR9Eze51JJ6qwOMytIU1O6HemQIemxqamqYjwcV7+K6AmtA74QEe8CJgOnSdoDmAXcHxETSXd2n5XzHwFMzD8zgcsgBRTgPGA/YF/gvJKgclnO27rflJzeUR1m1teammDmzHRX04j0OHNmVYHIQah+9fmvLSKWAEvy81WS5gFjganAwTnbNcADwJdy+rUREcAjkkZKGpPz3hcRywAk3QdMkfQAMCIifpXTrwWOAe7qpA4zq8Jrr8H++8Py5VXsvGwKbFjYNq0FmD4EzqysqOXL4ZBDqmiDFa7Q7w6SGoC9gUeBnXOAIiKWSNopZxsLLC7ZrTmndZbeXCadTupo366ZpJ4U48ePr/LdmQ18CxbA/Plw1FHphqQV+f6NQGyavkHw8c9U3JZjj614F+sHCgtCkrYBbgHOjIiV+bRN2axl0qKK9G6LiEagEWDSpEkV7Ws2mLz5Zno86yw49NAKd/7Jv6chuPYmTIDvVR6ErD4VMjtO0makANQUEbfm5FfyMBv58dWc3gzsWrL7OOClLtLHlUnvrA4zq8Jbb6XHLbesYufZs2H48LZpw4endBs0ipgdJ+AKYF5EfKtk0+1A6wy3GcBtJenT8yy5ycCKPKR2D3C4pFF5QsLhwD152ypJk3Nd09uVVa4OM6tCaxDaaqsqdp42DRobU89HSo+NjSndBo0ihuMOBE4CnpH0VE47B7gAuEnSKcAi4Li87U7gSGA+6bTlyQARsUzS14HHc77zWycpAKcCVwNbkSYk3JXTO6rDzKrQOhxXVU8IUsBx0BnUipgd9xDlz9sAHFYmfwCndVDWlcCVZdLnAHuVSX+9XB1mVp0eDceZ4RUTzKwHejQcZ4aDkNngVKOVCno8HGeDnq8xNhtsWlcqaGlJr1tXKoCKz894OM56ykHIrE5ddRU89VTX+TbdcQ20fKNtWgtw6hp4rLKiHn00PW6xRRXtMMNByKxuffaz6RYGFZ+PWXVMB+nAtZW344MfTDOszarhIGRWhyJg9Wr4l3+B88+vcOeGvTteqWDhwlo0z6zbPDHBrA699VYKRFtvXcXOXqnA+hEHIbM6tHp1emwfS7rFKxVYP+LhOLM61DqxraqeEHilAus33BMy62s1uEanRz0hs37EPSGzvlSja3Rad3cQsnrnIGRWoaVL4fHHu85X1ln3QcsH26a15PRR3Q9Cc+emx6qH48z6CQchswqdcQbceGO1e19dPvlV4KjKS9t552rbYdY/OAiZVeiVV2DvveEHP6hi56lT4eUlm6a/bQzcVtntrbbdFt71riraYNaPOAiZVWjlShg7Fvbdt4qdL/5423NCkE7sXPw5qKY8szrn2XFmFVq5EkaMqHJnX6Nj1oaDkA0eNbp9QY+CEKSAs3AhbNiQHh2AbBDzcJzVleXLYd26Kna8+WY462x4swXYHl78E/zz2bBqC/jYxyoqqsdByMz+wkHI6sYNN8CJJ1a798fyT4k3gVPzT4VGjaq2HWZWykHI6sbcuWkk7TvfqWLnM84AoswGwXe/W1FRw4bBccdV0QYz24SDkPW+piY491xYtAjGj0+rNVdxHmTpUthxRzj99CracPGPO759wemVBSEzqx1PTLDe1bpMzYsvpnsPtC5TU8WkgNYgVBXfvsCsX3JPaCCrUQ/k5Zdhv/1gxYoq2rDyaIg/tk1rAU4aAqdVVtSf/gQf+EAVbYCN77sGx8PMasdBqLfUKAD0qP4aLJQJ8Mgj6W2cdBJsv32F7fjOVZQ9FxOCf/xchYWlBQeq5tsXmPU7iih3stZaTZo0KebMmVPZTu0DAKShnwovSoyAY4+Fp5+urHoAFi8qP5d52DDYdXxFRa1cCa+/DqtWwTbbVNiOhgbfStpsEJL0RERM6iqfe0K94dxzWdCyM0fz441pLcDJm8E3ul/M+vXw3HNwyCEwblyFbfivB8qnrwPeP73CwmDPPasIQJB6gOUCss/FmBkOQr1j0SK2YAx78Gzb9LXAHn9VUVH775+mJFd8ceQvvtJxD+TayoNQ1Xwuxsw64eG4LlQ1HNcfhqBqNCRoZlaN7g7HeYp2b+gP04G9UKaZ1QEPx/WG/jIE5dlgZtbPOQj1FgcAM7MueTjOzMwKM+iCkKQpkp6TNF/SrKLbY2Y2mA2qICRpKPA94AhgD+BESXsU2yozs8FrUAUhYF9gfkQsiIg1wA1ATxaCMTOzHhhsQWgssLjkdXNOa0PSTElzJM1ZunRpnzXOzGywGWyz41QmbZOrdSOiEWgEkLRUUpkrT+vKjsBrRTeiH/Hx2MjHoi0fj416eiwmdCfTYAtCzcCuJa9pTLMzAAAFeElEQVTHAS91tkNEjO7VFvUBSXO6c+XyYOHjsZGPRVs+Hhv11bEYbMNxjwMTJe0maXPgBOD2gttkZjZoDaqeUESsk3Q6cA8wFLgyIuYW3Cwzs0FrUAUhgIi4E7iz6Hb0scaiG9DP+Hhs5GPRlo/HRn1yLLyKtpmZFWawnRMyM7N+xEHIzMwK4yA0gEnaVdLPJM2TNFfS54puU9EkDZX0a0l3FN2WokkaKelmSb/LfyP7F92mokj6fP4f+a2k6yVtWXSb+pKkKyW9Kum3JWnbS7pP0vP5cVRv1O0gNLCtA74QEe8CJgOnea08PgfMK7oR/cR3gLsj4p3A3zBIj4ukscBngUkRsRdp5uwJxbaqz10NTGmXNgu4PyImAvfn1zXnIDSARcSSiHgyP19F+pDZZJmiwULSOOAo4PKi21I0SSOAg4ArACJiTUQsL7ZVhRoGbCVpGDCcLi5iH2gi4hfAsnbJU4Fr8vNrgGN6o24HoUFCUgOwN/BosS0p1LeBLwIbim5IP/B2YClwVR6evFzS1kU3qggR8UfgYmARsARYERH3FtuqfmHniFgC6QstsFNvVOIgNAhI2ga4BTgzIlYW3Z4iSPoI8GpEPFF0W/qJYcB7gcsiYm9gNb003NLf5XMdU4HdgF2ArSX9Q7GtGjwchAY4SZuRAlBTRNxadHsKdCDwUUkLSbfwOFTSD4ttUqGageaIaO0Z30wKSoPRh4AXImJpRKwFbgUOKLhN/cErksYA5MdXe6MSB6EBTJJIY/7zIuJbRbenSBFxdkSMi4gG0knnn0bEoP22GxEvA4slvSMnHQY8W2CTirQImCxpeP6fOYxBOkmjnduBGfn5DOC23qhk0C3bM8gcCJwEPCPpqZx2Tl66yOwMoCkv5rsAOLng9hQiIh6VdDPwJGlG6a8ZZMv3SLoeOBjYUVIzcB5wAXCTpFNIgfq4Xqnby/aYmVlRPBxnZmaFcRAyM7PCOAiZmVlhHITMzKwwDkJmZlYYByGzKklaL+mpvPLyjyQNr6KMy1sXlZV0TrttD9eonVdL+lgtyurNMm1wchAyq96bEfGevPLyGuDTlRYQEZ+MiNaLRM9pt81X7duA5yBkVhsPArsDSDor945+K+nMnLa1pJ9I+k1OPz6nPyBpkqQLSKs4PyWpKW/7U36UpIvyfs+U7Htw3r/1nkBN+Yr/DknaR9LPJT0h6R5JYyS9S9JjJXkaJD3dUf7aHzobzLxiglkP5eX/jwDulrQPaeWB/QABj0r6OWnV6pci4qi8z3alZUTELEmnR8R7ylTxd8B7SPf82RF4XNIv8ra9gT1Jtx74JWmVjIc6aOdmwHeBqRGxNAez2RHxT5I2l/T2iFgAHE+6Ur5sfuCfqjlOZuU4CJlVb6uS5ZAeJK3Tdyrw3xGxGkDSrcAHgLuBiyVdCNwREQ9WUM/7gesjYj1pUcmfA+8DVgKPRURzruspoIEOghDwDmAv4L7cYRpKunUBwE3Ax0lLtRyffzrLb1YTDkJm1Xuzfc+lo+GwiPh97iUdCfybpHsj4vxu1tPZENufS56vp/P/aQFzI6LcbbxvBH6Ug2ZExPOS/rqT/GY14XNCZrX1C+CYvCLz1sCxwIOSdgFaIuKHpBuolbttwto8BFauzOMlDZU0mnRH1MfK5OvKc8BoSftDGp6TtCdARPyBFMS+TApIneY3qxX3hMxqKCKelHQ1G4PE5RHxa0kfBi6StAFYSxq2a68ReFrSkxExrST9v4H9gd8AAXwxIl6W9M4K27YmT6u+NJ+TGka62+zcnOVG4CLSzd26k9+sx7yKtpmZFcbDcWZmVhgHITMzK4yDkJmZFcZByMzMCuMgZGZmhXEQMjOzwjgImZlZYf4/Pp+rPa3Mjs0AAAAASUVORK5CYII=\\n\",\n \"text/plain\": [\n \"<Figure size 432x288 with 1 Axes>\"\n ]\n },\n \"metadata\": {\n \"needs_background\": \"light\"\n },\n \"output_type\": \"display_data\"\n }\n ],\n \"source\": [\n \"plt.scatter(X,y,color='red')\\n\",\n \"plt.plot(X_grid,regressor.predict(X_grid),color='blue')\\n\",\n \"plt.title('Truth vs Bluff(Decision tree regression)')\\n\",\n \"plt.xlabel('Position level')\\n\",\n \"plt.ylabel('Salary')\\n\",\n \"plt.show()\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": null,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": []\n }\n ],\n \"metadata\": {\n \"kernelspec\": {\n \"display_name\": \"Python 3\",\n \"language\": \"python\",\n \"name\": \"python3\"\n },\n \"language_info\": {\n \"codemirror_mode\": {\n \"name\": \"ipython\",\n \"version\": 3\n },\n \"file_extension\": \".py\",\n \"mimetype\": \"text/x-python\",\n \"name\": \"python\",\n \"nbconvert_exporter\": \"python\",\n \"pygments_lexer\": \"ipython3\",\n \"version\": \"3.7.3\"\n }\n },\n \"nbformat\": 4,\n \"nbformat_minor\": 2\n}"
}
{
"filename": "code/artificial_intelligence/src/decision_tree/decision_trees_information_gain.py",
"content": "from math import log\nimport numpy as np\nfrom collections import Counter\n\n\nclass Node:\n def __init__(self):\n self.split_column = None\n self.split_value = None\n self.left = None\n self.right = None\n self.node_def = True\n self.label = None\n\n\nclass decision_tree:\n def __init__(self):\n self.head = Node()\n self.max_depth = 3\n self.root_entropy = 1\n self.min_samples = 0\n\n def train(self, x_train, y_train):\n self.head = self.build(self.head, x_train, y_train, self.root_entropy, 1)\n\n def build(self, current_node, x_train, y_train, entropy_parent, cur_depth):\n if cur_depth > self.max_depth or len(y_train) <= self.min_samples:\n temp_node = Node()\n temp_node.node_def = False\n return temp_node\n\n row_length = len(x_train)\n col_length = len(x_train[0])\n count = {}\n prob = {}\n entropy = {}\n information_gain = {}\n weighted_avg = {}\n output_set = set(y_train)\n\n for i in range(0, col_length):\n count[i] = {}\n prob[i] = {}\n entropy[i] = {}\n s = set(x_train[:, i])\n for j in s:\n count[i][j] = 0\n entropy[i][j] = 0\n for k in output_set:\n count[i][j + \"and\" + k] = 0\n prob[i][j + \"and\" + k] = 0\n\n for i in range(0, col_length):\n for j in range(0, row_length):\n count[i][x_train[j][i]] = count[i][x_train[j][i]] + 1\n count[i][x_train[j][i] + \"and\" + y_train[j]] = (\n count[i][x_train[j][i] + \"and\" + y_train[j]] + 1\n )\n\n for i in range(0, col_length):\n s = set(x_train[:, i])\n weighted_avg[i] = 0\n temp_sum = 0\n for j in s:\n for k in output_set:\n prob[i][j + \"and\" + k] = count[i][j + \"and\" + k] / count[i][j]\n if prob[i][j + \"and\" + k]:\n entropy[i][j] = entropy[i][j] + prob[i][j + \"and\" + k] * (\n log(prob[i][j + \"and\" + k]) / log(2)\n )\n if entropy[i][j]:\n entropy[i][j] = entropy[i][j] * -1\n\n weighted_avg[i] = weighted_avg[i] + entropy[i][j] * count[i][j]\n temp_sum = temp_sum + count[i][j]\n weighted_avg[i] = weighted_avg[i] / temp_sum\n information_gain[i] = entropy_parent - weighted_avg[i]\n\n max_key = max(information_gain, key=information_gain.get)\n split_set = set(x_train[:, max_key])\n split_value = None\n\n for i in split_set:\n split_value = i\n break\n\n x_train_left = []\n x_train_right = []\n y_train_left = []\n y_train_right = []\n split_data_left = []\n split_data_right = []\n\n for j in range(0, row_length):\n if x_train[j, max_key] == split_value:\n split_data_left.append(j)\n else:\n split_data_right.append(j)\n\n x_train_left = x_train[split_data_left]\n y_train_left = y_train[split_data_left]\n x_train_right = x_train[split_data_right]\n y_train_right = y_train[split_data_right]\n current_node.split_column = max_key\n current_node.split_value = split_value\n temp_dict = {}\n\n for i in y_train:\n temp_dict[i] = 0\n for i in y_train:\n temp_dict[i] = temp_dict[i] + 1\n\n current_node.label = Counter(temp_dict).most_common(1)[0][0]\n current_node.left = Node()\n current_node.right = Node()\n current_node.left = self.build(\n current_node.left,\n x_train_left,\n y_train_left,\n entropy[current_node.split_column][current_node.split_value],\n cur_depth + 1,\n )\n current_node.right = self.build(\n current_node.right,\n x_train_right,\n y_train_right,\n entropy[current_node.split_column][current_node.split_value],\n cur_depth + 1,\n )\n\n return current_node\n\n def predict(self, test):\n temp_list = []\n for i in test:\n temp_list.append(self.test_fun(self.head, i))\n return temp_list\n\n def test_fun(self, cur_node, test):\n\n if cur_node.left.node_def is False and cur_node.right.node_def is False:\n return cur_node.label\n\n if test[cur_node.split_column] == cur_node.split_value:\n return self.test_fun(cur_node.left, test)\n else:\n return self.test_fun(cur_node.right, test)\n\n\nx_train = [\n [\"Steep\", \"Bumpy\", \"Yes\"],\n [\"Steep\", \"Smooth\", \"Yes\"],\n [\"Flat\", \"Bumpy\", \"No\"],\n [\"Steep\", \"Smooth\", \"No\"],\n]\ny_train = [\"Slow\", \"Slow\", \"Fast\", \"Fast\"]\n\nx_train = np.array(x_train)\ny_train = np.array(y_train)\n\nclf = decision_tree()\nclf.train(x_train, y_train)\nprint(clf.predict(x_train))"
}
{
"filename": "code/artificial_intelligence/src/decision_tree/Position_Salaries.csv",
"content": "Position,Level,Salary\nBusiness Analyst,1,45000\nJunior Consultant,2,50000\nSenior Consultant,3,60000\nManager,4,80000\nCountry Manager,5,110000\nRegion Manager,6,150000\nPartner,7,200000\nSenior Partner,8,300000\nC-level,9,500000\nCEO,10,1000000"
}
{
"filename": "code/artificial_intelligence/src/factorization_machines/matrix_factorization.py",
"content": "import numpy as np\n\n\ndef matrix_factorization(data, K, steps=5000, beta=0.0002, lamda=0.02):\n\n W = np.random.rand(data.shape[0], K)\n H = np.random.rand(data.shape[1], K)\n # W = np.random.normal(scale=1./K, size = (data.shape[0], K))\n # H = np.random.normal(scale=1./K, size = (data.shape[1], K))\n\n H = H.T\n\n b_u = np.zeros(data.shape[0])\n\n for i in range(len(data)):\n # print(\"len\",len(np.where(data[i] != 0)[0]))\n if len(np.where(data[i] != 0)[0]) == 0:\n b_u[i] = 0\n else:\n b_u[i] = np.mean(data[i][np.where(data[i] != 0)])\n # print(b_u[i])\n\n b_i = np.zeros(data.shape[1])\n for i in range(len(data.T)):\n if len(np.where(data.T[i] != 0)[0]) == 0:\n b_i[i] = 0\n else:\n b_i[i] = np.mean(data.T[i][np.where(data.T[i] != 0)])\n\n # b_i[i] = np.mean(data.T[i][np.where(data.T[i] != 0)])\n # print(b_i[i])\n\n b = np.mean(data[np.where(data != 0)])\n\n # i : user\n # j : item\n\n for step in range(steps):\n for i in range(len(data)):\n for j in range(len(data[i])):\n if data[i][j] > 0:\n p_bar = b + b_u[i] + b_i[j] + np.dot(W[i, :], H[:, j])\n\n eij = data[i][j] - p_bar\n\n b = b + beta * eij\n\n b_u[i] += beta * (eij - lamda * b_u[i])\n b_i[j] += beta * (eij - lamda * b_i[j])\n\n \"\"\"\n for k in range(K):\n P[i][k] = P[i][k] + beta * (2 * eij * Q[k][j] - lamda * P[i][k])\n Q[k][j] = Q[k][j] + beta * (2 * eij * P[i][k] - lamda * Q[k][j])\n \"\"\"\n W[i, :] += beta * (2 * eij * H[:, j] - lamda * W[i, :])\n H[:, j] += beta * (2 * eij * W[i, :] - lamda * H[:, j])\n\n edata = np.dot(W, H)\n e = 0\n for i in range(len(data)):\n for j in range(len(data[i])):\n if data[i][j] > 0:\n e = e + pow(data[i][j] - np.dot(W[i, :], H[:, j]), 2)\n for k in range(K):\n e = e + (lamda / 2) * (pow(W[i][k], 2) + pow(H[k][j], 2))\n\n if e < 0.001:\n print(\"Epsilon's exit\")\n break\n return W, H.T, b_u, b_i, b\n\n\ndef readFile(fileString):\n return np.loadtxt(fileString, delimiter=\" \", dtype=\"int\").tolist()\n\n\nif __name__ == \"__main__\":\n fileString = r\"/home/ledsinh/test.txt\"\n \"\"\"\n You can read the matrix in many dismension\n\n \"\"\"\n data = readFile(fileString)\n\n data = np.array(data)\n print(data)\n K = 2\n\n W, H, b_u, b_i, b = matrix_factorization(data, K)\n print(\"Matrix Factorization with Bias\")\n fitted = b + W.dot(H.T) + b_u[:, np.newaxis] + b_i[np.newaxis, :]\n\n for i in fitted:\n print(np.around(i, decimals=2))\n # print(fitted)"
}
{
"filename": "code/artificial_intelligence/src/factorization_machines/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# Factorization machines\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/artificial_intelligence/src/gaussian_mixture_model/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# Gaussian mixture model\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/artificial_intelligence/src/gaussian_naive_bayes/gaussian_naive_bayes_moons.py",
"content": "############################################################################\n############################################################################\n\n# Importing necessary packages\nimport numpy as np\nimport scipy\nimport matplotlib.pyplot as plt\nfrom sklearn.utils import check_random_state\nfrom sklearn.utils import shuffle as util_shuffle\nfrom sklearn.preprocessing import scale\nfrom pandas import DataFrame\n\n\n############################################################################\n############################################################################\ndef make_moons(n_samples=100, shuffle=True, noise=None, random_state=None):\n \"\"\"Make two interleaving half circles\n A simple toy dataset to visualize clustering and classification\n algorithms. Read more in the :ref:`User Guide <sample_generators>`.\n Parameters\n ----------\n n_samples : int, optional (default=100)\n The total number of points generated.\n shuffle : bool, optional (default=True)\n Whether to shuffle the samples.\n noise : double or None (default=None)\n Standard deviation of Gaussian noise added to the data.\n random_state : int, RandomState instance or None, optional (default=None)\n If int, random_state is the seed used by the random number generator;\n If RandomState instance, random_state is the random number generator;\n If None, the random number generator is the RandomState instance used\n by `np.random`.\n Returns\n -------\n X : array of shape [n_samples, 2]\n The generated samples.\n y : array of shape [n_samples]\n The integer labels (0 or 1) for class membership of each sample.\n \"\"\"\n n_samples_out = n_samples // 2 # Floor division\n n_samples_in = n_samples - n_samples_out # splitting it into two halves\n\n generator = check_random_state(random_state)\n\n outer_circ_x = np.cos(np.linspace(0, np.pi, n_samples_out))\n outer_circ_y = np.sin(np.linspace(0, np.pi, n_samples_out))\n inner_circ_x = 1 - np.cos(np.linspace(0, np.pi, n_samples_in))\n inner_circ_y = 1 - np.sin(np.linspace(0, np.pi, n_samples_in)) - 1\n\n X = np.vstack(\n (np.append(outer_circ_x, inner_circ_x), np.append(outer_circ_y, inner_circ_y))\n ).T\n y = np.hstack(\n [np.zeros(n_samples_out, dtype=np.intp), np.ones(n_samples_in, dtype=np.intp)]\n )\n\n if shuffle:\n X, y = util_shuffle(X, y, random_state=generator)\n\n if noise is not None:\n X += generator.normal(scale=noise, size=X.shape)\n\n return X, y\n\n\n############################################################################\n# generating 2D classification dataset\n\ncrts, Class = make_moons(n_samples=2000, noise=0.08, random_state=0)\n\nClass = Class + 1\n\ndf = DataFrame(dict(x=crts[:, 0], y=crts[:, 1], label=Class))\n\n\ncolors = {1: \"red\", 2: \"blue\"}\nfig, ax = plt.subplots()\n\n############################################################################\n############################################################################\n# Separating the co ordinates according to the classes CLASSES 1 followed by CLASSES 2\ngrouped = df.groupby(\"label\")\n\n\nu = [] # The MEAN\ngX = [] # Group\n\nfor key, group in grouped:\n gX.append(group.values)\n u.append(group.mean().values)\n\n group.plot(ax=ax, kind=\"scatter\", x=\"x\", y=\"y\", label=key, color=colors[key])\n\nplt.savefig(\"graph.png\")\n\n\ngX = np.array(gX)\nprint(gX)\n\n############################################################################\n\nX = gX[:, :, -2]\nY = gX[:, :, -1]\nclasses = gX[:, :, -3]\n\nprint(\"Classes\")\nclasses = np.hstack(classes)\nprint(classes)\n\nprint(\"Xh\")\nXh = np.hstack(X)\nprint(Xh)\n\nprint(\"Yh\")\nYh = np.hstack(Y)\nprint(Yh)\n\nprint(\"XY\")\nXY = np.column_stack((Xh, Yh))\nprint(XY)\n\n############################################################################\n# Calculating Covariance\nprint(\"Covariance\")\nC = np.cov(Xh, Yh)\nprint(C)\n\nprint(\"Cinv\")\nCinv = np.linalg.inv(C)\nprint(Cinv)\n\n############################################################################\n############################################################################\n\"\"\"\nThe Gaussain Bayes Classifier is:\n\nlog (Likelihood Ratio ^) = W' * X + b\n where\n\n W = (u1 - u2)' * Cinv * X\n b = 0.5 * ((u2' * Cinv * u2) - (u1' * Cinv * u1))\n\n\nNow, if the Likelihood Ratio is:\n Greater than 1? Class 0 Txt Book Class 1\n Lesser than 1? Class 1 Txt Book Class 2\n\nNow, if the Logarithm of the Likelihood Ratio is:\n Greater than log(1) = 0? Class 0 Txt Book Class 1\n Lesser than log(1) = 0? Class 1 Txt Book Class 2\n\n\"\"\"\n############################################################################\n############################################################################\n# Finding W\n\nmask = [False, True, True]\n\nu1 = u[0] # Mean of Class 1\nu2 = u[1] # Mean of Class 2\n\n\nu1 = u1[mask]\nu2 = u2[mask]\n\n\nprint(\"u1 - u2\")\nprint((u1 - u2).T.shape)\n############################################################################\n\nstage1W = np.asmatrix(np.dot((u1 - u2).T, Cinv))\nprint(\"stage1W\")\nprint(stage1W.shape)\n\nprint(\"W\")\nW = np.dot(stage1W, XY.T)\nprint(W)\n\nprint(\"Weight W: \")\nprint(W.shape)\n\n############################################################################\n############################################################################\n# Finding b\nu1 = np.asmatrix(u1)\n\nu2 = np.asmatrix(u2)\n\n\nprint(\"Stage 1b\")\nstage11b = np.dot(u2, Cinv)\nstage1b = np.dot(stage11b, u2.T)\nstage1b = np.asscalar(np.array(stage1b))\n\nprint(\"Stage 2b\")\nstage22b = np.dot(u1, Cinv)\nstage2b = np.dot(stage22b, u1.T)\nstage2b = np.asscalar(np.array(stage2b))\n\nb = 0.5 * (stage1b - stage2b)\n\nprint(\"Bias b: \")\nprint(b)\n\n############################################################################\n############################################################################\n# Calculating error rate\nprint(\"xtest\")\nxtest = XY[:, 0]\nxtest = np.asmatrix(xtest)\nprint(xtest.shape)\n\n\nytest = np.multiply(W, xtest)\nytest = np.add(ytest, b)\nprint(ytest)\n\n# ERROR CLASSIFICATION\nprint(\"CLASSIFICATION\")\nytest[ytest > 0] = 1\nytest[ytest < 1] = 2\nprint(ytest)\n\nprint(classes)\n\nerror = ytest - classes\n\nSQE = np.power(error, [2])\nERR = np.sum(SQE)\n\nprint(\"CLASSIFICATION ERROR RATE:\")\nMSE = (ERR / 2000) * 100\nprint(MSE)\n\n\nytest = np.subtract(ytest, [1])\nax.plot(xtest, ytest, marker=\"x\", linewidth=10)\n\nplt.show()\n\n############################################################################\n############################################################################"
}
{
"filename": "code/artificial_intelligence/src/gaussian_naive_bayes/gaussian_naive_bayes.py",
"content": "# example using iris dataset\n# Part of Cosmos by OpenGenus\nimport numpy as np\nimport matplotlib.pyplot as plt\nimport pandas as pd\nfrom sklearn.model_selection import train_test_split\nfrom sklearn.naive_bayes import GaussianNB\nfrom sklearn.metrics import classification_report, confusion_matrix\n\ndataset = pd.read_csv(\"iris1.csv\", header=0)\n\nX = dataset.iloc[:, :-1].values\ny = dataset.iloc[:, 4].values\n\n\nX_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.30, stratify=y)\n\n\nclassifier = GaussianNB()\nclassifier.fit(X_train, y_train)\n\ny_pred = classifier.predict(X_test)\n\n# labeled confusion matrix\nprint(\n pd.crosstab(y_test, y_pred, rownames=[\"True\"], colnames=[\"Predicted\"], margins=True)\n)\n\nfrom sklearn.model_selection import StratifiedKFold\nfrom sklearn.metrics import accuracy_score\n\nskf = StratifiedKFold(n_splits=10)\nskf.get_n_splits(X, y)\nStratifiedKFold(n_splits=10, random_state=None, shuffle=False)\na = 0\nfor train_index, test_index in skf.split(X, y):\n # print(\"TRAIN:\", train_index, \"TEST:\", test_index) #These are the mutually exclusive sets from the 10 folds\n X_train, X_test = X[train_index], X[test_index]\n y_train, y_test = y[train_index], y[test_index]\n y_pred = classifier.predict(X_test)\n accuracy = accuracy_score(y_test, y_pred)\n a += accuracy\nprint(\"\\nK-fold cross validation (10 folds): \" + str(a / 10))"
}
{
"filename": "code/artificial_intelligence/src/gaussian_naive_bayes/iris1.csv",
"content": "sepallength,sepalwidth,petallength,petalwidth,class\n5.1,3.5,1.4,0.2,Iris-setosa\n4.9,3,1.4,0.2,Iris-setosa\n4.7,3.2,1.3,0.2,Iris-setosa\n4.6,3.1,1.5,0.2,Iris-setosa\n5,3.6,1.4,0.2,Iris-setosa\n5.4,3.9,1.7,0.4,Iris-setosa\n4.6,3.4,1.4,0.3,Iris-setosa\n5,3.4,1.5,0.2,Iris-setosa\n4.4,2.9,1.4,0.2,Iris-setosa\n4.9,3.1,1.5,0.1,Iris-setosa\n5.4,3.7,1.5,0.2,Iris-setosa\n4.8,3.4,1.6,0.2,Iris-setosa\n4.8,3,1.4,0.1,Iris-setosa\n4.3,3,1.1,0.1,Iris-setosa\n5.8,4,1.2,0.2,Iris-setosa\n5.7,4.4,1.5,0.4,Iris-setosa\n5.4,3.9,1.3,0.4,Iris-setosa\n5.1,3.5,1.4,0.3,Iris-setosa\n5.7,3.8,1.7,0.3,Iris-setosa\n5.1,3.8,1.5,0.3,Iris-setosa\n5.4,3.4,1.7,0.2,Iris-setosa\n5.1,3.7,1.5,0.4,Iris-setosa\n4.6,3.6,1,0.2,Iris-setosa\n5.1,3.3,1.7,0.5,Iris-setosa\n4.8,3.4,1.9,0.2,Iris-setosa\n5,3,1.6,0.2,Iris-setosa\n5,3.4,1.6,0.4,Iris-setosa\n5.2,3.5,1.5,0.2,Iris-setosa\n5.2,3.4,1.4,0.2,Iris-setosa\n4.7,3.2,1.6,0.2,Iris-setosa\n4.8,3.1,1.6,0.2,Iris-setosa\n5.4,3.4,1.5,0.4,Iris-setosa\n5.2,4.1,1.5,0.1,Iris-setosa\n5.5,4.2,1.4,0.2,Iris-setosa\n4.9,3.1,1.5,0.1,Iris-setosa\n5,3.2,1.2,0.2,Iris-setosa\n5.5,3.5,1.3,0.2,Iris-setosa\n4.9,3.1,1.5,0.1,Iris-setosa\n4.4,3,1.3,0.2,Iris-setosa\n5.1,3.4,1.5,0.2,Iris-setosa\n5,3.5,1.3,0.3,Iris-setosa\n4.5,2.3,1.3,0.3,Iris-setosa\n4.4,3.2,1.3,0.2,Iris-setosa\n5,3.5,1.6,0.6,Iris-setosa\n5.1,3.8,1.9,0.4,Iris-setosa\n4.8,3,1.4,0.3,Iris-setosa\n5.1,3.8,1.6,0.2,Iris-setosa\n4.6,3.2,1.4,0.2,Iris-setosa\n5.3,3.7,1.5,0.2,Iris-setosa\n5,3.3,1.4,0.2,Iris-setosa\n7,3.2,4.7,1.4,Iris-versicolor\n6.4,3.2,4.5,1.5,Iris-versicolor\n6.9,3.1,4.9,1.5,Iris-versicolor\n5.5,2.3,4,1.3,Iris-versicolor\n6.5,2.8,4.6,1.5,Iris-versicolor\n5.7,2.8,4.5,1.3,Iris-versicolor\n6.3,3.3,4.7,1.6,Iris-versicolor\n4.9,2.4,3.3,1,Iris-versicolor\n6.6,2.9,4.6,1.3,Iris-versicolor\n5.2,2.7,3.9,1.4,Iris-versicolor\n5,2,3.5,1,Iris-versicolor\n5.9,3,4.2,1.5,Iris-versicolor\n6,2.2,4,1,Iris-versicolor\n6.1,2.9,4.7,1.4,Iris-versicolor\n5.6,2.9,3.6,1.3,Iris-versicolor\n6.7,3.1,4.4,1.4,Iris-versicolor\n5.6,3,4.5,1.5,Iris-versicolor\n5.8,2.7,4.1,1,Iris-versicolor\n6.2,2.2,4.5,1.5,Iris-versicolor\n5.6,2.5,3.9,1.1,Iris-versicolor\n5.9,3.2,4.8,1.8,Iris-versicolor\n6.1,2.8,4,1.3,Iris-versicolor\n6.3,2.5,4.9,1.5,Iris-versicolor\n6.1,2.8,4.7,1.2,Iris-versicolor\n6.4,2.9,4.3,1.3,Iris-versicolor\n6.6,3,4.4,1.4,Iris-versicolor\n6.8,2.8,4.8,1.4,Iris-versicolor\n6.7,3,5,1.7,Iris-versicolor\n6,2.9,4.5,1.5,Iris-versicolor\n5.7,2.6,3.5,1,Iris-versicolor\n5.5,2.4,3.8,1.1,Iris-versicolor\n5.5,2.4,3.7,1,Iris-versicolor\n5.8,2.7,3.9,1.2,Iris-versicolor\n6,2.7,5.1,1.6,Iris-versicolor\n5.4,3,4.5,1.5,Iris-versicolor\n6,3.4,4.5,1.6,Iris-versicolor\n6.7,3.1,4.7,1.5,Iris-versicolor\n6.3,2.3,4.4,1.3,Iris-versicolor\n5.6,3,4.1,1.3,Iris-versicolor\n5.5,2.5,4,1.3,Iris-versicolor\n5.5,2.6,4.4,1.2,Iris-versicolor\n6.1,3,4.6,1.4,Iris-versicolor\n5.8,2.6,4,1.2,Iris-versicolor\n5,2.3,3.3,1,Iris-versicolor\n5.6,2.7,4.2,1.3,Iris-versicolor\n5.7,3,4.2,1.2,Iris-versicolor\n5.7,2.9,4.2,1.3,Iris-versicolor\n6.2,2.9,4.3,1.3,Iris-versicolor\n5.1,2.5,3,1.1,Iris-versicolor\n5.7,2.8,4.1,1.3,Iris-versicolor\n6.3,3.3,6,2.5,Iris-virginica\n5.8,2.7,5.1,1.9,Iris-virginica\n7.1,3,5.9,2.1,Iris-virginica\n6.3,2.9,5.6,1.8,Iris-virginica\n6.5,3,5.8,2.2,Iris-virginica\n7.6,3,6.6,2.1,Iris-virginica\n4.9,2.5,4.5,1.7,Iris-virginica\n7.3,2.9,6.3,1.8,Iris-virginica\n6.7,2.5,5.8,1.8,Iris-virginica\n7.2,3.6,6.1,2.5,Iris-virginica\n6.5,3.2,5.1,2,Iris-virginica\n6.4,2.7,5.3,1.9,Iris-virginica\n6.8,3,5.5,2.1,Iris-virginica\n5.7,2.5,5,2,Iris-virginica\n5.8,2.8,5.1,2.4,Iris-virginica\n6.4,3.2,5.3,2.3,Iris-virginica\n6.5,3,5.5,1.8,Iris-virginica\n7.7,3.8,6.7,2.2,Iris-virginica\n7.7,2.6,6.9,2.3,Iris-virginica\n6,2.2,5,1.5,Iris-virginica\n6.9,3.2,5.7,2.3,Iris-virginica\n5.6,2.8,4.9,2,Iris-virginica\n7.7,2.8,6.7,2,Iris-virginica\n6.3,2.7,4.9,1.8,Iris-virginica\n6.7,3.3,5.7,2.1,Iris-virginica\n7.2,3.2,6,1.8,Iris-virginica\n6.2,2.8,4.8,1.8,Iris-virginica\n6.1,3,4.9,1.8,Iris-virginica\n6.4,2.8,5.6,2.1,Iris-virginica\n7.2,3,5.8,1.6,Iris-virginica\n7.4,2.8,6.1,1.9,Iris-virginica\n7.9,3.8,6.4,2,Iris-virginica\n6.4,2.8,5.6,2.2,Iris-virginica\n6.3,2.8,5.1,1.5,Iris-virginica\n6.1,2.6,5.6,1.4,Iris-virginica\n7.7,3,6.1,2.3,Iris-virginica\n6.3,3.4,5.6,2.4,Iris-virginica\n6.4,3.1,5.5,1.8,Iris-virginica\n6,3,4.8,1.8,Iris-virginica\n6.9,3.1,5.4,2.1,Iris-virginica\n6.7,3.1,5.6,2.4,Iris-virginica\n6.9,3.1,5.1,2.3,Iris-virginica\n5.8,2.7,5.1,1.9,Iris-virginica\n6.8,3.2,5.9,2.3,Iris-virginica\n6.7,3.3,5.7,2.5,Iris-virginica\n6.7,3,5.2,2.3,Iris-virginica\n6.3,2.5,5,1.9,Iris-virginica\n6.5,3,5.2,2,Iris-virginica\n6.2,3.4,5.4,2.3,Iris-virginica\n5.9,3,5.1,1.8,Iris-virginica"
}
{
"filename": "code/artificial_intelligence/src/getting_started_with_ml/README.md",
"content": "--- \n## A Beginner's roadmap to mastering Machine Learning \n---\nI decided to collect resources and concentrate them to form a basic roadmap, to help other beginners like myself, who would like to explore the field further and not feel lost.\n\n### Topics touched in the post:\n * Introduction to my background to give you an idea.\n * Why ML?\n * Essentials to get an extensive insight into machine learning.\n * \"What is ML\" and surrounding misconceptions. \n * A basic roadmap I devised, for anybody who wishes to pursue this art further. \n\n### Gist of the roadmap:\n 1. Learn and/or revise Math.\n 2. Learn Python.\n 3. Get acquainted to Python libraries.\n 4. Code, code, and code. \n \n<p>\n\tFor deeper insights, refer to <a href=\"https://iq.opengenus.org/how-i-mastered-ml-as-fresher/\">How I mastered ML as a freshman</a>\n</p>\n\n---\n<p align=\"center\">\n\tA massive collaborative effort by the <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/artificial_intelligence/src/gradient_boosting_trees/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# Gradient boosting trees\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/artificial_intelligence/src/hierachical_clustering/hierachical_clustering.cpp",
"content": "#include <fstream>\n#include <iostream>\n#include <cstdlib>\n#include <cmath>\n#include <string>\n#include <stdio.h>\n#include <assert.h>\n#include <float.h>\n#include <iomanip>\n\n#define MAX_DIMENSION 21705\n\nusing namespace std;\n\nclass TreeNode {\nprivate:\n double location[MAX_DIMENSION]; //location or the center\n double sum[MAX_DIMENSION]; //for cummulative adding\n int dataNum; //denominator\n TreeNode *left; //left treenode pointer\n TreeNode *right; //right treenode pointer\n TreeNode *next; //next for a list\n bool isLegit; //spam or legit corpus\n int serialNum; //ID in the \"string of leaves\"\n\npublic:\n TreeNode()\n {\n left = NULL;\n right = NULL;\n next = NULL;\n dataNum = 1;\n isLegit = false;\n for (int i = 0; i < MAX_DIMENSION; i++)\n {\n location[i] = 0;\n sum[i] = 0;\n }\n } // end of constructor\n\n void setLocation(int i, double j)\n {\n location[i] = j;\n sum[i] = j;\n } //for entering the data of the treenode\n\n TreeNode* getNext()\n {\n return next;\n }\n\n double getLocation(int i)\n {\n return location[i];\n }\n\n void setNext( TreeNode *input)\n {\n next = input;\n }\n\n void setLegit()\n {\n isLegit = true;\n }\n\n bool Legitimacy()\n {\n return isLegit;\n }\n\n friend class HierachicalTree;\n}; //end of TreeNode\n\nstruct Subtree\n{\n TreeNode *begin;\n int length;\n Subtree *next;\n};\nclass HierachicalTree {\nprivate:\n TreeNode *root;\n TreeNode *listHead; //for a sequence of temp subtrees\n TreeNode *listRear; //for a sequence of temp subtrees\n TreeNode *tmp;\n Subtree *subtreeHead, *subtreeHandle;\n\nprotected:\n void createTree (); //create tree from the sequence of treenodes\n void dfs (TreeNode *treePtr); //traverse the tree\n Subtree *atLevel(int lvl, TreeNode *parent);\n\npublic:\n HierachicalTree()\n {\n root = NULL;\n listHead = NULL;\n listRear = NULL;\n } // end of constructor\n\n void addToList(TreeNode *Item)\n {\n if (listHead == NULL && listRear == NULL)\n {\n listHead = Item;\n listRear = Item;\n }\n else\n {\n listRear->next = Item;\n listRear = Item;\n }\n } // put the data into the list\n\n void organise()\n {\n tmp = 0;\n int tok = 0;\n cout << \"root: \" << root << endl;\n system(\"pause\");\n dfs(root);\n tmp = listHead;\n\n while (tmp != NULL)\n {\n tmp->serialNum = tok++;\n tmp = tmp->next;\n }\n\n } //access the protected function\n\n void buildTree()\n {\n createTree();\n } //access the protected function\n\n Subtree *subtreeAt(int level)\n {\n subtreeHead = subtreeHandle = NULL;\n return atLevel(level, root);\n }\n\n int rightEnd(TreeNode *p)\n {\n if (p->right == NULL)\n return p->serialNum;\n else\n return rightEnd(p->right);\n }\n\n int leftEnd(TreeNode *p)\n {\n if (p->left == NULL)\n return p->serialNum;\n else\n return leftEnd(p->left);\n }\n\n};\n\nvoid HierachicalTree::createTree()\n{\n TreeNode *goal;\n TreeNode *temp, *temp2;\n double sum = 0;\n double minimum = MAX_DIMENSION;\n cout << \"Creating tree\" << endl;\n while (listHead != listRear)\n {\n temp = listHead->next;\n while (temp != NULL)\n {\n sum = 0;\n for (int i = 0; i < MAX_DIMENSION; i++)\n sum += abs(listHead->location[i] - temp->location[i]); //CAUTION: BIT STREAM ONLY\n //FOR NON-INT SCALING, USE EUCLIDEAN DISTANCE\n if (sum < minimum)\n {\n goal = temp;\n minimum = sum;\n }\n temp = temp->next;\n }\n cout << \"\t\tComparison between listHead and others completed\"<< endl;\n temp = new TreeNode;\n temp->dataNum = listHead->dataNum + goal->dataNum;\n\n for (int i = 0; i < MAX_DIMENSION; i++)\n {\n temp->sum[i] = listHead->sum[i] + goal->sum[i];\n temp->location[i] = temp->sum[i] / temp->dataNum;\n }\n cout << \"\t\tSubtree built\"<< endl;\n temp2 = listHead;\n cout << \"\t\t temp2 = \"<< temp2 << endl;\n cout << \"\t\t goal = \"<< goal << endl;\n while (temp2->next != goal)\n {\n cout << \"\t\t\t\ttemp2 to be set to: \"<< temp2->next << endl;\n temp2 = temp2->next;\n cout << \"\t\t\t\tdone.\"<< endl;\n }\n cout << \"\t\ttemp2 allocated\"<< endl;\n\n temp->left = listHead;\n temp->right = goal;\n\n if (goal == listRear && listHead->next != listRear)\n {\n cout << \"\t\t Subtree: head and rear\"<< endl;\n temp2->next = temp;\n listHead = listHead->next;\n }\n else if (listHead->next == listRear)\n {\n cout << \"\t\t Subtree: Now root found\"<< endl;\n listHead = temp;\n }\n else\n {\n cout << \"\t\t Subtree: head and someone in the middle\"<< endl;\n temp2->next = goal->next;\n listRear->next = temp;\n listHead = listHead->next;\n }\n cout << \"\t\tSubtree mounted to list\"<< endl;\n minimum = MAX_DIMENSION;\n listRear = temp;\n }\n\n root = listHead;\n listHead = NULL;\n listRear = NULL;\n temp = NULL;\n temp2 = NULL;\n goal = NULL;\n\n}\n\n\nvoid HierachicalTree::dfs(TreeNode *treePtr)\n{\n //=============\n // This method sequencially \"strings\" all leaf nodes\n //=============\n if (treePtr->left == NULL && treePtr->right == NULL) //Leaf\n {\n if (listHead)\n {\n treePtr->next = NULL;\n tmp->next = treePtr;\n tmp = treePtr;\n }\n else\n {\n listHead = treePtr;\n tmp = treePtr;\n }\n }\n else //2 children\n {\n dfs(treePtr->left);\n dfs(treePtr->right);\n }\n return;\n}\n\nSubtree *HierachicalTree::atLevel(int lvl, TreeNode *parent)\n{\n if (lvl == 0)\n {\n Subtree *subtreeTmp = new Subtree;\n subtreeTmp->begin = parent;\n subtreeTmp->length = rightEnd(parent) - leftEnd(parent);\n return subtreeTmp;\n }\n else\n return atLevel(lvl - 1, parent->left);\n}\n\nint main(void)\n{\n int axis, oldAxis;\n int fileSeq = 1;\n char buffer[10];\n string fileName;\n string str;\n ifstream inf;\n HierachicalTree hTree; //hierachical tree\n TreeNode *tempNode;\n\n fileName = sprintf(buffer, \"%d\", fileSeq);\n fileName += \".txt\";\n inf.open(fileName.c_str(), ios::in);\n\n while (inf)\n {\n tempNode = new TreeNode;\n inf >> str;\n oldAxis = -1; //init to a sub-zero number\n while (inf.eof())\n {\n axis = atof(str.c_str());\n if (axis < oldAxis) //reach the bottom of file\n {\n cout << axis << endl;\n if (axis)\n tempNode->setLegit();\n }\n else\n {\n tempNode->setLocation(axis, 1);\n oldAxis = axis;\n cout << axis << endl;\n }\n inf >> str;\n }\n system(\"pause\");\n hTree.addToList(tempNode);\n cout << fileName << \" read\" << endl;\n inf.close();\n fileName = sprintf(buffer, \"%d\", ++fileSeq);\n fileName += \".txt\";\n inf.open(fileName.c_str(), ios::in);\n }\n inf.close();\n system(\"pause\");\n hTree.buildTree();\n cout << \"Built\" << endl;\n system(\"pause\");\n hTree.organise();\n cout << \"Linked list built\" << endl;\n system(\"pause\");\n\n return 0;\n}"
}
{
"filename": "code/artificial_intelligence/src/hierachical_clustering/hierachical_clustering/hierarchical_clustering.ipynb",
"content": "{\n \"nbformat\": 4,\n \"nbformat_minor\": 0,\n \"metadata\": {\n \"colab\": {\n \"name\": \"Hierarchical Clustering\",\n \"version\": \"0.3.2\",\n \"provenance\": [],\n \"collapsed_sections\": []\n },\n \"kernelspec\": {\n \"name\": \"python3\",\n \"display_name\": \"Python 3\"\n }\n },\n \"cells\": [\n {\n \"metadata\": {\n \"id\": \"7lEU2B93ivBz\",\n \"colab_type\": \"text\"\n },\n \"cell_type\": \"markdown\",\n \"source\": [\n \"# Hierarchical Clustering\\n\",\n \"In this notebook we will give a basic example of how agglomerative hierarchical cluster works. \\n\",\n \"We use scipy and sklearn libraries. \"\n ]\n },\n {\n \"metadata\": {\n \"id\": \"beCEkyHzwL-5\",\n \"colab_type\": \"code\",\n \"colab\": {}\n },\n \"cell_type\": \"code\",\n \"source\": [\n \"from sklearn.metrics import normalized_mutual_info_score\\n\",\n \"import matplotlib.pyplot as plt\\n\",\n \"from scipy.cluster.hierarchy import dendrogram, linkage, fcluster\\n\",\n \"from sklearn.datasets.samples_generator import make_blobs\\n\",\n \"import numpy as np \"\n ],\n \"execution_count\": 0,\n \"outputs\": []\n },\n {\n \"metadata\": {\n \"id\": \"elEYgSyIjP8c\",\n \"colab_type\": \"text\"\n },\n \"cell_type\": \"markdown\",\n \"source\": [\n \"# Generating Sample data\\n\",\n \" `make_blobs` is used to generate sample data where:\\n\",\n \" \\n\",\n \"\\n\",\n \"`n_samples` : the total number of points equally divided among clusters.\\n\",\n \"\\n\",\n \"`centers` : the number of centers to generate, or the fixed center locations.\\n\",\n \"\\n\",\n \"`n_features` : the number of features for each sample. \\n\",\n \"\\n\",\n \"`random_state`: determines random number generation for dataset creation.\\n\",\n \"\\n\",\n \"\\n\",\n \"\\n\",\n \"This function returns two outputs: \\n\",\n \"\\n\",\n \"`X`: the generated samples. \\n\",\n \"\\n\",\n \"`y`: The integer labels for cluster membership of each sample. \\n\",\n \"\\n\",\n \"Then we use `plt.scatter` to plot the data points in the figure below.\\n\",\n \"\\n\"\n ]\n },\n {\n \"metadata\": {\n \"id\": \"Nxjz1FiSEl9Q\",\n \"colab_type\": \"code\",\n \"outputId\": \"3f6f6713-ab54-4250-df8a-68b7922d5313\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 347\n }\n },\n \"cell_type\": \"code\",\n \"source\": [\n \"X, y = make_blobs(n_samples=90, centers=4, n_features=3, random_state=4)\\n\",\n \"plt.scatter(X[:, 0], X[:, 1])\\n\",\n \"plt.show()\"\n ],\n \"execution_count\": 0,\n \"outputs\": [\n {\n \"output_type\": \"display_data\",\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAAe0AAAFKCAYAAAAwrQetAAAABHNCSVQICAgIfAhkiAAAAAlwSFlz\\nAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4yLCBo\\ndHRwOi8vbWF0cGxvdGxpYi5vcmcvOIA7rQAAIABJREFUeJzt3Xt0VOW9N/BvMsnMJORCJky4FFGu\\nuspFg6Bc5FIaSqXCwkJI4AS18taKQu1ZtKLoKvZVrLI8a6mFgkVQFwrEIEX09BSKBBflIlJSEM7B\\ncDmvhGsmyeRGkpnMZN4/aAYmmdlze2b23k++n7/M7Mnez4/E/Oa5/Z4Ej8fjAREREWleotoNICIi\\notAwaRMREekEkzYREZFOMGkTERHpBJM2ERGRTjBpExER6USS2g0IxmZrULsJMZGVlQq7vUntZggn\\na1yAvLHJGhcgb2yyxgXIG1s4cVmt6QGvsaetkqQkg9pNiAlZ4wLkjU3WuAB5Y5M1LkDe2ETFxaRN\\nRESkE0zaREREOsGkTUREpBNM2kRERDrBpE1ERKQTTNpEREQ6waRNRESkE0zaREREOiG0IlpJSQl2\\n7tzp/frkyZMoKyvzfj106FCMHDnS+/X7778Pg0G+jfSOVjfqGh3ITDPBlCxffEREpA6hSTs/Px/5\\n+fkAgCNHjuC//uu/fK6npaVh06ZNIh+pKe62NhTvPYuychtq6h2wZJiQO8SKgimDYEjkoAYREUUn\\nZplkzZo1eOqpp2J1e00q3nsWe45eRHW9Ax4A1fUO7Dl6EcV7zwq5v6PVjUp7ExytbiH3IyIifYnJ\\ngSEnTpxA7969YbVafV53Op1YunQpLl26hGnTpuFnP/tZLB6vCkerG2XlNr/XysqrMHvSwIiHytmD\\nJyIiIEZJe9u2bXj44Yc7vf7ss89i5syZSEhIQFFREUaNGoXhw4cr3isrK1UXBeSvVF1HTYPD7zV7\\nQwsMxmRYe3TzeV3pJJdbrd/xDfYcvej9ur0Hn5pixM9nKf/7qSHUuPRI1thkjQuQNzZZ4wLkjU1E\\nXDFJ2l999RVefPHFTq/PmzfP+99jxoxBeXl50KStlyPa3K1uWNJNqK7vnLiz0s1wO1t9jhm1WtND\\nOnbU0erGgeOX/F47cPwyHrzvNk0tdgs1Lj2SNTZZ4wLkjU3WuAB5YwsnrrgezXnt2jV069YNRqPR\\n5/Xz589j6dKl8Hg8cLlcOHbsGAYPHiz68aoxJRuQO8Tq91rukB4RJ9a6Rgdq/HwQAG704Osa/V8j\\nIiL5CO9p22w2WCwW79d/+tOfMHr0aOTm5qJXr16YM2cOEhMTMWXKFIwYMUL041VVMGUQgBtz2PaG\\nFmSlm5E7pIf39UhkpplgyQjcg89MM0V8byIi0pcEj8fjUbsRSvQ4TBLKPu1whko27yn3mdNulzeq\\nL+bnDYmqraLJOrQFyBubrHEB8sYma1yAvLGJGh6PyZx2V2dKNiAnK1XY/WLRgyciIv1h0tYBQ2Ii\\n5ucNwexJA1lpjYioC+MmX52Id2lUFnIhItIe9rQ1Lt6FVVjIhYhIu5i0NaZjj7q9NGq79sIqAGKy\\nCC3ezyMiotAxaWuEvx7uiIHZOHGu2u/7oy2N6k8sS7ESEVH0ON6pEf4OGyktu+x3fzYQm8IqLORC\\nRKRtTNoRErlQS6mHm5jg/3syuhmRYhI7UNJeyMUfFnIhIlIfh8fDFIuFWko93LYApW9qG534v+9/\\nLXSRWHspVn+FXAKVYo33qnYioq6MSTtMsViopVSq1JSciLHDeuGbczWorm/xuRaLRWKhFnIJ9OFl\\n8dxcIe0gIqLOmLTDEKuFWko9XEdrG5IMifjtY6Pw0savYfczryxykViohVwCfXhJTTFi1vg7om4H\\nERF1xjntMMRyodasCf1hNvpPumXlVai77kRtgPvHYpFYeylWfwm7yeHC309c9vt9h09eYUEWIqIY\\nYdIOQywXajU2tcLh9J/s7A0tgMejmUViW/5WjhZnm99rVbXNXGVORBQjTNphiNWZ2YDyB4LuaSZY\\ns1Jj9uxwOFrdOH3BHvB6diZXmRMRxUqXmtMWsdI5ViduKc1rNzlc+OTLc5gzeUCHZ5twV78szJow\\nIKpnh0NpigAARgyychU5EVGMdImkLXKbVixP3GpP/H8/cQUttwyVtzjdPqvEZ03oj81/O4PT39Xg\\n4MmrOH3BHrf64Eor3c1GA56YNQzXOTxORBQTXWJ43F+1sT1HL6J479mI76m0UCtShsREzJ40EN3M\\n/j9LlZVXwdHqxo79/4uDJ6+ipsEpLJ5QKU0RPDCiN1JTjDFvAxFRVyV90g62TUtrK52DrVC31Tar\\nHk/BlEHIG9UX2RlmJCYA2Rlm5I3qG/UUAY8DJSJSJv3weCjbtHKyUuPcqsCUhp+z0s2Ax6N6PKKn\\nCHgcKBFRaKT/i6jVetotTpffXmWwFerWrFTNxCNqiiAW0xdERDKSvqcdST3tWGrvVZ44Vw2bvdlv\\nr3LWhAFobnHh9AU77A0OnxXqhsRETcUTLR4HSkQUOumTNhC7bVqRUKpdXjBlkM8wcVa6EWOG9sL8\\nqYORakr2fo9a8cTicBC9TV8QEampSyTtWG7TCoej1Y1j31b6vVZWXgV3mwelxy55X6tpcOLgyatI\\nNSf5HAgS73hiOeccbA6fhVqIiG6Sfk77VrHYphUqd1sbPtz1LWoanH6v19S34J/lVX6vBVoVHq94\\nRM8537pKPJZV5oiIZNMletpaULz3LA6cvBrwemaaMeiBIGoME4uccw7UY+9c6U296QsiIi1j0o4D\\npcTXLndwD5w4V625YWKRc87BziJXe/qCiEjrhA6Pf/XVVxgzZgwWLFiABQsW4OWXX/a5fvDgQcyZ\\nMwcFBQVYs2aNyEdrWrB63eOG9cL8qUMUh4kBqFJ4RNSWuVCK3Kg5fUFEpAfCe9r33Xcf3n77bb/X\\nXnnlFWzYsAE9e/ZEUVERpk2bhkGD5B8CVVpslZ1hwoJpd8KQmOh3Vfg9g7PR5vHgxfWHVSk8ImrL\\nHFeJdw2x2GFARDfFbXi8oqICmZmZ6N27NwBg0qRJOHToUJdI2sqJ7+apWP5WhX/y5Tl8oTCkHA8i\\ntphxlbjcWNWOKD6EJ+2zZ8/iySefRF1dHRYvXozx48cDAGw2GywWi/d9FosFFRUVoh+vWR0TX4/u\\nKRgxMNtv4msfJtZK4RERW8y0VuSGxAq2XoGIxBCatO+44w4sXrwYDz74ICoqKvDII49g9+7dMBoj\\nP/kpKysVSUly/EF/Zt69aHG6YK93ICvDBLNR+Z//StV11DQEHlI2GJNh7dEtFk0NqG8I77Fa0/2+\\nvnhuLlJTjDh88gqqapvRo3sKxgzrjcdnDIXBoI/eWKDY9C6auFqcLpw4V+332olz1fjF7JSgv+ux\\nxJ+Z/sgam4i4hP6f1LNnT0yfPh0A0K9fP/To0QPXrl3DbbfdhpycHFRV3dyHfO3aNeTk5AS9p93e\\nJLKJmpAEwGxMgs3WoPg+d6sblvTAQ8puZ2vQe8Sb1Zqu2KZZ4+/Ag/fd5tNjr6m5HscWRi5YbHoV\\nbVyV9ibY7M1+r9lqm/E/Z23oa02L+P7R4M9Mf2SNLZy4lJK70O7Nzp07sWHDBgA3hsOrq6vRs2dP\\nAEDfvn3R2NiIixcvwuVyobS01Dt0Tv7JWniEq8TlorTDwOMB3vz4n9i0+1tcqb7OY1eJoiS0pz1l\\nyhT8+te/xhdffIHW1la89NJL+Pzzz5Geno6pU6fipZdewtKlSwEA06dPR//+/UU+XkpaqptO5I/S\\negXgRjne0mOXUHrsErK5QI0oKgkej8ejdiOUyDhMAoQ/BBRsK41WttrIOrQFyBubiLhuXT3ubzqn\\no7xRfeOyQC1YbFr5/yZcsv4uAvLGJmp4nBXRdKJ9SLkjbrUhUaJJYO07DCaO6I3fbvw66PvVPnaV\\n/9+QXjFp61ygrTbNLS4UTbtTV70HCo+oXqLb3YbNe8qFJDBrViqyA+zHv5XaBXW4RY30iklbx5T2\\ncR84eRX/810NRt6Zw96DZET3Ejd+dkpYAgs2v91OzYI6Wql/QBQJ/iXXsWA1zWsanFEdoUnaJPKo\\nVEerG4dPXvF7LdCRsMEUTBmEvFF9kZ1hDvieUHY/3HqEq0ihlNQl0ir2tHVMqTTordh7kIfIXqKj\\n1Y3zl+pQGWCPdaRD2LdW0Kupb8GeoxU4ca4m5N0PsZ5vZkld0jMmbR0LdShS7flDEkfEwSsdk2Ji\\nItDW1vl90SYwU7IBvbO7YcG0u8Kaf4/1fDNL6pKecXhc524ORQb+48regzyUCpkYkw1ISw1eMrjj\\n8Lq/hA0AqeYkJBkSomjtTaEW1AnlCFcRbh3CT0wAsjPMyBvVl/UPSPPY09a5W4ciN+36FgdPXu30\\nHvYe5KHUS2xxurFj/3nF3qhSUuyoorIRxXvPxnU1dbyOcBVxCA6RGtjTloQp2YCfTb+LvYcuYNaE\\n/jAb/SeYYL3RYIsXw72faEojCcFGjCJZuMaSuqQ37GlLhL2HrqGxqRUOp//EFKw3GurixVDvJ1ok\\n881KC9eIZMOetoTYe5BbNL1RpUNoIrlfLIQ73yxyCxyR1rGnTaQz0a5+7ngITY/uKTAlG1BR2Rjy\\n/WJZszucEaNgC9danC6hbSNSG5M2kQ5Fc/pbx6Q48I5s1Nqv/2uIWfl+4eyhjjaxB6q3f+u9na42\\nxYVr9noH/8iRVPj7TKQTHZNgtOsX2pOi2ZgUcu82lD3UsSyO0vHeWelGmIwGtPiZ489KNyMrw4SG\\nOv/FYyh+9HqamhYxaRNpnFISVOqNRiJY7zaUamyxLI7S8d41Dc6A780d0gNmYxLkO+RRP3iamnj8\\nVyPSOK0stAplD3W4xVHC2aaldG+z0YDsDBO3OmqMVn53ZcKeNpGGaelEqlBqdodaHCWSHpjSvZ2t\\nbiwvGgljsqHLDcFqdehZS7+7MmHSJtKweFUIC0Uoq9ZDPYwjkiH0YPe2drFtjlofetbS765M1P/J\\nElFA0ezJjoVge6iV9oG3J/ZI64uHcu+uROtDz6J+d2N1RKtesadNpGFaO5EqlFXmwbajRdMDi2ar\\nm0xanC7NDz1H+7ur9ZEEtTBpE2lcNIkqVvOdSqvMgyX2aM6zZqneG+z12h56bv+9mzVhAIDIfndj\\nfUSrXjFpE2lcsETlLzFroZcSKLGLGD0QvdVNb7IyIv/gE0uBfu9+t3A0GptaQ/6QxUVsgTFpE+lE\\nx0SllJiVeila6KVymDs6ZmOSpqZN2onqHXMRW2BM2kQ6FegPpNvdhhPnqv1+z99PXMGxbythb3DC\\nkmHCXf2ysKQwN15N9uIwd/S09sFHZO84mikU2TFpE+mQ4h/IM1Woa/RfKazF6faW/Kyud+DAyas4\\n9vJujB/eW5UFPl19mDsaWvvgI7J3rLUFmFrCpE2kQ0p/IOsaneieZoK9MbQzs5sdbi7w0TGtfPAR\\n3TvW2kiCVghP2qtWrcI//vEPuFwu/OIXv8CPfvQj77UpU6agV69eMBhufEp644030LNnT9FNIJKe\\n0h9IS4YZIwZlo/TYpbDu2dUX+FB0RPeOtTaSoBVCk/bhw4dx5swZFBcXw2634+GHH/ZJ2gCwfv16\\ndOvWTeRjibqcYH8gbwx1J6CsvAo1DS3I7GZEfZMTbW2B79nVF/hQ9GLRO9bKSIJWCE3ao0ePxogR\\nIwAAGRkZaG5uhtvt9vasiUgcpT+QhsREFEwZBLe7DWVnqlAbYI77Vl19gQ9Fz1/vGACq61rYUxZE\\naNI2GAxITb3xiWjbtm2YOHFip4S9YsUKXLp0Cffeey+WLl2KhIQEkU0g6jKCDR8W7z2L0rLLId+v\\nqy/wIXFMyQZkZ5pVrxUgowSPx+MRfdM9e/bgnXfewcaNG5Genu59fceOHZgwYQIyMzPx9NNP4+GH\\nH8aPf/xjxXu5XG4kJfEPCVE4WpwuPL1qLyrtzUHfm5gI/HjMHXhi1nAYDPxjKpMWpwv2egeyMkww\\nG+O77nj9jm+wc//5Tq/PnDAAP581PK5tkYnwn+L+/fuxbt06vPvuuz4JGwBmzZrl/e+JEyeivLw8\\naNK225tEN1ETrNZ02GwNajdDOFnjAvQVW6W9CbYQEjZwI2HPmTgANTXXY9yq+NPTzywcweJSuyKe\\no9WNA8f9L4Q8cPwyHrzvtoCjOl31Z9bxvYEI/ek1NDRg1apVeOedd9C9e/dO1xYuXAin88bc2tdf\\nf43BgweLfDwR/YvSCUuJCUDCLSd0PcFej3TUPgEslD3bFBmhPe2//OUvsNvt+NWvfuV97f7778ed\\nd96JqVOnYuLEiSgoKIDJZML3v//9oL1sIorcnf2ycPDk1U6vT7qnD6bd1887B84hcblooW43K5rF\\njtCkXVBQgIKCgoDXH330UTz66KMiH0lEt+g4LGo23vjj7HC6YcnwXV1OctJC3W5WNIsdVkQjkkjH\\neuTtJUvHD+uFoml38o9lF6CVXi4rmsUGkzaRJJSGRU9fqI1za0gtsejldjz+NZRz2lnRLDaYtIkk\\noYVhUdIGUb3cjtMtWelGdEsxoqmlNeRV6VqqaBbKhw2tY9ImkoRWhkVJfaJ6uR2nW2oanKhpuFld\\nL9LzsuNN7S1wIumrtUQUUPuwqD9c/NM1tfdyIx0SDzTd0lFZeRUcre6wnxEvam+BE4lJm0giBVMG\\nIW9UX2RnmJF4y15sLv7RN0erG5X2prgmRqXplo60vPc62BY4LX/Y8IfD40QS4eIfuQQa1l08Nzfm\\nz1aabulIy9Mvsq31YE+bSELRDIuSdgQa1t342amYP1tpuqUjLU+/KFUH1PKHjUCYtImINEhpWPfw\\nySs+w7qxGj7vON1iSTehb043mI03U4fZmIg2jwdupcPaVSTbWg8OjxMRaZDSsG5VbTPqGh0xP/7S\\n33TLJ1+ew57Km4fLtDjbsPcfl5CYkKDZFeQyFXph0iYi0iClOeUe3VOQmWbqtCUrVluw2qdbtFDX\\nPBIyrfXg8DgRkQYpDeuOGdYbAOK+Klrvp3fJsNaDSZuISKMCbeF7fMbQoAnUVtssfJ473ou61Njq\\npnUcHici0qhAw7oGQ6Li8Lkx2YA3P/4n7A1OofPc8Tq9S6YKZqIxaRMRaZy/+t1KCbTF6fae8CZ6\\nnjsei7riNVevR0zaREQ61TmBmnC9pRUtzs7br0QtFIv1oq4WpyuixW4yHAYSCiZtIiKd6phAna1u\\nrNj4td/3iq7+FavTu+z14VUw62pD6fJFRETUxbQnUGtWqu6rf2VlhLfYTabDQELBpE1EJAkZqn+Z\\njUkhxyDbYSCh4PA4EZFEZKj+FWoMsh0GEgombSIiichQ/SvUGJS2vellOiBcHB4nIpKQDNW/gsUg\\nw3RAuNjTJiLSEUerG1eqrsPd6pYyKYVLhumAcDBpExHpgM/WpgYHLOna29qkxl5pGaYDwsGkTUSk\\nA1quEuZua8Pmv5Wj7EwVahudyFZhr3Ss9o1rjTY+nhERUUBa3trkbmvD/33/KErLLqO20QlA/r3S\\nahKetF999VUUFBSgsLAQJ06c8Ll28OBBzJkzBwUFBVizZo3oRxMRSUnLR2Ju3nMGFZWNfq+p/YFC\\nRkKT9pEjR/Ddd9+huLgYK1euxMqVK32uv/LKK/jDH/6ALVu24MCBAzh7lp/CiIiCifeRmKFytLrx\\nz/KqgNdr6rV/xrbeCE3ahw4dQl5eHgBg4MCBqKurQ2PjjU9gFRUVyMzMRO/evZGYmIhJkybh0KFD\\nIh9PRCQlrW5tqmt0oFYhKWemGTW9V1qP53ULXYhWVVWFoUOHer+2WCyw2WxIS0uDzWaDxWLxuVZR\\nUSHy8URE0tLi1ial4iYAkDv45gcKLZ3CpedDRmK6etzj8UR9j6ysVCQlybl832pNV7sJMSFrXIC8\\nsckaFyBXbM/MuxctThfs9Q5kZZhgNqq/AWj83d/Dzv3nO70+oE8Gnpl3LwBg42encPjkFdhqm2Ht\\nnoIxw3rj8RlDYTD4T5Cx/pmt3/GN35X4qSlG/HzW8Jg9V0RcQn/iOTk5qKq6Ob9RWVkJq9Xq99q1\\na9eQk5MT9J52e5PIJmqG1ZoOm61B7WYIJ2tcgLyxyRoXIG9svf8VlxYimzG2H5qanSgrr0JNQwu6\\ndzPhniE9MD9vMGpqrmPznnKfBFlpb8bO/efR1Oz0u7c61j8zR6sbB45f8nvtwPHLePC+22IyEhBO\\nXErJXWjSHj9+PP7whz+gsLAQp06dQk5ODtLS0gAAffv2RWNjIy5evIhevXqhtLQUb7zxhsjHExFR\\nnCkVN1Haqvb3E1dw7NtK2BucPsPTsab3Q0aEJu2RI0di6NChKCwsREJCAlasWIHt27cjPT0dU6dO\\nxUsvvYSlS5cCAKZPn47+/fuLfDwREanEX3ETpQTZ4nSjxXljAdithWLah9RjRe+HjAifEPn1r3/t\\n8/Vdd93l/e/Ro0ejuLhY9COJiEiDgi1U66isvAotTldM29S+Ev/WIft2ejhkRNvL5IiISLeUtqr5\\nY29ogT3EBB+NgimDkDeqL7IzzEhMALIzzMgb1VcXh4yov/SQiIik1XmrmgnXW1rR4mzr9N6sdDOy\\nMkxoqGuOaZv0fMgIkzYREcWMvwT5yZfnAg5Pm41JcVsVr8dDRpi0iYgo5m5NkKEUitFSMRYtYdIm\\nIqK4UhqedrvbsHlPuS6rlcUDkzYREanC3/D0xs9OafbccC3gxxYiItIER6sbh09e8XuNx3zewKRN\\nRESaUNfogK3W/8pxtc8N1wombSIi0oTMNBOs3VP8XtNDtbJ4YNImIiJNMCUbMGZYb7/X9FCtLB64\\nEI2IiDTj8RlDvaeGaeXccC1h0iYioqiJ2ldtMOi3Wlk8MGkTEVHE3G1tKN57Vvi+aj1WK4sHJm0i\\nIopY8d6z3FcdR1yIRkREEXG0ulFWbvN7jfuqY4NJm4iIIlLX6EBNgKM0ua86Npi0iYgoIplpJlgy\\n/O+d5r7q2GDSJiKiiJiSDcgdYvV7jfuqY4ML0YiIKGKhHLNJ4jBpExFRxJSO2STxmLSJiChq3Fcd\\nH5zTJiIi0gkmbSIiIp1g0iYiItIJJm0iIiKdYNImIiLSCWGrx10uF1544QVcuHABbrcbzz77LEaN\\nGuXznqFDh2LkyJHer99//30YDNwaQEREFAphSfvTTz9FSkoKtmzZgjNnzuD555/Htm3bfN6TlpaG\\nTZs2iXokERFRlyIsac+cORMPPfQQAMBisaC2tlbUrYmIiAgC57STk5NhMt0oDv/BBx94E/itnE4n\\nli5disLCQrz33nuiHk1ERNQlJHg8Hk+431RSUoKSkhKf15YsWYIJEybgo48+wt69e7Fu3TokJyf7\\nvGfLli2YOXMmEhISUFRUhN/97ncYPny44rNcLjeSkjjvTUREFFHSDqSkpAR//etf8cc//tHb6w5k\\n1apVGDhwIGbPnq34PputQVTzNMVqTZcyNlnjAuSNTda4AHljkzUuQN7YwonLak0PeE3Y8HhFRQW2\\nbt2K1atX+03Y58+fx9KlS+HxeOByuXDs2DEMHjxY1OOJiIikJ2whWklJCWpra/HEE094X9uwYQPe\\nf/99jB49Grm5uejVqxfmzJmDxMRETJkyBSNGjBD1eCIiIukJHR6PBRmHSQAOAemRrLHJGhcgb2yy\\nxgXIG5vmhseJiIgotpi0iYiIdIJJm4iISCeYtImIiHSCSZuIiEgnmLSJiIh0gkmbiIhIJ5i0iYiI\\ndIJJm4iISCeYtImIiHSCSZuIiEgnmLSJiIh0gkmbiIhIJ5i0iYiIdIJJm4iISCeYtImIiHSCSZuI\\niEgnmLSJiIh0gkmbiIhIJ5i0iYiIdIJJm4iISCeYtImIiHSCSZuIiEgnmLSJiIh0gkmbiIhIJ5i0\\niYiIdCJJ1I22b9+Ot956C/369QMAjBs3DosWLfJ5z86dO/HBBx8gMTERc+fORX5+vqjHExERSU9Y\\n0gaA6dOnY9myZX6vNTU1Yc2aNdi2bRuSk5MxZ84cTJ06Fd27dxfZBCIiImnFbXj8+PHjGD58ONLT\\n02E2mzFy5EgcO3YsXo8nIiLSPaFJ+8iRI1i4cCEeffRR/Pd//7fPtaqqKlgsFu/XFosFNptN5OOJ\\niIikFtHweElJCUpKSnxe+8lPfoIlS5Zg8uTJKCsrw7Jly/DZZ58FvIfH4wnpWVlZqUhKMkTSTM2z\\nWtPVbkJMyBoXIG9sssYFyBubrHEB8sYmIq6IknZ+fr7iIrLc3FzU1NTA7XbDYLiRcHNyclBVVeV9\\nT2VlJe65556gz7LbmyJpouZZremw2RrUboZwssYFyBubrHEB8sYma1yAvLGFE5dSchc2PL5+/Xp8\\n/vnnAIDy8nJYLBZvwgaAu+++G9988w3q6+tx/fp1HDt2DKNGjRL1eCIiIukJWz0+Y8YM/OY3v8HW\\nrVvhcrmwcuVKAMCf/vQnjB49Grm5uVi6dCkWLlyIhIQEPP3000hPl3MIhIiIKBYSPKFOLqtExmES\\ngENAeiRrbLLGBcgbm6xxAfLGprnhcSIiIootJm0iIiKdYNImIiLSCSZtIiIinWDSJiIi0gkmbSIi\\nIp1g0iYiItIJJm0iIiKdYNImIiLSCSZtIiIinWDSJiIi0gkmbSIiIp1g0iYiItIJJm0iIiKdYNIm\\nIiLSCSZtIiIinWDSJiIi0gkmbSIiIp1g0iYiItIJJm0iIiKdYNImIiLSCSZtIiIinWDSJiIi0gkm\\nbSIiIp1g0iYiItIJJm0iIiKdSBJ1o7Vr1+LgwYMAgLa2NlRVVWHXrl3e6xcvXsSMGTMwbNgwAEBW\\nVhbefvttUY8nIiKSnrCkvWjRIixatAgA8Oc//xnV1dWd3tO/f39s2rRJ1COJiIi6FOHD4y6XC1u2\\nbEFRUZHoWxMREXVpwpP27t278cADD8BsNne6VlVVhV/+8pcoLCzEzp07RT+aiIhIagkej8cT7jeV\\nlJSgpKTE57UlS5ZgwoQJWLhwIX73u9+hb9++PtcbGxuxa9cuzJw5Ew0NDcjPz8eWLVuQk5Oj+CyX\\ny42kJEO4TSQi6hJanC7Y6x3IyjDBbBQ240kaFVHSDqSpqQn5+fn4z//8z6DvfeaZZzBv3jyMGTNG\\n8X02W4Oo5mmK1ZouZWyyxgVphYdYAAARk0lEQVTIG5uscQHyxma1puPqtToU7z2LsnIbauodsGSY\\nkDvEioIpg2BI1O/GIJl/ZqHGZbWmB7wm9Cd7+vRpDBgwwO+1w4cP4/e//z2AG8n99OnT6N+/v8jH\\nExF1GcV7z2LP0YuornfAA6C63oE9Ry+ieO9ZtZsWEUerG5X2JrQ4XWo3RdOEjqXYbDZYLBaf11au\\nXIlHHnkEo0aNwo4dO1BQUAC3240nnngCPXv2FPl4IqIuocXpQlm5ze+1svIqzJ40EKZkfUwrutva\\nfEYMrFkpGDEwW/cjBrEiNGlPmzYN06ZN83nthRde8P73a6+9JvJxRERdkr3egZp6h/9rDS2oa3Qg\\nJys1zq2KTPuIQbtKe7P36/l5Q9RqlmbxYwwRkc5kZZhgyTD5v5ZuRmaa/2ta42h1K44YOFrdcW6R\\n9jFpExHpjNmYhNwhVr/Xcof00M3QeF1j8BED8sX9AUREOlQwZRCAGz1Se0MLstLNyB3Sw/u6HmSm\\n3RgxqPaTuCMZMXC0ulHX6EBmmkk3H1zCxaRNRKRDhsREzM8bgtmTBuo2UZmSDcgdYvWZ024XzohB\\nx8Vssmx/84dJm4hIx0zJBt0sOvOn44hBj+43V4+HquNitvbtb4B8i9mYtImISDUdRwwG3pGNhrrm\\nkL8/2GI2PW1/C4Vc4wZERKRL7SMG4ZZi7WqL2Zi0iYhIt9oXs/mjp+1voWLSJiIi3WpfzOaPnra/\\nhYpz2kREpGsybH8LFZM2EREFFMu9z6LuLcP2t1AxaRMRUSex3PusdO9o6H37WyiYtImIqJNY7n1W\\nuvcz8+6N6t6y40I0IiLyEcuDPILdm+dpK2PSJiIiH3WNDr/1wIHo9z4H21dtD3CNbmDSJiIiL3db\\nG3Z9XYHEBP/Xg+19drS6UWlvCtgbD7avOivANbqBc9pERORVvPcsSo9dCng90N7nUBeuBTskxGxM\\nQoOYUKTEpE1ERACU55sTE4BJ9/QJuMJ7854zPsleaeFaV9pXLRqTNhERAVCeb/YAmHZfv07bvdxt\\nbdj8t3J8+c/Lfr/P36EdXWlftWic0yYiIgDK882WAHPZxXvPorTsMto8/u+ptHCtfV81E3bomLSJ\\niAhA+HW8lYbT28l4aIeaODxORCQJEWVBw5lvVhpObyfjoR1qYtImItI5kSVHw5lvbh9O97enOzEB\\nmJT7PS4uE4zD40REOtdeFrS63gEPbq7cLt57NuJ73jrfHGjvdZIhAanmZL/fP+mePljwozujrlMe\\na8H2lWsNe9pERDrT4nSh0t7knStWKgvaceV2OJR68C63Bx/u+hYVlY2dvu+2nDTMnxpdffJYi+WB\\nKLHEpE1EpBPtiebEuWrY7M2wZJhwZ78sxbKgdY2OiE++CnSwx7cXanG92YmaBqff72tqccHl9sCg\\n3dwX0wNRYknD/6RERHSr9kRTaW/2DoMfPHkVJqP/nnQ0K7eVVoZXVDYGTNhA9PXJYy2WB6LEWsRJ\\n+8iRIxg7dixKS0u9r50+fRqFhYUoLCzEihUrOn1Pa2srli5dinnz5qGoqAgVFRWRPp6IqEsJZXtV\\nR9Gs3A5lZXggWt/mFezQEi1/4IgoaV+4cAHvvfceRo4c6fP6ypUrsXz5cmzduhWNjY348ssvfa5/\\n/vnnyMjIwJYtW/Dkk0/iP/7jPyJvORFRF6KUaBxON8YP64XsDDMSE4DsDDPyRvWNauW2UqGVYLS+\\nzSvYoSXhfOCI90K2iOa0rVYrVq9ejRdeeMH7mtPpxKVLlzBixAgAwA9+8AMcOnQIkyZN8r7n0KFD\\nmDVrFgBg3LhxWL58eTRtJyLqMpS2V1kyzCiadicACCsLqnSwRyDZtyzm0rJgh5aE8m+n1kK2iJJ2\\nSkpKp9fsdjsyMjK8X2dnZ8Nm8x3KqaqqgsViAQAkJiYiISEBTqcTRqMx4LOyslKRlKTdT2zRsFrT\\n1W5CTMgaFyBvbLLGBcgV2/i7v4ed+8/7eb0P+vbpDgDoK+A5LU4X7PUO/HzWcKSmGHH45BVU1Taj\\nR/cUpKUk4/zl+k7f88NRt+HJ2SNgNka/vjkeP7PFc3M7xTZsYA/8n1nD0C0lcE5qt37HN34XsqWm\\nGPHzWcP9fo+IuIL+65aUlKCkpMTntSVLlmDChAmK3+fxBChEG+Z77PamoO/RI6s1HTabfAfQyRoX\\nIG9sssYFyBfbjLH90NTsxIlz1aiqbfZWK5sxtp+QOAP1Hn/72Gg0NjmRmWZCkiHhX+/pWDFtIBrq\\nmqM+VjPUn5mI6m+zxt+BH436Hjb/7QxOf1eD0qMVOF5eGbTH7Gh148Bx/8eXHjh+GQ/ed1unNoXz\\nu6iU3IMm7fz8fOTn5wd9iMViQW1trffra9euIScnx+c9OTk5sNlsuOuuu9Da2gqPx6PYyyYiopva\\nq5X9YnYKzv2/auGnY4W6DUrNE7pED0vv2P+/OHjyqvfrULZ+hbKQLdJtdsEIG3hPTk7GgAEDcPTo\\nUQDA7t27O/XGx48fj7/+9a8AgNLSUtx///2iHk9E1GWYjUnCT8cKZxuUiF5upERWf4t065fIhWzh\\nimjyYd++fdiwYQPOnz+PU6dOYdOmTdi4cSOWL1+O3/72t2hra8Pdd9+NcePGAQAWLVqEtWvXYvr0\\n6Th48CDmzZsHo9GI1157TWgwREQUmVB6j9mZZlWriAVLsuFWf4u0xyxiIVukIkrakydPxuTJkzu9\\nPmjQIGzevLnT62vXrgUAGAwG/P73v4/kkUREFENKq9Pbe49qVxETPSwdSsyBhHMamkgsY0pEREF7\\nj0DsapyHKpok6080PeZwTkMTiWVMiYgIwI3eY96ovn6LtGihilh7kvUn0mFppZhDbZPo9QVK2NMm\\nIiIAyr1H0b3cSIkellarxxwpJm0iIvLR3nvs+FqwoeR4rCqPVZL1F7MWMWkTEVFIAvVy50wegM17\\nyuO6qlwvSVY0Jm0iIgpJoF7u5j3lujybWo+4EI2IiMJy6+IrPZ9NrUdM2kREFDEtrCrvSpi0iYgo\\nYmqW9OyKmLSJiChisdg7HQpHqxuV9qYuN/zOhWhERBSVeJb0FH3Kl94waRMRUVTiWaBE7frnapP/\\nYwkREcVFrEt6cqU6kzYREekEV6ozaRMRkU5wpTqTNhER6YRaK9W1hAvRiIhIN6JdqR6PQ01iiUmb\\niIh0I9KV6rJsFWPSJiIi3Qn3lC9Ztorp5+MFERFRBGTaKsakTUREUpNpqxiTNhERSU2mrWJM2kRE\\nJDWZtopxIRoREUkvnoeaxBKTNhERSS+eh5rEEpM2ERF1GeFuFdOaiOe0jxw5grFjx6K0tNT72unT\\npzF//nwUFRXhqaeeQnNzs8/3bN++HZMmTcKCBQuwYMECrF27NvKWExERdTER9bQvXLiA9957DyNH\\njvR5/ZVXXsFzzz2HESNG4PXXX8f27dvxb//2bz7vmT59OpYtWxZ5i4mIiLqoiHraVqsVq1evRnp6\\nus/r69atw4gRIwAAFosFtbW10beQiIiIAETY005JSfH7elpaGgCgqakJn376Kd56661O7zly5AgW\\nLlwIl8uFZcuW4fvf/77is7KyUpGUpL/FAqGwWtODv0mHZI0LkDc2WeMC5I1N1rgAeWMTEVfQpF1S\\nUoKSkhKf15YsWYIJEyb4fX9TUxMWLVqExx9/HAMHDvS5dvfdd8NisWDy5MkoKyvDsmXL8Nlnnyk+\\n325vCtZEXbJa02GzNajdDOFkjQuQNzZZ4wLkjU3WuAB5YwsnLqXkHjRp5+fnIz8/P6QHuVwuPPXU\\nU3jooYfw05/+tNP1gQMHehN5bm4uampq4Ha7YTDI2ZMmIiISSWhFtPXr1+O+++4LmOTXr1+Pzz//\\nHABQXl4Oi8XChE1ERBSiiOa09+3bhw0bNuD8+fM4deoUNm3ahI0bN+Kjjz5C3759cejQIQDA/fff\\nj8WLF2PRokVYu3YtZsyYgd/85jfYunUrXC4XVq5cKTQYIiIimSV4PB6P2o0gIiKi4HhgCBERkU4w\\naRMREekEkzYREZFOMGkTERHpBJM2ERGRTjBpExER6YQuknZVVRVGjx6Nr776Su2mCNNee33evHmY\\nO3cujh49qnaTovbqq6+ioKAAhYWFOHHihNrNEWrVqlUoKCjA7NmzsXv3brWbI1RLSwvy8vKwfft2\\ntZsi1M6dOzFz5kz89Kc/xb59+9RujhDXr1/H4sWLsWDBAhQWFmL//v1qNylq5eXlyMvLw4cffggA\\nuHLlChYsWID58+fjmWeegdPpVLmFkfEX12OPPYaioiI89thjsNlsEd1XF0l71apVuO2229RuhlCf\\nfvopUlJSsGXLFqxcuRKvvfaa2k2KypEjR/Ddd9+huLgYK1eulKpwzuHDh3HmzBkUFxfj3Xffxauv\\nvqp2k4Rau3YtMjMz1W6GUHa7HWvWrMHmzZuxbt06fPHFF2o3SYg///nP6N+/PzZt2oS33npL9/+f\\nNTU14eWXX8bYsWO9r7399tuYP38+Nm/ejNtvvx3btm1TsYWR8RfXm2++iblz5+LDDz/E1KlT8d57\\n70V0b80n7UOHDqFbt24YMmSI2k0RaubMmXj++ecByHGM6aFDh5CXlwfgRo35uro6NDY2qtwqMUaP\\nHu09sS4jIwPNzc1wu90qt0qMc+fO4ezZs5g8ebLaTRHq0KFDGDt2LNLS0pCTk4OXX35Z7SYJkZWV\\n5f1bUV9fj6ysLJVbFB2j0Yj169cjJyfH+9pXX32FH/7whwCAH/zgB94Km3riL64VK1Zg2rRpAHx/\\njuHSdNJ2Op1Ys2YN/v3f/13tpgiXnJwMk8kEAPjggw/w0EMPqdyi6FRVVfn8AbFYLBEP/2iNwWBA\\namoqAGDbtm2YOHGiNDXzX3/9dTz33HNqN0O4ixcvoqWlBU8++STmz5+vyz/8/vzkJz/B5cuXMXXq\\nVBQVFWHZsmVqNykqSUlJMJvNPq81NzfDaDQCALKzs3X5d8RfXKmpqTAYDHC73di8eTNmzJgR2b1F\\nNFAEf0eATpw4Efn5+cjIyFCpVWIoHW/60Ucf4dSpU1i3bp1KrYsNGavj7tmzB9u2bcPGjRvVbooQ\\nO3bswD333CPd1FO72tparF69GpcvX8YjjzyC0tJSJCQkqN2sqHz66afo06cPNmzYgNOnT2P58uXS\\nrUW4lWx/R9xuN5599lmMGTPGZ+g8HJpJ2v6OAC0sLERbWxs++ugjXLhwASdOnMBbb72FwYMHq9TK\\nyAQ63rSkpAR79+7FH//4RyQnJ6vQMnFycnJQVVXl/bqyshJWq1XFFom1f/9+rFu3Du+++y7S06M/\\nyF4L9u3bh4qKCuzbtw9Xr16F0WhEr169MG7cOLWbFrXs7Gzk5uYiKSkJ/fr1Q7du3VBTU4Ps7Gy1\\nmxaVY8eO4YEHHgAA3HXXXaisrJTueOPU1FS0tLTAbDbj2rVrPkPMevf888/j9ttvx+LFiyO+h6aH\\nx7du3YqPP/4YH3/8MSZPnowVK1boLmEHUlFRga1bt2L16tXeYXI9Gz9+PHbt2gUAOHXqFHJycpCW\\nlqZyq8RoaGjAqlWr8M4776B79+5qN0eYN998E5988gk+/vhj5Ofn46mnnpIiYQPAAw88gMOHD6Ot\\nrQ12ux1NTU26n/8FgNtvvx3Hjx8HAFy6dAndunWTKmEDwLhx47x/S3bv3o0JEyao3CIxdu7cieTk\\nZPzyl7+M6j6a6Wl3NSUlJaitrcUTTzzhfW3Dhg3euRy9GTlyJIYOHYrCwkIkJCRgxYoVajdJmL/8\\n5S+w2+341a9+5X3t9ddfR58+fVRsFSnp2bMnpk2bhrlz5wIAXnzxRSQmarqPEpKCggIsX74cRUVF\\ncLlceOmll9RuUlROnjyJ119/HZcuXUJSUhJ27dqFN954A8899xyKi4vRp08fzJo1S+1mhs1fXNXV\\n1TCZTFiwYAGAGwt2I/n58WhOIiIindD/R08iIqIugkmbiIhIJ5i0iYiIdIJJm4iISCeYtImIiHSC\\nSZuIiEgnmLSJiIh0gkmbiIhIJ/4/0hVflg12a20AAAAASUVORK5CYII=\\n\",\n \"text/plain\": [\n \"<Figure size 576x396 with 1 Axes>\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n }\n }\n ]\n },\n {\n \"metadata\": {\n \"id\": \"Gd2x3DM3qiLi\",\n \"colab_type\": \"text\"\n },\n \"cell_type\": \"markdown\",\n \"source\": [\n \"# Performing Hierarchical clustering: \\n\",\n \"In this part, we are performing agglomerative hierarchical clustering using linkage function from scipy library:: \\n\",\n \"\\n\",\n \"`method`: is the linkage method, 'single' means the linkage method will be single linkage method. \\n\",\n \"\\n\",\n \"`metric`: is our similarity metric, 'euclidean' means the metric will be euclidean distance. \\n\",\n \"\\n\",\n \"\\\"A `(n-1)` by 4 matrix `Z` is returned. At the -th iteration, clusters with indices `Z[i, 0]` and `Z[i, 1]` are combined to form cluster with index `(n+i)` . A cluster with an index less than `n` corresponds to one of the `n` original observations. The distance between clusters `Z[i, 0]` and `Z[i, 1]` is given by `Z[i, 2]`. The fourth value `Z[i, 3]` represents the number of original observations in the newly formed cluster.\\n\",\n \"\\n\",\n \"The following linkage methods are used to compute the distance `d(s,t)`between two clusters `s`and `t`. The algorithm begins with a forest of clusters that have yet to be used in the hierarchy being formed. When two clusters `s` and `t`from this forest are combined into a single cluster `u`, `s`and `t` are removed from the forest, and `u` is added to the forest. When only one cluster remains in the forest, the algorithm stops, and this cluster becomes the root.\\n\",\n \"\\n\",\n \"A distance matrix is maintained at each iteration. The `d[i,j]`` entry corresponds to the distance between cluster `ii` and `j` in the original forest.\\n\",\n \"\\n\",\n \"At each iteration, the algorithm must update the distance matrix to reflect the distance of the newly formed cluster u with the remaining clusters in the forest.\\\"\\n\",\n \"\\n\",\n \"\\n\",\n \"For more details check the docmentation of linkage: https://docs.scipy.org/doc/scipy/reference/generated/scipy.cluster.hierarchy.linkage.html\\n\"\n ]\n },\n {\n \"metadata\": {\n \"id\": \"hrFUAgplFE8T\",\n \"colab_type\": \"code\",\n \"outputId\": \"fa9c51c8-3ef6-431b-dd36-15ba837f440a\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 1547\n }\n },\n \"cell_type\": \"code\",\n \"source\": [\n \"Z = linkage(X, method=\\\"single\\\", metric=\\\"euclidean\\\")\\n\",\n \"print(Z.shape)\\n\",\n \"Z\"\n ],\n \"execution_count\": 0,\n \"outputs\": [\n {\n \"output_type\": \"stream\",\n \"text\": [\n \"(89, 4)\\n\"\n ],\n \"name\": \"stdout\"\n },\n {\n \"output_type\": \"execute_result\",\n \"data\": {\n \"text/plain\": [\n \"array([[1.90000000e+01, 8.60000000e+01, 1.30442991e-01, 2.00000000e+00],\\n\",\n \" [1.00000000e+00, 2.90000000e+01, 1.82464805e-01, 2.00000000e+00],\\n\",\n \" [2.60000000e+01, 4.10000000e+01, 2.24296317e-01, 2.00000000e+00],\\n\",\n \" [3.40000000e+01, 9.10000000e+01, 2.58407755e-01, 3.00000000e+00],\\n\",\n \" [1.70000000e+01, 8.80000000e+01, 4.46261329e-01, 2.00000000e+00],\\n\",\n \" [3.00000000e+00, 2.80000000e+01, 4.59526997e-01, 2.00000000e+00],\\n\",\n \" [8.00000000e+00, 9.30000000e+01, 4.70577188e-01, 4.00000000e+00],\\n\",\n \" [6.40000000e+01, 7.00000000e+01, 4.85796818e-01, 2.00000000e+00],\\n\",\n \" [6.80000000e+01, 9.70000000e+01, 5.52673653e-01, 3.00000000e+00],\\n\",\n \" [2.20000000e+01, 7.10000000e+01, 5.67637608e-01, 2.00000000e+00],\\n\",\n \" [4.80000000e+01, 5.20000000e+01, 5.72218127e-01, 2.00000000e+00],\\n\",\n \" [2.70000000e+01, 9.20000000e+01, 5.83346355e-01, 3.00000000e+00],\\n\",\n \" [7.70000000e+01, 9.00000000e+01, 5.88123667e-01, 3.00000000e+00],\\n\",\n \" [4.50000000e+01, 9.50000000e+01, 6.06112007e-01, 3.00000000e+00],\\n\",\n \" [4.90000000e+01, 9.80000000e+01, 6.06134706e-01, 4.00000000e+00],\\n\",\n \" [3.80000000e+01, 8.50000000e+01, 6.54175526e-01, 2.00000000e+00],\\n\",\n \" [2.00000000e+01, 1.05000000e+02, 6.64553292e-01, 3.00000000e+00],\\n\",\n \" [9.00000000e+00, 4.60000000e+01, 6.70931992e-01, 2.00000000e+00],\\n\",\n \" [9.90000000e+01, 1.02000000e+02, 6.82628319e-01, 5.00000000e+00],\\n\",\n \" [7.00000000e+00, 1.20000000e+01, 6.95405759e-01, 2.00000000e+00],\\n\",\n \" [2.10000000e+01, 1.00000000e+02, 7.00137901e-01, 3.00000000e+00],\\n\",\n \" [7.50000000e+01, 8.40000000e+01, 7.18486705e-01, 2.00000000e+00],\\n\",\n \" [6.70000000e+01, 1.01000000e+02, 7.20982261e-01, 4.00000000e+00],\\n\",\n \" [6.50000000e+01, 1.04000000e+02, 7.30097372e-01, 5.00000000e+00],\\n\",\n \" [2.30000000e+01, 3.60000000e+01, 7.30257122e-01, 2.00000000e+00],\\n\",\n \" [5.60000000e+01, 8.30000000e+01, 7.31435561e-01, 2.00000000e+00],\\n\",\n \" [6.90000000e+01, 1.15000000e+02, 7.39908983e-01, 3.00000000e+00],\\n\",\n \" [3.20000000e+01, 7.90000000e+01, 7.52488630e-01, 2.00000000e+00],\\n\",\n \" [4.20000000e+01, 7.40000000e+01, 7.72963318e-01, 2.00000000e+00],\\n\",\n \" [9.40000000e+01, 1.06000000e+02, 7.75139691e-01, 5.00000000e+00],\\n\",\n \" [1.30000000e+01, 5.70000000e+01, 8.09976391e-01, 2.00000000e+00],\\n\",\n \" [4.70000000e+01, 1.10000000e+02, 8.17317663e-01, 4.00000000e+00],\\n\",\n \" [8.70000000e+01, 1.21000000e+02, 8.37778371e-01, 5.00000000e+00],\\n\",\n \" [7.20000000e+01, 1.18000000e+02, 8.40307889e-01, 3.00000000e+00],\\n\",\n \" [1.09000000e+02, 1.13000000e+02, 8.40561182e-01, 7.00000000e+00],\\n\",\n \" [1.10000000e+01, 4.40000000e+01, 8.47017348e-01, 2.00000000e+00],\\n\",\n \" [5.40000000e+01, 1.16000000e+02, 8.53348573e-01, 4.00000000e+00],\\n\",\n \" [6.10000000e+01, 1.24000000e+02, 8.60237379e-01, 8.00000000e+00],\\n\",\n \" [8.10000000e+01, 1.14000000e+02, 8.69649593e-01, 3.00000000e+00],\\n\",\n \" [1.60000000e+01, 3.50000000e+01, 8.71267240e-01, 2.00000000e+00],\\n\",\n \" [8.90000000e+01, 1.23000000e+02, 8.87225579e-01, 4.00000000e+00],\\n\",\n \" [1.40000000e+01, 5.10000000e+01, 8.97306320e-01, 2.00000000e+00],\\n\",\n \" [1.27000000e+02, 1.31000000e+02, 9.03391600e-01, 1.00000000e+01],\\n\",\n \" [7.60000000e+01, 1.30000000e+02, 9.42132535e-01, 5.00000000e+00],\\n\",\n \" [2.00000000e+00, 4.00000000e+00, 9.43591329e-01, 2.00000000e+00],\\n\",\n \" [6.00000000e+01, 1.32000000e+02, 9.52055045e-01, 1.10000000e+01],\\n\",\n \" [1.50000000e+01, 1.03000000e+02, 9.55858009e-01, 4.00000000e+00],\\n\",\n \" [6.20000000e+01, 1.36000000e+02, 9.55990815e-01, 5.00000000e+00],\\n\",\n \" [7.80000000e+01, 1.12000000e+02, 9.62094518e-01, 5.00000000e+00],\\n\",\n \" [1.26000000e+02, 1.33000000e+02, 9.70616432e-01, 9.00000000e+00],\\n\",\n \" [3.10000000e+01, 1.37000000e+02, 9.73453020e-01, 6.00000000e+00],\\n\",\n \" [3.00000000e+01, 1.38000000e+02, 9.76974714e-01, 6.00000000e+00],\\n\",\n \" [1.17000000e+02, 1.34000000e+02, 9.87111827e-01, 4.00000000e+00],\\n\",\n \" [6.00000000e+00, 4.00000000e+01, 9.88659025e-01, 2.00000000e+00],\\n\",\n \" [6.30000000e+01, 1.41000000e+02, 9.90928355e-01, 7.00000000e+00],\\n\",\n \" [1.80000000e+01, 1.35000000e+02, 9.94563563e-01, 1.20000000e+01],\\n\",\n \" [4.30000000e+01, 1.44000000e+02, 1.01672289e+00, 8.00000000e+00],\\n\",\n \" [1.19000000e+02, 1.40000000e+02, 1.02423556e+00, 1.10000000e+01],\\n\",\n \" [5.30000000e+01, 1.47000000e+02, 1.02433354e+00, 1.20000000e+01],\\n\",\n \" [0.00000000e+00, 5.80000000e+01, 1.04742170e+00, 2.00000000e+00],\\n\",\n \" [9.60000000e+01, 1.48000000e+02, 1.05140979e+00, 1.60000000e+01],\\n\",\n \" [1.11000000e+02, 1.29000000e+02, 1.05383364e+00, 4.00000000e+00],\\n\",\n \" [1.49000000e+02, 1.50000000e+02, 1.06811164e+00, 1.80000000e+01],\\n\",\n \" [1.43000000e+02, 1.52000000e+02, 1.13528499e+00, 2.00000000e+01],\\n\",\n \" [1.42000000e+02, 1.45000000e+02, 1.13534989e+00, 1.60000000e+01],\\n\",\n \" [1.00000000e+01, 1.54000000e+02, 1.13899133e+00, 1.70000000e+01],\\n\",\n \" [8.00000000e+01, 1.55000000e+02, 1.14020634e+00, 1.80000000e+01],\\n\",\n \" [1.08000000e+02, 1.39000000e+02, 1.16825903e+00, 1.40000000e+01],\\n\",\n \" [1.28000000e+02, 1.57000000e+02, 1.17114497e+00, 1.70000000e+01],\\n\",\n \" [3.30000000e+01, 1.46000000e+02, 1.18258513e+00, 9.00000000e+00],\\n\",\n \" [8.20000000e+01, 1.53000000e+02, 1.20489168e+00, 2.10000000e+01],\\n\",\n \" [5.00000000e+01, 7.30000000e+01, 1.26106706e+00, 2.00000000e+00],\\n\",\n \" [1.25000000e+02, 1.56000000e+02, 1.26262400e+00, 2.00000000e+01],\\n\",\n \" [1.51000000e+02, 1.59000000e+02, 1.26658643e+00, 1.30000000e+01],\\n\",\n \" [1.07000000e+02, 1.62000000e+02, 1.27731453e+00, 2.20000000e+01],\\n\",\n \" [1.58000000e+02, 1.61000000e+02, 1.30683670e+00, 1.90000000e+01],\\n\",\n \" [5.50000000e+01, 1.65000000e+02, 1.31086896e+00, 2.00000000e+01],\\n\",\n \" [1.20000000e+02, 1.63000000e+02, 1.32666243e+00, 1.50000000e+01],\\n\",\n \" [2.40000000e+01, 1.66000000e+02, 1.34505705e+00, 2.10000000e+01],\\n\",\n \" [2.50000000e+01, 1.67000000e+02, 1.35109125e+00, 1.60000000e+01],\\n\",\n \" [5.90000000e+01, 1.68000000e+02, 1.43253745e+00, 2.20000000e+01],\\n\",\n \" [3.70000000e+01, 1.70000000e+02, 1.45594208e+00, 2.30000000e+01],\\n\",\n \" [1.22000000e+02, 1.69000000e+02, 1.47692245e+00, 2.10000000e+01],\\n\",\n \" [6.60000000e+01, 1.60000000e+02, 1.55016373e+00, 2.20000000e+01],\\n\",\n \" [3.90000000e+01, 1.72000000e+02, 1.57569084e+00, 2.20000000e+01],\\n\",\n \" [5.00000000e+00, 1.73000000e+02, 1.70993648e+00, 2.30000000e+01],\\n\",\n \" [1.64000000e+02, 1.75000000e+02, 3.30049427e+00, 4.50000000e+01],\\n\",\n \" [1.74000000e+02, 1.76000000e+02, 1.07623457e+01, 6.70000000e+01],\\n\",\n \" [1.71000000e+02, 1.77000000e+02, 1.31670775e+01, 9.00000000e+01]])\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n },\n \"execution_count\": 3\n }\n ]\n },\n {\n \"metadata\": {\n \"id\": \"5KVO5Sb4wJNx\",\n \"colab_type\": \"text\"\n },\n \"cell_type\": \"markdown\",\n \"source\": [\n \"# Plotting dendrogram \\n\",\n \"The dedrogram function from scipy is used to plot dendrogram: \\n\",\n \"\\n\",\n \"\\n\",\n \"\\n\",\n \"* On the `x` axis we see the indexes of our samples. \\n\",\n \"* On the `y` axis we see the distances of our metric ('Euclidean'). \\n\",\n \"\\n\",\n \"\\n\",\n \"\\n\"\n ]\n },\n {\n \"metadata\": {\n \"id\": \"g5xM3EWJJBsH\",\n \"colab_type\": \"code\",\n \"outputId\": \"2006ee9b-4637-4cb3-c936-2c2e05196ab9\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 640\n }\n },\n \"cell_type\": \"code\",\n \"source\": [\n \"plt.figure(figsize=(25, 10))\\n\",\n \"plt.title(\\\"Hierarchical Clustering Dendrogram\\\")\\n\",\n \"plt.xlabel(\\\"Samples indexes\\\")\\n\",\n \"plt.ylabel(\\\"distance\\\")\\n\",\n \"dendrogram(Z, leaf_rotation=90., leaf_font_size=8. ) \\n\",\n \"plt.show()\"\n ],\n \"execution_count\": 0,\n \"outputs\": [\n {\n \"output_type\": \"display_data\",\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAABaEAAAJbCAYAAAD9kFiiAAAABHNCSVQICAgIfAhkiAAAAAlwSFlz\\nAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4yLCBo\\ndHRwOi8vbWF0cGxvdGxpYi5vcmcvOIA7rQAAIABJREFUeJzs3XmQ1/Vh//HXsisxcijQVUs0eCUe\\nNRRj2gQR8QB3QWhQqyEU49G08ZbRn+IRIxFjijFKJGrVycS70SpemQje8SYRUm2MVqNVQTw2ulwu\\nyLH7+4NxK3Kt6PuLC4/HTAfZ/X6+r/euDus8/fSTqpaWlpYAAAAAAEABHdb1AQAAAAAAWH+J0AAA\\nAAAAFCNCAwAAAABQjAgNAAAAAEAxIjQAAAAAAMWI0AAAAAAAFCNCAwBsoHbccce8+eaby31s0qRJ\\nOeKII5Ik119/fSZMmFDxc82cOTO77LLLSj/3Sc60uvdNkttvvz3Dhw9PfX199ttvv5xyyil56623\\nkiQTJ07MWWedtVa7SXLzzTd/7GveeuutDB06dK03P2rixIn52te+lvr6+uy7776pr6/PxIkTs2jR\\nok9tI0mOOOKITJo06VN9TwAA2readX0AAAA+m0aNGrWuj7CCUme68cYbc/XVV+fyyy/P9ttvn8WL\\nF+fyyy/PqFGj8utf//oTvffSpUtzwQUX5NBDD/1Y122xxRafePuj6urq8qMf/ShJ0tDQkHPPPTfH\\nHHNMfvGLX3yqOwAA8GHuhAYAYKU+fPfvm2++maOPPjp1dXWpq6vLb3/72yTL7i7ec889c/7557cG\\n4vvvvz/Dhg1LXV1dDjrooDz33HNJkqlTp2bEiBE56aSTcsoppyRZdvfxB+956qmnLndX7i233JJh\\nw4ZlwIABrTH2w2eaMWNG/umf/imDBg3KwQcfnGeffTZJ8vLLL+fb3/52Bg8enEGDBq0x5DY3N+fS\\nSy/ND37wg2y//fZJko022ignnnhixowZk6qqquVev+++++app55a4fdLlizJWWedlbq6ugwaNCjH\\nH3985s+fnyOPPDLz5s1LfX19ZsyY0ebv5Yfv3J40aVJOPPHEnHnmmamrq8uQIUPy4osvtl43fPjw\\n7LvvvvnBD36Q733ve226E7m2tjYXX3xx/vd//zePPvpokmTatGk5+OCDM2jQoBx66KGZMWPGGvdn\\nzJiRQw45JAMHDswpp5ySpUuXtm7suOOOueKKK1JXV5elS5fm+eefz4gRI1JfX59vfvObeeSRR1r/\\nHowbNy79+vXLt7/97Vx55ZU57LDDkiSnn356fvzjH2fYsGG5++67s2DBgowePTp1dXXZd999M378\\n+Na9ww47LFdeeWW+9a1v5Rvf+EZuuOGGXHbZZamvr8+QIUNavx4AACpLhAYAYI3GjBmTnXbaKVOm\\nTMmVV16Z0047LY2NjUmS2bNnZ+edd87111+fJUuW5PTTT8+4ceMyZcqUFSLhn/70p4wYMSI//elP\\nM3PmzIwfPz7XXnttJk+enAULFuTaa69NsixKLl68OHfddVfOOOOMlT6C4+yzz84BBxyQe++9N8cc\\nc0xOO+20JMkFF1yQffbZJ3fffXfOP//8nHXWWVm8ePEqv7aXX345c+bMSb9+/Vb43MCBA9OxY8c2\\nfY8effTRzJw5M5MnT84999yTHXbYIX/4wx9y/vnnp7q6OpMnT87WW2/d5u/lRz388MMZOXJkpkyZ\\nkq9//eu55pprWr/efv365YEHHshee+2Vxx9/vE3nTZKamprstddemTp1aubPn59jjjkmJ598cu69\\n99585zvfyUknnbTG/QsvvDB9+/bNfffdl8MPPzzTp09fbqOlpSVTpkxJVVVVTj755IwaNSqTJ0/O\\neeedl1NOOSXz58/Pb3/72zz88MO55557cvnll+e2225b7j2eeOKJ3HLLLRk8eHD+4z/+I++9914m\\nT56c2267LZMmTVruPwr8/ve/zw033JAf//jH+clPfpItt9wykydPzg477JBbb721zd8bAAA+PSI0\\nAMAG7LDDDkt9fX3r/1100UUrvKapqSlTp05tfVZ0r169svvuu7fewbt48eIMGjQoybKo+fjjj6dP\\nnz5Jkq997WvL3X268cYbp2/fvkmSxx57LLvttlu22GKLVFVV5ac//WnrRktLS4YPH54k2WWXXVZ4\\ndvX777+fqVOntj4zeb/99mt97vJll12Wf/7nf06S7L777nn//ffT0NCwyu/B7Nmz07179xXueP64\\nunfvnpdeein33ntv6926/fv3X+41H+d7+VHbb799dt111yTLvidvvPFGkuSpp55q/T4MHDgwm2++\\n+cc6d+fOnTNv3rxMmzYtW2yxRWuMHzp0aF577bXMmjVrjftDhgxJkvTu3Tvbbbfdcu+/9957J1l2\\nx/Zf/vKXHHDAAUmSr3zlK+nZs2f++7//O0899VT23nvvdOrUKZtttlnraz7Qt2/ffO5zn0uSHHXU\\nUbnssstSVVWVTTfdNF/60pcyc+bM1tfus88+qampyZe//OUsWLAgdXV1SZIvf/nLefvttz/W9wYA\\ngE+HZ0IDAGzArrvuumy55Zatv580aVLuvPPO5V4zb968tLS0ZMSIEa0fa2pqyje+8Y0kSXV1dTp3\\n7rzce952221ZtGhRFi1atFzc3XTTTVv/urGxMV27dm39/QeR8YP3/PznP58k6dChQ5qbm5c70+zZ\\ns9Pc3JwuXbokSaqqqtKpU6ckySOPPJLLL788jY2NqaqqSktLywrXf1i3bt3yzjvvZMmSJampWft/\\nPe7du3e+//3v57rrrsuYMWOy77775pxzzlnuNR/3e/lhH3ytH7zug8dezJ07d7nv6xZbbPGxzv36\\n669nu+22y9y5czNjxozU19e3fq5jx4559913V7s/Z86c5c784b+nSbLZZpslSd5999106dJluX8e\\nunbtmnfffTdz585d7twf/Ro+/PW98sor+bd/+7e8/PLL6dChQ958880cdNBBrZ//4J+D6urq5X6/\\nsn+OAACoDBEaAIDV6tGjR6qrq3Prrbe2Br0PfPgO1CSZPn16rrrqqvznf/5nttpqqzz22GM5++yz\\nV/q+3bp1yx/+8IfW38+fPz8LFy5s05m6deuWqqqqNDY2pnv37mlpaclrr72Wnj17ZvTo0ZkwYUIG\\nDBiQRYsWpXfv3qt9r2233Tbdu3fPAw88kP3333+5z/385z/PyJEjl/vYR2PmnDlzWv/6gzvKZ8+e\\nnTPPPDO/+MUvcsghh7R+/uN8L9uqU6dOaWpqav396u76/qh58+bl8ccfz2GHHZbFixdnu+22W+nz\\npF944YVVvkfXrl0zf/781t9/EK0/qkePHpkzZ05aWlpaQ/Ts2bPTo0ePdO7cuc1fw7nnnpu/+Zu/\\nyaWXXprq6urlgj4AAJ9NHscBAMBq1dTUZMCAAfnVr36VJFmwYEHOOOOM1scxfNi7776bHj16pGfP\\nnlmwYEFuu+22NDU1paWlZYXXDhgwINOnT8/MmTPT0tKSc845J7fcckubztSxY8f069ev9dnBjzzy\\nSP71X/81CxYsSFNTU+tjI6655ppstNFGywXOj+rQoUNGjx6d8847L88880ySZY/FuPjii3Pfffet\\ncGdybW1tnn/++STJb37zm7z//vtJkltvvTWXXnppkmV3/37wWIqNNtoozc3NmT9//sf6XrZV7969\\nc/fddydJHnzwwTY/cuLdd9/N//t//y9f//rX89WvfjV/+7d/m4aGhjz99NNJlv0PDp566qkr/Xv3\\nYX369Mm9996bZNl/hHjttddW+rqtttoqW265ZX7zm9+0vvYvf/lLevfuna985St56KGHsnDhwsyd\\nO7f161mZd955JzvvvHOqq6vz2GOP5dVXX13t318AANY9ERoAgDUaO3Zsfv/736e+vj4HHnhgtt56\\n6/z1X//1Cq/r379/Nt988wwcODBHHXVUDj/88HTp0iUnnnjiCq/dcsstc+655+bwww9vfW7vkUce\\n2eYz/ehHP8qDDz6Y/fbbLxMmTMiFF16Yrl275rvf/W6GDx+e4cOH54tf/GIGDhyYo48+OgsWLFjl\\nex188ME57bTTcvbZZ6euri7Dhg1LY2NjrrnmmhX+hwmPPfbYXH311Rk6dGheeuml7LDDDkmWPZf6\\n2Wefzf7775/Bgwfnz3/+c4488sjU1tZm9913zz777JPp06e3+XvZVqeeemruueee1NfX54knnkif\\nPn1W+XzrKVOmpL6+PgMHDsw//uM/ZqeddsqFF16YZNnzui+55JKMGzcugwcPznHHHZf6+vo1Piv7\\n1FNPzYMPPpiBAwfmhhtuyB577LHS11VVVeWiiy7K9ddfn8GDB+e8887Lz372s2yyySYZNGhQdt11\\n19TX1+eEE07I4MGDV7l3zDHHZPz48Rk6dGh+97vf5fjjj8/EiRMzbdq0Nn7HAACotKqWNd3aAAAA\\nfKZ9+BEXBx98cI455pgMHDhwHZ/q4/nw13DDDTfk8ccfb72zHACA9s2d0AAA0I6NHz8+P/zhD5Mk\\nL730Ul5++eXWx5G0F88991z222+/zJkzJ0uWLMk999yTPn36rOtjAQDwKXEnNAAAtGNvv/12Tjvt\\ntLz++uvp0KFDjj766Bx44IHr+lgf2yWXXJI77rgj1dXV6dOnT374wx/m85///Lo+FgAAnwIRGgAA\\nAACAYjyOAwAAAACAYkRoAAAAAACKqVnXB1idhoZ5q/18t26bpLGxaa3f/5Ncb9u2bdu2bdu2bdu2\\nbdu2bdu2bdu2bdu2l6mt7bLK69r1ndA1NdXr7Hrbtm3btm3btm3btm3btm3btm3btm3btr1m7TpC\\nAwAAAADw2SZCAwAAAABQjAgNAAAAAEAxIjQAAAAAAMWI0AAAAAAAFCNCAwAAAABQjAgNAAAAAEAx\\nIjQAAAAAAMWI0AAAAAAAFCNCAwAAAABQjAgNAAAAAEAxIjQAAAAAAMWI0AAAAAAAFCNCAwAAAABQ\\njAgNAAAAAEAxIjQAAAAAAMWI0AAAAAAAFCNCAwAAAABQjAgNAAAAAEAxIjQAAAAAAMWI0AAAAAAA\\nFCNCAwAAAABQjAgNAAAAAEAxNev6AJ9lY8d+LnfdtfJvUYcOSXNzp7V+709yvW3btm3btm3btu3/\\nM2zYkowd+/5abwAAAGW5E3o17rqrJrNmVa3rYwAAsAqzZlWt8qYBAADgs8G/sa9Bz54tmTbtvRU+\\nXlvbJQ0NK368rT7J9bZt27Zt27Zt27aX2X33tb/LGgAAqAx3QgMAAAAAUIwIDQAAAABAMSI0AAAA\\nAADFiNAAAAAAABQjQgMAAAAAUIwIDQAAAABAMSI0AAAAAADFiNAAAAAAABQjQgMAAAAAUIwIDQAA\\nAABAMSI0AAAAAADFiNAAAAAAABQjQgMAAAAAUIwIDQAAAABAMSI0AAAAAADFiNAAAAAAABQjQgMA\\nAAAAUIwIDQAAAABAMSI0AAAAAADFiNAAAAAAABQjQgMAAAAAUIwIDQAAAABAMSI0AAAAAADFiNAA\\nAAAAABQjQgMAAAAAUIwIDQAAAABAMSI0AAAAAADFiNAAAAAAABQjQgMAAAAAUIwIDQAAAABAMSI0\\nAAAAAADFiNAAAAAAABQjQgMAAAAAUIwIDQAAAABAMSI0AAAAAADFiNAAAAAAABQjQgMAAAAAUIwI\\nDQAAAABAMSI0AAAAAADFiNAAAAAAABRTNEK/8MILGThwYK6//vokyRtvvJEjjjgio0aNyhFHHJGG\\nhoaS8wAAAAAArGPFInRTU1PGjRuXvn37tn5swoQJOfTQQ3P99ddn0KBB+eUvf1lqHgAAAACAz4Bi\\nEbpjx4656qqrsvnmm7d+7JxzzkldXV2SpFu3bpk9e3apeQAAAAAAPgOKReiamppsvPHGy31sk002\\nSXV1dZYuXZobb7wxw4YNKzUPAAAAAMBnQFVLS0tLyYGJEyemW7duGTVqVJJk6dKlOe2007Ltttvm\\n+OOPX+21S5YsTU1NdcnjrdY22yz79ZVX1tkRAABYDf++BgAAn301lR4844wz0qtXrzUG6CRpbGxa\\n7edra7ukoWHeWp9lTdc3N3dKkjQ0vFfx7VLX2rZt27Zt27Ztr0/b/n3Ntm3btm3btm3btu3PxnZt\\nbZdVXlfscRwrc+edd2ajjTbKiSeeWMlZAAAAAADWkWJ3Qv/xj3/M+PHj8/rrr6empiZTpkzJO++8\\nk8997nM57LDDkiTbb799xo4dW+oIAAAAAACsY8Ui9K677prrrruu1NsDAAAAANAOVPRxHAAAAAAA\\nbFhEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAA\\nAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAA\\nAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAA\\nAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoA\\nAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQG\\nAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGh\\nAQAAAAAopmZdHwAAShk79nO5667V/6jr0CFpbu601huf5Hrbtm1/8utnzapKkuy++4qvWZ+/btu2\\nN7TtYcOWZOzY99d6CwBYt9wJDcB66667aloDFbB+6tmzJT17tqzrYwAFzZpVtcb/qAwAfLb5SQ7A\\neq1nz5ZMm/beKj9fW9slDQ2r/vyafJLrbdu2bdu2bdtrvn5l/58OAED74k5oAAAAAACKEaEBAAAA\\nAChGhAYAAAAAoBgRGgAAAACAYkRoAAAAAACKEaEBAAAAAChGhAYAAAAAoBgRGgAAAACAYkRoAAAA\\nAACKEaEBAAAAAChGhAYAAAAAoBgRGgAAAACAYkRoAAAAAACKEaEBAAAAAChGhAYAAAAAoBgRGgAA\\nAACAYkRoAAAAAACKEaEBAAAAAChGhAYAAAAAoBgRGgAAAACAYkRoAAAAAACKEaEBAAAAAChGhAYA\\nAAAAoBgRGgAAAACAYopG6BdeeCEDBw7M9ddfnyR54403cthhh2XkyJE56aSTsmjRopLzAAAAAACs\\nY8UidFNTU8aNG5e+ffu2fuySSy7JyJEjc+ONN6ZXr1655ZZbSs0DAAAAAPAZUCxCd+zYMVdddVU2\\n33zz1o9NnTo1++23X5Jkn332yRNPPFFqHgAAAACAz4CaYm9cU5OamuXffsGCBenYsWOSpEePHmlo\\naCg1DwAAAADAZ0BVS0tLS8mBiRMnplu3bhk1alT69u3bevfzq6++mjFjxuRXv/rVKq9dsmRpamqq\\nSx5vtbbZZtmvr7yyzo4AwCfgz3EAaP/8PAeA9q/YndArs8kmm2ThwoXZeOON89Zbby33qI6VaWxs\\nWu3na2u7pKFh3lqfZ03XNzd3SpI0NLxX8e1S19q2bdv2hrS9uj/HS2+Xvta2bdu2bdveULZX9vN8\\nQ/i6bdu2bdu27fa2XVvbZZXXFXsm9MrssccemTJlSpLknnvuSf/+/Ss5DwAAAABAhRW7E/qPf/xj\\nxo8fn9dffz01NTWZMmVKLrzwwpx++um56aab0rNnzwwfPrzUPAAAAAAAnwHFIvSuu+6a6667boWP\\n//KXvyw1CQAAAADAZ0xFH8cBAAAAAMCGRYQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAo\\nRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAA\\nihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAA\\ngGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAA\\nAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAA\\nAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAA\\nAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoA\\nAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQG\\nAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGh\\nAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJE\\naAAAAAAAihGhAQAAAAAopqaSY++9917GjBmTOXPmZPHixTnuuOPSv3//Sh4BAAAAAIAKqmiEvu22\\n27LtttvmlFNOyVtvvZXDDz88kydPruQRAAAAAACooIo+jqNbt26ZPXt2kmTu3Lnp1q1bJecBAAAA\\nAKiwit4JfcABB2TSpEkZNGhQ5s6dmyuuuKKS8wAAAAAAVFhVS0tLS6XG7rjjjjz11FMZN25cnn/+\\n+Zx55pmZNGnSKl+/ZMnS1NRUV+p4K9hmm2W/vvLKOjsCAJ+AP8cBoP3z8xwA2r+K3gk9ffr07Lnn\\nnkmSnXbaKW+//XaWLl2a6uqVh+bGxqbVvl9tbZc0NMxb6/Os6frm5k5JkoaG9yq+Xepa27Zt296Q\\ntlf353jp7dLX2rZt27Zt2xvK9sp+nm8IX7dt27Zt27bd3rZra7us8rqKPhO6V69eefrpp5Mkr7/+\\nejp16rTKAA0AAAAAQPtX0Tuhv/Wtb+XMM8/MqFGjsmTJkowdO7aS8wAAAAAAVFhFI3SnTp3ys5/9\\nrJKTAAAAAACsQxV9HAcAAAAAABsWERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoA\\nAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQG\\nAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGh\\nAQAAAAAopk0RetGiRbnhhhty4YUXJkmefvrpvP/++0UPBgAAAABA+9emCD127Ni89tprmTp1apLk\\n2Wefzemnn170YAAAAAAAtH9titAvv/xyzjjjjGy88cZJkpEjR+btt98uejAAAAAAANq/NkXompqa\\nJElVVVWSpKmpKQsXLix3KgAAAAAA1gs1bXlRfX19Dj/88MycOTPnnXdeHn744YwcObL02QAAAAAA\\naOfaFKFHjRqV3r1753e/+106duyYiy66KLvuumvpswEAAAAA0M61KUK//fbb+a//+q9897vfTZJc\\nfPHFqa2tzRZbbFH0cAAAAAAAtG9teib0GWeckb/6q79q/f2OO+6YM888s9ihAAAAAABYP7QpQi9a\\ntChDhgxp/f2QIUOyePHiYocCAAAAAGD90KYInSQPP/xwFi5cmKampkyZMqXkmQAAAAAAWE+06ZnQ\\n5513Xs4555ycdNJJqaqqyle/+tWMGzeu9NkAAAAAAGjn2hShe/XqlauvvrrwUQAAAAAAWN+0KUI/\\n+eSTue666zJnzpy0tLS0fvyGG24odjAAAAAAANq/NkXoc845J8ccc0x69uxZ+jwAAAAAAKxH2hSh\\nt9pqqwwfPrz0WQAAAAAAWM+0KUL3798/N910U/7+7/8+NTX/d8nWW29d7GAAAAAAALR/bYrQ1157\\nbZLkiiuuaP1YVVVV7r///jKnAgAAAABgvdCmCP3AAw+s8LFp06Z96ocBAAAAAGD90qYIPX/+/Nxx\\nxx1pbGxMkixevDi33nprHn300aKHAwAAAACgfevQlheNHj06//M//5NJkyblvffey4MPPpixY8cW\\nPhoAAAAAAO1dmyL0+++/n3PPPTdf+MIXMmbMmFx77bW5++67S58NAAAAAIB2rk0RevHixWlqakpz\\nc3MaGxuz2WabZcaMGaXPBgAAAABAO9emZ0J/85vfzM0335xDDjkkQ4YMSffu3dOrV6/SZwMAAAAA\\noJ1rU4Q+4IAD0rVr1yRJ3759884776RLly5FDwYAAAAAQPu3xsdxNDc357jjjktLS0uam5tTW1ub\\nHXbYIccee2wlzgcAAAAAQDu22juhf/3rX2fixIl59dVXs/POO6eqqiotLS2pqqpK//79K3VGAAAA\\nAADaqdVG6KFDh2bo0KGZOHFiTjjhhEqdCQAAAACA9cQaH8eRJAceeGCmTZuWJLn55ptz5pln5qWX\\nXip6MAAAAAAA2r82RegzzjgjG220Uf70pz/l5ptvTl1dXc4777zSZwMAAAAAoJ1rU4SuqqpK7969\\nc++992bUqFEZMGBAWlpaSp8NAAAAAIB2rk0RuqmpKc8880ymTJmSvfbaK4sWLcrcuXNLnw0AAAAA\\ngHauTRH6qKOOytlnn51DDz003bt3z8SJEzN06NDSZwMAAAAAoJ2racuLhgwZkiFDhrT+/uSTT05V\\nVVWxQwEAAAAAsH5YbYQePXp0JkyYkAEDBqw0Oj/00EOlzgUAAAAAwHpgtRF6l112ye23357Ro0dX\\n6jwAAAAAAKxHVhuhX3zxxbz44ouZPXt2nnvuufTp0ydLlizJM888k9122y0HHnhgpc4JAAAAAEA7\\ntNoI/ZOf/CRJcuKJJ+a+++7LxhtvnCSZP39+vv/975c/HQAAAAAA7VqHtrxo1qxZrQE6STp37pxZ\\ns2YVOxQAAAAAAOuH1d4J/YEvfelLGTFiRHbbbbd06NAhTz/9dHr16lX6bAAAAAAAtHNtitDnn39+\\nHn/88bzwwgtpaWnJv/zLv6RR89QFAAAgAElEQVR///6lzwYAAAAAQDvXpghdVVWVfv36pV+/fqXP\\nAwAAAADAeqRNz4QGAAAAAIC1IUIDAAAAAFCMCA0AAAAAQDEiNAAAAAAAxYjQAAAAAAAUI0IDAAAA\\nAFCMCA0AAAAAQDEiNAAAAAAAxYjQAAAAAAAUU/EIfeedd+Yf/uEfctBBB+Whhx6q9DwAAAAAABVU\\n0Qjd2NiYSy+9NDfeeGP+/d//Pffff38l5wEAAAAAqLCaSo498cQT6du3bzp37pzOnTtn3LhxlZwH\\nAAAAAKDCKnon9MyZM7Nw4cIcffTRGTlyZJ544olKzgMAAAAAUGFVLS0tLZUau/LKKzN9+vT8/Oc/\\nz6xZs/Kd73wnDz74YKqqqlb6+iVLlqamprpSx1vBNtss+/WVV9bZEQD4BPw5DgDtn5/nAND+VfRx\\nHD169Mhuu+2WmpqafPGLX0ynTp3y7rvvpkePHit9fWNj02rfr7a2Sxoa5q31edZ0fXNzpyRJQ8N7\\nFd8uda1t27Ztb0jbq/tzvPR26Wtt27Zt27btDWV7ZT/PN4Sv27Zt27Zt225v27W1XVZ5XUUfx7Hn\\nnnvmySefTHNzcxobG9PU1JRu3bpV8ggAAAAAAFRQRe+E3mKLLVJXV5dDDz00SfL9738/HTpUtIMD\\nAAAAAFBBFY3QSTJixIiMGDGi0rMAAAAAAKwDbkMGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAA\\nAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAA\\nAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoA\\nAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQG\\nAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGh\\nAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJE\\naAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAY\\nERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAo\\nRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAA\\nihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAA\\ngGJEaAAAAAAAihGhAQAAAAAoRoQGAAAAAKAYERoAAAAAgGLWSYReuHBhBg4cmEmTJq2LeQAAAAAA\\nKmSdROjLL788m2666bqYBgAAAACggioeoV966aX8+c9/zt57713paQAAAAAAKqziEXr8+PE5/fTT\\nKz0LAAAAAMA6UNXS0tJSqbHbb789s2bNyrHHHpuJEyfmC1/4Qg466KBVvn7JkqWpqamu1PFWsM02\\ny3595ZV1dgQAPgF/jgNA++fnOQC0fzWVHHvooYcyY8aMPPTQQ3nzzTfTsWPHbLnlltljjz1W+vrG\\nxqbVvl9tbZc0NMxb6/Os6frm5k5JkoaG9yq+Xepa27Zt296Qtlf353jp7dLX2rZt27Zt2xvK9sp+\\nnm8IX7dt27Zt27bd3rZra7us8rqKRugJEya0/vUHd0KvKkADAAAAAND+VfyZ0AAAAAAAbDgqeif0\\nh51wwgnrahoAAAAAgApxJzQAAAAAAMWI0AAAAAAAFCNCAwAAAABQjAgNAAAAAEAxIjQAAAAAAMWI\\n0AAAAAAAFCNCAwAAAABQjAgNAAAAAEAxIjQAAAAAAMWI0AAAAAAAFCNCAwAAAABQjAgNAAAAAEAx\\nIjQAAAAAAMWI0AAAAAAAFCNCAwAAAABQjAgNAAAAAEAxIjQAAAAAAMWI0AAAAAAAFCNCAwAAAABQ\\njAgNAAAAAEAxIjQAAAAAAMXUrOsDAAAAn01j35yZu+Y2tvn1Hf5clebmlrXe+yTX215/t2ct/mqS\\nZPcX/rvi25/2tbbb//awrt0ydsut1vosABsqd0IDAAArddfcxsxavGhdH4MNXM9bpqfnLdPX9TEg\\nsxYv+lj/YQ6A/+NOaAAAYJV6btQx0778lTa9tra2Sxoa5q311ie53rZt27ZLb3/4bnwAPh53QgMA\\nAAAAUIwIDQAAAABAMSI0AAAAAADFiNAAAAAAABQjQgMAAAAAUIwIDQAAAABAMSI0AAAAAADFiNAA\\nAAAAABQjQgMAAAAAUIwIDQAAAABAMSI0AAAAAADFiNAAAAAAABQjQgMAAAAAUIwIDQAAAABAMSI0\\nAAAAAADFiNAAAAAAABQjQgMAAAAAUIwIDQAAAABAMSI0AAAAAADFiNAAAAAAABQjQgMAAAAAUIwI\\nDQAAAABAMSI0AAAAAADFiNAAAAAAABQjQgMAAAAAUIwIDQAAAABAMSI0AAAAAADFiNAAAAAAABQj\\nQgMAAAAAUIwIDQAAAABAMSI0AAAAAADFiNAAAAAAABQjQgMAAAAAUIwIDQAAAABAMSI0AAAAAADF\\niNAAAAAAABQjQgMAAAAAUIwIDQAAAABAMSI0AAAAAADFiNAAAAAAABQjQgMAAAAAUIwIDQAAAABA\\nMSI0AAAAAADFiNAAAAAAABQjQgMAAAAAUExNpQcvuOCCTJs2LUuWLMn3vve97L///pU+AgAAAAAA\\nFVLRCP3kk0/mxRdfzE033ZTGxsYceOCBIjQAAAAAwHqsohH67/7u79K7d+8kSdeuXbNgwYIsXbo0\\n1dXVlTwGAAAAAAAVUtFnQldXV2eTTTZJktxyyy3Za6+9BGgAAP5/e3ceXkWV53/8k50tEdBACAEj\\nixuIQsRdcMYFRLYZF2zUbllaG3FBbVFp1DSigAKKEAUbheCoY+PYMAFbGh1bESFhk12BAEoSgUAS\\nQojZ8/sjv3u90JDcUyf33izv1/P4mJCcnHOrTp2q+tap7wEAAADQgAVVVlZW+rvSzz//XPPmzdO7\\n776ryMjIM/5eWVm5QkMDF6SOj6/6//79AWsCAMAC4zgA2Ilfs0aStP/qqwPcEgAIPMZEAHDO7wsT\\nrlq1SnPnztX8+fOrDUBLUm5uYbU/j46OVHb2ccdtqal8RUVzSVJ29gm/1+2rstRN3dRN3Y2p7urG\\ncV/X7euy1E3d1E3d/qi7oqJqvoq3f6+hfG7qpm7qpu7TlTUdE2uzbn+Xp27qpm7qdlI+OvrMsV6/\\nBqGPHz+uV155RQsXLlTLli39WTUAAAAAAAAAIAD8GoT+9NNPlZubq3Hjxrn/bdq0aYqNjfVnMwAA\\nAAAAAAAAfuLXIPSwYcM0bNgwf1YJAAAAAAAAAAig4EA3AAAAAAAAAADQcBGEBgAAAAAAAAD4DEFo\\nAAAAAAAAAIDPEIQGAAAAAAAAAPgMQWgAAAAAAAAAgM8QhAYAAAAAAAAA+ExooBsAAAAAAADgC4kH\\nM7R8T54qKiodlQ/eE+Qum1VaIklK2LXVUflTDYpqpcSYOEftAoD6hpnQAAAAAACgQUrJz1VGcXGt\\n/K3YsHDFhoXXyt/KKi1RSn5urfwtAKgPmAkNAAAAAAAarLiICK3r0t1R2ejoSGVnH3dc95nKm8ym\\nBoCGgJnQAAAAAAAAAACfIQgNAAAAAAAAAPAZ0nEAAKw1T5woLV+q1g4XfFFwkPOy1ZQPzvpGktQ6\\n4Tq/112T4kFDpaRZzusFAAAAAKCeIAgNALAWkbJEysqUYtsHuiknSY+tJvgcQMFZmVXbjCA0AABA\\nnZV4MEPL9+SpwmLCQvCeoNOWzyotkVR9bugzla3JoKhWSoyJMy4HAL5EEBoAUDvi4pSzztkCK9HR\\nkcqxXPDFaflA1N06wdnCOAAAAPCflPxcZZWWKDYsvNb/ti/+plQV3E7JzyUIDaDOIQgNAAAAAABw\\nGnEREVrXxfkEgujoSGVbTJYwLVvdzGoACCSC0ACAeqt54sSqtBY2eZ0DkBM6OCuz6ov4+IDko7Yt\\nSz5rAAAAAICJehuETvx2opbvW+o4N1NeUa4Kywqr/Z3y43skSe3e6uKoDm/FtjDLoRoc7CwvlDfl\\nB3UeqsRrJjv+2wDgTxEpS6oCunH163XDiv+fOzskwO1wgnzWAAAAAABT9TYInZK+RFkFmcYBXJfC\\nskKVV5YrJOjMIYCQx30bfK5rsgoylZK+hCA0gHqlIra9Qvbvr1c5oetz3eSzBgAAAACYqrdBaEmK\\ni4rTunuc5TtKeK+7goODHJe3yetkW95XdSe8R2ABAAAAAAAAQO0KDnQDAAAAAAAAAAANV72eCR0o\\ntvmoJbu8zr7KCZ1VULVQVnUzon2Zj9qXZQd1HqqkIeQvBdBwNE+cKC1f6veFCWtlUUWHdRcPGqoT\\niaSMAgAAAID6hiC0A7b5qL1ZFDGQXMHouqyiskKV8j6A8eZ3b2je5iQftgieXMdGXXzY0pjrZuHR\\nhiUiZYmUlSnFOjsXVScoL1dBhTWcpzIyrF6nMi0bVF6upvOSqj63wyB2VcUWZU9TnsA4AAAAANSM\\nILRDtvmoCwsKHQexG2qAzKRsVkFmjQtLAvgVC482UHFxylnnfG2DMy1M2Dqhu4IKC1VRTYA7xGI8\\ntylblwRnZSoiZQlBaAAAAACoAUHoALEJYjfERRFNy7pShmy4b5vf6/Z3eeqmbm+5UgWdSVZBZrXp\\ndoZ1v0vjez7vqG40PBWx7ZWz4cxjbHVB7JrYlK2ufPPEiVUzpf0oOCtTrRO8XNjXdhb2sLuk8Ryj\\njV3iwQyl5OdW+zvBeywnDXiUzyotkSQl7NqqvPIyFVZUOP673ooNC3dUzuRzD4pqpcSYOEf1AAAA\\nwBwLEwJAA5GSvkQZ+Rmn/Vlsi/bVvn2RVZCpxTsW+6ppgF9EpCz5NWe1H1TEtq92tnhtCs7KlBZz\\njEJKyc91B4b9ITYs3B0ULqyoULnfavadrNKSGgP5AAAAqF3MhAaABsTpWxbVzZAG6hNfzuC2LW9T\\ntnVCd5GACi6xYeHacP4lZ/y5r97oSdhVdX4JRN21Wdb1OQDA1xIPZmj5njznKSkdvNni+QaLzZsx\\npmV5wwRATQhCAwAA+FjzxInS8qWO03G4Znh7nfrjVKTyAADA71xvrzhNM+SEP+tycb1hQhAaQHUI\\nQjdwid9OVEr6yfkxG8rChNK/zt7MK8pVYVmh4/bVFZ5pE+rKNjcxqPNQFsADAA8RKUukrEzJYfqO\\nitj2CgkOkhyMye5UHgShAQDwu7iICK3r4uwhcn14u0TiDRMA3iEI3cClpC9RVkFmtblg66MzfZ7C\\nskKVV5YrJIiXlgMlqyBTKelLCEIDwKni4pSzzvlNmtN0HqTyQH1h89p6XnmZCrd7t2iiK691u+0b\\njOsxFRsW7rcFHb116ixJf76u72lQVCslRV/kqCwA3y9Ua1LWMwWIv+s+U/nbWrRkZjZQxxCEbgRi\\nW7TXhvt+zY/pr6ehtV3em7KumdGen9dfdfuqfH2rm9zCAADACZvX1l2LJnrzwMXfD2VM2tZYuF7d\\nTwp0Q4B6LBCpPs6kLrTBU0ZxsVIqSA8C1DUEoQEAAADUCU5fW0/YtVXBwUGOX3mXfPfg3tcLOta3\\nCQsSr+4DtcWXC9XWx7HFpfeebVYzqQH4BkFoAABQZzRPnFiVP7k6wUGnXeDPq8X7zlDWpXjQUJ1I\\nJJ0QAADAmdSlVCAunqmP/Jl2yanTzR6vjTQktb3NB0W1YkY5ag1BaAAAUGdEpCxRcFamKhws4Oek\\njKfgrExFpCwhCA0AABq1mnL0Z5WW1JhmqHlIiM4K9l8iIs/UR6Q/qh2u1EkEoVFbCEIDAIA6pSK2\\nvXI2/GtufxenC/TVVLbaGdQB1jxxorR8abWzuM/Eqxni1SgeNFRKmuWoLAAAqH9qyjddUw7orNIS\\ntQoNdZwiydHaRB6pj+pjGpLEgxlaXpDnuF5fySotcW/burTQrmcfZIHd+oMgNAAAQB0XkbJEysqU\\nHMz2rmzWTEGFhe5gtImg8nI1fWu2NC9J5xiX/v/i4hwFzyUC4KgbbF87zyotkVR9HuTqyvvyVeia\\nZjvWxOnNu2ubxK9ZQ+AAOANvZiNL1Y8tw/LbanxUG0f1O83RX1ObcHp1aaFJl1PbwkK7v2KBXWcI\\nQgMNUOK3E5WSXpVTNTjY4qmgg7JZBVVBjoT3uvu9bpdBnYcqaQhBCwANTFycctaZ39S1TuguFRY6\\nTlcSnJUplZdLIQ5uOSoqpIwMBTuoN6i8XE3nJTmaAU5ub9Qm28CATUDB169C23y2vPIyFZbazYjL\\nKC62Ku9EuaR5Rw9p+Rr/B99tyxM8b1xqYzby4sOHHQeh4X82gX/J94tBVrfQbmOcfZ5RWqLQf/7T\\nUdlmwcEq3O7sHOo69k3OJXUltzdBaKABSklfoqyCTMW2sMuP6kRt1JlXlKvCskJHZcsryzVvc5KW\\n71tqfHE/qPNQJV5D0AJAA+QwgC1VBbFDgoN0xGEAPMRhjm8X09A3ub3hC7Fh4ae96XaxuYGu6SbY\\n81Xo07GZ6Sg5D3ok7Nqqwgq7WXuBmrBQHzHrrnFiNjLgOzYPYmPDwr3KjX4m/pxVXpdyexOEBhqo\\n2BbtteG+bfXyiWTCe91VWFDo1yB6VkGmUtKXEIQGgNpmEQCXzHOA1+Xc3sDpVHcTXNdnOta1WXve\\npE6pz7JKS6xSmEiBy51aU/m6MksPQONi+6AnODjI8YNcp2VdvD2H1qUHUgShAdRJcVFxWnePs8Ey\\n8duJWr5vqXG5rIJMR2lEmEENAABs2MxGxq/qYk7V2lLfP1N16VtcKVKqe4BgO+MfABB4BKHrGVdw\\nzdsAmWd+XpfqAmwE0+oW0/3t4jQvc0PZ/07SkTiddc0MaqD+aJ44sdr8wq6F+6qdSTvsLmn8875o\\nHlAnVLcQlq8XwULj4otFEV19tLadOks3UG8L1ue6bdK3BHrGPwCgdhCErmdMg2smgTWCaXWP09zO\\nTgKqDW3/O51J7WQWtWsGtQsPeszUFBisjitoqPh4Fi5DjSJSlkhZmdIZ8hPXlLc4OCtTWryYIDQa\\ntPqcGgL1iy9mLbv+Vl55mQor7BZNdDndLF2btBSNeXFBZvwDQONGELoesklTIJ35CbZnEA11h6/2\\n96nY/1V40ON/NQUGq1MR215BebkKyshQsEG5oPJyNZ2XVFV3cJBRALshBK8b9Yxg2wX6ark5QF1E\\noAj+YpOLs7prXG9m3QZiYUMWFwQANGYEoQHgFL7MR33qrOlTDet+l8b3rIeBPVuWgUEVFtY4i7U2\\nBGdlKiJlSb0PQjMjGADQ0MWGhWvD+Zec8eeBSEvBgxoAgeDNoq3evOFRXVoukzdEWIi08SIIDQC1\\nqLqZ1DXNms4qyNTiHYsbZxDalkUQOzo6Ujle3khWOzO4vmFGMAC4kQsbANBQ1Vb6o9pIn+R6I4Qg\\ndONEELqRqW6hu9MtYniqRjtLs54yWdjw1P2fV5SrwrLCasvUFFRtrP3F6UxqUqIAwMmc5Iw/KZ1M\\nI0y3A2fIhQ1v2Syo6HqgEb9mjXF5Zg4CsOHLt0NMyvNGSONGELqRYZZm42KS3/jU3yksK1R5ZblC\\ngpzNd6S/oD4wDXCdNldyNUEuAlr/qlHno4YxJznjnabmaSjpduAcubDrj5oCwb6cvW4zo9DpLEJm\\nDgIAGgKC0I1QXZylmfjtRKWkL6n2d7xZPKS62dwmi48M6jy0wSweZ7O/g4ODHOdGZlYv6gPTAJdJ\\ncIuA1umRjxrGGmG6HVJDANWrKRDs69nrNgsqSuYzDnnQAQBoCAhCo04wmbFbHdvyUlUgOyV9SYMJ\\nQgOogUWASzpzkKsuBbTqHPJRA9UiNQRQM5tAMEFdAKg9JmmKTn2YnldepsLtFdWWqenah4fv9QdB\\naNQZsS3aa8N92874c7/lKGIGLwAACDBSQwAAgPrAJE3Rqb9TWFGhcsnxJBMevtcvBKEBAAAAAPAD\\nJwsbes4cDN7jfYpBiQUNAfiHzcPz4OAg3mwxZDP7XFKN5xJfnTsIQsNvEr+dqOX7lp4+v2E1uZxd\\nhnW/y/Eid9XV7U1bason3ZBySAPwXnWL7LHAHhBYLDwKoC5ysrAhCxoCADzZzD6viS/PHQSh4TfV\\n5X2uKZdzVkGmFu9Y7DgIbZJz2jSvNDmkgcarukX2WGAPCCwWHgVQVzmdMZh4MEPLC/KMymSVlng9\\n+41Z0wBQf9isjVBdulpfzi4nCA2/iouK07p7zDt0beRpdlq3VMMBSg5poHFzuMgeC+wBfsDCowAa\\nENNZ1Caz35g1DQDwNYLQAAAAAADUAzYz36QzT65prHlVAQD+QxAaAAAAAIAGrrqFrE63cNWphuW3\\n1fioNj5rHyA5W7xTcr6AJ2loAP8hCA0AAAAAQANXXTqPmlJ3ZJWWaPHhwwSh4XNOFu+UnC3g2ZDS\\n0PCQCd6q6UGPL/sLQWgAAAAAABoBp+k8SNcBf/JV2plTNaR+zUMmeKumBz2+7C8EoQEAAAAAAIB6\\njIdM8JbNgx6b/hLsuCQAAAAAAAAAADUgCA0AAAAAAAAA8BmC0AAAAAAAAAAAnyEIDQAAAAAAAADw\\nGYLQAAAAAAAAAACfCQ10AwAAAAAAAABbiQcztHxPnioqKmv83azSEklSwq6t7n8L3hNUbdlBUa2U\\nGBNn31CgESIIDQAAAJyieeJEaflStT7NjWhwVqYkqXVC9zP/gWF3SeOf91XzAADAaaTk5yqrtESx\\nYeE1/q43v+Mpq7REKfm5BKEBhwhCAwAAAKeISFkiZWVKse3/5WcVp/k3T8FZmdLixQShAQAIgLiI\\nCK3rUs2D4mpER0cqO/v4aX/mOWMagDmC0AAAAMDpxMUpZ535DWfrhO4K8UFzAACA79SUyuN06TtO\\nNSy/rcZHtfFJ+4D6joUJAQAAAAAA0Kil5Ocqo7j4jD+PDQuvNoVHVmmJFh8+7IumAQ0CM6GBGiR+\\nO1HL9y0989PQgqq8kAnvnfl1n2Hd79L4nrySCwAAAABAXWWTyoN0HUD1mAkN1CAlfYky8jPO+PPY\\nFu0V2+LMuSGzCjK1eMdiXzQNAAAAAAAAqPP8PhP65Zdf1ubNmxUUFKQJEyaoR48e/m4CYCwuKk7r\\n7nH2VLO6GdIAAAAAAABAQ+fXIHRaWpp+/PFHffTRR0pPT9eECRP00Ucf+bMJAAAAAAAAAAA/8ms6\\njjVr1uimm26SJHXu3FnHjh1TQUGBP5sAAAAAAAAAAPAjvwahjxw5olatWrm/b926tbKzs/3ZBAAA\\nAAAAAACAHwVVVlZW+quy5557Tn379nXPhv7Nb36jl19+Weedd56/mgAAAAAAAAAA8CO/zoRu06aN\\njhw54v7+8OHDio6O9mcTAAAAAAAAAAB+5Ncg9LXXXqsVK1ZIkrZv3642bdqoRYsW/mwCAAAAAAAA\\nAMCPQv1ZWa9evdStWzfdfffdCgoK0gsvvODP6gEAAAAAAAAAfubXnNAAAAAAAAAAgMbFr+k4AAAA\\nAAAAAACNC0FoAAAAAAAAAIDPEIQGAAAA6oiSkpJANwEBUFhY6KhcRkZGLbcEaFhycnJ0+PDhQDfD\\nkYqKCr/XWVZWptzcXL/X6+n48eMBrb+4uDig9QO+duLEiYDV7deFCQEAAAD8q8WLF+vzzz9XXl6e\\nmjRpot/+9re68cYbA90s+Mi0adO0e/duDR48WIMHD9acOXM0fvx4r8rOnTtXklRZWanU1FRdddVV\\n+sMf/uDL5tYJt956qx577DH1798/0E1BPfDmm2+qXbt2+uabb9SsWTPFxMRo7NixgW5Wjd577z3F\\nxcXp448/VpMmTXTJJZfo/vvv90vdH3zwgb788ktFRUUpPz9ft9xyi+68806/1P3aa69p8+bNuvTS\\nS7V3716dc845euGFF/xS96lmzZrl9XgM1BczZ87U1q1bde2112r9+vVq06aNJk2a5Pd2hCQmJib6\\nvVaH3n//fXXr1k3Bwc4mcB87dkwfffSRvvzyS2VlZalLly4KCQkx/juffPKJLrroIqMyW7duVWho\\nqGbOnKnPP/9c8fHxatWqlXHdkvTFF1+oU6dOXv/+3r17tXLlSsXExCgpKUlNmjRRbGysV2V//vln\\nhYSE6P3339fatWvVsWNHNW/e3Ou6d+/erb///e/q1KmTwsPDtXLlSnXu3Nnr8p7Wr1/vdbsl6auv\\nvlJ8fLyjumz7iu12c3HS18rKyvTll19q/fr1On78uOLi4rwua9tum75WUFCgsLAwffHFF9q+fbs6\\nduyosLAwr+u22WdHjhxRamqqOnbsqODgYKWlpal9+/Zela3NPv75558bHduSlJubq4ULF+qzzz5T\\nenq6OnXqpCZNmnhVNicnRxs3blSbNm3017/+VZGRkWrZsqXXddtsc5vx3PZccCqTMXXv3r369NNP\\n1aZNGyUlJSkiIsLrviLZ9xebc4nN8SnZbfcDBw4oPDxc77//vlJTU9WpUyc1bdrU+O9I5uOi7bgW\\nqG1uu79sj2/bsSUtLU3R0dH661//qubNm6t169Ze121z/rYdH2rr/C2ZX68tW7ZMU6dO1dGjR/XS\\nSy/pnXfe8ToIXZvtlszb7uLkusWmr9mUlezGZJvrLamqn0+fPl3ffvutKioqtG/fPl177bVelU1O\\nTlZsbKx69uypjIwM3XjjjUbnIptzWWlpqT799FOtXr1ahw8fVnx8vNX52OT6/qefflLbtm01d+5c\\nlZaWKiQkxGhssdnfNucx2/sK2zHVk+m1Zm3ubyfjgyfTcWnlypXKy8vTpEmT9O///u/66quvvD7G\\nbO9LbPraP/7xD2VnZz8OXeIAABu9SURBVOvFF19Uv379tGLFCl133XVelbUdE1NSUvTqq6+qX79+\\nGjx4sJYsWaI+ffp4Xd5mbFm3bp2mTJmiZcuWafr06dq6dauuvPJKr+v2ZNrXRo4cqaVLl2rp0qVa\\nsmSJNmzYoN/97ndel7c5xgN5fW57X2PT32zrDmR8zSZ2YNNu28/85ZdfasaMGZo/f77eeustff31\\n17rhhhu8Lm+7z1zqVTqOr7/+WhMmTNDatWsdlZ8xY4a6du0qSQoNDTV6sta7d2+NHDlSI0aM0Jtv\\nvqmRI0ca1Z2SkqK33npLv/nNbzR27FjNnz/f67IbN250/7dhwwZ9+umnRnUnJSUpMjJSjz76qPr1\\n66dFixZ5XXbu3LmaO3euLr74Yt1www167bXXjOp+88031aFDB7300ksqKSnRpk2bvC576NChk/5b\\ntmyZUd1vv/22nnvuOf34449G5SS7viLZbTfbvvbyyy8rJydHO3fu1MaNG/Xiiy/6pd2SXV+bOXOm\\nkpKSdPz4cTVr1kyTJ082qttmn7344ov6+eef9ec//1mS9M9//tPrsjZ9XJIGDhzo3t9Tp0413t/T\\npk1Tr169dN999+nCCy/U1KlTvS47efJk7dy5U2PHjlXLli01Y8YMo7pttrnNeG57LrAZU//yl7+o\\nbdu2euqppzRkyBClpKQY1W3bX2zOJTbHp2S33d977z298cYb6tSpky6//HJNnz7dqLzNuGg7rgVq\\nm9vuL9vj22ZsmTZtmvbt26eHHnpIMTExeuedd4zqtjl/244PNv3F9notLy9P6enpys3NVU5OjtHr\\n0Lb93Kbtttcttn3NaVnJbky2ud6SqgKLOTk5GjFihJYtW6Y9e/Z4XXb27NkKCQnR3r171b59e/Xu\\n3duobptz2QsvvKDS0lJdcMEFys/Pl+l8Jpvr+6CgIA0YMEBTpkxRcHCwFi9ebFS3zf62OY/Z3lfY\\njqk215q2+9tmfLAdU/fu3avMzEzl5+crJydHBw4c8Lqs7X2JTV8rKSlRaGiovvnmG33zzTc6ePCg\\n12Vtx8Rjx47p0KFDkqqO1fz8fKPyNmOL62FLRkaGDhw4YJwSxKavde/eXW+88YbeffddLViwQP36\\n9TOq2+YYD+T1ue19jU1/s607kPE1m9iBTbttykpSdna28vLy9Mgjj+iXX34xTgNmu89c6lU6jnPP\\nPVfjx4/Xf//3f2vhwoVq2rSp0UX2WWedpWuuuUbr1q3TkCFDtGXLFq/Lzps3T8uWLdPjjz+u5ORk\\nPfzww8btb9mypfsJqMnslKlTp570BDIrK8u43ltvvVX79u1Tjx49jJ6sNW/eXC1atNAVV1whSUZP\\nUiXp7LPPVt++fdWpUyfNnDnT6AndyJEj1aNHD/f327ZtM6q7W7duevjhhzVv3jxlZGSoQ4cO+uMf\\n/+hVWZu+ItltN9u+FhkZqTvvvFOzZ8/WQw89pJdeeskv7Zbs+1pYWJj+4z/+Q5KUlpZmVLfNPouJ\\nidHw4cO1Y8cOvfXWW0b12vRxSRo7dqx++OEHPfzww5o7d67x/m7durV7lkB8fLxWrVpl1PbRo0fr\\n4MGDGjBggLZu3WpUt802txnPbc8FNmNq+/btddNNN+nrr7/WhRdeqDZt2nhdVrLvL5Lzc0mrVq0c\\nH5+S/XZv1qyZe7t/8cUXRnXbjIu245rkfJvbjIk2ZSXpnHPOsTq+bcaWc889V/fff78OHDigm266\\nSd9//71R3Z7n7wMHDqhjx45en79t+6lNf7G9Xrv99tv14Ycf6ne/+50KCgr05JNPel3Wtp/btN32\\nusWmr9mUlezGZNf11pw5c4yvt6Sq839eXp5at26tCRMmKDk52aj8iBEjlJqaqvT0dKNykhQXF+f4\\nXNa6dWv953/+p/t704dFNtf33bp1kySFh4drwIABGjBggFHdtudgz/PY559/7nU52/uK+Ph4qzHV\\n5lrTdn/bjA+2Y+ptt93m/jojI0MPPvig12Vt70ts+tojjzyixYsXa+XKlYqOjtazzz7rdVnbMXH0\\n6NGaOXOmjh49qnbt2umBBx4wKm9znXzvvfdq9erVmjp1qpKTk3Xrrbca1W3T1x544AGVlZW5vzdN\\n+WNzjNte79lc99je19j0N9u6pcDF12xiB5LzdtuWffDBB3X06FFddNFF2rJli26//Xaj8rWxz6R6\\nFoQOCgpSaGio7r33Xg0dOtS4/PHjx/X444+ra9eu2rRpkyIiIrwu26tXL8XHx2v69OmqrKxUYWGh\\nmjVr5nX5tLQ0hYaGqm/fvkpPTzdaZGDMmDHq2rWr4uLijJ9GSlJERIQ2btyohx56SJs3bzZK9H/R\\nRRdp+fLluvvuu1VSUqIhQ4YY1R0ZGam0tDRdccUV6tu3r5577jmv8yuNHj1al19+uTp06CCp6kmb\\niaCgIEVFRempp55SSUmJ9u7d63VZV1/p1auXNm7cqPDwcKO6PbdbUVGR+wLGG66+NnXqVEfpYg4d\\nOqTp06eradOm2rZtmwoKCozavWzZMt18880KDg7W8OHDjeo+ta+ZPF2Ljo7W2rVr3Tfdpidg1z5L\\nSEjQpk2bjPZZSUmJtmzZoh49emjPnj1KSkryup969vEuXbpo7ty5RjnEbr31Vl100UWaNGmScT+T\\nqp62P/fccwoNDVVxcbFiYmK8LltUVKQdO3Zo4sSJ2rVrl44ePWpUt+c2X79+vdGY6jmeDx482PhG\\n0FW2b9++xq9eeY6ppiorKzV27Fh16dJFzzzzjFq0aGFU3tVfunTpom7duhnnnHOdS4YMGaIff/zR\\n6PgODw/Xhg0b3Men6YIUrn02YMAA3XzzzUYXPitXrlRYWJiGDh2q7OxsZWdnG9XtGhdnzJhhvEiP\\na1y7++67FRYW5vUrrS6e23z37t1G9XuOievXrzcaEyMiItz7a9OmTcazFQoLC93H9+7du5WTk2NU\\n3jW2tG3bVj///LPatm3rddnc3FxNmDBBlZWVmjVrlvHYIsnx+dtzbLn33nuNjhHp5PN3WFiYrrnm\\nGq/Leo4tBw4cUFBQkFHdl19+uS6//HKjMi6e/fzQoUPG+UPHjBmjLl26uK+5TNrueY3sZJE8z752\\n8OBBo5uaU8ueddZZRnVHRkZq3bp16t27t3r37q0pU6Z4PSZ7Xm9t3brVeCEtz1d/w8LCNHr0aKPy\\nknTllVc6el29oqLCfS57+umnjc7f+fn5euuttxQTE6ODBw8aj02e1/fl5eVGM4oHDx5sVNepbO5L\\nPM9jhw4dMhrXTr1GNdneUtUMUZsx1fNa0/TewnN///zzz8b7+9R76OLiYq8/v2tMbdasmUpLS43H\\nVM/7L88HH95w3Zc88cQTKi8vV8eOHY3Ke44tvXr10iuvvOJ1X4uKitKoUaOM6nPxHBPXr1+vXr16\\nGZXv2rWr8T23J9d1ctu2bTVq1Cidd955Xpft0qWLunTpIkl65plnjOv27GulpaVGZU+9nr/00kuN\\nynse46axA9f13t133+3oek+S4+sez/uaMWPGGKdWOPUcbHJf5Fn3o48+ahzQ9Lw+37Ztm+P4WkFB\\ngfHYUlxc7I4d7Nq1S0uWLPH6+E5NTVVYWJj7Xq6oqMjrej0/85o1a4zviS688EL316ZjonTyPnv6\\n6acdxaqkehaELi4u1ogRIxwn0j7nnHN07rnnatu2bfr555+NgjWuxUMGDhyoTp06GS0eIkk333yz\\n2rVrp4ULFyo8PNwo+PHdd98pOTn5pCT9JiorKzVnzhwNHTpUt9xyi9HgsHv3bhUWFuq6667Thg0b\\njGdbFBUVad68eTp48KAGDx5stMDOvHnz1LRpU/cNkbezoFySk5PVs2dP9e/fX+Hh4ScddDVJTEzU\\nsWPH3DczJrMdJGnPnj0qLCzUeeedp4KCAu3fv9/rsq5FNIqKipSdna2kpCSjRTQuvvhiHT9+XNu3\\nb9f333+vhIQEr8v+9NNPuvnmm5WSkqKOHTsa37yHhYVp1qxZuuyyy4z76gcffKAxY8bovPPOU+vW\\nrY0DhFFRUdq3b5+ys7P17rvvGtW9Zs0aXXbZZerRo4cGDx5sdEMXHh7ufpUnNTXV6IGD9OsiOxMm\\nTNC3335rVFaqunC59NJLlZKSotjYWKOTQVpamhISEnTxxRcrOjraeGGCqKgo7d27V0VFRZo/f77R\\nxUNJSYnuv/9+R4uPtGrVyr0o09q1a40XZEpLS9P777+vIUOGaNCgQUYXe6GhoerevbukqqfmV199\\ntddlXeUzMzP10UcfqVmzZsb95eqrr9auXbu0adMmDRgwQKtXr/a6rOc564ILLjB+eh4VFaW//e1v\\n+uabb9S8eXO1bdvW67EpIiJC48aNU4cOHXTWWWdpypQpRnW7Fqvp0aOHNm/erD//+c9e95dBgwbp\\ntttuU05OjqKiovT6668b1d2nTx9t2bJFK1as0Lp164yCsaGhoXr99dfVp08frVq1Sueee67XZSMj\\nI5Wamqp169Zp7dq17hmu3jr//PN18OBBJSUlqbCw0LivSlUz3mJiYpSZmWk0tqxatUrjxo1T//79\\n9cMPPxh9bqlqluaXX3550qJM3p7DT5w4odGjR7sXe3vzzTeNrtd27NihkpISDR8+XP379zfqLz/8\\n8IN++OEHSb+OTf6SmZmpSy65RCEhIVq7dq1++eUXo/L/9m//dtL3Jg+pXONxmzZttH//fs2dO9do\\nTA4KCtKPP/7onmFpknf1iSee0IEDB3T06FHFxMQYv8ZcXl6upKQkXXfddVq/fr3Rcfbdd9/pzjvv\\n1KhRo1RaWmr82nsgPfLII+6vXUFGb3Xp0kVxcXF69NFH1bt3b/Xt29eo7oKCAu3Zs0dTp05VkyZN\\ndPHFFxuVt/HYY4+5v7766quN7ktGjBihuLg4TZ06VUVFRUZjaps2bRQdHa3jx49r1qxZxmNDhw4d\\nFBUVpZUrV6qgoECXXHKJUXnXQneZmZnatm2b+20Vb6SlpWncuHEqLy/XVVddZRzUlKpmSyYmJmrr\\n1q1GD9937typvLy8k647/OmWW27RsmXLFBkZqbPPPtuobEVFhebMmeO+J7r++ut91MqTNW3aVOHh\\n4QoKClJFRYWjCS42goODdeONN2r16tWKjY11nKPXCc9F19LS0vT888/7bdG1pk2b6qefflJUVJR6\\n9eqllStXel22efPm2r9/vz744AP3ApomHnrooZO+N7nuKSsrc9/vnzhxQuXl5UZ1l5aWKjMzUzEx\\nMXr44YeNzsGlpaU6ceKEIiMjVVxcrJKSEqO6+/btq++++04ff/yx0tPTFR0d7XXZTZs2adGiRSfF\\nFU106tRJR48e1dixYxUREWE0UXPp0qXur9u1a2d0/+9KE7Ns2TKtXbvW0bW9jcLCQhUVFalz584a\\nNWqUo1ngUj3LCR0eHq4FCxZo48aNmjt3rqMnudnZ2ZoxY4ZefPFFo8BDWVmZ5s+fr9zcXOMna1JV\\nfqU9e/ZoxowZmjJlilHdISEhWrhwoY4cOaLZs2cbL0RRVlamd999V0ePHtX27duNZhwWFRVp0aJF\\n2rFjhxYsWGC8zcvKyvTOO+/o6NGj2rBhg1H5Pn36qKKiQk8++aSWLVtmFMiVpN/+9rcnlTcJoI8a\\nNUqPP/64O6/UZ599ZlR3SEiIFi1apODgYON9lpeXp927d2vGjBlatGiR8dPQQ4cOqbS0VG+++aZm\\nz57tzuvljWPHjmnv3r1KTk42Pkakqs+dnJzsqK/26dNHYWFhSk5O1nfffWf8wCM0NNS6bldfMQm+\\n79ixQ6GhoUpISFC7du2MA0XXX3+9Kioq9Kc//UmFhYXGn9tzn5mOLX379lV4eLiefPJJrV69WpmZ\\nmUZ1u8YHJ2Nyq1atHI9rrm3eq1cvtWvXzuhBi/TreH7kyBFt2LDBqOyOHTsUFhamhIQEtW/f3njW\\n4rFjx9zHt5NjzHNM3bx5s9GT+1GjRmnkyJEaOXKkxowZoxUrVjhu+6RJk4za7jmef/3118av87rO\\ng0ePHlVycrJRfxk1apRGjx6t8ePH68EHHzQez4uKirRw4UJt3LhR8+bNM+rnnsdIcnKyUdkdO3Yo\\nPDzc3c9Nc74eOnRIW7ZsUVJSkhYsWGCcW9HV9u3bt2vhwoWOz9+7d+82HlsOHTqkzZs3KykpSTNm\\nzDA6jzVt2lTz5893X3eY8rxmMk1hsn37dquxycb27dvVpEkTJSQkKDY21rhu19jg5LrH9bl79+7t\\nqG7XNVOHDh20aNEi4+P7hRde0KxZs/Tss89aHd9z5841SmPSp08ftWvXTk8++aRWrFjhKC1GoHie\\nDx577DGj84FrbNm2bZsWLFhgdHxK0sGDB086vo8cOWLafMc8P/eIESOMAkWeY6rrPOytnTt3KiIi\\nwn2taHqMHDp0SPv371dSUpJmz55tPJ67tvk777yj1NRUo33mGs//7//+T1lZWcb93LW9R40apdde\\ne83oGPW8JzK97rDl2ubz58/Xa6+9ZtzPT70nMg1iO7Vjxw41adJEvXv3Vvv27f16HpJOjnk4uc61\\nUVRU5I4Vvf32245naTrhee2wYcMGo+vzY8eOKT093fE2e+KJJxyfvz37qen5V/r1czdr1szRfaTT\\n62vp5LbPmTPHqO2efcVJXNHzOnXmzJlGb0J5nod+//vfG+0vz/tQJ9dbtjzvQ3fv3m08g9ylXgWh\\nbRNp2yxOYLN4SG3UbZOk36btttvcdrvZLD5is3hJt27drBYnsNlnNn1FslvMwrZu275qs78DVbft\\n4kC2i+zY7jObum3GB5v9ZbvNA7kYVG0cY07bbjuuBbKv2fQX289t089tytr2NZtzgW3bJbv9bdN2\\n2+uOQI4PNmzrtlmUqTbGZNvje8GCBbVyfJumKbLp54FkMy7aji225W0E6nMHejy3LW/Tz23GFtvr\\nDhu22+zUcc00JZZTgTwPSYHdZ7bXLTZsrh1st5nNMVbf40xO225bt834YHMeCvTxbXuN7RKSaLrE\\nbQDFx8eruLhY559/vnbu3Knzzz/fKG9NeXm54uPj1alTJ/3000/q3bu311Pvu3btqqKiIrVq1UrX\\nXnutjhw5YvQ6kk3dcXFx2rhxox588EG99957uuWWW4w+t03bbbe5Td25ubm64IILFBISoq5duxrn\\n8bQpf8kll6isrMw9IyYyMtLo1RibfWbTVyTpsssuc7+uf/z4cf3hD3/w+rV727ptPrft/g5k3T17\\n9tSJEye0c+fOkxY58EfdNvvMtm6b8cF2XLPZ5rbjuU3dtseYTdttx7VA9jWb/mL7uW36ue051Kav\\n2ZwLbNtuu79t2m57fAdyfLBlU7ftcWJTd309vm37eSDZbDfbscW2vI1Afu5Ajuc25W37uc02t71m\\nsmG7zW2vc20E8jwUyH1me81lw+bawXab2Rxj9TnOZNN227ptxodAXm/Zsr1GdgmqrKys9EH7AAAA\\nAAAAAACoX+k4AAAAAAAAAAD1C0FoAAAAAAAAAIDPEIQGAABAg/LVV1/pnnvu0X333ac77rhD48aN\\nU35+vs/q++STT/THP/7R6m/s3LlTL774ote/X1ZWpgsuuMCqTgAAAMBfQgPdAAAAAKC2lJSUaPz4\\n8UpJSXEv7vPqq6/q448/1siRIwPcujO76KKL9NxzzwW6GQAAAIBPEIQGAABAg1FcXKzCwkL98ssv\\n7n976qmn3F+vXLlS8+fPV3h4uMrLy/XKK68oLi5O9913ny6//HJt2bJF+/fv14QJE7RkyRLt2rVL\\nQ4cO1ZgxYzR79mwdOHBAubm5ys7O1lVXXaVnnnnmpPq///57TZs2TWVlZSotLdXzzz+viy++WMnJ\\nyfrf//1fNW3aVE2aNNGrr76qVq1auculpqbq9ddf14cffqj77rtPV199tTZt2qT9+/frkUce0eDB\\ng7V371499dRTatq0qa688kp32ZKSEk2aNEk//vijTpw4oYEDB2rkyJGaPHmyzj77bI0ZM0apqama\\nMWOGPvzwQ+3evdtRGwEAAACnCEIDAACgwYiMjNQjjzyioUOH6tJLL9WVV16pfv36qVOnTpKk/Px8\\nvfbaa4qNjdW8efP0/vvv6+mnn5YkVVZW6p133tHs2bM1ffp0LV26VIcPH3YHoSVp9+7dWrx4sSoq\\nKnTbbbdp6NChJ9X/1FNPKSkpSR07dtT333+vCRMm6JNPPtEbb7yhFStW6JxzztGqVat0+PDhagO8\\nhYWF+stf/qK0tDRNnjxZgwcPVlJSkm6//XYNHz5c//jHP9y/u2jRIrVp00aTJ09WeXm57rrrLl1z\\nzTV68sknddddd+mWW27RlClTNHPmTIWEhNRaGwEAAABvEYQGAABAg/LAAw/ozjvv1OrVq5Wamqq7\\n7rpLTzzxhIYPH65zzjlHTz/9tCorK5Wdna2ePXu6y/Xq1UuSFBMTo27duik8PFwxMTE6fvy4+3eu\\nuuoqhYZWXUJ3795d6enp7p8dPXpU+/bt05/+9Cf3vxUUFKiiokJ33HGHRo8erX79+ql///4677zz\\nqv0MV1xxhSQpNjZWx44dkyTt2rVLDzzwgLsdLqmpqTp48KDWrVsnqWpm9E8//aQLL7xQiYmJGj58\\nuMaMGaNOnTrVahsBAAAAbxGEBgAAQIPyyy+/qFWrVho4cKAGDhyo/v37a+rUqbrzzjs1btw4/e1v\\nf1N8fLz+67/+S9u2bXOXcwWXT/3aU0VFhfvryspKBQUFub8PDw9XWFiY3nvvvX8p9+yzzyozM1Nf\\nffWVxo4dq6efflp9+/Y942fwrL+ystL9/+DgqnXFy8vLT6p37Nix6t+//7/8nSNHjigqKkpZWVm1\\n3kYAAADAW8GBbgAAAABQW1atWqVhw4apoKDA/W8HDhzQueeeqxMnTig4OFjt27dXcXGxvvjiC5WU\\nlBj9/XXr1qm8vFwlJSXaunWrLrjgAvfPIiMjFRcXp6+++kqStG/fPs2ZM0fHjh3T7Nmz1a5dOw0f\\nPlz33HOPtm7davzZOnfurO+++06StGbNGve/JyQk6O9//7ukqiD5lClTlJeXp5ycHL3xxhv66KOP\\ntHXrVqWlpfm8jQAAAMDpMBMaAAAADcb111+v/fv36/7771fTpk1VWVmps88+W88//7xatmypgQMH\\n6o477lBsbKxGjRql8ePHuwO43ujQoYMee+wxZWRk6LbbblPnzp21efNm98+nTZumyZMn6+2331ZZ\\nWZmeeeYZnXXWWTpx4oTuuOMORUVFKTQ0VC+99JLxZ3PNTv7ss8/Us2dP92zpe+65R7t379awYcNU\\nXl6uG264QS1bttRjjz2m3//+92rdurUmTZqkhx9+WP/zP//j0zYCAAAApxNU6Xq/DwAAAMAZzZ49\\nW2VlZXr88ccD3RQAAACgXiEdBwAAAAAAAADAZ5gJDQAAAAAAAADwGWZCAwAAAAAAAAB8hiA0AAAA\\nAAAAAMBnCEIDAAAAAAAAAHyGIDQAAAAAAAAAwGcIQgMAAAAAAAAAfIYgNAAAAAAAAADAZ/4f0yJd\\nmghlpQQAAAAASUVORK5CYII=\\n\",\n \"text/plain\": [\n \"<Figure size 1800x720 with 1 Axes>\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n }\n }\n ]\n },\n {\n \"metadata\": {\n \"id\": \"kbERWste0pfM\",\n \"colab_type\": \"text\"\n },\n \"cell_type\": \"markdown\",\n \"source\": [\n \"# Retrive the clusters\\n\",\n \"`fcluster` is used to retrive clusters with some level of distance. \\n\",\n \"\\n\",\n \"The number two determines the distance in which we want to cut the dendrogram. The number of crossed line is equal to number of clusters. \"\n ]\n },\n {\n \"metadata\": {\n \"id\": \"vscUQI1hKYHc\",\n \"colab_type\": \"code\",\n \"outputId\": \"aeef37c7-347a-408a-8a70-0c3e23397308\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 102\n }\n },\n \"cell_type\": \"code\",\n \"source\": [\n \"cluster = fcluster(Z, 2, criterion=\\\"distance\\\")\\n\",\n \"cluster\"\n ],\n \"execution_count\": 0,\n \"outputs\": [\n {\n \"output_type\": \"execute_result\",\n \"data\": {\n \"text/plain\": [\n \"array([4, 4, 3, 4, 3, 4, 4, 3, 4, 3, 3, 3, 3, 2, 3, 4, 2, 4, 3, 1, 4, 2,\\n\",\n \" 1, 1, 1, 2, 2, 2, 4, 4, 2, 4, 3, 2, 4, 2, 1, 1, 4, 2, 4, 2, 1, 2,\\n\",\n \" 3, 4, 3, 2, 2, 3, 1, 3, 2, 4, 1, 1, 1, 2, 4, 1, 3, 3, 4, 2, 3, 3,\\n\",\n \" 4, 2, 3, 1, 3, 1, 1, 1, 1, 2, 1, 1, 2, 3, 3, 1, 4, 1, 2, 4, 1, 2,\\n\",\n \" 4, 1], dtype=int32)\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n },\n \"execution_count\": 5\n }\n ]\n },\n {\n \"metadata\": {\n \"id\": \"jXxmbM1i7cVT\",\n \"colab_type\": \"text\"\n },\n \"cell_type\": \"markdown\",\n \"source\": [\n \"# Plotting Clusters\\n\",\n \"Plotting the final result. Each color represents a different cluster (four clusters in total).\"\n ]\n },\n {\n \"metadata\": {\n \"id\": \"VMAFl7wiOOGt\",\n \"colab_type\": \"code\",\n \"outputId\": \"23188b59-f7a1-42bf-d30b-0a30aaf7d1c2\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 483\n }\n },\n \"cell_type\": \"code\",\n \"source\": [\n \"plt.figure(figsize=(10, 8))\\n\",\n \"plt.scatter(X[:, 0], X[:, 1], c=cluster, cmap=\\\"Accent\\\")\\n\",\n \"plt.savefig(\\\"clusters.png\\\")\\n\",\n \"plt.show()\"\n ],\n \"execution_count\": 0,\n \"outputs\": [\n {\n \"output_type\": \"display_data\",\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAAlwAAAHSCAYAAAA5ThWFAAAABHNCSVQICAgIfAhkiAAAAAlwSFlz\\nAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4yLCBo\\ndHRwOi8vbWF0cGxvdGxpYi5vcmcvOIA7rQAAIABJREFUeJzs3Xl4FNedL/xvVW9Sq7W11FoQqyQk\\nFrGL3WCbgWCDje0YjJNx7NzxxBNP7CT3kuXNTOYmmSTz3OubN89NbjLxm8SxHduXOHhJvAO2sY3Z\\nQZhVQiAB2ve1pd5qef8QNFS6JZBU1d1qfT/zzPNE55Sqfj5hhq/POXVKUFVVBREREREZRox2AURE\\nRETxjoGLiIiIyGAMXEREREQGY+AiIiIiMhgDFxEREZHBGLiIiIiIDGaOdgE30traG+0SYkZ6uh2d\\nnf3RLiNmcDxCcUy0OB5aHA8tjocWx0NrpOPhciWHbecM1xhiNpuiXUJM4XiE4phocTy0OB5aHA8t\\njoeW3uPBwEVERERkMAYuIiIiIoMxcBEREREZjIGLiIiIyGAMXEREREQGY+AiIiIiMhgDFxEREZHB\\nGLiIiIiIDMbARURERGQwBi4iIiIigzFwERERERmMgYuIiIjIYAxcRERERAZj4CIiIiIymFnPm+3Y\\nsQNvvPFG8OfTp0/j+PHjwZ9nz56NhQsXBn9+7rnnYDKZ9Cxh7FBVCH8+A/HdC4A7ABSkQ358ETA1\\nPdqVERERkc50DVxbtmzBli1bAACHDx/Gu+++q+l3OBx44YUX9HzkmCX++BOYfnsMgqQONHxaA+HT\\nGkh/uAcozohucURERKQrw5YUf/3rX+Of//mfjbr92FbXA9OfzlwLW1eIVZ0w/fpwlIoiIiIioxgS\\nuE6ePInc3Fy4XC5Nu9/vx7Zt2/Dggw/i2WefNeLRY4L4ViWEDk/YPuFUi3EPPt0C4Q/HIRyuN+4Z\\nREREFELXJcWrXnnlFdx3330h7d/5znewadMmCIKAhx56CKWlpZgzZ86Q90pPt8Nsjq99Xr7cFHgH\\n6TM7rEh3JQ/6u64h+gaj9vvR/8jrkHZeAPoCgM0E0+opSHzuPphyhn+/WDKS8Yh3HBMtjocWx0OL\\n46HF8dDSczwMCVyHDh3C97///ZD2L3zhC8H/vGzZMlRWVt4wcHV29uteX9Stz4c5Px1idWdIl3/J\\nBLS29ob9NZcredC+oZi27YLptfJrDT4Z8u5q9PzjXyE9e8+w7xcrRjoe8YxjosXx0OJ4aHE8tDge\\nWiMdj8FCmu5Lis3NzUhKSoLVatW0V1dXY9u2bVBVFZIkoaysDNOnT9f78WNDghnyv94CJe/afymq\\nWYCyLh/Kt1fq+yyfBOGTy2G7hE9rgAb+HxcREZHRdJ/ham1thdPpDP7829/+FosXL8aCBQuQk5OD\\nzZs3QxRFrFmzBnPnztX78WOGurEI0opJEF84CfT4oC6eAPVzBYAg6Psgtx9CV/gFTKHXD6GhB+oE\\nTiETEREZSVBVVb3xZdHD6c1rRjS9qagwb3gJ4mfNoV1TUiHteQSwW3SqMLI4/R2KY6LF8dDieGhx\\nPLQ4Hloxv6RIMUYUoHxhDtQE7WSmahKg3D9zzIYtIiKiscSQTfMUW5RH5kFNNEP88xkItT1Qs5Kg\\n3l0E5SsLb/zLRERENGoMXOOE+sBsyA/MjnYZRERE4xKXFImIiIgMxsBFREREZDAGLiIiIiKDMXAR\\nERERGYyb5seLPj+E18oBswnqvcVAIo+DICIiihQGrnFA/H0ZxKePQazrAQAovzwE5ZtLoWwtiXwx\\n/QGI//cU0OmBunwS1FsmR74GIiKiCGPginPC4XqY/uc+CL3+YJt4sQvCjz6BsjAXmJ4RuVo+ugTT\\nv3wY/Gi3aj0CZW0+5Kc3AlZTxOogIiKKNO7hihPCXytgemAHzIt/B/PnXoT4/x4AFHXgsNPrwlbw\\n+g4PTC+eilyBfhmmH3wUDFsAIPhlmN45D/GpfZGrg4iIKAo4wxUHhNfKYf7ObgjuwEBDbQ+Ek80Q\\n2vqBbt/gv9gT/qPWRhD+UgHxXHvYPnFfDZSIVUJERBR5nOGKA+KLp66FrSsEAOKb56DmOgb9PbU4\\n0+DKrqun0zN4Z58UsTqIiIiigYFrrFNUiBc7wnYJbR6oBelQZoUGK2VBDpRH5hld3bXnbSyCmp4Q\\ntk8NUx8REVE84ZKikVr7Ib5wAugLQF05CertUwFB0PcZogA1NRFCY19Il2ozQZ2XA+nZqTD9/CCE\\nssaB60snQP5/Vkb2aIiJKZC3zILp92UQrls/VCanQP7nxZGrg4iIKAoYuAwivHoW5h/vhdDkBgCo\\nvz0G5XMFA2/kWfR9I0/5u6kQK9pC2tWlE4H5OQAA+Rd3XNehQjjWABxvgrp6CpAQmT8Gyo9uA/LT\\nIeyqgtDjg1rohPzYImCWKyLPJyIiihYGLiP0+mD6j0+DYQsAhIAC09vnof7iEJRvrdD1ccr3VkFu\\n80DceQFClw+q1QR1SR6kn60NvfhwPcw//hjC8SYIkgplWhqUL8+D8k+lutYUliBA+fJ84Mvzh/+7\\nsgK09AEpCUASD20lIqKxhXu4DCD+31MQ63vD9gn7avV/oFmE/L/XQ35oLpQpqVAzEqGaBAgnmrXX\\n9Qdg3rYL4pFGCJI6UOvFLpj+xz4I757Xvy6diL8vg3n9i7As/wMsK/8A05PvAr1DvH1JREQUYzjD\\nZQTP4G/dCT5j3sgT//0TmJ4+CkG90tDohljWCCmgQP38zIFrXjoJ8XzoBnvBI0F8tQLyndMNqW00\\nxO2nYfrxJxB88kBDkxumHWchdHuBdx+ObnFEREQ3iTNcBlDuKICabA3bp5Zk6f/ALg9Mf6m4Frau\\nEHr9EP948lpDc+jG+qCOfv3r0oHwytlrYev69r01kI7UR6EiIiKi4WPgMsIMF5TNs6D+zegqxRmQ\\nn9D/jTxhfx2ERnfYPrGqA/APBBZ1pgvqYC9JTk7Vva7REt6vhljWGL7PI0E+VBfhioiIiEaGS4oG\\nkf9jDdSZmRDevwj0+4EZmZC/WgpMTNH9WerkVKg2U9iZIDXVBlgGkp96bzHUF05AOKidGVKzkyD/\\nlxFsZDeQsKsK5m+8B2GQ5VnVZoJpQU6EqyIiIhoZBi6jCAKUh+cBD0fgcNGSLKjLJkL4+HJIl3rb\\n1Gtnf5lESL/fBNO/fwLhYC0ErwSlJAvKV0uBebEVXsRnP4PQOfinh9QVk2BeOQVoDf9yAhERUSxh\\n4IoT0lNrYf6vOyEcaYAQUKAmmqBMS4eyIGfgSAXTlfXNTDvkX94BKCogKYBV3zPB9CJcCH96PgDI\\nJS7I/+fOCFZDREQ0OtzDFS+mpEF69QFIz2yCMjMTkAHT2TaYn3gX5o3bgfJW7fWiELNhCwCQagvb\\nrJoEKP99NZBpj3BBREREIze+Z7jc/oFP73T7oC7N0y6/jUWCAPGjSxDLr506L6iA8FkTzN/7ANLr\\nW0P/+cpbYXrxJNDlgzo1FcpXFgJpiREuPJRy+1SIp1tD2tVFuVBXTYl8QURERKMwbgOX8H4VTN/f\\nA/FSNwBAtYhQ1kyD/Nu7ANsYHRZVhbC3JmyXcKwRwpEGqEvyrrW9chbm//4RhA5PsE15+wKk398N\\nFDoNL3coyndWQm50Q9xZBaHXD1UE1IW5kJ5aO7ZDMRERjUtjNFmMkk+C6d/3BsMWcOXTOzuroD61\\nH8q/rY5icaOgAoI7/AnsQkAB6nsAXAlcfhmmXx7ShC0AECvaYPrZfshP32VwsTdgMUH+1QbI59og\\nfnIZ6pRUqOsKGLaIiGhMGpeBS/hLBcTK9rB94v4aKBGuRzeiAKUoE6bG0ANO1VwH1NunBn8WPrgI\\nsTL8xnThWCOgqrERboozoRRnRrsKIiKiURmfgatr8OMG4Ak9y2osUf5hAcRTzRA6rv0zqiYB8uaZ\\nN783KwZyVsScboHp/zsG4UIH1GQr1L/Lh/LYwtgIm0REFDfGZeBSNhZB/cXhkOU0AFBnje3ZFHV9\\nAaT/3AjxjycgXOqC6kyEeud0KP+gPdhU/btpUIoyws70qYsmjI/AcaIJ5n98E2JtT7BJ3VsD4WIn\\n5P+xNoqFERFRvBmXgQsTUyA/MAum35VBkK99gFCZkgr5a0uiWJg+1NumQr5t6tAXWU2Qv7EEwg8+\\ngtB23ab5GZmQv73C2AJjhOnpY5qwBQy81Sm+XgH5sUVAfnqUKiMiongzPgMXAOUHtwKFTgg7q4Ae\\nH1DohPxPi4CijGiXFpZwtB79r5+DqbYbyEuB/F/mAUWjm41T75+FwJxsmF44CXR6oE5Lg/KVRUBK\\n+DOw4o1Q0Ra+vdsHcWcVlMdLI1wRERHFq3EbuCAIUB6aCzw0N9qV3JDwajnM3/8QgU4vrh5VKu68\\nAOmXd0C95W/OpFJUoLoTsFuACck3vnlRBuQf3657zWOC3TJol+qM/llkREQUP3jSfKyTFZh+cyTk\\nu4JCgxvi/zmibXv1LMx3vAjL6mdhWfkHmLfuAM6EHh5KA5TVk8O3F2dAvW9GhKshIqJ4Nn5nuMYI\\n4WQzhDAnrgOAeLIZco8PSLFB2F8L8/f3XAtmHgnCxzUwP/E2pHf+HkgcfDYnFgh/PQfxlTMQmvqg\\n5jqgbC2BunG6oc9U/ttyyBe7IL5XBcErDbQVpEP+0W2x/dkjIiIacxi4YpyaaAYsIhAIczqYWQx+\\nlFrcfjpkFgwAxPJ2iC+dgvKPC40udcTEPxyH6Sd7IfQHBhpOtUDcXwu52wvli3OMe7DFBPnpu6Ac\\nrYfwSQ3gTISydXbMh1MiIhp7GLhiWX0PTNtPA0kWoCv0BHm1dMJAHwA0uwe9jVDXM2hf1EkKxBdO\\nXgtbVwjuAMTnT0B5sGTgQ9sGUkvzoJbm3fhCIiKiEWLgilUVrTA/+ibEqs6w3cosF6R/ueVaQ+7g\\nG+TVKWl6V6efS12Dvy1Y0QY09gJ5KaN7hqpCeO8CxN3VAABl9RSom4oND3JERERXMXDFKNP/PhQ2\\nbKkOC+Qnl0L5ykLNW3bKl+ZA/KBac6YWAChzsqB8scTwekcsNQFwWIFef2hfshVIHuURFaoK07d3\\nDyy5XjlzTdx+GsquKsi/2sDQRUREEcG3FGOUcKI5fLs7ADXLHnKkgVqaB+l/roO8dAJUuxlqegKU\\n9fmQfrMRsMVwrnbZoa6cFLZLWT5p1GeCCburIP7ptOaAW0EFxNcqIPz5zKjuTUREdLNi+G/icc48\\nRBYeZFO3unE65A2FkFv6BkJWWoJBxelL+skamDu9EI7UQ1AGvv2oLpsI+adrRn1vcfdFCJIa0i4A\\nEPfWQH4whmf/iIgobugauA4dOoRvfOMbmD594HX+oqIi/Nu//Vuwf//+/fj5z38Ok8mE1atX42tf\\n+5qej48r6pI84HxHSLtSlDH0cQmCAGQ7QptfL4f4egWEdg/UyalQHp4LdXn4maWIm5gC6S9bIbx7\\nAUJlO9RZmVDXFejzPUclNGwFqUP0ERER6Uj3Ga4lS5bgl7/8Zdi+n/zkJ3jmmWeQnZ2Nhx56COvX\\nr0dhYaHeJcQF+Xu3QKhsh3ikIdgm5CVD/u4KwDK8M6LEXx2G6X/th+CTBxqONULcexnSz9dD/VyB\\nnmWPnCBA3TAd6gZ9z95S1kyDuP0UhDCnaigrYiRwEhFR3IvYkmJtbS1SU1ORm5sLALj11ltx4MAB\\nBq7BZNohvfbAwGbv8jaoqTakfesW+Ib731h/AOKLJ6+FrSuENg/E35VBjpXAZRB1QyGUzbMg7jgL\\n4cqElgpAuWs61C9wOZFGRlEUnDlzCi0tzXC5XCgpmQdR5JZYIhqc7oHrwoUL+OpXv4ru7m488cQT\\nWLlyJQCgtbUVTqczeJ3T6URtba3ej48vFhOUh+cFfzS5koHW3mHdQjhYC/FSd9g+sbwNslcCEuJ4\\nK58gQP7FHVBunQrxo4uACigrJ0F9YHbw0Fii4eju7sLLL7+ImppLwbYDBz7Fli1fhNOZEb3CiCim\\n6fo37dSpU/HEE0/gzjvvRG1tLR5++GHs2rULVqt1xPdMT7fDbOZnVq5yuW7ig9TXkQpd6BvkpHox\\n2YrMCakQxnDwuOnx+OqSgf8dB4b7ZyTe6T0er776kiZsAUBt7WXs3v0WnnjiCV2fZQT++dDieGhx\\nPLT0HA9dA1d2djY2bNgAAJg8eTIyMzPR3NyMSZMmISsrC21t1w64bG5uRlZW1g3v2dnZr2eJY5rL\\nlYzWYc5wYZID5tIJEA/UhXRJS/LQ1tGnU3WRN6LxiHMcEy29x8Pj8eDcucqwfZWVlbh4sQEOR+z+\\nhcU/H1ocDy2Oh9ZIx2OwkKbr1MYbb7yBZ555BsDAEmJ7ezuys7MBABMnToTb7UZdXR0kScKePXuC\\ny41kIEGA9OPboJS4gk2qSYCyahLkf78tamURjUV+vw9+f+hntgDA5/PB4/GE7SMi0nWGa82aNfjW\\nt76FDz74AIFAAD/84Q/x1ltvITk5GevWrcMPf/hDbNu2DQCwYcMGTJs2Tc/H02BKsiG9+/cQdpyF\\nUN8DdU421PU6HbtANI4kJ6cgOzsX9fWh+09drmykpaVHoSoiGgsEVY3tw4g4vXkNp3u1OB6hOCZa\\nRoxHWdkRvP32X+HzeTXtoijC6czA7NlzsXbtHRBi8F9o+OdDi+OhxfHQ0ntJMY5fTyMi0t/ChYth\\ntyehrOwILl++iL4+N4CBoyLa2lrx8ccf4Pz5SgAqVFXBxImTcfvt65CSkhrdwokoqsbu62lERFEy\\nY8YsfP7zW2EyhX+DuqGhFg0NdWhsbMCRIwfx4ot/CJkRI6LxhYGLiGgE2tpa0dMT/oy7v9XQUI99\\n+/YaXBERxTIuKdI1sgJhx9mBIyQsJigbp0O9fWq0qyKKSRkZGUhKcgSXFG+kpaXJ4Ir0I8syFEWB\\nxWKJdilEcYOBiwYEZJgffRPCripc3eor/vkM5H9YAOWHt0a1NKKR6u3txe7d76KtrQUJCYmYP38R\\npk3T53NWiYl2FBfPRFnZkZu63maz6fJcI3V2dmDXrndw+fJFyLKMvLyJWL36dkydGt+fACOKBAYu\\nAgCIvy+DuKtK0yb4ZZie/wzqXYVQF03gMRI0pnR0tOPll/+I+vr6YNupUyewdu16rFixWpdnbNp0\\nPwRBwLlzFXC7e+BwJMPj6Ycsa79darFYMW/eIl2eaRRJkrB9+x/R0HDtkOTKygo0Nzfhy19+DC7X\\njQ+qJqLBMXARAEA4UB++3SPB/MArUHOSod4yCfIPbgOSuMxA+lAUBR5PP6xWm+7LV3v27NaELWDg\\n4NJ9+z7BokVLYLMljPoZZrMZ9933ADweD7q7O5Ge7sRnn5Xhk08+QHf3wP6u5OQUrFixCvn5sT1L\\ndPToIU3Yuqq7uwsHDnyKTZs+H4WqiOIHAxcNGGLySuiXIFR3AtWdEOp7Ib14H2e7aNQOHdqPY8cO\\no62tFXa7HQUFRdi48Z5RfXv1enV1NWHbu7u7cOLEcSxZslyX5wBAYmIiEhMTAQBLl67AvHkLcPz4\\nMaiqgnnzFiEpKUm3Zxmlra110L6uro4IVkIUnxi4CACglk4Adlbd8Dph72UIe2ugrp4SgaooXpWV\\nHcF7772JQCAAYGDm6dixQ/B4+vHFLz6iyzMEYfCXsAc7zkEvCQmJWL78llHdQ1EUSJIEi8USkUNU\\nHQ7HoH12e+wHRqJYx8BFAADlsYVQ9tdC3HNpyOsEvwKhrJGBi0bl+PGjwbB1vQsXzqGxsQG5uRNG\\n/YzJk6eEfTPQ6czA3LkLRn1/owQCAbz33pu4cKESXq8HmZlZKC1digULSg197pIlK1BWdgQdHe2a\\n9oSEBCxcuNjQZxONBwxcNMBmhvTHeyG+dArC4XoI+2shNvWFXKYCUCemRL4+iivd3Z1h2/1+Py5f\\nvjjqwKUoCtauvQPt7S24ePFisN1uT8Jtt62N6eMOXn31Tzh9+kTw576+i2hsrIfZbMGcOfMMe67d\\nbse9927B7t3voK6uFqqqIisrG8uXr0J+fqFhzyUaLxi46BqLCcqX5wNfng/xxVMQvvc+hICiuUSd\\nmwX13hlRKpDihcORgo6O0H1BJpMJubl5I7qnoijYs2c3ystPo6+vD+npTqxcuRwzZ85FXV0N3O4e\\nFBQUx/TsVmNjAyory0Pa/X4/jh07ZGjgAoD8/EI89tiTqK2tQSDgw9SpBYYvvxKNFwxcFJby0Byg\\nsQfin85ArO+FahGhLsyF9NPbATM/UECjM3v2XNTWXoaqqpp2QRBw+vRnmDhx0rD/on/nnb/i4MF9\\nwZ97e3vQ2FiP/PzpqK+vg9vdg+rqKhw/fhRr165HSYmx4WUkLl2qgt/vD9vX2RmZjeuCIGDyZG4Z\\nINIbAxcNSvn2SiiPL4aw5yKQ6+BZXKSbFStWwePx4MCBvZpvDEqShAMHPgUAbNx4703fr6+vD6dP\\nnwppDwQCOHfurKatra0F77zzBqZMyUdycvII/wmMkZWVA1EUoShKSF9S0uCb2oko9nGqgobmsEK9\\nuxhqaR7DFulGEASsXn07EhISw/ZXVJwZdKYnnKtLhjerp6cbR44cuOnrIyU/vxBTpkwLaRcEAbNm\\nzYlCRUSkFwYuIoqK3t4e9PR0he3r6uqC29170/fKyMiE1Tq8T+d4PJ5hXR8JgiDg85/fiunTi2E2\\nD2zsT01NxS233IaVK/U5HZ+IooNLikQUFcnJKUhNTUNXV+gbi2lpaXA4bn65LzPThfz8AlRUnL3x\\nxVfk5U266WsjKT3diUce+QpaWprR2dmBKVOmISFheKfiy7KM3t4eJCbax8Q3HInGAwYuIooKq9WK\\nmTNnB/dsXW/mzJJhnzh/771b8Prrf0Z1dRUCAT/s9iTMnDkDly5dRnt7m+ba/PwCzJ07f1T1Gy0r\\nKxtZWdnD/r29e/fg+PFj6OhoQ2KiHYWFRbj77vuGPQNIRPpi4CKiqLnjjrshCALKy0+jq6sLaWlp\\nmDmzBOvX3zXsezkcyfjSlx5FU1MjWlqaMHnyVEyfPhlnzpzHxx9/iIaGOphMZkydOg1r194JUYy/\\nHRUHD36K3bvfDW667+3twfHjR+Hz+fHFLz4c5eqIxjcGLiKKGpPJhA0b7sHatXfC7e6Fw5E86m8p\\n5uTkIicnN/hzVlYOtmz54mhLHRNOnvws7BuOFy6cQ3NzE1yu2Hork2g8YeAioqizWq1wOjOiXUaQ\\nLMs4fPgALl2qBgBMm1aAxYuXxfwhoN3d4V9C8Pt9qK29jJKS6RGuiIiuYuAiIrqOLMvYvv2PqKg4\\nE2w7c+Ykqqsv4MEHvxQzS5GyLKOrqxN2ux2JiXYAQEpKatjQZbFYMXHi5EiXSETXYeAiIrpOWdkR\\nTdi66uzZUzhxoizsR6S9Xi9qai4hLS19RBvdh2vv3o9w/PgRtLa2wG63Iz+/EHfd9XmUlMxDfX1t\\nyLJiQcF0zTIrEUUeAxcRGa6/vx+HDu1DT08P0tLSsGzZSthswzvqIFIuXbo4aF919QVN4FJVFbt2\\nvYOTJ8vQ3d0Ni8WKqVPzsWnT/UhPTzekvqNHD+L999+FLMsABk7ZP3XqBLxeHx5++FH4/T6cOFGG\\ntrZW2O1JKCiYjk2b7jekFopNHb52XOg7BwhAsWMWUi1p0S6JwMBFRAarqbmMV1/drjma4bPPjuGB\\nB/5+xB+qNpIoDv5FBVHU7uHat+9jfPrpR8FvQgYCfpw/X4G//OXP+PKXH4NgwNcZTpw4Hgxb17t4\\n8QJqay/j9tvXYdWq29HR0Q6HIxl2u133Gih2HejYi9M9n8GvDnyp4WTPccxPWYTS9GVRroxiYzMC\\nEcWt999/N+QcrNbWFuze/W6UKhpacfGssEFJFEXMnDlL03bmzKmQD3ADwKVL1bh4sdqQ+rq7u8O2\\nS5KE+vpaAIDZbEZWVjbD1jhzse8CPus+FgxbAOBTvDjWdQiN3vooVkYAAxcRGaizswM1NZfC9tXU\\nXEZ/f19kC7oJs2fPwaJFSzRvJJpMJpSWLkNxsTZw9fW5w95DlmW0tDSFtKuqivLy03j77b9i1663\\n0dbWOuz6UlNTw7ZbLJaYPT2fIqO6/wIUhM5+SpBQ6a6IQkV0PS4pEpFhZFkOey4UACiKMmhfNAmC\\ngHvu2YySkrkoLx/4VNCsWSUoKAg9UiEtzYmOjvaQdqvVhqlT8zVtsizj5ZdfRHn56eCs2JEjh7Bm\\nzTosX77qpuubN28hamouhSwrTptWiMmTp970fSj+SGpgiD4pgpVQOAxcRGSYjIxM5OVNRG1tTUjf\\nhAl5w/peYiQJgoDCwmIUFhYPeV1p6RLU1l5GIODXtBcXzwh5K/DTTz/G2bOnNG0eTz/27HkfM2fO\\nQVrazW1sLi1dCp/Pi7KygbcUExPtKCiYjrvvvu+mfp/C6/C1o8nXAJctGy5bVrTLGRGXNQcX+irD\\n9uXY+JZqtDFwEZFhBEHALbfchjfeeE2z/JaSkorVq9dEsTJ9zJ27AJIk4ejRQ2hra0ViYiIKCopw\\n5513h1xbXX0h7D36+/tQVnYEa9asu+nnrlx5K5YtuwXd3V2w2+1ISEgc8T/DeCcpAbzf+h5qPJcQ\\nUP0ww4y8xMlYm3kHEsxja1znpszHpf4qNPq0+7UmJUzBzOSSKFVFVzFwEZGhZs+ei/T0DBw5cgBu\\ndy+Sk1OxdOkKZGfnRLs0XSxcuBgLFpQiEPDDbLYMejCqooTurblKloe/3GMymWLqdP6x6pP2Pajq\\nvzYrJEHCZU81Pup4H3dkhQbnWGYWLdiYfR+OdR1Cs78JAoBcWx4WpS2BKHDLdrQxcBGR4SZMyMM9\\n92yOdhmGEQQBVqttyGsmTMjDxYtVIe0WiwUzZ3L2IRoCSgC1nkth+2o9l9Ev98NuGltvetpMNqzI\\nWK3LvdySG2d6TsCv+JFly0aRY6YhR52MFwxcREQRsHr1Gly6dDF4dAMwENTmz1+EiRP5dmE0eCUv\\nvLInbJ9f8cEt9Y65wKWX8+5z2Ne+B33KlTeJe4EK9xlsyL4XFtES3eLGKAYuIqIISEpy4JFH/hH7\\n9n2MxsYGWCxWFBXNwMKFi6Nd2riVZElCmjUdbf7Q4zlSzGlwWpxRqGp4OnztONd3BrIqY1LiFExO\\nnDbqWShJCeBQ56fXwtYVdd6zrnRpAAAgAElEQVQaHOz8FKsybh/V/ccrBi4iGhP8fh/Kyo7A7/dj\\n1qw5yMx0RbukYbPbk7Bu3YZol0FXiIKIYsdsdHTs1ZxfJUBAUdIMmGN8Jqes6wjKug7Bp/oADJwq\\nX5hUhLWuDaPas1XZV45uKfQj6ADQ6K0b8X3HOwYuIoq6zs4OfPrpx2huboTVasP06cVYtmxl8N/U\\nT548jt2730VnZwcA4JNPPsSCBaXYsOEe7imhUZmfughmwYRKdwV6pR4kmRwoSJqO+amhHymPJV2B\\nThzrPgT/lbAFACpUnO87h2xbLualLhrxvQPK4Od5yWrsnZ03VjBwEVFUdXR04IUXnkFra3OwrbKy\\nHC0tTbjnns3o6+vDzp1vaT5p4/V6cfDgPmRn56C0lN+Io9EpSZmPkpT50S5jWCp6T8Ov+ML21Xlq\\nRhW4pifNwLGuw/Ao/SF9Y/WMsljAwEVEUbV374easHXViRNlWLJkBc6dOxv2+4GqqqKiohzz55fi\\n4MFPUVNzGaIoIjs7GyUl88fkkiPRzVIw+EyTrA5+BMnNsJuTUJI8D8e6D2uWWtPN6ViUunRU9x7P\\nGLiIKKqamhrDtvv9fpSXn0YgMPjyhtfrwUsvPYvz588F206fBj74YBemTJmGzZs/j/R0nrBN8Wdy\\nYj5Odh+HHObbiS5b9qjvv8S5Ak5rJqr6zsGn+pFucWJ+yiIkW1JGfe/xiiehEVFUmc2D/3uf1WrF\\n1Kn5QxwmqmjC1vUuX76I559/Hm53ry51EsWSiYmTMN0xI6Q925qLhan6vPla6CjC+uy7sSnnfqzK\\nuJ1ha5QYuIgoqsJ9FBoAUlPTUFq6FEVFM1BcPCukPzMzC1ardch7t7W14eDBfbrUSRRr1mSux60Z\\na5FvL8SUxGlYlLoUd+fcD5spIdqlURhcUiSiqFq16nY0Nzfi7NnTkOWB5ZGUlFSsW7ch+I3ArVsf\\nwkcf7UZ1dRUkKYDc3DysWnU73n//vRvev7e3x9D6iaJFEASUpMxDScq8aJdCN0H3wPXUU0/h2LFj\\nkCQJ//RP/4TPfe5zwb41a9YgJycHJpMJAPCzn/0M2dmjX2smorHLZDJh69Yvobr6AqqqKmGxWLF4\\n8XIkJSUFrzGbzVi79s4R3T8tLV2vUomIRkzXwHXw4EGcP38eL7/8Mjo7O3HfffdpAhcA/O53v9P8\\nP1IiIgDIzy9Efn7hTV/f3Nw06P6tq3JycrB8+S2jLY2IaNR0DVyLFy/G3LlzAQApKSnweDyQZTk4\\no0VENBrV1Rdw+PABdHZ2oL/fDZ/PG/Y6URRRWFiEBx7YHFyWJCKKJl0Dl8lkgt0+8KHPV155BatX\\nrw4JWz/4wQ9QX1+PRYsWYdu2bTwlmohuSkXFGbz++p/R19d3w2sLCorw8MP/CJcrGa2tfEuRxg+/\\n4gcAWMWhXyihyDNk0/z777+PV155BX/4wx807V//+texatUqpKam4mtf+xp27tyJO+64Y8h7pafb\\nYTZzhuwqlys52iXEFI5HqHgdk5deOnhTYQsAiooKguMQr+MxUhwPrXgZjwZ3A/bU70F9Xz0AIC8p\\nD7fl3YY8R96w7hMv46EXPcdD98C1d+9ePP300/j973+P5GRtoffee2/wP69evRqVlZU3DFydnaGf\\nFhiv+G/rWhyPUPE6JrIso7b25j6am5c3EfPnL0Nra2/cjsdIcTy04mU8+qV+vNb4Z80Hp893n0dL\\nXys+n/sF2M32m7pPvIyHXkY6HoOFNF0DV29vL5566ik899xzSEtLC+n75je/id/85jewWq04cuQI\\n1q9fr+fjiShOCYIw6JlbgiAgN3cCzGYL8vIm4dZb/w6Jidy3Rfrpl/pxtOsAmn1NECEgO2EClqQt\\nh9Vki3ZpAICTPcc0YeuqbqkLJ3uOYZlzVRSqor+la+B655130NnZiW9+85vBtqVLl6K4uBjr1q3D\\n6tWrsXXrVthsNsyaNeuGs1tERMDAJvhp0wrQ0dEe0jdlyjQ8+ujj3A9KhvArfrzd/Dpa/E3BtiZ/\\nI1r9zdiUsxkmIfpbXnqlwc+aG6qPIkvXwLV161Zs3bp10P5HHnkEjzzyiJ6PJKJx4s4770Z3dxeq\\nqy9AUQY+3Jubm4cNG+5h2CLDnOg+pglbVzV461DeezomDh1NNA2+ZDhUH0UWT5onojEhISERjzzy\\nFZw/X4H6+jqkpqZj3rwFPHaGDNXubxu0r8XXBCD6gWtOygJccJ9Dn6J9qSRJTMKclAVRqor+FgMX\\nEcU0r9eL+vpapKc74XRmoKhoJoqKZka7LBonLKJliL7YOHoh1ZKGWzPX4WjXweBsXJY1B6Vpy5Bq\\nSbvBb1OkMHARUUxSVRXvvfcWTp/+DN3d3bDZbMjPL8Q992yGw8FX1ykyCpNm4Ly7AjJkTbtVsGKG\\nY3aUqgo1LakAU+35aPO3AlCRac3iUnuMEaNdABFROB9//AH27fsY3d3dAACfz4fy8jN47bWXo1wZ\\njSdT7FOxKG0pEsRrb77axSQsSV8Jly0ripWFEgQBLlsWXLZshq0YxBkuIopJ5eWnw7ZfvFiFhoY6\\nTJgwMcIV0Xi1OH05ZjpKUNlXDkEQMCNpNhJv8mwroqsYuIgoJrnd4Q8cDAQCaGxsYOCiiHJYkrEw\\nbUm0y6AxjEuKRBST0tKcYdsTEhIwZUp+hKshMpZH7ke7rw2yKgEY2MPY6K3DOfdZeCR+cSUecIaL\\niGLSggWLUF9fC0mSNO3FxbOQmZkZpaqI9OWVPPio433UeWrgU7xIM6djUuIUtPlb0eRrhAoFdjEJ\\n0x0zsNJ5K/dmjWEMXEQUk0pLl0GWFZSVHUFHRzvsdjumTy/GHXfcHe3SiHTzftu7uOy5GPy5S+pE\\nV2+n5pp+pQ8neo4hyeTAgrTSSJdomA5fG073nkC/3I9kczLmpCxEiiUl2mUZhoGLiGLW0qUrsGTJ\\ncvh8XlgsVh5ySnGlyduIOk/NTV9/sf9C3ASuKvd5fNzxPjzyteXSqv4LWOe6E7kJeVGszDjcw0VE\\nMU0QBCQkJDJsUdxp9TWHnO81FK/sMbCayFFVFWXdhzRhCwB6pW4c7ToYpaqMx8BFRERxTVVV1Htq\\nUd13HpISiHY5QTkJE2AexkJTvJwa3+ZvRYu/OWxfs68JPsUX4Yoig0uKREQUtxo8tdjf8Qmar3zy\\nJtWchpKU+ZifuijKlQEuWxYm2afiYv+FG15rExNi4kPZehCu/I8KNUzfQH88YuAiIqK45Ff8+LBt\\nN7qla5vQu6UuHOrYh1RzKlyu6H/YeW3mHfik/UPUeWvgk71ItaajOGkm3LIbdZ7L8Ck+pFsyMCdl\\nHqbY4+M4lAxrJrJtOWjyNYb05dgmwBoj36jUGwMXERHFpTM9JzRh6yoJAZxzl2MJoh+4rCYb1mbd\\niYASgE/xwm5KgigM7PaRVRlne06hLdCCBm890i1OpFszolzx6AmCgMVpK7CnbRfc8rUDjtPN6ViS\\nvjKKlRmLgYuIiOJSvzz4gaFeJfwGdFmVEVACsIm2iJ55ZREtsIiW4M8+2Yt3mv+KBl9dsK3CfQbL\\n01dhdsrciNVllMn2qbh/whdwquf4lWMhUjE3eT4SzIk3/uUxioGLiIjiUoZ18ANyU8zaDeiSEsCn\\nHXtQ67kMr+xFusWJmclzohZuDnXt04QtAPApXhztOoTpjhlxsezmMCdjuXN1tMuIGL6lSEREcanI\\nMRO5ttAznRwmB+akzNe07W59D2d6T6FH6oFf9aPZ34RP2/egovdspMrVaPKG7m8CALfcg3Pu6NRE\\no8PARUREcUkURNyRtQnFSbOQYk5FkikJUxLzsc61AS5bVvC6Vl8Laq477f0qCRIq3KcjWXKQCmXQ\\nPkW9+bO7KHZwSZGIiOKW3WzH2qw7oaoqVKjBDenXa/I2QFLDn8/VK/WGbTdaljUHbf7WkHa7mISi\\npJlRqIhGizNcREQU9wRBCBu2ACDDlgkR4b9kYDfZjSxrUKVpy5BhcWnaTDBjTsp8JJqjUxONDme4\\niIhoXJuQMBETEiaizntZ0y5AQIF9OgAMOUNmhGRLCu7N2YLPeo6hM9AOq2hDYdIMTLFPjcjzSX8M\\nXERENO6tdd2Bj9p2o95bh4DqR7I5FUVJMzAree5Au6cWfsUPpzUDc1IWIj+pwPCaEsyJWOa8xfDn\\nhONTfKjuO49EUyKmJOZH9IiMeMXARURE416S2YGNOfehJ9CNXqkHWbYcWEQL3mp6HZc91cHr+r19\\naPO3wCzchcn2KVGs2DhHOvfjbO/p4KGkLms2VjhvxcTESVGubGzjHi4iIqIrUiypyEucBItoQZ2n\\nBrWeyyHXeBUvzvaeiEJ1xjvnPoujXYc1J8C3+pvxcdtuBGLow99jEQMXERFRGM3eRigIfwRDt9Qd\\n4Woio6qvMuw/c5fUifLeU1GoKH4wcBEREYWRbE4ZtC9RjM9P0Hhl76B9fUN8KolujHu4iIiIwih0\\nFONEzzG0+Js17QJE5CdNj1JVxkq1pKHRVx/SLkBAti17yN9t8NTiZM9n6Ap0wGZKwNTEfMxPLeWG\\n+ysYuIiIiMIQBRG3Z67H3vYP0ehrgAoFDlMyZjhmoyRlXrTLM8TclIWo81yGW3Zr2icmTMY0e+Gg\\nv1fnqcHu1revfTA8ADR469Ar9WJ15hojSx4zGLiIiIgGkWlz4d7cB9Dka0Sf1IvJ9qmwirZol2UY\\nly0L61wb8VnPUbT5W2EWLJiQMBErnKuHnKk62VN2LWxd53xfBRakliLZMvjy7HjBwEVERDQEQRCQ\\nmzAh2mVEzITEiZiQOHFYv9Phbw/b7lU8uNRfjTmp88P2jyfcNE9ERESjYhWtg/YlmR0RrCR2MXAR\\nERHRqExKCH8IrMuajWl240/lHwsYuIiIiGhUljhXojCpGObrdiplWlxYnbGGbylewT1cRERENCom\\nwYT1WXeh2duEem8NkkwOTHfMiNjHvscCBi4iIiLSRXZCDrITcqJdRkxi4CIionGjK9CB0z0n4VO8\\nmBDIRoFp9pAbvon0wsBFRETjwnl3BfZ27IHnynlRFe4zOGE5hTuzNyHVkhbl6ijeMXAREVHck1UZ\\nR7sOBsPWVe2BVhzu3Id1WRujVNnwuCU3DnZ8imZvAyAAWQm5WJy6DGnW9GiXRjfAwEVERHHvcv9F\\ndATCH87Z5GuEqqox/zZdo7cBbzW9Br/qC7Z1uTvR5mvBfTkPIMEcnx/Ujhd8fYCIiOKeoiqD9qlQ\\nI1jJyPRJbrzT/BdN2LqqI9CGEz1lUaiKhoOBi4iI4t60pHykmZ1h+7KtOTE/u3Wi+xi8imfQ/i6p\\nM4LV0EjoHrj+4z/+A1u3bsWDDz6IkydPavr279+PzZs3Y+vWrfj1r3+t96OJiIjCMglmLExbDNvf\\nfHg63ezE4vQVUarq5nVL3UP2W4X4/aB2vNB1D9fhw4dx+fJlvPzyy6iqqsK//Mu/4OWXXw72/+Qn\\nP8EzzzyD7OxsPPTQQ1i/fj0KCwv1LIGIiCismcklyLBmorz3FLyKD7kpWZhuLkGi2R7t0m4oQRx8\\nf5YIETOSZ0ewGhoJXQPXgQMHsHbtWgBAQUEBuru74Xa74XA4UFtbi9TUVOTm5gIAbr31Vhw4cICB\\ni4iIIibLloMs28DBnC5XMlpbe6Nc0c2ZlVyCqr5K+FRvSN+itGXITZgQhapoOHRdUmxra0N6+rVX\\nU51OJ1pbWwEAra2tcDqdYfuIiIhocNkJuViRsRrp1+1DSzI5sC5zA5akL49iZfrrl/rQ7G2EX/FH\\nuxRdGXoshKqO/s2P9HQ7zGaTDtXEB5crOdolxBSORyiOiRbHQ4vjoTWWxuNW1wqsVJbgfPd5mGBC\\nQVoBTEL4vx/bPe040X4CqqpilnMWcpNyb+oZ0RwPn+TDm5feRFV3FTyyB6nWVMxyzsK6Seui9k1G\\nPcdD18CVlZWFtra24M8tLS1wuVxh+5qbm5GVlXXDe3Z29t/wmvFiLE1/RwLHIxTHRIvjocXx0Bqr\\n45GJiQCAjrbwfz8e7TyIz7qPwnflCIkDTQcxO3kOVjpvG/JtzGiPx3vNb6Cq/3zw525/Nw40HYDk\\nAZY4I/9iw0jHY7CQpmtkXLlyJXbu3AkAOHPmDLKysuBwOAAAEydOhNvtRl1dHSRJwp49e7By5Uo9\\nH09ERDSuNXubcKz7cDBsAYCkBnCy5ziq+iujWNnQugNdqPVcDttX3X9elxWzaNN1hmvhwoWYPXs2\\nHnzwQQiCgB/84Ad47bXXkJycjHXr1uGHP/whtm3bBgDYsGEDpk2bpufjiYiIxrVKdzkkNRDSrkLF\\nxb4qFCYVR6GqG2v3t8Gvht+z1Sf3QVZlmIWx/XEc3av/1re+pfl5xowZwf+8ePFizTERREREpB8Z\\n0qB9kirBr/hR3nsasiqhIKkYqZbUCFY3uCxbNhLExLCHuyabUwbdqzaWjO24SEREREG5tjyc6T05\\naP/2uufhlnsAAGXdRzDbMQfLM1ZHqrxBOczJmGrPR4X7jKZdgICipBkx/yWAm8FP+xAREcWJ6Y4Z\\nmJIYul3HZc1Gg6cuGLYAwKd4cbznGCrd5ZEscVC3Za7DnJT5SDGnwiJYkWHJxLL0WzAvdVG0S9MF\\nZ7iIiIjihCiIuDN7E453HUW9tw4qFGTbciErMk74j4Vcr0JBdd8FFDlmRqFaLZNgwuqMv4PslOBT\\n/EgQE6J2HIQRGLiIiIjiiEkwozR9GUqva9vbtmfQ6wODbFaPFpNght0Uf/EkfqIjERERhZUzxKd/\\nnJbMCFYyfjFwERERxbnCpCJMTpwa0u60ZGJ+amnoL5Du4m/OjoiIiDQEQcCdWZtwuPMAGr31kCHB\\nZc3GorSlSDInRbu8cYGBi4iIaBwwixasiIEjIMYrLikSERERGYwzXERERKTR4mtGk7cBLlsWchPy\\nol1OXGDgIiIiIgCAX/Fjd+s7qOu/DAkSTDBhQsIkrHXdCbvZHu3yxjQuKRIREREA4JP2D3GpvwrS\\nlW8yypBR672Ej9vfj3JlYx8DFxEREcEv+1HnuRy2r85Tg365P8IVxRcGLiIiIoJX9sIre8P2+VUf\\n+iV3hCuKLwxcREREBIfFgXSrM2xfmjkdaZbwfXRzGLiIiIgIoiBipqMEJpg07QIEFDlmwizyPbvR\\n4OgRERERAGBu6gKYRQsq3WfhlnphNyWhMKkYc1MXRLu0MY+Bi4iIiIJmJZdgVnJJtMuIO1xSJCIi\\nIjIYAxcRERGRwbikSERENEZc7KtCpbscXsWDVHMa5qQsQIYtM9pl0U1g4CIiIhoDPus+hkMd+yAh\\nAACoQw0uey7hc1kb+L3DMYBLikRERDEuoARwqud4MGxd5ZZ7cLz7SJSqouFg4CIiIopxl/ur0SN1\\nh+1r8TVDVdUIV0TDxcBFREQU46yibdA+s8DdQWMBAxcREVGMm5Q4BVnW7LB9ubY8CIIQ4YpouBi4\\niIiIYpwgCFiWvgop5lRNe64tD8udq6NUFQ0H5yGJiIjGgEn2Kdia8CWc7jmBfrkfmVYXihwzIQqc\\nOxkLGLiIiIjGCKtow8K0JdEug0aAsZiIiIjIYAxcRERERAZj4CIiIiIyGAMXERERkcEYuIiIiIgM\\nxsBFREREZDAGLiIiIiKDMXARERERGYyBi4iIiMhgDFxEREREBmPgIiIiIjIYAxcRERGRwRi4iIiI\\niAxm1utGkiThX//1X1FTUwNZlvGd73wHpaWlmmtmz56NhQsXBn9+7rnnYDKZ9CqBiIiIKCbpFrj+\\n+te/IjExEdu3b8f58+fxve99D6+88ormGofDgRdeeEGvRxIRERGNCboFrk2bNuGuu+4CADidTnR1\\ndel1ayIiIqIxTbc9XBaLBTabDQDw/PPPB8PX9fx+P7Zt24YHH3wQzz77rF6PJiIiIoppgqqq6nB/\\naceOHdixY4em7cknn8SqVavw0ksv4cMPP8TTTz8Ni8WiuWb79u3YtGkTBEHAQw89hB/96EeYM2fO\\nkM+SJBlmM/d5ERER0dg1osA1mB07duC9997Df/7nfwZnuwbz1FNPoaCgAPfff/+Q17W29upV3pjn\\nciVzPK7D8QjFMdHieGhxPLQ4HlocD62RjofLlRy2XbclxdraWvzpT3/Cr371q7Bhq7q6Gtu2bYOq\\nqpAkCWVlZZg+fbpejyciIiKKWbptmt+xYwe6urrw2GOPBdueeeYZPPfcc1i8eDEWLFiAnJwcbN68\\nGaIoYs2aNZg7d65ejyciIiKKWbouKRqB05vXcLpXi+MRimOixfHQ4nhocTy0OB5aMbukSERERETh\\nMXARERERGYyBi4iIiMhgDFxEREREBmPgIiIiIjIYAxcRERGRwRi4iIiIiAzGwEVERERkMAYuIiIi\\nIoMxcBEREREZjIGLiIiIyGAMXEREREQGY+AiIiIiMhgDFxEREZHBGLiIiIiIDMbARURERGQwBi4i\\nIiIigzFwERERERmMgYuIiIjIYAxcRERERAZj4CIiIiIyGAMXERERkcEYuIiIiIgMxsBFREREZDAG\\nLiIiIiKDMXARERERGYyBi4iIiMhgDFxEREREBmPgIiIiIjIYAxcRERGRwRi4iIiIiAzGwEVERERk\\nMAYuIiIiIoMxcBEREREZjIGLiIiIyGAMXEREREQGY+AiIiIiMhgDFxEREZHBGLiIiIiIDMbARURE\\nRGQwBi4iIiIigzFwERERERnMrNeNXnvtNfziF7/A5MmTAQArVqzA448/rrnmjTfewPPPPw9RFPHA\\nAw9gy5Ytej2eiIiIKGbpFrgAYMOGDfjud78btq+/vx+//vWv8corr8BisWDz5s1Yt24d0tLS9CyB\\niIiIKOZEbEnxxIkTmDNnDpKTk5GQkICFCxeirKwsUo8nIiIiihpdA9fhw4fx6KOP4pFHHsHZs2c1\\nfW1tbXA6ncGfnU4nWltb9Xw8ERERUUwa0ZLijh07sGPHDk3bxo0b8eSTT+K2227D8ePH8d3vfhdv\\nvvnmoPdQVfWmnpWebofZbBpJmXHJ5UqOdgkxheMRimOixfHQ4nhocTy0OB5aeo7HiALXli1bhtzw\\nvmDBAnR0dECWZZhMA2EpKysLbW1twWtaWlowf/78Gz6rs7N/JCXGJZcrGa2tvdEuI2ZwPEJxTLQ4\\nHlocDy2OhxbHQ2uk4zFYSNNtSfF3v/sd3nrrLQBAZWUlnE5nMGwBwLx583Dq1Cn09PSgr68PZWVl\\nKC0t1evxRERERDFLt7cU7777bnz729/Gn/70J0iShJ/+9KcAgN/+9rdYvHgxFixYgG3btuHRRx+F\\nIAj42te+huRkTl0SERFR/BPUm91MFSWc3ryG071aHI9QHBMtjocWx0OL46HF8dCK2SVFIiIiIgqP\\ngYuIiIjIYAxcRERERAZj4CIiIiIyGAMXERERkcEYuIiIiIgMxsBFREREZDAGLiIiIiKDMXARERER\\nGYyBi4iIiMhgDFxEREREBmPgIiIiIjIYAxcRERGRwRi4iIiIiAzGwEVERERkMAYuIiIiIoMxcBER\\nEREZjIGLiIiIyGAMXEREREQGY+AiIiIiMhgDFxEREZHBGLiIiIiIDMbARURERGQwBi4iIiIigzFw\\nERERERmMgYuIiIjIYAxcRERERAZj4CIiIiIyGAMXERERkcEYuIiIiIgMxsBFREREZDAGLiIiIiKD\\nMXARERERGYyBi4iIiMhgDFxEREREBmPgIiIiIjIYAxcRERGRwRi4iIiIiAzGwEVERERkMAYuIiIi\\nIoMxcBEREREZjIGLiIiIyGBmvW70m9/8Bvv37wcAKIqCtrY27Ny5M9hfV1eHu+++GyUlJQCA9PR0\\n/PKXv9Tr8UREREQxS7fA9fjjj+Pxxx8HALz++utob28PuWbatGl44YUX9HokERER0Zig+5KiJEnY\\nvn07HnroIb1vTURERDQm6R64du3ahVtuuQUJCQkhfW1tbfj617+OBx98EG+88YbejyYiIiKKSYKq\\nqupwf2nHjh3YsWOHpu3JJ5/EqlWr8Oijj+JHP/oRJk6cqOl3u93YuXMnNm3ahN7eXmzZsgXbt29H\\nVlbWkM+SJBlms2m4JRIRERHFjBEFrsH09/djy5YtePvtt2947Te+8Q184QtfwLJly4a8rrW1V6/y\\nxjyXK5njcR2ORyiOiRbHQ4vjoXX9eIj+Llh7LkAVzPCnzYJqska5usjjnw+tkY6Hy5Uctl23TfMA\\nUFFRgfz8/LB9Bw8exJ49e/C9730P/f39qKiowLRp0/R8PBER0fCoKuzN+2DrKoeo+AAAcvtn6Hct\\nhT99ZpSLo3ii6x6u1tZWOJ1OTdtPf/pT1NbWorS0FN3d3di6dSsefvhhPPbYY8jOztbz8URERMNi\\n7TqHhI4TwbAFACbJDXvLfggBdxQro3ij65KiETi9eQ2ne7U4HqE4JlocDy2Oh5bLlQxP2cuw9VaH\\n7e/PLIUna+htL/GEfz60YnpJkYiIaCwRlMDgnUP1xRNFgujvgRpgJDASR5eIiMYt2eYE+mpD2lUI\\nkOwTolBRBKkqElsPw9ZdCVOgG2ptIpISJ6Ev51bAbIt2dXGH31IkIqJxy5OxEAGbM6Td75iCQHL4\\nl8DiRUL7cSS2HYEp0D3QEPAgoacSjsYPoltYnOIMFxERjVuqJQm9k+5CYtsxmL2tgGBCwD4BHtcS\\nQBCiXZ6hrD0XEO6f0OKuhejthJKQHvGa4hkDFxERjWuqNQX9E26PdhmRpaoQpb6wXaIagNnbAj8D\\nl664pEhERDTeCAIUsyNslyJaIdl5bJPeGLiIiIjGIX/qdKhhFhX9jilQrGmjf4AiA7F98lREcUmR\\niIhoHPJmzAdUGbauczD5eyDYEuFNnDjwluIoWHouIKHjJEy+TkC0IpCUh76cVYBo0anysYmBi4iI\\naJzyZi6CN2MBRKkfzuwM9HX4bvxLQ7D0XoKjYc+1k/tlD0xd3RCkfrgn36VDxWMXlxSJiIjGM0GE\\nYnFA0OGD3bbOM5rPJH5G3QQAABeWSURBVF1lddfC3Fc/6vuPZQxcREREpAsxEP5TOAJkmD1NEa4m\\ntjBwERERkS5UU0L4dgCKNSWyxcQYBi4iIiLShT+lMOybj1JiNvzJhVGoKHZw0zwRERHpwucsgSj1\\nwdZVAZPUCxUmBJJy0ZezOu5P7r8RBi4iIiLSjSdrKTyZC2Dpq4dsdkBJdEW7pJjAwEVERET6Eq0I\\nJE+LdhUxhXu4iIiIiAzGwEVERERkMAYuIiIiIoNxDxcREVE0qPLA23zeVqiiDd702VCNPqtK9iOh\\n/TOYfR1QRQt8qUWQHJOMfSYBYOAiIiKKOEHyIrn2HVg8DcE2W9dZ9GXfgkBasTHPDPQhpfZtmL0t\\nwTZrz3l4MhfD61pkyDPpGi4pEhERRVhi60FN2Pr/27v34KjKuw/g33PbS/ZCsmEDBEnAaOUVG4Fa\\nNWgUKlRfRPS1Ewg0aR070xEG2s44Lch0hs5YnOK0jnZooYPIdBSEhtJinb7KqxKH9o1Gi/USB1BQ\\nLgFy3SR73z2X94+8Btfd3Hf37JLv5z+eZ885Px4yyzfPc85zAEDSwnB0NAO6mqFrvpMQtgBANFTY\\nut+HEA9n5Jp0GQMXERFRlsmhiynbpXgvLL0nM3PNSFvqa2ohWPpOZOSadBkDFxERUZYJhj54X4Zm\\nuJDilTsj66N0YOAiIiLKFkODtftDwNBSdmuyA7FJ12bk0mrB1EGu6USscHZGrkmX8aZ5IiKibNDj\\ncJ99GUqoNWW3ARmRoq/DkO0ZuXx48s2Qwh2whC8vZ+qiFeHJ34AhWTNyTbqMgYuIiCgL7B3vpAxb\\nBkREneWIFs2B6po5spMZBuTQRYixXsSdM2AozuEPkW3wlz8Aq68FcqQDhmhBpGg2dBvfdZgNDFxE\\nRERZoIQvpWwXoEOzl4w4bInRbjguvgkldLH/WMmOmPsahKbeAQjD3IslSogWVyI6ytpp/HgPFxER\\nUTYY6TiHAceFI7CEWiGg/8Z7SQvD5vsQts5/peEClCkMXERERFmg2qekbNdFK2KTvjaicyh9p6GE\\nk7eUEABY/J+NpzzKMAYuIiKiLAhNvgmxgmkJbQYkRDyV0C2Thj3e6muB4+Lrg27gIGhcKMxlvIeL\\niIgoG2Qr/GX3w9b9IeRIOwxRRtR9LVRn2bCHirFe2NubIOmxQT+jWTP8HkYaFwYuIiKibBFlRCbP\\nG/VhVl8LJC0yaL8uWhEtvGE8lVGGMXARERHlOEGPD9qniTYESxch7r46ixXRaPEeLiIiohyn2koG\\nfcgxWnQ94u6KrNZDo8cZLiIioi8R4gHYuj+EoEehWT2IFs4BRMnUmmKF1yHedxKW4LmE9rh1MiLF\\no1+ipOxj4CIiIvp/St9pOC69CUkNDrRZe07AP2MpDMVhYmUCYo5yiLFeiFoEhiAi7ihDaEpVxl4F\\nROnFJUUiIiIAMHTYO95OCFsAoETaUNDeZFJR/QouvglH+z8gx/sg6jFIWgRStMvUmjJKi8Ha/QGs\\nXf+GoIbNriYtOMNFREQTjqDFYIS6AV0AxP7/ChX/51AGCTFyis1G00EMd6Kg853+bSIEEap9GkIl\\nVZdn0wwDYrgd1t7jSftvKdEu2Dv/hdC0hRmpzSxWXwvsHe9AUgMAAK3zPUSKKxGZ/A2TKxsfBi4i\\nIpo4dLX/PYSBszC0IAoVN6LuaxEuuXXIJwEFQ097KULcD9f5VyDHewba5FgvpKgPfeUPwN7RDEvg\\ncwhxP0RDTXkOOdyZ9rrMJEa6UdD+vxC/tImrpAVh73gHqq0EqnOGidWNDwMXERFNGI4LR2DrOzHw\\nZyneB3vXvwBBQnjyPGgdbkjxvqTjBnstz3jYu/6dELa+oETa4Dp7CJZBXnb9ZYbJN/Onm62nJSFs\\nfUE0VFh7T+R14OI9XERENCEI8SAswc+T2wEo/lOAICNcPBe6oCT0a8okhIrTv5wlxnoH7ZPDHSM6\\nR9xxVbrKyQmCNsQs4xAzkPlgzIGrubkZVVVVOHLkyEDb8ePHUVtbi9raWmzevDnpmHg8jkcffRSr\\nVq1CXV0dzp07l/QZIiKiTJCjnSlnTwBAigcBPY6opxL+GUsRmTQbUedMhD03orf8fuh2b9rrMSTb\\noH0itKGPhYioqyLv72v6KnWIcdasRVmsJP3GFLjOnj2L3bt3Y/78+QntW7ZswaZNm7Bv3z4EAgG8\\n+eabCf0vv/wy3G43XnzxRTzyyCP4zW9+M/bKiYiIRkG1ToY2SMjRFAcg9s9sqc4ZCE5fjEDZMoSm\\nVsOwZOYdhdFJs5Nm04D+neN1IfUdP5pkR8gzD31lyxC46h5AuLIWqqJF1yNmn5bUrlonI+KZa0JF\\n6TOmfymv14tt27bB5XINtMViMbS2tqKyshIAsGjRIjQ1JT5G29TUhCVLlgAAFixYgGPHjo21biIi\\nolExFAfizpnJ7QBirmsA4avPAWaW6rwKoSlV0JTLgS5uLUaw9FtQHdNTHhMtvB7hqbf1v/A6y/Vm\\nhSAhMONehIu+jrjNi7h1MsKF16Ov7F4Y8uAzgsOeVouZvr3EmG6at9uTN1nz+Xxwuy//0BQXF6Oj\\nI3ENurOzEx6PBwAgiiIEQUAsFoPFYhlLGURERKMSnLYIECQogTOQ1BBUixsx9zWIeG8ypZ6opxLR\\nwuth8Z+CISiIu2YCggjVPhWOi29ACZ6HaKjQJDti7gqES24xpc5sMmQbQtPuTMu5xGgPCtr/CTl4\\nEQI0qDYvIsXzEHfNSsv5R2PYwNXQ0ICGhoaEtvXr16O6unrI4wxjsLc+je4zRUUFkOUr6ymM8fB6\\nXcN/aALheCTjmCTieCTieACYshyGFgPiISgWJyyiDKfpNX018LmA0lXQQ11AqAuSezocFgcyvdf9\\nlfTzYegqjPcOAIHLT3taQhdgifcCkydDdJcOe450jsewgaumpgY1NTXDnsjj8aCn5/LjrW1tbSgp\\nKUn4TElJCTo6OjB79mzE43EYhjHs7JbPFxr22hOF1+tCR4ff7DJyBscjGcckEccjEccjkddbmN3x\\nMDRYe45DjPuhWT2Iua8dwbKgBcA0oFcHkNlar7SfD2v3h3AGUmytEQ8i8lkzgqV3DXn8WMdjsJCW\\ntn24FEXB1VdfjXfffRc33XQTDh8+jPr6+oTP3HbbbXjllVdQXV2NI0eO4JZbrvypUSIiIjHSCVfr\\na5Cj/RuVGgDivg8RmH6Pye9ozF2K/zNYfR9DVAPQ5QLE3NchVvi1ER8vxpL3OBvoiwfSUeKojOmm\\n+cbGRtTX1+Po0aN46qmn8PDDDwMANm3ahKeeegq1tbUoKyvDggULAABr1qwBACxduhS6rmPVqlXY\\ns2cPHn300TT9NYiIiHKXo+0fA2EL6N/7yxK6iIK2f5hX1HgZBsSoD2I8/bNilp7jcLYehjXwGZRI\\nB6yBM3BeeAO2rvdGXp4y+HKgLhWko8xREYyR3EhloitpenO8rrTp3vHieCTjmCTieCTieCTK1niI\\nUR8KT+2DkGJvLU12oOea+oH3OZppNOOh9H4Ce9d7kCMdACTEC6YgNGUBtHTsyG8YcH92AEqkLalL\\ntRSht6IWEEZwb7cex6TTDZBj3YnNohX+Gf8JdZhNY9O9pHhlbeBBRESUYwQtkjJsAYCgqxCMoTc5\\nzTVS6BIcl96EEmmHAAMCVFhCrXC2/g+gxcZ9fkELQ4p2p+yTYz5IkdQvGE8iKghMvwuxgukwIPUv\\n49q8CE6tHjZsZYL5kZqIiOgKptlLoFqKIMd8SX2qrRiGZE1ok/1nYPN9ADnaDV20QHXMQGhK1chm\\ndbLA1vMxJC2S1C7HemDzfTju3e8NUYEhKYCa/CofXZBhSMlbUw1Gs0+Bf+Z/QYz2QjDi0KzFpu1f\\nxsBFRESUSYKEqOfrENuaIBqXQ4Qu2ZJ2T5eD5+C88BokrX+TTgmAEu2CGA8gMOOebFY9KCEeHLRP\\nHKJvxEQF8YKrIPWdTOpSC6ZDt4x+qwbdOmn8dY0TAxcREVGGRTyV0GQHrD0nIGph6IoTkaIbknaU\\nt3V/NBC2vkwJfA4p3Jaee6TGSVcG37VsLGEoldDU2yGqISihVggwYABQ7VMRnDr0HqC5jIGLiIgo\\nC+LuCsTdFUN+RoomLzsCgGioUIKtORG4Ip4bYPF/BklL3CdTtRYjUnRDWq5hyAXwl98POXAGcqQD\\nurXQlNcvpRMDFxERUY4wZBuQ4r5zA4A+xDYH2aTbvAiWfgu2rmOQw+2AIPXPPk1ZMPAC8LQQBKiu\\nmVBdM9N3ThMxcBEREeWImHMW5NAFfHUeR7N5ERtmdiyb4q6ZiLtmQlCDgCDBkMb+YumJgoGLiIgo\\nR0SK50JUA7D0noSkhfs3XbBPQXDqHYCQezs5GTJ3yR8pBi4iIqJcIQgITa1GuHgeLP7PoSkuqM6y\\nvL53ifoxcBEREeUYQ3Ei6knPDeiUGxi4iIiI8oWhwer7CErwIiAIiDumI1o4hzNgeYCBi4iIKB8Y\\nOpzn/hvWwOcDTZa+T6AEWxGY/m2GrhyXe3fgERERURKr76OEsAUAAvpDl8X/qSk10cgxcBEREeUB\\nJXQxZbsAQAmcy24xNGoMXERERHnAGGLJcKg+yg0MXERERHkg7iiDkaLdgIiYM3c2RaXUGLiIiIjy\\nQGzSdYhOmg3jS/vQGxARKZoD1TnDxMpoJPiUIhERUT4QBARL70LMVQEleAaAgJhrVv/GqJTzGLiI\\niIjyhSAg7p6FuHuW2ZXQKDFwERER0egZGiw9JyDFeqArLkQLrwdEyeyqchYDFxEREY2KGO2Fs/Uw\\nlEjbQJvN1wL/9MXQbZNNrCx38aZ5IiIiGpWC9n8mhC0AkKOdcLT906SKch8DFxEREY2YoEUhBy+k\\n7JNDFyDGA1muKD8wcBEREdHIGSoEQ03ZJRgaoMWyXFB+YOAiIiKiETOkAmiD3Kel2rzQrYVZrig/\\nMHARERHRyAkCwsVzoUu2hGZdtCBSVAkIjBap8ClFIiIiGpW4+xr4JTusvo8hqQHosgORwuugOsvN\\nLi1nMXARERHRqKmO6VAd080uI29w3o+IiIgowzjDRURERNln6LB1vw85eB6CoUO1lSBcPB+QrWZX\\nlhEMXERERJRdhgHn+Vdh9Z8aaLIEz0EOtcJffj8gKiYWlxlcUiQiIqKsUvynYfGfTmq3hC/B1vVv\\nEyrKPAYuIiIiyioleB4CjJR9crg9y9VkBwMXERERZZcgja0vjzFwERERUVZFCq+DnuI+LQNAzFmW\\n/YKygIGLiIiIskq3eREu/gZ00TLQZkBCtPB6xAr/w8TKModPKRIREVHWRbw3Iea6GtbeExCgI+ac\\nBdVRanZZGcPARURERKbQbR6EbVVml5EVXFIkIiIiyjAGLiIiIqIMY+AiIiIiyjAGLiIiIqIMG3Pg\\nam5uRlVVFY4cOTLQdvz4caxevRp1dXVYu3YtwuFwwjEHDx7EnXfeifr6etTX12P79u1jr5yIiIgo\\nT4zpKcWzZ89i9+7dmD9/fkL7L3/5S2zcuBGVlZXYunUrDh48iO9+97sJn1m6dCk2bNgw9oqJiIiI\\n8syYZri8Xi+2bdsGl8uV0L5jxw5UVlYCADweD3p6esZfIREREVGeG1PgstvtkKTkdx05nU4AQCgU\\nwqFDh3DPPfckfaa5uRk/+MEP8P3vfx8ff/zxWC5PRERElFeGXVJsaGhAQ0NDQtv69etRXV2d8vOh\\nUAhr1qzBww8/jIqKioS+G2+8ER6PBwsXLsR7772HDRs24G9/+9uQ1y8qKoAsX5kvshwLr9c1/Icm\\nEI5HMo5JIo5HIo5HIo5HIo5HonSOx7CBq6amBjU1NSM6maqqWLt2LZYtW4YHH3wwqb+iomIghM2b\\nNw/d3d3QNC3lbNkXfL7QiK49EXi9LnR0+M0uI2dwPJJxTBJxPBJxPBJxPBJxPBKNdTwGC2lp3RZi\\n586duPnmmwcNaDt37sTLL78MADh58iQ8Hs+QYYuIiIjoSjCmpxQbGxuxa9cunD59Gi0tLXj++efx\\n3HPPYc+ePbjqqqvQ1NQEALjllluwbt06rFmzBtu3b8d9992Hn/70p9i3bx9UVcWWLVvS+pchIiIi\\nykWCYRiG2UUMhdObl3G6NxHHIxnHJBHHIxHHIxHHIxHHI1FOLykSERERUTIGLiIiIqIMy/klRSIi\\nIqJ8xxkuIiIiogxj4CIiIiLKMAYuIiIiogxj4CIiIiLKMAYuIiIiogxj4CIiIiLKMAauPKKqKjZs\\n2IBVq1ZhxYoVePfdd80uyTRPPPEEVq5cidraWnzwwQdml2O6J598EitXrsR3vvMdHD582OxyckIk\\nEsHixYtx8OBBs0vJCS+99BKWL1+OBx98EI2NjWaXY6pgMIh169ahvr4etbW1OHr0qNklmeLkyZNY\\nvHgxXnjhBQDAxYsXUV9fj9WrV+PHP/4xYrGYyRVmV6rxeOihh1BXV4eHHnoIHR0d4zo/A1ceOXTo\\nEOx2O1588UVs2bIFv/rVr8wuyRTNzc04c+YM9u/fjy1btkz4d3K+9dZb+OSTT7B//348++yzeOKJ\\nJ8wuKSds374dkyZNMruMnODz+fC73/0Oe/fuxY4dO/D666+bXZKp/vKXv2DWrFl4/vnn8cwzz0zI\\n75BQKITHH38cVVVVA22//e1vsXr1auzduxfl5eU4cOCAiRVmV6rxePrpp7FixQq88MILWLJkCXbv\\n3j2uazBw5ZHly5fjscceAwB4PB709PSYXJE5mpqasHjxYgBARUUFent7EQgETK7KPN/85jfxzDPP\\nAADcbjfC4TA0TTO5KnOdOnUKn376KRYuXGh2KTmhqakJVVVVcDqdKCkpweOPP252SaYqKioa+P7s\\n6+tDUVGRyRVln8Viwc6dO1FSUjLQ9vbbb+Ouu+4CACxatAhNTU1mlZd1qcZj8+bNuPvuuwEk/syM\\nFQNXHlEUBVarFQDwxz/+EcuWLTO5InN0dnYmfEF6PJ5xT/XmM0mSUFBQAAA4cOAA7rjjDkiSZHJV\\n5tq6dSs2btxodhk54/z584hEInjkkUewevXqCfUfaSr33nsvLly4gCVLlqCurg4bNmwwu6Ssk2UZ\\nNpstoS0cDsNisQAAiouLJ9T3aqrxKCgogCRJ0DQNe/fuxX333Te+a4zraMqYhoYGNDQ0JLStX78e\\n1dXV2LNnD1paWrBjxw6TqsstfDtVv9deew0HDhzAc889Z3YppvrrX/+KuXPnYsaMGWaXklN6enqw\\nbds2XLhwAd/73vdw5MgRCIJgdlmmOHToEEpLS7Fr1y4cP34cmzZt4r1+X8Hv1X6apuFnP/sZbr31\\n1oTlxrFg4MpRNTU1qKmpSWpvaGjAG2+8gd///vdQFMWEysxXUlKCzs7OgT+3t7fD6/WaWJH5jh49\\nih07duDZZ5+Fy+UyuxxTNTY24ty5c2hsbMSlS5dgsVgwdepULFiwwOzSTFNcXIx58+ZBlmWUlZXB\\n4XCgu7sbxcXFZpdmimPHjuH2228HAMyePRvt7e3QNG3CzwwXFBQgEonAZrOhra0tYXltonrsscdQ\\nXl6OdevWjftcXFLMI+fOncO+ffuwbdu2gaXFiei2227Dq6++CgBoaWlBSUkJnE6nyVWZx+/348kn\\nn8Qf/vAHFBYWml2O6Z5++mn8+c9/xp/+9CfU1NRg7dq1EzpsAcDtt9+Ot956C7quw+fzIRQKTcj7\\nlr5QXl6O999/HwDQ2toKh8Mx4cMWACxYsGDgu/Xw4cOorq42uSJzvfTSS1AUBT/60Y/Scj7OcOWR\\nhoYG9PT04Ic//OFA265duwbW3CeK+fPnY86cOaitrYUgCNi8ebPZJZnq73//O3w+H37yk58MtG3d\\nuhWlpaUmVkW5ZMqUKbj77ruxYsUKAMDPf/5ziOLE/X175cqV2LRpE+rq6qCqKn7xi1+YXVLWffTR\\nR9i6dStaW1shyzJeffVV/PrXv8bGjRuxf/9+lJaW4oEHHjC7zKxJNR5dXV2wWq2or68H0P+Q1nh+\\nVgSDC7VEREREGTVxf8UhIiIiyhIGLiIiIqIMY+AiIiIiyjAGLiIiIqIMY+AiIiIiyjAGLiIiIqIM\\nY+AiIiIiyjAGLiIiIqIM+z+sn8Ds7/53bQAAAABJRU5ErkJggg==\\n\",\n \"text/plain\": [\n \"<Figure size 720x576 with 1 Axes>\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n }\n }\n ]\n },\n {\n \"metadata\": {\n \"id\": \"2GU4miqf-dLu\",\n \"colab_type\": \"text\"\n },\n \"cell_type\": \"markdown\",\n \"source\": [\n \"# Evaluting clusters: \\n\",\n \"Finally we will use Normalized Mutual Information (NMI) score to evaluate our clusters. Mutual information is a symmetric measure for the degree of dependency between the clustering and the manual classification. When NMI value is close to one, it indicates high similarity between clusters and actual labels. But if it was close to zero, it indicates high dissimilarity between them. \"\n ]\n },\n {\n \"metadata\": {\n \"id\": \"BirJIkyZOpfZ\",\n \"colab_type\": \"code\",\n \"outputId\": \"2c8f934f-0b98-474a-f7c5-610378c9f79b\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 88\n }\n },\n \"cell_type\": \"code\",\n \"source\": [\n \"normalized_mutual_info_score(y, cluster)\"\n ],\n \"execution_count\": 0,\n \"outputs\": [\n {\n \"output_type\": \"stream\",\n \"text\": [\n \"/usr/local/lib/python3.6/dist-packages/sklearn/metrics/cluster/supervised.py:844: FutureWarning: The behavior of NMI will change in version 0.22. To match the behavior of 'v_measure_score', NMI will use average_method='arithmetic' by default.\\n\",\n \" FutureWarning)\\n\"\n ],\n \"name\": \"stderr\"\n },\n {\n \"output_type\": \"execute_result\",\n \"data\": {\n \"text/plain\": [\n \"1.0\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n },\n \"execution_count\": 7\n }\n ]\n },\n {\n \"metadata\": {\n \"id\": \"b_TD3pKJbBkl\",\n \"colab_type\": \"code\",\n \"colab\": {}\n },\n \"cell_type\": \"code\",\n \"source\": [\n \"\"\n ],\n \"execution_count\": 0,\n \"outputs\": []\n }\n ]\n}"
}
{
"filename": "code/artificial_intelligence/src/hierachical_clustering/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# Hierachical Clustering\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/artificial_intelligence/src/image_processing/canny/canny.cpp",
"content": "//\n// canny.cpp\n// Canny Edge Detector\n//\n\n#include <iostream>\n#define _USE_MATH_DEFINES\n#include <cmath>\n#include <vector>\n#include \"canny.h\"\n#include \"opencv2/imgproc/imgproc.hpp\"\n#include \"opencv2/highgui/highgui.hpp\"\n\n\n\ncanny::canny(std::string filename)\n{\n img = cv::imread(filename);\n\n if (!img.data) // Check for invalid input\n cout << \"Could not open or find the image\" << std::endl;\n\n else\n {\n\n std::vector<std::vector<double>> filter = createFilter(3, 3, 1);\n\n //Print filter\n for (int i = 0; i < filter.size(); i++)\n for (int j = 0; j < filter[i].size(); j++)\n cout << filter[i][j] << \" \";\n\n grayscaled = cv::Mat(img.toGrayScale()); //Grayscale the image\n gFiltered = cv::Mat(useFilter(grayscaled, filter)); //Gaussian Filter\n sFiltered = cv::Mat(sobel()); //Sobel Filter\n\n non = cv::Mat(nonMaxSupp()); //Non-Maxima Suppression\n thres = cv::Mat(threshold(non, 20, 40)); //Double Threshold and Finalize\n\n cv::namedWindow(\"Original\");\n cv::namedWindow(\"GrayScaled\");\n cv::namedWindow(\"Gaussian Blur\");\n cv::namedWindow(\"Sobel Filtered\");\n cv::namedWindow(\"Non-Maxima Supp.\");\n cv::namedWindow(\"Final\");\n\n cv::imshow(\"Original\", img);\n cv::imshow(\"GrayScaled\", grayscaled);\n cv::imshow(\"Gaussian Blur\", gFiltered);\n cv::imshow(\"Sobel Filtered\", sFiltered);\n cv::imshow(\"Non-Maxima Supp.\", non);\n cv::imshow(\"Final\", thres);\n\n }\n}\n\nMat canny::toGrayScale()\n{\n grayscaled = Mat(img.rows, img.cols, CV_8UC1); //To one channel\n for (int i = 0; i < img.rows; i++)\n for (int j = 0; j < img.cols; j++)\n {\n int b = img.at<Vec3b>(i, j)[0];\n int g = img.at<Vec3b>(i, j)[1];\n int r = img.at<Vec3b>(i, j)[2];\n\n double newValue = (r * 0.2126 + g * 0.7152 + b * 0.0722);\n grayscaled.at<uchar>(i, j) = newValue;\n\n }\n return grayscaled;\n}\n\nstd::vector<std::vector<double>> canny::createFilter(int row, int column, double sigmaIn)\n{\n std::vector<std::vector<double>> filter;\n\n for (int i = 0; i < row; i++)\n {\n std::vector<double> col;\n for (int j = 0; j < column; j++)\n col.push_back(-1);\n filter.push_back(col);\n }\n\n float coordSum = 0;\n float constant = 2.0 * sigmaIn * sigmaIn;\n\n // Sum is for normalization\n float sum = 0.0;\n\n for (int x = -row / 2; x <= row / 2; x++)\n for (int y = -column / 2; y <= column / 2; y++)\n {\n coordSum = (x * x + y * y);\n filter[x + row / 2][y + column / 2] = (exp(-(coordSum) / constant)) / (M_PI * constant);\n sum += filter[x + row / 2][y + column / 2];\n }\n\n // Normalize the Filter\n for (int i = 0; i < row; i++)\n for (int j = 0; j < column; j++)\n filter[i][j] /= sum;\n\n return filter;\n\n}\n\ncv::Mat canny::useFilter(cv::Mat img_in, std::vector<std::vector<double>> filterIn)\n{\n int size = (int)filterIn.size() / 2;\n cv::Mat filteredImg = cv::Mat(img_in.rows - 2 * size, img_in.cols - 2 * size, CV_8UC1);\n for (int i = size; i < img_in.rows - size; i++)\n for (int j = size; j < img_in.cols - size; j++)\n {\n double sum = 0;\n\n for (int x = 0; x < filterIn.size(); x++)\n for (int y = 0; y < filterIn.size(); y++)\n sum += filterIn[x][y] * (double)(img_in.at<uchar>(i + x - size, j + y - size));\n\n\n filteredImg.at<uchar>(i - size, j - size) = sum;\n }\n\n return filteredImg;\n}\n\ncv::Mat canny::sobel()\n{\n\n //Sobel X Filter\n double x1[] = {-1.0, 0, 1.0};\n double x2[] = {-2.0, 0, 2.0};\n double x3[] = {-1.0, 0, 1.0};\n\n std::vector<std::vector<double>> xFilter(3);\n xFilter[0].assign(x1, x1 + 3);\n xFilter[1].assign(x2, x2 + 3);\n xFilter[2].assign(x3, x3 + 3);\n\n //Sobel Y Filter\n double y1[] = {1.0, 2.0, 1.0};\n double y2[] = {0, 0, 0};\n double y3[] = {-1.0, -2.0, -1.0};\n\n std::vector<std::vector<double>> yFilter(3);\n yFilter[0].assign(y1, y1 + 3);\n yFilter[1].assign(y2, y2 + 3);\n yFilter[2].assign(y3, y3 + 3);\n\n //Limit Size\n int size = (int)xFilter.size() / 2;\n\n cv::Mat filteredImg = cv::Mat(gFiltered.rows - 2 * size, gFiltered.cols - 2 * size, CV_8UC1);\n\n angles = cv::Mat(gFiltered.rows - 2 * size, gFiltered.cols - 2 * size, CV_32FC1); //AngleMap\n\n for (int i = size; i < gFiltered.rows - size; i++)\n for (int j = size; j < gFiltered.cols - size; j++)\n {\n double sumx = 0;\n double sumy = 0;\n\n for (int x = 0; x < xFilter.size(); x++)\n for (int y = 0; y < xFilter.size(); y++)\n {\n sumx += xFilter[x][y] *\n (double)(gFiltered.at<uchar>(i + x - size, j + y - size)); //Sobel_X Filter Value\n sumy += yFilter[x][y] *\n (double)(gFiltered.at<uchar>(i + x - size, j + y - size)); //Sobel_Y Filter Value\n }\n double sumxsq = sumx * sumx;\n double sumysq = sumy * sumy;\n\n double sq2 = sqrt(sumxsq + sumysq);\n\n if (sq2 > 255) //Unsigned Char Fix\n sq2 = 255;\n filteredImg.at<uchar>(i - size, j - size) = sq2;\n\n if (sumx == 0) //Arctan Fix\n angles.at<float>(i - size, j - size) = 90;\n else\n angles.at<float>(i - size, j - size) = atan(sumy / sumx);\n }\n\n return filteredImg;\n}\n\n\ncv::Mat canny::nonMaxSupp()\n{\n cv::Mat nonMaxSupped = cv::Mat(sFiltered.rows - 2, sFiltered.cols - 2, CV_8UC1);\n for (int i = 1; i < sFiltered.rows - 1; i++)\n for (int j = 1; j < sFiltered.cols - 1; j++)\n {\n float Tangent = angles.at<float>(i, j);\n\n nonMaxSupped.at<uchar>(i - 1, j - 1) = sFiltered.at<uchar>(i, j);\n //Horizontal Edge\n if (((-22.5 < Tangent) && (Tangent <= 22.5)) ||\n ((157.5 < Tangent) && (Tangent <= -157.5)))\n if ((sFiltered.at<uchar>(i,\n j) <\n sFiltered.at<uchar>(i,\n j + 1)) ||\n (sFiltered.at<uchar>(i, j) < sFiltered.at<uchar>(i, j - 1)))\n nonMaxSupped.at<uchar>(i - 1, j - 1) = 0;\n //Vertical Edge\n if (((-112.5 < Tangent) && (Tangent <= -67.5)) ||\n ((67.5 < Tangent) && (Tangent <= 112.5)))\n if ((sFiltered.at<uchar>(i,\n j) <\n sFiltered.at<uchar>(i + 1,\n j)) ||\n (sFiltered.at<uchar>(i, j) < sFiltered.at<uchar>(i - 1, j)))\n nonMaxSupped.at<uchar>(i - 1, j - 1) = 0;\n\n //-45 Degree Edge\n if (((-67.5 < Tangent) && (Tangent <= -22.5)) ||\n ((112.5 < Tangent) && (Tangent <= 157.5)))\n if ((sFiltered.at<uchar>(i,\n j) <\n sFiltered.at<uchar>(i - 1,\n j + 1)) ||\n (sFiltered.at<uchar>(i, j) < sFiltered.at<uchar>(i + 1, j - 1)))\n nonMaxSupped.at<uchar>(i - 1, j - 1) = 0;\n\n //45 Degree Edge\n if (((-157.5 < Tangent) && (Tangent <= -112.5)) ||\n ((22.5 < Tangent) && (Tangent <= 67.5)))\n if ((sFiltered.at<uchar>(i,\n j) <\n sFiltered.at<uchar>(i + 1,\n j + 1)) ||\n (sFiltered.at<uchar>(i, j) < sFiltered.at<uchar>(i - 1, j - 1)))\n nonMaxSupped.at<uchar>(i - 1, j - 1) = 0;\n }\n return nonMaxSupped;\n}\n\ncv::Mat canny::threshold(cv::Mat imgin, int low, int high)\n{\n if (low > 255)\n low = 255;\n if (high > 255)\n high = 255;\n\n cv::Mat EdgeMat = cv::Mat(imgin.rows, imgin.cols, imgin.type());\n\n for (int i = 0; i < imgin.rows; i++)\n for (int j = 0; j < imgin.cols; j++)\n {\n EdgeMat.at<uchar>(i, j) = imgin.at<uchar>(i, j);\n if (EdgeMat.at<uchar>(i, j) > high)\n EdgeMat.at<uchar>(i, j) = 255;\n else if (EdgeMat.at<uchar>(i, j) < low)\n EdgeMat.at<uchar>(i, j) = 0;\n else\n {\n bool anyHigh = false;\n bool anyBetween = false;\n for (int x = i - 1; x < i + 2; x++)\n {\n for (int y = j - 1; y < j + 2; y++)\n {\n if (x <= 0 || y <= 0 || EdgeMat.rows || y > EdgeMat.cols) //Out of bounds\n continue;\n else\n {\n if (EdgeMat.at<uchar>(x, y) > high)\n {\n EdgeMat.at<uchar>(i, j) = 255;\n anyHigh = true;\n break;\n }\n else if (EdgeMat.at<uchar>(x,\n y) <= high && EdgeMat.at<uchar>(x, y) >= low)\n anyBetween = true;\n }\n }\n if (anyHigh)\n break;\n }\n if (!anyHigh && anyBetween)\n for (int x = i - 2; x < i + 3; x++)\n {\n for (int y = j - 1; y < j + 3; y++)\n {\n if (x < 0 || y < 0 || x > EdgeMat.rows || y > EdgeMat.cols) //Out of bounds\n continue;\n else if (EdgeMat.at<uchar>(x, y) > high)\n {\n EdgeMat.at<uchar>(i, j) = 255;\n anyHigh = true;\n break;\n }\n }\n if (anyHigh)\n break;\n }\n if (!anyHigh)\n EdgeMat.at<uchar>(i, j) = 0;\n }\n }\n return EdgeMat;\n}"
}
{
"filename": "code/artificial_intelligence/src/image_processing/canny/canny.h",
"content": "//\n// canny.h\n// Canny Edge Detector\n//\n\n#ifndef _CANNY_\n#define _CANNY_\n#include \"opencv2/imgproc/imgproc.hpp\"\n#include \"opencv2/highgui/highgui.hpp\"\n#include <vector>\n\n\n\nclass canny {\nprivate:\n cv::Mat img; //Original Image\n cv::Mat grayscaled; // Grayscale\n cv::Mat gFiltered; // Gradient\n cv::Mat sFiltered; //Sobel Filtered\n cv::Mat angles; //Angle Map\n cv::Mat non; // Non-maxima supp.\n cv::Mat thres; //Double threshold and final\npublic:\n\n canny(std::string); //Constructor\n\tcv::Mat toGrayScale();\n\tstd::vector<std::vector<double> > createFilter(int, int, double); //Creates a gaussian filter\n\tcv::Mat useFilter(cv::Mat, std::vector<std::vector<double> >); //Use some filter\n cv::Mat sobel(); //Sobel filtering\n cv::Mat nonMaxSupp(); //Non-maxima supp.\n cv::Mat threshold(cv::Mat, int, int); //Double threshold and finalize picture\n};\n\n#endif"
}
{
"filename": "code/artificial_intelligence/src/image_processing/canny/main.cpp",
"content": "//\n// main.cpp\n// Canny Edge Detector\n//\n\n#include <iostream>\n#include <string.h>\n#define _USE_MATH_DEFINES\n#include <cmath>\n#include <vector>\n#include \"opencv2/imgproc/imgproc.hpp\"\n#include \"opencv2/highgui/highgui.hpp\"\n#include \"canny.h\"\n\n\nint main()\n{\n std::string filePath = \"lena.jpg\"; //Filepath of input image\n canny cny(filePath);\n\n return 0;\n}"
}
{
"filename": "code/artificial_intelligence/src/image_processing/Cell-Segmentation/img/blogdiagram.drawio",
"content": "<mxfile host=\"65bd71144e\">\r\n <diagram id=\"EOms3ZQl2FKXDm_uvD9-\" name=\"Page-1\">\r\n <mxGraphModel dx=\"924\" dy=\"585\" grid=\"1\" gridSize=\"10\" guides=\"1\" tooltips=\"1\" connect=\"1\" arrows=\"1\" fold=\"1\" page=\"1\" pageScale=\"1\" pageWidth=\"850\" pageHeight=\"1100\" background=\"#ffffff\" math=\"0\" shadow=\"0\">\r\n <root>\r\n <mxCell id=\"0\"/>\r\n <mxCell id=\"1\" parent=\"0\"/>\r\n <mxCell id=\"41\" value=\"\" style=\"rounded=0;whiteSpace=wrap;html=1;fillColor=none;dashed=1;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;strokeColor=#000000;strokeWidth=2;fontFamily=Helvetica;\" vertex=\"1\" parent=\"1\">\r\n <mxGeometry x=\"140\" y=\"600\" width=\"360\" height=\"150\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"28\" value=\"\" style=\"rounded=0;whiteSpace=wrap;html=1;fillColor=none;dashed=1;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;strokeColor=#000000;strokeWidth=2;fontFamily=Helvetica;\" vertex=\"1\" parent=\"1\">\r\n <mxGeometry x=\"140\" y=\"130\" width=\"560\" height=\"190\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"18\" value=\"\" style=\"edgeStyle=none;html=1;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;strokeColor=#000000;strokeWidth=2;fontFamily=Helvetica;\" edge=\"1\" parent=\"1\" source=\"2\" target=\"4\">\r\n <mxGeometry relative=\"1\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"2\" value=\"2018 Kaggle Data Science Bowl\" style=\"rounded=1;whiteSpace=wrap;html=1;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;fillColor=none;strokeWidth=2;strokeColor=#000000;fontStyle=1;fontSize=13;fontFamily=Helvetica;\" vertex=\"1\" parent=\"1\">\r\n <mxGeometry x=\"360\" y=\"30\" width=\"120\" height=\"70\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"26\" value=\"\" style=\"edgeStyle=none;html=1;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;strokeColor=#000000;exitX=0.5;exitY=1;exitDx=0;exitDy=0;strokeWidth=2;fontFamily=Helvetica;\" edge=\"1\" parent=\"1\" source=\"3\" target=\"24\">\r\n <mxGeometry relative=\"1\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"3\" value=\"Training Images\" style=\"rounded=1;whiteSpace=wrap;html=1;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;fillColor=none;strokeWidth=2;strokeColor=#000000;fontStyle=1;fontSize=13;fontFamily=Helvetica;\" vertex=\"1\" parent=\"1\">\r\n <mxGeometry x=\"160\" y=\"220\" width=\"120\" height=\"60\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"27\" value=\"\" style=\"edgeStyle=none;html=1;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;strokeColor=#000000;exitX=0.5;exitY=1;exitDx=0;exitDy=0;strokeWidth=2;fontFamily=Helvetica;\" edge=\"1\" parent=\"1\" source=\"4\" target=\"24\">\r\n <mxGeometry relative=\"1\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"4\" value=\"Masked Images\" style=\"rounded=1;whiteSpace=wrap;html=1;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;fillColor=none;strokeWidth=2;strokeColor=#000000;fontStyle=1;fontSize=13;fontFamily=Helvetica;\" vertex=\"1\" parent=\"1\">\r\n <mxGeometry x=\"360\" y=\"220\" width=\"120\" height=\"60\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"5\" value=\"Test Images\" style=\"rounded=1;whiteSpace=wrap;html=1;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;fillColor=none;strokeWidth=2;strokeColor=#000000;fontStyle=1;fontSize=13;fontFamily=Helvetica;\" vertex=\"1\" parent=\"1\">\r\n <mxGeometry x=\"560\" y=\"220\" width=\"120\" height=\"60\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"34\" value=\"\" style=\"edgeStyle=none;html=1;labelBackgroundColor=none;labelBorderColor=none;fontFamily=Helvetica;fontSize=13;fontColor=#000000;strokeColor=#000000;strokeWidth=2;\" edge=\"1\" parent=\"1\" source=\"24\" target=\"33\">\r\n <mxGeometry relative=\"1\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"24\" value=\"Image Augmentation\" style=\"rounded=1;whiteSpace=wrap;html=1;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;fillColor=none;strokeWidth=2;strokeColor=#000000;fontStyle=1;fontSize=13;fontFamily=Helvetica;\" vertex=\"1\" parent=\"1\">\r\n <mxGeometry x=\"240\" y=\"380\" width=\"160\" height=\"60\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"29\" value=\"Microscopy Cell Images Dataset\" style=\"text;strokeColor=none;fillColor=none;align=left;verticalAlign=middle;spacingLeft=4;spacingRight=4;overflow=hidden;points=[[0,0.5],[1,0.5]];portConstraint=eastwest;rotatable=0;dashed=1;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;fontStyle=1;fontSize=14;fontFamily=Helvetica;\" vertex=\"1\" parent=\"1\">\r\n <mxGeometry x=\"445\" y=\"140\" width=\"235\" height=\"30\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"10\" value=\"\" style=\"endArrow=none;html=1;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;strokeColor=#000000;strokeWidth=2;fontFamily=Helvetica;\" edge=\"1\" parent=\"1\">\r\n <mxGeometry width=\"50\" height=\"50\" relative=\"1\" as=\"geometry\">\r\n <mxPoint x=\"220\" y=\"180\" as=\"sourcePoint\"/>\r\n <mxPoint x=\"620\" y=\"180\" as=\"targetPoint\"/>\r\n </mxGeometry>\r\n </mxCell>\r\n <mxCell id=\"12\" value=\"\" style=\"endArrow=classic;html=1;entryX=0.5;entryY=0;entryDx=0;entryDy=0;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;strokeColor=#000000;strokeWidth=2;fontFamily=Helvetica;\" edge=\"1\" parent=\"1\" target=\"3\">\r\n <mxGeometry width=\"50\" height=\"50\" relative=\"1\" as=\"geometry\">\r\n <mxPoint x=\"220\" y=\"180\" as=\"sourcePoint\"/>\r\n <mxPoint x=\"470\" y=\"210\" as=\"targetPoint\"/>\r\n </mxGeometry>\r\n </mxCell>\r\n <mxCell id=\"13\" value=\"\" style=\"endArrow=classic;html=1;entryX=0.5;entryY=0;entryDx=0;entryDy=0;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;strokeColor=#000000;strokeWidth=2;fontFamily=Helvetica;\" edge=\"1\" parent=\"1\" target=\"5\">\r\n <mxGeometry width=\"50\" height=\"50\" relative=\"1\" as=\"geometry\">\r\n <mxPoint x=\"620\" y=\"180\" as=\"sourcePoint\"/>\r\n <mxPoint x=\"470\" y=\"210\" as=\"targetPoint\"/>\r\n </mxGeometry>\r\n </mxCell>\r\n <mxCell id=\"32\" value=\"\" style=\"edgeStyle=none;html=1;labelBackgroundColor=none;labelBorderColor=none;fontSize=13;fontColor=#000000;strokeColor=#000000;strokeWidth=2;fontFamily=Helvetica;\" edge=\"1\" parent=\"1\" source=\"31\" target=\"24\">\r\n <mxGeometry relative=\"1\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"31\" value=\"<b>Albumentations<br>Library</b>\" style=\"ellipse;whiteSpace=wrap;html=1;labelBackgroundColor=none;labelBorderColor=none;fontSize=13;fontColor=#000000;strokeColor=#000000;strokeWidth=2;fillColor=none;fontFamily=Helvetica;\" vertex=\"1\" parent=\"1\">\r\n <mxGeometry x=\"460\" y=\"370\" width=\"120\" height=\"80\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"36\" style=\"edgeStyle=none;html=1;exitX=0.5;exitY=1;exitDx=0;exitDy=0;entryX=0.5;entryY=0;entryDx=0;entryDy=0;labelBackgroundColor=none;labelBorderColor=none;fontFamily=Helvetica;fontSize=13;fontColor=#000000;strokeColor=#000000;strokeWidth=2;\" edge=\"1\" parent=\"1\" source=\"33\" target=\"35\">\r\n <mxGeometry relative=\"1\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"38\" style=\"edgeStyle=none;html=1;exitX=0.5;exitY=1;exitDx=0;exitDy=0;entryX=0.5;entryY=0;entryDx=0;entryDy=0;labelBackgroundColor=none;labelBorderColor=none;fontFamily=Helvetica;fontSize=13;fontColor=#000000;strokeColor=#000000;strokeWidth=2;\" edge=\"1\" parent=\"1\" source=\"33\" target=\"37\">\r\n <mxGeometry relative=\"1\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"44\" value=\"\" style=\"edgeStyle=none;html=1;labelBackgroundColor=none;labelBorderColor=none;fontFamily=Helvetica;fontSize=13;fontColor=#000000;strokeColor=#000000;strokeWidth=2;\" edge=\"1\" parent=\"1\" source=\"33\" target=\"43\">\r\n <mxGeometry relative=\"1\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"33\" value=\"UNet++ Model\" style=\"rounded=1;whiteSpace=wrap;html=1;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;fillColor=none;strokeWidth=2;strokeColor=#000000;fontStyle=1;fontSize=13;fontFamily=Helvetica;\" vertex=\"1\" parent=\"1\">\r\n <mxGeometry x=\"260\" y=\"500\" width=\"120\" height=\"60\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"35\" value=\"Predicted Masks\" style=\"rounded=1;whiteSpace=wrap;html=1;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;fillColor=none;strokeWidth=2;strokeColor=#000000;fontStyle=1;fontSize=13;fontFamily=Helvetica;\" vertex=\"1\" parent=\"1\">\r\n <mxGeometry x=\"180\" y=\"620\" width=\"120\" height=\"60\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"37\" value=\"Original Masks\" style=\"rounded=1;whiteSpace=wrap;html=1;fontColor=#000000;labelBackgroundColor=none;labelBorderColor=none;fillColor=none;strokeWidth=2;strokeColor=#000000;fontStyle=1;fontSize=13;fontFamily=Helvetica;\" vertex=\"1\" parent=\"1\">\r\n <mxGeometry x=\"340\" y=\"620\" width=\"120\" height=\"60\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"39\" value=\"\" style=\"shape=link;html=1;labelBackgroundColor=none;labelBorderColor=none;fontFamily=Helvetica;fontSize=13;fontColor=#000000;strokeColor=#000000;strokeWidth=2;exitX=1;exitY=0.5;exitDx=0;exitDy=0;entryX=0;entryY=0.5;entryDx=0;entryDy=0;\" edge=\"1\" parent=\"1\" source=\"35\" target=\"37\">\r\n <mxGeometry width=\"100\" relative=\"1\" as=\"geometry\">\r\n <mxPoint x=\"380\" y=\"730\" as=\"sourcePoint\"/>\r\n <mxPoint x=\"480\" y=\"730\" as=\"targetPoint\"/>\r\n </mxGeometry>\r\n </mxCell>\r\n <mxCell id=\"42\" value=\"Comparison and Precision Score and Accuracy Score\" style=\"text;strokeColor=none;fillColor=none;align=left;verticalAlign=middle;spacingLeft=4;spacingRight=4;overflow=hidden;points=[[0,0.5],[1,0.5]];portConstraint=eastwest;rotatable=0;labelBackgroundColor=none;labelBorderColor=none;fontFamily=Helvetica;fontSize=13;fontColor=#000000;fontStyle=1\" vertex=\"1\" parent=\"1\">\r\n <mxGeometry x=\"145\" y=\"700\" width=\"350\" height=\"30\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"43\" value=\"<b>Medical Image Segmentation</b>\" style=\"ellipse;whiteSpace=wrap;html=1;labelBackgroundColor=none;labelBorderColor=none;fontSize=13;fontColor=#000000;strokeColor=#000000;strokeWidth=2;fillColor=none;fontFamily=Helvetica;\" vertex=\"1\" parent=\"1\">\r\n <mxGeometry x=\"460\" y=\"490\" width=\"120\" height=\"80\" as=\"geometry\"/>\r\n </mxCell>\r\n <mxCell id=\"45\" value=\"\" style=\"endArrow=classic;html=1;labelBackgroundColor=none;labelBorderColor=none;fontFamily=Helvetica;fontSize=13;fontColor=#000000;strokeColor=#000000;strokeWidth=2;exitX=0.5;exitY=1;exitDx=0;exitDy=0;entryX=1;entryY=0.5;entryDx=0;entryDy=0;\" edge=\"1\" parent=\"1\" source=\"5\" target=\"37\">\r\n <mxGeometry width=\"50\" height=\"50\" relative=\"1\" as=\"geometry\">\r\n <mxPoint x=\"400\" y=\"470\" as=\"sourcePoint\"/>\r\n <mxPoint x=\"560\" y=\"650\" as=\"targetPoint\"/>\r\n <Array as=\"points\">\r\n <mxPoint x=\"620\" y=\"650\"/>\r\n </Array>\r\n </mxGeometry>\r\n </mxCell>\r\n </root>\r\n </mxGraphModel>\r\n </diagram>\r\n</mxfile>"
}
{
"filename": "code/artificial_intelligence/src/image_processing/Cell-Segmentation/README.md",
"content": "<h2> Cell Segmentation <a href=\"https://colab.research.google.com/github/Curovearth/Cell-Segmentation-and-Denoising/blob/main/Cell_Segmentation_.ipynb\"><img src='https://colab.research.google.com/assets/colab-badge.svg' align=right></a></h2>\r\n\r\n\r\n<p>The study aims to determine a solution for the automatic segmentation and localization of cells. I have tried to utilise UNet++ architecture over UNet to detect cell nuclei and perform segmentation into individual objects.</p>\r\n\r\n\r\n## Image sets and experiment description\r\n\r\n- **Dataset** which was used was extracted from the mentioned repo data: <a href=\"https://github.com/Subham2901/Nuclei-Cell-segmentaion/tree/master/Data\">Shubham2901/Nuclei Cell Segmentation</a>\r\n\r\n| Dataset | Images | Input Size | Modality | Provider |\r\n| --- | --- | --- | --- | --- | \r\n| Cell Nuclei | 670 | 96x96 | microscopy | Kaggle Data Science Bowl 2018|\r\n\r\n<p align=center>\r\n <img src='img/blogdiagram.png' width=400><br>\r\n <samp>Block diagram for the overall process</samp>\r\n</p>\r\n\r\n\r\n## Results Obtained\r\n\r\n<p>TP, FP, TN and FN are the numbers of true positive, false positive, true negative \r\nand false negative detections.</p>\r\n\r\n| | |\r\n| --- | --- |\r\n| <b>Precision Score</b> | 0.91 |\r\n| <b>Recall Score</b> | 0.85 |\r\n| <b>F-1 Score</b> | 0.88 |\r\n| <b>Sensitivity</b> | 0.85 |\r\n| <b>Specificity</b> | 0.99 |\r\n| <b>IOU</b> | 0.79 |\r\n| <b>AUC</b> | 0.92 |\r\n\r\n| | Precision | Recall | F-1 Score | Support |\r\n| --- | --- | --- | --- | --- |\r\n| False | 0.98 | 0.99 | 0.98 | 5707370 |\r\n| True | 0.91 | 0.85 | 0.88 | 846230 |\r\n| Accuracy ||| 0.97 | 6553600 |\r\n| Macro Avg | 0.95 | 0.92 | 0.93 | 6553600 |\r\n| Weighted Avg | 0.97 | 0.97 | 0.97 | 6553600 |\r\n\r\n\r\n## Final Result\r\n\r\n<p align=center>\r\n <img src='img/img2.png'>\r\n <img src='img/img3.png'>\r\n</p>\r\n\r\n## Future of Cell Segmentation\r\n\r\nWith the rise of size and complexity of cell images, the requirements for cells segmentation methods are also increasing. The basic image processing algo developed decades ago should not be the golden standards for dealing with these challenging cell segmentation problems any more.\r\n\r\nOn the contrary, development of more effective image processing algorithms is more promising for the progress of cell segmentation. In the meantime, comparison of these newly developed algorithms and teaching the biologists to use these newly developed algorithms are also very important. Hence, the open access and authoritative platforms are necessary for researchers all over the world to share, learn, and teach the data, codes, and algorithms. \r\n\r\n---\r"
}
{
"filename": "",
"content": ""
}
{
"filename": "",
"content": ""
}
{
"filename": "",
"content": ""
}
{
"filename": "code/artificial_intelligence/src/image_processing/connected_component_labeling/Connected-Component-Labeling.ipynb",
"content": "{\n \"cells\": [\n {\n \"cell_type\": \"code\",\n \"execution_count\": 38,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"# Importing the libraries \\n\",\n \"import cv2\\n\",\n \"import numpy as np\\n\",\n \"import matplotlib.pyplot as plt\\n\",\n \"\\n\",\n \"\\n\",\n \"def connected_component_label(path):\\n\",\n \" \\n\",\n \" # Getting the input image\\n\",\n \" img = cv2.imread(path, 0)\\n\",\n \" # Converting those pixels with values 1-127 to 0 and others to 1\\n\",\n \" img = cv2.threshold(img, 127, 255, cv2.THRESH_BINARY)[1]\\n\",\n \" # Applying cv2.connectedComponents() \\n\",\n \" num_labels, labels = cv2.connectedComponents(img)\\n\",\n \" \\n\",\n \" # Map component labels to hue val, 0-179 is the hue range in OpenCV\\n\",\n \" label_hue = np.uint8(179*labels/np.max(labels))\\n\",\n \" blank_ch = 255*np.ones_like(label_hue)\\n\",\n \" labeled_img = cv2.merge([label_hue, blank_ch, blank_ch])\\n\",\n \"\\n\",\n \" # Converting cvt to BGR\\n\",\n \" labeled_img = cv2.cvtColor(labeled_img, cv2.COLOR_HSV2BGR)\\n\",\n \"\\n\",\n \" # set bg label to black\\n\",\n \" labeled_img[label_hue==0] = 0\\n\",\n \" \\n\",\n \" \\n\",\n \" # Showing Original Image\\n\",\n \" plt.imshow(cv2.cvtColor(img, cv2.COLOR_BGR2RGB))\\n\",\n \" plt.axis(\\\"off\\\")\\n\",\n \" plt.title(\\\"Orginal Image\\\")\\n\",\n \" plt.show()\\n\",\n \" \\n\",\n \" #Showing Image after Component Labeling\\n\",\n \" plt.imshow(cv2.cvtColor(labeled_img, cv2.COLOR_BGR2RGB))\\n\",\n \" plt.axis('off')\\n\",\n \" plt.title(\\\"Image after Component Labeling\\\")\\n\",\n \" plt.show()\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 39,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAAOIAAAD3CAYAAAAAC4j/AAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADh0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uMy4yLjEsIGh0dHA6Ly9tYXRwbG90bGliLm9yZy+j8jraAAAgAElEQVR4nO2deVhV1f7/3+twmEFBETXDEJE0EiccUHPADL05PJb5Fa/dQMXhJo5p5UBOmRrmhAOVIjhU4s0cug5oOaDmxZwSEBVCGRJFZRYEz+f3B3h+EBxkOOfsfeDzep7383D2sPZ7H3iz9t5r7bUEEYFhGGlRSG2AYRgOIsPIAg4iw8gADiLDyAAOIsPIAA4iw8gADqIECCFaCiFyhBBGWihruxBimTZ8MdLBQawCQggfIcQfQog8IcQ9IcRmIYRNTcsjortEZEVEz7Tp8++U+I7U5TEY7cBBfAFCiNkAVgKYA6AhgB4AXgEQIYQw0bCPUn8OmboAB7EShBANACwG4E9ER4iokIgSAYxCcRjHlmy3SAixVwixUwiRBcBHCNFKCHFaCJEthDguhNgohNhZsr2jEIKeB1YIcVIIsVQIcbZk+2NCCLtSPsJLauLMkjJda3g+iUKIOUKIa0KIXCHEViFEUyHE4VI+batyXCFEYyHEQSFElhAiSgixrHTtK4RoK4SIEEI8EkLECSFG1cRzfYGDWDk9AZgB+LH0QiLKAXAYwMBSi4cD2AvABsAuALsB/A9AYwCLALz/gmONAeALwB6ACYCPSq07DKBNybpLJeXXlHdLfLsAGFpS9jwAdij+e5hWxeNuBJALoBmAD0oEABBCWAKIQPF3YA/AG8Cmmv4DqQ/wJVTl2AFIJ6KiCtb9BaBLqc/niegnABBCNAHQFcAAInoKIFIIceAFxwohopsl++8BMOz5CiLa9vxnIcQiAI+FEA2JKLMG57SBiNJKyjoD4D4RXS75vA/AgBcdF0AOigP9OhHlAYgRQoQC6Fey+RAAiUQUUvL5khDiPwBGAoiugec6D9eIlZMOwE7DPV/zkvXPSSr180sAHpX8kVa0viLulfo5D4AVAAghjIQQK4QQ8SWXvYkl29ihZqSV+vlJBZ+rctwmKP4nXvqcSv/8CoDuQoiM5wLwTxTXnkwFcBAr5zyAAgDvlF5Ycuk1GMCJUotLv8byF4BGQgiLUsscauhhDIove99E8cMix+c2alieNo77AEARgJdLbV/6/JIAnCIim1KyIqIpOvZssHAQK6Hk0m8xgA1CiEFCCGMhhCOAcADJAHZo2O8OgIsAFgkhTIQQHii+H6sJ1ij+Z/AQgAWA5TUsR2vHLWl2+RHF52chhGgL4F+l9j0EwEUI8X7Jd2YshOgqhGinJ+8GBwfxBRDRKhQ/zAgEkAXgAor/4w8gooJKdv0nAA8U/yEvA/ADiv+wq0sYgDsAUgDEAPitBmXUhBcddyqKa8p7KP6H9B1Kzo+IsgG8BWA0gNSSbVYCMNWHcUNE8IvB+kEI8QOAG0T0mdRedIEQYiWAZkT0wQs3ZsrBNaKOKLkUay2EUAghBqH4fusnqX1pi5J2QjdRTDcA4wHsk9qXocLNF7qjGYrvoxqj+H5yyvNmgjqCNYovR18CcB/AagD7JXVkwPClKcPIAL40ZRgZUOmlqRCCq0uG0SJEVGH7L9eIDCMDOIgMIwM4iAwjAziIDCMDOIgMIwM4iAwjAziIDCMDOIgMIwM4iAwjAziIDCMDOIgMIwM4iAwjAziIDCMDOIgMIwM4iAwjAziIDCMDOIgMIwM4iAwjAziIDCMDOIgMIwM4iAwjAziIdRwjIyMYGxvjzz//xN27d3H37l28//77UCp5bGk5wb8NmdOiRQsoFDX/f/nVV1+hc+fOcHFxwbNnzwAAy5Ytw61btzB8+HA8fvxYve3Dhw+Rl5enqShGh1Q60jePa6ofOnfujEaNGlW4burUqbCwsKhwXVX45JNPcOnSpQrX7dmzBzY2NurPP//8M6Kjiyf0zc3Nxfnz52t8XKZiNI1rykGUmL59++Lrr7/GyZMnkZOTU259QEAAcnNz9eJlzJgx6NKleDbywsJCpKUVTyZ88OBB3L59Wy8e6jocRJnh6OiIRYsW4datW0hKSsLevXtldVnYsGFDDB8+HADQvn17NGnSBABw584dfPZZnZxZTi9wEGWEtbU1Lly4gNu3b2PChAm4f/++1JYqxcnJCba2tgCAli1bYt68eep1RAQPDw/1/SdTORxEGWBiYoLz58/DwsICHh4eePr0qaxqwapgZGQEa2tr9ecLFy4AKK41nz59KpUtg0FTEEFEGgWAWLWXlZUVubi4UEREBMXHx5OpqanknrQlIQTFxsbSxYsXqWnTppL7kbs0ZY3bEXWMtbU1Fi5ciH379iE7Oxu9e/dGQUGB1La0xvNL09zcXGzfvh2Ojo5SWzJI+NJUh3z88ccwNTWFQqHAL7/8gtOnT0ttSWe0bNkSmzZtgkqlwocffoikpCSpLckSvkfUM4GBgZg0aRJGjBiB48ePS21HL7Rq1QqdOnXCwIED1U9Zn7NhwwacOnVKImfyQVMQuWeNDvjyyy/x4YcfolevXhob0+sif/75J/7880/8/vvvMDc3Vy8fN24cWrZsKaEzA4Af1mhXJiYmFB4eTt26dZPci1ykVCopJCSEevfuLbkXqcUPa/TEjBkz4O7uXqYPZ32nqKgISUlJOHr0KBo2bCi1HVnCl6Y64NNPP8WtW7ektiErAgICIITA0KFDsXPnTqntyA6uERm9sXDhQqxatUpqG7KEg8gwMoCDqEU8PT1hY2ODAwcOSG2FMTA4iFrC3d0dBw4cQF5ensH1H2VkADdf1F4mJiaUm5tLq1atktyL3HXgwAHq3Lmz5D6kksascRBrL19fX1qyZInkPgxB5ubmFBMTI7kPqaQpa9x8oQWWLl0KBwcHqW0wBgzfIzJ6paCgAPPmzcPnn38utRVZwUGsJT///DM8PT2fX8ozL0ClUiE2NhZt27aV2oqs4CDWEltbWzx69EhqGwZFYWEhcnNzubtbKTiIjN5JSEjAvn37MHPmTKmtyAYOIsPIAA4iw8gADmItWLBgAdzc3KS2YZD88ssvUKlU6N+/v9RWZAEHsYYolUq8/PLLGDp0KB4+fCi1HYMjMzMTAMoM+V+f4Qb9GjJp0iRcvHgRv/76q9RWmDoA14gMIwO4RqwBjo6OsLOze+HrTsbGxpg/f3655fn5+VixYoWu7NWaTz/9FKamptXa5+7du9i2bVu19jl58iT69++PM2fOID09vVr71jU4iDXgeRAvX75c4fpZs2ahd+/eKCoqwu7du8ut//vQ9IsWLYKbmxsyMjIwbtw4nXiuiJCQEDRs2BBXrlzBkiVLMH36dPTt2xffffcdCgsLq1WWvb09fvzxRwQHB+Po0aNV2uf06dOYPn067Ozs6n0Q+e2LGqhfv360YcOGCtdNmDCBJkyYQC4uLtSmTZtKyxkxYgTFxsbSe++9Ry4uLtS9e3eKjY2lhQsX0uLFiyk2NpauXbtGJePLakXe3t4UGxtLsbGxlJeXR23btqXRo0dTbGwsTZw4kVxcXEihUFS7XHNzcwoICKC//vqrWq85vfTSSxQREUFmZmaS/171IX4NSksSQtCgQYNo5cqVZZYrFAoaNmwYBQQE0I4dO6h9+/Yay7CxsaGsrCxav349mZmZlfnDHzhwIAUFBZGxsTGZmZmRtbU1JScnU0pKCqWkpFBGRgb16tWrWp5tbGwoIyODUlJSKC0tjfz8/MjMzEz9x+/p6UmBgYFkbm5eq+/GyMiI1q5dSzk5OeTs7Ew2NjZkaWn5wv1+++03unfvHllbW0v++9W1OIha0ltvvUW//PJLmWVCCPLy8qK1a9dS06ZNadOmTZXWhra2thQdHU2vvPJKmeVKpZL8/PwoKChI475Lly6l3NxcatCgQZX8tmnThlJTU+no0aOVbjdnzhxasGBBrcMIgL799luKioqiqKgoCgsLoy5dulCjRo0q3ef8+fN06dIlyX+/uha/j6gFFAoFwsPD8cUXX5RbPmDAAHz55ZeYOHEiQkNDKx1OsaCgAFFRUZg8eTJiY2PVy01MTNCrVy+cOHFC474LFy4EEVVpCjR3d3eEhIQgMjISo0ePrnTb6OhojBo1CuPGjUN2djYyMzNx+fJl9OvXr8x2Dx8+xM8//1xpWRMmTFD/7Orqiu3bt2P9+vXYsWOHxn28vLxw8uTJF55TXYXnvqgGCoUCiYmJGoePb9WqFVq0aIHIyMgqlffaa6/By8tL/TkvLw/BwcFa8QoUTwveuXNnfPvtt8jOzq7SPmPHjkWTJk1gbGyMBg0alOusYGJiAnt7+3L7/fnnnwgKCqqwzI8++ghpaWmVBrFBgwY4efIkOnfuXCWfhgrPfaEFwsLC8P7772tc/3zuh6oSExODmJgYbVirkFOnTlV74pfng/9aWFjA2dkZ165dK7Pe2toa3bt3L7PM1tYWwcHBUCiKm6WHDBkCAEhLS8OaNWvg5+eHZcuW1fQ06gd8j1h1JSYmkpGRkeQ+5CaFQkEODg40depUmjp1Kjk4OJCDgwN17dqV9uzZQw4ODmRhYVFpGUIIGjBgAK1du1by89Gl+GFNLWVra0u///47B7ESCSHKNbVU5/t6/fXX6dGjRzRr1izJz0VX4kloaoGTkxOOHTuGYcOG4dmzZ1LbkS2l/oGrqc73df36dfj7+6svcesT9e+Ma4C/vz9SUlKQm5srtZU6z82bN2Fubl7vRsXjhzVVZMOGDcjIyKhVGVOmTEHHjh3LLV+zZg1u3LhRq7J1yeTJk9GpU6cyy/bu3YuIiAitHysqKgrvvfce2rVrV6+m/+Yg6hAzM7MybYIhISHYvn17ue0++eQTtG7dGv369UP79u2xceNGrFy5UpI5NEJCQuDi4lJmWWhoaDnfQ4cOxaJFi0BE6Nu3L1+y1xIOog4wMjLCiRMn0KJFC3h4eKiXZ2VlVdgQHxMTgytXruDq1avo1KkT9u3bh7CwMAwePBjnz5/Xud+BAwciLCwMz549w+jRo8vVzhX5vnbtGr766isoFArEx8dDqVRi165dWLBgAYqKinh4yWrC94hapmHDhvjxxx/RunVrdOrUCenp6Wpp6g2TmZmJ/v37o2HDhjAyMkJISAg2btyIEydOwM7OTqd+HR0dsW/fPqxbtw4ODg6IjIyEpaUlrK2toVAoNPrOzc1Feno67t+/j1atWsHBwQFXr17FgwcP8O6779bLBy61gWtELTNp0iS0bNkSffr0QU5OTpX26dq1Kw4dOoTjx4/D2dkZY8eOxcaNG8t1L9MVkZGR6vcje/Xqhf/+97+4cuUKHjx4gJEjR75w/+e13+7du2FrawtfX1+oVCo8evSoXndbqw4cxBfQvn17ZGZmIjExscr7xMbGIicnBzNmzICJiUm59atXr1bfU3l6eiI4OBjh4eGYNm0aVCoVfvrpJ/j4+KBFixbaOo1KadWqFfr16wdLS0sEBwdjy5YtCAwMhL+/P3r27Ilz585VuayNGzdi48aNCAgIgI+PD5ycnHTovO7AQawEJycnfP311/j+++8RHx9fpX0OHjyI4cOHY/v27ejTpw8mTpxYZv3q1avRuXNndRBjYmIQEBCA/fv3Q6VSoW3btvD390e3bt2wbdu2KvcRrSkPHjzAokWL0Lt3b+Tn52POnDnYu3cvCgsLceXKFWzevBnjx4/HxYsXq1Xu8uXL4ePjoxvTdRAOYiU0btwYaWlp2LVrV5X3iY2Nhbe3N2xsbKBSqXD9+vUy6y9fvlymlkxNTS3zdnpSUhISExNx+/ZtBAYGoqCgoPYnUgm5ubn47rvv0KRJExQWFpZpojlx4gRu376NadOmwd3dHdnZ2XjjjTeqVO6xY8cgRIX9m5kK4CC+gEePHlV7GIe7d+/i7t27Fa57UXthbm4uEhMTUVRUpNeZhx88eFBuWWZmJq5duwZ/f38olUpYW1sjNTW1SuX17NmzwjKrwsKFCxEeHo4bN25o/B7rGhxEGfLNN99IbaEMT548AQBkZ2fjpZde0vnxCgoKYGJiUq+evNafM2UYGcNBZBgZwEFkZIePjw/279+PtLQ0qa3oD34fsXiApbi4uDKyt7enQ4cOkb29veT+6pvs7Ozou+++oxYtWkjuRdvi9xE1YGlpiStXruDcuXNwc3ODm5sbsrKycPXqVTRp0gT379+X2mK9Iz09Hba2tjA2Ni63ztraGnfu3EFKSkoZZWdnG/QMxPzUFMUz2Pr6+qo/d+3aFY0aNcLhw4cldMX8nVdeeQX79u3D/fv30bt37zJT4oWGhhp0uyUHkTEIXn31VWzatAl//fUXvL29YW9vj3379uHYsWMAgN9++61KQ0zKFQ4iYxD07dsXDx8+xIwZM5CTk4MFCxZg6tSp+Omnn6S2phXq/T0iYzj8+uuvSE1NhUKhwHvvvVdnQghwEDWSkZGhHp+TkRdFRUV49913q9UHWO5wEDWgUqlq3FeS0T0PHjzQ+UvT+oSDyMgOJycnJCUlIT8/X+M2BQUFSEpKQuvWrfXoTHdwEBnZMXnyZISHh+PevXsat7l//z5++OEH/Pvf/9ajM93BQWQMlpiYGDx+/LjMAF2GCgeRMRjmzJmDDh06qD8/H/R5x44dOH/+PKysrCR0Vzs4iIxBEBoaioiICPz6669lxvLZvHkzunfvDjMzMyiVhtssbrjOtQQRIS0tDU2bNq1fvf0NjIKCAkyaNAkNGjTArVu3YGVlBZVKhfz8fDRo0ABGRkZSW6wV9b5GzMvLw/Tp07F+/XqprTBVwNvbG+Hh4ejfv7962apVq5CRkYHCwkIJndWOel8jAsVtUjdu3Kj20IH6QKFQYO7cueoOzQcOHED37t2xc+dOjX0rmzVrVqYT+8aNG5GVlaUXv7XF1dUVeXl5lU74evnyZWzduhWOjo7o1q0b7ty5g3nz5hn0JEH1vkYEih+FR0dH621A3+rw9ddfY8aMGUhISEBCQgJ8fHwQGBiI3bt3IzAwsMJ9WrRoAS8vLyQkJGD06NEICwszmPFfOnbsiJycHNy6davC9Z999hmWLVuGadOmASgeEDk+Pr7Kg1rJFa4RSzh69Chef/119O3bt9rTXeuKsLAwjBw5Ep07d1aP/nb27FmEhoYCQKVDLSYmJuKHH37A+PHjsXHjRqhUKr141jUDBgzA22+/LZvfkbbgIJaQmZkJhUIBa2trqa0AKL7vGTp0KNq2bVtmSMHk5GQkJydXuu+zZ88wbNgwpKamwtzcHLGxsbq2qxXc3NywefNmLFiwoNw6pVKJNWvWYPHixTh9+jSA4sl+FAqFQd8bquGhMv6/li1bRkOGDJHcR32UQqGgIUOG0PLly8uts7CwoMWLF1NycjJ17txZvXzgwIG0evVqyb1XRzxUBiNrTE1NMWTIEOzfv7/c8tmzZ2PMmDHw9fXFpUuXJHKoWziIpYiIiEDXrl3RuHFjqa3UO548eYItW7bg22+/VQ/r/9FHH2HlypUYP348/P39dTJDsWzgS9OyOnDgADk7O0vuoz6qQYMGFBgYSNHR0XTw4EHKycmhYcOGUc+ePctta2dnR5s3b6Z27dpJ7rs60pQ1fljzN4jIoAchMmSysrKwaNEibN68GUDx7yIhIaHcdkZGRvjf//6HmzdvGsyDqBfCNWJZKZVKun79OpmamkruhVWxmjRpQpcvXyalUim5l+qKH9ZUkaKiIsTExKBjx45SW2E0cOnSJXTp0gVFRUVSW9EeXCNWrPT0dBo2bJjkPljllZSURAqFQnIfNRHXiNVkxYoV+PrrrzFmzBiprTD1AH5Yo4G1a9ciOzsbb775Jnbv3i21nTqPpaUlDh48qP788ccfIyoqqtx269atg62trT6t6Qe+NNUsY2NjmjRpEk2YMEHvx1YoFGRkZETXrl2jyMhIyb8LXSs+Pp6uXLlCzZs3p6CgIHrw4AG9/PLL6vVCCPriiy8oLy+P3NzcJPdbU2nMGgexco0aNYpmzZql16eolpaWFBYWRqmpqZSQkEBGRkY1LsvOzo5atGhBzZs3l/y71KSmTZvSpUuX1Pd9QgjauXMnubu7EwAyMTGhuXPn0sOHD6lHjx6S+62NOIi10Jo1a2jAgAE6P46JiQl5enrSunXrKDY2ll577bUal9WsWTPy9PSkb775ho4fP0779+8nT09PcnFxkfz7/Luio6PJ3Ny83PL4+HgaOHAg+fv7U3JyMg0dOlRyr7UVN+jLHCEE5syZg8mTJ2Pv3r0YN24cYmJialSWvb09AgMD4eDgAD8/P9y8eRPW1tbYunUrmjdvjilTpuD69etaPgPts337dvzjH/8AAMycObPMPWSdg2vEF0sfNeLq1avp0aNHNGjQoFqX1aVLF4qMjCRXV9cyy5s2bUphYWF09uxZWXXj01Qj1kVx84WMCQoKwpEjR+Dp6YkjR45g5MiRuHDhAtq3b49Dhw7VqMzbt28jOjq6zLK0tDTMnj0b9+7dw4EDB3D27FnJu/OtXLkSrVq1ktSDLOAa8cX68ssvycvLS2flW1tbk5mZGb388suUkpJCGRkZNGfOHLK0tKSEhAQyMzMjU1NTtYQQlZbXqVMnys7OpilTplS43sLCgmxtbSkpKYmuXLlCxsbGknyvRkZGtGfPHurWrZvkv2N9iR/W1FIRERE6ndP9zp07dPbsWQJA//73v0mlUtGdO3eoadOmdOPGDYqLi1PrrbfeImdn53IqHah//etftHz58kov+YyNjSk+Pp4OHz6s9+/T0tKS1q5dSyNGjJD8d6tP8cMamUNEWLZsGRo3boz27dvj2rVr+L//+z+kpaWhbdu2ZbZds2YNnJycypURHh6O9PR0HDlyBElJSQgICMCTJ08QGBiIJ0+elNu+sLAQHh4e+Oyzz9CuXTu9vMmgUCjw9ttvw9HREa1atcL333+v82MaAhxEmRAUFAQPDw+4u7sjPT0dEyZMQFxcXIXbzpw5s8Ll06dPx6ZNmzBz5kxs3boVU6dORa9evTB79uznVzj4/fffceTIEfU+BQUFuHfvHhwcHPQSxLlz52L27NmYNm0ahg8frvPjGQx8aVo19enTh0JCQnTa2djMzIz69u1bqzJGjBhR7gnvP/7xDxo5ciQtXLiQbt26RW+++SYBxfdoBw8epCNHjujlO1yxYgVlZ2fr9H5b7uJ7RC0oMTGxVr1cpJaFhQUtWbKEUlJSqGPHjnT69GlKSkqili1b6vzYy5cvp7y8vHr1YKYicRC1oOvXr1PDhg0l91EbKZVKsrS0pEOHDpGDg4Ne2u+mT59OWVlZBt89TRvSlDXx/N6hIkoekzMlKBQKJCYmomXLllJbMRisrKwwdepUXL9+vcZtonUJIqqw4ZYb9Bmd4uHhgUaNGnEIXwAHsRoQEYKCguDn5ye1FdmjUCjwxRdfYPz48VJbMQj40rSaNG/eHLt27YKnp6fUVtSMHTsW3t7eAIDc3FyMGjVKr8e3sbHBrl27yixTqVTYtGkTACAhIUFjU0x9Q9OlKbcjVpPK/nFJRZs2bXDgwAFERETg7Nmzej326dOnYW5uXi78RITExES9ejFo+Klp9eXp6UlbtmwhMzMzyb0AoI8//phSU1MpOTmZbt68qZdjWlpa0rFjx+j+/fv15s0JbYibL7SoTp06UXx8vCRDaMhBdnZ2tGvXLoqOjqZmzZpJ7seQxEHUsj788EMKCwsje3t7yb3oU40bN6atW7fS+fPnDW64ezmI30fUMqdPn4aTk1OFna/rKubm5ti0aRNcXV0xZcqUujPcvRzgGrHmatOmDe3cudPge9tURWFhYRQZGUlxcXH06quvSu7HUMWXpjrSuXPnyM7OTnIfutJHH31ESUlJ1L17d2rWrBk1btxYck+GLH4fUUekpaWhWbNmSE9Pl9qK1jE3N4eVlRUmTpyICxcuSG2nTsMN+logOTkZDg4OsmxjrCkWFhaYO3cuVCoVlixZIrWdOgP3NWWqjFKpREBAAMaMGYPz589LbadewJemTBkWL14MBwcHnDx5EocPH8apU6ektlQ/4Ic1tVdycnKFI6uZmZlRVFQURUVF0fjx4yX3+SItWbKEcnNz6Y033pDcS10VtyPqkI4dO+LixYvllhsZGcHS0hJr165F8+bNoVDI9+tWKpVo2bIlvLy8cObMGant1Dvk+5dhQGRlZaFBgwbllqtUKiQnJ+PXX3+FqakpJk2aBBcXF8kH9f07FhYWWL58OY4fP47IyEip7dRLOIg65MmTJ5gxYwbWrVuH2NhYDBw4ECtWrMA777yDvn37Sm0PQggMHz4cPj4+cHZ2RlJSktSW6i38sEZP7N69Wz3h6YIFC6BUKtG3b18cPXpUkjY6X19fODg4QKFQ4Pbt23jnnXf07oH5/3AQJWDZsmWwsbHB0qVLERwcjPfffx9//PFHtcowNTUt9zJuRSxZsgTXrl0DALRr1w5Lly4FAPTr1w+zZ89GaGho9U+A0TocRInIyMjAggULsG7dOhw8eBA9evTAvXv31OsXLVqE9957r8J9hRDIz8/HmDFjXniczz77DG5ubhBCICYmBgsWLFCvu3XrVu1PhNEKHEQJyczMhJ+fH5RKJSIjI9G8eXP1uvnz58Pd3b3S/SsaRv/v+Pj4qJ/WqlQqFBQU1M40oxM4iBJTWFiIwsJCdOnSRSflc/AMA35qyjAygIPIMDKAg8gwMoCDqAWEEJW+AjVo0CD4+/vr0RFjcHCn79orMTGx0umvhw8fTpmZmTR27FiDnk2KVXvxUBk6kpubGx08ePCF202YMIHS09PJ2dlZcs8s6cRB1JGqM2bN5MmTadq0aVwr1mPxmDUyYMuWLYiLi4OTkxNmzJghtR1GRnAQ9cyYMWNw6tQpWFpa8qxSjBoOop75/fff0alTJ1y9ehX5+fmYPn06VCqV1LYYieEgSsCtW7fg4eGBn376CYmJidi7dy8A4NmzZ0hOTpbYHSMFHESJuHr1Klq1aoWJEyciODgYQPHchs9/BoALFy4gMzNTKouMHuFxTWvJuXPnMGzYMK0MMGxtbY2FCz4drWkAAAcsSURBVBeqPz9+/BiFhYXIzMzEN998U+vyGenRNK4pB7GWaDOIf8fLywt2dnawsLBAnz59AACbN2/GuXPntH4sRj9oCiJfmsqYo0ePAgBMTEwQFRUFAJg4cSKCgoLU2xw/fhxz586VxB+jPbhGrCFKpRLr1q1DeHg4Tp06pbfh9s3NzWFsbKz+7OXlhXXr1qk/P336FI6Ojlo5Tmny8/Pr1JQCUqGpRuSeNTXUhx9+SKmpqdShQwfJvZRWSkpKjfd96aWXqG3bttShQweKjo5WKzMzk6di05K4Z40OCAgIwNWrV6W2USssLCwwaNAgAEDPnj3h6OiIgoICuLq6qrf5z3/+I5W9egMHsR4zZ84cWFpaqjsUhIaGVjia3A8//ABvb28sW7YMRUVF+rZZL+Ag1gBXV1f4+fmVeWgiF6ysrPDll19izpw56mVCCISHh5cb8n/Pnj14+PAhIiIiKi1zz549iI2Nxauvvgpvb+9y621sbLBt27Yyy3x9fbkNtBpwEGtAkyZN8McffyA8PFxqK+Vwd3dHmzZtysxvT0R499138ezZszLbxsfHl1umicGDB8PW1lZd7qpVqxASEoITJ07AwsICH3zwgXrb3bt3w9TUVAtnU4/ghzXVk4uLC+Xk5NDKlSsl96JJQggyMzMrI22V/by8JUuWUEpKCrVu3ZpMTU3LbHPmzBmyt7eX/HuQo/h9RC2oQ4cOlJeXR1u2bJHci5zFQdQsDqIWdPfuXVq/fr3kPuSs7t27U1xcHAdRgziItdSkSZPo8ePH/HZ9JfLw8KArV67Q+vXrycLCQnI/chQHsRaaPHkypaen0+jRoyucGZgFcnV1pZiYGFq7di3Z2NiUWz937lw6duxYlcb3qcviINZCa9asIW9vb64NK1Hv3r0pNDSUrKysKlwfGhpKgwcPpr/++ktyr1KKe9bUkvv371f5UX99o2nTpvj888/Rv3//cqMNmJiY4NNPP8WJEydw+PBh7q+qAR5gmKk1T58+xZ07d+Dk5KRe1qNHD7zxxhv45z//CU9PzzJTzjHl4RqRqTWPHz9GcHAwtm3bhu+//x4A4OLiAqVSidjYWFlMUy53OIiMVrh9+zZ27Nih/vzJJ58gPz+/zDbz588v93oVUwwHkdEKaWlpLxzO45133sHIkSP15Miw4CBWgWfPnsHIyEhqGwaLQqFAUFAQZs6ciTNnzkhtR55w80XVFBERQT169JDch6HJ3NycPv/8c0pLSyNXV1fJ/UgtbkespT7//HPKycnhtsRqyMzMjObNm0fx8fHk6ekpuR85SFPWeMyaanDnzh0EBgZiw4YNUluRPVOnToWVlRVsbGxw+vRp/Pe//5XakiwgHrOm9hoxYgRlZ2fT/PnzJfciZwUEBFB2djYNHz5cci9yE1+aakmenp6UnZ1Nn3zyieRe5Kj58+dTTk4O9evXT3IvchQHUUsSQpCXlxdlZWWRn58fKRQKyT3JQQqFgqZMmUJZWVk0YMAA7hyvQRxELcvb25sePXrEncEBMjIyorFjx9LDhw9p1KhRkvuRsziIOtCkSZMoPT2dfHx8JPcipcaNG0cPHjygCRMmSO5F7uK3L3RAcHAwCgoKsG7dOjRq1AhfffWV1Jb0ygcffABnZ2fk5+dj9uzZCAsLk9qS4cI1Yu319ttvU3Z2Ni1cuFByL/qSr68vpaWl0bhx4/h+sBrSlDV+DUoL/Pzzz+jUqRNu376NuLg4vP3221Jb0gnW1taIi4tDXFwcmjRpgl69eiEsLIzfMdQGXCNqTwqFgmbNmkX37t2jnJwccnBwkNyTNmRlZUWNGjWihIQEOnnyJJmamtb7B1Q1Ffes0TM7d+7EK6+8glmzZuHRo0eIj4+X2lK1MDMzQ/v27QEAfn5+6NChA/r06YOCggKJnRk2mnrWcBB1iImJCbZs2YL09HTExMQAAH788UdkZWVJ7Ewzw4YNQ6NGjWBhYQF3d3cAQFBQEC5duiSxs7oBB1FCnJ2d1feNLVq0gLGxMZKSkmT1lNXT0xNDhw7FmDFj8NVXXyE1NbXMi76MduAgyoQePXrAwsICzZs3h6+vr3q5SqXC4MGD9TpA1d69e2FjYwMA+O233/DLL78AAE6dOsUDZekIDqLMMDY2hr29vfqzQqHAyZMnq/QC8pAhQ9SXun+nYcOGuHz5cpU8DBkyBI8fPwYAZGdny/qSua7AQTQAqjoKwKFDh/Daa69VuC4rKwsdO3asUjlc6+kfDiLDyABNQeQGfYaRARxEhpEBHESGkQEcRIaRARxEhpEBHESGkQEcRIaRARxEhpEBHESGkQEcRIaRARxEhpEBHESGkQEcRIaRARxEhpEBHESGkQEcRIaRARxEhpEBHESGkQEcRIaRARxEhpEBHESGkQGVjuLGMIx+4BqRYWQAB5FhZAAHkWFkAAeRYWQAB5FhZAAHkWFkwP8DW/8Uhk8QM4gAAAAASUVORK5CYII=\\n\",\n \"text/plain\": [\n \"<Figure size 432x288 with 1 Axes>\"\n ]\n },\n \"metadata\": {\n \"needs_background\": \"light\"\n },\n \"output_type\": \"display_data\"\n },\n {\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAAOIAAAD3CAYAAAAAC4j/AAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADh0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uMy4yLjEsIGh0dHA6Ly9tYXRwbG90bGliLm9yZy+j8jraAAAgAElEQVR4nO2dd5gURfrHPzWbYZccd8lJgiAZyYgoKCBnFkxg9k4Uz/PUn2D2DIeiZwKzeJhQEUQliAKCiIB4KKySYQmSYZewuX5/VK8zuzszO7M7M927+36e532mu6u7+u3q/k5VV1dQWmsEQbAXl90OCIIgQhQERyBCFAQHIEIUBAcgQhQEByBCFAQHUGmFqJSqr5RaqpTKUEo9Y7c/QnAopZoppbRSKrqsxyqlFiulbrCWr1RKLQi1vyVRKiEqpbYrpYaE2pkIcxNwEKimtb7L82aEEqXUUA/BH1BKLVFKXRDq8ziVkp4VpdQgpdSuSPrkD631DK31uZE+b6XNEYGmwAYdohYNSqkoL9suAWYC04FGQH3gAWBkKM4pVCC01kEbsB0YYi2PBZYDU4CjwFagj7U9DdgPXOtx7HBgLZBuhT9UJO5rgB3AIWBSkXO5gHuBLVb4R0AtHz7WBOYCB4Aj1nIjK+xtIAfIBo5b/ucBmdb6i9Z+bYGFwGHgd+Ayj/jfBl4BvgROFPjoEa6AncDdftLRBUy0rnc/RrDVrbBmgAbGWel0BLgF6AGss9L6RY+4Cu7DC8Ax4DfgbI/wZGCOdS2bgRs9wh6y0nI6kAGsB7oXOfYTKy23AbcHcizwLpAPnLLS9Z9e0mAQsMtH+vh8VjzS5yZgD7AXuKtI2np9VjyOjbbWFwM3eKTjMo94tJXum6x78BKgrLAo4BlMyWobcJtnvEFpKkRCzLUemCjgMesBfAmIA861blCiR8J3tBKqE7AP+IsV1t66Yf2AWGAyRjAF55oA/IDJXeKAacD7PnysDVwMVAGSMDnTZ0WE9JjH+p83w1qvat38cUA00NVK8A4exx8D+lrXEl/k/G2tm9LcTzpehxFFCyAR+BR4t8jDMhWIt9IxE/gMqAekYMQ7sMh9uBOIAS63/Ct4+JYAL1txdcaI6mwPMWUC51v38AngB48Heg0mJ4+1fN0KDC3p2KLPio80GIRvIfp7VgrS533rXnW0rqnEZ4XghTgXqAE0sc4xzAq7BdhgnaMm8DU2C3GTR1hHy5n6HtsOAZ19xPUcMMVafgAPYWFElO1xrlQK/8s3xAi1xAu3Hr4jQQjxcuC7InFMAx70OH66n/P1tdIh3s8+i4C/eqyfVnA9Hg9LSpF0vNxj/RNggsd92IP1b21t+xG4GmiMyfGTPMKeAN72ENPXHmHtgVPWci9gZxG/7wPeKunYsgqxhGelIH3aeoQ/DbxR0rNC8ELs57H+EXCvtfwNcLNH2BBKKcSga5x8sM9j+RSA1rrotkQApVQv4EngdMw/bBwmtwJTBEorOEhrfVIpdcgjnqbALKVUvse2PMy7125Ph5RSVTDF5WGYfyuAJKVUlNY6L4Bragr0Ukod9dgWjSluFZCGbwr8bogptngjGVMsLWCHdY76HtuKpqPXdLXYra0nwiO+ZMsOa60zioR191j/w2P5JBBv1So2BZKLpEMU8F1Jx2qtcykDJTwrBXjegx2YjAD8PyvBUvT6CtK80POK/+fBL3ZU1ryHeVdprLWujil6KStsLyabB0AplYApYhaQBpynta7hYfFa60IitLgLk8P00lpXAwYUROvDr6KVNmnAkiLnStRa3+rnGE9+t+K42M8+ezAPTAFNMMXLfd53L5EUpZTn9TWxzrEHqKWUSioS5i3dipIGbCuSDkla6/MD9MlfGpWEv2elgMYeywXXW+B3oM9KaSn0vBbxJSjsEGIS5t85UynVExjjEfYxMFIp1UcpFQs8TOGEnwo8rpRqCqCUqquUGuXnPKeAo0qpWsCDJfi1D/P+U8BcoI1S6mqlVIxlPZRS7QK5SCtn+jswSSk1TilVTSnlUkr1U0q9au32PnCnUqq5UioR+BfwYRlyknrA7ZavlwLtgC+11mnA98ATSql4pVQn4HpgRgBx/gikK6XuUUolKKWilFKnK6V6BOhT0XT1iuWXpyn8PysFTFJKVVFKdcC8z39obQ/mWSktHwF3KKVSlFI1gHtKG5EdQvwr8IhSKgPzTvhRQYDWej0wHvgA82+TgamQyLJ2eR7zD7nAOv4HzDuMN54DEjAVLD8A80rw63ngEqXUEaXUf6xi3LnAFZh/2T+ApzDFo4DQWn+Mede8zopjH6Yya7a1y5uYou5STPE1E3P9pWUl0BpzzY8Dl2itC4rIozHvRnuAWZh33YUBXEMe5nNLZ8vHg8DrQPUAfXoCmKiUOqqU+oePfVIwf5qe1hI/z4oHSzAVXouAyVrrgo/xwTwrpeU1YAGmFnstpgY9F1MEDoqCalhHYuUSR4HWWmtf71kCoJQai6lw6Ge3L5UVpdR5wFStddMSdy6C4z7oK6VGWkWNqpjPF79gat4EwVFYRfXzlVLRSqkUzOvPrNLE5TghAqNwVzC0Bq7QTs62hcqMwtRjHMEUTVMxRejgI5JnXBDsx4k5oiBUOvx+0FdKSXYpCCFEa+31O7bkiILgAESIguAARIiC4ABEiILgAESIguAARIiC4ABEiILgAESIguAARIiC4ABEiILgAESIguAARIiC4ABEiILgAESIguAARIiC4ABEiILgAESIguAARIiC4ABEiILgAESIguAARIiC4ABCNS2b4FCiFLgUbLzcLAPcvwre3wy5MkafYxAhOpyUKkZIpeXZ3tC1DrT5EPKsmQIf6wGbroBR8+FIlnvfQ1lwskwzGgqlxe9I3zKuaWToWgdq+Zhj6rYOUKUMf5f3/gg/HfQe9tEQqBHrXv9iJ6w/YpZP5MKK0s7SKPjE17imIkSbGdgQXh0Ai/fA8Zzi4Q+sNqKIBGNaQbc6ZjknH/adMsuf74DN6ZHxoaIjQnQYzZLgoW6w6RikHYePtzmrWFg9FkZZk4t1rAV1E8zyjgx4cI19fpV3RIgOIikGVv7F5DI3LIX9p+z2yD8tkqCmVXRukgj/18UdpoHen0GePCkBIUJ0ALEuWPEX887X+zPIzndWLhgIUcr8kRSw8kLz23GmuR7BPyJEG0mMgeQq8FI/k7u0/wiyKshDq4ANl8GJHBg+z/1eKXhHJqGxiaQYmNQFZp0LGdnQb07FESG4i6YncuHtQebdVwgeyRHDyD1nQFyU+Q74zW5Y+ofdHoWPJonwcj/I1/C3ZZB2wm6PnIkUTSPM5DPh5nZw4QL4erfd3kSG5knQpTac08hdy1rAC7/Ckr32+OUkfAlRWtaEgX/3gr+1h75zfH9Mr4hsyzC25iAkeDxZ151mckzBD1prn4Z5BRALwmJd6JlD0D3r2u+LUyxaod8aiO7XwH5f7DZfWpPKmhAzoSN0rwtHsu32xDnkavPOOP9801BAKI4UTcPAfT+aFjOCmwdWm08dI5vCfzfZ7Y3zkBxRiBiTVsPTvez2wpmIEAXBAYgQQ8jgZNOtaM4Ouz0RyhsixBDRvS7MGWrajpa39qOC/YgQQ0CsC5aMhJc3wGNr7fbG2aw+YDpCC4WRljUhYFwbaF7N1AwK/kmIgjUXQfuZdntiD9KyJow82gMaz7DbC6E8I0VTIaJk5cH/rYLHe9jtibMQIZaRL4bB4Lmm/ZJQMvlA6hFoW8NuT5yFCLGM1IyDw1kl7ye4yck3HYmluZsbEaIQcbZmwKztcGdHuz1xDiJEQXAAIkRBcAAixDIwsQt0qmW3F+WTb/aYYTXOSrbbE2cgQiwl0QoaVYWR8+FQpt3elD+OWf01a0iFDSAf9EvNze1h9UH4do/dnggVAckRBcEBSI5YCpolQp14mLPd/34x0XD/zcW3Z2bCk2+ExbWQ0Oo+cPmYncoXp3ZC2pvBHbN4r3lH/O4POFjJi/cixFLQLAnqxMHaQ97D/34t9OsKubnw3hfFw7OLdJO6+iFo0QmOH4Vnrgu5uz454y2IqQ7HfoZNj0DzO6D2QNj9PuR7mZnKH3H1oPunsGMaHJgf2DFL98Idp5s/tcouRBnFrRQ2qCH6hT7ew2642FibZujWTfzH0/dC9Bup6AGXohu1QbftZdavnITm4URNal3NujoaFTrfk0ejB6UaO+8kumpbdPIVZr3JTeiqbdC4go/XlYBu/QD6nL3o6l2D8KcKeuFwdHyU/fc1EuZLa5IjBokC4qPgZF7h7S4FIwZBcj1o3RRWroNffAySlFgDZuyEBW/DrV0gJxu0NQz/S7dDn1HAncfhieMQoyCtnjkxQFUFww/D8sCzrOgacPZ2yDsBKhp+nwi73jVh+ZkQnwz7vjDb8ks5d0X+Kdj8OMTWgt5LYWlnyD4IOsec1xd7TpppCbaPhtYfQkaQOXGFQXLE4OzcRuhvRhTephR6aF/0c/ei69dGvzwJ3bqp7zgSa6JfW4+uX2SfqGj0eTeib3vRjw+PJmpONNBUUwH5W7U1esgedK/5/vdreTe69USTs5U1jTq9ju63yljn6ejq3dAxtfwfs2IU+qeL7L+/4TbJEUOAS8HMIfDEz0W2u+DsM+Hfb8JNl8I7s2GTn3FrcrJg4yoYcQvsSHVvj4mF0/vC2kV+nJh03NzSbF2iv9W7Q+e34PAy+OkK//tmrIeGl0Hj6yAvA3KOQfpaqD2o8H7Zh2C/l/deT9bd4F5O6gBnvA3b/gO73/V9zNAvYfFI//FWZKSHfhC4lClCNXnPe3jzFEipD8t+Ciy+pu2h+1D3euZJ+GJa2f0soNZAqN4V0l6H3IzAjkm5CmLrgisGoqsZ4XniijUVM0U5uQ22v+g9zhb/gKx9/oVYLcYIseungflZXpEe+iFg+iC4+lvf4dt2GwuUHRuMhYvDS4wFw+7/mt+oKlClFWSsKxwenQQ1ioxNGlMTOk3jz6/S9UeY36x9sG0KNLkRNj0WtPuVCskRg2D7aGj5gUxTXQwXxKdAg1Fm9Y/Z5jeuAbS8GzbcBTmHIO+k7ygUZjjKkU1hwoqwe2wbvnJEqawJ0GrGoddchI4K4aeECmeK4p9agvgscXpN9OFr0X/v6IBrCZPJJDRloEUSLDgfLpgvuaFfCh43T/K87eidX4/A+OXmXbyyIUIMgPGnw+4TZngHIbxsPGbmVmxc1W5PIotU1gTIC7/C0bJOtXbrmdC5YfHtU5bBbwfKGHkYGXALNO5SeNtPH0PqwpCfatUBuLQFtKtZuab/FiGGk/hoWHSje/2t1fD2muL73TsIWtaGQdOgYyd4aQo89QzMKeGDXTi49i2o16bwthXvwIq3C2/rNBJGPARaw7MDIT+IMqhQDBFiOIhywaIbIKU69H7ZvT09E7K9PLAb9sPPd8D/JkCXF2DWHJj+Opz3F1ixMvz+tjsHxk4HnQevXwF//FY4/FQ65BUpDuxaB7HPgnLBo1sgKhpWzoA5EyEvl+Ivi4JfpNa0ZJvSG312coD7V4/XzL5Gk3afJjE28PM0q2mOiY/T1K6tefxhzclDmjp1wnt9tZtpnj+uGXqvBqvZXK2mmtrNNUn1AoxHGes5RjPlqKbrJRrlKrVPT/cyTQntvu/hMGniFilu7gVNasCAqXA8wJfKHo1g7lj4ejO0agVXXQEvTYNB/cPq6p9sXgbznzTLLfvCbV/Crp8h4wC8ekkAEWjz8+N7UKUm9BkHOh9OHIaNi8PldYVChFgCHWuZ8VW2Hw/ioNT9RoQT+kFsVPHwZ76DPKu7xeCWMO1CmPkL3D7HjKj02ecw9mpIidDISnWaQ5tBEFcVrpwG302FhZNh0Hho2Qe2fB94XItfMjb8Aeg9Fia2CJfXFQoRoh9aJMGr/eGDLbAlPcCDPk+FUe3h7ctgQDO4qUjjyWdGQNdk9wfJDfvggYUwe4MRYdvTYPyt0LM7vDkdMgJsJFpaMg7A3IegVT/IyYRP7jY1onk5Jlcc8wpMvx52BDnV1Vf/MkIUAkKE6Ifa8bDvFMzYHMRBqfth9PtQIwHy8+HXfYXD1+6BWI9k35MOBz3q6dN2wfadsHkrTH4OssI8nn/2CVj1PiTWNd3yTx51h/22CPZvhsG3Q9PukJkBkwMsLt+xAFQl/DJfSkSIJXA4qxTDOOw8aswbJX0vPHECtu+A3Bw46adxZqg57sWvU8dg9zr4YLypFY1PgqcCHLbu6T7e4wyASatg5jnw21HYGcwrQTlGhOhEXgtyFKZwk3MKcjA54j3hf2/NyjezMFemZl+V6VoFwbGIEAXBAYgQBccxtg3M3mEqyioL8o4ItK4Oc4dZKxpQ0H8OPNgNrguyh7tQdubuhBf6wpwdptdLZaDSC7FqNPx8MXy0BW5ZZrYtuwD+d7Gpsdtfif6VncLBTKgZa0aSLEpSDPx6qZkEyJNqsdBohntym/JGpRciwNZ0GOeR8/WYBbXi4Kvz7PNJKE7TRJh1rvlz7Den8JR47wxyD/1aHhEhCuWC06rDy/1g70kYvQjqxRtRLthlwn/YD9n59vpYFkSIQrlgYDIcyoIJ38PxXJjYFW5bDp9tt9uz0CC1pkK54ds9Zoh+F6YXf0URIYgQfXI0C0bMs9sLwRu5Gi5eCDMG2+1J6BAh+iAfOFDZpwpzMAdOmencKgoiRMFxtEgyA0dl+hkGJysP0o5Dy2qR8yuciBAFx3FLe5i5Ff7w8w13fyZ8uAX+2j5yfoUTEaJQbtlwBI5kQe/6dntSdkSIQrnh7jPgjNru9d0n4UQuvHsWrBgFiTH2+VZWRIhCueCdjbBwF3w7AlI8RgF/ZQP0mmWGkC3a7K08UemFqDGt/Osn2O2J4I+sPLj5O5i/CzZd7n5wM/MgShkrz1R6IZ7MhTu+h//0sdsTIRBGLzIVOWeluLc9fab57psjTdzKNwcyzfgoferD9/tK3j+yuKD2P/mzSfPxOZDQC479F7T3rgYxNKA+4/5c38tL5BHoMHT20qGm+XPc5sfdtYfgjQHQ7H3oWRd2ZMD//WjeF8srlT5HBNOaf/0RGBShYUSDouGrUHsC5Gw1Vn0s1J8MKe+ZXy/EkkINhpLJVupwBa2ZTnm51Z1rw/Ec2ORDiA92g8e6w+3WUKt9G5ihLvdEcJytcCA5osX8XXB6LRjYEJbstdsbi+TpUO0S2NoVsq35KE4uh2PvmGXte6jFLLZzkA+pz/X8wUuYtkLln7OTYfg8B92jECFCtDiWbfKMJKdUgdd7GpJGwpa2kLPTvT13lzG/5FGLC+jBHlwkcJLUsLoaKjrVglf6w8RVxcOiFUzpAw+vgaWWCKOUmdS0PL8b/olMQuO2x7qjRzSx34/KaC5l0v5fPYqHVYlGP9wNvetKdNc67u3npKCfOdN+34MxmbpbcDRxLhjRxAwaVWh7FNzVCca0gnGL4aeDtrgXdkSIHizcDT3qQu04uz2pfJzKg6mp8PoA6N/AbPtHJ3iqJ1x/Goxfbu5PRUXeET1Ystf8+9aMM73BhciyNd1Umk3tb5bPSoYx38BHW4t/VqoTDxc1h//8ao+voUaEWASNzJ1iF+k58NAa02wNQGvY6mUyrCgFP/4FNh6DVB9TjJQ7pLKmsEUr9K+XouNc9vsi5t3qxqPXXmTuld2+BGtSWRMgudp0r+lcx25PBF/8dBF0+9Tcq4qCCNELl30NXwyDC5ra7YlQWRAh+uDJn81swWNa2e2JUBmQyhofPPcLZOTAkBR4L5gZg4VSUTUaPh/mXr9nJazyMs/p831MrXZFQ3JEH+RqePN3WLkfbmgb+fMrXLiIYjLreIRlkXcgwqy7xExzcOU35h39y/OgkUcHYAU80RNubAt9ZleUlrNuJEf0Q06+GROlUVXT8iMrQnc/jqrcyCt0ZAg5ZPJPupQ6rjrUIo5Y8slnL/tD6GXoqJ9g2vp2/9QIbPxyqBELDarArhNm9uAJHeGmdjB4Lqw7bLfHYUA+X5RsU3qjz04O/3miidWnM1iP5Xk9hVTdiPaljqsB9fRg+urX+Lf+mg/1bN7Sg+mr29DC9vQsausvRSdEFd++5QrTnnR8B9POdGRT+30tq/nSmuSIjkFxAXdzDrfwAx/zCtexiw2liqkedZjMJBqTzI3czUa2kkQibzCZhtTnVu7jV34Lsf+h5+2NcH4Ts3znCvh8h//9yzWSI5ZskcgRr+EZ/SaHdWeGlTmubnTUy/hMd6BNoe31qaOn87xezmzdima2p2uB+coRK6LJB30Hcx0v8jPzeITB/Mw8LuE0VnI1HanLXC4uVZyb2c56Nhbato+D3MUj/MF+5vA2y5mNsnlWwad6QfMkW11wBFI0DYDcfIgO41/We9wHZFKHeHbzV6oSw+OsYCtHaU9t4olGo//cP5s8j7Xi5KO5mPNZyVpe4Z1CYQc4xNXcThxxrONr1rKAHpxHDpEf8CVKGREO+tz0vqjMKKsI6j1QKX/3u1Kx8HwYuyR8c7rv4BZ2kUFfZvBXuvAiQ0gjg55MZwljCuVb4/marRzzEscxcqyK/Wu4lLa05FGe4xTeZ9OJIZrfWMpGtnEeV4bjsnxSNRoe72F6vMzaHtFT24rW2msRRHLEQAlzCU6jeYzvqU08HanLOg5wOXPYx0na8nqhfacwmBbUKBbHTH7jIKeYxzbS2M0DTOAUmUxmqlcx5pBLby7gQf5OO1qTyqawXV8BLgXDm0CzRJMbfrAl7KcsF0iOGCALh8PYxeHLEf9BD6oRRw75xOJiNptZzR9BxXEH3XiU/tzJIt7gF4ZxFn3pThbZfxZl17COeXz75zHVqcbtXM9KfmIBS0J4Rd65t7Pp83n7cni/EorQV44otaYB2oAG6LcGmrFVwnWOeKL0QBqXKY4Laa3Ppmmhbedztr6EEXoSE/Qmlush9NeAjiJKf847eh4zIpKGT/ZEZ4xDD21k//20y3xqTYQYuG0fjY4qh33gCqwKCfoR7ta7+Ul3poNeyiydxmrdhJSwn/tfPdAnr0P3rGt/OthpIsQQ2K+XoqvH2u9HWSyaaF2VBD2Xd3RjknUC8WE/5x2no9PHos+sZ//1222+tCbviEHgUrB9NDR5z25Pyg+JMXBbB/j1MMzdWfL+FR1f74jyQV8IK73rmV4VIkL/iBCDQGt4cb3piiP4x6VMt6XrJa0CQoqmQdKwCswYbLrjOIWrcDGaKABOAJeRE9Hz14g1aeJJvoaXrTbrW9Ph9+LtDyol8kE/RPj537KN1riYQz4LyWc5sRE999KRkBBtxvnxRGvYfjyirpRvpNY0eBucjJ7aHx3vkB4D9xCl9xCndxGnNxIbkXNWjUYvOB+9/+rK03MiFCa9L0LIkSw4JwWuam23J4anyCOZLBqRRRu8T14aSurEw6sDzFz2nT6WBtuhQIRYCtYegmd/gQENoF683d5EltpxputSiyS4ZCH8ccpujyoGIsRSsnQvtKhmrLKQEAUv9zPTa9+6rAINd+8ApNa0DLSuZqaS/ttyM/hRRWb6WSYXrBsPF8yXWtDSIrWmYWBTuskRYypwueIfneCOjqYYuiPDjGwnM2WFHhFiGdl3ChokwEHvfW/LNQlRponaTUvN+K5C+KjA/+WR4cIFMO/8sPcbjjhVouGezubD/FdpdntT8REhCsWIVvBAVzPvx4p9Je8vlB0pmgqFeLgbNE6ExXtMTrhkr90eVQ5EiGEkPgq+u8AsT90Ab/xurz8l8Uh3M4zFsC/hu+BG6RDKiBRNQ0DnT2D1RcW3RykzWtlzv5jG4i4Hv0hGK2iSCENFhLYgQgwB6dlQLab49nxtJlH5dg/ERcHN7aBNdedV7FSJhn/1hK93wTIRoS2IEMPIqTyYsMLM6Zd61LRPfbInXNQcBja02zvzhzCqKYxtA62qQVqYRqgTSkbeESPEe5vdE55O7GJGDh/YEObvsucb3bg2plLGpWBzOly0MPI+CG5EiDbw2FrTmfbR7jCtP1z9LfwS5Jx/cS6rM24J5dxH1rjnE2xXAx7tYZYHNYS7foB3Nvo+VogcIkSbOJoNE1ebYuvnQ+HMzwr3ZHioG1zawvuxCsjMgzHflHyeB7tCp9rmmA1HYeIqd9gmaS/qGESINnIsG25camosl40yNasF3L/KzKDrj0D6AY5d7K6tzQeypO+gIxEh2kxOPuQA3UoQXWmJ1HTjQtmQWlNBcAAiREFwACJEQXAAIsQQoJQZossXwxrD+A4Rc0coj8hwimW37aPRMS7f4aOaoo+NRV/VunzPJiVWdpPhFMNEp1rmY3yOn9rJ2TvMx/PneptZcgWhKDJ4VBn5fpQZTCmQoTJuaQexUfDSesiTlK2UyGxQDmBqKvytAzxzpt2eCE5DhBhhxiyCG9rCawPs9kRwEiLECLPmIHT5BK5sBS/0kRsgGKSJmw1sSofen8FnQ82MSR9vNdvztOlILFQ+RIg28b/D0Px9uKmd6QoFcCIXpqW691m5v+KPIC4YpNa0jARTa1oSSTEwqat7/UiW+SxyLBte+63s8Qv2I0PulwMycuCfK93rQxuZKdCqRMO7Z5ltr2yA72Ws0QqHCNHBzN9lfmNdsOqAWb6pHbzY173P17sLi1con0jRtJREK9O7fuZWMwhvpBIqIarwpDdDGxs/CsjOg2bvh+Y8nmTmRe4aKzK+iqYixFLytw5wfxc470tT8eIUdl8JKTNKd2xyFagWa4Z+fG+we3ujqtBzlkzFFgrkHTEMPLDaWSIsDVWiYVgjs9ynATRLMsNpdJjp3ueTc+zxrTIhQqzE3H2GGYk83yr3vLPR+2hyH26B0a3gsZ8gV8pIYUGEWAo61IQb28KL6+32pDiJMfDvXnC3RwWOAmYOKT7k/0db4VAmLNztP86PtkLqZXBadRjtZeS4GrHw5sDC28YtkW+gwSBCLAV1403OMXOr3Z4Up/ssM6V46mXubVrDxQuL9/jYkh54L5DzvoKase54n/4fvPU7LBpuirfXLnbv+95g854pBIF0DA7O2lRHHx+HfqqX/b74MgU6PqqwhSrugvge6Y7efSW6ZTV0XJH4v7sAXS/B/nRwovnUmggxcDujNvrkdeip/e33xckmQvRt0kM/BHw+FF7/DZ4LCwMAAARKSURBVG75zm5PnEuvelAv3m4vyh8ixAC5uZ1pC3rnCrs9cS6965sG7PN3wfEcu70pX0hlTQDc0g4e6wG3LnNX9QuF6VAT3hgAC3bBQ2vgZG7h8H+eAUNSzDfKkfPt8dHJSI4YAKfVgPHLTS2p6NA7NeNMe9iJq8wEO0XpUBOm/ALd60bet/KA5IgBsv+UDPjki/oJ8HgPOOtzM9GNJ7EuuK8LLNoNX6XJH5kvJEcUykx2PuzIgBbV3NvOrAf9G8CVrWFwcuEp54TiSI4olJkjWWZkgTcHwgdbzLY21c2syKlHYeDn9vpXHhAhCiFhczq8u8m9fu+PpuuUJ/d3Kd69SjCIEIWQsO9UycN5XNQcLlkYGX/KGyLEAMjTECVv06XGpcyoAneugO/22u2NM5HHKwD+8QPc3clUQAjBkRAFj3aHi5ubnh5Sa+odEWKA/HgAvh4OUV77VwveiI+COzvBFS1h9CJYf8Ruj5yLDJURBDvGwOT/wQsO7IfoNG7rYPpG1oiFpXvhyzS7PXIGvobKkN4XQdiFzdAZ49D3d7HfFyfbA11NOo1qar8vTjPpBhUiG5xsHrJ7O9vvixPt/i6mv+aghvb74kQTIYbIFOihjdDp49A3tkW7HOCTE8yl0Le2N+lydrJJJ7t9cqKJEENso1uiD19rfiv7dNxRCn1VK/Sha9CXtbDfHyebCDEMdnM79MFr0GPb2O+LnXbdaegD16BvaGu/L043X1qTD/plYFqq6V/3fB+oFQfP/mK3R5Hl2jbQqpppynbXCpi+qeRjBB9Ijlh2G97EVOBM6mq/L5GycW3Q+642uaG8DwZuvrQm3xFDRKtq0KMuPNQd/r4Cvthpt0ehJykGVl9klt/4DT7dBtszZNDhYJAh98PM5nTYmg4Nq5ghIxJjoN1HkFYBZgBOjIbYKFh9Iew8DkO/gtx86SgdSiRHDBP/PQuaJpnc8XCWGcy3PBEfBR1rmeUb28IZtWHAHMgq2gVfCAqZDcoGYl0wtb+ZTXiD1c7y022Q7uARzi5oaiqeqkS7x5d5cT38dNBevyoKIkQbaVUNhjcxyylVzfyGacedVcs6OBlGNoUxreDZdbDnZOGOvkJoECE6hDPrmdymYRUYd5p7e74280tE8r3r43NMo2yAH/bBN3vM8pK98v4XLkSIDiPGBfUS3OsuYPHIwLpZjZgHG456D6seA2svDsyHEfPgiDX0YUa2s4vMFQURYjkg0L6Oc4dB+5rew9KzofMngcUjuV7kESEKggPwJUTpoS8IDkCEKAgOQIQoCA5AhCgIDkCEKAgOQIQoCA5AhCgIDkCEKAgOQIQoCA5AhCgIDkCEKAgOQIQoCA5AhCgIDkCEKAgOQIQoCA5AhCgIDkCEKAgOQIQoCA5AhCgIDkCEKAgOQIQoCA7A7yhugiBEBskRBcEBiBAFwQGIEAXBAYgQBcEBiBAFwQGIEAXBAfw/uitk2O5ZHj4AAAAASUVORK5CYII=\\n\",\n \"text/plain\": [\n \"<Figure size 432x288 with 1 Axes>\"\n ]\n },\n \"metadata\": {\n \"needs_background\": \"light\"\n },\n \"output_type\": \"display_data\"\n }\n ],\n \"source\": [\n \"connected_component_label('C:/Users/Yash Joshi/OG_notebooks/face.jpg')\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 40,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAAOcAAAD3CAYAAADmIkO7AAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADh0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uMy4yLjEsIGh0dHA6Ly9tYXRwbG90bGliLm9yZy+j8jraAAAgAElEQVR4nO2de1hUdf7H319guIMoCEiAIZpXFFHErB7XVctSVlPLVdQ0N/NWVmplv0rL2lVTS9uQevJu7uatdXV3XdRMzUUELyCighhIKd64yn1mPr8/BnhA5zAwzMw5M3xez/N+HuZ8z/nO53s47znfc743QURgGEZ52MkdAMMw+mFzMoxCYXMyjEJhczKMQmFzMoxCYXMyjEJhc8qIECJYCHFfCGFvgrw2CyE+MUVcjDJgczYDIcQ0IcQFIUSZECJPCLFeCOFlbH5EdJ2I3IlIY8o4H6Qm7p/N+R2M6WFzNhEhxAIAKwAsAtAGwEAAHQEcEkI4ShzjYLkIGVuDzdkEhBCeAD4C8BoRHSSiaiLKBvAidAadXLPfUiHEbiHEdiFEMYBpQogQIcRxIUSJEOKwEOIrIcT2mv0fFUJQrYmFED8JIZYJIU7W7B8vhPCpF8eumjt2UU2ePY0sT7YQYpEQIlUIUSqE2CCE8BNC/KdenG2b8r1CCG8hxH4hRLEQIkkI8Un9u7QQopsQ4pAQIl8IcUUI8aIxMbdG2JxNYxAAZwB7628kovsA/gNgeL3NowHsBuAF4DsAOwCcBuANYCmAKQa+axKA6QB8ATgCWFgv7T8AutSkna3J31jG1cT9GIDomrzfA+AD3XXxehO/9ysApQD8AbxUIwCAEMINwCHozoEvgIkAYo39UWltcLWrafgAuEtEaj1pNwH0q/c5gYj+AQBCiPYAIgEMJaIqAD8LIf5p4Ls2EVFGzfE7AfyhNoGINtb+LYRYCqBACNGGiIqMKNOXRHSrJq8TAG4T0bmazz8AGGroewHch87kvYioDEC6EGILgN/V7D4KQDYRbar5fFYIsQfAeAAXjYi5VcF3zqZxF4CPxDNkh5r0WnLr/R0AIL/mwtWXro+8en+XAXAHACGEvRBiuRAiq6bKnF2zjw+M41a9v8v1fG7K97aH7ge+fpnq/90RQJQQorBWAGKgu8syBmBzNo0EAJUAxtbfWFNtexbAkXqb6w/zuQmgnRDCtd62ICNjmARdlXkYdC+kHq0Nw8j8TPG9dwCoAQTW279++XIBHCMir3pyJ6LZZo7ZJmBzNoGaauNHAL4UQowQQqiEEI8C2AXgVwDbJI7LAZAMYKkQwlEI8Th0z3fG4AHdD8Q9AK4A/mxkPib73pomoL3Qlc9VCNENwNR6xx4A8JgQYkrNOVMJISKFEN0tFLtVw+ZsIkS0EroXJqsAFANIhO7OMJSIKhs5NAbA49Bd3J8A+B66i725bAWQA+A3AOkAThmRhzEY+t550N1R86D7kfobaspHRCUAngbwRwA3avZZAcDJEoFbO4IHW1sWIcT3AC4T0RK5YzEHQogVAPyJ6CWDOzONwndOM1NTjQsVQtgJIUZA9/z2D7njMhU17Zi9hY4BAGYA+EHuuGwBbkoxP/7QPZd5Q/d8Oru2ycJG8ICuKhsA4DaA1QD2yRqRjcDVWoZRKFytZRiF0mi1VgjBt1WGMTNEpLetmu+cDKNQ2JwMo1DYnAyjUNicDKNQ2JwMo1DYnAyjUNicDKNQ2JwMo1DYnAyjUNicDKNQ2JwMo1DYnAyjUNicDKNQ2JwMo1DYnAyjUNicDKNQ2JwMo1DYnAyjUNicDKNQ2JwMo1DYnAyjUNicDKNQ2JwMo1Cs1pxPPPEEhDD30pQMIx+NLseg5Emls7KysH79emg0GqSmpuLIkSOGD2IYBSI1qTSISFLQrdKsKE2YMIE2b95MJSUlVEtsbKzscbFYxkrSf9ZmzpUrV9KD3L59m8aNGyd7bCyWMZLyn9U+c9anffv2aN++vdxhMIxJsSpzenp6ol27dnrT/Pz84OLiYuGIGMaMWFO1dvDgwZSRkfFQtbaWJ554QvYYWazmyiaqtceOHcM//iG9Yvv06dPh5uZmwYgYxnxYlTkNMWPGDDYnYzNYnTlXrVqFn3/+We4wGMbsWJ05b9++jdLSUsn0zMxM7jnE2ARWZ04AuHr1KiorK/WmGfvGtl27dggPD4eHhwd69OjRkvAYxjRY09va+rp27ZreN7bV1dX0/PPPNzmfP/7xjxQTE0NxcXFERPSXv/yFbt++TVOnTqXhw4fLXk6W7UvKf1bbt/batWsICQnRm5adnS2ZBgDDhw/H8OHDAQBvvvkmHBwc9O53/PhxDB48uOXBmpHIyEg4Ojri5MmTcofCGIlU31r9V6UVMG3aNBw5ckTSWA/i5OSEffv2AQBCQ0PRuXNnc4Zndjw8PLBr1y488sgjsLe3x/Xr1xETE4N79+6Z9Hvmzp2L6OhoaDQajBo1Co39mDMmxlqrtUIIqqiokKzabtu2jYQQ9Pnnn9Mvv/xC2dnZpNVqJTsw6KOsrIw++ugj2cuqTz4+Pg/Fm52dTfb29ibJv1+/fvTLL79QUVERERFptVpKSEigmtoUy4SS9J+1mhMABQQESBpLo9FQVVUVaTSaZhmyPsePHyc7OzvZy6lP9Ufl1KLVaiknJ4e8vLyMztfb25u6d+9O1dXVevM/fPgwubu7y15+W5KU/6zybW0tarVaMs3Ozg4qlQp2ds0vIhHh5MmTSElJgVarbUmIZkOlUj20TQiB4OBgHD58uNFnbil69OiB1NRUpKen631cEEIgIiIC06ZNMyZkprlY851zwYIFRt8V9XHy5EmKjY2lr776SrF3zFp98803jZblyJEj1LVr1ybnFxUVRRcuXDB4juLj42Uvu62JbKVaO3bsWIqNjaX169eTWq023ok1lJaW0qxZs2jWrFnUu3dv2cvXVHl7exss248//kgdO3Y0mFdkZCSdPXvWYH75+fk0atQo2ctuayJrNqebmxudOHGCTpw4Qbm5uca58AEWL15MTzzxBEVFRclePmPk4OBAr7zyisFynjt3jjw8PCTzCQ0NpcuXLxvMp7q6mgYMGCB7uW1RZE3mdHBwIAcHB9q4cSPl5eXRrVu3jHNgvQurqqqKLl68SL6+vuTr60tOTk6y/1NMcZ7mz59vsAaRm5tLDg4ODx3v5eVFd+/ebdL569mzp+zltVVJ+k8qgWQwZ1BQEA0ZMoQ0Gg1pNJpmN31IERERQUIIm20GWLp0KZWXl0uWX6vVUlZWFrVv377umICAAL1vfB/k7t27Vlu7sBZJ+k8qgSxszoEDBzbpYjGG8PBw2f8B5taKFSsaNSgRUUJCAnXu3Jn69OlD2dnZBs/b9evX+RnTAiKlmzMpKcl49xmgNZgTAH3wwQcG23UPHTpE58+fN3jOfv31V540zUIiJZtz5syZTXr2eZDCwkKKiYmhmJgYOnz4sOR+Bw4cIBcXF9n/CeaWEILmzZvX7PP4IEVFRfTMM8/IXp7WIlKyOYODg+nixYtNvnjmzJlD4eHh1KtXr7o8AgMDG20O8PT0lP2fYAmpVCqaOXOmca4k3fNp3759ZS9Ha5Kk/6QSyILm/OKLL6iqqkrvxVJWVkalpaV06tQp8vT0JE9PT71vHgHQiRMnJC+6mzdvyv5PsJTs7e3pnXfeaZYpKysraezYsa3mR0xJUrQ5AdCuXbvo0qVLpNVqqbi4mNLT0yk9PZ06derU5Dy2b9+ut08oka6zgdz/BEtqypQpVFBQ0CRjFhYW0owZM2SPubVK8eYEdFWy3bt309tvv210Hnl5eXovwIqKilY3eHrjxo1NMud3330ne6ytWVZhTlNIypxEROnp6bLHZykNHDiQzp071yRzpqam8py/MqrVmHPUqFGSzQmtxZxhYWGUkpLSJGPWkpaWRhEREbLH3hrVaszp4OAgac6Kigpat26d7DGaU35+fnT9+vVmGbOW3NxcCgwMlL0MrU1S/rPq8ZxSFBcX693u5OSEgIAAC0djGdzd3XHt2jVkZ2cjKCjIqDwCAwORkZEBHx8fE0fHGIPNmVOtViM8PFwyvW3btujQoYMFIzI/QUFBOHr0KEJCQuDs7Cy5X2FhIZKTk1FQUCC5j4uLCzIyMtC1a1dzhMo0B1ur1gK6sY7x8fGS1bcPP/xQ9hhNpc6dOzda1lqKiorojTfeIAA0d+5cg80sGRkZNHDgQNnL1xok6T9bNCcAGj58uM2bMzg4mP773/8aNGZVVRVNnz69wbFTp06VnCCtlpSUFBo0aJDs5bR1sTnrcfXqVatvOvD09KTExESDxiQiGj16tN48Ro4cafDYS5cuUVhYmOzltWW1OnM6OztTUFAQ7dmzp8HFptVqadWqVVbfET4zM9OgsTQaDY0aNUpyPiQhBD399NMGR7Lk5eWRn5+f7GW2VbU6c9bqyy+/pKqqKsrLy6MbN27Qhg0bFD95lyH5+PhQWFhYo4a6f/8+TZo0qUn5jRs3zuBY2vLycjaomdRqzQmAvv32W3Jzc5M9DlNpx44djd7t8vPzad68ec3K89VXXzU4bO/WrVtcxTWDWrU5bU2TJ0+W7OBfUlJCr732mlH5zp49u26Gd6m8m2t6lmFJ+c9q10ppzajV6tofzwZotVrMnj0b27dvNyrf9evXo7CwEFu3bpVcg6apa9MwJoDvnNapB5tB5s6da7J2yREjRui9c+bl5VGPHj1kL7utiau1NiYfHx96+umnafPmzeTj40MqlcqkeZ88eZLmz59PlZWVVFlZST169KB27drJXm5blJT/rHZ9TsYyCKFbOrKx64RpGWRr63MyloFNKR821/GdYWwFNifDKBQ2J6Mopk6divPnz+P8+fNwd3eXOxxZ4RdCjCLo2rUrTp8+DUdHx7oxqbdu3YK/v7/MkZkfqRdCbE5GdhwcHFBZWfnQKuRlZWVwc3OTKSrLIWVOrtYysjNq1Ci5Q1AkbE5GduLi4h66awKASqXC66+/LkNEyoDNycjOyy+/DK1W+9B2lUqFWbNmyRCRMmBzMrITHx8vdwiKhM3JMAqFzckoGmdnZ4SGhsodhiywORnZISLs3r1bb1pISAhWrFhh4YiUAZvTwgghsGTJEnTu3Bljx47FhAkT0LFjR7nDkhWNRoO3335b7jAUB49KsTBCCCxatAh/+MMf0L59e9jb2+PGjRsYOnSo5DISTOuEzWlB7OzskJiYCFdXV0RERNRtDwgIgJOTk4yRKRshBIQQRg1fs7Oz09tMYxXwTAiWU3x8PGm1Wr1TgGRlZcken5wKDAyUnP1Po9HQ+++/bzAPOzs76tChQwPl5ubSI488ouhZHHiCL5np3LkzvL2962YWeJABAwZYOCJl8euvv2LixIl62zzt7Owe6kHk7u6O/v37N9jWrl077NmzR2/eiYmJmDRpEq5du2bawM0Im9NCzJ07t0FVlmkeUVFRmDdvXl31Njg4GIsWLWrW8TNnzsS7775rxihNC5tTAfz5z3/G/fv35Q5D0Tz33HN47rnnWpTHiBEjsHfvXpw+fdpEUZkXNqcFGDlyJCZMmCCZ/s9//hOVlZUWjEhZ2Nvb48SJE/Dy8jLr9/Tp0wehoaFsTkaHEAIdOnSQXLD3jTfewJkzZywcleWws7NrMBG1j48PUlJSGuwjhEC7du0kn8dNhVqttq43t/y2tnF5enqSh4cHPfLII0YtgNS/f3/JN7RERDNmzJC9jOaQnZ0dhYaG0ltvvUVarbaBLIlWq6WrV69SZmYmLVq0SPbzok/Eb2ubh5OTE4YNG4aRI0dCo9Fg6NCh+OSTT1BUVITbt28jKSmpSfnUttHp45dffsH169dNGbYiGDx4MHx9fbFz506Lfm9FRQUOHz7cYJtarcbzzz9v0ThMBZtTgrZt2+LAgQMNtn333XcAgCtXrmDLli3Yt28f0tPTJfNwdnbGtGnTJNMPHDiAQ4cOmSRepTB27FisX78evr6+Zv+uCxcuYP/+/XWfS0pKsHz5crN/r6XgOYT0IITA7t27MXbs2Eb3S0hIQE5ODiorK/WasG3btsjPz9d77MWLFzFlyhScO3fOFCErht27d2PcuHFmy//NN9/EzZs3AehqHtbycqcxSGIOIX7mlHheqqqqavJzjUajodTUVEpNTaWZM2fW5dO2bVvJY3788UfZy2kO7d6925hHwybz2GOPyV5GU4sk/MejUkyAnZ0dwsLCEBYWhnXr1qG4uBi9e/dGZmam3v21Wi3Ky8stHKX5eeONN7Bjxw5cuXKl0f00Gg1KSkrqVFBQAE9PT3h4eMDDwwN///vfLRSxsuFnTj0QEdLS0tCmTRt06tSpWcc6OTnByckJ58+fl3wRlJeXh5EjR5oiVEXxxRdfANBNDN2lS5e6Lnc3b97E3bt36/Y7e/Zso8/iarXarHFaC2xOPRARIiIi0K1bNyxZsgQAMGzYMPj4+DQ5D3O32SmZMWPGYMuWLXB0dASge5H24Ms1Y3nuueeQlZUFjUZjkvwUjVR9l1rxM6c+TZkyhd5//32TtNMVFxfTn/70J9nLpFRFR0fTb7/9pvfcabVacnZ2lj1GU0rKf/y2thkIIer6d44fP77Rqpkh7t27h4SEBADAggULkJGRYYoQbYazZ8+ib9++D20nIri6uqKiokKGqMwD8dta08rLy4tCQ0Pp4sWLLe75kpubS5mZmXTkyBHZy6UUnT17ttXfOdmcLZRKpSJHR0cKCwujO3fuUGlpqdEm1Wq1VFFRQZ999hl5e3uTt7e37OWTS42ZMyAgQPb4TCk2p4W0bNkyo82pj9/97ncUFhYme7ksrS1btlB1dbXec5Kfny97fKYUm9MCCgkJof3795vUnES6au+QIUNkL5+lVVhY2KrNyZ0QTEi/fv0kV8zas2eP0S99AgMDERcXh3Xr1qFnz54tCZGxIticJuLRRx/F0qVLJdNPnDiBF198EUOGDMH48eObnf9jjz0GZ2fnun6lTCuAq7WmUY8ePSSrpRqNhl577bW6fWtniXvvvfeourqaqqurm/S2t6ioiIYOHSp7WS2lxqq1xoytVaok/cfmbLmEEDRkyBBJU23atIlq2owfOs7Ozo7s7OwoNTWVcnNz6c6dO5L5FBYWtipzXrp0Se+PllarpcTERNnjM5XYnGaUm5ub5J2vqKioWSPwo6Ki6PDhw5SXl/dQXosXL5a9rJaUk5MTJSQk6D2vSUlJssdnKkn5j/vWmpnLly/js88+a/L+iYmJGDZsGKZOnYq+fftCpVJhwoQJNjFusblUVlZi+vTpuHTpUoPtFRUV+Nvf/iZTVBaE75wt19dffy1552xp9cvBwYFGjx5NISEh1Lt3b9nLamm1adOGvvzyywZV2mnTpskelykl5T/uW2sCioqK4OnpqXf7oEGDGp3KhDFM27Zt0blz57rPycnJaOy6tTZIom8tm7OFHDt2DE899ZTeIWL5+fnw9vaWISrGmpAyJ7dztpA2bdroNSYRWdW6HIzyYHO2gIiICL3V2VqefPJJC0bD2BpszhYQGhoKV1dXvWmxsbE83QbTIticLaBDhw6Si95+8cUXVjuVRlxcnNwhMFCYOZ2cnHDhwgWsXbvWLPl36tQJn3/+ucnyi4qK0rv4jrW+SYyNjUVaWhpefvllpKWl1c2fxMiEEto5nZ2d6eTJk3T//n3SarVUXV1NJSUlVFJSQhERES3O39XVlW7cuEGlpaUN8s7LyyMnJyej863twVJUVNSgbfOFF17Q211Pyfr4448fGj9ZVVVFr7zyiuyx2boU3UMoLi4OgwYNqvvs4OAAd3d3ALo2rQEDBiA5OdmovIOCghAfH99gla/avGNiYlBVVWV03JWVlXj88cfh4uJSNx8QANy+fduq7p5eXl4IDAxssBoYAKhUKqhUKpmiYhRx59y8ebPe3jW1lJaW0ogRI5qdb7du3ejEiROS+drifDTGaOLEiZLnaM6cObLHZ+uS8p+injmlcHV1xebNmxtdgPZBunTpgtjYWG7OYKwWRZhzzZo1kksX1OLn5yc5y8CD+Pr6Yvv27RgyZEij+82cObNF1VqGMStKqNYCoICAACooKGi0eltSUmLwBYVKpaLs7OxG89FqtfTqq6+SSqWSvUqjBHG1Vl4pvlp748YNBAcH4969e5L7uLu7IzY2FuPHj69bh6N+mo+PD7KzsxEcHCyZR2VlJd5991188803qK6uNln8DGNqFGNOQLf4aUREBC5fviy5j4ODA3bt2oUxY8bU9Wn18/PD1q1bcefOHQQEBEiuU1JWVobVq1dj5cqVVvU2lWmlKKVaW1/h4eGUlJRksGr60ksvkZ+fH23fvr3RfYmIqquraenSpbJXYZQortbKK0n/KdGcAKh///507ty5Rg1XWlpKe/bsMWhMIqL58+fL/k9Qqtic8krKf4qq1tYnOTkZkyZNQk5OjuQ+rq6uBpeGB4AZM2bgr3/9qynDYxizo1hzAsClS5fQv39/lJSUGHW8VqvFnDlzsHXrVqvthM60XhRtTgC4e/cugoKCkJeX16zjysvL8eGHH2L9+vU8dIuxShRvTkA3F8+TTz6JtLS0Ju1fXl6OtWvX4tNPPzVzZAxjPqzCnABQXFyMlJSUJu1769YtLF682MwRMYx5UcSoFEO4uLhgw4YNiI6ObtL+Pj4+WLhwIVatWmXmyJSFEAIbNmx4qIOGIUJCQiTTpk6digEDBjQ7lvLycsyePbvZxzH1UGpTSn1JzfrdGEVFRfT666/LHrulFBcXR8nJyS1aYduUqNVqSkpKoo8//lj2c6N0kbW1cwK6frKJiYlGX3Dl5eUUExNjdQOfm6vly5dTVVVViw1lDiorK2nevHmynyMli6ytndPb2xv79+9HZGSkZHc8Qzg7O2Pbtm0YPXp0s6t61kKbNm0QEBCg2EHRjo6OcHZ2ljsMq0SRV2yHDh2wbt06PPPMM40as6KiAj/88APOnz8vuY8QAj/88ANeeOEFc4QqO5GRkQ1mkWBsCKlbKslUrfX29qYdO3YYrC5ptVp65513CNB19TPUF7eqqspmu6KtXbvW+HqnBVi4cKHs50jJImt45lSpVHTw4MEm/cNfffXVBs+S4eHhlJ6e3ugxRUVFNnmh9OnThy5cuGCcc8zMTz/9RF26dJH9HClZZA0LGSUmJhp8xiQizJw5E1u2bHloPGbHjh2RnJwMHx8fyWP37t1r1LLvSic4OFhygmtDjBo1SnKZwmXLlmHHjh1Gx1VcXIwbN24YfXxrgCTWSlHEndPFxYUSEhIafSurVqupsLCQ3nrrrUaXHPfw8NC7XLlWq6WkpCRydHSU/ZdSaeJRKfJKyn+KeCG0fv16DBw4UPKOqdFosHPnTnh5eWHNmjXQarWSeZWUlKB79+64evVqg+2nT59GZGQkzxnEWA2ym7N79+7o1KmTZDoRYcuWLZg0aVKT87x58yaef/55nD17FgDwr3/9y+BkXwyjNGTvvufq6goXFxfJ9FWrVuG9995rdr5paWmYNWsWBg8ejK1bt6K8vLwlYTKMxZHdnGfOnMHFixfRv3//h9KWLVuGFStWGD3kKykpCUlJSS0NkWFkQfZqLQAsWLAAKSkpdZNuERHWrl2L5cuXo7S0VOboGEYeZL9zAsC9e/fQr18/pKeno02bNjh48CDeeuutRl/8MIytowhzAro3sl27dpU7DIZRDIqo1jIM8zBsToZRKGxOhlEobE6GUShsToZRKGxOBjdv3sS1a9fkDoN5ADYng59++gm7du2SOwzmAdicDADg+++/f2i6l6NHj+Lw4cMyRcQoarA1Iy+dOnWCp6dn3ed79+4hNzdXxohaB1KDrdmcjM3i5OQEZ2dnHD9+HAMHDoRarVbkauZS5uRqLWOT+Pv7IyEhAQUFBQgLC0NpaSliY2Mb1AwUjxKmKWGxTK2vv/5a77QrEydOlD22B6XoaUqYhjz11FPo1auX3GFYLZGRkejXr5/cYbQYNqeC8Pf3x969exEXF4eePXvKHY7VYivmVMyQsdZOQkICfH19G51PiWld8J1TZmJjY1FWVoaoqKgGxty6dSvfPVs5bE6ZUalUcHFxeWhaUEdHR/Ts2dOoBZg8PDzQr1+/OqlUKja6FcLVWgXz/fffw8PDA/fv32/S/kIITJ48GT179sQ777xTt33+/PmYO3cuzzRhZbA5ZWbnzp0YPnw4OnbsqDd9yZIlWLRoUaN5jBkzBk899RSEEJg/f/5Dd9u1a9ciMzPTZDEzFoLbOeVXYyt3FxcXSx7XrVs3io+Pp5ycHMnjayktLW01q0zPmTNH8jxYUzsn3zkVwJgxY5Ceno527drpTRdC6FadEqLu2fTKlStwd3eHv79/k77D1dUV4eHhJouZMT/8QkgB3Lp1C3l5eXXz9tbH3d0dKSkp8Pf3x5o1a1BRUYGKigqEhoY22ZgAoFarUVBQYMqwGTPDd06F0LdvX1RUVDy0XQiBsLAw3Lx50+i8//e//yE7OxsvvfRSS0JkLAyb04Y5cuQILl++jLfffhtlZWVyh8M0EzanQlCr1Xj//ffx6aeftiifgoKCumaUY8eOISMjwxThMTLA5lQIBw4cgJ+fX4vyGD16NH777TecOXPGRFExcsKDrWVCpVIB0C1xOHHiRHh7ezerNxAR1Q0c/uCDD7Bx40bk5+fbzPoyDg4OcHV1RWZmpuSiylI4OzvDw8NDb1pxcTEqKyubHU9eXh769esHrVYLjUbT7OMbg5S87HxrUlBQEEVFRZFGoyGNRkNardZgG+WDXL9+nc6cOUNCCKr5AbUptW/fni5cuEAajabZ58ZcaLVa0mg0tGnTJnJ3dzdpeUnKf1IJxOY0qbp3707PPvss3blzp8UXSmhoqOzlMae2bdvW4nNkTmbMmGHS8hJ3QpAHf39/TJ06FdHR0XjyySflDkfxDBgwAD169JA7DEXA5jQjbm5u2Lx5M5555hmT5rtmzRqMGzfO6BW/lUxxcTE3+9TAPYTMxL59+3Dq1CmjjPntt9/i4MGDkunR0dFGDSWzBi5fvozs7Gy5w1AE/LbWTGRkZKBLly5N2re8vBwajQY5OTl4/PHHUVVVBSxNoBcAAALBSURBVCEEkpOT9Y7DJCLk5OQgJCTE1GErAmdnZxw9etToeZRUKhWcnJz0plVUVLSoxrFx40YsXLjQpFNsEr+ttawyMjIMvli4e/cupaWlUe/evfXmsXfvXlKr1XqPvXPnjuxlVKpsZVSKbdaNZGbQoEFwd3eXTC8vL8euXbuwaNEi9OrVC6mpqXr3Gzt2rOTzl5OTE37/+9+bJF5GmbA5zUBgYCBiY2P1Vp8+++wzLF68GC+++CI2bdpkMK+VK1fqHa3i4eHR4q5+jMLhaq35VF5eTkREhw4doujoaIqOjiZnZ+dm5eHu7i7ZUSE/P5/mzZsnezmVJlup1nJTihnp1asXhBAoLi7G7du3TZ5/27Zt0adPH5PnyygDrtaakaysLFy9erVFxrx//z4iIyMl06dPn47Zs2cbnT+jXNicVkBhYSGysrL0ptnb28Pe3t7CETGWgM1pBWRlZeH//u//JNMHDhzYrClLGOuAzWklnDt3DocOHdKbFhMTg27dulk4IsbcsDmthIyMDCQlJUmmr169Gt7e3haMiDE3bE4rYsWKFYiPj9fb7rlixQoUFhbKEBVjLticVkRxcTGeffZZXLt2rW5baWkpbty4gVWrVsHHx0fG6BhTw+2cVoZWq8WpU6dw/fp1AMC///1vrFq1SuaoGHPA5rRCJk+eLHcIjAXgai1jcxw9ehTHjh2TO4wWw+ZkbI5Lly7hypUrcofRcrjjO8sW5ebmRj///DNVVFTUafXq1eTo6Ch7bA9Kyn88EwLDyAxJzITA1VqGUShsToZRKGxOhlEobE6GUShsToZRKGxOhlEobE6GUShsToZRKGxOhlEobE6GUShsToZRKGxOhlEobE6GUShsToZRKGxOhlEobE6GUSiNDrZmGEY++M7JMAqFzckwCoXNyTAKhc3JMAqFzckwCoXNyTAK5f8BiBQh2ZfFgGkAAAAASUVORK5CYII=\\n\",\n \"text/plain\": [\n \"<Figure size 432x288 with 1 Axes>\"\n ]\n },\n \"metadata\": {\n \"needs_background\": \"light\"\n },\n \"output_type\": \"display_data\"\n },\n {\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAAOcAAAD3CAYAAADmIkO7AAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADh0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uMy4yLjEsIGh0dHA6Ly9tYXRwbG90bGliLm9yZy+j8jraAAAgAElEQVR4nO3dd5wU5f3A8c9zd3uNOzh6h9CkSxMwaqzYISLwk9hQjN0kNowpthiNDYkYBKxBEjFiwYIlWLFEqg1EiiBN+gkc5drezu+PZxaWZWd2b+uze983r32xNzP77Hdn57vzzDPPPKMsy0IIYZ6sVAcghAhNklMIQ0lyCmEoSU4hDCXJKYShJDmFMJQkJ6CUaq6U+lgptUcp9XCq4xG1o5T6mVLKUkrlxPpapdRHSqnL7ecXKqXmxDveSMWcnEqptUqpIfEIJoWuBHYA9S3LujnwC4onpdTpAT8C25VSc5VSv4z3+5gq3LailDpRKbUxmTG5sSzrOcuyTkvV+8ueU2sPLLPi1CNDKZUdYtoo4EVgOtAGaA7cAQyLx3uKDGRZVkwPYC0wxH5+KfAZ8HdgF7AGOMaevgHYBlwS8NqzgS+BMnv+XUFljwHWAaXA7UHvlQX8AVhtz58JNHKIsSEwG9gO7LSft7HnTQOqgSpgrx1/DVBh/z3JXq4b8C7wE7ACOC+g/GnAFOAtYJ8/xoD5ClgP3OKyHrOA2+zPuw2dxA3seT8DLGCsvZ52AlcDA4Fv7HU9KaAs//fwD2A3sBw4JWB+K+B1+7N8D1wRMO8ue11OB/YA3wJHBb32ZXtd/gD8LpLXAv8CfEC5vV5/H2IdnAhsdFg/jttKwPq5EtgEbAZuDlq3IbeVgNfm2H9/BFwesB4/DSjHstf7Kvs7eAxQ9rxs4GF0DewH4DeB5UaVWwlITq+9EWUD99gb5WNAHnCa/aUVBXwZve2VdySwFRhuz+thf4nHAbnAeHQS+d/rBmAeei+UBzwOPO8QY2NgJFAIFKP3YK8GJdc9AX8f+ILsv+vZG8RYIAfob38JPQNevxs41v4s+UHv383+ojq4rMfL0InSESgCXgH+FbQBTQXy7fVYAbwKNANaoxP6hKDv4UbAA4y24/NvkHOByXZZfdGJdkpAglUAZ9nf4X3AvICNfDF6j59rx7oGOD3ca4O3FYd1cCLOyem2rfjXz/P2d9Xb/kxhtxVqn5yzgRKgnf0eZ9jzrgaW2e/REHgPA5NzVcC83naAzQOmlQJ9Hcp6BPi7/fwOApINnVhVAe/1HYfuDVqikzfsyrA3yJ21SM7RwCdBZTwO3Bnw+uku73esvR7yXZZ5H7g24O+u/s8TsAG1DlqPowP+fhm4IeB72IT9q25PWwBcDLRF1wyKA+bdB0wLSLD3Aub1AMrt54OB9UFx/xH4Z7jXxpqcYbYV//rpFjD/QeDpcNsKtU/O4wL+ngn8wX7+AXBVwLwhxJictW7disDWgOflAJZlBU8rAlBKDQbuB3qhf4nz0Hs10NWnDf4XWZa1XylVGlBOe2CWUsoXMK0GfSz3Y2BASqlCdFX7DPSvGkCxUirbsqyaCD5Te2CwUmpXwLQcdFXNbwPO/HG3RFd5QmmFrtL6rbPfo3nAtOD1GHK92n607K0koLxW9uMny7L2BM07KuDvLQHP9wP5dmtme6BV0HrIBj4J91rLsrzEIMy24hf4HaxD7xzAfVupreDP51/nh2yvuG8PEUl1g9AM9LFPW8uyGqCrbcqetxldRQBAKVWArp76bQDOtCyrJOCRb1nWIYlpuxm9JxpsWVZ94Hh/sQ5xBTcMbQDmBr1XkWVZ17i8JtAKu4yRLstsQm9Efu3QVdOtoRcPq7VSKvDztbPfYxPQSClVHDQv1HoLtgH4IWg9FFuWdVaEMbmto3DcthW/tgHP/Z/XH3ek20q0Dtleg2KJSqqTsxj9K16hlBoEXBAw7yVgmFLqGKVULvAXDv0ypgL3KqXaAyilmiqlznF5n3Jgl1KqEXBnmLi2oo+n/GYDRyilLlZKeezHQKVU90g+pL0Huwm4XSk1VilVXymVpZQ6Tin1hL3Y88CNSqkOSqki4G/ACzHscZoBv7Nj/T+gO/CWZVkbgP8B9yml8pVSRwK/Bp6LoMwFQJlS6lalVIFSKlsp1UspNTDCmILXa0h2XIEPhfu24ne7UqpQKdUT3T7wgj29NttKtGYC1yulWiulSoBbYy0w1cl5LXC3UmoP+hhzpn+GZVnfAr8F/oP+VdqDbvSotBeZiP4lnWO/fh76mCiUR4ACdCPOPOCdMHFNBEYppXYqpR61q4CnAb9C/xpvAR5AV60iYlnWS+hj18vsMraiG8xesxd5Bl1N/hhd9a1Af/5ozQe6oD/zvcAoy7L81evz0cdam4BZ6GPndyP4DDXoUz997Rh3AE8BDSKM6T7gNqXULqXUOIdlWqN/SAMfnXDZVgLMRTeqvQ+MtyzL34GgNttKtJ4E5qBbz79Et9x70dXnqPibgY1n7012AV0sy3I6bhOAUupSdKPGcamOpa5SSp0JTLUsq33YhR2kes/pSik1zK6m1EOfSlmCbvETwih2Nf8spVSOUqo1+tBpVixlGp2cwDkcbMToAvzKSpddvahrFLpdZCe6WvsduvodfYGyrQthJtP3nELUWa6dEJRSslsVIsEsywp5vl32nEIYSpJTCENJcgphKElOIQwlySmEoSQ5hTCUJKcQhpLkFMJQkpxCGEqSUwhDSXIKYShJTiEMJckphKEkOYUwlCSnEIaS5BTCUJKcQhhKklMIQ0lyCmEoSU4hDCXJKYShJDmFMJQkpxCGStvkPLan8801hcgErrdjMHlQ6dXTYcobUOODb9bA+1+mOiIhouM0qHQibjufUKNPhDMHQrMSeOhKPW3KG5KcIvOkXbV2QBe45DQoKjg4bdQvYOQvUheTEImQdskZStMSaBrpvZWFSBNplZz1C6FRceh5zRtCQW5y4xEikdIqOft1huOPDD3vrjHQv0ty4xEikdIqOed+A69+5jx/7OlQLz958QiRSGmVnOH8+kxJTpE50i45x78Iny5NdRRCJF7aJee2XbCvwnn+qmmgpOuQyABpl5wA32+CyurQ8wryoiuzUTH07QTFBdCjffSxCREvadt9b82/oEOLw6d7a+C8v8Isl4ajQL86CbKz4Be94KqhcP9/9LHruMdh80/w7uL4xi1EMKfuexmXnABrt0KHi5xfe+oAOLW/fn7jSMjJDr3cx9/ACTfHFmeitRkI2bmwLsIfI2GejOlb63fpQ/D+g86JFSzPA6/9BVDQqRV0bpXQ8BIurxgueBEatAaVDbvWwwsXwv7S+L7P0ddB92Hgq4FnhwLG/lxnnrTdcyoF5W/qpAvmrYH/fAhjHoQJV8PwY/XlZe2a1a6xqLwKHnoB7pwet7Djpl4TuG37odN2roPxnXQixar1ALjwJShsBHn1wbJgw3yYcgySoHGWcdVagFaN4cf/hJ7ns6CmRh9PZkXZ7PXJEjhxHPh80ceYKH/ZA7lFh06zLNi9ASb2gYpd0ZVb2BjqNYPrv4HsoHqVZcGaD2D6cKjaG1354nBOyZmWrbV+Xpc9RJYCT050iWlZ8NlS+HqNmYkJkBWixqAUlLSDy9+Dhh1qX2azHjopb1p2eGL6y2/VHwZcWvuyRe2l7TEnwMVD4lve/77VCWlZ8NtJeu9rqi+mw6ArQs9rPQBGPgWvXgs7VkRWXtvBMOIpqB/mWHzjIvh8Uu1iFdFJu2rtiONgSH/9K37FWbraGov9FXDz4/r5/5bpURXSQWFjuH2H+zKrP4SXxsKude7LtRkI5z4Orfq5L1e+E2aOgeWzaxercJfWx5z18uGd+/Tzn7WANk1iL/NPz+hTJd4amL889vKSLSsHBoyFEU+4L7f5K3j8eKjcE3p+405wyZvQtKt7OT4vTD0WNiyILl7hLK2S03965Ikb4axBei/ZrCT68rw1uqq66kc4aZyetnufcy+jdJGVo091nP0wZLmcUtq9ER7soBMsUH4J3PK93gu78Xnh0b6w9dvYYxaHS4vkbNtUn39870H/+8enn+yAa+DL1fq5y8dNW0PuguNvBY/DFTmWBTt/gMlHwz779EtxKxi34vAW32D7S2Ha2fo0ikgM4zshHN0d3n3g0LGB4sVnZWZS+r13F3gK4JjfQU6IBFUKGnWEMa/DzIshtx5c/Fr4xNy9QTcqSWKmhjHJ+Y/fJCYx64q3b4XKvXovqhwaydodDcOnQL2m0DBM5/6yH2H2jdL4k0pGnOe88mznfrJudu+Di+7XD7ehMe8ZG/3VKunkg3vgjevdl+k8BFr2cV+mogxe+jUsfTl+sYnaM+KYs10zePtvkV+qdd2j+rSHtwaWrtXT2jSF1+/W4wyF0uAcKNsfl3CNluWBo8bqUyPRsCyYNAA2yTjASWP0MedNo6BL69Dzyiv1BrNkLZx2q562v/Lw3kEbt7tfhL3in9BydFzCNZqvGhY9DQUN4Yz7I39dTRU8fz58/x5UliUuPhE5I5LzhsnQujH06gBd28Decthon2Afehus2RxZOeu26oalUFeq1C+MX7ym89VA2SYo3wUFEZyCqtgNb94M376S+NhE5Iyo1vp5cuD5P8GC5fDgzOjK2DJTj2EbrLIaht0G734RW4zpZOQzuoobzlcz9OVmIjXSouN7tRdG3R19YrrJ88DE6+JfrqnaHh2+O55fi97Q/tjExiNqz6jkjIfLJ5jdYT0ZWvSGEY9Dq76RL3/u4/qKE2GOjEvOdxbieDFwx5bwaIbvPYua676yLRxGxnfSvCeMeQ0atElMXKL2Mi45wfmUSZ5HX6CdiXKL4JY18Pu1UNI2ujIatIGbV0JhHC4sELHLuOT01kDfq5znNyyClo2SF08yNGgLV3wIjTo4968F3Xq7cZG+9MuJpwDGrYQmYa5SEYmXcckJsLfCeUjLk/vp60AzRePOMPJpaHOU+3IVZfD+X+CxgTDndp2oTgoawiVv6O5+InUyMjlLy+ChF1MdReKVtINzHoMup7ovV1MNs2+Azx7Rf897DGZfD95K59c06QLDH4f2x8QvXlE7GZmc4Yw5FY7tmeooYpNXXw+N2eW08MvO+D9Y/M9Dp30xHf490v11LY+EEU9D897Rxymil7HJ+ck30O4CeOXTQ6dblr6N4BerUhNXvPx2MbQd5L6M5YNnh8F3b4Sev+IteOZ0vZyTZt3g8nd1K7BIroxNzopq2LAdNpXqzg1bd+rbK/zzv/D7J/WYtOmqXhP49wj3Zar2wQsX60u+HJPPglVzYMZ57kNdFjWHW9dKgiabUd33EuWpm+D6ye4d49PJr2bAkaOdr9ss3wnv3lG7UfIGXQWn3+s+ZMnebfDUENi6pHbxCndp0X0vUS6fkDmJCbD8LefxdCv3wnt31n74ygWP61bcCpcrUjyF0PGE2pUromfEVSmidnxeQvaCsnzw6jXw1b+jK3f+FD1S/HnT9eBhoThNF/FXJ6q1meivFZATMLrDa7+BTYth/bzYyz7iDBj79uHT926FJ0+Gbctifw9xUFqMviciV9gEWveHPhfA2+OgfLe+0DpeZY95DZbMhDPskRD/0Q/2bIHyn+LzHuIgSU4RHf9mI1tCwhg9TIkwmCRlytSJ1loh0pEkpxCGkuQUZhnTFL7qqx9FdXvzlAYhYYauBbDgSMjNgnw7KbdWQYuFqY0rCaRBSJgrR8Gyfvp25IGKXW6dVgfU7XqDMMPQEGOZCklOYYCpnQ7fawJ4FPyuZfLjMYQkp0i9y74PPZ6pJwuujuIOVxlCklOk3hyXAY3qMElOIQwlySnMlp8FnVzG+8xgkpwi9SwLXioNPa9DPjwQ4Y1bM4wkZ7IpBXf+CTp3hBHnwOhR0L5dqqNKrRr0UPXiENIJIdmUgltugl8OhaZNIDsbNm2CU86Csj2pjk4YRJIzmbIUzP8YCgugf8AtwFq1hLx8QJIzJKX0daXRdCbNAlyG/jSZVGuT6Z3XYUA/vbEFmzc3+fGYpMaCUoehHIY3gj9HcPuzLKCl59DHuqOgdS40Sr/9UPpFnK46d4TGjUMnJsCg45Ibj2k2VsH5K2FOiKH4s9ThPYiKsuCookOnNfLAy91ClD0Q5u+BC1bAGpd7UBhGkjNZrrv60KqsqJ3BRfCblgert+1y4ZZa3Ex0cDFc2QL+sC5REcadJKcJ/vYg7N2X6ijMdlYj/YjFGQ3hlVJY4DK8vUEkOZPh7DP1KRMnr78JlelT3Yq7bOCT3lCS4M2xTz3doUGSUwD6GLNlC/0I5YZbYPGXyY0pmbLQ12v6NfHA10HVe4VusHE6Ho8Xr5VWLbeSnOHUr697sNQvhs1bnO+D4GRAf3jC5d4Ie/eC1xtbjCbKQvfuOacRjP/ZofMSnYSBLAvWVOjj1Ce2wgs7kvfeMZLkdJKXC0NO1lXSGi+cchLccz/sLoNt22Ghw62zgymcN8Yf1sL6jfGK2Bwn1IdmHpgZouU0kSp88F7QFS5eC85dntw44kSS00nDhjD7lUOnPTdN/79iFTz7b3htNiz7zrmM/Hy49CLn+bPfgnffjzVSs4xoBFM66+RMtCX74I2AIej31MD9Pyb+fZNEBvgKRSl4aYbu++rm8/mwbr1uzLn0ysPnN2wIPzlsLN9+Bxf/Gr78KvZ4TfJSVxjZJHHl3/gDbLZvrvpDRdo07riRAb5qQykYdnb45X4+WD98voPnMCdNhSeeCf/abdszLzGT4a2fYGUG3c/RhXTfc1SLSkNWFvTupR+PPgxl2+DIXrDK4S6zPh+Ul8cnTJPc0Apm7IAV+92Xq7F0FdT/2OmF+vOg+HP9+M92lxcnsTEpxWTPGYplwdJl0KA+dOxQu9fm5enHV/OdG4K2bIGzz409TtM8skn/P6YpdCk42OVucxXsCOg3+8U+uHSVczletx/GunOkJckZimVB/59Dt6762kvQLbdNXO7JHiyZpwtMM3w5PNsFcu118Nx2mL0zPmWf1QhWb9LXgGY4aRCK1MUXQPu2cPcdsSfenr1w0+/hqWlxCS3jDGsIUztDq9zD51kWFM7Tp00yhNyfMx6UgrNO189HnQuXXhx9WaWl8PkC/fzmP8BKl2peXfRFH+hXdPh0SU57piSns5IG0LgRvP4ydO+qp0W7R934I1RUwvoNcMqZ8YsxnUlySmtt1HbthtU/QN/BkN8Q+gyCHaWwP0xLZShtWuvrPU86Hip2wkN/04nfOMarMDJVGl44HQ1JzlhVV0NVFSz5Fpq2hQmPRl+WUrqld9wNsGOjfpx4PPQOcQFypluyP3SrrVKwtF/y40kBSc546tAe+vaJb5kfvgNvvar3qnXJJatgXx1oknUhyRlPA/rDUIdjxpdfjb7Rp01rmDoJHh0PPbtHH59IK5Kc8fKzdnDXn53nf/IZnHcRnHQ6jLqg9uUf0RnyC2Dz1uhjFGlFkjNeCutBzx6h5/l8+i5aXy+Bjz6BWa9Dq07w57v0tZxer26FDGf0SOgX52pzuqoDW24d+IhJoBQ0b+Y8f/pzMGnKwb99Pti8Ge57CPJK9GPpt/qUyg6H2xKA3XOtDp3d2lwV+kerJBs+PzL58SSZJGc8FBbA+2+FnldWpq/5DLWRWZa9V/XBkYOgbRcYOgLe/wi2bjt8+Qce1vPqir5fwfwQl4SpEENlZqC6ccIolZavhIceiXz5+QthyFkw5kJdhfV49OBgCxYlLkZTVVowdhV81//Q6RU+eN7typXMIMkZDxMejH+Z05/Tj5wcPVrCN0uguDj+72O6zVUwabMesxZ0beOa1TAtRM0iw0j3vXjYvUUPBHbY9DI45kRYlp5j2BijYTZ0Ljj496K9GXXoLSMhJMrcOc57tBqvJGY87KyBhek/HEltSYNQrBrUD93h3bJgzdqkhyMyhyRnLPr3DV2d9TvulOTFIjKOJGcsOnWEwsLQ8yY/kZmDRYukkeSMRcsWevDpUB6ZBDVp2nG799RURyAwLDmzyeMylnAKExNSfgM6cjJ/j1+BgwdCScnh0yPpimeiXpPhhKXQ9jL9/xF3pjqiOs2IUynZ5HM+79OMPuRQiEUNXvTYpDM4ga18EVP5Hgq5ku/JowHZ5B4ou5p9TKE9NUR5h6+8XPhoDvToru+l4nfeRfDSrPRK0iPuhi5/BBXQgO+rhqXXwfonUxdXHWD0qZTTmUprjjnwtyKHXPQQFZewiOkMYgvR9ZAppi2jmUMRLQ9M85f9BhdSQ1X0gVdWwc9PhIJ8+Pyjg9O3bU+vxMwpgYI2hyYmQJYHVBJuqyBCMiI53SgUFzCXWYzkB96p1Wsb040zeJLGhL6hzgheZQKFB/akUSuvgL5Hx1ZGKjU7E9qOTXUUIohRx5xOPBRyNtPoxuiIX9OQLpzKZNpwXAIjEyJxjEjOhUxgJ+6jBNSjOZ0ZGlF5hTRjGP+mPSe5LvdfroytWitEAhmRnNv5hhmcSAW7XJfrwnD6cIXrMll4GMMCWjLIcRkLi/9yNUt4FiudbnUs6hQjkhNgL5uYQjvKcb7YOJciTmMyXRmFCgrdQxEFNOFq1lKfdo5leKlkLn/gK57AR7XjckKkmjHJCVDFHqbRn1KcO4tnkcNwXqQLw/HfcaqQ5gxlOr9jO8W0Qjnciaqa/SzkYebzIBl1WYPISEYlJ0AZ63md89kc5tTJcF6iF2OoR3NO5mGOwP2uXT68LOAhPsZlEC4hDGJccgJs4yvmcA1bcb65rEJxGpM5lcn05MKwZX7AOD7lrjhGKURiGZmcAFtYxBtcwG7WOS7joZCujAhb1tv8mi+YFM/whEg4Y5MToJTveJajqGJPVK+38DGHa1nKdKy6cENHkVGMTk6AcnYwmbbsZUutXuelnE+4gy+Zgg+5dEukH+OTE6CS3TzHcWxnaUTLeylnERP5nHsTHJkQiZMWyQlQRRnb+DqiZfexlbn8McERCZFYxnd8B8ihgDN5ms4Mi2j5ApowiHEsYHyCIzONgj5PU+vf3MIOzvPajIES595WjnzlsOSa2r9OHGDE9ZzhXMTntKZ2V31UUsYn3M5iYrhfZjrpPRUaHAUN+kd/h+14smpg95ew7W1YeUeqozFaWt52PgsPF/EpLRjo2OvHjZcK3uZyljGDjO4R1O1+6HiTvv7SNL4qWHYzrJVTWU7S7rbz+TRmFG9EnZgAOeQzlH/RhXMO64ubMXIaQH4rMxMTICsXsvJTHUVaMnKLrUdLTuVROnC6a2J6qWAls8L2JBrBLLryf4kINfVKBkLDY8IvJ9KOccmZT2NO5mF64H6DWQuLT7mLWYzgHa4I2xd3KP+iH9fGM1Qz7HgPtr2Z6ihEAhiVnFl4+CXP0YPzwy47h2vsq0t0V793uIJSvnNcPhsPJ3AfgxgXt3iNseEZ2BPZOeCkK50LW19LdRRpyagGoTHMD3uMaWHxDleylGcPux6zPu25lEUU0MTxtSt5hVcZFde4jVDQDrIdBrgOp9lQ6PFQ6Hkr/wqbZkQfV3UZVG6K/vV1gNGj7+VQwK/4wDUxfdRQzV4+426W8EzIEQzKWMdUOnItG8ijwSHzLCy2sJg3wlSX01b5+uhfW7+f87zKLbBXbsaUCkZUa09jCq052jUxlzOTRyhhIRNchxapYg9P0p2dfH/I9M0sYDoDZcwgkTZSnpyN6U4JHR3nW1gs5dla7fH2sZlXOJct9mDUq3mT58MM9iWEaVJerfVQiIcCx/kLGM/H/KnW5e5gKf/latpxAkuZjpfyWMIUIulSnpxbWMx2vqUFRx027zP+ynweiPqSry0sZAsLYw1RiJRIebUW4ENuZhtfY9ld7CwsFjGRedxPNftSHJ0QqWFEcpZTyjQGsJNV7GMr3zKdD7gJL/tTHZoQKZPyaq2fRQ1P0jXVYQhhDCP2nEKIw0lyCmEoSU4hDCXJKYShJDmFMJQkp4DKzbB/TaqjEEEkOQWUfgSbXkx1FCKIJKfQNr0Au4OGe9nxoR5pQaSEURdbixQr7Ag59Q/+XVUKFRtSF08dkZZDYwoRizxyySeXj3mCoxmLlxqqDbxvTtoNjSlELFrQmM95hp18QG86s49PmMyt1KdeqkOLmCSnyEh/4Sr60RUV8O9yhnM2x6U6tIhJchroFyh6RTmQtoCB9GAA3VIdRswkOQ3SAngFD1Px0FOSM2o6ObunOoyYGXPJWF33Obk0AzrK76WwyZaQYpPJYT95DEYdkpjTZe9Z50lyppgHKLAbLALlougZ5e2XioEBqAMPD0iipyGp1hrsBXIppoK9ES6vgIvIoidZ3Brw1V5PNdeRTVcZszetSHKm2Ex8nIpFe4c9253kcEuYE+fDyeIXZKGA68m2nx00EQ+rXAbiFmaSam2KvYuPzS439r2KbMd53VDMwcNEPNxEDjeSc1hi+rVGcbf8FqcV+bYMMJwqlpFHI4fEUuj7civ7AbCCXIpQtIjwWLIQRV857kwrsuc0wFZgC9aBcXsDFQFfk0sLYAI5VJBHBXl0qkViAnix2Bm3iEUyyJ7TEP2oooK8w6YrFL1RbCb6W7f/Dx9rsbgk6JaJwmySnBnsfWpYjsXvZXjutCTJaQgvcBte7sUTUzk7sbjVbt2di4+VLo1NwmySnIaYjYfmMTbYnEMVP2KxWBIyI8jF1ini3z+OJ4fzyaYxOJ4GCcXCOnAEeTtenqGGnyBjzmbmkE0h+axiVq1/svLJo5jCkPPK2EdlFJ0xtlDKAC7Gh4+aOK9lGQnBEG2BVij+Ry7gPz1Su81vAxbbsTjK3sgy7UtqSgkfMJUedKzVD1Yi+dvSpzOb3zKevXE8ipeREFKsO4ozyeIL8phHHlkoskL0qY3ESVQxgCosMi8xASZwI73oZExigv4BzUJxKcMYzZCkvKcccyZYC2AM2Qwjm+PktzCsQfSkBx1THYYRJDkTqB4wDQ+nu3TBi8YEchhp5FBVsStjH/upSHUYRpCf8gR5DQ/zyI0qMZ/CyzvUOM4fRlbGfnHLWctaNqU6DCNIg1CCrCSXLhGmUDkWNcA6LH5OFVXohqJF5NIzRBkWFuuADlTGM2Rj5JPLh0ylF52jer2HbPLsBrdgFVThdfnhC+cZXmMcE+Nab3FqEJJqbbtREBoAAAIZSURBVAqVYrEFiwuo5psQTTsrseiGRXZQw4hCUZSRTUFaBVX8nMuifv21jOIxbg057zLu5nn+G3XZyZSptaOUOgZFkUtLYzkWL1LDLXjpRVXIxAQYQbVjg30ecLJ8fRlNvt0EaINiMl68IZLuIbz8ES/nUc0/I6hePYg35NUqxSjulYpPRpPkTICZ+LiHmgNHJe9Rwy+p4pdUcQdeJtbimOcRl2W7ovhNnFuChTnkpzeBelGFAsqw2JaA8hui6GPQiXoRX7LnTKDVWHwfY2LuBQa69AUdSzbXyN4zI0lypoFdwGqHztbZKEnNDCXJmQZWY/Fnl/NqR5NFiyTGI5JDkjNNfInFuw6NQxeSTTf5KjOOfKNpYiUWC106HjxMDo2TGI9IPEnONPIAXuZQE/K85wN42ZWCmETiSHKmkTLgTKpZE5Cc+7DYhMV4PDRJXWgiAeQ8Z5rxAfOwWG8ff76Fj/ExdOQW5pLkTEMXyfizdYJUa0XG+ZDFzOWLVIcRM0lOkXG+4wdWsC7VYcRMklNkpJv4O5/xNZVUHXhM4Dle5oNUhxYxGQlBiBSToTGFSDOSnEIYSpJTCENJcgphKElOIQwlySmEoSQ5hTCUJKcQhpLkFMJQkpxCGEqSUwhDSXIKYShJTiEMJckphKEkOYUwlCSnEIZyvdhaCJE6sucUwlCSnEIYSpJTCENJcgphKElOIQwlySmEof4fsKpb0xI7vc4AAAAASUVORK5CYII=\\n\",\n \"text/plain\": [\n \"<Figure size 432x288 with 1 Axes>\"\n ]\n },\n \"metadata\": {\n \"needs_background\": \"light\"\n },\n \"output_type\": \"display_data\"\n }\n ],\n \"source\": [\n \"connected_component_label('C:/Users/Yash Joshi/OG_notebooks/crosses.jpg')\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 41,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAAQoAAAD3CAYAAADlsBq6AAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADh0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uMy4yLjEsIGh0dHA6Ly9tYXRwbG90bGliLm9yZy+j8jraAAAX4ElEQVR4nO3dfXAUdZoH8O8zyeQ9M0nEdW/LE+UMJERAMKBY4Au+gLuaxERBeRGClMounrt7bq2lfwiYvdJTyjKA1urK+oaCQIKetevK3e7prQveuWIpCZziAu55igqaZEICifPcH5lhA6bTmcnM/Lqnv5+qpwoyM93PdJgv/XTP9IiqgohoMD7TDRCR8zEoiMgWg4KIbDEoiMgWg4KIbDEoiMgWgyINiMgZIhISkYwELOspEWlIRF+UPhgUBojIIhF5X0SOiMhnIvKYiBTFuzxV/VhVC1T1m0T2ebJI339M5jrImRgUKSYi/wTgAQA/AxAEcAGAkQC2iUiWxWMyU9ch0bcxKFJIRAIAVgC4XVVfVdUeVd0PYDb6wmJ+5H7LRWSziDwnIu0AFonIWSLyhoh0iMi/ichaEXkucv8zRUSjgSIi/yEi94nIm5H7vyYiI/r1sSmyJ9MWWWZFnM9nv4j8TETeE5FOEXlSRE4Tkd/267N4KOsVkVNE5F9FpF1E/ltEGvrvvYhImYhsE5HDIvI/IjI7np4pPgyK1LoQQA6Apv4/VNUQgN8CuKLfj6sBbAZQBGA9gOcB/BeAUwAsB7DAZl1zAdQD+A6ALAB39rvttwBKI7e9E1l+vOoifY8GcE1k2XcDGIG+f1//OMT1rgXQCeC7ABZGCgAgIvkAtqFvG3wHwI0AHo034Ch23KVNrREAvlTV3gFu+xTAef3+vl1VtwKAiJwKYDKAy1T1GIA/isjLNuv6tap+EHn8iwCqojeo6rron0VkOYCvRCSoqm1xPKfVqnowsqz/BPC5qu6M/L0ZwGV26wUQQl/gnKOqRwC0isjTAC6J3P1qAPtV9deRv78jIlsAXAegJY6eKUbco0itLwGMsDjm8HeR26P+2u/P3wNwOPIiGuj2gXzW789HABQAgIhkiMj9IvJRZKzZH7nPCMTnYL8/dw3w96Gs91T0/afV/zn1//NIAOeLyNfRAjAPfXsflAIMitTaDuAogNr+P4zsWl8F4N/7/bj/x3o/BVAiInn9fvb3cfYwF31jzeXoO5h6ZrSNOJeXiPV+AaAXwOn97t//+f0VwOuqWtSvClR1aZJ7pggGRQpFdu1XAFgtIrNExC8iZwLYBOB/ATxr8bgDAN4GsFxEskRkKvqOB8SjEH1hdQhAHoB/jnM5CVtv5LRuE/qeX56IlAG4qd9jXwEwWkQWRLaZX0Qmi0h5inr3PAZFiqnqv6DvYN9DANoBvIW+/zEvU9Wjgzx0HoCp6HuhNQDYiL4XXqyeAXAAwCcAWgHsiGMZ8bBb7zL07Wl8hr7AfAGR56eqHQCuBHADgP+L3OcBANmpaJwA4YVr3ElENgLYo6r3mu4lGUTkAQDfVdWFtnempOMehUtEdrX/QUR8IjILffP+VtN9JUrkfRLjpc8UADcDaDbdF/Xh6VH3+C765vhT0Hc8Y2n0NGSaKETfuPE9AJ8DWAXgJaMd0XEcPYjIFkcPIrI16OghItzdIPIQVR3w/TTcoyAiWwwKIrLFoCAiWwwKIrLFoCAiWwwKIrLFoCAiWwwKIrLFoCAiWwwKIrLFoCAiWwwKIrLFoCAiWwwKIrLFoCAiWwwKIrLFoCAiWwwKIrLlyaDIzs7GnXfeiYKCAtOtELmCJ4Ni3LhxaGhoQE1NjelWiFzBk0Hxi1/8AllZWaivr0dJSYnpdogcz3NBMWvWLEyZMgUighkzZmDatGmmWyJyvEG/ACjdLtefnZ2N9evXo66u7vjP3n33XZx33nkIh8MGOyOny8zMhMiAV7J3FFVFb2/vcB4/4JP0VFBMnToV27ZtQ35+/vGfHTt2DLfccguefvppg52R023btg1lZWWm27B18OBBTJ06FT09PXE93ioooKqWBUDTpbKysvT555/Xgbz99ttaWFhovEeWc+u9994b8N+O03zyySealZUV9/NUiyzwzDGKCRMmYM6cOQPeNm7cOFRVVaW4IyL38ExQNDQ0wOcb+OlmZWVh8eLFPANCZMETQTFz5kxMmTJl0PvwDAiRtbQPipycHCxZsgRFRUW2973vvvtccWSbKNXSPigmTpyI73//+0O6b1lZGRYuXJjkjojcJ62DIisrC3fccQfy8vKGfP9ly5YhEAgkuTMid0nroJg0aRKuv/76mB4zfvx4XH311UnqiMid0jooVq5caXmmw4rf78fNN9+M4uLiJHVF5D5pGxSzZs3C5MmT43rsjBkzMH369AR3ROReaRkUOTk5WLx48ZDOdFhpaGjgGRCiiLQMinPPPXfYxxlGjx6NRYsWJaYhIpdLu6Dw+/34yU9+gtzc3GEtJzs7G0uXLkUwGExQZ0TulXZBUVlZecLHyIfj3HPPHfJ7MIjSWdoFxfLly5GRkZGQZfn9/iG/q5MonaVVUESvXpVIM2bMwMUXX5zQZRK5TdoERU5ODurr65Pyvz/PgJDXpU1QTJgwIWnXlCgtLUV9fX1Slk3kBmkRFH6/H3feeSdycnKSsvzs7GzcdtttPANCnpUWQTFlypSkf0fHxIkTcdVVVyV1HUROlRZBce+99yIzMzOp68jMzMQtt9zCMyDkSa4PipkzZ8b9mY5YXXrppbjkkktSsi4iJ0nuf8NJlpubm7QzHVYaGhrw0ksvRa9SnlbOOuss/OAHP4jpMa+++ir27t2bpI7IKVwdFOPGjUN1dXVK13n22Wejvr4e69atS+l6Ey0nJwcTJ07ExIkTceuttwIAAoEAzjzzzJiWc+DAAbS1tQEAnnzySbz99tvYuXMnurq6Et0ymWR1HX91+Pd6ZGZm6ubNmw18c4LqW2+9pUVFRca3Qazl8/n01FNP1cbGRm1qatJwOJzwbRMOh3Xr1q26Zs0aPe200zQjI8P4805Eef17PVwbFNOmTdNjx46Z+F1oT0+Pzpkzx/g2iKXOPvtsXb58uXZ0dOg333yT9G0UDoe1o6NDV65cqWPGjDH+/IdbDAqXBsXvfvc7E7+H437/+99rMBg0vh3sauzYsfrggw/qvn37jG2r/fv368MPP6wVFRXGt0e8xaBwYVDMnDlTv/rqKxO/hxPU1NQY3xZWFQgEtLGxUT/99FPTm+m4gwcP6po1a1w5tjEoXBYUubm5umHDBhO/g2/ZtWuX+nw+49vk5Kqurtbf/OY3KRkxYhUOh/W1117T2tpa49splmJQuCwoJk+erN3d3SZ+B9/S3d2tN998s/FtEi2fz6e33XabdnR0mN40tkKhkN5+++2uOdjJoHBRUGRkZGhTU5OJ7W9px44dWlxcbHzblJaW6rp167Srq8v0Jhmyo0eP6lNPPaXl5eXGtx+DIo2C4qKLLjJ2psNKb2+vzp492+h2GT16tO7atSsppztTYffu3VpWVmb83xeDwjooXPMWbhHB3XffDb/fb7qVE2RkZGDp0qXGvl2stLQUW7ZsQUVFhWuvmVFWVobm5maUlZWZboUsuCYorrjiCpx//vmm2xjQxRdfjMsuuyzl6y0tLUVzczPOOeeclK870aJhUV5ebroVGoArgsLEZzpiISJoaGhI2LU6h2L06NFobm7G2LFjU7bOZCsrK0NTUxP3LBzIFUFxzjnnoLa21nQbgxo1alTKvgfE5/PhhhtucPW4YaWsrAzz589PaejSEFgdvFCHHMzMyMjQrVu3mjguFLPt27drSUlJUreHz+fTJUuWOOYUcTIcO3ZMly5d6qj3qPBgpsNNnz7dNVeWmjx5Mi6//PKkrmPChAlYvXo1srOzk7oek/x+Px5++GFUVlaaboUiHB0UIoK77roLWVlZplsZkoyMDPzoRz9CYWFhUpZfUlKCFStWJO3aoE6SnZ2NFStW4JRTTjHdCsHhQeHkMx1Wpk+fjiuvvDIpy543b96wv1PVTWbOnMnvf3UIxwZFXl6eo890WBERrFy5MuEH44LBIO6+++60O3g5GBHBz3/+c5SUlJhuxfMce4WrsWPHJuw7RFMtegbkySefTNgyly1bhhEjRiRseW5RUlKCO+64A/fee6/RPj766CNXhPQXX3yRnMs0Wh3lVINnPXw+n7700kuufUuyquqf/vSnhJ0BGTlypO7atcv0UzKmtbVVR40aZfzMhxdK3XTW45JLLsHMmTNdkeBWpkyZkrB3a15zzTWoqKhIyLLcqLy8POnf20KDEx1kN0VErG9MouLiYowbN87EqhPq8OHD2LVr17CWUVxcjDfffNPzb23eu3cvLrjgAhw6dMh0K2lNVQf+39lqV0Md8oYrr9cPf/hDV49giRIOh/XHP/6x8d9Hupe6afSgPllZWZgwYYKrR7BEERGMHz8+rd9o5mSOHD2oT0lJCQ4cOICCggLTrThCV1cXRo4ciS+++MJ0K2lLLUYP7lE42NVXX+2ad6WmQmZmJqqqqky34UkMCgebMWMGg6Ifv99v5LofxKAgoiFgUDjUGWecgUmTJpluw3HGjx+Ps846y3QbnsOgcKjTTz89Ld5LkmgVFRUYOXKk6TY8h0FBRLYYFERki0FBRLYYFERki0HhUG65Tih5A4PCod566y3TLRAdx6BwqMOHD5tugeg4BgUR2WJQEJEtBgUR2WJQOJT+7Spj1A+3iRkMCofauXMnmpubTbfhOK+88gp27Nhhug3PYVA4VHd3Nzo6Oky34TihUAjd3d2m2/AcBoWDcfw4EbeHObxmpoONGTMG77zzDvLy8ky34gjd3d2orKxES0uL6VbSFq+Z6UIHDx5EW1ub6TYco729HZ999pnpNjyJQeFgX3/9Ne6//37TbTjGgw8+yC8AMoRB4XAHDhzgQU0AnZ2d2L9/v+k2PIvHKFzg9ddfx0UXXWS6DaN27NiBqVOnmm4j7fEYhYvdc889nj7ar6q45557TLfhaQwKF/jzn//s6Tdf8U1W5jEoXKCrqwtr165FV1eX6VZS7ujRo1izZg2OHDliuhVPY1C4xPbt2/GHP/zBdBsp98Ybb+DNN9803YbnMShcoqurC48//jhCoZDpVlKms7MTv/zlL9HZ2Wm6FYq+LXagAqAsZ9WyZcs0HA6rF/z0pz81vr29VmqRBdyjcJmXX34Zra2taX0WRFWxe/dubN261XQrFMGgcJmPP/4YNTU1af15hz179qC2thZ/+ctfTLdCEQwKF9q7dy8eeOAB9Pb2mm4l4Xp7e7Fq1Srs2bPHdCvUn9VMojxG4ejKzMzUVatWpdXxinA4rKtXr1a/3298+3q11CoLrG5QBsWgVVxcrBMmTDDaQ25urq5atUp7enpMvK4Tqre3VxsbGzUvL8/479bLpQyKxFV+fr5u3rxZ29ratK6uTiOfiTFSmZmZ2tjY6Oqw6O3t1ccee0yzsrKM/269XsqgSEwFAgFtbm7Wb775RlVVOzs7dfbs2UZ78vv9+tBDD7lyDAmHw9rY2MhxwyGlDIrhV3Fxsb744ovfekEePnxYb7zxRqO95ebm6oUXXqjvv/9+yl7kw9Xa2qrTpk3juOGgUgbF8Co/P1+3bNli+Y++vb1dr7vuOqNjCAAtLS3Vd999V7u7u5P6Ih+Oo0eP6q5du7SsrMz475V1YimDIv4KBoMnjBtWOjs7dc6cOcb7zc/P18WLF+uRI0eS9mKPV3d3t956661aUFBgfDuxvl3KoIivioqKdOPGjUOe/50whgBQn8+nkyZN0o0bN+rBgwcT/oKP1eeff66bN2/WSZMmqc/nM759WAOXMihir/z8fG1qaor5IKFTxpBoTZ06VR977DENhUIpPeAZDoc1FArpE088odOmTTO+HVj2pQyK2Gqo44aVUCjkiDEkWn6/X8eMGaNPPfWUdnZ2DjsE7HR1dekzzzyj5eXlPO3polIGxdAr1nHDyuHDh/WGG24w/nz6l4joeeedp9XV1bpz507dt2/fsJ5jf/v379edO3dqbW2tVlZWcsRwYalFFvDiuifJz8/Hs88+i5qaGogMeJ3RmIRCIdTX12PLli0YbFubMmrUKFx11VUAgLFjx+Kmm26K6fHr16/H+++/DwB47bXX8OGHHya8R0odtbi4LoOin6KiIqxbtw7V1dXw+RL3ebnOzk4sWbIEGzZsSNgyk8Hv96OwsDCmx4RCIRw7dixJHVGqWQUFR49IFRUVDfhmqkSJng1xygFOFmugsswCBkX8Zzdi1dHR4aizISzWycWgsKiioiJtamqK++xGrEKhkOMOcLJY0WJQDFDJHjescAxhObUYFCdVfn6+Njc3G/vEJccQlhPLMgusbtA0DopUjxtWQqGQI97uzWJF6+QMUK8GRTAYNDJuWIm+KYt7FiwnlDIozI8bVkKhEMcQliNKvR4UxcXFumXLFuPjhpWOjg6OISzjpV4Oiui44XQcQ1imS70aFAUFBbp161bHjRtWOIawTJZ6MShKSkoccXYjVh0dHTp37lzj24/lvVKvBUUgENBNmzaZeJ0nBMcQlolSLwWF28YNK52dnXrdddcZ354s75R6JSjcOm5Y4RjCSmWpRRak3ZcUh8Nh9PT0mG4jYdLt+ZBLWSWIunSPAoDm5eXppk2bXD96dHR0aHV1tfHtyfJOqVdGj2gVFBTo888/b+L1nRCHDh3S2tpaHsxkpbTUa0EB/O0iuW47XtHW1qbXX3+98e3H8l6pF4MCcN8YwnGDZbLUq0EB9I0h69evN/G6j8mhQ4e0rq6O4wbLWKmXgwLo+7zHhg0bHDuGtLW18T0TLOOlXg8KAJqbm+uoa1FEtbe3c9xgOaKUQdFXhYWFun79eseEBccNlpNKGRR/K6eMIdEvMza9PVisaJ2cAerloADMjyEcN1hOLMsssLpB0zwoAHNjCMcNllOLQWFRwWAwpW/Kam9v17q6OuPPm8UaqBgUg1SqxpDouME9CZZTi0FhU4FAIKljyKFDh3jgkuX4YlAMoQKBQFLOhnDcYLmlGBRDrESPIW1tbRw3WK4pBkUMFQgE9Lnnnht2WHz55Zfck2C5qhgUMVYgEBjW2ZD29natra01/jxYrFiKQRFH5ebm6saNG2Pes+C4wXJrMSjirFjHkOibqUz3HU9deumlumjRIuN9sMwVg2IYNdQxxO3jRlVVlXZ0dOi8efPU5/MZ74eV+mJQDLPsxpB0GDeqqqpUVbWrq0vnz59vvB9W6otBkYAKBoMDjiHpcnYjGhTR4OMY4r1iUCSoCgsLTxhD2tvb9dprrzXeVyKqf1CoKscQDxaDIoGVm5urGzZs0K+//lqrqqpcPW70r5ODIjqGLFiwwHhvrNQUgyLBFQwGtby83HgfiayBgqL/GJIugciyLqssyATFpa2tDW1tbabbSIlAIIA1a9agp6cHL7zwAsLhsOmWKMXS7rtHKTny8/Pxq1/9CvPnzzfdChnAPQqXu+uuu1BXV5eQZRUVFQ16e05ODhobGyEieOaZZ6LjKXkAg8LlzjjjDFRWVqZsfcFgEGvXrkVvby/HEA/h6EEx4xjiPQwKikt0DFm4cCFExHQ7lGQMCopbMBjEo48+irlz58Ln4z+ldMbfLg1LXl4ennjiCSxYsMB0K5REDAoattzcXDzyyCNYtGgRx5A0xaCghIieDZk3bx7HkDTE3yglTF5eHh5//HGOIWmIQUEJFR1DrrjiCtOtUAIxKCihVBWffPIJDhw4YLoVSiAGBSXUnj17cO211+KDDz4w3QolEIOCEqa1tZUhkab4WQ8aNlXF7t27GRJpjHsUNGwcN9Ifg4KGpaWlBTU1NQyJNMfRg+ISHTdqa2sZEh7APQqKC49JeAuDgmLW0tLCkPAYjh4u19zcjH379iVkWRUVFVi4cKHl7Rw3PIyX62dFy+py/VEtLS06evRo432ykldWWcDRg4aE44a3cfSgQXHcIIAHM8kGz24QwKCgQXDcoCiOHvQtqorW1laOG3Qcg4K+Jfop0A8//NB0K+QQHD3oBC0tLaitrWVI0AlksO+PjHzNPXnEmDFjoKocNzxMVQe8jDqDgoiOswoKjh5EZItBQUS2GBREZItBQUS2GBREZItBQUS2GBREZItBQUS2GBREZItBQUS2GBREZItBQUS2GBREZItBQUS2GBREZItBQUS2GBREZItBQUS2GBREZItBQUS2GBREZItBQUS2GBREZItBQUS2GBREZGvQbwojIgK4R0FEQ8CgICJbDAoissWgICJbDAoissWgICJb/w8bBbzEIHiwHQAAAABJRU5ErkJggg==\\n\",\n \"text/plain\": [\n \"<Figure size 432x288 with 1 Axes>\"\n ]\n },\n \"metadata\": {\n \"needs_background\": \"light\"\n },\n \"output_type\": \"display_data\"\n },\n {\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAAQoAAAD3CAYAAADlsBq6AAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADh0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uMy4yLjEsIGh0dHA6Ly9tYXRwbG90bGliLm9yZy+j8jraAAAZLUlEQVR4nO3dd5wU9f3H8df3+nENsCUWQBQ8kCJIMQIBERUriBAsQYqKFY2xdxMVNNEYDRqxJIoaFQvRn8nPhy1iicZETWKw/KzYIhbKHcdR7m5+f3xnYVlvd27vdndmdt7Px+Mej72d3ZnPzs6+dz4zszPGcRxERFIp8LsAEQk+BYWIeFJQiIgnBYWIeFJQiIgnBYWIeFJQZIgxZjtjzPPGmHpjzHV+1yPpMcb0MMY4xpiijj7XGPOcMeZ49/YxxpgnM11vrgUiKIwxHxtjxvldRwfNBr4Bqh3HOSt+YckkY8wBcYH0tTFmiTHmsExPJ6i8lhVjzBhjzGe5rCkVx3HudRxnf7/r6KhABEWe6A685WToCDZjTGEr900GHgQWAjsC2wGXAodmYpoiSTmO4/sf8DEwzr09A3gJuB5YBXwI7O3e/ynwFTA97rkHA28Ade7wyxPGfSywDPgWuCRhWgXA+cAH7vBFQNckNXYBHge+Bla6t3d0h90JbAQ2AGvc+puBde7/893H1QJPASuAd4EfxY3/TuC3wJ+BhliNccMN8AlwTor5WABc7L7er7CBUuMO6wE4wEx3Pq0ETgKGAv925/X8uHHF3offAKuBd4B944ZvDzzmvpb3gRPihl3uzsuFQD2wFBiS8NyH3Xn5EXB6W54L3A20AI3ufD23lXkwBvgsyfxJuqzEzZ/ZwBfAf4GzEuZtq8tK3HOL3P+fA46Pm48vxo3Hcef7e+57cBNg3GGFwHXYNdOPgNPix+vrZ9TvApIERZO7QBcCV7ofkJuAUmB/dwGqjFsw+rtv5ABgOTDRHdbXXaBGAiXAtdgPdGxaPwFewX47lwILgPuS1LgVcATQCajCfrP/MeGDfmXc/5sWFvf/CnfhnAkUAYPdBWL3uOevBka4r6UsYfq17kKzc4r5OAv7oe0JVAKPAHcnLMy3AGXufFwH/BHYFtgBGy6jE96HM4FiYKpbX+zDsQS42R3XHtgP/b5xH/Z1wEHuezgPeCXuA/cadk2oxK31Q+AAr+cmLitJ5sEYkgdFqmUlNn/uc9+r/u5r8lxWSD8oHgc6A93caYx3h50EvOVOowvwNAqKlEHxXtyw/u7M2i7uvm+BPZKM69fA9e7tS4n74GM/5BvipvU2W35Lfh8bJJ5vjPvhWBn3/52kDoqpwAsJ41gAXBb3/IUppjfCnQ9lKR7zDHBK3P+7xV5P3MK8Q8J8nBr3/8PAT+Lehy9wv+3c+14FpgE7YdeYquKGzQPudG9fDjwdN6wv0OjeHg58klD3BcDvvZ6buKwkmQdjSBIUHstKbP7Uxg3/BXCH17JC+kExMu7/RcD57u1ngRPjho0jIEGR9hbeHFked7sRwHGcxPsqAYwxw4GrgX7Yb6hS7Lc92FXcT2NPchxnrTHm27jxdAcWG2Na4u5rxvb+n8cXZIzphG2HxmPTHqDKGFPoOE5zG15Td2C4MWZV3H1F2NXpmE9JLlb397Grpa3ZHtt2xCxzp7Fd3H2J87HV+er63HGX2Ljxbe/+rXAcpz5h2JC4/7+Mu70WKHP3CnQHtk+YD4XAC17PdRyniQ7wWFZi4t+DZdgvKki9rKQr8fXF5vkWyyupl4ecyoeNmX/A9so7OY5Tg121Nu6w/2JX4wAwxpRjW4iYT4EDHcfpHPdX5jjOFiHhOgv7DT3ccZxq4Iex0SapK3Gj5qfAkoRpVTqOc3KK58R71x3HESke8wV2gY7phm0flrf+cE87GGPiX183dxpfAF2NMVUJw1qbb4k+BT5KmA9VjuMc1MaaUs0jL6mWlZid4m7HXm+s7rYuK+21xfKaUIuv8iEoqrDfbuuMMcOAo+OGPQQcaozZ2xhTAvyMLReMW4CrjDHdAYwx2xhjJqSYTiOwyhjTFbjMo67l2P475nGgtzFmmjGm2P0baozp05YX6X6z/xS4xBgz0xhTbYwpMMaMNMbc6j7sPuBMY8zOxphKYC7wQAe+ibcFTndrnQL0Af7sOM6nwF+BecaYMmPMAOA44N42jPNVoM4Yc54xptwYU2iM6WeMGdrGmhLna6vcuuL/DKmXlZhLjDGdjDG7Y7cnPeDen86y0l6LgDOMMTsYYzoD52V4/O2WD0FxCvBzY0w9dpvEotgAx3GWAnOA+7FpXY/dYLfefcgN2G+YJ93nv4LtoVvza6AcuwHyFeAJj7puACYbY1YaY250V9P3B47Efkt9CVyDXf1tE8dxHsJu65jljmM5dmPvo+5DfodtZZ7HtifrsK+/vf4G9MK+5quAyY7jxFqgo7C9+RfAYuy2lqfa8Bqasbtz93Br/Aa4HahpY03zgIuNMauMMWcnecwO2FCP/9uFFMtKnCXYDcLPANc6jhM7WCqdZaW9bgOexO6FegO7B6wJ2+L4KrZbJhLcb9lVQC/HcZL1+QIYY2ZgN8iN9LuWqDLGHAjc4jhOd88HZ1k+rFGkZIw51F2VrMDuHn0Tu+VcJFDcVuwgY0yRMWYHbHu72O+6IAJBAUxg8wa4XsCRTpRWoyRMDHY72kps6/E2tkXyXaRaDxFpnyisUYhIB6U84MoYo9UNkQhxHKfV44K0RiEinhQUIuJJQSEinhQUIuJJQSEinhQUIuJJQSEinhQUIuJJQSEinhQUIuJJQSEinhQUIuJJQSEinhQUIuJJQSEinhQUIuJJQSEinhQUIuIpkkFRUgozz4ZOld6PFZGIBkWv/jDnShg70e9KRMIhkkFx+lVQXAKHz4Sarn5XIxJ8kQuKEeNhwDAwBoaPhUG6YJ6Ip5Sn6883JaUw+Xio6rz5vtN+Bs8/Di0t/tUlIVBk7HW8gs4BmjJ/lY1IBUXfwTBy/Jb37dIXDp0Gj97lT00SEv+7L9RW+12Ft+Xr4AdPwMbMfvNFJiiKS+DoOVBe8d37j5kDTz8CDfX+1CYhsF0Z7Fjh/Ti/FWRnzScy2yh2Gwjjp7Y+rFd/GHNYbusRCZPIBMXpV0JBkldbXAKTZmkPiEgykQiKEQdA/2GpH6M9ICLJ5X1QlJTBEQl7OpKZc4XdbSoiW8r7oOgzCEYd1LbH9qyFCdOzW49IGOV1UBSXwLQzoLxT2x9/1GlQEYK9YCK5lNdB0Wcw7D8lvef0HgBjDslOPSJhlddBcdrPk+/pSKa4GCYdB9VdslOTSBjlbVCMGA/9h7bvucPHwp6jMluPSJjlZVCUltnjItqypyOZOVdqD4hITF4GxW57wOgObmfo0RsmzMhIOSKhl3dBUVQM08+EsvKOjaekFI48GSprMlOXSJjlXVDsPgTGHZGZcdXuAT9s4zEYIvks74Li1MuhsDAz4yoqtkd1VndgW4dIPsiroBgx3vs3HekaPhaGjM7sOEXCJm+CorQMJs3s2J6OZLQHRKIub4Jit4HZO6dE914wcWZ2xi0SBnkRFEXFMONsu1aRDSWlMPUkqNIeEImovAiK/sNg3yxfo6N2EIw8MLvTEAmqvAiKky+Dwiyf/bOoCKbMzs42EJGgC31QjDig/b/pSNewfWDomNxMSyRIQn0W7tJye7WvXH7Ln34l/OVRcDJ/6QT/Ve8MPQ5O7znLnoDV72enHgmMUAdF7/4wdkJup9ltVxtOj/wut9PNuMIy2GaQ/et/or2vpBqqe6Q3nrplsGG1vb30DvjqH/D1G9DUmNFyxV+hDYrCIph1rj0nZi6VlMKUE+GpR6B+VW6n3WGmAMq2gmGXQOWO0HNixw8Qqe6++fboG+yq1oePQcNn8OoV0PgNOM0dm4b4LrRBMXAv2Mena3H0HWy3jTzxgD/Tb5eaXaH2xzDoLCjuZEMjG4yBXSbYwOgzHd64Ht65F1a9m53pSU6ENihOusQeP+GHwiL40Ynw4hOwZrU/NbRZ177QdybsOjn9tqIjjIHiSrv2UnssfLAYlt4OK5bmrgbJmFDu9WjLdTqybdg+MHwff2tIqaQaRt8Ihz8Dg8/ObUgkqu4Og34Ck56F0fOhVPuYwyZ0QVHmw56OZOakuPqYr3pOgPH3w4BToeJ7flezWadtYcApcOAi2GWS39VIGoK4mKe0az8Ym+WjMNuq265B+w1IAfQ/Cfa/B3ocmL3tEB1hDHTbD/ZfCAPngMnQOQEkqwK4JCVXWAjHX2D3PARBSSlMPiEgZ+zu3AvG3Q6jroeSSr+r8VZcASOvhXF3QJc+flcjHkIVFINGdPxcmJm2+xDYez+fi+jcGw5eDH1mQFGO9xd3RGGJ3TNyyCPQpdbvaiSF8ASFgRMutNfdCJLCQph6MlT6dXWxml5w8MOw1e7hPWlGl1obdAqLwApNUOy9HwwY7ncVrRsyGobv68OEO/eCQxbDVv18mHiGdY2FhdqQIApFUARpT0drjLG/ASnI5Xa5WLvRtW8OJ5plXWvVhgRUKIJi134wLuB703bqCRNn5GhipgB6HxnudiOZLrX2CFLtDQmUwAdFQSHMvsheaTzISsrsGbtrumZ5QqYA+s6CoRdmeUI+Gnwu9JsdzN27ERX4d2LPUTAqJGeW6jcU9hqX5YlsPRBG/wYKA7KPOBsKi+GH18O2Q/yuRFyBDgpj4Pjzg782EVNYCEedChVVWZpAWVfY62fh2gXaXoWl9rWWbeV3JULAg+IHAd7Tkcyeo2Dv/bM08t2OgR4BO5Akm7odYI8NEd8FNijKOgV7T0cyxsBpP8/c1co2KamBIRfm38bLVIyBIedBabY3/IiXwP7MfJe+sF+GriGaazv1tFdCf+SODI504GlQvnUGRxgSpV1hjzPgb5f5W8cH9eEI6a/XQRZO02icFCd/NMb4cmbIggK4YTGMOTQc701r/vkynHoIrF6RgZFVdYfD/mR3h0bRirfhsUOg7kO/K8l7juO0+okLZOsxZIw950RYQwJgwDDYK1NHa+58aHRDAqBrH9glID8ZjqhArlFUd7Enzg271Svgvf90cCSlXWDKS/bDEmWr3odFe8G6b/2uJK8lW6MI5DaKupXwj+f9riIgeh+lQ5oBanaB2mnwz1/7XUkkBbL1EFdBCWwzMNw9WKYYA1sPyO8DzQJMQRFkxZWw29F+VxEcvY+05wKVnFNQBNnOh9i1CrEKimBnn67REHEKiiDbaaw9C5RYBcWwkx8n/hAFhYh4UlAEVVU32Gaw31UEz9YD7MWUJacUFEFVuSNsnQcHk2TaVrvbI1UlpxQUIuJJQSEinhQUIuJJQSEinhQUQdU9JCcKlUhQUATV8r/5XYHIJgqKoFqXiTPeiGSGgkJEPCkoRMSTgkJEPCkogspx7J9sSfPEFwqKoPr6Dfhgsd9VBM/Hj8OXr/hdReQoKIKqeR1srPe7iuDZsMbOG8kpBUWQqf3YkuOQlavbiCcFRZD942poavS7iuBoXg9/n+t3FZGkoAiyxuWwYbXfVQTHhjpY+6XfVUSSgiLI1q+yaxVivf5LXQDIJwqKoKtfBhu0UZONDVD3sd9VRJaCIug+fNTuKo26b96E9x/yu4rIUlCEwcsXRXvvh+PYeSC+UVCEwfLXon3wlQ6y8p2CIgyaG+HfN0VzV2nzevjXfGha63clkaagCIv/vgyf/cXvKnLv8+fhi5f8riLyFBRh0dwI/7nVHsIcFRsb4D8LoKnB70oiT0ERJh8+Cn+9IDobNl+5FN5/2O8qBAVF+Hz0GKx4K7/DwnFgxdvwwR/9rkRcCoqwqf8EHp8IK5b6XUn2rHwH/jQJ6j70uxJxKSjCaPX78No10NLkdyWZ19IEr19nw0ICQ0ERVv93P/zrxvxqQRwH3rwF3lnodyWSQEHRTlV0YVcG+ldASxO8fDG8cX1+rFm0NMO/58NL50HLRr+rkQQKinYoo4LzuI35PM8YjsBg/CmkqRH+eh68+dtwh0VLMyy9DV44WwdWBVSR3wWETQXVXMRdjOAwCijgIhZSQCHPssifglqa4IWzoHkDDPopGJ9Cq70cB9682b4GrUkElnFS9LjGmDxqgDuuii6cwwLGMHmLtYh6VvIrTuVp7vOvuKJy2GYQjF0AW/Xzr450rHgbnp0NX72uNYmAcByn1W8aBUUblVHBxSxkNJNaHb6WeuYxiyU8jOPneR0794IDH4SutVBY6l8dqTRvgFXvwZ8na+9GwCgoOqCCGi7izk3tRjLrWMs8ZvEsD+SwulYUV0CvqTBmvl3TCJLm9bDkDHj3XtgYocPRQ0JB0U6VdOYcFrAPU9q00TIQbQgABbDNHrDnebDjGOi0rb/lNH5tf+D197nwzT/BafG3HmmVgqIdyqjgEu5mFBPT2rMRmDYk5ns/gD7HQu00KOqUuw2ejmO3Pbx7H7xzF3zxYm6mK+2moEhTJTVcwJ2M9Gg3kllHA1dzHM/43YbEFBRDdU8YegH0mmIDI5ua1sF7D8Jr82DVB9CyIbvTk4xQUKQh3XYjGduGnMLT3J/B6jrKwLaDoXJHGH45lHaG6h6ZGXXdMli/El69wv4m5avXAbUYYaKgaKP2thvJrGUN85gZnDYkUXVP6HGgvd21r21R0vHOvfDtm/b2J0/avRkSWgqKNqikMxfwO0YyoV3tRjK2DTmeZwK1ZtGKgmIoqUrvORvWqK3IIwoKD5V05lxu/c7BVJmyeW/I/ej6mRJUCooUMt1uJNPIGuYGuQ2RyEsWFJH/UVglnbmEuxnJhKz/uKucSi7kTsYyNavTEcm0SP8oLNZuZHtNIl45FZzFzRiM2hAJjci2HrbduIdROViTaI3aEAkitR5xNrcbh/l2LolYG7IvR/oyfZF0RK71qKAm5+1GMuVU8FNuwsHhWR7QmoUEVqRajzIquJR7crLhMh2NNDCXGTzHw2ibhfgp8q1HFV24mIWM8LHdSKacCi7k94xTGyIBFYnWo4IazmFB0pPOBEE5lWpDJLDyvvUop5JLuMfXDZfpWEcDV6kNEZ9EsvWooqvbbhwaipAAux3lQn7Pfhzldykim+Rt61FBNeeygB9yuN+lpK2cSs5kPi20qA2RQMjL1iNs7UYy61jLVUznOR7yuxSJiMi0HmFsN5Ipo5PbhhztdykScXkXFA4tNJE/F5JpybPXI+GUl61HGZ24iLsY7efl/jKgkTVcwY95gUf9LkUiIjKtB9jefi4zg39GqRTqWMFVTOdFHvO7FJH83evRyBqu4xQKKGQMkzN6artsa6COazmJJTzidykiQJ62HvFK6cTFIWpD1G6InyLVesRb77Yh/l+5y5ttN2bwgtoNCZi8Dwqw39K/4hSe4QFaAnqdiQbq+CUnskSHbksA5X3rEa+Uci7irqydabu91lLPFUzjRbUb4rPIth7x1tPI1RzH09wXmMOi61jBXGZq74YEWqSCAuy393Wcwl9Y5HsbspZ6tRsSCpFqPeL53Yao3ZAgUuuRwM82pI4VzFO7ISES2aCA+DbkwZy1IbbdmK0T00ioRLb1iJerNmRzu/EYCgkJIrUeKaynkWs4PqttiG03ZrnbJBQSEi4KClcDdVzHyVnZG7KWen7BbJ2ARkJLrUeCTLchDdRxJceq3ZBQUOvRRrE25Cn+0OE2pI5v1W5IXlBQtKKBOn7Vwb0hsXbDHkwlEm55ez6KjmqgjrnMAGAfpqTVhmzZboiEn9YoUlhPI7/ghLTakDpWcHVI2419KGAGhX6XIQGkoPBg94a0rQ2x7cYJ7sFU4VMF/IYijgnV+cAkF7Q8tMFatw15joeSrlk0UMcVTGMJi3NcXWZVYridYo7WoiFxtDS0kW1DZrfahtTxbWjbjdaUYbiJYrUhsomCIg0NrHYPytrchqylnmtC3G4kU41RGyKbaK9HmtZSz1xm4OAwnPFcxbG8yP/4XVZWxNqQAjZyd0BPISi5oSMz26mCGrZhez7mbb9LyZjDKOBRSr5zfx0OZ9DEXTTnQWMlqSQ7MlNrFO3UwGoaWO13GTlRjWE+RWzE4T7fzwsmflD7KW1S4bYhP9YiE0laowi58ynkiAztnejsMbwMw40UY2hiodqQSFFQhFw3DENy+C1fg+EmimhSGxIpWo+UtKkNiR6909IusTZkOgUBupSSZIuCQtqtBsPN7uHeWpDym95f6ZBOGG6jmGlalPKa3l3psHIMN7i/DVEbkp8UFJIRsb0h+m1IftJ7KhnTCcOtakPykt5RyahYG7KfFq28ondTMsrB4XMclum4zbyioJCMegeHw9nI/yko8oqCQjLmLVoUEnlKv/WQDnNweFtrEnlNaxTSYWo38p+CQjpkKS1MVEjkPbUe0i6xdmOSQiIStEYh7aJtEtGioJC0LdXejchR6xFyi2nhIzZmZFy7U8D0FKfVU7sRXTpdv2yS7HT9MTpOIv8lO12/Wg9pE7Ub0abWQ1JSuyGgNQrxoL0bAgoKSUHthsSo9ZDvcHB4S+2GxFFQyHe85bYb7ykkxKXWQ7awlBYmKSQkgY6jkE12w+CA2o0IS3YchYJCRDbRAVci0m4KChHxpKAQEU8KChHxpKAQEU8KChHxpKAQEU8KChHxpKAQEU8KChHxpKAQEU8KChHxpKAQEU8KChHxpKAQEU8KChHxpKAQEU8KChHxpKAQEU8KChHxpKAQEU8KChHxpKAQEU8KChHxpKAQEU8prxQmIgJaoxCRNlBQiIgnBYWIeFJQiIgnBYWIeFJQiIin/weAsOfrQ7xDpQAAAABJRU5ErkJggg==\\n\",\n \"text/plain\": [\n \"<Figure size 432x288 with 1 Axes>\"\n ]\n },\n \"metadata\": {\n \"needs_background\": \"light\"\n },\n \"output_type\": \"display_data\"\n }\n ],\n \"source\": [\n \"connected_component_label('C:/Users/Yash Joshi/OG_notebooks/shapes.png')\"\n ]\n }\n ],\n \"metadata\": {\n \"kernelspec\": {\n \"display_name\": \"Python 3\",\n \"language\": \"python\",\n \"name\": \"python3\"\n },\n \"language_info\": {\n \"codemirror_mode\": {\n \"name\": \"ipython\",\n \"version\": 3\n },\n \"file_extension\": \".py\",\n \"mimetype\": \"text/x-python\",\n \"name\": \"python\",\n \"nbconvert_exporter\": \"python\",\n \"pygments_lexer\": \"ipython3\",\n \"version\": \"3.7.4\"\n }\n },\n \"nbformat\": 4,\n \"nbformat_minor\": 4\n}"
}
{
"filename": "code/artificial_intelligence/src/image_processing/erode_dilate/main.cpp",
"content": "#include \"opencv2/imgproc/imgproc.hpp\"\n#include \"opencv2/highgui/highgui.hpp\"\n#include \"highgui.h\"\n#include <stdlib.h>\n#include <stdio.h>\n\n/// Global variables\ncv::Mat src, erosion_dst, dilation_dst;\n\nint erosion_elem = 0;\nint erosion_size = 0;\nint dilation_elem = 0;\nint dilation_size = 0;\nint const max_elem = 2;\nint const max_kernel_size = 21;\n\n/** Function Headers */\nvoid Erosion( int, void* );\nvoid Dilation( int, void* );\n\n/** @function main */\nint main( int argc, char** argv )\n{\n /// Load an image\n src = cv::imread( argv[1] );\n\n if (!src.data)\n return -1;\n /// Create windows\n cv::namedWindow( \"Erosion Demo\", CV_WINDOW_AUTOSIZE );\n cv::namedWindow( \"Dilation Demo\", CV_WINDOW_AUTOSIZE );\n cvMoveWindow( \"Dilation Demo\", src.cols, 0 );\n\n /// Create Erosion Trackbar\n cv::createTrackbar( \"Element:\\n 0: Rect \\n 1: Cross \\n 2: Ellipse\", \"Erosion Demo\",\n &erosion_elem, max_elem,\n Erosion );\n\n cv::createTrackbar( \"Kernel size:\\n 2n +1\", \"Erosion Demo\",\n &erosion_size, max_kernel_size,\n Erosion );\n\n /// Create Dilation Trackbar\n cv::createTrackbar( \"Element:\\n 0: Rect \\n 1: Cross \\n 2: Ellipse\", \"Dilation Demo\",\n &dilation_elem, max_elem,\n Dilation );\n\n cv::createTrackbar( \"Kernel size:\\n 2n +1\", \"Dilation Demo\",\n &dilation_size, max_kernel_size,\n Dilation );\n\n /// Default start\n Erosion( 0, 0 );\n Dilation( 0, 0 );\n\n cv::waitKey(0);\n return 0;\n}\n\n/** @function Erosion */\nvoid Erosion( int, void* )\n{\n int erosion_type;\n if (erosion_elem == 0)\n erosion_type = cv::MORPH_RECT;\n else if (erosion_elem == 1)\n erosion_type = cv::MORPH_CROSS;\n else if (erosion_elem == 2)\n erosion_type = cv::MORPH_ELLIPSE;\n cv::Mat element = cv::getStructuringElement( erosion_type,\n cv::Size( 2 * erosion_size + 1,\n 2 * erosion_size + 1 ),\n cv::Point( erosion_size, erosion_size ) );\n\n /// Apply the erosion operation\n cv::erode( src, erosion_dst, element );\n cv::imshow( \"Erosion Demo\", erosion_dst );\n}\n\n/** @function Dilation */\nvoid Dilation( int, void* )\n{\n int dilation_type;\n if (dilation_elem == 0)\n dilation_type = cv::MORPH_RECT;\n else if (dilation_elem == 1)\n dilation_type = cv::MORPH_CROSS;\n else if (dilation_elem == 2)\n dilation_type = cv::MORPH_ELLIPSE;\n cv::Mat element = cv::getStructuringElement( dilation_type,\n cv::Size( 2 * dilation_size + 1,\n 2 * dilation_size + 1 ),\n cv::Point( dilation_size, dilation_size ) );\n /// Apply the dilation operation\n cv::dilate( src, dilation_dst, element );\n cv::imshow( \"Dilation Demo\", dilation_dst );\n}"
}
{
"filename": "code/artificial_intelligence/src/image_processing/houghtransform/main.cpp",
"content": "#include <opencv/cv.h>\n#include <opencv/highgui.h>\n#include <opencv2/core/types_c.h>\n#include <math.h>\n#include <stdlib.h>\n\n#define WINDOW_NAME \"Hough Transformation\"\n#define THETA_GRANULARITY (4 * 100)\n#define RHO_GRANULARITY 1\n\nIplImage *img_read;\nIplImage *img_edges;\nIplImage *img_sine;\nint *img_sine_arr;\nint threshold;\n\nCvSize img_attrib_dim;\nCvSize img_attrib_sine;\nint max_rho;\n\n/*****************************************************************\n* create and destroy gui items\n*****************************************************************/\nvoid create_gui()\n{\n cvNamedWindow(WINDOW_NAME);\n\n max_rho = sqrt(\n img_attrib_dim.width * img_attrib_dim.width + img_attrib_dim.height *\n img_attrib_dim.height);\n\n printf(\"Image diagonal size (max rho): %d\\n\", max_rho);\n img_attrib_sine.width = THETA_GRANULARITY;\n img_attrib_sine.height = RHO_GRANULARITY * max_rho * 2;\n\n img_sine_arr = (int *)malloc(img_attrib_sine.width * img_attrib_sine.height * sizeof(int));\n img_edges = cvCreateImage(img_attrib_dim, IPL_DEPTH_8U, 1);\n img_sine = cvCreateImage(img_attrib_sine, IPL_DEPTH_8U, 1);\n cvZero(img_sine);\n}\n\nvoid destroy_gui()\n{\n cvDestroyWindow(WINDOW_NAME);\n cvReleaseImage(&img_read);\n cvReleaseImage(&img_edges);\n cvReleaseImage(&img_sine);\n}\n\n/*****************************************************************\n* display a message and wait for escape key to continue\n*****************************************************************/\nvoid disp_msg(const char *msg)\n{\n printf(\"%s. Press escape to continue.\\n\", msg);\n char c;\n do\n c = cvWaitKey(500);\n while (27 != c);\n}\n\n/*****************************************************************\n* main.\n* 1. read image\n* 2. detect edges\n* 3. hough transform to detect lines\n*****************************************************************/\nint main(int argc, char **argv)\n{\n if (argc < 3)\n {\n printf(\"Usage: %s <image> <threshold>\\n\", argv[0]);\n return 1;\n }\n\n img_read = cvLoadImage(argv[1]);\n if (NULL == img_read)\n {\n printf(\"Error loading image %s\\n\", argv[1]);\n return -1;\n }\n img_attrib_dim = cvGetSize(img_read);\n threshold = atoi(argv[2]);\n\n create_gui();\n\n cvShowImage(WINDOW_NAME, img_read);\n printf(\"Original image size: %d x %d\\n\", img_attrib_dim.width, img_attrib_dim.height);\n disp_msg(\"This is the original image\");\n\n // detect edges\n cvCvtColor(img_read, img_edges, CV_BGR2GRAY);\n cvLaplace(img_edges, img_edges, 3);\n cvThreshold(img_edges, img_edges, 200, 255, CV_THRESH_BINARY);\n cvShowImage(WINDOW_NAME, img_edges);\n disp_msg(\"This is the edge detected image\");\n printf(\"working...\\n\");\n\n // for each pixel in the edge\n int max_dest = 0;\n int min_dest = INT_MAX;\n for (int y = 0; y < img_edges->height; y++)\n {\n uchar *row_ptr = (uchar*) (img_edges->imageData + y * img_edges->widthStep);\n for (int x = 0; x < img_edges->width; x++)\n {\n if (*(row_ptr + x) > 0)\n for (int theta_div = 0; theta_div < THETA_GRANULARITY; theta_div++)\n {\n double theta = (CV_PI * theta_div / THETA_GRANULARITY) - (CV_PI / 2);\n int rho = (int)round(cos(theta) * x + sin(theta) * y + max_rho);\n int *dest = img_sine_arr + rho * img_attrib_sine.width + theta_div;\n int d_val = *dest = *dest + 1;\n if (d_val > max_dest)\n max_dest = d_val;\n if (d_val < min_dest)\n min_dest = d_val;\n }\n }\n }\n\n // scale the intensity for contrast\n float div = ((float)max_dest) / 255;\n printf(\"max_dest: %d, min_dest: %d, scale: %f\\n\", max_dest, min_dest, div);\n for (int y = 0; y < img_sine->height; y++)\n {\n uchar *row_ptr = (uchar*) (img_sine->imageData + y * img_sine->widthStep);\n for (int x = 0; x < img_sine->width; x++)\n {\n int val = (*((img_sine_arr + y * img_attrib_sine.width) + x)) / div;\n if (val < 0)\n val = 0;\n *(row_ptr + x) = val;\n }\n }\n\n // find rho and theta at maximum positions (within the threshold)\n int possible_edge = round((float)max_dest / div) - threshold;\n printf(\"possible edges beyond %d\\n\", possible_edge);\n for (int y = 0; y < img_sine->height; y++)\n {\n uchar *row_ptr = (uchar*) (img_sine->imageData + y * img_sine->widthStep);\n for (int x = 0; x < img_sine->width; x++)\n {\n int val = *(row_ptr + x);\n if (possible_edge <= val)\n {\n float theta_rad = (CV_PI * x / THETA_GRANULARITY) - (CV_PI / 2);\n float theta_deg = theta_rad * 180 / CV_PI;\n printf(\"val: %d at rho %d, theta %f (%f degrees)\\n\", val, y - max_rho, theta_rad,\n theta_deg);\n }\n }\n }\n\n // display the plotted intensity graph of the hough space\n cvShowImage(WINDOW_NAME, img_sine);\n disp_msg(\"this is the hough space image\");\n\n destroy_gui();\n return 0;\n}"
}
{
"filename": "code/artificial_intelligence/src/image_processing/image_stitching/imagestitching.cpp",
"content": "#include <iostream>\n#include <stdlib.h>\n#include <stdio.h>\n#include <opencv2/opencv_modules.hpp>\n#include <opencv2/core/utility.hpp>\n#include <opencv2/imgcodecs.hpp>\n#include <opencv2/highgui.hpp>\n#include <opencv2/features2d.hpp>\n#include <opencv2/stitching/detail/blenders.hpp>\n#include <opencv2/stitching/detail/camera.hpp>\n#include <opencv2/stitching/detail/exposure_compensate.hpp>\n#include <opencv2/stitching/detail/matchers.hpp>\n#include <opencv2/stitching/detail/motion_estimators.hpp>\n#include <opencv2/stitching/detail/seam_finders.hpp>\n#include <opencv2/stitching/detail/util.hpp>\n#include <opencv2/stitching/detail/warpers.hpp>\n#include <opencv2/stitching/warpers.hpp>\n\nint main(int argc, char* argv[])\n{\n// Default parameters\n std::vector<std::string> img_names;\n double scale = 1;\n std::string features_type = \"orb\"; //\"surf\" or \"orb\" features type\n float match_conf = 0.3f;\n float conf_thresh = 1.f;\n std::string adjuster_method = \"ray\"; //\"reproj\" or \"ray\" adjuster method\n bool do_wave_correct = true;\n cv::detail::WaveCorrectKind wave_correct_type = cv::detail::WAVE_CORRECT_HORIZ;\n std::string warp_type = \"spherical\";\n int expos_comp_type = cv::detail::ExposureCompensator::GAIN_BLOCKS;\n std::string seam_find_type = \"gc_color\";\n\n float blend_strength = 5;\n int blend_type = cv::detail::Blender::MULTI_BAND;\n std::string result_name = \"img/panorama_result.jpg\";\n double start_time = cv::getTickCount();\n // 1-Input images\n if (argc > 1)\n for (int i = 1; i < argc; i++)\n img_names.push_back(argv[i]);\n else\n {\n img_names.push_back(\"./panorama1.jpg\");\n img_names.push_back(\"./panorama2.jpg\");\n }\n // Check if have enough images\n int num_images = static_cast<int>(img_names.size());\n if (num_images < 2)\n {\n std::cout << \"Need more images\" << std::endl; return -1;\n }\n // 2- Resize images and find features steps\n std::cout << \"Finding features...\" << std::endl;\n double t = cv::getTickCount();\n cv::Ptr<cv::detail::FeaturesFinder> finder;\n if (features_type == \"surf\")\n finder = cv::makePtr<cv::detail::SurfFeaturesFinder>();\n else if (features_type == \"orb\")\n finder = cv::makePtr<cv::detail::OrbFeaturesFinder>();\n else\n {\n std::cout << \"Unknown 2D features type: '\" << features_type << std::endl; return -1;\n }\n\n cv::Mat full_img, img;\n std::vector<cv::detail::ImageFeatures> features(num_images);\n std::vector<cv::Mat> images(num_images);\n\n std::vector<cv::Size> full_img_sizes(num_images);\n for (int i = 0; i < num_images; ++i)\n {\n full_img = cv::imread(img_names[i]);\n full_img_sizes[i] = full_img.size();\n if (full_img.empty())\n {\n std::cout << \"Can't open image \" << img_names[i] << std::endl; return -1;\n }\n resize(full_img, img, cv::Size(), scale, scale);\n images[i] = img.clone();\n (*finder)(img, features[i]);\n features[i].img_idx = i;\n std::cout << \"Features in image #\" << i + 1 << \" are : \" << features[i].keypoints.size() <<\n std::endl;\n }\n finder->collectGarbage();\n full_img.release();\n img.release();\n std::cout << \"Finding features, time: \" <<\n ((cv::getTickCount() - t) / cv::getTickFrequency()) << \" sec\" << std::endl;\n // 3- cv::Match features\n std::cout << \"Pairwise matching\" << std::endl;\n t = cv::getTickCount();\n std::vector<cv::detail::MatchesInfo> pairwise_matches;\n BestOf2Nearestcv::Matcher matcher(false, match_conf);\n matcher(features, pairwise_matches);\n matcher.collectGarbage();\n std::cout << \"Pairwise matching, time: \" <<\n ((cv::getTickCount() - t) / cv::getTickFrequency()) << \" sec\" << std::endl;\n // 4- Select images and matches subset to build panorama\n std::vector<int> indices = leaveBiggestComponent(features, pairwise_matches, conf_thresh);\n std::vector<cv::Mat> img_subset;\n std::vector<std::string> img_names_subset;\n std::vector<cv::Size> full_img_sizes_subset;\n\n for (size_t i = 0; i < indices.size(); ++i)\n {\n img_names_subset.push_back(img_names[indices[i]]);\n img_subset.push_back(images[indices[i]]);\n full_img_sizes_subset.push_back(full_img_sizes[indices[i]]);\n }\n\n images = img_subset;\n img_names = img_names_subset;\n full_img_sizes = full_img_sizes_subset;\n // Estimate camera parameters rough\n HomographyBasedEstimator estimator;\n std::cout << \"0\\n\";\n std::vector<CameraParams> cameras;\n estimator(features, pairwise_matches, cameras);\n // // if (!estimator(features, pairwise_matches, cameras))\n // // {std::cout <<\"Homography estimation failed.\" << std::endl; return -1; }\n std::cout << \"1\\n\";\n for (size_t i = 0; i < cameras.size(); ++i)\n {\n cv::Mat R;\n std::cout << \"2\\n\";\n cameras[i].R.convertTo(R, CV_32F);\n cameras[i].R = R;\n std::cout << \"Initial intrinsic #\" << indices[i] + 1 << \":\\n\" << cameras[i].K() <<\n std::endl;\n }\n // 5- Refine camera parameters globally\n cv::Ptr<BundleAdjusterBase> adjuster;\n if (adjuster_method == \"reproj\")\n // \"reproj\" method\n adjuster = cv::makePtr<BundleAdjusterReproj>();\n else // \"ray\" method\n adjuster = cv::makePtr<BundleAdjusterRay>();\n adjuster->setConfThresh(conf_thresh);\n if (!(*adjuster)(features, pairwise_matches, cameras))\n {\n std::cout << \"Camera parameters adjusting failed.\" << std::endl; return -1;\n }\n // Find median focal length\n std::vector<double> focals;\n\n\n for (size_t i = 0; i < cameras.size(); ++i)\n {\n std::cout << \"Camera #\" << indices[i] + 1 << \":\\n\" << cameras[i].K() << std::endl;\n focals.push_back(cameras[i].focal);\n }\n sort(focals.begin(), focals.end());\n float warped_image_scale;\n if (focals.size() % 2 == 1)\n warped_image_scale = static_cast<float>(focals[focals.size() / 2]);\n else\n warped_image_scale =\n static_cast<float>(focals[focals.size() / 2 - 1] + focals[focals.size() / 2]) * 0.5f;\n // 6- Wave correlation (optional)\n if (do_wave_correct)\n {\n std::vector<cv::Mat> rmats;\n for (size_t i = 0; i < cameras.size(); ++i)\n rmats.push_back(cameras[i].R.clone());\n waveCorrect(rmats, wave_correct_type);\n for (size_t i = 0; i < cameras.size(); ++i)\n cameras[i].R = rmats[i];\n }\n // 7- Warp images\n std::cout << \"Warping images (auxiliary)... \" << std::endl;\n t = cv::getTickCount();\n std::vector<Point> corners(num_images);\n std::vector<Ucv::Mat> masks_warped(num_images);\n std::vector<Ucv::Mat> images_warped(num_images);\n std::vector<cv::Size> sizes(num_images);\n std::vector<Ucv::Mat> masks(num_images);\n // Prepare images masks\n for (int i = 0; i < num_images; ++i)\n {\n masks[i].create(images[i].size(), CV_8U);\n masks[i].setTo(cv::Scalar::all(255));\n }\n // Map projections\n cv::Ptr<WarperCreator> warper_creator;\n if (warp_type == \"rectilinear\")\n warper_creator = cv::makePtr<cv::CompressedRectilinearWarper>(2.0f, 1.0f);\n else if (warp_type == \"cylindrical\")\n warper_creator = cv::makePtr<cv::CylindricalWarper>();\n else if (warp_type == \"spherical\")\n warper_creator = cv::makePtr<cv::SphericalWarper>();\n else if (warp_type == \"stereographic\")\n warper_creator = cv::makePtr<cv::StereographicWarper>();\n else if (warp_type == \"panini\")\n warper_creator = cv::makePtr<cv::PaniniWarper>(2.0f, 1.0f);\n\n if (!warper_creator)\n {\n std::cout << \"Can't create the following warper\" << warp_type << std::endl; return 1;\n }\n cv::Ptr<RotationWarper> warper =\n warper_creator->create(static_cast<float>(warped_image_scale * scale));\n\n for (int i = 0; i < num_images; ++i)\n {\n cv::Mat_<float> K;\n cameras[i].K().convertTo(K, CV_32F);\n float swa = (float)scale;\n K(0, 0) *= swa; K(0, 2) *= swa;\n K(1, 1) *= swa; K(1, 2) *= swa;\n corners[i] = warper->warp(images[i], K, cameras[i].R, INTER_LINEAR, BORDER_REFLECT,\n images_warped[i]);\n\n sizes[i] = images_warped[i].size();\n warper->warp(masks[i], K, cameras[i].R, cv::INTER_NEAREST, cv::BORDER_CONSTANT,\n masks_warped[i]);\n }\n std::vector<Ucv::Mat> images_warped_f(num_images);\n\n for (int i = 0; i < num_images; ++i)\n images_warped[i].convertTo(images_warped_f[i], CV_32F);\n\n std::cout << \"Warping images, time: \" << ((cv::getTickCount() - t) / cv::getTickFrequency()) <<\n \" sec\" << std::endl;\n // 8- Compensate exposure errors\n cv::Ptr<Ecv::detail::xposureCompensator> compensatcv::detail::or =\n ExposureCompensator::createDefault(expos_comp_type);\n compensator->feed(corners, images_warped, masks_warped);\n // 9- Find seam masks\n cv::Ptr<SeamFinder> seam_finder;\n if (seam_find_type == \"no\")\n seam_finder = cv::makePtr<NoSeamFinder>();\n else if (seam_find_type == \"voronoi\")\n seam_finder = cv::makePtr<VoronoiSeamFinder>();\n else if (seam_find_type == \"gc_color\")\n seam_finder = cv::makePtr<GraphCutSeamFinder>(GraphCutSeamFinderBase::COST_COLOR);\n else if (seam_find_type == \"gc_colorgrad\")\n seam_finder = cv::makePtr<GraphCutSeamFinder>(GraphCutSeamFinderBase::COST_COLOR_GRAD);\n else if (seam_find_type == \"dp_color\")\n seam_finder = cv::makePtr<DpSeamFinder>(DpSeamFinder::COLOR);\n else if (seam_find_type == \"dp_colorgrad\")\n seam_finder = cv::makePtr<DpSeamFinder>(DpSeamFinder::COLOR_GRAD);\n if (!seam_finder)\n {\n std::cout << \"Can't create the following seam finder\" << seam_find_type << std::endl;\n return 1;\n }\n\n\n seam_finder->find(images_warped_f, corners, masks_warped);\n // Release unused memory\n images.clear();\n images_warped.clear();\n images_warped_f.clear();\n masks.clear();\n // 10- Create a blender\n cv::Ptr<cv::detail::Blender> blender = cv::detail::Blender::createDefault(blend_type, false);\n cv::Size dst_sz = resultRoi(corners, sizes).size();\n float blend_width = sqrt(static_cast<float>(dst_sz.area())) * blend_strength / 100.f;\n\n if (blend_width < 1.f)\n blender = cv::detail::Blender::createDefault(cv::detail::Blender::NO, false);\n else if (blend_type == cv::detail::Blender::MULTI_BAND)\n {\n MultiBandcv::detail::Blender* mb =\n dynamic_cast<MultiBandcv::detail::Blender*>(blender.get());\n mb->setNumBands(static_cast<int>(ceil(log(blend_width) / log(2.)) - 1.));\n std::cout << \"Multi-band blender, number of bands: \" << mb->numBands() << std::endl;\n }\n else if (blend_type == cv::detail::Blender::FEATHER)\n {\n Feathercv::detail::Blender* fb = dynamic_cast<Feathercv::detail::Blender*>(blender.get());\n fb->setSharpness(1.f / blend_width);\n std::cout << \"Feather blender, sharpness: \" << fb->sharpness() << std::endl;\n }\n blender->prepare(corners, sizes);\n // 11- Compositing step\n std::cout << \"Compositing...\" << std::endl;\n t = cv::getTickCount();\n cv::Mat img_warped, img_warped_s;\n\n\n\n cv::Mat dilated_mask, seam_mask, mask, mask_warped;\n for (int img_idx = 0; img_idx < num_images; ++img_idx)\n {\n std::cout << \"Compositing image #\" << indices[img_idx] + 1 << std::endl;\n // 11.1- Read image and resize it if necessary\n full_img = cv::imread(img_names[img_idx]);\n if (abs(scale - 1) > 1e-1)\n resize(full_img, img, cv::Size(), scale, scale);\n else\n img = full_img;\n full_img.release();\n cv::Size img_size = img.size();\n cv::Mat K;\n cameras[img_idx].K().convertTo(K, CV_32F);\n // 11.2- Warp the current image\n warper->warp(img, K, cameras[img_idx].R, INTER_LINEAR, BORDER_REFLECT, img_warped);\n // Warp the current image mask\n mask.create(img_size, CV_8U);\n mask.setTo(cv::Scalar::all(255));\n warper->warp(mask, K, cameras[img_idx].R, cv::INTER_NEAREST, cv::BORDER_CONSTANT,\n mask_warped);\n // 11.3- Compensate exposure error step\n compensator->apply(img_idx, corners[img_idx], img_warped, mask_warped);\n img_warped.convertTo(img_warped_s, CV_16S);\n img_warped.release();\n img.release();\n mask.release();\n dilate(masks_warped[img_idx], dilated_mask, cv::Mat());\n resize(dilated_mask, seam_mask, mask_warped.size());\n\n\n mask_warped = seam_mask & mask_warped;\n // 11.4- Blending images step\n blender->feed(img_warped_s, mask_warped, corners[img_idx]);\n }\n cv::Mat result, result_mask;\n blender->blend(result, result_mask);\n std::cout << \"Compositing, time: \" << ((cv::getTickCount() - t) / cv::getTickFrequency()) <<\n \" sec\" << std::endl;\n imwrite(result_name, result);\n // imshow(result_name,result);\n // waitKey(8000);\n std::cout << \"Finished, total time: \" <<\n ((cv::getTickCount() - start_time) / cv::getTickFrequency()) << \" sec\" << std::endl;\n return 0;\n}"
}
{
"filename": "code/artificial_intelligence/src/image_processing/install_opencv.sh",
"content": "sudo apt-get -y update\nsudo apt-get -y upgrade\nsudo apt-get -y dist-upgrade\nsudo apt-get -y autoremove\n\n\n# INSTALL THE DEPENDENCIES\n\n# Build tools:\nsudo apt-get install -y build-essential cmake\n\n# GUI (if you want to use GTK instead of Qt, replace 'qt5-default' with 'libgtkglext1-dev' and remove '-DWITH_QT=ON' option in CMake):\nsudo apt-get install -y qt5-default libvtk6-dev\n\n# Media I/O:\nsudo apt-get install -y zlib1g-dev libjpeg-dev libwebp-dev libpng-dev libtiff5-dev libjasper-dev libopenexr-dev libgdal-dev\n\n# Video I/O:\nsudo apt-get install -y libdc1394-22-dev libavcodec-dev libavformat-dev libswscale-dev libtheora-dev libvorbis-dev libxvidcore-dev libx264-dev yasm libopencore-amrnb-dev libopencore-amrwb-dev libv4l-dev libxine2-dev\n\n# Parallelism and linear algebra libraries:\nsudo apt-get install -y libtbb-dev libeigen3-dev\n\n# Python:\nsudo apt-get install -y python-dev python-tk python-numpy python3-dev python3-tk python3-numpy\n\n# Java:\nsudo apt-get install -y ant default-jdk\n\n# Documentation:\nsudo apt-get install -y doxygen\n\n\n# INSTALL THE LIBRARY (YOU CAN CHANGE '2.4.13' FOR THE LAST STABLE VERSION)\n\nsudo apt-get install -y unzip wget\nwget https://github.com/opencv/opencv/archive/2.4.13.zip\nunzip 2.4.13.zip\nrm 2.4.13.zip\nmv opencv-2.4.13 OpenCV\ncd OpenCV\nmkdir build\ncd build\ncmake -DWITH_QT=ON -DWITH_OPENGL=ON -DFORCE_VTK=ON -DWITH_TBB=ON -DWITH_GDAL=ON -DWITH_XINE=ON -DBUILD_EXAMPLES=ON ..\nmake -j4\nsudo make install\nsudo ldconfig"
}
{
"filename": "code/artificial_intelligence/src/image_processing/prewittfilter/prewitt.cpp",
"content": "#include <iostream>\n#include <opencv2/imgproc/imgproc.hpp>\n#include <opencv2/highgui/highgui.hpp>\n\n\n\n\n// Computes the x component of the gradient vector\n// at a given point in a image.\n// returns gradient in the x direction\nint xGradient(cv::Mat image, int x, int y)\n{\n return image.at<uchar>(y - 1, x - 1)\n + image.at<uchar>(y, x - 1)\n + image.at<uchar>(y + 1, x - 1)\n - image.at<uchar>(y - 1, x + 1)\n - image.at<uchar>(y, x + 1)\n - image.at<uchar>(y + 1, x + 1);\n}\n\n// Computes the y component of the gradient vector\n// at a given point in a image\n// returns gradient in the y direction\n\nint yGradient(cv::Mat image, int x, int y)\n{\n return image.at<uchar>(y - 1, x - 1)\n + image.at<uchar>(y - 1, x)\n + image.at<uchar>(y - 1, x + 1)\n - image.at<uchar>(y + 1, x - 1)\n - image.at<uchar>(y + 1, x)\n - image.at<uchar>(y + 1, x + 1);\n}\n\nint main()\n{\n\n cv::Mat src, dst;\n int gx, gy, sum;\n\n // Load an image\n src = cv::imread(\"lena.jpg\", CV_LOAD_IMAGE_GRAYSCALE);\n dst = src.clone();\n if (!src.data)\n return -1;\n\n for (int y = 0; y < src.rows; y++)\n for (int x = 0; x < src.cols; x++)\n dst.at<uchar>(y, x) = 0.0;\n\n for (int y = 1; y < src.rows - 1; y++)\n for (int x = 1; x < src.cols - 1; x++)\n {\n gx = xGradient(src, x, y);\n gy = yGradient(src, x, y);\n sum = abs(gx) + abs(gy);\n sum = sum > 255 ? 255 : sum;\n sum = sum < 0 ? 0 : sum;\n dst.at<uchar>(y, x) = sum;\n }\n\n cv::namedWindow(\"final\");\n cv::imshow(\"final\", dst);\n\n cv::namedWindow(\"initial\");\n cv::imshow(\"initial\", src);\n\n cv::waitKey();\n\n\n return 0;\n}"
}
{
"filename": "code/artificial_intelligence/src/image_processing/README.md",
"content": "# OpenCv\nopencv in c++\nopencv in py\n\n# Install\n`sudo apt-get install libopencv-dev python-opencv`\n\n#Run \n```bash\n$ g++ -o out_file file.cpp `pkg-config opencv --cflags --libs`\n```"
}
{
"filename": "code/artificial_intelligence/src/image_processing/sobelfilter/sobel.cpp",
"content": "#include <iostream>\n#include <opencv2/imgproc/imgproc.hpp>\n#include <opencv2/highgui/highgui.hpp>\n\n\n\n// Computes the x component of the gradient vector\n// at a given point in a image.\n// returns gradient in the x direction\nint xGradient(cv::Mat image, int x, int y)\n{\n return image.at<uchar>(y - 1, x - 1)\n + 2 * image.at<uchar>(y, x - 1)\n + image.at<uchar>(y + 1, x - 1)\n - image.at<uchar>(y - 1, x + 1)\n - 2 * image.at<uchar>(y, x + 1)\n - image.at<uchar>(y + 1, x + 1);\n}\n\n// Computes the y component of the gradient vector\n// at a given point in a image\n// returns gradient in the y direction\n\nint yGradient(cv::Mat image, int x, int y)\n{\n return image.at<uchar>(y - 1, x - 1)\n + 2 * image.at<uchar>(y - 1, x)\n + image.at<uchar>(y - 1, x + 1)\n - image.at<uchar>(y + 1, x - 1)\n - 2 * image.at<uchar>(y + 1, x)\n - image.at<uchar>(y + 1, x + 1);\n}\n\nint main()\n{\n\n cv::Mat src, dst;\n int gx, gy, sum;\n\n // Load an image\n src = cv::imread(\"lena.jpg\", CV_LOAD_IMAGE_GRAYSCALE);\n dst = src.clone();\n if (!src.data)\n return -1;\n\n for (int y = 0; y < src.rows; y++)\n for (int x = 0; x < src.cols; x++)\n dst.at<uchar>(y, x) = 0.0;\n\n for (int y = 1; y < src.rows - 1; y++)\n for (int x = 1; x < src.cols - 1; x++)\n {\n gx = xGradient(src, x, y);\n gy = yGradient(src, x, y);\n sum = abs(gx) + abs(gy);\n sum = sum > 255 ? 255 : sum;\n sum = sum < 0 ? 0 : sum;\n dst.at<uchar>(y, x) = sum;\n }\n\n cv::namedWindow(\"final\");\n cv::imshow(\"final\", dst);\n\n cv::namedWindow(\"initial\");\n cv::imshow(\"initial\", src);\n\n cv::waitKey();\n\n\n return 0;\n}"
}
{
"filename": "code/artificial_intelligence/src/Inception_Pre-trained_Model/Inception_model.md",
"content": "The architecture of the Inception model is heavily engineered and quite complex to understand as it is huge both in terms of depth and width. \n\nThe visual representations below make it simpler to understand the entire architecture and thus make it easier to understand the subsequent versions \n\nLearn more: https://iq.opengenus.org/inception-pre-trained-cnn-model/"
}
{
"filename": "code/artificial_intelligence/src/Inception_Pre-trained_Model/README.md",
"content": "This article at opengenus about the Inception Pre-Trained cnn model gives an entire overview of the following :\n\n* Inception model\n* It's architecture\n* The various versions of this model\n* Their evolution history\n\nThe Inception model , introduced in 2014, is a deep CNN architecture. It has also won the ImageNet Large-Scale Visual Recognition Challenge (ILSVRC14). These networks are useful in computer vision and tasks where convolutional filters are used.\n\nHeretofore, inception has evolved through its four versions namely, **Inception v1** ,**Inception v2 and v3**, **Inception v4 and Resnet**.\nThese versions have showed iterative improvement over each other which has helped in pushing its speed and accuracy.\n\nAll of these, along with their architectures, have been discussed in detail in the above article."
}
{
"filename": "code/artificial_intelligence/src/isodata_clustering/isodata.py",
"content": "# Part of Cosmos by OpenGenus Foundation\nfrom math import sqrt\n\n# measure distance between two points\ndef distance_2point(x1, y1, x2, y2):\n return sqrt((x2 - x1) ** 2 + (y2 - y1) ** 2)\n\n\n# estimate volume of the cluster\ndef volume_estimation(cluster, center):\n num_of_points = len(cluster)\n distance = []\n for i in range(num_of_points):\n distance.append(\n distance_2point(center[0], center[1], cluster[i][0], cluster[i][1])\n )\n\n return sum(distance) / num_of_points\n\n\n# defining of new cluster center\ndef new_cluster_centers(cluster):\n s = list(map(sum, zip(*cluster)))\n length = len(cluster)\n return (s[0] / length, s[1] / length)\n\n\n# measure distances between each two pairs of cluster centers\ndef center_distance(centers):\n D_ij = {}\n # offset coeficient\n k = 0\n for i in range(len(centers)):\n for j in range(k, len(centers)):\n if i == j:\n pass\n else:\n D_ij[(i, j)] = distance_2point(\n centers[i][0], centers[i][1], centers[j][0], centers[j][1]\n )\n k += 1\n return D_ij\n\n\n# standart deviation vector for cluster\ndef standart_deviation(values, center):\n n = len(values)\n x_coord = []\n y_coord = []\n for i in range(n):\n x_coord.append((values[i][0] - center[0]) ** 2)\n y_coord.append((values[i][1] - center[1]) ** 2)\n\n x = sqrt(sum(x_coord) / n)\n y = sqrt(sum(y_coord) / n)\n\n return (x, y)\n\n\ndef cluster_points_distribution(centers, points):\n centers_len = len(centers)\n points_len = len(points)\n distances = []\n distance = []\n\n # define array for clusters\n clusters = [[] for i in range(centers_len)]\n\n # iteration throught all points\n for i in range(points_len):\n # iteration throught all centers\n for j in range(centers_len):\n distance.append(\n distance_2point(\n centers[j][0], centers[j][1], points[i][0], points[i][1]\n )\n )\n distances.append(distance)\n distance = []\n\n # distribution\n for i in range(points_len):\n ind = distances[i].index(min(distances[i]))\n clusters[ind].append(points[i])\n\n return clusters\n\n\ndef cluster_division(cluster, center, dev_vector):\n # divide only center of clusters\n\n # coeficient\n k = 0.5\n\n max_deviation = max(dev_vector)\n index = dev_vector.index(max(dev_vector))\n g = k * max_deviation\n\n # defining new centers\n center1 = list(center)\n center2 = list(center)\n center1[index] += g\n center2[index] -= g\n\n cluster1 = []\n cluster2 = []\n\n return tuple(center1), tuple(center2)\n\n\ndef cluster_union(cluster1, cluster2, center1, center2):\n x1 = center1[0]\n x2 = center2[0]\n y1 = center1[1]\n y2 = center2[1]\n n1 = len(cluster1)\n n2 = len(cluster2)\n\n x = (n1 * x1 + n2 * x2) / (n1 + n2)\n y = (n1 * y1 + n2 * y2) / (n1 + n2)\n center = (x, y)\n cluster = cluster1 + cluster2\n\n return center, cluster\n\n\ndef clusterize():\n # initial values\n K = 4 # max cluster number\n THETA_N = 1 # for cluster elimination\n THETA_S = 1 # for cluster division\n THETA_C = 3 # for cluster union\n L = 3 #\n I = 4 # max number of iterations\n N_c = 1 # number of primary cluster centers\n\n distance = [] # distances array\n centers = [] # clusters centers\n clusters = [] # array for clusters points\n iteration = 1 # number of current iteration\n\n centers.append(points[0]) # first cluster center\n\n while iteration <= I:\n # print (\"Iteration \", iteration)\n # step 2\n\n \"\"\"\n if there are one cluster center - all points goes to first cluster\n otherwise we distribute points between clusters\n \"\"\"\n if len(centers) <= 1:\n clusters.append(points)\n else:\n clusters = cluster_points_distribution(centers, points)\n\n # step 3\n # eliminating small clusters (unfinished!!!!!!)\n \"\"\"\n for i in range(len(clusters)):\n\n if len(clusters[i]) <= THETA_N:\n print(clusters[i][i])\n item = clusters[i][i]\n points.remove(item)\n #del clusters[i]\n break\n else:\n print(\"else\")\n break\t\n \"\"\"\n\n # step 4\n # erasing existing centers and defining a new ones\n centers = []\n for i in range(len(clusters)):\n centers.append(new_cluster_centers(clusters[i]))\n\n # step 5 - estimating volumes of all clusters\n # array for clusters volume\n D_vol = []\n for i in range(len(centers)):\n D_vol.append(volume_estimation(clusters[i], centers[i]))\n\n # step 6\n if len(clusters) <= 1:\n D = 0\n else:\n cluster_length = []\n vol_sum = []\n for i in range(len(centers)):\n cluster_length.append(len(clusters[i]))\n vol_sum.append(cluster_length[i] * D_vol[i])\n\n D = sum(vol_sum) / len(points)\n\n # step 7\n if iteration >= I:\n THETA_C = 0\n\n elif (N_c >= 2 * K) or (iteration % 2 == 0):\n pass\n\n else:\n # step 8\n # vectors of all clusters standart deviation\n vectors = []\n for i in range(len(centers)):\n vectors.append(standart_deviation(clusters[i], centers[i]))\n\n # step 9\n max_s = []\n for v in vectors:\n max_s.append(max(v[0], v[1]))\n\n # step 10 (cluster division)\n for i in range(len(max_s)):\n length = len(clusters[i])\n coef = 2 * (THETA_N + 1)\n\n if (max_s[i] > THETA_S) and (\n (D_vol[i] > D and length > coef) or N_c < float(K) / 2\n ):\n center1, center2 = cluster_division(\n clusters[i], centers[i], vectors[i]\n )\n del centers[i]\n centers.append(center1)\n centers.append(center2)\n N_c += 1\n\n else:\n pass\n\n # for i in clusters:\n # \tprint(i)\n\n # step 11\n D_ij = center_distance(centers)\n rang = {}\n for coord in D_ij:\n if D_ij[coord] < THETA_C:\n rang[coord] = D_ij[coord]\n else:\n pass\n\n \"\"\"\n # step 13 (cluster union)\n for key in rang.keys():\n cluster_union(clusters[key], clusters[key.next()], centers[key], centers[key.next()])\n N_c -= 1\n\n \"\"\"\n\n iteration += 1\n\n return clusters\n\n\nif __name__ == \"__main__\":\n # if file called as a script\n # cluster points\n points1 = [\n (2, 5),\n (2, 4),\n (2, 3),\n (8, 8),\n (7, 7),\n (6, 6),\n (-5, -4),\n (-6, -4),\n (-9, -4),\n (5, -6),\n (6, -5),\n (44, 49),\n (45, 50),\n ]\n points2 = [\n (-0.99, 1.09),\n (-0.98, 1.08),\n (-0.97, 1.07),\n (-0.96, 1.06),\n (-0.95, 1.05),\n (-0.94, 1.04),\n (-0.93, 1.04),\n (0.94, 1.04),\n (0.95, 1.05),\n (0.96, 1.06),\n (0.97, 1.07),\n (0.98, 1.08),\n (0.99, 1.09),\n ]\n points3 = [\n (-99, 109),\n (-98, 108),\n (-97, 107),\n (-96, 106),\n (-95, 105),\n (-94, 104),\n (-93, 104),\n (94, 104),\n (95, 105),\n (96, 106),\n (97, 107),\n (98, 108),\n (99, 109),\n ]\n points = points3\n cl = clusterize()\n for i in cl:\n print(\"Cl\", i)"
}
{
"filename": "code/artificial_intelligence/src/isodata_clustering/readme.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# ISODATA Clustering\nISODATA stands for The Iterative Self-Organizing Data Analysis Technique algorithm used for Multispectral pattern recognition was developed by Geoffrey H. Ball and David J. Hall, working in the Stanford Research Institute in Menlo Park, CA.\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/artificial_intelligence/src/k_means/k-means.c",
"content": "// Part of Cosmos by OpenGenus\n#include<stdio.h>\n#include<conio.h>\nint main()\n{\n int i,j,k,t1,t2;\n int a0[10];\n int a1[10];\n int a2[10];\n \n printf(\"\\nEnter 10 numbers:\\n\");\n for(i=0;i<10;i++)\n {\n scanf(\"%d\",&a0[i]);\n }\n \n //initial means\n int m1;\n int m2;\n printf(\"\\nEnter first mean: \");\n scanf(\"%d\",&m1);\n printf(\"\\nEnter second mean: \");\n scanf(\"%d\",&m2);\n \n int om1,om2; //previous means\n \n do\n {\n om1=m1;\n om2=m2;\n \n //creating clusters\n i=j=k=0;\n for(i=0;i<10;i++)\n {\n //calculating distance to means\n t1=a0[i]-m1;\n if(t1<0){t1=-t1;}\n t2=a0[i]-m2;\n if(t2<0){t2=-t2;}\n if(t1<t2)\n {\n //near to first mean\n a1[j]=a0[i];\n j++;\n }\n else\n {\n //near to second mean\n a2[k]=a0[i];\n k++;\n }\n }\n \n t2=0;\n //calculating new mean\n for(t1=0;t1<j;t1++)\n {\n t2=t2+a1[t1];\n }\n m1=t2/k;\n \n t2=0;\n for(t1=0;t1<k;t1++)\n {\n t2=t2+a2[t1];\n }\n m2=t2/k;\n \n //printing clusters\n printf(\"\\nCluster 1:\\n__________\\n\");\n for(t1=0;t1<j;t1++)\n {\n printf(\"%d \",a1[t1]);\n }\n printf(\"\\nm1= %d\",m1);\n \n printf(\"\\nCluster 2:\\n___________\\n\");\n for(t1=0;t1<k;t1++)\n {\n printf(\"%d \",a2[t1]);\n }\n printf(\"\\nm2= %d\",m2);\n }while(m1!=om1&&m2!=om2);\n getch();\n}"
}
{
"filename": "code/artificial_intelligence/src/k_means/k_means.cpp",
"content": "/*\n * Kmeans written in C++ for use on Images.\n * Requires several data structures from the user context\n *\n * The Image is represented by ByteImage\n * A point datastructure must exist with values, x, y, I (value from 0 to 255)\n *\n * main not included. pulled out of a larger project. Please add one, refine out ByteImage should be simple. :)\n *\n */\n#include <iostream> // Used for debugging & learning code\n#include <algorithm> // Use for sorting\n#include <cstdio>\n#include <cstdlib>\n#include <cmath>\n#include <cstring>\n#include <ctime>\n#include <vector>\n\n#define PI 4 * atan(1.0)\n\n// Point structure\nstruct point\n{\n int x;\n int y;\n int I;\n int radx;\n int rady;\n int area;\n double avg;\n double r;\n};\n\n// Kmeans with weight option\ntemplate<typename ByteImage, typename byte>\ndouble kmeans(ByteImage& bimg, const int K, double weight)\n{\n\n // Create k random points (centers)\n point * centers = new point[K];\n\n // Totals for updating\n point * totals = new point[K];\n\n // Initialize k points\n for (int k = 0; k < K; k++)\n {\n // Initiate centers\n centers[k].x = rand() % bimg.nr;\n centers[k].y = rand() % bimg.nc;\n centers[k].I = rand() % 256;\n\n // Initiate sumation for average\n totals[k].x = 0;\n totals[k].y = 0;\n totals[k].I = 0;\n totals[k].area = 0;\n }\n // Create label map\n byte * label = new byte[bimg.nr * bimg.nc];\n double shortestDistance, presentDistance;\n double error = bimg.nr * bimg.nr + bimg.nc * bimg.nc + 255 * 255, old_error;\n\n // Weights maybe? Set these to one for original kmeans.\n double wi = 1, wj = 1; //255.0*255/(double)bimg.nr/bimg.nr, wj = 255.0*255/(double)bimg.nc/bimg.nc;\n int closestK = 0;\n\n do {\n\n // Update previous error\n old_error = error;\n error = 0;\n // A. For each point in space, find distance to each center, assign to min center (map colors)\n for (int i = 0; i < bimg.nr; i++)\n for (int j = 0; j < bimg.nc; j++)\n {\n // Initialize shortestDistance to max possible.\n shortestDistance = sqrt(bimg.nr * bimg.nr + bimg.nc * bimg.nc + 255 * 255);\n // Check distance to each center\n for (int k = 0; k < K; k++)\n {\n // Calculate the distance\n presentDistance = sqrt((double)wi * (centers[k].x - i) * (centers[k].x - i) +\n (double)wj * (centers[k].y - j) * (centers[k].y - j) +\n (double)weight *\n (centers[k].I - bimg.image[i * bimg.nc + j]) *\n (centers[k].I - bimg.image[i * bimg.nc + j]));\n\n // Update shortest distance\n if (presentDistance < shortestDistance)\n {\n shortestDistance = presentDistance;\n closestK = k;\n label[i * bimg.nc + j] = (255.0 / K) * k;\n }\n }\n\n // Update error\n error += shortestDistance;\n\n // Update totals to compute new centroid\n totals[closestK].x += i;\n totals[closestK].y += j;\n totals[closestK].I += bimg.image[i * bimg.nc + j];\n totals[closestK].area += 1;\n }\n error = error / bimg.nr / bimg.nc;\n // Update centers\n for (int k = 0; k < K; k++)\n {\n // Compute new centers\n if (totals[k].area > 0)\n {\n centers[k].x = totals[k].x / totals[k].area;\n centers[k].y = totals[k].y / totals[k].area;\n centers[k].I = totals[k].I / totals[k].area;\n }\n\n // Reset totals\n totals[k].x = 0;\n totals[k].y = 0;\n totals[k].I = 0;\n totals[k].area = 0;\n }\n\n // B. Repeat A until centers do not move\n } while (error != old_error);\n\n // Extra credit code to find and \"average\" distance between the nodes, which must be less than user input\n std::cout << \"K=\" << K << std::endl;\n\n // Make label image\n bimg.image = label;\n delete [] totals;\n delete [] centers;\n\n std::cout << shortestDistance << std::endl;\n return shortestDistance;\n}"
}
{
"filename": "code/artificial_intelligence/src/k_means/k_means.py",
"content": "from random import random\n\n# sets and datas\nsets = [random() for i in range(10)]\ndata = [random() for i in range(1000)]\n\nparameter = 0.01 # This parameter change the mean changing speed must be Min: 0 Max: 1\n\nfor x in data: # O(data count)\n nearest_k = 0\n mininmum_error = 9999\n # At normal condition it must goes to infinite.\n for k in enumerate(sets): # O(mean count)\n error = abs(x - k[1])\n if error < mininmum_error:\n mininmum_error = error\n nearest_k = k[0]\n sets[nearest_k] = sets[nearest_k] * (1 - parameter) + x * (parameter)\n\nprint(sets)\n\n# Algorithmic Anlayze-->O(data*means) it means Data count = K and Set count = J // O(K.J) \\\\"
}
{
"filename": "code/artificial_intelligence/src/k_means/k_means.swift",
"content": "import Foundation\n\nclass KMeans<Label: Hashable> {\n let numCenters: Int\n let labels: [Label]\n private(set) var centroids = [Vector]()\n\n init(labels: [Label]) {\n assert(labels.count > 1, \"Exception: KMeans with less than 2 centers.\")\n self.labels = labels\n self.numCenters = labels.count\n }\n\n private func indexOfNearestCenter(_ x: Vector, centers: [Vector]) -> Int {\n var nearestDist = DBL_MAX\n var minIndex = 0\n\n for (idx, center) in centers.enumerated() {\n let dist = x.distanceTo(center)\n if dist < nearestDist {\n minIndex = idx\n nearestDist = dist\n }\n }\n return minIndex\n }\n\n func trainCenters(_ points: [Vector], convergeDistance: Double) {\n let zeroVector = Vector([Double](repeating: 0, count: points[0].length))\n\n var centers = reservoirSample(points, k: numCenters)\n\n var centerMoveDist = 0.0\n repeat {\n var classification: [[Vector]] = .init(repeating: [], count: numCenters)\n\n for p in points {\n let classIndex = indexOfNearestCenter(p, centers: centers)\n classification[classIndex].append(p)\n }\n\nlet newCenters = classification.map { assignedPoints in\n assignedPoints.reduce(zeroVector, +) / Double(assignedPoints.count)\n }\n\n centerMoveDist = 0.0\n for idx in 0..<numCenters {\n centerMoveDist += centers[idx].distanceTo(newCenters[idx])\n }\n\n centers = newCenters\n } while centerMoveDist > convergeDistance\n\n centroids = centers\n }\n\n func fit(_ point: Vector) -> Label {\n assert(!centroids.isEmpty, \"Exception: KMeans tried to fit on a non trained model.\")\n\n let centroidIndex = indexOfNearestCenter(point, centers: centroids)\n return labels[centroidIndex]\n }\n\n func fit(_ points: [Vector]) -> [Label] {\n assert(!centroids.isEmpty, \"Exception: KMeans tried to fit on a non trained model.\")\n\n return points.map(fit)\n }\n}\n\nfunc reservoirSample<T>(_ samples: [T], k: Int) -> [T] {\n var result = [T]()\n\n for i in 0..<k {\n result.append(samples[i])\n }\n\n for i in k..<samples.count {\n let j = Int(arc4random_uniform(UInt32(i + 1)))\n if j < k {\n result[j] = samples[i]\n }\n }\n return result\n}"
}
{
"filename": "code/artificial_intelligence/src/k_means/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# k Means\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/artificial_intelligence/src/k_nearest_neighbours/iris.data",
"content": "5.1,3.5,1.4,0.2,Iris-setosa\n4.9,3.0,1.4,0.2,Iris-setosa\n4.7,3.2,1.3,0.2,Iris-setosa\n4.6,3.1,1.5,0.2,Iris-setosa\n5.0,3.6,1.4,0.2,Iris-setosa\n5.4,3.9,1.7,0.4,Iris-setosa\n4.6,3.4,1.4,0.3,Iris-setosa\n5.0,3.4,1.5,0.2,Iris-setosa\n4.4,2.9,1.4,0.2,Iris-setosa\n4.9,3.1,1.5,0.1,Iris-setosa\n5.4,3.7,1.5,0.2,Iris-setosa\n4.8,3.4,1.6,0.2,Iris-setosa\n4.8,3.0,1.4,0.1,Iris-setosa\n4.3,3.0,1.1,0.1,Iris-setosa\n5.8,4.0,1.2,0.2,Iris-setosa\n5.7,4.4,1.5,0.4,Iris-setosa\n5.4,3.9,1.3,0.4,Iris-setosa\n5.1,3.5,1.4,0.3,Iris-setosa\n5.7,3.8,1.7,0.3,Iris-setosa\n5.1,3.8,1.5,0.3,Iris-setosa\n5.4,3.4,1.7,0.2,Iris-setosa\n5.1,3.7,1.5,0.4,Iris-setosa\n4.6,3.6,1.0,0.2,Iris-setosa\n5.1,3.3,1.7,0.5,Iris-setosa\n4.8,3.4,1.9,0.2,Iris-setosa\n5.0,3.0,1.6,0.2,Iris-setosa\n5.0,3.4,1.6,0.4,Iris-setosa\n5.2,3.5,1.5,0.2,Iris-setosa\n5.2,3.4,1.4,0.2,Iris-setosa\n4.7,3.2,1.6,0.2,Iris-setosa\n4.8,3.1,1.6,0.2,Iris-setosa\n5.4,3.4,1.5,0.4,Iris-setosa\n5.2,4.1,1.5,0.1,Iris-setosa\n5.5,4.2,1.4,0.2,Iris-setosa\n4.9,3.1,1.5,0.1,Iris-setosa\n5.0,3.2,1.2,0.2,Iris-setosa\n5.5,3.5,1.3,0.2,Iris-setosa\n4.9,3.1,1.5,0.1,Iris-setosa\n4.4,3.0,1.3,0.2,Iris-setosa\n5.1,3.4,1.5,0.2,Iris-setosa\n5.0,3.5,1.3,0.3,Iris-setosa\n4.5,2.3,1.3,0.3,Iris-setosa\n4.4,3.2,1.3,0.2,Iris-setosa\n5.0,3.5,1.6,0.6,Iris-setosa\n5.1,3.8,1.9,0.4,Iris-setosa\n4.8,3.0,1.4,0.3,Iris-setosa\n5.1,3.8,1.6,0.2,Iris-setosa\n4.6,3.2,1.4,0.2,Iris-setosa\n5.3,3.7,1.5,0.2,Iris-setosa\n5.0,3.3,1.4,0.2,Iris-setosa\n7.0,3.2,4.7,1.4,Iris-versicolor\n6.4,3.2,4.5,1.5,Iris-versicolor\n6.9,3.1,4.9,1.5,Iris-versicolor\n5.5,2.3,4.0,1.3,Iris-versicolor\n6.5,2.8,4.6,1.5,Iris-versicolor\n5.7,2.8,4.5,1.3,Iris-versicolor\n6.3,3.3,4.7,1.6,Iris-versicolor\n4.9,2.4,3.3,1.0,Iris-versicolor\n6.6,2.9,4.6,1.3,Iris-versicolor\n5.2,2.7,3.9,1.4,Iris-versicolor\n5.0,2.0,3.5,1.0,Iris-versicolor\n5.9,3.0,4.2,1.5,Iris-versicolor\n6.0,2.2,4.0,1.0,Iris-versicolor\n6.1,2.9,4.7,1.4,Iris-versicolor\n5.6,2.9,3.6,1.3,Iris-versicolor\n6.7,3.1,4.4,1.4,Iris-versicolor\n5.6,3.0,4.5,1.5,Iris-versicolor\n5.8,2.7,4.1,1.0,Iris-versicolor\n6.2,2.2,4.5,1.5,Iris-versicolor\n5.6,2.5,3.9,1.1,Iris-versicolor\n5.9,3.2,4.8,1.8,Iris-versicolor\n6.1,2.8,4.0,1.3,Iris-versicolor\n6.3,2.5,4.9,1.5,Iris-versicolor\n6.1,2.8,4.7,1.2,Iris-versicolor\n6.4,2.9,4.3,1.3,Iris-versicolor\n6.6,3.0,4.4,1.4,Iris-versicolor\n6.8,2.8,4.8,1.4,Iris-versicolor\n6.7,3.0,5.0,1.7,Iris-versicolor\n6.0,2.9,4.5,1.5,Iris-versicolor\n5.7,2.6,3.5,1.0,Iris-versicolor\n5.5,2.4,3.8,1.1,Iris-versicolor\n5.5,2.4,3.7,1.0,Iris-versicolor\n5.8,2.7,3.9,1.2,Iris-versicolor\n6.0,2.7,5.1,1.6,Iris-versicolor\n5.4,3.0,4.5,1.5,Iris-versicolor\n6.0,3.4,4.5,1.6,Iris-versicolor\n6.7,3.1,4.7,1.5,Iris-versicolor\n6.3,2.3,4.4,1.3,Iris-versicolor\n5.6,3.0,4.1,1.3,Iris-versicolor\n5.5,2.5,4.0,1.3,Iris-versicolor\n5.5,2.6,4.4,1.2,Iris-versicolor\n6.1,3.0,4.6,1.4,Iris-versicolor\n5.8,2.6,4.0,1.2,Iris-versicolor\n5.0,2.3,3.3,1.0,Iris-versicolor\n5.6,2.7,4.2,1.3,Iris-versicolor\n5.7,3.0,4.2,1.2,Iris-versicolor\n5.7,2.9,4.2,1.3,Iris-versicolor\n6.2,2.9,4.3,1.3,Iris-versicolor\n5.1,2.5,3.0,1.1,Iris-versicolor\n5.7,2.8,4.1,1.3,Iris-versicolor\n6.3,3.3,6.0,2.5,Iris-virginica\n5.8,2.7,5.1,1.9,Iris-virginica\n7.1,3.0,5.9,2.1,Iris-virginica\n6.3,2.9,5.6,1.8,Iris-virginica\n6.5,3.0,5.8,2.2,Iris-virginica\n7.6,3.0,6.6,2.1,Iris-virginica\n4.9,2.5,4.5,1.7,Iris-virginica\n7.3,2.9,6.3,1.8,Iris-virginica\n6.7,2.5,5.8,1.8,Iris-virginica\n7.2,3.6,6.1,2.5,Iris-virginica\n6.5,3.2,5.1,2.0,Iris-virginica\n6.4,2.7,5.3,1.9,Iris-virginica\n6.8,3.0,5.5,2.1,Iris-virginica\n5.7,2.5,5.0,2.0,Iris-virginica\n5.8,2.8,5.1,2.4,Iris-virginica\n6.4,3.2,5.3,2.3,Iris-virginica\n6.5,3.0,5.5,1.8,Iris-virginica\n7.7,3.8,6.7,2.2,Iris-virginica\n7.7,2.6,6.9,2.3,Iris-virginica\n6.0,2.2,5.0,1.5,Iris-virginica\n6.9,3.2,5.7,2.3,Iris-virginica\n5.6,2.8,4.9,2.0,Iris-virginica\n7.7,2.8,6.7,2.0,Iris-virginica\n6.3,2.7,4.9,1.8,Iris-virginica\n6.7,3.3,5.7,2.1,Iris-virginica\n7.2,3.2,6.0,1.8,Iris-virginica\n6.2,2.8,4.8,1.8,Iris-virginica\n6.1,3.0,4.9,1.8,Iris-virginica\n6.4,2.8,5.6,2.1,Iris-virginica\n7.2,3.0,5.8,1.6,Iris-virginica\n7.4,2.8,6.1,1.9,Iris-virginica\n7.9,3.8,6.4,2.0,Iris-virginica\n6.4,2.8,5.6,2.2,Iris-virginica\n6.3,2.8,5.1,1.5,Iris-virginica\n6.1,2.6,5.6,1.4,Iris-virginica\n7.7,3.0,6.1,2.3,Iris-virginica\n6.3,3.4,5.6,2.4,Iris-virginica\n6.4,3.1,5.5,1.8,Iris-virginica\n6.0,3.0,4.8,1.8,Iris-virginica\n6.9,3.1,5.4,2.1,Iris-virginica\n6.7,3.1,5.6,2.4,Iris-virginica\n6.9,3.1,5.1,2.3,Iris-virginica\n5.8,2.7,5.1,1.9,Iris-virginica\n6.8,3.2,5.9,2.3,Iris-virginica\n6.7,3.3,5.7,2.5,Iris-virginica\n6.7,3.0,5.2,2.3,Iris-virginica\n6.3,2.5,5.0,1.9,Iris-virginica\n6.5,3.0,5.2,2.0,Iris-virginica\n6.2,3.4,5.4,2.3,Iris-virginica\n5.9,3.0,5.1,1.8,Iris-virginica"
}
{
"filename": "code/artificial_intelligence/src/k_nearest_neighbours/k_nearest_neighbours.cpp",
"content": "// Part of Cosmos by OpenGenus\n#include <cmath>\n#include <iomanip>\n#include <iostream>\n#include <vector>\n\nstruct Point {\n float w, x, y, z; // w, x, y and z are the values of the dataset\n float distance; // distance will store the distance of dataset point from\n // the unknown test point\n int op; // op will store the class of the point\n};\n\nint main() {\n int n, k;\n Point p;\n std::cout << \"Number of data points: \";\n std::cin >> n;\n std::vector<Point> vec;\n std::cout << \"\\nEnter the dataset values: \\n\";\n std::cout << \"w\\tx\\ty\\tz\\top\\n\";\n\n // Taking dataset\n for (int i = 0; i < n; ++i) {\n float wCoordinate, xCoordinate, yCoordinate, zCoordinate;\n int opCoordinate;\n std::cin >> wCoordinate >> xCoordinate >> yCoordinate >> zCoordinate >>\n opCoordinate;\n vec.push_back(Point());\n vec[i].w = wCoordinate;\n vec[i].x = xCoordinate;\n vec[i].y = yCoordinate;\n vec[i].z = zCoordinate;\n vec[i].op = opCoordinate;\n }\n\n std::cout << \"\\nw\\tx\\ty\\tz\\top\\n\";\n\n for (int i = 0; i < n; ++i) {\n std::cout << std::fixed << std::setprecision(2) << vec[i].w << '\\t'\n << vec[i].x << '\\t' << vec[i].y << '\\t' << vec[i].z << '\\t'\n << vec[i].op << '\\n';\n }\n\n std::cout\n << \"\\nEnter the feature values of the unknown point: \\nw\\tx\\ty\\tz\\n\";\n std::cin >> p.w >> p.x >> p.y >> p.z;\n\n float euclideanDistance(Point p, Point v);\n for (int i = 0; i < n; ++i) {\n vec[i].distance = euclideanDistance(p, vec[i]);\n }\n\n // Sorting the training data with respect to distance\n for (int i = 1; i < n; ++i) {\n for (int j = 0; j < n - i; ++j) {\n if (vec[j].distance > vec[j + 1].distance) {\n Point temp;\n temp = vec[j];\n vec[j] = vec[j + 1];\n vec[j + 1] = temp;\n }\n }\n }\n\n std::cout << \"\\nw\\tx\\ty\\tz\\top\\tdistance\\n\";\n for (int i = 0; i < n; ++i) {\n std::cout << std::fixed << std::setprecision(2) << vec[i].w << '\\t'\n << vec[i].x << '\\t' << vec[i].y << '\\t' << vec[i].z << '\\t'\n << vec[i].op << '\\t' << vec[i].distance << '\\n';\n }\n\n // Taking the K nearest neighbors\n std::cout << \"\\nNumber of nearest neighbors(k): \";\n std::cin >> k;\n\n int freq[1000] = {0};\n int index = 0, maxfreq = 0;\n\n // Creating frequency array of the class of k nearest neighbors\n for (int i = 0; i < k; ++i) {\n freq[vec[i].op]++;\n }\n\n // Finding the most frequent occurring class\n for (int i = 0; i < 1000; ++i) {\n if (freq[i] > maxfreq) {\n maxfreq = freq[i];\n index = i;\n }\n }\n\n std::cout << \"The class of the unknown point is \" << index;\n return 0;\n}\n\n// Measuring the Euclidean distance\nfloat euclideanDistance(Point p, Point v) {\n float result;\n result = sqrt(((v.w - p.w) * (v.w - p.w)) + ((v.x - p.x) * (v.x - p.x)) +\n ((v.y - p.y) * (v.y - p.y)) + ((v.z - p.z) * (v.z - p.z)));\n return result;\n}\n\n// Sample Output\n/*\nNumber of data points: 5\n\nEnter the dataset values:\nw x y z op\n1 2 3 4 5\n10 20 30 40 50\n5 2 40 7 10\n40 30 100 28 3\n20 57 98 46 8\n\nw x y z op\n1.00 2.00 3.00 4.00 5\n10.00 20.00 30.00 40.00 50\n5.00 2.00 40.00 7.00 10\n40.00 30.00 100.00 28.00 3\n20.00 57.00 98.00 46.00 8\n\nEnter the feature values of the unknown point:\nw x y z\n40 30 80 30\n\nw x y z op distance\n40.00 30.00 100.00 28.00 3 20.10\n20.00 57.00 98.00 46.00 8 41.34\n10.00 20.00 30.00 40.00 50 60.00\n5.00 2.00 40.00 7.00 10 64.33\n1.00 2.00 3.00 4.00 5 94.39\n\nNumber of nearest neighbors(k): 3\nThe class of unknown point is 3\n*/"
}
{
"filename": "code/artificial_intelligence/src/k_nearest_neighbours/k_nearest_neighbours.py",
"content": "# k Nearest Neighbours implemented from Scratch in Python\n\nimport csv\nimport random\nimport math\nimport operator\n\n\ndef loadDataset(filename, split, trainingSet=[], testSet=[]):\n with open(filename, \"rt\") as csvfile:\n lines = csv.reader(csvfile)\n dataset = list(lines)\n for x in range(len(dataset) - 1):\n for y in range(4):\n dataset[x][y] = float(dataset[x][y])\n if random.random() < split:\n trainingSet.append(dataset[x])\n else:\n testSet.append(dataset[x])\n\n\ndef euclideanDistance(instance1, instance2, length):\n distance = 0\n for x in range(length):\n distance += pow((instance1[x] - instance2[x]), 2)\n return math.sqrt(distance)\n\n\ndef getNeighbors(trainingSet, testInstance, k):\n distances = []\n length = len(testInstance) - 1\n for x in range(len(trainingSet)):\n dist = euclideanDistance(testInstance, trainingSet[x], length)\n distances.append((trainingSet[x], dist))\n distances.sort(key=operator.itemgetter(1))\n neighbors = []\n for x in range(k):\n neighbors.append(distances[x][0])\n return neighbors\n\n\ndef getResponse(neighbors):\n classVotes = {}\n for x in range(len(neighbors)):\n response = neighbors[x][-1]\n if response in classVotes:\n classVotes[response] += 1\n else:\n classVotes[response] = 1\n sortedVotes = sorted(classVotes.items(), key=operator.itemgetter(1), reverse=True)\n return sortedVotes[0][0]\n\n\ndef getAccuracy(testSet, predictions):\n correct = 0\n for x in range(len(testSet)):\n if testSet[x][-1] == predictions[x]:\n correct += 1\n return (correct / float(len(testSet))) * 100.0\n\n\ndef main():\n # prepare data\n trainingSet = []\n testSet = []\n split = 0.67\n loadDataset(\"iris.data\", split, trainingSet, testSet)\n print(\"Train set: \" + repr(len(trainingSet)))\n print(\"Test set: \" + repr(len(testSet)))\n # generate predictions\n predictions = []\n k = 3\n for x in range(len(testSet)):\n neighbors = getNeighbors(trainingSet, testSet[x], k)\n result = getResponse(neighbors)\n predictions.append(result)\n print(\"> predicted=\" + repr(result) + \", actual=\" + repr(testSet[x][-1]))\n accuracy = getAccuracy(testSet, predictions)\n print(\"Accuracy: \" + repr(accuracy) + \"%\")\n\n\nmain()"
}
{
"filename": "code/artificial_intelligence/src/k_nearest_neighbours/KNN.c",
"content": "// Part of Cosmos by OpenGenus\n//Sample Input and Output:\n//\n//------------------------\n//Number of data points: 5\n//\n//Enter the dataset values:\n//w x y z op\n//1 2 4 3 1\n//1 3 2 1 1\n//8 7 6 9 2\n//7 8 6 9 2\n//6 9 7 5 2\n//w x y z op\n//1.00 2.00 4.00 3.00 1\n//1.00 3.00 2.00 1.00 1\n//8.00 7.00 6.00 9.00 2\n//7.00 8.00 6.00 9.00 2\n//6.00 9.00 7.00 5.00 2\n//\n//Enter the feature values of the unkown point:\n//w x y z\n//8 7 5 9\n//w x y z op distance\n//8.00 7.00 6.00 9.00 2 1.00\n//7.00 8.00 6.00 9.00 2 1.73\n//6.00 9.00 7.00 5.00 2 5.29\n//1.00 2.00 4.00 3.00 1 10.54\n//1.00 3.00 2.00 1.00 1 11.75\n//\n//Number of nearest neighbors(k): 2\n//\n//The class of unknown point is: 2\n//\n//---------Code---------------\n\n#include <stdio.h>\n#include <math.h>\n#include <string.h>\n\nstruct point {\n\tfloat w,x,y,z;\n\tfloat distance;\n\tint op;\t\n};\n\nint main(){\n\tint i,j,n,k;\n\tpoint p;\n\tpoint temp;\n\tprintf(\"Number of data points: \");\n\tscanf(\"%d\",&n);\n\tpoint arr[n];\n\tprintf(\"\\nEnter the dataset values: \\n\");\n\tprintf(\"w\\tx\\ty\\tz\\top\\n\");\n\t\n\t// Taking input data\n\tfor (i=0;i<n;i++){\n\t\tscanf(\"%f%f%f%f%d\",&arr[i].w,&arr[i].x,&arr[i].y,&arr[i].z,&arr[i].op);\t\n\t}\n\tprintf(\"w\\tx\\ty\\tz\\top\\n\");\n\tfor (i=0;i<n;i++){\n\t\tprintf(\"%.2f\\t%.2f\\t%.2f\\t%.2f\\t%d\\n\",arr[i].w,arr[i].x,arr[i].y,arr[i].z,arr[i].op);\n\t}\n\tprintf(\"\\nEnter the feature values of the unkown point: \\nw\\tx\\ty\\tz\\n\");\n\tscanf(\"%f%f%f%f\",&p.w,&p.x,&p.y,&p.z);\n\t\n\t//Measuring the Euclidean distance\n\tfor (i=0;i<n;i++){\n\t\tarr[i].distance=sqrt((arr[i].w - p.w) * (arr[i].w - p.w) + (arr[i].x - p.x) * (arr[i].x - p.x) + (arr[i].y - p.y) * (arr[i].y - p.y) + (arr[i].z - p.z) * (arr[i].z - p.z));\t\n\t}\n\t\n\t//Sorintg the training data w.r.t distance\t\n\tfor (i = 1; i < n; i++){\n\t for (j = 0; j < n - i; j++) {\n\t if (arr[j].distance > arr[j + 1].distance) {\n\t temp = arr[j];\n\t arr[j] = arr[j + 1];\n\t arr[j + 1] = temp;\n\t }\n\t }\n\t}\n\tprintf(\"w\\tx\\ty\\tz\\top\\tdistance\\n\");\n\tfor (i=0;i<n;i++){\n\t\tprintf(\"%.2f\\t%.2f\\t%.2f\\t%.2f\\t%d\\t%.2f\\n\",arr[i].w,arr[i].x,arr[i].y,arr[i].z,arr[i].op,arr[i].distance);\n\t}\n\t\n\t// Taking the K -nearest neighbors\n\tprintf(\"\\nNumber of nearest neighbors(k): \");\n\tscanf(\"%d\",&k);\n\tint freq1 = 0;\t// for class 1\n\tint freq2 = 0; // for class 2 \n\tint freq3 = 0; // for class 3\n\tint none = 0;\t\t\t\n\tfor (int i = 0; i < k; i++){ \n\t if (arr[i].op == 1) \n\t freq1++; \n\t else if (arr[i].op == 2) \n\t freq2++; \n\t else if(arr[i].op==3)\n\t \tfreq3++;\n\t else\n\t \tnone++;\n\t} \n\tif(freq1>= freq2 && freq1 >= freq3){\n\t\tprintf(\"\\nThe class of unknown point is: 1\");\n\t}\n\telse if(freq2>= freq1 && freq2 >= freq3){\n\t\tprintf(\"\\nThe class of unknown point is: 2\");\n\t}\n\telse{\n\t\tprintf(\"\\nThe class of unknown point is: 3\");\t\n\t}\n}"
}
{
"filename": "code/artificial_intelligence/src/lasso_regression/lasso_regression.ipynb",
"content": "{\n \"nbformat\": 4,\n \"nbformat_minor\": 0,\n \"metadata\": {\n \"colab\": {\n \"name\": \"lasso_regression.ipynb\",\n \"provenance\": []\n },\n \"kernelspec\": {\n \"name\": \"python3\",\n \"display_name\": \"Python 3\"\n }\n },\n \"cells\": [\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"-BknsqHEEqsI\",\n \"colab_type\": \"code\",\n \"colab\": {}\n },\n \"source\": [\n \"import pandas as pd\\n\",\n \"import numpy\\n\",\n \"import matplotlib.pyplot as plt\\n\",\n \"import seaborn as sns\\n\",\n \"sns.set()\"\n ],\n \"execution_count\": 0,\n \"outputs\": []\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"83xnU_AyFGT9\",\n \"colab_type\": \"code\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 212\n },\n \"outputId\": \"1501ef32-7099-4f1b-b853-e829c9c0c701\"\n },\n \"source\": [\n \"from pandas_datareader import data as pdr\\n\",\n \"import yfinance as yf\\n\",\n \"import datetime\\n\",\n \"start = datetime.datetime(2012,1,1)\\n\",\n \"end= datetime.datetime(2018,8,30)\\n\",\n \"yf.pdr_override()\\n\",\n \"df_full = yf.download(\\\"jpm\\\",start = start,end=end).reset_index()\\n\",\n \"df_full.to_csv('jpm.csv',index=False)\\n\",\n \"df_full.head()\"\n ],\n \"execution_count\": 5,\n \"outputs\": [\n {\n \"output_type\": \"stream\",\n \"text\": [\n \"\\r[*********************100%***********************] 1 of 1 completed\\n\"\n ],\n \"name\": \"stdout\"\n },\n {\n \"output_type\": \"execute_result\",\n \"data\": {\n \"text/html\": [\n \"<div>\\n\",\n \"<style scoped>\\n\",\n \" .dataframe tbody tr th:only-of-type {\\n\",\n \" vertical-align: middle;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe tbody tr th {\\n\",\n \" vertical-align: top;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe thead th {\\n\",\n \" text-align: right;\\n\",\n \" }\\n\",\n \"</style>\\n\",\n \"<table border=\\\"1\\\" class=\\\"dataframe\\\">\\n\",\n \" <thead>\\n\",\n \" <tr style=\\\"text-align: right;\\\">\\n\",\n \" <th></th>\\n\",\n \" <th>Date</th>\\n\",\n \" <th>Open</th>\\n\",\n \" <th>High</th>\\n\",\n \" <th>Low</th>\\n\",\n \" <th>Close</th>\\n\",\n \" <th>Adj Close</th>\\n\",\n \" <th>Volume</th>\\n\",\n \" </tr>\\n\",\n \" </thead>\\n\",\n \" <tbody>\\n\",\n \" <tr>\\n\",\n \" <th>0</th>\\n\",\n \" <td>2012-01-03</td>\\n\",\n \" <td>34.060001</td>\\n\",\n \" <td>35.189999</td>\\n\",\n \" <td>34.009998</td>\\n\",\n \" <td>34.980000</td>\\n\",\n \" <td>27.864342</td>\\n\",\n \" <td>44102800</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>1</th>\\n\",\n \" <td>2012-01-04</td>\\n\",\n \" <td>34.439999</td>\\n\",\n \" <td>35.150002</td>\\n\",\n \" <td>34.330002</td>\\n\",\n \" <td>34.950001</td>\\n\",\n \" <td>28.040850</td>\\n\",\n \" <td>36571200</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>2</th>\\n\",\n \" <td>2012-01-05</td>\\n\",\n \" <td>34.709999</td>\\n\",\n \" <td>35.919998</td>\\n\",\n \" <td>34.400002</td>\\n\",\n \" <td>35.680000</td>\\n\",\n \" <td>28.626539</td>\\n\",\n \" <td>38381400</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>3</th>\\n\",\n \" <td>2012-01-06</td>\\n\",\n \" <td>35.689999</td>\\n\",\n \" <td>35.770000</td>\\n\",\n \" <td>35.139999</td>\\n\",\n \" <td>35.360001</td>\\n\",\n \" <td>28.369787</td>\\n\",\n \" <td>33160600</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>4</th>\\n\",\n \" <td>2012-01-09</td>\\n\",\n \" <td>35.439999</td>\\n\",\n \" <td>35.680000</td>\\n\",\n \" <td>34.990002</td>\\n\",\n \" <td>35.299999</td>\\n\",\n \" <td>28.321667</td>\\n\",\n \" <td>23001800</td>\\n\",\n \" </tr>\\n\",\n \" </tbody>\\n\",\n \"</table>\\n\",\n \"</div>\"\n ],\n \"text/plain\": [\n \" Date Open High Low Close Adj Close Volume\\n\",\n \"0 2012-01-03 34.060001 35.189999 34.009998 34.980000 27.864342 44102800\\n\",\n \"1 2012-01-04 34.439999 35.150002 34.330002 34.950001 28.040850 36571200\\n\",\n \"2 2012-01-05 34.709999 35.919998 34.400002 35.680000 28.626539 38381400\\n\",\n \"3 2012-01-06 35.689999 35.770000 35.139999 35.360001 28.369787 33160600\\n\",\n \"4 2012-01-09 35.439999 35.680000 34.990002 35.299999 28.321667 23001800\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n },\n \"execution_count\": 5\n }\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"SE2zgaEFFOUV\",\n \"colab_type\": \"code\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 402\n },\n \"outputId\": \"92b4b3cc-45c8-4eef-ce8a-5bba35e0b87d\"\n },\n \"source\": [\n \"import pandas as pd\\n\",\n \"df = pd.read_csv('jpm.csv')\\n\",\n \"df\"\n ],\n \"execution_count\": 6,\n \"outputs\": [\n {\n \"output_type\": \"execute_result\",\n \"data\": {\n \"text/html\": [\n \"<div>\\n\",\n \"<style scoped>\\n\",\n \" .dataframe tbody tr th:only-of-type {\\n\",\n \" vertical-align: middle;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe tbody tr th {\\n\",\n \" vertical-align: top;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe thead th {\\n\",\n \" text-align: right;\\n\",\n \" }\\n\",\n \"</style>\\n\",\n \"<table border=\\\"1\\\" class=\\\"dataframe\\\">\\n\",\n \" <thead>\\n\",\n \" <tr style=\\\"text-align: right;\\\">\\n\",\n \" <th></th>\\n\",\n \" <th>Date</th>\\n\",\n \" <th>Open</th>\\n\",\n \" <th>High</th>\\n\",\n \" <th>Low</th>\\n\",\n \" <th>Close</th>\\n\",\n \" <th>Adj Close</th>\\n\",\n \" <th>Volume</th>\\n\",\n \" </tr>\\n\",\n \" </thead>\\n\",\n \" <tbody>\\n\",\n \" <tr>\\n\",\n \" <th>0</th>\\n\",\n \" <td>2012-01-03</td>\\n\",\n \" <td>34.060001</td>\\n\",\n \" <td>35.189999</td>\\n\",\n \" <td>34.009998</td>\\n\",\n \" <td>34.980000</td>\\n\",\n \" <td>27.864342</td>\\n\",\n \" <td>44102800</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>1</th>\\n\",\n \" <td>2012-01-04</td>\\n\",\n \" <td>34.439999</td>\\n\",\n \" <td>35.150002</td>\\n\",\n \" <td>34.330002</td>\\n\",\n \" <td>34.950001</td>\\n\",\n \" <td>28.040850</td>\\n\",\n \" <td>36571200</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>2</th>\\n\",\n \" <td>2012-01-05</td>\\n\",\n \" <td>34.709999</td>\\n\",\n \" <td>35.919998</td>\\n\",\n \" <td>34.400002</td>\\n\",\n \" <td>35.680000</td>\\n\",\n \" <td>28.626539</td>\\n\",\n \" <td>38381400</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>3</th>\\n\",\n \" <td>2012-01-06</td>\\n\",\n \" <td>35.689999</td>\\n\",\n \" <td>35.770000</td>\\n\",\n \" <td>35.139999</td>\\n\",\n \" <td>35.360001</td>\\n\",\n \" <td>28.369787</td>\\n\",\n \" <td>33160600</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>4</th>\\n\",\n \" <td>2012-01-09</td>\\n\",\n \" <td>35.439999</td>\\n\",\n \" <td>35.680000</td>\\n\",\n \" <td>34.990002</td>\\n\",\n \" <td>35.299999</td>\\n\",\n \" <td>28.321667</td>\\n\",\n \" <td>23001800</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>...</th>\\n\",\n \" <td>...</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>...</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>1671</th>\\n\",\n \" <td>2018-08-23</td>\\n\",\n \" <td>114.959999</td>\\n\",\n \" <td>115.150002</td>\\n\",\n \" <td>114.430000</td>\\n\",\n \" <td>114.730003</td>\\n\",\n \" <td>109.777924</td>\\n\",\n \" <td>9265400</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>1672</th>\\n\",\n \" <td>2018-08-24</td>\\n\",\n \" <td>114.980003</td>\\n\",\n \" <td>115.220001</td>\\n\",\n \" <td>114.449997</td>\\n\",\n \" <td>114.680000</td>\\n\",\n \" <td>109.730087</td>\\n\",\n \" <td>8845700</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>1673</th>\\n\",\n \" <td>2018-08-27</td>\\n\",\n \" <td>115.220001</td>\\n\",\n \" <td>117.279999</td>\\n\",\n \" <td>115.169998</td>\\n\",\n \" <td>116.709999</td>\\n\",\n \" <td>111.672455</td>\\n\",\n \" <td>13768000</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>1674</th>\\n\",\n \" <td>2018-08-28</td>\\n\",\n \" <td>117.000000</td>\\n\",\n \" <td>117.029999</td>\\n\",\n \" <td>115.970001</td>\\n\",\n \" <td>116.139999</td>\\n\",\n \" <td>111.127060</td>\\n\",\n \" <td>8302600</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>1675</th>\\n\",\n \" <td>2018-08-29</td>\\n\",\n \" <td>116.349998</td>\\n\",\n \" <td>116.370003</td>\\n\",\n \" <td>115.360001</td>\\n\",\n \" <td>115.760002</td>\\n\",\n \" <td>110.763466</td>\\n\",\n \" <td>7221700</td>\\n\",\n \" </tr>\\n\",\n \" </tbody>\\n\",\n \"</table>\\n\",\n \"<p>1676 rows × 7 columns</p>\\n\",\n \"</div>\"\n ],\n \"text/plain\": [\n \" Date Open High ... Close Adj Close Volume\\n\",\n \"0 2012-01-03 34.060001 35.189999 ... 34.980000 27.864342 44102800\\n\",\n \"1 2012-01-04 34.439999 35.150002 ... 34.950001 28.040850 36571200\\n\",\n \"2 2012-01-05 34.709999 35.919998 ... 35.680000 28.626539 38381400\\n\",\n \"3 2012-01-06 35.689999 35.770000 ... 35.360001 28.369787 33160600\\n\",\n \"4 2012-01-09 35.439999 35.680000 ... 35.299999 28.321667 23001800\\n\",\n \"... ... ... ... ... ... ... ...\\n\",\n \"1671 2018-08-23 114.959999 115.150002 ... 114.730003 109.777924 9265400\\n\",\n \"1672 2018-08-24 114.980003 115.220001 ... 114.680000 109.730087 8845700\\n\",\n \"1673 2018-08-27 115.220001 117.279999 ... 116.709999 111.672455 13768000\\n\",\n \"1674 2018-08-28 117.000000 117.029999 ... 116.139999 111.127060 8302600\\n\",\n \"1675 2018-08-29 116.349998 116.370003 ... 115.760002 110.763466 7221700\\n\",\n \"\\n\",\n \"[1676 rows x 7 columns]\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n },\n \"execution_count\": 6\n }\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"RoA46lkJFRRH\",\n \"colab_type\": \"code\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 218\n },\n \"outputId\": \"aeb7fe9b-a0cd-4f57-fb67-d5fc3659d6b1\"\n },\n \"source\": [\n \"close_px = df['Adj Close']\\n\",\n \"mavg = close_px.rolling(window=100).mean()\\n\",\n \"mavg\"\n ],\n \"execution_count\": 7,\n \"outputs\": [\n {\n \"output_type\": \"execute_result\",\n \"data\": {\n \"text/plain\": [\n \"0 NaN\\n\",\n \"1 NaN\\n\",\n \"2 NaN\\n\",\n \"3 NaN\\n\",\n \"4 NaN\\n\",\n \" ... \\n\",\n \"1671 105.630344\\n\",\n \"1672 105.676721\\n\",\n \"1673 105.728722\\n\",\n \"1674 105.801820\\n\",\n \"1675 105.858816\\n\",\n \"Name: Adj Close, Length: 1676, dtype: float64\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n },\n \"execution_count\": 7\n }\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"vk-AL5u4FU3r\",\n \"colab_type\": \"code\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 448\n },\n \"outputId\": \"7bf22cdc-6f47-4b94-beeb-963d9ee9f486\"\n },\n \"source\": [\n \"%matplotlib inline\\n\",\n \"import matplotlib.pyplot as plt\\n\",\n \"from matplotlib import style\\n\",\n \"\\n\",\n \"# Adjusting the size of matplotlib\\n\",\n \"import matplotlib as mpl\\n\",\n \"mpl.rc('figure', figsize=(8, 7))\\n\",\n \"mpl.__version__\\n\",\n \"\\n\",\n \"# Adjusting the style of matplotlib\\n\",\n \"style.use('ggplot')\\n\",\n \"\\n\",\n \"close_px.plot(label='AAPL')\\n\",\n \"mavg.plot(label='mavg')\\n\",\n \"plt.legend()\"\n ],\n \"execution_count\": 8,\n \"outputs\": [\n {\n \"output_type\": \"execute_result\",\n \"data\": {\n \"text/plain\": [\n \"<matplotlib.legend.Legend at 0x7fbc55745748>\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n },\n \"execution_count\": 8\n },\n {\n \"output_type\": \"display_data\",\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAAesAAAGeCAYAAAC0HCnTAAAABHNCSVQICAgIfAhkiAAAAAlwSFlz\\nAAALEgAACxIB0t1+/AAAADh0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uMy4xLjMsIGh0\\ndHA6Ly9tYXRwbG90bGliLm9yZy+AADFEAAAgAElEQVR4nOzdeZyVZf3/8dd1n2X2hWGZBRgYFkUQ\\nQRBURERFQL+pPzXTUjM1s4wyUysrUzNJcyky0kStjEwzSy3FBRc2RRQQBARZhgFmBph9nzPnnPv6\\n/XGfdebMvnDOzOf5ePQ4937um8l5z3Xd16K01hohhBBCRC3jWN+AEEIIIdomYS2EEEJEOQlrIYQQ\\nIspJWAshhBBRTsJaCCGEiHIS1kIIIUSUk7AWQgghopz9WN9AW4qKio71LfSonJwceaYY0R+fS54p\\ndvTH55Jn6tj1WiMlayGEECLKSVgLIYQQUU7CWgghhIhyUf3OujmtNY2NjZimiVLqWN9OpxUWFtLQ\\n0NCj19RaYxgG8fHxMflvIoQQon0xFdaNjY04HA7s9pi67QCHw9ErgerxeGhsbCQhIaHHry2EEOLY\\ni6lqcNM0Yzaoe5Pdbsc0zWN9G0IIIXpJTIW1VPO2Tv5thBCi/4qpsBZCCCEGIgnrbqqpqWHBggU8\\n9thjLfb96U9/4rzzzqOioiJs+5VXXsnXv/51brjhBq677jreffddAD799FNuuummPrlvIYQQsUPC\\nuptWrlzJxIkTeffdd3G73YHtXq+Xt99+m8mTJ/P222+3OO/ee+/l6aef5qc//SkPPvggVVVVfXnb\\nQgghYoiEdTetWLGCq6++mjFjxrBu3brA9o8++oicnByuu+46VqxY0er548ePJzExkeLi4r64XSGE\\nEDEopptWmx+8i163sleurc6YhzHrnDaP2bt3L9XV1UybNo3y8nJWrFjB3LlzASvEFy5cyOTJk/F4\\nPOzYsYMpU6a0uMbmzZtpampixIgR7NmzpzceRQghRIyL6bA+1l5//XXmz5+PUoo5c+bw2GOPUVJS\\ngt1u59NPP+XOO+8EYMGCBaxYsSIsrO+++26cTidJSUnce++9JCcnH6vHEEIIEeViOqyNWedAO6Xf\\n3uJ2u3nnnXdwOBy89dZbgDU4yZtvvonT6cTj8XD99dcD1vvrxsZGbr31VgzDevNw7733kpeXd0zu\\nXQghRGyJ6bA+ltatW8fIkSPDWoFv376dX//61zgcDn71q18xffr0wL477riD9957j3PPPfdY3K4Q\\nQogoordtQleUYpw5v0PHS1h30YoVK5g3b17YtkmTJlFYWAjAySefHLZv3rx5vPbaa+2G9b59+7j8\\n8ssD69OnT+cnP/lJD921EEIIP601+tV/oE6ZjRqe26ffbS65x1qQsO5dDz74YMTt7733XsTtCxYs\\n4Etf+hJut5vnn38+4jFTp06N2M1LCCFEL2ioR//vefTqN7A98myffa0O6ebbUdJ1SwghxMDk8YWm\\ny9WnX6s3fRBc9ng6dI6EtRBCiIGpyR/SGvOV5/Dee0uvf6U+lI9+5rfBDfW1aNOL+fqLbZ4nYS2E\\nEGJgcjdZn1qj//c8HMpHN/VuKdv85a0QOktiXS1s/xT9n7+1eZ6EtRBCiIHJH8xaB7eVl/Tud+pm\\n0xnX14LT2e5pEtZCCCEGJv+7am2Cf5rhxoa++W6br313XQ14239vLWEthBBiQNCuRszX/on2eq0N\\nIdXgOBzWcl+F9ZBM66vra6Gpqd3DJayFEEIMCPqVv6NfXo7esNraEFoNbvdVRTfW983NZAzxfV8D\\n+vMt7R4u/ayFEEL0e7quBv32K74V671xoDGZBhxWWOuGBlRv3UPZ0cCySk1Hg/WHw+4d7Z4rJWsh\\nhBD9nl71RnDFF8zBkrXZJ9XgeuWrwZXskdZnB4IaYrxk/e6+Kt7ZW9kr1z53bDrnjElr85izzz6b\\nG264gbVr11JdXc1tt93Gpk2b2LBhAx6Ph3vuuYdRo0ZRXl7OfffdR319PS6Xi9NOO41vf/vbNDY2\\ncsUVV/Dss8+SlmZ91+OPP05iYiLXXnstq1at4umnnyYuLo6zzjqLp59+mtdff52EhIReeWYhhOi3\\nKssCi8ruC+bQd8X+bb1ZDZ6abn2OOR614FL0y8s7fKqUrLspKSmJJ554gm9961v8/Oc/58QTT2TZ\\nsmXMnz+f5cutH0RycjKLFy/mmWee4amnnuKLL75gw4YNxMfHM3v2bFautObk9nq9vPPOOyxYsIDy\\n8nIeffRRFi9ezLJly4iLizuWjymEEDFNb/k4uGJ60YUH0Fs+Cm6z2azPgr29dxONjWAYGD9+EGUP\\nLyur69oekCWmS9bnjElrt/Tb6/dwjjVF5/jx41FKcfrppwNw3HHHsWbNGsAK4SeeeILt27ejtaa8\\nvJw9e/Ywc+ZMFi5cyGOPPcZll13GRx99RG5uLllZWaxbt47x48czYsQIAM4//3z++Mc/HpuHFEKI\\nWNcQLDFrtxt9z6Lw/UeLrX0b16EL9qBGjev5e6irhqQUlBFeTlY3/BDjtLltnhrTYR0NnL7O7Dab\\nDYf/nYdv3evrHvDiiy9SU1PDk08+iWEYPPzwwzT5ql8mT55MfX09+/bt44033mDhwoV9/xBCCNGP\\naa3D30V7Ikyk4Q6pEq+qaP+a7va7W7U4p7YaklMj3WC750o1eB+ora1l8ODBxMXFUVJSwgcffBC2\\nf8GCBfzzn/9k69atzJkzB4ATTjiB3bt3B6bcfPPNN/v8voUQol9oarIakY2dYK1HCusQ5r/bnoFL\\nHynCvPnL1L3/RpvHtVBbA8kpwfWppwGghmW3e6qEdR+49NJL2bZtG9dccw0PPfRQi7mu58+fz9tv\\nv80ZZ5xBfHw8ABkZGdx6663ceeed3HjjjVRVVWG32+XdtRBCdJbLKlWrE6db6yFhrWacGVy+4pvW\\nQmEB2mw2LGioI1Yhqvyhn3fuPmqrISlYsjZuvhPjvsdR/j8i2iDV4N0QOnd1VlYWr7zySmB96tSp\\n/OlPfwrse/zxx3E4HLgjzGOamZnJO++802L7zJkzmTt3LgArVqxgwoQJGIb8fSWEEJ2h17xlLfiH\\nFHV7wO5AnfslGJEHH6+BrOGocy9E/+dZqyTu9UJrv2+7UAUOQGMDKqQ3j1IKsoZ36FQJ6yj273//\\nm/fffx+v10tqaiq33377sb4lIYSIPb530GrSyVZ3KdNrla4dTpTTiQZQBkop1IVfRb/0V+sYHOjC\\nAvS2jRgLLg1cTtdUhS2rlA42dHY3Bft4d5KEdRS7+uqrufrqq4/1bQghRGyLiwe7HXLHWuv+/tV2\\nBziavVr0T7Dhm1zDXHw7NLnQ512MMnzdu1yNgcPNX/4AY/GfUB0J4W6EdUzVqeoOtJgbqOTfRggh\\nWlFbDXEJwS5T/pHL7I7gyGX+ma/8/a39k334j/WvQ3g1eGUZ5tOPduw+mpo6NB1mJDEV1iChFIn8\\nmwghRGTa60WvfduaihKs99ChYT18FADq9LOtbc1K1gGekHW3x6o2T0iy1jd92P59mF7rmvYBUA3u\\ndDpxuVyBFtPC4nK5Av29hRBChDi0P3zdNNGrfV2uHHZUShrG75+3qsoh2KjMa6JDBlIJC2tPEzgc\\naH+r8o4UmPyNi7v4uzqmwtrhcOD1eqmrq7Na0cWYhIQEGhp6dpB4rXWLAVmEEEJYzD89CIDx3Z+2\\n3OkbD1wlJAa3hZasQ/tje0OW3W7r3PpaauyJrMyegbGjjMEJdsZmxDMirWUXW/MPv7IWmr8j76CY\\nCmsgpkvVOTk5FBUVHevbEEKIgaPksPWZd3zLffYIhZyQd9bmD68JbNbr3oHzLkY54wItyfcmD+fX\\nk6+lPC4dNpcEjp0/Lo1vz8jCZoQUKndutT4HQslaCCGE6JKUlsN8Rupypex2qyuX6Q3brl9eDkUH\\nUTfeBm43h5KGcfeYr5LkaeChTY8x4oHfU1rn4Z19Vbz8eTmmhkWnZrWsBY7rWoEz5hqYCSGEEB02\\nJBN16lnBbleh0jNabvMf17yBGaA/s2buqvbA/aMvw2l6uG/zE4ytLybRYWNknJdvnJjGlycNZuXe\\nKt7aY/XHDm0ErOIiT3G8tqC6zceQsBZCCNF/uZtaL83655cO1bzrVgjdUM+nxXX8NOEMKuxJ3NXw\\nIcNcleDxoBvrMb9/JeaDP+aqKUOYkpXIsk+O8MGBaig6GLxIs/ZFWmuWf1rCQ2vbfkUqYS2EEKL/\\nanKBs5VGXc4IIe5vYNYUPqSoF8XvTvgqd797EDcGPyt9i9l33YO68lvWAf5APrAPQyluOT2bvEFx\\nPLimiBf2NRAoW4cMYaq15rmtpby4vYzzxrY9CpqEtRBCiP6rrbC2R2i25S9ZN9SGbV6ZPZM1mSdz\\n+XHJPHbgeSYb1Rhx8aiRowHQOz4NHKvdTQxOdHD/ebnMHZ3KPw5olo3/fzQZ9sB37itv5IE1hfxz\\nmxXUN5+a1eZjSAMzIYQQ/ZJ2u63q7FbCOmIXYN87a11fF9hUY0/k72MWMqlyL181U6CxDjUs09rp\\nL4kXHQheo7wUMnNw2gx+MCubtMYqXmEWn2SeRHZ+Ens2fkGDx8RpU1w6MYNrpg7FaKc7soS1EEKI\\n/qmyzPqM1JCsNf6SdX2wZL18zELq7fF8c/fL8OkR6113vK+hmD/cG4NjaOg9n6MycwDrD4Lrhrs5\\n6c1nWTbzBipdXs4ek8rwVCdz89JIdkZo+BaBhLUQQoj+qewoACpjaMfP8VVT6xeeBqAwYSjvZM/k\\n/MJ1jKo7AoOHQXUl+IcatTUbbxyCfyT4mV5OrviCJ6Zq1LgxXXoUeWcthBCif6r1dYeK1Oq7Nbbw\\nku5Lo87Gbnq5rOBdOHGaNeOWuwn8o55FmIkrLLgBvGb4sV0gYS2EEKJfCrx3Dh1OtD0hgVqcMJjV\\nmSezMK2edHcdKic3+AdAIKx9MeoPa2dci5bkgQFWbBLWQgghRDj/RBz+KuuOsAXfDv9z1Dzs2uSS\\n+dOxLXsVfO+hrWs2K1k3uawwjosHd/OStSf82C6QsBZCCNHvaK/X6k6ljM4N8ekr/e5NHs7qzJP5\\n0rgUMhKsAFfDRwcOU/GJYcdTWQ4oX8k6PKy1vxpcStZCCCFEkH7tBdixGbSJMjoRdTY7pXFp/Hry\\ntaQ31XLJhEGBXWrshOBxzUvWXo/1P2ccurw0/Jr+anApWQshhBAhig9F3p6T2+ZpdSb8avL1NNji\\n+fnWp0lJaeV9tz+sbc1itPgg7PoMveuz4DavvLMWQgghWoqPPGGGcc9jbZ72/O56ChOH8aPtz5JX\\nV4yKNI0mBN+DNy8t+wZg0YUFAJgrX0X/+XeRj+0ECWshhBD9j6+7lnHXb8M2Rxy1zMflMXn3QD2n\\nl3zGlIo9bV8/wT8oSjBG1YVXYix+EgC96g201uiX/ho8R0rWQgghRAiPG+LiUbljI+9Pbjm/9boD\\nNdS5NecVf9T+9SOUrNVpZ6PSBsGocdbwo7U1kBoyQYeEtRBCCBGiob7VVuDGD+7FuOt3Lba/ubuS\\nnBQHJ1butY77zk9anvv9X8D0WSiH09oQGsC+0c/UWQut9SYXZAwLObnrkSvDjQohhOg39P7d6Ldf\\nRW9YBeMmRjxGTTq5xbaCShc7Sxv4xslD8VeUq2mzWp47+RRsk08Jbgh9Dz1oiPXpnzikyYXKHoHe\\ns8Na70wXsmYkrIUQQvQb5hMPBsYEJ63jw4y+uacSu6E4d0zb80q3ENIa3P8+XMXFWfNXN7msblsZ\\nQ7A9+EznrtuMhLUQQoj+Izk1OIEHbU876efymLy/r4pZI1NIjbdjXvjViKXviFSEqm1/ydrVCB5P\\n2KhoXSVhLYQQIqbpz7dA1ggrqAuCrbi1Njt0vtWwzGTBeF8L8ou+2uHvjti63Omr7m5y9V1YP/vs\\ns3z00UeUlJTw8MMPk5trdSgvKipi6dKl1NbWkpyczKJFi8jOzm53nxBCCNGTzEfvirjdOP3sDp2/\\nan81mckOJg2L3De7Per8y1CTZwQ3BN5ZN6K93kDDs+5ot2nazJkzuffeexk6NHw+0GXLlrFgwQKW\\nLFnCggULePLJJzu0TwghhOgpWuuI223LXkVNPa3d8ysbPGw9XMeZo1Lb7IPdFuPSa1HjQxqzJSVb\\n91ZXaw1B2gMl63bDesKECQwZMiRsW1VVFfn5+cyePRuA2bNnk5+fT3V1dZv7hBBCiB7lcXfr9HUH\\najA1zBndst91lyWlWJ/7dsFnn/RIybpLVygrKyMjIwPD12fMMAwGDRpEaak1eHlr+1JTO/ePkZOT\\n0/5BMUaeKXb0x+eSZ4od/fG5euOZzJpqCpttizv5VIZ18LvWv1fM2CFJnD4xr0vfH+mZtNYcAvTa\\nt637SUjs8P20JqobmBUVFR3rW+hROTk58kwxoj8+lzxT7OiPz9Vbz6Qry1ts89z8sw5919FaN1uL\\nqrh6ypAu3VtHn8nl9XbouLb+mOnScCqDBw+mvLwc07Ra2pmmSUVFBUOGDGlznxBCCNGj3E1dPnVN\\ngfV69sxRPVgF7hcynaZK6WTf7Qi6FNZpaWmMHj2atWvXArB27Vry8vJITU1tc58QQgjRo7oZ1scP\\niScrxdmDN+QT2lhtSFa3L9duNfgzzzzDhg0bqKys5L777iMlJYVHH32UG2+8kaVLl/LSSy+RlJTE\\nokWLAue0tU8IIYToMV0M64NVLvIrXHxz+rD2D+6SkLBOTOr21doN6+uvv57rr7++xfbhw4ezePHi\\niOe0tU8IIYToMRVlXTpt9f5qDAWze6MKvBk1JLPb14jqBmZCCCFEW7RvxDJ11kIYNxGVPbL9c7Rm\\nTUE1J2YmMiihl2LQV7BWZy2Eaad3+3IS1kIIIWJXVQWkpmNcfXOHT/mirJHiGjeXTRzca7dlfOUG\\nzOWPoy6/vsuDrYSSsBZCCBGzdHUlpHZ8di2At/dUEm9XnDEqpZfuCtTo8dh+/miPXa/rM2ELIYQQ\\nx1pdbXDEsA5ocJusKahh9qhUEh229k+IEhLWQgghYpfHDQ5Hhw/fWFRLo8fknLzu933uSxLWQggh\\nYlcnp6DcVFRHktNgwtCuzbB1rEhYCyGEiF1eD8resZK11ppNxXVMzUrCZnS/0VdfkrAWQggRuzzu\\nDs9qtb/SRUWDh2k53R+kpK9JWAshhIhdHk+Hw3pjUR0A03KSe/OOeoWEtRBCiKinG+rRtdUtd3jc\\n0MFq8M1FteQNiiOjtwZC6UUS1kIIIaKe+atbMW+9GvOjVeE7PJ4OhXW928vnJQ1My469KnCQsBZC\\nCBHltNZwtNhafuoRdFlJcKfX3aHW4FuK6/Hq2KwCBwlrIYQQ0a62Jny9cH9wuYPvrDcV15Jgj70u\\nW34S1kIIIaKa3rklfL281Po0vWCa7VaDa63ZWFTHlOxE7DHWZctPwloIIUR084VzQE2V9enxWJ/t\\nlKzzK1yU1XuYMTw2q8BBwloIIUS0czUAoC6/zlp3u6xPf1i38856w6FaFHCKhLUQQgjRS1yN4IzD\\nmH8JJCRCU5O13esL63bGBt9QWMPxQxJIj4+9Llt+EtZCCCGiW2MjxMVbyw4nuH1h7XZbn22UrEvr\\n3ewtdzFzROyWqkHmsxZCCBHl9Oo3gisOJzT5qsG97b+z3nCoFiDmw1pK1kIIIaKW1jp8gzMO7a8G\\nDzQwa70afPX+akamORmR6uylO+wbEtZCCCGil6/KW5210Fr3VYPrfbswf3Gzta+VkvXhmiY+L2lg\\nbl4aSsVmly0/CWshhBDRq9FqCU5OrvXpjIMmF3rvzuAxtsgl6/f3V6OAs0an9u499gEJayGEENHL\\nH9bxvpHHEhKhoR79z6eDxzhalqy11ry3r4oTMxMZmtSxiT6imYS1EEKI6NVQD4DyhbVKSIIjReHH\\nqJZRtqu0kcO1bubmxX6pGiSshRBCRDFz6f3WQvZI6zMpKTBISkBjs3XgnX2VOG2KWbkpvXyHfUO6\\nbgkhhIhK2t0EFdZQo8of1gnBLljGbb9CH9oPU2aEnVfe4OHdfdWcMyaVRIetr263V0nJWgghRHTy\\n96fOHRvYpGbMDu5Pz8CYdxHKCA/kVz4vx9SaSycO7ou77BMS1kIIIaKO+dwTmD+4CgB11oLAdjVi\\nNOrri6yV9JZhXO3y8sbuCs4clUp2Smz3rQ4l1eBCCCGiii45jH7v9eAGR1zYfuPM+XDm/Ijn/ndn\\nOY0ezZcn9Z9SNUjJWgghRJQJNCrzUc6OlZDr3V5e+6KC00Ymk5se1/4JMUTCWgghRHQ5Why+7uhY\\nWL++q5K6JrPflapBwloIIUS08c+q5deBsG70mLyys5zpOUmMH5zQSzd27EhYCyGEiG4p7Q9s8ubu\\nSqpdXi4/sf+VqkEamAkhhIg2w0dBYQHG0hehsgw1LKfNw10ek//sKOOkzEROGJrYRzfZt6RkLYQQ\\nIro0NqBOPwfljGs3qAFW7q2iorH/lqpBwloIIUQU0aYJ9bXBiTva4fKYvLi9jIlDE5ic2T9L1SBh\\nLYQQIkporeHTj6zJO8ad0KFzXvm8nIoGD9dMHRrzc1a3Rd5ZCyGEiAr6P8+iV7wEgJo2q93j38+v\\n4h+flXL6yBQmDuu/pWqQkrUQQogood96JbCs7G2XJXeVNrDkw2ImDUvk1lnZvX1rx5yEtRBCiOjg\\ncHToMJfH5HcfFDM4wc5PzxpOnL3/R1n/f0IhhBBRQe/egXa5Wj/ANDt0neVbSiiqaeJ7p2f3mykw\\n2yNhLYQQokeZH76HufKVsG2eo4cxf/MT9PI/RjxH19cFp8Rsw46j9fx3ZwXnj09nSlZSj9xvLJAG\\nZkIIIXqMLjqAfua31sq8iwPb3ft2Wfs3r498Yl1N+9fWmj9vOkpGop1rTx7W7XuNJRLWQgghes7h\\nwsCi9npRNqua2ltRZm10NUQ+r74OAHXNzagpp0Y8ZGNRHV+UNXLzzCwSHAOrYnhgPa0QQohepUOq\\nss1bvmZtc7up+MPi4DFud8sT62sBUJkjUGmDIl77+c9KyUx2cO7YtB6849ggYS2EEKLnhFZnuxrQ\\n2zZi3n5t2CH6f8+3PK/BKlmTGPk99J6yRnaXNXLRhEHYjf47+ElrJKyFEEL0HH/o+phL7w+Umv30\\n6y+im72j1vVth/WbeyqIsynOzht4pWqQsBZCCNGTmlxgt0PuGGvd4wnuM0Iip7w0/Dx/WCe0DOu6\\nJi+r91dz5uhUkpwDo6tWcxLWQggxwOhP1uK98aIWpdse0dQEzjiMi65qsUt97dvBlSOFmGvesibu\\nAKtErlTECTxW7a+m0aNZOD695+83RkhYCyHEAGOufNVaKDzQ8xd3NYIzDlJbVlerrBHBe/jTb9DP\\n/gHztmvQe3ZYJeuERJQRHktaa97YXcnYjDjGZcT3/P3GCAlrIYQYaOy+YT09TT1/7SaXFdbJqS33\\njRjdclttDebv7oXaakhKabH7syP1FFS6WDBuUL+eVas9EtZCCDHQ+CbJMH97d49fWvvDOn1wy52J\\nSai550c6C71hNTSG98HWWvP3LaVkJNiZmxch/AcQCWshhBho7B2bMKNLXI3gcKIcDsg7ztqWkETO\\ncytRSqGu/FbkcwBqqsI2f1JYx87SBr5y4uABMVlHWwb20wshxEDk7oXqb6ySMAV7UdkjATDufAh1\\n4ZUYt9+PLc1qHOYf0QzAuOt3Yecbv1waWPaYmmc2HSUnxcm8sQO3YZmfhLUQQgwwKic3sKwbG9qe\\nCaszmpqsPtW+hmRKKYyLvobyd+Pyf/8Fl8PkU2BkHgzN8m1UkDk8cMxruyooqmni+mnDcNgG7rtq\\nPxkbXAghBjDze1eAYWD88aWwUm+X+Mf9jm+71bZxyTXBlZLD1qczPtASfF95I8u3lDBjeBKnDB84\\nM2u1RUrWQggx0DSvBjdNqKns/nX9757jutDFyhfUNS4vv15dSIrTxqLTsgd0C/BQEtZCCDGAmH9e\\ngl71RssdVT0R1lbJWsW1HNikXTYDr6l5ZF0R5Q0efjxnOOnxUvnrJ2EthBADhHY1oj94x1pRBsbv\\nnsP40QPWenUPhHVjF0rWx50IgEfZeXzDYTYX13HTjEyOH9KFwO/HJKyFEGKAMB/8cXBFm6ikZEjP\\nsFZ7Iqz91eDtvLMOZcy7iDpbPPePv5K391bx5UmDmT9OWn83J3UMQggxUBzMDyyqGWdaC6m+YKyu\\n6P71/Q3MOlEN/kljAo/P+CGVcSl877Qs6abVCglrIYQYKGx28HowvvMTOGkmACou3qq27oGSte5E\\nNbjH1Cz/tIT/7ItjlKec20tWMnHsz7t9D/2VhLUQQgwUhoGadwlq2qzw7c44a0zv7upgNXhJnZuH\\n1haxq7SBhePSuG77W8RdsKD739+PSVgLIcQAoN1uq8tWhPmicTjA7Y54jvnAjzAuuBw1fVbL85rr\\nQDX4hkM1LPmwGK8Jd8zOYfaoVDj1+x19jAFLwloIIQaChjrrMzG55T67EzxudNEBsDtQw7Kt7Xt2\\nwIG9mC//DVsbYa1NE73iX1BZZo1E5nCG79ea7cXV/HHVITYcqmXMoDh+dOZwslOcrVxRNCdhLYQQ\\nA0G9L6wTElvuczjQHjf67kUA2JZZ813rilJrv72dUD24D/3y8sD1lWFQVu/m/fxqvihr4IvSRsob\\nPCQ7Da46aQj/b2IGTpt0RuoMCWshhBgIfCVrlRihGtzugOKDLbf7B0rRpjVJx+b1mP/4E8bPHkGF\\nToEZOspYSjpv7K7gmY1HcXk12SkOThyWyOzjszkxzSTJ2c0hTQcoCWshhBgIAtXgkcLaDgWFLbfX\\nVfvOrUevfRv97B8A0Ds/Q50211p2NWI+a82WpYE3sk9j2YYjTMtO4lszMgNV3Tk5ORQVFfXkEw0o\\nEtZCCDEQBKrBWylZR+LxWJ/lJehn/4AGjsYPIikhDZvLS5LTQL/5H+oPHWRd9kxWZU5jR/oYpuck\\nceec4TikqrvHSFgLIcQAoNsKa0d4WJtr3sI4cz54rbD2ovhg2BReHnkW+SnDYRuwbTcjUp1MPJzE\\n+lN/TLUzmcyGMm607+eCuQswZAKOHtXtsN64cSMvvPCC9T4D+PKXv8ypp55KUVERS5cupba2luTk\\nZBYtWkR2dna3b1gIIUQXtACJhNYAACAASURBVFUNvmtb2Kp+9g/oM84Fj4eDmeN4dOSFFCRnM8Jb\\nzfW7X8GYfR5N2aPYVFTLysRxTKzM56ptf+H4yy/HmHa2zJTVC7oV1lpr/vCHP3DvvfeSm5tLQUEB\\nd911FzNmzGDZsmUsWLCAOXPmsHr1ap588knuvvvunrpvIYQQrdD7d0Pu2MD80ObKV9D/+ou1M8Lo\\nYuq0ueg1b4VvPFRApUdxz7ivYcbFc8c4xenZmbBmHSrtDIxJg7ls0mCa7vk+tsL9ABh541HOuF58\\nsoGr2yVrpRT19fUA1NXVMWjQIGpqasjPz+euu+4CYPbs2TzzzDNUV1eTmpra3a8UQggRgd7zOeZL\\nf4E9n6OuvBGmz8L8xzLY9EHgmEilXuPri9CnzIb6WvTBfPTrL+J67V885DiFOls8Dy0cx+hB8egj\\nRZgATS601iilsLldkDUcskbC4GF99qwDTbfCWinFrbfeykMPPURcXBwNDQ3ceeedlJWVkZGRgeH7\\nq84wDAYNGkRpaWmnwjonJ6c7txeV5JliR398Lnmm2NGZ59KmSeUzS6j9z9+D255fRnzBHhpCgtqW\\nNbz164ZsL/zsE5Y0jmJHag63Fb/FrEnzAfDYoBjQf1mCY/27ZD7yZ4q8HuKnnE7GD37Ro88UK/rq\\nmboV1l6vl5dffpk77riDCRMmsHPnTn7729/yve99r0durr818++PXRf64zNB/3wueabY0dnn0ls+\\nxgwJar+GmqqwdW/WiA5d9+njL+EDTxZf3/saZxxcFThHV5YFjmna+RmFWzdjNjRQ7/HQ2M51++PP\\nqqefqa3g71a7+v3791NeXs6ECRMAmDBhAvHx8TgcDsrLyzFNEwDTNKmoqGDIkCHd+TohhBARmO+/\\n3sqeZlXeTU3tXuvDAzX815PFBYfWcvHBVeE7beHlO/35Fmu8cXlP3eu6FdaDBw+mvLw88JfFoUOH\\nqKysJDs7m9GjR7N27VoA1q5dS15enryvFkKI3rD38/D1xCSITwh0vfJTx5/Y7qX+83k5OU4v1+39\\nX/OobxHWuBqssHZIWPe2blWDp6en881vfpNHHnkk8H76O9/5DsnJydx4440sXbqUl156iaSkJBYt\\nWtQjNyyEECJIb/oAGuoD6+ry66CsBL3+PairCW6ffwlq4WVtXiu/opFdpQ1cn+3Bps2WBzQP6wpf\\ntXhShO5gokd1uzX4mWeeyZlnntli+/Dhw1m8eHF3Ly+EEKIN5uMPWAt5x0H+F6hxE9FV66zRx+pq\\ngwdmZge6crXmzd2VOAzF2aNTghsnnRxctodEhjKgrMRaTpJa094mI5gJIUSMMv/3fGBZnfN/qOPv\\nRA0ajP70I/B6oTZYsm51SFGfereX9/KrmT0qhdQxOegfPwDZuaikkCk1Q8M+PgFdboW1Sk5B9C4J\\nayGEiEFaa/QrzwXWVWo6apBvJiy73XpfHfrOurK8zeut2V9Do8dk4fhB1vXGTWxxjFIKps1C2Wzo\\n3TvAF9YR58gWPUpGWRdCiFhUXxu+PiQruNz83TKghrU+3LPWmjd2VzA6PY7jh7Qc4SyU7Ts/wfjW\\nHdZIaFUV1sZkqQbvbVKyFkKIWFQXWsVth6EhYR1S5a2+dQdq7AkwKGT+6WZ2lzWyr8LFt2dkdnxc\\n77iQFuBSDd7rJKyFECIW+RqPqRlnor52U3jIul2BRZWUgspoe4yLd/dV4bQpzsrrRAn5wL7gckJi\\nx88TXSLV4EIIEYt8JWt17oWoZtXQeu/O4EpqepuXcXs1awuqOXVEMokOW5duRRldO090nIS1EELE\\nIF1yxFqIUL1tnHthYFmNGN3mdTYX11LTZDI3L61L92H88L4unSc6R6rBhRAiFh3Kt1phD2pZxa1O\\nnI6afR5ktj/JxIcHa0hyGkzN7trAJuqEKV06T3SOhLUQQsQgfWg/jMxrtUGYcW37Eyp5Tc3HhXWc\\nkpOM3ehgwzL/9e98CF18sFPniK6TsBZCiFhUVYE6blK3LrGztIEal5dTR3S+n7QaczxqzPHd+n7R\\ncfLOWgghYpGrwerr3A0bDtViN+DkHBnbO9pJWAshRCxyuXokrE/MTOpyK3DRdySshRAixmjT65tH\\nuuthfajaRVFNEzOHy1ChsUDCWgghYk2lb5jPbpSsNxyyBlWZ2YX31aLvSVgLIUSM0StetBYKC7p8\\njY8P1TJmUBxDk9qejUtEBwlrIYSINenWQChq3kVdOr260cPO0gYpVccQCWshhIg1/qkv2xmdrDUb\\ni+owNZwi76tjhoS1EELEmkar25YyuvYr/JOiWtLjbYzN6F5rctF3JKyFECLWNDZAfEKXTvWams3F\\ndUzPScbo6HSY4piTsBZCiFjT2ABxXQvrnaUN1DWZnDJcBkKJJRLWQggRY3Q3StafFNZiUzAlS8I6\\nlkhYCyFEDNBNLnSVr3+1q+thvbGwjonDEklyyqhlsUTCWgghYoD++xOYt1+LbqiHhvouhXVJnZuC\\nKpdUgccgCWshhIgBessGa+FwITQ2oLoQ1p8UWqOWnZIjXbZijYS1EELEAoc10pj56nNQcrhLQ41u\\nLKolK9nB8FRnT9+d6GUS1kIIEQv8faq3bbQ+PZ5OnV7v9rK5uJ4ZI5JR0mUr5khYCyFELFDNfl2n\\npHbq9A2HavGYmtm5nTtPRAcJayGEiAWho5WdMAV14Vc7dfraghoGJ9o5boiMWhaLJKyFECIWGMGu\\nVsb1t3aqgVldk5fNxXWckZsio5bFKAlrIYSIBSEhq9IzOnWqvwr8DKkCj1kS1kIIEQuccQAY37ur\\n06euO1DNEKkCj2kS1kIIEQtML0w9FXXSjE6dVuurAp8lVeAxTcJaCCGinHY3QWEBytn5kvHagmo8\\nJpw5SqrAY5mEtRBCRLuqCtAacsd0+tS391QxKi2O8YOlCjyWSVgLIUS08w+A0smGZfsrGtlT3si8\\ncWkyEEqMk7AWQoho57XCWtntnTrt7b1V2A3F3NFSBR7rJKyFECLa+UvWto6Htdtrsiq/ilNHJJMa\\n37mQF9FHwloIIaKdr2RNJ0rW6w/WUtNkct649F66KdGXJKyFECLadaFkvXJvJcOS7EzJSuylmxJ9\\nScJaCCGinbdzYV1W72bL4Xrm5qVJ3+p+QsJaCCGinadz1eBrCqrRwNy8tN67J9GnJKyFECLadbJk\\n/X5+NeMHxzM81dmLNyX6koS1EEJEu040MDtQ6SK/wsVZ0l2rX5GwFkKIKKc70cBs1f5qDCXDi/Y3\\nEtZCCBHtOliyNrVmVX4VJ2cnkZ4gfav7EwlrIYSIdh0sWe8saaCk3sMcqQLvdySshRAi2nWwZL2m\\noBqnTXHqiJQ+uCnRlySshRAi2nWgZO01NR8cqOGU4ckkOORXe38jP1EhhIh2Hei6tf1oPZWNXmaP\\nklJ1fyRhLYQQ0a4Dg6KsLagh3q44JSe5j25K9CUJayGEiHZeDygFRuRf2V5T8+HBGmYMTybOLr/W\\n+yP5qQohRLTzeMBmQ7UyzvdnR+qpdnmZLX2r+y0JayGEiHZeD9gcre5eW1BNvN1gWk5SH96U6EsS\\n1kIIEe08nlbfV3tMzfpDtcwckYzTJr/S+yv5yQohRLTzWtXgkWw7Uk+Ny8usXGkF3p9JWAshRLTz\\ntl6yXnfAVwWeLVXg/ZmEtRBCRDldVxuxj7XX1Kw/WMuM4UnSCryfk5+uEEJEu707UXnHt9i87ajV\\nCvyMXGkF3t/JtCxCCBGltOmFygpwNUL6oBb7rVbgSlqBDwAS1kIIEYW0aWLedElwg8MZtt/tNfng\\nQA2njUiRKvABQH7CQggRhfTbL4dvaBbWm4rqqG0yZTrMAULCWgghooQ+Woz3lq/hLtiL3rze2pjo\\nq+J2xoUdu2p/NWlxNqZIK/ABQcJaCCGihN78IdTXUvrAnVajsulnoKaeZu0MGWm03u3l48JazhiV\\ngt2IPASp6F8krIUQIlo01APgObAPADVnAQzNtPbVVAcOW5VfTZNXc3ZeWp/fojg2JKyFED1CN9Yf\\n61uIaVpr9KYPgxsSk1ATp0JahrXe2BDY9daeSvIGxTF+cHwf36U4ViSshRDdpjd+gPm9K9EH84/1\\nrcSuhjooPgh5x1nr9XUAqNPmouaej/q/rwCwp6yRfRUuzhub3uosXKL/kbAWQnSL9rgxV/zLWv58\\nyzG+m+inTRPd5Gq5o64WADVzTthm5XBiXPUdVGo6YJWqnTbFWXnSCnwgkX7WQohO0aVHoL4OlTvG\\nWv/3s1Cwx9rZUHcM7yw26L/8Hv3huzBqHMZPH4bDh9DrVqKmnwGAGjKMxAsuo37EmBbn1ru9rNpf\\nzexRqSQ7I0/sIfonCWshRKeYd94IgG3ZqwDozz4J7NOb16MnnIQ6fnK3v0dXlkFqOsroX6GkP3zX\\nWijYg37qEbTbDZ+uB6/X2p6YQsZ376SxqKjFuWsLamj0mMwfJw3LBppuh3VTUxN//etf+eyzz3A4\\nHBx33HHcdNNNFBUVsXTpUmpra0lOTmbRokVkZ2f3xD0LIaKA1tp6Z1pRHtxYWID58M8AUGctxLj6\\n5q5du64G847rUPMuRl1xQ0/cbvRITIZ6q8pbf7wGhmZZy1s2WPtTWg/ilXsrGZHqZMKQhF6/TRFd\\nuv3Oevny5TgcDpYsWcIjjzzCFVdcAcCyZctYsGABS5YsYcGCBTz55JPdvlkhRBTZ9AHa3QSuBtT/\\nuxpycsN261VvdP3avi5M+pO14desqkB7PF2/7jGmXY1QX4u65JrgxpLD1mfpEetzyLCI5x6odLGr\\ntJH546Rh2UDUrbBubGxk9erVXHnllYH/86Snp1NVVUV+fj6zZ88GYPbs2eTn51NdXd3W5YQQUUy7\\nXJivvxhYN594MNj3NzkVIgSI+e+/WgHVWf5ArixDHy5E53+BrqnCvP1a9J9/15XbPyZ0XS3mEw9a\\nVfoA5aXWZ8ZQVCu1DqrZsKJ+b+6pxG7AXGlYNiB1qxr88OHDpKSk8OKLL7J9+3bi4+O58sorcTqd\\nZGRkYBjW3wKGYTBo0CBKS0tJTZX/owkRi8w7vwk1VeHbXlgGgEpOheyR6MIC1IJL0G/+BwC94iXU\\n6ONg2umd+7KQ1tLmPd8Db7A0rTesxnukCDVhMnz/Z117mL6y6zP0xnXo+lqMs87H3LAKAJWTi8od\\ng3f5H8OPzx0b8TKVjR7e3lPJmaNSSYuXpkYDUbd+6qZpcuTIEfLy8rjmmmvYvXs3Dz74ID/84Q97\\n5OZycnJ65DrRRJ4pdvTH5+rqM5n1dRQ2C2oAfIN4DB6dh236qTTOmEXy/13O0YK9NO3cCkAqXlI6\\n+b2uqlKO+le8Eaq9C/agC/bgufIGhtkV9mHR2R6m4cAQSgE+34Lp69YWN2Umw06zah2PTJxC045g\\ndzdHXBxZvn8r/8/K1JrH/rsdj4bvnjORnIzEPn2GniT/TXVdt8J6yJAh2Gw2zjjD6nIwfvx4UlJS\\ncDqdlJeXY5omhmFgmiYVFRUMGTKkU9cvitAaMpbl5OTIM8WI/vhc3Xkm7X+v2oqyxiYUNpg2m5ri\\nYszxk8AX1lUF+6np5PfqosIOHVd83ZeAYMv0vqCrKqxW6h14b2wWhz+3Omsh7nMvDPwc9A23YRQW\\nWFX9zz2BZ84CioqKAj+rJq/JHz86zHv51Xx96lAcjZUUFVX2ynP1NvlvqmPXa0233lmnpqYyadIk\\ntm61/qMsKiqiurqa7OxsRo8ezdq1VuOQtWvXkpeXJ1XgQsQovW8XAOq6WyIHY3Kz/7aHZQWXIw0A\\n0p6unNPLtNeL+c7/MG+/Fvbu7NhJoc8xbiLG1TejskcGNqnUdNQJUzDOvgDbslcxTjs7sG/7kXpu\\nW7Gf9/Kr+epJQ7h0YkZPPYqIQd1++XHjjTfy+OOP8+yzz2K321m0aBFJSUnceOONLF26lJdeeomk\\npCQWLVrUE/crhDgG9FOPAKBaq25OSglbVafORY09AfPXd4C788HbvFGauvhr6Feea/34uhpUs3vo\\nafrpR62uVvhaqY+d0H7p2vcc6prvok49q0Pfc7imiYde2sLafWUMS7Lzi7kjmD48uVv3LmJft8M6\\nMzOTe+65p8X24cOHs3jx4u5eXghxDGiPG/Pu72Fc/o3gFI0Ag6xXWeqq76BffCZQclT28F8lSimr\\n/7Azrmul5PKS8PWkFNSsc9EfvBP5+MOFMHZC57+nE/xBDaDf+S+MOb7F0KAtznnhKQDU6We32so7\\n1LoD1fz+w8PYDMVVJw3h4hMyiLPLqNBCRjATQkRSchiOFmEuXYzxs0cCm9Vgqw+wMfd89LgJmPfe\\n0vZ1nHEccRt8uKOMo7VuDGUFud1QTMlKZGp2EkaE0qne8alVtV5rdQ1TZy5AV5a3OE4lJqHr66Cm\\nD97jxsUHSsqANcRqhLDWdbUQFxcIagDsjjYv7TU1z20t5V/byzh+SDwPXzYNs7bl84qBS8JaCNFS\\nSIMy8/7brIUTpoQfk5DU5iXcXpMHRlzEJ0mjYHMJKXE20BoTaPJoXv68nOGpTi6aMIiz89ICJUjv\\n4w/A51tQ51+GXvES4Cu5X/AV9BfbYM/nge9w5I6laedWdFMTvT5MyPBRVmD7JyuprWlxiPnBO+g/\\nLwnbps6Y12Z1+RelDfzt0xK2HqnnvLFp3DQjk6zUeIpqe/TuRYyTsBZCtGC+9s8W24zQUbcA4lvv\\nQuT2mjy4pohPkkZxec1WzvrqxYxMiwvb/8GBGl7ZWcHjG47wj62l/L8TMlgwPp24TR8AoEaPR93z\\nGGjTWo+Lw/jeXZi3fC1wnbiTpltdxHqoQZr2uK2+4XPPh8RklC1kXPK6WtTgYagf3of51CPoSF3Z\\n8r9oua2Ve9tb3sjfPi1hc3EdKU6Dm2dmMX9cmoxOJiKSsBZCtORr/R1K+edZ9ov3jU+dNihss9ur\\n+c3aIj4urOVbNR+zsHwLtrSvhB3jsBmclZfGnNGpbDtaz4vbyvjL5hKWbynhuKnf5qSK3eQ2ppCg\\nBjNucDxmg4fKRg9xdiehg3Ea/nG0eyqsP3wP/epz6FefQ806F3WdVc3vffzXcKQQTjgJdcIUGJkH\\nNVXoksOYv/guxi+WoLJHQGND8N/r9LPRH77XYhhWU2v+vqWUf+8oI8Vp4+tTh3L+cekkOvrXhCWi\\nZ0lYCyHCmK/+w1qYeDLs2AyAsbjl2P7KZkN98zZUSMMuU2uWfFjEhkO13DQjkwUrD7YZpEopJmcm\\nMTkziV2lDazPr+DTjU6ez1sA+UD+oRbnjJzxQ2Yd3coZJVsZlORrJe1u6voDh/KV4gH0B++gJ09H\\nnTI7MPiLv9W7Skm3+kavfx88bvRH78PFV1nrw7Ix7vsjyrChL/06JAcn5tBa8/TGo/xvVwXnjknj\\n+unDZKpL0SES1kKIMPq/Vlgbl1yNuvXeNo81Qrojaa15auNR1hTU8PWpQ7nguEGYq+LQHSz1Hj8k\\ngeOcLq5+8vfU2hMovWUxdelZ5Fe4sBuK9AQbFQ0e1q3cxz9Hz+OFvPnk7VVMz1vArFqTyAN1dpKp\\nw1ffehnbKbODG/zdw1JSraFX/c/mjIN6/1zeKjCtp0ofHHa9f+8o53+7KrhwwiBumDZMqrxFh0lY\\nCyEC/MGqZp+HGj2+U+e+8FkZr+2q4OIJg4IDeMTFQclhzP8+j/q/y9ufm9rX+jvZ00BqbiYqMYmT\\nssIbsp3/8BOUO1NZv+BbbEwbz79zz+ZfjQbf31vJuWPTO3XPLdT5Go2Nnwi7d4AR3m1KTZ9lLaSk\\nW0Htf2/tjAssqwuvjHjpd/dV8eynJcwZlcr1EtSik6QDnxACsErG5ncvt1aav59ux+r91fzjs1LO\\nGZPKdaFB5LQalelXn0NvtBqO6S+2of2zTzVj/vYXAKirb0YlRh4IxPj+3Qy+4houvGgOT351On9e\\n90umlH/B79cf5okNh6l1eTt172FqqyEuAeO7vjm5c3LRpnU9ddHXUBlDreNSrBHb9LqVwees9YV1\\n89HcsIL6sfXFTMlK5PunZ0fsriZEWySshRjAtMeD9g9A0hR87xspcFqzq7SB339YzMShCdw8Myu8\\nxOgMGQhk83q0aWI+9FPMB38U+WK+vtT+/tyRqMnTMeYsCKynzjufO3csZ97YNN7cU8ntb+5nf0Xn\\np+XUNVXola+Cw2GNhpY13KradvsmEnEE+0qrlGYleIcTqip8NxT+jvrFbaUs+bCYyZmJ3DlnBA6b\\nBLXoPAlrIQYw/Z+/Yf74BqsbUl1Iv+EhmR06/2itm8WrDpGRaOfOOcNx2Jr9SnEGu2tp0xsMtFZK\\n1qRlwJSZqBOndfwhUlJxehpZdFIKi8/LpcFtcsvr+3lg9SEOVXe8lbj2z9Xtq4onKQVdXwse3x8x\\noSOQpTT7Y8bjRh8qsOb0zhwOgMtj8ui6YpZvKWXO6FTumjuCBIf8yhVdI++shRjA9K7PrIVD+yG0\\n2rkDYV1S5+ZnKwtwezX3zRtBaqR5lkMDzuMJDiMaYehNrTXUVqGadXVql7+/d2MjJwwdwpIL8njt\\niwr+t6uCzSv2c9OMLM7ITWl/2E5vs+rzxGT47BPMZ37nu+eQUchS0sIO1c/+IbBdxcVTUmf9EZNf\\n4eKaKUO5bFKGvKMW3SJhLcRAlmg13tIVZehH7wJAfeMWVGLbo5N5TM2DawqpcZncf14uuSEDnoSx\\nhfyK2bIBc8sGa9ke4VdPSbEVmM2CsF3+oTw9bgDSE+xcNWUoC8en85s1RSz5sJjHNxxmSlYS549P\\n56SspMhV0f7GZJNPAXxDmQJs/dja7gh5xsHD4MRpqNRB4eOVJyZTUOnirncO4PZqfj53BKfIJByi\\nB0hYCzGQ+Up7+s+/C26aOrPd017bVcHuskbumJ3D2Iz41g+MFMoAIa3CtdbgasT82betDa00LGuV\\nv5TudodtHpzoYPF5uWw5XMem4jrW7K/m48Ja4u2KyZmJzMpNZfaoFJz+qntfQzLjph+HX9dHDQp2\\nw1KGge2We9DVlWFhXZSSxd3vHsSmFIsX5DKitT9ihOgkCWshBrLmVb9pGe1ONVla7+a5raWckpPE\\nGbntTEtpay2srYDURQcwl94fPs62xx35nFYoh90qAUc4z2YopuUkMy0nmWunDmNTUS2bi63w/vjD\\nYpZ/WsKtZ2QzOTPJCvu0DFScL2BTwxuRqQknRXi+4B8dnw4az5Lsi8DU/GqeBLXoWRLWQgxkdeGz\\nRajzLm73lGc2HsXUmhtPyWz/PWxrYe3brjd+AEeLg9uzR7Y77WQLdn/Juu1RzBw2xakjUzh1ZApa\\na7YeqeeJDUe4a+VBrpoyhEs97vAW3xdcjv58C2pIJkQKagCbjTJnKq+OnMN/R85hpLean5wnQS16\\nnoS1EDFGb1yHPnoY4/zLun+xuvCZo1Q7Dcs2FdWy7kANV500hKyU9udnjlgNPnZCMKADo35ZjO/d\\nhUpofYKQiBwdC+tQSimmZCXx6Pmj+eOGwyzfUopb5XGFY2/wmLh4bD99uNVrmFrzyu5alp/2EzyG\\nnflF67k+V5OQ1v5rBCE6S8JaiCin62rAZkP5Wj2bTzxobZ84FTWq64Nsaq2hrhpOnAY11ajM4dZy\\nK5q8Jk9+coScFCeX+Ecoa4ey+6qoQ7eNPQF9cB/a1Qj1tZAxJNiVK64LJVL/HwQeT6dPTXAY3Dor\\nG7uheGHfCZRlay6ucrXeYM6nrsnLA2sK2Xq4npnlO7ki/23y6opRU67r/P0L0QES1kJEOfOBH0NV\\nBcaS58Lmcjb//Sy2dsbu1k0u9P9eQJ3zJcjJCd9ZdhSamlAnzcQ4+4J27+Pf28sprnFz7zkjW/an\\nbo3NEb4elwANddDUhLnoKy2Pd7bRWK01XShZa63Rr/wddeJ0jHEnsOjULBK2beDNlONZ+b98Jg5N\\n4OwxacwamUJyXPgQqQ1uk1++d4jdZQ18d2Ym5/zmR8G5tNM69keMEJ0lYS1EtDvsm3mq9Aimb5IN\\nADXuhHZP1eveQa/4F1SWUbl+BKZXo879Eio+Ef35lg5fp6i6iX9tL+PMUSlMzW67W1eYkGpw49Z7\\nIe94q0FZa5wdqFpvzveeWbubaK8ns/Z6QWtresvX/ol+7Z/Ylr2KzVDcUPEhl9VsZdWCm3l7bxVL\\nPzrMnz4+zPScZOaMTmXG8GRcXs397x/ii7IG7pidw6zcVEKb6KlJJ3f+/oXoAAlrIaKI3r0DGhtQ\\nk6db66FVu9WV8PkW1Mw56I/XgLftal9dV4t+7glr+cP38L+d1js+xXbHYquUnpIGI0a3eZ2qRg+/\\nXn0Ih01x/fSOjWwW4A/r1HTURCvI1ORTgoOxAMbtizEf/qm1r72JPiKJ85XG25ndS5tezMW3gcOJ\\n8Y3vB7dv22Rdw+Mh3enh0kmDuWRiBnvLXazeX8Xqgho+OmR1+TK1lfW3+4I6lPGbP3dqmFYhOkPC\\nWogoYv7mJwDYlr1qbQhpAOYvCZOTa1UXu9oJp7VvR97xxTarGnj3dhg1rs0W3fsrGnlobRFH69zc\\nNXcEGQmd/JXh79oU8h3qvIvRr/49OBZ5ajdnykrwlfQbgo3VdG01JKWEP9vuz+HAPmu5ujKw2Vxy\\nj7WQkxu4F6UU4wbHM25wPNeePIztR+tZf7AGlGLemDTGROpb7pQW4KL3yEC1QvQRbZptzu2sQ/o8\\n+5f1K38PHlBhNcJSU2ZCfDy4Gtr+wopWxt8GzN//EkoOW9eKwOUx+dunJdz2xn5qm7zcffbIFlNV\\ndoj/nbVpBjYpw4DjQ7pCJSWh5l1kNTTrirh464+BBuvfQ5ccxrz1avR7r4UdposPBpbN5Y+3vE7R\\nAVRCy2e0GYqTspL41owsvnVKZuSghq41jhOigySshegj+h9/wvzu5eiQ4Arb//Ga4EpVBbr0CDok\\ncPXqN62FxGSrFNdeyfpgvtVNKoQ6Zba1sG2jtX78iS3OO1LbxB1vFvjeUafy+//L48TMTnan8hvq\\nqzb3T47hY3x9UXAl682CIwAAIABJREFUMRnjim9ie/CZLn2FMgxwxqP37bI2+CYL0evfDz+w7Ghw\\nOSS4wyR1fWhQZXe0f5AQXSRhLUQfMF/5O/r9FdaKq+X0jdrjRj/9aHDDkULMO2+EbZug+YhiSckQ\\nF4/esAodOqBIc3U1kDYI48cPBLdljwg7RGWPDCyX1Ln552el3Pr6fkrr3dx99gh+MCuHtEgTdHSQ\\nSk5Fzb8E4/u/CN+enmENwJI1vGdCztUAOzajy44Gx/iuseaXNte+jS4siFgToeYsDN/QzuhtQhwr\\n8s5aiD4QCGqAhnprSsq4eFTaIGtb0YGw403fpBoADMuGfN+7a5vdKlX7Gp6Zv7sb2+Inw7/L9KL/\\n9wIcPoTKHQsJvtKiUpAeMr71eRejtWbL4Xpe/6KCjwtrMTVMzU7i2zMyye7IoCcdYFweue+x8ZUb\\n0Jdf3yPfoeYsRK9+wwpofxeu0iPo+jr0Xx+z+nqPGmf9+/kb5qWmoy67FvWV64PdyLpRshaiN0lY\\nC9EXRo2F7ZsBMJ96GHbvAEIakpUcbv3clDRrsJJtmyAxyddoyjfUiK/0GGb35+j/Pm8tO5yBmbXs\\nZ3+JN71D2XXcZdQ4EqlMm0nJf/ZS3uAhNc7GpRMHs2BcOsOS+646t6emjVQzZqNXv4Hevtn6A8XH\\n/N3dwYMK9sDgYRi33GO1uM8bH3IBA7RpDQwjRBSSsBb9ni4+aA1vOX4i7N+Dmji1728idFhNX1CH\\n0r5W3+pr34b6Wits/SVAdxNqxGiri5Gv5Pd5XCavTZzL1kHjyVt5gFNHJHPayBSGJjmsVt7+6277\\nBHX1d9hy88M8cdDBkSIXqUMmkeauZZBdcVJmIlOyk8Jnn4pFcQkA6JeXo75zZ3B7/hfhx9kdqGav\\nAqwTfe0IOjuXthB9RMJa9Hvmkw/Bof2BdeOxF1DxCX3y3broADp/txUaocNqhh5jetF/+yMA6oxz\\nUc449AWXWzNifboeRo0LNj5zOHltVwVPjf4KSZ4GTq74goIh6Ty18ShPbTzKuIx4Zh62MzpjAk7T\\nw95xp7H69f0UVJrkDjK4Z24OJ73zLGroEIxz5/bJv0GfiA+20NZtjWTWXkk+vfMjkBn3PBaxHYIQ\\nPUnCWvR/IUENQHUF9HJYmytfhcOH0KveCGwzvnELeudW9Osvhh8c0khM+frqKqWsAUV8rbfrE9PY\\nkz6G94aexXufHGFGw0Fu/eRJ4k03xg+uoKjGzfqDNaw/WMNzCZPgpEmBa441FN89NYsrTz+e8pIj\\nENoSu78ImaqyzcFR/KPBNWP8cimUl3apWl4NH9Xpc4ToLAlrMfBUVcKwnPaP6yKtNfqFp8K2qXMv\\nRJ0wBbJHtgxrfxV5hHtyeUz+tb2MV4tH0jj12yit+fKkwXw13o3a4Ju/ee/nDB83kcsmDeaySYMp\\n/dVPKB08kqZLvsGItLjAQCbxji6MDhYrQhrOBd7Xd4LKHgkhLeOFiDYS1qJf0xVlLTdWV/TMtbXG\\n/MXNcLgQ4/GXgl2QqspbHpzle0/qb/3tv0ZDPXrDagCMb/4wsN1ratYUVPPc1lKO1Lo5Y7Bi7nvP\\nMM7eQMbVjwFD0T/5DeYDP4LakFHOqioYVLCDQYYHW1cGMYlRyhmHOv/L1jjozQeD8TUeEyKWxXCL\\nEjFQ6boadDsDggSEtJZW53wJsKaY1Ka3tTM6dg/uJtiyAQ4XWuv/+ktwp29QDhKSUNd81/pu31jf\\nSimM2+9HfeUG65iiA8FhQTOGorXmo4M13PJ6Pr/9oJgkh8F9547kjhmDmV6+k7SG4DCZ/lbeYaOi\\n+ar8VbPBUAYCddKM4PK8i1Fzz7dWbP24RkEMGFKyFjHH/MFVMCwH2/1PtH7MhtXo1f+/vTsNjKo6\\nGzj+P3cy2feVQAg7CSKCCwIKCkXcqr7aquVV6Wvr1gLuInWnVaxV1FpFC4pUrFqMba21WkFRcF8Q\\nRdYAYU1IyL4vk7nn/XAzM5lkshACmUme35e5+5wDN/eZe+6553kP46IrgKaMT5lj0WvetjYoLoSk\\nfl0vw9MPgmusbkB/8G+YeZ01vW2j9Z23LEANzUBPPssrQYXKGGMF5teXWYN1JKVCSAg7HKEsX72P\\nLYW19I8K5s7J/ZmUHoWhFNoZCsNHYfy4WVrJ4NYJLMwVz1jfcdZFXa5bwPLqh6AhIdmabHT0SHGE\\n6E4SrEXA0Ns2Qk2VNXMor/1t//qsNfhIdlN2p+BQa1hKlw4yVnXIFajDIqwEEs3STGrX60Ip1jNo\\nn5mkEpIhNp6Sf2XxXVgaHw49m03v7SUm1Mavxqdw9vBYbEaz5Bc2G7b5f/A+RlNnNP3S0zj/8zq2\\n3z9v9SAHiOviONuBrHmw1hqVPsz1Nrq7J74x7+GeKJkQR0yCtQgY5uP3dn5jV9By9bRuSqOorrsD\\n/fyiDtMptqRNE5Rq3Vs4IQliRnq/R11VCcNHodoZurKyweSPmVexPtR6lp1iVjNrXBLnj4wlvLMd\\nwZpneSoqsD7DI2B4pvcPk76iRbAmPsk9a9z1GBgGKjrOx45C+L8++BctApGvbFXa4Wne1KaJ86nf\\nWgOHgDsY6+JCa74pI5KKjPJa3+H3Nn2HedsszEX3oLdv8krEYVzxK1R4JFRXeXYqKmg37eOO4lpu\\ne3cPG0NSuXzPah775imeTS/k0tEJnQ/UAMHew4HqRgdUV7b7I6FXaxas1fQLvFoXVGyCBGoR0CRY\\ni8CQvbn1si0bPNMHdsOm9ZhPLUA3y1XM/t3Wp2t8bNfdaCeCtd6zA3P2TzHfWG4lxcjehLnobvTn\\nayAyGjVxKmrEcdbdbFMuZZ27F4oPeQ152dzqnWXctWofSsHD1euYuWc1w6pysZ1xToflaUkp5XV3\\nbT5wo5Wn2Ueax76geUIQldy/1Y8ZIQKZNIMLv2f+82XPu8mjT3SPsa13bHbnY26eDtF8yPMKFLXV\\nkDoQFRVtzbuCWyd6k7vu0vV7//Re/tG7VrB33amFR1pDhGrtTmOpTj7da5/KeicvflvAmpwKxvUL\\n5/bT+xP51xrrmWpyatfHyA4O8fzwcD3H78PJKIxmaTa7a9xxIfyBBGvh17Rpole96Z5XJ52GbgrW\\nzYfu1PtyPDu53rMNCbWGgWyWnOGHahuvjvs1tTsjiC/dT3SIjfjwIBLD7QxPCGVYfChBro5d1Z73\\nl73s2WF9BoegtaYoJAabEUpseSm4epsnp1rl0ppP9lby/PoCKuudXH58AjPHJGIzFKa96c4vKqZr\\n/zjgSQfZjJp6ftePF+BUfB/sWCf6BAnWwq+YrzwH/dIwpl9oLSgrtl69SR+Kcf5lMG4iatgozL8u\\ndjd366oKyN7U+mBDM2Dr96im8Z5X7yzjmW/rSA6JYZDNQUm9kwMV9ZTUOmk0rX7DEcEG04bEcOn4\\nSCL35bifEzn7DWT7rx9h06dfU5GTQ7SjmvyGoWz4+07K6zPg9AcI/ncuA06+mbF1uRyXV40C3ttR\\nxjd51QyPD2XBtIEMjfeMYY3darZVR5I8onmTP0DaEFRYeNePJ4TwSxKshd/Q+3e78z7rhGTUuAno\\n778GwLj8WlTG8daGA9Kt57KukcKKD1m9f0+cCBu+cB9PpfRHb/0e0KzbU8HiL/M5KTmEeW88Tujl\\nV2NMPwkAU2tKahvZVljLF/sr+e+OMt7e/g3RST9lePx0QswGtsUOofTDXBSphPWLoyYojCiHkxMH\\nRTAquBbnf7I4FBpHTmQa/04+lTfXWoOlhNgUvzwpmQsy4rxexbIK2PRToDvvBg/s7r5jCSH8hgRr\\n4TfcPbkBc/FCjAVPo12dyNK8kyWoiEgr9SWgm0btMn78M/TAoei3XrW2GTUW/dG7fJ10PH/8LI/j\\nksOYPzkF++sOaKhHFxWA1hhJ/UgMtzN5kJ3Jg6KprHeyq7SRNa+8wb600dSHRZMRG8zk9GhOrtlL\\nyJMP4FA2gmbfRdA4K2GGc+ln1ndOPZ+6y85lf7mV+WlgTHDbPbxd2aHC++4zZiFE50iwFv6jqsJr\\n1lzxjJXScOCQ1q8jhUW4B0jR778FAwZB2mBUYrI7WDtOmMjq655k+a5GhsSFcO/UNEKCDEyloK4O\\n8y5rxDE15WyMZpmookJszEh2MmbbStTpt2FM9OS/1vnxmIBdOzEGeJqvjXufxPzHCtQlVxFut5GR\\n2ImsXq5hMJ1HNvSpl5DQjrcRQgQceXVLHBatNbrZeNvdqroSYhMwbnrAms/ZDru2+d42PAJqqjE/\\n/A8cykONHI2y2aygnjqQg2EJzHtvL0t3OMhIDOWBHw0k3G5zv+6kt2/01OnjVehm+Yj1oTwO3WGN\\n3a1adP5S/QagppwNGWNQzYYrVYOGYbv1t9Y71501sqlZP6br7/8aD/0Z44E/eZrSm72+JIToPeTO\\nWhwW/eYr6HdeR02/EJyNmDfd0z3H1Rr96ftWz+jEFO+V+308h3UlsXh1iTUf5gmSn8xawJ+/LcFW\\n4+CuMwYwIS3S+zWe4JBWPwL091+hTj3Dmn4ny/ODJG1wq682uikftDF+CjoxBQaP6PIxVNOQpsZv\\nHsO88xdHNN55rzRkZE+XQIhuIcFadJo+lId+53Vr+oN/A1A38QwYNvrID+56DSshGTrTm7nlHWx4\\nBA6nZsnX+azeVU5GYhi3n55KSqSPgTGapUtUF/wM/fZKr+xcOs96Fq7OuQR1BHe9naG6KZiouATU\\ndXegMsd0y/F6C9vdi3q6CEJ0C2kGF53nGrqzmYZd27vn2Af3AWBcfg1Ex6AmTcO4+3EA1MRprTZX\\n4d6jdFWGRPG7D/ezelc5l45O4Pcz0n0HaoBQz48BdeFMa6KiHN00sAm5e4m8+AqMS3/RDRU7doxT\\nz5AhNYXopeTOWnReU7IKddZFVqcuoLEpn/OR0uVN7wvHxqMMG+qXtwJgPPcPnwN/0C8Nguw0mJpP\\nk07g5UNpVDlruGVSKtOGtj/IiDHnbsw3/oJxw3wrI1Z4BPqd163m/Z9dAw31BPUb0O4xhBDiWJJg\\nLQDrmTHZm2HkaJ/DNGqt0WXWe81q9InuYN2wYwvaNI88y5NrcI8WCTBUkO9TVKcO5N83LuWNr/ZQ\\naY9gRFQID0xI9R50pA0qbQi2W37rWdDoSZepVy4DIPSkSbQxfpkQQhxz0gwuANBfrfMkqfC1/qU/\\nof+21JoZeTzqjHNQZ5yDMz/Xk4bySBQXQHgkqhOvHtU4nDyyLpflGwoZVnmAezcu4w/nDO5UoPap\\nZVKPAYOwDziCUcWEEKKbyZ21sBQ0JYEoaB14dWE++tMPAFCnT0cFh6BmzUEf2I1e9x56zw7UYTQb\\nm6v+CTHxGBPO9HzHgT3Qf2CH+xbVOHjoowPsLavnulOSOV83QmU8RsvRwY5EsxSYQgjhDyRYC4u2\\nxsbGR9DTO7cCoGbNQZ1+lmdFajoE2dEfr4KJUzv3NTnb0VnLrelTz0AphZm1HHZtQ51/Wbv7bi+q\\n5Q/rcql2mNw3NY2T+kcC8Z363sNhXPXrbj+mEEIcCQnWAgC9d6c1UVqELsz3GvCDEqsXuJowFWXz\\nDJ2pbDYryUb2JnT2JpRrkI/2vmffLs9MRRnExKFXWSkoVbOczlprqhtMahtNthbW8sneCr46UEVC\\neBCPnJ3OkLjuG6nLuOl+9L4c1KDhmGv/C8OP67ZjCyFEd5BgLSyFVvO3/vQD9KcfoCb9CHX+ZVbz\\ndllJ0/PkkFa7xfzfHMpfWozeu8sdrLXWUJiPakoT6aXZSGEU5lujd0XHosZNgPgkNuZX8/b2UrYU\\n1lJZ7xmGMz4siEuOi+ey4xPaHmu7i9SYU1BjTgHAdvxJ3XpsIYToDhKshaXCewhR/fka9OdrsD3/\\nljUMaGSUz92iLrua8leWQnmpZ9/P1qD/8hTqFzejhmSgUtNoNDU7i+uoqgqmPnE0htaE7SshLLYW\\ne1As+20D+M+qfWwvqiUuLIgJaZGkx4QQZjcYGB1MRlIYho9e6kII0RdIsBaYX661kmIk9bPudlvQ\\nNVVtZoZSSkFkFHrVm3Dp1dbCpjSNevlT5IfG8c7Vj7Judznl9SYwHI4fbm13EDi4F06YDRpS6hr5\\n1fgUpg+LIdgmLyoIIYSLBGuBfqFppLAhI9EtgrUuKoDNGyDzhLYPkNgPKptlzLIFUW6PIGvQdN7r\\nPwmVXcr4+lxOz15DQn05waYDE4M6WzD1tmDqbMFEjZ/ECRf9WO6ehRDCBwnWAvqnQ94+1Pgp6K/W\\nea1ypZF09xb3QY0eh965Bd3YCDYbG0oa+dP426iwh3PWwa+ZOW00sUue8t7nnJ+g3/uHZz78ZAnU\\nQgjRBgnWAmqqUKdNhzGnoC6YiTrzHPSat9Hv/t29iXHWRW3vHxqOQ9n44LkVvJM+hQMRkxlQc4j7\\nv3+ewdX5YNvltbnxm0dRwzJxfvq+J4e1awQzIYQQrUiw7uN0ZbnV2zshycoH/T9XWCtGjfMK1rQz\\n6Mnm+lCePvUOCsISGFlXxQ3Z7zI1fz0hY0+GDfmw9Xuv7dWwzNYHad6MLoQQwosE6z5Ob/jCmmj5\\nmlWz96zVz65F9Utrta/DqXl67U5eLhtICsXcu3EZJyYGofI2Y9z2IGSegHn9/7T53eqiK9Cv/hl1\\n9iWoGe3cuQshRB8nwbqvq60BQI2d4L08Psk9qc48r9VuhdUOHv04l+ziOmYMi+XqfzxBWGkBlDX1\\n4k4Z4J0Q5LgTYcsGr2MY086Haed3Tz2EEKIXk/dj+rraalAGtEig0TyLlrLbvdZtzK/mtnf3sL+8\\ngUcuOp65E1OJfPR5SEyxxtUOCYW4BK99jDl3H706CCFELyd31n2c/uIj0J1PcfndwWoe/OgA/SLt\\n3HXmAE7NSCYvrykJSFi49ZmY0irNpgoOQV0/r91e5UIIIXyTYN3LaK2hphoV4XsQk1aKD3X62Otz\\nq3jk41wGxgTz4PR0okJaDPsZ3DQcaWV5650BY/yUTn+XEEIID2kG72X0R+9g3nJFq8FNfG5bUw2A\\nOvVMn+uNux7DuGMhWmtW7Sxj4doDpEUHs+BHA1sHasD4v5sg8wSMufd5FoaEda0iQggh3OTOuhcx\\nX12C/vA/1kxRgVePbp9Ki6zPcaf6XK2GZlBU4+DZjw6wPq+asf3C+c0ZA9pMpKFS07Dd/pDXMuOR\\n56G+/rDqIYQQwpsE617EHagBGho63iH/AAAqLrH1sbRm9a5yln97CKepufbkZM4fGYfNR77r9qjI\\naOhki7wQQgjfJFj3ErpFxy1dUUp7YVXv2IL55z9YM/HewbrR1Cz+8iBrcioYkxLO3An96BcV3M0l\\nFkII0VnyzDrA6cZGtGm6m7TVzOsgOBhy93q2KcjDzFqObtYcrbM3eQ4SE++erG80eWTdAdbkVDBz\\nTAK/mz5QArUQQvSwbruzzsrKIisri0WLFpGenk52djbPP/88DQ0NJCUlceONNxITE9NdXyewOoiZ\\nN/8vjDkFGh0AqIFD0QOHovfudG9nvrbEypw1eDhERGL+7QUIj3CvVzbrGXSNw8nCtblsLqjhV+NT\\nOG9k3LGtkBBCCJ+65c46JyeHHTt2kJRkjXplmiZPP/0011xzDU899RSjRo3ilVde6Y6vEk201lag\\nBvjhG8/42wMGoYZmwM6tVnpLgOoq6zNvP+bTD8HB/bBrGwBq0jQAnKbmsY/z2HKohltOS5VALYQQ\\nfuSIg7XD4WDZsmVce+217mU5OTkEBweTmWklbJgxYwaff/75kX6VaKIP7vc95nZcIioiEjVuImCl\\nt9TFh2DPDmu/t/8GNHu2nZiC8ctbAVjxXSHfHqzmhvEpTB0iLSBCCOFPjjhYr1y5kilTppCcnOxe\\nVlRURGKip9NSdHQ0WmuqqqqO9OsEoLdt9LncmN/UYSzB839hLvfOI01jo2c6ygrKa3LKeXNrCeeP\\njOXcEXJHLYQQ/uaInllnZ2eTk5PDlVde2V3l8dK/f/+jctye1B11qoyKwpX9OXrmtVT87QUAUkeM\\nxAgNQ6ckc8C18fYfWu2vwsLRtTUYpcUcdIbx3FfZnJIey30XjCPIdvi/33rj/xP0znpJnQJHb6yX\\n1KnrjihYb9myhdzcXObOnQtAcXExCxcu5LzzzqOoqMi9XUVFBUopIiMP74Vb95jTvUT//v27pU7O\\nJYsAMB5eSlVCMjQF64PFJe4xuY1n38Ccfal7H3XBz9Bvr7Smr5uH/tNv+WLASTyR9R3JEXZuPjWJ\\nQwUdj3rWUnfVyd/0xnpJnQJHb6yX1Klzx2vLEQXriy++mIsvvtg9P2fOHObPn09aWhoffPAB27Zt\\nIzMzk9WrVzNp0qQj+Srhg2oxQlnz5BnK7v26lUob7H5arYeO5K2f3stLxdEMjw3h/qlpRPsYPlQI\\nIYR/OCqDohiGwdy5c1m6dCkOh8P96pY4MrqxEfOpBQCo6Re6lxuPLYfCglbbq8t+gc5abs00jdFt\\n2kN4YXM1/y2OZtLAKG49LZWQIHndXggh/Fm3BuvFixe7pzMyMnj88ce78/B9mt7+A3rzBnB1Lhs8\\n3L1OxSZAbEKrfYyzL8HcvQNGHAex8Whg6dirWLWjjJ8cF8+scUkY6vCGDxVCCHHsyXCjAcJcdI/X\\nfFuZsloybrgTsN7LfvGCe1lVFc1Pjovn5+OSWuWcFkII4Z+k/TMA6H05XvPG7Q+hjM7/12mteWlD\\nIf+piubCzDgJ1EIIEWDkzjoAmA/eYk2EhKImTkVlntDpfbXWvLqxiH9uLeG8EbFcc1KyBGohhAgw\\nEqz9nHY63dPGA39q1QO8PU5Ts/SbAv67o4yzhsVw/fgUCdRCCBGAJFj7Ob38jwCoq2YfVqAuq23k\\nsU/z2FRQw0+Pi+cq6UwmhBABq9c9s9Z1tZhff9wqv3Mg0lqjv/sS+qejTvtRp/c7VOXgzlV7yS6q\\n5ZZJqfz8xGQJ1EIIEcB63Z21eePPANBLH8NY+q/AbvY9dBDq61ATp7Ua5KQtBVUN3Pv+PqodJg/P\\nSGdEQthRLqQQQoijrdfdWXs5dLCnS9Bl2nSiP37Pmolr/Q61L/mVDdyzeh81DpMHp0ugFkKI3qJX\\nBWvzg7e9FxQGZrDW2zZi3nAJes9OANT4KR3uc7CygXve30ddoxWoh8WHHu1iCiGEOEZ6TbDW9fXo\\nvy0FQM2wcj2bb72GmfViTxarS/TmDdbE9h8gqR/K1v643Qeb7qjrnZrfTU9nqARqIYToVXpPsP7s\\nfc/M4BHW5+5s9Ko3e6ZAR0D/9++emZD2A29uRQN3r96Hw9Q8NH2gBGohhOiFek+wfnWJe1oNHwUx\\ncT1YmtZ0fT1mXW3H27XsxV5S5HtDIK/C6kzWaGoenD6QwXESqIUQojfqNcGa2HgwDIwn/oqKT/J6\\nzmt++j7ml2sx33oV7XD0SPHMB+Zw8OoLOt6wvMT6jEu0PkN9dxLLr5RALYQQfUXveXVLgzptOioq\\n2ppPTvWs+sufPNs5nahLZh3bomkNxYcwAaOsBPOFx1FDRmL89P9ab/vlOgCMq29Cl5eiXE36zZTX\\nNbLgw/00OE0ePCtdArUQQvRyvSJY60YHVFVARJRnYRudsnRB7jEqVTPVle5Jc8UzsP0H9PYfoEWw\\nNte8jX5jOYSFQ/pQjMjoVodyOE1+vy6X4ppGfjd9IEMkUAshRK/XO5rBd20HZyNqaIZnmT3E97Z5\\n+49NmZrbs8MzXVrsnmz5fFq/ZvVmN257EOUjUGutWfJ1AVsLa7lpYiqjksKPTnmFEEL4ld4RrOub\\nOm41GzxEjZ+MOv8yjAeftRa4gl9tzTEtms7ZjvnUbz0LDuz2TDsavDeOirE+Bw33eax3sstYvauc\\nS0cnMGVw62AuhBCid+oVzeC4Oo3Z7e5FKsjufjZt3PJbGDIS/fbf0Gv/e8yKpQvzMX8/r+0NHA0Q\\n3KwFwB6MmvQjn0Okrs+t4oX1BYwfEMmVYxOPQmmFEEL4q15xZ60bm4J1kN3nejX6RFR4BIRHQkO9\\nZ/ujUZbvvkC77p6bv3blK2NWg3VnrU0n+rsvoKIUomNabZZTUsejn+QxODaE20/vL0k5hBCij+kl\\nd9ZNzckdJbsIjwBAb/iiU0N4Hi5dWYG5+GEA1NTzUBljrOnxU1DX3Ebsrs2UPHavV7n1rm2Yj9zp\\nXqSGj/I6ZmG1g999dIDIYIN7p6YRZu8Vv6+EEEIcht5x5e/gztpFjT/Dmti17eiUo7TQPak/ehdz\\nyaPW9/7v9SibjYip52I8vBQ1a7a1zbaNmH991vsYqenuybLaRhas2U99o8n90waSEN5+/YQQQvRO\\nveTOuvUza19UVDQMHII+Wtm4an2PUKaiPE3bKqkf5CeiAf3yYmthWAQ4G6GhHuKTACitbeTe9/dR\\nWO3g/mkDGRTbRu92IYQQvV6vCNb6HyusiQ7urAEwTfjhG3R9HaqDcbcPW53V09y4YyHmonsAULPm\\ntN5u5BjrPXCn09r+V/MhYwxUlqPsdspaBOrjU+QVLSGE6MsCuhlcm07Md9/odDM44Ol9fRTSZ+ra\\namsiNgGaOoGp037UajsVEoJxx0LPgsholM2Gio2npLaRez+QQC2EEMIjoIM1W7733FUDyui4OsaP\\nL7cmmu5qG03dztaHqaLM+gyPxHjyFYw/voJq6wdEYopnuukd8PzKBu5atZfCagf3TUuTQC2EEAII\\n8GZwnX/APe3qtNUhW1OVGxt5bWMhr28qZlh8KFMGRTNlcDTxYV3/J9FffwKJKZ7xydsT3SwrWFwC\\ne8vqeWDNfhqdJg9OT2dkou8EHkIIIfqegA7WzcfcVkMy2tmwGdeY4Y2NTB8ai6lhfV41L357iL9s\\nOMSYlHBGJoQxND6EjMSwTvfA1tmbvIcV7YAyDIx7HofEFL7Lr2HRJ7kE2wwenjGIdOlMJoQQopnA\\nDtblpZ7pZlm22uVqlnY2khxp58qxSVw5NokD5fWs3VPBZ/sq+XtBMa7W8eOSwpg2NIaJaZFEh7b9\\nz6X37zns4te/w+/yAAAL30lEQVQOGMprG4t4a1spA2OCuW9qGimRHbwrLoQQos8J2GCt6+vRX3xk\\nzSij8z27g5qq7Gz0WpwWE+IO3A6nye7SejYW1PDBrjIWf5nPsvWKCzLiuWRUPJEhPjJ6NfUEVxfO\\n7FQxPttXwZKvCyirc3LeiFh+cVIyIUGB3YVACCHE0RGwwZp9u8DRgPrlrRiTpnV+v2bPrNtitxmM\\nTAxjZGIYPz0unt2l9fx9SzFvbC7m3R2lXH58Av+TGQ9aQ3Wl9R51bQ0E2TEuuqLDIryxuZiXvytk\\nWHwI905NY0SCPJ8WQgjRtoAK1rqmCqqrYH8O5nOPAKAGDTu8g9h831m3RSnF0PhQ5k0ewKWj63j5\\nu0KWf1vI7pJ6Ztd/R9Brf7Yye9XXWnmoO7BqZxkvf1fIGYOiueW0VGyGjPMthBCifQETrPWhg5j3\\n3NB6RdxhZqBqagbXjY0cbpgcEhfKfVPTyNpUzCsbiyhsjOYuWwgR2zZad9ah7d8hf5pTzHNf5XNS\\nagQ3TZJALYQQonP8/iGpdjjQhfmQt6/VOjVxKqoTd7Ne2nhm3VlKKS5LrOOW0aFss8XxwNjrqVi5\\nAv3lWghpO1jvKa3j7rc2MSg2hDunDMBuk0AthBCic/z+zlr/4yX0+2+1XhGXiHHNbYd/wMNsBvcq\\ny6ZvITEF8/7ZnAGEJYxi0eiruOfEX/ObTS/Rv6mTWUultY08+NEBIkJskjlLCCHEYfP7qKG3bfSa\\nN+beB1gpKLvEFawdbQdr7XSi61on5TCfWoB536/d8+PjFfdnaMoj4rnz5BtZ+5M7W42IVt3g5KGP\\nDlBZ7+TJn4wlUTJnCSGEOEx+fWdtrnwBDuyB2HgoK0Fdcxtq7HiMZ7IguIvvI4c2veLVUOdztTad\\n6OV/RH+5FmPpv1BNY3zr+nqv7Yx5D6NGHs8JwJOjHfzh41yeyq7jL/t2MjIhlGHxoSRH2HlrWyn7\\ny+v5zRkDyEiJIi+v0se3CiGEEG3z62Dtav42rr7ZSowxaixgJcLoKmW3grxe/xmcd2mr9eYNl3i+\\n/8u1aGcjbPsBdfFV3humDnRPJkXYefScQazPrWbd3gp2l9bxTW41Gohuavo+qX9kl8sshBCib/Pr\\nYO02YBAqNr57j7l3J9p0ogwfA5w00cuecE+rsy70Wtc8RzWAoRTj0yIZn2YF5RqHk/I6J3FhQYTK\\nYCdCCCGOQGAE68Pt8d1ZFWVWOssmvp5Tu9fl5x7WocPtNsLtbf8QEEIIITorMG75grs3sYVyNX+X\\nlnivcKW49GXTt91aBiGEEKKzAiJYuzp5ddvxThhvTdRUeS3X337m2Wb8FO91X3wI0bEYNz+AseCZ\\nbi2PEEII0Z7AaAbvbq5m9RbvReu/vwSAcd+TUFON/vpj7/0Gj0Adf/KxKKEQQgjh5td31mriVOg3\\noPsPHGoFa11eijad1nTTJ2A9x46Mbl2eISO6vyxCCCFEB/z6zrpLI5R1Rpg1LKh+bSnk7kXNmgNN\\nHcjUT36Oio5Fh0W03i+idQAXQgghjja/DtZHTbOEG3rdezBrjvt5tRpzivVpt6OuvhkVHQPDMtHv\\n/xs1+aweKa4QQoi+rU8G65bvVutvPkH/61Vrpl+ae7lx+nTPPhf97zEpmxBCCNGSXz+zPlbMJY+6\\np1VQn/z9IoQQwo/13WCt+m7VhRBCBJa+G7HsTdmvUpr1Nh+W2TNlEUIIIdrRZ4O1+vlciEtETT3X\\nWhCfiO03j7a/kxBCCNED+uwDWmPCmTDhTMyv1jUt6d5R0oQQQoju0mfvrF2Ua/CTbh7SVAghhOgu\\nfT5YExnV0yUQQggh2iXB2jVASpC9Z8shhBBCtKHPPrN2S+yHmnoeauqPe7okQgghhE99Plgrw0Bd\\n+eueLoYQQgjRJmkGF0IIIfycBGshhBDCz0mwFkIIIfycBGshhBDCz0mwFkIIIfycBGshhBDCz0mw\\nFkIIIfycBGshhBDCz0mwFkIIIfycBGshhBDCz0mwFkIIIfycBGshhBDCz0mwFkIIIfycBGshhBDC\\nz0mwFkIIIfyc0lrrni6EEEIIIdomd9ZCCCGEn5NgLYQQQvg5CdZCCCGEn5NgLYQQQvg5CdZCCCGE\\nn5NgLYQQQvg5CdZCCCGEn5NgLYQQQvg5CdZCCCGEnwvq6QK0lJeXx+LFi6mqqiIyMpK5c+eSmpra\\n08VqV2VlJc888wz5+fkEBQWRmprK9ddfT3R0NNnZ2Tz//PM0NDSQlJTEjTfeSExMDEC76/xJVlYW\\nWVlZLFq0iPT09ICvU0NDAy+99BI//PADdrudkSNHcsMNN7R77vn7ebl+/XpWrlyJa0DCSy+9lAkT\\nJgRUnVasWMGXX35JYWGh+1zrqJyBUD9f9WrvmgHt/x35w99YW/9XLi2vGYFcp7auF3CMzz/tZxYs\\nWKDXrl2rtdZ67dq1esGCBT1coo5VVlbqTZs2uedXrFihn332We10OvXcuXP11q1btdZav/HGG3rx\\n4sVaa93uOn+ya9cuvXDhQj179my9d+/eXlGnZcuW6eXLl2vTNLXWWpeWlmqt2z/3/Pm8NE1TX331\\n1Xrv3r1aa6337NmjZ82apZ1OZ0DVaevWrbqwsNB9rrl0tQ7+Uj9f9WrrmqF1+39H/vI31tb/ldat\\nrxkdldvf69TW9ULrY3v++VUzeHl5Obt372by5MkATJ48md27d1NRUdHDJWtfZGQko0ePds+PGDGC\\noqIicnJyCA4OJjMzE4AZM2bw+eefA7S7zl84HA6WLVvGtdde614W6HWqq6tj3bp1zJw5E6UUALGx\\nse2ee4FwXiqlqKmpAaC6upq4uDgqKysDqk6ZmZkkJiZ6Levq/4s/1c9Xvdq6ZkBg/I35qhP4vmZA\\n4NapresFdP3c7Cq/agYvLi4mPj4ew7B+QxiGQVxcHEVFRe7mIX9nmiarV6/m5JNPpqioyOs/Pzo6\\nGq01VVVV7a6LjIzsiaK3snLlSqZMmUJycrJ7WaDXKT8/n6ioKLKysti8eTOhoaHMnDmT4ODgNs89\\nwK/PS6UUt956K4899hghISHU1tZy1113tfv3BP5dJ5eu1qG9df5UP/C+ZkBg/435umZA4NapretF\\nZmbmMf/78qs7697gxRdfJCQkhHPPPbeni3JEsrOzycnJ4ZxzzunponQr0zQpKChgyJAhPPLII1x5\\n5ZUsWrSIurq6ni5alzmdTt58803mzZvHs88+y/z583nyyScDuk59iVwz/Fdb1wtXK9ax5Fd31gkJ\\nCZSUlGCaJoZhYJompaWlPptb/NGKFSvIz89n/vz5GIZBYmKi+1cWQEVFBUopIiMj213nD7Zs2UJu\\nbi5z584FrDuchQsXct555wVsnQASExOx2WycfvrpgNX8GBUVRXBwcJvnntbar8/LPXv2UFJS4m5G\\nzMzMJDQ0FLvdHrB1cmnvmtBeHQKlfi2vGUDAXjfaumbMnj07YOvU1vXi4MGDJCYmHtPzz6/urGNi\\nYhg8eDCffPIJAJ988glDhgzxu2YrX1599VV2797NvHnzsNvtAAwdOpSGhga2bdsGwOrVq5k0aVKH\\n6/zBxRdfzJIlS1i8eDGLFy8mISGBe+65h4suuihg6wRWE9vo0aPZuHEjYPXYrKioIDU1tc1zz9/P\\nS1dAy8vLA+DAgQOUlZUFdJ1c2itnV9f5C1/XDAjc60Zb14yxY8cGbJ3aul7069fvmJ9/Suumdz38\\nRG5uLosXL6a6upqIiAjmzp1L//79e7pY7dq/fz+33347qampBAcHA5CcnMy8efPYvn07S5cuxeFw\\nuF9JcHVQaG+dv5kzZw7z588nPT094OtUUFDAc889R2VlJUFBQcycOZMTTzyx3XPP38/Ljz/+mDff\\nfNN9d3bZZZdx6qmnBlSdXnzxRb766ivKysqIiooiKiqKJ554ost18Jf6+arXrbfe2uY1A9r/O/KH\\nv7G2/q+aa37NCOQ6tXW9gGN7/vldsBZCCCGEN79qBhdCCCFEaxKshRBCCD8nwVoIIYTwcxKshRBC\\nCD8nwVoIIYTwcxKshRBCCD8nwVoIIYTwcxKshRBCCD/3/4XviCyLJ73OAAAAAElFTkSuQmCC\\n\",\n \"text/plain\": [\n \"<Figure size 576x504 with 1 Axes>\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n }\n }\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"ZDCC88yEFYYM\",\n \"colab_type\": \"code\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 448\n },\n \"outputId\": \"cafcf6ad-67e9-4b7d-bae6-7008acbf50e8\"\n },\n \"source\": [\n \"dfcomp = yf.download(['jpm','AAPL', 'GE', 'GOOG', 'IBM', 'MSFT'],start = start,end=end)['Adj Close']\\n\",\n \"dfcomp\"\n ],\n \"execution_count\": 9,\n \"outputs\": [\n {\n \"output_type\": \"stream\",\n \"text\": [\n \"[*********************100%***********************] 6 of 6 completed\\n\"\n ],\n \"name\": \"stdout\"\n },\n {\n \"output_type\": \"execute_result\",\n \"data\": {\n \"text/html\": [\n \"<div>\\n\",\n \"<style scoped>\\n\",\n \" .dataframe tbody tr th:only-of-type {\\n\",\n \" vertical-align: middle;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe tbody tr th {\\n\",\n \" vertical-align: top;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe thead th {\\n\",\n \" text-align: right;\\n\",\n \" }\\n\",\n \"</style>\\n\",\n \"<table border=\\\"1\\\" class=\\\"dataframe\\\">\\n\",\n \" <thead>\\n\",\n \" <tr style=\\\"text-align: right;\\\">\\n\",\n \" <th></th>\\n\",\n \" <th>AAPL</th>\\n\",\n \" <th>GE</th>\\n\",\n \" <th>GOOG</th>\\n\",\n \" <th>IBM</th>\\n\",\n \" <th>JPM</th>\\n\",\n \" <th>MSFT</th>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>Date</th>\\n\",\n \" <th></th>\\n\",\n \" <th></th>\\n\",\n \" <th></th>\\n\",\n \" <th></th>\\n\",\n \" <th></th>\\n\",\n \" <th></th>\\n\",\n \" </tr>\\n\",\n \" </thead>\\n\",\n \" <tbody>\\n\",\n \" <tr>\\n\",\n \" <th>2012-01-03</th>\\n\",\n \" <td>50.994907</td>\\n\",\n \" <td>13.639091</td>\\n\",\n \" <td>331.462585</td>\\n\",\n \" <td>139.934006</td>\\n\",\n \" <td>27.864342</td>\\n\",\n \" <td>22.020796</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>2012-01-04</th>\\n\",\n \" <td>51.268970</td>\\n\",\n \" <td>13.787668</td>\\n\",\n \" <td>332.892242</td>\\n\",\n \" <td>139.363144</td>\\n\",\n \" <td>28.040850</td>\\n\",\n \" <td>22.539021</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>2012-01-05</th>\\n\",\n \" <td>51.838169</td>\\n\",\n \" <td>13.780239</td>\\n\",\n \" <td>328.274536</td>\\n\",\n \" <td>138.702209</td>\\n\",\n \" <td>28.626539</td>\\n\",\n \" <td>22.769344</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>2012-01-06</th>\\n\",\n \" <td>52.380054</td>\\n\",\n \" <td>13.854527</td>\\n\",\n \" <td>323.796326</td>\\n\",\n \" <td>137.109772</td>\\n\",\n \" <td>28.369787</td>\\n\",\n \" <td>23.123066</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>2012-01-09</th>\\n\",\n \" <td>52.296970</td>\\n\",\n \" <td>14.010525</td>\\n\",\n \" <td>310.067780</td>\\n\",\n \" <td>136.396225</td>\\n\",\n \" <td>28.321667</td>\\n\",\n \" <td>22.818701</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>...</th>\\n\",\n \" <td>...</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>...</td>\\n\",\n \" <td>...</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>2018-08-23</th>\\n\",\n \" <td>211.061111</td>\\n\",\n \" <td>11.874558</td>\\n\",\n \" <td>1205.380005</td>\\n\",\n \" <td>135.556732</td>\\n\",\n \" <td>109.777924</td>\\n\",\n \" <td>105.249374</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>2018-08-24</th>\\n\",\n \" <td>211.717331</td>\\n\",\n \" <td>11.836680</td>\\n\",\n \" <td>1220.650024</td>\\n\",\n \" <td>136.181503</td>\\n\",\n \" <td>109.730087</td>\\n\",\n \" <td>106.071335</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>2018-08-27</th>\\n\",\n \" <td>213.460739</td>\\n\",\n \" <td>12.092353</td>\\n\",\n \" <td>1241.819946</td>\\n\",\n \" <td>136.787628</td>\\n\",\n \" <td>111.672455</td>\\n\",\n \" <td>107.245552</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>2018-08-28</th>\\n\",\n \" <td>215.184570</td>\\n\",\n \" <td>12.082885</td>\\n\",\n \" <td>1231.150024</td>\\n\",\n \" <td>136.694382</td>\\n\",\n \" <td>111.127060</td>\\n\",\n \" <td>107.891388</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>2018-08-29</th>\\n\",\n \" <td>218.397171</td>\\n\",\n \" <td>12.281741</td>\\n\",\n \" <td>1249.300049</td>\\n\",\n \" <td>137.580231</td>\\n\",\n \" <td>110.763466</td>\\n\",\n \" <td>109.613571</td>\\n\",\n \" </tr>\\n\",\n \" </tbody>\\n\",\n \"</table>\\n\",\n \"<p>1676 rows × 6 columns</p>\\n\",\n \"</div>\"\n ],\n \"text/plain\": [\n \" AAPL GE ... JPM MSFT\\n\",\n \"Date ... \\n\",\n \"2012-01-03 50.994907 13.639091 ... 27.864342 22.020796\\n\",\n \"2012-01-04 51.268970 13.787668 ... 28.040850 22.539021\\n\",\n \"2012-01-05 51.838169 13.780239 ... 28.626539 22.769344\\n\",\n \"2012-01-06 52.380054 13.854527 ... 28.369787 23.123066\\n\",\n \"2012-01-09 52.296970 14.010525 ... 28.321667 22.818701\\n\",\n \"... ... ... ... ... ...\\n\",\n \"2018-08-23 211.061111 11.874558 ... 109.777924 105.249374\\n\",\n \"2018-08-24 211.717331 11.836680 ... 109.730087 106.071335\\n\",\n \"2018-08-27 213.460739 12.092353 ... 111.672455 107.245552\\n\",\n \"2018-08-28 215.184570 12.082885 ... 111.127060 107.891388\\n\",\n \"2018-08-29 218.397171 12.281741 ... 110.763466 109.613571\\n\",\n \"\\n\",\n \"[1676 rows x 6 columns]\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n },\n \"execution_count\": 9\n }\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"2A201lhUFc_3\",\n \"colab_type\": \"code\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 225\n },\n \"outputId\": \"4cb7bc13-c5a9-4d51-b050-200148706a16\"\n },\n \"source\": [\n \"retscomp = dfcomp.pct_change()\\n\",\n \"\\n\",\n \"corr = retscomp.corr()\\n\",\n \"corr\"\n ],\n \"execution_count\": 10,\n \"outputs\": [\n {\n \"output_type\": \"execute_result\",\n \"data\": {\n \"text/html\": [\n \"<div>\\n\",\n \"<style scoped>\\n\",\n \" .dataframe tbody tr th:only-of-type {\\n\",\n \" vertical-align: middle;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe tbody tr th {\\n\",\n \" vertical-align: top;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe thead th {\\n\",\n \" text-align: right;\\n\",\n \" }\\n\",\n \"</style>\\n\",\n \"<table border=\\\"1\\\" class=\\\"dataframe\\\">\\n\",\n \" <thead>\\n\",\n \" <tr style=\\\"text-align: right;\\\">\\n\",\n \" <th></th>\\n\",\n \" <th>AAPL</th>\\n\",\n \" <th>GE</th>\\n\",\n \" <th>GOOG</th>\\n\",\n \" <th>IBM</th>\\n\",\n \" <th>JPM</th>\\n\",\n \" <th>MSFT</th>\\n\",\n \" </tr>\\n\",\n \" </thead>\\n\",\n \" <tbody>\\n\",\n \" <tr>\\n\",\n \" <th>AAPL</th>\\n\",\n \" <td>1.000000</td>\\n\",\n \" <td>0.231933</td>\\n\",\n \" <td>0.358248</td>\\n\",\n \" <td>0.284489</td>\\n\",\n \" <td>0.302823</td>\\n\",\n \" <td>0.361851</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>GE</th>\\n\",\n \" <td>0.231933</td>\\n\",\n \" <td>1.000000</td>\\n\",\n \" <td>0.311084</td>\\n\",\n \" <td>0.413162</td>\\n\",\n \" <td>0.469752</td>\\n\",\n \" <td>0.313180</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>GOOG</th>\\n\",\n \" <td>0.358248</td>\\n\",\n \" <td>0.311084</td>\\n\",\n \" <td>1.000000</td>\\n\",\n \" <td>0.338729</td>\\n\",\n \" <td>0.365240</td>\\n\",\n \" <td>0.486786</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>IBM</th>\\n\",\n \" <td>0.284489</td>\\n\",\n \" <td>0.413162</td>\\n\",\n \" <td>0.338729</td>\\n\",\n \" <td>1.000000</td>\\n\",\n \" <td>0.428698</td>\\n\",\n \" <td>0.408897</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>JPM</th>\\n\",\n \" <td>0.302823</td>\\n\",\n \" <td>0.469752</td>\\n\",\n \" <td>0.365240</td>\\n\",\n \" <td>0.428698</td>\\n\",\n \" <td>1.000000</td>\\n\",\n \" <td>0.423728</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>MSFT</th>\\n\",\n \" <td>0.361851</td>\\n\",\n \" <td>0.313180</td>\\n\",\n \" <td>0.486786</td>\\n\",\n \" <td>0.408897</td>\\n\",\n \" <td>0.423728</td>\\n\",\n \" <td>1.000000</td>\\n\",\n \" </tr>\\n\",\n \" </tbody>\\n\",\n \"</table>\\n\",\n \"</div>\"\n ],\n \"text/plain\": [\n \" AAPL GE GOOG IBM JPM MSFT\\n\",\n \"AAPL 1.000000 0.231933 0.358248 0.284489 0.302823 0.361851\\n\",\n \"GE 0.231933 1.000000 0.311084 0.413162 0.469752 0.313180\\n\",\n \"GOOG 0.358248 0.311084 1.000000 0.338729 0.365240 0.486786\\n\",\n \"IBM 0.284489 0.413162 0.338729 1.000000 0.428698 0.408897\\n\",\n \"JPM 0.302823 0.469752 0.365240 0.428698 1.000000 0.423728\\n\",\n \"MSFT 0.361851 0.313180 0.486786 0.408897 0.423728 1.000000\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n },\n \"execution_count\": 10\n }\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"emt_IgD8Fges\",\n \"colab_type\": \"code\",\n \"colab\": {}\n },\n \"source\": [\n \"import math\\n\",\n \"import numpy as np\\n\",\n \"from sklearn import preprocessing\\n\",\n \"dfreg = df.loc[:,['Adj Close','Volume']]\\n\",\n \"dfreg['HL_PCT'] = (df['High']-df['Low']) / df['Close'] * 100.0\\n\",\n \"dfreg['PCT_change'] = (df['Close']-df['Open']) / df['Open'] * 100.0\\n\",\n \"dfreg.fillna(value=-99999, inplace=True)\\n\",\n \"forecast_out = int(math.ceil(0.01 * len(dfreg)))\\n\",\n \"forecast_col = 'Adj Close'\\n\",\n \"dfreg['label'] = dfreg[forecast_col].shift(-forecast_out)\\n\",\n \"X = np.array(dfreg.drop(['label'], 1))\\n\",\n \"X = preprocessing.scale(X)\\n\",\n \"X_lately = X[-forecast_out:]\\n\",\n \"X = X[:-forecast_out]\\n\",\n \"y = np.array(dfreg['label'])\\n\",\n \"y = y[:-forecast_out]\"\n ],\n \"execution_count\": 0,\n \"outputs\": []\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"-WeqmxZMFpnr\",\n \"colab_type\": \"code\",\n \"colab\": {}\n },\n \"source\": [\n \"from sklearn.model_selection import train_test_split\\n\",\n \"from sklearn.linear_model import Lasso\\n\",\n \"X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.33, random_state=42)\"\n ],\n \"execution_count\": 0,\n \"outputs\": []\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"3lUYeEpQF3Eb\",\n \"colab_type\": \"code\",\n \"colab\": {}\n },\n \"source\": [\n \"clflasso = Lasso()\\n\",\n \"clflasso.fit(X_train, y_train)\\n\",\n \"confidencelasso = clflasso.score(X_test,y_test)\"\n ],\n \"execution_count\": 0,\n \"outputs\": []\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"dlSop_ESF61C\",\n \"colab_type\": \"code\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 34\n },\n \"outputId\": \"d4fcadeb-8b01-4c8e-f841-2c7f56898d6b\"\n },\n \"source\": [\n \"print(\\\"The Accuracy of our Model is %r\\\" %confidencelasso)\"\n ],\n \"execution_count\": 16,\n \"outputs\": [\n {\n \"output_type\": \"stream\",\n \"text\": [\n \"The Accuracy of our Model is 0.9806857462238061\\n\"\n ],\n \"name\": \"stdout\"\n }\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"WQEzaQ8NGH71\",\n \"colab_type\": \"code\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 101\n },\n \"outputId\": \"5db69305-95ff-4dfa-e1d5-4e0b5d023aee\"\n },\n \"source\": [\n \"forecast_set =clflasso.predict(X_lately)\\n\",\n \"dfreg['Forecast'] = np.nan\\n\",\n \"forecast_set\"\n ],\n \"execution_count\": 18,\n \"outputs\": [\n {\n \"output_type\": \"execute_result\",\n \"data\": {\n \"text/plain\": [\n \"array([111.34130043, 111.5623028 , 110.72433671, 109.66539761,\\n\",\n \" 107.97107304, 108.67090898, 107.79611405, 108.78140282,\\n\",\n \" 108.78140282, 108.64327267, 109.28785802, 108.96556167,\\n\",\n \" 108.74456664, 108.6985306 , 110.56779948, 110.04292987,\\n\",\n \" 109.69301924])\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n },\n \"execution_count\": 18\n }\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"ToRQqQbgGMRX\",\n \"colab_type\": \"code\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 448\n },\n \"outputId\": \"fa4b99e6-83da-45ed-8867-4dff5cd6afbc\"\n },\n \"source\": [\n \"next_unix = datetime.datetime.now() + datetime.timedelta(days=1)\\n\",\n \"\\n\",\n \"for i in forecast_set:\\n\",\n \" next_date = next_unix\\n\",\n \" next_unix += datetime.timedelta(days=1)\\n\",\n \" dfreg.loc[next_date] = [np.nan for _ in range(len(dfreg.columns)-1)]+[i]\\n\",\n \"dfreg['Adj Close'].tail(500).plot()\\n\",\n \"dfreg['Forecast'].tail(500).plot()\\n\",\n \"plt.legend(loc=4)\\n\",\n \"plt.xlabel('Date')\\n\",\n \"plt.ylabel('Price')\\n\",\n \"plt.show()\"\n ],\n \"execution_count\": 19,\n \"outputs\": [\n {\n \"output_type\": \"display_data\",\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAAfwAAAGvCAYAAAC+SGdKAAAABHNCSVQICAgIfAhkiAAAAAlwSFlz\\nAAALEgAACxIB0t1+/AAAADh0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uMy4xLjMsIGh0\\ndHA6Ly9tYXRwbG90bGliLm9yZy+AADFEAAAgAElEQVR4nOzdd5ycVb348c95tve+2ZKeDUkoSaSE\\nAAlIjYIFrt2LeuUaxUu8lmtDBUV+YgFEhFgIooJSrlIULwGUHqUmBEghdTdte9/ZvvOc3x/nmbY7\\nuzuzO7Mzu/N9v168ZuYpZ87OLvnOad+jtNYaIYQQQsxoVqwrIIQQQojok4AvhBBCJAAJ+EIIIUQC\\nkIAvhBBCJAAJ+EIIIUQCkIAvhBBCJAAJ+EIIIUQCSI51BaKttrY21lWY0SoqKuQzngLyOUeffMZT\\nQz7n6KqoqBj1nLTwhRBCiAQgAV8IIYRIABLwhRBCiAQgAV8IIYRIABLwhRBCiAQgAV8IIYRIABLw\\nhRBCiAQgAV8IIYRIABLwhRBCiAQgAV8IIYRIABLwhRBCiAQgAV8IIYRIABLwhRBCiAQgAV8IIYRI\\nABLwhRBCiAjTHW3Y992B7uuNdVW8JOALIYQQEWbfci366b/B7jdiXRUvCfhCCDGN6bojuL/wEXRj\\nbayrIhz6SDUcO2Sex9HvRQK+EEJMY/rwQejrhSM1sa6KcOhXXwDLMv/VHY11dbySY10BIYQQk9DR\\nCoBub0HFuCoC9OED6Feeh6XLYWgIXR8/AV9a+EIIMZ11tJnH9tbY1kOgbTf2T66G1mascy9Glc2G\\nuqNorWNdNUACvhBCTG/tYwd87XZj//0vcTVbfMZqboT+PtS/X4lauRrKZ0OPC7o6Yl0zQAK+EEJM\\na9qvSz+ofTvR//sb9O9vm8JaJQ77f3+De/37zIuGYwCoirnmsaTcHG9uGL+cJx/G/fPve1+39Azi\\ntiPbMyABXwghpjMn4I/apW+7AdCvbYmbruWZRP/9L+ZxcBBdbwI+ZZXmsbjUnGtpHL+cP/0W3noN\\n3eNiX0svVzx8gM372iJaVwn4QggxnXnG8DtGCfj9/b7ndUeiX58EEvAFytUJ9ccgMxuyc82xohLz\\nGELA99q3m99uM9fvaozsMIwEfCGEmKZ0fx/09pgg09sTdJxe9/f5XoQTeMT4/LvquzrQDcegrBKl\\nzHoJlZ4JWTmhfe5FTm/AnjdZPisLgLbeoYhWVwK+EEJMV57W/bxFga/9+QV83dY8BZVKHLpmv+9F\\nRxscOYiqnBd4UVEpujmEgD9gemL0m6/xkZOKWFeVz5GO/ogOw0jAF0KI6arbBYCaVWFeuzpHXuPf\\nwm+TpXsR5TdEot98BXq6USe8I/Ca4tJxW/haazObPzffTPw7uIc5eal0Ddh09LsjVl0J+EIIEQd0\\nj8s36StUvSbgU1JmHru7Rl4z4AT8nDwYbSa/mJjWRkg2+ev0v56GpCRYtjLgElVUCi0NY7fU+3vB\\n7Uad/S5ITUO/8TJz8tIAONzeP/p9YZKAL4QQccD+wVexr/l8eDf1dAOgik3A164gAb+/H5JToLBE\\nuvQjTDc3wpyFoCzTJb9oKSozK/Ci/EIYGDDpj0fj9NRQVIL139eizjyfipxUAOpdg+PW43B7P3e+\\n1jDuMr4pSa1799138/LLL9PU1MRNN93E3LlzxzwOUFtby8aNG3G5XGRnZ7NhwwbKy8unorpCCDH1\\nnE1WtNbeSV/j0U7Ap3SMFn5/L6SlQ0ExNNVFoqbCo7UJNX+xSZ/b24NasGTkNZ4Z+65OyMgMOKXr\\nj6HKKr2/N5WVg1pyEgBFtiZJQUMIAX/T1gberO/hrLk5zJk9+nVT0sJftWoV1113HSUlJSEdB9i0\\naRPr1q3j1ltvZd26ddxxxx1TUVUhhIitgTC6cHudgF80y7Qygwb8fkhLQxUUgrTwI0Jrjd6x1YzN\\nF5aYlRIA86pGXKv8A75/GXt2YF/zeeznH/e18LOyveeTLEVJVgoNroFx61OQbtruO5vGXsY3JQF/\\n6dKlFBcXh3y8o6OD6upq1qxZA8CaNWuorq6mszPIhBQhhJiGtKsT9w1fxX52c+D4rid4hKKn2+zI\\nlp4BWVmjBPw+SE2H/CLo6Q5cpicmZvcb2LdeB7btXU4HoOaPDPiMFvBr9prHp/7m+71l5QRcU5qd\\nElILP8mJ5G/Wd495XVyO4be0tFBYWIhlmepZlkVBQQHNzfLtVAgxM+jdb0L1XvQffwm7t/tOhBvw\\nM7PMEEBWLgQZw9cD/b4ufYA2mbg3Wf6Z81ShXw918ayRFzsBX3cNa7AeOmAeaw+jdzm//2EBvyzE\\ngN/lzOQfL1HPjN8et6KiItZVmPHkM54a8jlH31R+xm31h3E6csk8sNv7vDg7k7QQ69GCZiAnj/KK\\nChoKilCDA5QOu7cRG3Jzya06jiagKEmRHuO/pen+t9ze68Lz1WrWylOwf/4H3C3NZFRWjrjWzsvl\\nGJCXBDkVFbTe9gMG9uzEXb2XtJWrGKzZj/3CkwBUVC1GpaZ5760qH+DJ/R3kF5eSmTp6uO7TteRl\\npNDRO/aXg7gM+EVFRbS2tmLbNpZlYds2bW1tQbv/x1NbWxuFGgqPiooK+YyngHzO0TfVn7F7+6tQ\\ndTwceBvX1he9x5uPHEblFIZWRksTpKZTW1uLOzUNWptG/Azuzk7IzafFWc7dfGAPVknsAu5M+Ft2\\nH9wLsyqxvvFjGrUFGbkwO5e2ID+X1hqSkmi/82e0v7wFdmwFZwhnYF4VavW58KsfQ04edc2BvS8Z\\nthl+eWP/Eeblp40o26Olq48TS9KpdyWNWe+4DPh5eXnMnz+fLVu2cPbZZ7NlyxYWLFhAbm5urKsm\\nhBCT5v7pNXD4AOo9HzVL5Y4d8p3sC6NLv7fbO/NbZeWgj1aPvKa/z7QaC4rMa+nSn7zGOigpQ+WM\\nH5OUUqZbv6PNG+zVR9eDqxN15gWowmKsn90LgyMnaxZlmhDd1js0ZsDvGnCTm5bEv68YOQHe35QE\\n/LvuuotXXnmF9vZ2rr/+enJycvjpT3866nGA9evXs3HjRh588EGysrLYsGHDVFRVCCGiSg8Nwu43\\noHIe6oL3offtDMjEpnt7GG1Rnv3EQ3D0ENZ/ftkc6On27cyWlRN0DJ+BPkhPR6WlQ0YWtLWg9+8G\\npVCLlkb2h0sAWmtorEctPiH0m6wkz80AqMp5qKXLvadVVjaQPeK23DRzX0ffyJz6u5t6mJefRnqy\\nRfeAm+zUJCpzU8esxpQE/CuuuIIrrrgi5OMAlZWV3HDDDdGumhBCTK3OdgDUee9BZWWjikvRe4AV\\nq+CNV0adtKe1Rv/5d+b5pzagklNMKtcMJ9FLdg4M9KMHB1Apfv/w9/ebWfoABUXovTvQz/wfAEmb\\n/hqNn3Bm62o3uQ082Q1DMTzDYYj35jkBv3NYel1Xv5tvPnmYxUXpfO/cOdgactLG7s6HOJ2lL4QQ\\nM5azwY3Kc8bp+8w4rfKkZB2tS7/Wb2vb+qO4b/yWCSSezG6eGd7dXeiuDux7foF+/SVf4h0wS/P8\\nhg+0bUfkR0ooxw4DoCrmhH6P/7LLpGTf8Mo4stOSsNTIgL+3xczG39fSR52zTl8CvhBCxJt2ZwOb\\n/AIA1FkXmMeVp0Nq6ugt/B1bfc9fehb27jAvhszMbJXtBHxXF/ZdP0M//zj2A3fC0BCkOeO/npam\\nZxhAtssNmz5WY57Mnh/6TStXm8fkZCgqRVnjB2cASylyUpPo6DMB39aarn43e1t8uRTufr0J8HX/\\nj1le6DUWQggxWbrDCfhOC1+ddApJm/6KKioxY+yjrcNvazZfCJKS0E88bI7Nq0KdutY892vhU3/U\\nPPcE9LQM817nvwdS07A+st4crz+K7uqM6Bas4bDv34S96eaYvPeEHa2BnDxUbkHIt1if+zrWbfdD\\n+RwoHyP3bRC56Uk0dA9ysLWPjS/Xc/mf93Hfm80UZSZz3sJc3mwwfy+htPDjcpa+EELMWB1tJg1u\\nbt7Ic+mZo2+y0ttjZnunZZhtWRctJembP/Gd92Z064KuDtRpa9GvvmCOOSlbrbPfhV67zpvZzX70\\nfji0H3X5f6HWXhSpnzBk+qlHzePHP+dMXIt/+uih8Fr3gEpOhuRkrCu/CSljT6wbLjctie113exo\\n6PHO2gc4aVYmnz55Fp19bo52DlCeM365EvCFEGIqtbdCbn7wbt30DHRv8PSouq8H0jNRF38I3n4T\\ndcH7Ay9wWvi6rdmk0509H2vtRWYm//JTvZd5l4nl5EG1Se/qmUg4lfxT/No3Xo115TdQZeG1fqea\\ntm2oPYw6590Tul+Vhr8BXG6aCdNDtqbBNcj7lxbwrsUF5KUnkZWaxDXnhj6XQAK+EEJMEb1jG3rL\\n32HuouAXZI7Rpd/bAxmZWKefA6efM/K8Zwy/4Zh5zMlDLVsxal3Uxz5r6nTHjd55AFPKM+wAcOwQ\\n+omHUZ/6wtTXIxy9PTA4AIXhJ4GbqLz0wC+GZTmpVIyz/G40MoYvhBBTxP7tz8yT0ba/TcsYu0t/\\n2Paq/lRqGqSkoutMIB0vKYx12lqs09aaLubBqQ/42ll1YH3jx1A5D113ZJw74oB3k5upG34YcAfO\\nrwil6340EvCFEGKqeDYEc1rXw6mUFDOrPpi+HlT66AEfMN36npZzTn5odUpJCbuFr/t60J1tYd0z\\nQt0Rs0Rt/mKTxKb2CNp2j39fLPWYHQ/UsE1uoql3MHDpZFl2yoTLkoAvhBBTQLvd0NGOuuTDo2e4\\nS04ePfj29o7ZwgdMt76zzp8Q0r6a90wx3dRh0A/djf2z74V1zwjNDVBUYia0VcyB3m7sz10WsPww\\n7gTZtz7aPn1yCZcsKWBleRZJCkqzJOALIUR862gDbfu2qQ0meYzWtl/e/FH5tzyzg6wCCCYlNfyA\\n39rsyycwQbq3GzJN4FTlvoln9tP/N6lyo0l7uvQzpy7gz8pO5bOnzuK8BblcVJVPkjVa4uXxScAX\\nQoip0NYMgBory1pyStDxdO12w0C/WbY3Fs/EvaTk8b8ceIw1jDCavl6TwW8y/OckVM7zHd+xDd3R\\nhv3Xe3F/7dMxyxEQVI+nhT91Xfoe5yzI48pVYaTzDUICvhBCTAUn4I/dwk8Gd5Dg65nIl5Ex5luo\\nXGfc3j1klt+FIjkVHWYLn75eGBiY3Jh7T7dJNASonDysb/4E60vXmV6QYzXoR+83mQHrj038PSLN\\n06U/hS38SJKAL4QQUWb/4y/Yv3aS5Iy1pGu0Ln3P2vxxWvjqwkshNx9OOnXM6wKkhD+G7/0C0j9y\\nS9eQ9fagPPsAgJnXUGi2d9Vdnd7jeue2ib9HpHV3QVq6mVw5Dck6fCGEiCLd2YZ+5I++A2O1DpNT\\nwO1G2zbK8muPORvqeHfGG4UqKSPp5rvD2xQnJfgwQjB63y7sR+6Bbicg94cwkXA0weYkeLIFNtX5\\n3nPXdrjgfRN7j0jrcU3b1j1IwBdCiKjSLz1rMt8tXW72oB+rq93TchwaxP7nP1DLVpjsc72hdel7\\nBHxZGE9y6ug79A2j9+2EvTt9B/r6Rr94rHLcbvOZDP8Ck5UFSqE975GZDbWHJ/Qe0aC7XVM6Qz/S\\nJOALIUQUaK1h93Y4uBcKS0j6n/83/k1Jzj/J7S3oe3+NrphL0nW3+wLyeJP2JiIlBbpC7NL3jGF7\\n9E8s4Ht/nmEtfGUlmYDq7ASoTjwF/doLaNsd8g5z0aD37jBfurq7YjJhL1Ik4AshRDQc2I19y3fN\\n85PPCO0ep4WvDzo57pOd1z3OGP44XfoToZJT0KFm2usZHvAnOFN/rJ8nO9dsAJSSClXL4JXnoKM9\\n5D3ko8G+8VvmSeU8mFURs3pMlkzaE0KIaPBrDat5VaHd4wR4Du4x93m2UvXk108PrUs/LOOsw9d9\\nvbi//Tn0nh2+degeE23hO5MQ/SfteXnG8UvLzZbBAK1NE3ufSHN1oabxGL4EfCGEiALt8gVHVTE3\\ntJs8LXrPLnapaeax9jCkpUNeiOlywzHeOvymemisQx856GuZO7Qzhq+7OnH/90exX34utPfsDd6l\\nD/gSBpWUe1c06Nbm0MqNAu2/EqGrHXILYlaXyZKAL4QQ0eAyM9nVuz8IJ54S2j3JzihrzT7z6CzR\\n0zX7YF5VdMaxx0ut60nV29cTZAzf6dJvqoPeHvSdN4e2pr939C595UyKU7PKvcv0aIthC7+53vfc\\ntqGgMHZ1mSQJ+EIIEQ1dHZCcgrrsEyZffAhU8rD13YOD6KFBOHIQNX9x5OsIpkt/aIwufc8mOX29\\n0DNKl77/lr5vvTbuW/rmJARp4XvmE5SUmy8EaRkQwxa+/xJBAJUvAV8IIYQ/Vydk54ae8Q58Y/gO\\nPTQIR2tgaAi1IFoB36zDHzWFbUe7eezrhe7ALn1vAp5e33G9a/v47+nt0h/Zwtee8f2cPPPZFRaj\\nmxvGLE53tqNDXFoYDl13BPsXPwo8mBe7yYOTJQFfCCGiQDsBPyzDewIGB9ANteZ5qPMAwpWSClqD\\ne5Q0uZ4Wfrdr5Kx8p4WvPQF89oIQA/7oLXy18nTzZO5C83ruQji4Z9QvJHpwEPsHX8H+5Y/Hf98w\\n6VeeN6l+/UmXvhBCiACuztC3qPUY3qU/NGSGBsCkzI0Gz3uO1q3vjOHrlsaR5zxd+k4XvTr1LGiq\\nRzfWjbzWX7fLpKgNMtSh1l6EddsDqOJZ5sCSk8xnUHckaFH6pWdMl/+u19F7doz9vuFyhhesb9/s\\nVM6CnCj9HqaABHwhhIiGrk5U2C384WP4AybYWVb0Urp6svuNshZfeybtecbRU1PNY06er8Xf222y\\nCJ58prlnvFZ+Z8eoX2CUUii/5YdqyUmmzD1vBa/fs5vN+vjMbPSLT439vuEaHIDMLJhXZX43efmo\\npNglAJosCfhCCBENE+nSTxnW4h0aNAE/Jy+8dLlhvacTwEdLvuPp0u9oNY+zF5ggmJXjS63b22Oy\\nAJZVQmEJetfrY76l7moPvceipMx8jkeqR5bTVA+HD6DOPA8WH48+sCe0MkM1OAApaWYuQW4e5E3f\\n7nyQgC+EEBGn3W6TlW4yLXzLMpPpOttNazpaUsbr0m8PeGldejnW1TdBWjrav0s/M8u0zo9fCW+/\\nhR5rbX8YP5NSyiQcCvKFRG/7l7nm5DNRC5dA/VEzdyJSBgd8n0/FPNScBZErOwYk4AshRKR1OkEy\\n3EDtH/CzckwL39UZ1YDvXQoYLKAO9AfMwAdMBryySsgrgH27sO+8Gb37De8EPLXiNHPP22+M/qad\\n7ahw5iSMlg2wZj+UlKGKZ6EWLTPHDkaula8HBrw9INZV30L9++cjVnYsSMAXQohI86TGdWaah8x/\\nEltWjgnCne2oqLbwPV36QQKqZ/zekwJXKRPoAeuj62HJieiXnzPd/Z5rTjgFLAv71uuw7759RJHa\\nPWS+xIQT8JOTzRLF4WX1dvs2s5m/GDKzsJ94KLztgccyOODNdqiSU0LOpxCvJOALIUSE6b07TKAI\\nNYe+R0ALP9t0sztj+FHjtyXvCJ6eilJnw5jsXG+PgCopI+kL15htf8G7pl6lpKDWXASAfuHJEYHa\\n7mg3ywDDbuEHqV9fr69nIS0N9cFPm+17d449hyBkA/2+z2cGkIAvhBARpvfugEVLw28R+gf87Fwz\\nNt7XG92A78nff7QG9y9uCEyN67TwlWeHuCArBTwb/ATMrP/oZ1Af+6x5cbQm4Hq3p8xwA36wOQY9\\n3QHJe5STwlhHarOdoUFfD8gMIAFfCCEiSA8OwLFDvjHlcPgFfJWVY3K3Q/TW4IM3oOk//gpefwn8\\n1rJ70+p6WvjBUuGWm4RA/pvMqJRU1AqTQEcPG1O321vMk3C+xDjZAEfo7UFl+O0gmO1070dq4t5A\\nv28DoxlAAr4QQkRSa7Ppsi6ZFf69/mu8PcELUOEm8AnH8C5r9xB6+8vYW/5uZugrBaVl5lywzHhl\\nleaJZ9meR2GxWcY2LOC725zrwhrDH2XSXl9PYAs/JdUE6OHb+E7U4MDI/Q2msek9A0EIIeKNk5FO\\nFZWGfWtA3n3/7vPMnJEXR0rKsBbs0BD2849DSxNqyYmmJe7ZpS9YC3+WCfjebn+HUgoWHjeihe/2\\nZOwLY027SklBD2vha9sdMIbvlZ0DrkgF/EFfoqEZQAK+EEJEkDcFrWdr14lK8wvEWVEM+H5j7wC6\\nv9ekvu1xmSx7uQWmJQ2oYC38wmKsb/wYZs8feW7hEvTrL6G7Or29FEPHDkFeQdCyRpWcMnJSoWfj\\nnvRh5WTloCPVwh/oH/mFaBqTLn0hhIik1iaTc72geHLlBMzYH7mrXMSkpQe+7naZMfDuLmhvNelk\\nT10LK1ah3n950CJU1bKASXve4wuXmCfVvlb+4JFD3l6BkAVbh9/rBPwRLfzciHbpz6QWvgR8IYSI\\npJZGyC+c/Jpt/9nh0cqjD2bM238oocdlAqZtQ2MdKrcAlZlF0obvoArC3Bp2XhUohX3b9ei9OwHT\\nwldls8MrJ9ikPc82upmBX4ZUVoS79GVZnhBCiGB0azMUTbI7HwJn7EdxpriyLEj1a+V3tgfuc59f\\nMPGy09JR7/4QAPqV59BdndhdHSbnfjiSU4K08J0teYd36WfnQPfkZ+lr2w3uIenSF0II4WM/9Sju\\n733BvGiqR012/B4zUW3K+HXH66b6wHO5Ew/4ANZll0PVMnTtYWg4CvjN7A9VSioMDaJtGz04gL35\\nz9i//Zk5N7xLPysHursnn21vwPmCMYO69GXSnhBCTJK+f5N5rN5rxvAXvG/yhU7lcrD0DOhwng/f\\nyz5vcgEfQFXMRW/9F9pTdmnF2DcM53z5sT93KRy/Evy3380YNr8hOwe0Db3d6MzswJUP4fAMISTP\\nnIAvLXwhhJgsJzjbm/8MgFq2cuJlzauC498xtRne/CfueVYZONQkW/gAVMw18wKq95nXhWFOaPT/\\nLPyDPUDGsMmCWWY1gH7w99hfvyJoDv6QDDqJhKSFL4QQwiuvwATK118yCWUq5k64qKTv/BQAvW+X\\nOTAVgT/IDHuvvMln+VMVc9GAfus1rIIikyAnHMOHN1LTzJI5GNHCV3n55r1eeNIcOHQAFi0N6W20\\n1qYMpXxd+pJaVwghhJdnkhugVp098W5kf54u/akIOMOX5vkLI0HOqCqdL0AtjSSXlod///DhjXy/\\nOg2f0LhkOaw83ftSH3g75LexP/t+9H2/Ni+cSYJhfzmJYxLwhRBiEvTgoOmuzspBrT4X9YH/iEzB\\nnlbtFORyD7aGHqVMd/ZYrf9Qy88t8HbjJ00k4A8PuvlFWNf/AnXFl0d8uVJJSVj/9S2sn94DxbPQ\\nb76KHhoa9y08k/z0M4+ZA4Mzb9KeBHwhhJiMLrOFrPq3T2L955cjt2e6J6/+VAQcT1AvKfMdy8mD\\n3ILI9FaA2a8eSC4tG+fCkYavWFD5Raiy2VhnnBv8eqVQOXmoquNhz1vYX/gw9v/979hv4rfsT7vd\\n6O0vmRfSwhdCCAGYDWYAFYHZ7AHcTqt0+Cz0aEgzAV+tPhd1/nvNpMHM7IjM0Pfw7C0QtDdhPCNa\\n+KENM6gPfAr1if+CoSH0rtfHvri/z/tU//U+9OYHg7/3NCaT9oQQYjI8W8hGYja7v4p5qIsuRZ17\\nSWTLDcYThNPSsd73MQDsh/8AmZH7sqGWnIT++19ILp8T/s3Dx/ALQgz4+YWos9+Fe+d2qDsy9sX+\\nAf8xv94ACfhCCCEAdLuz3WsEZrP7U5aF+tAVES1zVOnOpD2/yXvWZcHz5k+UWrEK69pbyVx1Jh11\\ndePf4G/4LP288FL8qpxc9N6OsS/yC/gBZAxfCCGErj+KfuhusxQv0i38qZTma+FHk5qzYGJzAvxa\\n2WrtRahly8O7PycPurtMutzROAFffejTZoLhSaea49Hcx2CKSQtfCCFCYP/xV+iudpKu/Ca6rxf9\\nu59DTi70uLCuuz1yk/ViwenSV2lxmjfeL9ud9ckN4d+fnQdam50Ac/KCX+MJ+POPw/rxXWZNfk83\\nKksCvhBCJBRdvRcOHzCb4xzaj976TzOTPjMbNYlEO/FApaejIXATnXgy2X0Fckz2Pbo6Rg/4A06X\\nvtPLoZSCGRTsQQK+EEKEprMdtEa/8pzZJx7A7YZZYeaFj0ezZpv1/hNZIz8VJrmvgMrJM19oukbf\\nRU/3O5n74rWXIwIk4AshxDi01t719vqlZwPOqXgNkmFQ5bNJ2vinWFdjdJOdKe9p4bvGmLjX72RL\\nTJt8oqF4JQFfCCHG09sDQ0OmBXzskDmWnmFS6oa785sI32S79LNNN77u6mDUKYMJ0MKXWfpCiISk\\nuzqx/3b/mDO3dWMt9p/ugg7Tha/eebH3nLr0E+bJTOjSj3eeFv7cRRO7P9szhj96l763hR+v8xgi\\nQFr4QoiEpP/0G/SLz6DmLYaTTjHHtMb+f19GXfB+rDPOxf7fu+CNV7yzxFX5HNSXr4NZlWY8f8uT\\nJn2riCqVlIT11Rt8m/CEe39yskki5AzLBNXfD8nJ03u1xTim5Ce7++67efnll2lqauKmm25i7lzz\\nS6utrWXjxo24XC6ys7PZsGED5eXl454TQohJczZU0d2dvm7erg44fBD274IzzvWmt9UvPGHO5+ah\\n/FqZSd/9+RRWOLGpJSdOroCiUnRz4+jn+/tmdOsepqhLf9WqVVx33XWUlJQEHN+0aRPr1q3j1ltv\\nZd26ddxxxx0hnRNCiEnzpJPtdvmOtTQBoNtazOuGWvPY5Uz2yo1sNj0xddSsSqg/OvoF/X2+jIMz\\n1JQE/KVLl1JcXBxwrKOjg+rqatasWQPAmjVrqK6uprOzc8xzQggREcr556+1yXfM87ytGd3XC80N\\ngePGnrFgMf2UVUJzI3poMPh5aeFHT0tLC4WFhViWqYJlWRQUFNDc3DzmOSGEiIjebvPY4gv42hPw\\n21ug9jBojXXxB73n1STXg4sYmlUB2oameu8hvWs79v2bzPOB/qinFo61mTs7wVFRITNoo00+46kh\\nn3NkNbkH6QNSutqZVVHBUN1R0o8cpBfA1UVedwdtQOmKU+n/wrcZPHSAAvkdREQs/pb7T1xJI5D+\\n3GPkXHY57b++if63tgJQ/tb5404AACAASURBVIVv0aRtyM2ldAb/jmMW8IuKimhtbcW2bSzLwrZt\\n2traKC4uRms96rlw1dbWRqH2wqOiokI+4ykgn3PkuVtMj+FAQy21tbW4118acL59+2sANA4MoZaf\\nDstPp1d+B5MWq79lbZmVFj1P/R89z2wG2/aeq317F3ZXJ+TmT/v/z8b6MhWzLv28vDzmz5/Pli1b\\nANiyZQsLFiwgNzd3zHNCCBERPc5kvY420507jD6032yNmpE5xRUT0aAys1Cf+R/zwi/YA2YIx9WJ\\nmuFd+lMS8O+66y6uvPJKWlpauP766/nKV74CwPr163n88cf54he/yOOPP8769eu994x1TgghJq2n\\nG/KcLW0PH/QeVqetNU8O7Ye8wolt5yriknX6OUG3u9WvvwQtjTDZpX9xbkq69K+44gquuOKKEccr\\nKyu54YYbgt4z1jkhhJgMbdtm69NTz0K/+gLaGctV//HfqFVno1/7p5nglTeN97gXweXm+3p3HPqJ\\nhyAjE7X63BhVampIal0hROLp6zEBfV4VpGWg33oVAFVQjEpJ9e0aJwF/5vHPpZDq25RHnXQaKn3m\\nbpwDEvCFEInIk2wnOxdmz4Mj1eZ1gTMx2EnhqvIKY1A5EU0qx2ykw0mnYt34O9+JhcfFpD5TSQK+\\nECLx9Jg1+CorCzV3oe94QZE5XlxmXgcZ7xXTnNPCV7n5KL/fr1ogAV8IIWYcffBt8yS/CHXWBd7j\\n3i7d7Bzz2NczxTUTUefp0s/NCzw+Z+HIa2eYGZ94Rwgh/OmBfvTfHoDjToB5VWYWfl6BN7MngFp1\\nDvrJh1FrLoxhTUVUeAJ+jtPS//SX4NghVMrMz6IoAV8IkVhq9kFnO9YnrvIuubNuuIPy0hLq281+\\nHaqohKRb/hjLWoooUbn5aABnLN8687yY1mcqSZe+ECKh6CM15sn8xd5jKjUNS8brE0P5HEhKQlXM\\niXVNppy08IUQieXIQdO6kyV3CUnNqsD6+f2o1LRYV2XKSQtfCJFQ9NEamD1fMuglsEQM9iABXwiR\\nQLTthtrDqDkLYl0VIaacBHwhROLodsHgABSVxromQkw5CfhCiMTRaxLukJkV23oIEQMS8IUQicOT\\nYS9DZuSLxCMBXwiROJyAL3vci0QkAV8IkTikS18kMAn4QoiEoXsk4IvEJQFfCJE4PC38DAn4IvFI\\nwBdCJI6eblAWpKXHuiZCTDkJ+EKIxNHbAxmZKEv+6ROJR/7qhRCJo6dbxu9FwpKAL4RIGLq3W5bk\\niYQlu+UJIeKW3rsD+/GHUIuWYl3y4ckX2OMC2QZXJChp4Qsh4pb9+EPw1mvov9yL7mxHDw5i3/sr\\ndN2RiRXY2yMz9EXCkoAvhIhLWmuo2QcLjgNto//1FOzfhX7mMexrr0L39oRfaE83Srr0RYKSgC+E\\niE9tzdDVgTrjXJhXhd6xDX1wj/e0fvPV8Mvs7ZFJeyJhScAXQsSnmv0AqHlVqHmL4GgNunovlJab\\ndfQHdodVnNYa+ntlDb5IWBLwhRBxR7e3Yv/lj5CSCrPnm/+6u+CNV1CLlsHCJej94QV83ENg25Ca\\nFo0qCxH3JOALIeKOfu0FqD2M9bmvo1LTUJXzfSeXLjdB/+ih8Mbx+/vNo7TwRYKSgC+EiD/tbZCc\\nDMtPM69nz/eeUqetRc2eB9qGlobQy+zvM4/SwhcJStbhCyHiT2c75OajlAJAZWah1l5kWvcpKeis\\nHHNdtyv0MgecgC8tfJGgJOALIeKO7myD3IKAY9YnN/heeAK+qyv0Qp0ufZUmLXyRmKRLXwgRf5wW\\n/qicgK+7wwn4ni59aeGLxCQBXwgRfzrbUXkFo5+XLn0hwiYBXwgRV7Tthq6OsVv4qamQnALdnaEX\\nPOCZpS9d+iIxScAXQsQXV5dZLz9GwFdKQXZOWC187VmWJ136IkFJwBdCxA3d2Y79tf8AGLtLHyAr\\nZ4Jj+NLCF4lJAr4QIn4cqTate4CcMbr0AbKyTfa9UMkYvkhwEvCFEHFDt7eaJyefCQsWj31xVnhd\\n+r5Me9LCF4lJAr4QIn60twBg/eeXUSmpY16qsnLGbOHrQwdw33ItesdWc6C/D5JTUFZSxKorxHQi\\nAV8IET/aWyEzGxXKOHtW9pgtfL3tX7BrO/at16Gb6k2XvnTniwQmAV8IETd0ewsUFIV2cXYuDA6g\\n+4JvoKNrD0NGJiQno5982CzLk+58kcAkta4QIn60tUB+YWjXFpWax5YmqJznPayHBtEvPAnVe2HZ\\nSlR2DnrLP2DhcbIkTyQ0aeELIeJHRysqxICvisvMk6b6gOP62c3oe38NHW2oirmodf8Gbjfs3Sld\\n+iKhScAXQsQF7XZDRzvkh9ilXzzL3NfsC/h6cBD92J9818yqQJWWo04507y25J88kbjkr18IERf0\\ng78ze9yXVYZ2Q3YOpGdAc6PvWN1h6OowW+lmZKGOOwEAteZCc756b2QrLcQ0ImP4QoiY0q9tQdce\\nRv/9L6i1F6FWnR3SfUopKJ5lZuAD9kN3oxtqzbnz3xu4ne6y5ebRb6xfiEQjAV8IEVP2r3/ifa4u\\n+0R46+SLy2D7S9gvP4fe/GenEAtKKwIuU1YS1o9/AykyS18kLunSF0LEVqqTYOfkM1A5eWHdquZX\\nAaDvvNl3MC8flZIy8trCElRO7kRrKcS0Jy18IURsKQu1+lzU5f8V/q0Xfwh1+jnoR/6AbqwzY/Q9\\n3VGopBDTn7TwhRBTQmttHm03+thh83xw0KS8LatETSApjlIKVTwL6zP/g/Xl75uDzkQ9IUQgaeEL\\nIaJODw1iX/1Z1KlnQfls9D2/wPrva2HOAnNBVs6k30NlZGJdc4sZ1xdCjCAtfCFEVOn+fmhqgPYW\\n9D/+in78IQDsx/7kzYWvsicf8AHU3EWozKyIlCXETCMtfCFEVNkbPmRmznt4MuPt323y3UNEWvhC\\niLFJC18IETW6xUmKo23zWD7HPM5dZB737jCPEvCFiDoJ+EKIqNFvbQ14rc6+yDyeeZ45v8cJ+BHq\\n0hdCjE4CvhAiavTu7b4XmdmoNRei3vUB1FnnQ04e1B0x57JkfbwQ0SYBXwgRPY31vh3qCotR6ZlY\\nH/gUKj0TKuaa4ympE1qSJ4QIT1xM2tu2bRsPPPAAQ0NDZGdnc9VVV1FaWkptbS0bN27E5XKRnZ3N\\nhg0bKC8vj3V1hRCham+GpcvhjVegoDjglJpfhd7zFgwOxKZuQiSYmLfwXS4XGzdu5Itf/CI333wz\\n559/Pps2bQJg06ZNrFu3jltvvZV169Zxxx13xLi2QohQ6f5+cHWh5i+GjExUSeD6eHXJR2DRUlix\\nKjYVFCLBxDzg19fXk5eXR0WF2ezi5JNP5o033qCjo4Pq6mrWrFkDwJo1a6iurqazszOW1RVChKq9\\nxTwWlmD9zw9Ql3w44LTKyCTpmz/BuurbMaicEIkn5l36FRUVtLe3s3//fqqqqnjhhRcAaGlpobCw\\nEMsy30ksy6KgoIDm5mZyc0Of4OP5IiGiRz7jqTHdPue+plqagOLFS0hfcVqsqxOS6fYZT1fyOcdG\\nzAN+ZmYmX/rSl/j973/P4OAgK1euJCsri76+voiUX1tbG5FyRHAVFRXyGU+B6fg52/v3ANDiBjUN\\n6j4dP+PpSD7n6Brry1TMAz7A8uXLWb58OQDt7e08+uijlJSU0Nraim3bWJaFbdu0tbVRXFw8TmlC\\niLjQ1mweC+T/WSHiQczH8MEEeQDbtrnvvvu48MILKSkpYf78+WzZsgWALVu2sGDBgrC684UQsaHd\\nbvS+nZCVI0vuhIgTcdHCv//++9mzZw9DQ0MsX76cj3/84wCsX7+ejRs38uCDD5KVlcWGDRtiXFMh\\nRCj0vb+GHdtQ//bJWFdFCOGIi4B/5ZVXBj1eWVnJDTfcMMW1EUJMht79Bvr5x1HrLsN69wdjXR0h\\nhCMuuvSFENOXHhrCfd1/Y2/+s3ldvRcA9d6PxbJaQohh4qKFL4SYxt5+E47WoI/WoJecBB1tkJGF\\n8qTUFULEBWnhCyEmRb/2Aihlnm97Ed3eCnkFMa6VEGI4aeELISZMa41+41XUqrPR9cfQNftgaBDy\\nC2NdNSHEMNLCF0JMXGMduDrhuBNQ86vg8AFob0VJC1+IuCMBXwgxYfqgyaanFi6F+YuhtwdaGiFP\\nWvhCxBsJ+ELMMPaLz2Df+6upebODb0N6BlTMQS1a5jsuXfpCxB0J+ELEAX3sEPamm9GDg5Mrx+1G\\nP3wP+pnHzHh6lOma/TB/McpKgrJK7+Q9mbQnRPwJK+C/+eab/PKXv+RHP/oRAAcOHGDHjh1RqZgQ\\niUD392Hf9TPsB3+PfuU5qN4zuQLfes2bw97e/Ge01hGoZXBaa2ioRZXNBkApBStON88zsqL2vkKI\\niQk54G/evJlNmzZRXl7O7t27AUhNTeX++++PWuWEmPH27kC/+LQJ1IA+MLmAr/fsgNRU1Hs/Ctte\\nRD/+YCRqGZyrC3q7YVa595D1qQ2od38Ali6P3vsKISYk5ID/2GOPcc0113DppZd696ivrKyUbQ6F\\nmATdWBf4+uAkA35zAxTNMlnu5lWhd74+qfLG1Gj+31clvu04VXYu1r99CpWSEr33FUJMSMjr8Ht7\\ne0dsTTs0NERysizlF2LC6o8CThra2sPofTvRtg39fWAlhbzTnLbd0NQALQ1QPAulFKq0HH1of9Sq\\nrhucL/t+LXwhRPwKuYW/bNkyHnnkkYBjmzdv5oQTToh4pYRIFLruKCxcgvW+j8E7VkNnO+zajn3L\\nteg/bAytjL5e7G9fif2dK+FINaq41JwoKIa2luiN4zfWgrKgeFZ0yhdCRFTIAf+KK67glVde4aqr\\nrqKvr48vfvGLvPjii3zqU5+KZv2EmNnqj6LKnUlvp5wJeQXYj94H1XtH7d7X7a3o+mO+AzX7oLnB\\n97rICcAFRTA4AN1d0al7Yx0UlaCSpfteiOkg5P74goICfvjDH3LgwAGampooKiqiqqrKO54vhAiP\\n7u0xG83McgJ+cgrq7HehH73PXNBUjx7oR6UGduvb37kS+vtI2vRXU05LU8B55bS4VUERGkwrv60F\\ne9NNWBu+jSqtIBJ0axMUlUakLCFE9IUcrWtqamhpaaGqqoozzjiD4447jtbWVmpqaqJYPSFmsI42\\n81jgS1Kj3vkuSHK+h2sNdUdH3tffZ0739ZrXrU7Az8w2j4XOXJv8Iud8M/Zt10PdEfTrL0Wu/m3N\\nqILi8a8TQsSFkAP+bbfdhtvtDjg2NDTE7bffHvFKCZEQXJ2AmdnuoXILUOsug5NOBUxCnlF5vgw4\\nqWytK74EOXngDBHgBGO963Xv2nyqI5OMR9tuaG/1fbkQQsS9kAN+c3Mzs2YFTs4pKyujqalplDuE\\nEGNyAj5+AR/AuuwTWFd9G5JToDYw4PtPwNO1h81jaxMUFqNWrCLpp/eg0jPNBXkFoCz0nrfM61mV\\n6AO7IzOJr6MdbNv7pUIIEf9CDviFhYUcPHgw4NjBgwcpKJAUmsKw//HXwMlkYkzaE/Bz8kacU0lJ\\nUDYbfexw4Inebt9zJ+DT0oQKMpaukpKgqAScXgK1+hzTKve09ifDGUZQ0sIXYtoIOeBfcskl3Hjj\\njWzevJlt27axefNmbrrpJt7znvdEs35imtAD/egH7sT+8ddjXZXpoyt4C99DVc4d0cKno937VB8+\\nYNbstzZBYUnw95izwDxmZqEWLDHPh03y0wP9uH/5Q/SBt0Ovu+dLg7TwhZg2Qp6lf8EFF5CVlcXT\\nTz9NS0sLRUVFfPKTn2T16tXRrJ+YLjwTyFxRWgI2E7k6ISUVUkdJrlM5D15+Dt3j16rvaDWPVctg\\nzw6zW93Q4Khr4dXchWaiXkm5dwc73d6Ks8UN+q2t6H07YduL2G0tWFffiFIKvX83uqEW66zzg5ar\\nW52ALy18IaaNsNLknXHGGZxxxhnRqouYzjwBX4TO1Qk5uWbTmSBUxTyzrK7uCFQtBkA7M/utd38Q\\n+7brsTfdZK5dcmLwMuYsQgOqpMy3ZW1HCwD2X+/zLQEEqN4Lb78Jy1Zg//gb5tgoAZ/mBkhL960M\\nEELEvTED/vPPP8/ZZ58NwNNPPz3qdeedd15kayWmHwn4YdOuzlG78wGonGuuO1LtO+ZZyle1DE48\\nBXZsNfvRl88JXsbcheaxpMwE5+QUM44P6JeeMeUMDqLWXoR+4E70thdNwh5PHbUO+oVE1+yDeYtG\\n/bIihIg/Ywb8f/7zn96A/8ILL4x6nQR84VkbDuC+9XtYn9ggE7rG09UxdsAvLIGSMvTfH6G9z4Xd\\n22d6BVJTISML67JPYO/YCguXjt5LUFCE+sRVqBPeYa7JL4T2VvTgADQ3oFa/E+t9HwfA/cYr6Gcf\\nQz/7mK+A/l7wzPrHWSVwtAYOH0BdeGkkPgUhxBQZM+BfffXVgPmf/Morr6S4uJikpKQpqZiYZvxb\\n+Du2oXe9jlpzYezqMx24OlElo288oywLdeH70ff+mq4H73EOKlixygTvuQuxvnK9tydgNNbZ63wv\\n8gvNGH7DMZPYx69nQB2/Eu1s0+vV3R0Y8B/7E/qRP5jrFy0N8QcVQsSDkGbpK6X46le/Kt13YlR6\\neJf+8OVkIoC23aaFnzNGCx9QZ12AOv+9lN78O5hXBVpjnekbV1fLVqByQ18aq/KcFn6dWT6pymb7\\nzq29CPXRzwbe0OPy1bmrA735z75zEvCFmFZCnrQ3f/586urqqKysjGZ9xHTV1xPw0pMURgSn//kU\\n9PWiqpaNeZ1KTUN9dD1pFRVYH74C+6m/wUmnTPyN8wpg6z/Rd/zEvJ7lt5d9Wjrq/Pegyyuxf3+7\\nWe7nF/DZvxv6+0yvQmY2Kkj+ACFE/Ao54J9wwgnccMMNnHPOORQXB47Nyhi+oH9YC18C/pj04w+Z\\nFvIpZ4V8jzruRJKOCz4bP2R+LXoyskZszAOgjn8H1lXfxr7+S9Dt18JvqjdP5i5EZeVMrh5CiCkX\\ncsDfs2cPpaWl7N69e8Q5CfiCPjNpT138IejvQz/1KLrHhZJlWyNo24aWBtQpZ0z5MJlaeyFq2Qqw\\nFAwOjX5hlvm96R6Xd80+zfWQkSVL8YSYpsYN+P39/Tz44IOkpaWxcOFCLrvsMlJSZP9rMUxfLySn\\nYF32CfSu19FPPQoH9ozoftY7X4fZ81F5CZySuasD3G4oGCU7XhSp5BQoC2FYzhPU/cfwmxqgpEzm\\n8ggxTY07ae83v/kNW7duZfbs2bz88svcc889U1EvMd3095r14ACLT4DUNPRbr6Lrj6H37wJAb/0n\\n9s++i33N59H1QbZ9TRROWlrlt9497qRngGWh//Rb7Mf+ZI4110NJ8Ix+Qoj4N27A3759O9/5zne4\\n/PLLufrqq9m6detU1EtMN32+gK9SUmHZCvSbr2HfeTP2Ld9F73od+55fmDHk3h7T0k9UrfGfh14p\\nZZL0APrhe8wwRHMDqrgsxjUTQkzUuAG/v7/fuyNecXExPT0949whEpH2C/gA6uQzzT7th/bDQD/2\\nLd+F1DSs/74WMrMggVv4us2kto37PPQD/b7n7a0wNDRqzn4hRPwbdwzf7XazY8cO72vbtgNeA5x4\\n4iRnDotpR9cdQT//BO25efBuM1GPtHTvebX6nejnH4eDe1Dv+gB0daDe+1FUYYnZl70ucQM+bc2Q\\nnDx2lr14oizfMETR1M87EEJExrgBPy8vj1/+8pfe19nZ2QGvlVLcfvvt0amdiEt61+umxQ50Adb5\\n7zNd+plZ3muUZWF9/YfQ3Y0allxGlc1G79o+lVWOL23NUFAc/5Pf8ougvQW07VuSF8/zDoQQYxo3\\n4G/cuHEq6iGmEf3WNkhJRX3oCvS9vzJZ9fp6R3RRKyspeCa58tnw4tPo3h5URubI8zOcbm/x7VwX\\nx6zrbkc//zj6wd/D4QPmYL4EfCGmq5BS6woBYD//OHrHNvSRg2Zp3YknA6APH4AeF8pvDH8s3nSu\\niZqcp7M9rHS4saIys1BOrn196ICZxCcJd4SYtkJOvCMSm25tQt/zC7M/O6DOfhcUlaLSM9B/+IU5\\nGGoWuHlVpszqvYm5AUtXJ0yXtLSeLyaHDkBBUfwPQwghRiUtfBGahtrA15VzUZZFspPERa29CHVG\\naBkXVWGx2fp1/8isjTOddruhu2vcTXPiRl6+eezvlfF7IaY5aeGLkGgn4KuPrkffvwm1ZDkAhV/5\\nHk1734blp4XV+lNVy9B7d6C1TqxWo6vTPObkx7YeofKrp5LxeyGmNQn4IjSNtZCaijr3EtTZ60xy\\nHSB10VJUxgRaqwuXwCvPQ0fbtJjAFjFdHQAjVi7EK5WSYiZjtjZD7jT5kiKECEq69EVIdGMdlJSj\\nLMsb7CfFMzbc3TX5sqYTJ+BPmzF8wPr81bD4eNTy02JdFSHEJEgLX4Sm4RhUzI1YcSoj00wA7E2s\\nzI16GgZ8NX8xSV//UayrIYSYJGnhi3F586iXRDCPumf9fRQCvu52Yd+z0fRKxJsuzxj+9An4QoiZ\\nQQK+GJ+r0+RRj+R2rk7A132RDfhaa+zbr0c//wT6pWcjWnZEuDpAKe9+80IIMVUk4IvxtZvNXlRB\\nBCfXpXta+N1BT2tPSzhcrc2+5X4NxyZWRoTYLz+Hbm8NPNjZAdm5JguhEEJMIQn4YnxtTtCK5LIs\\nb5d+74hT+u03sb9yOXrHBLZiPuKkgM3ORR+tmXj9Jkn39aDvvBn7h18LPN4ms92FELEhAV+MSzst\\n/IgG/LR007UdpIWv3zKBXh/cG3ax+tBBUBZq9Tuh/ih6cHCyNZ0YTw9Fa1Pg8WM1qMp5U18fIUTC\\nk4AvxtfeYrZIzYtc/ndlWZCeYTbd8WM/9Sh6307zYij8YK0PHzCb8yxcArYNdUdCu++NV7Ef+1PY\\n7zcql2+5oW41W8vqbpcZcpi9IHLvI4QQIZKAL8bX1gK5+aikCI87Z2QGzNLXPS70/Zug2mnZtzSN\\ncuMYjtag5ixAzVtkyqwO3kugBwexH7ob3d6K7u0xE/0evif89xuNyzcHQe94zVs3ADV7fuTeRwgh\\nQiQBX4wratu5pmei/bv021oC37elIfwyu7tMT0RJOeQVwt6dwa/b8yZ685/R//sb9LOPhf8+Qei9\\nO7GffAStNdoT8C0L/eKz5rxnTsGc+RF5PyGECIck3hHja2+FSK7B98jIDOzSbzNd3ygLKudCS2NY\\nxWnbhv4+SMtAKYU67gT0K8+hz1kHVcvQzz0BaOh2me1eAf3qC7D7Db8y3BOaQa8HB7F/81MzZm8p\\nPNsKqgsvRT/xEHrXdvSu1808iLwESiUshIgbEvDFmLTW0NKIWro88oVnZPomtwHaaeFbP7wD/eLT\\n6L/cix4cNPncQ9HfZx7T083jcSfAqy9g3/gtOPlM2PavwOtLK2BowIyrl5ZDYx309/tWEIRIH63B\\nvvNmE+xLytCbH0SddT5YFuqi96O3/Qv7lmsBUO/+QGJtFiSEiBvSpS/G1t1lWuHFpREvWmVkjWzh\\nK2W65ItmAaD/8kfsX//EfPEYT79TVnqGKf+M81Hnv9cc2/YveMdqrM9/0wR/gJxcrI99FkorUGee\\n75TRF/bPod9+A44dQl38YdR7PgKd7ejdb0JWDiq3AOvaW2GWs43wWReGXb4QQkSCtPDF2JrMOLoq\\nnhX5stMzoLsT3eNCZWb7Jgcmp8CJJ6NTUtFPPGTe/wOfgvHq4PnykOYE/LQ0+Mhn0K9tgY421Mln\\noE4+E2vxidgH3sZ69wdRK1aRtHI19kvPmHsHwg/4dLtAKdT7Pw6uDtObX7MPyueYeqRnYF3zM2iu\\nR82qCL98IYSIAGnhizHpZmfiXDQCvmWBqwv7m+vRe3eapDTOWn+Vk4c66wLvpfaNV2M//Iexy3MC\\nvnJa+IAZy1+y3ATkE05xys4l6abfoVas8l2X6gwD9PeH/3O4uiAz2+wkmFsA86rMcb8tcFVamqy/\\nF0LEVFy08Ldu3coDDzzg7bb94Ac/yOmnn05tbS0bN27E5XKRnZ3Nhg0bKC8vj3FtZzZt22aNPKAH\\nB3yZ66IR8Mud3fcys7Bv/rZZN7/ydO9p9YFPot5xOvYt34XWZjOr/szzRm8le8fwMwIOq/d/HHXK\\nGWPvQZ/mCfgjM/+Nq7sLsnJ877f6nehD+6G9LfyyhBAiSmLewtdac/vtt7NhwwZuvPFGNmzYwMaN\\nG7Ftm02bNrFu3TpuvfVW1q1bxx133BHr6k4Luq0FvfP18O9rrMX+3KXoN181r//wS/TmBwFQ6eFN\\nZAuFOvdirJ/di3Xtz7yteTV/se98eibq+HdAkTN/IDkZvXmM5Dh9gWP43nJKy1GecfvRpKWZxwm0\\n8LWrE7L9Av7p7wwsUwgh4kDMAz6YbteeHpOApbu7m4KCArq6uqiurmbNmjUArFmzhurqajo7J7ip\\nygymt7+E7vS1JvWj92Hf9n3TQh/rvl3b0W+84jtQexgA+4E7nXJfNsdVdP5MlGWhsrJRmdlYn9yA\\n9etHsC758IjrrK/dgPWdW1Bnr0O/+Ay6qd73M9i277l3DD89/Mo44/4TmbRHtyuwhZ+Ti/WV67H+\\n61vhlyWEEFES8y59pRRf/vKXufHGG0lLS6O3t5err76alpYWCgsLsZzuZcuyKCgooLm5mdzcMbpm\\nh6momNmTpDofvIeOu24l6+IPUHjV1QDU1exjyO2m2NVOyqKlWOkjA2D/nh00OkvF5vyfyQTXczCX\\nFoDGOoraGmnJyoKcXIq++n3Sxvgco/4ZO+W7j1tK7fNPkPzHX1Dy/dvo37GNlh9/i7I7HyEprwBX\\nehptQNm8BSQVhbeV7yBu6oH8zHSyKirQWtPzzGMkV8wlbelJY95b29dDWtUSivw/hyh8JjP9bzke\\nyGc8NeRzjo2YB3y3280jjzzC1772NZYuXcrbb7/NLbfcwhe+8IWIlF9bWxuRcuKB7ulGP3IPuqke\\na8M1YFnY95phju7qyvuk2wAAIABJREFU/fTV1qK7u7CPVAPQ+PXPQGk5ST/49Yiy7Pt+431+rPog\\nKi0d+9hR77HGb6wHrVHv+Qgt+aUwyudYUVExpZ+x+vSXGNh0I7V33gpp6eiebur/+SwsW4GuOQhA\\nfUcHqj+8PPy6vQOAtvp6Ompr0bWHsW/+LgDWF7+HOvHkUe91d7TTayVH9XOY6s85EclnPDXkc46u\\nsb5MxbxLv6amhtbWVpYuXQrA0qVLSU9PJyUlhdbWVmyny9a2bdra2iguLo5ldWNG97iwb7kW/cxj\\nsGObycve1uIbtz5aYyY97n878MbGuuDl1ewza94B6p1A7+S1tzZcA55174XhtZSjzTptDbxjNfq5\\nx70/m67eh/79bei/3W8uSp1Al76nF8SzLK/R9w+S/dRf0W530Nv00KCZ6OfXpS+EEPEo5gG/qKiI\\n1tZW7ze+o0eP0t7eTnl5OfPnz2fLli0AbNmyhQULFoTVnT9T6P4+M1P9SDXq41eaY/t2QL2zE9zK\\n1dDVAR2t2H9/BDKzxy7P1QlN9agzzjOva51y+pyNbBYv816rwuwanwrWee+FHhf6lecB0DV70U6v\\nBqlp3lUGYUl1Jtj1mYCvnS8T6tyLYcc27K9+MviciG6XeZSAL4SIczHv0s/Pz+czn/kMN998s3e8\\n/vOf/zzZ2dmsX7+ejRs38uCDD5KVlcWGDRtiXNvY0A/+Hmr2YV31LdTK1biffBi9d6d3Mp1adTZ6\\n+0vYX/u0ef3vV8KhA+gtfw++6U3Nft99Lz/nnaxHbw+kZ5gkOB6Fkc+wN2memfye7XOr94EnGA+G\\nv6UuYPLnp6T6WvhN9WZt/aWXm8/62CGTCbB0WHeZZxvcbAn4Qoj4FvOAD7B27VrWrl074nhlZSU3\\n3HBDDGoUP3S3C/3sY6h3XoxauRoAtfgE9FuvQW4+ZGahlp+Krjoe3EOoU9egzl6HspKwU9PQLz49\\nsswjZqybBcdBaTnas2d8bzdkZAVeXBh/QygqLQ0Kik0ATk4x9fbQ9ug3jictzbssTzfWQUmZWUHw\\n4f80ufDbW0cG/G6zakRJC18IEefiIuCLMTTWmslzJ7zDd+y4E+DFp9Fb/wXlc1Bp6SR940cj703P\\nhL4+tNaBG7Y0N0J2Liozy6xxdzat0b093o1jrK/+AP3WVlRqnK4ln1VhAv6yFfDWa5EpMy0D+nvN\\nUsVd21GnOV9CnV4S3d7K8G1vdHureZKbH5k6CCFElMR8DF8Ep4eG0EOD3rFkSnwZBtVxJ5gnrk7U\\nkjGWjKWnmxbvsLFn3dbsbbmrgiLftrR+AV8tOQnrg/8RkZ8lGjzZ9tTxKya27j6Y1DR0by/27f/P\\nvPZk9PNsZ+sJ7v48k/tKJQOkECK+SQs/Tunf/NTszT5noTlQ4pfa1j/4OxPvgvKmi+3zTUoDs42r\\nJ1VufhF0dZjZ5r0902cs2ulaVwUl6LkL4cDbJjXvZKSlg2e4Y/lpqPOcnfYys8z4fkeQVLkNtVBY\\nEr89IUII4ZCAH6f04QNmAlp6JuQXBgQUpRTq3EvQtYdRZZWjF+LJHtfXCzl5vuNtzb5eggKzWQ0d\\nbdDbgyopi/BPEh1qzgKzK11pOWr1uSbonnWB+bwmKi3d7HIHWO//d2/ufaWU6dYP0sLXDbW+ngAh\\nhIhjEvDjiHa7UUlJJl1sazMMDaKPHYIgQdj6+OfGLU+lZ5ig6JcuVvf1QE83FJjldiq/yFzT1mKW\\n5Q3LQx+3li7Huu52VMVc1JwFcPa6SRep8gvRnhfO1rZeeQXojsCAr7WGhmOoVedM+r2FECLaZAw/\\nTujXtmBfeZnJE+/q8C05O7QfVTLB8WH/Ln2PVme83jP73mnh27+91bTyh8/Sj1NKKVTF3MiW+c6L\\nfc9TUgLP5QVp4bs6zZcnaeELIaYBaeHHCfsBk+pW79uJGt66nLNgYoWmB9ny1Qn4qiAw4Hsnn2VE\\nfle86UJVLYNTzkSVB/kikV9oMhz6azhm7pOAL4SYBiTgxwHd0gjtZmkcR2pGzDpXK1ZNrGDvGL5f\\nl35ro3niyaA3PCufK7F3I0y68pvBT+TkmSV7A/3e+RS6wfmSJAFfCDENSJd+PDh0wDwmJaEPH0C3\\nNAWcnvBEOueLg3fbWICGOpOsxmnZK6VQ7/2oSdmbkYk6+YyJvddM55n02OX3hajhGCQlQdGs4PcI\\nIUQckRZ+HNBNTt72U9egX34OvXcHWBbqY5/zdb1PRPrIMXzdWGtmtltJ3mPW+z5unpx7MSI4lZtn\\nJvS5Ory9I7qh1mTjS0oa814hhIgHEvDjQUOtyXy3+lz0vp2QlIyavxjrne+eXLlpzni8/xh+Qy2M\\ntZRPBJfjZNLr7EDbttmgp6EWZslnKYSYHiTgxwHdWAezKlAnnkzSj++KXMGpqaAU+omH0SecDJVz\\nobFu4nMCEpmzJt/++XUwrwrrWzeaz9I/5bEQQsQxGcOPB411E196NwallNnX3tWJffv10NwA7iGZ\\nZDYROX658g/tR9+9EQYHUAuOi12dhBAiDBLwY0wP9Jtc9rOinIu9tRn7h18HGLnsT4xvWEIi/c9/\\noM5/L5xyVowqJIQQ4ZEu/Vjb/SYAas6i6L+XqxNrwzWwcEn032uGCdhtMDkZddYFqA//Z+BxIYSI\\nYxLwY0y/+DRk58IJK6NSvvXTP0DtYeybvgXpGagVp0XlfRKJ9f1fTJs9B4QQwkMCfgzpbhf6jZf/\\nf3t3Ht9Ulf9//HVTErqkBUoptiBSBhFF2XTcgM6IS535oV/1oeKMMgNKQaHDuIzg8nXAn8I8BJcH\\nIiIyAhYHxLpvIIgLFBRcQEYBFaiKINJSujctac73j0Ck0kJL09yUvJ//NL25ufnkPA68e07uPRfr\\nd3/AauU8+guOgRWfgDn5VKzfXYqVfmmzvEfEaZ9sdwUiIo2mwLeR+TQXvN4j3+I2CCxHFNYNY5r1\\nPSKB9f+uxeR9478kT0SkhVHg28h8/D6kdoEu3ewuRRrAccUNdpcgInLMNFSx0448rFP76MQvERFp\\ndgp8m5gqj3/J2zbt7C5FREQigALfLiVF/p8JCnwREWl+Cny7HAh8K6HtUXYUERFpOgW+XQIjfAW+\\niIg0PwW+TYwCX0REQkiBb5eDgR/fxt46REQkIijw7VJSBHHxWK20FIKIiDQ/Bb4NfC9nYz54G2Lj\\n7C5FREQihALfBmbZK/4HB6f1RUREmpkC3w7tOwLgGHWnzYWIiEikUODboWQf1oWXYfXWrWpFRCQ0\\nFPghZqo84KnUkroiIhJSCvxQ05K6IiJiAwV+qGlJXRERsYECP9SK9/l/tlHgi4hI6CjwQ8yUHAh8\\nTemLiEgIKfBDraQILEtL6oqISEgp8EOteB+4E7CiouyuREREIogCP8TM3j2Q2MHuMkREJMIo8EOt\\nsADaK/BFRCS0FPhBYjyVmC0bMcbUv48xsHcPVmJyCCsTERFR4AeNWf0uvkf+F/PKgvp3KiuF6iqN\\n8EVEJOQU+MFSUQ6AWfIixuere5/CfAAsfYcvIiIhpsAPlqrKXx5XVtS9z949/p/tNaUvIiKh1cru\\nAo4blYcEfnkJxLkBML4azKrlWG0T/Wfog6b0RUQk5BT4weI5JPBLSyA5FQDz6WrMc09iANolQZtE\\niIu3pUQREYlcmtIPEuM5ZBq/vNS/zRjMslehbSI4XbCvAOuUM7Asy6YqRUQkUinwg8VTCYlJAJiy\\nEv+2nd/B91ux/ngNnNbXv+2U0+2pT0REIpoCP1g8FZDU0f/4QOCbDWvBsrD6n4/V7zxwOLBO7WNj\\nkSIiEqn0HX6wVFZgndAZExWF2bQBc3IvzPq10O0UrDbt4PzBWD16YXU4we5KRUQkAinwg8VTCTGx\\n/hPyvlqP76v1AFhDR/p/WhYo7EVExCaa0g8WTyVEx4B1SJNGx2ANuMi+mkRERA7QCD8IjNcL+6sh\\nOhaKC/0bk1Ow0i/Fiom1tzgREREU+MFxcJW9Q8LdMWkGltNlU0EiIiK1KfCD4eBSutExOLLuw+z+\\nUWEvIiJhRYEfDAdW2bOiY7H6/Barz29tLkhERKQ22wN/z549TJs2LfB7RUUFFRUVzJs3j127djFz\\n5kzKyspwu91kZWWRkpJiY7X18PwywhcREQlHtgd+cnJyrcCfP38+NTU1AMyZM4eMjAzS09NZuXIl\\nTz/9NBMnTrSr1DoZYzDrP/b/osAXEZEwFVaX5Xm9XlatWsUFF1xAcXExeXl5DBw4EICBAweSl5dH\\nSUmJzVX+yo48/3r58MtKeyIiImEmrAL/008/JTExkW7durF3714SExNxOPwlOhwO2rVrR0FBgc1V\\n/sqBG+U4bvv//hX1REREwpDtU/qHev/997nggguCeszU1NSgHu/XKr//hgKgQ9duuJr5vcJVc7ex\\n+Kmdm5/aODTUzvYIm8AvLCxk06ZNZGVlAdC+fXsKCwvx+Xw4HA58Ph/79u0jKSmpUcfdtWtXc5Qb\\n4Nv9EwD5JaVYzfxe4Sg1NbXZ21jUzqGgNg4NtXPzOtIfU2Ezpf/BBx/Qr18/4uPjAWjTpg1du3Yl\\nNzcXgNzcXNLS0khISLCzzMMdXHQnOtreOkRERI4gbEb4H374ISNGjKi1LTMzk5kzZ/LSSy8RFxcX\\nGP2Hlaoq/0+XAl9ERMJX2AT+9OnTD9vWqVMnpkyZYkM1jXBwhN9agS8iIuErbKb0WyyPB1o5saKi\\n7K5ERESkXgr8pqr26Pt7EREJewr8pvJ4oLVW2BMRkfCmwG8iU+0BV2u7yxARETkiBX5TVXm0hr6I\\niIQ9BX5TeTTCFxGR8KfAb6pqjfBFRCT8KfCbyuPB0ghfRETCnAK/qTTCFxGRFkCB31RVHq2yJyIi\\nYU+B3wTGmAMn7SnwRUQkvCnwm8K7H4xPK+2JiEjYU+A3hcfj/6kRvoiIhDkFflNUHwh8jfBFRCTM\\nKfCb4sAI39JJeyIiEuYU+E1RVen/qcAXEZEwp8BviqoDU/oKfBERCXMK/KZQ4IuISAuhwG8Co8AX\\nEZEWQoHfFFW6LE9ERFoGBX5T6LI8ERFpIRT4TaGFd0REpIVQ4DdFlQdatcJq1cruSkRERI5Igd8U\\nVR5orVvjiohI+FPgN0WVB1q3trsKERGRo1LgN4VG+CIi0kIo8JvAVHl0Db6IiLQICvymqKpU4IuI\\nSIugwG+KqioFvoiItAgK/Kao8ujWuCIi0iIo8JtC3+GLiEgLocBvCgW+iIi0EAr8Y2Q8lTppT0RE\\nWgwF/jHy/fsRAKxT+9hciYiIyNEp8I/VN19iDboEq2dvuysRERE5KgX+MTBeL1RWQJtEu0sRERFp\\nEAV+A5mqql9+qSz3/4xz21OMiIhII+m+rkfgW7MC8/oi6HACbNuC456HsTp3hbJS/w5x8bbWJyIi\\n0lAa4dfD7MjDzJsOXi9s2Qj7q/G9vtD/ZLk/8C0FvoiItBAa4dfB99KzmKUvgas1jvtnQEkR5pNV\\nmDeex3y/DcrL/Dsq8EVEpIWIuMA332+DVk6sTl3qfj5/tz/sAevCIf5RfFw8XPQ/mBVv4nt9IdaZ\\nA/w76zt8EZFajDF4PB58Ph+WZR32/M6dO6msrLShsuODMQYAl8uF0+ls1GsjKvCNMfgevA0Ax+xX\\nsBxRh++TuxwsB44HZ0FSx8B2KzYO65IrMK8+98tiOxrhi4jU4vF4cDqdtGpVd7w4nc46/xCQhjPG\\nUFVVRU1NDdHRDV/8LbK+w9+985fH69fWesps24LvP09hVrwBfc/GSk7BctRuHuvCIeCOx3yyCiwL\\nYmJDUbWISIvh8/nqDXsJDsuyiI6OpqamplGvi6jAN1995n/gcuFbNBtTWBB4zrf8VcwHbwMWjqGZ\\ndb7eio7FOuf3B47R+rA/CEREIp1G76HT2LaOmMQyXi8m911IORHHPY9CZTnmjUW/7LD9G/hNTxz3\\nPoLVvkO9x7F6nO5/UOVp5opFRCQYSktLycjIYMaMGUfcb/78+cyaNQuA119/nZycnDr3M8bw4osv\\nMnz4cIYPH05mZiYPP/wwZWVlbNiwgdGjRwf9MwRDxMy7mKUvws7vcYy5B6tTF6zfDsJ8kosZOtJ/\\n1v2+AqyMq7BSOh/5QCefFpqCRUQkKN59911OO+003nvvPW6++eYGnex2+eWX1/vc3Llz+eKLL3j0\\n0UdJTEzEGMOqVasoKSkJZtlBFxGBb7ZsxLy+COvsdKx+5wJgDbgYs3oFZtUySGjr39b91KMey4pv\\n43/QOqbZ6hURkeBZsmQJo0ePZuHChaxevZrf//73AJSVlTFt2jTy8vJITEwkOTmZdu3aAf7RfmVl\\nJbfcckutY1VWVvLCCy8wZ84cEhP9y6tblkV6ejoAe/bsqbX/O++8w+LFi7Esi9TUVG6//XbatWvH\\nl19+yeOPP47P58Pr9TJs2DAuvPBCysvLefLJJ9m+fTvV1dX07duXMWPGEBV1+EnmjRUZgb/xE3A6\\nsf76t182dj8VTj8T8+oC+M2pEBsHnbs26HiOh56BqIhoOhGRY+Zb8x5m9bu1t1lW4NKyprAGXITj\\n/MFH3W/btm2UlJTQv39/CgsLWbJkSSDws7OziY2NJTs7m+LiYkaNGhV4rj7fffcdTqeTLl3qvrT7\\nUHl5ecyZM4fZs2fTvn175s6dy+OPP87EiRNZtGgRQ4cO5cILL8QYQ3m5f8n2J598kj59+nDnnXfi\\n8/mYPHkyS5YsYciQIUd9v6OJiO/wzZ6fIOkELFfrwDbLsnAMGws1Ptj8BdZp/bAa+BeUldgBq027\\n5ipXRESC5O233+aSSy4JjMI3b95Mfn4+ABs2bOCPf/wjAG3atGHQoEFBfe/169dzzjnn0L59ewAu\\nu+wyPv/8cwD69evHggULWLBgAZs3b8bt9q/rsmbNGhYvXszIkSMZNWoU33zzDT/++GNQ6omMYWr+\\nbkhOOWyzlZiE9duBmI8/gNP7h74uEZHjmOP8wfCrUbjT6WT//v0hef/9+/ezYsUKnE4ny5YtA8Dr\\n9fLOO+9www03HNMxu3btSnV1NTt27ODEE0885tquvvpqzjvvPD777DNmzJjBWWedxU033YQxhgce\\neIDU1NRjPnZ9jvsRvjEGCnZjdTihzuetP1wNPXtj9Tk7xJWJiEhzWr16NSeeeCI5OTk8//zzPP/8\\n80ybNo2lS5cC/lH2wcfFxcXk5uYe9ZgxMTFcc801PPLII+zbtw/w50xubi67du2qtW+/fv1Yu3Yt\\nhYWFALz55puceeaZAOzYsYNOnTpx+eWXc9VVV7FlyxYAzj//fBYuXBi4xr64uJiffvopCK0RCSP8\\n4kKoroYOh4/wAazULkTd8WCIixIRkea2ZMkSLrroolrbevXqhTGGDRs2MGzYMKZOncpf/vIXEhMT\\n6d27d61967vOfeTIkeTk5HDbbf6VW40xnHHGGfTt27fWSXtpaWlkZmbyj3/8A8uySElJ4fbbbwfg\\n5ZdfZv369TidTpxOJ+PGjQMgKyuL2bNnM3LkSCzLwul0kpWVRUpK3RnWGJYJxtkTYWznB8vxTbsb\\nx98nYWnaPuhSU1MP+6tWgk/t3PzUxsFRUVFBbGz9q5CGckq/KR577DE6duzIn//8Z7tLqVddbX2k\\nrwKO/yn9n3b4H3QM/vchIiJy/Jk6dSqbN28+bHagpTv+p/S//i+0Tax1IxwREZH6jB8/3u4SmsXx\\nP8Lf+AlWzz5a31lERCJaWIzwq6urefbZZ/nvf/+L0+mkR48ejB49ml27djFz5kzKyspwu93HduJC\\nlQdO7X30/URERI5jYRH4zz33HE6nk+nTp2NZFkVFRQDMmTOHjIwM0tPTWblyJU8//TQTJ05s3MFP\\n7YPV/7xmqFpERKTlsH1K3+PxsHLlSq677rrAtHvbtm0pLi4mLy+PgQMHAjBw4EDy8vIafXMCR9b/\\nYkXrvvUiIhLZbB/h7969m/j4eHJycvjqq6+Ijo7muuuuw+VykZiYiOPAPecdDgft2rWjoKCAhISE\\nBh//0OV0RUREIpXtge/z+fj5559JS0tj2LBhfPvttzz00EOBxQmaqjmWJ5Ta1MahoXZufmrjptu5\\nc+dRbz/bkNvTBsvVV1+Ny+XC5XIB0L9//8AiN+Hk7bff5vTTT2/QTXkOiomJaVSftT3wk5KSiIqK\\nYsCAAQCcfPLJxMfH43K5KCwsxOfz4XA48Pl87Nu3j6SkpEYdXwtpNC8tVhIaaufmpzYOjsrKyiNe\\nFRXqhXeMMUyaNIm0tLTAtoa+f01NTVBuS9sQb731Fm63u1EnpldWVh7WZ4/0B4DtgZ+QkECvXr3Y\\nuHEjffr0YdeuXZSUlJCSkkLXrl3Jzc0lPT2d3Nxc0tLSGjWdLyIi8muFhYU89thj7Nq1C2MMQ4cO\\nJSMjA4DrrruOwYMHs379etLS0hg/fjxLly7ltddeo6amBrfbza233hoYif/nP/9hxYoVWJZFTEwM\\njz/+OEVFRTzwwAOUl5dTXV3Nueeey8033wxAbm4uc+fOxeFwUFNTw9///nd++uknvv76a2bMmMEz\\nzzzDLbfcElhzP5hsD3yAzMxMZs2aRXZ2Nq1atSIrK4u4uDgyMzOZOXMmL730EnFxcWRlZdldqoiI\\nNNB724tZsa2o1jbLcmCMr8nHvvA3bRncrU2D9p04cWJgSn/UqFEsWbKEtLQ0HnjgAfbu3cvo0aPp\\n0aNHYBagvLycWbNmAbBx40Y++OADpk+fjsvlYu3atUydOpUnnniCpUuXsmbNGp544gliY2MpLi7G\\n4XDgdruZMmUKMTExeL1exo8fz7p16zj77LOZN28ed9xxB7169aKmpgaPx0Pfvn155513GDp0KOed\\n13xXlYVF4Hfs2JFJkyYdtr1Tp05MmTIl9AWJiMhx4/777681pT958mTGjBkDQPv27TnnnHMCI3og\\nMNoH//3pt23bFtjfGENZWRkAH3/8MZdffnlgPfs2bfx/gNTU1PDUU0/x5ZdfAv4Zha1bt3L22WfT\\nv39/Zs6cSXp6Ouecc06tuppbWAS+iIgcfwZ3a3PYKLwl3DwnJiam1u9/+MMfuPHGGxv8+pycHEpL\\nS5k1axYul4uHH36Y6upqAMaOHcv27dv5/PPPmTRpEtdccw1DhgwJav31sf06fBERkVDq378/b775\\nJuAffa9du5Z+/frVue95553HsmXLyM/PB/yj96+//hqAc889l9dff52KigrAf+96gLKyMtq3b4/L\\n5SI/P581a9YEjvfDDz/QrVs3rr76ai6++GK2bNkCQFxcXGDmoLlohC8iIhHlb3/7G48++ig33XQT\\nxhgyMzPrnVrv06cPN910E/feey81NTV4vV5+97vfccopp5CRkUFBQQFjxoyhVatWxMTEMH36dK66\\n6iruv/9+RowYQYcOHWr9MTFnzhx+/PFHoqKicLvd3HnnnQAMGTKEWbNmsXjx4mY7ac8yxpigHzWM\\n6DKb5qVLmUJD7dz81MbBUdc92g/VEqb0W4q62vpIl+VpSl9ERCQCKPBFREQigAJfREQkAijwRUQk\\naI7z08LCSmPbWoEvIiJB43A48Hq9dpdxXDPG4PF4Gr3Ovy7LExGRoImOjsbj8VBVVVXnTXRiYmKo\\nrKy0obLjw8FRvcvlavRdBxX4IiISNAdvIlMfXf5oH03pi4iIRAAFvoiISARQ4IuIiESA435pXRER\\nEdEIX0REJCIo8EVERCKAAl9ERCQCKPBFREQigAJfREQkAijwRUREIoACX0REJAIo8EVERCKAAl9E\\nRCQCtJi75WVnZ7N27Vry8/N5+OGH6dKlyxG3A3z++ecsXrwYr9eL2+1m7NixJCcnAzB16lTy8/Ox\\nLIvo6GhuvPFGunbtasdHCxt1tWVpaSlPPPEEu3fvplWrVqSkpDBq1CgSEhIAeP/993nrrbfw+Xwk\\nJyeTlZWF2+3G5/Nx3333UV1dDUDbtm3JzMwMtH8kq6/PHqlPqi83zpH+XwDIyckhJyen1nPqy41X\\nXzuPHTsWp9MZuH3r9ddfT9++fQG1s61MC7F582aTn59vxowZY77//vujbi8tLTU33nij2blzpzHG\\nmA8//NA8+OCDgefLy8sDj9etW2fGjx8fgk8R3upqy9LSUvPll18G9snOzjZPPvmkMcaYHTt2mFGj\\nRpni4mJjjDEvvviimT17dmDfQ9v4rbfeMtOmTQvFxwh79fXZ+vqk+nLj1dfGxhizbds2M3ny5FrP\\nqS8fm/raua52N0btbLcWM6Xfs2dPkpKSGrx99+7dtGnThtTUVAD69+/PF198QUlJCQCxsbGBfSsq\\nKrAsq5kqbznqaku3202vXr0Cv5988skUFBQAsGPHDrp27RoY7ffr14/c3NzAvmrjutXXZ+trL/Xl\\nxquvjffv388zzzzDyJEja21XXz429bVzfdTO9moxU/qNlZqaSlFREVu3bqV79+6sWrUKgIKCgkBn\\ne+qpp/jiiy8AuOeee2yrtaXw+XwsX76cM888E4CTTjqJbdu2sWfPHjp06EBubi4ej4eysjLcbjcA\\n//rXv9i+fTsJCQnce++9dpbfItTVJ9WXg2fx4sUMGjTosGli9eXgmzFjBsYYevbsyZ/+9Cfi4uLU\\nzjY7bgM/NjaWW2+9lWeffZb9+/fTt29f4uLiiIqKCuxz8803A7By5Uqee+457r77brvKbRHmzp1L\\n69atufTSSwF/EI0YMYLHHnsMy7I466yzAHA4fpk4uvvuu/H5fLz66qu8/PLLh42spLa6+qT6cnB8\\n8803bN++neuvv/6w59SXg+v+++8nKSmJ/fv3M3/+fJ555hnGjRundrbZcRv4AL1796Z3794AFBUV\\n8cYbb9CxY8fD9ktPT2f27NmUlpYSHx8f6jJbhOzsbHbv3s2ECRNq/eMcMGAAAwYMAGDr1q0sW7as\\n1rQc+P8xDx48mHHjxukfbwP9uk+qLzfdpk2b2LlzJ1lZWQDs3buXyZMnM2bMGPr06aO+HEQHp/md\\nTicZGRk89NDNPMyDAAAENklEQVRDgefUzvY5rgO/qKiItm3b4vP5WLRoERdffDHR0dGBKaSDnfLT\\nTz/F7XYHppSktoULF5KXl8ddd90VOOv2oINtXF1dzQsvvMBll10GEPh++eCU80cffXTYmdLyi6P1\\nSfXlprviiiu44oorAr+PHTuWCRMmBPql+nJweDwefD4fsbGxGGNYvXp1ratG1M72sYwxxu4iGmLu\\n3LmsW7eOoqIi4uPjiY+P59FHH613O/i/1/z666/xer307t2bv/71r7hcLoqKipg2bRoejweHw4Hb\\n7WbYsGF069bN5k9pr7ra8rbbbuOOO+4gJSUFl8sFQHJyMnfeeScAU6ZMIT8/H6/Xy4ABA7j22mtx\\nOBz88MMPzJw5k5qaGowxJCcnM3z48DpHpZGmrnb+5z//ecQ+qb7cOEf6f+GgXwe++nLj1dXOEyZM\\n4JFHHsHn8+Hz+ejcuTMjRoygXbt2gNrZTi0m8EVEROTYtZjL8kREROTYKfBFREQigAJfREQkAijw\\nRUREIoACX0REJAIo8EVERCLAcb3wjog03dixYykqKiIqKgqHw0Hnzp1JT0/noosuqrXqYl327NlD\\nVlYWixYtqrUUsIiEngJfRI5qwoQJ9O7dm4qKCjZt2sS8efPYunUrY8aMsbs0EWkgBb6INFhsbCxn\\nnXUWbdu25d5772XIkCEUFBTw/PPP8/PPPxMbG8sFF1zAtddeC8DEiRMBGD58OAD33XcfPXr04L33\\n3uONN96gqKiI7t27M2rUKDp06GDXxxKJCPoOX0QarXv37iQmJrJlyxZat25NVlYW8+bN46677mL5\\n8uWsW7cO8N81DWD+/PksWLCAHj168Mknn/DKK69wxx138O9//5uePXsyffp0Oz+OSERQ4IvIMUlM\\nTKSsrIxevXrRpUsXHA4HJ510EgMGDGDTpk31vm758uVceeWVdO7cmaioKK688kq+++478vPzQ1i9\\nSOTRlL6IHJPCwkLcbjfffvstCxcu5IcffsDr9eL1ejn33HPrfV1+fj7z5s0jOzs7sM0YQ2Fhoab1\\nRZqRAl9EGm3r1q0UFhbSs2dPpk2bRkZGBnfffTcul4v58+cHbnVqWdZhr01KSuKqq65i0KBBoS5b\\nJKJpSl9EGqyiooLPPvuM6dOnM2jQILp06UJlZSVutxuXy8XWrVvJzc0N7J+QkIBlWfz888+BbRdf\\nfDGvvvoqO3bsCBzzo48+CvlnEYk0uj2uiBzRodfhW5ZF586dGTRoEJdccgkOh4OPP/6Y7OxsysrK\\nOO200+jQoQPl5eWMGzcOgMWLF7Ns2TJqamq455576NGjBytXruS1116joKCA2NhYzjjjDF3iJ9LM\\nFPgiIiIRQFP6IiIiEUCBLyIiEgEU+CIiIhFAgS8iIhIBFPgiIiIRQIEvIiISART4IiIiEUCBLyIi\\nEgEU+CIiIhHg/wCRSwDydCeP4QAAAABJRU5ErkJggg==\\n\",\n \"text/plain\": [\n \"<Figure size 576x504 with 1 Axes>\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n }\n }\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"ahKjg6KyGYnd\",\n \"colab_type\": \"code\",\n \"colab\": {}\n },\n \"source\": [\n \"\"\n ],\n \"execution_count\": 0,\n \"outputs\": []\n }\n ]\n}"
}
{
"filename": "code/artificial_intelligence/src/linear_regression/linear_regression.java",
"content": "// author @Yatharth Shah\n\npublic class LinearRegression { \n\n public static void main(String[] args) { \n int MAXN = 1000;\n int n = 0;\n double[] x = new double[MAXN];\n double[] y = new double[MAXN];\n\n // first pass: read in data, compute xbar and ybar\n double sumx = 0.0, sumy = 0.0, sumx2 = 0.0;\n while(!StdIn.isEmpty()) {\n x[n] = StdIn.readDouble();\n y[n] = StdIn.readDouble();\n sumx += x[n];\n sumx2 += x[n] * x[n];\n sumy += y[n];\n n++;\n }\n double xbar = sumx / n;\n double ybar = sumy / n;\n\n // second pass: compute summary statistics\n double xxbar = 0.0, yybar = 0.0, xybar = 0.0;\n for (int i = 0; i < n; i++) {\n xxbar += (x[i] - xbar) * (x[i] - xbar);\n yybar += (y[i] - ybar) * (y[i] - ybar);\n xybar += (x[i] - xbar) * (y[i] - ybar);\n }\n double beta1 = xybar / xxbar;\n double beta0 = ybar - beta1 * xbar;\n\n // print results\n StdOut.println(\"y = \" + beta1 + \" * x + \" + beta0);\n\n // analyze results\n int df = n - 2;\n double rss = 0.0; // residual sum of squares\n double ssr = 0.0; // regression sum of squares\n for (int i = 0; i < n; i++) {\n double fit = beta1*x[i] + beta0;\n rss += (fit - y[i]) * (fit - y[i]);\n ssr += (fit - ybar) * (fit - ybar);\n }\n double R2 = ssr / yybar;\n double svar = rss / df;\n double svar1 = svar / xxbar;\n double svar0 = svar/n + xbar*xbar*svar1;\n StdOut.println(\"R^2 = \" + R2);\n StdOut.println(\"std error of beta_1 = \" + Math.sqrt(svar1));\n StdOut.println(\"std error of beta_0 = \" + Math.sqrt(svar0));\n svar0 = svar * sumx2 / (n * xxbar);\n StdOut.println(\"std error of beta_0 = \" + Math.sqrt(svar0));\n\n StdOut.println(\"SSTO = \" + yybar);\n StdOut.println(\"SSE = \" + rss);\n StdOut.println(\"SSR = \" + ssr);\n }\n}"
}
{
"filename": "code/artificial_intelligence/src/linear_regression/linear_regression.js",
"content": "/**\n* Author: Daniel Hernández (https://github.com/DHDaniel)\n\n* Description: Multivariate linear regression that uses the normal equation\n* as an optimizer. The normal equation is fine to use\n* when n, the number of features being worked with, is\n* less than 10,000. Beyond that, it is best to use\n* other methods like gradient descent.\n\n* Requirements:\n* - mathjs (install using npm)\n*/\n\n// this is installed using npm: `npm install mathjs`\nconst math = require(\"mathjs\");\n\n/**\n * Solves the normal equation and returns the optimum thetas for\n * the model hypothesis.\n * @param {mathjs.matrix} X - a matrix of m x n (rows x columns), where m is the number of records and n is the number of features. It is common practice to add a leading 1 to each row m, in order to account for the y-intercept in regression.\n * @param {mathjs.matrix} y - a vector of m x 1 (rows x columns), where m is the number of records. Contains the labeled values for each corresponding X row.\n * @returns {mathjs.matrix} A n x 1 matrix containing the optimal thetas.\n */\nconst normalEqn = function normalEqn(X, y) {\n // computing the equation theta = (X^T * X)^-1 * X^T * y\n var thetas = math.multiply(math.transpose(X), X);\n thetas = math.multiply(math.inv(thetas), math.transpose(X));\n thetas = math.multiply(thetas, y);\n // returning a vector containing thetas for hypothesis\n return thetas;\n};\n\n/**\n * Trains and returns a function that serves as a model for predicting values y based on an input X\n * @param {mathjs.matrix} X - a matrix of m x n (rows x columns), where m is the number of records and n is the number of features. It is common practice to add a leading 1 to each row m, in order to account for the y-intercept in regression.\n * @param {mathjs.matrix} y - a vector of m x 1 (rows x columns), where m is the number of records. Contains the labeled values for each corresponding X row.\n * @returns {function} A function that accepts a matrix X, and returns predictions for each row.\n */\nconst train = function train(X, y) {\n // getting optimal thetas using normal equation\n var thetas = normalEqn(X, y);\n // creating a model that accepts\n const model = function(X) {\n // create predictions by multiplying theta^T * X, creating a model that looks like (theta_1 * x_1) + (theta_2 * x_2) + (theta_3 * x_3) etc.\n return math.multiply(math.transpose(thetas), X);\n };\n return model;\n};\n\n/* TESTS */\n\n// test values for X and y\nvar Xmatrix = math.matrix([[2, 1, 3], [7, 1, 9], [1, 8, 1], [3, 7, 4]]);\nvar ylabels = math.matrix([[2], [5], [5], [6]]);\n\n// should show thetas (in the _data part of object) to be 0.008385744234748138, 0.5681341719077577, 0.4863731656184376\nconsole.log(normalEqn(Xmatrix, ylabels));\n\n// test values #2\nXmatrix = math.matrix([[1], [2], [3], [4]]);\nylabels = math.matrix([[1], [2], [3], [4]]);\n\n// should show theta of 1 (which forms a perfectly diagonal line if plotted)\nconsole.log(normalEqn(Xmatrix, ylabels));\n\n// test values #3\nXmatrix = math.matrix([[1, 5], [1, 2], [1, 4], [1, 5]]);\nylabels = math.matrix([[1], [6], [4], [2]]);\n\n// should show thetas of 9.25 and -1.5\nconsole.log(normalEqn(Xmatrix, ylabels));"
}
{
"filename": "code/artificial_intelligence/src/linear_regression/linear_regression/linear_regression.js",
"content": "/**\n* Author: Daniel Hernández (https://github.com/DHDaniel)\n\n* Description: Multivariate linear regression that uses the normal equation\n* as an optimizer. The normal equation is fine to use\n* when n, the number of features being worked with, is\n* less than 10,000. Beyond that, it is best to use\n* other methods like gradient descent.\n\n* Requirements:\n* - mathjs (install using npm)\n*/\n\n// this is installed using npm: `npm install mathjs`\nconst math = require(\"mathjs\");\n\n/**\n * Solves the normal equation and returns the optimum thetas for\n * the model hypothesis.\n * @param {mathjs.matrix} X - a matrix of m x n (rows x columns), where m is the number of records and n is the number of features. It is common practice to add a leading 1 to each row m, in order to account for the y-intercept in regression.\n * @param {mathjs.matrix} y - a vector of m x 1 (rows x columns), where m is the number of records. Contains the labeled values for each corresponding X row.\n * @returns {mathjs.matrix} A n x 1 matrix containing the optimal thetas.\n */\nconst normalEqn = function normalEqn(X, y) {\n // computing the equation theta = (X^T * X)^-1 * X^T * y\n var thetas = math.multiply(math.transpose(X), X);\n thetas = math.multiply(math.inv(thetas), math.transpose(X));\n thetas = math.multiply(thetas, y);\n // returning a vector containing thetas for hypothesis\n return thetas;\n};\n\n/**\n * Trains and returns a function that serves as a model for predicting values y based on an input X\n * @param {mathjs.matrix} X - a matrix of m x n (rows x columns), where m is the number of records and n is the number of features. It is common practice to add a leading 1 to each row m, in order to account for the y-intercept in regression.\n * @param {mathjs.matrix} y - a vector of m x 1 (rows x columns), where m is the number of records. Contains the labeled values for each corresponding X row.\n * @returns {function} A function that accepts a matrix X, and returns predictions for each row.\n */\nconst train = function train(X, y) {\n // getting optimal thetas using normal equation\n var thetas = normalEqn(X, y);\n // creating a model that accepts\n const model = function(X) {\n // create predictions by multiplying theta^T * X, creating a model that looks like (theta_1 * x_1) + (theta_2 * x_2) + (theta_3 * x_3) etc.\n return math.multiply(math.transpose(thetas), X);\n };\n return model;\n};\n\n/* TESTS */\n\n// test values for X and y\nvar Xmatrix = math.matrix([[2, 1, 3], [7, 1, 9], [1, 8, 1], [3, 7, 4]]);\nvar ylabels = math.matrix([[2], [5], [5], [6]]);\n\n// should show thetas (in the _data part of object) to be 0.008385744234748138, 0.5681341719077577, 0.4863731656184376\nconsole.log(normalEqn(Xmatrix, ylabels));\n\n// test values #2\nXmatrix = math.matrix([[1], [2], [3], [4]]);\nylabels = math.matrix([[1], [2], [3], [4]]);\n\n// should show theta of 1 (which forms a perfectly diagonal line if plotted)\nconsole.log(normalEqn(Xmatrix, ylabels));\n\n// test values #3\nXmatrix = math.matrix([[1, 5], [1, 2], [1, 4], [1, 5]]);\nylabels = math.matrix([[1], [6], [4], [2]]);\n\n// should show thetas of 9.25 and -1.5\nconsole.log(normalEqn(Xmatrix, ylabels));"
}
{
"filename": "code/artificial_intelligence/src/linear_regression/linear_regression.py",
"content": "import numpy as np\nimport matplotlib.pyplot as plt\n\n\n# A basic implementation of linear regression with one variable\n# Part of Cosmos by OpenGenus Foundation\ndef estimate_coef(x, y):\n # number of observations/points\n n = np.size(x)\n\n # mean of x and y vector\n m_x, m_y = np.mean(x), np.mean(y)\n\n # calculating cross-deviation and deviation about x\n SS_xy = np.sum(y * x - n * m_y * m_x)\n SS_xx = np.sum(x * x - n * m_x * m_x)\n\n # calculating regression coefficients\n b_1 = SS_xy / SS_xx\n b_0 = m_y - b_1 * m_x\n\n return (b_0, b_1)\n\n\ndef plot_regression_line(x, y, b):\n # plotting the actual points as scatter plot\n plt.scatter(x, y, color=\"m\", marker=\"o\", s=30)\n\n # predicted response vector\n y_pred = b[0] + b[1] * x\n\n # plotting the regression line\n plt.plot(x, y_pred, color=\"r\")\n\n # putting labels\n plt.xlabel(\"x\")\n plt.ylabel(\"y\")\n\n # function to show plot\n plt.show()\n\n\ndef main():\n # observations\n x = np.array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])\n y = np.array([1, 3, 2, 5, 7, 8, 8, 9, 10, 12])\n\n # estimating coefficients\n b = estimate_coef(x, y)\n print(\n \"Estimated coefficients are:\\nb_0 = {} \\\n \\nb_1 = {}\".format(\n b[0], b[1]\n )\n )\n\n # plotting regression line\n plot_regression_line(x, y, b)\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/artificial_intelligence/src/linear_regression/linear_regression_scikit_learn.ipynb",
"content": "{\n \"cells\": [\n {\n \"cell_type\": \"code\",\n \"execution_count\": 3,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"import numpy as np\\n\",\n \"import pandas as pd\\n\",\n \"import matplotlib.pyplot as plt\\n\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 4,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"%matplotlib inline\\n\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 5,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"#importing the data set\\n\",\n \"dataset=pd.read_csv('Salary_Data.csv')\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 7,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/html\": [\n \"<div>\\n\",\n \"<style scoped>\\n\",\n \" .dataframe tbody tr th:only-of-type {\\n\",\n \" vertical-align: middle;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe tbody tr th {\\n\",\n \" vertical-align: top;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe thead th {\\n\",\n \" text-align: right;\\n\",\n \" }\\n\",\n \"</style>\\n\",\n \"<table border=\\\"1\\\" class=\\\"dataframe\\\">\\n\",\n \" <thead>\\n\",\n \" <tr style=\\\"text-align: right;\\\">\\n\",\n \" <th></th>\\n\",\n \" <th>YearsExperience</th>\\n\",\n \" <th>Salary</th>\\n\",\n \" </tr>\\n\",\n \" </thead>\\n\",\n \" <tbody>\\n\",\n \" <tr>\\n\",\n \" <th>count</th>\\n\",\n \" <td>30.000000</td>\\n\",\n \" <td>30.000000</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>mean</th>\\n\",\n \" <td>5.313333</td>\\n\",\n \" <td>76003.000000</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>std</th>\\n\",\n \" <td>2.837888</td>\\n\",\n \" <td>27414.429785</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>min</th>\\n\",\n \" <td>1.100000</td>\\n\",\n \" <td>37731.000000</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>25%</th>\\n\",\n \" <td>3.200000</td>\\n\",\n \" <td>56720.750000</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>50%</th>\\n\",\n \" <td>4.700000</td>\\n\",\n \" <td>65237.000000</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>75%</th>\\n\",\n \" <td>7.700000</td>\\n\",\n \" <td>100544.750000</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>max</th>\\n\",\n \" <td>10.500000</td>\\n\",\n \" <td>122391.000000</td>\\n\",\n \" </tr>\\n\",\n \" </tbody>\\n\",\n \"</table>\\n\",\n \"</div>\"\n ],\n \"text/plain\": [\n \" YearsExperience Salary\\n\",\n \"count 30.000000 30.000000\\n\",\n \"mean 5.313333 76003.000000\\n\",\n \"std 2.837888 27414.429785\\n\",\n \"min 1.100000 37731.000000\\n\",\n \"25% 3.200000 56720.750000\\n\",\n \"50% 4.700000 65237.000000\\n\",\n \"75% 7.700000 100544.750000\\n\",\n \"max 10.500000 122391.000000\"\n ]\n },\n \"execution_count\": 7,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"dataset.describe()\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 7,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"X=dataset.iloc[:,:-1].values\\n\",\n \"y=dataset.iloc[:,1].values\\n\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 8,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"array([[ 1.1],\\n\",\n \" [ 1.3],\\n\",\n \" [ 1.5],\\n\",\n \" [ 2. ],\\n\",\n \" [ 2.2],\\n\",\n \" [ 2.9],\\n\",\n \" [ 3. ],\\n\",\n \" [ 3.2],\\n\",\n \" [ 3.2],\\n\",\n \" [ 3.7],\\n\",\n \" [ 3.9],\\n\",\n \" [ 4. ],\\n\",\n \" [ 4. ],\\n\",\n \" [ 4.1],\\n\",\n \" [ 4.5],\\n\",\n \" [ 4.9],\\n\",\n \" [ 5.1],\\n\",\n \" [ 5.3],\\n\",\n \" [ 5.9],\\n\",\n \" [ 6. ],\\n\",\n \" [ 6.8],\\n\",\n \" [ 7.1],\\n\",\n \" [ 7.9],\\n\",\n \" [ 8.2],\\n\",\n \" [ 8.7],\\n\",\n \" [ 9. ],\\n\",\n \" [ 9.5],\\n\",\n \" [ 9.6],\\n\",\n \" [10.3],\\n\",\n \" [10.5]])\"\n ]\n },\n \"execution_count\": 8,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"X\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 9,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"array([ 39343., 46205., 37731., 43525., 39891., 56642., 60150.,\\n\",\n \" 54445., 64445., 57189., 63218., 55794., 56957., 57081.,\\n\",\n \" 61111., 67938., 66029., 83088., 81363., 93940., 91738.,\\n\",\n \" 98273., 101302., 113812., 109431., 105582., 116969., 112635.,\\n\",\n \" 122391., 121872.])\"\n ]\n },\n \"execution_count\": 9,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"y\\n\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 13,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"from sklearn.model_selection import train_test_split\\n\",\n \"X_train,X_test,y_train,y_test=train_test_split(X,y,test_size=1/3,random_state=0)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 14,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"LinearRegression(copy_X=True, fit_intercept=True, n_jobs=None,\\n\",\n \" normalize=False)\"\n ]\n },\n \"execution_count\": 14,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"from sklearn.linear_model import LinearRegression\\n\",\n \"regressor=LinearRegression()\\n\",\n \"regressor.fit(X_train,y_train)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 15,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"y_pred=regressor.predict(X_test)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 16,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"array([ 40835.10590871, 123079.39940819, 65134.55626083, 63265.36777221,\\n\",\n \" 115602.64545369, 108125.8914992 , 116537.23969801, 64199.96201652,\\n\",\n \" 76349.68719258, 100649.1375447 ])\"\n ]\n },\n \"execution_count\": 16,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"y_pred\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 17,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"array([ 37731., 122391., 57081., 63218., 116969., 109431., 112635.,\\n\",\n \" 55794., 83088., 101302.])\"\n ]\n },\n \"execution_count\": 17,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"y_test\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 19,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"Text(0, 0.5, 'Salary')\"\n ]\n },\n \"execution_count\": 19,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n },\n {\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAAZsAAAEWCAYAAACwtjr+AAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4yLCBodHRwOi8vbWF0cGxvdGxpYi5vcmcvOIA7rQAAIABJREFUeJzt3XucXdP9//HXO4kgitCEEiaTEre4m7qWqmsilG+/fNG0UtXm11Zb9a1+DalbCVEtvVCaolRDtCmtijtB3SXul1SCkQQVRFBxS/L5/bHXjHNOztySc2bP5f18PM5j9l577b0/5yRzPrP2XnstRQRmZmbV1CvvAMzMrPtzsjEzs6pzsjEzs6pzsjEzs6pzsjEzs6pzsjEzs6pzsrEOI6lB0l55x9EVSfqPpM/mHMP/k/TLKh17d0lPV+PY7YhBkqZJ2iTPOLorJxtrF0mfl3SfpLclzZd0r6TP5R1XNUi6TNJH6Yu+8fV4HrFExKci4oU8zg0gqS/wE+AcSbsWfB7vSYqSz6imvcePiDsjYljlI2+epD9JOrUghgDOBU7ryDh6ij55B2Bdh6TVgOuB7wB/BvoCuwIfVvm8fSJiUTXP0YKfRcRPcjp33u+90IHAjIh4GXgZ+BSApFrgRaB/c3FK6gUQEUs6JNLl8zfgAklrRcS8vIPpTtyysfbYCCAiroqIxRHxfkTcEhFPAEjaQNIdkt6U9IakiZL6lzuQpO0l3S9pgaRXJZ2f/npu3B6SjpY0E5gp6QJJvyg5xj8k/bDMsS+S9POSsr9L+t+0fLyklyW9K+lfkvZs7wch6VBJL6QEjKQRkv4taWBB/D9Idd6QdE7jl27a/g1Jz0p6S9LNkgY3994LyjZMyytK+rmk2ZJeS+935bRtd0lzJf1I0rz02R5ZcOyVJf1C0kupdXpPwb47plbrAkmPS9q94C2PAO5qx+dzj6TTJd0PvAfUSPpmes/vSnpe0jcL6u8lqaFgfa6k/5X0ZIrzKkkrNnOujSTdneq9IenKgm2bSbottcJnSPrvVP5d4FDgxNQauxYgIhYCjwF7t/W9WhtFhF9+tekFrAa8CVxO9uWzRsn2Dcl+SVcEBgJ3A78s2N4A7JWWtwN2JGtd1wLPAj8sqBvArcCawMrA9sArQK+0fQCwEFi7TJy7AXMApfU1gPeBdYGN07Z107ZaYINm3u9lwBktfB4TU51Pp9j2L4l/aoq/BngO+GbadhAwC9g0vf+fAPc1994LyjZMy78ErkvbVwX+AZyVtu0OLAJ+CqwA7Jc+pzXS9guAO4FBQG9g5/TvNSj92+5H9kfo3ml9YNrvYeCQMp9BbYqtT0n5Penfe9MURx/gAOCzgIA90r/Jlqn+XkBDwf5zgQeAz6TPt+nzKxPDX4DjU9wrAbuk8lXJWmFHpPNvl97Txmn7n4BTyxzvt2Qt2tx/57rTK/cA/Opar/TlcVn6MliUvvSW+sJPdQ8CHi1YbyAlmzJ1fwhcW7AewB4ldZ4F9k7L3wNuaOZYAmYDu6X1bwF3pOUNgXnpy22FVt7rZcAHwIKC1+UF2/un8zwJ/K5k3wCGF6x/F7g9Ld8IHFWwrRdZQhjcwnuPFLvIWgobFGzbCXgxLe+evsT7FGyfR5bYe6VtW5V5r8cDV5SU3QyMTsszC99PQZ1amk82J7fy+V4PHJ2WyyWbwwrWzwXOb+Y4VwIXAoNKykcBU0vKLgHGpuXmks3ZwIS8fse668uX0axdIuLZiPh6RKwHbE7WWvglgKS1JE1Kl6jeIftlHlDuOOnSx/Xp0tM7wJll6s4pWb8c+Gpa/ipwRTMxBjAJODwVfYWsFUJEzCJLbKcC81K867bwln8eEf0LXqMLzrOA7K/qzYFflNm3MP6XyD4rgMHAr9LlqgXAfLIkMqiZfQsNBPoB0wv2vymVN3oziu+fLCS7xzKA7C//58scdzBwSOMx03E/D6yTtr9F1lJoj6L3IGl/SQ+mS1oLgH1o5v9H8u8y76GcH5G1nqaly26N/0aDgV1K3tOhfPKemrMq2R8WVkFONrbMImIG2V//m6eis8j+yt0yIlYjSwhqZvcLgRnA0FT3xDJ1S4ck/xNwoKStyFpYf2shvKuAg9O9kB2AvxbEfWVEfJ7syyjI/pJtN0lbA99I5/p1mSrrFyzXkF1qg+xL+P+VJLGVI+K+gvrNDcf+BlnrZFjBvqtHRHNfxKX7fgBsUGbbHLKWTWFMq0TE+LT9CdI9u3Zoeg/pvtBksv8ja0dEf+AWmv//0faTRLwaEd+MiHWAo4EJkoak93R7yXv6VER8rzS+EpsCufQ67M6cbKzNJG2Sbjyvl9bXJ2s9PJCqrAr8B1ggaRDw4xYOtyrwDvAfZc81fKe180fEXLJ7B1cAf42I91uo+yjwOnAxcHNqhSBpY0l7pJvNH5B9cS9u7dylJK1ElvxOBI4EBqWbzoV+LGmN9DkdA1ydyi8CTpA0LB1rdUmHtOW8kfXo+j1wnqS10v6DJO3bxn0vBc6VtK6k3pJ2Sp/Fn4ADJO2byldKnQ3WS7vfAHyhLTE2Y0Wy3ouvA4sl7Q+0u2NGOZL+J/1/g6xFEmT/ptcBwyR9RdIK6bW9pI1T3dfI7iEVHmtlYGvgtkrEZp9wsrH2eJeslfCgpPfIksxTZJcxIHs+YVvgbWAKcE0LxzqO7PLWu2Rfnle3ULfQ5cAWNHMJrcRVZPcCriwoWxEYT/ZX/r+BtcgSRnP+T8XPkLyRys8C5kbEhRHxIVkr7gxJQwv2/Tswnax30xSy+wVExLVkralJ6RLiU2QdLtrqeLIOBg+k/W8j6/jQFseR3WN6mOzy3dlknS7mkHVvPpEsIcwh+2Oh8TviH8AmrVxybFZK9scC16bzHkx2z6YSdgAeTv8nryG7DzQ7It4G9iX7t3mV7N/7LLL/A5D9IbKVsh6Bk1PZQcCtEfFahWKzpLG3jlmXIGk3sr/Ca6MTP7chKcguEc7KO5ZKkTQG2Cwilupu3h1IElkS/lpEPJt3PN2Nk411GZJWILvx/3hE/DTveFrSHZON2fLwZTTrEiRtSnY9fh1S7zcz6zrcsjEzs6pzy8bMzKrOA3EmAwYMiNra2rzDMDPrUqZPn/5GRAxsrZ6TTVJbW8u0adPyDsPMrEuR9FJb6vkympmZVZ2TjZmZVZ2TjZmZVZ2TjZmZVZ2TjZmZVZ2TjZmZVZ2TjZmZVZ2TjZlZD3XNI3M54ZonOuRcfqjTzKyHefv9j9nqtFua1s/68pZVP6eTjZlZD3LRXc8z/sYZTet3//iLHXJeJxszsx5g3rsfsP2425vWx+z2WU7cb9MOO7+TjZlZN3fmDc8y4e4XmtYfGrsna626UofG4A4CZmbd1Ow3F1JbP6Up0Rw/fBMaxo/MEs3EiVBbC716ZT8nTqxqLG7ZmJl1Q8de/RjXPvpy0/rjp+zD6iuvkK1MnAhjxsDChdn6Sy9l6wCjRlUlHs/UmdTV1YWnGDCzru6ZV95hv1//s2n97P/egkM/V1NcqbY2SzClBg+GhoZ2nU/S9Iioa62eWzZmZt1ARPC1Sx7inllvALBK395MP2lvVlqh99KVZ88uf5DmyiugavdsJF0qaZ6kpwrKzpE0Q9ITkq6V1L9g2wmSZkn6l6R9C8qHp7JZkuoLyodIelDSTElXS+qbyldM67PS9tpqvUczs87g4Yb5DDnhhqZE87uvbcfTPx1ePtEA1NS0r7wCqtlB4DJgeEnZrcDmEbEl8BxwAoCkzYDDgGFpn99K6i2pN3ABMALYDDg81QU4GzgvIoYCbwFHpfKjgLciYkPgvFTPzKzbWbR4CfucdxeHXHQ/AEMGrMLMcSPYd9hnWt5x3Djo16+4rF+/rLxKqpZsIuJuYH5J2S0RsSitPgCsl5YPBCZFxIcR8SIwC9g+vWZFxAsR8REwCThQkoA9gMlp/8uBgwqOdXlangzsmeqbmXUbd8x4jQ3H3shzr/0HgKu+tSNTj9udFXq34Wt91CiYMCG7RyNlPydMqFrnAMj3ns03gKvT8iCy5NNobioDmFNSvgPwaWBBQeIqrD+ocZ+IWCTp7VT/jdIAJI0BxgDUVLH5aGZWKR98vJidzrqdtxZ+DMD2tWsyacyO9OrVzr+pR42qanIplUuykTQWWAQ0duwu9ykF5Vte0UL9lo61dGHEBGACZL3RWgjZzCx31zwyl//98+NN69d///NsPmj1HCNquw5PNpJGA/sDe8Yn/a7nAusXVFsPeCUtlyt/A+gvqU9q3RTWbzzWXEl9gNUpuZxnZtaVvPvBx2xx6icDZ47cch3OP3wbutIdgg4dQUDScOB44EsRsbBg03XAYakn2RBgKPAQ8DAwNPU860vWieC6lKSmAgen/UcDfy841ui0fDBwR/hhIjProi6558WiRDP1uN254CvbdqlEA1Vs2Ui6CtgdGCBpLnAKWe+zFYFb0wf1QER8OyKelvRn4Bmyy2tHR8TidJzvATcDvYFLI+LpdIrjgUmSzgAeBS5J5ZcAV0iaRdaiOaxa79HMrFre/M+HbHfGbU3rX9+5llO/NCzHiJaPRxBIPIKAmXUW59w8gwumPt+0/sAJe/KZ1Tt24My28ggCZmZdzNy3FvL5s6c2rf9o7434/p5Dc4yocpxszMw6geMnP8HV0z550uOxk/emf7++OUZUWU42ZmY5mvnau+x93t1N62cctDlf3XFwjhFVh5ONmVkOIoJvXj6N22fMA2CF3uLxU/ahX9/u+bXcPd+VmVkn9sjst/jyb+9rWj//K9uw/5br5hhR9XmmTjPrXjp4Bsr2WLwkOOA39zQlmkH9V+a5M0Z0+0QDbtmYWXeSwwyUbXXXc68z+tKHmtavOGp7dh06MMeIOpafs0n8nI1ZN1DBGSgr5aNFS9j1Z3fw2jsfArDV+v259js7t3/gzE7Kz9mYWc+TwwyULfnH46/w/asebVr/29G7sPX6/VvYo/tysjGz7qOmpnzLpoOnEHnvw0VscerNLEkXjvbebG0mfG27LjeeWSW5g4CZdR85zEBZ6or7Gxh2yieJ5rb/3Y3fH1HXoxMNuGVjZt1JYyeAsWOzS2c1NVmi6YDOAW+99xHbnH5r0/rh29dw1pe3qPp5uwonGzPrXjp4BkqAX902k/Nue65p/d76PRjUf+UOjaGzc7IxM1tGr779PjuddUfT+vf32JAf7bNxjhF1Xk42ZmbLoLZ+StH6IyftzZqrdJ+BMyvNHQTMzNrh9mdfK0o0+w5bm4bxI51oWuGWjZlZG0QEQ064oajsoRP3ZK3VOuekZp2Nk42ZWSuuemg2J1zzZNP6FzceyB+O3D7HiLoeJxszs2YsXhJscGJxa+bJU/dh1ZVWyCmirsvJxsysjF/c8i9+c8espvXROw3mtAM3zzGirs3JxsyswAcfL2aTk24qKnvujBH07eP+VMvDycbMLDn26se49tGXm9ZP3G8Txuy2QY4RdR9ONmbW45UONQPw4ln79fjxzCrJycbMerT/+u29PDp7QdP6rw7bmgO3HpRjRN2TL0KaWY80Z/5CauunFCWahvEj255oOvH0052RWzZm1uNseerNvPPBoqb1K7+1AztvMKDtB+jE0093Vm7ZmFmP8dTLb1NbP6Uo0TSMH9m+RAPZFAaNiabRwoVZuZXllo2Z9QilA2feeMyubLrOast2sE42/XRX4JaNmXVrdz/3elGiWWvVFWkYP3LZEw00P810B08/3ZW4ZWNm3VZpa+a++j1YtxKTmo0bV3zPBjp8+umuxi0bM+t2rn54dlGi2WHImjSMH1mZRANZJ4AJE2DwYJCynxMmuHNAC9yyMbNuo9zAmY+fvA+r96vCwJk5TD/dlTnZmFm3MPrSh7jrudeb1jdee1VuPna3HCOyQk42Ztalvf/RYjY9uXjgzGd+ui/9+vrrrTPxv4aZdVk7nHkbr73zYdP6rkMHcMVRO+QYkTXHycbMupzX3/2Qz427rajs+TP3o3cvD5zZWVWtN5qkSyXNk/RUQdmakm6VNDP9XCOVS9KvJc2S9ISkbQv2GZ3qz5Q0uqB8O0lPpn1+rTQ8a3PnMLPuobZ+SlGiOXKXWhrGj3Si6eSq2fX5MmB4SVk9cHtEDAVuT+sAI4Ch6TUGuBCyxAGcAuwAbA+cUpA8Lkx1G/cb3so5zKwLm/nau0s9N9MwfiSnHDAsp4isPap2GS0i7pZUW1J8ILB7Wr4cuBM4PpX/MSICeEBSf0nrpLq3RsR8AEm3AsMl3QmsFhH3p/I/AgcBN7ZwDjProkqTzGlfGsbonWvzCcaWSUffs1k7Il4FiIhXJa2VygcBcwrqzU1lLZXPLVPe0jmWImkMWeuIGg8zYdbp3Pf8G3zl9w8WlTWMH5lTNLY8OssIAuUutsYylLdLREyIiLqIqBs4cGB7dzczqNq8LrX1U4oSzSWj65xourCObtm8Jmmd1OJYB5iXyucC6xfUWw94JZXvXlJ+Zypfr0z9ls5hZpVWhXldJk+fy3F/ebyozEmm6+vols11QGOPstHA3wvKj0i90nYE3k6Xwm4G9pG0RuoYsA9wc9r2rqQdUy+0I0qOVe4cZlZpFZ7XpbZ+SlGiuf77n3ei6Saq1rKRdBVZq2SApLlkvcrGA3+WdBQwGzgkVb8B2A+YBSwEjgSIiPmSTgceTvV+2thZAPgOWY+3lck6BtyYyps7h5lVWoXmdTn3ln/x6ztmFZU5yXQvyjqAWV1dXUybNi3vMMy6ltra7NJZqcGDoaGh1d2XLAk+WzJw5r31ezCoUqMzW9VJmh4Rda3V6ywdBMysKxo3LpvHpVAb53U5euIjRYmmb+9eNIwf6UTTTXm4GjNbdo2dAMaOzS6d1dRkiaaFzgEfLlrMxj8pHjjziVP3YbWVqjANgHUaTjZmtnzaMa/Lnr+4k+dff69pfev1+/O3o3epVmTWiTjZmFnVvfXeR2xz+q1FZTPHjWCF3r6S31M42ZhZVZUONfM/devxs4O3yikay4v/rDDriqr01H4lNbzx3lKJ5sWz9nOi6aHcsjHraqrw1H6llSaZ+hGb8O0vbJBTNNYZ+DmbxM/ZWJexnM+2VNP0l+bz3xfeX1TmhzO7t7Y+Z+OWjVlXU6Gn9iuttDVzwVe2ZeSW6+QUjXU2TjZmXU1NTfmWTU7TZFz8zxc4Y8qzRWVuzVgpdxAw62qW46n9dmulI0Jt/ZSiRPPX7+zkRGNluWVj1tUsw1P7y6SFjgg/7rs5f5k+t6i6k4y1xMnGrCtqx1P7y6zM9AGxcCFDnuxP4US5N/xgVzZbd7XqxmJdnpONmZVX0uFg+JG/YcZaQ4rK3JqxtnKyMbPyUkeED3v3YePj/la06aET92St1VbKKTDripxszKy8ceOofbL/UsUNWywAJxprJ/dGM7OlzH/vo6USzbN/+WGWaDrJKAXWtbhlY2ZFSh/OHNR/Ze6t3wN8f8aWg5ONmQEwa95/2Ovcu4rKXjhzP3r1Uk4RWXfiZGNmS7VmRmz+GS786nY5RWPdkZONWQ9276w3GHXxg0Vl7s5s1eBkY9ZDlbZmjt1rI47Za2hO0Vh352Rj1sNc+eBsTrz2yaIyt2as2pxszHqQ0tbM+V/Zhv23XDenaKwnaVOykdQ7IhZXOxgzq47T/vE0f7i3oajMrRnrSG1t2cySNBn4Q0Q8U82AzKyySlsz13x3Z7atWSOnaKynamuy2RI4DLhYUi/gUmBSRLxTtcjMbLn8z0X381DD/KIyt2YsL21KNhHxLvB74PeSdgOuAs5LrZ3TI2JWFWM0s3ZYtHgJG469sajs3vo9GNR/5ZwiMmvHPRtgJHAkUAv8ApgI7ArcAGxUpfjMrB2Gjr2BjxdHUZlbM9YZtPUy2kxgKnBORNxXUD45tXTMLEfvfPAxW556S1HZU6fty6dWdIdT6xxa/Z+YWjWXRcRPy22PiB9UPCoza7PSDgCfWrEPT522b07RmJXXarKJiMWSvgiUTTZmlo/Zby5kt3OmFpU9f+Z+9PbAmdYJtbWNfZ+k84GrgfcaCyPikapEZWYtKm3N7Dp0AFcctUNO0Zi1rq3JZuf0s7B1E8AelQ3HzJo1cSJTz5/IkV84uqjYHQCsK2hr1+cvVjsQM2vBxInZzJkFiWb4rAe56L82zjEos7Zrc1cVSSOBYUDT5OPNdRows8q5+J8vcEbJFM0NZ++fLUwb7GmarUvo1ZZKki4CDgW+Dwg4BBi8rCeVdKykpyU9JekqSStJGiLpQUkzJV0tqW+qu2Jan5W21xYc54RU/i9J+xaUD09lsyTVL2ucZnmrrZ/CGVOebVr/8V2Xf5JoAGbPziEqs/ZrU7IBdo6II4C3IuI0YCdg/WU5oaRBwA+AuojYHOhNNhTO2cB5ETEUeAs4Ku1yVDrvhsB5qR6SNkv7DQOGA7+V1Dt11b4AGAFsBhye6pp1Gcde/dhSnQAazt6fox/4S3HFmpoOjMps2bU12byffi6UtC7wMTBkOc7bB1hZUh+gH/AqWWeDyWn75cBBafnAtE7avqckpfJJEfFhRLwIzAK2T69ZEfFCRHwETEp1zbqE2vopXPvoy03rE762HQ1bLIB+/Yor9usH48Z1cHRmy6at92yul9QfOAd4hKwn2sXLcsKIeFnSz4HZZEnsFmA6sCAiFqVqc4FBaXkQMCftu0jS28CnU/kDBYcu3GdOSXnZPqGSxgBjAGr8F6LlbNef3cGc+e8XlTX1NBuW7suMHZtdOqupyRKN79dYF9HW3minp8W/SroeWCki3l6WE0pag6ylMQRYAPyF7JLXUqdt3KWZbc2Vl2utRZkyImICMAGgrq6ubB2zalu8JNjgxBuKym48Zlc2XWe14oqjRjm5WJfVYrKR9OUWthER1yzDOfcCXoyI19NxriF7jqe/pD6pdbMe8EqqP5fs/tDcdNltdWB+QXmjwn2aKzfrVErvy4Cfm7HuqbWWzQEtbAtgWZLNbGBHSf3ILqPtCUwjG+jzYLJ7LKOBv6f616X1+9P2OyIiJF0HXCnpXGBdYCjwEFmLZ6ikIcDLZJ0IvrIMcZpVzbsffMwWJQNnTvvJXgz41Io5RWRWXS0mm4g4stInjIgH0zw4jwCLgEfJLmVNASZJOiOVXZJ2uQS4QtIsshbNYek4T0v6M/BMOs7RjVNXS/oecDNZT7dLI+LpSr8Ps2Xl1oz1RIpo262K7v5QZ11dXUybNi3vMKwbKzdw5r/OGM6KfXrnFJHZ8pM0PSLqWqvX1snTLiLrovxFsl5oB5NdsjKzNihtzfQSvHCWWzPWc7R5IM6I2FLSExFxmqRfsGz3a8x6lAdfeJNDJzxQVPbiWfuRPSpm1nO0NdmUPtQ5n+V7qNOs2yttzez02U9z1Zgdc4rGLF/tfajzZ2QPYMIyPtRp1t1d9dBsTrjmyaIydwCwnq6152w+B8xpfKhT0qeAJ4EZZOOUmVmB0tbMt7+wAfUjNskpGrPOo7WWze/IHsJE0m7AeLKRn7cm6658cFWjM+siTvrbU1zxwEtFZW7NmH2itWTTOyLmp+VDgQkR8VeyYWseq25oZl1DaWvml4duzUHbDGqmtlnP1GqyKRhCZk/SoJVt3NesW9v0pJt4/+PFRWVuzZiV11rCuAq4S9IbZD3S/gkgaUNgmQbiNOvqIoIhJxQPnHnlt3Zg5w0G5BSRWefX2nA14yTdDqwD3BKfDDfQi+zejVmP4qFmzJZNq5fCIuKBMmXPVSccs85p4UeL2Ozkm4vK7v7xF6n5dL9m9jCzQr7vYtYKt2bMlp+TjVkz5sxfyK4/Kx448+nT9mWVFf1rY9Ze/q0xK8OtGbPKcrIxK3D/829y+O+Lb1O+cOZ+9OrlgTPNloeTjVlS2prp27sXz40bkVM0Zt2Lk431eH+8v4GT/148masvmZlVlpON9WilrZkvbjyQPxy5fU7RmHVfTjbWI/3oz4/z10fmFpW5NWNWPb3yDsCso9XWTylKND/ae6PWE83EiVBbC716ZT8nTqxqjGbdjVs21mPsMv4OXl7wflFZm1ozEyfCmDGwcGG2/tJL2TrAqFEVjtKse9Inw531bHV1dTFt2rS8w7AqKDdw5sVH1LHXZmu37QC1tVmCKTV4MDQ0LHd8Zl2ZpOkRUddaPbdsrFuryMOZs2e3r9zMluJ7NtYtfbho8VKJ5tZjd1u2TgA1NS2X+36OWavcsrFup+JDzYwbV3zPBqBfv6zc93PM2sTJxrqNee98wPZn3l5U9tjJe9O/X9/lO3Bj0hg7Nrt0VlOTJZpRo7KWTGESgmx97FgnG7MCvoxmlZPj5aTa+ilLJZqG8SOXP9E0GjUq6wywZEn2szGR+H6OWZu4ZWOVkdPlpMfmLOCgC+4tKps1bgR9enfQ31E1NeV7qjV3n8esh3LLxipj7NjmLydVSW39lKUSTcP4kR2XaCC7nNavZLbOxvs5ZtbELRurjA68nHT9E6/wvSsfLSrLbaiZlu7nmFkTJxurjA66nFTa02ybmv5c+91dKnqOdhs1ysnFrBVONlYZLXUProBzbp7BBVOfLyrzwJlmXYeTjVVGFS8nlbZmjtlzKMfuvdFyH9fMOo6TjVVOhS8nffXiB7ln1htFZW7NmHVN7o1mnVJt/ZSiRHPhqG0rl2g8vIxZh3PLxjqVISdMoXQg8oq2Zjy8jFkucmnZSOovabKkGZKelbSTpDUl3SppZvq5RqorSb+WNEvSE5K2LTjO6FR/pqTRBeXbSXoy7fNrScrjfXZbVWgZLFq8hNr64kRzww92rfxlsxyeBzKz/Fo2vwJuioiDJfUF+gEnArdHxHhJ9UA9cDwwAhiaXjsAFwI7SFoTOAWoAwKYLum6iHgr1RkDPADcAAwHbuzIN9htVaFlUPGBM1vi4WXMctHhLRtJqwG7AZcARMRHEbEAOBC4PFW7HDgoLR8I/DEyDwD9Ja0D7AvcGhHzU4K5FRietq0WEfdHNjPcHwuOZcurgi2DBQs/WirRTPvJXtXtBNDadAFmVhV5tGw+C7wO/EHSVsB04Bhg7Yh4FSAiXpW0Vqo/CJhTsP/cVNZS+dwy5UuRNIasBUSNv2zapkItgw5tzRSq8vNAZlZeHvds+gDbAhdGxDbAe2SXzJpT7n5LLEP50oVW25ZaAAAPOUlEQVQREyKiLiLqBg4c2HLUllnOlsGsee8ulWieO2NEx3VpHjUKJkzIpnSWsp8TJrhzgFmV5dGymQvMjYgH0/pksmTzmqR1UqtmHWBeQf31C/ZfD3glle9eUn5nKl+vTH2rhOVoGeTWminl4WXMOlyHt2wi4t/AHEkbp6I9gWeA64DGHmWjgb+n5euAI1KvtB2Bt9PltpuBfSStkXqu7QPcnLa9K2nH1AvtiIJj2fJahpbB1Bnzlko0L561nx/QNOtB8nqo8/vARElPAFsDZwLjgb0lzQT2TuuQ9SZ7AZgF/B74LkBEzAdOBx5Or5+mMoDvABenfZ7HPdEqq7mJxMqorZ/CkZc93LS+1fr9aRg/krK90dvapdoPZZp1OYrSJ+h6qLq6upg2bVreYXReEye2a9yzi+56nvE3zigqa7ElU9qlGrLLc6WtprbWM7MOIWl6RNS1Ws/JJuNk04J2fsGXXjL7xi5DOPmAzVo+R21t+SkKBg/OWk/trWdmHcLJpp2cbFrQxi/4y+59kVP/8UxRlTbfl+nVi6XGqYHsvtCSJe2vZ2Ydoq3JxmOjWeva8GxNaWvm54dsxcHbrVe6R/PaOvlaB03SZmaV5VGfrXUtPFtz0t+eWirRNIwf2b5EA9k9oH79isvKdaluaz0z61ScbKx1Zb7go18/ag+7gCse+KSVcedG79Aw6ehl6yXW1i7VfijTrEvyPZvE92xaUdAb7ZAjz+PhgRsWbW7YYoF7iZn1QO4g0E5ONq374OPFbHLSTUVlj528N/379XUvMbMeyh0ErKK2OOVm3v1wUdN6/34r8NjJ+3xSwUP3m1kLnGysRQsWfsTWP721qOy5M0bQt0/J7T73EjOzFjjZWLNKe5l9eZtBnHvo1uUre+h+M2uBk40tZfabC9ntnKlFZS+etV/58cwaNXYCaMeQNmbWczjZWJHS1syP992Yo7+4YTO1S3jofjNrhpONAfDI7Lf48m/vKyrzFABmVilONrZUa+Y3h2/DAVutm1M0ZtYdOdn0YDc++SrfmfhIUZlbM2ZWDU42PVRpa2byt3eirnbNnKIxs+7OyaaH+d1dz3NWeyY1MzOrACebHiIiGHLCDUVlU4/bnSEDVskpIjPrSZxseoD6vz7BpIfnFJW5NWNmHcnJphv7ePESho69sajskZP2Zs1V+uYUkZn1VE423dSXf3svj8xe0LQ+ZMAqTD1u9/wCMrMezcmmm3n3g4/Z4tRbispmnD6clVbonVNEZmaeqbN6Jk7M5nhZllkrl9Exkx4tSjTDh32GhvEjnWjMLHdu2VTDxInFIyC/9FK2DlUZO2z+ex+x7enF0wC8cOZ+9OrVwsCZZmYdyDN1JhWdqbMDZ6088IJ7eXzOJ/dmfn34NnzJQ82YWQfxTJ156oBZK+fMX8iuPyueBsDdmc2ss3KyqYYqz1pZOkXzld/agZ03GFCRY5uZVYM7CFTDuHHZLJWFKjBr5VMvv01t/ZSiRNMwfqQTjZl1ek421TBqFEyYkN2jkbKfEyYsV+eA2vop7P+be5rWb7pxHA0/O6DDerqZmS0PX0arlgrNWnnXc68z+tKHmtbX7rOEB887rMN6upmZVYKTTSdWOg3AffV7sO7Wm36SaBotXAhjxzrZmFmn5WTTCU2ePpfj/vJ40/qOn12TSWN2ylY6oKebmVmlOdl0IkuWBJ89sXgagMdP2YfVV17hk4Iq93QzM6sGdxDoJM6/Y2ZRojnsc+vTMH5kcaKBqvV0MzOrJrdscvbBx4vZ5KSbispaHDiz8b7M2LHZpbOamizR+H6NmXViTjY5Kp3U7Lh9NuJ7ewxtfccK9XQzM+souV1Gk9Rb0qOSrk/rQyQ9KGmmpKsl9U3lK6b1WWl7bcExTkjl/5K0b0H58FQ2S1J9R7+31rz9/sfU1k8pSjQvnLlf2xKNmVkXlOc9m2OAZwvWzwbOi4ihwFvAUan8KOCtiNgQOC/VQ9JmwGHAMGA48NuUwHoDFwAjgM2Aw1PdTuHwCQ+w1WmfTANwzsFb0jB+ZGVGaM5hWgMzs7bIJdlIWg8YCVyc1gXsAUxOVS4HDkrLB6Z10vY9U/0DgUkR8WFEvAjMArZPr1kR8UJEfARMSnUrrx1f7q8seJ/a+inc/8KbTWUN40dySN36lYtlzJisp1rEJw97OuGYWSeQ1z2bXwL/B6ya1j8NLIiIxkG/5gKD0vIgYA5ARCyS9HaqPwh4oOCYhfvMKSnfoVwQksYAYwBq2tt1uB1z1uxw5m289s6HTeuXf2N7vrDRwPadrzVjx/phTzPrtDq8ZSNpf2BeREwvLC5TNVrZ1t7ypQsjJkREXUTUDRzYzi//lr7ckxn/fofa+ilFiaZh/MjKJxrww55m1qnl0bLZBfiSpP2AlYDVyFo6/SX1Sa2b9YBXUv25wPrAXEl9gNWB+QXljQr3aa68clr5ch9/4wwuuuv5puLrv/95Nh+0esXDaOKHPc2sE+vwlk1EnBAR60VELdkN/jsiYhQwFTg4VRsN/D0tX5fWSdvviGx60euAw1JvtSHAUOAh4GFgaOrd1jed47qKv5FmvsTnbLo1tfVTmhLNsHVXo2H8yOomGvDDnmbWqXWm52yOByZJOgN4FLgklV8CXCFpFlmL5jCAiHha0p+BZ4BFwNERsRhA0veAm4HewKUR8XTFox03rvieDXDcAT9i8mZfbFp//OR9WL3fCuX2rjw/7GlmnZiyRoLV1dXFtGnT2rfTxIkwdiwzForh3zi/qfisL2/B4dv78pWZdX+SpkdEXWv1OlPLpusZNYp5B/w3w8+8HYAV+/TisZP3YeW+zQw1Y2bWQznZLKeV+/bmCxsN5LDPrc+ILdbJOxwzs07JyWY5rbrSClz+je3zDsPMrFPzFANmZlZ1TjZmZlZ1TjZmZlZ1TjZmZlZ1TjZmZlZ1TjZmZlZ1TjZmZlZ1TjZmZlZ1HhstkfQ6UGaM/k5tAPBG3kHkqKe/f/Bn0NPfP+T/GQyOiFYn6XKy6cIkTWvLAHjdVU9//+DPoKe/f+g6n4Evo5mZWdU52ZiZWdU52XRtE/IOIGc9/f2DP4Oe/v6hi3wGvmdjZmZV55aNmZlVnZONmZlVnZNNFyNpfUlTJT0r6WlJx+QdUx4k9Zb0qKTr844lD5L6S5osaUb6v7BT3jF1NEnHpt+BpyRdJWmlvGOqNkmXSpon6amCsjUl3SppZvq5Rp4xNsfJputZBPwoIjYFdgSOlrRZzjHl4Rjg2byDyNGvgJsiYhNgK3rYZyFpEPADoC4iNgd6A4flG1WHuAwYXlJWD9weEUOB29N6p+Nk08VExKsR8UhafpfsS2ZQvlF1LEnrASOBi/OOJQ+SVgN2Ay4BiIiPImJBvlHlog+wsqQ+QD/glZzjqbqIuBuYX1J8IHB5Wr4cOKhDg2ojJ5suTFItsA3wYL6RdLhfAv8HLMk7kJx8Fngd+EO6lHixpFXyDqojRcTLwM+B2cCrwNsRcUu+UeVm7Yh4FbI/RoG1co6nLCebLkrSp4C/Aj+MiHfyjqejSNofmBcR0/OOJUd9gG2BCyNiG+A9Oumlk2pJ9yUOBIYA6wKrSPpqvlFZS5xsuiBJK5AlmokRcU3e8XSwXYAvSWoAJgF7SPpTviF1uLnA3IhobNFOJks+PclewIsR8XpEfAxcA+ycc0x5eU3SOgDp57yc4ynLyaaLkSSya/XPRsS5ecfT0SLihIhYLyJqyW4I3xERPeov2oj4NzBH0sapaE/gmRxDysNsYEdJ/dLvxJ70sE4SBa4DRqfl0cDfc4ylWX3yDsDabRfga8CTkh5LZSdGxA05xmQd7/vAREl9gReAI3OOp0NFxIOSJgOPkPXQfJQuMmzL8pB0FbA7MEDSXOAUYDzwZ0lHkSXhQ/KLsHkersbMzKrOl9HMzKzqnGzMzKzqnGzMzKzqnGzMzKzqnGzMzKzqnGys25O0WNJjBa+qPm0v6UvVPkcz562T9OuOPq9ZW7jrs3V7kv4TEZ/qoHP1iYhFHXGuzkpS74hYnHcc1rm4ZWM9kqTVJf2r8Sn8NB/Kt9LyfyT9QtIjkm6XNDCVbyDpJknTJf1T0iap/DJJ50qaCpwt6euSzk/bBkr6q6SH02uXVH5qmpvkTkkvSPpBQWxHSHpC0uOSrmjpOCXvaffG+X1aOn5B/aMknVew/i1J56blr0p6KLUEfyepdyq/UNK0NI/MaQX7Nkg6WdI9wCGSfiDpmfQ+Ji3fv5Z1CxHhl1/d+gUsBh4reB2ayvcG7icb9uamgvoBjErLJwPnp+XbgaFpeQeyoXIgm2PkeqB3Wv96wT5XAp9PyzVkwwwBnArcB6wIDADeBFYAhgH/Agakemu2dJyS97k7cH1Lxy+pvwrwfGN5qr8FsCnwj4Ly3wJHlMTTG7gT2DKtNwD/V3DsV4AV03L/vP8P+JX/y8PVWE/wfkRsXVoYEbdKOgS4gGwCskZLgKvT8p+Aa9Io2zsDf8mG4gKyL/JGf4nyl472AjYr2Gc1Saum5SkR8SHwoaR5wNrAHsDkiHgjxTi/peNENqdRc8odf27B+39P0h3A/pKeJUsuT0r6HrAd8HA638p8Mrjj/0gaQzbU1TrAZsATaVvjZ0Yqmyjpb8DfWojReggnG+uxJPUi+yv+fWBNCr6ISwTZJecF5ZJW8l4z5b2AnSLi/ZJzA3xYULSY7PdR6XxtOk4ryh2/1MXAicAM4A+N4QGXR8QJJTEPAY4DPhcRb0m6DCicirnwMxhJNsHbl4CTJA2LHn4vq6fzPRvryY4lGyn4cODSNHUDZL8XB6flrwD3RDZn0IupJYQyW5UesIxbgO81rkhqLlk1up2s9fDpVH/NZTxOm0Q2TcH6ZO/zqoIYDpa0VmMMkgYDq5EllLclrQ2MKHfMlMTXj4ipZJPc9Qc6pIOGdV5u2VhPsHLBCNkANwGXAt8Eto+IdyXdDfyEbBTd94BhkqYDbwOHpv1GARdK+gnZ/ZVJwOOtnPsHwAWSniD7fbsb+HZzlSPiaUnjgLskLSYbzfjr7T1OO/0Z2Doi3koxPJPe4y0pcXwMHB0RD0h6FHiabKTpe5s5Xm/gT5JWJ2slnRc9c9pqK+Cuz2YlOrKrdGeQerCdFxG35x2LdV++jGbWQ0nqL+k5sg4UTjRWVW7ZmJlZ1bllY2ZmVedkY2ZmVedkY2ZmVedkY2ZmVedkY2ZmVff/Ad1JwYuOA6AQAAAAAElFTkSuQmCC\\n\",\n \"text/plain\": [\n \"<Figure size 432x288 with 1 Axes>\"\n ]\n },\n \"metadata\": {\n \"needs_background\": \"light\"\n },\n \"output_type\": \"display_data\"\n }\n ],\n \"source\": [\n \"#Visualsing the training set results\\n\",\n \"plt.scatter(X_train,y_train,color='red')\\n\",\n \"plt.plot(X_train,regressor.predict(X_train),)\\n\",\n \"plt.title('Salary vs Experience(Train set)')\\n\",\n \"plt.xlabel('Experience in years')\\n\",\n \"plt.ylabel('Salary')\\n\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 22,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAAZsAAAEWCAYAAACwtjr+AAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4yLCBodHRwOi8vbWF0cGxvdGxpYi5vcmcvOIA7rQAAIABJREFUeJzt3XuYXFWZ7/HvjwQCAcItASG3hgFBQByg5aIOwwByERH0wAFth+DByRkVHW/jqPEGGh1HAQdFIIISNBIRUCJyPYHxDpgAIlcTJDcIEiBBQjCQ5D1/rNX0rurqTqfTVbu6+vd5nnq69tq3tyrpevtde9XaigjMzMzqaZOyAzAzs9bnZGNmZnXnZGNmZnXnZGNmZnXnZGNmZnXnZGNmZnXnZGOlkLRA0lFlxzHYSJogaaWkYSXH8RVJHy4zhoEiaaSkRyRtV3YsrczJxvpN0psk/VbSc5KelfQbSa8vO65WFhGLImKriFhbVgySxgCnA5dI6sjJb6WkFyWtKyyv3Ihz7CVpzcBFXXHsOyS9u3M5IlYBM4CP1+N8ljjZWL9IGgVcD3wT2B4YC5wNrK7zeYfX8/jNrIle+xnADRHxYkTMyMlvK+A44InO5dw2WMwAzmyi97jlONlYf70aICKujIi1+YPnloi4D0DS30m6TdIzkp6WNEPStrUOJOkgSb+TtELSUknfkrRZYX1I+oCkecA8SRdKOrfqGD+r1a0j6WJJX69qu07SR/Pz/5D0uKTnc1fKkT3EOELS1yUtkvSXfNwtCse4o/ODStL7JD0gaXNJbTn+yZKeyK/vY4XjbiLpk5Ieze/VVZK2z+s69z1T0iLgtkJb57m2kXRZPu7jkr7U2cUm6QxJv85xL5f0mKTjCufeXtL3clzLJf20sO6tku7N/ya/lbRf4e04DvhFrfeph/dufH7Pn5b0Z0n/Wlj3Rkn3SPqrpCclfSWv+iUwrFAl7V/juD3ti6R/kHRnjv9uSW/M7ecCrwcuzcc9FyAiHgVeBg7s6+uyDRQRfvixwQ9gFPAMMJ304bNd1frdgTcDI4AxpA+PbxTWLwCOys8PBA4BhgNtwEPAhwvbBnArqYLaAjgIeALYJK8fDawCdqoR52HAYkB5eTvgRWAXYM+8bpe8rg34ux5e7zeAWTmGrYGfAV/J6zbJr+8LwB7AcmD/wjEDuBLYEngtsKzw2j8M3AGMy+/VJcCVVftekffdotA2PG/z07zPlsCOwF3A/83rziB9gP4LMAx4X37fOt+LnwM/yu/JpsA/5vYDgKeAg/N+k/K/14i8fhnw+hrv0eHAkqq2YcAfgf8ANiP9kbKocK57gFPy862Bg/PzvYA16/k/2NO+baT/m0flf5u35Ji3y+vvAN5d43i3AJPL/t1q1UfpAfgxeB/Aa4DLgSXAmvxh3O0DP297EnBPYXlB5wdujW0/DPyksBzAEVXbPAS8OT8/i9StU+tYyh9uh+XlfwFuy893zx+qRwGb9vI6BbxAIREBhwKPFZbbgGdzXJ+qag9gr0LbfwGXFV7HkYV1O5MSxPDCvrvVON5wYCdSt+UWhfXvBG7Pz88A5hfWjcz7viqfZx1VfyTk7S4CvljV9ghdCeLl4uspbHM43ZPNPwLzqtrOBi7Kz+8CpgA7VG3Tl2TT076fB75T1fYL4NT8vKdkcw3wibJ/r1r14W4067eIeCgizoiIccC+pGrhGwCSdpQ0M3ft/BX4AakC6UbSqyVdn7tC/gp8uca2i6uWpwOdF3nfDXy/hxgDmEn6EAZ4F6l/noiYT0psXwCeyvHuUuMwY0gf1HNzt8wK4Kbc3nmeBcDtpGRwYY1jFONfSHqvACYCPykc9yFgLSmR1Nq3aCKpIlla2P8SUoXT6clCjKvy062A8cCzEbG8h+N+rPOY+bjjCzEvJ1USfTERaKs61kdJCQ9S1bQf8Kfc7XVMH4/b274TgXdXnbO9EH9PtgZWbMD5bQM42diAiIiHSVXOvrnpK6S/oveLiFGkhKAedr8IeBjYI2/76RrbVk9P/gPgREmvI1VYP6VnVwInS5pI6hq6phD3DyPiTaQPqAC+WmP/p0ldb/tExLb5sU0ULoBLegup2pkNfK3GMcYXnk8gdWdBSiTHFY67bURsHhGP9/LaKey7Ghhd2HdUROzT4ztRue/2qn0dbTEwtSqmkRFxZV5/H/maXR/P83DVsbaOiLfDK3+wnEpKkBcA1ypdr1vvdPS97LsYuLTqnFtGxPmdu/ZwyNcAf+jj67IN5GRj/aI0NPVjksbl5fGk6uGOvMnWwEpghaSxwL/3critgb8CKyXtRbq20KuIWAL8nlTRXBMRL/ay7T2kPvtLgZsjYkWOeU9JR0gaAfyNlFC6DSmOiHXAd4DzJe2Y9x3b+Ze0pNHAZcB7SX9tn5CTT9Fnlb7PsQ/wHtK1EoCLgak5ESJpjKQT1/f6c1xLSdcZzpU0Kg82+DtJ/9jHfW8Evi1pO0mbSjosr/4O8K+SDlaypaTjJXVWMzeQusf64tf5dX1YacDEcEn7STogt58uaYdIQ7mfIyWCdaTuzWGSJvR04F72nQ6cIulIScMkbZGfd1ZTfwF2qzrWbqRrSnP7+LpsQ5Xdj+fH4HyQhjpfBTxOup7xOKkLZ1Revw/pF3clcC/wMQr9+VQOEDiMVNmsBH4FnAP8urBtALvXiOHded0/9SHez+ZtTym07Ufq93+edL3levJggRr7b07q3vszKTE+BHwor7sWuLiw7XGkymUHuq6xTM5tT1K4LkD6g++jpGsizwOPAl/O6zr3HV7YvqIN2IZUGS4hfeDeA5yW151RfB+r30vSYIfppA/f5cC1he2OJSXzFcBS4MfA1nnd6Hy+LaqOfThV12xy+/j8f6XzPL+h6xraVaTK8XnSQIK3FPb7KumPhBXA39c4bm/7vpGU6JaTEtcsugaC/CMwP6/7r8L/jy+X/XvVyo/OUSlmg07+S/wHQFuk6qPpSGoDHiMNQKjLlxTLIOnLwFMR8Y2yY9lYkkaSkvShEfFs2fG0KicbG5QkbUq68P+HiDin7Hh60qrJxmxD+ZqNDTqSXkPqWtmZPPrNzJqbKxszM6s7VzZmZlZ3nnQuGz16dLS1tZUdhpnZoDJ37tynI2LM+rZzssna2tqYM2dO2WGYmQ0qkhb2ZTt3o5mZWd052ZiZWd052ZiZWd052ZiZWd052ZiZWd052ZiZWd052ZiZWd052ZiZDVF/+hN86Uvw8sv1P5eTjZnZEBMBp5wCe+4Jn/0sPPHE+vfZWJ5BwMxsCJk7F9rbu5a//32YOLH+53WyMTMbAtatg3/4B/jtb9PyTjvBwoUwYkRjzu9uNDOzVjZjBrN3ehfDhnUlmhtvhCefbFyiAScbM7OW9fL0H9L2z2/iqKd+CMD+3M2aLbbm2GdmNDwWJxszsxb04x/DZme8i4WRLsj8jkO4mwMZ9uJKmDKl4fH4mo2ZWQt54QXYbruu4czHcz0/4wRU3GjRoobH5crGzKxFXHQRbLVVV6J5YOejuL460QBMmNDo0OqXbCR9V9JTku4vtH1N0sOS7pP0E0nbFtZ9StJ8SY9IOqbQfmxumy/pk4X2XSXdKWmepB9J2iy3j8jL8/P6tnq9RjOzZvDMMyDB+9+flidPTt+l2ftr74GRIys3HjkSpk5teIz1rGwuB46tarsV2Dci9gP+BHwKQNLewGnAPnmfb0saJmkYcCFwHLA38M68LcBXgfMjYg9gOXBmbj8TWB4RuwPn5+3MzFrS2WfD6NFdywsXwiWX5IWODpg2LX2RRko/p01L7Q1Wt2QTEb8Enq1quyUi1uTFO4Bx+fmJwMyIWB0RjwHzgYPyY35E/DkiXgJmAidKEnAEcHXefzpwUuFY0/Pzq4Ej8/ZmZi1j8eKUP77whbT8uc+laqZbD1lHByxYkL5os2BBKYkGyr1m83+AG/PzscDiwrolua2n9h2AFYXE1dlecay8/rm8fTeSJkuaI2nOsmXLNvoFmZk1wvvfX5lUli1LFU4zKyXZSJoCrAE6B3vXqjyiH+29Hat7Y8S0iGiPiPYxY8b0HrSZWckeeihVMxddlJa/+c1UzRS70ZpVw4c+S5oEvBU4MiI6k8ASYHxhs3FA59RwtdqfBraVNDxXL8XtO4+1RNJwYBuquvPMzAaTCHj72+G669LyJpvAc8+lkWeDRUMrG0nHAv8BvC0iVhVWzQJOyyPJdgX2AO4Cfg/skUeebUYaRDArJ6nbgZPz/pOA6wrHmpSfnwzcVkhqZmaDyl13peTSmWhmzoS1awdXooH6Dn2+EvgdsKekJZLOBL4FbA3cKuleSRcDRMQDwFXAg8BNwAciYm2uWs4CbgYeAq7K20JKWh+VNJ90Teay3H4ZsENu/yjwynBpM7OmMWMGtLWlTNLWlpYL1q5NszMffHBaHj8eVq+GU09teKQDQv6jP2lvb485c+aUHYaZDQUzZqQvw6wqdPCMHPnKsOSbb4ZjC18cueUWePObGx9mX0iaGxHt693OySZxsjGzhmlrS1+IqfLShN1pe3keS5em5YMPTjM1b9LEc730Ndk08UswM2tRNeYmm8mpjFjUlWjuvBPuuKO5E82G8EScZmaNNmHCK5XNSrZka1a+surtb4drrklDnFtJi+RMM7NBZOpUGDmSC/hgRaJ5+L9mce21rZdowJWNmVnDLTu6gx1XdU0b8/6truDCi4eVNpVMIzjZmJk10Gc+Uznp8uLFMG7c6eUF1CDuRjMza4CFC1P3WGeiOeecNDPAuHG979cqXNmYmdXZe98Ll13WtfzMM7D99uXFUwZXNmZmdfLAA6ma6Uw0F1+cqpluiWY9swm0Alc2ZmYDLAKOPx5uzDdR2XzzVM1U3zQT6D6bwMKFaRlaasCAKxszswHU+Y3/zkRz9dXw4os9JBqAKVMqp62BtDxlSl3jbDRXNmZmA2DtWjjgALjvvrS8227w8MOw6abr2bHGbAK9tg9SrmzMzDbSDTfA8OFdiWb2bHj00T4kGqhxH+f1tA9STjZmZv20ejWMGZOuzwC86U2pwjniiA04SJ5NoMLIkZVfxmkBTjZmZv1wzDHpwv/TT6flOXPgV7/qx8SZHR3p1gITJ6ahaxMnvnKrgVbiZGNmVrSeYchLlqSccMstXW3r1sGBB27EOTs6YMGCdKAFC1ou0YCTjZlZl85hyAsXpvHLncOQc8IZNy7dMbPTDTekzVpx4syB5mRjZtaph2HIf/z3K5Dg8ce7miPguOMaG95g5qHPZmadagw3FgFLu5bnzNnILrMhypWNmVmnwnDj2RyREk02alSqZpxo+sfJxsysUx6GLIKjmP1K82Pn/5TnnisxrhbgZGNmlv0gOtCqF15ZPnTEXOIHM2j78EklRtUafM3GzIa8detg2LDKtnQbgAMB95sNBFc2ZjakffnLlYlm0qQebgNgG8WVjZkNSatXpxkAil58sXubDQxXNmY25Lz3vZVJ5eyzUzXjRFM/rmzMbMhYvrx799jatf2Yz8w2mN9iMxsSDjusMtFcfnmqZpxoGsOVjZm1tIUL03yaRRE1N7U6ck43s5a1ww6VieaWW5xoyuLKxsxazpw58PrXV7Y5yZTLycbMWkr1dP/33guve105sVgXd6OZWUu48MLuiSbCiaZZONmY2eDQyx00JTjrrK5N77/f3WbNxsnGzJpfD3fQ/NAxD9esZvbZp5wwrWe+ZmNmza/qDpprGMamq16AW7o2efJJ2GmnEmKzPqlbZSPpu5KeknR/oW17SbdKmpd/bpfbJekCSfMl3SfpgMI+k/L28yRNKrQfKOmPeZ8LpPT3TU/nMLNBrHAHzcO5nU1Z88ry2LGpmnGiaW717Ea7HDi2qu2TwOyI2AOYnZcBjgP2yI/JwEWQEgfweeBg4CDg84XkcVHetnO/Y9dzDjMbrCZM4Hm2QgS/4PBXml8YvxdLlpQXlvVd3ZJNRPwSeLaq+URgen4+HTip0H5FJHcA20raGTgGuDUino2I5cCtwLF53aiI+F1EBHBF1bFqncPMBqktn5zPKJ5/ZfltXEeM3JKRX/lsiVHZhmj0NZudImIpQEQslbRjbh8LLC5styS39da+pEZ7b+foRtJkUnXEhMK9x82sOSxeDOlXs+ujai3D2GTieJg6DTo6SovNNkyzjEZTjbboR/sGiYhpEdEeEe1jxozZ0N3NrI6kzkSTfPrTeeLMWAsLFjjRDDKNrmz+ImnnXHHsDDyV25cA4wvbjQOeyO2HV7X/T24fV2P73s5hZoPA3LnQ3l7Z5u/MDH6NrmxmAZ0jyiYB1xXaT8+j0g4BnstdYTcDR0vaLg8MOBq4Oa97XtIheRTa6VXHqnUOM2tyUmWi6bwNgA1+datsJF1JqkpGS1pCGlX2n8BVks4EFgGn5M1vAN4CzAdWAe8BiIhnJX0R+H3e7pyI6Bx08D7SiLctgBvzg17OYWZN6rrr4KSqoTxOMq1F4X9RANrb22POnDllh2E25FTPAHD77XD44aWEYv0gaW5EtK9vu2YZIGBmQ8y559aeONOJpjV5uhoza6hat2J++GHYc89y4rHGcGVjZg3z3vd2TzQRTjRDgSsbM6u7l1+GzTarbFu2DEaPLiceazxXNmZWVwcdVJlo9twzVTNONEOLKxszq4sVK2C7qjnX//Y3GDGinHisXK5szGzASZWJ5rTTUjXjRDN0ubIxswHz2GOw226VbevWdR/ibEOPKxszGxBSZaI555xUzTjRGLiyMbON9POfw1vfWtnmiUmsmisbM+s3qTLRXHmlE43V5mRjZhusp6lmTjutnHis+bkbzcw2SHWSmTULTjihnFhs8HBlY2Z98s//XLuacaKxvnBlY2a9qjVx5h/+APvtV048Njg52ZhZj179apg3r7LNAwCsP5xszKybF1+EkSMr2/7yF9hxx3LiscHPycbMKtT6EqarGdtYHiBgZgAsXdo90fztb040NjCcbMwMCXbZpWv5ta/1xJk2sJxszIawe+7pXs2sWwf33VdOPNa6nGzMhigJDjiga/nMMz1xptWPBwiYDTHXXAMnn1zZ5usyVm+ubMyGEKky0Xzzm0401hh9SjaShtU7EDOrny99qfZUM2edVU48NvT0tRttvqSrge9FxIP1DMjMBlZ1krn5Zjj66HJisaGrr91o+wF/Ai6VdIekyZJG1TEuM9tI73hH7WrGicbK0KdkExHPR8R3IuINwCeAzwNLJU2XtHtdIzSzDbJuXUoyP/lJV9tDD/najJWrT91o+ZrN8cB7gDbgXGAG8A/ADcCr6xSfmW2AV70qzWFW5CRjzaCv3WjzgBOBr0XE/hFxXkT8JSKuBm6qX3hmg9iMGdDWlubnb2tLy3WycmWqZoqJ5plnnGiseay3sslVzeURcU6t9RHxoQGPymywmzEDJk+GVavS8sKFaRmgo2NAT+WJM20wWG9lExFrgX9qQCxmrWPKlK5E02nVqtQ+QBYt6p5oXnrJicaaU1+HPv9W0reAHwEvdDZGxN11icpssFu0aMPaN1B1kjn0UPjtbwfk0GZ10ddk84b8s9iVFsARAxuOWYuYMCF1ndVq3wi33tp96HLn6DOzZtanZBMR7kYz2xBTp1Zes4F068upU/t9yOqE8pa3wM9/3u/DmTVUnyfilHQ8sA+weWdbT4MGzIa8zkEAU6akrrMJE1Ki6cfggPPPh49+tLLN12VssOnr3GgXA6cCHwQEnAJM7O9JJX1E0gOS7pd0paTNJe0q6U5J8yT9SNJmedsReXl+Xt9WOM6ncvsjko4ptB+b2+ZL+mR/4zTbKB0dsGBB6udasKBfiUaqTDRf/KITjQ1Off2ezRsi4nRgeUScDRwKjO/PCSWNBT4EtEfEvsAw4DTgq8D5EbEHsBw4M+9yZj7v7sD5eTsk7Z332wc4Fvi2pGF5qPaFwHHA3sA787Zmg8Y731l7qpnPfKaceMw2Vl+TzYv55ypJuwAvA7tuxHmHA1tIGg6MBJaSBhtcnddPB07Kz0/My+T1R0pSbp8ZEasj4jFgPnBQfsyPiD9HxEvAzLyt2aAgwcyZXcs//amrGRv8+nrN5npJ2wJfA+4mjUS7tD8njIjHJX0dWERKYrcAc4EVEbEmb7YEGJufjwUW533XSHoO2CG331E4dHGfxVXtB9eKRdJkYDLAhI0cJWS2sXbeGZ58srLNScZaRV8n4vxiRKyIiGtI12r2iojP9ueEkrYjVRq7ArsAW5K6vLqdtnOXHtZtaHv3xohpEdEeEe1jxoxZX+hmdbFmTapmionm/vudaKy19FrZSHpHL+uIiGv7cc6jgMciYlk+zrWk7/FsK2l4rm7GAU/k7ZeQrg8tyd1u2wDPFto7Fffpqd2sqXiqGRsq1teNdkIv6wLoT7JZBBwiaSSpG+1IYA5wO3Ay6RrLJOC6vP2svPy7vP62iAhJs4AfSjqPVCHtAdxFqmz2kLQr8DhpEMG7+hGnWd08+yzssENl29NPd28zaxW9JpuIeM9AnzAi7sx3/bwbWAPcA0wDfg7MlPSl3HZZ3uUy4PuS5pMqmtPycR6QdBXwYD7OB/I8bkg6C7iZNNLtuxHxwEC/DrP+cjVjQ5Gij//LW/1Lne3t7TFnzpyyw7AW9sADsO++lW0vvwzD+/zVarPmI2luRLSvb7u+3jztYtIQ5X8ijUI7mdRlZWZ9UF3N7Lhj95ucmbWyhn+p02womTWr9pcznWhsqOnvlzrXsHFf6jRreRKcWPg68amn+tqMDV19TTadX+r8L9IXMB8jjRozsypTp9auZmb6N8aGsPV9z+b1wOKI+GJe3gr4I/AwaZ4yMyuoTjLnntt9xmazoWh9lc0lwEsAkg4D/jO3PUcarmxmwAkn1K5mnGjMkvWNRhsWEc/m56cC0/KUNddIure+oZk1vwjYpOpPtptugmOOqb292VC13mRTmELmSPKklX3c16yl+cuZZn23vm60K4FfSLqONCLtVwCSdid1pZkNOatXd080993nRGPWm/VNVzNV0mxgZ+CW6JpuYBPSXTvNhhRXM2b9s96usIi4o0bbn+oTjllzevxxGDeusu2ZZ2D77cuJx2yw8XUXs/VwNWO28fr6pU6zIec3v+meaNascaIx6w9XNmY1uJoxG1iubMwKLr649pcznWjMNo4rG7OsOskceST8v/9XTixmrcaVjQ15kybVrmacaMwGjpONDWkSXHFF1/LZZ7vLzKwe3I1mQ9LOO8OTT1a2OcmY1Y8rGxtSIlI1U0w011/vRGNWb65sbMjwcGaz8riysZb3wgvdE80jjzjRmDWSKxtraa5mzJqDKxtrSQsWdE80zz3nRGNWFlc21nJczZg1H1c21jJmz+6eaNaudaIxawaubKwlVCeZLbaAVavKicXMunNlY4PaeefVnmrGicasubiysUGrOsm8/e1w7bXlxGJmvXNlY4PDjBnQ1gabbMIZW11ds5pxojFrXk421vxmzIDJk2HhQhTrmP7Cya+sOu88DwAwGwzcjWbNb8oUxq96mCWMr2iOiW3wkQWlhGRmG8aVjTW1detACxdUJJpf8SYCwaJFJUZmZhvClY01rZpfzqTQOGFC44Ixs43iysaazvPPd080izZ/dWWiGTkSpk5tbGBm1m9ONtZUJBg1qrItAsZf+nmYODFtMHEiTJsGHR3lBGlmG6yUZCNpW0lXS3pY0kOSDpW0vaRbJc3LP7fL20rSBZLmS7pP0gGF40zK28+TNKnQfqCkP+Z9LpBqdchYM3n00e7VzKpVhZFmHR1pds1169JPJxqzQaWsyua/gZsiYi/gdcBDwCeB2RGxBzA7LwMcB+yRH5OBiwAkbQ98HjgYOAj4fGeCyttMLux3bANek/WTBLvvXtkWkaacMbPW0PBkI2kUcBhwGUBEvBQRK4ATgel5s+nASfn5icAVkdwBbCtpZ+AY4NaIeDYilgO3AsfmdaMi4ncREcAVhWNZE7nllu7VzLp1/t6MWSsqo7LZDVgGfE/SPZIulbQlsFNELAXIP3fM248FFhf2X5LbemtfUqO9G0mTJc2RNGfZsmUb/8qszyQ45piu5de+NiUZd3iataYyks1w4ADgoojYH3iBri6zWmp9/EQ/2rs3RkyLiPaIaB8zZkzvUduA+PrXa0+ced995cRjZo1RRrJZAiyJiDvz8tWk5POX3AVG/vlUYfviV8fHAU+sp31cjXYrmQT//u9dyx/8oLvMzIaKhiebiHgSWCxpz9x0JPAgMAvoHFE2CbguP58FnJ5HpR0CPJe72W4Gjpa0XR4YcDRwc173vKRD8ii00wvHshK84x21q5kLLignHjNrvLJGo30QmCHpPuDvgS8D/wm8WdI84M15GeAG4M/AfOA7wPsBIuJZ4IvA7/PjnNwG8D7g0rzPo8CNDXhNVoMEP/lJ1/L06QNczRRmg6atLS2bWdNRuB8DgPb29pgzZ07ZYbSMrbeGlSsr2wb8v1rnbNDFO6WNHOkvfJo1kKS5EdG+vu08g4ANqLVrUzVTTDR33lmnazNTpnS/JeeqVandzJqKk40NmAMPhOFVU7tGwEEHDfCJOrvOFi6svd6zQZs1Hc/6bBvthRdgq60q25YuhVe9qg4nq9V1Vs2zQZs1HScb2yjVo8wmTOi54BgQtbrOijwbtFlTcjea9cvjj3dPNC+/XOdEA713kXk2aLOm5crGNlh1kvlf/wuuvrpBJ++pdJo4Mc0GbWZNyZWN9dndd9eeOLNhiQZSF9nIkZVt7joza3pONtYnUhpt1umcc0qaOLOjI3WV+UZqZoOKu9GsV7feCkcfXdlW+veAOzqcXMwGGVc21iOpMtHMmtUEicbMBiUnG+tm2rTaE2eecEI58ZjZ4OduNKtQnWTuvRde97pyYjGz1uHKxgD4+MdrVzNONGY2EFzZDHFr13afz+zxx2GXXcqJx8xakyubIezooysTzejRqZpxojGzgebKZghauTLdb6a6bcsty4nHzFqfK5shZvvtKxPNMcekasaJxszqyZXNEPHEEzB2bGXbmjUwbFg58ZjZ0OLKZgiQKhPNxz+eqhknGjNrFFc2Lezee2H//SvbPAOAmZXBlU2LkioTzXe+40RjZuVxZdNirr9MpTOXAAAKwklEQVS++7QyTjJmVjYnmxZSPQPArbfCUUeVE4uZWZG70VrAjTfWnmrGicbMmoUrm0EsAjap+nNh8WIYN66ceMzMeuLKZpC69NLKRHPUUSn5ONGYWTNyZTPI1Jo4c8UK2GabcuIxM+sLVzaDyOc+V5lo3ve+VM040ZhZs3NlMwisWtV97rLVq2GzzcqJx8xsQ7myaXIdHZWJ5mtfS9WME42ZDSaubJrU00/DmDGVbevWdR/ibGY2GLiyaUIHHFCZaGbOTNWME42ZDVaubJrIo4/C7rtXtnmqGTNrBa5smsSIEZWJ5he/cKIxs9bhyqZkd90FBx9c2eYkY2atxsmmRNXXYB54APbeu5xYzMzqqbRuNEnDJN0j6fq8vKukOyXNk/QjSZvl9hF5eX5e31Y4xqdy+yOSjim0H5vb5kv6ZKNf2/r87GeViWb33VM140RjZq2qzGs2/wY8VFj+KnB+ROwBLAfOzO1nAssjYnfg/LwdkvYGTgP2AY4Fvp0T2DDgQuA4YG/gnXnb0nWOKHvb27ranngC5s0rLyYzs0YoJdlIGgccD1yalwUcAVydN5kOnJSfn5iXyeuPzNufCMyMiNUR8RgwHzgoP+ZHxJ8j4iVgZt62VN/+duXEmSeckJLPzjuXF5OZWaOUdc3mG8AngK3z8g7AiohYk5eXAGPz87HAYoCIWCPpubz9WOCOwjGL+yyuaq+6BJ9ImgxMBpgwYcJGvJyerVkDm25a2fbXv8LWW9fe3sysFTW8spH0VuCpiJhbbK6xaaxn3Ya2d2+MmBYR7RHRPqb66/oD4BOfqEw0H/lIqmacaMxsqCmjG+2NwNskLSB1cR1BqnS2ldRZaY0DnsjPlwDjAfL6bYBni+1V+/TU3jAvvQQ77ZTmMSu2nXceMGMGtLWlPrW2trRsZtbiGp5sIuJTETEuItpIF/hvi4gO4Hbg5LzZJOC6/HxWXiavvy0iIreflker7QrsAdwF/B7YI49u2yyfY1YDXhoAP/pR+oLmU0+l5f/+71TNbLopKbFMngwLF6bGhQvTshOOmbW4ZvqezX8AMyV9CbgHuCy3XwZ8X9J8UkVzGkBEPCDpKuBBYA3wgYhYCyDpLOBmYBjw3Yh4oN7Br1yZ7iuzbl1aPuEEuO66qu/STJmS7hdQtGpVau/oqHeIZmalUfjr6gC0t7fHnDlz+rXvhRfCWWd1LT/4ILzmNTU23GST2tMDSF1ZysxsEJE0NyLa17ed50bbSJdd1pVoJk9OuaRmogHoacRbnUbCmZk1CyebjbTvvvCGN8CiRXDJJevZeOpUGDmysm3kyNRuZtbCnGw20sEHw29+A+PHr39bOjpg2jSYODF1nU2cmJZ9vcbMWlwzDRAYGjo6nFzMbMhxZWNmZnXnZGNmZnXnZGNmZnXnZGNmZnXnZGNmZnXnZGNmZnXnZGNmZnXnudEyScuAhWXHsQFGA0+XHUTJ/B74PQC/B2W//okRsd4bgjnZDFKS5vRl8rtW5vfA7wH4PRgsr9/daGZmVndONmZmVndONoPXtLIDaAJ+D/wegN+DQfH6fc3GzMzqzpWNmZnVnZONmZnVnZPNICNpvKTbJT0k6QFJ/1Z2TGWQNEzSPZKuLzuWMkjaVtLVkh7O/xcOLTumRpP0kfw7cL+kKyVtXnZM9Sbpu5KeknR/oW17SbdKmpd/bldmjD1xshl81gAfi4jXAIcAH5C0d8kxleHfgIfKDqJE/w3cFBF7Aa9jiL0XksYCHwLaI2JfYBhwWrlRNcTlwLFVbZ8EZkfEHsDsvNx0nGwGmYhYGhF35+fPkz5kxpYbVWNJGgccD1xadixlkDQKOAy4DCAiXoqIFeVGVYrhwBaShgMjgSdKjqfuIuKXwLNVzScC0/Pz6cBJDQ2qj5xsBjFJbcD+wJ3lRtJw3wA+AawrO5CS7AYsA76XuxIvlbRl2UE1UkQ8DnwdWAQsBZ6LiFvKjao0O0XEUkh/jAI7lhxPTU42g5SkrYBrgA9HxF/LjqdRJL0VeCoi5pYdS4mGAwcAF0XE/sALNGnXSb3k6xInArsCuwBbSnp3uVFZb5xsBiFJm5ISzYyIuLbseBrsjcDbJC0AZgJHSPpBuSE13BJgSUR0VrRXk5LPUHIU8FhELIuIl4FrgTeUHFNZ/iJpZ4D886mS46nJyWaQkSRSX/1DEXFe2fE0WkR8KiLGRUQb6YLwbRExpP6ijYgngcWS9sxNRwIPlhhSGRYBh0gamX8njmSIDZIomAVMys8nAdeVGEuPhpcdgG2wNwL/DPxR0r257dMRcUOJMVnjfRCYIWkz4M/Ae0qOp6Ei4k5JVwN3k0Zo3sMgmbZlY0i6EjgcGC1pCfB54D+BqySdSUrCp5QXYc88XY2ZmdWdu9HMzKzunGzMzKzunGzMzKzunGzMzKzunGzMzKzunGys5UlaK+newqOu37aX9LZ6n6OH87ZLuqDR5zXrCw99tpYnaWVEbNWgcw2PiDWNOFezkjQsItaWHYc1F1c2NiRJ2kbSI53fws/3Q/mX/HylpHMl3S1ptqQxuf3vJN0kaa6kX0naK7dfLuk8SbcDX5V0hqRv5XVjJF0j6ff58cbc/oV8b5L/kfRnSR8qxHa6pPsk/UHS93s7TtVrOrzz/j69Hb+w/ZmSzi8s/4uk8/Lzd0u6K1eCl0galtsvkjQn30fm7MK+CyR9TtKvgVMkfUjSg/l1zNy4fy1rCRHhhx8t/QDWAvcWHqfm9jcDvyNNe3NTYfsAOvLzzwHfys9nA3vk5weTpsqBdI+R64FhefmMwj4/BN6Un08gTTME8AXgt8AIYDTwDLApsA/wCDA6b7d9b8epep2HA9f3dvyq7bcEHu1sz9u/FngN8LNC+7eB06viGQb8D7BfXl4AfKJw7CeAEfn5tmX/H/Cj/Ienq7Gh4MWI+Pvqxoi4VdIpwIWkG5B1Wgf8KD//AXBtnmX7DcCP01RcQPog7/TjqN11dBSwd2GfUZK2zs9/HhGrgdWSngJ2Ao4Aro6Ip3OMz/Z2nEj3NOpJreMvKbz+FyTdBrxV0kOk5PJHSWcBBwK/z+fbgq7JHf+3pMmkqa52BvYG7svrOt8zctsMST8FftpLjDZEONnYkCVpE9Jf8S8C21P4IK4SpC7nFbWSVvZCD+2bAIdGxItV5wZYXWhaS/p9VD5fn46zHrWOX+1S4NPAw8D3OsMDpkfEp6pi3hX4OPD6iFgu6XKgeCvm4ntwPOkGb28DPitpnxji17KGOl+zsaHsI6SZgt8JfDffugHS78XJ+fm7gF9HumfQY7kSQsnrqg9Ywy3AWZ0LknpKVp1mk6qHHfL22/fzOH0S6TYF40mv88pCDCdL2rEzBkkTgVGkhPKcpJ2A42odMyfx8RFxO+kmd9sCDRmgYc3LlY0NBVsUZsgGuAn4LvBe4KCIeF7SL4HPkGbRfQHYR9Jc4Dng1LxfB3CRpM+Qrq/MBP6wnnN/CLhQ0n2k37dfAv/a08YR8YCkqcAvJK0lzWZ8xoYeZwNdBfx9RCzPMTyYX+MtOXG8DHwgIu6QdA/wAGmm6d/0cLxhwA8kbUOqks6PoXnbaivw0GezKo0cKt0M8gi28yNidtmxWOtyN5rZECVpW0l/Ig2gcKKxunJlY2ZmdefKxszM6s7JxszM6s7JxszM6s7JxszM6s7JxszM6u7/A/2um3VtCWiAAAAAAElFTkSuQmCC\\n\",\n \"text/plain\": [\n \"<Figure size 432x288 with 1 Axes>\"\n ]\n },\n \"metadata\": {\n \"needs_background\": \"light\"\n },\n \"output_type\": \"display_data\"\n }\n ],\n \"source\": [\n \"#Visualising the test set results\\n\",\n \"plt.scatter(X_test,y_test,color='red')\\n\",\n \"plt.plot(X_train,regressor.predict(X_train),color='blue')\\n\",\n \"plt.title('Salary vs experience(Test set)')\\n\",\n \"plt.xlabel('Experience in years')\\n\",\n \"plt.ylabel('Salary')\\n\",\n \"plt.show()\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": null,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": []\n }\n ],\n \"metadata\": {\n \"kernelspec\": {\n \"display_name\": \"Python 3\",\n \"language\": \"python\",\n \"name\": \"python3\"\n },\n \"language_info\": {\n \"codemirror_mode\": {\n \"name\": \"ipython\",\n \"version\": 3\n },\n \"file_extension\": \".py\",\n \"mimetype\": \"text/x-python\",\n \"name\": \"python\",\n \"nbconvert_exporter\": \"python\",\n \"pygments_lexer\": \"ipython3\",\n \"version\": \"3.7.1\"\n }\n },\n \"nbformat\": 4,\n \"nbformat_minor\": 2\n}"
}
{
"filename": "code/artificial_intelligence/src/linear_regression/linear_regression_scikit_learn.py",
"content": "import numpy as np\nimport pandas as pd\nimport matplotlib.pyplot as plt\n\n%matplotlib inline\n\n#importing the data set\ndataset=pd.read_csv('Salary_Data.csv')\n\ndataset.describe()\t\n\nX=dataset.iloc[:,:-1].values\ny=dataset.iloc[:,1].values\n \n#Splitting the dataset into train set and test set\nfrom sklearn.model_selection import train_test_split\nX_train,X_test,y_train,y_test=train_test_spit(X,y,test_size=1/3,random_state=0)\n\n#building the model\nfrom sklearn.linear_model import LinearRegression\nregressor=LinearRegression()\nregressor.fit(X_train,y_train)\n\n#Predicting the results\ny_pred=regressor.predict(X_test)\n\n\n#Visualsing the training set results\nplt.scatter(X_train,y_train,color='red')\nplt.plot(X_train,regressor.predict(X_train),)\nplt.title('Salary vs Experience(Train set)')\nplt.xlabel('Experience in years')\nplt.ylabel('Salary')\n\n#Visualising the test set results\nplt.scatter(X_test,y_test,color='red')\nplt.plot(X_train,regressor.predict(X_train),color='blue')\nplt.title('Salary vs experience(Test set)')\nplt.xlabel('Experience in years')\nplt.ylabel('Salary')\nplt.show()"
}
{
"filename": "code/artificial_intelligence/src/linear_regression/linear_regression.swift",
"content": "import Foundation\n\nlet cosmos_size: [Double] = [1, 2, 10, 3, 20, 11]\nlet cosmos_contributors: [Double] = [500, 1000, 5000, 1500, 10000, 5500]\n\nfunc average(_ input: [Double]) -> Double {\n return input.reduce(0, +) / Double(input.count)\n}\n\nfunc multiply(_ a: [Double], _ b: [Double]) -> [Double] {\n return zip(a, b).map(*)\n}\n\nfunc linearRegression(_ xs: [Double], _ ys: [Double]) -> (Double) -> Double {\n let sum1 = average(multiply(xs, ys)) - average(xs) * average(ys)\n let sum2 = average(multiply(xs, xs)) - pow(average(xs), 2)\n let slope = sum1 / sum2\n let intercept = average(ys) - slope * average(xs)\n return { x in intercept + slope * x }\n}\n\nlet result = linearRegression(cosmos_size, cosmos_contributors)(4)\n\nprint(\"Perdicted £\\(Int(result))\")"
}
{
"filename": "code/artificial_intelligence/src/linear_regression/README.md",
"content": "# Linear Regression\n\nThe **linear regression** is a statistical approach for modelling relationship between a dependent variable with a given set of independent variables.\n\nIn order to provide a basic understanding of linear regression, we start with the most basic version of linear regression, i.e. Simple linear regression.\n\nSimple Linear Regression\n------------------------\n\nSimple linear regression is an approach for predicting a response using a single feature.\n\nIt is assumed that the two variables are linearly related. Hence, we try to find a linear function that predicts the response value(y) as accurately as possible as a function of the feature or independent variable(x).\n\nMultiple linear regression\n--------------------------\n\nMultiple linear regression attempts to model the relationship between two or more features and a response by fitting a linear equation to observed data.\n\nClearly, it is nothing but an extension of Simple linear regression.\n\nApplications\n------------\n\n1. Trend lines: A trend line represents the variation in some quantitative data with passage of time (like GDP, oil prices, etc.). These trends usually follow a linear relationship. Hence, linear regression can be applied to predict future values. However, this method suffers from a lack of scientific validity in cases where other potential changes can affect the data.\n\n2. Economics: Linear regression is the predominant empirical tool in economics. For example, it is used to predict consumption spending, fixed investment spending, inventory investment, purchases of a country’s exports, spending on imports, the demand to hold liquid assets, labor demand, and labor supply.\n\n3. Finance: Capital price asset model uses linear regression to analyze and quantify the systematic risks of an investment.\n\n4. Biology: Linear regression is used to model causal relationships between parameters in biological systems.\n\n\n\nThis article is obtained as reference from [Geeksforgeeks](http://www.geeksforgeeks.org/linear-regression-python-implementation/)"
}
{
"filename": "code/artificial_intelligence/src/linear_regression/Salary_Data.csv",
"content": "YearsExperience,Salary\n1.1,39343.00\n1.3,46205.00\n1.5,37731.00\n2.0,43525.00\n2.2,39891.00\n2.9,56642.00\n3.0,60150.00\n3.2,54445.00\n3.2,64445.00\n3.7,57189.00\n3.9,63218.00\n4.0,55794.00\n4.0,56957.00\n4.1,57081.00\n4.5,61111.00\n4.9,67938.00\n5.1,66029.00\n5.3,83088.00\n5.9,81363.00\n6.0,93940.00\n6.8,91738.00\n7.1,98273.00\n7.9,101302.00\n8.2,113812.00\n8.7,109431.00\n9.0,105582.00\n9.5,116969.00\n9.6,112635.00\n10.3,122391.00\n10.5,121872.00"
}
{
"filename": "code/artificial_intelligence/src/logistic_regression/logistic_regression.py",
"content": "# Logistic regression implemented from Scratch in Python\n\nimport numpy as np\nimport matplotlib.pyplot as plt\n\n\ndef sigmoid(scores):\n return 1 / (1 + np.exp(-scores))\n\n\ndef log_likelihood(features, target, weights):\n scores = np.dot(features, weights)\n ll = np.sum(target * scores - np.log(1 + np.exp(scores)))\n return ll\n\n\ndef logistic_regression(\n features, target, num_steps, learning_rate, add_intercept=False\n):\n if add_intercept:\n intercept = np.ones((features.shape[0], 1))\n features = np.hstack((intercept, features))\n\n weights = np.zeros(features.shape[1])\n\n for step in range(num_steps):\n scores = np.dot(features, weights)\n predictions = sigmoid(scores)\n\n # Update weights with gradient\n output_error_signal = target - predictions\n gradient = np.dot(features.T, output_error_signal)\n weights += learning_rate * gradient\n\n # Print log-likelihood every so often\n if step % 10000 == 0:\n print(log_likelihood(features, target, weights))\n\n return weights\n\n\nnp.random.seed(12)\nnum_observations = 5000\n\nx1 = np.random.multivariate_normal([0, 0], [[1, 0.75], [0.75, 1]], num_observations)\nx2 = np.random.multivariate_normal([1, 4], [[1, 0.75], [0.75, 1]], num_observations)\n\nsimulated_separableish_features = np.vstack((x1, x2)).astype(np.float32)\nsimulated_labels = np.hstack((np.zeros(num_observations), np.ones(num_observations)))\n\nplt.figure(figsize=(12, 8))\nplt.scatter(\n simulated_separableish_features[:, 0],\n simulated_separableish_features[:, 1],\n c=simulated_labels,\n alpha=0.4,\n)\n\nplt.show()\n\n# Running the model\n\nweights = logistic_regression(\n simulated_separableish_features,\n simulated_labels,\n num_steps=300000,\n learning_rate=5e-5,\n add_intercept=True,\n)\n\nprint(weights)"
}
{
"filename": "code/artificial_intelligence/src/logistic_regression/README.md",
"content": "# Logistical Regression\n\nThe **logistical regression** algorithm build upon linear regression and is basically a supervised classification algorithm. In a classification problem, the target variable (or output), Y, can take only discrete values for given set of features (or inputs), X.\nWe can also say that the target variable is categorical. Based on the number of categories, Logistic regression can be classified as:\n\n1. binomial: target variable can have only 2 possible types: 0 or 1 which may represent \"win\" vs \"loss\", \"pass\" vs \"fail\", \"dead\" vs \"alive\", etc.\n2. multinomial: target variable can have 3 or more possible types which are not ordered(i.e. types have no quantitative significance) like “disease A” vs “disease B” vs “disease C”.\n3. ordinal: it deals with target variables with ordered categories. For example, a test score can be categorized as:“very poor”, “poor”, “good”, “very good”. Here, each category can be given a score like 0, 1, 2, 3.\n\nHere are some points about Logistic regression to ponder upon:\n--------------------------------------------------------------\n\n- Does NOT assume a linear relationship between the dependent variable and the independent variables, but it does assume linear relationship between the logit of the explanatory variables and the response.\n- Independent variables can be even the power terms or some other nonlinear transformations of the original independent variables.\n- The dependent variable does NOT need to be normally distributed, but it typically assumes a distribution from an exponential family (e.g. binomial, Poisson, multinomial, normal,…); binary logistic regression assume binomial distribution of the response.\n- The homogeneity of variance does NOT need to be satisfied.\n- Errors need to be independent but NOT normally distributed.\n- It uses maximum likelihood estimation (MLE) rather than ordinary least squares (OLS) to estimate the parameters, and thus relies on large-sample approximations.\n\n\nThis article is obtained as a reference from [Geeksforgeeks](http://www.geeksforgeeks.org/understanding-logistic-regression/)"
}
{
"filename": "code/artificial_intelligence/src/minimax/minimax.py",
"content": "import operator\nimport time\n\n\nclass IllegalMoveException(Exception):\n pass\n\n\ndef new_copy(in_list):\n if isinstance(in_list, list):\n return list(map(new_copy, in_list))\n else:\n return in_list\n\n\nclass GameState(object):\n def __init__(self, board, turn, move_count=0):\n self.board = board\n self.turn = turn\n self.move_count = move_count\n\n assert len(board) == len(board[0])\n self.n = len(board)\n\n # Once a move is made, these will record the x and y index of the move most recently made on the board,\n # as well as whose turn it was when the move was made.\n self.x = None\n self.y = None\n\n def switch_turn(self):\n self.turn = \"X\" if self.turn == \"O\" else \"O\"\n\n def move(self, x, y):\n if self.board[y][x] != None:\n raise IllegalMoveException(\n \"there is already a symbol placed at this location (x = {}, y = {})\".format(\n x, y\n )\n )\n # record move (for indication of most recent move when printing board)\n self.x = x\n self.y = y\n\n # make move and switch turn\n self.board[y][x] = self.turn\n self.switch_turn()\n\n # update move_count\n self.move_count += 1\n\n def __str__(self):\n ret = \"\\n\"\n for y in range(self.n):\n for x in range(self.n):\n if y == self.y and x == self.x:\n ret += \" ({}) |\".format(self.board[y][x])\n else:\n ret += \" {} |\".format(self.board[y][x] or \" \")\n ret = ret[:-1] + \"\\n\" + \"-\" * self.n * 6 + \"\\n\"\n\n result = self.check_winner()\n if result in (\"X\", \"O\"):\n ret += \"\\nWinner: {}\".format(result)\n elif result == \"draw\":\n ret += \"\\nThe game is a draw.\"\n else: # no winner yet\n ret += \"\\nTurn to move: {}\".format(self.turn)\n\n return ret\n\n def check_winner(self):\n # check row\n for y in range(self.n):\n win = self.board[y][0]\n if win == None:\n continue\n for x in range(self.n):\n if self.board[y][x] != win:\n break\n else:\n return win\n\n # check column\n for x in range(self.n):\n win = self.board[0][x]\n if win == None:\n continue\n for y in range(self.n):\n if self.board[y][x] != win:\n break\n else:\n return win\n\n # check forward diagonal\n win = self.board[0][0]\n if win != None:\n for z in range(1, self.n):\n if self.board[z][z] != win:\n break\n else:\n return win\n\n # check backward diagonal\n win = self.board[0][self.n - 1]\n if win != None:\n for y in range(1, self.n):\n if self.board[y][self.n - y - 1] != win:\n break\n else:\n return win\n\n if self.move_count == self.n ** 2:\n return \"draw\"\n\n return None\n\n def next_states_and_moves(self):\n \"\"\"\n\t\treturn a list of tuples, where each tuple contains:\n\t\t\t- a state immediately reachable from the current game state\n\t\t\t- another tuple with the x and y coordinates of the move required to create that state\n\t\t\"\"\"\n states = []\n for y in range(self.n):\n for x in range(self.n):\n if self.board[y][x] == None:\n new_state = GameState(\n new_copy(self.board), self.turn, move_count=self.move_count\n )\n new_state.move(x, y)\n states.append((new_state, (x, y)))\n return states\n\n def evaluate(self, symbol):\n \"\"\"\n\t\treturn an estimate of how desirable of a position this position is (for the player with the provided symbol)\n\t\tfor each player, this heuristic looks at each row, column, and diagonal; if the row/column/diagonal is not obstructed\n\t\tby pieces belonging to the other player, the player's value is increased according to how many pieces they have on that\n\t\trow/column/diagonal\n\t\tthe overall value is the player's value minus the opponent's value\n\t\t\"\"\"\n other_symbol = \"X\" if symbol == \"O\" else \"O\"\n\n player_total = 0\n opponent_total = 0\n\n for total, own, other in (\n (player_total, symbol, other_symbol),\n (opponent_total, other_symbol, symbol),\n ):\n\n # rows\n for y in range(self.n):\n benefit = 0\n for x in range(self.n):\n if self.board[y][x] == None:\n continue\n elif self.board[y][x] == own:\n benefit += 1\n elif self.board[y][x] == other: # this row is obstructed\n break\n else:\n total += benefit # row is unobstructed\n\n # ccolumns\n for x in range(self.n):\n benefit = 0\n for y in range(self.n):\n if self.board[y][x] == None:\n continue\n elif self.board[y][x] == own:\n benefit += 1\n elif self.board[y][x] == other: # this column is obstructed\n break\n else:\n total += benefit # column is unobstructed\n\n # forward diagonal\n benefit = 0\n for z in range(1, self.n):\n if self.board[z][z] == None:\n continue\n elif self.board[z][z] == own:\n benefit += 1\n elif self.board[z][z] == other: # this diagonal is obstructed\n break\n else:\n total += benefit # diagonal is unobstructed\n\n # backward diagonal\n benefit = 0\n for y in range(1, self.n):\n if self.board[y][self.n - y - 1] == None:\n continue\n elif self.board[y][self.n - y - 1] == own:\n benefit += 1\n elif (\n self.board[y][self.n - y - 1] == other\n ): # this diagonal is obstructed\n break\n else:\n total += benefit # diagonal is unobstructed\n\n return player_total - opponent_total\n\n\nclass GameDriver(object):\n def __init__(self):\n symbol = input(\"Choose your symbol (X/O)\\n\")\n while symbol != \"X\" and symbol != \"O\":\n symbol = input(\"Could not understand your answer. Please enter X or O.\\n\")\n\n self.player_symbol = symbol\n\n n = input(\n \"\\nChoose the size of your board (enter a positive integer, this will be the height and width of your board\\n\"\n )\n while not n.isdigit() or int(n) < 1:\n n = input(\"Please enter a positive integer\\n\")\n n = int(n)\n\n first = input(\"\\nWould you like to start? (Y/N)\\n\")\n while first != \"Y\" and first != \"N\":\n first = input(\"Could not understand your answer. Please enter Y or N.\\n\")\n\n print(\n \"\\nThank you. To enter your moves, enter the index of your move on the board, with indices defined as follows:\\n\"\n )\n\n col_width = n\n indices_str = \"\"\n\n all_n = range(1, n ** 2 + 1)\n rows = [all_n[i : i + n] for i in range(0, n ** 2, n)]\n\n for row in rows:\n row = [str(i) for i in row]\n indices_str += \"|\".join(word.center(col_width) for word in row) + \"\\n\"\n indices_str += \"-\" * (col_width + 1) * n + \"\\n\"\n indices_str = indices_str[: -1 * (col_width + 1) * n - 1]\n\n print(indices_str)\n\n input(\"\\nPress Enter to continue!\\n\")\n\n if first == \"Y\":\n self.game = GameState(\n [[None for _ in range(n)] for _ in range(n)], self.player_symbol\n )\n else:\n self.game = GameState(\n [[None for _ in range(n)] for _ in range(n)],\n \"X\" if self.player_symbol == \"O\" else \"O\",\n )\n\n self.start()\n\n def player_move(self):\n move = input(\"Enter the index of your move.\\n\")\n while True:\n while not move.isdigit() or int(move) > self.game.n ** 2 or int(move) < 1:\n move = input(\n \"Please enter an integer greater than or equal to 1 and less than or equal to {}.\\n\".format(\n self.game.n ** 2\n )\n )\n\n move = (\n int(move) - 1\n ) # easier to calculate x and y this way, since arrays representing game board are 0-indexed\n y = int(move / self.game.n)\n x = move % self.game.n\n\n if self.game.board[y][x] != None:\n print(\"There is already a piece in that location on the board.\")\n move = \"\"\n else:\n break\n self.game.move(x, y)\n\n def computer_move(self):\n \"\"\"\n\t\tpick first possible move from list of states\n\t\t\"\"\"\n\n delay = 0.5\n print(\"Thinking...\")\n time.sleep(delay)\n print(\".\")\n time.sleep(delay)\n print(\".\")\n time.sleep(delay)\n print(\".\\n\")\n time.sleep(delay)\n\n # TODO: how deep to look? maybe base this on a difficulty parameter specified by user?\n move = self.minimax(self.game, 6, True, float(\"-inf\"), float(\"+inf\"))[1]\n self.game.move(*move)\n\n def minimax(self, board, level, comp_turn, alpha, beta):\n winner = board.check_winner()\n if winner == \"draw\":\n return 0, None\n elif winner == self.player_symbol:\n return -1, None\n elif winner != None:\n return 1, None\n\n if level == 0:\n # TODO: normalize this between -1 and 1. Also come up with a better way to identify player/computer symbols\n return -1 * board.evaluate(self.player_symbol), None\n\n states_and_moves = board.next_states_and_moves()\n best_move = None\n if comp_turn:\n for state, move in states_and_moves:\n score = self.minimax(state, level - 1, False, alpha, beta)[0]\n if score > alpha:\n alpha = score\n best_move = move\n if alpha >= beta:\n break\n return alpha, best_move\n else:\n for state, move in states_and_moves:\n score = self.minimax(state, level - 1, True, alpha, beta)[0]\n if score < beta:\n beta = score\n best_move = move\n if alpha >= beta:\n break\n return beta, best_move\n\n def start(self):\n while True:\n if self.game.turn == self.player_symbol:\n self.player_move()\n else:\n self.computer_move()\n print(self.game)\n if self.game.check_winner():\n break\n\n\nif __name__ == \"__main__\":\n GameDriver()"
}
{
"filename": "code/artificial_intelligence/src/minimax/README.md",
"content": "# Minimax Algorithm\n\nAccording to wikipedia , Minimax is a \"decision rule used in decision theory, game theory, statistics and philosophy for\nminimizing the possible loss for a worst case (maximum loss) scenario.\" Put forward in simpler words, it is an algorithm \nwhich is generally used to analyze games mathematically and to find optimal moves for a player so that if the player takes\nthose moves , he/she is likely to win the game , given that the other player also plays optimally(does not make mistakes).\nIt is used widely in 2 player turn taking games such as tic-tac-toe , chess , etc.\n\n\n\n# Further Reading \n\nTo know more about this algorithm , follow these links :-\n\n1. [Minimax - Wikipedia , the free encyclopedia](https://en.wikipedia.org/wiki/Minimax)\n2. [Minimax Algoritm in Game Theory - GeeksforGeeks](https://www.geeksforgeeks.org/minimax-algorithm-in-game-theory-set-1-introduction/)\n3. [Minimax - Brilliant.org](https://brilliant.org/wiki/minimax/)\n4. [Minimax - Umichigan Teaching](https://web.eecs.umich.edu/~akamil/teaching/sp03/minimax.pdf)"
}
{
"filename": "code/artificial_intelligence/src/missionaries_and_cannibals.py",
"content": "# -*- coding: utf-8 -*-\n\"\"\"Missionaries_and_cannibals.ipynb\n\nAutomatically generated by Colaboratory.\n\nOriginal file is located at\n https://colab.research.google.com/drive/18FiKma_HxV25I73fzJk6HItVVk8Yyfqu\n\"\"\"\n\ndef get_boat_at_left_new_states(game_state): #generating further steps to be taken.\n new_states = [] #A new list to generate all the future states. \n for missionary in range(game_state[0] + 1): #traversing in loop for m+1 times and c+1 times\n for cannibal in range(game_state[1] + 1): \n if missionary + cannibal < 1 or missionary + cannibal > 2: #at max, only two or min. 1 can travel on a boat. rest all cases to be eliminated.\n continue\n new_state = (game_state[0] - missionary, game_state[1] - cannibal,'right') #If we have 1 or 2 people on the boat, we will consider them. \n if 0 < new_state[0] < new_state[1]: #if Cannibals outnumber Missionaries on the left side, we skip.\n print(\"Future State: \" + str(new_state) + \": Missionaries Killed\")\n continue\n if 0 < 3 - new_state[0] < 3 - new_state[1]: #if Cannibals outnumber Missionaries on the right side, we skip. \n print(\"Future State: \" + str(new_state) + \": Missionaries Killed\")\n continue\n new_states.append(new_state) #else, we can consider them a valid case and append it the list of future states. \n return new_states\n\ndef get_boat_at_right_new_states(game_state): #generating further steps to be taken.\n new_states = []\n for missionary in range(3 - game_state[0] + 1):\n for cannibal in range(3 - game_state[1] + 1):\n if missionary + cannibal < 1 or missionary + cannibal > 2:\n continue\n new_state = (game_state[0] + missionary, game_state[1] + cannibal,'left')\n if 0 < new_state[0] < new_state[1]:\n print(\"Future State: \" + str(new_state) + \": Missionaries Killed\")\n continue\n if 0 < 3 - new_state[0] < 3 - new_state[1]:\n print(\"Future State: \" + str(new_state) + \": Missionaries Killed\") \n continue\n new_states.append(new_state)\n return new_states\n\ndef get_future_states(game_state): #a function to generate future states. \n if game_state[2] == 'left': \n return get_boat_at_left_new_states(game_state)\n else:\n return get_boat_at_right_new_states(game_state)\n\ndef bfs():\n state_stack = list() #maintaining a stack for the states.\n visited_states = set() #maintaining a set for visited states. (allowing only unique states to consider)\n initial_state = (3, 3, 'left') #initialising our initial state. \n visited_states.add(initial_state) #visiting the initial state. \n states = [initial_state] #maintaining a list of states in consideration at a time.\n all_states = [states] #maintaining a list of all the states taken in consideration throughout the game. \n print(\"Initial state: \" + str(initial_state)) #initialising our initial state. \n\n win = False \n while not win: #looping over until we win\n print(\"States: \" + str(states)) #printing the current states in consideration.\n new_states = []\n for game_state in states: #for every state in the states taken in consideration, checking for goal test condition\n print(\"Current State: \" + str(game_state))\n state_stack.append(game_state) \n if game_state[0] == game_state[1] == 0: \n print(\"Win! Last state: \" + str(game_state))\n win = True\n break\n future_states = get_future_states(game_state) #if goal not achieved, moving ahead and generating its future states.(children)\n for future_state in future_states: #for every future state generated,\n if future_state not in visited_states: #ensuring, we have not visited it before\n new_states.append(future_state) #only then we will consider it as our next state. \n visited_states.add(future_state) #visiting the state. \n print(\"Future State: \" + str(future_state)) \n else:\n print(\"Future State: \" + str(future_state)) \n print(\"We have visited \" + str(future_state) + \" state before. let's move back\") \n print()\n if not win: \n states = new_states\n all_states.append(states)\n\nbfs()"
}
{
"filename": "code/artificial_intelligence/src/naive_bayes/iris1.csv",
"content": "sepallength,sepalwidth,petallength,petalwidth,class\n5.1,3.5,1.4,0.2,Iris-setosa\n4.9,3,1.4,0.2,Iris-setosa\n4.7,3.2,1.3,0.2,Iris-setosa\n4.6,3.1,1.5,0.2,Iris-setosa\n5,3.6,1.4,0.2,Iris-setosa\n5.4,3.9,1.7,0.4,Iris-setosa\n4.6,3.4,1.4,0.3,Iris-setosa\n5,3.4,1.5,0.2,Iris-setosa\n4.4,2.9,1.4,0.2,Iris-setosa\n4.9,3.1,1.5,0.1,Iris-setosa\n5.4,3.7,1.5,0.2,Iris-setosa\n4.8,3.4,1.6,0.2,Iris-setosa\n4.8,3,1.4,0.1,Iris-setosa\n4.3,3,1.1,0.1,Iris-setosa\n5.8,4,1.2,0.2,Iris-setosa\n5.7,4.4,1.5,0.4,Iris-setosa\n5.4,3.9,1.3,0.4,Iris-setosa\n5.1,3.5,1.4,0.3,Iris-setosa\n5.7,3.8,1.7,0.3,Iris-setosa\n5.1,3.8,1.5,0.3,Iris-setosa\n5.4,3.4,1.7,0.2,Iris-setosa\n5.1,3.7,1.5,0.4,Iris-setosa\n4.6,3.6,1,0.2,Iris-setosa\n5.1,3.3,1.7,0.5,Iris-setosa\n4.8,3.4,1.9,0.2,Iris-setosa\n5,3,1.6,0.2,Iris-setosa\n5,3.4,1.6,0.4,Iris-setosa\n5.2,3.5,1.5,0.2,Iris-setosa\n5.2,3.4,1.4,0.2,Iris-setosa\n4.7,3.2,1.6,0.2,Iris-setosa\n4.8,3.1,1.6,0.2,Iris-setosa\n5.4,3.4,1.5,0.4,Iris-setosa\n5.2,4.1,1.5,0.1,Iris-setosa\n5.5,4.2,1.4,0.2,Iris-setosa\n4.9,3.1,1.5,0.1,Iris-setosa\n5,3.2,1.2,0.2,Iris-setosa\n5.5,3.5,1.3,0.2,Iris-setosa\n4.9,3.1,1.5,0.1,Iris-setosa\n4.4,3,1.3,0.2,Iris-setosa\n5.1,3.4,1.5,0.2,Iris-setosa\n5,3.5,1.3,0.3,Iris-setosa\n4.5,2.3,1.3,0.3,Iris-setosa\n4.4,3.2,1.3,0.2,Iris-setosa\n5,3.5,1.6,0.6,Iris-setosa\n5.1,3.8,1.9,0.4,Iris-setosa\n4.8,3,1.4,0.3,Iris-setosa\n5.1,3.8,1.6,0.2,Iris-setosa\n4.6,3.2,1.4,0.2,Iris-setosa\n5.3,3.7,1.5,0.2,Iris-setosa\n5,3.3,1.4,0.2,Iris-setosa\n7,3.2,4.7,1.4,Iris-versicolor\n6.4,3.2,4.5,1.5,Iris-versicolor\n6.9,3.1,4.9,1.5,Iris-versicolor\n5.5,2.3,4,1.3,Iris-versicolor\n6.5,2.8,4.6,1.5,Iris-versicolor\n5.7,2.8,4.5,1.3,Iris-versicolor\n6.3,3.3,4.7,1.6,Iris-versicolor\n4.9,2.4,3.3,1,Iris-versicolor\n6.6,2.9,4.6,1.3,Iris-versicolor\n5.2,2.7,3.9,1.4,Iris-versicolor\n5,2,3.5,1,Iris-versicolor\n5.9,3,4.2,1.5,Iris-versicolor\n6,2.2,4,1,Iris-versicolor\n6.1,2.9,4.7,1.4,Iris-versicolor\n5.6,2.9,3.6,1.3,Iris-versicolor\n6.7,3.1,4.4,1.4,Iris-versicolor\n5.6,3,4.5,1.5,Iris-versicolor\n5.8,2.7,4.1,1,Iris-versicolor\n6.2,2.2,4.5,1.5,Iris-versicolor\n5.6,2.5,3.9,1.1,Iris-versicolor\n5.9,3.2,4.8,1.8,Iris-versicolor\n6.1,2.8,4,1.3,Iris-versicolor\n6.3,2.5,4.9,1.5,Iris-versicolor\n6.1,2.8,4.7,1.2,Iris-versicolor\n6.4,2.9,4.3,1.3,Iris-versicolor\n6.6,3,4.4,1.4,Iris-versicolor\n6.8,2.8,4.8,1.4,Iris-versicolor\n6.7,3,5,1.7,Iris-versicolor\n6,2.9,4.5,1.5,Iris-versicolor\n5.7,2.6,3.5,1,Iris-versicolor\n5.5,2.4,3.8,1.1,Iris-versicolor\n5.5,2.4,3.7,1,Iris-versicolor\n5.8,2.7,3.9,1.2,Iris-versicolor\n6,2.7,5.1,1.6,Iris-versicolor\n5.4,3,4.5,1.5,Iris-versicolor\n6,3.4,4.5,1.6,Iris-versicolor\n6.7,3.1,4.7,1.5,Iris-versicolor\n6.3,2.3,4.4,1.3,Iris-versicolor\n5.6,3,4.1,1.3,Iris-versicolor\n5.5,2.5,4,1.3,Iris-versicolor\n5.5,2.6,4.4,1.2,Iris-versicolor\n6.1,3,4.6,1.4,Iris-versicolor\n5.8,2.6,4,1.2,Iris-versicolor\n5,2.3,3.3,1,Iris-versicolor\n5.6,2.7,4.2,1.3,Iris-versicolor\n5.7,3,4.2,1.2,Iris-versicolor\n5.7,2.9,4.2,1.3,Iris-versicolor\n6.2,2.9,4.3,1.3,Iris-versicolor\n5.1,2.5,3,1.1,Iris-versicolor\n5.7,2.8,4.1,1.3,Iris-versicolor\n6.3,3.3,6,2.5,Iris-virginica\n5.8,2.7,5.1,1.9,Iris-virginica\n7.1,3,5.9,2.1,Iris-virginica\n6.3,2.9,5.6,1.8,Iris-virginica\n6.5,3,5.8,2.2,Iris-virginica\n7.6,3,6.6,2.1,Iris-virginica\n4.9,2.5,4.5,1.7,Iris-virginica\n7.3,2.9,6.3,1.8,Iris-virginica\n6.7,2.5,5.8,1.8,Iris-virginica\n7.2,3.6,6.1,2.5,Iris-virginica\n6.5,3.2,5.1,2,Iris-virginica\n6.4,2.7,5.3,1.9,Iris-virginica\n6.8,3,5.5,2.1,Iris-virginica\n5.7,2.5,5,2,Iris-virginica\n5.8,2.8,5.1,2.4,Iris-virginica\n6.4,3.2,5.3,2.3,Iris-virginica\n6.5,3,5.5,1.8,Iris-virginica\n7.7,3.8,6.7,2.2,Iris-virginica\n7.7,2.6,6.9,2.3,Iris-virginica\n6,2.2,5,1.5,Iris-virginica\n6.9,3.2,5.7,2.3,Iris-virginica\n5.6,2.8,4.9,2,Iris-virginica\n7.7,2.8,6.7,2,Iris-virginica\n6.3,2.7,4.9,1.8,Iris-virginica\n6.7,3.3,5.7,2.1,Iris-virginica\n7.2,3.2,6,1.8,Iris-virginica\n6.2,2.8,4.8,1.8,Iris-virginica\n6.1,3,4.9,1.8,Iris-virginica\n6.4,2.8,5.6,2.1,Iris-virginica\n7.2,3,5.8,1.6,Iris-virginica\n7.4,2.8,6.1,1.9,Iris-virginica\n7.9,3.8,6.4,2,Iris-virginica\n6.4,2.8,5.6,2.2,Iris-virginica\n6.3,2.8,5.1,1.5,Iris-virginica\n6.1,2.6,5.6,1.4,Iris-virginica\n7.7,3,6.1,2.3,Iris-virginica\n6.3,3.4,5.6,2.4,Iris-virginica\n6.4,3.1,5.5,1.8,Iris-virginica\n6,3,4.8,1.8,Iris-virginica\n6.9,3.1,5.4,2.1,Iris-virginica\n6.7,3.1,5.6,2.4,Iris-virginica\n6.9,3.1,5.1,2.3,Iris-virginica\n5.8,2.7,5.1,1.9,Iris-virginica\n6.8,3.2,5.9,2.3,Iris-virginica\n6.7,3.3,5.7,2.5,Iris-virginica\n6.7,3,5.2,2.3,Iris-virginica\n6.3,2.5,5,1.9,Iris-virginica\n6.5,3,5.2,2,Iris-virginica\n6.2,3.4,5.4,2.3,Iris-virginica\n5.9,3,5.1,1.8,Iris-virginica"
}
{
"filename": "code/artificial_intelligence/src/naive_bayes/naive_bayes.cpp",
"content": "#include <iostream>\n#include <string>\n#include <vector>\n#include <random>\n#include <set>\n#include <map>\n#include <unordered_map>\n\nusing namespace std;\nunordered_map<string, int> count(pair<vector<string>, vector<string>> &feature, vector<string> &position)\n{\n unordered_map<string, int> d;\n\n for (int i = 0; i < 2; i++) // because pairs only have 2 values\n {\n for (int j = 0; j < feature.first.size(); j++)\n {\n if (i == 0)\n {\n d.emplace(feature.first[j], 0);\n }\n else\n {\n d.emplace(feature.second[j], 0);\n }\n }\n }\n\n for (int i = 0; i < position.size(); i++)\n {\n d.emplace(position[i], 0);\n }\n\n for (int i = 0; i < 2; i++)\n {\n for (int j = 0; j < feature.first.size(); j++)\n {\n if (i == 0)\n {\n d[feature.first[j]] = d[feature.first[j]] + 1;\n }\n else\n {\n d[feature.second[j]] = d[feature.second[j]] + 1;\n }\n }\n }\n\n for (int i = 0; i < position.size(); i++)\n {\n d[position[i]] = d[position[i]] + 1;\n }\n\n for (int i = 0; i < 2; i++) // because pairs only have 2 values\n {\n for (int j = 0; j < feature.first.size(); j++)\n {\n for (int k = 0; k < position.size(); k++)\n {\n if (i == 0)\n {\n d.emplace(feature.first[j] + \"/\" + position[k], 0);\n }\n else\n {\n d.emplace(feature.second[j] + \"/\" + position[k], 0);\n }\n }\n }\n }\n\n for (int i = 0; i < position.size(); i++)\n {\n d[feature.first[i] + \"/\" + position[i]] = d[feature.first[i] + \"/\" + position[i]] + 1;\n d[feature.second[i] + \"/\" + position[i]] = d[feature.second[i] + \"/\" + position[i]] + 1;\n }\n\n return d;\n}\nunordered_map<string, double> calcProb(unordered_map<string, int> &d, pair<vector<string>, vector<string>> &feature, vector<string> &position)\n{\n int sum = 0;\n unordered_map<string, double> x;\n sum = position.size();\n\n for (int i = 0; i < position.size(); i++)\n {\n x.emplace(position[i], (double)d[position[i]] / (double)sum);\n }\n\n for (int i = 0; i < sum; i++)\n {\n x.emplace(feature.first[i] + \"/\" + position[i], (double)d[feature.first[i] + \"/\" + position[i]] / (double)d[position[i]]);\n x.emplace(feature.second[i] + \"/\" + position[i], (double)d[feature.second[i] + \"/\" + position[i]] / (double)d[position[i]]);\n }\n\n return x;\n}\n\nvector<string> finalPredictions(unordered_map<string, double> &x, pair<vector<string>, vector<string>> &feature, vector<string> &position)\n{\n vector<string> predict_array;\n vector<double> predict_array_prob;\n double temp;\n int size = position.size();\n\n for (int i = 0; i < size; i++)\n {\n predict_array.push_back(\"\");\n predict_array_prob.push_back(0.0);\n }\n\n for (int k = 0; k < size; k++)\n {\n for (int i = 0; i < size; i++)\n {\n temp = 1.0;\n for (int j = 0; j < 2; j++)\n {\n if (j == 0)\n {\n temp = temp * x[feature.first[i] + \"/\" + position[k]];\n }\n else\n {\n temp = temp * x[feature.second[i] + \"/\" + position[k]];\n }\n }\n if (predict_array_prob[i] < temp * x[position[k]])\n {\n predict_array[i] = position[k];\n predict_array_prob[i] = temp * x[position[k]];\n }\n }\n }\n\n return predict_array;\n}\n\nint main()\n{\n\n vector<string> weather = {\n \"sunny\",\n \"rainy\",\n \"sunny\",\n \"sunny\",\n \"sunny\",\n \"rainy\",\n \"rainy\",\n \"sunny\",\n \"sunny\",\n \"rainy\",\n };\n\n vector<string> car = {\n \"working\",\n \"broken\",\n \"working\",\n \"working\",\n \"working\",\n \"broken\",\n \"broken\",\n \"working\",\n \"broken\",\n \"broken\",\n\n };\n\n vector<string> position = {\n \"go-out\",\n \"go-out\",\n \"go-out\",\n \"go-out\",\n \"go-out\",\n \"stay-home\",\n \"stay-home\",\n \"stay-home\",\n \"stay-home\",\n \"stay-home\",\n\n };\n\n pair<vector<string>, vector<string>> feature;\n feature.first = weather;\n feature.second = car;\n unordered_map<string, int> d = count(feature, position);\n unordered_map<string, double> x = calcProb(d, feature, position);\n\n vector<string> predictions = finalPredictions(x, feature, position);\n\n for (int i = 0; i < weather.size(); i++)\n {\n cout << weather[i] << \" \" << car[i] << \" prediction is -> \" << predictions[i] << endl;\n }\n\n // test cases from naive_bayes.py file\n\n return 0;\n}"
}
{
"filename": "code/artificial_intelligence/src/naive_bayes/naive_bayes.py",
"content": "import numpy as np\nfrom collections import defaultdict\n\n\ndef count_words(features, output):\n my_set = []\n\n d = defaultdict(float)\n for i in range(0, len(features)):\n for j in range(0, len(features[0])):\n\n d[features[i][j]] = 0\n\n for i in range(0, len(output)):\n d[output[i]] = 0\n\n for i in range(0, len(features)):\n for j in range(0, len(features[0])):\n d[features[i][j]] = d[features[i][j]] + 1\n\n for i in range(0, len(output)):\n d[output[i]] = d[output[i]] + 1\n\n for i, j in enumerate(set(output)):\n for k in range(0, len(features)):\n for p, q in enumerate(set(features[k])):\n d[q + \"/\" + j] = 0\n\n for i, j in enumerate(output):\n for k in range(len(features)):\n d[features[k][i] + \"/\" + j] = d[features[k][i] + \"/\" + j] + 1\n\n return d\n\n\ndef calculate_probabilities(d, features, output):\n x = defaultdict(float)\n sumi = sum([d[j] for j in set(output)])\n for i in output:\n x[i] = (d[i] * 1.0) / (sumi)\n\n for i, j in enumerate(set(output)):\n for k in range(0, len(features)):\n for p, q in enumerate(set(features[k])):\n x[q + \"/\" + j] = d[q + \"/\" + j] / d[j]\n\n return x\n\n\ndef final_prediction(x, features, output_set):\n predict_array = []\n predict_array_prob = []\n for i in range(0, len(features[0])):\n predict_array.append(0)\n predict_array_prob.append(0)\n\n for k in output_set:\n for i in range(0, len(features[0])):\n temp = 1.0\n for j in range(0, len(features)):\n temp = temp * x[features[j][i] + \"/\" + k]\n if predict_array_prob[i] < temp * x[k]:\n predict_array[i] = k\n predict_array_prob[i] = temp * x[k]\n\n return predict_array\n\n\nWeather = [\n \"sunny\",\n \"rainy\",\n \"sunny\",\n \"sunny\",\n \"sunny\",\n \"rainy\",\n \"rainy\",\n \"sunny\",\n \"sunny\",\n \"rainy\",\n]\nCar = [\n \"working\",\n \"broken\",\n \"working\",\n \"working\",\n \"working\",\n \"broken\",\n \"broken\",\n \"working\",\n \"broken\",\n \"broken\",\n]\nClass = [\n \"go-out\",\n \"go-out\",\n \"go-out\",\n \"go-out\",\n \"go-out\",\n \"stay-home\",\n \"stay-home\",\n \"stay-home\",\n \"stay-home\",\n \"stay-home\",\n]\n\nd = count_words([Weather, Car], Class)\nx = calculate_probabilities(d, [Weather, Car], Class)\npredict_array = final_prediction(\n x, [Weather, Car], set(Class)\n) # Testing on the training set itself\n\nfor i in range(0, len(Weather)):\n print(Weather[i], \"\\t\", Car[i], \"\\t-->\\tprediction is \", predict_array[i])"
}
{
"filename": "code/artificial_intelligence/src/naive_bayes/naive_bayes.swift",
"content": "// Part of Cosmos by OpenGenus Foundation\nimport Foundation\n\nextension String: Error {}\n\nextension Array where Element == Double {\n\n func mean() -> Double {\n return self.reduce(0, +) / Double(count)\n }\n\n func standardDeviation() -> Double {\n let calculatedMean = mean()\n\n let sum = self.reduce(0.0) { (previous, next) in\n return previous + pow(next - calculatedMean, 2)\n }\n\n return sqrt(sum / Double(count - 1))\n }\n}\n\nextension Array where Element == Int {\n\n func uniques() -> Set<Element> {\n return Set(self)\n }\n\n}\n\nenum NBType {\n\n case gaussian\n case multinomial\n func calcLikelihood(variables: [Any], input: Any) -> Double? {\n\n if case .gaussian = self {\n\n guard let input = input as? Double else {\n return nil\n }\n\n guard let mean = variables[0] as? Double else {\n return nil\n }\n\n guard let stDev = variables[1] as? Double else {\n return nil\n }\n\n let eulerPart = pow(M_E, -1 * pow(input - mean, 2) / (2 * pow(stDev, 2)))\n let distribution = eulerPart / sqrt(2 * .pi) / stDev\n\n return distribution\n\n } else if case .multinomial = self {\n\n guard let variables = variables as? [(category: Int, probability: Double)] else {\n return nil\n }\n\n guard let input = input as? Int else {\n return nil\n }\n\n return variables.first { variable in\n return variable.category == input\n }?.probability\n\n }\n\n return nil\n }\n\n func train(values: [Any]) -> [Any]? {\n\n if case .gaussian = self {\n\n guard let values = values as? [Double] else {\n return nil\n }\n\n return [values.mean(), values.standardDeviation()]\n\n } else if case .multinomial = self {\n\n guard let values = values as? [Int] else {\n return nil\n }\n\n let count = values.count\n let categoryProba = values.uniques().map { value -> (Int, Double) in\n return (value, Double(values.filter { $0 == value }.count) / Double(count))\n }\n return categoryProba\n }\n\n return nil\n }\n}\n\nclass NaiveBayes<T> {\n\n var variables: [Int: [(feature: Int, variables: [Any])]]\n var type: NBType\n\n var data: [[T]]\n var classes: [Int]\n\n init(type: NBType, data: [[T]], classes: [Int]) throws {\n self.type = type\n self.data = data\n self.classes = classes\n self.variables = [Int: [(Int, [Any])]]()\n\n if case .gaussian = type, T.self != Double.self {\n throw \"When using Gaussian NB you have to have continuous features (Double)\"\n } else if case .multinomial = type, T.self != Int.self {\n throw \"When using Multinomial NB you have to have categorical features (Int)\"\n }\n }\n\n func train() throws -> Self {\n\n for `class` in classes.uniques() {\n variables[`class`] = [(Int, [Any])]()\n\n let classDependent = data.enumerated().filter { (offset, _) in\n return classes[offset] == `class`\n }\n\n for feature in 0..<data[0].count {\n\n let featureDependent = classDependent.map { $0.element[feature] }\n\n guard let trained = type.train(values: featureDependent) else {\n throw \"Critical! Data could not be casted even though it was checked at init\"\n }\n\n variables[`class`]?.append((feature, trained))\n }\n }\n\n return self\n }\n\n func classify(with input: [T]) -> Int {\n let likelihoods = classifyProba(with: input).max { (first, second) -> Bool in\n return first.1 < second.1\n }\n\n guard let `class` = likelihoods?.0 else {\n return -1\n }\n\n return `class`\n }\n\n func classifyProba(with input: [T]) -> [(Int, Double)] {\n\n var probaClass = [Int: Double]()\n let amount = classes.count\n\n classes.forEach { `class` in\n let individual = classes.filter { $0 == `class` }.count\n probaClass[`class`] = Double(individual) / Double(amount)\n }\n\n let classesAndFeatures = variables.map { (`class`, value) -> (Int, [Double]) in\n let distribution = value.map { (feature, variables) -> Double in\n return type.calcLikelihood(variables: variables, input: input[feature]) ?? 0.0\n }\n return (`class`, distribution)\n }\n\n let likelihoods = classesAndFeatures.map { (`class`, distribution) in\n return (`class`, distribution.reduce(1, *) * (probaClass[`class`] ?? 0.0))\n }\n\n let sum = likelihoods.map { $0.1 }.reduce(0, +)\n let normalized = likelihoods.map { (`class`, likelihood) in\n return (`class`, likelihood / sum)\n }\n\n return normalized\n }\n}"
}
{
"filename": "code/artificial_intelligence/src/naive_bayes/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# Naive bayes algorithm\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/artificial_intelligence/src/named_entity_recognition/ner_dataset.csv",
"content": "\r"
}
{
"filename": "code/artificial_intelligence/src/named_entity_recognition/NER.ipynb",
"content": "{\n \"cells\": [\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"colab_type\": \"text\",\n \"id\": \"q4gSqDnOaJ7W\"\n },\n \"source\": [\n \"<h2 align=center> NER using LSTMs with Keras</h2>\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 1,\n \"metadata\": {\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 52\n },\n \"colab_type\": \"code\",\n \"id\": \"oLK7Y1jiNXDa\",\n \"outputId\": \"0f319464-ee49-4035-f6fb-8396f488c41f\"\n },\n \"outputs\": [\n {\n \"name\": \"stdout\",\n \"output_type\": \"stream\",\n \"text\": [\n \"Tensorflow version: 2.1.0\\n\",\n \"GPU detected: [PhysicalDevice(name='/physical_device:GPU:0', device_type='GPU')]\\n\"\n ]\n }\n ],\n \"source\": [\n \"#Step 1: importing modules and project\\n\",\n \"%matplotlib inline\\n\",\n \"import matplotlib.pyplot as plt\\n\",\n \"import pandas as pd\\n\",\n \"import numpy as np\\n\",\n \"np.random.seed(0)\\n\",\n \"plt.style.use(\\\"ggplot\\\")\\n\",\n \"\\n\",\n \"import tensorflow as tf\\n\",\n \"print('Tensorflow version:', tf.__version__)\\n\",\n \"print('GPU detected:', tf.config.list_physical_devices('GPU'))\"\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {},\n \"source\": [\n \"*Essential info about tagged entities*:\\n\",\n \"- geo = Geographical Entity\\n\",\n \"- org = Organization\\n\",\n \"- per = Person\\n\",\n \"- gpe = Geopolitical Entity\\n\",\n \"- tim = Time indicator\\n\",\n \"- art = Artifact\\n\",\n \"- eve = Event\\n\",\n \"- nat = Natural Phenomenon\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 2,\n \"metadata\": {\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 363\n },\n \"colab_type\": \"code\",\n \"id\": \"mCKmz4SAbI_m\",\n \"outputId\": \"a03b1aed-dc60-4f03-cb06-19e440fa6367\"\n },\n \"outputs\": [\n {\n \"data\": {\n \"text/html\": [\n \"<div>\\n\",\n \"<style scoped>\\n\",\n \" .dataframe tbody tr th:only-of-type {\\n\",\n \" vertical-align: middle;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe tbody tr th {\\n\",\n \" vertical-align: top;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe thead th {\\n\",\n \" text-align: right;\\n\",\n \" }\\n\",\n \"</style>\\n\",\n \"<table border=\\\"1\\\" class=\\\"dataframe\\\">\\n\",\n \" <thead>\\n\",\n \" <tr style=\\\"text-align: right;\\\">\\n\",\n \" <th></th>\\n\",\n \" <th>Sentence #</th>\\n\",\n \" <th>Word</th>\\n\",\n \" <th>POS</th>\\n\",\n \" <th>Tag</th>\\n\",\n \" </tr>\\n\",\n \" </thead>\\n\",\n \" <tbody>\\n\",\n \" <tr>\\n\",\n \" <th>0</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>Thousands</td>\\n\",\n \" <td>NNS</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>1</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>of</td>\\n\",\n \" <td>IN</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>2</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>demonstrators</td>\\n\",\n \" <td>NNS</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>3</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>have</td>\\n\",\n \" <td>VBP</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>4</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>marched</td>\\n\",\n \" <td>VBN</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>5</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>through</td>\\n\",\n \" <td>IN</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>6</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>London</td>\\n\",\n \" <td>NNP</td>\\n\",\n \" <td>B-geo</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>7</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>to</td>\\n\",\n \" <td>TO</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>8</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>protest</td>\\n\",\n \" <td>VB</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>9</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>the</td>\\n\",\n \" <td>DT</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>10</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>war</td>\\n\",\n \" <td>NN</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>11</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>in</td>\\n\",\n \" <td>IN</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>12</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>Iraq</td>\\n\",\n \" <td>NNP</td>\\n\",\n \" <td>B-geo</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>13</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>and</td>\\n\",\n \" <td>CC</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>14</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>demand</td>\\n\",\n \" <td>VB</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>15</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>the</td>\\n\",\n \" <td>DT</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>16</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>withdrawal</td>\\n\",\n \" <td>NN</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>17</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>of</td>\\n\",\n \" <td>IN</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>18</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>British</td>\\n\",\n \" <td>JJ</td>\\n\",\n \" <td>B-gpe</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>19</th>\\n\",\n \" <td>Sentence: 1</td>\\n\",\n \" <td>troops</td>\\n\",\n \" <td>NNS</td>\\n\",\n \" <td>O</td>\\n\",\n \" </tr>\\n\",\n \" </tbody>\\n\",\n \"</table>\\n\",\n \"</div>\"\n ],\n \"text/plain\": [\n \" Sentence # Word POS Tag\\n\",\n \"0 Sentence: 1 Thousands NNS O\\n\",\n \"1 Sentence: 1 of IN O\\n\",\n \"2 Sentence: 1 demonstrators NNS O\\n\",\n \"3 Sentence: 1 have VBP O\\n\",\n \"4 Sentence: 1 marched VBN O\\n\",\n \"5 Sentence: 1 through IN O\\n\",\n \"6 Sentence: 1 London NNP B-geo\\n\",\n \"7 Sentence: 1 to TO O\\n\",\n \"8 Sentence: 1 protest VB O\\n\",\n \"9 Sentence: 1 the DT O\\n\",\n \"10 Sentence: 1 war NN O\\n\",\n \"11 Sentence: 1 in IN O\\n\",\n \"12 Sentence: 1 Iraq NNP B-geo\\n\",\n \"13 Sentence: 1 and CC O\\n\",\n \"14 Sentence: 1 demand VB O\\n\",\n \"15 Sentence: 1 the DT O\\n\",\n \"16 Sentence: 1 withdrawal NN O\\n\",\n \"17 Sentence: 1 of IN O\\n\",\n \"18 Sentence: 1 British JJ B-gpe\\n\",\n \"19 Sentence: 1 troops NNS O\"\n ]\n },\n \"execution_count\": 2,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"#Step 2: Load and Explore the NER Dataset\\n\",\n \"data = pd.read_csv(\\\"ner_dataset.csv\\\", encoding=\\\"latin1\\\")\\n\",\n \"data = data.fillna(method=\\\"ffill\\\")\\n\",\n \"data.head(20)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 4,\n \"metadata\": {\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 34\n },\n \"colab_type\": \"code\",\n \"id\": \"riOztP-8NXHT\",\n \"outputId\": \"200d251b-17c9-451b-9e96-523f458be47e\"\n },\n \"outputs\": [\n {\n \"name\": \"stdout\",\n \"output_type\": \"stream\",\n \"text\": [\n \"Unique words in corpus: 35178\\n\",\n \"Unique tags in corpus: 17\\n\"\n ]\n }\n ],\n \"source\": [\n \"print(\\\"Unique words in corpus:\\\", data['Word'].nunique())\\n\",\n \"print(\\\"Unique tags in corpus:\\\", data['Tag'].nunique())\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 3,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"words = list(set(data[\\\"Word\\\"].values))\\n\",\n \"words.append(\\\"ENDPAD\\\")\\n\",\n \"num_words = len(words)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 5,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"tags = list(set(data[\\\"Tag\\\"].values))\\n\",\n \"num_tags = len(tags)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 6,\n \"metadata\": {\n \"colab\": {},\n \"colab_type\": \"code\",\n \"id\": \"VdJst_g5NYY_\"\n },\n \"outputs\": [],\n \"source\": [\n \"#Step 3: Retrieve Sentences and Corresponsing Tags\\n\",\n \"class SentenceGetter(object):\\n\",\n \" def __init__(self, data):\\n\",\n \" self.n_sent = 1\\n\",\n \" self.data = data\\n\",\n \" self.empty = False\\n\",\n \" agg_func = lambda s: [(w, p, t) for w, p, t in zip(s[\\\"Word\\\"].values.tolist(),\\n\",\n \" s[\\\"POS\\\"].values.tolist(),\\n\",\n \" s[\\\"Tag\\\"].values.tolist())]\\n\",\n \" self.grouped = self.data.groupby(\\\"Sentence #\\\").apply(agg_func)\\n\",\n \" self.sentences = [s for s in self.grouped]\\n\",\n \" \\n\",\n \" def get_next(self):\\n\",\n \" try:\\n\",\n \" s = self.grouped[\\\"Sentence: {}\\\".format(self.n_sent)]\\n\",\n \" self.n_sent += 1\\n\",\n \" return s\\n\",\n \" except:\\n\",\n \" return None\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 7,\n \"metadata\": {\n \"colab\": {},\n \"colab_type\": \"code\",\n \"id\": \"nMUQLppspkPj\"\n },\n \"outputs\": [],\n \"source\": [\n \"getter = SentenceGetter(data)\\n\",\n \"sentences = getter.sentences\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 8,\n \"metadata\": {\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 434\n },\n \"colab_type\": \"code\",\n \"id\": \"GhiSTt2UdzYC\",\n \"outputId\": \"95d275f6-f386-46d8-a583-2861d64c6b72\"\n },\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"[('Thousands', 'NNS', 'O'),\\n\",\n \" ('of', 'IN', 'O'),\\n\",\n \" ('demonstrators', 'NNS', 'O'),\\n\",\n \" ('have', 'VBP', 'O'),\\n\",\n \" ('marched', 'VBN', 'O'),\\n\",\n \" ('through', 'IN', 'O'),\\n\",\n \" ('London', 'NNP', 'B-geo'),\\n\",\n \" ('to', 'TO', 'O'),\\n\",\n \" ('protest', 'VB', 'O'),\\n\",\n \" ('the', 'DT', 'O'),\\n\",\n \" ('war', 'NN', 'O'),\\n\",\n \" ('in', 'IN', 'O'),\\n\",\n \" ('Iraq', 'NNP', 'B-geo'),\\n\",\n \" ('and', 'CC', 'O'),\\n\",\n \" ('demand', 'VB', 'O'),\\n\",\n \" ('the', 'DT', 'O'),\\n\",\n \" ('withdrawal', 'NN', 'O'),\\n\",\n \" ('of', 'IN', 'O'),\\n\",\n \" ('British', 'JJ', 'B-gpe'),\\n\",\n \" ('troops', 'NNS', 'O'),\\n\",\n \" ('from', 'IN', 'O'),\\n\",\n \" ('that', 'DT', 'O'),\\n\",\n \" ('country', 'NN', 'O'),\\n\",\n \" ('.', '.', 'O')]\"\n ]\n },\n \"execution_count\": 8,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"sentences[0]\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 9,\n \"metadata\": {\n \"colab\": {},\n \"colab_type\": \"code\",\n \"id\": \"SvENHO18pkaQ\"\n },\n \"outputs\": [],\n \"source\": [\n \"#Step 4: Define Mappings between Sentences and Tags\\n\",\n \"word2idx = {w: i + 1 for i, w in enumerate(words)}\\n\",\n \"tag2idx = {t: i for i, t in enumerate(tags)}\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 10,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"{'Bychkova': 1,\\n\",\n \" 'victorious': 2,\\n\",\n \" 'struggled': 3,\\n\",\n \" 'ex-President': 4,\\n\",\n \" 'Rehnquist': 5,\\n\",\n \" 'Uganda': 6,\\n\",\n \" 're-tried': 7,\\n\",\n \" 'Welfare': 8,\\n\",\n \" 'intent': 9,\\n\",\n \" 'stance': 10,\\n\",\n \" 'Depending': 11,\\n\",\n \" 'pro-rebel': 12,\\n\",\n \" 'Catalonians': 13,\\n\",\n \" 'stalls': 14,\\n\",\n \" 'gored': 15,\\n\",\n \" 'jeopardy': 16,\\n\",\n \" 'epitomizes': 17,\\n\",\n \" 'Bernie': 18,\\n\",\n \" 'Price': 19,\\n\",\n \" 'sets': 20,\\n\",\n \" 'Cayes': 21,\\n\",\n \" 'however': 22,\\n\",\n \" \\\"'T\\\": 23,\\n\",\n \" 'immorality': 24,\\n\",\n \" 'climatic': 25,\\n\",\n \" 'touch': 26,\\n\",\n \" 'vivid': 27,\\n\",\n \" 'Shaffi': 28,\\n\",\n \" 'Sudan': 29,\\n\",\n \" '3,600': 30,\\n\",\n \" 'carmaker': 31,\\n\",\n \" 'runway': 32,\\n\",\n \" '(': 33,\\n\",\n \" 'due': 34,\\n\",\n \" 'Masorin': 35,\\n\",\n \" 'except': 36,\\n\",\n \" 'consultation': 37,\\n\",\n \" 'usually': 38,\\n\",\n \" 'rebuked': 39,\\n\",\n \" 'connect': 40,\\n\",\n \" 'defrauding': 41,\\n\",\n \" 'cease-fire': 42,\\n\",\n \" 'Husseindoust': 43,\\n\",\n \" 'hockey': 44,\\n\",\n \" 'hay': 45,\\n\",\n \" 'Elliott': 46,\\n\",\n \" 'genes': 47,\\n\",\n \" 'shareholders': 48,\\n\",\n \" 'foot': 49,\\n\",\n \" 'quails': 50,\\n\",\n \" 'decisions': 51,\\n\",\n \" 'Seche': 52,\\n\",\n \" 'Kane': 53,\\n\",\n \" 'representing': 54,\\n\",\n \" 'Nuristani': 55,\\n\",\n \" 'Monument': 56,\\n\",\n \" 'welcome': 57,\\n\",\n \" 'disappointment': 58,\\n\",\n \" 'Sunni-dominant': 59,\\n\",\n \" 'pegs': 60,\\n\",\n \" 'VanAllen': 61,\\n\",\n \" 'conspicuous': 62,\\n\",\n \" 'detainment': 63,\\n\",\n \" '454-seat': 64,\\n\",\n \" 'Luzon': 65,\\n\",\n \" 'burning': 66,\\n\",\n \" 'unintended': 67,\\n\",\n \" 'Lithuanians': 68,\\n\",\n \" 'amount': 69,\\n\",\n \" 'coasts': 70,\\n\",\n \" 'Cassini': 71,\\n\",\n \" 'Chase': 72,\\n\",\n \" '213': 73,\\n\",\n \" '46.3': 74,\\n\",\n \" 'jailed': 75,\\n\",\n \" '94.2': 76,\\n\",\n \" 'Incoming': 77,\\n\",\n \" 'Moallem': 78,\\n\",\n \" 'Meles': 79,\\n\",\n \" 'led': 80,\\n\",\n \" 'dismantle': 81,\\n\",\n \" 'preserving': 82,\\n\",\n \" 'Iran-Oman': 83,\\n\",\n \" '113.2': 84,\\n\",\n \" 'e-gaming': 85,\\n\",\n \" 'WTO': 86,\\n\",\n \" 'Challenge': 87,\\n\",\n \" 'cooperated': 88,\\n\",\n \" 'self-financing': 89,\\n\",\n \" 'Vince': 90,\\n\",\n \" 'IADB': 91,\\n\",\n \" 'give': 92,\\n\",\n \" 'Musical': 93,\\n\",\n \" 'enterprises': 94,\\n\",\n \" 'arsonists': 95,\\n\",\n \" '1933': 96,\\n\",\n \" 'dissipating': 97,\\n\",\n \" 'Zwelinzima': 98,\\n\",\n \" 'al-Shahristani': 99,\\n\",\n \" 'jumped': 100,\\n\",\n \" 'coward': 101,\\n\",\n \" 'stabilized': 102,\\n\",\n \" 'Albert': 103,\\n\",\n \" 'Muslim-Christian': 104,\\n\",\n \" 'unto': 105,\\n\",\n \" '42-year-old': 106,\\n\",\n \" 'GHANNOUCHI': 107,\\n\",\n \" 'recognition': 108,\\n\",\n \" 'Bruce': 109,\\n\",\n \" 'Al-Mansoorain': 110,\\n\",\n \" 'Mongolian': 111,\\n\",\n \" 'anfo': 112,\\n\",\n \" 'nurtured': 113,\\n\",\n \" 'soldiers': 114,\\n\",\n \" 'Railroad': 115,\\n\",\n \" 'Farmers': 116,\\n\",\n \" 'Sekouba': 117,\\n\",\n \" '58.5': 118,\\n\",\n \" 'Endeavour': 119,\\n\",\n \" 'termed': 120,\\n\",\n \" 'floats': 121,\\n\",\n \" 'art': 122,\\n\",\n \" 'Fadlallah': 123,\\n\",\n \" 'high-risk': 124,\\n\",\n \" 'broadly': 125,\\n\",\n \" 'malignant': 126,\\n\",\n \" 'exuberant': 127,\\n\",\n \" 'JEM': 128,\\n\",\n \" '1985': 129,\\n\",\n \" 'sponsor': 130,\\n\",\n \" 'fiercer': 131,\\n\",\n \" 'highly-enriched': 132,\\n\",\n \" 'cheered': 133,\\n\",\n \" 'drill': 134,\\n\",\n \" 'whatever': 135,\\n\",\n \" 'adopting': 136,\\n\",\n \" 'mar': 137,\\n\",\n \" 'wrathful': 138,\\n\",\n \" 'kicked': 139,\\n\",\n \" 'Triangle': 140,\\n\",\n \" 'Honduran': 141,\\n\",\n \" 'carriers': 142,\\n\",\n \" 'occured': 143,\\n\",\n \" 'professors': 144,\\n\",\n \" 'welfare-dependent': 145,\\n\",\n \" 'reinsurer': 146,\\n\",\n \" 'one-party': 147,\\n\",\n \" '275-members': 148,\\n\",\n \" 'Tomini': 149,\\n\",\n \" 'filmmaking': 150,\\n\",\n \" 'Crozet': 151,\\n\",\n \" 'Oleksiy': 152,\\n\",\n \" 'Byzantine': 153,\\n\",\n \" 'kidnapped': 154,\\n\",\n \" 'resemble': 155,\\n\",\n \" 'reductions': 156,\\n\",\n \" 'commemorated': 157,\\n\",\n \" 'mosaic': 158,\\n\",\n \" 'posthumous': 159,\\n\",\n \" 'doubtful': 160,\\n\",\n \" '77.95': 161,\\n\",\n \" 'Next': 162,\\n\",\n \" 'stiffly': 163,\\n\",\n \" 'native': 164,\\n\",\n \" 'warn': 165,\\n\",\n \" 'ensure': 166,\\n\",\n \" 'Bullitt': 167,\\n\",\n \" 'Sirisia': 168,\\n\",\n \" 'For': 169,\\n\",\n \" 'Hewitt': 170,\\n\",\n \" 'booed': 171,\\n\",\n \" 'Youn-jin': 172,\\n\",\n \" 'Alexeyeva': 173,\\n\",\n \" 'dioxin': 174,\\n\",\n \" 'seated': 175,\\n\",\n \" 'Sun': 176,\\n\",\n \" 'real': 177,\\n\",\n \" 'directors': 178,\\n\",\n \" 'Cali': 179,\\n\",\n \" 'reigning': 180,\\n\",\n \" 'conspirators': 181,\\n\",\n \" 'drift': 182,\\n\",\n \" 'Basic': 183,\\n\",\n \" '454-member': 184,\\n\",\n \" 'dry': 185,\\n\",\n \" '481': 186,\\n\",\n \" 'readies': 187,\\n\",\n \" 'subpoena': 188,\\n\",\n \" 'tempered': 189,\\n\",\n \" 'evaluate': 190,\\n\",\n \" 'rutile': 191,\\n\",\n \" 'refuge': 192,\\n\",\n \" 'unchallenged': 193,\\n\",\n \" 'Khazaee': 194,\\n\",\n \" 'wayward': 195,\\n\",\n \" 'Du': 196,\\n\",\n \" 'lender': 197,\\n\",\n \" '1,75,000': 198,\\n\",\n \" 'Banda': 199,\\n\",\n \" 'Fayyum': 200,\\n\",\n \" 'respond': 201,\\n\",\n \" 'by': 202,\\n\",\n \" 'pro-reform': 203,\\n\",\n \" 'Giant': 204,\\n\",\n \" 'Placid': 205,\\n\",\n \" 'capital-intensive': 206,\\n\",\n \" 'mixture': 207,\\n\",\n \" 'pointless': 208,\\n\",\n \" 'Nord': 209,\\n\",\n \" 'Alam': 210,\\n\",\n \" 'west-northwest': 211,\\n\",\n \" 'Russian': 212,\\n\",\n \" 'non-local': 213,\\n\",\n \" 'finalizing': 214,\\n\",\n \" 'parting': 215,\\n\",\n \" 'VimpelCom': 216,\\n\",\n \" 'heaviest': 217,\\n\",\n \" 'Soufriere': 218,\\n\",\n \" 'grandchild': 219,\\n\",\n \" 'NATO-led': 220,\\n\",\n \" '02-May': 221,\\n\",\n \" 'contract': 222,\\n\",\n \" 'hazards': 223,\\n\",\n \" 'Stefano': 224,\\n\",\n \" 'Khalilzad': 225,\\n\",\n \" 'ate': 226,\\n\",\n \" 'S-300': 227,\\n\",\n \" 'Hermitage': 228,\\n\",\n \" '55-nation': 229,\\n\",\n \" 'hot': 230,\\n\",\n \" 'Celia': 231,\\n\",\n \" 'Faizullah': 232,\\n\",\n \" 'Andre': 233,\\n\",\n \" 'reconstructed': 234,\\n\",\n \" 'damaging': 235,\\n\",\n \" 'Toronto': 236,\\n\",\n \" 'Dorsey': 237,\\n\",\n \" 'Najaf': 238,\\n\",\n \" 'Kim': 239,\\n\",\n \" 'Safwat': 240,\\n\",\n \" 'chase': 241,\\n\",\n \" 'Wolong': 242,\\n\",\n \" 'Jong-Woon': 243,\\n\",\n \" 'postponement': 244,\\n\",\n \" '321': 245,\\n\",\n \" 'Jolie': 246,\\n\",\n \" 'Sarah': 247,\\n\",\n \" 'Malindi': 248,\\n\",\n \" 'diagnosed': 249,\\n\",\n \" 'Al-Mukmin': 250,\\n\",\n \" 'insert': 251,\\n\",\n \" 'UNAMI': 252,\\n\",\n \" 'phone': 253,\\n\",\n \" 'Tbilisi': 254,\\n\",\n \" 'selling': 255,\\n\",\n \" 'Registration': 256,\\n\",\n \" 'execute': 257,\\n\",\n \" 'shorter-range': 258,\\n\",\n \" 'Goldman-Sachs': 259,\\n\",\n \" 'album-release': 260,\\n\",\n \" 'Buenos': 261,\\n\",\n \" 'disciplines': 262,\\n\",\n \" 'focused': 263,\\n\",\n \" 'Schlender': 264,\\n\",\n \" 'Dictatorship': 265,\\n\",\n \" 'car-free': 266,\\n\",\n \" '23.7': 267,\\n\",\n \" 'Ahmedou': 268,\\n\",\n \" 'Harmony': 269,\\n\",\n \" 'Slavic': 270,\\n\",\n \" 'Machakos': 271,\\n\",\n \" 'Government-sponsored': 272,\\n\",\n \" 'technocrat': 273,\\n\",\n \" 'drunken': 274,\\n\",\n \" 'Mint': 275,\\n\",\n \" 'functioning': 276,\\n\",\n \" 'debating': 277,\\n\",\n \" 'attributing': 278,\\n\",\n \" 'got': 279,\\n\",\n \" 'fairer': 280,\\n\",\n \" 'Christina': 281,\\n\",\n \" 'Zebari': 282,\\n\",\n \" 'multiple': 283,\\n\",\n \" 'dispose': 284,\\n\",\n \" 'crowd': 285,\\n\",\n \" 'Gloria': 286,\\n\",\n \" 'Filipino': 287,\\n\",\n \" 'shoot-out': 288,\\n\",\n \" 'emotional': 289,\\n\",\n \" 'civilian-led': 290,\\n\",\n \" 'bluegrass': 291,\\n\",\n \" 'Plateau': 292,\\n\",\n \" 'length': 293,\\n\",\n \" 'Chacahua': 294,\\n\",\n \" 'Shelter': 295,\\n\",\n \" 'Hellenic': 296,\\n\",\n \" 'Kurram': 297,\\n\",\n \" 'motions': 298,\\n\",\n \" 'necessities': 299,\\n\",\n \" '733': 300,\\n\",\n \" 'crest': 301,\\n\",\n \" '27th': 302,\\n\",\n \" 'Each': 303,\\n\",\n \" '1,44,000': 304,\\n\",\n \" 'Thing': 305,\\n\",\n \" 'oversee': 306,\\n\",\n \" 'shootouts': 307,\\n\",\n \" 'Danish': 308,\\n\",\n \" 'check': 309,\\n\",\n \" 'Altria': 310,\\n\",\n \" 'once': 311,\\n\",\n \" 'Scientists': 312,\\n\",\n \" 'Rood': 313,\\n\",\n \" 'Simmonds': 314,\\n\",\n \" 'Huthi': 315,\\n\",\n \" 'glimpse': 316,\\n\",\n \" 'Empress': 317,\\n\",\n \" 'anti-proliferation': 318,\\n\",\n \" 'non-oil': 319,\\n\",\n \" 'rigors': 320,\\n\",\n \" 'acquired': 321,\\n\",\n \" 'Silvestre': 322,\\n\",\n \" 'Arab': 323,\\n\",\n \" 'contrive': 324,\\n\",\n \" 'proportion': 325,\\n\",\n \" 'succumb': 326,\\n\",\n \" 'Haidallah': 327,\\n\",\n \" 'stalling': 328,\\n\",\n \" 'unexplored': 329,\\n\",\n \" 'Cannon': 330,\\n\",\n \" 'cruised': 331,\\n\",\n \" 'Rahho': 332,\\n\",\n \" 'crippling': 333,\\n\",\n \" 'confessing': 334,\\n\",\n \" 'Hammad': 335,\\n\",\n \" '1718': 336,\\n\",\n \" 'prompting': 337,\\n\",\n \" 'mistreated': 338,\\n\",\n \" 'deliverer': 339,\\n\",\n \" 'justly': 340,\\n\",\n \" 'dart': 341,\\n\",\n \" 'obesity': 342,\\n\",\n \" 'neighbor': 343,\\n\",\n \" 'Restoring': 344,\\n\",\n \" '13-point': 345,\\n\",\n \" 'hymns': 346,\\n\",\n \" 'rain-swept': 347,\\n\",\n \" \\\"O'Keefe\\\": 348,\\n\",\n \" 'Presiding': 349,\\n\",\n \" 'attempt': 350,\\n\",\n \" 'sea-based': 351,\\n\",\n \" 'congratulatory': 352,\\n\",\n \" 'Armored': 353,\\n\",\n \" 'Sirnak': 354,\\n\",\n \" 'Ouagadougou': 355,\\n\",\n \" 'Kids': 356,\\n\",\n \" 'Let': 357,\\n\",\n \" 'views': 358,\\n\",\n \" 'profound': 359,\\n\",\n \" 'Parliamentary': 360,\\n\",\n \" 'skeptical': 361,\\n\",\n \" 'Albright': 362,\\n\",\n \" 'colliding': 363,\\n\",\n \" 'Tayr': 364,\\n\",\n \" 'Jessye': 365,\\n\",\n \" 'proceeds': 366,\\n\",\n \" 'playoff': 367,\\n\",\n \" 'Azhari': 368,\\n\",\n \" 'stuff': 369,\\n\",\n \" 'slid': 370,\\n\",\n \" 'Stockholm': 371,\\n\",\n \" 'Apr-29': 372,\\n\",\n \" 'irrationality': 373,\\n\",\n \" 'wheel': 374,\\n\",\n \" 'porous': 375,\\n\",\n \" 'MORALES': 376,\\n\",\n \" 'Plum': 377,\\n\",\n \" 'stepped-up': 378,\\n\",\n \" 'pat-downs': 379,\\n\",\n \" 'firmly': 380,\\n\",\n \" 'indicated': 381,\\n\",\n \" 'grapes': 382,\\n\",\n \" 'Jaffer': 383,\\n\",\n \" 'Problems': 384,\\n\",\n \" 'Crawford': 385,\\n\",\n \" 'discharges': 386,\\n\",\n \" 'Liliput': 387,\\n\",\n \" 'growing': 388,\\n\",\n \" 'defeated': 389,\\n\",\n \" 'Karshi-Khanabad': 390,\\n\",\n \" 'predictor': 391,\\n\",\n \" 'Bilge': 392,\\n\",\n \" 'suicides': 393,\\n\",\n \" '500-million': 394,\\n\",\n \" 'Jarkko': 395,\\n\",\n \" 'NIGHTINGALE': 396,\\n\",\n \" 'Khartoum-backed': 397,\\n\",\n \" 'Micheletti': 398,\\n\",\n \" 'Little': 399,\\n\",\n \" 'Horbach': 400,\\n\",\n \" '28,000': 401,\\n\",\n \" 'applying': 402,\\n\",\n \" 'Summer': 403,\\n\",\n \" 'counting': 404,\\n\",\n \" 'breakdowns': 405,\\n\",\n \" 'Shyloh': 406,\\n\",\n \" 'exceeding': 407,\\n\",\n \" 'acclaim': 408,\\n\",\n \" 'Iger': 409,\\n\",\n \" 'suburban': 410,\\n\",\n \" 'linked': 411,\\n\",\n \" '1857': 412,\\n\",\n \" 'bail': 413,\\n\",\n \" 'Croatia': 414,\\n\",\n \" '2,400-kilometer': 415,\\n\",\n \" '16,000': 416,\\n\",\n \" 'ROUSSEFF': 417,\\n\",\n \" 'Technology': 418,\\n\",\n \" 'bioterrorism': 419,\\n\",\n \" 'Bitar': 420,\\n\",\n \" 'retire': 421,\\n\",\n \" 'Afghan-Pakistan': 422,\\n\",\n \" 'Walker': 423,\\n\",\n \" 'duffle': 424,\\n\",\n \" 'Study': 425,\\n\",\n \" 'Palpa': 426,\\n\",\n \" 'al-Hamash': 427,\\n\",\n \" 'Bundesbank': 428,\\n\",\n \" 'falcon': 429,\\n\",\n \" 'Press': 430,\\n\",\n \" 'helm': 431,\\n\",\n \" 'outlets': 432,\\n\",\n \" 'curriculum': 433,\\n\",\n \" 'slow-moving': 434,\\n\",\n \" 'co-defendents': 435,\\n\",\n \" 'renewal': 436,\\n\",\n \" 'sown': 437,\\n\",\n \" 'Sindh': 438,\\n\",\n \" 'condone': 439,\\n\",\n \" 'trod': 440,\\n\",\n \" 'intersection': 441,\\n\",\n \" 'slide': 442,\\n\",\n \" 'popes': 443,\\n\",\n \" 'Kong': 444,\\n\",\n \" 'slaughterhouse': 445,\\n\",\n \" 'barbarity': 446,\\n\",\n \" 'Diplomacy': 447,\\n\",\n \" '1950': 448,\\n\",\n \" 'W.': 449,\\n\",\n \" 'Plutonium': 450,\\n\",\n \" 'reshuffle': 451,\\n\",\n \" 'Various': 452,\\n\",\n \" 'memories': 453,\\n\",\n \" 'salivated': 454,\\n\",\n \" 'Men': 455,\\n\",\n \" 'code': 456,\\n\",\n \" 'fused': 457,\\n\",\n \" 'Weddings': 458,\\n\",\n \" 'airstrips': 459,\\n\",\n \" 'Jesper': 460,\\n\",\n \" 'hinges': 461,\\n\",\n \" 'Serious': 462,\\n\",\n \" 'copra': 463,\\n\",\n \" 'Alamzai': 464,\\n\",\n \" 'authorities': 465,\\n\",\n \" '48th': 466,\\n\",\n \" 'KENYATTA': 467,\\n\",\n \" 'Kuiper': 468,\\n\",\n \" 'interesting': 469,\\n\",\n \" 'excursion': 470,\\n\",\n \" 'Zimbabwe': 471,\\n\",\n \" '73,000': 472,\\n\",\n \" 'Tarantino': 473,\\n\",\n \" 'Aleppo': 474,\\n\",\n \" 'ventures': 475,\\n\",\n \" 'Haham': 476,\\n\",\n \" 'medications': 477,\\n\",\n \" 'Hawo': 478,\\n\",\n \" 'tune': 479,\\n\",\n \" 'Martin': 480,\\n\",\n \" 'Abdouramane': 481,\\n\",\n \" 'hemorrhagic': 482,\\n\",\n \" 'engine': 483,\\n\",\n \" 'SIHANOUK': 484,\\n\",\n \" 'ISSUED': 485,\\n\",\n \" 'Marcelo': 486,\\n\",\n \" 'Benghazi': 487,\\n\",\n \" '20-25': 488,\\n\",\n \" 'Conquer': 489,\\n\",\n \" 'considerations': 490,\\n\",\n \" 'photographic': 491,\\n\",\n \" 'Jib': 492,\\n\",\n \" 'thankful': 493,\\n\",\n \" 'overseen': 494,\\n\",\n \" 'herd': 495,\\n\",\n \" '79': 496,\\n\",\n \" 'overpowered': 497,\\n\",\n \" 'solicit': 498,\\n\",\n \" 'boarded': 499,\\n\",\n \" 'out': 500,\\n\",\n \" '25,000': 501,\\n\",\n \" 'Israeli-Palestinian': 502,\\n\",\n \" 'centrally-planned': 503,\\n\",\n \" '35-member': 504,\\n\",\n \" 'stimulant': 505,\\n\",\n \" 'Asgiriya': 506,\\n\",\n \" 'liturgy': 507,\\n\",\n \" 'injustice': 508,\\n\",\n \" 'anymore': 509,\\n\",\n \" 'Brent': 510,\\n\",\n \" 'capitol': 511,\\n\",\n \" 'Rubaie': 512,\\n\",\n \" 'differing': 513,\\n\",\n \" 'Heraldo': 514,\\n\",\n \" 'reluctant': 515,\\n\",\n \" 'idleness': 516,\\n\",\n \" 'Competition': 517,\\n\",\n \" 'bouncing': 518,\\n\",\n \" 'affiliates': 519,\\n\",\n \" 'surging': 520,\\n\",\n \" 'religion-based': 521,\\n\",\n \" 'crisis': 522,\\n\",\n \" 'mistakenly': 523,\\n\",\n \" 'Theo': 524,\\n\",\n \" '7,00,000': 525,\\n\",\n \" '4,218': 526,\\n\",\n \" 'possibilities': 527,\\n\",\n \" 'inquired': 528,\\n\",\n \" 'wanting': 529,\\n\",\n \" 'diluting': 530,\\n\",\n \" 'duped': 531,\\n\",\n \" 'symbolism': 532,\\n\",\n \" '592': 533,\\n\",\n \" 'Srebrenica': 534,\\n\",\n \" 'guise': 535,\\n\",\n \" 'Hong-Kong-based': 536,\\n\",\n \" 'wells': 537,\\n\",\n \" 'testifying': 538,\\n\",\n \" 'Jamrud': 539,\\n\",\n \" 'monasteries': 540,\\n\",\n \" 'Separatist': 541,\\n\",\n \" 'bicycle': 542,\\n\",\n \" 'narrated': 543,\\n\",\n \" 'Akhmad': 544,\\n\",\n \" 'LLC': 545,\\n\",\n \" 'hired': 546,\\n\",\n \" 'disproportionately': 547,\\n\",\n \" 'Escamilla': 548,\\n\",\n \" 'dismay': 549,\\n\",\n \" 'Venezuelan': 550,\\n\",\n \" 'Google-based': 551,\\n\",\n \" 'Tarongoy': 552,\\n\",\n \" '300-km': 553,\\n\",\n \" 'writings': 554,\\n\",\n \" 'Madagonians': 555,\\n\",\n \" 'backed-militias': 556,\\n\",\n \" 'railways': 557,\\n\",\n \" 'heyday': 558,\\n\",\n \" 'Petroleum': 559,\\n\",\n \" 'Kamal': 560,\\n\",\n \" 'ruler': 561,\\n\",\n \" 'counter-protesters': 562,\\n\",\n \" 'impressed': 563,\\n\",\n \" '132-seat': 564,\\n\",\n \" 'Hezbollah-led': 565,\\n\",\n \" 'Net': 566,\\n\",\n \" 'Norway': 567,\\n\",\n \" 'Washington-area': 568,\\n\",\n \" 'Sharon': 569,\\n\",\n \" 'Geodynamic': 570,\\n\",\n \" 'retreated': 571,\\n\",\n \" 'revived': 572,\\n\",\n \" 'Ramin': 573,\\n\",\n \" 'rebounding': 574,\\n\",\n \" 'choosing': 575,\\n\",\n \" 'WNBA': 576,\\n\",\n \" 'Bodies': 577,\\n\",\n \" '1278': 578,\\n\",\n \" 'force': 579,\\n\",\n \" 'author': 580,\\n\",\n \" 'ridicule': 581,\\n\",\n \" 'ranches': 582,\\n\",\n \" 'Sooden': 583,\\n\",\n \" 'whitewash': 584,\\n\",\n \" 'hiking': 585,\\n\",\n \" '49': 586,\\n\",\n \" 'crescent': 587,\\n\",\n \" 'conscious': 588,\\n\",\n \" 'Madeleine': 589,\\n\",\n \" 'caucuses': 590,\\n\",\n \" 'Hedi': 591,\\n\",\n \" 'forward': 592,\\n\",\n \" 'solution': 593,\\n\",\n \" '45-year-old': 594,\\n\",\n \" 'Chelsea': 595,\\n\",\n \" 'Avril': 596,\\n\",\n \" 'promising': 597,\\n\",\n \" 'anti-labor': 598,\\n\",\n \" 'Aref': 599,\\n\",\n \" 'Stanford': 600,\\n\",\n \" 'chemist': 601,\\n\",\n \" 'earth': 602,\\n\",\n \" '80.5': 603,\\n\",\n \" 'Dutch': 604,\\n\",\n \" 'pearls': 605,\\n\",\n \" 'vehicle': 606,\\n\",\n \" 'higher': 607,\\n\",\n \" 'Singer-songwriter': 608,\\n\",\n \" 'decorating': 609,\\n\",\n \" 'SOME': 610,\\n\",\n \" 'availability': 611,\\n\",\n \" 'unsatisfactory': 612,\\n\",\n \" 'condolences': 613,\\n\",\n \" 'Zulia': 614,\\n\",\n \" 'Glyn': 615,\\n\",\n \" 'soothe': 616,\\n\",\n \" 'catapulted': 617,\\n\",\n \" 'liberty': 618,\\n\",\n \" 'iron-ore': 619,\\n\",\n \" 'observatory': 620,\\n\",\n \" 'EAGLE': 621,\\n\",\n \" 'cot': 622,\\n\",\n \" 'Succeeding': 623,\\n\",\n \" 'racing': 624,\\n\",\n \" 'barreling': 625,\\n\",\n \" 'one-on-one': 626,\\n\",\n \" 'sponsorship': 627,\\n\",\n \" 'traveling': 628,\\n\",\n \" 'community': 629,\\n\",\n \" 'Collier': 630,\\n\",\n \" 'Obasanjo': 631,\\n\",\n \" 'Miro': 632,\\n\",\n \" '4000': 633,\\n\",\n \" 'Czech': 634,\\n\",\n \" 'wearily': 635,\\n\",\n \" 'Wanzhou': 636,\\n\",\n \" 'lakeside': 637,\\n\",\n \" 'confiscation': 638,\\n\",\n \" 'subsistence-based': 639,\\n\",\n \" 'massacres': 640,\\n\",\n \" 'Rugigana': 641,\\n\",\n \" 'prescribe': 642,\\n\",\n \" 'Alu': 643,\\n\",\n \" '1,32,000': 644,\\n\",\n \" 'Now': 645,\\n\",\n \" '2,46,000': 646,\\n\",\n \" 'Ohio-based': 647,\\n\",\n \" 'narcotics': 648,\\n\",\n \" 'base-closing': 649,\\n\",\n \" 'younger': 650,\\n\",\n \" 'Supposing': 651,\\n\",\n \" 'compounding': 652,\\n\",\n \" 'Sardinia': 653,\\n\",\n \" 'tarnishing': 654,\\n\",\n \" 'blocking': 655,\\n\",\n \" 'releases': 656,\\n\",\n \" 'G-8': 657,\\n\",\n \" 'medieval': 658,\\n\",\n \" 'Muslim-majority': 659,\\n\",\n \" 'KindHearts': 660,\\n\",\n \" 'balloting': 661,\\n\",\n \" 'Raz': 662,\\n\",\n \" 'aggressive': 663,\\n\",\n \" 'Chung': 664,\\n\",\n \" 'Serge': 665,\\n\",\n \" 'sharing': 666,\\n\",\n \" 'chain': 667,\\n\",\n \" 'reporting': 668,\\n\",\n \" 'Creation': 669,\\n\",\n \" 'block-to-block': 670,\\n\",\n \" 'captive': 671,\\n\",\n \" 'prepare': 672,\\n\",\n \" 'Jabel': 673,\\n\",\n \" 'mayhem': 674,\\n\",\n \" '1667': 675,\\n\",\n \" 'guarantee': 676,\\n\",\n \" 'Benedetti': 677,\\n\",\n \" 'Rostock': 678,\\n\",\n \" 'parties': 679,\\n\",\n \" 'Sandagiri': 680,\\n\",\n \" 'anti-terror': 681,\\n\",\n \" 'exchange-rate': 682,\\n\",\n \" 'Bells': 683,\\n\",\n \" 'meets': 684,\\n\",\n \" 'Nickelodeon': 685,\\n\",\n \" 'weblogs': 686,\\n\",\n \" 'modernity': 687,\\n\",\n \" 'Circuit': 688,\\n\",\n \" 'lobbying': 689,\\n\",\n \" 'prized': 690,\\n\",\n \" 'Fisheries': 691,\\n\",\n \" 'hood': 692,\\n\",\n \" 'farmyard': 693,\\n\",\n \" 'dubious': 694,\\n\",\n \" 'repealed': 695,\\n\",\n \" 'sizes': 696,\\n\",\n \" 'Neal': 697,\\n\",\n \" 'Izarra': 698,\\n\",\n \" 'heighten': 699,\\n\",\n \" 'wound': 700,\\n\",\n \" 'democratization': 701,\\n\",\n \" 'unclaimed': 702,\\n\",\n \" 'clusters': 703,\\n\",\n \" 'furthering': 704,\\n\",\n \" 'Blizzard-like': 705,\\n\",\n \" 'Venevision': 706,\\n\",\n \" 'convenient': 707,\\n\",\n \" 'sexually-suggestive': 708,\\n\",\n \" 'Naad': 709,\\n\",\n \" 'Singer': 710,\\n\",\n \" 'But': 711,\\n\",\n \" 'astronauts': 712,\\n\",\n \" 'Baghdadi': 713,\\n\",\n \" 'Campo': 714,\\n\",\n \" '92,000': 715,\\n\",\n \" 'Nsanje': 716,\\n\",\n \" 'tying': 717,\\n\",\n \" 'Monaf': 718,\\n\",\n \" 'Sperling': 719,\\n\",\n \" \\\"O'Keeffe\\\": 720,\\n\",\n \" 'Marjayoun': 721,\\n\",\n \" 'staff-reduction': 722,\\n\",\n \" 'Wilfred': 723,\\n\",\n \" 'sleeve': 724,\\n\",\n \" 'cows': 725,\\n\",\n \" 'route': 726,\\n\",\n \" 'historical': 727,\\n\",\n \" 'Ui-Chun': 728,\\n\",\n \" 'al-Mutairi': 729,\\n\",\n \" 'Carlsen': 730,\\n\",\n \" 'male': 731,\\n\",\n \" 'aiding': 732,\\n\",\n \" 'strapping': 733,\\n\",\n \" 'untapped': 734,\\n\",\n \" 'circulating': 735,\\n\",\n \" 'Poupard': 736,\\n\",\n \" 'Property': 737,\\n\",\n \" 'practice': 738,\\n\",\n \" 'Anita': 739,\\n\",\n \" 'Enforcement': 740,\\n\",\n \" 'Conakry': 741,\\n\",\n \" 'Jae': 742,\\n\",\n \" 'collateral': 743,\\n\",\n \" 'formalizing': 744,\\n\",\n \" 'Kaduna': 745,\\n\",\n \" 'Livio': 746,\\n\",\n \" 'Paradorn': 747,\\n\",\n \" \\\"d'Ivoire\\\": 748,\\n\",\n \" '100-thousand': 749,\\n\",\n \" 'derived': 750,\\n\",\n \" 'Gadahn': 751,\\n\",\n \" '1876': 752,\\n\",\n \" 'pacts': 753,\\n\",\n \" 'religion': 754,\\n\",\n \" 'uphill': 755,\\n\",\n \" 'raise': 756,\\n\",\n \" 'closure': 757,\\n\",\n \" 'chosen': 758,\\n\",\n \" 'Ono': 759,\\n\",\n \" 'alert': 760,\\n\",\n \" 'rogue': 761,\\n\",\n \" 'cubs': 762,\\n\",\n \" 'via': 763,\\n\",\n \" 'legislator': 764,\\n\",\n \" 'characterization': 765,\\n\",\n \" 'rescind': 766,\\n\",\n \" 'prostitutes': 767,\\n\",\n \" 'strong': 768,\\n\",\n \" 'earnestly': 769,\\n\",\n \" 'Gardez': 770,\\n\",\n \" 'righteous': 771,\\n\",\n \" 'resumes': 772,\\n\",\n \" 'appears': 773,\\n\",\n \" 'undecided': 774,\\n\",\n \" 'al-Salami': 775,\\n\",\n \" 'lawsuits': 776,\\n\",\n \" 'Stosur': 777,\\n\",\n \" 'GDP': 778,\\n\",\n \" 'Convergence': 779,\\n\",\n \" 'Bragg': 780,\\n\",\n \" 'Plummer': 781,\\n\",\n \" 'effects-laden': 782,\\n\",\n \" '10th': 783,\\n\",\n \" 'Chee-hwa': 784,\\n\",\n \" 'diabetes': 785,\\n\",\n \" 'deserts': 786,\\n\",\n \" 'Katif': 787,\\n\",\n \" 'Shiekh': 788,\\n\",\n \" 'exciting': 789,\\n\",\n \" 'Ill.': 790,\\n\",\n \" 'operate': 791,\\n\",\n \" 'mon': 792,\\n\",\n \" 'hill-top': 793,\\n\",\n \" 'struck': 794,\\n\",\n \" 'Sagues': 795,\\n\",\n \" 'drug-making': 796,\\n\",\n \" 'winding': 797,\\n\",\n \" 'Villaraigosa': 798,\\n\",\n \" 'desecrating': 799,\\n\",\n \" 'disinfected': 800,\\n\",\n \" 'whereby': 801,\\n\",\n \" 'furnish': 802,\\n\",\n \" 'Ajmal': 803,\\n\",\n \" 'Kahar': 804,\\n\",\n \" '87-run': 805,\\n\",\n \" 'firestorm': 806,\\n\",\n \" 'Preparations': 807,\\n\",\n \" 'opium-traffickers': 808,\\n\",\n \" 'Santos': 809,\\n\",\n \" 'port': 810,\\n\",\n \" 'Joey': 811,\\n\",\n \" 'Sarfraz': 812,\\n\",\n \" 'treat': 813,\\n\",\n \" 'Lutfullah': 814,\\n\",\n \" 'Salaam': 815,\\n\",\n \" 'poverty': 816,\\n\",\n \" 'VSV-30': 817,\\n\",\n \" 'Zurbriggen': 818,\\n\",\n \" 'Apure': 819,\\n\",\n \" 'NHK': 820,\\n\",\n \" 'Bradman': 821,\\n\",\n \" 'capitals': 822,\\n\",\n \" 'loans': 823,\\n\",\n \" 'town': 824,\\n\",\n \" 'Zahir': 825,\\n\",\n \" 'Bolivian': 826,\\n\",\n \" 'overrun': 827,\\n\",\n \" 'insulted': 828,\\n\",\n \" 'trigger': 829,\\n\",\n \" 'detriment': 830,\\n\",\n \" 'deficiencies': 831,\\n\",\n \" 'owed': 832,\\n\",\n \" 'blind': 833,\\n\",\n \" 'shies': 834,\\n\",\n \" 'backbone': 835,\\n\",\n \" 'Monte': 836,\\n\",\n \" 'dependence': 837,\\n\",\n \" 'clarified': 838,\\n\",\n \" 'Goats': 839,\\n\",\n \" 'sole': 840,\\n\",\n \" 'traditions': 841,\\n\",\n \" 'selective': 842,\\n\",\n \" 'military-run': 843,\\n\",\n \" 'approximation': 844,\\n\",\n \" 'competition': 845,\\n\",\n \" 'extracting': 846,\\n\",\n \" 'Tamiflu': 847,\\n\",\n \" 'king': 848,\\n\",\n \" '1930s': 849,\\n\",\n \" '124': 850,\\n\",\n \" 'umbrella-like': 851,\\n\",\n \" 'OLIVE-TREE': 852,\\n\",\n \" 'Paulos': 853,\\n\",\n \" 'land-for-peace': 854,\\n\",\n \" 'Al-Badri': 855,\\n\",\n \" 'circa': 856,\\n\",\n \" 'resignation': 857,\\n\",\n \" 'Colin': 858,\\n\",\n \" 'tyrannical': 859,\\n\",\n \" 'Mohaqiq': 860,\\n\",\n \" 'plant': 861,\\n\",\n \" 'revitalize': 862,\\n\",\n \" 'Al-Fasher': 863,\\n\",\n \" 'Proper': 864,\\n\",\n \" 'Smugglers': 865,\\n\",\n \" 'waistcoat': 866,\\n\",\n \" 'abolition': 867,\\n\",\n \" 'Tutwiler': 868,\\n\",\n \" 'cloak': 869,\\n\",\n \" 'tolls': 870,\\n\",\n \" 'underline': 871,\\n\",\n \" 'Rameez': 872,\\n\",\n \" 'Brigades': 873,\\n\",\n \" '336': 874,\\n\",\n \" '500-meters': 875,\\n\",\n \" 'undersecretary': 876,\\n\",\n \" 'Dinari': 877,\\n\",\n \" 'Sergei': 878,\\n\",\n \" 'traded': 879,\\n\",\n \" 'philanthropy': 880,\\n\",\n \" 'Vines': 881,\\n\",\n \" 'circular': 882,\\n\",\n \" 'Anambra': 883,\\n\",\n \" 'al-Nabaie': 884,\\n\",\n \" '5.3': 885,\\n\",\n \" 'Dera': 886,\\n\",\n \" 'twin-rotor': 887,\\n\",\n \" 'engaging': 888,\\n\",\n \" 'Republican-proposed': 889,\\n\",\n \" 'gullet-bag': 890,\\n\",\n \" 'Summits': 891,\\n\",\n \" 'Donald': 892,\\n\",\n \" 'Samo': 893,\\n\",\n \" 'Maxim': 894,\\n\",\n \" 'Departments': 895,\\n\",\n \" 'aim': 896,\\n\",\n \" 'Sibneft': 897,\\n\",\n \" 'lynx': 898,\\n\",\n \" 'Bashir': 899,\\n\",\n \" '170-meter': 900,\\n\",\n \" 'peninsula': 901,\\n\",\n \" 'disbursing': 902,\\n\",\n \" 'criticizes': 903,\\n\",\n \" 'IDF': 904,\\n\",\n \" 'expletive': 905,\\n\",\n \" 'Karadzic': 906,\\n\",\n \" 'Khushab': 907,\\n\",\n \" 'Loyalist': 908,\\n\",\n \" 'eagle': 909,\\n\",\n \" 'fodder': 910,\\n\",\n \" 'Lashkar-e-Taiba': 911,\\n\",\n \" 'breakdown': 912,\\n\",\n \" 'Homeland': 913,\\n\",\n \" 'accurate': 914,\\n\",\n \" 'Gode': 915,\\n\",\n \" 'Thuan': 916,\\n\",\n \" 'Soviet': 917,\\n\",\n \" 'Pandas': 918,\\n\",\n \" '752.2': 919,\\n\",\n \" 'Farms': 920,\\n\",\n \" 'Jones': 921,\\n\",\n \" 'biased': 922,\\n\",\n \" 'stimulating': 923,\\n\",\n \" 'co-chairman': 924,\\n\",\n \" 'Matambanadzo': 925,\\n\",\n \" 'Of': 926,\\n\",\n \" 'Bei': 927,\\n\",\n \" 'Film': 928,\\n\",\n \" 'Verheugen': 929,\\n\",\n \" '17,765': 930,\\n\",\n \" 'year-ago': 931,\\n\",\n \" 'restraint': 932,\\n\",\n \" '81': 933,\\n\",\n \" 'outgoing': 934,\\n\",\n \" 'prize': 935,\\n\",\n \" 'genders': 936,\\n\",\n \" 'Marthinus': 937,\\n\",\n \" 'wavered': 938,\\n\",\n \" 'emitted': 939,\\n\",\n \" 'Vocalist': 940,\\n\",\n \" 'Benazir': 941,\\n\",\n \" 'undertake': 942,\\n\",\n \" 'Dhahran': 943,\\n\",\n \" 'poverty-ridden': 944,\\n\",\n \" 'Vidar': 945,\\n\",\n \" 'Guenael': 946,\\n\",\n \" 'Vuk': 947,\\n\",\n \" 'Haruna': 948,\\n\",\n \" 'misquoted': 949,\\n\",\n \" 'Yuschenko': 950,\\n\",\n \" 'strongmen': 951,\\n\",\n \" 'about': 952,\\n\",\n \" 'newly-energized': 953,\\n\",\n \" 'arc': 954,\\n\",\n \" 'takes': 955,\\n\",\n \" 'Kutesa': 956,\\n\",\n \" 'life-spans': 957,\\n\",\n \" 'anti-pollution': 958,\\n\",\n \" '12.97': 959,\\n\",\n \" 'dogs': 960,\\n\",\n \" 'towering': 961,\\n\",\n \" 'Mexican-American': 962,\\n\",\n \" 'decades-long': 963,\\n\",\n \" 'Abdurrahman': 964,\\n\",\n \" 'Raziq': 965,\\n\",\n \" 'Peru': 966,\\n\",\n \" 'classes': 967,\\n\",\n \" 'left-wing': 968,\\n\",\n \" 'ATP': 969,\\n\",\n \" 'privileges': 970,\\n\",\n \" 'eater': 971,\\n\",\n \" 'Weisfield': 972,\\n\",\n \" 'disabling': 973,\\n\",\n \" 'insistence': 974,\\n\",\n \" 'Iranativu': 975,\\n\",\n \" 'Columbus': 976,\\n\",\n \" 'reopened': 977,\\n\",\n \" 'saints': 978,\\n\",\n \" 'After': 979,\\n\",\n \" 'Dixie': 980,\\n\",\n \" 'Undersecretary-General': 981,\\n\",\n \" 'Mekong': 982,\\n\",\n \" 'mad-cow': 983,\\n\",\n \" 'plebiscites': 984,\\n\",\n \" '28-point': 985,\\n\",\n \" 'winter': 986,\\n\",\n \" 'Siraj': 987,\\n\",\n \" 'Generalized': 988,\\n\",\n \" 'Then': 989,\\n\",\n \" 'assassins': 990,\\n\",\n \" 'unprepared': 991,\\n\",\n \" 'Aruban': 992,\\n\",\n \" 'cutthroat': 993,\\n\",\n \" 'Knives': 994,\\n\",\n \" 'phase-out': 995,\\n\",\n \" 'sci-fi': 996,\\n\",\n \" 'north-south': 997,\\n\",\n \" 'Apple': 998,\\n\",\n \" 'Edelist': 999,\\n\",\n \" 'Bears': 1000,\\n\",\n \" ...}\"\n ]\n },\n \"execution_count\": 10,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"word2idx\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 11,\n \"metadata\": {\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 265\n },\n \"colab_type\": \"code\",\n \"id\": \"R44g5T7NYp_H\",\n \"outputId\": \"135a85a6-7a85-4b16-a8eb-3ad7bd4da5b6\"\n },\n \"outputs\": [\n {\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAAX0AAAD4CAYAAAAAczaOAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADh0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uMy4xLjEsIGh0dHA6Ly9tYXRwbG90bGliLm9yZy8QZhcZAAAXgElEQVR4nO3df0zV1/3H8ecF1BauAvdeqQE1E3/8USrDeIlopjC925LafeNXjUk7l9g036Vlg6DZMmyTuWSrI20pRMTQVKNL26RZjJJ232VLbhiYjZhc5EdXuom2btGgIvdzZVzAivD5/mF2v9qKcBUucM/r8Vfv534+9573Pfjqueeee67Dtm0bERExQsJ0N0BERGJHoS8iYhCFvoiIQRT6IiIGUeiLiBhEoS8iYpCkiZz04x//mCeeeIKEhAQSExOpqKggHA5TVVXFjRs3WLhwIXv37sXpdGLbNsePH6etrY158+ZRXFxMdnY2AI2NjZw6dQqA7du3U1RUNO5zd3d3R1WQx+Oht7c3qmtmK9Uan1RrfIplrZmZmWPeN6HQBzhw4AALFiyI3K6vr2f16tVs27aN+vp66uvr2b17N21tbVy7do1Dhw5x4cIFjh49ysGDBwmHw5w8eZKKigoAysvL8Xq9OJ3OxyhNRESi8cjTO4FAgMLCQgAKCwsJBAIAtLS0sGnTJhwOB6tWrWJgYIBQKER7ezu5ubk4nU6cTie5ubm0t7dPThUiIjIhEx7pv/766wB85zvfwefz0dfXR3p6OgBpaWn09fUBYFkWHo8ncp3b7cayLCzLwu12R467XC4sy/ra8/j9fvx+PwAVFRX3PdaECkpKivqa2Uq1xifVGp9mSq0TCv1f/epXuFwu+vr6+PWvf/21+SKHw4HD4ZiUBvl8Pnw+X+R2tHNgmiOMT6o1PqnWqfGwOf0JTe+4XC4AUlNTyc/P5+LFi6SmphIKhQAIhUKR+X6Xy3VfYcFgEJfLhcvlIhgMRo5blhV5XBERiY1xQ//WrVsMDQ1F/vuTTz5h6dKleL1empqaAGhqaiI/Px8Ar9fLmTNnsG2brq4ukpOTSU9PJy8vj46ODsLhMOFwmI6ODvLy8qawNBER+apxp3f6+vp46623ABgZGeFb3/oWeXl5LF++nKqqKhoaGiJLNgHWrFlDa2srpaWlzJ07l+LiYgCcTic7duxg//79AOzcuVMrd0REYswx07dW1jr9sanW+KRa49OsmtMXEZH4MOElmzJzjPzPfwFw/SvHE9/9KPaNEZFZRSN9ERGDKPRFRAyi0BcRMYhCX0TEIAp9ERGDaPVOHPnPqp4H0coeEQGN9EVEjKLQFxExiEJfRMQgCn0REYMo9EVEDKLVOzPYw1bjiIg8Co30RUQMotAXETGIQl9ExCAKfRERg+iDXEOM9aGwtmcQMYtG+iIiBlHoi4gYRKEvImIQhb6IiEEU+iIiBlHoi4gYRKEvImIQhb6IiEEU+iIiBlHoi4gYRKEvImIQhb6IiEEU+iIiBlHoi4gYRKEvImKQCe+nPzo6Snl5OS6Xi/Lycnp6eqiurqa/v5/s7GxKSkpISkpieHiYw4cP88UXXzB//nzKysrIyMgA4PTp0zQ0NJCQkMCLL75IXl7elBUmIiJfN+GR/h/+8AeysrIit99//322bt1KTU0NKSkpNDQ0ANDQ0EBKSgo1NTVs3bqVDz74AIArV67Q3NzM22+/zWuvvcaxY8cYHR2d5HJERORhJhT6wWCQ1tZWtmzZAoBt23R2dlJQUABAUVERgUAAgJaWFoqKigAoKCjg008/xbZtAoEAGzZsYM6cOWRkZLBo0SIuXrw4BSWJiMhYJjS9c+LECXbv3s3Q0BAA/f39JCcnk5iYCIDL5cKyLAAsy8LtdgOQmJhIcnIy/f39WJbFypUrI4957zX38vv9+P1+ACoqKvB4PNEVlJQU9TUz1fUYPMdsea3iqV/Ho1rj00ypddzQP3fuHKmpqWRnZ9PZ2TnlDfL5fPh8vsjt3t7eqK73eDxRXzPdxvr92liYLa/VbOzXR6Va41Msa83MzBzzvnFD//z587S0tNDW1sbt27cZGhrixIkTDA4OMjIyQmJiIpZl4XK5gLsj+GAwiNvtZmRkhMHBQebPnx85/h/3XiMiIrEx7pz+Cy+8QF1dHbW1tZSVlfHMM89QWlpKTk4OZ8+eBaCxsRGv1wvA2rVraWxsBODs2bPk5OTgcDjwer00NzczPDxMT08PV69eZcWKFVNXmYiIfM2El2x+1Q9+8AOqq6v58MMPWbZsGZs3bwZg8+bNHD58mJKSEpxOJ2VlZQAsWbKE9evXs2/fPhISEnjppZdISNDXBEREYslh27Y93Y14mO7u7qjOn41zhNM5p5/47kfT9tzRmI39+qhUa3yaKXP6GmqLiBhEoS8iYpBHntOX+DDW1NJsmfYRkehopC8iYhCFvoiIQRT6IiIGUeiLiBhEoS8iYhCFvoiIQRT6IiIGUeiLiBhEoS8iYhCFvoiIQRT6IiIGUeiLiBhEG67F0HTumy8iAhrpi4gYRaEvImIQhb6IiEEU+iIiBlHoi4gYRKEvImIQhb6IiEEU+iIiBlHoi4gYRKEvImIQhb6IiEEU+iIiBlHoi4gYRKEvImIQhb6IiEEU+iIiBtGPqMgDjfWDL4nvfhTjlojIZNJIX0TEIOOO9G/fvs2BAwe4c+cOIyMjFBQUsGvXLnp6eqiurqa/v5/s7GxKSkpISkpieHiYw4cP88UXXzB//nzKysrIyMgA4PTp0zQ0NJCQkMCLL75IXl7elBcoIiL/b9yR/pw5czhw4ABvvvkmb7zxBu3t7XR1dfH++++zdetWampqSElJoaGhAYCGhgZSUlKoqalh69atfPDBBwBcuXKF5uZm3n77bV577TWOHTvG6Ojo1FYnIiL3GTf0HQ4HTzzxBAAjIyOMjIzgcDjo7OykoKAAgKKiIgKBAAAtLS0UFRUBUFBQwKeffopt2wQCATZs2MCcOXPIyMhg0aJFXLx4cYrKEhGRB5nQB7mjo6P8/Oc/59q1a3zve9/jqaeeIjk5mcTERABcLheWZQFgWRZutxuAxMREkpOT6e/vx7IsVq5cGXnMe6+5l9/vx+/3A1BRUYHH44muoKSkqK+JlevT3YBJMF2v7Uzu18mmWuPTTKl1QqGfkJDAm2++ycDAAG+99Rbd3d1T1iCfz4fP54vc7u3tjep6j8cT9TUycdP12prUr6o1PsWy1szMzDHvi2r1TkpKCjk5OXR1dTE4OMjIyAhwd3TvcrmAuyP4YDAI3J0OGhwcZP78+fcd/+o1IiISG+OG/r///W8GBgaAuyt5PvnkE7KyssjJyeHs2bMANDY24vV6AVi7di2NjY0AnD17lpycHBwOB16vl+bmZoaHh+np6eHq1ausWLFiisoSEZEHGXd6JxQKUVtby+joKLZts379etauXcvixYuprq7mww8/ZNmyZWzevBmAzZs3c/jwYUpKSnA6nZSVlQGwZMkS1q9fz759+0hISOCll14iIUFfExARiSWHbdv2dDfiYaL9/GAmzxGO9S3X2WS6vpE7k/t1sqnW+DQr5/RFRGR2U+iLiBhEG65NgXiYxhGR+KSRvoiIQRT6IiIGUeiLiBhEoS8iYhCFvoiIQRT6IiIGUeiLiBhEoS8iYhCFvoiIQRT6IiIGUeiLiBhEoS8iYhCFvoiIQRT6IiIGUeiLiBhE++lLVMb6rYDp+hlFEYmORvoiIgZR6IuIGEShLyJiEIW+iIhBFPoiIgZR6IuIGEShLyJiEIW+iIhBFPoiIgZR6IuIGEShLyJiEO298xjG2odGRGSm0khfRMQgCn0REYOMO73T29tLbW0tN2/exOFw4PP5ePbZZwmHw1RVVXHjxg0WLlzI3r17cTqd2LbN8ePHaWtrY968eRQXF5OdnQ1AY2Mjp06dAmD79u0UFRVNaXEiInK/cUM/MTGRH/7wh2RnZzM0NER5eTm5ubk0NjayevVqtm3bRn19PfX19ezevZu2tjauXbvGoUOHuHDhAkePHuXgwYOEw2FOnjxJRUUFAOXl5Xi9XpxO55QXKSIid407vZOenh4ZqT/55JNkZWVhWRaBQIDCwkIACgsLCQQCALS0tLBp0yYcDgerVq1iYGCAUChEe3s7ubm5OJ1OnE4nubm5tLe3T2FpIiLyVVGt3unp6eHSpUusWLGCvr4+0tPTAUhLS6Ovrw8Ay7LweDyRa9xuN5ZlYVkWbrc7ctzlcmFZ1teew+/34/f7AaioqLjvsSZUUFJS1Nc8qusxeZbZYapf81j263RTrfFpptQ64dC/desWlZWV7Nmzh+Tk5PvuczgcOByOSWmQz+fD5/NFbvf29kZ1vcfjifoaeXxT/Zqb1K+qNT7FstbMzMwx75vQ6p07d+5QWVnJxo0bWbduHQCpqamEQiEAQqEQCxYsAO6O4O8tLBgM4nK5cLlcBIPByHHLsnC5XNFXIyIij2zc0Ldtm7q6OrKysnjuuecix71eL01NTQA0NTWRn58fOX7mzBls26arq4vk5GTS09PJy8ujo6ODcDhMOBymo6ODvLy8KSpLREQeZNzpnfPnz3PmzBmWLl3Kz372MwCef/55tm3bRlVVFQ0NDZElmwBr1qyhtbWV0tJS5s6dS3FxMQBOp5MdO3awf/9+AHbu3KmVOyIiMeawbdue7kY8THd3d1Tnx3LeTNsw/L/Edz+a0sfX3G98Uq1T47Hn9EVEJD4o9EVEDKLQFxExiEJfRMQg2k9fptRYH3ZP9Qe/IvJgGumLiBhEoS8iYhBN78ik0HcWRGYHjfRFRAyi0BcRMYhCX0TEIAp9ERGDKPRFRAyi0BcRMYhCX0TEIAp9ERGDKPRFRAyi0BcRMYhCX0TEIAp9ERGDKPRFRAyi0BcRMYi2Vp4AbRssIvFCI30REYMo9EVEDKLQFxExiEJfRMQgCn0REYMo9EVEDKLQFxExiEJfRMQgCn0REYMo9EVEDKLQFxExyLh77xw5coTW1lZSU1OprKwEIBwOU1VVxY0bN1i4cCF79+7F6XRi2zbHjx+nra2NefPmUVxcTHZ2NgCNjY2cOnUKgO3bt1NUVDR1VYmIyAONO9IvKiri1Vdfve9YfX09q1ev5tChQ6xevZr6+noA2trauHbtGocOHeJHP/oRR48eBe7+T+LkyZMcPHiQgwcPcvLkScLh8BSUIyIiDzNu6D/99NM4nc77jgUCAQoLCwEoLCwkEAgA0NLSwqZNm3A4HKxatYqBgQFCoRDt7e3k5ubidDpxOp3k5ubS3t4+BeWIiMjDPNLWyn19faSnpwOQlpZGX18fAJZl4fF4Iue53W4sy8KyLNxud+S4y+XCsqwHPrbf78fv9wNQUVFx3+NNRFJSUtTXjOf6pD6aADOiX2cq1RqfZkqtj72fvsPhwOFwTEZbAPD5fPh8vsjt3t7eqK73eDxRXyOxp34dm2qNT7GsNTMzc8z7Hmn1TmpqKqFQCIBQKMSCBQuAuyP4e4sKBoO4XC5cLhfBYDBy3LIsXC7Xozy1iIg8hkcKfa/XS1NTEwBNTU3k5+dHjp85cwbbtunq6iI5OZn09HTy8vLo6OggHA4TDofp6OggLy9v8qoQEZEJGXd6p7q6ms8++4z+/n5efvlldu3axbZt26iqqqKhoSGyZBNgzZo1tLa2Ulpayty5cykuLgbA6XSyY8cO9u/fD8DOnTu/9uGwiIhMPYdt2/Z0N+Jhuru7ozp/KubN9Bu5ky/x3Y+iOl9zv/FJtU6NSZ/TFxGR2UmhLyJiEIW+iIhBFPoiIgZR6IuIGEShLyJiEIW+iIhBHnvvnXii9fgiEu800hcRMYhG+jItxnpXFe03dUUkOhrpi4gYRKEvImIQhb6IiEEU+iIiBlHoi4gYRKEvImIQhb6IiEG0Tl9mlDG/FX26ObYNEYlTGumLiBhEoS8iYhCFvoiIQRT6IiIGUeiLiBhEoS8iYhCFvoiIQbROX2aF6/+94YHHtf++SHQ00hcRMYhCX0TEIAp9ERGDGDmnP+b+LjLr6Ld2RaKjkb6IiEEU+iIiBjFyekfin6Z9RB5MI30REYPEfKTf3t7O8ePHGR0dZcuWLWzbti3WTRCDPcqH+Hp3IPEkpiP90dFRjh07xquvvkpVVRV//etfuXLlSiybICJitJiO9C9evMiiRYt46qmnANiwYQOBQIDFixdPyfNpaaZMhsn6OxrrHcNXH//6OOeLPI6Yhr5lWbjd7shtt9vNhQsX7jvH7/fj9/sBqKioIDMzM+rniVzzvy2P3liRWDH87/RR/o3PVjOh1hn3Qa7P56OiooKKiopHur68vHySWzRzqdb4pFrj00ypNaah73K5CAaDkdvBYBCXyxXLJoiIGC2mob98+XKuXr1KT08Pd+7cobm5Ga/XG8smiIgYLfGXv/zlL2P1ZAkJCSxatIiamhr++Mc/snHjRgoKCib9ebKzsyf9MWcq1RqfVGt8mgm1Omzbtqe7ESIiEhsz7oNcERGZOgp9ERGDxM2Ga/G8vUNvby+1tbXcvHkTh8OBz+fj2WefJRwOU1VVxY0bN1i4cCF79+7F6XROd3MnxejoKOXl5bhcLsrLy+np6aG6upr+/n6ys7MpKSkhKWn2//kODAxQV1fH5cuXcTgcvPLKK2RmZsZlv/7+97+noaEBh8PBkiVLKC4u5ubNm3HTr0eOHKG1tZXU1FQqKysBxvw3ats2x48fp62tjXnz5lFcXBy7+X47DoyMjNg/+clP7GvXrtnDw8P2T3/6U/vy5cvT3axJY1mW/fnnn9u2bduDg4N2aWmpffnyZfu9996zT58+bdu2bZ8+fdp+7733prOZk+rjjz+2q6ur7d/85je2bdt2ZWWl/Ze//MW2bdt+55137D/96U/T2bxJU1NTY/v9ftu2bXt4eNgOh8Nx2a/BYNAuLi62v/zyS9u27/bnn//857jq187OTvvzzz+39+3bFzk2Vl+eO3fOfv311+3R0VH7/Pnz9v79+2PWzriY3rl3e4ekpKTI9g7xIj09PTIKePLJJ8nKysKyLAKBAIWFhQAUFhbGTc3BYJDW1la2bNkCgG3bdHZ2RlZ6FRUVxUWtg4OD/P3vf2fz5s0AJCUlkZKSErf9Ojo6yu3btxkZGeH27dukpaXFVb8+/fTTX3tHNlZftrS0sGnTJhwOB6tWrWJgYIBQKBSTds7O91FfMZHtHeJFT08Ply5dYsWKFfT19ZGeng5AWloafX1909y6yXHixAl2797N0NAQAP39/SQnJ5OYmAjc/ZKfZVnT2cRJ0dPTw4IFCzhy5Aj/+te/yM7OZs+ePXHZry6Xi+9///u88sorzJ07l29+85tkZ2fHZb/ea6y+tCwLj8cTOc/tdmNZVuTcqRQXI31T3Lp1i8rKSvbs2UNycvJ99zkcDhwOxzS1bPKcO3eO1NTUGbGeeaqNjIxw6dIlvvvd7/LGG28wb9486uvr7zsnXvo1HA4TCASora3lnXfe4datW7S3t093s2JqpvRlXIz0Tdje4c6dO1RWVrJx40bWrVsHQGpqKqFQiPT0dEKhEAsWLJjmVj6+8+fP09LSQltbG7dv32ZoaIgTJ04wODjIyMgIiYmJWJYVF/3rdrtxu92sXLkSgIKCAurr6+OyX//2t7+RkZERqWXdunWcP38+Lvv1XmP1pcvlore3N3JeLDMrLkb68b69g23b1NXVkZWVxXPPPRc57vV6aWpqAqCpqYn8/PzpauKkeeGFF6irq6O2tpaysjKeeeYZSktLycnJ4ezZswA0NjbGRf+mpaXhdrvp7u4G7gbj4sWL47JfPR4PFy5c4Msvv8S27Uit8div9xqrL71eL2fOnMG2bbq6ukhOTo7J1A7E0TdyW1tb+e1vf8vo6Cjf/va32b59+3Q3adL84x//4Be/+AVLly6NvD18/vnnWblyJVVVVfT29sbV0r7/6Ozs5OOPP6a8vJzr169TXV1NOBxm2bJllJSUMGfOnOlu4mP75z//SV1dHXfu3CEjI4Pi4mJs247Lfv3d735Hc3MziYmJfOMb3+Dll1/Gsqy46dfq6mo+++wz+vv7SU1NZdeuXeTn5z+wL23b5tixY3R0dDB37lyKi4tZvnx5TNoZN6EvIiLji4vpHRERmRiFvoiIQRT6IiIGUeiLiBhEoS8iYhCFvoiIQRT6IiIG+T98/oDqOK9iUAAAAABJRU5ErkJggg==\\n\",\n \"text/plain\": [\n \"<Figure size 432x288 with 1 Axes>\"\n ]\n },\n \"metadata\": {},\n \"output_type\": \"display_data\"\n }\n ],\n \"source\": [\n \"#Step 5: Padding Input Sentences and Creating Train/Test Splits\\n\",\n \"plt.hist([len(s) for s in sentences], bins=50)\\n\",\n \"plt.show()\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 12,\n \"metadata\": {\n \"colab\": {},\n \"colab_type\": \"code\",\n \"id\": \"FS4u3CRkpkc1\"\n },\n \"outputs\": [],\n \"source\": [\n \"from tensorflow.keras.preprocessing.sequence import pad_sequences\\n\",\n \"\\n\",\n \"max_len = 50\\n\",\n \"\\n\",\n \"X = [[word2idx[w[0]] for w in s] for s in sentences]\\n\",\n \"X = pad_sequences(maxlen=max_len, sequences=X, padding=\\\"post\\\", value=num_words-1)\\n\",\n \"\\n\",\n \"y = [[tag2idx[w[2]] for w in s] for s in sentences]\\n\",\n \"y = pad_sequences(maxlen=max_len, sequences=y, padding=\\\"post\\\", value=tag2idx[\\\"O\\\"])\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 13,\n \"metadata\": {\n \"colab\": {},\n \"colab_type\": \"code\",\n \"id\": \"q7VfnnkXpkfS\"\n },\n \"outputs\": [],\n \"source\": [\n \"from sklearn.model_selection import train_test_split\\n\",\n \"x_train, x_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=1)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 14,\n \"metadata\": {\n \"colab\": {},\n \"colab_type\": \"code\",\n \"id\": \"Y2vM7IkXpkiH\"\n },\n \"outputs\": [],\n \"source\": [\n \"#Step 6: Build and Compile a Bidirectional LSTM\\n\",\n \"from tensorflow.keras import Model, Input\\n\",\n \"from tensorflow.keras.layers import LSTM, Embedding, Dense\\n\",\n \"from tensorflow.keras.layers import TimeDistributed, SpatialDropout1D, Bidirectional\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 15,\n \"metadata\": {\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 330\n },\n \"colab_type\": \"code\",\n \"id\": \"Aee3mCZ3pkkv\",\n \"outputId\": \"b7fb911b-21d1-43e6-adc9-bb2d8bdfb921\"\n },\n \"outputs\": [\n {\n \"name\": \"stdout\",\n \"output_type\": \"stream\",\n \"text\": [\n \"Model: \\\"model\\\"\\n\",\n \"_________________________________________________________________\\n\",\n \"Layer (type) Output Shape Param # \\n\",\n \"=================================================================\\n\",\n \"input_1 (InputLayer) [(None, 50)] 0 \\n\",\n \"_________________________________________________________________\\n\",\n \"embedding (Embedding) (None, 50, 50) 1758950 \\n\",\n \"_________________________________________________________________\\n\",\n \"spatial_dropout1d (SpatialDr (None, 50, 50) 0 \\n\",\n \"_________________________________________________________________\\n\",\n \"bidirectional (Bidirectional (None, 50, 200) 120800 \\n\",\n \"_________________________________________________________________\\n\",\n \"time_distributed (TimeDistri (None, 50, 17) 3417 \\n\",\n \"=================================================================\\n\",\n \"Total params: 1,883,167\\n\",\n \"Trainable params: 1,883,167\\n\",\n \"Non-trainable params: 0\\n\",\n \"_________________________________________________________________\\n\"\n ]\n }\n ],\n \"source\": [\n \"input_word = Input(shape=(max_len,))\\n\",\n \"model = Embedding(input_dim=num_words, output_dim=50, input_length=max_len)(input_word)\\n\",\n \"model = SpatialDropout1D(0.1)(model)\\n\",\n \"model = Bidirectional(LSTM(units=100, return_sequences=True, recurrent_dropout=0.1))(model)\\n\",\n \"out = TimeDistributed(Dense(num_tags, activation=\\\"softmax\\\"))(model)\\n\",\n \"model = Model(input_word, out)\\n\",\n \"model.summary()\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 16,\n \"metadata\": {\n \"colab\": {},\n \"colab_type\": \"code\",\n \"id\": \"kOBpQg26pkqh\"\n },\n \"outputs\": [],\n \"source\": [\n \"model.compile(optimizer=\\\"adam\\\",\\n\",\n \" loss=\\\"sparse_categorical_crossentropy\\\",\\n\",\n \" metrics=[\\\"accuracy\\\"])\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 17,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"#STep 7: Train Model\\n\",\n \"from tensorflow.keras.callbacks import ModelCheckpoint, EarlyStopping\\n\",\n \"from livelossplot.tf_keras import PlotLossesCallback\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 18,\n \"metadata\": {\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 536\n },\n \"colab_type\": \"code\",\n \"id\": \"Q9HWH06Ypkxh\",\n \"outputId\": \"e83ba281-0c14-4bca-dacb-cb708edacba5\"\n },\n \"outputs\": [\n {\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAA1gAAAE3CAYAAAC3ouAOAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADh0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uMy4xLjEsIGh0dHA6Ly9tYXRwbG90bGliLm9yZy8QZhcZAAAgAElEQVR4nOzdeVhUZfvA8e9zZhh2kGFx30VzFzElzS1NTc2sNJfS3NteSsvWX5Zlvdnb+qaVuVZuiZlL9ZqmppYa4pYa5pJmuYWAibLDeX5/jI4iIKjACN6f6/KCmTnLfR4HDvc897mP0lprhBBCCCGEEEJcM8PVAQghhBBCCCFEWSEJlhBCCCGEEEIUEUmwhBBCCCGEEKKISIIlhBBCCCGEEEVEEiwhhBBCCCGEKCKSYAkhhBBCCCFEEZEES5RaQ4YMoXPnzq4Ogy1btlCxYkWSk5NdHUouWVlZDBs2jMDAQJRSrF271tUhUaNGDV577bUi3aZpmjRo0ICvv/66SLcrhBBCCHGlJMES1+R6SXJcacyYMTzzzDN4e3uXyP6OHDlS6GRp0aJFzJs3j6+//prjx4/TunXr4g/wnBEjRtChQ4dcz8fExDBmzJgi3ZdhGIwfP56xY8dimmaRblsIIYQQ4kpIgiXENYiJiSEmJoYhQ4a4OpQ87d+/n8qVK9O6dWsqVKiAzWZzdUgEBwcXSzLau3dvEhIS+N///lfk2xZCCHFlMjIyXB2CEC4jCZYoVmfOnOGhhx4iODgYd3d3WrRowcqVK3Mss337diIiInB3dyc0NJSFCxdeVRmZ1pq3336bWrVqYbPZqF27Nu+//36OZZYuXUpYWBheXl6UK1eOli1bsn37dgAyMzN58sknqVKlCu7u7lSsWJH+/ftfdp9z586lXbt2BAQE5Hh+69atdOvWDT8/P3x8fGjZsiXR0dHO1z/77DMaNGiAzWajSpUqvPjii2RlZTlf/+mnn2jTpg2+vr74+vrStGlTVqxYAUDVqlUB6NixI0opatSokWdsHTp0YNy4cRw8eDDHch06dGDEiBE5ln3ttddybOf8zOTUqVOpXr06fn5+9OrVi7///jvHeqtWraJt27Z4eXnh7+9P+/bt+f333xk/fjwzZsxg3bp1KKVQSvHpp58CuUsEC3qP/PHHHyiliIqKomfPnnh5eVGrVi3n9s6z2Wz06NGDOXPm5DkeQghRVn3//fd06NABu93u/F28efNm5+tnz55l9OjRVK1aFXd3d2rUqMG///1v5+txcXEMHTqU8uXL4+HhQb169Zg5cyYAa9euRSnFkSNHcuzTarU6fw+f/z09d+5cunfvjre3N+PGjUNrzciRI6lduzaenp7UqlWLF154gfT09Bzbyu9csnbtWiwWC3/99VeO5T///HP8/f2vy9J8IUASLFHMhg0bxooVK5gzZw47duygTZs29OzZk99++w2AlJQUunfvTnBwMDExMcyePZv33nuPuLi4K97XRx99xLhx43juuef49ddfefrpp3nuueeYMWMGACdOnKBv374MGDCAX3/9lU2bNjF69GisVisAkyZNIioqijlz5rB//36WLVtGRETEZfe5bt06WrZsmeO5X3/91Zl0rVmzhu3btzNmzBhn6dq3337LsGHDGDRoELt37+add97hww8/5JVXXgEc10316tWLVq1asW3bNrZt28b48ePx8vICYNu2bYCj/O/48ePExMTkGdtXX33FU089RY0aNS67XH5iYmL44Ycf+Pbbb1mxYgW7du1i7NixztdXrVpF165dCQ8PZ9OmTURHRzN48GAyMzMZO3YsAwcO5JZbbuH48eMcP36cfv365bmfgt4j5z333HMMHjyYnTt30r9/f0aMGMG+fftyLNOqVSt++OGHKzpOIYQo7c6ePcujjz7Kpk2b2LhxI6GhoXTr1o2EhAS01vTs2ZNly5YxadIk9uzZw+eff05wcDAAqamptG/fnl9++YW5c+cSGxvLpEmTnOecK/Hss89y//33s3v3bh5++GG01oSEhDBv3jz27NnD+++/z6xZs3Ikd5c7l3To0IHQ0FBnsnfetGnTGDhwYImV5gtxxbQQ1+DBBx/UnTp1yvO1/fv3a0B/++23OZ4PCwvTQ4cO1VprPXXqVO3t7a3/+ecf5+t79uzRgJ4wYcIV7btKlSr66aefzrHM6NGjdc2aNbXWWm/btk0D+tChQ3lu7/HHH9cdO3bUpmledr8X8/f31x999FGO5x544AHdpEkTnZ2dnec6t956q+7bt2+O595//33t4eGh09PTdWJiogb0Dz/8kOf6f/3112Vfv9jLL7+sa9euneO59u3b6+HDh+d4bsKECbp69erOxw8++KAODg7WaWlpzucmTpyoK1SokOM4evToke++hw8frtu3b5/r+erVqzv/bwvzHjl06JAG9DvvvON8PSsrS/v4+OgpU6bkWG/p0qUa0GfPns03LiGEKOuys7N1uXLl9Jw5c/SqVas0oGNiYvJcdvr06drd3V3/9ddfeb7+ww8/aCDX6xaLRc+aNUtrfeH39KuvvlpgbO+++66uU6eO83FB55J33nlHV6tWzXlOPf83wrZt2wrclxCuIjNYotjExsYC0K5duxzPt2vXjl9//dW5TP369fH393e+ftNNN1GuXDnn43//+9/4+Pg4//3444+59pWUlMSRI0dy7at9+/b88ccfpKSk0KRJE7p27UqjRo24++67+e9//5uj7GDo0KHs2rWLOnXq8PDDD7No0aICa8hTU1Px8PDI8dzWrVvp1KkThpH3j9f5Ga5L40xLS+P3338nICCAESNG0LVrV+644w4mTpzI3r17LxtHcbjppptwd3d3Pq5UqVKOEsGtW7fSpUuXa9pHYd4j5zVr1sz5vcViISQkJFfJ4vn/i9TU1GuKSwghSpNDhw4xaNAg6tSpg5+fH35+fpw+fZrDhw+zdetWAgICaNGiRZ7rbt26lQYNGlClSpVrjuPSig5wzDa1atWK8uXL4+Pjw/PPP8/hw4dz7P9y55IHH3yQuLg4Z5n89OnTCQ8PJyws7JrjFaK4SIIlXE4pddnXH374YXbs2OH8l99JoiAWi4Xly5ezZs0abr75ZhYtWkTdunX55ptvAMcf8IcOHeLtt9/GZrPxxBNP0KxZM5KSkvLdZnBwMImJiVcVz+VMmzaNrVu3cvvtt7Nu3ToaNWrEJ598UiTbNgwDrXWO5zIzM3Mtd2lDDKVUrvVKUl7xXNoxMDExEYvFgt1uL8nQhBDCpXr27Mmff/7Jhx9+yM8//8yOHTsICQkpkkYT5z8svPj3f3Z2dp4dWy8t2Vu4cCGPPfYY/fr143//+x/bt2/npZdeyvOck5/AwED69OnDtGnTyMjI4PPPP2fUqFFXeTRClAxJsESxadiwIQDr16/P8fz69etp1KgRAA0aNGDPnj2cPn3a+frevXv5559/nI/tdjt16tRx/vP09My1Lz8/P6pUqZJrX+vWraNmzZrOWnKlFC1btuSFF15g/fr1tG/fnlmzZjmX9/Hx4e677+aDDz5gy5Yt7Nmzh3Xr1uV7jM2bN8810xIeHs7q1avzbRfesGHDPOP09PSkdu3azucaNWrEk08+yfLlyxk+fDhTp04FLiQa2dnZ+cZ1OSEhIRw7dizHc+ev67oS4eHhuRqWXMxmsxUYY2HeI1di165dhIWF5Tt7KIQQZU1CQgKxsbE899xzdO3alQYNGuDh4eG8ljk8PJxTp06xZcuWPNcPDw8nNjY2VxOL80JCQgBynDd27NhRqA/c1q9fT1hYGE8++STh4eGEhobyxx9/5Nr/5c4lAA899BBff/01n3zyCampqQwYMKDAfQvhSvJXiLhmZ8+ezTHDtGPHDn777Tdq165N3759efTRR1mxYgW//fYbTzzxBLt37+bpp58G4P7778fHx8fZvCA6Oprhw4fj6elZ4MzWpZ5//nkmTZrEtGnT2L9/P5988gkff/wxL7zwAgAbN25kwoQJREdH8+eff7J69Wp27txJgwYNAHjrrbeYO3cuv/76K4cOHWLmzJlYLBbq1q2b7z67d++eKzl45pln2L9/P/fffz9btmzh999/Z+HChWzatMkZ56JFi5g4cSL79u0jKiqK8ePH89RTT2Gz2Thw4ADPPvssP/30E4cPH2bTpk38+OOPzjiDgoLw8fFh5cqVnDhxglOnTl3ROHXu3JlVq1axcOFCDhw4wMSJE/MsuyzIuHHjWL58OaNHj2bnzp3s3buXTz/91FnOWLNmTX777Td+/fVX4uPjc3WNAgr1HrkSa9eupUePHle8nhBClFYBAQEEBwczbdo09u3bx6ZNmxgwYIDzw8jbbruNtm3b0q9fP5YuXcqhQ4fYsGED06dPB2DAgAFUr16dXr16sWrVKg4dOsTq1atZsGABAHXq1KF69eqMHz+e3377jZ9++okxY8YU6hxdr149du3axdKlS/n999/573//y1dffZVjmYLOJQC33nor9erVY+zYsfTv3x9fX9+iGj4hiodrLwETpd2DDz6ogVz/6tWrp7XW+vTp03rUqFE6KChI22w2HR4erlesWJFjG9u2bdOtWrXSNptN16lTRy9cuFAHBwfrt99+u8B9X9zkwjRN/Z///EfXqFFDW61WXbNmTf3ee+85X9+9e7e+4447dPny5bXNZtPVqlXTY8eO1enp6VprradMmaKbN2+ufX19tbe3t27RooVesmTJZWNISkrSvr6+esOGDTmej46O1p06ddJeXl7ax8dHt2rVSkdHRztf//TTT/VNN92k3dzcdKVKlfQLL7ygMzMztdZaHzt2TN999926cuXK2maz6YoVK+oRI0bkaATy2Wef6Ro1amiLxZKjOcWl8mpykZGRoZ944gkdHBys/f399aOPPqrHjRuXq8nFpc1LZs+erS/9lfHdd9/piIgI7eHhof38/HSHDh3077//rrXWOiEhQd9xxx3az89PA86LoS9ucqF1we+R8xdP//jjjzn2Xbt2bf3yyy87H//+++/aarXqP//8M9/xEEKIsmjt2rW6SZMm2t3dXdetW1d/+eWXOX5HJiUl6X/961+6QoUK2s3NTdeoUUO/8cYbzvWPHz+uBw0apAMDA7W7u7uuV6+e83e21lr//PPPunnz5trDw0M3adJEr1+/Ps8mF5f+ns7IyNCjRo3SAQEB2tfXVw8YMEBPmjTpis4l573//vsa0Js3by66gROimCitXXhRhRB5OHz4MDVq1GDZsmXceeedrg6nQBMmTGDr1q0sWbLE1aHc0B599FG01nz88ceuDkUIIUQRe+aZZ/j++++d964U4npmdXUAQsyZM4fKlStTs2ZNDh8+zDPPPEP16tWvuUNdSXn66ad56623SE5OlntyuIhpmlSpUkUufBZCiDLm9OnT7Nu3j6lTp/LBBx+4OhwhCkVmsITL/fe//+WDDz7g6NGj2O122rRpwzvvvEO1atVcHZoQQgghXKhDhw5ER0fTv39/ZsyYIU2MRKkgCZYQQgghhBBCFBH5GEAIIYQQQgghiogkWEIIIYQQQghRRCTBEkIIIYQQQogiUuq6CF58J3GRW1BQEPHx8a4O47on41Q4Mk6FI+NUOJUqVXJ1CCVOzlkFk5+fwpFxKhwZp8KRcSqcqz1vyQyWEEIIIYQQQhQRSbCEEEIIIYQQoohIgiWEEEIIIYQQRUQSLCGEEEIIIYQoIpJgCSGEEEIIIUQRkQRLCCGEEEIIIYqIJFhCCCGEEEIIUUQkwRJCCCGEEEKIi+h/Eq563VJ3o+FHlh0kyMtKoJeVIC+3nF+93fC1GSilXB2mEEIIIYQQopTRWVnoNV+jl30BX/14VdsodQlWzQB3ElKy2P13CgmpWZg65+s2iyLQy0qglxtBno6kK/CihCzIy4qfu0WSMCGEEEIIIYST/m0n5rxP4Phf0LjFVW+n1CVYz7St7Pw+29T8k5ZFQorjX3xKJvEpWSSkZJKQkkXsyRQSDmeRfUkS5maoHEnXpV+DvKz4eVgwJAkTQgghhBCiTNOnEtALZ6JjfoSg8hj/ehHVtOVVb6/UJVgXsxiKQC83Ar3c8l3G1JrTadm5kq/4lCzikzP5LT6VhJRMssyc61kNsHs6kq3zydf5MsSgczNk5SQJE0IIIYQQolTSWZnoVcvQ3ywA00TdOQDV7R6Uzf2atluqE6zCMJQiwNNKgKeV0MC8lzG1Jik9m/hkRwJ2PhE7/3VfQioJf2WReUk9okWB/aIyxAuzYOdKFL2slPOwYjEkCRNCCCGEEOJ6off84igHPHEEmrbE6DcCFVyhSLZd5hOswjCUopyHIxmqE+iR5zL6XBJ2vhTROQt27vuDiWlsPnKWjEvqEY1zSdj5hOviMsTAcwmZ3VOSMCGEEEIIIYqbTjyJjpqJ3roBgitgRI5DNbm5SPchCVYhKaXw97Di72Gllj3/JOxMhuksQzyZ7PiakOqYDfvjn3S2HD1Leh5JWICH9UJzDu9zs2CeFxIxu5cVqyRhQgghhBBCXDGdlYn+fqmjHFBr1F0DUV3vQbnZinxfpS7B0qdPofwDXB1GnpRS+Llb8HO3UDOfELXWJGeYec6CJaRk8tfpdLYfP0taVs4kTAHlPK35t6j3smL3zP9aNCGEEEIIIW5E+tftmPOnwt9HoVkERr/hqKDyxba/UpdgmS8+jLqzP+q2nihr6UsolFL4uFvwcbdQ4zJJWEqmmaszYvy5hOxoUgY7T6SQkmnmWtfudZAAD8u55hwXyhCDL2rU4WaR+0sLIUq/HTt2MGvWLEzTpFOnTvTu3TvH6ydPnuTjjz8mKSkJHx8fIiMjCQx0XIw7Z84ctm3bhtaaxo0bM3ToUJRS/PTTTyxevBilFAEBAURGRuLn50dUVBSrV6/Gz88PgAEDBtC8efMSP2YhhBCFpxNOYkZNh22bIKQixhMvoxqFF/t+S12CRZ0G6IWz0D9+j9F/JKphmKsjKnJKKbxtFrxtFqqVy7+LSUpm9rnk60ICdjbbwtHEs5w4k8nuuBSSM3InYf7uFoK8zyVfnueuCfO+MDNm97TibpUkTAhx/TJNkxkzZvDiiy8SGBjI888/T4sWLahSpYpzmdmzZ9OuXTs6dOjA7t27mTdvHpGRkezdu5e9e/fy9ttvAzBu3DhiY2O56aab+PTTT3n33Xfx8/Njzpw5fPfdd9x3330A9OjRg169ernkeIUQQhSezsxEr1yM/l8UAKr3A6gud6PcSmZyptQlWMbjL8HOLZgLpmG+/zI0a4Vx3/Ai6/pRmni5Wajmb6Ga/4UkLCgoiPj4eOfj1Ewzj86IjpmxuLOZxMalcDaPJMzXPfcs2MXNOYK8JAkTQrjOgQMHqFChAuXLO0o8WrduTUxMTI4E68iRIwwePBiAhg0b8tZbbwGOD7EyMjLIyspCa012djb+/v5ordFak56e7qgkSEmhQoUb79wihBClmd69FXP+NIg7Bs1vceQJgSElGkOpS7CUUtD0ZowGzdDfL0F/G4X50mOornej7uiLcr+2vvVljaebQRV/d6r45z8uaVlmjlmwi68Ji0/J4rf4NM6kZ+daz9dm5JF8Xdwx0Q1PN0nChBBFLzEx0VnuBxAYGMj+/ftzLFO9enU2b95M9+7d2bx5M6mpqZw5c4a6devSsGFDRo0ahdaabt26OROzkSNHMnbsWNzd3alYsSIjRoxwbm/FihWsX7+eWrVqMXjwYHx8fErmYIUQQhRIx/+NuWAG7PgZylfGGP2KyyrdCpVgFVTnnpmZyeTJkzl48CC+vr6MHj2akJAQ4uLiGDNmDJUqVQIgNDSUUaNGAXDw4EE+/PBDMjIyCAsLc9a/F5Zyc0N174uO6Ihe9Bn62yj0pjWoPsNQLdpc0bZudB5Wg8p+Nir75d9FJT3LJDH13DVhyRdmwRJSHTdsPpCQxuk8kjBvm0GQ57kkzDtn8nU+IfNysxTn4QkhblCDBg1i5syZrF27lvr162O32zEMgxMnTnD06FGmTJkCwIQJE9izZw+hoaGsXLmSN998k/LlyzNz5kwWL17MvffeS5cuXejTpw8ACxYs4PPPP+fRRx/Ntc9Vq1axatUqACZOnEhQUFDJHXApZbVaZZwKQcapcGScCqcsjZPOSCd5yTySF30GysDngYfx6tW/WLoDFlaBCVZh6tzXrFmDt7c3kyZNYsOGDcydO5cxY8YAUKFCBWdZxsWmTZvGQw89RGhoKG+88QY7duwgLOzKs0xlD0KNfArdvhvm/Knoqf9Br2vsuD6rSo0r3p7Im7vVoKKvjYq++b9ZM7JNEvPojHi+OcfBU2n8k5Y7CfNyM/K9UfP557zcDEmahRBOdrudhIQE5+OEhATsdnuuZcaOHQtAWloa0dHReHt7s3r1akJDQ/HwcNxyIywsjH379uF2rjb/fFngLbfcwtKlSwEoV66cc7udOnXizTffzDOuzp0707lzZ+fji0u2Rd4uLW0XeZNxKhwZp8IpK+Okd8ZgfjENTp5AhbdB3TeMVHswqaeTimT75yeJrlSBCVZh6ty3bNlC3759AYiIiGDmzJlorfPcHsCpU6dITU2lbt26ALRr146YmJirSrDOU3UbYox7F71+JXrJHMxXR6M63OHoce/te9XbFYVnsxhU8LVR4TJJWGb2+ZmwrFxdEhPO3Svsn9QsLn33eFiNy1wT5vje2yZJmBA3itq1a3P8+HHi4uKw2+1s3LiRxx9/PMcy57sHGobB4sWL6dixI+D4w2L16tVkZ2ejtSY2Npbu3btjt9s5cuQISUlJ+Pn5sXPnTipXrgw4zlsBAY7Wr5s3b6Zq1aole8BCCCGc9MkTmAumwy+boUJljDGvoho0c3VYTgUmWIWpc794GYvFgpeXF2fOnAEgLi6OZ555Bk9PT/r370/9+vXz3GZiYmKe+7/icos+gzC73sXZeVNJXbkEtvyE9wMP4dnpTpSl7JeilYYp34oFvJ6ZbZKQnEHc2QzizqYTdyaduLMZnDzr+LozLpWE5AzMS7IwTzeDYB93Qnxs5766E+JrI8THnWAfx1c/DytKqVIxTtcDGafCkXEqeRaLhWHDhvH6669jmiYdO3akatWqLFiwgNq1a9OiRQtiY2OZN28eSinq16/P8OHDAccHgbt373bObjVr1owWLVoA0KdPH15++WUsFgtBQUE89thjgKOt+x9//IFSiuDgYGe5uxBCiJKjM9LR332F/m4RGAbq3gdRnXtdd7duKtYmFwEBAXz00Uf4+vpy8OBB3nrrLd55550r2sZVl1vcOwSjZXvM+Z9w5uP/cObbLzEGjELVaXBF+y9tysqUrxWoZINKdgV2D8Ajx+tZpuZUalaezTniUzI4lJDMqdSsXEmYzaII8rJSwd8LPzedaxYs0MuKn7tFZsLOKSvvp+Im41Q4V1tqkZ/mzZvnuhdVv379nN9HREQQERGRaz3DMPJNkLp06UKXLl1yPR8ZGXmN0QohhLgW+pfNjnLA+L9RN7dF9RmKsl+fH24WmGAVts49ISGBwMBAsrOzSUlJwdfXF6WUs6a9Vq1alC9fnuPHjxdqm0VBVa2J8fQb6Jgf0QtnYb75HCqigyPbLRdY8AbEdctqKIK93Qj2dgM881wm29T8k3bpNWGO709nmPyamEpCHkmYm6FyXQuW46u3IwkzJAkTQgghhChWOu64I7HatQUqVsV4cgKqflNXh3VZBSZYhalzDw8PZ+3atdStW5eff/6Zhg0bopTKUf/+999/c/z4ccqXL4+Pjw+enp7s27eP0NBQ1q9fT7du3YrlAJVSqJbt0E1uRi//0nHTse0/o3r0c0wpltANx0TJsxjq3PVabtS7JAk7P+OQbWpOp2cTn5x5oTPiRV/3nEwlMTWTrEtuFWY9l4QFeloJ8s7doj7Iyw1/D0nChBBCCCGuhk5PR3/3Jfq7r8BiRfUdirrtTpT1+r/LVIERFqbO/bbbbmPy5MlERkbi4+PD6NGjAYiNjSUqKgqLxYJhGIwcOdJ535ARI0bw0UcfkZGRQbNmza6pwUVhKA9P1N2D0G06Y0bNQH/1Gfqn7zH6j0A1blGs+xbXL4uhsHtasXvm/6Ngas3ptOxcs2Dnm3Psi09lY0oWWZdMhVkUOUoPL+2MGOhlpZyHFYshSZgQQgghBOBolPdLNOYX0yEhDtWynSO5KkXVZ0pfrt3fdejYsWNFsh29a6uj+8jfR6HJzRj9hqNCivb6AFeQa0EKp6jHydSapPTsnLNg52fFUs9dJ5acReYlSZihwO6ZR4t67wvPBbgwCZP3U+HIOBVOUV+DVRoU1TmrLJOfn8KRcSocGafCuV7HSccdw5w/DXZvhUrVMAY+hKrX2GXxFFub9rJKNQ7HqN8Evfpr9NcLMF/+F+r2u1Dd70N55H1NjxD5MZSinIdjRqq23SPPZbTWnEnPzrdF/aFTacQczSIjO3cSFuCZs0V98EWzYEFebtg9ZSZMCCGEEKWTTk9H/28heuVXYHVD3Tcc1bFHqSgHzEvpjLqIKKsbqus96FYdHCWDyxehN/3g6ErSsp10khNFSimFn4cVPw8rtfLp6aK15myGmUdnRMf3h/9JZ+vRs6TnkYSV87A6Z8EuLUsM9LJi93TDzSLvaSGEEEJcH7TWsH0T5oIZkHjyXDO6IahyRd/8riTd0AnWeaqcHTVsDLr9HZjzp6Knv4Neu9zR1r1aLVeHJ24gSil83S34uluoEZD3MlprkjNNZwliQuq52bBkx2zYX6cz2H48hbRLOnMooJyH5cKNmr3dCPK8MAsW5O24Fs3NYhT/gQohhBDihqZPHMWcPxVit0Pl6hhPv4Gq29DVYRUJSbAuomrfhPHCW+gNq9FffY752pOodl1QvR9A+fi5OjwhAEcS5mOz4GPLPwkDSM7IztUZ8Xx54vEzGez6O4WUTDPXev4eFucsWGX7abyNrFzNOWyShAkhhBDiKuj0NPS3UeiVS8BmQ/Ub4SgHtFhcHVqRkQTrEsqwoNp2QTdvjf56PvqHb9ExPzmSrHZdy9R/vijbvG0WvG0WqpVzz3eZlMzsPDsjJqRkceJsJrEn4ziTnp1rPT93yyX3B7u4UYfjq7tVkuSmYkYAACAASURBVDAhhBBCOGitYdtGzKgZkBiPuqWjoxzQ/zKfFpdSkmDlQ3n7oPqPRN96O+YX09DzpqDXf4fRfxSqXiNXhydEkfBys+Dlb6Gqf95JWFBQEH8djyMh9ZLOiOcSsZPJmfx2MoUzGblnwnxtBkHebgR6XtSi3jtncw4PScKEEEKIMk8fP4I5/xPY8wtUqYkxciyqTgNXh1VsJMEqgKpSA+Op185l3DMx334BdXNbRyMMe5CrwxOi2Hm6GVRxc6eKX/4zYelZZp6zYOcf70tIIymPmTAfm3HZWbAgLzc83SQJE0IIIUojnZaK/mYBetUysLmjBoxCtb+jzFeESYJVCEopCG+D0agF+rtFjn+/bEZ174vq0hvlZnN1iEK4lLvVoJKfjUp++f8spGeZJKZe0hkxOZOEc/cJO5CYxum03EmYt5uR742azzfn8HIr27+ohRBCiNJEa43esgEdNQP+SUC16YS650GUXzlXh1YiJMG6AsrdHXXXQHTr2zC/nIVeMge9YRXGfcOhaUtp6y7EZbhbDSr62qjom38SlpFtkpjHNWHnm3P8cSqNU3kkYZ5W40KLeu+LZsE8Lzz2djPkZ1QIIYQoZvr4X5jzPoHfdkK1WhgPP4uqfZOrwypRkmBdBRVcAcsjz6Njt2N+MR3zw9ehUXOMfiNQFaq4OjwhSi2bxaCCr40Kl0nCMrM1iak57w+WcFEidvhYMv+kZqEvWc/Dqi4qR8xZlnj+OR+bJGFCCCHE1dBpKeivF6BXLwN3D9TAh1Htu6KMG6/KRBKsa6AahGG89F/02m/Ry+Zjjo9EdeqF6tkP5enl6vCEKJPcLIryPjbK++SfhGWZmlMX3x8s9cIsWHxyJr8cT+ZUWhbmJVmYu0XlSL4uLUsM8rLi626RJEwIIYQ4R2uN3rwe/eUs+CcRdevtqHsGo3z9XR2ay0iCdY2U1YrqfBe6ZTv0V7PRKxejo9c66kwjOqAMuUBfiJJmNRTB3m4Ee7tBcN7LZJuaU2lZzhs0X1yOGJ+Sxa6/U0hMzZ2E2SzqQvJ17kbNNUIycddpzkTMX5IwIYQQNwB99LDjZsF7d0H1OhiPPI+qVc/VYbmcJFhFRPkFoIY8jm7fDXP+VPSs98+1dR+JqhHq6vCEEJewGOpceaAb4JnnMtmm5p+0rEs6I14oS4w9mUJCShbZsYk51rMaKt9ZsEAvK8Febvh5WDAkCRNCCFEK6dQU9LL56DVfg4cX6oFHUW1vvyHLAfMiCVYRUzXrYjz3H/SmNehFn2H+e6xjqrT3AzdM5xQhygqL4SgZDCwgCbN4+7P/yN8XZsGSLzTq2BufysaUTLIuuVWY1QC7Z/6dEQO93PB3t2AxJAkTQghxfdBao6PXor/8FJL+cfyNe/dglK+fq0O7rkiCVQyUYaDadEaH3YL+5gv0mm/QWzag7hqI6tC9zPf+F+JGYjEUQd42CPQkNDDvZUytSUrLvqQM8UJzjn0JqcT/lUXWJfWIFgX28zdq9s4jEfOyUs7DKkmYEEKIYqePHHJ0B9wfCzVCMR57EVVTqrTyIglWMVJe3qj7hqPbdsH8Yhr6i2no9SscZYP1m7o6PCFECTGUopynlXKeVuoEeuS5jNaapPRsElKyOOlMvi60q/89MY3NR86Ska0v2TYEeF7cDTFnZ8RALyt2T0nChBBCXB2dkoxeNg/9w7fg5Y0a9Jhj5kr6DORLEqwSoCpWxRj9CuyIxlwwHfPdcRDeGqPvMFRgiKvDE0JcB5RS+HtY8fewUsuefxJ2JsN03KA55eKbNju+HjqVTszRfJIwj0uuCfO2Euh5IRGze1mxShImhBDiHK01etMP6EWfwpnTqHZdHZe8+Eg5YEEkwSohSikIi8BoGOboNLj8S8xdW1Dd+qC63o2yubs6RCHEdU4phZ+7BT93C7XseS+jteZshpnjBs0X37T5r9PpbD9+lrSsnEmYAsp5Wi/bot7u6YabRZIwIYQo6/RfhzDnTYEDe6BmXYzHX0JVr+PqsEoNSbBKmLK5o3r2R9/SCf3lLMeU64ZVGPcNg7BbpLWzEOKaKKXwdbfg626hRkDey2itSc40c9ygOf6iLolHkzL45XgKqZd25gDKeVguSr4ulCFeXKLoZimZspEdO3Ywa9YsTNOkU6dO9O7dO8frJ0+e5OOPPyYpKQkfHx8iIyMJDHRcKDdnzhy2bduG1prGjRszdOhQlFL89NNPLF68GKUUAQEBREZG4ufnx9mzZ3nvvfc4efIkwcHBjBkzBh8fnxI5TiGEKCk65Sx6yVz02uXg7YN6MBLVupOUA14hSbBcRAUGox56xtHW/YtpmB9PhPpNHddnVarm6vCEEGWYUgofmwUfm4Xq5fKfPU/JzM5xg+aLyxGPn8lg998pJGfmTsL83S2OpMvbjUDPC7Ng91eqVGTHYJomM2bM4MUXXyQwMJDnn3+eFi1aUKVKFecys2fPpl27dnTo0IHdu3czb948IiMj2bt3L3v37uXtt98GYNy4ccTGxnLTTTfx6aef8u677+Ln58ecOXP47rvvuO+++1iyZAmNGzemd+/eLFmyhCVLlvDAAw8U2fEIIYQradN0dsDm7BlUh26oux5AecsHSVdDEiwXUzc1wRj3PnrdcvTSuZivPI66rSfqzgEoL29XhyeEuIF5uVmo5m+hmv/lk7DES+4Pdj4R+/tsJrFxKZzNcCRh999av8hiO3DgABUqVKB8+fIAtG7dmpiYmBwJ1pEjRxg8eDAADRs25K233gIcCWZGRgZZWVlorcnOzsbf399xvYHWpKeno7UmJSWFChUqABATE8P48eMBaN++PePHj5cESwhRJujDv2PO/wR+/w1q34QxejyqWm1Xh1WqSYJ1HVAWC+q2nuib26KXzEGv/hodvQ51z2CZlhVCXNe83Cx4+VuocpkkLC3LJD4ls0j3m5iY6Cz3AwgMDGT//v05lqlevTqbN2+me/fubN68mdTUVM6cOUPdunVp2LAho0aNQmtNt27dnInZyJEjGTt2LO7u7lSsWJERI0YAcPr0aQICHDWX5cqV4/Tp03nGtWrVKlatWgXAxIkTCQoKKtLjLousVquMUyHIOBWOjFPhWK1W7B42zs6dSurKJSgfP3wj/w+PDnfI351FQBKs64jy9UcNegzdrivm/Knozyah132HMWAUqlY9V4cnhBBXxcNqUMWv5Bv5DBo0iJkzZ7J27Vrq16+P3W7HMAxOnDjB0aNHmTJlCgATJkxgz549hIaGsnLlSt58803Kly/PzJkzWbx4Mffee2+O7Sql8r1etnPnznTu3Nn5OD4+vvgOsIwICgqScSoEGafCkXEqmDZNfHZGk/TZh5B8FtWxO+qugSR7+ZCcmOjq8K4rla6ytL1QCVZBFxJnZmYyefJkDh48iK+vL6NHjyYk5EL78fj4eMaMGUPfvn3p1asXAN988w1r1qxBKUXVqlV59NFHsdlsV3UQZY2qXgfj2TfP3Sn7M8w3nnbMZN07GOWXz1XrQghxA7Hb7SQkJDgfJyQkYLfbcy0zduxYANLS0oiOjsbb25vVq1cTGhqKh4ejHX5YWBj79u3Dzc0NwFkWeMstt7B06VIA/P39OXXqFAEBAZw6dQo/P2lTLIQoffThA5hzp5B0aB/UaYAx8CFU1ZquDqvMKXAO8PyFxC+88ALvvfceGzZs4MiRIzmWWbNmDd7e3kyaNIkePXowd+7cHK9/9tlnhIWFOR8nJiayfPlyJk6cyDvvvINpmmzcuLGIDqlsUEphRHTEeO0jVNd70NHrMF98BHPlEnRWlqvDE0IIl6pduzbHjx8nLi6OrKwsNm7cSIsWLXIsk5SUhGk6rv9avHgxHTt2BByfcO/Zs4fs7GyysrKIjY2lcuXK2O12jhw5QlJSEgA7d+6kcuXKALRo0YJ169YBsG7dOm6++eaSOlQhhLhm+mwS5uyPMF9/ChLi8HtiHMYzb0hyVUwKnMEqzIXEW7ZsoW/fvgBEREQwc+ZMtNYopdi8eTMhISG4u+csDzFNk4yMDCwWCxkZGc7adpGT8vBC9RmCvvV2zAXT0Qtnon9ciTFgJKpBWMEbEEKIMshisTBs2DBef/11TNOkY8eOVK1alQULFlC7dm1atGhBbGws8+bNQylF/fr1GT58OOA4T+3evds5u9WsWTNnctanTx9efvllLBYLQUFBPPbYYwD07t2b9957jzVr1jjbtAshxPVOmyb6p+/Riz+HlGRUpztRdw7As1p1kqWUstgUmGAV5kLii5exWCx4eXlx5swZbDYbS5cuZdy4cSxbtsy5vN1u58477+SRRx7BZrPRtGlTmjZtmuf+5YLhc4KC0A0/IGPLRs7MfJ/s917GvVU7fIZEYq1Q2bmYXNxZODJOhSPjVDgyTq7RvHlzmjdvnuO5fv36Ob+PiIggIiIi13qGYTBq1Kg8t9mlSxe6dOmS63lfX19eeumla4xYCCFKjj6033Gz4D/2Q2gDjIEPo6rUcHVYN4RibXIRFRVFjx49nHXu5509e5aYmBg+/PBDvLy8ePfdd1m/fj3t2rXLtQ25YPgSNeuhX/oA9f0S0r+NIn3rJlS3e1Dd+qDc3eXizkKScSocGafCkXEqnKu9WFgIIUTh6TNJ6MWfo3/6HvzKoYY/iWrVPt/mPKLoFZhgFfZC4oSEBAIDA8nOziYlJQVfX18OHDhAdHQ0c+fOJTk5GaUUNpsNf39/QkJCnBcJt2rVin379uWZYInclJsbqntfdERH9KLP0N8sQG9cjeozDN3tLleHJ4QQQgghSpg2s9HrV6KXzIHUZFTnXo77qnp6uTq0G06BCdbFFxLb7XY2btzI448/nmOZ8PBw1q5dS926dfn5559p2LAhSileffVV5zJRUVF4eHjQrVs39u/fz/79+0lPT8dms7Fr1y5q15Ybml0pZQ9CjXwK3b6bo6371P9wauMq9L1DZApYCCGEEOIGoQ/uxZz3CRw+APUaYwx4CFW5mqvDumEVmGAV5kLi2267jcmTJxMZGYmPjw+jR4++7DZDQ0OJiIjg2WefxWKxUKNGjRxlgOLKqLoNMca9i16/gqyl89CvjkZ1uAN11/0obx9XhyeEEEIIIYqBPnMa/dW5csBydtTIsaib20o5oIsprbV2dRBX4tixY64O4bpmt7kRP8txg2K8vVF3D0LdejvKsLg6tOuKXDNTODJOhSPjVDg34jVYcs4qmPz8FI6MU+HcKOOkzWz0uhXoJbMhPQ3VqRfqzn4oj8KVA94o43StivVGw6L0MPz8Me5/GN22C+YXU9GzP0KvW4ExYBSqTn1XhyeEEEIIIa6B/v03R3fAPw/CTU0cf+NVknLA64kkWGWUqlYL4+k30DE/ohfOwnzzWVREB9S9D6LKBRa8ASGEEEIIcd3QSf84mpttXA3lAlGjnkG1aCPlgNchSbDKMKUUqmU7dJOb0cu/RK9cjN4ejep5n2Mq2c3N1SEKIYQQQojL0NnZ6LXL0UvnQkY6qtu9qB73oTw8XR2ayIckWDcA5eGJunsQuk1nzKgZjk8/fvweo/9IVONwV4cnhBBCCCHyoA/EYs79BI4cgvpNHd0BK1ZxdViiAJJg3UBUSEUs/3oRvWsr5oLpmB+8Ak1uxug3HBVy4118LoQQQghxPdJJp9Bffore9APYgzAefhaat5ZywFJCEqwbkGocjlG/CXr11+ivF2C+/C/U7Xehust0sxBCCCGEq+jsbPQP36KXzYOMDNQdfRzlgO4erg5NXAFJsG5QyuqG6noPulUHR8ng8kXoTT+g+gxFtWwnn5AIIYQQQpQgvW+342bBRw9DwzCM/qNQFSq7OixxFSTBusGpcnbU8DHo9t0w509FT38HvXa5o+VntVquDk8IIYQQokzT/ySiv5yFjl4H9mCMR56HsAj5sLsUkwRLAKDq1Mf4v7fRP61CL56N+dqTqPZdUXfdj/Lxc3V4QgghhBBlis7KulAOmJXpuFSje1+Uu7urQxPXSBIs4aQMC6pdV3R4G/TX8x0/9Jt/RPV+wJFsGRZXhyiEEEIIUerpvbsdNws+9ic0Cnd0di4vDcfKCkmwRC7K2wfVfyT61tsxv5iGnjcFvX4FxoCRqLqNXB2eEEIIIUSppP9JQC+chd68HgJDMB57AZq2knLAMkYSLJEvVaUGxlOvwbaNmFEzMd96AXVzW0cjDHuQq8MTQgghhCgVdFbWue7NX0B2Fqpnf9Qd96JsUg5YFkmCJS5LKQXhbTAatUB/t8jx75fNjhrhLr1RbjZXhyiEEEIIcd3Se37BnD8Vjv917v6jI1AhFV0dlihGkmCJQlHu7qi7BqJb34b55Sz0kjnoDasw7hsOTVvK1LYQQgghxEV0YryjO2DMjxBUHuNfL6KatnR1WKIESIIlrogKroDlkefRsdsxv5iO+eHr0Ki549OYClVcHZ4QQgghhEvprEz0qmXobxaAaaLuHIDqdo+UA95AJMESV0U1CMN46b+OToNfz8cc/ziq852oHv1Qnl6uDk8IIYQQosTp2B2OcsATR6BpS8cH0MEVXB2WKGGSYImrpqxW1O13oVu1Q3/1OXrFYvTPa1H3DkG1ao8yDFeHKIQow3bs2MGsWbMwTZNOnTrRu3fvHK+fPHmSjz/+mKSkJHx8fIiMjCQwMBCAOXPmsG3bNrTWNG7cmKFDh5KWlsZLL73kXD8xMZG2bdsyZMgQ1q5dy+zZs7Hb7QB069aNTp06ldzBCiGuazrxJGbUDNi6EYIrYDz+EqpxC1eHJVxEEixxzZRfAGrIE+j2d2DOn4qe+R563XKMAaNQ1eu4OjwhRBlkmiYzZszgxRdfJDAwkOeff54WLVpQpcqFUuXZs2fTrl07OnTowO7du5k3bx6RkZHs3buXvXv38vbbbwMwbtw4YmNjadiwIW+99ZZz/WeffZaWLS9cL9G6dWuGDx9ecgcphLju6cxM9PdL0N9GARp11/2orndLE7AbnEwxiCKjatbFeO4/qCGPQ9xxzNefwvx8MvrMaVeHJoQoYw4cOECFChUoX748VquV1q1bExMTk2OZI0eO0KiR4959DRs2ZMuWLYCjO2pGRgZZWVlkZmaSnZ2Nv79/jnWPHTtGUlIS9evXL5kDEkKUOnr3NszxkejFs6FBGMYrH2L07CfJlZAZLFG0lGGg2nRGh92C/uYL9Jpv0Fs2oO4aiOrQHWWxuDpEIUQZkJiY6Cz3AwgMDGT//v05lqlevTqbN2+me/fubN68mdTUVM6cOUPdunVp2LAho0aNQmtNt27dcsx8AWzcuJFbbrklR4fU6Oho9uzZQ8WKFXnwwQcJCpL7AQpxI9IJcZgLpsP2nyGkIsYTL6Mahbs6LHEdkQRLFAvl5Y26bzi6bRfML6ahv5iGXr8Co/9IVP2mrg5PCHEDGDRoEDNnzmTt2rXUr18fu92OYRicOHGCo0ePMmXKFAAmTJjAnj17csxWbdiwgcjISOfj8PBw2rRpg5ubG99//z0ffvghL7/8cq59rlq1ilWrVgEwceJEScIKwWq1yjgVgoxT4RTnOOmMdJKXziP5y88B8Ln/IbzuGlAqZ6zk/VS8JMESxUpVrIox+hXYEY25YDrmu+MgvDVG32GowBBXhyeEKKXsdjsJCQnOxwkJCc4GFBcvM3bsWADS0tKIjo7G29ub1atXExoaioeHBwBhYWHs27fPmWD98ccfmKZJrVq1nNvy9fV1ft+pUyfmzJmTZ1ydO3emc+fOzsfx8fHXeKRlX1BQkIxTIcg4FU5xjZPetRXzi6kQdxyat8a4bzipgcGknk4q8n2VBHk/FU6lSpWuar1CJVgFdWrKzMxk8uTJHDx4EF9fX0aPHk1IyIU/nuPj4xkzZgx9+/alV69eACQnJzNlyhT++usvlFI88sgj1K1b96oOQlzflFIQFoHRMAy9cjF6+ZeYu7aguvVxXAgq94UQQlyh2rVrc/z4ceLi4rDb7WzcuJHHH388xzLnuwcahsHixYvp2LEj4PjDYvXq1WRnZ6O1JjY2lu7duzvX27BhA23atMmxrVOnThEQEADAli1bcpUUCiHKJh3/t6MccEc0lK+MMfoVVMMwV4clrnMFJliF6dS0Zs0avL29mTRpEhs2bGDu3LmMGTPG+fpnn31GWFjON+OsWbNo1qwZTz31FFlZWaSnpxfhYYnrkbK5o3r2R9/SCb1wJnrZPPSGVRj3DYewiBzXOgghxOVYLBaGDRvG66+/jmmadOzYkapVq7JgwQJq165NixYtiI2NZd68eSilqF+/vrMDYEREBLt373bObjVr1owWLS60U960aRPPP/98jv0tX76cLVu2YLFY8PHx4dFHHy25gxVClDidmYH+7iv08i9BKdQ9g1Gd70K5ubk6NFEKFJhgXdypCXB2aro4wdqyZQt9+/YFHCeumTNnorVGKcXmzZsJCQnB3f3CLEVKSgp79uzhsccecwRhtWK1SrXijUIFBqMefhb9207ML6ZhfvwG1G/qaOtesaqrwxNClBLNmzenefPmOZ7r16+f8/uIiAgiIiJyrWcYBqNGjcp3u5MnT8713MCBAxk4cOA1RCuEKC30LzGYC6bByROo8Dao+4ah7MGuDkuUIgW2ac+rU1NiYmK+y1gsFry8vDhz5gxpaWksXbrUmXydFxcXh5+fHx999BHPPPMMU6ZMIS0trSiOR5Qi6qYmGOPeR/UfBYcPYL7yOOaCGeiUZFeHJoQQQogbjD55guxJEzAnTwCLFWPMqxgPPyvJlbhixTptFBUVRY8ePZwXEp+XnZ3NoUOHGDZsGKGhocyaNYslS5bQv3//XNuQjkxXplR2hek3BLPbXZydN5XU75ehYtbj/cAjeNzWHWUUz63aSuU4uYCMU+HIOAkhROmlM9LR3y1CL18EFguqzxBUpztRVikHFFenwASrsJ2aEhISCAwMJDs7m5SUFHx9fTlw4ADR0dHMnTuX5ORklFLYbDYiIiIIDAwkNDQUcJRxLFmyJM/9S0emK1Oqu8L0HY7Rsj3m/Kkkffhvkr5d6CgbrFWvyHdVqsepBMk4FY6MU+FcbTcmIYQoDlpr+GWzo4lF/N+om9ui+g5DBQQWvLIQl1FgglWYTk3h4eGsXbuWunXr8vPPP9OwYUOUUrz66qvOZaKiovDw8KBbt26Ao9Tw2LFjVKpUiV27dklHJgGAql4H49k30dFr0V9+hvnG06jWnVD3Dkb5Bbg6PCGEEEKUATruGOYX02HXFqhYFeOp11A3NXF1WKKMKDDBKkynpttuu43JkycTGRmJj48Po0ePLnDHw4YN44MPPiArK4uQkBDpyCSclFKoiI7oZq3Q30ShVy1Db9+E6tkfdVtPlDREEUIIIcRV0Onp6OUL0Su+AqubY8ZK/rYQRUxprbWrg7gSx44dc3UI17WyWKqkTxx1TN/v3goVqmAMGIlqcG33oCiL41QcZJwKR8apcG7EEkE5ZxVMfn4KR8apcPIbJ6017Ih2/D2REIdq1R7VZyiqnD2PrZR98n4qnGK90bAQrqQqVMZ4/CXYuQVzwTTM91523Li47zBUcAVXhyeEEEKI65j++xjmF1Nh9zaoXB1j7L9R9Rq5OixRhkmCJUoFpRQ0vRmjQTP090vQ30Zh7noM1e0eVLc+qIvusyaEEEIIodPT0P9biF65GNxsqH7DUR16SDmgKHbyDhOlinJzQ3Xvi47oiF70KfqbBeiNqzH6DoPwNo5ETAghhBA3LK01bNuEGTUdEuNRER0drdf9pVmWKBmSYIlSSdmDUCPHotvfgTl/KuYn/4F6jTH6j0RVqeHq8IQQQgjhAllHD2N+9CbE7oAqNTBGjEWFNnB1WOIGIwmWKNVU3YYY495Fr1+BXjIXc8JoVIfuqF4DUd4+rg5PCCGEECVAp6Wiv40iYdVSRzlg/1GoDnegLBZXhyZuQJJgiVJPGRZUh+7oFreil85D//A/9OZ1qLsHoW69HWXIL1chhBCiLNJaw9YNmFEz4VQ8Hh27k9Gzn9w7U7iUJFiizFA+fqj7H0a37YL5xVT07I/Q61ZgDBiFqlPf1eEJIYQQogjp439hzp8Ke36BqjUxRj2Nf0RbaT8uXE4SLFHmqGq1MJ5+Ax3zI3rhLMw3n0VFdEDdO+SGvd+FEEIIUVbotBRHk6tVy8DdAzXwIVT7blKxIq4bkmCJMkkphWrZDt3kZvTyL9ErF6O3R6Pu7IfqdKerwxNCCCHEFdJan/vwdCb8k4hq0xl1z2CUXzlXhyZEDpJgiTJNeXii7h6EbtMZM2oG+stP0T9+T/rIJ6F6qKvDE0IIIUQh6KN/Ys7/BPbugmq1MR5+DlX7JleHJUSeJMESNwQVUhHLv15E79qKuWA6/7z2FDS5GaPfcFRIJVeHJ4QQQog86LQU9NdfoFd/De6eqPsfRrXrKuWA4romCZa4oajG4Rj1m+C1aQ1nF8zEfPlfqNt7o7r3RXl4ujo8IYQQQnCuHHDzevTCWZB0ytEV+O7BKF8/V4cmRIEkwRI3HGV1w/vu+0lpfDN60WeOa7Q2/eC4y3vLdiilXB2iKGFaa9LS0jBN86r+///++2/S09OLIbLSR2uNYRh4eHjIz5IQ4qroo4cx530C+3ZD9ToYj72AqlnX1WFdN671nAVy3rpYcZy3JMESNyxVzo4aPgbdvhvm/Kno6e+g1y53tHWvVsvV4YkSlJaWhpubG1br1f1KtFqtWORmlk5ZWVmkpaXh6SmzwkKIwtMpyeiv56PXfAOe3qhBj8r9LPNwrecskPPWpYr6vCUJlrjhqTr1Mf7vbfRPq9CLZ2O+9iSqfVfUXfejfKQU4UZgmuY1nahETlarVT4ZFUIUmtYaHb3WUQ545jSqbVfU3Q/IOTgfcs4qekV93pL/HSEAZVhQ7bqiw9s4Pj374Vv05h9RvR9wJFvy6VmZJqVsRU/GVAhRGPrIIUc5PwZ7XQAAIABJREFU4P5YqFkXI3IcqoZ0+b0c+f1aPIpyXCXBEuIiytsH1X8k+tbbMb+Yhp43Bb1+BcaAkai6jVwdnhDiIjt27GDWrFmYpkmnTp3o3bt3jtdPnjzJxx9/TFJSEj4+PkRGRhIYGAjAnDlz2LZtG1prGjduzNChQ0lLS+Oll15yrp+YmEjbtm0ZMmQImZmZTJ48mYMHD+Lr68vo0aMJCQkp0eMVoizRKWfRyxwfaOLljRr8L8d9rQzD1aEJcc3kXSxEHlSVGhhPvYbx8LOQchbzrRcwp72NTox3dWiiDDp9+jSffvrpFa83aNCg/2fv3uNsrvbHj7/WZ8/9bs80ppAyKA3CTAwKw1STW3IJCSG6nREdXXQ4dSodv0P17cRJMSINBiWXCENSJsakm0YuJ6dyC+M2xtz2/qzfH5vNMMzGzOy5vJ+Ph0d79v7sz35/Vnv2mvdea70XJ06cuOwxkyZNYsOGDVcZWcVlmiZJSUm8+OKLvPXWW2zcuJG9e/cWOWbOnDm0a9eOyZMn07t3b+bOnQvAjh072LFjB5MnT+aNN97gv//9L5mZmfj6+jJp0iTnv7CwMFq2bAnAunXr8Pf355133qFLly4kJyeX+zULURVo08RMW4s57gn0uuWodvdivDYN4657JLmqRKTfujx5JwtxCUopVHRbjFf+g+raD731G8zxT2B+tgBdWODu8EQVcvLkST788MOL7rfZbJd93pw5cwgODr7sMc8++yzt2rW7pvgqot27dxMREUHNmjXx8PCgTZs2bNmypcgxe/fupXFjx8hzVFQUGRkZgON3u6CgAJvNRmFhIXa7/aJ23L9/PydPnqRRo0YAZGRk0KFDBwBiY2PZtm0bWusyvkohqhb9+6+Y/3oB/cHbcF0Ext/exBjwBMo/0N2hiSsk/dblyRRBIUqgvL1R9z+EbtMRc+FM9KcfoTemYvR9FJreIXOhxTV7/fXX+e2337j77rvx9PTE29ub4OBgdu/ezddff83QoUPZv38/+fn5DBs2jIcffhiAVq1asXLlSnJycnj44Ydp2bIlGRkZREREMHPmTHx9fRk1ahTx8fF07dqVVq1a0adPH9asWYPNZuO9996jfv36ZGVl8dRTT/Hnn38SHR3Nhg0b+Pzzz7FarW5umUs7evSoc7ofQGhoKLt27SpyTN26dUlPT6dz586kp6eTm5tLdnY2DRs2JCoqihEjRqC1JiEhgdq1axd5blpaGq1bt3b+fp//ehaLBT8/P7KzswkKKroIPzU1ldTUVAAmTpxIWFhYqV97VePh4SHt5ILK3E5mTjan5k4n9/NPUAFBBP7lRXziOpfJiFVlbidX/fnnn6VS5OJazvHPf/6T3377jXvuueeifuubb75h8ODBzn7r0UcfZdCgQQDExMSwatUqcnJyeOihh4r0W7Nnz8bX15eRI0dy9913061bN2JiYnjwwQdZvXo1hYWFzJgxgwYNGnDkyBGeeOKJIv3W6tWri/QLV8rb27vU3juSYAnhInVdBJYnX0Rnfoc5fwbmlNegcQuMvo+iImqXfAJRKZjzp6P/2HNlz1HqsqMZqs7NGP2GX/LxF198kR07drBmzRrS0tIYNGgQ69at48YbbwTgjTfeoEaNGuTm5tKlSxc6d+58UfKzZ88epk6dyqRJk3jsscdYsWIFvXr1uui1rFYrq1atYtasWUybNo3Jkyfz5ptv0rZtWxITE/niiy+YN2/eFV1/RTVw4EBmzpzJ+vXradSoEVarFcMwOHjwIPv27WPatGkAvPrqq2zfvt05WgWwceNGEhMTr/g14+PjiY+Pd/585IhMKy5JWFiYtJMLKmM7adNEf7MO/fFsOJWN6pCAuv9hcvwDyDl6tExeszK205XKz893lli/mj4LHCP5l+q3SuqzAMaOHcsvv/zC6tWrL+q3bDYbkydPLtJvJSQkYLVa0Vpjt9ux2+38+uuvTJkyhX/961889thjLF26lF69emGaJna7HZvNhtaakJAQPv/8c2bNmsXUqVOZPHkykyZNok2bNs5+a+7cuc7nXK38/PyL3js33HDDVZ1LEiwhrpC6rTnG3992VBpcNg/z5ZGo+G6oLn1Rvn7uDk9UAc2aNXMmVwAzZ85k5cqVgGPq2p49ey5KsOrUqeOcDte0aVP++OOPYs993333OY85e8709HSSkpIAiIuLIyQkpHQvqAxYrVaysrKcP2dlZV3UJlarlTFjxgCOfWM2b96Mv78/a9eupUGDBvj4+ADQvHlzdu7c6Uyw/ve//2GaJvXq1StyrqysLEJDQ7Hb7Zw+fZrAQJnWJMSl6N/+izl3Gvy6AyJvxRj1D9ljsgqTfqsoSbCEuArKwwN19/3oVu3Qn3yIXrUYvWk9qtcjqFbtZaFuJVbSt3bF8fDwuKZvzS7k53cuUU9LS+Orr75i2bJl+Pr60rt372L36vD29nbetlgs5OXlFXvus8dZLBbsdnupxVzeIiMjOXDgAIcOHcJqtZKWlsbIkSOLHHO2eqBhGCxevJi4uDjA8Q332rVrsdvtaK3JzMykc+fOzudt3LiRtm3bFjlXdHQ069evp2HDhmzatImoqCiZHixEMXROtmMq/ZefQ0AQasjTqNg46RfLyNX0WSD9Vllz6d3+/fff8/TTT5OYmMinn3560eOFhYW89dZbJCYm8uKLL3Lo0KEijx85coSBAweydOnSIvebpslzzz3HxIkTr+EShHAfFVQD45GnMV6cDNbr0DPfcizg/W23u0MTlYi/vz+nTp0q9rHs7GyCg4Px9fVl9+7dbN26tdRf/4477mDZsmUAfPnllxw/frzUX6O0WSwWhg4dyoQJExg9ejStW7emTp06pKSkOItZZGZmMmrUKJ5++mlOnDhBz549AUeRipo1azJmzBieffZZ6tatS0xMjPPc33zzzUUJVseOHTl16hSJiYksX76cAQMGlN/FClEJaNPE/Gq1ozrgl6tQHbtivPYuRptOklxVQdJvXV6JI1hnS+GOGzeO0NBQxo4dS0xMTJEFweeXr924cSPJycmMHj3a+fjs2bNp3rz5RedesWIFtWrVIjc3t5QuRwj3UDc3xHjhX8655uaEv6LuvBv1wEBU4OWr5QhhtVq544476NixIz4+PkUW2Xbo0IE5c+bQvn17IiMjadGiRam//jPPPMOTTz7Jxx9/THR0NOHh4fj7+5f665S2Fi1aXNQeffv2dd6OjY0lNjb2oucZhsGIESMued4pU6ZcdJ+XlxfPPPPMNUQrRNWl/7fLsVnwnp1Q/zaMhx5D1bnZ3WGJMiT91uUpXUKd2Z07d7Jw4UL+9re/AbB48WIAHnjgAecxEyZMoE+fPjRs2BC73c6IESOYMWMGSinS09PZsWMH3t7e+Pj40L17d8AxX37q1Kn07NmT5cuX88ILL7gU8P79+6/qQquL6rC4szSUZTvp0zno5fPR65aDtw+q+wBUh/tQZxakVibV5f10+vTpItMbrlRpT7Uob2cXTHt4eJCRkcHYsWNZs2bNNZ2zuDa92sXClZn0WSWrLp8z16oitpM+dRK9eA76q9UQGIzqPQQV28Gt02crYjuVtmvts0D6reKUZr9V4giWK6VwL1W+1svLiyVLljB+/PiLpgfOmjWLhx9+uMTRKyl5e2WqQ3nS0lC27RQGTz6PrVtfspPeomD++1jSUgl8dDReTaLL6DXLRnV5P5VGydvSKJnrLr///jvDhw/HNE28vLx48803r/l6SrPcrRCiYtGmHf3VGvTiOZCbg+rUDdWtP8qv4owgiKpt3759PP74485+a9KkSe4OqYgy/YtgwYIFdOnSxVmp6axvv/2W4OBg6tWrx88//3zZc0jJ2ytTHb65KQ3l0k6+AeinxmF8twnbgiSO/T0RFd0W1WcoKvS6sn3tUlJd3k/nl7y9GpX9m8Abb7yRVatWFbnvWq+nNMvdCiEqDr1nJ2byNPhtNzSMwuj/GKr2Te4OS1Qz9erVY/Xq1e4O45JKTLBcLYVbXPna3bt3s3nzZpKTk8nJyUEphZeXF0ePHiUjI4PvvvuOgoICcnNz+fe//31RBSghKjulFLRojdG4BXr1YvTKReiftqASeqPufQDl5V3ySYQQQgg309kn0Ys/RH+9BoJqoB79K6plO6mmKUQxSkywXCmFe6nyta+88orzmAULFuDj40NCQgIADz30EAA///wzy5Ytk+RKVGnKyxvVtR+6dUf0wg/QS+eiN6ZiPDgMmsdKByWEEKJC0qYdvWEVevFHkHcaFd/dMR1Q9n0U4pJKTLDOL4VrmiZxcXHOUriRkZHExMTQsWNHpkyZQmJiIgEBAYwaNao8Yhei0lGh4ajHn0f/8iPm/OmY7/4TGt2O0X8E6vo67g5PCCGEcNL//cVRHfD3/8ItTRzTAWvdWPIThajmSqwiWNFIRabLqy5rZq5VRWgnbbej169EL02G/DxUXFdUt34VapFwRWin8lDdqwiWBaki6CB9Vsmqy+fMtSrPdtInj6M/mY3euBZCrI61w3fcVSlmW1SH95NUESwbpdlvyc5vQriJslgwOnXFeG0aqm08eu1SzHGPY25MRZumu8MTFViDBg0AOHjwIMOHDy/2mN69e/PDDz9c9jzTp08vUsl14MCBnDhxovQCFUJUKtq0Y37xGeb4J9Cb1qPufQDj1f9gyForcY2qW78lCZYQbqYCgzEGPoXxtzcg/Hr0rH9jTnwOvWenu0MTFVxERATTp0+/6ufPmDGjSEc1Z84cgoNlY2whqiO9ezvma8+g574HdetjvPRvjN5DUD6y1kqUnurSb1XejVuEqGJU3foYz/8/9Ob16EWzMV8fg2rbCdVzECqohrvDE2Xo9ddf54YbbuCRRx4B4I033sBisZCWlsaJEyew2Ww899xz3HvvvUWe98cffzB48GDWrVtHbm4uzzzzDJmZmdSvX5+8vDzncS+88AI//PADeXl5dOnShTFjxpCUlMSff/5Jnz59qFGjBosWLaJVq1asXLkSq9XKe++9R0pKCgD9+/dn+PDh/PHHHzz88MO0bNmSjIwMIiIimDlzJr6+vuXWVkKI0qVPHkMvmo3+Zh2EhGI89hxEt5URK3FZ0m9dniRYQlQgSilUbBy6WSv08hR06jL01m8cFZviuqAq8Wa2lcWMjD/Zcyyv5APPo5TicstZb67hw6MxNS/5ePfu3XnppZecHdWyZctITk5m2LBhBAYGcvToUbp168Y999xzyT96PvzwQ3x9ffnyyy/JzMx0VmwFeP7556lRowZ2u52+ffuSmZnJsGHDeP/991m4cOFFW2/8+OOPLFiwgOXLl6O1pmvXrrRu3Zrg4GD27NnD1KlTmTRpEo899hgrVqygV69eV9BaQoiKwLEOeAV6yVwoyEfd1wvV+UGUj3xhUplcTZ8Fl++3SuqzQPqtkshfa0JUQMrHD9V7CPrOuzFTZqAXJKG/Wo3R71HUbc3dHZ4oZY0bN+bIkSMcPHiQrKwsgoODCQ8P5+WXX2bz5s0opTh48CCHDx8mPDy82HNs3ryZoUOHAnDbbbfRqFEj52NnOz673c6ff/7Jrl27uO222y4ZT3p6OgkJCc7Fvvfddx+bN2/mnnvuoU6dOjRu3BiApk2b8scff5RWMwghyone+TPmvPdg7//gtuYY/YejImq7OyxRiUi/dXmSYAlRgamI2hgjX4Ift2CmzMB86yVoHovRZyjqugh3h1cllfStXXFKoxpT165d+eyzzzh06BDdu3fnk08+ISsri5UrV+Lp6UmrVq3Iz8+/4vP+/vvvvPfee3z22WeEhIQwatSoItMwrpS397nNsS0WyzWdSwhRvvSJY+hFs9CbvgBrGMYTL0Dz1jIdsBK7mj4LpN8qa1LkQogKTimFur0lxj+moB4YCD9/h/n3pzCXJKOv4oNLVEzdu3dnyZIlfPbZZ3Tt2pXs7GzCwsLw9PRk48aN7N2797LPb9WqFZ9++ikAv/zyC9u3bwcgOzsbX19fgoKCOHz4MF988YXzOQEBAZw6darYc61atYrc3FxOnz7N559/TqtWrUrxaoUQ5Unb7ZipSxzVATO+QnXug/HKf1At2khyJa6a9FuXJiNYQlQSytML1bkPOjYO/fEsxxqttLUYfYbKguQq4JZbbiEnJ4eIiAhq1qxJz549GTx4MJ06daJp06bUr1//ss8fNGgQzzzzDO3bt6dBgwY0bdoUgKioKBo3bky7du244YYbuOOOO5zPGTBgAAMGDKBmzZosWrTIeX+TJk3o06cPXbp0ARyLhRs3bizTAYWohPTObY7Ngvf9Bo1bYPQbgapZ/fakE6VP+q1Lk42Gq5jqsMFeaagK7eSYQ/8+7N0DtzTB6D8CVatuqb5GVWgnV8hGw6VPNhp2kD6rZNXlc+ZaXWk76eNZ6IWz0OlfQmg4Rt9HoVmrKv9lXHV4P8lGw2WjNPstGcESopJSDaMwxr+J3rAK/Wky5itPozp0RnV/COUf4O7whBBCuIG22dDrlqGXzge7DdW1LyqhN+q8dShCiLIlCZYQlZgyLKgOndExd6KXzEV/sQKdvgH1wEDUnfEow+LuEIUQQpQTveMnzORpcOAPaBLjqDwbXv1GjoVwN0mwhKgCVEAQasDj6LvuwZz/PnrOVPSGVRj9hqPqNyr5BNVcJZspXSlImwpRfvSxLPTCmegtXzmmAz71N7i9ZZWfDlhdyedr2SjNdpUES4gqRN1YD+PZf6LTN6AXzcL8f8+jYuNQvQajQqwln6CaMgwDm82Gh2zkXCpsNhuGIUVqhShr2laIXrsMvSzFMR2wWz9UQi+Ul0wHrMqkzyp9pd1vyf8ZIaoYpRSqVXv07S3RKxai13yK/m4TqltfVKduKA9Pd4dY4fj4+JCXl0d+fv5VfePr7e19VXt9VEVaawzDwMfHx92hCFGl6e0/OKoDHtwLt7fE6Puo7I9YTVxrnwXSb52vLPotSbCEqKKUjy+q5yD0nfGYC2Y6Npf8ao2jE24S7e7wKhSlFL6+vlf9/OpQtUoIUTHoo0fQC5LQ326E6yIwEsejmt5R8hNFlXGtfRZIv1XWJMESoopT4Tdg+cs49E/fYqbMwPz3P6DpHRh9h8niZ1Gpff/993zwwQeYpkmnTp3o0aNHkccPHz7Mu+++y8mTJwkICCAxMZHQ0FAAPvroI7Zu3YrWmiZNmjBkyBCUUthsNpKSksjMzEQpRb9+/YiNjWX9+vXMmTMHq9Ux1TYhIYFOnTqV+zWL6ksXFmKu/Bj9WQqYpqNibEJPlKeXu0MTQlxAEiwhqgnVJBqjUVPnfH3zpb+g7nkAdV9vlM+1fRMmRHkzTZOkpCTGjRtHaGgoY8eOJSYmhtq1azuPmTNnDu3ataNDhw5s27aNuXPnkpiYyI4dO9ixYweTJ08GYPz48WRmZhIVFcUnn3xCcHAwb7/9NqZpcurUKef52rRpw7Bhw8r9WoXQmd+RtSAJve93aNYK48FhMh1QiApMViELUY0oD0+Me3tivPYuKuYu9IqFmOOfxNz8pVQlEpXK7t27iYiIoGbNmnh4eNCmTRu2bNlS5Ji9e/fSuHFjAKKiosjIyAAc02sKCgqw2WwUFhZit9sJDg4G4IsvvnCOhBmGQVBQUDlelRBF6azD2N+diPnWS2CaGCNfwvLU3yS5EqKCkxEsIaohFWJFDRuNbp+AOe999Iw30F+uxOj/GKrOze4OT4gSHT161DndDyA0NJRdu3YVOaZu3bqkp6fTuXNn0tPTyc3NJTs7m4YNGxIVFcWIESPQWpOQkEDt2rXJyckBICUlhczMTGrWrMnQoUMJCQkBYPPmzWzfvp3rr7+ewYMHExYWdlFcqamppKamAjBx4sRijxFFeXh4SDtdQBcWcHrJPE4tmg1a4//QCIJ7DcQuexuWSN5PrpF2KluSYAlRjan6jTD+Nhn9dSp68RzMV0ej2t+Lun8AKkC+uReV28CBA5k5cybr16+nUaNGWK1WDMPg4MGD7Nu3j2nTpgHw6quvsn37dmrVqkVWVha33HILgwcPZvny5cyZM4fExESio6Np27Ytnp6erFmzhqlTp/LSSy9d9Jrx8fHEx8c7f5ZF5CWTxfZF6W3fYs6bDof2Q4vWGA8OIy80nADDIu3kAnk/uUbayTU33HB1a9UlwRKimlOGBdXuXnR0W/TSuej1K9Bbvkb1GIB+YIC7wxOiWFarlaysLOfPWVlZzgIU5x8zZswYAPLy8ti8eTP+/v6sXbuWBg0aOEvyNm/enJ07d3Lrrbfi7e1Ny5YtAYiNjWXdunUABAYGOs/bqVMnPvroozK9PlH96KxDmCkz4LtNEH4DxtMvoxq3cHdYQoirIGuwhBAAKP8AjP4jMMb/H9S+CZ08jaNjhqJ3bnN3aEJcJDIykgMHDnDo0CFsNhtpaWnExMQUOebkyZOYpgnA4sWLiYuLAxzf3G7fvh273Y7NZiMzM5NatWqhlCI6OprMzEwAtm3b5iyacezYMed5MzIyihTTEOJa6MICzOUpmH9/En7+DvXAQIyX35HkSohKTEawhBBFqNo3Yfz1Nfh2I+bHszEnvYhq2Q7V6xGUVeZri4rBYrEwdOhQJkyYgGmaxMXFUadOHVJSUoiMjCQmJobMzEzmzp2LUopGjRo5KwDGxsaybds25+hWs2bNnMnZgAEDmDJlCrNmzSIoKIgnn3wSgJUrV5KRkYHFYiEgIMB5vxDXQv+UgTnvfTh8EKLbYPQZhgq9zt1hCSGukdIulA4raa+RwsJCpkyZwq+//kpgYCCjRo0iPDzc+fiRI0cYPXo0ffr0oXv37hw5coSpU6dy/PhxlFLEx8fTuXNnlwLev3//FV5i9SJzal0j7eSa0MAADn/0Pvrzj8EwUF0eRN3dA+Xp6e7QKhR5P7nmaueyV2bSZ5WsOv7+6MMHHdMBf0iHiFoY/Uegbmt+2edUx3a6GtJOrpF2ck2ZrcFyZa+RdevW4e/vzzvvvMPGjRtJTk5m9OjRzsdnz55N8+bnPjgsFgsDBw6kXr165Obm8sILL9C0aVOZciFEBaO8fTDufwjdpiPmwpnoxXPQX6/B6DscmsaglHJ3iEIIUWnognz055+c+9Kq12BUfHeUh3xpJURVUuIaLFf2GsnIyKBDhw7AuakXZwfG0tPTCQ8PL5I81ahRg3r16gHg6+tLrVq1OHr0aGldkxCilKnrIrA8+SLG6H+AhyfmlFcx//0K+uA+d4cmhBCVgv5hC+bLiehl81C3t8R45T8YCb0kuRKiCipxBMuVvUbOP8ZiseDn50d2djZeXl4sWbKE8ePHs3Tp0mLPf+jQIfbs2UP9+vWLfVz2FLkysq+Ba6SdXHNRO7W7G90mjtMrFpGTkoT5j0T8uvXFv88jGL7+7gvUzeT9JIS4FH34IOb86fDjFri+DsYzr6Ia3e7usIQQZahMi1wsWLCALl26OEvhXigvL4833niDRx55BD8/v2KPkT1FrozMqXWNtJNrLtlObeJRjaPhkw85vTiZ0+tWOIpgtGqPMqpfcVJ5P7mmOq7BEtWXLshHr/zYMR3Q4oHqPQTVqauMWAlRDZSYYLm610hWVhahoaHY7XZOnz5NYGAgu3fvZvPmzSQnJ5OTk4NSCi8vLxISErDZbLzxxhvcddddtGrVqvSvTAhRplRQDdQjT6Pb34c59z30zLfQX650LNauW/yItBBCVHVaa/hhM+b8GZB1yFGFtfcQVI3Qkp8shKgSSkywzt9rxGq1kpaWxsiRI4scEx0dzfr162nYsCGbNm0iKioKpRSvvPKK85gFCxbg4+NDQkICWmumTZtGrVq16Nq1a+lflRCi3KibG2KMnYT+Zh3649mYE/6KuvNu1AMDUYHB7g5PCCHKjT60H3PedNj2LdxwI8aYCahbmrg7LCFEOSsxwXJlr5GOHTsyZcoUEhMTCQgIYNSoUZc9544dO9iwYQM33ngjzz77LAD9+/enRQvZVE+IykgZBqptPLp5a/Sy+egvlqO/3YjqPgDV4T6UxeLuEIUQoszo/Hz0ioXo1Z+Ahyeqz1BUx64oD9luVIjqyKV9sCoS2VPk8mQtiGuknVxzte2k9//uWNS9/QeoVRej33DUrU3LIMKKQd5PrqmOa7CkzypZZf790VrDd99gpiTB0cOOdai9h6BCrCU/+QpV5nYqT9JOrpF2ck2Z7YMlhBBXSt1wI8boV+C7TZgLkjDfGIeKbuv4Vjf0OneHJ4QQ10wf3Ic5733I/M7xRdKzr6MaNnZ3WEKICkASLCFEmVBKQYvWGI1boFcvRq9chP5pC+q+3qh7HkB5ebs7RCGEuGI6Pw/92QL06k/BywvV91FUXBeZCi2EcJIESwhRppSXN6prP3TrjuiFH6CXzEV/nYrx4DBoHutIxIQQooLTWsPWNMwFSXD0CKp1nGN7iuAa7g5NCFHBSIIlhCgXKjQc9fjz6F9+xJw/HfPdf8JtzRzrs66v4+7whBDikvSBvZjz3nOsK619E8ajY1ANbnN3WEKICkoSLCFEuVK3NsUY/3/o9SvRS5Mx/zESFdcV1a0fys/f3eEJIYSTzstFL09Bpy4FL29UvxFSGVUIUSJJsIQQ5U5ZLKhOXdEt70IvnoNeuxS9eT2q12BU644ow3B3iEKIakxrjc7YiF6QBMezUG06oXoNQgXJdEAhRMkkwRJCuI0KDEYN+gu6fQLmvPfRs/6N/vJzjP4jUDc3dHd4QohqSB/4A3Pue/DLj1DnZozHnkPVb+TusIQQlYgkWEIIt1N162M8NxG9+Uv0x7MwXx+DatsJ1VO+MRZClA+ddxq9LAW9dil4+6AeehzV/l6UIdMBhRBXRhIsIUSFoAwD1ToO3bzVmTUPy9Bbv0F16+8ogewhH1dCiNKntUanb0Av+gCOH0W1jXdMVw4MdndoQohKSv5iEUJUKMrHD9V7CPrOuzFTZqDEWnr5AAAgAElEQVQXJKG/Wu2oNnhbM3eHJ4SoQvS+3x3VAXf8BDdGYjz+AiryVneHJYSo5CTBEkJUSCqiNsbIl+DHLZgpMzDf+js0j8XoMxR1XYS7wxNCVGI69zR62Tz02mXg44ca8ASq3T0yHVAIUSokwRJCVFhKKbi9JcZtzdBrlqA/W4C57S+oe3uiEnqhvL3dHaIQohLRWjvWei76AE4eR915N+qBQajAIHeHJoSoQiTBEkJUeMrTC9W5Dzo2Dv3xLPTy+ei0VIw+QyG6rSMRE0KIy9B7/+eYDrjzZ7ipAcZT41A3N3B3WEKIKkgSLCFEpaGsYajhY9Dt78Oc9z7me/+CW5o4yrrXquvu8EQ5+/777/nggw8wTZNOnTrRo0ePIo8fPnyYd999l5MnTxIQEEBiYiKhoaEAfPTRR2zduhWtNU2aNGHIkCEopbDZbCQlJZGZmYlSin79+hEbG0thYSFTpkzh119/JTAwkFGjRhEeHu6OyxZXSJ/OQS+di/7iM/DzRw18yjFyJfvtCSHKiCRYQohKRzWMwhj/JnrDKvSnyZivPI3q0BnV/SGUf4C7wxPlwDRNkpKSGDduHKGhoYwdO5aYmBhq167tPGbOnDm0a9eODh06sG3bNubOnUtiYiI7duxgx44dTJ48GYDx48eTmZlJVFQUn3zyCcHBwbz99tuYpsmpU6cAWLduHf7+/rzzzjts3LiR5ORkRo8e7ZZrF67RWqO/+QL98SzIPoFqdy+qx8OoAJkOKIQoW/L1jRCiUlKGBaNDZ4zX3kW1uxf9xQrMcY9jbliFNu3uDk+Usd27dxMREUHNmjXx8PCgTZs2bNmypcgxe/fupXHjxgBERUWRkZEBONb2FRQUYLPZKCwsxG63ExzsKMn9xRdfOEfCDMMgKMjxx3hGRgYdOnQAIDY2lm3btqG1Lo9LFVdB/7EH818voD/4PwgNx3hxMsbDT0pyJYQoFzKCJYSo1FRAEGrAE+i77sWc/z56zlT0hlWOaYNSbrnKOnr0qHO6H0BoaCi7du0qckzdunVJT0+nc+fOpKenk5ubS3Z2Ng0bNiQqKooRI0agtSYhIYHatWuTk5MDQEpKCpmZmdSsWZOhQ4cSEhJS5PUsFgt+fn5kZ2c7E7CzUlNTSU1NBWDixImEhYWVZTNUCR4eHqXWTmZONqfmTSd35SeogCACnxqLT8cuVWI6YGm2U1Um7eQaaaeyJQmWEKJKUDfWw3j2n2c2DJ2FOfE5VGycY8PQEKu7wxNuMHDgQGbOnMn69etp1KgRVqsVwzA4ePAg+/btY9q0aQC8+uqrbN++nVq1apGVlcUtt9zC4MGDWb58OXPmzCExMdHl14yPjyc+Pt7585EjR0r9uqqasLCwa24nbZrnpgOeyka1T0D1GECOfyA5R4+WTqBuVhrtVB1IO7lG2sk1N9xww1U9TxIsIUSVoZRCtWqPvr0lesVC9JpP0d9tQnXri+rUDeXh6e4QRSmxWq1kZWU5f87KysJqtV50zJgxYwDIy8tj8+bN+Pv7s3btWho0aICPjw8AzZs3Z+fOndx66614e3vTsmVLwDEVcN26dUVeLzQ0FLvdzunTpwkMDCyPSxUl0L//F3Pue/DfXyDyVoxRL6NujHR3WEKIaqzyj5kLIcQFlI8vRs9BGP+YArc2cYxovTwSve1bd4cmSklkZCQHDhzg0KFD2Gw20tLSiImJKXLMyZMnMU0TgMWLFxMXFwc4vrndvn07drsdm81GZmYmtWrVQilFdHQ0mZmZAGzbts1ZNCM6Opr169cDsGnTJqKiomR7ADfTOacwk6dhvvZXOHQA9cjTGM9NlORKCOF2SleyVbr79+93dwgVmgz5ukbayTVVpZ30T99ipsyAP/c5Ni5+cCgq/OqG/YtTVdqprF3tVItL2bp1K7Nnz8Y0TeLi4ujZsycpKSlERkYSExPDpk2bmDt3LkopGjVqxLBhw/D09MQ0TWbMmMH27dsBaNasGYMHDwYcpd2nTJlCTk4OQUFBPPnkk4SFhVFQUMCUKVPYs2cPAQEBjBo1ipo1a5YYo/RZJbvS3x9tmui0teiPZ0POKVRcZ9T9D6H8qnYFUfmccY20k2uknVxztf2WJFhVjPzCuEbayTVVqZ20rRC9dhl6WQrYC1H3PIC6rzfKx/eaz12V2qkslXaCVRlIn1WyK/n90b/txkyeBnt2Qv1GGP0fQ91Yr4wjrBjkc8Y10k6ukXZyTZmuwSppM8eSNmA8cuQIo0ePpk+fPnTv3t2lcwohRGlSHp6oe3uiW7VHf/yhY41W2jpU70dQLdvJdC8hKjCdk41ePAe9YRUEBqOGjEK1jpPfWyFEhVTiGqyzmzm++OKLvPXWW2zcuJG9e/cWOeb8DRi7dOlCcnJykcdnz55N8+bNr+icQghRFlRIKMaw0RjPT4SgEPSMNzAnjUX/scfdoQkhLqBNE3PDKsxxj6O/Wo3q2BXj1Xcx2nSU5EoIUWGVmGC5spnj5TZgTE9PJzw83LlQ2NVzCiFEWVL1b8P422TUwKfgwF7MV0djJk9Dnzrp7tCEEIDeswvzn8+i50yF6+tgjH8Lo99wlJ+/u0MTQojLKnGKoCubOV5qA0YvLy+WLFnC+PHjWbp06RWd8yzZtPHKyMZxrpF2ck21aKeeAzDv6cap+TPIXbkYMr7Gf8AIfO++H2WxuHSKatFOQpQTfeqkYzrgV6shKAQ17BlUq/YyYiWEqDTKdB+sBQsW0KVLF+deI1dDNm28MrJo0TXSTq6pVu3UYxBGTDvM+dPJfm8y2Z99jNF/BKphVIlPrVbtdA2qY5EL4Tpt2tFfrUEvngO5OahO3VHd+6N8/dwdmhBCXJESEyxXN3MsbgPG3bt3s3nzZpKTk8nJyUEphZeXF/Xq1SvxnEIIUd5U7Zsw/voafLsRc+FMzEljHQUweg9B1Qgt+QRCiKuif93h2Cz4t93QsDHGQ4+hatV1d1hCCHFVSkywzt/M0Wq1kpaWxsiRI4scc3YDxoYNGxbZgPGVV15xHrNgwQJ8fHxISEjAbreXeE4hhHAHpRTE3InR5A705x87/v2QjurcB3V3D5Snp7tDFKLK0NknOJEyHTN1GQRbUY/+Vap6CiEqvRITLIvFwtChQ5kwYYJzM8c6deoU2cyxY8eOTJkyhcTEROcGjFdzTiGEqCiUtzfq/ofQbTpiLpzpWBPy9RqMvsOhaYz8ASjENdCmHf3lKvSnH5GXn+vYl65bX5SPTAcUQlR+stFwFSNrQVwj7eQaaadzdOZ3mPNnwIE/oHE0Rt9HURG1AGknV1XHNVjSZ11M//cXx3TA3/8LtzQh9KkXOO4b6O6wKjz5nHGNtJNrpJ1cU6YbDQshRHWnbmuO8fe30V98hl42D/PlRFR8d1TXB90dmhCVgj55HP3JbPTGtRASihrxLCrmTjyuuw7kDz0hRBUiCZYQQrhIeXig7r4f3bIdevGH6FWfoDetJ/eRv6CjomXaoBDF0HY7+suV6CXJkJ+HurcnqmtflI+vu0MTQogyIQmWEEJcIRVcA/XI0+h2CZjz3ufk269A5K0Y/R9D1Y10d3hCVBh6dyZm8nuwdw80ut3xO3J9bXeHJYQQZUoSLCGEuEqq3i0YYycR8FM6J2dPxZzwDOque1A9HkYFBrs7PCHcRp88hl40C/3NF1AjDOPx56FFGxnlFUJUC5JgCSHENVCGgW+nrpxq0AS9bD76i+XojK9R3QegOtyHsljcHaIQ5Ubb7Y51ikvnQkEB6r5eqC59Ud4+7g5NCCHKjSRYQghRCpSfP6rvMPRdd2POn46e/z76q1UY/Yajbm3q7vCEKHN65zZHdcB9v8FtzTH6D0dFyHRAIUT1IwmWEEKUInXDjRijX4HvNmEuSMJ8Yxwqui2qz1BU6HXuDk+IUqePH0Uv+gC9+UuwXofxxAvQvLVMBxRCVFuSYAkhRClTSkGL1hiNW6BXL0avXIT+aQvqvt6ODVW9vN0dohDXTNts56YD2gpRnR9Ede6D8pb3txCiepMESwghyojy8kZ17Ydu3RG98AP0krnor1Mx+j4KzVrJN/yi0tI7tmHOnQb7f3dsvN1vOKpm9dtIWgghiiMJlhBClDEVGo56/Hn09h8w50/H/M/rjjUq/R5FXV/H3eEJ4TJ9PMvxZUH6BggNx3jqRbhdviwQQojzSYIlhBDlRDW6HePvb6PXr0QvTcb8x0hUx66orv1Qfv7uDk+IS9I2G3rtMvSy+WC3OTYKTugt0wGFEKIYkmAJIUQ5UhYLqlNXdMu70IvnoFOXojd/ieo5GNU6DmUY7g5RiCL09h8w570PB/6AJjGO6YDh17s7LCGEKDWFdpPjeXaO59k4cea/x3PtjLzh6qY+S4IlhBBuoAKDUYP+gm6fgDnvffSst9FfrsToPwJ1c0N3hycE+ugRR3XALV9BWE2Mv4xD3d7S3WEJIYRLCuwmx3PPJEt5NmcCdTzPzvHcovflFJjFnmPkPVf32pJgCSGEG6m69TGem4je/CX641mYr49BtY1H9RyICqrh7vAqtO+//54PPvgA0zTp1KkTPXr0KPL44cOHeffddzl58iQBAQEkJiYSGhoKwEcffcTWrVvRWtOkSROGDBmCUoqXX36ZY8eO4eXlBcC4ceMIDg5m/fr1zJkzB6vVCkBCQgKdOnUq3wsuJ9pW6BhZXZ4Cponq1h+V0FOqXwoh3C7fZl6QJF2QQJ133+nC4pMmf0+DEF8PQnws3BTiTYiPHyE+HoT4ehDsY3HcPvPfqyUJlhBCuJkyDFTrOHTzVujlKejUZeitaY4/bOO6oDzko/pCpmmSlJTEuHHjCA0NZezYscTExFC79rmNbefMmUO7du3o0KED27ZtY+7cuSQmJrJjxw527NjB5MmTARg/fjyZmZlERUUBMHLkSCIjIy96zTZt2jBs2LDyuUA30ZnfO6YDHtwLt7fE6Pso6roId4clhKjC8mxmkcToWO550/TOH3nKtZNrKz5pCvAynIlRPas3IT7+ziTJkTw5bgf7WPCylP1UfOm1hRCiglA+fqjeQ9B33o2ZMgO9IAn91WrHmpfbmrk7vApl9+7dREREULNmTcCR/GzZsqVIgrV3714GDRoEQFRUFJMmTQIc+5QVFBRgs9nQWmO32wkODi7/i6hA9NHDmAuS4Ns0uC4CI3E8qukd7g5LCFEJaa3JtZmOJOmCUaZjueem6Z04c1+eTRd7nkBvizNJqm/1cY4yXZg4BXt74GmpWJVMJcESQogKRkXUxhj5Evy4BTNlBuZbf4fmsRgPDkOF1XR3eBXC0aNHndP9AEJDQ9m1a1eRY+rWrUt6ejqdO3cmPT2d3NxcsrOzadiwIVFRUYwYMQKtNQkJCUUSs//85z8YhkGrVq3o1auXswT55s2b2b59O9dffz2DBw8mLCzsorhSU1NJTU0FYOLEicUeU5HowgJOL53PqYWzQJv49x+Of4+HynU6oIeHR4Vvp4pA2sk10k6uudJ20lqTU2Dn6OlCjp4u4NiZ/x49Xei8nXW6kGNn7ssvZqRJAcG+nlj9PLH6eXFjaIDzdg0/x/2hZ27X8PXEoxxGmsqKJFhCCFEBKaUcU7Rua4Ze/Sl6xULMvz+FurcnKqGXlMd2wcCBA5k5cybr16+nUaNGWK1WDMPg4MGD7Nu3j2nTpgHw6quvsn37dho1asTIkSOxWq3k5ubyxhtvsGHDBtq3b090dDRt27bF09OTNWvWMHXqVF566aWLXjM+Pp74+Hjnz0eOHCm3671S+ufvHNMB/9wHzWIx+g4jL6wmeSezgexyiyMsLKxCt1NFIe3kGmkn14SFhXH48GFyCk3n9DvnlLzzRpkcVfUctwvsF480Gcox0lTjzPS8W6zehNTyv2gtU4ivB8HeFizG5UaaTCAP8vI4nldml35FbpAqgkIIUfUoTy9UlwfRrTuiP56FXj4fnbYW48Gh0KJNtd3g1Wq1kpWV5fw5KyvLWYDi/GPGjBkDQF5eHps3b8bf35+1a9fSoEEDfHx8AGjevDk7d+50JmEAvr6+3HnnnezevZv27dsTGBjoPG+nTp346KOPyvoSy4zOOoy5YAZs/QbCr8d4+iVU42h3hyWEKAVaa04VmJdMls5O2csu2EPW6QJsZvFJU7C35UzRBw9qB3kVWcd0fuIUWGLSVD1JgiWEEJWAsoahho9xlnU3p/0/uLWpY31WrbruDq/cRUZGcuDAAQ4dOoTVaiUtLY2RI0cWOeZs9UDDMFi8eDFxcXGA45vbtWvXYrfb0VqTmZlJ586dsdvt5OTkEBQUhM1m49tvv6VJkyYAHDt2jBo1HFUdMzIyikwprCx0YSF69WL0igUAqB4Po+55AOXp6ebIhBCXY2rNqXx70TLjZ5KlY+etZTqea+dEvo3i6kBYFASflxg1qOmPr7KdS5Z8zyVOgd4WjGr65V1pkQRLCCEqEdWwMca4t9BfrUJ/moz5ytOOSoPd+qP8A9wdXrmxWCwMHTqUCRMmYJomcXFx1KlTh5SUFCIjI4mJiSEzM5O5c+eilKJRo0bOCoCxsbFs27bNObrVrFkzYmJiyMvLY8KECdjtdkzTpEmTJs7pfitXriQjIwOLxUJAQABPPvmk2679auifvsWc/z4cOgAtWjvW84WGuzssIaotU2tO5hctAlGkcl6unWNnkqmTeTaKmZ2Hh3E2aXL8uynE56Jk6eztAC+jSNIkUynLltJaF1+6o4Lav3+/u0Oo0OQXxjXSTq6RdnKNu9pJnzqJXpKM/nIV+Aegeg5Cte2EMizlHosrrnYue2Xm7j5LH/kTM2UGfL8ZatZybGQd1dytMV1IPmdcI+3kGne2k93UZOefKTVezGa2zsp5uTZO5NspZnYeHoYixMdCjTPV8oIvmJJ3/jS9AC/jqqeJy/vJNbIGSwghqhkVEIQa8AT6rnsx57+P/nAK+svPHX9ER97q7vCEG+nCAvSqT9ArFoFSjuQ7/n6ZDijEFbKbmhP5FyRLxSROx/NsZF8iafKyKGeyFObnSYNQn4vWMgWfSZz8Pa8+aRIVh0sJ1vfff88HH3yAaZp06tSJHj16FHm8sLCQKVOm8OuvvxIYGMioUaMIDw9n9+7dvPfee87j+vTpQ8uWLQFYvnw569atQylFnTp1ePLJJ/Hy8irFSxNCiOpB3VgP49l/otM3oBfNwpz4HCo2DtVrMCrEWvIJRJWif9yCOX86HD6Iim6LenAoynqdu8MSosKwmdpZGe+iZCm36Aa32fl2ipvq5W1Rzj2ZIgI8uTXMl+DzRp7O36fJ10OSpuqmxATLNE2SkpIYN24coaGhjB07lpiYmCILfNetW4e/vz/vvPMOGzduJDk5mdGjR1OnTh0mTpyIxWLh2LFjPPvss0RHR3PixAlWrlzJW2+9hZeXF2+++SZpaWl06NChLK9VCCGqLKUUqlV79O0t0SsWotd8iv5uE6pbX1SnbigPGbmo6vThg47pgD+kQ0RtjNGvyAbVotootGuO59k4bM/mt4Onik2WjuU6So5nFxRTBQLw8TCcydENQV7cFn5BsnTemiZfz8q7R5MoeyUmWLt37yYiIoKaNR2bW7Zp04YtW7YUSbAyMjLo06cP4Fg8PHPmTLTWeJ+3T0thYWGR7N00TQoKCrBYLBQUFDirMwkhhLh6yscX1XMQ+s54zJQk9KJZ6K/WYPR7VEpxV1G6IB/9+cfolR+DxYLq/Ygk1aJKKLSbzpGlY7kXTMnLPbc/0/E8G6cukTT5ehiE+Dr2aaoT7E2Tmn7OkacLEycfD0maROkoMcE6evQooaGhzp9DQ0PZtWvXJY+xWCz4+fmRnZ1NUFAQu3bt4t133+Xw4cMkJiZisViwWq1069aNJ554Ai8vL26//XZuv/32Yl8/NTWV1NRUACZOnCi7c5dAdjB3jbSTa6SdXFMh2yksDP7xNvnfppGd9Db2t/+B9x13EjBkJB7XV74S46J4+od0x3TAI3+i7rgL1WcoqkZoyU8Uwk3ybSZFN7G1n0mezp+y5ygGkVNYfNLk72k4S47fGOLN7T5+zs1s64TXwCg47UygvCVpEm5Q5kUuGjRowJtvvsnevXuZOnUqzZo1o6CggC1btjB16lT8/Px488032bBhA+3atbvo+fHx8c4yuYBUPCmBVIVxjbSTa6SdXFOh26luQ/Tf/w+VupT85QvIH/mQY++jzn1Q3j7lGkp1rCJYVvShA47E6qcMuL4Oxl9fQ93a1N1hiWoqz2YWKTd+yWIQuXZyi9ukCQjwMpyjSTfX8CbE19+ZJNU4r3pesI8FL8ulk6awsFCOHKlUBbJFFVRigmW1WsnKynL+nJWV5dzp/sJjQkNDsdvtnD59usiu9wC1a9fGx8eHP/74g0OHDhEeHk5QUBAArVq1YufOncUmWEIIIa6N8vBEJfRCx3ZAf/yhY41W2jpUnyGOUQ9ZfF1p6Px89OeL0J9/AhYPx//Djt1QHlIUWJSu3ELTuZntRYnTBeub8mzFJzSB3hZnklTf6lOk8MPZZKmGrwfB3h54WuRzSFQdJX4iR0ZGcuDAAQ4dOoTVaiUtLY2RI0cWOSY6Opr169fTsGFDNm3aRFRUFEopDh06RGhoKBaLhcOHD7N//36uu+46TNNk165d5Ofn4+XlxU8//URkZGSZXaQQQghQIaGoYaPR7e/FnDcdPX0yev0KjP6Poerc7O7wxGVoreH7zY4iFlmHUC3bo/o8ggqR6YDCNVprcm3meRvYnkuSThQz8pRfzM62ivOSJl8PGob5nreWyeKcpne2JLmHIUmTqJ5KTLAsFgtDhw5lwoQJmKZJXFwcderUISUlhcjISGJiYujYsSNTpkwhMTGRgIAARo0aBcAvv/zCp59+isViwTAMhg0bRlBQEEFBQcTGxvL8889jsVi46aabikwDFEIIUXZU/dsw/jYZ/XUqevEczFdHo9onoO5/CBUQ5O7wxAX0n/sx578P27ZCrboYY15H3dLY3WGJCkBrTc6ZkaYTuecKQBy7YGre2WIQBZdImoLOS5JuDfNyjCoVkzgFe1uwSNIkRImU1rpSTVTdv3+/u0Oo0Cr0WpAKRNrJNdJOrqnM7aRzTqGXzkWvXwG+/qgeA1Dt7kUZllJ/req4Buta+iydn+eYzrl6MXh4OhLgDl2q3HTAyvz7Uxa01pwqMM+NKJ1JnAoML/YfzS4yZe9Enp3CYna2NRQEeztGmYLPT5Kc+zSduy+wiiVN8n5yjbSTa66236pan9JCCCGuiPIPQPUfgb7rHsz509HJ09BfrsLoPwLVMMrd4VVLWmv47hvMlCQ4ehgV2wHV6xHZNLoSM7XmVL69aJnxi9Y3nSk9nm+juDoQFkWRZOnGEC/nmqbgCxKoQG8LhqytFMJtJMESQgiBqn0Txl9fg283Yi6ciTlprGOdT+9HpOx3OdIH92HOex8yv3NMB3z2n5LoVlCm1mSflzQ5NrG1XzTydLbkeDGz8/AwINj7XNGHm0K8i2xme/7I0021anL0vKJjQoiKSxIsIYQQAI5qgjF3YjS5w1mpTv+wGdXlQVT8/ShP2bi2rOj8PPRnKejVS8DLC9VvOKpDZ5Sl9Kdqikuzm2eTpqKV845dkCw5RprsFDM7Dw9DORMjq68H9ZzV886uZTq3wW2Al+FyFU8ZkRKi8pAESwghRBHK2xt1/wB0m06YC2aiP/kQ/dVqjL7DUbff4e7wqhSttWPUcMFMOHYE1bojqvdgVFANd4dWZdhNzYn8i/dkOnFmSt6x8+7LvkTS5GVRzsp4YX6e50qO+57bpyn4zG1/T9eTJiFE1SQJlhBCiGKp6yKwPPUi+ufvMOdPx5zyKjSJwXhwGCqilrvDq/T0gb2Y896D7T9AnZsxRoxB1b/N3WFVCjZTOyvjXbSZ7dkE6kw58ux8O8VV8/KyqDMFHyxEBHhya5jvubVM540y1fC14OshSZMQwnWSYAkhhLgsFdUc46V/o9ctRy+bh/lyIiq+O6rrgygfP3eHV+novFz08hR06lLw8kb1H4Fqf1+1nw5YaNecyC+6gW2R2+clUNn59mLP4eOhzhR98OD6IE8ahZ+/T5OHc31TsI8kTUKIsiMJlhBCiBIpDw/UPT3QrdqjF3+IXvUJetN6x3S2Vh3kD1UXaK3RGV+jF8yE41motp1QPQejgkLcHVqZKbSb51XMO29N09mKeXk2sgt/Iysnn1MFxZTOA3w9DOeIUu0gLxqHn9vM9sLEycfDKOcrFEKIi0mCJYQQwmUquAbqkafR7RIw572PTnoLvX4lRv/HUHUj3R1ehaX3/+6oDvjLj3BjPYzHn0dF3urusK5Kvs0sdi1T0U1uHcUgcgqLT5r8PQ1nyfF6oX5EXed9UbJ0NoHylqRJCFHJSIIlhBDiiql6t2CMnYT+Zh3649mYE55B3XUPqsdAVGCQu8OrMHTeafSy+ei1y8DbFzXg8TLbyPla5NnMC/ZkOn9905nKeWeq6eUWt0kT4O9lUONMgnRzDW9CfP2LlBl3JlC+Frws55Im2fBUCFHVSIIlhBDiqijDQLWNRzdv7UgivliOzvgadf8AWVN0hjnuSThx1JF8PjAQFRhcbq+dW2gWkyzZipmyZyfvEklToNeZkSZfDyKd5cbPFYE4f4NbT4uMNAkhBEiCJYQQ4hopP39U32Hou+7GnD8dPe999IZVGP1HoG5p4u7w3CvEivHkWFS9W675VFprcm1m8YUfLrwv10Z+MTvbKiDQ2+IcUWoY6kuwr8U58hTic259U5C3B54WWVsnhBBXShIsIYQQpULdcCPG6Ffgu02YC5IwJ/8NFXMnqvcQVOh1pf5633//PR988AGmadKpUyd69OhR5PHDhw/z7rvvcvLkSQICAkhMTCQ0NBSAjz76iK1bt6K1pkmTJgwZMgSlFC+//DLHjh3Dy8sLgHHjxhEcHExhYSFTpmISOUUAAA8DSURBVEzh119/JTAwkFGjRhEeHl5ijMaLky47HVBrTc6ZkaYTuRdvcHt2TdPZkuQFl0iags4bSbo1zOuiZOns7WBvCxZDkiYhhChLkmAJIYQoNUopaNEao3EL9KrF6JWL0D+mo+7rDSOeKbXXMU2TpKQkxo0bR2hoKGPHjiUmJobatWs7j5kzZw7t2rWjQ4cObNu2jblz55KYmMiOHTvYsWMHkydPBmD8+PFkZmYSFRUFwMiRI4mMLFqwY926dfj7+/POO++wceNGkpOTGT16dIlxbjucV2QqnqMoRNH1TYXF7GxrKAjytlDD11FyvFaQV9G1TOclTkGSNAkhRIUiCZYQQohSp7y8Ud36odt0xFw4E71kbqkmWLt37yYiIoKaNWsC0KZNG7Zs2VIkwdq7dy+DBg0CICoqikmTJjliU4qCggJsNhtaa+x2O8HBl18blZGRQZ8+fQCIjY1l5syZaK1LLE8/LvUP521D4aycF+LjQZ1gL+eapvPXMoX4ehDoJUmTEEJUVpJgCSGEKDMqNBzL4y+gt/9Qquc9evSoc7ofQGhoKLt27SpyTN26dUlPT6dz586kp6eTm5tLdnY2DRs2JCoqihEjRqC1JiEhoUhi9p///AfDMGjVqhW9evVCKVXk9SwWC35+fmRnZxMUVLRiYmpqKqmpqQBMnDiRf/dsTA0/T6z/v737j4m6/uMA/rwD5Idcxd2FCBMZSI6RfTNugjM1lLkmtlwzm1kbGVvljLFVS2YarWg5ok0GDTZFR5sFy1X+Yf1TkDKFBqEFgsiPEEWG3EEgeHH3+by/f7jvfePrV3l/+X7uPnfyfGxu3vFh9/o8B/fc++7N5yIW4IGwYBj5eWF3CA4OhtVq1XsMv8ec5DAnOczJu7jAIiIirzOk/MPnj/nyyy+jqqoK9fX1SElJgdlshtFoxNDQEK5du4aKigoAwIcffoiOjg6kpKQgLy8PZrMZt27dQklJCU6fPo3169dLP2ZWVhaysrI8t5eGuwHhhnvyFhyTmp/ifYGXaZfDnOQwJznMSU5sbOycvo8LLCIiCjhmsxl2u91z2263w2w233HM22+/DQBwOp1oamrCwoUL8eOPPyI5ORlhYWEAgJUrV6Krq8uzCAOA8PBwPPnkk+ju7sb69es9j2exWKAoCqampmAymXx0tkREFEj4oRVERBRwkpKScP36dQwPD8PtduPs2bOw2WwzjhkfH4eq3v58p2+++QaZmZkAbr9y29HRAUVR4Ha7cfHiRcTFxUFRFIyPjwMA3G43WlpasGTJEgBAWloa6uvrAQCNjY1ITU2d9e+viIhofuI7WEREFHCCgoKwa9cuFBUVQVVVZGZmYsmSJaipqUFSUhJsNhsuXryI48ePw2AwICUlBa+++iqA2xepaGtr87y79fjjj8Nms8HpdKKoqAiKokBVVaxYscKz3W/Dhg0oKyvDm2++icjISOTn5+t27kRE5N8MQog7rw/rxwYHB/Uewa9xT60c5iSHOclhTnLmupc9kLGzZsffHznMSQ5zksOc5My1t7hFkIiIiIiISCNcYBEREREREWmECywiIiIiIiKNcIFFRERERESkES6wiIiIiIiINBJwVxEkIiIiIiLyVwH1DtbevXv1HsHvMSM5zEkOc5LDnOTMt5zm2/nOFXOSw5zkMCc5zEnOXHMKqAUWERERERGRP+MCi4iIiIiISCNBhYWFhXoP8b9ITEzUewS/x4zkMCc5zEkOc5Iz33Kab+c7V8xJDnOSw5zkMCc5c8mJF7kgIiIiIiLSCLcIEhERERERaSRY7wH+bmRkBOXl5RgbG4PBYEBWVhY2b9484xghBI4ePYrW1laEhoZi9+7d8+4tTpmczpw5g++++w5CCISHhyM3NxcJCQn6DKwTmZz+pbu7G++99x7y8/ORkZHh40n1JZtTe3s7jh07BkVRYDKZ8MEHH+gwrX5kcpqamkJpaSnsdjsURcEzzzyDzMxMnSbWx/T0NN5//3243W4oioKMjAxs3759xjEulwtlZWXo7e2FyWRCfn4+oqOjdZr4/8PeksPeksPeksPeksPekuOV3hJ+xOFwiJ6eHiGEEFNTUyIvL08MDAzMOKalpUUUFRUJVVXFpUuXREFBgR6j6komp87OTjExMSGEEOLXX39lTnfJSQghFEURhYWF4uOPPxbnzp3z9Zi6k8np5s2bIj8/X9y4cUMIIcTY2JjP59SbTE4nTpwQX3zxhRBCiD///FPk5OQIl8vl81n1pKqquHXrlhBCCJfLJQoKCsSlS5dmHPPDDz+IyspKIYQQDQ0N4rPPPvP5nFphb8lhb8lhb8lhb8lhb8nxRm/51RbBqKgoz6t64eHhiIuLg8PhmHFMc3Mz1q1bB4PBgEceeQSTk5MYHR3VY1zdyOS0fPlyREZGAgCSk5Nht9t9PqfeZHICgO+//x7p6el44IEHfD2iX5DJqaGhAenp6bBarQCABx980Odz6k0mJ4PBAKfTCSEEnE4nIiMjYTT61dOs1xkMBoSFhQEAFEWBoigwGAwzjmlubsZTTz0FAMjIyEBbWxtEgP45MHtLDntLDntLDntLDntLjjd6y28THB4eRl9fH5YtWzbjfofD4fllAQCLxfJfn3zmi7vl9Hc//fQTVq5c6cOp/M+9fp5++eUXbNq0SafJ/Mvdcrp+/Tpu3ryJwsJCvPvuu/j55591mtA/3C2np59+GteuXcNrr72Gt956C6+88sq8KyoAUFUV77zzDnJzc7FixQokJyfP+LrD4YDFYgEABAUFISIiAhMTE3qMqin2lhz2lhz2lhz2lhz21r1p3Vt+maDT6URJSQlycnIQERGh9zh+SyantrY21NXVYefOnT6ezn/cK6djx45h586d8/LJ5D/dKydFUdDX14e9e/di3759OHHiBAYHB3WaVF/3yunChQtYunQpKisrUVxcjCNHjmBqakqnSfVjNBpRXFyMiooK9PT04MqVK3qP5HXsLTnsLTnsLTnsLTnsrdlp3Vt+dZELAHC73SgpKcHatWuRnp5+x9fNZjNGRkY8t+12O8xmsy9H9Auz5QQA/f39qKysREFBAUwmk48n9A+z5dTT04NDhw4BAMbHx9Ha2gqj0YhVq1b5elRdzZaTxWKByWRCWFgYwsLCkJKSgv7+fsTGxuowrX5my6murg5bt26FwWBATEwMoqOjMTg4eM9X6u9nCxcuRGpqKs6fP4/4+HjP/WazGXa7HRaLBYqiYGpqKqCfo9hbcthbcthbcthbcthb/xutesuvXv4QQqCiogJxcXHYsmXLfz3GZrPh9OnTEEKgq6sLERERiIqK8vGk+pLJaWRkBJ9++in27Nkz755M/kUmp/Lycs+/jIwM5ObmzruSkv296+zshKIo+Ouvv9Dd3Y24uDgfT6ovmZysVit+//13AMDY2BgGBwcD9up4czU+Po7JyUkAt6/M9Ntvv93xs5KWlob6+noAQGNjI1JTU+/Y7x4o2Fty2Fty2Fty2Fty2FtyvNFbfvVBw52dnThw4ADi4+M9Q+/YscPzyt+mTZsghMCRI0dw4cIFLFiwALt370ZSUpKeY/ucTE4VFRVoamry7PsPCgrCJ598otvMepDJ6e/Ky8uRlpY27y53K5vTyZMnUVdXB6PRiA0bNiA7O1u3mfUgk5PD4cDnn3/uuYDBs88+i3Xr1uk2sx76+/tRXl4OVVUhhMDq1auxbds21NTUICkpCTabDdPT0ygrK0NfXx8iIyORn5+PRYsW6T36nLC35LC35LC35LC35LC35Hijt/xqgUVERERERBTI/GqLIBERERERUSDjAouIiIiIiEgjXGARERERERFphAssIiIiIiIijXCBRUREREREpBEusIgC0PDwMLZv3w5FUfQehYiIaFbsLZpPuMAiIiIiIiLSCBdYREREREREGgnWewCi+4XD4UBVVRU6OjoQFhaG7OxsbN68GbW1tRgYGIDRaERraysWL16MN954AwkJCQCAq1ev4vDhw/jjjz9gNpvx4osvwmazAQCmp6fx1VdfobGxEZOTk4iPj8f+/fs9j3nmzBnU1NRgenoa2dnZeO655/Q4dSIiCkDsLSLv4DtYRBpQVRUHDx5EQkICKisrceDAAZw6dQrnz58HADQ3N2P16tWoqqrCmjVrUFxcDLfbDbfbjYMHD+Kxxx7D4cOHsWvXLpSWlmJwcBAAUF1djd7eXnz00Uc4evQoXnrpJRgMBs/jdnZ24tChQ9i/fz++/vprXL16VZfzJyKiwMLeIvIeLrCINNDT04Px8XFs27YNwcHBWLRoETZu3IizZ88CABITE5GRkYHg4GBs2bIFLpcLly9fxuXLl+F0OrF161YEBwfj0UcfxRNPPIGGhgaoqoq6ujrk5OTAbDbDaDRi+fLlCAkJ8Tzu888/jwULFiAhIQFLly5Ff3+/XhEQEVEAYW8ReQ+3CBJp4MaNGxgdHUVOTo7nPlVVkZKSAqvVCovF4rnfaDTCYrFgdHQUAGC1WmE0/vu1jocffhgOhwMTExNwuVyIiYm56+M+9NBDnv+HhobC6XRqeFZERHS/Ym8ReQ8XWEQasFqtiI6ORmlp6R1fq62thd1u99xWVRV2ux1RUVEAgJGREaiq6imrkZERLF68GCaTCSEhIRgaGvLseyciItICe4vIe7hFkEgDy5YtQ3h4OL799ltMT09DVVVcuXIF3d3dAIDe3l40NTVBURScOnUKISEhSE5ORnJyMkJDQ3Hy5Em43W60t7ejpaUFa9asgdFoRGZmJqqrq+FwOKCqKrq6uuByuXQ+WyIiCnTsLSLvMQghhN5DEN0PHA4Hqqur0d7eDrfbjdjYWLzwwgvo7OyccTWmmJgYvP7660hMTAQADAwMzLga044dO7Bq1SoAt6/GdPz4cZw7dw5OpxMJCQnYt28fxsbGsGfPHnz55ZcICgoCABQWFmLt2rXYuHGjbhkQEVHgYG8ReQcXWEReVltbi6GhIeTl5ek9ChER0azYW0T/H24RJCIiIiIi0ggXWERERERERBrhFkEiIiIiIiKN8B0sIiIiIiIijXCBRUREREREpBEusIiIiIiIiDTCBRYREREREZFGuMAiIiIiIiLSCBdYREREREREGvknaBRHCyUXmn8AAAAASUVORK5CYII=\\n\",\n \"text/plain\": [\n \"<Figure size 864x576 with 2 Axes>\"\n ]\n },\n \"metadata\": {},\n \"output_type\": \"display_data\"\n },\n {\n \"name\": \"stdout\",\n \"output_type\": \"stream\",\n \"text\": [\n \"Log-loss (cost function):\\n\",\n \"training (min: 0.037, max: 0.181, cur: 0.037)\\n\",\n \"validation (min: 0.048, max: 0.066, cur: 0.048)\\n\",\n \"\\n\",\n \"accuracy:\\n\",\n \"training (min: 0.958, max: 0.989, cur: 0.989)\\n\",\n \"validation (min: 0.981, max: 0.986, cur: 0.986)\\n\",\n \"\\n\",\n \"Epoch 00003: val_loss improved from 0.05093 to 0.04835, saving model to model_weights.h5\\n\",\n \"38367/38367 [==============================] - 228s 6ms/sample - loss: 0.0368 - accuracy: 0.9887 - val_loss: 0.0484 - val_accuracy: 0.9856\\n\",\n \"CPU times: user 15min 48s, sys: 27.2 s, total: 16min 15s\\n\",\n \"Wall time: 11min 41s\\n\"\n ]\n }\n ],\n \"source\": [\n \"%%time\\n\",\n \"#View Loss/Accuracy graph at each interation\\n\",\n \"chkpt = ModelCheckpoint(\\\"model_weights.h5\\\", monitor='val_loss',verbose=1, save_best_only=True, save_weights_only=True, mode='min')\\n\",\n \"\\n\",\n \"early_stopping = EarlyStopping(monitor='val_accuracy', min_delta=0, patience=1, verbose=0, mode='max', baseline=None, restore_best_weights=False)\\n\",\n \"\\n\",\n \"callbacks = [PlotLossesCallback(), chkpt, early_stopping]\\n\",\n \"\\n\",\n \"history = model.fit(\\n\",\n \" x=x_train,\\n\",\n \" y=y_train,\\n\",\n \" validation_data=(x_test,y_test),\\n\",\n \" batch_size=32, \\n\",\n \" epochs=3,\\n\",\n \" callbacks=callbacks,\\n\",\n \" verbose=1\\n\",\n \")\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 19,\n \"metadata\": {\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 52\n },\n \"colab_type\": \"code\",\n \"id\": \"6euqX7UHplG7\",\n \"outputId\": \"7222c24c-52c5-454b-a5d4-03d6df4173f0\"\n },\n \"outputs\": [\n {\n \"name\": \"stdout\",\n \"output_type\": \"stream\",\n \"text\": [\n \"9592/9592 [==============================] - 12s 1ms/sample - loss: 0.0484 - accuracy: 0.9856\\n\"\n ]\n },\n {\n \"data\": {\n \"text/plain\": [\n \"[0.04835232572507321, 0.9855588]\"\n ]\n },\n \"execution_count\": 19,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"#Step 8: Evaluate model\\n\",\n \"model.evaluate(x_test, y_test)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 29,\n \"metadata\": {\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 920\n },\n \"colab_type\": \"code\",\n \"id\": \"Tyg4mKOVplJ-\",\n \"outputId\": \"59e897c3-cb77-4dc2-a239-dea2c94eec42\"\n },\n \"outputs\": [\n {\n \"name\": \"stdout\",\n \"output_type\": \"stream\",\n \"text\": [\n \"Word True \\t Pred\\n\",\n \"\\n\",\n \"------------------------------\\n\",\n \"The O\\tO\\n\",\n \"United B-org\\tB-org\\n\",\n \"Nations I-org\\tI-org\\n\",\n \"has O\\tO\\n\",\n \"been O\\tO\\n\",\n \"under O\\tO\\n\",\n \"fire O\\tO\\n\",\n \"for O\\tO\\n\",\n \"failing O\\tO\\n\",\n \"to O\\tO\\n\",\n \"stop O\\tO\\n\",\n \"ongoing O\\tO\\n\",\n \"ethnic O\\tO\\n\",\n \"violence O\\tO\\n\",\n \"in O\\tO\\n\",\n \"Ituri B-geo\\tB-geo\\n\",\n \". O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\",\n \"Surrey O\\tO\\n\"\n ]\n }\n ],\n \"source\": [\n \"i = np.random.randint(0, x_test.shape[0]) #659\\n\",\n \"p = model.predict(np.array([x_test[i]]))\\n\",\n \"p = np.argmax(p, axis=-1)\\n\",\n \"y_true = y_test[i]\\n\",\n \"print(\\\"{:15}{:5}\\\\t {}\\\\n\\\".format(\\\"Word\\\", \\\"True\\\", \\\"Pred\\\"))\\n\",\n \"print(\\\"-\\\" *30)\\n\",\n \"for w, true, pred in zip(x_test[i], y_true, p[0]):\\n\",\n \" print(\\\"{:15}{}\\\\t{}\\\".format(words[w-1], tags[true], tags[pred]))\\n\",\n \"#Padding value of \\\"Surrey\\\" at the end till max length is reached\"\n ]\n }\n ],\n \"metadata\": {\n \"accelerator\": \"GPU\",\n \"colab\": {\n \"collapsed_sections\": [],\n \"name\": \"NER.ipynb\",\n \"provenance\": []\n },\n \"kernelspec\": {\n \"display_name\": \"Python 3\",\n \"language\": \"python\",\n \"name\": \"python3\"\n },\n \"language_info\": {\n \"codemirror_mode\": {\n \"name\": \"ipython\",\n \"version\": 3\n },\n \"file_extension\": \".py\",\n \"mimetype\": \"text/x-python\",\n \"name\": \"python\",\n \"nbconvert_exporter\": \"python\",\n \"pygments_lexer\": \"ipython3\",\n \"version\": \"3.7.3\"\n }\n },\n \"nbformat\": 4,\n \"nbformat_minor\": 1\n}"
}
{
"filename": "code/artificial_intelligence/src/named_entity_recognition/README.md",
"content": "# Named Entity Recognition\n**Named Entity Recognition** is a key task in Natural Language Processing. The process of detecting a word or a group of words that can be classified into known categories like person name, geographical location, date, numerical values, units of measurement, product names, etc. \n\nFor eg. in the sentence, \"Rahul is looking at Taj Mahal, which is one of the 7 wonders of the world.\" Rahul is a person's name, Taj Mahal is a monument, 7 is a numerical value. So, these 3 known categories can be detected as part of Named Entity Recognition.\n\n# Packages used\n* Tensorflow - for Named Entity Recognition task\n* Numpy - for scientific calculations\n* Pandas - loading CSV dataset and data wrangling\n* Matplotlib - plotting and visualization\n\nOne can replicate the same results by following these steps:\n1. Downloading this jupyter notebook on Google Colab. Alternatively, they can also load the dataset to their own computers instead of using Google Drive (as done in this notebook).\n2. Running all the cells **sequentially** in the order as in the notebook.\n3. It's done! You can go ahead and try the same algorithm on different datasets."
}
{
"filename": "code/artificial_intelligence/src/nearest_sequence_memory/nsm_matlab/main.m",
"content": "% change as desired\n% runtime approx (i7 @3 GHz)\n% 100 episodes -- < 90 s\n% 1000 episodes -- 2 - 3 min\nnum_trials = 1000;\n\n% gather data -- may take time\nnsmAgent = nsm_agent();\n[steps_learn, ~] = nsmAgent.Trials(num_trials);\n\n% plot nice output graphs\nplot(steps_learn);\nylim([0 500]);\ntitle('NSM Trials');\nylabel('Number of Steps until terminal');\nxlabel('Episodes');"
}
{
"filename": "code/artificial_intelligence/src/nearest_sequence_memory/nsm_matlab/nsm_agent.m",
"content": "classdef nsm_agent < handle\n % The agent keeps a history of all past episodes (max. 20 steps).\n % This information is used to make decisions about the current action,\n % by comparing chains of previous observations and actions.\n \n properties(Access=private)\n episodes_stored = 0;\n world = simulator();\n STM = zeros(20,3);\n LTM = zeros(20,3,0);\n end\n \n methods\n function [steps, ret_LTM] = Trials(this, num_trials)\n % gather num_trials many episodes using NSM action\n % selection. For each Episode reccord the total number of steps\n % and the last 20 visited nodes.\n % Input: num_trials --- the number of trials to be run\n % Output: steps --- 1D array where steps(idx) corresponds to\n % the number of steps needed to reach the\n % goal in episode idx.\n % LTM --- A reccord of the last 20 reccorded\n % observations, actions and rewards.\n % LTM(20,:) is the last observed state.\n % If an episode requires less than 20\n % steps, the top rows are zero padded\n % until size(LTM) = [20 3];\n \n this.LTM = zeros(20,3,num_trials);\n \n steps = zeros(1,num_trials);\n for idx = 1:num_trials\n [step, foo] = this.NSMEpisode();\n steps(idx) = step;\n this.LTM(:,:,idx) = foo;\n this.episodes_stored = this.episodes_stored + 1;\n end\n ret_LTM = this.LTM;\n end\n\n end\n methods(Access = private)\n function [num_steps, ret_mat] = NSMEpisode(this)\n % Generates an episode using previously observed experience\n % stored in LTM.\n % Output: num_steps --- The total number of steps until the\n % goal was reached.\n % ret_mat --- The last 20 observations, actions\n % and rewards, stored in LTM format.\n gamma = 0.9;\n num_steps = 0;\n \n % make sure the world is in a random state and we have no\n % current memory\n this.world.reset();\n this.STM = zeros(20,3);\n \n % traverse the world and try to pick a good action in each step\n while ~this.world.isGoal()\n o = this.world.observe();\n a = this.NSMSelectAction(o);\n r = this.world.take_action(a);\n \n % after each step, reccord what happned\n this.STM = circshift(this.STM,[-1 0]);\n this.STM(20,:) = [o a r];\n num_steps = num_steps + 1;\n end\n \n % calculate the discounted reward\n this.STM(:,3) = this.STM(20,3) * gamma.^(19:-1:0);\n this.STM(1:(20-num_steps),3) = 0;\n \n ret_mat = this.STM;\n end\n \n function a = NSMSelectAction(this, o)\n % Select an action based on actions and observations \n % (STM) made in this episode and past experiences (LTM).\n % The action selection is epsilon-greedy, with epsilon = 10 %.\n % Input: o --- the current observation\n % Output: a --- action chosen\n \n if rand < 0.1\n % pick a random action\n a = randi(4);\n \n else\n % select an action based on past experiences\n \n % find the 10 closest POMDP states observed in the past and\n % average their discounted reward with respect to the first\n % action taken.\n kNN = this.kNearest(o);\n rewards = zeros(1,4);\n for idx = 1:4\n same_action = (kNN(:,2) == idx);\n rewards(idx) = mean(kNN(same_action,3));\n end\n rewards(isnan(rewards)) = 0;\n \n % select the best action. If tie for multiple, pick random\n % witin those.\n max_val = max(rewards);\n available_actions = 1:4;\n available_actions = ...\n (available_actions(abs(rewards-max_val) < 0.01));\n rand_action = mod(randi(12),size(available_actions,1)) + 1;\n a = available_actions(rand_action);\n end\n end\n \n function score = proximity(this, episode, step, o)\n % Calculate how close a state in the LTM is to the current\n % state.\n % Input: (episode,step) --- The state in the LTM\n % o --- The current observation\n % Output: score --- The closeness of the two\n % states. Higher is closer. \n \n % if observation differs from LTM's present observation,\n % score is 0\n if this.LTM(step,1,episode) ~= o\n score = 0;\n return;\n end\n \n % local copy of LTM stripped from everything unneeded\n past = this.LTM(1:step-1,1:2,episode);\n zero_rows = all(past == 0,2);\n past(zero_rows,:) = [];\n \n % local copy of STM stripped from everything unneeded\n local_STM = this.STM(:,1:2);\n zero_rows = all(local_STM == 0,2);\n local_STM(zero_rows,:) = [];\n \n % compare LTM's past to STM past to see how close it is\n STM_size = size(local_STM,1);\n past_size = size(past, 1);\n common = (min(STM_size,past_size)-1):-1:0;\n past = past(end - common,:);\n local_STM = this.STM(end - common,1:2);\n \n %find out how many (o,a) pairs match\n matches = flipud(all(past == local_STM,2));\n num_matches = find([matches; 0] == 0, 1);\n score = 1 + (num_matches-1);\n end\n \n function kNN = kNearest(this, o)\n % find and return the 10 nearest neighbours in the LTM, given \n % the STM and a current observation\n % Input: o --- The current observation\n % Output: kNN --- A list of the 10 nearest states and\n % their score.\n \n kNN = zeros(10,4);\n lowest_score = 0;\n \n for step = 20:-1:2\n for episode = 1:this.episodes_stored\n % this entire for loop could be vectorized. But this\n % would require to refactor and remove proximity() thus\n % it has been omitted in this implementation.\n \n score = this.proximity(episode,step,o);\n if lowest_score <= score && score > 0\n [~,idx] = min(kNN(:,4));\n kNN(idx,:) = [this.LTM(step,:,episode) score];\n lowest_score = min(kNN(:,4));\n end\n end\n end\n end\n end\nend"
}
{
"filename": "code/artificial_intelligence/src/nearest_sequence_memory/nsm_matlab/simulator.m",
"content": "classdef simulator < handle\n % A small simulation of a variation of McCallum's grid-world.\n % Initialized in a random state. The world is only partially\n % observable.\n %\n % reset() -- reset the world to a random starting state\n % observe() -- get the observation of the current goal state\n % take_action(a) -- execute action a, returns the reward associated\n % with taking this action in the current state\n % isGoal(a) -- check if in the goal state\n %\n % (c) 2016 Sebastian Wallkoetter\n \n properties(Access=private)\n s = randi(11); % current state\n T = ones(4,11); % transition matrix\n observations = ones(11,1);\n reward_function\n end\n \n methods\n function obj = simulator(~)\n % construct a POMDP based on McCallum's grid world. This\n % routine creates the world by setting the transition function\n % T as well as the observations for each state.\n \n obj.T = [\n 4 2 6 7 9 11 7 8 9 10 11\n 1 2 3 4 5 6 8 9 10 11 11\n 1 2 3 1 2 4 4 8 5 10 11\n 1 2 3 4 5 6 7 7 8 9 10\n ];\n obj.observations = [14 14 14 10 10 10 9 5 1 5 3];\n end\n \n function r = take_action(this, a)\n % execute action a. This will result in a new current state and\n % a reward\n % Input: a --- The action to be executed\n % Output: r --- The reward obtained from taking action a in\n % state s\n \n r = this.reward(a);\n this.s = this.T(a,this.s);\n end\n \n function o = observe(this)\n % Resturns what can be seen in the current state\n % Output: o --- The current observation\n \n o = this.observations(this.s);\n end\n \n function reset(this)\n % Reset the world, placing the agent in a random state ~= 2\n \n possible_states = 1:11;\n possible_states(2) = [];\n this.s = randsample(possible_states,1);\n end\n \n function b = isGoal(this)\n % returns true if the current state is the goal state\n % Output: b --- True, if goal has been reached. False\n % otherwise.\n \n b = (this.s == 2);\n end\n \n end\n \n methods(Access = private)\n function r = reward(this, a)\n % the reward for taking action a in the current state\n % Input: a --- the action taken\n % Output: r --- reward for taking that action in the\n % current state\n \n if this.s == 5 && a == 3\n r = 10;\n else\n r = 0;\n end\n end\n end\nends"
}
{
"filename": "code/artificial_intelligence/src/neural_network/keras_nn.py",
"content": "import numpy as np\r\n'''numpy stands for numerical python library. It is used for scientific computing in python '''\r\nimport keras.utils\r\nfrom keras.datasets import mnist\r\n''' mnist is a data set which contains images of numbers from 1-8 in 28*28input size'''\r\nfrom keras.models import Sequential\r\nfrom keras.layers import Dense, Dropout\r\n''' Demse indicates layers of connected neurons'''\r\nfrom keras.optimizers import Adam\r\n# Adam is a special optimizing algorithm\r\n\r\n\r\n\"\"\" To run this code install keras, numpy and tensorflow.\r\n\"\"\"\r\n\r\nbatch_size = 32\r\ncategories = 10\r\nepochs = 50 #epochs means no. of times data will be trained\r\n\r\n(x_train, y_train), (x_test, y_test) = mnist.load_data()\r\n\r\n# reshape function is used to reshape matrix in python.\r\n\r\nx_train = x_train.reshape(60000, 784)\r\nx_test = x_test.reshape(10000, 784)\r\n\r\n\r\n''' The below process is called noramalization. in this we try to scale the\r\ndata so that the process could be fast.'''\r\n\r\nx_train /= 255\r\nx_test /= 255\r\n\r\n\r\nprint(x_train.shape[0], \"train samples\")\r\nprint(x_test.shape[0], \"test samples\")\r\n\r\n# convert class vectors to binary class matrices\r\ny_train = keras.utils.to_categorical(y_train, categories)\r\ny_test = keras.utils.to_categorical(y_test, categories)\r\n\r\nmodel = Sequential()\r\nmodel.add(Dense(128, activation=\"relu\", input_shape=(784,)))\r\nmodel.add(Dropout(0.2))\r\nmodel.add(Dense(128, activation=\"relu\")) \r\n''' relu stands for rectified linear unit. it is used as an activation function when output is expected not to be 0 or 1'''\r\nmodel.add(Dropout(0.2))\r\nmodel.add(Dense(128, activation=\"relu\"))\r\nmodel.add(Dropout(0.2))\r\nmodel.add(Dense(categories, activation=\"softmax\")) #it is an activation function which returns the highest value\r\n\r\n\r\nmodel.compile(loss=\"categorical_crossentropy\", optimizer=Adam(), metrics=[\"accuracy\"])\r\n\r\nmodel.fit(\r\n x_train,\r\n y_train,\r\n batch_size=batch_size,\r\n epochs=epochs,\r\n verbose=1,\r\n validation_data=(x_test, y_test),\r\n)\r\nmodel.evaluate(x_test, y_test, verbose=1)\r"
}
{
"filename": "code/artificial_intelligence/src/neural_network/neural_network.py",
"content": "# The following is a from scratch implementation of a neural network in Python without any deep learning libraries\n\n# Import necessary packages\nimport numpy as np\nimport dill\n\n# Create a class \nclass neural_network:\n def __init__(self, num_layers, num_nodes, activation_function, cost_function):\n self.num_layers = num_layers\n self.num_nodes = num_nodes\n self.layers = []\n self.cost_function = cost_function\n\n for i in range(num_layers):\n if i != num_layers - 1:\n layer_i = layer(num_nodes[i], num_nodes[i + 1], activation_function[i])\n else:\n layer_i = layer(num_nodes[i], 0, activation_function[i])\n self.layers.append(layer_i)\n\n # Function to train\n def train(self, batch_size, inputs, labels, num_epochs, learning_rate, filename):\n self.batch_size = batch_size\n self.learning_rate = learning_rate\n for j in range(num_epochs):\n i = 0\n print(\"== EPOCH: \", j, \" ==\")\n while i + batch_size != len(inputs):\n self.error = 0\n self.forward_pass(inputs[i : i + batch_size])\n self.calculate_error(labels[i : i + batch_size])\n self.back_pass(labels[i : i + batch_size])\n i += batch_size\n print(\"Error: \", self.error)\n dill.dump_session(filename)\n\n # Feedforward function \n def forward_pass(self, inputs):\n self.layers[0].activations = inputs\n for i in range(self.num_layers - 1):\n self.layers[i].add_bias(\n self.batch_size, self.layers[i + 1].num_nodes_in_layer\n )\n temp = np.add(\n np.matmul(self.layers[i].activations, self.layers[i].weights_for_layer),\n self.layers[i].bias_for_layer,\n )\n if self.layers[i + 1].activation_function == \"sigmoid\":\n self.layers[i + 1].activations = self.sigmoid(temp)\n elif self.layers[i + 1].activation_function == \"softmax\":\n self.layers[i + 1].activations = self.softmax(temp)\n elif self.layers[i + 1].activation_function == \"relu\":\n self.layers[i + 1].activations = self.relu(temp)\n elif self.layers[i + 1].activation_function == \"tanh\":\n self.layers[i + 1].activations = self.tanh(temp)\n else:\n self.layers[i + 1].activations = temp\n\n # Create mathematical relu function\n def relu(self, layer):\n layer[layer < 0] = 0\n return layer\n \n # Create mathematical softmax function\n def softmax(self, layer):\n exp = np.exp(layer)\n return exp / np.sum(exp, axis=1, keepdims=True)\n\n # Create mathematical sigmoid function\n def sigmoid(self, layer):\n return np.divide(1, np.add(1, np.exp(layer)))\n\n # Use the tanh function in numpy\n def tanh(self, layer):\n return np.tanh(layer)\n\n # Function to calculate the error (mean squared error, cross-entropy)\n def calculate_error(self, labels):\n if len(labels[0]) != self.layers[self.num_layers - 1].num_nodes_in_layer:\n print(\"Error: Label is not of the same shape as output layer.\")\n print(\"Label: \", len(labels), \" : \", len(labels[0]))\n print(\n \"Out: \",\n len(self.layers[self.num_layers - 1].activations),\n \" : \",\n len(self.layers[self.num_layers - 1].activations[0]),\n )\n return\n\n if self.cost_function == \"mean_squared\":\n self.error = np.mean(\n np.divide(\n np.square(\n np.subtract(\n labels, self.layers[self.num_layers - 1].activations\n )\n ),\n 2,\n )\n )\n elif self.cost_function == \"cross_entropy\":\n self.error = np.negative(\n np.sum(\n np.multiply(\n labels, np.log(self.layers[self.num_layers - 1].activations)\n )\n )\n )\n\n # Back propagation function \n # NOTE: This is the most important part of a neural network by application of calculus and the chain rule\n def back_pass(self, labels):\n # if self.cost_function == \"cross_entropy\" and self.layers[self.num_layers-1].activation_function == \"softmax\":\n targets = labels\n i = self.num_layers - 1\n y = self.layers[i].activations\n deltaw = np.matmul(\n np.asarray(self.layers[i - 1].activations).T,\n np.multiply(y, np.multiply(1 - y, targets - y)),\n )\n new_weights = self.layers[i - 1].weights_for_layer - self.learning_rate * deltaw\n for i in range(i - 1, 0, -1):\n y = self.layers[i].activations\n deltaw = np.matmul(\n np.asarray(self.layers[i - 1].activations).T,\n np.multiply(\n y,\n np.multiply(\n 1 - y,\n np.sum(\n np.multiply(new_weights, self.layers[i].weights_for_layer),\n axis=1,\n ).T,\n ),\n ),\n )\n self.layers[i].weights_for_layer = new_weights\n new_weights = (\n self.layers[i - 1].weights_for_layer - self.learning_rate * deltaw\n )\n self.layers[0].weights_for_layer = new_weights\n\n def predict(self, filename, input):\n dill.load_session(filename)\n self.batch_size = 1\n self.forward_pass(input)\n a = self.layers[self.num_layers - 1].activations\n a[np.where(a == np.max(a))] = 0.9\n a[np.where(a != np.max(a))] = 0.1\n return a\n\n # Check model accuracy\n def check_accuracy(self, filename, inputs, labels):\n dill.load_session(filename)\n self.batch_size = len(inputs)\n self.forward_pass(inputs)\n a = self.layers[self.num_layers - 1].activations\n num_classes = 10\n targets = np.array([a]).reshape(-1)\n a = np.asarray(a)\n one_hot_labels = np.eye(num_classes)[a.astype(int)]\n total = 0\n correct = 0\n for i in range(len(a)):\n total += 1\n if np.equal(one_hot_labels[i], labels[i]).all():\n correct += 1\n print(\"Accuracy: \", correct * 100 / total)\n\n def load_model(self, filename):\n dill.load_session(filename)\n\n# Class for each layer used in the network\nclass layer:\n def __init__(\n self, num_nodes_in_layer, num_nodes_in_next_layer, activation_function\n ):\n self.num_nodes_in_layer = num_nodes_in_layer\n self.activation_function = activation_function\n self.activations = np.zeros([num_nodes_in_layer, 1])\n if num_nodes_in_next_layer != 0:\n self.weights_for_layer = np.random.normal(\n 0, 0.001, size=(num_nodes_in_layer, num_nodes_in_next_layer)\n )\n else:\n self.weights_for_layer = None\n self.bias_for_layer = None\n\n def add_bias(self, batch_size, num_nodes_in_next_layer):\n if num_nodes_in_next_layer != 0:\n self.bias_for_layer = np.random.normal(\n 0, 0.01, size=(batch_size, num_nodes_in_next_layer)\n )"
}
{
"filename": "code/artificial_intelligence/src/number_recogniser/python/driver.py",
"content": "import nn\r\nimport numpy as np\r\nimport os\r\nimport matplotlib.pyplot as plt\r\n\r\npath = os.path.join(os.path.dirname(os.path.realpath(__file__)), \"mnist.npz\")\r\nwith np.load(path) as data:\r\n training_images = data[\"training_images\"]\r\n training_labels = data[\"training_labels\"]\r\n\r\nlayer_sizes = (784, 5, 10)\r\n\r\nnet = nn.neural_network(layer_sizes)\r\nnet.accuracy(training_images, training_labels)\r"
}
{
"filename": "code/artificial_intelligence/src/number_recogniser/python/nn.py",
"content": "import numpy as np\r\n\r\n\r\nclass neural_network:\r\n def __init__(self, layer_sizes):\r\n weight_shapes = [(a, b) for a, b in zip(layer_sizes[1:], layer_sizes[:-1])]\r\n self.weights = [\r\n np.random.standard_normal(s) / s[1] ** 0.5 for s in weight_shapes\r\n ]\r\n self.biases = [np.zeros((s, 1)) for s in layer_sizes[1:]]\r\n\r\n def predict(self, a):\r\n for w, b in zip(self.weights, self.biases):\r\n a = self.activation(np.matmul(w, a) + b)\r\n return a\r\n\r\n def accuracy(self, images, labels):\r\n np.set_printoptions(suppress=True)\r\n predictions = self.predict(images)\r\n num_correct = sum(\r\n [np.argmax(a) == np.argmax(b) for a, b in zip(predictions, labels)]\r\n )\r\n print(\r\n \"{0}/{1} accuracy: {2}%\".format(\r\n num_correct, len(images), (num_correct / len(images)) * 100\r\n )\r\n )\r\n\r\n @staticmethod\r\n def activation(x):\r\n return 1 / (1 + np.exp(-x))\r"
}
{
"filename": "code/artificial_intelligence/src/particle_swarm_optimization/gbestPSO/Gbest2D.py",
"content": "import numpy as np\nfrom matplotlib.pyplot import subplots\n\n\ndef f(x):\n return -15 * np.sin(x - 5) / (abs(x) + 1)\n\n\nparticles = 10\n\npositions = np.random.uniform(-10, 10, particles)\nvelocities = np.random.uniform(-1, 1, particles)\nbest_locals = positions\nnum_iters = 20\n\nw_min = 0.01\nw_max = 0.1\nc1 = 0\nc2 = 0\n\ngbest = positions[0]\n\nfor p in positions:\n if f(p) < f(gbest):\n gbest = p\n\n\nfig, ax = subplots()\nfig.show()\n\nfor k in range(num_iters):\n for i in range(particles):\n\n c1 = 2.5 - 2 * (k / num_iters)\n c2 = 0.5 + 2 * (k / num_iters)\n w = w_max - ((w_max - w_min) * k) / num_iters\n\n r1, r2 = np.random.uniform(0, 1, 1), np.random.uniform(0, 1, 1)\n\n velocities[i] = (\n w * velocities[i]\n + c1 * r1 * (best_locals[i] - positions[i])\n + c2 * r2 * (gbest - positions[i])\n )\n positions[i] += velocities[i]\n\n if f(positions[i]) < f(best_locals[i]):\n best_locals[i] = positions[i]\n\n if f(best_locals[i]) < f(gbest):\n gbest = best_locals[i]\n\n ax.clear()\n ax.axis([-20, 15, -17, 15])\n ax.plot(np.arange(-10, 10, 0.1), f(np.arange(-10, 10, 0.1)), \"-\")\n ax.plot(positions, f(positions), \"ro\", markersize=5)\n\n fig.canvas.draw()\n print(\"Iter: {}\".format(k))\n\nlowest = np.argmin(positions)\nprint(positions[lowest])\nlocal_lowest = np.argmin(best_locals)\nprint(best_locals[local_lowest])"
}
{
"filename": "code/artificial_intelligence/src/particle_swarm_optimization/gbestPSO/Gbest3D.py",
"content": "import numpy as np\nimport matplotlib.pyplot as plt\nfrom mpl_toolkits.mplot3d import Axes3D\n\n\ndef f(args):\n return np.sum([x ** 2 for x in args])\n\n\ndimensions = 2\nboundary = (-10, 10)\nparticles = 20\npositions = []\nvelocities = []\n\nw_min = 0.01\nw_max = 0.1\nc1 = 0\nc2 = 0\nnum_iters = 20\n\nfor i in range(particles):\n positions.append(np.random.uniform(boundary[0], boundary[1], dimensions))\n\npositions = np.array(positions)\n\nfor i in range(particles):\n velocities.append(np.random.uniform(-1, 1, dimensions))\n\nvelocities = np.array(velocities)\n\nbest_locals = positions\ngbest = positions[0]\n\nfor p in positions:\n if f(p) < f(gbest):\n gbest = p\n\nfig = plt.figure()\nax = fig.add_subplot(111, projection=\"3d\")\n\nfig.show()\n\nx = y = np.arange(boundary[0], boundary[1], 0.1)\nsurface_x, surface_y = np.meshgrid(x, y)\nsurface_z = np.array(\n [f([x, y]) for x, y in zip(np.ravel(surface_x), np.ravel(surface_y))]\n).reshape(surface_x.shape)\n\npositions_x = [p[0] for p in positions]\npositions_y = [p[1] for p in positions]\npositions_z = [f([x, y]) for x, y in zip(positions_x, positions_y)]\n\nfor k in range(num_iters):\n for p in range(particles):\n for d in range(dimensions):\n c1 = 2.5 - 2 * (k / num_iters)\n c2 = 0.5 + 2 * (k / num_iters)\n w = w_max - ((w_max - w_min) * k) / num_iters\n\n r1, r2 = np.random.rand(), np.random.rand()\n velocities[p][d] = (\n w * velocities[p][d]\n + c1 * r1 * (best_locals[p][d] - positions[p][d])\n + c2 * r2 * (gbest[d] - positions[p][d])\n )\n positions[p][d] += velocities[p][d]\n\n if f(positions[p]) < f(best_locals[p]):\n best_locals[p] = positions[p]\n\n if f(best_locals[p]) < f(gbest):\n gbest = best_locals[p]\n\n ax.clear()\n ax.plot_surface(surface_x, surface_y, surface_z, alpha=0.3)\n\n positions_x = [p[0] for p in positions]\n positions_y = [p[1] for p in positions]\n positions_z = [f([x, y]) for x, y in zip(positions_x, positions_y)]\n\n ax.scatter(positions_x, positions_y, positions_z, c=\"#FF0000\")\n fig.canvas.draw()\n print(\"Iter: {}\".format(k))"
}
{
"filename": "code/artificial_intelligence/src/particle_swarm_optimization/lbestPSO/Lbest2D.py",
"content": "import numpy as np\nfrom matplotlib.pyplot import subplots\n\n\ndef f(x):\n return -15 * np.sin(x - 5) / (abs(x) + 1)\n\n\ndef distance(p1, p2):\n return np.sqrt((p2 - p1) ** 2 + (f(p2) - f(p1)) ** 2)\n\n\nparticles = 15\n\npositions = np.random.uniform(-20, 20, particles)\nvelocities = np.random.uniform(-1, 1, particles)\nbest_locals = positions\nnum_iters = 50\n\nw_min = 0.01\nw_max = 0.1\nc1 = 0\nc2 = 0\n\nfig, ax = subplots()\nfig.show()\n\nfor k in range(num_iters):\n for i in range(particles):\n\n c1 = 2.5 - 2 * (k / num_iters)\n c2 = 0.5 + 2 * (k / num_iters)\n w = w_max - ((w_max - w_min) * k) / num_iters\n\n distances = list(\n zip(\n [\n distance(positions[i], other)\n for other in positions\n if (other != positions[i]).any()\n ],\n range(particles),\n )\n )\n distances.sort()\n\n neighbours_index = list(zip(*distances[:2]))[1]\n neighbours_index += (i,)\n\n neighbours = [positions[n] for n in neighbours_index]\n neighbours_best = neighbours[0]\n\n for n in neighbours:\n if f(n) < f(neighbours_best):\n neighbours_best = n\n\n r1, r2 = np.random.rand(), np.random.rand()\n\n velocities[i] = (\n w * velocities[i]\n + c1 * r1 * (best_locals[i] - positions[i])\n + c2 * r2 * (neighbours_best - positions[i])\n )\n\n positions[i] += velocities[i]\n\n if f(positions[i]) < f(best_locals[i]):\n best_locals[i] = positions[i]\n\n if f(best_locals[i]) < f(neighbours_best):\n neighbours_best = best_locals[i]\n\n ax.clear()\n ax.axis([-20, 15, -17, 15])\n ax.plot(np.arange(-10, 10, 0.1), f(np.arange(-10, 10, 0.1)), \"-\")\n ax.plot(positions, f(positions), \"ro\", markersize=5)\n\n fig.canvas.draw()\n print(\"Iter: {}\".format(k))"
}
{
"filename": "code/artificial_intelligence/src/particle_swarm_optimization/lbestPSO/LBest3D.py",
"content": "import numpy as np\nimport matplotlib.pyplot as plt\nfrom mpl_toolkits.mplot3d import Axes3D\n\n\ndef f(args):\n return np.sum([x ** 2 for x in args])\n\n\ndef distance(p1, p2):\n return np.sqrt((p2[0] - p1[0]) ** 2 + (p2[1] - p1[1]) ** 2 + (f(p2) - f(p1)) ** 2)\n\n\ndimensions = 2\nboundary = (-10, 10)\nparticles = 30\npositions = []\nvelocities = []\n\nw_min = 0.01\nw_max = 0.1\nc1 = 0\nc2 = 0\nnum_iters = 10\n\nfor i in range(particles):\n positions.append(np.random.uniform(boundary[0], boundary[1], dimensions))\n\npositions = np.array(positions)\nbest_positions = positions\n\nfor i in range(particles):\n velocities.append(np.random.uniform(-1, 1, dimensions))\n\nvelocities = np.array(velocities)\n\nfig = plt.figure()\nax = fig.add_subplot(111, projection=\"3d\")\n\nfig.show()\n\nx = y = np.arange(boundary[0], boundary[1], 0.1)\nsurface_x, surface_y = np.meshgrid(x, y)\nsurface_z = np.array(\n [f([x, y]) for x, y in zip(np.ravel(surface_x), np.ravel(surface_y))]\n).reshape(surface_x.shape)\n\nfor k in range(num_iters):\n for p in range(particles):\n c1 = 2.5 - 2 * (k / num_iters)\n c2 = 0.5 + 2 * (k / num_iters)\n w = w_max - ((w_max - w_min) * k) / num_iters\n\n distances = list(\n zip(\n [\n distance(positions[p], other)\n for other in positions\n if (other != positions[p]).any()\n ],\n range(particles),\n )\n )\n distances.sort()\n\n neighbours = [positions[i] for i in list(zip(*distances[:5]))[1]]\n neighbours_best = neighbours[0]\n\n for n in neighbours:\n if f(n) < f(neighbours_best):\n neighbours_best = n\n\n for d in range(2):\n r1, r2 = np.random.rand(), np.random.rand()\n velocities[p][d] = (\n w * velocities[p][d]\n + c1 * r1 * (best_positions[p][d] - positions[p][d])\n + c1 * r2 * (neighbours_best[d] - positions[p][d])\n )\n\n positions[p][d] += velocities[p][d]\n\n if f(positions[p]) < f(best_positions[p]):\n best_positions[p] = positions[p]\n\n if f(best_positions[p]) < f(neighbours_best):\n neighbours_best = best_positions[p]\n\n ax.clear()\n ax.plot_surface(surface_x, surface_y, surface_z, alpha=0.3)\n\n positions_x = [p[0] for p in positions]\n positions_y = [p[1] for p in positions]\n positions_z = [f([x, y]) for x, y in zip(positions_x, positions_y)]\n\n ax.scatter(positions_x, positions_y, positions_z, c=\"#FF0000\")\n fig.canvas.draw()\n print(\"Iter: {}\".format(k))\nlowest = np.argmin(f(positions))\nprint(positions[lowest])"
}
{
"filename": "code/artificial_intelligence/src/perceptron/perceptron.py",
"content": "# Function that essentially involves the calculation inside the perceptron\ndef predict(row, weights):\n activation = weights[0]\n for i in range(len(row) - 1):\n activation += weights[i + 1] * row[i]\n return 1.0 if activation >= 0.0 else 0.0\n\n\n# Stochastic Gradient Descent\n\n\ndef train_weights(train, l_rate, n_epoch):\n weights = [0.0 for i in range(len(train[0]))]\n for epoch in range(n_epoch):\n sum_error = 0.0\n for row in train:\n prediction = predict(row, weights)\n error = row[-1] - prediction\n sum_error += error ** 2\n weights[0] = weights[0] + l_rate * error\n for i in range(len(row) - 1):\n weights[i + 1] = weights[i + 1] + l_rate * error * row[i]\n print(\"epoch=%d, lrate=%.3f, error=%.3f\" % (epoch, l_rate, sum_error))\n return weights\n\n\ndataset = [\n [2.7810836, 2.550537003, 0],\n [1.465489372, 2.362125076, 0],\n [3.396561688, 4.400293529, 0],\n [1.38807019, 1.850220317, 0],\n [3.06407232, 3.005305973, 0],\n [7.627531214, 2.759262235, 1],\n [5.332441248, 2.088626775, 1],\n [6.922596716, 1.77106367, 1],\n [8.675418651, -0.242068655, 1],\n [7.673756466, 3.508563011, 1],\n]\nl_rate = 0.1\nn_epoch = 5\nweights = train_weights(dataset, l_rate, n_epoch)\nprint(weights)"
}
{
"filename": "code/artificial_intelligence/src/principal_component_analysis/pca.py",
"content": "\"\"\"\nSolution to Principal Component Analysis Inspired by http://sebastianraschka.com/Articles/2014_pca_step_by_step.html\nSolution is proposed for Python 3, using numpy and matplotlib\n\nThe PCA approach may be summerized according to these steps:\n1. Take the whole dataset consisting of dd-dimensional samples ignoring the class labels\n2. Compute the dd-dimensional mean vector (i.e., the means for every dimension of the whole dataset)\n3. Compute the scatter matrix (alternatively, the covariance matrix) of the whole data set\n4. Compute eigenvectors and corresponding eigenvalues\n5. Sort the eigenvectors by decreasing eigenvalues and choose kk eigenvectors with the largest eigenvalues.\n6. Use this d×kd×k eigenvector matrix to transform the samples onto the new subspace.\n\"\"\"\n\nimport numpy as np\n\n# Visualization ------------------------------------------------------------\nfrom matplotlib import pyplot as plt\nfrom mpl_toolkits.mplot3d import Axes3D\nfrom mpl_toolkits.mplot3d import proj3d\nfrom matplotlib.patches import FancyArrowPatch\n\n\ndef visualize_data(data1, data2):\n \"\"\" Create a 3D plot for data visualization \"\"\"\n fig = plt.figure(figsize=(8, 8))\n ax = fig.add_subplot(111, projection=\"3d\")\n plt.rcParams[\"legend.fontsize\"] = 10\n ax.plot(\n data1[0, :],\n data1[1, :],\n data1[2, :],\n \"o\",\n markersize=8,\n color=\"blue\",\n alpha=0.5,\n label=\"class1\",\n )\n ax.plot(\n data2[0, :],\n data2[1, :],\n data2[2, :],\n \"^\",\n markersize=8,\n alpha=0.5,\n color=\"red\",\n label=\"class2\",\n )\n ax.set_xlabel(\"x_values\")\n ax.set_ylabel(\"y_values\")\n ax.set_zlabel(\"z_values\")\n\n plt.title(\"Samples for class 1 and class 2\")\n ax.legend(loc=\"upper right\")\n\n plt.show()\n\n\nclass Arrow3D(FancyArrowPatch):\n \"\"\" Arrow graphics class \"\"\"\n\n def __init__(self, xs, ys, zs, *args, **kwargs):\n FancyArrowPatch.__init__(self, (0, 0), (0, 0), *args, **kwargs)\n self._verts3d = xs, ys, zs\n\n def draw(self, renderer):\n xs3d, ys3d, zs3d = self._verts3d\n xs, ys, zs = proj3d.proj_transform(xs3d, ys3d, zs3d, renderer.M)\n self.set_positions((xs[0], ys[0]), (xs[1], ys[1]))\n FancyArrowPatch.draw(self, renderer)\n\n\ndef visualize_eigen(data, eig_vec_sc):\n \"\"\" Create 3D representation of the eigenvectors computed \"\"\"\n # separate mean vector components\n mean_x = np.mean(data[0, :])\n mean_y = np.mean(data[1, :])\n mean_z = np.mean(data[2, :])\n # create figure\n fig = plt.figure(figsize=(7, 7))\n ax = fig.add_subplot(111, projection=\"3d\")\n # plot eigen vectors centered at the mean value of the dataset\n ax.plot(\n data[0, :], data[1, :], data[2, :], \"o\", markersize=8, color=\"green\", alpha=0.2\n )\n ax.plot([mean_x], [mean_y], [mean_z], \"o\", markersize=10, color=\"red\", alpha=0.5)\n for v in eig_vec_sc.T:\n a = Arrow3D(\n [mean_x, v[0]],\n [mean_y, v[1]],\n [mean_z, v[2]],\n mutation_scale=20,\n lw=3,\n arrowstyle=\"-|>\",\n color=\"r\",\n )\n ax.add_artist(a)\n ax.set_xlabel(\"x_values\")\n ax.set_ylabel(\"y_values\")\n ax.set_zlabel(\"z_values\")\n\n plt.title(\"Eigenvectors\")\n\n plt.show()\n\n\ndef visulize_pca(transformed):\n \"\"\"\n 2D visualization of the principal components to evidence the dimensionality reduction.\n The markers shows different classes of the original normalized_distributed_data().\n Its valuable to notice that class classification is not made by the PCA.\n \"\"\"\n plt.plot(\n transformed[0, 0:20],\n transformed[1, 0:20],\n \"o\",\n markersize=7,\n color=\"blue\",\n alpha=0.5,\n label=\"class1\",\n )\n plt.plot(\n transformed[0, 20:40],\n transformed[1, 20:40],\n \"^\",\n markersize=7,\n color=\"red\",\n alpha=0.5,\n label=\"class2\",\n )\n plt.xlim([-4, 4])\n plt.ylim([-4, 4])\n plt.xlabel(\"PC 1\")\n plt.ylabel(\"PC 2\")\n plt.legend()\n plt.title(\"Transformed samples with class labels\")\n\n plt.show()\n\n\n# Data Generation ------------------------------------------------------------\ndef normalized_distributed_data(mean_value):\n \"\"\" Generate 3x20 datasets for PCA \"\"\"\n # mean vector\n mu_vec1 = mean_value * np.ones(3)\n # covariance matrix\n cov_mat1 = np.array([[1, 0, 0], [0, 1, 0], [0, 0, 1]])\n # generate uniformed distributed samples over the mean mu_vec\n class_sample = np.random.multivariate_normal(mu_vec1, cov_mat1, 20).T\n assert class_sample.shape == (3, 20), \"The matrix has not the dimensions 3x20\"\n return class_sample\n\n\n# PCA subroutines -----------------------------------------------------------\ndef generate_data():\n \"\"\"\n Combine two 3x20 datasets ignoring their labels.\n This is the data which will be analyzed by the PCA algorithm\n \"\"\"\n # generate a mean 0 data\n class1_sample = normalized_distributed_data(0)\n # generate a mean 1 data\n class2_sample = normalized_distributed_data(1)\n # visualize data\n visualize_data(class1_sample, class2_sample)\n # combine them into one structure\n data = np.concatenate((class1_sample, class2_sample), axis=1)\n assert data.shape == (3, 40), \"The matrix has not the dimensions 3x40\"\n return data\n\n\ndef compute_mean_vector(data):\n \"\"\" Compute the mean vector for the dataset \"\"\"\n mean_x = np.mean(data[0, :])\n mean_y = np.mean(data[1, :])\n mean_z = np.mean(data[2, :])\n\n mean_vector = np.array([[mean_x], [mean_y], [mean_z]])\n print(\"Mean Vector:\\n\", mean_vector)\n\n return mean_vector\n\n\ndef compute_scatter_matrix(data, mean_vector):\n \"\"\" Compute scatter matrix values \"\"\"\n scatter_matrix = np.zeros((3, 3))\n for i in range(data.shape[1]):\n scatter_matrix += (data[:, i].reshape(3, 1) - mean_vector).dot(\n (data[:, i].reshape(3, 1) - mean_vector).T\n )\n print(\"Scatter Matrix:\\n\", scatter_matrix)\n return scatter_matrix\n\n\ndef compute_covariance_matrix(data):\n \"\"\" Compute covariance matrix, an alternative to scatter matrix \"\"\"\n cov_mat = np.cov([data[0, :], data[1, :], data[2, :]])\n print(\"Covariance Matrix:\\n\", cov_mat)\n return cov_mat\n\n\ndef verify_eigen_values(scatter_matrix, eig_val_sc, eig_vec_sc):\n \"\"\"\n Verify if the eigen values of the scatter matrix were made correctly.\n Test consists in asserting that the covariance and eigen vector product equals the eigen value and eigen vector.\n \"\"\"\n for i in range(len(eig_val_sc)):\n eigv = eig_vec_sc[:, i].reshape(1, 3).T\n np.testing.assert_array_almost_equal(\n scatter_matrix.dot(eigv),\n eig_val_sc[i] * eigv,\n decimal=6,\n err_msg=\"\",\n verbose=True,\n )\n # test eigen vectors module to equal one\n for ev in eig_vec_sc:\n np.testing.assert_array_almost_equal(1.0, np.linalg.norm(ev))\n return True\n\n\ndef compute_eigen(scatter_matrix, cov_mat):\n \"\"\" Compute eigen vectors and corresponding eigen values for the scatter and covariance matrix \"\"\"\n # eigenvectors and eigenvalues for the from the scatter matrix\n eig_val_sc, eig_vec_sc = np.linalg.eig(scatter_matrix)\n\n # eigenvectors and eigenvalues for the from the covariance matrix\n eig_val_cov, eig_vec_cov = np.linalg.eig(cov_mat)\n\n for i in range(len(eig_val_sc)):\n eigvec_sc = eig_vec_sc[:, i].reshape(1, 3).T\n eigvec_cov = eig_vec_cov[:, i].reshape(1, 3).T\n # assert if the scatter_matrix and covariance matrix eigen vectors are identical\n assert eigvec_sc.all() == eigvec_cov.all(), \"Eigenvectors are not identical\"\n\n print(\"Eigenvector {}: \\n{}\".format(i + 1, eigvec_sc))\n print(\"Eigenvalue {} from scatter matrix: {}\".format(i + 1, eig_val_sc[i]))\n print(\"Eigenvalue {} from covariance matrix: {}\".format(i + 1, eig_val_cov[i]))\n print(\"Scaling factor: \", eig_val_sc[i] / eig_val_cov[i])\n print(40 * \"-\")\n\n verify_eigen_values(scatter_matrix, eig_val_sc, eig_vec_sc)\n\n return eig_val_sc, eig_vec_sc\n\n\ndef sort_eigen_vectors(eigen_vec, eigen_val):\n \"\"\"\n Sort eigen vectors by decreasing eigenvalues.\n Eigen vectors with lower eigenvalues hold less information about the data distribution.\n \"\"\"\n # Make a list of (eigenvalue, eigenvector) tuples\n eig_pairs = [(np.abs(eigen_val[i]), eigen_vec[:, i]) for i in range(len(eigen_val))]\n\n # Sort the (eigenvalue, eigenvector) tuples from high to low\n eig_pairs.sort(key=lambda x: x[0], reverse=True)\n\n # Visually confirm that the list is correctly sorted by decreasing eigenvalues\n print(\"Eigen values ordered in decreasing order:\")\n for pair in eig_pairs:\n print(pair[0])\n\n return eig_pairs\n\n\ndef get_2_largest_eigen_vectors(eigen_vec, eigen_val):\n \"\"\"\n Return 2 principal components eigen vectors.\n The decision of which vectors are principals is made by their sorting\n \"\"\"\n eig_pairs = sort_eigen_vectors(eigen_vec, eigen_val)\n # combine the two highest eigen vectors into matrix_w\n matrix_w = np.hstack((eig_pairs[0][1].reshape(3, 1), eig_pairs[1][1].reshape(3, 1)))\n print(\"Matrix W:\\n\", matrix_w)\n return matrix_w\n\n\ndef principal_subspace_transformation(data, matrix_w):\n \"\"\" Transform data into a new subspace through the equation: transformed = matrix_w' * data \"\"\"\n transformed = matrix_w.T.dot(data)\n assert transformed.shape == (2, 40), \"The matrix is not 2x40 dimensional.\"\n return transformed\n\n\ndef main():\n \"\"\" Main function to perform Principal Component Analysis\"\"\"\n # 1. Take the whole dataset consisting of dd-dimensional samples ignoring the class labels\n all_samples = generate_data()\n # 2. Compute the dd-dimensional mean vector (i.e., the means for every dimension of the whole dataset)\n mean_vector = compute_mean_vector(all_samples)\n # 3. Compute the scatter matrix (alternatively, the covariance matrix) of the whole data set\n scatter_matrix = compute_scatter_matrix(all_samples, mean_vector)\n cov_mat = compute_covariance_matrix(all_samples)\n # 4. Compute eigenvectors and corresponding eigenvalues\n eigen_val, eigen_vec = compute_eigen(scatter_matrix, cov_mat)\n visualize_eigen(all_samples, eigen_vec)\n # 5. Sort the eigenvectors by decreasing eigenvalues and choose kk eigenvectors with the largest eigenvalues.\n matrix_w = get_2_largest_eigen_vectors(eigen_vec, eigen_val)\n # 6. Use this d×kd×k eigenvector matrix to transform the samples onto the new subspace.\n transformed = principal_subspace_transformation(all_samples, matrix_w)\n visulize_pca(transformed)\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/artificial_intelligence/src/principal_component_analysis/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# Principal component analysis\nSolution to Principal Component Analysis Inspired by [Sebastian Raschka](http://sebastianraschka.com/Articles/2014_pca_step_by_step.html)\n\nThe PCA approach may be summarized according to these steps:\n1. Take the whole dataset consisting of dd-dimensional samples ignoring the class labels\n2. Compute the dd-dimensional mean vector (i.e., the means for every dimension of the whole dataset)\n3. Compute the scatter matrix (alternatively, the covariance matrix) of the whole data set\n4. Compute eigenvectors and corresponding eigenvalues\n5. Sort the eigenvectors by decreasing eigenvalues and choose eigenvectors with the largest eigenvalues.\n6. Use eigenvector matrix to transform the samples onto the new subspace.\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a>\n</p>\n\n---"
}
{
"filename": "code/artificial_intelligence/src/q_learning/q_learning.ipynb",
"content": "{\n \"nbformat\": 4,\n \"nbformat_minor\": 0,\n \"metadata\": {\n \"colab\": {\n \"name\": \"Q-Learning_OpenGenus.ipynb\",\n \"provenance\": [],\n \"collapsed_sections\": []\n },\n \"kernelspec\": {\n \"name\": \"python3\",\n \"display_name\": \"Python 3\"\n },\n \"accelerator\": \"GPU\"\n },\n \"cells\": [\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"Nrcytm9HnDkI\",\n \"colab_type\": \"text\"\n },\n \"source\": [\n \"# I. Q-Learning - Table approach\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"fC9d2GXQnAvp\",\n \"colab_type\": \"code\",\n \"colab\": {}\n },\n \"source\": [\n \"import random\\n\",\n \"import numpy as np\\n\",\n \"import pandas as pd\\n\",\n \"import tensorflow as tf\\n\",\n \"import matplotlib.pyplot as plt\\n\",\n \"import gym\\n\",\n \"\\n\",\n \"from collections import deque\\n\",\n \"from time import time\"\n ],\n \"execution_count\": 0,\n \"outputs\": []\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"x5TH5NPrnfyI\",\n \"colab_type\": \"code\",\n \"outputId\": \"6cc3d02f-29f4-4b68-aeb3-2daca6d4e95d\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 35\n }\n },\n \"source\": [\n \"seed = 2205\\n\",\n \"random.seed(seed)\\n\",\n \"\\n\",\n \"print('Seed: {}'.format(seed))\"\n ],\n \"execution_count\": 0,\n \"outputs\": [\n {\n \"output_type\": \"stream\",\n \"text\": [\n \"Seed: 2205\\n\"\n ],\n \"name\": \"stdout\"\n }\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"PG6dN6trnqdF\",\n \"colab_type\": \"text\"\n },\n \"source\": [\n \"##Game Design\"\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"DjE-BXUPntvI\",\n \"colab_type\": \"text\"\n },\n \"source\": [\n \"There exists a board with 4 cells. The Q-agent will receive a +1 reward every time it fills a vacant cell, and will receive a -1 penalty when it tries to fill an already filled cell.\\n\",\n \"\\n\",\n \"The game ends when the board is full.\\n\",\n \"\\n\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"J-i-4Vw7nogO\",\n \"colab_type\": \"code\",\n \"colab\": {}\n },\n \"source\": [\n \"class Game:\\n\",\n \" \\n\",\n \" board = None\\n\",\n \" board_size = 0\\n\",\n \" \\n\",\n \" def __init__(self, board_size = 4):\\n\",\n \" self.board_size = board_size\\n\",\n \" self.reset()\\n\",\n \" \\n\",\n \" def reset(self):\\n\",\n \" self.board = np.zeros(self.board_size)\\n\",\n \" \\n\",\n \" def play(self, cell):\\n\",\n \" ## Returns a tuple: (reward, game_over?)\\n\",\n \" if self.board[cell] == 0:\\n\",\n \" self.board[cell] = 1\\n\",\n \" game_over = len(np.where(self.board == 0)[0]) == 0\\n\",\n \" return (1, game_over)\\n\",\n \" else:\\n\",\n \" return (-1, False)\"\n ],\n \"execution_count\": 0,\n \"outputs\": []\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"VhMs6jaPos5M\",\n \"colab_type\": \"code\",\n \"outputId\": \"8b3f7745-001c-4466-bbf6-655c3b776e99\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 321\n }\n },\n \"source\": [\n \"def state_to_str(state):\\n\",\n \" return str(list(map(int, state.tolist())))\\n\",\n \"\\n\",\n \"all_states = list()\\n\",\n \"for i in range(2):\\n\",\n \" for j in range(2):\\n\",\n \" for k in range(2):\\n\",\n \" for l in range(2):\\n\",\n \" s = np.array([i, j, k, l])\\n\",\n \" all_states.append(state_to_str(s))\\n\",\n \"\\n\",\n \"print('All possible states are: ')\\n\",\n \"for s in all_states:\\n\",\n \" print(s)\"\n ],\n \"execution_count\": 0,\n \"outputs\": [\n {\n \"output_type\": \"stream\",\n \"text\": [\n \"All possible states are: \\n\",\n \"[0, 0, 0, 0]\\n\",\n \"[0, 0, 0, 1]\\n\",\n \"[0, 0, 1, 0]\\n\",\n \"[0, 0, 1, 1]\\n\",\n \"[0, 1, 0, 0]\\n\",\n \"[0, 1, 0, 1]\\n\",\n \"[0, 1, 1, 0]\\n\",\n \"[0, 1, 1, 1]\\n\",\n \"[1, 0, 0, 0]\\n\",\n \"[1, 0, 0, 1]\\n\",\n \"[1, 0, 1, 0]\\n\",\n \"[1, 0, 1, 1]\\n\",\n \"[1, 1, 0, 0]\\n\",\n \"[1, 1, 0, 1]\\n\",\n \"[1, 1, 1, 0]\\n\",\n \"[1, 1, 1, 1]\\n\"\n ],\n \"name\": \"stdout\"\n }\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"13rUr5OVpTge\",\n \"colab_type\": \"text\"\n },\n \"source\": [\n \"Initializing the game:\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"b3oYifvnpGAU\",\n \"colab_type\": \"code\",\n \"colab\": {}\n },\n \"source\": [\n \"game = Game()\"\n ],\n \"execution_count\": 0,\n \"outputs\": []\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"YTcYkolgpbNr\",\n \"colab_type\": \"text\"\n },\n \"source\": [\n \"## Q-Learning\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"FCBsp5cgpW_o\",\n \"colab_type\": \"code\",\n \"colab\": {}\n },\n \"source\": [\n \"num_of_games = 2000\\n\",\n \"epsilon = 0.1\\n\",\n \"gamma = 1\"\n ],\n \"execution_count\": 0,\n \"outputs\": []\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"My1XivF3ppli\",\n \"colab_type\": \"text\"\n },\n \"source\": [\n \"Initializing the Q-Table:\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"ArHwI7kEpowo\",\n \"colab_type\": \"code\",\n \"colab\": {}\n },\n \"source\": [\n \"q_table = pd.DataFrame(0, \\n\",\n \" index = np.arange(4),\\n\",\n \" columns = all_states)\"\n ],\n \"execution_count\": 0,\n \"outputs\": []\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"nXwaRzbUp4Eu\",\n \"colab_type\": \"text\"\n },\n \"source\": [\n \"Letting the agent play and learn:\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"yW--SweBpzuV\",\n \"colab_type\": \"code\",\n \"outputId\": \"e775e65f-0dd5-4a6b-8ead-0c03b96d5c04\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 247\n }\n },\n \"source\": [\n \"r_list = [] ##Stores the total reward of each game so we can plot it later\\n\",\n \"\\n\",\n \"for g in range(num_of_games):\\n\",\n \" game_over = False\\n\",\n \" game.reset()\\n\",\n \" total_reward = 0\\n\",\n \" \\n\",\n \" while not game_over:\\n\",\n \" state = np.copy(game.board)\\n\",\n \" if random.random() < epsilon:\\n\",\n \" action = random.randint(0, 3)\\n\",\n \" else:\\n\",\n \" action = q_table[state_to_str(state)].idxmax()\\n\",\n \" \\n\",\n \" reward, game_over = game.play(action)\\n\",\n \" total_reward += reward\\n\",\n \" if np.sum(game.board) == 4: ##Terminal state\\n\",\n \" next_state_max_q_value = 0\\n\",\n \" else:\\n\",\n \" next_state = np.copy(game.board)\\n\",\n \" next_state_max_q_value = q_table[state_to_str(next_state)].max()\\n\",\n \" q_table.loc[action, state_to_str(state)] = reward + gamma * next_state_max_q_value\\n\",\n \" r_list.append(total_reward)\\n\",\n \"q_table\"\n ],\n \"execution_count\": 0,\n \"outputs\": [\n {\n \"output_type\": \"execute_result\",\n \"data\": {\n \"text/html\": [\n \"<div>\\n\",\n \"<style scoped>\\n\",\n \" .dataframe tbody tr th:only-of-type {\\n\",\n \" vertical-align: middle;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe tbody tr th {\\n\",\n \" vertical-align: top;\\n\",\n \" }\\n\",\n \"\\n\",\n \" .dataframe thead th {\\n\",\n \" text-align: right;\\n\",\n \" }\\n\",\n \"</style>\\n\",\n \"<table border=\\\"1\\\" class=\\\"dataframe\\\">\\n\",\n \" <thead>\\n\",\n \" <tr style=\\\"text-align: right;\\\">\\n\",\n \" <th></th>\\n\",\n \" <th>[0, 0, 0, 0]</th>\\n\",\n \" <th>[0, 0, 0, 1]</th>\\n\",\n \" <th>[0, 0, 1, 0]</th>\\n\",\n \" <th>[0, 0, 1, 1]</th>\\n\",\n \" <th>[0, 1, 0, 0]</th>\\n\",\n \" <th>[0, 1, 0, 1]</th>\\n\",\n \" <th>[0, 1, 1, 0]</th>\\n\",\n \" <th>[0, 1, 1, 1]</th>\\n\",\n \" <th>[1, 0, 0, 0]</th>\\n\",\n \" <th>[1, 0, 0, 1]</th>\\n\",\n \" <th>[1, 0, 1, 0]</th>\\n\",\n \" <th>[1, 0, 1, 1]</th>\\n\",\n \" <th>[1, 1, 0, 0]</th>\\n\",\n \" <th>[1, 1, 0, 1]</th>\\n\",\n \" <th>[1, 1, 1, 0]</th>\\n\",\n \" <th>[1, 1, 1, 1]</th>\\n\",\n \" </tr>\\n\",\n \" </thead>\\n\",\n \" <tbody>\\n\",\n \" <tr>\\n\",\n \" <th>0</th>\\n\",\n \" <td>4</td>\\n\",\n \" <td>3</td>\\n\",\n \" <td>3</td>\\n\",\n \" <td>2</td>\\n\",\n \" <td>3</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>2</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>2</td>\\n\",\n \" <td>-1</td>\\n\",\n \" <td>1</td>\\n\",\n \" <td>-1</td>\\n\",\n \" <td>1</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>1</th>\\n\",\n \" <td>4</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>3</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>2</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>3</td>\\n\",\n \" <td>2</td>\\n\",\n \" <td>2</td>\\n\",\n \" <td>1</td>\\n\",\n \" <td>1</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>2</th>\\n\",\n \" <td>4</td>\\n\",\n \" <td>3</td>\\n\",\n \" <td>2</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>3</td>\\n\",\n \" <td>2</td>\\n\",\n \" <td>1</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>2</td>\\n\",\n \" <td>1</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" </tr>\\n\",\n \" <tr>\\n\",\n \" <th>3</th>\\n\",\n \" <td>4</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>2</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>3</td>\\n\",\n \" <td>1</td>\\n\",\n \" <td>2</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>2</td>\\n\",\n \" <td>0</td>\\n\",\n \" <td>1</td>\\n\",\n \" <td>0</td>\\n\",\n \" </tr>\\n\",\n \" </tbody>\\n\",\n \"</table>\\n\",\n \"</div>\"\n ],\n \"text/plain\": [\n \" [0, 0, 0, 0] [0, 0, 0, 1] ... [1, 1, 1, 0] [1, 1, 1, 1]\\n\",\n \"0 4 3 ... 0 0\\n\",\n \"1 4 0 ... 0 0\\n\",\n \"2 4 3 ... 0 0\\n\",\n \"3 4 0 ... 1 0\\n\",\n \"\\n\",\n \"[4 rows x 16 columns]\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n },\n \"execution_count\": 9\n }\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"edwSIGCoryCL\",\n \"colab_type\": \"text\"\n },\n \"source\": [\n \"Let's verify that the agent indeed learnt a correct strategy by seeing what action it will choose in each one of the possible states\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"Gwo52TJ7rtPJ\",\n \"colab_type\": \"code\",\n \"outputId\": \"27e7bc3a-e34d-4455-ad7a-a9647acbf90b\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 305\n }\n },\n \"source\": [\n \"for i in range(2):\\n\",\n \" for j in range(2):\\n\",\n \" for k in range(2):\\n\",\n \" for l in range(2):\\n\",\n \" b = np.array([i, j, k, l])\\n\",\n \" if len(np.where(b == 0)[0]) != 0:\\n\",\n \" action = q_table[state_to_str(b)].idxmax()\\n\",\n \" pred = q_table[state_to_str(b)].tolist()\\n\",\n \" print('Board: {b}\\\\tPredicted Q-values: {p}\\\\tBest action: {a}\\\\tCorrect action? {s}'\\n\",\n \" .format(b = b, p = pred, a = action, s = b[action]==0))\"\n ],\n \"execution_count\": 0,\n \"outputs\": [\n {\n \"output_type\": \"stream\",\n \"text\": [\n \"Board: [0 0 0 0]\\tPredicted Q-values: [4, 4, 4, 4]\\tBest action: 0\\tCorrect action? True\\n\",\n \"Board: [0 0 0 1]\\tPredicted Q-values: [3, 0, 3, 0]\\tBest action: 0\\tCorrect action? True\\n\",\n \"Board: [0 0 1 0]\\tPredicted Q-values: [3, 3, 2, 2]\\tBest action: 0\\tCorrect action? True\\n\",\n \"Board: [0 0 1 1]\\tPredicted Q-values: [2, 0, 0, 0]\\tBest action: 0\\tCorrect action? True\\n\",\n \"Board: [0 1 0 0]\\tPredicted Q-values: [3, 2, 0, 0]\\tBest action: 0\\tCorrect action? True\\n\",\n \"Board: [0 1 0 1]\\tPredicted Q-values: [0, 0, 0, 0]\\tBest action: 0\\tCorrect action? True\\n\",\n \"Board: [0 1 1 0]\\tPredicted Q-values: [2, 0, 0, 0]\\tBest action: 0\\tCorrect action? True\\n\",\n \"Board: [0 1 1 1]\\tPredicted Q-values: [0, 0, 0, 0]\\tBest action: 0\\tCorrect action? True\\n\",\n \"Board: [1 0 0 0]\\tPredicted Q-values: [2, 3, 3, 3]\\tBest action: 1\\tCorrect action? True\\n\",\n \"Board: [1 0 0 1]\\tPredicted Q-values: [-1, 2, 2, 1]\\tBest action: 1\\tCorrect action? True\\n\",\n \"Board: [1 0 1 0]\\tPredicted Q-values: [1, 2, 1, 2]\\tBest action: 1\\tCorrect action? True\\n\",\n \"Board: [1 0 1 1]\\tPredicted Q-values: [-1, 1, 0, 0]\\tBest action: 1\\tCorrect action? True\\n\",\n \"Board: [1 1 0 0]\\tPredicted Q-values: [1, 1, 2, 2]\\tBest action: 2\\tCorrect action? True\\n\",\n \"Board: [1 1 0 1]\\tPredicted Q-values: [0, 0, 1, 0]\\tBest action: 2\\tCorrect action? True\\n\",\n \"Board: [1 1 1 0]\\tPredicted Q-values: [0, 0, 0, 1]\\tBest action: 3\\tCorrect action? True\\n\"\n ],\n \"name\": \"stdout\"\n }\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"qFYAnBc1tteR\",\n \"colab_type\": \"text\"\n },\n \"source\": [\n \"We can improve the performance of our algorithm by increasing the <math xmlns=\\\"http://www.w3.org/1998/Math/MathML\\\"><mi>ε</mi></math> (epsilon) value (which controls how much the algorithm can explore), or by letting the agent play more games.\"\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"id\": \"xu13o5eduKvC\",\n \"colab_type\": \"text\"\n },\n \"source\": [\n \"Let's plot the total reward the agent received per game:\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"metadata\": {\n \"id\": \"ex2SsbBvtK2k\",\n \"colab_type\": \"code\",\n \"outputId\": \"50e81ebd-138a-4440-9540-aa6e2c109846\",\n \"colab\": {\n \"base_uri\": \"https://localhost:8080/\",\n \"height\": 466\n }\n },\n \"source\": [\n \"plt.figure(figsize = (14, 7))\\n\",\n \"plt.plot(range(len(r_list)), r_list)\\n\",\n \"plt.xlabel('Games played')\\n\",\n \"plt.ylabel('Reward')\\n\",\n \"plt.show()\"\n ],\n \"execution_count\": 0,\n \"outputs\": [\n {\n \"output_type\": \"display_data\",\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAA0IAAAGtCAYAAAA708QKAAAABHNCSVQICAgIfAhkiAAAAAlwSFlz\\nAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4zLCBo\\ndHRwOi8vbWF0cGxvdGxpYi5vcmcvnQurowAAIABJREFUeJzs3Xm8JUVh9/9vnbvMnX2YGWaDGWZY\\nB2bYZAAFcRdBXKJxjVlMVB4TTZ6YxEQ0Mdtjkidmf3zyM8QnJnHBRJRE4xaNG8YFAQFBUfYdYRy2\\n2efe278/zj3ndPepqq5eztL3fN6vlzK3T3d1dXd1dVXX0iaKIgEAAADAKGkMOgIAAAAA0G9UhAAA\\nAACMHCpCAAAAAEYOFSEAAAAAI4eKEAAAAICRQ0UIAAAAwMihIgQAAABg5FARAgAAADByqAgBAAAA\\nGDnjg45AHqtXr442b9486GgAAAAAGFLXXHPNziiKDs9ar1YVoc2bN+vqq68edDQAAAAADCljzF0h\\n69E1DgAAAMDIoSIEAAAAYORQEQIAAAAwcqgIAQAAABg5VIQAAAAAjBwqQgAAAABGDhUhAAAAACOH\\nihAAAACAkUNFCAAAAMDIoSIEAAAAYORQEQIAAAAwcqgIAQAAABg5A68IGWPGjDHfMcb8x6DjAgAA\\nAGA0DLwiJOl/Svr+oCMBAAAAYHSMD3LnxpgjJV0k6V2Sfm2Qccnr0b0H9aPHD0iSJsaMJOnQTKSF\\nE2M6MD2j2UgaaxiNNYzGG0azUaRDM7MyxsjMreuzasmkHtt3SNOx9SbHG5qZjTQz273toskx7T04\\n4/w7vlySlk6Na6xhtO/gjB7Ze6j9275DM1q9ZIF+vPuA0rtZNDmmQzOziqREvNLi5yAeD1ec0oxp\\nhiFJew/OZIbRWtY6ttbv65ZNaeeeA2oYo4PTs9Z9+eK0YLyhRQvG9Pi+Q5oYa2jBePP4XeuvWz6l\\nBx/bn1h21KpFuv/RfTo0E7WPq7V9wzSv6f5Ds+24xOPvEj9e27mQpH2HZhRF3ctt2y1fOKGpiUY7\\nPcd/X7dsSg8+3jwmY6Sp8TEZ447juuVTenzfIW/aWzQ5pkf2HtLMbNTe1+R4Q0sWjGvXnoMaa0gT\\nY53zsmSqmU3Nzkbt43Kdg6NWLdIDj+1vX+/4cTeMtGB8TLNRpOnZWc3MJo+vZdPKRXr4iQNdxxly\\nvVYunpQx0r6DM5oYa+ixfYes5ykd7/g9k15vejbSmDGanp1t5xtbVi/W3bv2amY2ShzDosnm9WkY\\noyf2Tzv3F9cw0voVC7Vr90HtO+ROV61zuHrJAu2fntH0TOROB7E4LRhvaNqRb6XjlnUPxPOoXXsO\\naCZ2W7fO4dREJ67x65Xeny/PbOXdtnyjYaSpufzp0Myslk5NaNeeg9a4Tow1dGB6RgenZ9vXdunU\\nuGZmI0WRtGCioUf32tNIy5qlC7TTkh+3jI8ZTTQaihSpYYzGx4we3zetFYsmtP/QjPYfmp27BrNa\\nMN48R637q3W+D0zPaLzRUMMYGSMdmJ5NXK9Fk2Mam3uO7TkwI2Oa8frR4wfaz7+JsUbiOh6Ynm2f\\nw6mJhg7NNPcfP+fx+z6+bPGCMT2y55AOWzyhMWPUMEY7dx9oX1uXeLxs13fh5Jj2HmguixRp/6FZ\\nLZ0a15KpcT2291Ai/un92OLaWlfq5K3xf9vCmI2ixPMz61md596wxf+wxRPae2BGByxpufVMWjg5\\n1i5vLJwY0/7p5POjlXfG8wdbfhiP2+olk+18vpVvPPTEgcT+l0yNa8nkuB7evT9RNvLdy4smx7Rg\\noqE9B2YS9+fKxZPad3BGs1HUPtaJMaNI0uRc2gwpg7TWWbZwXIsmxvXg4/u9eWKrrHJwZqadvtPP\\neldeEz8uW9qIrxv/b7wcFlquch2nizHSqsWTemTvwXY+6yubtaxdtkArFk3mjs8gDbQiJOmvJP2m\\npKUDjkduL3zP13TPrn2DjgaG3NIF43riwHT2iqhEFee7DtesDnEEAIyWP3npyXrVWZsGHY1cBlYR\\nMsa8QNJDURRdY4x5hme9iyVdLEmbNg3PyX10zyE984TDdf62dbrk49+VJL32nM36x6/fKUn6y1ee\\nqrf8y/XO7f/wJ7Zr1WJ7rfkzNz6oT15/vyTpT192ipYsGNePHt+v3//k99phLxgfa69/5S07ddlV\\nd2vhxJj+/BWn6pc+dK0k6efP3awzN69sr/fmD19rfau446jDtOfgjL7/wOOJ5X/7mie1/33Fd+7T\\n57/3o/bf//snT9bSqYmusG59aLf+4vM/lCQdsWKh7nt0n156+hF6bN8h/dfND+mMow7T6566xXle\\nJLXjL0nbNizTTfc343XRKev1qRse0LYNy/SmZx7btW7LBdvW6bp7Hk286f/9F23T4UsXJNb78Lfu\\n1tdu3amnHrtaP3V2Mm09/MQB/e4nbrLG7/VP3aInHXVYYtmffe4Hun3nHm1etUi/ecFWSdIff+b7\\n7cryu16yXe+44kZJ0v942tE6deOKdtzf+rwT9N4v39Yu2L7o1A26YPu6zHPTOicXnbxekvTuz/1A\\nd+zc0/6tdf3e+5XbdMO9jyW2a6WNy666W1feslOSdOrGFTKSrrvnUT1v21pdf89jevDx/Tpp/TK9\\n+VnHJvZ9ypHL9canH5MI88//8we67eHu/UvS//vaHbrmrkcS68fvl5Zj1yzRrQ/tliT91gVbdetD\\nu/Wxa+9NrPMTp23Qv113f/vvC7ev0wtP3aA//ezNuvPHeyVJf/jibfrarTv1uZuaabaVFuNaaWvN\\n0gX6vRdtkyT9zX/dopsffKK9TjxtuK5X61i/8L0f6ePfuS+xj/OOW61Xpx4Kn7z+fn3mxgd18hHL\\n9YvPOEa3/Gi3/vILP+w6Z5dfc6++ePNDiW3/8MXb9JdfuKX9Vrp1DCsXT+qpx67WJ67vnJeLTl6v\\ni05Zr1/71+u0/9CsXn3WRp133OFK++XLvtPVWvPKHRv19BOa6/7KZd/R9Gyknzp7kz569T2J1uyf\\nfcpRevLRqxLbts7h+uVT+oVzt+hdn272fP7rV52mibFkb+yr7tjVTgMXbFunz970oKTm9XrHRScm\\n1v2/X7q1nRe0tM5X/BxK0s88+Sh94Jt3SWpery2rF0vqToenblyh//G0o/XBb96lr9/2Y5133Gr9\\nxGlH6Nc/2sy7f++FJ2nNsqnEPm15zk8+6Ug9+8Q11uOKx/W6ex7VpV+9PbH8555ylM5OncOW1v05\\nOdbQX73qtK7f9xyY1lsvv6FreTzftN1nknTS+mX6XirPj2tdr3Q6fOWOjfrE9fdr36GZxH5c0vs/\\nfOkC/f6Ltun+R/fpf32qmTY2LJ/Sb7/gJN29a6/+5DM3e8NrrWvz1o9erz2xN9StNG+7ZpK88X/D\\neVt0+qZmPn/vI3v1R59uxmvL6sV66/NOkCR96oYH9KnvPuCM61GrFum35p4HO3cf0Dv/vflM+bOX\\nn6pFk2P6xHX367M3PdhOhy3x+C6eHNOZW1bqyz94WJLa+Ubc127dqQ9/625Njjf01GNX64s3P6Qz\\nNx+mx/Yd0g9/1MxP33bhVm1auSixXfq8xK9VPC9qrdc6X5tWLtLdu5p57W+cf7z+/so79Ni+Q+18\\nIx7uX73yNP3m5Tfo4MxsIj/83v2P6z1furXrnKXTy08/eZM++M27ref3D168TauXLNAXvv8jffza\\nTt772xedqAUTY/qdf7uxa5uztqzUa8/ZbA3v2rse0fu+dof1N6lzTr5+205nnF52xpG6/JrmM+vN\\nzzxWn7nxAd328J72c711buLHFT/md7/sFC1e0Cya/+pHrtPBmVlnOm0tf8YJh+sVOzY645329iu+\\nq0f3HtIrdhypZ5ywxrpO/Br+n1efrrGGsd5HLzx1gy6MlVdOPmJ5cDyGxSBbhM6V9CJjzPMlTUla\\nZoz5YBRFPx1fKYqiSyVdKkk7duzw9yfrsy2rlyQefqdvWqF//Hrz3xdsW6+3yF0RevbWNdqwYqH1\\ntzt27tEn5zZ93rZ1Wr5wQnfs3NOuCF24fX27a4YkPbbvkC67SlqxaELPnysUS9IZRx2W+HvZwglr\\nN4wjD1uoR/Ye0vdT+Xl82+/e91iiInT+Set0mKUi9+07d0mfb/571ZJJ3ffoPp20YVm7y9iGFQsT\\n4WZZv3xhOwM4Ye1SfUoPaM3SBd4wjl+7RLfv3K0HY/nGM044XEetWpxY779v3amv3dp8WKXDu/vH\\ne/W7sleEzjjqMF2YWv/9/32Hbt+5RysXT7bD+tsv36p71Cx8P+fEte2K0JmbV+o5J61tb/uUY1bp\\nsqvubhest65f6jy+ybGGDsb6A21d21n3/f99R6Ii1Fp+xXfuk5SsCD1pUzNtfPP2H7crQkesaBb4\\nrrtHOn7tUt2xc48efLzZ1J2Oz9plU13L/unrdyYqQvHfP3Pjg10VoR2bD+sqoK1ZuqBdETrvuNVa\\nMN7Qx1J574nrlyUqQsfPnYP3XXl7uyL0rBPX6qEnDrQrQqvn0mLcumVTuun+x7V0arwd18uuujtR\\nEbKljXOPXa2PfLtzvSbHGnr+yet1/6P7uipCm1Z2b3/zA4/rM+qc128v3aW//EL3Obv2rkf0xeSh\\n61knrtWlV96uXXOnuXV/LF4wpq3rl+oTsSznuLVL9PyT1+sPPvk9PXhov045coU1Xf3qv1zXVRE6\\n+cjl7XV/+99u1K49B3XakSt0xbX36dBMp6B5qiXMj3z7Ht384BNaNjWhpx63ur38wu3rNTmerAgd\\nmpnVP3692YXl+LVL9Nm5W27VksmucD969T1dd2Rrnfg5lKTTNq5oV4TOPXa1Ttu4QlJ3OtywvJmO\\nr7zlYX39th9ry+rFumD7unZF6Flb12rTqmTh0WbbhmWJ+LaOKx3XsYbp2vb0TYc57/ev37ZTV97S\\nPD+2dR7bd8haEYrnm2cc1X2fSc1urL6KUOt6fefuR/TFWN1k+5HL9dmbHtS+QzOJ/bQsGG8kumGl\\n7/NVc3nkLT96ol0ROnwuP7nxvmQ+ZbNqiTv//51/uzFRETr5CHuab0nHf7xhND13L5xx1Mr2C6nm\\nS8LmSYg/f2750W5vRWh1LK737NorzaXg521bq6VTE7r5gcf12Zs66bBlaqLT5WzFokkdvXpJuyJk\\ny3uf2H9IH/5Ws5vzMYcv1hdvljYetkgLxg+0K0LnHbda2zb4C6nxtGI7b63ztXrJZLsidO6xq3X5\\nNffqsX2HtD2Wb7RcsH2d3n7Fd3VwRtq8anH796VT43rPl/xxkKTTNh7mrHQ8a+saHXlYs/v5x9XJ\\ne59xwuGJMlKcLU9uaRg5K0Jjjc49uOfAtD4oe5y2b1imy69p/vvso1fq23fu0m0P79GJ65t5xLKp\\ncT2+fzpxXPF75Hnb12nZ3Evmd/77Tdq5+4DWL5+yVoRa1+Po1Utylave/bkf6NG9h3TyEd3Xy+YF\\np6yXMd15lyRtXecur9TFwCZLiKLokiiKjoyiaLOkV0n6YroSVDfxhOJIM8G/p9fzrd76LYrSy5Nb\\npX/v7KPZj9YbD0e8fOu19mfm+p3bwskSf4HcPs6sjYzpOvb033OrJf5r+80RfPeyufATaUDxf7u3\\nN0peG1tcW6LU0cfDcl1fG9v1MOqcNyP7MYWEaf3NsmwsINyQa9O5jsnznUiL1rAt1ywVuP1aJ891\\n+ppkbd9ZaGL/H7Zt/LpInfsjfu0665pE/Jz7ydh31L6R3efeFp5Jre+73FGUXMGWln3J25c/maD1\\nYuk+MM6u/XnXy7lt1j3o2jaeb9oqX1JzHJmP7b5qxqnz25ilBJG+Tun7vPNcSIaZXuaS537LCi8d\\n/3jIIekgK/wolpCT4SUfPulwup7lid+7j799r0fxsO3r+LjSSuf37hjEyw+u9G27vq74pOPgLftY\\n8vB26K57xhOe79fktXSvly4DtM/N3GLbuRpLbJPYqyT3vdrO/3MWrCLbTegRWgaoq2GYNa6WOom5\\nk0Di929WuvE9hOK/tf5tW5YVVkae1haSxtP7yPNgbph4hhUWp5Z4ptgIPKBGYIHNdm5968d+da7v\\nSgPGc/3S5zL0utm2DdVoX494JUDtQ4tXXkPj40vT1vOfEXBjbpB01n461zG5LOvcNCzHl45SyP59\\ny60V8NZ/HQXNrH3H49i6PxqmO+7pzfPE273vdNq1hRf73fEywLafPOk+rfsest9v3S8hkmnHpI4x\\n9PYKz5NteYfvWeCPh7uA5M5v0mG72AqvrfBaYWYVnCX3fW57cZhVOcuSNy/1xd/3zA0N3x1evu0z\\nr5XludOVJgNKe1nn33a+0vmuLUx7OSYsDr54u85jw7irNP6yl3tfoeu5niddcWzE/+1Pa650Gs//\\niyiT57bMhzrSoCdLkCRFUfRlSV8ecDRKC8k4W3w/224e47mh3G+rQisOAQ8zS0bj2Ks1fOP81W8s\\nllvkKZSEHVN35pz+zcZXqUq3SnR+d2+fDi5PYaBowaHzAEmm2Zmo8wbKdkxF42L7ZTyrItSwpzNX\\nYcekHrJZ56boSwZ3odQW1+xlrtNgO+/p42rdH9ZKSkBcXPt3LXNVJJLrddKN62VAet30v/Py5YmJ\\nfzu376SFZF4bmvbt4XWtZynU+W4DY0mjIdvG803XfZZViXHtO17pHgsoXbv2n6hwWpYV0Z0Osgr2\\n7vj7CrGh4SfD607r4ekrLC9Lhp0vDCk7T7adr6zKTfyeysoPbHEIeWlsy7ed+Z23YlXBsy5RwQ97\\ntsSPOV9FqJP/F1H2fpPCWhqHHS1CJZhUwSDPQz13i5DnRukUPNLhdMfXvr/sCorvjatrH503U0q0\\nNORhyyCyQmiY7szOVyD1VWxsbL+09pc45470YHur7iq4de/bXgnwbWdb3CnkJMMysX9bj8kbpn3/\\n8f3F2d4Up+8nV2XAFnbyXCRbTnzXLF2BcsUnGa942N33qG+/8e6HrfBsXJXA+OpjsTTcVUlp/dZu\\n9cgukMb3k/53aItQu/VXyeO3XoNY/LPSsu++9xUyGpZjSQcaf7NcpHIW2hKRVRl0/Rb69rwlnm+O\\njTmue1ZFqL2P7n22jsNWcE4v6erqlDrnzWX+40yGH/bstP2dlo5//C9XuslTUXblLelz0NWt1ZHH\\ndcdyLpxYF6n4S65kXL1RleROKy2t85V+zPnysuazpDsPclbix9LnwvMstqSl1n5c6cTbAuspEYcO\\nfUgfY+uveL6YDi9+jyTDdt9n8eV5y1V57rfssMqHMWhUhCoU8gap83v2zR3/t6+Q4Lp5q3rbZFvH\\nWRGyLYtlSnnvmWQXj7Bt0t1bWsvS/C1C/vBDwnI9VLvf3Od7eBdd17adq4LdaNi7MxSNi70Q7A/X\\n1arjSovpB1BmQc9RgcqKo+t+s6UL+7JUeDkqKPFzkvx3dpp3xzts3+nKiku8cpNVwfeNz8rDWxGy\\njDN0xSOe7pvhFNt/vu6OvnDd2zX3Y98unm86W4QyC/H2fcfTQUjXuHFHwsuqgBdha73y8cU/pMKT\\npyBpax0Ib23P+j077JB9ZbcI+fNj1z1uq9C78ufuFiF3fNzH6n/x62I7R7Zz4n95Efu3p/wS/zt+\\nj9hbhOz3UNmucVWM/SkfwuBRESqoNdgsngiStfrsQp77t+5MzfdWM083DJuQe6FMd7zEm6mcd01W\\nk7GNrSXBXhBP/jexfs5Cva0bSaIQGO8PnHEu8z1cw9e17SNZUE3GPatbjitMK2th2x9eulXHtZ2t\\nsOjrGhFfp3u77DiWLRSlV8vzwI5XeOLpvOE4V+ltrcutD3r/vlt8LwWa//HnicmXR8Ufqb57KBGu\\nJd3H10lX9ooWVN0tON3L/G+o/QXmoBYhZ7ca/7G5BqLH00FWwdm3H3sFPDM4r9CXDC2++IdUiPNU\\nUG1psqqXlfYW3HxhSNlpIqtSkJU3BrUIdVWEsq9RVwW44W43DO2N44pPnjB8+Vv876wXvlktQmVf\\niJZRQRADR0WohPT1z5OoQgbIxsMsktbC+25mr5cuLDkrQrZlJtYcnPNI4vvNM3tZSGHTV9D3vzWy\\nLLP8Ziy/x/frDj/8HJVuEUpVfqLY743ORSsdF/ukAd3Lkl8zd4z1cjxQ4ovT29rmmbJVBsMK+vZZ\\nzVytKFnL3IUp+wPYxOKdbH2x36PtWeNc+7Huu3tZ6AuGdvwUXhltznblXzcknHQcmr/Flju6CTYS\\ncc4urKWFXlNrGvGFmxGPkMptnhYZXxw6YcfyjwIVofhsokXiVOWscb74+/KFrPCzWrLT1zV9TP5Z\\n4yzxSOzHHk5IWnallXYYc4HEo+frrdJZ3r7BupdlxKHI/WE8G4aEl4xPK4/qHHVo+SA+o541jnPc\\nk5s0t87q3pr3BULUHgucbzsbxgghuN9o93aBYVqWpcULE759uKdX9j1a/Pv0ae0vJKN0sU0rmRXX\\nhgm7wdtBeypJ3u1S+0xv5xooads+9IFVZPps6+Lu51KqwBh/yFgqLLYgc17bzBah9v/5tzOWcy+T\\nXX+z3VthBalUwc4zPbV/KnTfPhzLYxWe+HghV+tZalOrrEJbu/XbhJ0fV/dQa5wcFZbc02d3xSu+\\noPv6tvcVOZZbw/Ht36T+dqxnWeZ9gWCp5GeFJyXzTdsU1839OnebWi91bLG92rrXdU2fHbD/eBor\\nI7SLYkvX1N6esNrrxD914KzwttZ1TZ/dvcwnuZ/uOyHxQsE1NiagwOq6Vu3fHS974i/Q7Pvu/t2Z\\ndrvG92aXfUJfRISGl1hmuVHylt/iG0WW9RKfCbGE7erG2nlZWezGKXu/zRdUhCqU5+18aB9T1wM6\\nJKwqmj1dYeV5Q5Ye4J1HkTFC1i48tgGmrbea1oJgjkgmwvL/btufvxAXtt+87N3Ckv/OOqa0vJXH\\n7O6jYdNnuyZ+yDo3tuMLGiMUcI19gru2OvYd79IZP4bu1ppy96xv3z55Cnkh3WpC+PKnkO6Nzi42\\nBSsLuVr5gqYHDr92Uuj02WEHZ3vx0IpzyBgh1/iGkHs7r7zXL3iMkGO9PHmBawKSENndXrPXzXs/\\n2tjOV8jLYFteG5ouva0vlglvWtsUaamw7SvrmH1h+PKh+N/J2XG7w3Z2b835jE6ja1wTFaGC7F1t\\n8hRg3b/lzTDLFsxChPaBd1U4ik6WkBgjFHi32ydL6F4vXqC0heEM39bNy3KdTOL37v26wqsqHYVs\\n56oEmEQhu/zD2vZTZguGo+DtSovpQnVWtG3XLOQhnK9C4Y+Dax++fcevS54Z3dzn217p6Vor5wsG\\no+zKbvzncpMluP+23ZPdM5nZCxRFxwi5C4Tdy0LeUOepWEnJfNPZNS4w80ivF09rIWOEXOsUvV98\\nutO8P8BejRHK+t5OS3j6yrqPsp+TRboxplU7RsiVdpPp1V/2sd8fRV++VjNZgv0YfS9LikxSIeWv\\nULu2H3VUhEowJlmECJ2cQAq/kULWd3WfCH04h7w7Ce1ykCzYdOKR5y1xXLxvbHr6SZf4/trbWtdz\\nZyLefMlTOHd1A0x2jesusPoqSsldN39sZYwhb+N8x54eD2Fiv/uumS9Maxxs5yyjy0F6Bq/OOvYH\\nSrrwm9UNw3bNugvU9nsxkc4d405c++1KmzkKuc3jsv3bVsFOxi/kno3vJx2P5guNkG07P4bmK+lK\\nb2iaS8cxHW76361/truapNKA7c1yiNAWIfvEFL5nQSteYfFoieeb7skSwsKyVS5acbaNXUgvcU+f\\nbU9jmfHxpITcLULpqZodYdnSkC/8sXa6toeR3j5r+uzkfjzheJ61IWk5s0VorPsZnH5u2Ngm3nDt\\nqmuyhIo/qOo7RF+LUOjYwXQZqHNu5pa1/o4dl7sC2lye9TIh7wtR14ufIuZDZWooPqg6X+Splee9\\nGU1AZtC9UXB0MpVJ6/HMIG9z9Vhg5pPYnyzXwnZOLZlzZ1/undkL1ZaM3jI2obluOh7+v31xKP4m\\nqHv7dBw7b/bD9uE/Z+6Hd1x6rFRWIT0eTneLVixcT3zjcetu8ejmOkzbA9teoA8rrNkrgckXAvF0\\nV7xFyBZHW3wslXjrep34ZSXP1rpRVDwtt+Lmipi10uoonNsK/SFCt7Pm7d5wWwWW4vnmuGOgddb0\\n2S22+62rQumLS+qgO2NH8+W77e09o8Xytuj54p8u0NrXcVznwApvO/1njHwN6UbcFWbkXickHJux\\ndnzt27jP09x/A/aVTq8hFd/QF7WSb6y0/bq1WqiSkyVkxyn9b1/X5aItQq6wQ1XSNa50CINHi1BB\\nUWqQrRTWX7olb4tQyMMyzz7yKlogaG/bLrDl229IX/eu/TW6m8V9mamvMGdd31FAbW5nD9jXRN79\\nNjD7OIu+CUrHId2K0h702jDeD6r64hT+W/bDPeResLUUNozJ7AoS/wihM562wkvD5Jg1zp1WfNs1\\nl9v3EX/7m3gj6kjzrUKWaz+2Ywl9OWANMha/rEp0VitQKF+LTHLmyeZ/XXl1aMtOWmhLUu6XLo4K\\nWpb48TmPNbSrcVec8n1jzNlKYF03KEpOebsZ+7vGZYfjCt4+rsS/j7jcs8Yl4pq9jktWrxbrB3Rz\\n7Dt5X9rXdbUg+the+hRJSrZtQq9lZ9/JY3RNJJE4b8502NzalU59LxV8qpg1rmjeNIyoCFWo7BtX\\n348h3Sey3gCVmTUuNLHHH0TxaVJbS8s80Fv/yo5r2Bghfxie3yzLbK0LyYK5O2xjuqeNdklPhRxf\\nNc+scbbrke5K5uvyZZ81Lt9JzrpfjOzXIf0AtT1kTdCDsPv4Qlq/0mt4p/MNWJb1FjW9rN1K0DCK\\nt2pmpfk8lyfRmta+ka2xtGxr2r9k7jNxX3TnHXF5Zo1L3G+W9dq/R8n9hV6bNF+LVNZi333geruf\\nJVERchxE0Rah+L1lbdVN/e0qxDUS19uXxsJ1vwDzSxdA43F3zf4Vkld3nsn2WeP8C23hJWLg/L3s\\nrHGZLy6slQJjnQktzjqDrGNf6XQZ8tK4u7t5dWOE8k6WYBzHmC6/xI8r617M/ji492fflkU3jPXy\\nqT8qQiU0C/gm8XeoKscI5XmjXFS5FqHiN2qxD6rmfyCmeStCjgKqlCykJ1sa3MeRDi6sL3d3uHnY\\nPtSYjq/tmELiFByHjLjbCvfSBTb9AAAgAElEQVS27dpvpxvJZdndSWTZzruJdf/e5Z60UiS89Bih\\neME+K+553hqG5kG+t9whaTPZUhocPW843eGG56dFK4+hLUmhrYad9cP2nxbyQdXwyWfScUpO257F\\nOdDbkq+U7cXQdR0KtHDYwsr7jA1Nc+Fj0Py/J/PuYmGErGOfOCD+7/ACvXN8VXqMUMD9YR8jlD8t\\n2Vvhw5bZfkv8u5FeL/sebf8e+CzLq4o8dz6MEaIiVKE8mbhvVWs/8pwF8+by6hJome5RjVSFMY/k\\ntJJh29i6VOVvrXCv750py9K6kHdMUJ7uJkULDq5uV/F/583o8sYl86HpKNy7uiGlB7Nmh999DsPO\\nvT+8RFwtaSVrYHRnub3QER/oGr9GWWk+z+WxFSBDx7fkaRHKe+5dfPeULd6h3ZjzvHwJ2S5vYbho\\nHh7PN0O7Abp0V/L8rcVprjFKeV/4hehuLc4oYHpqSmFd4+zL7bPGFT/e0LzMt27IvoqcrzwvM1wv\\nBxPhdVWE3OG5noMNYwo1VdjOkW3WRX93VmP9t+0+au/DcY+0uD6oGhKfXmwX33Ye1IOoCBXV7g4T\\nSwShXQ2krLeA+TJMV4LMKoDHfsnMM0IfyLYJApItNPnuGluLkC2E9PeGuo7dGtdkPONC+u0n1+/e\\nzliW2cNOzULmKxjNxdr2/QBngdq2zFXIjv3b9bbNHaZ9/67fspa5WnW603X3w7BZMfDH11qBShek\\nLNulu124KrzNdd3LOufaVXCx7zse7/g16qoMdMXPVZiz7yf979DWjHi3zdBWOWOy07IvJN89loxD\\n6lhi++9eN7ygED5GqHuZr2WmaGEjOX22fePQWeNslbx43p6WXuSeNS6+zH0PdYcf/uzMun7pcxP/\\nK5GGHC39WZWOrJnG2uk/8OVIdyzT+0veUyFxtcXHpXW+EndU4rnhz2NCKk2u/N0fbjoMd9c432mw\\nxT/PDK3pMOLP1PRY1nh47rJjc3nWrHF5X5h0ulfn2iyhPca2eBBDg4pQhaqqGYcWGrN+q7ZFKLRA\\nYF1a+GZJNKUHzpxn1F2AtsXL1+3e+5bWtsxTMemuoPrjFnTdLA+WPFyF2nj/5U7FM2wfeeNivSap\\n/vchrSzpCrKtUuob02Qsy3zbNUz4BAMhZyTvG9z48cbfiLrSfKHJEizrhVZO4oW7rONvxdk3tiFE\\n1z1liU+cq5UkSp2M4Jbw9N85rqlvF0Wz8Hi+mfUxxiy+vDQkiPR+OsOB7HlQFu+YvMAKafv3ki2D\\nrq1Dx5W003/qmPKOCUtUeBqdeyqxTkBpL7Rrm2vWuKxbOHlfht0j/rJPdyWltY1rM9+5te2r1VoT\\nPmucEutFluXpfWVNluBquSw6tK51LGXKiJ2XSfWvClERKsEomQbyzBrnU7RFqHt5JdHJFZarYFT0\\nXhlLZSrO/abe1nS3CBV7Y2L/0bZ+87+Jt4iyZ9JZrVVhb0WT+80rq/Wi+Taxu3KXN8yW0FnWEvFp\\n+N+ipv9OV4Cyzk3Dcnxh/ejD7zf7S42wwpor/rbKT7PSmNo+IH4uoXmQ96VNwH0f0qIZoitNWFqS\\nfeu7FO0W6nzbbZ1iPaxQlUc83yz7QVXfi5y848B84TbXDYqSU3ea9wdY+oOqjhJUeJ5ZzUPaVRcJ\\nnYQndJ2iH1TNs27Wi8OscFthFCnk2zYJ7eZo+813vHnOW8iHi4uopGtcVZEZICpCFaoqU8vfj7y3\\n8SkbVpltx8biY4TCCgyuLlVV8Y8Riq2XKqB3loc/LJzrNDoF4CKyCrrxgvXgxgjl/aCq/b/u8Lvj\\nUaawENJ6ZZO/W0Nrf7HWFxNQscyxnzIVIdvLgJD9lMknfJXLvC+WivANhM5aHjIGIq94vukaX1BF\\n3pEnrwrZf9nrEt4dvMn38jI9+Yp9f+XObVXl22SrjCtO2eEUOV95XiQFRDP4pYJvnSqfi1kTRKS5\\njtH1Ai8rPKm6l+xpZYLtYRGr76gIFWRrkqwqrYa8RY5r3bxZ3x4oM312aLU/vs/2lJmN4k2w8Uyo\\n9S/7dNCxh7OtJcGz+zLdENr7bBVIPZUk1/bGmNSbO/e+092cbOe7e5sw6bfznQJ3d4TsXc0Cd2TZ\\nn41p/59/u9Z+22nMJJc7w7dU9IIOIX1ftac0d+/Ds7kznq7rGe8OEj+GrAp3yPWxVd473Sgs6/te\\nCgTsM1FQiD2Nyk6fnTh2WxpqHWBq+uyiQs+1bXFIS3fe+CVmjXOEX7RwZYx9OuSWdFRDxoL40lge\\n3V0k/QF2fezVE1Z7ndhKWQP+010t04odr3/6bFdiCXsp4//d2uVPxjoldJztezeh90ie7+q1NEyx\\njrZlXgB1fnMd49w1sq2XM52mFc2+ynRHbm05HypEVIQqVFUrRN7nU9m3UiHCQ7JlGvFl+W7Z0A+q\\nJt+8lP+OkI+vMGMfAGys67rCC+tuYg8rVFiLUPcx+RQdsOniahHqvrbJeIaeG98182/nDy8RN8t6\\nrdWyCg9Z+29W+DvHkFUYD9lP5+FmO++eDSz7NSY736iqRcjf7ST+y1yf+4qf3ln3eIv9vLrjUrhr\\nXMDYyqKnIJ3XZsnXOlbuuuRtTfC2CAUcp2vzot9oKqqK1p5mOPkL5K6JJOzhZ++rSIuQrUW4yKkN\\nHU+Yp3eKK+zEcyejJF51fmWLQ16dMbb1rwlRESrDk7BLBVuwYJRV8HEHW+Gscab73w3jnsElS3LW\\nuLlwLevFMwrrVMK2uKbiWYZ/Zq3k37YWjdDCRXoGsJAxFtaKm2OsQvyctPdhfQtoCTPneQwZp2Qd\\nI9TVDan13+S5yWgUsF6zkHSTTs+dsWCWlW377QrPsV5GhSveBdRa+U/FL2Q/rYe+rXKYt4tknjfQ\\nzXvAdC1PrOvdp/tva4E7lZl04lEsMwjtkhU6jqyzfr601RLUIhSap1viZFLnLWv9xO+WbU2O4/Sl\\nq668NiNT8s0a55rxLSSvtn2nzcZVmExvZpv50BqO6Wxc5EVI6FiV+Frx1hf3C4C53wNebIa+VPCt\\n03yW+eNi/637R9uscf6XF8n10ufGdq7c4ZlEHLrjG18rXN483cZV7qwjKkIlJaZArKgmlPctkbMA\\nPIAEai1wqvhbg/g5DZlGsx0H74OkqXBzsqeyk/igauq/LVndN0LOVPmCm+UYlGy2z5vJ5k+3lmtS\\nZtY4k/67s55r9rf4dvFl/u1M+OQP1rTS/dDOI16Gj/+7K5S5cDtd9+z7sXX1CW25sIWYOK8Zhxbv\\n0lMmu+o6p/GustZjsYeT1ZXJuX9HmuzerzvvsIZb8KSEtKSHPq+6u7qF5cmddVLhtbqVB6ax7vi4\\nr1GVLULpwn7WOslw/fvthNv8b9ascUW62KU3KTLWJq11vpJdCGPxyAg/eU4d63R9CyojUEdYru1y\\nzxpn6eYYOkaoYWIt/57jcofX3Nr1naGiXXpbx1KmyJqu2NUZFaGCXAWkKuRNnO6pWiuITE6uh1vR\\nU+NrWk6sl/ox5M1+UbZj6RT+TNd66bh1v+1LhRVwV+b5oKGNtaUlHvfY372bLCH795C6Rfrch1bg\\n0i1IUvYbZNv+0+El1g3Yvuj9nq4oZn0DKU9ByJ4+3HGxLTMm+wVIr/LMZGtD9/pVvyTqbpEKrwiF\\njBHKH5/ufCjPfv1h2//tkmc3ZdNDd97qD8/fNS77HOaZJt26fUVPpipae6Ri5ytP/pl8Poadu6qO\\nLYQtnKyPyPp+8x1vnm7BPZssoUS4eVpxhx0VoRLSja9lElVc/gKl423VAFKobY8NU7wiYvugqk3W\\nm8AqT4X9GFuF0+718mbs+cYIZa4avA9X96LwMUL54hAyhies4Jg896H3j61FKOzto+sB3r3MWggP\\nDM+9/07YvvFN3RWu8AJFmRahTkU0+wVIVc93/xghW7yrzRvLTIneizFCiUlmSrYIpYV2EcqzTmfd\\nQlFy7iu0q1dWWK5zWHUls6jqxgj5f7edr7CWjdbv8bQTFoeQeFf1ssMWJ98xZ71Q9HXRzfPc6d30\\n2eW3ZYwQEqobI1TNfvudGUuuDKl4i1Cya5x7vaw3slXerL7CjO3hmRm3AgVW35ikEN5xE6l1wisW\\n+eKStXqzoB9WII8vD6+4dRf6wwp2/vASy3yzCeTYp239+BghWzih3bXsYXf/FlrYiHdVzNpjdRPM\\npI41MfWxbb+V7Na5j6zZxHzbJn8rFtGQSk7RF3d5W4TyHEPZ9NCdl/rXd32o0haWdR3H8n73xqii\\ntScknKzWkewJcLLjU1WLUJFnvrVFyNItzfdsdFV+fOWAIue9CtV8ULWiyAwQFaGSjCPRlwuzWMEo\\nq09wmemzQ/vOxzOf+BSrRSsitr6xrslBE3/leKuUv5+t+w2RsayV9aa+0PTZljs3z/TZtl3E4xXF\\nVrLFJzRMn5CWMXtB2x6bdBe5rMvqu2ZZ8Uruvd/TZzf/G+9yGin7TWqegl382rSnNrale8uykMJO\\nVpzKTp+dVThrL6lo+uys7q9d+02s6z5H7TEkeafPdowpsIWdVzy/Chl7GbKfomOz0vK+AEuP5UmM\\nfXFEPCSqnWeyf+WAD1dYt0oz8XRS4lxm5RG2sU8mFiNXnm6bPjs0/QVV8mzLCqRv2za2SUXaz46M\\nPD/xbyWfS+mu6D5ZY86KT59d3DyqB1ERKsyS8qp6y5h/zIArnP4nUWvBtVGmRSgsiXYV+np4e/rf\\ngpuu9TILqI6wfMpe25CWltxjhHIm3KKtL+5uKq23dMX3X6ZFqOgYobyXMt432/dWMbTCZdvI3mJo\\ni0v3sni6ydpldS+P0uHa/91Zv9r8odwYIXe4ReMZkm8WzSN93X2s++njcyhvt6rQaZDzx6O/z95+\\nvYTNahHKOmfJSkLYPRLU6mjrvpa9Wea+Jf+4KOvLIcdLGFfXc9d+s+JVhTLh5i0fDDMqQiUYk7wR\\nqprrPXcXI3UKRt71nL9XN322bX/+4plfclpsdwhZ5973c+6CqGdZ8trZM0tb17jQOHRNkuB4+5QZ\\nX1vhMPVvE7hu1v6dYQQcs2+GvnRsOm/pTGypW+iEAFlx8k1PbT93yfi5x/jZ9x9/ADW6k4Fz+5B8\\npTNVbDycVJqL78O6387xZe0yz1gaX1CucWPx+MSXtxe104wvliHCCm95K2W+e9An5FlU9JjjLZF5\\nr5M7Lr15dma2Onv+LjL9cnqdzBbRYmfLsz8lIpj3tGZ2JXTkx53byX/OfBUD1/JkXhS2TXpfoWyb\\n2CtCc/+1lKCdFcNUXh1yXC3jGS82it49pSpCjXL7HiZUhCrUq7ebmft1XMWqJm/Iw17gNYUfdKFd\\nYzPfRFU6RsiSMTa6C8Ot1Xoxfqns0djHKthDrWKMkK23RkiwoYXv+Lp5xwhlLQsVGl6RCkpy/c5/\\nfduWGSNkb+mx7sS7qH+zxoVVRHoltEUoZJKSkHAy4xM082Qx8SgN4BHj1XVvlSjhlDm2fr8kz+qS\\nVjaclqyPi+Z58RH6vEmE7wjX2/01B1uc7JMltJ41/vs52f3Nc1wFznsVyqRT37OibqgIFWQbFzBs\\ns8YNy0OqTDyy3oS09LN51loebL8h6s60Q2eUyhWHkmHkGUAe3tUsbxxCCubdy7Kmiy9TcSvXVaA/\\n27kmS6hiP75zGDoZRHt8RHMFr6pu29CKSK9kTYDSWS9728RvBZ/QoflmEXkGx/ebb4rikPVDf8uM\\nR5/fk/dq9sW0rAJ5ni5eoS3h/egq7tq35O8al/UMSd4r7vWyhIz5K6KKrnHzARWhCvUrMwrd72DG\\nCNkLUEVjEvomJOu5X+WpsL8t73470qkcpbcPKzT59GOMUEvvviNU7QPO93Cyrx+2LO/+s6TXylvg\\nirc0euOb+i3s45dhD/h0XJLrNf8bRVHAG+KqCjA5C8CV7NW9vzytq76oFj0/Iflm0VMfUpgdFN84\\njLJhDbOqKqRZwWQN2s/zHHbtq8iLw6pmhgxtEepMzOPfr//eDo9X774jVHxb4zkHdUNFqISyBRpn\\nuDmDac+SFaWXJ//uz6xx3fszlriEimdCrfDsM5YVL/jk7T7gmykr/pvro6fpc2FyxKE9a1wrkNh2\\nZWeNi+e1kfwzAVUza1zODZxarbPGstQt6yEWvnf3rHGh+7WG65o1rj3OpZPaQq5HyH5bqyTSQnvW\\nuOx9SPZWUef+HCvknTUuNNzO78n7p+ykZV33tLOQ173M273RkbdnCfnuSNHXU8YkZwRNKzQPWo9m\\njctKgen9JmaNc1yXPFHt16xxjXg66eGscVkxyjNrXOi+4tu4jixr0oJQ9kliLGF70lWiDJDIR+f+\\n69mXS9aYv+KzxhV/CJv2f+tfE6IiNISq6mI0mBYhx/KC4YW+CQku+FTAV5ixjxHKeFtcIGplD8c3\\nzqlreXABP1+kwloowsPzDWC1r1/Nw9MXnk3ZB0dijJDn4uRtJWluY9/WtX3WGKjs74r06k1n1n4r\\n3l/guQ49r53fisUnKN8sGHbeWeP6qcqxYsN2bD6D6o2SNx5FWhODXuBUdvzdy3zTZ1vDSHzDzJc/\\nh8erZy1CFdwfNbpNnKgIFVTRCyyr3AXK9nb25XL8Hl8z+81t/kKevUCU78TZPqhqi0nmrHG+3yq4\\nkTtv0rPj2zUrjsKnF69q1jhrV4LUv13pyh1m3opQwDpBpbVkhtzOoDP3n69Q6t57zgfC3HpFWqyk\\n+AOoM0bItmpWurMZszzcjOeB53spEEXFW4RC05wzXOcvqW8itSt+OQK37S99TzuvXfa2id/ypq05\\nQV3j8gXZ2c4M76xx6Z3nn4HVHVZ7cY4g+zZrXDyd9PRljqOCn/W7Jb2EV4SyQq+wi61lD9YPqrZm\\n18wIwzfZT540n3U/l7mXi5oPFaAWKkIlJQoMFYVZ3WQJ/U+peQo2IaprESq2/1B5CouhA6v9+8u/\\njS8OzTDLpaNeTJZQJLy6jRHKvZ9Ga3/++GalO/s27nMYOhlMa62Qbj89axHqc97XuzFCxeITNkao\\nWOBDPVlC6u8ykyXUa4xQNeGUPebsFqH8+wr7jlBYWFmMJRzfGCFrGI5j7M6Pw+MV0tW1iGpahGp0\\nozhQESqhV9c/b+LMenPcT649Fo1J8GQJJR54efkqO/Gf8owRymsoJ0vImXCrztt9lVH7/sPPQdHw\\nbMqmRRN7AOXpehGyW9+3IbJaETvrNZdGUfY+e1cRylih4t2GzlqXN80V/6BqQEWoUMjJ7Ya9slAm\\nedWqa1xFF6KqvCnk9yon4anqWtnCyfqIrI+/oh0e595Nn1083NDv9dUBFaGSXF3BysjdIuS4SWxv\\nN3rOWRMqdnJC34T086FlHZhpO/C5RVlvi4sN6sy9SXKf1g/BldtX3sOovkVoLh45148rV3AKW6/s\\nUSe/I+TbT1grhW0bX5e3xPqec9jsGuffZ68K0tldknq7P9dxhZ7XrHCyBE2WUDDsIt2b+qXrOpRI\\nYMN2bD7DXiFtKdT1OOQFTmVlr+5l9u8Ild9XnjB6OR1+Ub6Z8+pm+M5uTfRwiFDuhOVafTAtQo5K\\nWcHwquoaV6XQfbVWy+waVygO/WsR6tUkAFVfs/xjlKptEQoeR1fyuONdAP3fQal2v/bt3ecwUvb0\\n2b3qVpGVbVSf9tLh52kR8oXbwxahCs7BsBXA09EpE706FfDqUmkrkl7ydOkty94iVO1zokgYtnFK\\ng9YZizt8ccuLilBJ8bRcVYKoKpxBPKRc93bRfGMoP6ga3ELSKbAmlhcML650i5Bt2TxpEQrla80o\\nIjxdFN+H1Ll2DZMvpyibr9inqO1eL8916NVtm5W2ejU+rSVPPujtPtPDD6pW8ZwZtvEBefMA3/TW\\n5V6KFN602P76u7vCipzTsJbsath2ZasIFdlfOqlVOX32INAiBEm9qwlXNvBvANmja4+duOSLU/hU\\nyLmCLSX0vLpahLr+LnCdejFGyFmoqSCnsxYAS4ea3kfOtFVxi1DelrOie4qPherF+BJ3eJZllvVa\\n3ZEGOUbIHWx/9lfVZAlF8/CQfLOS7j3lg6hUr8eC1s3QHUOB+IRs0tNZ4yp+TnT2FW7YWl6l2HNo\\nwPGoAhWhkuKJoKpMp7KbeiAtQvadFvtwXPibkL6OEcr55j9zTFCRh0PZVoUcb/KryIRtL16rvmb5\\nW6TClpUJz6aqrnHGGG+Bt/KKpm2ZrWA/999I2QX5QY0RqvrzB6Hfr7GnOV9ltlh8+vUGedi6ZOWN\\nTVWD2dN6+XmNPIYlHi29GyNUTTq0fkeoojFCpboqD9dtJokWoUoYY6aMMVcZY643xtxkjPn9QcWl\\niKq+hG0zLB9HGwZDOUYo53q9KOwN4xihvKofp5EvQPvHQHvfIlRWcrKEwd7jvspRFGWPEapL2sq7\\nvzwzMPZijFC/BlcP4RjuygzjW/i6K3JOQ1pFe/kSetwyPqeSbqU5whjGcTiDfvZUaXyA+z4g6VlR\\nFO02xkxI+pox5jNRFH1zgHHKxZje9JGubuBfJcHkUvUuq5oWtEp5xwhlTymaPw69+N5D2TFCeVV9\\n71TxHaM6jAto3RN5J0voBXvLYqsilJ0fzJdnaZlvg/lnjSt2gsK6xlVQmBu2C1hhdIbu2OaBnk2X\\nX9n04d3LejV1dd11rmX9z8/AKkJRs0ll99yfE3P/G7KG3MHo5Zz4vVb1LofzFgtspZr7b+YMVoVi\\n0L8WoWF8G2WT/0PEYcuq3n/5b3W09uePbz+um20frThFCngJUJO0laV7QpQcb3u9LULVxMe632JB\\n595PP82X9DRf9Sq5VFVXseVXvfqYad21TtWQZQGFDLRh2xgzZoy5TtJDkj4fRdG3BhmfIuJpoLrm\\n2WrCyTMUJSvu4d3BTOzfTf2o3abjNxbyRrTovgJbU2bnuk+GfGQuvoq312U684niP7m647iXxX8z\\nJnlOWm/Cio7v8pmaqDLrCTvPaZ1pqDvL8o7/soWXuW3YLgKmxe98UNX2RrR9jeW/jgsnO9eitU2U\\nSFdzy9R93fwtQlGlLUJ5rm/ensulZ9RL57V5jsuz79YxLxjPd7+ETTkcFlZ6tSiSJufiYwtikAWj\\n9L7r+ma1Oz3lqFhXHJegfcbueZup8bHmegViF8+38kxLX5W8z5X0KTCp5e1zFZQ6/esWPeys6xUW\\nxtx/C4cwPAbZNU5RFM1IOs0Ys0LSFcaY7VEU3RhfxxhzsaSLJWnTpk0DiKWdK/lccuFWnbRhmSTp\\n//7Uk3T3rr26/eHd2rx6sbauW6rdB6Z1YHrWG/b65Qt10Snr9YzjD08s/6OXnKwViyYs60/pdU/d\\noleeuVGS9Ik3n6vP3vhg1w384Tc8WX/5+R9qejbSzt0H9IMHn9CKRRN66/NO0IHpGf3Nf92q55+8\\nTm/5l+v07pedmtj2olPW66o7dunowxdr7bIpd+Rju/ybV5+u9115h04+Yrluuv8x7zHHXf7Gp+jS\\nr96uLasXa8mCcb35mcfqOSet1bYNy/TqszbqV559XHvdj//SOfryzQ/pJ884Un/86Zu1dGpc529b\\nqyMPW6iduw/qizc/5NzP68/bovse3afXPXWL9fffe+FJuuG+x3TBtnW65aHdevfnfpA+xLbzt63V\\ndfc8qgu2r28v27X3kCRp4cRY+7iuvGVn17ZG0vtfe6be/bkfaOXiBXry0Sudcf74L56jT95wv157\\nzmb9zX/doqcet7r925+/4lS99yu3aeXiST09lnb+4MXbNdYwmhxr6FVnbdJXfvhwO2387FM261+/\\nfY8OX7pAp21cobO2rNTiyc45fOiJAzpryypJ0offcLauumOXHnxsv97y3OO74vbsrWt01R27JClx\\njdLOPXaVXrGjmVZ/5VnH6uknrNF19zyqv/vKbXr3y0/VzQ88rrt+vFeStHXd0q7tzzjqML3m7E16\\n6ZOO0EevvlfP2rpWknTOMat03nGr9dyTmn+fv22tXn7GkXpi/7Quef5W3fnjvfrhg09ocryhDSsW\\n6oS1S/WsrWv0M085qh32aRsPa//7/JPW6g3nddLG+3/+TN32ULMR+/2vPVMf/ObdWjDR0AtP2SBJ\\nWr1kUi86dYO+ePND2n1g2nn8tofXJRdu1YnrlyWWveyMjbrh3sf00WvulST96U+eIkl65glrdMO9\\nj+qFp67XqRtX6Nlb1+j1T92iM446TMeuWaJb5+LY2s0/v+4sfeSqe7TOcd/+zkUn6a2X36BX7DhS\\nrzxzkz74zbu0aeWi9u8fesPZuvzqe7Vq8aQuufBE/dM37rSmY9vxZT2oxxtGb3z6MXrBKet13Nol\\nieuV9q6XbNff/Nctev7J66151CUXNrf58Z6DWjY1nrheaedvW6ujVi3Sr82l44uffrR+9Ph+vfbc\\n5vV+z0+drt377dfwg687W//8jTu1df0yrV22QFfdsUurlyzoOq6WDcun9EcvPbn99y+cu0VLp8a1\\ncHJMd/14ryY83wjZum6pnnPiWr36rI3Odf7m1afrP66/X+ccs0qP7ZvWI3sPamqioVfu2Khj1yyR\\nJP3Bi7dp/6EZ3ffIPp133OG6e9feRKH07c/fqqmJMe07OKM//szNifBfdsZGfefuR3XT/Y9ry+rF\\n2rRykX79/BP0gW/cqZM2LNNfvvJUzc5Kd+3aq6cff7gWjDf0yRvu18lHLNcTc+fwd15wkjavWqSv\\n/vBh/dw5m9th//6Ltuk9X7pVf/XK09rLfvuiE/X3V96uv33Nk/T6f7pax65Zoomxhn7unM3671t3\\n6hfOtefXUifNv+yMI7VsakIbljfT/Md+8Sn6u6/crmvvfkRP2tS8x8/aslLnHLNKT9q0QnsPzmjD\\nioV6y3OO1/TsrPW58c4XnKT3fuU2/dnLO+nuGSes0caVC3XPrn1avWSBdu4+IEk6fdMKHbNmsf7H\\n045JhPGnP3mKpibH2n8/96S1etWZG9vpsOXyN56jT15/vw7NRHr1WRt1xGELdeN9j+nA9Ize+YJt\\nXXE7fOkCvf6pW/TyHTPuDEsAACAASURBVBu1aeUi3XDvo/r180/Qr1z2HUnNvNHm8jc+RV/+wcO6\\n88d79LTjms+Mtz7vhPY5avng687Wjfc/ptM2rtBPP3mTLj7vGN2+c3c7r/n7n92hD37zLm1etViS\\ndMUvnaPX/9PV+sOf2C5JetuFW3XZVfdY83NJ+uOXnqxFk2PtstHbn79VUSTt3H1AyxdO6B9//kzd\\n+tBunb1lld75iRv1nbsfTWy/eskCvfi0DXpk76F2/h/3pmceo/OOO1wfu+Ze/epz3M8mSfrlZx2r\\nc45ZrXf++41aMjWus7es1C8+4xg9P/ZsX7ZwXBc/7Wj9xGlH6Ku3PKxr73pEZxx1mO5/dJ+OW7NE\\nl73hybrmruaz8M9fcar+9su36czNzXP6kYufrI9fe2/iuKTutCFJH3r92frXq+/R4UsW6FeefZye\\nvGWl/u26+3TM4Uu0bvmUnn784dq9f1qv8uQPNu/7uR36wDc618vm8jc+RW/84DX6o5d08q6PXPxk\\n/cPX7tCxa5bo2rsfkTQ/upCaXg76z8MY805Je6Mo+jPXOjt27IiuvvrqPsbKbcsln9IvP/NY/epz\\njtfRb/+0JOkH/+sCLRgfy9hyfnt8/yGd8nv/KUm6808uai//0Lfu0juuuFGvPmuT/jhWKOi1zW/7\\nVFdcyob1pd94hrasdmcgLT/z/76lK2/ZqT96ycn6qbO7K/Gt8L73B8/TosmBvpPoubd97AZ95Nv3\\n6I9ferJefVa+Fxovf+/X9e07H2n/XcW1dDk4Pavjf/szkqTr33m+lltePIT435+9Wf/fl2/TW593\\ngt70zGMTv33qhgf0pg9fq/NPWqtLf3ZHZlh50vBVd+zSK/7uG5Kk9/70kxIV8yod/47P6ODMrD71\\nK0/Vtg3LE79dfs29+o2PXq/TN63QFb90bqX3YFmXfPy7uuyqu/Wul2zXa84+KnuDCvb1Oy84yfmy\\nZZA+/d0H9EsfulbPOXGt3vdznXQ4TNcrr9+8/Hr969X36u3P36qLU5WQXvnvW3fqNe/7lk5Yu1QX\\nnbJef/H5H+qXn3Wsfv38E/qyf5+fft+39LVbd+rSnzlD529bN+jodGmltW+9/dn+F6wx37z9x3rV\\npc2h5FlptM5pedi96tJv6Ju379L7fnaHnmOpfA4DY8w1URRlPmQHOWvc4XMtQTLGLJT0XEk3+7ca\\nPom3n/OikbCc7DMwHBXvMvJe5ewxQqSbYZF4udWjy9LuftbDsOf+6sEeUvvzjREaylu9/5Ea1rt7\\nWONVhX7mqXU4j8P+1j5P7Ib7SEZHp6vfYONRhUG+hl4v6Z+MMWNqVsj+NYqi/xhgfHIZzof84A17\\nhttPrTFCWf2XOWXDoxdj/nz76GXYg0pX7TFCg9n90BnW+3tY41XGQF4q1eA8Dn0UezRWEL03Hy7H\\nIGeNu0HS6YPaf1XiN+V8SBBljcIpCL3O8+mNyajox0O2l7tItlD3nnfiEN4WDbn5lzENYiarOrTo\\nD/szqKpZFtF/dUj/Webx59D6gDuyyyicktAbv1UOpEWoPvrQM66nIcfDHlSljhahpGG9vedjvjOI\\nY6rDeRz2OOabZRFDZR5cECpCFZoH6aG0+fB2IEveh0r21OTz/5zVRXLGs/pdl763CFnHCNXvvPXS\\nsKaj4YxVOaEfsa50n33bU3HD/ozJNUZouA9l5MyHy0FFCJVyZVKdjHg+3DZhWnP/0yJUn2PsZytK\\nr/fUj3Pu6xo3nD3japIQ+2BYK2hljN5Txm8eXmIMiU431PonMipCFZoPCaJXevFRzkFhjFB+w1ko\\n9uvVZevlueh3UrPtrzVr3GwdL3oPDOv9PyyfzuiFvo4RGtYLrFheM7xRlJT3HA75waB2qAiVwO04\\nmkIz7dYzKLNFqGR8gJZ+T95ibxGaGyM0f8vZGFKD+Nr9ENeD2oY9inSNq6/5cDmoCFVoPiSIskYh\\nkwo+xODJEkbgpNVQHS9L/6PsHiNEPaiphsmotlpdsBkjlDTszxgmS6ivIU9aQagIFTCfuxSUNeyD\\nMqsQ3DWuPUYoI7yS8UFv1DEt9/uhZJ81rvlf8sk586GkUBNmAGmvDpd32KOYb/rsVmW3V7FBHnV8\\nTqZREaoQN+ZonIO8N37mrHEjcM7QH/1+KNnHCJGgMRiDSXnDn96H/pYs0CI07Ic0KoY+bQWgIlTC\\nfEgAVRuFU5J/sgS6xtVRHS9L/1uEundYx/PWS5yO/jED6JZJei8vV9e4eTRb2XwwH64CFSFUahQy\\np9AjDJ0sAagrW8pmsgQMWj/THrl7efnmjDO5t0EPzYMLQUWoAFcmOwqVgCzZZ2AelI6CW4TCxghh\\nNPXiThiqMUJDea/3P048Fvqvvy1CXOCy8pxDTvdwmE8vuqgIlTAfBolVjUyqYzZw1jgMpzpetmHI\\nkxq0CGFABjJZQt/2NH8VOYd1zJ8xnKgIoVKj8HYstLDZ/pbd/D8lGBJ9bxGy3Avtwmh/owK002Nf\\nu8aRv5dWaIwQVVBUhIoQkFNwpj33NB6FymGoIgWUQbUslHnQDizOfUpqrW5v9q5xrcLoqFeFmsc/\\n/Kdh6CMYzAygW2Y8nxjWaz2s8WrJNX222jUhoBJUhAoY8jwFPZY3/2WMEEYJXUExKOS1o4NrjapQ\\nESqB530R9T9poS08zBrXrcipGNTpK7PfkG17cVj96i7SnrnJsrvh7hrXz8RUlw8/Dn0Egw1ixsL4\\n9R3+az2c6BpXP/MprVMRAnLK2TNuXmUYo6SOl20YviPUnjVuOGtCGAEkvfmL5ymqRkWoAPq+j7bg\\nD6qqNX02OTf6o98pzf8dIfJJ9FcrPTJZQr3kahGqTUsr6oKKEJBT8KxxTJ9da3Wc5GI4viM0VxHq\\nb1SAdk1oUJMlDKthz8pyTZbAXAmoGBWhErgRR1TwB1Wb/2VQZz3V87L1N9a2Agxd4zAoTJ9dT/la\\nhFrbcOJRDSpCQE5581/ya/TLMLUIAf02iKRHci8vzymkRQhVoyJUAC86R1vwZAmt9XlS1lIdL9sw\\njBFq6Wf3JECicFxX+Z6R1IRQLSpCQE7B02dHTJaA/up7pdv7QdX+RgVoYaKOeinSIgRUhYpQCdyQ\\no4kPqo6GOrbk9b9FyDJGaO6pQlkU/db+hhVpr1YKjRHqSUwwiqgIoc/q/4QKnj6bWePg0Ys7gTFC\\nWeqf/8CtPVnCgOOBfPK8dGqtW8cXVfPJfHrZQEWogPmUANA7s3MJhfwa/TIMU/l2Zo0jo0R/tfLa\\nWdLevNWZNW6g0cA8QkUIyClvYXMYCqcYDUMwRIg3tRgY0p7dfKoXti7xcLc8o06oCJVApltE/c9Z\\ncNe4uf82uMtgUf87wZ4HtpYMZ9lrPpx1uLTT3nAmPlSIO3mw5lPxlyIa0CPMGof5zpayW+md7kkY\\nFFLe/EUPC1SNilABfB9jtOVuESLfxjzlmyyBehD6zdAkZDWf3sW1P6g6j44Jg0VFCMgp+I1U+1lM\\njo35yXYvtKcw7nNcAGaNGyU8V1ENKkJATnlbhHhzhXnL1iLUoEUIg8F3hOY/WoRQNSpCQE5581/y\\na8xX9q5x/Y8HIJHXjoL2d4QGHA/MH1SECuBt02gLnS2Q76hgvrNOnz3k88Zh/up0yyTtzVd8RwhV\\noyJUAjfiaAq97J2ucSQUzE+2tN2gexIGjLQ3f/E4RdWoCAE5BY8RmnsYk29jvvJ9UJWyKPqNtDf/\\ndZ6rPFlRDSpCQE7BXePmHse8wcJ85RsjRNdQDApJb/5iEiJUjYoQ0CO8ucJ8Z58+m7fyGAzGCM1/\\nrRcsPFVRFSpCJVDALWJ0HlDtihDJBBbz4U6wpe3h/qblUEYKFWk/k7nM81bnucqDdZCGM38vhooQ\\nAKAyhq5xGBA+5gsgLypCBfB8L2N03uK0m/BH55CRw3xNFq238sOZTc7Xsw4p3ho5nKkP5dHTYjjM\\np/M/sIqQMWajMeZLxpjvGWNuMsb8z0HFBegFps/GfGdN2nxGCAPSaY0cbDzQO0xChKqND3Df05J+\\nPYqia40xSyVdY4z5fBRF3xtgnHLhRoQP02djFNE9CYMy3K2RqAKTEKFqA2sRiqLogSiKrp379xOS\\nvi/piEHFB6gab64wiuiehEGhRWj+Y/psVG0oxggZYzZLOl3Styy/XWyMudoYc/XDDz/c76hZMTVn\\ntidtWpH4+5Qjmn+fd9zqvsZj9ZIFlYX1olM35Fr/JacfKUlaNjVh/f2UI5eXjlNdPO34wyVJ2zfk\\nP+YLtq+vOjo99+SjV0mSzjjqsK7fjlu7RJL07BPXBIe3eslk0HrLF3XS2tGrlwSHn9crzmym7TFL\\naWTB+Jgk6eU7NraXbV61qGdxyeNpc/nPyUeMzr3ncuyaZvp4jiUdDsv1yuv0Tc377ZxjVvVtn2uW\\nTkmSXnDKeu3Y3Nz/WVtW9m3/Ps/btlaStHnV4gHHxO6ZJxyee5uVi5p54YsDnscNIy2bGmTHp/nr\\ngm3rJEmbVtYzr4gzg35rZ4xZIukrkt4VRdHHfevu2LEjuvrqq/sTMY+9B6d10js/p7dduFVvfPox\\n2vy2T0mS7vyTiwYcs+Gw/9CMxhpGE2PJevbuA9NasqC/mdLB6VlFitqFszJmZiMdnJ7VwsmwsGZn\\nI+07NKPFjmM+NDOrmdlIUxPl41YHRa9/FEW695F9Ou9PvySp9/dZVfez73h3H5jW4smxoPFjB6Zn\\nZGQ0OR723mrPgWnNRpGWOirgVZiZjXRgekaLJu3Ht/fgtKbGx9RoGO0/NKOGCY9/r/UrH7rk49/V\\nZVfdrXe9ZLtec/ZRPd9fEbZ0OGzXK69BPGf2HJjWornzOIj9u0RRpD0HZ4YmPmnTM7M6NBMFP1Nb\\n9hyY1sKJZv7ikzfvRLhhT1uSZIy5JoqiHVnrDfQIjDETkj4m6UNZlaBhRMusnatgP4gbpsoMcKxh\\ncmXYjYZxVoIkaWKsoRGpA0kqfv2NMUOd2br44pznePJW4n1pripjDeOsBElK/DZsFf06pqVesZ2L\\nYbteeQ3i+sbvuWFKX8Oed46PNVTkHWVoHlfFC1DYDXvaymOQs8YZSf9P0vejKPqLQcUDwPCjPzgA\\nAKjaINsLz5X0M5KeZYy5bu5/zx9gfIIxEBMAAACot4G1a0VR9DXVvHcZb6mB/mCqVAAAUDVGkAEY\\nftSDAABAxagIAQAAABg5VIQKYIgQ0F90QwUAAFWjIlQC4xYAAACAeqIiBGDo8coBAABUjYoQgKFn\\n6BsHAAAqRkWogIgPCQEAAAC1RkWoBF5SA/3BrQYAAKpGRQjA0OOlAwAAqBoVoQLoGAcAAADUGxUh\\nAEOPqeoBAEDVqAgBAAAAGDlUhAAMPcYIAQCAqlERKoDZswEAAIB6oyIEAAAAYORQESqBr90D/cGt\\nBgAAqkZFCAAAAMDIoSJUBGOEgL5i+mwAAFA1KkIlUDQD+oOucQAAoGpUhAAAAACMHCpCAIYeDUIA\\nAKBqVIQKiBgkBAAAANQaFaESGLcA9AdT1QMAgKpREQIw9KgGAQCAqlERAgAAADByqAgVEDFECOgr\\nesYBAICqUREqgbIZ0B+MEQIAAFWjIgQAAABg5FARKoCecQAAAEC9URECAAAAMHKoCJXAuAUAAACg\\nnqgIAQAAABg5VIQKiJg/GwAAAKg1KkIAAAAARg4VoRIYIgQAAADUExUhAAAAACOHilABjBACAAAA\\n6o2KUAn0jAMAAADqiYoQAAAAgJFDRQgAAADAyBloRcgY8w/GmIeMMTcOMh558RkhAAAAoN4G3SL0\\nj5IuGHAcimP+bAAAAKCWBloRiqLoq5J2DTIOAAAAAEbPoFuEACDYWINWWNTDsqlxSdLCibEBxwQA\\n4DI+6AhkMcZcLOliSdq0adOAY9MU8SUhoO/++lWn6eQjlvd8P//2pnP1yJ6DPd8P5re3PPd4rVoy\\nqRefdsSgowIAcBj6ilAURZdKulSSduzYMVQ1EN5NA/3TrwLlaRtX9GU/mN+mJsZ08dOOGXQ0AAAe\\ndI0DAAAAMHIGPX32ZZK+IekEY8y9xpjXDTI+wYaqXQoAAABAXgPtGhdF0asHuX8AAAAAo4mucSXw\\nGSEAAACgnqgIAQAAABg5VIQKYIgQAAAAUG9UhAAAAACMHO9kCcaY78rTABJF0SmVx6hGDF8SAgAA\\nAGopa9a4F8z9901z//3A3H9f05voAAAAAEDveStCURTdJUnGmOdGUXR67Ke3GWOulfS2XkZuWEUM\\nEgIAAABqLXSMkDHGnBv745wc285bTJ8NAAAA1FPoB1V/QdL7jTHL5/5+dG4ZAAAAANROZkXIGNOQ\\ndGwURae2KkJRFD3W85gBAAAAQI9kdm+LomhW0m/O/fsxKkFSxJeEAAAAgFoLHefzBWPMbxhjNhpj\\nVrb+19OY1QBDhAAAAIB6Ch0j9Mq5/74ptiySdHS10QEAAACA3guqCEVRtKXXEQEAAACAfgltEZIx\\nZrukkyRNtZZFUfTPvYjUsOM7QgAAAEC9BVWEjDG/K+kZalaEPi3pQklfkzSSFaEWviMEAAAA1FPo\\nZAkvk/RsSQ9GUfTzkk6VtNy/CQAAAAAMp9CK0L65abSnjTHLJD0kaWPvogUAAAAAvRM6RuhqY8wK\\nSX8v6RpJuyV9o2exGnIMEQIAAADqLXTWuF+a++d7jTGflbQsiqIbehetejB8SQgAAACopdDJEj4g\\n6auSroyi6ObeRgkAAAAAeit0jNA/SFov6f8YY243xnzMGPM/exivoRYxfzYAAABQa6Fd475kjPmq\\npDMlPVPSGyVtk/TXPYwbAAAAAPREaNe4/5K0WM0JEq6UdGYURQ/1MmK1wBAhAAAAoJZCu8bdIOmg\\npO2STpG03RizsGexAgAAAIAeCu0a9xZJMsYslfRaSe+XtE7Sgp7FbIgxRAgAAACot9CucW+WdJ6k\\nMyTdqebkCVf2Llr1QM84AAAAoJ5CP6g6JekvJF0TRdF0D+MDAAAAAD0XNEYoiqI/kzQh6WckyRhz\\nuDFmSy8jBgAAAAC9ElQRMsb8rqTfknTJ3KIJSR/sVaQAAAAAoJdCZ417iaQXSdojSVEU3S9paa8i\\nVRfGMEoIAAAAqKPQitDBKIoiSZEkGWMW9y5KAAAAANBboRWhfzXG/J2kFcaYN0j6gqT39S5aAAAA\\nANA7od8R+jNjzHMlPS7pBEnvjKLo8z2N2RDjO0IAAABAvYVOn625is/nJckY0zDGvCaKog/1LGY1\\nwAghAAAAoJ68XeOMMcuMMZcYY95jjDnfNL1Z0u2SXtGfKAIAAABAtbJahD4g6RFJ35D0eklvV7Mh\\n5CeiKLqux3EDAAAAgJ7IqggdHUXRyZJkjHmfpAckbYqiaH/PYzbEIjFICAAAAKizrFnjDrX+EUXR\\njKR7R70SFMdnhAAAAIB6ymoROtUY8/jcv42khXN/G0lRFEXLeho7AAAAAOgBb0UoiqKxfkWkTpg+\\nGwAAAKi30A+q9oQx5gJjzA+MMbcaY942yLgAAAAAGB0DqwgZY8Yk/V9JF0o6SdKrjTEnDSo+RTBG\\nCAAAAKinQbYInSXp1iiKbo+i6KCkj0h68QDjAwAAAGBEZE2W0EtHSLon9ve9ks5Or2SMuVjSxZK0\\nadOm/sQsQ3qI0HnHrdbB6dmBxAUAAABAfoOsCAWJouhSSZdK0o4dO4ZymoIPvK6r/gYAAABgiA2y\\na9x9kjbG/j5yblltGDFICAAAAKijQVaEvi3pOGPMFmPMpKRXSfrEAOMDAAAAYEQMrGtcFEXTxpg3\\nS/qcpDFJ/xBF0U2Dik8eER8SAgAAAGptoGOEoij6tKRPDzIOZTB9NgAAAFBPA/2gKgAAAAAMAhUh\\nAAAAACOHilABjBACAAAA6o2KEAAAAICRQ0UIAAAAwMihIgQAAABg5FARKoDPCAEAAAD1RkWoBMOH\\nhAAAAIBaoiIEAAAAYORQESqEvnEAAABAnVERAgAAADByqAiVwAghAAAAoJ6oCAEAAAAYOVSECmD6\\nbAAAAKDeqAgBAAAAGDlUhErgM0IAAABAPVERAgAAADByqAgVwBAhAAAAoN6oCJVgmEAbAAAAqCUq\\nQgAAAABGDhUhAAAAACOHilABfEcIAAAAqDcqQiUwfTYAAABQT1SEAAAAAIwcKkIAAAAARg4VoQIi\\nviQEAAAA1BoVoRIYIgQAAADUExUhAAAAACOHilABTJ8NAAAA1BsVIQAAAAAjh4pQCXxHCAAAAKgn\\nKkIAAAAARg4VoQIYIwQAAADUGxUhAAAAACOHilApDBICAAAA6oiKEAAAAICRQ0WogEgMEgIAAADq\\njIpQCUyfDQAAANQTFSEAAAAAI4eKEAAAAICRM5CKkDHm5caYm4wxs8aYHYOIQxl8RwgAAACot0G1\\nCN0o6aWSvjqg/VeCIUIAAABAPY0PYqdRFH1fkgyzDQAAAAAYAMYIAQAAABg5PWsRMsZ8QdI6y0/v\\niKLo33OEc7GkiyVp06ZNFcUOAAAAwCjrWUUoiqLnVBTOpZIulaQdO3YM1TQFdO0DAAAA6omucQAA\\nAABGzqCmz36JMeZeSU+R9CljzOcGEQ8AAAAAo2lQs8ZdIemKQey7CnxHCAAAAKg3usaVwAghAAAA\\noJ6oCAEAAAAYOVSECohE3zgAAACgzqgIAQAAABg5VIRK4DNCAAAAQD1REQIAAAAwcqgIFcD02QAA\\nAEC9UREqga5xAAAAQD1REQIAAAAwcqgIAQAAABg5VIQKYIgQAAAAUG9UhEowYpAQAAAAUEdUhAAA\\nAACMHCpCAAAAAEYOFaECIj4kBAAAANQaFaEyGCIEAAAA1BIVIQAAAAAjh4oQAAAAgJFDRagARggB\\nAAAA9UZFqASGCAEAAAD1REUIAAAAwMihIlQAs2cDAAAA9UZFCAAAAMDIoSJUgjGMEgIAAADqiIoQ\\nAAAAgJFDRagQBgkBAAAAdUZFqAQ6xgEAAAD1REUIAAAAwMihIgQAAABg5FARKoDvCAEAAAD1RkWo\\nBGbPBgAAAOqJihAAAACAkUNFCAAAAMDIoSJUAEOEAAAAgHqjIlSC4UtCAAAAQC1REQIAAAAwcqgI\\nAQAAABg5VIQK4DtCAAAAQL1RESqB7wgBAAAA9URFCAAAAMDIoSJUQETfOAAAAKDWBlIRMsa82xhz\\nszHmBmPMFcaYFYOIBwAAAIDRNKgWoc9L2h5F0SmSfijpkgHFoxSGCAEAAAD1NJCKUBRF/xlF0fTc\\nn9+UdOQg4gEAAABgNA3DGKFfkPQZ14/GmIuNMVcbY65++OGH+xgtN0YIAQAAAPU23quAjTFfkLTO\\n8tM7oij697l13iFpWtKHXOFEUXSppEslaceOHcNVB6FvHAAAAFBLPasIRVH0HN/vxpjXSnqBpGdH\\nTMMGAAAAoI96VhHyMcZcIOk3JT09iqK9g4gDAAAAgNE1qDFC75G0VNLnjTHXGWPeO6B4FEL7FQAA\\nAFBvA2kRiqLo2EHst2qGQUIAAABALQ3DrHEAAAAA0FdUhAAAAACMHCpCBUR8SQgAAACoNSpCJRiG\\nCAEAAAC1REUIAAAAwMihIgQAAABg5FARKoIhQgAAAECtUREqgSFCAAAAQD1REQIAAAAwcqgIFUDP\\nOAAAAKDeqAgBAAAAGDlUhEowfEgIAAAAqCUqQgAAAABGDhWhAiIGCQEAAAC1RkWoBHrGAQAAAPVE\\nRQgAAADAyKEiBAAAAGDkUBEqIOJLQgAAAECtUREqgSFCAAAAQD1REQIAAAAwcqgIAQAAABg5VIQK\\n4DtCAAAAQL1RESqB7wgBAAAA9URFCAAAAMDIoSIEAAAAYORQESqAIUIAAABAvVERKoVBQgAAAEAd\\nURECAAAAMHKoCAEAAAAYOVSECoj4kBAAAABQa1SESuA7QgAAAEA9URECAAAAMHKoCBVAxzgAAACg\\n3qgIlUDPOAAAAKCeqAgBAAAAGDlUhAAAAP7/9u4/VtKqvuP4+yOgpMjPQpCCywLlh9RShKtZK6i1\\nyK9U8UdisbagbUJoaS21psXQWJumCdTUphaFFEVRAQ0qQrGmYGu1JSIsy8KCu7jLj43FBawYtqEW\\npPvtH8+5MNzce3fnXobnzs77lTy5z5w5M3PmO2fPPt8553lG0sQxEVoITxKSJEmSxpqJ0CLE62dL\\nkiRJY8lESJIkSdLEMRGSJEmSNHF6SYSS/GWSO5OsTnJDkp/rox0LVZ4kJEmSJI21vmaEPlxVR1XV\\n0cD1wAd7aseieIaQJEmSNJ56SYSqavPAzV3wOmySJEmSnkc79vXCSf4KOAN4DPiVvtohSZIkafKk\\najSTMUm+DrxklrvOr6prB+p9ANi5qv58juc5CzgLYNmyZcdu3LhxFM0dyqOPP8m6hzbzi/vvzq47\\n79R3cyRJkiQ1SW6rqqmt1htVIrStkiwD/qmqXr61ulNTU7Vy5crnoVWSJEmSxtG2JkJ9XTXu0IGb\\npwHr+miHJEmSpMnU1zlCFyQ5HNgCbATO7qkdkiRJkiZQL4lQVb29j9eVJEmSJOjvd4QkSZIkqTcm\\nQpIkSZImjomQJEmSpIljIiRJkiRp4pgISZIkSZo4JkKSJEmSJo6JkCRJkqSJYyIkSZIkaeKYCEmS\\nJEmaOCZCkiRJkiaOiZAkSZKkiWMiJEmSJGnipKr6bsM2S/JDYGPf7Wj2Bv6r70Zs54zx6Bnj0TPG\\no2eMR88Yj5bxHT1jPHpLKcYHVtU+W6s0VonQUpJkZVVN9d2O7ZkxHj1jPHrGePSM8egZ49EyvqNn\\njEdvHGPs0jhJkiRJE8dESJIkSdLEMRFauH/ouwETwBiPnjEePWM8esZ49IzxaBnf0TPGozd2MfYc\\nIUmSJEkTxxkhSZIkSRPHRGgBkpyc5J4kG5Kc13d7xlWSlyb5RpLvJrk7yR+28g8leTDJ6radOvCY\\nD7S435PkpP5aPx6SPJBkTYvjyla2V5Ibk6xvf/ds5Uny0RbfO5Mc02/rl74khw/009VJNic51z68\\nOEkuS/JIkrsGvnqxWQAACFNJREFUyobut0nObPXXJzmzj/eyVM0R4w8nWdfieE2SPVr58iQ/GejP\\nlww85tg2xmxon0P6eD9L0RwxHnps8JhjbnPE+AsD8X0gyepWbj8e0jzHadvPeFxVbkNswA7AvcDB\\nwAuBO4Aj+27XOG7AfsAxbX9X4HvAkcCHgPfPUv/IFu8XAQe1z2GHvt/HUt6AB4C9Z5T9NXBe2z8P\\nuLDtnwp8DQiwAvhO3+0fp62NDQ8BB9qHFx3L1wLHAHcNlA3Vb4G9gPva3z3b/p59v7elss0R4xOB\\nHdv+hQMxXj5Yb8bz3NLinvY5nNL3e1sq2xwxHmps8Jhj+BjPuP9vgA+2ffvx8PGd6zhtuxmPnREa\\n3quADVV1X1U9CXweOK3nNo2lqtpUVava/n8Da4H953nIacDnq+qJqrof2ED3eWg4pwGXt/3LgbcM\\nlH+mOjcDeyTZr48GjqlfBe6tqvl+9Nk+vA2q6lvAozOKh+23JwE3VtWjVfVj4Ebg5NG3fjzMFuOq\\nuqGqnmo3bwYOmO85Wpx3q6qbqzva+QzPfC4Tb45+PJe5xgaPOeYxX4zbrM47gKvmew778dzmOU7b\\nbsZjE6Hh7Q98f+D2fzL/wbu2QZLlwCuA77Si32/TqpdNT7li7BeigBuS3JbkrFa2b1VtavsPAfu2\\nfeO7OKfz7P9w7cPPrWH7rbFenN+m+2Z32kFJbk/yzSTHt7L96eI6zRhvm2HGBvvxwh0PPFxV6wfK\\n7McLNOM4bbsZj02E1LskLwa+BJxbVZuBi4FDgKOBTXRT21qY46rqGOAU4Jwkrx28s3375aUjFynJ\\nC4E3A1e3IvvwCNlvRyvJ+cBTwBWtaBOwrKpeAbwPuDLJbn21b8w5Njx/3smzv5yyHy/QLMdpTxv3\\n8dhEaHgPAi8duH1AK9MCJNmJ7h/XFVX1ZYCqeriq/q+qtgCX8szSIWM/pKp6sP19BLiGLpYPTy95\\na38fadWN78KdAqyqqofBPjwiw/ZbY70ASd4N/BrwrnaAQ1uu9aO2fxvdOSuH0cVzcPmcMd6KBYwN\\n9uMFSLIj8DbgC9Nl9uOFme04je1oPDYRGt6twKFJDmrfAp8OXNdzm8ZSW7/7SWBtVX1koHzwvJS3\\nAtNXg7kOOD3Ji5IcBBxKd4KjZpFklyS7Tu/TnQh9F10cp6/YciZwbdu/DjijXfVlBfDYwNS35ves\\nbx7twyMxbL/9Z+DEJHu25UcntjLNIcnJwJ8Ab66q/xko3yfJDm3/YLp+e1+L8+YkK9p4fgbPfC6a\\nxQLGBo85FuYEYF1VPb3kzX48vLmO09iexuO+r9YwjhvdVTG+R/dtwvl9t2dcN+A4uunUO4HVbTsV\\n+CywppVfB+w38JjzW9zvwau6bC2+B9NdYegO4O7pvgr8LPAvwHrg68BerTzAx1p81wBTfb+HcdiA\\nXYAfAbsPlNmHFxfTq+iWsfyUbi357yyk39Kd57Khbe/p+30tpW2OGG+gW8c/PR5f0uq+vY0hq4FV\\nwJsGnmeK7mD+XuAi2g+1u80Z46HHBo85hotxK/80cPaMuvbj4eM713HadjMepzVOkiRJkiaGS+Mk\\nSZIkTRwTIUmSJEkTx0RIkiRJ0sQxEZIkSZI0cUyEJEmSJE0cEyFJ0tCS7JvkyiT3JbktybeTvLXv\\nds0lyb8lmRrh878+yfWjen5J0nPPREiSNJT2I3tfAb5VVQdX1bF0P/R4wPyPlCRp6TARkiQN6w3A\\nk1V1yXRBVW2sqr8HSLI8yb8nWdW2X27lr0/yzSTXtpmkC5K8K8ktSdYkOaTV2yfJl5Lc2rbXtPLX\\nJVndttuT7DrYqPa665JckWRtki8m+ZmZjU9ycZKVSe5O8het7A1JvjJQ541Jrmn7J7YZr1VJrk7y\\n4lZ+cnu9VcDbntsQS5JGzURIkjSsX6D7Zfa5PAK8saqOAX4d+OjAfb8EnA28DPgt4LCqehXwCeAP\\nWp2/A/62ql5J92vwn2jl7wfOqaqjgeOBn8zy2ocDH6+qlwGbgd+bpc75VTUFHAW8LslRwDeAI5Ls\\n0+q8B7gsyd7AnwEntPezEnhfkp2BS4E3AccCL5knHpKkJchESJK0KEk+luSOJLe2op2AS5OsAa4G\\njhyofmtVbaqqJ4B7gRta+Rpgeds/AbgoyWrgOmC3NgtzE/CRJO8F9qiqp2Zpzver6qa2/znguFnq\\nvKPN4txOl9QdWVUFfBb4zSR7AK8GvgasaO2/qbXnTOBA4Ajg/qpa3x77uW2LliRpqdix7wZIksbO\\n3XQzNQBU1Tlt5mRlK/oj4GG62Z8XAP878NgnBva3DNzewjP/J70AWFFVg48DuCDJV4FT6RKTk6pq\\n3Yw6Nd/tJAfRzSy9sqp+nOTTwM7t7k8B/9jae3VVPdXOh7qxqt4543mORpI01pwRkiQN61+BnZP8\\n7kDZ4Lk4uwObqmoL3fK3HYZ8/ht4Zpnc00lHkkOqak1VXQjcSjcrM9OyJK9u+78B/MeM+3cDHgce\\nS7IvcMr0HVX1A+AHdEvhPtWKbwZek+TnWxt2SXIYsA5YPn1eE/CsREmStPSZCEmShtKWgr2F7vya\\n+5PcAlwO/Gmr8nHgzCR30CUrjw/5Eu8FppLcmeS7dOcUAZyb5K4kdwI/pVu6NtM9wDlJ1gJ7AhfP\\naPsddEvi1gFX0i23G3QF3fK6ta3+D4F3A1e11/02cESbrToL+GpbZvfIkO9RktSzdP+fSZI03pIs\\nB66vqpcv4jkuAm6vqk8+V+2SJC1NniMkSRKQ5Da62as/7rstkqTRc0ZIkiRJ0sTxHCFJkiRJE8dE\\nSJIkSdLEMRGSJEmSNHFMhCRJkiRNHBMhSZIkSRPHREiSJEnSxPl/kRd1HRA212gAAAAASUVORK5C\\nYII=\\n\",\n \"text/plain\": [\n \"<Figure size 1008x504 with 1 Axes>\"\n ]\n },\n \"metadata\": {\n \"tags\": []\n }\n }\n ]\n }\n ]\n}"
}
{
"filename": "code/artificial_intelligence/src/q_learning/q_learning.js",
"content": "// Q Learning Introduction: https://en.wikipedia.org/wiki/Q-learning\n// Algorithm: Q(s,a):=Q(s,a)+α[r+γ*maxQ(s′,a′)−Q(s,a)]\n\nconst TERMINAL = \"terminal\";\nconst TOTAL_EPISODE = 10;\n\nfunction State(stateName, actions) {\n this.name = stateName;\n // Actions of State\n this.actions = {};\n actions.forEach(action => {\n this.actions[action] = 0;\n });\n}\n\nclass QLearning {\n constructor(\n actions,\n learningRate = 0.01,\n discountFactor = 0.9,\n eGreedy = 0.9\n ) {\n this.actions = actions;\n this.lr = learningRate;\n this.gamma = discountFactor;\n this.epsilon = eGreedy;\n // Q Table Structure\n this.states = {};\n this.statesList = [];\n }\n\n addState(name, actions) {\n const state = new State(name, actions);\n this.states[name] = state;\n this.statesList.push(state);\n return state;\n }\n\n static bestAction(stateActions) {\n return new Promise(resolve => {\n let bestAction = null;\n let bestActionReward = null;\n Object.keys(stateActions).forEach(actionName => {\n if (!bestActionReward) {\n bestActionReward = stateActions[actionName];\n }\n if (stateActions[actionName] > bestActionReward) {\n bestAction = actionName;\n bestActionReward = stateActions[actionName];\n } else if (\n stateActions[actionName] === bestActionReward &&\n Math.random() > 0.5\n ) {\n bestAction = actionName;\n } else {\n bestAction = bestAction || actionName;\n }\n });\n resolve(bestAction);\n });\n }\n\n checkStateExist(stateName) {\n if (!this.states[stateName]) this.addState(stateName, this.actions);\n }\n\n async chooseAction(stateName) {\n this.checkStateExist(stateName);\n\n console.log(`Q Value: ${JSON.stringify(this.states[stateName].actions)}`);\n\n let nextAction = null;\n if (Math.random() < this.epsilon) {\n // choose next action based on Q Value\n const stateActions = this.states[stateName].actions;\n nextAction = await QLearning.bestAction(stateActions);\n console.log(`pickAction: ${nextAction}`);\n } else {\n // choose next action randomly\n nextAction = this.actions[\n Math.floor(Math.random() * this.actions.length)\n ];\n console.log(`randomAction: ${nextAction}`);\n }\n return nextAction;\n }\n\n learn(stateName, actionName, reward, nextStateName) {\n this.checkStateExist(nextStateName);\n\n const qPredict = this.states[stateName].actions[actionName];\n\n // γ*maxQ(s′,a′)\n const maxQValue =\n this.gamma *\n Math.max(...Object.values(this.states[nextStateName].actions));\n const qTarget = stateName !== TERMINAL ? reward + maxQValue : reward;\n\n this.states[stateName].actions[actionName] +=\n this.lr * (qTarget - qPredict);\n }\n}\n\n//\n// SAMPLE Command Line Environment\n//\n\nclass RoadEnv {\n constructor() {\n this.totalActions = [\"LEFT\", \"RIGHT\"];\n this.resetStateName = \"0\";\n }\n\n static render(stateName) {\n return new Promise(resolve => {\n const road = [\"-\", \"-\", \"-\", \"-\", \"-\", \"-\"];\n road[Number(stateName)] = \"0\";\n console.log(`\\n ${road.join(\"\")}`);\n resolve();\n });\n }\n\n static step(stateName, action) {\n return {\n \"0-LEFT\": {\n nextStateName: TERMINAL,\n reward: -1000,\n done: true\n },\n \"0-RIGHT\": RoadEnv.normalTransition(\"1\"),\n \"1-LEFT\": RoadEnv.normalTransition(\"0\"),\n \"1-RIGHT\": RoadEnv.normalTransition(\"2\"),\n \"2-LEFT\": RoadEnv.normalTransition(\"1\"),\n \"2-RIGHT\": RoadEnv.normalTransition(\"3\"),\n \"3-LEFT\": RoadEnv.normalTransition(\"2\"),\n \"3-RIGHT\": RoadEnv.normalTransition(\"4\"),\n \"4-LEFT\": RoadEnv.normalTransition(\"3\"),\n \"4-RIGHT\": {\n nextStateName: TERMINAL,\n reward: 100,\n done: true\n }\n }[`${stateName}-${action}`];\n }\n\n static normalTransition(stateName) {\n return {\n nextStateName: stateName,\n reward: 0,\n done: false\n };\n }\n}\n\nconst update = (RL, stateName, totalSteps) => {\n return new Promise(resolve => {\n setTimeout(async () => {\n RoadEnv.render(stateName);\n\n console.log(`stateName: ${stateName}`);\n const action = await RL.chooseAction(stateName);\n\n const step = RoadEnv.step(stateName, action);\n\n let nextStateName = null;\n let reward = null;\n let done = null;\n ({ nextStateName, reward, done } = step);\n\n RL.learn(stateName, action, reward, nextStateName);\n const currentSteps = totalSteps + 1;\n\n if (!done) {\n resolve(await update(RL, nextStateName, currentSteps));\n } else {\n console.log(`endReward: ${reward}`);\n resolve(totalSteps);\n }\n }, 100);\n });\n};\n\n// Run Q Learning For 10 Episode\nconst run = async () => {\n const env = new RoadEnv();\n const RL = new QLearning(env.totalActions);\n\n for (let episode = 1; episode <= TOTAL_EPISODE; episode += 1) {\n const stateName = env.resetStateName;\n let totalSteps = 1;\n console.log(`\\nEpisode: ${episode} =============================== `);\n totalSteps = await update(RL, stateName, totalSteps);\n console.log(`\\nTotalSteps: ${totalSteps}`);\n }\n};\n\nrun();"
}
{
"filename": "code/artificial_intelligence/src/q_learning/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# Q Learning\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/artificial_intelligence/src/random_forests/Position_Salaries.csv",
"content": "Position,Level,Salary\nBusiness Analyst,1,45000\nJunior Consultant,2,50000\nSenior Consultant,3,60000\nManager,4,80000\nCountry Manager,5,110000\nRegion Manager,6,150000\nPartner,7,200000\nSenior Partner,8,300000\nC-level,9,500000\nCEO,10,1000000"
}
{
"filename": "code/artificial_intelligence/src/random_forests/Random_Forest_Regression_Model.ipynb",
"content": "{\n \"cells\": [\n {\n \"cell_type\": \"code\",\n \"execution_count\": 1,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"#importing the libraries\\n\",\n \"import numpy as np\\n\",\n \"import pandas as pd\\n\",\n \"import matplotlib.pyplot as plt\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 2,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"%matplotlib inline\\n\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 3,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"#importing the dataset\\n\",\n \"dataset=pd.read_csv('Position_Salaries.csv')\\n\",\n \"X=dataset.iloc[:,1:2].values\\n\",\n \"y=dataset.iloc[:,2].values\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 4,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"array([[ 1],\\n\",\n \" [ 2],\\n\",\n \" [ 3],\\n\",\n \" [ 4],\\n\",\n \" [ 5],\\n\",\n \" [ 6],\\n\",\n \" [ 7],\\n\",\n \" [ 8],\\n\",\n \" [ 9],\\n\",\n \" [10]], dtype=int64)\"\n ]\n },\n \"execution_count\": 4,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"X\\n\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 5,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"array([ 45000, 50000, 60000, 80000, 110000, 150000, 200000,\\n\",\n \" 300000, 500000, 1000000], dtype=int64)\"\n ]\n },\n \"execution_count\": 5,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"y\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 15,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"RandomForestRegressor(bootstrap=True, criterion='mse', max_depth=None,\\n\",\n \" max_features='auto', max_leaf_nodes=None,\\n\",\n \" min_impurity_decrease=0.0, min_impurity_split=None,\\n\",\n \" min_samples_leaf=1, min_samples_split=2,\\n\",\n \" min_weight_fraction_leaf=0.0, n_estimators=300, n_jobs=None,\\n\",\n \" oob_score=False, random_state=0, verbose=0, warm_start=False)\"\n ]\n },\n \"execution_count\": 15,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"#fitting the random forest regression to the dataset\\n\",\n \"from sklearn.ensemble import RandomForestRegressor\\n\",\n \"regressor=RandomForestRegressor(n_estimators=300,random_state=0)\\n\",\n \"regressor.fit(X,y)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 16,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": [\n \"#predicting the results\\n\",\n \"from numpy import array\\n\",\n \"y_pred=regressor.predict(array([[6.5]]))\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 17,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"text/plain\": [\n \"array([160333.33333333])\"\n ]\n },\n \"execution_count\": 17,\n \"metadata\": {},\n \"output_type\": \"execute_result\"\n }\n ],\n \"source\": [\n \"y_pred\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 18,\n \"metadata\": {},\n \"outputs\": [\n {\n \"data\": {\n \"image/png\": \"iVBORw0KGgoAAAANSUhEUgAAAaEAAAEWCAYAAADPZygPAAAABHNCSVQICAgIfAhkiAAAAAlwSFlzAAALEgAACxIB0t1+/AAAADl0RVh0U29mdHdhcmUAbWF0cGxvdGxpYiB2ZXJzaW9uIDMuMC4yLCBodHRwOi8vbWF0cGxvdGxpYi5vcmcvOIA7rQAAIABJREFUeJzt3XmcXFWd9/HPNxtJgBCWiJCdIaJBZWshiI8woOwQZkRBoyDCk5GBEUFfCsQRXKIwoAwuA08EBMYWiKCCDOuAbIpAAggERCIknRYIgZAQ0iFJd37PH/c0qTTVS1VX1+1Kfd+vV7+q6txz7/ndW939q3PvqXMVEZiZmeVhQN4BmJlZ/XISMjOz3DgJmZlZbpyEzMwsN05CZmaWGychMzPLjZOQ9YikHSX1q/H8kgZJCkkTelj//0iaL+lNSYdL2k7SA5JWSDo/1fmApIf6Mu4u4vuYpAV5tG2VJ2k/SfMqsJ1hkp6VtHUl4upvnIQ2AumfavvPOkmrCl5PK3ObzZL2q3CopcbwsbQ/7fvSLOmbvdjkd4GLImKziLgZ+CLwIjAiIr5eUOeCghiaC47ny5Iul7RpL2LIXUHyXllwbF+tcgzdJlxJv5C0JsW3VNIdkt5TpRB7LSLuiYidK7CdVcBVwNd6H1X/4yS0EUj/VDeLiM2AJuCIgrLGjvUlDap+lGVrKti3fYGTJR1e5rbGA/M6vH460je2JY0BPgL8rsN6h6T2dwf2YuP5Z7Bzwe/JNqWuXKXfo++lYz8aeAX4WV80UgN/E43ACZIG5x1IpTkJ1QFJ35V0naRrJK0APps+ZZ5bUOftT6aSrgG2B25Nn0LPKKh3XOodLJF0ZiftfUTS3yUNKCj7pKRH0/Mpkh6V9IakxZIuKLadjiLib8CDwORO2n1A0ucLXp8k6Z70fAEwrmCf/huYBpydXu8HHAg8EhGrO2n/ReAOYNeCNo6U9Hg6pdck6d8Llu2YehxFj5mk4ZL+W9Lr6bTNHh32Z2dJ90paJulJSYcVLPuFpJ9Iuj3Ff5+kbSX9ONV/RtIuPTmuRY7jF9Npy9ck/VbSdqm8vQf1r5LmA39J5ZMl/W/qrfxF0icKtnV4imVFOganS9qCLNGPK+iJvaurmFJvYHbhsU/bPym1+bqkWyWNLVh2iKS/Slqejssf2n8/0nr3SfqRpKXAN7ranqQBqe4raXtPSJrc2T6m8g16ez14P3+U2lwh6UFJEwv2fyGwEtizp+9jzYgI/2xEP8AC4GMdyr4LrAGOIPvgMQz4BXBuQZ2PAQsKXjcD+xW83hEI4FJgKFmvYDUwqUgMSnH8Y0HZb4CvpuePAJ9OzzcH9upkXzrGtBPwErBvej0oxTQhvX4A+HxB/ZOAe7rYp47H4CLg4g4xvL0OMBZ4GvhBwfL9gfen47oL8CpweE+OGXAhcA+wJalX1r6/wBDgBbJe1+B0LN4EdiyI/RVgt7Tte1P9zwADgfOAOzs5rhsctw7LDkzb3TVt97+Auzusd1uKeVh6//4OHJeW7wG8BuyU1lkCfDg93wrYvdh720mcb78/wGbANcDcguVHA8+m34tBwLnA/WnZu4AVwNR0/M4A1rb/fqTfjVbg5HS8hnWzvcOAh4Et0ns9GXh3T/exh+/nq0BDWn4d8IsOx+MW4F/z/h9T6R/3hOrHAxHxu4hYF9mnynKdGxFvRcSjZKe23vFpO7K/mGuBTwNIGgkclMog+2cwSdLWEbEiIroaCDAufXJ8g+yT9wPAH3sRf1dGkv3j6uhmZT3IJrKk9O32BRFxd0Q8lY7rn8n2cd8O63d2zD4FfDciXo/sk+5PCtbZh+wf1wURsTYi/he4FTi2oM4NEfFYRLwF/BZ4MyJ+GRFtZP/Edutmf59Ix3aZpB+msmnAZRHxeNrumcC+yk5VtvteinkVcCTw14i4OiJaI2JuiuXoVHctMFnS5hGxNB2DUpwpaRnZ+7IXcHzBsn9JsTwbEa1kH7b2lDQaOBx4PCJujIi1ZB8wOl73aoqISyKiLe1LV9tbC4wA3gsQEU9HxMsl7GNP3s/rI2JOireRDr2+dAxG9uio1RAnofqxqBIbKfjDA2gh+4RazC+BTyg7h/0J4KGIaE7LTiD7JPmspIclHdpFk00RMTIiRpB9+l4HXNGrnejc62Sf7Ds6PCI2Bw4Adib7tAuApL0l3ZNOtS0n+4S9wfWVLo7Zdmz4viwseL492b5Hh+WjC14vLni+qsjrzt6bdh9Mx3ZkRLSfct2+MI6IeIPsuBS2WxjzeGCfgmS2DDgm7RvAP5ElqqZ0nPbqJqaOzouIkcBEst78pA5t/7Sg3VfJfj/GpP14O850HJvZUMe/iU63FxF3kPVoLwEWS7pUUvvvSk/2sSfvZ3d/W5sDy4psu6Y5CdWPjsOrVwLDC16/u5v6pTUW8QTZqbODyE4R/bJg2bMRcSzZKZMfADdIGtqDbS5L2zmikyrd7VN3ngA6HX0VEXeTnTYpvIZ1LXADMDYitgAuIzsd2RMvk53iazeu4PmLwFhJ6rD87z3cdrleJPtnDED6R7tlh3YLfzcWAXcVJLORkQ10OBUgIh6KiCPJ3uubWd8bLun3KyIWAKcDP5a0SUHbJ3Zoe1jqWb9Eloza90Ns+A+/WAxdbY+I+M+I2J3s9OtkslN8Xe1joUq8n+8D/lxC/ZrgJFS/HgcOk7RluvD8pQ7LFwM79LKNa8j+cewNXN9eKOlzkraJiHXAcrJ/Buu621j6h3gMG45wK/Q4We9rmLKhvF8oMd47gA9JGtJFnYuAQyW9P73eHFgaEW9JmsKGp1e6M5tsYMRISeOAUwuW/ZHsmsVXJA2WtD9waFqnL10DnCjpg+mf/ffJrot07EW0uwnYWdJnUpyDJe0paaf0PnxG0oh0imkF0JbWWwxsU9Cb6FZE3Ep2/eWkVHQpMEPS+yA77Sup/TTgzcDuko5QNvLtNGBUN010ur20T3umba0k65W1dbOPhXr1fqbfj83IrqduVJyE6teVwDNkpwRu452f3r4HfCudmvhymW38kuzC/Z0R8XpB+aHAM+k6y4XAMRGxppNtvD2CKsW6OfC5TupeSJbQXiE7ZfeLUoKNbPTb/XTe02o/tdYItI+COxn4ftqXsyktSZxD9ol9Adn1gasL2lmd4phKdlroR8BnIuKvJWy/ZBFxG9k1r9+k2MaRXSfqrP5yst7uZ1P9l8kSV3tv5XhgYbqmdyLpvYuIp8h6kAvS71iXo+MKXAh8XdKQiPgV8EPgV2n7T6RYiIjFZB9Yfkg2UOIfgMfIBoZ0ti+dbo/sWszlZKfDFqR9vairfeyw7d6+n9OAn3fxd1KztOEpSrP6JukDwM8iYkresVjlSBpIdkrs6Ii4P+94SiFpGFkvf5+IqOqXiqvBScjMNkqSDib7XtlbwFnA/wV2iE6+B2b58Ok4M9tYfQR4nuz018HAUU5A/Y97QmZmlhv3hMzMLDf9fdK+3G2zzTYxYcKEvMMwM6spc+fOfTUiuhsW7yTUnQkTJjBnzpy8wzAzqymSFnZfy6fjzMwsR05CZmaWGychMzPLjZOQmZnlxknIzMxy02dJSNIVym6F+1RB2VaS7pT0XHrcMpUr3dp2vrLb5u5esM7xqf5zko4vKN9D2S1y56d1VW4bZmaWNDbChAkwYED22NjYp831ZU/oSrKpMgqdSXbvkUnAXek1wCFkN6uaBEwnu3EUkrYim2l4L7J7q5/TnlRSnekF6x1cThtmZpY0NsL06bBwIURkj9On92ki6rMkFBH3AUs7FE8FrkrPrwKOKii/OjJ/Akame9wcRHYbgKXpVgB3AgenZSMi4sF0p8KrO2yrlDbMzAxgxgxoadmwrKUlK+8j1b4mtG1EvASQHtvvITKaDW+125zKuipvLlJeThvvIGm6pDmS5ixZsqSkHTQzq1lNTaWVV0B/GZhQ7HbIUUZ5OW28szBiVkQ0RETDqFHdzjphZrZxGDeutPIKqHYSWtx+Ciw9vpLKm4GxBfXGkN2AqqvyMUXKy2nDzMwAZs6E4cM3LBs+PCvvI9VOQjeR3QqX9HhjQflxaQTbFGB5OpV2O3CgpC3TgIQDgdvTshWSpqRRccd12FYpbZiZGcC0aTBrFowfD1L2OGtWVt5H+mwCU0nXAPsB20hqJhvldh4wW9KJQBPwyVT9FuBQYD7QApwAEBFLJX0HeCTV+3ZEtA92OJlsBN4w4Nb0Q6ltmJlZgWnT+jTpdOSb2nWjoaEhPIu2mVlpJM2NiIbu6vWXgQlmZlaHnITMzCw3TkJmZpYbJyEzM8uNk5CZmeXGScjMzHLjJGRmZrlxEjIzs9w4CZmZWW6chMzMLDdOQmZmlhsnITMzy42TkJmZ5cZJyMzMcuMkZGZmuXESMjOz3DgJmZlZbpyEzMwsN05CZmaWGychMzPLjZOQmZnlxknIzMxy4yRkZma5cRIyM7PcOAmZmVlunITMzCw3TkJmZpYbJyEzM8uNk5CZmeXGScjMzHLjJGRmZrlxEjIzs9zkkoQknS5pnqSnJF0jaaikiZIekvScpOskDUl1N0mv56flEwq2c1Yqf1bSQQXlB6ey+ZLOLCgv2oaZmeVjULUblDQa+BIwOSJWSZoNHAscClwUEddKuhQ4EbgkPb4eETtKOhY4HzhG0uS03s7A9sD/SnpPauanwMeBZuARSTdFxNNp3WJtmJltNG68EZ54onfbGDsWPv/5ioTTpaonoYJ2h0laCwwHXgL2Bz6Tll8FnEuWIKam5wDXAz+RpFR+bUSsBl6QNB/YM9WbHxHPA0i6Fpgq6Zku2jAz22h84QuwdGnvtrHPPtVJQlU/HRcRfwcuBJrIks9yYC6wLCJaU7VmYHR6PhpYlNZtTfW3LizvsE5n5Vt30YaZ2UZj7Vr48pehtbX8n3vvrU6seZyO25KsFzMRWAb8CjikSNVoX6WTZZ2VF0usXdUvFuN0YDrAuHHjilUxM+u31q2DQYNg4MC8I+leHgMTPga8EBFLImIt8Gvgw8BISe1JcQzwYnreDIwFSMu3AJYWlndYp7PyV7toYwMRMSsiGiKiYdSoUb3ZVzOzqmtrgwE1MvY5jzCbgCmShqdrOwcATwO/B45OdY4HbkzPb0qvScvvjohI5cem0XMTgUnAw8AjwKQ0Em4I2eCFm9I6nbVhZrbRWLfOSahTEfEQ2QCDR4EnUwyzgK8DZ6QBBlsDl6dVLge2TuVnAGem7cwDZpMlsNuAUyKiLV3zORW4HXgGmJ3q0kUbZmYbjVpKQso6CNaZhoaGmDNnTt5hmJn12MCBcPbZ8J3v5BeDpLkR0dBdvRrJlWZm1lO11BOqkTDNzKwn2k9uOQmZmVnVtbVlj7UwPBuchMzMNirr1mWP7gmZmVnVOQmZmVlunITMzCw3TkJmZpYbJyEzM8tN++g4JyEzM6u69p6Qh2ibmVnV+XScmZnlxknIzMxy4yRkZma5cRIyM7PceHScmZnlxj0hMzPLjYdom5lZbtwTMjOz3DgJmZlZbpyEzMwsNx4dZ2ZmuXFPyMzMcuMkZGZmufEQbTMzy417QmZmlhsnITMzy41Hx5mZWW7cEzIzs9w4CZmZWW6chMzMLDdOQmZmlpta+57QoLwDMDOz9R54AO6/v/z158/PHmulJ5RLEpI0ErgMeD8QwBeAZ4HrgAnAAuBTEfG6JAEXA4cCLcDnI+LRtJ3jgW+kzX43Iq5K5XsAVwLDgFuA0yIiJG1VrI2+3Vszs5477TR49NHebWPYMBg9ujLx9LW8cuXFwG0R8V5gF+AZ4EzgroiYBNyVXgMcAkxKP9OBSwBSQjkH2AvYEzhH0pZpnUtS3fb1Dk7lnbVhZtYvrF4NU6fCW2+V//PGGzBxYt570jNVT0KSRgAfBS4HiIg1EbEMmApclapdBRyVnk8Fro7Mn4CRkrYDDgLujIilqTdzJ3BwWjYiIh6MiACu7rCtYm2YmfULbW0wZAhsskn5P4Nq6EJLHj2hHYAlwM8lPSbpMkmbAttGxEsA6fFdqf5oYFHB+s2prKvy5iLldNHGBiRNlzRH0pwlS5aUv6dmZiVqa6udQQWVkEcSGgTsDlwSEbsBK+n6tJiKlEUZ5T0WEbMioiEiGkaNGlXKqmZmveIk1PeageaIeCi9vp4sKS1Op9JIj68U1B9bsP4Y4MVuyscUKaeLNszM+gUnoSIkVeyQRMTLwCJJO6WiA4CngZuA41PZ8cCN6flNwHHKTAGWp1NptwMHStoyDUg4ELg9LVshaUoaWXdch20Va8PMrF+otyTU08tX8yVdD/w8Ip6uQLv/BjRKGgI8D5xAlhBnSzoRaAI+mereQjY8ez7ZEO0TACJiqaTvAI+ket+OiKXp+cmsH6J9a/oBOK+TNszM+oXW1toaWNBbPd3VDwLHApdJGgBcAVwbEW+U02hEPA40FFl0QJG6AZzSyXauSLF0LJ9D9h2kjuWvFWvDzKy/qLeeUI9Ox0XEioj4WUR8GPga2fdzXpJ0laQd+zRCM7M64iRUhKSBko6U9BuyL5r+gGyo9e/ITpeZmVkF1FsS6unpuOeA3wMXRMQfC8qvl/TRyodlZlafnIQ6SCPjroyIbxdbHhFfqnhUZmZ1qt6SULen4yKiDfjHKsRiZlb32to8Oq6YP0r6CdkM1CvbC9tnszYzs8poba2vnlBPk9CH02PhKbkA9q9sOGZm9Ssiuymdk1AHEeHTcWZmfazW7opaCT0+8yjpMGBnYGh7WWeDFczMrHRtbdljPSWhnn5P6FLgGLLpdkQ23c34PozLzKzutCehehqY0NNZtD8cEccBr0fEt4C92XAGazMz6yX3hDq3Kj22SNoeWAvUyM1jzcxqg5NQ526WNBK4AHgUWABc21dBmZnVo9ZrfgXAwDNOgwkToLEx34CqoKej476Tnt4g6WZgaEQs77uwzMzqTGMjbWfMAD7JQFph4UKYPj1bNm1arqH1pS6TkKR/7mIZEfHryodkZlaHZsygbdVqAAaSzsu1tMCMGfWbhIAjulgWgJOQmVklNDXRxvYADKJ1g/KNWZdJKCJOqFYgZmZ1bdw42hYGUNATSuUbM39Z1cysP5g5k7aTvgdvFSSh4cNh5sx84+pjPUpC6cuqw8lm074MOBp4uA/jMjOrOd/9LlxwQblrT6MtPgXAYFph/PgsAW3E14OghAlMI+KDkp6IiG9J+gG+HmRmtoGHH4ZNNulN3hjM0KFw4Fd/CVtXMrL+q6dJqOOXVZfiL6uamW2gtTX7es9FF+UdSe3oaRJq/7LqfwBzU9llfROSmVltqre7olZCd98T+hCwqP3LqpI2A54E/gI415uZFWhtra/JRyuhu2l7/h+wBkDSR4HzUtlyYFbfhmZmVlvq7a6oldBdzh4YEUvT82OAWRFxA9n0PY/3bWhmZrWlrQ2GDu2+nq3XXU9ooKT2RHUAcHfBMnc6zcwK+HRc6bo7XNcA90p6lWyE3P0AknYkOyVnZmaJByaUrrtpe2ZKugvYDrgjIiItGkB2l1UzM0vcEypdt4crIv5UpOyvfROOmVnt8sCE0vX0pnZmZtaNtjb3hErlJGRmViE+HVc6JyEzswrxwITS5ZaEJA2U9Fi6XTiSJkp6SNJzkq6TNCSVb5Jez0/LJxRs46xU/qykgwrKD05l8yWdWVBetA0zs0pwT6h0efaETgOeKXh9PnBRREwCXgdOTOUnAq9HxI5kUwWdDyBpMnAs2T2ODgb+KyW2gcBPgUOAycCnU92u2jAz6zX3hEqXSxKSNAY4jDQJqiQB+wPXpypXAUel51PTa9LyA1L9qcC1EbE6Il4A5gN7pp/5EfF8RKwBrgWmdtOGmVmvuSdUurx6Qv8JfA1Yl15vDSyLiPYbqzcDo9Pz0cAigLR8ear/dnmHdTor76qNDUiaLmmOpDlLliwpdx/NrM54iHbpqp6EJB0OvBIRcwuLi1SNbpZVqvydhRGzIqIhIhpGjRpVrIqZ2Tt4iHbp8jhc+wBHSjoUGAqMIOsZjZQ0KPVUxgAvpvrNwFigOc1jtwXZTfXay9sVrlOs/NUu2jAz6zWfjitd1XtCEXFWRIyJiAlkAwvujohpwO+Bo1O144Eb0/Ob0mvS8rvT9EE3Acem0XMTgUnAw8AjwKQ0Em5IauOmtE5nbZiZ9ZoHJpSuP31P6OvAGZLmk12/uTyVXw5sncrPAM4EiIh5wGzgaeA24JSIaEu9nFOB28lG381Odbtqw8ys19wTKl2uhysi7gHuSc+fJxvZ1rHOW8AnO1l/JjCzSPktwC1Fyou2YWZWCR6YULr+1BMyM6tZ69ZBhHtCpfLhMjMD/ud/4FvfyhJJOdrXc0+oNE5CZmbAbbfB44/Dxz9e/jaOOAIOO6xyMdUDJyEzM2DNGth666xHZNXja0JmZmRJaIinNK46JyEzM2DtWiehPDgJmZnhnlBenITMzHASyouTkJkZWRIaPDjvKOqPk5CZGe4J5cVJyMwMJ6G8OAmZmeEklBcnITOzxkbWPvYkQ26/CSZMgMbGvCOqG05CZlbfGhth+vSsJ8QaWLgQpk93IqoSJyEzq28zZkBLC2sYkiUhgJaWrNz6nOeOM7ONwooV2Z1NS7ZwObAFbzGUwaxdX97UVKnQrAtOQmZW8264AY4+uty1X3/72XBa1hePG9ermKxnnITMrOb97W/Z4/nnlzHCbe4cuG42WruaqdyYlQ0fDjPfcdNm6wNOQmZW89akSzlnnFHOnU0b4OBns2tATU0wbnyWgKZNq3SYVoSTkJnVvNWrYcCAXtxae9o0J52ceHScmdW81athk03yjsLK4SRkZjXPSah2OQmZWc1bvdpT7tQqJyEzq3nuCdUuJyEzq3lOQrXLScjMat6aNU5CtcpJyMxqnq8J1S4nITOreT4dV7v8ZVUzy9XatfC738GqVeVvY9Ei2HbbysVk1eMkZGa5uvNO+MQner+dXXft/Tas+pyEzCxXr6dJrO+4I7upabnGj69IOFZlTkJmlquVK7PHyZNh9Oh8Y7Hq88AEM8tVS7qFz6ab5huH5aPqSUjSWEm/l/SMpHmSTkvlW0m6U9Jz6XHLVC5JP5I0X9ITknYv2Nbxqf5zko4vKN9D0pNpnR9JUldtmFlOGhtp+dZ/ADB8l0nQ2JhzQFZtefSEWoGvRMT7gCnAKZImA2cCd0XEJOCu9BrgEGBS+pkOXAJZQgHOAfYC9gTOKUgql6S67esdnMo7a8PMqq2xEaZPZ+WytQyklcFN82H6dCeiOlP1JBQRL0XEo+n5CuAZYDQwFbgqVbsKOCo9nwpcHZk/ASMlbQccBNwZEUsj4nXgTuDgtGxERDwYEQFc3WFbxdows2qbMQNaWmhhOJuyEkF2bm7GjLwjsyrK9ZqQpAnAbsBDwLYR8RJkiQp4V6o2GlhUsFpzKuuqvLlIOV200TGu6ZLmSJqzZMmScnfPzLrS1ARAC8MZTss7yq0+5DY6TtJmwA3AlyPijXTZpmjVImVRRnmPRcQsYBZAQ0NDSeua1ZOXX85GtS1bVsbK0Zo9MIAdeW59+bhxlQnOakIuSUjSYLIE1BgRv07FiyVtFxEvpVNqr6TyZmBswepjgBdT+X4dyu9J5WOK1O+qDTMrw/PPZ9/z+exnYeLEEld+ch7cfDO0rmVvHszKhg+HmTMrHqf1X1VPQmmk2uXAMxHxw4JFNwHHA+elxxsLyk+VdC3ZIITlKYncDnyvYDDCgcBZEbFU0gpJU8hO8x0H/LibNsysDG+8kT2ecgpMmVLq2h+Axieya0BNTTBufJaApk2rdJjWj+XRE9oH+BzwpKTHU9nZZIlhtqQTgSbgk2nZLcChwHygBTgBICWb7wCPpHrfjoil6fnJwJXAMODW9EMXbZhZGdqT0Oabl7mBadOcdOpc1ZNQRDxA8es2AAcUqR/AKZ1s6wrgiiLlc4D3Fyl/rVgbZlae9iQ0YkS+cVjt8owJZlY2JyHrLc8dZ1aPGhtZd/Y3OKXp6zQNew+8Z6eyJm6bPz973GyzCsdndcNJyKzepJkKXmzZkkv5IhNWvcA2T74MbwyFrbcuaVMjRsAJJ8DAgX0Uq230nITM6k2aqWAx7wXgIk7nqHU3wrrx8MiCfGOzuuNrQmb1Js1I8EqaMGRbFm9QblZN7gmZ1aipU+Ghh8pYUYsh2niLoUBBEvJMBZYDJyGzGrRuXTbZwG67QUNDiSs/twzuuxdaW9mOl5jIC56pwHLjJGRWg5YvzxLRtGlw+umlrj0JGh/2TAXWLzgJmdWgV1/NHrfZpswNeKYC6yechMyqrbGRR7/6Sx5+eRxstVV2cWfPPUvaxMKF2WOJI6rN+h0nIbNqSt/ROa7lIebxflgK/Dz9lGjgQNhxx0oHaFZdTkJm1TRjBtHSwvPswBe5hHP4VlY+Ziw88kjX63YwbBhssUUfxGhWRU5CZiW6+easQ1OWhd+nlUGsYjiTeZp3tw+P/vsr8O6KhWhWM5yEzEp08cXwhz/A2LHd132HQXtCaysf4An245715f6OjtUpJyGzEi1eDAceCL/9bRkrN/4Jpk+Hlpb1Zf6OjtUxT9tjVqKXX4Ztty1z5WnTYNYsGD8epOxx1iwPl7a65Z6Q1Y/GRn72pSf5ytKzCQ2ATTaBQYNL3sybb8K7e3P9xt/RMXubk5DVhzQ0+o6WK9mE1RwXV0PrYPjHj8P73lfSpgYOzG5fYGa95yRkNWPpUrj2WmhtLWPlc5+DlhOZQwMNzOEHfBVagafHwy0LKhypmfWUk5DVjFmz4Kyzyl373LefHcfV64t9+wKzXDkJWd9rbIQZM1i58FXWjt0BvvEN+NSnSt7MvHnZtZh588qIYZddoHkRIhjJsvXlHhptlisnIetb6VrMPS0fYn+eJxYNgH8h+ynDvvtm062V7LyveWi0WT/kJLQxSz2QbLr+cWVP179kCRx+eHb7gJL9bQq0zuU1tmYobzGTGYiALbeCb36z5M0dcEAZMcD6/a7A8TCzylFE5B1Dv9bQ0BBz5swpfcUKJYDWVli5svTmmT0bTjuNdave4jJOopkx2XDk/feH9763pE09/3w2Vc2RR2bzlZXkumvffrov93Iyl2YvpOyGOGa2UZI0NyK6veWik1BHwhUWAAAHBUlEQVQ3ykpCjY28eNI3Oeutgk/6AwfB3nvDDjv0eDPr1sGtt8Jrr5XWfDGDWMtmvAkDBsCI0me9nDwZ7r8/W70kEyasv+9AofHjYcGCkuMws9rQ0yTk03F9YcYMVr01kPv46PqyNuDBQdBc2qbGjIFTToGRI0uM4YwzgOwDxlgW8QluQAAheL2KPZCZM30txsw65STUF5qa+AeCF+jQ61kneKFKCeDiXxfvgVR7NJivxZhZFzx3XF/o7B99NRPAzJlZj6NQXj2QadOyU2/r1mWPTkBmljgJ9YX+kAA8UaaZ1QCfjusL/eUUlCfKNLN+zkmorzgBmJl1y6fjzMwsN3WXhCQdLOlZSfMlnZl3PGZm9ayukpCkgcBPgUOAycCnJU3ONyozs/pVV0kI2BOYHxHPR8Qa4Fpgas4xmZnVrXpLQqOBRQWvm1PZBiRNlzRH0pwlS5ZULTgzs3pTb6PjVKTsHZPnRcQsYBaApCWSikw9UFO2AV7NO4h+xMdjPR+LDfl4rNfbYzG+J5XqLQk1A2MLXo8BXuxqhYgY1acRVYGkOT2ZSLBe+His52OxIR+P9ap1LOrtdNwjwCRJEyUNAY4Fbso5JjOzulVXPaGIaJV0KnA7MBC4IiLKuVm0mZlVQF0lIYCIuAW4Je84qmxW3gH0Mz4e6/lYbMjHY72qHAvf1M7MzHJTb9eEzMysH3ESMjOz3DgJbcQkjZX0e0nPSJon6bS8Y8qbpIGSHpN0c96x5E3SSEnXS/pL+h3ZO++Y8iLp9PQ38pSkayQNzTumapJ0haRXJD1VULaVpDslPZcet+yLtp2ENm6twFci4n3AFOAUz5XHacAzeQfRT1wM3BYR7wV2oU6Pi6TRwJeAhoh4P9nI2WPzjarqrgQO7lB2JnBXREwC7kqvK85JaCMWES9FxKPp+QqyfzLvmKaoXkgaAxwGXJZ3LHmTNAL4KHA5QESsiYhl+UaVq0HAMEmDgOF08yX2jU1E3Acs7VA8FbgqPb8KOKov2nYSqhOSJgC7AQ/lG0mu/hP4GrAu70D6gR2AJcDP0+nJyyRtmndQeYiIvwMXAk3AS8DyiLgj36j6hW0j4iXIPtAC7+qLRpyE6oCkzYAbgC9HxBt5x5MHSYcDr0TE3Lxj6ScGAbsDl0TEbsBK+uh0S3+XrnVMBSYC2wObSvpsvlHVDyehjZykwWQJqDEifp13PDnaBzhS0gKyW3jsL+kX+YaUq2agOSLae8bXkyWlevQx4IWIWBIRa4FfAx/OOab+YLGk7QDS4yt90YiT0EZMksjO+T8TET/MO548RcRZETEmIiaQXXS+OyLq9tNuRLwMLJK0Uyo6AHg6x5Dy1ARMkTQ8/c0cQJ0O0ujgJuD49Px44Ma+aKTupu2pM/sAnwOelPR4Kjs7TV1k9m9AY5rM93nghJzjyUVEPCTpeuBRshGlj1Fn0/dIugbYD9hGUjNwDnAeMFvSiWSJ+pN90ran7TEzs7z4dJyZmeXGScjMzHLjJGRmZrlxEjIzs9w4CZmZWW6chMzKJKlN0uNp5uVfSRpexjYua59UVtLZHZb9sUJxXinp6Epsqy+3afXJScisfKsiYtc08/Ia4IulbiAiToqI9i+Jnt1hmb+1bxs9JyGzyrgf2BFA0hmpd/SUpC+nsk0l/Y+kP6fyY1L5PZIaJJ1HNovz45Ia07I306MkXZDWe7Jg3f3S+u33BGpM3/jvlKQ9JN0raa6k2yVtJ+l9kh4uqDNB0hOd1a/8obN65hkTzHopTf9/CHCbpD3IZh7YCxDwkKR7yWatfjEiDkvrbFG4jYg4U9KpEbFrkSb+GdiV7J4/2wCPSLovLdsN2Jns1gN/IJsl44FO4hwM/BiYGhFLUjKbGRFfkDRE0g4R8TxwDNk35YvWB75QznEyK8ZJyKx8wwqmQ7qfbJ6+k4HfRMRKAEm/Bv4PcBtwoaTzgZsj4v4S2vkIcE1EtJFNKnkv8CHgDeDhiGhObT0OTKCTJATsBLwfuDN1mAaS3boAYDbwKbKpWo5JP13VN6sIJyGz8q3q2HPp7HRYRPw19ZIOBb4v6Y6I+HYP2+nqFNvqgudtdP03LWBeRBS7jfd1wK9S0oyIeE7SB7qob1YRviZkVln3AUelGZk3Bf4JuF/S9kBLRPyC7AZqxW6bsDadAiu2zWMkDZQ0iuyOqA8XqdedZ4FRkvaG7PScpJ0BIuJvZEns38kSUpf1zSrFPSGzCoqIRyVdyfokcVlEPCbpIOACSeuAtWSn7TqaBTwh6dGImFZQ/htgb+DPQABfi4iXJb23xNjWpGHVP0rXpAaR3W12XqpyHXAB2c3delLfrNc8i7aZmeXGp+PMzCw3TkJmZpYbJyEzM8uNk5CZmeXGScjMzHLjJGRmZrlxEjIzs9z8f7ZM682SeDVNAAAAAElFTkSuQmCC\\n\",\n \"text/plain\": [\n \"<Figure size 432x288 with 1 Axes>\"\n ]\n },\n \"metadata\": {\n \"needs_background\": \"light\"\n },\n \"output_type\": \"display_data\"\n }\n ],\n \"source\": [\n \"#visualising the Regression results\\n\",\n \"X_grid=np.arange(min(X),max(X),0.01)\\n\",\n \"X_grid=X_grid.reshape(len(X_grid),1)\\n\",\n \"plt.scatter(X,y,color='red')\\n\",\n \"plt.plot(X_grid,regressor.predict(X_grid),color='blue')\\n\",\n \"plt.title('Truth vs Bluff(Random Forest Regression)')\\n\",\n \"plt.xlabel('Position level')\\n\",\n \"plt.ylabel('Salary')\\n\",\n \"plt.show()\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": null,\n \"metadata\": {},\n \"outputs\": [],\n \"source\": []\n }\n ],\n \"metadata\": {\n \"kernelspec\": {\n \"display_name\": \"Python 3\",\n \"language\": \"python\",\n \"name\": \"python3\"\n },\n \"language_info\": {\n \"codemirror_mode\": {\n \"name\": \"ipython\",\n \"version\": 3\n },\n \"file_extension\": \".py\",\n \"mimetype\": \"text/x-python\",\n \"name\": \"python\",\n \"nbconvert_exporter\": \"python\",\n \"pygments_lexer\": \"ipython3\",\n \"version\": \"3.7.1\"\n }\n },\n \"nbformat\": 4,\n \"nbformat_minor\": 2\n}"
}
{
"filename": "code/artificial_intelligence/src/random_forests/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# Random forests\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/artificial_intelligence/src/residual_convolutional_neural_network/.gitignore",
"content": "data"
}
{
"filename": "code/artificial_intelligence/src/residual_convolutional_neural_network/main.py",
"content": "import torch\nimport torch.nn as nn\nimport torch.nn.functional as F\nfrom torch.utils.tensorboard import SummaryWriter\nfrom torchvision import datasets, transforms\nfrom sklearn import metrics\nimport numpy as np\nfrom tqdm import tqdm\n\n\nclass params:\n batch_size = 1024\n epochs = 100\n lr = 1e-3\n\n\nclass Block(nn.Module):\n def __init__(self, in_channels, out_channels):\n super(Block, self).__init__()\n self.conv1 = nn.Conv2d(in_channels, out_channels, 3, 1, 1)\n self.bn1 = nn.BatchNorm2d(out_channels)\n\n self.conv2 = nn.Conv2d(out_channels, out_channels, 3, 1, 1)\n self.bn2 = nn.BatchNorm2d(out_channels)\n\n self.shortcut = (\n nn.Sequential()\n if in_channels == out_channels\n else nn.Sequential(\n nn.Conv2d(in_channels, out_channels, 1, bias=False),\n nn.BatchNorm2d(out_channels),\n )\n )\n\n def forward(self, x):\n out = F.relu(self.bn1(self.conv1(x)))\n out = self.bn2(self.conv2(out))\n\n out += self.shortcut(x)\n\n out = F.relu(out)\n return out\n\n\nclass ConvNet(nn.Module):\n def __init__(self, num_classes=10):\n super(ConvNet, self).__init__()\n structure = [\n (3, 64),\n 64,\n \"p\",\n (64, 128),\n 128,\n \"p\",\n (128, 256),\n 256,\n \"p\",\n (256, 512),\n 512,\n ]\n\n layers = []\n\n for layer in structure:\n if layer == \"p\":\n layers.append(nn.MaxPool2d(2))\n elif isinstance(layer, tuple):\n layers.append(Block(layer[0], layer[1]))\n else:\n layers.append(Block(layer, layer))\n\n self.conv = nn.Sequential(*layers)\n self.avgpool = nn.AdaptiveAvgPool2d((1, 1))\n self.dropout = nn.Dropout()\n self.fc = nn.Linear(512, num_classes)\n\n def forward(self, x):\n x = self.conv(x)\n x = self.avgpool(x)\n\n x = torch.flatten(x, 1)\n x = self.dropout(x)\n\n x = self.fc(x)\n x = F.log_softmax(x, 1)\n\n return x\n\n\nis_cuda = torch.cuda.is_available()\nprint(f\"Using {'GPU' if is_cuda else 'CPU'}\")\ndevice = torch.device(\"cuda\" if is_cuda else \"cpu\")\n\nkwargs = {\"batch_size\": params.batch_size}\nif is_cuda:\n kwargs.update({\"num_workers\": 1, \"pin_memory\": True, \"shuffle\": True})\n\ntransform = transforms.Compose([transforms.ToTensor()])\n\ntrain_set = datasets.CIFAR10(\"./data\", train=True, download=True, transform=transform)\ntest_set = datasets.CIFAR10(\"./data\", train=False, transform=transform)\n\ntrain_loader = torch.utils.data.DataLoader(train_set, **kwargs)\ntest_loader = torch.utils.data.DataLoader(test_set, **kwargs)\n\nlabel_names = [\n \"airplane\",\n \"automobile\",\n \"bird\",\n \"cat\",\n \"deer\",\n \"dog\",\n \"frog\",\n \"horse\",\n \"ship\",\n \"truck\",\n]\nmetric_names = [\"loss\", \"accuracy\"]\n\nmodel = ConvNet(num_classes=10)\nmodel.to(device)\n\nnum_params = sum(p.numel() for p in model.parameters() if p.requires_grad)\nprint(f\"Parameters: {num_params}\")\n\noptimizer = torch.optim.Adam(model.parameters(), lr=params.lr)\n\nwriter = SummaryWriter(log_dir=\"./logs\")\ntemp_ipt = torch.rand(5, 3, 32, 32, device=device)\n\nwriter.add_graph(model, temp_ipt)\n\nfor epoch in range(params.epochs):\n model.train()\n\n train_metrics = [0, 0]\n\n for idx, (data, target) in tqdm(\n enumerate(train_loader), f\"Epoch: {epoch + 1}\", total=len(train_loader)\n ):\n data, target = data.to(device), target.to(device)\n\n optimizer.zero_grad()\n output = model(data)\n\n loss = F.nll_loss(output, target)\n loss.backward()\n optimizer.step()\n\n train_metrics[0] += loss.item()\n\n output = output.cpu().detach().numpy()\n target = target.cpu().detach().numpy()\n output = np.argmax(output, 1)\n\n train_metrics[1] += metrics.accuracy_score(y_true=target, y_pred=output)\n\n train_metrics = np.array(train_metrics) / len(train_loader)\n for name, val in zip(metric_names, train_metrics):\n writer.add_scalar(\"train/\" + name, val, epoch)\n\n test_metrics = [0, 0]\n model.eval()\n with torch.no_grad():\n for data, target in test_loader:\n data, target = data.to(device), target.to(device)\n output = model(data)\n\n test_metrics[0] += F.nll_loss(output, target).item()\n\n output = output.cpu().detach().numpy()\n target = target.cpu().detach().numpy()\n output = np.argmax(output, 1)\n\n test_metrics[1] += metrics.accuracy_score(y_true=target, y_pred=output)\n\n test_metrics = np.array(test_metrics) / len(test_loader)\n for name, val in zip(metric_names, test_metrics):\n writer.add_scalar(\"test/\" + name, val, epoch)"
}
{
"filename": "code/artificial_intelligence/src/restricted_boltzmann_machine/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# Restricted boltzmann machine\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/artificial_intelligence/src/sat/togasat.cpp",
"content": "/************************************************************\n* MiniSat -- Copyright (c) 2003-2006, Niklas Een, Niklas Sorensson\n* Copyright (c) 2007-2010 Niklas Sorensson\n* Permission is hereby granted, free of charge, to any person obtaining a\n* copy of this software and associated documentation files (the\n* \"Software\"), to deal in the Software without restriction, including\n* without limitation the rights to use, copy, modify, merge, publish,\n* distribute, sublicense, and/or sell copies of the Software, and to\n* permit persons to whom the Software is furnished to do so, subject to\n* the following conditions:\n* The above copyright notice and this permission notice shall be included\n* in all copies or substantial portions of the Software.\n* THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS\n* OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF\n* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND\n* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE\n* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION\n* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION\n* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.\n************************************************************/\n#include <algorithm>\n#include <cassert>\n#include <fstream>\n#include <iostream>\n#include <list>\n#include <queue>\n#include <sstream>\n#include <stdio.h>\n#include <string>\n#include <vector>\n#include <set>\n#include <cmath>\n\n#include <unordered_map>\n#include <unordered_set>\n// SAT Solver\n// CDCL Solver\n// Author togatoga\n// https://github.com/togatoga/Togasat\nnamespace togasat {\nusing Var = int;\nusing CRef = int;\nusing lbool = int;\nconst CRef CRef_Undef = -1;\nclass Solver {\n\nprivate:\n const lbool l_True = 0;\n const lbool l_False = 1;\n const lbool l_Undef = 2;\n\n const int var_Undef = -1;\n\n // Literal\n struct Lit\n {\n int x;\n inline bool operator==(Lit p) const\n {\n return x == p.x;\n }\n inline bool operator!=(Lit p) const\n {\n return x != p.x;\n }\n inline bool operator<(Lit p) const\n {\n return x < p.x;\n }\n inline Lit operator~()\n {\n Lit q;\n q.x = x ^ 1;\n return q;\n }\n };\n\n inline Lit mkLit(Var var, bool sign)\n {\n Lit p;\n p.x = var + var + sign;\n return p;\n }\n inline bool sign(Lit p) const\n {\n return p.x & 1;\n }\n inline int var(Lit p) const\n {\n return p.x >> 1;\n }\n inline int toInt(Var v)\n {\n return v;\n }\n inline int toInt(Lit p)\n {\n return p.x;\n }\n inline Lit toLit(int x)\n {\n Lit p;\n p.x = x;\n return p;\n }\n const Lit lit_Undef = {-2};\n\n // lifted boolean\n // VarData\n struct VarData\n {\n CRef reason;\n int level;\n };\n inline VarData mkVarData(CRef cr, int l)\n {\n VarData d = {cr, l};\n return d;\n }\n // Watcher\n struct Watcher\n {\n CRef cref;\n Lit blocker;\n Watcher()\n {\n }\n Watcher(CRef cr, Lit p) : cref(cr), blocker(p)\n {\n }\n bool operator==(const Watcher &w) const\n {\n return cref == w.cref;\n }\n bool operator!=(const Watcher &w) const\n {\n return cref != w.cref;\n }\n };\n\n // Clause\n class Clause {\n public:\n struct\n {\n bool learnt;\n int size;\n } header;\n std::vector<Lit> data; //(x1 v x2 v not x3)\n Clause()\n {\n }\n Clause(const std::vector<Lit> &ps, bool learnt)\n {\n header.learnt = learnt;\n header.size = ps.size();\n //data = move(ps);\n data.resize(header.size);\n for (size_t i = 0; i < ps.size(); i++)\n data[i] = ps[i];\n // //data.emplace_back(ps[i]);\n }\n\n int size() const\n {\n return header.size;\n }\n bool learnt() const\n {\n return header.learnt;\n }\n Lit &operator[](int i)\n {\n return data[i];\n }\n Lit operator[](int i) const\n {\n return data[i];\n }\n };\n\n CRef allocClause(std::vector<Lit> &ps, bool learnt = false)\n {\n static CRef res = 0;\n ca[res] = Clause(ps, learnt);\n return res++;\n }\n\n Var newVar(bool sign = true, bool dvar = true)\n {\n int v = nVars();\n\n assigns.emplace_back(l_Undef);\n vardata.emplace_back(mkVarData(CRef_Undef, 0));\n activity.emplace_back(0.0);\n seen.push_back(false);\n polarity.push_back(sign);\n decision.push_back(0);\n setDecisionVar(v, dvar);\n return v;\n }\n\n bool addClause_(std::vector<Lit> &ps)\n {\n //std::sort(ps.begin(), ps.end());\n // empty clause\n if (ps.size() == 0)\n return false;\n else if (ps.size() == 1)\n uncheckedEnqueue(ps[0]);\n else\n {\n CRef cr = allocClause(ps, false);\n //clauses.insert(cr);\n attachClause(cr);\n }\n\n return true;\n }\n void attachClause(CRef cr)\n {\n const Clause &c = ca[cr];\n\n assert(c.size() > 1);\n\n watches[(~c[0]).x].emplace_back(Watcher(cr, c[1]));\n watches[(~c[1]).x].emplace_back(Watcher(cr, c[0]));\n }\n\n // Input\n void readClause(const std::string &line, std::vector<Lit> &lits)\n {\n lits.clear();\n int var;\n var = 0;\n std::stringstream ss(line);\n while (ss)\n {\n int val;\n ss >> val;\n if (val == 0)\n break;\n var = abs(val) - 1;\n while (var >= nVars())\n newVar();\n lits.emplace_back(val > 0 ? mkLit(var, false) : mkLit(var, true));\n }\n }\n\n std::unordered_map<CRef, Clause> ca; // store clauses\n std::unordered_set<CRef> clauses; // original problem;\n std::unordered_set<CRef> learnts;\n std::unordered_map<int, std::vector<Watcher>> watches;\n std::vector<VarData> vardata; // store reason and level for each variable\n std::vector<bool> polarity; // The preferred polarity of each variable\n std::vector<bool> decision;\n std::vector<bool> seen;\n // Todo\n size_t qhead;\n std::vector<Lit> trail;\n std::vector<int> trail_lim;\n // Todo rename(not heap)\n std::set<std::pair<double, Var>> order_heap;\n std::vector<double> activity;\n double var_inc;\n std::vector<Lit> model;\n std::vector<Lit> conflict;\n int nVars() const\n {\n return vardata.size();\n }\n int decisionLevel() const\n {\n return trail_lim.size();\n }\n void newDecisionLevel()\n {\n trail_lim.emplace_back(trail.size());\n }\n\n inline CRef reason(Var x) const\n {\n return vardata[x].reason;\n }\n inline int level(Var x) const\n {\n return vardata[x].level;\n }\n inline void varBumpActivity(Var v)\n {\n std::pair<double, Var> p = std::make_pair(activity[v], v);\n activity[v] += var_inc;\n if (order_heap.erase(p) == 1)\n order_heap.emplace(std::make_pair(activity[v], v));\n\n if (activity[v] > 1e100)\n {\n //Rescale\n std::set<std::pair<double, Var>> tmp_order;\n tmp_order = std::move(order_heap);\n order_heap.clear();\n for (int i = 0; i < nVars(); i++)\n activity[i] *= 1e-100;\n for (auto &val : tmp_order)\n order_heap.emplace(std::make_pair(activity[val.second], val.second));\n var_inc *= 1e-100;\n }\n\n }\n bool satisfied(const Clause &c) const\n {\n for (int i = 0; i < c.size(); i++)\n if (value(c[i]) == l_True)\n return true;\n\n return false;\n }\n lbool value(Var p) const\n {\n return assigns[p];\n }\n lbool value(Lit p) const\n {\n if (assigns[var(p)] == l_Undef)\n return l_Undef;\n return assigns[var(p)] ^ sign(p);\n }\n void setDecisionVar(Var v, bool b)\n {\n decision[v] = b;\n order_heap.emplace(std::make_pair(0.0, v));\n }\n void uncheckedEnqueue(Lit p, CRef from = CRef_Undef)\n {\n assert(value(p) == l_Undef);\n assigns[var(p)] = sign(p);\n vardata[var(p)] = mkVarData(from, decisionLevel());\n trail.emplace_back(p);\n }\n // decision\n Lit pickBranchLit()\n {\n Var next = var_Undef;\n while (next == var_Undef or value(next) != l_Undef)\n {\n if (order_heap.empty())\n {\n next = var_Undef;\n break;\n }\n else\n {\n auto p = *order_heap.rbegin();\n next = p.second;\n order_heap.erase(p);\n }\n }\n return next == var_Undef ? lit_Undef : mkLit(next, polarity[next]);\n }\n // clause learning\n void analyze(CRef confl, std::vector<Lit> &out_learnt, int &out_btlevel)\n {\n int pathC = 0;\n Lit p = lit_Undef;\n int index = trail.size() - 1;\n out_learnt.emplace_back(mkLit(0, false));\n do {\n assert(confl != CRef_Undef);\n Clause &c = ca[confl];\n for (int j = (p == lit_Undef) ? 0 : 1; j < c.size(); j++)\n {\n Lit q = c[j];\n if (not seen[var(q)] and level(var(q)) > 0)\n {\n varBumpActivity(var(q));\n seen[var(q)] = 1;\n if (level(var(q)) >= decisionLevel())\n pathC++;\n else\n out_learnt.emplace_back(q);\n }\n }\n while (not seen[var(trail[index--])])\n ;\n p = trail[index + 1];\n confl = reason(var(p));\n seen[var(p)] = 0;\n pathC--;\n } while (pathC > 0);\n\n out_learnt[0] = ~p;\n\n // unit clause\n if (out_learnt.size() == 1)\n out_btlevel = 0;\n else\n {\n int max_i = 1;\n for (size_t i = 2; i < out_learnt.size(); i++)\n if (level(var(out_learnt[i])) > level(var(out_learnt[max_i])))\n max_i = i;\n\n Lit p = out_learnt[max_i];\n out_learnt[max_i] = out_learnt[1];\n out_learnt[1] = p;\n out_btlevel = level(var(p));\n }\n\n for (size_t i = 0; i < out_learnt.size(); i++)\n seen[var(out_learnt[i])] = false;\n }\n\n // backtrack\n void cancelUntil(int level)\n {\n if (decisionLevel() > level)\n {\n for (int c = trail.size() - 1; c >= trail_lim[level]; c--)\n {\n Var x = var(trail[c]);\n assigns[x] = l_Undef;\n polarity[x] = sign(trail[c]);\n order_heap.emplace(std::make_pair(activity[x], x));\n }\n qhead = trail_lim[level];\n trail.erase(trail.end() - (trail.size() - trail_lim[level]), trail.end());\n trail_lim.erase(trail_lim.end() - (trail_lim.size() - level),\n trail_lim.end());\n }\n }\n CRef propagate()\n {\n CRef confl = CRef_Undef;\n int num_props = 0;\n while (qhead < trail.size())\n {\n Lit p = trail[qhead++]; // 'p' is enqueued fact to propagate.\n std::vector<Watcher> &ws = watches[p.x];\n std::vector<Watcher>::iterator i, j, end;\n num_props++;\n\n for (i = j = ws.begin(), end = i + ws.size(); i != end;)\n {\n // Try to avoid inspecting the clause:\n Lit blocker = i->blocker;\n if (value(blocker) == l_True)\n {\n *j++ = *i++;\n continue;\n }\n\n CRef cr = i->cref;\n Clause &c = ca[cr];\n Lit false_lit = ~p;\n if (c[0] == false_lit)\n c[0] = c[1], c[1] = false_lit;\n assert(c[1] == false_lit);\n i++;\n\n Lit first = c[0];\n Watcher w = Watcher(cr, first);\n if (first != blocker && value(first) == l_True)\n {\n *j++ = w;\n continue;\n }\n\n // Look for new watch:\n for (int k = 2; k < c.size(); k++)\n if (value(c[k]) != l_False)\n {\n c[1] = c[k];\n c[k] = false_lit;\n watches[(~c[1]).x].emplace_back(w);\n goto NextClause;\n }\n *j++ = w;\n if (value(first) == l_False) // conflict\n {\n confl = cr;\n qhead = trail.size();\n while (i < end)\n *j++ = *i++;\n }\n else\n uncheckedEnqueue(first, cr);\n NextClause :;\n }\n int size = i - j;\n ws.erase(ws.end() - size, ws.end());\n }\n return confl;\n }\n\n static double luby(double y, int x)\n {\n\n // Find the finite subsequence that contains index 'x', and the\n // size of that subsequence:\n int size, seq;\n for (size = 1, seq = 0; size < x + 1; seq++, size = 2 * size + 1)\n ;\n\n while (size - 1 != x)\n {\n size = (size - 1) >> 1;\n seq--;\n x = x % size;\n }\n\n return std::pow(y, seq);\n }\n\n lbool search(int nof_conflicts)\n {\n int backtrack_level;\n std::vector<Lit> learnt_clause;\n learnt_clause.emplace_back(mkLit(-1, false));\n int conflictC = 0;\n while (true)\n {\n CRef confl = propagate();\n\n if (confl != CRef_Undef)\n {\n // CONFLICT\n conflictC++;\n if (decisionLevel() == 0)\n return l_False;\n learnt_clause.clear();\n analyze(confl, learnt_clause, backtrack_level);\n cancelUntil(backtrack_level);\n if (learnt_clause.size() == 1)\n uncheckedEnqueue(learnt_clause[0]);\n else\n {\n CRef cr = allocClause(learnt_clause, true);\n //learnts.insert(cr);\n attachClause(cr);\n uncheckedEnqueue(learnt_clause[0], cr);\n }\n //varDecay\n var_inc *= 1.05;\n }\n else\n {\n // NO CONFLICT\n if ((nof_conflicts >= 0 and conflictC >= nof_conflicts))\n {\n cancelUntil(0);\n return l_Undef;\n }\n Lit next = pickBranchLit();\n\n if (next == lit_Undef)\n return l_True;\n newDecisionLevel();\n uncheckedEnqueue(next);\n }\n }\n }\n\npublic:\n std::vector<lbool> assigns; // The current assignments (ex assigns[0] = 0 ->\n // X1 = True, assigns[1] = 1 -> X2 = False)\n lbool answer; // SATISFIABLE 0 UNSATISFIABLE 1 UNKNOWN 2\n Solver()\n {\n qhead = 0;\n }\n void parseDimacsProblem(std::string problem_name)\n {\n std::vector<Lit> lits;\n int vars = 0;\n int clauses = 0;\n std::string line;\n std::ifstream ifs(problem_name, std::ios_base::in);\n while (ifs.good())\n {\n getline(ifs, line);\n if (line.size() > 0)\n {\n if (line[0] == 'p')\n sscanf(line.c_str(), \"p cnf %d %d\", &vars, &clauses);\n else if (line[0] == 'c' or line[0] == 'p')\n continue;\n else\n {\n readClause(line, lits);\n if (lits.size() > 0)\n addClause_(lits);\n }\n }\n }\n ifs.close();\n }\n lbool solve()\n {\n model.clear();\n conflict.clear();\n lbool status = l_Undef;\n answer = l_Undef;\n var_inc = 1.01;\n int curr_restarts = 0;\n double restart_inc = 2;\n double restart_first = 100;\n while (status == l_Undef)\n {\n double rest_base = luby(restart_inc, curr_restarts);\n status = search(rest_base * restart_first);\n curr_restarts++;\n }\n answer = status;\n return status;\n }\n\n void addClause(std::vector<int> &clause)\n {\n std::vector<Lit> lits;\n lits.resize(clause.size());\n for (size_t i = 0; i < clause.size(); i++)\n {\n int var = abs(clause[i]) - 1;\n while (var >= nVars())\n newVar();\n lits[i] = clause[i] > 0 ? mkLit(var, false) : mkLit(var, true);\n }\n addClause_(lits);\n }\n void printAnswer()\n {\n if (answer == 0)\n {\n std::cout << \"SAT\" << std::endl;\n for (size_t i = 0; i < assigns.size(); i++)\n {\n if (assigns[i] == 0)\n std::cout << (i + 1) << \" \";\n else\n std::cout << -(i + 1) << \" \";\n }\n std::cout << \"0\" << std::endl;\n }\n else\n std::cout << \"UNSAT\" << std::endl;\n }\n};\n} // togasat"
}
{
"filename": "code/artificial_intelligence/src/shufflenet_v1/README.md",
"content": "# ShuffeNet architecture\nImplementation of ShuffleNet architecture using CIFAR 10 dataset using Pytorch. The architecture is inspired from the original [ShuffleNet paper](https://arxiv.org/abs/1707.01083).\n\nOne can replicate the same results by following these steps:\n1. Downloading this jupyter notebook on Google Colab. Alternatively, they can also load the dataset to their own computers instead of using Google Drive (as done in this notebook).\n2. Using GPU instead of CPU in google colab or similarly for your personal computer. In Google Colab, this can be done by going to Edit -> Notebook Settings -> Select GPU -> Save. The training happens faster with GPU as compared to CPU.\n3. Running all the cells **sequentially** in the order as in the notebook.\n4. It's done! You can go ahead and try the same architecture on different datasets."
}
{
"filename": "code/artificial_intelligence/src/skull_stripping/data.py",
"content": "#downloading necessary files\n!wget --header 'Host: fcp-indi.s3.amazonaws.com' --user-agent 'Mozilla/5.0 (X11; Ubuntu; Linux x86_64; rv:87.0) Gecko/20100101 Firefox/87.0' --header 'Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8' --header 'Accept-Language: en-US,en;q=0.5' --referer 'http://preprocessed-connectomes-project.org/' --header 'Upgrade-Insecure-Requests: 1' 'https://fcp-indi.s3.amazonaws.com/data/Projects/RocklandSample/NFBS_Dataset.tar.gz' --output-document 'NFBS_Dataset.tar.gz'\n!wget --header 'Host: doc-08-2g-docs.googleusercontent.com' --user-agent 'Mozilla/5.0 (X11; Ubuntu; Linux x86_64; rv:87.0) Gecko/20100101 Firefox/87.0' --header 'Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8' --header 'Accept-Language: en-US,en;q=0.5' --referer 'https://drive.google.com/' --header 'Cookie: AUTH_9k7m8g8qrj7at7i0b1sl7f8s8gafrr77_nonce=fchj71prkosgs' --header 'Upgrade-Insecure-Requests: 1' 'https://doc-08-2g-docs.googleusercontent.com/docs/securesc/5baff4djp5n2ak1832to2m6lltldb4gl/lld851i2e7pqe774volddlu45niullkl/1621202400000/00677992938550103544/12785117600840412092/1N1Qx-R5tLCX5EhXPoPdyg6YvEkDtf-cD?e=download&authuser=0&nonce=fchj71prkosgs&user=12785117600840412092&hash=0lccq4nigav75jop1timeo66ook4s4q1' --output-document 'ANTs-28-03-2019.7z'\n!wget --header 'Host: doc-00-48-docs.googleusercontent.com' --user-agent 'Mozilla/5.0 (X11; Ubuntu; Linux x86_64; rv:87.0) Gecko/20100101 Firefox/87.0' --header 'Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8' --header 'Accept-Language: en-US,en;q=0.5' --referer 'https://drive.google.com/' --header 'Cookie: AUTH_9k7m8g8qrj7at7i0b1sl7f8s8gafrr77=12785117600840412092|1621202400000|urd0fgd911j76j00e1g3sfrlmgno1864' --header 'Upgrade-Insecure-Requests: 1' 'https://doc-00-48-docs.googleusercontent.com/docs/securesc/5baff4djp5n2ak1832to2m6lltldb4gl/andn6io8vgl8gpaplbqeg39eum6c4c3k/1621202475000/15988357211529156717/12785117600840412092/1y8N6JpKhyoLDQcscdudwMCgqubjQyfd1?e=download&authuser=0' --output-document 'T1w_MRI_test_data.zip'\n!unzip '/content/T1w_MRI_test_data.zip'\n\n#for handling MRI images\n!pip install nipype\n!pip install antspyx\n!pip install nilearn\n!pip install SimpleITK\n\n#for segmentation\n!pip install q keras==2.3.1\n!pip install segmentation_models\n\n#for running ANTS library in colab\n!7z x ANTs-28-03-2019.7z\n!cp bin/* /usr/local/bin\n\n#Extracting nfbs dataset\nimport tarfile\nfile = tarfile.open('/content/NFBS_Dataset.tar.gz')\nfile.extractall('/content/')\nfile.close()\n\nprint('Each folder contains..')\nprint(os.listdir('/content/NFBS_Dataset/A00028185'))\nimg=nib.load('/content/NFBS_Dataset/A00028185/sub-A00028185_ses-NFB3_T1w.nii.gz')\nprint('Shape of image=',img.shape)\n\n#storing the address of 3 types of files\nimport os\nbrain_mask=[]\nbrain=[]\nraw=[]\nfor subdir, dirs, files in os.walk('/content/NFBS_Dataset'):\n for file in files:\n #print os.path.join(subdir, file)y\n filepath = subdir + os.sep + file\n\n if filepath.endswith(\".gz\"):\n if '_brainmask.' in filepath:\n brain_mask.append(filepath)\n elif '_brain.' in filepath:\n brain.append(filepath)\n else:\n raw.append(filepath)\n\n\n#creating a dataframe for ease of use..\ndata=pd.DataFrame({'brain_mask':brain_mask,'brain':brain,'raw':raw})\ndata.head()"
}
{
"filename": "code/artificial_intelligence/src/skull_stripping/model.py",
"content": "#Import Libraries\nimport json\nimport os\nimport matplotlib.pyplot as plt\nimport numpy as np\n!pip install segmentation_models\n%env SM_FRAMEWORK=tf.keras\nenv: SM_FRAMEWORK=tf.keras\nimport segmentation_models\nfrom segmentation_models import Unet\nimport keras\nfrom segmentation_models.losses import dice_loss\nfrom keras.callbacks import ModelCheckpoint\n\nfrom tensorflow.keras.layers import Input\nfrom tensorflow.keras.models import Model\n\nfrom tensorflow.keras.optimizers import Adam\nfrom tensorflow.keras import backend as K\nimport tensorflow as tf\n\n#load the required dataset\n\n#check data\nprint(len(os.listdir('.../train/images/images')))\n\nimage = plt.imread('../images/train_image_0_slice_112_.png')\n\nprint(image.shape)\nprint(image.max())\nprint(image.min())\nplt.imshow(image)\n\n#Data Augmentation\naugmentations=dict(\n horizontal_flip=True,\n vertical_flip=True\n)\n\nimages_datagen = tf.keras.preprocessing.image.ImageDataGenerator(\n rescale = 1/255,\n # **augmentations,\n validation_split=0.2\n )\n\nmask_datagen = tf.keras.preprocessing.image.ImageDataGenerator(\n rescale = 1/255,\n # **augmentations,\n validation_split=0.2\n )\nBATCH_SIZE = 128\n\ntrain_images_generator = images_datagen.flow_from_directory(\n '/content/content/Images/train/images',\n target_size=(128, 128),\n batch_size=BATCH_SIZE,\n seed=42,\n interpolation='bilinear',\n color_mode='grayscale',\n class_mode=None,\n subset = 'training'\n )\n\n\ntrain_mask_generator = mask_datagen.flow_from_directory(\n '/content/content/Images/train/masks',\n target_size=(128, 128),\n batch_size=BATCH_SIZE,\n seed=42,\n class_mode=None,\n color_mode='grayscale',\n interpolation='bilinear',\n subset = 'training'\n )\n\nval_images_generator = images_datagen.flow_from_directory(\n '/content/content/Images/valid/images',\n target_size=(128, 128),\n batch_size=BATCH_SIZE,\n seed=42,\n interpolation='bilinear',\n color_mode='grayscale',\n class_mode=None,\n subset = 'validation'\n )\n\n\nval_mask_generator = mask_datagen.flow_from_directory(\n '/content/content/Images/valid/masks',\n target_size=(128, 128),\n batch_size=BATCH_SIZE,\n interpolation ='bilinear',\n color_mode='grayscale',\n seed=42,\n class_mode=None,\n subset = 'validation'\n )\n\ntrain_combined_generator = zip(train_images_generator, train_mask_generator)\nval_combined_generator = zip(val_images_generator, val_mask_generator)\n\nimages,masks = next(train_combined_generator)\n# print(images[0])\n\nprint(images[0].shape)\nprint(images[0].max())\nprint(images[0].min())\nprint(images[0].squeeze().shape)\n\nfig = plt.figure()\nfig.add_subplot(1, 2, 1)\nplt.imshow(images[0].squeeze())\nfig.add_subplot(1, 2, 2)\nplt.imshow(masks[0].squeeze())\n\n#Model for skull stripping\n\nmodel_base = Unet('efficientnetb1', classes=1, activation='sigmoid', encoder_weights='imagenet', encoder_freeze=False)\n\n\ninput_ = Input(shape=(None, None, 1))\nlayer_1 = tf.keras.layers.Conv2D(3, (1, 1))(input_)\nout = model_base(layer_1)\nmodel = Model(input_, out, name=\"Model1\")\n\noptimizer = tf.keras.optimizers.Adam(lr=2e-4)\n\nmodel.compile(optimizer=optimizer,\n loss=dice_loss,\n metrics=[segmentation_models.metrics.FScore(threshold=0.5)])\n\nsave_path = '/content/drive/MyDrive/ZPO_projekt/models/'\n\ncallbacks = [\n keras.callbacks.EarlyStopping(\n monitor=\"val_f1-score\",\n patience=2,\n verbose=1,\n mode=\"max\"\n ),\n keras.callbacks.ModelCheckpoint(\n monitor=\"val_f1-score\",\n save_best_only=1,\n verbose=1,\n mode=\"max\",\n filepath=save_path+\"model_{epoch}\"\n ),\n keras.callbacks.ReduceLROnPlateau(\n monitor=\"val_f1-score\",\n factor=0.5,\n patience=2,\n mode=\"max\"\n ),\n keras.callbacks.History()\n]\nmodel.summary()\n\nBATCH_SIZE = 1024\ntraining_samples = train_images_generator.n\nvalidation_samples = val_images_generator.n\n\n\nhistory = model.fit(\n train_combined_generator,\n steps_per_epoch=training_samples // BATCH_SIZE,\n validation_data = val_combined_generator,\n validation_steps = validation_samples // BATCH_SIZE,\n epochs=20,\n callbacks=callbacks,\n # workers=2,\n # use_multiprocessing=True,\n verbose=1\n)\n\n#Grapth the results\n\n# list all data in history\nprint(history.history.keys())\n# summarize history for accuracy\nplt.plot(history.history['f1-score'])\nplt.plot(history.history['val_f1-score'])\nplt.title('model score')\nplt.ylabel('score')\nplt.xlabel('epoch')\nplt.legend(['train', 'val'], loc='upper left')\nplt.show()\n\n# summarize history for loss\nplt.plot(history.history['loss'])\nplt.plot(history.history['val_loss'])\nplt.title('model loss')\nplt.ylabel('loss')\nplt.xlabel('epoch')\nplt.legend(['train', 'val'], loc='upper left')\nplt.show()"
}
{
"filename": "code/artificial_intelligence/src/support_vector_machine/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# Support Vector Machines\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/artificial_intelligence/src/support_vector_machine/support_vector_machine.cpp",
"content": "#include <iostream>\n#include <vector>\nusing namespace std;\n\nclass SVMModel {\npublic:\n vector<double> weights;\n double bias;\n\n SVMModel(int numFeatures) : weights(numFeatures, 0), bias(0) {}\n};\n\nclass SVM {\nprivate:\n static const double LEARNING_RATE;\n static const int MAX_ITERATIONS;\n\npublic:\n static SVMModel train(const vector<vector<double>>& features, const vector<int>& labels) {\n int numFeatures = features[0].size();\n SVMModel model(numFeatures);\n\n for (int iteration = 0; iteration < MAX_ITERATIONS; iteration++) {\n for (size_t i = 0; i < features.size(); i++) {\n const vector<double>& x = features[i];\n int y = labels[i];\n\n double prediction = predict(model.weights, x, model.bias);\n double hingeLoss = max(0.0, 1 - y * prediction);\n\n // Update weights and bias using gradient descent\n if (hingeLoss > 0) {\n for (int j = 0; j < numFeatures; j++) {\n model.weights[j] -= LEARNING_RATE * (-y * x[j]);\n }\n model.bias -= LEARNING_RATE * (-y);\n }\n }\n }\n\n return model;\n }\n\n static double predict(const vector<double>& weights, const vector<double>& x, double bias) {\n double result = bias;\n for (size_t i = 0; i < weights.size(); i++) {\n result += weights[i] * x[i];\n }\n return result;\n }\n};\n\nconst double SVM::LEARNING_RATE = 0.01;\nconst int SVM::MAX_ITERATIONS = 1000;\n\nint main() {\n vector<vector<double>> features = {{1, 2}, {2, 3}, {3, 4}};\n // Add more training examples as needed\n\n vector<int> labels = {1, 1, -1};\n // Add corresponding labels for each training example\n\n SVMModel model = SVM::train(features, labels);\n\n // Test the trained model\n vector<double> testExample = {4, 5};\n double prediction = SVM::predict(model.weights, testExample, model.bias);\n cout << \"Prediction: \" << prediction << endl;\n\n return 0;\n}"
}
{
"filename": "code/artificial_intelligence/src/support_vector_machine/svm.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n#include <time.h>\n#define EPS 0.01\n#define DIM 2\n#define TAM 8\n#define TOL 0.001 /* Error tolerance */\n#define C 10000 /* Maximun value apsumed for the alphas */\n\nfloat target[] = {1, 1, -1, -1, 1, -1, 1, 1}; /*desire output vector*/\nfloat point[][DIM] = {{1,1}, {2,1.5}, {1,3}, {2.1,3.5}, {1.5,0}, {1.3,2}, {1.5,1.2}, {3,2.8}}; /*trainig point matrix*/\nfloat* alphas; /* Lagrange multipliers */\nfloat* E; /*actual error vector*/\nfloat* w; /* weigth vector solution */\nfloat b; /* bias */\n\nvoid smo();\nint examineExample(int i2);\nint takeStep(int i1, int i2);\nvoid initializeVariables();\nvoid desalocatePointers();\nint nonzerosNonCAlphasRandomLoop(int i2);\nint allAlphasRandomLoop(int i2);\nint nonvalueAlphasCount(float value);\nint alphasVectorCount(float* alphas);\nfloat* nonzeroNonCAlphasPos();\nfloat* nonvalueAlphasPos(float value);\nint* randomPermutation(int n);\nint classifyNewPoint(float* point);\nfloat linearKernel(int pos1, int pos2);\nvoid updateErrorCache();\nint maxVectorIndex(float* vector);\nint minVectorIndex(float* vector);\nfloat absolute(float x);\nfloat max(float num1, float num2);\nfloat min(float num1, float num2);\n\nint\nmain(int argc, char *argv[])\n{\n int i;\n\n smo(); \n\n printf(\"Success!!\\n\");\n printf(\"w: %.5f %.5f\\n\", w[0], w[1]);\n printf(\"b: %.5f\\n\", b);\n\n desalocatePointers();\n return 0;\n}\n\nvoid \nsmo()\n{\n int i, n;\n int numChanged = 0,\n examineAll = 1;\n float* nonzeroNonCAlphasVector;\n\n initializeVariables();\n\n while (numChanged > 0 || examineAll) {\n numChanged = 0;\n if (examineAll) {\n for (i = 0; i < TAM; i++) {\n numChanged += examineExample(i);\n }\n } else {\n nonzeroNonCAlphasVector = nonzeroNonCAlphasPos();\n n = alphasVectorCount(nonzeroNonCAlphasVector);\n\n for (i = 0; i < n; i++) {\n numChanged += examineExample(nonzeroNonCAlphasVector[i]);\n }\n free(nonzeroNonCAlphasVector);\n }\n\n /* validation to continue the loop */\n if (examineAll) {\n examineAll = 0;\n } else if (!numChanged) {\n examineAll = 1;\n }\n }\n \n}\n\nint \nexamineExample(int i2)\n{\n float y2, alpha2, E2, r2;\n int i1, i;\n\n y2 = target[i2];\n alpha2 = alphas[i2];\n E2 = E[i2];\n r2 = y2 * E2;\n\n if ((r2 < -TOL && alpha2 < C) || (r2 > TOL && alpha2 > 0)) {\n /* Pick i1 */\n if (nonvalueAlphasCount(0) & nonvalueAlphasCount(C) > 1) {\n /* x1 must have the biggest error over x2 */\n if (E2 < 0) {\n i1 = maxVectorIndex(E);\n } else {\n i1 = minVectorIndex(E);\n }\n\n\n if (takeStep(i1, i2)) {\n return 1;\n }\n }\n }\n\n /* loop over all nonzeros and non-C alphas, start random */\n if (nonzerosNonCAlphasRandomLoop(i2)) {\n return 1;\n }\n\n /* Loop over all possible i1, start random */\n if (allAlphasRandomLoop(i2)) {\n return 1;\n }\n\n return 0;\n}\n\nint\ntakeStep(int i1, int i2) //Break function\n{\n float alpha1, y1, E1, alpha2, y2, E2;\n float s, L, H, k11, k12, k22, a1,\n a2, f1, f2, L1, H1, Lobj, Hobj, \n eta, b1, b2, bnew;\n int i;\n\n if (i1 == i2) return 0;\n \n alpha1 = alphas[i1];\n y1 = target[i1];\n E1 = E[i1];\n y2 = target[i2];\n alpha2 = alphas[i2];\n E2 = E[i2];\n s = y1 * y2;\n\n /* Calculate upper and lower limits */\n if (y1 == y2) {\n L = max(0, alpha2 + alpha1 - C);\n H = min(C, alpha2 + alpha1);\n } else {\n L = max(0, alpha2 - alpha1);\n H = min(C, C + alpha2 - alpha1);\n }\n\n k11 = linearKernel(i1, i1);\n k12 = linearKernel(i1, i2);\n k22 = linearKernel(i2, i2);\n\n eta = k11 + k22 - 2*k12;\n\n /* Calculate new alpha2 */\n if (eta > 0) {\n a2 = alpha2 + y2 * (E1 - E2) / eta;\n a2 = min(H, max(L, a2));\n } else {\n /* If eta is non-negative, move new a2 to bound \n with greater objective function value */\n f1 = y1*(E1 + b) - alpha1*k11 - s*alpha2*k12;\n f2 = y2*(E2 + b) - alpha2*k22 - s*alpha1*k12;\n L1 = alpha1 + s*(alpha2 - L);\n H1 = alpha1 + s*(alpha2 - H);\n Lobj = L1*f1 + 0.5*L1*L1*k11 + 0.5*L*L*k22 + s*L*L1*k12;\n Hobj = H1*f1 + 0.5*H1*H1*k11 + 0.5*H*H*k22 + s*H*H1*k12;\n\n if (Lobj < Hobj - EPS)\n a2 = L;\n else if (Lobj > Hobj + EPS)\n a2 = H;\n else\n a2 = alpha2;\n }\n\n /* Checking a2 */\n if (absolute(a2 - alpha2) < EPS*(a2 + alpha2 + EPS)) \n return 0;\n\n /* Calculate new alpha1 */\n a1 = alpha1 + s*(alpha2 - a2);\n\n /* Update threshold b */\n b1 = E1 + y1*(a1 - alpha1)*k11 + y2*(a2 - alpha2)*k12 + b;\n b1 = E2 + y1*(a1 - alpha1)*k12 + y2*(a2 - alpha2)*k22 + b;\n\n if (0 < a1 && a1 < C)\n bnew = b1;\n else if (0 < a2 && a2 < C)\n bnew = b2;\n else\n bnew = (b1 + b2) / 2;\n\n /* Update weigth vector w */\n for (i = 0; i < DIM; i++) {\n w[i] = w[i] + y1*(a1 - alpha1)*point[i1][i] + \n y2*(a2 - alpha2)*point[i2][i];\n }\n\n /* Update alphas and error cache*/\n alphas[i1] = a1;\n alphas[i2] = a2;\n b = bnew;\n updateErrorCache();\n\n return 1;\n}\n\nvoid\ninitializeVariables()\n{\n int i;\n b = 0;\n\n /* create zero alphas vecto */\n alphas = malloc(sizeof(float) * TAM);\n for (i = 0; i < TAM; i++) {\n alphas[i] = 0;\n }\n\n /* create zero w vector */\n w = malloc(sizeof(float) * DIM);\n for (i = 0; i < DIM; i++) {\n w[i] = 0;\n }\n\n /* Error Cache start */\n E = malloc(sizeof(float) *TAM);\n for (i = 0; i < TAM; i++) {\n E[i] = -target[i];\n }\n}\n\nvoid\ndesalocatePointers()\n{\n int i;\n free(E);\n free(w);\n free(alphas);\n}\n\nint\nnonzerosNonCAlphasRandomLoop(int i2)\n{\n int i, n;\n int* randomAlphas;\n float* nonzerosNonCAlphasVector;\n\n nonzerosNonCAlphasVector = nonzeroNonCAlphasPos();\n n = alphasVectorCount(nonzerosNonCAlphasVector);\n\n randomAlphas = randomPermutation(n);\n\n for (i = 0; i < n; i++) {\n if (takeStep(randomAlphas[i], i2)) {\n free(randomAlphas);\n free(nonzerosNonCAlphasVector);\n return 1;\n }\n }\n\n free(randomAlphas);\n free(nonzerosNonCAlphasVector);\n return 0;\n}\n\nint\nallAlphasRandomLoop(int i2)\n{\n int i;\n int* randomAlphas = randomPermutation(TAM);\n\n for (i = 0; i < TAM; i++) {\n if (takeStep(randomAlphas[i], i2)) {\n free(randomAlphas);\n return 1;\n }\n }\n\n free(randomAlphas);\n return 0;\n}\n\nint\nnonvalueAlphasCount(float value)\n{\n int i;\n float* nonvalueVector = nonvalueAlphasPos(value);\n\n for (i = 0; i < TAM; i++) {\n if (nonvalueVector[i] == -1) {\n break;\n }\n }\n\n free(nonvalueVector);\n return i;\n}\n\nint\nalphasVectorCount(float* alphas) \n{\n int i;\n\n for (i = 0; i < TAM; i++) {\n if (alphas[i] == -1) {\n break;\n }\n }\n\n return i;\n}\n\nfloat*\nnonzeroNonCAlphasPos()\n{\n int i, j;\n float* nonvalueVectorPos;\n\n nonvalueVectorPos = malloc(sizeof(float) * TAM);\n\n for (i = 0, j = 0; i < TAM; i++) {\n if (alphas[i] != 0 && alphas[i] != C ) {\n nonvalueVectorPos[j] = alphas[i];\n j++;\n }\n }\n\n for (; j < TAM; j++) {\n nonvalueVectorPos[j] = -1;\n }\n\n return nonvalueVectorPos;\n}\n\nfloat* \nnonvalueAlphasPos(float value)\n{\n int i, j;\n float* nonvalueVectorPos = malloc(sizeof(float) * TAM);\n\n for (i = 0, j = 0; i < TAM; i++) {\n if (alphas[i] != value) {\n nonvalueVectorPos[j] = i;\n j++;\n }\n }\n\n for (j++; j < TAM; j++) {\n nonvalueVectorPos[j] = -1;\n }\n\n return nonvalueVectorPos;\n}\n\nint*\nrandomPermutation(int n)\n{\n int i, j, temp;\n int* permutation = malloc(sizeof(int) * n);\n\n for (i = 0; i < n; i++) {\n permutation[i] = i;\n }\n // shuffle\n for(i = 0; i < n; i++) {\n j = rand() % (i +1);\n\n temp = permutation[i];\n permutation[i] = permutation[j];\n permutation[j] = temp;\n }\n\n return permutation;\n\n}\n\nint\nclassifyNewPoint(float* point)\n{\n int i;\n float res = -b;\n\n for (i = 0; i < DIM; i++) {\n res += w[i] * point[i];\n }\n\n return res >=0 ? 1 : -1;\n}\n\nfloat\nlinearKernel(int pos1, int pos2)\n{\n int i;\n float res = 0.0;\n for (i = 0; i < DIM; i++) {\n res += point[pos1][i] * point[pos2][i];\n }\n\n return res;\n}\n\nvoid\nupdateErrorCache()\n{\n int i, j;\n for (i = 0; i < TAM; i++) {\n E[i] = - b - target[i];\n for (j = 0; j < DIM; j++) {\n E[i] += w[j]*point[i][j];\n }\n }\n}\n\nint\nmaxVectorIndex(float* vector)\n{\n int i;\n int maxValueIndex = 0;\n for (i = 1; i < TAM; i++) {\n if (vector[i] > vector[maxValueIndex])\n maxValueIndex = i;\n }\n\n return maxValueIndex;\n}\n\nint\nminVectorIndex(float* vector)\n{\n int i;\n int minValueIndex = 0;\n for (i = 1; i < TAM; i++) {\n if (vector[i] < vector[minValueIndex])\n minValueIndex = i;\n }\n\n return minValueIndex;\n}\n\nfloat\nabsolute(float x)\n{\n return x >= 0 ? x : -x;\n}\n\nfloat\nmax(float num1, float num2)\n{\n return num1 > num2 ? num1 : num2;\n}\n\nfloat\nmin(float num1, float num2)\n{\n return num1 < num2 ? num1 : num2;\n}"
}
{
"filename": "code/artificial_intelligence/src/support_vector_machine/svm.py",
"content": "import numpy as np\n\n\nclass svm:\n def __init__(self):\n self.b = None\n self.t = None\n self.count = None\n self._lambda = None\n\n def train(self, xtrain, ytrain, count, _lambda):\n self.count = count\n self.b = []\n for i in range(0, len(xtrain[0])):\n self.b.append(0.0)\n self.t = 1\n self._lambda = _lambda\n for i in range(self.count):\n for j in range(len(xtrain)):\n output = ytrain[j] * xtrain[j][0] * self.b[0]\n for k in range(1, len(xtrain[0])):\n output = output + xtrain[j][k] * self.b[k]\n\n if output > 1:\n for k in range(0, len(xtrain[0])):\n self.b[k] = self.b[k] * (\n 1 - (1 / self.t)\n ) # /(self._lambda*self.t)\n else:\n for k in range(0, len(xtrain[0])):\n self.b[k] = self.b[k] * (1 - (1 / self.t)) + (\n ytrain[j] * xtrain[j][k]\n ) / (self._lambda * self.t)\n self.t = self.t + 1\n\n def predict(self, xtest):\n p_array = []\n for i in range(len(xtest)):\n output = 0\n for j in range(len(xtest[0])):\n output = output + self.b[j] * xtest[i][j]\n if output < 0:\n p_array.append(-1)\n else:\n p_array.append(1)\n\n return p_array\n\n\nxtrain = [\n [2.327868056, 2.458016525],\n [3.032830419, 3.170770366],\n [4.485465382, 3.696728111],\n [3.684815246, 3.846846973],\n [2.283558563, 1.853215997],\n [7.807521179, 3.290132136],\n [6.132998136, 2.140563087],\n [7.514829366, 2.107056961],\n [5.502385039, 1.404002608],\n [7.432932365, 4.236232628],\n]\nytrain = [-1, -1, -1, -1, -1, 1, 1, 1, 1, 1]\n\nclf = svm()\nclf.train(xtrain, ytrain, 16, 0.5)\nprint(clf.predict(xtrain))"
}
{
"filename": "code/artificial_intelligence/src/t_distributed_stochastic_neighbor_embedding/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# t-Distributed stochastic neighbor embedding\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/artificial_intelligence/src/tsp/algo.md",
"content": "### Building and running the executable\n\n1) Run `make` to get the executable tsp\n2) Run `./tsp < input >` output to get the results\n\n### Algorithm\n\n1) Used simulated annealing to first direct the path towards the random walk when temperature is high and then towards hill climbing when temperature is low.\n\n2) Randomly picked up two edges and swapped to get a suggested path, if cost of suggested path is better than current path we move towards it. In case the suggested path is worse in comparison to current path we still move towards it with some probability when temperature is high.\n\n3) Called the optimization function to get rid of long edges by using a series of swap operations.\n\n4) Kept a tabu tenure counter to make the current path equal to best path in case we are not able to find better results.\n\n5) Repeated the above process till the best_path became static for a large duration."
}
{
"filename": "code/artificial_intelligence/src/tsp.c",
"content": "/*\n * Implement any scheme to find the optimal solution for the\n * Traveling Salesperson problem and then solve the same problem\n * instance using any approximation algorithm and determine\n * the error in the approximation.\n */\n\n\n#include <stdio.h>\n\nint a[10][10], visited[10], no, cost, sum, vs[10];\nint \nleast(int c)\n{\n\tint i, nc = 999, min = 999, kmin;\n\tfor(i = 1; i <= no; i++)\n\t{\n\t\tif((a[c][i] != 0) && (visited[i] == 0))\n\t\t\tif(a[c][i] < min)\n\t\t\t{\n\t\t\t\tmin = a[i][1] + a[c][i];\n\t\t\t\tkmin = a[c][i];\n\t\t\t\tnc = i;\n\t\t\t}\n\t}\n\tif(min != 999)\n\t\tcost += kmin;\n\treturn nc;\n}\n\nvoid \ntsp(int city)\n{\n\tint ncity;\n\tvisited[city] = 1;\n\tprintf(\"%d ->\",city);\n\tncity = least(city);\n\tif(ncity == 999)\n\t{\n\t\tcost += a[city][1];\n\t\tprintf(\"1\\n\");\n\t\treturn;\n\t}\n\ttsp(ncity);\n}\n\nvoid \nnearest_n(int city)\n{\n\tint min, j, i, u;\n\tvs[city] = 1;\n\tprintf(\"%d -> \", city);\n\tu = city;\n\tfor(j = 1; j <= no; j++)\n\t{\n\t\tmin = 999;\n\t\tfor (i = 0; i <= no; ++i)\n\t\t\tif((vs[i] == 0) && (a[city][i] != 0))\n\t\t\t\tif(a[city][i] < min)\n\t\t\t\t{\n\t\t\t\t\tmin = a[city][i];\n\t\t\t\t\tu = i;\n\t\t\t\t}\n\t\tvs[u] = 1;\n\t\tif(min != 999)\n\t\t{\n\t\t\tsum += min;\n\t\t\tprintf(\"%d -> \", u);\n\t\t}\n\t\tcity = u;\n\t}\n\tprintf(\"1\\n\");\n\tsum += a[u][1];\n}\n\nint \nmain()\n{\n\tint i, j;\n\n\tprintf(\"Enter the No of Cities\\n\");\n\tscanf(\"%d\", &no);\n\n\tprintf(\"\\nEnter the Adjacency Matrix\\n\");\n\tfor(i = 1; i <= no; i++)\n\t\tfor(j = 1; j <=no; j++)\n\t\t\tscanf(\"%d\", &a[i][j]);\n\n\tprintf(\"Using Dynamic Prog\\n\");\n\ttsp(1);\n\tprintf(\"Cost is %d\\n\", cost);\n\tprintf(\"Using approx method\\n\");\n\tnearest_n(1);\n\tprintf(\"Cost is %d\\n\", sum);\n\tprintf (\"\\nRatio is %f\\n\", (float)sum/cost);\n\treturn 0;\n}"
}
{
"filename": "code/artificial_intelligence/src/tsp/makefile",
"content": "target: salesman.cpp\n\tg++ -o tsp salesman.cpp\nclean:\n\trm -rf *.o"
}
{
"filename": "code/artificial_intelligence/src/tsp/salesman.cpp",
"content": "#include <climits>\n#include <vector>\n#include <iostream>\n#include <algorithm>\n#include <cmath>\n\nusing namespace std;\n#define fio std::ios_base::sync_with_stdio(false); cin.tie(NULL)\n#define ll long long\n#define vi vector<int>\n#define all(a) a.begin(), a.end()\n#define pb push_back\nconst ll mod = 1e9 + 7;\nconst ll N = 1e5;\nconst ll MAX = 1e18;\n#define epsilon 1e-6\nvector<vector<long double>> adjacency_matrix;\n// Initial Temperature\nlong double temperature = 10000000000000;\nlong long num_cities;\nlong double answer = INT_MAX;\nvoid initialize_vectors(vector<long long> &best_path, vector<long long> ¤t_path,\n vector<long long> &suggested_path)\n{\n for (long long i = 0; i < num_cities; i++)\n {\n current_path[i] = i;\n suggested_path[i] = i;\n best_path[i] = i;\n }\n random_shuffle(current_path.begin(), current_path.end());\n}\nlong double evaluation(vector<long long> vec)\n{\n long double cost = 0;\n for (long long i = 0; i < num_cities - 1; i++)\n cost += adjacency_matrix[vec[i]][vec[i + 1]];\n cost += adjacency_matrix[vec[vec.size() - 1]][vec[0]];\n return cost;\n}\n// swaps random edges\npair<long, long> random_edge()\n{\n long long start_ind = rand() % num_cities;\n long long end_ind = (rand() % num_cities) + 1;\n if (start_ind > end_ind)\n swap(start_ind, end_ind);\n return make_pair(start_ind, end_ind);\n}\n// Uses triangle law to swap longer edges\nvoid Optimization(vector<long long> &auxiliary_best)\n{\n for (size_t i = 0; i < auxiliary_best.size() - 3; i++)\n {\n long double AB = adjacency_matrix[auxiliary_best[i]][auxiliary_best[i + 1]];\n long double CD = adjacency_matrix[auxiliary_best[i + 2]][auxiliary_best[i + 3]];\n long double AC = adjacency_matrix[auxiliary_best[i]][auxiliary_best[i + 2]];\n long double BD = adjacency_matrix[auxiliary_best[i + 1]][auxiliary_best[i + 3]];\n if (AB + CD > AC + BD)\n {\n swap(auxiliary_best[i + 1], auxiliary_best[i + 2]);\n i += 3;\n }\n else\n i++;\n }\n}\nvoid simulated_annealing()\n{\n vector<long long> best_path(num_cities, 0), current_path(num_cities, 0), suggested_path(\n num_cities, 0);\n initialize_vectors(best_path, current_path, suggested_path);\n int limit = 10000000;\n long double best_path_cost = 0;\n long long tabu_tenure = 0, break_counter = 0;\n while (limit-- && break_counter <= 200000)\n {\n suggested_path = current_path;\n pair<long long, long long> edges = random_edge();\n long long start = edges.first, end = edges.second;\n reverse(suggested_path.begin() + start, suggested_path.begin() + end);\n Optimization(suggested_path);\n long double current_path_cost = evaluation(current_path);\n long double suggested_path_cost = evaluation(suggested_path);\n long double net_gain = suggested_path_cost - current_path_cost;\n long double rand_num = (double) (rand() / (double) RAND_MAX);\n long double probability = 1 / (1 + std::pow(M_E, (net_gain / temperature)));\n best_path_cost = evaluation(best_path);\n if (probability > rand_num)\n current_path = suggested_path;\n if (suggested_path_cost < best_path_cost)\n {\n best_path = suggested_path;\n answer = min(answer, suggested_path_cost);\n tabu_tenure = 0;\n current_path = suggested_path;\n break_counter = 0;\n for (size_t i = 0; i < best_path.size(); i++)\n cout << best_path[i] + 1 << \" \";\n cout << best_path[0] + 1 << endl;\n }\n else\n tabu_tenure++;\n if (tabu_tenure >= 500)\n {\n Optimization(best_path);\n if (best_path_cost > evaluation(best_path))\n {\n best_path_cost = evaluation(best_path);\n answer = best_path_cost;\n current_path = best_path;\n break_counter = 0;\n for (size_t i = 0; i < best_path.size(); i++)\n cout << best_path[i] + 1 << \" \";\n cout << best_path[0] + 1 << endl;\n }\n\n tabu_tenure = 0;\n }\n cout << \"Best_Path_till now \" << best_path_cost << endl;\n // Cooling Rate\n temperature *= 0.999;\n break_counter++;\n }\n cout << \"Final Path:\\n\";\n for (size_t i = 0; i < best_path.size(); i++)\n cout << best_path[i] + 1 << \" \";\n cout << best_path[0] + 1 << endl;\n cout << endl;\n\n}\nvoid processing_data()\n{\n vector<pair<long double, long double>> location;\n string type;\n cin >> type;\n long double x, y;\n cin >> num_cities;\n srand (time(NULL));\n for (long long i = 0; i < num_cities; i++)\n {\n cin >> x >> y;\n location.push_back(make_pair(x, y));\n }\n for (long long i = 0; i < num_cities; i++)\n {\n vector<long double> temp;\n for (long long j = 0; j < num_cities; j++)\n {\n cin >> x;\n temp.push_back(x);\n }\n adjacency_matrix.push_back(temp);\n }\n}\nint main()\n{\n processing_data();\n simulated_annealing();\n cout << \"Minimum Path found so far \" << answer << endl;\n return 0;\n}"
}
{
"filename": "",
"content": ""
}
{
"filename": "code/artificial_intelligence/src/Vaccum_Cleaner/VC.md",
"content": "This is the code for simple reflex, goal based agent for vaccum cleaner."
}
{
"filename": "code/artificial_intelligence/src/VGG-11/README.md",
"content": "The article at OpenGenus elaborted about VGG-11, its architecture, number of layers it comprise of along with its application and importnance.\n\nIt is a pre-trained model, on a dataset and contains the weights that represent the features of whichever dataset it was trained on. \nVGG-11, it attributes to 11 weighted layers, Weights represent the strength of connections between units\nin adjacent network layers,are used to connect the each neurons in one layer to the every neurons in the next layer,\nweights near zero mean changing this input will not change the output.\n\nVGG incorporates 1x1 convolutional layers to make the decision function more non-linear without changing the receptive fields.\nLabeled as deep CNN, VGG surpasses datasets outside of ImageNet.\nThe architecture of VGG-11 and the ctivation functions insight to different applications and importance whose \ndetialed account has ben provided.\n\nLearn more: https://iq.opengenus.org/vgg-11/"
}
{
"filename": "code/artificial_intelligence/src/VGG-11/vgg11.md",
"content": "11 Layers f VGG-11 are as follows: \nThe detialed explanation has been provided in order to get better insight of how the model should be incorporated. \n\nConvolution using 64 filters + Max Pooling\nConvolution using 128 filters + Max Pooling\nConvolution using 256 filters\nConvolution using 256 filters + Max Pooling\nConvolution using 512 filters\nConvolution using 512 filters + Max Pooling\nConvolution using 512 filters\nConvolution using 512 filters + Max Pooling\nFully connected with 4096 nodes\nFully connected with 4096 nodes\nOutput layer with Softmax activation with 1000 nodes.\nNote: Here the max-pooling layer that is shown in the image is not counted among the 11 layers of VGG, \nthe reason for which is listed above. In accordance with the Softmax Activation function, it can be easily verified \nfrom the VGG-11 architecture.\nOne major conclusion that can be drawn from the whole study is VGG-11 prospers with an appreciable number of layers due to small size \nof convolution filter. This accounts for good accuracy of the model and decent performance outcomes\n\nLearn more: https://iq.opengenus.org/vgg-11/"
}
{
"filename": "code/artificial_intelligence/src/yolo_v1/README.md",
"content": "# YOLO \nYOLO, abbreviated from You Only Look Once is an mult-object detection CNN architecture developed by Joseph Redmon, Santosh Divvala, Ross Girshick and Ali Farhadi. You can find more about it on [this link](https://arxiv.org/abs/1506.02640)\nI have implemented the same algorithm using Pytorch.\n\n# Required Packages\n1. pytorch\n2. torch vision\n3. pandas\n4. numpy\n5. PIL\n6. matplotlib\nRest of them are common python libraries\n\n# Steps for replication\n1. Downloading this jupyter notebook on Google Colab. Alternatively, they can also load the dataset to their own computers instead of using Google Drive or Kaggle (as done in this notebook).\n2. Running all the cells **sequentially** in the order as in the notebook.\n3. It's done! You can go ahead and try the same algorithm on different datasets. "
}
{
"filename": "code/artificial_intelligence/src/yolo_v1/yolo-v1-implementation.ipynb",
"content": "{\"metadata\":{\"kernelspec\":{\"language\":\"python\",\"display_name\":\"Python 3\",\"name\":\"python3\"},\"language_info\":{\"name\":\"python\",\"version\":\"3.7.10\",\"mimetype\":\"text/x-python\",\"codemirror_mode\":{\"name\":\"ipython\",\"version\":3},\"pygments_lexer\":\"ipython3\",\"nbconvert_exporter\":\"python\",\"file_extension\":\".py\"}},\"nbformat_minor\":4,\"nbformat\":4,\"cells\":[{\"cell_type\":\"markdown\",\"source\":\"# **IMPORTING PACKAGES**\",\"metadata\":{}},{\"cell_type\":\"code\",\"source\":\"import torch.nn as nn\\nimport torch\\n\\n#for dataset loading\\nimport os\\nimport pandas as pd\\nfrom PIL import Image\\n\\n#for training\\nimport torchvision.transforms as transforms\\nimport torch.optim as optim\\nimport torchvision.transforms.functional as FT\\nfrom tqdm import tqdm #for progress bar\\nfrom torch.utils.data import DataLoader\\n\\n#for visualization\\nimport numpy as np\\nimport matplotlib.pyplot as plt\\nimport matplotlib.patches as patches\\nfrom collections import Counter\\n\\n#For checkpoint\\nimport time\\nimport sys\\nimport datetime\",\"metadata\":{\"execution\":{\"iopub.status.busy\":\"2021-10-25T17:12:58.694312Z\",\"iopub.execute_input\":\"2021-10-25T17:12:58.694669Z\",\"iopub.status.idle\":\"2021-10-25T17:12:58.700624Z\",\"shell.execute_reply.started\":\"2021-10-25T17:12:58.694632Z\",\"shell.execute_reply\":\"2021-10-25T17:12:58.699683Z\"},\"trusted\":true},\"execution_count\":2,\"outputs\":[]},{\"cell_type\":\"markdown\",\"source\":\"# **DEFINING CONSTANTS**\",\"metadata\":{}},{\"cell_type\":\"code\",\"source\":\"seed = 123\\ntorch.manual_seed(seed)\\n\\n# Hyperparameters \\nLEARNING_RATE = 2e-5\\nDEVICE = \\\"cuda\\\" if torch.cuda.is_available else \\\"cpu\\\"\\nBATCH_SIZE = 16 # 64 in original paper but setting it to 16 due to low computation power\\nWEIGHT_DECAY = 0\\nEPOCHS = 1000\\nNUM_WORKERS = 2\\nPIN_MEMORY = True\\nLOAD_MODEL = False #True, if you wanna use existing trained model at LOAD_MODEL_FILE path, else set to False\\nLOAD_MODEL_FILE = \\\"/kaggle/working/best_model.pth.tar\\\"\\nIS_TRAIN = True #True, if you wanna train model else set to False for testing\\n\\n#dataset paths\\n\\\"\\\"\\\"\\nChange root directory according to your file directory. \\nFor kaggle keep as default. \\nFor google colab, mount desired google drive, and set path to 'gdrive/My Drive/<your-dataset-file>'\\nFor local PC, set value according to your file directory after downloading the dataset from https://www.kaggle.com/dataset/734b7bcb7ef13a045cbdd007a3c19874c2586ed0b02b4afc86126e89d00af8d2.\\n\\\"\\\"\\\"\\nROOT_DIR = '/kaggle/input/pascalvoc-yolo/' \\nIMG_DIR = ROOT_DIR + \\\"images\\\"\\nLABEL_DIR = ROOT_DIR + \\\"labels\\\"\\nFULL_DATASET_FILEPATH = ROOT_DIR + \\\"train.csv\\\"\\nEIGHT_EXAMPLE_DATASET_FILEPATH = ROOT_DIR + \\\"8examples.csv\\\"\\nHUNDRED_EXAMPLE_DATASET_FILEPATH = ROOT_DIR + \\\"100examples.csv\\\"\\nTEST_DATASET_FILEPATH = ROOT_DIR + \\\"test.csv\\\"\\n\\n#class labels\\nCLASS_LABELS = [\\\"aeroplane\\\", \\\"bicycle\\\", \\\"bird\\\", \\\"boat\\\", \\\"bottle\\\", \\\"bus\\\", \\\"car\\\", \\\"cat\\\", \\\"chair\\\", \\\"cow\\\", \\\"table\\\", \\\"dog\\\", \\\"horse\\\", \\\"motorbike\\\", \\\"person\\\", \\\"potted plant\\\", \\\"sheep\\\", \\\"sofa\\\", \\\"train\\\", \\\"tv/monitor\\\"]\\nassert len(CLASS_LABELS) == 20, \\\"Some classes are missing from class labels!\\\"\\n\\\"Class labels are correct!\\\"\",\"metadata\":{\"execution\":{\"iopub.status.busy\":\"2021-10-25T18:19:05.168345Z\",\"iopub.execute_input\":\"2021-10-25T18:19:05.168627Z\",\"iopub.status.idle\":\"2021-10-25T18:19:05.182824Z\",\"shell.execute_reply.started\":\"2021-10-25T18:19:05.168597Z\",\"shell.execute_reply\":\"2021-10-25T18:19:05.181934Z\"},\"trusted\":true},\"execution_count\":19,\"outputs\":[]},{\"cell_type\":\"markdown\",\"source\":\"# **ARCHITECTURE CONFIG**\",\"metadata\":{}},{\"cell_type\":\"code\",\"source\":\"#refer YOLO V1 paper for the architecture\\n#architecture excluding fully connected layer output\\narchitecture_config = [\\n #Tuple: (kernel_size, num_filters, stride, padding)\\n (7, 64, 2, 3),\\n #String: stands for maxpool layer\\n \\\"M\\\",\\n (3, 192, 1, 1),\\n \\\"M\\\",\\n (1,128, 1, 0),\\n (3,256, 1, 1),\\n (1,256, 1, 0),\\n (3,512, 1, 1),\\n \\\"M\\\",\\n #List: [#Tuple,.., int represents no of repeats]\\n [(1, 256, 1, 0), (3, 512, 1, 1), 4],\\n (1, 512, 1, 0),\\n (3, 1024, 1, 1),\\n \\\"M\\\",\\n [(1, 512, 1, 0), (3, 1024, 1, 1), 2],\\n (3, 1024, 1, 1),\\n (3, 1024, 2, 1),\\n (3, 1024, 1, 1),\\n (3, 1024, 1, 1)\\n]\",\"metadata\":{\"execution\":{\"iopub.status.busy\":\"2021-10-25T17:13:04.879059Z\",\"iopub.execute_input\":\"2021-10-25T17:13:04.879354Z\",\"iopub.status.idle\":\"2021-10-25T17:13:04.887056Z\",\"shell.execute_reply.started\":\"2021-10-25T17:13:04.879321Z\",\"shell.execute_reply\":\"2021-10-25T17:13:04.886367Z\"},\"trusted\":true},\"execution_count\":4,\"outputs\":[]},{\"cell_type\":\"markdown\",\"source\":\"# **YOLO NETWORK DEFINATION**\",\"metadata\":{}},{\"cell_type\":\"code\",\"source\":\"#represents each convolution layer with a conv layer, batch norm and a relu\\nclass CNNBlock(nn.Module):\\n def __init__(self, in_channels, out_channels, **kwargs):\\n super(CNNBlock, self).__init__()\\n self.conv = nn.Conv2d(in_channels, out_channels, bias = False, **kwargs)\\n self.batchnorm = nn.BatchNorm2d(out_channels) #wasn't used in YOLO V1, but this one gives better results\\n self.leakyrelu = nn.LeakyReLU(0.1)\\n \\n def forward(self, x):\\n return self.leakyrelu(self.batchnorm(self.conv(x))) \",\"metadata\":{\"execution\":{\"iopub.status.busy\":\"2021-10-25T17:13:05.999073Z\",\"iopub.execute_input\":\"2021-10-25T17:13:05.999669Z\",\"iopub.status.idle\":\"2021-10-25T17:13:06.005350Z\",\"shell.execute_reply.started\":\"2021-10-25T17:13:05.999629Z\",\"shell.execute_reply\":\"2021-10-25T17:13:06.004501Z\"},\"trusted\":true},\"execution_count\":5,\"outputs\":[]},{\"cell_type\":\"code\",\"source\":\"class Yolov1(nn.Module):\\n def __init__(self, in_channels= 3, **kwargs):\\n super(Yolov1, self).__init__()\\n self.architecture = architecture_config\\n self.in_channels = in_channels\\n #the entire convolution section is referred as darknet, so darknet + fully connected layer = YOLO\\n self.darknet = self._create_conv_layers(self.architecture)\\n self.fcs = self._create_fcs(**kwargs)\\n \\n def forward(self, x):\\n x = self.darknet(x)\\n return self.fcs(torch.flatten(x, start_dim = 1)) # starting from 1 because 0th dimension contains no of examples\\n \\n def _create_conv_layers(self, architecture):\\n layers = [] # add all layers to thsi list\\n in_channels = self.in_channels\\n \\n for x in architecture:\\n if(type(x) == tuple):\\n # Tuple structure for ref: (kernel_size(0), num_filters(1), stride(2), padding(3))\\n layers += [CNNBlock(\\n in_channels,\\n x[1], \\n kernel_size = x[0], \\n stride = x[2], \\n padding = x[3]\\n )]\\n in_channels = x[1]\\n \\n elif (type(x) == str):\\n layers += [nn.MaxPool2d(kernel_size = 2, stride = 2)]\\n \\n elif (type(x) == list):\\n #List structure for ref: [#Tuple,.., int represents no of repeats]\\n num_repeats = x[2] #int\\n num_conv = len(x) - 1\\n for _ in range(num_repeats):\\n for i in range(num_conv): \\n layers += [CNNBlock(\\n in_channels, \\n x[i][1], \\n kernel_size = x[i][0], \\n stride = x[i][2], \\n padding = x[i][3], \\n )]\\n in_channels = x[i][1]\\n \\n return nn.Sequential(*layers)\\n \\n def _create_fcs(self, split_size, num_boxes, num_classes):\\n S, B, C = split_size, num_boxes, num_classes\\n return nn.Sequential(\\n nn.Flatten(),\\n nn.Linear(1024 * S * S, 496), #originally its 4096, instead of 496 \\n nn.Dropout(0.0), #originally 0.5\\n nn.LeakyReLU(0.1),\\n nn.Linear(496, S * S * (C + B * 5)), #originally its 4096, instead of 496, (S , S, 30) C+B*5 = 30 \\n ) \",\"metadata\":{\"execution\":{\"iopub.status.busy\":\"2021-10-25T17:13:06.972619Z\",\"iopub.execute_input\":\"2021-10-25T17:13:06.973068Z\",\"iopub.status.idle\":\"2021-10-25T17:13:06.987365Z\",\"shell.execute_reply.started\":\"2021-10-25T17:13:06.973032Z\",\"shell.execute_reply\":\"2021-10-25T17:13:06.986622Z\"},\"trusted\":true},\"execution_count\":6,\"outputs\":[]},{\"cell_type\":\"markdown\",\"source\":\"# **TEST CASE FOR TESTING YOLO MODEL**\",\"metadata\":{}},{\"cell_type\":\"code\",\"source\":\"#DO NOT EDIT THIS, USE THIS FOR CONFIRMING THE YOLO MODEL\\ndef test(S = 7, B = 2, C = 20):\\n model = Yolov1(split_size = S, num_boxes = B, num_classes = C)\\n x = torch.randn((2, 3, 448, 448))\\n assert model(x).shape == torch.Tensor(2, 1470).shape, \\\"Something wrong with the model!\\\"\\n print('Model is correct!')\\ntest()\",\"metadata\":{\"execution\":{\"iopub.status.busy\":\"2021-10-25T17:13:08.578905Z\",\"iopub.execute_input\":\"2021-10-25T17:13:08.579325Z\",\"iopub.status.idle\":\"2021-10-25T17:13:10.626877Z\",\"shell.execute_reply.started\":\"2021-10-25T17:13:08.579290Z\",\"shell.execute_reply\":\"2021-10-25T17:13:10.626130Z\"},\"trusted\":true},\"execution_count\":7,\"outputs\":[]},{\"cell_type\":\"markdown\",\"source\":\"# **UTILITY METHODS**\",\"metadata\":{}},{\"cell_type\":\"code\",\"source\":\"#Calculates intersection over union(iou)\\ndef intersection_over_union(boxes_preds, boxes_labels, box_format=\\\"midpoint\\\"): \\n\\n if box_format == \\\"midpoint\\\":\\n box1_x1 = boxes_preds[..., 0:1] - boxes_preds[..., 2:3] / 2\\n box1_y1 = boxes_preds[..., 1:2] - boxes_preds[..., 3:4] / 2\\n box1_x2 = boxes_preds[..., 0:1] + boxes_preds[..., 2:3] / 2\\n box1_y2 = boxes_preds[..., 1:2] + boxes_preds[..., 3:4] / 2\\n box2_x1 = boxes_labels[..., 0:1] - boxes_labels[..., 2:3] / 2\\n box2_y1 = boxes_labels[..., 1:2] - boxes_labels[..., 3:4] / 2\\n box2_x2 = boxes_labels[..., 0:1] + boxes_labels[..., 2:3] / 2\\n box2_y2 = boxes_labels[..., 1:2] + boxes_labels[..., 3:4] / 2\\n\\n if box_format == \\\"corners\\\":\\n box1_x1 = boxes_preds[..., 0:1]\\n box1_y1 = boxes_preds[..., 1:2]\\n box1_x2 = boxes_preds[..., 2:3]\\n box1_y2 = boxes_preds[..., 3:4] # (N, 1)\\n box2_x1 = boxes_labels[..., 0:1]\\n box2_y1 = boxes_labels[..., 1:2]\\n box2_x2 = boxes_labels[..., 2:3]\\n box2_y2 = boxes_labels[..., 3:4]\\n\\n x1 = torch.max(box1_x1, box2_x1)\\n y1 = torch.max(box1_y1, box2_y1)\\n x2 = torch.min(box1_x2, box2_x2)\\n y2 = torch.min(box1_y2, box2_y2)\\n\\n # .clamp(0) is for the case when they do not intersect\\n intersection = (x2 - x1).clamp(0) * (y2 - y1).clamp(0)\\n\\n box1_area = abs((box1_x2 - box1_x1) * (box1_y2 - box1_y1))\\n box2_area = abs((box2_x2 - box2_x1) * (box2_y2 - box2_y1))\\n\\n return intersection / (box1_area + box2_area - intersection + 1e-6)\\n\\n#For Non Max Suppression given bboxes\\ndef non_max_suppression(bboxes, iou_threshold, threshold, box_format=\\\"corners\\\"):\\n\\n assert type(bboxes) == list\\n\\n bboxes = [box for box in bboxes if box[1] > threshold]\\n bboxes = sorted(bboxes, key=lambda x: x[1], reverse=True)\\n bboxes_after_nms = []\\n\\n while bboxes:\\n chosen_box = bboxes.pop(0)\\n\\n bboxes = [\\n box\\n for box in bboxes\\n if box[0] != chosen_box[0]\\n or intersection_over_union(\\n torch.tensor(chosen_box[2:]),\\n torch.tensor(box[2:]),\\n box_format=box_format,\\n )\\n < iou_threshold\\n ]\\n\\n bboxes_after_nms.append(chosen_box)\\n\\n return bboxes_after_nms\\n\\n#for calculating mean average precision\\ndef mean_average_precision(\\n pred_boxes, true_boxes, iou_threshold=0.5, box_format=\\\"midpoint\\\", num_classes=20):\\n # list storing all AP for respective classes\\n average_precisions = []\\n\\n # used for numerical stability later on\\n epsilon = 1e-6\\n\\n for c in range(num_classes):\\n detections = []\\n ground_truths = []\\n\\n # Go through all predictions and targets,\\n # and only add the ones that belong to the\\n # current class c\\n for detection in pred_boxes:\\n if detection[1] == c:\\n detections.append(detection)\\n\\n for true_box in true_boxes:\\n if true_box[1] == c:\\n ground_truths.append(true_box)\\n\\n # find the amount of bboxes for each training example\\n # Counter here finds how many ground truth bboxes we get\\n # for each training example, so let's say img 0 has 3,\\n # img 1 has 5 then we will obtain a dictionary with:\\n # amount_bboxes = {0:3, 1:5}\\n amount_bboxes = Counter([gt[0] for gt in ground_truths])\\n\\n # We then go through each key, val in this dictionary\\n # and convert to the following (w.r.t same example):\\n # ammount_bboxes = {0:torch.tensor[0,0,0], 1:torch.tensor[0,0,0,0,0]}\\n for key, val in amount_bboxes.items():\\n amount_bboxes[key] = torch.zeros(val)\\n\\n # sort by box probabilities which is index 2\\n detections.sort(key=lambda x: x[2], reverse=True)\\n TP = torch.zeros((len(detections)))\\n FP = torch.zeros((len(detections)))\\n total_true_bboxes = len(ground_truths)\\n \\n # If none exists for this class then we can safely skip\\n if total_true_bboxes == 0:\\n continue\\n\\n for detection_idx, detection in enumerate(detections):\\n # Only take out the ground_truths that have the same\\n # training idx as detection\\n ground_truth_img = [\\n bbox for bbox in ground_truths if bbox[0] == detection[0]\\n ]\\n\\n num_gts = len(ground_truth_img)\\n best_iou = 0\\n\\n for idx, gt in enumerate(ground_truth_img):\\n iou = intersection_over_union(\\n torch.tensor(detection[3:]),\\n torch.tensor(gt[3:]),\\n box_format=box_format,\\n )\\n\\n if iou > best_iou:\\n best_iou = iou\\n best_gt_idx = idx\\n\\n if best_iou > iou_threshold:\\n # only detect ground truth detection once\\n if amount_bboxes[detection[0]][best_gt_idx] == 0:\\n # true positive and add this bounding box to seen\\n TP[detection_idx] = 1\\n amount_bboxes[detection[0]][best_gt_idx] = 1\\n else:\\n FP[detection_idx] = 1\\n\\n # if IOU is lower then the detection is a false positive\\n else:\\n FP[detection_idx] = 1\\n\\n TP_cumsum = torch.cumsum(TP, dim=0)\\n FP_cumsum = torch.cumsum(FP, dim=0)\\n recalls = TP_cumsum / (total_true_bboxes + epsilon)\\n precisions = torch.divide(TP_cumsum, (TP_cumsum + FP_cumsum + epsilon))\\n precisions = torch.cat((torch.tensor([1]), precisions))\\n recalls = torch.cat((torch.tensor([0]), recalls))\\n # torch.trapz for numerical integration\\n average_precisions.append(torch.trapz(precisions, recalls))\\n\\n return sum(average_precisions) / len(average_precisions)\\n\\n#Plots bounding boxes on the image\\ndef plot_image(image, boxes):\\n im = np.array(image)\\n height, width, _ = im.shape\\n\\n # Create figure and axes\\n fig, ax = plt.subplots(1)\\n # Display the image\\n ax.imshow(im)\\n\\n # box[0] is x midpoint, box[2] is width\\n # box[1] is y midpoint, box[3] is height\\n\\n # Create a Rectangle potch\\n for box in boxes:\\n class_label = CLASS_LABELS[int(box[0])]\\n score = box[1]\\n box = box[2:]\\n assert len(box) == 4, \\\"Got more values than in x, y, w, h, in a box!\\\" #to ensure there are only 4 elements in the box dimensions\\n upper_left_x = box[0] - box[2] / 2\\n upper_left_y = box[1] - box[3] / 2\\n rect = patches.Rectangle(\\n (upper_left_x * width, upper_left_y * height),\\n box[2] * width,\\n box[3] * height,\\n linewidth=1,\\n edgecolor=\\\"r\\\",\\n facecolor=\\\"none\\\",\\n )\\n label = \\\"%s (%.3f)\\\" % (class_label, score)\\n plt.text(upper_left_x * width, upper_left_y * height, label, color='white', bbox = dict(facecolor='red', alpha=0.5, edgecolor='red'))\\n # Add the patch to the Axes\\n ax.add_patch(rect)\\n \\n plt.tight_layout()\\n plt.show()\\n\\ndef get_bboxes(\\n loader,\\n model,\\n iou_threshold,\\n threshold,\\n pred_format=\\\"cells\\\",\\n box_format=\\\"midpoint\\\",\\n device=\\\"cuda\\\",\\n):\\n all_pred_boxes = []\\n all_true_boxes = []\\n\\n # make sure model is in eval before get bboxes\\n model.eval()\\n train_idx = 0\\n\\n for batch_idx, (x, labels) in enumerate(loader):\\n x = x.to(device)\\n labels = labels.to(device)\\n\\n with torch.no_grad():\\n predictions = model(x)\\n\\n batch_size = x.shape[0]\\n true_bboxes = cellboxes_to_boxes(labels)\\n bboxes = cellboxes_to_boxes(predictions)\\n\\n for idx in range(batch_size):\\n nms_boxes = non_max_suppression(\\n bboxes[idx],\\n iou_threshold=iou_threshold,\\n threshold=threshold,\\n box_format=box_format,\\n )\\n\\n\\n #if batch_idx == 0 and idx == 0:\\n # plot_image(x[idx].permute(1,2,0).to(\\\"cpu\\\"), nms_boxes)\\n # print(nms_boxes)\\n\\n for nms_box in nms_boxes:\\n all_pred_boxes.append([train_idx] + nms_box)\\n\\n for box in true_bboxes[idx]:\\n # many will get converted to 0 pred\\n if box[1] > threshold:\\n all_true_boxes.append([train_idx] + box)\\n\\n train_idx += 1\\n\\n model.train()\\n return all_pred_boxes, all_true_boxes\\n\\n\\n#for output of YOLO \\ndef convert_cellboxes(predictions, S=7):\\n\\n predictions = predictions.to(\\\"cpu\\\")\\n batch_size = predictions.shape[0]\\n predictions = predictions.reshape(batch_size, 7, 7, 30)\\n bboxes1 = predictions[..., 21:25]\\n bboxes2 = predictions[..., 26:30]\\n scores = torch.cat(\\n (predictions[..., 20].unsqueeze(0), predictions[..., 25].unsqueeze(0)), dim=0\\n )\\n best_box = scores.argmax(0).unsqueeze(-1)\\n best_boxes = bboxes1 * (1 - best_box) + best_box * bboxes2\\n cell_indices = torch.arange(7).repeat(batch_size, 7, 1).unsqueeze(-1)\\n x = 1 / S * (best_boxes[..., :1] + cell_indices)\\n y = 1 / S * (best_boxes[..., 1:2] + cell_indices.permute(0, 2, 1, 3))\\n w_y = 1 / S * best_boxes[..., 2:4]\\n converted_bboxes = torch.cat((x, y, w_y), dim=-1)\\n predicted_class = predictions[..., :20].argmax(-1).unsqueeze(-1)\\n best_confidence = torch.max(predictions[..., 20], predictions[..., 25]).unsqueeze(\\n -1\\n )\\n converted_preds = torch.cat(\\n (predicted_class, best_confidence, converted_bboxes), dim=-1\\n )\\n\\n return converted_preds\\n\\n#convert cellboxes to box for whole image\\ndef cellboxes_to_boxes(out, S=7):\\n converted_pred = convert_cellboxes(out).reshape(out.shape[0], S * S, -1)\\n converted_pred[..., 0] = converted_pred[..., 0].long()\\n all_bboxes = []\\n\\n for ex_idx in range(out.shape[0]):\\n bboxes = []\\n\\n for bbox_idx in range(S * S):\\n bboxes.append([x.item() for x in converted_pred[ex_idx, bbox_idx, :]])\\n all_bboxes.append(bboxes)\\n\\n return all_bboxes\\n\\n#for saving checkpoint of image\\ndef save_checkpoint(state, filename=\\\"my_checkpoint.pth.tar\\\"):\\n print(\\\"=> Saving checkpoint\\\")\\n torch.save(state, filename)\\n\\n#for loading the same checkpoint\\ndef load_checkpoint(checkpoint, model, optimizer):\\n print(\\\"=> Loading checkpoint\\\")\\n model.load_state_dict(checkpoint[\\\"state_dict\\\"])\\n optimizer.load_state_dict(checkpoint[\\\"optimizer\\\"])\\n\",\"metadata\":{\"execution\":{\"iopub.status.busy\":\"2021-10-25T17:13:10.628713Z\",\"iopub.execute_input\":\"2021-10-25T17:13:10.629133Z\",\"iopub.status.idle\":\"2021-10-25T17:13:10.675020Z\",\"shell.execute_reply.started\":\"2021-10-25T17:13:10.629095Z\",\"shell.execute_reply\":\"2021-10-25T17:13:10.674316Z\"},\"trusted\":true},\"execution_count\":8,\"outputs\":[]},{\"cell_type\":\"markdown\",\"source\":\"# **LOSS FUNCTION**\",\"metadata\":{}},{\"cell_type\":\"code\",\"source\":\"class YoloLoss(nn.Module):\\n def __init__(self, S=7, B=2, C=20):\\n super(YoloLoss, self).__init__()\\n self.mse = nn.MSELoss(reduction=\\\"sum\\\")\\n\\n \\\"\\\"\\\"\\n S is split size of image (in paper 7),\\n B is number of boxes (in paper 2),\\n C is number of classes (in paper and VOC dataset is 20),\\n \\\"\\\"\\\"\\n self.S = S\\n self.B = B\\n self.C = C\\n\\n # These are from Yolo paper, signifying how much we should\\n # pay loss for no object (noobj) and the box coordinates (coord)\\n self.lambda_noobj = 0.5\\n self.lambda_coord = 5\\n\\n def forward(self, predictions, target):\\n # predictions are shaped (BATCH_SIZE, S*S(C+B*5) when inputted\\n predictions = predictions.reshape(-1, self.S, self.S, self.C + self.B * 5)\\n\\n # Calculate IoU for the two predicted bounding boxes with target bbox\\n # 0-19 will be class probabilities, 20 will be class score, 21-25 will be bounding values and box conv1, 26-30 will be bounding values and box conv2\\n iou_b1 = intersection_over_union(predictions[..., 21:25], target[..., 21:25])\\n iou_b2 = intersection_over_union(predictions[..., 26:30], target[..., 21:25])\\n ious = torch.cat([iou_b1.unsqueeze(0), iou_b2.unsqueeze(0)], dim=0)\\n\\n # Take the box with highest IoU out of the two prediction\\n # Note that bestbox will be indices of 0, 1 for which bbox was best\\n iou_maxes, bestbox = torch.max(ious, dim=0)\\n exists_box = target[..., 20].unsqueeze(3) # in paper this is Iobj_i\\n\\n # ======================== #\\n # FOR BOX COORDINATES #\\n # ======================== #\\n\\n # Set boxes with no object in them to 0. We only take out one of the two \\n # predictions, which is the one with highest Iou calculated previously.\\n box_predictions = exists_box * (\\n (\\n bestbox * predictions[..., 26:30]\\n + (1 - bestbox) * predictions[..., 21:25]\\n )\\n )\\n\\n box_targets = exists_box * target[..., 21:25]\\n\\n # Take sqrt of width, height of boxes to ensure that\\n box_predictions[..., 2:4] = torch.sign(box_predictions[..., 2:4]) * torch.sqrt(\\n torch.abs(box_predictions[..., 2:4] + 1e-6)\\n )\\n box_targets[..., 2:4] = torch.sqrt(box_targets[..., 2:4])\\n\\n box_loss = self.mse(\\n torch.flatten(box_predictions, end_dim=-2),\\n torch.flatten(box_targets, end_dim=-2),\\n )\\n\\n # ==================== #\\n # FOR OBJECT LOSS #\\n # ==================== #\\n\\n # pred_box is the confidence score for the bbox with highest IoU\\n pred_box = (\\n bestbox * predictions[..., 25:26] + (1 - bestbox) * predictions[..., 20:21]\\n )\\n\\n object_loss = self.mse(\\n torch.flatten(exists_box * pred_box),\\n torch.flatten(exists_box * target[..., 20:21]),\\n )\\n\\n # ======================= #\\n # FOR NO OBJECT LOSS #\\n # ======================= #\\n #(N, S, S, 1) > (N, S*S)\\n no_object_loss = self.mse(\\n torch.flatten((1 - exists_box) * predictions[..., 20:21], start_dim=1),\\n torch.flatten((1 - exists_box) * target[..., 20:21], start_dim=1),\\n )\\n\\n no_object_loss += self.mse(\\n torch.flatten((1 - exists_box) * predictions[..., 25:26], start_dim=1),\\n torch.flatten((1 - exists_box) * target[..., 20:21], start_dim=1)\\n )\\n\\n # ================== #\\n # FOR CLASS LOSS #\\n # ================== #\\n #(N, S, S, 20) > ( N*N*S , 20)\\n class_loss = self.mse(\\n torch.flatten(exists_box * predictions[..., :20], end_dim=-2,),\\n torch.flatten(exists_box * target[..., :20], end_dim=-2,),\\n )\\n\\n loss = (\\n self.lambda_coord * box_loss # first two rows in paper\\n + object_loss # third row in paper\\n + self.lambda_noobj * no_object_loss # forth row\\n + class_loss # fifth row\\n )\\n\\n return loss\",\"metadata\":{\"execution\":{\"iopub.status.busy\":\"2021-10-25T17:13:11.700682Z\",\"iopub.execute_input\":\"2021-10-25T17:13:11.703535Z\",\"iopub.status.idle\":\"2021-10-25T17:13:11.735600Z\",\"shell.execute_reply.started\":\"2021-10-25T17:13:11.703486Z\",\"shell.execute_reply\":\"2021-10-25T17:13:11.734194Z\"},\"trusted\":true},\"execution_count\":9,\"outputs\":[]},{\"cell_type\":\"markdown\",\"source\":\"# **DATASET**\",\"metadata\":{}},{\"cell_type\":\"code\",\"source\":\"#Common for Kaggle, Colab and Local, send paths for directories and train csv accordingly\\nclass VOCDataset(torch.utils.data.Dataset):\\n def __init__(\\n self, csv_file, img_dir, label_dir, S=7, B=2, C=20, transform=None,\\n ):\\n self.annotations = pd.read_csv(csv_file)\\n self.img_dir = img_dir\\n self.label_dir = label_dir\\n self.transform = transform\\n self.S = S\\n self.B = B\\n self.C = C\\n\\n def __len__(self):\\n return len(self.annotations)\\n\\n def __getitem__(self, index):\\n label_path = os.path.join(self.label_dir, self.annotations.iloc[index, 1])\\n boxes = []\\n with open(label_path) as f:\\n for label in f.readlines():\\n class_label, x, y, width, height = [\\n float(x) if float(x) != int(float(x)) else int(x)\\n for x in label.replace(\\\"\\\\n\\\", \\\"\\\").split()\\n ]\\n\\n boxes.append([class_label, x, y, width, height])\\n\\n img_path = os.path.join(self.img_dir, self.annotations.iloc[index, 0])\\n image = Image.open(img_path)\\n boxes = torch.tensor(boxes)\\n\\n if self.transform:\\n # image = self.transform(image)\\n image, boxes = self.transform(image, boxes)\\n\\n # Convert To Cells\\n label_matrix = torch.zeros((self.S, self.S, self.C + 5 * self.B))\\n for box in boxes:\\n class_label, x, y, width, height = box.tolist()\\n class_label = int(class_label)\\n\\n # i,j represents the cell row and cell column\\n i, j = int(self.S * y), int(self.S * x)\\n x_cell, y_cell = self.S * x - j, self.S * y - i\\n\\n #Calculating the width and height of cell of bounding box,relative to the cell\\n \\n width_cell, height_cell = (\\n width * self.S,\\n height * self.S,\\n )\\n\\n # If no object already found for specific cell i,j\\n if label_matrix[i, j, 20] == 0:\\n # Set that there exists an object\\n label_matrix[i, j, 20] = 1\\n\\n # Box coordinates\\n box_coordinates = torch.tensor(\\n [x_cell, y_cell, width_cell, height_cell]\\n )\\n\\n label_matrix[i, j, 21:25] = box_coordinates\\n\\n label_matrix[i, j, class_label] = 1\\n\\n return image, label_matrix\",\"metadata\":{\"execution\":{\"iopub.status.busy\":\"2021-10-25T17:13:12.534572Z\",\"iopub.execute_input\":\"2021-10-25T17:13:12.535036Z\",\"iopub.status.idle\":\"2021-10-25T17:13:12.550829Z\",\"shell.execute_reply.started\":\"2021-10-25T17:13:12.534994Z\",\"shell.execute_reply\":\"2021-10-25T17:13:12.549573Z\"},\"trusted\":true},\"execution_count\":10,\"outputs\":[]},{\"cell_type\":\"code\",\"source\":\"#user-defined class for transforms\\nclass Compose(object):\\n def __init__(self, transforms):\\n self.transforms = transforms\\n\\n def __call__(self, img, bboxes):\\n for t in self.transforms:\\n img, bboxes = t(img), bboxes\\n\\n return img, bboxes\\n\\ntransform = Compose([transforms.Resize((448, 448)), transforms.ToTensor(),]) #normalization can also be added\",\"metadata\":{\"execution\":{\"iopub.status.busy\":\"2021-10-25T17:13:12.919487Z\",\"iopub.execute_input\":\"2021-10-25T17:13:12.919721Z\",\"iopub.status.idle\":\"2021-10-25T17:13:12.925149Z\",\"shell.execute_reply.started\":\"2021-10-25T17:13:12.919695Z\",\"shell.execute_reply\":\"2021-10-25T17:13:12.924225Z\"},\"trusted\":true},\"execution_count\":11,\"outputs\":[]},{\"cell_type\":\"markdown\",\"source\":\"# **TRAINING MODEL**\",\"metadata\":{}},{\"cell_type\":\"code\",\"source\":\"def train_fn(train_loader, model, optimizer, loss_fn):\\n loop = tqdm(train_loader, leave=True) #for progress bar\\n mean_loss = []\\n\\n for batch_idx, (x, y) in enumerate(loop):\\n x, y = x.to(DEVICE), y.to(DEVICE)\\n out = model(x)\\n loss = loss_fn(out, y)\\n mean_loss.append(loss.item())\\n optimizer.zero_grad()\\n loss.backward()\\n optimizer.step()\\n\\n # update progress bar\\n loop.set_postfix(loss=loss.item())\\n\\n print(f\\\"Mean loss : {sum(mean_loss)/len(mean_loss)}\\\")\",\"metadata\":{\"execution\":{\"iopub.status.busy\":\"2021-10-25T17:13:13.763720Z\",\"iopub.execute_input\":\"2021-10-25T17:13:13.764205Z\",\"iopub.status.idle\":\"2021-10-25T17:13:13.770323Z\",\"shell.execute_reply.started\":\"2021-10-25T17:13:13.764166Z\",\"shell.execute_reply\":\"2021-10-25T17:13:13.769328Z\"},\"trusted\":true},\"execution_count\":12,\"outputs\":[]},{\"cell_type\":\"markdown\",\"source\":\"# **WHERE IT ALL COMES TOGETHER!**\",\"metadata\":{}},{\"cell_type\":\"code\",\"source\":\"model = Yolov1(split_size=7, num_boxes=2, num_classes=20).to(DEVICE)\\noptimizer = optim.Adam(\\n model.parameters(), lr=LEARNING_RATE, weight_decay=WEIGHT_DECAY\\n)\\nloss_fn = YoloLoss()\\n\\nif LOAD_MODEL:\\n load_checkpoint(torch.load(LOAD_MODEL_FILE), model, optimizer)\\n\\ntrain_dataset = VOCDataset(\\n HUNDRED_EXAMPLE_DATASET_FILEPATH,\\n transform=transform,\\n img_dir=IMG_DIR,\\n label_dir=LABEL_DIR,\\n)\\n\\n\\ntest_dataset = VOCDataset(\\n TEST_DATASET_FILEPATH, transform=transform, img_dir=IMG_DIR, label_dir=LABEL_DIR\\n)\\n\\n\\ntrain_loader = DataLoader(\\n dataset=train_dataset,\\n batch_size=BATCH_SIZE,\\n num_workers=NUM_WORKERS,\\n pin_memory=PIN_MEMORY,\\n shuffle=True,\\n drop_last=True,\\n)\\nprint(\\\"Training set loaded...\\\")\\n\\ntest_loader = DataLoader(\\n dataset=test_dataset,\\n batch_size=BATCH_SIZE,\\n num_workers=NUM_WORKERS,\\n pin_memory=PIN_MEMORY,\\n shuffle=True,\\n drop_last=True,\\n)\\nprint(\\\"Testing set loaded...\\\")\\n\\nbest_acc = 0.0\\nif IS_TRAIN:\\n start_training = datetime.datetime.now()\\n for epoch in range(EPOCHS):\\n print(f\\\"Running epoch : {epoch + 1}/{EPOCHS}\\\")\\n \\n pred_boxes_train, target_boxes_train = get_bboxes(\\n train_loader, model, iou_threshold=0.5, threshold=0.4\\n )\\n\\n mean_avg_prec_train = mean_average_precision(\\n pred_boxes_train, target_boxes_train, iou_threshold=0.5, box_format=\\\"midpoint\\\"\\n )\\n\\n print(f\\\"Train mAP: {mean_avg_prec_train}\\\")\\n \\n if mean_avg_prec > best_acc:\\n best_acc = mean_avg_prec\\n \\n# if mean_avg_prec >0.9 and mean_avg_prec == best_acc:\\n# checkpoint = {\\n# \\\"state_dict\\\": model.state_dict(),\\n# \\\"optimizer\\\": optimizer.state_dict(),\\n# }\\n# save_checkpoint(checkpoint, filename=LOAD_MODEL_FILE)\\n# time.sleep(10)\\n \\n train_fn(train_loader, model, optimizer, loss_fn)\\n\\n print(f\\\"Total training time {(datetime.datetime.now()-start_training).seconds/60} minutes.\\\")\",\"metadata\":{\"execution\":{\"iopub.status.busy\":\"2021-10-25T18:19:16.023684Z\",\"iopub.execute_input\":\"2021-10-25T18:19:16.023940Z\",\"iopub.status.idle\":\"2021-10-25T19:12:14.010246Z\",\"shell.execute_reply.started\":\"2021-10-25T18:19:16.023912Z\",\"shell.execute_reply\":\"2021-10-25T19:12:14.009434Z\"},\"trusted\":true},\"execution_count\":20,\"outputs\":[]},{\"cell_type\":\"code\",\"source\":\"#for testing\\n# load_checkpoint(torch.load(LOAD_MODEL_FILE), model, optimizer)\\nfor x, y in train_loader:\\n x = x.to(DEVICE)\\n for idx in range(2):\\n bboxes_pred = cellboxes_to_boxes(model(x))\\n bboxes_pred = non_max_suppression(bboxes_pred[idx], iou_threshold=0.5, threshold=0.4, box_format=\\\"midpoint\\\")\\n plot_image(x[idx].permute(1,2,0).to(\\\"cpu\\\"), bboxes_pred)\",\"metadata\":{\"execution\":{\"iopub.status.busy\":\"2021-10-25T19:12:14.012418Z\",\"iopub.execute_input\":\"2021-10-25T19:12:14.017437Z\",\"iopub.status.idle\":\"2021-10-25T19:12:19.714810Z\",\"shell.execute_reply.started\":\"2021-10-25T19:12:14.017394Z\",\"shell.execute_reply\":\"2021-10-25T19:12:19.714029Z\"},\"trusted\":true},\"execution_count\":21,\"outputs\":[]},{\"cell_type\":\"code\",\"source\":\"\",\"metadata\":{},\"execution_count\":null,\"outputs\":[]}]}"
}
{
"filename": "code/artificial_intelligence/test/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/backtracking/src/algorithm_x/algorithm_x.cpp",
"content": "/*\n * algo-x.cpp\n * by Charles (@c650)\n *\n * This code is from my repo at https://github.com/c650/knuth-algorithm-x\n *\n * Path of Cosmos by OpenGenus Foundation\n */\n#include <iostream>\n\n#include <vector>\n\n#include <algorithm>\n#include <limits>\n\ntypedef std::vector<bool> matrix_row;\ntypedef std::vector<matrix_row> incidence_matrix;\n\n/* implementing Knuth's Algorithm X */\nbool\nalgo_x(const incidence_matrix& m, std::vector<bool> available_rows,\n std::vector<bool> available_cols, std::vector<size_t>& p_solution)\n{\n\n if (available_rows.empty())\n available_rows.resize(m.size(), true);\n if (available_cols.empty())\n available_cols.resize(m[0].size(), true);\n\n /* if the current matrix has no columns, the current partial\n * solution is a valid solution. terminate successfully */\n /* recursion terminating condition */\n if (available_cols.end() == std::find(available_cols.begin(), available_cols.end(), true))\n {\n /* we should do some printing or something. */\n std::cout << \"Solution: \\n\";\n for (const size_t& st : p_solution)\n std::cout << char(st + 'A') << \" \";\n std::cout << std::endl;\n\n return true;\n }\n\n /* debug */\n for (size_t i = 0; i < available_rows.size(); i++)\n if (available_rows[i])\n std::cout << \"\\t\" << char(i + 'A') << \" is still an available row.\\n\";\n for (size_t i = 0; i < available_cols.size(); i++)\n if (available_cols[i])\n std::cout << \"\\t\" << i + 1 << \" is still an available column.\\n\";\n\n /* Otherwise, deterministically choose a column. */\n size_t col_w_min_ones,\n min_ones,\n ones_in_col,\n num_col;\n num_col = m[0].size();\n min_ones = std::numeric_limits<size_t>::max();\n for (size_t i = 0; i < num_col; i++)\n {\n\n if (!available_cols[i])\n continue;\n\n ones_in_col = 0;\n for (size_t j = 0; j < m.size(); j++)\n {\n if (!available_rows[j])\n continue; /* skip over deleted rows */\n\n if (m[j][i])\n ones_in_col++;\n }\n if (ones_in_col < min_ones)\n {\n min_ones = ones_in_col;\n col_w_min_ones = i;\n }\n\n /* if we don't have a min >= 1, we won't get\n * an exact cover! :O */\n if (min_ones == 0)\n return false;\n }\n\n std::cout << \"\\tLeast ones in column: \" << col_w_min_ones + 1\n << \" with \" << min_ones << \" ones.\\n\";\n\n /* Choose the first row with a 1 in col_w_min_ones. */\n std::vector<bool> local_a_row, local_a_col;\n size_t curr_p_sol_len = p_solution.size();\n\n for (size_t i = 0; i < m.size(); i++)\n /* find the first row with the min number of ones*/\n if (m[i][col_w_min_ones] && available_rows[i])\n {\n\n std::cout << \"\\tPicking the row: \" << char(i + 'A') << std::endl;\n\n /* include the row in the partial solution. */\n p_solution.push_back( i );\n\n local_a_row = available_rows;\n local_a_col = available_cols;\n\n /* \"delete\" all cols in row with value of 1 */\n for (size_t j = 0; j < m[i].size(); j++)\n if (m[i][j])\n {\n\n local_a_col[j] = false;\n std::cout << \"\\tDeleting column: \" << j + 1 << std::endl;\n\n /* all rows with a 1 in col j must go. */\n for (size_t k = 0; k < m.size(); k++)\n {\n if (!available_rows[k])\n continue;\n\n if (m[k][j])\n {\n local_a_row[k] = false;\n std::cout << \"\\tDeleting row: \" << char(k + 'A') << std::endl;\n }\n }\n\n } /* end if */\n /* end for */\n\n /* call the function with the new subset in the partial solution\n * and the deleted rows and cols updated accordingly. */\n if (algo_x(m, local_a_row, local_a_col, p_solution) )\n return true;\n std::cout << char(i + 'A') << \" did not work!\\n\";\n p_solution.resize(curr_p_sol_len);\n\n } /* end if */\n /* end for */\n\n /* default to return FALSE */\n return false;\n}\n\nint main()\n{\n incidence_matrix m = {\n { 1, 0, 0, 1, 0, 0, 1 },\n { 1, 0, 0, 1, 0, 0, 0 },\n { 0, 0, 0, 1, 1, 0, 1 },\n { 0, 0, 1, 0, 1, 1, 0 },\n { 0, 1, 1, 0, 0, 1, 1 },\n { 0, 1, 0, 0, 0, 0, 1 }\n };\n\n std::vector<size_t> solution;\n algo_x(m, std::vector<bool>{}, std::vector<bool>{}, solution);\n return 0;\n}"
}
{
"filename": "code/backtracking/src/algorithm_x/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/backtracking/src/crossword_puzzle/crossword_puzzle.cpp",
"content": "#include<iostream>\nusing namespace std;\n\n\nint ways = 0;\n\n\nvoid printMatrix(vector<string>& matrix, int n)\n{\n\tfor (int i = 0; i < n; i++)\n\t\tcout << matrix[i] << endl;\n}\n\n\nvector<string> checkHorizontal(int x, int y,\n\t\t\t\t\t\t\tvector<string> matrix,\n\t\t\t\t\t\t\tstring currentWord)\n{\n\tint n = currentWord.length();\n\n\tfor (int i = 0; i < n; i++) {\n\t\tif (matrix[x][y + i] == '#' ||\n\t\t\tmatrix[x][y + i] == currentWord[i]) {\n\t\t\tmatrix[x][y + i] = currentWord[i];\n\t\t}\n\t\telse {\n\n\t\t\t\n\t\t\tmatrix[0][0] = '@';\n\t\t\treturn matrix;\n\t\t}\n\t}\n\n\treturn matrix;\n}\n\n\nvector<string> checkVertical(int x, int y,\n\t\t\t\t\t\t\tvector<string> matrix,\n\t\t\t\t\t\t\tstring currentWord)\n{\n\tint n = currentWord.length();\n\n\tfor (int i = 0; i < n; i++) {\n\t\tif (matrix[x + i][y] == '#' ||\n\t\t\tmatrix[x + i][y] == currentWord[i]) {\n\t\t\tmatrix[x + i][y] = currentWord[i];\n\t\t}\n\t\telse {\n\n\t\t\t\n\t\t\tmatrix[0][0] = '@';\n\t\t\treturn matrix;\n\t\t}\n\t}\n\treturn matrix;\n}\n\n\nvoid solvePuzzle(vector<string>& words,\n\t\t\t\tvector<string> matrix,\n\t\t\t\tint index, int n)\n{\n\tif (index < words.size()) {\n\t\tstring currentWord = words[index];\n\t\tint maxLen = n - currentWord.length();\n\n\t\tfor (int i = 0; i < n; i++) {\n\t\t\tfor (int j = 0; j <= maxLen; j++) {\n\t\t\t\tvector<string> temp = checkVertical(j, i,\n\t\t\t\t\t\t\t\t\t\tmatrix, currentWord);\n\n\t\t\t\tif (temp[0][0] != '@') {\n\t\t\t\t\tsolvePuzzle(words, temp, index + 1, n);\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\n\t\tfor (int i = 0; i < n; i++) {\n\t\t\tfor (int j = 0; j <= maxLen; j++) {\n\t\t\t\tvector<string> temp = checkHorizontal(i, j,\n\t\t\t\t\t\t\t\t\tmatrix, currentWord);\n\n\t\t\t\tif (temp[0][0] != '@') {\n\t\t\t\t\tsolvePuzzle(words, temp, index + 1, n);\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t}\n\telse {\n\t\n\t\tcout << (ways + 1) << \" way to solve the puzzle \"\n\t\t\t<< endl;\n\t\tprintMatrix(matrix, n);\n\t\tcout << endl;\n\n\t\tways++;\n\t\treturn;\n\t}\n}\n\nint main()\n{\n\tint n1 = 10;\n\n\tvector<string> matrix;\n\n\n\tmatrix.push_back(\"*#********\");\n\tmatrix.push_back(\"*#********\");\n\tmatrix.push_back(\"*#****#***\");\n\tmatrix.push_back(\"*##***##**\");\n\tmatrix.push_back(\"*#****#***\");\n\tmatrix.push_back(\"*#****#***\");\n\tmatrix.push_back(\"*#****#***\");\n\tmatrix.push_back(\"*#*######*\");\n\tmatrix.push_back(\"*#********\");\n\tmatrix.push_back(\"***#######\");\n\n\tvector<string> words;\n\n\n\twords.push_back(\"PUNJAB\");\n\twords.push_back(\"JHARKHAND\");\n\twords.push_back(\"MIZORAM\");\n\twords.push_back(\"MUMBAI\");\n\n\n\tways = 0;\n\n\t\n\tsolvePuzzle(words, matrix, 0, n1);\n\tcout << \"Number of ways to fill the grid is \"\n\t\t<< ways << endl;\n\n\treturn 0;\n}"
}
{
"filename": "code/backtracking/src/crossword_puzzle/crossword_puzzle.java",
"content": "import java.io.*;\nimport java.util.*;\n\npublic class CrosswordPuzzle {\n public static void display(String[][] arr){\n for(int i = 0; i < arr.length; i++){\n for(int j = 0; j < arr[i].length; j++){\n System.out.print(arr[i][j]);\n }\n System.out.println();\n }\n }\n\n public static void main(String[] args) {\n Scanner scn = new Scanner(System.in);\n\n String[][] grid = new String[10][10];\n for(int i = 0; i < 10; i++){\n grid[i] = scn.nextLine().split(\"\");\n }\n\n String[] words = scn.nextLine().split(\";\");\n\n for(int i = 0; i < words.length; i++){\n if(puzzleSolved(grid, words, words[i], 0, 0)){\n break;\n }\n }\n\n display(grid);\n }\n\n public static boolean puzzleSolved(String[][] grid, String[] words, String word, int row, int col){\n\n if(col >= grid.length){\n col = 0;\n row += 1;\n }\n\n while(row < grid.length && !grid[row][col].equals(\"-\")){\n col += 1;\n if(col >= grid.length){\n col = 0;\n row += 1;\n }\n }\n\n if(row == grid.length){\n return false;\n }\n\n boolean isPlacedHoz = false, isPlacedVer = false;\n while(row - 1 >= 0 && !grid[row - 1][col].equals(\"+\")){\n row -= 1;\n }\n\n isPlacedVer = placeVertical(grid, row, col, word);\n if(!isPlacedVer){\n while(col - 1 >= 0 && !grid[row][col - 1].equals(\"+\")){\n col -= 1;\n }\n\n isPlacedHoz = placeHorizontal(grid, row, col, word);\n }\n if( isPlacedVer || isPlacedHoz){\n String[] remainingWords = new String[words.length - 1];\n\n if(remainingWords.length == 0){\n return true;\n }\n\n int i = 0, j = 0;\n while(i < words.length){\n if(!words[i].equals(word)){\n remainingWords[j] = words[i];\n j++;\n }\n i++;\n }\n\n String pword = word;\n for(int k = 0; k < remainingWords.length; k++){\n word = remainingWords[k];\n if(puzzleSolved(grid, remainingWords, word, row, col + 1)){\n return true;\n }\n }\n if(isPlacedHoz){\n clearHorizontal(grid, row, col, pword);\n }\n if(isPlacedVer){\n clearVertical(grid, row, col, pword);\n }\n }\n return false;\n }\n\n public static boolean placeHorizontal(String[][] grid, int row, int col, String word){\n if(word.length() == 0 && (col == grid.length || grid[row][col].equals(\"+\"))){\n return true;\n }else if(col == grid.length || word.length() == 0){\n return false;\n }\n\n if(grid[row][col].equals(\"-\") || grid[row][col].equals(word.charAt(0)+\"\")){\n String prev = grid[row][col];\n grid[row][col] = word.charAt(0) + \"\";\n if(placeHorizontal(grid, row, col + 1, word.substring(1))){\n return true;\n }\n grid[row][col] = prev;\n }\n return false;\n }\n\n public static boolean placeVertical(String[][] grid, int row, int col,String word){\n if(word.length() == 0 && (row == grid.length || grid[row][col].equals(\"+\"))){\n return true;\n } else if(row == grid.length || word.length() == 0){\n return false;\n }\n if(grid[row][col].equals(\"-\") || grid[row][col].equals(word.charAt(0)+\"\")){\n String prev = grid[row][col];\n grid[row][col] = word.charAt(0) + \"\";\n if(placeVertical(grid, row + 1, col, word.substring(1))){\n return true;\n }\n grid[row][col] = prev;\n }\n return false;\n }\n\n public static void clearHorizontal(String[][] grid, int row, int col, String word){\n int pcol = col;\n while(pcol < grid.length && pcol < col + word.length()){\n grid[row][pcol] = \"-\";\n pcol++;\n }\n }\n\n public static void clearVertical(String[][] grid, int row, int col, String word){\n int prow = row;\n while(prow < grid.length && prow < row + word.length()){\n grid[prow][col] = \"-\";\n prow++;\n }\n }\n}"
}
{
"filename": "code/backtracking/src/crossword_puzzle/crossword_puzzle.js",
"content": "\nfunction printMatrix(matrix, n) {\nfor (let i = 0; i < n; i++) {\n\tconsole.log(matrix[i]);\n}\n}\n\n\nfunction checkHorizontal(x, y, matrix, currentWord) {\nconst n = currentWord.length;\n\nfor (let i = 0; i < n; i++) {\n\tif (matrix[x][y + i] === '#' || matrix[x][y + i] === currentWord[i]) {\n\tmatrix[x] = matrix[x].slice(0, y + i) + currentWord[i] + matrix[x].slice(y + i + 1);\n\t} else {\n\n\n\tmatrix[0] = \"@\";\n\treturn matrix;\n\t}\n}\nreturn matrix;\n}\n\n\n\nfunction checkVertical(x, y, matrix, currentWord) {\nconst n = currentWord.length;\n\nfor (let i = 0; i < n; i++) {\n\tif (matrix[x + i][y] === '#' || matrix[x + i][y] === currentWord[i]) {\n\tmatrix[x + i] = matrix[x + i].slice(0, y) + currentWord[i] + matrix[x + i].slice(y + 1);\n\t} else {\n\n\t\n\tmatrix[0] = \"@\";\n\treturn matrix;\n\t}\n}\nreturn matrix;\n}\n\n\n\nfunction solvePuzzle(words, matrix, index, n) {\nif (index < words.length) {\n\tconst currentWord = words[index];\n\tconst maxLen = n - currentWord.length;\n\n\tfor (let i = 0; i < n; i++) {\n\tfor (let j = 0; j <= maxLen; j++) {\n\t\tconst temp = checkVertical(j, i, [...matrix], currentWord);\n\t\tif (temp[0] !== \"@\") {\n\t\tsolvePuzzle(words, temp, index + 1, n);\n\t\t}\n\t}\n\t}\n\n\tfor (let i = 0; i < n; i++) {\n\tfor (let j = 0; j <= maxLen; j++) {\n\t\tconst temp = checkHorizontal(i, j, [...matrix], currentWord);\n\t\tif (temp[0] !== \"@\") {\n\t\tsolvePuzzle(words, temp, index + 1, n);\n\t\t}\n\t}\n\t}\n} else {\n\n\t\n\tconsole.log(`${ways + 1} way to solve the puzzle`);\n\tprintMatrix(matrix, n);\n\tconsole.log();\n\n\tways += 1;\n\treturn;\n}\n}\n\nconst n1 = 10;\n\nconst matrix = [];\n\n\nmatrix.push(\"*#********\");\nmatrix.push(\"*#********\");\nmatrix.push(\"*#****#***\");\nmatrix.push(\"*##***##**\");\nmatrix.push(\"*#****#***\");\nmatrix.push(\"*#****#***\");\nmatrix.push(\"*#****#***\");\nmatrix.push(\"*#*######*\");\nmatrix.push(\"*#********\");\nmatrix.push(\"***#######\");\n\n\nconst words = [];\nwords.push(\"PUNJAB\");\nwords.push(\"JHARKHAND\");\nwords.push(\"MIZORAM\");\nwords.push(\"MUMBAI\");\n\n\nways = 0;\n\t\n\n\nsolvePuzzle(words, matrix, 0, n1);\nconsole.log(\"Number of ways to fill the grid is \" + ways);"
}
{
"filename": "code/backtracking/src/generate_parentheses/generate_parentheses.cpp",
"content": "/*\nInput: n = 3\nOutput: [\"((()))\",\"(()())\",\"(())()\",\"()(())\",\"()()()\"] \n\n\nApproach:\nonly add open paraenthesis if open < n\nonly add close parenthesis if close < open\nstop- valid parenthesis if close == open == n\nTC/SC - O(4^n)\n\n\n*/\n#include<bits/stdc++.h>\nusing namespace std;\nvector<string> res;\n \nvoid backtrack(int open, int close, string s,int n)\n {\n if(open == n and close == n)\n {\n res.push_back(s);\n return;\n }\n \n if(open < n)\n {\n backtrack(open+1, close, s+'(', n);\n }\n if(close < open)\n {\n backtrack(open, close+1, s +')', n);\n }\n \n }\nvector<string> generateParenthesis(int n) \n{\n backtrack(0, 0, \"\", n);\n return res;\n}\n\nint main()\n{\n vector<string> res;\n int n = 3;\n res = generateParenthesis(n);\n for(auto x : res)\n cout << x << \" \";\n\n return 0;\n}"
}
{
"filename": "code/backtracking/src/knight_tour/knight_tour.c",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n#include <stdio.h>\n#include <stdlib.h>\n#include <string.h>\n#include <unistd.h>\n \ntypedef unsigned char cell;\nint dx[] = { -2, -2, -1, 1, 2, 2, 1, -1 };\nint dy[] = { -1, 1, 2, 2, 1, -1, -2, -2 };\n \nvoid init_board(int w, int h, cell **a, cell **b)\n{\n\tint i, j, k, x, y, p = w + 4, q = h + 4;\n\t/* b is board; a is board with 2 rows padded at each side */\n\ta[0] = (cell*)(a + q);\n\tb[0] = a[0] + 2;\n \n\tfor (i = 1; i < q; i++) {\n\t\ta[i] = a[i-1] + p;\n\t\tb[i] = a[i] + 2;\n\t}\n memset(a[0], 255, p * q);\n\tfor (i = 0; i < h; i++) {\n\t\tfor (j = 0; j < w; j++) {\n\t\t\tfor (k = 0; k < 8; k++) {\n\t\t\t\tx = j + dx[k], y = i + dy[k];\n\t\t\t\tif (b[i+2][j] == 255) b[i+2][j] = 0;\n\t\t\t\tb[i+2][j] += x >= 0 && x < w && y >= 0 && y < h;\n\t\t\t}\n\t\t}\n\t}\n}\n \n#define E \"\\033[\"\nint walk_board(int w, int h, int x, int y, cell **b)\n{\n\tint i, nx, ny, least;\n\tint steps = 0;\n\tprintf(E\"H\"E\"J\"E\"%d;%dH\"E\"32m[]\"E\"m\", y + 1, 1 + 2 * x);\n \n\twhile (1) {\n\t\tb[y][x] = 255;\n\n\t\tfor (i = 0; i < 8; i++)\n\t\t\tb[ y + dy[i] ][ x + dx[i] ]--;\n\t\tleast = 255;\n\t\tfor (i = 0; i < 8; i++) {\n\t\t\tif (b[ y + dy[i] ][ x + dx[i] ] < least) {\n\t\t\t\tnx = x + dx[i];\n\t\t\t\tny = y + dy[i];\n\t\t\t\tleast = b[ny][nx];\n\t\t\t}\n\t\t}\n if (least > 7) {\n\t\t\tprintf(E\"%dH\", h + 2);\n\t\t\treturn steps == w * h - 1;\n\t\t}\n \n\t\tif (steps++) printf(E\"%d;%dH[]\", y + 1, 1 + 2 * x);\n\t\tx = nx, y = ny;\n\t\tprintf(E\"%d;%dH\"E\"31m[]\"E\"m\", y + 1, 1 + 2 * x);\n\t\tfflush(stdout);\n\t\tusleep(120000);\n\t}\n}\n \nint solve(int w, int h)\n{\n\tint x = 0, y = 0;\n\tcell **a, **b;\n\ta = malloc((w + 4) * (h + 4) + sizeof(cell*) * (h + 4));\n\tb = malloc((h + 4) * sizeof(cell*));\n \n\twhile (1) {\n\t\tinit_board(w, h, a, b);\n\t\tif (walk_board(w, h, x, y, b + 2)) {\n\t\t\tprintf(\"Success!\\n\");\n\t\t\treturn 1;\n\t\t}\n\t\tif (++x >= w) x = 0, y++;\n\t\tif (y >= h) {\n\t\t\tprintf(\"Failed to find a solution\\n\");\n\t\t\treturn 0;\n\t\t}\n\t\tprintf(\"Any key to try next start position\");\n\t\tgetchar();\n\t}\n}\n \nint main(int c, char **v)\n{\n\tint w, h;\n\tif (c < 2 || (w = atoi(v[1])) <= 0) w = 8;\n\tif (c < 3 || (h = atoi(v[2])) <= 0) h = w;\n\tsolve(w, h);\n \n\treturn 0;\n} "
}
{
"filename": "code/backtracking/src/knight_tour/knight_tour.cpp",
"content": "// Part of Cosmos by OpenGenus Foundation\n// C program for Knight Tour problem\n#include <stdio.h>\n#define N 8\n\nint solveKTUtil(int x, int y, int movei, int sol[N][N],\n int xMove[], int yMove[]);\n\n/* A utility function to check if i,j are valid indexes\n * for N*N chessboard */\nbool isSafe(int x, int y, int sol[N][N])\n{\n return x >= 0 && x < N && y >= 0 &&\n y < N && sol[x][y] == -1;\n}\n\n/* A utility function to print solution matrix sol[N][N] */\nvoid printSolution(int sol[N][N])\n{\n for (int x = 0; x < N; x++)\n {\n for (int y = 0; y < N; y++)\n printf(\" %2d \", sol[x][y]);\n printf(\"\\n\");\n }\n}\n\n/* This function solves the Knight Tour problem using\n * Backtracking. This function mainly uses solveKTUtil()\n * to solve the problem. It returns false if no complete\n * tour is possible, otherwise return true and prints the\n * tour.\n * Please note that there may be more than one solutions,\n * this function prints one of the feasible solutions. */\nbool solveKT()\n{\n int sol[N][N];\n\n /* Initialization of solution matrix */\n for (int x = 0; x < N; x++)\n for (int y = 0; y < N; y++)\n sol[x][y] = -1;\n\n /* xMove[] and yMove[] define next move of Knight.\n * xMove[] is for next value of x coordinate\n * yMove[] is for next value of y coordinate */\n int xMove[8] = { 2, 1, -1, -2, -2, -1, 1, 2 };\n int yMove[8] = { 1, 2, 2, 1, -1, -2, -2, -1 };\n\n // Since the Knight is initially at the first block\n sol[0][0] = 0;\n\n /* Start from 0,0 and explore all tours using\n * solveKTUtil() */\n if (solveKTUtil(0, 0, 1, sol, xMove, yMove) == false)\n {\n printf(\"Solution does not exist\");\n return false;\n }\n else\n printSolution(sol);\n\n return true;\n}\n\n/* A recursive utility function to solve Knight Tour\n * problem */\nint solveKTUtil(int x, int y, int movei, int sol[N][N],\n int xMove[N], int yMove[N])\n{\n int k, next_x, next_y;\n if (movei == N * N)\n return true;\n\n /* Try all next moves from the current coordinate x, y */\n for (k = 0; k < 8; k++)\n {\n next_x = x + xMove[k];\n next_y = y + yMove[k];\n if (isSafe(next_x, next_y, sol))\n {\n sol[next_x][next_y] = movei;\n if (solveKTUtil(next_x, next_y, movei + 1, sol,\n xMove, yMove) == true)\n return true;\n else\n sol[next_x][next_y] = -1; // backtracking\n }\n }\n return false;\n}\n\n/* Driver program to test above functions */\nint main()\n{\n solveKT();\n return 0;\n}"
}
{
"filename": "code/backtracking/src/knight_tour/knight_tour.go",
"content": "// Part of Cosmos by OpenGenus Foundation\npackage main\n\nimport \"fmt\"\n\n/* Expect Result\n\n 0 59 38 33 30 17 8 63\n37 34 31 60 9 62 29 16\n58 1 36 39 32 27 18 7\n35 48 41 26 61 10 15 28\n42 57 2 49 40 23 6 19\n47 50 45 54 25 20 11 14\n56 43 52 3 22 13 24 5\n51 46 55 44 53 4 21 12\n*/\ntype Move struct {\n\tX int\n\tY int\n}\n\nvar boardSize int\n\nfunc isSafe(nextX, nextY int) bool {\n\treturn nextX >= 0 && nextX < boardSize && nextY >= 0 && nextY < boardSize\n}\n\nfunc solveKT(board [][]int, currentStep, currentX, currentY int, moves []Move) bool {\n\tif currentStep == boardSize*boardSize {\n\t\treturn true\n\t}\n\n\tfor _, v := range moves {\n\t\tnextX := currentX + v.X\n\t\tnextY := currentY + v.Y\n\t\tif isSafe(nextX, nextY) && board[nextX][nextY] == -1 {\n\t\t\tboard[nextX][nextY] = currentStep\n\t\t\tif solveKT(board, currentStep+1, nextX, nextY, moves) {\n\t\t\t\treturn true\n\t\t\t} else {\n\n\t\t\t\tboard[nextX][nextY] = -1\n\t\t\t}\n\n\t\t}\n\t}\n\treturn false\n}\n\nfunc main() {\n\tboardSize = 8\n\tboard := make([][]int, boardSize)\n\tfor i := range board {\n\t\tboard[i] = make([]int, boardSize)\n\t\tfor j := range board[i] {\n\t\t\tboard[i][j] = -1\n\t\t}\n\t}\n\n\t//Init how knight can walk\n\tmoves := []Move{\n\t\t{X: 2, Y: 1},\n\t\t{X: 1, Y: 2},\n\t\t{X: -1, Y: 2},\n\t\t{X: -2, Y: 1},\n\t\t{X: -2, Y: -1},\n\t\t{X: -1, Y: -2},\n\t\t{X: 1, Y: -2},\n\t\t{X: 2, Y: -1},\n\t}\n\n\t//Knight start from (0,0)\n\tboard[0][0] = 0\n\tif !solveKT(board, 1, 0, 0, moves) {\n\t\tfmt.Println(\"solutions don't exist\")\n\t} else {\n\t\tfor _, row := range board {\n\t\t\tfor _, v := range row {\n\t\t\t\tfmt.Printf(\"%4d\", v)\n\t\t\t}\n\t\t\tfmt.Printf(\"\\n\")\n\t\t}\n\t}\n}"
}
{
"filename": "code/backtracking/src/knight_tour/knight_tour.java",
"content": "// Part of Cosmos by OpenGenus Foundation\nimport java.util.*;\n \npublic class KnightsTour {\n private final static int base = 12;\n private final static int[][] moves = {{1,-2},{2,-1},{2,1},{1,2},{-1,2},\n {-2,1},{-2,-1},{-1,-2}};\n private static int[][] grid;\n private static int total;\n \n public static void main(String[] args) {\n grid = new int[base][base];\n total = (base - 4) * (base - 4);\n \n for (int r = 0; r < base; r++)\n for (int c = 0; c < base; c++)\n if (r < 2 || r > base - 3 || c < 2 || c > base - 3)\n grid[r][c] = -1;\n \n int row = 2 + (int) (Math.random() * (base - 4));\n int col = 2 + (int) (Math.random() * (base - 4));\n \n grid[row][col] = 1;\n \n if (solve(row, col, 2))\n printResult();\n else System.out.println(\"no result\");\n \n }\n \n private static boolean solve(int r, int c, int count) {\n if (count > total)\n return true;\n \n List<int[]> nbrs = neighbors(r, c);\n \n if (nbrs.isEmpty() && count != total)\n return false;\n \n Collections.sort(nbrs, new Comparator<int[]>() {\n public int compare(int[] a, int[] b) {\n return a[2] - b[2];\n }\n });\n \n for (int[] nb : nbrs) {\n r = nb[0];\n c = nb[1];\n grid[r][c] = count;\n if (!orphanDetected(count, r, c) && solve(r, c, count + 1))\n return true;\n grid[r][c] = 0;\n }\n \n return false;\n }\n \n private static List<int[]> neighbors(int r, int c) {\n List<int[]> nbrs = new ArrayList<>();\n \n for (int[] m : moves) {\n int x = m[0];\n int y = m[1];\n if (grid[r + y][c + x] == 0) {\n int num = countNeighbors(r + y, c + x);\n nbrs.add(new int[]{r + y, c + x, num});\n }\n }\n return nbrs;\n }\n \n private static int countNeighbors(int r, int c) {\n int num = 0;\n for (int[] m : moves)\n if (grid[r + m[1]][c + m[0]] == 0)\n num++;\n return num;\n }\n \n private static boolean orphanDetected(int cnt, int r, int c) {\n if (cnt < total - 1) {\n List<int[]> nbrs = neighbors(r, c);\n for (int[] nb : nbrs)\n if (countNeighbors(nb[0], nb[1]) == 0)\n return true;\n }\n return false;\n }\n \n private static void printResult() {\n for (int[] row : grid) {\n for (int i : row) {\n if (i == -1) \n continue;\n System.out.printf(\"%2d \", i);\n }\n System.out.println();\n }\n }\n}"
}
{
"filename": "code/backtracking/src/knight_tour/knight_tour.py",
"content": "import copy\n\n# Part of Cosmos by OpenGenus Foundation\nboardsize = 6\n_kmoves = ((2, 1), (1, 2), (-1, 2), (-2, 1), (-2, -1), (-1, -2), (1, -2), (2, -1))\n\n\ndef chess2index(chess, boardsize=boardsize):\n \"Convert Algebraic chess notation to internal index format\"\n chess = chess.strip().lower()\n x = ord(chess[0]) - ord(\"a\")\n y = boardsize - int(chess[1:])\n return (x, y)\n\n\ndef boardstring(board, boardsize=boardsize):\n r = range(boardsize)\n lines = \"\"\n for y in r:\n lines += \"\\n\" + \",\".join(\n \"%2i\" % board[(x, y)] if board[(x, y)] else \" \" for x in r\n )\n return lines\n\n\ndef knightmoves(board, P, boardsize=boardsize):\n Px, Py = P\n kmoves = set((Px + x, Py + y) for x, y in _kmoves)\n kmoves = set(\n (x, y)\n for x, y in kmoves\n if 0 <= x < boardsize and 0 <= y < boardsize and not board[(x, y)]\n )\n return kmoves\n\n\ndef accessibility(board, P, boardsize=boardsize):\n access = []\n brd = copy.deepcopy(board)\n for pos in knightmoves(board, P, boardsize=boardsize):\n brd[pos] = -1\n access.append((len(knightmoves(brd, pos, boardsize=boardsize)), pos))\n brd[pos] = 0\n return access\n\n\ndef knights_tour(start, boardsize=boardsize, _debug=False):\n board = {(x, y): 0 for x in range(boardsize) for y in range(boardsize)}\n move = 1\n P = chess2index(start, boardsize)\n board[P] = move\n move += 1\n if _debug:\n print(boardstring(board, boardsize=boardsize))\n while move <= len(board):\n P = min(accessibility(board, P, boardsize))[1]\n board[P] = move\n move += 1\n if _debug:\n print(boardstring(board, boardsize=boardsize))\n input(\"\\n%2i next: \" % move)\n return board\n\n\nif __name__ == \"__main__\":\n while 1:\n boardsize = int(input(\"\\nboardsize: \"))\n if boardsize < 5:\n continue\n start = input(\"Start position: \")\n board = knights_tour(start, boardsize)\n print(boardstring(board, boardsize=boardsize))"
}
{
"filename": "code/backtracking/src/knight_tour/knight_tour.rs",
"content": "extern crate nalgebra as na;\nextern crate num;\n\nuse na::DMatrix;\nuse na::Scalar;\nuse na::dimension::Dynamic;\nuse std::fmt::Display;\nuse num::FromPrimitive;\n\nconst N: usize = 5; //map size N x N\ntrait ChessFunc {\n fn isSafe(&self, x: isize, y: isize) -> bool;\n fn solveKTUtil(\n &mut self,\n x: isize,\n y: isize,\n movei: usize,\n moves: &Vec<(isize, isize)>,\n ) -> bool;\n fn printSolution(&self);\n fn solveKT(&mut self);\n}\nimpl<S> ChessFunc for DMatrix<S>\nwhere\n S: Scalar + Display + FromPrimitive,\n{\n fn solveKT(&mut self) {\n let moves = [\n (2, 1),\n (1, 2),\n (-1, 2),\n (-2, 1),\n (-2, -1),\n (-1, -2),\n (1, -2),\n (2, -1),\n ].to_vec(); //knight movement\n\n if self.solveKTUtil(0, 0, 1, &moves) {\n self.printSolution();\n } else {\n println!(\"Solution does not exist\");\n }\n }\n fn solveKTUtil(\n &mut self,\n x: isize,\n y: isize,\n movei: usize,\n moves: &Vec<(isize, isize)>,\n ) -> bool {\n if movei == N * N {\n true\n } else {\n let mut result = false;\n for cnt in 0..moves.len() {\n let next_x: isize = x + moves[cnt].0;\n let next_y: isize = y + moves[cnt].1;\n\n if self.isSafe(next_x, next_y) {\n unsafe {\n *self.get_unchecked_mut(next_x as usize, next_y as usize) =\n FromPrimitive::from_usize(movei).unwrap();\n }\n if self.solveKTUtil(next_x, next_y, movei + 1, moves) {\n result = true;\n break;\n } else {\n unsafe {\n *self.get_unchecked_mut(next_x as usize, next_y as usize) =\n FromPrimitive::from_isize(-1).unwrap();\n }\n }\n }\n }\n result\n }\n }\n fn printSolution(&self) {\n for (idx, item) in self.iter().enumerate() {\n print!(\"{position:>3}\", position = item);\n if idx % N == (N - 1) {\n println!();\n }\n }\n }\n fn isSafe(&self, x: isize, y: isize) -> bool {\n let mut result = false;\n //println!(\"({},{})\", x, y);\n match ((x + 1) as usize, (y + 1) as usize) {\n (1...N, 1...N) => {\n match self.iter().nth((y * N as isize + x) as usize) {\n Some(sc) => {\n let temp: S = FromPrimitive::from_isize(-1).unwrap();\n if temp == *sc {\n result = true;\n }\n }\n None => {}\n };\n }\n _ => {}\n }\n //println!(\"({})\", result);\n result\n }\n}\n\nfn main() {\n let DimN = Dynamic::new(N); //from nalgebra make dynamic dimension in here make N dimension\n let mut chessmap = DMatrix::from_fn_generic(DimN, DimN, |r, c| match (r, c) {\n (0, 0) => 0,\n _ => -1,\n }); //initialize matrix (0,0) set 0 and the others set -1\n //chessmap.printSolution();\n chessmap.solveKT(); //solve problum\n}"
}
{
"filename": "code/backtracking/src/knight_tour/knight_tour_withoutbt.c",
"content": "/*\n** Reimua\n** Part of Cosmos by OpenGenus Foundation\n*/\n\n#include <stdio.h>\n#include <string.h>\n#include <stdlib.h>\n#include <unistd.h>\n\nstatic const int move_nbr = 8;\nstatic const int move_x[] = {2, 2, 1, 1, -2, -2, -1, -1};\nstatic const int move_y[] = {1, -1, 2, -2, 1, -1, 2, -2};\n\n/*\n** Check if a case is safe for our dear knight.\n** 1 : The position is in the map.\n** 2 : We didn't use this position.\n*/\n\nint is_safe(char **map, int x, int y, int pos_x, int pos_y)\n{\n\tif (pos_x < 0 || pos_x >= x || pos_y < 0 || pos_y >= y)\n\t\treturn (0);\n\tif (map[pos_y][pos_x] != '0')\n\t\treturn (0);\n\treturn (1);\n}\n\n/*\n** Order the moveset so we prioritize the case with the less possibility.\n*/\n\nvoid order_moveset(int *move_set, int *combination)\n{\n\tint i = 0;\n\tint k = 0;\n\tint j = 0;\n\n\twhile (i < move_nbr)\n\t{\n\t\twhile (j < move_nbr)\n\t\t{\n\t\t\tif (combination[j] == k)\n\t\t\t{\n\t\t\t\tmove_set[i++] = j;\n\t\t\t\tcombination[j] = -1;\n\t\t\t}\n\t\t\tj++;\n\t\t}\n\t\tj = 0;\n\t\tk++;\n\t}\n}\n\n/*\n** Generate an effective move_set instead of blindly use backtracking.\n*/\n\nint *generate_moveset(char **map, int sol[][2], int x, int y, int depth)\n{\n\tint i = 0;\n\tint j = 0;\n\tint combination[move_nbr];\n\tint *move_set = malloc(sizeof(int) * move_nbr);\n\n\tif (!move_set)\n\t\treturn (NULL); \n\tmemset(combination, '\\0', 8 * sizeof(int));\n\twhile (i < move_nbr)\n\t{\n\t\tint pos_x = sol[depth][0] + move_x[i];\n\t\tint pos_y =\tsol[depth][1] + move_y[i];\n\n\t\tif (is_safe(map, x, y, pos_x, pos_y))\n\t\t{\n\t\t\twhile (j < move_nbr)\n\t\t\t{\n\t\t\t\tint new_pos_x = pos_x + move_x[j];\n\t\t\t\tint new_pos_y = pos_y + move_y[j];\n\n\t\t\t\tif (is_safe(map, x, y, new_pos_x, new_pos_y))\n\t\t\t\t\tcombination[i]++;\n\t\t\t\tj++;\n\t\t\t}\n\t\t}\n\t\tj = 0;\n\t\ti++;\n\t}\n\torder_moveset(move_set, combination);\n\treturn (move_set);\n}\n\n/*\n** Solve the knight tour recursively.\n** \n** The first time i did it, it took a stupid amount of time\n** compared to the other on the repositories.\n** \n** It was due to the order of the movement in my \"move_x\" and \"move_y\" arrays.\n** Simply by changing it, I managed to complete a knight tour in less that 1s.\n** \n** So, in order to not be \"order\" dependant, I select my moveset by using the Warnsdorf's rule.\n** But now I'm not even sure if backtracking is needed. --(apparently it's not)\n*/\n\nint kt_solve(char **map, int sol[][2], int x, int y, int depth)\n{\n\tif (depth == x * y - 1)\n\t\treturn (1);\n\n\tint i = 0;\n\tint *move_set = generate_moveset(map, sol, x, y, depth);\n\tmap[sol[depth][1]][sol[depth][0]] = 'x';\n\n\tif (!move_set)\n\t\treturn (0);\n\twhile (i < move_nbr)\n\t{\n\t\tint pos_x = sol[depth][0] + move_x[move_set[i]];\n\t\tint pos_y =\tsol[depth][1] + move_y[move_set[i]];\n\t\t\n\t\tif (is_safe(map, x, y, pos_x, pos_y))\n\t\t{\n\n\t\t\tsol[depth + 1][0] = pos_x;\n\t\t\tsol[depth + 1][1] = pos_y;\n\t\t\tif (kt_solve(map, sol, x, y, depth + 1))\n\t\t\t{\n\t\t\t\tfree(move_set);\n\t\t\t\treturn (1);\n\t\t\t}\n\t\t\telse\n\t\t\t{\n\t\t\t\tfree(move_set);\n\t\t\t\treturn (0);\n\t\t\t}\n\t\t}\n\t\ti++;\n\t}\n\tfree(move_set);\n\tmap[sol[depth][1]][sol[depth][0]] = '0';\n\treturn (0);\n}\n\n/*\n** Display the solution step by step.\n*/\n\nint print_solution(char **map, int sol[][2], int x, int y)\n{\n\tint i = 0;\n\tint j = 0;\n\t\n\twhile (i < y)\n\t\tmemset(map[i++], '.', x);\n\twhile (j < x * y)\n\t{\n\t\ti = 0;\n\t\tmap[sol[j][1]][sol[j][0]] = 'K';\n\t\tprintf(\"\\n\");\n\t\twhile (i < y)\n\t\t\tprintf(\"%s\\n\", map[i++]);\n\t\tusleep(500000);\n\t\tmap[sol[j][1]][sol[j][0]] = 'x';\n\t\tj++;\n\t}\n\treturn (1);\n}\n\n/*\n** Set up the knight tour.\n*/\n\nint knight_tour(int x, int y, int start_x, int start_y)\n{\n\tint i = 0;\n\tint sol[y * x][2];\n\tchar **map = malloc(sizeof(char*) * y);\n\n\tif (!map)\n\t\treturn (1);\n\twhile (i < y)\n\t{\n\t\tmap[i] = malloc(sizeof(char) * (x + 1));\n\t\tmemset(map[i], '0', x);\n\t\tmap[i++][x] = '\\0';\t\t\n\t}\n\tsol[0][0] = start_x,\n\tsol[0][1] = start_y;\n\tmap[sol[0][1]][sol[0][0]] = 'K';\n\tif (kt_solve(map, sol, x, y, 0))\n\t\tprint_solution(map, sol, x, y);\n\telse\n\t\tprintf(\"%s\\n\", \"The knight tour is not possible.\\n\");\n\ti = 0;\n\twhile (i < y)\n\t\tfree(map[i++]);\n\tfree(map);\n\treturn (1);\n}\n\n/*\n** Check size and start params.\n**\n*/\n\nint check_input(int x, int y, int start_x, int start_y)\n{\n\tif (start_x >= x || start_x < 0 || start_y >= y || start_y < 0)\n\t{\n\t\tprintf(\"Starting position is out of range.\\n\");\n\t\treturn (1);\n\t}\n\tif (x > 20 || y > 20) // no one like stack overflox.\n\t{\n\t\tprintf(\"Size can't go above 20\");\n\t\treturn (1);\n\t}\n\treturn (0);\n}\t\n\n/*\n** Rmq: If i take a long time, it's probably because a knight tour is not possible.\n*/\n\nint main(int argc, char **argv)\n{\n\tint size_x;\n\tint size_y;\n\tint start_x;\n\tint start_y;\n\n\tif (argc != 5)\n\t{\n\t\tprintf(\"Usage: %s : [size_x] [size_y] [pos_x] [pos_y]\\n\\n\", argv[0]);\n\t\tprintf(\"size_x & size_y : Size of the map.\\n\");\n\t\tprintf(\"pos_x & pos_y : Knight starting position\\n\");\n\t\treturn (1);\n\t}\n\tsize_x = atoi(argv[1]);\n\tsize_y = atoi(argv[2]);\n\tstart_x = atoi(argv[3]);\n\tstart_y = atoi(argv[4]);\n\tif (check_input(size_x, size_y, start_x, start_y))\n\t\treturn (1);\n\tknight_tour(size_x, size_y, start_x, start_y);\n\treturn (0);\n}"
}
{
"filename": "code/backtracking/src/knight_tour/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/backtracking/src/kth_grammar/kth-Grammar.cpp",
"content": "//https://leetcode.com/problems/k-th-symbol-in-grammar/submissions/\n\nclass Solution {\npublic:\n int kthGrammar(int n, int k) \n {\n if(n==1)\n {\n return 0;\n }\n \n int mid=(1<<(n-1))/2;\n \n if(k<=mid) \n {\n return kthGrammar(n-1,k);\n }\n \n return !kthGrammar(n-1,k-mid);\n }\n};"
}
{
"filename": "code/backtracking/src/m_coloring_problem/m_coloring_problem.py",
"content": "# Given m colors and a graph tell whether it is possible to assign colors\n# to all the nodes such that no 2 connected nodes have the same color\n\n\ndef isSafe(graph, m, v): # For checking whether this node can be colored with m or not\n for i in range(len(graph[v])):\n if graph[v][i] == 1 and col[i] == m:\n return False\n return True\n\n\ndef solve(graph, m, v):\n if v >= 4:\n return True\n else:\n for i in range(m):\n if isSafe(graph, i, v):\n col[v] = i\n if solve(graph, m, v + 1):\n return True\n col[v] = -1\n return False\n\n\n# Can consider any graph\ngraph = [[0, 1, 1, 1], [1, 0, 1, 0], [1, 1, 0, 1], [1, 0, 1, 0]]\n\n# initialise color with -1\ncol = [-1 for i in range(4)]\n\n# Number of different colors allowed\nm = int(input(\"Enter number of different colors\"))\n\nif solve(graph, m, 0):\n print(\"Solution Exists: Following are the assigned colors\")\n for i in col:\n print(i),\n print()\nelse:\n print(\"No Solution Exists\")"
}
{
"filename": "code/backtracking/src/Min_Max/alphabetaprune.cpp",
"content": "#include <iostream>\n#include <algorithm>\n#include <cmath>\n#include <climits>\n#define SIZE(arr) (sizeof(arr) / sizeof(arr[0]))\nusing namespace std;\nint getHeight(int n) {\n return (n == 1) ? 0 : 1 + log2(n / 2);\n}\nint minmax(int height, int depth, int nodeIndex,\nbool maxPayer, int values[], int alpha, int beta) {\n if (depth == height) {\n return values[nodeIndex];\n }\n if (maxPayer) {\n int bestValue = INT_MIN;\n for (int i = 0; i < height - 1; i++) {\n int val = minmax(height, depth + 1, nodeIndex * 2 + i, false, values, alpha, beta);\n bestValue = max(bestValue, val);\n alpha = max(alpha, bestValue);\n if (beta <= alpha)\n break;\n }\n return bestValue;\n } else {\n int bestValue = INT_MAX;\n for (int i = 0; i < height - 1; i++) {\n int val = minmax(height, depth + 1, nodeIndex * 2 + i, true, values, alpha, beta);\n bestValue = min(bestValue, val);\n beta = min(beta, bestValue);\n if (beta <= alpha)\n break;\n }\n return bestValue;\n }\n}\nint main() {\n int values[] = {13, 8, 24, -5, 23, 15, -14, -20};\n int height = getHeight(SIZE(values));\n int result = minmax(height, 0, 0, true, values, INT_MIN, INT_MAX);\n cout <<\"Result : \" << result << \"\\n\";\n return 0;\n}"
}
{
"filename": "code/backtracking/src/Min_Max/aphabetapruning.java",
"content": "\n// Part of cosmos repository\n// Implementing alpha beta prruning in java\nimport java.io.*;\nimport java.util.*;\nclass AlphaBetaPruningJava {\n\nstatic int MAXIMUM = 1000;\nstatic int MINIMUM = -1000;\n\nstatic int minimaxAlphaBeta(int values[],\n int depth, //Minimax function\n int node,\n\t\t\t\tBoolean maxPlayer,\n\t\t\t\tint alpha,\n\t\t\t\tint beta)\n{\n //We know the depth here , hence a constant value. Otherwise it can be found out.(logn)\n\tif (depth == 3)\n\t\treturn values[node];\n\n\tif (maxPlayer==true)\n\t{\n\t\tint ans = MINIMUM;\n\t\tfor (int i = 0; i < 2; i++)\n\t\t{\n\t\t\tint currValue = minimaxAlphaBeta(values,depth + 1, node * 2 + i,\n\t\t\t\t\t\t\tfalse, alpha, beta);\n\t\t\tans = Math.max(ans, currValue);\n\t\t\talpha = Math.max(alpha, ans);\n\n\t\t\t// Alpha Beta Pruning\n\t\t\tif (beta <= alpha)\n\t\t\t\tbreak;\n\t\t}\n\t\treturn ans;\n\t}\n\telse\n\t{\n\t\tint ans = MAXIMUM;\n\t\tfor (int i = 0; i < 2; i++)\n\t\t{\n\t\t\t\n\t\t\tint currValue = minimaxAlphaBeta(values,depth + 1, node * 2 + i,true, alpha, beta);\n\t\t\tans = Math.min(ans, currValue);\n\t\t\tbeta = Math.min(beta, ans);\n\n\t\t\tif (beta <= alpha)\n\t\t\t\tbreak;\n\t\t}\n\t\treturn ans;\n\t}\n}\n\tpublic static void main (String[] args)\n\t{\n\t\tint values[] = {13, 8, 24, -5, 23, 15, -14, -20};\n\t\tSystem.out.println(minimaxAlphaBeta(values,0,0, true, MINIMUM, MAXIMUM));\n\t}\n}"
}
{
"filename": "code/backtracking/src/Min_Max/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/backtracking/src/n_queen/n_queen_backtracking.cpp",
"content": "#include <iostream>\n// Part of Cosmos by OpenGenus Foundation\nusing namespace std;\n\nbool isSafe(char board[][100], int row, int column, int n)\n{\n\n //check that whole row should not have a queen\n for (int i = 0; i < n; i++)\n if (board[row][i] == 'Q')\n return false;\n\n //check that whole column should not have a queen\n for (int i = 0; i < n; i++)\n if (board[i][column] == 'Q')\n return false;\n\n //check that left diagonal to the current cell should not have queen\n int p = row, q = column;\n while (p >= 0 && q >= 0)\n {\n if (board[p][q] == 'Q')\n return false;\n p--; q--;\n }\n\n //check that right diagonal to the current cell should not have queen\n p = row; q = column;\n while (p >= 0 && q <= n - 1)\n {\n if (board[p][q] == 'Q')\n return false;\n p--; q++;\n }\n\n return true;\n}\n\nbool nqueen(char board[][100], int n, int i = 0)\n{\n if (i == n)\n {\n //board is filled with the n-queens, just print the board\n for (int i = 0; i < n; i++)\n {\n for (int j = 0; j < n; j++)\n cout << board[i][j] << \" \";\n cout << endl;\n }\n return true; //hack :: if to print all possible combination just make it false\n }\n\n for (int j = 0; j < n; j++)\n if (isSafe(board, i, j, n)) //check that cell is safe or not\n {\n board[i][j] = 'Q'; //if it's safe, then place a queen at that cell\n if (nqueen(board, n, i + 1)) //now searching place for queen in next row\n return true;\n board[i][j] = '.'; //since, not safe, so again mark that cell with '.'\n }\n return false;\n}\n\nint main()\n{\n int n;\n cin >> n;\n\n char board[100][100];\n for (int i = 0; i < n; i++)\n for (int j = 0; j < n; j++)\n board[i][j] = '.';\n\n if (!nqueen(board, n))\n cout << \"No state possible!\";\n\n return 0;\n}"
}
{
"filename": "code/backtracking/src/n_queen/n_queen_backtracking.rs",
"content": "extern crate nalgebra as na;\nextern crate num;\n#[macro_use]\nextern crate lazy_static;\n\nuse na::DMatrix;\nuse na::Scalar;\nuse na::dimension::Dynamic;\nuse num::FromPrimitive;\nuse std::sync::{Arc, Mutex};\nuse std::io;\nuse std::fmt::Display;\n\nconst BK: isize = 0;\nconst Q: isize = 1;\n\ntrait Nqueen {\n fn isSafe(&self, x: isize, y: isize) -> bool;\n fn solveNqueenRec(&mut self, i: isize) -> bool;\n fn printSolution(&self);\n fn solveNqueen(&mut self);\n fn between(&self, pos: isize) -> bool {\n pos >= 0 && pos < *N.lock().unwrap() as isize\n }\n}\nimpl<S> Nqueen for DMatrix<S>\nwhere\n S: Scalar + Display + FromPrimitive,\n{\n fn isSafe(&self, x: isize, y: isize) -> bool {\n let mut result = true;\n let n = *N.lock().unwrap();\n if self.between(x) && self.between(y) {\n result = self.iter()\n .enumerate()\n .filter(|ci| {\n let temp: S = FromPrimitive::from_isize(Q).unwrap();\n *ci.1 == temp\n })\n .filter(|cw| {\n let diffx = x - (cw.0 % n) as isize;\n let diffy = y - (cw.0 / n) as isize;\n match (diffx, diffy) {\n (0, 0) => false,\n (0, _) => true,\n (_, 0) => true,\n (t_x, t_y) => {\n if t_x == t_y || t_x == -t_y {\n true\n } else {\n false\n }\n }\n }\n })\n .count() == 0;\n }\n result\n }\n fn solveNqueen(&mut self) {\n if self.solveNqueenRec(0) {\n self.printSolution();\n } else {\n println!(\"Solution does not exist\");\n }\n }\n fn solveNqueenRec(&mut self, i: isize) -> bool {\n let n = *N.lock().unwrap() as isize;\n if i == n {\n true\n } else {\n let mut result = false;\n for j in 0..n {\n if self.isSafe(i, j) {\n unsafe {\n *self.get_unchecked_mut(i as usize, j as usize) =\n FromPrimitive::from_isize(Q).unwrap();\n }\n if self.solveNqueenRec(i + 1) {\n result = true;\n break;\n }\n unsafe {\n *self.get_unchecked_mut(i as usize, j as usize) =\n FromPrimitive::from_isize(BK).unwrap();\n }\n }\n }\n result\n }\n }\n fn printSolution(&self) {\n let n = *N.lock().unwrap();\n for (idx, item) in self.iter().enumerate() {\n print!(\"{position:>1}\", position = item);\n if idx % n == (n - 1) {\n println!();\n }\n }\n }\n}\n\nlazy_static! {\n static ref N: Arc<Mutex<usize>> = Arc::new(Mutex::new(0));\n}\nfn main() {\n println!(\"Please Enter the size of the Map\");\n let mut input_str = String::new();\n io::stdin().read_line(&mut input_str).unwrap();\n if let Ok(t) = input_str.trim().parse::<usize>() {\n *N.lock().unwrap() = t;\n let DimN = Dynamic::new(t);\n let mut map = DMatrix::from_element_generic(DimN, DimN, BK);\n map.solveNqueen();\n }\n}"
}
{
"filename": "code/backtracking/src/n_queen/n_queen_bit.go",
"content": "// Part of Cosmos by OpenGenus Foundation\npackage main\n\nimport \"fmt\"\n\n/*\nExpected output\n\nTotal solutions 73712 for bordsize 13\n*/\n\nvar totalSol int\nvar limit uint\nvar boardSize uint\n\nfunc nQueen(boardSize, position, left, right, depth uint) {\n\tif boardSize == depth {\n\t\ttotalSol++\n\t\treturn\n\t}\n\n\tvar newPos uint\n\tcurrentPos := position | left | right\n\n\tfor currentPos < limit {\n\t\tnewPos = (currentPos + 1) & ^currentPos\n\t\tnQueen(boardSize, position|newPos, limit&((left|newPos)<<1), limit&((right|newPos)>>1), depth+1)\n\t\tcurrentPos = currentPos | newPos\n\n\t}\n\treturn\n}\n\nfunc main() {\n\tboardSize = 13\n\n\tlimit = (1 << (boardSize)) - 1\n\ttotalSol = 0\n\tnQueen(boardSize, 0, 0, 0, 0)\n\tfmt.Printf(\"Total solutions %d for bordsize %d\\n\", totalSol, boardSize)\n}"
}
{
"filename": "code/backtracking/src/n_queen/n_queen_bitimp.cpp",
"content": "#include <iostream>\n// Part of Cosmos by OpenGenus Foundation\nusing namespace std;\nint limit;\nint N;\nint counter;\nvoid Backtracking(int position, int left, int right, int depth)\n{\n if (depth == N)\n {\n counter++;\n return;\n }\n int currentPos = position | left | right;\n int newPos;\n while (currentPos < limit)\n {\n newPos = (currentPos + 1) & ~currentPos;\n Backtracking( position | newPos, limit & ((left | newPos) << 1),\n limit & ((right | newPos) >> 1), depth + 1);\n currentPos = currentPos | newPos;\n }\n}\n\nint main()\n{\n cout << \"Please Enter the size of board\" << endl;\n cin >> N;\n counter = 0;\n limit = (1 << (N)) - 1;\n Backtracking(0, 0, 0, 0);\n cout << \"There are \" << counter << \" ways\" << endl;\n}"
}
{
"filename": "code/backtracking/src/n_queen/nqueen_bitmask.c",
"content": "#include <stdio.h>\n\nint ans = 0;\nvoid solve(int rowMask, int ld, int rd, int done) {\n if (rowMask == done) {\n ++ans;\n return;\n }\n int safe = done & (~(rowMask | ld | rd));\n while (safe) {\n int p = safe & (-safe);\n safe -= p;\n solve(rowMask | p, (ld | p) << 1, (rd | p) >> 1, done);\n }\n}\n\nint main() {\n int n,done;\n scanf(\"%d\", &n);\n done = (1 << n) - 1;\n solve(0, 0, 0, done);\n printf(\"%d\", ans);\n}"
}
{
"filename": "code/backtracking/src/n_queen/n_queen_bitset.cpp",
"content": "///GIVES TOTAL NUMBER OF POSSIBLE WAYS TO SOLVE N QUEEN PROBLEM USING BITSET\n// Part of Cosmos by OpenGenus Foundation\n#include <iostream>\n#include <bitset>\nusing namespace std;\n\nbitset<30> column, diag1, diag2;\n\nvoid solveNQueen(int r, int n, int &ans)\n{\n if (r == n)\n {\n ans++;\n return;\n }\n for (int c = 0; c < n; c++)\n if (!column[c] && !diag1[r - c + n - 1] && !diag2[r + c])\n {\n column[c] = diag1[r - c + n - 1] = diag2[r + c] = 1;\n solveNQueen(r + 1, n, ans);\n //backtrack\n column[c] = diag1[r - c + n - 1] = diag2[r + c] = 0;\n }\n\n}\n\nint main()\n{\n int n;\n cout << \"Enter N: \";\n cin >> n;\n int ans = 0;\n solveNQueen(0, n, ans);\n cout << endl << ans << endl;\n\n return 0;\n}"
}
{
"filename": "code/backtracking/src/n_queen/n_queen.c",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include<stdio.h>\n#include<stdlib.h>\n#define SIZE 20\n\nstruct stack\n{\n int top;\n int arr[SIZE];\n} s;\n\nint \nis_empty()\n{\n if(s.top==-1)\n return (1);\n return (0);\n}\n\nvoid \npush(int v)\n{\n if(s.top == SIZE - 1)\n {\n printf(\"stack overflow\\n\");\n return;\n }\n else\n {\n s.top+=1;\n s.arr[s.top]=v;\n }\n}\n\nint \npop()\n{\n if(is_empty())\n {\n printf(\"stack underflow\\n\");\n return (-1);\n }\n else\n {\n int q = s.arr[s.top];\n s.top -= 1;\n return (q);\n }\n}\n\nint \nsafe(int r, int c)\n{\n\tint sum = r+c;\n\tint diff = r-c;\n\n\tfor(int i=s.top;i>=0;i--)\n\t{\n\t\tif(sum == i + s.arr[i] || diff == i - s.arr[i] || r == i \n\t\t|| c == s.arr[i])\n\t\t{\n\t\t\treturn (0);\n\t\t}\n\t}\n\n\treturn (1);\n}\n\nvoid \ndisplay(int n)\n{\n\tint *a[n];\n\tfor(int i=0; i<n; i++)\n\t{\n a[i] = (int *)calloc(n,sizeof(int));\n\t}\n\n\tfor(int i=s.top;i>=0;i--)\n\t{\n\t\ta[i][s.arr[i]] = 1;\n\t}\n\n\tfor(int i=0;i<n;i++)\n\t{\n\t\tfor(int j=0;j<n;j++)\n\t\t{\n\t\t\tif(a[i][j])\n\t\t\t\tprintf(\"Q\\t\");\n\t\t\telse\n\t\t\t\tprintf(\"_\\t\");\n\t\t}\n\t\tprintf(\"\\n\");\n\t}\n\n\tprintf(\"\\n\\n\");\n}\n\nvoid \nsol()\n{\n int currRow = 0, currCol = 0;\n int flag = 0, n, counter = 0;\n scanf(\"%d\",&n);\n while(1)\n {\n \tfor(int i = currCol; i < n; i++)\n \t{\n \t\tif(safe(currRow, i))\n \t\t{\n\t\t\t\tcurrCol = i;\n\t\t\t\tflag=1;\n\t\t\t\tbreak;\n \t\t}\n \t}\n\n \tif(flag)\n \t{\n\t\t \tpush(currCol);\n\t\t \tcurrRow += 1;\n\t\t \tcurrCol = 0;\n \t}\n \telse\n \t{\n \t\tif(is_empty())\n \t\t{\n \t\t if(!counter)\n printf(\"No soln\\n\");\n\t\t \tbreak;\n \t\t}\n \t\telse\n\t\t\t{\n\t\t \tcurrRow -= 1;\n \t\t currCol = pop() + 1;\n\t\t\t}\n \t}\n\n\n \tif(s.top == n-1)\n \t{\n \t\tprintf(\"Solution No. : %d\\n\", ++counter);\n \t\tdisplay(n);\n \t\tcurrCol++;\n \t}\n \tflag = 0;\n }\n}\n\n\nint main()\n{\n\ts.top = -1;\n\tsol();\n\treturn (0);\n}"
}
{
"filename": "code/backtracking/src/n_queen/n_queen.go",
"content": "// Part of Cosmos by OpenGenus Foundation\npackage main\n\nimport \"fmt\"\n\n/*\nExpected output\n\nSolutions 1\n-O--\n---O\nO---\n--O-\nSolutions 2\n--O-\nO---\n---O\n-O--\n\n*/\n\nvar totalSol int\n\nfunc abs(number int) int {\n\tif number < 0 {\n\t\treturn number * -1\n\t}\n\treturn number\n}\n\n//Check could we put a queen on specific row,col\nfunc checkPlace(board []int, row, col int) bool {\n\tfor i := 0; i < row; i++ {\n\t\t//check same col\n\t\tif board[i] == col {\n\t\t\treturn false\n\t\t}\n\t\t//check diag, if the different count between rows is same as the different count between col\n\t\tif abs(board[i]-col) == abs(i-row) {\n\t\t\treturn false\n\t\t}\n\t}\n\n\treturn true\n\n}\n\nfunc nQueen(board []int, currentRow int) {\n\tif currentRow == len(board) {\n\t\ttotalSol++\n\t\tfmt.Printf(\"Solutions %d\\n\", totalSol)\n\t\tfor i := 0; i < len(board); i++ {\n\t\t\tfor j := 0; j < len(board); j++ {\n\t\t\t\tif board[i] == j {\n\t\t\t\t\tfmt.Printf(\"O\")\n\t\t\t\t} else {\n\t\t\t\t\tfmt.Printf(\"-\")\n\t\t\t\t}\n\t\t\t}\n\t\t\tfmt.Printf(\"\\n\")\n\t\t}\n\t\treturn\n\t}\n\n\tfor i := 0; i < len(board); i++ {\n\t\tif checkPlace(board, currentRow, i) {\n\t\t\tboard[currentRow] = i\n\t\t\tnQueen(board, currentRow+1)\n\t\t}\n\t}\n\n}\n\nfunc main() {\n\tboardSize := 4\n\tboard := make([]int, boardSize)\n\n\ttotalSol = 0\n\tnQueen(board, 0)\n}"
}
{
"filename": "code/backtracking/src/n_queen/n_queen.hs",
"content": "import Data.Maybe (listToMaybe)\n\ntype Board = [Int]\n\nnQueens :: Int -> Maybe Board\nnQueens = listToMaybe . nQueens'\n\nnQueens' :: Int -> [Board]\nnQueens' n = go n []\n where\n go 0 bs = [bs]\n go k bs = concatMap (go (k - 1)) [ x : bs | x <- [1..n], placeable x bs]\n\n placeable n xs = null [ i | (i,x) <- zip [1..] xs, n == x || abs (n - x) == i]"
}
{
"filename": "code/backtracking/src/n_queen/n_queen.java",
"content": "import java.util.Scanner;\n/*\n *\tPart of Cosmos by OpenGenus Foundation\n*/\n class NQueen {\n\n\tpublic static int[][] array;\n\t\n\tpublic static boolean solveNQueen(int queens, int i){\n\t\tif(i == queens){\n\t\t\treturn true;\n\t\t}\n\t\t\n\t\tfor (int j =0; j <queens; j++) {\n\t\t\tif(isSafe(i, j, queens)) {\n\t\t\t\tarray[i][j] = 1;\n\t\t\t\tif(solveNQueen(queens, i+1))\t\t\t\t\n\t\t\t\t\treturn true;\n\t\t\t\tarray[i][j] = -1;\n\t\t\t}\n\t\t}\n\t\treturn false;\n\t}\n\t\n\tpublic static boolean isSafe(int row, int column, int n){\n\t\tfor(int i = 0; i < n; i++) {\n\t\t\tif(array[row][i] == 1)\n\t\t\t\treturn false;\n\t\t}\n\t \n\t\tfor(int i = 0; i < n; i++) {\n\t\t\tif(array[i][column] == 1)\n\t\t\t\treturn false;\n\t\t}\n\t \n\t\tint copyRow = row, copyColumn = column;\n\t\twhile(copyRow >= 0 && copyColumn >= 0) {\n\t\t\tif(array[copyRow][copyColumn] == 1)\n\t\t\t\treturn false;\n\t\t\tcopyRow--; \n\t\t\tcopyColumn--;\n\t\t}\n\t \n\t\tcopyRow = row; copyColumn = column;\n\t\twhile(copyRow >= 0 && copyColumn <= n-1) {\n\t\t\tif(array[copyRow][copyColumn] == 1)\n\t\t\t\treturn false;\n\t\t\tcopyRow--; \n\t\t\tcopyColumn++;\n\t\t}\n\t \n\t\treturn true;\n\t}\n\n\tpublic static void initField(int queens) {\n\t\tarray = new int[queens][queens];\n\t\tfor (int i=0;i < queens ; i++) {\n\t\t\tfor (int j = 0;j < queens ; j++)\n\t\t\t\tarray[i][j] = 0;\n\t\t}\n\t}\n\n\tpublic static void main(String[] args) {\n\t\tScanner sc = new Scanner(System.in);\n\t\tSystem.out.print(\"Type in the number of queens: \");\n\t\tint queens = sc.nextInt();\n\t\tinitField(queens);\n\t\tif(!solveNQueen(queens, 0))\n\t\t\tSystem.out.println(\"No combination possible!\");\n\t\telse\n\t\t\tfor (int k = 0; k < queens ;k++ ){\n\t\t\t\tfor (int j = 0; j < queens ;j++ )\n\t\t\t\t\tSystem.out.print(array[k][j] > 0 ? \"Q \" : \". \");\n\t\t\t\tSystem.out.println();\n\t\t\t}\n\t}\n}"
}
{
"filename": "code/backtracking/src/n_queen/n_queen.py",
"content": "# Part of Cosmos by OpenGenus Foundation\ndef place(k, i):\n for l in range(1, k):\n if (x[l] == i) or (abs(x[l] - i) == abs(l - k)):\n return False\n return True\n\n\ndef nqueens(k):\n global x, n, flag\n\n for j in range(1, n + 1): # iterate over all columns\n if place(k, j):\n x[k] = j\n if k == n:\n print(x[1 : n + 1])\n flag = 1\n else:\n nqueens(k + 1)\n\n\ndef main():\n global n, x, flag\n flag = 0\n n = input(\"Enter n for n-queen problem solution:\")\n x = [i for i in range(n + 1)]\n for m in range(1, n + 1):\n print(\"\\nFirst queen is placed in column:\", m, \"\\n\")\n x[1] = m\n nqueens(2)\n if flag == 0:\n print(\"No solution exists for queen placed in column:\", m)\n print(\"\\n\")\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/backtracking/src/n_queen/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/backtracking/src/number_of_ways_in_maze/number_of_ways_in_maze.c",
"content": "#include<stdio.h>\n#define MAX_SIZE 10\n/*\n * Part of Cosmos by OpenGenus Foundation\n*/\n\n///i,j = current cell, m,n = destination\nvoid solveMaze(char maze[][10],int sol[][10],int i,int j,int m,int n,int *ways){\n ///Base Case\n if(i==m && j==n){\n sol[m][n] = 1;\n (*ways)++;\n ///Print the soln\n for(int i=0;i<=m;i++){\n for(int j=0;j<=n;j++){\n printf(\"%d \", sol[i][j]);\n }\n printf(\"\\n\");\n }\n printf(\"\\n\");\n return ;\n }\n\n if (sol[i][j]) {\n return;\n }\n ///Rec Case\n ///Assume there is a way from this cell\n sol[i][j] = 1;\n\n /// Try going Right\n if(j+1<=n && maze[i][j+1]!='X'){\n solveMaze(maze,sol,i,j+1,m,n,ways);\n }\n /// Try going Left\n if(j-1>=0 && maze[i][j-1]!='X'){\n solveMaze(maze,sol,i,j-1,m,n,ways);\n }\n /// Try going Up\n if(i-1>=0 && maze[i-1][j]!='X'){\n solveMaze(maze,sol,i-1,j,m,n,ways);\n }\n /// Try going Down\n if(i+1<=m && maze[i+1][j]!='X'){\n solveMaze(maze,sol,i+1,j,m,n,ways);\n }\n\n ///Backtracking!\n sol[i][j] =0;\n return;\n}\n\n\nint main(){\n\n char maze[MAX_SIZE][MAX_SIZE] = {\n \"00XXX\",\n \"00000\",\n \"0XX00\",\n \"000X0\",\n \"0X000\"\n };\n\n int m = 4,n=4;\n\n int sol[MAX_SIZE][MAX_SIZE];\n\n int i,j;\n for (i = 0; i < MAX_SIZE; i++) {\n for (j = 0; j <MAX_SIZE; j++) {\n sol[i][j]=0;\n }\n }\n\n int ways=0;\n solveMaze(maze,sol,0,0,m,n,&ways);\n if(ways){\n printf(\"Total ways %d\\n\", ways);\n }\n else{\n printf(\"No ways\\n\");\n }\nreturn 0;\n}"
}
{
"filename": "code/backtracking/src/number_of_ways_in_maze/number_of_ways_in_maze.cpp",
"content": "#include <iostream>\nusing namespace std;\n\n\n///i,j = current cell, m,n = destination\nbool solveMaze(char maze[][10], int sol[][10], int i, int j, int m, int n, int &ways)\n{\n ///Base Case\n if (i == m && j == n)\n {\n sol[m][n] = 1;\n ways++;\n ///Print the soln\n for (int i = 0; i <= m; i++)\n {\n for (int j = 0; j <= n; j++)\n cout << sol[i][j] << \" \";\n cout << endl;\n\n }\n cout << endl;\n\n return true;\n }\n\n ///Rec Case\n ///Assume jis cell pe khada hai vaha se rasta hai\n sol[i][j] = 1;\n\n /// Right mein X nahi hai\n if (j + 1 <= n && maze[i][j + 1] != 'X')\n {\n\n bool rightSeRastaHai = solveMaze(maze, sol, i, j + 1, m, n, ways);\n if (rightSeRastaHai)\n {\n // return true;\n }\n }\n /// Down jake dekho agr rasta nahi mila right se\n if (i + 1 <= m && maze[i + 1][j] != 'X')\n {\n\n bool downSeRastaHai = solveMaze(maze, sol, i + 1, j, m, n, ways);\n if (downSeRastaHai)\n {\n //return true;\n }\n }\n\n\n ///Agr code is line mein aagya ! - Backtracking\n sol[i][j] = 0;\n return false;\n}\n\n\nint main()\n{\n\n char maze[10][10] = {\n \"00XXX\",\n \"00000\",\n \"0XX00\",\n \"000X0\",\n \"0X000\"\n };\n\n int m = 4, n = 4;\n\n int sol[10][10] = {0};\n int ways = 0;\n solveMaze(maze, sol, 0, 0, m, n, ways);\n if (ways != 0)\n cout << \"Total ways \" << ways << endl;\n else\n cout << \"Koi rasta nahi hai \" << endl;\n\n\n\n return 0;\n}"
}
{
"filename": "code/backtracking/src/number_of_ways_in_maze/number_of_ways_in_maze.go",
"content": "// Part of Cosmos by OpenGenus Foundation\npackage main\n\nimport \"fmt\"\n\ntype Point struct {\n\tX int\n\tY int\n}\n\nvar totalWays int\n\nfunc SolveMaze(maze [][]byte, sol [][]byte, current, end Point, limitH, limitW int) {\n\tif maze[current.X][current.Y] == '1' {\n\t\treturn\n\t}\n\n\tif sol[current.X][current.Y] == '1' {\n\t\treturn\n\t}\n\n\tsol[current.X][current.Y] = '1'\n\tif current == end {\n\t\ttotalWays++\n\t\tfor _, v := range sol {\n\t\t\tfor _, k := range v {\n\t\t\t\tfmt.Printf(\"%c \", k)\n\t\t\t}\n\t\t\tfmt.Print(\"\\n\")\n\t\t}\n\t\tfmt.Print(\"\\n\")\n\t}\n\n\tif current.X+1 < limitH {\n\t\tcurrent.X += 1\n\t\tSolveMaze(maze, sol, current, end, limitH, limitW)\n\t\tcurrent.X -= 1\n\t}\n\n\tif current.X-1 >= 0 {\n\t\tcurrent.X -= 1\n\t\tSolveMaze(maze, sol, current, end, limitH, limitW)\n\t\tcurrent.X += 1\n\t}\n\n\tif current.Y+1 < limitW {\n\t\tcurrent.Y += 1\n\t\tSolveMaze(maze, sol, current, end, limitH, limitW)\n\t\tcurrent.Y -= 1\n\t}\n\n\tif current.Y-1 >= 0 {\n\t\tcurrent.Y -= 1\n\t\tSolveMaze(maze, sol, current, end, limitH, limitW)\n\t\tcurrent.Y += 1\n\t}\n\n\tsol[current.X][current.Y] = '0'\n\treturn\n}\n\nfunc main() {\n\tmaze := [][]byte{\n\t\t{'0', '0', '1', '1', '1'},\n\t\t{'0', '0', '0', '0', '0'},\n\t\t{'0', '1', '1', '0', '0'},\n\t\t{'0', '0', '0', '1', '0'},\n\t\t{'0', '1', '0', '0', '0'},\n\t}\n\n\tsolution := [][]byte{\n\t\t{'0', '0', '0', '0', '0'},\n\t\t{'0', '0', '0', '0', '0'},\n\t\t{'0', '0', '0', '0', '0'},\n\t\t{'0', '0', '0', '0', '0'},\n\t\t{'0', '0', '0', '0', '0'},\n\t}\n\n\ttotalWays = 0\n\tstart := Point{X: 0, Y: 0}\n\tend := Point{X: 4, Y: 4}\n\tSolveMaze(maze, solution, start, end, 5, 5)\n\tfmt.Printf(\"There are %d ways to escape from (%d:%d) to (%d:%d)\\n\", totalWays, start.X, start.Y, end.X, end.Y)\n}"
}
{
"filename": "code/backtracking/src/number_of_ways_in_maze/number_of_ways_in_maze.java",
"content": "\n/*\n * Part of Cosmos by OpenGenus Foundation\n*/\npublic class RatMaze\n{\n final int N = 4;\n \n /* A function to print solution matrix\n sol[N][N] */\n void printSolution(int sol[][])\n {\n for (int i = 0; i < N; i++)\n {\n for (int j = 0; j < N; j++)\n System.out.print(\" \" + sol[i][j] +\n \" \");\n System.out.println();\n }\n }\n \n /* A function to check if x,y is valid\n index for N*N maze */\n boolean isSafe(int maze[][], int x, int y)\n {\n // if (x,y outside maze) return false\n return (x >= 0 && x < N && y >= 0 &&\n y < N && maze[x][y] == 1);\n }\n \n boolean solveMaze(int maze[][])\n {\n int sol[][] = {{0, 0, 0, 0},\n {0, 0, 0, 0},\n {0, 0, 0, 0},\n {0, 0, 0, 0}\n };\n \n if (solveMazeUtil(maze, 0, 0, sol) == false)\n {\n System.out.print(\"Solution doesn't exist\");\n return false;\n }\n \n printSolution(sol);\n return true;\n }\n \n boolean solveMazeUtil(int maze[][], int x, int y,\n int sol[][])\n {\n // if (x,y is goal) return true\n if (x == N - 1 && y == N - 1)\n {\n sol[x][y] = 1;\n return true;\n }\n \n // Check if maze[x][y] is valid\n if (isSafe(maze, x, y) == true)\n {\n // mark x,y as part of solution path\n sol[x][y] = 1;\n \n /* Move forward in x direction */\n if (solveMazeUtil(maze, x + 1, y, sol))\n return true;\n \n /* If moving in x direction doesn't give\n solution then Move down in y direction */\n if (solveMazeUtil(maze, x, y + 1, sol))\n return true;\n \n /* If none of the above movements work then\n BACKTRACK: unmark x,y as part of solution\n path */\n sol[x][y] = 0;\n return false;\n }\n \n return false;\n }\n \n public static void main(String args[])\n {\n RatMaze rat = new RatMaze();\n int maze[][] = {{1, 0, 0, 0},\n {1, 1, 0, 1},\n {0, 1, 0, 0},\n {1, 1, 1, 1}\n };\n rat.solveMaze(maze);\n }\n}"
}
{
"filename": "code/backtracking/src/number_of_ways_in_maze/number_of_ways_in_maze.rs",
"content": "extern crate nalgebra as na;\nextern crate num;\n\n\nuse na::DMatrix;\nuse na::Scalar;\nuse na::dimension::Dynamic;\nuse num::FromPrimitive;\nuse std::fmt::Display;\n\ntrait MazeSolve {\n fn solveMaze(&mut self, end: (usize, usize));\n fn solveMazeRec(&mut self, pos: (usize, usize), end: (usize, usize), ways: &mut usize);\n fn printSolution(&self, end: (usize, usize));\n}\n\nconst BK: usize = 0;\nconst BLOCK: usize = 1;\nconst PASSED: usize = 2;\n\nstatic START: (usize, usize) = (0, 0);\n\nimpl<S> MazeSolve for DMatrix<S>\nwhere\n S: Scalar + Display + FromPrimitive,\n{\n fn solveMaze(&mut self, end: (usize, usize)) {\n let mut ways: usize = 0;\n\n self.solveMazeRec(START, end, &mut ways);\n if ways != 0 {\n println!(\"Total ways: {}\", ways);\n } else {\n println!(\"No way\");\n }\n }\n fn solveMazeRec(&mut self, pos: (usize, usize), end: (usize, usize), ways: &mut usize) {\n unsafe {\n *self.get_unchecked_mut(pos.0, pos.1) = FromPrimitive::from_usize(PASSED).unwrap();\n }\n let check_move = [(0, 1), (1, 0)].to_vec();\n if pos == end {\n *ways += 1;\n println!(\"Solution {}\", *ways);\n self.printSolution(end);\n } else {\n for (m_x, m_y) in check_move {\n let after_mov = (pos.0 + m_x, pos.1 + m_y);\n if after_mov.0 <= end.0 && after_mov.1 <= end.1 {\n let self_at: S = FromPrimitive::from_usize(BLOCK).unwrap();\n if self.iter()\n .enumerate()\n .filter(|i| {\n i.0 == after_mov.0 + after_mov.1 * (end.0 + 1) && *i.1 == self_at\n })\n .count() == 0\n {\n self.solveMazeRec(after_mov, end, ways);\n }\n }\n }\n }\n unsafe {\n *self.get_unchecked_mut(pos.0, pos.1) = FromPrimitive::from_usize(BK).unwrap();\n }\n }\n fn printSolution(&self, end: (usize, usize)) {\n for (idx, item) in self.iter().enumerate() {\n let bk: S = FromPrimitive::from_usize(BK).unwrap();\n let block: S = FromPrimitive::from_usize(BLOCK).unwrap();\n let passed: S = FromPrimitive::from_usize(PASSED).unwrap();\n let charector = if *item == bk {\n \"O\"\n } else if *item == block {\n \"X\"\n } else if *item == passed {\n \"*\"\n } else {\n \"O\"\n };\n print!(\"{position:>1}\", position = charector);\n if idx % (end.0 + 1) == end.0 {\n println!();\n }\n }\n println!();\n }\n}\n\nfn main() {\n let map_sample = [\n [0, 0, 1, 1, 1],\n [0, 0, 0, 0, 0],\n [0, 1, 1, 0, 0],\n [0, 0, 0, 1, 0],\n [0, 1, 0, 0, 0],\n ];\n let mut map =\n DMatrix::from_fn_generic(Dynamic::new(5), Dynamic::new(5), |r, c| map_sample[c][r]);\n println!(\"Print Map\");\n map.printSolution((4, 4));\n map.solveMaze((4, 4));\n}"
}
{
"filename": "code/backtracking/src/number_of_ways_in_maze/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/backtracking/src/partitions_of_number/partitions_of_number.cpp",
"content": "/// Part of Cosmos by OpenGenus Foundation\n#include <stdio.h>\n#include <vector>\nvoid backtrack(int level);\nvoid print_sol(int length);\nstd::vector<int> solution;\nint N, S = 0;\nint solsFound = 0;\nint main()\n{\n scanf(\"%d\", &N);\n solution.reserve(N + 1);\n solution[0] = 1;\n backtrack(1);\n return 0;\n}\nvoid backtrack(int level)\n{\n if (S == N)\n print_sol(level - 1);\n else\n for (int i = solution[level - 1]; i <= N - S; i++)\n {\n solution[level] = i;\n S += i;\n backtrack(level + 1);\n S -= i;\n }\n}\nvoid print_sol(int length)\n{\n printf(\"SOLUTION %d\\n\", ++solsFound);\n printf(\"%d=\", N);\n for (int i = 1; i <= length - 1; i++)\n printf(\"%d+\", solution[i]);\n printf(\"%d\\n\", solution[length]);\n}"
}
{
"filename": "code/backtracking/src/partitions_of_number/partitions_of_number.go",
"content": "/// Part of Cosmos by OpenGenus Foundation\npackage main\n\nimport \"fmt\"\n\nvar totalSol int\n\nfunc partitions(input int, sol []int, start int) {\n\tif input == 0 {\n\t\tfmt.Println(sol)\n\t\ttotalSol++\n\t\treturn\n\t}\n\n\tfor i := start; i <= input; i++ {\n\t\tsol = append(sol, i)\n\t\tpartitions(input-i, sol, i)\n\t\tsol = sol[:len(sol)-1]\n\n\t}\n}\n\nfunc main() {\n\tinput := 10\n\tsol := []int{}\n\ttotalSol = 0\n\tpartitions(input, sol, 1)\n\tfmt.Printf(\"There are %d ways to combine %d\\n\", totalSol, input)\n}"
}
{
"filename": "code/backtracking/src/partitions_of_number/partitions_of_number.rs",
"content": "#[macro_use]\nextern crate text_io;\n\nfn main() {\n let n: usize = read!();\n let mut s: usize = 0;\n let mut solution: Vec<usize> = Vec::new();\n solution.push(1);\n for _ in 0..n {\n solution.push(0);\n }\n backtrack(&mut solution, n, &mut s, 1);\n}\nfn backtrack(vec: &mut Vec<usize>, n: usize, s: &mut usize, level: usize) {\n //println!(\"{:?}\", vec);\n if n == *s {\n printsol(vec, n, level - 1);\n } else {\n let mut i = vec[level - 1];\n while i <= n - *s {\n if let Some(elem) = vec.get_mut(level) {\n *elem = i;\n }\n *s += i;\n backtrack(vec, n, s, level + 1);\n *s -= i;\n i += 1;\n }\n }\n}\nfn printsol(map: &Vec<usize>, num: usize, level: usize) {\n println!(\"Solution\");\n print!(\"{} = \", num);\n for idx in 1..level + 1 {\n if idx == 1 {\n print!(\"{:?}\", map[idx]);\n } else {\n print!(\" + {:?}\", map[idx]);\n }\n }\n println!();\n}"
}
{
"filename": "code/backtracking/src/partitions_of_number/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/backtracking/src/partitions_of_set/partitions_of_set.cpp",
"content": "/// Part of Cosmos by OpenGenus Foundation\n#include <stdio.h>\n#include <vector>\nvoid backtrack(int level);\nvoid print_sol();\nstd::vector<int> v;\nstd::vector<int> solution;\nint maxfromSol = 0;\nint N;\nint solsFound = 0;\nint main()\n{\n scanf(\"%d\", &N);\n v.reserve(N + 1);\n solution.reserve(N + 1);\n for (int i = 1; i <= N; i++)\n scanf(\"%d\", &v[i]);\n backtrack(1);\n return 0;\n}\nvoid backtrack(int level)\n{\n if (level == N + 1)\n print_sol();\n else\n {\n for (int i = 1; i <= maxfromSol; i++)\n {\n solution[level] = i;\n backtrack(level + 1);\n }\n solution[level] = maxfromSol + 1;\n maxfromSol++;\n backtrack(level + 1);\n maxfromSol--;\n }\n}\nvoid print_sol()\n{\n printf(\"SOLUTION %d\\n\", ++solsFound);\n for (int i = 1; i <= maxfromSol; i++)\n {\n printf(\"Partition %d:\", i);\n for (int j = 1; j <= N; j++)\n if (solution[j] == i)\n printf(\"%d \", v[j]);\n\n printf(\"\\n\");\n }\n}"
}
{
"filename": "code/backtracking/src/partitions_of_set/partitions_of_set.go",
"content": "/// Part of Cosmos by OpenGenus Foundation\npackage main\n\nimport \"fmt\"\n\nvar totalSol int\n\n/*\nexpected output:\nsolution 1\n[1 2 3]\nsolution 2\n[1 2]\n[3]\nsolution 3\n[1 3]\n[2]\nsolution 4\n[1]\n[2 3]\nsolution 5\n[1]\n[2]\n[3]\n*/\nfunc setPartition(data []int, indexArr []int, index, currentLevel int) {\n\tif index == len(data) {\n\t\ttotalSol++\n\t\tfmt.Printf(\"solution %d\\n\", totalSol)\n\t\tfor level := 0; level <= currentLevel; level++ {\n\t\t\tans := []int{}\n\t\t\tfor i, v := range indexArr {\n\t\t\t\tif v == level {\n\t\t\t\t\tans = append(ans, data[i])\n\t\t\t\t}\n\t\t\t}\n\t\t\tfmt.Println(ans)\n\t\t}\n\t\treturn\n\t}\n\n\t//Try eveny level within currentLevel for current index\n\tfor i := 0; i <= currentLevel; i++ {\n\t\tindexArr[index] = i\n\t\tsetPartition(data, indexArr, index+1, currentLevel)\n\t}\n\n\t//Move to next level\n\tindexArr[index] = currentLevel + 1\n\tcurrentLevel++\n\tsetPartition(data, indexArr, index+1, currentLevel)\n\tcurrentLevel--\n}\n\nfunc main() {\n\tdata := []int{1, 2, 3}\n\n\t//Use index array to indicate which partition the element belong to\n\tindex := make([]int, len(data))\n\n\t//Since {1},{2,3} is equal to {2,3},{1},\n\t//We can always put first element in first partition and generate the partition of the rest of elements.\n\tindex[0] = 0\n\ttotalSol = 0\n\tsetPartition(data, index, 1, 0)\n}"
}
{
"filename": "code/backtracking/src/partitions_of_set/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/backtracking/src/permutations_of_string/permutations_of_string.c",
"content": "#include <stdio.h>\n#include <string.h>\n/* Function to swap values at two pointers */\n// Part of Cosmos by OpenGenus Foundation\nvoid swap(char *x, char *y)\n{\n char temp;\n temp = *x;\n *x = *y;\n *y = temp;\n}\n\n/* Function to print permutations of string\n This function takes three parameters:\n 1. String\n 2. Starting index of the string\n 3. Ending index of the string. */\nvoid permute(char *a, int l, int r)\n{\n int i;\n if (l == r) {\n printf(\"%s\\n\", a);\n }\n else\n {\n for (i = l; i <= r; i++)\n {\n swap((a+l), (a+i));\n permute(a, l+1, r);\n swap((a+l), (a+i)); //backtrack\n }\n }\n}\n\n/* Driver program to test above functions */\nint main() {\n char str[] = \"ABC\";\n int n = strlen(str);\n permute(str, 0, n - 1);\n return 0;\n}\n/*OUTPUT\nABC\nACB\nBAC\nBCA\nCBA\nCAB*/"
}
{
"filename": "code/backtracking/src/permutations_of_string/permutations_of_string.go",
"content": "// Part of Cosmos by OpenGenus Foundation\npackage main\n\nimport \"fmt\"\n\nfunc permutation(data []byte, start, end int) {\n\tif start == end {\n\t\tfmt.Println(string(data))\n\t} else {\n\t\tfor i := start; i <= end; i++ {\n\t\t\tdata[start], data[i] = data[i], data[start]\n\t\t\tpermutation(data, start+1, end)\n\t\t\tdata[start], data[i] = data[i], data[start]\n\t\t}\n\t}\n}\n\nfunc main() {\n\tdata := string(\"ABC\")\n\tbyteArray := []byte(data)\n\n\tpermutation(byteArray, 0, len(byteArray)-1)\n}"
}
{
"filename": "code/backtracking/src/permutations_of_string/permutations_of_string_itertools.py",
"content": "# Part of Cosmos by OpenGenus Foundation\nimport itertools\nimport typing\n\n\ndef permute(string: str) -> typing.Iterator[str]:\n \"\"\"\n >>> list(permute('ABC'))\n ['ABC', 'ACB', 'BAC', 'BCA', 'CAB', 'CBA']\n \"\"\"\n yield from map(\"\".join, itertools.permutations(string))"
}
{
"filename": "code/backtracking/src/permutations_of_string/permutations_of_string.kt",
"content": "// Part of Cosmos by OpenGenus Foundation\n\nfun permute(s: String, l: Int = 0, r: Int = s.length - 1) {\n val builder = StringBuilder(s)\n var i: Int\n if (l == r) {\n println(s)\n } else {\n i = l\n while (i <= r) {\n var tmp = builder[l]\n builder[l] = builder[i]\n builder[i] = tmp\n\n permute(builder.toString(), l + 1, r)\n\n tmp = builder[l]\n builder[l] = builder[i]\n builder[i] = tmp\n\n i++\n }\n }\n}\n\nfun main(args: Array<String>) {\n permute(\"ABC\")\n /*\n Output:\n ABC\n ACB\n BAC\n BCA\n CBA\n CAB\n */\n}"
}
{
"filename": "code/backtracking/src/permutations_of_string/permutations_of_string.py",
"content": "# Part of Cosmos by OpenGenus Foundation\nimport typing\n\n\ndef permute(s: str, i: int = 0) -> typing.Iterator[str]:\n \"\"\"\n >>> list(permute('ABC'))\n ['ABC', 'ACB', 'BAC', 'BCA', 'CBA', 'CAB']\n \"\"\"\n if i == len(s) - 1:\n yield \"\".join(s)\n else:\n for j in range(i, len(s)):\n yield from permute(s[:i] + s[i : j + 1][::-1] + s[j + 1 :], i + 1)"
}
{
"filename": "code/backtracking/src/permutations_of_string/permutations_of_string_stl.cpp",
"content": "// Part of Cosmos by OpenGenus Foundation\n#include <algorithm>\n#include <iostream>\n#include <string>\nusing namespace std;\nint main()\n{\n string s;\n cin >> s;\n sort(s.begin(), s.end());\n do\n cout << s << endl;\n while (next_permutation(s.begin(), s.end()));\n return 0;\n}"
}
{
"filename": "code/backtracking/src/permutations_of_string/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/backtracking/src/power_set/power_set.c",
"content": "#include <stdio.h>\n#include <math.h>\n\nvoid\nfind_power_set(char *set, int set_size)\n{\n\tunsigned int power_set_size = 1 << set_size;\n\tint counter, j;\n\n\tfor (counter = 0; counter < power_set_size; counter++) {\n\t\tfor (j = 0; j < set_size; j++) {\n\t\t\tif (counter & (1 << j))\n\t\t\t\tprintf(\"%c\", set[j]);\n\t\t}\n\t\tprintf(\"\\n\");\n\t}\n}\n\nint\nmain()\n{\n\tchar set[] = {'a', 'b', 'c'};\n\tfind_power_set(set, sizeof(set) / sizeof(set[0]));\n\treturn (0);\n}"
}
{
"filename": "code/backtracking/src/power_set/power_set.go",
"content": "//Part of Open Genus foundation\npackage main\n\n/*\n{1 2 3 }\n{1 2 }\n{1 3 }\n{1 }\n{2 3 }\n{2 }\n{3 }\n{}\n*/\n\nimport \"fmt\"\n\nfunc powerSet(input, record []int, index int) {\n\tif index == len(input) {\n\t\tfmt.Printf(\"{\")\n\t\tfor i, v := range record {\n\t\t\tif v == 1 {\n\t\t\t\tfmt.Printf(\" %v\", input[i])\n\t\t\t}\n\t\t}\n\t\tfmt.Printf(\" }\\n\")\n\t\treturn\n\t}\n\n\trecord[index] = 1\n\tpowerSet(input, record, index+1)\n\trecord[index] = 0\n\tpowerSet(input, record, index+1)\n\n}\n\nfunc main() {\n\tinput := []int{1, 2, 3}\n\trecord := make([]int, len(input))\n\n\tpowerSet(input, record, 0)\n}"
}
{
"filename": "code/backtracking/src/power_set/power_set.java",
"content": "/**\n * Part of Cosmos by OpenGenus Foundation\n * This code snippet shows how to generate the powerset of a set which is the \n * set of all subsets of a set. There are two common ways of doing this which\n * are to use the binary representation of numbers on a computer or to \n * do it recursively. Both methods are shown here, pick your flavor! \n * \n * Time Complexity: O( 2^n )\n *\n * @author William Fiset, [email protected]\n **/\n\npublic class PowerSet {\n\n // Use the fact that numbers represented in binary can be\n // used to generate all the subsets of an array\n static void powerSetUsingBinary(int[] set) {\n\n final int N = set.length;\n final int MAX_VAL = 1 << N;\n\n for (int subset = 0; subset < MAX_VAL; subset++) {\n System.out.print(\"{ \");\n for (int i = 0; i < N; i++) {\n int mask = 1 << i;\n if ((subset & mask) == mask)\n System.out.print(set[i] + \" \");\n }\n System.out.println(\"}\");\n }\n\n }\n\n // Recursively generate the powerset (set of all subsets) of an array by maintaining\n // a boolean array used to indicate which element have been selected\n static void powerSetRecursive(int at, int[] set, boolean[] used) {\n\n if (at == set.length) {\n\n // Print found subset!\n System.out.print(\"{ \");\n for (int i = 0; i < set.length; i++)\n if (used[i])\n System.out.print(set[i] + \" \");\n System.out.println(\"}\");\n\n } else {\n\n // Include this element\n used[at] = true;\n powerSetRecursive(at + 1, set, used);\n\n // Backtrack and don't include this element\n used[at] = false;\n powerSetRecursive(at + 1, set, used);\n\n }\n\n }\n\n public static void main(String[] args) {\n \n // Example usage:\n int[] set = {1, 2, 3 };\n\n powerSetUsingBinary(set);\n // prints:\n // { }\n // { 1 }\n // { 2 }\n // { 1 2 }\n // { 3 }\n // { 1 3 }\n // { 2 3 }\n // { 1 2 3 }\n\n System.out.println();\n\n powerSetRecursive(0, set, new boolean[set.length]);\n // prints:\n // { 1 2 3 }\n // { 1 2 }\n // { 1 3 }\n // { 1 }\n // { 2 3 }\n // { 2 }\n // { 3 }\n // { }\n\n }\n\n}"
}
{
"filename": "code/backtracking/src/rat_in_a_maze/rat_in_a_maze.cpp",
"content": "/* C/C++ program to solve Rat in a Maze problem using\n * backtracking */\n#include <stdio.h>\n\n// Maze size\n#define N 4\n\nbool solveMazeUtil(int maze[N][N], int x, int y, int sol[N][N]);\n\n/* A utility function to print solution matrix sol[N][N] */\nvoid printSolution(int sol[N][N])\n{\n for (int i = 0; i < N; i++)\n {\n for (int j = 0; j < N; j++)\n printf(\" %d \", sol[i][j]);\n printf(\"\\n\");\n }\n}\n\n/* A utility function to check if x,y is valid index for N*N maze */\nbool isSafe(int maze[N][N], int x, int y)\n{\n // if (x,y outside maze) return false\n if (x >= 0 && x < N && y >= 0 && y < N && maze[x][y] == 1)\n return true;\n\n return false;\n}\n\n/* This function solves the Maze problem using Backtracking. It mainly\n * uses solveMazeUtil() to solve the problem. It returns false if no\n * path is possible, otherwise return true and prints the path in the\n * form of 1s. Please note that there may be more than one solutions,\n * this function prints one of the feasible solutions.*/\nbool solveMaze(int maze[N][N])\n{\n int sol[N][N] = { {0, 0, 0, 0},\n {0, 0, 0, 0},\n {0, 0, 0, 0},\n {0, 0, 0, 0}\n };\n\n if (solveMazeUtil(maze, 0, 0, sol) == false)\n {\n printf(\"Solution doesn't exist\");\n return false;\n }\n\n printSolution(sol);\n return true;\n}\n\n/* A recursive utility function to solve Maze problem */\nbool solveMazeUtil(int maze[N][N], int x, int y, int sol[N][N])\n{\n // if (x,y is goal) return true\n if (x == N - 1 && y == N - 1)\n {\n sol[x][y] = 1;\n return true;\n }\n\n // Check if maze[x][y] is valid\n if (isSafe(maze, x, y) == true)\n {\n // mark x,y as part of solution path\n sol[x][y] = 1;\n\n /* Move forward in x direction */\n if (solveMazeUtil(maze, x + 1, y, sol) == true)\n return true;\n\n /* If moving in x direction doesn't give solution then\n * Move down in y direction */\n if (solveMazeUtil(maze, x, y + 1, sol) == true)\n return true;\n\n /* If none of the above movements work then BACKTRACK:\n * unmark x,y as part of solution path */\n sol[x][y] = 0;\n return false;\n }\n\n return false;\n}\n\n// driver program to test above function\nint main()\n{\n int maze[N][N] = { {1, 0, 0, 0},\n {1, 1, 0, 1},\n {0, 1, 0, 0},\n {1, 1, 1, 1}\n };\n\n solveMaze(maze);\n return 0;\n}"
}
{
"filename": "code/backtracking/src/rat_in_a_maze/rat_in_a_maze.py",
"content": "# Maze size\nN = 4\n\ndef solve_maze_util(maze, x, y, sol):\n # if (x,y is goal) return True\n if x == N - 1 and y == N - 1:\n sol[x][y] = 1\n return True\n\n # Check if maze[x][y] is valid\n if is_safe(maze, x, y):\n # Mark x,y as part of solution path\n sol[x][y] = 1\n\n # Move forward in x direction\n if solve_maze_util(maze, x + 1, y, sol):\n return True\n\n # If moving in x direction doesn't give a solution then move down in y direction\n if solve_maze_util(maze, x, y + 1, sol):\n return True\n\n # If none of the above movements work, then BACKTRACK: unmark x,y as part of the solution path\n sol[x][y] = 0\n return False\n\n return False\n\ndef is_safe(maze, x, y):\n # if (x,y outside maze) return False\n if 0 <= x < N and 0 <= y < N and maze[x][y] == 1:\n return True\n return False\n\ndef print_solution(sol):\n for row in sol:\n for val in row:\n print(val, end=' ')\n print()\n\ndef solve_maze(maze):\n sol = [[0 for _ in range(N)] for _ in range(N)]\n\n if not solve_maze_util(maze, 0, 0, sol):\n print(\"Solution doesn't exist\")\n return False\n\n print_solution(sol)\n return True\n\n# Driver program to test above function\nif __name__ == \"__main__\":\n maze = [[1, 0, 0, 0],\n [1, 1, 0, 1],\n [0, 1, 0, 0],\n [1, 1, 1, 1]]\n\n solve_maze(maze)"
}
{
"filename": "code/backtracking/src/rat_in_a_maze/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/backtracking/src/README.md",
"content": "# Backtracking\nBacktracking is a general algorithm for finding all (or some) solutions to some computational problems, notably constraint satisfaction problems, that incrementally builds candidates to the solutions, and abandons each partial candidate (\"backtracks\") as soon as it determines that the candidate cannot possibly be completed to a valid solution.\n\nThe classic textbook example of the use of backtracking is the eight queens puzzle, that asks for all arrangements of eight chess queens on a standard chessboard so that no queen attacks any other. In the common backtracking approach, the partial candidates are arrangements of k queens in the first k rows of the board, all in different rows and columns. Any partial solution that contains two mutually attacking queens can be abandoned.\n\nBacktracking can be applied only for problems which admit the concept of a \"partial candidate solution\" and a relatively quick test of whether it can possibly be completed to a valid solution. It is useless, for example, for locating a given value in an unordered table. When it is applicable, however, backtracking is often much faster than brute force enumeration of all complete candidates, since it can eliminate a large number of candidates with a single test.\n# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/backtracking/src/subset_sum/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\nSubsetSum is to compute a sum S using a selected subset of a given set of N weights.\n\nIt works by backtracking, returning all possible solutions one at a time, keeping track of the selected weights using a 0/1 mask vector of size N.\n\nA={1,2,3,4,7}\nS=4\nsubets={1,3},{4}"
}
{
"filename": "code/backtracking/src/subset_sum/subset_sum.c",
"content": "// Part of Cosmos by OpenGenus Foundation\n\n#include <stdio.h>\n// Recursive Solution\n// Driver program to test above function\n\n\n// Returns true if there is a subset of set[] with sun equal to given sum\nint isSubsetSum(int set[], int n, int sum)\n{\n // Base Cases\n if (sum == 0)\n return 1;\n if (n == 0 && sum != 0)\n return 0;\n \n // If last element is greater than sum, then ignore it\n if (set[n-1] > sum)\n return isSubsetSum(set, n-1, sum);\n \n /* else, check if sum can be obtained by any of the following\n (a) including the last element\n (b) excluding the last element */\n return isSubsetSum(set, n-1, sum) || isSubsetSum(set, n-1, sum-set[n-1]);\n}\n\nint main()\n{\n int n,i,sum;\n printf(\"Input number of elements\\n\");\n scanf(\"%d\",&n);\n int set[n];\n printf(\"Input array elements\\n\");\n for(i = 0; i < n;i++)\n scanf(\"%d\",&set[i]);\n printf(\"Enter Sum to check\\n\");\n scanf(\"%d\",&sum);\n if (isSubsetSum(set, n, sum) == 1)\n printf(\"Found a subset with given sum\\n\");\n else\n printf(\"No subset with given sum\\n\");\n return 0;\n}"
}
{
"filename": "code/backtracking/src/subset_sum/subset_sum.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <iostream>\n#include <vector>\n\nusing namespace std;\n\n/*\n * Generate all possible subset sums\n * of array v that sum up to targetSum\n */\nvector< vector<int>> generateSubsetSums(int v[], size_t size, int targetSum)\n{\n\n vector< vector<int>> solutions;\n\n for (int i = 0; i < (1 << size); ++i)\n {\n\n int currentSum = 0;\n for (size_t j = 0; j < size; ++j)\n if (i & (1 << j))\n currentSum += v[j];\n\n if (currentSum == targetSum)\n {\n vector<int> subsetSum;\n for (size_t j = 0; j < size; ++j)\n if (i & (1 << j))\n subsetSum.push_back(v[j]);\n\n solutions.push_back(subsetSum);\n }\n }\n\n return solutions;\n}\n\nint main()\n{\n\n int v[] = {5, 12, -3, 10, -2, 7, -1, 8, 11};\n int targetSum = 7;\n\n vector< vector<int>> subsetSums = generateSubsetSums(v, sizeof(v) / sizeof(v[0]), targetSum);\n\n cout << \"Subset sums:\\n\";\n for (size_t i = 0; i < subsetSums.size(); ++i)\n {\n cout << subsetSums[i][0];\n for (size_t j = 1; j < subsetSums[i].size(); ++j)\n cout << \", \" << subsetSums[i][j];\n cout << '\\n';\n }\n\n return 0;\n}"
}
{
"filename": "code/backtracking/src/subset_sum/subset_sum_duplicates.py",
"content": "def combinationSum(candidates, target):\n \"\"\"\n Problem description:\n\n Given a set of candidate numbers (C) (without duplicates) and a target number (T), \n find all unique combinations in C where the candidate numbers sums to T.\n\n The same repeated number may be chosen from C unlimited number of times.\n \n Args:\n candidates: list of pickable integers\n target: target sum\n\n Returns:\n List of subsets that sum to the target sum of target\n \"\"\"\n result_set = []\n backtrack(0, sorted(candidates), target, 0, result_set, [])\n return result_set\n\n\ndef backtrack(pos, candidates, target, currentSum, result_set, sequence):\n \"\"\"\n Helper function for backtracking. Initially does a DFS traversal on each\n index to check all combinations of subsets to see if they sum to the target\n sum. \n\n Args:\n pos: current pivot index\n candidates: candidates list (main list of pickable integers)\n currentSum: current sum of the list\n result_set: list to store all result_set\n sequence: list being used to contain elements relevent to the current DFS\n\n Returns:\n None\n \"\"\"\n if currentSum == target:\n result_set.append(sequence[:])\n elif currentSum < target:\n for i in range(pos, len(candidates)):\n newSum = currentSum + candidates[i]\n if newSum > target:\n break\n sequence.append(candidates[i])\n backtrack(i, candidates, target, newSum, result_set, sequence)\n sequence.pop()\n\n\nif __name__ == \"__main__\":\n arr = [2, 3, 6, 7]\n print(combinationSum(arr, 7))"
}
{
"filename": "code/backtracking/src/subset_sum/subset_sum.go",
"content": "// Part of Cosmos by OpenGenus Foundation\n\npackage main\n\nimport \"fmt\"\n\nvar totalSol int\n\n/*\n\nExpected output:\n[1 2 3]\n[1 5]\n[2 4]\n[6]\nThere're 4 ways to combine 6 from [1 2 3 4 5 6 7 8 9 10 11 12 13 14]\n\n*/\n\nfunc subSetSum(data []int, sol []int, index, target int) {\n\tif target == 0 {\n\t\ttotalSol++\n\t\tfmt.Println(sol)\n\t\treturn\n\t}\n\n\tif target < 0 || index >= len(data) {\n\t\treturn\n\t}\n\n\tsol = append(sol, data[index])\n\tsubSetSum(data, sol, index+1, target-data[index])\n\tsol = sol[:len(sol)-1]\n\tsubSetSum(data, sol, index+1, target)\n\n}\n\nfunc main() {\n\tdata := []int{1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14}\n\tsol := []int{}\n\ttarget := 6\n\n\ttotalSol = 0\n\tsubSetSum(data, sol, 0, target)\n\tfmt.Printf(\"There're %d ways to combine %d from %v\\n\", totalSol, target, data)\n}"
}
{
"filename": "code/backtracking/src/subset_sum/subset_sum.java",
"content": "import java.util.ArrayList;\nimport java.util.Scanner;\n\npublic class SubsetSum {\n public static ArrayList<ArrayList<Integer>> SubsetGenerate(int arr[], int requiredSum){\n\n ArrayList<ArrayList<Integer>> RequiredSubsets = new ArrayList<ArrayList<Integer>>();\n int temp,size = arr.length;\n\n for(int i=0;i<(1<<size);i++){\n\n temp = 0;\n\n for(int j=0;j<size;j++)\n if((i & (1<<j)) > 0)\n temp += arr[j];\n\n if(requiredSum == temp){\n ArrayList<Integer> subset = new ArrayList<>();\n\n for(int j=0;j<size;j++)\n if((i & (1<<j)) > 0)\n subset.add(arr[j]);\n\n RequiredSubsets.add(subset);\n }\n }\n\n return RequiredSubsets;\n }\n public static void main(String[] args) {\n Scanner sc = new Scanner(System.in);\n\n System.out.print(\"Enter n >> \");\n int n = sc.nextInt();\n int arr[] = new int[n];\n\n System.out.print(\"Enter n array elements >> \");\n for(int i=0;i<n;i++)\n arr[i] = sc.nextInt();\n\n System.out.print(\"Enter required Subset Sum >> \");\n int requiredSum = sc.nextInt();\n\n ArrayList<ArrayList<Integer>> RequiredSubsets = SubsetGenerate(arr,requiredSum);\n\n int temp;\n System.out.println(\"Subsets with sum = \" + requiredSum + \" : \");\n\n for(int i=0;i<RequiredSubsets.size();i++){\n temp = RequiredSubsets.get(i).size();\n for(int j=0;j<temp;j++){\n System.out.print(RequiredSubsets.get(i).get(j) + \" \");\n }\n System.out.println();\n }\n\n }\n}"
}
{
"filename": "code/backtracking/src/subset_sum/subset_sum.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n# subsetsum function below\ndef subsetsum(cs, k, r, x, w, d):\n x[k] = 1\n if cs + w[k] == d:\n for i in range(0, k + 1):\n\n if x[i] == 1:\n print(w[i], end=\" \")\n print()\n\n elif cs + w[k] + w[k + 1] <= d:\n subsetsum(cs + w[k], k + 1, r - w[k], x, w, d)\n\n if (cs + r - w[k] >= d) and (cs + w[k] <= d):\n x[k] = 0\n subsetsum(cs, k + 1, r - w[k], x, w, d)\n\n\n# driver for the above code\n# Main array w\nw = [2, 3, 4, 5, 0]\nx = [0 for i in range(len(w))]\nprint(\"Enter the no u want to get the subsets sum for\")\nnum = int(input())\nif num <= sum(w):\n print(\"The subsets are:-\\n\")\n\n subsetsum(0, 0, sum(w), x, w, num)\nelse:\n print(\n \"Not possible The no is greater than the sum of all the elements in the array\"\n )"
}
{
"filename": "code/backtracking/src/sudoku_solve/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/backtracking/src/sudoku_solve/sudoku_solve.c",
"content": "/*\n** Reimua\n** Part of Cosmos by OpenGenus Foundation\n*/\n\n#include <stdio.h>\n#include <string.h>\n#include <stdlib.h>\n#include <stdbool.h>\n\nstatic const int map_size = 9;\n\n/*\n** Check a number's existance on a line.\n*/\n\nint exist_on_line(char map[map_size][map_size + 2], const int pos_y, const char c)\n{\n\tint i = 0;\n\n\twhile (i < map_size)\n\t{\n\t\tif (map[pos_y][i++] == c)\n\t\t\treturn (1);\n\t}\n\treturn (0);\n}\n\n/*\n** Check a number's existance on a column.\n*/\n\nint exist_on_column(char map[map_size][map_size + 2], const int pos_x, const char c)\n{\n\tint i = 0;\n\n\twhile (i < map_size)\n\t{\n\t\tif (map[i++][pos_x] == c)\n\t\t\treturn (1);\n\t}\n\treturn (0);\n}\n\n/*\n** Check a number's existance on a block.\n*/\n\nint exist_on_block(char map[map_size][map_size + 2], const int pos_x, const int pos_y, const char c)\n{\n\tint i = pos_y - (pos_y % 3);\n\tint j = pos_x - (pos_x % 3);\n\tint m = i;\n\tint n = j;\n\n\twhile (m < i + 3)\n\t{\n\t\twhile (n < j + 3)\n\t\t{\n\t\t\tif (map[m][n] == c)\n\t\t\t\treturn (1);\n\t\t\tn++;\n\t\t}\n\t\tm++;\n\t\tn = j;\n\t}\n\treturn (0);\n}\n\n/*\n** Solve the sudoku by using backtracking.\n** \n** The following could be improved by changing\n** the order of completion instead of going\n** from 0 to map_size².\n*/\n\nint resolve_sudoku(char map[map_size][map_size + 2], const int pos)\n{\n\tif (pos == map_size * map_size)\n\t\treturn (1);\n\n\tint pos_x = pos % 9;\n\tint pos_y = pos / 9;\n\n\tif (map[pos_y][pos_x] != '0')\n\t\treturn (resolve_sudoku(map, pos + 1));\n\n\tchar c = '1';\n\n\twhile (c <= map_size + '0')\n\t\t{\n\t\t\tif (!exist_on_line(map, pos_y, c) &&\n\t\t\t\t!exist_on_column(map, pos_x, c) &&\n\t\t\t\t!exist_on_block(map, pos_x, pos_y, c))\n\t\t\t{\n\t\t\t\tmap[pos_y][pos_x] = c;\n\t\t\t\tif (resolve_sudoku(map, pos + 1))\n\t\t\t\t\treturn (1);\n\t\t\t}\n\t\t\tc++;\n\t\t}\n\n\tmap[pos_y][pos_x] = '0';\n\treturn (0);\n}\n\n/*\n** Read from stdin.\n*/\n\nint read_map(char map[map_size][map_size + 2])\n{\n\tint i = 0;\n\n\twhile (i < map_size)\n \t{\n \t\tif (!fgets(map[i], map_size + 2, stdin) ||\n \t\t\t(int)strlen(map[i]) < map_size)\n \t\t\treturn (1);\n \t\tmap[i++][map_size] = '\\0';\n \t}\n \treturn (0);\n}\n\nint main(void)\n{\n\tint i = 0;\n\tchar map[map_size][map_size + 2];\n \n\tif (read_map(map))\n\t\treturn (1); \n \tresolve_sudoku(map, 0);\n \twhile (i < map_size)\n \t\tprintf(\"%s\\n\", map[i++]);\n \treturn (0);\n}"
}
{
"filename": "code/backtracking/src/sudoku_solve/sudoku_solve.cpp",
"content": "#include <iostream>\nusing namespace std;\n\n/*\n * Part of Cosmos by OpenGenus Foundation\n */\nint n = 9;\n\nbool isPossible(int mat[][9], int i, int j, int no)\n{\n ///Row or col should not have no\n for (int x = 0; x < n; x++)\n if (mat[x][j] == no || mat[i][x] == no)\n return false;\n\n /// Subgrid should not have no\n int sx = (i / 3) * 3;\n int sy = (j / 3) * 3;\n\n for (int x = sx; x < sx + 3; x++)\n for (int y = sy; y < sy + 3; y++)\n if (mat[x][y] == no)\n return false;\n\n return true;\n}\nvoid printMat(int mat[][9])\n{\n\n for (int i = 0; i < n; i++)\n {\n for (int j = 0; j < n; j++)\n {\n cout << mat[i][j] << \" \";\n if ((j + 1) % 3 == 0)\n cout << '\\t';\n\n }\n if ((i + 1) % 3 == 0)\n cout << endl;\n cout << endl;\n }\n}\n\n\nbool solveSudoku(int mat[][9], int i, int j)\n{\n ///Base Case\n if (i == 9)\n {\n printMat(mat);\n return true;\n }\n\n ///Crossed the last Cell in the row\n if (j == 9)\n return solveSudoku(mat, i + 1, 0);\n\n ///Skip if filled cell\n if (mat[i][j] != 0)\n return solveSudoku(mat, i, j + 1);\n ///White Cell\n ///Try to place every possible no\n for (int no = 1; no <= 9; no++)\n if (isPossible(mat, i, j, no))\n {\n ///Place the no - assuming solution is possible with this\n mat[i][j] = no;\n bool isSolve = solveSudoku(mat, i, j + 1);\n if (isSolve)\n return true;\n ///loop will place the next no.\n }\n ///Backtracking\n mat[i][j] = 0;\n return false;\n}\n\nint main()\n{\n\n int mat[9][9] =\n {{5, 3, 0, 0, 7, 0, 0, 0, 0},\n {6, 0, 0, 1, 9, 5, 0, 0, 0},\n {0, 9, 8, 0, 0, 0, 0, 6, 0},\n {8, 0, 0, 0, 6, 0, 0, 0, 3},\n {4, 0, 0, 8, 0, 3, 0, 0, 1},\n {7, 0, 0, 0, 2, 0, 0, 0, 6},\n {0, 6, 0, 0, 0, 0, 2, 8, 0},\n {0, 0, 0, 4, 1, 9, 0, 0, 5},\n {0, 0, 0, 0, 8, 0, 0, 7, 9}};\n printMat(mat);\n cout << \"Solution \" << endl;\n solveSudoku(mat, 0, 0);\n return 0;\n}"
}
{
"filename": "code/backtracking/src/sudoku_solve/sudoku_solveNxN.cpp",
"content": "#include<iostream>\n\nusing std ::cout;\nusing std ::cin;\n\nconst int n = 9;\n\nint grid[n][n];\n\nvoid input_grid() {\n //int i, j;\n for (int i = 0; i < n; i++) {\n for (int j = 0; j < n; j++) {\n cin >> grid[i][j];\n }\n }\n}\n\nvoid print_grid() {\n //int i, j;\n for (int i = 0; i < n; i++) {\n for (int j = 0; j < n; j++) {\n cout << grid[i][j] << \" \";\n }\n cout << '\\n';\n }\n}\n\nbool can_be_placed(int row, int col, int num) {\n int ii = 0, jj = col;\n // row check\n while (ii < n) {\n if (grid[ii][jj] == num) {\n return false;\n }\n ii++;\n }\n // col check\n ii = row; jj = 0;\n while (jj < n) {\n if (grid[ii][jj] == num) {\n return false;\n }\n jj++;\n }\n // 3*3 check\n int startrow, startcol;\n startrow = (row / 3) * 3;\n startcol = (col / 3) * 3;\n for (ii = startrow; ii < startrow + 3; ii++) {\n for (jj = startcol; jj < startcol + 3; jj++) {\n if (grid[ii][jj] == num) {\n return false;\n }\n }\n }\n return true;\n}\n\nvoid sudoku_solver(int row, int col) {\n // cout<<row<<\" \"<<col<<'\\n';\n if (row == n && col == 0) {\n print_grid();\n return ;\n }\n if (grid[row][col] == 0) {\n for (int num = 1; num <= n; num++) {\n if ((can_be_placed(row, col, num))) {\n grid[row][col] = num;\n if (col == n - 1) {\n sudoku_solver(row + 1, 0);\n }\n else {\n sudoku_solver(row, col + 1);\n }\n grid[row][col] = 0;\n }\n }\n }\n else {\n if (col == n - 1) {\n sudoku_solver(row + 1, 0);\n }\n else {\n sudoku_solver(row, col + 1);\n }\n }\n}\n\nint main()\n{\n input_grid();\n sudoku_solver(0, 0);\n}\n//created by aryan "
}
{
"filename": "code/backtracking/src/sudoku_solve/sudoku_solve.py",
"content": "# A Backtracking program in Pyhton to solve Sudoku problem\n\n\n# A Utility Function to print the Grid\ndef print_grid(arr):\n for i in range(9):\n for j in range(9):\n print(arr[i][j], end=\" \"),\n print(end=\"\\n\")\n\n\n# Function to Find the entry in the Grid that is still not used\n# Searches the grid to find an entry that is still unassigned. If\n# found, the reference parameters row, col will be set the location\n# that is unassigned, and true is returned. If no unassigned entries\n# remain, false is returned.\n# 'l' is a list variable that has been passed from the solve_sudoku function\n# to keep track of incrementation of Rows and Columns\ndef find_empty_location(arr, l):\n for row in range(9):\n for col in range(9):\n if arr[row][col] == 0:\n l[0] = row\n l[1] = col\n return True\n return False\n\n\n# Returns a boolean which indicates whether any assigned entry\n# in the specified row matches the given number.\ndef used_in_row(arr, row, num):\n for i in range(9):\n if arr[row][i] == num:\n return True\n return False\n\n\n# Returns a boolean which indicates whether any assigned entry\n# in the specified column matches the given number.\ndef used_in_col(arr, col, num):\n for i in range(9):\n if arr[i][col] == num:\n return True\n return False\n\n\n# Returns a boolean which indicates whether any assigned entry\n# within the specified 3x3 box matches the given number\ndef used_in_box(arr, row, col, num):\n for i in range(3):\n for j in range(3):\n if arr[i + row][j + col] == num:\n return True\n return False\n\n\n# Checks whether it will be legal to assign num to the given row,col\n# Returns a boolean which indicates whether it will be legal to assign\n# num to the given row,col location.\ndef check_location_is_safe(arr, row, col, num):\n\n # Check if 'num' is not already placed in current row,\n # current column and current 3x3 box\n return (\n not used_in_row(arr, row, num)\n and not used_in_col(arr, col, num)\n and not used_in_box(arr, row - row % 3, col - col % 3, num)\n )\n\n\n# Takes a partially filled-in grid and attempts to assign values to\n# all unassigned locations in such a way to meet the requirements\n# for Sudoku solution (non-duplication across rows, columns, and boxes)\ndef solve_sudoku(arr):\n\n # 'l' is a list variable that keeps the record of row and col in find_empty_location Function\n l = [0, 0]\n\n # If there is no unassigned location, we are done\n if not find_empty_location(arr, l):\n return True\n\n # Assigning list values to row and col that we got from the above Function\n row = l[0]\n col = l[1]\n\n # consider digits 1 to 9\n for num in range(1, 10):\n\n # if looks promising\n if check_location_is_safe(arr, row, col, num):\n\n # make tentative assignment\n arr[row][col] = num\n\n # return, if sucess, ya!\n if solve_sudoku(arr):\n return True\n\n # failure, unmake & try again\n arr[row][col] = 0\n\n # this triggers backtracking\n return False\n\n\n# Driver main function to test above functions\nif __name__ == \"__main__\":\n\n # creating a 2D array for the grid\n grid = [[0 for x in range(9)] for y in range(9)]\n\n # assigning values to the grid\n grid = [\n [3, 0, 6, 5, 0, 8, 4, 0, 0],\n [5, 2, 0, 0, 0, 0, 0, 0, 0],\n [0, 8, 7, 0, 0, 0, 0, 3, 1],\n [0, 0, 3, 0, 1, 0, 0, 8, 0],\n [9, 0, 0, 8, 6, 3, 0, 0, 5],\n [0, 5, 0, 0, 9, 0, 6, 0, 0],\n [1, 3, 0, 0, 0, 0, 2, 5, 0],\n [0, 0, 0, 0, 0, 0, 0, 7, 4],\n [0, 0, 5, 2, 0, 6, 3, 0, 0],\n ]\n\n # if sucess print the grid\n if solve_sudoku(grid):\n print_grid(grid)\n else:\n print(\"No solution exists\")"
}
{
"filename": "code/backtracking/src/sudoku_solve/sudoku_solver.java",
"content": "public static boolean isSafe(int[][] board,\n int row, int col,\n int num)\n{\n \n for (int d = 0; d < board.length; d++)\n {\n if (board[row][d] == num) {\n return false;\n }\n }\n\n for (int r = 0; r < board.length; r++)\n {\n if (board[r][col] == num)\n {\n return false;\n }\n }\n\n int sqrt = (int)Math.sqrt(board.length);\n int boxRowStart = row - row % sqrt;\n int boxColStart = col - col % sqrt;\n\n for (int r = boxRowStart;\n r < boxRowStart + sqrt; r++)\n {\n for (int d = boxColStart;\n d < boxColStart + sqrt; d++)\n {\n if (board[r][d] == num)\n {\n return false;\n }\n }\n }\n return true;\n}\n\npublic static boolean solveSudoku(\n int[][] board, int n)\n{\n int row = -1;\n int col = -1;\n boolean isEmpty = true;\n for (int i = 0; i < n; i++)\n {\n for (int j = 0; j < n; j++)\n {\n if (board[i][j] == 0)\n {\n row = i;\n col = j;\n\n isEmpty = false;\n break;\n }\n }\n if (!isEmpty) {\n break;\n }\n }\n\n if (isEmpty)\n {\n return true;\n }\n \n for (int num = 1; num <= n; num++)\n {\n if (isSafe(board, row, col, num))\n {\n board[row][col] = num;\n if (solveSudoku(board, n))\n {\n return true;\n }\n else\n {\n board[row][col] = 0;\n }\n }\n }\n return false;\n}\n\npublic static void print(\n int[][] board, int N)\n{\n\n for (int r = 0; r < N; r++)\n {\n for (int d = 0; d < N; d++)\n {\n System.out.print(board[r][d]);\n System.out.print(\" \");\n }\n System.out.print(\"\\n\");\n\n if ((r + 1) % (int)Math.sqrt(N) == 0)\n {\n System.out.print(\"\");\n }\n }\n}\n\npublic static void main(String args[])\n{\n\n int[][] board = new int[][] {\n { 3, 0, 6, 5, 0, 8, 4, 0, 0 },\n { 5, 2, 0, 0, 0, 0, 0, 0, 0 },\n { 0, 8, 7, 0, 0, 0, 0, 3, 1 },\n { 0, 0, 3, 0, 1, 0, 0, 8, 0 },\n { 9, 0, 0, 8, 6, 3, 0, 0, 5 },\n { 0, 5, 0, 0, 9, 0, 6, 0, 0 },\n { 1, 3, 0, 0, 0, 0, 2, 5, 0 },\n { 0, 0, 0, 0, 0, 0, 0, 7, 4 },\n { 0, 0, 5, 2, 0, 6, 3, 0, 0 }\n };\n int N = board.length;\n\n if (solveSudoku(board, N))\n {\n print(board, N);\n }\n else {\n System.out.println(\"No solution\");\n }\n}"
}
{
"filename": "code/backtracking/src/sudoku_solve/sudoku_solve.rs",
"content": "// Part of Cosmos by OpenGenus\nconst N: usize = 9;\nconst UNASSIGNED: u8 = 0;\n\ntype Board = [[u8; N]; N];\n\nfn solve_sudoku(grid: &mut Board) -> bool {\n let row;\n let col;\n\n if let Some((r, c)) = find_unassigned_cells(&grid) {\n row = r;\n col = c;\n } else {\n // SOLVED\n return true;\n }\n for num in 1..=9 {\n if is_safe(&grid, row, col, num) {\n grid[row][col] = num;\n\n if solve_sudoku(grid) {\n return true;\n }\n\n // Failed, try again\n grid[row][col] = UNASSIGNED;\n }\n }\n false\n}\n\nfn used_in_row(grid: &Board, row: usize, num: u8) -> bool {\n for col in 0..N {\n if grid[row][col] == num {\n return true;\n }\n }\n false\n}\n\nfn used_in_col(grid: &Board, col: usize, num: u8) -> bool {\n for row in grid.iter().take(N) {\n if row[col] == num {\n return true;\n }\n }\n false\n}\n\nfn used_in_box(grid: &Board, row_start: usize, col_start: usize, num: u8) -> bool {\n for row in 0..3 {\n for col in 0..3 {\n if grid[row + row_start][col + col_start] == num {\n return true;\n }\n }\n }\n false\n}\n\nfn is_safe(grid: &Board, row: usize, col: usize, num: u8) -> bool {\n !used_in_row(grid, row, num)\n && !used_in_col(grid, col, num)\n && !used_in_box(grid, row - (row % 3), col - (col % 3), num)\n}\n\nfn find_unassigned_cells(grid: &Board) -> Option<(usize, usize)> {\n for (row, _) in grid.iter().enumerate().take(N) {\n for col in 0..N {\n if grid[row][col] == UNASSIGNED {\n return Some((row, col));\n }\n }\n }\n None\n}\n\nfn print_grid(grid: &Board) {\n for row in grid.iter().take(N) {\n for col in 0..N {\n print!(\"{} \", row[col]);\n }\n println!();\n }\n}\n\n\n#[test]\nfn test1() {\n let mut board = [\n [3, 0, 6, 5, 0, 8, 4, 0, 0],\n [5, 2, 0, 0, 0, 0, 0, 0, 0],\n [0, 8, 7, 0, 0, 0, 0, 3, 1],\n [0, 0, 3, 0, 1, 0, 0, 8, 0],\n [9, 0, 0, 8, 6, 3, 0, 0, 5],\n [0, 5, 0, 0, 9, 0, 6, 0, 0],\n [1, 3, 0, 0, 0, 0, 2, 5, 0],\n [0, 0, 0, 0, 0, 0, 0, 7, 4],\n [0, 0, 5, 2, 0, 6, 3, 0, 0],\n ];\n // Can solve\n assert!(solve_sudoku(&mut board));\n let solved_board = [\n [3, 1, 6, 5, 7, 8, 4, 9, 2],\n [5, 2, 9, 1, 3, 4, 7, 6, 8],\n [4, 8, 7, 6, 2, 9, 5, 3, 1],\n [2, 6, 3, 4, 1, 5, 9, 8, 7],\n [9, 7, 4, 8, 6, 3, 1, 2, 5],\n [8, 5, 1, 7, 9, 2, 6, 4, 3],\n [1, 3, 8, 9, 4, 7, 2, 5, 6],\n [6, 9, 2, 3, 5, 1, 8, 7, 4],\n [7, 4, 5, 2, 8, 6, 3, 1, 9],\n ];\n assert_eq!(board, solved_board)\n}\n\n#[test]\nfn test2() {\n let mut board = [\n [2, 0, 0, 9, 0, 0, 1, 0, 0],\n [6, 0, 0, 0, 5, 1, 0, 0, 0],\n [0, 9, 5, 2, 0, 0, 0, 0, 0],\n [0, 0, 0, 0, 0, 0, 2, 0, 0],\n [5, 0, 9, 1, 0, 2, 4, 0, 3],\n [0, 0, 8, 0, 0, 0, 0, 0, 0],\n [0, 0, 0, 0, 0, 5, 6, 3, 0],\n [0, 0, 0, 4, 6, 0, 0, 0, 9],\n [0, 0, 3, 0, 0, 9, 0, 0, 2],\n ];\n // Can solve\n assert!(solve_sudoku(&mut board));\n let solved = [\n [2, 3, 4, 9, 7, 6, 1, 8, 5],\n [6, 8, 7, 3, 5, 1, 9, 2, 4],\n [1, 9, 5, 2, 4, 8, 3, 7, 6],\n [4, 1, 6, 5, 3, 7, 2, 9, 8],\n [5, 7, 9, 1, 8, 2, 4, 6, 3],\n [3, 2, 8, 6, 9, 4, 7, 5, 1],\n [9, 4, 1, 8, 2, 5, 6, 3, 7],\n [7, 5, 2, 4, 6, 3, 8, 1, 9],\n [8, 6, 3, 7, 1, 9, 5, 4, 2],\n ];\n assert_eq!(board, solved);\n}"
}
{
"filename": "code/backtracking/test/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/bit_manipulation/src/addition_using_bits/addition_using_bits.c",
"content": "/*\nProblem Statement :\nAdd two numbers using bitwise operators\n(i.e without using arithmetic operators)\n*/\n\n#include <stdio.h>\n#include <limits.h>\n\n// Function to add two numbers using bitwise operators\nint bitwiseAddition(int n, int m)\n{\n while (m != 0)\n {\n int carry = n & m;\n n = n ^ m;\n m = carry << 1;\n\n // Check for overflow (positive to negative or vice versa)\n if ((n < 0 && m > 0) || (n > 0 && m < 0))\n {\n fprintf(stderr, \"Overflow detected. Cannot add numbers.\\n\");\n return INT_MAX; // Return the maximum possible value\n }\n }\n return n;\n}\n\n// recursive function\nint bitwiseAdditionRecursive(int n, int m)\n{\n if (m == 0)\n return n;\n else\n {\n int carry = n & m;\n return bitwiseAdditionRecursive(n ^ m, carry << 1);\n }\n}\n\nint main()\n{\n int a, b;\n printf(\"Enter two numbers: \");\n if (scanf(\"%d %d\", &a, &b) != 2)\n {\n fprintf(stderr, \"Invalid input. Please enter two integers.\\n\");\n return 1; // Exit with an error code\n }\n\n int result = bitwiseAddition(a, b);\n if (result == INT_MAX)\n {\n return 1; // Exit with an error code\n }\n\n printf(\"\\nBitwise addition using iterative function : %d\", bitwiseAddition(a, b));\n printf(\"\\nBitwise addition using recursive function : %d\", bitwiseAdditionRecursive(a, b));\n return 0;\n}\n\n/*\nEnter two numbers : 3 5\n\nBitwise addition using iterative function : 8\nBitwise addition using recursive function : 8\n*/"
}
{
"filename": "code/bit_manipulation/src/addition_using_bits/addition_using_bits.cpp",
"content": "#include <iostream>\n\n// Part of Cosmos by OpenGenus Foundation\nint bitwiseAddition(int n, int m)\n{\n while (m != 0)\n {\n int carry = n & m; // Variable carry keeps track of bits that carry over\n n = n ^ m; // This adds up the individual bits\n m = carry << 1; // Shift carry over bits so they can be added\n }\n return n;\n}\n\nint bitwiseAdditionRecursive(int n, int m)\n{\n if (m == 0)\n return n;\n else\n {\n int carry = n & m;\n return bitwiseAdditionRecursive(n ^ m, carry << 1);\n }\n}"
}
{
"filename": "code/bit_manipulation/src/addition_using_bits/addition_using_bits.cs",
"content": "using System;\n\nnamespace BitwiseAddition\n{\n class Program\n {\n static internal int bitwiseRecursiveAddition(int n, int m)\n {\n if(m == 0)\n return n;\n \n var carry = n & m;\n return bitwiseRecursiveAddition(n ^ m, carry << 1);\n }\n\n static internal int bitwiseIterativeAddition(int n, int m)\n {\n while(m != 0) {\n var carry = n & m;\n n ^= m;\n m = carry << 1;\n }\n return n;\n }\n \n static public void Main()\n {\n Console.WriteLine(bitwiseRecursiveAddition(10, 20));\n Console.WriteLine(bitwiseIterativeAddition(50, 20));\n }\n }\n}"
}
{
"filename": "code/bit_manipulation/src/addition_using_bits/addition_using_bits.java",
"content": "/*\nProblem Statement : \nAdd two numbers using bitwise operators\n(i.e without using arithmetic operators)\n*/\n\nimport java.util.Scanner;\n\nclass Addition_Using_Bits\n{\n // iterative function\n static int bitwiseAddition(int n, int m)\n {\n while (m != 0)\n {\n int carry = n & m; \n n = n ^ m; \n m = carry << 1; \n }\n return n;\n }\n \n // recursive function\n static int bitwiseAdditionRecursive(int n, int m)\n {\n if (m == 0)\n return n;\n else\n {\n int carry = n & m;\n return bitwiseAdditionRecursive(n ^ m, carry << 1);\n }\n }\n \n public static void main(String args[])\n {\n Scanner in = new Scanner(System.in);\n \n int a, b;\n System.out.print(\"Enter two numbers : \");\n a = in.nextInt();\n b = in.nextInt();\n \n System.out.println(\"\\nBitwise addition using iterative function : \" + bitwiseAddition(a, b));\n System.out.println(\"Bitwise addition using recursive function : \" + bitwiseAdditionRecursive(a, b));\n }\n}\n\n/*\nEnter two numbers : 3 5\n\nBitwise addition using iterative function : 8\nBitwise addition using recursive function : 8\n*/"
}
{
"filename": "code/bit_manipulation/src/addition_using_bits/addition_using_bits.js",
"content": "function bitwiseRecursiveAddition(n, m) {\n if (m == 0) return n;\n\n let carry = n & m;\n return bitwiseRecursiveAddition(n ^ m, carry << 1);\n}\n\nfunction bitwiseIterativeAddition(n, m) {\n while (m != 0) {\n let carry = n & m;\n n ^= m;\n m = carry << 1;\n }\n return n;\n}\n\nconsole.log(bitwiseIterativeAddition(10, 20));\nconsole.log(bitwiseRecursiveAddition(30, 20));"
}
{
"filename": "code/bit_manipulation/src/addition_using_bits/addition_using_bits.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n# Function adds 2 numbers a and b without using + operator\ndef addition(a, b):\n while b != 0:\n c = a & b # carry contains set bits of both a and b\n a = a ^ b # a ^ b is equal to 1 if, the bits of a and b are different\n b = c << 1 # carry is right-shifted by 1\n return a\n\n\n# Driver code\nif __name__ == \"__main__\":\n print(addition(5, 9))\n print(addition(4, 3))"
}
{
"filename": "code/bit_manipulation/src/bit_division/bit_division.c",
"content": "#include <stdio.h>\n// Part of Cosmos by OpenGenus Foundation\nunsigned bitDivision(unsigned numerator, unsigned denominator) {\n\n unsigned current = 1;\n unsigned answer=0;\n\n if ( denominator > numerator)\n return 0;\n\n else if ( denominator == numerator)\n return 1;\n\n while (denominator < numerator) {\n denominator <<= 1;\n current <<= 1;\n }\n\n denominator >>= 1;\n current >>= 1;\n\n while (current!=0) {\n if ( numerator >= denominator) {\n numerator -= denominator;\n answer |= current;\n }\n current >>= 1;\n denominator >>= 1;\n }\n return answer;\n}\n\nvoid test(){\n int numerator = 23, denominator = 5;\n printf(\"%d\\n\", bitDivision(numerator,denominator));\n}\n\nint main() {\n test();\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/bit_division/bit_division.cpp",
"content": "/*\nProblem Statement : \nDivide two numbers using bitwise operators\n(i.e without using arithmetic operators)\n*/\n\n#include <iostream>\nusing namespace std;\n\nint bitDivision(int numerator, int denominator) \n{\n int current = 1;\n int answer = 0;\n\n if (denominator > numerator)\n return 0;\n else if (denominator == numerator)\n return 1;\n\n while (denominator < numerator) \n {\n denominator <<= 1;\n current <<= 1;\n }\n\n denominator >>= 1;\n current >>= 1;\n\n while (current != 0) \n {\n if (numerator >= denominator) \n {\n numerator -= denominator;\n answer |= current;\n }\n\n current >>= 1;\n denominator >>= 1;\n }\n\n return answer;\n}\n\nint main() \n{\n int numerator, denominator;\n // numerator -> represents dividend\n // denominator -> represents divisor\n\n cout << \"Enter numerator and denominator : \";\n cin >> numerator >> denominator;\n\n cout << \"\\nQuotient after bitwise division : \" << bitDivision(numerator, denominator) << endl;\n\n return 0;\n}\n\n/*\nEnter numerator and denominator : 10 4\n\nQuotient after bitwise division : 2\n*/"
}
{
"filename": "code/bit_manipulation/src/bit_division/bit_division.go",
"content": "package main\n// Part of Cosmos by OpenGenus Foundation\nimport (\n\t\"fmt\"\n\t\"math\"\n\t\"errors\"\n)\n\nfunc divide(dividend int, divisor int) (int, error) {\n\tvar positive bool = false\n\tvar answer int = 0\n\n\tif divisor == 0 {\n\t\treturn 0, errors.New(\"cannot divide by zero\")\n\t}\n\n\tif dividend > 0 && divisor > 0 {\n\t\tpositive = true\n\t}\n\n\tdividend = int(math.Abs(float64(dividend)))\n\tdivisor = int(math.Abs(float64(divisor)))\n\n\tfor dividend >= divisor {\n\t\ttemp, i := divisor, 1\n\t\tfor dividend >= temp {\n\t\t\tdividend -= temp\n\t\t\tanswer += i\n\t\t\ti <<= 1\n\t\t\ttemp <<= 1\n\t\t}\n\t}\n \n\tif !positive {\n\t\tanswer = -answer\n\t}\n\n\treturn answer, nil\n}"
}
{
"filename": "code/bit_manipulation/src/bit_division/bit_division.java",
"content": "import java.util.Scanner;\n// Part of Cosmos by OpenGenus Foundation\nclass BitDivision\n{\n\tstatic int division(int divident, int divisor)\n\t{\n\t\tint curr=1;\n\t\tint result=0;\t\n\t\tif ( divisor >divident)\n \t return 0;\n\n \telse if ( divisor ==divident)\n \t return 1;\n\n \twhile (divisor <divident) \n \t{\n \t divisor <<= 1;\n \t curr <<= 1;\n \t}\n\n \tdivisor >>= 1;\n \tcurr >>= 1;\n\n \t \twhile (curr!=0) \n \t \t{\n \tif (divident >= divisor) \n \t{\n \t divident -= divisor;\n \t \tresult |= curr;\n \t}\n \tcurr >>= 1;\n \tdivisor >>= 1;\n \t}\n \treturn result;\n\t}\n\n\tpublic static void main(String[]args)\n\t{\n\t\tScanner scan=new Scanner(System.in);\n\t\t//a -> represents divident\n\t\tint a=scan.nextInt();\n\t\t//b -> represents divisor\n\t\tint b=scan.nextInt();\n\t\tSystem.out.println(division(a,b));\n\t}\n}"
}
{
"filename": "code/bit_manipulation/src/bit_division/bit_division.js",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\nfunction divide(dividend, divisor) {\n if (divisor === 0) {\n return \"undefined\";\n }\n\n const isPositive = dividend > 0 && divisor > 0;\n dividend = Math.abs(dividend);\n divisor = Math.abs(divisor);\n\n var answer = 0;\n while (dividend >= divisor) {\n let temp = divisor;\n let i = 1;\n while (dividend >= temp) {\n dividend -= temp;\n answer += i;\n i <<= 1;\n temp <<= 1;\n }\n }\n\n if (!isPositive) {\n answer = -answer;\n }\n\n return answer;\n}\n\nfunction test() {\n var testCases = [[9, 4], [-10, 3], [103, -10], [-9, -4], [0, -3], [2, 0]];\n\n testCases.forEach(test =>\n console.log(`${test[0]} / ${test[1]} = ${divide(test[0], test[1])}`)\n );\n}\n\ntest();"
}
{
"filename": "code/bit_manipulation/src/bit_division/bit_division.py",
"content": "\"\"\" Part of Cosmos by OpenGenus Foundation \"\"\"\n\n\ndef divide(dividend, divisor):\n\n if divisor == 0:\n return \"undefined\"\n\n isPositive = (dividend > 0) is (divisor > 0)\n dividend = abs(dividend)\n divisor = abs(divisor)\n\n answer = 0\n while dividend >= divisor:\n temp, i = divisor, 1\n while dividend >= temp:\n dividend -= temp\n answer += i\n i <<= 1\n temp <<= 1\n\n if not isPositive:\n answer = -answer\n\n return answer\n\n\ndef test():\n\n testCases = [[9, 4], [-10, 3], [103, -10], [-9, -4], [0, -3], [2, 0]]\n\n for test in testCases:\n print(\"{:3} / {:<3} = {:<3}\".format(test[0], test[1], divide(test[0], test[1])))\n\n\ntest()"
}
{
"filename": "code/bit_manipulation/src/bit_division/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/bit_manipulation/src/byte_swapper/byte_swapper.java",
"content": "// Part of Cosmos by OpenGenus Foundation\npublic class ByteSwapper {\n\n private ByteSwapper() {\n throw new AssertionError();\n }\n\n public static short swap (short value) {\n int b1 = value & 0xFF;\n int b2 = value >> 8 & 0xFF;\n return (short) (b1 << 8 | b2 << 0);\n }\n\n public static int swap (int value) {\n int b1 = value >> 0 & 0xff;\n int b2 = value >> 8 & 0xff;\n int b3 = value >> 16 & 0xff;\n int b4 = value >> 24 & 0xff;\n return b1 << 24 | b2 << 16 | b3 << 8 | b4 << 0;\n }\n\n public static long swap (long value) {\n long b1 = value >> 0 & 0xff;\n long b2 = value >> 8 & 0xff;\n long b3 = value >> 16 & 0xff;\n long b4 = value >> 24 & 0xff;\n long b5 = value >> 32 & 0xff;\n long b6 = value >> 40 & 0xff;\n long b7 = value >> 48 & 0xff;\n long b8 = value >> 56 & 0xff;\n return b1 << 56 | b2 << 48 | b3 << 40 | b4 << 32 | b5 << 24 | b6 << 16 | b7 << 8 | b8 << 0;\n }\n\n public static float swap (float value) {\n int intValue = Float.floatToIntBits(value);\n intValue = swap(intValue);\n return Float.intBitsToFloat(intValue);\n }\n\n public static double swap (double value) {\n long longValue = Double.doubleToLongBits(value);\n longValue = swap(longValue);\n return Double.longBitsToDouble(longValue);\n }\n\n public static void swap (final short[] array) {\n if (array == null || array.length <= 0) {\n throw new IllegalArgumentException(\"Array can't be null or empty\");\n }\n for (int i = 0; i < array.length; i++) {\n array[i] = swap(array[i]);\n }\n }\n\n public static void swap (int[] array) {\n if (array == null || array.length <= 0) {\n throw new IllegalArgumentException(\"Array can't be null or empty\");\n }\n for (int i = 0; i < array.length; i++) {\n array[i] = swap(array[i]);\n }\n }\n\n public static void swap (long[] array) {\n if (array == null || array.length <= 0) {\n throw new IllegalArgumentException(\"Array can't be null or empty\");\n }\n for (int i = 0; i < array.length; i++) {\n array[i] = swap(array[i]);\n }\n }\n\n public static void swap (float[] array) {\n if (array == null || array.length <= 0) {\n throw new IllegalArgumentException(\"Array can't be null or empty\");\n }\n for (int i = 0; i < array.length; i++) {\n array[i] = swap(array[i]);\n }\n }\n\n public static void swap (double[] array) {\n if (array == null || array.length <= 0) {\n throw new IllegalArgumentException(\"Array can't be null or empty\");\n }\n for (int i = 0; i < array.length; i++) {\n array[i] = swap(array[i]);\n }\n }\n}"
}
{
"filename": "code/bit_manipulation/src/clear_bits_from_msb/clear_bits_from_msb.c",
"content": "/*\nProblem Statement :\nClear all MSB's except for the first i LSB's of a number n.\nMSB -> Most Significant Bit\nLSB -> Most Significant Bit\n*/\n\n#include <stdio.h>\n\nint clearBitsFromMsb(int n, int i)\n{\n\tint mask = (1 << i) - 1;\n\treturn n & mask;\n}\n\nint main()\n{\n\tint n, i;\n\tprintf(\"Enter n and i : \");\n\tscanf(\"%d %d\", &n, &i);\n\n\tn = clearBitsFromMsb(n, i);\n\tprintf(\"\\nResult : %d\", n);\n\n\treturn 0;\n}\n\n/*\nEnter n and i : 14 3\n\nResult : 6\n*/"
}
{
"filename": "code/bit_manipulation/src/clear_bits_from_msb/clear_bits_from_msb.cpp",
"content": "// Part of Cosmos (OpenGenus)\n#include <iostream>\n#include <bits/stdc++.h>\nusing namespace std;\n\nint clearAllBits(int n, int i){\n int mask = (1 << i) - 1;\n n = n & mask;\n\n return n;\n}\n\nint main() {\n\tint n, i;\n\tcin >> n >> i;\n\tcout<< clearAllBits(n, i) <<endl;\n\n\treturn 0;\n}"
}
{
"filename": "code/bit_manipulation/src/clear_bits_from_msb/clear_bits_from_msb.java",
"content": "/*\nProblem Statement :\nClear all MSB's except for the first i LSB's of a number n.\nMSB -> Most Significant Bit\nLSB -> Most Significant Bit \n*/\n\nimport java.util.*;\n\nclass ClearBitsFromMsb\n{ \n static int clearBitsFromMsb(int n, int i)\n {\n int mask = (1 << i) - 1;\n return n & mask;\n }\n\n public static void main(String args[])\n { \n Scanner sc = new Scanner(System.in); \n\n System.out.print(\"Enter n and i : \");\n int n = sc.nextInt(); \n int i = sc.nextInt();\n\n n = clearBitsFromMsb(n, i);\n System.out.println(\"\\nResult : \" + n); \n } \n} \n\n/*\nEnter n and i : 14 3\n\nResult : 6\n*/"
}
{
"filename": "code/bit_manipulation/src/clear_bits_from_msb/clear_bits_from_msb.py",
"content": "\"\"\"\nProblem Statement :\nClear all MSB's except for the first i LSB's of a number n.\nMSB -> Most Significant Bit\nLSB -> Most Significant Bit \n\"\"\"\n\ndef clear_bits_from_msb(n, i):\n mask = (1 << i) - 1\n return n & mask\n\nif __name__ == \"__main__\":\n print(\"Enter n and i : \")\n n, i = map(int, input().split())\n\n n = clear_bits_from_msb(n, i)\n print(\"Result : \" + str(n))\n\n\"\"\"\nEnter n and i :\n14 3\nResult : 6\n\"\"\""
}
{
"filename": "code/bit_manipulation/src/convert_number_binary/binary_to_integer.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n\ndef binary_to_int(binary_input):\n \"\"\"\n Parameters\n ----------\n binary_input : String\n Returns\n -------\n integer_output : Integer\n \"\"\"\n integer_output = 0\n \"\"\"\n it reads from the left most to the right most.\n Eventually the left-most is going to have the largest value added \n to the integer output.\n \"\"\"\n for digit in binary_input:\n integer_output = integer_output * 2 + int(digit)\n\n return integer_output\n\n\n# test\n\nsample_binary_input = \"01111101\"\nprint(\n \"Integer value of \"\n + sample_binary_input\n + \" is \"\n + str(binary_to_int(sample_binary_input))\n)"
}
{
"filename": "code/bit_manipulation/src/convert_number_binary/convert_number_binary.c",
"content": "#include <stdio.h>\n\nint main(void)\n{\n long num, decimal_num, remainder, base = 1, binary = 0, no_of_1s = 0;\n\n printf(\"Enter a decimal integer \\n\");\n scanf(\"%ld\", &num);\n decimal_num = num;\n while (num > 0)\n {\n remainder = num % 2;\n /* To count the number of ones */\n if (remainder == 1)\n {\n no_of_1s++;\n }\n binary = binary + remainder * base;\n num = num / 2;\n base = base * 10;\n }\n printf(\"Input number is = %ld\\n\", decimal_num);\n printf(\"Its binary equivalent is = %ld\\n\", binary);\n printf(\"No.of 1's in the binary number is = %ld\\n\", no_of_1s);\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/convert_number_binary/convert_number_binary.cpp",
"content": "#include <iostream>\n#include <string.h>\nusing namespace std;\n// Part of Cosmos by OpenGenus Foundation\nstring to_binary(int n)\n{\n string binary = \"\";\n while (n > 0)\n {\n if ((n & 1) == 0)\n binary = '0' + binary;\n else\n binary = '1' + binary;\n\n n >>= 1;\n }\n return binary;\n}\n\nint to_number(string s)\n{\n int number = 0;\n int n = s.length();\n for (int i = 0; i < n; i++)\n if (s[i] == '1')\n number += (1 << (n - 1 - i));\n\n return number;\n}\n\nint main()\n{\n //sample test\n string binary = to_binary(10);\n cout << binary << endl;\n\n int number = to_number(\"111\");\n cout << number << endl;\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/convert_number_binary/convert_number_binary.hs",
"content": "-- convert_number_binary.hs\n--\n-- @author Sidharth Mishra\n-- @description Program to convert Decimal numbers into Binary numbers\n-- @created Thu Oct 12 2017 17:15:23 GMT-0700 (PDT)\n-- @last-modified Thu Oct 12 2017 17:15:38 GMT-0700 (PDT)\n\nmodule NumConv (\n convDecToBin\n)\n\nwhere\n\n-- Binary is used as an type alias for [Char] or String\n-- [MSD......LSD]\ntype Binary = String\n\nnotEnoughBits :: String\nnotEnoughBits = \"Need atleast 1 bit to represent the binary nbr :)\" \n\nneedMoreBits :: String\nneedMoreBits = \"Needs more bits to convert, partially converted\"\n\nnegativeNumberNotSupported :: String\nnegativeNumberNotSupported = \"Negative Ints not supported yet\"\n\n\nconvDecToBin :: Int -> Int -> Binary\n-- Converts a Decimal positive number to its Binary equivalent\n-- first param -- number of bits in the Binary number\n-- second param -- the decimal number\n-- result -- the binary number\nconvDecToBin 0 _ = notEnoughBits\nconvDecToBin k n\n | n >= 0 = case convDecToBin' k n \"\" of \n Left s -> s\n Right b -> b\n | otherwise = negativeNumberNotSupported\n\nconvDecToBin' :: Int -> Int -> Binary -> Either String Binary\n-- private helper\nconvDecToBin' 0 n b\n | n >= 1 = Left needMoreBits\n | otherwise = return b\nconvDecToBin' k n b = convDecToBin' (k - 1) n' b'\n where n' = n `quot` 2\n r' = n `mod` 2\n b' = show r' ++ b\n\n\n-- For testing\nprocessInput :: [String] -> Maybe (Int, Int)\n-- processes the input from the console/stdin\nprocessInput [] = Nothing\nprocessInput [s] = return (32, read s::Int)\nprocessInput (s:ss) = return (k,n)\n where [k,n] = map (\\x -> read x :: Int) (s:ss)\n\nassert :: String -> String -> Bool\n-- asserts if expected and actual are equal\nassert ex ac = ex == ac\n\nmain :: IO()\nmain = do\n t <- getLine\n let (k,n) = case processInput $ words t of\n Nothing -> (32,0)\n Just (x,y) -> (x,y)\n b = convDecToBin k n\n putStrLn $ \"\\\"\" ++ b ++ \"\\\"\"\n print $ assert (convDecToBin 3 3) \"011\"\n print $ assert (convDecToBin 3 4) \"100\"\n print $ assert (convDecToBin 32 32) \"00000000000000000000000000100000\"\n print $ assert (convDecToBin 10 743) \"1011100111\"\n print $ assert (convDecToBin 2 4) needMoreBits\n print $ assert (convDecToBin 2 (-4)) negativeNumberNotSupported"
}
{
"filename": "code/bit_manipulation/src/convert_number_binary/convert_number_binary.java",
"content": "// Part of Cosmos by OpenGenus Foundation\npublic class ConvertNumberBinary {\n\tpublic static void main(String[] args) {\n\t\tString binary = toBinary(20);\n\t\tSystem.out.println(binary);\n\n\t\tint number = toNumber(\"10101\");\n\t\tSystem.out.println(number);\n\t}\n\n\tpublic static String toBinary(int n) {\n\t\tStringBuilder binary = new StringBuilder(\"\");\n\t\twhile (n > 0) {\n\t\t\tif ((n&1) == 0)\n\t\t\t\tbinary.append('0');\n\t\t\telse\n\t\t\t\tbinary.append('1');\n\n\t\t\tn >>= 1;\n\t\t}\n\t\treturn binary.toString();\n\t}\n\n\tpublic static int toNumber(String s) {\n\t\tint number = 0;\n\t\tint n = s.length();\n\t\tfor (int i = 0; i < n; i++) {\n\t\t\tif (s.charAt(i) == '1') {\n\t\t\t\tnumber += (1 << (n - 1 - i));\n\t\t\t}\n\t\t}\n\t\treturn number;\n\t}\n}"
}
{
"filename": "code/bit_manipulation/src/convert_number_binary/convert_number_binary.js",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n//Using built in functions:\n\nfunction toBinary(val) {\n return val.toString(2);\n}\n\nfunction fromBinary(bitString) {\n return parseInt(bitString, 2);\n}\n\n//Using bitshifts\n\nfunction toBinary_B(val) {\n let out = \"\";\n while (val > 0) {\n out = (val & 1) + out;\n val >>= 1;\n }\n return out;\n}\n\nfunction fromBinary_B(bitString) {\n let out = 0;\n let l = bitString.length;\n let i = 0;\n for (; i < l; i++) {\n out += +bitString[i] ? 1 << (l - i - 1) : 0;\n }\n return out;\n}"
}
{
"filename": "code/bit_manipulation/src/convert_number_binary/convert_number_binary.php",
"content": "<?php\n\n/**\n * Part of Cosmos by OpenGenus Foundation\n */\n\n/**\n * Converts binary number to decimal number\n *\n * @param int $bin binary number\n * @return int decimal number\n */\nfunction binary_to_decimal(int $bin)\n{\n $arr = array_map('intval', str_split($bin));\n $cnt = count($arr);\n $dec = 0;\n for ($i = 1; $i < $cnt + 1; $i++) {\n $dec += $arr[$i - 1] * (2 ** ($cnt - $i));\n }\n return $dec;\n}\n\n\n/**\n * Converts decimal number to binary number\n *\n * @param int decimal number\n * @return int $bin binary number\n */\nfunction decimal_to_binary(int $dec)\n{\n $i = 1;\n $bin = 0;\n while ($dec > 0) {\n $rem = $dec % 2;\n $bin = $bin + ($i * $rem);\n $dec = floor($dec / 2);\n $i *= 10;\n }\n return $bin;\n}\n\necho \"binary_to_decimal\\n\";\n$test_data = [\n [0, 0],\n [1, 1],\n [10, 2],\n [101, 5],\n [1001, 9],\n [1010, 10],\n [1001001010100, 4692],\n [1001011110100, 4852],\n];\nforeach ($test_data as $test_case) {\n $input = $test_case[0];\n $expected = $test_case[1];\n $result = binary_to_decimal($input);\n printf(\n \" input %d, expected %s, got %s: %s\\n\",\n $input,\n var_export($expected, true),\n var_export($result, true),\n ($expected === $result) ? 'OK' : 'FAIL'\n );\n}\n\necho \"decimal_to_binary\\n\";\n$test_data = [\n [0, 0],\n [1, 1],\n [2, 10],\n [5, 101],\n [9, 1001],\n [10, 1010],\n [4692, 1001001010100],\n [4852, 1001011110100],\n];\nforeach ($test_data as $test_case) {\n $input = $test_case[0];\n $expected = $test_case[1];\n $result = decimal_to_binary($input);\n printf(\n \" input %d, expected %s, got %s: %s\\n\",\n $input,\n var_export($expected, true),\n var_export($result, true),\n ($expected === $result) ? 'OK' : 'FAIL'\n );\n}"
}
{
"filename": "code/bit_manipulation/src/convert_number_binary/convert_number_binary.py",
"content": "# part of Cosmos by OpenGenus Foundation\n\nimport math\n\n\ndef intToBinary(i):\n if i == 0:\n return \"0\"\n s = \"\"\n while i:\n if i % 2 == 1:\n s = \"1\" + s\n else:\n s = \"0\" + s\n i /= 2\n return s\n\n\n# sample test\nn = 741\nprint(intToBinary(n))"
}
{
"filename": "code/bit_manipulation/src/convert_number_binary/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\n## Package: Convert number to binary\nPrograms in this folder are about converting numbers from `base 10`(decimal) to `base 2`(binary)\n\n### Examples:\n\n----------------\n| Decimal | Binary |\n| :---: | :---: |\n| 0 | 0 |\n| 1 | 1 |\n| 2 | 10 |\n| 3 | 11 |\n| 4 | 100 |\n| 16 | 10000 |\n----------------\n\n### Main idea:\nBinary numbers have `2` permissible digits `0` **and** `1`.\n\n### How to convert?\nLet `n` be a decimal number.\n\nLet `k` denote the `k` number of bits in the binary number `b`.\n\n`mod` is the modulus function, divides the left operand with right operand and provides just the remainder.\n\n\n`quot` is the quotient function, divides left operand by right operand and provides just the quotient.\n\n* step 1\n\n n `mod` 2 = b<sub>k - 1</sub>\n\n n `quot` 2 = n<sub>1</sub>\n\n* step 2\n\n n<sub>1</sub> `mod` 2 = b<sub>k-2</sub>\n\n n<sub>1</sub> `quot` 2 = n<sub>2</sub>\n\n... (continue till final step)\n\n* final step\n\n n<sub>k - 1</sub> `mod` 2 = b<sub>0</sub>\n\n n<sub>k - 1</sub> `quot` 2 = 0\n\n\nLets walk with example of converting number `5` into a `8-bit` binary number:\n\nn = 5, k = 8\n\n---\n\n5 `mod` 2 = 1 -- b<sub>7</sub>\n\n5 `quot` 2 = 2\n\n---\n\n2 `mod` 2 = 0 -- b<sub>6</sub>\n\n2 `quot` 2 = 1\n\n---\n\n1 `mod` 2 = 1 -- b<sub>5</sub>\n\n1 `quot` 2 = 0 -- calc ends(end), all 0s after this point\n\n---\n\n0 `mod` 2 = 0 -- b<sub>4</sub>\n\n0 `quot` 2 = 0\n\n---\n\n0 `mod` 2 = 0 -- b<sub>3</sub>\n\n0 `quot` 2 = 0\n\n---\n\n0 `mod` 2 = 0 -- b<sub>2</sub>\n\n0 `quot` 2 = 0\n\n---\n\n0 `mod` 2 = 0 -- b<sub>1</sub>\n\n0 `quot` 2 = 0\n\n---\n\n0 `mod` 2 = 0 -- b<sub>0</sub>\n\n0 `quot` 2 = 0\n\n---\n\nb = [0, 0, 0, 0, 0, 1, 0, 1] -- \"00000101\"\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/bit_manipulation/src/count_set_bits/brian_kernighan_algo/brian_kernighan_algorithm.cpp",
"content": "//Brian Kernighan’s Algorithm. This programme uses O(logn) to count set bits.\n\n#include <iostream> \n \nint countSetBits(int n) \n{ \n\t// base case \n\tif (n == 0)\n\t{ \n\t\treturn 0; \n \t}\n\telse\n\t{\n\t\t// if last bit is set, add 1 else add 0 \n\t\treturn (n & 1) + countSetBits(n >> 1);\n\t} \n} \n\nint main() \n{ \n\tint n;\n\tstd::cout << \"Enter a positive integer : \";\n\tstd::cin >> n;\n\tstd::cout << countSetBits(n); \n\n\treturn 0; \n}"
}
{
"filename": "code/bit_manipulation/src/count_set_bits/count_set_bits.c",
"content": "#include <stdio.h>\n// Part of Cosmos by OpenGenus Foundation\nint count_set_bits(int n){\n\tint c = 0;\n\twhile (n) {\n\t\tc++;\n\t\tn&=(n-1);\n\t}\n\treturn c;\n}\n\nint main() {\t\n\tint n;\n\tscanf(\"%d\", &n);\n\tprintf(\"%d\\n\", count_set_bits(n));\n\treturn 0;\n}"
}
{
"filename": "code/bit_manipulation/src/count_set_bits/count_set_bits.cpp",
"content": "#include <iostream>\nusing namespace std;\n// Part of Cosmos by OpenGenus Foundation\nint count(int n)\n{\n int c = 0;\n while (n)\n {\n c++;\n n &= (n - 1);\n }\n return c;\n}\n\nint main()\n{\n\n int n;\n cin >> n;\n\n #ifdef BUILTIN\n cout << __builtin_popcount(n); // use builtin popcount\n #else\n cout << count(n); // manual\n #endif\n\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/count_set_bits/count_set_bits.cs",
"content": "using System;\n\nnamespace CountSetBits\n{\n class Program\n {\n static internal int countSetBits(int number)\n {\n string bin = Convert.ToString(number, 2); // getting binary number\n int count = 0;\n foreach (char bit in bin)\n {\n if(bit == '1')\n count++;\n }\n return count;\n }\n\n static public void Main()\n {\n Console.WriteLine(countSetBits(13));\n }\n }\n}"
}
{
"filename": "code/bit_manipulation/src/count_set_bits/count_set_bits.java",
"content": "// Part of Cosmos by OpenGenus Foundation \n\n/* \n**\n** @AUTHOR: VINAY BADHAN\n** @GREEDY PROBLEM: COUNT SET BITS\n** @GITHUB LINK: https://github.com/vinayb21\n*/\n\nimport java.util.*;\nimport java.io.*;\n\nclass CountSetBits {\n \n public static int countSetBits(int n)\n {\n int count = 0,bit;\n while(n>0)\n {\n bit = n&1;\n if(bit==1)\n count++;\n n >>= 1;\n }\n return count;\n }\n \n /**\n * Counts the number of set bits in parallel.\n * @param n 32-bit integer whose set bits are to be counted\n * @return the number of set bits in (n)\n * @see <a href=\"https://graphics.stanford.edu/~seander/bithacks.html#CountBitsSetParallel\">Bit Twiddling Hacks</a>\n */\n public static int countSetBitsParallel(int n)\n {\n final int[] S = {\n 1,\n 2,\n 4,\n 8,\n 16\n };\n\n final int[] B = {\n 0x55555555,\n 0x33333333,\n 0x0F0F0F0F,\n 0x00FF00FF,\n 0x0000FFFF\n };\n\n int C = n - ((n >>> 1) & B[0]);\n C = ((C >>> S[1]) & B[1]) + (C & B[1]);\n C = ((C >>> S[2]) + C) & B[2];\n C = ((C >>> S[3]) + C) & B[3];\n C = ((C >>> S[4]) + C) & B[4];\n return C;\n }\n \n\n public static void main(String[] args) throws IOException {\n int n;\n \tInputStreamReader in = new InputStreamReader(System.in);\n \tBufferedReader buffer = new BufferedReader(in);\n \tPrintWriter out = new PrintWriter(System.out, true);\n \tString line = buffer.readLine().trim();\n \tn = Integer.parseInt(line);\n \tint ans = countSetBitsParallel(n);\n \tout.println(\"No. of set bits in \"+n+\" is \"+ans);\n }\n}"
}
{
"filename": "code/bit_manipulation/src/count_set_bits/count_set_bits.js",
"content": "// Part of Cosmos by OpenGenus Foundation\n\nexport default function countSetBits(n) {\n n = +n;\n\n if (!(n >= 0 || n < 0)) {\n return 0;\n } else if (n < 0) {\n throw new Error(\"negtive numbers are not supported\");\n }\n\n let cnt = 0;\n while (n) {\n cnt++;\n n &= n - 1;\n }\n\n return cnt;\n}"
}
{
"filename": "code/bit_manipulation/src/count_set_bits/count_set_bits_lookup_table.cpp",
"content": "// Part of Cosmos by OpenGenus Foundation\n#include <vector>\n#include <iostream>\n#include <sstream>\n#include <algorithm>\n#include <random>\n#include <chrono>\n\n// I'm not crazy, I generated this lookup table with a Ruby script ;)\nconst unsigned bits[] = {\n 0, // 0\n 1, // 1\n 1, // 10\n 2, // 11\n 1, // 100\n 2, // 101\n 2, // 110\n 3, // 111\n 1, // 1000\n 2, // 1001\n 2, // 1010\n 3, // 1011\n 2, // 1100\n 3, // 1101\n 3, // 1110\n 4, // 1111\n 1, // 10000\n 2, // 10001\n 2, // 10010\n 3, // 10011\n 2, // 10100\n 3, // 10101\n 3, // 10110\n 4, // 10111\n 2, // 11000\n 3, // 11001\n 3, // 11010\n 4, // 11011\n 3, // 11100\n 4, // 11101\n 4, // 11110\n 5, // 11111\n 1, // 100000\n 2, // 100001\n 2, // 100010\n 3, // 100011\n 2, // 100100\n 3, // 100101\n 3, // 100110\n 4, // 100111\n 2, // 101000\n 3, // 101001\n 3, // 101010\n 4, // 101011\n 3, // 101100\n 4, // 101101\n 4, // 101110\n 5, // 101111\n 2, // 110000\n 3, // 110001\n 3, // 110010\n 4, // 110011\n 3, // 110100\n 4, // 110101\n 4, // 110110\n 5, // 110111\n 3, // 111000\n 4, // 111001\n 4, // 111010\n 5, // 111011\n 4, // 111100\n 5, // 111101\n 5, // 111110\n 6, // 111111\n 1, // 1000000\n 2, // 1000001\n 2, // 1000010\n 3, // 1000011\n 2, // 1000100\n 3, // 1000101\n 3, // 1000110\n 4, // 1000111\n 2, // 1001000\n 3, // 1001001\n 3, // 1001010\n 4, // 1001011\n 3, // 1001100\n 4, // 1001101\n 4, // 1001110\n 5, // 1001111\n 2, // 1010000\n 3, // 1010001\n 3, // 1010010\n 4, // 1010011\n 3, // 1010100\n 4, // 1010101\n 4, // 1010110\n 5, // 1010111\n 3, // 1011000\n 4, // 1011001\n 4, // 1011010\n 5, // 1011011\n 4, // 1011100\n 5, // 1011101\n 5, // 1011110\n 6, // 1011111\n 2, // 1100000\n 3, // 1100001\n 3, // 1100010\n 4, // 1100011\n 3, // 1100100\n 4, // 1100101\n 4, // 1100110\n 5, // 1100111\n 3, // 1101000\n 4, // 1101001\n 4, // 1101010\n 5, // 1101011\n 4, // 1101100\n 5, // 1101101\n 5, // 1101110\n 6, // 1101111\n 3, // 1110000\n 4, // 1110001\n 4, // 1110010\n 5, // 1110011\n 4, // 1110100\n 5, // 1110101\n 5, // 1110110\n 6, // 1110111\n 4, // 1111000\n 5, // 1111001\n 5, // 1111010\n 6, // 1111011\n 5, // 1111100\n 6, // 1111101\n 6, // 1111110\n 7, // 1111111\n 1, // 10000000\n 2, // 10000001\n 2, // 10000010\n 3, // 10000011\n 2, // 10000100\n 3, // 10000101\n 3, // 10000110\n 4, // 10000111\n 2, // 10001000\n 3, // 10001001\n 3, // 10001010\n 4, // 10001011\n 3, // 10001100\n 4, // 10001101\n 4, // 10001110\n 5, // 10001111\n 2, // 10010000\n 3, // 10010001\n 3, // 10010010\n 4, // 10010011\n 3, // 10010100\n 4, // 10010101\n 4, // 10010110\n 5, // 10010111\n 3, // 10011000\n 4, // 10011001\n 4, // 10011010\n 5, // 10011011\n 4, // 10011100\n 5, // 10011101\n 5, // 10011110\n 6, // 10011111\n 2, // 10100000\n 3, // 10100001\n 3, // 10100010\n 4, // 10100011\n 3, // 10100100\n 4, // 10100101\n 4, // 10100110\n 5, // 10100111\n 3, // 10101000\n 4, // 10101001\n 4, // 10101010\n 5, // 10101011\n 4, // 10101100\n 5, // 10101101\n 5, // 10101110\n 6, // 10101111\n 3, // 10110000\n 4, // 10110001\n 4, // 10110010\n 5, // 10110011\n 4, // 10110100\n 5, // 10110101\n 5, // 10110110\n 6, // 10110111\n 4, // 10111000\n 5, // 10111001\n 5, // 10111010\n 6, // 10111011\n 5, // 10111100\n 6, // 10111101\n 6, // 10111110\n 7, // 10111111\n 2, // 11000000\n 3, // 11000001\n 3, // 11000010\n 4, // 11000011\n 3, // 11000100\n 4, // 11000101\n 4, // 11000110\n 5, // 11000111\n 3, // 11001000\n 4, // 11001001\n 4, // 11001010\n 5, // 11001011\n 4, // 11001100\n 5, // 11001101\n 5, // 11001110\n 6, // 11001111\n 3, // 11010000\n 4, // 11010001\n 4, // 11010010\n 5, // 11010011\n 4, // 11010100\n 5, // 11010101\n 5, // 11010110\n 6, // 11010111\n 4, // 11011000\n 5, // 11011001\n 5, // 11011010\n 6, // 11011011\n 5, // 11011100\n 6, // 11011101\n 6, // 11011110\n 7, // 11011111\n 3, // 11100000\n 4, // 11100001\n 4, // 11100010\n 5, // 11100011\n 4, // 11100100\n 5, // 11100101\n 5, // 11100110\n 6, // 11100111\n 4, // 11101000\n 5, // 11101001\n 5, // 11101010\n 6, // 11101011\n 5, // 11101100\n 6, // 11101101\n 6, // 11101110\n 7, // 11101111\n 4, // 11110000\n 5, // 11110001\n 5, // 11110010\n 6, // 11110011\n 5, // 11110100\n 6, // 11110101\n 6, // 11110110\n 7, // 11110111\n 5, // 11111000\n 6, // 11111001\n 6, // 11111010\n 7, // 11111011\n 6, // 11111100\n 7, // 11111101\n 7, // 11111110\n 8, // 11111111\n};\n\nunsigned long long popcount_naive(unsigned size, uint16_t* data)\n{\n unsigned long long acc = 0;\n\n for (unsigned i = 0; i < size; ++i)\n {\n uint16_t num = data[i];\n while (num)\n {\n // Naive approach: Get the last bit and shift the number\n acc += num & 1;\n num = num >> 1;\n }\n }\n\n return acc;\n}\n\nunsigned long long popcount_lookup_table(unsigned size, uint8_t* data)\n{\n unsigned long long acc = 0;\n\n for (unsigned i = 0; i < size; ++i)\n // Look it up!\n acc += bits[data[i]];\n\n return acc;\n}\n\nunsigned long long popcount_builtin(unsigned size, uint64_t* data)\n{\n unsigned long long acc = 0;\n\n for (unsigned i = 0; i < size; ++i)\n // https://gcc.gnu.org/onlinedocs/gcc/Other-Builtins.html\n // This is really fast if your CPU has a dedicated instruction (and it should)\n acc += __builtin_popcountll(data[i]);\n\n return acc;\n}\n\n// Usage:\n// ./count_set_bits_lookup_table <amount of random numbers>, e.g:\n// ./count_set_bits_lookup_table 2017\n// -> Naive approach: 7962 in 107μs\n// -> Lookup table: 7962 in 20μs\n// -> GCC builtin: 7962 in 5μs\n\nint main(int argc, char* argv[])\n{\n if (argc < 2)\n return -1;\n\n unsigned amount;\n std::stringstream ss;\n ss << argv[1];\n ss >> amount;\n\n // we want length divisible by 4\n unsigned length = amount + (-amount) % 4;\n\n uint16_t* numbers = new uint16_t[length];\n\n std::default_random_engine generator;\n std::uniform_int_distribution<uint16_t> dist(0, 255);\n\n for (unsigned n = 0; n < amount; ++n)\n numbers[n] = dist(generator);\n // pad with zeros\n for (unsigned n = amount; n < length; ++n)\n numbers[n] = 0;\n\n unsigned long long count;\n\n std::chrono::high_resolution_clock a_clock;\n auto start = a_clock.now();\n count = popcount_naive(amount, numbers);\n auto end = a_clock.now();\n std::cout << \"Naive approach: \" << count << \" in \" <<\n std::chrono::duration_cast<std::chrono::microseconds>(end - start).count() << \"μs\" <<\n std::endl;\n\n start = a_clock.now();\n count = popcount_lookup_table(2 * amount, (uint8_t*)(numbers));\n end = a_clock.now();\n std::cout << \"Lookup table: \" << count << \" in \" <<\n std::chrono::duration_cast<std::chrono::microseconds>(end - start).count() << \"μs\" <<\n std::endl;\n\n start = a_clock.now();\n count = popcount_builtin(length / 4, (uint64_t*)(numbers));\n end = a_clock.now();\n std::cout << \"GCC builtin: \" << count << \" in \" <<\n std::chrono::duration_cast<std::chrono::microseconds>(end - start).count() << \"μs\" <<\n std::endl;\n}"
}
{
"filename": "code/bit_manipulation/src/count_set_bits/count_set_bits.py",
"content": "# Part of Cosmos by OpenGenus Foundation\ndef count_set_bit(number):\n count = 0\n\n while number:\n count = count + (number & 1)\n number = number >> 1\n\n return count\n\n\nprint(count_set_bit(9))"
}
{
"filename": "code/bit_manipulation/src/count_set_bits/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "",
"content": ""
}
{
"filename": "code/bit_manipulation/src/first_set_bit/first_set_bit.cpp",
"content": "// Part of Cosmos (OpenGenus)\n#include <bits/stdc++.h>\nusing namespace std;\n\nint returnFirstSetBit(int n)\n{\n if(n == 0)\n return 0;\n int position = 1;\n int m = 1;\n\n while (!(n & m))\n {\n m = m << 1;\n position++;\n }\n return (1 << (position - 1));\n}\n\nint main()\n{\n int n;\n cin >> n;\n cout << returnFirstSetBit(n) << endl;\n\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/flip_bits/flipbits.java",
"content": "// Part of Cosmos by OpenGenus Foundation \n\n/* \n**\n** @AUTHOR: VINAY BADHAN\n** @BIT MANIPULATION PROBLEM: FLIP BITS\n** @GITHUB LINK: https://github.com/vinayb21\n*/\n\nimport java.util.*;\nimport java.io.*;\n\nclass FlipBits {\n \n public static int flipBits(int n)\n {\n int flipN = 0;\n int pow = 0;\n\n while(n>0)\n {\n if(n%2==0)\n flipN += (1 << pow);\n n/=2;\n pow++;\n }\n return flipN;\n }\n\n public static void main(String[] args) throws IOException {\n \tInputStreamReader in = new InputStreamReader(System.in);\n \tBufferedReader buffer = new BufferedReader(in);\n \tString line = buffer.readLine().trim();\n \tint n = Integer.parseInt(line);\n \tint ans = flipBits(n);\n \tSystem.out.println(\"Number obtained by flipping bits of \"+n +\" is \"+ans);\n }\n}"
}
{
"filename": "code/bit_manipulation/src/flip_bits/flippingbits.c",
"content": "#include <stdio.h>\n// Part of Cosmos by OpenGenus Foundation\nint main() {\n int count;\n unsigned int to_flip;\n unsigned int flipped;\n printf(\"How many flips? \");\n scanf(\"%d\", &count);\n\n while(count--) {\n printf(\"To flip: \");\n scanf(\"%d\", &to_flip);\n flipped = ~to_flip;\n printf(\"Flipped: %d\\n\", flipped);\n }\n}"
}
{
"filename": "code/bit_manipulation/src/flip_bits/flippingbits.cpp",
"content": "#include <iostream>\n// Part of Cosmos by OpenGenus Foundation\nusing namespace std;\n\n\nint main()\n{\n int T;\n unsigned int n;\n cin >> T;\n\n while (T--)\n {\n cin >> n;\n cout << ~n << endl;\n }\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/flip_bits/flippingbits.py",
"content": "#! /usr/bin/env python3\n# Part of Cosmos by OpenGenus Foundation\ndef main():\n count = int(input(\"How many flips? \"))\n\n while count > 0:\n to_flip = int(input(\"To flip: \"))\n flipped = ~to_flip\n print(\"Flipped:\", flipped)\n count -= 1\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/bit_manipulation/src/flip_bits/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/bit_manipulation/src/hamming_distance/hamming_distance2.py",
"content": "def main():\n num = input(\"enter a number\")\n return (\"{0:08b}\".format(x)).count(\"1\")\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/bit_manipulation/src/hamming_distance/hamming_distance.c",
"content": "#include<stdio.h>\n#define BITS 8\n\nvoid hamming(int ar1[],int ar2[]);\nvoid input(int ar1[]);\nint count_ham(int ar[]);\nint n;\n\nint main(){\n\tint ar1[BITS],ar2[BITS];\n\tprintf(\"Enter the number of bits(max 8-bits):\");\n\tscanf(\"%d\",&n);\n\tprintf(\"Enter a binary number(space between each bit and MAX 8-bit):\");\n\tinput(ar1);\n\tprintf(\"Enter a binary number(space between each bit and MAX 8-bit):\");\n\tinput(ar2);\n\thamming(ar1,ar2);\n\treturn 0;\n}\n\nvoid input(int ar1[]){\n\tint i;\n\tfor(i=0;i<n;i++){\n\t\tscanf(\"%d\",&ar1[i]);\n\t}\n\n}\nint count_ham(int ar[]){\n\tint i,count=0;\n\tfor(i=0;i<n;i++){\n\t\tif(ar[i]==1)\n\t\t\tcount++;\n\t}\n\treturn count;\n\n}\nvoid hamming(int ar1[],int ar2[]){\n\tint i,count;\n\tint res[BITS];\n\tfor(i=0;i<n;i++){\n\t\tif((ar1[i]==1 && ar2[i]==0)||(ar1[i]==0 && ar2[i]==1)){\n\t\t\tres[i] = 1;\n\t\t}\n\t\telse{\n\t\t\tres[i] = 0;\n\t\t}\n\t}\n\tcount = count_ham(res);\n\tprintf(\"Hamming distance will be: %d\",count);\n\tprintf(\"\\n\");\n}"
}
{
"filename": "code/bit_manipulation/src/hamming_distance/hamming_distance.cpp",
"content": "/*\n *\n * Part of Cosmos by OpenGenus Foundation\n *\n * The Hamming distance between two integers is the number of positions\n * at which the corresponding bits are different.\n *\n * Given two integers x and y, calculate the Hamming distance.\n */\n\nint hammingDistance(int x, int y)\n{\n int temp = x ^ y;\n int count = 0;\n //count the number of set bits in the xor of two numbers\n while (temp)\n {\n temp = temp & (temp - 1);\n count++;\n }\n return count;\n}"
}
{
"filename": "code/bit_manipulation/src/hamming_distance/hamming_distance.go",
"content": "package main\n\nimport \"fmt\"\n\n// Hamming distance between two integers is the number of positions\n// at which the corresponding bits are different.\nfunc HammingDistance(x, y int) (count int) {\n\tt := x ^ y\n\n\t//count the number of set bits in the xor of two numbers\n\tfor t != 0 {\n\t\tt = t & (t - 1)\n\t\tcount++\n\t}\n\treturn\n}\n\nfunc main() {\n\tfmt.Printf(\"Hamming distance beetwen (%d) and (%d) is (%d)\\n\", 1, 2, HammingDistance(1, 2))\n}"
}
{
"filename": "code/bit_manipulation/src/hamming_distance/hamming_distance.java",
"content": "// Part of Cosmos by OpenGenus Foundation\n\n// The Hamming distance between two integers is the number of positions \n// at which the corresponding bits are different.\n\nimport java.io.*;\nimport java.lang.*;\nimport java.math.*;\nimport java.util.*;\n\nclass HammingDistance {\n\tprivate int hammingDistance(int x, int y) {\n\t\tint temp = x^y;\n\t\tint count = 0;\n\t\t\n\t\t//count the number of set bits in the xor of two numbers\n\t\twhile(temp != 0){\n\t\t\ttemp = temp&(temp-1);\n\t\t\tcount++;\n\t\t}\n\t\treturn count;\n\t}\n\t\n\tpublic static void main(String args[]) {\n\t\tHammingDistance ob = new HammingDistance();\n\t\tint testHammingDistance = ob.hammingDistance(2,5);\n\t\tSystem.out.println(testHammingDistance);\n\t}\n}"
}
{
"filename": "code/bit_manipulation/src/hamming_distance/hamming_distance.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n# The Hamming distance between two integers is the number of positions\n# at which the corresponding bits are different.\n\n\ndef hammingDistance(x, y):\n res = x ^ y\n ans = 0\n while res:\n ans += 1\n res = res & (res - 1)\n return ans\n\n\nprint(hammingDistance(2, 1))"
}
{
"filename": "code/bit_manipulation/src/hamming_distance/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/bit_manipulation/src/invert_bit/invert_bit.c",
"content": "#include <stdio.h>\n\n\n//Part of Cosmos by OpenGenus Foundation\nint\ninvert_bit(int n, int number_bit)\n{\n n ^= 1 << number_bit;\n return (n);\n}\n\nint\nmain()\n{\n int numeric, number_bit, res;\n printf(\"Enter numeric: \");\n scanf(\"%d\", &numeric);\n printf(\"Enter number bit: \");\n scanf(\"%d\", &number_bit);\n res = invert_bit(numeric, number_bit);\n printf(\"Result: %d\\n\", res);\n return (0);\n}"
}
{
"filename": "code/bit_manipulation/src/invert_bit/invert_bit.cpp",
"content": "#include <iostream>\n#include <bitset>\n\n\n//Part of Cosmos by OpenGenus Foundation\nint invertBit(int n, int numBit)\n{\n n ^= 1 << numBit;\n return n;\n}\n\nint main()\n{\n int numeric, numberBit, res;\n std::cout << \"Enter numeric: \" << std::endl;\n std::cin >> numeric;\n std::bitset<32> bs(numeric);\n std::cout << bs << '\\n';\n std::cout << \"Enter number bit: \" << std::endl;\n std::cin >> numberBit;\n res = invertBit(numeric, numberBit);\n std::bitset<32> bsRes(res);\n std::cout << res << std::endl;\n std::cout << bsRes << std::endl;\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/invert_bit/invert_bit.js",
"content": "const invertBit = (num, bit) => num ^ (1 << bit);\n\nconsole.log(invertBit(10, 5)); // 42"
}
{
"filename": "code/bit_manipulation/src/invert_bit/invert_bit.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n\ndef invert_bit(digit, number_bit):\n digit ^= 1 << number_bit\n return digit\n\n\nnumber = int(input(\"Enter numeric: \"))\nnum_bit = int(input(\"Enter number bit: \"))\nres = invert_bit(number, num_bit)\nprint(res)"
}
{
"filename": "code/bit_manipulation/src/invert_bit/invert_bit.rs",
"content": "// Part of Cosmos by OpenGenus Foundation\n\nuse std::{io::{self, Write}, process};\n\n/// Invert a bit at a given place\n///\n/// # Arguments\n///\n/// * `bits` - The value/bits you want to modify\n///\n/// * `place` - The position of the bit you want to invert\n///\n/// # Examples\n/// ```\n/// let value = 50; // 110010\n/// let place = 2; // ^ 000100\n/// let new_value = invert_bit(value, place); // = 110110\n/// ```\nfn invert_bit(bits: u32, place: u8) -> u32 {\n bits ^ 1 << place\n}\n\nfn main() {\n // Get value\n print!(\"enter a value: \");\n io::stdout().flush().unwrap();\n\n let mut value = String::new();\n io::stdin().read_line(&mut value).unwrap_or_else(|_| {\n eprintln!(\"error: unable to read input (value)\");\n process::exit(1);\n });\n let value = value.trim();\n\n let value = value.parse::<u32>().unwrap_or_else(|_| {\n eprintln!(\"error: invalid input (value must be unsigned 32-bit integer)\");\n process::exit(1);\n });\n\n // Get place\n print!(\"enter a place: \");\n io::stdout().flush().unwrap();\n\n let mut place = String::new();\n io::stdin().read_line(&mut place).unwrap_or_else(|_| {\n eprintln!(\"error: unable to read input (place)\");\n process::exit(1);\n });\n let place = place.trim();\n\n let place = place.parse::<u8>().unwrap_or_else(|_| {\n eprintln!(\"error: invalid input (place must be unsigned 8-bit integer)\");\n process::exit(1);\n });\n\n if place > 31 {\n eprintln!(\"error: left bit shift overflow (place must be between 0 inclusive and 31 inclusive)\");\n process::exit(1);\n }\n\n // Invert bit\n let new_value = invert_bit(value, place);\n println!(\"{:>8}: {:032b}\", \"input\", value);\n println!(\"{:>8}: {:032b}\", \"output\", new_value);\n}"
}
{
"filename": "code/bit_manipulation/src/lonely_integer/lonely_integer.c",
"content": "/*\n *Part of Cosmos by OpenGenus Foundation\n *The Lonely Integer Problem\n *Given an array in which all the no. are present twice except one, find that lonely integer.\n */\n\n#include <stdio.h>\n\nint a[7] = {0,0,1,1,3,3,2};\n\n\nint \nlonely(int n) {\n\tint no=0;\n\n\tfor(int i=0;i<n;i++) {\n\t\tno^=a[i];\n\t}\n\n\treturn (no);\n}\n\nint \nmain() \n{\n\tint n=sizeof(a)/sizeof(int);\n\tprintf(\"Lonely Integer is %d\\n\",lonely(n));\n\n\treturn (0);\n}"
}
{
"filename": "code/bit_manipulation/src/lonely_integer/lonely_integer.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n * The Lonely Integer Problem\n * Given an array in which all the no. are present twice except one, find that lonely integer.\n */\n\n#include <iostream>\nusing namespace std;\n\nint LonelyInteger(int *a, int n)\n{\n int lonely = 0;\n //finds the xor sum of the array.\n for (int i = 0; i < n; i++)\n lonely ^= a[i];\n return lonely;\n}\n\nint main()\n{\n int a[] = {2, 3, 4, 5, 3, 2, 4};\n cout << LonelyInteger(a, sizeof(a) / sizeof(a[0]));\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/lonely_integer/lonely_integer.go",
"content": "/*\nPart of Cosmos by OpenGenus Foundation\nThe Lonely Integer Problem\nGiven an array in which all the no. are present twice except one, find that lonely integer.\n*/\n\npackage main\n\nimport \"fmt\"\n\nfunc LonelyInteger(a []int) int{\n\tlonely := 0\n\tfor i := 0; i < len(a); i++ {\n\t\tlonely ^= a[i]\n\t}\n\treturn lonely\n}\n\nfunc main() {\n\ta := []int{2,3,4,5,3,2,4}\n\tfmt.Println(LonelyInteger(a))\n}"
}
{
"filename": "code/bit_manipulation/src/lonely_integer/lonely_integer.java",
"content": "/*\n* Part of Cosmos by OpenGenus Foundation\n* The Lonely Integer Problem\n* Given an array in which all the no. are present twice except one, find that lonely integer.\n*/\n\npublic class LonelyInt{\n public static void main(String args[]){\n int[] testArr = {1,1,2,4,2};\n System.out.println(lonelyInt(testArr));\n }\n public static int lonelyInt(int[] arr){\n int lonely = 0;\n for(int i : arr){\n lonely ^= i;\n }\n return lonely;\n }\n}"
}
{
"filename": "code/bit_manipulation/src/lonely_integer/lonely_integer.js",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n * The Lonely Integer Problem\n * Given an array in which all the no. are present twice except one, find that lonely integer.\n */\nfunction lonelyInt(arr) {\n var lonely = 0;\n arr.forEach(number => {\n lonely ^= number;\n });\n return lonely;\n}\n\nfunction test() {\n var test = [1, 1, 2, 4, 2];\n console.log(lonelyInt(test));\n}\n\ntest();"
}
{
"filename": "code/bit_manipulation/src/lonely_integer/lonely_integer.py",
"content": "\"\"\"\nPart of Cosmos by OpenGenus Foundation\nThe Lonely Integer Problem\nGiven an array in which all the no. are present twice except one, find that lonely integer.\n\"\"\"\n\n\ndef LonelyInteger(a):\n lonely = 0\n # finds the xor sum of the array.\n for i in a:\n lonely ^= i\n return lonely\n\n\na = [2, 3, 4, 5, 3, 2, 4]\nprint(LonelyInteger(a))"
}
{
"filename": "code/bit_manipulation/src/lonely_integer/lonely_integer.rs",
"content": "/*\n* Part of Cosmos by OpenGenus Foundation\n*/\nfn lonely_integer(a: &[i64]) -> i64 {\n let mut lonely = 0;\n \n for i in a {\n lonely ^= *i;\n }\n \n lonely\n}\n\nfn main() {\n let a = [2, 3, 4, 5, 3, 2, 4];\n println!(\"{}\", lonely_integer(&a));\n}"
}
{
"filename": "code/bit_manipulation/src/lonely_integer/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/bit_manipulation/src/magic_number/magic_number.c",
"content": "#include <stdio.h>\n\n// Find the nth magic number where n is a positive number\n// @param n: the nth number in the sequence to be calculated and returned\n// @param fac: factor level. \n// @return: an integer where it is the nth number that can be expressed as a power or sum of the factor level\nint \nmagicNumber(int n, int fac)\n{\n int answer = 0;\n int expo = 1;\n\n while (n != 0)\n {\n expo = expo * fac;\n if (n & 1)\n answer += expo;\n n = n >> 1; \n }\n return answer;\n}\n\nint \nmain()\n{\n int n, f;\n printf(\"n: \\n>> \");\n scanf(\"%d\", &n);\n printf(\"factor: \\n>> \");\n scanf(\"%d\", &f);\n \n if (n > 0 && f > 0) {\n printf(\"The magic number is: %d\\n\", magicNumber(n, f));\n }\n else {\n printf(\"n and f must be positive and nonzero.\\n\");\n }\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/magic_number/magic_number.cpp",
"content": "//Part of Cosmos by OpenGenus Foundation\n#include <iostream>\n#include <cmath>\nusing namespace std;\n\n// Function to find nth magic numebr\nint nthMagicNo(int n)\n{\n int pow = 1, answer = 0;\n\n // Go through every bit of n\n while (n)\n {\n pow = pow * 5;\n\n // If last bit of n is set\n if (n & 1)\n answer += pow;\n\n // proceed to next bit\n n >>= 1; // or n = n/2\n }\n return answer;\n}\n\nint main()\n{\n int n;\n cin >> n;\n cout << \"nth magic number is \" << nthMagicNo(n) << endl;\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/magic_number/magic_number.java",
"content": "// Part of Cosmos by OpenGenus Foundation \n\n/* \n**\n** @AUTHOR: VINAY BADHAN\n** @BIT MANIPULATION PROBLEM: Nth MAGIC NUMBER\n** @GITHUB LINK: https://github.com/vinayb21\n*/\n\nimport java.util.*;\nimport java.io.*;\n\nclass MagicNumber {\n \n public static int magicNumber(int n)\n {\n int pow = 1, ans = 0;\n while(n > 0)\n {\n pow = pow*5;\n \n if (n%2==1)\n ans += pow;\n n >>= 1; \n }\n return ans;\n }\n \n\n public static void main(String[] args) throws IOException {\n int n;\n \tInputStreamReader in = new InputStreamReader(System.in);\n \tBufferedReader buffer = new BufferedReader(in);\n \tPrintWriter out = new PrintWriter(System.out, true);\n \tString line = buffer.readLine().trim();\n \tn = Integer.parseInt(line);\n \tint ans = magicNumber(n);\n \tout.println(n+\"th Magic Number is \"+ans);\n }\n}"
}
{
"filename": "code/bit_manipulation/src/magic_number/magic_number.py",
"content": "# Python Program to Find nth Magic Number.\n# A magic number is defined as a number which can be expressed as a power of 5 or sum of unique powers of 5\n# Part of Cosmos by OpenGenus Foundation\n# Function to find nth magic numebr\ndef nthMagicNo(n):\n pow = 1\n ans = 0\n while n:\n pow = pow * 5\n\n # If last bit of n is set\n if n & 1:\n ans += pow\n\n # proceed to next bit\n n >>= 1\n return ans\n\n\nn = int(input())\nprint(nthMagicNo(n))"
}
{
"filename": "code/bit_manipulation/src/magic_number/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/bit_manipulation/src/maximum_xor_value/maximum_xor_value.cpp",
"content": "#include <iostream>\nusing namespace std;\n\nint maxXor(int l, int r)\n{\n int num = l ^ r, max = 0;\n while (num > 0)\n {\n max <<= 1;\n max |= 1;\n num >>= 1;\n }\n return max;\n}\n\nint main()\n{\n int res, _l, _r;\n\n cin >> _l;\n cin >> _r;\n\n res = maxXor(_l, _r);\n cout << res;\n\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/maximum_xor_value/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/bit_manipulation/src/multiply_by_2/multiply_by_2.cpp",
"content": "#include<iostream>\n\nusing namespace std;\n\nunsigned multiplyWith2(unsigned n) { //Since C++ 11 signed shift left of a negative int is undefined. \n return (n << 1); //To avoid unexpected results always use unsigned when doing a multiply by 2 \n}\n\nint main(){\n cout << multiplyWith2(8) << endl;\n cout << multiplyWith2(15) << endl;\n\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/multiply_by_2/README.md",
"content": "# Mutliply by 2\n\n- A simple Program to multiply a number with 2 by using bit manipulation"
}
{
"filename": "code/bit_manipulation/src/power_of_2/power_of_2.c",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n * @Author: Ayush Garg \n * @Date: 2017-10-13 18:46:12 \n * @Last Modified by: Ayush Garg\n * @Last Modified time: 2017-10-13 18:50:15\n */\n\n#include <stdio.h>\n\nint isPowerOf2(long long n)\n{\n return n && (!(n & (n - 1)));\n}\nint main()\n{\n long long n;\n printf(\"Enter number to check\\n\");\n scanf(\"%lld\", &n);\n if (isPowerOf2(n))\n printf(\"%lld Is a power of 2\\n\",n);\n else\n printf(\"%lld Not a power of 2\\n\",n);\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/power_of_2/power_of_2.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n/* Created by Shubham Prasad Singh on 12/10/2017 */\n\n/* Check if a number is a power of 2 */\n#include <iostream>\nusing namespace std;\ntypedef long long ll;\n\n/*\n * If a power of two number is subtracted by 1\n * then all the unset bits after the set bit become set,\n * and the set bit become unset\n * \n * For Example : 4(power of 2) --> 100\n * 4-1 --> 3 --> 011 \n * \n * So,if a number n is a power of 2 then\n * (n&(n-1)) = 0\n * \n * For Example: 4 & 3 = 0 \n * and 4 is a power of 2\n *\n * The expression (n & (n-1)) will not work when n=0\n * To handle this case , the expression is modified to\n * (n & (n&(n-1)))\n */\nbool isPowerOf2(ll n)\n{\n return n && (!(n & (n - 1)));\n} \nint main()\n{\n ll n;\n cout << \"Enter a number\\n\";\n cin >> n;\n if (isPowerOf2(n))\n cout << \"Is a power of 2\" << endl;\n else\n cout << \"Not a power of 2\" << endl;\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/power_of_2/power_of_2.cs",
"content": "using System;\n\nclass MainClass {\n public static void Main(String[] args){\n \t\tint num = Convert.ToInt32(Console.ReadLine());\n\n\t\tif(((num & (num-1))==0) && (num != 0))\n\t\t\tConsole.WriteLine(\"Yes, the number is a power of 2\");\n\t\telse\n\t\t\tConsole.WriteLine(\"No, the number is not a power of 2\");\n }\n}"
}
{
"filename": "code/bit_manipulation/src/power_of_2/power_of_2.go",
"content": "// Part of Cosmos by OpenGenus Foundation\n// @Author: Chanwit Piromplad\n// @Date: 2017-10-15 20:57\n\npackage main\n\nimport (\n\t\"fmt\"\n)\n\nfunc isPowerOf2(n int) bool {\n\treturn n > 0 && (n&(n-1) == 0)\n}\n\nfunc main() {\n\tfmt.Println(isPowerOf2(64))\n}"
}
{
"filename": "code/bit_manipulation/src/power_of_2/power_of_2.java",
"content": "import java.io.*;\nimport java.lang.*;\nimport java.math.*;\nimport java.util.*;\n\nclass PowerOf2{\n\tpublic static void main(String args[]){\n\t\tScanner sc = new Scanner(System.in);\n\t\tint num = sc.nextInt();\n\n\t\tif(((num & (num-1))==0) && (num != 0))\n\t\t\tSystem.out.println(\"Yes, the number is a power of 2\");\n\t\telse\n\t\t\tSystem.out.println(\"No, the number is not a power of 2\");\n\t}\n}"
}
{
"filename": "code/bit_manipulation/src/power_of_2/power_of_2.jl",
"content": "print(\"Enter a number to check if it is a power of 2: \")\nn = parse(Int64, chomp(readline()))\n\nif (n != 0) && (n & (n-1) == 0)\n println(\"YES\")\nelse\n println(\"NO\")\nend"
}
{
"filename": "code/bit_manipulation/src/power_of_2/power_of_2.js",
"content": "/*\n\n Part of Cosmos by OpenGenus Foundation\n Created by Jiraphapa Jiravaraphan on 14/10/2017 \n Check if a number is a power of 2 - javascript implementation\n\n*/\n\nfunction isPowerOf2(num) {\n if (typeof num === \"number\") return num && (num & (num - 1)) === 0;\n}\n\nconsole.log(isPowerOf2(64));"
}
{
"filename": "code/bit_manipulation/src/power_of_2/power_of_2.php",
"content": "<?php\nfunction isPowerOf2($x)\n{\n if (($x & ($x - 1)) != 0)\n \t\treturn \"$x is not power of 2\";\n else\n {\n \t\treturn \"$x is power of 2\";\n }\n}\nprint_r(isPowerOf2(32).\"\\n\");\nprint_r(isPowerOf2(31).\"\\n\");"
}
{
"filename": "code/bit_manipulation/src/power_of_2/power_of_2.py",
"content": "def isPowerOf2(num):\n return ((num & (num - 1)) == 0) and (num != 0)\n\n\nn = int(input(\"Enter a number: \"))\n\nif isPowerOf2(n):\n print(\"Power of 2 spotted!\")\nelse:\n print(\"Not a power of 2\")"
}
{
"filename": "code/bit_manipulation/src/power_of_2/power_of_2.rs",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n/* Created by Luke Diamond on 13/10/2017 */\n\nfn is_power_of_2(x: i32) -> bool {\n\tx != 0 && ((x & (x - 1)) == 0)\n}\n\nfn main() {\n\tlet mut input_text = String::new();\n\tprintln!(\"Input Number:\");\n\tstd::io::stdin().read_line(&mut input_text);\n\tlet number = input_text.trim().parse::<i32>().unwrap();\n\n\tif is_power_of_2(number) {\n\t\tprintln!(\"{} Is a power of 2\", number);\n\t} else {\n\t\tprintln!(\"{} Is not a power of 2\", number);\n\t}\n}"
}
{
"filename": "code/bit_manipulation/src/power_of_4/Main.java",
"content": "public class Main {\n public static void main(String[] args) {\n int number = 16; // Change this number to check if it's a power of 4\n boolean result = isPowerOfFour(number);\n if (result) {\n System.out.println(number + \" is a power of 4.\");\n } else {\n System.out.println(number + \" is not a power of 4.\");\n }\n }\n\n public static boolean isPowerOfFour(int num) {\n if (num <= 0) {\n return false;\n }\n // A number is a power of 4 if it is a power of 2 and has only one set bit in its binary representation.\n // Count the number of set bits and check if it's odd.\n int countSetBits = 0;\n int temp = num;\n while (temp > 0) {\n temp = temp >> 1;\n countSetBits++;\n }\n // Check if there is only one set bit and it's at an odd position (0-based index)\n return (countSetBits == 1) && ((countSetBits - 1) % 2 == 0);\n }\n}"
}
{
"filename": "code/bit_manipulation/src/power_of_4/power_of_4.cpp",
"content": "// part of cosmos repository\n#include <iostream>\nusing namespace std ;\n\n// Function to check if the number is a power of 4\nbool isPowerOfFour(int num) {\n // First if the num is +ve ,\n // then it is a power of 2 ,\n // then (4^n - 1) % 3 == 0\n // another proof:\n // (1) 4^n - 1 = (2^n + 1) * (2^n - 1)\n // (2) among any 3 consecutive numbers, there must be one that is a multiple of 3\n // among (2^n-1), (2^n), (2^n+1), one of them must be a multiple of 3, and (2^n) cannot be the one, therefore either (2^n-1) or (2^n+1) must be a multiple of 3, and 4^n-1 must be a multiple of 3 as well.\n return num > 0 && (num & (num - 1)) == 0 && (num - 1) % 3 == 0;\n}\n\nint main()\n{\n int n ;\n cin>>n ;\n \n isPowerOfFour(n)?cout<<\"YES\":cout<<\"NO\" ;\n \n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/bit_manipulation/src/set_ith_bit/set_ith_bit.cpp",
"content": "/*\nYou are given two integers N and i. You need to make ith bit of binary representation of N to 1 and return the updated N.\nCounting of bits start from 0 from right to left.\nInput Format :\nTwo integers N and i (separated by space)\nOutput Format :\nUpdated N\nSample Input 1 :\n4 1\nSample Output 1 :\n6\nSample Input 2 :\n4 4\nSample Output 2 :\n20\n*/\n#include <iostream>\nusing namespace std;\n\nint turnOnIthBit(int n, int i){\n n = (n | (1 << i));\n return n;\n}\n\nint main() {\n\tint n, i;\n\tcin >> n >> i;\n\tcout<< turnOnIthBit(n, i) <<endl;\n\treturn 0;\n}"
}
{
"filename": "code/bit_manipulation/src/set_ith_bit/set_ith_bit.py",
"content": "def setithBit(n,i):\n \n # ith bit of n is being\n # set by this operation\n return ((1 << i) | n)\n \n# Driver code\n \nn = 10\ni = 2\n \nprint(\"Kth bit set number = \", setithBit(n, i))\n "
}
{
"filename": "code/bit_manipulation/src/subset_generation/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/bit_manipulation/src/subset_generation/subset_generator_using_bit.cpp",
"content": "#include <iostream>\n#include <cstring>\nusing namespace std;\ntypedef long long int ll;\n\nvoid filter(char *a, int no)\n{\n int i = 0;\n while (no > 0)\n {\n (no & 1) ? cout << a[i] : cout << \"\";\n i++;\n no = no >> 1;\n }\n cout << endl;\n}\n\nvoid generateSub(char *a)\n{\n int n = strlen(a);\n int range = (1 << n) - 1;\n for (int i = 0; i <= range; i++)\n filter(a, i);\n}\nint main()\n{\n char a[4] = {'a', 'b', 'c', 'd'};\n generateSub(a);\n}"
}
{
"filename": "code/bit_manipulation/src/subset_generation/subset_mask_generator.cpp",
"content": "#include <iostream>\n\ntypedef unsigned long long ll;\n\n//Loops over all subsets of the bits in to_mask. Except to_mask itself\n//For test input (111)\n//110\n//101\n//100\n//010\n//001\n//000\n\nvoid generate_masks(ll to_mask)\n{\n for (int mask = to_mask; mask;)\n {\n --mask &= to_mask;\n std::cout << mask << std::endl;\n }\n}\n\nint main()\n{\n generate_masks(7);\n}"
}
{
"filename": "code/bit_manipulation/src/subset_generation/subset_sum.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n#include <iostream>\n#include <vector>\nusing namespace std;\n\n//To print all subset sums\nvoid printSums(int arr[], int n)\n{\n long long total = 1 << n; //total = 2^n\n for (long long i = 0; i < total; i++) //We are iterating from 0 to 2^n-1\n {\n long long sum = 0;\n for (int j = 0; j < n; j++)\n if (i & (1 << j))\n sum += arr[j];\n //Adding elements to set for which the bit is set\n cout << sum << \" \";\n }\n}\n\n/*\n * We are not generating all subsets, but we are calculating all\n * subset sums based on all combinations of set bits\n * which can be obtained by iterating through 0 to 2^n-1\n */\n\n//Driver code\nint main()\n{\n int arr[] = {4, 9, 3, 12, 6, 9};\n int n = sizeof(arr) / sizeof(int);\n printSums(arr, n);\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/sum_binary_numbers/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/bit_manipulation/src/sum_binary_numbers/sum_binary_numbers.c",
"content": "#include <stdio.h>\n \nint main()\n{\n \n long bin1, bin2;\n int i = 0, remainder = 0, sum[20];\n \n printf(\"Enter the first binary number: \");\n scanf(\"%ld\", &bin1);\n printf(\"Enter the second binary number: \");\n scanf(\"%ld\", &bin2);\n while (bin1 != 0 || bin2 != 0)\n {\n sum[i++] =(bin1 % 10 + bin2 % 10 + remainder) % 2;\n remainder =(bin1 % 10 + bin2 % 10 + remainder) / 2;\n bin1 = bin1 / 10;\n bin2 = bin2 / 10;\n }\n if (remainder != 0)\n sum[i++] = remainder;\n --i;\n printf(\"Sum of two binary numbers: \");\n while (i >= 0)\n printf(\"%d\", sum[i--]);\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/sum_binary_numbers/sum_binary_numbers.cpp",
"content": "#include <iostream>\nusing namespace std;\n \nint main()\n{\n \n long bin1, bin2;\n int i = 0, remainder = 0, sum[20];\n \n cout<<\"Enter the first binary number: \";\n cin>>bin1;\n cout<<\"Enter the second binary number: \";\n cin>>bin2;\n while (bin1 != 0 || bin2 != 0)\n {\n sum[i++] =(bin1 % 10 + bin2 % 10 + remainder) % 2;\n remainder =(bin1 % 10 + bin2 % 10 + remainder) / 2;\n bin1 = bin1 / 10;\n bin2 = bin2 / 10;\n }\n if (remainder != 0)\n sum[i++] = remainder;\n --i;\n cout<<\"Sum of two binary numbers: \";\n while (i >= 0)\n cout<<sum[i--];\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/sum_equals_xor/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/bit_manipulation/src/sum_equals_xor/sum_equals_xor.c",
"content": "#include <math.h>\n#include <stdio.h>\n#include <string.h>\n#include <stdlib.h>\n#include <assert.h>\n#include <limits.h>\n#include <stdbool.h>\n/*\nCounts the number of values between 0 and a given number that satisfy the condition x+n = x^n (0<=x<=n)\n*/\nlong int solve(long int n) {\n long c = 0;\n while(n){\n c += n%2?0:1;\n n/=2; \n }\n c=pow(2,c);\n return c;\n}\n\nint main() {\n long int n; \n scanf(\"%li\", &n);\n long int result = solve(n);\n printf(\"%ld\\n\", result);\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/sum_equals_xor/sum_equals_xor.cpp",
"content": "/*\n * Counts the number of values between 0 and a given number that satisfy the condition x+n = x^n (0<=x<=n)\n */\n#include <iostream>\n#include <cmath>\nusing namespace std;\n\nlong solve(long n)\n{\n long c = 0;\n while (n)\n {\n c += n % 2 ? 0 : 1;\n n /= 2;\n }\n c = pow(2, c);\n return c;\n}\n\nint main()\n{\n long n;\n cin >> n;\n long result = solve(n);\n cout << result << endl;\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/sum_equals_xor/sum_equals_xor.py",
"content": "# Counts the number of values between 0 and a given number that satisfy the condition x+n = x^n (0<=x<=n)\n\n# Works for both python 2 and python 3\n\nn = int(input(\"Enter number n\"))\n# x^n = x+n means that x can contain one's only in its binary representation where it is 0 in n and vice versa.\n# To Implement it we calculate number of 0's in the binary representation of n and return the answer as pow(2,n) as there will be two possibilities for each of the 0's in n i.e. 1 and 0.\nprint(int(pow(2, (bin(n)[2:]).count(\"0\"))))"
}
{
"filename": "code/bit_manipulation/src/thrice_unique_number/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/bit_manipulation/src/thrice_unique_number/thrice_unique_number.cpp",
"content": "/*\n *\n * Part of Cosmos by OpenGenus Foundation\n * Find unique number in an array where every element occours thrice except one.Find that unique Number\n *\n */\n#include <iostream>\nusing namespace std;\nint n;\nint a[104] = {1, 1, 1, 3, 3, 2, 3};\nint f()\n{\n int count[65] = {0};\n for (int i = 0; i < n; i++)\n {\n int j = 0;\n int temp = a[i];\n while (temp)\n {\n count[j] += (temp & 1);\n j++;\n temp = temp >> 1;\n }\n }\n int p = 1, ans = 0;\n for (int i = 0; i <= 64; i++)\n {\n count[i] %= 3;\n ans += (count[i] * p);\n p = p << 1;\n }\n return ans;\n}\nint main()\n{\n n = sizeof(a) / sizeof(a[0]);\n cout << f();\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/thrice_unique_number/thrice_unique_number.java",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n*/\nimport java.util.*;\n\npublic class ThriceUniqueNumber {\n \n final public static int BITS=32;\n \n public static int ConvertToDec(int bitArr[]){\n int decNum=0;\n for(int i=0;i<BITS;++i){\n decNum+=bitArr[i]* (1<<i);\n }\n return decNum;\n }\n public static void SetArray(int bitArr[], int num){\n // update the bitArr to binary code of num\n \n int i=0;\n while(num!=0){\n if((num&1)!=0){\n bitArr[i]++;\n }\n num>>=1;\n i++;\n }\n}\n public static int thriceUniqueNumber(int arr[], int size){\n \n // at max the numeber of bits can be 32 in a binary no.\n int[] bitArr=new int[BITS];\n \n for(int i=0;i<size;++i){\n SetArray(bitArr,arr[i]);\n }\n //by doing this all the numbers appearing thrice will be reomved from\n //the binary form\n for(int i=0;i<BITS;++i){\n bitArr[i] %= 3;\n }\n \n int ans=ConvertToDec(bitArr);\n \n return ans;\n }\n \n \n \n public static void main(String args[]) {\n \n Scanner s=new Scanner(System.in);\n //size of the array ( Number of elements to input)\n int size=s.nextInt();\n int[] arr=new int[size];\n //input size number of elements\n for(int i=0;i<size;i++){\n arr[i]=s.nextInt();\n }\n //function call\n System.out.println(thriceUniqueNumber(arr,size));\n\n /*Example\n Input\n 10\n 1 1 1 2 2 2 9 9 9 8\n\n OutPut\n 8*/\n }\n}"
}
{
"filename": "code/bit_manipulation/src/thrice_unique_number/thrice_unique_number.js",
"content": "// Part of Cosmos by OpenGenus Foundation\n\nlet numbers = [\n 1,\n 1,\n 1,\n 2,\n 2,\n 2,\n 3,\n 3,\n 3,\n 4,\n 5,\n 5,\n 5,\n 6,\n 6,\n 6,\n 7,\n 7,\n 7,\n 8,\n 8,\n 8,\n 9,\n 9,\n 9\n];\nlet counter = {};\n\nfor (var index = 0; index < numbers.length; index++) {\n let current = counter[numbers[index]];\n\n if (current === undefined) {\n counter[numbers[index]] = 1;\n } else {\n counter[numbers[index]] = current + 1;\n }\n}\n\nfor (let num in counter) {\n let count = counter[num];\n\n if (count === 1) {\n console.log(`Unique number is ${num}`);\n }\n}"
}
{
"filename": "code/bit_manipulation/src/thrice_unique_number/thrice_unique_number.py",
"content": "# we define the function\ndef uniqueNumber(array):\n d = {}\n result = array[0]\n # case multiple elements\n if len(array) > 1:\n for x in array:\n # fill the dictionary in O(n)\n if d.has_key(x):\n d[x] += 1\n else:\n d[x] = 1\n # case 1 element\n else:\n return result\n keys = d.keys()\n # find the result key in O(1/3 * n)\n for k in keys:\n if d.get(k) == 1:\n return k\n\n\n# asking for the parameters\narray_lenght = input(\"Enter Size of array: \")\nvariable = []\nfor i in range(array_lenght):\n array_index = input(\"Enter element: \")\n variable.append(array_index)\n\nresult = uniqueNumber(variable)\n# printing result\nprint(result)"
}
{
"filename": "code/bit_manipulation/src/twice_unique_number/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/bit_manipulation/src/twice_unique_number/twice_unique_number.c",
"content": "#include <stdio.h>\n\nvoid findUnique2(int *a,int n){\n \n int res=0;\n for(int i=0;i<n;i++){\n res = res^a[i];\n }\n // find the rightmost set bit in res\n int i=0;\n int temp=res;\n while(temp>0){\n if(temp&1){\n break;\n }\n i++;\n temp = temp>>1;\n }\n \n int mask = (1<<i);\n \n int firstNo = 0;\n for(int i=0;i<n;i++){\n if((mask&a[i])!=0){\n firstNo = firstNo^a[i];\n }\n }\n \n int secondNo = res^firstNo;\n printf(\"%d\\n\",firstNo);\n printf(\"%d\\n\",secondNo);\n \n}\nint main(){\n \n int n;\n int a[] = {1,3,5,6,3,2,1,2};\n n = sizeof(a)/sizeof(int);\n findUnique2(a,n);\n \n return 0; \n}"
}
{
"filename": "code/bit_manipulation/src/twice_unique_number/twice_unique_number.cpp",
"content": "#include <iostream>\nusing namespace std;\n\n//Part of Cosmos by OpenGenus Foundation\n\nvoid findUnique2(int *a, int n)\n{\n int res = 0;\n for (int i = 0; i < n; i++)\n res = res ^ a[i];\n // find the rightmost set bit in res\n int i = 0;\n int temp = res;\n while (temp > 0)\n {\n if (temp & 1)\n break;\n i++;\n temp = temp >> 1;\n }\n\n int mask = (1 << i);\n\n int firstNo = 0;\n for (int i = 0; i < n; i++)\n if ((mask & a[i]) != 0)\n firstNo = firstNo ^ a[i];\n\n int secondNo = res ^ firstNo;\n cout << firstNo << endl;\n cout << secondNo << endl;\n}\n\n\nint main()\n{\n\n int n;\n int a[] = {1, 3, 5, 6, 3, 2, 1, 2};\n n = sizeof(a) / sizeof(int);\n findUnique2(a, n);\n\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/xor_swap/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\n## Xor Swap\nIn computer programming, the XOR swap is an algorithm that uses the XOR \nbitwise operation to swap values of distinct \nvariables having the same data type without using a temporary variable.\n\n## Proof of correctness\nLet's say we have two distinct registers R1 and R2 as in the table below, \nwith initial values A and B respectively.\n\n| Step\t| Operation\t| R1\t| R2\t|\n|---\t|---\t|---\t|---\t|\n| 0\t| Initial\t| A\t| B\t|\n| 1\t| R1 := R1 ^ R2\t| A ^ B\t| B\t|\n| 2\t| R2 := R1 ^ R2\t| A ^ B\t| (A ^ B) ^ B => A \t|\n| 3\t| R1 := R1 ^ R2\t| (A ^ B) ^ A => B\t| A\t|"
}
{
"filename": "code/bit_manipulation/src/xor_swap/xor_swap.c",
"content": "#include <stdio.h>\n\n/*\n * This can be similarly implemented for other data types\n */\nvoid\nxor_swap(int *a, int *b)\n{\n\t*a = *a ^ *b;\n\t*b = *a ^ *b;\n\t*a = *a ^ *b;\n\n\treturn;\n}\n\nint main()\n{\n\tint a = 10, b = 15;\n\n\tprintf(\"Before swapping: A = %d and B = %d\\n\", a, b);\n\n\txor_swap(&a, &b);\n\n\tprintf(\"After swapping: A = %d and B = %d\\n\", a, b);\n\n\treturn 0;\n}"
}
{
"filename": "code/bit_manipulation/src/xor_swap/xor_swap.cpp",
"content": "#include <iostream>\nusing namespace std;\n\nvoid xor_swap(int * a, int * b)\n{\n *a = *a ^ *b;\n *b = *a ^ *b;\n *a = *a ^ *b;\n}\n\nint main()\n{\n int a = 10, b = 15;\n\n cout << \"Before swapping: A = \" << a << \" and B = \" << b << \"\\n\";\n\n xor_swap(&a, &b);\n\n cout << \"After swapping: A = \" << a << \" and B = \" << b << \"\\n\";\n\n return 0;\n}"
}
{
"filename": "code/bit_manipulation/src/xor_swap/xor_swap.go",
"content": "package main\n\nimport \"fmt\"\n\nfunc XorSwap(r1 *int, r2 *int) {\n\t*r1 = *r1 ^ *r2\n\t*r2 = *r1 ^ *r2\n\t*r1 = *r1 ^ *r2\n\n\treturn\n}\n\nfunc main() {\n\tA := 10\n\tB := 15\n\n\tfmt.Printf(\"Before swapping: A = %d and B = %d\\n\", A, B)\n\n\tXorSwap(&A, &B)\n\n\tfmt.Printf(\"After swapping: A = %d and B = %d\\n\", A, B)\n}"
}
{
"filename": "code/bit_manipulation/src/xor_swap/xor_swap.js",
"content": "// Part of Cosmos by OpenGenus Foundation\n\nfunction xorSwap(a, b) {\n console.log(`Before swap: a = ${a}, b = ${b}`);\n \n a = a ^ b; // Step 1: a now contains the XOR of both\n b = a ^ b; // Step 2: b gets the original value of a\n a = a ^ b; // Step 3: a gets the original value of b\n\n console.log(`After swap: a = ${a}, b = ${b}`);\n}\n\n// Example usage:\nlet x = 5;\nlet y = 10;\nxorSwap(x, y);"
}
{
"filename": "code/bit_manipulation/src/xor_swap/xor_swap.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n# Swaps two given numbers making use of xor\n\n# Works for both python 2 and python 3\n\n\ndef xorswap(n, m):\n n = m ^ n\n m = n ^ m\n n = m ^ n\n return n, m\n\n\nn = 10\nm = 15\nprint(\"Earlier A was equal to \", n, \" and B was equal to \", m)\nn, m = xorswap(n, m)\nprint(\"Now A is equal to \", n, \" and B is equal to \", m)"
}
{
"filename": "code/bit_manipulation/test/addition_using_bits_test.cpp",
"content": "#include <assert.h>\n#include \"./../src/addition_using_bits/addition_using_bits.cpp\"\n\n// Part of Cosmos by OpenGenus Foundation\nint main()\n{\n // Testing bitwiseAddition function\n assert(bitwiseAddition(10, 5) == (10 + 5));\n assert(bitwiseAddition(5, 10) == (5 + 10));\n assert(bitwiseAddition(0, 1) == (0 + 1));\n assert(bitwiseAddition(2, 0) == (2 + 0));\n assert(bitwiseAddition(-27, 3) == (-27 + 3));\n assert(bitwiseAddition(27, -3) == (27 + -3));\n assert(bitwiseAddition(-1, -1) == (-1 + -1));\n\n // Testing bitwiseAdditionRecursive function\n assert(bitwiseAdditionRecursive(2, 3) == (2 + 3));\n assert(bitwiseAdditionRecursive(3, 2) == (3 + 2));\n assert(bitwiseAdditionRecursive(1, 0) == (1 + 0));\n assert(bitwiseAdditionRecursive(0, 3) == (0 + 3));\n assert(bitwiseAdditionRecursive(25, -50) == (25 + -50));\n assert(bitwiseAdditionRecursive(-25, 50) == (-25 + 50));\n assert(bitwiseAdditionRecursive(-5, -10) == (-5 + -10));\n std::cout << \"Testing Complete\" << \"\\n\";\n}"
}
{
"filename": "code/bit_manipulation/test/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/blockchain/Application.java",
"content": "import java.util.*;\nimport java.util.List;\npublic class Application {\n\tstatic List<Block> blockchain=new ArrayList<Block>();\n\tfinal static int difficulty=4;\n\t\n\t\n\tpublic static void main(String[] args) {\n\t\t// TODO Auto-generated method stub\n\t\tSystem.out.println(\"Create Genesis Block\");\n\t\taddBlock(new Block(\"0\",\"this is first transaction\"));\n\t\taddBlock(new Block(blockchain.get(blockchain.size()-1).currentHashValue(),\"Second Transaction\"));\n\n\t\tSystem.out.println(blockchain.toString());\n\t\tSystem.out.println(UtilityClass.getJSONData(blockchain));\n\t\tSystem.out.println(isChainValid(blockchain));\n\t\t\n\t\t\n\t}\n\tpublic static void addBlock(Block newBlock) {\n\t\tnewBlock.mineBlock(difficulty);\n\t\tblockchain.add(newBlock);\n\t\tSystem.out.println(\"Block added to blockchain\");\n\t}\n\tpublic static Boolean isChainValid(List<Block> blockchain) {\n\t\tBlock currentBlock; \n\t\tBlock previousBlock;\n\t\t\n\t\t//loop through blockchain to check hashes:\n\t\tfor(int i=1; i < blockchain.size(); i++) {\n\t\t\tcurrentBlock = blockchain.get(i);\n\t\t\tpreviousBlock = blockchain.get(i-1);\n\t\t\t//compare registered hash and calculated hash:\n\t\t\tif(!currentBlock.currentHashValue().equals(currentBlock.calculateHash()) ){\n\t\t\t\tSystem.out.println(\"Current Hashes not equal\");\t\t\t\n\t\t\t\treturn false;\n\t\t\t}\n\t\t\t//compare previous hash and registered previous hash\n\t\t\tif(!previousBlock.currentHashValue().equals(currentBlock.prevHashValue()) ) {\n\t\t\t\tSystem.out.println(\"Previous Hashes not equal\");\n\t\t\t\treturn false;\n\t\t\t}\n\t\t}\n\t\treturn true;\n\n\n}\n}"
}
{
"filename": "code/blockchain/Block.java",
"content": "import java.util.Date;\n\npublic class Block {\n\n\n\tprivate String previousHash;\n\tprivate String currentHash;\n\tprivate String data;\n\tprivate Long timestamp;\n\tprivate int nonce=0;\n\tpublic Block(String previousHash,String data) {\n\t\tthis.previousHash=previousHash;\n\t\tthis.data=data;\n\t\tthis.timestamp=new Date().getTime();\n\t\tthis.currentHash=calculateHash();\n\t}\n\tpublic String currentHashValue()\n\t{\n\t\treturn currentHash;\n\t}\n\tpublic String prevHashValue()\n\t{\n\t\treturn previousHash;\n\t}\n\tpublic String calculateHash() {\n\t\tString calculateHash=UtilityClass.getSHA256Hash(\n\t\t\t\tpreviousHash+data+Long.toString(timestamp)+\n\t\t\t\tInteger.toString(nonce)\n\t\t\t\t);\n\t\t\t\treturn calculateHash;\n\t}\n\tpublic void mineBlock(int difficulty) {\n\t\tString target=UtilityClass.getDifficultyString(difficulty);\n\t\twhile(!currentHash.substring(0,difficulty).equals(target)) {\n\t\t\tnonce=nonce+1;\n\t\t\tcurrentHash=calculateHash();\n\t\t}\n\t\tSystem.out.println(\"Block is mined \"+currentHash);\n\t\t\n\t}\n\n\n}"
}
{
"filename": "code/blockchain/explainBlockchain.md",
"content": "### Explanation of blockchain\r\n\r\nPlease read article below for more details.\r\n\r\n[Explaining Blockchain intuitively to a Five year old!](https://iq.opengenus.org/explaining-blockchain-to-a-5-year-old/)\r\n\r\nBlockchain Technology basically helps in Trade. Because of this technology, you can trade with anyone around the globe, without knowing about him personally and without any intermediate party (like banks or other companies).\r\nFor eg: Let’s say you want to sell your smartphone. Currently you will use platform like ebay which acts as an intermediate between you and the person to whom you want to sell. \r\n\r\nThe payments might be processed via some bank which will cost you or/and the other person some money. Now because of blockchain, you will be able to sell your smartphone to that person without actually involving ebay or any other bank in between. That’s blockchain explained on very basic level.\r\n\r\nLets level up a bit. Blockchain is basically a network of computers called nodes which all have same history of transactions. So instead of one company or a database which holds all the information, now the information is spread across whole of the network. Whenever a transaction occurs, that is validated by everyone and is put ahead in that history of transactions in that network. All the transactions are encoded using a technique called cryptography.\r\n\r\nPlease note that blockchain is not only about Bitcoin. Because it is in news all over, people think blockchain means bitcoin. Bitcoin is simply a digital currency which is build using blockchain technology. There are many use cases being build over which we can basically trade any asset using blockchain.\r\n\r\nThe bottom line is that the basic explanation of a blockchain ledger includes the following concepts:\r\n\r\n It's maintained by every user on the blockchain.\r\n It's decentralized, meaning every user has a complete copy.\r\n It can track the entire transaction history of any given item or currency."
}
{
"filename": "code/blockchain/UtilityClass.java",
"content": "import java.security.MessageDigest;\nimport java.util.List;\n\nimport com.google.gson.GsonBuilder;\n\npublic class UtilityClass {\n\n\tpublic static String getDifficultyString(int difficulty) {\n\t\t\n\t\treturn new String(new char[difficulty]).replace('\\0','0');\n\t}\n\n\tpublic static String getSHA256Hash(String inputString) {\n\t\ttry {\n\t\t\tMessageDigest message=MessageDigest.getInstance(\"SHA-256\");\n\t\t\tbyte[] hash=message.digest(inputString.getBytes(\"UTF-8\"));\n\t\t\tStringBuffer hexString=new StringBuffer();\n\t\t\tfor(int i=0;i<hash.length;i++) {\n\t\t\t\tString hex=Integer.toHexString(0xff&hash[i]);\n\t\t\t\tif(hex.length()==1) {\n\t\t\t\t\t\n\t\t\t\t\thexString.append('0');\n\t\t\t\t}\n\t\t\t\thexString.append(hex);\n\t\t\t}\n\t\t\t\n\t\t\n\t\treturn hexString.toString();\n\t\t}\n\t\tcatch(Exception e) {\n\t\t\tSystem.out.println(\"Exception occured\");\n\t\t}\n\t\treturn null;\n\t}\n\t\tpublic static String getJSONData(List<Block> blockchain) {\n\t\t\treturn new GsonBuilder().setPrettyPrinting().create().toJson(blockchain);\n\t\t}\n\t\t\n\t}"
}
{
"filename": "code/cellular_automaton/src/brians_brain/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# Cellular Automaton\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/cellular_automaton/src/conways_game_of_life/conway.java",
"content": "import java.awt.*;\nimport java.awt.event.*;\nimport javax.swing.*;\n\npublic class Conway extends JFrame {\n\n\tprivate int size = 80, gen =0, kill = 1;\n\tprivate JButton grid[][] = new JButton[size][size], toggle, wow, gun, clear;\n\tprivate JButton exit; // for termination of the program\n\tprivate int grid_num[][] = new int[size][size];\n\tprivate JLabel status;\n\tConway() {\n\t\tContainer cp = this.getContentPane();\n\t\tJPanel gd = new JPanel(new GridLayout(size, size));\n\t\tfor (int i = 0;i<size;i++)\n\t\t\tfor (int j = 0;j<size;j++) {\n\t\t\t\tgrid[i][j] = new JButton();\n\t\t\t\tgrid[i][j].setBackground(Color.WHITE);\n\t\t\t\tgd.add(grid[i][j]);\n\t\t\t\tgrid[i][j].addActionListener(new Listen());\t\t\t\t\n\t\t\t\tgrid[i][j].setBorder(null);\n\t\t\t}\n\n\t\tJPanel st_bar = new JPanel(new FlowLayout(FlowLayout.CENTER, 10, 10));\n\t\tstatus = new JLabel(\"Status\");\n\t\ttoggle = new JButton(\"Start\");\n\t\twow = new JButton(\"Benchmark\");\n\t\tgun = new JButton(\"Glider Gun\");\n\t\tclear = new JButton(\"Clear\");\n\t\texit = new JButton(\"Exit\");\n\n\t\tst_bar.add(status);\n\t\tst_bar.add(toggle);\n\t\tst_bar.add(wow);\n\t\tst_bar.add(gun);\n\t\tst_bar.add(clear);\n\t\tst_bar.add(exit);\n\t\ttoggle.addActionListener(new Listen());\n\t\twow.addActionListener(new Listen());\n\t\tgun.addActionListener(new Listen());\n\t\tclear.addActionListener(new Listen());\n\t\texit.addActionListener(new Listen());\n\n\t\tcp.setLayout(new BorderLayout());\n\t\tcp.add(gd, BorderLayout.CENTER);\n\t\tcp.add(st_bar, BorderLayout.SOUTH);\n\n\t\tsetDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);\n\t\tsetTitle(\"Game of Life!\");\n\t\tsetLocationRelativeTo(null);\n\t\tsetSize(600, 600);\n\t\tsetVisible(true);\n\t\ttry {\n\t\t\tUIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());\n\t\t}\n\t\tcatch (Exception e) {}\n\t}\n\n\n//A function that returns Live neighbours...\n//---------------------------------LOGIC-------------------------\n\n\t\tpublic int neigh(int x, int y) {\n\t\t\tint ctr = 0;\n\t\t\tint startPosX = (x - 1 < 0) ? x : x-1;\n\t\t\tint startPosY = (y - 1 < 0) ? y : y-1;\n\t\t\tint endPosX = (x + 1 > size-1) ? x : x+1;\n\t\t\tint endPosY = (y + 1 > size-1) ? y : y+1;\n\t\t\tfor (int i = startPosX; i<=endPosX; i++)\n\t\t\t\tfor (int j = startPosY; j<=endPosY; j++)\n\t\t\t\t\tif (grid_num[i][j] == 1)\n\t\t\t\t\t\tctr++;\n\t\t\treturn ctr;\n\t\t}\n//-------------------------------LOGIC ENDS---------------------\n\n\tpublic void setGrid() {\n\t\tfor (int i=0;i<size;i++) \n\t\t\tfor (int j = 0;j<size;j++) \n\t\t\t\tif (grid_num[i][j] == 1)\n\t\t\t\t\tgrid[i][j].setBackground(Color.BLACK);\n\t\t\t\telse\n\t\t\t\t\tgrid[i][j].setBackground(Color.WHITE);\n\t}\n\t\n\tpublic void loop() {\n\t\tint tmp = 0;\n\t\tint tmp_grid[][] = new int[size][size];\n\t\tfor (int i=0;i<size;i++) \n\t\t\tfor (int j = 0;j<size;j++) {\n\t\t\t\ttmp = neigh(i, j);\n\t\t\t\ttmp = (grid_num[i][j] == 1) ? tmp-1 : tmp;\n\t\t\t\tif (tmp < 2 || tmp > 3)\n\t\t\t\t\t\ttmp_grid[i][j] = 0;\n\t\t\t\telse if(tmp == 3)\n\t\t\t\t\t\ttmp_grid[i][j] = 1;\n\t\t\t\telse\n\t\t\t\t\t\ttmp_grid[i][j] = grid_num[i][j];\n\t\t\t}\n\t\t\tgrid_num = tmp_grid;\n\t\t\tsetGrid();\n\t\t}\n//----------------------------------------------------------------------------------------------------------------------------------\n\tpublic static void main(String[] args) {\n\t\tSwingUtilities.invokeLater(new Jobs());\n\t}\n\n\tpublic static class Jobs implements Runnable {\n\t\tpublic void run() {\n\t\t\tnew Conway();\n\t\t}\n\t}\n//-----------------------------------------------------------------------------------------------------------------------------------\n\tpublic class Seed extends Thread {\n\t\tpublic void run() {\n\t\t\tfor (int i =0;i<10000;i++){\n\t\t\t\tif (kill == 1) break;\n\t\t\t\tloop();\n\t\t\t\tstatus.setText(\"Generation: \"+(gen++));\n\t\t\t\ttry {\n\t\t\t\t\tThread.sleep(100);\n\t\t\t\t}\n\t\t\t\tcatch(InterruptedException e) {}\n\t\t\t}\n\t\t}\n\t}\n\tpublic class Listen implements ActionListener {\n\t\tpublic void actionPerformed(ActionEvent ae) {\n\t\t\tif (ae.getSource() == toggle){\n\t\t\t\tif (kill == 0) {\n\t\t\t\t\ttoggle.setText(\"Start\");\n\t\t\t\t\tkill = 1;\n\t\t\t\t}\n\t\t\t\telse {\n\t\t\t\t\ttoggle.setText(\"Stop\");\n\t\t\t\t\tkill = 0;\n\t\t\t\t\tSeed t = new Seed();\n\t\t\t\t\tt.start();\n\t\t\t\t}\n\t\t\t}\n\t\t\telse if (ae.getSource() == wow) {\n\t\t\t\tfor (int i = 0;i<size;i++) {\n\t\t\t\t\tgrid[size/2][i].setBackground(Color.BLACK);\n\t\t\t\t\tgrid_num[size/2][i] = 1;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\telse if (ae.getSource() == gun) {\n\t\t\t\tint i_fill[] = {4, 4, 5, 5, 2, 2, 3, 4, 5, 6, 7, 8, 8, 5, 3, 4, 5, 6, 5, 2, 3, 4 , 2, 3, 4, 1, 5, 0, 1, 5, 6, 2, 2, 3, 3,7};\n\t\t\t\tint j_fill[] = {0,1,0,1,12,13,11,10,10,10,11,12,13,14,15,16,16,16,17,20,20,20,21,21,21,22,22,24,24,24,24,34,35,34,35, 15};\n\t\t\t\tint shift = 4;\n\t\t\t\tfor (int i = 0;i<36;i++){\n\t\t\t\t\t\t\tgrid_num[i_fill[i]+shift][j_fill[i]+shift] = 1;\n\t\t\t\t\t\t\tgrid[i_fill[i]][j_fill[i]].setBackground(Color.BLACK);\n\t\t\t\t\t\t}\n\t\t\t}\n\t\t\telse if (ae.getSource() == clear) {\n\t\t\t\tfor (int i = 0;i<size;i++)\n\t\t\t\t\tfor(int j=0;j<size;j++) {\n\t\t\t\t\t\tgrid_num[i][j] = 0;\n\t\t\t\t\t\tgrid[i][j].setBackground(Color.GRAY);\n\t\t\t\t\t\t}\n\t\t\t}\n\t\t\telse if (ae.getSource() == exit) {\n\t\t\t\tSystem.exit(0);\n\t\t\t}\n\t\t\telse {\n\t\t\t\tfor (int i = 0;i<size;i++)\n\t\t\t\t\tfor(int j=0;j<size;j++) {\n\t\t\t\t\t\tif (ae.getSource() == grid[i][j]) {\n\t\t\t\t\t\t\tif (grid_num[i][j] == 0) {\n\t\t\t\t\t\t\t\tgrid_num[i][j] = 1;\n\t\t\t\t\t\t\t\tgrid[i][j].setBackground(Color.BLACK);\n\t\t\t\t\t\t\t\treturn;\n\t\t\t\t\t\t\t} else if (grid_num[i][j] == 1) {\n\t\t\t\t\t\t\t\tgrid_num[i][j] = 0;\n\t\t\t\t\t\t\t\tgrid[i][j].setBackground(Color.WHITE);\n\t\t\t\t\t\t\t\treturn;\n\t\t\t\t\t\t\t}\n\t\t\t\t\t\t\t\n\t\t\t\t\t\t}\n\t\t\t\t\t}\n\t\t\t}\n\t\t}\n\t}\n}"
}
{
"filename": "code/cellular_automaton/src/conways_game_of_life/conways_game_of_life.rb",
"content": "# Part of Cosmos by OpenGenus Foundation\nclass GameOfLife\n attr_accessor :matrix, :rows, :columns\n\n def initialize(rows, columns)\n @rows = rows\n @columns = columns\n @matrix = []\n rows.times do |row|\n @matrix[row] ||= []\n columns.times do |column|\n @matrix[row][column] = false\n end\n end\n end\n\n def next_tick\n new_matrix = []\n rows.times do |row|\n new_matrix[row] ||= []\n columns.times do |column|\n alive_neighbours_count = neighbours(row, column).count(true)\n if !matrix[row][column] && alive_neighbours_count == 3\n new_matrix[row][column] = true\n elsif matrix[row][column] && alive_neighbours_count != 2 && alive_neighbours_count != 3\n new_matrix[row][column] = false\n else\n new_matrix[row][column] = matrix[row][column]\n end\n end\n end\n self.matrix = new_matrix\n end\n\n def print_cells\n rows.times do |row|\n columns.times do |column|\n matrix[row][column] ? print('O') : print('-')\n end\n print \"\\n\"\n end\n print \"\\n\"\n end\n\n def neighbours(row, column)\n neighbours = []\n rows_limit = matrix.count - 1\n columns_limit = matrix[0].count - 1\n ([0, row - 1].max..[rows_limit, row + 1].min).to_a.each do |row_index|\n ([0, column - 1].max..[columns_limit, column + 1].min).to_a.each do |column_index|\n neighbours << matrix[row_index][column_index] unless row_index == row && column_index == column\n end\n end\n neighbours\n end\nend\n\ngame = GameOfLife.new(100, 100)\n\ngame.matrix[0][0] = true\ngame.matrix[0][1] = true\ngame.matrix[1][0] = true\ngame.matrix[1][2] = true\ngame.matrix[2][1] = true\ngame.matrix[2][2] = true\n\ngame.print_cells\n\n5.times do\n game.next_tick\n game.print_cells\nend"
}
{
"filename": "code/cellular_automaton/src/conways_game_of_life/game_of_life_c_sdl.c",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n * This program uses SDL 1.2\n * Author : ABDOUS Kamel\n */\n\n#include <stdlib.h>\n#include <time.h>\n#include <SDL/SDL.h>\n\n\n/* -------------------------------------------------------------------- */\n/* Change automaton state delay */ \n#define RESPAWN_DELAY 25\n\n/*\n * When SIMPLE_MODE = 1, the program displays dead cels in black, & alive cels in green.\n * When SIMPLE_MODE = 0, the program also displays the reborn cells & cells that are going to die.\n */\n#define SIMPLE_MODE 1\n\nint X_NB_CELLS = 800, Y_NB_CELLS = 600, /* Number of cels */\n X_CELL_SIZE = 1, Y_CELL_SIZE = 1, /* Size of cels */\n WINDOW_WIDTH, WINDOW_HEIGHT; /* Calculated in main */\n/* -------------------------------------------------------------------- */\n\n/* This struct is passed as parameter to the SDL timer. */\ntypedef struct\n{\n int **cels, /* Cels matrix */\n **buffer; /* This buffer is used to calculate the new state of the automaton */\n\n} UpdateCelsTimerParam;\n\ntypedef enum\n{\n DEAD,\n ALIVE,\n REBORN,\n DYING\n\n} CelsState;\n\n/* Function to call to launch the automaton */\nint \nlaunch_app(SDL_Surface* screen);\n\n/* Updates the state of the automaton.\n * That's a SDL timer callback function.\n */\nUint32 \nupdate_cels(Uint32 interval, void* param);\n\n/* Inc neighborhood according to game of life rules. */\nvoid \ninc_neighborhood(int cel, int* neighborhood);\n\n/* Returns the new state of cel regarding the value of neighborhood */\nint \nlive_cel(int cel, int neighborhood);\n\nvoid \ndisplay_cels(int** cels, SDL_Surface* screen);\n\nvoid \ndisplay_one_cel(int cel, int x, int y, SDL_Surface* screen);\n\n/* Function used to initialise the two matrices cels & buffer as size_x * size_y matrices. */\nvoid \nalloc_cels(int*** cels, int*** buffer, int size_x, int size_y);\n\nvoid \nfree_cels(int** cels, int** buffer, int size_x);\n\n/* Helper function that colores one pixel. */\nvoid \nset_pixel(SDL_Surface* surf, int x, int y, Uint32 color);\n\nint \nmain()\n{\n srand(time(NULL));\n\n if (SDL_Init(SDL_INIT_VIDEO | SDL_INIT_TIMER) != 0) {\n fprintf(stderr, \"Erreur lors de l'initialisation de la SDL : %s\\n\", SDL_GetError());\n return (EXIT_FAILURE);\n }\n\n WINDOW_WIDTH = X_NB_CELLS * X_CELL_SIZE;\n WINDOW_HEIGHT = Y_NB_CELLS * Y_CELL_SIZE;\n SDL_Surface* screen = SDL_SetVideoMode(WINDOW_WIDTH, WINDOW_HEIGHT, 32, SDL_HWSURFACE | SDL_DOUBLEBUF);\n\n if (screen == NULL) {\n fprintf(stderr, \"Can't create application window : %s\\n\", SDL_GetError());\n SDL_Quit();\n return (EXIT_FAILURE);\n }\n\n int exit_code = launch_app(screen);\n\n SDL_FreeSurface(screen);\n SDL_Quit();\n return (exit_code);\n}\n\n/* Function to call to launch the automaton */\nint \nlaunch_app(SDL_Surface* screen)\n{\n int **cels = NULL, **buffer;\n alloc_cels(&cels, &buffer, X_NB_CELLS, Y_NB_CELLS);\n\n SDL_Event event;\n int stop = 0;\n\n /* The SDL timer params */\n UpdateCelsTimerParam timer_param = {cels, buffer};\n SDL_AddTimer(RESPAWN_DELAY, update_cels, &timer_param);\n\n while (!stop) {\n SDL_PollEvent(&event);\n switch (event.type) {\n case SDL_QUIT:\n stop = 1;\n break;\n }\n\n SDL_FillRect(screen, NULL, SDL_MapRGB(screen->format, 0, 0, 0));\n display_cels(cels, screen);\n SDL_Flip(screen);\n }\n\n free_cels(cels, buffer, X_NB_CELLS);\n return (EXIT_SUCCESS);\n}\n\n/* Updates the state of the automaton.\n * That's a SDL timer callback function.\n */\nUint32 \nupdate_cels(Uint32 interval, void* param)\n{\n UpdateCelsTimerParam* param_struct = param;\n\n int **cels = param_struct->cels,\n **buffer = param_struct->buffer;\n\n int i = 0, j = 0, neighborhood;\n\n /* Here we update the automaton state in the buffer */\n while (i < X_NB_CELLS) {\n j = 0;\n \n /* Conditions in this loop are just an application of the game of life rules */\n while (j < Y_NB_CELLS) {\n if (cels[i][j] == REBORN)\n buffer[i][j] = ALIVE;\n\n else if (cels[i][j] == DYING)\n buffer[i][j] = DEAD;\n\n /* Number of useful cels in the neighborhood */\n neighborhood = 0;\n if (i != 0) {\n inc_neighborhood(cels[i - 1][j], &neighborhood);\n \n if (j != 0)\n inc_neighborhood(cels[i - 1][j - 1], &neighborhood);\n\n if (j != Y_NB_CELLS - 1)\n inc_neighborhood(cels[i - 1][j + 1], &neighborhood);\n }\n\n if (i != X_NB_CELLS - 1) {\n inc_neighborhood(cels[i + 1][j], &neighborhood);\n \n if(j != 0)\n inc_neighborhood(cels[i + 1][j - 1], &neighborhood);\n\n if(j != Y_NB_CELLS - 1)\n inc_neighborhood(cels[i + 1][j + 1], &neighborhood);\n }\n\n if (j != 0)\n inc_neighborhood(cels[i][j - 1], &neighborhood);\n\n if (j != Y_NB_CELLS - 1)\n inc_neighborhood(cels[i][j + 1], &neighborhood);\n\n buffer[i][j] = live_cel(cels[i][j], neighborhood);\n j++;\n }\n\n ++i;\n }\n\n /* Here we copy the new state in the cels matrix */\n for (i = 0; i < X_NB_CELLS; ++i) {\n for (j = 0; j < Y_NB_CELLS; ++j)\n cels[i][j] = buffer[i][j];\n }\n\n return (interval);\n}\n\n/* Inc neighborhood according to game of life rules. */\nvoid \ninc_neighborhood(int cel, int* neighborhood)\n{\n if (cel == ALIVE || cel == REBORN)\n (*neighborhood)++;\n}\n\n/* Returns the new state of cel regarding the value of neighborhood */\nint \nlive_cel(int cel, int neighborhood)\n{\n if (!SIMPLE_MODE) {\n if (cel == ALIVE || cel == REBORN) {\n if(neighborhood == 2 || neighborhood == 3)\n return (ALIVE);\n\n else\n return (DYING);\n }\n\n else {\n if(neighborhood == 3)\n return (REBORN);\n\n else\n return (DEAD);\n }\n }\n\n else {\n if (cel == ALIVE)\n return (neighborhood == 2 || neighborhood == 3);\n\n else\n return (neighborhood == 3);\n }\n}\n\nvoid \ndisplay_cels(int** cels, SDL_Surface* screen)\n{\n int i = 0, j = 0;\n \n while (i < X_NB_CELLS) {\n j = 0;\n while (j < Y_NB_CELLS) {\n display_one_cel(cels[i][j], i, j, screen);\n ++j;\n }\n\n ++i;\n }\n}\n\nvoid \ndisplay_one_cel(int cel, int x, int y, SDL_Surface* screen)\n{\n int i = 0, j = 0,\n r = 0, g = 0, b = 0;\n\n if (!SIMPLE_MODE) {\n if (cel == ALIVE)\n g = 255;\n else if (cel == DEAD)\n r = 255;\n else if (cel == REBORN)\n b = 255;\n else {\n r = 255;\n g = 140;\n }\n }\n\n else {\n if (cel == DEAD)\n return;\n\n g = 255;\n }\n\n while (i < X_CELL_SIZE) {\n j = 0;\n while (j < Y_CELL_SIZE) {\n set_pixel(screen, x * X_CELL_SIZE + i, y * Y_CELL_SIZE + j, SDL_MapRGB(screen->format, r, g, b));\n ++j;\n }\n\n ++i;\n }\n}\n\n/* Function used to initialise the two matrices cels & buffer as size_x * size_y matrices. */\nvoid \nalloc_cels(int*** cels, int*** buffer, int size_x, int size_y)\n{\n *cels = malloc(sizeof(int*) * size_x);\n *buffer = malloc(sizeof(int*) * size_x);\n\n int i = 0, j = 0;\n while (i < size_x) {\n (*cels)[i] = malloc(sizeof(int) * size_y);\n (*buffer)[i] = malloc(sizeof(int) * size_y);\n\n j = 0;\n while (j < size_y) {\n /* The initial state is picked randomly */\n (*cels)[i][j] = rand() % 2;\n ++j;\n }\n\n i++;\n }\n}\n\nvoid \nfree_cels(int** cels, int** buffer, int size_x)\n{\n int i = 0;\n while (i < size_x) {\n free(cels[i]);\n free(buffer[i]);\n ++i;\n }\n\n free(cels);\n free(buffer);\n}\n\n/* --------------------------------------------------------------------------------- */\n/* Helper function that colores one pixel. */\nvoid \nset_pixel(SDL_Surface* surf, int x, int y, Uint32 color)\n{\n int bpp = surf->format->BytesPerPixel;\n Uint8* p = (Uint8*)surf->pixels + y * surf->pitch + x * bpp;\n\n switch (bpp) {\n case 1:\n *p = color;\n break;\n\n case 2:\n *(Uint16*)p = color;\n break;\n\n case 3:\n if (SDL_BYTEORDER == SDL_BIG_ENDIAN) {\n p[0] = (color >> 16) & 0xff;\n p[1] = (color >> 8) & 0xff;\n p[2] = color & 0xff;\n }\n\n else {\n p[0] = color & 0xff;\n p[1] = (color >> 8) & 0xff;\n p[2] = (color >> 16) & 0xff;\n }\n break;\n\n case 4:\n *(Uint32*)p = color;\n break;\n }\n}"
}
{
"filename": "code/cellular_automaton/src/conways_game_of_life/gameoflife.hs",
"content": "{-# LANGUAGE DeriveFunctor #-}\n\nmodule GameOfLife where\n\nimport Control.Comonad\nimport Graphics.Gloss\nimport Data.List\nimport Control.Monad\nimport System.Random\n\ndata Zipper a = Zipper [a] a Int [a] deriving (Functor)\n -- Zipper ls x n rs represents the doubly-infinite list (reverse ls ++\n -- [x] ++ rs) viewed at offset n\ninstance (Show a) => Show (Zipper a) where\n show (Zipper ls x n rs) =\n show (reverse (take 3 ls)) ++ \" \" ++ show (x,n) ++ \" \" ++ show (take 3 rs)\n\ninstance Comonad Zipper\n where\n extract (Zipper _ x _ _) = x\n duplicate z = Zipper (tail $ iterate back z) z 0 (tail $ iterate forth z)\n\nback, forth :: Zipper a -> Zipper a\nback (Zipper (l:ls) x n rs) = Zipper ls l (n-1) (x:rs)\nforth (Zipper ls x n (r:rs)) = Zipper (x:ls) r (n+1) rs\n\nnewtype Grid a = Grid (Zipper (Zipper a)) deriving (Functor)\ninstance Show a => Show (Grid a) where\n show (Grid (Zipper ls x n rs)) =\n unlines $ zipWith (\\a b -> a ++ \" \" ++ b)\n (map show [n-3..n+3])\n (map show (reverse (take 3 ls) ++ [x] ++ (take 3 rs)))\n\ninstance Comonad Grid\n where\n extract = get\n duplicate (Grid z) = fmap Grid $ Grid $ roll $ roll z\n where\n roll g = Zipper (tail $ iterate (fmap back) g) g 0 (tail $ iterate (fmap forth) g)\n\nup, down, right, left :: Grid a -> Grid a\nup (Grid g) = Grid (back g)\ndown (Grid g) = Grid (forth g)\nleft (Grid g) = Grid (fmap back g)\nright (Grid g) = Grid (fmap forth g)\n\nset :: a -> Grid a -> Grid a\nset y (Grid (Zipper ls row n rs)) = (Grid (Zipper ls (set' row) n rs))\n where set' (Zipper ls' x m rs') = Zipper ls' y m rs'\n\nget :: Grid a -> a\nget (Grid (Zipper _ (Zipper _ x _ _) _ _)) = x\n\nresetH :: Grid a -> Grid a\nresetH g@(Grid (Zipper _ (Zipper _ _ m _) n _))\n | m > 0 = resetH (left g)\n | m < 0 = resetH (right g)\n | otherwise = g\n\nresetV :: Grid a -> Grid a\nresetV g@(Grid (Zipper _ (Zipper _ _ m _) n _))\n | n > 0 = resetV (up g)\n | n < 0 = resetV (down g)\n | otherwise = g\n\nrecenter :: Grid a -> Grid a\nrecenter = resetH . resetV\n\nfalseGrid :: Grid Bool\nfalseGrid =\n let falseRow = Zipper falses False 0 falses\n falses = repeat False\n falseRows = repeat falseRow\n in Grid (Zipper falseRows falseRow 0 falseRows)\n\ncopyBox :: [[a]] -> Grid a -> Grid a\ncopyBox [] g = recenter g\ncopyBox (xs:xss) g = copyBox xss (down $ copyArray xs g)\n where\n copyArray [] g = resetH g\n copyArray (x:xs) g = copyArray xs (right $ set x g)\n\nextractBox :: Int -> Int -> Grid a -> [[a]]\nextractBox m n (Grid g) = map (f n) $ (f m) g\n where\n f m (Zipper _ x _ xs) = x : take (m - 1) xs\n\nliveNeighborCount :: Grid Bool -> Int\nliveNeighborCount grid = length . filter (True == ) $ map (\\direction -> get $ direction grid) directions\n where\n directions = [left, right, up, down, up . left, up . right, down . left, down . right]\n\nstepCell :: Grid Bool -> Bool\nstepCell grid\n | cell == True && numNeighbors < 2 = False\n | cell == True && numNeighbors < 4 = True\n | cell == True && numNeighbors > 3 = False\n | cell == False && numNeighbors == 3 = True\n | otherwise = cell\n where\n cell = extract grid\n numNeighbors = liveNeighborCount grid\n\nmakeRandomGrid :: Int -> Int -> IO (Grid Bool)\nmakeRandomGrid m n = do\n bools <- (replicateM m $ replicateM n (randomIO :: IO Bool))\n return $ copyBox bools falseGrid\n\ntoPicture :: Int -> Int -> Grid Bool -> Picture\ntoPicture m n grid =\n let bools = extractBox m n grid\n (width, height) = (fromIntegral m , fromIntegral n)\n indexed = map (zip [0.. ] . sequence) . zip [0..]\n cell = rectangleSolid 10 10\n draw (x, (y, s)) = color (if s then black else white) . translate ((x-width/2)*10+5) ((y-height/2)*10+5)\n in pictures . map (pictures . map (\\s -> draw s cell)) . indexed $ bools\n\nmain :: IO ()\nmain = do\n grid <- makeRandomGrid 100 100\n simulate FullScreen blue 1 grid (toPicture 100 100) (const $ const (=>> stepCell))"
}
{
"filename": "code/cellular_automaton/src/conways_game_of_life/life.c",
"content": "#include <time.h>\n#include <stdlib.h>\n#include <stdio.h>\n#include <string.h>\n// Part of Cosmos by OpenGenus Foundation\n#define width 40\n#define height 30\n\nconst char* clear = \"\\e[1;1H\\e[2J\";\n\nint main(){\n srand(time(NULL));\n\n int board[height][width];\n int temp[height][width];\n\n for (int i = 0; i < height; ++i) {\n for (int j = 0; j < width; ++j) {\n board[i][j] = rand() % 2;\n }\n }\n\n memcpy(temp, board, sizeof(int) * width * height);\n\n while (1) {\n for (int i = 0; i < height; ++i) {\n for (int j = 0; j < width; ++j) {\n putc(32 + 16 * board[i][j], stdout);\n }\n putc('\\n', stdout);\n }\n for (int i = 0; i < height; ++i) {\n for (int j = 0; j < width; ++j) {\n int sum = board[(i + 1) % height][(j + 1) % width] +\n board[(i + 1) % height][j] +\n board[(i + 1) % height][(j + width - 1) % width] +\n board[i][(j + 1) % width] +\n board[i][(j + width - 1) % width] +\n board[(i + height - 1) % height][(j + 1) % width] +\n board[(i + height - 1) % height][j] +\n board[(i + height - 1) % height][(j + width - 1) % width];\n\n temp[i][j] = (temp[i][j] && sum >= 2 && sum <= 3) || sum == 3;\n }\n }\n memcpy(board, temp, sizeof(int) * width * height);\n getchar();\n printf(\"%s\", clear);\n }\n return 0;\n}"
}
{
"filename": "code/cellular_automaton/src/conways_game_of_life/life.cpp",
"content": "#include <iostream>\n#include <fstream>\n#include <cstdlib>\nusing namespace std;\n// Part of Cosmos by OpenGenus Foundation\n// Defines the world size parameters\nconst int SIZE_X = 50;\nconst int SIZE_Y = 160;\n// Number of random cells are filled\nconst int number = SIZE_X * SIZE_Y / 8;\n\nvoid loadConfig(char world[][SIZE_Y]);\n// Initializes world and loads up several default values\n\nvoid generation(char world[][SIZE_Y], int& gen);\n// Takes the world array and decides whether cells live, die, or multiply.\n\nvoid copyGeneration(char world[][SIZE_Y], char worldCopy[][SIZE_Y]);\n// Copies the current generation from world to worldCopy.\n\nvoid updateGeneration(char world[][SIZE_Y], char worldCopy[][SIZE_Y]);\n// Updates the current generation in worldCopy to world.\n\nvoid display(const char world[][SIZE_Y], ofstream& fout, const int gen);\n// Outputs the world as text.\n// Records each successive world generation in a text file.\n\nint getNeighbors(const char world[][SIZE_Y], int posX, int posY);\n// Retrieves the amount of neighbors a cell has\n\nint main()\n{\n int gen(0);\n char world[SIZE_X][SIZE_Y];\n\n ofstream fout;\n fout.open(\"life.txt\");\n if (fout.fail())\n {\n cout << \"Opening file for output failed!\\n\";\n exit(1);\n }\n\n loadConfig(world);\n display(world, fout, gen);\n char c;\n cout << \"\\nPress return to create a new generation and press x to exit!\\n\";\n cin.get(c);\n while (c == '\\n') {\n generation(world, gen);\n display(world, fout, gen);\n cout << \"\\nPress return to create a new generation and press x to exit!\\n\";\n cin.get(c);\n }\n\n return 0;\n}\n\nvoid loadConfig(char world[][SIZE_Y])\n{\n for (int i = 0; i < SIZE_X; i++)\n for (int j = 0; j < SIZE_Y; j++)\n world[i][j] = '-';\n\n srand(time(NULL));\n\n for (int i = 0; i < number; i++)\n {\n int randX = rand() % SIZE_X;\n int randY = rand() % SIZE_Y;\n world[randX][randY] = '*';\n }\n}\n\nint getNeighbors(const char world[][SIZE_Y], int posX, int posY)\n{\n int neighbors(0);\n\n int row = posX - 1, column = posY - 1;\n // Top-left\n if (column >= 0 && row >= 0)\n if (world[row][column] == '*')\n neighbors++;\n // Top-middle\n column++;\n if (row >= 0)\n if (world[row][column] == '*')\n neighbors++;\n // Top-right\n column++;\n if (column < SIZE_Y && row >= 0)\n if (world[row][column] == '*')\n neighbors++;\n\n row = posX, column = posY; // Reset\n\n // Left\n column--;\n if (column >= 0)\n if (world[row][column] == '*')\n neighbors++;\n\n // Right\n column = posY + 1;\n if (column < SIZE_Y)\n if (world[row][column] == '*')\n neighbors++;\n\n row = posX + 1, column = posY - 1; // Reset\n\n // Bottom-left\n if (row < SIZE_X && column >= 0)\n if (world[row][column] == '*')\n neighbors++;\n column++;\n if (row < SIZE_X)\n if (world[row][column] == '*')\n neighbors++;\n column++;\n if (row < SIZE_X && column < SIZE_Y)\n if (world[row][column] == '*')\n neighbors++;\n\n return neighbors;\n}\n\nvoid generation(char world[][SIZE_Y], int& gen)\n{\n char worldCopy[SIZE_X][SIZE_Y];\n copyGeneration(world, worldCopy);\n for (int i = 0; i < SIZE_X; i++)\n for (int j = 0; j < SIZE_Y; j++)\n {\n int neighbors = getNeighbors(world, i, j);\n if (neighbors <= 1 || neighbors > 3)\n worldCopy[i][j] = '-';\n else if (neighbors == 3 && worldCopy[i][j] == '-')\n worldCopy[i][j] = '*';\n }\n updateGeneration(world, worldCopy);\n gen++;\n}\n\nvoid copyGeneration(char world[][SIZE_Y], char worldCopy[][SIZE_Y])\n{\n for (int i = 0; i < SIZE_X; i++)\n for (int j = 0; j < SIZE_Y; j++)\n worldCopy[i][j] = world[i][j];\n\n}\n\nvoid updateGeneration(char world[][SIZE_Y], char worldCopy[][SIZE_Y])\n{\n for (int i = 0; i < SIZE_X; i++)\n for (int j = 0; j < SIZE_Y; j++)\n world[i][j] = worldCopy[i][j];\n\n}\n\nvoid display(const char world[][SIZE_Y], ofstream& fout, const int gen)\n{\n cout << \"Generation \" << gen << endl;\n fout << 'G' << ' ' << gen << '\\n';\n for (int i = 0; i < SIZE_X; i++)\n {\n for (int j = 0; j < SIZE_Y; j++)\n {\n char cell = world[i][j];\n cout << cell;\n fout << cell;\n }\n cout << endl;\n fout << '\\n';\n }\n cout << endl;\n fout << '\\n';\n}"
}
{
"filename": "code/cellular_automaton/src/conways_game_of_life/life.go",
"content": "package main\n\nimport (\n\t\"os\"\n\t\"os/exec\"\n\t\"bytes\"\n\t\"fmt\"\n\t\"math/rand\"\n\t\"time\"\n)\n\ntype Game struct {\n\twidth int\n\theight int\n\tdata [][]bool\n}\n\nfunc NewGame(width int, height int) *Game {\n\tdata := make([][]bool, height)\n\tfor i := range data {\n\t\tdata[i] = make([]bool, width)\n\t}\n\treturn &Game{\n\t\twidth: width,\n\t\theight: height,\n\t\tdata: data,\n\t}\n}\n\nfunc (g *Game) Init(n int) {\n\tfor i := 0; i < n; i ++ {\n\t\tg.Set(rand.Intn(g.width), rand.Intn(g.height), true)\n\t}\n}\n\nfunc (g *Game) Set(i int, j int, b bool) {\n\tg.data[j][i] = b\n}\n\n// Check the cell is alive\nfunc (g *Game) Alive(i int, j int) bool {\n\ti = (i + g.width) % g.width\n\tj = (j + g.height) % g.height\n\treturn g.data[j][i]\n}\n\n// Return next state of a cell, false = dead, true = alive\nfunc (g *Game) NextState(x int, y int) bool {\n\talive := 0\n\tfor i := -1; i <= 1; i++ {\n\t\tfor j := -1; j <= 1; j++ {\n\t\t\tif !((i == 0) && (j == 0)) && g.Alive(x + i, y + j) {\n\t\t\t\talive++\n\t\t\t}\n\t\t}\n\t}\n\treturn alive == 3 || alive == 2 && g.Alive(x, y)\n}\n\nfunc (g *Game) Next() *Game {\n\tng := NewGame(g.width, g.height)\n\tfor i := 0; i < g.width; i ++ {\n\t\tfor j := 0; j < g.height; j ++ {\n\t\t\tng.Set(i, j, g.NextState(i, j))\n\t\t}\n\t}\n\treturn ng\n}\n\nfunc (g *Game) String() string {\n\tvar buf bytes.Buffer\n\tfor j := 0; j < g.height; j ++ {\n\t\tbuf.WriteString(\"'\")\n\t\tfor i := 0; i < g.width; i ++ {\n\t\t\tif g.Alive(i, j) {\n\t\t\t\tbuf.WriteString(\"o\")\n\t\t\t} else {\n\t\t\t\tbuf.WriteString(\" \")\n\t\t\t}\n\t\t}\n\t\tbuf.WriteString(\"'\\n\")\n\t}\n\treturn buf.String()\n}\n\nfunc main() {\n\tg := NewGame(10, 10)\n\tg.Init(30)\n\tfor i := 0; i < 100; i ++ {\n\t\t// Clear screen\n\t\tc := exec.Command(\"clear\")\n\t\tc.Stdout = os.Stdout\n\t\tc.Run()\n\t\tfmt.Print(g)\n\t\tg = g.Next()\n\t\ttime.Sleep(100 * time.Millisecond)\n\t}\n\tfmt.Println(\"vim-go\")\n}"
}
{
"filename": "code/cellular_automaton/src/conways_game_of_life/life.py",
"content": "from tkinter import *\nimport random\n\n\nclass Game:\n def __init__(self):\n self.createButtons()\n\n def createButtons(self):\n self.buttons = {}\n for i in range(0, 400):\n status = random.choice([1, 0])\n self.buttons[i] = [\n Button(root, bg=(\"yellow\" if status == 1 else \"black\")),\n status,\n ]\n self.buttons[i][0].grid(row=i // 20, column=i % 20)\n\n def run(self):\n for k in range(0, 400):\n row = k // 20\n column = k % 20\n c = 0\n for i in [row - 1, row, row + 1]:\n for j in [column - 1, column, column + 1]:\n if self.validButton(i, j):\n c = c + self.buttons[i * 20 + j][1]\n\n print(c)\n c = c - self.buttons[k][1]\n if c <= 1 or c >= 4:\n self.buttons[k][0].configure(bg=\"black\")\n elif self.buttons[k][1] == 1 or c == 3:\n self.buttons[k][0].configure(bg=\"yellow\")\n else:\n self.buttons[k][0].configure(bg=\"black\")\n for k in range(0, 400):\n self.buttons[k][1] = 0 if self.buttons[k][0].cget(\"bg\") == \"black\" else 1\n if k % 20 == 0:\n print(\" \")\n root.after(500, self.run)\n\n def validButton(self, i, j):\n if i < 0 or j < 0 or j > 19 or i > 19:\n return False\n else:\n return True\n\n\ndef main():\n global root\n root = Tk()\n root.title(\"Game Of Life\")\n game = Game()\n root.after(500, game.run)\n root.mainloop()\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/cellular_automaton/src/conways_game_of_life/life.rb",
"content": "#!/usr/bin/env ruby\n# Part of Cosmos by OpenGenus Foundation\n\nclass GameOfLife\n attr_accessor :board\n\n def initialize(board = [])\n self.board = board.empty? ? Array.new(rand(1..10)) { Array.new(rand(1..10), rand(0..1)) } : board\n end\n\n def play(turns = 1)\n for turn in 1..turns\n next_board = Array.new(board.size) { Array.new(board.first.size, 0) }\n next_board.each_index do |row|\n next_board[row].each_index { |col| next_board[row][col] = next_state(row, col) }\n end\n self.board = next_board\n pretty_print\n end\n end\n\n def pretty_print\n board.each do |row|\n puts row.join(' ')\n end\n puts '**' * board.size\n end\n\n private\n\n def next_state(row, col)\n alive = count_alive_neighbors(row, col)\n return 1 if (alive > 1) && (alive < 4) && (board[row][col] == 1)\n return 1 if (alive == 3) && (board[row][col] == 0)\n 0\n end\n\n def count_alive_neighbors(row, col)\n neighbors = 0\n for i in -1..1\n for j in -1..1\n next if (i == 0) && (j == 0)\n neighbors += 1 if alive_neighbor?(row + i, col + j)\n end\n end\n neighbors\n end\n\n def alive_neighbor?(row, col)\n (row >= 0) && (row < board.size) && (col >= 0) && (col < board.first.size) && (board[row][col] == 1)\n end\nend\n\nboard = [[0, 0, 0], [1, 1, 1], [0, 0, 0]]\ngame = GameOfLife.new(board)\ngame.pretty_print\ngame.play(3)"
}
{
"filename": "code/cellular_automaton/src/conways_game_of_life/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# Cellular Automaton\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/cellular_automaton/src/elementary_cellular_automata/elementarycellularautomaton.java",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\npublic class ElementaryCellularAutomaton {\n\tprivate static final int SIZE = 200;\n\tprivate static final int HISTORY = 200;\n\t\n\tprivate final byte rule;\n\n\tprivate boolean[][] grid = new boolean[HISTORY][SIZE];\n\tprivate int step = 0;\n\n\tpublic static void main(String[] args) {\n\t\tElementaryCellularAutomaton elCell = new ElementaryCellularAutomaton((byte)2);\n\t\tdo {\n\t\t\tSystem.out.println(elCell);\n\t\t} while(elCell.step());\n\t}\n\n\t/**\n\t * This is the main class for an elementary cellular automaton\n\t * @param ruleNr Identifier for which of the 256 rules should be used\n\t */\n\tpublic ElementaryCellularAutomaton(byte ruleNr) {\n\t\trule = ruleNr;\n\t\t/*\n\t\t * Initialize center to 1\n\t\t * (other initializations are possible and will result in different behavior)\n\t\t */\n\t\tgrid[0][SIZE / 2] = true;\n\t}\n\n\tpublic boolean step() {\n\t\tif (step < (HISTORY-1)) {\n\t\t\tfor (int i = 0; i < SIZE; i++) {\n\t\t\t\tbyte neighbors = getNeighbors(i);\n\t\t\t\tboolean nextValue = (((rule >> neighbors) & 1) == 1);\n\t\t\t\tgrid[step+1][i] = nextValue;\n\t\t\t}\n\t\t\tstep++;\n\t\t\treturn true;\n\t\t} else {\n\t\t\treturn false;\n\t\t}\n\t}\n\t\n\t/*\n\t * Convert the cell and its neighbors to a 3-bit number\n\t */\n\tprivate byte getNeighbors(int cell) {\n\t\treturn (byte) ((grid[step][getCellIndex(cell-1)] ? 4 : 0) + \n\t\t\t\t(grid[step][getCellIndex(cell)] ? 2 : 0) +\n\t\t\t\t(grid[step][getCellIndex(cell+1)] ? 1 : 0));\n\t}\n\t\n\t/*\n\t * Convert the cell number to the index of the grid array\n\t */\n\tprivate int getCellIndex(int cell) {\n\t\tint result = cell%SIZE;\n\t\tif(result < 0) {\n\t\t\tresult += SIZE;\n\t\t}\n\t\treturn result;\n\t}\n\t\n\t@Override\n\tpublic String toString() {\n\t\tStringBuilder builder = new StringBuilder();\n\t\tboolean[] array = grid[step];\n\t\tfor (int i = 0; i < array.length; i++) {\n\t\t if (array[i]) builder.append(\"1\"); else builder.append(\"0\");\n\t\t}\n\t\treturn builder.toString();\n\t}\n}"
}
{
"filename": "code/cellular_automaton/src/elementary_cellular_automata/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# Cellular Automaton\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/cellular_automaton/src/genetic_algorithm/genetic_algorithm2.py",
"content": "import math\nimport random\n\n\ndef poblacion_inicial(max_poblacion, num_vars):\n # Crear poblacion inicial aleatoria\n poblacion = []\n for i in range(max_poblacion):\n gen = []\n for j in range(num_vars):\n if random.random() > 0.5:\n gen.append(1)\n else:\n gen.append(0)\n poblacion.append(gen[:])\n return poblacion\n\n\ndef adaptacion_3sat(gen, solucion):\n # contar clausulas correctas\n n = 3\n cont = 0\n clausula_ok = True\n for i in range(len(gen)):\n n = n - 1\n if gen[i] != solucion[i]:\n clausula_ok = False\n if n == 0:\n if clausula_ok:\n cont = cont + 1\n n = 3\n clausula_ok = True\n if n > 0:\n if clausula_ok:\n cont = cont + 1\n return cont\n\n\ndef evalua_poblacion(poblacion, solucion):\n # evalua todos los genes de la poblacion\n adaptacion = []\n for i in range(len(poblacion)):\n adaptacion.append(adaptacion_3sat(poblacion[i], solucion))\n return adaptacion\n\n\ndef seleccion(poblacion, solucion):\n adaptacion = evalua_poblacion(poblacion, solucion)\n # suma de todas las puntuaciones\n total = 0\n for i in range(len(adaptacion)):\n total = total + adaptacion[i]\n # escogemos dos elementos\n val1 = random.randint(0, total)\n val2 = random.randint(0, total)\n sum_sel = 0\n for i in range(len(adaptacion)):\n sum_sel = sum_sel + adaptacion[i]\n if sum_sel >= val1:\n gen1 = poblacion[i]\n break\n sum_sel = 0\n for i in range(len(adaptacion)):\n sum_sel = sum_sel + adaptacion[i]\n if sum_sel >= val2:\n gen2 = poblacion[i]\n break\n return gen1, gen2\n\n\ndef cruce(gen1, gen2):\n # creuza 2 genes y obtiene 2 descendientes\n nuevo_gen1 = []\n nuevo_gen2 = []\n corte = random.randint(0, len(gen1))\n nuevo_gen1[0:corte] = gen1[0:corte]\n nuevo_gen1[corte:] = gen2[corte:]\n nuevo_gen2[0:corte] = gen2[0:corte]\n nuevo_gen2[corte:] = gen1[corte:]\n return nuevo_gen1, nuevo_gen2\n\n\ndef mutacion(prob, gen):\n # muta un gen con una probabilidad prob\n if random.random() < prob:\n cromosoma = random.randint(0, len(gen))\n if gen[cromosoma - 1] == 0:\n gen[cromosoma - 1] = 1\n else:\n gen[cromosoma - 1] = 0\n return gen\n\n\ndef elimina_peores_genes(poblacion, solucion):\n # elimina los dos peores genes\n adaptacion = evalua_poblacion(poblacion, solucion)\n i = adaptacion.index(min(adaptacion))\n del poblacion[i]\n del adaptacion[i]\n i = adaptacion.index(min(adaptacion))\n del poblacion[i]\n del adaptacion[i]\n\n\ndef mejor_gen(poblacion, solucion):\n # devuelve el mejor gen de la poblacion\n adaptacion = evalua_poblacion(poblacion, solucion)\n return poblacion[adaptacion.index(max(adaptacion))]\n\n\ndef algoritmo_genetico():\n max_iter = 10\n max_poblacion = 50\n num_vars = 10\n fin = False\n solucion = poblacion_inicial(1, num_vars)[0]\n poblacion = poblacion_inicial(max_poblacion, num_vars)\n\n iteraciones = 0\n while not fin:\n iteraciones = iteraciones + 1\n for i in range(len(poblacion) // 2):\n gen1, gen2 = seleccion(poblacion, solucion)\n nuevo_gen1, nuevo_gen2 = cruce(gen1, gen2)\n nuevo_gen1 = mutacion(0.1, nuevo_gen1)\n nuevo_gen2 = mutacion(0.1, nuevo_gen2)\n poblacion.append(nuevo_gen1)\n poblacion.append(nuevo_gen2)\n elimina_peores_genes(poblacion, solucion)\n\n if max_iter < iteraciones:\n fin = True\n\n print(\"Solucion: \" + str(solucion))\n mejor = mejor_gen(poblacion, solucion)\n return mejor, adaptacion_3sat(mejor, solucion)\n\n\nif __name__ == \"__main__\":\n random.seed()\n mejor_gen = algoritmo_genetico()\n print(\"Mejor gen encontrado:\" + str(mejor_gen[0]))\n print(\"Funcion de adaptacion: \" + str(mejor_gen[1]))"
}
{
"filename": "code/cellular_automaton/src/genetic_algorithm/genetic_algorithm.go",
"content": "package main\n\nimport (\n \"fmt\";\n \"math/rand\";\n \"time\"\n)\n\nfunc main() {\n rand.Seed(time.Now().UnixNano())\n const geneSet = \" abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ!.\"\n target := \"Not all those who wander are lost.\"\n calc := func (candidate string) int {\n return getFitness(target, candidate)\n }\n\n start := time.Now()\n\n disp := func (candidate string) {\n fmt.Print(candidate)\n fmt.Print(\"\\t\")\n fmt.Print(calc(candidate))\n fmt.Print(\"\\t\")\n fmt.Println(time.Since(start))\n }\n\n var best = getBest(calc, disp, geneSet, len(target))\n println(best)\n\n fmt.Print(\"Total time: \")\n fmt.Println(time.Since(start))\n}\n\nfunc getBest(getFitness func(string) int, display func(string), geneSet string, length int) string {\n var bestParent = generateParent(geneSet, length)\n value := getFitness(bestParent)\n var bestFitness = value\n\n for bestFitness < length {\n child := mutateParent(bestParent, geneSet)\n fitness := getFitness(child)\n if fitness > bestFitness {\n display(child)\n bestFitness = fitness\n bestParent = child\n }\n }\n\n return bestParent\n}\n\nfunc mutateParent(parent, geneSet string) string {\n geneIndex := rand.Intn(len(geneSet))\n parentIndex := rand.Intn(len(parent))\n candidate := \"\"\n if parentIndex > 0 {\n candidate += parent[:parentIndex]\n }\n candidate += geneSet[geneIndex:1+geneIndex]\n if parentIndex+1 < len(parent) {\n candidate += parent[parentIndex+1:]\n }\n return candidate\n}\n\nfunc generateParent(geneSet string, length int) string {\n s := \"\"\n for i := 0; i < length; i++ {\n index := rand.Intn(len(geneSet))\n s += geneSet[index:1+index]\n }\n return s\n}\n\nfunc getFitness(target, candidate string) int {\n differenceCount := 0\n for i := 0; i < len(target); i++ {\n if target[i] != candidate[i] {\n differenceCount++\n }\n }\n return len(target) - differenceCount\n}"
}
{
"filename": "code/cellular_automaton/src/genetic_algorithm/genetic_algorithm.java",
"content": "import javax.swing.*;\nimport java.awt.*;\nimport java.util.*;\n\npublic class GeneticAlgorithm extends JFrame {\n\tRandom rnd = new Random(1);\n\tint n = rnd.nextInt(300) + 250;\n\n\tint generation;\n\tdouble[] x = new double[n];\n\tdouble[] y = new double[n];\n\tint[] bestState;\n\n\t{\n\t\tfor (int i = 0; i < n; i++) {\n\t\t\tx[i] = rnd.nextDouble();\n\t\t\ty[i] = rnd.nextDouble();\n\t\t}\n\t}\n\n\tpublic void geneticAlgorithm() {\n\t\tbestState = new int[n];\n\t\tfor (int i = 0; i < n; i++)\n\t\t\tbestState[i] = i;\n\t\tfinal int populationLimit = 100;\n\t\tfinal Population population = new Population(populationLimit);\n\t\tfinal int n = x.length;\n\t\tfor (int i = 0; i < populationLimit; i++)\n\t\t\tpopulation.chromosomes.add(new Chromosome(optimize(getRandomPermutation(n))));\n\n\t\tfinal double mutationRate = 0.3;\n\t\tfinal int generations = 10_000;\n\n\t\tfor (generation = 0; generation < generations; generation++) {\n\t\t\tint i = 0;\n\t\t\twhile (population.chromosomes.size() < population.populationLimit) {\n\t\t\t\tint i1 = rnd.nextInt(population.chromosomes.size());\n\t\t\t\tint i2 = (i1 + 1 + rnd.nextInt(population.chromosomes.size() - 1)) % population.chromosomes.size();\n\n\t\t\t\tChromosome parent1 = population.chromosomes.get(i1);\n\t\t\t\tChromosome parent2 = population.chromosomes.get(i2);\n\n\t\t\t\tint[][] pair = crossOver(parent1.p, parent2.p);\n\n\t\t\t\tif (rnd.nextDouble() < mutationRate) {\n\t\t\t\t\tmutate(pair[0]);\n\t\t\t\t\tmutate(pair[1]);\n\t\t\t\t}\n\n\t\t\t\tpopulation.chromosomes.add(new Chromosome(optimize(pair[0])));\n\t\t\t\tpopulation.chromosomes.add(new Chromosome(optimize(pair[1])));\n\t\t\t}\n\t\t\tpopulation.nextGeneration();\n\t\t\tbestState = population.chromosomes.get(0).p;\n\t\t\trepaint();\n\t\t}\n\t}\n\n\tint[][] crossOver(int[] p1, int[] p2) {\n\t\tint n = p1.length;\n\t\tint i1 = rnd.nextInt(n);\n\t\tint i2 = (i1 + 1 + rnd.nextInt(n - 1)) % n;\n\n\t\tint[] n1 = p1.clone();\n\t\tint[] n2 = p2.clone();\n\n\t\tboolean[] used1 = new boolean[n];\n\t\tboolean[] used2 = new boolean[n];\n\n\t\tfor (int i = i1; ; i = (i + 1) % n) {\n\t\t\tn1[i] = p2[i];\n\t\t\tused1[n1[i]] = true;\n\t\t\tn2[i] = p1[i];\n\t\t\tused2[n2[i]] = true;\n\t\t\tif (i == i2) {\n\t\t\t\tbreak;\n\t\t\t}\n\t\t}\n\n\t\tfor (int i = (i2 + 1) % n; i != i1; i = (i + 1) % n) {\n\t\t\tif (used1[n1[i]]) {\n\t\t\t\tn1[i] = -1;\n\t\t\t} else {\n\t\t\t\tused1[n1[i]] = true;\n\t\t\t}\n\t\t\tif (used2[n2[i]]) {\n\t\t\t\tn2[i] = -1;\n\t\t\t} else {\n\t\t\t\tused2[n2[i]] = true;\n\t\t\t}\n\t\t}\n\n\t\tint pos1 = 0;\n\t\tint pos2 = 0;\n\t\tfor (int i = 0; i < n; i++) {\n\t\t\tif (n1[i] == -1) {\n\t\t\t\twhile (used1[pos1])\n\t\t\t\t\t++pos1;\n\t\t\t\tn1[i] = pos1++;\n\t\t\t}\n\t\t\tif (n2[i] == -1) {\n\t\t\t\twhile (used2[pos2])\n\t\t\t\t\t++pos2;\n\t\t\t\tn2[i] = pos2++;\n\t\t\t}\n\t\t}\n\t\treturn new int[][]{n1, n2};\n\t}\n\n\tvoid mutate(int[] p) {\n\t\tint n = p.length;\n\t\tint i = rnd.nextInt(n);\n\t\tint j = (i + 1 + rnd.nextInt(n - 1)) % n;\n\t\treverse(p, i, j);\n\t}\n\n\t// http://en.wikipedia.org/wiki/2-opt\n\tstatic void reverse(int[] p, int i, int j) {\n\t\tint n = p.length;\n\t\t// reverse order from i to j\n\t\twhile (i != j) {\n\t\t\tint t = p[j];\n\t\t\tp[j] = p[i];\n\t\t\tp[i] = t;\n\t\t\ti = (i + 1) % n;\n\t\t\tif (i == j) break;\n\t\t\tj = (j - 1 + n) % n;\n\t\t}\n\t}\n\n\tdouble eval(int[] state) {\n\t\tdouble res = 0;\n\t\tfor (int i = 0, j = state.length - 1; i < state.length; j = i++)\n\t\t\tres += dist(x[state[i]], y[state[i]], x[state[j]], y[state[j]]);\n\t\treturn res;\n\t}\n\n\tstatic double dist(double x1, double y1, double x2, double y2) {\n\t\tdouble dx = x1 - x2;\n\t\tdouble dy = y1 - y2;\n\t\treturn Math.sqrt(dx * dx + dy * dy);\n\t}\n\n\tint[] getRandomPermutation(int n) {\n\t\tint[] res = new int[n];\n\t\tfor (int i = 0; i < n; i++) {\n\t\t\tint j = rnd.nextInt(i + 1);\n\t\t\tres[i] = res[j];\n\t\t\tres[j] = i;\n\t\t}\n\t\treturn res;\n\t}\n\n\t// try all 2-opt moves\n\tint[] optimize(int[] p) {\n\t\tint[] res = p.clone();\n\t\tfor (boolean improved = true; improved; ) {\n\t\t\timproved = false;\n\t\t\tfor (int i = 0; i < n; i++) {\n\t\t\t\tfor (int j = 0; j < n; j++) {\n\t\t\t\t\tif (i == j || (j + 1) % n == i) continue;\n\t\t\t\t\tint i1 = (i - 1 + n) % n;\n\t\t\t\t\tint j1 = (j + 1) % n;\n\t\t\t\t\tdouble delta = dist(x[res[i1]], y[res[i1]], x[res[j]], y[res[j]])\n\t\t\t\t\t\t\t+ dist(x[res[i]], y[res[i]], x[res[j1]], y[res[j1]])\n\t\t\t\t\t\t\t- dist(x[res[i1]], y[res[i1]], x[res[i]], y[res[i]])\n\t\t\t\t\t\t\t- dist(x[res[j]], y[res[j]], x[res[j1]], y[res[j1]]);\n\t\t\t\t\tif (delta < -1e-9) {\n\t\t\t\t\t\treverse(res, i, j);\n\t\t\t\t\t\timproved = true;\n\t\t\t\t\t}\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\treturn res;\n\t}\n\n\tclass Chromosome implements Comparable<Chromosome> {\n\t\tfinal int[] p;\n\t\tprivate double cost = Double.NaN;\n\n\t\tpublic Chromosome(int[] p) {\n\t\t\tthis.p = p;\n\t\t}\n\n\t\tpublic double getCost() {\n\t\t\treturn Double.isNaN(cost) ? cost = eval(p) : cost;\n\t\t}\n\n\t\t@Override\n\t\tpublic int compareTo(Chromosome o) {\n\t\t\treturn Double.compare(getCost(), o.getCost());\n\t\t}\n\t}\n\n\tstatic class Population {\n\t\tList<Chromosome> chromosomes = new ArrayList<>();\n\t\tfinal int populationLimit;\n\n\t\tpublic Population(int populationLimit) {\n\t\t\tthis.populationLimit = populationLimit;\n\t\t}\n\n\t\tpublic void nextGeneration() {\n\t\t\tCollections.sort(chromosomes);\n\t\t\tchromosomes = new ArrayList<>(chromosomes.subList(0, (chromosomes.size() + 1) / 2));\n\t\t}\n\t}\n\n\t// visualization code\n\tpublic GeneticAlgorithm() {\n\t\tsetContentPane(new JPanel() {\n\t\t\tprotected void paintComponent(Graphics g) {\n\t\t\t\tsuper.paintComponent(g);\n\t\t\t\t((Graphics2D) g).setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON);\n\t\t\t\t((Graphics2D) g).setStroke(new BasicStroke(3));\n\t\t\t\tg.setColor(Color.BLUE);\n\t\t\t\tint w = getWidth() - 5;\n\t\t\t\tint h = getHeight() - 30;\n\t\t\t\tfor (int i = 0, j = n - 1; i < n; j = i++)\n\t\t\t\t\tg.drawLine((int) (x[bestState[i]] * w), (int) ((1 - y[bestState[i]]) * h),\n\t\t\t\t\t\t\t(int) (x[bestState[j]] * w), (int) ((1 - y[bestState[j]]) * h));\n\t\t\t\tg.setColor(Color.RED);\n\t\t\t\tfor (int i = 0; i < n; i++)\n\t\t\t\t\tg.drawOval((int) (x[i] * w) - 1, (int) ((1 - y[i]) * h) - 1, 3, 3);\n\t\t\t\tg.setColor(Color.BLACK);\n\t\t\t\tg.drawString(String.format(\"length: %.3f\", eval(bestState)), 5, h + 20);\n\t\t\t\tg.drawString(String.format(\"generation: %d\", generation), 150, h + 20);\n\t\t\t}\n\t\t});\n\t\tsetSize(new Dimension(600, 600));\n\t\tsetDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);\n\t\tsetVisible(true);\n\t\tnew Thread(this::geneticAlgorithm).start();\n\t}\n\n\tpublic static void main(String[] args) {\n\t\tnew GeneticAlgorithm();\n\t}\n}"
}
{
"filename": "code/cellular_automaton/src/genetic_algorithm/genetic_algorithm.js",
"content": "var Gene = function(code) {\n if (code) this.code = code;\n this.cost = 9999;\n};\nGene.prototype.code = \"\";\nGene.prototype.random = function(length) {\n while (length--) {\n this.code += String.fromCharCode(Math.floor(Math.random() * 255));\n }\n};\nGene.prototype.mutate = function(chance) {\n if (Math.random() > chance) return;\n\n var index = Math.floor(Math.random() * this.code.length);\n var upOrDown = Math.random() <= 0.5 ? -1 : 1;\n var newChar = String.fromCharCode(this.code.charCodeAt(index) + upOrDown);\n var newString = \"\";\n for (var i = 0; i < this.code.length; i++) {\n if (i == index) newString += newChar;\n else newString += this.code[i];\n }\n\n this.code = newString;\n};\nGene.prototype.mate = function(gene) {\n var pivot = Math.round(this.code.length / 2) - 1;\n\n var child1 = this.code.substr(0, pivot) + gene.code.substr(pivot);\n var child2 = gene.code.substr(0, pivot) + this.code.substr(pivot);\n\n return [new Gene(child1), new Gene(child2)];\n};\nGene.prototype.calcCost = function(compareTo) {\n var total = 0;\n for (var i = 0; i < this.code.length; i++) {\n total +=\n (this.code.charCodeAt(i) - compareTo.charCodeAt(i)) *\n (this.code.charCodeAt(i) - compareTo.charCodeAt(i));\n }\n this.cost = total;\n};\nvar Population = function(goal, size) {\n this.members = [];\n this.goal = goal;\n this.generationNumber = 0;\n while (size--) {\n var gene = new Gene();\n gene.random(this.goal.length);\n this.members.push(gene);\n }\n};\nPopulation.prototype.display = function() {\n document.body.innerHTML = \"\";\n document.body.innerHTML +=\n \"<h2>Generation: \" + this.generationNumber + \"</h2>\";\n document.body.innerHTML += \"<ul>\";\n for (var i = 0; i < this.members.length; i++) {\n document.body.innerHTML +=\n \"<li>\" + this.members[i].code + \" (\" + this.members[i].cost + \")\";\n }\n document.body.innerHTML += \"</ul>\";\n};\nPopulation.prototype.sort = function() {\n this.members.sort(function(a, b) {\n return a.cost - b.cost;\n });\n};\nPopulation.prototype.generation = function() {\n for (var i = 0; i < this.members.length; i++) {\n this.members[i].calcCost(this.goal);\n }\n\n this.sort();\n this.display();\n var children = this.members[0].mate(this.members[1]);\n this.members.splice(this.members.length - 2, 2, children[0], children[1]);\n\n for (var i = 0; i < this.members.length; i++) {\n this.members[i].mutate(0.5);\n this.members[i].calcCost(this.goal);\n if (this.members[i].code == this.goal) {\n this.sort();\n this.display();\n return true;\n }\n }\n this.generationNumber++;\n var scope = this;\n setTimeout(function() {\n scope.generation();\n }, 20);\n};\n\nvar population = new Population(\"Hello, world!\", 20);\npopulation.generation();"
}
{
"filename": "code/cellular_automaton/src/genetic_algorithm/genetic_algorithm.py",
"content": "import random\n\n#\n# Global variables\n# Setup optimal string and GA input variables.\n#\n\nOPTIMAL = \"Hello, World\"\nDNA_SIZE = len(OPTIMAL)\nPOP_SIZE = 20\nGENERATIONS = 5000\n\n#\n# Helper functions\n# These are used as support, but aren't direct GA-specific functions.\n#\n\n\ndef weighted_choice(items):\n \"\"\"\n Chooses a random element from items, where items is a list of tuples in\n the form (item, weight). weight determines the probability of choosing its\n respective item. Note: this function is borrowed from ActiveState Recipes.\n \"\"\"\n weight_total = sum((item[1] for item in items))\n n = random.uniform(0, weight_total)\n for item, weight in items:\n if n < weight:\n return item\n n = n - weight\n return item\n\n\ndef random_char():\n \"\"\"\n Return a random character between ASCII 32 and 126 (i.e. spaces, symbols,\n letters, and digits). All characters returned will be nicely printable.\n \"\"\"\n return chr(int(random.randrange(32, 126, 1)))\n\n\ndef random_population():\n \"\"\"\n Return a list of POP_SIZE individuals, each randomly generated via iterating\n DNA_SIZE times to generate a string of random characters with random_char().\n \"\"\"\n pop = []\n for i in range(POP_SIZE):\n dna = \"\"\n for c in range(DNA_SIZE):\n dna += random_char()\n pop.append(dna)\n return pop\n\n\n#\n# GA functions\n# These make up the bulk of the actual GA algorithm.\n#\n\n\ndef fitness(dna):\n \"\"\"\n For each gene in the DNA, this function calculates the difference between\n it and the character in the same position in the OPTIMAL string. These values\n are summed and then returned.\n \"\"\"\n fitness = 0\n for c in range(DNA_SIZE):\n fitness += abs(ord(dna[c]) - ord(OPTIMAL[c]))\n return fitness\n\n\ndef mutate(dna):\n \"\"\"\n For each gene in the DNA, there is a 1/mutation_chance chance that it will be\n switched out with a random character. This ensures diversity in the\n population, and ensures that is difficult to get stuck in local minima.\n \"\"\"\n dna_out = \"\"\n mutation_chance = 100\n for c in range(DNA_SIZE):\n if int(random.random() * mutation_chance) == 1:\n dna_out += random_char()\n else:\n dna_out += dna[c]\n return dna_out\n\n\ndef crossover(dna1, dna2):\n \"\"\"\n Slices both dna1 and dna2 into two parts at a random index within their\n length and merges them. Both keep their initial sublist up to the crossover\n index, but their ends are swapped.\n \"\"\"\n pos = int(random.random() * DNA_SIZE)\n return (dna1[:pos] + dna2[pos:], dna2[:pos] + dna1[pos:])\n\n\n#\n# Main driver\n# Generate a population and simulate GENERATIONS generations.\n#\n\nif __name__ == \"__main__\":\n # Generate initial population. This will create a list of POP_SIZE strings,\n # each initialized to a sequence of random characters.\n population = random_population()\n\n # Simulate all of the generations.\n for generation in range(GENERATIONS):\n print(\"Generation %s... Random sample: '%s'\" % (generation, population[0]))\n weighted_population = []\n\n # Add individuals and their respective fitness levels to the weighted\n # population list. This will be used to pull out individuals via certain\n # probabilities during the selection phase. Then, reset the population list\n # so we can repopulate it after selection.\n for individual in population:\n fitness_val = fitness(individual)\n\n # Generate the (individual,fitness) pair, taking in account whether or\n # not we will accidently divide by zero.\n if fitness_val == 0:\n pair = (individual, 1.0)\n else:\n pair = (individual, 1.0 / fitness_val)\n\n weighted_population.append(pair)\n\n population = []\n\n # Select two random individuals, based on their fitness probabilites, cross\n # their genes over at a random point, mutate them, and add them back to the\n # population for the next iteration.\n for _ in range(POP_SIZE // 2):\n # Selection\n ind1 = weighted_choice(weighted_population)\n ind2 = weighted_choice(weighted_population)\n\n # Crossover\n ind1, ind2 = crossover(ind1, ind2)\n\n # Mutate and add back into the population.\n population.append(mutate(ind1))\n population.append(mutate(ind2))\n\n # Display the highest-ranked string after all generations have been iterated\n # over. This will be the closest string to the OPTIMAL string, meaning it\n # will have the smallest fitness value. Finally, exit the program.\n fittest_string = population[0]\n minimum_fitness = fitness(population[0])\n\n for individual in population:\n ind_fitness = fitness(individual)\n if ind_fitness <= minimum_fitness:\n fittest_string = individual\n minimum_fitness = ind_fitness\n\n print(\"Fittest String: %s\" % fittest_string)\n exit(0)"
}
{
"filename": "code/cellular_automaton/src/genetic_algorithm/genetic.cpp",
"content": "/**\n * Root finding using genetic algorithm (Uses C++11).\n * Reference: https://arxiv.org/abs/1703.03864\n */\n\n#include <algorithm>\n#include <cmath>\n#include <iostream>\n#include <random>\n#include <vector>\n\nstruct StopCondition\n{\n StopCondition(double tol, long iter)\n : tolerance(tol)\n , iterations(iter)\n {\n }\n double tolerance;\n long iterations;\n};\n\nstruct GAConfig\n{\n GAConfig(double learning_rate, double variance, long generation_size)\n : lr(learning_rate)\n , var(variance)\n , size(generation_size)\n {\n factor = lr / (var * size);\n }\n double lr;\n double var;\n long size;\n double factor;\n};\n\ntemplate <typename UnaryFunc>\ndouble solve(UnaryFunc f, double initial, GAConfig conf, StopCondition cond)\n{\n std::default_random_engine gen;\n std::normal_distribution<double> normal(0, 1);\n\n std::vector<double> noise(conf.size);\n std::vector<double> generation(conf.size);\n std::vector<double> fitness(conf.size);\n\n double x = initial, error = 0;\n long iter = 0;\n\n do {\n double delta = 0;\n for (int i = 0; i < conf.size; ++i)\n {\n noise[i] = normal(gen);\n generation[i] = x + conf.var * noise[i];\n fitness[i] = exp(-abs(f(generation[i])));\n delta += fitness[i] * noise[i];\n }\n x = x + conf.factor * delta;\n error = std::abs(f(x));\n std::cout << iter + 1 << \"\\tx = \" << x << \"\\t error = \" << error\n << std::endl;\n } while ((error > cond.tolerance) && (++iter < cond.iterations));\n\n return x;\n}\n\nint main()\n{\n auto f = [](double x)\n {\n return (x - 1.0) * (x - 2.0);\n };\n\n auto config = GAConfig{ 0.2, 0.1, 10 };\n auto stop_condition = StopCondition{ 1e-3, 10000 };\n double solution = solve(f, 0.0, config, stop_condition);\n\n std::cout << solution << std::endl;\n}"
}
{
"filename": "code/cellular_automaton/src/langtons_ant/langtons_ant.cpp",
"content": "#include <iostream>\n#include <vector>\n#include <array>\n\nusing std::cout;\nusing std::cin;\nusing std::vector;\nusing std::array;\n\nclass Direction\n{\npublic:\n int x;\n int y;\n const static Direction north;\n const static Direction east;\n const static Direction south;\n const static Direction west;\n const static array<Direction, 4> directions;\n\nprivate:\n int i;\n\nprivate:\n Direction(int x_, int y_, int i_)\n {\n x = x_;\n y = y_;\n i = i_;\n };\npublic:\n Direction() : Direction(north)\n {\n };\n\n Direction right() const\n {\n return directions.at((this->i + 1) % 4);\n }\n\n Direction left() const\n {\n return directions.at((this->i + 3) % 4);\n }\n};\n\nconst Direction Direction::north{0, 1, 0};\nconst Direction Direction::east{1, 0, 1};\nconst Direction Direction::south{0, -1, 2};\nconst Direction Direction::west{-1, 0, 3};\n\nconst array<Direction, 4> Direction::directions({\n north, east, south, west,\n});\n\nclass LangtonAnt\n{\nprivate:\n vector<vector<unsigned char>> board;\n int ant_x;\n int ant_y;\n int width;\n int height;\n Direction direction;\n\npublic:\n LangtonAnt(int width_, int height_)\n {\n board.assign(width_, vector<unsigned char>(height_, 0));\n width = width_;\n height = height_;\n ant_x = width / 2;\n ant_y = height / 2;\n }\n\n void show()\n {\n for (int i = 0; i < height; ++i)\n {\n for (int j = 0; j < width; ++j)\n cout << (board[j][i] ? '#' : ' ');\n cout << '\\n';\n }\n }\n\n void step()\n {\n if (board[ant_x][ant_y])\n {\n board[ant_x][ant_y] = 0;\n direction = direction.left();\n }\n else\n {\n board[ant_x][ant_y] = 1;\n direction = direction.right();\n }\n ant_x = (ant_x + direction.x + width) % width;\n ant_y = (ant_y + direction.y + height) % height;\n }\n};\n\nint main()\n{\n const int width = 100;\n const int height = 50;\n\n const int max_step = 10000;\n\n LangtonAnt langtonAnt(width, height);\n\n for (int step = 0; step <= max_step; ++step)\n {\n cout << \"Step \" << step << '\\n';\n langtonAnt.show();\n cin.get();\n langtonAnt.step();\n }\n}"
}
{
"filename": "code/cellular_automaton/src/langtons_ant/langtons_ant.html",
"content": "<!DOCTYPE html>\n<html>\n\n<head>\n <style>\n canvas {\n position: absolute;\n left: 0;\n top: 0;\n border: 1px solid #000000;\n }\n div {\n position: relative;\n }\n </style>\n <script>\n //Size of the pixel to display (1 is too small to really see)\n var scale = 10;\n\n function fillPixel(x, y) {\n var canvas = document.getElementById(\"gridCanvas\").getContext(\"2d\");\n canvas.fillRect(x * scale, y * scale, scale, scale);\n }\n\n function clearPixel(x, y) {\n var canvas = document.getElementById(\"gridCanvas\").getContext(\"2d\");\n canvas.clearRect(x * scale, y * scale, scale, scale);\n }\n\n function isFilled(x, y) {\n var canvas = document.getElementById(\"gridCanvas\").getContext(\"2d\");\n var imageData = canvas.getImageData(x * scale, y * scale, scale, scale).data;\n\n //Don't need to check them all but doesn't hurt\n for (var i = 0; i < imageData.length; i++) {\n if (imageData[i] > 0) {\n return true;\n }\n }\n\n return false;\n }\n\n function drawAnt(x, y) {\n var canvas = document.getElementById(\"antCanvas\").getContext(\"2d\");\n canvas.clearRect(0, 0, document.getElementById(\"antCanvas\").width, document.getElementById(\"antCanvas\").height);\n\n canvas.strokeStyle = \"#FF0000\";\n canvas.strokeRect(x * scale + 2, y * scale + 2, scale - 4, scale - 4);\n }\n\n var direction = [{\n dx: -1,\n dy: 0\n }, //Left\n {\n dx: 0,\n dy: 1\n }, //Up\n {\n dx: 1,\n dy: 0\n }, //Right\n {\n dx: 0,\n dy: -1\n } //Down\n ];\n\n var directionIndex = 0;\n\n function turnLeft() {\n directionIndex--;\n\n //Should only iterate once\n while (directionIndex < 0) {\n directionIndex = directionIndex + 4;\n }\n }\n\n function turnRight() {\n directionIndex = (directionIndex + 1) % 4;\n }\n\n //height/width is 200 with a scale of 10 so 20 coordinates. 10 is half\n var x = 10;\n var y = 10;\n\n\n function nextMove() {\n //Flip current\n if (isFilled(x, y)) {\n clearPixel(x, y);\n } else {\n fillPixel(x, y);\n }\n\n //Step forward\n x += direction[directionIndex].dx;\n y += direction[directionIndex].dy;\n\n //Turn for next move\n if (isFilled(x, y)) {\n turnLeft();\n } else {\n turnRight();\n }\n\n drawAnt(x, y);\n }\n </script>\n</head>\n\n<body>\n\n <h2>Langton Ant</h2>\n\n <button type=\"button\" onclick=\"nextMove()\">Step</button><br><br>\n\n <div>\n <canvas id=\"gridCanvas\" width=\"200\" height=\"200\" style=\"z-index: 0;\"></canvas>\n <canvas id=\"antCanvas\" width=\"200\" height=\"200\" style=\"z-index: 1;\"></canvas>\n </div>\n\n</body>\n\n</html>"
}
{
"filename": "code/cellular_automaton/src/langtons_ant/langtons_ant.java",
"content": "import java.awt.Color;\nimport java.awt.Dimension;\nimport java.awt.Graphics;\nimport java.awt.Graphics2D;\nimport java.awt.Point;\nimport java.util.ArrayList;\n\nimport javax.swing.JFrame;\nimport javax.swing.JPanel;\n\npublic class LangtonAnt {\n private static final int STEPS = 11000;\n \n private Point position = new Point(0, 0);\n private Direction direction = Direction.LEFT;\n private ArrayList<Point> blackTiles = new ArrayList<>();\n\n private void step() {\n\tif (blackTiles.contains(position)) {\n\t direction = direction.turnRight();\n\t} else {\n\t direction = direction.turnLeft();\n\t}\n\tint index;\n\tif((index = blackTiles.indexOf(position)) != -1) {\n\t blackTiles.remove(index);\n\t} else {\n\t blackTiles.add((Point)position.clone());\n\t}\n\tswitch (direction) {\n\tcase LEFT:\n\t position.translate(-1, 0);\n\t break;\n\tcase RIGHT:\n\t position.translate(1, 0);\n\t break;\n\tcase UP:\n\t position.translate(0, 1);\n\t break;\n\tcase DOWN:\n\t position.translate(0, -1);\n\t break;\n\t}\n }\n\n public static void main(String[] args) {\n\tLangtonAnt ant = new LangtonAnt();\n\tJFrame frame = new JFrame(\"Langtons Ant\");\n\tGridPanel gridPanel = ant.new GridPanel();\n\tframe.getContentPane().add(gridPanel);\n\tframe.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);\n\tframe.pack();\n\tframe.setVisible(true);\n\tfor (int steps = 0; steps < STEPS; steps++) {\n\t ant.step();\n\t try {\n\t\tThread.sleep(10);\n\t } catch (InterruptedException e) {\n\t\te.printStackTrace();\n\t }\n\t frame.repaint();\n\t}\n }\n\n private enum Direction {\n\tLEFT, RIGHT, UP, DOWN;\n\n\tpublic Direction turnLeft() {\n\t switch (this) {\n\t case LEFT:\n\t\treturn DOWN;\n\t case RIGHT:\n\t\treturn UP;\n\t case UP:\n\t\treturn LEFT;\n\t case DOWN:\n\t\treturn RIGHT;\n\t default:\n\t\t// Should never happen\n\t\treturn UP;\n\t }\n\t}\n\n\tpublic Direction turnRight() {\n\t switch (this) {\n\t case LEFT:\n\t\treturn UP;\n\t case RIGHT:\n\t\treturn DOWN;\n\t case UP:\n\t\treturn RIGHT;\n\t case DOWN:\n\t\treturn LEFT;\n\t default:\n\t\t// Should never happen\n\t\treturn UP;\n\t }\n\t}\n }\n \n private class GridPanel extends JPanel {\n\tprivate static final int GRID_SIZE = 10;\n\tprivate static final int DISPLAY_TILES = 60;\n\tprivate static final int PIXEL_SIZE = GRID_SIZE*DISPLAY_TILES;\n\tprivate static final int CENTER = PIXEL_SIZE/2;\n\t\n\tpublic GridPanel() {\n\t setPreferredSize(new Dimension(DISPLAY_TILES*GRID_SIZE, DISPLAY_TILES*GRID_SIZE));\n\t}\n\t\n\t@Override\n\tprotected void paintComponent(Graphics g) {\n\t Graphics2D g2d = (Graphics2D) g;\n\t g2d.setColor(Color.BLACK);\n\t for (Point tile : blackTiles) {\n\t\tg2d.fillRect(tile.x*GRID_SIZE+CENTER, tile.y*GRID_SIZE+CENTER, GRID_SIZE, GRID_SIZE);\n\t }\n\t g2d.setColor(Color.RED);\n\t g2d.fillOval(position.x*GRID_SIZE+CENTER, position.y*GRID_SIZE+CENTER, GRID_SIZE, GRID_SIZE);\n\t}\n }\n}"
}
{
"filename": "code/cellular_automaton/src/langtons_ant/langtons_ant.py",
"content": "import turtle\nimport numpy\n\n\ndef update_maps(graph, turtle, color):\n graph[turtle_pos(turtle)] = color\n return None\n\n\ndef turtle_pos(turtle):\n return (round(turtle.xcor()), round(turtle.ycor()))\n\n\ndef langton_move(turtle, pos, maps, step):\n if pos not in maps or maps[pos] == \"white\":\n turtle.fillcolor(\"black\")\n turtle.stamp()\n update_maps(maps, turtle, \"black\")\n turtle.right(90)\n turtle.forward(step)\n pos = turtle_pos(turtle)\n elif maps[pos] == \"black\":\n turtle.fillcolor(\"white\")\n update_maps(maps, turtle, \"white\")\n turtle.stamp()\n turtle.left(90)\n turtle.forward(step)\n pos = turtle_pos(turtle)\n return pos\n\n\ndef move():\n # Screen\n BACKGROUND_COLOR = \"white\"\n WIDTH = 2000\n HEIGHT = 2000\n # Ant\n SHAPE = \"square\"\n SHAPE_SIZE = 0.5\n SPEED = -1\n STEP = 11\n\n # Data structure ex. {(x, y): \"black\", ..., (x_n, y_n): \"white\"}\n maps = {}\n\n # Initialize Window\n window = turtle.Screen()\n window.bgcolor(BACKGROUND_COLOR)\n window.screensize(WIDTH, HEIGHT)\n\n # Initialize Ant\n ant = turtle.Turtle()\n ant.shape(SHAPE)\n ant.shapesize(SHAPE_SIZE)\n ant.penup()\n ant.speed(SPEED)\n ant.right(180)\n pos = turtle_pos(ant)\n\n moves = 0\n while True:\n pos = langton_move(ant, pos, maps, STEP)\n moves += 1\n print(\"Movements:\", moves)\n\n\nmove()"
}
{
"filename": "code/cellular_automaton/src/langtons_ant/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# Cellular Automaton\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/cellular_automaton/src/nobili_cellular_automata/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# Cellular Automaton\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/cellular_automaton/src/von_neumann_cellular_automata/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\n# Cellular Automaton\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/cellular_automaton/src/von_neumann_cellular_automata/von_neumann_cellular_automata.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n#include <assert.h>\n#define WIDTH 20\n#define HEIGHT 30\n\nconst char* clear = \"\\e[1;1H\\e[2J\";\n\nenum states{ \tU,\t\t\t\t\t\t\t\t/*Empty state (__)*/\n\t\tS, S0, S00, S000, S01, S1, S10, S11,\t//Transition states (??)*/\n\t\tC00, C01, C10, C11, \t\t\t\t\t/*Confluent states ([])*/\n\t\tC_NORTH, C_EAST, C_SOUTH, C_WEST, \t\t/*Common Transmition states (/\\, ->, \\/, <-)*/\n\t\tS_NORTH, S_EAST, S_SOUTH, S_WEST\t\t/*Special Transition states (/\\, ->, \\/, <-)*/\n\t};\t\t\t\t\t\t\n\nstruct cell{\n\tshort state;\n\tshort active; \n};\n\nvoid \ntransmition(struct cell board[][WIDTH], struct cell temp[][WIDTH], int i, int j){\n\tassert(board[i][j].active <= 1);\n\tif(board[i][j].active == 1){\n\t\tswitch(board[i][j].state){\n\t\t/*Common States*/\n\t\t\tcase C_NORTH:\n\t\t\t\tif(i > 0 && board[i -1][j].state <= C_WEST){\n\t\t\t\t\tif(board[i -1][j].state == U)\n\t\t\t\t\t\ttemp[i -1][j].state = S;\n\t\t\t\t\telse\t\t\t\t\t\n\t\t\t\t\t\ttemp[i -1][j].active++;\n\t\t\t\t}\n\t\t\t\telse if(i > 0)\n\t\t\t\t\ttemp[i -1][j].state = U;\n\t\t\t\t\t\n\t\t\t\ttemp[i][j].active--;\n\t\t\t\tbreak;\n\t\t\t\t\n\t\t\tcase C_EAST:\n\t\t\t\tif(j < WIDTH && board[i][j +1].state <= C_WEST){\t\t\t\t\t\n\t\t\t\t\tif(board[i][j +1].state == U)\n\t\t\t\t\t\ttemp[i][j +1].state = S;\n\t\t\t\t\telse\n\t\t\t\t\t\ttemp[i][j +1].active++;\n\t\t\t\t}\n\t\t\t\telse if(j < WIDTH)\n\t\t\t\t\ttemp[i][j +1].state = U;\n\t\t\t\ttemp[i][j].active--;\n\t\t\t\tbreak;\n\t\t\t\t\n\t\t\tcase C_SOUTH:\n\t\t\t\tif(i < HEIGHT && board[i +1][j].state <= C_WEST){\n\t\t\t\t\tif(board[i +1][j].state == U)\n\t\t\t\t\t\ttemp[i +1][j].state = S;\n\t\t\t\t\telse\t\t\t\t\t\n\t\t\t\t\t\ttemp[i +1][j].active++;\n\t\t\t\t}\n\t\t\t\telse if(i < HEIGHT)\n\t\t\t\t\ttemp[i][j +1].state = U;\n\t\t\t\ttemp[i][j].active--;\n\t\t\t\tbreak;\n\t\t\t\n\t\t\tcase C_WEST:\n\t\t\t\tif(j > 0 && board[i][j -1].state <= C_WEST){\n\t\t\t\t\tif(board[i][j -1].state == U)\n\t\t\t\t\t\ttemp[i][j -1].state = S;\n\t\t\t\t\telse\t\t\t\t\t\n\t\t\t\t\t\ttemp[i][j -1].active++;\n\t\t\t\t}\n\t\t\t\telse if(j > 0)\n\t\t\t\t\ttemp[i][j -1].state = U;\n\t\t\t\ttemp[i][j].active--;\n\t\t\t\tbreak;\n\n\t\t/*Special states*/\n\t\t\tcase S_NORTH:\n\t\t\t\tif(i > 0 && (board[i -1][j].state >= S_NORTH || board[i -1][j].state < C_NORTH)){\n\t\t\t\t\tif(board[i -1][j].state == U)\n\t\t\t\t\t\ttemp[i -1][j].state = S;\n\t\t\t\t\telse if(board[i -1][j].state >= C00 && board[i -1][j].state <= C11)\n\t\t\t\t\t\ttemp[i -1][j].state = U;\n\t\t\t\t\telse\n\t\t\t\t\t\ttemp[i -1][j].active++;\n\t\t\t\t}\n\t\t\t\tif(i > 0)\n\t\t\t\t\ttemp[i -1][j].state = U;\n\t\t\t\t\t\n\t\t\t\ttemp[i][j].active--;\n\t\t\t\tbreak;\n\t\t\t\t\n\t\t\tcase S_EAST:\n\t\t\t\tif(j < WIDTH && (board[i][j +1].state >= S_NORTH || board[i][j +1].state < C_NORTH)){\n\t\t\t\t\tif(board[i][j +1].state == U)\n\t\t\t\t\t\ttemp[i][j +1].state = S;\n\t\t\t\t\telse if(board[i][j + 1].state >= C00 && board[i][j +1].state <= C11)\n\t\t\t\t\t\ttemp[i][j +1].state = U;\n\t\t\t\t\telse\t\t\t\t\t\n\t\t\t\t\t\ttemp[i][j +1].active++;\n\t\t\t\t}\n\t\t\t\telse if(j < WIDTH)\n\t\t\t\t\ttemp[i][j +1].state = U;\n\t\t\t\ttemp[i][j].active--;\n\t\t\t\tbreak;\n\t\t\t\t\n\t\t\tcase S_SOUTH:\n\t\t\t\tif(i < HEIGHT && (board[i +1][j].state >= S_NORTH || board[i +1][j].state < C_NORTH)){\n\t\t\t\t\tif(board[i +1][j].state == U)\n\t\t\t\t\t\ttemp[i +1][j].state = S;\n\t\t\t\t\telse if(board[i +1][j].state >= C00 && board[i +1][j].state <= C11)\n\t\t\t\t\t\ttemp[i +1][j].state = U;\n\t\t\t\t\telse\t\t\t\t\t\n\t\t\t\t\t\ttemp[i +1][j].active++;\n\t\t\t\t}\n\t\t\t\telse if(i < HEIGHT)\n\t\t\t\t\ttemp[i][j +1].state = U;\n\t\t\t\ttemp[i][j].active--;\n\t\t\t\tbreak;\n\t\t\t\n\t\t\tcase S_WEST:\n\t\t\t\tif(j > 0 && (board[i][j -1].state >= S_NORTH || board[i][j -1].state < C_NORTH)){\n\t\t\t\t\tif(board[i][j -1].state == U)\n\t\t\t\t\t\ttemp[i][j -1].state = S;\n\t\t\t\t\telse if(board[i][j -1].state >= C00 && board[i][j -1].state <= C11)\n\t\t\t\t\t\ttemp[i][j -1].state = U;\n\t\t\t\t\telse\t\t\t\t\t\n\t\t\t\t\t\ttemp[i][j -1].active++;\n\t\t\t\t}\n\t\t\t\telse if(j > 0)\n\t\t\t\t\ttemp[i][j -1].state = U;\n\t\t\t\ttemp[i][j].active--;\n\t\t\t\tbreak;\n\t\t\t\t\n\t\t}\n\t}\n}\n\nvoid \nconfluent(struct cell board[][WIDTH], struct cell temp[][WIDTH], int i, int j){\n\tint aux[4];\t\t/*Neighborhood vector [up, left, down, right]*/\n\tint in = 0, out = 0;\n\n\taux[0] = (i > 0 && board[i -1][j].state == C_SOUTH && ++in) - \n\t\t\t\t(i > 0 && (board[i -1][j].state == C_NORTH || board[i -1][j].state == S_NORTH) && ++out); \n\taux[1] = (j > 0 && board[i][j -1].state == C_EAST && ++in) -\n\t\t\t\t(j > 0 && (board[i][j -1].state == C_WEST || board[i][j -1].state == S_WEST)&& ++out);\n\taux[2] = (i < HEIGHT && board[i +1][j].state == C_NORTH && ++in) - \n\t\t\t\t(i < HEIGHT && (board[i +1][j].state == C_SOUTH || board[i +1][j].state == S_SOUTH) && ++out);\n\taux[3] = (j < WIDTH && board[i][j +1].state == C_WEST && ++in) - \n\t\t\t\t(j < WIDTH && (board[i][j +1].state == C_EAST || board[i][j +1].state == S_EAST) && ++out);\n\t\n\tswitch(board[i][j].state){\n\t\tcase C00:\n\t\t\tif(temp[i][j].active >= in)\n\t\t\t\ttemp[i][j].state = C01;\n\t\t\ttemp[i][j].active = 0;\n\t\t\tbreak;\n\n\t\tcase C01:\n\t\t\tif(out > 0){\t\t\t/*Transfering data for neighborhood*/\n\t\t\t\tif(aux[0] == -1)\ttemp[i -1][j].active++;\n\t\t\t\tif(aux[1] == -1)\ttemp[i][j -1].active++;\n\t\t\t\tif(aux[2] == -1)\ttemp[i +1][j].active++;\n\t\t\t\tif(aux[3] == -1)\ttemp[i][j +1].active++;\n\t\t\t\t\n\t\t\t}\n\t\t\tif(temp[i][j].active >= in)\n\t\t\t\ttemp[i][j].state = C11;\n\t\t\telse\n\t\t\t\ttemp[i][j].state = C10;\n\t\t\ttemp[i][j].active = 0;\n\t\t\tbreak;\n\n\t\tcase C10:\n\t\t\tif(temp[i][j].active >= in)\n\t\t\t\ttemp[i][j].state = C01;\n\t\t\telse\n\t\t\t\ttemp[i][j].state = C00;\n\t\t\ttemp[i][j].active = 0;\n\t\t\tbreak;\n\n\t\tcase C11:\n\t\t\tif(out > 0){\n\t\t\t\tif(aux[0] == -1)\ttemp[i -1][j].active++;\n\t\t\t\tif(aux[1] == -1)\ttemp[i][j -1].active++;\n\t\t\t\tif(aux[2] == -1)\ttemp[i +1][j].active++;\n\t\t\t\tif(aux[3] == -1)\ttemp[i][j +1].active++;\n\t\t\t\t\n\t\t\t}\n\t\t\tif(temp[i][j].active >= in)\n\t\t\t\ttemp[i][j].state = C11;\n\t\t\telse \n\t\t\t\ttemp[i][j].state = C10;\n\t\t\ttemp[i][j].active = 0;\n\t\t\tbreak;\n\t}\n}\n\nvoid \ntransition(struct cell board[][WIDTH], struct cell temp[][WIDTH], int i, int j){\n\t\n\tswitch(board[i][j].state){\n\t\tcase S:\n\t\t\tif(temp[i][j].active == 1)\n\t\t\t\ttemp[i][j].state = S1;\n\t\t\telse\n\t\t\t\ttemp[i][j].state = S0;\n\t\t\ttemp[i][j].active--;\n\t\t\tbreak;\n\n\t\tcase S0:\n\t\t\tif(temp[i][j].active == 1)\n\t\t\t\ttemp[i][j].state = S01;\n\t\t\telse\n\t\t\t\ttemp[i][j].state = S00;\n\t\t\ttemp[i][j].active--;\n\t\t\tbreak;\n\n\t\tcase S00:\n\t\t\tif(temp[i][j].active == 1)\n\t\t\t\ttemp[i][j].state = C_WEST;\n\t\t\telse\n\t\t\t\ttemp[i][j].state = S000;\n\t\t\ttemp[i][j].active--;\n\t\t\tbreak;\n\n\t\tcase S000:\n\t\t\tif(temp[i][j].active == 1)\n\t\t\t\ttemp[i][j].state = C_NORTH;\n\t\t\telse\n\t\t\t\ttemp[i][j].state = C_EAST;\n\t\t\ttemp[i][j].active--;\n\t\t\tbreak;\n\n\t\tcase S01:\n\t\t\tif(temp[i][j].active == 1)\n\t\t\t\ttemp[i][j].state = S_EAST;\n\t\t\telse\n\t\t\t\ttemp[i][j].state = C_SOUTH;\n\t\t\ttemp[i][j].active--;\n\t\t\tbreak;\n\n\t\tcase S1:\n\t\t\tif(temp[i][j].active == 1)\n\t\t\t\ttemp[i][j].state = S11;\n\t\t\telse\n\t\t\t\ttemp[i][j].state = S10;\n\t\t\ttemp[i][j].active--;\n\t\t\tbreak;\n\n\t\tcase S10:\n\t\t\tif(temp[i][j].active == 1)\n\t\t\t\ttemp[i][j].state = S_WEST;\n\t\t\telse\n\t\t\t\ttemp[i][j].state = S_NORTH;\n\t\t\ttemp[i][j].active--;\n\t\t\tbreak;\n\n\t\tcase S11:\n\t\t\tif(temp[i][j].active == 1)\n\t\t\t\ttemp[i][j].state = C00;\n\t\t\telse\n\t\t\t\ttemp[i][j].state = S_SOUTH;\n\t\t\ttemp[i][j].active--;\n\t\t\tbreak;\n\n\t}\n}\n\nvoid \nupdate(struct cell board[][WIDTH], struct cell temp[][WIDTH]){\n\tint i, j;\n\n\tfor(i = 0; i < HEIGHT; i++){\n\t\tfor(j = 0; j < WIDTH; j++){\n\t\t\t\n\t\t\ttemp[i][j].active = temp[i][j].active > 0;\n\t\t\tboard[i][j].state = temp[i][j].state;\n\t\t\tboard[i][j].active = temp[i][j].active;\n\t\t}\n\t}\n\n\tfor(i = 0; i < HEIGHT; i++){\n\t\t\tfor(j = 0; j < WIDTH; j++){\n\t\t\t\tif(board[i][j].state >= C_NORTH)\t/*Transmition states*/\n\t\t\t\t\ttransmition(board, temp, i, j);\n\t\t\t}\n\t}\n\n\tfor(i = 0; i < HEIGHT; i++){\n\t\t\tfor(j = 0; j < WIDTH; j++){\n\t\t\t\t\n\t\t\t\tif(board[i][j].state == U)\t\t\t\t/*Empty state*/\n\t\t\t\t\tcontinue;\n\n\t\t\t\telse if(board[i][j].state < C00) \t\t/*Transition states*/\n\t\t\t\t\ttransition(board, temp, i, j);\n\n\t\t\t\telse if(board[i][j].state < C_NORTH) \t/*Confluent states*/\n\t\t\t\t\tconfluent(board, temp, i, j);\n\t\t\t\t\n\t\t\t}\n\t\t}\n\n\t\n}\n\nvoid print_board(struct cell board[][WIDTH]){\n\tint i, j;\n\tfor(i = 0; i < HEIGHT; i++){\n\t\tfor(j = 0; j < WIDTH; j++){\n\t\t\tif(board[i][j].active == 1)\n\t\t\t\tprintf(\"++\");\n\t\t\telse if(board[i][j].state == U )\n\t\t\t\tprintf(\"__\");\n\t\t\telse if(board[i][j].state < C00)\n\t\t\t\tprintf(\"??\");\n\t\t\telse if(board[i][j].state < C_NORTH)\n\t\t\t\tprintf(\"<>\");\n\t\t\telse{ \n\t\t\t\tif((board[i][j].state - C_NORTH) % 4 == 0)\n\t\t\t\t\tprintf(\"/\\\\\");\n\t\t\t\telse if((board[i][j].state - C_NORTH) % 4 == 1)\n\t\t\t\t\tprintf(\"->\");\n\t\t\t\telse if((board[i][j].state - C_NORTH) % 4 == 2)\n\t\t\t\t\tprintf(\"\\\\/\");\n\t\t\t\telse if((board[i][j].state - C_NORTH) % 4 == 3)\n\t\t\t\t\tprintf(\"<-\");\n\t\t\t}\n\t\t\tprintf(\".\");\n\t\t}\n\t\tprintf(\"\\n\");\n\t}\n}\n\nvoid clear_board(struct cell board[][WIDTH], struct cell temp[][WIDTH]){\n\tint i, j;\t\n\tfor(i = 0; i < HEIGHT; i++){\n\t\tfor(j = 0; j < WIDTH; j++){\n\t\t\tboard[i][j].state = U;\n\t\t\tboard[i][j].active = 0;\n\t\t\ttemp[i][j].state = U;\n\t\t\ttemp[i][j].active = 0;\n\t\t}\n\t}\n}\n\nvoid initial_board(struct cell board[][WIDTH], struct cell temp[][WIDTH]){\n\tint i;\n\t/*Create loop*/\n\tfor(i = 5; i <= 10; i++){\n\t\tboard[5][i].state = C_EAST;\n\t\tboard[10][i].state = C_WEST;\n\t\tboard[i][5].state = C_NORTH;\n\t\tboard[i][10].state = C_SOUTH;\n\n\t\ttemp[5][i].state = C_EAST;\n\t\ttemp[10][i].state = C_WEST;\n\t\ttemp[i][5].state = C_NORTH;\n\t\ttemp[i][10].state = C_SOUTH;\n\n\t}\n\tboard[5][5].state = C_EAST;\n\tboard[5][10].state = C_SOUTH;\n\tboard[10][10].state = C_WEST;\n\tboard[10][5].state = C_NORTH;\n\ttemp[5][5].state = C_EAST;\n\ttemp[5][10].state = C00;\n\ttemp[5][11].state = S_EAST;\n\ttemp[10][10].state = C_WEST;\n\ttemp[10][5].state = C_NORTH;\n\t\n\t/*initial condition*/\n\ttemp[10][5].active = 1;\n\ttemp[10][7].active = 1;\n\ttemp[10][8].active = 1; \n}\n\nint main(){\n\tstruct cell board[HEIGHT][WIDTH];\n\tstruct cell temp[HEIGHT][WIDTH];\n\t\n\tclear_board(board, temp);\n\tinitial_board(board, temp);\t\n\n\tdo{\n\t\t\n\t\t/*Update boards*/\n\t\tupdate(board, temp);\n\t\tprint_board(board);\t\t\n\n\t\tgetchar();\n\t\tprintf(\"%s\", clear);\n\t}while(1);\n}"
}
{
"filename": "code/cellular_automaton/test/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/compression/src/lossless_compression/huffman/huffman.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n *\n * huffman compression synopsis\n *\n * template<typename _Tp>\n * struct BinaryTreeNode {\n * _Tp value;\n * std::shared_ptr<BinaryTreeNode<_Tp> > left, right;\n * BinaryTreeNode(_Tp v,\n * std::shared_ptr<BinaryTreeNode<_Tp> > l = {},\n * std::shared_ptr<BinaryTreeNode<_Tp> > r = {});\n * };\n *\n * template<typename _Tp>\n * struct Comp {\n * public:\n * bool operator()(_Tp a, _Tp b) const;\n * };\n *\n * class HuffmanTest;\n *\n * class Huffman {\n * private:\n * typedef size_t size_type;\n * typedef unsigned long long base_type;\n * typedef std::pair<char, size_type> freq_node_type;\n * typedef std::unordered_map<char, size_type> freq_type;\n * typedef std::pair<size_type, std::pair<char, std::string> > value_type;\n * typedef BinaryTreeNode<value_type> tree_node_type;\n * typedef std::priority_queue<std::shared_ptr<tree_node_type>,\n * std::vector<std::shared_ptr<tree_node_type> >,\n * Comp<std::shared_ptr<tree_node_type> > > tree_type;\n * typedef std::unordered_map<char, std::string> dictionary_type;\n * typedef std::unordered_map<std::string, char> reverse_dictionary_type;\n *\n * public:\n * Huffman()\n * :frequency_(), tree_(), dictionary_(), reverse_dictionary_(), sentinel_(), binary_bit_();\n *\n * std::string compression(std::string const &in);\n *\n * std::string compression(std::istream &in);\n *\n * void compression(std::string const &in, std::ostream &out);\n *\n * void compression(std::istream &in, std::ostream &out);\n *\n * std::string decompression(std::string const &in);\n *\n * std::string decompression(std::istream &in);\n *\n * void decompression(std::string const &in, std::ostream &out);\n *\n * void decompression(std::istream &in, std::ostream &out);\n *\n * private:\n * freq_type frequency_;\n * tree_type tree_;\n * dictionary_type dictionary_;\n * reverse_dictionary_type reverse_dictionary_;\n * std::shared_ptr<tree_node_type> sentinel_;\n * size_type binary_bit_;\n * std::vector<size_type> power_;\n * const char DELIMITER = ' ';\n * const size_type GUARANTEE_BIT = sizeof(base_type) * 8;\n * const int HEX_BIT = 16;\n *\n * void calculateFrequency(std::string const &input);\n *\n * // no cover test\n * void buildForest();\n *\n * // no cover test\n * void initialTree();\n *\n * // no cover test\n * void initialForest();\n *\n * // no cover test\n * void appendDegree();\n *\n * // no cover test\n * void buildDictionary();\n *\n * std::pair<std::string, std::string> seperateHeaderAndCode(std::string const &str);\n *\n * // no cover test\n * std::string restore(std::string const &input);\n *\n * std::string exportHeader();\n *\n * std::string exportDictionary();\n *\n * void importHeader(std::string const &str);\n *\n * void importDictionary(std::string const &str);\n *\n * std::string addSeperateCode(std::string const &str);\n *\n * std::string removeSeperateCode(std::string const &str);\n *\n * std::string stringToHex(std::string const &str);\n *\n * std::string stringToBinary(std::string const &input);\n *\n * // return string always fill 0 while size is not N * GUARANTEE_BIT\n * std::string binaryToHex(std::string const &str);\n *\n * std::string hexToBinary(std::string const &str);\n * };\n */\n\n#include <functional>\n#include <algorithm>\n#include <iomanip>\n#include <sstream>\n#include <memory>\n#include <cmath>\n#include <stack>\n#include <string>\n#include <vector>\n#include <queue>\n#include <unordered_map>\n#include <cassert>\n#include <iostream>\n\ntemplate<typename _Tp>\nstruct BinaryTreeNode\n{\n _Tp value;\n std::shared_ptr<BinaryTreeNode<_Tp>> left, right;\n BinaryTreeNode(_Tp v,\n std::shared_ptr<BinaryTreeNode<_Tp>> l = {},\n std::shared_ptr<BinaryTreeNode<_Tp>> r = {}) : value(v), left(l), right(r)\n {\n };\n};\n\ntemplate<typename _Tp>\nstruct Comp\n{\npublic:\n bool operator()(_Tp a, _Tp b) const\n {\n return a->value.first > b->value.first;\n }\n};\n\nclass HuffmanTest;\n\nclass Huffman {\nprivate:\n typedef size_t size_type;\n typedef unsigned long long base_type;\n typedef std::pair<char, size_type> freq_node_type;\n typedef std::unordered_map<char, size_type> freq_type;\n typedef std::pair<size_type, std::pair<char, std::string>> value_type;\n typedef BinaryTreeNode<value_type> tree_node_type;\n typedef std::priority_queue<std::shared_ptr<tree_node_type>,\n std::vector<std::shared_ptr<tree_node_type>>,\n Comp<std::shared_ptr<tree_node_type>>> tree_type;\n typedef std::unordered_map<char, std::string> dictionary_type;\n typedef std::unordered_map<std::string, char> reverse_dictionary_type;\n\npublic:\n Huffman()\n : frequency_(), tree_(), dictionary_(), reverse_dictionary_(), sentinel_(), binary_bit_()\n {\n power_.resize(GUARANTEE_BIT);\n for (auto i = GUARANTEE_BIT; i > 0; --i)\n power_.at(i - 1) = pow(2, GUARANTEE_BIT - i);\n };\n\n std::string compression(std::string const &in)\n {\n calculateFrequency(in);\n buildForest();\n buildDictionary();\n\n // don't change order on this and next line\n // because stringToHex will calc. binary length\n std::string res = stringToHex(in);\n res = addSeperateCode(exportHeader()) + res;\n\n return res;\n }\n\n std::string compression(std::istream &in)\n {\n return compression(readFile(in));\n }\n\n void compression(std::string const &in, std::ostream &out)\n {\n out << compression(in);\n }\n\n void compression(std::istream &in, std::ostream &out)\n {\n out << compression(in);\n }\n\n std::string decompression(std::string const &in)\n {\n std::pair<std::string, std::string> header_and_code = seperateHeaderAndCode(in);\n importHeader(header_and_code.first);\n std::string res = hexToBinary(header_and_code.second);\n res = restore(res);\n\n return res;\n }\n\n std::string decompression(std::istream &in)\n {\n return decompression(readFile(in));\n }\n\n void decompression(std::string const &in, std::ostream &out)\n {\n out << decompression(in);\n }\n\n void decompression(std::istream &in, std::ostream &out)\n {\n out << decompression(in);\n }\n\nprivate:\n freq_type frequency_;\n tree_type tree_;\n dictionary_type dictionary_;\n reverse_dictionary_type reverse_dictionary_;\n std::shared_ptr<tree_node_type> sentinel_;\n unsigned long long binary_bit_;\n std::vector<size_type> power_;\n const char DELIMITER = ' ';\n const unsigned long long GUARANTEE_BIT = sizeof(base_type) * 8;\n const unsigned long long HEX_BIT = 16;\n\n std::string readFile(std::istream &in)\n {\n std::string s{};\n char c;\n while (in >> std::noskipws >> c)\n s.push_back(c);\n\n return s;\n }\n\n void calculateFrequency(std::string const &input)\n {\n char c;\n std::stringstream in(input);\n while (in >> std::noskipws >> c)\n {\n if (frequency_.find(c) == frequency_.end())\n frequency_.insert(std::make_pair(c, 1));\n else\n frequency_.at(c) += 1;\n }\n }\n\n// no cover test\n void buildForest()\n {\n initialTree();\n initialForest();\n appendDegree();\n }\n\n// no cover test\n void initialTree()\n {\n for_each(frequency_.begin(),\n frequency_.end(),\n [&] (freq_node_type freq_node)\n {\n value_type n{std::make_pair(freq_node.second, std::make_pair(freq_node.first, \"\"))};\n tree_.push({std::make_shared<tree_node_type>(tree_node_type((n),\n sentinel_,\n sentinel_))});\n });\n if (frequency_.size() == 1)\n tree_.push({std::make_shared<tree_node_type>(tree_node_type({}))});\n }\n\n// no cover test\n void initialForest()\n {\n while (tree_.size() > 1)\n {\n std::shared_ptr<tree_node_type> left{}, right{}, parent{};\n left = tree_.top();\n tree_.pop();\n right = tree_.top();\n tree_.pop();\n if (Comp<std::shared_ptr<tree_node_type>>()(left, right))\n swap(left, right);\n parent = std::make_shared<tree_node_type>(tree_node_type({}, left, right));\n parent->value.first = left->value.first + right->value.first;\n tree_.push(parent);\n }\n }\n\n// no cover test\n void appendDegree()\n {\n if (!tree_.empty())\n {\n std::string degree{};\n std::stack<std::shared_ptr<tree_node_type>> nodes;\n nodes.push(tree_.top());\n\n // post order travel\n while (!nodes.empty())\n {\n while (nodes.top()->left)\n {\n nodes.push(nodes.top()->left);\n degree += '0';\n }\n while (!nodes.empty() && nodes.top()->right == sentinel_)\n {\n if (nodes.top()->left == sentinel_)\n nodes.top()->value.second.second = degree;\n nodes.pop();\n while (!degree.empty() && degree.back() == '1')\n degree.pop_back();\n if (!degree.empty())\n degree.pop_back();\n }\n if (!nodes.empty())\n {\n auto right = nodes.top()->right;\n nodes.pop();\n nodes.push(right);\n degree += '1';\n }\n }\n }\n }\n\n// no cover test\n void buildDictionary()\n {\n if (tree_.size() == 1)\n {\n std::stack<std::shared_ptr<tree_node_type>> nodes;\n nodes.push(tree_.top());\n tree_.pop();\n\n // post order travel\n while (!nodes.empty())\n {\n while (nodes.top()->left != sentinel_)\n nodes.push(nodes.top()->left);\n\n while (!nodes.empty() && nodes.top()->right == sentinel_)\n {\n if (nodes.top()->left == sentinel_)\n dictionary_.insert(make_pair(nodes.top()->value.second.first,\n nodes.top()->value.second.second));\n nodes.pop();\n }\n if (!nodes.empty())\n {\n auto right = nodes.top()->right;\n nodes.pop();\n nodes.push(right);\n }\n }\n }\n }\n\n std::pair<std::string, std::string> seperateHeaderAndCode(std::string const &str)\n {\n auto it = --str.begin();\n size_type separate_count{};\n while (++it != str.end())\n if (separate_count == 4 && it + 1 != str.end() && *(it + 1) != ' ')\n break;\n else if (*it == ' ')\n ++separate_count;\n else\n separate_count = 0;\n\n\n return std::make_pair(std::string{str.begin(), it - 4}, std::string{it, str.end()});\n }\n\n// no cover test\n std::string restore(std::string const &input)\n {\n std::stringstream ss{input};\n std::string res{};\n char c;\n std::string k;\n while (ss >> c)\n {\n k = {};\n ss.putback(c);\n while (reverse_dictionary_.find(k) == reverse_dictionary_.end())\n {\n if (ss >> c)\n k.push_back(c);\n else\n break;\n }\n if (reverse_dictionary_.find(k) != reverse_dictionary_.end())\n res.push_back(reverse_dictionary_.at(k));\n else\n break;\n }\n\n return res;\n }\n\n std::string exportHeader()\n {\n return std::to_string(binary_bit_) + DELIMITER + exportDictionary();\n }\n\n std::string exportDictionary()\n {\n std::string res{};\n for_each(dictionary_.begin(),\n dictionary_.end(),\n [&] (std::pair<char, std::string> value)\n {\n res.push_back(value.first);\n res += DELIMITER;\n res.append(value.second);\n res += DELIMITER;\n });\n if (!res.empty())\n res.erase(--res.end());\n\n return res;\n }\n\n void importHeader(std::string const &str)\n {\n auto it = str.begin();\n while (it != str.end() && *it != DELIMITER)\n ++it;\n binary_bit_ = stoull(std::string{str.begin(), it});\n if (it != str.end())\n ++it;\n importDictionary({it, str.end()});\n }\n\n void importDictionary(std::string const &str)\n {\n char key{}, delimiter{};\n std::stringstream ss{str};\n std::string value{};\n while (ss >> std::noskipws >> key)\n {\n ss >> std::noskipws >> delimiter;\n ss >> std::noskipws >> value;\n ss >> std::noskipws >> delimiter;\n reverse_dictionary_.insert(make_pair(value, key));\n }\n }\n\n std::string addSeperateCode(std::string const &str)\n {\n return str + DELIMITER + DELIMITER + DELIMITER + DELIMITER;\n }\n\n std::string removeSeperateCode(std::string const &str)\n {\n if (str.size() >= 4)\n {\n auto it = str.end();\n if (*--it == DELIMITER\n && *--it == DELIMITER\n && *--it == DELIMITER\n && *--it == DELIMITER)\n return std::string(str.begin(), str.end() - 4);\n }\n\n return str;\n }\n\n std::string stringToHex(std::string const &str)\n {\n return binaryToHex(stringToBinary(str));\n }\n\n std::string stringToBinary(std::string const &input)\n {\n std::string res{};\n char c{};\n std::stringstream in(input);\n while (in >> std::noskipws >> c)\n res.append(dictionary_.at(c));\n binary_bit_ = res.size();\n\n return res;\n }\n\n// return string always fill 0 while size is not N * GUARANTEE_BIT\n std::string binaryToHex(std::string const &str)\n {\n if (str.empty())\n return {};\n\n std::stringstream res{};\n size_type seat{};\n base_type c{};\n while (true)\n {\n if (seat < str.size())\n c |= (str.at(seat) == '1') ? 1 : 0;\n ++seat;\n if ((seat % GUARANTEE_BIT) == 0)\n {\n res << std::hex << c << DELIMITER;\n c = {};\n if (seat >= str.size())\n break;\n }\n c <<= 1;\n }\n\n return res.str();\n }\n\n std::string hexToBinary(std::string const &str)\n {\n std::stringstream in_ss{str};\n std::string res{}, s{};\n size_type seat{}, g{};\n base_type c{};\n seat = 0 - 1;\n while (true)\n {\n if (++seat < str.size())\n {\n in_ss >> s;\n c = static_cast<base_type>(stoull(s, 0, HEX_BIT));\n g = 0 - 1;\n }\n else if (g % GUARANTEE_BIT == 0)\n break;\n while (++g < GUARANTEE_BIT)\n if (c >= power_.at(g))\n {\n c -= power_.at(g);\n res.push_back('1');\n }\n else\n res.push_back('0');\n\n }\n res.erase(res.begin() + binary_bit_, res.end());\n\n return res;\n }\n\n// for test\n friend HuffmanTest;\n};"
}
{
"filename": "code/compression/src/lossless_compression/huffman/README.md",
"content": "# cosmos #\nYour personal library of every algorithm and data structure code that you will ever encounter\n\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/compression/src/lossless_compression/lempel_ziv_welch/lzw.cpp",
"content": "#include <string>\n#include <map>\n#include <iostream>\n#include <iterator>\n#include <vector>\n\n/* Compress a string to a list of output symbols.\n * The result will be written to the output iterator\n * starting at \"result\"; the final iterator is returned.\n */\ntemplate <typename Iterator>\nIterator compress(const std::string &uncompressed, Iterator result)\n{\n // Build the dictionary.\n int dictSize = 256;\n std::map<std::string, int> dictionary;\n for (int i = 0; i < 256; i++)\n dictionary[std::string(1, i)] = i;\n\n std::string w;\n for (std::string::const_iterator it = uncompressed.begin();\n it != uncompressed.end(); ++it)\n {\n char c = *it;\n std::string wc = w + c;\n \n if (dictionary.count(wc)) w = wc;\n else\n {\n *result++ = dictionary[w];\n // Add wc to the dictionary.\n dictionary[wc] = dictSize++;\n w = std::string(1, c);\n }\n }\n\n // Output the code for w.\n if (!w.empty())\n *result++ = dictionary[w];\n\n return result;\n}\n\n/*\n * Decompress a list of output ks to a string.\n * \"begin\" and \"end\" must form a valid range of ints\n */\ntemplate <typename Iterator>\nstd::string decompress(Iterator begin, Iterator end)\n{\n // Build the dictionary.\n int dictSize = 256;\n std::map<int, std::string> dictionary;\n for (int i = 0; i < 256; i++)\n dictionary[i] = std::string(1, i);\n\n std::string w(1, *begin++);\n std::string result = w;\n std::string entry;\n for (; begin != end; begin++)\n {\n int k = *begin;\n if (dictionary.count(k))\n entry = dictionary[k];\n else if (k == dictSize)\n entry = w + w[0];\n else\n throw \"Bad compressed k\";\n\n result += entry;\n\n // Add w+entry[0] to the dictionary.\n dictionary[dictSize++] = w + entry[0];\n\n w = entry;\n }\n return result;\n}\n\nint main()\n{\n //Test Case 1\n std::vector<int> compressed;\n compress(\"Hello world !!\", std::back_inserter(compressed));\n copy(compressed.begin(), compressed.end(), std::ostream_iterator<int>(std::cout, \", \"));\n std::cout << std::endl;\n std::string decompressed = decompress(compressed.begin(), compressed.end());\n std::cout << decompressed << std::endl;\n\n return 0;\n}"
}
{
"filename": "code/compression/src/lossless_compression/lempel_ziv_welch/lzw.py",
"content": "#!/usr/bin/env python3\n\n\ndef compress(input_string):\n \"\"\"Compresses the given input string\"\"\"\n\n # Build the dictionary\n dict_size = 256 # All ASCII characters\n dictionary = {chr(i): i for i in range(dict_size)}\n\n buffer = \"\"\n result = []\n for ch in input_string:\n tmp = buffer + ch\n if tmp in dictionary:\n buffer = tmp\n else:\n result.append(dictionary[buffer])\n dictionary[tmp] = dict_size\n dict_size += 1\n buffer = ch\n if buffer:\n result.append(dictionary[buffer])\n return result\n\n\ndef decompress(compressed):\n \"\"\"Given a list of numbers given by compress to a string\"\"\"\n\n dict_size = 256 # ASCII characters\n dictionary = {i: chr(i) for i in range(dict_size)}\n\n result = \"\"\n buffer = chr(compressed.pop(0))\n result += buffer\n for ch in compressed:\n if ch in dictionary:\n entry = dictionary[ch]\n elif ch == dict_size:\n entry = ch + ch[0]\n else:\n raise ValueError(\"Bad Compression for %s\" % ch)\n result += entry\n\n dictionary[dict_size] = buffer = entry[0]\n dict_size += 1\n\n buffer = entry\n return result\n\n\nif __name__ == \"__main__\":\n \"\"\"Test cases for LZW\"\"\"\n\n # Test 1\n test_case_1 = \"Hello World!!\"\n compressed = compress(test_case_1)\n print(compressed)\n decompressed = decompress(compressed)\n print(decompressed)"
}
{
"filename": "code/compression/src/lossless_compression/lempel_ziv_welch/README.md",
"content": "# Lempel-Ziv-Welch compression\n\nThe **Lempel-Ziv-Welch** (LZW) algorithm is a dictionary-based lossless compressor. The algorithm uses a dictionary to map a sequence of symbols into codes which take up less space. LZW performs especially well on files with frequent repetitive sequences.\n\n## Encoding algorithm\n\n1. Start with a dictionary of size 256 or 4096 (where the key is an ASCII character and the values are consecutive)\n2. Find the longest entry W in the dictionary that matches the subsequent values.\n3. Output the index of W and remove W from the input.\n4. Add (W + (the next value)) to the dictionary as a key with value (dictionary size + 1)\n5. Repeat steps 2 to 4 until the input is empty\n\n**Example:** the input \"ToBeOrNotToBeABanana\" (length of 20) would be encoded in 17.\n```\n[T][o][B][e][O][r][N][o][t][To][Be][A][B][a][n][an][a]\n 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17\n```\nThe brackets separate the values (e.g. `[To]` is encoded as one value). The LZW algorithm replaces the second occurences of `To`, `Be`, and `an` with one value, eliminating data redundancy.\n\nNote that there is **no need to store the dictionary** because the LZW uncompression process reconstructs the dictionary as it goes along.\n\n### Encoding example\n\n\n> Image credits: https://slideplayer.com/slide/5049613/\n\n## Decoding algorithm\n\n1. Start with a dictionary of size 256 or 4096 (must be the same as what the encoding process used in step 1)\n2. Variable PREV = \"\"\n3. Is the next code V in the dictionary?\n - Yes:\n 1. Variable T = dictionary key whose value = V\n 2. Output T\n 3. Add (PREV + (first character of T)) to the dictionary\n - No:\n 1. Variable T = PREV + (first character of PREV)\n 2. Output T\n 3. Add T to the dictionary\n4. Repeat step 3 until the end of the input is reached\n\n---\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n---"
}
{
"filename": "code/compression/src/lossless_compression/README.md",
"content": "# cosmos #\nYour personal library of every algorithm and data structure code that you will ever encounter\n\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/compression/src/lossy_compression/README.md",
"content": "# cosmos #\nYour personal library of every algorithm and data structure code that you will ever encounter\n\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/compression/src/README.md",
"content": "# cosmos #\nYour personal library of every algorithm and data structure code that you will ever encounter\n\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/compression/test/lossless_compression/huffman/test_huffman.cpp",
"content": "#include \"../../../src/lossless_compression/huffman/huffman.cpp\"\n#include <iostream>\n#include <fstream>\n#include <iterator>\nusing namespace std;\n\n// test only for Huffman::base_type is 64bit\nclass HuffmanTest {\npublic:\n HuffmanTest()\n {\n if (sizeof(Huffman::base_type) != 8)\n cout << \"the test require that Huffman::base_type based on 64bit\\n\";\n\n testCalculateFrequency();\n testImportDictionary();\n testExportDictionary();\n testSeperateHeaderAndCode();\n testSeperateCode();\n testStringToBinary();\n testBinaryToHex();\n testHexToBinary();\n }\n\n void testCalculateFrequency()\n {\n Huffman huf;\n\n assert(huf.frequency_.empty() == true);\n\n huf.frequency_.clear();\n huf.calculateFrequency(\"a\");\n assert(huf.frequency_.size() == 1\n && huf.frequency_.find('a') != huf.frequency_.end()\n && huf.frequency_.find('a')->second == 1);\n\n huf.frequency_.clear();\n huf.calculateFrequency(\"aba\");\n assert(huf.frequency_.size() == 2\n && huf.frequency_.find('a') != huf.frequency_.end()\n && huf.frequency_.find('a')->second == 2\n && huf.frequency_.find('b') != huf.frequency_.end()\n && huf.frequency_.find('b')->second == 1\n && huf.frequency_.find('c') == huf.frequency_.end());\n }\n\n void testImportDictionary()\n {\n Huffman huf;\n std::string parameter{};\n\n assert(huf.reverse_dictionary_.empty() == true);\n\n parameter.clear();\n huf.reverse_dictionary_.clear();\n parameter = \"\";\n huf.importDictionary(parameter);\n assert(huf.reverse_dictionary_.empty() == true);\n\n parameter.clear();\n huf.reverse_dictionary_.clear();\n parameter.append(\"c\");\n parameter += huf.DELIMITER;\n parameter.append(\"123\");\n parameter += huf.DELIMITER;\n huf.importDictionary(parameter);\n assert(huf.reverse_dictionary_.size() == 1\n && huf.reverse_dictionary_.find(\"123\")->second == 'c');\n }\n\n void testExportDictionary()\n {\n Huffman huf;\n std::string res{};\n\n assert(huf.dictionary_.empty() == true);\n\n huf.dictionary_.insert(std::make_pair('c', \"123\"));\n assert(huf.dictionary_.size() == 1\n && huf.dictionary_.find('c')->second == \"123\");\n }\n\n void testSeperateHeaderAndCode()\n {\n Huffman huf;\n std::pair<std::string, std::string> res;\n res = huf.seperateHeaderAndCode(huf.addSeperateCode(\"\"));\n assert(res.first == \"\" && res.second == \"\");\n\n res = huf.seperateHeaderAndCode(huf.addSeperateCode(\"123\"));\n assert(res.first == \"123\" && res.second == \"\");\n\n res = huf.seperateHeaderAndCode(huf.addSeperateCode(\"123\").append(\"456\"));\n assert(res.first == \"123\" && res.second == \"456\");\n }\n\n void testSeperateCode()\n {\n Huffman huf;\n std::string res;\n res = huf.removeSeperateCode(huf.addSeperateCode(\"\"));\n assert(res == \"\");\n\n res = huf.removeSeperateCode(huf.addSeperateCode(huf.addSeperateCode(\"\")));\n assert(res == huf.addSeperateCode(\"\"));\n\n res = huf.removeSeperateCode(huf.addSeperateCode(\"123\"));\n assert(res == \"123\");\n\n res = huf.removeSeperateCode( \\\n huf.addSeperateCode(huf.removeSeperateCode(huf.addSeperateCode(\"456\"))));\n assert(res == \"456\");\n }\n\n void testStringToBinary()\n {\n Huffman huf;\n std::string res;\n huf.dictionary_.insert(std::make_pair('a', \"0\"));\n huf.dictionary_.insert(std::make_pair('b', \"10\"));\n huf.dictionary_.insert(std::make_pair('c', \"11\"));\n\n res = huf.stringToBinary(\"\");\n assert(res == \"\");\n\n res = huf.stringToBinary(\"a\");\n assert(res == \"0\");\n\n res = huf.stringToBinary(\"ab\");\n assert(res == \"010\");\n\n res = huf.stringToBinary(\"abc\");\n assert(res == \"01011\");\n\n res = huf.stringToBinary(\"abca\");\n assert(res == \"010110\");\n\n res = huf.stringToBinary(\"cbabc\");\n assert(res == \"111001011\");\n }\n\n void testBinaryToHex()\n {\n Huffman huf;\n std::string res, expect;\n std::string delim{}, bin{};\n\n bin = \"\";\n huf.binary_bit_ = bin.size();\n res = huf.binaryToHex(bin);\n expect = \"\";\n while (expect.size() % (int)(huf.GUARANTEE_BIT / sqrt(huf.HEX_BIT)))\n expect.push_back('0');\n assert(res == \"\");\n\n bin = \"1011\";\n huf.binary_bit_ = bin.size();\n res = huf.binaryToHex(bin);\n expect = \"b000000000000000 \";\n assert(res == expect);\n\n bin = \"10111110\";\n huf.binary_bit_ = bin.size();\n res = huf.binaryToHex(bin);\n expect = \"be00000000000000 \";\n assert(res == expect);\n\n bin = \"101111101001\";\n huf.binary_bit_ = bin.size();\n res = huf.binaryToHex(bin);\n expect = \"be90000000000000 \";\n assert(res == expect);\n\n bin = \"1011111010010111\";\n huf.binary_bit_ = bin.size();\n res = huf.binaryToHex(bin);\n expect = \"be97000000000000 \";\n assert(res == expect);\n\n bin = \"10111110100101111010011100101000\";\n huf.binary_bit_ = bin.size();\n res = huf.binaryToHex(bin);\n expect = \"be97a72800000000 \";\n assert(res == expect);\n\n bin = (\"10111110100101111010011100101000111\");\n huf.binary_bit_ = bin.size();\n res = huf.binaryToHex(bin);\n expect = \"be97a728e0000000 \";\n assert(res == expect);\n\n bin = (\"1011111010010111101001110010100011100100111010001011111110110101\" \\\n \"1011111010010111101001110010100011100100111010001011111110110101\");\n huf.binary_bit_ = bin.size();\n res = huf.binaryToHex(bin); expect = \"be97a728e4e8bfb5 be97a728e4e8bfb5 \";\n assert(res == expect);\n }\n\n void testHexToBinary()\n {\n Huffman huf;\n std::string hex, res;\n std::string delim{};\n\n hex = \"\";\n huf.binary_bit_ = hex.size() * 4;\n while (hex.size() % huf.GUARANTEE_BIT)\n hex.push_back('0');\n res = huf.hexToBinary(hex);\n assert(res == \"\");\n\n hex = \"b00000000000000 \";\n huf.binary_bit_ = hex.size() * 4;\n res = huf.hexToBinary(hex);\n assert(res == \"0000101100000000000000000000000000000000000000000000000000000000\");\n\n hex = \"be97a728e4e8bfb5 be97a728e4e8bfb5 \";\n huf.binary_bit_ = hex.size() * 4;\n res = huf.hexToBinary(hex);\n assert(res == (\"1011111010010111101001110010100011\" \\\n \"1001001110100010111111101101011011\" \\\n \"1110100101111010011100101000111001\" \\\n \"0011101000101111111011010110111110\"));\n }\n};\n\nint main()\n{\n HuffmanTest test;\n\n Huffman huf;\n if (huf.decompression(huf.compression(huf.decompression(huf.compression(\"\")))) != \"\")\n cout << \"error empty\\n\";\n\n Huffman huf2;\n if (huf2.decompression(huf2.compression(huf2.decompression(huf2.compression(\" \"))))\n != \" \")\n cout << \"error separate\\n\";\n\n Huffman huf6;\n if (huf6.decompression(huf6.compression(huf6.decompression(\n huf6.compression(\"COMPRESSION_TESTCOMPRESSION_TEST\"))))\n != \"COMPRESSION_TESTCOMPRESSION_TEST\")\n cout << \"error binary6\\n\";\n\n Huffman huf7;\n if (huf7.decompression(huf7.compression(huf7.decompression(huf7.compression(\" \")))) != \" \")\n cout << \"error delimiter\\n\";\n\n // Huffman huf8;\n // fstream in, out;\n // in.open(\"input.png\");\n // out.open(\"output.png\", ios::out | ios::trunc);\n // if (in.fail() || out.fail())\n // cout << \"error find file\\n\";\n // else\n // huf8.decompression(huf8.compression(in), out);\n // in.close();\n // out.close();\n\n return 0;\n}"
}
{
"filename": "code/computational_geometry/src/2d_line_intersection/2d_line_intersection.c",
"content": "// computational geometry | 2D line intersecton | C\n// Part of Cosmos by OpenGenus Foundation\n\n// main.c\n// forfun\n//\n// Created by Daniel Farley on 10/10/17.\n// Copyright © 2017 Daniel Farley. All rights reserved.\n\n#include <stdio.h>\n#include <stdlib.h>\n\n#define min(a,b) ((a) < (b) ? (a) : (b))\n#define max(a,b) ((a) > (b) ? (a) : (b))\n\ntypedef struct{\n double x;\n double y;\n} vec2;\n\n/* return point of intersection, in parent's coordinate space of its parameters */\nvec2 fintersection(double x1, double y1, double x2, double y2, double x3, double y3, double x4, double y4){\n double x12 = x1 - x2;\n double x34 = x3 - x4;\n double y12 = y1 - y2;\n double y34 = y3 - y4;\n \n double c = x12 * y34 - y12 * x34;\n \n double a = x1 * y2 - y1 * x2;\n double b = x3 * y4 - y3 * x4;\n \n double x = (a * x34 - b * x12) / c;\n double y = (a * y34 - b * y12) / c;\n \n vec2 ret;\n ret.x = x;\n ret.y = y;\n return ret;\n}\n\n/* line segments defined by 2 points a-b, and c-d */\nvec2 intersection(vec2 a, vec2 b, vec2 c, vec2 d){\n return fintersection(a.x, a.y, b.x, b.y, c.x, c.y, d.x, d.y);\n}\n\nint main() {\n //example set 1\n vec2 a; a.x=10; a.y=3;\n vec2 b; b.x=20; b.y=10;\n vec2 c; c.x=10; c.y=10;\n vec2 d; d.x=20; d.y=3;\n \n vec2 intersectionpoint = intersection(a,b,c,d);\n if(intersectionpoint.x >= min(a.x, b.x) && intersectionpoint.x <= max(a.x, b.x) &&\n intersectionpoint.y >= min(a.y, b.y) && intersectionpoint.y <= max(a.y, b.y)){\n printf(\"intersection: %f %f\", intersectionpoint.x, intersectionpoint.y);\n } else {\n printf(\"no intersection\");\n }\n return 0;\n}"
}
{
"filename": "code/computational_geometry/src/2d_line_intersection/2d_line_intersection.cpp",
"content": "// computational geometry | 2D line intersecton | C++\n// main.cpp\n// forfun\n//\n// Created by Ivan Reinaldo Liyanto on 10/5/17.\n// Copyright © 2017 Ivan Reinaldo Liyanto. All rights reserved.\n// Path of Cosmos by OpenGenus Foundation\n\n#include <iostream>\n#include <vector>\n#include <cmath>\nusing namespace std;\n\nstruct vec2\n{\n double x;\n double y;\n vec2(double x, double y) : x(x), y(y)\n {\n }\n};\n\n/* return point of intersection, in parent's coordinate space of its parameters */\nvec2 intersection(double x1, double y1, double x2, double y2, double x3, double y3, double x4,\n double y4)\n{\n double x12 = x1 - x2;\n double x34 = x3 - x4;\n double y12 = y1 - y2;\n double y34 = y3 - y4;\n\n double c = x12 * y34 - y12 * x34;\n\n double a = x1 * y2 - y1 * x2;\n double b = x3 * y4 - y3 * x4;\n\n double x = (a * x34 - b * x12) / c;\n double y = (a * y34 - b * y12) / c;\n return vec2(x, y);\n}\n\n/* line segments defined by 2 points a-b, and c-d */\nvec2 intersection(vec2 a, vec2 b, vec2 c, vec2 d)\n{\n return intersection(a.x, a.y, b.x, b.y, c.x, c.y, d.x, d.y);\n}\n\nint main()\n{\n //example set 1\n vec2 a(10, 3);\n vec2 b(20, 10);\n vec2 c(10, 10);\n vec2 d(20, 3);\n\n //example set 2\n// vec2 a(10, 3);\n// vec2 b(20, 3);\n// vec2 c(10, 5);\n// vec2 d(20, 5);\n\n vec2 intersectionpoint = intersection(a, b, c, d);\n if (intersectionpoint.x >= min(a.x, b.x) && intersectionpoint.x <= max(a.x, b.x) &&\n intersectionpoint.y >= min(a.y, b.y) && intersectionpoint.y <= max(a.y, b.y))\n cout << \"intersection: \" << intersectionpoint.x << \" \" << intersectionpoint.y;\n else\n cout << \"no intersection\";\n\n return 0;\n}"
}
{
"filename": "code/computational_geometry/src/2d_line_intersection/2d_line_intersection.cs",
"content": "// computational geometry | 2D line intersecton | C#\nusing System;\n\n/**\nModel class which represents a Vector.\n**/\nclass Vector {\n\n public int x { get; set; }\n public int y { get; set; }\n\n public Vector(int x, int y) {\n this.x = x;\n this.y = y;\n }\n\n}\n\n/**\nModel class which represents a Line in form Ax + By = C\n**/\nclass Line {\n\n public int A { get; set; }\n public int B { get; set; }\n public int C { get; set; }\n\n public Line(int a, int b, int c) {\n this.A = a;\n this.B = b;\n this.C = c;\n }\n}\n\n/**\nClass which provides useful helper methods for straight lines.\n**/\nclass LineOperation {\n\n /**\n Returns point of intersection, in parent's coordinate space of its parameters\n **/\n public Vector getIntersection(int x1, int y1, int x2, int y2, int x3, int y3, int x4, int y4) {\n int x12 = x1 - x2;\n int x34 = x3 - x4;\n int y12 = y1 - y2;\n int y34 = y3 - y4;\n\n int c = x12 * y34 - y12 * x34;\n\n int a = x1 * y2 - y1 * x2;\n int b = x3 * y4 - y3 * x4;\n\n int x = (a * x34 - b * x12) / c;\n int y = (a * y34 - b * y12) / c;\n\n return new Vector(x, y);\n }\n\n /**\n Line segments defined by 2 points a-b and c-d\n **/\n public Vector getIntersection(Vector vec1, Vector vec2, Vector vec3, Vector vec4) {\n return getIntersection(vec1.x, vec1.y, vec2.x, vec2.y, vec3.x, vec3.y, vec4.x, vec4.y);\n }\n\n // Accepts line in Ax + By = C format\n public Vector getIntersection(Line line1, Line line2) {\n int A1 = line1.A;\n int B1 = line1.B;\n int C1 = line1.C;\n int A2 = line2.A;\n int B2 = line2.B;\n int C2 = line2.C;\n\n int delta = A1*B2 - A2*B1;\n if (delta == 0)\n Console.WriteLine(\"Lines are parallel\");\n\n int x = (B2*C1 - B1*C2) / delta;\n int y = (A1*C2 - A2*C1) / delta;\n\n return new Vector(x, y);\n }\n}\n\n// Driver Program\nclass MainClass {\n\n static void printVector(Vector intersectionPoint) {\n Console.WriteLine(String.Format(\"Lines intersect at x:{0}, y:{1}\", intersectionPoint.x, intersectionPoint.y ) );\n }\n public static void Main(string[] args) {\n // 3x + 4y = 1\n Line line1 = new Line(3, 4, 1);\n // 2x + 5y = 3\n Line line2 = new Line(2, 5, 3);\n\n LineOperation lineOperation = new LineOperation();\n\n Vector intersectionPoint = lineOperation.getIntersection(line1, line2);\n\n Console.WriteLine(\"Test using line equation\");\n printVector(intersectionPoint);\n Console.WriteLine(\"Test using points\");\n\n intersectionPoint = lineOperation.getIntersection(new Vector(2, 5), new Vector(5, 3), new Vector(7, 4), new Vector(1, 6) );\n\n printVector(intersectionPoint);\n }\n}"
}
{
"filename": "code/computational_geometry/src/2d_line_intersection/2d_line_intersection.hs",
"content": "-- computational geometry | 2D line intersecton | Haskell\n-- line2dintersection.hs\n-- @author Sidharth Mishra\n-- @description 2 dimensional line intersection program in Haskell\n-- @copyright 2017 Sidharth Mishra\n-- @created Fri Oct 13 2017 20:42:25 GMT-0700 (PDT)\n-- @last-modified Fri Oct 13 2017 20:43:52 GMT-0700 (PDT)\n--\n\nmodule Lines (\n Line(..),\n Point,\n getIntersectionPoint,\n convertToSlopeIntercept,\n getLineFromPoints\n) where\n\n-- Line can be represented in the following ways\n-- SlopeIntercept (y = mx + c)\n-- GeneralForm (ax + by = c)\n-- XAxis (y = c)\n-- YAxis (x = c)\ndata Line = SlopeIntercept { \n slope :: Double,\n intercept :: Double\n } \n | GeneralForm {\n xcoef :: Double,\n ycoef :: Double,\n constant :: Double\n }\n | XAxis {\n yintercept :: Double\n }\n | YAxis {\n xintercept :: Double\n }\n deriving (Show)\n\n\n-- Point in 2-D plane\ndata Point = Point {\n x::Double, \n y::Double\n }\n deriving (Show)\n\n\nconvertToSlopeIntercept :: Line -> Line\n-- converts the line from General form to Slope Intercept form\n-- XAxis and YAxis and lines parallel to the axes remain unchanged\nconvertToSlopeIntercept l1 = case l1 of\n (GeneralForm a b c) -> SlopeIntercept {\n slope = (-1) * (a/b),\n intercept = c/b\n }\n (SlopeIntercept s i) -> SlopeIntercept {\n slope = s,\n intercept = i\n }\n (YAxis c) -> YAxis c\n (XAxis c) -> SlopeIntercept {\n slope = 0,\n intercept = c\n }\n\ngetIntersectionPoint :: Line -> Line -> Maybe Point\n-- Finds the intersection point of the 2 lines\n-- first, convert each line into slope-intercept form,\n-- then, if m1 == m2, lines are parallel -- return Nothing\n-- otherwise, finds the intersection point\ngetIntersectionPoint l1 l2 = getIntersectionPoint' (convertToSlopeIntercept l1) (convertToSlopeIntercept l2)\n\ngetIntersectionPoint' :: Line -> Line -> Maybe Point\n-- function for internal usage\n-- y = m1x + c1 :: Line #1\n-- y = m2x + c2 :: Line #2\n-- --------------\n-- x = (c2 - c1) / (m1 - m2)\n-- y = (m1(c2 - c1) / (m1 - m2)) + c1\ngetIntersectionPoint' (SlopeIntercept m c) (YAxis c') = getIntersectionPoint' (YAxis c') (SlopeIntercept m c)\ngetIntersectionPoint' (YAxis c') (SlopeIntercept m c) =\n -- intersection between YAxis and the line -- x = c', y = (m*c' + c)\n return Point {\n x = c',\n y = (m * c') + c\n }\ngetIntersectionPoint' (SlopeIntercept m1 c1) (SlopeIntercept m2 c2) = \n if m1 == m2 \n then \n Nothing -- lines are parallel\n else\n return Point {\n x = (c2 - c1) / (m1 - m2),\n y = (m1 * (c2 - c1) / (m1 - m2)) + c1\n }\ngetIntersectionPoint' _ _ = Nothing -- catch all condition, this should not be possible though\n\ngetLineFromPoints :: Point -> Point -> Line\n-- creates a line in slope intercept form from the given 2 points\n-- (x1,y1) ------ (x2,y2)\n-- given the line is of form y = mx + b\n-- slope = m = (y1 - y2) / (x1 - x2)\n-- intercept = b = y1 - (m * x1)\ngetLineFromPoints (Point x1 y1) (Point x2 y2)\n | x1 == x2 = YAxis { xintercept = x1 } -- m = Infinity\n | y1 == y2 = XAxis { yintercept = y1 } -- m = 0\n | otherwise = SlopeIntercept {\n slope = m,\n intercept = b\n }\n where m = (y1 - y2) / (x1 - x2) \n b = y1 - (m * x1)\n\n\n-- For testing, Testing harness\nline1 :: Line\n-- example line :: y = 0.7x -4\nline1 = getLineFromPoints (Point 10 3) (Point 20 10)\n\nline2 :: Line\n-- example line :: y = -0.7x + 17\nline2 = getLineFromPoints (Point 10 10) (Point 20 3)\n\nline3 :: Line\n-- example line :: y = x + 3\nline3 = SlopeIntercept { slope = 1, intercept = 3 }\n\nline4 :: Line\n-- example line :: y = x + 4\nline4 = SlopeIntercept { slope = 1, intercept = 4 }\n\nline5 :: Line\n-- example line :: y = 0 -- X Axis\nline5 = SlopeIntercept { slope = 0, intercept = 0 }\n\nline6 :: Line\n-- example line :: x = 1 -- Y Axis\nline6 = getLineFromPoints (Point 1 5) (Point 1 8)\n\ntestIntersection :: Line -> Line -> String\n-- for testing intersection of 2 lines\ntestIntersection l1 l2 = \"Intersection point:: \" ++ case getIntersectionPoint l1 l2 of\n Nothing -> \"None, Lines are parallel\"\n Just i -> show i\n\nmain :: IO()\n-- main driver for testing logic\n{- Sample output\n *Lines Data.Maybe> main\n Line1:: SlopeIntercept {slope = 0.7, intercept = -4.0}\n Line2:: SlopeIntercept {slope = -0.7, intercept = 17.0}\n Line3:: SlopeIntercept {slope = 1.0, intercept = 3.0}\n Line4:: SlopeIntercept {slope = 1.0, intercept = 4.0}\n Line5:: SlopeIntercept {slope = 0.0, intercept = 0.0}\n Line6:: YAxis {xintercept = 1.0}\n Line1 & Line2:: Intersection point:: Point {x = 15.000000000000002, y = 6.5}\n Line2 & Line3:: Intersection point:: Point {x = 8.23529411764706, y = 11.235294117647058}\n Line3 & Line4:: Intersection point:: None, Lines are parallel\n Line1 & Line4:: Intersection point:: Point {x = -26.666666666666664, y = -22.666666666666664}\n Line1 & Line3:: Intersection point:: Point {x = -23.33333333333333, y = -20.33333333333333}\n Line2 & Line4:: Intersection point:: Point {x = 7.647058823529412, y = 11.647058823529413}\n Line2 & Line5:: Intersection point:: Point {x = 24.28571428571429, y = 0.0}\n Line5 & Line6:: Intersection point:: Point {x = 1.0, y = 0.0}\n Line6 & Line5:: Intersection point:: Point {x = 1.0, y = 0.0}\n *Lines Data.Maybe>\n-}\nmain = do\n putStrLn $ \"Line1:: \" ++ show line1\n putStrLn $ \"Line2:: \" ++ show line2\n putStrLn $ \"Line3:: \" ++ show line3\n putStrLn $ \"Line4:: \" ++ show line4\n putStrLn $ \"Line5:: \" ++ show line5\n putStrLn $ \"Line6:: \" ++ show line6\n putStrLn $ \"Line1 & Line2:: \" ++ testIntersection line1 line2\n putStrLn $ \"Line2 & Line3:: \" ++ testIntersection line2 line3\n putStrLn $ \"Line3 & Line4:: \" ++ testIntersection line3 line4\n putStrLn $ \"Line1 & Line4:: \" ++ testIntersection line1 line4\n putStrLn $ \"Line1 & Line3:: \" ++ testIntersection line1 line3\n putStrLn $ \"Line2 & Line4:: \" ++ testIntersection line2 line4\n putStrLn $ \"Line2 & Line5:: \" ++ testIntersection line2 line5\n putStrLn $ \"Line5 & Line6:: \" ++ testIntersection line5 line6\n putStrLn $ \"Line6 & Line5:: \" ++ testIntersection line6 line5"
}
{
"filename": "code/computational_geometry/src/2d_line_intersection/2d_line_intersection.java",
"content": "/**\n computational geometry | 2D line intersecton | C\n Model class which represents a Vector.\n**/\nclass Vector {\n\n int x;\n int y;\n\n public Vector(int x, int y) {\n this.x = x;\n this.y = y;\n }\n\n}\n\n/**\nModel class which represents a Line in form Ax + By = C\n**/\nclass Line {\n\n int A;\n int B;\n int C;\n\n public Line(int a, int b, int c) {\n this.A = a;\n this.B = b;\n this.C = c;\n }\n}\n\n/**\nClass which provides useful helper methods for straight lines.\n**/\nclass LineOperation {\n\n /**\n Returns point of intersection, in parent's coordinate space of its parameters\n **/\n public Vector getIntersection(int x1, int y1, int x2, int y2, int x3, int y3, int x4, int y4) {\n int x12 = x1 - x2;\n int x34 = x3 - x4;\n int y12 = y1 - y2;\n int y34 = y3 - y4;\n\n int c = x12 * y34 - y12 * x34;\n\n int a = x1 * y2 - y1 * x2;\n int b = x3 * y4 - y3 * x4;\n\n int x = (a * x34 - b * x12) / c;\n int y = (a * y34 - b * y12) / c;\n\n return new Vector(x, y);\n }\n\n /**\n Line segments defined by 2 points a-b and c-d\n **/\n public Vector getIntersection(Vector vec1, Vector vec2, Vector vec3, Vector vec4) {\n return getIntersection(vec1.x, vec1.y, vec2.x, vec2.y, vec3.x, vec3.y, vec4.x, vec4.y);\n }\n\n // Accepts line in Ax + By = C format\n public Vector getIntersection(Line line1, Line line2) {\n int A1 = line1.A;\n int B1 = line1.B;\n int C1 = line1.C;\n int A2 = line2.A;\n int B2 = line2.B;\n int C2 = line2.C;\n\n int delta = A1*B2 - A2*B1;\n if (delta == 0)\n System.out.println(\"Lines are parallel\");\n\n int x = (B2*C1 - B1*C2) / delta;\n int y = (A1*C2 - A2*C1) / delta;\n\n return new Vector(x, y);\n }\n}\n\n// Driver Program\nclass Main {\n\n static void printVector(Vector intersectionPoint) {\n System.out.println(String.format(\"Lines intersect at x:%d, y:%d\", intersectionPoint.x, intersectionPoint.y ) );\n }\n public static void main(String[] args) {\n // 3x + 4y = 1\n Line line1 = new Line(3, 4, 1);\n // 2x + 5y = 3\n Line line2 = new Line(2, 5, 3);\n\n LineOperation lineOperation = new LineOperation();\n\n Vector intersectionPoint = lineOperation.getIntersection(line1, line2);\n\n System.out.println(\"Test using line equation\");\n printVector(intersectionPoint);\n System.out.println(\"Test using points\");\n\n intersectionPoint = lineOperation.getIntersection(new Vector(2, 5), new Vector(5, 3), new Vector(7, 4), new Vector(1, 6) );\n\n printVector(intersectionPoint);\n }\n}"
}
{
"filename": "code/computational_geometry/src/2d_line_intersection/2d_line_intersection.js",
"content": "// computational geometry | 2D line intersecton | JavaScript\nfunction intersection_by_points(x1, y1, x2, y2, x3, y3, x4, y4) {\n var x12 = x1 - x2;\n var x34 = x3 - x4;\n var y12 = y1 - y2;\n var y34 = y3 - y4;\n\n var c = x12 * y34 - y12 * x34;\n\n var a = x1 * y2 - y1 * x2;\n var b = x3 * y4 - y3 * x4;\n\n var x = (a * x34 - b * x12) / c;\n var y = (a * y34 - b * y12) / c;\n\n // Keeping points integers. Change according to requirement\n x = parseInt(x);\n y = parseInt(y);\n\n return { x: x, y: y };\n}\n\n// Line segments defined by 2 points a-b and c-d\nfunction intersection_by_vectors(vec1, vec2, vec3, vec4) {\n return intersection_by_points(\n vec1[\"x\"],\n vec1[\"y\"],\n vec2[\"x\"],\n vec2[\"y\"],\n vec3[\"x\"],\n vec3[\"y\"],\n vec4[\"x\"],\n vec4[\"y\"]\n );\n}\n\n// Accepts line in Ax+By = C format\nfunction intersection_by_line_euqation(line1, line2) {\n var A1 = line1[\"A\"];\n var B1 = line1[\"B\"];\n var C1 = line1[\"C\"];\n var A2 = line2[\"A\"];\n var B2 = line2[\"B\"];\n var C2 = line2[\"C\"];\n\n var delta = A1 * B2 - A2 * B1;\n if (delta === 0) console.log(\"Lines are parallel\");\n\n var x = (B2 * C1 - B1 * C2) / delta;\n var y = (A1 * C2 - A2 * C1) / delta;\n\n // Keeping points integers. Change according to requirement\n x = parseInt(x);\n y = parseInt(y);\n\n return { x: x, y: y };\n}\n\n// Driver Program\nfunction main() {\n // 3x + 4y = 1\n var line1 = { A: 3, B: 4, C: 1 };\n // 2x + 5y = 3\n var line2 = { A: 2, B: 5, C: 3 };\n\n var intersection_point = intersection_by_line_euqation(line1, line2);\n\n console.log(\"Test using line equation\");\n console.log(\n `Lines intersect at x:${intersection_point[\"x\"]}, y:${\n intersection_point[\"y\"]\n }`\n );\n console.log(\"Test using points\");\n\n intersection_point = intersection_by_vectors(\n { x: 2, y: 5 },\n { x: 5, y: 3 },\n { x: 7, y: 4 },\n { x: 1, y: 6 }\n );\n console.log(\n `Lines intersect at x:${intersection_point[\"x\"]}, y:${\n intersection_point[\"y\"]\n }`\n );\n}\n\nmain();"
}
{
"filename": "code/computational_geometry/src/2d_line_intersection/2d_line_intersection.py",
"content": "# computational geometry | 2D line intersecton | Python\ndef parallel_int(verticeX, verticeY):\n k = (verticeY[2] - verticeY[0]) * (verticeX[1] - verticeX[0]) - (\n verticeX[2] - verticeX[0]\n ) * (verticeY[1] - verticeY[0])\n if k == 0:\n return \"infinite\"\n else:\n return \"no\"\n\n\ndef line_int(verticeX, verticeY):\n x12 = verticeX[0] - verticeX[1]\n x34 = verticeX[2] - verticeX[3]\n y12 = verticeY[0] - verticeY[1]\n y34 = verticeY[2] - verticeY[3]\n\n c = x12 * y34 - y12 * x34\n\n if c == 0:\n return (\"NoInt\", None)\n\n a = verticeX[0] * verticeY[1] - verticeY[0] * verticeX[1]\n b = verticeX[2] * verticeY[3] - verticeY[2] * verticeX[3]\n\n x = (a * x34 - b * x12) / c\n y = (a * y34 - b * y12) / c\n\n return (x, y)\n\n\nX = []\nY = []\ntry:\n input = raw_input\nexcept NameError:\n pass\n\nprint(\"Enter 2-point form of each line\")\n\nX.append(float(input(\"X of point 1, line 1: \")))\nY.append(float(input(\"Y of point 1, line 1: \")))\n\nX.append(float(input(\"X of point 2, line 1: \")))\nY.append(float(input(\"Y of point 2, line 1: \")))\n\nX.append(float(input(\"X of point 1, line 2: \")))\nY.append(float(input(\"Y of point 1, line 2: \")))\n\nX.append(float(input(\"X of point 2, line 2: \")))\nY.append(float(input(\"Y of point 2, line 2: \")))\n\nintersectn = line_int(X, Y)\nif intersectn[0] == \"NoInt\":\n print(\"Parallel lines found with \" + parallel_int(X, Y) + \" intersections\")\nelse:\n print(\n \"Point of intersection : (\"\n + str(intersectn[0])\n + \", \"\n + str(intersectn[1])\n + \")\"\n )"
}
{
"filename": "code/computational_geometry/src/2d_line_intersection/2d_line_intersection.rb",
"content": "class LineInterserction\n def initialize(x1, y1, x2, y2, x3, y3, x4, y4)\n @x1 = x1\n @x2 = x2\n @x3 = x3\n @y1 = y1\n @y2 = y2\n @y3 = y3\n @x4 = x4\n @y4 = y4\n @denom = ((@x1 - @x2) * (@y3 - @y4)) - ((@y1 - @y2) * (@x3 - @x4))\n end\n\n def is_parallel\n @denom == 0\n end\n\n def intersection_point\n p1 = ((@x1 * @y2 - @y1 * @x2) * (@x3 - @x4) - (@x1 - @x2) * (@x3 * @y4 - @y3 * @x4)) / @denom\n p2 = ((@x1 * @y2 - @y1 * @x2) * (@y3 - @y4) - (@y1 - @y2) * (@x3 * @y4 - @y3 * @x4)) / @denom\n \"#{p1},#{p2}\"\n end\nend\n\nobj = LineInterserction.new(10, 3, 20, 3, 10, 5, 20, 5) #=> Cordinate points (x1,y1,x2,y2,x3,y3,x4,y4)\nif obj.is_parallel == false\n puts \"Intersection Point: #{obj.intersection_point}\"\nelse\n puts \"Line Parallel : #{obj.is_parallel}\"\nend"
}
{
"filename": "code/computational_geometry/src/2d_line_intersection/line_determinant_method.cpp",
"content": "#include <iostream>\nclass TwoDimensionalLineIntersection\n{\npublic:\n bool determinantMethod();\n\n void setCoordinatesOfLines(double x1_, double y1_, double x2_, double y2_, double x3_, double y3_, double x4_, double y4_);\n\n void printIntersectionPoints();\nprivate:\n double x1_, y1_, x2_, y2_, x3_, y3_, x4_, y4_, xin_, yin_;\n};\nvoid TwoDimensionalLineIntersection :: setCoordinatesOfLines(double x1_, double y1_, double x2_, double y2_, double x3_,\n double y3_, double x4_, double y4_)\n{\n this->x1_ = x1_;\n this->x2_ = x2_;\n this->x3_ = x3_;\n this->x4_ = x4_;\n this->y1_ = y1_;\n this->y2_ = y2_;\n this->y3_ = y3_;\n this->y4_ = y4_;\n}\nbool TwoDimensionalLineIntersection :: determinantMethod()\n{\n double slopeOfLine1;\n double slopeOfLine2;\n if(x2_ - x1_ != 0)\n slopeOfLine1 = (y2_ - y1_)/(x2_ - x1_);\n else\n slopeOfLine1 = 0;\n if(x4_ - x3_ != 0)\n slopeOfLine2 = (y4_ - y3_)/(x4_ - x3_);\n else\n slopeOfLine1 = 0;\n\n if(slopeOfLine1 != slopeOfLine2)\n {\n xin_ = ((x1_*y2_ - y1_*x2_)*(x3_ - x4_) - (x3_*y4_ - y3_*x4_)*(x1_ - x2_) )/( ((x1_ - x2_)*(y3_ - y4_))- ((y1_ - y2_)*(x3_ - x4_)));\n yin_ = ((x1_*y2_ - y1_*x2_)*(y3_ - y4_) - (x3_*y4_ - y3_*x4_)*(y1_ - y2_) )/( ((x1_ - x2_)*(y3_ - y4_))- ((y1_ - y2_)*(x3_ - x4_)));\n return true;\n } else\n return false;\n\n}\nvoid TwoDimensionalLineIntersection ::printIntersectionPoints()\n{\n if(determinantMethod())\n {\n std::cout << \"\\nIntersection Coordinate : \";\n std::cout << \"\\nX-coordinate : \" << xin_;\n std::cout << \"\\nY-coordinate : \" << yin_;\n } else\n std::cout << \"\\nLines are Parallel.\";\n}\nint main()\n{\n TwoDimensionalLineIntersection t;\n double x1, y1, x2, y2, x3, y3, x4, y4;\n\n std::cout << \"\\nEnter the Coordinates for Line-1 : \";\n std::cout << \"\\nLine-1 | x1-coordinate : \";\n std::cin >> x1;\n std::cout << \"\\nLine-1 | y1-coordinate : \";\n std::cin >> y1;\n std::cout << \"\\nLine-1 | x2-coordinate : \";\n std::cin >> x2;\n std::cout << \"\\nLine-1 | y2-coordinate : \";\n std::cin >> y2;\n std::cout << \"\\nEnter the Coordinates for Line-2 : \";\n std::cout << \"\\nLine-2 | x3-coordinate : \";\n std::cin >> x3;\n std::cout << \"\\nLine-2 | y3-coordinate : \";\n std::cin >> y3;\n std::cout << \"\\nLine-2 | x4-coordinate : \";\n std::cin >> x4;\n std::cout << \"\\nLine-2 | y4-coordinate : \";\n std::cin >> y4;\n\n t.setCoordinatesOfLines(x1, y1, x2, y2, x3, y3, x4, y4);\n t.printIntersectionPoints();\n}"
}
{
"filename": "code/computational_geometry/src/2d_line_intersection/line_elemination_method.cpp",
"content": "#include <iostream>\n#include <vector>\nclass EliminationMethd2DLineIntersection\n{\npublic:\n void acceptTheCoordinates(double x1, double y1, double x2, double y2, double x3, double y3, double x4, double y4);\n void intersection1(double x1, double y1, double x2, double y2, double x3, double y3, double x4, double y4);\n\nprivate:\n std::vector<double> a, b, c, d; //four coordinates constituting 2 Dimensional Lines.\n};\nvoid EliminationMethd2DLineIntersection::acceptTheCoordinates(double x1, double y1, double x2, double y2, double x3, double y3, double x4, double y4)\n{\n a.push_back(x1);\n a.push_back(y1);\n b.push_back(x1);\n b.push_back(y1);\n c.push_back(x1);\n c.push_back(y1);\n d.push_back(x1);\n d.push_back(y1);\n intersection1(a[0], a[1], b[0], b[1], c[0], c[1], d[0], d[1]);\n}\nvoid EliminationMethd2DLineIntersection::intersection1(double x1, double y1, double x2, double y2, double x3, double y3, double x4, double y4)\n{\n double x12 = x1 - x2;\n double x34 = x3 - x4;\n double y12 = y1 - y2;\n double y34 = y3 - y4;\n double c = x12 * y34 - y12 * x34;\n double a = x1 * y2 - y1 * x2;\n double b = x3 * y4 - y3 * x4;\n if(c != 0)\n {\n double x = (a * x34 - b * x12) / c;\n double y = (a * y34 - b * y12) / c;\n std::cout << \"Intersection point coordinates : \\n\";\n std::cout << \"Xin : \" << x << std::endl;\n std::cout << \"Yin : \" << y << std::endl;\n } \n else\n {\n std::cout << \"Lines are parallel\";\n }\n}\nint main()\n{\n EliminationMethd2DLineIntersection obj;\n int value;\n double x1, y1, x2, y2, x3, y3, x4, y4, x5, y5, x6, y6, x7, y7, x8, y8;\n std::cout << \"\\nEnter the Coordinates for Line-1\";\n std::cout << \"\\nEnter the X-coordinate for Point-1: \";\n std::cin >> x1;\n std::cout << \"\\nEnter the Y-coordinate for Point-1: \";\n std::cin >> y1;\n std::cout << \"\\nEnter the X-coordinate for Point-2: \";\n std::cin >> x2;\n std::cout << \"\\nEnter the Y-coordinate for Point-2: \";\n std::cin >> y2;\n std::cout << \"\\nEnter the Coordinates for Line-2\";\n std::cout << \"\\nEnter the X-coordinate for Point-1: \";\n std::cin >> x3;\n std::cout << \"\\nEnter the Y-coordinate for Point-1: \";\n std::cin >> y3;\n std::cout << \"\\nEnter the X-coordinate for Point-2: \";\n std::cin >> x4;\n std::cout << \"\\nEnter the Y-coordinate for Point-2: \";\n std::cin >> y4;\n obj.acceptTheCoordinates(x1,y1, x2, y2, x3, y3, x4, y4);\n return 0;\n}"
}
{
"filename": "code/computational_geometry/src/2d_line_intersection/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\n## 2 Dimensional line intersection\n\nThis package contains programs for 2D line intersections.\n\nThe program checks if two lines are `parallel` or `intersecting`. If they intersect, it gives the point of intersection.\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>"
}
{
"filename": "code/computational_geometry/src/2d_separating_axis_test/2d_separating_axis_test.cpp",
"content": "// computational geometry | Two convex polygon intersection 2d seperating axis test | C++\n//Ivan Reinaldo Liyanto\n// open genus - cosmos\n#include <cmath>\n#include <iostream>\n#include <vector>\n\nusing namespace std;\nstruct vec2\n{\n double x, y;\n vec2(double x, double y) : x(x), y(y)\n {\n }\n friend vec2 operator+(vec2 lhs, const vec2& vec)\n {\n return vec2(lhs.x + vec.x, lhs.y + vec.y);\n }\n friend vec2 operator-(vec2 lhs, const vec2& vec)\n {\n return vec2(lhs.x - vec.x, lhs.y - vec.y);\n }\n\n};\n\ndouble dot(vec2 a, vec2 b)\n{\n return a.x * b.x + a.y * b.y;\n}\n\nvector<vec2> inputPolygon1;\nvector<vec2> inputPolygon2;\n\nbool sat()\n{\n //project every points onto every axis\n for (size_t i = 1; i < inputPolygon1.size(); i++)\n {\n vec2 axis = inputPolygon1[i] - inputPolygon1[i - 1];\n\n double leftMostPolygonA = __FLT_MAX__;\n double rightMostPolygonA = __FLT_MIN__;\n for (size_t j = 0; j < inputPolygon1.size(); j++)\n {\n double d = dot(inputPolygon1[j], axis);\n if (d > rightMostPolygonA)\n rightMostPolygonA = d;\n if (d < leftMostPolygonA)\n leftMostPolygonA = d;\n }\n\n double leftMostPolygonB = __FLT_MAX__;\n double rightMostPolygonB = __FLT_MIN__;\n for (size_t j = 0; j < inputPolygon2.size(); j++)\n {\n double d = dot(inputPolygon2[j], axis);\n if (d > rightMostPolygonB)\n rightMostPolygonB = d;\n if (d < leftMostPolygonB)\n leftMostPolygonB = d;\n }\n\n if ((leftMostPolygonB < rightMostPolygonA && rightMostPolygonB > rightMostPolygonA) ||\n (leftMostPolygonA < rightMostPolygonB && rightMostPolygonA > rightMostPolygonB) )\n return true;\n }\n\n //false = no intersection\n return false;\n}\n\nint main()\n{\n //given 2 convex polygons defined by set of points, determine if the two polygon intersects using Separating Axis Test\n //example set 1\n inputPolygon1.push_back(vec2(5, 5));\n inputPolygon1.push_back(vec2(7, 10));\n inputPolygon1.push_back(vec2(15, 10));\n inputPolygon1.push_back(vec2(4, 4));\n\n inputPolygon2.push_back(vec2(6, 6));\n inputPolygon2.push_back(vec2(0, 0));\n inputPolygon2.push_back(vec2(7, 0));\n\n cout << \"The two polygons \" << (sat() ? \"\" : \"do not \") << \"intersect\\n\";\n\n return 0;\n}"
}
{
"filename": "code/computational_geometry/src/area_of_polygon/area_of_polygon.c",
"content": "/* computational geometry | area of polygon | C\n * @Author: Ayush Garg \n * @Date: 2017-10-13 01:20:26 \n * @Last Modified by: Ayush Garg\n * @Last Modified time: 2017-10-13 01:31:03\n * Part of Cosmos by OpenGenus Foundation\n */\n\n#include <stdio.h>\n\ntypedef struct\n{\n double x, y;\n} point;\n\ndouble calcArea(point a, point b, point c)\n{\n double tmp = ((a.x - b.x) * (c.y - b.y) - (a.y - b.y) * (c.x - b.x)) / 2;\n return (tmp < 0) ? (-tmp) : tmp;\n}\n\nint main()\n{\n double ans = 0;\n int n;\n printf(\"Enter number of vertices \\n\");\n scanf(\"%d\", &n);\n point points[n];\n\n for (int i = 0; i < n; i++)\n {\n scanf(\"%lf %lf\", &points[i].x, &points[i].y);\n }\n\n for (int i = 2; i < n; i++)\n {\n ans += calcArea(points[0], points[i - 1], points[i]);\n }\n\n printf(\"\\n Answer is: %lf\\n\", ans);\n}"
}
{
"filename": "code/computational_geometry/src/area_of_polygon/area_of_polygon.cpp",
"content": "#include <iostream>\ntypedef std::pair<double, double> point;\n\nconst int size = 100000;\n\npoint points[size];\n\n#define x first\n#define y second\n\ndouble calcArea(point a, point b, point c)\n{\n return abs( (a.first - b.first) * (c.second - b.second) - (a.second - b.second) *\n (c.first - b.first) ) / 2;\n}\n\nint main ()\n{\n double answer = 0;\n\n int n;\n std::cin >> n;\n\n for (int i = 0; i < n; i++)\n std::cin >> points[i].x >> points[i].y;\n\n for (int i = 2; i < n; i++)\n answer += calcArea(points[0], points[i - 1], points[i]);\n\n std::cout << answer;\n}"
}
{
"filename": "code/computational_geometry/src/area_of_polygon/area_of_polygon.java",
"content": "public class AreaOfPolygon {\n\n public static void main(String []args){\n // input points {xPoints[i],yPoints[j]}\n int[] xPoints = {4, 4, 8, 8, -4, -4};\n int[] yPoints = {6, -4, -4, -8, -8, 6};\n \n double result = 0;\n int j = xPoints.length - 1;\n \n for (int i=0; i< xPoints.length; i++){\n result += (xPoints[j] + xPoints[i]) * (yPoints[j] - yPoints[i]); \n j = i;\n }\n \n result = result/2;\n \n System.out.println(result);\n }\n}"
}
{
"filename": "code/computational_geometry/src/area_of_polygon/area_of_polygon.py",
"content": "def area_of_polygon(verticeX, verticeY):\n length = len(verticeX)\n area = 0.0\n for a in range(0, length):\n b = (a + 1) % length\n area += verticeX[a] * verticeY[b] - verticeX[b] * verticeY[a]\n area = abs(area) / 2.0\n return area\n\n\nX = []\nY = []\ntry:\n input = raw_input\nexcept NameError:\n pass\n\nn = int(input(\"Enter number of vertices of the polygon:\"))\n\nfor i in range(0, n):\n X.append(float(input(\"X of vertex \" + str(i + 1) + \": \")))\n Y.append(float(input(\"Y of vertex \" + str(i + 1) + \": \")))\n\nprint(\"Area of polygon : \" + str(area_of_polygon(X, Y)))"
}
{
"filename": "code/computational_geometry/src/area_of_triangle/area_of_triangle.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n\nfloat \narea(float x1, float x2, float y1, float y2,\n\tfloat z1, float z2)\n{\n\treturn (fabsf((x1 - y1) * (z2 - y2) - (x2 - y2) * (z1 - y1)) / 2.0);\n}\n\nint \nmain()\n{\n\tfloat x1, x2, y1, y2, z1, z2;\n\tprintf(\"Enter x1 = \");\n\tscanf(\"%f\", &x1);\n\tprintf(\"Enter x2 = \");\n\tscanf(\"%f\", &x2);\n\tprintf(\"Enter y1 = \");\n\tscanf(\"%f\", &y1);\n\tprintf(\"Enter y2 = \");\n\tscanf(\"%f\", &y2);\n\tprintf(\"Enter z1 = \");\n\tscanf(\"%f\", &z1);\n\tprintf(\"Enter z2 = \");\n\tscanf(\"%f\", &z2);\n\n\tprintf(\"Area of the Triangle = %f \\n\", area(x1, x2, y1, y2, z1, z2));\n\n\treturn (0);\n}"
}
{
"filename": "code/computational_geometry/src/area_of_triangle/area_of_triangle.cpp",
"content": "#include <iostream>\ntypedef std::pair<double, double> point;\n\ndouble calcArea(point a, point b, point c)\n{\n return abs( (a.first - b.first) * (c.second - b.second) - (a.second - b.second) *\n (c.first - b.first) ) / 2;\n}\n\nint main ()\n{\n point a, b, c;\n std::cin >> a.first >> a.second >> b.first >> b.second >> c.first >> c.second;\n std::cout << calcArea(a, b, c);\n}"
}
{
"filename": "code/computational_geometry/src/area_of_triangle/area_of_triangle.go",
"content": "package main\n// Part of Cosmos by OpenGenus Foundation\n\nimport (\n\t\"fmt\"\n\t\"math\"\n)\n\ntype vector struct {\n\tx, y float64\n}\n\nfunc calculateArea(a, b, c vector) float64 {\n\treturn math.Abs((a.x - b.x) * (c.y - b.y) - (a.y - b.y) * (c.x - b.x)) / 2\n}\n\nfunc main() {\n\ta := vector{x: 5, y: 5}\n\tb := vector{x: 5, y: 10}\n\tc := vector{x: 10, y: 5}\n\n\t// 12.5\n\tfmt.Println(calculateArea(a, b, c))\n\n\ta = vector{x: 2, y: 4}\n\tb = vector{x: 3, y: 12}\n\tc = vector{x: 8, y: 5}\n\n\t// 23.5\n\tfmt.Println(calculateArea(a, b, c))\n}"
}
{
"filename": "code/computational_geometry/src/area_of_triangle/area_of_triangle_herons_formula.cpp",
"content": "#include <iostream>\n#include <cmath>\n\nclass AreaOfTriangle\n{\npublic:\n AreaOfTriangle(double a, double b, double c) : a_(a), b_(b), c_(c) {}\n\n double calculateArea();\n\nprivate:\n double a_, b_, c_;\n\n};\n\ndouble AreaOfTriangle::calculateArea()\n{\n /*\n * As magnitude of length of sides must be positive.\n * Given length of sides of triangle must follow the following result :\n * \"Sum of any two sides of triangle must be smaller than the third side of triangle\".\n */\n if (a_ < 0 || b_ < 0 || c_ < 0 || a_+ b_ <= c_ || a_+ c_ <= b_ || b_+ c_ <= a_)\n return 0.0;\n double s = (a_ + b_ + c_) / 2; //semi-perimeter of triangle\n return sqrt(s * (s - a_) * (s - b_) * (s - c_)); //Heron's Formula\n}\n\nint main()\n{\n double ta, tb, tc;\n std::cout << \"\\nEnter the length of side-1 : \";\n std::cin >> ta;\n std::cout << \"\\nEnter the length of side-2 : \";\n std::cin >> tb;\n std::cout << \"\\nEnter the length of side-3 : \";\n std::cin >> tc;\n AreaOfTriangle a(ta, tb, tc);\n if (a.calculateArea() == 0.0)\n std::cout << \"\\nInvalid Triangle\";\n else\n std::cout << \"\\nArea of Triangle : \" << a.calculateArea() << \" square units.\";\n\n}"
}
{
"filename": "code/computational_geometry/src/area_of_triangle/area_of_triangle.java",
"content": "import java.lang.Math;\n\npublic class AreaOfTriangle {\n\n public static void main(String []args){\n // input points {x,y}\n int[] pointA = {15,15};\n int[] pointB = {23,30};\n int[] pointC = {50,25};\n \n double result = Math.abs(((pointA[0]*(pointB[1] - pointC[1])) + (pointB[0]*(pointC[1] - pointA[1])) + (pointC[0]*(pointA[1] - pointB[1])))/2);\n \n System.out.println(result);\n }\n}"
}
{
"filename": "code/computational_geometry/src/area_of_triangle/area_of_triangle.js",
"content": "// Part of Cosmos by OpenGenus Foundation\n// Author: Igor Antun\n// Github: @IgorAntun\n// Function to find area of a triangle using three different vertices.\n\n/* Function */\nconst area_of_triangle = (a, b, c) =>\n Math.abs(\n a.x * b.y + a.y * c.x + b.x * c.y - a.y * b.x - a.x * c.y - b.y * c.x\n ) / 2;\n\n/* Test */\narea_of_triangle({ x: 3, y: 50 }, { x: -6, y: 8 }, { x: 8, y: 0 }); // should return 330"
}
{
"filename": "code/computational_geometry/src/area_of_triangle/area_of_triangle.py",
"content": "#! /usr/local/bin/python3\n\n# Part of Cosmos by OpenGenus Foundation\n# Programmer: Amariah Del Mar\n# Date Written: October 6th, 2017\n# Function to find area of a triangle using three different vertices.\n\n\nclass MyPoint:\n def __init__(self, x=0, y=0):\n self.x = x\n self.y = y\n\n\ndef area_of_triangle(a, b, c):\n area = 0.5 * abs(\n (a.x * b.y)\n + (b.x * c.y)\n + (c.x * a.y)\n - (a.x * c.y)\n - (c.x * b.y)\n - (b.x * a.y)\n )\n return area\n\n\ndef test(a, b, c):\n a.x, a.y = 3, 50\n b.x, b.y = -6, 8\n c.x, c.y = 8, 0\n return a, b, c\n\n\nif __name__ == \"__main__\":\n pt1 = MyPoint()\n pt2 = MyPoint()\n pt3 = MyPoint()\n pt1, pt2, pt3 = test(pt1, pt2, pt3)\n tri_area = area_of_triangle(pt1, pt2, pt3)\n print(\n \"The area of a triangle with vertices ({},{}), ({},{}) and ({},{}) is {}.\".format(\n pt1.x, pt1.y, pt2.x, pt2.y, pt3.x, pt3.y, tri_area\n )\n )"
}
{
"filename": "code/computational_geometry/src/area_of_triangle/area_of_triangle.rs",
"content": "struct Point {\n x: f64,\n y: f64\n}\n\nstruct Triangle {\n a: Point,\n b: Point,\n c: Point\n}\n\nimpl Triangle {\n fn area(&self) -> f64 {\n // TODO: How can i destructure properly?\n let ref a = self.a;\n let ref b = self.b;\n let ref c = self.c;\n \n ((a.x * b.y) + (a.y * c.x) + (b.x * c.y) \n - (a.y * b.x) - (a.x * c.y) - (b.y * c.x)).abs() / 2.0\n }\n}\n\n#[test]\nfn test_tri_area() {\n let tri = Triangle {\n a: Point {\n x: 3.0,\n y: 50.0\n },\n b: Point {\n x: -6.0,\n y: 8.0\n },\n c: Point {\n x: 8.0,\n y: 0.0\n }\n };\n \n assert_eq!(tri.area(), 330.0);\n}"
}
{
"filename": "code/computational_geometry/src/axis_aligned_bounding_box_collision/axis_aligned_bounding_box_collision.cpp",
"content": "#include <iostream>\n// Part of Cosmos by OpenGenus Foundation\nstruct Vector\n{\n int x;\n int y;\n};\n\nstruct Shape\n{\n Vector center;\n int width;\n int height;\n};\n\nbool checkAABBCollision(Shape &a, Shape &b)\n{\n // change '<' to '<=' if you want to include edge touching as a collision\n return (abs(a.center.x - b.center.x) * 2 < (a.width + b.width)) &&\n (abs(a.center.y - b.center.y) * 2 < (a.height + b.height));\n}\n\nint main()\n{\n Shape a = { Vector {3, 3}, 4, 5 };\n Shape b = { Vector {9, 3}, 6, 4 };\n\n // 0 - no collision\n std::cout << checkAABBCollision(a, b) << std::endl;\n\n a = { Vector {3, 3}, 4, 5 };\n b = { Vector {7, 3}, 6, 4 };\n\n // 1 - collision\n std::cout << checkAABBCollision(a, b) << std::endl;\n\n a = { Vector {3, 10}, 4, 6 };\n b = { Vector {3, 5}, 6, 6 };\n\n // 1 - collision\n std::cout << checkAABBCollision(a, b) << std::endl;\n}"
}
{
"filename": "code/computational_geometry/src/axis_aligned_bounding_box_collision/axis_aligned_bounding_box_collision.go",
"content": "package main\n// Part of Cosmos by OpenGenus Foundation\n\nimport (\n\t\"fmt\"\n\t\"math\"\n)\n\ntype vector struct {\n\tx, y float64\n}\n\ntype shape struct {\n\tcenter vector\n\theight, width float64\n}\n\nfunc checkAABBCollision(a, b shape) bool {\n\t// change '<' to '<=' if you want to include edge touching as a collision\n\treturn (math.Abs(a.center.x - b.center.x) *2 < (a.width + b.width)) &&\n\t\t(math.Abs(a.center.y - b.center.y) * 2 < (a.height + b.height))\n}\n\nfunc main() {\n\tshapeA := shape{center: vector{3, 3}, height: 5, width: 4}\n\tshapeB := shape{center: vector{9, 3}, height: 4, width: 6}\n\n\t// 0 - no collision\n\tfmt.Println(checkAABBCollision(shapeA, shapeB))\n\n\tshapeA = shape{center: vector{3, 3}, height: 5, width: 4}\n\tshapeB = shape{center: vector{7, 3}, height: 4, width: 6}\n\n\t// 1 - collision\n\tfmt.Println(checkAABBCollision(shapeA, shapeB))\n\n\tshapeA = shape{center: vector{3, 10}, height: 6, width: 4}\n\tshapeB = shape{center: vector{3, 5}, height: 6, width: 6}\n\n\t// 1 - collision\n\tfmt.Println(checkAABBCollision(shapeA, shapeB))\n}"
}
{
"filename": "code/computational_geometry/src/bresenham_circle/bresenham_circle.cpp",
"content": "#include <iostream>\r\n#include \"graphics.h\"\r\n\r\nclass BresenhamCircle\r\n{\r\n\r\npublic:\r\n BresenhamCircle(int radius_) : radius_(radius_) { }\r\n\r\n void getRadiusCenter();\r\n\r\n void drawBresenhamCircle();\r\n\r\n void displayBresenhmCircle(int xc_, int yc_, int x, int y);\r\n\r\nprivate:\r\n int radius_;\r\n int xc_;\r\n int yc_;\r\n\r\n};\r\n\r\nvoid BresenhamCircle::drawBresenhamCircle()\r\n{\r\n int x = 0, y = radius_;\r\n int decesionParameter = 3 - 2 * radius_;\r\n displayBresenhmCircle(xc_, yc_, x, y);\r\n while (y >= x)\r\n {\r\n x++;\r\n if (decesionParameter > 0)\r\n {\r\n y--;\r\n decesionParameter = decesionParameter + 4 * (x - y) + 10;\r\n }\r\n else\r\n decesionParameter = decesionParameter + 4 * x + 6;\r\n displayBresenhmCircle(xc_, yc_, x, y); //displaying all the Eight Pixels of (x,y)\r\n delay(30);\r\n }\r\n}\r\n\r\nvoid BresenhamCircle::getRadiusCenter()\r\n{\r\n std::cout << \"\\nEnter Radius of the Circle : \";\r\n std::cin >> radius_;\r\n std::cout << \"\\nEnter X-Coordinate of Center of Circle : \";\r\n std::cin >> xc_;\r\n std::cout << \"\\nEnter Y-Coordinate of Center of Circle : \";\r\n std::cin >> yc_;\r\n}\r\n\r\nvoid BresenhamCircle::displayBresenhmCircle(int xc_,int yc_, int x, int y)\r\n{\r\n //displaying all 8 coordinates of(x,y) residing in 8-octants\r\n putpixel(xc_+x, yc_+y, WHITE);\r\n putpixel(xc_-x, yc_+y, WHITE);\r\n putpixel(xc_+x, yc_-y, WHITE);\r\n putpixel(xc_-x, yc_-y, WHITE);\r\n putpixel(xc_+y, yc_+x, WHITE);\r\n putpixel(xc_-y, yc_+x, WHITE);\r\n putpixel(xc_+y, yc_-x, WHITE);\r\n putpixel(xc_-y, yc_-x, WHITE);\r\n}\r\n\r\nint main()\r\n{\r\n int gd = DETECT, gm;\r\n initgraph(&gd, &gm, NULL);\r\n BresenhamCircle b(0);\r\n //accepting the radius and the centre coordinates of the circle\r\n b.getRadiusCenter();\r\n /*\r\n * selecting the nearest pixel and displaying all the corresponding\r\n * points of the nearest pixel point lying in 8-octants.\r\n *\r\n */\r\n b.drawBresenhamCircle();\r\n delay(200);\r\n closegraph();\r\n\r\n}\r"
}
{
"filename": "code/computational_geometry/src/bresenham_circle/graphics.h",
"content": "// The winbgim library, Version 6.0, August 9, 2004\r\n// Written by:\r\n// Grant Macklem ([email protected])\r\n// Gregory Schmelter ([email protected])\r\n// Alan Schmidt ([email protected])\r\n// Ivan Stashak ([email protected])\r\n// Michael Main ([email protected])\r\n// CSCI 4830/7818: API Programming\r\n// University of Colorado at Boulder, Spring 2003\r\n\r\n\r\n// ---------------------------------------------------------------------------\r\n// Notes\r\n// ---------------------------------------------------------------------------\r\n// * This library is still under development.\r\n// * Please see http://www.cs.colorado.edu/~main/bgi for information on\r\n// * using this library with the mingw32 g++ compiler.\r\n// * This library only works with Windows API level 4.0 and higher (Windows 95, NT 4.0 and newer)\r\n// * This library may not be compatible with 64-bit versions of Windows\r\n// ---------------------------------------------------------------------------\r\n\r\n\r\n// ---------------------------------------------------------------------------\r\n// Macro Guard and Include Directives\r\n// ---------------------------------------------------------------------------\r\n#ifndef WINBGI_H\r\n#define WINBGI_H\r\n#include <windows.h> // Provides the mouse message types\r\n#include <limits.h> // Provides INT_MAX\r\n#include <sstream> // Provides std::ostringstream\r\n// ---------------------------------------------------------------------------\r\n\r\n\r\n\r\n// ---------------------------------------------------------------------------\r\n// Definitions\r\n// ---------------------------------------------------------------------------\r\n// Definitions for the key pad extended keys are added here. When one\r\n// of these keys are pressed, getch will return a zero followed by one\r\n// of these values. This is the same way that it works in conio for\r\n// dos applications.\r\n#define KEY_HOME 71\r\n#define KEY_UP 72\r\n#define KEY_PGUP 73\r\n#define KEY_LEFT 75\r\n#define KEY_CENTER 76\r\n#define KEY_RIGHT 77\r\n#define KEY_END 79\r\n#define KEY_DOWN 80\r\n#define KEY_PGDN 81\r\n#define KEY_INSERT 82\r\n#define KEY_DELETE 83\r\n#define KEY_F1 59\r\n#define KEY_F2 60\r\n#define KEY_F3 61\r\n#define KEY_F4 62\r\n#define KEY_F5 63\r\n#define KEY_F6 64\r\n#define KEY_F7 65\r\n#define KEY_F8 66\r\n#define KEY_F9 67\r\n\r\n// Line thickness settings\r\n#define NORM_WIDTH 1\r\n#define THICK_WIDTH 3\r\n\r\n// Character Size and Direction\r\n#define USER_CHAR_SIZE 0\r\n#define HORIZ_DIR 0\r\n#define VERT_DIR 1\r\n\r\n\r\n// Constants for closegraph\r\n#define CURRENT_WINDOW -1\r\n#define ALL_WINDOWS -2\r\n#define NO_CURRENT_WINDOW -3\r\n\r\n// The standard Borland 16 colors\r\n#define MAXCOLORS 15\r\nenum colors { BLACK, BLUE, GREEN, CYAN, RED, MAGENTA, BROWN, LIGHTGRAY, DARKGRAY,\r\n LIGHTBLUE, LIGHTGREEN, LIGHTCYAN, LIGHTRED, LIGHTMAGENTA, YELLOW, WHITE };\r\n\r\n// The standard line styles\r\nenum line_styles { SOLID_LINE, DOTTED_LINE, CENTER_LINE, DASHED_LINE, USERBIT_LINE };\r\n\r\n// The standard fill styles\r\nenum fill_styles { EMPTY_FILL, SOLID_FILL, LINE_FILL, LTSLASH_FILL, SLASH_FILL,\r\n BKSLASH_FILL, LTBKSLASH_FILL, HATCH_FILL, XHATCH_FILL, INTERLEAVE_FILL,\r\n WIDE_DOT_FILL, CLOSE_DOT_FILL, USER_FILL };\r\n\r\n// The various graphics drivers\r\nenum graphics_drivers { DETECT, CGA, MCGA, EGA, EGA64, EGAMONO, IBM8514, HERCMONO,\r\n ATT400, VGA, PC3270 };\r\n\r\n// Various modes for each graphics driver\r\nenum graphics_modes { CGAC0, CGAC1, CGAC2, CGAC3, CGAHI, \r\n MCGAC0 = 0, MCGAC1, MCGAC2, MCGAC3, MCGAMED, MCGAHI,\r\n EGALO = 0, EGAHI,\r\n EGA64LO = 0, EGA64HI,\r\n EGAMONOHI = 3,\r\n HERCMONOHI = 0,\r\n ATT400C0 = 0, ATT400C1, ATT400C2, ATT400C3, ATT400MED, ATT400HI,\r\n VGALO = 0, VGAMED, VGAHI,\r\n PC3270HI = 0,\r\n IBM8514LO = 0, IBM8514HI };\r\n\r\n// Borland error messages for the graphics window.\r\n#define NO_CLICK -1 // No mouse event of the current type in getmouseclick\r\nenum graph_errors { grInvalidVersion = -18, grInvalidDeviceNum = -15, grInvalidFontNum,\r\n grInvalidFont, grIOerror, grError, grInvalidMode, grNoFontMem,\r\n grFontNotFound, grNoFloodMem, grNoScanMem, grNoLoadMem,\r\n grInvalidDriver, grFileNotFound, grNotDetected, grNoInitGraph,\r\n grOk };\r\n\r\n// Write modes\r\nenum putimage_ops{ COPY_PUT, XOR_PUT, OR_PUT, AND_PUT, NOT_PUT };\r\n\r\n// Text Modes\r\nenum horiz { LEFT_TEXT, CENTER_TEXT, RIGHT_TEXT };\r\nenum vertical { BOTTOM_TEXT, VCENTER_TEXT, TOP_TEXT }; // middle not needed other than as seperator\r\nenum font_names { DEFAULT_FONT, TRIPLEX_FONT, SMALL_FONT, SANS_SERIF_FONT,\r\n GOTHIC_FONT, SCRIPT_FONT, SIMPLEX_FONT, TRIPLEX_SCR_FONT,\r\n\t\t\t COMPLEX_FONT, EUROPEAN_FONT, BOLD_FONT };\r\n// ---------------------------------------------------------------------------\r\n\r\n\r\n\r\n// ---------------------------------------------------------------------------\r\n// Structures\r\n// ---------------------------------------------------------------------------\r\n// This structure records information about the last call to arc. It is used\r\n// by getarccoords to get the location of the endpoints of the arc.\r\nstruct arccoordstype\r\n{\r\n int x, y; // Center point of the arc\r\n int xstart, ystart; // The starting position of the arc\r\n int xend, yend; // The ending position of the arc.\r\n};\r\n\r\n\r\n// This structure defines the fill style for the current window. Pattern is\r\n// one of the system patterns such as SOLID_FILL. Color is the color to\r\n// fill with\r\nstruct fillsettingstype\r\n{\r\n int pattern; // Current fill pattern\r\n int color; // Current fill color\r\n};\r\n\r\n\r\n// This structure records information about the current line style.\r\n// linestyle is one of the line styles such as SOLID_LINE, upattern is a\r\n// 16-bit pattern for user defined lines, and thickness is the width of the\r\n// line in pixels.\r\nstruct linesettingstype\r\n{\r\n int linestyle; // Current line style\r\n unsigned upattern; // 16-bit user line pattern\r\n int thickness; // Width of the line in pixels\r\n};\r\n\r\n\r\n// This structure records information about the text settings.\r\nstruct textsettingstype\r\n{\r\n int font; // The font in use\r\n int direction; // Text direction\r\n int charsize; // Character size\r\n int horiz; // Horizontal text justification\r\n int vert; // Vertical text justification\r\n};\r\n\r\n\r\n// This structure records information about the viewport\r\nstruct viewporttype\r\n{\r\n int left, top, // Viewport bounding box\r\n right, bottom;\r\n int clip; // Whether to clip image to viewport\r\n};\r\n\r\n\r\n// This structure records information about the palette.\r\nstruct palettetype\r\n{\r\n unsigned char size;\r\n signed char colors[MAXCOLORS + 1];\r\n};\r\n// ---------------------------------------------------------------------------\r\n\r\n\r\n\r\n// ---------------------------------------------------------------------------\r\n// API Entries\r\n// ---------------------------------------------------------------------------\r\n#ifdef __cplusplus\r\nextern \"C\" {\r\n#endif\r\n\r\n// Drawing Functions\r\nvoid arc( int x, int y, int stangle, int endangle, int radius );\r\nvoid bar( int left, int top, int right, int bottom );\r\nvoid bar3d( int left, int top, int right, int bottom, int depth, int topflag );\r\nvoid circle( int x, int y, int radius );\r\nvoid cleardevice( );\r\nvoid clearviewport( );\r\nvoid drawpoly(int n_points, int* points);\r\nvoid ellipse( int x, int y, int stangle, int endangle, int xradius, int yradius );\r\nvoid fillellipse( int x, int y, int xradius, int yradius );\r\nvoid fillpoly(int n_points, int* points);\r\nvoid floodfill( int x, int y, int border );\r\nvoid line( int x1, int y1, int x2, int y2 );\r\nvoid linerel( int dx, int dy );\r\nvoid lineto( int x, int y );\r\nvoid pieslice( int x, int y, int stangle, int endangle, int radius );\r\nvoid putpixel( int x, int y, int color );\r\nvoid rectangle( int left, int top, int right, int bottom );\r\nvoid sector( int x, int y, int stangle, int endangle, int xradius, int yradius );\r\n\r\n// Miscellaneous Functions\r\nint getdisplaycolor( int color );\r\nint converttorgb( int color );\r\nvoid delay( int msec );\r\nvoid getarccoords( arccoordstype *arccoords );\r\nint getbkcolor( );\r\nint getcolor( );\r\nvoid getfillpattern( char *pattern );\r\nvoid getfillsettings( fillsettingstype *fillinfo );\r\nvoid getlinesettings( linesettingstype *lineinfo );\r\nint getmaxcolor( );\r\nint getmaxheight( );\r\nint getmaxwidth( );\r\nint getmaxx( );\r\nint getmaxy( );\r\nbool getrefreshingbgi( );\r\nint getwindowheight( );\r\nint getwindowwidth( );\r\nint getpixel( int x, int y );\r\nvoid getviewsettings( viewporttype *viewport );\r\nint getx( );\r\nint gety( );\r\nvoid moverel( int dx, int dy );\r\nvoid moveto( int x, int y );\r\nvoid refreshbgi(int left, int top, int right, int bottom);\r\nvoid refreshallbgi( ); \r\nvoid setbkcolor( int color );\r\nvoid setcolor( int color );\r\nvoid setfillpattern( char *upattern, int color );\r\nvoid setfillstyle( int pattern, int color );\r\nvoid setlinestyle( int linestyle, unsigned upattern, int thickness );\r\nvoid setrefreshingbgi(bool value);\r\nvoid setviewport( int left, int top, int right, int bottom, int clip );\r\nvoid setwritemode( int mode );\r\n\r\n// Window Creation / Graphics Manipulation\r\nvoid closegraph( int wid=ALL_WINDOWS );\r\nvoid detectgraph( int *graphdriver, int *graphmode );\r\nvoid getaspectratio( int *xasp, int *yasp );\r\nchar *getdrivername( );\r\nint getgraphmode( );\r\nint getmaxmode( );\r\nchar *getmodename( int mode_number );\r\nvoid getmoderange( int graphdriver, int *lomode, int *himode );\r\nvoid graphdefaults( );\r\nchar *grapherrormsg( int errorcode );\r\nint graphresult( );\r\nvoid initgraph( int *graphdriver, int *graphmode, char *pathtodriver );\r\nint initwindow\r\n ( int width, int height, const char* title=\"Windows BGI\", int left=0, int top=0, bool dbflag=false, bool closeflag=true );\r\nint installuserdriver( char *name, int *fp ); // Not available in WinBGI\r\nint installuserfont( char *name ); // Not available in WinBGI\r\nint registerbgidriver( void *driver ); // Not available in WinBGI\r\nint registerbgifont( void *font ); // Not available in WinBGI\r\nvoid restorecrtmode( );\r\nvoid setaspectratio( int xasp, int yasp );\r\nunsigned setgraphbufsize( unsigned bufsize ); // Not available in WinBGI\r\nvoid setgraphmode( int mode );\r\nvoid showerrorbox( const char *msg = NULL );\r\n\r\n// User Interaction\r\nint getch( );\r\nint kbhit( );\r\n\r\n// User-Controlled Window Functions (winbgi.cpp)\r\nint getcurrentwindow( );\r\nvoid setcurrentwindow( int window );\r\n \r\n// Double buffering support (winbgi.cpp)\r\nint getactivepage( );\r\nint getvisualpage( );\r\nvoid setactivepage( int page );\r\nvoid setvisualpage( int page );\r\nvoid swapbuffers( );\r\n\r\n// Image Functions (drawing.cpp)\r\nunsigned imagesize( int left, int top, int right, int bottom );\r\nvoid getimage( int left, int top, int right, int bottom, void *bitmap );\r\nvoid putimage( int left, int top, void *bitmap, int op );\r\nvoid printimage(\r\n const char* title=NULL,\t\r\n double width_inches=7, double border_left_inches=0.75, double border_top_inches=0.75,\r\n int left=0, int top=0, int right=INT_MAX, int bottom=INT_MAX,\r\n bool active=true, HWND hwnd=NULL\r\n );\r\nvoid readimagefile(\r\n const char* filename=NULL,\r\n int left=0, int top=0, int right=INT_MAX, int bottom=INT_MAX\r\n );\r\nvoid writeimagefile(\r\n const char* filename=NULL,\r\n int left=0, int top=0, int right=INT_MAX, int bottom=INT_MAX,\r\n bool active=true, HWND hwnd=NULL\r\n );\r\n\r\n// Text Functions (text.cpp)\r\nvoid gettextsettings(struct textsettingstype *texttypeinfo);\r\nvoid outtext(char *textstring);\r\nvoid outtextxy(int x, int y, char *textstring);\r\nvoid settextjustify(int horiz, int vert);\r\nvoid settextstyle(int font, int direction, int charsize);\r\nvoid setusercharsize(int multx, int divx, int multy, int divy);\r\nint textheight(char *textstring);\r\nint textwidth(char *textstring);\r\nextern std::ostringstream bgiout; \r\nvoid outstream(std::ostringstream& out=bgiout);\r\nvoid outstreamxy(int x, int y, std::ostringstream& out=bgiout); \r\n \r\n// Mouse Functions (mouse.cpp)\r\nvoid clearmouseclick( int kind );\r\nvoid clearresizeevent( );\r\nvoid getmouseclick( int kind, int& x, int& y );\r\nbool ismouseclick( int kind );\r\nbool isresizeevent( );\r\nint mousex( );\r\nint mousey( );\r\nvoid registermousehandler( int kind, void h( int, int ) );\r\nvoid setmousequeuestatus( int kind, bool status=true );\r\n\r\n// Palette Functions\r\npalettetype *getdefaultpalette( );\r\nvoid getpalette( palettetype *palette );\r\nint getpalettesize( );\r\nvoid setallpalette( palettetype *palette );\r\nvoid setpalette( int colornum, int color );\r\nvoid setrgbpalette( int colornum, int red, int green, int blue );\r\n\r\n// Color Macros\r\n#define IS_BGI_COLOR(v) ( ((v) >= 0) && ((v) < 16) )\r\n#define IS_RGB_COLOR(v) ( (v) & 0x03000000 )\r\n#define RED_VALUE(v) int(GetRValue( converttorgb(v) ))\r\n#define GREEN_VALUE(v) int(GetGValue( converttorgb(v) ))\r\n#define BLUE_VALUE(v) int(GetBValue( converttorgb(v) ))\r\n#undef COLOR\r\nint COLOR(int r, int g, int b); // No longer a macro\r\n\r\n#ifdef __cplusplus\r\n}\r\n#endif\r\n// ---------------------------------------------------------------------------\r\n\r\n#endif // WINBGI_H\r\n\r"
}
{
"filename": "code/computational_geometry/src/bresenham_line/bresenham_line2.py",
"content": "# Import graphics library\nfrom graphics import GraphWin\n\nimport time\n\ndef bresenham_line(xa, ya, xb, yb):\n dx = abs(xb - xa)\n dy = abs(yb - ya)\n\n slope = dy / float(dx)\n\n x, y = xa, ya\n\n # creating the window\n win = GraphWin('Bresenham Line', 600, 480)\n\n # checking the slope if slope > 1\n # then interchange the role of x and y\n if slope > 1:\n dx, dy = dy, dx\n x, y = y, x\n xa, ya = ya, xa\n xb, yb = yb, xb\n # initialization of the inital disision parameter\n p = 2 * dy - dx\n\n Put_Pixel(win, x, y) # Plot Pixels To Draw Line\n\n for k in range(2, dx):\n if p > 0:\n y += 1 if y < yb else y - 1\n p += 2 * (dy - dx)\n else:\n p += 2 * dy\n x += 1 if x < xb else x - 1\n\n time.sleep(0.01) # delay for 0.01 secs\n Put_Pixel(win, x, y) # Plot Pixels To Draw Line\n\ndef Put_Pixel(win, x, y):\n \"\"\"Plot a pixel In the window at point (x, y)\"\"\"\n pt = Point(x, y)\n pt.draw(win)\n\ndef main():\n # Taking coordinates from User\n xa = int(input(\"Enter Start X: \"))\n ya = int(input(\"Enter Start Y: \"))\n xb = int(input(\"Enter End X: \"))\n yb = int(input(\"Enter End Y: \"))\n\n # Calling Out The function\n Bresenham_Line(xa, ya, xb, yb)\n\n# Driver Function\nif __name__ == \"__main__\":\n main()\n\n# Input - Enter Start X : 100\n# Enter Start Y : 100\n# Enter End X : 300\n# Enter End Y : 300\n\n# Sample output - https://ibb.co/4fzGM6W"
}
{
"filename": "code/computational_geometry/src/bresenham_line/bresenham_line.cpp",
"content": "// Part of Cosmos by OpenGenus Foundation\n#include <functional>\n\ntemplate<typename Color, typename fabs>\nvoid Line(float x1, float y1, float x2, float y2, const Color& color )\n{\n const bool steep = (fabs(y2 - y1) > fabs(x2 - x1));\n if (steep)\n {\n std::swap(x1, y1);\n std::swap(x2, y2);\n }\n\n if (x1 > x2)\n {\n std::swap(x1, x2);\n std::swap(y1, y2);\n }\n\n const float dx = x2 - x1;\n const float dy = fabs(y2 - y1);\n\n float error = dx / 2.0f;\n const int ystep = (y1 < y2) ? 1 : -1;\n int y = (int)y1;\n\n const int maxX = (int)x2;\n\n for (int x = (int)x1; x < maxX; x++)\n {\n if (steep)\n SetPixel(y, x, color);\n else\n SetPixel(x, y, color);\n\n error -= dy;\n if (error < 0)\n {\n y += ystep;\n error += dx;\n }\n }\n}"
}
{
"filename": "code/computational_geometry/src/bresenham_line/bresenham_line.java",
"content": "package applet;\n\nimport java.awt.Color;\nimport java.awt.Dimension;\nimport java.awt.Graphics;\nimport java.awt.event.MouseEvent;\nimport java.awt.event.MouseListener;\nimport java.awt.event.MouseMotionListener;\n\npublic class bresenhamLine extends java.applet.Applet implements MouseListener, MouseMotionListener {\n\n private static final long serialVersionUID = 1L;\n int width, height;\n int xa = 0;\n int ya = 0;\n int xb = 0;\n int yb = 0;\n int pixelsize = 2;\n\n\n /**\n * This method does initialization\n * @param no parameters used .\n */\n\n public void init() {\n this.width = getSize().width;\n this.height = getSize().height;\n this.addMouseListener(this);\n this.addMouseMotionListener(this);\n }\n\n /**\n * This method is used to Draw Line.\n * @param xa first coordinate\n * @param ya first Coordinate\n * @param xb Second Coordinate\n * @param yb Second Coordinate\n * @return will not return anything .\n */\n public void bresenhamLineDraw(int xa, int ya, int xb, int yb) {\n\n // if point xa, ya is on the right side of point xb, yb, change them\n if ((xa - xb) > 0) {\n bresenhamLineDraw(xb, yb, xa, ya);\n return;\n }\n\n // test inclination of line\n // function Math.abs(y) defines absolute value y\n if (Math.abs(yb - ya) > Math.abs(xb - xa)) {\n // line and y axis angle is less then 45 degrees\n // thats why go on the next procedure\n bresteepLine(ya, xa, yb, xb);\n return;\n }\n\n // line and x axis angle is less then 45 degrees, so x is guiding\n // auxiliary variables\n int x = xa, y = ya, sum = xb - xa, Dx = 2 * (xb - xa), Dy = Math.abs(2 * (yb - ya));\n int delta_Dy = ((yb - ya) > 0) ? 1 : -1;\n\n // draw line\n for (int i = 0; i <= xb - xa; i++) {\n setpix(x, y);\n x++;\n sum -= Dy;\n if (sum < 0) {\n y += delta_Dy;\n sum += Dx;\n }\n }\n }\n\n /**\n * This method is used to Draw Steeper Line.\n * @param xc first coordinate\n * @param yc first Coordinate\n * @param xd Second Coordinate\n * @param yd Second Coordinate\n * @return will not return anything .\n */\n\n public void bresteepLine(int xc, int yc, int xd, int yd) {\n\n /** if point xc, yc is on the right side\n of point xd yd,\n change them\n **/\n if ((xc - xd) > 0) {\n bresteepLine(xd, yd, xc, yc);\n return;\n }\n\n int x = xc, y = yc, sum = xd - xc, Dx = 2 * (xd - xc), Dy = Math.abs(2 * (yd - yc));\n int delta_Dy = ((yd - yc) > 0) ? 1 : -1;\n\n for (int i = 0; i <= xd - xc; i++) {\n setPix(y, x);\n x++;\n sum -= Dy;\n if (sum < 0) {\n y += delta_Dy;\n sum += Dx;\n }\n }\n }\n\n /**\n * This method is used to Set pixel for Line.\n * @param x first coordinate\n * @param y first Coordinate\n * @return will not return anything .\n */\n public void setPix(int x, int y) {\n Graphics g = getGraphics();\n g.setColor(Color.green);\n g.fillRect(pixelsize * x, pixelsize * y, pixelsize, pixelsize);\n }\n\n /**\n * This method is used to paint the line on screen.\n * @param g of graphics library\n * @return will not return anything .\n */\n public void paint(Graphics g) {\n Dimension d = getSize();\n g.drawLine(0, 0, d.width, 0);\n g.drawLine(0, 0, 0, d.height);\n g.drawLine(d.width - 1, d.height - 1, d.width - 1, 0);\n g.drawLine(d.width - 1, d.height - 1, 0, d.height - 1);\n bresenhamLineDraw(xa, ya, xb, yb);\n }\n\n public void mousePressed(MouseEvent e) {\n xa = e.getX() / pixelsize;\n ya = e.getY() / pixelsize;\n }\n\n public void mouseDragged(MouseEvent e) {\n xb = e.getX() / pixelsize;\n yb = e.getY() / pixelsize;\n repaint();\n }\n\n public void mouseReleased(MouseEvent e) {\n }\n public void mouseClicked(MouseEvent e) {\n }\n public void mouseEntered(MouseEvent e) {\n }\n public void mouseExited(MouseEvent e) {\n }\n public void mouseMoved(MouseEvent e) {\n }\n\n}\n\n/**\n * Sample Input - Enter Start X: 100\n * Enter Start Y: 100\n * Enter End X: 300\n * Enter End Y: 300\n * Sample output - https://ibb.co/NxYdXqd\n *\n*/"
}
{
"filename": "code/computational_geometry/src/bresenham_line/bresenham_line.py",
"content": "def calc_del(x1, x2, y1, y2):\n \"\"\"\n Calculate the delta values for x and y co-ordinates\n \"\"\"\n return x2 - x1, y2 - y1\n\n\ndef bresenham(x1, y1, x2, y2):\n \"\"\"\n Calculate the co-ordinates for Bresehnam lines\n bresenham(x1, y1, x2, y2)\n returns a list of tuple of points\n \"\"\"\n swap = False\n\n # Calculate delta for x and y\n delX, delY = calc_del(x1, x2, y1, y2)\n\n # Swap x and y if y > x\n if abs(delY) > abs(delX):\n x1, y1 = y1, x1\n x2, y2 = y2, x2\n\n # Swap x and y co-ordinates if x1 > x2\n if x1 > x2:\n swap = True\n x1, x2 = x2, x1\n y1, y2 = y2, y1\n\n # Recalculate delta for x and y\n delX, delY = calc_del(x1, x2, y1, y2)\n\n # calculate step and error\n step = 1 if y1 < y2 else -1\n error = int(delX / 2.0)\n\n y = y1\n co_ords = []\n\n # Calculate co-ordinates for the bresenham line\n for x in range(x1, x2 + 1):\n point = (x, y) if abs(delX) > abs(delY) else (y, x)\n error -= abs(delY)\n if error < 0:\n y += step\n error += delX\n co_ords.append(point)\n\n return co_ords if abs(delX) > abs(delY) else co_ords[::-1]\n\n\ndef main():\n x1, y1, x2, y2 = int(input())\n print(bresenham(x1, y1, x2, y2))"
}
{
"filename": "code/computational_geometry/src/chans_algorithm/chans_algorithm.cpp",
"content": " #include <iostream>\n #include <stdlib.h>\n #include <vector>\n #include <algorithm> // For qsort() algorithm\n #include <utility> // For pair() STL\n #define RIGHT_TURN -1 // CW\n #define LEFT_TURN 1 // CCW\n #define COLLINEAR 0 // Collinear\n\nusing namespace std;\n\n/*\n * Class to handle the 2D Points!\n */\nclass Point\n{\npublic:\n\n int x;\n int y;\n Point (int newx = 0, int newy = 0)\n {\n x = newx;\n y = newy;\n }\n /*\n * Overloaded == operator to check for equality between 2 objects of class Point\n */\n friend bool operator== (const Point& p1, const Point& p2)\n {\n return p1.x == p2.x && p1.y == p2.y;\n }\n /*\n * Overloaded != operator to check for non-equality between 2 objects of class Point\n */\n friend bool operator!= (const Point& p1, const Point& p2)\n {\n return !(p1.x == p2.x && p1.y == p2.y);\n }\n /*\n * Overloaded ostream << operator to check for print object of class Point to STDOUT\n */\n friend ostream& operator<<(ostream& output, const Point& p)\n {\n output << \"(\" << p.x << \",\" << p.y << \")\";\n return output;\n }\n\n} p0; // Global Point class object\n\n/*\n * Returns square of the distance between the two Point class objects\n * @param p1: Object of class Point aka first Point\n * @param p2: Object of class Point aka second Point\n */\nint dist(Point p1, Point p2)\n{\n return (p1.x - p2.x) * (p1.x - p2.x) + (p1.y - p2.y) * (p1.y - p2.y);\n}\n\n/*\n * Returns orientation of the line joining Points p and q and line joining Points q and r\n * Returns -1 : CW orientation\n +1 : CCW orientation\n * 0 : Collinear\n * @param p: Object of class Point aka first Point\n * @param q: Object of class Point aka second Point\n * @param r: Object of class Point aka third Point\n */\nint orientation(Point p, Point q, Point r)\n{\n int val = (q.y - p.y) * (r.x - q.x) - (q.x - p.x) * (r.y - q.y);\n if (val == 0)\n return 0; // Collinear\n return (val > 0) ? -1 : 1; // CW: -1 or CCW: 1\n}\n\n/*\n * Predicate function used while sorting the Points using qsort() inbuilt function in C++\n * @param p: Object of class Point aka first Point\n * @param p: Object of class Point aka second Point\n */\nint compare(const void *vp1, const void *vp2)\n{\n Point *p1 = (Point *)vp1;\n Point *p2 = (Point *)vp2;\n int orient = orientation(p0, *p1, *p2);\n if (orient == 0)\n return (dist(p0, *p2) >= dist(p0, *p1)) ? -1 : 1;\n return (orient == 1) ? -1 : 1;\n}\n\n/*\n * Returns the index of the Point to which the tangent is drawn from Point p.\n * Uses a modified Binary Search Algorithm to yield tangent in O(log n) complexity\n * @param v: vector of objects of class Points representing the hull aka the vector of hull Points\n * @param p: Object of class Point from where tangent needs to be drawn\n */\nint tangent(vector<Point> v, Point p)\n{\n int l = 0;\n int r = v.size();\n int l_before = orientation(p, v[0], v[v.size() - 1]);\n int l_after = orientation(p, v[0], v[(l + 1) % v.size()]);\n while (l < r)\n {\n int c = ((l + r) >> 1);\n int c_before = orientation(p, v[c], v[(c - 1) % v.size()]);\n int c_after = orientation(p, v[c], v[(c + 1) % v.size()]);\n int c_side = orientation(p, v[l], v[c]);\n if (c_before != RIGHT_TURN and c_after != RIGHT_TURN)\n return c;\n else if ((c_side == LEFT_TURN) and (l_after == RIGHT_TURN or l_before ==\n l_after) or (c_side == RIGHT_TURN and c_before ==\n RIGHT_TURN))\n r = c;\n else\n l = c + 1;\n l_before = -c_after;\n l_after = orientation(p, v[l], v[(l + 1) % v.size()]);\n }\n return l;\n}\n\n/*\n * Returns the pair of integers representing the Hull # and the Point in that Hull which is the extreme amongst all given Hull Points\n * @param hulls: Vector containing the hull Points for various hulls stored as individual vectors.\n */\npair<int, int> extreme_hullpt_pair(vector<vector<Point>>& hulls)\n{\n int h = 0, p = 0;\n for (int i = 0; i < hulls.size(); ++i)\n {\n int min_index = 0, min_y = hulls[i][0].y;\n for (int j = 1; j < hulls[i].size(); ++j)\n if (hulls[i][j].y < min_y)\n {\n min_y = hulls[i][j].y;\n min_index = j;\n }\n if (hulls[i][min_index].y < hulls[h][p].y)\n {\n h = i;\n p = min_index;\n }\n }\n return make_pair(h, p);\n}\n\n/*\n * Returns the pair of integers representing the Hull # and the Point in that Hull to which the Point lPoint will be joined\n * @param hulls: Vector containing the hull Points for various hulls stored as individual vectors.\n * @param lPoint: Pair of the Hull # and the leftmost extreme Point contained in that hull, amongst all the obtained hulls\n */\npair<int, int> next_hullpt_pair(vector<vector<Point>>& hulls, pair<int, int> lPoint)\n{\n Point p = hulls[lPoint.first][lPoint.second];\n pair<int, int> next = make_pair(lPoint.first, (lPoint.second + 1) % hulls[lPoint.first].size());\n for (int h = 0; h < hulls.size(); h++)\n if (h != lPoint.first)\n {\n int s = tangent(hulls[h], p);\n Point q = hulls[next.first][next.second];\n Point r = hulls[h][s];\n int t = orientation(p, q, r);\n if (t == RIGHT_TURN || (t == COLLINEAR) && dist(p, r) > dist(p, q))\n next = make_pair(h, s);\n }\n return next;\n}\n\n/*\n * Constraint to find the outermost boundary of the Points by checking if the Points lie to the left otherwise adding the given Point p\n * Returns the Hull Points\n * @param v: Vector of all the Points\n * @param p: New Point p which will be checked to be in the Hull Points or not\n */\nvector<Point> keep_left (vector<Point>& v, Point p)\n{\n while (v.size() > 1 && orientation(v[v.size() - 2], v[v.size() - 1], p) != LEFT_TURN)\n v.pop_back();\n if (!v.size() || v[v.size() - 1] != p)\n v.push_back(p);\n return v;\n}\n\n/*\n * Graham Scan algorithm to find convex hull from the given set of Points\n * @param Points: List of the given Points in the cluster (as obtained by Chan's Algorithm grouping)\n * Returns the Hull Points in a vector\n */\nvector<Point> GrahamScan(vector<Point>& Points)\n{\n if (Points.size() <= 1)\n return Points;\n qsort(&Points[0], Points.size(), sizeof(Point), compare);\n vector<Point> lower_hull;\n for (int i = 0; i < Points.size(); ++i)\n lower_hull = keep_left(lower_hull, Points[i]);\n reverse(Points.begin(), Points.end());\n vector<Point> upper_hull;\n for (int i = 0; i < Points.size(); ++i)\n upper_hull = keep_left(upper_hull, Points[i]);\n for (int i = 1; i < upper_hull.size(); ++i)\n lower_hull.push_back(upper_hull[i]);\n return lower_hull;\n}\n\n/*\n * Implementation of Chan's Algorithm to compute Convex Hull in O(nlogh) complexity\n */\nvector<Point> chansalgorithm(vector<Point> v)\n{\n for (int t = 0; t < v.size(); ++t)\n for (int m = 1; m < (1 << (1 << t)); ++m)\n {\n vector<vector<Point>> hulls;\n for (int i = 0; i < v.size(); i = i + m)\n {\n vector<Point> chunk;\n if (v.begin() + i + m <= v.end())\n chunk.assign(v.begin() + i, v.begin() + i + m);\n else\n chunk.assign(v.begin() + i, v.end());\n hulls.push_back(GrahamScan(chunk));\n }\n cout << \"\\nM (Chunk Size): \" << m << \"\\n\";\n for (int i = 0; i < hulls.size(); ++i)\n {\n cout << \"Convex Hull for Hull #\" << i << \" (Obtained using Graham Scan!!)\\n\";\n for (int j = 0; j < hulls[i].size(); ++j)\n cout << hulls[i][j] << \" \";\n cout << \"\\n\";\n }\n vector<pair<int, int>> hull;\n hull.push_back(extreme_hullpt_pair(hulls));\n for (int i = 0; i < m; ++i)\n {\n pair<int, int> p = next_hullpt_pair(hulls, hull[hull.size() - 1]);\n vector<Point> output;\n if (p == hull[0])\n {\n for (int j = 0; j < hull.size(); ++j)\n output.push_back(hulls[hull[j].first][hull[j].second]);\n return output;\n }\n hull.push_back(p);\n }\n }\n}\n\nint main()\n{\n int T = 0, x = 0, y = 0;\n cout << \"Enter Total Number of points\";\n cin >> T;\n if (T <= 0)\n return -1;\n Point Points[T];\n for (int i = 0; i < T; ++i)\n {\n cin >> x >> y;\n Points[i].x = x;\n Points[i].y = y;\n }\n vector<Point> v(Points, Points + T);\n vector<Point> output = chansalgorithm(v);\n cout << \" \\ n-------------------- After Using Chan\n 's Algorithm --------------------\\n\";\n cout << \"\\n******************** CONVEX HULL ********************\\n\";\n for (int i = 0; i < output.size(); ++i)\n cout << output[i] << \" \";\n cout << \"\\n\";\n return 0;\n}"
}
{
"filename": "code/computational_geometry/src/cohen_sutherland_lineclip/cohen_sutherland_lineclip.c",
"content": "#include<stdio.h>\n#include<stdlib.h>\n#include<math.h>\n#include<graphics.h>\n#include<dos.h>\n \ntypedef struct coordinate\n{\n int x,y;\n char code[4];\n}PT;\n \nvoid drawwindow();\nvoid drawline(PT p1,PT p2);\nPT setcode(PT p);\nint visibility(PT p1,PT p2);\nPT resetendpt(PT p1,PT p2);\n \nint main()\n{\n int gd=DETECT,v,gm;\n PT p1,p2,p3,p4,ptemp;\n \n printf(\"\\nEnter x1 and y1\\n\");\n scanf(\"%d %d\",&p1.x,&p1.y);\n printf(\"\\nEnter x2 and y2\\n\");\n scanf(\"%d %d\",&p2.x,&p2.y);\n \n initgraph(&gd,&gm,\"c:\\\\turboc3\\\\bgi\");\n drawwindow();\n delay(500);\n \n drawline(p1,p2);\n delay(500);\n cleardevice();\n \n delay(500);\n p1=setcode(p1);\n p2=setcode(p2);\n v=visibility(p1,p2);\n delay(500);\n \n switch(v)\n {\n case 0: drawwindow();\n delay(500);\n drawline(p1,p2);\n break;\n \n case 1: drawwindow();\n delay(500);\n break;\n \n case 2: p3=resetendpt(p1,p2);\n p4=resetendpt(p2,p1);\n drawwindow();\n delay(500);\n drawline(p3,p4);\n break;\n }\n \n delay(5000);\n closegraph();\n}\n \nvoid drawwindow()\n{\n line(150,100,450,100);\n line(450,100,450,350);\n line(450,350,150,350);\n line(150,350,150,100);\n}\n \nvoid drawline(PT p1,PT p2)\n{\n line(p1.x,p1.y,p2.x,p2.y);\n}\n \nPT setcode(PT p) //for setting the 4 bit code\n{\n PT ptemp;\n \n if(p.y<100)\n ptemp.code[0]='1'; //Top\n else\n ptemp.code[0]='0';\n \n if(p.y>350)\n ptemp.code[1]='1'; //Bottom\n else\n ptemp.code[1]='0';\n \n if(p.x>450)\n ptemp.code[2]='1'; //Right\n else\n ptemp.code[2]='0';\n \n if(p.x<150)\n ptemp.code[3]='1'; //Left\n else\n ptemp.code[3]='0';\n \n ptemp.x=p.x;\n ptemp.y=p.y;\n \n return(ptemp);\n}\n \nint visibility(PT p1,PT p2)\n{\n int i,flag=0;\n \n for(i=0;i<4;i++)\n {\n if((p1.code[i]!='0') || (p2.code[i]!='0'))\n flag=1;\n }\n \n if(flag==0)\n return(0);\n \n for(i=0;i<4;i++)\n {\n if((p1.code[i]==p2.code[i]) && (p1.code[i]=='1'))\n flag='0';\n }\n \n if(flag==0)\n return(1);\n \n return(2);\n}\n \nPT resetendpt(PT p1,PT p2)\n{\n PT temp;\n int x,y,i;\n float m,k;\n \n if(p1.code[3]=='1')\n x=150;\n \n if(p1.code[2]=='1')\n x=450;\n \n if((p1.code[3]=='1') || (p1.code[2]=='1'))\n {\n m=(float)(p2.y-p1.y)/(p2.x-p1.x);\n k=(p1.y+(m*(x-p1.x)));\n temp.y=k;\n temp.x=x;\n \n for(i=0;i<4;i++)\n temp.code[i]=p1.code[i];\n \n if(temp.y<=350 && temp.y>=100)\n return (temp);\n }\n \n if(p1.code[0]=='1')\n y=100;\n \n if(p1.code[1]=='1')\n y=350;\n \n if((p1.code[0]=='1') || (p1.code[1]=='1'))\n {\n m=(float)(p2.y-p1.y)/(p2.x-p1.x);\n k=(float)p1.x+(float)(y-p1.y)/m;\n temp.x=k;\n temp.y=y;\n \n for(i=0;i<4;i++)\n temp.code[i]=p1.code[i];\n \n return(temp);\n }\n else\n return(p1);\n}"
}
{
"filename": "code/computational_geometry/src/cohen_sutherland_lineclip/cohen_sutherland_lineclip.cpp",
"content": "#include <iostream>\n\nclass CohenSutherLandAlgo\n{\n \npublic:\n CohenSutherLandAlgo() : x1_(0.0), x2_(0.0), y1_(0.0), y2_(0.0) { }\n\n void setCoordinates(double x1, double y1, double x2, double y2);\n\n void setClippingRectangle(double x_max, double y_max, double x_min, double y_min);\n\n int generateCode(double x, double y);\n\n void cohenSutherland();\n\nprivate:\n double x1_, y1_, x2_, y2_;\n double xMax_, yMax_, xMin_, yMin_;\n const int Inside = 0;\t// 0000\n const int Left = 1;\t// 0001\n const int Right = 2;\t// 0010\n const int Bottom = 4;\t// 0100\n const int Top = 8;\t// 1000\n\n};\n\nvoid CohenSutherLandAlgo::setCoordinates(double x1, double y1, double x2, double y2)\n{\n this->x1_ = x1;\n this->y1_ = y1;\n this->x2_ = x2;\n this->y2_ = y2;\n}\n\nvoid CohenSutherLandAlgo::setClippingRectangle(double x_max, double y_max, double x_min, double y_min)\n{\n this->xMax_ = x_max;\n this->yMax_ = y_max;\n this->xMin_ = x_min;\n this->yMin_ = y_min;\n}\n\nint CohenSutherLandAlgo::generateCode(double x, double y)\n{\n int code = Inside; // intially initializing as being inside\n if (x < xMin_) // lies to the left of rectangle\n code |= Left;\n else if (x > xMax_) // lies to the right of rectangle\n code |= Right;\n if (y < yMin_) // lies below the rectangle\n code |= Bottom;\n else if (y > yMax_) // lies above the rectangle\n code |= Top;\n return code;\n}\n\nvoid CohenSutherLandAlgo::cohenSutherland()\n{\n int code1 = generateCode(x1_, y1_); // Compute region codes for P1.\n int code2 = generateCode(x2_, y2_); // Compute region codes for P2.\n bool accept = false; // Initialize line as outside the rectangular window.\n while (true)\n {\n if ((code1 == 0) && (code2 == 0))\n {\n // If both endpoints lie within rectangle.\n accept = true;\n break;\n }\n else if (code1 & code2)\n {\n break; // If both endpoints are outside rectangle,in same region.\n }\n else\n {\n // Some segment of line lies within the rectangle.\n int codeOut;\n double x, y;\n // At least one endpoint lies outside the rectangle, pick it.\n if (code1 != 0)\n codeOut = code1;\n else\n codeOut = code2;\n /*\n * Find intersection point by using formulae :\n y = y1 + slope * (x - x1)\n x = x1 + (1 / slope) * (y - y1)\n */\n if (codeOut & Top)\n {\n // point is above the clip rectangle\n x = x1_ + (x2_ - x1_) * (yMax_ - y1_) / (y2_ - y1_);\n y = yMax_;\n }\n else if (codeOut & Bottom)\n {\n // point is below the rectangle\n x = x1_ + (x2_ - x1_) * (yMin_ - y1_) / (y2_ - y1_);\n y = yMin_;\n }\n else if (codeOut & Right)\n {\n // point is to the right of rectangle\n y = y1_ + (y2_ - y1_) * (xMax_ - x1_) / (x2_ - x1_);\n x = xMax_;\n }\n else if (codeOut & Left)\n {\n // point is to the left of rectangle\n y = y1_ + (y2_ - y1_) * (xMin_ - x1_) / (x2_ - x1_);\n x = xMin_;\n }\n /*\n * Intersection point x,y is found.\n Replace point outside rectangle by intersection point.\n */\n if (codeOut == code1)\n {\n x1_ = x;\n y1_ = y;\n code1 = generateCode(x1_, y1_);\n }\n else\n {\n x2_ = x;\n y2_ = y;\n code2 = generateCode(x2_, y2_);\n }\n }\n }\n if (accept)\n {\n std::cout <<\"Line accepted from \" <<\"(\"<< x1_ << \", \"\n << y1_ << \")\" << \" to \"<< \"(\" << x2_ << \", \" << y2_ << \")\" << std::endl;\n }\n else\n std::cout << \"Line rejected\" << std::endl;\n}\n\nint main() {\n\n CohenSutherLandAlgo c;\n double x1, y1, x2, y2, x_max, y_max, x_min, y_min;\n\n std::cout << \"\\nEnter Co-ordinates of P1(X1,Y1) of Line Segment : \";\n std::cout << \"\\nEnter X1 Co-ordinate : \";\n std::cin >> x1;\n std::cout << \"\\nEnter Y1 Co-ordinate : \";\n std::cin >> y1;\n std::cout << \"\\nEnter Co-ordinates of P2(X2,Y2) of Line Segment : \";\n std::cout << \"\\nEnter X2 Co-ordinate : \";\n std::cin >> x2;\n std::cout << \"\\nEnter Y2 Co-ordinate : \";\n std::cin >> y2;\n c.setCoordinates(x1, y1, x2, y2);\n\n std::cout << \"\\nEnter the Co-ordinates of Interested Rectangle.\";\n std::cout << \"\\nEnter the X_MAX : \";\n std::cin >> x_max;\n std::cout << \"\\nEnter the Y_MAX : \";\n std::cin >> y_max;\n std::cout << \"\\nEnter the X_MIN : \";\n std::cin >> x_min;\n std::cout << \"\\nEnter the Y_MIN : \";\n std::cin >> y_min;\n c.setClippingRectangle(x_max, y_max, x_min, y_min);\n\n c.cohenSutherland();\n}"
}
{
"filename": "code/computational_geometry/src/cohen_sutherland_lineclip/README.md",
"content": "# Working of Cohem Sutherland Line-clipping\n\n## Enter the co-ordinates\nEnter x1 and y1 \n## `100` `100`\n\nEnter x2 and y2\n## `200` `200`\n\n\n\n## Before Clipping -\n\n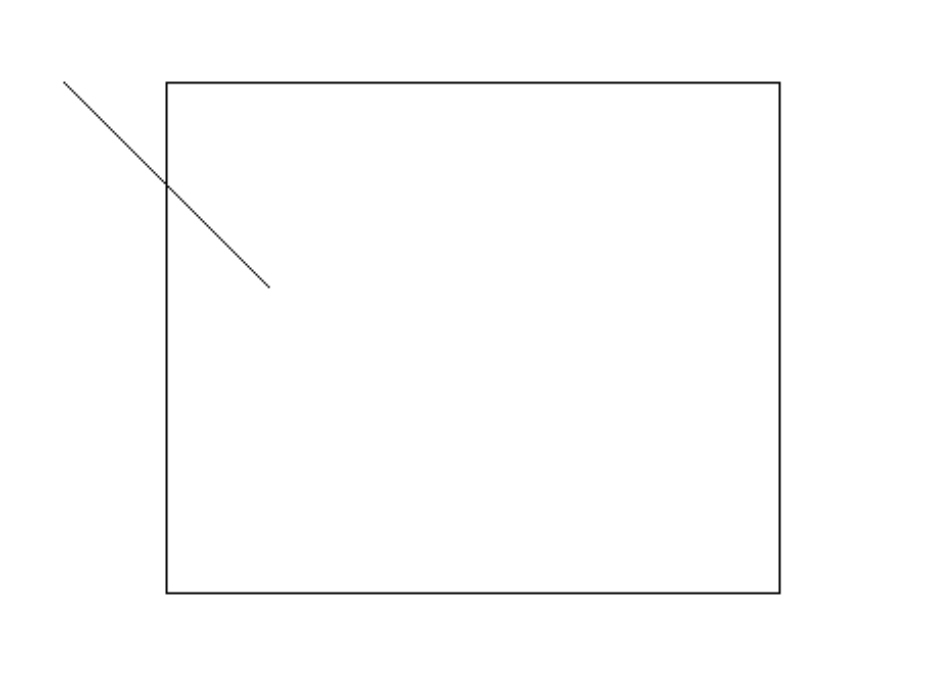\n\n\n## After Clipping -\n\n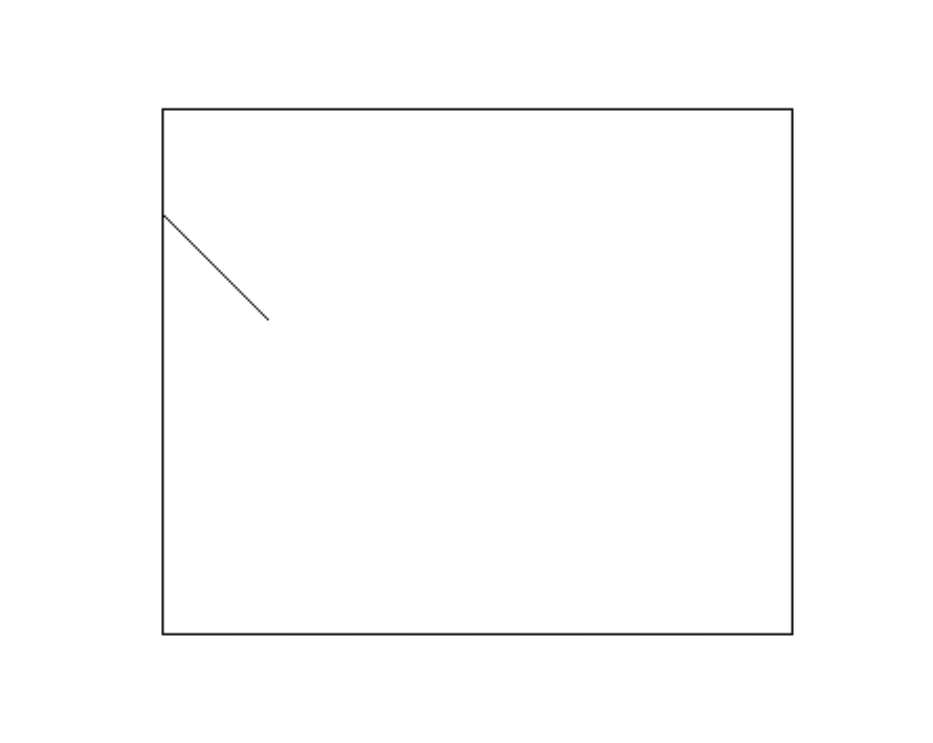\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/computational_geometry/src/dda_line/dda_line.cpp",
"content": "#include <iostream>\r\n\r\nclass DDALineDrawingAlgorithm\r\n{\r\npublic:\r\n void ddaLineDrawing();\r\n\r\n void getCoordinates();\r\n\r\nprivate:\r\n int x1_, x2_, y1_, y2_;\r\n\r\n};\r\n\r\nvoid DDALineDrawingAlgorithm::ddaLineDrawing()\r\n{\r\n //calculating range for line between start and end point\r\n int dx = x2_ - x1_;\r\n int dy = y2_ - y1_;\r\n // calculate steps required for creating pixels\r\n int step = abs(abs(dx) > abs(dy) ? dx : dy);\r\n // calculate increment in x & y for each steps\r\n float xIncrement = (dx) / (float)step;\r\n float yIncrement = (dy) / (float)step;\r\n // drawing pixel for each step\r\n float x = x1_;\r\n float y = y1_;\r\n putpixel((x), (y),GREEN); //this putpixel is for very first pixel of the line\r\n for(int i = 1; i <= step; ++i)\r\n {\r\n x = x + xIncrement; // increment in x at each step\r\n y = y + yIncrement; // increment in y at each step\r\n putpixel(round(x), round(y),GREEN); // display pixel at coordinate (x, y)\r\n delay(200); //delay introduced for visualization at each step\r\n }\r\n delay(500);\r\n}\r\n\r\nvoid DDALineDrawingAlgorithm::getCoordinates()\r\n{\r\n std::cout << \"\\nEnter the First Coordinate \";\r\n std::cout << \"\\nEnter X1 : \";\r\n std::cin >> x1_;\r\n std::cout << \"\\nEnter Y1 : \";\r\n std::cin >> y1_;\r\n std::cout << \"\\nEnter the Second Coordinate \";\r\n std::cout << \"\\nEnter X2 : \";\r\n std::cin >> x2_;\r\n std::cout << \"\\nEnter Y2 : \";\r\n std::cin >> y2_;\r\n}\r\n\r\nint main()\r\n{\r\n DDALineDrawingAlgorithm d;\r\n d.getCoordinates();\r\n int gd=DETECT,gm;\r\n initgraph(&gd,&gm,NULL);\r\n d.ddaLineDrawing();\r\n}\r"
}
{
"filename": "code/computational_geometry/src/distance_between_points/distance_between_points.c",
"content": "#include <stdio.h>\n#include <math.h>\n\nstruct Point {\n double x;\n double y;\n};\n\ndouble distanceBetweenPoints(Point a, Point b) {\n return sqrt(pow(b.x - a.x, 2) + pow(b.y - a.y, 2));\n}\n\nint main() {\n Point a, b;\n\n printf(\"First point (x y): \");\n scanf(\"%lg %lg\", &a.x, &a.y);\n printf(\"Second point (x y): \");\n scanf(\"%lg %lg\", &b.x, &b.y);\n\n printf(\"%lg\", distanceBetweenPoints(a, b));\n\n return 0;\n}"
}
{
"filename": "code/computational_geometry/src/distance_between_points/distance_between_points.cpp",
"content": "#include <iostream>\n#include <cmath>\n\ntypedef std::pair<double, double> point;\n\n#define x first\n#define y second\n\ndouble calcDistance (point a, point b)\n{\n return sqrt(pow(a.x - b.x, 2) + pow(a.y - b.y, 2));\n}\n\nint main ()\n{\n point a, b, c;\n std::cin >> a.x >> a.y >> b.x >> b.y;\n std::cout << calcDistance(a, b);\n}"
}
{
"filename": "code/computational_geometry/src/distance_between_points/distance_between_points.go",
"content": "package main\n// Part of Cosmos by OpenGenus Foundation\n\nimport (\n\t\"fmt\"\n\t\"math\"\n)\n\ntype vector struct {\n\tx, y float64\n}\n\nfunc calculateDistance(a, b vector) float64 {\n\treturn math.Sqrt((a.x - b.x) * (a.x - b.x) + (a.y - b.y) * (a.y - b.y))\n}\n\nfunc main() {\n\ta := vector{x: 5, y: 5}\n\tb := vector{x: 5, y: 10}\n\n\t// 5\n\tfmt.Println(calculateDistance(a, b))\n\n\ta = vector{x: 2, y: 4}\n\tb = vector{x: 4, y: -4}\n\n\t// 8.246\n\tfmt.Println(calculateDistance(a, b))\n}"
}
{
"filename": "code/computational_geometry/src/distance_between_points/distance_between_points.java",
"content": "import java.lang.Math;\n\npublic class DistanceBetweenPoints {\n\n public static void main(String []args){\n // input points {x,y}\n int[] pointA = {0,1};\n int[] pointB = {0,4};\n \n double result = Math.sqrt(Math.pow((pointB[0] - pointA[0]), 2) + Math.pow((pointB[1] - pointA[1]), 2));\n \n System.out.println(result);\n }\n}"
}
{
"filename": "code/computational_geometry/src/distance_between_points/distance_between_points.js",
"content": "function distanceBetweenPoints(x1, x2, y1, y2) {\n return Math.hypot(x1, x2, y1, y2);\n}\n\nconsole.log(distanceBetweenPoints(0, 3, 0, 4));"
}
{
"filename": "code/computational_geometry/src/distance_between_points/distance_between_points.py",
"content": "def calc_distance(x1, y1, x2, y2):\n return (((x1 - x2) ** 2) + ((y1 - y2) ** 2)) ** 0.5\n\n\nx1 = float(input(\"Enter X coordinate of point 1: \"))\ny1 = float(input(\"Enter Y coordinate of point 1: \"))\nx2 = float(input(\"Enter X coordinate of point 2: \"))\ny2 = float(input(\"Enter Y coordinate of point 2: \"))\n\nprint(\"Distance between (x1, y1) and (x2, y2) : \" + str(calc_distance(x1, y1, x2, y2)))"
}
{
"filename": "code/computational_geometry/src/distance_between_points/distance_between_points.rs",
"content": "struct Point {\n x: f64,\n y: f64\n}\n\nimpl Point {\n fn distance_to(&self, b: &Point) -> f64 {\n ((self.x - b.x) * (self.x - b.x) + (self.y - b.y) * (self.y - b.y)).sqrt()\n } \n}\n\n#[test]\nfn test_point_distance() {\n // Make 2 points\n // (0,0), (3,4), distance should be 5\n let a = Point { x: 0.0, y: 0.0 };\n let b = Point { x: 3.0, y: 4.0 };\n\n assert_eq!(a.distance_to(&b), 5.0);\n}"
}
{
"filename": "code/computational_geometry/src/distance_between_points/README.md",
"content": "# Distance Between two Points\n\nCalculating the distance between two points is done by creating a right-angled triangle using the two points. The line between the two points is the hypotenuse (the longest side, opposite to the 90° angle). Lines drawn along the X and Y-axes complete the other sides of the triangle, whose lengths can be found by finding the change in X and change in Y between the two points. We then use the Pythagorean theorem to find the length of the hypotenuse, which is the distance between the two points. If you didn't quite get that, the diagram below should help:\n\n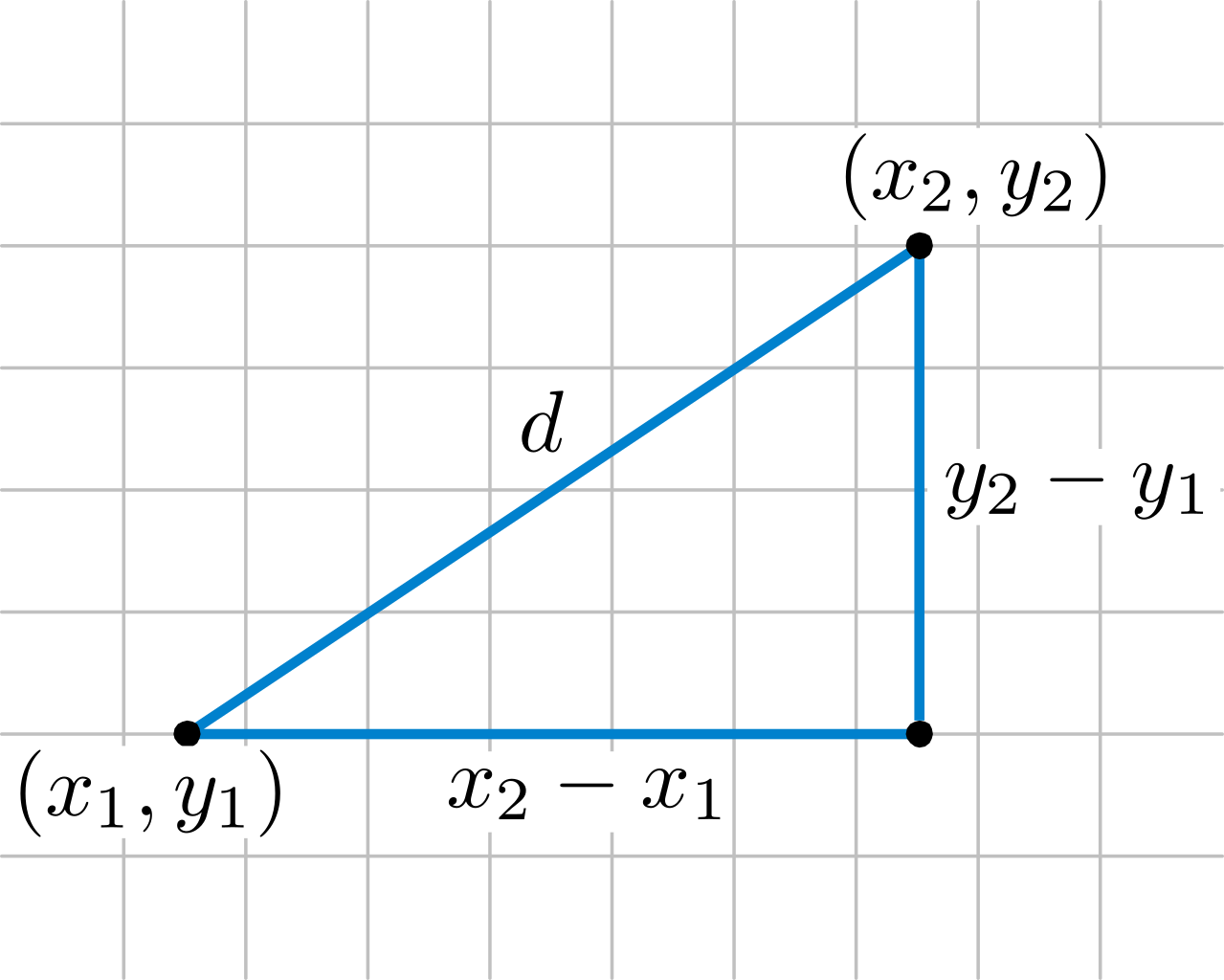\n\nImage credit: `By Jim.belk, public domain, https://commons.wikimedia.org/wiki/File:Distance_Formula.svg`\n\nThus, using the Pythagorean theorem, the equation for the length of `d` would be:\n\n`d = sqrt((x2 - x1)^2 + (y2 - y1)^2)`\n\n## Sources and more info:\n\n- https://www.mathsisfun.com/algebra/distance-2-points.html\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/computational_geometry/src/graham_scan/graham_scan2.cpp",
"content": "#include <iostream>\n#include <stack>\n#include <stdlib.h>\nusing namespace std;\n// Part of Cosmos by OpenGenus Foundation\n// A C++ program to find convex hull of a set of points.\nstruct Point\n{\n int x, y;\n};\n\n// A globle point needed for sorting points with reference\n// to the first point Used in compare function of qsort()\nPoint p0;\n\n// A utility function to find next to top in a stack\nPoint nextToTop(stack<Point> &S)\n{\n Point p = S.top();\n S.pop();\n Point res = S.top();\n S.push(p);\n return res;\n}\n\n// A utility function to swap two points\nint swap(Point &p1, Point &p2)\n{\n Point temp = p1;\n p1 = p2;\n p2 = temp;\n}\n\n// A utility function to return square of distance\n// between p1 and p2\nint distSq(Point p1, Point p2)\n{\n return (p1.x - p2.x) * (p1.x - p2.x) +\n (p1.y - p2.y) * (p1.y - p2.y);\n}\n\n// To find orientation of ordered triplet (p, q, r).\n// The function returns following values\n// 0 --> p, q and r are colinear\n// 1 --> Clockwise\n// 2 --> Counterclockwise\nint orientation(Point p, Point q, Point r)\n{\n int val = (q.y - p.y) * (r.x - q.x) -\n (q.x - p.x) * (r.y - q.y);\n\n if (val == 0)\n return 0; // colinear\n return (val > 0) ? 1 : 2; // clock or counterclock wise\n}\n\n// A function used by library function qsort() to sort an array of\n// points with respect to the first point\nint compare(const void *vp1, const void *vp2)\n{\n Point *p1 = (Point *)vp1;\n Point *p2 = (Point *)vp2;\n\n // Find orientation\n int o = orientation(p0, *p1, *p2);\n if (o == 0)\n return (distSq(p0, *p2) >= distSq(p0, *p1)) ? -1 : 1;\n\n return (o == 2) ? -1 : 1;\n}\n\n// Prints convex hull of a set of n points.\nvoid convexHull(Point points[], int n)\n{\n // Find the bottommost point\n int ymin = points[0].y, min = 0;\n for (int i = 1; i < n; i++)\n {\n int y = points[i].y;\n\n // Pick the bottom-most or chose the left\n // most point in case of tie\n if ((y < ymin) || (ymin == y &&\n points[i].x < points[min].x))\n ymin = points[i].y, min = i;\n }\n\n // Place the bottom-most point at first position\n swap(points[0], points[min]);\n\n // Sort n-1 points with respect to the first point.\n // A point p1 comes before p2 in sorted ouput if p2\n // has larger polar angle (in counterclockwise\n // direction) than p1\n p0 = points[0];\n qsort(&points[1], n - 1, sizeof(Point), compare);\n\n // If two or more points make same angle with p0,\n // Remove all but the one that is farthest from p0\n // Remember that, in above sorting, our criteria was\n // to keep the farthest point at the end when more than\n // one points have same angle.\n int m = 1; // Initialize size of modified array\n for (int i = 1; i < n; i++)\n {\n // Keep removing i while angle of i and i+1 is same\n // with respect to p0\n while (i < n - 1 && orientation(p0, points[i],\n points[i + 1]) == 0)\n i++;\n\n\n points[m] = points[i];\n m++; // Update size of modified array\n }\n\n // If modified array of points has less than 3 points,\n // convex hull is not possible\n if (m < 3)\n return;\n\n // Create an empty stack and push first three points\n // to it.\n stack<Point> S;\n S.push(points[0]);\n S.push(points[1]);\n S.push(points[2]);\n\n // Process remaining n-3 points\n for (int i = 3; i < m; i++)\n {\n // Keep removing top while the angle formed by\n // points next-to-top, top, and points[i] makes\n // a non-left turn\n while (orientation(nextToTop(S), S.top(), points[i]) != 2)\n S.pop();\n S.push(points[i]);\n }\n\n // Now stack has the output points, print contents of stack\n while (!S.empty())\n {\n Point p = S.top();\n cout << \"(\" << p.x << \", \" << p.y << \")\" << endl;\n S.pop();\n }\n}\n\n// Driver program to test above functions\nint main()\n{\n Point points[] = {{0, 3}, {1, 1}, {2, 2}, {4, 4},\n {0, 0}, {1, 2}, {3, 1}, {3, 3}};\n int n = sizeof(points) / sizeof(points[0]);\n convexHull(points, n);\n return 0;\n}"
}
{
"filename": "code/computational_geometry/src/graham_scan/graham_scan.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <iostream>\n#include <algorithm>\n#include <vector>\nusing namespace std;\n\nstruct point\n{\n double x, y;\n point(double x, double y) : x(x), y(y)\n {\n }\n point()\n {\n }\n};\n\n// custom compare for sorting points\nbool cmp(point a, point b)\n{\n return a.x < b.x || (a.x == b.x && a.y < b.y);\n}\n\n// check clockwise orientation of points\nbool cw(point a, point b, point c)\n{\n return a.x * (b.y - c.y) + b.x * (c.y - a.y) + c.x * (a.y - b.y) < 0;\n}\n\n// check counter-clockwise orientation of points\nbool ccw(point a, point b, point c)\n{\n return a.x * (b.y - c.y) + b.x * (c.y - a.y) + c.x * (a.y - b.y) > 0;\n}\n\n// graham scan with Andrew improvements\nvoid convex_hull(vector<point> &points)\n{\n if (points.size() == 1)\n return;\n sort(points.begin(), points.end(), &cmp);\n point p1 = points[0], p2 = points.back();\n vector<point> up, down;\n up.push_back(p1);\n down.push_back(p1);\n for (size_t i = 1; i < points.size(); ++i)\n {\n if (i == points.size() - 1 || cw(p1, points[i], p2))\n {\n while (up.size() >= 2 && !cw(up[up.size() - 2], up[up.size() - 1], points[i]))\n up.pop_back();\n up.push_back(points[i]);\n }\n if (i == points.size() - 1 || ccw(p1, points[i], p2))\n {\n while (down.size() >= 2 &&\n !ccw(down[down.size() - 2], down[down.size() - 1], points[i]))\n down.pop_back();\n down.push_back(points[i]);\n }\n }\n cout << \"Convex hull is:\" << endl;\n for (size_t i = 0; i < up.size(); ++i)\n cout << \"x: \" << up[i].x << \" y: \" << up[i].y << endl;\n for (int i = down.size() - 2; i > 0; --i)\n cout << \"x: \" << down[i].x << \" y: \" << down[i].y << endl;\n}\n\nint main()\n{\n int n;\n cout << \"Enter number of points followed by points\" << endl;\n cin >> n;\n vector<point> points(n);\n for (int i = 0; i < n; i++)\n cin >> points[i].x >> points[i].y;\n convex_hull(points);\n return 0;\n}"
}
{
"filename": "code/computational_geometry/src/graham_scan/graham_scan.java",
"content": "import java.util.*;\n\npublic class GrahamScan {\n\n public static class Point {\n private final int x;\n private final int y;\n private final int pos;\n public Point(final int x, final int y, final int p) {\n this.x = x;\n this.y = y;\n this.pos = p;\n }\n }\n\n public static double dist(Point p1, Point p2) {\n return Math.sqrt((p1.x-p2.x)*(p1.x-p2.x)+(p1.y-p2.y)*(p1.y-p2.y));\n }\n\n public static int ccw(Point a, Point b, Point c) {\n int cross = ((b.x - a.x) * (c.y - a.y)) - ((b.y - a.y) * (c.x - a.x));\n\n if(cross > 0) {\n return -1;\n } else if(cross < 0) {\n return 1;\n } else {\n return 0;\n }\n }\n\n public static Point getBottomMostLeftPoint(List<Point> points) {\n\n Point lowest = points.get(0);\n for(int i = 1; i < points.size(); i++) {\n if(points.get(i).y < lowest.y || (points.get(i).y == lowest.y && points.get(i).x < lowest.x)) {\n lowest = points.get(i);\n }\n }\n\n return lowest;\n }\n\n public static void sortByPolarAngle(ArrayList<Point> points) {\n final Point bottomMostLeft = getBottomMostLeftPoint(points);\n Collections.sort(points, new Comparator<Point>() {\n @Override\n public int compare(Point a, Point b) {\n double angleA = Math.atan2(a.y - bottomMostLeft.y, a.x - bottomMostLeft.x);\n double angleB = Math.atan2(b.y - bottomMostLeft.y, b.x - bottomMostLeft.x);\n\n if(angleA < angleB) {\n return 1;\n } else if(angleA > angleB) {\n return -1;\n } else {\n if(dist(a, bottomMostLeft) < dist(b, bottomMostLeft)) {\n return 1;\n } else {\n return -1;\n }\n }\n }\n });\n }\n\n public static ArrayList<Point> getConvexHull(ArrayList<Point> points) {\n if(points.size() == 1) {\n return points;\n }\n\n sortByPolarAngle(points);\n Collections.reverse(points);\n\n ArrayList<Point> hull = new ArrayList<>();\n hull.add(points.get(0));\n hull.add(points.get(1));\n for(int i = 2; i < points.size(); i++) {\n Point c = points.get(i);\n Point b = hull.get(hull.size()-1);\n Point a = hull.get(hull.size()-2);\n\n switch (ccw(a, b, c)) {\n case -1:\n hull.add(c);\n break;\n case 1:\n hull.remove(hull.size()-1);\n i--;\n break;\n case 0:\n hull.remove(hull.size()-1);\n hull.add(c);\n break;\n }\n }\n\n hull.add(hull.get(0));\n return hull;\n }\n\n public static void main(String[] args) {\n Scanner in = new Scanner(System.in);\n int N = in.nextInt();\n\n ArrayList<Point> points = new ArrayList<>();\n\n for (int i = 0; i < N; i++) {\n int x = in.nextInt(), y = in.nextInt();\n Point p = new Point(x, y, i + 1);\n points.add(p);\n }\n\n Collections.sort(points, new Comparator<Point>() {\n @Override\n public int compare(Point o1, Point o2) {\n if (o1.x == o2.x) {\n return o1.y - o2.y;\n } else {\n return o1.x - o2.x;\n }\n }\n });\n\n\n ArrayList<Point> temp = new ArrayList<>();\n temp.add(points.get(0));\n for(int i = 1; i < N; i++) {\n if(points.get(i).x != points.get(i-1).x || points.get(i).y != points.get(i-1).y) {\n temp.add(points.get(i));\n }\n }\n\n points = temp;\n points = getConvexHull(points);\n\n double ans = 0;\n for (int i = 1; i < points.size(); i++) {\n ans += dist(points.get(i - 1), points.get(i));\n }\n\n System.out.println(ans);\n System.out.println(\"AntiClockwise starting from Bottom-most left point: \");\n\n for(int i = 0; i < points.size()-1; i++) {\n System.out.print(points.get(i).pos + \" \");\n }\n\n if(points.size() == 1) {\n System.out.print(\"1\");\n }\n\n System.out.println(\"\\n\");\n }\n}"
}
{
"filename": "code/computational_geometry/src/graham_scan/graham_scan.py",
"content": "from functools import cmp_to_key\n\n\nclass Point:\n def __init__(self, x, y):\n self.x = x\n self.y = y\n\n\ndef orientation(p1, p2, p3):\n # Slope of line segment (p1, p2): σ = (y2 - y1)/(x2 - x1)\n # Slope of line segment (p2, p3): τ = (y3 - y2)/(x3 - x2)\n #\n # If σ > τ, the orientation is clockwise (right turn)\n #\n # Using above values of σ and τ, we can conclude that,\n # the orientation depends on sign of below expression:\n #\n # (y2 - y1)*(x3 - x2) - (y3 - y2)*(x2 - x1)\n #\n # Above expression is negative when σ < τ, i.e., counterclockwise\n val = (float(p2.y - p1.y) * (p3.x - p2.x)) - (float(p2.x - p1.x) * (p3.y - p2.y))\n if val > 0:\n # Clockwise orientation\n return 1\n\n elif val < 0:\n # Counterclockwise orientation\n return 2\n\n else:\n # Collinear orientation\n return 0\n\n\ndef distSquare(p1, p2):\n return ((p1.x - p2.x) ** 2 +\n (p1.y - p2.y) ** 2)\n\n\ndef compare(p1, p2):\n # Find orientation\n o = orientation(p0, p1, p2)\n if o == 0:\n if distSquare(p0, p2) >= distSquare(p0, p1):\n return -1\n else:\n return 1\n else:\n if o == 2:\n return -1\n else:\n return 1\n\n\ndef ConvexHull(points, n):\n # Find the bottommost point\n ymin = points[0].y\n minimum = 0\n for i in range(1, n):\n y = points[i].y\n\n # Pick the bottom-most or choose the left\n # most point in case of tie\n if ((y < ymin) or\n (ymin == y and points[i].x < points[minimum].x)):\n ymin = points[i].y\n minimum = i\n\n # Place the bottom-most point at first position\n points[0], points[minimum] = points[minimum], points[0]\n\n # Sort n-1 points with respect to the first point.\n # A point p1 comes before p2 in sorted output if p2\n # has larger polar angle (in counterclockwise\n # direction) than p1\n p0 = points[0]\n sorted_points = sorted(points, key=cmp_to_key(compare))\n\n # If two or more points make same angle with p0,\n # Remove all but the one that is farthest from p0\n # Remember that, in above sorting, our criteria was\n # to keep the farthest point at the end when more than\n # one points have same angle.\n m = 1 # Initialize size of modified array\n for i in range(1, n):\n # Keep removing i while angle of i and i+1 is same\n # with respect to p0\n while ((i < n - 1) and\n (orientation(p0, sorted_points[i], sorted_points[i + 1]) == 0)):\n i += 1\n\n sorted_points[m] = sorted_points[i]\n m += 1 # Update size of modified array\n\n # If modified array of points has less than 3 points,\n # convex hull is not possible\n if m < 3:\n return\n\n S = [sorted_points[0], sorted_points[1], sorted_points[2]]\n\n # Process remaining n-3 points\n for i in range(3, m):\n # Keep removing top while the angle formed by\n # points next-to-top, top, and points[i] makes\n # a non-left turn\n while ((len(S) > 1) and\n (orientation(S[-2], S[-1], sorted_points[i]) != 2)):\n S.pop()\n S.append(sorted_points[i])\n\n S2 = S.copy()\n # Now stack has the output points,\n # print contents of stack\n while S:\n p = S[-1]\n print(\"(\" + str(p.x) + \", \" + str(p.y) + \")\")\n S.pop()\n\n return S2\n\n\ndef visualize(points, convex_hull_points):\n from matplotlib import pyplot as plt\n import numpy as np\n x_co = []\n y_co = []\n for point in points:\n x_co.append(point.x)\n y_co.append(point.y)\n plt.scatter(np.array(x_co), np.array(y_co))\n\n convex_x_co = []\n convex_y_co = []\n for point in convex_hull_points:\n convex_x_co.append(point.x)\n convex_y_co.append(point.y)\n plt.plot(np.array(convex_x_co), np.array(convex_y_co))\n\n plt.show()\n\n\nif __name__ == \"__main__\":\n n = int(input(\"Enter number of points followed by points: \"))\n\n points = [] # List of Points\n\n p0 = Point(0, 0)\n\n for i in range(n):\n x, y = list(map(int, input().split()))\n points.append(Point(x, y))\n\n convex_hull_points = ConvexHull(points, n)\n\n # Optional, please comment this line if you don't want to visualize\n visualize(points, convex_hull_points)"
}
{
"filename": "code/computational_geometry/src/halfplane_intersection/halfplane_intersection.cpp",
"content": "#include <cmath>\n#include <iostream>\n#include <vector>\n\nusing namespace std;\n\nconst double INF = 1e9;\nconst double EPS = 1e-8;\n\nclass Point {\npublic:\n double x, y;\n Point()\n {\n }\n Point(double x, double y) : x(x), y(y)\n {\n }\n bool operator==(const Point &other) const\n {\n return abs(x - other.x) < EPS && abs(y - other.y) < EPS;\n }\n Point operator-(const Point &a) const\n {\n return Point(x - a.x, y - a.y);\n }\n double operator^(const Point &a) const\n {\n return x * a.y - a.x * y;\n }\n void scan()\n {\n cin >> x >> y;\n }\n void print() const\n {\n cout << x << \" \" << y << \"\\n\";\n }\n};\n\nclass Line {\npublic:\n double a, b, c;\n Line()\n {\n }\n Line(double a, double b, double c) : a(a), b(b), c(c)\n {\n }\n Line(const Point &A, const Point &B) : a(A.y - B.y), b(B.x - A.x),\n c(A.x * (B.y - A.y) + A.y * (A.x - B.x))\n {\n }\n bool operator==(const Line &other) const\n {\n return a == other.a && b == other.b && c == other.c;\n }\n bool contains_point(const Point &A) const\n {\n return a * A.x + b * A.y + c == 0;\n }\n bool intersects(const Line &l) const\n {\n return !(a * l.b == b * l.a);\n }\n Point intersect(const Line &l) const\n {\n return Point(-(c * l.b - l.c * b) / (a * l.b - l.a * b),\n -(a * l.c - l.a * c) / (a * l.b - l.a * b));\n }\n};\n\nclass Segment {\nprivate:\n Point A, B;\npublic:\n Segment()\n {\n }\n Segment(Point A, Point B) : A(A), B(B)\n {\n }\n bool intersects(const Line &l) const\n {\n return l.intersects(Line(A, B)) &&\n l.intersect(Line(A, B)).x <= max(A.x, B.x) + EPS &&\n l.intersect(Line(A, B)).x >= min(A.x, B.x) - EPS &&\n l.intersect(Line(A, B)).y <= max(A.y, B.y) + EPS &&\n l.intersect(Line(A, B)).y >= min(A.y, B.y) - EPS;\n }\n\n double length2() const\n {\n return (A - B).x * (A - B).x + (A - B).y * (A - B).y;\n }\n\n double length() const\n {\n return sqrt(length2());\n }\n};\n\nclass HalfPlane {\nprivate:\n double a, b, c;\npublic:\n HalfPlane()\n {\n }\n HalfPlane(Line l, Point A)\n {\n if (l.a * A.x + l.b * A.y + l.c < 0)\n {\n a = -l.a;\n b = -l.b;\n c = -l.c;\n }\n else\n {\n a = l.a;\n b = l.b;\n c = l.c;\n }\n }\n bool contains_point(const Point &p) const\n {\n return a * p.x + b * p.y + c >= 0;\n }\n Line line() const\n {\n return Line(a, b, c);\n }\n};\n\nclass HalfPlaneIntersector {\nprivate:\n vector <Point> points;\npublic:\n HalfPlaneIntersector()\n {\n points.push_back(Point(-INF, INF));\n points.push_back(Point(INF, INF));\n points.push_back(Point(INF, -INF));\n points.push_back(Point(-INF, -INF));\n }\n\n void intersect(const HalfPlane &h)\n {\n vector <Point> result;\n for (unsigned int i = 0; i < points.size(); i++)\n {\n Line a = Line(points[i], points[(i + 1) % points.size()]);\n Segment a_seg = Segment(points[i], points[(i + 1) % points.size()]);\n if (h.contains_point(points[i]))\n result.push_back(points[i]);\n if (a_seg.intersects(h.line()))\n result.push_back(a.intersect(h.line()));\n }\n points = result;\n }\n\n double perimeter() const\n {\n double res = 0;\n for (unsigned int i = 0; i < points.size(); i++)\n res += Segment(points[i], points[(i + 1) % points.size()]).length();\n return res;\n }\n};\n\n// Calculate perimeter of 2 triangle intersection for examle.\nint main()\n{\n HalfPlaneIntersector hpi;\n for (int i = 0; i < 2; i++)\n {\n Point p1, p2, p3;\n p1.scan();\n p2.scan();\n p3.scan();\n hpi.intersect(HalfPlane(Line(p1, p2), p3));\n hpi.intersect(HalfPlane(Line(p1, p3), p2));\n hpi.intersect(HalfPlane(Line(p2, p3), p1));\n }\n cout << hpi.perimeter();\n}"
}
{
"filename": "code/computational_geometry/src/jarvis_march/jarvis_march2.cpp",
"content": "// A C++ program to find convex hull of a set of points\n#include <bits/stdc++.h>\nusing namespace std;\n\nstruct Point // Structure to store the co-ordinates of every point\n{\n int x, y;\n};\n\n// To find orientation of ordered triplet (p, q, r).\n// The function returns following values\n// 0 --> p, q and r are colinear\n// 1 --> Clockwise\n// 2 --> Counterclockwise\n\nint orientation(Point p, Point q, Point r)\n{\n /*\n * Finding slope ,s = (y2 - y1)/(x2 - x1) ;\n * s1 = (y3 - y2)/(x3 - x2) ;\n * if s == s1\tpoints are collinear\n * s < s1 orientation towards left (left turn)\n * s > s1 orientation towards right (right turn)\n */\n\n int val = (q.y - p.y) * (r.x - q.x) -\n (q.x - p.x) * (r.y - q.y);\n\n if (val == 0)\n return 0; // colinear\n return (val > 0) ? 1 : 2; // clock or counterclock wise\n\n}\n\n// Prints convex hull of a set of n points.\n\nvoid convexHull(Point points[], int n)\n{\n // There must be at least 3 points\n if (n < 3)\n return;\n\n // Initialize Result\n vector<Point> hull;\n\n // Find the leftmost point\n\n int l = 0;\n for (int i = 1; i < n; i++)\n if (points[i].x < points[l].x)\n l = i;\n\n // Start from leftmost point, keep moving counterclockwise\n // until reach the start point again. This loop runs O(h)\n // times where h is number of points in result or output.\n\n int p = l, q;\n do\n {\n // Add current point to result\n hull.push_back(points[p]);\n\n // Search for a point 'q' such that orientation(p, x,\n // q) is counterclockwise for all points 'x'. The idea\n // is to keep track of last visited most counterclock-\n // wise point in q. If any point 'i' is more counterclock-\n // wise than q, then update q.\n\n q = (p + 1) % n;\n for (int i = 0; i < n; i++)\n // If i is more counterclockwise than current q, then\n // update q\n if (orientation(points[p], points[i], points[q]) == 2)\n q = i;\n\n // Now q is the most counterclockwise with respect to p\n // Set p as q for next iteration, so that q is added to\n // result 'hull'\n p = q;\n\n } while (p != l); // While we don't come to first point\n\n // Print Result\n for (int i = 0; i < hull.size(); i++)\n cout << \"(\" << hull[i].x << \", \"\n << hull[i].y << \")\\n\";\n}\n\n// Driver program to test above functions\nint main()\n{\n Point points[] = {{0, 3}, {2, 2}, {1, 1}, {2, 1},\n {3, 0}, {0, 0}, {3, 3}};\n int n = sizeof(points) / sizeof(points[0]);\n convexHull(points, n);\n return 0;\n}"
}
{
"filename": "code/computational_geometry/src/jarvis_march/jarvis_march.cpp",
"content": "#include <iostream>\n#include <vector>\n#include <cmath>\n\nusing namespace std;\n\nstruct Point\n{\n int x;\n int y;\n int pos;\n Point(int x, int y, int p)\n {\n this->x = x;\n this->y = y;\n this->pos = p;\n }\n};\n\nint orientation(Point p, Point q, Point r)\n{\n int cross = (q.y - p.y) * (r.x - q.x) - (q.x - p.x) * (r.y - q.y);\n if (cross == 0)\n return 0;\n return (cross > 0) ? 1 : -1;\n}\n\nvector<Point> getConvexHull(vector<Point> points)\n{\n if (points.size() < 3)\n return points;\n\n int left = 0;\n for (size_t i = 1; i < points.size(); i++)\n if (points[i].x < points[left].x)\n left = i;\n\n // Starting from left move counter-clockwise\n vector<Point> hull;\n int tail = left, middle;\n do {\n middle = (tail + 1) % points.size();\n for (size_t i = 0; i < points.size(); i++)\n if (orientation(points[tail], points[i], points[middle]) == -1)\n middle = i;\n\n hull.push_back(points[middle]);\n tail = middle;\n } while (tail != left);\n\n return hull;\n}\n\ndouble dist(Point p1, Point p2)\n{\n return sqrt((p1.x - p2.x) * (p1.x - p2.x) + (p1.y - p2.y) * (p1.y - p2.y));\n}\n\nint main()\n{\n int N;\n cin >> N;\n vector<Point> points;\n for (int i = 0; i < N; i++)\n {\n int x, y;\n cin >> x >> y;\n points.push_back(Point(x, y, i + 1));\n }\n\n vector<Point> result = getConvexHull(points);\n result.push_back(result[0]);\n double ans = 0;\n for (size_t i = 1; i < result.size(); i++)\n ans += dist(result[i], result[i - 1]);\n\n cout << ans << '\\n';\n cout << \"Points lying on hull\\n\";\n for (size_t i = 0; i < result.size(); i++)\n cout << result[i].pos << ' ';\n}"
}
{
"filename": "code/computational_geometry/src/jarvis_march/jarvis_march.py",
"content": "import matplotlib.pyplot as plt\nimport numpy as np\nimport math\n\n\nclass Point:\n def __init__(self, x, y):\n self.x = x\n self.y = y\n\n def equal(self, pt):\n return self.x == pt.x and self.y == pt.y\n\n def __str__(self):\n return \"(\" + str(self.x) + \",\" + str(self.y) + \")\"\n\n\ndef left_most_point(pts):\n if not isinstance(pts, list):\n return False\n curr = pts[0]\n for pt in pts[1:]:\n if pt.x < curr.x or (pt.x == curr.x and pt.y < curr.y):\n curr = pt\n return curr\n\n\ndef cross_product(p, p1, p2):\n return (p.y - p2.y) * (p.x - p1.x) - (p.y - p1.y) * (p.x - p2.x)\n\n\ndef distance(p, p1, p2):\n return math.hypot(p.x - p1.x, p.y - p1.y) - math.hypot(p.x - p2.x, p.y - p2.y)\n\n\ndef iter(pts):\n colinear = list()\n curr_pt = left_most_point(pts)\n convex_list = [curr_pt]\n\n for pt1 in pts:\n if curr_pt.equal(pt1):\n continue\n target = pt1\n for pt2 in pts:\n if pt2.equal(target) or pt2.equal(curr_pt):\n continue\n\n res = cross_product(curr_pt, target, pt2)\n if res > 0:\n colinear = list()\n target = pt2\n elif res == 0:\n if distance(curr_pt, target, pt2) > 0:\n if pt2 not in colinear:\n colinear.append(pt2)\n else:\n if target not in colinear:\n colinear.append(target)\n target = pt2\n\n if target not in convex_list:\n convex_list.append(target)\n\n for colinear_pt in colinear:\n if colinear_pt not in convex_list:\n convex_list.append(colinear_pt)\n\n curr_pt = target\n return convex_list\n\n\ndef main():\n pts = [Point(x, y) for x, y in np.random.rand(100, 2)]\n convex_lst = iter(pts)\n len_convex_pts = len(convex_lst)\n\n print(*convex_lst)\n\n plt.plot(\n [p.x for p in convex_lst] + [convex_lst[0].x],\n [p.y for p in convex_lst] + [convex_lst[0].y],\n \"-\",\n )\n plt.scatter([p.x for p in pts], [p.y for p in pts], color=\"red\")\n plt.title(\n \"Jarvis March Algorithm (Total \"\n + str(len_convex_pts)\n + \"/\"\n + str(len(pts))\n + \" points)\"\n )\n plt.grid()\n plt.show()\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/computational_geometry/src/jarvis_march/README.md",
"content": "# Jarvis March Algorithm\n## python version 1.0\n\nHow to start? \n * Run \"python jarvis_march.py\" and see what happens (hint: MAGIC)\n * Change the points in the main function.\n\nThese are some results:\n#### 10 random points\n<p align=\"center\">\n <img src=\"https://i.imgur.com/VP3VDuG.png\" width=\"350\"/>\n <img src=\"https://i.imgur.com/YVyaEaW.png\" width=\"350\"/>\n</p>\n\n#### 100 random points\n<p align=\"center\">\n <img src=\"https://i.imgur.com/Dvvy5De.png\" width=\"350\"/>\n <img src=\"https://i.imgur.com/IHdmDjv.png\" width=\"350\"/>\n</p>\n\n#### 1000 random points\n<p align=\"center\">\n <img src=\"https://i.imgur.com/hcuFKzi.png\" width=\"350\"/>\n <img src=\"https://i.imgur.com/z8YGVEy.png\" width=\"350\"/>\n</p>\n\n### Useful Links for further reading:\n\n * [jarvis_march on youtube](https://www.youtube.com/watch?v=Vu84lmMzP2o) - great explenation (demo+code) \n * [convex hull + jarvis theory](http://jeffe.cs.illinois.edu/teaching/373/notes/x05-convexhull.pdf) - Theoretical explenation\n * [Gift wrapping algorithm](https://en.wikipedia.org/wiki/Gift_wrapping_algorithm) - wikipedia overview"
}
{
"filename": "code/computational_geometry/src/liang_barsky_algo/liang_barsky_algo.c",
"content": "/* Header files included */\n#include <stdio.h>\n#include <conio.h>\n#include <graphics.h>\n\nint\nmain()\n{\n int gd = DETECT, gm;\n /* \n * x1, x2, y1 & y2 are line coordinates\n * xmax, xmin, ymax & ymin are window's lower and upper corner coordinates\n * xx1, yy1, xx2 & yy2 are the new coordinates of clipped line\n */\n int x1, y1, x2, y2;\n int xmax, xmin, ymax, ymin;\n int xx1, yy1, xx2, yy2;\n int dx, dy, i;\n int p[4], q[4];\n float t1, t2, t[4];\n\n initgraph (& gd, & gm, \"C:\\\\TC\\\\BGI\");\n /* Enter the coordinates for window*/\n printf (\"Enter the lower co-ordinates of window\");\n scanf (\"%d\", &xmin, &ymin);\n printf (\"Enter the upper co-ordinates of window\");\n scanf (\"%d\", &xmax, &ymax);\n\n /* Graphics function to draw rectangle/clipping window */\n setcolor (RED);\n rectangle (xmin, ymin, xmax, ymax);\n\n /* Enter the coordinates of line */\n printf (\"Enter x1:\");\n scanf(\"%d\", &x1);\n printf(\"Enter y1:\");\n scanf(\"%d\", &y1);\n printf(\"Enter x2:\");\n scanf(\"%d\", &x2);\n printf(\"Enter y2:\");\n scanf(\"%d\", &y2);\n\n /* Graphics Function to draw line */\n line (x1, y1, x2, y2);\n\n dx = x2 - x1;\n dy = y2 - y1;\n p[0] = - dx;\n p[1] = dx;\n p[2] = - dy;\n p[3] = dy;\n q[0] = x1 - xmin;\n q[1] = xmax - x1;\n q[2] = y1 - ymin;\n q[3] = ymax - y1;\n\n for (i=0; i < 4; i++)\n {\n\n if (p[i] != 0) {\n t[i] = (float) q[i] / p[i];\n }\n\n else {\n if (p[i] == 0 && q[i] < 0)\n printf (\"line completely outside the window\");\n\n else\n if (p[i] == 0 && q[i] >= 0)\n printf (\"line completely inside the window\");\n }\n }\n\n if (t[0] > t[2])\n t1 = t[0];\n\n else\n t1 = t[2];\n\n if (t[1] < t[3])\n t2 = t[1];\n\n else\n t2 = t[3];\n\n if (t1 < t2) {\n xx1 = x1 + t1 * dx;\n xx2 = x1 + t2 * dx;\n yy1 = y1 + t1 * dy;\n yy2 = y1 + t2 * dy;\n\n /* Draw the clipped line */\n printf (\"line after clipping:\");\n setcolor (WHITE);\n line (xx1, yy1, xx2, yy2);\n }\n\n else\n printf (\"line lies out of the window\");\n\n getch();\n return (0);\n}"
}
{
"filename": "code/computational_geometry/src/liang_barsky_algo/liang_barsky_algo.cpp",
"content": "// Header files included\n#include <iostream.h>\n#include <conio.h>\n#include <graphics.h>\nusing namespace std;\n\nint main()\n{\n int gd = DETECT, gm;\n /* \n * x1, x2, y1 & y2 are line coordinates\n * xmax, xmin, ymax & ymin are window's lower and upper corner coordinates\n * xx1, yy1, xx2 & yy2 are the new coordinates of clipped line\n */\n int x1, y1, x2, y2;\n int xmax, xmin, ymax, ymin;\n int xx1, yy1, xx2, yy2;\n int dx, dy, i;\n int p[4], q[4];\n float t1, t2, t[4];\n\n initgraph (& gd, & gm, \"C:\\\\TC\\\\BGI\");\n // Enter the coordinates for window\n cout << \"Enter the lower co-ordinates of window\";\n cin >> xmin >> ymin;\n cout << \"Enter the upper co-ordinates of window\";\n cin >> xmax >> ymax;\n\n // Graphics function to draw rectangle/clipping window\n setcolor (RED);\n rectangle (xmin, ymin, xmax, ymax);\n\n // Enter the coordinates of line\n cout << \"Enter x1:\";\n cin >> x1;\n cout << \"Enter y1:\";\n cin >> y1;\n cout << \"Enter x2:\";\n cin >> x2;\n cout << \"Enter y2:\";\n cin >> y2;\n\n // Graphics Function to draw line\n line (x1, y1, x2, y2);\n\n dx = x2 - x1;\n dy = y2 - y1;\n p[0] = - dx;\n p[1] = dx;\n p[2] = - dy;\n p[3] = dy;\n q[0] = x1 - xmin;\n q[1] = xmax - x1;\n q[2] = y1 - ymin;\n q[3] = ymax - y1;\n\n for (i=0; i < 4; i++)\n {\n\n if (p[i] != 0)\n {\n t[i] = (float) q[i] / p[i];\n }\n\n else\n {\n if (p[i] == 0 && q[i] < 0)\n cout << \"line completely outside the window\";\n\n else\n if (p[i] == 0 && q[i] >= 0)\n cout << \"line completely inside the window\";\n }\n }\n if (t[0] > t[2])\n t1 = t[0];\n\n else\n t1 = t[2];\n\n if (t[1] < t[3])\n t2 = t[1];\n\n else\n t2 = t[3];\n\n if (t1 < t2)\n {\n xx1 = x1 + t1 * dx;\n xx2 = x1 + t2 * dx;\n yy1 = y1 + t1 * dy;\n yy2 = y1 + t2 * dy;\n cout << \"line after clipping:\";\n\n setcolor (WHITE);\n line (xx1, yy1, xx2, yy2);\n }\n else\n cout << \"line lies out of the window\";\n\n getch();\n return (0);\n}"
}
{
"filename": "code/computational_geometry/src/mandelbrot_fractal/mandelbrot_fractal.py",
"content": "import numpy\r\nimport matplotlib.pyplot as plt\r\n\r\n\r\ndef mandelbrot(Re, Im, max_iter):\r\n c = complex(Re, Im)\r\n z = 0.0j\r\n\r\n for i in range(max_iter):\r\n z = z * z + c\r\n if (z.real * z.real + z.imag * z.imag) >= 4:\r\n return i\r\n\r\n return max_iter\r\n\r\n\r\ncolumns = 2000\r\nrows = 2000\r\n\r\nresult = numpy.zeros([rows, columns])\r\nfor row_index, Re in enumerate(numpy.linspace(-2, 1, num=rows)):\r\n for column_index, Im in enumerate(numpy.linspace(-1, 1, num=columns)):\r\n result[row_index, column_index] = mandelbrot(Re, Im, 100)\r\n\r\nplt.figure(dpi=100)\r\nplt.imshow(result.T, cmap=\"plasma\", interpolation=\"bilinear\", extent=[-2, 1, -1, 1])\r\nplt.xlabel(\"Re\")\r\nplt.ylabel(\"Im\")\r\nplt.show()\r"
}
{
"filename": "code/computational_geometry/src/quick_hull/quick_hull2.cpp",
"content": "#include <bits/stdc++.h>\nusing namespace std;\n// Part of Cosmos by OpenGenus Foundation\n// C++ program to implement Quick Hull algorithm to find convex hull.\n\n\n// iPair is integer pairs\n#define iPair pair<int, int>\n\n// Stores the result (points of convex hull)\nset<iPair> hull;\n\n// Returns the side of point p with respect to line\n// joining points p1 and p2.\nint findSide(iPair p1, iPair p2, iPair p)\n{\n int val = (p.second - p1.second) * (p2.first - p1.first) -\n (p2.second - p1.second) * (p.first - p1.first);\n\n if (val > 0)\n return 1;\n if (val < 0)\n return -1;\n return 0;\n}\n\n// Returns the square of distance between\n// p1 and p2.\n\nint dist(iPair p, iPair q)\n{\n return (p.second - q.second) * (p.second - q.second) +\n (p.first - q.first) * (p.first - q.first);\n}\n\n// returns a value proportional to the distance\n// between the point p and the line joining the\n// points p1 and p2\n\nint lineDist(iPair p1, iPair p2, iPair p)\n{\n return abs ((p.second - p1.second) * (p2.first - p1.first) -\n (p2.second - p1.second) * (p.first - p1.first));\n}\n\n// End points of line L are p1 and p2. side can have value\n// 1 or -1 specifying each of the parts made by the line L\nvoid quickHull(iPair a[], int n, iPair p1, iPair p2, int side)\n{\n int ind = -1;\n int max_dist = 0;\n\n // finding the point with maximum distance\n // from L and also on the specified side of L.\n for (int i = 0; i < n; i++)\n {\n int temp = lineDist(p1, p2, a[i]);\n if (findSide(p1, p2, a[i]) == side && temp > max_dist)\n {\n ind = i;\n max_dist = temp;\n }\n }\n\n // If no point is found, add the end points\n // of L to the convex hull.\n if (ind == -1)\n {\n hull.insert(p1);\n hull.insert(p2);\n return;\n }\n\n // Recur for the two parts divided by a[ind]\n quickHull(a, n, a[ind], p1, -findSide(a[ind], p1, p2));\n quickHull(a, n, a[ind], p2, -findSide(a[ind], p2, p1));\n}\n\nvoid printHull(iPair a[], int n)\n{\n // a[i].second -> y-coordinate of the ith point\n if (n < 3)\n {\n cout << \"Convex hull not possible\\n\";\n return;\n }\n\n // Finding the point with minimum and\n // maximum x-coordinate\n int min_x = 0, max_x = 0;\n for (int i = 1; i < n; i++)\n {\n if (a[i].first < a[min_x].first)\n min_x = i;\n if (a[i].first > a[max_x].first)\n max_x = i;\n }\n\n // Recursively find convex hull points on\n // one side of line joining a[min_x] and\n // a[max_x].\n\n quickHull(a, n, a[min_x], a[max_x], 1);\n\n // Recursively find convex hull points on\n // other side of line joining a[min_x] and\n // a[max_x]\n\n quickHull(a, n, a[min_x], a[max_x], -1);\n\n cout << \"The points in Convex Hull are:\\n\";\n while (!hull.empty())\n {\n cout << \"(\" << ( *hull.begin()).first << \", \"\n << (*hull.begin()).second << \") \";\n hull.erase(hull.begin());\n }\n}\n\n// Driver code\nint main()\n{\n iPair a[] = {{0, 3}, {1, 1}, {2, 2}, {4, 4},\n {0, 0}, {1, 2}, {3, 1}, {3, 3}};\n int n = sizeof(a) / sizeof(a[0]);\n printHull(a, n);\n return 0;\n}"
}
{
"filename": "code/computational_geometry/src/quick_hull/quick_hull.cpp",
"content": "#include <vector>\n#include <iostream>\n#include <cmath>\nusing namespace std;\n\nstruct vec2\n{\n float x, y;\n vec2(float x, float y) : x(x), y(y)\n {\n }\n vec2()\n {\n }\n};\n\nvector<vec2> input;\nvector<vec2> output;\n\n\nfloat lineToPointSupport(vec2 l1, vec2 l2, vec2 p)\n{\n return abs ((p.y - l1.y) * (l2.x - l1.x) -\n (l2.y - l2.y) * (p.x - l1.x));\n}\n\nfloat dot(vec2 A, vec2 B)\n{\n return A.x * B.x + A.y * B.y;\n}\n\n\nvoid findHull(int a, int b)\n{\n float dy = input[b].y - input[a].y;\n float dx = input[b].x - input[a].x;\n vec2 normal(-dy, dx);\n float biggestLinePointDistance = -1;\n int c = 0;\n\n for (size_t i = 0; i < input.size(); i++)\n {\n vec2 aToPoint(input[i].x - input[a].x, input[i].y - input[a].y);\n if (dot(aToPoint, normal) < 0 || static_cast<int>(i) == a || static_cast<int>(i) == b)\n continue;\n float lineToPoint = lineToPointSupport(input[a], input[b], input[i]);\n if (lineToPoint > biggestLinePointDistance)\n {\n c = i;\n biggestLinePointDistance = lineToPoint;\n }\n }\n\n if (biggestLinePointDistance == -1)\n return;\n output.push_back(input[c]);\n findHull(a, c);\n findHull(c, b);\n}\n\n\nvoid quickhull()\n{\n if (input.size() < 3)\n return;\n\n float mini = 0;\n float maxi = 0;\n for (size_t i = 0; i < input.size(); i++)\n {\n if (input[i].x < input[mini].x)\n mini = i;\n if (input[i].x > input[maxi].x)\n maxi = i;\n }\n output.push_back(input[mini]);\n output.push_back(input[maxi]);\n findHull(mini, maxi);\n findHull(maxi, mini);\n}\n\nint main()\n{\n\n //example set 1\n input.push_back(vec2(5, 10));\n input.push_back(vec2(10, 10));\n input.push_back(vec2(7, 15));\n input.push_back(vec2(7, 13));\n\n //example set 2\n// input.push_back(vec2(0, 15));\n// input.push_back(vec2(10, 15));\n// input.push_back(vec2(10, 20));\n// input.push_back(vec2(0, 20));\n// input.push_back(vec2(5, 17));\n quickhull();\n for (size_t i = 0; i < output.size(); i++)\n cout << \"x: \" << output[i].x << \"y: \" << output[i].y << endl;\n return 0;\n}"
}
{
"filename": "code/computational_geometry/src/quick_hull/quick_hull.hs",
"content": "-- Part of Cosmos by OpenGenus\n-- Point in 2-D plane\ndata Point = Point {\n x::Double,\n y::Double\n }\n deriving (Show)\n\ninstance Eq Point where\n (==) (Point x1 y1) (Point x2 y2) = x1 == x2 && y1 == y2\n\ninstance Ord Point where\n compare (Point x1 y1) (Point x2 y2) = compare x1 x2\n (<=) (Point x1 y1) (Point x2 y2) = x1 <= x2\n\npplus :: Point -> Point -> Point\npplus (Point x1 y1) (Point x2 y2) = Point (x1+x2) (y1+y2)\n\npminus :: Point -> Point -> Point\npminus (Point x1 y1) (Point x2 y2) = Point (x1-x2) (y1-y2)\n\n-- Compute the dot product AB ⋅ BC\ndot :: Point -> Point -> Double -- we use point type to represent vectors\ndot (Point ab1 ab2) (Point bc1 bc2) = ab1 * bc1 + ab2 * bc2\n\n-- Compute the cross product AB x AC\n -- we use point type to represent vectors\ncross :: Point -> Point -> Double -- 2 dimensional so return scalar\ncross (Point ab1 ab2) (Point ac1 ac2) = ab1 * ac2 - ab2 * ac1\n\ndistance :: Point -> Point -> Double\ndistance (Point a1 a2) (Point b1 b2) = sqrt (d1*d1+d2*d2)\n where d1 = a1 - b1\n d2 = a2 - b2\n\nquickHull :: [Point] -> [Point]\nquickHull [] = []\nquickHull l = trace (\"A: \" ++ show pA ++ \" and B: \" ++ show pB ++ \"\\n\") $ pA : pB : findHull rhs pA pB ++ findHull lhs pB pA\n where (lhs, rhs) = partition l pA pB\n pA = maximum l\n pB = minimum l\n\npartition :: [Point] -> Point -> Point -> ([Point], [Point])\n-- returns ([points on LHS of line AB], [points on RHS of line AB])\npartition l pA pB = (partition' (\\x -> snd x > 0) , partition' (\\x -> snd x < 0))\n where pointOPMap = zip l (map _ABCrossAP l)\n _ABCrossAP p = (pB `pminus` pA) `cross` (p `pminus` pA)\n partition' f = map fst (filter f pointOPMap)\n\n-- Triangle PQM looks like this:\n-- M\n-- ·\n-- · ·\n-- S2 · · S1\n-- · S0 ·\n-- · ·\n-- · · · · · · · ·\n-- Q P\nfindHull :: [Point] -> Point -> Point -> [Point]\nfindHull [] _ _ = []\nfindHull s p q = trace (\"M: \" ++ show m ++ \" P: \" ++ show p ++ \"Q: \" ++ show q ++ \"\\n\") $ m : findHull s1 p m ++ findHull s2 m q\n where m = s!!fromJust (elemIndex max_dist dists) -- point of maximum distance on RHS of PQ\n max_dist = maximum dists\n dists = map (linePointDist p q) s\n s1 = snd $ partition s p m -- rhs of line PM (outside triangle)\n s2 = fst $ partition s q m -- lhs of line QM (outside triangle)\n\n\n-- Compute the distance from line segment AB to C\nlinePointDist :: Point -> Point -> Point -> Double\nlinePointDist a b c | dot1 > 0 = distbc\n | dot2 > 0 = distac\n | otherwise = abs dist\n where dot1 = dot (c `pminus` b) (b `pminus` a)\n dot2 = dot (c `pminus` a) (a `pminus` b)\n distbc = distance b c\n distac = distance a c\n dist = cross (b `pminus` a) (c `pminus` a) / distance a b"
}
{
"filename": "code/computational_geometry/src/quick_hull/quick_hull.java",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\nimport java.util.ArrayList;\nimport java.util.Scanner;\n\npublic class quickHull {\n public static class Point {\n private final int x;\n private final int y;\n private final int pos;\n public Point(final int x, final int y, final int p) {\n this.x = x;\n this.y = y;\n this.pos = p;\n }\n }\n\n public static double dist(Point p1, Point p2) {\n return Math.sqrt((p1.x-p2.x)*(p1.x-p2.x)+(p1.y-p2.y)*(p1.y-p2.y));\n }\n\n public static Point getLeftMostBottomPoint(ArrayList<Point> points) {\n\n Point result = points.get(0);\n for(int i = 1; i < points.size(); i++) {\n if(points.get(i).x < result.x || (points.get(i).x == result.x && points.get(i).y < result.y)) {\n result = points.get(i);\n }\n }\n\n return result;\n }\n\n public static Point getRightMostUpperPoint(ArrayList<Point> points) {\n\n Point result = points.get(0);\n for(int i = 1; i < points.size(); i++) {\n if(points.get(i).x > result.x || (points.get(i).x == result.x && points.get(i).y > result.y)) {\n result = points.get(i);\n }\n }\n\n return result;\n }\n\n\n // Partition P with respect to line joining A and B\n public static int partition(Point A, Point B, Point P) {\n int cp1 = (B.x - A.x) * (P.y - A.y) - (B.y - A.y) * (P.x - A.x);\n if (cp1 > 0) return 1;\n else if (cp1 == 0) return 0;\n else return -1;\n }\n\n // https://brilliant.org/wiki/distance-between-point-and-line/\n public static int distance(Point A, Point B, Point C) {\n return Math.abs((B.x - A.x) * (A.y - C.y) - (B.y - A.y) * (A.x - C.x));\n }\n\n public static ArrayList<Point> quickHull(ArrayList<Point> points) {\n ArrayList<Point> convexHull = new ArrayList<Point>();\n if (points.size() < 3) return (new ArrayList<Point> (points));\n\n Point leftMostBottom = getLeftMostBottomPoint(points);\n Point rightMostUpper = getRightMostUpperPoint(points);\n points.remove(leftMostBottom);\n points.remove(rightMostUpper);\n convexHull.add(leftMostBottom);\n convexHull.add(rightMostUpper);\n\n ArrayList<Point> leftPart = new ArrayList<>();\n ArrayList<Point> rightPart = new ArrayList<>();\n\n for(int i = 0; i < points.size(); i++) {\n Point p = points.get(i);\n if(partition(leftMostBottom, rightMostUpper,p) == -1) {\n leftPart.add(p);\n } else if(partition(leftMostBottom, rightMostUpper, p) == 1) {\n rightPart.add(p);\n }\n }\n\n hullSet(leftMostBottom, rightMostUpper, rightPart, convexHull);\n hullSet(rightMostUpper, leftMostBottom, leftPart, convexHull);\n\n return convexHull;\n }\n\n public static void hullSet(Point a, Point b, ArrayList<Point> set, ArrayList<Point> hull) {\n int insertPosition = hull.indexOf(b);\n if (set.size() == 0) return;\n if (set.size() == 1) {\n Point p = set.get(0);\n set.remove(p);\n hull.add(insertPosition, p);\n return;\n }\n\n int furthestDist = 0;\n Point furthestPoint = null;\n for (int i = 0; i < set.size(); i++) {\n Point p = set.get(i);\n int distance = distance(a, b, p);\n if (distance > furthestDist) {\n furthestDist = distance;\n furthestPoint = p;\n }\n }\n\n set.remove(furthestPoint);\n hull.add(insertPosition, furthestPoint);\n\n // Determine who's to the left of line joining a and furthestPoint\n ArrayList<Point> leftSet = new ArrayList<Point>();\n for (int i = 0; i < set.size(); i++) {\n Point current = set.get(i);\n if (partition(a, furthestPoint, current) == 1) {\n leftSet.add(current);\n }\n }\n\n hullSet(a, furthestPoint, leftSet, hull);\n\n // Determine who's to the left of line joining furthestPoint and b\n leftSet = new ArrayList<>();\n for (int i = 0; i < set.size(); i++) {\n Point current = set.get(i);\n if (partition(furthestPoint, b, current) == 1) {\n leftSet.add(furthestPoint);\n }\n }\n\n hullSet(furthestPoint, b, leftSet, hull);\n }\n\n public static void main(String[] args) {\n Scanner in = new Scanner(System.in);\n int N = in.nextInt();\n ArrayList<Point> points = new ArrayList<>();\n for(int i = 0; i < N; i++) {\n int x = in.nextInt(), y = in.nextInt();\n points.add(new Point(x, y, i+1));\n }\n\t\n ArrayList<Point> hull = quickHull(points);\n double ans = 0;\n for(int i = 1; i < hull.size(); i++) {\n ans += dist(hull.get(i), hull.get(i-1));\n }\n \n ans += dist(hull.get(0), hull.get(hull.size()-1));\n if(hull.size() == 1) ans = 0;\n System.out.println(ans);\n System.out.println(\"Points on the hull are:\");\n for(Point p: hull) {\n System.out.print(p.pos+\" \");\n }\n }\n}"
}
{
"filename": "code/computational_geometry/src/quick_hull/README.md",
"content": "# Quickhull\nFor theoretical background see [Quickhull](https://en.wikipedia.org/wiki/Quickhull).\nTest data can be found in `test_data.csv`. \n\nExample of convex hull of a set of points:\n\n"
}
{
"filename": "code/computational_geometry/src/quick_hull/test_data.csv",
"content": "x,y\r0.321534855,0.036295831\r0.024023581,-0.23567288\r0.045908512,-0.415640992\r0.3218384,0.13798507\r0.115064798,-0.105952147\r0.262254,-0.297028733\r-0.161920957,-0.405533972\r0.190537863,0.369860101\r0.238709092,-0.016298271\r0.074958887,-0.165982511\r0.331934184,-0.18218141\r0.077036358,-0.249943064\r0.2069243,-0.223297076\r0.046040795,-0.192357319\r0.050542958,0.475492946\r-0.390058917,0.279782952\r0.312069339,-0.050632987\r0.011388127,0.40025047\r0.00964515,0.10602511\r-0.035979332,0.295363946\r0.181829087,0.001454398\r0.444056063,0.250249717\r-0.053017525,-0.065539216\r0.482389623,-0.477617\r-0.308922685,-0.063561122\r-0.271780741,0.18108106\r0.429362652,0.298089796\r-0.004796652,0.382663813\r0.430695573,-0.29950735\r0.179966839,-0.297346747\r0.493216685,0.492809416\r-0.352148791,0.43526562\r-0.490736801,0.186582687\r-0.104792472,-0.247073392\r0.437496186,-0.00160628\r0.003256208,-0.272919432\r0.043103782,0.445260405\r0.491619838,-0.345391701\r0.001675087,0.153183767\r-0.440428957,-0.289485599"
}
{
"filename": "code/computational_geometry/src/quick_hull/test_data_soln.txt",
"content": "-0.161920957418085 -0.4055339716426413 \n0.05054295812784038 0.4754929463150845 \n0.4823896228171788 -0.4776170002088109 \n0.4932166845474547 0.4928094162538735 \n-0.3521487911717489 0.4352656197131292 \n-0.4907368011686362 0.1865826865533206 \n0.4916198379282093 -0.345391701297268 \n-0.4404289572876217 -0.2894855991839297 "
}
{
"filename": "code/computational_geometry/src/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\nComputational geometry is a branch of computer science devoted to the study of algorithms which can be formulated in geometric terms. While modern computational geometry is a recent development, it is one of the oldest fields of computing with history stretching back to antiquity. \n\nThe primary goal of research in combinatorial computational geometry is to develop efficient algorithms and data structures for solving problems stated in terms of basic geometrical objects: points, line segments, polygons, polyhedra, etc.\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/computational_geometry/src/sphere_tetrahedron_intersection/luvector.hpp",
"content": "/*\n * N dimensional loop unrolled vector class.\n * \n * https://github.com/RedBlight/LoopUnrolledVector\n * \n * Refactored a little bit for \"cosmos\" repository.\n *\n */\n\n#ifndef LU_VECTOR_INCLUDED\n#define LU_VECTOR_INCLUDED\n\n#include <sstream>\n#include <string>\n#include <complex>\n#include <vector>\n#include <iostream>\n\nnamespace LUV\n{\n // LuVector.hpp\n\n template<std::size_t N, class T>\n class LuVector;\n\n template<class T>\n using LuVector2 = LuVector<2, T>;\n\n template<class T>\n using LuVector3 = LuVector<3, T>;\n\n using LuVector2f = LuVector<2, float>;\n using LuVector2d = LuVector<2, double>;\n using LuVector2c = LuVector<2, std::complex<double>>;\n\n using LuVector3f = LuVector<3, float>;\n using LuVector3d = LuVector<3, double>;\n using LuVector3c = LuVector<3, std::complex<double>>;\n \n // Added for cosmos\n const double pi = 3.14159265358979323846;\n \n // LuVector_Op.hpp\n\t\n template<std::size_t N>\n struct LoopIndex\n {\n\n };\n\n template<class T, class S>\n struct OpCopy\n {\n inline void operator ()(T& lhs, const S& rhs)\n {\n lhs = rhs;\n }\n };\n\n //////////////////////////////////////////////////////////////\n\n template<class T, class S>\n struct OpAdd\n {\n inline void operator ()(T& lhs, const S& rhs)\n {\n lhs += rhs;\n }\n };\n\n template<class T, class S>\n struct OpSub\n {\n inline void operator ()(T& lhs, const S& rhs)\n {\n lhs -= rhs;\n }\n };\n\n template<class T, class S>\n struct OpMul\n {\n inline void operator ()(T& lhs, const S& rhs)\n {\n lhs *= rhs;\n }\n };\n\n template<class T, class S>\n struct OpDiv\n {\n inline void operator ()(T& lhs, const S& rhs)\n {\n lhs /= rhs;\n }\n };\n\n //////////////////////////////////////////////////////////////\n\n template<class T, class S>\n struct OpAbs\n {\n inline void operator ()(T& lhs, const S& rhs)\n {\n lhs = std::abs(rhs);\n }\n };\n\n template<class T, class S>\n struct OpArg\n {\n inline void operator ()(T& lhs, const S& rhs)\n {\n lhs = std::arg(rhs);\n }\n };\n\n template<std::size_t N, class T, class S>\n struct OpMin\n {\n inline void operator ()(LuVector<N, T>& result, const LuVector<N, T>& lhs, const LuVector<N, S>& rhs, const std::size_t& I)\n {\n result[I] = lhs[I] < rhs[I] ? lhs[I] : rhs[I];\n }\n };\n\n template<std::size_t N, class T, class S>\n struct OpMax\n {\n inline void operator ()(LuVector<N, T>& result, const LuVector<N, T>& lhs, const LuVector<N, S>& rhs, const std::size_t& I)\n {\n result[I] = lhs[I] > rhs[I] ? lhs[I] : rhs[I];\n }\n };\n\n template<std::size_t N, class T, class S>\n struct OpDot\n {\n inline void operator ()(T& result, const LuVector<N, T>& lhs, const LuVector<N, S>& rhs, const std::size_t& I)\n {\n result += lhs[I] * rhs[I];\n }\n };\n\n //////////////////////////////////////////////////////////////\n\n template<class T>\n struct OpOstream\n {\n inline void operator ()(std::ostream& lhs, const T& rhs, const std::string& delimiter)\n {\n lhs << rhs << delimiter;\n }\n };\n \n // LuVector_Unroll.hpp\n\n // VECTOR OP SCALAR\n template<std::size_t I, std::size_t N, class T, class S, template<class, class> class OP>\n inline void Unroll(LuVector<N, T>& lhs, const S& rhs, OP<T, S> operation, LoopIndex<I>)\n {\n Unroll(lhs, rhs, operation, LoopIndex<I-1>());\n operation(lhs[I], rhs);\n }\n\n template<std::size_t N, class T, class S, template<class, class> class OP>\n inline void Unroll(LuVector<N, T>& lhs, const S& rhs, OP<T, S> operation, LoopIndex<0>)\n {\n operation(lhs[0], rhs);\n }\n\n // SCALAR OP VECTOR\n template<std::size_t I, std::size_t N, class T, class S, template<class, class> class OP>\n inline void Unroll(S& lhs, const LuVector<N, T>& rhs, OP<T, S> operation, LoopIndex<I>)\n {\n Unroll(lhs, rhs, operation, LoopIndex<I-1>());\n operation(lhs, rhs[I]);\n }\n\n template<std::size_t N, class T, class S, template<class, class> class OP>\n inline void Unroll(S& lhs, const LuVector<N, T>& rhs, OP<T, S> operation, LoopIndex<0>)\n {\n operation(lhs, rhs[0]);\n }\n\n // VECTOR OP VECTOR\n template<std::size_t I, std::size_t N, class T, class S, template<class, class> class OP>\n inline void Unroll(LuVector<N, T>& lhs, const LuVector<N, S>& rhs, OP<T, S> operation, LoopIndex<I>)\n {\n Unroll(lhs, rhs, operation, LoopIndex<I-1>());\n operation(lhs[I], rhs[I]);\n }\n\n template<std::size_t N, class T, class S, template<class, class> class OP>\n inline void Unroll(LuVector<N, T>& lhs, const LuVector<N, S>& rhs, OP<T, S> operation, LoopIndex<0>)\n {\n operation(lhs[0], rhs[0]);\n }\n\n // SCALAR = VECTOR OP VECTOR (could be cross-element)\n template<std::size_t I, std::size_t N, class T, class S, template<std::size_t, class, class> class OP>\n inline void Unroll(T& result, const LuVector<N, T>& lhs, const LuVector<N, S>& rhs, OP<N, T, S> operation, LoopIndex<I>)\n {\n Unroll(result, lhs, rhs, operation, LoopIndex<I-1>());\n operation(result, lhs, rhs, I);\n }\n\n template<std::size_t N, class T, class S, template<std::size_t, class, class> class OP>\n inline void Unroll(T& result, const LuVector<N, T>& lhs, const LuVector<N, S>& rhs, OP<N, T, S> operation, LoopIndex<0>)\n {\n operation(result, lhs, rhs, 0);\n }\n\n // VECTOR = VECTOR OP VECTOR (could be cross-element)\n template<std::size_t I, std::size_t N, class T, class S, template<std::size_t, class, class> class OP>\n inline void Unroll(LuVector<N, T>& result, const LuVector<N, T>& lhs, const LuVector<N, S>& rhs, OP<N, T, S> operation, LoopIndex<I>)\n {\n Unroll(result, lhs, rhs, operation, LoopIndex<I-1>());\n operation(result, lhs, rhs, I);\n }\n\n template<std::size_t N, class T, class S, template<std::size_t, class, class> class OP>\n inline void Unroll(LuVector<N, T>& result, const LuVector<N, T>& lhs, const LuVector<N, S>& rhs, OP<N, T, S> operation, LoopIndex<0>)\n {\n operation(result, lhs, rhs, 0);\n }\n\n // OSTREAM OP VECTOR\n template<std::size_t I, std::size_t N, class T, template<class> class OP>\n inline void Unroll(std::ostream& lhs, const LuVector<N, T>& rhs, const std::string& delimiter, OP<T> operation, LoopIndex<I>)\n {\n Unroll(lhs, rhs, delimiter, operation, LoopIndex<I-1>());\n operation(lhs, rhs[I], delimiter);\n }\n\n template<std::size_t N, class T, template<class> class OP>\n inline void Unroll(std::ostream& lhs, const LuVector<N, T>& rhs, const std::string& delimiter, OP<T> operation, LoopIndex<0>)\n {\n operation(lhs, rhs[0], delimiter);\n }\n \n // LuVector_Body.hpp\n \n template<std::size_t N, class T>\n class LuVector\n {\n public:\n LuVector() = default;\n\n ~LuVector() = default;\n\n template<class... S> \n LuVector(const S&... elem) : _{ static_cast<T>(elem) ...}\n {\n\n }\n\n LuVector(const LuVector<N, const T>& other) : _{ other._ }\n {\n\n }\n\n template<class S> \n LuVector(const LuVector<N, S>& other)\n {\n Unroll(*this, other, OpCopy<T, S>(), LoopIndex<N-1>());\n }\n\n template<class S> \n LuVector(const S& scalar)\n {\n Unroll(*this, scalar, OpCopy<T, S>(), LoopIndex<N-1>());\n }\n\n template<class S>\n inline LuVector<N, T>& operator =(const LuVector<N, S>& other)\n {\n Unroll(*this, other, OpCopy<T, S>(), LoopIndex<N-1>());\n return *this;\n }\n\n inline const T& operator [](const std::size_t& idx) const\n {\n return _[idx];\n }\n\n inline T& operator [](const std::size_t& idx)\n {\n return _[idx];\n }\n\n inline std::string ToString() const\n {\n std::stringstream ss;\n ss << \"(\";\n Unroll(ss, *this, \",\", OpOstream<T>(), LoopIndex<N-2>());\n ss << _[N-1] << \")\";\n return std::string(ss.str());\n }\n\n private:\n T _[N];\n };\n \n // LuVector_Overload.hpp\n\n // OSTREAM << VECTOR\n template<std::size_t N, class T>\n std::ostream& operator <<(std::ostream& lhs, const LuVector<N, T>& rhs)\n {\n lhs << \"(\";\n Unroll(lhs, rhs, \",\", OpOstream<T>(), LoopIndex<N-2>());\n lhs << rhs[N-1] << \")\";\n return lhs;\n }\n\n // VECTOR += SCALAR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T>& operator +=(LuVector<N, T>& lhs, const S& rhs)\n {\n Unroll(lhs, rhs, OpAdd<T, S>(), LoopIndex<N-1>());\n return lhs;\n }\n\n // VECTOR -= SCALAR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T>& operator -=(LuVector<N, T>& lhs, const S& rhs)\n {\n Unroll(lhs, rhs, OpSub<T, S>(), LoopIndex<N-1>());\n return lhs;\n }\n\n // VECTOR *= SCALAR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T>& operator *=(LuVector<N, T>& lhs, const S& rhs)\n {\n Unroll(lhs, rhs, OpMul<T, S>(), LoopIndex<N-1>());\n return lhs;\n }\n\n // VECTOR /= SCALAR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T>& operator /=(LuVector<N, T>& lhs, const S& rhs)\n {\n Unroll(lhs, rhs, OpDiv<T, S>(), LoopIndex<N-1>());\n return lhs;\n }\n\n //////////////////////////////////////////////////////////////\n\n // VECTOR += VECTOR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T>& operator +=(LuVector<N, T>& lhs, const LuVector<N, S>& rhs)\n {\n Unroll(lhs, rhs, OpAdd<T, S>(), LoopIndex<N-1>());\n return lhs;\n }\n\n // VECTOR -= VECTOR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T>& operator -=(LuVector<N, T>& lhs, const LuVector<N, S>& rhs)\n {\n Unroll(lhs, rhs, OpSub<T, S>(), LoopIndex<N-1>());\n return lhs;\n }\n\n // VECTOR *= VECTOR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T>& operator *=(LuVector<N, T>& lhs, const LuVector<N, S>& rhs)\n {\n Unroll(lhs, rhs, OpMul<T, S>(), LoopIndex<N-1>());\n return lhs;\n }\n\n // VECTOR /= VECTOR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T>& operator /=(LuVector<N, T>& lhs, const LuVector<N, S>& rhs)\n {\n Unroll(lhs, rhs, OpDiv<T, S>(), LoopIndex<N-1>());\n return lhs;\n }\n\n //////////////////////////////////////////////////////////////\n\n // VECTOR + SCALAR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T> operator +(const LuVector<N, T>& lhs, const S& rhs)\n {\n LuVector<N, T> result(lhs);\n return result += rhs;\n }\n\n // SCALAR + VECTOR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T> operator +(const T& lhs, const LuVector<N, S>& rhs)\n {\n LuVector<N, T> result(lhs);\n return result += rhs;\n }\n\n // VECTOR - SCALAR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T> operator -(const LuVector<N, T>& lhs, const S& rhs)\n {\n LuVector<N, T> result(lhs);\n return result -= rhs;\n }\n\n // SCALAR - VECTOR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T> operator -(const T& lhs, const LuVector<N, S>& rhs)\n {\n LuVector<N, T> result(lhs);\n return result -= rhs;\n }\n\n // VECTOR * SCALAR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T> operator *(const LuVector<N, T>& lhs, const S& rhs)\n {\n LuVector<N, T> result(lhs);\n return result *= rhs;\n }\n\n // SCALAR * VECTOR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T> operator *(const T& lhs, const LuVector<N, S>& rhs)\n {\n LuVector<N, T> result(lhs);\n return result *= rhs;\n }\n\n // VECTOR / SCALAR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T> operator /(const LuVector<N, T>& lhs, const S& rhs)\n {\n LuVector<N, T> result(lhs);\n return result /= rhs;\n }\n\n // SCALAR / VECTOR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T> operator /(const T& lhs, const LuVector<N, S>& rhs)\n {\n LuVector<N, T> result(lhs);\n return result /= rhs;\n }\n\n //////////////////////////////////////////////////////////////\n\n // VECTOR + VECTOR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T> operator +(const LuVector<N, T>& lhs, const LuVector<N, S>& rhs)\n {\n LuVector<N, T> result(lhs);\n return result += rhs;\n }\n\n // VECTOR - VECTOR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T> operator -(const LuVector<N, T>& lhs, const LuVector<N, S>& rhs)\n {\n LuVector<N, T> result(lhs);\n return result -= rhs;\n }\n\n // VECTOR * VECTOR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T> operator *(const LuVector<N, T>& lhs, const LuVector<N, S>& rhs)\n {\n LuVector<N, T> result(lhs);\n return result *= rhs;\n }\n\n // VECTOR / VECTOR\n template<std::size_t N, class T, class S>\n inline LuVector<N, T> operator /(const LuVector<N, T>& lhs, const LuVector<N, S>& rhs)\n {\n LuVector<N, T> result(lhs);\n return result /= rhs;\n }\n\n //////////////////////////////////////////////////////////////\n\n // - VECTOR\n template<std::size_t N, class T>\n inline LuVector<N, T> operator -(const LuVector<N, T>& vec)\n {\n return T(0) - vec;\n }\n\n //////////////////////////////////////////////////////////////\n \n // LuVector_Math.hpp\n \n template<std::size_t N, class T>\n inline T Sum(const LuVector<N, T>& vec)\n {\n T result = 0;\n Unroll(result, vec, OpAdd<T, T>(), LoopIndex<N-1>());\n return result;\n }\n\n // Abs\n template<std::size_t N, class T>\n inline LuVector<N, T> Abs(const LuVector<N, T>& vec)\n {\n LuVector<N, T> absVec;\n Unroll(absVec, vec, OpAbs<T, T>(), LoopIndex<N-1>());\n return absVec;\n }\n\n // Length\n template<std::size_t N, class T>\n inline T Length(const LuVector<N, T>& vec)\n {\n T result = 0;\n Unroll(result, vec, vec, OpDot<N, T, T>(), LoopIndex<N-1>());\n return sqrt(result);\n }\n\n // Unit\n template<std::size_t N, class T>\n inline LuVector<N, T> Unit(const LuVector<N, T>& vec)\n {\n return vec / Length(vec);\n }\n\n //////////////////////////////////////////////////////////////\n\n // Abs Complex\n template<std::size_t N, class T>\n inline LuVector<N, T> Abs(const LuVector<N, std::complex<T>>& vec)\n {\n LuVector<N, T> absVec;\n Unroll(absVec, vec, OpAbs<T, std::complex<T>>(), LoopIndex<N-1>());\n return absVec;\n }\n\n // Arg Complex\n template<std::size_t N, class T>\n inline LuVector<N, T> Arg(const LuVector<N, std::complex<T>>& vec)\n {\n LuVector<N, T> argVec;\n Unroll(argVec, vec, OpArg<T, std::complex<T>>(), LoopIndex<N-1>());\n return argVec;\n }\n\n // Length Complex\n template<std::size_t N, class T>\n inline T Length(const LuVector<N, std::complex<T>>& vec)\n {\n return Length(Abs(vec)); \n }\n\n // Unit Complex\n template<std::size_t N, class T>\n inline LuVector<N, std::complex<T>> Unit(const LuVector<N, std::complex<T>>& vec)\n {\n return vec / Length(vec);\n }\n\n //////////////////////////////////////////////////////////////\n\n // Min\n template<std::size_t N, class T, class S>\n inline LuVector<N, T> Min(const LuVector<N, T>& lhs, const LuVector<N, S>& rhs)\n {\n LuVector<N, T> result;\n Unroll(result, lhs, rhs, OpMin<N, T, S>(), LoopIndex<N-1>());\n return result;\n }\n\n // Max\n template<std::size_t N, class T, class S>\n inline LuVector<N, T> Max(const LuVector<N, T>& lhs, const LuVector<N, S>& rhs)\n {\n LuVector<N, T> result;\n Unroll(result, lhs, rhs, OpMax<N, T, S>(), LoopIndex<N-1>());\n return result;\n }\n\n // Dot\n template<std::size_t N, class T, class S>\n inline T Dot(const LuVector<N, T>& lhs, const LuVector<N, S>& rhs)\n {\n T result = 0;\n Unroll(result, lhs, rhs, OpDot<N, T, S>(), LoopIndex<N-1>());\n return result;\n }\n\n // Cross\n template<class T, class S>\n inline LuVector<3, T> Cross(const LuVector<3, T>& lhs, const LuVector<3, S>& rhs)\n {\n return LuVector<3, T>(\n lhs[1] * rhs[2] - lhs[2] * rhs[1],\n lhs[2] * rhs[0] - lhs[0] * rhs[2],\n lhs[0] * rhs[1] - lhs[1] * rhs[0]\n );\n }\n\n //////////////////////////////////////////////////////////////\n\n // Reflect\n template<std::size_t N, class T, class S>\n inline LuVector<N, T> Reflect(const LuVector<N, T>& vec, const LuVector<N, S>& normal)\n {\n return vec - normal * Dot(vec, normal) * 2;\n }\n\n // Rotate...\n\n //////////////////////////////////////////////////////////////\n\n // CtsToSph\n template<class T>\n inline LuVector<3, T> CtsToSph(const LuVector<3, T>& cts)\n {\n T len = Length(cts);\n return LuVector<3, T>(\n len,\n cts[0] == 0 && cts[1] == 0 ? 0 : std::atan2(cts[1], cts[0]),\n std::acos(cts[2] / len)\n );\n }\n\n // SphToCts\n template<class T>\n inline LuVector<3, T> SphToCts(const LuVector<3, T>& sph)\n {\n T planarVal = sph[0] * std::sin(sph[2]);\n return LuVector<3, T>(\n planarVal * std::cos(sph[1]),\n planarVal * std::sin(sph[1]),\n sph[0] * std::cos(sph[2])\n );\n }\n\n // CtsToPol\n template<class T>\n inline LuVector<2, T> CtsToPol(const LuVector<2, T>& cts)\n {\n T len = Length(cts);\n return LuVector<2, T>(\n len,\n cts[0] == 0 && cts[1] == 0 ? 0 : std::atan2(cts[1], cts[0])\n );\n }\n\n // PolToCts\n template<class T>\n inline LuVector<2, T> PolToCts(const LuVector<2, T>& pol)\n {\n return LuVector<2, T>(\n pol[0] * std::cos(pol[1]),\n pol[0] * std::sin(pol[1])\n );\n }\n\n //////////////////////////////////////////////////////////////\n\n // OrthonormalSet N=3\n // N: looking outside the sphere at given angles\n // U: up vector\n // R: right vector, parallel to XY plane\n // N = U X R \n template<class T>\n inline void OrthonormalSet(const T& angP, const T& angT, LuVector<3, T>& dirN, LuVector<3, T>& dirU, LuVector<3, T>& dirR)\n {\n T cp = std::cos(angP);\n T sp = std::sin(angP);\n T ct = std::cos(angT);\n T st = std::sin(angT);\n\n dirN[0] = st * cp;\n dirN[1] = st * sp;\n dirN[2] = ct;\n\n dirR[0] = sp;\n dirR[1] = -cp;\n dirR[2] = 0;\n\n dirU = Cross(dirR, dirN);\n }\n\n // OrthonormalSet N=2\n template<class T>\n inline void OrthonormalSet(const T& ang, LuVector<2, T>& dirN, LuVector<2, T>& dirR)\n {\n T c = std::cos(ang);\n T s = std::sin(ang);\n\n dirN[0] = c;\n dirN[1] = s;\n\n dirR[0] = s;\n dirR[1] = -c;\n }\n\n // OrthogonalR N=3\n template<class T>\n inline LuVector<3, T> OrthonormalR(const LuVector<3, T>& dirN)\n {\n T ang = dirN[0] == 0 && dirN[1] == 0 ? 0 : std::atan2(dirN[1], dirN[0]);\n T c = std::cos(ang);\n T s = std::sin(ang);\n\n return LuVector<3, T>(s, -c, 0); \n }\n\n // OrthogonalR N=2\n template<class T>\n inline LuVector<2, T> OrthonormalR(const LuVector<2, T>& dirN)\n {\n return LuVector<2, T>(dirN[1], -dirN[0]); \n }\n\n // OrthogonalU N=3\n template<class T>\n inline LuVector<3, T> OrthonormalU(const LuVector<3, T>& dirN)\n {\n return Cross(OrthogonalR(dirN), dirN); \n }\n \n // LuVector_Geometry.hpp\n\n // ProjLine N=3\n template<class T>\n inline LuVector<3, T> ProjLine(const LuVector<3, T>& vec, const LuVector<3, T>&v1, const LuVector<3, T>& v2) \n {\n LuVector<3, T> v12 = Unit(v2 - v1);\n return v1 + Dot(v12, vec - v1) * v12;\n }\n\n // ProjLine N=2\n template<class T>\n inline LuVector<2, T> ProjLine(const LuVector<2, T>& vec, const LuVector<2, T>&v1, const LuVector<2, T>& v2) \n {\n LuVector<2, T> v12 = Unit(v2 - v1);\n return v1 + Dot(v12, vec - v1) * v12;\n }\n\n // ProjLineL N=3\n template<class T>\n inline LuVector<3, T> ProjLineL(const LuVector<3, T>& vec, const LuVector<3, T>&v1, const LuVector<3, T>& lineDir) \n {\n return v1 + Dot(lineDir, vec - v1) * lineDir;\n }\n\n // ProjLineL N=2\n template<class T>\n inline LuVector<2, T> ProjLineL(const LuVector<2, T>& vec, const LuVector<2, T>&v1, const LuVector<2, T>& lineDir) \n {\n return v1 + Dot(lineDir, vec - v1) * lineDir;\n }\n\n // LineNormal N=3\n template<class T>\n inline LuVector<3, T> LineNormal(const LuVector<3, T>& v1, const LuVector<3, T>& v2) \n {\n LuVector<3, T> v12 = Unit(v2 - v1);\n return OrthogonalR(v12);\n }\n\n // LineNormal N=2\n template<class T>\n inline LuVector<2, T> LineNormal(const LuVector<2, T>& v1, const LuVector<2, T>& v2) \n {\n LuVector<2, T> v12 = Unit(v2 - v1);\n return OrthogonalR(v12);\n }\n\n // LineNormalL N=3\n template<class T>\n inline LuVector<3, T> LineNormalL(const LuVector<3, T>& lineDir) \n {\n return OrthogonalR(lineDir);\n }\n\n // LineNormalL N=2\n template<class T>\n inline LuVector<2, T> LineNormalL(const LuVector<2, T>& lineDir) \n {\n return OrthogonalR(lineDir);\n }\n\n // LineNormalP N=3\n template<class T>\n inline LuVector<3, T> LineNormalP(const LuVector<3, T>& vec, const LuVector<3, T>& v1, const LuVector<3, T>& v2) \n {\n LuVector<3, T> v12 = Unit(v2 - v1);\n LuVector<3, T> ort = vec - ProjLineL(vec, v1, v12);\n T len = Length(ort);\n return len > 0 ? ort / len : LineNormalL(v12);\n }\n\n // LineNormalP N=2\n template<class T>\n inline LuVector<2, T> LineNormalP(const LuVector<2, T>& vec, const LuVector<2, T>& v1, const LuVector<2, T>& v2) \n {\n LuVector<2, T> v12 = Unit(v2 - v1);\n LuVector<2, T> ort = vec - ProjLineL(vec, v1, v12);\n T len = Length(ort);\n return len > 0 ? ort / len : LineNormalL(v12);\n }\n\n // LineNormalPL N=3\n template<class T>\n inline LuVector<3, T> LineNormalPL(const LuVector<3, T>& vec, const LuVector<3, T>& v1, const LuVector<3, T>& lineDir) \n {\n LuVector<3, T> ort = vec - ProjLineL(vec, v1, lineDir);\n T len = Length(ort);\n return len > 0 ? ort / len : LineNormalL(lineDir);\n }\n\n // LineNormalPL N=2\n template<class T>\n inline LuVector<2, T> LineNormalPL(const LuVector<2, T>& vec, const LuVector<2, T>& v1, const LuVector<2, T>& lineDir) \n {\n LuVector<2, T> ort = vec - ProjLineL(vec, v1, lineDir);\n T len = Length(ort);\n return len > 0 ? ort / len : LineNormalL(lineDir);\n }\n\n //////////////////////////////////////////////////////////////\n\n // ProjPlane\n template<class T>\n inline LuVector<3, T> ProjPlane(const LuVector<3, T>& vec, const LuVector<3, T>& v1, const LuVector<3, T>& n) \n {\n return vec - Dot(vec - v1, n) * n;\n }\n\n // PlaneNormal\n template<class T>\n inline LuVector<3, T> PlaneNormal(const LuVector<3, T>& v1, const LuVector<3, T>& v2, const LuVector<3, T>& v3)\n {\n return Unit(Cross(v2 - v1, v3 - v1));\n }\n\n // PlaneNormalP\n template<class T>\n inline LuVector<3, T> PlaneNormalP( const LuVector<3, T>& vec, const LuVector<3, T>& v1, const LuVector<3, T>& v2, const LuVector<3, T>& v3) \n {\n LuVector<3, T> n = PlaneNormal(v1, v2, v3);\n return Dot(n, vec - v1) > 0 ? n : -n;\n }\n\n // PlaneNormalPN\n template<class T>\n inline LuVector<3, T> PlaneNormalPN( const LuVector<3, T>& vec, const LuVector<3, T>& v1, const LuVector<3, T>& n) \n {\n return Dot(n, vec - v1)> 0 ? n : -n;\n }\n\n};\n#endif"
}
{
"filename": "code/computational_geometry/src/sphere_tetrahedron_intersection/sphere_tetrahedron_intersection.cpp",
"content": "/*\n * Analytical calculations for sphere-tetrahedron intersections.\n *\n * See here for it's mathematical description:\n * https://www.quora.com/What-are-some-equations-that-you-made-up/answer/Mahmut-Akku%C5%9F\n *\n * - RedBlight\n *\n */\n\n#include <cmath>\n#include \"LuVector.hpp\"\nusing Vec3 = LUV::LuVector<3, double>;\n\n// Returns the solid angle of sphere cap contended by a cone.\n// Apex of the cone is center of the sphere.\ndouble SolidAngleCap(double apexAngle)\n{\n return 2.0 * LUV::pi * (1.0 - std::cos(apexAngle));\n}\n\n// Returns the solid angle of a spherical triangle.\n// v1, v2, v3 are vertices of the triangle residing on a unit sphere.\ndouble SolidAngleSphtri(const Vec3& v1, const Vec3& v2, const Vec3& v3)\n{\n double ang0 = std::acos(LUV::Dot(v1, v2));\n double ang1 = std::acos(LUV::Dot(v2, v3));\n double ang2 = std::acos(LUV::Dot(v3, v1));\n double angSum = (ang0 + ang1 + ang2) / 2.0;\n return 4.0 * std::atan(std::sqrt(\n std::tan(angSum / 2.0) *\n std::tan((angSum - ang0) / 2.0) *\n std::tan((angSum - ang1) / 2.0) *\n std::tan((angSum - ang2) / 2.0)\n ));\n}\n\n// Returns the volume of spherical cap.\ndouble VolumeCap(double radius, double height)\n{\n return radius * radius * height * LUV::pi * (2.0 / 3.0);\n}\n\n// Returns the volume of cone.\ndouble VolumeCone(double radius, double height)\n{\n return radius * radius * height * LUV::pi * (1.0 / 3.0);\n}\n\n// Returns the volume of tetrahedron given it's 4 vertices.\ndouble VolumeTetrahedron(const Vec3& v1, const Vec3& v2, const Vec3& v3, const Vec3& v4)\n{\n return std::abs(LUV::Dot(v1 - v4, LUV::Cross(v2 - v4, v3 - v4))) / 6.0;\n}\n\n// Returns the volume of triangular slice taken from a sphere.\ndouble VolumeSphtri(const Vec3& v1, const Vec3& v2, const Vec3& v3, double radius)\n{\n return SolidAngleSphtri(v1, v2, v3) * radius * radius * radius / 3.0;\n}\n\n/*\n * Observation tetrahedra (OT) are special tetrahedra constructed for analytical calculations.\n * OTs have right angles in many of their corners, and one of their vertices are the center of the sphere.\n * For this reason, their intersections with the sphere can be calculated analytically.\n * When 24 OTs contructed for each vertex, of each edge, of each face, of any arbitrary tetrahedron,\n * their combinations can be used to analytically calculate intersections of any arbitrary sphere and tetrahedron.\n *\n */\nclass ObservationTetrahedron\n{\npublic:\n\n // Cartesian coordinates of vertices:\n Vec3 posR, posA, posB, posC;\n\n // Distances from posR:\n double lenA, lenB, lenC;\n\n // Direction of sphere-edge intersection points as radius increases:\n Vec3 prdA, prdB, prdC, prdD, prdE, prdF;\n\n // Some angles between lines:\n double angBAC, angERA, angFAE;\n\n // Max. solid angle contended by OT\n double solidAngleFull;\n\n // Constructor\n ObservationTetrahedron(const Vec3& vR, const Vec3& vA, const Vec3& vB, const Vec3& vC)\n {\n posR = vR;\n posA = vA;\n posB = vB;\n posC = vC;\n\n Vec3 AmR = vA - vR;\n Vec3 BmR = vB - vR;\n Vec3 CmR = vC - vR;\n Vec3 BmA = vB - vA;\n Vec3 CmA = vC - vA;\n Vec3 CmB = vC - vB;\n\n lenA = LUV::Length(AmR);\n lenB = LUV::Length(BmR);\n lenC = LUV::Length(CmR);\n\n prdA = AmR / lenA;\n prdB = BmR / lenB;\n prdC = CmR / lenC;\n prdD = BmA / LUV::Length(BmA);\n prdE = CmA / LUV::Length(CmA);\n prdF = CmB / LUV::Length(CmB);\n\n angBAC = std::acos(LUV::Dot(prdD, prdE));\n solidAngleFull = SolidAngleSphtri(prdA, prdB, prdC);\n }\n\n // Solid angle of the sphere subtended by OT as a function of sphere radius.\n double GetSolidAngle(double radius)\n {\n RecalculateForRadius(radius);\n\n if (radius >= lenC)\n return 0;\n\n else if (radius >= lenB)\n return SolidAngleSphtri(dirA, dirC, dirF) -\n SolidAngleCap(angERA) * angFAE / (2.0 * LUV::pi);\n\n else if (radius >= lenA)\n return solidAngleFull -\n SolidAngleCap(angERA) * angBAC / (2.0 * LUV::pi);\n\n return solidAngleFull;\n }\n\n // Surface area of the sphere subtended by OT as a function of sphere radius.\n double GetSurfaceArea(double radius)\n {\n return GetSolidAngle(radius) * radius * radius;\n }\n\n // Volume of OT-sphere intersection, as a function of sphere radius.\n double GetVolume(double radius)\n {\n RecalculateForRadius(radius);\n\n if (radius >= lenC)\n return VolumeTetrahedron(posR, posA, posB, posC);\n\n else if (radius >= lenB)\n return VolumeSphtri(dirA, dirC, dirF, radius) - (\n VolumeCap(radius, LUV::Length(prpA - posA)) -\n VolumeCone(prlE, lenA)\n ) * angFAE / (2.0 * LUV::pi) +\n VolumeTetrahedron(posR, posA, posB, prpF);\n\n else if (radius >= lenA)\n return VolumeSphtri(dirA, dirB, dirC, radius) - (\n VolumeCap(radius, LUV::Length(prpA - posA)) -\n VolumeCone(prlE, lenA)\n ) * angBAC / (2.0 * LUV::pi);\n\n return VolumeSphtri(dirA, dirB, dirC, radius);\n }\n\nprivate:\n\n // Angles of RBF triangle\n double angRBF, angBFR, angFRB;\n\n // Distance of sphere-edge intersections:\n double prlA, prlB, prlC, prlD, prlE, prlF;\n //R->A, R->B, R->C, A->B, A->C, B->C\n\n // Positions of sphere-edge intersections:\n Vec3 prpA, prpB, prpC, prpD, prpE, prpF;\n\n // Positions relative to posR:\n // All have the length = radius\n Vec3 vecA, vecB, vecC, vecD, vecE, vecF;\n\n // Directions from posR:\n Vec3 dirA, dirB, dirC, dirD, dirE, dirF;\n\n // OT vertices are not actually dependent on the radius of the sphere, only the center.\n // But some values need to be calculated for each radius.\n void RecalculateForRadius(double radius)\n {\n angRBF = std::acos(LUV::Dot(posR - posB, posC - posB));\n angBFR = std::asin(lenB * std::sin(angRBF) / radius);\n angFRB = LUV::pi - (angRBF + angBFR);\n\n prlA = radius;\n prlB = radius;\n prlC = radius;\n prlD = std::sqrt(radius * radius - lenA * lenA);\n prlE = prlD;\n prlF = lenB * std::sin(angFRB) / sin(angBFR);\n\n prpA = posR + prdA * prlA;\n prpB = posR + prdB * prlB;\n prpC = posR + prdC * prlC;\n prpD = posA + prdD * prlD;\n prpE = posA + prdE * prlE;\n prpF = posB + prdF * prlF;\n\n vecA = prpA - posR;\n vecB = prpB - posR;\n vecC = prpC - posR;\n vecD = prpD - posR;\n vecE = prpE - posR;\n vecF = prpF - posR;\n\n dirA = vecA / LUV::Length(vecA);\n dirB = vecB / LUV::Length(vecB);\n dirC = vecC / LUV::Length(vecC);\n dirD = vecD / LUV::Length(vecD);\n dirE = vecE / LUV::Length(vecE);\n dirF = vecF / LUV::Length(vecF);\n\n angERA = std::acos(LUV::Dot(dirE, dirA));\n Vec3 vecAF = prpF - posA;\n Vec3 vecAE = prpE - posA;\n angFAE = std::acos(LUV::Dot(vecAF, vecAE) / (LUV::Length(vecAF) * LUV::Length(vecAE)));\n }\n\n};\n\n// Main class for the intersection.\nclass SphereTetrahedronIntersection\n{\n\npublic:\n\n // Constructor,\n // vecTetA..D are vertices of the tetrahedron.\n // vecSphCenter is the center of the sphere.\n SphereTetrahedronIntersection(const Vec3& vecTetA, const Vec3& vecTetB, const Vec3& vecTetC,\n const Vec3& vecTetD, const Vec3& vecSphCenter)\n {\n // Adding OTs for each face of the tetrahedron.\n AddOtForFace(vecTetA, vecTetB, vecTetC, vecTetD, vecSphCenter);\n AddOtForFace(vecTetB, vecTetC, vecTetD, vecTetA, vecSphCenter);\n AddOtForFace(vecTetC, vecTetD, vecTetA, vecTetB, vecSphCenter);\n AddOtForFace(vecTetD, vecTetA, vecTetB, vecTetC, vecSphCenter);\n\n // Calculating OT signs.\n for (int idf = 0; idf < 4; ++idf)\n for (int idl = 0; idl < 3; ++idl)\n {\n int ids = 3 * idf + idl;\n int idp = 2 * ids;\n int idm = idp + 1;\n\n obsTetSgn.push_back(\n LUV::Dot(obsTet[idp].prdA, dirN[idf]) *\n LUV::Dot(obsTet[idp].prdF, dirL[ids]) *\n LUV::Dot(obsTet[idp].prdD, dirU[ids])\n );\n\n obsTetSgn.push_back(\n -1 *\n LUV::Dot(obsTet[idm].prdA, dirN[idf]) *\n LUV::Dot(obsTet[idm].prdF, dirL[ids]) *\n LUV::Dot(obsTet[idm].prdD, dirU[ids])\n );\n }\n }\n\n // Solid angle subtended by tetrahedron, as a function of sphere radius.\n double GetSolidAngle(double radius)\n {\n double solidAngle = 0;\n for (int idx = 0; idx < 24; ++idx)\n {\n double solidAngleOt = obsTet[idx].GetSolidAngle(radius) * obsTetSgn[idx];\n solidAngle += std::isnan(solidAngleOt) ? 0 : solidAngleOt;\n }\n return solidAngle;\n }\n\n // Surface area subtended by tetrahedron, as a function of sphere radius.\n double GetSurfaceArea(double radius)\n {\n return GetSolidAngle(radius) * radius * radius;\n }\n\n // Sphere-tetrahedron intersection volume, as a function of sphere radius.\n double GetVolume(double radius)\n {\n double volume = 0;\n for (int idx = 0; idx < 24; ++idx)\n {\n double volumeOt = obsTet[idx].GetVolume(radius) * obsTetSgn[idx];\n volume += std::isnan(volumeOt) ? 0 : volumeOt;\n }\n return volume;\n }\n\nprivate:\n\n std::vector<ObservationTetrahedron> obsTet; //24 in total\n std::vector<double> obsTetSgn;\n\n std::vector<Vec3> dirN;\n std::vector<Vec3> dirU;\n std::vector<Vec3> dirL;\n\n void AddOtForEdge(const Vec3& vecM, const Vec3& vecP, const Vec3& vecT, const Vec3& vecR)\n {\n Vec3 PmM = vecP - vecM;\n dirL.push_back(PmM / LUV::Length(PmM));\n Vec3 vecU = vecT - LUV::ProjLine(vecT, vecM, vecP);\n dirU.push_back(vecU / LUV::Length(vecU));\n Vec3 vecA = LUV::ProjPlane(vecR, vecM, LUV::PlaneNormal(vecM, vecP, vecT));\n Vec3 vecB = LUV::ProjLine(vecA, vecM, vecP);\n obsTet.push_back(ObservationTetrahedron(vecR, vecA, vecB, vecP));\n obsTet.push_back(ObservationTetrahedron(vecR, vecA, vecB, vecM));\n }\n\n void AddOtForFace(const Vec3& vecA, const Vec3& vecB, const Vec3& vecC, const Vec3& vecU,\n const Vec3& vecR)\n {\n Vec3 vecN = vecU - LUV::ProjPlane(vecU, vecA, LUV::PlaneNormal(vecA, vecB, vecC));\n dirN.push_back(vecN / LUV::Length(vecN));\n AddOtForEdge(vecA, vecB, vecC, vecR);\n AddOtForEdge(vecB, vecC, vecA, vecR);\n AddOtForEdge(vecC, vecA, vecB, vecR);\n }\n\n};\n\n// An example usage...\nint main()\n{\n // Tetrahedron with a volume of 1000\n Vec3 vecTetA(0, 0, 0);\n Vec3 vecTetB(10, 0, 0);\n Vec3 vecTetC(0, 20, 0);\n Vec3 vecTetD(0, 0, 30);\n\n // Center of the sphere\n Vec3 vecSphCenter(0, 0, 0);\n\n // Intersection class\n SphereTetrahedronIntersection testIntersection(vecTetA, vecTetB, vecTetC, vecTetD,\n vecSphCenter);\n\n // Demonstrating that numerical integral of surface area is equal to analytical calculation of volume:\n int stepCount = 10000;\n double radiusStart = 0;\n double radiusEnd = 30;\n double radiusDelta = (radiusEnd - radiusStart) / stepCount;\n double volumeNumerical = 0;\n for (double radius = radiusStart; radius < radiusEnd; radius += radiusDelta)\n volumeNumerical += testIntersection.GetSurfaceArea(radius) * radiusDelta;\n double volumeAnalytical = testIntersection.GetVolume(radiusEnd);\n\n // These 2 values must be almost equal:\n std::cout << volumeAnalytical << \", \" << volumeNumerical << std::endl;\n\n return 0;\n}"
}
{
"filename": "code/computational_geometry/src/sutherland_hodgeman_clipping/README.md",
"content": "## Working of Sutherland Hodgeman Polygon Clipping\n\n**Coordinates of rectangular clip window** :\n\nxmin,ymin :`200 200`\n\nxmax,ymax :`400 400`\n\n\n**Polygon to be clipped** :\n\nNumber of sides :`3`\n\nEnter the coordinates :\n\n`150 300`\n\n`300 150` \n\n`300 300`\n\n\nBefore clipping:\n\n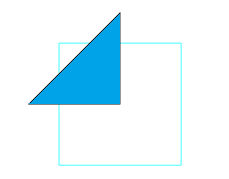\n\nAfter clipping:\n\n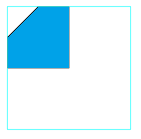\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/computational_geometry/src/sutherland_hodgeman_clipping/sutherland_hodgeman_clipping.c",
"content": "#include<stdio.h>\n#include<math.h>\n#include<graphics.h>\n#define round(a) ((int)(a+0.5))\n// Part of Cosmos by OpenGenus Foundation\nint k;\nfloat xmin,ymin,xmax,ymax,arr[20],m;\n\nvoid clipl(float x1,float y1,float x2,float y2) {\n\tif(x2-x1)\n\t\tm=(y2-y1)/(x2-x1);\n\telse\n\t\tm=100000;\n\tif(x1 >= xmin && x2 >= xmin) {\n\t\tarr[k]=x2;\n\t\tarr[k+1]=y2;\n\t\tk+=2;\n\t}\n\tif(x1 < xmin && x2 >= xmin) {\n\t\tarr[k]=xmin;\n\t\tarr[k+1]=y1+m*(xmin-x1);\n\t\tarr[k+2]=x2;\n\t\tarr[k+3]=y2;\n\t\tk+=4;\n\t}\n\tif(x1 >= xmin && x2 < xmin) {\n\t\tarr[k]=xmin;\n\t\tarr[k+1]=y1+m*(xmin-x1);\n\t\tk+=2;\n\t}\n}\n\nvoid clipt(float x1,float y1,float x2,float y2) {\n\tif(y2-y1)\n\t\tm=(x2-x1)/(y2-y1);\n\telse\n\t\tm=100000;\n\tif(y1 <= ymax && y2 <= ymax) {\n\t\tarr[k]=x2;\n\t\tarr[k+1]=y2;\n\t\tk+=2;\n\t}\n\tif(y1 > ymax && y2 <= ymax) {\n\t\tarr[k]=x1+m*(ymax-y1);\n\t\tarr[k+1]=ymax;\n\t\tarr[k+2]=x2;\n\t\tarr[k+3]=y2;\n\t\tk+=4;\n\t}\n\tif(y1 <= ymax && y2 > ymax) {\n\t\tarr[k]=x1+m*(ymax-y1);\n\t\tarr[k+1]=ymax;\n\t\tk+=2;\n\t}\n}\n\nvoid clipr(float x1,float y1,float x2,float y2) {\n\tif(x2-x1)\n\t\tm=(y2-y1)/(x2-x1);\n\telse\n\t\tm=100000;\n\tif(x1 <= xmax && x2 <= xmax) {\n\t\tarr[k]=x2;\n\t\tarr[k+1]=y2;\n\t\tk+=2;\n\t}\n\tif(x1 > xmax && x2 <= xmax) {\n\t\tarr[k]=xmax;\n\t\tarr[k+1]=y1+m*(xmax-x1);\n\t\tarr[k+2]=x2;\n\t\tarr[k+3]=y2;\n\t\tk+=4;\n\t}\n\tif(x1 <= xmax && x2 > xmax) {\n\t\tarr[k]=xmax;\n\t\tarr[k+1]=y1+m*(xmax-x1);\n\t\tk+=2;\n\t}\n}\n\nvoid clipb(float x1,float y1,float x2,float y2) {\n\tif(y2-y1)\n\t\tm=(x2-x1)/(y2-y1);\n\telse\n\t\tm=100000;\n\tif(y1 >= ymin && y2 >= ymin) {\n\t\tarr[k]=x2;\n\t\tarr[k+1]=y2;\n\t\tk+=2;\n\t}\n\tif(y1 < ymin && y2 >= ymin) {\n\t\tarr[k]=x1+m*(ymin-y1);\n\t\tarr[k+1]=ymin;\n\t\tarr[k+2]=x2;\n\t\tarr[k+3]=y2;\n\t\tk+=4;\n\t}\n\tif(y1 >= ymin && y2 < ymin) {\n\t\tarr[k]=x1+m*(ymin-y1);\n\t\tarr[k+1]=ymin;\n\t\tk+=2;\n\t}\n}\n\nvoid main() {\n\nint gdriver=DETECT,gmode,n,poly[20],i=0;\nfloat xi,yi,xf,yf,polyy[20];\n\nprintf(\"Coordinates of rectangular clip window :\\nxmin,ymin :\");\nscanf(\"%f%f\", &xmin, &ymin);\nprintf(\"xmax,ymax :\");\nscanf(\"%f%f\",&xmax,&ymax);\nprintf(\"\\n\\nPolygon to be clipped :\\nNumber of sides :\");\nscanf(\"%d\",&n);\nprintf(\"Enter the coordinates :\");\nfor(i=0;i < 2*n;i++)\n\tscanf(\"%f\",&polyy[i]);\n\npolyy[i]=polyy[0];\npolyy[i+1]=polyy[1];\n\nfor(i=0;i < 2*n+2;i++)\n\tpoly[i]=round(polyy[i]);\n\ninitgraph(&gdriver,&gmode,NULL);\nsetcolor(RED);\nrectangle(xmin,ymax,xmax,ymin);\nprintf(\"\\t\\tUNCLIPPED POLYGON\");\nsetcolor(WHITE);\nfillpoly(n,poly);\ngetch();\ncleardevice();\n\nk=0;\nfor(i=0;i < 2*n;i+=2)\n\tclipl(polyy[i],polyy[i+1],polyy[i+2],polyy[i+3]);\nn=k/2;\n\nfor(i=0;i < k;i++)\n\tpolyy[i]=arr[i];\npolyy[i]=polyy[0];\npolyy[i+1]=polyy[1];\nk=0;\n\nfor(i=0;i < 2*n;i+=2)\n\tclipt(polyy[i],polyy[i+1],polyy[i+2],polyy[i+3]);\nn=k/2;\n\nfor(i=0;i < k;i++)\n\tpolyy[i]=arr[i];\npolyy[i]=polyy[0];\npolyy[i+1]=polyy[1];\nk=0;\n\nfor(i=0;i < 2*n;i+=2)\n\tclipr(polyy[i],polyy[i+1],polyy[i+2],polyy[i+3]);\nn=k/2;\n\nfor(i=0;i < k;i++)\n\tpolyy[i]=arr[i];\npolyy[i]=polyy[0];\npolyy[i+1]=polyy[1];\nk=0;\n\nfor(i=0;i < 2*n;i+=2)\n\tclipb(polyy[i],polyy[i+1],polyy[i+2],polyy[i+3]);\n\nfor(i=0;i < k;i++)\n\tpoly[i]=round(arr[i]);\nif(k)\n\tfillpoly(k/2,poly);\n\nsetcolor(RED);\nrectangle(xmin,ymax,xmax,ymin);\nprintf(\"\\tCLIPPED POLYGON\");\ngetch();\nclosegraph();\n}"
}
{
"filename": "code/computational_geometry/src/sutherland_hodgeman_clipping/sutherland_hodgeman_clipping.cpp",
"content": "//\n// main.cpp\n// forfun\n//\n// Created by Ivan Reinaldo Liyanto on 10/5/17.\n// Copyright © 2017 Ivan Reinaldo Liyanto. All rights reserved.\n// Path of Cosmos by OpenGenus Foundation\n\n#include <iostream>\n#include <vector>\n#include <cmath>\nusing namespace std;\n\n\nstruct coor2d\n{\n float x, y;\n coor2d(float a, float b) : x(a), y(b)\n {\n }\n coor2d()\n {\n }\n};\n\nstruct edge\n{\n float x1, y1, x2, y2;\n float xnormal, ynormal;\n edge(float x1, float y1, float x2, float y2) : x1(x1), y1(y1), x2(x2), y2(y2)\n {\n float dx = x2 - x1;\n float dy = y2 - y1;\n xnormal = -dy;\n ynormal = dx;\n }\n};\n\nstruct polygon\n{\n vector<coor2d> points;\n\n polygon(const vector<coor2d> &p)\n {\n for (size_t i = 0; i < p.size(); i++)\n points.push_back(p[i]);\n }\n\n};\n\nbool inside(coor2d input, edge clipedge);\ncoor2d ComputeIntersection(coor2d a, coor2d b, edge c);\n\n\n\n/*\n * 100\n *\n * y\n *\n * 0 x 100\n */\nint main()\n{\n //since there's normal computing involved, polygon points must be defined in clockwise manner\n vector<coor2d> clipperCoords;\n //example set 1\n clipperCoords.push_back(coor2d(2, 5));\n clipperCoords.push_back(coor2d(5, 5));\n clipperCoords.push_back(coor2d(5, 1));\n clipperCoords.push_back(coor2d(2, 1));\n\n// example set 2\n// clipperCoords.push_back(coor2d(5,10));\n// clipperCoords.push_back(coor2d(10,1));\n// clipperCoords.push_back(coor2d(0,1));\n\n vector<coor2d> clippedCoords;\n // example set 1\n clippedCoords.push_back(coor2d(2, 7));\n clippedCoords.push_back(coor2d(3, 7));\n clippedCoords.push_back(coor2d(3, 3));\n clippedCoords.push_back(coor2d(2, 3));\n\n// example set 2\n// clippedCoords.push_back(coor2d(5,5));\n// clippedCoords.push_back(coor2d(15,7));\n// clippedCoords.push_back(coor2d(13,1));\n\n\n polygon clipper = polygon(clipperCoords);\n polygon clipped = polygon(clippedCoords);\n\n vector<coor2d> outputCoords;\n vector<coor2d> inputList;\n outputCoords = clipped.points;\n for (size_t i = 0; i < clipper.points.size() - 1; i++)\n {\n inputList = outputCoords;\n outputCoords.clear();\n coor2d a = clipper.points[i];\n coor2d b = clipper.points[i + 1];\n edge clipedge = edge(a.x, a.y, b.x, b.y);\n coor2d s = inputList[inputList.size() - 1];\n for (size_t j = 0; j < inputList.size(); j++)\n {\n if (inside(inputList[j], clipedge))\n {\n if (!inside(s, clipedge))\n outputCoords.push_back(ComputeIntersection(inputList[j], s, clipedge));\n outputCoords.push_back(inputList[j]);\n }\n else if (inside(s, clipedge))\n outputCoords.push_back(ComputeIntersection(inputList[j], s, clipedge));\n s = inputList[j];\n }\n }\n\n cout << \"Clipped area: \" << endl;\n for (size_t i = 0; i < outputCoords.size(); i++)\n cout << \"X: \" << outputCoords[i].x << \" Y: \" << outputCoords[i].y << endl;\n return 0;\n}\n\nbool inside(coor2d input, edge clipedge)\n{\n coor2d a = coor2d(input.x - clipedge.x1, input.y - clipedge.y1);\n float dot = a.x * clipedge.xnormal + a.y * clipedge.ynormal;\n if (dot <= 0)\n return true;\n return false;\n}\n\n\ncoor2d ComputeIntersection(coor2d ca, coor2d cb, edge edg)\n{\n float x1 = ca.x;\n float x2 = cb.x;\n float x3 = edg.x1;\n float x4 = edg.x2;\n float y1 = ca.y;\n float y2 = cb.y;\n float y3 = edg.y1;\n float y4 = edg.y2;\n\n float x12 = x1 - x2;\n float x34 = x3 - x4;\n float y12 = y1 - y2;\n float y34 = y3 - y4;\n\n float c = x12 * y34 - y12 * x34;\n\n\n float a = x1 * y2 - y1 * x2;\n float b = x3 * y4 - y3 * x4;\n\n float x = (a * x34 - b * x12) / c;\n float y = (a * y34 - b * y12) / c;\n return coor2d(x, y);\n\n}"
}
{
"filename": "code/computational_geometry/test/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "",
"content": ""
}
{
"filename": "code/computer_graphics/src/circle_drawing_algorithm/mid_point_algorithm/mid_point_algorithm.c",
"content": "#include<stdio.h>\n#include<graphics.h>\n#include<conio.h>\nint main(){\n int gd=DETECT,gm;\n int xc,yc,x=0,y=0,r,p;\n initgraph(&gd,&gm,\"C://TURBOC3//BGI\");\n printf(\"Enter center and radious:\\t\");\n scanf(\"%d%d%d\",&xc,&yc,&r);\n p=1-r;\n y=r;\n cleardevice();\n while(x<=y){\n if(p<0){\n x=x+1;\n y=y;\n p=p+2*x+1;\n }\n else{\n x=x+1;\n y=y-1;\n p=p+2*x+1-2*y;\n }\n putpixel(x+xc,y+yc,WHITE);\n putpixel(x+xc,-y+yc,WHITE);\n putpixel(-x+xc,y+yc,WHITE);\n putpixel(-x+xc,-y+yc,WHITE);\n putpixel(y+xc,x+yc,WHITE);\n putpixel(y+xc,-x+yc,WHITE);\n putpixel(-y+xc,x+yc,WHITE);\n putpixel(-y+xc,-x+yc,WHITE);\n\n }\n getch();\n closegraph();\n}"
}
{
"filename": "code/computer_graphics/src/diamond_square/diamondsquare.java",
"content": "import java.util.Scanner;\n\n/**\n * @author Samy Metadjer\n * Diamond Square algorithm is a method allowing to generate\n * heightmaps for computer graphics by using a 2D Grid.\n */\npublic class DiamondSquare{\n public static void main(String[] args){\n Scanner sc = new Scanner(System.in);\n\n System.out.println(\"The size of your matrix needs to be 2^n+1. Enter the number n please : \");\n double size = Math.pow(2, sc.nextInt())+1;\n double[][] matrix = new double[(int)size][(int)size];\n diamondSquare(matrix);\n\n for(int i = 0; i < matrix.length; i++){\n for(int j = 0; j < matrix.length; j++){\n System.out.println(\"matrix[\" + i + \"][\" + j + \"] = \" + matrix[i][j]);\n }\n }\n }\n\n /**\n * Generates random value for each cell of the given array.\n * @param matrix the array to be manipulated\n */\n public static void diamondSquare(double[][] matrix){\n int height = matrix.length;\n int boundary = 40;\n int i = height - 1;\n int id;\n int shift;\n\n /*\n ** Set a random value to each corner of the matrix (Seeds values).\n ** It's the first square step of the algorithm\n */\n matrix[0][0] = (Math.random() * (boundary - (-boundary) )) + (-boundary);\n matrix[0][height-1] = (Math.random() * (boundary - (-boundary) )) + (-boundary);\n matrix[height-1][0] = (Math.random() * (boundary - (-boundary) )) + (-boundary);\n matrix[height-1][height-1] = (Math.random() * (boundary - (-boundary) )) + (-boundary);\n\n while (i > 1) {\n id = i / 2;\n for (int xIndex = id; xIndex < height; xIndex += i) { // Beginning of the Diamond Step\n for (int yIndex = id; yIndex < height; yIndex += i) {\n double moyenne = (matrix[xIndex - id][yIndex - id]\n + matrix[xIndex - id][yIndex + id]\n + matrix[xIndex + id][yIndex + id]\n + matrix[xIndex + id][yIndex - id]) / 4;\n matrix[xIndex][yIndex] = moyenne + (Math.random() * (height - (-height) )) + (-height);\n }\n }\n for (int xIndex = 0; xIndex < height; xIndex += id) { // Beginning of the Square Step\n if (xIndex % i == 0) {\n shift = id;\n } else {\n shift = 0;\n }\n for (int yIndex = shift; yIndex < height; yIndex += i) {\n int somme = 0;\n int n = 0;\n if (xIndex >= id) {\n somme += matrix[xIndex - id][yIndex];\n n += 1;\n }\n if (xIndex + id < height) {\n somme += matrix[xIndex + id][yIndex];\n n += 1;\n }\n if (yIndex >= id) {\n somme += matrix[xIndex][yIndex - id];\n n += 1;\n }\n if (yIndex + id < height) {\n somme += matrix[xIndex][yIndex + id];\n n += 1;\n }\n matrix[xIndex][yIndex] = somme / n + (Math.random() * (height - (-height) )) + (-height);\n }\n }\n i = id;\n }\n }\n}"
}
{
"filename": "code/computer_graphics/src/diamond_square/diamond_square.py",
"content": "import numpy as np\n\n\ndef show_as_height_map(height, mat):\n from mpl_toolkits.mplot3d import Axes3D\n import matplotlib.pyplot as plt\n\n x, y = np.meshgrid(np.arange(height), np.arange(height))\n fig = plt.figure()\n ax = fig.add_subplot(111, projection=\"3d\")\n ax.plot_surface(x, y, mat)\n plt.title(\"height map\")\n plt.show()\n\n\ndef update_pixel(pixel, mean, magnitude):\n return mean + (2 * pixel * magnitude) - magnitude\n\n\ndef main(n, smooth_factor, plot_enable):\n height = (1 << n) + 1\n mat = np.random.random((height, height))\n\n i = height - 1\n\n magnitude = 1\n\n # seeds init\n mat[0, 0] = update_pixel(mat[0, 0], 0, magnitude)\n mat[0, height - 1] = update_pixel(mat[0, height - 1], 0, magnitude)\n mat[height - 1, height - 1] = update_pixel(\n mat[height - 1, height - 1], 0, magnitude\n )\n mat[0, height - 1] = update_pixel(mat[0, height - 1], 0, magnitude)\n\n while i > 1:\n id_ = i >> 1\n magnitude *= smooth_factor\n for xIndex in range(id_, height, i): # Beginning of the Diamond Step\n for yIndex in range(id_, height, i):\n mean = (\n mat[xIndex - id_, yIndex - id_]\n + mat[xIndex - id_, yIndex + id_]\n + mat[xIndex + id_, yIndex + id_]\n + mat[xIndex + id_, yIndex - id_]\n ) / 4\n mat[xIndex, yIndex] = update_pixel(mat[xIndex, yIndex], mean, magnitude)\n for xIndex in range(0, height, id_): # Beginning of the Square Step\n if xIndex % i == 0:\n shift = id_\n else:\n shift = 0\n\n for yIndex in range(shift, height, i):\n sum_ = 0\n n = 0\n if xIndex >= id_:\n sum_ += mat[xIndex - id_, yIndex]\n n += 1\n\n if xIndex + id_ < height:\n sum_ += mat[xIndex + id_, yIndex]\n n += 1\n\n if yIndex >= id_:\n sum_ += mat[xIndex, yIndex - id_]\n n += 1\n\n if yIndex + id_ < height:\n sum_ += mat[xIndex, yIndex + id_]\n n += 1\n\n mean = sum_ / n\n mat[xIndex, yIndex] = update_pixel(mat[xIndex, yIndex], mean, magnitude)\n i = id_\n\n if plot_enable:\n show_as_height_map(height, mat)\n\n return mat\n\n\ndef check_smooth_factor(value):\n fvalue = float(value)\n if fvalue < 0 or fvalue > 1:\n raise argparse.ArgumentTypeError(\"%s is an invalid smooth factor value\" % value)\n return fvalue\n\n\ndef check_positive(value):\n ivalue = int(value)\n if ivalue <= 0:\n raise argparse.ArgumentTypeError(\"%s is an invalid positive int value\" % value)\n return ivalue\n\n\nif __name__ == \"__main__\":\n import argparse\n\n parser = argparse.ArgumentParser(description=\"machine generated calculation\")\n parser.add_argument(\n \"-n\",\n help=\"the size of the image will be 2**n + 1\",\n required=False,\n default=8,\n type=check_positive,\n )\n\n parser.add_argument(\n \"-s\",\n help=\"smooth factor, needs to be in range of [0, 1], value of 0 means image is very smooth,\"\n \"value of 1 means image is very rough\",\n required=False,\n default=0.5,\n type=check_smooth_factor,\n )\n\n parser.add_argument(\"-p\", help=\"plot with matplotlib\", action=\"store_true\")\n\n args = parser.parse_args()\n\n main(args.n, args.s, args.p)"
}
{
"filename": "code/computer_graphics/src/line_drawing_alrogrithm/dda/dda.c",
"content": "#include<stdio.h>\n#include<graphics.h>\n#include<conio.h>\n#include<math.h>\nint main(){\n int gd=DETECT, gm;\n float x1,y1,x2,y2;\n float x,y,i;\n float slope;\n float dx,dy;\n initgraph(&gd,&gm,\"C://TURBOC3//BGI\");\n scanf(\"%f %f %f %f\", &x1,&y1,&x2,&y2);\n\n dx=x2-x1;\n dy=y2-y1;\n printf(\"%f %f\", dx,dy);\n if(dx>=dy) slope=dx;\n else slope=dy;\n dx=dx/slope;\n dy=dy/slope;\n x=x1;\n y=y1;\n i=1;\n while(i<=slope){\n putpixel((int)(x),(int)y,YELLOW);\n x=x+dx;\n y=y+dy;\n i=i+1;\n delay(10);\n }\n getch();\n closegraph();\n}"
}
{
"filename": "code/computer_graphics/src/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\nComputer Graphics is creation of pictures with the help of a computer. The end product of the computer graphics is a picture it may be a business graph, drawing, and engineering.\n\n> Computer Graphics\n\nComputer graphics algorithm is collectio of algorithm used to draw diffent kind on shape on the screen.\n\n***\n<p align=\"center\">\nA massive colaborative effort by \n<a href=\"https://github.com/OpenGenus\">OpenGenus Foundation</a>\n</p>\n\n\n***"
}
{
"filename": "",
"content": ""
}
{
"filename": "",
"content": ""
}
{
"filename": "",
"content": ""
}
{
"filename": "code/computer_graphics/src/Transformation/README.md",
"content": "# Transformation\n> Transformatino is process of modifying and re-arranging the grapics.\n\n\n## Types of transformation:-\n2D Transformation\n3D Transformation\n\n\n### 2D Transformation\n> In 2d Transformation we modify 2D graphics.\n\n#### Types of 2D Transformation\n1. Translation\n2. Rotation\n3. Scaling\n4. Reflection\n5. Shearing\n\n***\n<p align=\"center\">\nA massive colaborative effort by \n<a href=\"https://github.com/OpenGenus\">OpenGenus Foundation</a>\n</p>\n\n\n***"
}
{
"filename": "code/cryptography/src/aes_128/aes_128.cpp",
"content": "/*\n * National University from Colombia\n * Author:Andres Vargas, Jaime\n * Gitub: https://github.com/jaavargasar\n * webpage: https://jaavargasar.github.io/\n * Language: C++\n *\n * Description: Given an array 1 ( outpu1 ), an array2 (output2) and a key\n * we gotta decipher the messege and read what the messege is as a plane text.\n * we're gonna do this with the Algorithm AES 128\n *\n * original plane text:Paranoia is our profession\n */\n\n#include <iostream>\nusing namespace std;\n\n\n//S-box\nconst unsigned char sbox[256] =\n{0x63, 0x7c, 0x77, 0x7b, 0xf2, 0x6b, 0x6f, 0xc5, 0x30, 0x01, 0x67, 0x2b, 0xfe, 0xd7, 0xab, 0x76,\n 0xca, 0x82, 0xc9, 0x7d, 0xfa, 0x59, 0x47, 0xf0, 0xad, 0xd4, 0xa2, 0xaf, 0x9c, 0xa4, 0x72, 0xc0,\n 0xb7, 0xfd, 0x93, 0x26, 0x36, 0x3f, 0xf7, 0xcc, 0x34, 0xa5, 0xe5, 0xf1, 0x71, 0xd8, 0x31, 0x15,\n 0x04, 0xc7, 0x23, 0xc3, 0x18, 0x96, 0x05, 0x9a, 0x07, 0x12, 0x80, 0xe2, 0xeb, 0x27, 0xb2, 0x75,\n 0x09, 0x83, 0x2c, 0x1a, 0x1b, 0x6e, 0x5a, 0xa0, 0x52, 0x3b, 0xd6, 0xb3, 0x29, 0xe3, 0x2f, 0x84,\n 0x53, 0xd1, 0x00, 0xed, 0x20, 0xfc, 0xb1, 0x5b, 0x6a, 0xcb, 0xbe, 0x39, 0x4a, 0x4c, 0x58, 0xcf,\n 0xd0, 0xef, 0xaa, 0xfb, 0x43, 0x4d, 0x33, 0x85, 0x45, 0xf9, 0x02, 0x7f, 0x50, 0x3c, 0x9f, 0xa8,\n 0x51, 0xa3, 0x40, 0x8f, 0x92, 0x9d, 0x38, 0xf5, 0xbc, 0xb6, 0xda, 0x21, 0x10, 0xff, 0xf3, 0xd2,\n 0xcd, 0x0c, 0x13, 0xec, 0x5f, 0x97, 0x44, 0x17, 0xc4, 0xa7, 0x7e, 0x3d, 0x64, 0x5d, 0x19, 0x73,\n 0x60, 0x81, 0x4f, 0xdc, 0x22, 0x2a, 0x90, 0x88, 0x46, 0xee, 0xb8, 0x14, 0xde, 0x5e, 0x0b, 0xdb,\n 0xe0, 0x32, 0x3a, 0x0a, 0x49, 0x06, 0x24, 0x5c, 0xc2, 0xd3, 0xac, 0x62, 0x91, 0x95, 0xe4, 0x79,\n 0xe7, 0xc8, 0x37, 0x6d, 0x8d, 0xd5, 0x4e, 0xa9, 0x6c, 0x56, 0xf4, 0xea, 0x65, 0x7a, 0xae, 0x08,\n 0xba, 0x78, 0x25, 0x2e, 0x1c, 0xa6, 0xb4, 0xc6, 0xe8, 0xdd, 0x74, 0x1f, 0x4b, 0xbd, 0x8b, 0x8a,\n 0x70, 0x3e, 0xb5, 0x66, 0x48, 0x03, 0xf6, 0x0e, 0x61, 0x35, 0x57, 0xb9, 0x86, 0xc1, 0x1d, 0x9e,\n 0xe1, 0xf8, 0x98, 0x11, 0x69, 0xd9, 0x8e, 0x94, 0x9b, 0x1e, 0x87, 0xe9, 0xce, 0x55, 0x28, 0xdf,\n 0x8c, 0xa1, 0x89, 0x0d, 0xbf, 0xe6, 0x42, 0x68, 0x41, 0x99, 0x2d, 0x0f, 0xb0, 0x54, 0xbb, 0x16};\n\n//Inverted S-box\nconst unsigned char isbox[256] =\n{0x52, 0x09, 0x6a, 0xd5, 0x30, 0x36, 0xa5, 0x38, 0xbf, 0x40, 0xa3, 0x9e, 0x81, 0xf3, 0xd7, 0xfb,\n 0x7c, 0xe3, 0x39, 0x82, 0x9b, 0x2f, 0xff, 0x87, 0x34, 0x8e, 0x43, 0x44, 0xc4, 0xde, 0xe9, 0xcb,\n 0x54, 0x7b, 0x94, 0x32, 0xa6, 0xc2, 0x23, 0x3d, 0xee, 0x4c, 0x95, 0x0b, 0x42, 0xfa, 0xc3, 0x4e,\n 0x08, 0x2e, 0xa1, 0x66, 0x28, 0xd9, 0x24, 0xb2, 0x76, 0x5b, 0xa2, 0x49, 0x6d, 0x8b, 0xd1, 0x25,\n 0x72, 0xf8, 0xf6, 0x64, 0x86, 0x68, 0x98, 0x16, 0xd4, 0xa4, 0x5c, 0xcc, 0x5d, 0x65, 0xb6, 0x92,\n 0x6c, 0x70, 0x48, 0x50, 0xfd, 0xed, 0xb9, 0xda, 0x5e, 0x15, 0x46, 0x57, 0xa7, 0x8d, 0x9d, 0x84,\n 0x90, 0xd8, 0xab, 0x00, 0x8c, 0xbc, 0xd3, 0x0a, 0xf7, 0xe4, 0x58, 0x05, 0xb8, 0xb3, 0x45, 0x06,\n 0xd0, 0x2c, 0x1e, 0x8f, 0xca, 0x3f, 0x0f, 0x02, 0xc1, 0xaf, 0xbd, 0x03, 0x01, 0x13, 0x8a, 0x6b,\n 0x3a, 0x91, 0x11, 0x41, 0x4f, 0x67, 0xdc, 0xea, 0x97, 0xf2, 0xcf, 0xce, 0xf0, 0xb4, 0xe6, 0x73,\n 0x96, 0xac, 0x74, 0x22, 0xe7, 0xad, 0x35, 0x85, 0xe2, 0xf9, 0x37, 0xe8, 0x1c, 0x75, 0xdf, 0x6e,\n 0x47, 0xf1, 0x1a, 0x71, 0x1d, 0x29, 0xc5, 0x89, 0x6f, 0xb7, 0x62, 0x0e, 0xaa, 0x18, 0xbe, 0x1b,\n 0xfc, 0x56, 0x3e, 0x4b, 0xc6, 0xd2, 0x79, 0x20, 0x9a, 0xdb, 0xc0, 0xfe, 0x78, 0xcd, 0x5a, 0xf4,\n 0x1f, 0xdd, 0xa8, 0x33, 0x88, 0x07, 0xc7, 0x31, 0xb1, 0x12, 0x10, 0x59, 0x27, 0x80, 0xec, 0x5f,\n 0x60, 0x51, 0x7f, 0xa9, 0x19, 0xb5, 0x4a, 0x0d, 0x2d, 0xe5, 0x7a, 0x9f, 0x93, 0xc9, 0x9c, 0xef,\n 0xa0, 0xe0, 0x3b, 0x4d, 0xae, 0x2a, 0xf5, 0xb0, 0xc8, 0xeb, 0xbb, 0x3c, 0x83, 0x53, 0x99, 0x61,\n 0x17, 0x2b, 0x04, 0x7e, 0xba, 0x77, 0xd6, 0x26, 0xe1, 0x69, 0x14, 0x63, 0x55, 0x21, 0x0c, 0x7d};\n\n//E Table\nconst unsigned char etable[256] =\n{0x01, 0x03, 0x05, 0x0F, 0x11, 0x33, 0x55, 0xFF, 0x1A, 0x2E, 0x72, 0x96, 0xA1, 0xF8, 0x13, 0x35,\n 0x5F, 0xE1, 0x38, 0x48, 0xD8, 0x73, 0x95, 0xA4, 0xF7, 0x02, 0x06, 0x0A, 0x1E, 0x22, 0x66, 0xAA,\n 0xE5, 0x34, 0x5C, 0xE4, 0x37, 0x59, 0xEB, 0x26, 0x6A, 0xBE, 0xD9, 0x70, 0x90, 0xAB, 0xE6, 0x31,\n 0x53, 0xF5, 0x04, 0x0C, 0x14, 0x3C, 0x44, 0xCC, 0x4F, 0xD1, 0x68, 0xB8, 0xD3, 0x6E, 0xB2, 0xCD,\n 0x4C, 0xD4, 0x67, 0xA9, 0xE0, 0x3B, 0x4D, 0xD7, 0x62, 0xA6, 0xF1, 0x08, 0x18, 0x28, 0x78, 0x88,\n 0x83, 0x9E, 0xB9, 0xD0, 0x6B, 0xBD, 0xDC, 0x7F, 0x81, 0x98, 0xB3, 0xCE, 0x49, 0xDB, 0x76, 0x9A,\n 0xB5, 0xC4, 0x57, 0xF9, 0x10, 0x30, 0x50, 0xF0, 0x0B, 0x1D, 0x27, 0x69, 0xBB, 0xD6, 0x61, 0xA3,\n 0xFE, 0x19, 0x2B, 0x7D, 0x87, 0x92, 0xAD, 0xEC, 0x2F, 0x71, 0x93, 0xAE, 0xE9, 0x20, 0x60, 0xA0,\n 0xFB, 0x16, 0x3A, 0x4E, 0xD2, 0x6D, 0xB7, 0xC2, 0x5D, 0xE7, 0x32, 0x56, 0xFA, 0x15, 0x3F, 0x41,\n 0xC3, 0x5E, 0xE2, 0x3D, 0x47, 0xC9, 0x40, 0xC0, 0x5B, 0xED, 0x2C, 0x74, 0x9C, 0xBF, 0xDA, 0x75,\n 0x9F, 0xBA, 0xD5, 0x64, 0xAC, 0xEF, 0x2A, 0x7E, 0x82, 0x9D, 0xBC, 0xDF, 0x7A, 0x8E, 0x89, 0x80,\n 0x9B, 0xB6, 0xC1, 0x58, 0xE8, 0x23, 0x65, 0xAF, 0xEA, 0x25, 0x6F, 0xB1, 0xC8, 0x43, 0xC5, 0x54,\n 0xFC, 0x1F, 0x21, 0x63, 0xA5, 0xF4, 0x07, 0x09, 0x1B, 0x2D, 0x77, 0x99, 0xB0, 0xCB, 0x46, 0xCA,\n 0x45, 0xCF, 0x4A, 0xDE, 0x79, 0x8B, 0x86, 0x91, 0xA8, 0xE3, 0x3E, 0x42, 0xC6, 0x51, 0xF3, 0x0E,\n 0x12, 0x36, 0x5A, 0xEE, 0x29, 0x7B, 0x8D, 0x8C, 0x8F, 0x8A, 0x85, 0x94, 0xA7, 0xF2, 0x0D, 0x17,\n 0x39, 0x4B, 0xDD, 0x7C, 0x84, 0x97, 0xA2, 0xFD, 0x1C, 0x24, 0x6C, 0xB4, 0xC7, 0x52, 0xF6, 0x01};\n\n//L ,Table\nconst unsigned char ltable[256] =\n{0x00, 0x00, 0x19, 0x01, 0x32, 0x02, 0x1A, 0xC6, 0x4B, 0xC7, 0x1B, 0x68, 0x33, 0xEE, 0xDF, 0x03,\n 0x64, 0x04, 0xE0, 0x0E, 0x34, 0x8D, 0x81, 0xEF, 0x4C, 0x71, 0x08, 0xC8, 0xF8, 0x69, 0x1C, 0xC1,\n 0x7D, 0xC2, 0x1D, 0xB5, 0xF9, 0xB9, 0x27, 0x6A, 0x4D, 0xE4, 0xA6, 0x72, 0x9A, 0xC9, 0x09, 0x78,\n 0x65, 0x2F, 0x8A, 0x05, 0x21, 0x0F, 0xE1, 0x24, 0x12, 0xF0, 0x82, 0x45, 0x35, 0x93, 0xDA, 0x8E,\n 0x96, 0x8F, 0xDB, 0xBD, 0x36, 0xD0, 0xCE, 0x94, 0x13, 0x5C, 0xD2, 0xF1, 0x40, 0x46, 0x83, 0x38,\n 0x66, 0xDD, 0xFD, 0x30, 0xBF, 0x06, 0x8B, 0x62, 0xB3, 0x25, 0xE2, 0x98, 0x22, 0x88, 0x91, 0x10,\n 0x7E, 0x6E, 0x48, 0xC3, 0xA3, 0xB6, 0x1E, 0x42, 0x3A, 0x6B, 0x28, 0x54, 0xFA, 0x85, 0x3D, 0xBA,\n 0x2B, 0x79, 0x0A, 0x15, 0x9B, 0x9F, 0x5E, 0xCA, 0x4E, 0xD4, 0xAC, 0xE5, 0xF3, 0x73, 0xA7, 0x57,\n 0xAF, 0x58, 0xA8, 0x50, 0xF4, 0xEA, 0xD6, 0x74, 0x4F, 0xAE, 0xE9, 0xD5, 0xE7, 0xE6, 0xAD, 0xE8,\n 0x2C, 0xD7, 0x75, 0x7A, 0xEB, 0x16, 0x0B, 0xF5, 0x59, 0xCB, 0x5F, 0xB0, 0x9C, 0xA9, 0x51, 0xA0,\n 0x7F, 0x0C, 0xF6, 0x6F, 0x17, 0xC4, 0x49, 0xEC, 0xD8, 0x43, 0x1F, 0x2D, 0xA4, 0x76, 0x7B, 0xB7,\n 0xCC, 0xBB, 0x3E, 0x5A, 0xFB, 0x60, 0xB1, 0x86, 0x3B, 0x52, 0xA1, 0x6C, 0xAA, 0x55, 0x29, 0x9D,\n 0x97, 0xB2, 0x87, 0x90, 0x61, 0xBE, 0xDC, 0xFC, 0xBC, 0x95, 0xCF, 0xCD, 0x37, 0x3F, 0x5B, 0xD1,\n 0x53, 0x39, 0x84, 0x3C, 0x41, 0xA2, 0x6D, 0x47, 0x14, 0x2A, 0x9E, 0x5D, 0x56, 0xF2, 0xD3, 0xAB,\n 0x44, 0x11, 0x92, 0xD9, 0x23, 0x20, 0x2E, 0x89, 0xB4, 0x7C, 0xB8, 0x26, 0x77, 0x99, 0xE3, 0xA5,\n 0x67, 0x4A, 0xED, 0xDE, 0xC5, 0x31, 0xFE, 0x18, 0x0D, 0x63, 0x8C, 0x80, 0xC0, 0xF7, 0x70, 0x07};\n\nconst unsigned char Rcon[11] = {\n 0x8d, 0x01, 0x02, 0x04, 0x08, 0x10, 0x20, 0x40, 0x80, 0x1b, 0x36};\n\nconst unsigned char invMix[4][4] =\n{ {0x0e, 0x0b, 0x0d, 0x09},\n {0x09, 0x0e, 0x0b, 0x0d},\n {0x0d, 0x09, 0x0e, 0x0b},\n {0x0b, 0x0d, 0x09, 0x0e} };\n\nunsigned char ini_keys[4][4] =\n{ {0x2b, 0x28, 0xab, 0x09},\n {0x7e, 0xae, 0xf7, 0xcf},\n {0x15, 0xd2, 0x15, 0x4f},\n {0x16, 0xa6, 0x88, 0x3c} };\n\nunsigned char output1[4][4] =\n{ {0x11, 0xe1, 0xa4, 0x50},\n {0x04, 0x63, 0x26, 0x4b},\n {0x14, 0x67, 0x92, 0x7d},\n {0xd9, 0xce, 0x82, 0xae} };\n\nunsigned char output2[4][4] =\n{ {0xb2, 0x51, 0x2b, 0x48},\n {0x7e, 0x0c, 0x11, 0xc1},\n {0x32, 0x0f, 0xee, 0x38},\n {0xee, 0x1a, 0x9d, 0x06} };\n\nunsigned char keys[44][4];\n\n// ----------------------------------- KEYS --------------------------------(begin)\n//inicialize the key arrays\nvoid init()\n{\n for (int i = 0; i < 4; i++)\n for (int j = 0; j < 4; j++)\n keys[i][j] = ini_keys[i][j];\n\n}\n\n//rotate the keys through the left\nvoid shitrow(int pos )\n{\n int aux = pos + 4;\n for (int i = aux; i < aux + 3; i++)\n keys[i][3] = keys[++pos][3];\n\n keys[aux + 3][3] = keys[pos - 3][3];\n}\n\n//do the subword\nvoid subword(const int& pos)\n{\n int aux = pos + 4;\n for (int i = aux; i < aux + 4; i++)\n keys[i][3] = sbox[ keys[i][3] ];\n}\n\n//do the rcon step\nvoid rcon(const int& pos, const int& index )\n{\n int aux = pos + 4;\n keys[aux][3] ^= Rcon[ index];\n}\n\n//create a new round key\nvoid newKey(int pos)\n{\n int org = pos;\n int aux = pos + 4, ini = 3;\n for (int k = 0; k < 4; k++)\n {\n ini = (ini + 1) % 4;\n pos = org;\n for (int i = aux; i < aux + 4; i++)\n keys[i][ini] = ( keys[pos++][ini] ^ keys[i][ (ini + 4 - 1) % 4 ] );\n }\n}\n\n//find all the posible keys\nvoid expandkeys()\n{\n int cnt = 1;\n for (int i = 0; i < 44; i = i + 4)\n {\n shitrow(i);\n subword(i);\n rcon(i, cnt++);\n newKey(i);\n }\n\n}\n\nvoid findAllKeys()\n{\n //we gotta find first all the keys\n init();\n expandkeys();\n\n}\n// ----------------------------------- KEYS --------------------------------(end)\n\n\n//---------------------------------Decrypt--------------------------------(begin)\n//do the add round key\nvoid addRoundKey(int pos)\n{\n for (int i = 0; i < 4; i++)\n for (int j = 0; j < 4; j++)\n {\n output1[i][j] ^= ( keys[pos + i][j] );\n output2[i][j] ^= ( keys[pos + i][j] );\n }\n\n}\n\n//roate the state through the right by rows\nvoid shifrigth( )\n{\n for (int i = 1; i <= 2; i++)\n for (int j = 3; j >= i; j--)\n {\n swap( output1[i][j], output1[i][j - i] );\n swap( output2[i][j], output2[i][j - i] );\n }\n\n for (int j = 0; j < 3; j++)\n {\n swap(output1[3][j], output1[3][j + 1] );\n swap(output2[3][j], output2[3][j + 1] );\n }\n\n}\n\n// do the inverse of the subword\nvoid invsubWord( )\n{\n for (int i = 0; i < 4; i++)\n for (int j = 0; j < 4; j++)\n {\n output1[i][j] = isbox[ output1[i][j] ];\n output2[i][j] = isbox[ output2[i][j] ];\n }\n\n}\n//do galois field\nunsigned char gaz(unsigned char a, unsigned char b)\n{\n return etable [ (ltable[a] + ltable[b]) % 0xFF ];\n}\n\n//do mix colums step\nvoid mixColums()\n{\n unsigned char aux[4][4];\n unsigned char aux2[4][4];\n\n for (int i = 0; i < 4; i++)\n for (int j = 0; j < 4; j++)\n {\n aux[j][i] = gaz( output1[0][i], invMix[j][0]) ^ gaz( output1[1][i], invMix[j][1]) ^ gaz(\n output1[2][i], invMix[j][2]) ^ gaz( output1[3][i], invMix[j][3]);\n aux2[j][i] =\n gaz( output2[0][i],\n invMix[j][0]) ^\n gaz( output2[1][i],\n invMix[j][1]) ^\n gaz( output2[2][i], invMix[j][2]) ^ gaz( output2[3][i], invMix[j][3]);\n }\n\n for (int i = 0; i < 4; i++)\n for (int j = 0; j < 4; j++)\n output1[i][j] = aux[i][j];\n\n for (int i = 0; i < 4; i++)\n for (int j = 0; j < 4; j++)\n output2[i][j] = aux2[i][j];\n\n}\n\n//print the state in hexadecimal\nvoid print()\n{\n\n for (int i = 0; i < 4; i++)\n {\n cout << endl;\n for (int j = 0; j < 4; j++)\n cout << hex << output1[i][j] << \" \";\n //cout<<hex<<output2[i][j]<<\" \";\n }\n cout << endl;\n\n}\n\n//Decipher Algorithm\nvoid decrypt( )\n{\n addRoundKey(40);\n shifrigth();\n invsubWord();\n addRoundKey(36);\n for (int i = 32; i >= 0; i = i - 4)\n {\n mixColums();\n shifrigth();\n invsubWord();\n addRoundKey(i);\n }\n}\n\n//---------------------------------Decrypt--------------------------------(end)\n\n\n//main\nint main()\n{\n\n findAllKeys(); //given a initial key\n decrypt(); //AES 128\n\n //print the plan text of the output1\n for (int i = 0; i < 4; i++)\n {\n cout << endl;\n for (int j = 0; j < 4; j++)\n cout << output1[j][i] << \" \";\n }\n cout << endl;\n\n //print plan text of output2\n for (int i = 0; i < 4; i++)\n {\n cout << endl;\n for (int j = 0; j < 4; j++)\n cout << output2[j][i] << \" \";\n }\n\n return 0;\n}"
}
{
"filename": "code/cryptography/src/aes_128/aes_128.py",
"content": "# AES 128\n# By: David Beltrán (github.com/debeltranc)\n# National university of Colombia\n# Language: python\n# This code demonstrates how AES works internally, for academic purposes only.\n# If you want to use AES in your production software use robust libraries\n# like PyCrypto\n\n\n# Lookup tables\n# Using lookup tables can help you to reduce time complexity, at cost of space complexity.\n\n# S-box\n# Used by the subBytes transformation\nsBox = [\n 0x63,\n 0x7C,\n 0x77,\n 0x7B,\n 0xF2,\n 0x6B,\n 0x6F,\n 0xC5,\n 0x30,\n 0x01,\n 0x67,\n 0x2B,\n 0xFE,\n 0xD7,\n 0xAB,\n 0x76,\n 0xCA,\n 0x82,\n 0xC9,\n 0x7D,\n 0xFA,\n 0x59,\n 0x47,\n 0xF0,\n 0xAD,\n 0xD4,\n 0xA2,\n 0xAF,\n 0x9C,\n 0xA4,\n 0x72,\n 0xC0,\n 0xB7,\n 0xFD,\n 0x93,\n 0x26,\n 0x36,\n 0x3F,\n 0xF7,\n 0xCC,\n 0x34,\n 0xA5,\n 0xE5,\n 0xF1,\n 0x71,\n 0xD8,\n 0x31,\n 0x15,\n 0x04,\n 0xC7,\n 0x23,\n 0xC3,\n 0x18,\n 0x96,\n 0x05,\n 0x9A,\n 0x07,\n 0x12,\n 0x80,\n 0xE2,\n 0xEB,\n 0x27,\n 0xB2,\n 0x75,\n 0x09,\n 0x83,\n 0x2C,\n 0x1A,\n 0x1B,\n 0x6E,\n 0x5A,\n 0xA0,\n 0x52,\n 0x3B,\n 0xD6,\n 0xB3,\n 0x29,\n 0xE3,\n 0x2F,\n 0x84,\n 0x53,\n 0xD1,\n 0x00,\n 0xED,\n 0x20,\n 0xFC,\n 0xB1,\n 0x5B,\n 0x6A,\n 0xCB,\n 0xBE,\n 0x39,\n 0x4A,\n 0x4C,\n 0x58,\n 0xCF,\n 0xD0,\n 0xEF,\n 0xAA,\n 0xFB,\n 0x43,\n 0x4D,\n 0x33,\n 0x85,\n 0x45,\n 0xF9,\n 0x02,\n 0x7F,\n 0x50,\n 0x3C,\n 0x9F,\n 0xA8,\n 0x51,\n 0xA3,\n 0x40,\n 0x8F,\n 0x92,\n 0x9D,\n 0x38,\n 0xF5,\n 0xBC,\n 0xB6,\n 0xDA,\n 0x21,\n 0x10,\n 0xFF,\n 0xF3,\n 0xD2,\n 0xCD,\n 0x0C,\n 0x13,\n 0xEC,\n 0x5F,\n 0x97,\n 0x44,\n 0x17,\n 0xC4,\n 0xA7,\n 0x7E,\n 0x3D,\n 0x64,\n 0x5D,\n 0x19,\n 0x73,\n 0x60,\n 0x81,\n 0x4F,\n 0xDC,\n 0x22,\n 0x2A,\n 0x90,\n 0x88,\n 0x46,\n 0xEE,\n 0xB8,\n 0x14,\n 0xDE,\n 0x5E,\n 0x0B,\n 0xDB,\n 0xE0,\n 0x32,\n 0x3A,\n 0x0A,\n 0x49,\n 0x06,\n 0x24,\n 0x5C,\n 0xC2,\n 0xD3,\n 0xAC,\n 0x62,\n 0x91,\n 0x95,\n 0xE4,\n 0x79,\n 0xE7,\n 0xC8,\n 0x37,\n 0x6D,\n 0x8D,\n 0xD5,\n 0x4E,\n 0xA9,\n 0x6C,\n 0x56,\n 0xF4,\n 0xEA,\n 0x65,\n 0x7A,\n 0xAE,\n 0x08,\n 0xBA,\n 0x78,\n 0x25,\n 0x2E,\n 0x1C,\n 0xA6,\n 0xB4,\n 0xC6,\n 0xE8,\n 0xDD,\n 0x74,\n 0x1F,\n 0x4B,\n 0xBD,\n 0x8B,\n 0x8A,\n 0x70,\n 0x3E,\n 0xB5,\n 0x66,\n 0x48,\n 0x03,\n 0xF6,\n 0x0E,\n 0x61,\n 0x35,\n 0x57,\n 0xB9,\n 0x86,\n 0xC1,\n 0x1D,\n 0x9E,\n 0xE1,\n 0xF8,\n 0x98,\n 0x11,\n 0x69,\n 0xD9,\n 0x8E,\n 0x94,\n 0x9B,\n 0x1E,\n 0x87,\n 0xE9,\n 0xCE,\n 0x55,\n 0x28,\n 0xDF,\n 0x8C,\n 0xA1,\n 0x89,\n 0x0D,\n 0xBF,\n 0xE6,\n 0x42,\n 0x68,\n 0x41,\n 0x99,\n 0x2D,\n 0x0F,\n 0xB0,\n 0x54,\n 0xBB,\n 0x16,\n]\n\n# Inverted S-box\n# Used by the invSubBytes transformation\nisBox = [\n 0x52,\n 0x09,\n 0x6A,\n 0xD5,\n 0x30,\n 0x36,\n 0xA5,\n 0x38,\n 0xBF,\n 0x40,\n 0xA3,\n 0x9E,\n 0x81,\n 0xF3,\n 0xD7,\n 0xFB,\n 0x7C,\n 0xE3,\n 0x39,\n 0x82,\n 0x9B,\n 0x2F,\n 0xFF,\n 0x87,\n 0x34,\n 0x8E,\n 0x43,\n 0x44,\n 0xC4,\n 0xDE,\n 0xE9,\n 0xCB,\n 0x54,\n 0x7B,\n 0x94,\n 0x32,\n 0xA6,\n 0xC2,\n 0x23,\n 0x3D,\n 0xEE,\n 0x4C,\n 0x95,\n 0x0B,\n 0x42,\n 0xFA,\n 0xC3,\n 0x4E,\n 0x08,\n 0x2E,\n 0xA1,\n 0x66,\n 0x28,\n 0xD9,\n 0x24,\n 0xB2,\n 0x76,\n 0x5B,\n 0xA2,\n 0x49,\n 0x6D,\n 0x8B,\n 0xD1,\n 0x25,\n 0x72,\n 0xF8,\n 0xF6,\n 0x64,\n 0x86,\n 0x68,\n 0x98,\n 0x16,\n 0xD4,\n 0xA4,\n 0x5C,\n 0xCC,\n 0x5D,\n 0x65,\n 0xB6,\n 0x92,\n 0x6C,\n 0x70,\n 0x48,\n 0x50,\n 0xFD,\n 0xED,\n 0xB9,\n 0xDA,\n 0x5E,\n 0x15,\n 0x46,\n 0x57,\n 0xA7,\n 0x8D,\n 0x9D,\n 0x84,\n 0x90,\n 0xD8,\n 0xAB,\n 0x00,\n 0x8C,\n 0xBC,\n 0xD3,\n 0x0A,\n 0xF7,\n 0xE4,\n 0x58,\n 0x05,\n 0xB8,\n 0xB3,\n 0x45,\n 0x06,\n 0xD0,\n 0x2C,\n 0x1E,\n 0x8F,\n 0xCA,\n 0x3F,\n 0x0F,\n 0x02,\n 0xC1,\n 0xAF,\n 0xBD,\n 0x03,\n 0x01,\n 0x13,\n 0x8A,\n 0x6B,\n 0x3A,\n 0x91,\n 0x11,\n 0x41,\n 0x4F,\n 0x67,\n 0xDC,\n 0xEA,\n 0x97,\n 0xF2,\n 0xCF,\n 0xCE,\n 0xF0,\n 0xB4,\n 0xE6,\n 0x73,\n 0x96,\n 0xAC,\n 0x74,\n 0x22,\n 0xE7,\n 0xAD,\n 0x35,\n 0x85,\n 0xE2,\n 0xF9,\n 0x37,\n 0xE8,\n 0x1C,\n 0x75,\n 0xDF,\n 0x6E,\n 0x47,\n 0xF1,\n 0x1A,\n 0x71,\n 0x1D,\n 0x29,\n 0xC5,\n 0x89,\n 0x6F,\n 0xB7,\n 0x62,\n 0x0E,\n 0xAA,\n 0x18,\n 0xBE,\n 0x1B,\n 0xFC,\n 0x56,\n 0x3E,\n 0x4B,\n 0xC6,\n 0xD2,\n 0x79,\n 0x20,\n 0x9A,\n 0xDB,\n 0xC0,\n 0xFE,\n 0x78,\n 0xCD,\n 0x5A,\n 0xF4,\n 0x1F,\n 0xDD,\n 0xA8,\n 0x33,\n 0x88,\n 0x07,\n 0xC7,\n 0x31,\n 0xB1,\n 0x12,\n 0x10,\n 0x59,\n 0x27,\n 0x80,\n 0xEC,\n 0x5F,\n 0x60,\n 0x51,\n 0x7F,\n 0xA9,\n 0x19,\n 0xB5,\n 0x4A,\n 0x0D,\n 0x2D,\n 0xE5,\n 0x7A,\n 0x9F,\n 0x93,\n 0xC9,\n 0x9C,\n 0xEF,\n 0xA0,\n 0xE0,\n 0x3B,\n 0x4D,\n 0xAE,\n 0x2A,\n 0xF5,\n 0xB0,\n 0xC8,\n 0xEB,\n 0xBB,\n 0x3C,\n 0x83,\n 0x53,\n 0x99,\n 0x61,\n 0x17,\n 0x2B,\n 0x04,\n 0x7E,\n 0xBA,\n 0x77,\n 0xD6,\n 0x26,\n 0xE1,\n 0x69,\n 0x14,\n 0x63,\n 0x55,\n 0x21,\n 0x0C,\n 0x7D,\n]\n\n# E Table\n# Used to perform Galois Field multiplications\neTable = [\n 0x01,\n 0x03,\n 0x05,\n 0x0F,\n 0x11,\n 0x33,\n 0x55,\n 0xFF,\n 0x1A,\n 0x2E,\n 0x72,\n 0x96,\n 0xA1,\n 0xF8,\n 0x13,\n 0x35,\n 0x5F,\n 0xE1,\n 0x38,\n 0x48,\n 0xD8,\n 0x73,\n 0x95,\n 0xA4,\n 0xF7,\n 0x02,\n 0x06,\n 0x0A,\n 0x1E,\n 0x22,\n 0x66,\n 0xAA,\n 0xE5,\n 0x34,\n 0x5C,\n 0xE4,\n 0x37,\n 0x59,\n 0xEB,\n 0x26,\n 0x6A,\n 0xBE,\n 0xD9,\n 0x70,\n 0x90,\n 0xAB,\n 0xE6,\n 0x31,\n 0x53,\n 0xF5,\n 0x04,\n 0x0C,\n 0x14,\n 0x3C,\n 0x44,\n 0xCC,\n 0x4F,\n 0xD1,\n 0x68,\n 0xB8,\n 0xD3,\n 0x6E,\n 0xB2,\n 0xCD,\n 0x4C,\n 0xD4,\n 0x67,\n 0xA9,\n 0xE0,\n 0x3B,\n 0x4D,\n 0xD7,\n 0x62,\n 0xA6,\n 0xF1,\n 0x08,\n 0x18,\n 0x28,\n 0x78,\n 0x88,\n 0x83,\n 0x9E,\n 0xB9,\n 0xD0,\n 0x6B,\n 0xBD,\n 0xDC,\n 0x7F,\n 0x81,\n 0x98,\n 0xB3,\n 0xCE,\n 0x49,\n 0xDB,\n 0x76,\n 0x9A,\n 0xB5,\n 0xC4,\n 0x57,\n 0xF9,\n 0x10,\n 0x30,\n 0x50,\n 0xF0,\n 0x0B,\n 0x1D,\n 0x27,\n 0x69,\n 0xBB,\n 0xD6,\n 0x61,\n 0xA3,\n 0xFE,\n 0x19,\n 0x2B,\n 0x7D,\n 0x87,\n 0x92,\n 0xAD,\n 0xEC,\n 0x2F,\n 0x71,\n 0x93,\n 0xAE,\n 0xE9,\n 0x20,\n 0x60,\n 0xA0,\n 0xFB,\n 0x16,\n 0x3A,\n 0x4E,\n 0xD2,\n 0x6D,\n 0xB7,\n 0xC2,\n 0x5D,\n 0xE7,\n 0x32,\n 0x56,\n 0xFA,\n 0x15,\n 0x3F,\n 0x41,\n 0xC3,\n 0x5E,\n 0xE2,\n 0x3D,\n 0x47,\n 0xC9,\n 0x40,\n 0xC0,\n 0x5B,\n 0xED,\n 0x2C,\n 0x74,\n 0x9C,\n 0xBF,\n 0xDA,\n 0x75,\n 0x9F,\n 0xBA,\n 0xD5,\n 0x64,\n 0xAC,\n 0xEF,\n 0x2A,\n 0x7E,\n 0x82,\n 0x9D,\n 0xBC,\n 0xDF,\n 0x7A,\n 0x8E,\n 0x89,\n 0x80,\n 0x9B,\n 0xB6,\n 0xC1,\n 0x58,\n 0xE8,\n 0x23,\n 0x65,\n 0xAF,\n 0xEA,\n 0x25,\n 0x6F,\n 0xB1,\n 0xC8,\n 0x43,\n 0xC5,\n 0x54,\n 0xFC,\n 0x1F,\n 0x21,\n 0x63,\n 0xA5,\n 0xF4,\n 0x07,\n 0x09,\n 0x1B,\n 0x2D,\n 0x77,\n 0x99,\n 0xB0,\n 0xCB,\n 0x46,\n 0xCA,\n 0x45,\n 0xCF,\n 0x4A,\n 0xDE,\n 0x79,\n 0x8B,\n 0x86,\n 0x91,\n 0xA8,\n 0xE3,\n 0x3E,\n 0x42,\n 0xC6,\n 0x51,\n 0xF3,\n 0x0E,\n 0x12,\n 0x36,\n 0x5A,\n 0xEE,\n 0x29,\n 0x7B,\n 0x8D,\n 0x8C,\n 0x8F,\n 0x8A,\n 0x85,\n 0x94,\n 0xA7,\n 0xF2,\n 0x0D,\n 0x17,\n 0x39,\n 0x4B,\n 0xDD,\n 0x7C,\n 0x84,\n 0x97,\n 0xA2,\n 0xFD,\n 0x1C,\n 0x24,\n 0x6C,\n 0xB4,\n 0xC7,\n 0x52,\n 0xF6,\n 0x01,\n]\n\n# L Table\n# Used to perform Galois Field multiplications\nlTable = [\n None,\n 0x00,\n 0x19,\n 0x01,\n 0x32,\n 0x02,\n 0x1A,\n 0xC6,\n 0x4B,\n 0xC7,\n 0x1B,\n 0x68,\n 0x33,\n 0xEE,\n 0xDF,\n 0x03,\n 0x64,\n 0x04,\n 0xE0,\n 0x0E,\n 0x34,\n 0x8D,\n 0x81,\n 0xEF,\n 0x4C,\n 0x71,\n 0x08,\n 0xC8,\n 0xF8,\n 0x69,\n 0x1C,\n 0xC1,\n 0x7D,\n 0xC2,\n 0x1D,\n 0xB5,\n 0xF9,\n 0xB9,\n 0x27,\n 0x6A,\n 0x4D,\n 0xE4,\n 0xA6,\n 0x72,\n 0x9A,\n 0xC9,\n 0x09,\n 0x78,\n 0x65,\n 0x2F,\n 0x8A,\n 0x05,\n 0x21,\n 0x0F,\n 0xE1,\n 0x24,\n 0x12,\n 0xF0,\n 0x82,\n 0x45,\n 0x35,\n 0x93,\n 0xDA,\n 0x8E,\n 0x96,\n 0x8F,\n 0xDB,\n 0xBD,\n 0x36,\n 0xD0,\n 0xCE,\n 0x94,\n 0x13,\n 0x5C,\n 0xD2,\n 0xF1,\n 0x40,\n 0x46,\n 0x83,\n 0x38,\n 0x66,\n 0xDD,\n 0xFD,\n 0x30,\n 0xBF,\n 0x06,\n 0x8B,\n 0x62,\n 0xB3,\n 0x25,\n 0xE2,\n 0x98,\n 0x22,\n 0x88,\n 0x91,\n 0x10,\n 0x7E,\n 0x6E,\n 0x48,\n 0xC3,\n 0xA3,\n 0xB6,\n 0x1E,\n 0x42,\n 0x3A,\n 0x6B,\n 0x28,\n 0x54,\n 0xFA,\n 0x85,\n 0x3D,\n 0xBA,\n 0x2B,\n 0x79,\n 0x0A,\n 0x15,\n 0x9B,\n 0x9F,\n 0x5E,\n 0xCA,\n 0x4E,\n 0xD4,\n 0xAC,\n 0xE5,\n 0xF3,\n 0x73,\n 0xA7,\n 0x57,\n 0xAF,\n 0x58,\n 0xA8,\n 0x50,\n 0xF4,\n 0xEA,\n 0xD6,\n 0x74,\n 0x4F,\n 0xAE,\n 0xE9,\n 0xD5,\n 0xE7,\n 0xE6,\n 0xAD,\n 0xE8,\n 0x2C,\n 0xD7,\n 0x75,\n 0x7A,\n 0xEB,\n 0x16,\n 0x0B,\n 0xF5,\n 0x59,\n 0xCB,\n 0x5F,\n 0xB0,\n 0x9C,\n 0xA9,\n 0x51,\n 0xA0,\n 0x7F,\n 0x0C,\n 0xF6,\n 0x6F,\n 0x17,\n 0xC4,\n 0x49,\n 0xEC,\n 0xD8,\n 0x43,\n 0x1F,\n 0x2D,\n 0xA4,\n 0x76,\n 0x7B,\n 0xB7,\n 0xCC,\n 0xBB,\n 0x3E,\n 0x5A,\n 0xFB,\n 0x60,\n 0xB1,\n 0x86,\n 0x3B,\n 0x52,\n 0xA1,\n 0x6C,\n 0xAA,\n 0x55,\n 0x29,\n 0x9D,\n 0x97,\n 0xB2,\n 0x87,\n 0x90,\n 0x61,\n 0xBE,\n 0xDC,\n 0xFC,\n 0xBC,\n 0x95,\n 0xCF,\n 0xCD,\n 0x37,\n 0x3F,\n 0x5B,\n 0xD1,\n 0x53,\n 0x39,\n 0x84,\n 0x3C,\n 0x41,\n 0xA2,\n 0x6D,\n 0x47,\n 0x14,\n 0x2A,\n 0x9E,\n 0x5D,\n 0x56,\n 0xF2,\n 0xD3,\n 0xAB,\n 0x44,\n 0x11,\n 0x92,\n 0xD9,\n 0x23,\n 0x20,\n 0x2E,\n 0x89,\n 0xB4,\n 0x7C,\n 0xB8,\n 0x26,\n 0x77,\n 0x99,\n 0xE3,\n 0xA5,\n 0x67,\n 0x4A,\n 0xED,\n 0xDE,\n 0xC5,\n 0x31,\n 0xFE,\n 0x18,\n 0x0D,\n 0x63,\n 0x8C,\n 0x80,\n 0xC0,\n 0xF7,\n 0x70,\n 0x07,\n]\n\n# Lookup Functions =============================================================\n\n# Function getInvsBox (decypher process)\n# input: numerical (integer) position t look up in the Inverted S-box table.\n# output: number at the position <<num>> inside the Inverted S-box table.\ndef getInvsBox(num):\n return isBox[num]\n\n\n# Function getsBox\n# input: numerical (integer) position t look up in the S-box table.\n# output: number at the position <<num>> inside the S-box table.\ndef getsBox(num):\n return sBox[num]\n\n\n# ===============================================================================\n\n\n# Galois field multiplication =================================================\n# The multiplication is simply the result of a lookup of the L-Table, followed\n# by the addition of the results, followed by a lookup to the E-Table.\ndef gMult(a, b):\n # special case check: mutiplying by zero\n if a == 0x00 or b == 0x00:\n return 0x00\n # special case check: multiplying by one\n elif a == 0x01 or b == 0x01:\n return a * b\n # if no special conditions, then lookup on the E-Table the result of adding\n # the lookup of both operands on the L-Table modulo 255\n else:\n return eTable[(lTable[a] + lTable[b]) % 0xFF]\n\n\n# ===============================================================================\n\n\n# Functions part of the cypher and decypher processes ==========================\n\n# SubBytes Transformation (cypher process)\n# Uses an S-Box to perform byte-by-byte substitution of the State matrix (stmt).\n# Purpose: (high) non-linearity, confusion by non-linear substitution.\ndef subBytes(stmt):\n rt = []\n for i in range(4):\n rt.append([])\n for j in range(4):\n rt[i].append(getsBox(stmt[i][j]))\n return rt\n\n\n# InvSubBytes Transformation (decypher process)\n# The InvSubBytes Transformation is another lookup table using the Inverse S-Box.\ndef invSubBytes(stmt):\n rt = []\n for i in range(4):\n rt.append([])\n for j in range(4):\n rt[i].append(getInvsBox(stmt[i][j]))\n return rt\n\n\n# ShiftRow transformation (cypher process)\n# The four rows of the state matrix (stmt) are shifted cyclically to the left as follows\n# row 0 is not shifted\n# row 1 is shifted cyclically by 1 position to the left\n# row 2 is shifted cyclically by 2 positions to the left\n# row 3 is shifted cyclically by 3 positions to the left\n# Purpose: high diffusion through linear operation.\ndef shiftRows(stmt):\n return [\n [stmt[0][0], stmt[0][1], stmt[0][2], stmt[0][3]],\n [stmt[1][1], stmt[1][2], stmt[1][3], stmt[1][0]],\n [stmt[2][2], stmt[2][3], stmt[2][0], stmt[2][1]],\n [stmt[3][3], stmt[3][0], stmt[3][1], stmt[3][2]],\n ]\n\n\n# InvShiftRow Transformation (decypher process)\n# The inverse of ShiftRow is obtained by shifting the rows to the right instead of the left.\ndef invShiftRows(stmt):\n return [\n [stmt[0][0], stmt[0][1], stmt[0][2], stmt[0][3]],\n [stmt[1][3], stmt[1][0], stmt[1][1], stmt[1][2]],\n [stmt[2][2], stmt[2][3], stmt[2][0], stmt[2][1]],\n [stmt[3][1], stmt[3][2], stmt[3][3], stmt[3][0]],\n ]\n\n\n# MixColumn Transformation (cypher process)\n# Each column is treated as a polynomial over GF(2^8) and is then multiplied\n# modulo (x^4)+1 with a fixed polynomial 3(x^3)+(x^2)+x+2.\n# Purpose: high diffusion through linear operation.\ndef mixColumns(stmt):\n rtmt = []\n m = [\n [0x02, 0x03, 0x01, 0x01],\n [0x01, 0x02, 0x03, 0x01],\n [0x01, 0x01, 0x02, 0x03],\n [0x03, 0x01, 0x01, 0x02],\n ]\n for i in range(4):\n rtmt.append([])\n for j in range(4):\n rtmt[i].append(\n gMult(m[i][0], stmt[0][j])\n ^ gMult(m[i][1], stmt[1][j])\n ^ gMult(m[i][2], stmt[2][j])\n ^ gMult(m[i][3], stmt[3][j])\n )\n return rtmt\n\n\n# InvMixColumn Transformation (decypher process)\n# The inverse of MixColumn exists because the 4×4 matrix used in MixColumn is invetible.\ndef InvMixColumns(stmt):\n rtmt = []\n # The transformation InvMixColumn is given by multiplying by the following matrix.\n m = [\n [0x0E, 0x0B, 0x0D, 0x09],\n [0x09, 0x0E, 0x0B, 0x0D],\n [0x0D, 0x09, 0x0E, 0x0B],\n [0x0B, 0x0D, 0x09, 0x0E],\n ]\n for i in range(4):\n rtmt.append([])\n for j in range(4):\n rtmt[i].append(\n gMult(m[i][0], stmt[0][j])\n ^ gMult(m[i][1], stmt[1][j])\n ^ gMult(m[i][2], stmt[2][j])\n ^ gMult(m[i][3], stmt[3][j])\n )\n return rtmt\n\n\n# AddRoundKey Transformation (cypher and decypher process)\n# The Round Key is bitwise XORed to the State.\ndef addRoundKey(stmt, rk):\n rt = [[], [], [], []]\n for i in range(4):\n for j in range(4):\n rt[i].append(stmt[i][j] ^ rk[i][j])\n return rt\n\n\n# ==============================================================================\n\n# Key generation functions =====================================================\n# AES-128 must first create Nr (10) subkeys as follows:\n# * From a given key k arranged into a 4×4 matrix of bytes, we label the\n# first four columns W[0], W[1], W[2], W[3].\n# * This matrix is expanded by adding 40 more columns W[4], ... , W[43]\n# which are computed recursively as follows:\n# W[i] = W[i − 4] ⊕ W[i − 1] , if i ≡ 0 (mod 4) for i ∈ [4..43]\n# W[i − 4] ⊕ T(W[i − 1]), otherwise for i ∈ [4..43]\n# where T is the transformation of W[i − 1] obtained as follows:\n# Let the elements of the column W[i − 1] be a, b, c, d. Shift these\n# cyclically to obtain b, c, d, a. Now replace each of these bytes\n# with the corresponding element in the S-Box from the ByteSub\n# transformation to get 4 bytes e, f, g, h. Finally, compute the\n# round constant r[i] = 00000010^((i−4)/4) in GF(2^8) then T(W[i − 1])\n# is the columnvector (e ⊕ r[i], f, g, h)\n# * The round key for the ith round consist of the columns W[4i], W[4i+1],\n# W[4i + 2], W[4i + 3].\n\n# rotWord\n# The rotate operation takes a 32-bit word and rotates it eight bits to the left\n# such that the high eight bits \"wrap around\" and become the low eight bits of the result.\ndef rotWord(rw):\n return [rw[1], rw[2], rw[3], rw[0]]\n\n\n# subWord\n# The key schedule uses Rijndael's S-box.\ndef subWord(rw):\n ret = []\n for i in rw:\n ret.append(getsBox(i))\n return ret\n\n\n# rcon\n# Rcon is what the Rijndael documentation calls the exponentiation of 2 to a user-specified value.\ndef rcon(r, rw):\n if 1 <= r <= 8:\n return [rw[0] ^ (1 << (r - 1)), rw[1], rw[2], rw[3]]\n elif r == 9:\n return [rw[0] ^ 0x1B, rw[1], rw[2], rw[3]]\n elif r == 10:\n return [rw[0] ^ 0x36, rw[1], rw[2], rw[3]]\n\n\n# transpose\n# not really a key generation function, just a matrix transposition operation\ndef transpose(matrix):\n return list(zip(*matrix))\n\n\n# Key generation\n# This function returns 10 subkeys following the Rijndael key schedule\ndef keyGen(keymt):\n roundKeys = {\n 0: keymt,\n 1: [],\n 2: [],\n 3: [],\n 4: [],\n 5: [],\n 6: [],\n 7: [],\n 8: [],\n 9: [],\n 10: [],\n }\n for i in range(1, 11):\n lrk = transpose(roundKeys[i - 1])\n tr = rcon(i, subWord(rotWord(lrk[-1])))\n rk = []\n for j in range(4):\n rk.append([])\n for k in range(4):\n if j == 0:\n rk[j].append(tr[k] ^ lrk[j][k])\n else:\n rk[j].append(rk[j - 1][k] ^ lrk[j][k])\n roundKeys[i] = transpose(rk)\n return roundKeys\n\n\n# ===============================================================================\n\n# Single block (128 bit text) cypher and decypher operations ===================\n\n# decypher\n# inputs: cypher text as a 128-bit hex string, 128-bit hex key (as string)\n# (optional) set process to true if you want to see\n# all the steps of this process\n# output: matrix containing clear message in hexadecimal ASCII\ndef decypher(ct, ky, process=False):\n if process:\n print(\"decipher process:\")\n c1 = ct.replace(\" \", \"\")\n k1 = ky.replace(\" \", \"\")\n keymatrix = [\n [\n int(k1[0] + k1[1], 16),\n int(k1[8] + k1[9], 16),\n int(k1[16] + k1[17], 16),\n int(k1[24] + k1[25], 16),\n ],\n [\n int(k1[2] + k1[3], 16),\n int(k1[10] + k1[11], 16),\n int(k1[18] + k1[19], 16),\n int(k1[26] + k1[27], 16),\n ],\n [\n int(k1[4] + k1[5], 16),\n int(k1[12] + k1[13], 16),\n int(k1[20] + k1[21], 16),\n int(k1[28] + k1[29], 16),\n ],\n [\n int(k1[6] + k1[7], 16),\n int(k1[14] + k1[15], 16),\n int(k1[22] + k1[23], 16),\n int(k1[30] + k1[31], 16),\n ],\n ]\n stamatrix = [\n [\n int(c1[0] + c1[1], 16),\n int(c1[8] + c1[9], 16),\n int(c1[16] + c1[17], 16),\n int(c1[24] + c1[25], 16),\n ],\n [\n int(c1[2] + c1[3], 16),\n int(c1[10] + c1[11], 16),\n int(c1[18] + c1[19], 16),\n int(c1[26] + c1[27], 16),\n ],\n [\n int(c1[4] + c1[5], 16),\n int(c1[12] + c1[13], 16),\n int(c1[20] + c1[21], 16),\n int(c1[28] + c1[29], 16),\n ],\n [\n int(c1[6] + c1[7], 16),\n int(c1[14] + c1[15], 16),\n int(c1[22] + c1[23], 16),\n int(c1[30] + c1[31], 16),\n ],\n ]\n rkeys = keyGen(keymatrix)\n if process:\n print(\"\\n====first Stage====\")\n print(\"keys (10)\")\n printMatrix(rkeys[10])\n print(\"Add round key (10)\")\n state = addRoundKey(stamatrix, rkeys[10])\n printMatrix(state)\n print(\"Inverse Shift Rows\")\n state = invShiftRows(state)\n printMatrix(state)\n print(\"Inverse Sub Bytes\")\n state = invSubBytes(state)\n printMatrix(state)\n print(\"\\n====Second Stage====\")\n else:\n state = invSubBytes(invShiftRows(addRoundKey(stamatrix, rkeys[10])))\n for i in range(9, 0, -1):\n if process:\n print(\"keys (\" + str(i) + \")\")\n printMatrix(rkeys[i])\n print(\"Add round key (\" + str(i) + \")\")\n state = addRoundKey(state, rkeys[i])\n printMatrix(state)\n print(\"Inverse Mix Columns\")\n state = InvMixColumns(state)\n printMatrix(state)\n print(\"Inverse Shift Rows\")\n state = invShiftRows(state)\n printMatrix(state)\n print(\"Inverse Sub Bytes\")\n state = invSubBytes(state)\n printMatrix(state)\n else:\n state = invSubBytes(\n invShiftRows(InvMixColumns(addRoundKey(state, rkeys[i])))\n )\n if process:\n print(\"====Third Stage====\")\n print(\"keys (0\")\n printMatrix(rkeys[0])\n print(\"Add round key (0)\")\n state = addRoundKey(state, rkeys[0])\n printMatrix(state)\n print(\"====Result====\")\n return state\n else:\n return addRoundKey(state, rkeys[0])\n\n\n# cypher\n# inputs: clear text message as an ASCII hexadecimal 128-bit string\n# \t\t 128-bit hex key (as string)\n# (optional) set process to true if you want to see\n# all the steps of this process\n# output: matrix containing the cypher text in hexadecimal representation\ndef cypher(mt, ky, process=False):\n if process:\n print(\"cipher process:\")\n m1 = mt.replace(\" \", \"\")\n k1 = ky.replace(\" \", \"\")\n keymatrix = [\n [\n int(k1[0] + k1[1], 16),\n int(k1[8] + k1[9], 16),\n int(k1[16] + k1[17], 16),\n int(k1[24] + k1[25], 16),\n ],\n [\n int(k1[2] + k1[3], 16),\n int(k1[10] + k1[11], 16),\n int(k1[18] + k1[19], 16),\n int(k1[26] + k1[27], 16),\n ],\n [\n int(k1[4] + k1[5], 16),\n int(k1[12] + k1[13], 16),\n int(k1[20] + k1[21], 16),\n int(k1[28] + k1[29], 16),\n ],\n [\n int(k1[6] + k1[7], 16),\n int(k1[14] + k1[15], 16),\n int(k1[22] + k1[23], 16),\n int(k1[30] + k1[31], 16),\n ],\n ]\n stamatrix = [\n [\n int(m1[0] + m1[1], 16),\n int(m1[8] + m1[9], 16),\n int(m1[16] + m1[17], 16),\n int(m1[24] + m1[25], 16),\n ],\n [\n int(m1[2] + m1[3], 16),\n int(m1[10] + m1[11], 16),\n int(m1[18] + m1[19], 16),\n int(m1[26] + m1[27], 16),\n ],\n [\n int(m1[4] + m1[5], 16),\n int(m1[12] + m1[13], 16),\n int(m1[20] + m1[21], 16),\n int(m1[28] + m1[29], 16),\n ],\n [\n int(m1[6] + m1[7], 16),\n int(m1[14] + m1[15], 16),\n int(m1[22] + m1[23], 16),\n int(m1[30] + m1[31], 16),\n ],\n ]\n rkeys = keyGen(keymatrix)\n state = stamatrix\n if process:\n print(\"\\n====first Stage====\")\n print(\"keys (0)\")\n printMatrix(rkeys[0])\n print(\"add round key (0)\")\n state = addRoundKey(state, rkeys[0])\n printMatrix(state)\n print(\"\\n====Second Stage====\")\n else:\n state = addRoundKey(state, rkeys[0])\n for i in range(1, 10):\n if process:\n print(\"Sub Bytes\")\n state = subBytes(state)\n printMatrix(state)\n print(\"Shift Rows\")\n state = shiftRows(state)\n printMatrix(state)\n print(\"Mix Columns\")\n state = mixColumns(state)\n printMatrix(state)\n print(\"keys (\" + str(i) + \")\")\n printMatrix(rkeys[i])\n print(\"add round key (\" + str(i) + \")\")\n state = addRoundKey(state, rkeys[i])\n printMatrix(state)\n else:\n state = addRoundKey(mixColumns(shiftRows(subBytes(state))), rkeys[i])\n if process:\n print(\"\\n====third Stage====\")\n print(\"Sub Bytes\")\n state = subBytes(state)\n printMatrix(state)\n print(\"Shift Rows\")\n state = shiftRows(state)\n printMatrix(state)\n print(\"keys (10)\")\n printMatrix(rkeys[10])\n print(\"add round key (10)\")\n state = addRoundKey(state, rkeys[10])\n printMatrix(state)\n print(\"====Result====\")\n return transpose(zip(*state))\n else:\n return transpose(addRoundKey(shiftRows(subBytes(state)), rkeys[10]))\n\n\n# ===============================================================================\n\n# multi block text cypher and decypher =========================================\n\n# encryption\n# inputs: clear text message and 128-bit key (as hex string)\n# (optional) set process to true if you want to see\n# all the steps of this process\n# output: cypher text as an hexadecimal string\ndef encryption(message, key, process=False):\n # split the array in 16 bit chunks\n messageArray = hexStringChunks(message.strip())\n # now encrypt the message and store it in a result matrix\n cyphertextArray = list()\n for msg in messageArray:\n cyphertextArray.append(matrixToLinearString(cypher(msg, key, process)))\n # return a string containing an hexadecimal cyphertext\n return \"\".join(cyphertextArray)\n\n\n# decryption\n# inputs: cypher text and 128-bit key (as hex string)\n# (optional) set process to true if you want to see\n# all the steps of this process\n# output: clear text as string\ndef decryption(cyphertext, key, process=False):\n # split the array in 16 bit ckunks\n # and unhandled error should appear if the text given is not a cyphertext\n # not during this step but while the actual decypher is ocurring\n cyphertextArray = stringChunks(cyphertext.replace(\" \", \"\"))\n # get the cleartext and store it in a result matrix\n messageArray = list()\n for ct in cyphertextArray:\n messageArray.append(matrixToText(decypher(ct, key, process)))\n # return the message, as we fill the last matrix with spaces to have full\n # 128 bit blocks, strip the string before returning it\n return (\"\".join(messageArray)).strip()\n\n\n# ===============================================================================\n\n# Formatting functions =========================================================\n\n# Function printMatrix\n# input: two-dimensional array of integers\n# output: prints a matrix in console\ndef printMatrix(matrix):\n for i in range(len(matrix)):\n print(\"|\", end=\" \")\n for j in range(len(matrix[i])):\n print(format(matrix[i][j], \"#04x\"), end=\" \")\n print(\"|\\n\", end=\"\")\n\n\n# Function matrixToLinearString\n# input: two-dimensional array of integers\n# output: string containing byte-formatted hexadecimal numbers\ndef matrixToLinearString(matrix):\n string = \"\"\n for i in range(len(matrix)):\n for j in range(len(matrix[i])):\n string += format(matrix[i][j], \"02x\")\n return string\n\n\n# Function hexStringChunks\n# input: string of text\n# output: array containing hexadecimal representation of 16 character\n# chunks of the original string, if a chunk is smaller than\n# 16 characters, is filled with spaces\ndef hexStringChunks(string):\n return [\n str(string[i : 16 + i].ljust(16)[:16]).encode(\"utf-8\").hex()\n for i in range(0, len(string), 16)\n ]\n\n\ndef stringChunks(string):\n return [string[i : 32 + i] for i in range(0, len(string), 32)]\n\n\ndef printHexArray(array):\n print(\"[\" + \" \".join(\"0x{:02x}\".format(x) for x in array) + \"]\")\n\n\ndef matrixToText(matrix):\n nm = transpose(matrix)\n ret = \"\"\n for i in nm:\n for j in i:\n ret += format(j, \"#04x\")[2:]\n return bytes.fromhex(ret).decode(\"utf-8\")\n\n\n# ===============================================================================\n\n# Test use cases ===============================================================\nif __name__ == \"__main__\":\n # This case uses the following message \"AES es muy facil\"\n # Chosen because has exactly 16 characters (128-bit string)\n # translated into ASCII Hex code gives us 414553206573206d757920666163696c\n # the key to use is 2b7e151628aed2a6abf7158809cf4f3c\n # our expected cypher text is e448e574a374d90cc33c22af9b8eab7f\n\n m = \"41 45 53 20 65 73 20 6d 75 79 20 66 61 63 69 6c\"\n c = \"e4 48 e5 74 a3 74 d9 0c c3 3c 22 af 9b 8e ab 7f\"\n k = \"2b 7e 15 16 28 ae d2 a6 ab f7 15 88 09 cf 4f 3c\"\n newc = matrixToLinearString(cypher(m, k))\n print(\"First use case:\")\n print(\"cypher-->\\n\\t\" + newc)\n print(\"decypher the result-->\\n\\t\" + matrixToText(decypher(newc, k)))\n print(\"check the expected cypher text-->\\n\\t\" + matrixToText(decypher(c, k)))\n\n # This case uses the phrase \"Paranoia is our profession\"\n # Chosen because it has more than 16 characters (32 characters)\n # the given cypher text is 110414d9e16367cea4269282504b7daeb27e32ee510c0f1a2b11ee9d48c13806\n # the given key is 2b7e151628aed2a6abf7158809cf4f3c\n\n m = \"Paranoia is our profession\"\n c1 = \"11 04 14 d9 e1 63 67 ce a4 26 92 82 50 4b 7d ae\"\n c2 = \"b2 7e 32 ee 51 0c 0f 1a 2b 11 ee 9d 48 c1 38 06\"\n k = \"2b 7e 15 16 28 ae d2 a6 ab f7 15 88 09 cf 4f 3c\"\n\n print(\"\\n\\nSecond use case:\")\n print(\n \"using block functions-->\\n\\t\"\n + matrixToText(decypher(c1, k))\n + matrixToText(decypher(c2, k))\n )\n print(\"decryption using decryption function-->\\n\\t\" + decryption(c1 + c2, k))\n print(\"check te cypher-->\\n\\t\" + encryption(m, k))"
}
{
"filename": "code/cryptography/src/aes_128/aes_csharp/aescipher.cs",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation.\n * Author : ABDOUS Kamel\n */\n \nusing System;\nusing System.Collections.Generic;\nusing System.Linq;\nusing System.Text;\nusing System.Threading.Tasks;\n\nnamespace AES\n{\n public partial class AESCipher\n {\n // Size of MainKey will determine which version of AES to use.\n // An ArgumentException is thrown in case of unknown size.\n public AESCipher(byte[] MainKey)\n {\n this.MainKey = MainKey;\n }\n\n // Cipher a block of size AESCipher.BlockSize (see AESConsts.cs).\n // The array CipherText must be of size AESCipher.BlockSize.\n public void DoCipher(byte[] PlainText, byte[] CipherText)\n {\n byte[][] State = new byte[4][];\n int i, j;\n\n for(i = 0; i < 4; ++i)\n {\n State[i] = new byte[StateNB];\n for (j = 0; j < StateNB; ++j)\n State[i][j] = PlainText[i + StateNB * j];\n }\n\n AddRoundKey(State, 0);\n\n for(i = 1; i < NR; ++i)\n {\n SubBytes(State, SubByte_SBox);\n ShiftRows(State);\n\n MixColumns(State);\n AddRoundKey(State, i);\n }\n\n SubBytes(State, SubByte_SBox);\n ShiftRows(State);\n AddRoundKey(State, NR);\n\n for(i = 0; i < 4; ++i)\n {\n for (j = 0; j < StateNB; ++j)\n CipherText[i + StateNB * j] = State[i][j];\n }\n }\n\n protected void SubBytes(byte[][] State, byte[,] sbox)\n {\n for(int i = 0; i < 4; ++i)\n Helpers.SubBytes(State[i], sbox);\n }\n\n protected void ShiftRows(byte[][] State)\n {\n for(int i = 0; i < StateNB; ++i)\n {\n for (int j = 0; j < i; ++j)\n Helpers.ShiftBytesLeft(State[i]);\n }\n }\n\n protected void MixColumns(byte[][] State)\n {\n byte[][] buff = new byte[4][];\n int i, j;\n\n for(i = 0; i < 4; ++i)\n {\n buff[i] = new byte[StateNB];\n State[i].CopyTo(buff[i], 0);\n }\n\n for(i = 0; i < StateNB; ++i)\n {\n State[0][i] = (byte)(GF_MulArray[buff[0][i], 0] ^ GF_MulArray[buff[1][i], 1] ^ buff[2][i] ^ buff[3][i]);\n State[1][i] = (byte)(buff[0][i] ^ GF_MulArray[buff[1][i], 0] ^ GF_MulArray[buff[2][i], 1] ^ buff[3][i]);\n State[2][i] = (byte)(buff[0][i] ^ buff[1][i] ^ GF_MulArray[buff[2][i], 0] ^ GF_MulArray[buff[3][i], 1]);\n State[3][i] = (byte)(GF_MulArray[buff[0][i], 1] ^ buff[1][i] ^ buff[2][i] ^ GF_MulArray[buff[3][i], 0]);\n }\n }\n\n protected void AddRoundKey(byte[][] State, int round)\n {\n uint rkey;\n int cpt = 24;\n\n for(int i = 0; i < 4; ++i)\n {\n rkey = RoundKeys[StateNB * round + i];\n for(int j = 0; j < StateNB; ++j)\n {\n State[j][i] ^= (byte)((rkey >> cpt) & 0x000000ff);\n cpt -= 8;\n }\n }\n }\n }\n}"
}
{
"filename": "code/cryptography/src/aes_128/aes_csharp/aesconsts.cs",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation.\n * Author : ABDOUS Kamel\n */\n\nusing System;\nusing System.Collections.Generic;\nusing System.Linq;\nusing System.Text;\nusing System.Threading.Tasks;\n\nnamespace AES\n{\n public partial class AESCipher\n {\n const int StateNB = 4;\n\n public const int BlockSize = StateNB * 4;\n\n public static readonly byte[,] SubByte_SBox =\n {\n {0x63, 0x7c, 0x77, 0x7b, 0xf2, 0x6b, 0x6f, 0xc5, 0x30, 0x01, 0x67, 0x2b, 0xfe, 0xd7, 0xab, 0x76},\n {0xca, 0x82, 0xc9, 0x7d, 0xfa, 0x59, 0x47, 0xf0, 0xad, 0xd4, 0xa2, 0xaf, 0x9c, 0xa4, 0x72, 0xc0},\n {0xb7, 0xfd, 0x93, 0x26, 0x36, 0x3f, 0xf7, 0xcc, 0x34, 0xa5, 0xe5, 0xf1, 0x71, 0xd8, 0x31, 0x15},\n {0x04, 0xc7, 0x23, 0xc3, 0x18, 0x96, 0x05, 0x9a, 0x07, 0x12, 0x80, 0xe2, 0xeb, 0x27, 0xb2, 0x75},\n {0x09, 0x83, 0x2c, 0x1a, 0x1b, 0x6e, 0x5a, 0xa0, 0x52, 0x3b, 0xd6, 0xb3, 0x29, 0xe3, 0x2f, 0x84},\n {0x53, 0xd1, 0x00, 0xed, 0x20, 0xfc, 0xb1, 0x5b, 0x6a, 0xcb, 0xbe, 0x39, 0x4a, 0x4c, 0x58, 0xcf},\n {0xd0, 0xef, 0xaa, 0xfb, 0x43, 0x4d, 0x33, 0x85, 0x45, 0xf9, 0x02, 0x7f, 0x50, 0x3c, 0x9f, 0xa8},\n {0x51, 0xa3, 0x40, 0x8f, 0x92, 0x9d, 0x38, 0xf5, 0xbc, 0xb6, 0xda, 0x21, 0x10, 0xff, 0xf3, 0xd2},\n {0xcd, 0x0c, 0x13, 0xec, 0x5f, 0x97, 0x44, 0x17, 0xc4, 0xa7, 0x7e, 0x3d, 0x64, 0x5d, 0x19, 0x73},\n {0x60, 0x81, 0x4f, 0xdc, 0x22, 0x2a, 0x90, 0x88, 0x46, 0xee, 0xb8, 0x14, 0xde, 0x5e, 0x0b, 0xdb},\n {0xe0, 0x32, 0x3a, 0x0a, 0x49, 0x06, 0x24, 0x5c, 0xc2, 0xd3, 0xac, 0x62, 0x91, 0x95, 0xe4, 0x79},\n {0xe7, 0xc8, 0x37, 0x6d, 0x8d, 0xd5, 0x4e, 0xa9, 0x6c, 0x56, 0xf4, 0xea, 0x65, 0x7a, 0xae, 0x08},\n {0xba, 0x78, 0x25, 0x2e, 0x1c, 0xa6, 0xb4, 0xc6, 0xe8, 0xdd, 0x74, 0x1f, 0x4b, 0xbd, 0x8b, 0x8a},\n {0x70, 0x3e, 0xb5, 0x66, 0x48, 0x03, 0xf6, 0x0e, 0x61, 0x35, 0x57, 0xb9, 0x86, 0xc1, 0x1d, 0x9e},\n {0xe1, 0xf8, 0x98, 0x11, 0x69, 0xd9, 0x8e, 0x94, 0x9b, 0x1e, 0x87, 0xe9, 0xce, 0x55, 0x28, 0xdf},\n {0x8c, 0xa1, 0x89, 0x0d, 0xbf, 0xe6, 0x42, 0x68, 0x41, 0x99, 0x2d, 0x0f, 0xb0, 0x54, 0xbb, 0x16}\n };\n\n public static readonly byte[,] InvSubBytes_SBox =\n {\n {0x52, 0x09, 0x6a, 0xd5, 0x30, 0x36, 0xa5, 0x38, 0xbf, 0x40, 0xa3, 0x9e, 0x81, 0xf3, 0xd7, 0xfb},\n {0x7c, 0xe3, 0x39, 0x82, 0x9b, 0x2f, 0xff, 0x87, 0x34, 0x8e, 0x43, 0x44, 0xc4, 0xde, 0xe9, 0xcb},\n {0x54, 0x7b, 0x94, 0x32, 0xa6, 0xc2, 0x23, 0x3d, 0xee, 0x4c, 0x95, 0x0b, 0x42, 0xfa, 0xc3, 0x4e},\n {0x08, 0x2e, 0xa1, 0x66, 0x28, 0xd9, 0x24, 0xb2, 0x76, 0x5b, 0xa2, 0x49, 0x6d, 0x8b, 0xd1, 0x25},\n {0x72, 0xf8, 0xf6, 0x64, 0x86, 0x68, 0x98, 0x16, 0xd4, 0xa4, 0x5c, 0xcc, 0x5d, 0x65, 0xb6, 0x92},\n {0x6c, 0x70, 0x48, 0x50, 0xfd, 0xed, 0xb9, 0xda, 0x5e, 0x15, 0x46, 0x57, 0xa7, 0x8d, 0x9d, 0x84},\n {0x90, 0xd8, 0xab, 0x00, 0x8c, 0xbc, 0xd3, 0x0a, 0xf7, 0xe4, 0x58, 0x05, 0xb8, 0xb3, 0x45, 0x06},\n {0xd0, 0x2c, 0x1e, 0x8f, 0xca, 0x3f, 0x0f, 0x02, 0xc1, 0xaf, 0xbd, 0x03, 0x01, 0x13, 0x8a, 0x6b},\n {0x3a, 0x91, 0x11, 0x41, 0x4f, 0x67, 0xdc, 0xea, 0x97, 0xf2, 0xcf, 0xce, 0xf0, 0xb4, 0xe6, 0x73},\n {0x96, 0xac, 0x74, 0x22, 0xe7, 0xad, 0x35, 0x85, 0xe2, 0xf9, 0x37, 0xe8, 0x1c, 0x75, 0xdf, 0x6e},\n {0x47, 0xf1, 0x1a, 0x71, 0x1d, 0x29, 0xc5, 0x89, 0x6f, 0xb7, 0x62, 0x0e, 0xaa, 0x18, 0xbe, 0x1b},\n {0xfc, 0x56, 0x3e, 0x4b, 0xc6, 0xd2, 0x79, 0x20, 0x9a, 0xdb, 0xc0, 0xfe, 0x78, 0xcd, 0x5a, 0xf4},\n {0x1f, 0xdd, 0xa8, 0x33, 0x88, 0x07, 0xc7, 0x31, 0xb1, 0x12, 0x10, 0x59, 0x27, 0x80, 0xec, 0x5f},\n {0x60, 0x51, 0x7f, 0xa9, 0x19, 0xb5, 0x4a, 0x0d, 0x2d, 0xe5, 0x7a, 0x9f, 0x93, 0xc9, 0x9c, 0xef},\n {0xa0, 0xe0, 0x3b, 0x4d, 0xae, 0x2a, 0xf5, 0xb0, 0xc8, 0xeb, 0xbb, 0x3c, 0x83, 0x53, 0x99, 0x61},\n {0x17, 0x2b, 0x04, 0x7e, 0xba, 0x77, 0xd6, 0x26, 0xe1, 0x69, 0x14, 0x63, 0x55, 0x21, 0x0c, 0x7d}\n };\n\n // - This table stores pre-calculated values for all possible GF(2^8) calculations.This\n // table is only used by the (Inv)MixColumns steps.\n // USAGE: The second index (column) is the coefficient of multiplication. Only 7 different\n // coefficients are used: 0x01, 0x02, 0x03, 0x09, 0x0b, 0x0d, 0x0e, but multiplication by\n // 1 is negligible leaving only 6 coefficients. Each column of the table is devoted to one\n // of these coefficients, in the ascending order of value, from values 0x00 to 0xFF.\n // (Columns are listed double-wide to conserve vertical space.)\n //\n // This table was taken from : https://github.com/B-Con/crypto-algorithms/blob/master/aes.c\n public static readonly byte[,] GF_MulArray =\n {\n {0x00,0x00,0x00,0x00,0x00,0x00},{0x02,0x03,0x09,0x0b,0x0d,0x0e},\n {0x04,0x06,0x12,0x16,0x1a,0x1c},{0x06,0x05,0x1b,0x1d,0x17,0x12},\n {0x08,0x0c,0x24,0x2c,0x34,0x38},{0x0a,0x0f,0x2d,0x27,0x39,0x36},\n {0x0c,0x0a,0x36,0x3a,0x2e,0x24},{0x0e,0x09,0x3f,0x31,0x23,0x2a},\n {0x10,0x18,0x48,0x58,0x68,0x70},{0x12,0x1b,0x41,0x53,0x65,0x7e},\n {0x14,0x1e,0x5a,0x4e,0x72,0x6c},{0x16,0x1d,0x53,0x45,0x7f,0x62},\n {0x18,0x14,0x6c,0x74,0x5c,0x48},{0x1a,0x17,0x65,0x7f,0x51,0x46},\n {0x1c,0x12,0x7e,0x62,0x46,0x54},{0x1e,0x11,0x77,0x69,0x4b,0x5a},\n {0x20,0x30,0x90,0xb0,0xd0,0xe0},{0x22,0x33,0x99,0xbb,0xdd,0xee},\n {0x24,0x36,0x82,0xa6,0xca,0xfc},{0x26,0x35,0x8b,0xad,0xc7,0xf2},\n {0x28,0x3c,0xb4,0x9c,0xe4,0xd8},{0x2a,0x3f,0xbd,0x97,0xe9,0xd6},\n {0x2c,0x3a,0xa6,0x8a,0xfe,0xc4},{0x2e,0x39,0xaf,0x81,0xf3,0xca},\n {0x30,0x28,0xd8,0xe8,0xb8,0x90},{0x32,0x2b,0xd1,0xe3,0xb5,0x9e},\n {0x34,0x2e,0xca,0xfe,0xa2,0x8c},{0x36,0x2d,0xc3,0xf5,0xaf,0x82},\n {0x38,0x24,0xfc,0xc4,0x8c,0xa8},{0x3a,0x27,0xf5,0xcf,0x81,0xa6},\n {0x3c,0x22,0xee,0xd2,0x96,0xb4},{0x3e,0x21,0xe7,0xd9,0x9b,0xba},\n {0x40,0x60,0x3b,0x7b,0xbb,0xdb},{0x42,0x63,0x32,0x70,0xb6,0xd5},\n {0x44,0x66,0x29,0x6d,0xa1,0xc7},{0x46,0x65,0x20,0x66,0xac,0xc9},\n {0x48,0x6c,0x1f,0x57,0x8f,0xe3},{0x4a,0x6f,0x16,0x5c,0x82,0xed},\n {0x4c,0x6a,0x0d,0x41,0x95,0xff},{0x4e,0x69,0x04,0x4a,0x98,0xf1},\n {0x50,0x78,0x73,0x23,0xd3,0xab},{0x52,0x7b,0x7a,0x28,0xde,0xa5},\n {0x54,0x7e,0x61,0x35,0xc9,0xb7},{0x56,0x7d,0x68,0x3e,0xc4,0xb9},\n {0x58,0x74,0x57,0x0f,0xe7,0x93},{0x5a,0x77,0x5e,0x04,0xea,0x9d},\n {0x5c,0x72,0x45,0x19,0xfd,0x8f},{0x5e,0x71,0x4c,0x12,0xf0,0x81},\n {0x60,0x50,0xab,0xcb,0x6b,0x3b},{0x62,0x53,0xa2,0xc0,0x66,0x35},\n {0x64,0x56,0xb9,0xdd,0x71,0x27},{0x66,0x55,0xb0,0xd6,0x7c,0x29},\n {0x68,0x5c,0x8f,0xe7,0x5f,0x03},{0x6a,0x5f,0x86,0xec,0x52,0x0d},\n {0x6c,0x5a,0x9d,0xf1,0x45,0x1f},{0x6e,0x59,0x94,0xfa,0x48,0x11},\n {0x70,0x48,0xe3,0x93,0x03,0x4b},{0x72,0x4b,0xea,0x98,0x0e,0x45},\n {0x74,0x4e,0xf1,0x85,0x19,0x57},{0x76,0x4d,0xf8,0x8e,0x14,0x59},\n {0x78,0x44,0xc7,0xbf,0x37,0x73},{0x7a,0x47,0xce,0xb4,0x3a,0x7d},\n {0x7c,0x42,0xd5,0xa9,0x2d,0x6f},{0x7e,0x41,0xdc,0xa2,0x20,0x61},\n {0x80,0xc0,0x76,0xf6,0x6d,0xad},{0x82,0xc3,0x7f,0xfd,0x60,0xa3},\n {0x84,0xc6,0x64,0xe0,0x77,0xb1},{0x86,0xc5,0x6d,0xeb,0x7a,0xbf},\n {0x88,0xcc,0x52,0xda,0x59,0x95},{0x8a,0xcf,0x5b,0xd1,0x54,0x9b},\n {0x8c,0xca,0x40,0xcc,0x43,0x89},{0x8e,0xc9,0x49,0xc7,0x4e,0x87},\n {0x90,0xd8,0x3e,0xae,0x05,0xdd},{0x92,0xdb,0x37,0xa5,0x08,0xd3},\n {0x94,0xde,0x2c,0xb8,0x1f,0xc1},{0x96,0xdd,0x25,0xb3,0x12,0xcf},\n {0x98,0xd4,0x1a,0x82,0x31,0xe5},{0x9a,0xd7,0x13,0x89,0x3c,0xeb},\n {0x9c,0xd2,0x08,0x94,0x2b,0xf9},{0x9e,0xd1,0x01,0x9f,0x26,0xf7},\n {0xa0,0xf0,0xe6,0x46,0xbd,0x4d},{0xa2,0xf3,0xef,0x4d,0xb0,0x43},\n {0xa4,0xf6,0xf4,0x50,0xa7,0x51},{0xa6,0xf5,0xfd,0x5b,0xaa,0x5f},\n {0xa8,0xfc,0xc2,0x6a,0x89,0x75},{0xaa,0xff,0xcb,0x61,0x84,0x7b},\n {0xac,0xfa,0xd0,0x7c,0x93,0x69},{0xae,0xf9,0xd9,0x77,0x9e,0x67},\n {0xb0,0xe8,0xae,0x1e,0xd5,0x3d},{0xb2,0xeb,0xa7,0x15,0xd8,0x33},\n {0xb4,0xee,0xbc,0x08,0xcf,0x21},{0xb6,0xed,0xb5,0x03,0xc2,0x2f},\n {0xb8,0xe4,0x8a,0x32,0xe1,0x05},{0xba,0xe7,0x83,0x39,0xec,0x0b},\n {0xbc,0xe2,0x98,0x24,0xfb,0x19},{0xbe,0xe1,0x91,0x2f,0xf6,0x17},\n {0xc0,0xa0,0x4d,0x8d,0xd6,0x76},{0xc2,0xa3,0x44,0x86,0xdb,0x78},\n {0xc4,0xa6,0x5f,0x9b,0xcc,0x6a},{0xc6,0xa5,0x56,0x90,0xc1,0x64},\n {0xc8,0xac,0x69,0xa1,0xe2,0x4e},{0xca,0xaf,0x60,0xaa,0xef,0x40},\n {0xcc,0xaa,0x7b,0xb7,0xf8,0x52},{0xce,0xa9,0x72,0xbc,0xf5,0x5c},\n {0xd0,0xb8,0x05,0xd5,0xbe,0x06},{0xd2,0xbb,0x0c,0xde,0xb3,0x08},\n {0xd4,0xbe,0x17,0xc3,0xa4,0x1a},{0xd6,0xbd,0x1e,0xc8,0xa9,0x14},\n {0xd8,0xb4,0x21,0xf9,0x8a,0x3e},{0xda,0xb7,0x28,0xf2,0x87,0x30},\n {0xdc,0xb2,0x33,0xef,0x90,0x22},{0xde,0xb1,0x3a,0xe4,0x9d,0x2c},\n {0xe0,0x90,0xdd,0x3d,0x06,0x96},{0xe2,0x93,0xd4,0x36,0x0b,0x98},\n {0xe4,0x96,0xcf,0x2b,0x1c,0x8a},{0xe6,0x95,0xc6,0x20,0x11,0x84},\n {0xe8,0x9c,0xf9,0x11,0x32,0xae},{0xea,0x9f,0xf0,0x1a,0x3f,0xa0},\n {0xec,0x9a,0xeb,0x07,0x28,0xb2},{0xee,0x99,0xe2,0x0c,0x25,0xbc},\n {0xf0,0x88,0x95,0x65,0x6e,0xe6},{0xf2,0x8b,0x9c,0x6e,0x63,0xe8},\n {0xf4,0x8e,0x87,0x73,0x74,0xfa},{0xf6,0x8d,0x8e,0x78,0x79,0xf4},\n {0xf8,0x84,0xb1,0x49,0x5a,0xde},{0xfa,0x87,0xb8,0x42,0x57,0xd0},\n {0xfc,0x82,0xa3,0x5f,0x40,0xc2},{0xfe,0x81,0xaa,0x54,0x4d,0xcc},\n {0x1b,0x9b,0xec,0xf7,0xda,0x41},{0x19,0x98,0xe5,0xfc,0xd7,0x4f},\n {0x1f,0x9d,0xfe,0xe1,0xc0,0x5d},{0x1d,0x9e,0xf7,0xea,0xcd,0x53},\n {0x13,0x97,0xc8,0xdb,0xee,0x79},{0x11,0x94,0xc1,0xd0,0xe3,0x77},\n {0x17,0x91,0xda,0xcd,0xf4,0x65},{0x15,0x92,0xd3,0xc6,0xf9,0x6b},\n {0x0b,0x83,0xa4,0xaf,0xb2,0x31},{0x09,0x80,0xad,0xa4,0xbf,0x3f},\n {0x0f,0x85,0xb6,0xb9,0xa8,0x2d},{0x0d,0x86,0xbf,0xb2,0xa5,0x23},\n {0x03,0x8f,0x80,0x83,0x86,0x09},{0x01,0x8c,0x89,0x88,0x8b,0x07},\n {0x07,0x89,0x92,0x95,0x9c,0x15},{0x05,0x8a,0x9b,0x9e,0x91,0x1b},\n {0x3b,0xab,0x7c,0x47,0x0a,0xa1},{0x39,0xa8,0x75,0x4c,0x07,0xaf},\n {0x3f,0xad,0x6e,0x51,0x10,0xbd},{0x3d,0xae,0x67,0x5a,0x1d,0xb3},\n {0x33,0xa7,0x58,0x6b,0x3e,0x99},{0x31,0xa4,0x51,0x60,0x33,0x97},\n {0x37,0xa1,0x4a,0x7d,0x24,0x85},{0x35,0xa2,0x43,0x76,0x29,0x8b},\n {0x2b,0xb3,0x34,0x1f,0x62,0xd1},{0x29,0xb0,0x3d,0x14,0x6f,0xdf},\n {0x2f,0xb5,0x26,0x09,0x78,0xcd},{0x2d,0xb6,0x2f,0x02,0x75,0xc3},\n {0x23,0xbf,0x10,0x33,0x56,0xe9},{0x21,0xbc,0x19,0x38,0x5b,0xe7},\n {0x27,0xb9,0x02,0x25,0x4c,0xf5},{0x25,0xba,0x0b,0x2e,0x41,0xfb},\n {0x5b,0xfb,0xd7,0x8c,0x61,0x9a},{0x59,0xf8,0xde,0x87,0x6c,0x94},\n {0x5f,0xfd,0xc5,0x9a,0x7b,0x86},{0x5d,0xfe,0xcc,0x91,0x76,0x88},\n {0x53,0xf7,0xf3,0xa0,0x55,0xa2},{0x51,0xf4,0xfa,0xab,0x58,0xac},\n {0x57,0xf1,0xe1,0xb6,0x4f,0xbe},{0x55,0xf2,0xe8,0xbd,0x42,0xb0},\n {0x4b,0xe3,0x9f,0xd4,0x09,0xea},{0x49,0xe0,0x96,0xdf,0x04,0xe4},\n {0x4f,0xe5,0x8d,0xc2,0x13,0xf6},{0x4d,0xe6,0x84,0xc9,0x1e,0xf8},\n {0x43,0xef,0xbb,0xf8,0x3d,0xd2},{0x41,0xec,0xb2,0xf3,0x30,0xdc},\n {0x47,0xe9,0xa9,0xee,0x27,0xce},{0x45,0xea,0xa0,0xe5,0x2a,0xc0},\n {0x7b,0xcb,0x47,0x3c,0xb1,0x7a},{0x79,0xc8,0x4e,0x37,0xbc,0x74},\n {0x7f,0xcd,0x55,0x2a,0xab,0x66},{0x7d,0xce,0x5c,0x21,0xa6,0x68},\n {0x73,0xc7,0x63,0x10,0x85,0x42},{0x71,0xc4,0x6a,0x1b,0x88,0x4c},\n {0x77,0xc1,0x71,0x06,0x9f,0x5e},{0x75,0xc2,0x78,0x0d,0x92,0x50},\n {0x6b,0xd3,0x0f,0x64,0xd9,0x0a},{0x69,0xd0,0x06,0x6f,0xd4,0x04},\n {0x6f,0xd5,0x1d,0x72,0xc3,0x16},{0x6d,0xd6,0x14,0x79,0xce,0x18},\n {0x63,0xdf,0x2b,0x48,0xed,0x32},{0x61,0xdc,0x22,0x43,0xe0,0x3c},\n {0x67,0xd9,0x39,0x5e,0xf7,0x2e},{0x65,0xda,0x30,0x55,0xfa,0x20},\n {0x9b,0x5b,0x9a,0x01,0xb7,0xec},{0x99,0x58,0x93,0x0a,0xba,0xe2},\n {0x9f,0x5d,0x88,0x17,0xad,0xf0},{0x9d,0x5e,0x81,0x1c,0xa0,0xfe},\n {0x93,0x57,0xbe,0x2d,0x83,0xd4},{0x91,0x54,0xb7,0x26,0x8e,0xda},\n {0x97,0x51,0xac,0x3b,0x99,0xc8},{0x95,0x52,0xa5,0x30,0x94,0xc6},\n {0x8b,0x43,0xd2,0x59,0xdf,0x9c},{0x89,0x40,0xdb,0x52,0xd2,0x92},\n {0x8f,0x45,0xc0,0x4f,0xc5,0x80},{0x8d,0x46,0xc9,0x44,0xc8,0x8e},\n {0x83,0x4f,0xf6,0x75,0xeb,0xa4},{0x81,0x4c,0xff,0x7e,0xe6,0xaa},\n {0x87,0x49,0xe4,0x63,0xf1,0xb8},{0x85,0x4a,0xed,0x68,0xfc,0xb6},\n {0xbb,0x6b,0x0a,0xb1,0x67,0x0c},{0xb9,0x68,0x03,0xba,0x6a,0x02},\n {0xbf,0x6d,0x18,0xa7,0x7d,0x10},{0xbd,0x6e,0x11,0xac,0x70,0x1e},\n {0xb3,0x67,0x2e,0x9d,0x53,0x34},{0xb1,0x64,0x27,0x96,0x5e,0x3a},\n {0xb7,0x61,0x3c,0x8b,0x49,0x28},{0xb5,0x62,0x35,0x80,0x44,0x26},\n {0xab,0x73,0x42,0xe9,0x0f,0x7c},{0xa9,0x70,0x4b,0xe2,0x02,0x72},\n {0xaf,0x75,0x50,0xff,0x15,0x60},{0xad,0x76,0x59,0xf4,0x18,0x6e},\n {0xa3,0x7f,0x66,0xc5,0x3b,0x44},{0xa1,0x7c,0x6f,0xce,0x36,0x4a},\n {0xa7,0x79,0x74,0xd3,0x21,0x58},{0xa5,0x7a,0x7d,0xd8,0x2c,0x56},\n {0xdb,0x3b,0xa1,0x7a,0x0c,0x37},{0xd9,0x38,0xa8,0x71,0x01,0x39},\n {0xdf,0x3d,0xb3,0x6c,0x16,0x2b},{0xdd,0x3e,0xba,0x67,0x1b,0x25},\n {0xd3,0x37,0x85,0x56,0x38,0x0f},{0xd1,0x34,0x8c,0x5d,0x35,0x01},\n {0xd7,0x31,0x97,0x40,0x22,0x13},{0xd5,0x32,0x9e,0x4b,0x2f,0x1d},\n {0xcb,0x23,0xe9,0x22,0x64,0x47},{0xc9,0x20,0xe0,0x29,0x69,0x49},\n {0xcf,0x25,0xfb,0x34,0x7e,0x5b},{0xcd,0x26,0xf2,0x3f,0x73,0x55},\n {0xc3,0x2f,0xcd,0x0e,0x50,0x7f},{0xc1,0x2c,0xc4,0x05,0x5d,0x71},\n {0xc7,0x29,0xdf,0x18,0x4a,0x63},{0xc5,0x2a,0xd6,0x13,0x47,0x6d},\n {0xfb,0x0b,0x31,0xca,0xdc,0xd7},{0xf9,0x08,0x38,0xc1,0xd1,0xd9},\n {0xff,0x0d,0x23,0xdc,0xc6,0xcb},{0xfd,0x0e,0x2a,0xd7,0xcb,0xc5},\n {0xf3,0x07,0x15,0xe6,0xe8,0xef},{0xf1,0x04,0x1c,0xed,0xe5,0xe1},\n {0xf7,0x01,0x07,0xf0,0xf2,0xf3},{0xf5,0x02,0x0e,0xfb,0xff,0xfd},\n {0xeb,0x13,0x79,0x92,0xb4,0xa7},{0xe9,0x10,0x70,0x99,0xb9,0xa9},\n {0xef,0x15,0x6b,0x84,0xae,0xbb},{0xed,0x16,0x62,0x8f,0xa3,0xb5},\n {0xe3,0x1f,0x5d,0xbe,0x80,0x9f},{0xe1,0x1c,0x54,0xb5,0x8d,0x91},\n {0xe7,0x19,0x4f,0xa8,0x9a,0x83},{0xe5,0x1a,0x46,0xa3,0x97,0x8d}\n };\n }\n}"
}
{
"filename": "code/cryptography/src/aes_128/aes_csharp/aesdecipher.cs",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation.\n * Author : ABDOUS Kamel\n */\n\nusing System;\nusing System.Collections.Generic;\nusing System.Linq;\nusing System.Text;\nusing System.Threading.Tasks;\n\nnamespace AES\n{\n public partial class AESCipher\n {\n public void DoDecipher(byte[] CipherText, byte[] PlainText)\n {\n byte[][] State = new byte[4][];\n int i, j;\n\n for(i = 0; i < 4; ++i)\n {\n State[i] = new byte[StateNB];\n for (j = 0; j < StateNB; ++j)\n State[i][j] = CipherText[i + StateNB * j];\n }\n\n AddRoundKey(State, NR);\n\n for(i = NR - 1; i > 0; --i)\n {\n InvShiftRows(State);\n SubBytes(State, InvSubBytes_SBox);\n\n AddRoundKey(State, i);\n InvMixColumns(State);\n }\n\n InvShiftRows(State);\n SubBytes(State, InvSubBytes_SBox);\n\n AddRoundKey(State, 0);\n\n for(i = 0; i < 4; ++i)\n {\n for (j = 0; j < StateNB; ++j)\n PlainText[i + StateNB * j] = State[i][j];\n }\n }\n\n protected void InvShiftRows(byte[][] State)\n {\n for(int i = 0; i < 4; ++i)\n {\n for(int j = 0; j < i; ++j)\n Helpers.ShiftBytesRight(State[i]);\n }\n }\n\n protected void InvMixColumns(byte[][] State)\n {\n byte[][] buff = new byte[4][];\n int i, j;\n\n for(i = 0; i < 4; ++i)\n {\n buff[i] = new byte[StateNB];\n State[i].CopyTo(buff[i], 0);\n }\n\n for(i = 0; i < StateNB; ++i)\n {\n State[0][i] = (byte)(GF_MulArray[buff[0][i], 5] ^ GF_MulArray[buff[1][i], 3] ^ GF_MulArray[buff[2][i], 4] ^ GF_MulArray[buff[3][i], 2]);\n State[1][i] = (byte)(GF_MulArray[buff[0][i], 2] ^ GF_MulArray[buff[1][i], 5] ^ GF_MulArray[buff[2][i], 3] ^ GF_MulArray[buff[3][i], 4]);\n State[2][i] = (byte)(GF_MulArray[buff[0][i], 4] ^ GF_MulArray[buff[1][i], 2] ^ GF_MulArray[buff[2][i], 5] ^ GF_MulArray[buff[3][i], 3]);\n State[3][i] = (byte)(GF_MulArray[buff[0][i], 3] ^ GF_MulArray[buff[1][i], 4] ^ GF_MulArray[buff[2][i], 2] ^ GF_MulArray[buff[3][i], 5]);\n }\n }\n }\n}"
}
{
"filename": "code/cryptography/src/aes_128/aes_csharp/aeskeygen.cs",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation.\n * Author : ABDOUS Kamel\n */\n\nusing System;\nusing System.Collections.Generic;\nusing System.Linq;\nusing System.Text;\nusing System.Threading.Tasks;\n\nnamespace AES\n{\n public partial class AESCipher\n {\n byte[] vMainKey;\n public byte[] MainKey\n {\n get\n {\n return vMainKey;\n }\n\n set\n {\n switch(value.Length)\n {\n case 16:\n GenRoundKeys(value, 4);\n break;\n\n case 24:\n GenRoundKeys(value, 6);\n break;\n\n case 32:\n GenRoundKeys(value, 8);\n break;\n\n default:\n throw new ArgumentException(\"Unknown main key size.\");\n }\n vMainKey = value;\n }\n }\n\n public uint[] RoundKeys { get; protected set; }\n\n public int NK { get; protected set; }\n\n public int NR { get; protected set; }\n\n protected void GenRoundKeys(byte[] MainKey, int nk)\n {\n int nr = Helpers.GetAESNbRounds(nk),\n i, j,\n rk_size = AESCipher.StateNB * (nr + 1);\n uint tmp;\n byte rcon = 0x01;\n\n RoundKeys = new uint[rk_size];\n\n for(i = 0; i < nk; ++i)\n RoundKeys[i] = (uint)((MainKey[i * 4] << 24) +\n (MainKey[i * 4 + 1] << 16) +\n (MainKey[i * 4 + 2] << 8) +\n (MainKey[i * 4 + 3]));\n\n while(i < rk_size)\n {\n tmp = RoundKeys[i - 1];\n\n if (i % nk == 0)\n {\n tmp = Helpers.ShiftWordLeft(tmp);\n tmp = Helpers.SubWord(tmp, AESCipher.SubByte_SBox);\n\n tmp ^= ((uint)rcon) << 24;\n rcon = Helpers.X_Time(rcon);\n }\n\n else if (nk > 6 && i % nk == 4)\n tmp = Helpers.SubWord(tmp, AESCipher.SubByte_SBox);\n\n RoundKeys[i] = tmp ^ RoundKeys[i - nk];\n ++i;\n }\n\n this.NK = nk;\n this.NR = nr;\n }\n }\n}"
}
{
"filename": "code/cryptography/src/aes_128/aes_csharp/example/README.md",
"content": "# Example of how to cipher or decipher a stream with AES\n\nThis provides an example of how to cipher or decipher a stream with AES. Just create an instance of StreamCipher with a key, and call DoCipher or DoDecipher with streams."
}
{
"filename": "code/cryptography/src/aes_128/aes_csharp/example/streamcipher.cs",
"content": "using System;\nusing System.Collections.Generic;\nusing System.Linq;\nusing System.Text;\nusing System.Threading.Tasks;\nusing System.IO;\n\nnamespace AES\n{\n public class StreamCipher\n {\n AESCipher TheCipher;\n\n public StreamCipher(byte[] MainKey)\n {\n TheCipher = new AESCipher(MainKey);\n }\n\n // Abstract stream ciphering\n public void DoCipher(Stream Src, Stream Dest)\n {\n DoTheJob(Src, Dest, true);\n }\n\n public void DoDecipher(Stream Src, Stream Dest)\n {\n DoTheJob(Src, Dest, false);\n }\n\n private void DoTheJob(Stream Src, Stream Dest, bool Cipher)\n {\n if (!Src.CanRead)\n throw new StreamCipherException(StreamCipherException.Code.CantRead);\n\n if (!Dest.CanWrite)\n throw new StreamCipherException(StreamCipherException.Code.CantWrite);\n\n byte[] SrcBuff = new byte[AES.AESCipher.BlockSize],\n DestBuff = new byte[AES.AESCipher.BlockSize];\n\n int n, i;\n while((n = Src.Read(SrcBuff, 0, AESCipher.BlockSize)) != 0)\n {\n // If we read less caracters then the size of an AES block,\n // we fill remaining boxes with zeros.\n for (i = n; i < AESCipher.BlockSize; ++i)\n SrcBuff[i] = 0;\n\n if (Cipher)\n TheCipher.DoCipher(SrcBuff, DestBuff);\n\n else\n TheCipher.DoDecipher(SrcBuff, DestBuff);\n\n Dest.Write(DestBuff, 0, AESCipher.BlockSize);\n }\n }\n }\n}"
}
{
"filename": "code/cryptography/src/aes_128/aes_csharp/example/streamcipherexception.cs",
"content": "using System;\nusing System.Collections.Generic;\nusing System.Linq;\nusing System.Text;\nusing System.Threading.Tasks;\n\nnamespace AES\n{\n public class StreamCipherException : Exception\n {\n public enum Code\n {\n CantRead, CantWrite\n }\n\n public Code ErrorCode { get; set; }\n\n public StreamCipherException(Code ErrorCode) : base()\n {\n this.ErrorCode = ErrorCode;\n }\n }\n}"
}
{
"filename": "code/cryptography/src/aes_128/aes_csharp/helpers.cs",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation.\n * Author : ABDOUS Kamel\n */\n\nusing System;\nusing System.Collections.Generic;\nusing System.Linq;\nusing System.Text;\nusing System.Threading.Tasks;\n\nnamespace AES\n{\n public class Helpers\n {\n // Shift all bytes left by one position.\n public static void ShiftBytesLeft(byte[] bytes)\n {\n byte tmp = bytes[0];\n int i;\n\n for(i = 0; i < bytes.Length - 1; ++i)\n bytes[i] = bytes[i + 1];\n\n bytes[i] = tmp;\n }\n\n // Shift all bytes right by one position\n public static void ShiftBytesRight(byte[] bytes)\n {\n byte tmp = bytes.Last();\n int i;\n\n for (i = bytes.Length - 1; i > 0; --i)\n bytes[i] = bytes[i - 1];\n\n bytes[0] = tmp;\n }\n\n public static uint ShiftWordLeft(uint word)\n {\n byte[] b = new byte[4];\n\n b[0] = (byte)((word & 0xff000000) >> 24);\n b[1] = (byte)((word & 0x00ff0000) >> 16);\n b[2] = (byte)((word & 0x0000ff00) >> 8);\n b[3] = (byte)(word & 0x000000ff);\n\n return (uint)(b[0] + (b[3] << 8) + (b[2] << 16) + (b[1] << 24));\n }\n\n public static void SubBytes(byte[] bytes, byte[,] sbox)\n {\n byte row, col;\n\n for(int i = 0; i < bytes.Length; ++i)\n {\n row = (byte)((bytes[i] & 0xf0) >> 4);\n col = (byte)(bytes[i] & 0x0f);\n\n bytes[i] = sbox[row, col];\n }\n }\n\n public static uint SubWord(uint word, byte[,] sbox)\n {\n int i, row, col, cpt = 24;\n uint mask = 0xff000000, ret = 0;\n byte b;\n\n for(i = 0; i < 4; ++i)\n {\n b = (byte)((word & mask) >> cpt);\n row = (b & 0xf0) >> 4;\n col = b & 0x0f;\n\n ret += (uint)(sbox[row, col] << cpt);\n\n mask >>= 8;\n cpt -= 8;\n }\n\n return ret;\n }\n\n public static byte X_Time(byte a)\n {\n int tmp = a & 0x80;\n a <<= 1;\n\n if (tmp == 0x80)\n a ^= 0x1b;\n\n return a;\n }\n\n public static int GetAESNbRounds(int nk)\n {\n return 10 + nk - 4;\n }\n }\n}"
}
{
"filename": "code/cryptography/src/aes_128/aes_csharp/README.md",
"content": "# Implementation of AES-128, AES-192 and AES-256 in C#.\n\nThe implementation chooses the version of AES to use according to the size of the provided key.\n\nSee folder example for an example of how to cipher streams."
}
{
"filename": "code/cryptography/src/affine_cipher/affine_cipher.py",
"content": "import sys, pyperclip, cryptomath, random\n\nSYMBOLS = \"\"\" !\"#$%&'()*+,-./0123456789:;<=>?@ABCDEFGHIJKLMNOPQRSTUVWXYZ[\\] ^_`abcdefghijklmnopqrstuvwxyz{|}~\"\"\" # note the space at the front\n\n\ndef main():\n myMessage = \"\"\"\"A computer would deserve to be called intelligent if it could deceive a human into believing that it was human.\" -Alan Turing\"\"\"\n myKey = 2023\n myMode = \"encrypt\" # set to 'encrypt' or 'decrypt'\n\n if myMode == \"encrypt\":\n translated = encryptMessage(myKey, myMessage)\n elif myMode == \"decrypt\":\n translated = decryptMessage(myKey, myMessage)\n print(\"Key: %s\" % (myKey))\n print(\"%sed text:\" % (myMode.title()))\n print(translated)\n pyperclip.copy(translated)\n print(\"Full %sed text copied to clipboard.\" % (myMode))\n\n\ndef getKeyParts(key):\n keyA = key // len(SYMBOLS)\n keyB = key % len(SYMBOLS)\n return (keyA, keyB)\n\n\ndef checkKeys(keyA, keyB, mode):\n if keyA == 1 and mode == \"encrypt\":\n sys.exit(\n \"The affine cipher becomes incredibly weak when key A is set to 1. Choose a different key.\"\n )\n if keyB == 0 and mode == \"encrypt\":\n sys.exit(\n \"The affine cipher becomes incredibly weak when key B is set to 0. Choose a different key.\"\n )\n if keyA < 0 or keyB < 0 or keyB > len(SYMBOLS) - 1:\n sys.exit(\n \"Key A must be greater than 0 and Key B must be between 0 and %s.\"\n % (len(SYMBOLS) - 1)\n )\n if cryptomath.gcd(keyA, len(SYMBOLS)) != 1:\n sys.exit(\n \"Key A (%s) and the symbol set size (%s) are not relatively prime. Choose a different key.\"\n % (keyA, len(SYMBOLS))\n )\n\n\ndef encryptMessage(key, message):\n keyA, keyB = getKeyParts(key)\n checkKeys(keyA, keyB, \"encrypt\")\n ciphertext = \"\"\n for symbol in message:\n if symbol in SYMBOLS:\n # encrypt this symbol\n symIndex = SYMBOLS.find(symbol)\n ciphertext += SYMBOLS[(symIndex * keyA + keyB) % len(SYMBOLS)]\n else:\n ciphertext += symbol # just append this symbol unencrypted\n return ciphertext\n\n\ndef decryptMessage(key, message):\n keyA, keyB = getKeyParts(key)\n checkKeys(keyA, keyB, \"decrypt\")\n plaintext = \"\"\n modInverseOfKeyA = cryptomath.findModInverse(keyA, len(SYMBOLS))\n\n for symbol in message:\n if symbol in SYMBOLS:\n # decrypt this symbol\n symIndex = SYMBOLS.find(symbol)\n plaintext += SYMBOLS[(symIndex - keyB) * modInverseOfKeyA % len(SYMBOLS)]\n else:\n plaintext += symbol # just append this symbol undecrypted\n return plaintext\n\n\ndef getRandomKey():\n while True:\n keyA = random.randint(2, len(SYMBOLS))\n keyB = random.randint(2, len(SYMBOLS))\n if cryptomath.gcd(keyA, len(SYMBOLS)) == 1:\n return keyA * len(SYMBOLS) + keyB"
}
{
"filename": "code/cryptography/src/affine_cipher/affine.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n#include <iostream>\n#include <string>\n\n/*\n * Affine Cipher: each alphabet letter is maped by a linear function to its numeric equivalent\n * and converted to letter again.\n *\n * Let x, y, a, b ∈ Z(26)\n * >> Encryption: Eĸ(x) = y ≡ a * x + b mod 26\n * >> Decryption: Dĸ(y) = x ≡ a-¹ * ( y - b) mod 26\n *\n * with the key ĸ = (a,b), which has the restriction: gcd(a, 26) = 1.\n *\n */\n\n/*\n * getChar returns the char letter in alphabet with the modulo's position\n * in the ring\n */\nchar getChar(int n)\n{\n char a = 'a';\n int n_ = n % 26;\n if (n_ < 0)\n n_ += 26; // It's necessary because e.i. -1 % 26 = -1. (In Python it works better)\n return a + n_;\n}\n\n/*\n * a and b are the ĸey pair (a, b)\n * Recall: Encryption: Eĸ(x) = y ≡ a * x + b mod 26\n */\nvoid crypter(std::string *plain_text, int a, int b)\n{\n for (auto &letter:*plain_text)\n {\n int letter_ = letter - 97; // 97 is the 'a' position in char\n letter = getChar((a * letter_) + b);\n }\n}\n\n/*\n * Recall: Decryption: Dĸ(y) = x ≡ a-¹ * ( y - b) mod 26\n */\nvoid decrypter(std::string *cipher_text, int a, int b)\n{\n // Supose that (a) is inversible. Let's find (a)-¹\n // There is a number in the open-closed range [0, 26) such that a-¹ * (invertor) ≡ 1 mod 26\n int invertor = 0;\n for (auto i(0ul); i < 26; i++)\n if ( (a * i) % 26 == 1)\n {\n invertor = i;\n break;\n }\n\n for (auto &letter:*cipher_text)\n {\n int letter_ = letter - 97;\n letter = getChar(invertor * (letter_ - b) % 26);\n }\n}\n\nint main(void)\n{\n int a = 3, b = 6; // Remember that gcd(a, 26) must be equals to 1.\n\n std::string text = \"palavraz\";\n\n std::cout << \">>> Original plain text: \" << text << std::endl;\n // Encryption pass\n std::cout << \">>> Encryption function with the key pair (\" << a << \", \" << b << \")\" <<\n std::endl;\n crypter(&text, a, b);\n std::cout << \">>> Generated cipher text: \" << text << std::endl;\n\n // Decryption pass\n std::cout << \">>> Decryption function with the key pair (\" << a << \", \" << b << \")\" <<\n std::endl;\n decrypter(&text, a, b);\n std::cout << \">>> Decrypted plain text: \" << text << std::endl;\n}"
}
{
"filename": "code/cryptography/src/affine_cipher/affine.htm",
"content": "<!DOCTYPE html>\n<html>\n<title>Affine Cipher</title>\n<head>\n<!--Part of Cosmos by OpenGenus Foundation\nAffine Cipher: each alphabet letter is maped by a linear function to its numeric equivalent\nand converted to letter again. -->\n<script>\nfunction encryption(a, b) {\n for (var x = 0; x < 26; x++){ \n var uchar = String.fromCharCode(65 + x);\n var cchar = String.fromCharCode(((a*x+b)%26) + 65);\n document.write(uchar + \":\" + cchar);\n document.write(\"<br>\");\n }\n}\nencryption(5, 8);\n</script>\n</head>\n<body>\n</body>\n</html>"
}
{
"filename": "code/cryptography/src/affine_cipher/affine.java",
"content": "import java.util.Scanner;\n\n/**\n * Created by Phuwarin on 10/23/2017.\n */\npublic class Affine {\n\n private static final Scanner SCANNER = new Scanner(System.in);\n private static final int ALPHABET_SIZE = 26;\n\n public static void main(String[] args) {\n\n System.out.print(\"Type 1 for encryption and 2 for decryption then Enter >> \");\n int command = getCommand();\n switch (command) {\n case 1:\n encryption();\n break;\n case 2:\n decryption();\n break;\n default:\n System.out.println(\"Command error, pls try again.\");\n main(null);\n }\n }\n\n private static void decryption() {\n int a, aInverse = 0, b;\n boolean isAOk = false;\n\n System.out.print(\"Enter ciphertext which want to decryption >> \");\n String ciphertext = SCANNER.next();\n\n while (!isAOk) {\n System.out.print(\"Enter key 'a' >> \");\n a = SCANNER.nextInt();\n if (gcd(a, ALPHABET_SIZE) == 1) {\n isAOk = true;\n aInverse = findInverse(a, ALPHABET_SIZE);\n } else {\n System.out.println(\"'a' is not ok, pls try again.\");\n }\n }\n\n System.out.print(\"Enter key 'b' >> \");\n b = SCANNER.nextInt();\n\n decrypt(aInverse, b, ciphertext);\n }\n\n private static void decrypt(int aInverse, int b, String ciphertext) {\n if (ciphertext == null || ciphertext.length() <= 0) {\n System.out.println(\"Plaintext has a problem. Bye bye :)\");\n return;\n }\n\n ciphertext = ciphertext.toLowerCase();\n StringBuilder plaintext = new StringBuilder();\n int z, j;\n\n for (int i = 0; i < ciphertext.length(); i++) {\n char agent = ciphertext.charAt(i);\n z = aInverse * ((agent - 97) - b);\n j = z < 0 ? minusMod(z, ALPHABET_SIZE) : z % ALPHABET_SIZE;\n plaintext.append((char) ('A' + j));\n }\n System.out.println(\"Plaintext: \" + plaintext);\n }\n\n private static int minusMod(int minus, int mod) {\n int a = Math.abs(minus);\n return (mod * ((a / mod) + 1)) - a;\n }\n\n private static int findInverse(double firstNumber, double anotherNumber) {\n int a1, b1, a2, b2, r, q, temp_a2, temp_b2, n1, n2, max;\n\n if (firstNumber > anotherNumber) {\n max = (int) firstNumber;\n n1 = (int) firstNumber;\n n2 = (int) anotherNumber;\n } else {\n max = (int) anotherNumber;\n n1 = (int) anotherNumber;\n n2 = (int) firstNumber;\n }\n\n a1 = b2 = 1;\n b1 = a2 = 0;\n temp_a2 = a2;\n temp_b2 = b2;\n\n r = n1 % n2;\n q = n1 / n2;\n\n while (r != 0) {\n n1 = n2;\n n2 = r;\n a2 = a1 - q * a2;\n b2 = b1 - q * b2;\n a1 = temp_a2;\n b1 = temp_b2;\n temp_a2 = a2;\n temp_b2 = b2;\n r = n1 % n2;\n q = n1 / n2;\n }\n\n int result;\n if (firstNumber == max) {\n if (a2 < 0) {\n result = (int) (a2 - anotherNumber * Math.floor(a2 / anotherNumber));\n } else {\n result = a2;\n }\n } else {\n if (b2 < 0) {\n result = (int) (b2 - anotherNumber * Math.floor(b2 / anotherNumber));\n } else\n result = b2;\n }\n return result;\n }\n\n private static void encryption() {\n boolean isAOk = false, isBOk = false;\n int a = 0, b = 0;\n\n System.out.print(\"Enter plaintext which want to encryption >> \");\n String plaintext = SCANNER.next();\n\n while (!isAOk) {\n System.out.print(\"Choose 'a' but gcd (a,26) must equal 1\\n\" +\n \"'a' might be {1, 3, 5, 7, 9, 11, 15, 17, 19, 21, 23, 25} >> \");\n a = SCANNER.nextInt();\n if (gcd(a, ALPHABET_SIZE) == 1) {\n isAOk = true;\n } else {\n System.out.println(\"'a' is not ok, pls try again.\");\n }\n }\n\n while (!isBOk) {\n System.out.print(\"Choose 'b' which number you want but not equal 1 >> \");\n b = SCANNER.nextInt();\n if (b != 1) {\n isBOk = true;\n } else {\n System.out.println(\"'b' is not ok, pls try again.\");\n }\n }\n encrypt(a, b, plaintext);\n }\n\n private static int gcd(int a, int b) {\n return b == 0 ? a : gcd(b, a % b);\n }\n\n private static int getCommand() {\n return SCANNER.nextInt();\n }\n\n private static void encrypt(int a, int b, String plaintext) {\n if (plaintext == null || plaintext.length() <= 0) {\n System.out.println(\"Plaintext has a problem. Bye bye :)\");\n return;\n }\n\n plaintext = plaintext.toLowerCase();\n StringBuilder ciphertext = new StringBuilder();\n\n for (int i = 0; i < plaintext.length(); i++) {\n char agent = plaintext.charAt(i);\n int value = ((a * (agent - 97) + b) % ALPHABET_SIZE);\n ciphertext.append((char) (value + 97));\n }\n System.out.println(\"Ciphertext: \" + ciphertext);\n }\n}"
}
{
"filename": "code/cryptography/src/affine_cipher/affinekotlin.kt",
"content": "import java.util.*\n\n/**\n * Created by Phuwarin on 10/1/2018\n * Part of Cosmos by OpenGenus\n */\n\nobject AffineKotlin {\n\n private val SCANNER = Scanner(System.`in`)\n private const val ALPHABET_SIZE = 26\n\n private val command: Int\n get() = SCANNER.nextInt()\n\n @JvmStatic\n fun main(args: Array<String>) {\n do {\n print(\"Type 1 for encryption and 2 for decryption then Enter >> \")\n val command = command\n when (command) {\n 1 -> encryption()\n 2 -> decryption()\n else -> {\n println(\"Command error, pls try again.\")\n }\n }\n } while (command != 1 && command != 2)\n }\n\n private fun decryption() {\n var a: Int\n var aInverse = 0\n var isAOk = false\n\n print(\"Enter cipherText which want to decryption >> \")\n val cipherText = SCANNER.next()\n\n while (!isAOk) {\n print(\"Enter key 'a' >> \")\n a = SCANNER.nextInt()\n if (gcd(a, ALPHABET_SIZE) == 1) {\n isAOk = true\n aInverse = findInverse(a.toDouble(), ALPHABET_SIZE.toDouble())\n } else {\n println(\"'a' is not ok, pls try again.\")\n }\n }\n\n print(\"Enter key 'b' >> \")\n val b: Int = SCANNER.nextInt()\n decrypt(aInverse, b, cipherText)\n }\n\n private fun decrypt(aInverse: Int, b: Int, cipherText: String?) {\n if (cipherText == null || cipherText.isEmpty()) {\n println(\"Plaintext has a problem. Bye bye :)\")\n return\n }\n\n val cipherText = cipherText.toLowerCase()\n val plaintext = StringBuilder()\n var z: Int\n var j: Int\n\n for (i in 0 until cipherText.length) {\n val agent = cipherText[i]\n z = aInverse * (agent.toInt() - 97 - b)\n j = if (z < 0) minusMod(z, ALPHABET_SIZE) else z % ALPHABET_SIZE\n plaintext.append(('A'.toInt() + j).toChar())\n }\n println(\"Plaintext: $plaintext\")\n }\n\n private fun minusMod(minus: Int, mod: Int): Int {\n val a = Math.abs(minus)\n return mod * (a / mod + 1) - a\n }\n\n private fun findInverse(firstNumber: Double, anotherNumber: Double): Int {\n var a1: Int\n var b1: Int\n var a2: Int\n var b2: Int\n var r: Int\n var q: Int\n var a2Temp: Int\n var b2Temp: Int\n var n1: Int\n var n2: Int\n val max: Int\n\n if (firstNumber > anotherNumber) {\n max = firstNumber.toInt()\n n1 = firstNumber.toInt()\n n2 = anotherNumber.toInt()\n } else {\n max = anotherNumber.toInt()\n n1 = anotherNumber.toInt()\n n2 = firstNumber.toInt()\n }\n\n b2 = 1\n a1 = b2\n a2 = 0\n b1 = a2\n a2Temp = a2\n b2Temp = b2\n\n r = n1 % n2\n q = n1 / n2\n\n while (r != 0) {\n n1 = n2\n n2 = r\n a2 = a1 - q * a2\n b2 = b1 - q * b2\n a1 = a2Temp\n b1 = b2Temp\n a2Temp = a2\n b2Temp = b2\n r = n1 % n2\n q = n1 / n2\n }\n\n val result: Int\n result = if (firstNumber == max.toDouble()) {\n if (a2 < 0) {\n (a2 - anotherNumber * Math.floor(a2 / anotherNumber)).toInt()\n } else {\n a2\n }\n } else {\n if (b2 < 0) {\n (b2 - anotherNumber * Math.floor(b2 / anotherNumber)).toInt()\n } else\n b2\n }\n return result\n }\n\n private fun encryption() {\n var isAOk = false\n var isBOk = false\n var a = 0\n var b = 0\n\n print(\"Enter plaintext which want to encryption >> \")\n val plaintext = SCANNER.next()\n\n while (!isAOk) {\n print(\"Choose 'a' but gcd (a,26) must equal 1\\n\" + \"'a' might be {1, 3, 5, 7, 9, 11, 15, 17, 19, 21, 23, 25} >> \")\n a = SCANNER.nextInt()\n if (gcd(a, ALPHABET_SIZE) == 1) {\n isAOk = true\n } else {\n println(\"'a' is not ok, pls try again.\")\n }\n }\n\n while (!isBOk) {\n print(\"Choose 'b' which number you want but not equal 1 >> \")\n b = SCANNER.nextInt()\n if (b != 1) {\n isBOk = true\n } else {\n println(\"'b' is not ok, pls try again.\")\n }\n }\n encrypt(a, b, plaintext)\n }\n\n private fun gcd(a: Int, b: Int): Int {\n return if (b == 0) a else gcd(b, a % b)\n }\n\n private fun encrypt(a: Int, b: Int, plaintext: String?) {\n if (plaintext == null || plaintext.isEmpty()) {\n println(\"Plaintext has a problem. Bye bye :)\")\n return\n }\n\n val plaintext = plaintext.toLowerCase()\n val cipherText = StringBuilder()\n\n for (i in 0 until plaintext.length) {\n val agent = plaintext[i]\n val value = (a * (agent.toInt() - 97) + b) % ALPHABET_SIZE\n cipherText.append((value + 97).toChar())\n }\n println(\"cipherText: $cipherText\")\n }\n}"
}
{
"filename": "code/cryptography/src/affine_cipher/affine.py",
"content": "\"\"\"\nPart of Cosmos by OpenGenus Foundation\n Affine Cipher: each alphabet letter is maped by a linear function to its numeric equivalent\n and converted to letter again. \n\"\"\"\n\n\ndef encryption(a, b):\n for x in range(26):\n print(chr(x + 65) + \":\" + chr((a * x + b) % 26 + 65))\n\n\nencryption(5, 8)"
}
{
"filename": "code/cryptography/src/atbash_cipher/atbash_cipher.cpp",
"content": "#include <iostream>\n#include <string>\n#include <cctype>\nusing namespace std;\n\n//Atbash Cipher modified for English\n\nstring atbash(string s);\nint main()\n{\n string s;\n cout << \"Enter message to encrypt/decrypt: \";\n getline(cin, s);\n cout << endl << atbash(s) << endl;\n}\n\nstring atbash(string s)\n{\n string newstr = \"\";\n for (size_t i = 0; i < s.length(); i++)\n {\n if (!isalpha(s[i]))\n newstr += s[i];\n else if (isupper(s[i]))\n newstr += (char)('Z' - (s[i] - 'A'));\n else\n newstr += (char)('z' - (s[i] - 'a'));\n }\n return newstr;\n}"
}
{
"filename": "code/cryptography/src/atbash_cipher/atbash_cipher.java",
"content": "import java.util.Scanner;\n\npublic class atbash_cipher {\n\t\n\tpublic static void main( String[] args) {\n\t\n\t\tScanner scan = new Scanner(System.in);\n\t\n\t\tString newStr,s;\n\t \tchar alpha;\n\t\n\t\ts = scan.nextLine();\n\t\n\t\tnewStr = s;\n\t\n\t // Converting string to character array\n\t\tchar[] n = newStr.toCharArray();\n\t\n\t\tfor(int i = 0; i < s.length(); i++) {\n\t\t\talpha = s.charAt(i);\n\t\t\n\t\t\tif(Character.isUpperCase(alpha) == true ) { \n\t\t\t\tn[i] = (char) ('Z' - ( alpha - 'A' ));\n\t\t\n\t\t\t} else if(Character.isLowerCase(alpha) == true ) {\n\t\t\t\tn[i] = (char) ( 'z' -( alpha - 'a' ));\n\t\t\n\t\t\t} else { \n\t\t\t\tn[i] = alpha;\n\t\t \t}\n\t \t}\n\t\t// Converting character array back to string\n\t\tString cipher = new String(n);\n\t\n\t\tSystem.out.println(cipher);\n\t}\n}"
}
{
"filename": "code/cryptography/src/atbash_cipher/atbash_cipher.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n\ndef cipher(plaintext):\n \"\"\"\n Function to encode/decode given string.\n \"\"\"\n cipher = list(plaintext)\n for i, c in enumerate(plaintext):\n if ord(c) in range(ord(\"a\"), ord(\"z\") + 1):\n cipher[i] = chr(ord(\"a\") + ord(\"z\") - ord(c))\n return \"\".join(cipher)\n\n\nif __name__ == \"__main__\":\n import sys\n\n print cipher(sys.argv[1])"
}
{
"filename": "code/cryptography/src/atbash_cipher/README.md",
"content": "# Atbash Cipher\nAtbash cipher is a method of encrypting messages. It is a type of monoalphabetic cipher where every letter in the original message (also known as plaintext) is mapped to it's reverse. The first letter becomes the last, the second letter becomes the second last and so on. In the latin alphabet the mapping would be as follows.\n\n| Plain | A | B | C | D | E | F | G | H | I | J | K | L | M | N | O | P | Q | R | S | T | U | V | W | X | Y | Z |\n| ------ |---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|\n| Cipher | Z | Y | X | W | V | U | T | S | R | Q | P | O | N | M | L | K | J | I | H | G | F | E | D | C | B | A |\n\n_We only try to implement atbash cipher encryption/decryption algorithms for latin alphabet._\n\n#### Algorithm\n> For encryption\n1. Read the first character of the input string.\n\n2. If it's value is an upper case alphabet then, find out it's distance from 'A' and subtract that from 'Z'. Copy the calculated value to the corrosponding index in a new character array.\n\n3. If it's value is a lower case alphabet then, repeat the above step with 'a' and 'z'.\n\n4. Else copy the read character unchanged at the corrosponding index in the new character array.\n\n5. Repeat the above steps until you read null from input string.\n\n6. The character array at the end is the encrypted message.\n\n> For decryption.\n1. Read the first character of the input string.\n\n2. If it's value is an upper case alphabet then, find out it's distance from 'Z' and subtract that from 'A'. Copy the calculated value to the corrosponding index in a new character array.\n\n3. If it's value is a lower case alphabet then, repeat the above step with 'z' and 'a'.\n\n4. Else copy the read character unchanged at the corrosponding index in the new character array.\n\n5. Repeat the above steps until you read null from input string.\n\n6. The character array at the end is the decrypted message.\n\n\n\n#### Time Complexity\nThe time complexity for the above algorithm would be O(n), where n is the length of the input string. "
}
{
"filename": "code/cryptography/src/autokey_cipher/autokey_cipher.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n#include <string.h>\n\nvoid flush_stdin()\n{\n while (fgetc(stdin) != '\\n')\n ;\n}\n\nvoid autokeycipher(char **text, char key, int encrypt)\n{\n int textsize;\n\n textsize = strlen((*text));\n\n for (int i = 0; i < textsize; ++i)\n {\n if (isalpha((*text)[i]))\n {\n (*text)[i] = toupper((*text)[i]);\n }\n }\n\n int nextkey, keyvalue, result;\n nextkey = toupper(key) - 'A';\n\n for (int i = 0; i < textsize; ++i)\n {\n if (isalpha((*text)[i]))\n {\n keyvalue = nextkey;\n if (encrypt)\n {\n nextkey = (*text)[i] - 'A';\n (*text)[i] = ((*text)[i] - 'A' + keyvalue) % 26 + 'A';\n }\n else\n {\n result = ((*text)[i] - 'A' - keyvalue) % 26 + 'A';\n (*text)[i] = result < 'A' ? (result + 26) : (result);\n nextkey = (*text)[i] - 'A';\n }\n }\n }\n}\n\nint main(int argc, char **argv)\n{\n char key, *plaintext;\n\n printf(\"Enter the Single Key word: \");\n scanf(\"%c\", &key);\n\n int length;\n printf(\"Enter the length of Plain Text: \");\n scanf(\"%d\", &length);\n\n plaintext = (char *)malloc((length + 1) * sizeof(char));\n flush_stdin();\n\n printf(\"Enter the Plain Text: \");\n fgets(plaintext, length + 1, stdin);\n flush_stdin();\n\n printf(\"\\nThe Plain Text is: %s\", plaintext);\n /* 3rd argument decides whither to do 1-Encryption or 0-Decryption. */\n autokeycipher(&plaintext, key, 1);\n printf(\"\\nThe Text after Encryption(Cipher text) is: %s\", plaintext);\n\n autokeycipher(&plaintext, key, 0);\n printf(\"\\nAfter Decryption the Text is: %s\", plaintext);\n\n free(plaintext);\n return 0;\n}\n\n/*\nSample Input:\nEnter the Single Key word: L //In A-Z, L will be at 11 index.\nEnter the length of Plain Text: 5\nEnter the Plain Text: hello\nSample Output:\nThe Plain Text is: hello\nThe Text after Encryption(Cipher text) is: slpwz\nAfter Decryption the Text is: hello\n*/"
}
{
"filename": "code/cryptography/src/autokey_cipher/autokey_cipher.cpp",
"content": "#include <iostream>\n\nstd::string autokeycipher(std::string text, char key, int encrypt)\n{\n for (int i = 0; i < text.length(); ++i)\n {\n if (isalpha(text[i]))\n {\n text[i] = toupper(text[i]);\n }\n }\n\n int nextkey, keyvalue, result;\n nextkey = toupper(key) - 'A';\n\n for (int i = 0; i < text.length(); ++i)\n {\n if (isalpha(text[i]))\n {\n keyvalue = nextkey;\n if (encrypt)\n {\n nextkey = text[i] - 'A';\n text[i] = (text[i] - 'A' + keyvalue) % 26 + 'A';\n }\n else\n {\n result = (text[i] - 'A' - keyvalue) % 26 + 'A';\n text[i] = result < 'A' ? (result + 26) : (result);\n nextkey = text[i] - 'A';\n }\n }\n }\n return text;\n}\n\nint main(int argc, char **argv)\n{\n char key;\n std::string plaintext;\n\n std::cout << \"Enter the Single Key word: \";\n std::cin >> key;\n\n std::cout << \"Enter the Plain Text: \";\n std::cin >> plaintext;\n\n std::cout << std::endl\n << \"The Plain Text is: \" << plaintext;\n\n /* 3rd argument decides whither to do 1-Encryption or 0-Decryption. */\n std::string ciphertext = autokeycipher(plaintext, key, 1);\n std::cout << std::endl\n << \"The Text after Encryption(Cipher text) is: \" << ciphertext;\n\n std::string decryptedtext = autokeycipher(ciphertext, key, 0);\n std::cout << std::endl\n << \"After Decryption the Text is: \" << decryptedtext;\n\n return 0;\n}\n\n/*\nSample Input:\nEnter the Single Key word: L //In A-Z, L will be at 11 index.\nEnter the Plain Text: hello\n\nSample Output:\nThe Plain Text is: hello\nThe Text after Encryption(Cipher text) is: slpwz\nAfter Decryption the Text is: hello\n*/"
}
{
"filename": "code/cryptography/src/autokey_cipher/autokey_cipher.java",
"content": "import java.io.BufferedReader;\nimport java.io.IOException;\nimport java.io.InputStreamReader;\nimport java.util.HashMap;\n\npublic class autokey\n{\n public static String createkey(String plaintext, int keycount, HashMap<Character, Integer> hashmap1, HashMap<Integer, Character> hashmap2)\n {\n char keytextarray[] = plaintext.toLowerCase().toCharArray();\n int temp = keycount % 26;\n \n for(int i = 0; i < plaintext.length(); ++i)\n {\n int a = hashmap1.get(plaintext.charAt(i));\n if(i == 0)\n {\n a = (a + temp) % 26;\n }\n else\n {\n a = (a + hashmap1.get(plaintext.charAt(i - 1))) % 26;\n }\n keytextarray[i] = hashmap2.get(a);\n }\n return new String(keytextarray);\n }\n \n public static String encryption(String plaintext, String keytext, HashMap<Character, Integer> hashmap1, HashMap<Integer, Character> hashmap2)\n {\n char plaintextarray[] = plaintext.toLowerCase().toCharArray();\n for(int i = 0; i < plaintext.length(); ++i)\n {\n int a = hashmap1.get(plaintext.charAt(i));\n int b = hashmap1.get(keytext.charAt(i));\n a = (a + b) % 26;\n plaintextarray[i] = hashmap2.get(a);\n }\n return new String(plaintextarray);\n }\n \n public static String decryption(String encrypted, String keytext, HashMap<Character, Integer> hashmap1, HashMap<Integer, Character> hashmap2)\n {\n char encryptedarray[] = encrypted.toLowerCase().toCharArray();\n for(int i = 0; i < encrypted.length(); ++i)\n {\n int a = hashmap1.get(encrypted.charAt(i));\n int b = hashmap1.get(keytext.charAt(i));\n a -= b;\n if(a < 0)\n {\n int x;\n x = Math.abs(a) % 26;\n a = 26 - x;\n }\n else\n {\n a %= 26;\n }\n encryptedarray[i] = hashmap2.get(a);\n }\n return new String(encryptedarray);\n }\n \n public static void main(String[] args) throws IOException\n {\n int keycount = 0;\n String plaintext = null, keytext = null, encrypted = null, decrypted = null;\n HashMap<Character, Integer> hashmap1 = new HashMap<Character, Integer>();\n HashMap<Integer, Character> hashmap2 = new HashMap<Integer, Character>();\n BufferedReader bufferedreader = new BufferedReader(new InputStreamReader(System.in));\n \n for(int i = 0; i < 26; ++i)\n {\n hashmap1.put((char)(i + 97), i);\n hashmap2.put(i,(char)(i + 97));\n }\n \n System.out.print(\"Enter the Plain Text : \");\n plaintext = bufferedreader.readLine();\n \n System.out.print(\"Enter Key: \");\n keycount = Integer.parseInt(bufferedreader.readLine());\n \n System.out.println(\"Plain Text is: \" + plaintext);\n keytext = createkey(plaintext, keycount, hashmap1, hashmap2);\n encrypted = encryption(plaintext, keytext, hashmap1, hashmap2);\n System.out.println(\"Encrypted Text: \" + keytext);\n \n decrypted = decryption(encrypted, keytext, hashmap1, hashmap2);\n System.out.println(\"Decrypted Text: \" + decrypted);\n \n bufferedreader.close();\n }\n}\n\n/*\nSample Input:\nEnter the Plain Text : hello\nEnter Key: 11(L)\nSample Output:\nPlain Text is: hello\nEncrypted Text : slpwz\nDecrypted Text : hello\n*/"
}
{
"filename": "code/cryptography/src/autokey_cipher/autokey.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n# It uses the following Table called Tabula Recta to find the\n# ciphertext. The simple intersection of the row and column\n# is the ciphertext. Let's say for eg. T is the Key and E is\n# the original Plaintext, then the intersection of T column\n# and E row is the ciphertext i.e. X. The plaintext and key-\n# length must be the same. Autokey Cipher is similar to\n# Vigenere Cipher. The only difference lies in how the key is\n# chosen. In Vigenere, any random key is chosen which is\n# repeated till the length of the plaintext. In Autokey,\n# the key chosen contains some part of the plaintext itself.\n\n# A B C D E F G H I J K L M N O P Q R S T U V W X Y Z\n# ---------------------------------------------------\n# A A B C D E F G H I J K L M N O P Q R S T U V W X Y Z\n# B B C D E F G H I J K L M N O P Q R S T U V W X Y Z A\n# C C D E F G H I J K L M N O P Q R S T U V W X Y Z A B\n# D D E F G H I J K L M N O P Q R S T U V W X Y Z A B C\n# E E F G H I J K L M N O P Q R S T U V W X Y Z A B C D\n# F F G H I J K L M N O P Q R S T U V W X Y Z A B C D E\n# G G H I J K L M N O P Q R S T U V W X Y Z A B C D E F\n# H H I J K L M N O P Q R S T U V W X Y Z A B C D E F G\n# I I J K L M N O P Q R S T U V W X Y Z A B C D E F G H\n# J J K L M N O P Q R S T U V W X Y Z A B C D E F G H I\n# K K L M N O P Q R S T U V W X Y Z A B C D E F G H I J\n# L L M N O P Q R S T U V W X Y Z A B C D E F G H I J K\n# M M N O P Q R S T U V W X Y Z A B C D E F G H I J K L\n# N N O P Q R S T U V W X Y Z A B C D E F G H I J K L M\n# O O P Q R S T U V W X Y Z A B C D E F G H I J K L M N\n# P P Q R S T U V W X Y Z A B C D E F G H I J K L M N O\n# Q Q R S T U V W X Y Z A B C D E F G H I J K L M N O P\n# R R S T U V W X Y Z A B C D E F G H I J K L M N O P Q\n# S S T U V W X Y Z A B C D E F G H I J K L M N O P Q R\n# T T U V W X Y Z A B C D E F G H I J K L M N O P Q R S\n# U U V W X Y Z A B C D E F G H I J K L M N O P Q R S T\n# V V W X Y Z A B C D E F G H I J K L M N O P Q R S T U\n# W W X Y Z A B C D E F G H I J K L M N O P Q R S T U V\n# X X Y Z A B C D E F G H I J K L M N O P Q R S T U V W\n# Y Y Z A B C D E F G H I J K L M N O P Q R S T U V W X\n# Z Z A B C D E F G H I J K L M N O P Q R S T U V W X Y\n\nplaintext = \"DEFENDTHEEASTWALLOFTHECASTLE\"\nkey = \"FORTIFICATIONDEFENDTHEEASTWA\"\nciphertext = \"\"\n\nif __name__ == \"__main__\":\n for index in range(len(plaintext)):\n sum_key_plaintext = (ord(key[index]) - 64) + (ord(plaintext[index]) - 64)\n if sum_key_plaintext > 27:\n ciphertext += chr(sum_key_plaintext - 26 - 1 + 64)\n else:\n ciphertext += chr(sum_key_plaintext - 1 + 64)\n print(ciphertext)"
}
{
"filename": "code/cryptography/src/autokey_cipher/README.md",
"content": "# Auto Key Cipher\n\nAuto Key Cipher is a Symmetric Polyalphabetic Substitution Cipher. This algorithm is about changing plaintext letters based on secret key letters. Each letter of the message is shifted along some alphabet positions. The number of positions is equal to the place in the alphabet of the current key letter.\n\n## How this Algorithm works\n\nFormula used for Encryption:\n\n> Ei = (Pi + Ki) mod 2\n\nFormula used for Decryption:\n\n> Di = (Ei - Ki + 26) mod 26\n\n**Pseudo Code**\n\n1. Convert the Plain Text or Cipher Text to either Upper or lower(must be in one format).\n2. Encryption:\n3. for 0 to length(text), use above formula for Dncryption,\n4. nextkey = text[i] - 'A';\n5. text[i] = (text[i] - 'A' + keyvalue) % 26 + 'A';\n6. Decryption:\n7. for 0 to length(text), use above formula for Decryption,\n8. result = (text[i] - 'A' - keyvalue) % 26 + 'A';\n9. text[i] = result < 'A' ? (result + 26) : (result);\n10. nextkey = text[i] - 'A';\n11. Display the Results\n\n<p align=\"center\">\n\tFor more insights, refer to <a href=\"https://iq.opengenus.org/auto-key-cipher/\">Auto Key Cipher</a>\n</p>\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/ravireddy07\">me</a> at <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/cryptography/src/baconian_cipher/baconian_cipher.c",
"content": "#include <stdio.h>\n#include <string.h>\n#include <stdlib.h>\n \nconst char *codes[] = {\n \"AAAAA\", \"AAAAB\", \"AAABA\", \"AAABB\", \"AABAA\",\n \"AABAB\", \"AABBA\", \"AABBB\", \"ABAAA\", \"ABAAB\",\n \"ABABA\", \"ABABB\", \"ABBAA\", \"ABBAB\", \"ABBBA\",\n \"ABBBB\", \"BAAAA\", \"BAAAB\", \"BAABA\", \"BAABB\",\n \"BABAA\", \"BABAB\", \"BABBA\", \"BABBB\", \"BBAAA\",\n \"BBAAB\", \"BBBAA\"\n};\n\n/*Function that takes a char and returns its code*/\nchar*\nget_code(const char c) \n{\n (c >= 97 && c <= 122) ? return (codes[c - 97]) : return (codes[26]);\n}\n\n/*Function that takes a code and returns the corresponding char*/\nchar \nget_char(const char *code) \n{\n int i;\n if (!strcmp(codes[26], code)) return ' ';\n for (i = 0; i < 26; ++i) {\n if (strcmp(codes[i], code) == 0) return 97 + i;\n }\n printf(\"\\nCode \\\"%s\\\" is invalid\\n\", code);\n exit(1);\n}\n \nvoid \nstr_tolower(char s[]) \n{\n int i;\n for (i = 0; i < strlen(s); ++i) s[i] = tolower(s[i]);\n}\n \nchar*\nbacon_encode(char plain_text[], char message[]) \n{\n int i, count;\n int plen = strlen(plain_text), mlen = strlen(message);\n int elen = 5 * plen;\n char c;\n char *p, *et = malloc(elen + 1), *mt;\n str_tolower(plain_text);\n for (i = 0, p = et; i < plen; ++i, p += 5) {\n c = plain_text[i];\n strncpy(p, get_code(c), 5);\n }\n *++p = '\\0';\n \n /* 'A's to be in lower case, 'B's in upper case */\n str_tolower(message);\n mt = calloc(mlen + 1, 1);\n for (i = 0, count = 0; i < mlen; ++i) {\n c = message[i];\n if (c >= 'a' && c <= 'z') {\n if (et[count] == 'A')\n mt[i] = c;\n else\n mt[i] = c - 32; /* upper case equivalent */\n if (++count == elen) break;\n }\n else mt[i] = c;\n }\n free(et); \n return (mt);\n}\n \nchar*\nbacon_decode(char cipher_text[]) \n{\n int i, count, clen = strlen(cipher_text);\n int plen;\n char *p, *ct = calloc(clen + 1, 1), *pt;\n char c, quintet[6];\n for (i = 0, count = 0; i < clen; ++i) {\n c = cipher_text[i];\n if (c >= 'a' && c <= 'z')\n ct[count++] = 'A';\n else if (c >= 'A' && c <= 'Z')\n ct[count++] = 'B';\n }\n \n plen = strlen(ct) / 5; \n pt = malloc(plen + 1);\n for (i = 0, p = ct; i < plen; ++i, p += 5) {\n strncpy(quintet, p, 5);\n quintet[5] = '\\0';\n pt[i] = get_char(quintet);\n }\n pt[plen] = '\\0';\n free(ct);\n return (pt);\n} \n \nint \nmain() \n{\n char plain_text[] = \"this is plain text\";\n char message[] = \"this is a message\";\n char *cipher_text, *hidden_text;\n cipher_text = bacon_encode(plain_text, message);\n printf(\"Cipher text is\\n\\n%s\\n\", cipher_text);\n hidden_text = bacon_decode(cipher_text);\n printf(\"\\nHidden text is\\n\\n%s\\n\", hidden_text);\n free(cipher_text);\n free(hidden_text);\n return (0);\n}"
}
{
"filename": "code/cryptography/src/baconian_cipher/baconian_cipher.cpp",
"content": "#include <map>\n#include <string>\n\n// Part of Cosmos by OpenGenus Foundation\n// This function generates a baconian map\nstd::map<char, std::string> createBaconianMap()\n{\n std::map<char, std::string> cipher;\n std::string static alphabet = \"ABCDEFGHIJKLMNOPQRSTUVWXYZ\";\n std::string value;\n for (std::size_t i = 0; i < alphabet.length(); ++i)\n {\n for (std::size_t j = 0; j < 5; ++j)\n ((i & (1 << j)) == 0) ? value = 'A' + value : value = 'B' + value;\n char key = alphabet[i];\n cipher[key] = value;\n value.clear();\n }\n\n return cipher;\n}\n\nstd::string encrypt(std::map<char, std::string>& cipher, std::string message)\n{\n std::string newMessage;\n for (std::size_t i = 0; i < message.length(); ++i)\n (message[i] !=\n ' ') ? newMessage.append(cipher[char(toupper(message[i]))]) : newMessage.append(\" \");\n\n return newMessage;\n}\n\nstd::string decrypt(std::map <char, std::string>& cipher, std::string message)\n{\n std::string newMessage;\n std::string currentString;\n int currentStringLength = 0;\n for (std::size_t i = 0; i < message.length() + 1; ++i)\n {\n if (currentStringLength == 5)\n {\n std::map<char, std::string>::iterator it = cipher.begin();\n while (it->second != currentString)\n ++it;\n newMessage += it->first;\n currentString.clear();\n currentStringLength = 0;\n }\n\n if (message[i] == ' ')\n newMessage.append(\" \");\n else\n {\n currentString += message[i];\n currentStringLength++;\n }\n }\n\n return newMessage;\n}"
}
{
"filename": "code/cryptography/src/baconian_cipher/baconian_cipher.exs",
"content": "defmodule Cryptography do\n @baconian_encryption_table %{\n a: \"AAAAA\",\n b: \"AAAAB\",\n c: \"AAABA\",\n d: \"AAABB\",\n e: \"AABAA\",\n f: \"AABAB\",\n g: \"AABBA\",\n h: \"AABBB\",\n i: \"ABAAA\",\n j: \"ABAAB\",\n k: \"ABAAB\",\n l: \"ABABB\",\n m: \"ABBAA\",\n n: \"ABBAB\",\n o: \"ABBBA\",\n p: \"ABBBB\",\n q: \"BAAAA\",\n r: \"BAAAB\",\n s: \"BAABA\",\n t: \"BAABB\",\n u: \"BABAA\",\n v: \"BABAB\",\n w: \"BABBA\",\n x: \"BABAB\",\n y: \"BBAAA\",\n z: \"BBAAB\"\n }\n def baconian_encrypt(content) do\n content\n |> String.downcase()\n |> String.split(\"\", trim: true)\n |> Enum.map(&Map.get(@baconian_encryption_table, :\"#{&1}\", \"\"))\n |> Enum.join()\n end\n\n def baconian_decrypt(baconian) do\n baconian_decryption_table =\n Enum.map(@baconian_encryption_table, fn {k, v} -> {v, k} end) |> Enum.into(%{})\n\n baconian\n |> String.upcase()\n |> String.split(\"\", trim: true)\n |> Enum.chunk_every(5)\n |> Enum.map(fn x ->\n key = Enum.join(x)\n\n Map.get(baconian_decryption_table, key)\n |> Atom.to_string()\n end)\n |> Enum.join()\n end\nend\n\nmsg = \"Hello world\"\n\nCryptography.baconian_encrypt(msg)\n|> IO.inspect(label: \"Encrypting #{msg}\")\n|> Cryptography.baconian_decrypt()\n|> IO.inspect(label: \"Decrypting encrypted #{msg}\")"
}
{
"filename": "code/cryptography/src/baconian_cipher/baconian_cipher.java",
"content": "import java.util.HashMap;\nimport java.util.Map;\n\npublic class Baconian {\n\n private Map<String ,String> cipherMap = new HashMap<>();\n\n public Baconian() {\n cipherMap.put(\"a\", \"aaaaa\");\n cipherMap.put(\"b\", \"aaaab\");\n cipherMap.put(\"c\", \"aaaba\");\n cipherMap.put(\"d\", \"aaabb\");\n cipherMap.put(\"e\", \"aabaa\");\n cipherMap.put(\"f\", \"aabab\");\n cipherMap.put(\"g\", \"aabba\");\n cipherMap.put(\"h\", \"aabbb\");\n cipherMap.put(\"i\", \"abaaa\");\n cipherMap.put(\"j\", \"abaab\");\n cipherMap.put(\"k\", \"ababa\");\n cipherMap.put(\"l\", \"ababb\");\n cipherMap.put(\"m\", \"abbaa\");\n cipherMap.put(\"n\", \"abbab\");\n cipherMap.put(\"o\", \"abbba\");\n cipherMap.put(\"p\", \"abbbb\");\n cipherMap.put(\"q\", \"baaaa\");\n cipherMap.put(\"r\", \"baaab\");\n cipherMap.put(\"s\", \"baaba\");\n cipherMap.put(\"t\", \"baabb\");\n cipherMap.put(\"u\", \"babaa\");\n cipherMap.put(\"v\", \"babab\");\n cipherMap.put(\"w\", \"babba\");\n cipherMap.put(\"x\", \"babbb\");\n cipherMap.put(\"y\", \"bbaaa\");\n cipherMap.put(\"z\", \"bbaab\");\n }\n\n public String encode(String word) {\n StringBuilder results = new StringBuilder();\n word = word.toLowerCase();\n for (Character letter : word.toCharArray()) {\n results.append(this.cipherMap.get(letter.toString()));\n }\n return results.toString();\n }\n\n public String decode(String word) {\n StringBuilder results = new StringBuilder();\n word = word.toLowerCase();\n for (int start = 0; start < word.length() / 5; ++start) {\n StringBuilder code = new StringBuilder();\n int startIndex = start * 5;\n for (int index = startIndex; index < startIndex + 5; ++index) {\n code.append(word.charAt(index));\n }\n String letter = getKeyFromValue(this.cipherMap, code.toString());\n results.append(letter);\n }\n return results.toString();\n }\n\n public static String getKeyFromValue(Map hashmap, Object value) {\n for (Object key: hashmap.keySet()) {\n if (hashmap.get(key).equals(value)) {\n return key.toString();\n }\n }\n return null;\n }\n}"
}
{
"filename": "code/cryptography/src/baconian_cipher/baconian_cipher.js",
"content": "function baconianEncrypt(plainText) {\n let baconianCodes = {\n a: \"AAAAA\",\n b: \"AAAAB\",\n c: \"AAABA\",\n d: \"AAABB\",\n e: \"AABAA\",\n f: \"AABAB\",\n g: \"AABBA\",\n h: \"AABBB\",\n i: \"ABAAA\",\n j: \"BBBAA\",\n k: \"ABAAB\",\n l: \"ABABA\",\n m: \"ABABB\",\n n: \"ABBAA\",\n o: \"ABBAB\",\n p: \"ABBBA\",\n q: \"ABBBB\",\n r: \"BAAAA\",\n s: \"BAAAB\",\n t: \"BAABA\",\n u: \"BAABB\",\n v: \"BBBAB\",\n w: \"BABAA\",\n x: \"BABAB\",\n y: \"BABBA\",\n z: \"BABBB\"\n };\n\n let cipherText = \"\";\n [...plainText].forEach(\n char => (cipherText += baconianCodes[char.toLowerCase()])\n );\n\n return cipherText;\n}\n\nconsole.log(baconianEncrypt(\"THISISASECRETTEST\"));\nconsole.log(baconianEncrypt(\"SAVETHECASTLE\"));"
}
{
"filename": "code/cryptography/src/baconian_cipher/baconian_cipher.php",
"content": "<?php\n/**\n* Bacon's (aka Baconian) cipher (PHP)\n* based on https://en.wikipedia.org/wiki/Bacon%27s_cipher\n* implemented by Stefano Amorelli <[email protected]>\n* Part of Cosmos by OpenGenus Foundation\n*/\n\nclass BaconianCipher\n{\n private $mapping;\n\n function __construct() {\n $this->mapping = [];\n $this->initMapping();\n }\n\n public function encrypt($plainText) {\n if (!ctype_alpha($plainText)) {\n throw new Exception('Plaintext should be alphabetical strings (no spaces, symbols and numbers).');\n }\n $plainText = strtoupper($plainText);\n $encryptedText = [];\n foreach (str_split($plainText) as $character) {\n array_push($encryptedText, $this->mapping[$character]);\n }\n return implode(' ', $encryptedText);\n }\n\n public function decrypt($encryptedText) {\n $plainText = [];\n foreach (explode(' ', $encryptedText) as $character) {\n foreach ($this->mapping as $key => $value) {\n if ($value === $character) {\n $plainCharacter = $key;\n continue;\n }\n }\n array_push($plainText, $plainCharacter);\n }\n return implode('', $plainText);\n }\n\n private function initMapping() {\n $this->mapping['A'] = 'aaaaa';\n $this->mapping['B'] = 'aaaab';\n $this->mapping['C'] = 'aaaba';\n $this->mapping['D'] = 'aaabb';\n $this->mapping['E'] = 'aabaa';\n $this->mapping['F'] = 'aabab';\n $this->mapping['G'] = 'aabba';\n $this->mapping['H'] = 'aabbb';\n $this->mapping['I'] = 'abaaa';\n $this->mapping['J'] = 'abaab';\n $this->mapping['K'] = 'ababa';\n $this->mapping['L'] = 'ababb';\n $this->mapping['M'] = 'abbaa';\n $this->mapping['N'] = 'abbab';\n $this->mapping['O'] = 'abbba';\n $this->mapping['P'] = 'abbbb';\n $this->mapping['Q'] = 'baaaa';\n $this->mapping['R'] = 'baaab';\n $this->mapping['S'] = 'baaba';\n $this->mapping['T'] = 'baabb';\n $this->mapping['U'] = 'babaa';\n $this->mapping['V'] = 'babab';\n $this->mapping['W'] = 'babba';\n $this->mapping['X'] = 'babbb';\n $this->mapping['Y'] = 'bbaaa';\n $this->mapping['Z'] = 'bbaab';\n }\n}\n\n\n/* Example usage: \n\n$cipher = new BaconianCipher();\n \n$encrypted = $cipher->encrypt('LOREMIPSUMDOLOR');\n\n$decrypted = $cipher->decrypt($encrypted);\n\necho $decrypted; // output: LOREMIPSUMDOLOR\n\n*/"
}
{
"filename": "code/cryptography/src/baconian_cipher/baconian_cipher.py",
"content": "# Part of Cosmos by OpenGenus Foundation\nbaconian_codes = {\n \"a\": \"AAAAA\",\n \"b\": \"AAAAB\",\n \"c\": \"AAABA\",\n \"d\": \"AAABB\",\n \"e\": \"AABAA\",\n \"f\": \"AABAB\",\n \"g\": \"AABBA\",\n \"h\": \"AABBB\",\n \"i\": \"ABAAA\",\n \"j\": \"BBBAA\",\n \"k\": \"ABAAB\",\n \"l\": \"ABABA\",\n \"m\": \"ABABB\",\n \"n\": \"ABBAA\",\n \"o\": \"ABBAB\",\n \"p\": \"ABBBA\",\n \"q\": \"ABBBB\",\n \"r\": \"BAAAA\",\n \"s\": \"BAAAB\",\n \"t\": \"BAABA\",\n \"u\": \"BAABB\",\n \"v\": \"BBBAB\",\n \"w\": \"BABAA\",\n \"x\": \"BABAB\",\n \"y\": \"BABBA\",\n \"z\": \"BABBB\",\n}\n\nif __name__ == \"__main__\":\n plaintext = \"Text to be encrypted\"\n ciphertext = \"\"\n for ch in plaintext:\n if ch != \" \":\n ciphertext += baconian_codes[ch.lower()]\n else:\n ciphertext += \" \"\n print(ciphertext)"
}
{
"filename": "code/cryptography/src/baconian_cipher/baconian_cipher.rb",
"content": "# Written by Michele Riva\n\nBACONIAN_CODE = {\n 'a' => 'AAAAA', 'b' => 'AAAAB', 'c' => 'AAABA',\n 'd' => 'AAABB', 'e' => 'AABAA', 'f' => 'AABAB',\n 'g' => 'AABBA', 'h' => 'AABBB', 'i' => 'ABAAA',\n 'j' => 'BBBAA', 'k' => 'ABAAB', 'l' => 'ABABA',\n 'm' => 'ABABB', 'n' => 'ABBAA', 'o' => 'ABBAB',\n 'p' => 'ABBBA', 'q' => 'ABBBB', 'r' => 'BAAAA',\n 's' => 'BAAAB', 't' => 'BAABA', 'u' => 'BAABB',\n 'v' => 'BBBAB', 'w' => 'BABAA', 'x' => 'BABAB',\n 'y' => 'BABBA', 'z' => 'BABBB'\n}.freeze\n\ndef encode(string)\n text = string.downcase.split('')\n encoded_text = []\n\n text.each do |c|\n bac_char = BACONIAN_CODE[c]\n encoded_text.push(bac_char)\n end\n\n encoded_text.join('')\nend\n\n# TEST!\nputs encode('Hello World') # => AABBBAABAAABABAABABAABBABBABAAABBABBAAAAABABAAAABB"
}
{
"filename": "code/cryptography/src/baconian_cipher/README.md",
"content": "# Bacon's Cipher\n\nBacon's cipher (or Baconian cipher) is actually a form of steganography rather than a cipher. This means that it's a method to hide a message in plain sight. It works by utilizing two different typefaces. One would be 'A', the other 'B'. You then write some text using both typefaces mixed together, following a simple pattern corresponding to each letter of the message you want to hide:\n\n| Letter | Code | Letter | Code |\n|--------|-------|--------|-------|\n| A | aaaaa | N | abbab |\n| B | aaaab | O | abbba |\n| C | aaaba | P | abbbb |\n| D | aaabb | Q | baaaa |\n| E | aabaa | R | baaab |\n| F | aabab | S | baaba |\n| G | aabba | T | baabb |\n| H | aabbb | U | babaa |\n| I | abaaa | V | babab |\n| J | abaab | W | babba |\n| K | ababa | X | babbb |\n| L | ababb | Y | bbaaa |\n| M | abbaa | Z | bbaab |\n\n\\* There is also another version of this cipher where I and J, and U and V correspond to the same code. This version, where each letter has it's own distinct code, is more popular and is generally the one used in implementations.\n\nFor example, \"Hello world\" would become \"AABBBAABAAABABBABABBABBBABABBAABBBABAAABABABBAAABB\" (spaces are ignored). If we wanted to hide this in some Lorem Ipsum, making typeface A normal and typeface B bold, it would look like the following:\n\nLo**rem** ip**s**um d**o**l**or** s**i**t **am**e**t** **co**n**s**e**ct**et**ur** **a**d**i**pis**c**i**n**g **el**it s**ed**\n\n## Sources and more detailed information:\n\n- https://en.wikipedia.org/wiki/Bacon's_cipher\n- http://rumkin.com/tools/cipher/baconian.php\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/cryptography/src/caesar_cipher/caesar_cipher.c",
"content": "// A C program to illustrate Caesar Cipher Technique\n\n#include <stdio.h>\n#include <stdlib.h>\n#include <string.h>\n#include <ctype.h>\n\n//argc gives the number of arguments and argv[1] gives the key of the cipher\nint main(int argc, char* argv[])\n{\n if(argc == 2)\n {\n int r = atoi(argv[1]); //Converts key from string type to integer type and stores in r\n printf(\"plaintext: \");\n char s[100]; //Stores plaintext\n scanf(\"%s\", s);\n \n printf(\"ciphertext: \\n\");\n \n //Traverses through all characters of plaintext\n for(int i = 0; i < strlen(s); i++)\n {\n if(isalpha(s[i])) //Checks if the character is an alphabet\n {\n if(isupper(s[i])) //Checks if the character is an uppercase alphabet\n {\n printf(\"%c\",((s[i] - 65 + r) % 26) + 65); //Performs encryption\n }\n \n if(islower(s[i])) //Checks if the character is an lowercase alphabet\n { \n printf(\"%c\",((s[i] - 97 + r) % 26) + 97); //Performs encryption\n }\n }\n \n else\n \n printf(\"%c\",s[i]); //Simply prints the character if it is not an alphabet\n \n }\n printf(\"\\n\");\n return 0;\n }\n \n else //If key is not given as argument argv[1]\n {\n printf(\"Invalid key Entered.\\n\");\n \n return 1;\n\n }\n}"
}
{
"filename": "code/cryptography/src/caesar_cipher/caesar_cipher.cpp",
"content": "// Part of Cosmos by OpenGenus Foundation\n\n// A C++ program to illustrate Caesar Cipher Technique\n#include <iostream>\nusing namespace std;\n\n// returns the encrypted text\nstring caesarcipher(string text, int s)\n{\n string result = \"\";\n\n // traverse text\n for (size_t i = 0; i < text.length(); i++)\n {\n //Takes care of spaces\n if (int(text[i]) == 32)\n {\n result += char(32);\n continue;\n }\n\n // Encrypt Uppercase letters\n if (isupper(text[i]))\n result += char(int(text[i] + s - 65) % 26 + 65);\n\n // Encrypt Lowercase letters\n else\n result += char(int(text[i] + s - 97) % 26 + 97);\n }\n\n // Return the resulting string\n return result;\n}\n\nint main()\n{\n string text;\n cout << \"\\nEnter string to encrypt\\n\";\n getline(cin, text);\n int s;\n cout << \"\\nEnter no of shifts:\\n\";\n cin >> s;\n cout << \"\\nText : \" << text;\n cout << \"\\nShift: \" << s;\n text = caesarcipher(text, s);\n cout << \"\\nEncrypted: \" << text;\n cout << \"\\nDecrypted: \" << caesarcipher(text, 26 - s);\n return 0;\n}"
}
{
"filename": "code/cryptography/src/caesar_cipher/caesar_cipher.cs",
"content": "// CAESAR CIPHER USING C#\n// Sample Input:\n// qwertyuiopasdfghjklzxcvbnm@QWERTYUIOPASDFGHJKLZXCVBNM\n// 3\n// Sample Output:\n// Encrypted value is: rxfsuzvjpqbteghiklmaydwcon?RXFSUZVJPQBTEGHIKLMAYDWCON\n// Decrypted value is: qwertyuiopasdfghjklzxcvbnm?QWERTYUIOPASDFGHJKLZXCVBNM\n// Part of Cosmos by OpenGenus Foundation\nusing System.IO;\nusing System;\n\nclass Program\n{\n const int NUM_ALPHABETS = 26;\n static char addValue(char character, int valueToShiftBy){\n if(character >= 'a' && character <= 'z'){\n return Convert.ToChar((((character - 'a') + valueToShiftBy) % NUM_ALPHABETS) + 'a');\n }\n else if(character >= 'A' && character <= 'Z'){\n return Convert.ToChar((((character - 'A') + valueToShiftBy) % NUM_ALPHABETS) + 'A');\n }\n // Not sure if Caesar's happy with this :)\n return '?';\n }\n \n static char subtractValue(char character, int valueToShiftBy){\n return addValue(character, NUM_ALPHABETS-(valueToShiftBy % NUM_ALPHABETS));\n }\n\n static string DecryptByCaesarCipher(string stringToEncrypt, int keyToShiftBy){\n string result = string.Empty;\n foreach(var character in stringToEncrypt){\n result += subtractValue(character, keyToShiftBy);\n }\n return result;\n }\n\n static string EncryptByCaesarCipher(string stringToEncrypt, int keyToShiftBy){\n string result = string.Empty;\n foreach(var character in stringToEncrypt){\n result += addValue(character, keyToShiftBy);\n }\n return result;\n }\n\n static void Main()\n {\n Console.WriteLine(\"Enter string and key!\");\n var stringToEncrypt = Console.ReadLine();\n var keyToShiftBy = int.Parse(Console.ReadLine());\n var encryptedValue = EncryptByCaesarCipher(stringToEncrypt, keyToShiftBy);\n Console.WriteLine($\"Encrypted value is: {encryptedValue}\");\n var decryptedValue = DecryptByCaesarCipher(encryptedValue, keyToShiftBy);\n Console.WriteLine($\"Decrypted value is: {decryptedValue}\");\n }\n}"
}
{
"filename": "code/cryptography/src/caesar_cipher/caesar_cipher.go",
"content": "package main\n\nimport (\n\t\"fmt\"\n\t\"strings\"\n)\n\nfunc Encrypt(str string, key int) string {\n\tif err := checkKey(key); err != nil {\n\t\tfmt.Println(\"Error: \", err.Error())\n\t\treturn \"\"\n\t}\n\tshift := rune(key)\n\tdec := strings.Map(func(r rune) rune {\n\t\tif r >= 'a' && r <= 'z'-shift || r >= 'A' && r <= 'Z'-shift {\n\t\t\treturn r + shift\n\t\t} else if r > 'z'-shift && r <= 'z' || r > 'Z'-shift && r <= 'Z' {\n\t\t\treturn r + shift - 26\n\t\t}\n\t\treturn r\n\t}, str)\n\treturn dec\n}\n\nfunc Decrypt(str string, key int) string {\n\tif err := checkKey(key); err != nil {\n\t\tfmt.Println(\"Error: \", err.Error())\n\t\treturn \"\"\n\t}\n\tshift := rune(key)\n\tdec := strings.Map(func(r rune) rune {\n\t\tif r >= 'a'+shift && r <= 'z' || r >= 'A'+shift && r <= 'Z' {\n\t\t\treturn r - shift\n\t\t} else if r >= 'a' && r < 'a'+shift || r >= 'A' && r < 'A'+shift {\n\t\t\treturn r - shift + 26\n\t\t}\n\t\treturn r\n\t}, str)\n\treturn dec\n}\n\nfunc checkKey(k int) error {\n\tif k <= 26 {\n\t\treturn nil\n\t}\n\treturn fmt.Errorf(\"Invalid key\\n 0 <= Key <= 26\")\n}\n\nfunc main() {\n\tvar msg string\n\tvar key int\n\tvar mode rune\n\tfmt.Println(\"Enter text to encrypt\\\\decrypt\")\n\tfmt.Scanf(\"%s\\n\", &msg)\n\tfmt.Println(\"Enter key\")\n\tfmt.Scanf(\"%d\\n\", &key)\n\tfmt.Println(\"Enter 'e' to encrypt or 'd' to decrypt\")\n\tfmt.Scanf(\"%c\", &mode)\n\tif mode == rune('e') {\n\t\tfmt.Println(\"Encrypted Message: \", Encrypt(msg, key))\n\t} else if mode == rune('d') {\n\t\tfmt.Println(\"Deccrypted Message: \", Decrypt(msg, key))\n\t} else {\n\t\tfmt.Println(\"Unknown mode\")\n\t}\n}"
}
{
"filename": "code/cryptography/src/caesar_cipher/caesar_cipher.hs",
"content": "import Data.Char (ord, chr, isUpper, isAlpha)\n \ncaesar, uncaesar :: Int -> String -> String\ncaesar = (<$>) . tr\n \nuncaesar = caesar . negate\n \ntr :: Int -> Char -> Char\ntr offset c\n | isAlpha c = chr $ intAlpha + mod ((ord c - intAlpha) + offset) 26\n | otherwise = c\n where\n intAlpha =\n ord\n (if isUpper c\n then 'A'\n else 'a')\n \nmain :: IO ()\nmain = mapM_ print [encoded, decoded]\n where\n encoded = caesar (-114) \"Veni, vidi, vici\"\n decoded = uncaesar (-114) encoded"
}
{
"filename": "code/cryptography/src/caesar_cipher/caesar_cipher.java",
"content": "import java.util.Scanner;\n\npublic final class CaesarCipher {\n private final static int MIN_KEY = 1;\n private final static int MAX_KEY = 26;\n\n public static void main(String[] args){\n final Scanner scanner = new Scanner(System.in);\n String ans = \"\";\n\n System.out.print(\"Enter a message: \");\n String message = scanner.nextLine();\n\n System.out.print(\"Encrypt or Decrypt: \");\n char type = scanner.next().charAt(0);\n int key = 0;\n\n do {\n System.out.print(\"Enter a key: \");\n key = scanner.nextInt();\n } while (key < MIN_KEY || key > MAX_KEY);\n \n key = (type == 'd' || type == 'D') ? -key : key;\n\n for (char c : message.toCharArray()) {\n int num = (int) c;\n\n if (Character.isAlphabetic(c)) {\n num += key;\n if (Character.isUpperCase(c)) {\n if (num > 'Z') {\n num -= MAX_KEY;\n }\n else if (num < 'A') {\n num += MAX_KEY;\n }\n }\n else if (Character.isLowerCase(c)) {\n if (num > 'z') {\n num -= MAX_KEY;\n }\n else if (num < 'a') {\n num += MAX_KEY;\n }\n }\n }\n\n ans += (char) num;\n }\n\n System.out.println(ans);\n }\n}"
}
{
"filename": "code/cryptography/src/caesar_cipher/caesar_cipher.js",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n// Caesar Cipher in javascript\n\n//Use the 'Ref' string to set the range of characters that can be encrypted. Characters not in 'Ref' will not be encoded\nconst Ref = \"abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789\";\n\n// text = the string to be encrypted/decrypted\n// shift = the amount of shift for encryption/decryption\n\nfunction caesar_cipher(text, shift) {\n shift %= Ref.length;\n if (shift < 0) {\n shift += Ref.length;\n }\n\n return text\n .split(\"\")\n .map(cv => {\n let loc = Ref.indexOf(cv);\n return loc !== -1\n ? Ref.charAt(\n loc + shift < Ref.length ? loc + shift : loc + shift - Ref.length\n )\n : cv;\n })\n .join(\"\");\n}\n/*\n -Sample use:\n let encrypted = caesar_cipher( \"Hello World\", 10 );\n -Then to decrypt\n let decrypted = caesar_cipher( encrypted, -10 );\n */"
}
{
"filename": "code/cryptography/src/caesar_cipher/caesar_cipher.php",
"content": "<?php\n/* Part of Cosmos by OpenGenus Foundation */\n\nconst REF = 'AaBbCcDdEeFfGgHhIiJjKkLlMmNnOoPpQqRrSsTtUuVvWwXxYyZz';\n\nfunction caesar_cipher(string $message, int $shift): string {\n $shift %= 26;\n $ref_shifted = substr(REF, $shift * 2) . substr(REF, 0, $shift * 2);\n return strtr($message, REF, $ref_shifted);\n}\n\necho 'Encoding \"Hello World\", using a right shift of 10:' . PHP_EOL;\necho caesar_cipher('Hello World', 10) . PHP_EOL . PHP_EOL;\n\necho 'Decoding \"byFFI qILFx\", using a left shift of 6:' . PHP_EOL;\necho caesar_cipher('byFFI qILFx', -6);"
}
{
"filename": "code/cryptography/src/caesar_cipher/caesar_cipher.py",
"content": "MAX_KEY_SIZE = 26\n\n# Mode to select (e)ncryption or (d)ecryption\ndef get_mode():\n while True:\n print(\"Do you wish to encrypt or decrypt a message?\")\n mode = input().lower()\n if mode in \"encrypt e decrypt d\".split():\n return mode\n else:\n print('Enter either \"encrypt\" or \"e\" or \"decrypt\" or \"d\".')\n\n\n# Gets message from the user\ndef get_message():\n print(\"Enter your message:\")\n return input()\n\n\n# Gets key from the user\ndef get_key():\n key = 0\n while key < 1 or key > MAX_KEY_SIZE:\n print(\"Enter the key number (1-%s)\" % (MAX_KEY_SIZE))\n key = int(input())\n return key\n\n\n# Translation logic here\ndef get_translated_message(mode, message, key):\n if mode[0] == \"d\":\n key = -key\n translated = \"\"\n for symbol in message:\n if symbol.isalpha():\n num = ord(symbol)\n num += key\n if symbol.isupper():\n if num > ord(\"Z\"):\n num -= 26\n elif num < ord(\"A\"):\n num += 26\n elif symbol.islower():\n if num > ord(\"z\"):\n num -= 26\n elif num < ord(\"a\"):\n num += 26\n translated += chr(num)\n else:\n translated += symbol\n return translated\n\n\nmode = get_mode()\nmessage = get_message()\nkey = get_key()\n\nprint(\"Your translated text is:\")\nprint(get_translated_message(mode, message, key))"
}
{
"filename": "code/cryptography/src/caesar_cipher/caesar_cipher.rb",
"content": "# Part of Cosmos by OpenGenus Foundation\n# Written by Michele Riva\n\nCHARS_HASH = Hash[('a'..'z').to_a.zip((0..25).to_a)]\n\ndef caesar(string, shift)\n shift = 25 if shift > 25\n\n text = string.downcase.split('')\n encoded = []\n\n text.each do |c|\n orig_num = CHARS_HASH[c]\n\n if orig_num - shift > 0\n encoded_num = orig_num - shift\n else\n pre = shift - orig_num\n encoded_num = 26 - pre\n end\n\n encoded_char = CHARS_HASH.key(encoded_num)\n encoded.push(encoded_char)\n end\n\n encoded.join('')\nend\n\n# Test!\n# Input your string as first argument and the shift as second argument.\nputs caesar('cosmos', 5) # => xjnhjn"
}
{
"filename": "code/cryptography/src/caesar_cipher/decryption.cpp",
"content": "#include <iostream>\n\nusing namespace std;\n\nint main()\n{\n char message[100], ch;\n int i, key;\n\n cout << \"Enter a message to decrypt: \";\n cin.getline(message, 100);\n cout << \"Enter key: \";\n cin >> key;\n\n //Traverses the ciphertext\n for (i = 0; message[i] != '\\0'; ++i)\n {\n ch = message[i];\n\n if (ch >= 'a' && ch <= 'z') //Checks if the character of ciphertext is a lowercase alphabet\n {\n ch = ch - key; //Performs decryption\n\n if (ch < 'a') //If character goes below lowercase a\n ch = ch + 'z' - 'a' + 1; //then we bring it within the range of lowercase a to z\n\n message[i] = ch;\n }\n else if (ch >= 'A' && ch <= 'Z') //Checks if the character of ciphertext is a uppercase alphabet\n {\n ch = ch - key; //Performs decryption\n\n if (ch > 'a') //If character goes above lowercase a\n ch = ch + 'Z' - 'A' + 1; //then we bring it within the range of uppercase A to Z\n\n message[i] = ch;\n }\n }\n\n cout << \"Decrypted message: \" << message;\n\n return 0;\n}"
}
{
"filename": "code/cryptography/src/caesar_cipher/encryption.cpp",
"content": "#include <iostream>\n\nusing namespace std;\n\nint main()\n{\n char message[100], ch;\n int i, key;\n\n cout << \"Enter a message to encrypt: \";\n cin.getline(message, 100);\n cout << \"Enter key: \";\n cin >> key;\n\n for (i = 0; message[i] != '\\0'; ++i)\n {\n ch = message[i];\n\n if (ch >= 'a' && ch <= 'z')\n {\n ch = ch + key;\n\n if (ch > 'z')\n ch = ch - 'z' + 'a' - 1;\n\n message[i] = ch;\n }\n else if (ch >= 'A' && ch <= 'Z')\n {\n ch = ch + key;\n\n if (ch > 'Z')\n ch = ch - 'Z' + 'A' - 1;\n\n message[i] = ch;\n }\n }\n\n cout << \"Encrypted message: \" << message;\n\n return 0;\n}"
}
{
"filename": "code/cryptography/src/caesar_cipher/README.md",
"content": "# Caesar Cipher\nThe Caesar Cipher (also known as shift cipher) is a method of encrypting messages. In this cipher, the original message (plaintext) is replaced with a letter corresponding to the original letter shifted by N places according to the English alphabet. It is therefore a Substitution cipher where letter at ‘L th’ position is shifted to ‘L+N th’ position, where **N is the same** for all the letters.\n\nUsing this method, the original message that is understandable to any English speaking user, needs to be deciphered before arriving at context. \n\n\n## Example\n\nThis example uses a right shift of 3.\n\n```text\nA B C D E F G H I J K L M N O P Q R S T U V W X Y Z\n| | | | | | | | | | | | | | | | | | | | | | | | | |\nV V V V V V V V V V V V V V V V V V V V V V V V V V\nD E F G H I J K L M N O P Q R S T U V W X Y Z A B C\n```\n#### Plain text:\nI LOVE WORKING ON GITHUB\n\n#### Cipher text:\nL ORYH ZRUNLQJ RQ JLWKXE\n\n## Further Reading\n[Caesar Cipher](https://en.wikipedia.org/wiki/Caesar_cipher)\n\n#### Fun Trivia:\nThis cipher is named after Julius Caesar, who used this in his private correspondence."
}
{
"filename": "code/cryptography/src/columnar_transposition_cipher/columnar_transposition_cipher.cpp",
"content": "// CPP program for\n// Columnar Transposition Cipher\n#include <string>\n#include <iostream>\n#include <map>\nusing namespace std;\n\nmap<int, int> keyMap;\n\nvoid setPermutationOrder(string key)\n{\n // Add the permutation\n for (size_t i = 0; i < key.length(); i++)\n keyMap[key[i]] = i;\n}\n\n// Encryption code for cipher\nstring encrypt(string object, string key)\n{\n int row, col, j;\n string cipher = \"\";\n\n /* calculate column of the matrix*/\n col = key.length();\n\n /* calculate Maximum row of the matrix*/\n row = object.length() / col;\n\n if (object.length() % col)\n row += 1;\n\n char matrix[row][col];\n\n for (int i = 0, k = 0; i < row; i++)\n for (int j = 0; j < col;)\n {\n if (object[k] == '\\0')\n {\n /* Adding the padding character '_' */\n matrix[i][j] = '_';\n j++;\n }\n\n if (isalpha(object[k]) || object[k] == ' ')\n {\n /* Adding only space and alphabet into matrix*/\n matrix[i][j] = object[k];\n j++;\n }\n k++;\n }\n\n for (map<int, int>::iterator ii = keyMap.begin(); ii != keyMap.end(); ++ii)\n {\n j = ii->second;\n\n // getting cipher text from matrix column wise using permuted key\n for (int i = 0; i < row; i++)\n if (isalpha(matrix[i][j]) || matrix[i][j] == ' ' || matrix[i][j] == '_')\n cipher += matrix[i][j];\n }\n\n return cipher;\n}\n\n// Decryption code for cipher\nstring decrypt(string cipher, string key)\n{\n /* calculate row and column for cipher Matrix */\n int col = key.length();\n\n int row = cipher.length() / col;\n char cipherMat[row][col];\n\n /* add character into matrix column wise */\n for (int j = 0, k = 0; j < col; j++)\n for (int i = 0; i < row; i++)\n cipherMat[i][j] = cipher[k++];\n\n /* update the order of key for decryption */\n int index = 0;\n for (map<int, int>::iterator ii = keyMap.begin(); ii != keyMap.end(); ++ii)\n ii->second = index++;\n\n /* Arrange the matrix column wise according\n * to permutation order by adding into new matrix */\n char decCipher[row][col];\n int k = 0;\n for (int l = 0, j; key[l] != '\\0'; k++)\n {\n j = keyMap[key[l++]];\n for (int i = 0; i < row; i++)\n decCipher[i][k] = cipherMat[i][j];\n }\n\n /* getting Message using matrix */\n string msg = \"\";\n for (int i = 0; i < row; i++)\n for (int j = 0; j < col; j++)\n if (decCipher[i][j] != '_')\n msg += decCipher[i][j];\n\n return msg;\n}\n\n// Driver Program for columnar transposition\nint main()\n{\n /* message */\n string msg = \"defend the east wall of the castle\";\n string key = \"GERMAN\";\n setPermutationOrder(key);\n\n\n string cipher = encrypt(msg, key);\n cout << \"Encrypted Message: \" << cipher << endl;\n\n\n cout << \"Decrypted Message: \" << decrypt(cipher, key) << endl;\n\n return 0;\n}"
}
{
"filename": "code/cryptography/src/des_cipher/des_java/DES.java",
"content": "// Java code for the above approach\n\nimport java.util.*;\n\npublic class DES {\n\n // CONSTANTS\n // Initial Permutation Table\n int[] IP = {58, 50, 42, 34, 26, 18, 10, 2, 60, 52, 44, 36, 28, 20, 12, 4, 62, 54, 46, 38, 30,\n 22, 14, 6, 64, 56, 48, 40, 32, 24, 16, 8, 57, 49, 41, 33, 25, 17, 9, 1, 59, 51, 43, 35,\n 27, 19, 11, 3, 61, 53, 45, 37, 29, 21, 13, 5, 63, 55, 47, 39, 31, 23, 15, 7};\n\n // Inverse Initial Permutation Table\n int[] IP1 = {40, 8, 48, 16, 56, 24, 64, 32, 39, 7, 47, 15, 55, 23, 63, 31, 38, 6, 46, 14, 54,\n 22, 62, 30, 37, 5, 45, 13, 53, 21, 61, 29, 36, 4, 44, 12, 52, 20, 60, 28, 35, 3, 43, 11,\n 51, 19, 59, 27, 34, 2, 42, 10, 50, 18, 58, 26, 33, 1, 41, 9, 49, 17, 57, 25};\n\n // first key-hePermutation Table\n int[] PC1 = {57, 49, 41, 33, 25, 17, 9, 1, 58, 50, 42, 34, 26, 18, 10, 2, 59, 51, 43, 35, 27,\n 19, 11, 3, 60, 52, 44, 36, 63, 55, 47, 39, 31, 23, 15, 7, 62, 54, 46, 38, 30, 22, 14, 6,\n 61, 53, 45, 37, 29, 21, 13, 5, 28, 20, 12, 4};\n\n // second key-Permutation Table\n int[] PC2 = {14, 17, 11, 24, 1, 5, 3, 28, 15, 6, 21, 10, 23, 19, 12, 4, 26, 8, 16, 7, 27, 20,\n 13, 2, 41, 52, 31, 37, 47, 55, 30, 40, 51, 45, 33, 48, 44, 49, 39, 56, 34, 53, 46, 42,\n 50, 36, 29, 32};\n\n // Expansion D-box Table\n int[] EP = {32, 1, 2, 3, 4, 5, 4, 5, 6, 7, 8, 9, 8, 9, 10, 11, 12, 13, 12, 13, 14, 15, 16, 17,\n 16, 17, 18, 19, 20, 21, 20, 21, 22, 23, 24, 25, 24, 25, 26, 27, 28, 29, 28, 29, 30, 31,\n 32, 1};\n\n // Straight Permutation Table\n int[] P = {16, 7, 20, 21, 29, 12, 28, 17, 1, 15, 23, 26, 5, 18, 31, 10, 2, 8, 24, 14, 32, 27, 3,\n 9, 19, 13, 30, 6, 22, 11, 4, 25};\n\n // S-box Table\n int[][][] sbox = {\n {{14, 4, 13, 1, 2, 15, 11, 8, 3, 10, 6, 12, 5, 9, 0, 7},\n {0, 15, 7, 4, 14, 2, 13, 1, 10, 6, 12, 11, 9, 5, 3, 8},\n {4, 1, 14, 8, 13, 6, 2, 11, 15, 12, 9, 7, 3, 10, 5, 0},\n {15, 12, 8, 2, 4, 9, 1, 7, 5, 11, 3, 14, 10, 0, 6, 13}},\n\n {{15, 1, 8, 14, 6, 11, 3, 4, 9, 7, 2, 13, 12, 0, 5, 10},\n {3, 13, 4, 7, 15, 2, 8, 14, 12, 0, 1, 10, 6, 9, 11, 5},\n {0, 14, 7, 11, 10, 4, 13, 1, 5, 8, 12, 6, 9, 3, 2, 15},\n {13, 8, 10, 1, 3, 15, 4, 2, 11, 6, 7, 12, 0, 5, 14, 9}},\n {{10, 0, 9, 14, 6, 3, 15, 5, 1, 13, 12, 7, 11, 4, 2, 8},\n {13, 7, 0, 9, 3, 4, 6, 10, 2, 8, 5, 14, 12, 11, 15, 1},\n {13, 6, 4, 9, 8, 15, 3, 0, 11, 1, 2, 12, 5, 10, 14, 7},\n {1, 10, 13, 0, 6, 9, 8, 7, 4, 15, 14, 3, 11, 5, 2, 12}},\n {{7, 13, 14, 3, 0, 6, 9, 10, 1, 2, 8, 5, 11, 12, 4, 15},\n {13, 8, 11, 5, 6, 15, 0, 3, 4, 7, 2, 12, 1, 10, 14, 9},\n {10, 6, 9, 0, 12, 11, 7, 13, 15, 1, 3, 14, 5, 2, 8, 4},\n {3, 15, 0, 6, 10, 1, 13, 8, 9, 4, 5, 11, 12, 7, 2, 14}},\n {{2, 12, 4, 1, 7, 10, 11, 6, 8, 5, 3, 15, 13, 0, 14, 9},\n {14, 11, 2, 12, 4, 7, 13, 1, 5, 0, 15, 10, 3, 9, 8, 6},\n {4, 2, 1, 11, 10, 13, 7, 8, 15, 9, 12, 5, 6, 3, 0, 14},\n {11, 8, 12, 7, 1, 14, 2, 13, 6, 15, 0, 9, 10, 4, 5, 3}},\n {{12, 1, 10, 15, 9, 2, 6, 8, 0, 13, 3, 4, 14, 7, 5, 11},\n {10, 15, 4, 2, 7, 12, 9, 5, 6, 1, 13, 14, 0, 11, 3, 8},\n {9, 14, 15, 5, 2, 8, 12, 3, 7, 0, 4, 10, 1, 13, 11, 6},\n {4, 3, 2, 12, 9, 5, 15, 10, 11, 14, 1, 7, 6, 0, 8, 13}},\n {{4, 11, 2, 14, 15, 0, 8, 13, 3, 12, 9, 7, 5, 10, 6, 1},\n {13, 0, 11, 7, 4, 9, 1, 10, 14, 3, 5, 12, 2, 15, 8, 6},\n {1, 4, 11, 13, 12, 3, 7, 14, 10, 15, 6, 8, 0, 5, 9, 2},\n {6, 11, 13, 8, 1, 4, 10, 7, 9, 5, 0, 15, 14, 2, 3, 12}},\n {{13, 2, 8, 4, 6, 15, 11, 1, 10, 9, 3, 14, 5, 0, 12, 7},\n {1, 15, 13, 8, 10, 3, 7, 4, 12, 5, 6, 11, 0, 14, 9, 2},\n {7, 11, 4, 1, 9, 12, 14, 2, 0, 6, 10, 13, 15, 3, 5, 8},\n {2, 1, 14, 7, 4, 10, 8, 13, 15, 12, 9, 0, 3, 5, 6, 11}}};\n\n int[] shiftBits = {1, 1, 2, 2, 2, 2, 2, 2, 1, 2, 2, 2, 2, 2, 2, 1};\n\n\n // hexadecimal to binary conversion\n String hextoBin(String input) {\n int n = input.length() * 4;\n input = Long.toBinaryString(Long.parseUnsignedLong(input, 16));\n while (input.length() < n)\n input = \"0\" + input;\n return input;\n }\n\n // binary to hexadecimal conversion\n String binToHex(String input) {\n int n = (int) input.length() / 4;\n input = Long.toHexString(Long.parseUnsignedLong(input, 2));\n while (input.length() < n)\n input = \"0\" + input;\n return input;\n }\n\n /***\n * per-mutate input hexadecimal according to specified sequence\n * \n * @param sequence permutation table\n * @param input\n * @return\n */\n String permutation(int[] sequence, String input) {\n String output = \"\";\n input = hextoBin(input);\n for (int i = 0; i < sequence.length; i++)\n output += input.charAt(sequence[i] - 1);\n output = binToHex(output);\n return output;\n }\n\n // xor 2 hexadecimal strings\n String xor(String a, String b) {\n\n // hexadecimal to decimal(base 10)\n long t_a = Long.parseUnsignedLong(a, 16);\n\n // hexadecimal to decimal(base 10)\n long t_b = Long.parseUnsignedLong(b, 16);\n\n // xor\n t_a = t_a ^ t_b;\n\n // decimal to hexadecimal\n a = Long.toHexString(t_a);\n\n // prepend 0's to maintain length\n while (a.length() < b.length())\n a = \"0\" + a;\n return a;\n }\n\n // left Circular Shifting bits\n String leftCircularShift(String input, int numBits) {\n int n = input.length() * 4;\n int perm[] = new int[n];\n for (int i = 0; i < n - 1; i++)\n perm[i] = (i + 2);\n perm[n - 1] = 1;\n while (numBits-- > 0)\n input = permutation(perm, input);\n return input;\n }\n\n // preparing 16 keys for 16 rounds\n String[] getKeys(String key) {\n String keys[] = new String[16];\n\n // first key permutation\n key = permutation(PC1, key);\n for (int i = 0; i < 16; i++) {\n key = leftCircularShift(key.substring(0, 7), shiftBits[i])\n + leftCircularShift(key.substring(7, 14), shiftBits[i]);\n\n // second key permutation\n keys[i] = permutation(PC2, key);\n }\n return keys;\n }\n\n // s-box lookup\n String sBox(String input) {\n String output = \"\";\n input = hextoBin(input);\n for (int i = 0; i < 48; i += 6) {\n String temp = input.substring(i, i + 6);\n int num = i / 6;\n int row = Integer.parseInt(temp.charAt(0) + \"\" + temp.charAt(5), 2);\n int col = Integer.parseInt(temp.substring(1, 5), 2);\n output += Integer.toHexString(sbox[num][row][col]);\n }\n return output;\n }\n\n /***\n * Round function that takes 64-bit and 48-bit key Gives a 64-bit output\n * \n * There will be 16 rounds in DES\n * \n * @param input 64-bit\n * @param key 48-bit\n * @param num Round Number\n * @return String 64-bit\n */\n String round(String input, String key, int num) {\n\n // fk\n String left = input.substring(0, 8);\n String temp = input.substring(8, 16);\n String right = temp;\n\n // Expansion permutation\n temp = permutation(EP, temp);\n\n // xor temp and round key\n temp = xor(temp, key);\n\n // lookup in s-box table\n temp = sBox(temp);\n\n // Straight D-box\n temp = permutation(P, temp);\n\n // xor\n left = xor(left, temp);\n\n // System.out.println(\"Round \" + (num + 1) + \" \" + right.toUpperCase() + \" \"\n // + left.toUpperCase() + \" \" + key.toUpperCase());\n\n // swapper\n return right + left;\n }\n\n /***\n * Whole DES encryption process.\n * \n * @param plainText String 64-bit\n * @param key String 64-bit\n * @return String 64-bit encrypted cipher text\n */\n public String encrypt(String plainText, String key) {\n int i;\n\n // get round keys\n String keys[] = getKeys(key);\n\n // Initial permutation\n plainText = permutation(IP, plainText);\n\n // System.out.println(\"After initial permutation: \" + plainText.toUpperCase());\n // System.out.println(\"After splitting: L0=\" + plainText.substring(0, 8).toUpperCase() +\n // \"R0=\"\n // + plainText.substring(8, 16).toUpperCase() + \"\\n\");\n\n // 16 rounds\n for (i = 0; i < 16; i++) {\n plainText = round(plainText, keys[i], i);\n }\n\n // 32-bit swap\n plainText = plainText.substring(8, 16) + plainText.substring(0, 8);\n\n // Final permutation\n plainText = permutation(IP1, plainText);\n return plainText;\n }\n\n /***\n * Whole DES decryption process.\n * \n * @param cipherText String 64-bit\n * @param key String 64-bit\n * @return String 64-bit encrypted cipher text\n */\n String decrypt(String cipherText, String key) {\n int i;\n\n // get round keys\n String keys[] = getKeys(key);\n\n // Initial permutation\n cipherText = permutation(IP, cipherText);\n\n // System.out.println(\"After initial permutation: \" + cipherText.toUpperCase());\n // System.out.println(\"After splitting: L0=\" + cipherText.substring(0, 8).toUpperCase()\n // + \" R0=\" + cipherText.substring(8, 16).toUpperCase() + \"\\n\");\n\n // 16-rounds\n for (i = 15; i > -1; i--) {\n cipherText = round(cipherText, keys[i], 15 - i);\n }\n\n // 32-bit swap\n cipherText = cipherText.substring(8, 16) + cipherText.substring(0, 8);\n\n // Final permutation\n cipherText = permutation(IP1, cipherText);\n // System.out.println(\"After final permutation: \" + cipherText.toUpperCase());\n\n return cipherText;\n }\n\n}"
}
{
"filename": "code/cryptography/src/des_cipher/des_java/files/cipher.txt",
"content": "84727383327383328385808079836968328479326669326978678289808469683287697676326578683265768379326869678289808469680000003287697676"
}
{
"filename": "code/cryptography/src/des_cipher/des_java/files/key.txt",
"content": "AABB09182736CCDD"
}
{
"filename": "code/cryptography/src/des_cipher/des_java/files/msg.txt",
"content": "this is supposed to be encrypted well and also decrypted well"
}
{
"filename": "code/cryptography/src/des_cipher/des_java/.gitignore",
"content": "*.class"
}
{
"filename": "code/cryptography/src/des_cipher/des_java/Main.java",
"content": "import java.io.File;\nimport java.io.FileNotFoundException;\nimport java.io.FileWriter;\nimport java.io.IOException;\nimport java.util.Arrays;\nimport java.util.Scanner;\n\npublic class Main {\n\n public static void writeToCipherFile(String file_path, String cipher_text) {\n try {\n FileWriter myWriter = new FileWriter(file_path);\n myWriter.write(cipher_text);\n myWriter.close();\n } catch (IOException e) {\n System.out.println(\"An error occurred.\");\n e.printStackTrace();\n }\n }\n\n /***\n * Parsing the text from the file Converting it to byte arrays Converting it ot 64-bit blocks\n * \n * @param file_path\n * @param block_size\n * @return\n */\n public String[] desPlaintextBlock(String file_path, int block_size) {\n\n String plain_text = \"\";\n try {\n File myObj = new File(file_path);\n Scanner myReader = new Scanner(myObj);\n\n while (myReader.hasNextLine()) {\n plain_text += myReader.nextLine().toUpperCase();\n }\n myReader.close();\n } catch (FileNotFoundException e) {\n System.out.println(\"An error occurred.\");\n e.printStackTrace();\n }\n\n\n byte[] byte_array = plain_text.getBytes();\n plain_text = \"\";\n\n for (byte value : byte_array) {\n plain_text += value;\n }\n\n // System.out.println(plain_text);\n\n return split(plain_text, block_size);\n }\n\n /***\n * Converting blocks of decrypted text back into byte array then into String\n * \n * @param blocks\n * @return String\n */\n public static String blocksToPlainText(String[] blocks) {\n\n String decrypted_blocks = String.join(\"\", blocks);\n writeToCipherFile(\"files/cipher.txt\", decrypted_blocks);\n\n String[] decrypted_blocks_array = decrypted_blocks.split(\"(?<=\\\\G.{2})\");\n\n byte[] byte_array = new byte[decrypted_blocks_array.length];\n\n for (int i = 0; i < byte_array.length; i++) {\n byte_array[i] = (byte) Integer.parseInt(decrypted_blocks_array[i]);\n }\n\n return new String(byte_array);\n }\n\n /***\n * If a block size is less than 64-bit padding it with zeros\n * \n * @param inputString\n * @param length\n * @return\n */\n public String padLeftZeros(String inputString, int length) {\n if (inputString.length() >= length) {\n return inputString;\n }\n StringBuilder sb = new StringBuilder();\n while (sb.length() < length - inputString.length()) {\n sb.append('0');\n }\n sb.append(inputString);\n\n return sb.toString();\n }\n\n /**\n * Splitting the string into desired blocks\n */\n public String[] split(String src, int len) {\n String[] result = new String[(int) Math.ceil((double) src.length() / (double) len)];\n\n for (int i = 0; i < result.length; i++)\n result[i] = src.substring(i * len, Math.min(src.length(), (i + 1) * len));\n\n result[result.length - 1] = padLeftZeros(result[result.length - 1], len);\n return result;\n }\n\n // Driver code\n public static void main(String args[]) {\n\n // READING KEY\n String key = \"\";\n try {\n File myObj = new File(\"files/key.txt\");\n Scanner myReader = new Scanner(myObj);\n\n while (myReader.hasNextLine()) {\n key += myReader.nextLine().toUpperCase();\n }\n myReader.close();\n } catch (FileNotFoundException e) {\n System.out.println(\"An error occurred.\");\n e.printStackTrace();\n }\n\n Main driver = new Main();\n\n // PARSING TEXT\n String[] blocks = driver.desPlaintextBlock(\"files/msg.txt\", 16);\n String[] cipher_blocks = blocks;\n\n\n System.out.println(\"\\n== ENCRYPTION ==\");\n System.out.println(\"\\n== 64-bit blocks of file ==\\n\");\n System.out.println(Arrays.toString(blocks));\n System.out.println();\n\n // ENCRYPTING\n DES cipher = new DES();\n\n for (int i = 0; i < blocks.length; i++) {\n cipher_blocks[i] = cipher.encrypt(blocks[i], key);\n }\n\n System.out.println(\"\\n== CIPHERED BLOCKS ==\\n\");\n System.out.println(Arrays.toString(cipher_blocks));\n System.out.println(\"\\n== DECRYPTION ==\");\n\n\n // DECRYPTING\n for (int i = 0; i < cipher_blocks.length; i++) {\n blocks[i] = cipher.decrypt(blocks[i], key);\n }\n\n System.out.println(\"\\n== DECRYPTED BLOCKS ==\\n\");\n System.out.println(Arrays.toString(blocks));\n System.out.println();\n\n // BYTE ARR TO STRING\n System.out.println(\"\\n== PLAIN TEXT ==\\n\");\n System.out.println(blocksToPlainText(blocks));\n }\n}"
}
{
"filename": "code/cryptography/src/des_cipher/des_java/README.MD",
"content": "# Explanation\n\n- KeyFile = _files:/key.txt_\n- MsgFile = _files:/msg.txt_\n- CphFile = _files:/cipher.txt_\n\n**1. `desPlaintextBlock()` that takes the plaintext as input and splits it up into 64 bit blocks. If the last block is less than 64 bits, pad it with 0s.**\n\n```\n public String[] desPlaintextBlock(String file_path, int block_size) {\n\n String plain_text = \"\";\n try {\n File myObj = new File(file_path);\n Scanner myReader = new Scanner(myObj);\n\n while (myReader.hasNextLine()) {\n plain_text += myReader.nextLine().toUpperCase();\n }\n myReader.close();\n } catch (FileNotFoundException e) {\n System.out.println(\"An error occurred.\");\n e.printStackTrace();\n }\n\n return split(plain_text, block_size);\n }\n\n public String padLeftZeros(String inputString, int length) {\n if (inputString.length() >= length) {\n return inputString;\n }\n StringBuilder sb = new StringBuilder();\n while (sb.length() < length - inputString.length()) {\n sb.append('0');\n }\n sb.append(inputString);\n\n return sb.toString();\n }\n\n public String[] split(String src, int len) {\n String[] result = new String[(int) Math.ceil((double) src.length() / (double) len)];\n\n for (int i = 0; i < result.length; i++)\n result[i] = src.substring(i * len, Math.min(src.length(), (i + 1) * len));\n\n result[result.length - 1] = padLeftZeros(result[result.length - 1], len);\n return result;\n }\n\n```\n\n**2. `permutation()` that takes a 64-bit block, performs permutation according to the 8 x 8 IP table and returns the permuted block.**\n\nCONSTANTS:\n\n```\n// CONSTANTS\n// Initial Permutation Table\n\nint[] IP = {58, 50, 42, 34, 26, 18, 10, 2, 60, 52, 44, 36, 28, 20, 12, 4, 62, 54, 46, 38, 30,\n 22, 14, 6, 64, 56, 48, 40, 32, 24, 16, 8, 57, 49, 41, 33, 25, 17, 9, 1, 59, 51, 43, 35,\n 27, 19, 11, 3, 61, 53, 45, 37, 29, 21, 13, 5, 63, 55, 47, 39, 31, 23, 15, 7};\n```\n\nMETHOD:\n\n```\n /***\n * per-mutate input hexadecimal\n * according to specified sequence\n *\n * @param sequence permutation table\n * @param input\n * @return\n */\n String permutation(int[] sequence, String input) {\n String output = \"\";\n input = hextoBin(input);\n for (int i = 0; i < sequence.length; i++)\n output += input.charAt(sequence[i] - 1);\n output = binToHex(output);\n return output;\n }\n\n```\n\nUSAGE:\n\n```\n// Initial permutation\n plainText = permutation(IP, plainText);\n\n```\n\n**3. FinalPermutation that takes a 64-bit block, performs permutation according to the 8 x 8 FP table and returns the permuted block.**\n\n```\n // Inverse Initial Permutation Table\n int[] IP1 = {40, 8, 48, 16, 56, 24, 64, 32, 39, 7, 47, 15, 55, 23, 63, 31, 38, 6, 46, 14, 54,\n 22, 62, 30, 37, 5, 45, 13, 53, 21, 61, 29, 36, 4, 44, 12, 52, 20, 60, 28, 35, 3, 43, 11,\n 51, 19, 59, 27, 34, 2, 42, 10, 50, 18, 58, 26, 33, 1, 41, 9, 49, 17, 57, 25};\n\n\n\nUSAGE:\n\n// Final permutation\n plainText = permutation(IP1, plainText);\n\n```"
}
{
"filename": "code/cryptography/src/elgamal/elgamal.py",
"content": "# ElGamal encryption\n\n# pip install pycryptodome\nfrom Crypto.Util import number\n\n# Key generation algorithm\ndef elgamal_gen_key(bits):\n # Prime number p\n while True:\n q = number.getPrime(bits - 1)\n p = 2 * q + 1\n if number.isPrime(p):\n break\n # Primitive element g\n while True:\n g = number.getRandomRange(3, p)\n # Primitive element judgment\n if pow(g, 2, p) == 1:\n continue\n if pow(g, q, p) == 1:\n continue\n break\n # Secret value x\n x = number.getRandomRange(2, p - 1)\n # Public value y\n y = pow(g, x, p)\n return (p, g, y), x\n\n\n# Encryption algorithm\ndef elgamal_encrypt(m, pk):\n p, g, y = pk\n assert 0 <= m < p\n r = number.getRandomRange(2, p - 1)\n c1 = pow(g, r, p)\n c2 = (m * pow(y, r, p)) % p\n return (c1, c2)\n\n\n# Decryption algorithm\ndef elgamal_decrypt(c, pk, sk):\n p, g, y = pk\n c1, c2 = c\n return (c2 * pow(c1, p - 1 - sk, p)) % p\n\n\npk, sk = elgamal_gen_key(bits=20)\nprint(\"pk:\", pk) # Public key\nprint(\"sk:\", sk) # Private key\nprint()\n\nm = 314159 # Plain text\nprint(\"m:\", m)\nc = elgamal_encrypt(m, pk) # Encryption\nprint(\"c:\", c)\nd = elgamal_decrypt(c, pk, sk) # Decryption\nprint(\"d:\", d)"
}
{
"filename": "code/cryptography/src/elgamal/README.md",
"content": "# ElGamal encryption\nThe ElGamal encryption system is an asymmetric key encryption algorithm for public-key cryptography .\nElGamal encryption can be defined over any cyclic group $G$, like multiplicative group of integers modulo n. Its security depends upon the difficulty of a certain problem in $G$ related to computing discrete logarithms.\n\n\n## Sources and more detailed information:\nhttps://en.wikipedia.org/wiki/ElGamal_encryption"
}
{
"filename": "code/cryptography/src/hill_cipher/hill_cipher.py",
"content": "# Python Hill Cipher\nkeyMatrix = [[0] * 3 for i in range(3)]\n\nmessageVector = [[0] for i in range(3)]\n\ncipherMatrix = [[0] for i in range(3)]\n\ndef getKeyMatrix(key):\n\tk = 0\n\tfor i in range(3):\n\t\tfor j in range(3):\n\t\t\tkeyMatrix[i][j] = ord(key[k]) % 65\n\t\t\tk += 1\n\ndef encrypt(messageVector):\n\tfor i in range(3):\n\t\tfor j in range(1):\n\t\t\tcipherMatrix[i][j] = 0\n\t\t\tfor x in range(3):\n\t\t\t\tcipherMatrix[i][j] += (keyMatrix[i][x] *\n\t\t\t\t\t\t\t\t\tmessageVector[x][j])\n\t\t\tcipherMatrix[i][j] = cipherMatrix[i][j] % 26\n\ndef HillCipher(message, key):\n\tgetKeyMatrix(key)\n\n\tfor i in range(3):\n\t\tmessageVector[i][0] = ord(message[i]) % 65\n\n\tencrypt(messageVector)\n\n\tCipherText = []\n\tfor i in range(3):\n\t\tCipherText.append(chr(cipherMatrix[i][0] + 65))\n\n\tprint(\"Ciphertext: \", \"\".join(CipherText))\n\n# Driver Code\ndef main():\n\tmessage = \"ACT\"\n\tkey = \"GYBNQKURP\"\n\n\tHillCipher(message, key)\n\nif __name__ == \"__main__\":\n\tmain()"
}
{
"filename": "code/cryptography/src/huffman_encoding/huffman_encoding.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n\ntypedef struct node{\n\t\n\tint freq;\n\tchar alpha[26];\n\tstruct node *next;\n\tstruct node *left;\n\tstruct node *right;\n\tstruct node *parent;\n}node;\n\nnode* getnode(){\n\n\tnode* newnode = (node*)malloc(sizeof(node));\n\tnewnode->freq = -1;\n\tint i;\n\tfor(i=0;i<26;i++)newnode->alpha[i] = '$';\n\tnewnode->next = NULL;\n\tnewnode->left = NULL;\n\tnewnode->right = NULL;\n\tnewnode->parent = NULL;\n\treturn newnode;\n}\n\nnode* adds(node* headl,node* temp){\n\n\tif(headl == NULL){\n\n\t\theadl = temp;\n\t\treturn headl;\n\t}\n\telse{\n\t\ttemp->next = headl;\n\t\theadl = temp;\n\t\treturn headl;\n\t}\n}\n\nnode* addl(node* taill,node* temp){\n\n\tif(taill == NULL){\n\n\t\ttaill = temp;\n\t\treturn taill;\n\t}\n\telse{\n\t\ttaill->next = temp;\n\t\ttaill = temp;\n\t\treturn taill;\n\t}\n}\n\n\nnode* addbw(node* smaller,node* temp,node* greater){\n\n\ttemp->next = greater;\n\tsmaller->next = temp;\n\treturn temp;\n}\n\nnode* addafterp(node* p, node* temp){\n\n\tnode *te = p->next;\n\ttemp = addbw(p,temp,te);\n\treturn temp;\n}\n\nvoid printl(node* headl){\n\n\twhile(headl != NULL){\n\t\tprintf(\"%c%d \",headl->alpha[0],headl->freq);\n\t\theadl = headl->next;\n\t}\n}\n\nnode* search(node* headl,node *temp){\n\n\tnode* t1 = headl;\n\tnode* t2 = headl;\n\tif(t2->next != NULL){\n\t\tt2 = t2->next;\n\t}\n\tif(t2 == headl){\n\t\tif(t2->freq > temp->freq){\n\t\t\theadl = adds(t2,temp);\n\t\t\treturn headl;\n\t\t}\n\t\telse if(t2->freq < temp->freq){\n\t\t\ttemp = addl(t2,temp);\n\t\t\treturn headl;\n\t\t}\n\t\telse if(t2->freq == temp->freq){\n\t\t\tif(t2->alpha[0] > temp->alpha[0]){\n\t\t\t\ttemp = addl(t2,temp);\n\t\t\t\treturn headl;\n\t\t\t}\n\t\t\telse if(t2->alpha[0] < temp->alpha[0]){\n\t\t\t\ttemp = adds(t2,temp);\n\t\t\t\treturn headl;\n\t\t\t}\n\t\t}\n\t}\n\twhile(t1 != t2){\n\t\t\n\t\tif(temp->freq < t1->freq){\n\t\t\t\ttemp->next = t1;\n\t\t\t\theadl = temp;\n\t\t\t\treturn headl;\n\t\t}\n\t\telse if(temp->freq >t2->freq){\n\t\t\tif(t2->next == NULL){\n\t\t\t\ttemp = addl(t2,temp);\n\t\t\t\treturn headl;\n\t\t\t}\n\t\t\telse if(temp->freq > t2->freq && temp->freq < (t2->next)->freq){\n\t\t\t\ttemp = addafterp(t2,temp);\n\t\t\t\treturn headl;\n\t\t\t}\n\t\t}\n\t\telse if(t1->freq < temp->freq && t2->freq > temp->freq){\n\n\t\t\ttemp = addbw(t1,temp,t2);\n\t\t\t\n\t\t\treturn headl;\n\t\t}\n\t\telse if(t1->freq < temp->freq && t2->freq == temp->freq){\n\n\t\t\tif(temp->alpha[0] < t2->alpha[0]){\n\t\t\t\ttemp = addbw(t1,temp,t2);\n\t\t\t\treturn headl;\n\t\t\t}\n\t\t\tif(t2->next == NULL){\n\t\t\t\ttemp = addl(t2,temp);\n\t\t\t\treturn headl;\n\t\t\t}\n\t\t}\n\t\telse if(t1->freq == temp->freq && t2->freq == temp->freq){\n\n\t\t\tif(temp->alpha[0] < t2->alpha[0] && temp->alpha[0] > t1->alpha[0]){\n\t\t\t\ttemp = addbw(t1,temp,t2);\n\t\t\t\treturn headl;\n\t\t\t}\n\t\t\tif(t2->next == NULL){\n\t\t\t\ttemp = addl(t2,temp);\n\t\t\t\treturn headl;\n\t\t\t}\n\t\t}\n\t\telse if(t1->freq >temp->freq && t2->next == NULL){\n\t\t\theadl = adds(t1,temp);\n\t\t\treturn headl;\n\t\t}\n\t\telse if(t1->freq == temp->freq && t2->freq > temp->freq){\n\t\t\tif(temp->alpha[0] > t1->alpha[0]){\n\t\t\t\ttemp = addbw(t1,temp,t2);\n\t\t\t\treturn headl;\n\t\t\t}\n\t\t\telse{\n\t\t\t\theadl = adds(t1,temp);\n\t\t\t\treturn headl;\n\t\t\t}\n\t\t}\n\t\tif(t1->next != NULL){\n\t\t\tt1 = t1->next;\n\t\t}\n\t\tif(t2->next != NULL){\n\t\t\tt2 = t2->next;\n\t\t}\n\t}\n\n}\n\n\nnode* pop(node *headl){\n\n\tnode* temp = headl;\n\theadl = headl->next;\n\ttemp->next = NULL;\n\treturn temp;\n}\n\nnode* buildtree(node* headl){\n\n\t\twhile(headl->next != NULL){\n\t\t\n\t\tnode* t2 = headl;\n\t\theadl = headl->next;\n\t\tt2->next = NULL;\n\t\tnode* t1 = headl;\n\t\theadl = headl->next;\n\t\tt1->next = NULL;\n\t\tnode* par = getnode();\n\t\tpar->left = t2;\n\t\tpar->right = t1;\n\t\tpar->alpha[0] = (par->left)->alpha[0];\n\t\tpar->freq = t1->freq + t2->freq;\n\t\tt1->parent = par;\n\t\tt2->parent = par;\n\t\tif(headl == NULL){\n\t\t\theadl = par;\n\t\t}\n\t\telse{\n\t\t\theadl = search(headl,par);\n\t\t}\t\n\t}\n\t\n\treturn headl;\n\n}\n\nvoid printleaf(node *headl){\n\n\tif(headl == NULL)return;\n\telse\n\t{\n\t\tif(headl->left == NULL && headl->right == NULL)printf(\"%c \",headl->alpha[0]);\n\t\telse{\n\t\t\tprintleaf(headl->left);\n\t\t\tprintleaf(headl->right);\n\t\t}\n\t}\n\n}\n\nvoid printarr(int *arr, int n)\n{\n int i;\n for (i = 0; i < n; ++i)printf(\"%d\", arr[i]);\n printf(\"\\n\");\n}\n\nvoid printcodes(node* headl, int *arr, int top)\n{\n if (headl->left)\n {\n arr[top] = 0;\n printcodes(headl->left, arr, top + 1);\n }\n if (headl->right)\n {\n arr[top] = 1;\n printcodes(headl->right, arr, top + 1);\n }\n if (headl->left == NULL && headl->right == NULL)\n {\n printf(\"%c: \", headl->alpha[0]);\n printarr(arr, top);\n }\n}\n\nint main(){\n\n\tnode *head = getnode();\n\tchar input[100];\n\tint top =0,result[20];\n\tprintf(\"Enter the string to be coded: \");\n\tscanf(\"%s\",input);\n\tint frequency[26] = {0};\n\tint d,i;\n\tfor(i=0;input[i] != '\\0';i++){\n\n\t\td = input[i];\n\t\td -= 97;\n\t\tfrequency[d]++;\n\t}\n\tint f=0;\n\tfor(i=0;i<26;i++){\n\n\t\tif(frequency[i] != 0){\n\t\t\tif(f==0){\n\t\t\t\thead->alpha[0] = i+97;\n\t\t\t\thead->freq = frequency[i];\n\t\t\t\tf=1;\n\t\t\t}\n\t\t\telse\n\t\t\t{\n\t\t\t\tnode *t1 = getnode();\n\t\t\t\tt1->alpha[0] = i+97;\n\t\t\t\tt1->freq = frequency[i];\n\t\t\t\thead = search(head,t1);\n\t\t\t}\n\t\t}\n\t}\n\thead = buildtree(head);\n\tprintf(\"List of codes: \\n\");\n\tprintcodes(head,result,top);\n\treturn 0;\n}"
}
{
"filename": "code/cryptography/src/huffman_encoding/huffman.java",
"content": "import java.io.File;\nimport java.io.FileNotFoundException;\nimport java.util.PriorityQueue;\nimport java.util.Scanner;\nimport java.util.TreeMap;\n\n/* Huffman coding , decoding */\n\npublic class Huffman {\n static final boolean readFromFile = false;\n static final boolean newTextBasedOnOldOne = false;\n\n static PriorityQueue<Node> nodes = new PriorityQueue<>((o1, o2) -> (o1.value < o2.value) ? -1 : 1);\n static TreeMap<Character, String> codes = new TreeMap<>();\n static String text = \"\";\n static String encoded = \"\";\n static String decoded = \"\";\n static int ASCII[] = new int[128];\n\n public static void main(String[] args) throws FileNotFoundException {\n Scanner scanner = (readFromFile) ? new Scanner(new File(\"input.txt\")) : new Scanner(System.in);\n int decision = 1;\n while (decision != -1) {\n if (handlingDecision(scanner, decision)) continue;\n decision = consoleMenu(scanner);\n }\n }\n\n private static int consoleMenu(Scanner scanner) {\n int decision;\n System.out.println(\"\\n---- Menu ----\\n\" +\n \"-- [-1] to exit \\n\" +\n \"-- [1] to enter new text\\n\" +\n \"-- [2] to encode a text\\n\" +\n \"-- [3] to decode a text\");\n decision = Integer.parseInt(scanner.nextLine());\n if (readFromFile)\n System.out.println(\"Decision: \" + decision + \"\\n---- End of Menu ----\\n\");\n return decision;\n }\n\n private static boolean handlingDecision(Scanner scanner, int decision) {\n if (decision == 1) {\n if (handleNewText(scanner)) return true;\n } else if (decision == 2) {\n if (handleEncodingNewText(scanner)) return true;\n } else if (decision == 3) {\n handleDecodingNewText(scanner);\n }\n return false;\n }\n\n private static void handleDecodingNewText(Scanner scanner) {\n System.out.println(\"Enter the text to decode:\");\n encoded = scanner.nextLine();\n System.out.println(\"Text to Decode: \" + encoded);\n decodeText();\n }\n\n private static boolean handleEncodingNewText(Scanner scanner) {\n System.out.println(\"Enter the text to encode:\");\n text = scanner.nextLine();\n System.out.println(\"Text to Encode: \" + text);\n\n if (!IsSameCharacterSet()) {\n System.out.println(\"Not Valid input\");\n text = \"\";\n return true;\n }\n encodeText();\n return false;\n }\n\n private static boolean handleNewText(Scanner scanner) {\n int oldTextLength = text.length();\n System.out.println(\"Enter the text:\");\n text = scanner.nextLine();\n if (newTextBasedOnOldOne && (oldTextLength != 0 && !IsSameCharacterSet())) {\n System.out.println(\"Not Valid input\");\n text = \"\";\n return true;\n }\n ASCII = new int[128];\n nodes.clear();\n codes.clear();\n encoded = \"\";\n decoded = \"\";\n System.out.println(\"Text: \" + text);\n calculateCharIntervals(nodes, true);\n buildTree(nodes);\n generateCodes(nodes.peek(), \"\");\n\n printCodes();\n System.out.println(\"-- Encoding/Decoding --\");\n encodeText();\n decodeText();\n return false;\n\n\n\n }\n\n private static boolean IsSameCharacterSet() {\n boolean flag = true;\n for (int i = 0; i < text.length(); i++)\n if (ASCII[text.charAt(i)] == 0) {\n flag = false;\n break;\n }\n return flag;\n }\n\n private static void decodeText() {\n decoded = \"\";\n Node node = nodes.peek();\n for (int i = 0; i < encoded.length(); ) {\n Node tmpNode = node;\n while (tmpNode.left != null && tmpNode.right != null && i < encoded.length()) {\n if (encoded.charAt(i) == '1')\n tmpNode = tmpNode.right;\n else tmpNode = tmpNode.left;\n i++;\n }\n if (tmpNode != null)\n if (tmpNode.character.length() == 1)\n decoded += tmpNode.character;\n else\n System.out.println(\"Input not Valid\");\n\n }\n System.out.println(\"Decoded Text: \" + decoded);\n }\n\n private static void encodeText() {\n encoded = \"\";\n for (int i = 0; i < text.length(); i++)\n encoded += codes.get(text.charAt(i));\n System.out.println(\"Encoded Text: \" + encoded);\n }\n\n private static void buildTree(PriorityQueue<Node> vector) {\n while (vector.size() > 1)\n vector.add(new Node(vector.poll(), vector.poll()));\n }\n\n private static void printCodes() {\n System.out.println(\"--- Printing Codes ---\");\n codes.forEach((k, v) -> System.out.println(\"'\" + k + \"' : \" + v));\n }\n\n private static void calculateCharIntervals(PriorityQueue<Node> vector, boolean printIntervals) {\n if (printIntervals) System.out.println(\"-- intervals --\");\n\n for (int i = 0; i < text.length(); i++)\n ASCII[text.charAt(i)]++;\n\n for (int i = 0; i < ASCII.length; i++)\n if (ASCII[i] > 0) {\n vector.add(new Node(ASCII[i] / (text.length() * 1.0), ((char) i) + \"\"));\n if (printIntervals)\n System.out.println(\"'\" + ((char) i) + \"' : \" + ASCII[i] / (text.length() * 1.0));\n }\n }\n\n private static void generateCodes(Node node, String s) {\n if (node != null) {\n if (node.right != null)\n generateCodes(node.right, s + \"1\");\n\n if (node.left != null)\n generateCodes(node.left, s + \"0\");\n\n if (node.left == null && node.right == null)\n codes.put(node.character.charAt(0), s);\n }\n }\n}\n\nclass Node {\n Node left, right;\n double value;\n String character;\n\n public Node(double value, String character) {\n this.value = value;\n this.character = character;\n left = null;\n right = null;\n }\n\n public Node(Node left, Node right) {\n this.value = left.value + right.value;\n character = left.character + right.character;\n if (left.value < right.value) {\n this.right = right;\n this.left = left;\n } else {\n this.right = left;\n this.left = right;\n }\n }\n}"
}
{
"filename": "code/cryptography/src/huffman_encoding/huffman.py",
"content": "# A Huffman Tree Node\nclass Node:\n def __init__(self, prob, symbol, left=None, right=None):\n # probability of symbol\n self.prob = prob\n\n # symbol \n self.symbol = symbol\n\n # left node\n self.left = left\n\n # right node\n self.right = right\n\n # tree direction (0/1)\n self.code = ''\n\n\"\"\" A helper function to print the codes of symbols by traveling Huffman Tree\"\"\"\ncodes = dict()\n\ndef Calculate_Codes(node, val=''):\n # huffman code for current node\n newVal = val + str(node.code)\n\n if(node.left):\n Calculate_Codes(node.left, newVal)\n if(node.right):\n Calculate_Codes(node.right, newVal)\n\n if(not node.left and not node.right):\n codes[node.symbol] = newVal\n \n return codes \n\n\"\"\" A helper function to calculate the probabilities of symbols in given data\"\"\"\ndef Calculate_Probability(data):\n symbols = dict()\n for element in data:\n if symbols.get(element) == None:\n symbols[element] = 1\n else: \n symbols[element] += 1 \n return symbols\n\n\"\"\" A helper function to obtain the encoded output\"\"\"\ndef Output_Encoded(data, coding):\n encoding_output = []\n for c in data:\n # print(coding[c], end = '')\n encoding_output.append(coding[c])\n \n string = ''.join([str(item) for item in encoding_output]) \n return string\n \n\"\"\" A helper function to calculate the space difference between compressed and non-compressed data\"\"\" \ndef Total_Gain(data, coding):\n before_compression = len(data) * 8 # total bit space to stor the data before compression\n after_compression = 0\n symbols = coding.keys()\n for symbol in symbols:\n count = data.count(symbol)\n after_compression += count * len(coding[symbol]) # calculate how many bit is required for that symbol in total\n print(\"Space usage before compression (in bits):\", before_compression) \n print(\"Space usage after compression (in bits):\", after_compression) \n\ndef Huffman_Encoding(data):\n symbol_with_probs = Calculate_Probability(data)\n symbols = symbol_with_probs.keys()\n probabilities = symbol_with_probs.values()\n print(\"symbols: \", symbols)\n print(\"probabilities: \", probabilities)\n \n nodes = []\n \n # converting symbols and probabilities into huffman tree nodes\n for symbol in symbols:\n nodes.append(Node(symbol_with_probs.get(symbol), symbol))\n \n while len(nodes) > 1:\n # sort all the nodes in ascending order based on their probability\n nodes = sorted(nodes, key=lambda x: x.prob)\n \n # pick 2 smallest nodes\n right = nodes[0]\n left = nodes[1]\n \n left.code = 0\n right.code = 1\n \n # combine the 2 smallest nodes to create new node\n newNode = Node(left.prob+right.prob, left.symbol+right.symbol, left, right)\n \n nodes.remove(left)\n nodes.remove(right)\n nodes.append(newNode)\n \n huffman_encoding = Calculate_Codes(nodes[0])\n print(\"symbols with codes\", huffman_encoding)\n Total_Gain(data, huffman_encoding)\n encoded_output = Output_Encoded(data,huffman_encoding)\n return encoded_output, nodes[0] \n \n \ndef Huffman_Decoding(encoded_data, huffman_tree):\n tree_head = huffman_tree\n decoded_output = []\n for x in encoded_data:\n if x == '1':\n huffman_tree = huffman_tree.right \n elif x == '0':\n huffman_tree = huffman_tree.left\n try:\n if huffman_tree.left.symbol == None and huffman_tree.right.symbol == None:\n pass\n except AttributeError:\n decoded_output.append(huffman_tree.symbol)\n huffman_tree = tree_head\n \n string = ''.join([str(item) for item in decoded_output])\n return string "
}
{
"filename": "code/cryptography/src/huffman_encoding/README.md",
"content": "# Huffman Encoding\n\nHuffman Encoding is a famous greedy algorithm that is used for the loseless compression of file/data. It uses variable length encoding where variable length codes are assigned to all the characters depending on how frequently they occur in the given text. The character which occurs most frequently gets the smallest code and the character which occurs least frequently gets the largest code.\n\n\n## Sources and more detailed information:\n\n- https://iq.opengenus.org/huffman-encoding/\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/cryptography/src/monoalphabetic_cipher/monoalphabeticCipher.py",
"content": "keyDict = {}\n\n# Enter a custom map for the monoalphabetic encryption mapping\ndef create_Map():\n print(\"Enter the monoalphabetic map as a string(lenght 26)\")\n inputMap = input()\n cur = \"a\"\n curIndex = 0\n while curIndex < 26:\n keyDict[cur] = inputMap[curIndex]\n cur = chr(ord(cur) + 1)\n curIndex += 1\n\n\n# Return the corresponding decrypted char for a given input char\ndef get_key(value):\n for key, val in keyDict.items():\n if val == value:\n return key\n\n\n# Encrypt the input text\ndef monoalphabetic_encrypt():\n plainText = input(\"Enter plain text-\")\n wordList = plainText.split(\" \")\n cipherText = \"\"\n for word in wordList:\n c = \"\"\n for i in word:\n i = keyDict[i]\n c += i\n cipherText += c + \" \"\n cipherText = cipherText[:-1]\n return cipherText\n\n\n# De-crypt the input text\ndef monoalphabetic_decrypt():\n cipherText = input(\"Enter cipher text-\")\n wordList = cipherText.split(\" \")\n plainText = \"\"\n for word in wordList:\n c = \"\"\n for i in word:\n i = get_key(i)\n c += i\n plainText += c + \" \"\n plainText = plainText[:-1]\n return plainText\n\n\ncreate_Map()\nprint(monoalphabetic_encrypt())\nprint(monoalphabetic_decrypt())"
}
{
"filename": "code/cryptography/src/monoalphabetic_cipher/monoalphabetic.py",
"content": "import random\nalpha = \"abcdefghijklmnopqrstuvwxyz\"\n\n#Encrypts the plain text message\n\ndef encrypt(original, key=None):\n\n if key is None:\n\n l = list(alpha)\n\n random.shuffle(l)\n\n key = \"\".join(l)\n\n new = []\n\n for letter in original:\n\n new.append(key[alpha.index(letter)])\n\n return [\"\".join(new), key]\n\n#Decrypts the encrypted message\n\ndef decrypt(cipher, key=None):\n\n if key is not None:\n\n new = []\n\n for letter in cipher:\n\n new.append(alpha[key.index(letter)])\n\n return \"\".join(new)\n\n \n\ne = encrypt('monoalphabetic', None)\n\nprint(e) #Prints encrypted message\n\nprint(decrypt(e[0], e[1])) #Decodes the message and prints it"
}
{
"filename": "code/cryptography/src/morse_cipher/morse_code_generator.bf",
"content": "Morse Code Generator\n\nstore slash\n++++++[>+++++++<-]>+++++>\n\nstore space\n++++[>++++++++<-]>>\n\nstore dash & dot\n+++++[>+++++++++>+++++++++<<-]>+>>\n\n, Prime loop\nMain loop\n[\n --------------------------------\n\n space [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<<< <<.>> .>>>>> [-]]\n <\n\n ---------------- Shift so that 48 (char '0') is now 0\n\n 0 [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.....>> <<<<<.>>>>> [-]]\n <-\n\n 1 [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<.>....>> <<<<<.>>>>> [-]]\n <-\n\n 2 [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<..>...>> <<<<<.>>>>> [-]]\n <-\n\n 3 [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<...>..>> <<<<<.>>>>> [-]]\n <-\n\n 4 [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<....>.>> <<<<<.>>>>> [-]]\n <-\n\n 5 [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<.....>>> <<<<<.>>>>> [-]]\n <-\n\n 6 [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.<....>>> <<<<<.>>>>> [-]]\n <-\n\n 7 [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<..<...>>> <<<<<.>>>>> [-]]\n <-\n\n 8 [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<...<..>>> <<<<<.>>>>> [-]]\n <-\n\n 9 [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<....<.>>> <<<<<.>>>>> [-]]\n <\n\n -------- Uppercase letters\n\n a [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<.>.>> <<<<<.>>>>> [-]]\n <-\n\n b [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.<...>>> <<<<<.>>>>> [-]]\n <-\n\n c [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.<.>.<.>>> <<<<<.>>>>> [-]]\n <-\n\n d [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.<..>>> <<<<<.>>>>> [-]]\n <-\n\n e [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<.>>> <<<<<.>>>>> [-]]\n <-\n\n f [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<..>.<.>>> <<<<<.>>>>> [-]]\n <-\n\n g [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<..<.>>> <<<<<.>>>>> [-]]\n <-\n\n h [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<....>>> <<<<<.>>>>> [-]]\n <-\n\n i [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<..>>> <<<<<.>>>>> [-]]\n <-\n\n j [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<.>...>> <<<<<.>>>>> [-]]\n <-\n\n k [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.<.>.>> <<<<<.>>>>> [-]]\n <-\n\n l [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<.>.<..>>> <<<<<.>>>>> [-]]\n <-\n\n m [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<..>> <<<<<.>>>>> [-]]\n <-\n\n n [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.<.>>> <<<<<.>>>>> [-]]\n <-\n\n o [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<...>> <<<<<.>>>>> [-]]\n <-\n\n p [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<.>..<.>>> <<<<<.>>>>> [-]]\n <-\n\n q [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<..<.>.>> <<<<<.>>>>> [-]]\n <-\n\n r [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<.>.<.>>> <<<<<.>>>>> [-]]\n <-\n\n s [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<...>>> <<<<<.>>>>> [-]]\n <-\n\n t [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.>> <<<<<.>>>>> [-]]\n <-\n\n u [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<..>.>> <<<<<.>>>>> [-]]\n <-\n\n v [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<...>.>> <<<<<.>>>>> [-]]\n <-\n\n w [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<.>..>> <<<<<.>>>>> [-]]\n <-\n\n x [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.<..>.>> <<<<<.>>>>> [-]]\n <-\n\n y [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.<.>..>> <<<<<.>>>>> [-]]\n <-\n\n z [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<..<..>>> <<<<<.>>>>> [-]]\n <\n\n -------\n\n a [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<.>.>> <<<<<.>>>>> [-]]\n <-\n\n b [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.<...>>> <<<<<.>>>>> [-]]\n <-\n\n c [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.<.>.<.>>> <<<<<.>>>>> [-]]\n <-\n\n d [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.<..>>> <<<<<.>>>>> [-]]\n <-\n#\n e [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<.>>> <<<<<.>>>>> [-]]\n <-\n\n f [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<..>.<.>>> <<<<<.>>>>> [-]]\n <-\n\n g [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<..<.>>> <<<<<.>>>>> [-]]\n <-\n\n h [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<....>>> <<<<<.>>>>> [-]]\n <-\n\n i [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<..>>> <<<<<.>>>>> [-]]\n <-\n\n j [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<.>...>> <<<<<.>>>>> [-]]\n <-\n\n k [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.<.>.>> <<<<<.>>>>> [-]]\n <-\n\n l [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<.>.<..>>> <<<<<.>>>>> [-]]\n <-\n\n m [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<..>> <<<<<.>>>>> [-]]\n <-\n\n n [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.<.>>> <<<<<.>>>>> [-]]\n <-\n\n o [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<...>> <<<<<.>>>>> [-]]\n <-\n\n p [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<.>..<.>>> <<<<<.>>>>> [-]]\n <-\n\n q [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<..<.>.>> <<<<<.>>>>> [-]]\n <-\n\n r [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<.>.<.>>> <<<<<.>>>>> [-]]\n <-\n\n s [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<...>>> <<<<<.>>>>> [-]]\n <-\n\n t [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.>> <<<<<.>>>>> [-]]\n <-\n\n u [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<..>.>> <<<<<.>>>>> [-]]\n <-\n\n v [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<...>.>> <<<<<.>>>>> [-]]\n <-\n\n w [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<<.>..>> <<<<<.>>>>> [-]]\n <-\n\n x [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.<..>.>> <<<<<.>>>>> [-]]\n <-\n\n y [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<.<.>..>> <<<<<.>>>>> [-]]\n <-\n\n z [->+>+<<]>>[-<<+>>]<<\n >\n [->[-]+<]+>[-<->]<\n [ <<..<..>>> <<<<<.>>>>> [-]]\n <-\n\n [-]>[-]>[-]<<, Take input\n]"
}
{
"filename": "code/cryptography/src/morse_cipher/morse_code_generator.c",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n//C implementation of morse code generator\n#include <stdio.h>\n#include <stdlib.h>\n#include <string.h>\n\nvoid \nencrypt(char* msg)\n{\n int size = strlen(msg);\n char * morse_code = (char*) malloc(size * 5);\n\n // Morse Code for the numbers [0-10]\n char* num[] = {\"-----\", \".----\", \"..---\", \"...--\", \"....-\", \".....\", \"-....\", \"--...\", \"---..\", \"----.\"};\n // Morse Code for the alphabet\n char* letters[] = {\".-\", \"-...\", \"-.-.\", \"-..\", \".\", \"..-.\", \"--.\", \"....\", \"..\", \".---\", \"-.-\", \".-..\",\n \"--\", \"-.\", \"---\", \".--.\", \"--.-\", \".-.\", \"...\", \"-\", \"..-\", \"...-\", \".--\", \"-..-\", \"-.--\", \"--..\"};\n\n int i;\n for (i = 0;i < size; i++)\n if (msg[i] != ' ')\n if (msg[i] >= '0' && msg[i] <= '9')\n strcat(morse_code, num[msg[i] - 48]);\n else\n strcat(morse_code, letters[msg[i] - 65]);\n\n else\n strcat(morse_code, \" \");\n\n printf(\"%s\\n\", morse_code);\n\n free(morse_code);\n}\n\nint \nmain()\n{\n char* msg = \"CONVERT THIS MORSE CODE\";\n encrypt(msg);\n\n return (0);\n}"
}
{
"filename": "code/cryptography/src/morse_cipher/morse_code_generator.cpp",
"content": "//C++ implemention of morse code generator\n#include <iostream>\nusing namespace std;\n#include <map> //For map data structure\n\n// Encryption Function\nvoid encryption(string msg)\n{\n // Decalaring map structure and Defination of map\n map<char, string> encrypt;\n encrypt['A'] = \".-\"; encrypt['B'] = \"-...\"; encrypt['C'] = \"-.-.\"; encrypt['D'] = \"-..\";\n encrypt['E'] = \".\"; encrypt['F'] = \"..-.\"; encrypt['G'] = \"--.\"; encrypt['H'] = \"....\";\n encrypt['I'] = \"..\"; encrypt['J'] = \".---\"; encrypt['K'] = \"-.-\"; encrypt['L'] = \".-..\";\n encrypt['M'] = \"--\"; encrypt['N'] = \"-.\"; encrypt['O'] = \"---\"; encrypt['P'] = \".--.\";\n encrypt['Q'] = \"--.-\"; encrypt['R'] = \".-.\"; encrypt['S'] = \"...\"; encrypt['T'] = \"-\";\n encrypt['U'] = \"..-\"; encrypt['V'] = \"...-\"; encrypt['W'] = \".--\"; encrypt['X'] = \"-..-\";\n encrypt['Y'] = \"-.--\"; encrypt['Z'] = \"--..\";\n encrypt['1'] = \".----\"; encrypt['2'] = \"..---\"; encrypt['3'] = \"...--\"; encrypt['4'] = \"....-\";\n encrypt['5'] = \".....\"; encrypt['6'] = \"-....\"; encrypt['7'] = \"--...\";\n encrypt['8'] = \"---..\"; encrypt['9'] = \"----.\"; encrypt['0'] = \"-----\";\n\n string cipher;\n for (size_t i = 0; i < msg.size(); i++)\n {\n if (msg[i] != ' ')\n cipher = cipher + encrypt[msg[i]] + \" \";\n else\n cipher = cipher + \" \";\n }\n cout << cipher;\n}\n//Driver program\nint main()\n{\n string key = \"CONVERT THIS TO MORSE CODE\";\n //Calling Function\n encryption(key);\n return 0;\n}"
}
{
"filename": "code/cryptography/src/morse_cipher/morse_code_generator.rb",
"content": "MORSE_CODES = {\n 'a' => '.-', 'b' => '-...', 'c' => '-.-.',\n 'd' => '-..', 'e' => '.', 'f' => '..-.',\n 'g' => '--.', 'h' => '....', 'i' => '..',\n 'j' => '.---', 'k' => '-.-', 'l' => '.-..',\n 'm' => '--', 'n' => '-.', 'o' => '---',\n 'p' => '.--.', 'q' => '--.-', 'r' => '.-.',\n 's' => '...', 't' => '-', 'u' => '..-',\n 'v' => '...-', 'w' => '.--', 'x' => '-..-',\n 'y' => '-.--', 'z' => '--..', ' ' => ' ',\n '1' => '.----', '2' => '..---', '3' => '...--',\n '4' => '....-', '5' => '.....', '6' => '-....',\n '7' => '--...', '8' => '---..', '9' => '----.',\n '0' => '-----'\n}.freeze\n\ndef encode(string)\n text = string.downcase.split('')\n encoded_text = []\n\n text.each do |c|\n morse_char = MORSE_CODES[c]\n encoded_text.push(morse_char)\n end\n\n encoded_text.join('')\nend\n\n# TEST!\nputs encode('Hello World') # => ......-...-..--- .-----.-..-..-.."
}
{
"filename": "code/cryptography/src/morse_cipher/morse_code.java",
"content": "// Part of Cosmos by OpenGenus Foundation\nimport java.util.HashMap;\nimport java.util.Map;\n\npublic class MorseCode {\n \n private Map<String, String> engToMorse;\n private Map<String, String> morseToEng;\n private static final String rawMorseCodes = \"A:.-|B:-...|C:-.-.|D:-..|E:.|F:..-.|G:--.|H:....|I:..|J:.---|K:-.-|L:.-..|M:--|N:-.|O:---|P:.--.|Q:--.-|R:.-.|S:...|T:-|U:..-|V:...-|W:.--|X:-..-|Y:-.--|Z:--..|1:.----|2:..---|3:...--|4:....-|5:.....|6:-....|7:--...|8:---..|9:----.|0:-----|,:--..--|.:.-.-.-|?:..--..|/:-..-.|-:-....-|(:-.--.|):-.--.-\";\n \n public MorseCode() {\n engToMorse = new HashMap<String, String>();\n morseToEng = new HashMap<String, String>();\n String[] morseCodes = rawMorseCodes.split(\"\\\\|\");\n for (String morseCode: morseCodes) {\n String[] keyValue = morseCode.split(\":\");\n String english = keyValue[0];\n String morse = keyValue[1];\n engToMorse.put(english, morse);\n morseToEng.put(morse, english);\n }\n }\n\n public String encrypt(String english) {\n String cipherText = \"\";\n english = english.toUpperCase();\n for (int i = 0; i < english.length(); i++) {\n if (english.charAt(i) != ' ') {\n cipherText += engToMorse.get(String.valueOf(english.charAt(i)));\n cipherText += ' ';\n }\n else {\n cipherText += ' ';\n }\n }\n return cipherText;\n }\n\n public String decrypt(String morse) {\n String plainText = \"\";\n String[] words = morse.split(\"\\\\s{2}\");\n for (String word : words) {\n String[] letters = word.split(\"\\\\s\");\n for (String letter : letters) {\n plainText += morseToEng.get(letter);\n }\n plainText += \" \";\n }\n return plainText;\n }\n\n public static void main(String[] args) {\n String msg = \"alice killed bob\";\n String morseMsg = \".- .-.. .. -.-. . -.- .. .-.. .-.. . -.. -... --- -...\";\n MorseCode mCode = new MorseCode();\n System.out.println(mCode.encrypt(msg));\n System.out.println(mCode.decrypt(morseMsg));\n }\n}"
}
{
"filename": "code/cryptography/src/morse_cipher/morse_code.sh",
"content": "#!/bin/bash\n#get the file and be ready to decrypt one time internet file download is required\n\n\n\n\npwd=$PWD\n#check if file doesnt exist\nif ! [[ -f \"$pwd/morse_code.txt\" ]]\nthen\ncurl http://www.qsl.net/we6w/text/morse.txt >> morse.txt 2>/dev/null\nsed -i '17,367d' morse.txt\ncat morse.txt|grep -vi morse > morse_code.txt\nrm -f morse.txt\nfi\n\n\n\n#function to encrypt the msg\n\n\n\n\nfunction encrypt(){\ncol1=`cat morse_code.txt|awk '{print $1,$2}'`\ncol2=`cat morse_code.txt|awk '{print $3,$4}'`\ncol3=`cat morse_code.txt|awk '{print $5,$6}'`\ncol4=`cat morse_code.txt|awk '{print $7,$8}'`\nwhile read -n1 char\ndo\necho \"$col1\"|grep -i $char 2>/dev/null\necho \"$col2\"|grep -i $char 2>/dev/null\necho \"$col3\"|grep -i $char 2> /dev/null\necho \"$col4\"|grep -i $char 2> /dev/null\ndone<$pwd/msg.txt\n}\n\n\n#Program begins\n\nread -p \"Enter The Message \" MS\necho \"$MS\" > $pwd/msg.txt\nencrypt\nrm -f $pwd/msg.txt"
}
{
"filename": "code/cryptography/src/morse_cipher/morse_code_translator.cpp",
"content": "//\n// Created by miszk on 10/4/2017.\n//\n\n#include <map>\n#include <iostream>\nusing std::string;\nusing std::cin;\nusing std::cout;\nusing std::endl;\n\nstd::map<std::string,\n string> dictionary =\n{ {\"A\", \".-\"}, { \"B\", \"-...\"}, { \"C\", \"-.-.\"}, { \"D\", \"-..\"}, { \"E\", \".\"}, { \"F\", \"..-.\"},\n { \"G\", \"--.\"}, { \"H\", \"....\"}, { \"I\", \"..\"}, { \"J\", \".---\"}, { \"K\", \"-.-\"}, { \"L\", \".-..\"},\n { \"M\", \"--\"}, { \"N\", \"-.\"}, { \"O\", \"---\"}, { \"P\", \".--.\"}, { \"Q\", \"--.-\"}, { \"R\", \".-.\"},\n { \"S\", \"...\"}, { \"T\", \"-\"}, { \"U\", \"..-\"}, { \"V\", \"...-\"}, { \"W\", \".--\"}, { \"X\", \"-..-\"},\n { \"Y\", \"-.--\"}, { \"Z\", \"--..\"}, { \"1\", \".----\"}, { \"2\", \"..---\"}, { \"3\", \"...--\"},\n { \"4\", \"....-\"}, { \"5\", \".....\"}, { \"6\", \"-....\"}, { \"7\", \"--...\"}, { \"8\", \"---..\"},\n { \"9\", \"----.\"}, { \"0\", \"-----\"}, { \"}, {\", \"--..--\"}, { \".\", \".-.-.-\"}, { \"?\", \"..--..\"},\n { \"/\", \"-..-.\"}, { \"-\", \"-....-\"}, { \"(\", \"-.--.\"}, { \")\", \"-.--.-\"}};\n\n// Function to encrypt the string according to the morse code chart\nstring encrytp(string message)\n{\n string encrypted = \"\";\n string to_string;\n\n for (char c : message)\n {\n //check if char is a letter\n if (c != ' ')\n {\n //add morse letter (first make it uppercase letter 'cause we'll search for it in our dictionary)\n to_string = toupper(c);\n encrypted += dictionary.find(string(to_string))->second;\n //add a space to separate morse letters\n encrypted += \" \";\n }\n else\n encrypted += \" \";\n }\n\n return encrypted;\n}\n\n\n// Function to decrypt the string from morse to english\nstring decrypt (string message)\n{\n string morse_letter = \"\";\n string decrypted = \"\";\n\n for (char i : message)\n {\n // check for space or the end of current morse letter\n if (i == ' ')\n {\n\n if (morse_letter.length() != 0)\n {\n // it's the end of morse letter\n //search for key in dictionary\n for (auto o : dictionary)\n if (o.second == morse_letter)\n {\n decrypted += o.first;\n break;\n }\n morse_letter = \"\";\n }\n else\n //it's just a space -- we copy it\n decrypted += \" \";\n }\n else\n morse_letter += i;\n }\n return decrypted;\n}\n\n\nint main()\n{\n\n string msg = \"alice killed bob\";\n string morse_msg = \".- .-.. .. -.-. . -.- .. .-.. .-.. . -.. -... --- -... \";\n cout << encrytp(msg) << endl << decrypt(morse_msg) << endl;\n\n return 0;\n}"
}
{
"filename": "code/cryptography/src/morse_cipher/morse_code_translator.exs",
"content": "defmodule Cryptography do\n @morse_encryption_table %{\n A: \".-\",\n B: \"-...\",\n C: \"-.-.\",\n D: \"-..\",\n E: \".\",\n F: \"..-.\",\n G: \"--.\",\n H: \"....\",\n I: \"..\",\n J: \".---\",\n K: \"-.-\",\n L: \".-..\",\n M: \"--\",\n N: \"-.\",\n O: \"---\",\n P: \".--.\",\n Q: \"--.-\",\n R: \".-.\",\n S: \"...\",\n T: \"-\",\n U: \"..-\",\n V: \"...-\",\n W: \".--\",\n X: \"-..-\",\n Y: \"-.--\",\n Z: \"--..\",\n \"1\": \".----\",\n \"2\": \"..---\",\n \"3\": \"...--\",\n \"4\": \"....-\",\n \"5\": \".....\",\n \"6\": \"-....\",\n \"7\": \"--...\",\n \"8\": \"---..\",\n \"9\": \"----.\",\n \"0\": \"-----\",\n \",\": \"--..--\",\n \".\": \".-.-.-\",\n \"?\": \"..--..\",\n /: \"-..-.\",\n -: \"-....-\",\n \"(\": \"-.--.\",\n \")\": \"-.--.-\"\n }\n\n def morse_encrypt(content) do\n content\n |> String.upcase()\n |> String.split(\"\", trim: true)\n |> Enum.map(fn x ->\n @morse_encryption_table[:\"#{x}\"]\n end)\n |> Enum.join(\" \")\n end\n\n def morse_decrypt(morse) do\n translation_table =\n Enum.map(@morse_encryption_table, fn {k, v} -> {v, k} end)\n |> Enum.into(%{})\n\n morse\n |> String.split(\" \")\n |> Enum.map(fn\n \"\" ->\n \" \"\n\n x ->\n \"#{Map.get(translation_table, x)}\"\n end)\n |> Enum.join(\"\")\n end\nend\n\nmsg = \"Hello world\"\n\nCryptography.morse_encrypt(msg)\n|> IO.inspect(label: \"Encrypting #{msg} in morse\")\n|> Cryptography.morse_decrypt()\n|> IO.inspect(label: \"Decrypting #{msg}\")"
}
{
"filename": "code/cryptography/src/morse_cipher/morse_code_translator.js",
"content": "// Javascript program to implement morse code translator\n\n/*\n * VARIABLE KEY\n * 'cipher' -> 'stores the morse translated form of the english string'\n * 'decipher' -> 'stores the english translated form of the morse string'\n * 'citext' -> 'stores morse code of a single character'\n * 'i' -> 'keeps count of the spaces between morse characters'\n * 'message' -> 'stores the string to be encoded or decoded'\n */\n\n// Object representing the morse code chart\nconst MORSE_CODES = {\n A: \".-\",\n B: \"-...\",\n C: \"-.-.\",\n D: \"-..\",\n E: \".\",\n F: \"..-.\",\n G: \"--.\",\n H: \"....\",\n I: \"..\",\n J: \".---\",\n K: \"-.-\",\n L: \".-..\",\n M: \"--\",\n N: \"-.\",\n O: \"---\",\n P: \".--.\",\n Q: \"--.-\",\n R: \".-.\",\n S: \"...\",\n T: \"-\",\n U: \"..-\",\n V: \"...-\",\n W: \".--\",\n X: \"-..-\",\n Y: \"-.--\",\n Z: \"--..\",\n \"1\": \".----\",\n \"2\": \"..---\",\n \"3\": \"...--\",\n \"4\": \"....-\",\n \"5\": \".....\",\n \"6\": \"-....\",\n \"7\": \"--...\",\n \"8\": \"---..\",\n \"9\": \"----.\",\n \"0\": \"-----\",\n \",\": \"--..--\",\n \".\": \".-.-.-\",\n \"?\": \"..--..\",\n \"/\": \"-..-.\",\n \"-\": \"-....-\",\n \"(\": \"-.--.\",\n \")\": \"-.--.-\"\n};\n\n// Array of values from above\nconst CODE_VALS = Object.keys(MORSE_CODES).map(key => MORSE_CODES[key]);\n\n// Function to encrypt the string according to the morse code chart\nfunction encrypt(message) {\n var cipher = \"\";\n message.split(\"\").forEach(letter => {\n if (letter !== \" \") {\n // looks up the dictionary and adds the correspponding morse code\n // along with a space to separate morse codes for different characters\n cipher += MORSE_CODES[letter] + \" \";\n } else {\n // 1 space indicates different characters & 2 indicates different words\n cipher += \" \";\n }\n });\n\n return cipher;\n}\n\n// Function to decrypt the string from morse to english\nfunction decrypt(message) {\n message += \" \"; // extra space added at the end to access the last morse code\n var decipher = \"\";\n var citext = \"\";\n var i = 0; // counter to keep track of space\n message.split(\"\").forEach(letter => {\n // checks for space\n if (letter !== \" \") {\n i = 0;\n citext += letter; // storing morse code of a single character\n }\n // in case of space\n else {\n // if i = 1 that indicates a new character\n i += 1;\n // if i = 2 that indicates a new word\n if (i === 2) {\n decipher += \" \"; // adding space to separate words\n } else {\n // accessing the keys using their values (reverse of encryption via array of values)\n decipher += Object.keys(MORSE_CODES)[CODE_VALS.indexOf(citext)];\n citext = \"\";\n }\n }\n });\n\n return decipher;\n}\n\n//===================RUN IT====================\nvar message1 = \"ALICE KILED BOB\";\nvar result1 = encrypt(message1.toUpperCase());\nconsole.log(result1);\n\nvar message2 =\n \"-.- . ...- .. -. ... .--. .- -.-. . -.-- .. ... -.- . -.-- ... . .-. ... --- --.. .\";\nvar result2 = decrypt(message2);\nconsole.log(result2);"
}
{
"filename": "code/cryptography/src/morse_cipher/morse_code_translator.lua",
"content": "-- Lua code to convert text to morse code // tested on Lua 5.3.3\n\nfunction enc(message)\n -- Lua dictionary containing the morse alphabets\n change = {\n A = \".-\", B = \"-...\", C = \"-.-.\", D = \"-..\", E = \".\",\n F = \"..-.\", G = \"--.\", H = \"....\", I = \"..\", J = \".---\",\n K = \"-.-\", L = \".-..\", M = \"--\", N = \"-.\", O = \"---\",\n P = \".--.\", Q = \"--.-\", R = \".-.\", S = \"...\", T = \"-\",\n U = \"..-\", V = \"...-\", W = \".--\", X = \"-..-\", Y = \"-.--\",\n Z = \"--..\", [1]=\".----\", [2]=\"..---\", [3]=\"...--\",\n [4]=\"....-\", [5]=\".....\", [6]=\"-....\", [7]=\"--...\",\n [8]=\"---..\", [9]=\"----.\", [0]=\"-----\"\n }\n cipherText = \"\"\n for i=1, string.len(message) do\n -- (s.sub(s, i, i))\n str = string.sub(message, i, i)\n if str ~= \" \" then\n -- one space after every letter\n cipherText = cipherText .. change[str] .. \" \"\n else\n -- two spaces after every word\n cipherText = cipherText .. \" \"\n end\n end\n -- print the final cipher text\n print(cipherText)\nend\n-- user input from STDIN\nstr = io.read()\n-- calling main translation function\nenc(str)"
}
{
"filename": "code/cryptography/src/morse_cipher/morse_code_translator.php",
"content": "<?php\n/* Part of Cosmos by OpenGenus Foundation */\n\nconst LATIN_TO_MORSE_DICTIONARY = [\n 'A' => '.-',\n 'B' => '-...',\n 'C' => '-.-.',\n 'D' => '-..',\n 'E' => '.',\n 'F' => '..-.',\n 'G' => '--.',\n 'H' => '....',\n 'I' => '..',\n 'J' => '.---',\n 'K' => '-.-',\n 'L' => '.-..',\n 'M' => '--',\n 'N' => '-.',\n 'O' => '---',\n 'P' => '.--.',\n 'Q' => '--.-',\n 'R' => '.-.',\n 'S' => '...',\n 'T' => '-',\n 'U' => '..-',\n 'V' => '...-',\n 'W' => '.--',\n 'X' => '-..-',\n 'Y' => '-.--',\n 'Z' => '--..',\n '0' => '-----',\n '1' => '.----',\n '2' => '..---',\n '3' => '...--',\n '4' => '....-',\n '5' => '.....',\n '6' => '-....',\n '7' => '--...',\n '8' => '---..',\n '9' => '----.',\n ',' => '--..--',\n '.' => '.-.-.-',\n '?' => '..--..',\n '/' => '-..-.',\n '-' => '-....-',\n '(' => '-.--.',\n ')' => '-.--.-',\n ' ' => ' ',\n];\n\n// Converts $message to morse code\nfunction encrypt(string $message): string {\n $chars = str_split(strtoupper(trim($message)));\n $morse = '';\n foreach ($chars AS $char) {\n if (array_key_exists($char, LATIN_TO_MORSE_DICTIONARY)) {\n $morse .= LATIN_TO_MORSE_DICTIONARY[$char];\n }\n $morse .= ' ';\n }\n return rtrim($morse);\n}\n\n// Decrypts morse code\nfunction decrypt(string $morse): string {\n $tokens = explode(' ', $morse);\n $message = '';\n $morse_to_latin_dictionary = array_flip(LATIN_TO_MORSE_DICTIONARY);\n foreach ($tokens AS $token) {\n if (array_key_exists($token, $morse_to_latin_dictionary)) {\n $message .= $morse_to_latin_dictionary[$token];\n }\n else if ('' === $token){\n $message .= ' ';\n }\n }\n return rtrim($message);\n}\n\n\necho 'Encoding \"Alice\":' . PHP_EOL;\necho encrypt('Alice') . PHP_EOL . PHP_EOL;\n\necho 'Decoding \".... .. -... --- -...\":' . PHP_EOL;\necho decrypt('.... .. -... --- -...');"
}
{
"filename": "code/cryptography/src/morse_cipher/morse_code_translator.py",
"content": "# Python program to implement Morse Code Translator\n\n\"\"\"\nVARIABLE KEY\n'cipher' -> 'stores the morse translated form of the english string'\n'decipher' -> 'stores the english translated form of the morse string'\n'citext' -> 'stores morse code of a single character'\n'i' -> 'keeps count of the spaces between morse characters'\n'message' -> 'stores the string to be encoded or decoded'\n\"\"\"\n\n# Dictionary representing the morse code chart\nMORSE_CODE_DICT = {\n \"A\": \".-\",\n \"B\": \"-...\",\n \"C\": \"-.-.\",\n \"D\": \"-..\",\n \"E\": \".\",\n \"F\": \"..-.\",\n \"G\": \"--.\",\n \"H\": \"....\",\n \"I\": \"..\",\n \"J\": \".---\",\n \"K\": \"-.-\",\n \"L\": \".-..\",\n \"M\": \"--\",\n \"N\": \"-.\",\n \"O\": \"---\",\n \"P\": \".--.\",\n \"Q\": \"--.-\",\n \"R\": \".-.\",\n \"S\": \"...\",\n \"T\": \"-\",\n \"U\": \"..-\",\n \"V\": \"...-\",\n \"W\": \".--\",\n \"X\": \"-..-\",\n \"Y\": \"-.--\",\n \"Z\": \"--..\",\n \"1\": \".----\",\n \"2\": \"..---\",\n \"3\": \"...--\",\n \"4\": \"....-\",\n \"5\": \".....\",\n \"6\": \"-....\",\n \"7\": \"--...\",\n \"8\": \"---..\",\n \"9\": \"----.\",\n \"0\": \"-----\",\n \",\": \"--..--\",\n \".\": \".-.-.-\",\n \"?\": \"..--..\",\n \"/\": \"-..-.\",\n \"-\": \"-....-\",\n \"(\": \"-.--.\",\n \")\": \"-.--.-\",\n}\n\n# Function to encrypt the string according to the morse code chart\ndef encrypt(message):\n cipher = \"\"\n for letter in message:\n if letter != \" \":\n # looks up the dictionary and adds the correspponding morse code\n # along with a space to separate morse codes for different characters\n cipher += MORSE_CODE_DICT[letter] + \" \"\n else:\n # 1 space indicates different characters & 2 indicates different words\n cipher += \" \"\n\n return cipher\n\n\n# Function to decrypt the string from morse to english\ndef decrypt(message):\n message += \" \" # extra space added at the end to access the last morse code\n decipher = \"\"\n citext = \"\"\n for letter in message:\n # checks for space\n if letter != \" \":\n i = 0 # couunter to keep track of space\n citext += letter # storing morse code of a single character\n # in case of space\n else:\n # if i = 1 that indicates a new character\n i += 1\n # if i = 2 that indicates a new word\n if i == 2:\n decipher += \" \" # adding space to separate words\n else:\n # accessing the keys using their values (reverse of encryption)\n decipher += list(MORSE_CODE_DICT.keys())[\n list(MORSE_CODE_DICT.values()).index(citext)\n ]\n citext = \"\"\n\n return decipher\n\n\n# Hard-coded driver function to run the program\ndef main():\n message = \"ALICE KILED BOB\"\n result = encrypt(message.upper())\n print(result)\n\n message = \"-.- . ...- .. -. ... .--. .- -.-. . -.-- .. ... -.- . -.-- ... . .-. ... --- --.. .\"\n result = decrypt(message)\n print(result)\n\n\n# Executes the main function\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/cryptography/src/morse_cipher/morse_code_translator.ts",
"content": "// Program to convert morse code to ASCII and vice-versa\n\nconst MORSE_MAP = {\n A: '.-',\n B: '-...',\n C: '-.-.',\n D: '-..',\n E: '.',\n F: '..-.',\n G: '--.',\n H: '....',\n I: '..',\n J: '.---',\n K: '-.-',\n L: '.-..',\n M: '--',\n N: '-.',\n O: '---',\n P: '.--.',\n Q: '--.-',\n R: '.-.',\n S: '...',\n T: '-',\n U: '..-',\n V: '...-',\n W: '.--',\n X: '-..-',\n Y: '-.--',\n Z: '--..',\n '1': '.----',\n '2': '..---',\n '3': '...--',\n '4': '....-',\n '5': '.....',\n '6': '-....',\n '7': '--...',\n '8': '---..',\n '9': '----.',\n '0': '-----',\n ',': '--..--',\n '.': '.-.-.-',\n '?': '..--..',\n '/': '-..-.',\n '-': '-....-',\n '(': '-.--.',\n ')': '-.--.-',\n}\n\n/**\n * Encrypt a text string into morse code. One space implies\n * a different character, while two spaces implies a different word.\n * \n * @param str The ASCII input string\n */\nexport function encrypt(str: string): string {\n let encrypted = '';\n str.toUpperCase().split('').forEach((char: string) => {\n if (char !== ' ') {\n encrypted += MORSE_MAP[char] + ' ';\n } else {\n encrypted += ' ';\n }\n })\n\n return encrypted;\n}\n\n/**\n * Convert a morse code string back into regular text.\n * \n * @param str The Morse code input string\n */\nexport function decrypt(str: string): string {\n let decrypted = '';\n str.split(' ').forEach((word: string) => {\n word.split(' ').forEach((letter: string) => {\n decrypted += Object.keys(MORSE_MAP).filter(key => MORSE_MAP[key] === letter)[0];\n })\n // Add a space to split words\n decrypted += ' ';\n })\n return decrypted;\n}\n\nconst input1 = 'Hello world.';\nconst input2 = '.... . .-.. .-.. --- .-- --- .-. .-.. -.. .-.-.-';\nconst result1 = encrypt(input1);\nconst result2 = decrypt(input2);\n\nconsole.log(`Encrypted text: ${result1}`);\nconsole.log(`Decrypted text: ${result2}`);"
}
{
"filename": "code/cryptography/src/morse_cipher/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/cryptography/src/password_generator/strongpasswordgenerator.py",
"content": "# This program will generate a strong password using cryptography principles\n\n# Import secrets and string module\n# The string module defines the alphabet, and the secret module generates cryptographically sound random numbers\nimport secrets\nimport string\n\n# Define the alphabet to be digits, letters, and special characters\nletters = string.ascii_letters\ndigits = string.digits\nspecial = string.punctuation\nalphabet = letters + digits + special\n\n# Set the password length\nwhile True: \n\ttry:\n\t\tpassword_length = int(input(\"Please enter the length of your password: \"))\n\t\tbreak\n\texcept ValueError: \n\t\tprint(\"Please enter an integer length.\")\n\n# Generates strong password with at least one special character and one digit\nwhile True:\n\tpassword = ''\n\tfor i in range(password_length):\n\t\tpassword += ''.join(secrets.choice(alphabet))\n\tif (any(char in special for char in password) and any(char in digits for char in password)):\n\t\tbreak\n \nprint(\"--------Your Password Has Been Generated---------\")\nprint(password)\nprint(\"--------Make Sure To Keep Your Password Safe---------\")"
}
{
"filename": "code/cryptography/src/playfair_cipher/playfair_cipher.c",
"content": "// C program to implement Playfair Cipher\n\n#include <stdio.h>\n#include <stdlib.h>\n#include <string.h>\n\n#define SIZE 30\n\n// function to convert the string to lowercase\nvoid toLowerCase(char arr[], int ps)\n{\n\tint i;\n\tfor (i = 0; i < ps; i++) {\n\t\tif (arr[i] > 64 && arr[i] < 91)\n\t\t\tarr[i] += 32;\n\t}\n}\n\n// function to remove all spaces in a string\nint removeSpaces(char* arr, int ps)\n{\n\tint i, count = 0;\n\tfor (i = 0; i < ps; i++)\n\t\tif (arr[i] != ' ')\n\t\t\tarr[count++] = arr[i];\n\tarr[count] = '\\0';\n\treturn count;\n}\n\n// function to generate the 5x5 square matrix key\nvoid generateKeyTable(char arr[], int ks, char key_M[5][5])\n{\n\tint i, j, k, flag = 0, *arr1;\n\n\t// hashmap to store count of the alphabet\n\tarr1 = (int*)calloc(26, sizeof(int));\n\tfor (i = 0; i < ks; i++) {\n\t\tif (arr[i] != 'j')\n\t\t\tarr1[arr[i] - 97] = 2;\n\t}\n\n\tarr1['j' - 97] = 1;\n\n\ti = 0;\n\tj = 0;\n\n\tfor (k = 0; k < ks; k++) {\n\t\tif (arr1[arr[k] - 97] == 2) {\n\t\t\tarr1[arr[k] - 97] -= 1;\n\t\t\tkey_M[i][j] = arr[k];\n\t\t\tj++;\n\t\t\tif (j == 5) {\n\t\t\t\ti++;\n\t\t\t\tj = 0;\n\t\t\t}\n\t\t}\n\t}\n\n\tfor (k = 0; k < 26; k++) {\n\t\tif (arr1[k] == 0) {\n\t\t\tkey_M[i][j] = (char)(k + 97);\n\t\t\tj++;\n\t\t\tif (j == 5) {\n\t\t\t\ti++;\n\t\t\t\tj = 0;\n\t\t\t}\n\t\t}\n\t}\n}\n\n// Function to search for the characters of a digraph\n// in the key square and return their position\nvoid search(char key_M[5][5], char a, char b, int arr[])\n{\n\tint i, j;\n\n\tif (a == 'j')\n\t\ta = 'i';\n\telse if (b == 'j')\n\t\tb = 'i';\n\n\tfor (i = 0; i < 5; i++) {\n\n\t\tfor (j = 0; j < 5; j++) {\n\n\t\t\tif (key_M[i][j] == a) {\n\t\t\t\tarr[0] = i;\n\t\t\t\tarr[1] = j;\n\t\t\t}\n\t\t\telse if (key_M[i][j] == b) {\n\t\t\t\tarr[2] = i;\n\t\t\t\tarr[3] = j;\n\t\t\t}\n\t\t}\n\t}\n}\n\n// function to find the modulus with 5\nint mod5(int a)\n{\n\treturn (a % 5);\n}\n\n// function to make the plain text length to be even\nint prepare(char arr[], int n)\n{\n\tif (n % 2 != 0) {\n\t\tarr[n++] = 'z';\n\t\tarr[n] = '\\0';\n\t}\n\treturn n;\n}\n\n// function for assistance in encryption of the data\nvoid encrypt(char arr[], char key_M[5][5], int ps)\n{\n\tint i, arr1[4];\n\n\tfor (i = 0; i < ps; i += 2) {\n\n\t\tsearch(key_M, arr[i], arr[i + 1], arr1);\n\n\t\tif (arr1[0] == arr1[2]) {\n\t\t\tarr[i] = key_M[arr1[0]][mod5(arr1[1] + 1)];\n\t\t\tarr[i + 1] = key_M[arr1[0]][mod5(arr1[3] + 1)];\n\t\t}\n\t\telse if (arr1[1] == arr1[3]) {\n\t\t\tarr[i] = key_M[mod5(arr1[0] + 1)][arr1[1]];\n\t\t\tarr[i + 1] = key_M[mod5(arr1[2] + 1)][arr1[1]];\n\t\t}\n\t\telse {\n\t\t\tarr[i] = key_M[arr1[0]][arr1[3]];\n\t\t\tarr[i + 1] = key_M[arr1[2]][arr1[1]];\n\t\t}\n\t}\n}\n\n// function to encrypt using Playfair Cipher\nvoid encryptByPlayfairCipher(char arr[], char key[])\n{\n\tchar ps, ks, key_M[5][5];\n\n\t// Key\n\tks = strlen(key);\n\tks = removeSpaces(key, ks);\n\ttoLowerCase(key, ks);\n\n\t// Plaintext\n\tps = strlen(arr);\n\ttoLowerCase(arr, ps);\n\tps = removeSpaces(arr, ps);\n\n\tps = prepare(arr, ps);\n\n\tgenerateKeyTable(key, ks, key_M);\n\n\tencrypt(arr, key_M, ps);\n}\n\n// Main function that gets executed first\nint main()\n{\n\tchar arr[SIZE], key[SIZE];\n\n\t// Key to be encrypted\n\tstrcpy(key, \"Germany\");\n\tprintf(\"Key: %s\\n\", key);\n\n\t// Plaintext to be encrypted\n\tstrcpy(arr, \"Dusseldorf\");\n\tprintf(\"Text to be encrypted: %s\\n\", arr);\n\n\t// encrypt using Playfair Cipher\n\tencryptByPlayfairCipher(arr, key);\n\n\tprintf(\"Cipher: %s\\n\", arr);\n\n\treturn 0;\n}\n\n// C program to implement Playfair Cipher\n\n#include <stdio.h>\n#include <stdlib.h>\n#include <string.h>\n\n#define SIZE 30\n\n// function to convert the string to lowercase\nvoid toLowerCase(char arr[], int ps)\n{\n\tint i;\n\tfor (i = 0; i < ps; i++) {\n\t\tif (arr[i] > 64 && arr[i] < 91)\n\t\t\tarr[i] += 32;\n\t}\n}\n\n// function to remove all spaces in a string\nint removeSpaces(char* arr, int ps)\n{\n\tint i, count = 0;\n\tfor (i = 0; i < ps; i++)\n\t\tif (arr[i] != ' ')\n\t\t\tarr[count++] = arr[i];\n\tarr[count] = '\\0';\n\treturn count;\n}\n\n// function to generate the 5x5 square matrix key\nvoid generateKeyTable(char arr[], int ks, char key_M[5][5])\n{\n\tint i, j, k, flag = 0, *arr1;\n\n\t// hashmap to store count of the alphabet\n\tarr1 = (int*)calloc(26, sizeof(int));\n\tfor (i = 0; i < ks; i++) {\n\t\tif (arr[i] != 'j')\n\t\t\tarr1[arr[i] - 97] = 2;\n\t}\n\n\tarr1['j' - 97] = 1;\n\n\ti = 0;\n\tj = 0;\n\n\tfor (k = 0; k < ks; k++) {\n\t\tif (arr1[arr[k] - 97] == 2) {\n\t\t\tarr1[arr[k] - 97] -= 1;\n\t\t\tkey_M[i][j] = arr[k];\n\t\t\tj++;\n\t\t\tif (j == 5) {\n\t\t\t\ti++;\n\t\t\t\tj = 0;\n\t\t\t}\n\t\t}\n\t}\n\n\tfor (k = 0; k < 26; k++) {\n\t\tif (arr1[k] == 0) {\n\t\t\tkey_M[i][j] = (char)(k + 97);\n\t\t\tj++;\n\t\t\tif (j == 5) {\n\t\t\t\ti++;\n\t\t\t\tj = 0;\n\t\t\t}\n\t\t}\n\t}\n}\n\n// Function to search for the characters of a digraph\n// in the key square and return their position\nvoid search(char key_M[5][5], char a, char b, int arr[])\n{\n\tint i, j;\n\n\tif (a == 'j')\n\t\ta = 'i';\n\telse if (b == 'j')\n\t\tb = 'i';\n\n\tfor (i = 0; i < 5; i++) {\n\n\t\tfor (j = 0; j < 5; j++) {\n\n\t\t\tif (key_M[i][j] == a) {\n\t\t\t\tarr[0] = i;\n\t\t\t\tarr[1] = j;\n\t\t\t}\n\t\t\telse if (key_M[i][j] == b) {\n\t\t\t\tarr[2] = i;\n\t\t\t\tarr[3] = j;\n\t\t\t}\n\t\t}\n\t}\n}\n\n// function to find the modulus with 5\nint mod5(int a)\n{\n\treturn (a % 5);\n}\n\n// function to make the plain text length to be even\nint prepare(char arr[], int n)\n{\n\tif (n % 2 != 0) {\n\t\tarr[n++] = 'z';\n\t\tarr[n] = '\\0';\n\t}\n\treturn n;\n}\n\n// function for assistance in encryption of the data\nvoid encrypt(char arr[], char key_M[5][5], int ps)\n{\n\tint i, arr1[4];\n\n\tfor (i = 0; i < ps; i += 2) {\n\n\t\tsearch(key_M, arr[i], arr[i + 1], arr1);\n\n\t\tif (arr1[0] == arr1[2]) {\n\t\t\tarr[i] = key_M[arr1[0]][mod5(arr1[1] + 1)];\n\t\t\tarr[i + 1] = key_M[arr1[0]][mod5(arr1[3] + 1)];\n\t\t}\n\t\telse if (arr1[1] == arr1[3]) {\n\t\t\tarr[i] = key_M[mod5(arr1[0] + 1)][arr1[1]];\n\t\t\tarr[i + 1] = key_M[mod5(arr1[2] + 1)][arr1[1]];\n\t\t}\n\t\telse {\n\t\t\tarr[i] = key_M[arr1[0]][arr1[3]];\n\t\t\tarr[i + 1] = key_M[arr1[2]][arr1[1]];\n\t\t}\n\t}\n}\n\n// function to encrypt using Playfair Cipher\nvoid encryptByPlayfairCipher(char arr[], char key[])\n{\n\tchar ps, ks, key_M[5][5];\n\n\t// Key\n\tks = strlen(key);\n\tks = removeSpaces(key, ks);\n\ttoLowerCase(key, ks);\n\n\t// Plaintext\n\tps = strlen(arr);\n\ttoLowerCase(arr, ps);\n\tps = removeSpaces(arr, ps);\n\n\tps = prepare(arr, ps);\n\n\tgenerateKeyTable(key, ks, key_M);\n\n\tencrypt(arr, key_M, ps);\n}\n\n// Main function that gets executed first\nint main()\n{\n\tchar arr[SIZE], key[SIZE];\n\n\t// Key to be encrypted\n\tstrcpy(key, \"Germany\");\n\tprintf(\"Key: %s\\n\", key);\n\n\t// Plaintext to be encrypted\n\tstrcpy(arr, \"Dusseldorf\");\n\tprintf(\"Text to be encrypted: %s\\n\", arr);\n\n\t// encrypt using Playfair Cipher\n\tencryptByPlayfairCipher(arr, key);\n\n\tprintf(\"Cipher: %s\\n\", arr);\n\n\treturn 0;\n}\n\n\n// OUTPUT\n// Key: Germany\n// Text to be encrypted: Dusseldorf\n// Cipher: nzoxahntgi\n\n// Code curated by Subhadeep Das(username:- Raven1233)"
}
{
"filename": "code/cryptography/src/polybius_cipher/polybius_cipher.py",
"content": "from pycipher.base import Cipher\nimport re\n\n####################################################################################\nclass PolybiusSquare(Cipher):\n \"\"\"The Polybius square is a simple substitution cipher that outputs 2 characters of ciphertext for each character of plaintext. It has a key consisting\n which depends on 'size'. By default 'size' is 5, and the key is 25 letters (5^2). For a size of 6 a 36 letter key required etc.\n For a more detailed look at how it works see http://www.practicalcryptography.com/ciphers pycipher.polybius-square-cipher/.\n \n :param key: The keysquare, each row one after the other. The key must by size^2 characters in length.\n :param size: The size of the keysquare, if size=5, the keysquare uses 5^2 or 25 characters.\n :param chars: the set of characters to use. By default ABCDE are used, this parameter should have the same length as size.\n \"\"\"\n\n def __init__(self, key=\"phqgiumeaylnofdxkrcvstzwb\", size=5, chars=None):\n self.key = \"\".join([k.upper() for k in key])\n self.chars = chars or \"ABCDEFGHIJKLMNOPQRSTUVWXYZ\"[:size]\n self.size = size\n assert (\n len(self.key) == size * size\n ), \"invalid key in init: must have length size*size, has length \" + str(\n len(key)\n )\n assert (\n len(self.chars) == size\n ), \"invalid chars in init: must have length=size, has length \" + str(len(chars))\n\n def encipher_char(self, ch):\n row = (int)(self.key.index(ch) / self.size)\n col = self.key.index(ch) % self.size\n return self.chars[row] + self.chars[col]\n\n def decipher_pair(self, pair):\n row = self.chars.index(pair[0])\n col = self.chars.index(pair[1])\n return self.key[row * self.size + col]\n\n def encipher(self, string):\n \"\"\"Encipher string using Polybius square cipher according to initialised key.\n Example::\n ciphertext = Polybius('APCZWRLFBDKOTYUQGENHXMIVS',5,'MKSBU').encipher(plaintext) \n :param string: The string to encipher.\n :returns: The enciphered string. The ciphertext will be twice the length of the plaintext.\n \"\"\"\n string = self.remove_punctuation(string) # ,filter='[^'+self.key+']')\n ret = \"\"\n for c in range(0, len(string)):\n ret += self.encipher_char(string[c])\n return ret\n\n def decipher(self, string):\n \"\"\"Decipher string using Polybius square cipher according to initialised key.\n Example::\n plaintext = Polybius('APCZWRLFBDKOTYUQGENHXMIVS',5,'MKSBU').decipher(ciphertext) \n :param string: The string to decipher.\n :returns: The deciphered string. The plaintext will be half the length of the ciphertext.\n \"\"\"\n string = self.remove_punctuation(string) # ,filter='[^'+self.chars+']')\n ret = \"\"\n for i in range(0, len(string), 2):\n ret += self.decipher_pair(string[i : i + 2])\n return ret\n\n\nif __name__ == \"__main__\":\n print('use \"import pycipher\" to access functions')"
}
{
"filename": "code/cryptography/src/porta_cipher/porta_cipher.py",
"content": "from pycipher.base import Cipher\n\n####################################################################################\nclass Porta(Cipher):\n \"\"\"The Porta Cipher is a polyalphabetic substitution cipher, and has a key consisting of a word e.g. 'FORTIFICATION'.\n \n :param key: The keyword, any word or phrase will do. Must consist of alphabetical characters only, no punctuation of numbers. \n \"\"\"\n\n def __init__(self, key=\"FORTIFICATION\"):\n self.key = [k.upper() for k in key]\n\n def encipher(self, string):\n \"\"\"Encipher string using Porta cipher according to initialised key. Punctuation and whitespace\n are removed from the input. \n Example::\n ciphertext = Porta('HELLO').encipher(plaintext) \n :param string: The string to encipher.\n :returns: The enciphered string.\n \"\"\"\n string = self.remove_punctuation(string)\n ret = \"\"\n for (i, c) in enumerate(string):\n i = i % len(self.key)\n if self.key[i] in \"AB\":\n ret += \"NOPQRSTUVWXYZABCDEFGHIJKLM\"[self.a2i(c)]\n elif self.key[i] in \"YZ\":\n ret += \"ZNOPQRSTUVWXYBCDEFGHIJKLMA\"[self.a2i(c)]\n elif self.key[i] in \"WX\":\n ret += \"YZNOPQRSTUVWXCDEFGHIJKLMAB\"[self.a2i(c)]\n elif self.key[i] in \"UV\":\n ret += \"XYZNOPQRSTUVWDEFGHIJKLMABC\"[self.a2i(c)]\n elif self.key[i] in \"ST\":\n ret += \"WXYZNOPQRSTUVEFGHIJKLMABCD\"[self.a2i(c)]\n elif self.key[i] in \"QR\":\n ret += \"VWXYZNOPQRSTUFGHIJKLMABCDE\"[self.a2i(c)]\n elif self.key[i] in \"OP\":\n ret += \"UVWXYZNOPQRSTGHIJKLMABCDEF\"[self.a2i(c)]\n elif self.key[i] in \"MN\":\n ret += \"TUVWXYZNOPQRSHIJKLMABCDEFG\"[self.a2i(c)]\n elif self.key[i] in \"KL\":\n ret += \"STUVWXYZNOPQRIJKLMABCDEFGH\"[self.a2i(c)]\n elif self.key[i] in \"IJ\":\n ret += \"RSTUVWXYZNOPQJKLMABCDEFGHI\"[self.a2i(c)]\n elif self.key[i] in \"GH\":\n ret += \"QRSTUVWXYZNOPKLMABCDEFGHIJ\"[self.a2i(c)]\n elif self.key[i] in \"EF\":\n ret += \"PQRSTUVWXYZNOLMABCDEFGHIJK\"[self.a2i(c)]\n elif self.key[i] in \"CD\":\n ret += \"OPQRSTUVWXYZNMABCDEFGHIJKL\"[self.a2i(c)]\n return ret\n\n def decipher(self, string):\n \"\"\"Decipher string using Porta cipher according to initialised key. Punctuation and whitespace\n are removed from the input. For the Porta cipher, enciphering and deciphering are the same operation.\n Example::\n plaintext = Porta('HELLO').decipher(ciphertext) \n :param string: The string to decipher.\n :returns: The deciphered string.\n \"\"\"\n return self.encipher(string)\n\n\nif __name__ == \"__main__\":\n print('use \"import pycipher\" to access functions')"
}
{
"filename": "code/cryptography/src/rail_fence_cipher/rail_fence_cipher.cpp",
"content": "#include <iostream>\n#include <cstring>\n#include <string>\n// Part of Cosmos by OpenGenus Foundation\n// Rails fence cipher - Cryptography\nusing namespace std;\n\nstring Encryptor(string msg, int key)\n{\n int msgLen = msg.size();\n bool direction = true;\n char enc[key][msgLen];\n for (int i = 0; i < key; i++)\n for (int j = 0; j < msgLen; j++)\n enc[i][j] = '\\n';\n\n for (int i = 0, row = 0, col = 0; i < msgLen; i++)\n {\n if (row == 0 || row == key - 1)\n direction = !direction;\n enc[row][col++] = msg[i];\n direction ? row-- : row++;\n }\n string cipher;\n for (int i = 0; i < key; i++)\n for (int j = 0; j < msgLen; j++)\n if (enc[i][j] != '\\n')\n cipher.push_back(enc[i][j]);\n\n return cipher;\n}\n\nstring Decryptor(string cipher, int key)\n{\n int cipLen = cipher.size();\n bool direction;\n char dec[key][cipLen];\n for (int i = 0; i < key; i++)\n for (int j = 0; j < cipLen; j++)\n dec[i][j] = '\\n';\n\n for (int i = 0, row = 0, col = 0; i < cipLen; i++)\n {\n if (row == 0)\n direction = false;\n else if (row == key - 1)\n direction = true;\n dec[row][col++] = '*';\n direction ? row-- : row++;\n }\n int index = 0;\n for (int i = 0; i < key; i++)\n for (int j = 0; j < cipLen; j++)\n if (dec[i][j] == '*' && index < cipLen)\n dec[i][j] = cipher[index++];\n\n string msg;\n for (int i = 0, row = 0, col = 0; i < cipLen; i++)\n {\n if (row == 0)\n direction = false;\n else if (row == key - 1)\n direction = true;\n if (dec[row][col] != '*')\n msg.push_back(dec[row][col++]);\n direction ? row-- : row++;\n }\n return msg;\n}\n\n\nint main()\n{\n int key, choice;\n std::string cip, msg;\n cout << \"1. Encrypt \\n2. Decrypt \\n>>\";\n cin >> choice;\n\n if (choice == 1)\n {\n cout << \"Enter Message\\n>>\";\n cin.ignore();\n std::getline(std::cin, msg);\n cout << \"Enter Key\\n>>\";\n cin >> key;\n cout << \"Encrypted Message\\n>>\" << Encryptor(msg, key);\n }\n else if (choice == 2)\n {\n cout << \"Enter Encrypted Message\\n>>\";\n cin.ignore();\n std::getline(std::cin, cip);\n cout << \"Enter Key\\n>>\";\n cin >> key;\n cout << \"Original Message\\n>>\" << Decryptor(cip, key);\n }\n return 0;\n}"
}
{
"filename": "code/cryptography/src/rail_fence_cipher/rail_fence_cipher.py",
"content": "string = \"Aditya Krishnakumar\"\nkey = 2\nmode = 0\n\n\ndef cipher(s, k, m):\n w = len(s)\n h = k\n matrix = [[0 for x in range(w)] for y in range(h)]\n output = []\n replace = []\n\n def c(string):\n cur_x, cur_y, not_0 = 0, 0, 0\n for char in string:\n matrix[cur_y][cur_x] = char\n cur_x += 1\n if cur_y < h - 1 and not_0 == 0:\n cur_y += 1\n else:\n if cur_y > 0:\n cur_y -= 1\n not_0 = 1\n else:\n cur_y += 1\n not_0 = 0\n return matrix\n\n def d(string):\n pointer = 0\n for i in range(len(string)):\n replace.append(\"r\")\n for row in c(\"\".join(replace)):\n for pos, char in enumerate(row):\n if char == \"r\":\n row[pos] = list(string)[pointer]\n pointer += 1\n return matrix\n\n if m == 0:\n for row in c(string):\n print(row)\n for char in row:\n if char is not 0:\n output.append(char)\n return \"\".join(output)\n if m == 1:\n cur_x, cur_y, not_0 = 0, 0, 0\n matrix = d(string)\n for row in matrix:\n print(row)\n while len(output) < len(list(string)):\n output.append(matrix[cur_y][cur_x])\n cur_x += 1\n if cur_y < h - 1 and not_0 == 0:\n cur_y += 1\n else:\n if cur_y > 0:\n cur_y -= 1\n not_0 = 1\n else:\n cur_y += 1\n not_0 = 0\n return \"\".join(output)\n\n\nprint(string, \"->\", cipher(string, key, mode))"
}
{
"filename": "code/cryptography/src/rail_fence_cipher/rail_fence_cipher.rb",
"content": "# Part of Cosmos by OpenGenus Foundation\n\nrequire 'optparse'\n\noptions = {}\nOptionParser.new do |opt|\n opt.banner = 'Usage: rail_fence.rb [options]'\n\n opt.on('-e', '--encipher KEY') do |o|\n options[:encipher] = o\n end\n\n opt.on('-d', '--decipher KEY') do |o|\n options[:decipher] = o\n end\nend.parse!\n\n##\n# A class for enciphering and deciphering messages using the Rail-fence cipher.\n#\n# See: http://practicalcryptography.com/ciphers/rail-fence-cipher/\nclass RailFence\n ##\n # Encipher a +message+ with the Rail-fence result.\n #\n # Params:\n # +message+::Message to encipher.\n # +key+::Key to encipher message with.\n def self.encipher(message, key)\n message.tr! ' ', '_'\n\n # Handle case where key = 1\n return message if key == 1\n\n # Initialize a matrix of characters\n matrix = Array.new(key.to_i) do\n Array.new(message.length, '.')\n end\n\n down = false\n row = 0\n col = 0\n\n # Iterate over characters in message to create matrix\n message.split('').each do |char|\n # Swap direction at the top or bottom row\n down = !down if (row == 0) || (row == (key - 1))\n\n # Fill corresponding letter in matrix\n matrix[row][col] = char\n col += 1\n\n # Increment or decrement row\n down ? row += 1 : row -= 1\n end\n\n # Construct result from matrix\n cipher = []\n\n matrix.each do |array|\n array.each do |char|\n cipher.push(char) unless char == '.'\n end\n end\n\n cipher.join('')\n end\n\n ##\n # Decipher a +message+ with the Rail-fence result.\n #\n # Params:\n # +message+::Message to decipher.\n # +key+::Key to decipher message.\n def self.decipher(message, key)\n message.tr! ' ', '_'\n\n # Handle case where key = 1\n return message if key == 1\n\n # Initialize a matrix of characters\n matrix = Array.new(key.to_i) do\n Array.new(message.length, '.')\n end\n\n down = false\n row = 0\n col = 0\n\n # Iterate over characters in message to create matrix\n message.split('').each do |_char|\n # Determine direction\n down = true if row == 0\n down = false if row == key - 1\n\n # Fill corresponding position with marker\n matrix[row][col] = '*'\n col += 1\n\n # Increment or decrement row\n down ? row += 1 : row -= 1\n end\n\n index = 0\n characters = message.split('')\n\n # Fill the matrix with the message\n matrix.each_with_index do |array, i|\n array.each_with_index do |char, j|\n if char == '*'\n matrix[i][j] = characters[index]\n index += 1\n end\n end\n end\n\n # Construct result from matrix\n result = []\n\n down = false\n row = 0\n col = 0\n\n while col < message.length\n\n # Determine which direction to move in\n down = true if row == 0\n down = false if row == key - 1\n\n result.push matrix[row][col]\n col += 1\n\n # Increment or decrement row\n down ? row += 1 : row -= 1\n\n end\n\n result.join('')\n end\nend\n\nif options.key? :encipher\n key = options[:encipher]\n puts RailFence.encipher ARGV.join(' '), key.to_i\nend\n\nif options.key? :decipher\n key = options[:decipher]\n puts RailFence.decipher ARGV.join(' '), key.to_i\nend"
}
{
"filename": "code/cryptography/src/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\nSecuring the Internet presents great challenges and research opportunities. Potential applications such as Internet voting, universally available medical records, and ubiquitous e-commerce are all being hindered because of serious security and privacy concerns. The epidemic of hacker attacks on personal computers and web sites only highlights the inherent vulnerability of the current computer and network infrastructure. \n\n> This is where Cryptographic algorithms step in. \n\nCryptographic algorithms are designed around the idea of _computational hardness assumptions_, making such algorithms hard to break in practice. It is theoretically possible to break such a system but it is infeasible to do so by any known practical means. These schemes are therefore termed **computationally secure** such as improvements in integer factorization algorithms, and faster computing technology require these solutions to be continually adapted. There exist information-theoretically secure schemes that provably cannot be broken even with unlimited computing power—an example is the one-time pad—but these schemes are more difficult to implement than the best theoretically breakable but computationally secure mechanisms.\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/cryptography/src/rot13_cipher/README.md",
"content": "# ROT-13 Cipher\n\nROT-13 is a simple substitution cipher in which each letter is shifted by 13 letters in the alphabet, wrapping around when neccesary. An example is shown below:\n\n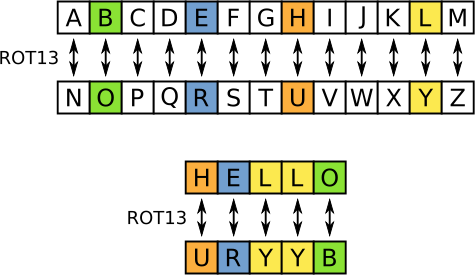\n\nImage credit: `By Benjamin D. Esham (bdesham) - Based upon ROT13.png by en:User:Matt Crypto. This version created by bdesham in Inkscape.This vector image was created with Inkscape., Public Domain, https://commons.wikimedia.org/w/index.php?curid=2707244`\n\n## ROT-N\n\nROT-N, more commonly known as a Caesar cipher, is similar to ROT-13, although letters can be shifted by any number of spaces. This makes ROT-13 a subset of a Caesar cipher where letters are shifted by 13 spaces.\n\n## Sources and more detailed information:\n\n- https://en.wikipedia.org/wiki/ROT13\n- https://en.wikipedia.org/wiki/Caesar_cipher\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/cryptography/src/rot13_cipher/rot13_cipher2.cpp",
"content": "#include <iostream>\n#include <string>\n#include <cctype>\n\nusing namespace std;\n\n/// @brief Run rotation cipher on a string\n/// @param text Text to encrypt\n/// @param n Amount to shift by\n/// @brief Encoded text\nstd::string rotN(const std::string &text, int n)\n{\n std::string output;\n\n // Loop through input text\n for (auto c : text)\n {\n // Check if character is a letter\n if (isalpha(c))\n {\n // Subtract 'A' if uppercase, 'a' if lowercase\n char sub = (c >= 65 && c <= 90) ? 'A' : 'a';\n // Convert character to alphabet index starting from zero (0 - 25)\n c -= sub;\n // Shift\n c += n;\n // Wrap around\n c %= 26;\n // Convert back into ASCII and add to output\n output += (c + sub);\n }\n else\n output += c;\n }\n\n return output;\n}\n\nint main()\n{\n // Example\n cout << rotN(\"Hello, world!\", 13) << endl; // Outputs \"Uryyb, jbeyq!\"\n\n return 0;\n}"
}
{
"filename": "code/cryptography/src/rot13_cipher/rot13_cipher2.js",
"content": "/**\n * Run rotation cipher on a string\n * @param {string} text - Text to encrypt\n * @param {number} n - Amount to shift by\n * @return {string} Encoded string\n */\nconst rotN = function rot(text, n) {\n let output = \"\";\n\n // Loop through input\n for (let char of text) {\n // Get ASCII code\n let ascii = char.charCodeAt(0);\n // Check if character is not a letter\n if (ascii < 65 || ascii > 122) {\n output += String(char);\n } else {\n // Subtract 'A' if uppercase, 'a' if lowercase\n let sub = ascii >= 65 && ascii <= 90 ? \"A\" : \"a\";\n // Convert to alphabet index starting from zero (0 - 25)\n ascii -= sub.charCodeAt(0);\n // Shift\n ascii += n;\n // Wrap around\n ascii = ascii % 26;\n // Convert back to ASCII code and add to output\n output += String.fromCharCode(ascii + sub.charCodeAt(0));\n }\n }\n\n return output;\n};\n\n// Examples\nconsole.log(rotN(\"Hello, world!\", 13)); // Outputs \"Uryyb, jbeyq!\""
}
{
"filename": "code/cryptography/src/rot13_cipher/rot13_cipher.c",
"content": "#include <stdio.h>\n#include <string.h>\n#include <ctype.h>\n\n\n// Run rotation cipher on a string\n// The string will be modified\nvoid rotN(char * string, int n) {\n char *p;\n char sub;\n\n // Loop through input text\n for(p = string ; *p != 0 ; p++) {\n // Check if character is a letter\n if (isalpha(*p)) {\n // Subtract 'A' if uppercase, 'a' if lowercase\n sub = (*p >= 65 && *p <= 90) ? 'A' : 'a';\n // Convert character to alphabet index starting from zero (0 - 25)\n *p -= sub;\n // Shift\n *p += n;\n // Wrap around\n *p %= 26;\n // Convert back into ASCII\n *p += sub;\n }\n }\n}\n\nint main() {\n // Example\n char str[] = \"Hello, world!\";\n rotN(str, 13);\n puts(str); // Outputs \"Uryyb, jbeyq!\"\n\n return 0;\n}"
}
{
"filename": "code/cryptography/src/rot13_cipher/rot13_cipher.cpp",
"content": "#include <string>\n#include <iostream>\n#include <cctype>\n\nstd::string rot13(const std::string &text)\n{\n std::string output;\n\n // Loop through input text\n for (auto c : text)\n {\n // Check if character is a letter\n if (isalpha(c))\n {\n // Subtract 'A' if uppercase, 'a' if lowercase\n char sub = (c >= 65 && c <= 90) ? 'A' : 'a';\n // Convert character to alphabet index starting from zero (0 - 25)\n c -= sub;\n // Shift\n c += 13;\n // Wrap around\n c %= 26;\n // Convert back into ASCII and add to output\n output += (c + sub);\n }\n else\n output += c;\n }\n return output;\n}\n\nint main()\n{\n // Example\n std::cout << rot13(\"Hello, world!\") << \"\\n\"; // Outputs \"Uryyb, jbeyq!\"\n std::cout << rot13(\"Uryyb, jbeyq!\") << \"\\n\"; // Output \"Hello, world!\"\n\n return 0;\n}"
}
{
"filename": "code/cryptography/src/rot13_cipher/rot13_cipher.java",
"content": "// Part of Cosmos by OpenGenus Foundation\npublic class RotN {\n\n public static void main(String[] args) {\n String test = \"test\";\n\t\t\n System.out.println(rot(test, 13)); // prints 'grfg'\n System.out.println(rot(test, 26)); // prints 'test'\n }\n \n public static String rot(String str, int n) {\n n = Math.max(0, n);\n \n String result = \"\";\n \n for (char c : str.toCharArray()) {\n if (!Character.isLetter(c))\n result += c;\n else {\n int b = c - 'a';\n int m = (b + n) % 26;\n result += (char) (m + 'a');\n }\n }\n \n return result;\n }\n}"
}
{
"filename": "code/cryptography/src/rot13_cipher/rot13_cipher.js",
"content": "function rot13(text) {\n return text.replace(/[a-z]/gi, function(char) {\n return String.fromCharCode(\n (char <= \"Z\" ? 90 : 122) >= (char = char.charCodeAt(0) + 13)\n ? char\n : char - 26\n );\n });\n}"
}
{
"filename": "code/cryptography/src/rot13_cipher/rot13_cipher.py",
"content": "# Part of Cosmos by OpenGenus Foundation\nif __name__ == \"__main__\":\n plaintext = \"This is unencrypted sample text\"\n ciphertext = \"\"\n for ch in plaintext:\n ascii_value = ord(ch)\n if ch == \" \":\n ciphertext += \" \"\n elif ascii_value >= 65 and ascii_value <= 90:\n if ascii_value + 13 > 90:\n ciphertext += chr(ascii_value - 13)\n else:\n ciphertext += chr(ascii_value + 13)\n elif ascii_value >= 97 and ascii_value <= 122:\n if ascii_value + 13 > 122:\n ciphertext += chr(ascii_value - 13)\n else:\n ciphertext += chr(ascii_value + 13)\n print(ciphertext)"
}
{
"filename": "code/cryptography/src/rot13_cipher/rot13_cipher.rb",
"content": "# Part of Cosmos by OpenGenus Foundation\nputs 'Enter text you want to encrypt: '\ntext = gets.chomp\n\nputs text.tr('A-Za-z', 'N-ZA-Mn-za-m')"
}
{
"filename": "code/cryptography/src/rot13_cipher/rot13_cipher.sh",
"content": "#!/bin/sh\n\n# Run rot13 on a string\nrot13()\n{\n str=$1\n result=$(echo -n \"$str\" | tr '[A-Za-z]' '[N-ZA-Mn-za-m]')\n echo $result\n}\n\n# Example\n# $ ./rot13.sh \"Hello world!\"\n# $ Uryyb jbeyq!\n\nif [ \"$#\" -ne 1 ] ; then\n echo \"Usage: $0 <string>\"\n exit 1\nfi\n\nrot13 \"$1\""
}
{
"filename": "code/cryptography/src/rot13_cipher/rotn_cipher.exs",
"content": "defmodule Cryptography do\n # Its rotn, but defaults to rot13\n def rotn(input, n \\\\ 13) do\n alphabet = \"abcdefghijklmnopqrstuvwxyz\"\n\n input\n |> String.downcase()\n |> String.split(\"\", trim: true)\n |> Enum.map(fn\n \" \" ->\n \" \"\n\n a ->\n alphabet_ind =\n String.split(alphabet, \"\", trim: true) |> Enum.find_index(fn x -> x == a end)\n\n if !is_nil(alphabet_ind) do\n rot = alphabet_ind + n\n\n rot_ind =\n cond do\n rot < 0 -> rem(rot, 26) * -1\n true -> rem(rot, 26)\n end\n\n String.at(alphabet, rot_ind)\n else\n a\n end\n end)\n |> Enum.join(\"\")\n end\nend\n\nmsg = \"Hello world\"\nn = 13\n\nCryptography.rotn(msg, n)\n|> IO.inspect(label: \"Encrypting '#{msg}' in rot#{n}\") # uryyb jbeyq"
}
{
"filename": "code/cryptography/src/rsa_digital_signature/rsa_digital_signature.ipynb",
"content": "{\n \"cells\": [\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"deletable\": true,\n \"editable\": true\n },\n \"source\": [\n \"# RSA Digital Signature\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 59,\n \"metadata\": {\n \"collapsed\": true\n },\n \"outputs\": [],\n \"source\": [\n \"from math import sqrt\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 1,\n \"metadata\": {\n \"collapsed\": true,\n \"deletable\": true,\n \"editable\": true\n },\n \"outputs\": [],\n \"source\": [\n \"def exp_mod(a, b, n):\\n\",\n \" \\\"\\\"\\\"\\n\",\n \" a to the power of b, mod n\\n\",\n \" >>> exp_mod(2, 3, 6)\\n\",\n \" 2\\n\",\n \" \\\"\\\"\\\"\\n\",\n \" return (a**b) % n\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 2,\n \"metadata\": {\n \"collapsed\": true,\n \"deletable\": true,\n \"editable\": true\n },\n \"outputs\": [],\n \"source\": [\n \"def gcd(a, b):\\n\",\n \" \\\"\\\"\\\"\\n\",\n \" Greatest common divisor\\n\",\n \" >>> gcd(72, 5)\\n\",\n \" 1\\n\",\n \" \\\"\\\"\\\"\\n\",\n \" if b > a:\\n\",\n \" return gcd(b, a)\\n\",\n \" if a % b == 0:\\n\",\n \" return b\\n\",\n \" return gcd(b, a % b)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 3,\n \"metadata\": {\n \"collapsed\": false,\n \"deletable\": true,\n \"editable\": true\n },\n \"outputs\": [],\n \"source\": [\n \"def gcd_qs(a, b):\\n\",\n \" \\\"\\\"\\\"\\n\",\n \" List of q values from gcd(a, b)\\n\",\n \" >>> gcd(72, 5)\\n\",\n \" [14, 2]\\n\",\n \" \\\"\\\"\\\"\\n\",\n \" if b > a:\\n\",\n \" a, b = b, a\\n\",\n \" assert a > b\\n\",\n \" assert gcd(a, b) == 1\\n\",\n \" \\n\",\n \" rem = None\\n\",\n \" while rem != 1:\\n\",\n \" q = a // b # 72 // 5 = 14 5 // 2 = 2\\n\",\n \" rem = a - q * b # 72 - 5*14 = 2 5 - 2*2 = 1\\n\",\n \" a = b # a is now 5 a is now 2\\n\",\n \" b = rem # b is now 2 b is now 1\\n\",\n \" yield q\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 4,\n \"metadata\": {\n \"collapsed\": true,\n \"deletable\": true,\n \"editable\": true\n },\n \"outputs\": [],\n \"source\": [\n \"def mod_inv(a, n):\\n\",\n \" \\\"\\\"\\\" \\n\",\n \" Modular inverse\\n\",\n \" >>> inv_mod(72, 5)\\n\",\n \" 29\\n\",\n \" \\\"\\\"\\\"\\n\",\n \" t0, t1 = 0, 1\\n\",\n \" for q in gcd_qs(a, n):\\n\",\n \" t = t0 - q * t1\\n\",\n \" t0, t1 = t1, t # move them all back one space\\n\",\n \" return t\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 63,\n \"metadata\": {\n \"collapsed\": true,\n \"deletable\": true,\n \"editable\": true\n },\n \"outputs\": [],\n \"source\": [\n \"def is_prime(n):\\n\",\n \" \\\"\\\"\\\"\\n\",\n \" Check whether a number is prime\\n\",\n \" >>> is_prime(5)\\n\",\n \" True\\n\",\n \" \\\"\\\"\\\"\\n\",\n \" return all(n % i != 0 \\n\",\n \" for i in range(2, int(sqrt(n))))\"\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"deletable\": true,\n \"editable\": true\n },\n \"source\": [\n \"## 1) Generate Keys\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 47,\n \"metadata\": {\n \"collapsed\": true\n },\n \"outputs\": [],\n \"source\": [\n \"from random import shuffle\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 68,\n \"metadata\": {\n \"collapsed\": false,\n \"deletable\": true,\n \"editable\": true\n },\n \"outputs\": [],\n \"source\": [\n \"# pick two big prime numbers\\n\",\n \"p = 101\\n\",\n \"q = 499\\n\",\n \"\\n\",\n \"assert is_prime(p)\\n\",\n \"assert is_prime(q)\\n\",\n \"\\n\",\n \"n = p * q # first part of public key\\n\",\n \"phi_n = (p-1)*(q-1)\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 55,\n \"metadata\": {\n \"collapsed\": false,\n \"deletable\": true,\n \"editable\": true,\n \"scrolled\": true\n },\n \"outputs\": [\n {\n \"name\": \"stdout\",\n \"output_type\": \"stream\",\n \"text\": [\n \"v = 167\\n\"\n ]\n }\n ],\n \"source\": [\n \"# pick v, second part of public key\\n\",\n \"valid_range = list(range(p+1, q))\\n\",\n \"shuffle(valid_range) # shuffles in place\\n\",\n \"\\n\",\n \"for v in valid_range: # search for a prime in (p, q)\\n\",\n \" if not is_prime(v): # need v to be prime\\n\",\n \" continue\\n\",\n \" if gcd(v, phi_n) == 1: # found one that fits\\n\",\n \" break\\n\",\n \" \\n\",\n \"print(f'v = {v}')\"\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"deletable\": true,\n \"editable\": true\n },\n \"source\": [\n \"## 2) Sign Message\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 56,\n \"metadata\": {\n \"collapsed\": false,\n \"deletable\": true,\n \"editable\": true,\n \"scrolled\": true\n },\n \"outputs\": [],\n \"source\": [\n \"# compute s\\n\",\n \"s = mod_inv(v, phi_n) # private key\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 57,\n \"metadata\": {\n \"collapsed\": false,\n \"deletable\": true,\n \"editable\": true\n },\n \"outputs\": [\n {\n \"name\": \"stdout\",\n \"output_type\": \"stream\",\n \"text\": [\n \"signed message = 16437\\n\"\n ]\n }\n ],\n \"source\": [\n \"# desired message to send\\n\",\n \"msg = 321 \\n\",\n \"assert 1 < msg < n\\n\",\n \"\\n\",\n \"signed_msg = exp_mod(msg, s, n)\\n\",\n \"print(f'signed message = {signed_msg}')\"\n ]\n },\n {\n \"cell_type\": \"markdown\",\n \"metadata\": {\n \"deletable\": true,\n \"editable\": true\n },\n \"source\": [\n \"## 3) Verify Authenticity\"\n ]\n },\n {\n \"cell_type\": \"code\",\n \"execution_count\": 69,\n \"metadata\": {\n \"collapsed\": false,\n \"deletable\": true,\n \"editable\": true\n },\n \"outputs\": [\n {\n \"name\": \"stdout\",\n \"output_type\": \"stream\",\n \"text\": [\n \"decrypted message = 321\\n\",\n \"same as original message: True\\n\"\n ]\n }\n ],\n \"source\": [\n \"decrypted = exp_mod(signed_msg, v, n)\\n\",\n \"print(f'decrypted message = {decrypted}')\\n\",\n \"print(f'same as original message: {decrypted == msg}')\"\n ]\n }\n ],\n \"metadata\": {\n \"kernelspec\": {\n \"display_name\": \"Python 3\",\n \"language\": \"python\",\n \"name\": \"python3\"\n },\n \"language_info\": {\n \"codemirror_mode\": {\n \"name\": \"ipython\",\n \"version\": 3\n },\n \"file_extension\": \".py\",\n \"mimetype\": \"text/x-python\",\n \"name\": \"python\",\n \"nbconvert_exporter\": \"python\",\n \"pygments_lexer\": \"ipython3\",\n \"version\": \"3.6.0\"\n }\n },\n \"nbformat\": 4,\n \"nbformat_minor\": 2\n}"
}
{
"filename": "code/cryptography/src/rsa/rsa.c",
"content": "/* Part of Cosmos by OpenGenus Foundation \n * RSA(Rivest–Shamir–Adleman) Public Key Cryptosystem\n*/\n#include <stdio.h>\n#include <math.h>\n#include <stdlib.h>\n#include<stdbool.h>\n\nlong long p, q, n, t, d, e, m[100], en[100];\n/*\n p: prime 1\n q: prime 2\n n: p*q\n t: euler totient function phi(n)\n e: encryption key\n d: decryption key\n\n Public Key: n, e\n Private Key: n, d (or p, q, t)\n*/\nchar msg[100];\n\nbool prime(int n)\n{\n\tif ( n == 1 )\n\t\treturn 0;\n\tint sq = sqrt(n);\n\tfor ( int i = 2 ; i <= sq ; i++ )\n\t{\n\t\tif ( n % i == 0 )\n\t\t\treturn 0;\n\t}\n\treturn 1;\n}\n\nvoid chooseED()\n{\n\tfor ( int i = 2 ; i < t ; i++ )\n\t{\n\t\tif ( t % i == 0 )\n\t\t\tcontinue;\n\t\tif ( !prime(i) )\n\t\t\tcontinue;\n\t\tint res = 1;\n\n\t\twhile ( 1 )\n\t\t{\n\t\t\tres += t;\n\t\t\tif ( res % i == 0 )\n\t\t\t{\n\t\t\t\te = i;\n\t\t\t\td = res / i;\n\t\t\t\treturn;\n\t\t\t}\n\t\t}\n\t}\n}\n\n// fast modular exponentiation, O(log n)\nlong long modular_pow(long long base, long long exponent, long long modulus)\n{\n long long result = 1;\n while (exponent > 0)\n {\n if (exponent % 2 == 1)\n result = (result * base) % modulus;\n exponent = exponent >> 1;\n base = (base * base) % modulus;\n }\n return result;\n}\n\nint main()\n{\n\tfreopen(\"rsa_input.in\", \"r\", stdin);\n\tprintf(\"Enter first prime number:\\n\");\n\tscanf(\"%lld\", &p);\n\n\tif ( !prime(p) )\n\t{\n\t\tprintf(\"Error: \\'p\\' is not prime\\n\");\n\t\texit(0);\n\t}\n\n\tprintf(\"Enter second prime number:\\n\");\n\tscanf(\"%lld\", &q);\n\n\tif ( !prime(q) )\n\t{\n\t\tprintf(\"Error: \\'q\\' is not prime\\n\");\n\t\texit(0);\n\t}\n\n\tn = p * q;\n\tt = (p-1) * (q-1);\n\tchooseED();\n\n\tprintf(\"Enter your secret message:\\n\");\n\tscanf(\"%s\", msg);\n\n\tfor ( int i = 0 ; msg[i] != '\\0' ; i++ )\n\t{\n\t\tm[i] = msg[i];\n\t\ten[i] = modular_pow(m[i], e, n);\n\t\ten[i+1] = -1;\n\t}\n\n\tprintf(\"Encrypted Message:\\n\");\n\tfor ( int i = 0 ; en[i] != -1 ; i++ )\n\t{\n\t\tprintf(\"%lld \", en[i]);\n\t}\n\tprintf(\"\\n\");\n\n\tlong long ans;\n\tprintf(\"Decrypted Message:\\n\");\n\tfor ( int i = 0 ; en[i] != -1 ; i++ )\n\t{\n\t\tans = modular_pow(en[i], d, n);\n\t\tprintf(\"%c\", (char)ans);\n\t}\n}"
}
{
"filename": "code/cryptography/src/rsa/rsa.cs",
"content": "/*\n * Bailey Thompson\n * github.com/bkthomps\n *\n * Simple implementation of RSA Encryption.\n */\n\nusing System;\nusing System.Numerics;\nusing System.Collections.Generic;\n\nnamespace rsa\n{\n /*\n * Entry point of the program, display information to user on console.\n */\n internal static class Program\n {\n private static void Main()\n {\n var primeGen = new Primes();\n var key = primeGen.GetKey();\n const string message = \"Hello World!\";\n Console.WriteLine(\"Original Text: \\\"\" + message + \"\\\"\");\n var cypherText = key.Encrypt(message);\n Console.Write(\"Cypher Text: \");\n var isFirstLetter = true;\n foreach (var place in cypherText)\n {\n if (isFirstLetter)\n {\n isFirstLetter = false;\n Console.Write(place);\n continue;\n }\n Console.Write(\", \" + place);\n }\n Console.WriteLine();\n var decryptedText = key.Decrypt(cypherText);\n Console.WriteLine(\"Decrypted Text: \\\"\" + decryptedText + \"\\\"\");\n }\n }\n\n /*\n * Create a list of primes, with ability to create keys using the list.\n */\n internal class Primes\n {\n private const int MaxValue = 25000;\n private readonly bool[] isPrime = new bool[MaxValue + 1];\n private readonly List<int> primes = new List<int>();\n\n internal Primes()\n {\n for (var i = 2; i <= MaxValue; i++)\n {\n if (!isPrime[i])\n {\n primes.Add(i);\n for (var j = i * i; j <= MaxValue; j += i)\n {\n isPrime[j] = true;\n }\n }\n }\n }\n\n internal Key GetKey()\n {\n var end = primes.Count - 1;\n var start = end / 4;\n var random = new Random();\n var primeOne = primes[random.Next(start, end)];\n var primeTwo = primes[random.Next(start, end)];\n while (primeTwo == primeOne)\n {\n primeTwo = primes[random.Next(start, end)];\n }\n return new Key(primeOne, primeTwo, primes);\n }\n }\n\n /*\n * Create a key using two primes. The variable n is both primes multiplied, phi is each prime minus one multiplied\n * together. The variable e has to satisfy gcd(e, phi) = 1, so just another prime. The variable d has to satisfy\n * ed = 1 mod phi. The public key is (e, n) and the private key is (d, n).\n */\n internal class Key\n {\n private readonly int n;\n private readonly int e;\n private int d;\n\n internal Key(int primeOne, int primeTwo, List<int> primes)\n {\n n = primeOne * primeTwo;\n var phi = (primeOne - 1) * (primeTwo - 1);\n var end = primes.Count - 1;\n var start = end / 4;\n var random = new Random();\n do\n {\n do\n {\n e = primes[random.Next(start, end)];\n } while (e == primeOne || e == primeTwo);\n } while (!IsFoundD(phi));\n Console.WriteLine(\"Public Key: (e, n) = (\" + e + \", \" + n + \")\");\n }\n\n private bool IsFoundD(int phi)\n {\n for (var i = phi - 1; i > 1; i--)\n {\n var mul = BigInteger.Multiply(e, i);\n var result = BigInteger.Remainder(mul, phi);\n if (result.Equals(1))\n {\n d = i;\n Console.WriteLine(\"Private Key: (d, n) = (\" + d + \", \" + n + \")\");\n return true;\n }\n }\n return false;\n }\n\n internal int[] Encrypt(string message)\n {\n var charArray = message.ToCharArray();\n var array = new int[charArray.Length];\n for (var i = 0; i < array.Length; i++)\n {\n array[i] = (int) BigInteger.ModPow(charArray[i], e, n);\n }\n return array;\n }\n\n internal string Decrypt(int[] cyphertext)\n {\n var array = new char[cyphertext.Length];\n for (var i = 0; i < array.Length; i++)\n {\n array[i] = (char) BigInteger.ModPow(cyphertext[i], d, n);\n }\n return new string(array);\n }\n }\n}"
}
{
"filename": "code/cryptography/src/rsa/rsa_input.in",
"content": "103 347\nhellohowareyou"
}
{
"filename": "code/cryptography/src/rsa/rsa.java",
"content": "import java.math.BigInteger;\nimport java.util.Random;\nimport java.util.Scanner;\n/**\n *\n * @author thompson naidu\n */\n \n \npublic class RSA {\n private BigInteger p,q,N,phi,e,d;\n private int bitlength=1024;\n private Random rand;\n \n \n public RSA(){\n rand=new Random();\n p=BigInteger.probablePrime(bitlength, rand);\n q=BigInteger.probablePrime(bitlength, rand);\n N=p.multiply(q);\n phi= p.subtract(BigInteger.ONE).multiply(q.subtract(BigInteger.ONE));\n e=BigInteger.probablePrime(bitlength/2, rand);\n while(phi.gcd(e).compareTo(BigInteger.ONE)>0 && e.compareTo(phi)< 0){\n e.add(BigInteger.ONE);\n }\n d=e.modInverse(phi);\n }\n \n public static String bytesToString(byte[] bytes){\n String text=\"\";\n for(byte b:bytes){\n text+=Byte.toString(b);\n }\n return text;\n }\n \n //encrypt the message\n public byte[] encrypt(byte[] message){\n return (new BigInteger(message)).modPow(e,N).toByteArray();\n }\n \n //decrypt the message\n public byte[] decrypt(byte[] message){\n return (new BigInteger(message)).modPow(d,N).toByteArray();\n }\n \n public static void main(String[] args) {\n RSA rsa=new RSA();\n Scanner sc=new Scanner(System.in);\n System.out.println(\"Enter the plain text: \");\n String plaintext=sc.nextLine();\n System.out.println(\"Encryption in progress..\");\n System.out.println(\"String in bytes : \"+bytesToString(plaintext.getBytes()));\n \n // encrypt\n byte[] encrypted = rsa.encrypt(plaintext.getBytes());\n // decrypt\n byte[] decrypted = rsa.decrypt(encrypted);\n System.out.println(\"Decrypting Bytes: \" + bytesToString(decrypted));\n System.out.println(\"Decrypted String: \" + new String(decrypted));\n }\n}"
}
{
"filename": "code/cryptography/src/rsa/rsa.py",
"content": "#!/usr/bin/env python\n\nfrom Crypto.PublicKey import RSA\n\n# Convert string to number\ndef strToNum(s):\n return int(s.encode(\"hex\"), 16)\n\n\n# Convert number to string\ndef numToStr(n):\n s = \"%x\" % n\n if len(s) % 2 != 0:\n s = \"0\" + s\n return s.decode(\"hex\")\n\n\n# Encrypt s using public key (e,n)\ndef encrypt(s, e, n):\n return numToStr(pow(strToNum(s), e, n))\n\n\n# Decrypt s using private key (d,n)\ndef decrypt(s, d, n):\n return numToStr(pow(strToNum(s), d, n))\n\n\n# Example\n# Generate random key\nkey = RSA.generate(2048)\nn = key.n\nd = key.d\ne = key.e\n\n# decrypt(encrypt(m)) = m\n# Output: Hello world!\nprint(decrypt(encrypt(\"Hello world!\", e, n), d, n))"
}
{
"filename": "code/cryptography/src/runningkey_cipher/README.md",
"content": "# cosmos\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\nPart of Cosmos by OpenGenus Foundation\n\nIt uses the following Table called Tabula Recta to find the ciphertext. The simple intersection of the row and column is the ciphertext. Let's say for eg. T is the Key and E is the original Plaintext, then the intersection of T column and E row is the ciphertext i.e. X. The plaintext and key-length must be the same. Running Key Cipher is similar to Vigenere Cipher. The difference lies in how the key is chosen; the Vigenere cipher uses a short key that repeats, whereas the running key cipher uses a long key such as an excerpt from a book. This means the key does not repeat, making cryptanalysis more difficult. \n\n A B C D E F G H I J K L M N O P Q R S T U V W X Y Z\n ---------------------------------------------------\n A A B C D E F G H I J K L M N O P Q R S T U V W X Y Z\n B B C D E F G H I J K L M N O P Q R S T U V W X Y Z A\n C C D E F G H I J K L M N O P Q R S T U V W X Y Z A B\n D D E F G H I J K L M N O P Q R S T U V W X Y Z A B C\n E E F G H I J K L M N O P Q R S T U V W X Y Z A B C D\n F F G H I J K L M N O P Q R S T U V W X Y Z A B C D E\n G G H I J K L M N O P Q R S T U V W X Y Z A B C D E F\n H H I J K L M N O P Q R S T U V W X Y Z A B C D E F G\n I I J K L M N O P Q R S T U V W X Y Z A B C D E F G H\n J J K L M N O P Q R S T U V W X Y Z A B C D E F G H I\n K K L M N O P Q R S T U V W X Y Z A B C D E F G H I J\n L L M N O P Q R S T U V W X Y Z A B C D E F G H I J K\n M M N O P Q R S T U V W X Y Z A B C D E F G H I J K L\n N N O P Q R S T U V W X Y Z A B C D E F G H I J K L M\n O O P Q R S T U V W X Y Z A B C D E F G H I J K L M N\n P P Q R S T U V W X Y Z A B C D E F G H I J K L M N O\n Q Q R S T U V W X Y Z A B C D E F G H I J K L M N O P\n R R S T U V W X Y Z A B C D E F G H I J K L M N O P Q\n S S T U V W X Y Z A B C D E F G H I J K L M N O P Q R\n T T U V W X Y Z A B C D E F G H I J K L M N O P Q R S\n U U V W X Y Z A B C D E F G H I J K L M N O P Q R S T\n V V W X Y Z A B C D E F G H I J K L M N O P Q R S T U\n W W X Y Z A B C D E F G H I J K L M N O P Q R S T U V\n X X Y Z A B C D E F G H I J K L M N O P Q R S T U V W\n Y Y Z A B C D E F G H I J K L M N O P Q R S T U V W X\n Z Z A B C D E F G H I J K L M N O P Q R S T U V W X Y\n\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/cryptography/src/runningkey_cipher/runningkey_cipher.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n#\n# It uses the following Table called Tabula Recta to find the\n# ciphertext. The simple intersection of the row and column\n# is the ciphertext. Let's say for eg. T is the Key and E is\n# the original Plaintext, then the intersection of T column\n# and E row is the ciphertext i.e. X. The plaintext and key-\n# length must be the same. Running Key Cipher is similar to\n# Vigenere Cipher. The difference lies in how the key is chosen;\n# the Vigenere cipher uses a short key that repeats, whereas the\n# running key cipher uses a long key such as an excerpt from\n# a book. This means the key does not repeat, making cryptanalysis\n# more difficult.\n\n# A B C D E F G H I J K L M N O P Q R S T U V W X Y Z\n# ---------------------------------------------------\n# A A B C D E F G H I J K L M N O P Q R S T U V W X Y Z\n# B B C D E F G H I J K L M N O P Q R S T U V W X Y Z A\n# C C D E F G H I J K L M N O P Q R S T U V W X Y Z A B\n# D D E F G H I J K L M N O P Q R S T U V W X Y Z A B C\n# E E F G H I J K L M N O P Q R S T U V W X Y Z A B C D\n# F F G H I J K L M N O P Q R S T U V W X Y Z A B C D E\n# G G H I J K L M N O P Q R S T U V W X Y Z A B C D E F\n# H H I J K L M N O P Q R S T U V W X Y Z A B C D E F G\n# I I J K L M N O P Q R S T U V W X Y Z A B C D E F G H\n# J J K L M N O P Q R S T U V W X Y Z A B C D E F G H I\n# K K L M N O P Q R S T U V W X Y Z A B C D E F G H I J\n# L L M N O P Q R S T U V W X Y Z A B C D E F G H I J K\n# M M N O P Q R S T U V W X Y Z A B C D E F G H I J K L\n# N N O P Q R S T U V W X Y Z A B C D E F G H I J K L M\n# O O P Q R S T U V W X Y Z A B C D E F G H I J K L M N\n# P P Q R S T U V W X Y Z A B C D E F G H I J K L M N O\n# Q Q R S T U V W X Y Z A B C D E F G H I J K L M N O P\n# R R S T U V W X Y Z A B C D E F G H I J K L M N O P Q\n# S S T U V W X Y Z A B C D E F G H I J K L M N O P Q R\n# T T U V W X Y Z A B C D E F G H I J K L M N O P Q R S\n# U U V W X Y Z A B C D E F G H I J K L M N O P Q R S T\n# V V W X Y Z A B C D E F G H I J K L M N O P Q R S T U\n# W W X Y Z A B C D E F G H I J K L M N O P Q R S T U V\n# X X Y Z A B C D E F G H I J K L M N O P Q R S T U V W\n# Y Y Z A B C D E F G H I J K L M N O P Q R S T U V W X\n# Z Z A B C D E F G H I J K L M N O P Q R S T U V W X Y\n\nplaintext = \"DEFENDTHEEASTWALLOFTHECASTLE\"\nkey = \"HOWDOESTHEDUCKKNOWTHATSAIDVI\"\nciphertext = \"\"\n\nif __name__ == \"__main__\":\n for index in range(len(plaintext)):\n sum_key_plaintext = (ord(key[index]) - 64) + (ord(plaintext[index]) - 64)\n if sum_key_plaintext > 27:\n ciphertext += chr(sum_key_plaintext - 26 - 1 + 64)\n else:\n ciphertext += chr(sum_key_plaintext - 1 + 64)\n print(ciphertext)"
}
{
"filename": "code/cryptography/src/sha/sha_256/sha_256.py",
"content": "initial_hash_values = [\n \"6a09e667\",\n \"bb67ae85\",\n \"3c6ef372\",\n \"a54ff53a\",\n \"510e527f\",\n \"9b05688c\",\n \"1f83d9ab\",\n \"5be0cd19\",\n]\n\nsha_256_constants = [\n \"428a2f98\",\n \"71374491\",\n \"b5c0fbcf\",\n \"e9b5dba5\",\n \"3956c25b\",\n \"59f111f1\",\n \"923f82a4\",\n \"ab1c5ed5\",\n \"d807aa98\",\n \"12835b01\",\n \"243185be\",\n \"550c7dc3\",\n \"72be5d74\",\n \"80deb1fe\",\n \"9bdc06a7\",\n \"c19bf174\",\n \"e49b69c1\",\n \"efbe4786\",\n \"0fc19dc6\",\n \"240ca1cc\",\n \"2de92c6f\",\n \"4a7484aa\",\n \"5cb0a9dc\",\n \"76f988da\",\n \"983e5152\",\n \"a831c66d\",\n \"b00327c8\",\n \"bf597fc7\",\n \"c6e00bf3\",\n \"d5a79147\",\n \"06ca6351\",\n \"14292967\",\n \"27b70a85\",\n \"2e1b2138\",\n \"4d2c6dfc\",\n \"53380d13\",\n \"650a7354\",\n \"766a0abb\",\n \"81c2c92e\",\n \"92722c85\",\n \"a2bfe8a1\",\n \"a81a664b\",\n \"c24b8b70\",\n \"c76c51a3\",\n \"d192e819\",\n \"d6990624\",\n \"f40e3585\",\n \"106aa070\",\n \"19a4c116\",\n \"1e376c08\",\n \"2748774c\",\n \"34b0bcb5\",\n \"391c0cb3\",\n \"4ed8aa4a\",\n \"5b9cca4f\",\n \"682e6ff3\",\n \"748f82ee\",\n \"78a5636f\",\n \"84c87814\",\n \"8cc70208\",\n \"90befffa\",\n \"a4506ceb\",\n \"bef9a3f7\",\n \"c67178f2\",\n]\n\n\ndef dec_to_bin(dec):\n return str(format(dec, \"b\"))\n\n\ndef dec_to_bin_8_bit(dec):\n return str(format(dec, \"08b\"))\n\n\ndef dec_to_bin_32_bit(dec):\n return str(format(dec, \"032b\"))\n\n\ndef dec_to_bin_64_bit(dec):\n return str(format(dec, \"064b\"))\n\n\ndef dec_to_hex(dec):\n return str(format(dec, \"x\"))\n\n\ndef bin_to_dec(bin_string):\n return int(bin_string, 2)\n\n\ndef hex_to_dec(hex_string):\n return int(hex_string, 16)\n\n\ndef L_P(SET, n):\n to_return = []\n j = 0\n k = n\n while k < len(SET) + 1:\n to_return.append(SET[j:k])\n j = k\n k += n\n return to_return\n\n\ndef string_to_list(bit_string):\n bit_list = []\n for i in range(len(bit_string)):\n bit_list.append(bit_string[i])\n return bit_list\n\n\ndef list_to_string(bit_list):\n bit_string = \"\"\n for i in range(len(bit_list)):\n bit_string += bit_list[i]\n return bit_string\n\n\ndef rotate_right(bit_string, n):\n bit_list = string_to_list(bit_string)\n for i in range(n):\n last_elem = list(bit_list.pop(-1))\n bit_list = last_elem + bit_list\n return list_to_string(bit_list)\n\n\ndef shift_right(bit_string, n):\n len_bit_string = len(bit_string)\n bit_string = bit_string[: len_bit_string - n :]\n front = \"0\" * n\n return front + bit_string\n\n\ndef mod_32_addition(input_set):\n return sum(input_set) % 4294967296\n\n\ndef xor_2str(string_1, string_2):\n xor_string = \"\"\n for i in range(len(string_1)):\n if string_1[i] == string_2[i]:\n xor_string += \"0\"\n else:\n xor_string += \"1\"\n return xor_string\n\n\ndef and_2str(string_1, string_2):\n and_list = []\n for i in range(len(string_1)):\n if string_1[i] == \"1\" and string_2[i] == \"1\":\n and_list.append(\"1\")\n else:\n and_list.append(\"0\")\n return list_to_string(and_list)\n\n\ndef or_2str(string_1, string_2):\n or_list = []\n for i in range(len(string_1)):\n if string_1[i] == \"0\" and string_2[i] == \"0\":\n or_list.append(\"0\")\n else:\n or_list.append(\"1\")\n return list_to_string(or_list)\n\n\ndef not_str(bit_string):\n not_string = \"\"\n for i in range(len(bit_string)):\n if bit_string[i] == \"0\":\n not_string += \"1\"\n else:\n not_string += \"0\"\n return not_string\n\n\n\"\"\"\nSHA-256 Specific Functions:\n\"\"\"\n\n\ndef Ch(x, y, z):\n return xor_2str(and_2str(x, y), and_2str(not_str(x), z))\n\n\ndef Maj(x, y, z):\n return xor_2str(xor_2str(and_2str(x, y), and_2str(x, z)), and_2str(y, z))\n\n\ndef e_0(x):\n return xor_2str(\n xor_2str(rotate_right(x, 2), rotate_right(x, 13)), rotate_right(x, 22)\n )\n\n\ndef e_1(x):\n return xor_2str(\n xor_2str(rotate_right(x, 6), rotate_right(x, 11)), rotate_right(x, 25)\n )\n\n\ndef s_0(x):\n return xor_2str(\n xor_2str(rotate_right(x, 7), rotate_right(x, 18)), shift_right(x, 3)\n )\n\n\ndef s_1(x):\n return xor_2str(\n xor_2str(rotate_right(x, 17), rotate_right(x, 19)), shift_right(x, 10)\n )\n\n\ndef message_pad(bit_list):\n pad_one = bit_list + \"1\"\n pad_len = len(pad_one)\n k = 0\n while ((pad_len + k) - 448) % 512 != 0:\n k += 1\n back_append_0 = \"0\" * k\n back_append_1 = dec_to_bin_64_bit(len(bit_list))\n return pad_one + back_append_0 + back_append_1\n\n\ndef message_bit_return(string_input):\n bit_list = []\n for i in range(len(string_input)):\n bit_list.append(dec_to_bin_8_bit(ord(string_input[i])))\n return list_to_string(bit_list)\n\n\ndef message_pre_pro(input_string):\n bit_main = message_bit_return(input_string)\n return message_pad(bit_main)\n\n\ndef message_parsing(input_string):\n return L_P(message_pre_pro(input_string), 32)\n\n\ndef message_schedule(index, w_t):\n new_word = dec_to_bin_32_bit(\n mod_32_addition(\n [\n bin_to_dec(s_1(w_t[index - 2])),\n bin_to_dec(w_t[index - 7]),\n bin_to_dec(s_0(w_t[index - 15])),\n bin_to_dec(w_t[index - 16]),\n ]\n )\n )\n return new_word\n\n\ndef sha_256(input_string):\n w_t = message_parsing(input_string)\n a = dec_to_bin_32_bit(hex_to_dec(initial_hash_values[0]))\n b = dec_to_bin_32_bit(hex_to_dec(initial_hash_values[1]))\n c = dec_to_bin_32_bit(hex_to_dec(initial_hash_values[2]))\n d = dec_to_bin_32_bit(hex_to_dec(initial_hash_values[3]))\n e = dec_to_bin_32_bit(hex_to_dec(initial_hash_values[4]))\n f = dec_to_bin_32_bit(hex_to_dec(initial_hash_values[5]))\n g = dec_to_bin_32_bit(hex_to_dec(initial_hash_values[6]))\n h = dec_to_bin_32_bit(hex_to_dec(initial_hash_values[7]))\n for i in range(0, 64):\n if i <= 15:\n t_1 = mod_32_addition(\n [\n bin_to_dec(h),\n bin_to_dec(e_1(e)),\n bin_to_dec(Ch(e, f, g)),\n hex_to_dec(sha_256_constants[i]),\n bin_to_dec(w_t[i]),\n ]\n )\n t_2 = mod_32_addition([bin_to_dec(e_0(a)), bin_to_dec(Maj(a, b, c))])\n h = g\n g = f\n f = e\n e = mod_32_addition([bin_to_dec(d), t_1])\n d = c\n c = b\n b = a\n a = mod_32_addition([t_1, t_2])\n a = dec_to_bin_32_bit(a)\n e = dec_to_bin_32_bit(e)\n else:\n w_t.append(message_schedule(i, w_t))\n t_1 = mod_32_addition(\n [\n bin_to_dec(h),\n bin_to_dec(e_1(e)),\n bin_to_dec(Ch(e, f, g)),\n hex_to_dec(sha_256_constants[i]),\n bin_to_dec(w_t[i]),\n ]\n )\n t_2 = mod_32_addition([bin_to_dec(e_0(a)), bin_to_dec(Maj(a, b, c))])\n h = g\n g = f\n f = e\n e = mod_32_addition([bin_to_dec(d), t_1])\n d = c\n c = b\n b = a\n a = mod_32_addition([t_1, t_2])\n a = dec_to_bin_32_bit(a)\n e = dec_to_bin_32_bit(e)\n hash_0 = mod_32_addition([hex_to_dec(initial_hash_values[0]), bin_to_dec(a)])\n hash_1 = mod_32_addition([hex_to_dec(initial_hash_values[1]), bin_to_dec(b)])\n hash_2 = mod_32_addition([hex_to_dec(initial_hash_values[2]), bin_to_dec(c)])\n hash_3 = mod_32_addition([hex_to_dec(initial_hash_values[3]), bin_to_dec(d)])\n hash_4 = mod_32_addition([hex_to_dec(initial_hash_values[4]), bin_to_dec(e)])\n hash_5 = mod_32_addition([hex_to_dec(initial_hash_values[5]), bin_to_dec(f)])\n hash_6 = mod_32_addition([hex_to_dec(initial_hash_values[6]), bin_to_dec(g)])\n hash_7 = mod_32_addition([hex_to_dec(initial_hash_values[7]), bin_to_dec(h)])\n final_hash = [\n dec_to_hex(hash_0),\n dec_to_hex(hash_1),\n dec_to_hex(hash_2),\n dec_to_hex(hash_3),\n dec_to_hex(hash_4),\n dec_to_hex(hash_5),\n dec_to_hex(hash_6),\n dec_to_hex(hash_7),\n ]\n return \"\".join(final_hash)\n\n\nassert (\n sha_256(\"cosmos\")\n) == \"4cbe19716b1aa73a67dc4b28c34391879b503259fc76852082b4dafcf0de85b2\""
}
{
"filename": "code/cryptography/src/simple_des/sdes.py",
"content": "def p_10(k):\n p_list=[2,4,1,6,3,9,0,8,7,5]\n for i in range(len(k)):\n p_list[i]=k[p_list[i]]\n return p_list\ndef p_8(k):\n p_list=[5,2,6,3,7,4,9,8]\n for i in range(len(p_list)):\n p_list[i]=k[p_list[i]]\n return p_list\ndef p_4(k):\n permutation_list=[1,3,2,0]\n for i in range(len(permutation_list)):\n permutation_list[i]=k[permutation_list[i]]\n return permutation_list\ndef left_shift_by_1(k):\n k.append(k[0])\n k.pop(0)\n return k\ndef left_shift_by_2(k):\n k.append(k[0])\n k.append(k[1])\n k.pop(0)\n k.pop(0)\n return k\ndef generate_keys(key):\n p_10_list=p_10(key)\n l=p_10_list[:5]\n r=p_10_list[5:]\n l1=left_shift_by_1(l)\n r1=left_shift_by_1(r)\n key1=l1+r1\n key1=p_8(key1)\n l2=left_shift_by_2(l)\n r2=left_shift_by_2(r)\n key2=l2+r2\n key2=p_8(key2)\n print(\"Key1 Generated : \",\"\".join(key1))\n print(\"Key2 Generated : \",\"\".join(key2))\n keys=[]\n keys.append(key1)\n keys.append(key2)\n return keys\ndef i_p(pt):\n p_list=[1,5,2,0,3,7,4,6]\n for i in range(len(p_list)):\n p_list[i]=pt[p_list[i]]\n return p_list\ndef inverse_i_p(k):\n i_p_list=[3,0,2,4,6,1,7,5]\n for i in range(len(i_p_list)):\n i_p_list[i]=k[i_p_list[i]]\n return i_p_list\ndef e_p(k):\n e_list=[3,0,1,2,1,2,3,0]\n for i in range(len(e_list)):\n e_list[i]=k[e_list[i]]\n return e_list\ndef xor(l1,l2):\n xor_list=[]\n for i in range(len(l1)):\n xor_list.append(str(int(l1[i])^int(l2[i])))\n return xor_list\ndef Part_1(pt):\n return i_p(pt)\ndef Part_2(pt,key,sbox1,sbox2):\n l=pt[:4]\n r=pt[4:]\n expanded_right_half=e_p(r)\n xor_out=xor(expanded_right_half,key)\n xor_left_half=xor_out[:4]\n xor_right_half=xor_out[4:]\n left_row_value=2*(int(xor_left_half[0]))+int(xor_left_half[3])\n left_column_value=2*(int(xor_left_half[1]))+int(xor_left_half[2])\n right_row_value=2*(int(xor_right_half[0]))+int(xor_right_half[3])\n right_column_value=2*(int(xor_right_half[1]))+int(xor_right_half[2])\n s0b=sbox1[left_row_value][left_column_value]\n s1b=sbox2[right_row_value][right_column_value]\n s0b=bin(s0b).replace(\"0b\",\"\") \n s1b=bin(s1b).replace(\"0b\",\"\") \n if(len(s0b)<2):\n s0b=\"0\"+s0b\n if(len(s1b)<2):\n s1b=\"0\"+s1b\n combined_s_value=s0b+s1b\n combined_s_value = p_4(combined_s_value)\n xcs=xor(l,combined_s_value)\n xcs = xcs+r\n return xcs\ndef encrypt(pt,keys,sbox1,sbox2):\n initial_permutation_list=Part_1(pt)\n xcs_r1 = Part_2(initial_permutation_list ,keys[0],sbox1,sbox2)\n xcs_r2 = Part_2(xcs_r1,keys[1],sbox1,sbox2)\n xcs_r2=xcs_r2[4:]+xcs_r2[:4] \n inverse_initial_permutation_value=inverse_i_p(xcs_r2)\n encrypted_text=\"\"\n for i in range(len(inverse_initial_permutation_value)):\n encrypted_text=encrypted_text+inverse_initial_permutation_value[i]\n return encrypted_text\ndef decrypt(dt,keys,sbox1,sbox2):\n initial_permutation_list=Part_1(dt)\n initial_permutation_list = initial_permutation_list[4:]+initial_permutation_list[:4]\n xcs_r1 = Part_2(initial_permutation_list,keys[1],sbox1,sbox2)\n xcs_r2 = Part_2(xcs_r1,keys[0],sbox1,sbox2)\n inverse_initial_permutation_value=inverse_i_p(xcs_r2)\n decrypted_text=\"\"\n for i in range(len(inverse_initial_permutation_value)):\n decrypted_text=decrypted_text+inverse_initial_permutation_value[i]\n return decrypted_text\n# key = list(input(\"Enter the 10-bit Key Value : \"))\n# pt = list(input(\"Enter the 8-bit Plain Text : \"))\nkey = list(\"1010000010\")\npt = list(\"10010101\")\nsbox1=[[1,0,3,2],\n [3,2,1,0],\n [0,2,1,3],\n [3,1,3,2]]\nsbox2=[[0,1,2,3],\n [2,0,1,3],\n [3,0,1,0],\n [2,1,0,3]]\nprint(\"Entered Plain text : \",\"\".join(pt))\nkeys=generate_keys(key)\nencrypted_text=encrypt(pt,keys,sbox1,sbox2)\nprint(\"After Encryption, Cipher Text : \",encrypted_text)\ndecrypted_text = decrypt(encrypted_text,keys,sbox1,sbox2)\nprint(\"After Decryption, Original Plain Text : \",decrypted_text)"
}
{
"filename": "code/cryptography/src/vernam_cipher/vernam_cipher.py",
"content": "mappingsDict = {}\n\ndef vernamEncryption(plaintext, key):\n ciphertext = ''\n\n for i in range(len(plaintext)):\n ptLetter = plaintext[i]\n keyLetter = key[i]\n sum = mappingsDict[ptLetter] + mappingsDict[keyLetter]\n if sum >= 26:\n sum -= 26\n ciphertext += chr(sum + 65)\n\n return ciphertext\n\ndef vernamDecryption(ciphertext, key):\n plaintext = ''\n\n for i in range(len(ciphertext)):\n ctLetter = ciphertext[i]\n keyLetter = key[i]\n diff = mappingsDict[ctLetter] - mappingsDict[keyLetter]\n if diff < 0:\n diff += 26\n plaintext += chr(diff + 65)\n\n return plaintext\n\nplaintext = input(\"Enter the plaintext : \")\nkey = input(\"Enter the key : \")\n\nalphabets = \"abcdefghijklmnopqrstuvwxyz\".upper()\nfor alphabet in alphabets:\n mappingsDict[alphabet] = ord(alphabet) - 65\n\nplaintext = plaintext.upper()\nkey = key.upper()\n\nif len(key) < len(plaintext):\n print(\"Length of Key Must be >= Length of Plaintext\")\nelse:\n ciphertext = vernamEncryption(plaintext, key)\n plaintext = vernamDecryption(ciphertext, key)\n\n print(\"Encrypted ciphertext is : \", ciphertext)\n print(\"Decrypted plaintext is : \", plaintext)"
}
{
"filename": "code/cryptography/src/vigenere_cipher/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/cryptography/src/vigenere_cipher/vigenere_cipher.c",
"content": "// Vigenere Cipher\n\n// Author: Rishav Pandey\n\n#include <stdio.h>\n#include <string.h>\n#include <stdlib.h>\n#include <ctype.h>\n\nint main(int argc, char* argv[])\n{\n //check if user give more or less than two arguments.\n if(argc != 2)\n {\n printf(\"Enter the Valid Key\");\n return 1;\n }\n \n else\n {\n \n for(int i =0, n = strlen(argv[1]); i<n ;i++)\n {\n //check weather the key is only alphabet or not\n if(!isalpha(argv[1][i]))\n {\n printf(\"Enter only Alphabets\");\n return 1;\n \n }\n }\n }\n \n printf(\"plaintext: \");\n char s[100];\n scanf(\"%s\", s);\n char* k = argv[1];\n \n int klen = strlen(k);\n \n \n printf(\"ciphertext: \");\n \n for(int i = 0, j = 0, n = strlen(s); i<n ; i++)\n {\n //wrap key for plaintext.\n int key = tolower(k[j % klen]) - 97;\n \n if(isalpha(s[i]))\n {\n //for upper case letters.\n if(isupper(s[i]))\n {\n printf(\"%c\",((s[i]-65+key)%26)+65); \n \n //increase key for next char.\n j++;\n \n } \n //for lower case letters.\n else if(islower(s[i]))\n {\n printf(\"%c\",((s[i]-97+key)%26)+97); \n //for lower case letters.\n j++; \n //increase key for next char.\n \n }\n \n }\n \n //for non alphabetical values.\n else\n \n {\n printf(\"%c\",s[i]); \n \n }\n }\n printf(\"\\n\");\n return 0;\n}"
}
{
"filename": "code/cryptography/src/vigenere_cipher/vigenere_cipher.cpp",
"content": "//C++ code for Vigenere Ciphere\n#include <iostream>\n#include <string>\n\nclass Vigenere\n{\npublic:\n Vigenere(const std::string& key)\n { //Traverses through the key\n for (size_t i = 0; i < key.size(); ++i)\n {\n if (key[i] >= 'A' && key[i] <= 'Z') //Check if the character of key is an uppercase alphabet\n this->key += key[i];\n else if (key[i] >= 'a' && key[i] <= 'z') //Check if the character of key is a lowercase alphabet\n this->key += key[i] + 'A' - 'a';\n }\n }\n\n //A function to perform encryption\n std::string encrypt(const std::string& text)\n {\n std::string result;\n //Traverses the plaintext\n for (size_t i = 0, j = 0; i < text.length(); ++i) \n {\n char c = text[i];\n\n if (c >= 'a' && c <= 'z') //Check if the character is a lowercase alphabet\n c += 'A' - 'a'; //Converts lowercase to uppercase characters\n else if (c < 'A' || c > 'Z') //Check if the character is not an alphabet\n continue;\n\n result += (c + key[j] - 2 * 'A') % 26 + 'A'; //Encryption of character is put into result by adding key to plaintext\n j = (j + 1) % key.length(); //Increment j within length of key\n }\n\n return result;\n }\n\n //A function to perform decryption\n std::string decrypt(const std::string& text)\n {\n std::string result;\n\n //Traverses the ciphertext\n for (size_t i = 0, j = 0; i < text.length(); ++i)\n {\n char c = text[i];\n\n if (c >= 'a' && c <= 'z') //Check if the character is a lowercase alphabet\n c += 'A' - 'a'; //Converts lowercase to uppercase characters\n else if (c < 'A' || c > 'Z') //Check if the character is not an alphabet\n continue;\n\n result += (c - key[j] + 26) % 26 + 'A'; //Decryption of character is put into result by Subtracting key from ciphertext\n j = (j + 1) % key.length(); //Increment j within length of key\n }\n\n return result;\n }\n\nprivate:\n std::string key;\n};\n\nint main()\n{\n Vigenere cipher{\"VIGENERECIPHER\"}; //key is VIGENERECIPHER of length 14\n\n std::string original =\n \"Beware the Jabberwock, my son! The jaws that bite, the claws that catch!\"; //Plaintext\n std::string encrypted = cipher.encrypt(original);\n std::string decrypted = cipher.decrypt(encrypted);\n\n std::cout << original << \"\\n\";\n std::cout << \"Encrypted: \" << encrypted << \"\\n\";\n std::cout << \"Decrypted: \" << decrypted << \"\\n\";\n}"
}
{
"filename": "code/cryptography/src/vigenere_cipher/vigenere_cipher.go",
"content": "package main\n\nimport (\n\t\"fmt\"\n)\n\nfunc Encrypt(text string, key string) string {\n\tif err := checkKey(key); err != nil {\n\t\tfmt.Println(\"Error: \", err.Error())\n\t\treturn \"Err\"\n\t}\n\tj := 0\n\tn := len(text)\n\tres := \"\"\n\tfor i := 0; i < n; i++ {\n\t\tr := text[i]\n\t\tif r >= 'a' && r <= 'z' {\n\t\t\tres += string((r+key[j]-2*'a')%26 + 'a')\n\t\t} else if r >= 'A' && r <= 'Z' {\n\t\t\tres += string((r+key[j]-2*'A')%26 + 'A')\n\t\t} else {\n\t\t\tres += string(r)\n\t\t}\n\n\t\tj = (j + 1) % len(key)\n\t}\n\treturn res\n}\n\nfunc Decrypt(text string, key string) string {\n\tif err := checkKey(key); err != nil {\n\t\tfmt.Println(\"Error: \", err.Error())\n\t\treturn \"Err\"\n\t}\n\tres := \"\"\n\tj := 0\n\tn := len(text)\n\tfor i := 0; i < n; i++ {\n\t\tr := text[i]\n\t\tif r >= 'a' && r <= 'z' {\n\t\t\tres += string((r-key[j]+26)%26 + 'a')\n\t\t} else if r >= 'A' && r <= 'Z' {\n\t\t\tres += string((r-key[j]+26)%26 + 'A')\n\t\t} else {\n\t\t\tres += string(r)\n\t\t}\n\n\t\tj = (j + 1) % len(key)\n\t}\n\treturn res\n}\n\nfunc checkKey(key string) error {\n\tif len(key) == 0 {\n\t\treturn fmt.Errorf(\"Key length is 0\")\n\t}\n\tfor _, r := range key {\n\t\tif !(r >= 'a' && r <= 'z' || r >= 'A' && r <= 'Z') {\n\t\t\treturn fmt.Errorf(\"Invalid Key\")\n\t\t}\n\t}\n\treturn nil\n}\n\nfunc main() {\n\tvar (\n\t\tmsg, key string\n\t\tmode rune\n\t)\n\tfmt.Println(\"Enter text to encrypt\\\\decrypt\")\n\tfmt.Scanf(\"%s\\n\", &msg)\n\tfmt.Println(\"Enter key\")\n\tfmt.Scanf(\"%s\\n\", &key)\n\tfmt.Println(\"Enter 'e' to encrypt or 'd' to decrypt\")\n\tfmt.Scanf(\"%c\", &mode)\n\tif mode == rune('e') {\n\t\tfmt.Println(\"Encrypted Message: \", Encrypt(msg, key))\n\t} else if mode == rune('d') {\n\t\tfmt.Println(\"Deccrypted Message: \", Decrypt(msg, key))\n\t} else {\n\t\tfmt.Println(\"Unknown mode\")\n\t}\n}"
}
{
"filename": "code/cryptography/src/vigenere_cipher/vigenere_cipher.hs",
"content": "--Vigenere cipher\nmodule Vigenere where\n\nimport Data.Char\n\nchrRg :: Int\nchrRg = length ['A'..'Z']\n\nencode :: Char -> Int\nencode = subtract (ord 'A') . ord . toUpper\n\ndecode :: Int -> Char\ndecode = chr . (ord 'A' +)\n\nshiftr :: Char -> Char -> Char\nshiftr c k = decode $ mod (encode c + encode k) chrRg\n\nshiftl :: Char -> Char -> Char\nshiftl c k = decode $ mod (encode c - encode k) chrRg\n\nvigenere :: String -> String -> String\nvigenere = vigProc shiftr \n\nunVigenere :: String -> String -> String\nunVigenere = vigProc shiftl \n\nvigProc :: (Char -> Char -> Char) -> (String -> String -> String)\nvigProc s cs = vigProc' s cs . cycle\n\nvigProc' :: (Char -> Char -> Char) -> (String -> String -> String)\nvigProc' _ [] _ = []\nvigProc' s (c:cs) (k:ks)\n | isLetter c = s c k : vigProc' s cs ks\n | otherwise = c : vigProc' s cs (k:ks)\n\nencrypt :: IO ()\nencrypt = do\n putStrLn \"Enter message to be encrypted: \"\n mes <- getLine\n putStrLn \"Enter key: \"\n key <- getLine\n putStrLn \"Encrypted message: \"\n putStrLn $ vigenere mes key\n{- Ex.\n encrypt\n Enter message to be encrypted:\n \"Hello, World!\"\n Enter key:\n \"World\"\n Encrypted message:\n \"CAZCZ, ZJMHR!\" -} \n\ndecrypt :: IO ()\ndecrypt = do\n putStrLn \"Enter message to be decrypted: \"\n mes <- getLine\n putStrLn \"Enter key: \"\n key <- getLine\n putStrLn \"Decrypted message: \"\n putStrLn $ unVigenere mes key\n{- Ex.\n decrypt\n Enter message to be decrypted:\n \"CAZCZ, ZJMHR!\"\n Enter key:\n \"World\"\n Decrypted message:\n \"HELLO, WORLD!\" -}\n "
}
{
"filename": "code/cryptography/src/vigenere_cipher/vigenere_cipher.java",
"content": "/**\n * Modified code from: https://rosettacode.org/wiki/Vigen%C3%A8re_cipher#Java\n * Part of Cosmos by Open Genus Foundation\n */\n\nimport java.util.Scanner;\n\npublic class vigenere_cipher{\n public static void main(String[] args) {\n System.out.println(\"Enter key: \");\n Scanner scanner = new Scanner(System.in);\n String key = scanner.nextLine();\n \n System.out.println(\"Enter text to encrypt: \");\n String text = scanner.nextLine();\n\n String enc = encrypt(text, key);\n System.out.println(enc);\n System.out.println(decrypt(enc, key));\n }\n \n static String encrypt(String text, final String key) {\n String res = \"\";\n for (int i = 0, j = 0; i < text.length(); i++) {\n char c = text.charAt(i);\n if (Character.isUpperCase(c)){\n res += (char)((c + key.charAt(j) - 2 * 'A') % 26 + 'A');\n j = ++j % key.length();\n }\n if (Character.isLowerCase(c)){\n res += (char)((c + Character.toLowerCase(key.charAt(j)) - 2 * 'a') % 26 + 'a');\n j = ++j % key.length();\n }\n }\n return res;\n }\n \n static String decrypt(String text, final String key) {\n String res = \"\";\n for (int i = 0, j = 0; i < text.length(); i++) {\n char c = text.charAt(i);\n if (Character.isUpperCase(c)) {\n res += (char)((c - key.charAt(j) + 26) % 26 + 'A');\n j = ++j % key.length();\n }\n if (Character.isLowerCase(c)) {\n res += (char)((c - Character.toLowerCase(key.charAt(j)) + 26) % 26 + 'a');\n j = ++j % key.length();\n }\n }\n return res;\n }\n}"
}
{
"filename": "code/cryptography/src/vigenere_cipher/vigenere_cipher.jl",
"content": "###!/* Part of Cosmos by OpenGenus Foundation */\n###Vigenere cipher implementation in Julia\n\nfunction encrypt(msg::AbstractString, key::AbstractString)\n msg = uppercase(join(filter(isalpha, collect(msg))))\n key = uppercase(join(filter(isalpha, collect(key))))\n msglen = length(msg)\n keylen = length(key)\n \n if keylen < msglen\n key = repeat(key, div(msglen - keylen, keylen) + 2)[1:msglen]\n end\n \n enc = Vector{Char}(msglen)\n \n @inbounds for i in 1:length(msg)\n enc[i] = Char((Int(msg[i]) + Int(key[i]) - 130) % 26 + 65)\n end\n \n return join(enc)\nend\n \nfunction decrypt(enc::AbstractString, key::AbstractString)\n enc = uppercase(join(filter(isalpha, collect(enc))))\n key = uppercase(join(filter(isalpha, collect(key))))\n msglen = length(enc)\n keylen = length(key)\n \n if keylen < msglen\n key = repeat(key, div(msglen - keylen, keylen) + 2)[1:msglen]\n end\n \n msg = Vector{Char}(msglen)\n \n @inbounds for i in 1:length(enc)\n msg[i] = Char((Int(enc[i]) - Int(key[i]) + 26) % 26 + 65)\n end\n \n return join(msg)\nend\n \nconst messages = (\"Attack at dawn.\", \"Don't attack.\", \"The war is over.\", \"Happy new year!\")\nconst key = \"LEMON\"\n \nfor msg in messages\n enc = encrypt(msg, key)\n dec = decrypt(enc, key)\n println(\"Original: $msg\\n -> encrypted: $enc\\n -> decrypted: $dec\")\nend"
}
{
"filename": "code/cryptography/src/vigenere_cipher/vigenere_cipher.js",
"content": "\"use strict\";\n\nclass Viegenere {\n static alphabetLen_() {\n return Viegenere.ALPHABET.length;\n }\n static encript(text, key) {\n let result = \"\";\n const preparedText = text.replace(new RegExp(\"\\\\s\", \"g\"), \"\").toLowerCase();\n const preparedKey = key.toLowerCase();\n const alphabetLen = Viegenere.alphabetLen_();\n for (let i = 0; i < preparedText.length; i++) {\n const char = preparedText[i];\n const keyChar = preparedKey[i % preparedKey.length];\n const shift = Viegenere.ALPHABET.indexOf(keyChar) % alphabetLen;\n const indexInAlphabet = Viegenere.ALPHABET.indexOf(char);\n result += Viegenere.ALPHABET[(indexInAlphabet + shift) % alphabetLen];\n }\n return result;\n }\n\n static decript(encripted, key) {\n let result = \"\";\n const preparedKey = key.toLowerCase();\n const alphabetLen = Viegenere.alphabetLen_();\n for (let i = 0; i < encripted.length; i++) {\n const char = encripted[i];\n const keyChar = preparedKey[i % preparedKey.length];\n let shift =\n Viegenere.ALPHABET.indexOf(char) - Viegenere.ALPHABET.indexOf(keyChar);\n shift = shift >= 0 ? shift : alphabetLen + shift;\n result += Viegenere.ALPHABET[shift];\n }\n return result;\n }\n}\n\nViegenere.ALPHABET = \"abcdefghijklmnopqrstuvwxyz\";\n\nconst key = \"LEMONLEMONLE\";\nconst text = \"Lorem ipsum dolor sit amet\";\nconst encripted = Viegenere.encript(text, key);\nconst decripted = Viegenere.decript(encripted, key);\n\nconsole.log(encripted);\nconsole.log(decripted);"
}
{
"filename": "code/cryptography/src/vigenere_cipher/vigenere_cipher.php",
"content": "<?php\n/**\n* Vigenère cipher (PHP)\n* based on https://en.wikipedia.org/wiki/Vigen%C3%A8re_cipher\n* implemented by Stefano Amorelli <[email protected]>\n* Part of Cosmos by OpenGenus Foundation\n*/\n\nclass VigenereCipher\n{\n public function encrypt($plainText, $key) {\n\n if (!ctype_alpha($plainText) || !ctype_alpha($key)) {\n throw new Exception('Plaintext and key should be alphabetical strings (no spaces, symbols and numbers).');\n }\n\n $plainText = strtolower($plainText);\n $key = strtolower($key);\n $encryptedText = [];\n $i = 0;\n\n foreach (str_split($plainText) as $character) {\n array_push($encryptedText, $this->encryptCharacter($character, $key[$i]));\n $i++;\n $i = $i % strlen($key);\n }\n return implode('', $encryptedText);\n\n }\n\n public function decrypt($encryptedText, $key) {\n\n if (!ctype_alpha($encryptedText) || !ctype_alpha($key)) {\n throw new Exception('Encrypted text and key should be alphabetical strings (no spaces, symbols and numbers).');\n }\n\n $encryptedText = strtolower($encryptedText);\n $key = strtolower($key);\n $plainText = [];\n $i = 0;\n\n foreach (str_split($encryptedText) as $character) {\n array_push($plainText, $this->decryptCharacter($character, $key[$i]));\n $i++;\n $i = $i % strlen($key);\n }\n return implode('', $plainText);\n\n }\n\n private function normalizeAscii($character) {\n return ord($character) - ord('a');\n }\n\n private function returnAscii($normalizedAscii) {\n return chr($normalizedAscii + ord('a'));\n }\n\n private function encryptCharacter($plainCharacter, $keyCharacter) {\n return $this->returnAscii(($this->normalizeAscii($plainCharacter) + $this->normalizeAscii($keyCharacter)) % 26);\n }\n\n private function decryptCharacter($encryptedCharacter, $keyCharacter) {\n return $this->returnAscii(($this->normalizeAscii($encryptedCharacter) - $this->normalizeAscii($keyCharacter) + 26) % 26);\n }\n}\n\n/* Example usage: \n\n$cipher = new VigenereCipher();\n\n$secret = 'mysecret';\n \n$encrypted = $cipher->encrypt('loremipsumdolor', $secret);\n\n$plain = $cipher->decrypt($encrypted, $secret);\n\necho $plain;\n\n*/"
}
{
"filename": "code/cryptography/src/vigenere_cipher/vigenere_cipher.py",
"content": "#Method for encryption that takes key and plaintext as arguments\ndef encrypt(key, text):\n\n alfa = \"abcdefghijklmnopqrstuvwxyz\"\n msg_to_be_decrypted = text.replace(\" \", \"\") #Removes spaces from plaintext\n msg_to_be_decrypted = msg_to_be_decrypted.lower() #Converts all characters to lowercase\n encrypted = \"\"\n\n #Traverses the plaintext\n for i in range(len(msg_to_be_decrypted)):\n letter_in_key = key[i % len(key)] #Puts the required character of the key in variable letter_in_key\n shift = (alfa.find(letter_in_key) + 1) % 26 #Puts the amount to be shifted in variable shift\n index_of_letter = alfa.find(msg_to_be_decrypted[i]) #Finds the character of plaintext to be encrypted\n encrypted += alfa[(index_of_letter + shift) % 26]\n\n return encrypted\n\n#Method for encryption that takes key and ciphertext as arguments\ndef decrypt(key, encrypted):\n\n alfa = \"abcdefghijklmnopqrstuvwxyz\"\n decrypted = \"\"\n\n #Traverses the ciphertext\n for i in range(len(encrypted)):\n letter_in_key = key[i % len(key)] #Puts the required character of the key in variable letter_in_key\n shift = (alfa.find(encrypted[i]) - alfa.find(letter_in_key) - 1) % 26 #Puts the amount to be shifted in variable shift\n decrypted += alfa[shift] #Performs decryption\n\n return decrypted\n\n\nkey = \"abc\"\ntext = \"ZZAla ma kota\"\ncipher = encrypt(key, text)\nprint(cipher)\nmsg = decrypt(key, cipher)\nprint(msg)"
}
{
"filename": "code/cryptography/src/vigenere_cipher/vigenere_cipher.rb",
"content": "class Vigenere\n @@alphabet = 'abcdefghijklmnopqrstuvwxyz'\n def initialize(key)\n @key = key.downcase\n end\n\n def encrypt(message)\n message = message.downcase\n result = ''\n for i in 0..message.length - 1\n letterNum = @@alphabet.index(message[i])\n keyNum = @@alphabet.index(@key[i % @key.length]) + 1\n resultNum = (letterNum + keyNum) % @@alphabet.length\n result << @@alphabet[resultNum]\n end\n result\n end\n\n def decrypt(cipher)\n cipher = cipher.downcase\n result = ''\n for i in 0..cipher.length - 1\n letterNum = @@alphabet.index(cipher[i])\n keyNum = @@alphabet.index(@key[i % @key.length]) + 1\n resultNum = (letterNum - keyNum) % @@alphabet.length\n result << @@alphabet[resultNum]\n end\n result\n end\n\n def self.encryptMessage(key, message)\n message = message.downcase\n result = ''\n for i in 0..message.length - 1\n letterNum = @@alphabet.index(message[i])\n keyNum = @@alphabet.index(key[i % key.length]) + 1\n resultNum = (letterNum + keyNum) % @@alphabet.length\n result << @@alphabet[resultNum]\n end\n result\n end\n\n def self.decryptMessage(key, cipher)\n cipher = cipher.downcase\n result = ''\n for i in 0..cipher.length - 1\n letterNum = @@alphabet.index(cipher[i])\n keyNum = @@alphabet.index(key[i % key.length]) + 1\n resultNum = (letterNum - keyNum) % @@alphabet.length\n result << @@alphabet[resultNum]\n end\n result\n end\nend\n\n# vig = Vigenere.new(\"abc\")\n# cipher = vig.encrypt(\"hello\")\n# puts cipher\n# puts vig.decrypt(cipher)\n# cipher2 = Vigenere.encryptMessage(\"abc\", \"hello\")\n# puts cipher2\n# puts Vigenere.decryptMessage(\"abc\", cipher2)"
}
{
"filename": "code/cryptography/test/baconian_cipher/baconian_test.cpp",
"content": "#include <assert.h>\n#include <iostream>\n#include \"./../../src/baconian_cipher/baconian.cpp\"\n\n// Part of Cosmos by OpenGenus Foundation\nint main()\n{\n std::string baconianString = \"AABBBAABAAABABBABABBABBBA BABBAABBBABAAABABABBAAABB\";\n std::string englishString = \"HELLO WORLD\";\n std::map<char, std::string> generatedCipher = createBaconianMap();\n assert(encrypt(generatedCipher, englishString) == baconianString);\n assert(decrypt(generatedCipher, baconianString) == englishString);\n std::cout << \"Testing Complete\\n\";\n}"
}
{
"filename": "code/cryptography/test/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/data_structures/src/2d_array/rotate2darray.java",
"content": "public class RotateMatrix {\n // function to rotate the matrix by 90 degrees clockwise\n static void rightRotate(int matrix[][], int n) {\n // determines the transpose of the matrix\n for (int i = 0; i < n; i++) {\n for (int j = i; j < n; j++) {\n int temp = matrix[i][j];\n matrix[i][j] = matrix[j][i];\n matrix[j][i] = temp;\n }\n }\n\n // then we reverse the elements of each row\n for (int i = 0; i < n; i++) {\n // logic to reverse each row i.e 1D Array.\n int low = 0, high = n - 1;\n while (low < high) {\n int temp = matrix[i][low];\n matrix[i][low] = matrix[i][high];\n matrix[i][high] = temp;\n low++;\n high--;\n }\n }\n\n System.out.println(\"The 90 degree Rotated Matrix is: \");\n for (int i = 0; i < 3; i++) {\n for (int j = 0; j < 3; j++) {\n System.out.print(matrix[i][j] + \" \" + \"\\t\");\n }\n System.out.println();\n }\n }\n // driver code\npublic static void main(String args[]) {\n int n = 3;\n // initializing a 3*3 matrix with an illustration\n int matrix[][] = new int[][]{{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};\n System.out.println(\"The Original Matrix is: \");\n for (int i = 0; i < 3; i++) {\n for (int j = 0; j < 3; j++) {\n System.out.print(matrix[i][j] + \" \" + \"\\t\");\n }\n System.out.println();\n }\n // function calling\n rightRotate(matrix, n);\n }\n}"
}
{
"filename": "code/data_structures/src/2d_array/rotate_matrix.cpp",
"content": "//#rotate square matrix by 90 degrees\n\n#include <bits/stdc++.h>\n#define n 5\nusing namespace std;\n\nvoid displayimage(\n int arr[n][n]);\n\n/* A function to\n * rotate a n x n matrix\n * by 90 degrees in\n * anti-clockwise direction */\nvoid rotateimage(int arr[][n])\n{ // Performing Transpose\n for (int i = 0; i < n; i++)\n {\n for (int j = i; j < n; j++)\n swap(arr[i][j], arr[j][i]);\n }\n\n // Reverse every row\n for (int i = 0; i < n; i++)\n reverse(arr[i], arr[i] + n);\n}\n\n// Function to print the matrix\nvoid displayimage(int arr[n][n])\n{\n for (int i = 0; i < n; i++)\n {\n for (int j = 0; j < n; j++)\n cout << arr[i][j] << \" \";\n\n cout << \"\\n\";\n }\n cout << \"\\n\";\n}\n\nint main()\n{\n\n int arr[n][n] = {\n {1, 2, 3, 4, 5},\n {6, 7, 8, 9, 10},\n {11, 12, 13, 14, 15},\n {16, 17, 18, 19, 20},\n {21, 22, 23, 24, 25}};\n\n // displayimage(arr);\n rotateimage(arr);\n\n // Print rotated matrix\n displayimage(arr);\n\n return 0;\n}\n\n/*-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------*/\n\n/* Test Case 2\n * int arr[n][n] = {\n * {1, 2, 3, 4},\n * {5, 6, 7, 8},\n * {9, 10, 11, 12},\n * {13, 14, 15, 16}\n * };\n */\n\n/* Tese Case 3\n *int mat[n][n] = {\n * {1, 2},\n * {3, 4}\n * };*/\n\n /*\nTime complexity : O(n*n)\nSpace complexity: O(1)\n */"
}
{
"filename": "code/data_structures/src/2d_array/set_matrix_zero.cpp",
"content": "#include <iostream>\n#include <string>\n#include <vector>\n#include <map>\n#include <cmath>\n#include <set>\n#include <unordered_map>\n#include <numeric>\n#include <algorithm>\n//#include <bits/stdc++.h>\nusing namespace std;\ntypedef long long ll;\n#define int int64_t\n\nint gcd(int a, int b)\n{\n if (b == 0)\n return a;\n return gcd(b, a % b);\n}\n\nvoid setneg(vector<vector<int>> &matrix, int row, int col)\n{\n for (int i = 0; i < matrix.size(); ++i)\n if (matrix[i][col] != 0)\n matrix[i][col] = -2147483636;\n for (int j = 0; j < matrix[0].size(); ++j)\n if (matrix[row][j] != 0)\n matrix[row][j] = -2147483636;\n}\n\nint32_t main()\n{\n ios_base::sync_with_stdio(false);\n cin.tie(0);\n cout.tie(0);\n int t = 1;\n cin >> t;\n while (t--)\n {\n // taking input of number of rows & coloums\n int n, m;\n cin >> n >> m;\n // creating a matrix of size n*m and taking input\n vector<vector<int>> matrix(n, vector<int>(m));\n for (int i = 0; i < n; i++)\n {\n for (int j = 0; j < m; j++)\n {\n cin >> matrix[i][j];\n }\n }\n for (int i = 0; i < n; i++)\n {\n for (int j = 0; j < m; j++)\n {\n if (!matrix[i][j])\n {\n setneg(matrix, i, j);\n }\n }\n }\n for (int i = 0; i < n; i++)\n {\n for (int j = 0; j < m; j++)\n {\n if (matrix[i][j]==-2147483636)\n {\n matrix[i][j]=0;\n }\n }\n }\n cout<<endl;\n for (int i = 0; i < n; i++)\n {\n for (int j = 0; j < m; j++)\n {\n cout<<matrix[i][j]<<\" \";\n }\n cout<<endl;\n }\n cout<<endl;\n }\n return 0;\n}"
}
{
"filename": "code/data_structures/src/2d_array/SpiralMatrix.cpp",
"content": "#include <iostream>\n#include <vector>\nusing namespace std;\n\nvector<vector<int>> generateSpiralMatrix(int n) {\n vector<vector<int>> matrix(n, vector<int>(n));\n\n int left = 0;\n int right = n - 1;\n int top = 0;\n int bottom = n - 1;\n\n int direction = 0;\n int value = 1;\n\n while (left <= right && top <= bottom) {\n if (direction == 0) {\n for (int i = left; i <= right; i++) {\n matrix[top][i] = value++;\n }\n top++;\n } else if (direction == 1) {\n for (int j = top; j <= bottom; j++) {\n matrix[j][right] = value++;\n }\n right--;\n } else if (direction == 2) {\n for (int i = right; i >= left; i--) {\n matrix[bottom][i] = value++;\n }\n bottom--;\n } else {\n for (int j = bottom; j >= top; j--) {\n matrix[j][left] = value++;\n }\n left++;\n }\n direction = (direction + 1) % 4;\n }\n return matrix;\n}\n\nint main() {\n int n;\n cin >> n;\n\n vector<vector<int>> spiralMatrix = generateSpiralMatrix(n);\n\n for (int i = 0; i < n; i++) {\n for (int j = 0; j < n; j++) {\n cout << spiralMatrix[i][j] << \" \";\n }\n cout << endl;\n }\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/3d_array/ThreeDArray.java",
"content": "/**\n * This Java program demonstrates the creation and manipulation of a 3D array.\n * We will create a 3D array, initialize its elements, and print them.\n */\n\n public class ThreeDArray {\n public static void main(String[] args) {\n // Define the dimensions of the 3D array\n int xSize = 3; // Size in the x-direction\n int ySize = 4; // Size in the y-direction\n int zSize = 2; // Size in the z-direction\n\n // Create a 3D array with the specified dimensions\n int[][][] threeDArray = new int[xSize][ySize][zSize];\n\n // Initialize the elements of the 3D array\n int value = 1;\n for (int x = 0; x < xSize; x++) {\n for (int y = 0; y < ySize; y++) {\n for (int z = 0; z < zSize; z++) {\n threeDArray[x][y][z] = value;\n value++;\n }\n }\n }\n\n // Print the 3D array\n for (int x = 0; x < xSize; x++) {\n for (int y = 0; y < ySize; y++) {\n for (int z = 0; z < zSize; z++) {\n System.out.println(\"threeDArray[\" + x + \"][\" + y + \"][\" + z + \"] = \" + threeDArray[x][y][z]);\n }\n }\n }\n }\n}"
}
{
"filename": "code/data_structures/src/bag/bag.cpp",
"content": "//Needed for random and vector\r\n#include <vector>\r\n#include <string>\r\n#include <cstdlib>\r\n\r\nusing std::string;\r\nusing std::vector;\r\n\r\n\r\n//Bag Class Declaration\r\nclass Bag\r\n{\r\nprivate: \r\n\tvector<string> items;\r\n\tint bagSize;\r\n\r\npublic:\r\n\tBag();\r\n\tvoid add(string);\r\n\tbool isEmpty();\r\n\tint sizeOfBag();\r\n\tstring remove();\r\n};\r\n\r\n/******************************************************************************\r\n * \t\t\t\t\t\t\t\tBag::Bag()\r\n * This is the constructor for Bag. It initializes the size variable.\r\n ******************************************************************************/\r\nBag::Bag()\r\n{\r\n\tbagSize = 0;\r\n}\r\n\r\n/******************************************************************************\r\n * \t\t\t\t\t\t\t\tBag::add\r\n * This function adds an item to the bag.\r\n ******************************************************************************/\r\nvoid Bag::add(string item)\r\n{\r\n\t//Store item in last element of vector\r\n\titems.push_back(item);\r\n\t//Increase the size\r\n\tbagSize++;\r\n}\r\n\r\n/******************************************************************************\r\n * \t\t\t\t\t\t\t\tBag::isEmpty\r\n * This function returns true if the bag is empty and returns false if the \r\n * bag is not empty\r\n ******************************************************************************/\r\nbool Bag::isEmpty(){\r\n\t//True\r\n\tif(bagSize == 0){\r\n\t\treturn true;\r\n\t}\r\n\t//False\r\n\telse{\r\n\t\treturn false;\r\n\t}\r\n}\r\n\r\n/******************************************************************************\r\n * \t\t\t\t\t\t\t\tBag::size\r\n * This function returns the current number of items in the bag\r\n ******************************************************************************/\r\nint Bag::sizeOfBag(){\r\n\treturn bagSize;\r\n}\r\n\r\n/******************************************************************************\r\n * \t\t\t\t\t\t\t\tBag::remove\r\n * This function removes a random item from the bag and returns which item was\r\n * removed from the bag\r\n ******************************************************************************/\r\nstring Bag::remove(){\r\n\t//Get random item\r\n\tint randIndx = rand() % bagSize;\r\n\tstring removed = items.at(randIndx);\r\n\t//Remove from bag\r\n\titems.erase(items.begin() + randIndx);\r\n\tbagSize--;\r\n\t//Return removed item\r\n\treturn removed;\r\n}\r\n\r"
}
{
"filename": "code/data_structures/src/bag/bag.java",
"content": "package bag.java;\n// Part of Cosmos by OpenGenus Foundation\nimport java.util.Iterator;\nimport java.util.NoSuchElementException;\n/**\n * Collection which does not allow removing elements (only collect and iterate)\n *\n * @param <Element> - the generic type of an element in this bag\n */\npublic class Bag<Element> implements Iterable<Element> {\n\n\tprivate Node<Element> firstElement; // first element of the bag\n\tprivate int size; // size of bag\n\n\tprivate static class Node<Element> {\n\t\tprivate Element content;\n\t\tprivate Node<Element> nextElement;\n\t}\n\n\t/**\n\t * Create an empty bag\n\t */\n\tpublic Bag() {\n\t\tfirstElement = null;\n\t\tsize = 0;\n\t}\n\n\t/**\n\t * @return true if this bag is empty, false otherwise\n\t */\n\tpublic boolean isEmpty() {\n\t\treturn firstElement == null;\n\t}\n\n\t/**\n\t * @return the number of elements\n\t */\n\tpublic int size() {\n\t\treturn size;\n\t}\n\n\t/**\n\t * @param element - the element to add\n\t */\n\tpublic void add(Element element) {\n\t\tNode<Element> oldfirst = firstElement;\n\t\tfirstElement = new Node<>();\n\t\tfirstElement.content = element;\n\t\tfirstElement.nextElement = oldfirst;\n\t\tsize++;\n\t}\n\n\t/**\n\t * Checks if the bag contains a specific element\n\t *\n\t * @param element which you want to look for\n\t * @return true if bag contains element, otherwise false\n\t */\n\tpublic boolean contains(Element element) {\n\t\tIterator<Element> iterator = this.iterator();\n\t\twhile(iterator.hasNext()) {\n\t\t\tif (iterator.next().equals(element)) {\n\t\t\t\treturn true;\n\t\t\t}\n\t\t}\n\t\treturn false;\n\t}\n\n\t/**\n\t * @return an iterator that iterates over the elements in this bag in arbitrary order\n\t */\n\tpublic Iterator<Element> iterator() {\n\t\treturn new ListIterator<>(firstElement);\n\t}\n\n\t@SuppressWarnings(\"hiding\")\n\tprivate class ListIterator<Element> implements Iterator<Element> {\n\t\tprivate Node<Element> currentElement;\n\n\t\tpublic ListIterator(Node<Element> firstElement) {\n\t\t\tcurrentElement = firstElement;\n\t\t}\n\n\t\tpublic boolean hasNext() {\n\t\t\treturn currentElement != null;\n\t\t}\n\n\t\t/**\n\t\t * remove is not allowed in a bag\n\t\t */\n\t\t@Override\n\t\tpublic void remove() {\n\t\t\tthrow new UnsupportedOperationException();\n\t\t}\n\n\t\tpublic Element next() {\n\t\t\tif (!hasNext())\n\t\t\t\tthrow new NoSuchElementException();\n\t\t\tElement element = currentElement.content;\n\t\t\tcurrentElement = currentElement.nextElement;\n\t\t\treturn element;\n\t\t}\n\t}\n\n\t/**\n\t * main-method for testing\n\t */\n\tpublic static void main(String[] args) {\n\t\tBag<String> bag = new Bag<>();\n\n\t\tbag.add(\"1\");\n\t\tbag.add(\"1\");\n\t\tbag.add(\"2\");\n\n\t\tSystem.out.println(\"size of bag = \" + bag.size());\n\t\tfor (String s : bag) {\n\t\t\tSystem.out.println(s);\n\t\t}\n\n\t\tSystem.out.println(bag.contains(null));\n\t\tSystem.out.println(bag.contains(\"1\"));\n\t\tSystem.out.println(bag.contains(\"3\"));\n\t}\n}"
}
{
"filename": "code/data_structures/src/bag/bag.js",
"content": "function Bag() {\n let bag = [];\n this.size = () => bag.length;\n\n this.add = item => bag.push(item);\n\n this.isEmpty = () => bag.length === 0;\n}\n\nlet bag1 = new Bag();\nconsole.log(bag1.size()); // 0\n\nbag1.add(\"Blue ball\");\nconsole.log(bag1.size()); // 1\n\nbag1.add(\"Red ball\");\nconsole.log(bag1.size()); // 2"
}
{
"filename": "code/data_structures/src/bag/bag.py",
"content": "class Bag:\n \"\"\"\n Create a collection to add items and iterate over it.\n There is no method to remove items.\n \"\"\"\n\n # Initialize a bag as a list\n def __init__(self):\n self.bag = []\n\n # Return the size of the bag\n def __len__(self):\n return len(self.bag)\n\n # Test if a item is in the bag\n def __contains__(self, item):\n return item in self.bag\n\n # Return a item in the bag by its index\n def __getitem__(self, key):\n return self.bag[key]\n\n # Add item to the bag\n def add(self, item):\n self.bag.append(item)\n\n # Return the bag showing all its items\n def items(self):\n return self.bag\n\n # Test if the bag is empty\n def isEmpty(self):\n return len(self.bag) == 0\n\n\n## Tests ##\nbag1 = Bag()\n\nprint(len(bag1))\n\nbag1.add(\"test\")\nbag1.add(\"shamps\")\n\nprint(len(bag1))\n\nprint(\"bag1 is empty?\", bag1.isEmpty())\n\nprint(\"test is in bag1\", \"test\" in bag1)\nprint(\"shampz is in bag1\", \"shampz\" in bag1)\n\nfor item in bag1:\n print(item)\n\nprint(bag1.items())"
}
{
"filename": "code/data_structures/src/binary_heap/binary_heap.cpp",
"content": "/* Binary Heap in C++ */\n\n#include <iostream>\n#include <cstdlib>\n#include <vector>\n#include <iterator>\n\n\nclass BinaryHeap{\n\n private:\n std::vector <int> heap;\n int left(int parent);\n int right(int parent);\n int parent(int child);\n void heapifyup(int index);\n void heapifydown(int index);\n public:\n BinaryHeap()\n {}\n void Insert(int element);\n void DeleteMin();\n int ExtractMin();\n void DisplayHeap();\n int Size();\n};\n\n// Return Heap Size\nint BinaryHeap::Size(){\n return heap.size();\n}\n \n// Insert Element into a Heap \nvoid BinaryHeap::Insert(int element){\n heap.push_back(element);\n heapifyup(heap.size() -1);\n}\n\n// Delete Minimum Element \nvoid BinaryHeap::DeleteMin(){\n \n if (heap.empty()){\n std::cout << \"Heap is Empty\\n\";\n return;\n }\n heap[0] = heap.at(heap.size() - 1);\n heap.pop_back();\n heapifydown(0);\n std::cout << \"Element Deleted\\n\";\n}\n \n// Extract Minimum Element\nint BinaryHeap::ExtractMin(){\n if (heap.empty())\n return -1;\n\n return heap.front();\n}\n \n// Display Heap\nvoid BinaryHeap::DisplayHeap(){\n std::vector <int>::iterator pos = heap.begin();\n\n std::cout << \"Heap --> \";\n while (pos != heap.end()){\n std::cout << *pos << \" \";\n pos++;\n }\n std::cout << \"\\n\";\n}\n \n// Return Left Child\nint BinaryHeap::left(int parent){\n int l = 2 * parent + 1;\n\n if (l < heap.size())\n return l;\n else\n return -1;\n}\n \n// Return Right Child\nint BinaryHeap::right(int parent){\n int r = 2 * parent + 2;\n\n if (r < heap.size())\n return r;\n else\n return -1;\n}\n \n// Return Parent\nint BinaryHeap::parent(int child){\n int p = (child - 1)/2;\n\n if (child == 0)\n return -1;\n else\n return p;\n}\n \n// Heapify- Maintain Heap Structure bottom up\nvoid BinaryHeap::heapifyup(int in){\n if (in >= 0 && parent(in) >= 0 && heap[parent(in)] > heap[in]){\n int temp = heap[in];\n heap[in] = heap[parent(in)];\n heap[parent(in)] = temp;\n heapifyup(parent(in));\n }\n}\n \n// Heapify- Maintain Heap Structure top down\nvoid BinaryHeap::heapifydown(int in){\n \n int child = left(in);\n int child1 = right(in);\n\n if (child >= 0 && child1 >= 0 && heap[child] > heap[child1]){\n child = child1;\n }\n if (child > 0 && heap[in] > heap[child]){\n int temp = heap[in];\n heap[in] = heap[child];\n heap[child] = temp;\n heapifydown(child);\n }\n}\n\nint main(){\n\n int choice, element;\n BinaryHeap h;\n while (true){\n std::cout << \"------------------\\n\";\n std::cout << \"Operations on Heap\\n\";\n std::cout << \"------------------\\n\";\n std::cout << \"1.Insert Element\\n\";\n std::cout << \"2.Delete Minimum Element\\n\";\n std::cout << \"3.Extract Minimum Element\\n\";\n std::cout << \"4.Print Heap\\n\";\n std::cout << \"5.Exit\\n\";\n\n std::cout << \"Enter your choice: \";\n std::cin >> choice;\n\n switch(choice){\n case 1:\n std::cout << \"Enter the element to be inserted: \";\n std::cin >> element;\n h.Insert(element);\n break;\n case 2:\n h.DeleteMin();\n break;\n case 3:\n std::cout << \"Minimum Element: \";\n if (h.ExtractMin() == -1){\n std::cout << \"Heap is Empty \\n\" ;\n }\n else\n std::cout << \"Minimum Element: \"<< h.ExtractMin() << \"\\n\";\n break;\n case 4:\n std::cout << \"Displaying elements of Hwap: \";\n h.DisplayHeap();\n break;\n case 5:\n return 1;\n default:\n std::cout << \"Enter Correct Choice \\n\";\n }\n }\n return 0;\n}"
}
{
"filename": "code/data_structures/src/binary_heap/binary_heap.dart",
"content": "class Node {\n int priority;\n dynamic value;\n\n Node(this.priority, this.value);\n}\n\nclass BinaryHeap {\n late List<Node> heap;\n\n BinaryHeap() {\n heap = [];\n }\n\n void insert(int priority, dynamic value) {\n final newNode = Node(priority, value);\n heap.add(newNode);\n _bubbleUp(heap.length - 1);\n }\n\n Node extractMin() {\n if (heap.isEmpty) {\n throw Exception(\"Heap is empty\");\n }\n\n final min = heap[0];\n final last = heap.removeLast();\n if (heap.isNotEmpty) {\n heap[0] = last;\n _heapify(0);\n }\n\n return min;\n }\n\n void _bubbleUp(int index) {\n while (index > 0) {\n final parentIndex = (index - 1) ~/ 2;\n if (heap[index].priority < heap[parentIndex].priority) {\n // Swap the elements if the current element has higher priority than its parent.\n final temp = heap[index];\n heap[index] = heap[parentIndex];\n heap[parentIndex] = temp;\n index = parentIndex;\n } else {\n break;\n }\n }\n }\n\n void _heapify(int index) {\n final leftChild = 2 * index + 1;\n final rightChild = 2 * index + 2;\n int smallest = index;\n\n if (leftChild < heap.length &&\n heap[leftChild].priority < heap[index].priority) {\n smallest = leftChild;\n }\n if (rightChild < heap.length &&\n heap[rightChild].priority < heap[smallest].priority) {\n smallest = rightChild;\n }\n\n if (smallest != index) {\n final temp = heap[index];\n heap[index] = heap[smallest];\n heap[smallest] = temp;\n _heapify(smallest);\n }\n }\n}\n\nvoid main() {\n final minHeap = BinaryHeap();\n\n minHeap.insert(4, \"A\");\n minHeap.insert(9, \"B\");\n minHeap.insert(2, \"C\");\n minHeap.insert(1, \"D\");\n minHeap.insert(7, \"E\");\n\n print(\"Extracted Min: ${minHeap.extractMin().value}\"); // Should print \"D\"\n print(\"Extracted Min: ${minHeap.extractMin().value}\"); // Should print \"C\"\n print(\"Extracted Min: ${minHeap.extractMin().value}\"); // Should print \"A\"\n}"
}
{
"filename": "code/data_structures/src/binary_heap/binary_heap.py",
"content": "class BinaryHeap:\n def __init__(self, size):\n self.List = (size) * [None]\n self.heapSize = 0\n self.maxSize = size\n\ndef peek(root):\n if not root:\n return\n else:\n return root.List[1]\n\ndef sizeof(root):\n if not root:\n return\n else:\n return root.heapSize\n\ndef heapifyTreeInsert(root, index):\n parentIndex = int(index/2)\n if index <= 1:\n return\n if root.List[index] < root.List[parentIndex]:\n temp = root.List[index]\n root.List[index] = root.List[parentIndex]\n root.List[parentIndex] = temp\n heapifyTreeInsert(root, parentIndex)\n\ndef insert(root, nodeValue):\n if root.heapSize + 1 == root.maxSize:\n return \"The Binary Heap is full.\"\n root.List[root.heapSize + 1] = nodeValue\n root.heapSize += 1\n heapifyTreeInsert(root, root.heapSize)\n return \"The node has been successfully inserted.\"\n\ndef heapifyTreeExtract(root, index):\n leftIndex = index * 2\n rightIndex = index * 2 + 1\n swapChild = 0\n\n if root.heapSize < leftIndex:\n return\n elif root.heapSize == leftIndex:\n if root.List[index] > root.List[leftIndex]:\n temp = root.List[index]\n root.List[index] = root.List[leftIndex]\n root.List[leftIndex] = temp\n return\n \n else:\n if root.List[leftIndex] < root.List[rightIndex]:\n swapChild = leftIndex\n else:\n swapChild = rightIndex\n if root.List[index] > root.List[swapChild]:\n temp = root.List[index]\n root.List[index] = root.List[swapChild]\n root.List[swapChild] = temp\n heapifyTreeExtract(root, swapChild)\n\ndef extract(root):\n if root.heapSize == 0:\n return \"The Binary Heap is empty.\"\n else:\n extractedNode = root.List[1]\n root.List[1] = root.List[root.heapSize]\n root.List[root.heapSize] = None\n root.heapSize -= 1\n heapifyTreeExtract(root, 1)\n return extractedNode\n\ndef DisplayHeap(root):\n print(root.List)\n\nB = BinaryHeap(5)\ninsert(B, 70)\nDisplayHeap(B)\ninsert(B, 40)\nDisplayHeap(B)\nextract(B)\nDisplayHeap(B)\ninsert(B, 30)\nDisplayHeap(B)\ninsert(B, 60)\nDisplayHeap(B)\nextract(B)\nDisplayHeap(B)\ninsert(B, 5)\nDisplayHeap(B)"
}
{
"filename": "code/data_structures/src/CircularLinkedList/circularLinkedList.cpp",
"content": "#include <iostream>\nusing namespace std;\n\n/**\n * A node will be consist of a int data &\n * a reference to the next node.\n * The node object will be used in making linked list\n */\nclass Node\n{\npublic:\n int data;\n Node *next;\n};\n\n/**\n * Using nodes for creating a circular linked list.\n *\n */\nclass CircularList\n{\npublic:\n /**\n * stores the reference to the last node\n */\n Node *last;\n\n /**\n * keeping predecessor reference while searching\n */\n\n Node *preLoc;\n\n /**\n * current node reference while searching\n */\n Node *loc;\n\n CircularList()\n {\n last = NULL;\n loc = NULL;\n preLoc = NULL;\n }\n\n /**\n * Checking if list empty\n */\n bool isEmpty()\n {\n return last == NULL;\n }\n\n /**\n * Inserting new node in front\n */\n void insertAtFront(int value)\n {\n Node *newnode = new Node();\n newnode->data = value;\n\n if (isEmpty())\n {\n newnode->next = newnode;\n last = newnode;\n }\n else\n {\n newnode->next = last->next;\n last->next = newnode;\n }\n }\n\n /**\n * Inserting new node in last\n */\n void insertAtLast(int value)\n {\n Node *newnode = new Node();\n newnode->data = value;\n\n if (isEmpty())\n {\n newnode->next = newnode;\n last = newnode;\n }\n else\n {\n newnode->next = last->next;\n last->next = newnode;\n last = newnode;\n }\n }\n\n /**\n * Printing whole list iteratively\n */\n void printList()\n {\n if (!isEmpty())\n {\n Node *temp = last->next;\n do\n {\n cout << temp->data;\n if (temp != last)\n cout << \" -> \";\n temp = temp->next;\n } while (temp != last->next);\n cout << endl;\n }\n else\n cout << \"List is empty\" << endl;\n }\n\n /**\n * Searching a value\n */\n void search(int value)\n {\n loc = NULL;\n preLoc = NULL;\n if (isEmpty())\n return;\n loc = last->next;\n preLoc = last;\n while (loc != last && loc->data < value)\n {\n preLoc = loc;\n loc = loc->next;\n }\n if (loc->data != value)\n {\n loc = NULL;\n // if(value > loc->data)\n // preLoc = last;\n }\n }\n\n /**\n * Inserting the value in its sorted postion in the list.\n */\n void insertSorted(int value)\n {\n Node *newnode = new Node();\n newnode->data = value;\n\n search(value);\n\n if (loc != NULL)\n {\n cout << \"Value already exist!\" << endl;\n return;\n }\n else\n {\n if (isEmpty())\n insertAtFront(value);\n else if (value > last->data)\n insertAtLast(value);\n else if (value < last->next->data)\n insertAtFront(value);\n else\n {\n newnode->next = preLoc->next;\n preLoc->next = newnode;\n }\n }\n }\n\n void deleteValue(int value)\n {\n search(value);\n if (loc != NULL)\n {\n if (loc->next == loc)\n {\n last = NULL;\n }\n else if (value == last->data)\n {\n preLoc->next = last->next;\n last = preLoc;\n }\n else\n {\n preLoc->next = loc->next;\n }\n delete loc;\n }\n else\n cout << \"Value not found\" << endl;\n }\n\n void destroyList()\n {\n Node *temp = last->next;\n while (last->next != last)\n {\n temp = last->next;\n last->next = last->next->next;\n delete temp;\n }\n delete last; // delete the only remaining node\n last = NULL;\n }\n};\n\n/**\n * Driver Code\n */\nint main()\n{\n\n CircularList *list1 = new CircularList();\n\n cout << \"Initializing the list\" << endl;\n list1->insertAtLast(2);\n list1->insertAtLast(3);\n list1->insertAtLast(4);\n list1->insertAtLast(6);\n list1->insertAtLast(8);\n\n list1->printList();\n\n cout << \"Inserting 5 in the list sorted\" << endl;\n list1->insertSorted(5);\n list1->printList();\n\n cout << \"Deleting 3 from the list\" << endl;\n list1->deleteValue(3);\n list1->printList();\n\n cout << \"Destroying the whole list\" << endl;\n list1->destroyList();\n list1->printList();\n}"
}
{
"filename": "code/data_structures/src/CircularLinkedList/src_java/circularlinkedlist.java",
"content": "class Node {\n int data;\n Node next;\n\n Node(int data) {\n this.data = data;\n this.next = null;\n }\n}\n\nclass CircularLinkedList {\n private Node head;\n\n // Constructor to create an empty circular linked list\n CircularLinkedList() {\n head = null;\n }\n\n // Function to insert a node at the end of the circular linked list\n void append(int data) {\n Node newNode = new Node(data);\n if (head == null) {\n head = newNode;\n head.next = head; // Point back to itself for a single node circular list\n } else {\n Node temp = head;\n while (temp.next != head) {\n temp = temp.next;\n }\n temp.next = newNode;\n newNode.next = head;\n }\n }\n\n // Function to delete a node by value from the circular linked list\n void delete(int data) {\n if (head == null)\n return;\n\n // If the head node contains the value to be deleted\n if (head.data == data) {\n Node temp = head;\n while (temp.next != head)\n temp = temp.next;\n if (head == head.next) {\n head = null; // If there is only one node\n } else {\n head = head.next;\n temp.next = head;\n }\n return;\n }\n\n // Search for the node to delete\n Node current = head;\n Node prev = null;\n while (current.next != head && current.data != data) {\n prev = current;\n current = current.next;\n }\n\n // If the node with the given data was found\n if (current.data == data) {\n prev.next = current.next;\n } else {\n System.out.println(\"Node with data \" + data + \" not found in the list.\");\n }\n }\n\n // Function to display the circular linked list\n void display() {\n if (head == null)\n return;\n\n Node temp = head;\n do {\n System.out.print(temp.data + \" \");\n temp = temp.next;\n } while (temp != head);\n System.out.println();\n }\n\n public static void main(String[] args) {\n CircularLinkedList cll = new CircularLinkedList();\n cll.append(1);\n cll.append(2);\n cll.append(3);\n\n System.out.print(\"Circular Linked List: \");\n cll.display(); // Output: 1 2 3\n\n cll.delete(2);\n System.out.print(\"After deleting 2: \");\n cll.display(); // Output: 1 3\n }\n}"
}
{
"filename": "",
"content": ""
}
{
"filename": "code/data_structures/src/disjoint_set/DisjointSet_DS.py",
"content": "f = []\nf = [0 for x in range(100)]\n\ndef find(f, node):\n\tif(f[node] == node):\n\t\treturn node\n\telse:\n\t\tf[node] = find(f, f[node])\n\t\treturn f[node]\n\ndef union_set(f, x, y):\n\tf[find(f, x)] = find(f, y)\n\treturn f\n\narr = []\narr = [0 for x in range(10)]\nfor i in range(1,11):\n\tarr[i-1] = i\n\tf[i] = i\nunion_set(f,1,3)\nunion_set(f,4,5)\nunion_set(f,1,2)\nunion_set(f,1,5)\nunion_set(f,2,8)\nunion_set(f,9,10)\nunion_set(f,1,9)\nunion_set(f,2,7)\nunion_set(f,3,6)\n\nfor i in arr:\n\tprint(f\"{i}->{f[i]}\\n\")"
}
{
"filename": "code/data_structures/src/disjoint_set/README.md",
"content": "<h1>DISJOINT SET</h1>\n\n<h2>Description</h2>\n<h3>Disjoint set is a data structure that stores a collection of disjoint (non-overlapping) sets. Equivalently, it stores a partition of a set into disjoint subsets. It provides operations for adding new sets, merging sets (replacing them by their union), and finding a representative member of a set.</h3>\n\n<h2>Functions</h2>\n<h3>Union() : Join two subsets into a single subset.</h3>\n<h3>Find() : Determine which subset a particular element is in. This can be used for determining if two elements are in the same subset.</h3>\n\n<h2>Time Complexity</h2>\n<h3>Union() : O(n) </h3>\n<h3>Find() : O(n) [Without path compression]</h3>\n<h3>Find() : O(log n) [With path compression]</h3>"
}
{
"filename": "",
"content": ""
}
{
"filename": "code/data_structures/src/DoubleLinkedList/lru_cache_with_dll/src_go/main.go",
"content": "/*\n- LRU cache implementation in Go\n- Change the 'capacity' variable to adjust the size of cache\n- Each node in the DLL can contain a key and a value\n- There are 2 main functions:\n 1. update(key, value): If the key-value pair is absent, then it will add it to the cache. If the key is already present, then it will update its value\n 2. get(key): It will print the key's value. If the key doesn't exist then it will print -1\n- traverse() is an additional function that can be used to visualize the DLL\n- The attach() function is used to attach a node just after 'head'\n- The detach() function is used to detach a node\n\n*/\npackage main\n\nimport \"fmt\"\n\nconst capacity int = 2 // Capacity of the cache. Change it accordingly\n\ntype node struct{\n data_key int\n data_val int\n next *node\n prev *node\n}\n\nvar head *node\nvar tail *node\nvar tracker map[int]*node\n\n\n\n///////////////////////////////////////\n// Helper functions //\n///////////////////////////////////////\nfunc attach(block *node){\n // Update the DLL\n block.next = head.next\n (head.next).prev = block\n head.next = block\n block.prev = head\n \n}\nfunc detach(block *node){\n //Detach the block\n (block.prev).next = block.next\n (block.next).prev = block.prev\n}\n\n\nfunc update(key int, value int){\n // If key is already present, then update the value\n v, found := tracker[key]\n if found{\n v.data_val = value\n detach(v)\n attach(v)\n return\n }\n\n // If key is absent\n nodeToAdd := &node{ data_key: key, data_val:value } // The node/block to be inserted\n if len(tracker) == capacity{\n\n nodeToRemove := tail.prev\n detach(nodeToRemove)\n delete(tracker, nodeToRemove.data_key) // Update the map accordingly\n attach(nodeToAdd)\n tracker[key] = nodeToAdd // Add the item to the map\n\n\n } else {\n attach(nodeToAdd)\n tracker[key] = nodeToAdd // Add the item to the map\n }\n\n}\n\nfunc get(key int){\n // Return the value\n reqdNode, found := tracker[key]\n if found{\n fmt.Printf(\"For key = %d, value = %d\\n\",key,reqdNode.data_val)\n } else {\n fmt.Printf(\"For key = %d, value = %d\\n\", key, -1)\n return\n }\n // Update the DLL\n detach(reqdNode)\n attach(reqdNode)\n\n}\n\n// Visualize the DLL\nfunc traverse(){\n fmt.Println(\"\\nHere's your DLL...\")\n temp:=head.next\n for temp != tail{\n fmt.Printf(\"(%d,%d) \", temp.data_key, temp.data_val)\n temp=temp.next\n }\n fmt.Println()\n}\n\nfunc main(){\n\n head = &node{ data_key: 0, data_val: 0 }\n tail = &node{ data_key: 0, data_val: 0, prev: head }\n head.next = tail\n tracker = make(map[int]*node)\n\n // Sample Operations\n update(1, 1)\n update(2, 2)\n get(1) // prints 1\n update(3,3)\n get(2) // prints -1\n update(4,4) \n get(1) // prints -1\n get(3) // prints 3\n get(4) // prints 4\n\n traverse()\n}"
}
{
"filename": "code/data_structures/src/DoubleLinkedList/src_c++/Doubly_LL.cpp",
"content": "#include <iostream>\r\nusing namespace std;\r\n\r\n\r\nstruct Node {\r\n int data;\r\n Node* prev;\r\n Node* next;\r\n \r\n Node(int value) : data(value), prev(nullptr), next(nullptr) {}\r\n};\r\n\r\nclass DoublyLinkedList {\r\nprivate:\r\n Node* head;\r\n Node* tail;\r\n\r\npublic:\r\n DoublyLinkedList() : head(nullptr), tail(nullptr) {}\r\n\r\n\r\n void insertAtTail(int value) {\r\n Node* newNode = new Node(value);\r\n if (tail == nullptr) {\r\n head = tail = newNode;\r\n } else {\r\n tail->next = newNode;\r\n newNode->prev = tail;\r\n tail = newNode;\r\n }\r\n }\r\n\r\n void insertAtHead(int value) {\r\n Node* newNode = new Node(value);\r\n if (head == nullptr) {\r\n head = tail = newNode;\r\n } else {\r\n head->prev = newNode;\r\n newNode->next = head;\r\n head = newNode;\r\n }\r\n }\r\n\r\n void printForward() {\r\n Node* current = head;\r\n while (current != nullptr) {\r\n std::cout << current->data << \" \";\r\n current = current->next;\r\n }\r\n std::cout << std::endl;\r\n }\r\n\r\n void printBackward() {\r\n Node* current = tail;\r\n while (current != nullptr) {\r\n std::cout << current->data << \" \";\r\n current = current->prev;\r\n }\r\n std::cout << std::endl;\r\n }\r\n};\r\n\r\nint main() {\r\n DoublyLinkedList dll;\r\n int n;\r\n\r\n cout << \"Enter the number of nodes in linked list: \";\r\n cin >> n;\r\n\r\n int x;\r\n cout << \"Enter element no. 0: \";\r\n cin >> x;\r\n dll.insertAtHead(x);\r\n\r\n for(int i=1; i<n; i++)\r\n {\r\n int x;\r\n cout << \"Enter element no. \" << i << \": \";\r\n cin >> x;\r\n dll.insertAtTail(x);\r\n }\r\n\r\n std::cout << \"Forward: \";\r\n dll.printForward(); // Output: 0 1 2 3\r\n\r\n std::cout << \"Backward: \";\r\n dll.printBackward(); // Output: 3 2 1 0\r\n\r\n return 0;\r\n}\r"
}
{
"filename": "code/data_structures/src/DoubleLinkedList/src_cpp/doublylinkedlist.cpp",
"content": "#include <iostream>\n\nclass Node {\npublic:\n int data;\n Node* next;\n Node* prev;\n \n Node(int val) {\n data = val;\n next = prev = nullptr;\n }\n};\n\nclass DoublyLinkedList {\nprivate:\n Node* head;\n Node* tail;\n\npublic:\n DoublyLinkedList() {\n head = tail = nullptr;\n }\n\n // Insert at the end of the list\n void append(int val) {\n Node* newNode = new Node(val);\n if (!head) {\n head = tail = newNode;\n } else {\n tail->next = newNode;\n newNode->prev = tail;\n tail = newNode;\n }\n }\n\n // Insert at the beginning of the list\n void prepend(int val) {\n Node* newNode = new Node(val);\n if (!head) {\n head = tail = newNode;\n } else {\n head->prev = newNode;\n newNode->next = head;\n head = newNode;\n }\n }\n\n // Delete a node by its value\n void remove(int val) {\n Node* current = head;\n while (current) {\n if (current->data == val) {\n if (current == head) {\n head = head->next;\n if (head) head->prev = nullptr;\n } else if (current == tail) {\n tail = tail->prev;\n if (tail) tail->next = nullptr;\n } else {\n current->prev->next = current->next;\n current->next->prev = current->prev;\n }\n delete current;\n return;\n }\n current = current->next;\n }\n }\n\n // Display the list from head to tail\n void display() {\n Node* current = head;\n while (current) {\n std::cout << current->data << \" \";\n current = current->next;\n }\n std::cout << std::endl;\n }\n};\n\nint main() {\n DoublyLinkedList dll;\n dll.append(1);\n dll.append(2);\n dll.append(3);\n dll.prepend(0);\n dll.display(); // Output: 0 1 2 3\n dll.remove(1);\n dll.display(); // Output: 0 2 3\n return 0;\n}"
}
{
"filename": "code/data_structures/src/DoubleLinkedList/src_java/DoubleLinkedLists.java",
"content": "public class DoubleLinkedLists {\n Node head;\n int size;\n public DoubleLinkedLists(){\n head = null;\n size = 0;\n }\n public boolean isEmpty(){\n return head == null;\n }\n public void addFirst(int item){\n if(isEmpty()){\n head = new Node(null, item, null);\n }\n else{\n Node newNode = new Node(null, item, head);\n head.prev = newNode;\n head = newNode;\n }\n size++;\n }\n public void addLast(int item){\n if(isEmpty()){\n addFirst(item);\n }\n else{\n Node current = head;\n while (current.next != null){\n current = current.next;\n }\n Node newNode = new Node(current, item, null);\n current.next = newNode;\n size++;\n }\n }\n public void add(int item, int index) throws Exception {\n if(isEmpty()){\n addFirst(item);\n } else if (index < 0 || index > size){\n throw new Exception(\"Nilai indeks di luar batas\");\n } else{\n Node current = head;\n int i = 0;\n while(i<index){\n current = current.next;\n i++;\n }\n if(current.prev == null){\n Node newNode = new Node(null, item, current);\n current.prev = newNode;\n head = newNode;\n }\n else{\n Node newNode = new Node(current.prev, item, current);\n newNode.prev = current.prev;\n newNode.next = current;\n current.prev.next = newNode;\n current.prev = newNode;\n }\n }\n size++;\n }\n public int size(){\n return size;\n }\n public void clear(){\n head = null;\n size = 0;\n }\n public void print(){\n if(!isEmpty()){\n Node tmp = head;\n while(tmp != null){\n System.out.print(tmp.data+\"\\t\");\n tmp = tmp.next;\n }\n System.out.println(\"\\nberhasil diisi\");\n } else{\n System.out.println(\"Linked Lists Kosong\");\n }\n }\n}"
}
{
"filename": "code/data_structures/src/DoubleLinkedList/src_java/DoubleLinkedListsMain.java",
"content": "public class DoubleLinkedListsMain {\n public static void main(String[] args) throws Exception {\n DoubleLinkedLists dll = new DoubleLinkedLists();\n dll.print();\n System.out.println(\"Size : \"+dll.size());\n System.out.println(\"=====================================\");\n dll.addFirst(3);\n dll.addLast(4);\n dll.addFirst(7);\n dll.print();\n System.out.println(\"Size : \"+dll.size());\n System.out.println(\"=====================================\");\n dll.add(40, 1);\n dll.print();\n System.out.println(\"Size : \"+dll.size());\n System.out.println(\"=====================================\");\n dll.clear();\n dll.print();\n System.out.println(\"Size : \"+dll.size());\n }\n}"
}
{
"filename": "code/data_structures/src/DoubleLinkedList/src_java/Node.java",
"content": "public class Node{\n int data;\n Node prev, next;\n Node(Node prev, int data, Node next){\n this.prev= prev;\n this.data= data;\n this.next= next;\n }\n}"
}
{
"filename": "code/data_structures/src/graph/graph.py",
"content": "class Graph:\n def __init__(self):\n #dictionary where keys are vertices and values are lists of adjacent vertices\n self.vertDict = {}\n def insert_vertex(self, vertex):\n if vertex not in self.vertDict:\n self.vertDict[vertex] = []\n\n def add_edge(self, vertex1, vertex2, directed=False):\n if vertex1 not in self.vertDict:\n self.insert_vertex(vertex1)\n if vertex2 not in self.vertDict:\n self.insert_vertex[vertex2]\n\n self.vertDict[vertex1].append(vertex2)\n #if the graph is undirected, add to both's adjacency lists\n if not directed:\n self.vertDict[vertex2].append(vertex1)\n\n def delete_edge(self, vertex1, vertex2, directed=False):\n if vertex1 in self.vertDict and vertex2 in self.vertDict[vertex1]:\n self.vertDict[vertex1].remove(vertex2)\n if not directed and vertex2 in self.vertDict and vertex1 in self.vertDict[vertex2]:\n self.vertDict[vertex2].remove(vertex1)\n\n def delete_vertex(self, vertex):\n if vertex in self.vertDict:\n for i in self.vertDict:\n if vertex in self.vertDict[i]:\n self.vertDict[i].remove(vertex)\n del self.vertDict[vertex]\n \n def get_neighbors(self, vertex):\n return self.vertDict.get(vertex, [])\n \n def printGraph(self):\n for i, j in self.vertDict.items():\n print(\"{vertex}: {neighbors}\")\n \n\ntester = Graph()\n\ntester.insert_vertex(\"A\")\ntester.insert_vertex(\"B\")\ntester.insert_vertex(\"C\")\n\ntester.add_edge(\"A\", \"B\")\ntester.add_edge(\"A\", \"C\")\ntester.add_edge(\"B\", \"C\")\n\ntester.printGraph()\n\nprint(\"Neighbors of A:\", tester.get_neighbors(\"A\"))\n\ntester.delete_edge(\"A\", \"B\")\n\ntester.printGraph()\n\ntester.delete_vertex(\"C\")\n\nprint(\"next\")\n\ntester.printGraph()"
}
{
"filename": "code/data_structures/src/hashs/bloom_filter/bloom_filter.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n\n\ntypedef struct st_BloomFilter\n{\n\tint size;\n\tchar *bits;\n} BloomFilter;\n\n// Declare interface\nBloomFilter* bl_init(int size);\nvoid bl_add(BloomFilter *bl, int value);\nint bl_contains(BloomFilter *bl, int value);\nvoid bl_destroy(BloomFilter *bl);\n\n// Implementation\nBloomFilter* bl_init(int size)\n{\n\tBloomFilter *bl = (BloomFilter*)malloc(sizeof(BloomFilter));\n\tbl->size = size;\n\tbl->bits = (char*)malloc(size/8 + 1);\n\treturn bl;\n}\n\nvoid bl_add(BloomFilter *bl, int value)\n{\n\tint hash = value % bl->size;\n\tbl->bits[hash / 8] |= 1 << (hash % 8);\n}\n\nint bl_contains(BloomFilter *bl, int value)\n{\n\tint hash = value % bl->size;\n\treturn (bl->bits[hash / 8] & (1 << (hash % 8))) != 0;\n}\n\nvoid bl_destroy(BloomFilter *bl)\n{\n\tfree(bl->bits);\n\tfree(bl);\n}\n\nint main()\n{\n\tBloomFilter *bl = bl_init(1000);\n\tbl_add(bl, 1);\n\tbl_add(bl, 2);\n\tbl_add(bl, 1001);\n\tbl_add(bl, 1004);\n\n\tif(bl_contains(bl, 1))\n\t\tprintf(\"bloomfilter contains 1\\n\");\n\n\tif(bl_contains(bl, 2))\n\t\tprintf(\"bloomfilter contains 2\\n\");\n\n\tif(!bl_contains(bl, 3))\n\t\tprintf(\"bloomfilter not contains 3\\n\");\n\n\tif(bl_contains(bl, 4))\n\t\tprintf(\"bloomfilter not contains 4, but return false positive\\n\");\n}"
}
{
"filename": "code/data_structures/src/hashs/bloom_filter/bloom_filter.cpp",
"content": "#include <iostream>\nusing namespace std;\n\nclass BloomFilter\n{\npublic:\n BloomFilter(int size)\n {\n size_ = size;\n bits_ = new char[size_ / 8 + 1];\n }\n ~BloomFilter()\n {\n delete [] bits_;\n }\n\n void Add(int value)\n {\n int hash = value % size_;\n bits_[hash / 8] |= 1 << (hash % 8);\n }\n\n bool Contains(int value)\n {\n int hash = value % size_;\n return (bits_[hash / 8] & (1 << (hash % 8))) != 0;\n }\n\nprivate:\n char* bits_;\n int size_;\n};\n\nint main()\n{\n BloomFilter bloomFilter(1000);\n bloomFilter.Add(1);\n bloomFilter.Add(2);\n bloomFilter.Add(1001);\n bloomFilter.Add(1004);\n\n if (bloomFilter.Contains(1))\n cout << \"bloomFilter contains 1\" << endl;\n\n if (bloomFilter.Contains(2))\n cout << \"bloomFilter contains 2\" << endl;\n\n if (!bloomFilter.Contains(3))\n cout << \"bloomFilter not contains 3\" << endl;\n\n if (bloomFilter.Contains(4))\n cout << \"bloomFilter not contains 4, but return false positive\" << endl;\n}"
}
{
"filename": "code/data_structures/src/hashs/bloom_filter/bloom_filter.go",
"content": "package main\n\nimport (\n\t\"fmt\"\n\t\"hash\"\n\t\"hash/fnv\"\n\n\t\"github.com/spaolacci/murmur3\"\n)\n\n// Minimal interface that the Bloom filter must implement\ntype Interface interface {\n\tAdd(item []byte) // Adds the item into the Set\n\tCheck(item []byte) bool // Performs probabilist test if the item exists in the set or not.\n}\n\n// BloomFilter probabilistic data structure definition\ntype BloomFilter struct {\n\tbitset []bool // The bloom-filter bitset\n\tk uint // Number of hash values\n\tn uint // Number of elements in the filter\n\tm uint // Size of the bloom filter\n\thashfns []hash.Hash64 // The hash functions\n}\n\n// Returns a new BloomFilter object,\nfunc New(size, numHashValues uint) *BloomFilter {\n\treturn &BloomFilter{\n\t\tbitset: make([]bool, size),\n\t\tk: numHashValues,\n\t\tm: size,\n\t\tn: uint(0),\n\t\thashfns: []hash.Hash64{murmur3.New64(), fnv.New64(), fnv.New64a()},\n\t}\n}\n\n// Adds the item into the bloom filter set by hashing in over the hash functions\nfunc (bf *BloomFilter) Add(item []byte) {\n\thashes := bf.hashValues(item)\n\ti := uint(0)\n\n\tfor {\n\t\tif i >= bf.k {\n\t\t\tbreak\n\t\t}\n\n\t\tposition := uint(hashes[i]) % bf.m\n\t\tbf.bitset[uint(position)] = true\n\n\t\ti += 1\n\t}\n\n\tbf.n += 1\n}\n\n// Test if the item into the bloom filter is set by hashing in over the hash functions\nfunc (bf *BloomFilter) Check(item []byte) (exists bool) {\n\thashes := bf.hashValues(item)\n\ti := uint(0)\n\texists = true\n\n\tfor {\n\t\tif i >= bf.k {\n\t\t\tbreak\n\t\t}\n\n\t\tposition := uint(hashes[i]) % bf.m\n\t\tif !bf.bitset[uint(position)] {\n\t\t\texists = false\n\t\t\tbreak\n\t\t}\n\n\t\ti += 1\n\t}\n\n\treturn\n}\n\n// Calculates all the hash values by hashing in over the hash functions\nfunc (bf *BloomFilter) hashValues(item []byte) []uint64 {\n\tvar result []uint64\n\n\tfor _, hashFunc := range bf.hashfns {\n\t\thashFunc.Write(item)\n\t\tresult = append(result, hashFunc.Sum64())\n\t\thashFunc.Reset()\n\t}\n\n\treturn result\n}\n\nfunc main() {\n\tbf := New(1024, 3)\n\n\tbf.Add([]byte(\"hello\"))\n\tbf.Add([]byte(\"world\"))\n\tbf.Add([]byte(\"1234\"))\n\tbf.Add([]byte(\"hello-world\"))\n\n\tfmt.Printf(\"hello in bloom filter = %t \\n\", bf.Check([]byte(\"hello\")))\n\tfmt.Printf(\"world in bloom filter = %t \\n\", bf.Check([]byte(\"world\")))\n\tfmt.Printf(\"helloworld in bloom filter = %t \\n\", bf.Check([]byte(\"helloworld\")))\n\tfmt.Printf(\"1 in bloom filter = %t \\n\", bf.Check([]byte(\"1\")))\n\tfmt.Printf(\"2 in bloom filter = %t \\n\", bf.Check([]byte(\"2\")))\n\tfmt.Printf(\"3 in bloom filter = %t \\n\", bf.Check([]byte(\"3\")))\n\n}"
}
{
"filename": "code/data_structures/src/hashs/bloom_filter/bloom_filter.java",
"content": "/**\n * Project: Cosmos\n * Package: main\n * File: BloomFilter.java\n * \n * @author sidmishraw\n * Last modified: Oct 18, 2017 6:25:58 PM\n */\npackage main;\n\nimport java.util.ArrayList;\nimport java.util.List;\n\n/**\n * <p>\n * {@link BloomFilter} This is a unoptimized version of a Bloom filter. A Bloom\n * filter is a data structure designed to tell you, rapidly and\n * memory-efficiently, whether an element is present in a set. The price paid\n * for this efficiency is that a Bloom filter is a <i>probabilistic data\n * structure</i>: it tells us that the element either definitely is not in the\n * set or may be in the set.\n * \n * For more information see:\n * <a href=\"https://llimllib.github.io/bloomfilter-tutorial/\"> Bloom filter\n * tutorial</a>\n * \n * This implementation is going to have 2 hash functions:\n * FNV and FNV-1a hashes.\n * \n * @author sidmishraw\n *\n * Qualified Name:\n * main.BloomFilter\n *\n */\npublic class BloomFilter {\n\n /**\n * Default bit vector size for the {@link BloomFilter}\n */\n private static final int BIT_VECTOR_SIZE = 16;\n\n /**\n * <p>\n * This is the base data structure of a bloom filter.\n * \n * <p>\n * To add an element to the Bloom filter, we simply hash it a few times and\n * set the bits in the bit vector at the index of those hashes to true.\n * \n * <p>\n * To test for membership, you simply hash the object with the same hash\n * functions, then see if those values are set in the bit vector. If they\n * aren't, you know that the element isn't in the set. If they are, you only\n * know that it might be, because another element or some combination of\n * other elements could have set the same bits.\n */\n private boolean[] bitvector;\n\n /**\n * The list of hash functions used by the bloom filter\n */\n private List<HashFunction> hashFunctions;\n\n /**\n * The number of elements added to the bloom filter, this influences the\n * probability of membership of an element, when checking membership.\n */\n private int nbrElements;\n\n /**\n * \n */\n public BloomFilter() {\n\n this.bitvector = new boolean[BIT_VECTOR_SIZE];\n this.nbrElements = 0;\n this.hashFunctions = new ArrayList<>();\n\n this.hashFunctions.add(BloomFilter::fnv); // passing in the method\n // reference\n // this.hashFunctions.add(this::fvn1a); // passing in the method\n // reference,\n // // for the instance\n }\n\n /**\n * Creates and initializes a new Bloom filter with `m` slots in the bit\n * vector table\n * \n * @param m\n * The size of the bit vector of the bloom filter\n * @param hashFunctions\n * The hash functions to be used by the bloom filter for\n */\n public BloomFilter(int m, HashFunction... hashFunctions) {\n\n this.bitvector = new boolean[m];\n\n this.nbrElements = 0;\n\n this.hashFunctions = new ArrayList<>();\n\n for (HashFunction func : hashFunctions) {\n\n this.hashFunctions.add(func);\n }\n\n if (this.hashFunctions.size() == 0) {\n\n this.hashFunctions.add(BloomFilter::fnv);\n }\n }\n\n /**\n * <p>\n * Adds the element into the bloom filter. To get added, it must pass\n * through the k hash functions of the bloom filter.\n * \n * T:: O(k); where k is the number of hash functions\n * \n * @param element\n * The element\n */\n public void add(Stringable element) {\n\n byte[] toBeHashed = element.stringValue().getBytes();\n\n for (HashFunction hFunc : this.hashFunctions) {\n\n long hash = hFunc.hash(toBeHashed);\n\n this.bitvector[(int) (hash % this.bitvector.length)] = true;\n }\n\n this.nbrElements++;\n }\n\n /**\n * <p>\n * Checks if the element is a member of the bloom filter.\n * T:: O(k); where k is the number of hash functions\n * \n * @param element\n * The element that needs to be checked for membership\n * @return The result containing the probability of membership and if it is\n * a member. The result is a valid JSON string of form\n * <code>\n * {\n * \"probability\": \"1\",\n * \"isMember\": \"false\"\n * }\n * </code>\n */\n public String check(Stringable element) {\n\n byte[] toBeHashed = element.stringValue().getBytes();\n\n boolean isMember = true;\n\n for (HashFunction hFunc : this.hashFunctions) {\n\n long hash = hFunc.hash(toBeHashed);\n\n isMember = isMember && this.bitvector[(int) (hash % this.bitvector.length)];\n }\n\n if (isMember) {\n\n return calcProbability();\n } else {\n\n return \"{\\\"probability\\\": 1, \\\"isMember\\\": false}\";\n }\n }\n\n /**\n * Computes the probability of membership using the formula : (1-e^-kn/m)^k\n * where ^ is exponentation and\n * m = number of bits in the bit vector table\n * n = number of elements in the bloom filter\n * k = number of hash functions\n * \n * @return The membership probability, json\n */\n private final String calcProbability() {\n\n Double prob = Math.pow(\n (1 - Math.exp(((-1) * this.hashFunctions.size() * this.nbrElements) / (double) this.bitvector.length)),\n this.hashFunctions.size());\n\n return String.format(\"{\\\"probability\\\": %s, \\\"isMember\\\": true}\", prob);\n }\n\n /**\n * <p>\n * Fetches m of the bloom filter\n * \n * @return The number of bits in the bit vector backing the bloom filter\n */\n public int getM() {\n\n return this.bitvector.length;\n }\n\n /**\n * <p>\n * Fetches k of the bloom filter\n * \n * @return The number of hash functions of the bloom filter\n */\n public int getK() {\n\n return this.hashFunctions.size();\n }\n\n /**\n * <p>\n * Fetches n of the bloom filter\n * \n * @return The number of elements added to the bloom filter\n */\n public int getN() {\n\n return this.nbrElements;\n }\n\n /**\n * Just for testing\n */\n @Override\n public String toString() {\n\n StringBuffer buf = new StringBuffer();\n\n if (this.bitvector.length > 0) {\n\n buf.append(\"[\");\n buf.append(String.format(\"(%d,%s)\", 0, this.bitvector[0]));\n }\n\n for (int i = 1; i < this.bitvector.length; i++) {\n\n buf.append(String.format(\",(%d,%s)\", i, this.bitvector[i]));\n }\n\n buf.append(\"]\");\n\n return buf.toString();\n }\n\n /**\n * <p>\n * {@link Stringable} are things that can give be made into a String.\n * \n * @author sidmishraw\n *\n * Qualified Name:\n * main.Stringable\n *\n */\n public static interface Stringable {\n\n public String stringValue();\n }\n\n /**\n * <p>\n * The hashfunctions that can be used by the bloom filter\n * \n * @author sidmishraw\n *\n * Qualified Name:\n * main.HashFunction\n *\n */\n @FunctionalInterface\n public static interface HashFunction {\n\n /**\n * Hashes the element(byte buffer) to an int\n * \n * @param buffer\n * The byte buffer of the element to be hashed\n * @return The hashed number\n */\n public long hash(byte[] buffer);\n }\n\n /**\n * The FNV hash function:\n * Uses the 32 bit FNV prime and FNV_OFFSET\n * \n * FNV hashing using the FNVHash - v1 see\n * <a href=\"http://isthe.com/chongo/tech/comp/fnv/#history\">link</a>,\n * uses:\n * 32 bit offset basis = 2166136261\n * 32 bit FNV_prime = 224 + 28 + 0x93 = 16777619\n * \n * @param buffer\n * The element to be hashed in bytes\n * @return The 32 bit FNV hash of the element\n */\n public static final long fnv(byte[] buffer) {\n\n final long OFFSET_BASIS_32 = 2166136261L;\n final long FNV_PRIME_32 = 16777619L;\n\n long hash = OFFSET_BASIS_32;\n\n for (byte b : buffer) {\n\n hash = hash * FNV_PRIME_32;\n hash = hash ^ b;\n }\n\n return Math.abs(hash);\n }\n\n /**\n * The FNV-1a hash function:\n * USes the 32 bit FNV prime and FNV_Offset\n * \n * @param buffer\n * The element to be hased, in bytes\n * @return The 32 bit FNV-1a hash of the element\n */\n public static final long fnv1a(byte[] buffer) {\n\n final long OFFSET_BASIS_32 = 2166136261L;\n final long FNV_PRIME_32 = 16777619L;\n\n long hash = OFFSET_BASIS_32;\n\n for (byte b : buffer) {\n\n hash = hash ^ b;\n hash = hash * FNV_PRIME_32;\n }\n\n return Math.abs(hash);\n }\n\n /**\n * For testing out the library\n * \n * @param args\n */\n public static void main(String[] args) {\n\n BloomFilter b = new BloomFilter(15);\n\n System.out.println(\"FNV hash of 'hello' = \" + BloomFilter.fnv(\"hello\".getBytes()) % b.bitvector.length);\n\n System.out.println(\"FNV-1a hash of 'hello' = \" + BloomFilter.fnv1a(\"hello\".getBytes()) % b.bitvector.length);\n\n b.add(() -> \"hello\");\n b.add(() -> \"helloWorld\");\n b.add(() -> \"helloDear\");\n b.add(() -> \"her\");\n b.add(() -> \"3456\");\n\n System.out.println(\"hello in bloom filter = \" + b.check(() -> \"hello\"));\n System.out.println(\"helloWorld in bloom filter = \" + b.check(() -> \"helloWorld\"));\n System.out.println(\"helloDear in bloom filter = \" + b.check(() -> \"helloDear\"));\n System.out.println(\"dear in bloom filter = \" + b.check(() -> \"dear\"));\n System.out.println(\"3456 in bloom filter = \" + b.check(() -> \"3456\"));\n System.out.println(\"3 in bloom filter = \" + b.check(() -> \"3\"));\n System.out.println(\"4 in bloom filter = \" + b.check(() -> \"4\"));\n System.out.println(\"5 in bloom filter = \" + b.check(() -> \"5\"));\n System.out.println(\"6 in bloom filter = \" + b.check(() -> \"6\"));\n }\n}"
}
{
"filename": "code/data_structures/src/hashs/bloom_filter/bloom_filter.js",
"content": "/**\n * bloom_filter.js\n * @author Sidharth Mishra\n * @description Bloom filter in Javascript(Node.js), to be used with Node.js for testing. For `OpenGenus/cosmos`\n * @created Wed Oct 18 2017 19:31:29 GMT-0700 (PDT)\n * @copyright 2017 Sidharth Mishra\n * @last-modified Wed Oct 18 2017 19:31:32 GMT-0700 (PDT)\n */\n\n/**\n * bf -- Bloom Filter utilities, uses FNV and FNV-1a hash algorithms for now.\n * Future work: Added hash mix with Murmur3 hash\n *\n * Addition into bloom filter: Time Complexity : O(k) where k = number of hash functions\n * Checking membership: Time Complexity : O(k) where k = number of hash functions\n */\n(function(bfilter) {\n /**\n * Makes a new bloom filter. Uses FNV Hash as the hash function. This implementation uses only\n * FNV Hash functions for now.\n * FNV hash versions:\n * -> FNV\n * -> FNV 1a\n *\n * :Future work:\n * Add Murmur3 hash function.\n *\n * @param {number} m The number of bits in the Bit Vector backing the bloom filter\n * @param {[Function]} k the hash functions to be used\n *\n * @returns {bfilter.BloomFilter} a bloom filter with m bits and k hash functions\n */\n bfilter.BloomFilter = function BloomFilter(m, k) {\n if (\n arguments.length < 2 ||\n undefined == m ||\n undefined == k ||\n undefined == k.length ||\n k.length == 0 ||\n typeof k !== \"object\"\n ) {\n throw new Error(\"Couldn't construct a bloom filter, bad args\");\n }\n // count of number of elements inserted into the bloom filter\n this.nbrElementsInserted = 0;\n this.bitVector = []; // the bit vector\n this.hashFunctions = k; // the list of hash functions to use for adding and testing membership\n // make the bit vector with m bits\n for (let i = 0; i < m; i++) {\n this.bitVector.push(false);\n }\n for (let i = 0; i < this.hashFunctions.length; i++) {\n if (typeof this.hashFunctions[i] !== \"function\") {\n throw new Error(\"Hash function is not a function\");\n }\n }\n };\n\n /**\n * FNV hashing using the FNVHash - v1a see link: http://isthe.com/chongo/tech/comp/fnv/#history,\n * uses:\n * 32 bit offset basis = 2166136261\n * 32 bit FNV_prime = 224 + 28 + 0x93 = 16777619\n * @param {Buffer} toBeHashed The element to be hashed, Node.js buffer\n */\n bfilter.fnvHash1a = function(toBeHashed) {\n const OFFSET_BASIS_32 = 2166136261;\n const FNV_PRIME = 16777619;\n let hash = OFFSET_BASIS_32; // 32 bit offset basis\n // or each octet_of_data to be hashed\n // toBeHashed is a Buffer that has octets of data to be hashed\n for (let i = 0; i < toBeHashed.length; i++) {\n hash = hash ^ toBeHashed[i];\n hash = hash * FNV_PRIME;\n }\n // since hash being returned is negative most of the times\n return Math.abs(hash);\n };\n\n /**\n * FNV hashing using the FNVHash - v1 see link: http://isthe.com/chongo/tech/comp/fnv/#history,\n * uses:\n * 32 bit offset basis = 2166136261\n * 32 bit FNV_prime = 224 + 28 + 0x93 = 16777619\n * @param {Buffer} toBeHashed The element to be hashed, Node.js buffer\n */\n bfilter.fnvHash = function(toBeHashed) {\n const OFFSET_BASIS_32 = 2166136261;\n const FNV_PRIME = 16777619;\n let hash = OFFSET_BASIS_32; // 32 bit offset basis\n // or each octet_of_data to be hashed\n // toBeHashed is a Buffer that has octets of data to be hashed\n for (let i = 0; i < toBeHashed.length; i++) {\n hash = hash * FNV_PRIME;\n hash = hash ^ toBeHashed[i];\n }\n // since hash being returned is negative most of the times\n return Math.abs(hash);\n };\n\n /**\n * Adds the element to the bloom filter. When an element is added to the bloom filter, it gets through\n * the k different hash functions; i.e it gets hashed k times.\n * T:: O(k) operation\n * @param {string} element The element is converted bits and then hashed k times before getting added\n * to the bit vector.\n */\n bfilter.BloomFilter.prototype.add = function(element) {\n // console.log(JSON.stringify(this));\n if (typeof element !== \"string\") {\n element = String(element);\n }\n let buffE = Buffer.from(element); // get the element buffer\n let hash = null;\n for (let i = 0; i < this.hashFunctions.length; i++) {\n hash = this.hashFunctions[i](buffE);\n // update the bit vector's index\n // depending on the hash value\n this.bitVector[hash % this.bitVector.length] = true;\n }\n this.nbrElementsInserted += 1;\n };\n\n /**\n * Check if the element is in the bloom filter.\n * T:: O(k) operation\n * @param {string} element The element that needs to get its membership verified\n */\n bfilter.BloomFilter.prototype.check = function(element) {\n if (typeof element !== \"string\") {\n element = String(element);\n }\n let buffE = Buffer.from(element); // get the element buffer\n let hash = null;\n let prob = true;\n for (let i = 0; i < this.hashFunctions.length; i++) {\n hash = this.hashFunctions[i](buffE);\n // checking membership by ANDing the bits in the bitVector\n prob = prob && this.bitVector[hash % this.bitVector.length];\n }\n if (prob) {\n return {\n probability: calcProb(\n this.bitVector.length,\n this.hashFunctions.length,\n this.nbrElementsInserted\n ),\n isMember: prob\n };\n } else {\n return {\n probability: 1,\n isMember: prob\n };\n }\n };\n\n /**\n * * Computes the probabilty of membership in the bloom filter = (1-e^-kn/m)^k\n * where ^ is exponentation\n *\n * @param {*} m The number of bits in the bit vector\n * @param {*} k The number of hash functions\n * @param {*} n The number of elements added to the bloom filter\n *\n * @returns {number} The probability of membership\n */\n function calcProb(m, k, n) {\n return Math.pow(1 - Math.exp((-1 * k * n) / m), k);\n }\n})((bf = {}));\n\n// exports the bf -- bloom filter utilities\nmodule.exports.bf = bf;\n\n/**\n * Tests\n *\n * @param {number} m The size of bit vector backing the bloom filter\n * @param {[Function]} k The nunber of times the element needs to be hashed\n */\nfunction test(m, k) {\n let bloomFilter = new bf.BloomFilter(m, k);\n bloomFilter.add(\"hello\");\n bloomFilter.add(\"helloWorld\");\n bloomFilter.add(\"helloDear\");\n bloomFilter.add(\"her\");\n bloomFilter.add(\"3456\");\n console.log(\n `hello in bloom filter = ${JSON.stringify(bloomFilter.check(\"hello\"))}`\n );\n console.log(\n `helloWorld in bloom filter = ${JSON.stringify(\n bloomFilter.check(\"helloWorld\")\n )}`\n );\n console.log(\n `helloDear in bloom filter = ${JSON.stringify(\n bloomFilter.check(\"helloDear\")\n )}`\n );\n console.log(\n `world in bloom filter = ${JSON.stringify(bloomFilter.check(\"world\"))}`\n );\n console.log(\n `123 in bloom filter = ${JSON.stringify(bloomFilter.check(\"123\"))}`\n );\n console.log(\n `Number(3456) in bloom filter = ${JSON.stringify(bloomFilter.check(3456))}`\n );\n console.log(\n `Number(3) in bloom filter = ${JSON.stringify(bloomFilter.check(3))}`\n );\n console.log(\n `Number(4) in bloom filter = ${JSON.stringify(bloomFilter.check(4))}`\n );\n console.log(\n `Number(5) in bloom filter = ${JSON.stringify(bloomFilter.check(5))}`\n );\n console.log(\n `Number(6) in bloom filter = ${JSON.stringify(bloomFilter.check(6))}`\n );\n}\n\n// some tests\nconsole.log(\"---------- test 1 ----------\");\ntry {\n test(32);\n} catch (e) {\n console.log(\"Test 1 passed!\");\n}\n\nconsole.log(\"---------- test 2 ----------\");\ntry {\n test(4, [bf.fnvHash, 55]);\n} catch (e) {\n console.log(\"Test 2 passed!\");\n}\n\nconsole.log(\"---------- test 3 ----------\");\ntry {\n test(15, [bf.fnvHash1a]);\n console.log(\"Test 3a passed! ---\");\n test(15, [bf.fnvHash]);\n console.log(\"Test 3b passed!\");\n} catch (e) {\n console.log(e);\n}\n\nconsole.log(\"---------- test 4 ----------\");\ntry {\n test(64, [bf.fnvHash1a]);\n console.log(\"Test 4 passed!\");\n} catch (e) {\n console.log(e);\n}\n\nconsole.log(\"---------- test 5 ----------\");\ntry {\n test(32, [bf.fnvHash1a, bf.fnvHash1a, bf.fnvHash, bf.fnvHash1a]);\n console.log(\"Test 5 passed!\");\n} catch (e) {\n console.log(e);\n}\n\nconsole.log(\"---------- test 6 ----------\");\ntry {\n test(32, 6);\n} catch (e) {\n console.log(\"Test 6 passed!\");\n}\n\nconsole.log(\"---------- test 7 ----------\");\ntry {\n test(32, [bf.fnvHash1a, bf.fnvHash1a, bf.fnvHash1a, bf.fnvHash1a]);\n console.log(\"Test 7 passed!\");\n} catch (e) {\n console.log(e);\n}\n\n// console.log(bf.fnvHash(Buffer.from(\"hello\")) % 15);"
}
{
"filename": "code/data_structures/src/hashs/bloom_filter/bloom_filter.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n# A Bloom filter implementation in python\n\n\nclass bloomFilter:\n def __init__(self, size=1000, hashFunctions=None):\n \"\"\" Construct a bloom filter with size bits(default: 1000) and the associated hashFunctions.\n Default hash function is i.e. hash(e)%size.\n \"\"\"\n self.bits = 0\n self.M = size\n if hashFunctions is None:\n self.k = 1\n self.hashFunctions = [lambda e, size: hash(e) % size]\n else:\n self.k = len(hashFunctions)\n self.hashFunctions = hashFunctions\n\n def add(self, value):\n \"\"\" Insert value in bloom filter\"\"\"\n for hf in self.hashFunctions:\n self.bits |= 1 << hf(value, self.M)\n\n def __contains__(self, value):\n \"\"\"\n Determine whether a value is present. A false positive might be returned even if the element is\n not present. However, a false negative will never be returned.\n \"\"\"\n for hf in self.hashFunctions:\n if self.bits & 1 << hf(value, self.M) == 0:\n return False\n return True\n\n\n# Example of usage\nbf = bloomFilter()\n\nbf.add(\"Francis\")\nbf.add(\"Claire\")\nbf.add(\"Underwood\")\n\nif \"Francis\" in bf:\n print(\"Francis is in BloomFilter :)\")\n\nif \"Zoe\" not in bf:\n print(\"Zoe is not in BloomFilter :(\")"
}
{
"filename": "code/data_structures/src/hashs/bloom_filter/bloom_filter.scala",
"content": "import collection.immutable.BitSet\n\nobject BloomFilter {\n def apply[T](size: Int): Option[BloomFilter[T]] = {\n if (size < 0) None\n Some(BloomFilter[T](size, BitSet.empty))\n }\n}\n\ncase class BloomFilter[T](size: Int, bitSet: BitSet) {\n\n def defaultIndicies(item: T): (Int, Int) = {\n val hash = item.hashCode()\n val hash2 = (hash >> 16) | (hash << 16)\n (Math.floorMod(hash, size), Math.floorMod(hash2, size))\n }\n\n def add(item: T): BloomFilter[T] = {\n val (h1, h2) = defaultIndicies(item)\n BloomFilter(size, bitSet.+(h1, h2))\n }\n\n def contains(item: T): Boolean = {\n val (h1, h2) = defaultIndicies(item)\n bitSet.contains(h1) && bitSet.contains(h2)\n }\n}\n\nobject Main {\n def main(args: Array[String]): Unit = {\n println(BloomFilter[Int](100).get.add(5))\n println(BloomFilter[Int](100).get.add(5).contains(5))\n println(BloomFilter[Int](100).get.add(5).contains(6))\n println(BloomFilter[Int](100).get.add(5).add(7))\n println(BloomFilter[Int](100).get.add(5).add(7).contains(5))\n println(BloomFilter[Int](100).get.add(5).add(7).contains(7))\n println(BloomFilter[Int](100).get.add(5).add(7).contains(6))\n }\n}"
}
{
"filename": "code/data_structures/src/hashs/bloom_filter/bloom_filter.swift",
"content": "public class BloomFilter<T> {\n private var array: [Bool]\n private var hashFunctions: [(T) -> Int]\n\n public init(size: Int = 1024, hashFunctions: [(T) -> Int]) {\n self.array = [Bool](repeating: false, count: size)\n self.hashFunctions = hashFunctions\n }\n\n private func computeHashes(_ value: T) -> [Int] {\n return hashFunctions.map { hashFunc in abs(hashFunc(value) % array.count) }\n }\n\n public func insert(_ element: T) {\n for hashValue in computeHashes(element) {\n array[hashValue] = true\n }\n }\n\n public func insert(_ values: [T]) {\n for value in values {\n insert(value)\n }\n }\n\n public func query(_ value: T) -> Bool {\n let hashValues = computeHashes(value)\n let results = hashValues.map { hashValue in array[hashValue] }\n let exists = results.reduce(true, { $0 && $1 })\n return exists\n }\n\n public func isEmpty() -> Bool {\n return array.reduce(true) { prev, next in prev && !next }\n }\n}"
}
{
"filename": "code/data_structures/src/hashs/bloom_filter/README.md",
"content": "Bloom Filter\n---\nA space-efficient probabilistic data structure that is used to test whether an element is a member of \na set. False positive matches are possible, but false negatives are not; i.e. a query returns either \"possibly in set\" \nor \"definitely not in set\". Elements can be added to the set, but not removed.\n\n## Api\n\n* `Add(item)`: Adds the item into the set\n* `Check(item) bool`: Check if the item possibly exists into the set\n\n## Complexity\n\n**Time**\n\nIf we are using a bloom filter with bits and hash function, \ninsertion and search will both take time. \nIn both cases, we just need to run the input through all of \nthe hash functions. Then we just check the output bits.\n\n| Operation | Complexity |\n|---|---|\n| insertion | O(k) |\n| search | O(k) |\n\n**Space**\nThe space of the actual data structure (what holds the data).\n\n| Complexity |\n|---|\n| O(m) |\n\nWhere `m` is the size of the slice."
}
{
"filename": "code/data_structures/src/hashs/hash_table/double_hashing.c",
"content": "// Part of Cosmos by OpenGenus Foundation\n\n#include<stdio.h>\n\nint hash_fn(int k, int i)\n{\n int h1,h2;\n h1 = k % 9;\n h2 = k % 8;\n return ((h1 + (i*h2))% 9);\n}\n\nvoid insert(int arr[])\n{\n int k,i=1,id;\n printf(\"Enter the element \\n\");\n scanf(\"%d\",&k);\n id = k % 9;\n while(arr[id]!=-1)\n {\n id = hash_fn(k,i);\n i++;\n }\n arr[id] = k; \n}\n\nvoid search(int arr[])\n{\n int q;\n printf(\"Enter query\\n\");\n scanf(\"%d\",&q);\n int id = q % 9 , i;\n for(i=0;i<9;i++)\n {\n if(arr[id] == q)\n {\n printf(\"Found\\n\");\n break;\n }\n }\n printf(\"Not Found\\n\");\n}\n\nvoid display(int arr[])\n{\n int i ;\n for (i=0;i<9;i++)\n {\n printf(\"%d \",arr[i]);\n }\n printf(\"\\n\");\n}\n\nint main()\n{\n int arr[9],i;\n for(i =0;i<9;i++)\n {\n arr[i] = -1;\n }\n\n for(i=0;i<9;i++)\n {\n insert(arr);\n }\n display(arr);\n search(arr);\n}"
}
{
"filename": "code/data_structures/src/hashs/hash_table/hash_table.c",
"content": "#include <stdio.h>\n#include <string.h>\n#include <stdlib.h>\n#include <stdbool.h>\n// Part of Cosmos by OpenGenus Foundation\n#define SIZE 20\n\nstruct DataItem {\n int data; \n int key;\n};\n\nstruct DataItem* hashArray[SIZE]; \nstruct DataItem* dummyItem;\nstruct DataItem* item;\n\nint hashCode(int key) {\n return key % SIZE;\n}\n\nstruct DataItem *search(int key) {\n //get the hash \n int hashIndex = hashCode(key); \n \n //move in array until an empty \n while(hashArray[hashIndex] != NULL) {\n \n if(hashArray[hashIndex]->key == key)\n return hashArray[hashIndex]; \n \n //go to next cell\n ++hashIndex;\n \n //wrap around the table\n hashIndex %= SIZE;\n } \n \n return NULL; \n}\n\nvoid insert(int key,int data) {\n\n struct DataItem *item = (struct DataItem*) malloc(sizeof(struct DataItem));\n item->data = data; \n item->key = key;\n\n //get the hash \n int hashIndex = hashCode(key);\n\n //move in array until an empty or deleted cell\n while(hashArray[hashIndex] != NULL && hashArray[hashIndex]->key != -1) {\n //go to next cell\n ++hashIndex;\n \n //wrap around the table\n hashIndex %= SIZE;\n }\n \n hashArray[hashIndex] = item;\n}\n\nstruct DataItem* delete(struct DataItem* item) {\n int key = item->key;\n\n //get the hash \n int hashIndex = hashCode(key);\n\n //move in array until an empty\n while(hashArray[hashIndex] != NULL) {\n \n if(hashArray[hashIndex]->key == key) {\n struct DataItem* temp = hashArray[hashIndex]; \n \n //assign a dummy item at deleted position\n hashArray[hashIndex] = dummyItem; \n return temp;\n }\n \n //go to next cell\n ++hashIndex;\n \n //wrap around the table\n hashIndex %= SIZE;\n } \n \n return NULL; \n}\n\nvoid display() {\n int i = 0;\n \n for(i = 0; i<SIZE; i++) {\n \n if(hashArray[i] != NULL)\n printf(\" (%d,%d)\",hashArray[i]->key,hashArray[i]->data);\n else\n printf(\" ~~ \");\n }\n \n printf(\"\\n\");\n}\n\nint main() {\n dummyItem = (struct DataItem*) malloc(sizeof(struct DataItem));\n dummyItem->data = -1; \n dummyItem->key = -1; \n\n insert(1, 20);\n insert(2, 70);\n insert(42, 80);\n insert(4, 25);\n insert(12, 44);\n insert(14, 32);\n insert(17, 11);\n insert(13, 78);\n insert(37, 97);\n\n display();\n item = search(37);\n\n if(item != NULL) {\n printf(\"Element found: %d\\n\", item->data);\n } else {\n printf(\"Element not found\\n\");\n }\n\n delete(item);\n item = search(37);\n\n if(item != NULL) {\n printf(\"Element found: %d\\n\", item->data);\n } else {\n printf(\"Element not found\\n\");\n }\n}"
}
{
"filename": "code/data_structures/src/hashs/hash_table/hash_table.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n *\n * hash_table synopsis\n *\n * template<typename _Tp, typename _HashFunc = std::hash<_Tp> >\n * class hash_table {\n * public:\n * typedef _Tp value_type;\n * typedef decltype (_HashFunc ().operator()(_Tp())) key_type;\n * typedef std::map<key_type, value_type> container_type;\n * typedef typename container_type::const_iterator const_iterator;\n * typedef typename container_type::size_type size_type;\n *\n * // Initialize your data structure here.\n * hash_table() :_container({}) {}\n *\n * // Inserts a value to the set. Returns true if the set did not already contain the specified\n * element.\n * std::pair<const_iterator, bool> insert(const _Tp val);\n *\n * template<typename _InputIter>\n * void insert(_InputIter first, _InputIter last);\n *\n * void insert(std::initializer_list<_Tp> init_list);\n *\n * // Removes a value from the set. Returns true if the set contained the specified element.\n * const_iterator erase(const _Tp val);\n *\n * const_iterator erase(const_iterator pos);\n *\n * // Find a value from the set. Returns true if the set contained the specified element.\n *\n * const_iterator find(const _Tp val) const;\n *\n * const_iterator end() const;\n *\n * bool empty() const;\n *\n * size_type size() const;\n *\n * private:\n * container_type _container;\n *\n * key_type hash(const value_type &val) const;\n *\n * template<typename _InputIter>\n * void _insert(_InputIter first, _InputIter last, std::input_iterator_tag);\n * };\n */\n\n#include <map>\ntemplate<typename _Tp, typename _HashFunc = std::hash<_Tp>>\nclass hash_table {\npublic:\n typedef _Tp value_type;\n typedef decltype (_HashFunc ().operator()(_Tp())) key_type;\n typedef std::map<key_type, value_type> container_type;\n typedef typename container_type::const_iterator const_iterator;\n typedef typename container_type::size_type size_type;\n\n/** Initialize your data structure here. */\n hash_table() : _container({})\n {\n }\n\n/** Inserts a value to the set. Returns true if the set did not already contain the specified\n * element. */\n std::pair<const_iterator, bool> insert(const _Tp val)\n {\n key_type key = hash(val);\n if (_container.find(key) == _container.end())\n {\n _container.insert(std::make_pair(key, val));\n\n return make_pair(_container.find(key), true);\n }\n\n return make_pair(_container.end(), false);\n }\n\n template<typename _InputIter>\n void insert(_InputIter first, _InputIter last)\n {\n _insert(first, last, typename std::iterator_traits<_InputIter>::iterator_category());\n }\n\n void insert(std::initializer_list<_Tp> init_list)\n {\n insert(init_list.begin(), init_list.end());\n }\n\n/** Removes a value from the set. Returns true if the set contained the specified element. */\n const_iterator erase(const _Tp val)\n {\n const_iterator it = find(val);\n if (it != end())\n return erase(it);\n\n return end();\n }\n\n const_iterator erase(const_iterator pos)\n {\n return _container.erase(pos);\n }\n\n/** Find a value from the set. Returns true if the set contained the specified element. */\n const_iterator find(const _Tp val)\n {\n key_type key = hash(val);\n\n return _container.find(key);\n }\n\n const_iterator find(const _Tp val) const\n {\n key_type key = hash(val);\n\n return _container.find(key);\n }\n\n const_iterator end() const\n {\n return _container.end();\n }\n\n bool empty() const\n {\n return _container.empty();\n }\n\n size_type size() const\n {\n return _container.size();\n }\n\nprivate:\n container_type _container;\n\n key_type hash(const value_type &val) const\n {\n return _HashFunc()(val);\n }\n\n template<typename _InputIter>\n void _insert(_InputIter first, _InputIter last, std::input_iterator_tag)\n {\n _InputIter pos = first;\n while (pos != last)\n insert(*pos++);\n }\n};\n\n/*\n * // for test\n *\n * // a user-defined hash function\n * template<typename _Tp>\n * struct MyHash {\n * // hash by C++ boost\n * // phi = (1 + sqrt(5)) / 2\n * // 2^32 / phi = 0x9e3779b9\n * void hash(std::size_t &seed, const _Tp &i) const\n * {\n * seed ^= std::hash<_Tp>()(i) + 0x87654321 * (seed << 1) + (seed >> 2);\n * seed <<= seed % 6789;\n * }\n *\n * std::size_t operator()(const _Tp &d) const\n * {\n * std::size_t seed = 1234;\n * hash(seed, d);\n *\n * return seed;\n * }\n * };\n *\n #include <iostream>\n #include <vector>\n * using namespace std;\n *\n * int main() {\n * hash_table<int, MyHash<int> > ht;\n *\n * // test find\n * if (ht.find(9) != ht.end())\n * cout << __LINE__ << \" error\\n\";\n *\n * // test insert(1)\n * if (!ht.insert(3).second) // return true\n * cout << __LINE__ << \" error\\n\";\n *\n * if (ht.insert(3).second) // return false\n * cout << __LINE__ << \" error\\n\";\n *\n * // test insert(2)\n * vector<int> input{10, 11, 12};\n * ht.insert(input.begin(), input.end());\n * if (ht.find(10) == ht.end())\n * cout << __LINE__ << \" error\\n\";\n * if (ht.find(11) == ht.end())\n * cout << __LINE__ << \" error\\n\";\n * if (ht.find(12) == ht.end())\n * cout << __LINE__ << \" error\\n\";\n *\n * // test insert(3)\n * ht.insert({13, 14, 15, 16});\n *\n * // test erase(1)\n * ht.erase(13);\n *\n * // test erase(2)\n * auto it = ht.find(14);\n * ht.erase(it);\n *\n * // test empty\n * if (ht.empty())\n * cout << __LINE__ << \" error\\n\";\n *\n * // test size\n * if (ht.size() != 6)\n * cout << __LINE__ << \" error\\n\";\n *\n * return 0;\n * }\n *\n * // */"
}
{
"filename": "code/data_structures/src/hashs/hash_table/hash_table.cs",
"content": "using System;\nusing System.Collections; // namespace for hashtable\nusing System.Linq;\nusing System.Text;\nusing System.Threading.Tasks;\n/*\n * Part of Cosmos by OpenGenus Foundation'\n *\n * The Hashtable class represents a collection of key-and-value pairs that are organized\n * based on the hash code of the key. It uses the key to access the elements in the collection.\n * */\nnamespace hashtables\n{\n sealed class hTable // a class\n {\n static int key; // key of the hastable\n public bool uniqueness { get; set; } // setting to check if allow uniqueness or not\n Hashtable hashtable = new Hashtable();\n internal void add(string value)\n {\n if(uniqueness)\n {\n if(hashtable.ContainsValue(value))\n {\n Console.WriteLine(\"Entry exists\");\n }\n else\n {\n hashtable.Add(++key,value);\n }\n }\n else\n {\n hashtable.Add(++key, value);\n }\n\n }\n internal void delete(int key)\n {\n if (hashtable.ContainsKey(key))\n {\n Console.WriteLine(\"Deleted value : \" + hashtable[key].ToString());\n hashtable.Remove(key);\n }\n else\n {\n Console.WriteLine(\"Key not found\");\n }\n }\n internal void show()\n {\n foreach(var key in hashtable.Keys)\n {\n Console.WriteLine(key.ToString() + \" : \" + hashtable[key]);\n }\n }\n\n }\n class Program\n {\n static void Main(string[] args)\n {\n hTable obj = new hTable();\n\n // i want uniqueness therefore making property true\n obj.uniqueness = true;\n\n // adding 3 values\n Console.WriteLine(\"ADDING EXAMPLE\");\n obj.add(\"terabyte\");\n obj.add(\"sandysingh\");\n obj.add(\"adichat\");\n\n // adding terabyte again to see the exists message\n Console.WriteLine(\"ADDING 'terabyte' AGAIN\");\n obj.add(\"terabyte\");\n\n // showing all original\n Console.WriteLine(\"Original Table\");\n obj.show();\n\n // removing value at key = 2\n Console.WriteLine(\"Deleting value\");\n obj.delete(2);\n\n // showing all new\n Console.WriteLine(\"New Table\");\n obj.show();\n\n\n Console.ReadKey(); // in ordr to pause the program\n }\n }\n}"
}
{
"filename": "code/data_structures/src/hashs/hash_table/hash_table.go",
"content": "package main\n\nimport (\n\t\"errors\"\n\t\"fmt\"\n\t\"container/list\"\n)\n\nvar (\n\tErrKeyNotFound = errors.New(\"key not found\")\n)\n\ntype Item struct {\n\tkey int \n\tvalue interface{}\n}\n\ntype Hashtable struct{\n\tsize int\n\tdata []*list.List\n}\n\nfunc (h *Hashtable) Insert(key int, value interface{}) {\n\titem := &Item{key: key, value: value}\n\thashed := key % h.size\n\tl :=\th.data[hashed]\n\tif l == nil {\n\t\tl = list.New()\n\t\th.data[hashed] = l\n\t}\n\tfor elem := l.Front(); elem != nil; elem = elem.Next() {\n\t\t// If found the element -> update\n\t\ti, _ := elem.Value.(*Item)\n\t\tif i.key == item.key {\n\t\t\ti.value = value\n\t\t\treturn\n\t\t}\n\t}\n\tl.PushFront(item)\n\treturn\n}\n\nfunc (h *Hashtable) Get(key int) (interface{}, error) {\n\thashed := key % h.size\n\tl := h.data[hashed]\n\tif l == nil {\n\t\treturn nil, ErrKeyNotFound\n\t}\n\tfor elem := l.Front(); elem != nil; elem = elem.Next() {\n\t\titem := elem.Value.(*Item)\n\t\tif item.key == key {\n\t\t\treturn item.value, nil\n\t\t}\n\t}\n\n\treturn nil, ErrKeyNotFound\n}\n\nfunc NewHashtable(size int) *Hashtable {\n\treturn &Hashtable{\n\t\tsize: size,\n\t\tdata: make([]*list.List, size),\n\t}\n}\n\nfunc main() {\n\th := NewHashtable(10)\n\th.Insert(1, \"abc\")\n\tvalue, _ := h.Get(1)\n\tfmt.Printf(\"h[1] = %s\\n\", value) // Print \"abc\"\n\n\th.Insert(11, \"def\")\n\tvalue, _ = h.Get(11)\n\tfmt.Printf(\"h[11] = %s\\n\", value) // Print \"def\"\n\n\tvalue, err := h.Get(2)\n\tfmt.Printf(\"Err = %#v\\n\", err) // ErrKeyNotFound\n}"
}
{
"filename": "code/data_structures/src/hashs/hash_table/hash_table.java",
"content": "// Part of Cosmos by OpenGenus Foundation\npublic class HashTable<K, V> {\n\tprivate class HTPair {\n\t\tK key;\n\t\tV value;\n\n\t\tpublic HTPair(K key, V value) {\n\t\t\tthis.key = key;\n\t\t\tthis.value = value;\n\t\t}\n\n\t\tpublic boolean equals(Object other) {\n\t\t\tHTPair op = (HTPair) other;\n\t\t\treturn this.key.equals(op.key);\n\t\t}\n\n\t\tpublic String toString() {\n\t\t\treturn \"{\" + this.key + \"->\" + this.value + \"}\";\n\t\t}\n\t}\n\n\tprivate LinkedList<HTPair>[] bucketArray;\n\tprivate int size;\n\n\tpublic static final int DEFAULT_CAPACITY = 5;\n\n\tpublic HashTable() {\n\t\tthis(DEFAULT_CAPACITY);\n\t}\n\n\tpublic HashTable(int capacity) {\n\t\tthis.bucketArray = (LinkedList<HTPair>[]) new LinkedList[capacity];\n\t\tthis.size = 0;\n\t}\n\n\tpublic int size() {\n\t\treturn this.size;\n\t}\n\n\tprivate int HashFunction(K key) {\n\t\tint hc = key.hashCode();\n\t\thc = Math.abs(hc);\n\t\tint bi = hc % this.bucketArray.length;\n\t\treturn bi;\n\t}\n\n\tpublic void put(K key, V value) throws Exception {\n\t\tint bi = HashFunction(key);\n\t\tHTPair data = new HTPair(key, value);\n\t\tif (this.bucketArray[bi] == null) {\n\t\t\tLinkedList<HTPair> bucket = new LinkedList<>();\n\t\t\tbucket.addLast(data);\n\t\t\tthis.size++;\n\t\t\tthis.bucketArray[bi] = bucket;\n\t\t} else {\n\t\t\tint foundAt = this.bucketArray[bi].find(data);\n\t\t\tif (foundAt == -1) {\n\t\t\t\tthis.bucketArray[bi].addLast(data);\n\t\t\t\tthis.size++;\n\t\t\t} else {\n\t\t\t\tHTPair obj = this.bucketArray[bi].getAt(foundAt);\n\t\t\t\tobj.value = value;\n\t\t\t\tthis.size++;\n\t\t\t}\n\t\t}\n\t\tdouble lambda = (this.size) * 1.0;\n\t\tlambda = this.size / this.bucketArray.length;\n\t\tif (lambda > 0.75) {\n\t\t\trehash();\n\t\t}\n\t}\n\n\tpublic void display() {\n\t\tfor (LinkedList<HTPair> list : this.bucketArray) {\n\t\t\tif (list != null && !list.isEmpty()) {\n\t\t\t\tlist.display();\n\t\t\t} else {\n\t\t\t\tSystem.out.println(\"NULL\");\n\t\t\t}\n\t\t}\n\t}\n\n\tpublic V get(K key) throws Exception {\n\t\tint index = this.HashFunction(key);\n\t\tLinkedList<HTPair> list = this.bucketArray[index];\n\t\tHTPair ptf = new HTPair(key, null);\n\t\tif (list == null) {\n\t\t\treturn null;\n\t\t} else {\n\t\t\tint findAt = list.find(ptf);\n\t\t\tif (findAt == -1) {\n\t\t\t\treturn null;\n\t\t\t} else {\n\t\t\t\tHTPair pair = list.getAt(findAt);\n\t\t\t\treturn pair.value;\n\t\t\t}\n\t\t}\n\t}\n\n\tpublic V remove(K key) throws Exception {\n\t\tint index = this.HashFunction(key);\n\t\tLinkedList<HTPair> list = this.bucketArray[index];\n\t\tHTPair ptf = new HTPair(key, null);\n\t\tif (list == null) {\n\t\t\treturn null;\n\t\t} else {\n\t\t\tint findAt = list.find(ptf);\n\t\t\tif (findAt == -1) {\n\t\t\t\treturn null;\n\t\t\t} else {\n\t\t\t\tHTPair pair = list.getAt(findAt);\n\t\t\t\tlist.removeAt(findAt);\n\t\t\t\tthis.size--;\n\t\t\t\treturn pair.value;\n\t\t\t}\n\t\t}\n\t}\n\n\tpublic void rehash() throws Exception {\n\t\tLinkedList<HTPair>[] oba = this.bucketArray;\n\t\tthis.bucketArray = (LinkedList<HTPair>[]) new LinkedList[2 * oba.length];\n\t\tthis.size = 0;\n\t\tfor (LinkedList<HTPair> ob : oba) {\n\t\t\twhile (ob != null && !ob.isEmpty()) {\n\t\t\t\tHTPair pair = ob.removeFirst();\n\t\t\t\tthis.put(pair.key, pair.value);\n\t\t\t}\n\t\t}\n\t}\n}"
}
{
"filename": "code/data_structures/src/hashs/hash_table/hash_table.js",
"content": "var HashTable = function() {\n this._storage = [];\n this._count = 0;\n this._limit = 8;\n};\n\nHashTable.prototype.insert = function(key, value) {\n //create an index for our storage location by passing it through our hashing function\n var index = this.hashFunc(key, this._limit);\n //retrieve the bucket at this particular index in our storage, if one exists\n //[[ [k,v], [k,v], [k,v] ] , [ [k,v], [k,v] ] [ [k,v] ] ]\n var bucket = this._storage[index];\n //does a bucket exist or do we get undefined when trying to retrieve said index?\n if (!bucket) {\n //create the bucket\n var bucket = [];\n //insert the bucket into our hashTable\n this._storage[index] = bucket;\n }\n\n var override = false;\n //now iterate through our bucket to see if there are any conflicting\n //key value pairs within our bucket. If there are any, override them.\n for (var i = 0; i < bucket.length; i++) {\n var tuple = bucket[i];\n if (tuple[0] === key) {\n //overide value stored at this key\n tuple[1] = value;\n override = true;\n }\n }\n\n if (!override) {\n //create a new tuple in our bucket\n //note that this could either be the new empty bucket we created above\n //or a bucket with other tupules with keys that are different than\n //the key of the tuple we are inserting. These tupules are in the same\n //bucket because their keys all equate to the same numeric index when\n //passing through our hash function.\n bucket.push([key, value]);\n this._count++;\n //now that we've added our new key/val pair to our storage\n //let's check to see if we need to resize our storage\n if (this._count > this._limit * 0.75) {\n this.resize(this._limit * 2);\n }\n }\n return this;\n};\n\nHashTable.prototype.remove = function(key) {\n var index = this.hashFunc(key, this._limit);\n var bucket = this._storage[index];\n if (!bucket) {\n return null;\n }\n //iterate over the bucket\n for (var i = 0; i < bucket.length; i++) {\n var tuple = bucket[i];\n //check to see if key is inside bucket\n if (tuple[0] === key) {\n //if it is, get rid of this tuple\n bucket.splice(i, 1);\n this._count--;\n if (this._count < this._limit * 0.25) {\n this._resize(this._limit / 2);\n }\n return tuple[1];\n }\n }\n};\n\nHashTable.prototype.retrieve = function(key) {\n var index = this.hashFunc(key, this._limit);\n var bucket = this._storage[index];\n\n if (!bucket) {\n return null;\n }\n\n for (var i = 0; i < bucket.length; i++) {\n var tuple = bucket[i];\n if (tuple[0] === key) {\n return tuple[1];\n }\n }\n\n return null;\n};\n\nHashTable.prototype.hashFunc = function(str, max) {\n var hash = 0;\n for (var i = 0; i < str.length; i++) {\n var letter = str[i];\n hash = (hash << 5) + letter.charCodeAt(0);\n hash = (hash & hash) % max;\n }\n return hash;\n};\n\nHashTable.prototype.resize = function(newLimit) {\n var oldStorage = this._storage;\n\n this._limit = newLimit;\n this._count = 0;\n this._storage = [];\n\n oldStorage.forEach(\n function(bucket) {\n if (!bucket) {\n return;\n }\n for (var i = 0; i < bucket.length; i++) {\n var tuple = bucket[i];\n this.insert(tuple[0], tuple[1]);\n }\n }.bind(this)\n );\n};\n\nHashTable.prototype.retrieveAll = function() {\n console.log(this._storage);\n};"
}
{
"filename": "code/data_structures/src/hashs/hash_table/hash_table.py",
"content": "\"\"\"\nHash Table implementation in Python using linear probing. \nThis code is based on the c/c++ implementation that I used to study hashing.\nhttps://github.com/jwasham/practice-c/tree/master/hash_table \n\"\"\"\n\n# Maximum number of elements.\nMAX_SIZE = 8 # Small size helps in checking for collission response\n\n# Key-Value pairs\nclass HashObject(object):\n def get_dummy_key():\n return \"<Dummy>\"\n\n def get_null_key():\n return \"<Null>\"\n\n def __init__(self):\n self.key_ = None\n self.value_ = None\n\n def set_key(self, key):\n self.key_ = key\n\n def set_value(self, value):\n self.value_ = value\n\n def get_key(self):\n return self.key_\n\n def get_value(self):\n return self.value_\n\n def set_as_dummy(self):\n self.set_key(HashObject.get_dummy_key())\n self.set_value(\"\")\n\n\n# HashTable class\nclass HashTable(object):\n\n # init method\n def __init__(self, n):\n self.size_ = n\n self.data_ = [HashObject() for _ in range(n)]\n\n # print method\n def __str__(self):\n output = \"\"\n for i in range(self.size_):\n if self.data_[i].get_key() != None:\n output += (\n str(i)\n + \" => \"\n + str(\n self.data_[i].get_key()\n + \" : \"\n + str(self.data_[i].get_value())\n + \"\\n\"\n )\n )\n\n return output\n\n # hash method\n def s_hash(self, key):\n hash_val = 0\n for i in key:\n hash_val = (hash_val * 31) + ord(i)\n\n return hash_val % self.size_\n\n # insert a key-value pair into the table\n def add(self, key_val_pair):\n index = self.s_hash(key_val_pair.get_key())\n original_index = index\n found = False\n dummy_index = -1\n\n while self.data_[index].get_key() != None:\n if self.data_[index].get_key() == key_val_pair.get_key():\n found = True\n break\n\n if (\n dummy_index == -1\n and self.data_[index].get_key() == HashObject.get_dummy_key()\n ):\n dummy_index = index\n\n index = (index + 1) % self.size_\n if index == original_index:\n return\n\n if not found and dummy_index != -1:\n index = dummy_index\n\n self.data_[index].set_key(key_val_pair.get_key())\n self.data_[index].set_value(key_val_pair.get_value())\n\n # check whether the key eists in the table\n def exists(self, key):\n index = self.s_hash(key)\n original_index = index\n\n while self.data_[index].get_key() != None:\n if self.data_[index].get_key() == key:\n return True\n\n index = (index + 1) % self.size_\n if index == original_index:\n return False\n\n return False\n\n # Get value corresponding to the key\n def get(self, key):\n index = self.s_hash(key)\n original_index = index\n\n while self.data_[index].get_key() != None:\n if self.data_[index].get_key() == key:\n return self.data_[index].get_value()\n\n index = (index + 1) % self.size_\n if index == original_index:\n return HashObject.get_null_key()\n\n return HashObject.get_null_key()\n\n # Remove the entry with the given key\n def remove(self, key):\n index = self.s_hash(key)\n original_index = index\n\n while self.data_[index].get_key() != None:\n if self.data_[index].get_key() == key:\n self.data_[index].set_as_dummy()\n break\n\n index = (index + 1) % self.size_\n if index == original_index:\n break\n\n\nif __name__ == \"__main__\":\n state = HashObject()\n table = HashTable(MAX_SIZE)\n\n state.set_key(\"Bihar\")\n state.set_value(\"Patna\")\n table.add(state)\n\n state.set_key(\"Jharkhand\")\n state.set_value(\"Ranchi\")\n table.add(state)\n\n state.set_key(\"Kerala\")\n state.set_value(\"Thiruvanantpuram\")\n table.add(state)\n\n state.set_key(\"WB\")\n state.set_value(\"Kolkata\")\n table.add(state)\n\n print(table)\n\n table.remove(\"Jharkhand\")\n\n table.remove(\"WB\")\n\n print(table)\n\n state.set_key(\"WB\")\n state.set_value(\"Kolkata\")\n table.add(state)\n\n print(table)"
}
{
"filename": "code/data_structures/src/hashs/hash_table/hash_table.rs",
"content": "use std::hash::{Hash, Hasher};\nuse std::collections::hash_map::DefaultHasher;\nuse std::fmt;\n\ntrait HashFunc {\n type Key;\n type Value;\n fn new(key: Self::Key, value: Self::Value) -> Self;\n fn get_value(&self) -> &Self::Value;\n fn get_key(&self) -> &Self::Key;\n}\nstruct HashTable<K, V> {\n list: Vec<Vec<HashPair<V, K>>>,\n mindex: u64,\n size: u64,\n}\nimpl<K, V> HashTable<K, V>\nwhere\n K: Hash + PartialEq + fmt::Display,\n V: fmt::Display,\n{\n fn new(index: u64) -> Self {\n let mut empty_vec = Vec::new();\n for _ in 0..index {\n empty_vec.push(Vec::new());\n }\n HashTable {\n list: empty_vec,\n size: 0,\n mindex: index,\n }\n }\n fn hash(&self, key: &K) -> u64 {\n let mut hasher = DefaultHasher::new();\n key.hash(&mut hasher);\n hasher.finish() % self.mindex\n }\n fn get(&self, key: K) -> Option<&V> {\n let index = self.hash(&key);\n if let Some(list) = self.list.get(index as usize) {\n if let Some(ele) = list.iter().find(|x| *x.get_key() == key) {\n Some(ele.get_value())\n } else {\n None\n }\n } else {\n None\n }\n }\n fn put(&mut self, key: K, value: V) {\n let index = self.hash(&key);\n if let Some(lin_list) = self.list.get_mut(index as usize) {\n let insert_value = HashPair::new(key, value);\n if let Some(index) = lin_list.iter().position(\n |x| *x.get_key() == insert_value.key,\n )\n {\n lin_list.remove(index);\n }\n lin_list.push(insert_value);\n self.size += 1;\n }\n }\n fn remove(&mut self, key: K) {\n let index = self.hash(&key);\n if let Some(lin_list) = self.list.get_mut(index as usize) {\n lin_list.retain(|x| *x.get_key() != key);\n self.size -= 1;\n }\n }\n fn size(&self) -> u64 {\n self.size\n }\n fn print(&self) {\n for index in 0..self.mindex {\n if let Some(lin_list) = self.list.get(index as usize) {\n for inner_index in 0..lin_list.len() {\n print!(\"{} \", lin_list.get(inner_index).unwrap());\n }\n }\n }\n println!();\n }\n}\nstruct HashPair<T, K> {\n key: K,\n value: T,\n}\nimpl<T, K> fmt::Display for HashPair<T, K>\nwhere\n T: fmt::Display,\n K: fmt::Display,\n{\n fn fmt(&self, f: &mut fmt::Formatter) -> fmt::Result {\n write!(f, \"({}, {})\", self.key, self.value)\n }\n}\nimpl<T, K> HashFunc for HashPair<T, K> {\n type Key = K;\n type Value = T;\n fn get_value(&self) -> &Self::Value {\n &self.value\n }\n fn get_key(&self) -> &Self::Key {\n &self.key\n }\n fn new(key: Self::Key, value: Self::Value) -> Self {\n HashPair {\n key: key,\n value: value,\n }\n }\n}\n\nconst MINDEX: u64 = 128;\n\nfn main() {\n let mut test = HashTable::new(MINDEX);\n test.put(\"hello\", \"bye\");\n test.put(\"what's up\", \"dude\");\n test.remove(\"hell\");\n test.print();\n}"
}
{
"filename": "code/data_structures/src/hashs/hash_table/hash_table.swift",
"content": "class HashElement<T: Hashable, U> {\n var key: T\n var value: U?\n \n init(key: T, value: U?) {\n self.key = key\n self.value = value\n }\n}\n\nstruct HashTable<Key: Hashable, Value: CustomStringConvertible> {\n private typealias Element = HashElement<Key, Value>\n private typealias Bucket = [Element]\n private var buckets: [Bucket]\n \n /// Number of key-value pairs in the hash table.\n private(set) public var count = 0\n \n /// A Boolean value that indicates whether the hash table is empty.\n public var isEmpty: Bool { return count == 0 }\n \n /// A string that represents the contents of the hash table.\n public var description: String {\n let pairs = buckets.flatMap { b in b.map { e in \"\\(e.key) = \\(e.value)\" } }\n return pairs.joined(separator: \", \")\n }\n \n /// A string that represents the contents of the hash table, suitable for debugging.\n public var debugDescription: String {\n var str = \"\"\n for (i, bucket) in buckets.enumerated() {\n let pairs = bucket.map { e in \"\\(e.key) = \\(e.value)\" }\n str += \"bucket \\(i): \" + pairs.joined(separator: \", \") + \"\\n\"\n }\n return str\n }\n \n /// Initialise hash table with the given capacity.\n ///\n /// - Parameter capacity: hash table capacity.\n init(capacity: Int) {\n assert(capacity > 0)\n buckets = Array<Bucket>(repeatElement([], count: capacity))\n }\n \n \n /// Returns an array index for a given key\n private func index(forKey key: Key) -> Int {\n return abs(key.hashValue) % buckets.count\n }\n \n /// Returns the value for the given key\n func value(for key: Key) -> Value? {\n let index = self.index(forKey: key)\n for element in buckets[index] {\n if element.key == key {\n return element.value\n }\n }\n return nil // key not in the hash table\n }\n \n /// Accesses element's value (get,set) in hash table for a given key.\n subscript(key: Key) -> Value? {\n get {\n return value(for: key)\n }\n set {\n if let value = newValue {\n updateValue(value, forKey: key)\n } else {\n removeValue(for: key)\n }\n }\n }\n \n /// Updates element's value in the hash table for the given key, or adds a new element if the key doesn't exists.\n @discardableResult\n mutating func updateValue(_ value: Value, forKey key: Key) -> Value? {\n let itemIndex = self.index(forKey: key)\n for (i, element) in buckets[itemIndex].enumerated() {\n if element.key == key {\n let oldValue = element.value\n buckets[itemIndex][i].value = value\n return oldValue\n }\n }\n buckets[itemIndex].append(HashElement(key: key, value: value)) // Adding element to the chain.\n count += 1\n return nil\n }\n \n /// Removes element from the hash table for a given key.\n @discardableResult\n mutating func removeValue(for key: Key) -> Value? {\n let index = self.index(forKey: key)\n // Search and destroy\n for (i, element) in buckets[index].enumerated() {\n if element.key == key {\n buckets[index].remove(at: i)\n count -= 1\n return element.value\n }\n }\n return nil // key not in the hash table\n }\n \n \n /// Removes all elements from the hash table.\n public mutating func removeAll() {\n buckets = Array<Bucket>(repeatElement(nil, count: buckets.count))\n count = 0\n }\n}"
}
{
"filename": "code/data_structures/src/hashs/hash_table/README.md",
"content": "# Definiton\n\nIn computing, a hash table (hash map) is a data structure which implements an associative array abstract data type, a structure that can map keys to values. A hash table uses a hash function to compute an index into an array of buckets or slots, from which the desired value can be found.</br>\nIdeally, the hash function will assign each key to a unique bucket, but most hash table designs employ an imperfect hash function, which might cause hash collisions where the hash function generates the same index for more than one key. Such collisions must be accommodated in some way.</br>\nIn many situations, hash tables turn out to be more efficient than search trees or any other table lookup structure.\n\n# Further Reading\n\nhttps://en.wikipedia.org/wiki/Hash_table"
}
{
"filename": "code/data_structures/src/Linked_List/Add_one_to_LL.cpp",
"content": "class Solution\n{\n public:\n Node* reverse( Node *head)\n {\n // code here\n // return head of reversed list\n Node *prevNode=NULL,*currNode=head,*nextNode=head;\n while(currNode!=NULL){\n nextNode=currNode->next;\n currNode->next=prevNode;\n prevNode=currNode;\n currNode=nextNode;\n }\n return prevNode;\n }\n Node* addOne(Node *head) \n {\n // Your Code here\n // return head of list after adding one\n if(head==NULL)\n return NULL;\n head=reverse(head);\n \n Node* node=head;int carry=1;\n while(node!=NULL){\n int sum=carry+node->data;\n node->data=(sum%10);\n carry=sum/10;\n \n if(node->next==NULL) break;\n \n node=node->next;\n }\n \n if(carry) node->next=new Node(carry);\n \n return reverse(head);\n \n }\n};"
}
{
"filename": "code/data_structures/src/Linked_List/add_two_numbers_represented_by_linked_lists.cpp",
"content": "// { Driver Code Starts\n// driver\n\n#include <bits/stdc++.h>\nusing namespace std;\n\n/* Linked list Node */\nstruct Node {\n int data;\n struct Node* next;\n Node(int x) {\n data = x;\n next = NULL;\n }\n};\n\nstruct Node* buildList(int size)\n{\n int val;\n cin>> val;\n \n Node* head = new Node(val);\n Node* tail = head;\n \n for(int i=0; i<size-1; i++)\n {\n cin>> val;\n tail->next = new Node(val);\n tail = tail->next;\n }\n \n return head;\n}\n\nvoid printList(Node* n)\n{\n while(n)\n {\n cout<< n->data << \" \";\n n = n->next;\n }\n cout<< endl;\n}\n\n\n // } Driver Code Ends\n/* node for linked list:\n\nstruct Node {\n int data;\n struct Node* next;\n Node(int x) {\n data = x;\n next = NULL;\n }\n};\n\n*/\n\nclass Solution\n{\n public:\n //Function to add two numbers represented by linked list.\n Node* reverse(Node* head){\n Node* prev = NULL;\n Node* next = NULL;\n Node* current = head;\n while(current != NULL){\n next = current->next;\n current->next = prev;\n prev = current;\n current = next;\n }\n \n return prev;\n }\n \n struct Node* addTwoLists(struct Node* first, struct Node* second){\n first = reverse(first);\n second = reverse(second);\n \n Node* temp = NULL;\n Node* res = NULL;\n Node* cur = NULL;\n \n int carry = 0, sum = 0;\n \n while(first != NULL || second != NULL){\n sum = carry + (first ? first->data : 0) + (second ? second->data : 0);\n carry = (sum>=10) ? 1 : 0;\n sum %= 10;\n \n temp = new Node(sum);\n if(res == NULL) res = temp;\n else cur->next = temp;\n cur = temp;\n \n if(first) first = first->next;\n if(second) second = second->next;\n }\n\n if(carry > 0){\n temp = new Node(carry);\n cur->next = temp;\n cur = temp;\n }\n \n res = reverse(res);\n \n return res;\n \n }\n};\n\n\n// { Driver Code Starts.\n\nint main()\n{\n int t;\n cin>>t;\n while(t--)\n {\n int n, m;\n \n cin>>n;\n Node* first = buildList(n);\n \n cin>>m;\n Node* second = buildList(m);\n Solution ob;\n Node* res = ob.addTwoLists(first,second);\n printList(res);\n }\n return 0;\n}\n // } Driver Code Ends"
}
{
"filename": "code/data_structures/src/Linked_List/Count_nodes_of_Linked_List.cpp",
"content": "// count the Number of Nodes n a Linked List\nint getCount(struct Node* head){\n \n int cnt=0;\n Node *curr = head;\n while(curr != NULL){\n cnt++;\n curr = curr->next;\n }\n return cnt;\n }"
}
{
"filename": "code/data_structures/src/Linked_List/creating_linked_list.cpp",
"content": "#include <iostream>\n\nusing namespace std;\n\ntypedef struct node Node;\nstruct node {\n int data;\n Node *next_pointer;\n};\n\nNode *create_node();\nvoid insert_in_beginning(int value, Node **start);\nvoid insert_in_ending(int value, Node **start);\nvoid insert_in_specific_position(int value, Node *start);\nvoid linked_list_print(Node *start);\n\nint main() {\n int arr[] = {5, 3, 9, 42, 0, 10};\n int length = 6;\n Node *start = NULL;\n\n for (int i = 0; i < length; i++) {\n // insert_in_beginning(arr[i], &start);\n insert_in_ending(arr[i], &start);\n }\n linked_list_print(start);\n}\n\nNode *create_node() {\n Node *node = (Node *)malloc(sizeof(Node));\n\n if (node == NULL) {\n cout << \"Error while creating new node\" << endl;\n }\n return node;\n}\n\nvoid insert_in_beginning(int value, Node **start) {\n Node *new_node = create_node();\n\n new_node->data = value;\n new_node->next_pointer = *start;\n *start = new_node;\n}\n\nvoid linked_list_print(Node *start) {\n if (start == NULL) {\n cout << \"Empty Linked List\" << endl;\n exit(1);\n }\n Node *current_node = start;\n while (current_node != NULL) {\n cout << current_node->data << \" \";\n current_node = current_node->next_pointer;\n }\n cout << endl;\n}\n\nvoid insert_in_ending(int value, Node **start) {\n Node *current_node = *start;\n Node *new_node = create_node();\n\n if (current_node == NULL) {\n *start = new_node;\n current_node = new_node;\n current_node->next_pointer = NULL;\n }\n while (current_node->next_pointer != NULL) {\n current_node = current_node->next_pointer;\n }\n new_node->data = value;\n current_node->next_pointer = new_node;\n new_node->next_pointer = NULL;\n}\n\n/*\n Creating and inserting should be always insert_in_ending() for exam\n*/"
}
{
"filename": "code/data_structures/src/Linked_List/deleting_a_node.cpp",
"content": "#include <iostream>\n\nusing namespace std;\n\ntypedef struct node Node;\nstruct node {\n int data;\n Node *next_pointer;\n};\n\nNode *create_node();\nvoid insert_in_ending(int value, Node **start);\nvoid insert_in_specific_position(int value, Node *start);\nvoid linked_list_print(Node *start);\nvoid deleting(int value, Node **start);\n\nint main() {\n int arr[] = {5, 3, 9, 42, 0, 10};\n int length = 6;\n Node *start = NULL;\n\n for (int i = 0; i < length; i++) {\n insert_in_ending(arr[i], &start);\n }\n linked_list_print(start);\n deleting(5, &start);\n linked_list_print(start);\n}\n\nNode *create_node() {\n Node *node = (Node *)malloc(sizeof(Node));\n\n if (node == NULL) {\n cout << \"Error while creating new node\" << endl;\n }\n return node;\n}\n\nvoid linked_list_print(Node *start) {\n if (start == NULL) {\n cout << \"Empty Linked List\" << endl;\n exit(1);\n }\n Node *current_node = start;\n while (current_node != NULL) {\n cout << current_node->data << \" \";\n current_node = current_node->next_pointer;\n }\n cout << endl;\n}\n\nvoid insert_in_ending(int value, Node **start) {\n Node *current_node = *start;\n Node *new_node = create_node();\n\n if (current_node == NULL) {\n *start = new_node;\n current_node = new_node;\n current_node->next_pointer = NULL;\n }\n while (current_node->next_pointer != NULL) {\n current_node = current_node->next_pointer;\n }\n new_node->data = value;\n current_node->next_pointer = new_node;\n new_node->next_pointer = NULL;\n}\n\n/*\n Deleting\n*/\nvoid deleting(int value, Node **start) {\n Node *current_node = *start;\n Node *next_node = current_node->next_pointer;\n\n if (current_node == NULL) {\n cout << \"Underflow\" << endl;\n exit(1);\n } else if (current_node->data == value) {\n *start = current_node->next_pointer;\n return;\n }\n while (current_node->next_pointer != NULL && next_node->data != value) {\n current_node = current_node->next_pointer;\n next_node = current_node->next_pointer;\n }\n\n current_node->next_pointer = next_node->next_pointer;\n free(next_node);\n}"
}
{
"filename": "code/data_structures/src/Linked_List/inserting_a_node.cpp",
"content": "#include <iostream>\n\nusing namespace std;\n\ntypedef struct node Node;\nstruct node {\n int data;\n Node *next_pointer;\n};\n\nNode *create_node();\nvoid insert_in_beginning(int value, Node **start);\nvoid insert_in_ending(int value, Node **start);\nvoid insert_in_specific_position(int value, Node *start);\nvoid linked_list_print(Node *start);\n\nint main() {\n int arr[] = {5, 3, 9, 42, 0, 10};\n int length = 6;\n Node *start = NULL;\n\n for (int i = 0; i < length; i++) {\n // insert_in_beginning(arr[i], &start);\n insert_in_ending(arr[i], &start);\n }\n linked_list_print(start);\n}\n\nNode *create_node() {\n Node *node = (Node *)malloc(sizeof(Node));\n\n if (node == NULL) {\n cout << \"Error while creating new node\" << endl;\n }\n return node;\n}\n\nvoid insert_in_beginning(int value, Node **start) {\n Node *new_node = create_node();\n\n new_node->data = value;\n new_node->next_pointer = *start;\n *start = new_node;\n}\n\nvoid linked_list_print(Node *start) {\n if (start == NULL) {\n cout << \"Empty Linked List\" << endl;\n exit(1);\n }\n Node *current_node = start;\n while (current_node != NULL) {\n cout << current_node->data << \" \";\n current_node = current_node->next_pointer;\n }\n cout << endl;\n}\n\nvoid insert_in_ending(int value, Node **start) {\n Node *current_node = *start;\n Node *new_node = create_node();\n\n if (current_node == NULL) {\n *start = new_node;\n current_node = new_node;\n current_node->next_pointer = NULL;\n }\n while (current_node->next_pointer != NULL) {\n current_node = current_node->next_pointer;\n }\n new_node->data = value;\n current_node->next_pointer = new_node;\n new_node->next_pointer = NULL;\n}"
}
{
"filename": "code/data_structures/src/Linked_List/Intersection_of_two_sorted_lists.cpp",
"content": "Node* findIntersection(Node* head1, Node* head2)\n{\n \n if(head1==NULL or head2==NULL) return NULL;\n \n if(head1->data==head2->data) \n {\n head1->next=findIntersection(head1->next,head2->next);\n return head1;\n }\n \n else if(head1->data>head2->data) \n return findIntersection(head1,head2->next);\n \n else return findIntersection(head1->next,head2);\n}"
}
{
"filename": "code/data_structures/src/Linked_List/linked_list_palindrome.cpp",
"content": "// Check if the liked list is palindrome or not \n bool checkpalindrome(vector<int> arr){\n int s = 0;\n int e = arr.size() -1;\n while(s<=e){\n if(arr[s] != arr[e]){\n return 0;\n }\n s++;\n e--;\n }\n return 1;\n }\n \n bool isPalindrome(Node *head)\n {\n vector<int>arr;\n Node* temp = head;\n while(temp != NULL){\n arr.push_back(temp -> data);\n temp = temp -> next;\n }\n return checkpalindrome(arr);\n }"
}
{
"filename": "code/data_structures/src/Linked_List/pairwise_swap_on_linked_list.cpp",
"content": "// Swaps the data of linked list in pairs\nstruct Node* pairwise_swap(struct Node* head)\n{\n \n Node* curr = head;\n while(curr!= NULL && curr->next != NULL){\n swap(curr->data, curr->next->data);\n curr = curr->next->next;\n }\n return head;\n}"
}
{
"filename": "code/data_structures/src/Linked_List/remove_duplicate_element_from_sorted_linked_list.cpp",
"content": "// { Driver Code Starts\n#include <bits/stdc++.h>\nusing namespace std;\n\nstruct Node {\n int data;\n struct Node *next;\n Node(int x) {\n data = x;\n next = NULL;\n }\n};\n\n\nvoid print(Node *root)\n{\n Node *temp = root;\n while(temp!=NULL)\n {\n cout<<temp->data<<\" \";\n temp=temp->next;\n }\n}\nNode* removeDuplicates(Node *root);\nint main() {\n\t// your code goes here\n\tint T;\n\tcin>>T;\n\n\twhile(T--)\n\t{\n\t\tint K;\n\t\tcin>>K;\n\t\tNode *head = NULL;\n Node *temp = head;\n\n\t\tfor(int i=0;i<K;i++){\n\t\tint data;\n\t\tcin>>data;\n\t\t\tif(head==NULL)\n\t\t\thead=temp=new Node(data);\n\t\t\telse\n\t\t\t{\n\t\t\t\ttemp->next = new Node(data);\n\t\t\t\ttemp=temp->next;\n\t\t\t}\n\t\t}\n\t\t\n\t\tNode *result = removeDuplicates(head);\n\t\tprint(result);\n\t\tcout<<endl;\n\t}\n\treturn 0;\n}// } Driver Code Ends\n\n\n/*\nstruct Node {\n int data;\n struct Node *next;\n Node(int x) {\n data = x;\n next = NULL;\n }\n};*/\n\n//Function to remove duplicates from sorted linked list.\nNode *removeDuplicates(Node *head)\n{\n Node* current = head;\n Node* n;\n \n if (current == NULL) return head;\n \n while(current->next != NULL){\n if(current->data == current->next->data){\n n = current->next->next;\n free(current->next);\n current->next = n;\n }\n else current = current->next;\n }\n \n return head;\n}"
}
{
"filename": "code/data_structures/src/Linked_List/Remove_duplicates_in_unsorted_linked_list.cpp",
"content": "// Removethe duplicate elements in the linked list\nNode * removeDuplicates( Node *head) \n {\n Node* temp1=head;\n\n Node* temp2=head->next;\n unordered_set<int> s;\n\n while(temp2!=NULL)\n\n {\n s.insert(temp1->data);\n if(s.find(temp2->data)!=s.end())\n {\n Node* del=temp2;\n temp2=temp2->next;\n temp1->next=temp2;\n delete del;\n \n }\n\n else\n\n {\n temp1=temp1->next;\n temp2=temp2->next;\n\n }\n\n }\n\n return head;\n }"
}
{
"filename": "code/data_structures/src/Linked_List/reverse_linked_list_in_k_groups.cpp",
"content": "#include <iostream>\n\nusing namespace std;\n\n// Definition for singly-linked list.\nstruct ListNode {\n int val;\n ListNode* next;\n ListNode(int x) : val(x), next(nullptr) {}\n};\n\nclass Solution {\npublic:\n ListNode* reverseKGroup(ListNode* head, int k) {\n if (k <= 1 || !head) {\n return head; // No need to reverse if k <= 1 or the list is empty.\n }\n\n ListNode* dummy = new ListNode(0);\n dummy->next = head;\n ListNode* prev_group_tail = dummy;\n ListNode* current = head;\n\n while (true) {\n int count = 0;\n ListNode* group_start = current;\n ListNode* group_end = current;\n\n // Find the kth node in the group.\n while (count < k && group_end) {\n group_end = group_end->next;\n count++;\n }\n\n if (count < k || !group_end) {\n // If the remaining group has fewer than k nodes, stop.\n break;\n }\n\n ListNode* next_group_start = group_end->next;\n\n // Reverse the current group.\n ListNode* prev = next_group_start;\n ListNode* curr = group_start;\n while (curr != next_group_start) {\n ListNode* temp = curr->next;\n curr->next = prev;\n prev = curr;\n curr = temp;\n }\n\n prev_group_tail->next = group_end;\n group_start->next = next_group_start;\n prev_group_tail = group_start;\n current = next_group_start;\n }\n\n ListNode* new_head = dummy->next;\n delete dummy;\n return new_head;\n }\n};\n\n// Helper function to create a linked list from an array.\nListNode* createLinkedList(int arr[], int n) {\n if (n == 0) {\n return nullptr;\n }\n ListNode* head = new ListNode(arr[0]);\n ListNode* current = head;\n for (int i = 1; i < n; i++) {\n current->next = new ListNode(arr[i]);\n current = current->next;\n }\n return head;\n}\n\n// Helper function to print a linked list.\nvoid printLinkedList(ListNode* head) {\n ListNode* current = head;\n while (current != nullptr) {\n cout << current->val << \" -> \";\n current = current->next;\n }\n cout << \"nullptr\" << endl;\n}\n\nint main() {\n int arr[] = {1, 2, 3, 4, 5};\n int k = 2;\n\n ListNode* head = createLinkedList(arr, 5);\n\n Solution solution;\n ListNode* reversed = solution.reverseKGroup(head, k);\n\n printLinkedList(reversed);\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/Linked_List/reversing_linkedlist.cpp",
"content": "#include<bits/stdc++.h>\nusing namespace std;\nstruct Node{\n int value;\n struct Node* next;\n Node(int value){\n this->value=value;\n next=NULL;\n }\n};\n\nstruct LL{\nNode* head;\n LL(){\n head=NULL;\n }\n void reverse(){\n Node* curr=head;\n Node* prev=NULL,*next=NULL;\n while(curr!=NULL){\n next=curr->next;\n curr->next=prev;\n prev=current;\n current=next;\n }\n head=prev;\n }\n void print(){\n struct Node* temp=head;\n while(temp!=NULL){\n cout<<temp->value<<\" \";\n temp=temp->next;\n }\n }\n void push(int value){\n Node* temp=new Node(value);\n temp->next=head;\n head=temp;\n }\n};\nint main(){\nLL obj;\nobj.push(5);\nobj.push(60);\nobj.push(4);\nobj.push(9);\ncout<<\"Entered linked list is: \"<<endl;\nobj.print();\nobj.reverse();\ncout<<\"Reversed linked list is: \"<<endl;\nobj.print();\n return 0;\n}\n\n\n}"
}
{
"filename": "code/data_structures/src/Linked_List/sort_a_linked_list.cpp",
"content": "#include <iostream>\n\nusing namespace std;\n\ntypedef struct node Node;\nstruct node {\n int data;\n Node *next_pointer;\n};\n\nNode *create_node();\nvoid insert_in_beginning(int value, Node **start);\nvoid insert_in_ending(int value, Node **start);\nvoid insert_in_specific_position(int value, Node *start);\nvoid linked_list_print(Node *start);\nvoid sort_linked_lis(Node **start);\nvoid insert_sorted_linked_list(int value, Node *start);\n\nint main() {\n int arr[] = {5, 3, 9, 42, 0, 10};\n int length = 6;\n Node *start = NULL;\n\n for (int i = 0; i < length; i++) {\n // insert_in_beginning(arr[i], &start);\n insert_in_ending(arr[i], &start);\n }\n linked_list_print(start);\n}\n\nNode *create_node() {\n Node *node = (Node *)malloc(sizeof(Node));\n\n if (node == NULL) {\n cout << \"Error while creating new node\" << endl;\n }\n return node;\n}\n\nvoid linked_list_print(Node *start) {\n if (start == NULL) {\n cout << \"Empty Linked List\" << endl;\n exit(1);\n }\n Node *current_node = start;\n while (current_node != NULL) {\n cout << current_node->data << \" \";\n current_node = current_node->next_pointer;\n }\n cout << endl;\n}\n\nvoid insert_in_ending(int value, Node **start) {\n Node *current_node = *start;\n Node *new_node = create_node();\n\n if (current_node == NULL) {\n *start = new_node;\n current_node = new_node;\n current_node->next_pointer = NULL;\n }\n while (current_node->next_pointer != NULL) {\n current_node = current_node->next_pointer;\n }\n new_node->data = value;\n current_node->next_pointer = new_node;\n new_node->next_pointer = NULL;\n}\n\nvoid sort_linked_lis(Node **start) {\n Node *current_node = *start;\n Node *new_node = create_node();\n *start = new_node;\n new_node->data = current_node->data;\n current_node = current_node->next_pointer;\n while (current_node != NULL) {\n insert_sorted_linked_list(current_node->data, *start);\n current_node = current_node->next_pointer;\n }\n}\n\nvoid insert_sorted_linked_list(int value, Node *start) {\n Node *current_node = start;\n while (current_node->next_pointer != NULL && current_node->data) {\n current_node = current_node->next_pointer;\n }\n}"
}
{
"filename": "code/data_structures/src/Linked_List/swap_nodes_in_pairs.cpp",
"content": "ListNode* swapPairs(ListNode* head) {\n ListNode **pp = &head, *a, *b;\n while ((a = *pp) && (b = a->next)) {\n a->next = b->next;\n b->next = a;\n *pp = b;\n pp = &(a->next);\n }\n return head;\n}"
}
{
"filename": "code/data_structures/src/Linked_List/traverse_a_linked_list.cpp",
"content": "#include <iostream>\n\nusing namespace std;\n\ntypedef struct node Node;\nstruct node {\n int data;\n Node *next_pointer;\n};\n\nNode *create_node();\nvoid insert_in_beginning(int value, Node **start);\nvoid insert_in_ending(int value, Node **start);\nvoid insert_in_specific_position(int value, Node *start);\nvoid linked_list_print(Node *start);\n\nint main() {\n int arr[] = {5, 3, 9, 42, 0, 10};\n int length = 6;\n Node *start = NULL;\n\n for (int i = 0; i < length; i++) {\n // insert_in_beginning(arr[i], &start);\n insert_in_ending(arr[i], &start);\n }\n linked_list_print(start);\n}\n\nNode *create_node() {\n Node *node = (Node *)malloc(sizeof(Node));\n\n if (node == NULL) {\n cout << \"Error while creating new node\" << endl;\n }\n return node;\n}\n\nvoid insert_in_beginning(int value, Node **start) {\n Node *new_node = create_node();\n\n new_node->data = value;\n new_node->next_pointer = *start;\n *start = new_node;\n}\n\n/*\n Traversing\n*/\n\nvoid linked_list_print(Node *start) {\n if (start == NULL) {\n cout << \"Empty Linked List\" << endl;\n exit(1);\n }\n Node *current_node = start;\n while (current_node != NULL) {\n cout << current_node->data << \" \";\n current_node = current_node->next_pointer;\n }\n cout << endl;\n}\n\nvoid insert_in_ending(int value, Node **start) {\n Node *current_node = *start;\n Node *new_node = create_node();\n\n if (current_node == NULL) {\n *start = new_node;\n current_node = new_node;\n current_node->next_pointer = NULL;\n }\n while (current_node->next_pointer != NULL) {\n current_node = current_node->next_pointer;\n }\n new_node->data = value;\n current_node->next_pointer = new_node;\n new_node->next_pointer = NULL;\n}"
}
{
"filename": "code/data_structures/src/list/circular_linked_list/circular_linked_list.c",
"content": "#include <stdio.h>\r\n#include <stdlib.h>\r\n#include <time.h>\r\n\r\ntypedef struct Node node;\r\nvoid display(node *tail);\r\nnode *insert_at_end(node *tail);\r\nnode *insert_at_front(node *tail);\r\nnode *delete_at_begin(node *tail);\r\nnode *delete_at_end(node *tail);\r\n\r\nstruct Node\r\n{\r\n\tint Data;\r\n\tstruct Node *next;\r\n};\r\n/*Traversing circular linked list*/\r\n\r\nnode *random_list(node *tail)\r\n{\r\n\tint t;\r\n\tprintf(\"Enter number of elements: \");\r\n\tscanf(\"%d\",&t);\r\n\tfree(tail);\r\n\ttail=(node*)malloc(sizeof(node));\r\n\tnode* head=tail;\r\n\ttail->Data=rand()%20 +1;\r\n\ttail->next=tail;\r\n\tfor (int i = 0; i < t-1; i++)\r\n\t{\r\n\t\tnode* new=(node*)malloc(sizeof(node));\r\n\t\tnew->Data=rand()%20 + 1;\r\n\t\ttail->next=new;\r\n\t\tnew->next=head;\r\n\t\ttail=new;\r\n\t}\r\n\treturn head;\r\n}\r\n\r\nvoid display(node *tail)\r\n{\r\n\tnode *root, *ptr;\r\n\tif (tail == NULL)\r\n\t\tprintf(\"\\nList is empty\\n\\n\");\r\n\telse\r\n\t{\r\n\t\troot = tail->next;\r\n\t\tptr = root;\r\n\t\twhile (ptr != tail)\r\n\t\t{\r\n\t\t\tprintf(\"%d \", ptr->Data);\r\n\t\t\tptr = ptr->next;\r\n\t\t}\r\n\t\tprintf(\"%d\\n\", ptr->Data);\r\n\t}\r\n}\r\n/*Inserting node at front of the list*/\r\nnode\r\n\t*\r\n\tinsert_at_front(node *tail)\r\n{ /*Creating new node*/\r\n\tnode *temp = (node *)malloc(sizeof(node));\r\n\tprintf(\"\\nEnter Data:\");\r\n\tscanf(\"%d\", &temp->Data);\r\n\t/*Check initial condition for list ,it is empty or not*/\r\n\tif (tail == NULL)\r\n\t{\r\n\t\ttail = temp;\r\n\t\ttail->next = temp;\r\n\t}\r\n\telse\r\n\t{\r\n\t\ttemp->next = tail->next;\r\n\t\ttail->next = temp;\r\n\t}\r\n\treturn (tail);\r\n}\r\n/*Inserting node at the end of list*/\r\nnode\r\n\t*\r\n\tinsert_at_end(node *tail)\r\n{\r\n\tnode *temp = (node *)malloc(sizeof(node));\r\n\tprintf(\"\\nEnter Data:\");\r\n\tscanf(\"%d\", &temp->Data);\r\n\tif (tail == NULL)\r\n\t{\r\n\t\ttail = temp;\r\n\t\ttail->next = temp;\r\n\t}\r\n\telse\r\n\t{\r\n\t\tnode *root;\r\n\t\troot = tail->next;\r\n\t\ttail->next = temp;\r\n\t\ttemp->next = root;\r\n\t\ttail = temp;\r\n\t}\r\n\treturn (tail);\r\n}\r\n/*Deleting node at the front of list*/\r\nnode\r\n\t*\r\n\tdelete_at_begin(node *tail)\r\n{\r\n\tif (tail == NULL)\r\n\t\tprintf(\"\\nList is Empty\\n\\n\");\r\n\telse\r\n\t{\r\n\t\tif (tail->next == tail)\r\n\t\t{\r\n\t\t\tfree(tail);\r\n\t\t\ttail = NULL;\r\n\t\t}\r\n\t\telse\r\n\t\t{\r\n\t\t\tnode *root, *ptr;\r\n\t\t\tptr = root = tail->next;\r\n\t\t\troot = root->next;\r\n\t\t\ttail->next = root;\r\n\t\t\tfree(ptr);\r\n\t\t}\r\n\t}\r\n\treturn (tail);\r\n}\r\n/*Deleting node at the end of list*/\r\nnode\r\n\t*\r\n\tdelete_at_end(node *tail)\r\n{\r\n\tif (tail == NULL)\r\n\t\tprintf(\"\\nList is empty\\n\\n\");\r\n\telse\r\n\t{\r\n\t\tif (tail->next == tail)\r\n\t\t{\r\n\t\t\tfree(tail);\r\n\t\t\ttail = NULL;\r\n\t\t}\r\n\t\telse\r\n\t\t{\r\n\t\t\tnode *root;\r\n\t\t\troot = tail->next;\r\n\t\t\twhile (root->next != tail)\r\n\t\t\t{\r\n\t\t\t\troot = root->next;\r\n\t\t\t}\r\n\t\t\troot->next = tail->next;\r\n\t\t\tfree(tail);\r\n\t\t\ttail = root;\r\n\t\t}\r\n\t}\r\n\treturn (tail);\r\n}\r\n\r\nint main(int argc, char *argv[])\r\n{ /*Declaring tail pointer to maintain last node address*/\r\n\tsrand(time(0));\r\n\tnode *tail = NULL;\r\n\tint choice;\r\n\twhile (1)\r\n\t{ /*We can also write print function outside of while loop */\r\n\t\tprintf(\"1.Create a random list\\n2.Insert at front\\n3.Insert at end \\n4.Display\\n5.Delete from front\\n6.Delete from End\\n7.Exit\\nEnter choice:\");\r\n\t\tscanf(\"%d\", &choice);\r\n\t\tswitch (choice)\r\n\t\t{\r\n\r\n\t\tcase 1:\r\n\t\t\ttail = random_list(tail);\r\n\t\t\tbreak;\r\n\t\tcase 2:\r\n\t\t\ttail = insert_at_front(tail);\r\n\t\t\tbreak;\r\n\t\tcase 3:\r\n\t\t\ttail = insert_at_end(tail);\r\n\t\t\tbreak;\r\n\t\tcase 4:\r\n\t\t\tdisplay(tail);\r\n\t\t\tbreak;\r\n\t\tcase 5:\r\n\t\t\ttail = delete_at_begin(tail);\r\n\t\t\tbreak;\r\n\t\tcase 6:\r\n\t\t\ttail = delete_at_end(tail);\r\n\t\t\tbreak;\r\n\t\tcase 7:\r\n\t\t\treturn (0);\r\n\t\t}\r\n\t}\r\n}\r"
}
{
"filename": "code/data_structures/src/list/circular_linked_list/circular_linked_list.cpp",
"content": "#include <iostream>\n// Part of Cosmos by OpenGenus Foundation\nusing namespace std;\n\nint main()\n{\n\n struct node\n {\n int info;\n node *next;\n }*ptr, *head, *start;\n\n int c = 1, data;\n\n ptr = new node;\n ptr->next = NULL;\n\n start = head = ptr;\n\n while (c < 3 && c > 0)\n {\n cout << \"1.Insert\\n2.Link List\\n\";\n cin >> c;\n\n switch (c){\n case 1:\n cout << \"Enter Data\\n\";\n cin >> data;\n\n ptr = new node;\n ptr->next = start;\n ptr->info = data;\n\n head->next = ptr;\n head = ptr;\n\n break;\n\n case 2:\n ptr = start->next;\n\n while (ptr != start && ptr != NULL)\n {\n cout << ptr->info << \"->\";\n ptr = ptr->next;\n }\n cout << \"\\n\";\n break;\n\n default:\n cout << \"Wrong Choice\\nExiting...\\n\";\n }\n\n }\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/circular_linked_list/circular_linked_list.java",
"content": "import java.util.Scanner;\n\n// Part of Cosmos by OpenGenus Foundation\npublic class CircularLinkedList {\n\n Node head, tail;\n\n // Node Class\n class Node {\n int value;\n Node next;\n\n public Node(int value, Node next) {\n this.value = value;\n this.next = next;\n }\n }\n\n // Manipulation Methods\n public void Add(int value) {\n Node n = new Node(value, head);\n if (head == null) {\n head = n;\n tail = n;\n n.next = n;\n }\n else {\n tail.next = n;\n tail = n;\n }\n }\n\n public void Remove(int value) {\n Node aux = head;\n Node prev = tail;\n boolean found = false;\n\n // Find the node with the value\n if (aux == null) return;\n if (aux == head && aux.value == value) { found = true; }\n else {\n prev = aux;\n aux = aux.next;\n while (aux != head) {\n if (aux.value == value) {\n found = true;\n break;\n }\n prev = aux;\n aux = aux.next;\n }\n }\n\n // If found, remove it\n if (!found) return;\n if (aux == head && aux == tail) { head = tail = null; }\n else {\n if (aux == head) head = aux.next;\n if (aux == tail) tail = prev;\n prev.next = aux.next;\n }\n }\n\n public void Print() {\n if (head == null) return;\n System.out.print(head.value + \" \");\n Node aux = head.next;\n while (aux != head) { System.out.print(aux.value + \" \"); aux = aux.next; }\n System.out.println();\n System.out.println(\"HEAD is \" + head.value);\n System.out.println(\"TAIL is \" + tail.value);\n }\n\n // Example Usage\n public static void main(String[] args) {\n int selection = 0;\n Scanner input = new Scanner(System.in);\n CircularLinkedList list = new CircularLinkedList();\n\n do {\n System.out.print(\"1. Insert\\n2. Remove\\n3. Print\\n> \");\n selection = input.nextInt();\n\n if (selection == 1) {\n System.out.print(\"Enter Value to Insert: \");\n int value = input.nextInt();\n list.Add(value);\n list.Print();\n System.out.print(\"\\n\");\n }\n else if (selection == 2) {\n System.out.print(\"Enter Value to Remove: \");\n int value = input.nextInt();\n list.Remove(value);\n list.Print();\n System.out.println(\"\\n\");\n }\n else if (selection == 3) {\n list.Print();\n System.out.println(\"\\n\");\n }\n else {\n System.out.println(\"Invalid Selection. Exiting\");\n }\n }\n while (selection > 0 && selection < 4);\n input.close();\n }\n}"
}
{
"filename": "code/data_structures/src/list/circular_linked_list/circular_linked_list.py",
"content": "class Node:\n\n # Constructor to create a new node\n def __init__(self, data):\n self.data = data\n self.next = None\n\n\nclass CircularLinkedList:\n\n # Constructor to create a empty circular linked list\n def __init__(self):\n self.head = None\n\n # Function to insert a node at the beginning of a\n # circular linked list\n def push(self, data):\n ptr1 = Node(data)\n temp = self.head\n\n ptr1.next = self.head\n\n # If linked list is not None then set the next of\n # last node\n if self.head is not None:\n while temp.next != self.head:\n temp = temp.next\n temp.next = ptr1\n\n else:\n ptr1.next = ptr1 # For the first node\n\n self.head = ptr1\n\n # Function to print nodes in a given circular linked list\n def printList(self):\n temp = self.head\n if self.head is not None:\n while True:\n print(\"%d\" % (temp.data), end=\" \")\n temp = temp.next\n if temp == self.head:\n break\n\n\n# Driver program to test above function\n\n# Initialize list as empty\ncllist = CircularLinkedList()\n\n# Created linked list will be 11->2->56->12\ncllist.push(12)\ncllist.push(56)\ncllist.push(2)\ncllist.push(11)\n\nprint(\"Contents of circular Linked List\")\ncllist.printList()"
}
{
"filename": "code/data_structures/src/list/circular_linked_list/operations/FloydAlgo_circular_ll.cpp",
"content": "#include <iostream>\nusing namespace std;\n\nstruct node{\n int data;\n struct node* next;\n};\nbool isCircular(node *head)\n{ //fast ptr is ahead of slow pointer\n node* slow,*fast;\n slow=head;\n fast=head->next;\n //if linked list is empty it returns true as its entirely null.\n if(head==NULL)\n return true;\n while(true)\n { //fast ptr traverses quickly so If its not circular then it reaches or points to null.\n if(fast==NULL||fast->next==NULL)\n {\n return false;\n }\n //when circular fast meets or points to slow pointer while traversing\n else if(slow==fast||fast->next==slow)\n {\n return true;\n }\n //for moving forward through linked list.\n else\n {\n slow=slow->next;\n //fast traverses way ahead so it distinguishes loops out of circular list.\n fast=fast->next->next;\n }\n }\n}\n\nnode *newNode(int data){\n struct node *temp=new node;\n temp->data=data;\n temp->next=NULL;\n}\nint main() {\n struct node* head=newNode(1);\n head->next=newNode(2);\n head->next->next=newNode(12);\n head->next->next->next=newNode(122);\n head->next->next->next->next=head;\n if(isCircular(head))\n cout<<\"yes\"<<endl;\n else\n cout<<\"no\"<<endl;\n struct node* head1=newNode(1);\n head1->next=newNode(2);\n head1->next->next=newNode(3);\n head1->next->next->next=newNode(7);\n\n if(isCircular(head1))\n cout<<\"yes\";\n else\n cout<<\"no\";\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/circular_linked_list/operations/has_loop.py",
"content": "import collections\n\n\nclass LinkedList(collections.Iterable):\n class Node(object):\n def __init__(self, data, next=None):\n super(LinkedList.Node, self).__init__()\n self.data = data\n self.next = next\n\n class LinkedListIterator(collections.Iterator):\n def __init__(self, head):\n self._cur = head\n\n def __iter__(self):\n return self\n\n def next(self):\n if self._cur is None:\n raise StopIteration\n\n retval = self._cur\n self._cur = self._cur.next\n\n return retval\n\n def __init__(self):\n super(LinkedList, self).__init__()\n self.head = None\n\n def __iter__(self):\n it = LinkedList.LinkedListIterator(self.head)\n return iter(it)\n\n def add(self, data):\n # add new node to head of list\n new = LinkedList.Node(data, self.head)\n self.head = new\n return new\n\n def add_node(self, node):\n node.next = self.head\n self.head = node\n return node\n\n def rm(self, node):\n # del node from list\n it = iter(self)\n prev = self.head\n try:\n while True:\n n = it.next()\n if n is node:\n if n is self.head:\n self.head = self.head.next\n else:\n prev.next = n.next\n return\n prev = n\n except StopIteration:\n raise KeyError\n\n def has_loop(self):\n class Skip2Iterator(LinkedList.LinkedListIterator):\n def next(self):\n super(Skip2Iterator, self).next()\n return super(Skip2Iterator, self).next()\n\n it = LinkedList.LinkedListIterator(self.head)\n it2 = Skip2Iterator(self.head)\n try:\n while True:\n n1 = it.next()\n n2 = it2.next()\n if n1 is n2:\n return True\n except StopIteration:\n return False"
}
{
"filename": "code/data_structures/src/list/circular_linked_list/operations/is_circular_linked_list.cpp",
"content": "#include <iostream>\nusing namespace std;\n//node structure\nstruct node{\n int data;\n struct node* next;\n};\n//function to find the circular linked list.\nbool isCircular(node *head){\n node *temp=head;\n while(temp!=NULL)\n { //if temp points to head then it has completed a circle,thus a circular linked list.\n if(temp->next==head)\n return true;\n temp=temp->next;\n }\n\n return false;\n\n}\n//function for inserting new nodes.\nnode *newNode(int data){\n struct node *temp=new node;\n temp->data=data;\n temp->next=NULL;\n}\nint main() {\n //first case\n struct node* head=newNode(1);\n head->next=newNode(2);\n head->next->next=newNode(3);\n head->next->next->next=newNode(4);\n head->next->next->next->next=head;\n if(isCircular(head))\n cout<<\"yes\"<<endl;\n else\n cout<<\"no\"<<endl;\n //second case\n struct node* head1=newNode(1);\n head1->next=newNode(2);\n\n if(isCircular(head1))\n cout<<\"yes\";\n else\n cout<<\"no\";\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/circular_linked_list/operations/is_circular.py",
"content": "# uses try block so dont have to check if reached the end, provides faster runtime. credit-leetcode\ndef is_circular(self, head):\n \"\"\"\n\tfunction checks if a link list has a cycle or not\n\t\"\"\"\n try:\n slow = head\n fast = head.next\n\n while slow is not fast:\n slow = slow.next\n fast = fast.next.next\n return True\n except:\n return False"
}
{
"filename": "code/data_structures/src/list/doubly_linked_list/c/doubly_linked_list.c",
"content": "#include <stdio.h>\n#include <stdint.h>\n#include <stdlib.h>\n#include \"doubly_linked_list.h\"\n\ndllist_t *dl_create() {\n dllist_t *l = (dllist_t *) calloc(1, sizeof(dllist_t));\n return l;\n}\n\nint8_t dl_destroy(dllist_t **l) {\n if (*l == (dllist_t *) NULL)\n\treturn ERR_NO_LIST;\n\n if ((*l)->head == (node_t *) NULL)\n\treturn ERR_EMPTY_LIST;\n\n node_t *p = (*l)->head;\n\n while (p != (node_t *) NULL) {\n\t(*l)->head = p->next;\n\tfree(p);\n\tp = (*l)->head;\n }\n\n (*l)->tail = (node_t *) NULL;\n *l = (dllist_t *) NULL;\n\n return SUCCESS;\n}\n\nint8_t dl_insert_beginning(dllist_t *l, int32_t value) {\n if (l == (dllist_t *) NULL)\n\treturn ERR_NO_LIST;\n\n node_t *nn = (node_t *) calloc(1, sizeof(node_t));\n\n if (nn == (node_t *) NULL)\n\treturn ERR_MALLOC_FAIL;\n\n nn->id = l->tail_id++;\n nn->data = value;\n nn->prev = (node_t *) NULL;\n\n node_t *p = l->head;\n\n if (p == (node_t *) NULL) {\n\tl->tail = nn;\n } else {\n\tnn->next = p;\n\tp->prev = nn;\n }\n l->head = nn;\n l->len++;\n\n return SUCCESS;\n}\n\nint8_t dl_insert_tail(dllist_t *l, int32_t value) {\n if (l == (dllist_t *) NULL)\n\treturn ERR_NO_LIST;\n\n node_t *nn = (node_t *) calloc(1, sizeof(node_t));\n\n if (nn == (node_t *) NULL)\n\treturn ERR_MALLOC_FAIL;\n\n nn->id = l->tail_id++;\n nn->data = value;\n node_t *p = l->tail;\n\n if (l->head == (node_t *) NULL) {\n\tl->head = nn;\n\tl->tail = nn;\n } else {\n\tp->next = nn;\n\tnn->prev = p;\n\tl->tail = nn;\n }\n l->len++;\n\n return SUCCESS;\n}\n\nint8_t dl_insert_after(dllist_t *l, int32_t key, int32_t value) {\n if (l == (dllist_t *) NULL)\n\treturn ERR_NO_LIST;\n\n if (l->head == (node_t *) NULL)\n\treturn ERR_EMPTY_LIST;\n\n node_t *nn = (node_t *) calloc(1, sizeof(node_t));\n\n if (nn == (node_t *) NULL)\n\treturn ERR_MALLOC_FAIL;\n\n nn->id = l->tail_id++;\n nn->data = value;\n\n node_t *p = l->head;\n\n while (p != (node_t *) NULL) {\n\tif (p->data == key) {\n\t nn->next = p->next;\n\t p->next = nn;\n\t nn->prev = p;\n\t if (p == l->tail) {\n\t\tl->tail = nn;\n\t }\n\t break;\n\t}\n\tp = p->next;\n }\n\n return SUCCESS;\n}\n\nint8_t dl_insert_before(dllist_t *l, int32_t key, int32_t value) {\n if (l == (dllist_t *) NULL)\n\treturn ERR_NO_LIST;\n\n if (l->head == (node_t *) NULL)\n\treturn ERR_EMPTY_LIST;\n\n node_t *nn = (node_t *) calloc(1, sizeof(node_t));\n\n if (nn == (node_t *) NULL)\n\treturn ERR_MALLOC_FAIL;\n\n nn->id = l->tail_id++;\n nn->data = value;\n\n node_t *p = l->head;\n\n while (p != (node_t *) NULL) {\n\tif (p->data == key) {\n\t if (p == l->head) {\n\t\tl->head = nn;\n\t } else {\n\t\tp->prev->next = nn;\n\t }\n\t nn->next = p;\n\t nn->prev = p->prev;\n\n\t break;\n\t}\n\tp = p->next;\n }\n\n return SUCCESS;\n}\n\nint8_t dl_remove_beginning(dllist_t *l) {\n if (l == (dllist_t *) NULL)\n\treturn ERR_NO_LIST;\n\n if (l->head == (node_t *) NULL)\n\treturn ERR_EMPTY_LIST;\n\n node_t *p = l->head;\n\n l->head = p->next;\n p->next = (node_t *) NULL;\n\n free(p);\n\n l->len--;\n\n return SUCCESS;\n}\n\nint8_t dl_remove_tail(dllist_t *l) {\n if (l == (dllist_t *) NULL)\n\treturn ERR_NO_LIST;\n\n if (l->head == (node_t *) NULL)\n\treturn ERR_EMPTY_LIST;\n\n node_t *p = l->tail;\n\n if (p == l->head) {\n\tl->head = (node_t *) NULL;\n } else {\n\tp->prev->next = (node_t *) NULL;\n\tl->tail = p->prev;\n }\n\n free(p);\n\n l->len--;\n\n return SUCCESS;\n\n}\n\nint8_t dl_remove_next(dllist_t *l, int32_t key) {\n if (l == (dllist_t *) NULL)\n\treturn ERR_NO_LIST;\n\n if (l->head == (node_t *) NULL)\n\treturn ERR_EMPTY_LIST;\n\n node_t *p = l->head;\n node_t *r = (node_t *) NULL;\n\n while (p->next != (node_t *) NULL) {\n\tif (p->id == key) {\n\t r = p->next;\n\n\t if (r->next == (node_t *) NULL) {\n\t\tl->tail = p;\n\t\tp->next = (node_t *) NULL;\n\t } else {\n\t\tr->next->prev = p;\n\t\tp->next = r->next;\n\t }\n\n\t free(r);\n\t l->len--;\n\t r = (node_t *) NULL;\n\t break;\n\t}\n\tp = p->next;\n }\n\n return SUCCESS;\n}\n\nint8_t dl_remove_prev(dllist_t *l, int32_t key) {\n if (l == (dllist_t *) NULL)\n\treturn ERR_NO_LIST;\n\n if (l->head == (node_t *) NULL)\n\treturn ERR_EMPTY_LIST;\n\n node_t *p = l->head->next;\n node_t *r = (node_t *) NULL;\n\n while (p != (node_t *) NULL) {\n\tif (p->id == key) {\n\t r = p->prev;\n\t if (r == l->head) {\n\t\tl->head = p;\n\t\tp->prev = (node_t *) NULL;\n\t } else {\n\t\tp->prev = r->prev;\n\t\tr->prev->next = p;\n\t }\n\t free(r);\n\t l->len--;\n\t r = (node_t *) NULL;\n\t break;\n\t}\n\tp = p->next;\n }\n\n return SUCCESS;\n}"
}
{
"filename": "code/data_structures/src/list/doubly_linked_list/c/doubly_linked_list.h",
"content": "#ifndef _D_L_LIST_H_\n#define _D_L_LIST_H_\n\n#include <stdint.h>\n\n#define SUCCESS 0\n#define ERR_NO_LIST -1\n#define ERR_EMPTY_LIST -2\n#define ERR_MALLOC_FAIL -3\n\ntypedef struct node_s {\n int32_t id;\n int32_t data;\n struct node_s *next;\n struct node_s *prev;\n} node_t ;\n\ntypedef struct dllist_s {\n int32_t len;\n int32_t tail_id;\n node_t *head;\n node_t *tail;\n} dllist_t;\n\ndllist_t * dl_create ();\nint8_t dl_destroy (dllist_t **l);\n\nint8_t dl_insert_beginning (dllist_t *l, int32_t value);\nint8_t dl_insert_end (dllist_t *l, int32_t value);\nint8_t dl_insert_after (dllist_t *l, int32_t key, int32_t value);\nint8_t dl_insert_before (dllist_t *l, int32_t key, int32_t value);\n\nint8_t dl_remove_beginning (dllist_t *l);\nint8_t dl_remove_end (dllist_t *l);\nint8_t dl_remove_next (dllist_t *l, int32_t key);\nint8_t dl_remove_prev (dllist_t *l, int32_t key);\n\n#endif"
}
{
"filename": "code/data_structures/src/list/doubly_linked_list/doubly_linked_list.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n/* Contributed by Vaibhav Jain (vaibhav29498) */\n#include <iostream>\n#include <cstdlib>\nusing namespace std;\n\ntemplate <typename T>\nstruct node\n{\n T info;\n node* pre; // Holds the pointer to the previous node\n node* next; // Holds the pointer to the next node\n node();\n};\n\ntemplate <typename T> node<T>::node()\n{\n info = 0;\n pre = nullptr;\n next = nullptr;\n}\n\ntemplate <class T>\nclass doubleLinkedList\n{\n node<T>* head;\npublic:\n doubleLinkedList();\n int listSize();\n void insertNode(T, int);\n void deleteNode(int);\n void print();\n void reversePrint();\n void insertionSort();\n};\n\ntemplate <class T> doubleLinkedList<T>::doubleLinkedList()\n{\n head = nullptr;\n}\n\ntemplate <class T> int doubleLinkedList<T>::listSize()\n{\n int i = 0;\n node<T> *ptr = head;\n\n // The loop below traverses the list to find out the size\n while (ptr != nullptr)\n {\n ++i;\n ptr = ptr->next;\n }\n return i;\n}\n\ntemplate <class T> void doubleLinkedList<T>::insertNode(T i, int p)\n{\n node<T> *ptr = new node<T>, *cur = head;\n ptr->info = i;\n if (cur == nullptr)\n head = ptr; // New node becomes head if the list is empty\n else if (p == 1)\n {\n // Put the node at the beginning of the list and change head accordingly\n ptr->next = head;\n head->pre = ptr;\n head = ptr;\n }\n else if (p > listSize())\n {\n // Navigate to the end of list and add the node to the end of the list\n while (cur->next != nullptr)\n cur = cur->next;\n cur->next = ptr;\n ptr->pre = cur;\n }\n else\n {\n // Navigate to 'p'th element of the list and insert the new node before it\n int n = p - 1;\n while (n--)\n cur = cur->next;\n ptr->pre = cur->pre;\n ptr->next = cur;\n cur->pre->next = ptr;\n cur->pre = ptr;\n }\n cout << \"Node inserted!\" << endl;\n}\n\ntemplate <class T> void doubleLinkedList<T>::deleteNode(int p)\n{\n if (p > listSize())\n {\n // List does not have a 'p'th node\n cout << \"Underflow!\" << endl;\n return;\n }\n node<T> *ptr = head;\n if (p == 1)\n {\n // Update head when the first node is being deleted\n head = head->next;\n head->pre = nullptr;\n delete ptr;\n }\n else if (p == listSize())\n {\n // Navigate to the end of the list and delete the last node\n while (ptr->next != nullptr)\n ptr = ptr->next;\n ptr->pre->next = nullptr;\n delete ptr;\n }\n else\n {\n // Navigate to 'p'th element of the list and delete that node\n int n = p - 1;\n while (n--)\n ptr = ptr->next;\n ptr->pre->next = ptr->next;\n ptr->next->pre = ptr->pre;\n delete ptr;\n }\n cout << \"Node deleted!\" << endl;\n}\n\ntemplate <class T> void doubleLinkedList<T>::print()\n{\n // Traverse the entire list and print each node\n node<T> *ptr = head;\n while (ptr != nullptr)\n {\n cout << ptr->info << \" -> \";\n ptr = ptr->next;\n }\n cout << \"NULL\" << endl;\n}\n\ntemplate <class T> void doubleLinkedList<T>::reversePrint()\n{\n node<T> *ptr = head;\n\n // First, traverse to the last node of the list\n while (ptr->next != nullptr)\n ptr = ptr->next;\n\n // From the last node, use `pre` attribute to traverse backwards and print each node in reverse order\n while (ptr != nullptr)\n {\n cout << ptr->info << \" <- \";\n ptr = ptr->pre;\n }\n cout << \"NULL\" << endl;\n}\n\ntemplate <class T> void doubleLinkedList<T>::insertionSort() \n{\n if (!head || !head->next) \n {\n // The list is empty or has only one element, which is already sorted.\n return;\n }\n\n node<T> *sorted = nullptr; // Initialize a sorted sublist\n\n node<T> *current = head;\n while (current) {\n node<T> *nextNode = current->next;\n\n if (!sorted || current->info < sorted->info) \n {\n // If the sorted list is empty or current node's value is smaller than the \n // sorted list's head,insert current node at the beginning of the sorted list.\n current->next = sorted;\n current->pre = nullptr;\n if (sorted) {\n sorted->pre = current;\n }\n sorted = current;\n } \n else \n {\n // Traverse the sorted list to find the appropriate position for the current node.\n node<T> *temp = sorted;\n while (temp->next && current->info >= temp->next->info) \n {\n temp = temp->next;\n }\n // Insert current node after temp.\n current->next = temp->next;\n current->pre = temp;\n if (temp->next) \n {\n temp->next->pre = current;\n }\n temp->next = current;\n }\n\n current = nextNode; // Move to the next unsorted node\n }\n\n // Update the head of the list to point to the sorted sublist.\n head = sorted;\n cout<<\"Sorting Complete\"<<endl;\n}\n\nint main()\n{\n doubleLinkedList<int> l;\n int m, i, p;\n while (true)\n {\n system(\"cls\");\n cout << \"1.Insert\" << endl\n << \"2.Delete\" << endl\n << \"3.Print\" << endl\n << \"4.Reverse Print\" << endl\n << \"5.Sort\" << endl\n << \"6.Exit\" << endl;\n cin >> m;\n switch (m)\n {\n case 1:\n cout << \"Enter info and position \";\n cin >> i >> p;\n l.insertNode(i, p);\n break;\n case 2:\n cout << \"Enter position \";\n cin >> p;\n l.deleteNode(p);\n break;\n case 3:\n l.print();\n break;\n case 4:\n l.reversePrint();\n break;\n case 5:\n l.insertionSort();\n break;\n case 6:\n exit(0);\n default:\n cout << \"Invalid choice!\" << endl;\n }\n system(\"pause\");\n }\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/doubly_linked_list/doubly_linked_list.go",
"content": "// Part of Cosmos by OpenGenus Foundation\n\npackage main\n\nimport (\n\t\"fmt\"\n)\n\ntype Node struct {\n\tkey interface{}\n\tnext *Node\n\tprev *Node\n}\n\ntype DoubleLinkedList struct {\n\thead *Node\n\ttail *Node\n}\n\nfunc (l *DoubleLinkedList) Insert(key interface{}) {\n\tnewNode := &Node{key, l.head, nil}\n\tif l.head != nil {\n\t\tl.head.prev = newNode\n\t}\n\tl.head = newNode\n}\n\nfunc (l *DoubleLinkedList) Delete(key interface{}) {\n\ttemp := l.head\n\tprev := &Node{}\n\tif temp != nil && temp.key == key {\n\t\tl.head = temp.next\n\t\ttemp.next.prev = l.head\n\t\treturn\n\t}\n\tfor temp != nil && temp.key != key {\n\t\tprev = temp\n\t\ttemp = temp.next\n\t}\n\tif temp == nil {\n\t\treturn\n\t}\n\tprev.next = temp.next\n}\n\nfunc (l *DoubleLinkedList) Show() {\n\tlist := l.head\n\tfor list != nil {\n\t\tfmt.Printf(\"%+v <-> \", list.key)\n\t\tlist = list.next\n\t}\n\tfmt.Println()\n}\n\nfunc main() {\n\tl := DoubleLinkedList{}\n\tl.Insert(1)\n\tl.Insert(2)\n\tl.Insert(3)\n\tl.Insert(4)\n\tl.Insert(5)\n\tl.Insert(6)\n\tl.Delete(1)\n\tl.Delete(9)\n\tl.Show()\n}"
}
{
"filename": "code/data_structures/src/list/doubly_linked_list/doubly_linked_list.java",
"content": "import java.util.*;\n\n/* An implementation of Doubly Linked List of integer type */\n\n/* Methods : \n 1. Insert a particular value at a particular position\n 2. Delete node at a particular position\n 3. Traverse the list in forward direction\n 4. Traverse the list in reverse direction\n */\n\n\nclass Node {\n\tpublic Node next;\n\tpublic Node prev;\n\tint data;\n\n\tpublic Node(int data){\n\t\tthis.data = data;\n\t}\n}\n\nclass DoublyLinkedList {\n\tprivate Node first;\n\tprivate Node last;\n\tprivate int n = 0; //size\n\n\t/*-----Insert Method-----*/\n\tpublic void insert(int pos, int data){\n\t\tif (pos > n + 1){\n\t\t\tpos = n + 1;\n\t\t}\n\n\t\tNode newNode = new Node(data);\n\n\t\tif (first == null) {\n\t\t\tfirst = newNode;\n\t\t\tlast = first;\n\t\t\tn++;\n\t\t\treturn;\n\t\t}\n\n\t\tif (pos == 1){\n\t\t\tnewNode.next = first;\n\t\t\tfirst.prev = newNode;\n\t\t\tfirst = newNode;\n\t\t\tn++;\n\t\t\treturn;\n\t\t}\n\n\t\tif (pos == n + 1){\n\t\t\tnewNode.prev = last;\n\t\t\tlast.next = newNode;\n\t\t\tlast = newNode;\n\t\t\tn++;\n\t\t\treturn;\n\t\t}\n\n\t\tint c = 1;\n\t\tNode cur = first;\n\n\t\twhile (c != pos){\n\t\t\tc++;\n\t\t\tcur = cur.next;\n\t\t}\n\n\t\tnewNode.next = cur;\n\t\tnewNode.prev = cur.prev;\n\t\tcur.prev.next = newNode;\n\t\tcur.prev = newNode;\n\t\tn++;\n\n\t}\n\n\t/*-----Delete Method-----*/\n\tpublic void delete(int pos){\n\t\tif (n == 0) {\n\t\t\tSystem.out.println(\"List is Empty!\");\n\t\t\treturn;\n\t\t}\n\n\t\tif(pos > n) {\n\t\t\tpos = n;\n\t\t}\n\n\t\tif (pos == 1) {\n\t\t\tfirst = first.next;\n\t\t\tfirst.prev = null;\n\t\t\treturn;\n\t\t}\n\n\t\tint c = 1;\n\t\tNode cur = first;\n\n\t\twhile (c != pos) {\n\t\t\tc++;\n\t\t\tcur = cur.next;\n\t\t}\n\n\t\tif (cur.prev != null) {\n\t\t\tcur.prev.next = cur.next;\n\t\t}\n\t\tif (cur.next != null) {\n\t\t\tcur.next.prev = cur.prev;\n\t\t}\n\t\tn--;\n\t}\n\n\t/*-----Print in forward direction-----*/\n\tpublic void display() {\n\t\tNode cur = first;\n\n\t\twhile (cur != null) {\n\t\t\tSystem.out.print(cur.data + \"->\");\n\t\t\tcur = cur.next;\n\t\t}\n\n\t\tSystem.out.println(\"null\");\n\t}\n\n\t/*-----Print in reverse direction-----*/\n\tpublic void reverseDisplay() {\n\t\tNode cur = last;\n\n\t\twhile (cur != null) {\n\t\t\tSystem.out.print(cur.data + \"->\");\n\t\t\tcur = cur.prev;\n\t\t}\n\n\t\tSystem.out.println(\"null\");\n\t}\n}\n\nclass App {\n\tpublic static void main(String[] args) {\n\t\tScanner sc = new Scanner(System.in);\n\t\tDoublyLinkedList l = new DoublyLinkedList();\n\n\t\twhile (true) {\n\t\t\tSystem.out.println(\"1.Insert\");\n\t\t\tSystem.out.println(\"2.Delete\");\n\t\t\tSystem.out.println(\"3.Print\");\n\t\t\tSystem.out.println(\"4.Print in reverse order\");\n\t\t\tSystem.out.println(\"5.Exit\");\n\n\t\t\tint c = sc.nextInt();\n\n\t\t\tswitch(c) {\n\t\t\t\tcase 1:\n\t\t\t\t\tSystem.out.println(\"Enter insertion position\");\n\t\t\t\t\tint pos = sc.nextInt();\n\t\t\t\t\tSystem.out.println(\"Enter value\");\n\t\t\t\t\tint val = sc.nextInt();\n\t\t\t\t\tl.insert(pos, val);\n\t\t\t\t\tbreak;\n\t\t\t\tcase 2:\n\t\t\t\t\tSystem.out.println(\"Enter deletion position\");\n\t\t\t\t\tpos = sc.nextInt();\n\t\t\t\t\tl.delete(pos);\n\t\t\t\t\tbreak;\n\t\t\t\tcase 3:\n\t\t\t\t\tl.display();\n\t\t\t\t\tbreak;\n\t\t\t\tcase 4:\n\t\t\t\t\tl.reverseDisplay();\n\t\t\t\t\tbreak;\n\t\t\t\tcase 5:\n\t\t\t\t\treturn;\n\t\t\t\tdefault:\n\t\t\t\t\treturn;\n\t\t\t}\n\t\t}\n\t}\n}"
}
{
"filename": "code/data_structures/src/list/doubly_linked_list/doubly_linked_list.js",
"content": "/** Source: https://github.com/brunocalou/graph-theory-js/blob/master/src/data_structures/linked_list.js */\n\n/**@module dataStructures */\n\n\"use strict\";\n\n/**\n * Node class\n * @constructor\n * @public\n * @param {any} value - The value to be stored\n */\nfunction Node(value) {\n this.value = value;\n this.next = null;\n this.prev = null;\n}\n\n/**\n * Linked list class\n * @constructor\n */\nfunction LinkedList() {\n /**@private\n * @type {node}\n */\n this._front = null;\n\n /**@private\n * @type {node}\n */\n this._back = null;\n\n /**@private\n * @type{number}\n */\n this._length = 0;\n\n Object.defineProperty(this, \"length\", {\n get: function() {\n return this._length;\n }\n });\n}\n\n/**\n * Inserts the element at the specified position in the list. If the index is not specified, will append\n * it to the end of the list\n * @param {any} element - The element to be added\n * @param {number} index - The index where the element must be inserted\n */\nLinkedList.prototype.add = function(element, index) {\n if (index > this._length || index < 0) {\n throw new Error(\"Index out of bounds\");\n } else {\n if (index === undefined) {\n //Add the element to the end\n index = this._length;\n }\n\n var node = new Node(element);\n\n if (index === 0) {\n //Add the element to the front\n if (this._length !== 0) {\n node.next = this._front;\n this._front.prev = node;\n } else {\n //Add the element to the back if the list is empty\n this._back = node;\n }\n this._front = node;\n } else if (index === this._length) {\n //Add the element to the back\n if (this._length !== 0) {\n node.prev = this._back;\n this._back.next = node;\n }\n this._back = node;\n } else {\n //Add the element on the specified index\n var current_node = this._front;\n var i;\n\n //Check if the index is closer to the front or to the back and performs the\n //insertion accordingly\n if (index < this._length / 2) {\n for (i = 0; i < this._length; i += 1) {\n if (i === index) {\n break;\n }\n //Get the next node\n current_node = current_node.next;\n }\n } else {\n current_node = this._back;\n\n for (i = this._length - 1; i > 0; i -= 1) {\n if (i === index) {\n break;\n }\n //Get the previous node\n current_node = current_node.prev;\n }\n }\n\n node.prev = current_node.prev;\n node.next = current_node;\n\n current_node.prev.next = node;\n\n current_node.prev = node;\n }\n\n this._length += 1;\n }\n};\n\n/**\n * Inserts the element at the begining of the list\n * @param {any} element - The element to be added\n */\nLinkedList.prototype.addFirst = function(element) {\n this.add(element, 0);\n};\n\n/**\n * Inserts the element at the end of the list\n * @param {any} element - The element to be added\n */\nLinkedList.prototype.addLast = function(element) {\n this.add(element);\n};\n\n/**\n * Removes all the elements of the list\n */\nLinkedList.prototype.clear = function() {\n while (this._length) {\n this.pop();\n }\n};\n\n/**\n * Returns if the list contains the element\n * @param {any} element - The element to be checked\n * @returns {boolean} True if the list contains the element\n */\nLinkedList.prototype.contains = function(element) {\n var found_element = false;\n\n this.every(function(elem, index) {\n if (elem === element) {\n found_element = true;\n return false;\n }\n return true;\n });\n\n return found_element;\n};\n\n/**\n * The function called by the forEach method.\n * @callback LinkedList~iterateCallback\n * @function\n * @param {any} element - The current element\n * @param {number} [index] - The index of the current element\n * @param {LinkedList} [list] - The linked list it is using\n */\n\n/**\n * Performs the fn function for all the elements of the list, untill the callback function returns false\n * @param {iterateCallback} fn - The function the be executed on each element\n * @param {object} [this_arg] - The object to use as this when calling the fn function\n */\nLinkedList.prototype.every = function(fn, this_arg) {\n var current_node = this._front;\n var index = 0;\n\n while (current_node) {\n if (!fn.call(this_arg, current_node.value, index, this)) {\n break;\n }\n current_node = current_node.next;\n index += 1;\n }\n};\n\n/**\n * Performs the fn function for all the elements of the list\n * @param {iterateCallback} fn - The function the be executed on each element\n * @param {object} [this_arg] - The object to use as this when calling the fn function\n */\nLinkedList.prototype.forEach = function(fn, this_arg) {\n var current_node = this._front;\n var index = 0;\n\n while (current_node) {\n fn.call(this_arg, current_node.value, index, this);\n current_node = current_node.next;\n index += 1;\n }\n};\n\n/**\n * Returns the element at the index, if any\n * @param {number} index - The index of the element to be returned\n * @returns {any} The element at the specified index, if any. Returns undefined otherwise\n */\nLinkedList.prototype.get = function(index) {\n if (index < 0 || index >= this._length) {\n return undefined;\n }\n\n var node = this._front;\n\n for (var i = 0; i < this._length; i += 1) {\n if (i === index) {\n return node.value;\n }\n\n node = node.next;\n }\n};\n\n/**\n * Returns the first element in the list\n * @returns {any} The first element in the list\n */\nLinkedList.prototype.getFirst = function() {\n return this.get(0);\n};\n\n/**\n * Returns the last element in the list\n * @returns {any} The last element in the list\n */\nLinkedList.prototype.getLast = function() {\n if (this._back != null) {\n return this._back.value;\n }\n};\n\n/**\n * Returns the index of the element in the list\n * @param {any} element - The element to search\n * @returns {number} The index of the element in the list, or -1 if not found\n */\nLinkedList.prototype.indexOf = function(element) {\n var node = this._front;\n\n for (var i = 0; i < this._length; i += 1) {\n if (node.value === element) {\n return i;\n }\n\n node = node.next;\n }\n\n return -1;\n};\n\n/**\n * Returns if the list is empty\n * @returns {boolean} If the list if empty\n */\nLinkedList.prototype.isEmpty = function() {\n return this._length === 0;\n};\n\n/**\n * Returns the last index of the element in the list\n * @param {any} element - The element to search\n * @returns {number} The last index of the element in the list, -1 if not found\n */\nLinkedList.prototype.lastIndexOf = function(element) {\n var current_node = this._back;\n var index = this._length - 1;\n\n while (current_node) {\n if (current_node.value === element) {\n break;\n }\n current_node = current_node.prev;\n index -= 1;\n }\n\n return index;\n};\n\n/**\n * Retrieves the first element without removing it.\n * This method is equivalent to getFirst\n * @see getFirst\n * @returns {any} The first element\n */\nLinkedList.prototype.peek = function() {\n return this.get(0);\n};\n\n/**\n * Removes and returns the first element of the list.\n * This method is equivalent to removeFirst\n * @see removeFirst\n * @returns {any} The removed element\n */\nLinkedList.prototype.pop = function() {\n return this.removeFirst();\n};\n\n/**\n * Inserts the element to the end of the list\n * This method is equivalent to addFirst\n * @see addFirst\n */\nLinkedList.prototype.push = function(element) {\n this.addFirst(element);\n};\n\n/**\n * Removes and retrieves the element at the specified index.\n * If not specified, it will remove the first element of the list\n * @param {number} index - The index of the element to be removed\n * @returns {any} The removed element\n */\nLinkedList.prototype.remove = function(index) {\n if (index > this._length - 1 || index < 0 || this._length === 0) {\n throw new Error(\"Index out of bounds\");\n } else {\n var removed_node;\n\n if (index === 0) {\n //Remove the first element\n removed_node = this._front;\n this.removeNode(this._front);\n } else if (index === this._length - 1) {\n //Remove the last element\n removed_node = this._back;\n this.removeNode(this._back);\n } else {\n //Remove the element at the specified index\n var node = this._front;\n var i;\n\n if (index < this._length / 2) {\n for (i = 0; i < this._length; i += 1) {\n if (i === index) {\n removed_node = node;\n this.removeNode(removed_node);\n break;\n }\n node = node.next;\n }\n } else {\n node = this._back;\n\n for (i = this._length - 1; i > 0; i -= 1) {\n if (i === index) {\n removed_node = node;\n this.removeNode(removed_node);\n break;\n }\n node = node.prev;\n }\n }\n }\n this._length -= 1;\n return removed_node.value;\n }\n};\n\n/**\n * Removes and retrieves the first element of the list\n * @returns {any} The removed element\n */\nLinkedList.prototype.removeFirst = function() {\n if (this._length > 0) {\n return this.remove(0);\n }\n};\n\n/**\n * Removes and retrieves the first occurrence of the element in the list\n * @returns {any} The removed element\n */\nLinkedList.prototype.removeFirstOccurrence = function(element) {\n var node = this._front;\n var index = 0;\n\n while (node) {\n if (node.value === element) {\n this.removeNode(node);\n return node.value;\n }\n\n node = node.next;\n index += 1;\n }\n};\n\n/**\n * Removes and retrieves the last element of the list\n * @returns {any} The removed element\n */\nLinkedList.prototype.removeLast = function() {\n if (this._length > 0) {\n return this.remove(this._length - 1);\n }\n};\n\n/**\n * Removes and retrieves the last occurrence of the element in the list\n * @returns {any} The removed element\n */\nLinkedList.prototype.removeLastOccurrence = function(element) {\n var node = this._back;\n var index = this._length - 1;\n\n while (node) {\n if (node.value === element) {\n this.removeNode(node);\n return node.value;\n }\n\n node = node.prev;\n index -= 1;\n }\n};\n\n/**\n * Removes the node from the list\n * @returns {any} The removed node\n */\nLinkedList.prototype.removeNode = function(node) {\n if (node === this._front) {\n if (this._length > 1) {\n this._front.next.prev = null;\n this._front = this._front.next;\n } else {\n this._front = null;\n this._back = null;\n }\n node.next = null;\n } else if (node === this._back) {\n this._back.prev.next = null;\n this._back = this._back.prev;\n node.prev = null;\n } else {\n var prev_node = node.prev;\n var next_node = node.next;\n\n prev_node.next = next_node;\n next_node.prev = prev_node;\n node.next = null;\n node.prev = null;\n }\n};\n\n/**\n * Replaces the element at the specified position in the list.\n * @param {any} element - The new element\n * @param {number} index - The index where the element must be replaced\n * @returns {any} The element previously at the specified position\n */\nLinkedList.prototype.set = function(element, index) {\n if (index > this._length - 1 || index < 0 || this._length === 0) {\n throw new Error(\"Index out of bounds\");\n } else {\n var node = this._front;\n var old_element;\n\n for (var i = 0; i < this._length; i += 1) {\n if (i === index) {\n old_element = node.value;\n node.value = element;\n return old_element;\n }\n node = node.next;\n }\n }\n};\n\n/**\n * Returns the size of the list\n * @returns {number} The size of the list\n */\nLinkedList.prototype.size = function() {\n return this._length;\n};\n\n/**\n * Converts the list to an array\n * @returns {array} The converted list in an array format\n */\nLinkedList.prototype.toArray = function() {\n var array = new Array(this._length);\n\n this.forEach(function(element, index) {\n array[index] = element;\n });\n\n return array;\n};\n\nmodule.exports = LinkedList;"
}
{
"filename": "code/data_structures/src/list/doubly_linked_list/doubly_linked_list.py",
"content": "# Part of Cosmos by OpenGenus Foundation\nclass DoublyLinkedList:\n class Node:\n def __init__(self, data, prevNode, nextNode):\n self.data = data\n self.prevNode = prevNode\n self.nextNode = nextNode\n\n def __init__(self, data=None):\n self.first = None\n self.last = None\n self.count = 0\n\n def addFirst(self, data):\n if self.count == 0:\n self.first = self.Node(data, None, None)\n self.last = self.first\n elif self.count > 0:\n # create a new node pointing to self.first\n node = self.Node(data, None, self.first)\n # have self.first point back to the new node\n self.first.prevNode = node\n # finally point to the new node as the self.first\n self.first = node\n self.current = self.first\n self.count += 1\n\n def popFirst(self):\n if self.count == 0:\n raise RuntimeError(\"Cannot pop from an empty linked list\")\n result = self.first.data\n if self.count == 1:\n self.first = None\n self.last = None\n else:\n self.first = self.first.nextNode\n self.first.prevNode = None\n self.current = self.first\n self.count -= 1\n return result\n\n def popLast(self):\n if self.count == 0:\n raise RuntimeError(\"Cannot pop from an empty linked list\")\n result = self.last.data\n if self.count == 1:\n self.first = None\n self.last = None\n else:\n self.last = self.last.prevNode\n self.last.nextNode = None\n self.count -= 1\n return result\n\n def addLast(self, data):\n if self.count == 0:\n self.addFirst(0)\n else:\n self.last.nextNode = self.Node(data, self.last, None)\n self.last = self.last.nextNode\n self.count += 1\n\n def __repr__(self):\n result = \"\"\n if self.count == 0:\n return \"...\"\n cursor = self.first\n for i in range(self.count):\n result += \"{}\".format(cursor.data)\n cursor = cursor.nextNode\n if cursor is not None:\n result += \" --- \"\n return result\n\n def __iter__(self):\n # lets Python know this class is iterable\n return self\n\n def __next__(self):\n # provides things iterating over class with next element\n if self.current is None:\n # allows us to re-iterate\n self.current = self.first\n raise StopIteration\n else:\n result = self.current.data\n self.current = self.current.nextNode\n return result\n\n def __len__(self):\n return self.count\n\n\ndll = DoublyLinkedList()\ndll.addFirst(\"days\")\ndll.addFirst(\"dog\")\ndll.addLast(\"of summer\")\n\nassert list(dll) == [\"dog\", \"days\", \"of summer\"]\nassert dll.popFirst() == \"dog\"\nassert list(dll) == [\"days\", \"of summer\"]\nassert dll.popLast() == \"of summer\"\nassert list(dll) == [\"days\"]"
}
{
"filename": "code/data_structures/src/list/doubly_linked_list/doubly_linked_list.swift",
"content": "public class DoublyLinkedList<T> {\n \n public class Node<T> {\n var value: T\n var next: Node?\n weak var previous: Node? // weak is used to avoid ownership cycles\n \n public init(value: T) {\n self.value = value\n }\n }\n \n fileprivate var head: Node<T>?\n private var tail: Node<T>?\n \n public var isEmpty: Bool {\n return head == nil\n }\n \n public var first: Node<T>? {\n return head\n }\n \n public var last: Node<T>? {\n return tail\n }\n \n public func append(value: T) {\n let newNode = Node(value: value)\n \n if let tailNode = tail {\n newNode.previous = tailNode\n tailNode.next = newNode\n } else {\n head = newNode\n }\n tail = newNode\n }\n \n public func nodeAt(_ index: Int) -> Node<T>? {\n if index >= 0 {\n var node = head\n var i = index\n while node != nil {\n if i == 0 { return node }\n i -= 1\n node = node!.next\n }\n }\n return nil\n }\n \n // e.g. list[0]\n public subscript(index: Int) -> T {\n let node = nodeAt(index)\n assert(node != nil)\n return node!.value\n }\n \n public func insert(value: T, atIndex index: Int) {\n let (prev, next) = nodesBeforeAndAfter(index: index) // 1\n \n let newNode = Node(value: value) // 2\n newNode.previous = prev\n newNode.next = next\n prev?.next = newNode\n next?.previous = newNode\n \n if prev == nil { // 3\n head = newNode\n }\n }\n \n public var count: Int {\n if var node = head {\n var c = 1\n while let next = node.next {\n node = next\n c += 1\n }\n return c\n } else {\n return 0\n }\n }\n \n public func remove(node: Node<T>) -> String {\n let prev = node.previous\n let next = node.next\n \n if let prev = prev {\n prev.next = next\n } else {\n head = next\n }\n next?.previous = prev\n \n if next == nil {\n tail = prev\n }\n \n node.previous = nil\n node.next = nil\n \n return node.value as! String\n }\n public func removeAll() {\n head = nil\n tail = nil\n }\n \n // Helper functions\n private func nodesBeforeAndAfter(index: Int) -> (Node<T>?, Node<T>?) {\n assert(index >= 0)\n \n var i = index\n var next = head\n var prev: Node<T>?\n \n while next != nil && i > 0 {\n i -= 1\n prev = next\n next = next!.next\n }\n assert(i == 0)\n \n return (prev, next)\n }\n}\n\nextension DoublyLinkedList {\n public func reverse() {\n var node = head\n tail = node\n while let currentNode = node {\n node = currentNode.next\n swap(¤tNode.next, ¤tNode.previous)\n head = currentNode\n }\n }\n}\n\nextension DoublyLinkedList {\n public func map<U>(transform: (T) -> U) -> DoublyLinkedList<U> {\n let result = DoublyLinkedList<U>()\n var node = head\n while node != nil {\n result.append(value: transform(node!.value))\n node = node!.next\n }\n return result\n }\n \n public func filter(predicate: (T) -> Bool) -> DoublyLinkedList<T> {\n let result = DoublyLinkedList<T>()\n var node = head\n while node != nil {\n if predicate(node!.value) {\n result.append(value: node!.value)\n }\n node = node!.next\n }\n return result\n }\n}\n\nextension DoublyLinkedList: CustomStringConvertible {\n public var description: String {\n var text = \"[\"\n var node = head\n while node != nil {\n text += \"\\(node!.value)\"\n node = node!.next\n if node != nil { text += \", \" }\n }\n return text + \"]\"\n }\n}\n\nextension DoublyLinkedList {\n convenience init(array: Array<T>) {\n self.init()\n \n for element in array {\n self.append(element)\n }\n }\n}"
}
{
"filename": "code/data_structures/src/list/doubly_linked_list/menu_interface/dlink.h",
"content": "#include <stdio.h>\n#include <stdlib.h>\n#include <string.h>\n#include <dirent.h>\n\n#define MAX_LENGTH 20\n\nvoid insert_beg(int num);\nvoid insert_end(int num);\nvoid insert_at_loc(int num, int loc);\nvoid insert_after_value(int num, int val);\nvoid delete_beg();\nvoid delete_end();\nvoid delete_node(int loc);\nvoid delete_value(int num);\nvoid replace_value(int num, int val);\nvoid replace_node(int num, int loc);\nvoid sortlist();\nvoid delete_dup_vals();\nint length();\nvoid display();\nvoid clear(void);\n\ntypedef struct dlist\n{\n\tint info;\n\tstruct dlist* next;\n\tstruct dlist* prev;\n}dlist;\n\ndlist *start = NULL, *curr = NULL, *node = NULL, *pre = NULL;\n\n\nvoid\ninsert_beg(int num)\n{\n\tnode = (dlist*) malloc(sizeof(dlist*));\n\tnode->info = num;\n\tnode->prev = NULL;\n\n\tif (start == NULL)\n\t{\n\t\tnode->next = NULL;\n\t\tstart = node;\n\t}\n\telse\n\t{\n\t\tnode->next = start;\n\t\tstart->prev = node;\n\t\tstart = node;\n\t}\n}\n\nvoid\ninsert_end(int num)\n{\n\tnode = (dlist*) malloc(sizeof(dlist*));\n\tnode->info = num;\n\tnode->next = NULL;\n\n\tif (start == NULL)\n\t{\n\t\tnode->prev = NULL;\n\t\tstart = node;\n\t}\n\telse\n\t{\n\t\tcurr = start;\n\t\twhile (curr->next != NULL)\n\t\t\tcurr = curr->next;\n\t\tcurr->next = node;\n\t\tnode->prev = curr;\n\t}\n}\n\nvoid\ninsert_at_loc(int num, int loc)\n{\n\tint n = length();\n\tif (loc > n || loc < 0)\n\t{\n\t\tprintf(\"Location is invalid!!\\n\");\n\t\treturn;\n\t}\n\n\tnode = (dlist*) malloc(sizeof(dlist*));\n\tnode->info = num;\n\n\tif (start == NULL)\n\t{\n\t\tnode->next = NULL;\n\t\tnode->prev = NULL;\n\t\tstart = node;\n\t}\n\telse\n\t{\n\t\tcurr = start;\n\t\tint i = 1;\n\t\twhile (curr != NULL && i < loc)\n\t\t{\n\t\t\tpre = curr;\n\t\t\tcurr = curr->next;\n\t\t\ti++;\n\t\t}\n\t\tif (pre != NULL)\n\t\t\tpre->next = node;\n\t\tnode->next = curr;\n\t\tnode->prev = pre;\n\t\tif(curr != NULL)\n\t\t\tcurr->prev = node;\n\t}\n}\n\nvoid\ninsert_after_value(int num, int val)\n{\n\tnode = (dlist*) malloc(sizeof(dlist*));\n\tnode->info = num;\n\n\tif (start == NULL)\n\t{\n\t\tnode->next = NULL;\n\t\tnode->prev = NULL;\n\t\tstart = node;\n\t}\n\telse\n\t{\n\t\tcurr = start;\n\t\twhile (curr != NULL && curr->info != val)\n\t\t\tcurr = curr->next;\n\t\tif (curr == NULL)\n\t\t{\n\t\t\tprintf(\"Value not found!! Please enter again\\n\");\n\t\t\treturn;\n\t\t}\n\t\tnode->next = curr->next;\n\t\tcurr->next->prev = node;\n\t\tcurr->next = node;\n\t\tnode->prev = curr;\n\t}\n}\n\nvoid\ndelete_beg()\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\n\tcurr = start;\n\tstart = start->next;\n\tstart->prev = NULL;\n\tfree(curr);\n}\n\nvoid\ndelete_end()\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\n\tcurr = start;\n\twhile (curr->next != NULL)\n\t{\n\t\tpre = curr;\n\t\tcurr = curr->next;\n\t}\n\tpre->next = NULL;\n\tfree(curr);\n}\n\nvoid\ndelete_node(int loc)\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\n\tint n = length();\n\n\tif (loc > n || loc < 0)\n\t{\n\t\tprintf(\"Location is invalid\\n\");\n\t\treturn;\n\t}\n\n\tcurr = start;\n\tint i = 1;\n\twhile (curr != NULL && i<loc)\n\t{\n\t\tpre = curr;\n\t\tcurr = curr->next;\n\t\ti++;\n\t}\n\tpre->next = curr->next;\n\tcurr->next->prev = pre;\n\tfree(curr);\n}\n\nvoid\ndelete_value(int num)\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\tcurr = start;\n\tif (start->info == num)\n\t{\n\t\tstart = start->next;\n\t\tstart->prev = NULL;\n\t\tfree(curr);\n\t}\n\tcurr = start;\n\twhile (curr != NULL && curr->info != num)\n\t{\n\t\tpre = curr;\n\t\tcurr = curr->next;\n\t}\n\n\tif (curr == NULL)\n\t{\n\t\tprintf(\"Value not found! Please try again\\n\");\n\t\treturn;\n\t}\n\n\tpre->next = curr->next;\n\tcurr->next->prev = pre;\n\tfree(curr);\n}\n\nvoid\nreplace_value(int num,int val)\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\n\tcurr = start;\n\twhile (curr != NULL && curr->info != num)\n\t\tcurr = curr->next;\n\n\tif (curr == NULL)\n\t{\n\t\tprintf(\"Value not found! Please try again\\n\");\n\t\treturn;\n\t}\n\tcurr->info = val;\n}\n\nvoid\nreplace_node(int loc, int num)\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\n\tint n = length();\n\n\tif (loc > n || loc < 0)\n\t{\n\t\tprintf(\"Location is invalid!! Try again\\n\");\n\t\treturn;\n\t}\n\tcurr = start;\n\tint i = 1;\n\twhile (curr != NULL && i<loc)\n\t{\n\t\tcurr = curr->next;\n\t\ti++;\n\t}\n\tcurr->info = num;\n}\n\nvoid\nsortlist()\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\n\tfor (curr=start; curr!= NULL; curr=curr->next)\n\t{\n\t\tfor(pre=curr->next; pre!=NULL; pre=pre->next)\n\t\t{\n\t\t\tif ((curr->info > pre->info))\n\t\t\t{\n\t\t\t\tint temp = curr->info;\n\t\t\t\tcurr->info = pre->info;\n\t\t\t\tpre->info = temp;\n\t\t\t}\n\t\t}\n\t}\n}\n\nvoid\ndelete_dup_vals()\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\n\tsortlist();\n\tcurr = start;\n\n\twhile (curr->next != NULL)\n\t{\n\t\tif(curr->info == curr->next->info)\n\t\t{\n\t\t\tpre = curr->next->next;\n\t\t\tfree(curr->next);\n\t\t\tcurr->next = pre;\n\t\t\tpre->prev = curr;\n\t\t}\n\t\telse\n\t\t\tcurr = curr->next;\n\t}\n}\n\nint\nlength()\n{\n\tif (start == NULL)\n\t\treturn 0;\n\tint i = 1;\n\tcurr = start;\n\twhile (curr != NULL)\n\t{\n\t\tcurr = curr->next;\n\t\ti++;\n\t}\n\treturn i;\n}\n\nvoid\ndisplay()\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"The list is empty\\n\");\n\t\treturn;\n\t}\n\tcurr = start;\n\twhile (curr != NULL)\n\t{\n\t\tprintf(\"%d\\n\", curr->info);\n\t\tcurr = curr->next;\n\t}\n}\n\nvoid\ndisplay_reverse()\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"The list is empty\\n\");\n\t\treturn;\n\t}\n\tcurr = start;\n\twhile (curr->next != NULL)\n\t\tcurr = curr->next;\n\n\twhile (curr != NULL)\n\t{\n\t\tprintf(\"%d\\n\", curr->info);\n\t\tcurr = curr->prev;\n\t}\n}\n\nvoid\nwritefile(char *filename)\n{\n\tchar path[MAX_LENGTH] = \"./files/\";\n\tstrcat(path,filename);\n\n\tFILE *fp;\n\tfp = fopen(path,\"w\");\n\tcurr = start;\n\twhile (curr != NULL)\n\t{\n\t\tfprintf(fp,\"%d\\n\", curr->info);\n\t\tcurr = curr->next;\n\t}\n\tfclose(fp);\n}\n\nvoid\nreadfile(char *filename)\n{\n\tstart = NULL;\n\tchar path[MAX_LENGTH] = \"./files/\";\n\tstrcat(path,filename);\n\n\tFILE *fp;\n\tint num;\n\tfp = fopen(path, \"r\");\n\twhile(!feof(fp))\n\t{\n\t\tfscanf(fp, \"%i\\n\", &num);\n\t\tinsert_end(num);\n\t}\n\n\tfclose(fp);\n}\n\nvoid\nlistfile()\n{\n\tDIR *d = opendir(\"./files/\");\n\tstruct dirent *dir;\n\tif (d)\n\t{\n\t\tprintf(\"Current list of files\\n\");\n\t\twhile ((dir = readdir(d)) != NULL)\n\t\t{\n\t\t\tif (strcmp(dir->d_name, \"..\") == 0 || strcmp(dir->d_name,\".\") == 0)\n\t\t\t\tcontinue;\n\t\t\tprintf(\"%s\\n\", dir->d_name);\n\t\t}\n\n\t\tclosedir(d);\n\t}\n}"
}
{
"filename": "code/data_structures/src/list/doubly_linked_list/menu_interface/doubly_link.c",
"content": "/* This project aims to demonstrate various operations on linked lists. It uses\na clean command line interface and provides a baseline to make linked list\napplications in C. There are two files. The C file containing main() function and\ninvoking functions. The dlink.h header file where all functions are implemented.\n*/\n\n#include \"dlink.h\"\n\n/* For clearing screen in Windows and UNIX-based OS */\n#ifdef _WIN32\n\t#define CLEAR \"cls\"\n#else \t\t/* In any other OS */\n\t#define CLEAR \"clear\"\n#endif\n\n/* For cross-platform delay function for better UI transition */\n#ifdef _WIN32\n\t#include <Windows.h>\n#else\n\t#include <unistd.h>\t\t/* for sleep() function */\n#endif\n\nvoid\nmsleep(int ms)\n{\n#ifdef _WIN32\n Sleep(ms);\n#else\n usleep(ms * 1000);\n#endif\n}\n\nint menu();\nvoid print();\n\nint\nmain()\n{\n\tint n;\n\tprintf(\"WELCOME TO THE LINKED LIST APPLICATION\\n\");\n\tclear();\n\tusleep(500000);\n\n\tdo\n\t{\n\t\tclear();\n\t\tmsleep(500);\n\t\tprint();\n\t\tn = menu();\n\n\t}while(n!=17);\n\tclear();\n}\n\nvoid\nprint()\n{\n\tprintf(\"1. Insert at beginning\\n\");\n\tprintf(\"2. Insert at end\\n\");\n\tprintf(\"3. Insert at a particular location\\n\");\n\tprintf(\"4. Insert after a particular value\\n\");\n\tprintf(\"5. Delete a number from beginning of list\\n\");\n\tprintf(\"6. Delete a number from end of list\\n\");\n\tprintf(\"7. Delete a particular node in the list\\n\");\n\tprintf(\"8. Delete a particular value in the list\\n\");\n\tprintf(\"9. Replace a particular value with another in a list\\n\");\n\tprintf(\"10. Replace a particular node in the list\\n\");\n\tprintf(\"11. Sort the list in ascending order\\n\");\n\tprintf(\"12. Delete all duplicate values and sort\\n\");\n\tprintf(\"13. Display\\n\");\n\tprintf(\"14. Display in reverse\\n\");\n\tprintf(\"15. Write to File\\n\");\n\tprintf(\"16. Read from a File\\n\");\n\tprintf(\"17. Quit\\n\");\n\n}\n\nint\nmenu()\n{\n\tint n, a, b;\n\tchar *filename = (char *) malloc(MAX_LENGTH*sizeof(char));\n\n\tprintf(\"Select the operation you want to perform (17 to Quit): \");\n\tscanf(\"%d\", &n);\n\tswitch(n)\n\t{\n\t\tcase 1:\n\t\t\tprintf(\"Enter the number you want to insert: \");\n\t\t\tscanf(\"%d\", &a);\n\t\t\tinsert_beg(a);\n\t\t\tbreak;\n\t\tcase 2:\n\t\t\tprintf(\"Enter the number you want to insert: \");\n\t\t\tscanf(\"%d\", &a);\n\t\t\tinsert_end(a);\n\t\t\tbreak;\n\t\tcase 3:\n\t\t\tprintf(\"Enter the number you want to insert: \");\n\t\t\tscanf(\"%d\", &a);\n\t\t\tprintf(\"Enter the location in which to insert: \");\n\t\t\tscanf(\"%d\", &b);\n\t\t\tinsert_at_loc(a, b);\n\t\t\tbreak;\n\t\tcase 4:\n\t\t\tprintf(\"Enter the number you want to insert: \");\n\t\t\tscanf(\"%d\", &a);\n\t\t\tprintf(\"Enter the value after which to insert: \");\n\t\t\tscanf(\"%d\", &b);\n\t\t\tinsert_after_value(a, b);\n\t\t\tbreak;\n\t\tcase 5:\n\t\t\tdelete_beg();\n\t\t\tbreak;\n\t\tcase 6:\n\t\t\tdelete_end();\n\t\t\tbreak;\n\t\tcase 7:\n\t\t\tprintf(\"Enter the location of node to delete: \");\n\t\t\tscanf(\"%d\", &a);\n\t\t\tdelete_node(a);\n\t\t\tbreak;\n\t\tcase 8:\n\t\t\tprintf(\"Enter the value you want to delete: \");\n\t\t\tscanf(\"%d\", &a);\n\t\t\tdelete_value(a);\n\t\t\tbreak;\n\t\tcase 9:\n\t\t\tprintf(\"Enter the value you want to replace: \");\n\t\t\tscanf(\"%d\", &a);\n\t\t\tprintf(\"Enter the new value: \");\n\t\t\tscanf(\"%d\",&b);\n\t\t\treplace_value(a, b);\n\t\t\tbreak;\n\t\tcase 10:\n\t\t\tprintf(\"Enter the node location you want to replace: \");\n\t\t\tscanf(\"%d\", &a);\n\t\t\tprintf(\"Enter the new number: \");\n\t\t\tscanf(\"%d\", &b);\n\t\t\treplace_node(a, b);\n\t\t\tbreak;\n\t\tcase 11:\n\t\t\tsortlist();\n\t\t\tbreak;\n\t\tcase 12:\n\t\t\tdelete_dup_vals();\n\t\t\tbreak;\n\t\tcase 13:\n\t\t\tdisplay();\n\t\t\tusleep(5000);\n\t\t\tbreak;\n\t\tcase 14:\n\t\t\tdisplay_reverse();\n\t\t\tusleep(5000);\n\t\t\tbreak;\n\t\tcase 15:\n\t\t\tprintf(\"Enter the name of file to write to: \");\n\t\t\tscanf(\"%s\", filename);\n\t\t\twritefile(filename);\n\t\t\tbreak;\n\t\tcase 16:\n\t\t\tlistfile();\n\t\t\tprintf(\"Enter the name of file to read from: \");\n\t\t\tscanf(\"%s\", filename);\n\t\t\treadfile(filename);\n\t\t\tbreak;\n\t\tcase 17:\n\t\t\treturn n;\n\t\t\tbreak;\n\t}\n}\n\nvoid\nclear(void)\n{\n\tsystem(CLEAR);\n}"
}
{
"filename": "code/data_structures/src/list/merge_two_sorted_lists/Merging_two_sorted.cpp",
"content": "//Write a SortedMerge function that takes two lists, each of which is sorted in increasing order, and merges the two together into one list which is in increasing order\r\n\r\n#include <bits/stdc++.h>\r\n#define mod 1000000007\r\n#define lli long long int\r\nusing namespace std;\r\n\r\nclass node{\r\n public:\r\n int data;\r\n node* next;\r\n\r\n node(int x){\r\n data=x;\r\n next=NULL;\r\n\r\n }\r\n};\r\n\r\nvoid insertATHead(node* &head,int val){\r\n node* n=new node(val);\r\n n->next=head;\r\n head=n;\r\n}\r\nvoid InsertAtTail(node* &head,int val){\r\n node* n=new node(val);\r\n node* temp=head;\r\n if(temp==NULL){\r\n head=n;\r\n return;\r\n }\r\n \r\n while(temp->next!=NULL){\r\n temp=temp->next;\r\n }\r\n temp->next=n;\r\n}\r\nvoid display(node* head){\r\n node* temp=head;\r\n while(temp!=NULL){\r\n cout<<temp->data<<\" \";\r\n temp=temp->next;\r\n }\r\n cout<<endl;\r\n}\r\nnode* mergerecur(node* &head1,node* &head2){\r\n node* result;\r\n if(head1==NULL){\r\n return head2;\r\n }\r\n if(head2==NULL){\r\n return head1;\r\n }\r\n if(head1->data<head2->data){\r\n result=head1;\r\n result->next=mergerecur(head1->next,head2);\r\n }\r\n else{\r\n result=head2;\r\n result->next=mergerecur(head1,head2->next);\r\n }\r\n return result;\r\n}\r\nnode* merge(node* &head1,node*&head2){\r\n node* p1=head1;\r\n node* p2=head2;\r\n node* dummynode=new node(-1);\r\n node* p3=dummynode;\r\n while(p1!=NULL && p2!=NULL){\r\n if(p1->data<p2->data){\r\n p3->next=p1;\r\n p1=p1->next;\r\n }\r\n else{\r\n p3->next=p2;\r\n p2=p2->next;\r\n }\r\n p3=p3->next;\r\n \r\n }\r\n while(p1!=NULL){\r\n p3->next=p1;\r\n p1=p1->next;\r\n p3=p3->next;\r\n }\r\n while(p2!=NULL){\r\n p3->next=p2;\r\n p2=p2->next;\r\n p3=p3->next;\r\n }\r\n return dummynode;\r\n}\r\n\r\n\r\nint main(){\r\n node* head1=NULL;\r\n node* head2=NULL;\r\n InsertAtTail(head1,1);\r\n InsertAtTail(head1,4);\r\n InsertAtTail(head1,5);\r\n InsertAtTail(head1,7);\r\n \r\n InsertAtTail(head2,3);\r\n InsertAtTail(head2,4);\r\n InsertAtTail(head2,6);\r\n display(head1);\r\n display(head2);\r\n node* newhead=mergerecur(head1,head2);\r\n display(newhead);\r\nreturn 0;\r\n}\r"
}
{
"filename": "code/data_structures/src/list/Queue_using_Linked_list/Queue_using_Linked_List.c",
"content": "#include<stdio.h>\n#include <stdlib.h>\n\nstruct node\n{\n int data;\n struct node *next;\n};\n\nstruct node * rear=0;\nstruct node * front=0;\n\n//insertion\nvoid enqueue(int x)\n{\n struct node * temp=0;\n temp=(struct node *)malloc(sizeof(struct node));\n\n if(temp==0)\n {\n printf(\"\\nQueue Overflow\");\n return;\n }\n\n temp->data=x;\n temp->next=0;\n\n if(front==0 && rear==0)\n {\n front=temp;\n rear=temp;\n }\n\n else\n {\n rear->next=temp;\n rear=temp;\n }\n}\n\n//deletion\nvoid dequeue()\n{\n if(front==0 && rear==0)\n {\n printf(\"\\nQueue underflow\");\n return;\n }\n\n struct node * temp=front;\n temp=(struct node *)malloc(sizeof(struct node));\n\n if(front==rear)\n {\n front=0;\n rear=0;\n }\n\n else\n {\n front=front->next;\n free(temp);\n }\n}\n\n//printing the elements\nvoid print()\n{\n if(front==0 && rear==0)\n {\n printf(\"\\nQueue underflow\");\n return;\n }\n\n printf(\"\\nQueue: \");\n struct node *temp = front;\n while(temp!=0)\n {\n printf(\"%d \",temp->data);\n temp=temp->next;\n }\n}\n\n\nint main()\n{\n print();\n enqueue(10);\n print();\n enqueue(20);\n enqueue(30);\n print();\n dequeue();\n dequeue();\n print();\n dequeue();\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/README.md",
"content": "# Linked List\n\nA linked list is a data structure containing a series of data that's linked together. Each node of a linked list contains a piece of data and a link to the next node, as shown in the diagram below:\n\n\n\nImage credit: `By Lasindi - Own work, Public Domain, https://commons.wikimedia.org/w/index.php?curid=2245162`\n\n## Types of Linked Lists\n\nThere are several types of linked lists. The most common ones are:\n\n### Singly linked list\n\nThe simplest type. Each node has a data field and a link to the next node, with the last node's link being empty or pointing to `null`.\n\n### Double linked list\n\n\n\nImage credit: `By\tLasindi, Public Domain, https://commons.wikimedia.org/wiki/File:Doubly-linked-list.svg`\n\nEach node of a doubly linked list contains, in addition to the data field and a link to the next code, a link to the previous node.\n\n## Circularly linked list\n\n\n\nImage credit: `By\tLasindi, Public Domain, https://commons.wikimedia.org/wiki/File:Circularly-linked-list.svg`\n\nA singly linked list, except that the last item links back to the first item, as opposed to a singly linked list which links to nothing or `null`.\n\n## Sources and more detailed information:\n\n- https://en.wikipedia.org/wiki/Linked_list\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/menu_interface/link.h",
"content": "#include <stdio.h>\n#include <stdlib.h>\n#include <string.h>\n#include <dirent.h>\n\n#define MAX_LENGTH 20\n\ntypedef struct list\n{\n\tint info;\n\tstruct list* next;\n}list;\n\nlist *start=NULL, *node=NULL, *curr=NULL, *prev=NULL;\n\nvoid insert_beg(int);\nvoid insert_end(int);\nvoid insert_at_loc(int, int);\nvoid insert_after_value(int, int);\nvoid delete_beg();\nvoid delete_end();\nvoid delete_node(int);\nvoid delete_value(int);\nvoid replace_node(int, int);\nvoid replace_value(int, int);\nvoid delete_dup_vals();\nint length();\nvoid display();\nvoid reverse();\nvoid clear(void);\nvoid sortlist();\nvoid writefile(char *filename);\nvoid readfile();\n\nvoid\ninsert_beg(int num)\n{\n\tnode = (list*)malloc(sizeof(list*));\n\tnode->info = num;\n\n\tif (start == NULL)\n\t{\n\t\tnode->next = NULL;\n\t\tstart = node;\n\t}\n\telse {\n\t\tnode->next = start;\n\t\tstart = node;\n\t}\n}\n\nvoid\ninsert_end(int num)\n{\n\tnode = (list*)malloc(sizeof(list*));\n\tnode->info = num;\n\tnode->next = NULL;\n\n\tif (start == NULL)\n\t\tstart = node;\n\telse {\n\t\tcurr = start;\n\t\twhile (curr->next != NULL)\n\t\t\tcurr=curr->next;\n\n\t\tcurr->next=node;\n\t}\n}\n\nvoid\ninsert_at_loc(int num, int loc)\n{\n\tint n = length();\n\tif (loc > n || loc < 0)\n\t{\n\t\tprintf(\"Location is invalid!! Try again\\n\");\n\t\treturn;\n\t}\n\tnode = (list*)malloc(sizeof(list*));\n\tnode->info = num;\n\tnode->next = NULL;\n\n\tif (start == NULL)\n\t\tstart = node;\n\telse {\n\t\tcurr = start;\n\t\tint i = 1;\n\n\t\twhile (curr != NULL && i<loc)\n\t\t{\n\t\t\tprev = curr;\n\t\t\tcurr = curr->next;\n\t\t\ti++;\n\t\t}\n\n\t\tnode->next = curr;\n\t\tprev->next = node;\n\t}\n}\n\nvoid\ninsert_after_value(int num, int val)\n{\n\tnode = (list*)malloc(sizeof(list*));\n\tnode->info = num;\n\n\tif (start == NULL)\n\t\tstart = node;\n\telse {\n\t\tcurr = start;\n\t\twhile(curr != NULL && curr->info != val)\n\t\t\tcurr=curr->next;\n\n\t\tif (curr == NULL)\n\t\t{\n\t\t\tprintf(\"Value was not found!! Try again\\n\");\n\t\t\treturn;\n\t\t}\n\t\tnode->next = curr->next;\n\t\tcurr->next = node;\n\t}\n}\n\nvoid\ndelete_beg()\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\tcurr = start;\n\tstart = start->next;\n\tfree(curr);\n}\n\nvoid\ndelete_end()\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\tcurr = start;\n\twhile (curr->next != NULL)\n\t{\n\t\tprev = curr;\n\t\tcurr = curr->next;\n\t}\n\tprev->next = NULL;\n\tfree(curr);\n}\n\nvoid\ndelete_node(int num)\n{\n\tint n = length();\n\n\tif (num > n || num < 0)\n\t{\n\t\tprintf(\"Location is invalid!! Try again\\n\");\n\t\treturn;\n\t}\n\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\tcurr = start;\n\tint i = 1;\n\twhile (curr != NULL && i < num)\n\t{\n\t\tprev = curr;\n\t\tcurr = curr->next;\n\t\ti++;\n\t}\n\tprev->next = curr->next;\n\tfree(curr);\n}\n\nvoid\ndelete_value(int num)\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\tcurr = start;\n\tif (start->info == num)\n\t{\n\t\tstart = start->next;\n\t\tfree(curr);\n\t}\n\tcurr = start;\n\twhile (curr != NULL && curr->info != num)\n\t{\n\t\tprev = curr;\n\t\tcurr = curr->next;\n\t}\n\n\tif (curr == NULL)\n\t{\n\t\tprintf(\"Value not found! Try again\\n\");\n\t\treturn;\n\t}\n\tprev->next = curr->next;\n\tfree(curr);\n}\n\nvoid\nreplace_node(int num, int loc)\n{\n\tint n = length();\n\n\tif (loc > n || loc < 0)\n\t{\n\t\tprintf(\"Location is invalid!! Try again\\n\");\n\t\treturn;\n\t}\n\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\tcurr = start;\n\tint i = 1;\n\n\twhile (curr != NULL && i < loc)\n\t{\n\t\tcurr = curr->next;\n\t\ti++;\n\t}\n\tcurr->info = num;\n}\n\nvoid\nreplace_value(int num, int val)\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\tcurr = start;\n\twhile (curr != NULL && curr->info != val)\n\t\tcurr = curr->next;\n\tif (curr == NULL)\n\t{\n\t\tprintf(\"Value not found! Try again\\n\");\n\t\treturn;\n\t}\n\tcurr->info = num;\n}\n\nvoid\ndelete_dup_vals()\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\tsortlist();\n\tcurr = start;\n\n\twhile (curr->next != NULL)\n\t{\n\t\tif (curr->info == curr->next->info)\n\t\t{\n\t\t\tprev = curr->next->next;\n\t\t\tfree(curr->next);\n\t\t\tcurr->next = prev;\n\t\t}\n\t\telse\n\t\t\tcurr = curr->next;\n\t}\n}\n\nint\nlength()\n{\n\tif (start == NULL)\n\t\treturn (0);\n\tint i = 1;\n\tcurr = start;\n\twhile (curr != NULL)\n\t{\n\t\tcurr = curr->next;\n\t\ti++;\n\t}\n\treturn (i);\n}\n\nvoid\ndisplay()\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\tcurr = start;\n\twhile (curr!=NULL)\n\t{\n\t\tprintf(\"%d\\n\", curr->info);\n\t\tcurr = curr->next;\n\t}\n}\n\nvoid\nreverse()\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\tcurr = start;\n\tprev = curr->next;\n\tcurr->next = NULL;\n\twhile (prev->next != NULL)\n\t{\n\t\tnode = prev->next;\n\t\tprev->next = curr;\n\t\tcurr = prev;\n\t\tprev = node;\n\t}\n\tprev->next = curr;\n\tstart = prev;\n}\n\nvoid\nsortlist()\n{\n\tif (start == NULL)\n\t{\n\t\tprintf(\"List is empty\\n\");\n\t\treturn;\n\t}\n\tfor (curr=start; curr!= NULL; curr=curr->next)\n\t{\n\t\tfor (prev=curr->next; prev!=NULL; prev=prev->next)\n\t\t{\n\t\t\tif ((curr->info > prev->info))\n\t\t\t{\n\t\t\t\tint temp = curr->info;\n\t\t\t\tcurr->info = prev->info;\n\t\t\t\tprev->info = temp;\n\t\t\t}\n\t\t}\n\t}\n}\n\nvoid\nwritefile(char *filename)\n{\n\tchar path[MAX_LENGTH] = \"./files/\";\n\tstrcat(path, filename);\n\n\tFILE *fp;\n\tfp = fopen(path, \"w\");\n\tcurr = start;\n\twhile (curr != NULL)\n\t{\n\t\tfprintf(fp,\"%d\\n\", curr->info);\n\t\tcurr = curr->next;\n\t}\n\tfclose(fp);\n}\n\nvoid\nreadfile(char *filename)\n{\n\tstart = NULL;\n\tchar path[MAX_LENGTH] = \"./files/\";\n\tstrcat(path, filename);\n\n\tFILE *fp;\n\tint num;\n\tfp = fopen(path, \"r\");\n\twhile (!feof(fp))\n\t{\n\t\tfscanf(fp, \"%i\\n\", &num);\n\t\tinsert_end(num);\n\t}\n\n\tfclose(fp);\n}\n\nvoid\nlistfile()\n{\n\tDIR *d = opendir(\"./files/\");\n\tstruct dirent *dir;\n\tif (d)\n\t{\n\t\tprintf(\"Current list of files\\n\");\n\t\twhile((dir = readdir(d)) != NULL)\n\t\t{\n\t\t\tif(strcmp(dir->d_name, \"..\") == 0 || strcmp(dir->d_name,\".\") == 0)\n\t\t\t\tcontinue;\n\t\t\tprintf(\"%s\\n\", dir->d_name);\n\t\t}\n\n\t\tclosedir(d);\n\t}\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/menu_interface/singly_list.c",
"content": "/* This project aims to demonstrate various operations on linked lists. It uses\na clean command line interface and provides a baseline to make linked list\napplications in C. There are two files. The C file containing main() function and\ninvoking functions. The link.h header file where all functions are implemented.\n*/\n\n#include \"link.h\" /* custom header file to implement functions */\n\n/* For clearing screen in Windows and UNIX-based OS */\n#ifdef _WIN32\n\t#define CLEAR \"cls\"\n#else \t\t/* In any other OS */\n\t#define CLEAR \"clear\"\n#endif\n\n/* For cross-platform delay function for better UI transition */\n#ifdef _WIN32\n\t#include <Windows.h>\n#else\n\t#include <unistd.h>\t\t/* for sleep() function */\n#endif\n\nvoid\nmsleep(int ms)\n{\n#ifdef _WIN32\n Sleep(ms);\n#else\n usleep(ms * 1000);\n#endif\n}\n\nvoid print();\nvoid clear();\nint menu();\n\nint\nmain()\n{\n\tint c;\n\n\tdo\n\t{\n\t\tc = menu();\n\t}while(c!=17);\n}\n\nvoid\nprint()\n{\n\tprintf(\"1. Insert at beginning\\n\");\n\tprintf(\"2. Insert at end\\n\");\n\tprintf(\"3. Insert at a particular location\\n\");\n\tprintf(\"4. Insert after a particular value\\n\");\n\tprintf(\"5. Delete from beginning\\n\");\n\tprintf(\"6. Delete from end\\n\");\n\tprintf(\"7. Delete a particular node\\n\");\n\tprintf(\"8. Delete a particular value\\n\");\n\tprintf(\"9. Replace a value at a particular node\\n\");\n\tprintf(\"10. Replace a particular value in the list\\n\");\n\tprintf(\"11. Delete all duplicate values and sort\\n\");\n\tprintf(\"12. Display\\n\");\n\tprintf(\"13. Reverse\\n\");\n\tprintf(\"14. Sort(Ascending)\\n\");\n\tprintf(\"15. Write to File\\n\");\n\tprintf(\"16. Read from a file\\n\");\n\tprintf(\"17. Quit\\n\");\n}\n\nint\nmenu()\n{\n\tclear();\n\tmsleep(500);\t\t/* for delay effect 500ms */\n\n\tprint();\n\n\tint n, a, b;\n\tchar *filename = (char *) malloc(MAX_LENGTH*sizeof(char));\t\t/* For writing and reading linked list data from file */\n\tprintf(\"Select the operation you want to perform (17 to Quit): \");\n\tscanf(\"%d\", &n);\n\n\tswitch(n)\n\t{\n\t\tcase 1:\n\t\t\tprintf(\"Enter the number you want to insert: \");\n\t\t\tscanf(\"%d\", &a);\n\t\t\tinsert_beg(a);\n\t\t\tbreak;\n\t\tcase 2:\n\t\t\tprintf(\"Enter the number you want to insert: \");\n\t\t\tscanf(\"%d\", &a);\n\t\t\tinsert_end(a);\n\t\t\tbreak;\n\t\tcase 3:\n\t\t\tprintf(\"Enter the number you want to insert: \");\n\t\t\tscanf(\"%d\", &a);\n\t\t\tprintf(\"Enter the location in which you want to insert: \");\n\t\t\tscanf(\"%d\", &b);\n\t\t\tinsert_at_loc(a, b);\n\t\t\tbreak;\n\t\tcase 4:\n\t\t\tprintf(\"Enter the number you want to insert: \");\n\t\t\tscanf(\"%d\", &a);\n\t\t\tprintf(\"Enter the value after which to insert: \");\n\t\t\tscanf(\"%d\", &b);\n\t\t\tinsert_after_value(a, b);\n\t\t\tbreak;\n\t\tcase 5:\n\t\t\tdelete_beg();\n\t\t\tbreak;\n\t\tcase 6:\n\t\t\tdelete_end();\n\t\t\tbreak;\n\t\tcase 7:\n\t\t\tprintf(\"Enter the location of node to delete: \");\n\t\t\tscanf(\"%d\", &a);\n\t\t\tdelete_node(a);\n\t\t\tbreak;\n\t\tcase 8:\n\t\t\tprintf(\"Enter the value you want to delete: \");\n\t\t\tscanf(\"%d\", &a);\n\t\t\tdelete_value(a);\n\t\t\tbreak;\n\t \tcase 9:\n\t\t\tprintf(\"Enter the location of node you want to replace: \");\n\t\t\tscanf(\"%d\", &b);\n\t\t\tprintf(\"Enter the new number: \");\n\t\t\tscanf(\"%d\", &a);\n\t\t\treplace_node(a, b);\n\t\t\tbreak;\n\t\tcase 10:\n\t\t\tprintf(\"Enter the value you want to replace: \");\n\t\t\tscanf(\"%d\", &a);\n\t\t\tprintf(\"Enter the new number: \");\n\t\t\tscanf(\"%d\", &b);\n\t\t\treplace_value(b, a);\n\t\t\tbreak;\n\t\tcase 11:\n\t\t\tdelete_dup_vals();\n\t\t\tbreak;\n\t\tcase 12:\n\t\t\tdisplay();\n\t\t\tmsleep(5000);\n\t\t\tbreak;\n\t case 13:\n\t\t\treverse();\n\t\t\tbreak;\n\t\tcase 14:\n\t\t\tsortlist();\n\t\t\tbreak;\n\t\tcase 15:\n\t\t\tprintf(\"Enter the name of file to write to: \");\n\t\t\tscanf(\"%s\", filename);\n\t\t\twritefile(filename);\n\t\t\tbreak;\n\t\tcase 16:\n\t\t\tlistfile();\n\t\t\tprintf(\"Enter the name of file to read from: \");\n\t\t\tscanf(\"%s\", filename);\n\t\t\treadfile(filename);\n\t\t\tbreak;\n\t\tcase 17:\n\t\t\treturn (n);\n\t}\n\treturn (0);\n}\n\nvoid\nclear(void)\n{\n\tsystem(CLEAR);\t\t/* Cross-platform clear screen */\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/delete/delete_node_with_key.java",
"content": "package linkedlist;\n\nclass LinkedList {\n Node head;\n \n static class Node {\n int data;\n Node next;\n \n Node(int data) {\n this.data = data;\n next = null;\n }\n }\n \n public void printList() {\n Node n = head;\n while(n!=null){\n System.out.print(n.data+\" \");\n n = n.next;\n }\n }\n \n public void delete(int key) {\n Node temp = head,prev = null;\n while(temp!=null && temp.data == key) {\n head = temp.next;\n return;\n }\n while(temp != null && temp.data != key) {\n prev = temp;\n temp = temp.next;\n }\n \n if(head == null)return;\n \n prev.next = temp.next;\n }\n \n public void push(int data) {\n Node new_node = new Node(data);\n new_node.next = head;\n head = new_node;\n }\n \n public static void main(String args[]) {\n LinkedList ll = new LinkedList();\n ll.push(1);\n ll.push(2);\n ll.printList();\n System.out.println();\n ll.delete(1);\n ll.printList();\n }\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/delete/delete_node_without_head_linked_list.cpp",
"content": "#include <bits/stdc++.h>\nusing namespace std;\n\n// Initializing a Linked List\nclass Node{\npublic:\n int data;\n Node *next;\n // Constructor for Linked List for a deafult value\n Node(int data){\n this -> data=data;\n this -> next=NULL;\n }\n};\n\n// Print Linked List Function\nvoid printList(Node* &head){\n Node* temphead = head;\n while(temphead != NULL){\n cout << temphead -> data << \" \";\n temphead = temphead -> next;\n }\n cout<<endl;\n}\n\n// Appending elements in the Linked List \nvoid insertattail(Node* &tail, int data){\n Node *hue = new Node(data);\n tail->next=hue;\n tail=hue;\n}\n\n// Deleting a single Node without head Pointer in Linked List\n// Time Complexity: O(1)\n// Auxilliary Space: O(1) \nvoid delwohead(Node* del){\n del->data=del->next->data;\n del->next=del->next->next;\n}\n\n// Driver Function\nint main(){\n Node* mainode=new Node(1);\n Node* head=mainode;\n Node* tail=mainode;\n Node* nodetobedeleted=mainode;\n\n insertattail(tail,3);\n insertattail(tail,4);\n insertattail(tail,7);\n insertattail(tail,9);\n\n // Our Linked List right now => 1 3 4 7 9\n cout<<\"<= Original Linked List =>\"<<endl;\n printList(head);\n\n // Let assume we want to delete the 2nd element from the Linked List\n for(int i=0;i<2;++i){\n nodetobedeleted=head;\n head=head->next;\n }\n // This will delete the second element from the Linked List i.e. 3\n delwohead(nodetobedeleted);\n cout<<endl<<\"<= New Linked List after deleting second element =>\"<<endl;\n printList(mainode);\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/delete/delete_nth_node.c",
"content": "/* delete the nth node from the link list */\n/* Part of Cosmos by OpenGenus Foundation */\n\n#include <stdio.h>\n#include <stdlib.h>\n\nstruct node{ /* defining a structure named node */\n int data; /* stores data */\n struct node* next; /* stores the address of the next node*/\n};\n\n\nvoid\ninsert(struct node **head , int data) /* inserts the value at the beginning of the list */\n{\n struct node* temp = (struct node*)malloc(sizeof(struct node*));\n temp->data = data;\n temp->next = *head;\n *head = temp;\n}\n\nvoid\nDelete(struct node **head , int n , int *c) /* delete the node at nth position */\n{\n if ( n <= *c ){\n struct node* temp1 = *head;\n if ( n == 1 ){\n *head = temp1->next;\n free(temp1); /* delete temp1 */\n return;\n }\n int i;\n for (i = 0 ; i < n-2 ; i++)temp1 = temp1->next;\n\n /* temp1 will point to (n-1)th node */\n\n struct node* temp2 = temp1->next; /* nth node */\n temp1->next = temp2->next; /* (n+1)th node */\n free(temp2); /* delete temp2 */\n }\n else printf(\"Position does not exists!\\n\");\n}\n/* Counts no. of nodes in linked list */\nint getCount(struct node **head)\n{\n int count = 0; /* Initialize count */\n struct node* current = *head; /* Initialize current */\n while (current != NULL)\n {\n count++;\n current = current->next;\n }\n return count;\n}\n\nvoid\nresult(struct node **head) /* print all the elements of the list */\n{\n struct node* temp = *head;\n printf(\"list:\");\n while ( temp != NULL ){\n printf(\"%d \",temp->data);\n temp = temp->next;\n }\n printf(\"\\n\");\n}\n\nint\nmain()\n{\n struct node* head = NULL; /* empty list */\n insert ( &head , 2 );\n insert ( &head , 4 );\n insert ( &head , 5 );\n insert ( &head , 7 );\n insert ( &head , 8 ); /* List:2 4 5 7 8 */\n result ( &head );\n int n , c;\n c = getCount( &head );\n printf (\"Enter the position:\");\n scanf ( \"%d\" , &n );\n Delete ( &head , n , &c );\n result ( &head );\n return (0);\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/detect_cycle/detect_cycle.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n */\n#include <iostream>\nusing namespace std;\n// Singly-Linked List Defined\nstruct Node\n{\n int data;\n Node* next;\n Node(int val)\n {\n data = val;\n next = NULL;\n }\n};\n//Function to add nodes to the linked list\nNode* TakeInput(int n)\n{\n\n Node* head = NULL;\n //head of the Linked List\n Node* tail = NULL;\n //Tail of the Linked List\n\n while (n--)\n {\n\n int value;\n //data value(int) of the node\n cin >> value;\n\n //creating new node\n Node* newNode = new Node(value);\n\n //if the is no elements/nodes in the linked list so far.\n if (head == NULL)\n head = tail = newNode; //newNode is the only node in the LL\n else // there are some elements/nodes in the linked list\n {\n tail->next = newNode; // new Node added at the end of the LL\n tail = newNode; // last node is tail node\n }\n }\n return head;\n}\nNode* DetectCycle(Node* head)\n{\n Node* slow = head;\n Node* fast = head;\n\n while (fast->next->next)\n {\n slow = slow->next;\n fast = fast->next->next;\n if (slow == fast)\n return fast;\n }\n\n return NULL;\n}\nvoid RemoveCycle(Node* head, Node* intersect_Node)\n{\n\n Node* slow = head;\n Node* prev = NULL;\n\n while (slow != intersect_Node)\n {\n slow = slow->next;\n prev = intersect_Node;\n intersect_Node = intersect_Node->next;\n }\n\n prev->next = NULL; //cycle removed\n}\nvoid PrintLL(Node* head)\n{\n Node* tempPtr = head;\n // until it reaches the end\n while (tempPtr != NULL)\n {\n //print the data of the node\n cout << tempPtr->data << \" \";\n tempPtr = tempPtr->next;\n }\n cout << endl;\n}\nint main()\n{\n int n;\n //size of the linked list\n cin >> n;\n Node* head = TakeInput(n);\n //creating a cycle in the linked list\n // For n=5 and values 1 2 3 4 5\n head->next->next->next->next->next = head->next->next; // change this according tp your input\n // 1-->2-->3-->4-->5-->3-->4-->5-->3--> ... and so on\n // PrintLL(head); Uncomment this to check cycle\n RemoveCycle(head, DetectCycle(head));\n PrintLL(head);\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/detect_cycle/detect_cycle_hashmap.py",
"content": "class Node:\n def __init__(self, data, next=None):\n self.data = data\n self.next = next\n\n\nclass LinkedList:\n def __init__(self):\n self.head = None\n\n def append(self, element):\n new_node = Node(data=element)\n\n if self.head is None:\n self.head = new_node\n return\n\n current_node = self.head\n while current_node.next is not None:\n current_node = current_node.next\n\n current_node.next = new_node\n\n def findLoop(self):\n hash_map = set()\n current_node = self.head\n while current_node is not None:\n if current_node in hash_map:\n return True\n\n hash_map.add(current_node)\n current_node = current_node.next\n\n return False\n\n def __repr__(self):\n all_nodes = []\n current_node = self.head\n while current_node is not None:\n all_nodes.append(str(current_node.data))\n current_node = current_node.next\n if len(all_nodes) > 0:\n return \" -> \".join(all_nodes)\n else:\n return \"\""
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/find/find.c",
"content": "/* Check weather element is present in link list (iterative method)*/\n/* Part of Cosmos by OpenGenus Foundation */\n\n#include <stdio.h>\n#include <stdlib.h>\nstruct node{ /* defining a structure named node */\n int data; struct node* next; \n};\n\nvoid\ninsert(struct node **head , int data) /* insert an integer to the beginning of the list */\n{\n struct node* temp = (struct node*)malloc(sizeof(struct node*));\n temp->data = data;\n temp->next = *head;\n *head = temp;\n\n}\n\nvoid\nfind(struct node **head , int n) /* delete the node at nth position */\n{\n struct node* temp1 = *head;\n while (temp1 != NULL)\n {\n if (temp1->data == n){\n printf (\"Element found\\n\");\n return;}\n temp1 = temp1->next;\n }\n printf (\"Element Not found \\n\");\n return ;\n}\n\nvoid\nresult(struct node **head) /* print all the elements of the list */\n{\n struct node* temp = *head;\n printf (\"list:\");\n while (temp != NULL){\n printf (\"%d \" , temp->data);\n temp = temp->next;\n }\n printf (\"\\n\");\n}\n\nint\nmain()\n{\n struct node* head = NULL; /* empty list */\n insert (&head , 2);\n insert (&head , 4);\n insert (&head , 5);\n insert (&head , 7);\n insert (&head , 8); /* List:2 4 5 7 8 */\n result (&head);\n int n;\n printf (\"Enter the element:\");\n scanf (\"%d\" , &n);\n find (&head , n);\n return (0);\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/find/find.java",
"content": "package linkedlist;\n\nclass LinkedList {\n Node head;\n static class Node {\n int data;\n Node next;\n \n Node(int data) {\n this.data = data;\n next = null;\n }\n }\n \n public void push(int data) {\n Node new_node = new Node(data);\n new_node.next = head;\n head = new_node;\n }\n public void printList() {\n Node n = head;\n while(n != null) {\n System.out.print(n.data+\" \");\n n = n.next;\n }\n }\n public boolean search(Node head, int x) {\n Node current = head;\n while(current != null) {\n if(current.data == x) {\n return true;\n }\n current = current.next;\n }\n return false; // not found\n }\n public static void main(String args[]) {\n LinkedList ll = new LinkedList();\n ll.push(5);\n ll.push(4);\n ll.push(3);\n ll.push(2);\n if(ll.search(ll.head,1)) {\n System.out.print(\" Yes \");\n } else {\n System.out.print(\" No \");\n }\n \n }\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/find/find.py",
"content": "class Node:\n \"\"\"\n A Node in a singly-linked list\n\n Parameters\n -------------------\n data :\n The data to be stored in the node\n next:\n The link to the next node in the singly-linked list\n \"\"\"\n\n def __init__(self, data=None, next=None):\n self.data = data\n self.next = next\n\n def __repr__(self):\n \"\"\" Node representation as required\"\"\"\n return self.data\n\n\nclass NodeAdapter1(Node):\n def __bool__(self):\n return False\n\n\nclass NodeAdapter2(Node):\n def __bool__(self):\n return True\n\n\nclass SinglyLinkedList:\n def __init__(self, NodeType):\n self.head = None\n self._NodeType = NodeType\n\n def append(self, data):\n \"\"\"\n Inserts New data at the ending of the Linked List\n Takes O(n) time\n \"\"\"\n new_node = self._NodeType(data=data)\n if self.head is None:\n self.head = new_node\n return\n\n current_node = self.head\n while current_node.next is not None:\n current_node = current_node.next\n\n current_node.next = new_node\n\n def find(self, data):\n \"\"\"\n Returns the first index at which the element is found\n Returns -1 if not found\n Takes O(n) time\n \"\"\"\n\n if self.head is not None:\n current_node = self.head\n index = 0\n while current_node is not None:\n if current_node.data == data:\n return index\n current_node = current_node.next\n index += 1\n return -1\n\n def __repr__(self):\n \"\"\"\n Gives a string representation of the list in the\n given format:\n a -> b -> c -> d\n \"\"\"\n all_nodes = []\n current_node = self.head\n while current_node is not None:\n all_nodes.append(str(current_node.data))\n current_node = current_node.next\n if len(all_nodes) > 0:\n return \" -> \".join(all_nodes)\n else:\n return \"\"\n\n\ndef main():\n lst1 = SinglyLinkedList(Node)\n lst1.append(10)\n lst1.append(20)\n lst1.append(30)\n lst1.find(10)\n\n lst2 = SinglyLinkedList(NodeAdapter1)\n lst2.append(10)\n lst2.append(20)\n lst2.append(30)\n lst2.find(20)\n\n lst3 = SinglyLinkedList(NodeAdapter2)\n lst3.append(10)\n lst3.append(20)\n lst3.append(30)\n lst3.find(30)\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/insertion/insertion_at_end.py",
"content": "# Python Utility to add element at the end of the Singly Linked List\r\n# Part of Cosmos by OpenGenus Foundation\r\n\r\n\r\nclass Node:\r\n \"\"\"\r\n A Node in a singly-linked list\r\n\r\n Parameters\r\n -------------------\r\n\r\n data :\r\n The data to be stored in the node\r\n\r\n next:\r\n The link to the next node in the singly-linked list\r\n \"\"\"\r\n\r\n def __init__(self, data=None, next=None):\r\n \"\"\" Initializes node structure\"\"\"\r\n self.data = data\r\n self.next = next\r\n\r\n def __repr__(self):\r\n \"\"\" Node representation as required\"\"\"\r\n return self.data\r\n\r\n\r\nclass SinglyLinkedList:\r\n \"\"\"\r\n A structure of singly linked lists\r\n \"\"\"\r\n\r\n def __init__(self):\r\n \"\"\"Creates a Singly Linked List in O(1) Time\"\"\"\r\n self.head = None\r\n\r\n def insert_in_end(self, data):\r\n \"\"\"\r\n Inserts New data at the ending of the Linked List\r\n Takes O(n) time\r\n \"\"\"\r\n if not self.head:\r\n self.head = Node(data=data)\r\n return\r\n\r\n current_node = self.head\r\n while current_node.next:\r\n current_node = current_node.next\r\n\r\n new_node = Node(data=data, next=None)\r\n current_node.next = new_node\r\n\r\n def __repr__(self):\r\n \"\"\"\r\n Gives a string representation of the list in the\r\n given format:\r\n\r\n a -> b -> c -> d\r\n \"\"\"\r\n all_nodes = []\r\n current_node = self.head\r\n while current_node:\r\n all_nodes.append(str(current_node.data))\r\n current_node = current_node.next\r\n if len(all_nodes) > 0:\r\n return \" -> \".join(all_nodes)\r\n else:\r\n return \"Linked List is empty\"\r"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/insertion/insertion_at_front.py",
"content": "# Python Utility to add element at the beginning of the Singly Linked List\n# Part of Cosmos by OpenGenus Foundation\n\n\nclass Node:\n \"\"\"\n A Node in a singly-linked list\n\n Parameters\n -------------------\n\n data :\n The data to be stored in the node\n\n next:\n The link to the next node in the singly-linked list\n \"\"\"\n\n def __init__(self, data=None, next=None):\n \"\"\" Initializes node structure\"\"\"\n self.data = data\n self.next = next\n\n def __repr__(self):\n \"\"\" Node representation as required\"\"\"\n return self.data\n\n\nclass SinglyLinkedList:\n \"\"\"\n A structure of singly linked lists\n \"\"\"\n\n def __init__(self):\n \"\"\"Creates a Singly Linked List in O(1) Time\"\"\"\n self.head = None\n\n def insert_in_front(self, data):\n \"\"\"\n Inserts New data at the beginning of the Linked List\n Takes O(1) time\n \"\"\"\n self.head = Node(self, data=data, next=self.head)\n\n def __repr__(self):\n \"\"\"\n Gives a string representation of the list in the\n given format:\n\n a -> b -> c -> d\n \"\"\"\n all_nodes = []\n current_node = self.head\n while current_node:\n all_nodes.append(str(current_node.data))\n current_node = current_node.next\n if len(all_nodes) > 0:\n return \" -> \".join(all_nodes)\n else:\n return \"Linked List is empty\""
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/insertion/insertion_at_nth_node.py",
"content": "# Python Utility to add element after the nth node of the Singly Linked List\r\n# Part of Cosmos by OpenGenus Foundation\r\n\r\n\r\nclass Node:\r\n \"\"\"\r\n A Node in a singly-linked list\r\n\r\n Parameters\r\n -------------------\r\n\r\n data :\r\n The data to be stored in the node\r\n\r\n next:\r\n The link to the next node in the singly-linked list\r\n \"\"\"\r\n\r\n def __init__(self, data=None, next=None):\r\n \"\"\" Initializes node structure\"\"\"\r\n self.data = data\r\n self.next = next\r\n\r\n def __repr__(self):\r\n \"\"\" Node representation as required\"\"\"\r\n return self.data\r\n\r\n\r\nclass SinglyLinkedList:\r\n \"\"\"\r\n A structure of singly linked lists\r\n \"\"\"\r\n\r\n def __init__(self):\r\n \"\"\"Creates a Singly Linked List in O(1) Time\"\"\"\r\n self.head = None\r\n\r\n def insert_nth(self, data, n):\r\n \"\"\"\r\n Inserts New data at the ending of the Linked List\r\n Takes O(n) time\r\n \"\"\"\r\n if not self.head:\r\n self.head = Node(data=data)\r\n return\r\n\r\n i = 1\r\n\r\n current_node = self.head\r\n while current_node.next and i != n:\r\n current_node = current_node.next\r\n i += 1\r\n # If nth node doesn't exist, add a node at the end\r\n if i != n and not current_node.next:\r\n current_node.next = Node(data, next=None)\r\n\r\n if i == n:\r\n rest_of_array = current_node.next\r\n new_node = Node(data, rest_of_array)\r\n current_node.next = new_node\r\n\r\n def __repr__(self):\r\n \"\"\"\r\n Gives a string representation of the list in the\r\n given format:\r\n\r\n a -> b -> c -> d\r\n \"\"\"\r\n all_nodes = []\r\n current_node = self.head\r\n while current_node:\r\n all_nodes.append(str(current_node.data))\r\n current_node = current_node.next\r\n if len(all_nodes) > 0:\r\n return \" -> \".join(all_nodes)\r\n else:\r\n return \"Linked List is empty\"\r"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/merge_sorted/merge_sorted.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n */\n\n\n\n\n#include <iostream>\nusing namespace std;\n//Definition for singly-linked list.\nstruct ListNode\n{\n int val;\n ListNode *next;\n ListNode(int x) : val(x), next(NULL)\n {\n }\n};\n\n\n\n//Merge Function for linkedlist\n\nListNode* merge(ListNode* head1, ListNode* head2)\n{\n\n ListNode* head3 = NULL;\n ListNode* temp3 = NULL;\n while (head1 && head2)\n {\n if (head1->val < head2->val)\n {\n if (head3 == NULL)\n head3 = head1;\n if (temp3)\n temp3->next = head1;\n temp3 = head1;\n head1 = head1->next;\n }\n else\n {\n if (head3 == NULL)\n head3 = head2;\n if (temp3)\n temp3->next = head2;\n temp3 = head2;\n head2 = head2->next;\n }\n }\n if (head1)\n {\n if (head3)\n temp3->next = head1;\n else\n return head1;\n }\n if (head2)\n {\n if (head3)\n temp3->next = head2;\n else\n return head2;\n }\n return head3;\n}\n\n\n//Sort function for linkedlist following divide and conquer approach\nListNode* merge_sort(ListNode* A)\n{\n if (A == NULL || A->next == NULL)\n return A;\n ListNode* temp = A;\n int i = 1;\n while (temp->next)\n {\n i++;\n temp = temp->next;\n\n }\n int d = i / 2;\n int k = 0;\n temp = A;\n while (temp)\n {\n k++;\n if (k == d)\n break;\n temp = temp->next;\n }\n ListNode* head1 = A;\n ListNode* head2 = temp->next;\n temp->next = NULL;\n head1 = merge_sort(head1);\n head2 = merge_sort(head2);\n ListNode* head3 = merge(head1, head2);\n return head3;\n}\n\n//function used for calling divide and xonquer algorithm\nListNode* sortList(ListNode* A)\n{\n ListNode* head = merge_sort(A);\n return head;\n\n}\n\nint main()\n{\n ListNode* head = new ListNode(5);\n ListNode* temp = head; // Creating a linkedlist\n int i = 4;\n while (i > 0) //Adding values to the linkedlist\n {\n ListNode* t = new ListNode(i);\n temp->next = t;\n temp = temp->next;\n i--;\n }\n head = sortList(head); //calling merge_sort function\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/nth_node_linked_list/nth_node_linked_list.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n#define NUM_NODES 100 \n// Part of Cosmos by OpenGenus Foundation\ntypedef struct node {\n\t\tint value;\n\t\tstruct node* next;\n\t}node;\n\nnode* head = NULL;\n\nnode* create_node(int val)\n{\n node *tmp = (node *) malloc(sizeof(node));\n tmp->value = val;\n tmp->next = NULL;\n return tmp;\n}\n\nvoid create_list(int val)\n{\n static node* tmp = NULL;\n if (!head)\n {\n head = create_node(val);\n tmp = head;\n }\n else\n {\n tmp->next = create_node(val);\n tmp = tmp->next;\n }\n}\n\nnode* ptr = NULL;\nvoid get_nth_node(int node_num,node* curr)\n{\n\tstatic int ctr = 0;\n if (curr->next)\n {\n get_nth_node(node_num,curr->next );\n }\n ctr++;\n if (ctr == node_num)\n ptr = curr;\n}\n\nvoid cleanup_list()\n{\n node* tmp = NULL;\n while (head->next)\n {\n tmp = head;\n head = head->next;\n free(tmp);\n }\n free(head);\n head = NULL;\n}\n\n\nint main()\n{\n int node_num,ctr;\n node* tmp = NULL;\n printf(\"Enter node number\\n\");\n scanf(\"%d\",&node_num);\n for (ctr = 0; ctr < NUM_NODES; ctr++)\n create_list(ctr); \n get_nth_node(node_num, head);\n if ((node_num > 0) && (node_num <= NUM_NODES))\n printf(\"curr->value = %d\\n\",ptr->value);\n else\n printf(\"node number has to be greater than 0\\n\");\n cleanup_list();\n return 0;\n}\n\n\n\n\t"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/nth_node_linked_list/nth_node_linked_list.cpp",
"content": "///\n/// Part of Cosmos by OpenGenus Foundation\n/// Contributed by: Pranav Gupta (foobar98)\n/// Print Nth node of a singly linked list in the reverse manner\n///\n\n#include <iostream>\nusing namespace std;\n\n// Linked list node\nstruct Node\n{\npublic:\n\n int data;\n Node *next;\n\n Node(int data)\n {\n this->data = data;\n next = NULL;\n }\n};\n\n// Create linked list of n nodes\nNode* takeInput(int n)\n{\n // n is the number of nodes in linked list\n\n Node *head = NULL, *tail = NULL;\n\n int i = n;\n cout << \"Enter elements: \";\n while (i--)\n {\n int data;\n cin >> data;\n Node *newNode = new Node(data);\n if (head == NULL)\n {\n head = newNode;\n tail = newNode;\n }\n else\n {\n tail->next = newNode;\n tail = newNode;\n }\n }\n return head;\n}\n\n\n// To find length of linked list\nint length(Node *head)\n{\n int l = 0;\n while (head != NULL)\n {\n head = head->next;\n l++;\n }\n return l;\n}\n\nvoid printNthFromEnd(Node *head, int n)\n{\n // Get length of linked list\n int len = length(head);\n\n // check if n is greater than length\n if (n > len)\n return;\n\n // nth from end = (len-n+1)th node from beginning\n for (int i = 1; i < len - n + 1; i++)\n head = head->next;\n\n cout << \"Nth node from end: \" << head->data << endl;\n}\n\nint main()\n{\n cout << \"Enter no. of nodes in linked list: \";\n int x;\n cin >> x;\n Node *head = takeInput(x);\n\n cout << \"Enter n (node from the end): \";\n int n;\n cin >> n;\n printNthFromEnd(head, n);\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/print_reverse/print_reverse.py",
"content": "# Part of Cosmos by OpenGenus Foundation\nclass Node(object):\n def __init__(self, data=None, next_node=None):\n self.data = data\n self.next_node = next_node\n\n size = 0\n\n\ndef returnsize(root):\n i = 0\n while root:\n root = root.next_node\n i += 1\n return i\n\n\ndef insertatbeg(d, root):\n newnode = Node(d, None)\n if root:\n newnode.next_node = root\n root.size += 1\n return newnode\n\n\ndef insertatend(d, root):\n pre = root\n newnode = Node(d, None)\n if root:\n root.size += 1\n\n i = 0\n while root:\n pre = root\n root = root.next_node\n\n i += 1\n pre.next_node = newnode\n else:\n root = newnode\n\n\nroot = insertatbeg(910, None)\n\nroot = insertatbeg(90, root)\nroot = insertatbeg(80, root)\ninsertatend(2, root)\ninsertatend(27, root)\ninsertatend(17, root)\ninsertatend(37, root)\ninsertatend(47, root)\ninsertatend(57, root)\nroot = insertatbeg(90, root)\nroot = insertatbeg(80, root)\ninsertatend(47, root)\ninsertatend(57, root)\n\nprint(returnsize(root))\nprint(root.size)\n\n\ndef printlinklist(root):\n while root:\n print(root.data, end=\" \")\n root = root.next_node\n\n\ndef printlinklist_in_reverse_order(root):\n if root != None:\n\n printlinklist_in_reverse_order(root.next_node)\n print(root.data, end=\" \")\n\n\nprintlinklist(root)\nprint()\nprintlinklist_in_reverse_order(root)"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/print_reverse/print_reverse.scala",
"content": "//Part of Cosmos by OpenGenus Foundation\n\nobject PrintReverse {\n\n case class Node[T](value: T, next: Option[Node[T]]) {\n // append new node at the end\n def :~>(tail: Node[T]): Node[T] = next match {\n case None => new Node(value, Some(tail))\n case Some(x) => new Node(value, Some(x :~> tail))\n }\n }\n\n object Node {\n def apply[T](value: T): Node[T] = new Node(value, None)\n }\n\n def printReverse[T](node: Node[T]): Unit = {\n node.next.foreach(printReverse)\n println(node.value)\n }\n\n def main(args: Array[String]): Unit = {\n val integerLinkedList = Node(1) :~> Node(2) :~> Node(3)\n val stringLinkedList = Node(\"hello\") :~> Node(\"world\") :~> Node(\"good\") :~> Node(\"bye\")\n printReverse(integerLinkedList)\n printReverse(stringLinkedList)\n }\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/push/push.cpp",
"content": "#include <iostream>\nusing namespace std;\n\n// Everytime you work with Append or Push function, there is a corner case.\n// When a NULL Node is passed you have to return a Node that points to the given value.\n// There are two ways to achieve this:\n// 1. Receive the double pointer as an argument.\n// 2. Return the new Node.\n\nstruct Node\n{\n int data;\n Node* next;\n};\n\nvoid printList( Node *head)\n{\n while (head)\n {\n cout << head->data << \" \";\n head = head->next;\n }\n cout << endl;\n}\n\nvoid push( Node** headref, int x)\n{\n Node* head = *headref;\n Node* newNode = new Node();\n newNode->data = x;\n newNode->next = head;\n *headref = newNode;\n}\n\n\nvoid pushAfter(Node* prev_Node, int data)\n{\n Node* newNode = new Node();\n newNode->data = data;\n newNode->next = prev_Node->next;\n prev_Node->next = newNode;\n}\n\nvoid append( Node** headref, int x)\n{\n Node* head = *headref;\n if (head == nullptr)\n {\n Node* newNode = new Node();\n newNode->data = x;\n newNode->next = nullptr;\n *headref = newNode;\n return;\n }\n while (head->next)\n head = head->next;\n Node *temp = new Node();\n head->next = temp;\n temp->data = x;\n}\n\nint main()\n{\n Node *head = nullptr;\n append(&head, 5);\n append(&head, 10);\n push(&head, 2);\n pushAfter(head->next, 4);\n printList(head);\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/reverse/reverse.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n#include <unistd.h>\n\ntypedef struct node {\n\t\t\tint value;\n\t\t\tstruct node* next;\n\t\t}node;\n\nnode* head = NULL;\n\nnode* create_node(int val)\n{\n node *tmp = (node *) malloc(sizeof(node));\n tmp->value = val;\n tmp->next = NULL;\n return tmp;\n}\n\nvoid create_list(int val)\n{\n static node* tmp = NULL; \n\tif (!head)\n {\n\t head = create_node(val);\n tmp = head;\n }\n else\n {\n\t tmp->next = create_node(val);\n tmp = tmp->next;\n\t}\n}\n\n\nvoid print_list(node* tmp)\n{\n while (tmp)\n {\n printf(\"%d \", tmp->value);\n tmp = tmp->next;\n }\n printf(\"\\n\");\n}\n\nvoid reverse_list()\n{\n node* trail_ptr = head;\n node* tmp = trail_ptr->next;\n node* lead_ptr = tmp;\n if (!tmp)\n return;\n while (lead_ptr->next)\n {\n\tlead_ptr = lead_ptr->next;\n if (lead_ptr)\n {\n tmp->next = trail_ptr;\n if (trail_ptr == head)\n head->next = NULL;\n trail_ptr = tmp;\n tmp = lead_ptr;\n }\n }\n tmp->next = trail_ptr;\n if (trail_ptr == head)\n trail_ptr->next = NULL;\n trail_ptr = tmp;\n if (lead_ptr != tmp)\n lead_ptr->next = tmp;\n if (lead_ptr)\n head = lead_ptr;\n}\n\nvoid cleanup_list()\n{\n node* tmp = NULL;\n while (head->next)\n {\n tmp = head;\n head = head->next;\n free(tmp);\n }\n free(head);\n head = NULL;\n} \n \nint main()\n{\n int num_nodes, value, ctr;\n printf(\"Enter number of nodes\\n\");\n scanf(\"%d\",&num_nodes);\n for (ctr = 0; ctr < num_nodes; ctr++)\n {\n printf(\"Enter value\\n\");\n scanf(\"%d\",&value);\n create_list(value);\n }\n print_list(head);\n reverse_list();\n print_list(head);\n cleanup_list();\n\treturn 0;\n} "
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/reverse/reverse.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n */\n\n\n#include <iostream>\nusing namespace std;\n\n//Definition for singly-linked list.\nstruct ListNode\n{\n int val;\n ListNode *next;\n ListNode(int x) : val(x), next(NULL)\n {\n }\n};\n// Reverse function for linkedlist\nListNode* reverseList(ListNode* A)\n{\n ListNode* prev = NULL;\n while (A && A->next)\n {\n ListNode* temp = A->next;\n A->next = prev;\n prev = A;\n A = temp;\n }\n A->next = prev;\n return A;\n}\n\nint main()\n{\n ListNode* head = new ListNode(5);\n ListNode* temp = head; // Creating a linkedlist\n int i = 4;\n while (i > 0) //Adding values to the linkedlist\n {\n ListNode* t = new ListNode(i);\n temp->next = t;\n temp = temp->next;\n i--;\n }\n head = reverseList(head); //calling reverse function\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/reverse/reverse_iteration.cpp",
"content": "#include <iostream>\nusing namespace std;\n\nstruct node\n{\n int data;\n struct node * next;\n}*head;\n\n\nvoid reverse()\n{\n node *prev = NULL;\n node *cur = head;\n node *next;\n\n while (cur != NULL)\n {\n next = cur->next;\n cur->next = prev;\n prev = cur;\n cur = next;\n }\n\n head = prev;\n}\n\nvoid push(int x)\n{\n node *ptr = new node;\n ptr->data = x;\n ptr->next = NULL;\n\n if (head == NULL)\n head = ptr;\n\n else\n {\n ptr->next = head;\n head = ptr;\n }\n}\n\nvoid print()\n{\n node *ptr = head;\n\n while (ptr != NULL)\n {\n cout << ptr->data << \" \";\n ptr = ptr->next;\n }\n}\n\nint main()\n{\n push(2);\n push(3);\n push(4);\n push(5);\n\n cout << \"\\nOriginal list is : \";\n print();\n\n cout << \"\\nReversed list is : \";\n reverse();\n\n print();\n cout << \"\\n\";\n return 0;\n\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/reverse/reverse_recursion2.cpp",
"content": "#include <iostream>\nusing namespace std;\n\nstruct Node\n{\n int data;\n struct Node* next;\n};\n\nvoid printReverse(struct Node* head)\n{\n if (head == NULL)\n return;\n printReverse(head->next);\n cout << head->data << \" \";\n}\n\nvoid push(struct Node** head_ref, char new_data)\n{\n struct Node* new_node = new Node;\n\n new_node->data = new_data;\n\n new_node->next = (*head_ref);\n\n (*head_ref) = new_node;\n}\n\nint main()\n{\n struct Node* head = NULL;\n for (int i = 0; i < 10; i++)\n push(&head, i);\n\n printReverse(head);\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/reverse/reverse_recursion.cpp",
"content": "#include <iostream>\n// Part of Cosmos by OpenGenus Foundation\nusing namespace std;\n\nclass node {\npublic:\n int data;\n node* next;\n\npublic:\n node(int d)\n {\n data = d;\n next = nullptr;\n }\n};\n\nvoid print(node*head)\n{\n while (head != nullptr)\n {\n cout << head->data << \"-->\";\n head = head->next;\n }\n}\n\nvoid insertAtHead(node*&head, int d)\n{\n node* n = new node(d);\n n->next = head;\n head = n;\n}\n\nvoid insertInMiddle(node*&head, int d, int p)\n{\n if (p == 0)\n insertAtHead(head, d);\n else\n {\n //assuming p<=length of LL\n node*temp = head;\n for (int jump = 1; jump < p; jump++)\n temp = temp->next;\n node*n = new node(d);\n n->next = temp->next;\n temp->next = n;\n }\n}\n\nvoid takeInput(node*&head)\n{\n int d;\n cin >> d;\n while (d != -1)\n {\n insertAtHead(head, d);\n cin >> d;\n }\n}\n\n///overloading to print LL\nostream& operator<<(ostream&os, node*head)\n{\n print(head);\n cout << endl;\n return os;\n}\nistream& operator>>(istream&is, node*&head)\n{\n takeInput(head);\n return is;\n}\n\n\n///optimized recursive reverse\nnode*recReverse2(node*head)\n{\n if (head == nullptr || head->next == nullptr)\n return head;\n node*newHead = recReverse2(head->next);\n head->next->next = head;\n head->next = nullptr;\n return newHead;\n}\n\nint main()\n{\n node*head = nullptr;\n cin >> head;\n cout << head;\n head = recReverse2(head);\n cout << \"Reversed linked list: \" << endl;\n cout << head;\n\n\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/rotate_a_linked_list_by_k_nodes/rotate_a_linked_list_by_k_nodes.cpp",
"content": "// C++ program to rotate a linked list counter clock wise by k Nodes\r\n// where k can be greater than length of linked list\r\n\r\n#include <iostream>\r\n\r\nclass Node {\r\npublic:\r\n int data;\r\n Node *next;\r\n\r\n Node(int d): next(nullptr),data(d) {\r\n }\r\n};\r\n\r\nNode *insert() {\r\n // no. of values to insert\r\n std::cout << \"Enter no. of Nodes you want to insert in linked list: \\n\";\r\n int n;\r\n std::cin >> n;\r\n Node *head = nullptr;\r\n Node *temp = head;\r\n\r\n std::cout << \"Enter \" << n << \" values of linked list : \\n\";\r\n for (int i = 0; i < n; ++i) {\r\n int value;\r\n std::cin >> value;\r\n if (i == 0) {\r\n // insert at head\r\n head = new Node(value);\r\n temp = head;\r\n continue;\r\n } else {\r\n temp->next = new Node(value);\r\n temp = temp->next;\r\n }\r\n }\r\n return head;\r\n}\r\n\r\nNode *rotate(Node *head, int k) {\r\n // first check whether k is small or greater than length of linked list\r\n // so first find length of linked list\r\n int len = 0;\r\n Node *temp = head;\r\n while (temp != nullptr) {\r\n temp = temp->next;\r\n len++;\r\n }\r\n // length of linked list = len\r\n\r\n // update k according to length of linked list\r\n // because k can be greater than length of linked list\r\n k %= len;\r\n\r\n if (k == 0) {\r\n // since when k is multiple of len its mod becomes zero\r\n // so we have to correct it\r\n k = len;\r\n }\r\n if (k == len) {\r\n // since if k==len then even after rotating it linked list will remain same\r\n return head;\r\n }\r\n\r\n int count = 1;\r\n temp = head;\r\n while (count < k and temp != nullptr) {\r\n temp = temp->next;\r\n count++;\r\n }\r\n\r\n Node *newHead = temp->next;\r\n temp->next = nullptr;\r\n temp = newHead;\r\n\r\n while (temp->next != nullptr) {\r\n temp = temp->next;\r\n }\r\n temp->next = head;\r\n return newHead;\r\n}\r\n\r\nvoid printList(Node *head) {\r\n Node *temp = head;\r\n while (temp != nullptr) {\r\n std::cout << temp->data << \" --> \";\r\n temp = temp->next;\r\n }\r\n std::cout << \"nullptr \\n\";\r\n return;\r\n}\r\n\r\nint main() {\r\n Node *head = insert();\r\n printList(head);\r\n std::cout << \"Enter value of k: \\n\";\r\n int k;\r\n std::cin >> k;\r\n head = rotate(head, k);\r\n std::cout << \"After rotation : \\n\";\r\n printList(head);\r\n return 0;\r\n}\r\n\r\n// Input and Output :\r\n/*\r\nEnter no. of Nodes you want to insert in linked list:\r\n9\r\nEnter 9 values of linked list :\r\n1 2 3 4 5 6 7 8 9\r\n1 --> 2 --> 3 --> 4 --> 5 --> 6 --> 7 --> 8 --> 9 --> nullptr\r\nEnter value of k:\r\n3\r\nAfter rotation :\r\n4 --> 5 --> 6 --> 7 --> 8 --> 9 --> 1 --> 2 --> 3 --> nullptr\r\n*/\r"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/rotate/rotate.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n */\n\n#include <iostream>\n\n//Definition for singly-linked list.\nstruct ListNode\n{\n int val;\n ListNode *next;\n ListNode(int x) : val(x), next(NULL)\n {\n }\n};\n\nListNode* rotate(ListNode* A, int B)\n{\n int t = 0;\n ListNode* temp = A;\n ListNode* prev2 = NULL;\n while (temp)\n {\n ++t;\n prev2 = temp;\n temp = temp->next;\n }\n B = B % t; //count by which the list is to be rotated\n if (B == 0)\n return A;\n int p = t - B;\n ListNode* prev = NULL;\n ListNode* head = A;\n temp = A;\n for (int i = 1; i < p; i++) //reaching that point from where the list is to be rotated\n {\n prev = temp;\n temp = temp->next;\n }\n prev = temp;\n if (temp && temp->next) //rotating the list\n temp = temp->next;\n if (prev2)\n prev2->next = A;\n if (prev)\n prev->next = NULL;\n head = temp;\n\n return head;\n}\n\nint main()\n{\n using namespace std;\n ListNode* head = new ListNode(5);\n ListNode* temp = head; // Creating a linkedlist\n int i = 4;\n while (i > 0) //Adding values to the linkedlist\n {\n ListNode* t = new ListNode(i);\n temp->next = t;\n temp = temp->next;\n --i;\n }\n\n head = rotate(head, 2); //calling rotate function\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/sort/bubble_sort.cpp",
"content": "///Bubble sort a linked list\n#include <iostream>\n#include <stack>\nusing namespace std;\n\nclass node {\npublic:\n int data;\n node* next;\n\npublic:\n node(int d)\n {\n data = d;\n next = NULL;\n }\n};\n\nvoid print(node*head)\n{\n while (head != NULL)\n {\n cout << head->data << \"-->\";\n head = head->next;\n }\n}\n\nvoid insertAtHead(node*&head, int d)\n{\n node* n = new node(d);\n n->next = head;\n head = n;\n}\n\nvoid insertInMiddle(node*&head, int d, int p)\n{\n if (p == 0)\n insertAtHead(head, d);\n else\n {\n //assuming p<=length of LL\n int jump = 1;\n node*temp = head;\n while (jump <= p - 1)\n {\n jump++;\n temp = temp->next;\n }\n node*n = new node(d);\n n->next = temp->next;\n temp->next = n;\n }\n}\n\nvoid takeInput(node*&head)\n{\n int d;\n cin >> d;\n while (d != -1)\n {\n insertAtHead(head, d);\n cin >> d;\n }\n}\n\n///overloading to print LL\nostream& operator<<(ostream&os, node*head)\n{\n print(head);\n cout << endl;\n return os;\n}\nistream& operator>>(istream&is, node*&head)\n{\n takeInput(head);\n return is;\n}\n\nint length(node*head)\n{\n int len = 0;\n while (head != NULL)\n {\n len++;\n head = head->next;\n }\n return len;\n}\n\nvoid bubbleSort(node*&head)\n{\n int n = length(head);\n for (int i = 0; i < n - 1; i++) //n-1 times\n {\n node*current = head;\n node*prev = NULL;\n node*next;\n\n while (current != NULL && current->next != NULL)\n {\n if (current->data > current->next->data)\n {\n //swapping\n if (prev == NULL)\n {\n next = current->next;\n current->next = next->next;\n next->next = current;\n\n head = next;\n prev = next;\n }\n else\n {\n next = current->next;\n prev->next = next;\n current->next = next->next;\n next->next = current;\n prev = next;\n }\n }\n else\n {\n prev = current;\n current = current->next;\n }\n }\n }\n}\n\n\nint main()\n{\n node*head = NULL;\n cin >> head;\n cout << head;\n bubbleSort(head);\n cout << \"BUBBLE SORTED LINKED LIST\" << endl;\n cout << head;\n\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/unclassified/linked_list_example.java",
"content": "import java.io.InputStreamReader;\nimport java.util.LinkedList;\nimport java.util.Scanner;\n\n\npublic class LinkedListeg {\n\n\tclass Node {\n\t\tint value;\n\t\tNode next = null;\n\t\tNode(int value) {\n\t\t\tthis.value = value;\n\t\t}\n\t}\n\n\tprotected Node head = null;\n\tprotected Node tail = null;\n\n\tpublic void addToFront(int value) {\n\t\tNode newNode = new Node(value);\n\t\tnewNode.next = head;\n\t\thead = newNode;\n\t\tif(newNode.next == null)\n\t\t{\n\t\t\ttail = newNode;\n\t\t}\n\t}\n\n\tpublic void addToBack(int value) {\n\t\tNode newNode = new Node(value);\n\t\tif(tail == null)\n\t\t{\n\t\t\thead = newNode;\n\t\t}\n\t\telse\n\t\t{\n\t\t\ttail.next = newNode;\n\t\t}\n\t\ttail = newNode;\n\t}\n\n\tpublic void addAtIndex(int index,int value) {\n\t\tif(index < 0)\n\t\t{\n\t\t\tthrow new IndexOutOfBoundsException();\n\t\t}\n\t\telse if(index == 0)\n\t\t{\n\t\t\taddToFront(value);\n\t\t}\n\t\telse\n\t\t{\n\t\t\tNode newNode = new Node(value);\n\t\t\tNode current = head;\n\t\t\tfor(int i = 0; i < index-1; i++) {\n\t\t\t\tif(current == null)\n\t\t\t\t{\n\t\t\t\t\tthrow new IndexOutOfBoundsException();\n\t\t\t\t}\n\t\t\tcurrent = current.next;\n\t\t}\n\t\tif(current.next == null)\n\t\t{\n\t\t\ttail = newNode;\n\t\t}\n\t\telse\n\t\t{\n\t\t\tnewNode.next = current.next;\n\t\t\tcurrent.next = newNode;\n\t\t}\n\t\t}\n\t}\n\n\tpublic void removeFromFront() {\n\t\tif(head != null)\n\t\t{\n\t\t\thead = head.next;\n\t\t}\n\t\tif(head == null)\n\t\t{\n\t\t\ttail = null;\n\t\t}\n\t}\n\n\tpublic void removeFromBack() {\n\t\tif(head == null)\n\t\t{\n\t\t\treturn;\n\t\t}\n\t\telse if(head.equals(tail))\n\t\t{\n\t\t\thead = null;\n\t\t\ttail = null;\n\t\t}\n\t\telse\n\t\t{\n\t\t\tNode current = head;\n\t\t\twhile(current.next != tail) {\n\t\t\t\tcurrent = current.next;\n\t\t\t}\n\t\t\ttail = current;\n\t\t\tcurrent.next = null;\n\t\t}\n\t}\n\n\tpublic void removeAtIndex(int index) {\n\t\tif(index < 0)\n\t\t{\n\t\t\tthrow new IndexOutOfBoundsException();\n\t\t}\n\t\telse if(index == 0)\n\t\t{\n\t\t\tremoveFromFront();\n\t\t}\n\t\telse\n\t\t{\n\t\t\tNode current = head;\n\t\t\tfor(int i = 0; i < index-1; i++) {\n\t\t\t\tif(current == null)\n\t\t\t\t{\n\t\t\t\t\tthrow new IndexOutOfBoundsException();\n\t\t\t\t}\n\t\t\t\tcurrent = current.next;\n\t\t\t}\n\t\t\tcurrent.next = current.next.next;\n\t\t\tif(current.next == null)\n\t\t\t{\n\t\t\t\ttail = current;\n\t\t\t}\n\t\t}\n\t}\n\n\tpublic void printList() {\n\t\tNode newNode = head;\n\t\tif(head != null)\n\t\t{\n\t\t\tSystem.out.println(\"The Linked List contains :\");\n\t\t\twhile(newNode != null) {\n\t\t\t\tSystem.out.println(newNode.value);\n\t\t\t\tnewNode = newNode.next;\n\t\t\t}\n\t\t}\n\t\telse\n\t\t{\n\t\t\tSystem.out.println(\"The list contains no elements\");\n\t\t}\n\t}\n\n\tpublic void count() {\n\t\tNode newNode=head;\n\t\tint counter = 0;\n\t\twhile(newNode != null ) {\n\t\t\tcounter++;\n\t\t\tnewNode = newNode.next;\n\t\t}\n\t\tSystem.out.println(\"The list contains \"+counter+\" elements\");\n\t}\n\tpublic static void main(String[] args) {\n\t\tScanner in=new Scanner(new InputStreamReader(System.in));\n\t\tLinkedListeg list = new LinkedListeg();\n\t\tint ch = 0;\n\t\tdo {\n\t\t\tSystem.out.println(\"Choose form the following\");\n\t\t\tSystem.out.println(\"1.Add to Front\\n2.Add to Back\\n3.Add at index\\n4.Remove from front\");\n\t\t\tSystem.out.println(\"5.Remove from back\\n6.remove at index\\n7.Print elements in the Linked List\");\n\t\t\tSystem.out.println(\"8.Count number of elements in the list\\n9.Exit\");\n\t\t\tch = in.nextInt();\n\t\t\tint value = 0;\n\t\t\tint index = 0;\n\t\t\tswitch (ch) {\n\t\t\tcase 1:\n\t\t\t\tSystem.out.println(\"Enter a value\");\n\t\t\t\tvalue = in.nextInt();\n\t\t\t\tlist.addToFront(value);\n\t\t\t\tbreak;\n\t\t\tcase 2:\n\t\t\t\tSystem.out.println(\"Enter a value\");\n\t\t\t\tvalue = in.nextInt();\n\t\t\t\tlist.addToBack(value);\n\t\t\t\tbreak;\n\t\t\tcase 3:\n\t\t\t\tSystem.out.println(\"Enter a value\");\n\t\t\t\tvalue = in.nextInt();\n\t\t\t\tSystem.out.println(\"Enter index\");\n\t\t\t\tindex = in.nextInt();\n\t\t\t\tlist.addAtIndex(index,value);\n\t\t\t\tbreak;\n\t\t\tcase 4:\n\t\t\t\tlist.removeFromFront();\n\t\t\t\tbreak;\n\t\t\tcase 5:\n\t\t\t\tlist.removeFromBack();\n\t\t\t\tbreak;\n\t\t\tcase 6:\n\t\t\t\tSystem.out.println(\"Enter the index\");\n\t\t\t\tindex = in.nextInt();\n\t\t\t\tlist.removeAtIndex(index);\n\t\t\t\tbreak;\n\t\t\tcase 7:\n\t\t\t\tlist.printList();\n\t\t\t\tbreak;\n\t\t\tcase 8:\n\t\t\t\tlist.count();\n\t\t\t\tbreak;\n\t\t\tcase 9:\n\t\t\t\tSystem.exit(0);\n\t\t\t\tbreak;\n\t\t\tdefault:\n\t\t\t\tSystem.out.println(\"Wrong Choice\");\n\t\t\t\tbreak;\n\t\t\t}\n\t\t\tSystem.out.println();\n\t\t} while (ch != 9);\n\t}\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/unclassified/linked_list.java",
"content": "// Part of Cosmos by OpenGenus Foundation\npublic class LinkedList {\n\tprivate class Node {\n\t\tint data;\n\t\tNode next;\n\n\t\tpublic Node() {\n\n\t\t}\n\n\t}\n\n\tprivate Node head;\n\tprivate Node tail;\n\tprivate int size;\n\n\tpublic int size() {\n\t\treturn this.size;\n\n\t}\n\n\tpublic boolean isEmpty() {\n\t\treturn this.size == 0;\n\t}\n\n\tpublic void display() {\n\t\tNode temp;\n\t\ttemp = head;\n\t\tSystem.out.println(\"------------------------------------------\");\n\t\twhile (temp != null) {\n\t\t\tSystem.out.print(temp.data + \"\\t\");\n\t\t\ttemp = temp.next;\n\n\t\t}\n\t\tSystem.out.println(\".\");\n\t\tSystem.out.println(\"--------------------------------------------\");\n\t}\n\n\tpublic int getfirst() throws Exception {\n\t\tif (this.size == 0) {\n\t\t\tthrow new Exception(\"LinkedList is empty\");\n\n\t\t}\n\t\treturn this.head.data;\n\n\t}\n\n\tpublic int getLast() throws Exception {\n\t\tif (this.size == 0) {\n\t\t\tthrow new Exception(\"LinkedList is empty\");\n\t\t}\n\n\t\treturn this.tail.data;\n\t}\n\n\tpublic int getAt(int idx) throws Exception {\n\t\tif (this.size == 0) {\n\t\t\tthrow new Exception(\"LinkedList is empty\");\n\t\t}\n\t\tif (idx < 0 || idx >= this.size) {\n\t\t\tthrow new Exception(\"index out of bound\");\n\t\t}\n\t\tNode temp = head;\n\t\tfor (int i = 0; i < idx; i++) {\n\t\t\ttemp = temp.next;\n\t\t}\n\t\treturn temp.data;\n\t}\n\n\tpublic void addLast(int data) {\n\t\tNode nn = new Node();\n\t\tnn.data = data;\n\t\tnn.next = null;\n\n\t\tif (this.size == 0) {\n\t\t\tthis.head = nn;\n\t\t\tthis.tail = nn;\n\n\t\t} else {\n\n\t\t\tthis.tail.next = nn;\n\t\t\tthis.tail = nn;\n\t\t}\n\n\t\tthis.size++;\n\t}\n\n\tpublic void addfirst(int data) {\n\t\tNode nn = new Node();\n\t\tnn.data = data;\n\n\t\tif (this.size == 0) {\n\t\t\tthis.head = nn;\n\t\t\tthis.tail = nn;\n\t\t} else {\n\t\t\tnn.next = this.head;\n\t\t\tthis.head = nn;\n\t\t}\n\t\tthis.size++;\n\n\t}\n\n\tprivate Node getnodeAt(int idx) throws Exception {\n\t\tif (this.size == 0) {\n\t\t\tthrow new Exception(\"LinkedList is empty\");\n\t\t}\n\t\tif (idx < 0 || idx >= this.size) {\n\t\t\tthrow new Exception(\"index out of bound\");\n\t\t}\n\t\tNode temp = head;\n\t\tfor (int i = 0; i < idx; i++) {\n\t\t\ttemp = temp.next;\n\t\t}\n\t\treturn temp;\n\t}\n\n\tpublic void addAt(int data, int idx) throws Exception {\n\t\tNode nn = new Node();\n\t\tnn.data = data;\n\n\t\tif (idx < 0 || idx > this.size) {\n\t\t\tthrow new Exception(\"index out of bound\");\n\t\t}\n\t\tif (idx == 0) {\n\t\t\tthis.addfirst(data);\n\t\t} else if (idx == this.size) {\n\t\t\tthis.addLast(data);\n\t\t}\n\n\t\telse {\n\n\t\t\tNode nm = this.getnodeAt(idx - 1);\n\t\t\tNode np1 = nm.next;\n\n\t\t\tnn.next = np1;\n\t\t\tnm.next = nn;\n\n\t\t}\n\t\tthis.size++;\n\t}\n\n\tpublic int removeFirst() throws Exception {\n\t\tif (this.size == 0) {\n\t\t\tthrow new Exception(\"LinkedList is empty\");\n\t\t}\n\n\t\tint rv = this.head.data;\n\n\t\tif (this.size == 1) {\n\t\t\tthis.head = null;\n\t\t\tthis.tail = null;\n\t\t} else {\n\t\t\tthis.head = this.head.next;\n\t\t}\n\t\tthis.size--;\n\t\treturn rv;\n\t}\n\n\tpublic int removelast() throws Exception {\n\t\tif (this.size == 0) {\n\t\t\tthrow new Exception(\"LinkedList is empty\");\n\t\t}\n\n\t\tint rv = this.tail.data;\n\n\t\tif (this.size == 1) {\n\t\t\tthis.head = null;\n\t\t\tthis.tail = null;\n\n\t\t} else {\n\t\t\tNode nm = this.getnodeAt(this.size - 2);\n\t\t\tnm.next = null;\n\t\t\tthis.tail = nm;\n\n\t\t}\n\t\tthis.size--;\n\n\t\treturn rv;\n\t}\n\n\tpublic int removeAt(int idx) throws Exception {\n\t\t{\n\t\t\tif (this.size == 0) {\n\t\t\t\tthrow new Exception(\"LinkedList is empty\");\n\t\t\t}\n\n\t\t\tif (idx < 0 || idx > this.size) {\n\t\t\t\tthrow new Exception(\"index out of bound\");\n\t\t\t}\n\t\t\tint rv = 0;\n\n\t\t\tif (this.size == 1) {\n\t\t\t\tthis.head = null;\n\t\t\t\tthis.tail = null;\n\t\t\t}\n\t\t\tif (idx == 0) {\n\t\t\t\tthis.removeFirst();\n\n\t\t\t} else if (idx == this.size) {\n\t\t\t\tthis.removelast();\n\t\t\t} else {\n\t\t\t\tNode nm = this.getnodeAt(idx - 1);\n\t\t\t\tNode n = nm.next;\n\t\t\t\trv = n.data;\n\t\t\t\tNode nm1 = this.getnodeAt(idx + 1);\n\n\t\t\t\tnm.next = nm1;\n\n\t\t\t}\n\t\t\tthis.size--;\n\t\t\treturn rv;\n\n\t\t}\n\n\t}\n\n\tpublic void displayreverse() throws Exception {\n\t\tint i = this.size - 1;\n\n\t\twhile (i >= 0) {\n\t\t\tSystem.out.print(this.getAt(i) + \"\\t\");\n\t\t\ti--;\n\t\t}\n\n\t}\n\n\t// o(n^2)\n\tpublic void reverselistDI() throws Exception {\n\t\tint left = 0;\n\t\tint right = this.size - 1;\n\t\twhile (left < right) {\n\t\t\tNode leftnode = this.getnodeAt(left);\n\t\t\tNode rightnode = this.getnodeAt(right);\n\n\t\t\tint temp = leftnode.data;\n\t\t\tleftnode.data = rightnode.data;\n\t\t\trightnode.data = temp;\n\t\t\tleft++;\n\t\t\tright--;\n\n\t\t}\n\n\t}\n\n\t// o(n)\n\tpublic void reverselistPI() {\n\t\tNode prev = this.head;\n\t\tNode curr = prev.next;\n\n\t\twhile (curr != null) {\n\t\t\tNode tc = curr;\n\t\t\tNode tp = prev;\n\n\t\t\tprev = curr;\n\t\t\tcurr = curr.next;\n\t\t\ttc.next = tp;\n\n\t\t}\n\t\tNode temp = this.head;\n\t\tthis.head = this.tail;\n\t\tthis.tail = temp;\n\n\t}\n\n\t// o(n)\n\tpublic void reverselistPR() {\n\n\t\treverselistPR_RH(this.head, this.head.next);\n\t\tNode temp = this.head;\n\t\tthis.head = this.tail;\n\t\tthis.tail = temp;\n\n\t\tthis.tail.next = null;\n\n\t}\n\n\tprivate void reverselistPR_RH(Node prev, Node curr) {\n\t\tif (curr == null) {\n\n\t\t\treturn;\n\t\t}\n\t\treverselistPR_RH(curr, curr.next);\n\t\tcurr.next = prev;\n\n\t}\n\n\t// o(n)\n\tpublic void reverselistDR() {\n\t\t// this.reverselistDR(this.head,this.head,0);\n\t\tHeapMover left = new HeapMover();\n\t\tleft.node = this.head;\n\t\treverselistDR1(left, head, 0);\n\n\t}\n\n\tprivate Node reverselistDR(Node left, Node right, int floor) {\n\t\tif (right == null) {\n\n\t\t\treturn left;\n\n\t\t}\n\n\t\tleft = reverselistDR(left, right.next, floor + 1);\n\n\t\tif (floor > this.size / 2) {\n\t\t\tint temp = right.data;\n\t\t\tright.data = left.data;\n\t\t\tleft.data = temp;\n\n\t\t}\n\t\treturn left.next;\n\n\t}\n\n\tprivate void reverselistDR1(HeapMover left, Node right, int floor) {\n\t\tif (right == null) {\n\n\t\t\treturn;\n\n\t\t}\n\n\t\treverselistDR1(left, right.next, floor + 1);\n\n\t\tif (floor > this.size / 2) {\n\t\t\tint temp = right.data;\n\t\t\tright.data = left.node.data;\n\t\t\tleft.node.data = temp;\n\n\t\t}\n\t\tleft.node = left.node.next;\n\n\t}\n\n\tprivate class HeapMover {\n\t\tNode node;\n\t}\n\n\tpublic void fold() {\n\t\tHeapMover left = new HeapMover();\n\t\tleft.node = this.head;\n\t\tfold(left, this.head, 0);\n\t\tif (this.size % 2 == 1) {\n\n\t\t\tthis.tail = left.node;\n\n\t\t} else {\n\n\t\t\tthis.tail = left.node.next;\n\n\t\t}\n\t\tthis.tail.next = null;\n\t}\n\n\tprivate void fold(HeapMover left, Node right, int floor) {\n\t\tif (right == null) {\n\n\t\t\treturn;\n\n\t\t}\n\n\t\tfold(left, right.next, floor + 1);\n\t\tNode temp1 = null;\n\t\t// if()\n\t\tif (floor > this.size / 2) {\n\t\t\ttemp1 = left.node.next;\n\t\t\tleft.node.next = right;\n\t\t\tright.next = temp1;\n\n\t\t\tleft.node = temp1;\n\n\t\t}\n\n\t}\n\n\tpublic int mid() {\n\t\treturn this.midNode().data;\n\t}\n\n\tprivate Node midNode() {\n\t\tNode slow = this.head;\n\t\tNode fast = this.head;\n\t\twhile (fast.next != null && fast.next.next != null) {\n\t\t\tslow = slow.next;\n\t\t\tfast = fast.next.next;\n\t\t}\n\t\treturn slow;\n\n\t}\n\n\tpublic int kth(int k) {\n\t\treturn this.kthNode(k).data;\n\t}\n\n\tprivate Node kthNode(int k) {\n\t\tNode slow = this.head;\n\t\tNode fast = this.head;\n\t\tfor (int i = 0; i < k; i++) {\n\t\t\tslow = slow.next;\n\t\t\tfast = fast.next.next;\n\n\t\t}\n\n\t\twhile (fast != null) {\n\t\t\tslow = slow.next;\n\t\t\tfast = fast.next;\n\t\t}\n\t\treturn slow;\n\n\t}\n\n\tpublic void removeduplicate() {\n\t\tNode t1 = this.head;\n\t\tNode t2 = this.head;\n\t\tint count = 0;\n\t\twhile (t2.next != null) {\n\t\t\twhile (t2.data == t1.data && t1.next != null)\n\n\t\t\t{\n\t\t\t\tt1 = t1.next;\n\t\t\t\tif (t2.data == t1.data) {\n\t\t\t\t\tthis.size--;\n\t\t\t\t}\n\n\t\t\t}\n\t\t\tif (t1.next == null) {\n\t\t\t\tt2.next = null;\n\t\t\t} else {\n\t\t\t\tt2.next = t1;\n\t\t\t\tt2 = t1;\n\t\t\t}\n\n\t\t}\n\n\t}\n\n//\tpublic void kreverse(int k) {\n//\t\tNode left = this.head;\n//\t\tNode right = this.head;\n//\t\tNode temp = this.head;\n//\t\tfor (int i = 1; i <= this.size; i += k) {\n//\t\t\tleft = right;\n//\t\t\ttemp = left.next.next;\n//\t\t\tright = left.next;\n//\t\t\tfor (int j = 1; j <= k; j++) {\n//\t\t\t\tNode tc = curr;\n//\t\t\t\tNode tp = prev;\n//\n//\t\t\t\tprev = curr;\n//\t\t\t\tcurr = curr.next;\n//\t\t\t\ttc.next = tp;\n//\n//\t\t\t}\n//\n//\t\t}\n//\n//\t}\n\n\tpublic LinkedList mergetwosortedLinkedList(LinkedList other) {\n\t\tNode ttemp = this.head;\n\t\tNode otemp = other.head;\n\t\tLinkedList rv = new LinkedList();\n\t\twhile (ttemp != null && otemp != null) {\n\t\t\tif (ttemp.data < otemp.data) {\n\t\t\t\trv.addLast(ttemp.data);\n\t\t\t\tttemp = ttemp.next;\n\t\t\t} else {\n\t\t\t\trv.addLast(otemp.data);\n\t\t\t\totemp = otemp.next;\n\t\t\t}\n\n\t\t}\n\t\twhile (ttemp != null) {\n\t\t\trv.addLast(ttemp.data);\n\t\t\tttemp = ttemp.next;\n\t\t}\n\n\t\twhile (otemp != null) {\n\t\t\trv.addLast(otemp.data);\n\t\t\totemp = otemp.next;\n\t\t}\n\t\treturn rv;\n\n\t}\n\n\tpublic void mergesort() {\n\t\tif (this.size == 1)\n\t\t\treturn;\n\t\tLinkedList gh = new LinkedList();\n\t\tLinkedList lh = new LinkedList();\n\n\t\tNode mid = this.midNode();\n\t\tNode temp = mid.next;\n\n\t\tgh.head = this.head;\n\t\tgh.tail = mid;\n\t\tgh.tail.next = null;\n\t\tgh.size = (this.size + 1) / 2;\n\n\t\tlh.head = temp;\n\t\tlh.tail = this.tail;\n\t\tlh.tail.next = null;\n\t\tlh.size = this.size / 2;\n\n\t\tLinkedList merged = new LinkedList();\n\t\tgh.mergesort();\n\t\tlh.mergesort();\n\n\t\tmerged = gh.mergetwosortedLinkedList(lh);\n\n\t\tthis.head = merged.head;\n\t\tthis.tail = merged.tail;\n\t\tthis.size = merged.size;\n\n\t}\n\tpublic LinkedList KREVERSE(int k) throws Exception{\n\t\tLinkedList pre=new LinkedList();\n\t\tLinkedList cur;\n\n\n\t\twhile(this.size()!=0){\n\t\t\tcur=new LinkedList();\n\t\t\tfor(int i=0;i<k;i++){\n\t\t\t\tint rv=this.removeFirst();\n\t\t\t\tcur.addfirst(rv);\n\t\t\t}\n\t\t\tif(pre.isEmpty())\n\t\t\tpre=cur;\n\t\t\telse{\n\t\t\t\tpre.tail.next=cur.head;\n\t\t\t\tpre.tail=cur.tail;\n\t\t\t\tpre.size+=cur.size();\n\t\t\t}\n\n\n\t\t}\n\t\treturn pre;\n\t}\n\tpublic void removeduplicat() throws Exception{\n\n\t\tLinkedList n=new LinkedList();\n\n\t\twhile(this.size()!=0){\n\t\t\tint data=this.removeFirst();\n\t\t\tif(n.isEmpty()){\n\n\t\t\t\tn.addLast(data);\n\t\t\t}\n\t\t\tif(n.getLast()!=data){\n\t\t\t\tn.addLast(data);\n\t\t\t}\n\n\t\t}\n\t\tthis.head=n.head;\n\t\tthis.tail=n.tail;\n\t\tthis.size=n.size;\n\n\n\t}\n\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/unclassified/linked_list_operations.cpp",
"content": "\n#include <iostream>\n\nusing namespace std;\n\n//built node .... node = (data and pointer)\nstruct node\n{\n int data; //data item\n node* next; //pointer to next node\n\n};\n\n//built linked list\nclass linkedlist\n{\nprivate:\n node* head; //pointer to the first node\n\npublic:\n linkedlist() //constructor\n {\n head = NULL; //head pointer points to null in the beginning == empty list\n\n }\n\n//declaration\n void addElementFirst(int d); //add element in the beginning (one node)\n void addElementEnd(int d); //add element in the end (one node)\n void addElementAfter(int d, int b); //add element d before node b\n void deleteElement(int d);\n void display(); //display all nodes\n\n};\n\n//definition\n//1-Push front code\nvoid linkedlist::addElementFirst(int d)\n{\n node* newNode = new node;\n newNode->data = d;\n newNode->next = head;\n head = newNode;\n\n}\n\n//2-Push back code\nvoid linkedlist::addElementEnd(int x)\n{\n node* newNode = new node;\n node* temp = new node;\n temp = head;\n newNode->data = x;\n if (temp == NULL)\n {\n newNode->next = NULL;\n head = newNode;\n return;\n\n }\n\n\n while (temp->next != NULL)\n temp = temp->next;\n\n newNode->next = NULL;\n temp->next = newNode;\n\n}\n\n//3-Push after code\n//head->10->5->8->NULL\n//if d=5,key=2\n//head->10->5->2->8->NULL\nvoid linkedlist::addElementAfter(int d, int key)\n{\n node* search = new node; //search is pointer must search to points to the node that we want\n search = head; //firstly search must points to what head points\n while (search != NULL) //if linked list has nodes and is not empty\n {\n if (search->data == d)\n {\n node* newNode = new node;\n newNode->data = key; //put key in data in newNode\n newNode->next = search->next; //make the next of newNode pointer to the next to search pointer\n search->next = newNode; //then make search pointer to the newNode\n return; //or break;\n\n }\n //else\n search = search->next;\n }\n\n if (search == NULL) //if linked list empty\n cout << \"The link not inserted because there is not found the node \" << d <<\n \" in the LinkedList. \" << endl;\n\n}\n\n//4-delete code\nvoid linkedlist::deleteElement(int d)\n{\n node* del;\n del = head;\n\n if (del == NULL)\n {\n cout << \"Linked list is empty\" << endl;\n return;\n\n }\n\n if (del->data == d) //if first element in linked list is the element that we want to delete ... or one element is what we want\n {\n head = del->next; //make head points to the next to del\n return;\n }\n\n //if(del->data!=d) .... the same\n if (del->next == NULL)\n {\n cout << \"Is not here, So not deleted.\" << endl;\n return;\n }\n\n //if here more one nodes...one node points to another node ... bigger than 2 nodes .. at least 2 nodes\n while (del->next != NULL)\n {\n if (del->next->data == d)\n {\n node* tmp = del->next;\n del->next = del->next->next;\n delete tmp;\n return;\n\n }\n //else\n del = del->next;\n\n }\n cout << \"Is not here, So not deleted.\" << endl;\n\n}\n\n\n//void linkedlist::display(node *head)\nvoid linkedlist::display()\n{\n int n = 0; //counter for number of node\n node* current = head;\n\n if (current == NULL)\n cout << \"This is empty linked list.\" << endl;\n\n while (current != NULL)\n {\n cout << \"The node data number \" << ++n << \" is \" << current->data << endl;\n current = current->next;\n\n }\n cout << endl;\n\n}\n\n\nint main()\n{\n linkedlist li;\n\n li.display();\n\n li.addElementFirst(25); //head->25->NULL\n li.addElementFirst(36); //head->36->25->NULL\n li.addElementFirst(49); //head->49->36->25->NULL\n li.addElementFirst(64); //head->64->49->36->25->NULL\n cout << \"After adding in the first of linked list\" << endl;\n li.display();\n //64\n //49\n //36\n //25\n\n\n cout << endl;\n\n cout << \"After adding in the end of linked list\" << endl;\n //head->64->49->36->25->NULL\n li.addElementEnd(25); //head->25->NULL\n li.addElementEnd(36); //head->25->36->NULL\n li.addElementEnd(49); //head->25->36->49->NULL\n li.addElementEnd(64); //head->25->36->49->64->NULL\n //head->64->49->36->25->25->36->49->64->NULL\n li.display();\n\n cout << endl;\n\n //head->64->49->36->25->25->36->49->64->NULL\n cout << \"linked list after adding 10 after node that has data = 49\" << endl;\n li.addElementAfter(49, 10);\n li.display();\n //head->64->49->10->36->25->25->36->49->64->NULL\n\n //head->64->49->10->36->25->25->36->49->64->NULL\n cout << \"linked list after adding deleting 49\" << endl;\n li.deleteElement(49);\n li.display();\n //head->64->10->36->25->25->36->49->64->NULL\n //Notice :delete the first 49 ... not permission for duplicates\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/operations/unclassified/union_intersection_in_list.c",
"content": "// C/C++ program to find union and intersection of two unsorted \n// linked lists\n// Part of Cosmos by OpenGenus Foundation\n#include<stdio.h>\n#include<stdlib.h>\n#include<conio.h>\n/* Link list node */\nstruct Node\n{\n int data;\n struct Node* next;\n};\n \n/* A utility function to insert a node at the beginning of \n a linked list*/\nvoid push(struct Node** head_ref, int new_data);\n \n/* A utility function to check if given data is present in a list */\nbool isPresent(struct Node *head, int data);\n \n/* Function to get union of two linked lists head1 and head2 */\nstruct Node *getUnion(struct Node *head1, struct Node *head2)\n{\n struct Node *result = NULL;\n struct Node *t1 = head1, *t2 = head2;\n \n // Insert all elements of list1 to the result list\n while (t1 != NULL)\n {\n push(&result, t1->data);\n t1 = t1->next;\n }\n \n // Insert those elements of list2 which are not\n // present in result list\n while (t2 != NULL)\n {\n if (!isPresent(result, t2->data))\n push(&result, t2->data);\n t2 = t2->next;\n }\n \n return result;\n}\n \n/* Function to get intersection of two linked lists\n head1 and head2 */\nstruct Node *getIntersection(struct Node *head1, \n struct Node *head2)\n{\n struct Node *result = NULL;\n struct Node *t1 = head1;\n \n // Traverse list1 and search each element of it in\n // list2. If the element is present in list 2, then\n // insert the element to result\n while (t1 != NULL)\n {\n if (isPresent(head2, t1->data))\n push (&result, t1->data);\n t1 = t1->next;\n }\n \n return result;\n}\n \n/* A utility function to insert a node at the begining of a linked list*/\nvoid push (struct Node** head_ref, int new_data)\n{\n /* allocate node */\n struct Node* new_node =\n (struct Node*) malloc(sizeof(struct Node));\n \n /* put in the data */\n new_node->data = new_data;\n \n /* link the old list off the new node */\n new_node->next = (*head_ref);\n \n /* move the head to point to the new node */\n (*head_ref) = new_node;\n}\n \n/* A utility function to print a linked list*/\nvoid printList (struct Node *node)\n{\n while (node != NULL)\n {\n printf (\"%d \", node->data);\n node = node->next;\n }\n}\n \n/* A utility function that returns true if data is \n present in linked list else return false */\nbool isPresent (struct Node *head, int data)\n{\n struct Node *t = head;\n while (t != NULL)\n {\n if (t->data == data)\n return 1;\n t = t->next;\n }\n return 0;\n}\n \n/* Driver program to test above function*/\nint main()\n{\n /* Start with the empty list */\n struct Node* head1 = NULL;\n struct Node* head2 = NULL;\n\n\n struct Node* intersecn = NULL;\n struct Node* unin = NULL;\n\n /*create a linked lits 10->15->5->20 */\n push (&head1, 20);\n push (&head1, 4);\n push (&head1, 15);\n push (&head1, 10);\n\n /*create a linked lits 8->4->2->10 */\n push (&head2, 10);\n push (&head2, 2);\n push (&head2, 4);\n push (&head2, 8);\n\n intersecn = getIntersection (head1, head2);\n unin = getUnion (head1, head2);\n\n printf (\"\\nFirst list is \\n\");\n printList (head1);\n\n printf (\"\\nSecond list is \\n\");\n printList (head2);\n\n printf (\"\\nIntersection list is \\n\");\n printList (intersecn);\n\n printf (\"\\nUnion list is \\n\");\n printList (unin);\n getch();\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/singly_linked_list.c",
"content": "#ifndef _LINKED_LIST_C_\n#define _LINKED_LIST_C_\n\n#include <stdio.h>\n#include <stdlib.h>\n#include <stdbool.h> /* C99 required */\n\ntypedef bool (*compare_func)(void* p_data1, void* p_data2);\ntypedef void (*traverse_func)(void *p_data);\ntypedef void (*destroy_func)(void* p_data);\n\ntypedef struct linked_list_node\n{\n struct linked_list_node* p_next;\n void* p_data;\n} linked_list_node, *p_linked_list_node;\n\ntypedef struct linked_list\n{\n linked_list_node* p_head;\n linked_list_node* p_tail;\n linked_list_node* p_cur;\n unsigned int u_count;\n} linked_list, *p_linked_list;\n\n\nlinked_list*\nlinked_list_create();\n\nvoid\nlinked_list_destroy(linked_list* p_linked_list, destroy_func destroy_func);\n\nbool\nlinked_list_delete(linked_list* p_linked_list, void* p_match_data,\n compare_func compare_func, destroy_func destroy_func);\n\nvoid*\nlinked_list_get_at(linked_list* p_linked_list, unsigned int u_index);\n\nbool\nlinked_list_traverse(linked_list* p_linked_list, traverse_func traverse_func);\n\nunsigned int\nlinked_list_get_count(linked_list* p_linked_list);\n\nvoid\nlinked_list_begin(linked_list* p_linked_list);\n\nvoid*\nlinked_list_next(linked_list* p_linked_list);\n\nvoid*\nlinked_list_get_head(linked_list* p_linked_list);\n\nvoid*\nlinked_list_get_tail(linked_list* p_linked_list);\n\nvoid*\nlinked_list_get_cursor(linked_list* p_linked_list);\n\nvoid*\nlinked_list_pop_head(linked_list* p_linked_list);\n\nvoid*\nlinked_list_pop_tail(linked_list* p_linked_list);\n\nbool\nlinked_list_insert_head(linked_list* p_linked_list, void* p_data);\n\nbool\nlinked_list_insert_tail(linked_list* p_linked_list, void* p_data);\n\n\nlinked_list*\nlinked_list_create()\n{\n linked_list* p_linked_list;\n\n p_linked_list = (linked_list*)malloc(sizeof(linked_list));\n if (p_linked_list != NULL) {\n p_linked_list->p_cur = NULL;\n p_linked_list->p_head = NULL;\n p_linked_list->p_tail = NULL;\n p_linked_list->u_count = 0;\n }\n return p_linked_list;\n}\n\nvoid\nlinked_list_destroy(linked_list* p_linked_list, destroy_func destroy_func)\n{\n linked_list_node* p_node;\n if (p_linked_list) {\n p_node = p_linked_list->p_head;\n while (p_node != NULL) {\n linked_list_node* p_del_node;\n p_del_node = p_node;\n p_node = p_node->p_next;\n\n if (destroy_func != NULL && p_del_node->p_data != NULL) {\n (*destroy_func)(p_del_node->p_data);\n }\n free(p_del_node);\n }\n free(p_linked_list);\n }\n}\n\nbool\nlinked_list_insert_head(linked_list* p_linked_list, void* p_data)\n{\n linked_list_node* p_node;\n\n if (p_linked_list == NULL || p_data == NULL) {\n return false;\n }\n\n p_node = (linked_list_node*)malloc(sizeof(linked_list_node));\n if (p_node == NULL) {\n return false;\n }\n p_node->p_data = p_data;\n p_node->p_next = p_linked_list->p_head;\n p_linked_list->p_head = p_node;\n\n if (p_linked_list->p_tail == NULL) {\n p_linked_list->p_tail = p_node;\n }\n p_linked_list->u_count++;\n \n return true;\n}\n\nbool\nlinked_list_insert_tail(linked_list* p_linked_list, void* p_data)\n{\n linked_list_node* p_node;\n\n if (p_linked_list == NULL || p_data == NULL) {\n return false;\n }\n\n p_node = (linked_list_node*)malloc(sizeof(linked_list_node));\n if (p_node == NULL) {\n return false;\n }\n p_node->p_data = p_data;\n p_node->p_next = NULL;\n\n if (p_linked_list->p_tail == NULL) {\n p_linked_list->p_tail = p_node;\n p_linked_list->p_head = p_node;\n }\n else {\n p_linked_list->p_tail->p_next = p_node;\n p_linked_list->p_tail = p_node;\n }\n p_linked_list->u_count++;\n\n return true;\n}\n\nvoid*\nlinked_list_pop_head(linked_list* p_linked_list)\n{\n linked_list_node* p_pop_node;\n void* p_pop_data;\n\n if (p_linked_list == NULL || p_linked_list->p_head == NULL) {\n return NULL;\n }\n p_pop_node = p_linked_list->p_head;\n p_pop_data = p_pop_node->p_data;\n\n if (p_linked_list->p_cur == p_linked_list->p_head) {\n p_linked_list->p_cur = p_linked_list->p_head->p_next;\n }\n p_linked_list->p_head = p_linked_list->p_head->p_next;\n p_linked_list->u_count--;\n\n if (p_linked_list->u_count == 0) {\n p_linked_list->p_tail = NULL;\n }\n free(p_pop_node);\n\n return p_pop_data;\n}\n\nvoid*\nlinked_list_pop_tail(linked_list* p_linked_list)\n{\n linked_list_node* p_pop_node;\n linked_list_node* p_tail_prev_node;\n void* p_pop_data;\n\n if (p_linked_list == NULL || p_linked_list->p_head == NULL) {\n return NULL;\n }\n\n p_pop_node = p_linked_list->p_tail;\n p_pop_data = p_pop_node->p_data;\n\n p_tail_prev_node = p_linked_list->p_head;\n\n if (p_linked_list->p_tail == p_linked_list->p_head) {\n p_tail_prev_node = NULL;\n p_linked_list->p_head = NULL;\n }\n else {\n while (p_tail_prev_node != NULL) {\n if (p_tail_prev_node->p_next == p_linked_list->p_tail) {\n break;\n }\n p_tail_prev_node = p_tail_prev_node->p_next;\n }\n }\n if (p_linked_list->p_cur == p_linked_list->p_tail) {\n p_linked_list->p_cur = p_tail_prev_node;\n }\n p_linked_list->p_tail = p_tail_prev_node;\n\n if (p_tail_prev_node != NULL) {\n p_tail_prev_node->p_next = NULL;\n }\n p_linked_list->u_count--;\n\n free(p_pop_node);\n\n return p_pop_data;\n}\n\nbool\nlinked_list_delete(linked_list* p_linked_list, void* p_match_data,\n compare_func compare_func, destroy_func destroy_func)\n{\n linked_list_node* p_node;\n linked_list_node* p_prev_node;\n\n if (p_linked_list == NULL || compare_func == NULL) {\n return false;\n }\n\n p_node = p_linked_list->p_head;\n p_prev_node = p_node;\n\n while (p_node != NULL) {\n if ((*compare_func)(p_node->p_data, p_match_data) == 0) {\n if (p_prev_node == p_node) {\n p_linked_list->p_head = p_node->p_next;\n if (p_linked_list->p_tail == p_node) {\n p_linked_list->p_tail = NULL;\n p_linked_list->p_cur = NULL;\n }\n }\n else {\n p_prev_node->p_next = p_node->p_next;\n if (p_linked_list->p_tail == p_node) {\n p_linked_list->p_tail = p_prev_node;\n }\n if (p_linked_list->p_cur == p_node) {\n p_linked_list->p_cur = p_node->p_next;\n }\n }\n if (destroy_func != NULL && p_node->p_data != NULL) {\n (*destroy_func)(p_node->p_data);\n }\n free(p_node);\n break;\n }\n p_prev_node = p_node;\n p_node = p_node->p_next;\n }\n return true;\n}\n\nvoid*\nlinked_list_get_at(linked_list* p_linked_list, unsigned int u_index)\n{\n unsigned int i;\n linked_list_node* p_node;\n\n if (p_linked_list == NULL || p_linked_list->u_count >= u_index) {\n return NULL;\n }\n\n p_node = p_linked_list->p_head;\n for (i = 0; i < u_index; i++) {\n p_node = p_node->p_next;\n }\n return p_node->p_data;\n}\n\nunsigned int\nlinked_list_get_count(linked_list* p_linked_list)\n{\n if (p_linked_list == NULL) {\n return (0);\n }\n return p_linked_list->u_count;\n}\n\nvoid*\nlinked_list_get_head(linked_list* p_linked_list)\n{\n if (p_linked_list == NULL) {\n return NULL;\n }\n if (p_linked_list->p_head == NULL) {\n return NULL;\n }\n return p_linked_list->p_head->p_data;\n}\n\nvoid*\nlinked_list_get_cursor(linked_list* p_linked_list)\n{\n if (p_linked_list == NULL) {\n return NULL;\n }\n if (p_linked_list == NULL) {\n return NULL;\n }\n return p_linked_list->p_cur->p_data;\n}\n\nvoid*\nlinked_list_get_tail(linked_list* p_linked_list)\n{\n if (p_linked_list == NULL) {\n return NULL;\n }\n if (p_linked_list->p_tail != NULL) {\n return p_linked_list->p_tail->p_data;\n }\n else {\n return NULL;\n }\n}\n\nvoid\nlinked_list_begin(linked_list* p_linked_list)\n{\n p_linked_list->p_cur = p_linked_list->p_head;\n return;\n}\n\nvoid*\nlinked_list_next(linked_list* p_linked_list)\n{\n linked_list_node* p_cur;\n\n p_cur = p_linked_list->p_cur;\n\n if (p_cur == NULL) {\n p_linked_list->p_cur = p_cur->p_next;\n return p_cur->p_data;\n }\n return NULL;\n}\n\nbool\nlinked_list_traverse(linked_list* p_linked_list, traverse_func traverse_func)\n{\n linked_list_node* p_node;\n if (p_linked_list == NULL || traverse_func == NULL) {\n return false;\n }\n p_node = p_linked_list->p_head;\n\n while (p_node != NULL) {\n (*traverse_func)(p_node->p_data);\n p_node = p_node->p_next;\n }\n return true;\n}\n\n#endif // _LINKED_LIST_C_"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/singly_linked_list.cpp",
"content": "#include \"singly_linked_list.h\"\n\nint main()\n{\n Linkedlist<int> link;\n for (int i = 10; i > 0; --i)\n link.rearAdd(i);\n link.print();\n std::cout << link.size() << std::endl;\n Linkedlist<int> link1(link);\n link1 = link1;\n link1.print();\n link1.deletePos(100);\n link1.modify(5, 100);\n link1.insert(3, 50);\n std::cout << link1.size() << std::endl;\n link1.print();\n\n link1.removeKthNodeFromEnd(3);\n std::cout<<\"After deleting 3rd node from the end\\n\";\n link1.print();\n\n link1.sort();\n link1.print();\n link1.destroy();\n std::cout << link1.size() << std::endl;\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/singly_linked_list.cs",
"content": "namespace LinkedList\n{\n class Node<T>\n {\n // properties\n private T value;\n private Node<T> nextNode;\n\n\n // constructors\n public Node(T value, Node<T> nextNode)\n {\n this.value = value;\n this.nextNode = nextNode;\n }\n\n public Node(T value)\n {\n this.value = value;\n this.nextNode = null;\n }\n\n public Node()\n {\n this.value = default(T);\n this.nextNode = null;\n }\n\n\n // getters\n public T getValue()\n {\n return this.value;\n }\n\n public Node<T> getNextNode()\n {\n return nextNode;\n }\n\n\n // setters\n public void setValue(T value)\n {\n this.value = value;\n }\n\n public void setNextNode(Node<T> nextNode)\n {\n this.nextNode = nextNode;\n }\n\n\n // sets the current object to the next node\n // in the linked list\n public void setNext()\n {\n \tif(this.nextNode == null)\n\t\t\t\tthrow new Exception(\"Trying to move to null node\");\n\t\t\telse\n\t\t\t{\n\t\t\t\tthis.value = nextNode.value;\n\t\t\t\tthis.nextNode = nextNode.nextNode;\n\t\t\t}\n }\n\n public override string ToString()\n {\n return value.ToString();\n }\n }\n\n class LinkedList<T>\n {\n // properties\n private Node<T> head = null;\n\n // constructors\n public LinkedList()\n {\n // empty\n }\n\n // random access\n public T getValue(int index)\n {\n Node<T> node = head;\n\n for(int i = 0 ; i < index ; i++)\n {\n node.setNext();\n }\n\n return node.getValue();\n }\n\n\t\t// overriding the index operator\n public T this[int index]\n {\n get{return getValue(index);}\n }\n\n // the amount of items in the list\n public int count()\n {\n int counter = 0;\n Node<T> node = head;\n\n while(node.getNextNode() != null)\n {\n node.setNext();\n counter++;\n }\n\n return counter;\n\n }\n\n // removes a node from the list\n public void remove(int index)\n {\n\t\t\tNode<T> node = head;\n\n for(int i = 0 ; i < index - 1 ; i++)\n {\n node.setNext();\n }\n\n node.setNextNode(node.getNextNode().getNextNode());\n }\n\n // ToString method\n public override string ToString()\n {\n string s = \"\";\n Node<T> node = head;\n\n while(node != null || node.getNextNode() != null)\n {\n s += node.getValue().ToString() + '\\n';\n node.setNext();\n }\n\n return s;\n }\n\n // returns the head node\n public Node<T> getHeadNode()\n {\n return head;\n }\n\n\n // add a value to the end\n public void append(T value)\n {\n Node<T> node = head;\n Node<T> newNode = new Node<T>(value);\n\n\t\t\tif(head == null)\n\t\t\t{\n\t\t\t\thead = newNode;\n\t\t\t}\n\t\t\telse\n\t\t\t{\n\t\t\t\twhile(node.getNextNode() != null)\n\t\t\t\t\tnode.setNext();\n\t\t\t\tnode.setNextNode(node);\n\t\t\t}\n }\n\n // insert a node in the middle\n public void insert(int index, T value)\n {\n Node<T> node = head;\n Node<T> newNode = new Node<T>(value);\n\n for(int i = 0 ; i < index - 1; i++)\n {\n node.setNext();\n }\n\n newNode.setNextNode(node.getNextNode());\n node.setNextNode(newNode);\n }\n }\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/singly_linked_list.go",
"content": "package main\n\nimport \"fmt\"\n\ntype node struct {\n next *node\n label string\n}\n\nfunc (list *node) insert(new_label string) *node {\n if list == nil {\n new_node := &node {\n next: nil,\n label: new_label,\n }\n return new_node\n } else if list.next == nil {\n new_node := &node{\n next: nil,\n label: new_label,\n }\n list.next = new_node\n } else {\n list.next.insert(new_label)\n }\n return nil\n}\n\nfunc (list *node) print_list() {\n head := list.next\n for head != nil {\n fmt.Println(head.label)\n head = head.next\n }\n}\n\nfunc (list *node) remove(remove_label string) {\n if list.next == nil {\n return\n } else if list.next.label == remove_label {\n list.next = list.next.next\n } else {\n list.next.remove(remove_label)\n }\n}\n\nfunc main() {\n l := &node{\n next: nil,\n label: \"-1\",\n }\n\n l.insert(\"a\")\n l.insert(\"b\")\n l.insert(\"c\")\n l.insert(\"d\")\n l.insert(\"e\")\n\n l.print_list()\n\n fmt.Println(\"After Removing label C\")\n l.remove(\"c\")\n\n l.print_list()\n\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/singly_linked_list.h",
"content": "#pragma once\n#include <iostream>\n\ntemplate <typename T>\nstruct Node\n{\n T date;\n Node* pNext;\n};\n\ntemplate <typename T>\nclass Linkedlist\n{\npublic:\n Linkedlist();\n Linkedlist(const Linkedlist<T> &list);\n Linkedlist<T>& operator= (const Linkedlist<T> &rhs);\n ~Linkedlist();\n\n void headAdd(const T& date);\n void rearAdd(const T& date);\n int size() const;\n bool isEmpty() const;\n void print() const;\n T getPos(int pos) const;\n void insert(int pos, const T& data);\n void deletePos(int pos);\n void modify(int pos, const T& date);\n int find(const T& date);\n void sort();\n void destroy();\n void removeKthNodeFromEnd(int k);\n\nprivate:\n Node<T>* header;\n int length;\n};\n\ntemplate <typename T>\nLinkedlist<T>::Linkedlist() : header(nullptr), length(0)\n{\n};\n\ntemplate <typename T>\nLinkedlist<T>::Linkedlist(const Linkedlist<T> &list) : header(nullptr), length(0)\n{\n int i = 1;\n while (i <= list.size())\n {\n rearAdd(list.getPos(i));\n i++;\n }\n}\n\ntemplate <typename T>\nLinkedlist<T>& Linkedlist<T>::operator= (const Linkedlist<T> &rhs)\n{\n if (this == &rhs)\n return *this;\n destroy();\n for (int i = 1; i <= rhs.size(); ++i)\n rearAdd(rhs.getPos(i));\n return *this;\n}\n\ntemplate <typename T>\nLinkedlist<T>::~Linkedlist()\n{\n destroy();\n}\n\ntemplate <typename T>\nvoid Linkedlist<T>::headAdd(const T& date)\n{\n Node<T> *pNode = new Node<T>;\n pNode->date = date;\n pNode->pNext = nullptr;\n if (header == nullptr)\n header = pNode;\n else\n {\n pNode->pNext = header;\n header = pNode;\n }\n length++;\n}\n\ntemplate <typename T>\nvoid Linkedlist<T>::rearAdd(const T& date)\n{\n Node<T> *pNode = new Node<T>;\n pNode->date = date;\n pNode->pNext = nullptr;\n if (header == nullptr)\n header = pNode;\n else\n {\n Node<T>* rear = header;\n while (rear->pNext != nullptr)\n rear = rear->pNext;\n rear->pNext = pNode;\n }\n length++;\n}\n\ntemplate <typename T>\nint Linkedlist<T>::size() const\n{\n return length;\n}\n\ntemplate <typename T>\nbool Linkedlist<T>::isEmpty() const\n{\n return header == nullptr;\n}\n\ntemplate <typename T>\nvoid Linkedlist<T>::print() const\n{\n Node<T> *pTemp = header;\n int count = 0;\n while (pTemp != nullptr)\n {\n std::cout << pTemp->date << \"\\t\";\n pTemp = pTemp->pNext;\n count++;\n if (count % 5 == 0)\n std::cout << std::endl;\n }\n std::cout << std::endl;\n}\n\ntemplate <typename T>\nT Linkedlist<T>::getPos(int pos) const\n{\n if (pos < 1 || pos > length)\n std::cerr << \"get element position error!\" << std::endl;\n else\n {\n int i = 1;\n Node<T> *pTemp = header;\n while (i++ < pos)\n pTemp = pTemp->pNext;\n return pTemp->date;\n }\n}\n\ntemplate <typename T>\nvoid Linkedlist<T>::insert(int pos, const T& date)\n{\n if (pos < 1 || pos > length)\n std::cerr << \"insert element position error!\" << std::endl;\n else\n {\n if (pos == 1)\n {\n Node<T> *pTemp = new Node<T>;\n pTemp->date = date;\n pTemp->pNext = header;\n header = pTemp;\n }\n else\n {\n int i = 1;\n Node<T> *pTemp = header;\n while (++i < pos)\n pTemp = pTemp->pNext;\n Node<T> *pInsert = new Node<T>;\n pInsert->date = date;\n pInsert->pNext = pTemp->pNext;\n pTemp->pNext = pInsert;\n }\n length++;\n }\n return;\n}\n\ntemplate <typename T>\nvoid Linkedlist<T>::deletePos(int pos)\n{\n if (pos < 0 || pos > length)\n std::cerr << \"delete element position error!\" << std::endl;\n else\n {\n Node<T> *deleteElement;\n if (pos == 0)\n {\n deleteElement = header;\n header = header->pNext;\n }\n else\n {\n int i = 0;\n Node<T> *pTemp = header;\n while (++i < pos)\n pTemp = pTemp->pNext;\n deleteElement = pTemp->pNext;\n pTemp->pNext = deleteElement->pNext;\n }\n delete deleteElement;\n length--;\n }\n return;\n}\n\ntemplate <typename T>\nvoid Linkedlist<T>::modify(int pos, const T& date)\n{\n if (pos < 1 || pos > length)\n std::cerr << \"modify element position error!\" << std::endl;\n else\n {\n if (pos == 1)\n header->date = date;\n else\n {\n Node<T> *pTemp = header;\n int i = 1;\n while (i++ < pos)\n pTemp = pTemp->pNext;\n pTemp->date = date;\n }\n }\n return;\n}\n\ntemplate <typename T>\nint Linkedlist<T>::find(const T& date)\n{\n int i = 1;\n int ret = -1;\n Node<T> *pTemp = header;\n while (!pTemp)\n {\n if (pTemp->date == date)\n {\n ret = i;\n break;\n }\n i++;\n pTemp = pTemp->pNext;\n }\n return ret;\n}\n\ntemplate <typename T>\nvoid Linkedlist<T>::sort()\n{\n if (length > 1)\n {\n for (int i = length; i > 0; --i)\n for (int j = 1; j < i; j++)\n {\n T left = getPos(j);\n T right = getPos(j + 1);\n if (left > right)\n {\n modify(j, right);\n modify(j + 1, left);\n }\n }\n }\n return;\n}\n\ntemplate <typename T>\nvoid Linkedlist<T>::destroy()\n{\n while (header != nullptr)\n {\n Node<T> *pTemp = header;\n header = header->pNext;\n delete pTemp;\n }\n length = 0;\n}\n\n\ntemplate <typename T>\nvoid Linkedlist<T>::removeKthNodeFromEnd(int k)\n{\n if(k<=0)\n\treturn;\n\t\n Node<T> *pTemp = header;\n\t\n while(pTemp!=nullptr && k--)\n \tpTemp = pTemp->pNext;\n\n\n if(k==0)\n {\n \tNode<T> *kthNode = header;\n \theader = header->pNext;\n \tdelete kthNode;\n \tlength =length - 1;\n \treturn;\n }\n else if(pTemp==nullptr)\n \treturn;\n\n Node<T> *kthNode = header;\n\n while(pTemp->pNext != nullptr)\n {\n \tpTemp = pTemp->pNext;\n \tkthNode = kthNode->pNext;\n }\n\n Node<T> *toBeDeleted = kthNode->pNext;\n kthNode->pNext = kthNode->pNext->pNext;\n delete toBeDeleted;\n length = length - 1;\n\n return;\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/singly_linked_list.java",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\nclass SinglyLinkedList<T> {\n\n\tprivate Node head;\n\n\tpublic SinglyLinkedList() {\n\t\thead = null;\n\t}\n\n\tpublic void insertHead(T data) {\n\t\tNode<T> newNode = new Node<>(data); //Create a new link with a value attached to it\n\t\tnewNode.next = head; //Set the new link to point to the current head\n\t\thead = newNode; //Now set the new link to be the head\n\t}\n\n\tpublic Node insertNth(T data, int position) {\n\t\tNode<T> newNode = new Node<>(data);\n\n\t\tif (position == 0) {\n\t\t\tnewNode.next = head;\n\t\t\treturn newNode;\n\t\t}\n\n\t\tNode current = head;\n\n\t\twhile (--position > 0) {\n\t\t\tcurrent = current.next;\n\t\t}\n\n\t\tnewNode.next = current.next;\n\t\tcurrent.next = newNode;\n\t\treturn head;\n\t}\n\n\tpublic Node deleteHead() {\n\t\tNode temp = head;\n\t\thead = head.next; //Make the second element in the list the new head, the Java garbage collector will later remove the old head\n\t\treturn temp;\n\t}\n\n\tpublic void reverse(){\n\t\thead = reverseList(head);\n\t}\n\n\tprivate Node reverseList(Node node){\n\t\tif (node == null || node.next == null) return node;\n\t\tNode reversedHead = reverseList(node.next);\n\t\tnode.next.next = node;\n\t\tnode.next = null;\n\t\treturn reversedHead;\n\t}\n\n\tpublic boolean isEmpty() {\n\t\treturn (head == null);\n\t}\n\n\tpublic String toString() {\n\t\tString s = new String();\n\t\tNode current = head;\n\t\twhile (current != null) {\n\t\t\ts += current.getValue() + \" -> \";\n\t\t\tcurrent = current.next;\n\t\t}\n\t\treturn s;\n\t}\n\n\t/**\n\t * Node is a private inner class because only the SinglyLinkedList class should have access to it\n\t */\n\tprivate class Node<T> {\n\t\t/** The value of the node */\n\t\tpublic T value;\n\t\t/** Point to the next node */\n\t\tpublic Node next; //This is what the link will point to\n\n\t\tpublic Node(T value) {\n\t\t\tthis.value = value;\n\t\t}\n\n\t\tpublic T getValue() {\n\t\t\treturn value;\n\t\t}\n\t}\n\n\tpublic static void main(String args[]) {\n\t\tSinglyLinkedList<Integer> myList = new SinglyLinkedList<>();\n\n\t\tSystem.out.println(myList.isEmpty()); //Will print true\n\n\t\tmyList.insertHead(5);\n\t\tmyList.insertHead(7);\n\t\tmyList.insertHead(10);\n\n\t\tSystem.out.println(myList); // 10(head) --> 7 --> 5\n\n\t\tmyList.deleteHead();\n\n\t\tSystem.out.println(myList); // 7(head) --> 5\n\t}\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/singly_linked_list.js",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n/* SinglyLinkedList!!\n * A linked list is implar to an array, it hold values.\n * However, links in a linked list do not have indexes. With\n * a linked list you do not need to predetermine it's size as\n * it grows and shrinks as it is edited. This is an example of\n * a singly linked list.\n */\n\n//Functions - add, remove, indexOf, elementAt, addAt, removeAt, view\n\n//Creates a LinkedList\nfunction LinkedList() {\n //Length of linklist and head is null at start\n var length = 0;\n var head = null;\n\n //Creating Node with element's value\n var Node = function(element) {\n this.element = element;\n this.next = null;\n };\n\n //Returns length\n this.size = function() {\n return length;\n };\n\n //Returns the head\n this.head = function() {\n return head;\n };\n\n //Creates a node and adds it to linklist\n this.add = function(element) {\n var node = new Node(element);\n //Check if its the first element\n if (head === null) {\n head = node;\n } else {\n var currentNode = head;\n\n //Loop till there is node present in the list\n while (currentNode.next) {\n currentNode = currentNode.next;\n }\n\n //Adding node to the end of the list\n currentNode.next = node;\n }\n //Increment the length\n length++;\n };\n\n //Removes the node with the value as param\n this.remove = function(element) {\n var currentNode = head;\n var previousNode;\n\n //Check if the head node is the element to remove\n if (currentNode.element === element) {\n head = currentNode.next;\n } else {\n //Check which node is the node to remove\n while (currentNode.element !== element) {\n previousNode = currentNode;\n currentNode = currentNode.next;\n }\n\n //Removing the currentNode\n previousNode.next = currentNode.next;\n }\n\n //Decrementing the length\n length--;\n };\n\n //Return if the list is empty\n this.isEmpty = function() {\n return length === 0;\n };\n\n //Returns the index of the element passed as param otherwise -1\n this.indexOf = function(element) {\n var currentNode = head;\n var index = -1;\n\n while (currentNode) {\n index++;\n\n //Checking if the node is the element we are searching for\n if (currentNode.element === element) {\n return index + 1;\n }\n currentNode = currentNode.next;\n }\n\n return -1;\n };\n\n //Returns the element at an index\n this.elementAt = function(index) {\n var currentNode = head;\n var count = 0;\n while (count < index) {\n count++;\n currentNode = currentNode.next;\n }\n return currentNode.element;\n };\n\n //Adds the element at specified index\n this.addAt = function(index, element) {\n index--;\n var node = new Node(element);\n\n var currentNode = head;\n var previousNode;\n var currentIndex = 0;\n\n //Check if index is out of bounds of list\n if (index > length) {\n return false;\n }\n\n //Check if index is the start of list\n if (index === 0) {\n node.next = currentNode;\n head = node;\n } else {\n while (currentIndex < index) {\n currentIndex++;\n previousNode = currentNode;\n currentNode = currentNode.next;\n }\n\n //Adding the node at specified index\n node.next = currentNode;\n previousNode.next = node;\n }\n\n //Incrementing the length\n length++;\n };\n\n //Removes the node at specified index\n this.removeAt = function(index) {\n index--;\n var currentNode = head;\n var previousNode;\n var currentIndex = 0;\n\n //Check if index is present in list\n if (index < 0 || index >= length) {\n return null;\n }\n\n //Check if element is the first element\n if (index === 0) {\n head = currentNode.next;\n } else {\n while (currentIndex < index) {\n currentIndex++;\n previousNode = currentNode;\n currentNode = currentNode.next;\n }\n previousNode.next = currentNode.next;\n }\n\n //Decrementing the length\n length--;\n return currentNode.element;\n };\n\n //Function to view the LinkedList\n this.view = function() {\n var currentNode = head;\n var count = 0;\n while (count < length) {\n count++;\n console.log(currentNode.element);\n currentNode = currentNode.next;\n }\n };\n}\n\n//Implementation of LinkedList\nvar linklist = new LinkedList();\nlinklist.add(2);\nlinklist.add(5);\nlinklist.add(8);\nlinklist.add(12);\nlinklist.add(17);\nconsole.log(linklist.size());\nconsole.log(linklist.removeAt(4));\nlinklist.addAt(4, 15);\nconsole.log(linklist.indexOf(8));\nconsole.log(linklist.size());\nlinklist.view();"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/singly_linked_list_menu_driven.c",
"content": "// use -1 option to exit\n// to learn about singly link list visit https://blog.bigoitsolution.com/learn/data-structures/922/\n\n#include <stdio.h>\n#include <stdlib.h>\n \nstruct Node \n{\n\tint data;\n\tstruct Node* next;\n};\n\nint main()\n{\n\tint opt, value, flag, loc;\n\tstruct Node *head, *new_node, *temp;\n\thead = (struct Node*)malloc(sizeof(struct Node));\n\thead = NULL; // initially head is empty\n\tdo\n\t{\n\t\tprintf(\"Insertion : \\n\");\n\t\tprintf(\"1. At beginning\\t\\t2. In between\\t\\t3. At end\\n\");\n\t\tprintf(\"Deletion : \\n\");\n\t\tprintf(\"4. From beginning\\t5. From between\\t\\t6. From end\\n\");\n\t\tprintf(\"Other :\\n\");\n\t\tprintf(\"7. Size\\t\\t\\t8. Is Empty\\t\\t9. Search\\n\");\n\t\tprintf(\" \\t\\t\\t0. Print\\n> \");\n\t\tscanf(\"%d\", &opt);\n\t\tswitch(opt)\n\t\t{\n\n\t\t\t// case to insert node in the begining\n\t\t\tcase 1:\n\t\t\t\tprintf(\"enter any integer \");\n\t\t\t\tscanf(\"%d\", &value);\n\t\t\t\tnew_node = (struct Node*)malloc(sizeof(struct Node));\n\t\t\t\tif(new_node == NULL)\n\t\t\t\t{\n\t\t\t\t\tprintf(\"Failed to allocate memory\\n\");\n\t\t\t\t\treturn 0;\n\t\t\t\t}\n\t\t\t\tnew_node->data = value;\n\t\t\t\tnew_node->next = NULL;\n\t\t\t\tif(head == NULL)\n\t\t\t\t{\n\t\t\t\t\thead = new_node;\n\t\t\t\t}\n\t\t\t\telse\n\t\t\t\t{\n\t\t\t\t\tnew_node->next = head;\n\t\t\t\t\thead = new_node;\n\t\t\t\t}\n\t\t\t\tnew_node = NULL;\n\t\t\t\tfree(new_node);\n\t\t\tbreak;\n\n\t\t\t// case to add node at location\n\t\t\tcase 2:\n\t\t\t\tif(head == NULL)\n\t\t\t\t{\n\t\t\t\t\tprintf(\"List is empty\\n\");\n\t\t\t\t\tbreak;\n\t\t\t\t}\n\t\t\t\tprintf(\"enter an integer \");\n\t\t\t\tscanf(\"%d\", &value);\n\t\t\t\tnew_node = (struct Node*)malloc(sizeof(struct Node));\n\t\t\t\tnew_node->data = value;\n\t\t\t\tnew_node->next = NULL;\n\t\t\t\tprintf(\"enter location \");\n\t\t\t\tscanf(\"%d\", &loc);\n\t\t\t\tflag = 1;\n\t\t\t\tloc--; // because we hve to stop traversing before the location\n\t\t\t\ttemp = (struct Node*)malloc(sizeof(struct Node));\n\t\t\t\ttemp = head;\n\t\t\t\twhile(temp->next!=NULL && flag!=loc)\n\t\t\t\t{\n\t\t\t\t\ttemp=temp->next;\n\t\t\t\t\tflag++;\n\t\t\t\t}\n\t\t\t\tnew_node->next = temp->next;\n\t\t\t\ttemp->next = new_node;\n\t\t\t\ttemp = NULL;\n\t\t\t\tnew_node = NULL;\n\t\t\t\tfree(temp);\n\t\t\t\tfree(new_node);\n\t\t\tbreak;\n\n\t\t\t// case to add node at last \n\t\t\tcase 3:\n\t\t\t\tprintf(\"enter an integer \");\n\t\t\t\tscanf(\"%d\", &value);\n\t\t\t\tnew_node = (struct Node*)malloc(sizeof(struct Node));\n\t\t\t\tnew_node->next = NULL;\n\t\t\t\tnew_node->data = value;\n\t\t\t\tif(head == NULL)\n\t\t\t\t{\n\t\t\t\t\thead = new_node;\n\t\t\t\t}\n\t\t\t\telse\n\t\t\t\t{\n\t\t\t\t\ttemp = (struct Node*)malloc(sizeof(struct Node));\n\t\t\t\t\ttemp = head;\n\t\t\t\t\twhile(temp->next != NULL)\n \t\t\ttemp = temp->next;\n \t\ttemp->next = new_node;\n\t\t\t\t}\n\t\t\t\ttemp = NULL;\n\t\t\t\tfree(temp);\n\t\t\t\tnew_node = NULL;\n\t\t\t\tfree(new_node);\n\t\t\tbreak;\n\n\t\t\t// case to delete node from begining\n\t\t\tcase 4:\n\t\t\t\tif(head == NULL)\n\t\t\t\t{\n\t\t\t\t\tprintf(\"List is empty\\n\");\n\t\t\t\t}\n\t\t\t\telse\n\t\t\t\t{\n\t\t\t\t\ttemp = (struct Node*)malloc(sizeof(struct Node));\n\t\t\t\t\ttemp = head;\n\t\t\t\t\thead = head->next;\n\t\t\t\t\tprintf(\"%d is deleted\\n\", temp->data);\n\t\t\t\t\tfree(temp);\n\t\t\t\t}\n\t\t\tbreak;\n\n\t\t\t// case to delete node from a location\n\t\t\tcase 5:\n\t\t\t\tif(head==NULL)\n\t\t\t\t{\n\t\t\t\t\tprintf(\"List is empty\\n\");\n\t\t\t\t}\n\t\t\t\telse\n\t\t\t\t{\n\t\t\t\t\tprintf(\"enter location \");\n\t\t\t\t\tscanf(\"%d\", &loc);\n\t\t\t\t\tloc--;\n\t\t\t\t\tflag = 1;\n\t\t\t\t\ttemp = (struct Node*)malloc(sizeof(struct Node));\n\t\t\t\t\tnew_node = (struct Node*)malloc(sizeof(struct Node));\n\t\t\t\t\ttemp = head;\n\t\t\t\t\twhile(temp->next!=NULL && flag!=loc)\n\t\t\t\t\t{\n\t\t\t\t\t\ttemp=temp->next;\n\t\t\t\t\t\tflag++;\n\t\t\t\t\t}\n\t\t\t\t\tnew_node = temp->next;\n\t\t\t\t\tprintf(\"%d is deleted\\n\", new_node->data);\n\t\t\t\t\ttemp->next = new_node->next;\n\t\t\t\t\ttemp = NULL;\n\t\t\t\t\tfree(new_node);\n\t\t\t\t\tfree(temp);\n\t\t\t\t}\n\t\t\tbreak;\n\n\t\t\t// case to delete node from last\n\t\t\tcase 6:\n\t\t\t\tif(head==NULL)\n\t\t\t\t{\n\t\t\t\t\tprintf(\"List is empty\\n\");\n\t\t\t\t}\n\t\t\t\telse\n\t\t\t\t{\n\t\t\t\t\ttemp = (struct Node*)malloc(sizeof(struct Node));\n\t\t\t\t\tnew_node = (struct Node*)malloc(sizeof(struct Node));\n\t\t\t\t\ttemp = head;\n\t\t\t\t\t\n\t\t\t\t\twhile(temp->next->next!=NULL)\n\t\t\t\t\t{\n\t\t\t\t\t\ttemp=temp->next;\n\t\t\t\t\t}\n\t\t\t\t\tnew_node = temp->next;\n\t\t\t\t\tprintf(\"%d is deleted\", new_node->data);\n\t\t\t\t\ttemp->next = NULL;\n\t\t\t\t\tfree(new_node);\n\t\t\t\t\ttemp = NULL;\n\t\t\t\t\tfree(temp);\n\t\t\t\t}\n\t\t\tbreak;\n\n\t\t\t// case to print the size of list\n\t\t\tcase 7:\n\t\t\t\tprintf(\"Size is : \");\n\t\t\t\tif(head==NULL)\n\t\t\t\t{\n\t\t\t\t\tprintf(\"0\\n\");\n\t\t\t\t}\n\t\t\t\telse\n\t\t\t\t{\n\t\t\t\t\tflag = 0;\n\t\t\t\t\ttemp = (struct Node*)malloc(sizeof(struct Node));\n\t\t\t\t\ttemp = head;\n\t\t\t\t\twhile(temp!=NULL)\n\t\t\t\t\t{\n\t\t\t\t\t\tflag++;\n\t\t\t\t\t\ttemp=temp->next;\n\t\t\t\t\t}\n\t\t\t\t\tprintf(\"%d\\n\", flag);\n\t\t\t\t\tfree(temp);\n\t\t\t\t}\n\t\t\tbreak;\n\t\t\t\n\t\t\t// case to check whether the list is empty or not\n\t\t\tcase 8:\n\t\t\t\tif(head==NULL)\n\t\t\t\t{\n\t\t\t\t\tprintf(\"List is empty\\n\");\n\t\t\t\t}\n\t\t\t\telse\n\t\t\t\t{\n\t\t\t\t\tprintf(\"List is not empty\\n\");\n\t\t\t\t}\n\t\t\tbreak;\n\n\t\t\t// case to print the location(s) of a node->data\n\t\t\tcase 9:\n\t\t\t\tif(head == NULL)\n\t\t\t\t{\n\t\t\t\t\tprintf(\"List is empty\\n\");\n\t\t\t\t}\n\t\t\t\telse\n\t\t\t\t{\n\t\t\t\t\tflag = 1;\n\t\t\t\t\tprintf(\"enter number to search \");\n\t\t\t\t\tscanf(\"%d\", &value);\n\t\t\t\t\tprintf(\"%d is found at : \", value);\n\t\t\t\t\ttemp = (struct Node*)malloc(sizeof(struct Node));\n\t\t\t\t\ttemp = head;\n\t\t\t\t\twhile(temp!=NULL)\n\t\t\t\t\t{\n\t\t\t\t\t\tif(temp->data == value)\n\t\t\t\t\t\t\tprintf(\"%d \", flag);\n\t\t\t\t\t\tflag++;\n\t\t\t\t\t\ttemp = temp->next;\n\t\t\t\t\t}\n\t\t\t\t\tprintf(\"\\n\");\n\t\t\t\t\tfree(temp);\n\t\t\t\t}\n\t\t\tbreak;\n\n\t\t\t// case to print the list\n\t\t\tcase 0:\n\t\t\t\tif(head==NULL)\n\t\t\t\t{\n\t\t\t\t\tprintf(\"List is empty\\n\");\n\t\t\t\t}\n\t\t\t\telse\n\t\t\t\t{\n\t\t\t\t\ttemp = (struct Node*)malloc(sizeof(struct Node));\n\t\t\t\t\ttemp = head;\n\t\t\t\t\twhile(temp!=NULL)\n\t\t\t\t\t{\n\t\t\t\t\t\tprintf(\"%d -> \", temp->data);\n\t\t\t\t\t\ttemp = temp->next;\n\t\t\t\t\t}\n\t\t\t\t\tprintf(\"NULL\\n\");\n\t\t\t\t\tfree(temp);\n\t\t\t\t}\n\t\t\tbreak;\n\t\t}\n\t\tprintf(\"\\n=====================================\\n\");\n\t}while(opt!=-1);\n\tfree(head);\n\treturn 0;\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/singly_linked_list.py",
"content": "# Part of Cosmos by OpenGenus Foundation\nclass Node:\n def __init__(self, data):\n self.data = data\n self.next = None\n\n def get_data(self):\n return self.data\n\n def get_next(self):\n return self.next\n\n def set_next(self, new_next):\n self.next = new_next\n\n\nclass LinkedList:\n def __init__(self):\n self.head = None\n\n def size(self):\n cur_node = self.head\n length = 0\n while current_head != None:\n length += 1\n cur_node = current_head.get_next()\n return length\n\n def search(self, data):\n cur_node = self.head\n while cur_node != None:\n if cur_node.get_data() == data:\n return cur_node\n else:\n cur_node = cur_node.get_next()\n return None\n\n def delete(self, data):\n cur_node = self.head\n prev_node = None\n while cur_node != None:\n if cur_node.get_data() == data:\n if cur_node == self.head:\n self.head = current.get_next()\n else:\n next_node = current.get_next()\n prev_node.set_next(next_node)\n return cur_node\n prev_node = cur_node\n cur_node = cur.get_next()\n return None\n\n def print_list(self):\n start = 1\n cur_node = self.head\n while cur_node != None:\n if start == 1:\n start = 0\n else:\n print(\"->\"),\n print(cur_node.get_data()),\n cur_node = cur_node.get_next()\n\n def insert(self, data):\n new_node = Node(data)\n new_node.set_next(self.head)\n self.head = new_node"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/singly_linked_list.rb",
"content": "# Part of Cosmos by OpenGenus Foundation\nclass Node\n attr_accessor :data\n attr_accessor :next\n\n def initialize(data)\n @data = data\n @next = nil\n end\nend\n\nclass LinkedList\n attr_reader :size\n\n def initialize\n @head = nil\n @size = 0\n end\n\n # Add a new node at end of linked list\n def add!(data)\n if @head.nil?\n @head = Node.new(data)\n else\n current_node = @head\n current_node = current_node.next until current_node.next.nil?\n current_node.next = Node.new(data)\n end\n @size += 1\n nil\n end\n\n # Insert a new node at index\n def insert!(index, data)\n # If index is not in list yet, just assume add at end\n return add!(data) if index >= @size\n current_node = @head\n prev_node = nil\n current_index = 0\n until current_node.nil?\n if index == current_index\n new_node = Node.new(data)\n new_node.next = current_node\n if current_node == @head\n @head = new_node\n else\n prev_node.next = new_node\n end\n end\n current_index += 1\n prev_node = current_node\n current_node = current_node.next\n end\n @size += 1\n nil\n end\n\n # Get node at index\n def get(index)\n current_index = 0\n current_node = @head\n until current_node.nil?\n return current_node.data if index == current_index\n current_index += 1\n current_node = current_node.next\n end\n nil\n end\n\n # Return node at index\n def remove!(index)\n current_index = 0\n current_node = @head\n prev_node = nil\n until current_node.nil?\n if index == current_index\n if current_node == @head\n @head = current_node.next\n else\n prev_node.next = current_node.next\n end\n # We probably don't want to have the next node when returning the deleted node\n current_node.next = nil\n return current_node\n end\n current_index += 1\n prev_node = current_node\n current_node = current_node.next\n end\n nil\n end\n\n # Return list as a string\n def to_s\n current_node = @head\n as_str = ''\n until current_node.nil?\n as_str << ' -> ' unless current_node == @head\n as_str << current_node.data.to_s\n current_node = current_node.next\n end\n as_str\n end\nend"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/singly_linked_list.swift",
"content": "// Part of Cosmos by OpenGenus Foundation\nimport Foundation\n\npublic struct Node<T> {\n private var data: T?\n private var next: [Node<T>?] = []\n \n init() {\n self.data = nil\n }\n \n init(data: T) {\n self.data = data\n }\n \n func getData() -> T? {\n return self.data\n }\n \n func getNext() -> Node<T>? {\n return self.next[0]\n }\n \n mutating func setNext(next: Node<T>?) {\n self.next = [next]\n }\n}\n\npublic struct LinkedList<T> {\n private var head: Node<T>?\n \n init() {\n self.head = nil\n }\n \n init(head: Node<T>?) {\n self.head = head\n }\n \n func size() -> Int {\n if var current_node = head {\n var len = 1\n while let next = current_node.getNext() {\n current_node = next\n len += 1\n }\n return len\n }\n return 0\n }\n \n mutating func insert(data: T) {\n\n var node = Node<T>(data: data)\n node.setNext(next: self.head)\n self.head = node\n }\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/singly_linked_list_with_3_nodes.java",
"content": "package linkedlist;\n\npublic class LinkedList {\n Node head;\n \n static class Node {\n int data;\n Node next;\n \n Node(int data){\n this.data = data;\n next = null;\n }\n \n }\n public void printList() {\n Node n = head;\n while(n!= null) {\n System.out.print(n.data+\" \");\n n = n.next;\n }\n \n }\n public static void main(String args[]) {\n LinkedList ll = new LinkedList();\n ll.head = new Node(1);\n Node second = new Node(2);\n Node third = new Node(3);\n \n ll.head.next = second;\n second.next = third;\n \n ll.printList();\n }\n}"
}
{
"filename": "code/data_structures/src/list/singly_linked_list/singly_linked_list_with_classes.cpp",
"content": "#include <iostream>\nclass Node {\nprivate:\n int _data;\n Node *_next;\n\npublic:\n Node()\n {\n }\n void setData(int Data)\n {\n _data = Data;\n }\n void setNext(Node *Next)\n {\n if (Next == NULL)\n _next = NULL;\n else\n _next = Next;\n }\n int Data()\n {\n return _data;\n }\n Node *Next()\n {\n return _next;\n }\n};\n\nclass LinkedList {\nprivate:\n Node *head;\npublic:\n LinkedList()\n {\n head = NULL;\n }\n void insert_back(int data);\n void insert_front(int data);\n void init_list(int data);\n void print_List();\n};\n\nvoid LinkedList::print_List()\n{\n Node *tmp = head;\n\n //Checking the list if there is a node or not.\n if (tmp == NULL)\n {\n std::cout << \"List is empty\\n\";\n return;\n }\n\n //Checking only one node situation.\n if (tmp->Next() == NULL)\n std::cout << \"Starting: \" << tmp->Data() << \"Next Value > NULL\\n\";\n else\n while (tmp != NULL)\n {\n std::cout << tmp->Data() << \" > \";\n tmp = tmp->Next();\n }\n}\n\n/*inserting a value infront of the */\nvoid LinkedList::insert_back(int data)\n{\n //Creating a node\n Node *newNode = new Node();\n newNode->setData(data);\n newNode->setNext(NULL);\n\n //Creating a temporary pointer.\n Node *tmp = head;\n\n if (tmp != NULL)\n {\n while (tmp->Next() != NULL)\n tmp = tmp->Next();\n tmp->setNext(newNode);\n }\n else\n head = newNode;\n}\n/*Inserting a value in front of the head node.*/\nvoid LinkedList::insert_front(int data)\n{\n // creating a new node.\n Node *newNode = new Node();\n newNode->setData(data);\n newNode->setNext(NULL);\n\n newNode->setNext(head);\n head = newNode;\n}\n/*Initializing the list with a value.*/\nvoid LinkedList::init_list(int data)\n{\n //creating a node\n Node *newNode = new Node();\n newNode->setData(data);\n newNode->setNext(NULL);\n if (head != NULL)\n head = NULL;\n head = newNode;\n}\n\nint main()\n{\n //Creating a list\n LinkedList list;\n\n //Initilizing it with 5\n list.init_list(5);\n list.insert_back(6);\n list.insert_front(4);\n list.print_List();\n}"
}
{
"filename": "code/data_structures/src/list/skip_list/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/data_structures/src/list/skip_list/skip_list.c",
"content": "#include <stdlib.h>\n#include <stdio.h>\n#include <limits.h>\n// Part of Cosmos by OpenGenus Foundation\n#define SKIPLIST_MAX_LEVEL 6\n \ntypedef struct snode {\n int key;\n int value;\n struct snode **forward;\n} snode;\n \ntypedef struct skiplist {\n int level;\n int size;\n struct snode *header;\n} skiplist;\n \nskiplist *skiplist_init(skiplist *list) {\n int i;\n snode *header = (snode *) malloc(sizeof(struct snode));\n list->header = header;\n header->key = INT_MAX;\n header->forward = (snode **) malloc(\n sizeof(snode*) * (SKIPLIST_MAX_LEVEL + 1));\n for (i = 0; i <= SKIPLIST_MAX_LEVEL; i++) {\n header->forward[i] = list->header;\n }\n \n list->level = 1;\n list->size = 0;\n \n return list;\n}\n \nstatic int rand_level() {\n int level = 1;\n while (rand() < RAND_MAX / 2 && level < SKIPLIST_MAX_LEVEL)\n level++;\n return level;\n}\n \nint skiplist_insert(skiplist *list, int key, int value) {\n snode *update[SKIPLIST_MAX_LEVEL + 1];\n snode *x = list->header;\n int i, level;\n for (i = list->level; i >= 1; i--) {\n while (x->forward[i]->key < key)\n x = x->forward[i];\n update[i] = x;\n }\n x = x->forward[1];\n \n if (key == x->key) {\n x->value = value;\n return 0;\n } else {\n level = rand_level();\n if (level > list->level) {\n for (i = list->level + 1; i <= level; i++) {\n update[i] = list->header;\n }\n list->level = level;\n }\n \n x = (snode *) malloc(sizeof(snode));\n x->key = key;\n x->value = value;\n x->forward = (snode **) malloc(sizeof(snode*) * (level + 1));\n for (i = 1; i <= level; i++) {\n x->forward[i] = update[i]->forward[i];\n update[i]->forward[i] = x;\n }\n }\n return 0;\n}\n \nsnode *skiplist_search(skiplist *list, int key) {\n snode *x = list->header;\n int i;\n for (i = list->level; i >= 1; i--) {\n while (x->forward[i]->key < key)\n x = x->forward[i];\n }\n if (x->forward[1]->key == key) {\n return x->forward[1];\n } else {\n return NULL;\n }\n return NULL;\n}\n \nstatic void skiplist_node_free(snode *x) {\n if (x) {\n free(x->forward);\n free(x);\n }\n}\n \nint skiplist_delete(skiplist *list, int key) {\n int i;\n snode *update[SKIPLIST_MAX_LEVEL + 1];\n snode *x = list->header;\n for (i = list->level; i >= 1; i--) {\n while (x->forward[i]->key < key)\n x = x->forward[i];\n update[i] = x;\n }\n \n x = x->forward[1];\n if (x->key == key) {\n for (i = 1; i <= list->level; i++) {\n if (update[i]->forward[i] != x)\n break;\n update[i]->forward[1] = x->forward[i];\n }\n skiplist_node_free(x);\n \n while (list->level > 1 && list->header->forward[list->level]\n == list->header)\n list->level--;\n return 0;\n }\n return 1;\n}\n \nstatic void skiplist_dump(skiplist *list) {\n snode *x = list->header;\n while (x && x->forward[1] != list->header) {\n printf(\"%d[%d]->\", x->forward[1]->key, x->forward[1]->value);\n x = x->forward[1];\n }\n printf(\"NIL\\n\");\n}\n \nint main() {\n int arr[] = { 3, 6, 9, 2, 11, 1, 4 }, i;\n skiplist list;\n skiplist_init(&list);\n \n printf(\"Insert:--------------------\\n\");\n for (i = 0; i < sizeof(arr) / sizeof(arr[0]); i++) {\n skiplist_insert(&list, arr[i], arr[i]);\n }\n skiplist_dump(&list);\n \n printf(\"Search:--------------------\\n\");\n int keys[] = { 3, 4, 7, 10, 111 };\n \n for (i = 0; i < sizeof(keys) / sizeof(keys[0]); i++) {\n snode *x = skiplist_search(&list, keys[i]);\n if (x) {\n printf(\"key = %d, value = %d\\n\", keys[i], x->value);\n } else {\n printf(\"key = %d, not fuound\\n\", keys[i]);\n }\n }\n \n printf(\"Search:--------------------\\n\");\n skiplist_delete(&list, 3);\n skiplist_delete(&list, 9);\n skiplist_dump(&list);\n \n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/skip_list/skip_list.cpp",
"content": "/**\n * skip list C++ implementation\n *\n * Average Worst-case\n * Space O(n) O(n log n)\n * Search O(log n) 0(n)\n * Insert O(log n) 0(n)\n * Delete O(log n) 0(n)\n * Part of Cosmos by OpenGenus Foundation\n */\n\n#include <algorithm> // std::less, std::max\n#include <cassert> // assert\n#include <iostream> // std::cout, std::endl, std::ostream\n#include <map> // std::map\n#include <stdlib.h> // rand, srand\n#include <time.h> // time\n\ntemplate <typename val_t> class skip_list;\ntemplate <typename val_t>\nstd::ostream & operator<<(std::ostream &os, const skip_list<val_t> &jls);\n\ntemplate <typename val_t>\nclass skip_list\n{\nprivate:\n/**\n * skip_node\n */\n struct skip_node\n {\n val_t data;\n skip_node **forward;\n int height;\n\n skip_node(int ht)\n : forward{new skip_node*[ht]}, height{ht}\n {\n for (int i = 0; i < ht; ++i)\n forward[i] = nullptr;\n }\n\n skip_node(const val_t &ele, int ht)\n : skip_node(ht)\n {\n data = ele;\n }\n\n ~skip_node()\n {\n if (forward[0])\n delete forward[0];\n delete[] forward;\n }\n\n };\n\n/* member variables */\n skip_node *head_;\n int size_,\n cur_height_;\n constexpr const static int MAX_HEIGHT = 10;\n constexpr const static float PROB = 0.5f;\n/* private functions */\n\n bool coin_flip()\n {\n return ((float) rand() / RAND_MAX) < PROB;\n }\n\n int rand_height()\n {\n int height = 1;\n for (; height < MAX_HEIGHT && coin_flip(); ++height)\n {\n }\n return height;\n }\n\n skip_node ** find(const val_t &ele)\n {\n auto comp = std::less<val_t>();\n skip_node **result = new skip_node*[cur_height_],\n *cur_node = head_;\n for (int lvl = cur_height_ - 1; lvl >= 0; --lvl)\n {\n while (cur_node->forward[lvl]\n && comp(cur_node->forward[lvl]->data, ele))\n cur_node = cur_node->forward[lvl];\n result[lvl] = cur_node;\n }\n return result;\n }\n\n void print(std::ostream &os) const\n {\n int i;\n for (skip_node *n = head_; n != nullptr; n = n->forward[0])\n {\n os << n->data << \": \";\n for (i = 0; i < cur_height_; ++i)\n {\n if (i < n->height)\n os << \"[ ] \";\n else\n os << \" | \";\n }\n os << std::endl;\n os << \" \";\n for (i = 0; i < cur_height_; ++i)\n os << \" | \";\n os << std::endl;\n }\n }\n\npublic:\n/* Default C'tor */\n skip_list()\n : head_{new skip_node(MAX_HEIGHT)}, size_{0}, cur_height_{0}\n {\n srand((unsigned)time(0));\n }\n\n/* D'tor */\n ~skip_list()\n {\n delete head_;\n }\n\n/**\n * size\n * @return - the number of elements in the list\n */\n int size()\n {\n return size_;\n }\n\n/**\n * insert\n * @param ele - the element to be inserted into the list\n */\n void insert(const val_t &ele)\n {\n int new_ht = rand_height();\n skip_node *new_node = new skip_node(ele, new_ht);\n cur_height_ = std::max(new_ht, cur_height_);\n skip_node **pre = find(ele);\n for (int i = 0; i < new_ht; ++i)\n {\n new_node->forward[i] = pre[i]->forward[i];\n pre[i]->forward[i] = new_node;\n }\n ++size_;\n delete[] pre;\n }\n\n/**\n * contains\n * @param ele - the element to search for\n * @retrun - true if the element is in the list, false otherwise\n */\n bool contains(const val_t &ele)\n {\n skip_node **pre = find(ele);\n bool result = pre[0] &&\n pre[0]->forward[0] &&\n pre[0]->forward[0]->data == ele;\n delete[] pre;\n return result;\n }\n\n/**\n * remove\n * @param ele - the element to delete if found\n */\n void remove(const val_t &ele)\n {\n if (!contains(ele))\n {\n std::cout << ele << \" not found!\" << std::endl;\n return;\n }\n skip_node *tmp, **pre = find(ele), *del = pre[0]->forward[0];\n for (int i = 0; i < cur_height_; ++i)\n {\n tmp = pre[i]->forward[i];\n if (tmp != nullptr && tmp->data == ele)\n {\n pre[i]->forward[i] = tmp->forward[i];\n tmp->forward[i] = nullptr;\n }\n }\n --size_;\n delete del;\n delete[] pre;\n }\n\n friend std::ostream & operator<< <val_t>(std::ostream &os,\n const skip_list<val_t> &ls);\n}; // skip_node\n\ntemplate<typename val_t>\nstd::ostream & operator<<(std::ostream &os, const skip_list<val_t> &ls)\n{\n ls.print(os);\n return os;\n}\n\nint main()\n{\n auto ints = { 1, 4, 2, 7, 9, 3, 5, 8, 6 };\n skip_list<int> isl;\n for (auto i : ints)\n {\n isl.insert(i);\n std::cout << isl << std::endl;\n }\n for (auto i : ints)\n {\n assert(isl.contains(i));\n std::cout << \"removing \" << i << std::endl;\n isl.remove(i);\n std::cout << isl << std::endl;\n }\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/skip_list/skip_list.java",
"content": "// Part of Cosmos by OpenGenus Foundation\nimport java.util.*;\npublic class SkipList<E> {\n /** Initialize the random variable */\n static private Random value = new Random(); // Hold the Random class object\n \n /** Create a random number function from the standard Java Random\n class. Turn it into a uniformly distributed value within the\n range 0 to n-1 by taking the value mod n.\n @param n The upper bound for the range.\n @return A value in the range 0 to n-1.\n */\n static int random(int n) {\n return Math.abs(value.nextInt()) % n;\n }\n \n // SkipNode class is a fairly passive data structure\n // that holds the key and value of a dictionary entry\n // and an array of pointers to help implement the skip list.\n private class SkipNode<E> {\n private int key;\n private E element;\n private SkipNode<E>[] forward;\n \n // make a skip node with data, but don't wire it in yet\n public SkipNode( int key, E element, int levels ) {\n this.key = key;\n this.element = element;\n this.forward = (SkipNode<E>[]) new SkipNode[levels+1];\n for (int i = 0; i <= levels; i++)\n this.forward[i] = null;\n }\n \n // make a skip node without data (designed for head node)\n public SkipNode( int levels ) {\n this.key = 0;\n this.element = null;\n this.forward = new SkipNode[levels+1];\n for (int i = 0; i <= levels; i++)\n this.forward[i] = null;\n }\n \n // adjust this node to have newLevel forward pointers.\n // assumes newLevel >= this.forward.length\n public void adjustLevel( int newLevel ) {\n SkipNode[] oldf = this.forward;\n this.forward = new SkipNode[newLevel+1];\n for (int i = 0; i < oldf.length; i++)\n this.forward[i] = oldf[i];\n for (int i = oldf.length; i <= newLevel; i++)\n this.forward[i] = null; // there aren't any nodes this tall yet, so there is nothing to point to\n }\n\n // Print the contents of the forward vector (I.e. write out who\n // each level is pointing to).\n public void printForward( ) {\n for (int i = this.forward.length-1; i >= 0; i--) {\n if (this.forward[i] == null)\n System.out.println( \"level : \" + i + \" ----> null\" );\n else\n System.out.println( \"level : \" + i + \" ----> (\" + this.forward[i].key() + \" : \" + this.forward[i].element() + \")\");\n }\n }\n\n // accessors \n public int key() { return this.key; }\n public E element() { return this.element; }\n }\n \n // Fields!\n private SkipNode head; // first node\n private int level; // max number of levels in the list\n private int size; // number of data elements in list\n \n public SkipList() {\n // it will start life as a humble linked list.\n this.level = 1;\n // make the head. It will contain no data\n this.head = new SkipNode( this.level );\n this.size = 0;\n }\n \n /** Pick a level using a geometric distribution */\n int randomLevel() {\n //return 1;\n int lev;\n for (lev=0; random(2) == 0; lev++); // Do nothing\n return lev;\n }\n \n // adjust the head to have an array of newLevel levesl\n public void adjustHead(int newLevel) { \n this.head.adjustLevel( newLevel );\n }\n \n /** Insert a record into the skiplist */\n public void insert(int k, E newValue) {\n int newLevel = randomLevel(); // New node’s level\n if (newLevel > level) // If new node is deeper\n adjustHead(newLevel); // adjust the header\n this.level = newLevel; // and record new distance.\n // Track end of level\n SkipNode<E>[] update = (SkipNode<E>[]) new SkipNode[level+1];\n SkipNode<E> x = this.head; // Start at header node\n for (int i=level; i>=0; i--) { // Find insert position\n while((x.forward[i] != null) &&\n (k > x.forward[i].key()))\n x = x.forward[i];\n update[i] = x; // Track end at level i\n }\n x = new SkipNode<E>(k, newValue, newLevel);\n for (int i=0; i <= newLevel; i++) { // Splice into list\n x.forward[i] = update[i].forward[i]; // Who x points to\n update[i].forward[i] = x; // Who y points to\n }\n this.size++; // Increment dictionary size\n }\n\n /** Skiplist Search */\n public E find(int searchKey) {\n SkipNode<E> x = this.head; // Dummy header node\n for (int i=level; i>=0; i--) // For each level...\n while ((x.forward[i] != null) && // go forward\n (searchKey > x.forward[i].key()))\n x = x.forward[i]; // Go one last step\n x = x.forward[0]; // Move to actual record, if it exists\n if ((x != null) && (searchKey == x.key()))\n return x.element(); // Got it\n else return null; // Its not there\n }\n \n public void printContents() {\n SkipNode ptr = this.head;\n while (true) {\n if (ptr.forward[0] == null)\n break;\n ptr = ptr.forward[0];\n System.out.println( ptr.key() + \" : \" + ptr.element() );\n }\n }\n \n public void printEverything() {\n SkipNode ptr = this.head;\n System.out.println( \"Head Node \" );\n ptr.printForward();\n ptr = ptr.forward[0];\n while (ptr != null) {\n System.out.println( \"Node (\" + ptr.key() + \" : \" + ptr.element() + \")\" );\n ptr.printForward();\n ptr = ptr.forward[0];\n }\n }\n \n public static void main( String[] args ) {\n SkipList<String> sl = new SkipList<String>();\n sl.insert( 1, \"One\" );\n System.out.println( \"\\nPrinting the list \");\n //System.out.println( \"Printing the header array: \");\n //sl.head.printForward();\n sl.printEverything();\n sl.insert( 4, \"Four\" );\n sl.insert( 10, \"Ten\" );\n sl.insert( 3, \"Three\" );\n sl.insert( 11, \"Eleven\" );\n System.out.println( \"\\nPrinting the list \");\n sl.printEverything();\n \n System.out.println( \"\\nPrinting just the traversal\" );\n sl.printContents();\n }\n\n}"
}
{
"filename": "code/data_structures/src/list/skip_list/skip_list.rb",
"content": "class Node\n attr_accessor :key\n attr_accessor :value\n attr_accessor :forward\n \n def initialize(k, v = nil)\n @key = k \n @value = v.nil? ? k : v \n @forward = []\n end\nend\n \nclass SkipList\n attr_accessor :level\n attr_accessor :header\n \n def initialize\n @header = Node.new(1) \n @level = 0\n @max_level = 3\n @p = 0.5\n @node_nil = Node.new(1000000)\n @header.forward[0] = @node_nil\n end\n \n def search(search_key)\n x = @header\n @level.downto(0) do |i|\n while x.forward[i].key < search_key do\n x = x.forward[i]\n end\n end \n x = x.forward[0]\n if x.key == search_key\n return x.value\n else\n return nil\n end\n end\n \n def random_level\n v = 0\n while rand < @p && v < @max_level\n v += 1\n end\n v\n end\n \n def insert(search_key, new_value = nil)\n new_value = search_key if new_value.nil? \n update = []\n x = @header\n @level.downto(0) do |i|\n while x.forward[i].key < search_key do\n x = x.forward[i]\n end\n update[i] = x\n end \n x = x.forward[0]\n if x.key == search_key\n x.value = new_value\n else\n v = random_level\n if v > @level\n (@level + 1).upto(v) do |i|\n update[i] = @header\n @header.forward[i] = @node_nil\n end\n @level = v\n end\n x = Node.new(search_key, new_value) \n 0.upto(v) do |i|\n x.forward[i] = update[i].forward[i]\n update[i].forward[i] = x\n end\n end\n end\nend"
}
{
"filename": "code/data_structures/src/list/skip_list/skip_list.scala",
"content": "import java.util.Random\nimport scala.collection.Searching._\nimport scala.reflect.ClassTag\n\nobject SkipList {\n val MAX_LEVELS = 32;\n val random = new Random()\n}\n\nclass SkipList[T](private var nextLists: Array[SkipList[T]], private var data: T) {\n\n def this(firstElement: T) = this(Array.ofDim(SkipList.MAX_LEVELS), firstElement)\n\n private def randomNumberOfLevels(maxLevels: Int): Int = {\n var i = SkipList.random.nextInt()\n var count = 1\n while ((i & 1) == 1 && count < maxLevels) {\n count += 1\n i = i >> 1\n }\n count\n }\n\n def contains(target: T)(implicit ord: Ordering[T]): Boolean = {\n return contains(target, nextLists.length - 1)\n }\n\n def contains(target: T, level: Int)(implicit ord: Ordering[T]): Boolean = {\n // println(s\"contains: level ${level}, target ${target}\")\n if (level == 0) {\n return (\n if (target == data) {\n true\n }\n else if (ord.lt(target, data)) {\n false\n }\n else if (nextLists(0) != null) {\n nextLists(0).contains(target, level)\n }\n else {\n false\n })\n }\n else if (nextLists(level) == null || ord.lt(target, nextLists(level).data)) {\n return contains(target, level - 1)\n }\n else if (ord.gteq(target, nextLists(level).data)) {\n return nextLists(0).contains(target, level)\n }\n throw new RuntimeException(\"Shouldn't get here.\")\n }\n\n def insert(newElement: T)(implicit ord: Ordering[T]): Unit = {\n insert(newElement, nextLists.length - 1, this)\n }\n\n def insert(newElement: T, level: Int, headOfList: SkipList[T])(implicit ord: Ordering[T]): Unit = {\n // println(s\"insert: level ${level}, newElement ${newElement}, current element: ${data}.\")\n if (level == 0) {\n if (newElement == data) {\n return\n }\n else if (nextLists(0) != null && ord.gteq(newElement, nextLists(0).data)) {\n return insert(newElement, level, headOfList)\n }\n else {\n // Create next node with random height and elements that don't fit into this node\n val numLevelsForNewNode = randomNumberOfLevels(SkipList.MAX_LEVELS)\n val nextNode = new SkipList(Array.ofDim[SkipList[T]](numLevelsForNewNode), newElement)\n // Fix bottom links, this -> new -> next\n nextNode.nextLists(0) = nextLists(0)\n nextLists(0) = nextNode\n\n if (ord.gt(data, newElement)) {\n nextNode.data = data\n data = newElement\n }\n\n return headOfList.insertNewNode(nextNode, nextNode.nextLists.length - 1)\n }\n }\n else if (nextLists(level) == null) {\n return insert(newElement, level - 1, headOfList)\n }\n else if (ord.gteq(newElement, nextLists(level).data)) {\n return nextLists(level).insert(newElement, level, headOfList)\n }\n throw new RuntimeException(\"Shouldn't get here.\")\n }\n\n def insertNewNode(newNode: SkipList[T], startLevel: Int)(implicit ord: Ordering[T]): Unit = {\n var currentNode = this\n for (level <- startLevel until 0 by -1) {\n while (currentNode.nextLists(level) != null && ord.gteq(newNode.data, currentNode.nextLists(level).data)) {\n currentNode = currentNode.nextLists(level)\n }\n newNode.nextLists(level) = currentNode.nextLists(level)\n currentNode.nextLists(level) = newNode\n }\n }\n\n override def toString(): String = {\n data + (if (nextLists(0) == null) \"\" else \", \" + nextLists(0))\n }\n}\n\nobject Main {\n def main(args: Array[String]): Unit = {\n val list = new SkipList(Array.ofDim[SkipList[String]](5), \"aab\")\n println(list)\n println(list.contains(\"aaa\"))\n println(list.contains(\"aab\"))\n list.insert(\"aaa\")\n println(list)\n println(list.contains(\"aaa\"))\n println(list.contains(\"aab\"))\n list.insert(\"aac\")\n println(list)\n println(list.contains(\"aaa\"))\n println(list.contains(\"aab\"))\n println(list.contains(\"aac\"))\n }\n}"
}
{
"filename": "code/data_structures/src/list/skip_list/skip_list.swift",
"content": "import Foundation\n\npublic struct Stack<T> {\n fileprivate var array: [T] = []\n\n public var isEmpty: Bool {\n return array.isEmpty\n }\n\n public var count: Int {\n return array.count\n }\n\n public mutating func push(_ element: T) {\n array.append(element)\n }\n\n public mutating func pop() -> T? {\n return array.popLast()\n }\n\n public func peek() -> T? {\n return array.last\n }\n}\n\nextension Stack: Sequence {\n public func makeIterator() -> AnyIterator<T> {\n var curr = self\n return AnyIterator { curr.pop() }\n }\n}\n\nprivate func coinFlip() -> Bool {\n return arc4random_uniform(2) == 1\n}\n\npublic class DataNode<Key: Comparable, Payload> {\n public typealias Node = DataNode<Key, Payload>\n\n var data: Payload?\n fileprivate var key: Key?\n var next: Node?\n var down: Node?\n\n public init(key: Key, data: Payload) {\n self.key = key\n self.data = data\n }\n\n public init(asHead head: Bool) {}\n\n}\n\nopen class SkipList<Key: Comparable, Payload> {\n public typealias Node = DataNode<Key, Payload>\n\n fileprivate(set) var head: Node?\n\n public init() {}\n\n}\n\nextension SkipList {\n\n func findNode(key: Key) -> Node? {\n var currentNode: Node? = head\n var isFound: Bool = false\n\n while !isFound {\n if let node = currentNode {\n\n switch node.next {\n case .none:\n\n currentNode = node.down\n case .some(let value) where value.key != nil:\n\n if value.key == key {\n isFound = true\n break\n } else {\n if key < value.key! {\n currentNode = node.down\n } else {\n currentNode = node.next\n }\n }\n\n default:\n continue\n }\n\n } else {\n break\n }\n }\n\n if isFound {\n return currentNode\n } else {\n return nil\n }\n\n }\n\n func search(key: Key) -> Payload? {\n guard let node = findNode(key: key) else {\n return nil\n }\n\n return node.next!.data\n }\n\n}\n\nextension SkipList {\n private func bootstrapBaseLayer(key: Key, data: Payload) {\n head = Node(asHead: true)\n var node = Node(key: key, data: data)\n\n head!.next = node\n\n var currentTopNode = node\n\n while coinFlip() {\n let newHead = Node(asHead: true)\n node = Node(key: key, data: data)\n node.down = currentTopNode\n newHead.next = node\n newHead.down = head\n head = newHead\n currentTopNode = node\n }\n\n }\n\n private func insertItem(key: Key, data: Payload) {\n var stack = Stack<Node>()\n var currentNode: Node? = head\n\n while currentNode != nil {\n\n if let nextNode = currentNode!.next {\n if nextNode.key! > key {\n stack.push(currentNode!)\n currentNode = currentNode!.down\n } else {\n currentNode = nextNode\n }\n\n } else {\n stack.push(currentNode!)\n currentNode = currentNode!.down\n }\n\n }\n\n let itemAtLayer = stack.pop()\n var node = Node(key: key, data: data)\n node.next = itemAtLayer!.next\n itemAtLayer!.next = node\n var currentTopNode = node\n\n while coinFlip() {\n if stack.isEmpty {\n let newHead = Node(asHead: true)\n\n node = Node(key: key, data: data)\n node.down = currentTopNode\n newHead.next = node\n newHead.down = head\n head = newHead\n currentTopNode = node\n\n } else {\n let nextNode = stack.pop()\n\n node = Node(key: key, data: data)\n node.down = currentTopNode\n node.next = nextNode!.next\n nextNode!.next = node\n currentTopNode = node\n }\n }\n }\n\n func insert(key: Key, data: Payload) {\n if head != nil {\n if let node = findNode(key: key) {\n var currentNode = node.next\n while currentNode != nil && currentNode!.key == key {\n currentNode!.data = data\n currentNode = currentNode!.down\n }\n } else {\n insertItem(key: key, data: data)\n }\n\n } else {\n bootstrapBaseLayer(key: key, data: data)\n }\n }\n\n}\n\nextension SkipList {\n public func remove(key: Key) {\n guard let item = findNode(key: key) else {\n return\n }\n\n var currentNode = Optional(item)\n\n while currentNode != nil {\n let node = currentNode!.next\n\n if node!.key != key {\n currentNode = node\n continue\n }\n\n let nextNode = node!.next\n\n currentNode!.next = nextNode\n currentNode = currentNode!.down\n\n }\n\n }\n}\n\nextension SkipList {\n\n public func get(key: Key) -> Payload? {\n return search(key: key)\n }\n}"
}
{
"filename": "code/data_structures/src/list/stack_using_linked_list/stack_using_linked_list.c",
"content": "#include<stdio.h>\n\nstruct node\n{\n int data;\n struct node *next;\n};\n\nstruct node * head=0;\n\n//inserting an element\nvoid push(int x)\n{\n struct node * temp=0;\n temp=(struct node *)malloc(sizeof(struct node));\n\n if(temp==0)\n {\n printf(\"\\nOverflow\");\n return;\n }\n\n temp->data=x;\n temp->next=head;\n head=temp;\n}\n\n//delete an element\nvoid pop()\n{\n if(head==0)\n {\n printf(\"\\nUnderflow\");\n return;\n }\n\n struct node * temp;\n temp=head;\n head=head->next;\n free(temp);\n}\n\n//printing the elements\nvoid print()\n{\n if(head==0)\n {\n printf(\"\\nUnderflow\");\n return;\n }\n\n struct node *temp = head;\n printf(\"\\n\");\n while(temp!=0)\n {\n printf(\"%d \",temp->data);\n temp=temp->next;\n }\n}\n\n\nint main()\n{\n print();\n push(10);\n print();\n push(20);\n push(30);\n print();\n pop();\n pop();\n pop();\n print();\n pop();\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/list/xor_linked_list/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/data_structures/src/list/xor_linked_list/xor_linked_list.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n *\n * xor linked list synopsis\n *\n *** incomplete ***\n ***\n ***Begin *** Iterator invalidation rules are NOT applicable. ***\n ***[x] Insertion: all iterators and references unaffected.\n ***[x] Erasure: only the iterators and references to the erased element is invalidated.\n ***[o] Resizing: as per insert/erase.\n ***\n ***Refer to: https://stackoverflow.com/questions/6438086/iterator-invalidation-rules\n ***End *** Iterator invalidation rules are NOT applicable. ***\n */\n\n#ifndef XOR_LINKED_LIST_CPP\n#define XOR_LINKED_LIST_CPP\n\n#include <iterator>\n#include <algorithm>\n#include <exception>\n#include <cstddef>\n\ntemplate<typename _Type>\nclass XorLinkedList;\n\ntemplate<class _Type>\nclass ListIter;\n\ntemplate<class _Type>\nclass ListConstIter;\n\ntemplate<typename _Type>\nclass __Node\n{\nprivate:\n using value_type = _Type;\n using SPNode = __Node<value_type> *;\n\npublic:\n explicit __Node(value_type value) : value_(value), around_(nullptr)\n {\n }\n\n inline value_type &value()\n {\n return value_;\n }\n\n inline const value_type &value() const\n {\n return value_;\n }\n\n inline void value(value_type v)\n {\n value_ = v;\n }\n\nprivate:\n value_type value_;\n void *around_;\n\n inline SPNode around(const SPNode &around)\n {\n return reinterpret_cast<SPNode>(reinterpret_cast<uintptr_t>(around) ^\n reinterpret_cast<uintptr_t>(around_));\n }\n\n inline void around(const SPNode &prev, const SPNode &next)\n {\n around_ = reinterpret_cast<void *>(reinterpret_cast<uintptr_t>(prev) ^\n reinterpret_cast<uintptr_t>(next));\n }\n\n friend XorLinkedList<value_type>;\n friend ListIter<value_type>;\n friend ListConstIter<value_type>;\n};\n\ntemplate<class _Type>\nclass ListIter : public std::iterator<std::bidirectional_iterator_tag, _Type>\n{\npublic:\n using value_type = _Type;\n using difference_type = std::ptrdiff_t;\n using pointer = _Type *;\n using reference = _Type &;\n using iterator_category = std::bidirectional_iterator_tag;\n\nprivate:\n using NodePtr = __Node<value_type> *;\n using Self = ListIter<value_type>;\n\npublic:\n ListIter()\n {\n }\n\n explicit ListIter(NodePtr prev, NodePtr curr) : prev_(prev), curr_(curr)\n {\n }\n\n ListIter(const Self &it) : prev_(it.prev_), curr_(it.curr_)\n {\n }\n\n reference operator*()\n {\n return curr_->value();\n }\n\n pointer operator->()\n {\n return &curr_->value();\n }\n\n Self &operator++()\n {\n auto next = curr_->around(prev_);\n prev_ = curr_;\n curr_ = next;\n\n return *this;\n }\n\n Self operator++(int)\n {\n auto temp = *this;\n ++*this;\n\n return temp;\n }\n\n Self &operator--()\n {\n auto prevOfprev = prev_->around(curr_);\n curr_ = prev_;\n prev_ = prevOfprev;\n\n return *this;\n }\n\n Self operator--(int)\n {\n auto temp = *this;\n --*this;\n\n return temp;\n }\n\n bool operator==(const Self &other) const\n {\n return curr_ == other.curr_;\n }\n\n bool operator!=(const Self &other) const\n {\n return !(*this == other);\n }\n\nprivate:\n NodePtr prev_, curr_;\n\n friend XorLinkedList<value_type>;\n friend ListConstIter<value_type>;\n};\n\ntemplate<class _Type>\nclass ListConstIter : public std::iterator<std::bidirectional_iterator_tag, _Type>\n{\npublic:\n using value_type = _Type;\n using difference_type = std::ptrdiff_t;\n using pointer = const _Type *;\n using reference = const _Type &;\n using iterator_category = std::bidirectional_iterator_tag;\n\nprivate:\n using NodePtr = __Node<value_type> *;\n using Self = ListConstIter<value_type>;\n using Iter = ListIter<value_type>;\n\npublic:\n ListConstIter()\n {\n }\n\n explicit ListConstIter(NodePtr prev, NodePtr curr) : prev_(prev), curr_(curr)\n {\n }\n\n ListConstIter(const Iter &it) : prev_(it.prev_), curr_(it.curr_)\n {\n }\n\n reference operator*() const\n {\n return curr_->value();\n }\n\n pointer operator->() const\n {\n return &curr_->value();\n }\n\n Self &operator++()\n {\n auto next = curr_->around(prev_);\n prev_ = curr_;\n curr_ = next;\n\n return *this;\n }\n\n Self operator++(int)\n {\n auto temp = *this;\n ++*this;\n\n return temp;\n }\n\n Self &operator--()\n {\n auto prevOfprev = prev_->around(curr_);\n curr_ = prev_;\n prev_ = prevOfprev;\n\n return *this;\n }\n\n Self operator--(int)\n {\n auto temp = *this;\n --*this;\n\n return temp;\n }\n\n bool operator==(const Self &other) const\n {\n return curr_ == other.curr_;\n }\n\n bool operator!=(const Self &other) const\n {\n return !(*this == other);\n }\n\nprivate:\n NodePtr prev_, curr_;\n\n Iter constCast()\n {\n return Iter(prev_, curr_);\n }\n\n friend XorLinkedList<value_type>;\n};\n\ntemplate<typename _Type>\nclass XorLinkedList\n{\nprivate:\n using Node = __Node<_Type>;\n using NodePtr = __Node<_Type> *;\n using Self = XorLinkedList<_Type>;\n\npublic:\n using value_type = _Type;\n using size_type = size_t;\n using difference_type = std::ptrdiff_t;\n using pointer = const value_type *;\n using const_pointer = const value_type *;\n using reference = value_type &;\n using const_reference = const value_type &;\n\n using iterator = ListIter<value_type>;\n using const_iterator = ListConstIter<value_type>;\n using reverse_iterator = std::reverse_iterator<iterator>;\n using const_reverse_iterator = std::reverse_iterator<const_iterator>;\n\n explicit XorLinkedList() : sz_(0)\n {\n construct();\n }\n\n XorLinkedList(const Self &list) : sz_(0)\n {\n construct();\n std::for_each(list.begin(), list.end(), [&](value_type v)\n {\n push_back(v);\n });\n };\n\n XorLinkedList(std::initializer_list<value_type> &&vs) : sz_(0)\n {\n construct();\n std::for_each(vs.begin(), vs.end(), [&](value_type v)\n {\n push_back(v);\n });\n }\n\n ~XorLinkedList()\n {\n clear();\n destruct();\n }\n\n// element access\n inline reference back();\n\n inline const_reference back() const;\n\n inline reference front();\n\n inline const_reference front() const;\n\n// modifiers\n void clear();\n\n template<class ... Args>\n reference emplace_back(Args&& ... args);\n\n template<class ... Args>\n reference emplace_front(Args&& ... args);\n\n iterator erase(const_iterator pos);\n\n iterator erase(const_iterator begin, const_iterator end);\n\n iterator insert(const_iterator pos, const value_type &v);\n\n iterator insert(const_iterator pos, size_type size, const value_type &v);\n\n iterator insert(const_iterator pos, value_type &&v);\n\n iterator insert(const_iterator pos, std::initializer_list<value_type> il);\n\n template<typename _InputIt>\n iterator insert(const_iterator pos, _InputIt first, _InputIt last);\n\n void pop_back();\n\n void pop_front();\n\n void push_back(const value_type &v);\n\n void push_front(const value_type &v);\n\n void resize(size_type count);\n\n void resize(size_type count, const value_type&value);\n\n void swap(Self&other);\n\n// capacity\n inline bool empty() const;\n\n inline size_type max_size() const;\n\n inline size_type size() const;\n\n// iterators\n iterator begin();\n\n const_iterator begin() const;\n\n const_iterator cbegin() const;\n\n iterator end();\n\n const_iterator end() const;\n\n const_iterator cend() const;\n\n reverse_iterator rbegin();\n\n const_reverse_iterator rbegin() const;\n\n const_reverse_iterator crbegin() const;\n\n reverse_iterator rend();\n\n const_reverse_iterator rend() const;\n\n const_reverse_iterator crend() const;\n\n// operations\n void merge(Self&other);\n\n void merge(Self&&other);\n\n template<class Compare>\n void merge(Self&other, Compare comp);\n\n template<class Compare>\n void merge(Self&&other, Compare comp);\n\n void reverse();\n\n void sort();\n\n template<class Compare>\n void sort(Compare comp);\n\n void splice(const_iterator pos, Self&other);\n\n void splice(const_iterator pos, Self&&other);\n\n void splice(const_iterator pos, Self&other, const_iterator it);\n\n void splice(const_iterator pos, Self&&other, const_iterator it);\n\n void splice(const_iterator pos, Self&other, const_iterator first, const_iterator last);\n\n void splice(const_iterator pos, Self&&other, const_iterator first, const_iterator last);\n\n void unique();\n\n template<class BinaryPredicate>\n void unique(BinaryPredicate p);\n\nprivate:\n NodePtr prevOfBegin_, end_, nextOfEnd_;\n size_type sz_;\n\n inline void construct();\n\n inline void destruct();\n\n inline iterator insertImpl(const_iterator pos, const value_type &v);\n\n inline iterator eraseImpl(const_iterator pos);\n};\n\n// element access\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::back()->reference\n{\n if (empty())\n throw std::out_of_range(\"access to empty list !\");\n\n return end_->around(nextOfEnd_)->value();\n}\n\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::back() const->const_reference\n{\n return const_cast<Self *>(this)->back();\n}\n\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::front()->reference\n{\n if (empty())\n throw std::out_of_range(\"access to empty list !\");\n\n return prevOfBegin_->around(nextOfEnd_)->value();\n}\n\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::front() const->const_reference\n{\n return const_cast<Self *>(this)->front();\n}\n\n// modifiers\ntemplate<typename _Type>\ninline void\nXorLinkedList<_Type>::clear()\n{\n NodePtr begin = prevOfBegin_, nextOfBegin;\n begin = begin->around(nextOfEnd_);\n\n while (begin != end_)\n {\n nextOfBegin = begin->around(prevOfBegin_);\n\n prevOfBegin_->around(nextOfEnd_, nextOfBegin);\n nextOfBegin->around(prevOfBegin_, nextOfBegin->around(begin));\n\n delete begin;\n begin = nextOfBegin;\n }\n\n sz_ = 0;\n}\n\ntemplate<typename _Type>\ntemplate<class ... Args>\nauto\nXorLinkedList<_Type>::emplace_back(Args&& ... args)->reference\n{\n}\n\ntemplate<typename _Type>\ntemplate<class ... Args>\nauto\nXorLinkedList<_Type>::emplace_front(Args&& ... args)->reference\n{\n}\n\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::erase(const_iterator pos)->iterator\n{\n return eraseImpl(pos);\n}\n\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::erase(const_iterator first, const_iterator last)->iterator\n{\n auto diff = std::distance(first, last);\n if (diff == 0)\n return first.constCast(); // check what is std return\n\n auto firstAfterEraseIter = first;\n while (diff--)\n firstAfterEraseIter = eraseImpl(firstAfterEraseIter);\n\n return firstAfterEraseIter.constCast();\n}\n\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::insert(const_iterator pos, const value_type &v)->iterator\n{\n return insertImpl(pos, v);\n}\n\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::insert(const_iterator pos, size_type size, const value_type &v)\n->iterator\n{\n if (size == 0)\n return pos.constCast();\n\n auto curr = insert(pos, v);\n auto firstOfInsert = curr;\n ++curr;\n while (--size)\n curr = ++insert(curr, v);\n\n return firstOfInsert;\n}\n\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::insert(const_iterator pos, value_type &&v)->iterator\n{\n return insertImpl(pos, v);\n}\n\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::insert(const_iterator pos, std::initializer_list<value_type> il)\n->iterator\n{\n if (il.begin() == il.end())\n return pos.constCast();\n\n auto curr = insert(pos, *il.begin());\n auto firstOfInsert = curr;\n ++curr;\n auto begin = il.begin();\n ++begin;\n std::for_each(begin, il.end(), [&](value_type v)\n {\n curr = ++insert(curr, v);\n });\n\n return firstOfInsert;\n}\n\ntemplate<typename _Type>\ntemplate<typename _InputIt>\ninline auto\nXorLinkedList<_Type>::insert(const_iterator pos, _InputIt first, _InputIt last)\n->iterator\n{\n if (first < last)\n {\n auto curr = insert(pos, *first);\n auto firstOfInsert = curr;\n ++curr;\n auto begin = first;\n ++begin;\n std::for_each(begin, last, [&](value_type it)\n {\n curr = ++insert(curr, it);\n });\n\n return firstOfInsert;\n }\n return pos.constCast();\n}\n\ntemplate<typename _Type>\nvoid\nXorLinkedList<_Type>::pop_back()\n{\n // Calling pop_back on an empty container is undefined.\n erase(--end());\n}\n\ntemplate<typename _Type>\nvoid\nXorLinkedList<_Type>::pop_front()\n{\n // Calling pop_front on an empty container is undefined.\n erase(begin());\n}\n\ntemplate<typename _Type>\nvoid\nXorLinkedList<_Type>::push_back(const value_type &v)\n{\n insert(end(), v);\n}\n\ntemplate<typename _Type>\nvoid\nXorLinkedList<_Type>::push_front(const value_type &v)\n{\n insert(begin(), v);\n}\n\ntemplate<typename _Type>\nvoid\nXorLinkedList<_Type>::resize(size_type count)\n{\n}\n\ntemplate<typename _Type>\nvoid\nXorLinkedList<_Type>::resize(size_type count, const value_type&value)\n{\n}\n\ntemplate<typename _Type>\nvoid\nXorLinkedList<_Type>::swap(Self&other)\n{\n}\n\n// capacity\ntemplate<typename _Type>\ninline bool\nXorLinkedList<_Type>::empty() const\n{\n return prevOfBegin_->around(nextOfEnd_) == end_;\n}\n\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::max_size() const->size_type\n{\n return static_cast<size_type>(-1) / sizeof(value_type);\n}\n\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::size() const->size_type\n{\n return sz_;\n}\n\n// iterators\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::begin()->iterator\n{\n return iterator(prevOfBegin_, prevOfBegin_->around(nextOfEnd_));\n}\n\ntemplate<typename _Type>\nauto\nXorLinkedList<_Type>::begin() const->const_iterator\n{\n return const_iterator(prevOfBegin_, prevOfBegin_->around(nextOfEnd_));\n}\n\ntemplate<typename _Type>\nauto\nXorLinkedList<_Type>::cbegin() const->const_iterator\n{\n return const_iterator(prevOfBegin_, prevOfBegin_->around(nextOfEnd_));\n}\n\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::end()->iterator\n{\n return iterator(end_->around(nextOfEnd_), end_);\n}\n\ntemplate<typename _Type>\nauto\nXorLinkedList<_Type>::end() const->const_iterator\n{\n return const_iterator(end_->around(nextOfEnd_), end_);\n}\n\ntemplate<typename _Type>\nauto\nXorLinkedList<_Type>::cend() const->const_iterator\n{\n return const_iterator(end_->around(nextOfEnd_), end_);\n}\n\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::rbegin()->reverse_iterator\n{\n return reverse_iterator(end());\n}\n\ntemplate<typename _Type>\nauto\nXorLinkedList<_Type>::rbegin() const->const_reverse_iterator\n{\n return const_reverse_iterator(end());\n}\n\ntemplate<typename _Type>\nauto\nXorLinkedList<_Type>::crbegin() const->const_reverse_iterator\n{\n return const_reverse_iterator(cend());\n}\n\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::rend()->reverse_iterator\n{\n return reverse_iterator(begin());\n}\n\ntemplate<typename _Type>\nauto\nXorLinkedList<_Type>::rend() const->const_reverse_iterator\n{\n return const_reverse_iterator(begin());\n}\n\ntemplate<typename _Type>\nauto\nXorLinkedList<_Type>::crend() const->const_reverse_iterator\n{\n return const_reverse_iterator(cbegin());\n}\n\n// operations\ntemplate<typename _Type>\ninline void\nXorLinkedList<_Type>::merge(Self&other)\n{\n}\n\ntemplate<typename _Type>\ninline void\nXorLinkedList<_Type>::merge(Self&&other)\n{\n}\n\ntemplate<typename _Type>\ntemplate<class Compare>\ninline void\nXorLinkedList<_Type>::merge(Self&other, Compare comp)\n{\n}\n\ntemplate<typename _Type>\ntemplate<class Compare>\ninline void\nXorLinkedList<_Type>::merge(Self&&other, Compare comp)\n{\n}\n\ntemplate<typename _Type>\ninline void\nXorLinkedList<_Type>::reverse()\n{\n std::swap(prevOfBegin_, end_);\n}\n\ntemplate<typename _Type>\ninline void\nXorLinkedList<_Type>::sort()\n{\n}\n\ntemplate<typename _Type>\ntemplate<class Compare>\ninline void\nXorLinkedList<_Type>::sort(Compare comp)\n{\n}\n\ntemplate<typename _Type>\ninline void\nXorLinkedList<_Type>::splice(const_iterator pos, Self&other)\n{\n}\n\ntemplate<typename _Type>\ninline void\nXorLinkedList<_Type>::splice(const_iterator pos, Self&&other)\n{\n}\n\ntemplate<typename _Type>\ninline void\nXorLinkedList<_Type>::splice(const_iterator pos, Self&other, const_iterator it)\n{\n}\n\ntemplate<typename _Type>\ninline void\nXorLinkedList<_Type>::splice(const_iterator pos, Self&&other, const_iterator it)\n{\n}\n\ntemplate<typename _Type>\ninline void\nXorLinkedList<_Type>::splice(const_iterator pos,\n Self&other,\n const_iterator first,\n const_iterator\n last)\n{\n}\n\ntemplate<typename _Type>\ninline void\nXorLinkedList<_Type>::splice(const_iterator pos,\n Self&&other,\n const_iterator first,\n const_iterator\n last)\n{\n}\n\ntemplate<typename _Type>\ninline void\nXorLinkedList<_Type>::unique()\n{\n}\n\ntemplate<typename _Type>\ntemplate<class BinaryPredicate>\ninline void\nXorLinkedList<_Type>::unique(BinaryPredicate p)\n{\n}\n\n// private functions\ntemplate<typename _Type>\ninline void\nXorLinkedList<_Type>::construct()\n{\n end_ = new Node(0);\n prevOfBegin_ = new Node(0);\n nextOfEnd_ = new Node(0);\n\n end_->around(prevOfBegin_, nextOfEnd_);\n prevOfBegin_->around(nextOfEnd_, end_);\n nextOfEnd_->around(end_, prevOfBegin_);\n}\n\ntemplate<typename _Type>\ninline void\nXorLinkedList<_Type>::destruct()\n{\n delete prevOfBegin_;\n delete end_;\n delete nextOfEnd_;\n}\n\ntemplate<typename _Type>\ninline auto\nXorLinkedList<_Type>::insertImpl(const_iterator pos, const value_type &v)\n->iterator\n{\n auto curr = pos.curr_;\n auto prev = pos.prev_;\n\n auto nextOfNext = curr->around(prev);\n auto prevOfPrev = prev->around(curr);\n auto newCurr = new Node(v);\n newCurr->around(prev, curr);\n curr->around(newCurr, nextOfNext);\n prev->around(prevOfPrev, newCurr);\n ++sz_;\n\n return iterator(prev, prev->around(prevOfPrev));\n}\n\ntemplate<typename _Type>\nauto\nXorLinkedList<_Type>::eraseImpl(const_iterator pos)->iterator\n{\n auto curr = pos.curr_;\n auto prev = pos.prev_;\n\n auto nextOfCurr = curr->around(prev);\n auto nextOfNext = nextOfCurr->around(curr);\n auto prevOfPrev = prev->around(curr);\n nextOfCurr->around(prev, nextOfNext);\n prev->around(prevOfPrev, nextOfCurr);\n delete curr;\n --sz_;\n\n return iterator(prev, nextOfCurr);\n}\n\n#endif // XOR_LINKED_LIST_CPP"
}
{
"filename": "code/data_structures/src/maxHeap/maxHeap.cpp",
"content": "\n\n#include \"maxHeap.h\"\nusing namespace std;\nvoid maxHeap::heapifyAdd(int idx)\n{\n int root = (idx-1)/2;\n if(heap[idx]>heap[root])\n {\n int temp = heap[root];\n heap[root] = heap[idx];\n heap[idx] = temp;\n heapifyAdd(root);\n }\n\n}\nvoid maxHeap::heapifyRemove(int idx)\n{\n int max = idx;\n int leftIdx = idx*2+1;\n int rightIdx = idx*2+2;\n if(leftIdx<currSize&& heap[leftIdx]>heap[idx])\n {\n max=leftIdx;\n }\n if(rightIdx<currSize&& heap[rightIdx]>heap[max])\n {\n max=rightIdx;\n }\n if(max!=idx) // swap\n {\n int temp = heap[max];\n heap[max] = heap[idx];\n heap[idx] = temp;\n heapifyRemove(max);\n }\n\n}\nmaxHeap::maxHeap(int capacity)\n{\n heap = new int[capacity] ;\n currSize = 0;\n this->capacity = capacity;\n}\nvoid maxHeap::insert(int itm)\n{\n if(currSize==capacity)\n return;\n heap[currSize] = itm;\n currSize++;\n heapifyAdd(currSize-1);\n}\nvoid maxHeap::remove()\n{\n if (currSize==0)\n return;\n heap[0] = heap[currSize-1];\n currSize--;\n heapifyRemove(0);\n}\nvoid maxHeap::print()\n{\n for(int i =0; i<currSize;i++)\n {\n cout<< heap[i];\n }\n cout<<endl;\n}"
}
{
"filename": "code/data_structures/src/maxHeap/maxHeap.h",
"content": "\n#ifndef COSMOS_MAXHEAP_H\n#define COSMOS_MAXHEAP_H\n#include <iostream>\n\n\nclass maxHeap {\n\nprivate :\n int* heap;\n int capacity;\n int currSize;\n void heapifyAdd(int idx);\n void heapifyRemove(int idx);\n\npublic:\n maxHeap(int capacity);\n void insert(int itm);\n void remove();\n void print();\n\n\n};\n\n\n#endif //COSMOS_MAXHEAP_H"
}
{
"filename": "code/data_structures/src/maxHeap/maxHeap.java",
"content": "public class MaxHeap {\r\n private int[] heap;\r\n private int currSize;\r\n private int capacity;\r\n\r\n public MaxHeap(int capacity) {\r\n this.heap = new int[capacity];\r\n this.currSize = 0;\r\n this.capacity = capacity;\r\n }\r\n\r\n private void heapifyAdd(int idx) {\r\n int root = (idx - 1) / 2;\r\n if(heap[idx] > heap[root]) {\r\n int temp = heap[root];\r\n heap[root] = heap[idx];\r\n heap[idx] = temp;\r\n heapifyAdd(root);\r\n }\r\n }\r\n\r\n private void heapifyRemove(int idx) {\r\n int max = idx;\r\n int leftIdx = idx * 2 + 1;\r\n int rightIdx = idx * 2 + 2;\r\n if(leftIdx < currSize && heap[leftIdx] > heap[idx]) {\r\n max = leftIdx;\r\n }\r\n if(rightIdx < currSize && heap[rightIdx] > heap[max]) {\r\n max = rightIdx;\r\n }\r\n if(max != idx) { // swap\r\n int temp = heap[max];\r\n heap[max] = heap[idx];\r\n heap[idx] = temp;\r\n heapifyRemove(max);\r\n }\r\n }\r\n\r\n public void insert(int itm) {\r\n if(currSize == capacity) {\r\n return;\r\n }\r\n heap[currSize] = itm;\r\n currSize++;\r\n heapifyAdd(currSize - 1);\r\n }\r\n\r\n public void remove() {\r\n if (currSize == 0) {\r\n return;\r\n }\r\n heap[0] = heap[currSize - 1];\r\n currSize--;\r\n heapifyRemove(0);\r\n }\r\n\r\n public void print() {\r\n for(int i = 0; i < currSize; i++) {\r\n System.out.print(heap[i] + \" \");\r\n }\r\n System.out.println();\r\n }\r\n\r\n public static void main(String[] args) {\r\n MaxHeap maxHeap = new MaxHeap(10);\r\n maxHeap.insert(3);\r\n maxHeap.insert(1);\r\n maxHeap.insert(6);\r\n maxHeap.insert(5);\r\n maxHeap.insert(9);\r\n maxHeap.insert(8);\r\n\r\n maxHeap.print(); // Expected output: 9 5 8 1 3 6\r\n\r\n maxHeap.remove();\r\n\r\n maxHeap.print(); // Expected output: 8 5 6 1 3\r\n }\r\n}\r"
}
{
"filename": "code/data_structures/src/maxHeap/maxheap.py",
"content": "# Python3 implementation of Max Heap\nimport sys\n \nclass MaxHeap:\n \n def __init__(self, maxsize):\n \n self.maxsize = maxsize\n self.size = 0\n self.Heap = [0] * (self.maxsize + 1)\n self.Heap[0] = sys.maxsize\n self.FRONT = 1\n \n # Function to return the position of\n # parent for the node currently\n # at pos\n def parent(self, pos):\n \n return pos // 2\n \n # Function to return the position of\n # the left child for the node currently\n # at pos\n def leftChild(self, pos):\n \n return 2 * pos\n \n # Function to return the position of\n # the right child for the node currently\n # at pos\n def rightChild(self, pos):\n \n return (2 * pos) + 1\n \n # Function that returns true if the passed\n # node is a leaf node\n def isLeaf(self, pos):\n \n if pos >= (self.size//2) and pos <= self.size:\n return True\n return False\n \n # Function to swap two nodes of the heap\n def swap(self, fpos, spos):\n \n self.Heap[fpos], self.Heap[spos] = (self.Heap[spos],\n self.Heap[fpos])\n \n # Function to heapify the node at pos\n def maxHeapify(self, pos):\n \n # If the node is a non-leaf node and smaller\n # than any of its child\n if not self.isLeaf(pos):\n if (self.Heap[pos] < self.Heap[self.leftChild(pos)] or\n self.Heap[pos] < self.Heap[self.rightChild(pos)]):\n \n # Swap with the left child and heapify\n # the left child\n if (self.Heap[self.leftChild(pos)] >\n self.Heap[self.rightChild(pos)]):\n self.swap(pos, self.leftChild(pos))\n self.maxHeapify(self.leftChild(pos))\n \n # Swap with the right child and heapify\n # the right child\n else:\n self.swap(pos, self.rightChild(pos))\n self.maxHeapify(self.rightChild(pos))\n \n # Function to insert a node into the heap\n def insert(self, element):\n \n if self.size >= self.maxsize:\n return\n self.size += 1\n self.Heap[self.size] = element\n \n current = self.size\n \n while (self.Heap[current] >\n self.Heap[self.parent(current)]):\n self.swap(current, self.parent(current))\n current = self.parent(current)\n \n # Function to print the contents of the heap\n def Print(self):\n \n for i in range(1, (self.size // 2) + 1):\n print(\"PARENT : \" + str(self.Heap[i]) +\n \"LEFT CHILD : \" + str(self.Heap[2 * i]) +\n \"RIGHT CHILD : \" + str(self.Heap[2 * i + 1]))\n \n # Function to remove and return the maximum\n # element from the heap\n def extractMax(self):\n \n popped = self.Heap[self.FRONT]\n self.Heap[self.FRONT] = self.Heap[self.size]\n self.size -= 1\n self.maxHeapify(self.FRONT)\n \n return popped"
}
{
"filename": "",
"content": ""
}
{
"filename": "",
"content": ""
}
{
"filename": "",
"content": ""
}
{
"filename": "code/data_structures/src/minHeap/minHeap.py",
"content": "import sys\r\n\r\nclass MinHeap:\r\n\r\n def __init__(self, maxsize):\r\n self.maxsize = maxsize\r\n self.size = 0\r\n self.Heap = [0] * (self.maxsize + 1)\r\n self.Heap[0] = -1 * sys.maxsize\r\n self.FRONT = 1\r\n\r\n def parent(self, pos):\r\n return pos // 2\r\n\r\n def leftChild(self, pos):\r\n return 2 * pos\r\n\r\n def rightChild(self, pos):\r\n return (2 * pos) + 1\r\n\r\n def isLeaf(self, pos):\r\n if pos >= (self.size//2) and pos <= self.size:\r\n return True\r\n return False\r\n\r\n def swap(self, fpos, spos):\r\n self.Heap[fpos], self.Heap[spos] = (self.Heap[spos],\r\n self.Heap[fpos])\r\n\r\n def minHeapify(self, pos):\r\n if not self.isLeaf(pos):\r\n if (self.Heap[pos] > self.Heap[self.leftChild(pos)] or\r\n self.Heap[pos] > self.Heap[self.rightChild(pos)]):\r\n\r\n # Swap with the left child and heapify the left child\r\n if (self.Heap[self.leftChild(pos)] <\r\n self.Heap[self.rightChild(pos)]):\r\n self.swap(pos, self.leftChild(pos))\r\n self.minHeapify(self.leftChild(pos))\r\n\r\n # Swap with the right child and heapify the right child\r\n else:\r\n self.swap(pos, self.rightChild(pos))\r\n self.minHeapify(self.rightChild(pos))\r\n\r\n def insert(self, element):\r\n if self.size >= self.maxsize:\r\n return\r\n self.size += 1\r\n self.Heap[self.size] = element\r\n\r\n current = self.size\r\n\r\n while (self.Heap[current] <\r\n self.Heap[self.parent(current)]):\r\n self.swap(current, self.parent(current))\r\n current = self.parent(current)\r\n\r\n def Print(self):\r\n for i in range(1, (self.size // 2) + 1):\r\n print(\" PARENT : \" + str(self.Heap[i]) +\r\n \" LEFT CHILD : \" + str(self.Heap[2 * i]) +\r\n \" RIGHT CHILD : \" + str(self.Heap[2 * i + 1]))\r\n\r\n def extractMin(self):\r\n popped = self.Heap[self.FRONT]\r\n self.Heap[self.FRONT] = self.Heap[self.size]\r\n self.size -= 1\r\n self.minHeapify(self.FRONT)\r\n return popped\r"
}
{
"filename": "code/data_structures/src/minqueue/minqueue.cpp",
"content": "#include <deque>\n#include <iostream>\n\nusing namespace std;\n\n//this is so the minqueue can be used with different types\n//check out the templeting documentation for more info about this\n\n//This version of minqueue is built upon the deque data structure\ntemplate <typename T>\nclass MinQueue {\n\n public:\n\n MinQueue() {}\n \n bool is_empty() const{\n //utilizes the size parameter that deque provides\n if (q.size() > 0){\n return false; //queue is not empty\n }\n return true; //queue is empty\n }\n\n int size() const{\n return q.size();\n }\n\n void pop(){ //Typically pop() should only change the queue state. It should not return the item\n //pop should only be called when there is an item in the \n //minQueue or else a deque exception will be raised\n if (q.size() > 0) { //perform check for an empty queue\n q.pop_front();\n } \n }\n\n const T & top(){\n return q.front();\n }\n\n void push(const T &item){\n q.push_back(item);\n //this forloop is to determine where in the minQueue the \n //new element should be placed\n for (int i = q.size()-1; i > 0; --i){\n if (q[i-1] > q[i]){\n swap(q[i-1], q[i]);\n }\n else{\n break;\n }\n }\n }\n\nprivate:\n deque<T> q;\n};\n\nint main(){\n MinQueue<int> minQ;\n //Here is a testing of the algorithim\n cout << minQ.size() << endl;\n minQ.push(2);\n cout << minQ.size() << endl;\n cout << minQ.top() << endl;\n minQ.push(1);\n cout << minQ.top() << endl;\n minQ.pop();\n cout << minQ.top() << endl;\n minQ.pop();\n cout << minQ.size() << endl;\n minQ.push(10);\n cout << minQ.top() << endl;\n minQ.push(8);\n cout << minQ.top() << endl;\n minQ.push(7);\n cout << minQ.top() << endl;\n minQ.push(6);\n cout << minQ.top() << endl;\n minQ.push(1);\n cout << minQ.top() << endl;\n\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/other/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/data_structures/src/other/ways_to_swap.cpp",
"content": "//DIFFERENT WAYS TO SWAP 2 NUMBERS:-\r\n\r\n#include<bits/stdc++.h> //it includes most of the headers files needed for basic programming\r\nusing namespace std;\r\n\r\n\r\nint main()\r\n{\r\n int a = 10, b = 5, temp;\r\n\r\n // 1. USING THIRD VARIABLE\r\n \r\n temp = a;\r\n a = b;\r\n b = temp;\r\n\r\n // 2. USING ADDITION AND SUBTRACTION\r\n\r\n a = a + b;\r\n b = a - b;\r\n a = a - b;\r\n\r\n // 3. USING MULTIPLICATION AND DIVISON\r\n\r\n a = a * b;\r\n b = a / b;\r\n a = a / b;\r\n\r\n // 4. USING BITWISE XOR OPERATOR\r\n\r\n a = a ^ b;\r\n b = a ^ b;\r\n a = a ^ b;\r\n\r\n cout<<\"after swapping a = \"<<a<<\" b = \"<<b;\r\n\r\n \r\n //There are few more ways u can find by using the arithmetic operators \r\n //differently or by using XOR in single line like this (a ^= b ^= a ^= b;) \r\n //try on your and keep learning , good luck ! :)\r\n\r\n \r\n return 0;\r\n}\r"
}
{
"filename": "code/data_structures/src/prefix_sum_array/prefix_sum_array.py",
"content": "def maximum_sum_subarray(arr, n):\n min_sum = 0\n res = 0\n prefixSum = []\n prefixSum.append(arr[0])\n for i in range(1, n):\n prefixSum.append(prefixSum[i-1] + arr[i])\n for i in range(n):\n res = max(res, prefixSum[i] - min_sum)\n min_sum = min(min_sum, prefixSum[i])\n return res\n\nn = int(input(\"Enter the size of the array: \"))\na = []\nfor i in range(n):\n a.append(int(input(\"Enter element no. \"+ str(i+1)+\": \")))\n\nprint(\"Result: \", maximum_sum_subarray(a, n))"
}
{
"filename": "code/data_structures/src/prefix_sum_array/prefix_sum_subarray.cpp",
"content": "// Part of Cosmos by OpenGenus\n//Max Sum Subarray in O(n)\n#include <bits/stdc++.h>\nusing namespace std;\nint maximumSumSubarray(int *arr, int n){\n int minPrefixSum = 0;\n int res = numeric_limits<int>::min();\n int prefixSum[n];\n prefixSum[0] = arr[0];\n for (int i = 1; i < n; i++) {\n prefixSum[i] = prefixSum[i - 1] + arr[i];\n }\n for (int i = 0; i < n; i++) {\n res = max(res, prefixSum[i] - minPrefixSum);\n minPrefixSum = min(minPrefixSum, prefixSum[i]);\n }\n return res;\n}\nint main(){\n\tint n;\n\tcin>>n; // size of the array\n int arr[n];\n for(int i=0;i<n;i++){ //input array\n \tcin>>arr[i];\n }\n\tcout << \"Result = \" << maximumSumSubarray(arr, n) <<endl;\n\treturn 0;\n}"
}
{
"filename": "code/data_structures/src/queue/circular_buffer/circular_buffer.cpp",
"content": "#include <iostream>\n#include <vector>\n\ntemplate <typename T, long SZ>\nclass circular_buffer\n{\nprivate:\n T* m_buffer;\n long m_index;\n\npublic:\n circular_buffer()\n : m_buffer {new T[SZ]()}\n , m_index {0}\n {\n }\n\n ~circular_buffer()\n {\n delete m_buffer;\n }\n\n std::vector<T> get_ordered() noexcept\n {\n std::vector<T> vec;\n for (long i = 0; i < SZ; ++i)\n vec.push_back(m_buffer[(i + m_index) % SZ]);\n return vec;\n }\n\n void push(T x) noexcept\n {\n m_buffer[m_index] = x;\n m_index = (m_index + 1) % SZ;\n }\n};\n\nint main()\n{\n circular_buffer<int, 4> buf;\n\n buf.push(1);\n buf.push(2);\n buf.push(3);\n buf.push(4);\n buf.push(5);\n buf.push(6);\n\n for (auto x : buf.get_ordered())\n std::cout << x << std::endl;\n}"
}
{
"filename": "code/data_structures/src/queue/circular_buffer/circular_buffer.py",
"content": "class CircularBuffer:\n __array = []\n __size = 0\n __idx = 0\n\n def __init__(self, size):\n self.__array = [[] for _ in range(0, size)]\n self.__size = size\n\n def push(self, x):\n self.__array[self.__idx] = x\n self.__idx = (self.__idx + 1) % self.__size\n\n def get_ordered(self):\n retarr = []\n for i in range(0, self.__size):\n retarr += [self.__array[(i + self.__idx) % self.__size]]\n return retarr\n\n\nbuf = CircularBuffer(2)\n\nbuf.push(1)\nbuf.push(2)\nbuf.push(3)\n\nfor n in buf.get_ordered():\n print(n)"
}
{
"filename": "code/data_structures/src/queue/double_ended_queue/deque_library_function.cpp",
"content": "#include <iostream>\n#include <deque>\n\nusing namespace std;\n\nvoid showdq (deque <int> g)\n{\n deque <int> :: iterator it; // Iterator to iterate over the deque .\n\n for (it = g.begin(); it != g.end(); ++it)\n cout << '\\t' << *it;\n\n cout << \"\\n\";\n}\n\nint main ()\n{\n deque <int> que;\n int option, x;\n do\n {\n\n\n cout << \"\\n\\n DEQUEUE USING STL C++ \";\n cout << \"\\n 1.Insert front\";\n cout << \"\\n 2.Insert Back\";\n cout << \"\\n 3.Delete front\";\n cout << \"\\n 4.Delete Back\";\n cout << \"\\n 5.Display \";\n\n cout << \"\\n Enter your option : \";\n cin >> option;\n\n switch (option)\n {\n case 1: cout << \"\\n Enter number : \";\n cin >> x;\n que.push_front(x);\n break;\n\n case 2: cout << \"\\n Enter number : \";\n cin >> x;\n que.push_back(x);\n break;\n\n case 3: que.pop_front();\n break;\n\n case 4: que.pop_back();\n break;\n case 5: showdq(que);\n break;\n }\n } while (option != 6);\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/queue/double_ended_queue/double_ended_queue.c",
"content": "#include <stdio.h>\n#include <conio.h> \n#define MAX 10 \n\nint deque[MAX];\nint left =-1 ;\nint right = -1 ;\n\nvoid inputdeque(void);\nvoid outputdeque(void); \nvoid insertleft(void);\nvoid insertright(void); \nvoid deleteleft(void); \nvoid deleteright(void); \nvoid display(void); \n\nint main( ) \n{ \n int option; \n printf(\"\\n *****MAIN MENU*****\"); \n printf(\"\\n 1.Input restricted deque\"); \n printf(\"\\n 2.Output restricted deque\"); \n printf(\"\\n Enter your option : \"); \n scanf(\"%d\",&option); \n\t\n switch (option) \n { \n case 1 : inputdeque(); \n\t \t break; \n\t case 2 : outputdeque(); \n\t\t\t break; \n } return 0;\n} \n\nvoid inputdeque( ) \n{ \n int option;\n\tdo \n\t{ \n\t printf(\"\\n\\n INPUT RESTRICTED DEQUE\"); \n\t\tprintf(\"\\n 1.Insert at right\"); \n\t\tprintf(\"\\n 2.Delete from left\"); \n\t\tprintf(\"\\n 3.Delete from right\"); \n\t\tprintf(\"\\n 4.Display\"); \n\t\tprintf(\"\\n 5.Quit\"); \n\t\tprintf(\"\\n Enter your option : \"); \n\t\t\n\t\tscanf(\"%d\",&option); \n\t\tswitch (option) \n\t\t{ \n\t\t\tcase 1 : insertright(); \n\t\t\t\t\t break; \n\t\t\tcase 2 : deleteleft(); \n\t\t\t\t\t break; \n\t\t\tcase 3 : deleteright(); \n\t\t\t\t\t break; \n\t\t\tcase 4 : display(); \n\t\t\t\t\t break; \n\t\t}\n\t\t \n\t} while (option!=5);\n} \n\nvoid outputdeque( ) \n{ \n\tint option; \n\tdo \n\t{ \n\t\tprintf(\"\\n\\n OUTPUT RESTRICTED DEQUE\"); \n\t\tprintf(\"\\n 1.Insert at right\"); \n\t\tprintf(\"\\n 2.Insert at left\"); \n\t\tprintf(\"\\n 3.Delete from left\"); \n\t\tprintf(\"\\n 4.Display\"); \n\t\tprintf(\"\\n 5.Quit\"); \n\t\tprintf(\"\\n Enter your option : \"); \n\t\n\t\tscanf(\"%d\",&option); \n\t\tswitch(option) \n\t\t\t{ \n\t\t\t\tcase 1 : insertright();\n\t\t\t\t \t break; \n\t\t\t\tcase 2 : insertleft(); \n\t\t\t\t\t\t break; \n\t\t\t\tcase 3 : deleteleft(); \n\t\t\t\t \t\t break; \n\t\t\t\tcase 4 : display(); \n\t\t\t\t\t break; \n\t\t\t}\n\t} while (option!=5); \n} \n\nvoid insertright( ) \n{ \n\tint val; \n\t\n\tprintf(\"\\n Enter the value to be added:\"); \n\tscanf(\"%d\", &val); \n\t\n\tif ( (left == 0 && right == MAX-1 ) || (left == right+1) )\n\t{ \n\t \tprintf(\"\\n OVERFLOW\");\n\t\treturn; \n\t}\n\tif (left == -1) // Queue is Empty Inititally\n\t{ \n\t\tleft = 0; \n\t\tright = 0;\n } \n\telse \n\t{\n \t\tif (right == MAX-1) \t\t\t\t\t\t\t//right is at last position of queue \n\t\t\tright = 0;\n\t \telse \n\t \t\tright = right+1; \n\t} \n\tdeque[right] = val ; \n} \n\nvoid insertleft( ) \n{ \n int val;\n\tprintf(\"\\n Enter the value to be added:\"); \n\tscanf(\"%d\", &val); \n\t \n\tif( (left ==0 && right == MAX-1) || (left == right+1) )\n\t{ \n\t \tprintf(\"\\n OVERFLOW\"); \n\t\treturn; \n\t}\n\tif (left == -1)\t\t\t\t\t\t\t\t\t\t\t//If queue is initially empty\n\t{ \n\t\tleft = 0; \n\t\tright = 0; \n\t} \n\telse \n\t{ \n\t\tif(left == 0)\n\t\t left = MAX - 1 ; \n\t\telse \n\t\t left = left - 1 ; \n\t} \n\t\n\tdeque[left] = val; \n}\n \nvoid deleteleft( ) \n{ \n\tif ( left == -1 ) \n\t{ \n\t\tprintf(\"\\n UNDERFLOW\"); \n\t\treturn ; \n\t} \n\n\tprintf(\"\\n The deleted element is : %d\", deque[left]);\n\n\tif (left == right) \t\t\t\t\t\t\t\t\t\t/*Queue has only one element */ \n\t{ \n\t\tleft = -1 ; \n\t\tright = -1 ; \n\t} \n\telse \n\t{ \n\t\tif ( left == MAX - 1 ) \n\t\tleft = 0; \n\t\telse \n\t\tleft = left+1; \n\t} \n} \t\n\nvoid deleteright() \n{ \n\tif ( left == -1 ) \n\t{ \n\t\tprintf(\"\\n UNDERFLOW\");\n\t\treturn ; \n\t} \n\t\n\tprintf(\"\\n The element deleted is : %d\", deque[right]); \n\t\n\tif (left == right) /*queue has only one element*/ \n\t{ \n\t\tleft = -1 ; \n\t\tright = -1 ; \n\t} \n\telse \n\t{ \n\t\tif (right == 0) \n\t\t\tright = MAX - 1 ; \n\t\telse \n\t\t\tright = right - 1 ; \n\t} \n} \n\nvoid display( ) \n{ \n\tint front = left, rear = right;\n\tif ( front == -1 ) \n\t{ \n\t \tprintf(\"\\n QUEUE IS EMPTY\"); \n\t\treturn; \n\t} \n\tprintf(\"\\n The elements of the queue are : \");\n\tif (front <= rear ) \n\t{ \n\t\twhile (front <= rear) \n\t\t{ \n\t\t\tprintf(\"%d \",deque[front]); \n\t\t\tfront++; \n\t\t} \n\t} \n\telse \n\t{ \n\t\twhile (front <= MAX - 1) \n\t\t{ \n\t\t\tprintf(\"%d \", deque[front]); \n\t\t\tfront++; \n\t\t} \n\t\tfront = 0; \n\t\t\n\t\twhile (front <= rear) \n\t\t{ \n\t\t\tprintf(\"%d \",deque[front]); \n\t\t\tfront++; \n\t\t} \n\t} \n\tprintf(\"\\n\"); \n}"
}
{
"filename": "code/data_structures/src/queue/double_ended_queue/double_ended_queue.cpp",
"content": "#include <iostream>\nusing namespace std;\n#define MAX 10\n\nint deque[MAX];\nint leftt = -1;\nint rightt = -1;\n\nvoid inputdeque(void);\nvoid outputdeque(void);\nvoid insertleft(void);\nvoid insertright(void);\nvoid deleteleft(void);\nvoid deleteright(void);\nvoid display(void);\n\nint main( )\n{\n int option;\n cout << \"\\n *****MAIN MENU*****\";\n cout << \"\\n 1.Input restricted deque\";\n cout << \"\\n 2.Output restricted deque\";\n cout << \"\\n Enter your option : \";\n cin >> option;\n\n switch (option)\n {\n case 1: inputdeque();\n break;\n case 2: outputdeque();\n break;\n } return 0;\n}\n\nvoid inputdeque( )\n{\n int option;\n do\n {\n cout << \"\\n\\n INPUT RESTRICTED DEQUE\";\n cout << \"\\n 1.Insert at right\";\n cout << \"\\n 2.Delete from left\";\n cout << \"\\n 3.Delete from right\";\n cout << \"\\n 4.Display\";\n cout << \"\\n 5.Quit\";\n cout << \"\\n Enter your option : \";\n\n cin >> option;\n switch (option)\n {\n case 1: insertright();\n break;\n case 2: deleteleft();\n break;\n case 3: deleteright();\n break;\n case 4: display();\n break;\n }\n\n } while (option != 5);\n}\n\nvoid outputdeque( )\n{\n int option;\n do\n {\n cout << \"\\n\\n OUTPUT RESTRICTED DEQUE\";\n cout << \"\\n 1.Insert at right\";\n cout << \"\\n 2.Insert at left\";\n cout << \"\\n 3.Delete from left\";\n cout << \"\\n 4.Display\";\n cout << \"\\n 5.Quit\";\n cout << \"\\n Enter your option : \";\n\n cin >> option;\n switch (option)\n {\n case 1: insertright();\n break;\n case 2: insertleft();\n break;\n case 3: deleteleft();\n break;\n case 4: display();\n break;\n }\n } while (option != 5);\n}\n\nvoid insertright( )\n{\n int val;\n cout << \"\\n Enter the value to be added:\";\n cin >> val;\n if ( (leftt == 0 && rightt == MAX - 1 ) || (leftt == rightt + 1) )\n {\n cout << \"\\n OVERFLOW\";\n return;\n }\n if (leftt == -1) // Queue is Empty Inititally\n {\n leftt = 0;\n rightt = 0;\n }\n else\n {\n if (rightt == MAX - 1) //rightt is at last position of queue\n rightt = 0;\n else\n rightt = rightt + 1;\n }\n deque[rightt] = val;\n}\n\nvoid insertleft( )\n{\n int val;\n cout << \"\\n Enter the value to be added:\";\n cin >> val;\n\n if ( (leftt == 0 && rightt == MAX - 1) || (leftt == rightt + 1) )\n {\n cout << \"\\n OVERFLOW\";\n return;\n }\n if (leftt == -1) //If queue is initially empty\n {\n leftt = 0;\n rightt = 0;\n }\n else\n {\n if (leftt == 0)\n leftt = MAX - 1;\n else\n leftt = leftt - 1;\n }\n deque[leftt] = val;\n}\n\nvoid deleteleft( )\n{\n if (leftt == -1)\n {\n cout << \"\\n UNDERFLOW\";\n return;\n }\n\n cout << \"\\n The deleted element is : \" << deque[leftt];\n\n if (leftt == rightt) /*Queue has only one element */\n {\n leftt = -1;\n rightt = -1;\n }\n else\n {\n if (leftt == MAX - 1)\n leftt = 0;\n else\n leftt = leftt + 1;\n }\n}\n\nvoid deleteright()\n{\n if (leftt == -1)\n {\n cout << \"\\n UNDERFLOW\";\n return;\n }\n\n cout << \"\\n The element deleted is : \" << deque[rightt];\n\n if (leftt == rightt) /*queue has only one element*/\n {\n leftt = -1;\n rightt = -1;\n }\n else\n {\n if (rightt == 0)\n rightt = MAX - 1;\n else\n rightt = rightt - 1;\n }\n}\n\nvoid display( )\n{\n int front = leftt, rear = rightt;\n if (front == -1)\n {\n cout << \"\\n QUEUE IS EMPTY\";\n return;\n }\n cout << \"\\n The elements of the queue are : \";\n if (front <= rear)\n while (front <= rear)\n {\n cout << deque[front] << \" \";\n front++;\n }\n else\n {\n while (front <= MAX - 1)\n {\n cout << deque[front] << \" \";\n front++;\n }\n front = 0;\n while (front <= rear)\n {\n cout << deque[front] << \" \";\n front++;\n }\n }\n cout << endl; //NEW LINE\n}"
}
{
"filename": "code/data_structures/src/queue/double_ended_queue/double_ended_queue.py",
"content": "\"\"\" python programme for showing dequeue data sturcture in python \"\"\"\n\n\n\"\"\" class for queue type data structure having following methods\"\"\"\n\n\nclass Queue:\n def __init__(self):\n self.array = [] # ''' initialise empty array '''\n\n def front_enqueue(self, data): # \t''' adding element from front '''\n self.array.insert(0, data)\n\n def front_dequeue(self): # ''' deleting element from front '''\n k = self.array[0]\n del self.array[0]\n\n def rear_dequeue(self): # \t''' deleting element from rear '''\n k = self.array.pop()\n print(k)\n\n def rear_enqueue(self, data): # ''' adding element from rear '''\n self.array.append(data)\n\n def traverse(self): # \t''' priting all array '''\n print(self.array)\n\n\nqueue = Queue() # \t''' initialise Queue instance '''\nqueue.front_enqueue(5)\nqueue.front_enqueue(4)\nqueue.front_enqueue(3)\nqueue.front_enqueue(2)\n\nqueue.traverse()\n\nqueue.front_dequeue()\n\nqueue.traverse()\n\nqueue.rear_enqueue(5)\nqueue.rear_enqueue(4)\nqueue.rear_enqueue(3)\nqueue.rear_enqueue(2)\n\nqueue.traverse()\n\nqueue.rear_dequeue()\nqueue.rear_dequeue()\n\nqueue.traverse()"
}
{
"filename": "code/data_structures/src/queue/queue/queue.c",
"content": "/*\n * C Program to Implement a Queue using an Array\n * Part of Cosmos by OpenGenus Foundation\n */\n#include <stdio.h>\n#include <stdlib.h>\n\n#define MAX 50\nint queue_array[MAX];\nint rear = -1;\nint front = -1;\n\nvoid insert() {\n int add_item;\n if (rear == MAX - 1) {\n printf(\"Queue Overflow \\n\");\n } else {\n\t\t/*If queue is initially empty */\n if (front == -1) {\n front = 0;\n\t\t}\n printf(\"Insert the element in queue: \");\n scanf(\"%d\", &add_item);\n rear = rear + 1;\n queue_array[rear] = add_item;\n }\n\t\n\treturn;\n}\n\nvoid delete() {\n if (front == -1 || front > rear) {\n printf(\"Queue Underflow\\n\");\n } else {\n printf(\"Element deleted from queue is: %d\\n\", queue_array[front]);\n front = front + 1;\n }\n\t\n\treturn;\n}\n\nvoid display() {\n int i;\n if (front == -1) {\n printf(\"Queue is empty\\n\");\n } else {\n printf(\"Queue is: \\n\");\n for (i = front; i <= rear; i++) {\n printf(\"%d \", queue_array[i]);\n }\n printf(\"\\n\");\n }\n\t\n\treturn;\n}\n\nint main(void) {\n int choice;\n while (1) {\n printf(\"1. Insert element to queue\\n\");\n printf(\"2. Delete element from queue\\n\");\n printf(\"3. Display all elements of queue\\n\");\n printf(\"4. Quit \\n\");\n printf(\"Enter your choice: \");\n scanf(\"%d\", &choice);\n switch (choice) {\n case 1:\n insert();\n break;\n case 2:\n delete();\n break;\n case 3:\n display();\n break;\n case 4:\n exit(1);\n break;\n default:\n printf(\"Wrong choice\\n\");\n break;\n }\n }\n\t\n\treturn 0;\n}"
}
{
"filename": "code/data_structures/src/queue/queue/queue.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <iostream>\n#include <cassert>\n\ntemplate <typename T>\nclass Queue {\n\nprivate:\n int m_f;\n int m_r;\n std::size_t m_size;\n T * m_data;\n\npublic:\n/*! \\brief Constructs queue object with size.\n * \\param sz Size of the queue.\n */\n Queue( std::size_t sz = 10) : m_f( -1)\n , m_r( -1)\n , m_size( sz)\n , m_data( new T[sz])\n {\n /* empty */\n }\n\n\n/*! \\brief Destructor\n */\n ~Queue()\n {\n delete [] m_data;\n }\n\n/*! \\brief Insert element at the end of the queue.\n * \\param value Value to be inserted.\n */\n void enqueue( const T& value )\n {\n int prox_r = (m_r + 1) % m_size;\n\n if (prox_r == 0 or prox_r != m_f)\n {\n (m_f == -1) ? m_f = 0 : m_f = m_f;\n m_data[prox_r] = value;\n m_r = prox_r;\n\n }\n else\n assert(false);\n }\n\n/*! \\brief Remove element at the end of the queue.\n */\n void dequeue()\n {\n if (m_f != -1)\n {\n // If there is only 1 element, f = r = -1\n if (m_f == m_r)\n m_f = m_r = -1;\n // If size > 1, f goes forward\n else\n m_f = (m_f + 1) % m_size;\n }\n else\n assert(false);\n }\n\n/*! \\brief Get element at the beginning of the queue.\n * \\return First element at the queue.\n */\n T front()\n {\n if (m_f != -1)\n return m_data[m_f];\n\n assert(false);\n }\n\n/*! \\brief Check if queue is empty.\n * \\return True if queue is empty, false otherwise.\n */\n bool isEmpty()\n {\n return m_f == -1;\n }\n\n/*! \\brief Clear content.\n */\n void makeEmpty()\n {\n reserve(m_size);\n m_f = m_r = -1;\n m_size = 0;\n }\n\n/*! \\brief Get size of the queue.\n * \\return Size of the queue.\n */\n T size() const\n {\n if (m_r == m_f)\n return 1;\n else if (m_r > m_f)\n return m_r - m_f + 1;\n else\n return m_f - m_r + 1;\n }\n\n/*! \\brief Increase the storage capacity of the array to a value\n * that’s is greater or equal to 'new_cap'.\n * \\param new_cap New capacity.\n */\n void reserve( std::size_t new_cap )\n {\n if (new_cap >= m_size)\n {\n //<! Allocates new storage area\n T *temp = new T[ new_cap ];\n\n //<! Copy content to the new list\n for (auto i(0u); i < m_size; i++)\n temp[i] = m_data[i];\n\n delete [] m_data; //<! Deallocate old storage area\n\n m_data = temp; //<! Pointer pointing to the new adress\n m_size = new_cap; //<! Updates size\n }\n }\n};\n\nint main()\n{\n\n Queue<int> queue(3);\n\n queue.enqueue(5);\n assert(queue.front() == 5);\n assert(queue.size() == 1);\n\n queue.enqueue(4);\n queue.enqueue(3);\n\n //remove 2 elements\n queue.dequeue();\n queue.dequeue();\n assert( queue.size() == 1);\n\n //Insert one more\n queue.enqueue(2);\n assert( queue.size() == 3);\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/queue/queue/queue.cs",
"content": "/**\n * Queue implementation using a singly-linked link.\n * Part of the OpenGenus/cosmos project. (https://github.com/OpenGenus/cosmos)\n * \n * A queue is a first-in first-out (FIFO) data structure.\n * Elements are manipulated by adding elements to the back of the queue and removing them from \n * the front.\n */\nusing System;\n\nnamespace Cosmos_Data_Structures\n{\n public class Queue<T>\n {\n //Node is a element that holds the data of the current element plus a reference to the next element.\n //Used to implement the singly-linked list.\n private class Node\n {\n public T data;\n public Node next;\n\n public Node(T data, Node next)\n {\n this.data = data;\n this.next = next;\n }\n }\n\n //Queue variables\n //This queue is implemented as a singly-linked list, where the\n //front of the queue is the first element of the linked list, \n //and the back of the queue is the last element of the linked list.\n private Node front;\n private Node rear;\n public int Size { get; private set; }\n\n public Queue()\n {\n front = null;\n rear = null;\n Size = 0;\n }\n\n //Adds an element to the back of the queue.\n public void Enqueue(T element)\n {\n Node tmp = new Node(element, null);\n\n if(IsEmpty())\n {\n front = tmp;\n rear = tmp;\n }\n else\n {\n rear.next = tmp;\n rear = tmp;\n }\n\n Size++;\n }\n\n //Removes and returns the element at the front of the queue.\n //Throws an exception if the queue is empty.\n public T Dequeue()\n {\n if(IsEmpty())\n {\n throw new InvalidOperationException(\"Cannot dequeue on an empty queue!\");\n }\n\n Node tmp = front;\n front = tmp.next;\n Size--;\n\n if(front == null)\n {\n rear = null;\n }\n\n return tmp.data;\n }\n\n //Returns the element at the front of the queue.\n //Throws an exception if the queue is empty.\n public T Front()\n {\n if (IsEmpty())\n {\n throw new InvalidOperationException(\"The queue is empty!\");\n }\n\n return front.data;\n }\n\n //Returns true if queue contains no elements, false otherwise.\n public bool IsEmpty()\n {\n return front == null && rear == null;\n }\n\n //Returns a string representation of the queue.\n public override string ToString()\n {\n Node tmp = front;\n string result = \"Queue([Front] \";\n\n while(tmp != null)\n {\n result += tmp.data;\n tmp = tmp.next;\n\n if (tmp != null)\n {\n result += \" -> \";\n }\n }\n\n result += \" [Back])\";\n return result;\n }\n }\n\n //Stack testing methods/class.\n public class QueueTest\n {\n static void Main(string[] args)\n {\n var strQueue = new Queue<string>();\n\n Console.WriteLine(\"Setting up queue...\");\n strQueue.Enqueue(\"Marth\");\n strQueue.Enqueue(\"Ike\");\n strQueue.Enqueue(\"Meta Knight\");\n Console.WriteLine(strQueue.ToString());\n\n Console.WriteLine(\"Removing first element...\");\n strQueue.Dequeue();\n Console.WriteLine(strQueue.ToString());\n Console.ReadKey();\n }\n }\n}"
}
{
"filename": "code/data_structures/src/queue/queue/queue.exs",
"content": "defmodule Queue do\n def init, do: loop([])\n def loop(queue) do\n receive do\n {:enqueue, {sender_pid, item}} ->\n queue = enqueue(queue, item)\n send sender_pid, {:ok, item}\n loop(queue)\n {:queue_next, {sender_pid}} ->\n {queue, next} = queue_next(queue)\n send sender_pid, {:ok, next}\n loop(queue)\n {:is_empty, {sender_pid}} ->\n is_empty = is_empty(queue)\n send sender_pid, {:ok, is_empty}\n loop(queue)\n {:size, {sender_pid}} ->\n size = size(queue)\n send sender_pid, {:ok, size}\n loop(queue)\n {:first_item, {sender_pid}} ->\n first_item = first_item(queue)\n send sender_pid, {:ok, first_item}\n loop(queue)\n {:last_item, {sender_pid}} ->\n last_item = last_item(queue)\n send sender_pid, {:ok, last_item}\n loop(queue)\n _ -> \n IO.puts \"Invalid message\"\n end\n end\n\n def enqueue(queue, item) do\n queue = queue ++ [item]\n queue\n end\n\n def queue_next([head | tail]) do\n {tail, head}\n end\n\n def is_empty(queue) do\n if queue |> length === 0 do true end\n false\n end\n\n def size(queue) do\n queue |> length\n end\n \n def first_item([head | _]) do\n head\n end\n\n def last_item(queue) do\n queue |> List.last\n end\nend\n\ndefmodule RunQueue do\n def initialize_queue do\n queue = spawn(Queue, :init, [])\n send queue, {:enqueue, {self(), \"hello\"}}\n send queue, {:size, {self()}}\n send queue, {:enqueue, {self(), \"message\"}}\n send queue, {:queue_next, {self()}}\n send queue, {:enqueue, {self(), \"another\"}}\n send queue, {:is_empty, {self()}}\n send queue, {:first_item, {self()}}\n send queue, {:last_item, {self()}}\n manage()\n end\n\n def manage do\n receive do\n {:ok, message} ->\n IO.puts message\n _ ->\n IO.puts \"Invalid message\"\n end\n manage()\n end\nend\n\nRunQueue.initialize_queue"
}
{
"filename": "code/data_structures/src/queue/queue/queue.go",
"content": "// Part of Cosmos by OpenGenus Foundation\npackage main\n\nimport \"fmt\"\n\ntype Queue []int\n\nfunc (s *Queue) Push(v int) Queue {\n\treturn append(*s, v)\n}\n\nfunc (s *Queue) Pop() Queue {\n\tlength := len(*s)\n\tif length != 0 {\n\t\treturn (*s)[1:]\n\t}\n\treturn *s\n}\n\nfunc (s *Queue) Top() int {\n\treturn (*s)[0]\n}\n\nfunc (s *Queue) Empty() bool {\n\tif len(*s) == 0 {\n\t\treturn true\n\t}\n\n\treturn false\n}\n\nfunc main() {\n\tqueue := Queue{}\n\tfmt.Printf(\"Current Queue is %s, Is Queue empty ? %v \\n\", queue, queue.Empty())\n\tfmt.Printf(\"Try to push 30 into Queue\\n\")\n\tqueue = queue.Push(30)\n\tfmt.Printf(\"Current Queue is %v, top value is %v\\n\", queue, queue.Top())\n\tfmt.Printf(\"Try to push 12 into Queue\\n\")\n\tqueue = queue.Push(12)\n\tfmt.Printf(\"Try to push 34 into Queue\\n\")\n\tqueue = queue.Push(34)\n\tfmt.Printf(\"Current Queue is %v, top value is %v\\n\", queue, queue.Top())\n\tfmt.Printf(\"Current Queue is %v, Is Queue empty ? %v \\n\", queue, queue.Empty())\n\tqueue = queue.Pop()\n\tfmt.Printf(\"Current Queue is %v, top value is %v\\n\", queue, queue.Top())\n\tqueue = queue.Pop()\n\tqueue = queue.Pop()\n\tfmt.Printf(\"Current Queue is %v, Is Queue empty ? %v\\n\", queue, queue.Empty())\n}"
}
{
"filename": "code/data_structures/src/queue/queue/queue.java",
"content": "\n/* Part of Cosmos by OpenGenus Foundation */\nimport java.util.ArrayList;\n\nclass Queue<T> {\n\tprivate int maxSize;\n\tprivate ArrayList<T> queueArray;\n\tprivate int front;\n\tprivate int rear;\n\tprivate int nItems;\n\n\tpublic Queue(int size) {\n\t\tmaxSize = size;\n\t\tqueueArray = new ArrayList<>();\n\t\tfront = 0;\n\t\trear = -1;\n\t\tnItems = 0;\n\t}\n\n\tpublic boolean insert(T data) {\n\t\tif (isFull())\n\t\t\treturn false;\n\t\tif (rear == maxSize - 1) //If the back of the queue is the end of the array wrap around to the front\n\t\t\trear = -1;\n\t\trear++;\n\t\tif(queueArray.size() <= rear)\n\t\t\tqueueArray.add(rear, data);\n\t\telse\n\t\t\tqueueArray.set(rear, data);\n\n\t\tnItems++;\n\t\treturn true;\n\t}\n\n\tpublic T remove() { //Remove an element from the front of the queue\n\t\tif (isEmpty()) {\n\t\t\tthrow new NullPointerException(\"Queue is empty\");\n\t\t}\n\t\tT temp = queueArray.get(front);\n\t\tfront++;\n\t\tif (front == maxSize) //Dealing with wrap-around again\n\t\t\tfront = 0;\n\t\tnItems--;\n\t\treturn temp;\n\t}\n\n\tpublic T peekFront() {\n\t\treturn queueArray.get(front);\n\t}\n\n\tpublic T peekRear() {\n\t\treturn queueArray.get(rear);\n\t}\n\n\tpublic boolean isEmpty() {\n\t\treturn (nItems == 0);\n\t}\n\n\tpublic boolean isFull() {\n\t\treturn (nItems == maxSize);\n\t}\n\n\tpublic int getSize() {\n\t\treturn nItems;\n\t}\n}\n\npublic class Queues {\n\n\tpublic static void main(String args[]) {\n\t\tQueue<Integer> myQueue = new Queue<>(4);\n\t\tmyQueue.insert(10);\n\t\tmyQueue.insert(2);\n\t\tmyQueue.insert(5);\n\t\tmyQueue.insert(3);\n\t\t//[10(front), 2, 5, 3(rear)]\n\n\t\tSystem.out.println(myQueue.isFull()); //Will print true\n\n\t\tmyQueue.remove(); //Will make 2 the new front, making 10 no longer part of the queue\n\t\t//[10, 2(front), 5, 3(rear)]\n\n\t\tmyQueue.insert(7); //Insert 7 at the rear which will be index 0 because of wrap around\n\t\t// [7(rear), 2(front), 5, 3]\n\n\t\tSystem.out.println(myQueue.peekFront()); //Will print 2\n\t\tSystem.out.println(myQueue.peekRear()); //Will print 7 \t\n\t}\n}"
}
{
"filename": "code/data_structures/src/queue/queue/queue.js",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n *\n */\n\nfunction Queue(size_max) {\n data = new Array(size_max + 1);\n max = (size_max + 1);\n start = 0;\n end = 0;\n\n this.empty = () => {\n return start == end;\n }\n\n this.full = () => {\n return start == (end + 1) % max;\n }\n\n this.enqueue = (x) => {\n if (this.full()) {\n console.log(\"Queue full\");\n return false;\n }\n data[end] = x;\n end = (end + 1) % max;\n return true;\n }\n\n this.dequeue = () => {\n if (this.empty()) {\n console.log(\"Queue empty\");\n return;\n }\n x = data[start];\n start = (start + 1) % max;\n return x;\n }\n\n this.top = () => {\n if (this.empty()) {\n console.log(\"Queue empty\");\n return;\n }\n return data[start];\n }\n\n this.display = () => {\n cur = start\n while (cur != end) {\n console.log(data[cur])\n cur = (cur + 1) % max\n }\n }\n}\n\nlet q = new Queue(3);\nq.enqueue(\"a\");\nq.enqueue(\"b\");\nq.enqueue(\"c\");\nq.enqueue(\"d\"); // Queue full\nq.display(); // a, b, c\nq.dequeue();\nq.display(); // b, c\nq.dequeue();\nq.dequeue();\nq.dequeue(); // Queue empty\nq.enqueue(\"z\");\nq.display() // z"
}
{
"filename": "code/data_structures/src/queue/queue/queue.py",
"content": "# Part of Cosmos by OpenGenus Foundation\nclass Queue:\n def __init__(self, size_max):\n self.data = [None] * (size_max + 1)\n self.max = (size_max + 1)\n self.head = 0\n self.tail = 0\n\n def empty(self):\n return self.head == self.tail\n\n def full(self):\n return self.head == (self.tail + 1) % self.max\n\n def enqueue(self, x):\n if self.full():\n print(\"Queue full\")\n return False\n self.data[self.tail] = x\n self.tail = (self.tail + 1) % self.max\n return True\n\n def dequeue(self):\n if self.empty():\n print(\"Queue empty\")\n return None\n x = self.data[self.head]\n self.head = (self.head + 1) % self.max\n return x\n\n def top(self):\n if self.empty():\n print(\"Queue empty\")\n return None\n return self.data[self.head]\n\n def display(self):\n cur = self.head\n while cur != self.tail:\n print(self.data[cur])\n cur = (cur + 1) % self.max\n\nprint(\"Enter the size of Queue\")\nn = int(input())\nq = Queue(n)\nwhile True:\n print(\"Press E to enqueue an element\")\n print(\"Press D to dequeue an element\")\n print(\"Press P to display all elements of the queue\")\n print(\"Press T to show top element of the queue\")\n print(\"Press X to exit\")\n opt = input().strip()\n if opt == \"E\":\n if q.full():\n print(\"Queue is full\")\n continue\n print(\"Enter the element\")\n ele = int(input())\n q.enqueue(ele)\n continue\n if opt == \"D\":\n ele = q.dequeue()\n if ele == None:\n print(\"Queue is empty\")\n else:\n print(\"Element is\", ele)\n if opt == \"P\":\n q.display()\n if opt == \"T\":\n print(q.top())\n if opt == \"X\":\n break"
}
{
"filename": "code/data_structures/src/queue/queue/queue.rb",
"content": "# Part of Cosmos by OpenGenus Foundation\nclass Queue\n attr_accessor :items\n\n def initialize\n @items = []\n end\n\n def enqueue(element)\n @items.push(element)\n end\n\n def dequeue\n @items.delete_at(0)\n end\n\n def empty?\n @items.empty?\n end\n\n def front\n @items.first\n end\n\n def back\n @items.last\n end\nend"
}
{
"filename": "code/data_structures/src/queue/queue/queue.swift",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\npublic struct Queue<T> {\n \n private var elements: Array<T>;\n \n init() {\n self.elements = [];\n }\n \n init(arrayLiteral elements: T...) {\n self.elements = elements\n }\n \n public var front: T? {\n return elements.first\n }\n \n public var back: T? {\n return elements.last\n }\n \n public var size: Int {\n return elements.count\n }\n \n public var isEmpty: Bool {\n return elements.isEmpty\n }\n \n mutating public func enqueue(_ element: T) {\n elements.append(element)\n }\n \n mutating public func dequeue() -> T? {\n return self.isEmpty ? nil : elements.removeFirst()\n }\n}"
}
{
"filename": "code/data_structures/src/queue/queue/queue_vector.cpp",
"content": "#include <iostream>\n#include <vector>\n\nusing namespace std;\n// Part of Cosmos by OpenGenus Foundation\nclass Queue\n{\nprivate:\n// Vector for storing all of the values\n vector<int> _vec;\n\npublic:\n void enqueue(int & p_put_obj); // Puts object at the end of the queue\n void dequeue(int & p_ret_obj); // Returns object at the start of the queue and removes from queue\n void peek(int & p_peek_obj); // Returns object at the start of the queue but doesn't remove it\n bool is_empty(); // Checks if the queue is full\n};\n\n// Purpose: Put a value onto the end of the queue\n// Params : p_put_obj - reference to the object you want to enqueue\nvoid Queue::enqueue(int & p_put_obj)\n{\n // Use the inbuild push_back vector method\n _vec.push_back(p_put_obj);\n}\n\n// Purpose: Gets the value from the front of the queue\n// Params : p_ret_obj - Reference to an empty object to be overwritten by whatever is dequeued\nvoid Queue::dequeue(int & p_ret_obj)\n{\n // Set the value to what's in the start of the vector\n p_ret_obj = _vec[0];\n // Delete the first value in the vector\n _vec.erase(_vec.begin());\n}\n\n// Purpose: Checks if the queue is empty\n// Returns: True if the queue is empty\nbool Queue::is_empty()\n{\n return _vec.empty();\n}\n\n// Purpose: Returns the value at the front of the queue, without popping it\n// Params : p_peek_obj - reference to the object to be overwritten by the data in the front of the queue\nvoid Queue::peek(int &p_peek_obj)\n{\n p_peek_obj = _vec[0];\n}\n\nint main()\n{\n Queue q;\n\n int i = 0;\n int j = 1;\n\n q.enqueue(i);\n cout << \"Enqueueing \" << i << \" to the queue\" << endl;\n q.enqueue(j);\n cout << \"Enqueueing \" << j << \" to the queue\" << endl;\n\n int k, l, m;\n\n q.peek(m);\n cout << \"Peeking into the queue, found \" << m << endl;\n q.dequeue(k);\n cout << \"Dequeueing, found \" << k << endl;\n q.dequeue(l);\n cout << \"Dequeueing, found \" << l << endl;\n if (q.is_empty())\n cout << \"The queue is now empty\" << endl;\n else\n cout << \"The queue is not empty\" << endl;\n}"
}
{
"filename": "code/data_structures/src/queue/queue/README.md",
"content": "# Cosmos\n\nCollaborative effort by [OpenGenus](https://github.com/OpenGenus/cosmos)"
}
{
"filename": "code/data_structures/src/queue/queue_stream/queue_stream.cs",
"content": "using System;\n\nnamespace Cosmos_Data_Structures\n{\n /// <summary>\n /// QueueStream provides an alternative Queue implementation to High speed \n /// IO/Stream operations that works on multitheaded environment.\n /// Internally use 'circular array' in the implementation.\n /// </summary>\n\t[Serializable]\n public class QueueStream\n {\n byte[] _array = new byte[64]; // array in memory\n int _head; // head index\n int _tail; // tail index\n int _size; // Number of elements.\n\n /// <summary>\n /// Buffer size (maximum limit) for single stream operation (Default is 64 KB)\n /// </summary>\n const int BufferBlock = 1024 * 64;\n\n /// <summary>\n /// Get total bytes in queue\n /// </summary>\n public int Count\n {\n get { return _size; }\n }\n\n /// <summary>\n /// Remove all bytes in queue\n /// </summary>\n public virtual void Clear()\n {\n _head = 0;\n _tail = 0;\n _size = 0;\n }\n\n /// <summary>\n /// Remove all bytes in queue and discard waste memory to GC.\n /// </summary>\n public void Clean()\n {\n _head = 0;\n _tail = 0;\n _size = 0;\n _array = new byte[64];\n }\n\n /// <summary>\n /// Adds a byte to the back of the queue.\n /// </summary>\n public virtual void Enqueue(byte obj)\n {\n if (_size == _array.Length)\n {\n SetCapacity(_size << 1);\n }\n\n _array[_tail] = obj;\n _tail = (_tail + 1) % _array.Length;\n _size++;\n }\n\n /// <summary>\n /// Removes and returns the byte at the front of the queue.\n /// </summary>\n /// <returns></returns>\n public byte Dequeue()\n {\n if (_size == 0)\n throw new InvalidOperationException(\"Invalid operation. Queue is empty!\");\n\n byte removed = _array[_head];\n _head = (_head + 1) % _array.Length;\n _size--;\n return removed;\n }\n\n /// <summary>\n /// Returns the byte at the front of the queue.\n /// </summary>\n /// <returns></returns>\n public byte Front()\n {\n if (_size == 0)\n throw new InvalidOperationException(\"Invalid operation. Queue is empty!\");\n\n return _array[_head];\n }\n\n /// <summary>\n /// Read and push stream bytes to queue. Can be multi-threaded.\n /// </summary>\n /// <param name=\"stream\">The stream to push</param>\n /// <param name=\"maxSize\">Maximum bytes size for this operation</param>\n /// <returns>Size of pushed bytes</returns>\n public int Enqueue(Stream stream, long maxSize = int.MaxValue)\n {\n if ((_size + BufferBlock) > _array.Length)\n {\n SetCapacity(Math.Max((_size + BufferBlock), _size << 1));\n }\n\n var count = Math.Min(Math.Min((int)maxSize, BufferBlock), _array.Length - _tail);\n\n lock (_array)\n {\n count = stream.Read(_array, _tail, count);\n _tail = (_tail + count) % _array.Length;\n _size += count;\n }\n\n return count;\n }\n\n /// <summary>\n /// Pop and write stream bytes to queue. Can be multi-threaded.\n /// </summary>\n /// <param name=\"stream\">The stream to pop</param>\n /// <param name=\"maxSize\">Maximum bytes size for this operation</param>\n /// <returns>Size of popped bytes</returns>\n public int Dequeue(Stream stream, long maxSize = int.MaxValue)\n {\n if (_size == 0)\n return 0; // It's okay to be empty\n\n int count = Math.Min(Math.Min(BufferBlock, _size), Math.Min((int)maxSize, _array.Length - _head));\n\n lock (_array)\n {\n stream.Write(_array, _head, count);\n _head = (_head + count) % _array.Length;\n _size -= count;\n }\n\n return count;\n }\n\n /// <summary>\n /// Set new capacity\n /// </summary>\n private void SetCapacity(int capacity)\n {\n lock (_array)\n {\n byte[] newarray = new byte[capacity];\n\n if (_size > 0)\n {\n if (_head < _tail)\n {\n Array.Copy(_array, _head, newarray, 0, _size);\n }\n else\n {\n Array.Copy(_array, _head, newarray, 0, _array.Length - _head);\n Array.Copy(_array, 0, newarray, _array.Length - _head, _tail);\n }\n }\n\n _array = newarray;\n _head = 0;\n _tail = (_size == capacity) ? 0 : _size;\n }\n }\n }\n}"
}
{
"filename": "code/data_structures/src/queue/queue_using_linked_list/queue_using_linked_list.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n\n/* @author AaronDills\n An implementation of a queue data structure\n using C and Linked Lists*/\n\n/* Structure for a Node containing an integer value and reference to another Node*/\nstruct Node {\n int value;\n struct Node *next;\n\n};\n\n/* Initalize global Nodes for the head and tail of the queue */\nstruct Node* head = NULL;\nstruct Node* tail = NULL;\n\n/* Function declarations */\nstruct Node* createNode();\nvoid enqueue();\nvoid dequeue();\nvoid printQueue();\n\nint main(){\n int choice = 0;\n while(1){\n printf(\"1.Insert element to queue \\n\");\n printf(\"2.Delete element from queue \\n\");\n printf(\"3.Display all elements of queue \\n\");\n printf(\"4.Quit \\n\");\n printf(\"Please make a selection:\");\n scanf(\"%d\", &choice);\n switch(choice){\n case 1:\n enqueue();\n break;\n case 2:\n dequeue();\n break;\n case 3:\n printQueue();\n break;\n case 4:\n exit(0);\n default:\n printf(\"Please enter a valid input\\n\");\n }\n }\n\n}\n\n/* Creates a Node object with a value provided from user input */\nstruct Node* createNode(){\n int userValue;\n printf(\"Enter the value you want to add: \");\n scanf(\"%d\", &userValue);\n\n struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));\n newNode->value = userValue;\n newNode->next = NULL;\n\n return newNode;\n}\n\n\n/* Add a Node to the end of the queue */\nvoid enqueue(){\n\n if(head == NULL && tail == NULL){\n head = tail = createNode();\n }else{\n tail-> next = createNode();\n tail = tail->next;\n }\n}\n\n/* Remove front Node from queue */\nvoid dequeue(){\n struct Node* current = head;\n if(current == NULL){\n printf(\"The queue is empty\\n\");\n }\n if(current == tail){\n head = NULL;\n tail = NULL;\n }\n else{\n head = head->next;\n }\n free(current);\n}\n\n/* Iterate through queue and print Node values*/\nvoid printQueue(){\n struct Node* temp = head;\n while(temp != NULL){\n printf(\"%d \", temp->value);\n temp = temp->next;\n }\n printf(\"\\n\");\n}"
}
{
"filename": "code/data_structures/src/queue/queue_using_linked_list/queue_using_linked_list.cpp",
"content": "#include <cassert>\n#include <iostream>\n// Part of Cosmos by OpenGenus Foundation\nusing namespace std;\n\ntemplate <class T>\nclass Queue\n{\nprivate:\n class Node\n {\n public:\n Node(const T &data, Node *next)\n : data(data), next(next)\n {\n }\n\n T data;\n Node *next;\n };\n\npublic:\n Queue(void);\n ~Queue();\n\n void enqueue(const T &data);\n T dequeue(void);\n\n bool empty(void) const;\n int size(void) const;\n T &front(void);\n\nprivate:\n Node *head;\n Node *back;\n int items;\n};\n\ntemplate <class T>\nQueue<T>::Queue(void) : head(NULL)\n{\n items = 0;\n}\n\ntemplate <class T>\nQueue<T>::~Queue()\n{\n while (head != NULL)\n {\n Node *tmpHead = head;\n head = head->next;\n delete tmpHead;\n }\n}\n\ntemplate <class T>\nvoid Queue<T>::enqueue(const T &data)\n{\n Node *newNode = new Node(data, NULL);\n if (empty())\n head = (back = newNode);\n else\n {\n back->next = newNode;\n back = back->next;\n }\n\n items++;\n}\n\ntemplate <class T>\nT Queue<T>::dequeue(void)\n{\n assert(!empty());\n Node *tmpHead = head;\n head = head->next;\n T data = tmpHead->data;\n items--;\n delete tmpHead;\n return data;\n}\n\ntemplate <class T>\nbool Queue<T>::empty(void) const\n{\n return head == NULL;\n}\n\ntemplate <class T>\nint Queue<T>::size(void) const\n{\n return items;\n}\n\ntemplate <class T>\nT &Queue<T>::front(void)\n{\n assert(!empty());\n return head->data;\n}\n\nint main()\n{\n Queue<int> queue;\n queue.enqueue(34);\n queue.enqueue(54);\n queue.enqueue(64);\n queue.enqueue(74);\n queue.enqueue(84);\n\n cout << queue.front() << endl;\n cout << queue.size() << endl;\n\n while (!queue.empty())\n cout << queue.dequeue() << endl;\n\n cout << queue.size() << endl;\n\n cout << endl;\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/queue/queue_using_linked_list/queue_using_linked_list.java",
"content": "class Node<T> //NODE CLASS\n{\n T data;\n Node<T> next;\n}\n// Part of Cosmos by OpenGenus Foundation\n//Queue Using linked list\npublic class QueueUsingLL<T> {\n\n\tprivate Node<T> front; // Front Node\n\tprivate Node<T> rear; // Rear Node\n\tprivate int size;\n\n\n\tpublic int size(){\n\t\treturn size;\n\t}\n\n //Returns TRUE if queue is empty\n\tpublic boolean isEmpty(){\n\t\treturn size() == 0;\n\t}\n\n\tpublic T front() {\n\n\t\treturn front.data;\n\t}\n\n\tpublic void enqueue(T element){ //to add an element in queue\n\t\tNode<T> newNode = new Node<>();\n\t\tnewNode.data = element;\n\t\tif(rear == null){\n\t\t\tfront = newNode;\n\t\t\trear = newNode;\n\t\t}\n\t\telse{\n\t\t\trear.next = newNode;\n\t\t\trear = newNode;\n\t\t}\n\t\tsize++;\n\n\t}\n\n\tpublic T dequeue() { //to remove an element from queue\n\n\t\tT temp = front.data;\n\t\tif(front.next == null){\n\t\t\tfront = null;\n\t\t\trear = null;\n\t\t}\n\t\telse{\n\t\t\tfront = front.next;\n\t\t}\n\t\tsize--;\n\t\treturn temp;\n\n\t}\n\n\tpublic static class QueueUse\n {\n //Main Function to implement Queue\n public static void main(String[] args) {\n QueueUsingLL<Integer> queueUsingLL=new QueueUsingLL<>();\n for(int i=1;i<=10;i++) // Creates a dummy queue which contains integers from 1-10\n {\n queueUsingLL.enqueue(i);\n }\n\n System.out.println(\"QUEUE :\");\n\n while (!queueUsingLL.isEmpty())\n {\n System.out.println(queueUsingLL.dequeue());\n }\n }\n }\n\n\n}"
}
{
"filename": "code/data_structures/src/queue/queue_using_linked_list/queue_using_linked_list.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\nQueue = {\"front\": None, \"back\": None}\n\n\nclass Node:\n def __init__(self, data, next):\n self.data = data\n self.next = next\n\n\ndef Enqueue(Queue, element):\n N = Node(element, None)\n if Queue[\"back\"] == None:\n Queue[\"front\"] = N\n Queue[\"back\"] = N\n else:\n Queue[\"back\"].next = N\n Queue[\"back\"] = Queue[\"back\"].next\n\n\ndef Dequeue(Queue):\n if Queue[\"front\"] != None:\n first = Queue[\"front\"]\n Queue[\"front\"] = Queue[\"front\"].next\n return first.data\n else:\n if Queue[\"back\"] != None:\n Queue[\"back\"] = None\n return \"Cannot dequeue because queue is empty\"\n\n\nEnqueue(Queue, \"a\")\nEnqueue(Queue, \"b\")\nEnqueue(Queue, \"c\")\nprint(Dequeue(Queue))"
}
{
"filename": "code/data_structures/src/queue/queue_using_linked_list/queue_using_linked_list.rb",
"content": "# Part of Cosmos by OpenGenus Foundation\n\nclass LinkedQueue\n attr_reader :size\n\n def initialize\n @head = nil\n @tail = nil\n @size = 0\n end\n\n def enqueue(element)\n new_node = Node.new(element)\n @size += 1\n if @head\n @tail.next = new_node\n @tail = new_node\n else\n @head = new_node\n @tail = new_node\n end\n end\n\n def dequeue\n return nil unless @head\n removed = @head\n @head = @head.next\n @size -= 1\n removed.element\n end\n\n def empty?\n @size == 0\n end\n\n def front\n @head.element\n end\nend\n\nclass Node\n attr_accessor :element\n attr_accessor :next\n\n def initialize(element)\n @element = element\n @next = nil\n end\nend\n\nqueue = LinkedQueue.new\nputs queue.empty?\nqueue.enqueue(1)\nputs queue.front\nqueue.enqueue(3)\nqueue.enqueue(4)\nqueue.enqueue(2)\nqueue.enqueue(0)\nputs queue.dequeue\nputs queue.dequeue\nputs queue.front\nputs format('Size: %d', queue.size)"
}
{
"filename": "code/data_structures/src/queue/queue_using_linked_list/README.md",
"content": "# Cosmos\n\nCollaborative effort by [OpenGenus](https://github.com/OpenGenus/cosmos)"
}
{
"filename": "code/data_structures/src/queue/queue_using_stack/queue_using_stack.c",
"content": "#include<stdio.h>\n#include<stdlib.h>\n#include<assert.h>\n\ntypedef struct stack \n{\n int top;\n unsigned capacity;\n int* A;\n} stack;\n\ntypedef struct queue \n{\n struct stack* S1;\n struct stack* S2;\n} queue;\n\n/*STACK*/\nstack* \ncreateStack(unsigned capacity) \n{\n stack* newStack = (stack*)malloc(sizeof(stack));\n newStack->capacity = capacity;\n newStack->top = -1;\n newStack->A = (int*)malloc(capacity*sizeof(int));\n return (newStack);\n}\n\nint \nisFull(stack* S)\n{\n return (S->top == S->capacity - 1);\n}\n\nint \nisEmpty(stack* S)\n{\n return (S->top == -1);\n}\n\nvoid \npush(stack* S, int item)\n{\n assert(!isFull(S));\n S->A[++S->top] = item;\n}\n\nint \npop(stack* S)\n{\n assert(!isEmpty(S));\n return (S->A[S->top--]);\n}\n\nvoid \ndeleteStack(stack* S)\n{\n\tfree(S->A);\n\tfree(S);\n}\n\n/*QUEUE*/\nqueue* \ncreateQueue(stack* S1, stack* S2)\n{\n queue* newQueue = (queue*)malloc(2*sizeof(stack*));\n newQueue->S1 = S1;\n newQueue->S2 = S2;\n return (newQueue);\n}\n\nvoid \nenqueue(queue* Q, int item)\n{\n push(Q->S1, item);\n}\n\nint \ndequeue(queue* Q)\n{\n if (!isEmpty(Q->S2)) {\n return (pop(Q->S2));\n }\n while (!isEmpty(Q->S1)) {\n push(Q->S2, pop(Q->S1));\n }\n return (pop(Q->S2));\n}\n\nvoid \ndeleteQueue(queue* Q)\n{\n\tfree(Q->S1);\n\tfree(Q->S2);\n\tfree(Q);\n}\n\n/*driver program*/\nint \nmain()\n{\n stack* S1 = createStack(100);\n stack* S2 = createStack(100);\n queue* Q = createQueue(S1, S2);\n enqueue(Q, 10);\n enqueue(Q, 20);\n enqueue(Q, 30);\n enqueue(Q, 40);\n /*Current appearance of Q : | 10 | 20 | 30 | 40 | */\n printf(\"%d\\n\", dequeue(Q));\n printf(\"%d\\n\", dequeue(Q));\n printf(\"%d\\n\", dequeue(Q));\n /*Current appearance of Q : | 40 | 10, 20, 30 have been dequeued*/\n enqueue(Q, 5);\n enqueue(Q, 8);\n /*Current appearance of Q : | 40 | 5 | 8 | */\n printf(\"%d\\n\", dequeue(Q));\n printf(\"%d\\n\", dequeue(Q));\n /*Current appearance of Q : | 8 | */\n deleteQueue(Q);\n return 0;\n}"
}
{
"filename": "code/data_structures/src/queue/queue_using_stack/queue_using_stack.cpp",
"content": "#include <iostream>\n#include <stack>\nusing namespace std;\n// Part of Cosmos by OpenGenus Foundation\n// queue data structure using two stacks\nclass queue {\nprivate:\n stack<int> s1, s2;\npublic:\n void enqueue(int element);\n int dequeue();\n void displayQueue();\n};\n\n// enqueue an element to the queue\nvoid queue :: enqueue(int element)\n{\n s1.push(element);\n}\n\n// dequeue the front element\nint queue :: dequeue()\n{\n // transfer all elements of s1 into s2\n if (s1.empty() && s2.empty())\n return 0;\n\n while (!s1.empty())\n {\n s2.push(s1.top());\n s1.pop();\n }\n // pop and store the top element from s2\n\n int ret = s2.top();\n s2.pop();\n // transfer all elements of s2 back to s1\n while (!s2.empty())\n {\n s1.push(s2.top());\n s2.pop();\n }\n return ret;\n}\n\n//display the elements of the queue\nvoid queue :: displayQueue()\n{\n cout << \"\\nDisplaying the queue :-\\n\";\n while (!s1.empty())\n {\n s2.push(s1.top());\n s1.pop();\n }\n while (!s2.empty())\n {\n cout << s2.top() << \" \";\n s1.push(s2.top());\n s2.pop();\n }\n}\n\n// main\nint main()\n{\n queue q;\n int exit = 0;\n int enqueue;\n char input;\n while (!exit)\n {\n cout << \"Enter e to enqueue,d to dequeue,s to display queue,x to exit: \";\n cin >> input;\n if (input == 'e')\n {\n cout << \"Enter number to enqueue: \";\n cin >> enqueue;\n q.enqueue(enqueue);\n }\n\n if (input == 'd')\n q.dequeue();\n\n if (input == 's')\n {\n q.displayQueue();\n cout << endl;\n }\n if (input == 'x')\n break;\n }\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/queue/queue_using_stack/queue_using_stack.java",
"content": "import java.util.NoSuchElementException;\nimport java.util.Stack;\n\n/**\n * Implements https://docs.oracle.com/javase/8/docs/api/java/util/Queue.html\n * Big O time complexity: O(n)\n * Part of Cosmos by OpenGenus Foundation\n */\nclass Queue<T> {\n Stack<T> temp = new Stack<>();\n Stack<T> value = new Stack<>();\n\n public Queue<T> enqueue(T element) {\n add(element);\n return this;\n }\n\n public T dequeue() {\n return remove();\n }\n\n /**\n *\n * Inserts the specified element into this queue if it is possible to do so immediately without violating capacity\n * restrictions, returning true upon success and throwing an IllegalStateException if no space is currently available.\n * @param item the element to add\n * @return true upon success, throwing an IllegalStateException if no space is currently available.\n */\n public boolean add(T item) {\n if (value.isEmpty()) {\n value.push(item);\n } else {\n while (!value.isEmpty()) {\n temp.push(value.pop());\n }\n\n value.push(item);\n\n while (!temp.isEmpty()) {\n value.push(temp.pop());\n }\n }\n return true;\n }\n\n /**\n * Inserts the specified element into this queue if it is possible to do so immediately without violating capacity\n * restrictions.\n * @param element the element to add\n * @return true if the element was added to this queue, else false\n */\n public boolean offer(T element) {\n try {\n return add(element);\n } catch (Exception ex) {\n //failed to add, return false\n return false;\n }\n }\n\n /**\n * Retrieves and removes the head of this queue.\n * This method differs from poll only in that it throws an exception if this queue is empty.\n * @return the head of this queue.\n * @throws NoSuchElementException if this queue is empty\n */\n public T remove() throws NoSuchElementException{\n try {\n return value.pop();\n } catch (Exception ex) {\n throw new NoSuchElementException();\n }\n }\n\n /**\n * Retrieves and removes the head of this queue, or returns null if this queue is empty.\n * This method differs from peek only in that it throws an exception if this queue is empty.\n * @return the head of this queue, or returns null if this queue is empty.\n */\n public T poll() {\n try {\n return remove();\n } catch (Exception ex) {\n //failed to remove, return null\n return null;\n }\n }\n\n /**\n * Retrieves, but does not remove, the head of this queue.\n * @return the head of this queue\n * @throws NoSuchElementException if this queue is empty\n */\n public T element() throws NoSuchElementException{\n try {\n return value.peek();\n } catch (Exception ex) {\n throw new NoSuchElementException();\n }\n }\n\n /**\n * Retrieves, but does not remove, the head of this queue, or returns null if this queue is empty.\n * @return the head of this queue, or returns null if this queue is empty.\n */\n public T peek() {\n try {\n return value.peek();\n } catch (Exception ex) {\n //failed to remove, return null\n return null;\n }\n }\n\n /**\n * Methods standard queue inherits from interface java.util.Collection\n */\n\n public boolean isEmpty() {\n return value.isEmpty();\n }\n}\n\npublic class QueueStacks\n{\n /**\n * Main function to show the use of the queue\n */\n public static void main(String[] args) {\n Queue<Integer> queueStacks = new Queue<>();\n for(int i=1; i<=10; i++) // Creates a dummy queue which contains integers from 1-10\n {\n queueStacks.enqueue(i);\n }\n\n System.out.println(\"QUEUE :\");\n\n while (!queueStacks.isEmpty())\n {\n System.out.println(queueStacks.dequeue());\n }\n }\n}"
}
{
"filename": "code/data_structures/src/queue/queue_using_stack/queue_using_stack.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n\nclass Stack:\n def __init__(self):\n self.items = []\n\n def push(self, x):\n self.items.append(x)\n\n def pop(self):\n return self.items.pop()\n\n def size(self):\n return len(self.items)\n\n def is_empty(self):\n return self.size() == 0\n\n\nclass Queue:\n def __init__(self):\n self.s1 = Stack()\n self.s2 = Stack()\n\n def enqueue(self, p):\n self.s1.push(p)\n\n def dequeue(self):\n # pop all elements in s1 onto s2\n while not self.s1.is_empty():\n self.s2.push(self.s1.pop())\n\n # pop all elements of s1\n return_value = self.s2.pop()\n\n # re pop all elements of s2 onto s1\n while not self.s2.is_empty():\n self.s1.push(self.s2.pop())\n\n return return_value\n\n\nif __name__ == \"__main__\":\n from random import choice\n\n a = Queue()\n\n l = [choice(range(1, 500)) for i in range(1, 10)]\n print(l)\n\n for i in l:\n a.enqueue(i)\n\n for i in range(len(l)):\n print(\"Dequeued:\", a.dequeue())"
}
{
"filename": "code/data_structures/src/queue/queue_using_stack/queue_using_stack.sh",
"content": "#!/bin/bash\n\n#Function to start the Program\n\n\nfunction start(){\necho -e \"\\t\\t1.[E]nter in Queue\"\necho -e \"\\t\\t2. [D]elete from Queue\"\necho -e \"\\t\\t3. [T]erminate the Program\"\nread OP\n}\n\n\n\n# Function to Insert Values Into Queue\n\n\nfunction insert(){\nread -p \"How many Elements would you like to Enter \" NM\nfor ((a=0;$a<$NM;a++))\ndo\n\tread -p \"Enter The value : \" arr[$a]\ndone\necho -e \"New Queue is ${arr[@]}\\n\"\n}\n\n\n#Function to Delete Values From Queue\n#Here arr is one stack and arr2 is another stack\n\nfunction delete(){\nread -p \"How Many Elements to be Deleted : \" ED\nc=`echo \"${arr[@]}\"`\nint=`echo \"${#arr[@]}\"`\nint=$[$int -1]\nif [[ -z \"$c\" ]]\nthen\necho -e \"Queue is Empty\\n\"\nfi\nif [[ \"$ED\" -gt \"$int\" ]]\nthen\necho -e \"The Number of Elements to be Deleted is Greater than the Number of elements in Queue\\n\"\nelse\nif [[ -n \"$c\" ]]\nthen\ned=$[$int-$ED]\nq=0\nwhile [ $ed -ne 0 ]\ndo\narr2[q]=`echo \"${arr[$int]}\"`\nq=$[$q+1]\nint=$[$int-1]\ned=$[$ed-1]\ndone\nfor((i=0;$i<=$int;i++))\ndo\necho -e \"Delete Elements are ${arr[$i]}\\n\"\nunset arr[$i]\ndone\necho -e \"New Queue ${arr2[@]}\\n \"\nelse\necho -e \"Empty Queue\\n\"\nfi\nfi\n}\nwhile :\ndo\nstart\ncase $OP in\n\"E\"|\"e\")\n\tclear\n\tinsert\n;;\n\"D\"|\"d\") \n\tclear\n\tdelete\n;;\n\"T\"|\"t\")\n\tclear\n\tbreak\n;;\n*)\nclear\necho -e \"Please Choose Again\\nEnter The First Letter of each Choice that is either 'E','D' or 'T'\\n\"\n;;\nesac\t\ndone"
}
{
"filename": "code/data_structures/src/queue/README.md",
"content": "# Queue\n\nDescription\n---\nA queue or FIFO (first in, first out) is an abstract data type that serves as a collection of elements, with two principal operations: enqueue, the process of adding an element to the collection.(The element is added from the rear side) and dequeue, the process of removing the first element that was added. (The element is removed from the front side). It can be implemented by using both array and linked list.\n\nFunctions\n---\n- dequeue\n--Removes an item from the queue\n- enqueue()\n--Adds an item to the queue\n- front()\n--Returns the front element from the queue\n- size()\n--Returns the size of the queue\n\n## Time Complexity\n- Enqueue - O(1)\n- Dequeue - O(1)\n- size - O(1)\n- front - O(n)"
}
{
"filename": "code/data_structures/src/queue/reverse_queue/reverse_queue.cpp",
"content": "#include <iostream>\n#include <stack>\n#include <queue>\nusing namespace std;\n// Part of Cosmos by OpenGenus Foundation\nvoid print(queue<int> q)\n{\n\n while (!q.empty())\n {\n cout << q.front() << \" \";\n q.pop();\n }\n cout << endl;\n}\n\nint main()\n{\n queue<int> q;\n stack<int> s;\n\n //build the queue\n for (int i = 0; i <= 5; i++)\n q.push(i);\n\n //print the queue\n print(q);\n\n //empty the queue into stack\n while (!q.empty())\n {\n s.push(q.front());\n q.pop();\n }\n //empty stack into queue\n while (!s.empty())\n {\n q.push(s.top());\n s.pop();\n }\n\n //print the reversed queue\n print(q);\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/queue/reverse_queue/reverse_queue.go",
"content": "// Part of Cosmos by OpenGenus Foundation\npackage main\n\n/*\nExptected output:\nCurrent Queue is [], Is Queue empty ? true\nTry to push 30 into Queue\nTry to push 12 into Queue\nTry to push 34 into Queue\nTry to push 2 into Queue\nTry to push 17 into Queue\nTry to push 88 into Queue\nReverse Queue\n88 17 2 34 12 30\n\n\n*/\nimport \"fmt\"\n\ntype Queue []int\n\nfunc (q *Queue) Push(v int) {\n\t*q = append(*q, v)\n}\n\nfunc (q *Queue) Pop() {\n\tlength := len(*q)\n\tif length != 0 {\n\t\t(*q) = (*q)[1:]\n\t}\n}\n\nfunc (q *Queue) Top() int {\n\treturn (*q)[0]\n}\n\nfunc (q *Queue) Empty() bool {\n\tif len(*q) == 0 {\n\t\treturn true\n\t}\n\n\treturn false\n}\n\nfunc (q *Queue) Reverse() {\n\tfor i, j := 0, len(*q)-1; i < j; i, j = i+1, j-1 {\n\t\t(*q)[i], (*q)[j] = (*q)[j], (*q)[i]\n\t}\n}\n\nfunc main() {\n\tqueue := Queue{}\n\tfmt.Printf(\"Current Queue is %s, Is Queue empty ? %v \\n\", queue, queue.Empty())\n\tfmt.Printf(\"Try to push 30 into Queue\\n\")\n\tqueue.Push(30)\n\tfmt.Printf(\"Try to push 12 into Queue\\n\")\n\tqueue.Push(12)\n\tfmt.Printf(\"Try to push 34 into Queue\\n\")\n\tqueue.Push(34)\n\tfmt.Printf(\"Try to push 2 into Queue\\n\")\n\tqueue.Push(2)\n\tfmt.Printf(\"Try to push 17 into Queue\\n\")\n\tqueue.Push(17)\n\tfmt.Printf(\"Try to push 88 into Queue\\n\")\n\tqueue.Push(88)\n\n\tfmt.Println(\"Reverse Queue\")\n\tqueue.Reverse()\n\tfor !queue.Empty() {\n\t\tfmt.Printf(\"%d \", queue.Top())\n\t\tqueue.Pop()\n\t}\n\tfmt.Printf(\"\\n\")\n\n}"
}
{
"filename": "code/data_structures/src/queue/reverse_queue/reverse_queue.java",
"content": "import java.util.*;\n\npublic class reverse_queue {\n// Part of Cosmos by OpenGenus Foundation\n //method to print contents of queue\n public static void print(Queue<Integer> queue) {\n for(int x : queue) {\n System.out.print(x + \" \");\n }\n System.out.print(\"\\n\");\n }\n\npublic static void main (String[] args) {\n Queue<Integer> queue = new LinkedList<Integer>();\n Stack<Integer> stack = new Stack<Integer>();\n\n //build the queue with 1..5\n for(int i=0; i<=5; i++) {\n queue.add(i);\n }\n //print the queue\n print(queue);\n\n //move queue contents to stack\n while(!queue.isEmpty()) {\n stack.push(queue.poll());\n }\n\n //move stack contents back to queue\n while(!stack.isEmpty()) {\n queue.add(stack.pop());\n }\n //reprint reversed queue\n print(queue);\n }\n}"
}
{
"filename": "code/data_structures/src/queue/reverse_queue/reverse_queue.py",
"content": "# Part of Cosmos by OpenGenus Foundation.\n\n# Make a new Queue class with list.\nclass Queue:\n def __init__(self, size_max):\n self.__max = size_max\n self.__data = []\n\n # Adds an item to the end of the queue.\n def enqueue(self, x):\n if len(self.__data) == self.__max:\n print(\"Queue is full\")\n else:\n self.__data.append(x)\n\n # Removes the first item of the queue without returning it.\n def dequeue(self):\n if len(self.__data) == 0:\n print(\"Queue is empty\")\n else:\n self.__data.pop(0)\n\n # Returns the first item of the queue.\n def front(self):\n if len(self.__data) == 0:\n print(\"Queue is empty\")\n return False\n return self.__data[0]\n\n # Returns the size of the queue, not the maximum size.\n def size(self):\n return len(self.__data)\n\n # Returns the maximum size that the queue can hold\n def capacity(selfs):\n return len(self.__max)\n\n\n# Return all items from queue in list.\ndef get(queue):\n lst = []\n while queue.size() > 0:\n lst.append(queue.front())\n queue.dequeue()\n\n # I don't the items to be removed, so I add them again.\n # But if you want, you can remove/comment it out.\n for item in lst:\n queue.enqueue(item)\n\n return lst\n\n\n# Return reversed queue.\ndef reverse(queue):\n # Reverse the return value, which is in list type, from get() method\n temp = get(queue)[::-1]\n\n # Add all items from reversed list to rev\n rev = Queue(len(temp))\n for item in temp:\n rev.enqueue(item)\n return rev\n\n\n# Print formatted output.\ndef display(queue):\n # First, it will show you the original queue.\n print(\"Original queue: {}\".format(get(queue)))\n # Then, it will show the reversed one.\n print(\"Reversed queue: {}\\n\".format(get(reverse(queue))))\n\n\n# Add all item in list to queue.\ndef listToQueue(lst):\n queue = Queue(len(lst))\n for item in lst:\n queue.enqueue(item)\n return queue\n\n\n# Examples.\nint_arr = [1, 3, 2, 5, 4]\nstring_arr = [\"alpha\", \"beta\", \"gamma\", \"echo\", \"delta\"]\ndouble_arr = [1.1, 3.2, 1.2, 5.4, 4.3]\nmix_arr = [1, \"alpha\", 2.1, True, \"D\"]\n\n# Collect all example in one list.\nexamples = [int_arr, string_arr, double_arr, mix_arr]\n\n# First, each example will be transfered from list to queue,\n# then it will be printed as output.\nfor item in examples:\n display(listToQueue(item))"
}
{
"filename": "code/data_structures/src/queue/reverse_queue/reverse_queue.swift",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n// Queue implementation\npublic struct Queue<T> {\n \n private var elements: Array<T>;\n \n init() {\n self.elements = [];\n }\n \n init(arrayLiteral elements: T...) {\n self.elements = elements\n }\n \n public var front: T? {\n return elements.first\n }\n \n public var back: T? {\n return elements.last\n }\n \n public var size: Int {\n return elements.count\n }\n \n public var isEmpty: Bool {\n return elements.isEmpty\n }\n \n mutating public func enqueue(_ element: T) {\n elements.append(element)\n }\n \n mutating public func dequeue() -> T? {\n return self.isEmpty ? nil : elements.removeFirst()\n }\n}\n\n// Reverse method implementation\nextension Queue {\n \n public func printElements() {\n \n for x in elements {\n print(x, terminator: \" \")\n }\n print()\n }\n \n mutating public func reverse() {\n \n var items = Array<T>()\n \n while !self.isEmpty {\n items.append(self.dequeue()!)\n }\n \n while !items.isEmpty {\n self.enqueue(items.removeLast())\n }\n \n }\n\n}\n\nfunc test() {\n \n var queue = Queue<Int>(arrayLiteral: 1, 3, 2, 5)\n \n print(\"Original queue:\")\n queue.printElements()\n \n queue.reverse()\n \n print(\"Reversed queue:\")\n queue.printElements()\n}\n\ntest()"
}
{
"filename": "code/data_structures/src/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/data_structures/src/sparse_table/sparse_table.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <iostream>\n#include <cassert>\n#include <functional>\n#include <iomanip>\n#include <cmath>\n\ntemplate<typename T, std::size_t S>\nclass SparseTable\n{\npublic:\n /*!\n * \\brief Builds a sparse table from a set of static data. It is the user responsibility to delete any allocated memory\n * \\param data The static data\n * \\param f The function to be applied on the ranges\n * \\param defaultValue The default value for the function (e.g. INFINITY for Range Minimum Queries)\n */\n SparseTable(const T* const data, const std::function<T(T, T)>& f, T defaultValue) : f_{f}, data_{data}, defaultValue_{defaultValue} \n { \n initializeSparseTable();\n }\n\n /*!\n * \\returns The number of elements in the set of static data\n */\n constexpr std::size_t size() const { return S; }\n\n /*!\n * \\brief Queries the range [l, r] in 0(1) time\n * It can only be used if the function is idempotent(e.g. Range Minimum Queries)\n * \\param l The starting index of the range to be queried\n * \\param r The end index of the range to be queried\n * \\returns The result of the function f applied to the range [l, r]\n */\n const T queryRangeIdempotent(int l, int r) \n {\n assert(l >= 0 && r < S);\n\n if (l > r)\n return defaultValue_;\n \n std::size_t length = r - l + 1;\n return f_(sparseTable_[logs_[length]][l], sparseTable_[logs_[length]][r - (1 << logs_[length]) + 1]);\n }\n\n /*!\n * \\brief Queries the range [l, r] in O(log(n)) time\n * It can be used for any function(e.g. Range Sum Queries)\n * \\param l The starting index of the range to be queried\n * \\param r The end index of the range to be queried\n * \\returns The result of the function f applied to the range [l, r]\n */\n const T queryRange(int l, int r) \n {\n assert(l >= 0 && r < S);\n\n T ans = defaultValue_;\n while (l < r) \n {\n ans = f_(ans, sparseTable_[logs_[r - l + 1]][l]);\n l += (1 << logs_[r - l + 1]);\n }\n return ans;\n }\n\nprivate:\n T sparseTable_ [((int)std::log2(S)) + 1][S];\n const T* const data_;\n const std::function<T(T, T)> f_;\n T defaultValue_;\n int logs_ [S + 1];\n\n /*\n * Computes and stores log2(i) for all i from 1 to S\n */\n void precomputeLogs() \n {\n logs_[1] = 0;\n\n for (int i = 2; i <= S; i++) \n logs_[i] = logs_[i / 2] + 1;\n \n }\n\n /*\n * Creates a sparse table from a set of static data\n * This precomputation takes O(nlog(n)) time\n */\n void initializeSparseTable() \n {\n\n precomputeLogs();\n\n for (int i = 0; i <= logs_[S] + 1; i++) \n {\n for (int j = 0; j + (1 << i) <= S; j++) \n {\n if (i == 0)\n sparseTable_[i][j] = data_[j];\n else\n sparseTable_[i][j] = f_(sparseTable_[i-1][j], sparseTable_[i-1][j + (1 << (i - 1))]);\n }\n }\n }\n};\n\n\nint main() \n{\n // Sparse table for range sum queries with integers\n {\n int data [] = { 4, 4, 6, 7, 8, 10, 22, 33, 5, 7 };\n std::function<int(int, int)> f = [](int a, int b) { return a + b; };\n SparseTable<int, 10> st(data, f, 0);\n\n std::cout << st.queryRange(3, 6) << std::endl;\n std::cout << st.queryRange(6, 9) << std::endl;\n std::cout << st.queryRange(0, 9) << std::endl;\n }\n\n // Sparse table for range minimum queries with doubles\n {\n\n double data [] = { 3.4, 5.6, 2.3, 9.4, 4.2 };\n std::function<double(double, double)> f = [](double a, double b) { return std::min(a, b); };\n SparseTable<double, 5> st(data, f, 10e8);\n\n std::setprecision(4);\n\n std::cout << st.queryRangeIdempotent(0, 3) << std::endl;\n std::cout << st.queryRangeIdempotent(3, 4) << std::endl;\n std::cout << st.queryRangeIdempotent(0, 4) << std::endl;\n\n }\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/stack/abstract_stack/cpp/array_stack/array_stack.cpp",
"content": "\n#include <iostream>\n\n#include \"../istack.h\"\n#include \"arraystack.h\"\n\nint main()\n{\n int s;\n IStack<int> *stack = new ArrayStack<int>();\n\n try {\n stack->peek();\n } catch (char const *e)\n {\n std::cout << e << std::endl << std::endl;\n }\n\n stack->push(20);\n std::cout << \"Added 20\" << std::endl;\n\n IStack<int> *stack2 = stack;\n std::cout << \"1 \" << std::endl;\n std::cout << stack->toString() << std::endl;\n std::cout << \"2 \" << std::endl;\n std::cout << stack2->toString() << std::endl;\n\n stack->push(30);\n std::cout << \"Added 30\" << std::endl;\n\n std::cout << std::endl << std::endl << stack->toString() << std::endl << std::endl;\n\n s = stack->peek();\n std::cout << \"First element is now: \" << s << std::endl;\n std::cout << \"removed: \" << stack->pop() << std::endl;\n\n s = stack->peek();\n std::cout << \"First element is now: \" << s << std::endl;\n\n delete stack;\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/stack/abstract_stack/cpp/array_stack/array_stack.h",
"content": "#ifndef ARRAYSTACH_H\n#define ARRAYSTACK_H\n\n#include <string>\n#include <sstream>\n\n#include \"../istack.h\"\n\ntemplate<typename T>\nclass ArrayStack : public IStack<T> {\n private:\n int capacity;\n int top;\n T **elements;\n void expandArray();\n public:\n ArrayStack(int capacity = 10);\n ArrayStack(const ArrayStack& stack);\n ~ArrayStack();\n ArrayStack& operator=(const ArrayStack& right);\n void push(const T& element);\n T pop();\n T peek() const;\n bool isEmpty() const;\n std::string toString() const;\n};\n\ntemplate<typename T>\nvoid ArrayStack<T>::expandArray() {\n if (this->capacity <= this->top) {\n T ** temp = this->elements;\n this->capacity = this->capacity * 2;\n this->elements = new T*[this->capacity];\n for (int i = 0; i < this->top; i++) {\n this->elements[i] = temp[i];\n }\n for (int i = this->top; i < this->capacity; i++) {\n this->elements[i] = nullptr;\n }\n delete [] temp;\n temp = nullptr;\n }\n}\n\ntemplate<typename T>\nArrayStack<T>::ArrayStack(int capacity) {\n this->capacity = capacity;\n this->top = 0;\n this->elements = new T*[this->capacity];\n for (int i = 0; i < this->capacity; i++) {\n this->elements[i] = nullptr;\n }\n}\n\ntemplate<typename T>\nArrayStack<T>::ArrayStack(const ArrayStack& stack) {\n this->top = stack.top;\n this->capacity = stack.capacity;\n\n if (stack.elements != nullptr) {\n this->elements = new T*[this->capacity];\n for (int i = 0; i < this->top; i++) {\n this->elements[i] = new T(*stack.elements[i]);\n }\n for (int i = this->top; i < this->capacity; i++) {\n this->elements[i] = nullptr;\n }\n }\n}\n\ntemplate<typename T>\nArrayStack<T>::~ArrayStack() {\n for (int i = 0; i < this->top; i++) {\n delete this->elements[i];\n }\n delete [] this->elements;\n}\n\ntemplate<typename T>\nArrayStack<T>& ArrayStack<T>::operator=(const ArrayStack& right) {\n if (this == &right) {\n throw \"Self-assigning at operator= \";\n }\n this->top = right.top;\n this->capacity = right.capacity;\n for (int i = 0; i < this->top; i++) {\n delete this->elements[i];\n }\n delete [] this->elements;\n if (right.elements != nullptr) {\n this->elements = new T*[this->capacity];\n for (int i = 0; i < this->top; i++) {\n this->elements[i] = new T(*right.elements[i]);\n }\n for (int i = this->top; i < this->capacity; i++) {\n this->elements[i] = nullptr;\n }\n }\n\n return *this;\n}\n\ntemplate<typename T>\nvoid ArrayStack<T>::push(const T& element) {\n this->expandArray();\n this->elements[this->top] = new T(element);\n this->top++;\n}\n\ntemplate<typename T>\nT ArrayStack<T>::pop() {\n if (this->isEmpty()) {\n throw \"The stack is empty.\"; \n } \n T temp = *this->elements[this->top - 1];\n delete this->elements[this->top - 1];\n this->elements[this->top - 1] = nullptr;\n this->top--;\n return temp;\n \n}\n\ntemplate<typename T>\nT ArrayStack<T>::peek() const {\n if (this->isEmpty()) {\n throw \"The stack is empty.\"; \n }\n return *this->elements[this->top - 1];\n}\n\ntemplate<typename T>\nbool ArrayStack<T>::isEmpty() const {\n return this->elements[0] == nullptr;\n}\n\ntemplate<typename T>\nstd::string ArrayStack<T>::toString() const {\n std::stringstream stream;\n if (!this->isEmpty()) {\n stream << \"The stack looks like; \" << std::endl;\n for (int i = 0; i < this->top; i++) {\n stream << i << \" : \" << '[' << *this->elements[i] << ']' << std::endl;\n }\n } else {\n stream << \"The stack is empty\" << std::endl;\n }\n return stream.str();\n}\n#endif"
}
{
"filename": "code/data_structures/src/stack/abstract_stack/cpp/array_stack.java",
"content": "import java.util.*;\nimport java.lang.*;\nimport java.io.*;\n\n/* Name of the class has to be \"Main\" only if the class is public. */\n class Stack<E> {\n\tprivate E[] arr = null;\n\tprivate int CAP;\n\tprivate int top = -1;\n\tprivate int size = 0;\n \n\t@SuppressWarnings(\"unchecked\")\n\tpublic Stack(int cap) {\n\t\tthis.CAP = cap;\n\t\tthis.arr = (E[]) new Object[cap];\n\t}\n \n\tpublic E pop() {\n\t\tif(this.size == 0)\n\t\t\treturn null;\n\t\t\n\t\tthis.size--;\n\t\tE result = this.arr[top];\n\t\t//prevent memory leaking\n\t\tthis.arr[top] = null;\n\t\tthis.top--; \n\t\treturn result;\n\t}\n \n\tpublic boolean push(E e) {\n\t\tif (!isFull())\n\t\t\treturn false; \n\t\tthis.size++;\n\t\tthis.arr[++top] = e;\n\t\treturn false;\n\t}\n \n\tpublic boolean isFull() {\n\t\tif (this.size == this.CAP)\n\t\t\treturn false;\n\t\treturn true;\n\t}\n \n\tpublic String toString() {\n\t\tif(this.size == 0)\n\t\t\treturn null;\n\t\t\n\t\tStringBuilder sb = new StringBuilder();\n\t\tfor(int i = 0; i < this.size; i++)\n\t\t\tsb.append(this.arr[i] + \", \");\n\t\t\n\t\tsb.setLength(sb.length()-2);\n\t\treturn sb.toString();\t\n\t}\n }\n\n class Stack1 {\n\t public static void main(String[] args) { \n\t\t Stack<String> stack = new Stack<String>(11);\n\t\t stack.push(\"hello\");\n\t\t stack.push(\"world\");\n \t\t System.out.println(stack);\n \t\t stack.pop();\n\t\t System.out.println(stack);\n \t\t stack.pop();\n\t\t System.out.println(stack);\n\t}\n}"
}
{
"filename": "code/data_structures/src/stack/abstract_stack/cpp/is_stack.h",
"content": "#ifndef ISTACK_H\n#define ISTACK_H\n\n#include <string>\n#include <sstream>\n\ntemplate <typename T>\nclass IStack {\n public:\n virtual ~IStack() {};\n /**\n * Add an element to stack\n */\n virtual void push(const T& element) = 0;\n /**\n * Remove an element from stack\n */ \n virtual T pop() = 0;\n /**\n * Retuns the fist element in the stack\n */\n virtual T peek() const = 0;\n /**\n * Returns if the stack is empty\n */ \n virtual bool isEmpty() const = 0;\n /**\n * Gets a string-representation of the stack\n */\n virtual std::string toString() const = 0;\n};\n \n#endif"
}
{
"filename": "code/data_structures/src/stack/abstract_stack/is_stack.h",
"content": "#ifndef ISTACK_H\n#define ISTACK_H\n\n#include <string>\n#include <sstream>\n\ntemplate <typename T>\nclass IStack {\n public:\n virtual ~IStack() {};\n /**\n * Add an element to stack\n */\n virtual void push(const T& element) = 0;\n /**\n * Remove an element from stack\n */ \n virtual T pop() = 0;\n /**\n * Retuns the fist element in the stack\n */\n virtual T peek() const = 0;\n /**\n * Returns if the stack is empty\n */ \n virtual bool isEmpty() const = 0;\n /**\n * Gets a string-representation of the stack\n */\n virtual std::string toString() const = 0;\n};\n \n#endif"
}
{
"filename": "code/data_structures/src/stack/abstract_stack/README.md",
"content": "# Stack\nDescription\n---\nStacks are an abstract data type (ADT) that function to store and manipulate data. Their order is last-in-first-out (LIFO). To implement a stack, an array or [linked list](../linked_list) can be used. A stack is similar to a [Tower of Hanoi](https://en.wikipedia.org/wiki/Tower_of_Hanoi) in that the first object pushed onto the stack cannot be removed until every other object pushed on top of it has also been removed. It is also similar to a stack of plates in a cafeteria, where the bottom plate cannot be removed until all of the plates above it have been removed as well.\n\nFunctions\n---\n- Pop()\n --Removes an item from the stack\n- Push()\n--Adds an item to the stack\n- Peek()\n--Returns the top element of the stack\n- isEmpty()\n--Returns true if the stack is empty\n\nTime Complexity\n---\nAll the functions are O(1).\n\n"
}
{
"filename": "code/data_structures/src/stack/balanced_expression/balanced_expression.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n#include <string.h>\n\ntypedef struct stack {\n\tchar data;\n\tstruct stack* next;\n} stack;\n\nvoid\npush(stack** top, char data) {\n stack* new = (stack*) malloc(sizeof(stack));\n new->data = data;\n new->next = *top;\n *top = new;\n}\n\nchar\npeek(stack* top) {\n return ( top -> data );\n}\n\nint\nempty(stack* top) {\n return (top == NULL);\n}\n\nvoid\npop(stack** top) {\n stack* temp = *top;\n *top = (*top)->next;\n free(temp);\n temp = NULL; \n}\n\nint\ncheckBalanced(char *s) {\n\tint len = strlen(s);\n\tif (len % 2 != 0) return 0;\n\tstack *head = NULL;\n\tfor (int i = 0;i < len ;i++) {\n\t\tif (s[i] == '{' || s[i] == '[' || s[i] == '(') {\n\t\t\tpush(&head, s[i]);\n\t\t}\n\t\telse {\n\t\t\tchar temp = peek(head);\n\t\t\tif (s[i] == '}' && temp == '{') pop(&head);\n\t\t\telse if (s[i] == ']' && temp == '[') pop(&head);\n\t\t\telse if (s[i] == ')' && temp == '(') pop(&head);\n\t\t\telse return 0;\n\t\t}\n\t}\n\treturn (empty(head));\n}\n\nint\nmain()\n{\n\tchar *s = (char*) malloc(sizeof(char) * 100);\n\tprintf(\"Enter the expression\\n\");\n\tscanf(\"%[^\\n]s\" , s);\n\tint res = checkBalanced(s);\n\tif (res) printf(\"Expression is valid\\n\");\n\telse printf(\"Expression is not valid\\n\");\n\treturn 0;\n}"
}
{
"filename": "code/data_structures/src/stack/balanced_expression/balanced_expression.cpp",
"content": "// Stack | Balance paraenthesis | C++\n// Part of Cosmos by OpenGenus Foundation\n\n#include <iostream>\n#include <stack>\n\nbool checkBalanced(string s)\n{\n // if not in pairs, then not balanced\n if (s.length() % 2 != 0)\n return false;\n std::stack <char> st;\n for (const char: s)\n {\n //adding opening brackets to stack\n if (s[i] == '{' || s[i] == '[' || s[i] == '(')\n st.push(s[i]); //if opening brackets encounter, push into stack\n else\n {\n // checking for each closing bracket, if there is an opening bracket in stack\n char temp = st.top();\n if (s[i] == '}' && temp == '{')\n st.pop();\n else if (s[i] == ']' && temp == '[')\n st.pop();\n else if (s[i] == ')' && temp == '(')\n st.pop();\n else\n return false;\n }\n }\n return st.empty();\n}\n\nint main()\n{\n std::string s;\n std::cin >> s;\n bool res = checkBalanced(s);\n if (res)\n std::cout << \"Expression is balanced\";\n else\n std::cout << \"Expression is not balanced\";\n return 0;\n}"
}
{
"filename": "code/data_structures/src/stack/balanced_expression/balanced_expression.java",
"content": "import java.util.*;\n\nclass BalancedExpression {\n\tpublic static void main(String args[]) {\n\t\tSystem.out.println(\"Balanced Expression\");\n\t\tScanner in = new Scanner(System.in);\n\t\tString input = in.next();\n\t\tif (isExpressionBalanced(input)) {\n\t\t\tSystem.out.println(\"The expression is balanced\");\n\t\t}\n\t\telse {\n\t\t\tSystem.out.println(\"The expression is not balanced\");\n\t\t}\n\n\t\tstatic boolean isExpressionBalanced(String input) {\n\t\t\tStack stack = new Stack();\n\t\t\tfor (int i=0; i<input.length(); i++) {\n\t\t\t\tif (input.charAt(i) == '(' || input.charAt(i) == '{'|| input.charAt(i) == '[') {\n\t\t\t\t\tstack.push(input.charAt(i));\n\t\t\t\t}\n\t\t\t\tif (input.charAt(i) == ')' || input.charAt(i) == '}'|| input.charAt(i) == ']') {\n\t\t\t\t\tif (stack.empty()) {\n\t\t\t\t\t\treturn false;\n\t\t\t\t\t}\n\t\t\t\t\tchar top_char = (char) stack.pop();\n\t\t\t\t\t\n\t\t\t\t\tif ( (top_char == '(' && input.charAt(i) != ')') || (top_char == '{' && input.charAt(i) != '}') || (top_char == '[' && input.charAt(i) != ']') ) {\n\t\t\t\t\t\treturn false;\n\t\t\t\t\t}\t \n\t\t\t\t}\n\t\t\t}\n\t\t\treturn stack.empty();\n\t\t}\n\t}\n}"
}
{
"filename": "code/data_structures/src/stack/balanced_expression/balanced_expression.py",
"content": "OPENING = '('\n\ndef is_balanced(parentheses):\n stack = []\n for paren in parentheses:\n if paren == OPENING:\n stack.append(paren)\n else:\n try:\n stack.pop()\n except IndexError: # too many closing parens\n return False\n return len(stack) == 0 # false if too many opening parens\n\n\ns = '((()))'\nif is_balanced(s):\n print(\"string is balanced\")\nelse:\n print(\"string is unbalanced\")"
}
{
"filename": "code/data_structures/src/stack/infix_to_postfix/infix_to_postfix2.cpp",
"content": "#include <iostream>\n#include <stack>\n\nclass InfixToPostfix\n{\npublic:\n InfixToPostfix(const std::string &expression) : expression_(expression) {}\n\n int getPrecedenceOfOperators(char);\n\n std::string convertInfixToPostfix();\n\nprivate:\n std::string expression_;\n\n};\n\nint InfixToPostfix::getPrecedenceOfOperators(char ch)\n{\n if (ch == '+' || ch == '-')\n return 1;\n if (ch == '*' || ch == '/')\n return 2;\n if (ch == '^')\n return 3;\n else\n return 0;\n}\n\nstd::string InfixToPostfix::convertInfixToPostfix()\n{\n std::stack <char> stack1;\n std::string infixToPostfixExp = \"\";\n int i = 0;\n while (expression_[i] != '\\0')\n {\n //if scanned character is open bracket push it on stack\n if (expression_[i] == '(' || expression_[i] == '[' || expression_[i] == '{')\n stack1.push(expression_[i]);\n\n //if scanned character is opened bracket pop all literals from stack till matching open bracket gets poped\n else if (expression_[i] == ')' || expression_[i] == ']' || expression_[i] == '}')\n {\n if (expression_[i] == ')')\n {\n while (stack1.top() != '(')\n {\n infixToPostfixExp = infixToPostfixExp + stack1.top();\n stack1.pop();\n }\n }\n if (expression_[i] == ']')\n {\n while (stack1.top() != '[')\n {\n infixToPostfixExp = infixToPostfixExp + stack1.top();\n stack1.pop();\n }\n }\n if (expression_[i] == '}')\n {\n while (stack1.top() != '{')\n {\n infixToPostfixExp = infixToPostfixExp + stack1.top();\n stack1.pop();\n }\n }\n stack1.pop();\n }\n //if scanned character is operator\n else if (expression_[i] == '+' || expression_[i] == '-' || expression_[i] == '*' || expression_[i] == '/' || expression_[i] == '^')\n {\n //very first operator of expression is to be pushed on stack\n if (stack1.empty()) {\n stack1.push(expression_[i]);\n }\n else{\n /*\n * check the precedence order of instack(means the one on top of stack) and incoming operator,\n * if instack operator has higher priority than incoming operator pop it out of stack&put it in\n * final postifix expression, on other side if precedence order of instack operator is less than i\n * coming operator, push incoming operator on stack.\n */\n if (getPrecedenceOfOperators(stack1.top()) >= getPrecedenceOfOperators(expression_[i]))\n {\n infixToPostfixExp = infixToPostfixExp + stack1.top();\n stack1.pop();\n stack1.push(expression_[i]);\n }\n else\n {\n stack1.push(expression_[i]);\n }\n }\n }\n else\n {\n //if literal is operand, put it on to final postfix expression\n infixToPostfixExp = infixToPostfixExp + expression_[i];\n }\n i++;\n }\n\n //poping out all remainig operator literals & adding to final postfix expression\n if (!stack1.empty())\n {\n while (!stack1.empty())\n {\n infixToPostfixExp = infixToPostfixExp + stack1.top();\n stack1.pop();\n }\n }\n\n return infixToPostfixExp;\n\n}\n\nint main()\n{\n std::string expr;\n std::cout << \"\\nEnter the Infix Expression : \";\n std::cin >> expr;\n InfixToPostfix p(expr);\n std::cout << \"\\nPostfix expression : \" << p.convertInfixToPostfix();\n}"
}
{
"filename": "code/data_structures/src/stack/infix_to_postfix/infix_to_postfix.c",
"content": "#include <stdio.h>\n#include <string.h>\n#include <stdlib.h>\n// Part of Cosmos by OpenGenus Foundation\n// Stack type\nstruct Stack\n{\n int top;\n unsigned capacity;\n int* array;\n};\n \n// Stack Operations\nstruct Stack* createStack( unsigned capacity )\n{\n struct Stack* stack = (struct Stack*) malloc(sizeof(struct Stack));\n \n if (!stack) \n return NULL;\n \n stack->top = -1;\n stack->capacity = capacity;\n \n stack->array = (int*) malloc(stack->capacity * sizeof(int));\n \n if (!stack->array)\n return NULL;\n return stack;\n}\nint isEmpty(struct Stack* stack)\n{\n return stack->top == -1 ;\n}\nchar peek(struct Stack* stack)\n{\n return stack->array[stack->top];\n}\nchar pop(struct Stack* stack)\n{\n if (!isEmpty(stack))\n return stack->array[stack->top--] ;\n return '$';\n}\nvoid push(struct Stack* stack, char op)\n{\n stack->array[++stack->top] = op;\n}\n \n \n// A utility function to check if the given character is operand\nint isOperand(char ch)\n{\n return (ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z');\n}\n \n// A utility function to return precedence of a given operator\n// Higher returned value means higher precedence\nint Prec(char ch)\n{\n switch (ch)\n {\n case '+':\n case '-':\n return 1;\n \n case '*':\n case '/':\n return 2;\n \n case '^':\n return 3;\n }\n return -1;\n}\n \n \n// The main function that converts given infix expression\n// to postfix expression. \nint infixToPostfix(char* exp)\n{\n int i, k;\n \n // Create a stack of capacity equal to expression size \n struct Stack* stack = createStack(strlen(exp));\n if(!stack) // See if stack was created successfully \n return -1 ;\n \n for (i = 0, k = -1; exp[i]; ++i)\n {\n // If the scanned character is an operand, add it to output.\n if (isOperand(exp[i]))\n exp[++k] = exp[i];\n \n // If the scanned character is an ‘(‘, push it to the stack.\n else if (exp[i] == '(')\n push(stack, exp[i]);\n \n // If the scanned character is an ‘)’, pop and output from the stack \n // until an ‘(‘ is encountered.\n else if (exp[i] == ')')\n {\n while (!isEmpty(stack) && peek(stack) != '(')\n exp[++k] = pop(stack);\n if (!isEmpty(stack) && peek(stack) != '(')\n return -1; // invalid expression \n else\n pop(stack);\n }\n else // an operator is encountered\n {\n while (!isEmpty(stack) && Prec(exp[i]) <= Prec(peek(stack)))\n exp[++k] = pop(stack);\n push(stack, exp[i]);\n }\n \n }\n \n // pop all the operators from the stack\n while (!isEmpty(stack))\n exp[++k] = pop(stack );\n \n exp[++k] = '\\0';\n printf( \"%sn\", exp );\n \n return 0;\n}\n \n// Driver program to test above functions\nint main()\n{\n char exp[] = \"a+b*(c^d-e)^(f+g*h)-i\";\n infixToPostfix(exp);\n return 0;\n}"
}
{
"filename": "code/data_structures/src/stack/infix_to_postfix/infix_to_postfix.cpp",
"content": "#include <stdio.h>\n#include <string.h>\n#include <stdlib.h>\n// Part of Cosmos by OpenGenus Foundation\n// Stack type\nstruct Stack\n{\n int top;\n unsigned capacity;\n int* array;\n};\n\n// Stack Operations\nstruct Stack* createStack( unsigned capacity )\n{\n struct Stack* stack = (struct Stack*) malloc(sizeof(struct Stack));\n\n if (!stack)\n return NULL;\n\n stack->top = -1;\n stack->capacity = capacity;\n\n stack->array = (int*) malloc(stack->capacity * sizeof(int));\n\n if (!stack->array)\n return NULL;\n return stack;\n}\nint isEmpty(struct Stack* stack)\n{\n return stack->top == -1;\n}\nchar peek(struct Stack* stack)\n{\n return stack->array[stack->top];\n}\nchar pop(struct Stack* stack)\n{\n if (!isEmpty(stack))\n return stack->array[stack->top--];\n return '$';\n}\nvoid push(struct Stack* stack, char op)\n{\n stack->array[++stack->top] = op;\n}\n\n\n// A utility function to check if the given character is operand\nint isOperand(char ch)\n{\n return (ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z');\n}\n\n// A utility function to return precedence of a given operator\n// Higher returned value means higher precedence\nint Prec(char ch)\n{\n switch (ch)\n {\n case '+':\n case '-':\n return 1;\n\n case '*':\n case '/':\n return 2;\n\n case '^':\n return 3;\n }\n return -1;\n}\n\n\n// The main function that converts given infix expression\n// to postfix expression.\nint infixToPostfix(char* exp)\n{\n int i, k;\n\n // Create a stack of capacity equal to expression size\n struct Stack* stack = createStack(strlen(exp));\n if (!stack) // See if stack was created successfully\n return -1;\n\n for (i = 0, k = -1; exp[i]; ++i)\n {\n // If the scanned character is an operand, add it to output.\n if (isOperand(exp[i]))\n exp[++k] = exp[i];\n\n // If the scanned character is an ‘(‘, push it to the stack.\n else if (exp[i] == '(')\n push(stack, exp[i]);\n\n // If the scanned character is an ‘)’, pop and output from the stack\n // until an ‘(‘ is encountered.\n else if (exp[i] == ')')\n {\n while (!isEmpty(stack) && peek(stack) != '(')\n exp[++k] = pop(stack);\n if (!isEmpty(stack) && peek(stack) != '(')\n return -1; // invalid expression\n else\n pop(stack);\n }\n else // an operator is encountered\n {\n while (!isEmpty(stack) && Prec(exp[i]) <= Prec(peek(stack)))\n exp[++k] = pop(stack);\n push(stack, exp[i]);\n }\n\n }\n\n // pop all the operators from the stack\n while (!isEmpty(stack))\n exp[++k] = pop(stack );\n\n exp[++k] = '\\0';\n printf( \"%sn\", exp );\n\n return 0;\n}\n\n// Driver program to test above functions\nint main()\n{\n char exp[] = \"a+b*(c^d-e)^(f+g*h)-i\";\n infixToPostfix(exp);\n return 0;\n}"
}
{
"filename": "code/data_structures/src/stack/infix_to_postfix/infix_to_postfix.java",
"content": "import java.util.Scanner;\nimport java.util.Stack;\n \npublic class InfixToPostfix {\n \n /**\n * Checks if the input is operator or not\n * @param c input to be checked\n * @return true if operator\n */\n private boolean isOperator(char c){\n if(c == '+' || c == '-' || c == '*' || c =='/' || c == '^')\n return true;\n return false;\n }\n \n /**\n * Checks if c2 has same or higher precedence than c1\n * @param c1 first operator\n * @param c2 second operator\n * @return true if c2 has same or higher precedence\n */\n private boolean checkPrecedence(char c1, char c2){\n if((c2 == '+' || c2 == '-') && (c1 == '+' || c1 == '-'))\n return true;\n else if((c2 == '*' || c2 == '/') && (c1 == '+' || c1 == '-' || c1 == '*' || c1 == '/'))\n return true;\n else if((c2 == '^') && (c1 == '+' || c1 == '-' || c1 == '*' || c1 == '/'))\n return true;\n else\n return false;\n }\n \n /**\n * Converts infix expression to postfix\n * @param infix infix expression to be converted\n * @return postfix expression\n */\n public String convert(String infix){\n System.out.printf(\"%-8s%-10s%-15s\\n\", \"Input\",\"Stack\",\"Postfix\");\n String postfix = \"\"; //equivalent postfix is empty initially\n Stack<Character> s = new Stack<>(); //stack to hold symbols\n s.push('#'); //symbol to denote end of stack\n \n System.out.printf(\"%-8s%-10s%-15s\\n\", \"\",format(s.toString()),postfix); \n \n for(int i = 0; i < infix.length(); i++){\n char inputSymbol = infix.charAt(i); //symbol to be processed\n if(isOperator(inputSymbol)){ //if a operator\n //repeatedly pops if stack top has same or higher precedence\n while(checkPrecedence(inputSymbol, s.peek()))\n postfix += s.pop();\n s.push(inputSymbol);\n }\n else if(inputSymbol == '(')\n s.push(inputSymbol); //push if left parenthesis\n else if(inputSymbol == ')'){\n //repeatedly pops if right parenthesis until left parenthesis is found\n while(s.peek() != '(') \n postfix += s.pop();\n s.pop();\n }\n else\n postfix += inputSymbol;\n System.out.printf(\"%-8s%-10s%-15s\\n\", \"\"+inputSymbol,format(s.toString()),postfix); \n }\n \n //pops all elements of stack left\n while(s.peek() != '#'){\n postfix += s.pop();\n System.out.printf(\"%-8s%-10s%-15s\\n\", \"\",format(s.toString()),postfix); \n \n }\n \n return postfix;\n }\n \n /**\n * Formats the input stack string\n * @param s It is a stack converted to string\n * @return formatted input\n */\n private String format(String s){\n s = s.replaceAll(\",\",\"\"); //removes all , in stack string\n s = s.replaceAll(\" \",\"\"); //removes all spaces in stack string\n s = s.substring(1, s.length()-1); //removes [] from stack string\n \n return s;\n }\n \n public static void main(String[] args) {\n InfixToPostfix obj = new InfixToPostfix();\n Scanner sc = new Scanner(System.in);\n System.out.print(\"Infix : \\t\");\n String infix = sc.next();\n System.out.print(\"Postfix : \\t\"+obj.convert(infix));\n }\n}"
}
{
"filename": "code/data_structures/src/stack/infix_to_postfix/infix_to_postfix.py",
"content": "# Part of Cosmos by OpenGenus Foundation\nclass Stack:\n def __init__(self):\n self.items = []\n\n def is_empty(self):\n return self.items == []\n\n def push(self, item):\n self.items.append(item)\n\n def pop(self):\n return self.items.pop()\n\n def peek(self):\n return self.items[len(self.items) - 1]\n\n def size(self):\n return len(self.items)\n\n\ndef infix_to_postfix(exp):\n prec = {}\n prec[\"*\"] = 3\n prec[\"/\"] = 3\n prec[\"+\"] = 2\n prec[\"-\"] = 2\n prec[\"(\"] = 1\n\n s = Stack()\n postfix_list = []\n token_list = exp.split()\n\n for i in token_list:\n if i in \"ABCDEFGHIJKLMNOPQRSTUVWXYZ\" or i in \"1234567890\":\n postfix_list.append(i)\n elif i == \"(\":\n s.push(i)\n elif i == \")\":\n top_token = s.pop()\n while top_token != \"(\":\n postfix_list.append(top_token)\n top_token = s.pop()\n else:\n while (not s.is_empty()) and (prec[s.peek()] >= prec[i]):\n postfix_list.append(s.pop())\n s.push(i)\n\n while not s.is_empty():\n postfix_list.append(s.pop())\n return \" \".join(postfix_list)\n\n\nprint(infix_to_postfix(\"A * B + C * D\"))\nprint(infix_to_postfix(\"( A + B ) * C - ( D - E ) * ( F + G )\"))"
}
{
"filename": "code/data_structures/src/stack/infix_to_postfix/README.md",
"content": "# Stack\nDescription\n---\nStacks are an abstract data type (ADT) that function to store and manipulate data. Their order is last-in-first-out (LIFO). To implement a stack, an array or [linked list](../linked_list) can be used. A stack is similar to a [Tower of Hanoi](https://en.wikipedia.org/wiki/Tower_of_Hanoi) in that the first object pushed onto the stack cannot be removed until every other object pushed on top of it has also been removed. It is also similar to a stack of plates in a cafeteria, where the bottom plate cannot be removed until all of the plates above it have been removed as well.\n\nFunctions\n---\n- Pop()\n --Removes an item from the stack\n- Push()\n--Adds an item to the stack\n- Peek()\n--Returns the top element of the stack\n- isEmpty()\n--Returns true if the stack is empty\n\nTime Complexity\n---\nAll the functions are O(1).\n\n"
}
{
"filename": "code/data_structures/src/stack/infix_to_prefix/infix_to_prefix.cpp",
"content": "// Including Library\n#include <iostream>\n#include <ctype.h>\n#include <string.h>\n#include <stack>\n\n/** Function to check if given character is\n an operator or not. **/\nbool isOperator(char c)\n{\n return (!isalpha(c) && !isdigit(c));\n}\n\n/** Function to find priority of given\n operator.**/\nint getPriority(char c)\n{\n if (c == '-' || c == '+')\n return 1;\n else if (c == '*' || c == '/')\n return 2;\n else if (c == '^')\n return 3;\n return 0;\n}\n\n/** Function that converts infix\n expression to prefix expression. **/\nstd::string infixToPrefix(std::string infix)\n{\n // stack for operators.\n std::stack<char> operators;\n // stack for operands.\n std::stack<std::string> operands;\n\n for (int i = 0; i < infix.length(); i++)\n {\n /** If current character is an\n opening bracket, then\n push into the operators stack. **/\n if (infix[i] == '(')\n operators.push(infix[i]);\n\n\n /** If current character is a\n closing bracket, then pop from\n both stacks and push result\n in operands stack until\n matching opening bracket is\n not found. **/\n else if (infix[i] == ')')\n {\n while (!operators.empty() && operators.top() != '(')\n {\n // operand 1\n std::string op1 = operands.top();\n operands.pop();\n // operand 2\n std::string op2 = operands.top();\n operands.pop();\n // operator\n char op = operators.top();\n operators.pop();\n /** Add operands and operator\n in form operator +\n operand1 + operand2. **/\n std::string tmp = op + op2 + op1;\n operands.push(tmp);\n }\n\n /** Pop opening bracket from stack. **/\n operators.pop();\n }\n\n /** If current character is an\n operand then push it into\n operands stack. **/\n else if (!isOperator(infix[i]))\n operands.push(std::string(1, infix[i]));\n\n\n /** If current character is an\n operator, then push it into\n operators stack after popping\n high priority operators from\n operators stack and pushing\n result in operands stack. **/\n else\n {\n while (!operators.empty() && getPriority(infix[i]) <= getPriority(operators.top())) {\n std::string op1 = operands.top();\n operands.pop();\n std::string op2 = operands.top();\n operands.pop();\n char op = operators.top();\n operators.pop();\n std::string tmp = op + op2 + op1;\n operands.push(tmp);\n }\n operators.push(infix[i]);\n }\n }\n\n /** Pop operators from operators stack\n until it is empty and add result\n of each pop operation in\n operands stack. **/\n while (!operators.empty())\n {\n std::string op1 = operands.top();\n operands.pop();\n\n std::string op2 = operands.top();\n operands.pop();\n\n char op = operators.top();\n operators.pop();\n\n std::string tmp = op + op2 + op1;\n operands.push(tmp);\n }\n\n /** Final prefix expression is\n present in operands stack. **/\n return operands.top();\n}\n\n// Driver code\nint main()\n{\n std::string s;\n std::cin >> s;\n std::cout << infixToPrefix(s);\n return 0;\n}\n\n// Input - (A-B/C)*(A/K-L)\n// Output - *-A/BC-/AKL"
}
{
"filename": "code/data_structures/src/stack/infix_to_prefix/infix_to_prefix.java",
"content": "// To include java library\nimport java.util.*;\n\npublic class infixPrefix {\n\n /**\n * This method checks if given character is\n * an operator or not.\n * @param c character input\n */\n static boolean isOperator(char c) {\n return (!(c >= 'a' && c <= 'z') &&\n !(c >= '0' && c <= '9') &&\n !(c >= 'A' && c <= 'Z'));\n }\n\n /**\n * This method checks priority of given operator.\n * @param c character input\n */\n static int getPriority(char C) {\n if (C == '-' || C == '+')\n return 1;\n else if (C == '*' || C == '/')\n return 2;\n else if (C == '^')\n return 3;\n return 0;\n }\n\n /**\n * This method converts infix\n * expression to prefix expression.\n * @param infix string input\n */\n static String infixToPrefix(String infix) {\n // stack for operators.\n Stack<Character> operators = new Stack<Character>();\n\n // stack for operands.\n Stack<String> operands = new Stack<String>();\n for (int i = 0; i < infix.length(); i++) {\n // If current character is an\n // opening bracket, then\n // push into the operators stack.\n if (infix.charAt(i) == '(') {\n operators.push(infix.charAt(i));\n }\n\n // If current character is a\n // closing bracket, then pop from\n // both stacks and push result\n // in operands stack until\n // matching opening bracket is\n // not found.\n else if (infix.charAt(i) == ')') {\n while (!operators.empty() && operators.peek() != '(') {\n // operand 1\n String op1 = operands.peek();\n operands.pop();\n // operand 2\n String op2 = operands.peek();\n operands.pop();\n // operator\n char op = operators.peek();\n operators.pop();\n // Add operands and operator\n // in form operator +\n // operand1 + operand2.\n String tmp = op + op2 + op1;\n operands.push(tmp);\n }\n // Pop opening bracket\n // from stack.\n operators.pop();\n }\n\n // If current character is an\n // operand then push it into\n // operands stack.\n else if (!isOperator(infix.charAt(i))) {\n operands.push(infix.charAt(i) + \"\");\n }\n\n // If current character is an\n // operator, then push it into\n // operators stack after popping\n // high priority operators from\n // operators stack and pushing\n // result in operands stack.\n else {\n while (!operators.empty() && getPriority(infix.charAt(i)) <= getPriority(operators.peek())) {\n String op1 = operands.peek();\n operands.pop();\n String op2 = operands.peek();\n operands.pop();\n char op = operators.peek();\n operators.pop();\n String tmp = op + op2 + op1;\n operands.push(tmp);\n }\n operators.push(infix.charAt(i));\n }\n }\n\n // Pop operators from operators\n // stack until it is empty and\n // operation in add result of\n // each pop operands stack.\n while (!operators.empty()) {\n String op1 = operands.peek();\n operands.pop();\n String op2 = operands.peek();\n operands.pop();\n char op = operators.peek();\n operators.pop();\n String tmp = op + op2 + op1;\n operands.push(tmp);\n }\n\n // Final prefix expression is\n // present in operands stack.\n return operands.peek();\n }\n\n}\n\n// Driver Code\npublic class infixConversion {\n public static void main(String args[]) {\n infixPrefix g = new infixPrefix();\n // Using Scanner for Getting Input from User\n Scanner in = new Scanner(System.in);\n String s = in.nextLine();\n // String s = \"(A-B/C)*(A/K-L)\";\n System.out.println(g.infixToPrefix(s));\n }\n}\n\n// Input - (A-B/C)*(A/K-L)\n// Output - *-A/BC-/AKL"
}
{
"filename": "code/data_structures/src/stack/infix_to_prefix/README.md",
"content": "# What are Prefix Notations?\nPolish notation (PN), also known as Normal Polish notation (NPN), Łukasiewicz notation, Warsaw notation, Polish prefix notation or simply prefix notation, is a mathematical notation in which operators precede their operands, in contrast to the more common infix notation, in which operators are placed between operands, as well as reverse Polish notation (RPN), in which operators follow their operands.\n\n# Application\n Prefix and Postfix notations are applied by computers while solving an expression, by converting that expression into either of those forms.\n\n# Steps in converting Infix to Prefix\nAt first infix expression is reversed. Note that for reversing the opening and closing parenthesis will also be reversed.\n\n```\nfor an example: The expression: A + B * (C - D)\nafter reversing the expression will be: ) D – C ( * B + A\n```\n\n**so we need to convert opening parenthesis to closing parenthesis and vice versa.**\n\nAfter reversing, the expression is converted to postfix form by using infix to postfix algorithm. After that again the postfix expression is reversed to get the prefix expression.\n\n# Algorithm\n* Push “)” onto STACK, and add “(“ to end of the A\n* Scan A from right to left and repeat step 3 to 6 for each element of A until the STACK is empty\n* If an operand is encountered add it to B\n* If a right parenthesis is encountered push it onto STACK\n* If an operator is encountered then:\n\t * Repeatedly pop from STACK and add to B each operator (on the top of STACK) which has same\n or higher precedence than the operator.\n * Add operator to STACK\n* If left parenthesis is encountered then\n\t * Repeatedly pop from the STACK and add to B (each operator on top of stack until a left parenthesis is encounterd)\n\t * Remove the left parenthesis\n* Exit "
}
{
"filename": "code/data_structures/src/stack/postfix_evaluation/postfix_evaluation.c",
"content": "#include <stdio.h>\n#include <string.h>\n#include <ctype.h>\n#include <stdlib.h>\n\n// Stack type\nstruct Stack\n{\n int top;\n unsigned capacity;\n int* array;\n};\n\n// Stack Operations\nstruct Stack* createStack( unsigned capacity )\n{\n struct Stack* stack = (struct Stack*) malloc(sizeof(struct Stack));\n\n if (!stack) return NULL;\n\n stack->top = -1;\n stack->capacity = capacity;\n stack->array = (int*) malloc(stack->capacity * sizeof(int));\n\n if (!stack->array) return NULL;\n\n return stack;\n}\n\nint isEmpty(struct Stack* stack)\n{\n return stack->top == -1 ;\n}\n\nchar peek(struct Stack* stack)\n{\n return stack->array[stack->top];\n}\n\nchar pop(struct Stack* stack)\n{\n if (!isEmpty(stack))\n return stack->array[stack->top--] ;\n return '$';\n}\n\nvoid push(struct Stack* stack, char op)\n{\n stack->array[++stack->top] = op;\n}\n\n\n// The main function that returns value of a given postfix expression\nint evaluatePostfix(char* exp)\n{\n // Create a stack of capacity equal to expression size\n struct Stack* stack = createStack(strlen(exp));\n int i;\n\n // See if stack was created successfully\n if (!stack) return -1;\n\n // Scan all characters one by one\n for (i = 0; exp[i]; ++i)\n {\n // If the scanned character is an operand (number here),\n // push it to the stack.\n if (isdigit(exp[i]))\n push(stack, exp[i] - '0');\n\n // If the scanned character is an operator, pop two\n // elements from stack apply the operator\n else\n {\n int val1 = pop(stack);\n int val2 = pop(stack);\n switch (exp[i])\n {\n case '+': push(stack, val2 + val1); break;\n case '-': push(stack, val2 - val1); break;\n case '*': push(stack, val2 * val1); break;\n case '/': push(stack, val2/val1); break;\n }\n }\n }\n return pop(stack);\n}\n\n// Driver program to test above functions\nint main()\n{\n char exp[] = \"231*+9-\";\n printf (\"Value of %s is %d\", exp, evaluatePostfix(exp));\n return 0;\n}"
}
{
"filename": "code/data_structures/src/stack/postfix_evaluation/postfix_evaluation.cpp",
"content": "/* In this Input will be Postfix Expression on which particular operations will be \nperformed using stacks and output will be the result of that expression.\nEg. Input => 4 6 + 2 / 5 * 7 + Output => 32\nEvaluation =>((((4+6)/2)*5)+7) = 32\n*/\n\n//code by Sanskruti Shahu\n\n#include<bits/stdc++.h>\nusing namespace std;\n\nint postfixEval (string s)\n{\n\tstack<int> st;\n\tint operand1,operand2;\n\tfor(int i=0;i<s.size();i++)\n\t{\n\t\tif(s[i]>=48)\n {\n\t\t\tst.push(s[i]-48);\n\t\t}\n\t\telse\n {\n\t\t\toperand2=st.top(); \n\t\t\tst.pop(); \n\t\t\toperand1=st.top(); \n\t\t\tst.pop();\n\t\t\tif(s[i]=='+')\n {\n\t\t\t\tst.push(operand1+operand2);\n\t\t\t}\n\t\t\telse if(s[i]=='-')\n {\n\t\t\t\tst.push(operand1-operand2);\n\t\t\t}\n\t\t\telse if(s[i]=='*')\n {\n\t\t\t\tst.push(operand1*operand2);\n\t\t\t}\n\t\t\telse if(s[i]=='/')\n {\n\t\t\t\tst.push(operand1/operand2);\n\t\t\t}\n\t\t\telse if(s[i]=='^')\n {\n\t\t\t\tst.push(operand1^operand2);\n\t\t\t}\n\t\t}\n\t}\n\treturn st.top();\n}\n\nint main()\n{\n string s;\n cin>>s;\n cout<<\"Result = \"<<postfixEval(s)<<endl;\n}\n\n/* Time Complexity is O(n). (where n is length of string)\n Space Complexity is O(1) */"
}
{
"filename": "code/data_structures/src/stack/postfix_evaluation/postfix_evaluation.java",
"content": "// Part of Cosmos by OpenGenus\n// Java proram to evaluate value of a postfix expression \n \nimport java.util.Scanner;\nimport java.util.Stack; \n \npublic class Evaluate \n{ \n // Method to evaluate value of a postfix expression \n static int evaluatePostfix(String s) \n { \n //create a stack \n Stack<Integer> stack=new Stack<>(); \n \n // Scan all characters one by one \n for(int i=0;i<s.length();i++) \n { \n char c=s.charAt(i); \n \n // If the scanned character is an operand (number here), \n // push it to the stack. \n if(Character.isDigit(c)) \n stack.push(c - '0'); \n \n // If the scanned character is an operator, pop two \n // elements from stack apply the operator \n else\n { \n int a = stack.pop(); \n int b = stack.pop(); \n \n switch(c) \n { \n case '+': \n stack.push(b+a); \n break; \n \n case '-': \n stack.push(b-a); \n break; \n \n case '/': \n stack.push(b/a); \n break; \n \n case '*': \n stack.push(b*a); \n break; \n } \n } \n } \n return stack.pop(); \n } \n \n // Driver program to test above functions \n public static void main(String[] args) \n { \n Scanner sc=new Scanner();\n String s=sc.next(); \n System.out.println(evaluatePostfix(s)); \n } \n\n /* Input : \n 231*+9-\n\n Output :\n -4\n */\n} "
}
{
"filename": "code/data_structures/src/stack/postfix_evaluation/postfix_evaluation.py",
"content": "# special feature verbose mode -> to trace what is happening\nimport sys\n\n\nclass postfix:\n def __init__(self, verbose=False):\n self._stack = []\n self._verbose = verbose\n pass\n\n def evaluate(self, post_fix_string):\n if self._verbose:\n print(\"[!] Postfix Evaluation Started For :\", post_fix_string)\n\n for x in post_fix_string:\n try:\n self._stack.append(int(x))\n if self._verbose:\n print(\"[!] Pushed \", x, \" in stack -> \", self._stack)\n except ValueError:\n self._act(x)\n\n if self._verbose:\n print(\"[!] Answer is : \", self._stack[0])\n return self._stack[0]\n else:\n return self._stack[0]\n\n def _act(self, operand):\n b = self._stack.pop()\n a = self._stack.pop()\n\n if self._verbose:\n print(\n \"[!] Popped last two values from stack, a = {} and b = {} -> \".format(\n a, b\n ),\n self._stack,\n )\n\n if operand == \"+\":\n self._stack.append(a + b)\n if self._verbose:\n print(\n \"[!] Performed {} + {} and Pushed in stack -> \".format(a, b),\n self._stack,\n )\n elif operand == \"/\":\n try:\n self._stack.append(a // b)\n if self._verbose:\n print(\n \"[!] Performed {} // (integer division) {} and Pushed in stack -> \".format(\n a, b\n ),\n self._stack,\n )\n except ZeroDivisionError:\n if self._verbose:\n print(\n \"[x] Error : Divide By Zero at a = {} and b = {} with current stack state -> \".format(\n a, b\n ),\n self._stack,\n )\n sys.exit(1)\n\n elif operand == \"-\":\n self._stack.append(a - b)\n if self._verbose:\n print(\n \"[!] Performed {} - {} and Pushed in stack -> \".format(a, b),\n self._stack,\n )\n elif operand == \"*\":\n self._stack.append(a * b)\n if self._verbose:\n print(\n \"[!] Performed {} * {} and Pushed in stack -> \".format(a, b),\n self._stack,\n )\n\n pass\n\n pass\n\n\np_fix1 = postfix()\np_fix2 = postfix(verbose=True) # with verbose mode\nprint(\"------ Without Verbose Mode ------\")\nprint(\"Answer is\", p_fix1.evaluate(\"12+3*1-\"))\n\nprint(\"\\n\\n------ With Verbose Mode ------\")\np_fix2.evaluate(\"1234+-/2+0/3-\") # raising exception intentionally"
}
{
"filename": "code/data_structures/src/stack/postfix_evaluation/postfix_evaluation.sh",
"content": "#!/bin/bash\n#Read the Expression\n\n\nread -p \"Enter The Exppression \" EX\n\n\n#Here q variable will act as top pointer in stack pointing to topmost element\n\nq=-1\n\n#cnt variable will count so that we an read each character\n\ncnt=1\n\n#Here re is the variable for regular expression check \n\n\n \nre='^-?[0-9]+([.][0-9]+)?$'\n\n\n#function to make required calculations\nfunction calculate(){\n\tupr=${arr[q]}\n\tk=$[$q-1]\n\tlwr=${arr[k]}\n\tres=$[$lwr $c $upr]\n\tarr[k]=$res\n\tq=$k\n}\n\n#Here The operations are performed\n\nwhile :\ndo\nc=`echo \"$EX\"|cut -d \" \" -f \"$cnt\"`\n\n#Here check if length of c is nonzero\n\n\nif [[ -n $c ]]\nthen\n\n#Here c is checked if not a number\n\nif ! [[ $c =~ $re ]]\nthen\n\tcalculate #This is a function defined Above \nelse\n\tq=$[$q+1]\n\tarr[q]=$c\nfi\nelse\nbreak\nfi\ncnt=$[$cnt+1]\ndone\necho -e \"Result of Expression is ${arr[0]}\\n\""
}
{
"filename": "code/data_structures/src/stack/postfix_evaluation/README.md",
"content": "# Stack\nDescription\n---\nStacks are an abstract data type (ADT) that function to store and manipulate data. Their order is last-in-first-out (LIFO). To implement a stack, an array or [linked list](../linked_list) can be used. A stack is similar to a [Tower of Hanoi](https://en.wikipedia.org/wiki/Tower_of_Hanoi) in that the first object pushed onto the stack cannot be removed until every other object pushed on top of it has also been removed. It is also similar to a stack of plates in a cafeteria, where the bottom plate cannot be removed until all of the plates above it have been removed as well.\n\nFunctions\n---\n- Pop()\n --Removes an item from the stack\n- Push()\n--Adds an item to the stack\n- Peek()\n--Returns the top element of the stack\n- isEmpty()\n--Returns true if the stack is empty\n\nTime Complexity\n---\nAll the functions are O(1).\n\n"
}
{
"filename": "code/data_structures/src/stack/postfix_to_prefix/postfix_to_prefix.cpp",
"content": "// Part of Cosmos by OpenGenus Foundation\n\n#include <bits/stdc++.h>\nusing namespace std;\n\nbool ValidOperator(char x)\n{\n switch (x)\n {\n case '+':\n case '-':\n case '/':\n case '*':\n return true;\n }\n return false;\n}\n\nstring PostfixToPrefix(string post_exp)\n{\n stack<string> s;\n\n int length = post_exp.size();\n\n for (int i = 0; i < length; i++)\n {\n\n if (ValidOperator(post_exp[i]))\n {\n\n string op1 = s.top();\n s.pop();\n string op2 = s.top();\n s.pop();\n\n string temp = post_exp[i] + op2 + op1;\n\n s.push(temp);\n }\n\n else\n {\n\n s.push(string(1, post_exp[i]));\n }\n }\n\n return s.top();\n}\n\nint main()\n{\n ios_base::sync_with_stdio(false);\n cin.tie(NULL);\n cout.tie(NULL);\n string post_exp = \"HACKT+O*BER/-COS/MOS-*\";\n cout << \"Prefix : \" << PostfixToPrefix(post_exp);\n return 0;\n}"
}
{
"filename": "code/data_structures/src/stack/postfix_to_prefix/README.md",
"content": "# Stack\nDescription\n---\nStacks are an abstract data type (ADT) that function to store and manipulate data. Their order is last-in-first-out (LIFO). To implement a stack, an array or [linked list](../linked_list) can be used. A stack is similar to a [Tower of Hanoi](https://iq.opengenus.org/tower-of-hanoi/) in that the first object pushed onto the stack cannot be removed until every other object pushed on top of it has also been removed. It is also similar to a stack of plates in a cafeteria, where the bottom plate cannot be removed until all of the plates above it have been removed as well.\n\nFunctions\n---\n- Pop()\n --Removes an item from the stack\n- Push()\n--Adds an item to the stack\n- Peek()\n--Returns the top element of the stack\n- isEmpty()\n--Returns true if the stack is empty\n\nTime Complexity\n---\nAll the functions are O(1)."
}
{
"filename": "code/data_structures/src/stack/prefix_to_postfix/prefix_to_postfix.py",
"content": "# Converting prefix to its equivalent postfix notation.\n# Part of Cosmos by OpenGenus Foundation\npostfix = []\noperator = -10\noperand = -20\nempty = -50\n\n\ndef typeof(s):\n if s is \"(\" or s is \")\":\n return operator\n elif s is \"+\" or s is \"-\" or s is \"*\" or s is \"%\" or s is \"/\":\n return operator\n elif s is \" \":\n return empty\n else:\n return operand\n\n\nprefix = input(\"Enter the prefix notation : \")\nprefix = prefix[::-1]\nprint(prefix)\nfor i in prefix:\n type = typeof(i)\n if type is operand:\n postfix.append(i)\n elif type is operator:\n op_first = postfix.pop()\n op_second = postfix.pop()\n postfix.append(op_first + op_second + i)\n elif type is empty:\n continue\n\nprint(\"It's postfix notation is \", \"\".join(postfix))"
}
{
"filename": "code/data_structures/src/stack/prefix_to_postfix/README.md",
"content": "# Stack\nDescription\n---\nStacks are an abstract data type (ADT) that function to store and manipulate data. Their order is last-in-first-out (LIFO). To implement a stack, an array or [linked list](../linked_list) can be used. A stack is similar to a [Tower of Hanoi](https://en.wikipedia.org/wiki/Tower_of_Hanoi) in that the first object pushed onto the stack cannot be removed until every other object pushed on top of it has also been removed. It is also similar to a stack of plates in a cafeteria, where the bottom plate cannot be removed until all of the plates above it have been removed as well.\n\nFunctions\n---\n- Pop()\n --Removes an item from the stack\n- Push()\n--Adds an item to the stack\n- Peek()\n--Returns the top element of the stack\n- isEmpty()\n--Returns true if the stack is empty\n\nTime Complexity\n---\nAll the functions are O(1).\n\n"
}
{
"filename": "code/data_structures/src/stack/Quick_sort_usingSack/quick_sort.cpp",
"content": "#include <iostream>\n#include <stack>\nusing namespace std;\n\nint arr[] = {4, 1, 5, 3, 50, 30, 70, 80, 28, 22};\nstack<int> higherStack, lowerStack;\n\nvoid displayArray();\nvoid quick_sort(int lower, int higher);\nvoid partition(int lower, int higher);\n\nint main()\n{\n lowerStack.push(0);\n higherStack.push(9);\n quick_sort(0,9);\n displayArray();\n}\n\nvoid quick_sort(int lower, int higher)\n{\n if (sizeof(arr) / 4 <= 1)\n {\n return;\n }\n\n while (!lowerStack.empty())\n {\n partition(lower, higher);\n\n quick_sort(lowerStack.top(), higherStack.top());\n lowerStack.pop();\n higherStack.pop();\n }\n}\n\nvoid displayArray()\n{\n for (int i = 0; i < 10; i++)\n {\n cout << arr[i] << \" \";\n }\n cout << endl;\n}\n\nvoid partition(int lower, int higher)\n{\n int i = lower;\n int j = higher;\n int pivot = arr[lower];\n while (i <= j)\n {\n while (pivot <= arr[i] && i<=higher)\n {\n i++;\n }\n while (pivot > arr[j] && j<=lower)\n {\n j--;\n }\n\n if(i>j) swap(arr[i], arr[j]);\n }\n swap(arr[j], arr[lower]);\n\n lowerStack.push(lower);\n lowerStack.push(j + 1);\n higherStack.push(j - 1);\n higherStack.push(higher);\n}"
}
{
"filename": "code/data_structures/src/stack/README.md",
"content": "# Stack\nDescription\n---\nStacks are an abstract data type (ADT) that function to store and manipulate data. Their order is last-in-first-out (LIFO). To implement a stack, an array or [linked list](../linked_list) can be used. A stack is similar to a [Tower of Hanoi](https://en.wikipedia.org/wiki/Tower_of_Hanoi) in that the first object pushed onto the stack cannot be removed until every other object pushed on top of it has also been removed. It is also similar to a stack of plates in a cafeteria, where the bottom plate cannot be removed until all of the plates above it have been removed as well.\n\nFunctions\n---\n- Pop()\n --Removes an item from the stack\n- Push()\n--Adds an item to the stack\n- Peek()\n--Returns the top element of the stack\n- isEmpty()\n--Returns true if the stack is empty\n\nTime Complexity\n---\nAll the functions are O(1).\n\n"
}
{
"filename": "code/data_structures/src/stack/reverse_array_using_stack/reverse_array_using_stack.cpp",
"content": "#include<bits/stdc++.h>\nusing namespace std; \nint main(){\n int n; \n cin>>n; \n int arr[n];\n for(int i=0;i<n;i++){\n cin>>arr[i];\n }\n stack<int>s;\n for(int i=0;i<n;i++){\n s.push(arr[i]);\n }\n while(!s.empty()){\n cout<<s.top()<<\" \";\n s.pop();\n }\n cout<<endl; \n return 0;\n }"
}
{
"filename": "code/data_structures/src/stack/reverse_stack/README.md",
"content": "# Stack\nDescription\n---\nStacks are an abstract data type (ADT) that function to store and manipulate data. Their order is last-in-first-out (LIFO). To implement a stack, an array or [linked list](../linked_list) can be used. A stack is similar to a [Tower of Hanoi](https://en.wikipedia.org/wiki/Tower_of_Hanoi) in that the first object pushed onto the stack cannot be removed until every other object pushed on top of it has also been removed. It is also similar to a stack of plates in a cafeteria, where the bottom plate cannot be removed until all of the plates above it have been removed as well.\n\nFunctions\n---\n- Pop()\n --Removes an item from the stack\n- Push()\n--Adds an item to the stack\n- Peek()\n--Returns the top element of the stack\n- isEmpty()\n--Returns true if the stack is empty\n\nTime Complexity\n---\nAll the functions are O(1).\n\n"
}
{
"filename": "code/data_structures/src/stack/reverse_stack/reverse_stack.c",
"content": "// C program to reverse a stack using recursion\n// Part of Cosmos by OpenGenus Foundation\n\n#include<stdio.h>\n#include<stdlib.h>\n#define bool int\n \n/* structure of a stack node */\nstruct sNode\n{\n char data;\n struct sNode *next;\n};\n \n/* Function Prototypes */\nvoid push(struct sNode** top_ref, int new_data);\nint pop(struct sNode** top_ref);\nbool isEmpty(struct sNode* top);\nvoid print(struct sNode* top);\nvoid insertAtBottom(struct sNode** top_ref, int item);\nvoid reverse(struct sNode** top_ref);\n/* Driver program to test above functions */\nint main()\n{\n struct sNode *s = NULL;\n push(&s, 4);\n push(&s, 3);\n push(&s, 2);\n push(&s, 1);\n \n printf(\"\\n Original Stack \");\n print(s);\n reverse(&s);\n printf(\"\\n Reversed Stack \");\n print(s);\n return 0;\n}\n\n// Below is a recursive function that inserts an element\n// at the bottom of a stack.\nvoid insertAtBottom(struct sNode** top_ref, int item)\n{\n if (isEmpty(*top_ref))\n push(top_ref, item);\n else\n {\n \n /* Hold all items in Function Call Stack until we\n reach end of the stack. When the stack becomes\n empty, the isEmpty(*top_ref)becomes true, the\n above if part is executed and the item is inserted\n at the bottom */\n int temp = pop(top_ref);\n insertAtBottom(top_ref, item);\n \n /* Once the item is inserted at the bottom, push all\n the items held in Function Call Stack */\n push(top_ref, temp);\n }\n}\n \n// Below is the function that reverses the given stack using\n// insertAtBottom()\nvoid reverse(struct sNode** top_ref)\n{\n if (!isEmpty(*top_ref))\n {\n /* Hold all items in Function Call Stack until we\n reach end of the stack */\n int temp = pop(top_ref);\n reverse(top_ref);\n \n /* Insert all the items (held in Function Call Stack)\n one by one from the bottom to top. Every item is\n inserted at the bottom */\n insertAtBottom(top_ref, temp);\n }\n}\n\n \n/* Driveer program to test above functions */\nint main()\n{\n struct sNode *s = NULL;\n push(&s, 4);\n push(&s, 3);\n push(&s, 2);\n push(&s, 1);\n \n printf(\"\\n Original Stack \");\n print(s);\n reverse(&s);\n printf(\"\\n Reversed Stack \");\n print(s);\n return 0;\n}\n\n \n/* Function to check if the stack is empty */\nbool isEmpty(struct sNode* top)\n{\n return (top == NULL)? 1 : 0;\n}\n \n/* Function to push an item to stack*/\nvoid push(struct sNode** top_ref, int new_data)\n{\n /* allocate node */\n struct sNode* new_node =\n (struct sNode*) malloc(sizeof(struct sNode));\n \n if (new_node == NULL)\n {\n printf(\"Stack overflow \\n\");\n exit(0);\n }\n \n /* put in the data */\n new_node->data = new_data;\n \n /* link the old list off the new node */\n new_node->next = (*top_ref);\n \n /* move the head to point to the new node */\n (*top_ref) = new_node;\n}\n \n/* Function to pop an item from stack*/\nint pop(struct sNode** top_ref)\n{\n char res;\n struct sNode *top;\n \n /*If stack is empty then error */\n if (*top_ref == NULL)\n {\n printf(\"Stack overflow \\n\");\n exit(0);\n }\n else\n {\n top = *top_ref;\n res = top->data;\n *top_ref = top->next;\n free(top);\n return res;\n }\n}\n \n/* Functrion to pront a linked list */\nvoid print(struct sNode* top)\n{\n printf(\"\\n\");\n while (top != NULL)\n {\n printf(\" %d \", top->data);\n top = top->next;\n }\n}"
}
{
"filename": "code/data_structures/src/stack/reverse_stack/reverse_stack.cs",
"content": "using System;\nusing System.Collections.Generic;\nusing System.Linq;\nusing System.Text;\nusing System.Threading.Tasks;\n// Part of Cosmos by OpenGenus Foundation\nnamespace reversed_stack\n{\n class Program\n {\n /// <summary>\n /// This will print all elements in stack \n /// Format :: [element1] [element2] ... \n /// </summary>\n /// <param name=\"STK\">an object of Stack collection of type int is accepted</param>\n static void print(Stack<int> STK)\n {\n foreach(int val in STK)\n {\n Console.Write(val.ToString() + \" \");\n }\n Console.WriteLine(); // printing new line\n }\n /// <summary>\n /// This will take a stack collection object of int type and returns the reverse of the same\n /// </summary>\n /// <param name=\"STK\"></param>\n /// <returns></returns>\n static Stack<int> reverse(Stack<int> STK)\n {\n Stack<int> ret = new Stack<int>(); // a temp object of stack\n while(STK.Count > 0) // if stack has some value it will execute\n {\n ret.Push(STK.Pop()); // STK.pop() returns the top most value which we have to push in temp / reversed stack\n }\n return ret;\n }\n\n static void Main(string[] args)\n {\n Stack<int> stk = new Stack<int>(); // creating a object of inbuilt collection Stack\n \n // here i am pushing 5 numbers in stack for demo\n stk.Push(5);\n stk.Push(4);\n stk.Push(3);\n stk.Push(2);\n stk.Push(1);\n \n // printing original elements in stack \n Console.WriteLine(\"ORIGINAL STACK\");\n print(stk);\n\n // reversing the stack now\n stk = reverse(stk);\n\n // printing original elements in stack \n Console.WriteLine(\"REVERSED STACK\");\n print(stk);\n\n Console.ReadKey(); // It has stopped till key has not been pressed\n }\n }\n}"
}
{
"filename": "code/data_structures/src/stack/reverse_stack/reverse_stack.go",
"content": "// Part of Cosmos by OpenGenus Foundation\npackage main\n\n/*\nExpect output\nCurrent Stack is [], Is Stack empty ? true\nTry to push 30 into Stack\nTry to push 12 into Stack\nTry to push 34 into Stack\nTry to push 2 into Stack\nTry to push 17 into Stack\nTry to push 88 into Stack\nReverse Stack\n30 12 34 2 17 88\n\n*/\nimport \"fmt\"\n\ntype Stack []int\n\nfunc (s *Stack) Push(v int) {\n\t*s = append(*s, v)\n}\n\nfunc (s *Stack) Pop() {\n\tlength := len(*s)\n\tif length != 0 {\n\t\t(*s) = (*s)[:length-1]\n\t}\n}\n\nfunc (s *Stack) Top() int {\n\treturn (*s)[len(*s)-1]\n}\n\nfunc (s *Stack) Empty() bool {\n\tif len(*s) == 0 {\n\t\treturn true\n\t}\n\n\treturn false\n}\n\nfunc (s *Stack) Reverse() {\n\tfor i, j := 0, len(*s)-1; i < j; i, j = i+1, j-1 {\n\t\t(*s)[i], (*s)[j] = (*s)[j], (*s)[i]\n\t}\n}\n\nfunc main() {\n\tstack := Stack{}\n\tfmt.Printf(\"Current Stack is %s, Is Stack empty ? %v \\n\", stack, stack.Empty())\n\tfmt.Printf(\"Try to push 30 into Stack\\n\")\n\tstack.Push(30)\n\tfmt.Printf(\"Try to push 12 into Stack\\n\")\n\tstack.Push(12)\n\tfmt.Printf(\"Try to push 34 into Stack\\n\")\n\tstack.Push(34)\n\tfmt.Printf(\"Try to push 2 into Stack\\n\")\n\tstack.Push(2)\n\tfmt.Printf(\"Try to push 17 into Stack\\n\")\n\tstack.Push(17)\n\tfmt.Printf(\"Try to push 88 into Stack\\n\")\n\tstack.Push(88)\n\n\tfmt.Println(\"Reverse Stack\")\n\tstack.Reverse()\n\tfor !stack.Empty() {\n\t\tfmt.Printf(\"%d \", stack.Top())\n\t\tstack.Pop()\n\t}\n\tfmt.Printf(\"\\n\")\n}"
}
{
"filename": "code/data_structures/src/stack/reverse_stack/reverse_stack.java",
"content": "// Part of Cosmos by OpenGenus Foundation\nimport java.util.Stack;\n// Assuming that you already know how the Stack works,\n// if not, see here (code/data_structures/stack/stack)\n\n\nclass reverseStack{\n\tpublic static void main(String[] args){\n\t\t// Examples\n\t\tInteger[] intArr = {1, 3, 2, 5, 4};\n\t\tString[] stringArr = {\"alpha\", \"beta\", \"gamma\", \"echo\", \"delta\"};\n\t\tDouble[] doubleArr = {1.1, 3.2, 1.2, 4.3, 5.4};\n\t\tCharacter[] charArr = {'D', 'E', 'L', 'T', 'A'};\n\t\tObject[] mixArr = {1, \"alpha\", 2.1, 'D', true};\n\t\t\n\t\t// Collecting all examples in single array\n\t\tObject[][] examples = {intArr, stringArr, doubleArr, charArr, mixArr};\n\t\t\n\t\t// Convert each array of examples to stack\n\t\t// and store them in single stack\n\t\tStack<Stack<Object>> stacks = new Stack<Stack<Object>>();\n\t\tfor(int i=examples.length-1; i>=0; i--)\n\t\t\tstacks.push(arrayToStack(examples[i]));\n\t\t\n\t\t// Print formatted output for each example\n\t\twhile(!stacks.isEmpty())\n\t\t\tprint(stacks.pop());\n\t\t\n\t\t// Print each example without storing it first\n\t\tSystem.out.println(\"\\nWithout Storing:\");\n\t\tfor(Object[] item: examples)\n\t\t\tprint(arrayToStack(item));\n\t}\n\t\n\tpublic static <T> void print(Stack<T> stack){\n\t\tSystem.out.println(\"Original stack: \"+stack);\n\t\t// Print stack after reverse\n\t\tSystem.out.println(\"Reversed stack: \"+reverse(stack)+\"\\n\");\n\t}\n\t\n\tpublic static <T> Stack<T> arrayToStack(T[] arr){\n\t\t// Push each item into stack\n\t\tStack<T> stack = new Stack<T>();\n\t\tfor(T item: arr)\n\t\t\tstack.push(item);\n\t\treturn stack;\n\t}\n\t\n\tpublic static <T> Stack<T> reverse(Stack<T> ori){\n\t\t// Push each item from last to first item of ori into rev\n\t\tStack<T> rev = new Stack<T>();\n\t\twhile(!ori.isEmpty())\n\t\t\trev.push(ori.pop());\n\t\treturn rev;\n\t}\n}"
}
{
"filename": "code/data_structures/src/stack/reverse_stack/reverse_stack.py",
"content": "\"\"\"\nPart of Cosmos by OpenGenus Foundation\n\"\"\"\n\n# stack class\nclass Stack:\n def __init__(self):\n self.items = []\n\n # check if the stack is empty\n def isEmpty(self):\n return self.items == []\n\n # push item into the stack\n def push(self, item):\n self.items.append(item)\n\n # pop item from the stack\n def pop(self):\n return self.items.pop()\n\n # get latest item in the stack\n def peek(self):\n return self.items[len(self.items) - 1]\n\n # get stack size\n def size(self):\n return len(self.items)\n\n\n# reverse stack function\ndef reverse(stack):\n # temp list\n items = []\n\n # pop items in the stack and append to the list\n # this will reverse items in the stack\n while not stack.isEmpty():\n items.append(stack.pop())\n\n # push reversed item back to the stack\n for item in items:\n stack.push(item)\n\n # return\n return stack\n\n\nif __name__ == \"__main__\":\n # init the stack\n inputStack = Stack()\n\n print(\n \"Enter the item to push into the stack and press Enter (type 'rev' to reverse the stack)\"\n )\n while True:\n # get input item\n inputItem = input(\"input item: \")\n\n if inputItem == \"rev\" and inputStack.isEmpty():\n # if stack is empty, return message\n print(\"The stack is empty\")\n print(\"========== +++++ ===========\")\n elif inputItem == \"rev\":\n # reverse the stack\n reverseStack = reverse(inputStack)\n print(\"reversed stack: \", reverseStack.items)\n break\n else:\n # push item into the stack\n inputStack.push(inputItem)\n print(\"current stack:\", inputStack.items)"
}
{
"filename": "code/data_structures/src/stack/reverse_stack/reverse_stack.swift",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n// Basic stack implementation\npublic struct Stack<T> {\n \n private var elements: Array<T>\n \n init() {\n self.elements = []\n }\n \n init(arrayLiteral elements: T...) {\n self.elements = elements\n }\n \n public var top: T? {\n return elements.last\n }\n \n public var size: Int {\n return elements.count\n }\n \n public var isEmpty: Bool {\n return elements.isEmpty\n }\n \n mutating public func push(_ element: T) {\n elements.append(element)\n }\n \n mutating public func pop() -> T? {\n return self.isEmpty ? nil : elements.removeLast()\n }\n \n}\n\n// Reverse method implementation\nextension Stack {\n \n public func printElements() {\n \n for x in self.elements.reversed() {\n print(x, terminator: \" \")\n }\n print()\n }\n \n mutating private func insertAtEnd(_ element: T) {\n \n guard let top = self.pop() else {\n self.push(element)\n return\n }\n \n self.insertAtEnd(element)\n self.push(top)\n }\n \n mutating public func reverse() {\n \n guard let top = self.pop() else { return }\n \n self.reverse()\n self.insertAtEnd(top)\n }\n \n}\n\nfunc test() {\n \n var stack = Stack<Int>(arrayLiteral: 1, 3, 2, 5)\n \n print(\"Original stack:\")\n stack.printElements()\n \n stack.reverse()\n \n print(\"Reversed stack:\")\n stack.printElements()\n}\n\ntest()"
}
{
"filename": "code/data_structures/src/stack/reverse_stack/reverse_stack_without_extra_space.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n */\n#include <iostream>\n#include <stack>\nusing namespace std;\nvoid InputElements(stack<int> &s)\n{\n\n //no. of elements to be inserted\n int size;\n cin >> size;\n\n while (size--)\n {\n int num;\n cin >> num;\n s.push(num);\n }\n}\nvoid InsertInStack(stack<int> &s, int x)\n{\n\n if (s.empty())\n {\n s.push(x);\n return;\n }\n\n int cur = s.top();\n s.pop();\n InsertInStack(s, x);\n s.push(cur);\n}\nvoid ReverseStack(stack<int> &s)\n{\n\n if (s.empty())\n return;\n\n int cur = s.top();\n s.pop();\n ReverseStack(s);\n\n InsertInStack(s, cur);\n}\nvoid PrintStack(stack<int> s)\n{\n while (!s.empty())\n {\n cout << s.top() << \" \";\n s.pop();\n }\n cout << endl;\n}\nint main()\n{\n //Stack Decalared\n stack<int> s;\n //Inserting elements in to the stack\n InputElements(s);\n //Print stack\n PrintStack(s);\n //Reverse Stack without using any extra space\n ReverseStack(s);\n //Print again\n PrintStack(s);\n return 0;\n}"
}
{
"filename": "code/data_structures/src/stack/sort_stack/README.md",
"content": "# Stack\nDescription\n---\nStacks are an abstract data type (ADT) that function to store and manipulate data. Their order is last-in-first-out (LIFO). To implement a stack, an array or [linked list](../linked_list) can be used. A stack is similar to a [Tower of Hanoi](https://en.wikipedia.org/wiki/Tower_of_Hanoi) in that the first object pushed onto the stack cannot be removed until every other object pushed on top of it has also been removed. It is also similar to a stack of plates in a cafeteria, where the bottom plate cannot be removed until all of the plates above it have been removed as well.\n\nFunctions\n---\n- Pop()\n --Removes an item from the stack\n- Push()\n--Adds an item to the stack\n- Peek()\n--Returns the top element of the stack\n- isEmpty()\n--Returns true if the stack is empty\n\nTime Complexity\n---\nAll the functions are O(1).\n\n"
}
{
"filename": "code/data_structures/src/stack/sort_stack/sort_stack.c",
"content": "// C program to sort a stack using recursion\n// Part of Cosmos by OpenGenus Foundation\n#include <stdio.h>\n#include <stdlib.h>\n \n// Stack is represented using linked list\nstruct stack\n{\n int data;\n struct stack *next;\n};\n \n// Utility function to initialize stack\nvoid initStack(struct stack **s)\n{\n *s = NULL;\n}\n \n// Utility function to chcek if stack is empty\nint isEmpty(struct stack *s)\n{\n if (s == NULL)\n return 1;\n return 0;\n}\n \n// Utility function to push an item to stack\nvoid push(struct stack **s, int x)\n{\n struct stack *p = (struct stack *)malloc(sizeof(*p));\n \n if (p == NULL)\n {\n fprintf(stderr, \"Memory allocation failed.\\n\");\n return;\n }\n \n p->data = x;\n p->next = *s;\n *s = p;\n}\n \n// Utility function to remove an item from stack\nint pop(struct stack **s)\n{\n int x;\n struct stack *temp;\n \n x = (*s)->data;\n temp = *s;\n (*s) = (*s)->next;\n free(temp);\n \n return x;\n}\n \n// Function to find top item\nint top(struct stack *s)\n{\n return (s->data);\n}\n \n// Recursive function to insert an item x in sorted way\nvoid sortedInsert(struct stack **s, int x)\n{\n // Base case: Either stack is empty or newly inserted\n // item is greater than top (more than all existing)\n if (isEmpty(*s) || x > top(*s))\n {\n push(s, x);\n return;\n }\n \n // If top is greater, remove the top item and recur\n int temp = pop(s);\n sortedInsert(s, x);\n \n // Put back the top item removed earlier\n push(s, temp);\n}\n \n// Function to sort stack\nvoid sortStack(struct stack **s)\n{\n // If stack is not empty\n if (!isEmpty(*s))\n {\n // Remove the top item\n int x = pop(s);\n \n // Sort remaining stack\n sortStack(s);\n \n // Push the top item back in sorted stack\n sortedInsert(s, x);\n }\n}\n \n// Utility function to print contents of stack\nvoid printStack(struct stack *s)\n{\n while (s)\n {\n printf(\"%d \", s->data);\n s = s->next;\n }\n printf(\"\\n\");\n}\n \n// Driver Program\nint main(void)\n{\n struct stack *top;\n \n initStack(&top);\n push(&top, 30);\n push(&top, -5);\n push(&top, 18);\n push(&top, 14);\n push(&top, -3);\n \n printf(\"Stack elements before sorting:\\n\");\n printStack(top);\n \n sortStack(&top);\n printf(\"\\n\\n\");\n \n printf(\"Stack elements after sorting:\\n\");\n printStack(top);\n \n return 0;\n}"
}
{
"filename": "code/data_structures/src/stack/sort_stack/sort_stack.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n/* Sort a stack */\n\n#include <iostream>\n#include <stack>\nusing namespace std;\nvoid InputElements(stack<int> &s) //////////// Function to insert the elements in stack\n{ //no. of elements to be inserted\n int size;\n cin >> size;\n\n while (size--)\n {\n int num;\n cin >> num;\n s.push(num);\n }\n}\nvoid PrintStack(stack<int> s) //////////// Function to print the contents of stack\n{\n while (!s.empty())\n {\n cout << s.top() << \" \";\n s.pop();\n }\n cout << endl;\n}\nvoid SortedInsert(stack<int> &s, int num) ////////// Function to insert a element at its right position in sorted way\n{\n if (s.empty() || s.top() > num)\n {\n s.push(num);\n return;\n }\n int top_element = s.top();\n s.pop();\n SortedInsert(s, num);\n s.push(top_element);\n}\nvoid SortStack(stack<int> &s) //////////// Function to sort the stack\n{\n if (s.empty())\n return;\n int top_element = s.top();\n s.pop();\n SortStack(s);\n SortedInsert(s, top_element);\n}\nint main()\n{\n stack<int> s;\n //Inserting elements in to the stack\n InputElements(s);\n //Print stack before sorting\n PrintStack(s);\n //Sort the stack\n SortStack(s);\n //Print stack after sorting\n PrintStack(s);\n return 0;\n}"
}
{
"filename": "code/data_structures/src/stack/sort_stack/sort_stack.py",
"content": "class stack:\n def __init__(self):\n self.__stack = []\n pass\n\n # method to parse_stack if have already\n def parse_stack(self, stack):\n self.__stack = stack\n pass\n\n def push(self, value):\n self.__stack.append(value)\n pass\n\n def pop(self):\n self.__stack.pop()\n pass\n\n def get_elements(self):\n return self.__stack\n\n def sort(self):\n self.__stack = sorted(self.__stack)\n\n pass\n\n\n# defining stack class instance\nstk = stack()\n\n# pushing elements in stack\nstk.push(10)\nstk.push(2)\nstk.push(-1)\n\n# viewing\nprint(\"Original Stack : \", stk.get_elements())\n\n# sorting stack\nstk.sort()\n\n# viewing again\nprint(\"Sorted Stack : \", stk.get_elements())"
}
{
"filename": "code/data_structures/src/stack/stack/README.md",
"content": "# Stack\nDescription\n---\nStacks are an abstract data type (ADT) that function to store and manipulate data. Their order is last-in-first-out (LIFO). To implement a stack, an array or [linked list](../../linked_list) can be used. A stack is similar to a [Tower of Hanoi](https://en.wikipedia.org/wiki/Tower_of_Hanoi) in that the first object pushed onto the stack cannot be removed until every other object pushed on top of it has also been removed. It is also similar to a stack of plates in a cafeteria, where the bottom plate cannot be removed until all of the plates above it have been removed as well.\n\nFunctions\n---\n- Pop()\n --Removes an item from the stack\n- Push()\n--Adds an item to the stack\n- Peek()\n--Returns the top element of the stack\n- isEmpty()\n--Returns true if the stack is empty\n\nTime Complexity\n---\nAll the functions are O(1).\n\n"
}
{
"filename": "code/data_structures/src/stack/stack/stack.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n#include <limits.h>\n#include <assert.h>\n \nstruct Stack\n{\n int top;\n unsigned capacity;\n int* array;\n};\n \nstruct Stack* \ncreate_stack(unsigned capacity)\n{\n struct Stack* stack = (struct Stack*) malloc(sizeof(struct Stack));\n stack->capacity = capacity;\n stack->top = -1;\n stack->array = (int*) malloc(stack->capacity * sizeof(int));\n return (stack);\n}\n\nvoid \ndelete_stack(struct Stack * stack)\n{ \n /* completely deletes stack */\n free(stack->array);\n free(stack);\n}\n \nint \nis_full(struct Stack* stack)\n{ \n /* checks for overflow error */\n return (stack->top == stack->capacity - 1); \n}\n \nint \nis_empty(struct Stack* stack)\n{ \n /* checks for underflow error */\n return (stack->top == -1); \n}\n \nvoid \npush(struct Stack* stack, int item)\n{\n assert(!is_full(stack));\n stack->array[++stack->top] = item;\n}\n\nint \npop(struct Stack* stack)\n{ \n assert(!is_empty(stack));\n return (stack->array[stack->top--]);\n}\n\nint \nmain()\n{\n struct Stack* stack = create_stack(100);\n \n push(stack, 10);\n push(stack, 20);\n push(stack, 30);\n \n printf(\"%d popped from stack\\n\", pop(stack));\n printf(\"%d popped from stack\\n\", pop(stack));\n printf(\"%d popped from stack\\n\", pop(stack));\n\n delete_stack(stack);\n \n return (0);\n}"
}
{
"filename": "code/data_structures/src/stack/stack/stack.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <iostream>\n#include <cstdlib>\n#include <climits>\nusing namespace std;\n\ntemplate <typename T>\nstruct Node\n{\n T n;\n Node* next;\n};\n\ntemplate <class T>\nclass Stack\n{\nprivate:\n Node<T>* top;\npublic:\n Stack();\n ~Stack();\n void push(T);\n T pop();\n void display();\n};\n\ntemplate <class T> Stack<T>::Stack()\n{\n top = nullptr;\n}\n\ntemplate <class T> Stack<T>::~Stack()\n{\n if (top)\n {\n Node<T>* ptr = top;\n while (ptr)\n {\n Node<T>* tmp = ptr;\n ptr = ptr->next;\n delete tmp;\n }\n }\n}\n\ntemplate <class T> void Stack<T>::push(T n)\n{\n Node<T>* ptr = new Node<T>;\n ptr->n = n;\n ptr->next = top;\n top = ptr;\n}\n\ntemplate <class T> T Stack<T>::pop()\n{\n if (top == nullptr)\n return INT_MIN;\n Node<T>* ptr = top;\n T n = ptr->n;\n top = top->next;\n delete ptr;\n return n;\n}\n\ntemplate <class T> void Stack<T>::display()\n{\n Node<T>* ptr = top;\n while (ptr != nullptr)\n {\n cout << ptr->n << \"-> \";\n ptr = ptr->next;\n }\n cout << \"NULL\" << endl;\n}\n\nint main()\n{\n Stack<int> S;\n int n, choice;\n while (choice != 4)\n {\n cout << \"1.Push\" << endl\n << \"2.Pop\" << endl\n << \"3.Display\" << endl\n << \"4.Exit\" << endl;\n cin >> choice;\n switch (choice)\n {\n case 1:\n cout << \"Enter the element to be entered. \";\n cin >> n;\n S.push(n);\n break;\n case 2:\n S.pop();\n break;\n case 3:\n S.display();\n break;\n default:\n break;\n }\n }\n return 0;\n}"
}
{
"filename": "code/data_structures/src/stack/stack/stack.cs",
"content": "/**\n * Stack implementation using a singly-linked link.\n * Part of the OpenGenus/cosmos project. (https://github.com/OpenGenus/cosmos)\n * \n * A stack is a first-in first-out (FIFO) data structure.\n * Elements are manipulated by adding and removing elements of the top of the stack.\n */\nusing System;\n\nnamespace Cosmos_Data_Structures\n{\n public class Stack<T>\n {\n //Node is a element that holds the data of the current element plus a reference to the next element.\n //Used to implement the singly-linked list.\n private class Node\n {\n public T data;\n public Node next;\n\n public Node(T data, Node next)\n {\n this.data = data;\n this.next = next;\n }\n }\n\n private Node top;\n public int Size { get; private set; }\n\n public Stack()\n {\n top = null;\n Size = 0;\n }\n\n //Add element to the top of the stack.\n public void Push(T element)\n {\n var newNode = new Node(element, top);\n top = newNode;\n Size++;\n }\n\n //Gets element at the top of the stack.\n //Throws an exception if the stack is empty.\n public T Peek()\n {\n if(IsEmpty())\n {\n throw new InvalidOperationException(\"Cannot peek on an empty stack!\");\n }\n\n return top.data;\n }\n\n //Removes and returns the element at the top of the stack.\n //Throws an exception if the stack is empty.\n public T Pop()\n {\n if(IsEmpty())\n {\n throw new InvalidOperationException(\"Cannot pop on an empty stack!\");\n }\n\n var oldTop = top;\n top = top.next;\n Size--;\n return oldTop.data;\n }\n\n //Returns true if stack contains no elements, false otherwise.\n public bool IsEmpty()\n {\n return top == null;\n }\n\n //Returns a string representation of the stack.\n public override string ToString()\n {\n Node tmp = top;\n string result = \"Stack([Top] \";\n\n while(tmp != null)\n {\n result += tmp.data;\n tmp = tmp.next;\n\n if(tmp != null)\n {\n result += \" -> \";\n }\n }\n\n result += \")\";\n return result;\n }\n }\n\n //Stack testing methods/class.\n public class StackTest\n {\n static void Main(string[] args)\n {\n Console.Write(\"Creating stack...\");\n var intStack = new Stack<int>();\n\n for (int i = 0; i < 10; i++)\n {\n intStack.Push(i);\n }\n Console.WriteLine(\"done\");\n\n Console.WriteLine(intStack.ToString());\n Console.WriteLine(\"Size of stack: \" + intStack.Size);\n Console.WriteLine(\"Topmost element is \" + intStack.Peek() + \".\\n\");\n\n Console.Write(\"Removing elements...\");\n for (int i = 0; i < 3; i++)\n {\n intStack.Pop();\n }\n Console.WriteLine(\"done\");\n\n Console.WriteLine(intStack.ToString());\n Console.WriteLine(\"Size of stack: \" + intStack.Size);\n Console.WriteLine(\"Topmost element is \" + intStack.Peek() + \".\\n\");\n\n Console.WriteLine(\"Press any key to continue.\");\n Console.ReadKey();\n }\n }\n}"
}
{
"filename": "code/data_structures/src/stack/stack/stack.erl",
"content": "% Part of Cosmos by OpenGenus Foundation\n\n% Pattern matching\n% H -> head\n% T -> tail\n-module(stack).\n-export([new/0, push/2, pop/1, empty/1, peek/1]).\n\nnew() -> [].\n\npush(H, T) -> [H, T].\n\npop([H, T]) -> {H, T}.\n\nempty([]) -> true;\nempty(_) -> false.\n\npeek([H, _]) -> H."
}
{
"filename": "code/data_structures/src/stack/stack/stack.ex",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n# Pattern matching\n# new_element = head\n# items = tail\ndefmodule Stack do\n def new, do: [] \n \n def push(new_element, items), do: [new_element | items]\n \n def pop([element | items]), do: {element, items}\n \n def empty?([]), do: true\n def empty?(_), do: false\n \n def peek([head | _]), do: head\nend"
}
{
"filename": "code/data_structures/src/stack/stack/stack.go",
"content": "// Part of Cosmos by OpenGenus Foundation\npackage main\n\nimport \"fmt\"\n\ntype Stack []int\n\nfunc (s *Stack) Push(v int) Stack {\n\treturn append(*s, v)\n}\n\nfunc (s *Stack) Pop() Stack {\n\tlength := len(*s)\n\tif length != 0 {\n\t\treturn (*s)[:length-1]\n\t}\n\treturn *s\n}\n\nfunc (s *Stack) Top() int {\n\treturn (*s)[len(*s)-1]\n}\n\nfunc (s *Stack) Empty() bool {\n\tif len(*s) == 0 {\n\t\treturn true\n\t}\n\n\treturn false\n}\n\nfunc main() {\n\tstack := Stack{}\n\tfmt.Printf(\"Current Stack is %s, Is Stack empty ? %v \\n\", stack, stack.Empty())\n\tfmt.Printf(\"Try to push 30 into Stack\\n\")\n\tstack = stack.Push(30)\n\tfmt.Printf(\"Current Stack is %v, top value is %v\\n\", stack, stack.Top())\n\tfmt.Printf(\"Try to push 12 into Stack\\n\")\n\tstack = stack.Push(12)\n\tfmt.Printf(\"Try to push 34 into Stack\\n\")\n\tstack = stack.Push(34)\n\tfmt.Printf(\"Current Stack is %v, top value is %v\\n\", stack, stack.Top())\n\tfmt.Printf(\"Current Stack is %v, Is Stack empty ? %v \\n\", stack, stack.Empty())\n\tstack = stack.Pop()\n\tfmt.Printf(\"Current Stack is %v, top value is %v\\n\", stack, stack.Top())\n\tstack = stack.Pop()\n\tstack = stack.Pop()\n\tfmt.Printf(\"Current Stack is %v, Is Stack empty ? %v\\n\", stack, stack.Empty())\n}"
}
{
"filename": "code/data_structures/src/stack/stack/stack_in_dart.dart",
"content": "class StackNode<T> {\n T data;\n\n StackNode<T>? below;\n\n StackNode(this.data);\n}\n\nclass Stack<T> {\n StackNode<T>? top;\n\n int size;\n\n Stack()\n : top = null,\n size = 0;\n\n bool get isEmpty => top == null;\n\n T pop() {\n if (isEmpty) throw InvalidIndexError();\n var output = top!;\n top = top?.below;\n size--;\n\n return output.data;\n }\n\n T? peek() => top?.data;\n\n void push(T data) {\n var newNode = StackNode(data);\n if (isEmpty) {\n top = newNode;\n } else {\n newNode.below = top;\n top = newNode;\n }\n size++;\n }\n}\n\nclass InvalidIndexError extends Error {\n @override\n String toString() => 'Invalid Index for this operation';\n}\n\nmixin BinaryHeapIndex {\n int parentOf(int idx) =>\n idx >= 0 ? ((idx - 1) / 2).truncate() : throw InvalidIndexError();\n\n int leftOf(int idx) => 2 * idx + 1;\n\n int rightOf(int idx) => 2 * idx + 2;\n}\n\ntypedef Comparer<T> = bool Function(T parent, T child);\n\nabstract class HeapBase<T> {\n bool get isEmpty;\n\n int get length;\n\n void insert(T item);\n\n void insertMany(List<T> items);\n\n void heapify(int rootIndex);\n\n T pop();\n\n T peek();\n}"
}
{
"filename": "code/data_structures/src/stack/stack/stack.java",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\nimport java.util.ArrayList;\nclass Stack<T>{\n\tprivate int maxSize;\n\tprivate ArrayList<T> stackArray;\n\tprivate int top;\n\n\tpublic Stack(int size){\n\t\tmaxSize = size;\n\t\tstackArray = new ArrayList<>();\n\t\ttop = -1;\n\t}\n\n\tpublic void push(T value){\n\t\tif(!isFull()){ //Checks for a full stack\n\t\t\ttop++;\n\t\t\tif(stackArray.size() <= top)\n\t\t\t\tstackArray.add(top, value);\n\t\t\telse\n\t\t\t\tstackArray.set(top, value);\n\t\t\t//stackArray[top] = value;\n\t\t}else{\n\t\t\tSystem.out.println(\"The stack is full, can't insert value\");\n\t\t}\n\t}\n\n\tpublic T pop(){\n\t\tif(!isEmpty()){ //Checks for an empty stack\n\t\t\treturn stackArray.get(top--);\n\t\t}else{\n\t\t\tthrow new IndexOutOfBoundsException();\n\t\t}\n\t}\n\n\tpublic T peek(){\n\t\tif(!isEmpty()){ //Checks for an empty stack\n\t\t\treturn stackArray.get(top);\n\t\t}else{\n\t\t\tthrow new IndexOutOfBoundsException();\n\t\t}\n\t}\n\n\tpublic boolean isEmpty(){\n\t\treturn(top == -1);\n\t}\n\n\tpublic boolean isFull(){\n\t\treturn(top+1 == maxSize);\n\t}\n\n\tpublic void makeEmpty(){ //Doesn't delete elements in the array but if you call\n\t\ttop = -1;\t\t\t //push method after calling makeEmpty it will overwrite previous values\n\t}\n}\n\npublic class Stacks{\n\t/**\n\t * Main method\n\t *\n\t * @param args Command line arguments\n\t */\n\tpublic static void main(String args[]){\n\t\tStack<Integer> myStack = new Stack<>(4); //Declare a stack of maximum size 4\n \t\t//Populate the stack\n \t\tmyStack.push(5);\n \t\tmyStack.push(8);\n \t\tmyStack.push(2);\n \t\tmyStack.push(9);\n\n\t\tSystem.out.println(\"*********************Stack Array Implementation*********************\");\n \t\tSystem.out.println(myStack.isEmpty()); //will print false\n \t\tSystem.out.println(myStack.isFull()); //will print true\n \t\tSystem.out.println(myStack.peek()); //will print 9\n \t\tSystem.out.println(myStack.pop()); //will print 9\n \t\tSystem.out.println(myStack.peek()); // will print 2\n\t}\n}"
}
{
"filename": "code/data_structures/src/stack/stack/stack.js",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n/* Stack!!\n * A stack is exactly what it sounds like. An element gets added to the top of\n * the stack and only the element on the top may be removed. This is an example\n * of an array implementation of a Stack. So an element can only be added/removed\n * from the end of the array.\n */\n\n// Functions: push, pop, peek, view, length\n\n//Creates a stack\nvar Stack = function() {\n //The top of the Stack\n this.top = 0;\n //The array representation of the stack\n this.stack = {};\n\n //Adds a value onto the end of the stack\n this.push = function(value) {\n this.stack[this.top] = value;\n this.top++;\n };\n\n //Removes and returns the value at the end of the stack\n this.pop = function() {\n if (this.top === 0) {\n return \"Stack is Empty\";\n }\n\n this.top--;\n var result = this.stack[this.top];\n delete this.stack[this.top];\n return result;\n };\n\n //Returns the size of the stack\n this.size = function() {\n return this.top;\n };\n\n //Returns the value at the end of the stack\n this.peek = function() {\n return this.stack[this.top - 1];\n };\n\n //To see all the elements in the stack\n this.view = function() {\n for (var i = 0; i < this.top; i++) console.log(this.stack[i]);\n };\n};\n\n//Implementation\nvar myStack = new Stack();\n\nmyStack.push(1);\nmyStack.push(5);\nmyStack.push(76);\nmyStack.push(69);\nmyStack.push(32);\nmyStack.push(54);\nconsole.log(myStack.size());\nconsole.log(myStack.peek());\nconsole.log(myStack.pop());\nconsole.log(myStack.peek());\nconsole.log(myStack.pop());\nconsole.log(myStack.peek());\nmyStack.push(55);\nconsole.log(myStack.peek());\nmyStack.view();"
}
{
"filename": "code/data_structures/src/stack/stack/stack.php",
"content": "<?php\n\n/**\n * Stack implementation\n * Part of Cosmos by OpenGenus Foundation\n * Note: To run this script, you need PHP >= 7.1\n * \n * @author Lucas Soares Candalo <[email protected]>\n */\nclass Stack\n{\n /**\n * @var array\n */\n private $elements = [];\n\n /**\n * Removes an item from the stack\n *\n * @return void\n */\n public function pop(): void\n {\n if (!$this->isEmpty()) {\n array_pop($this->elements);\n }\n }\n\n /**\n * Adds an item to the stack\n *\n * @param int $element\n * @return void\n */\n public function push(int $element): void\n {\n array_push($this->elements, $element);\n }\n\n /**\n * Gets the top element of the stack\n *\n * @return int\n */\n public function peek(): int\n {\n return end($this->elements);\n }\n\n /**\n * Checks if the stack is empty\n *\n * @return bool true if is empty, false otherwise\n */\n public function isEmpty(): bool\n {\n return empty($this->elements);\n }\n}\n\nclass StackPrinter\n{\n /**\n * Print stack elements\n *\n * @param Stack $stack\n * @return void\n */\n public function print(Stack $stack): void\n {\n $stackClone = clone $stack;\n\n echo \"Stack elements:\\n\\n\";\n while(!$stackClone->isEmpty()) {\n echo $stackClone->peek().\"\\n\";\n $stackClone->pop();\n }\n echo \"\\n\\n\";\n }\n}\n\n$stack = new Stack();\n$stackPrinter = new StackPrinter();\n\n// Add a few elements to the stack\n$stack->push(10);\n$stack->push(20);\n$stack->push(30);\n\n// Print stack elements\n$stackPrinter->print($stack);\n\n// Remove top element from the stack\n$stack->pop();\n\n// Print stack elements again\n$stackPrinter->print($stack);\n\n// Remove top element from the stack\n$stack->pop();\n\n// Print stack elements again\n$stackPrinter->print($stack);"
}
{
"filename": "code/data_structures/src/stack/stack/stack.py",
"content": "# Author: Alex Day\n# Purpose: Stack implementation with array in python\n# Date: October 2 2017\n\n\n# Part of Cosmos by OpenGenus Foundation\nclass Stack:\n # Quasi-Constructor\n def __init__(self):\n # Object data members\n self.stack_arr = [] # Just an array\n\n # When the client requests a push simply add the data to the list\n def push(self, data):\n self.stack_arr.append(data)\n\n def is_empty(self):\n return len(self.stack_arr) == 0\n\n # When the client requests a pop just run the pop function on the array\n def pop(self):\n assert len(self.stack_arr) > 0, \"The stack is empty!\"\n return self.stack_arr.pop()\n\n # When the client requests a peek just return the top value\n def peek(self):\n assert len(self.stack_arr) > 0, \"The stack is empty!\"\n return self.stack_arr[-1]\n\n\ndef main():\n stk = Stack()\n stk.push(1)\n stk.push(2)\n\n print(stk.peek())\n\n print(stk.pop())\n print(stk.pop())\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/data_structures/src/stack/stack/stack.rb",
"content": "# Part of Cosmos by OpenGenus Foundation\nclass Stack\n attr_accessor :items\n\n def initialize\n @items = []\n end\n\n def push(element)\n @items.push(element)\n end\n\n def pop\n @items.pop\n end\n\n def empty?\n @items.empty?\n end\n\n def peek\n @items.last\n end\nend"
}
{
"filename": "code/data_structures/src/stack/stack/stack.rs",
"content": "pub struct Stack<T> {\n elements: Vec<T>\n}\n\nimpl<T> Stack<T> {\n pub fn new() -> Stack<T> {\n Stack {\n elements: Vec::new()\n }\n }\n\n pub fn push(&mut self, elem: T) {\n self.elements.push(elem);\n }\n\n pub fn pop(&mut self) -> T {\n let last = self.elements.len() - 1;\n self.elements.remove(last)\n }\n\n pub fn peek(&mut self) -> &T {\n let last = self.elements.len() - 1;\n &self.elements[last]\n }\n\n pub fn peek_mut(&mut self) -> &mut T {\n let last = self.elements.len() - 1;\n &mut self.elements[last]\n }\n\n pub fn len(&self) -> usize {\n self.elements.len()\n }\n\n pub fn is_empty(&self) -> bool {\n self.elements.is_empty()\n }\n}\n\nfn main() {\n let mut stack = Stack::<i32>::new();\n stack.push(1);\n stack.push(2);\n println!(\"{}\", stack.pop());\n stack.push(-5);\n println!(\"{}\", stack.peek());\n}"
}
{
"filename": "code/data_structures/src/stack/stack/stack.swift",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\npublic struct Stack<T> {\n \n private var elements: Array<T>\n \n init() {\n self.elements = []\n }\n \n init(arrayLiteral elements: T...) {\n self.elements = elements\n }\n \n public var top: T? {\n return elements.last\n }\n \n public var size: Int {\n return elements.count\n }\n \n public var isEmpty: Bool {\n return elements.isEmpty\n }\n \n mutating public func push(_ element: T) {\n elements.append(element)\n }\n \n mutating public func pop() -> T? {\n return self.isEmpty ? nil : elements.removeLast()\n }\n \n}"
}
{
"filename": "code/data_structures/src/stack/stack/stack_using_array.py",
"content": "stack=[]\r\ndef push(item):\r\n stack.append(item)\r\ndef pop():\r\n if isEmpty(stack):\r\n return(\"STACK UNDERFLOW\")\r\n else:\r\n itempopped=stack[-1]\r\n stack.pop\r\n display()\r\n return int(itempopped)\r\ndef isEmpty(stack):\r\n return stack==[]\r\ndef display():\r\n print(stack)\r\nwhile True:\r\n choice=int(input(\"1.Push\\n2.Pop\\n3.Isempty\\n4.Exit\\n\"))\r\n if choice==1:\r\n element=int(input(\"Enter the element to be inserted: \"))\r\n push(element)\r\n if choice==2:\r\n print(\"Item deleted is %s\"%pop())\r\n if choice==3:\r\n print(isEmpty(stack))\r\n if choice==4:\r\n break\r"
}
{
"filename": "code/data_structures/src/stack/stack/stack_using_linked_list.py",
"content": "class node:\r\n def __init__(self,value):\r\n self.data=value\r\n self.next=None\r\nclass stack:\r\n def __init__(self,value):\r\n self.ptr=None\r\n def display(self):\r\n temp=self.ptr\r\n while temp:\r\n print (temp.data,end='->')\r\n temp=temp.next\r\n print('NULL')\r\n def insertnode(self,data):\r\n if self.ptr==None:\r\n self.ptr=node(data)\r\n else:\r\n newnode=node(data)\r\n newnode.next=self.ptr\r\n self.ptr=newnode\r\n def deletenode(self):\r\n if self.ptr==None:\r\n print(\"Stack is empty\")\r\n return\r\n else:\r\n print(\"Item deleted is %s\"%self.ptr.data)\r\n self.ptr=self.ptr.next\r\n def isEmpty(self):\r\n return self.ptr==None\r\n\r\ntop=stack()\r\nwhile True:\r\n choice=int(input(\"1.Push\\n2.Pop\\n3.Isempty\\n4.Exit\\n\"))\r\n if choice==1:\r\n element=int(input(\"Enter the element to be inserted: \"))\r\n top.insertnode(element)\r\n top.display()\r\n if choice==2:\r\n top.deletenode()\r\n top.display()\r\n if choice==3:\r\n print(top.isEmpty())\r\n if choice==4:\r\n break\r\n \r"
}
{
"filename": "code/data_structures/src/stack/stack_using_queue/stack_using_queue.cpp",
"content": "/**\n * @brief Stack Data Structure Using the Queue Data Structure\n * @details\n * Using 2 Queues inside the Stack class, we can easily implement Stack\n * data structure with heavy computation in push function.\n * \n * Part of Cosmos by OpenGenus Foundation\n */\n#include <iostream> \n#include <queue> \n#include <cassert>\nusing namespace std;\n\nclass Stack\n{\nprivate:\n queue<int> main_q; // stores the current state of the stack\n queue<int> auxiliary_q; // used to carry out intermediate operations to implement stack\n int current_size = 0; // stores the current size of the stack\npublic:\n int top();\n void push(int val);\n void pop();\n int size();\n};\n\n/**\n * Returns the top most element of the stack\n * @returns top element of the queue\n */\nint Stack :: top()\n{\n return main_q.front();\n}\n\n/**\n * @brief Inserts an element to the top of the stack.\n * @param val the element that will be inserted into the stack\n * @returns void\n */\nvoid Stack :: push(int val)\n{\n auxiliary_q.push(val);\n while(!main_q.empty())\n {\n auxiliary_q.push(main_q.front());\n main_q.pop();\n }\n swap(main_q, auxiliary_q);\n current_size++;\n}\n\n/**\n * @brief Removes the topmost element from the stack\n * @returns void\n */\nvoid Stack :: pop()\n{\n if(main_q.empty()) {\n return;\n }\n main_q.pop();\n current_size--;\n}\n\n/**\n * @brief Utility function to return the current size of the stack\n * @returns current size of stack\n */\nint Stack :: size()\n{\n return current_size;\n}\n\nint main()\n{\n Stack s;\n s.push(1); // insert an element into the stack\n s.push(2); // insert an element into the stack\n s.push(3); // insert an element into the stack\n \n assert(s.size()==3); // size should be 3\n \n assert(s.top()==3); // topmost element in the stack should be 3\n \n s.pop(); // remove the topmost element from the stack\n assert(s.top()==2); // topmost element in the stack should now be 2\n \n s.pop(); // remove the topmost element from the stack\n assert(s.top()==1);\n \n s.push(5); // insert an element into the stack\n assert(s.top()==5); // topmost element in the stack should now be 5\n \n s.pop(); // remove the topmost element from the stack\n assert(s.top()==1); // topmost element in the stack should now be 1\n \n assert(s.size()==1); // size should be 1\n return 0;\n}"
}
{
"filename": "code/data_structures/src/stack/stack_using_queue/stack_using_queue.py",
"content": "## Part of Cosmos by OpenGenus Foundation\n\nclass Stack:\n\n def __init__(self):\n self.main_q = []\n self.auxiliary_q = []\n self.current_size = 0\n\n def top(self):\n return self.main_q[0]\n\n def push(self, val) -> None:\n self.auxiliary_q.append(val)\n \n while len(self.main_q) > 0:\n self.auxiliary_q.append(self.main_q.pop(0))\n\n self.main_q, self.auxiliary_q = self.auxiliary_q, self.main_q\n\n self.current_size+=1\n \n def pop(self):\n if len(self.main_q) == 0:\n return \n self.main_q.pop(0)\n self.current_size-=1\n \n def size(self) -> int:\n return self.current_size\n\nif __name__=='__main__':\n s = Stack()\n s.push(1) # insert an element into the stack\n s.push(2) # insert an element into the stack\n s.push(3) # insert an element into the stack\n \n assert(s.size()==3) # size should be 3\n \n assert(s.top()==3) # topmost element in the stack should be 3\n \n s.pop() # remove the topmost element from the stack\n assert(s.top()==2) # topmost element in the stack should now be 2\n \n s.pop() # remove the topmost element from the stack\n assert(s.top()==1)\n \n s.push(5) # insert an element into the stack\n assert(s.top()==5) # topmost element in the stack should now be 5\n \n s.pop() # remove the topmost element from the stack\n assert(s.top()==1) # topmost element in the stack should now be 1\n \n assert(s.size()==1) # size should be 1"
}
{
"filename": "code/data_structures/src/tree/binary_tree/aa_tree/aa_tree.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n * arne andersson tree synopsis\n *\n * template<typename _Derive, typename _Tp, typename _Comp = std::less<_Tp> >\n * struct BinaryTreeNode {\n * _Tp value;\n * std::shared_ptr<_Derive> left, right;\n * BinaryTreeNode(_Tp v,\n * std::shared_ptr<_Derive> l = nullptr,\n * std::shared_ptr<_Derive> r = nullptr);\n * };\n *\n * template<typename _Tp, typename _Comp = std::less<_Tp> >\n * struct AABinaryTreeNode :public BinaryTreeNode<AABinaryTreeNode<_Tp, _Comp>, _Tp, _Comp> {\n * size_t level;\n * AABinaryTreeNode(_Tp v,\n * std::shared_ptr<AABinaryTreeNode> l = nullptr,\n * std::shared_ptr<AABinaryTreeNode> r = nullptr);\n * };\n *\n * template<typename _Tp,\n * typename _Comp = std::less<_Tp>,\n * typename _NodeType = BinaryTreeNode<_Tp, _Comp> >\n * class BinaryTree {\n * public:\n * typedef _Tp value_type;\n * typedef value_type & reference;\n * typedef value_type const &const_reference;\n * typedef std::ptrdiff_t difference_type;\n * typedef size_t size_type;\n *\n * protected:\n * typedef BinaryTree<_Tp, _Comp, _NodeType> self;\n * typedef _NodeType node_type;\n * typedef std::shared_ptr<node_type> p_node_type;\n *\n * public:\n * BinaryTree(p_node_type r = nullptr) :root_(r), sz_(0), comp_(_Comp()), release_(true);\n *\n * p_node_type const maximum() const;\n *\n * p_node_type const minimum() const;\n *\n * size_type size() const;\n *\n * bool empty() const;\n *\n * void inOrder(std::ostream &output) const;\n *\n * void preOrder(std::ostream &output) const;\n *\n * void postOrder(std::ostream &output) const;\n *\n * protected:\n * p_node_type root_;\n * size_type sz_;\n * _Comp comp_;\n * bool release_;\n * p_node_type nil_;\n *\n * p_node_type get(const_reference value);\n *\n * p_node_type const maximum(p_node_type n) const;\n *\n * p_node_type const minimum(p_node_type n) const;\n *\n * void inOrder(std::ostream &output, p_node_type const n) const;\n *\n * void preOrder(std::ostream &output, p_node_type const n) const;\n *\n * void postOrder(std::ostream &output, p_node_type const n) const;\n * };\n *\n * template<typename _Tp, typename _Comp = std::less<_Tp> >\n * class AATree :public BinaryTree<_Tp, _Comp, AABinaryTreeNode<_Tp, _Comp> > {\n * private:\n * typedef BinaryTree<_Tp, _Comp, AABinaryTreeNode<_Tp, _Comp> > base;\n * typedef AATree<_Tp, _Comp> self;\n *\n * public:\n * using typename base::size_type;\n * using typename base::value_type;\n * using typename base::reference;\n * using typename base::const_reference;\n * using typename base::difference_type;\n *\n * protected:\n * using typename base::p_node_type;\n * using typename base::node_type;\n * using base::root_;\n * using base::comp_;\n * using base::nil_;\n * using base::sz_;\n *\n * public:\n * AATree() :base();\n *\n * void insert(const_reference value);\n *\n * void erase(const_reference value);\n *\n * p_node_type const find(const_reference value);\n *\n * private:\n * // implement by recursive\n * void insert(p_node_type &n, const_reference value);\n *\n * void erase(p_node_type &n, const_reference value);\n *\n * // input: T, a node representing an AA tree that needs to be rebalanced.\n * // output: Another node representing the rebalanced AA tree.\n * p_node_type skew(p_node_type n);\n *\n * // input: T, a node representing an AA tree that needs to be rebalanced.\n * // output: Another node representing the rebalanced AA tree\n * p_node_type split(p_node_type n);\n *\n * void makeNode(p_node_type &n, value_type value);\n * };\n */\n\n#include <algorithm>\n#include <functional>\n#include <memory>\n#include <stack>\n#include <cstddef>\n\ntemplate<typename _Derive, typename _Tp, typename _Comp = std::less<_Tp>>\nstruct BinaryTreeNode\n{\n _Tp value;\n std::shared_ptr<_Derive> left, right;\n BinaryTreeNode(_Tp v,\n std::shared_ptr<_Derive> l = nullptr,\n std::shared_ptr<_Derive> r = nullptr)\n : value(v), left(l), right(r)\n {\n };\n};\n\ntemplate<typename _Tp, typename _Comp = std::less<_Tp>>\nstruct AABinaryTreeNode : public BinaryTreeNode<AABinaryTreeNode<_Tp, _Comp>, _Tp, _Comp>\n{\n size_t level;\n AABinaryTreeNode(_Tp v,\n std::shared_ptr<AABinaryTreeNode> l = nullptr,\n std::shared_ptr<AABinaryTreeNode> r = nullptr)\n : BinaryTreeNode<AABinaryTreeNode<_Tp, _Comp>, _Tp, _Comp>(v, l, r), level(1)\n {\n };\n};\n\ntemplate<typename _Tp,\n typename _Comp = std::less<_Tp>,\n typename _NodeType = BinaryTreeNode<_Tp, _Comp>>\nclass BinaryTree {\npublic:\n typedef _Tp value_type;\n typedef value_type & reference;\n typedef value_type const &const_reference;\n typedef std::ptrdiff_t difference_type;\n typedef size_t size_type;\n\nprotected:\n typedef BinaryTree<_Tp, _Comp, _NodeType> self;\n typedef _NodeType node_type;\n typedef std::shared_ptr<node_type> p_node_type;\n\npublic:\n BinaryTree(p_node_type r = nullptr) : root_(r), sz_(0), comp_(_Comp()), release_(true)\n {\n };\n\n p_node_type const maximum() const\n {\n auto f = maximum(root_);\n if (f == nil_)\n return nullptr;\n\n return f;\n }\n\n p_node_type const minimum() const\n {\n auto f = minimum(root_);\n if (f == nil_)\n return nullptr;\n\n return f;\n }\n\n size_type size() const\n {\n return sz_;\n }\n\n bool empty() const\n {\n return sz_ == 0;\n }\n\n void inOrder(std::ostream &output) const\n {\n inOrder(output, root_);\n }\n\n void preOrder(std::ostream &output) const\n {\n preOrder(output, root_);\n }\n\n void postOrder(std::ostream &output) const\n {\n postOrder(output, root_);\n }\n\nprotected:\n p_node_type root_;\n size_type sz_;\n _Comp comp_;\n bool release_;\n p_node_type nil_;\n\n p_node_type get(const_reference value)\n {\n p_node_type n = root_;\n while (n != nil_)\n {\n if (comp_(value, n->value))\n n = n->left;\n else if (comp_(n->value, value))\n n = n->right;\n else\n break;\n }\n\n return n;\n }\n\n p_node_type const maximum(p_node_type n) const\n {\n if (n != nil_)\n while (n->right != nil_)\n n = n->right;\n\n return n;\n }\n\n p_node_type const minimum(p_node_type n) const\n {\n if (n != nil_)\n while (n->left != nil_)\n n = n->left;\n\n return n;\n }\n\n void inOrder(std::ostream &output, p_node_type const n) const\n {\n if (n != nil_)\n {\n inOrder(output, n->left);\n output << n->value << \" \";\n inOrder(output, n->right);\n }\n }\n\n void preOrder(std::ostream &output, p_node_type const n) const\n {\n if (n != nil_)\n {\n output << n->value << \" \";\n preOrder(output, n->left);\n preOrder(output, n->right);\n }\n }\n\n void postOrder(std::ostream &output, p_node_type const n) const\n {\n if (n != nil_)\n {\n postOrder(output, n->left);\n output << n->value << \" \";\n postOrder(output, n->right);\n }\n }\n};\n\ntemplate<typename _Tp, typename _Comp = std::less<_Tp>>\nclass AATree : public BinaryTree<_Tp, _Comp, AABinaryTreeNode<_Tp, _Comp>> {\nprivate:\n typedef BinaryTree<_Tp, _Comp, AABinaryTreeNode<_Tp, _Comp>> base;\n typedef AATree<_Tp, _Comp> self;\n\npublic:\n using typename base::size_type;\n using typename base::value_type;\n using typename base::reference;\n using typename base::const_reference;\n using typename base::difference_type;\n\nprotected:\n using typename base::p_node_type;\n using typename base::node_type;\n using base::root_;\n using base::comp_;\n using base::nil_;\n using base::sz_;\n\npublic:\n AATree() : base()\n {\n nil_ = std::make_shared<node_type>(0);\n nil_->left = nil_;\n nil_->right = nil_;\n nil_->level = 0;\n root_ = nil_;\n }\n\n void insert(const_reference value)\n {\n insert(root_, value);\n }\n\n void erase(const_reference value)\n {\n erase(root_, value);\n }\n\n p_node_type const find(const_reference value)\n {\n auto f = base::get(value);\n if (f == nil_)\n return nullptr;\n\n return f;\n }\n\nprivate:\n// implement by recursive\n void insert(p_node_type &n, const_reference value)\n {\n if (n == nil_)\n {\n makeNode(n, value);\n ++sz_;\n }\n else\n {\n if (comp_(value, n->value))\n insert(n->left, value);\n else if (comp_(n->value, value))\n insert(n->right, value);\n else // depend on implement\n n->value = value;\n }\n n = skew(n);\n n = split(n);\n }\n\n void erase(p_node_type &n, const_reference value)\n {\n if (n != nil_)\n {\n if (comp_(value, n->value))\n erase(n->left, value);\n else if (comp_(n->value, value))\n erase(n->right, value);\n else\n {\n if (n->left != nil_ && n->right != nil_)\n {\n p_node_type leftMax = n->left;\n while (leftMax->right != nil_)\n leftMax = leftMax->right;\n n->value = leftMax->value;\n erase(n->left, n->value);\n }\n else // 3 way, n is leaf then nullptr, otherwise n successor\n {\n p_node_type successor = n->left == nil_ ? n->right : n->left;\n n = successor;\n --sz_;\n }\n }\n }\n\n if (n != nil_\n && (n->left->level < n->level - 1 || n->right->level < n->level - 1))\n {\n --n->level;\n if (n->right->level > n->level)\n n->right->level = n->level;\n n = skew(n);\n if (n->right != nil_)\n n->right = skew(n->right);\n if (n->right != nil_ && n->right != nil_)\n n->right->right = skew(n->right->right);\n n = split(n);\n if (n->right != nil_)\n n->right = split(n->right);\n }\n }\n\n// input: T, a node representing an AA tree that needs to be rebalanced.\n// output: Another node representing the rebalanced AA tree.\n p_node_type skew(p_node_type n)\n {\n if (n != nil_\n && n->left != nil_\n && n->left->level == n->level)\n {\n p_node_type left = n->left;\n n->left = left->right;\n left->right = n;\n n = left;\n }\n\n return n;\n }\n\n// input: T, a node representing an AA tree that needs to be rebalanced.\n// output: Another node representing the rebalanced AA tree\n p_node_type split(p_node_type n)\n {\n if (n != nil_\n && n->right != nil_\n && n->right->right != nil_\n && n->level == n->right->right->level)\n {\n p_node_type right = n->right;\n n->right = right->left;\n right->left = n;\n n = right;\n ++n->level;\n }\n\n return n;\n }\n\n void makeNode(p_node_type &n, value_type value)\n {\n n = std::make_shared<node_type>(value, nil_, nil_);\n }\n};\n\n/*\n * // for test\n * // test insert/erase/size function\n #include <iostream>\n * using namespace std;\n *\n * int main() {\n * std::shared_ptr<AATree<int> > aat = make_shared<AATree<int> >();\n *\n * if (!aat->empty())\n * cout << \"error\";\n *\n * auto f = aat->find(3);\n * if (f != nullptr)\n * cout << \"error\";\n *\n * f = aat->maximum();\n * if (f != nullptr)\n * cout << \"error\";\n *\n * f = aat->minimum();\n * if (f != nullptr)\n * cout << \"error\";\n *\n * aat->insert(0);\n * f = aat->find(0);\n * if (f == nullptr)\n * cout << \"error\";\n *\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->insert(1);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->insert(2);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->insert(3);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->insert(4);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->insert(5);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->insert(6);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->erase(0);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->erase(3);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->erase(1);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->insert(7);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->insert(3);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->erase(7);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->erase(3);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->insert(3);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->insert(1);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->erase(7);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n * aat->erase(8);\n * aat->inOrder(cout); cout << \"\\n\"; aat->preOrder(cout); cout << \"\\n\";\n * cout << aat->size() << \"\\n\\n\";\n *\n * f = aat->maximum();\n * if (f == nullptr || f->value != 6)\n * cout << \"error\";\n *\n * f = aat->minimum();\n * if (f == nullptr || f->value != 1)\n * cout << \"error\";\n *\n * if (aat->empty())\n * cout << \"error\";\n *\n * return 0;\n * }\n *\n *\n * expected:\n * 0\n * 0\n * 1\n *\n * 0 1\n * 0 1\n * 2\n *\n * 0 1 2\n * 1 0 2\n * 3\n *\n * 0 1 2 3\n * 1 0 2 3\n * 4\n *\n * 0 1 2 3 4\n * 1 0 3 2 4\n * 5\n *\n * 0 1 2 3 4 5\n * 1 0 3 2 4 5\n * 6\n *\n * 0 1 2 3 4 5 6\n * 3 1 0 2 5 4 6\n * 7\n *\n * 1 2 3 4 5 6\n * 3 1 2 5 4 6\n * 6\n *\n * 1 2 4 5 6\n * 2 1 5 4 6\n * 5\n *\n * 2 4 5 6\n * 4 2 5 6\n * 4\n *\n * 2 4 5 6 7\n * 4 2 6 5 7\n * 5\n *\n * 2 3 4 5 6 7\n * 4 2 3 6 5 7\n * 6\n *\n * 2 3 4 5 6\n * 4 2 3 5 6\n * 5\n *\n * 2 4 5 6\n * 4 2 5 6\n * 4\n *\n * 2 3 4 5 6\n * 4 2 3 5 6\n * 5\n *\n * 1 2 3 4 5 6\n * 2 1 4 3 5 6\n * 6\n *\n * 1 2 3 4 5 6\n * 2 1 4 3 5 6\n * 6\n *\n * 1 2 3 4 5 6\n * 2 1 4 3 5 6\n * 6\n *\n */"
}
{
"filename": "code/data_structures/src/tree/binary_tree/aa_tree/README.md",
"content": "# Cosmos\n\nCollaborative effort by [OpenGenus](https://github.com/OpenGenus/cosmos)"
}
{
"filename": "code/data_structures/src/tree/binary_tree/avl_tree/avl_tree.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n\nstruct node\n{\n\tstruct node *lcptr;\n\tint data;\n\tint height;\n\tstruct node *rcptr;\n};\n\nstruct node *rptr = NULL;\nint c = 0,f = 0;\n\nint\nheight(struct node *rptr)\n{\n\tif (rptr == NULL)\n\t\treturn (-1);\n\telse\n\t\treturn (rptr->height);\n}\n\nvoid\nheightUpdate(struct node *rptr)\n{\n\tint leftHeight = height(rptr->lcptr);\n\tint rightHeight = height(rptr->rcptr);\n\n\tif (leftHeight > rightHeight)\n\t\trptr->height = leftHeight + 1;\n\telse\n\t\trptr->height = rightHeight + 1;\n}\n\nint\ngetBalance(struct node *rptr)\n{\n\tif (rptr == NULL)\n\t\treturn (0);\n\telse\n\t\treturn (height(rptr->lcptr) - height(rptr->rcptr));\n}\n\nstruct node *\nRightRotate(struct node *rptr)\n{\n\tstruct node *jptr = rptr;\n\tstruct node *kptr = rptr->lcptr;\n\tjptr->lcptr = kptr->rcptr;\n\tkptr->rcptr = jptr;\n\n\theightUpdate(jptr);\n\theightUpdate(kptr);\n\n\treturn (kptr);\n}\n\nstruct node *\nLeftRotate(struct node *rptr)\n{\n\tstruct node *jptr = srptr;\n\tstruct node *kptr = rptr->rcptr;\n\tjptr->rcptr = kptr->lcptr;\n\tkptr->lcptr = jptr;\n\n\theightUpdate(jptr);\n\theightUpdate(kptr);\n\n\treturn (kptr);\n}\n\nstruct node *\nLeftRightRotate(struct node *rptr)\n{\n\tstruct node *jptr = rptr;\n\tstruct node *kptr = rptr->lcptr;\n\tjptr->lcptr = LeftRotate(kptr);\n\n\treturn RightRotate(jptr);\n}\n\nstruct node *\nRightLeftRotate(struct node *rptr)\n{\n\tstruct node *jptr = rptr;\n\tstruct node *kptr = rptr->rcptr;\n\tjptr->rcptr = RightRotate(kptr);\n\n\treturn LeftRotate(jptr);\n}\n\nstruct node *\ninsert(struct node *rptr, int x)\n{\n\tif (rptr == NULL) {\n\t\trptr = (struct node *)malloc(sizeof(struct node));\n\t\trptr->data = x;\n\t\trptr->lcptr = rptr->rcptr = NULL;\n\t\trptr->height = 0;\n\t}\n\telse {\n\t\tif (x < rptr->data) {\n\t\t\trptr->lcptr = insert(rptr->lcptr,x);\n\n\t\t\tif (getBalance(rptr) == 2 && x < (rptr->lcptr)->data)\n\t\t\t\trptr = RightRotate(rptr);\n\t\t\telse if (getBalance(rptr) == 2 && x >= (rptr->lcptr)->data)\n\t\t\t\trptr = LeftRightRotate(rptr);\n\t\t}\n\t\telse {\n\t\t\trptr->rcptr = insert(rptr->rcptr,x);\n\n\t\t\tif (getBalance(rptr) == -2 && x < (rptr->rcptr)->data)\n\t\t\t\trptr = RightLeftRotate(rptr);\n\t\t\telse if (getBalance(rptr) == -2 && x >= (rptr->rcptr)->data)\n\t\t\t\trptr = LeftRotate(rptr);\n\t\t}\n\t\theightUpdate(rptr);\n\t}\n\n\treturn (rptr);\n}\n\nstruct node *\ninorder_successor(struct node *rptr)\n{\n\tstruct node *t = rptr->rcptr;\n\n\twhile (t->lcptr != NULL)\n\t\tt = t->lcptr;\n\n\treturn (t);\n}\n\nstruct node *\ndelete(struct node *rptr, int x)\n{\n\tif (rptr == NULL) \n \treturn rptr;\n\n if (x < rptr->data)\n rptr->lcptr = delete(rptr->lcptr, x);\n \n else if (x > rptr->data)\n rptr->rcptr = delete(rptr->rcptr, x);\n\n else {\n if (rptr->lcptr == NULL) {\n struct node *temp = rptr->rcptr;\n free(rptr);\n f = 1;\n return (temp);\n }\n else if (rptr->rcptr == NULL) {\n struct node *temp = rptr->lcptr;\n free(rptr);\n f = 1;\n return (temp);\n }\n \n struct node *temp = inorder_successor(rptr); \n rptr->data = temp->data; \n rptr->rcptr = delete(rptr->rcptr, temp->data); \n }\n\n if (rptr == NULL) \n return (rptr);\n \n heightUpdate(rptr); \n \n if (getBalance(rptr) > 1 && getBalance(rptr->lcptr) >= 0)\n return (RightRotate(rptr));\n \n if (getBalance(rptr) > 1 && getBalance(rptr->lcptr) < 0)\n return (LeftRightRotate(rptr));\n \n if (getBalance(rptr) < -1 && getBalance(rptr->rcptr) <= 0)\n return (LeftRotate(rptr));\n \n if (getBalance(rptr) < -1 && getBalance(rptr->rcptr) > 0)\n return (RightLeftRotate(rptr));\n \n return (rptr);\n\n}\n\nvoid\ninorder(struct node *rptr)\n{\n\tif (rptr != NULL) {\n\t\tinorder(rptr->lcptr);\n\t\tprintf(\"%d \",rptr->data);\n\t\tinorder(rptr->rcptr);\n\t}\n}\n\nvoid \npreorder(struct node *rptr)\n{\n\tif (rptr != NULL) {\n\t\tprintf(\"%d \",rptr->data);\n\t\tpreorder(rptr->lcptr);\n\t\tpreorder(rptr->rcptr);\n\t}\n}\n\nvoid \npostorder(struct node *rptr)\n{\n\tif (rptr != NULL) {\n\t\tpostorder(rptr->lcptr);\n\t\tpostorder(rptr->rcptr);\n\t\tprintf(\"%d \",rptr->data);\n\t}\n}\n\nvoid \nsearch(int x)\n{\n\tstruct node *t = rptr;\n\tint f = 0;\n\twhile (t != NULL) {\n\t\tif (x < t->data)\n\t\t\tt = t->lcptr;\n\t\telse if (x > t->data)\n\t\t\tt = t->rcptr;\n\t\telse {\n\t\t\tf = 1;\n\t\t\tbreak;\n\t\t}\n\t}\n\tif (f == 1)\n\t\tprintf(\"%d is found in AVL\\n\",x);\n\telse\n\t\tprintf(\"%d is not found in AVL\\n\",x);\n}\n\nvoid \ncount(struct node *rptr)\n{\n\tif (rptr != NULL) {\n\t\tcount(rptr->lcptr);\n\t\tcount(rptr->rcptr);\n\t\tc++;\n\t}\n}\n\nvoid\navlcheck(struct node *rptr)\n{\n\tif (rptr != NULL) {\n\t\tavlcheck(rptr->lcptr);\n\t\tavlcheck(rptr->rcptr);\n\t\tprintf(\"Data: %d Balance Factor: %d\\n\",rptr->data,getBalance(rptr));\n\t}\n}\n\nint main()\n{\n\tint t;\n\twhile (1) {\n\t\tprintf(\"1.Insert\\n2.Delete\\n3.Search\\n4.Height\\n5.Count\\n6.Inorder\\n7.Preorder\\n8.Postorder\\n9.AVL Check\\n10.Exit\\n\");\n\t\tscanf(\"%d\",&t);\n\t\tswitch(t) {\n\t\t\t\n\t\t\tcase 1 :\n\t\t\t\tint x;\n\t\t\t\tprintf(\"Enter integer to be inserted\\n\");\n\t\t\t\tscanf(\"%d\",&x);\n\t\t\t\trptr = insert(rptr,x);\n\t\t\t\tprintf(\"%d is successfully inserted in AVL\\n\",x);\n\t\t\t\tbreak;\n\n\t\t\tcase 2 :\n\t\t\t\tint x;\n\t\t\t\tprintf(\"Enter integer to be deleted\\n\");\n\t\t\t\tscanf(\"%d\",&x);\n\t\t\t\tf = 0;\n\t\t\t\trptr = delete(rptr,x);\n\t\t\t\tif (f == 1)\n\t\t\t\t\tprintf(\"%d is successfully deleted from AVL\\n\",x);\n\t\t\t\telse\n\t\t\t\t\tprintf(\"%d is not found in AVL.Hence,cannot be deleted\\n\",x);\n\t\t\t\tbreak;\n\t\t\t\n\t\t\tcase 3 :\n\t\t\t\tint x;\n\t\t\t\tprintf(\"Enter integer to be searched\\n\");\n\t\t\t\tscanf(\"%d\",&x);\n\t\t\t\tsearch(x);\n\t\t\t\tbreak;\n\t\t\t\n\t\t\tcase 4 :\n\t\t\t\tprintf(\"Height of AVL: %d\\n\",height(rptr));\n\t\t\t\tbreak;\n\t\t\t\n\t\t\tcase 5 :\n\t\t\t\tc = 0;\n\t\t\t\tcount(rptr);\n\t\t\t\tprintf(\"Number of nodes present AVL:%d\\n\",c);\n\t\t\t\tbreak;\n\t\t\t\n\t\t\tcase 6 :\n\t\t\t\tprintf(\"Inorder sequence:-\\n\");\n\t\t\t\tinorder(rptr);\n\t\t\t\tprintf(\"\\n\");\n\t\t\t\tbreak;\n\t\t\t\n\t\t\tcase 7 :\n\t\t\t\tprintf(\"Preorder sequence:-\\n\");\n\t\t\t\tpreorder(rptr);\n\t\t\t\tprintf(\"\\n\");\n\t\t\t\tbreak;\n\t\t\t\n\t\t\tcase 8 :\n\t\t\t\tprintf(\"Postorder sequence:-\\n\");\n\t\t\t\tpostorder(rptr);\n\t\t\t\tprintf(\"\\n\");\n\t\t\t\tbreak;\n\n\t\t\tcase 9 :\n\t\t\t\tprintf(\"Balance Factor for each node:-\\n\");\n\t\t\t\tavlcheck(rptr);\n\t\t\t\tbreak;\n\n\t\t\tcase 10 :\n\t\t\t\treturn (0);\n\n\t\t}\n\t}\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/avl_tree/avl_tree.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n * avl tree synopsis\n *\n * template<typename _Tp, typename _Comp = std::less<_Tp> >\n * class avl_tree {\n * private:\n * struct AVLNode {\n * _Tp data;\n * std::shared_ptr<AVLNode> left;\n * std::shared_ptr<AVLNode> right;\n * int height;\n * };\n *\n * typedef _Tp value_type;\n * typedef AVLNode node_type;\n * typedef std::shared_ptr<AVLNode> p_node_type;\n *\n * public:\n * avl_tree() :root_(nullptr);\n *\n * // function to insert a node into the AVL tree\n * void insert(int data);\n *\n * // function to delete a node from the AVL tree\n * void erase(int data);\n *\n * // helper function to return height of a node\n * int getHeight(p_node_type node);\n *\n * // function to find the minimum element in the tree (lefftmost node)\n * p_node_type findMin(p_node_type root_);\n *\n * // function to find the maximum element in the tree (rightmost node)\n * p_node_type findMax(p_node_type root_);\n *\n * // preorder traversal of the AVL tree\n * void preOrder(std::ostream &out) const;\n *\n * // inorder traversal of the AVL tree\n * void inOrder(std::ostream &out) const;\n *\n * // postorder traversal of the AVL tree\n * void postOrder(std::ostream &out) const;\n *\n * private:\n * p_node_type root_;\n * _Comp comp_;\n *\n * // LL rotation root_ed at X\n * p_node_type rotateLL(p_node_type &X);\n *\n * // RR rotation root_ed at X\n * p_node_type rotateRR(p_node_type &X);\n *\n * // LR rotation root_ed at X\n * p_node_type rotateLR(p_node_type &X);\n *\n * // RL rotation root_ed at X\n * p_node_type rotateRL(p_node_type &X);\n *\n * // function to insert a node into the AVL tree\n * p_node_type insert(p_node_type root_, int data);\n *\n * // function to delete a node from the AVL tree\n * p_node_type erase(p_node_type root_, int data);\n *\n * // preorder traversal of the AVL tree\n * void preOrder(p_node_type root_, std::ostream &out) const;\n *\n * // inorder traversal of the AVL tree\n * void inOrder(p_node_type root_, std::ostream &out) const;\n *\n * // postorder traversal of the AVL tree\n * void postOrder(p_node_type root_, std::ostream &out) const;\n * };\n */\n\n#include <algorithm>\n#include <memory>\n#include <ostream>\n\ntemplate<typename _Tp, typename _Comp = std::less<_Tp>>\nclass avl_tree {\nprivate:\n struct AVLNode\n {\n _Tp data;\n std::shared_ptr<AVLNode> left;\n std::shared_ptr<AVLNode> right;\n int height;\n };\n\n typedef _Tp value_type;\n typedef AVLNode node_type;\n typedef std::shared_ptr<AVLNode> p_node_type;\n\npublic:\n avl_tree() : root_(nullptr)\n {\n ;\n }\n\n// function to insert a node into the AVL tree\n void insert(int data)\n {\n root_ = insert(root_, data);\n }\n\n// function to delete a node from the AVL tree\n void erase(int data)\n {\n root_ = erase(root_, data);\n }\n\n// helper function to return height of a node\n int getHeight(p_node_type node)\n {\n if (node)\n return node->height;\n\n return -1;\n }\n\n// function to find the minimum element in the tree (lefftmost node)\n p_node_type findMin(p_node_type root_)\n {\n if (root_ != nullptr)\n while (root_->left != nullptr)\n root_ = root_->left;\n\n return root_;\n }\n\n// function to find the maximum element in the tree (rightmost node)\n p_node_type findMax(p_node_type root_)\n {\n if (root_ != nullptr)\n while (root_->right != nullptr)\n root_ = root_->right;\n\n return root_;\n }\n\n// preorder traversal of the AVL tree\n void preOrder(std::ostream &out) const\n {\n preOrder(root_, out);\n }\n\n// inorder traversal of the AVL tree\n void inOrder(std::ostream &out) const\n {\n inOrder(root_, out);\n }\n\n// postorder traversal of the AVL tree\n void postOrder(std::ostream &out) const\n {\n postOrder(root_, out);\n }\n\nprivate:\n p_node_type root_;\n _Comp comp_;\n\n// LL rotation root_ed at X\n p_node_type rotateLL(p_node_type &X)\n {\n p_node_type W = X->left;\n X->left = W->right;\n W->right = X;\n X->height = std::max(getHeight(X->left), getHeight(X->right)) + 1;\n W->height = std::max(getHeight(W->left), getHeight(X)) + 1;\n\n return W; // new root_\n }\n\n// RR rotation root_ed at X\n p_node_type rotateRR(p_node_type &X)\n {\n p_node_type W = X->right;\n X->right = W->left;\n W->left = X;\n X->height = std::max(getHeight(X->left), getHeight(X->right)) + 1;\n W->height = std::max(getHeight(X), getHeight(W->right));\n\n return W; // new root_\n }\n\n// LR rotation root_ed at X\n p_node_type rotateLR(p_node_type &X)\n {\n X->left = rotateRR(X->left);\n\n return rotateLL(X);\n }\n\n// RL rotation root_ed at X\n p_node_type rotateRL(p_node_type &X)\n {\n X->right = rotateLL(X->right);\n\n return rotateRR(X);\n }\n\n// function to insert a node into the AVL tree\n p_node_type insert(p_node_type root_, int data)\n {\n if (root_ == nullptr)\n {\n p_node_type newNode = std::make_shared<node_type>();\n newNode->data = data;\n newNode->height = 0;\n newNode->left = newNode->right = nullptr;\n root_ = newNode;\n }\n else if (comp_(data, root_->data))\n {\n root_->left = insert(root_->left, data);\n if (getHeight(root_->left) - getHeight(root_->right) == 2)\n {\n if (comp_(data, root_->left->data))\n root_ = rotateLL(root_);\n else\n root_ = rotateLR(root_);\n }\n }\n else if (comp_(root_->data, data))\n {\n root_->right = insert(root_->right, data);\n if (getHeight(root_->right) - getHeight(root_->left) == 2)\n {\n if (comp_(root_->right->data, data))\n root_ = rotateRR(root_);\n else\n root_ = rotateRL(root_);\n }\n }\n root_->height = std::max(getHeight(root_->left), getHeight(root_->right)) + 1;\n\n return root_;\n }\n\n// function to delete a node from the AVL tree\n p_node_type erase(p_node_type root_, int data)\n {\n if (root_ == nullptr)\n return nullptr;\n else if (comp_(data, root_->data))\n {\n root_->left = erase(root_->left, data);\n if (getHeight(root_->right) - getHeight(root_->left) == 2)\n {\n if (getHeight(root_->right->right) > getHeight(root_->right->left))\n root_ = rotateRR(root_);\n else\n root_ = rotateRL(root_);\n }\n }\n else if (comp_(root_->data, data))\n {\n root_->right = erase(root_->right, data);\n if (getHeight(root_->left) - getHeight(root_->right) == 2)\n {\n if (getHeight(root_->left->left) > getHeight(root_->left->right))\n root_ = rotateLL(root_);\n else\n root_ = rotateLR(root_);\n }\n }\n else\n {\n p_node_type temp = nullptr;\n if (root_->left && root_->right)\n {\n temp = findMin(root_->right);\n root_->data = temp->data;\n root_->right = erase(root_->right, root_->data);\n if (getHeight(root_->left) - getHeight(root_->right) == 2)\n {\n if (getHeight(root_->left->left) > getHeight(root_->left->right))\n root_ = rotateLL(root_);\n else\n root_ = rotateLR(root_);\n }\n }\n else if (root_->left)\n {\n temp = root_;\n root_ = root_->left;\n }\n else if (root_->right)\n {\n temp = root_;\n root_ = root_->right;\n }\n else\n return nullptr;\n }\n\n return root_;\n }\n\n// preorder traversal of the AVL tree\n void preOrder(p_node_type root_, std::ostream &out) const\n {\n if (root_ != nullptr)\n {\n out << (root_)->data << \" \";\n preOrder((root_)->left, out);\n preOrder((root_)->right, out);\n }\n }\n\n// inorder traversal of the AVL tree\n void inOrder(p_node_type root_, std::ostream &out) const\n {\n if (root_ != nullptr)\n {\n inOrder((root_)->left, out);\n out << (root_)->data << \" \";\n inOrder((root_)->right, out);\n }\n }\n\n// postorder traversal of the AVL tree\n void postOrder(p_node_type root_, std::ostream &out) const\n {\n if (root_ != nullptr)\n {\n postOrder((root_)->left, out);\n postOrder((root_)->right, out);\n out << (root_)->data << \" \";\n }\n }\n};\n\n/*\n * // for test\n #include <iostream>\n * using namespace std;\n * int main() {\n * int ch, data;\n * shared_ptr<avl_tree<int> > avlt = make_shared<avl_tree<int> >();\n * while (1)\n * {\n * cout << \"1. Insert 2. Delete 3. Preorder 4. Inorder 5. Postorder 6. Exit\\n\";\n * cin >> ch;\n * switch (ch) {\n * case 1: cout << \"Enter data\\n\";\n * cin >> data;\n * avlt->insert(data);\n * break;\n * case 2: cout << \"Enter data\\n\";\n * cin >> data;\n * avlt->erase(data);\n * break;\n * case 3: avlt->preOrder(cout);\n * cout << endl;\n * break;\n * case 4: avlt->inOrder(cout);\n * cout << endl;\n * break;\n * case 5: avlt->postOrder(cout);\n * cout << endl;\n * break;\n * }\n * if (ch == 6)\n * break;\n * }\n *\n * return 0;\n * }\n *\n * // */"
}
{
"filename": "code/data_structures/src/tree/binary_tree/avl_tree/avl_tree.java",
"content": "/*AVL tree is a self-balancing Binary Search Tree (BST) where the difference between heights of left and right subtrees \ncannot be more than one for all nodes.\n*/\nclass Node\n{\n int key, height;\n Node left, right;\n \n Node(int d)\n {\n key = d;\n height = 1;\n }\n}\n \nclass AVLTree\n{\n Node root;\n // get height\n int height(Node N)\n {\n if (N == null)\n return 0;\n return N.height;\n }\n \n // get maximum of two integers\n int max(int a, int b)\n {\n return (a > b) ? a : b;\n }\n\n Node rightRotate(Node y)\n {\n Node x = y.left;\n Node T2 = x.right;\n\n x.right = y;\n y.left = T2;\n\n //Update heights\n y.height = max(height(y.left), height(y.right)) + 1;\n x.height = max(height(x.left), height(x.right)) + 1;\n\n return x;\n }\n \n Node leftRotate(Node x)\n {\n Node y = x.right;\n Node T2 = y.left;\n \n y.left = x;\n x.right = T2;\n \n // Update heights\n x.height = max(height(x.left), height(x.right)) + 1;\n y.height = max(height(y.left), height(y.right)) + 1;\n\n return y;\n }\n \n int getBalance(Node N)\n {\n if (N == null)\n return 0;\n return height(N.left) - height(N.right);\n }\n \n Node insert(Node node, int key)\n {\n //Perform the normal BST rotation\n if (node == null)\n return (new Node(key));\n \n if (key < node.key)\n node.left = insert(node.left, key);\n else if (key > node.key)\n node.right = insert(node.right, key);\n else \n return node;\n \n //Update height of this ancestor node\n node.height = 1 + max(height(node.left),\n height(node.right));\n \n // Get the balance factor of this ancestor node\n int balance = getBalance(node);\n \n // If this node becomes unbalanced, 4 criteria \n if (balance > 1 && key < node.left.key)\n return rightRotate(node);\n \n // RR criteria\n if (balance < -1 && key > node.right.key)\n return leftRotate(node);\n \n // LR criteria\n if (balance > 1 && key > node.left.key)\n {\n node.left = leftRotate(node.left);\n return rightRotate(node);\n }\n \n // RL criteria\n if (balance < -1 && key < node.right.key)\n {\n node.right = rightRotate(node.right);\n return leftRotate(node);\n }\n return node;\n }\n \n // Given a non-empty BST, return the node with min. key value\n Node minValueNode(Node node)\n {\n Node current = node;\n \n while (current.left != null)\n current = current.left;\n \n return current;\n }\n \n Node deleteNode(Node root, int key)\n {\n //implementing bst delete\n if (root == null)\n return root;\n \n // If the key is smaller than root's key, left subtree\n if (key < root.key)\n root.left = deleteNode(root.left, key);\n \n // If the key is greater than root's key, right subtree\n else if (key > root.key)\n root.right = deleteNode(root.right, key);\n \n // if key is same as root's key, the node is deleted\n else\n {\n if ((root.left == null) || (root.right == null))\n {\n Node temp = null;\n if (temp == root.left)\n temp = root.right;\n else\n temp = root.left;\n if (temp == null)\n {\n temp = root;\n root = null;\n }\n else \n root = temp; \n }\n else\n {\n Node temp = minValueNode(root.right);\n root.key = temp.key;\n root.right = deleteNode(root.right, temp.key);\n }\n }\n \n // If the tree had only one node then return\n if (root == null)\n return root;\n \n // update height of current node\n root.height = max(height(root.left), height(root.right)) + 1;\n \n // find the balance factor\n int balance = getBalance(root);\n \n // If this node becomes unbalanced, then there are 4 criteria\n // LL criteria\n if (balance > 1 && getBalance(root.left) >= 0)\n return rightRotate(root);\n \n // LR criteria\n if (balance > 1 && getBalance(root.left) < 0)\n {\n root.left = leftRotate(root.left);\n return rightRotate(root);\n }\n \n // RR criteria\n if (balance < -1 && getBalance(root.right) <= 0)\n return leftRotate(root);\n \n // RiL criteria\n if (balance < -1 && getBalance(root.right) > 0)\n {\n root.right = rightRotate(root.right);\n return leftRotate(root);\n }\n \n return root;\n }\n \n void preOrder(Node node)\n {\n if (node != null)\n {\n System.out.print(node.key + \" \");\n preOrder(node.left);\n preOrder(node.right);\n }\n }\n \n public static void main(String[] args)\n {\n AVLTree tree = new AVLTree();\n\n /* Given for instance :\n 10\n / \\\n 2 11\n / \\ \\\n 1 6 12\n / / \\\n -2 3 7\n */\n tree.root = tree.insert(tree.root, 10);\n tree.root = tree.insert(tree.root, 6);\n tree.root = tree.insert(tree.root, 11);\n tree.root = tree.insert(tree.root, 1);\n tree.root = tree.insert(tree.root, 7);\n tree.root = tree.insert(tree.root, 12);\n tree.root = tree.insert(tree.root, -2);\n tree.root = tree.insert(tree.root, 2);\n tree.root = tree.insert(tree.root, 3);\n \n System.out.println(\"Preorder traversal of \"+\n \"constructed tree is : \");\n tree.preOrder(tree.root);\n tree.root = tree.deleteNode(tree.root, 11);\n System.out.println(\"\");\n System.out.println(\"Preorder traversal after \"+\n \"deletion of 10 :\");\n tree.preOrder(tree.root);\n }\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/avl_tree/avl_tree.py.py",
"content": "class node:\r\n def __init__(self, num):\r\n self.value = num\r\n self.left = None\r\n self.right = None\r\n self.height = 1\r\n\r\nclass AVL:\r\n\r\n def height(self, Node):\r\n if Node is None:\r\n return 0\r\n else:\r\n return Node.height\r\n\r\n def balance(self, Node):\r\n if Node is None:\r\n return 0\r\n else:\r\n return self.height(Node.left) - self.height(Node.right)\r\n\r\n def rotateR(self, Node):\r\n a = Node.left\r\n b = a.right\r\n\t\t\t\t\t\r\n a.right = Node\r\n Node.left = b\r\n Node.height = 1 + max(self.height(Node.left), self.height(Node.right))\r\n a.height = 1 + max(self.height(a.left), self.height(a.right))\r\n return a\r\n\r\n def rotateL(self, Node):\r\n a = Node.right\r\n b = a.left\r\n a.left = Node\r\n Node.right = b\r\n Node.height = 1 + max(self.height(Node.left), self.height(Node.right))\r\n a.height = 1 + max(self.height(a.left), self.height(a.right))\r\n return a\r\n\r\n def insert(self, val, root):\r\n if root is None:\r\n return node(val)\r\n elif val <= root.value:\r\n print(\"\\n%s Inserted as Left Child of %s\" % (val, root.value))\r\n root.left = self.insert(val, root.left)\r\n \r\n elif val > root.value:\r\n print(\"\\n%s Inserted as Right Child of %s\" % (val, root.value))\r\n root.right = self.insert(val, root.right)\r\n \r\n \r\n root.height = 1 + max(self.height(root.left), self.height(root.right))\r\n balance = self.balance(root)\r\n\r\n if balance > 1 and root.left.value > val:\r\n print(\"\\nROTATE RIGHT of \", root.value)\r\n return self.rotateR(root)\r\n \r\n if balance < -1 and val > root.right.value:\r\n print(\"\\nROTATE LEFT of \", root.value)\r\n return self.rotateL(root)\r\n \r\n if balance > 1 and val > root.left.value:\r\n print(\"\\nROTATE LEFT of \", root.value)\r\n root.left = self.rotateL(root.left)\r\n return self.rotateR(root)\r\n \r\n if balance < -1 and val < root.right.value:\r\n print(\"\\nROTATE RIGHT of \", root.value)\r\n root.right = self.rotateR(root.right)\r\n return self.rotateL(root)\r\n \r\n return root\r\n \r\n def inorder(self, root):\r\n if root is None:\r\n return\r\n self.inorder(root.left)\r\n print(root.value, end = '->')\r\n self.inorder(root.right)\r\n\r\n \r\nTree = AVL()\r\nrt = None\r\n\r\nwhile True:\r\n choice = int(input(\"To Continue Insertion Enter 1 Else 0: \"))\r\n if choice == 0:\r\n break\r\n item = int(input(\"Enter the item to be inserted : \"))\r\n\r\n rt = Tree.insert(item, rt)\r\n Tree.inorder(rt)\r"
}
{
"filename": "code/data_structures/src/tree/binary_tree/avl_tree/avl_tree.swift",
"content": "public class TreeNode<Key: Comparable, Payload> {\n public typealias Node = TreeNode<Key, Payload>\n\n var payload: Payload?\n\n fileprivate var key: Key\n internal var leftChild: Node?\n internal var rightChild: Node?\n fileprivate var height: Int\n fileprivate weak var parent: Node?\n\n public init(key: Key, payload: Payload?, leftChild: Node?, rightChild: Node?, parent: Node?, height: Int) {\n self.key = key\n self.payload = payload\n self.leftChild = leftChild\n self.rightChild = rightChild\n self.parent = parent\n self.height = height\n\n self.leftChild?.parent = self\n self.rightChild?.parent = self\n }\n\n public convenience init(key: Key, payload: Payload?) {\n self.init(key: key, payload: payload, leftChild: nil, rightChild: nil, parent: nil, height: 1)\n }\n\n public convenience init(key: Key) {\n self.init(key: key, payload: nil)\n }\n\n var isRoot: Bool {\n return parent == nil\n }\n\n var isLeaf: Bool {\n return rightChild == nil && leftChild == nil\n }\n\n var isLeftChild: Bool {\n return parent?.leftChild === self\n }\n\n var isRightChild: Bool {\n return parent?.rightChild === self\n }\n\n var hasLeftChild: Bool {\n return leftChild != nil\n }\n\n var hasRightChild: Bool {\n return rightChild != nil\n }\n\n var hasAnyChild: Bool {\n return leftChild != nil || rightChild != nil\n }\n\n var hasBothChildren: Bool {\n return leftChild != nil && rightChild != nil\n }\n}\n\nopen class AVLTree<Key: Comparable, Payload> {\n public typealias Node = TreeNode<Key, Payload>\n\n fileprivate(set) var root: Node?\n fileprivate(set) var size = 0\n\n public init() { }\n}\n\nextension TreeNode {\n public func minimum() -> TreeNode? {\n return leftChild?.minimum() ?? self\n }\n\n public func maximum() -> TreeNode? {\n return rightChild?.maximum() ?? self\n }\n}\n\nextension AVLTree {\n subscript(key: Key) -> Payload? {\n get { return search(input: key) }\n set { insert(key: key, payload: newValue) }\n }\n\n public func search(input: Key) -> Payload? {\n return search(key: input, node: root)?.payload\n }\n\n fileprivate func search(key: Key, node: Node?) -> Node? {\n if let node = node {\n if key == node.key {\n return node\n } else if key < node.key {\n return search(key: key, node: node.leftChild)\n } else {\n return search(key: key, node: node.rightChild)\n }\n }\n return nil\n }\n}\n\nextension AVLTree {\n public func insert(key: Key, payload: Payload? = nil) {\n if let root = root {\n insert(input: key, payload: payload, node: root)\n } else {\n root = Node(key: key, payload: payload)\n }\n size += 1\n }\n\n private func insert(input: Key, payload: Payload?, node: Node) {\n if input < node.key {\n if let child = node.leftChild {\n insert(input: input, payload: payload, node: child)\n } else {\n let child = Node(key: input, payload: payload, leftChild: nil, rightChild: nil, parent: node, height: 1)\n node.leftChild = child\n balance(node: child)\n }\n } else {\n if let child = node.rightChild {\n insert(input: input, payload: payload, node: child)\n } else {\n let child = Node(key: input, payload: payload, leftChild: nil, rightChild: nil, parent: node, height: 1)\n node.rightChild = child\n balance(node: child)\n }\n }\n }\n}\n\nextension AVLTree \n{\n fileprivate func updateHeightUpwards(node: Node?) \n {\n if let node = node \n {\n let lHeight = node.leftChild?.height ?? 0\n let rHeight = node.rightChild?.height ?? 0\n node.height = max(lHeight, rHeight) + 1\n updateHeightUpwards(node: node.parent)\n }\n }\n\n fileprivate func lrDifference(node: Node?) -> Int \n {\n let lHeight = node?.leftChild?.height ?? 0\n let rHeight = node?.rightChild?.height ?? 0\n return lHeight - rHeight\n }\n\n fileprivate func balance(node: Node?) \n {\n guard let node = node else {\n return\n }\n\n updateHeightUpwards(node: node.leftChild)\n updateHeightUpwards(node: node.rightChild)\n\n var nodes = [Node?](repeating: nil, count: 3)\n var subtrees = [Node?](repeating: nil, count: 4)\n let nodeParent = node.parent\n\n let lrFactor = lrDifference(node: node)\n if lrFactor > 1 \n {\n if lrDifference(node: node.leftChild) > 0 \n {\n nodes[0] = node\n nodes[2] = node.leftChild\n nodes[1] = nodes[2]?.leftChild\n\n subtrees[0] = nodes[1]?.leftChild\n subtrees[1] = nodes[1]?.rightChild\n subtrees[2] = nodes[2]?.rightChild\n subtrees[3] = nodes[0]?.rightChild\n } \n else \n {\n nodes[0] = node\n nodes[1] = node.leftChild\n nodes[2] = nodes[1]?.rightChild\n\n subtrees[0] = nodes[1]?.leftChild\n subtrees[1] = nodes[2]?.leftChild\n subtrees[2] = nodes[2]?.rightChild\n subtrees[3] = nodes[0]?.rightChild\n }\n } \n else if lrFactor < -1 \n {\n if lrDifference(node: node.rightChild) < 0 \n {\n nodes[1] = node\n nodes[2] = node.rightChild\n nodes[0] = nodes[2]?.rightChild\n\n subtrees[0] = nodes[1]?.leftChild\n subtrees[1] = nodes[2]?.leftChild\n subtrees[2] = nodes[0]?.leftChild\n subtrees[3] = nodes[0]?.rightChild\n } \n else \n { \n nodes[1] = node\n nodes[0] = node.rightChild\n nodes[2] = nodes[0]?.leftChild\n\n subtrees[0] = nodes[1]?.leftChild\n subtrees[1] = nodes[2]?.leftChild\n subtrees[2] = nodes[2]?.rightChild\n subtrees[3] = nodes[0]?.rightChild\n }\n } \n else \n {\n balance(node: node.parent)\n return\n }\n\n if node.isRoot \n {\n root = nodes[2]\n root?.parent = nil\n } \n else if node.isLeftChild \n {\n nodeParent?.leftChild = nodes[2]\n nodes[2]?.parent = nodeParent\n } \n else if node.isRightChild \n {\n nodeParent?.rightChild = nodes[2]\n nodes[2]?.parent = nodeParent\n }\n\n nodes[2]?.leftChild = nodes[1]\n nodes[1]?.parent = nodes[2]\n nodes[2]?.rightChild = nodes[0]\n nodes[0]?.parent = nodes[2]\n\n nodes[1]?.leftChild = subtrees[0]\n subtrees[0]?.parent = nodes[1]\n nodes[1]?.rightChild = subtrees[1]\n subtrees[1]?.parent = nodes[1]\n\n nodes[0]?.leftChild = subtrees[2]\n subtrees[2]?.parent = nodes[0]\n nodes[0]?.rightChild = subtrees[3]\n subtrees[3]?.parent = nodes[0]\n\n updateHeightUpwards(node: nodes[1]) \n updateHeightUpwards(node: nodes[0]) \n\n balance(node: nodes[2]?.parent)\n }\n}\n\nextension AVLTree \n{\n fileprivate func display(node: Node?, level: Int) \n {\n if let node = node \n {\n display(node: node.rightChild, level: level + 1)\n print(\"\")\n if node.isRoot \n {\n print(\"Root -> \", terminator: \"\")\n }\n for _ in 0..<level \n {\n print(\" \", terminator: \"\")\n }\n print(\"(\\(node.key):\\(node.height))\", terminator: \"\")\n display(node: node.leftChild, level: level + 1)\n }\n }\n\n public func display(node: Node) \n {\n display(node: node, level: 0)\n print(\"\")\n }\n}\n\nextension AVLTree \n{\n public func delete(key: Key) \n {\n if size == 1 \n {\n root = nil\n size -= 1\n } \n else if let node = search(key: key, node: root) \n {\n delete(node: node)\n size -= 1\n }\n }\n\n private func delete(node: Node) \n {\n if node.isLeaf \n {\n if let parent = node.parent \n {\n guard node.isLeftChild || node.isRightChild else \n {\n fatalError(\"Error: tree is invalid.\")\n }\n\n if node.isLeftChild \n {\n parent.leftChild = nil\n } \n else if node.isRightChild \n {\n parent.rightChild = nil\n }\n\n balance(node: parent)\n } \n else \n {\n root = nil\n }\n } \n else \n {\n if let replacement = node.leftChild?.maximum(), replacement !== node {\n node.key = replacement.key\n node.payload = replacement.payload\n delete(node: replacement)\n } \n else if let replacement = node.rightChild?.minimum(), replacement !== node \n {\n node.key = replacement.key\n node.payload = replacement.payload\n delete(node: replacement)\n }\n }\n }\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_search_tree/BST_Operations.cpp",
"content": "#include<iostream>\n#include<algorithm>\nusing namespace std;\nclass BST{\nprivate:\n int data;\n BST *left,*right;\npublic:\n //Constructors:\n BST(){\n data=0;\n left=right=NULL;\n }\n BST(int val){\n data=val;\n left=right=NULL;\n }\n //Inserting a Node into BST:\n BST* InsertNode(BST*,int);\n //Delete a Node from BST:\n BST* DeletNode(BST*,int);\n //Traversals:\n void InOrder(BST*);\n void PreOrder(BST*);\n void PostOrder(BST*);\n //Searching\n BST* SearchNode(BST*,int);\n};\nBST* BST::InsertNode(BST *root,int key){\n if(root==NULL){\n root=new BST(key);\n return root;\n }\n else if(key<=root->data){\n if(root->left==NULL){\n root->left=new BST(key);\n return root;\n }\n else{\n root->left=InsertNode(root->left,key);\n return root;\n }\n }\n else{\n if(root->right==NULL){\n root->right=new BST(key);\n return root;\n }\n else {\n root->right=InsertNode(root->right,key);\n return root;\n }\n }\n}\nBST* BST::DeletNode(BST *root,int key){\n // Base case \n if (root == NULL) \n return root; \n //If root->data is greater than k then we delete the root's subtree\n if(root->data > key){ \n root->left = DeletNode(root->left, key); \n return root; \n } \n else if(root->data < key){ \n root->right = DeletNode(root->right, key); \n return root; \n } \n // If one of the children is empty \n if (root->left == NULL) { \n BST* temp = root->right;\n delete root; \n return temp; \n } \n else if (root->right == NULL) { \n BST* temp = root->left; \n delete root; \n return temp; \n } \n else {\n BST* Parent = root;\n // Find successor of the Node\n BST *succ = root->right; \n while (succ->left != NULL) { \n Parent = succ; \n succ = succ->left; \n } \n\n if (Parent != root) \n Parent->left = succ->right; \n else\n Parent->right = succ->right; \n\n // Copy Successor Data \n root->data = succ->data; \n\n // Delete Successor and return root \n delete succ;\n return root; \n } \n}\nBST* BST::SearchNode(BST *root,int key){\n //Base Case\n if(root==NULL||root->data==key)\n return root;\n if(root->data>key){\n return SearchNode(root->left,key);\n }\n return SearchNode(root->right,key);\n}\nvoid BST::InOrder(BST *root){\n //InOrder traversal of a Binary Search Tree gives sorted order.\n if(root==NULL)return;\n InOrder(root->left);\n cout<<root->data<<\" \";\n InOrder(root->right);\n}\nvoid BST::PreOrder(BST *root){\n if(root==NULL)return;\n cout<<root->data<<\" \";\n PreOrder(root->left);\n PreOrder(root->right);\n}\nvoid BST::PostOrder(BST *root){\n if(root==NULL)return;\n PostOrder(root->left);\n PostOrder(root->right);\n cout<<root->data<<\" \";\n}\nint main(){\n int choice,element;\n BST B,*root=NULL;\n while(true){\n cout << \"------------------\\n\";\n cout << \"Operations on Binary Search Tree\\n\";\n cout << \"------------------\\n\";\n cout << \"1.Insert Element\\n\";\n cout << \"2.Delete Element\\n\";\n cout << \"3.Search Element\\n\";\n cout << \"4.Traversals\\n\";\n cout << \"5.Exit\\n\";\n\n cout << \"Enter your choice: \";\n cin >> choice;\n\n switch(choice){\n case 1:\n cout << \"Enter the element to be inserted: \";\n cin >> element;\n root=B.InsertNode(root,element);\n break;\n case 2:\n cout << \"Enter the element to be deleted: \";\n cin >> element;\n root=B.DeletNode(root,element);\n break;\n case 3:\n cout << \"Search Element: \";\n cout << \"Enter the element to be Searched: \";\n cin >> element;\n if (B.SearchNode(root,element)){\n cout << \"Element Found \\n\" ;\n }\n else\n cout << \"Element Not Found\\n\";\n break;\n case 4:\n cout << \"Displaying elements of BST: \";\n cout <<\"\\nInORder: \";\n B.InOrder(root);\n cout <<\"\\nPreORder: \";\n B.PreOrder(root);\n cout <<\"\\nPostORder: \";\n B.PostOrder(root);\n cout<<endl;\n break;\n case 5:\n return 1;\n default:\n cout << \"Enter Correct Choice \\n\";\n }\n }\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_search_tree/README.md",
"content": "# Binary Search Tree\n\nDescription\n---\nBinary Search Tree is basically a binary tree which has the following properties:\n\n* The left subtree of a node contains only nodes with values **lesser** than the node’s value.\n* The right subtree of a node contains only nodes with value **greater** than the node’s value.\n* The left and right subtree each must also be a binary search tree.\n\nOperations performed on binary search tree are:\n1. Insertion\n2. Deletion\n3. Searching\n\nTraversals of a Binary Search tree:\n\n* Inorder: `Left, Root, Right`\n* Preorder: `Root, Left, Right`\n* Postorder: `Left, Right, Root`<br>\n\nNote: In Binary Search Tree inorder traversal gives the sorted order of the elements in BST."
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/balance_binary_tree/balance_bst_dsw.cpp",
"content": "#include <stdio.h>\n#include <stdlib.h>\n#include <iostream>\n\nusing namespace std;\n\nstruct node\n{\n int key;\n struct node *left, *right;\n};\n\nstruct node *newNode(int value)\n{\n struct node *temp = new node;\n temp->key = value;\n temp->left = temp->right = NULL;\n return temp;\n}\n\nstruct node* insert(struct node* node, int key)\n{\n if (node == NULL)\n return newNode(key);\n if (key < node->key)\n node->left = insert(node->left, key);\n else if (key > node->key)\n node->right = insert(node->right, key);\n return node;\n}\n\n//Inorder traversal\nvoid inorder(struct node *root)\n{\n if (root)\n {\n inorder(root->left);\n cout << root->key << \" \";\n inorder(root->right);\n }\n}\n\n//Preorder traversal\nvoid preorder(struct node *root)\n{\n if (root)\n {\n cout << root->key << \" \";\n preorder(root->left);\n preorder(root->right);\n }\n}\n\n\nstruct node* leftRotate(struct node * root)\n{\n struct node *right, *right_left;\n if ( (root == NULL) || (root->right ==NULL) )\n return root;\n right = root->right;\n right_left = right->left;\n right->left = root;\n root->right = right_left;\n return right;\n}\n\nstruct node* rightRotate(struct node * root){\n struct node *left, *left_right;\n if ( (root == NULL) || (root->left == NULL) )\n return root;\n left = root->left;\n left_right = left->right;\n left->right = root;\n root->left = left_right;\n return left;\n}\n\n/*\nMake a vine structure rooted at a dummy root and \nbranching only towards right\n*/\n\nstruct node* createBackbone(struct node* root)\n{\n struct node *parent, *r;\n r = newNode(-1);\n parent = r;\n r->right = root;\n // making tree skewed towards right;\n while (parent->right)\n {\n if (parent->right->left == NULL)\n parent = parent->right;\n else\n parent->right = rightRotate(parent->right); \n }\n return r;\n};\n\n\n/*\nCompress the tree using series of left rotations on\nalternate nodes provided by the count.\n*/\nstruct node* compress(struct node* root, int cnt)\n{\n struct node *temp;\n temp = root;\n while (cnt && temp)\n {\n temp->right = leftRotate(temp->right);\n temp = temp->right;\n cnt -= 1;\n }\n return root;\n}\n\n// To calculate height of the tree\nint height(struct node* root)\n{\n int left, right;\n if (root == NULL)\n return -1;\n left = height(root->left);\n right = height(root->right);\n left = left >= right ? left : right;\n return 1 + left;\n}\n\nstruct node* balanceTree(struct node* root)\n{\n root = createBackbone(root);\n \n /*\n Now a dummy root is added so we get original\n root from root->right;\n */\n cout << \"Backbone Tree structure\" << endl;\n cout << \"Inorder traversal: \";\n inorder(root->right);\n cout << \"\\nPreorder traversal: \";\n preorder(root->right);\n cout << endl;\n\n // h = total number of nodes\n int h = height(root);\n int left_count, temp, l = 0;\n temp = h + 1;\n\n // l = log(n+1) (base 2)\n while (temp > 1)\n {\n temp /= 2;\n l += 1; \n }\n \n /*\n make n-m rotations starting from the top of backbone\n where m = 2^( floor(lg(n+1)))-1 \n */\n left_count = h + 1 - (1 << l);\n if (left_count == 0)\n {\n left_count = (1 << (l - 1));\n }\n\n root = compress(root, left_count);\n h -= left_count ;\n\n while (h > 1)\n {\n h /= 2;\n root = compress(root, h);\n }\n\n return root;\n};\n\nint main()\n{\n /* Let us create following BST\n * 50\n * \\\n * 70\n * /\n * 60 */\n\n struct node *root = NULL;\n root = insert(root, 50);\n insert(root, 70);\n insert(root, 60);\n // insert(root, 80);\n \n root = balanceTree(root);\n root = root->right;\n\n /* Back-bone structure of above BST\n * 50\n * \\\n * 60 \n * \\ \n * 70 */\n\n\n /* Balanced BST\n * 60\n * / \\\n * 50 70 */\n\n cout << endl;\n \n cout << \"Balanced Tree Structure \" << endl;\n cout << \"Inorder traversal: \";\n inorder(root);\n\n cout << \"\\nPreorder traversal: \";\n preorder(root);\n \n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/balance_binary_tree/BST.py",
"content": "class BinaryTree:\r\n def __init__(self,value):\r\n self.left=None\r\n self.right=None\r\n self.value=value\r\n def insert(self,data):\r\n if self.value:\r\n if data<self.value:\r\n if self.left is None:\r\n self.left=BinaryTree(data)\r\n else:\r\n self.left.insert(data)\r\n elif data > self.value:\r\n if self.right is None:\r\n self.right=BinaryTree(data)\r\n else:\r\n self.right.insert(data)\r\n else:\r\n self.value=data\r\n def inorderprint(self):\r\n if self.left:\r\n self.left.inorderprint()\r\n print(self.value)\r\n if self.right:\r\n self.right.inorderprint()\r\n def Postorderprint(self):\r\n if self.left:\r\n self.left.Postorderprint()\r\n if self.right:\r\n self.right.Postorderprint()\r\n print(self.value)\r\n def Preorderprint(self):\r\n print(self.value)\r\n if self.left:\r\n self.left.Preorderprint()\r\n if self.right:\r\n self.right.Preorderprint()\r\n\r\nchoice=\"s\"\r\nrootvalue=int(input(\"Enter a value for the root: \"))\r\nroot=BinaryTree(rootvalue)\r\nwhile(choice==\"s\"):\r\n e=int(input(\"Enter element to be inserted: \"))\r\n root.insert(e)\r\n choice=input(\"Do you wish to insert another element(s/n)\")\r\nwhile(True):\r\n traversal=int(input(\"1.Preorder\\n2.Postorder\\n3.Inorder\\n4.Exit\\n\"))\r\n if traversal==1:\r\n root.Preorderprint()\r\n if traversal==2:\r\n root.Postorderprint()\r\n if traversal==3:\r\n root.inorderprint()\r\n if traversal==4:\r\n break\r"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/convert_to_doubly_linked_list/convert_to_doubly_linked_list.cpp",
"content": "/* Structure for tree and linked list\n *\n * struct Node\n * {\n * int data;\n * Node *left, *right;\n * };\n *\n */\n\n// root --> Root of Binary Tree\n// head_ref --> Pointer to head node of created doubly linked list\n\ntemplate<typename Node>\nvoid BToDLL(Node *root, Node **head_ref)\n{\n if (!root)\n return;\n\n BToDLL(root->right, head_ref);\n root->right = *head_ref;\n\n if (*head_ref)\n (*head_ref)->left = root;\n *head_ref = root;\n\n BToDLL(root->left, head_ref);\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/count_universal_subtree/count_universal_subtrees.cpp",
"content": "#include <iostream>\nusing namespace std;\n//binary tree structure\nstruct node{\n int data;\n struct node* left,*right;\n\n};\nbool findUniValueST(node *root , int &count)\n{\n if(root==NULL)\n return true;\n bool right=findUniValueST(root->right,count);\n bool left=findUniValueST(root->left,count);\n //if left or right subtree is not univalued then that subtree is not univalued\n if(right==false||left==false)\n return false;\n //if right node exists and the value is not equal to root's value,again not univalued\n if(root->right && root->right->data!=root->data)\n return false;\n //same for left node also.\n if(root->left && root->left->data!=root->data)\n return false;\n /*if above possible conditions not satisified then its a univalued subtree.\n Like this we increment as and when there is a univalued subtree*/\n count++;\n /*and return true*/\n return true;\n\n\n}\n//to return count of the univalued subtrees.\nint countUniValueST(node *root ){\n int count=0;\n findUniValueST(root,count);\n return count;\n}\n//for inserting a new node\nnode *newNode(int data){\n node *temp=new node;\n temp->data=data;\n temp->right=NULL;\n temp->left=NULL;\n return (temp);\n}\nint main() {\n//sample input of the binary tree\n struct node* root=newNode(1);\n root->left=newNode(3);\n root->right=newNode(3);\n root->left->right=newNode(3);\n root->left->left=newNode(3);\n cout<<\"Number of univalued subtrees:\"<<\" \"<<countUniValueST(root)<<endl;\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/diameter/diameter2.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n\n#define MAX(x, y) (((x) > (y)) ? (x) : (y))\n\ntypedef struct node{\n int data; \n struct node *left;\n struct node *right;\n}node;\n\nnode* newnode(int d){\n node* temp = (node*)malloc(sizeof(node));\n temp->data = d;\n temp->left = NULL;\n temp->right = NULL;\n}\n\nnode *buildTree(){\n int d;\n printf(\"\\nEnter data:\");\n scanf(\"%d\", &d);\n\n if(d==-1){\n return NULL;\n }\n\n ///rec case\n node *n =newnode(d);\n n->left = buildTree();\n n->right = buildTree();\n return n;\n}\n\nint height(node *root){\n if(root==NULL){\n return 0;\n }\n\n return (MAX./(height(root->left),height(root->right))+1);\n}\n\nint diameter(node*root){\n if(root==NULL){\n return 0;\n }\n\n int cp1 = height(root->left) + height(root->right);\n int cp2 = diameter(root->left);\n int cp3 = diameter(root->right);\n\n return MAX(cp1,MAX(cp2,cp3));\n}\n\nint main(){\n\n node *root = NULL;\n root = buildTree();\n printf(\"\\nDiameter of Binary Tree: \");\n printf(\"%d\", diameter(root));\n \n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/diameter/diameter2.cpp",
"content": "/*\n * Data Structures : Finding Diameter of a binary tree\n *\n * Description : Diameter of tree is defined as the longest path or route between any two nodes in a tree.\n * This path may or may not be through the root. The below algorithm computes the height of\n * the tree and uses it recursivley in the calculation of diameter of the specified tree.\n *\n * A massive collaborative effort by OpenGenus Foundation\n */\n\n#include <iostream>\nusing namespace std;\n\n// get the max of two no.s\nint max(int a, int b)\n{\n return (a > b) ? a : b;\n}\n\ntypedef struct node\n{\n int value;\n struct node *left, *right;\n} node;\n\n// create a new node\nnode *getNewNode(int value)\n{\n node *new_node = new node;\n new_node->value = value;\n new_node->left = NULL;\n new_node->right = NULL;\n return new_node;\n}\n\n// compute height of the tree\nint getHeight(node *root)\n{\n if (root == NULL)\n return 0;\n\n // find the height of each subtree\n int lh = getHeight(root->left);\n int rh = getHeight(root->right);\n\n return 1 + max(lh, rh);\n}\n\n// compute tree diameter recursively\nint getDiameter(node *root)\n{\n if (root == NULL)\n return 0;\n\n // get height of each subtree\n int lh = getHeight(root->left);\n int rh = getHeight(root->right);\n\n // compute diameters of each subtree\n int ld = getDiameter(root->left);\n int rd = getDiameter(root->right);\n\n return max(lh + rh + 1, max(ld, rd));\n}\n\n// create the tree\nnode *createTree()\n{\n node *root = getNewNode(31);\n root->left = getNewNode(16);\n root->right = getNewNode(52);\n root->left->left = getNewNode(7);\n root->left->right = getNewNode(24);\n root->left->right->left = getNewNode(19);\n root->left->right->right = getNewNode(29);\n return root;\n}\n\n// main\nint main()\n{\n node *root = createTree();\n cout << \"\\nDiameter of the tree is \" << getDiameter(root);\n cout << endl;\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/diameter/diameter.c",
"content": "#include <stdio.h>\n#include <unistd.h>\n#include <stdlib.h>\n\ntypedef struct node {\n\t\t\tint value;\n\t\t\tstruct node *left, *right;\n\t}node;\n\nnode* create_node(int );\nvoid create_tree(int );\nvoid cleanup_tree(node* );\nint maximum(int , int );\nint height_tree(node* );\nint diameter_tree(node* );\n\nnode* root = NULL;\nnode* create_node(int val)\n{\n\tnode* tmp = (node *) malloc(sizeof(node));\n\ttmp->value = val;\n\ttmp->left = tmp->right = NULL;\n\treturn tmp;\n}\n\nvoid create_tree(int val)\n{\n\tnode* tmp = root;\n\tif (!root)\n\t root = create_node(val);\n\telse\n {\n while (1)\n\t {\n\t if (val > tmp->value)\n {\n if (!tmp->right)\n {\n\t\t tmp->right = create_node(val);\n break;\n }\n else\n tmp = tmp->right;\n }\n\t else\n {\n if (!tmp->left)\n {\n\t\t tmp->left= create_node(val);\n break;\n }\n else\n tmp = tmp->left;\n }\t\n\t }\n\t}\n\t\n}\n\n int maximum(int left_ht, int right_ht)\n {\n return (left_ht > right_ht ? left_ht : right_ht);\n }\n\nint height_tree(node* tmp)\n{\n int left_ht, right_ht;\n if (!tmp)\n return 0;\n left_ht = height_tree(tmp->left);\n right_ht = height_tree(tmp->right);\n return 1+maximum(left_ht, right_ht);\n}\n\n//Prints the longest path between the leaf nodes\nint diameter_tree(node* tmp)\n{\n int leaf_depth, left_subtree_height, right_subtree_height, max_depth_between_left_and_right_subtree;\n if (!tmp)\n return 0;\n leaf_depth = height_tree(tmp->left) + height_tree(tmp->right);\n left_subtree_height = diameter_tree(tmp->left);\n right_subtree_height = diameter_tree(tmp->right);\n max_depth_between_left_and_right_subtree = maximum(left_subtree_height, right_subtree_height);\n return maximum(leaf_depth, max_depth_between_left_and_right_subtree);\n}\n\nvoid cleanup_tree(node* tmp)\n{\n if (tmp)\n {\n\tcleanup_tree(tmp->left);\n\tcleanup_tree(tmp->right);\n if (tmp->left)\n {\n free(tmp->left);\n \t tmp->left = NULL;\n }\n if (tmp->right)\n {\n free(tmp->right);\n \t tmp->right= NULL;\n }\n }\n if (tmp == root)\n {\n free(root);\n root = NULL;\n }\n}\n\n\nint main()\n{\n int val, num, ctr,longest_path_between_leaf_nodes;\n node tmp;\n\tprintf(\"Enter number of nodes\\n\");\n scanf(\"%d\",&num);\n for (ctr = 0; ctr < num; ctr++)\n {\n\t printf(\"Enter values\\n\");\n\t scanf(\"%d\",&val);\n\t create_tree(val);\n }\n longest_path_between_leaf_nodes = diameter_tree(root);\n printf(\"diameter_tree = %d\\n\",longest_path_between_leaf_nodes);\n cleanup_tree(root);\n return 0; \n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/diameter/diameter.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n *\n * diameter of tree synopsis\n *\n * template<typename _TreeNode>\n * size_t\n * diameter(_TreeNode node);\n *\n * template<typename _TreeNode>\n * void\n * diameterIterative(_TreeNode const &node, size_t &maximum);\n *\n * template<typename _TreeNode>\n * size_t\n * getDiameter(_TreeNode const &node);\n *\n * template<typename _TreeNode>\n * size_t\n * getDeep(_TreeNode const &node);\n *\n * template<typename _TreeNode>\n * size_t\n * diameterRecursive(_TreeNode node, size_t &maximum);\n */\n\n#include <algorithm>\n#include <memory>\n#include <stack>\n#include \"../node/node.cpp\"\n\ntemplate<typename _TreeNode>\nsize_t\ndiameter(_TreeNode node)\n{\n size_t res{};\n diameterIterative(node, res);\n\n return res;\n}\n\ntemplate<typename _TreeNode>\nvoid\ndiameterIterative(_TreeNode const &node, size_t &maximum)\n{\n maximum = 0;\n if (node != nullptr)\n {\n std::stack<_TreeNode> diameters;\n diameters.push(node);\n\n // DFS\n while (!diameters.empty())\n {\n while (diameters.top()->left() != nullptr)\n diameters.push(diameters.top()->left());\n while (!diameters.empty()\n && (diameters.top() == nullptr\n || diameters.top()->right() == nullptr))\n {\n if (diameters.top() == nullptr) // if back from right hand\n diameters.pop();\n auto top = diameters.top();\n maximum = std::max(maximum, static_cast<size_t>(getDiameter(top)));\n top->value(static_cast<int>(getDeep(top)));\n diameters.pop();\n }\n if (!diameters.empty())\n {\n auto right = diameters.top()->right();\n diameters.push(nullptr); // prevent visit two times when return to parent\n diameters.push(right);\n }\n }\n }\n}\n\ntemplate<typename _TreeNode>\nsize_t\ngetDiameter(_TreeNode const &node)\n{\n size_t res = 1;\n res += node->left() ? node->left()->value() : 0;\n res += node->right() ? node->right()->value() : 0;\n\n return res;\n}\n\ntemplate<typename _TreeNode>\nsize_t\ngetDeep(_TreeNode const &node)\n{\n size_t res = 1;\n res += std::max(node->left() ? node->left()->value() : 0,\n node->right() ? node->right()->value() : 0);\n\n return res;\n}\n\ntemplate<typename _TreeNode>\nsize_t\ndiameterRecursive(_TreeNode node, size_t &maximum)\n{\n if (node != nullptr)\n {\n size_t leftMax{}, rightMax{};\n\n // DFS\n size_t leftHeight = diameterRecursive(node->left(), leftMax);\n size_t rightHeight = diameterRecursive(node->right(), rightMax);\n\n maximum = leftHeight + rightHeight + 1;\n maximum = std::max(maximum, leftMax);\n maximum = std::max(maximum, rightMax);\n\n return std::max(leftHeight, rightHeight) + 1;\n }\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/diameter/diameter.hs",
"content": "data Tree = Nil | Tree `Fork` Tree\n deriving Show\n\nleaf :: Tree\nleaf = Fork Nil Nil\n\ndiameter :: Tree -> Int\ndiameter = snd . go\n where\n -- go keeps track of the diameter as well as the depth of the tree\n go :: Tree -> (Int, Int)\n go Nil = (0, 0)\n go (Fork t1 t2) = (ht, dt)\n where\n (h1, d1) = go t1\n (h2, d2) = go t2\n ht = 1 + max h1 h2\n dt = maximum [(h1 + h2), d1, d2]"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/diameter/diameter.java",
"content": "//Diameter of binary tree using java\nclass Node\n{\n\tint data;\n\tNode left, right;\n\n\tNode(int i)\n\t{\n\t\tdata = i;\n\t\tleft = right = null;\n\t}\n}\n\nclass Height\n{\n\tint h;\n}\n\nclass BinaryTreeDiameter\n{\n\tNode root;\n\n\t//Function to calculate diameter\n\tint calldiameter(Node root, Height height)\n\t{\n\t\tHeight l = new Height();//height of left sub-tree\n\t\tHeight r = new Height();//height of right sub-tree\n\n\t\tif (root == null)\n\t\t{\n\t\t\theight.h = 0;\n\t\t\treturn 0; \n\t\t}\n\t\n\t\tint ld = calldiameter(root.left, l);//diameter of left-subtree\n\t\tint rd = calldiameter(root.right, r);//diameter of right-subtree\n\n\t\t//Height of current node is max(left height,right height)+1\n\t\theight.h = Math.max(l.h,r.h) + 1;\n\n\t\treturn Math.max(l.h + r.h + 1, Math.max(ld, rd));\n\t}\n\n\n\tint diameter()\n\t{\n\t\tHeight height = new Height();\n\t\treturn calldiameter(root, height);\n\t}\n\n\t//Function to calculate height of tree\n\tstatic int height(Node node)\n\t{\n\t\t//tree is empty\n\t\tif (node == null)\n\t\t\treturn 0;\n\n\t\t//If not empty then height = 1 + max(left height,right height)\n\t\treturn (1 + Math.max(height(node.left), height(node.right)));\n\t}\n\n\tpublic static void main(String args[])\n\t{\n\t\t//Creating a binary tree\n\t\tBinaryTreeDiameter t = new BinaryTreeDiameter();\n\t\tt.root = new Node(1);\n\t\tt.root.left = new Node(3);\n\t\tt.root.right = new Node(2);\n\t\tt.root.left.left = new Node(7);\n\t\tt.root.left.right = new Node(6);\n t.root.right.left = new Node(5);\n\t\tt.root.right.right = new Node(4);\n\t\tt.root.left.left.right = new Node(10);\n t.root.right.left.left = new Node(9);\n\t\tt.root.right.left.right = new Node(8);\n\t\t\n\n\t\tSystem.out.println(\"The Diameter of given binary tree is : \"+ t.diameter());\n\t}\n}\n\n/*Input:\n 1\n / \\ \n 3 2 \n / \\ / \\\n 7 6 5 4\n \\ / \\\n 10 9 8\n*/\n/*Output:\nThe Diameter of given binary tree is : 7\n*/"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/diameter/diameter.py",
"content": "# Time: O(n)\n# Space: O(h)\n\n# Given a binary tree, you need to compute the length of the diameter of the tree.\n# The diameter of a binary tree is the length of the longest path between\n# any two nodes in a tree. This path may or may not pass through the root.\n#\n# Example:\n# Given a binary tree\n# 1\n# / \\\n# 2 3\n# / \\\n# 4 5\n# Return 3, which is the length of the path [4,2,1,3] or [5,2,1,3].\n#\n# Note: The length of path between two nodes is represented by the number of edges between them.\n\n# Definition for a binary tree node.\nclass TreeNode(object):\n def __init__(self, x):\n self.val = x\n self.left = None\n self.right = None\n\n\n# The function diameter_height returns the diameter and the height of the tree.\n# And the function find_tree_diameter uses it to just compute the diameter (by discarding the height).\nclass Solution:\n def diameter_height(node):\n if node is None:\n return 0, 0\n ld, lh = diameter_height(node.left)\n rd, rh = diameter_height(node.right)\n return max(lh + rh + 1, ld, rd), 1 + max(lh, rh)\n\n def find_tree_diameter(node):\n d, _ = diameter_height(node)\n return d\n\n\ndiameter = Solution()"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/diameter/README.md",
"content": "# Cosmos\n\nCollaborative effort by [OpenGenus](https://github.com/OpenGenus/cosmos)"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/is_balance/is_balance.java",
"content": "\n\nclass Node \n{\n int data;\n Node left, right;\n Node(int d) \n {\n data = d;\n left = right = null;\n }\n}\n \nclass BinaryTree \n{\n Node root;\n \n /* Returns true if binary tree with root as root is height-balanced */\n boolean isBalanced(Node node) \n {\n int lh; /* for height of left subtree */\n \n int rh; /* for height of right subtree */\n \n /* If tree is empty then return true */\n if (node == null)\n return true;\n \n /* Get the height of left and right sub trees */\n lh = height(node.left);\n rh = height(node.right);\n \n if (Math.abs(lh - rh) <= 1\n && isBalanced(node.left)\n && isBalanced(node.right)) \n return true;\n \n /* If we reach here then tree is not height-balanced */\n return false;\n }\n \n /* UTILITY FUNCTIONS TO TEST isBalanced() FUNCTION */\n /* The function Compute the \"height\" of a tree. Height is the\n number of nodes along the longest path from the root node\n down to the farthest leaf node.*/\n int height(Node node) \n {\n /* base case tree is empty */\n if (node == null)\n return 0;\n \n /* If tree is not empty then height = 1 + max of left\n height and right heights */\n return 1 + Math.max(height(node.left), height(node.right));\n }\n \n public static void main(String args[]) \n {\n BinaryTree tree = new BinaryTree();\n tree.root = new Node(1);\n tree.root.left = new Node(2);\n tree.root.right = new Node(3);\n tree.root.left.left = new Node(4);\n tree.root.left.right = new Node(5);\n tree.root.left.left.left = new Node(8);\n \n if(tree.isBalanced(tree.root))\n System.out.println(\"Tree is balanced\");\n else\n System.out.println(\"Tree is not balanced\");\n }\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/is_balance/README.md",
"content": "# Cosmos\n\nCollaborative effort by [OpenGenus](https://github.com/OpenGenus/cosmos)"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/is_binary_tree/is_binary_tree.cpp",
"content": "/* A binary tree node has data, pointer to left child\n * and a pointer to right child\n *\n * struct Node {\n * int data;\n * Node* left, * right;\n * };\n *\n */\n\n\n\n/* Should return true if tree represented by root is BST.\n * For example, return value should be 1 for following tree.\n * 20\n * / \\\n * 10 30\n * and return value should be 0 for following tree.\n * 10\n * / \\\n * 20 30 */\n\ntemplate<typename Node>\nNode *findmax(Node *root)\n{\n if (!root)\n return nullptr;\n\n while (root->right)\n root = root->right;\n\n return root;\n}\n\ntemplate<typename Node>\nNode *findmin(Node *root)\n{\n if (!root)\n return nullptr;\n\n while (root->left)\n root = root->left;\n\n return root;\n}\n\ntemplate<typename Node>\nbool isBST(Node* root)\n{\n if (!root)\n return 1;\n\n if (root->left && findmax(root->left)->data > (root->data))\n return 0;\n\n if (root->right && findmin(root->right)->data < (root->data))\n return 0;\n\n if (!isBST(root->left) || !isBST(root->right))\n return 0;\n\n return 1;\n}\n\n\n// Another variation\n\n// Utility function\n\n//another function for the same but need to provide the min and max in the the tree beforehand\ntemplate<typename Node>\nint isBSTUtil(Node *root, int min, int max)\n{\n if (!root)\n return 1;\n\n if (root->data < min || root->data > max)\n return 0;\n\n return isBSTUtil(root->left, min, root->data - 1) &&\n isBSTUtil(root->right, root->data + 1, max);\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/is_same/is_same.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n *\n * tree comparer synopsis\n *\n * template<typename _Tp, typename _Comp = std::equal_to<_Tp> >\n * class TreeComparer\n * {\n * public:\n * using NodeType = TreeNode<_Tp>;\n * using PNodeType = std::shared_ptr<NodeType>;\n *\n * bool isSameTree(PNodeType p, PNodeType q) const;\n *\n * private:\n * _Comp comp_;\n * };\n */\n\n#include <stack>\n#include <functional>\n#include <memory>\n#include \"../node/node.cpp\"\n\n#ifndef TREE_COMPARER\n#define TREE_COMPARER\n\ntemplate<typename _Tp, typename _Comp = std::equal_to<_Tp>>\nclass TreeComparer\n{\npublic:\n using NodeType = TreeNode<_Tp>;\n using PNodeType = std::shared_ptr<NodeType>;\n\n bool isSameTree(PNodeType const &f, PNodeType const &s) const\n {\n std::stack<PNodeType> first, second;\n first.push(f);\n second.push(s);\n\n // DFS\n while (!first.empty() || !second.empty())\n {\n // mining left\n while (first.top() != nullptr\n || second.top() != nullptr)\n {\n // check not same node and not same value\n if (first.top() == nullptr\n || second.top() == nullptr\n || !comp_(first.top()->value(), second.top()->value()))\n return false;\n\n first.push(first.top()->left());\n second.push(second.top()->left());\n }\n\n // escape if top is empty or right is empty\n while (!first.empty()\n && ((first.top() == nullptr && second.top() == nullptr)\n || (first.top()->right() == nullptr && second.top()->right() == nullptr)))\n {\n first.pop();\n second.pop();\n }\n\n if (!first.empty())\n {\n auto first_right = first.top()->right(),\n second_right = second.top()->right();\n first.pop();\n second.pop();\n first.push(first_right);\n second.push(second_right);\n }\n }\n\n return true;\n }\n\nprivate:\n _Comp comp_;\n};\n\n#endif // TREE_COMPARER"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/make_binary_tree/from_inorder_and_postorder/make_tree_from_inorder_and_postorder.c",
"content": "#include <stdlib.h>\n#include <stdio.h>\n\ntypedef struct btree {\n\tint data;\n\tstruct btree *left;\n\tstruct btree *right;\n}btree;\n\nbtree *makeTree(int *in , int *post , int start , int end , int *index) {\n\tif (start > end) return NULL;\n\n\tbtree *nn = (btree*)malloc(sizeof(btree));\n\tnn -> data = post[*index];\n\tint i;\n\tfor (i = start;i <= end;i++) {\n\t\tif (post[*index] == in[i]) break;\n\t}\n\t(*index)--;\n\tnn -> right = makeTree(in, post, i+1, end, index);\n\tnn -> left = makeTree(in, post, start, i-1, index);\n\treturn nn;\n}\n\nvoid printPostOrderTree(btree *root) {\n\tif(root == NULL) return;\n\tprintPostOrderTree(root -> left);\n\tprintPostOrderTree(root -> right);\n\tprintf(\"%d \",root -> data);\n}\n\nint main()\n{\n\tint post[] = {4,5,2,6,7,3,1};\n\tint in[] = {4,2,5,1,6,3,7};\n\tint index = 6;\n\tbtree *root = makeTree (in, post, 0, 6, &index);\n\n\tprintPostOrderTree(root);\n\treturn 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/make_binary_tree/from_inorder_and_postorder/make_tree_from_inorder_and_postorder.cpp",
"content": "#include <iostream>\n#include <vector>\n#include <algorithm>\n\nusing namespace std;\n\ntemplate<typename T>\nclass TreeNode\n{\n using Treetype = TreeNode<T>;\n\npublic:\n T data;\n Treetype * left;\n Treetype * right;\n\n TreeNode(T data) : data(data), left(NULL), right(NULL)\n {\n }\n};\n\ntemplate <typename BidiIt>\nTreeNode<int>* buildTreeHelper(BidiIt in_first, BidiIt in_last,\n BidiIt post_first, BidiIt post_last);\n\nTreeNode<int>* buildTree(vector<int> &inorder, vector<int> &postorder)\n{\n return buildTreeHelper(begin(inorder), end(inorder),\n begin(postorder), end(postorder));\n}\n\ntemplate <typename BidiIt>\nTreeNode<int>* buildTreeHelper(BidiIt in_first, BidiIt in_last,\n BidiIt post_first, BidiIt post_last)\n{\n if (in_first == in_last)\n return nullptr;\n if (post_first == post_last)\n return nullptr;\n const auto val = *prev(post_last);\n TreeNode<int>* root = new TreeNode<int>(val);\n auto in_root_pos = find(in_first, in_last, val);\n auto left_size = distance(in_first, in_root_pos);\n auto post_left_last = next(post_first, left_size);\n root->left = buildTreeHelper(in_first, in_root_pos, post_first, post_left_last);\n root->right = buildTreeHelper(next(in_root_pos), in_last, post_left_last,\n prev(post_last));\n return root;\n}\n\nvoid postorderPrint(TreeNode<int>* root)\n{\n if (root == NULL)\n return;\n\n postorderPrint(root->left);\n postorderPrint(root->right);\n cout << root->data << \" \";\n\n return;\n}\n\nint main()\n{\n vector<int> postorder = {7, 8, 6, 4, 2, 5, 3, 1};\n vector<int> inorder = {4, 7, 6, 8, 2, 1, 3, 5};\n\n TreeNode<int>* root = buildTree(inorder, postorder);\n\n postorderPrint(root); // 7 8 6 4 2 5 3 1\n\n return 0;\n}\n\n/*\n * // test tree is\n * 1\n * / \\\n * 2 3\n * / \\\n * 4 5\n \\\n \\ 6\n \\ / \\\n \\ 7 8\n */"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/make_binary_tree/from_inorder_and_preorder/make_tree_from_inorder_and_preorder.c",
"content": "#include<stdio.h>\n#include<stdlib.h> \n \n// Part of Cosmos by OpenGenus Foundation\nint search(int arr[], int x, int n)\n{\n for (int i = 0; i < n; i++)\n if (arr[i] == x)\n return i;\n return -1;\n}\n \n// Prints postorder traversal from given inorder and preorder traversals\nvoid printPostOrder(int in[], int pre[], int n)\n{\n // The first element in pre[] is always root, search it\n // in in[] to find left and right subtrees\n int root = search(in, pre[0], n);\n \n // If left subtree is not empty, print left subtree\n if (root != 0)\n printPostOrder(in, pre+1, root);\n \n // If right subtree is not empty, print right subtree\n if (root != n-1)\n printPostOrder(in+root+1, pre+root+1, n-root-1);\n \n \n printf(\"%d \",pre[0]);\n}\n \n\nint main()\n{\n int n;\n printf(\"Enter the no. of element in the tree\");\n printf(\"\\n\");\n scanf(\"%d\",&n);\n int in[10000];\n int pre[10000];\n printf(\"Enter elements of inorder traversal seprated by single space\");\n printf(\"\\n\");\n for(int x=0;x< n;x++){\n scanf(\"%d\",&in[x]);\n }\n printf(\"Enter elements of preorder traversal by single space\");\n printf(\"\\n\");\n for(int x=0;x< n;x++){\n scanf(\"%d\",&pre[x]);\n }\n printf(\"Postorder traversal \");\n printf(\"\\n\");\n printPostOrder(in, pre, n);\n printf(\"\\n\");\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/make_binary_tree/from_inorder_and_preorder/make_tree_from_inorder_and_preorder.cpp",
"content": "#include <iostream>\n#include <cmath>\n#include <queue>\n#include <cmath>\nusing namespace std;\n\ntemplate<typename T>\nclass Binarytreenode {\n\npublic:\n T data;\n Binarytreenode<T> * left;\n Binarytreenode<T> * right;\n\n\n Binarytreenode(T data)\n {\n this->data = data;\n left = NULL;\n right = NULL;\n }\n\n};\n\n\nvoid postorder(Binarytreenode<int>* root)\n{\n if (root == NULL)\n return;\n\n postorder(root->left);\n postorder(root->right);\n\n cout << root->data << \" \";\n return;\n}\nBinarytreenode<int> * create(int *preorder, int*inorder, int ps, int pe, int is, int ie)\n{\n if (ps > pe || is > ie)\n return NULL;\n\n int rootdata = preorder[ps];\n Binarytreenode<int> * root = new Binarytreenode<int>(rootdata);\n int k;\n for (int i = is; i <= ie; i++)\n if (inorder[i] == rootdata)\n {\n k = i;\n break;\n }\n\n root->left = create(preorder, inorder, ps + 1, ps + k - is, is, k - 1);\n root->right = create(preorder, inorder, ps + k - is + 1, pe, k + 1, ie);\n\n return root;\n}\nint main()\n{\n int preorder[100], inorder[100];\n int size;\n cin >> size;\n for (int i = 0; i < size; i++)\n cin >> preorder[i];\n\n for (int i = 0; i < size; i++)\n cin >> inorder[i];\n\n Binarytreenode<int> * root = create(preorder, inorder, 0, size - 1, 0, size - 1);\n\n\n postorder(root);\n\n\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/make_binary_tree/from_inorder_and_preorder/make_tree_from_inorder_and_preorder.java",
"content": "//createdBy arora-72\n//5-10-2017\n// Part of Cosmos by OpenGenus Foundation\n\npublic class MakeTreefromInorderAndPreorder {\n\tpublic static int pIndex=0;\n\tpublic Node makeBTree(int [] inOrder, int [] preOrder, int iStart, int iEnd ){\n\t\tif(iStart>iEnd){\n\t\t\treturn null;\n\t\t}\n\t\tNode root = new Node(preOrder[pIndex]);pIndex++;\n\n\t\tif(iStart==iEnd){\n\t\t\treturn root;\n\t\t}\n\t\tint index = getInorderIndex(inOrder, iStart, iEnd, root.data);\n\t\t\troot.left = makeBTree(inOrder,preOrder,iStart, index-1);\n\t\t\troot.right = makeBTree(inOrder,preOrder,index+1, iEnd);\n\t\t//}\n\t\treturn root;\n\t}\n\tpublic int getInorderIndex(int [] inOrder, int start, int end, int data){\n\t\tfor(int i=start;i<=end;i++){\n\t\t\tif(inOrder[i]==data){\n\t\t\t\treturn i;\n\t\t\t}\n\t\t}\n\t\treturn -1;\n\t}\n\tpublic void printINORDER(Node root){\n\t\tif(root!=null){\n\t\t\tprintINORDER(root.left);\n\t\t\tSystem.out.print(\" \" + root.data);\n\t\t\tprintINORDER(root.right);\n\t\t}\n\t}\n\tpublic static void main (String[] args) throws java.lang.Exception\n\t{\n\t\tint [] inOrder = {2,5,6,10,12,14,15};\n\t\tint [] preOrder = {10,5,2,6,14,12,15};\n\t\tMakeTreefromInorderAndPreorder i = new MakeTreefromInorderAndPreorder();\n\t\tNode x = i.makeBTree(inOrder, preOrder, 0, inOrder.length-1);\n\t\tSystem.out.println(\"Constructed Tree : \");\n\t\ti.printINORDER(x);\n\t}\n}\nclass Node{\n\tint data;\n\tNode left;\n\tNode right;\n\tpublic Node (int data){\n\t\tthis.data = data;\n\t\tleft = null;\n\t\tright = null;\n\t}\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/make_binary_tree/from_inorder_and_preorder/README.md",
"content": "# Cosmos\n\nCollaborative effort by [OpenGenus](https://github.com/OpenGenus/cosmos)"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/make_binary_tree/README.md",
"content": "# Tree\n\nDescription\n---\nA tree is a data structure that is comprised of various **nodes**. Each node has at least one parent node, and can have multiple child nodes. The only node that does not have a parent node is a unique node called the **root**, or sometimes base node. If a node has no children, it can be called a **leaf**."
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/make_mirror_tree/make_mirror_tree.c",
"content": "#include <stdio.h>\n#include <unistd.h>\n#include <stdlib.h>\n\ntypedef struct node {\n\t\t\tint value;\n\t\t\tstruct node *left, *right;\n\t}node;\n\nnode* create_node(int );\nvoid create_tree(int );\nvoid cleanup_tree(node* );\nvoid mirror_image(node* );\n\nnode* root = NULL;\nnode* create_node(int val)\n{\n\tnode* tmp = (node *) malloc(sizeof(node));\n\ttmp->value = val;\n\ttmp->left = tmp->right = NULL;\n\treturn tmp;\n}\n\nvoid create_tree(int val)\n{\n\tnode* tmp = root;\n\tif (!root)\n\t root = create_node(val);\n\telse\n {\n while (1)\n\t {\n\t if (val > tmp->value)\n {\n if (!tmp->right)\n {\n\t\t tmp->right = create_node(val);\n break;\n }\n else\n tmp = tmp->right;\n }\n\t else\n {\n if (!tmp->left)\n {\n\t\t tmp->left= create_node(val);\n break;\n }\n else\n tmp = tmp->left;\n }\t\n\t }\n\t}\n\t\n}\n\nvoid mirror_image(node* tmp)\n{\n node* tmp1 = NULL;\n if (tmp)\n {\n tmp1 = tmp->left;\n tmp->left = tmp->right;\n tmp->right = tmp1;\n mirror_image(tmp->left);\n mirror_image(tmp->right);\n }\n}\n\nvoid print_mirror_image(node* tmp)\n{\n if (tmp)\n {\n printf(\"%d\\n\",tmp->value);\n print_mirror_image(tmp->left);\n print_mirror_image(tmp->right);\n }\n}\n\nvoid cleanup_tree(node* tmp)\n{\n if (tmp)\n {\n\tcleanup_tree(tmp->left);\n\tcleanup_tree(tmp->right);\n if (tmp->left)\n {\n free(tmp->left);\n \t tmp->left = NULL;\n }\n if (tmp->right)\n {\n free(tmp->right);\n \t tmp->right= NULL;\n }\n }\n if (tmp == root)\n {\n free(root);\n root = NULL;\n }\n}\n\n\nint main()\n{\n int val, num, ctr;\n node tmp;\n\tprintf(\"Enter number of nodes\\n\");\n scanf(\"%d\",&num);\n for (ctr = 0; ctr < num; ctr++)\n {\n\t printf(\"Enter values\\n\");\n\t scanf(\"%d\",&val);\n\t create_tree(val);\n }\n \tmirror_image(root);\n print_mirror_image(root);\n cleanup_tree(root);\n return 0; \n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/make_mirror_tree/make_mirror_tree.cpp",
"content": "#include <iostream>\nusing namespace std;\n\ntypedef struct tree_node\n{\n int value;\n struct tree_node *left, *right;\n}node;\n\n// create a new node\nnode *getNewNode(int value)\n{\n node *new_node = new node;\n new_node->value = value;\n new_node->left = NULL;\n new_node->right = NULL;\n return new_node;\n}\n\n// create the tree\nnode *createTree()\n{\n node *root = getNewNode(31);\n root->left = getNewNode(16);\n root->right = getNewNode(45);\n root->left->left = getNewNode(7);\n root->left->right = getNewNode(24);\n root->left->right->left = getNewNode(19);\n root->left->right->right = getNewNode(29);\n return root;\n}\n\n// Inorder traversal of a tree\nvoid inorderTraversal(node *ptr)\n{\n if (ptr == NULL)\n return;\n else\n {\n inorderTraversal(ptr->left);\n cout << ptr->value << \"\\t\";\n inorderTraversal(ptr->right);\n }\n}\n\n// create mirror tree\nnode* mirror(node* root)\n{\n node* m_root = NULL;\n if (!root)\n return NULL;\n m_root = getNewNode(root->value);\n m_root->left = mirror(root->right);\n m_root->right = mirror(root->left);\n return m_root;\n}\n\n// main\nint main()\n{\n node *root = createTree();\n cout << \"\\n Inorder traversal before conversion \";\n inorderTraversal(root);\n node *m_root = mirror(root);\n cout << \"\\n Inorder traversal after conversion \";\n inorderTraversal(m_root);\n cout << endl;\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/make_mirror_tree/make_mirror_tree.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n\nclass Node(object):\n def __init__(self, data=-1, path=\"\", left=None, right=None):\n self.data = data\n self.path = path\n self.left = left\n self.right = right\n\n\ndef inorder(root):\n if root:\n print(root.data, \" path\", root.path, end=\" \\n\")\n\n inorder(root.left)\n inorder(root.right)\n\n\ndef insertbydata(root, d, char, newd):\n global x\n if root:\n if root.data == d:\n x = root\n if char == \"L\":\n\n x.left = Node(newd, x.path + \"1\")\n # x=x.left\n\n else:\n x.right = Node(newd, x.path + \"0\")\n # x=x.right\n # print(x.data)\n insertbydata(root.left, d, char, newd)\n insertbydata(root.right, d, char, newd)\n\n\ndef searchbypath(root, pat):\n global x\n if root:\n\n # print(root.path)\n if root.path == pat:\n x = root\n # print( x.data)\n searchbypath(root.left, pat)\n\n searchbypath(root.right, pat)\n if x == None:\n return -1\n return x.data\n\n\ndef mirror_path(st):\n k = \"\"\n for i in st:\n if i == \"0\":\n k += \"1\"\n else:\n k += \"0\"\n return k\n\n\ndef searchbydata(root, d):\n global x\n if root:\n if root.data == d:\n x = root\n searchbydata(root.left, d)\n\n searchbydata(root.right, d)\n if x != None:\n\n return x\n return -1\n\n\nhead = Node(1)\nt = [int(i) for i in input().split()]\nfor i in range(t[0] - 1):\n li = [j for j in input().split()]\n insertbydata(head, int(li[0]), li[2], int(li[1]))\n# inorder(head)\nfor i in range(t[1]):\n inputd = int(input())\n mirrorreflecttionofinputd = searchbypath(\n head, (mirror_path(searchbydata(head, inputd).path))\n )\n if inputd != head.data:\n\n if inputd == mirrorreflecttionofinputd:\n print(-1)\n else:\n print(mirrorreflecttionofinputd)\n else:\n\n print(head.data)\n\n\"\"\"\ninput should be like this\n10 8\n1 2 R\n1 3 L\n2 4 R\n2 5 L\n3 6 R\n3 7 L\n5 8 R\n5 9 L\n7 10 R\n2\n5\n3\n6\n1\n10\n9\n4\n\nFirst line of input is N and Q.\nNext N-1 line consists of two integers and one character first of whose is parent node , second is child node and character \"L\" representing Left child and \"R\" representing right child.\nNext Q lines represents qi.\nOutput:\nFor each qi printing the mirror node if it exists else printing -1.\n\"\"\""
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/make_mirror_tree/README.md",
"content": "# Cosmos\n\nCollaborative effort by [OpenGenus](https://github.com/OpenGenus/cosmos)"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/maximum_height/maximum_height2.cpp",
"content": "#include <iostream>\nusing namespace std;\n\nclass node {\nprivate:\n int value;\n node* left, *right;\npublic:\n node()\n {\n };\n ~node()\n {\n };\n node* create_node(int& val);\n void create_tree(int& val);\n int height_tree(node* tmp);\n void cleanup_tree(node* tmp);\n inline int maximum(int left_ht, int right_ht)\n {\n return left_ht > right_ht ? left_ht : right_ht;\n }\n};\n\nstatic node* root = NULL;\nnode* node::create_node(int& val)\n{\n node* tmp = new node;\n tmp->value = val;\n tmp->left = tmp->right = NULL;\n return tmp;\n}\n\nvoid node::create_tree(int& val)\n{\n node* tmp = root;\n if (!root)\n root = create_node(val);\n else\n while (1)\n {\n if (val > tmp->value)\n {\n if (!tmp->right)\n {\n tmp->right = create_node(val);\n break;\n }\n else\n tmp = tmp->right;\n }\n else\n {\n if (!tmp->left)\n {\n tmp->left = create_node(val);\n break;\n }\n else\n tmp = tmp->left;\n }\n }\n\n}\n\nint node::height_tree(node* tmp)\n{\n if (!tmp)\n return 0;\n int left_ht = height_tree(tmp->left);\n int right_ht = height_tree(tmp->right);\n return 1 + max(left_ht, right_ht);\n}\n\nvoid node::cleanup_tree(node* tmp)\n{\n if (tmp)\n {\n cleanup_tree(tmp->left);\n cleanup_tree(tmp->right);\n if (tmp->left)\n delete tmp->left;\n if (tmp->right)\n delete tmp->right;\n }\n if (tmp == root)\n delete root;\n}\n\n\nint main()\n{\n int val, num;\n node tmp;\n cout << \"Enter number of nodes\" << endl;\n cin >> num;\n for (int ctr = 0; ctr < num; ctr++)\n {\n cout << \"Enter values\" << endl;\n cin >> val;\n tmp.create_tree(val);\n }\n int tree_ht = tmp.height_tree(root);\n cout << \"Height of tree is \" << tree_ht << endl;\n tmp.cleanup_tree(root);\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/maximum_height/maximum_height.cpp",
"content": "#include <iostream>\n// #include<bits/stdc++.h>\nusing namespace std;\n\n// Part of Cosmos by OpenGenus Foundation\n\n// #include <iostream>\n// using namespace std;\n\n// max function\nint max(int a, int b)\n{\n return (a > b) ? a : b;\n}\n\nstruct node\n{\n int data;\n node* left;\n node* right;\n};\n\n// create a new node\nstruct node* getNode(int data)\n{\n node* newNode = new node();\n newNode->data = data;\n newNode->left = NULL;\n newNode->right = NULL;\n return newNode;\n}\n\n// Inorder Traversal\nvoid inorder(struct node* root)\n{\n if (root != NULL)\n {\n inorder(root->left);\n cout << root->data << \" \";\n inorder(root->right);\n }\n}\n\n//Insert new node into Binary Tree\nstruct node* Insert(struct node* root, int data)\n{\n if (root == NULL)\n return getNode(data);\n\n if (data < root->data)\n root->left = Insert(root->left, data);\n else if (data > root->data)\n root->right = Insert(root->right, data);\n\n return root;\n}\n\n// compute height of the tree\nint getHeight(node* root)\n{\n int l;\n int r;\n if(root){\n l = getHeight(root->left);\n r = getHeight(root->right);\n \n if(l>r){\n return l+1;\n }\n else{\n return r+1;\n }\n }\n}\n\nint main()\n{\n node* root = NULL;\n int a[30];\n int n;\n cout << \"\\nEnter no. of nodes: \";\n cin >> n;\n for (int i = 0; i < n; i++)\n {\n cin >> a[i];\n root = Insert(root, a[i]);\n }\n\n cout << \"\\n\\nInorder: \";\n inorder(root);\n cout << \"\\n\\nHeight of the tree is \" << getHeight(root);\n\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/maximum_height/maximum_height.java",
"content": "import java.util.*;\nimport java.lang.*;\nimport java.io.*;\n\n// Java program to find height of Binary Search Tree\n// A binary tree node\nclass Node \n{\n int data;\n Node left, right;\n \n Node(int item) \n {\n data = item;\n left = right = null;\n }\n}\n \nclass BinaryTreeHeight \n{\n Node root;\n \n /* Compute the \"maxDepth\" of a tree -- the number of \n nodes along the longest path from the root node \n down to the farthest leaf node.*/\n int maxDepth(Node node) \n {\n if (node == null)\n return 0;\n else\n {\n /* compute the depth of each subtree */\n int lDepth = maxDepth(node.left);\n int rDepth = maxDepth(node.right);\n \n /* use the larger one */\n if (lDepth > rDepth)\n return (lDepth + 1);\n else\n return (rDepth + 1);\n }\n }\n \n //The main function\n public static void main(String[] args) \n {\n BinaryTreeHeight tree = new BinaryTreeHeight();\n \n tree.root = new Node(1);\n tree.root.left = new Node(2);\n tree.root.right = new Node(3);\n tree.root.left.left = new Node(4);\n tree.root.left.right = new Node(5);\n \n System.out.println(\"Height of tree is : \" + tree.maxDepth(tree.root));\n }\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/maximum_height/maximum_height.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n\nclass Node:\n def __init__(self, data):\n self.data = data\n self.left = None\n self.right = None\n\n\n# Compute the HEIGHT of a tree\ndef height(node):\n if node is None:\n return 0\n else:\n # Compute the height of each subtree\n lh = height(node.left)\n rh = height(node.right)\n return max(lh, rh) + 1\n\n\nroot = Node(11)\nroot.left = Node(22)\nroot.right = Node(33)\nroot.left.left = Node(44)\nroot.left.right = Node(66)\n\nprint(\"Height of tree is %d\" % (height(root)))"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/maximum_height/README.md",
"content": "# Cosmos\n\nCollaborative effort by [OpenGenus](https://github.com/OpenGenus/cosmos)"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/minimum_height/minimum_height.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n// Part of Cosmos by OpenGenus Foundation\ntypedef struct node {\n\t\t\tint value;\n\t\t\tstruct node *left, *right;\n\t\t }node;\n\nstatic node* root = NULL;\nnode* create_node(int val)\n{\n node* tmp = (node *) malloc(sizeof(node));\n tmp->value = val;\n tmp->left = tmp->right = NULL;\n return tmp;\n}\n\nvoid create_tree(int val)\n{\n node* tmp = root;\n if (!root)\n root = create_node(val);\n else\n {\n while (1)\n {\n if (val > tmp->value)\n {\n if (!tmp->right)\n {\n tmp->right = create_node(val);\n break;\n }\n else\n tmp = tmp->right;\n }\n else\n {\n if (!tmp->left)\n {\n tmp->left= create_node(val);\n break;\n }\n else\n tmp = tmp->left;\n }\n }\n }\n\n}\n\nint min(int left_ht, int right_ht)\n{\n return (left_ht < right_ht ? left_ht : right_ht);\n}\n\nint min_depth_tree(node* tmp)\n{\n if (!tmp)\n return 0;\n if (!tmp->left && !tmp->right)\n return 1;\n if (!tmp->left) \n return min_depth_tree(tmp->right)+1;\n if (!tmp->right) \n return min_depth_tree(tmp->left)+1;\n\n return min(min_depth_tree(tmp->left),min_depth_tree(tmp->right))+1;\n}\n\nint main()\n{\n int ctr, num_nodes, value,min_depth;\n printf(\"Enter number of nodes\\n\");\n scanf(\"%d\",&num_nodes);\n for (ctr = 0; ctr < num_nodes; ctr++)\n {\n printf(\"Enter values\\n\");\n scanf(\"%d\",&value);\n create_tree(value);\n }\n min_depth = min_depth_tree(root);\n printf(\"minimum depth = %d\\n\",min_depth);\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/minimum_height/minimum_height.cpp",
"content": "#include <iostream>\nusing namespace std;\n\ntypedef struct tree_node\n{\n int value;\n struct tree_node *left, *right;\n}node;\n\n// create a new node\nnode *getNewNode(int value)\n{\n node *new_node = new node;\n new_node->value = value;\n new_node->left = NULL;\n new_node->right = NULL;\n return new_node;\n}\n\n// create the tree\nnode *createTree()\n{\n node *root = getNewNode(31);\n root->left = getNewNode(16);\n root->right = getNewNode(45);\n root->left->left = getNewNode(7);\n root->left->right = getNewNode(24);\n root->left->right->left = getNewNode(19);\n root->left->right->right = getNewNode(29);\n return root;\n}\n\nint minDepth(node* A)\n{\n if (A == NULL)\n return 0;\n\n int l = minDepth(A->left);\n int r = minDepth(A->right);\n\n if (l && r)\n return min(l, r) + 1;\n\n if (l)\n return l + 1;\n return r + 1;\n}\n\n\n// main\nint main()\n{\n node *root = createTree();\n\n cout << minDepth(root);\n cout << endl;\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/minimum_height/minimum_height.java",
"content": "class TreeNode<T>\n{\n\tT data;\n\tTreeNode<T> left,right;\n\tpublic TreeNode(T data)\n\t{\n\t\tthis.data = data;\n\t}\n}\n\npublic class BinaryTree {\n\n\tpublic static <T> int minHeight(TreeNode<T> root)\n\t{\n\t\tif(root == null)\n\t\t\treturn 0;\n\t\t\n\t\treturn Math.min(minHeight(root.left), minHeight(root.right)) + 1;\n\t}\n\t\n\tpublic static void main(String[] args) {\n\t\tTreeNode<Integer> root = new TreeNode<Integer>(1);\n\t\troot.left = new TreeNode<Integer>(2);\n\t\troot.right = new TreeNode<Integer>(3);\n\t\troot.left.left = new TreeNode<Integer>(4);\n\t\troot.left.right = new TreeNode<Integer>(5);\n\t\troot.right.left = new TreeNode<Integer>(6);\n\t\t\n\t\tSystem.out.println(minHeight(root));\n\n\t}\n\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/minimum_height/minimum_height.py",
"content": "# Python program to find minimum depth of a given Binary Tree\n# Part of Cosmos by OpenGenus Foundation\n# Tree node\nclass Node:\n def __init__(self, key):\n self.data = key\n self.left = None\n self.right = None\n\n\ndef minDepth(root):\n # Corner Case.Should never be hit unless the code is\n # called on root = NULL\n if root is None:\n return 0\n\n # Base Case : Leaf node.This acoounts for height = 1\n if root.left is None and root.right is None:\n return 1\n\n # If left subtree is Null, recur for right subtree\n if root.left is None:\n return minDepth(root.right) + 1\n\n # If right subtree is Null , recur for left subtree\n if root.right is None:\n return minDepth(root.left) + 1\n\n return min(minDepth(root.left), minDepth(root.right)) + 1\n\n\n# Driver Program\nroot = Node(1)\nroot.left = Node(2)\nroot.right = Node(3)\nroot.left.left = Node(4)\nroot.left.right = Node(5)\nprint(minDepth(root))"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/minimum_height/README.md",
"content": "# Cosmos\n\nCollaborative effort by [OpenGenus](https://github.com/OpenGenus/cosmos)"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/node/node.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n *\n * tree node synopsis\n *\n * // for normal binary tree\n * template<typename _Type>\n * class TreeNode\n * {\n * protected:\n * using SPNodeType = std::shared_ptr<TreeNode>;\n * using ValueType = _Type;\n *\n * public:\n * TreeNode(ValueType v, SPNodeType l = nullptr, SPNodeType r = nullptr)\n * :value_(v), left_(l), right_(r) {}\n *\n * ValueType value() {\n * return value_;\n * }\n *\n * void value(ValueType v) {\n * value_ = v;\n * }\n *\n * SPNodeType left() {\n * return left_;\n * }\n *\n * void left(SPNodeType l) {\n * left_ = l;\n * }\n *\n * SPNodeType right() {\n * return right_;\n * }\n *\n * void right(SPNodeType r) {\n * right_ = r;\n * }\n *\n * private:\n * ValueType value_;\n * SPNodeType left_;\n * SPNodeType right_;\n * };\n *\n * // for derivative binary tree (e.g., avl tree, splay tree)\n * template<typename _Type, class _Derivative>\n * class __BaseTreeNode\n * {\n * protected:\n * using SPNodeType = std::shared_ptr<_Derivative>;\n * using ValueType = _Type;\n *\n * public:\n * __BaseTreeNode(ValueType v, SPNodeType l = nullptr, SPNodeType r = nullptr)\n * :value_(v), left_(l), right_(r) {}\n *\n * ValueType value() {\n * return value_;\n * }\n *\n * void value(ValueType v) {\n * value_ = v;\n * }\n *\n * SPNodeType left() {\n * return left_;\n * }\n *\n * void left(SPNodeType l) {\n * left_ = l;\n * }\n *\n * SPNodeType right() {\n * return right_;\n * }\n *\n * void right(SPNodeType r) {\n * right_ = r;\n * }\n *\n * private:\n * ValueType value_;\n * SPNodeType left_;\n * SPNodeType right_;\n * };\n *\n * template<typename _Type>\n * class DerivativeTreeNode :public __BaseTreeNode<_Type, DerivativeTreeNode<_Type>>\n * {\n * private:\n * using BaseNode = __BaseTreeNode<_Type, DerivativeTreeNode<_Type>>;\n * using SPNodeType = typename BaseNode::SPNodeType;\n * using ValueType = typename BaseNode::ValueType;\n *\n * public:\n * DerivativeTreeNode(_Type v, SPNodeType l = nullptr, SPNodeType r = nullptr)\n * :__BaseTreeNode<_Type, DerivativeTreeNode<_Type>>(v, l, r) {}\n * };\n */\n\n#include <memory>\n\n#ifndef TREE_NODE_POLICY\n#define TREE_NODE_POLICY\n\ntemplate<typename _Type>\nclass TreeNode\n{\nprotected:\n using SPNodeType = std::shared_ptr<TreeNode>;\n using ValueType = _Type;\n\npublic:\n TreeNode(ValueType v, SPNodeType l = nullptr, SPNodeType r = nullptr)\n : value_(v), left_(l), right_(r)\n {\n }\n\n ValueType value()\n {\n return value_;\n }\n\n void value(ValueType v)\n {\n value_ = v;\n }\n\n SPNodeType left()\n {\n return left_;\n }\n\n void left(SPNodeType l)\n {\n left_ = l;\n }\n\n SPNodeType right()\n {\n return right_;\n }\n\n void right(SPNodeType r)\n {\n right_ = r;\n }\n\nprivate:\n ValueType value_;\n SPNodeType left_;\n SPNodeType right_;\n};\n\ntemplate<typename _Type, class _Derivative>\nclass __BaseTreeNode\n{\nprotected:\n using SPNodeType = std::shared_ptr<_Derivative>;\n using ValueType = _Type;\n\npublic:\n __BaseTreeNode(ValueType v, SPNodeType l = nullptr, SPNodeType r = nullptr)\n : value_(v), left_(l), right_(r)\n {\n }\n\n ValueType value()\n {\n return value_;\n }\n\n void value(ValueType v)\n {\n value_ = v;\n }\n\n SPNodeType left()\n {\n return left_;\n }\n\n void left(SPNodeType l)\n {\n left_ = l;\n }\n\n SPNodeType right()\n {\n return right_;\n }\n\n void right(SPNodeType r)\n {\n right_ = r;\n }\n\nprivate:\n ValueType value_;\n SPNodeType left_;\n SPNodeType right_;\n};\n\ntemplate<typename _Type>\nclass DerivativeTreeNode : public __BaseTreeNode<_Type, DerivativeTreeNode<_Type>>\n{\nprivate:\n using BaseNode = __BaseTreeNode<_Type, DerivativeTreeNode<_Type>>;\n using SPNodeType = typename BaseNode::SPNodeType;\n using ValueType = typename BaseNode::ValueType;\n\npublic:\n DerivativeTreeNode(_Type v, SPNodeType l = nullptr, SPNodeType r = nullptr)\n : __BaseTreeNode<_Type, DerivativeTreeNode<_Type>>(v, l, r)\n {\n }\n};\n\n#endif // TREE_NODE_POLICY"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/path_sum/path_sum.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n */\n\n#ifndef path_sum_cpp\n#define path_sum_cpp\n\n#include \"path_sum.hpp\"\n#include <queue>\n#include <stack>\n#include <set>\n#include <vector>\n#include <memory>\n#include <algorithm>\n#include <utility>\n#include <functional>\n\n// public\ntemplate<typename _Ty, typename _Compare, class _TreeNode>\nauto\nPathSum<_Ty, _Compare, _TreeNode>::countPathsOfSum(PNode<_Ty> root, _Ty sum)->size_type\n{\n if (path_type_ == PathType::Whole)\n return getPathsOfSum(root, sum).size();\n else // path_type_ == PathType::Part\n {\n if (root == nullptr)\n {\n return {};\n }\n\n return getPathsOfSum(root, sum).size()\n + countPathsOfSum(root->left(), sum)\n + countPathsOfSum(root->right(), sum);\n }\n}\n\ntemplate<typename _Ty, typename _Compare, class _TreeNode>\nstd::vector<std::vector<_Ty>>\nPathSum<_Ty, _Compare, _TreeNode>::getPathsOfSum(PNode<_Ty> root, _Ty sum)\n{\n std::vector<std::vector<_Ty>> res{};\n getPathsOfSumUp(root, {}, {}, sum, res);\n\n return res;\n}\n\n// public end\n\n// private\n\ntemplate<typename _Ty, typename _Compare, class _TreeNode>\nvoid\nPathSum<_Ty, _Compare, _TreeNode>::getPathsOfSumUp(PNode<_Ty> root,\n std::vector<_Ty> prev,\n _Ty prev_sum,\n _Ty const &sum,\n std::vector<std::vector<_Ty>> &res)\n{\n if (root != nullptr)\n {\n auto &curr = prev;\n curr.push_back(root->value());\n\n auto &curr_sum = prev_sum;\n curr_sum += root->value();\n\n if (path_type_ == PathType::Whole)\n {\n if (root->left() == nullptr\n && root->right() == nullptr\n && compare_(curr_sum, sum))\n res.push_back(curr);\n }\n else // path_type_ == PathType::Part\n if (compare_(curr_sum, sum))\n res.push_back(curr);\n\n\n getPathsOfSumUp(root->left(), curr, curr_sum, sum, res);\n getPathsOfSumUp(root->right(), curr, curr_sum, sum, res);\n }\n}\n\n// private end\n\n#endif /* path_sum_cpp */"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/path_sum/path_sum.hpp",
"content": "/*\n Part of Cosmos by OpenGenus Foundation\n */\n\n#ifndef path_sum_hpp\n#define path_sum_hpp\n\n#include <memory>\n#include <vector>\n#include <functional>\n#include \"../node/node.cpp\"\n\ntemplate<typename _Ty, typename _Compare = std::equal_to<_Ty>, class _TreeNode = TreeNode<_Ty>>\nclass PathSum\n{\nprivate:\n template<typename _T>\n using Node = _TreeNode;\n template<typename _T>\n using PNode = std::shared_ptr<Node<_T>>;\n using size_type = size_t;\n\npublic:\n enum class PathType\n {\n Whole,\n Part\n };\n\n PathSum(PathType py = PathType::Whole) :compare_(_Compare()), path_type_(py) {};\n\n ~PathSum() = default;\n\n size_type countPathsOfSum(PNode<_Ty> root, _Ty sum);\n\n std::vector<std::vector<_Ty>> getPathsOfSum(PNode<_Ty> root, _Ty sum);\n\nprivate:\n _Compare compare_;\n PathType path_type_;\n\n void getPathsOfSumUp(PNode<_Ty> root,\n std::vector<_Ty> prev,\n _Ty prev_sum,\n _Ty const &sum,\n std::vector<std::vector<_Ty>> &res);\n};\n\n#include \"path_sum.cpp\"\n\n#endif /* path_sum_hpp */"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/path_sum/sum_left/left_sum.py",
"content": "# Sum of left leaves in a binary tree\r\n\r\n# Class for individual nodes\r\nclass Node(object):\r\n def __init__(self, val):\r\n self.data = val\r\n self.left = None\r\n self.right = None\r\n\r\n\r\nclass BinaryTree(object):\r\n def __init__(self):\r\n self.head = None\r\n\r\n def insert(self, item):\r\n if self.head == None:\r\n self.head = Node(item)\r\n else:\r\n self.add(self.head, item)\r\n\r\n def add(self, node, val):\r\n # Compare parent's value with new value\r\n if val < node.data:\r\n if node.left == None:\r\n node.left = Node(val)\r\n else:\r\n self.add(node.left, val)\r\n else:\r\n if node.right == None:\r\n node.right = Node(val)\r\n else:\r\n self.add(node.right, val)\r\n\r\n\r\ndef preorder(hash_map, level, head):\r\n if head != None:\r\n if level not in hash_map.keys():\r\n hash_map[level] = head.data\r\n preorder(hash_map, level + 1, head.left)\r\n preorder(hash_map, level + 1, head.right)\r\n\r\n\r\nif __name__ == \"__main__\":\r\n print(\"Enter number of nodes: \")\r\n num = int(input())\r\n root = BinaryTree()\r\n for _ in range(num):\r\n print(\"Enter element #{}:\".format(_ + 1))\r\n item = int(input())\r\n root.insert(item)\r\n left_elements = dict()\r\n preorder(left_elements, 0, root.head)\r\n print(sum([*left_elements.values()]))\r"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/path_sum/sum_left/README.md",
"content": "# Cosmos\n\nCollaborative effort by [OpenGenus](https://github.com/OpenGenus/cosmos)"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/path_sum/sum_left/sum_left.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n\ntypedef struct node {\n\t\t\tint value;\n int left_flag;\n\t\t\tstruct node *left, *right;\n\t\t }node;\n\nstatic node* root = NULL;\nnode* create_node(int val)\n{\n node* tmp = (node *) malloc(sizeof(node));\n tmp->value = val;\n tmp->left_flag = 0;\n tmp->left = tmp->right = NULL;\n return tmp;\n}\n\nvoid create_tree(int val)\n{\n node* tmp = root;\n if (!root)\n root = create_node(val);\n else\n {\n while (1)\n {\n if (val > tmp->value)\n {\n if (!tmp->right)\n {\n tmp->right = create_node(val);\n break;\n }\n else\n tmp = tmp->right;\n }\n else\n {\n if (!tmp->left)\n {\n tmp->left= create_node(val);\n tmp->left->left_flag=1;\n break;\n }\n else\n tmp = tmp->left;\n }\n }\n }\n\n}\n\nint sum_left_leaves(node* tmp)\n{\n static int sum = 0;\n if(tmp) \n {\n if (!tmp->left && !tmp->right && tmp->left_flag)\n sum+=tmp->value;\n sum_left_leaves(tmp->left);\n sum_left_leaves(tmp->right);\n }\n return sum;\n}\n\nint main()\n{\n int ctr, sum, num_nodes, value,min_depth;\n printf(\"Enter number of nodes\\n\");\n scanf(\"%d\",&num_nodes);\n for (ctr = 0; ctr < num_nodes; ctr++)\n {\n printf(\"Enter values\\n\");\n scanf(\"%d\",&value);\n create_tree(value);\n }\n sum = sum_left_leaves(root);\n printf(\"sum = %d\\n\",sum);\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/README.md",
"content": "# Tree\n\nDescription\n---\nA tree is a data structure that is comprised of various **nodes**. Each node has at least one parent node, and can have multiple child nodes. The only node that does not have a parent node is a unique node called the **root**, or sometimes base node. If a node has no children, it can be called a **leaf**."
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/serializer/serializer.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n *\n * tree serializer synopsis\n *\n * class TreeSerializer\n * {\n * public:\n * using NodeType = TreeNode<int>;\n * using PNodeType = std::shared_ptr<NodeType>;\n *\n * // Encodes a tree to a single string.\n * std::string serialize(PNodeType root);\n *\n * // Decodes your encoded data to tree.\n * PNodeType deserialize(std::string data);\n *\n * private:\n * std::vector<std::string> splitToVector(std::string s);\n * };\n */\n\n#ifndef TREE_SERIALIZER\n#define TREE_SERIALIZER\n\n#include <vector>\n#include <stack>\n#include <queue>\n#include <iterator>\n#include <memory>\n#include <string>\n#include <sstream>\n#include \"../node/node.cpp\"\n\nclass TreeSerializer\n{\npublic:\n using NodeType = TreeNode<int>;\n using PNodeType = std::shared_ptr<NodeType>;\n\n// Encodes a tree to a single string.\n std::string serialize(PNodeType root)\n {\n if (root)\n {\n std::string ret {};\n std::stack<PNodeType> st {};\n PNodeType old {};\n\n st.push(root);\n ret.append(std::to_string(root->value()) + \" \");\n\n while (!st.empty())\n {\n while (st.top() && st.top()->left())\n {\n st.push(st.top()->left());\n ret.append(std::to_string(st.top()->value()) + \" \");\n }\n if (!st.top()->left())\n ret.append(\"# \");\n while (!st.empty())\n {\n old = st.top();\n st.pop();\n if (old->right())\n {\n st.push(old->right());\n if (st.top())\n ret.append(std::to_string(st.top()->value()) + \" \");\n break;\n }\n else\n ret.append(\"# \");\n }\n }\n\n return ret;\n }\n else\n return \"# \";\n }\n\n// Decodes your encoded data to tree.\n PNodeType deserialize(std::string data)\n {\n if (data.at(0) == '#')\n return nullptr;\n\n auto nodes = splitToVector(data);\n std::stack<PNodeType> st{};\n PNodeType ret = std::make_shared<NodeType>(stoi(nodes.at(0))), old{};\n st.push(ret);\n size_t i{}, sz {nodes.size()};\n i++;\n bool check_l{true};\n while (!st.empty())\n {\n while (i < sz && nodes.at(i) != \"#\" && check_l)\n {\n st.top()->left(std::make_shared<NodeType>(stoi(nodes.at(i++))));\n st.push(st.top()->left());\n }\n st.top()->left(nullptr);\n i++;\n check_l = false;\n while (!st.empty())\n {\n old = st.top();\n st.pop();\n check_l = true;\n if (nodes.at(i) != \"#\")\n {\n old->right(std::make_shared<NodeType>(stoi(nodes.at(i++))));\n st.push(old->right());\n break;\n }\n else\n {\n old->right(nullptr);\n i++;\n }\n }\n }\n\n return ret;\n }\n\nprivate:\n std::vector<std::string> splitToVector(std::string s)\n {\n std::stringstream ss(s);\n std::istream_iterator<std::string> begin(ss);\n std::istream_iterator<std::string> end;\n std::vector<std::string> vstrings(begin, end);\n\n return vstrings;\n }\n};\n\n#endif // TREE_SERIALIZER"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/Subtree_sum/subtreesum_recursive.cpp",
"content": "#include<iostream>\nusing namespace std;\n//Binary tree structure\nstruct node\n{\n int data;\n struct node* left;\n struct node* right;\n};\n//for inserting a new node\nnode *newNode(int data){\n node *temp=new node;\n temp->data=data;\n temp->right=NULL;\n temp->left=NULL;\n return (temp);\n}\n//helper function\n//Function to return sum of subtrees and count subtrees with sum=x\nint countSubtreesWithSumX(node* root, int x ,int& count)\n{ if(root==NULL)\n return 0;\n int ls= countSubtreesWithSumX(root->left,x,count);\n int rs= countSubtreesWithSumX(root->right,x,count);\n int sum=ls+rs+root->data;\n if(sum==x)\n count++;\n return sum;\n}\n//main function\n//Function to return the count of subtrees whose sum=x\nint countSubtreescheckroot(node* root, int x)\n{\n if(root==NULL)\n return 0;\n int count=0;\n //return sum of left and right subtrees respectively\nint ls= countSubtreesWithSumX(root->left,x,count);\nint rs= countSubtreesWithSumX(root->right,x,count);\n//checks if the root value added to sums of left and right subtrees equals x\nif(x==ls+rs+root->data)\ncount++;\n//returns the count of subtrees with their sum=x\nreturn count;\n}\nint main() {\n// input of sum to check for\n int x;\n cout<<\"Enter the sum to check for\"<<endl;\n cin>>x;\n//sample input of the binary tree\n\tstruct node* root=newNode(7);\n\troot->left=newNode(3);\n\troot->right=newNode(2);\n\troot->left->right=newNode(6);\n\troot->left->left=newNode(2);\n root->right->right=newNode(11);\n\tcout<<\"Number of subtrees with specific sum :\"<<\" \"<<countSubtreescheckroot(root, x)<<endl;\n\n\treturn 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/traversal/inorder/right_threaded/right_threaded.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n/*\n * Right-Threaded Binary Tree implementation in C++\n */\n//Author: Arpan Konar\n#include <iostream>\nusing namespace std;\ntypedef long long ll;\n#define REP(i, n) for (long long i = 0; i < (n); i++)\n#define FOR(i, a, b) for (long long i = (a); i <= (b); i++)\n#define FORD(i, a, b) for (int i = (a); i >= (b); i--)\n//Structure of a node\nstruct node\n{\n int data;\n node * left;\n node * right;\n bool isThreaded;\n};\n//Function to create a new node with value d\nnode * newnode(int d)\n{\n node *temp = new node();\n temp->data = d;\n temp->left = NULL;\n temp->right = NULL;\n temp->isThreaded = false;\n\n return temp; // the node will not be deleted\n}\n//Function to find the leftmost node of a subtree\nnode * leftmost(node * root)\n{\n node * temp = root;\n while (temp != NULL && temp->left != NULL)\n temp = temp->left;\n return temp;\n}\n//Function to find a node with value b\nnode * findNode(int b, node * root)\n{\n if (root->data == b)\n return root;\n root = leftmost(root);\n while (1)\n {\n if (root->data == b)\n return root;\n while (root->isThreaded)\n {\n root = root->right;\n if (root->data == b)\n return root;\n }\n if (root->right != NULL)\n root = leftmost(root->right);\n else\n break;\n }\n return NULL;\n}\n//Function to set the new node at left of node x\nnode * setLeftNode(node * x, int a)\n{\n if (x->left != NULL)\n {\n cout << a << \" is ignored\" << endl;\n return x;\n }\n node * temp = newnode(a);\n temp->right = x;\n x->left = temp;\n temp->isThreaded = true;\n return x;\n}\n//Function to set the new node at right of node x\nnode * setRightNode(node * x, int a)\n{\n if (x->right != NULL && !x->isThreaded)\n {\n cout << a << \" is ignored\" << endl;\n return x;\n }\n node * temp = newnode(a);\n if (x->isThreaded)\n {\n node *q = x->right;\n x->isThreaded = false;\n x->right = temp;\n temp->right = q;\n temp->isThreaded = true;\n }\n else\n x->right = temp;\n return x;\n}\n//Function to take input and create threaded tree\n/*Input is of the form number L/R number\n * where a is entered at the left or right position of b\n * if b is found\n * Input ends when a is -1\n */\nnode * createTree()\n{\n node * root = NULL;\n while (1)\n {\n int a, b;\n char c;\n cin >> a;\n if (a == -1)\n break;\n cin >> c >> b;\n if (root == NULL)\n root = newnode(a);\n else\n {\n node * x = findNode(b, root);\n if (x == NULL)\n cout << \"Node not found\" << endl;\n else\n {\n\n if (c == 'L')\n x = setLeftNode(x, a);\n else if (c == 'R')\n x = setRightNode(x, a);\n }\n }\n }\n return root;\n}\n//Function for inorder traversal of threaded binary tree\nvoid inOrder(node * root)\n{\n root = leftmost(root);\n while (1)\n {\n cout << root->data << \" \";\n while (root->isThreaded)\n {\n root = root->right;\n cout << root->data << \" \";\n }\n if (root->right != NULL)\n root = leftmost(root->right);\n else\n break;\n }\n}\n//Driver function to implement the above functions\nint main()\n{\n node * root = createTree();\n cout << \"Inorder:\";\n inOrder(root);\n cout << endl;\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/traversal/preorder/left_view/left_view.java",
"content": "//Java Program to print left View of a binary Tree\n\n/* Class containing left and right child of current\nnode and key value*/\n\nclass Node\n{\n int data;\n Node left, right;\n \n public Node(int item)\n {\n data = item;\n left = right = null;\n }\n}\n \n/* Class to print the left view */\nclass BinaryTree\n{\n Node root;\n static int max_level = 0;\n \n // recursive function to print left view\n void leftViewUtil(Node node, int level)\n {\n // Base Case\n if (node==null) return;\n \n // If this is the first node of its level\n if (max_level < level)\n {\n System.out.print(\" \" + node.data);\n max_level = level;\n }\n \n // Recur for left and right subtrees\n leftViewUtil(node.left, level+1);\n leftViewUtil(node.right, level+1);\n }\n \n // A wrapper over leftViewUtil()\n void leftView()\n {\n leftViewUtil(root, 1);\n }\n \n /* testing for example nodes */\n public static void main(String args[])\n {\n /* creating a binary tree and entering the nodes */\n BinaryTree tree = new BinaryTree();\n tree.root = new Node(12);\n tree.root.left = new Node(10);\n tree.root.right = new Node(30);\n tree.root.right.left = new Node(25);\n tree.root.right.right = new Node(40);\n \n tree.leftView();\n }\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/traversal/preorder/right_view/README.md",
"content": "# Cosmos\n\nCollaborative effort by [OpenGenus](https://github.com/OpenGenus/cosmos)"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/traversal/preorder/right_view/right_view2.cpp",
"content": "#include <iostream>\nusing namespace std;\n// Part of Cosmos by OpenGenus Foundation\nstruct node\n{\n int d;\n node *left;\n node *right;\n};\n\nstruct node* newnode(int num)\n{\n node *temp = new node;\n temp->d = num;\n temp->left = temp->right = NULL;\n return temp;\n}\n\nvoid rightview(struct node *root, int level, int *maxlevel)\n{\n if (root == NULL)\n return;\n\n\n if (*maxlevel < level)\n {\n cout << root->d << \" \";\n *maxlevel = level;\n }\n\n rightview(root->right, level + 1, maxlevel);\n rightview(root->left, level + 1, maxlevel);\n\n}\n\nint main()\n{\n struct node *root = newnode(1);\n root->left = newnode(2);\n root->right = newnode(3);\n root->left->left = newnode(4);\n root->left->right = newnode(5);\n root->right->left = newnode(6);\n root->right->right = newnode(7);\n root->right->left->right = newnode(8);\n\n\n int maxlevel = 0;\n\n cout << \"\\nRight view : \";\n rightview(root, 1, &maxlevel);\n\n cout << endl;\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/traversal/preorder/right_view/right_view.cpp",
"content": "#include <iostream>\nusing namespace std;\n// Part of Cosmos by OpenGenus Foundation\nstruct node\n{\n int d;\n node *left;\n node *right;\n};\n\nstruct node* newnode(int num)\n{\n node *temp = new node;\n temp->d = num;\n temp->left = temp->right = NULL;\n return temp;\n}\n\nvoid rightview(struct node *root, int level, int *maxlevel)\n{\n if (root == NULL)\n return;\n\n\n if (*maxlevel < level)\n {\n cout << root->d << \" \";\n *maxlevel = level;\n }\n\n rightview(root->right, level + 1, maxlevel);\n rightview(root->left, level + 1, maxlevel);\n\n}\n\nint main()\n{\n struct node *root = newnode(1);\n root->left = newnode(2);\n root->right = newnode(3);\n root->left->left = newnode(4);\n root->left->right = newnode(5);\n root->right->left = newnode(6);\n root->right->right = newnode(7);\n root->right->left->right = newnode(8);\n\n\n int maxlevel = 0;\n\n cout << \"\\nRight view : \";\n rightview(root, 1, &maxlevel);\n\n cout << endl;\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/traversal/preorder/right_view/right_view.py",
"content": "# Python program to print right view of Binary Tree\n# Part of Cosmos by OpenGenus Foundation\n# A binary tree node\nclass Node:\n # A constructor to create a new Binary tree Node\n def __init__(self, item):\n self.data = item\n self.left = None\n self.right = None\n\n\n# Recursive function to print right view of Binary Tree\n# used max_level as reference list ..only max_level[0]\n# is helpful to us\ndef rightViewUtil(root, level, max_level):\n\n # Base Case\n if root is None:\n return\n\n # If this is the last node of its level\n if max_level[0] < level:\n print(\"%d \" % (root.data)),\n max_level[0] = level\n\n # Recur for right subtree first, then left subtree\n rightViewUtil(root.right, level + 1, max_level)\n rightViewUtil(root.left, level + 1, max_level)\n\n\ndef rightView(root):\n max_level = [0]\n rightViewUtil(root, 1, max_level)\n\n\n# Driver program to test above function\nroot = Node(1)\nroot.left = Node(2)\nroot.right = Node(3)\nroot.left.left = Node(4)\nroot.left.right = Node(5)\nroot.right.left = Node(6)\nroot.right.right = Node(7)\nroot.right.left.right = Node(8)\n\nrightView(root)"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/traversal/zigzag/zigzag.cpp",
"content": "#include <iostream>\n#include <stack>\n\nclass Node\n{\npublic:\n int info;\n Node* left_child;\n Node* right_child;\n Node (int info) : info{info}, left_child{nullptr}, right_child{nullptr}\n {\n }\n};\n\nclass BinaryTree\n{\npublic:\n Node* root;\n BinaryTree() : root{nullptr}\n {\n }\n void zigzag_traversal();\n};\n\nvoid BinaryTree :: zigzag_traversal()\n{\n std::stack<Node*> st1, st2;\n st2.push (root);\n while (!st1.empty() || !st2.empty())\n {\n while (!st1.empty())\n {\n Node* curr = st1.top();\n st1.pop();\n if (curr->right_child)\n st2.push (curr->right_child);\n if (curr->left_child)\n st2.push (curr->left_child);\n std::cout << curr->info << \" \";\n }\n while (!st2.empty())\n {\n Node* curr = st2.top();\n st2.pop();\n if (curr->left_child)\n st1.push (curr->left_child);\n if (curr->right_child)\n st1.push (curr->right_child);\n std::cout << curr->info << \" \";\n }\n }\n}\n\nint main()\n{\n BinaryTree binary_tree;\n binary_tree.root = new Node (1);\n binary_tree.root->left_child = new Node (2);\n binary_tree.root->right_child = new Node (3);\n binary_tree.root->left_child->left_child = new Node (4);\n binary_tree.root->left_child->right_child = new Node (5);\n binary_tree.root->right_child->left_child = new Node (6);\n binary_tree.root->right_child->right_child = new Node (7);\n binary_tree.zigzag_traversal();\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/tree/bottom_view_binary_tree/bottom_view_tree.cpp",
"content": "#include <iostream>\n#include <map>\n#include <queue>\n#include <climits>\nusing namespace std;\n// Part of Cosmos by OpenGenus Foundation\n// Tree node class\nstruct Node\n{\n int data; //data of the node\n int hd; //horizontal distance of the node\n Node *left, *right; //left and right references\n\n // Constructor of tree node\n Node(int key)\n {\n data = key;\n hd = INT_MAX;\n left = right = NULL;\n }\n};\n\n// Method that prints the bottom view.\nvoid bottomView(Node *root)\n{\n if (root == NULL)\n return;\n\n // Initialize a variable 'hd' with 0\n // for the root element.\n int hd = 0;\n\n // TreeMap which stores key value pair\n // sorted on key value\n map<int, int> m;\n\n // Queue to store tree nodes in level\n // order traversal\n queue<Node *> q;\n\n // Assign initialized horizontal distance\n // value to root node and add it to the queue.\n root->hd = hd;\n q.push(root);\n\n // Loop until the queue is empty (standard\n // level order loop)\n while (!q.empty())\n {\n Node *temp = q.front();\n q.pop();\n\n // Extract the horizontal distance value\n // from the dequeued tree node.\n hd = temp->hd;\n\n // Put the dequeued tree node to TreeMap\n // having key as horizontal distance. Every\n // time we find a node having same horizontal\n // distance we need to replace the data in\n // the map.\n m[hd] = temp->data;\n\n // If the dequeued node has a left child, add\n // it to the queue with a horizontal distance hd-1.\n if (temp->left != NULL)\n {\n temp->left->hd = hd - 1;\n q.push(temp->left);\n }\n\n // If the dequeued node has a right child, add\n // it to the queue with a horizontal distance\n // hd+1.\n if (temp->right != NULL)\n {\n temp->right->hd = hd + 1;\n q.push(temp->right);\n }\n }\n\n // Traverse the map elements using the iterator.\n for (auto i = m.begin(); i != m.end(); ++i)\n cout << i->second << \" \";\n}\n\n// Driver Code\nint main()\n{\n Node *root = new Node(20);\n root->left = new Node(8);\n root->right = new Node(22);\n root->left->left = new Node(5);\n root->left->right = new Node(3);\n root->right->left = new Node(4);\n root->right->right = new Node(25);\n root->left->right->left = new Node(10);\n root->left->right->right = new Node(14);\n cout << \"Bottom view of the given binary tree :\\n\";\n bottomView(root);\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/tree/bottom_view_binary_tree/bottom_view_tree.java",
"content": "// Java Program to print Bottom View of Binary Tree\nimport java.util.*;\nimport java.util.Map.Entry;\n \n// Tree node class\nclass Node\n{\n int data; //data of the node\n int hd; //horizontal distance of the node\n Node left, right; //left and right references\n \n // Constructor of tree node\n public Node(int key)\n {\n data = key;\n hd = Integer.MAX_VALUE;\n left = right = null;\n }\n}\n \n//Tree class\nclass Tree\n{\n Node root; //root node of tree\n \n // Default constructor\n public Tree() {}\n \n // Parameterized tree constructor\n public Tree(Node node)\n {\n root = node;\n }\n \n // Method that prints the bottom view.\n public void bottomView()\n {\n if (root == null)\n return;\n \n // Initialize a variable 'hd' with 0 for the root element.\n int hd = 0;\n \n // TreeMap which stores key value pair sorted on key value\n Map<Integer, Integer> map = new TreeMap<>();\n \n // Queue to store tree nodes in level order traversal\n Queue<Node> queue = new LinkedList<Node>();\n \n // Assign initialized horizontal distance value to root\n // node and add it to the queue.\n root.hd = hd;\n queue.add(root);\n \n // Loop until the queue is empty (standard level order loop)\n while (!queue.isEmpty())\n {\n Node temp = queue.remove();\n \n // Extract the horizontal distance value from the\n // dequeued tree node.\n hd = temp.hd;\n \n // Put the dequeued tree node to TreeMap having key\n // as horizontal distance. Every time we find a node\n // having same horizontal distance we need to replace\n // the data in the map.\n map.put(hd, temp.data);\n \n // If the dequeued node has a left child add it to the\n // queue with a horizontal distance hd-1.\n if (temp.left != null)\n {\n temp.left.hd = hd-1;\n queue.add(temp.left);\n }\n // If the dequeued node has a left child add it to the\n // queue with a horizontal distance hd+1.\n if (temp.right != null)\n {\n temp.right.hd = hd+1;\n queue.add(temp.right);\n }\n }\n \n // Extract the entries of map into a set to traverse\n // an iterator over that.\n Set<Entry<Integer, Integer>> set = map.entrySet();\n \n // Make an iterator\n Iterator<Entry<Integer, Integer>> iterator = set.iterator();\n \n // Traverse the map elements using the iterator.\n while (iterator.hasNext())\n {\n Map.Entry<Integer, Integer> me = iterator.next();\n System.out.print(me.getValue()+\" \");\n }\n }\n}\n \n// Main driver class\npublic class BottomView\n{\n public static void main(String[] args)\n {\n Node root = new Node(20);\n root.left = new Node(8);\n root.right = new Node(22);\n root.left.left = new Node(5);\n root.left.right = new Node(3);\n root.right.left = new Node(4);\n root.right.right = new Node(25);\n root.left.right.left = new Node(10);\n root.left.right.right = new Node(14);\n Tree tree = new Tree(root);\n System.out.println(\"Bottom view of the given binary tree:\");\n tree.bottomView();\n }\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/tree/README.md",
"content": "# Binary tree\n\nDescription\n---\nA [binary tree](https://en.wikipedia.org/wiki/Binary_tree) is a variation of the [tree](..) data structure, the change being that each node can have a maximum of two children. It is useful for performing [binary searches](../../../search/binary_search), or generating [binary heaps](../../heap) to prepare for [sorting](../../../sorting)."
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/tree/tree2.java",
"content": "public class binary_search_tree {\n\tprivate int value;\n\tprivate binary_search_tree left;\n\tprivate binary_search_tree right;\n\n\tpublic binary_search_tree(int value) {\n\t\tthis.value = value;\n\t\tthis.left = null;\n\t\tthis.right = null;\n\t}\n\n\tpublic String printInorder(binary_search_tree root) {\n\t\tString result = \"\";\n\t\t\n\t\tif(root.left != null) {\n\t\t\tresult = result + root.printInorder(root.left);\n\t\t}\n\n\t\tresult = result + root.value;\n\n\t\tif(root.right != null) {\n\t\t\tresult = result + root.printInorder(root.right);\n\t\t}\n\n\t\treturn result;\n\t}\n\n\tpublic int height(binary_search_tree root) {\n\t\tif(root == null) {\n\t\t\treturn 0;\n\t\t}\n\n\t\tint left = 1 + root.height(root.left);\n\t\tint right = 1 + root.height(root.right);\n\n\t\tif(left > right) {\n\t\t\treturn left;\n\t\t} else {\n\t\t\treturn right;\n\t\t}\n\t}\n\n\tpublic String printPreorder(binary_search_tree root) {\n\t\tString result = \"\";\n\t\t\n\t\tresult = result + root.value;\n\t\t\n\t\tif(root.left != null) {\n\t\t\tresult = result + root.printPreorder(root.left);\n\t\t}\n\n\t\tif(root.right != null) {\n\t\t\tresult = result + root.printPreorder(root.right);\n\t\t}\n\n\t\treturn result;\n\t}\n\n\tpublic String printPostorder(binary_search_tree root) {\n\t\tString result = \"\";\n\t\t\n\t\tif(root.left != null) {\n\t\t\tresult = result + root.printPostorder(root.left);\n\t\t}\n\n\t\tif(root.right != null) {\n\t\t\tresult = result + root.printPostorder(root.right);\n\t\t}\n\n\t\tresult = result + root.value;\n\t\t\n\t\treturn result;\n\t}\n\n\tpublic binary_search_tree makeNode(int value) {\n\t\tbinary_search_tree node = new binary_search_tree(value);\n\t\treturn node;\n\t}\n\n\tpublic binary_search_tree addNode(binary_search_tree root, int value) {\n\t\tif(root == null) {\n\t\t\troot = root.makeNode(value);\n\t\t} else if(root.value == value) {\n\t\t\treturn root;\n\t\t} else if(root.value > value) {\n\t\t\tif(root.left == null) {\n\t\t\t\troot.left = root.makeNode(value);\n\t\t\t} else {\n\t\t\t\troot.left = root.addNode(root.left, value);\n\t\t\t}\n\t\t} else if(root.value < value) {\n\t\t\tif(root.right == null) {\n\t\t\t\troot.right = root.makeNode(value);\n\t\t\t} else {\n\t\t\t\troot.right = root.addNode(root.right, value);\n\t\t\t}\n\t\t}\n\n\t\treturn root;\n\t}\n\n\tpublic static void main(String []args) {\n\t\tbinary_search_tree tree = null;\n\t\ttree = new binary_search_tree(5);\n\t\ttree = tree.addNode(tree, 3);\n\t\ttree = tree.addNode(tree, 2);\n\t\ttree = tree.addNode(tree, 4);\n\t\ttree = tree.addNode(tree, 7);\n\t\ttree = tree.addNode(tree, 6);\n\t\ttree = tree.addNode(tree, 8);\n\n\t\tSystem.out.println(\"Inorder: \" + tree.printInorder(tree));\n\t\tSystem.out.println(\"Postorder: \" + tree.printPostorder(tree));\n\t\tSystem.out.println(\"Preorder: \" + tree.printPreorder(tree));\n\t\tSystem.out.println(\"Height: \" + tree.height(tree));\n\t}\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/tree/tree2.swift",
"content": "public indirect enum BinaryTree<T> {\n case empty\n case node(BinaryTree, T, BinaryTree)\n}\n\nextension BinaryTree: CustomStringConvertible {\n public var description: String {\n switch self {\n case let .node(left, value, right):\n return \"value: \\(value), left = [\\(left.description)], right = [\\(right.description)]\"\n case .empty:\n return \"\"\n }\n }\n}\n\nextension BinaryTree {\n public func traverseInOrder(process: (T) -> Void) {\n if case let .node(left, value, right) = self {\n left.traverseInOrder(process: process)\n process(value)\n right.traverseInOrder(process: process)\n }\n }\n \n public func traversePreOrder(process: (T) -> Void) {\n if case let .node(left, value, right) = self {\n process(value)\n left.traversePreOrder(process: process)\n right.traversePreOrder(process: process)\n }\n }\n \n public func traversePostOrder(process: (T) -> Void) {\n if case let .node(left, value, right) = self {\n left.traversePostOrder(process: process)\n right.traversePostOrder(process: process)\n process(value)\n }\n }\n}\n\nextension BinaryTree {\n func invert() -> BinaryTree {\n if case let .node(left, value, right) = self {\n return .node(right.invert(), value, left.invert())\n } else {\n return .empty\n }\n }\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/tree/tree.c",
"content": "//Author: Praveen Gupta\n// Part of Cosmos by OpenGenus Foundation\n#include<stdio.h>\n#include<stdlib.h>\n\n//Defining Node\ntypedef struct node{\n\tint data;\n\tstruct node *left;\n\tstruct node *right;\n\n}node;\n\n//Creates a new Node\nnode *newNode(int data){\n\tnode *temp=(node *)malloc(sizeof(node));\n\ttemp->data=data;\n\ttemp->left=temp->right=NULL;\n\n\treturn temp;\n}\n\n//Adds the new node to the appropriate position\nnode *create(node *head,int data){\n\n\tif (head==NULL){\n\t\thead = newNode(data);\n\t}\n\telse if(data<=head->data){\n\t\thead->left = create(head->left,data);\n\t}\n\telse{\n\t\thead->right = create(head->right,data);\n\t}\n\treturn head;\n}\n\n//Prints tree\nvoid print(node *head){\n\tif(head==NULL)\n\t{\n\t\treturn;\n\t}\n\tprintf(\"%d(\",head->data );\n\tprint(head->left);\n\tprintf(\" , \");\n\tprint(head->right);\n\tprintf(\")\");\n}\n\n//Searches for an element in the tree\nint search(node *head, int data){\n\tif(head==NULL)\n\t\treturn 0;\n\tif(head->data==data)\n\t\treturn 1;\n\tif(data<head->data)\n\t\treturn search(head->left, data);\n\telse\n\t\treturn search(head->right, data);\n}\n\n//Deletes a subtree with root as the parameter\nnode *deleteTree(node *head){\n\tif(head==NULL)\n\t\treturn NULL;\n\n\tdeleteTree(head->left);\n\tdeleteTree(head->right);\n\tfree(head);\n\treturn NULL;\n}\n\n//Deletes the node and its children's\nnode *delete(node *head, int data){\n\tif(head==NULL){\n\t\tprintf(\"Nor found\\n\");\t\t\n\t}\n\n\tif(head->data==data){\n\t\thead = deleteTree(head);\n\t}\n\t\n\telse if(data<head->data)\n\t\thead->left = delete(head->left, data);\n\t\n\telse\n\t\thead->right = delete(head->right, data);\n\n\treturn head;\n}\n//Finds the height of the tree\nint height(node *head) \n{\n if (head==NULL) \n return 0;\n else\n {\n /* compute the depth of each subtree */\n int left_height = height(head->left);\n int right_height = height(head->right);\n \n /* use the larger one */\n if (left_height > right_height) \n return(left_height+1);\n else return(right_height+1);\n }\n} \nint main(){\nnode *head=NULL;\n\n\tprintf(\"Binary Search Tree\\n\");\n\tint c,data;\n\n\tagain:\n\tprintf(\"\\n1. Insert Node 2. Delete Node\t\t 3.Search\t\t4.Find height\\n\");\n\tscanf(\"%d\",&c);\n\n\tswitch(c){\n\t\tcase 1:\tprintf(\"Enter data\\n\");\n\t\t\t\tscanf(\"%d\",&data);\n\t\t\t\thead=create(head,data);\n\t\t\t\tbreak;\n\n\t\tcase 2:\tprintf(\"Enter data to delete\\n\");\n\t\t\t\tscanf(\"%d\",&data);\n\t\t\t\thead = delete(head,data);\n\t\t\t\tbreak;\t\t\n\n\t\tcase 3: printf(\"Enter data to search\\n\");\n\t\t\t\tscanf(\"%d\",&data);\n\t\t\t\tsearch(head,data) ? printf(\"Found in tree\\n\"):printf(\"Not Found in tree\\n\");\n\t\tcase 4: printf(\"The height of BST is: %d \",height(head));\n\t}\n\tprint(head);\n\tgoto again;\nreturn 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/tree/tree.cpp",
"content": "#include <iostream>\nusing namespace std;\n\nstruct node\n{\n int key;\n struct node *left, *right;\n};\n\nstruct node *newNode(int item)\n{\n struct node *temp = new node;\n temp->key = item;\n temp->left = temp->right = NULL;\n return temp;\n}\n\n//Inorder traversal\nvoid inorder(struct node *root)\n{\n if (root != NULL)\n {\n inorder(root->left);\n cout << root->key << \" \";\n inorder(root->right);\n }\n}\n\n//Preorder traversal\nvoid preorder(struct node *root)\n{\n if (root == NULL)\n return;\n cout << root->key << \" \";\n preorder(root->left);\n preorder(root->right);\n}\n\n//Postorder traversal\nvoid postorder(struct node *root)\n{\n if (root == NULL)\n return;\n postorder(root->left);\n postorder(root->right);\n cout << root->key << \" \";\n}\n\n//Insert key\nstruct node* insert(struct node* node, int key)\n{\n if (node == NULL)\n return newNode(key);\n if (key < node->key)\n node->left = insert(node->left, key);\n else if (key > node->key)\n node->right = insert(node->right, key);\n return node;\n}\n\n// Driver Code\nint main()\n{\n /* Let us create following BST\n * 50\n * / \\\n * 30 70\n * / \\ / \\\n * 20 40 60 80 */\n struct node *root = NULL;\n root = insert(root, 50);\n insert(root, 30);\n insert(root, 20);\n insert(root, 40);\n insert(root, 70);\n insert(root, 60);\n insert(root, 80);\n\n cout << \"Inorder traversal: \";\n inorder(root);\n\n cout << \"\\nPreorder traversal: \";\n preorder(root);\n\n cout << \"\\nPostorder traversal: \";\n postorder(root);\n cout << endl;\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/tree/tree.go",
"content": "package main\n\n// Part of Cosmos by OpenGenus Foundation\nimport \"fmt\"\n\ntype Node struct {\n\tval int\n\tlchild *Node\n\trchild *Node\n}\n\nfunc (ref *Node) insert(newNode *Node) {\n\n\tif ref.val == newNode.val {\n\t\treturn\n\t}\n\n\tif ref.val < newNode.val {\n\t\tif ref.rchild == nil {\n\t\t\tref.rchild = newNode\n\t\t} else {\n\t\t\tref.rchild.insert(newNode)\n\t\t}\n\t} else {\n\t\tif ref.lchild == nil {\n\t\t\tref.lchild = newNode\n\t\t} else {\n\t\t\tref.lchild.insert(newNode)\n\t\t}\n\t}\n}\n\nfunc (ref *Node) inOrder(fn func(n *Node)) {\n\n\tif ref.lchild != nil {\n\t\tref.lchild.inOrder(fn)\n\t}\n\n\tfn(ref)\n\n\tif ref.rchild != nil {\n\t\tref.rchild.inOrder(fn)\n\t}\n\n}\n\nfunc max(a, b int) int {\n\tif a > b {\n\t\treturn a\n\t}\n\treturn b\n}\n\nfunc getHeight(node *Node) int {\n\tif node == nil {\n\t\treturn 0\n\t}\n\n\treturn max(getHeight(node.lchild), getHeight(node.rchild)) + 1\n}\n\ntype BSTree struct {\n\tref *Node\n\tlength int\n}\n\nfunc (BSTree *BSTree) insert(value int) {\n\tnode := &Node{val: value}\n\n\tif BSTree.ref == nil {\n\t\tBSTree.ref = node\n\t} else {\n\t\tBSTree.ref.insert(node)\n\t}\n}\n\nfunc (BSTree *BSTree) inorderPrint() {\n\tBSTree.ref.inOrder(func(node *Node) {\n\t\tfmt.Println(node.val)\n\t})\n}\n\nfunc (BSTree *BSTree) height() int {\n\treturn getHeight(BSTree.ref)\n}\n\nfunc main() {\n\n\ttree := new(BSTree)\n\ttree.insert(1)\n\ttree.insert(0)\n\ttree.insert(20)\n\ttree.insert(4)\n\ttree.insert(5)\n\ttree.insert(6)\n\tfmt.Println(\"Height \", tree.height())\n\n\ttree.inorderPrint()\n\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/tree/tree.java",
"content": "/* Part of Cosmos by OpenGenus Foundation */\nimport java.util.ArrayList;\n\nclass BinaryTree<T extends Comparable<T>>{\n\tprivate Node<T> root;\n\n\tpublic BinaryTree(){\n\t\troot = null;\n\t}\n\t\n\tprivate Node<T> find(T key){\n\t\tNode<T> current = root;\n\t\tNode<T> last = root;\n\t\twhile(current != null){\n\t\t\tlast = current;\n\t\t\tif(key.compareTo(current.data) < 0)\n\t\t\t\tcurrent = current.left;\n\t\t\telse if(key.compareTo(current.data) > 0)\n\t\t\t\tcurrent = current.right;\n\t\t\t//If you find the value return it\n\t\t\telse\n\t\t\t\treturn current;\n\t\t}\n\t\treturn last;\n\t}\n\n\tpublic void put(T value){\n\t\tNode<T> newNode = new Node<>(value);\n\t\tif(root == null)\n\t\t\troot = newNode;\n\t\telse{\n\t\t\t//This will return the soon to be parent of the value you're inserting\n\t\t\tNode<T> parent = find(value);\n\n\t\t\t//This if/else assigns the new node to be either the left or right child of the parent\n\t\t\tif(value.compareTo(parent.data) < 0){\n\t\t\t\tparent.left = newNode;\n\t\t\t\tparent.left.parent = parent;\n\t\t\t\treturn;\n\t\t\t}\n\t\t\telse{\n\t\t\t\tparent.right = newNode;\n\t\t\t\tparent.right.parent = parent;\n\t\t\t\treturn;\n\t\t\t}\n\t\t}\n\t}\n\n\tpublic boolean remove(T value){\n\t\t//temp is the node to be deleted\n\t\tNode<T> temp = find(value);\n\n\t\t//If the value doesn't exist\n\t\tif(temp.data != value)\n\t\t\treturn false;\n\n\t\t//No children\n\t\tif(temp.right == null && temp.left == null){\n\t\t\tif(temp == root)\n\t\t\t\troot = null;\n\n\t\t\t//This if/else assigns the new node to be either the left or right child of the parent\n\t\t\telse if(temp.parent.data.compareTo(temp.data) < 0)\n\t\t\t\ttemp.parent.right = null;\n\t\t\telse\n\t\t\t\ttemp.parent.left = null;\n\t\t\treturn true;\n\t\t}\n\n\t\t//Two children\n\t\telse if(temp.left != null && temp.right != null){\n\t\t\tNode<T> successor = findSuccessor(temp);\n\n\t\t\t//The left tree of temp is made the left tree of the successor\n\t\t\tsuccessor.left = temp.left;\n\t\t\tsuccessor.left.parent = successor;\n\n\t\t\t//If the successor has a right child, the child's grandparent is it's new parent\n\t\t\tif(successor.right != null && successor.parent != temp){\n\t\t\t\tsuccessor.right.parent = successor.parent;\n\t\t\t\tsuccessor.parent.left = successor.right;\n\t\t\t\tsuccessor.right = temp.right;\n\t\t\t\tsuccessor.right.parent = successor;\n\t\t\t}\n\t\t\tif(temp == root){\n\t\t\t\tsuccessor.parent = null;\n\t\t\t\troot = successor;\n\t\t\t\treturn true;\n\t\t\t}\n\n\t\t\t//If you're not deleting the root\n\t\t\telse{\n\t\t\t\tsuccessor.parent = temp.parent;\n\n\t\t\t\t//This if/else assigns the new node to be either the left or right child of the parent\n\t\t\t\tif(temp.parent.data.compareTo(temp.data) < 0)\n\t\t\t\t\ttemp.parent.right = successor;\n\t\t\t\telse\n\t\t\t\t\ttemp.parent.left = successor;\n\t\t\t\treturn true;\n\t\t\t}\n\t\t}\n\t\t//One child\n\t\telse{\n\t\t\t//If it has a right child\n\t\t\tif(temp.right != null){\n\t\t\t\tif(temp == root){\n\t\t\t\t\troot = temp.right; return true;}\n\n\t\t\t\ttemp.right.parent = temp.parent;\n\n\t\t\t\t//Assigns temp to left or right child\n\t\t\t\tif(temp.data.compareTo(temp.parent.data) < 0)\n\t\t\t\t\ttemp.parent.left = temp.right;\n\t\t\t\telse\n\t\t\t\t\ttemp.parent.right = temp.right;\n\t\t\t\treturn true;\n\t\t\t}\n\t\t\t//If it has a left child\n\t\t\telse{\n\t\t\t\tif(temp == root){\n\t\t\t\t\troot = temp.left; return true;}\n\n\t\t\t\ttemp.left.parent = temp.parent;\n\n\t\t\t\t//Assigns temp to left or right side\n\t\t\t\tif(temp.data.compareTo(temp.parent.data) < 0)\n\t\t\t\t\ttemp.parent.left = temp.left;\n\t\t\t\telse\n\t\t\t\t\ttemp.parent.right = temp.left;\n\t\t\t\treturn true;\n\t\t\t}\n\t\t}\n\t}\n\n\tprivate Node<T> findSuccessor(Node<T> n){\n\t\tif(n.right == null)\n\t\t\treturn n;\n\t\tNode<T> current = n.right;\n\t\tNode<T> parent = n.right;\n\t\twhile(current != null){\n\t\t\tparent = current;\n\t\t\tcurrent = current.left;\n\t\t}\n\t\treturn parent;\n\t}\n\n\tpublic T getRoot(){\n\t\t\treturn root.data;\n\t}\n\n\tpublic String toString()\n\t{\n\t\treturn inOrder(root).toString();\n\t}\n\n\tprivate ArrayList<T> inOrder(Node<T> localRoot){\n\t\tArrayList<T> temp = new ArrayList<>();\n\t\tif(localRoot != null){\n\t\t\tinOrder(localRoot.left);\n\t\t\ttemp.add(localRoot.data);\n\t\t\t//System.out.print(localRoot.data + \" \");\n\t\t\tinOrder(localRoot.right);\n\t\t}\n\t\treturn temp;\n\t}\n\t\n\tprivate void preOrder(Node<T> localRoot){\n\t\tif(localRoot != null){\n\t\t\tSystem.out.print(localRoot.data + \" \");\n\t\t\tpreOrder(localRoot.left);\n\t\t\tpreOrder(localRoot.right);\n\t\t}\n\t}\n\t\n\tprivate void postOrder(Node<T> localRoot){\n\t\tif(localRoot != null){\n\t\t\tpostOrder(localRoot.left);\n\t\t\tpostOrder(localRoot.right);\n\t\t\tSystem.out.print(localRoot.data + \" \");\n\t\t}\n\t}\n\n\tprivate class Node<T extends Comparable<T>>{\n\t\tpublic T data;\n\t\tpublic Node<T> left;\n\t\tpublic Node<T> right;\n\t\tpublic Node<T> parent;\n\n\t\tpublic Node(T value){\n\t\t\tdata = value;\n\t\t\tleft = null;\n\t\t\tright = null;\n\t\t\tparent = null;\n\t\t}\n\t}\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/tree/tree.js",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n/*Binary Search Tree!!\n *\n * Nodes that will go on the Binary Tree.\n * They consist of the data in them, the node to the left, the node\n * to the right, and the parent from which they came from.\n *\n * A binary tree is a data structure in which an element\n * has two successors(children). The left child is usually\n * smaller than the parent, and the right child is usually\n * bigger.\n */\n\n// Node in the tree\nfunction Node(val) {\n this.value = val;\n this.left = null;\n this.right = null;\n}\n\n// Search the tree for a value\nNode.prototype.search = function(val) {\n if (this.value == val) {\n return this;\n } else if (val < this.value && this.left != null) {\n return this.left.search(val);\n } else if (val > this.value && this.right != null) {\n return this.right.search(val);\n }\n return null;\n};\n\n// Visit a node\nNode.prototype.visit = function() {\n // Recursively go left\n if (this.left != null) {\n this.left.visit();\n }\n // Print out value\n console.log(this.value);\n // Recursively go right\n if (this.right != null) {\n this.right.visit();\n }\n};\n\n// Add a node\nNode.prototype.addNode = function(n) {\n if (n.value < this.value) {\n if (this.left == null) {\n this.left = n;\n } else {\n this.left.addNode(n);\n }\n } else if (n.value > this.value) {\n if (this.right == null) {\n this.right = n;\n } else {\n this.right.addNode(n);\n }\n }\n};\n\nfunction Tree() {\n // Just store the root\n this.root = null;\n}\n\n// Inorder traversal\nTree.prototype.traverse = function() {\n this.root.visit();\n};\n\n// Start by searching the root\nTree.prototype.search = function(val) {\n var found = this.root.search(val);\n if (found === null) {\n console.log(val + \" not found\");\n } else {\n console.log(\"Found:\" + found.value);\n }\n};\n\n// Add a new value to the tree\nTree.prototype.addValue = function(val) {\n var n = new Node(val);\n if (this.root == null) {\n this.root = n;\n } else {\n this.root.addNode(n);\n }\n};\n\n//Implementation of BST\nvar bst = new Tree();\nbst.addValue(6);\nbst.addValue(3);\nbst.addValue(9);\nbst.addValue(2);\nbst.addValue(8);\nbst.addValue(4);\nbst.traverse();\nbst.search(8);"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/tree/tree.py",
"content": "## BST operation\n## functionalities included are:\n\"\"\"\n*insert node\n*delete node\n*find successor of a node\n*find minimum in BST\n*find maximum in BST\n*traverse in BST(level order traversal)\n*find height of the BST\n*search in BST\n*get node path in BST\n*find Lowest Common Ancestor in BST(LCA)\n\"\"\"\n\n\n## class for node creation\nclass Node(object):\n def __init__(self, data=None):\n self.left = None\n self.right = None\n self.data = data\n\n def __str__(self):\n return str(self.data)\n\n\n## the Binary Search Tree class\nclass BST(object):\n def __init__(self):\n self.root = None\n\n def insert(self, val):\n if self.root == None:\n print(\"root!\")\n self.root = Node(val)\n else:\n root = self.root\n while True:\n if val < root.data:\n if not root.left:\n print(\"left\")\n root.left = Node(val)\n break\n else:\n print(\"left\")\n root = root.left\n else:\n if not root.right:\n print(\"right\")\n root.right = Node(val)\n break\n else:\n print(\"right\")\n root = root.right\n\n def insert_recursive(self, root, val):\n if root == None:\n return Node(val)\n else:\n if val < root.data:\n node = self.insert_recursive(root.left, val)\n root.left = node\n else:\n node = self.insert_recursive(root.right, val)\n root.right = node\n\n def traverse(self): ## level order traversals\n nodes = []\n nodes.append(self.root)\n print(\"\\n\\nBST representation\")\n while nodes:\n current = nodes.pop(0)\n print(current.data)\n if current.left:\n nodes.append(current.left)\n if current.right:\n nodes.append(current.right)\n\n def find_max(self):\n if self.root is None:\n return None\n else:\n current = self.root\n while True:\n if current.right is not None:\n current = current.right\n else:\n break\n return current.data\n\n def find_min(self):\n if self.root is None:\n return None\n else:\n current = self.root\n while True:\n if current.left is not None:\n current = current.left\n else:\n break\n return current.data\n\n def height_(self, root): ## the height driver function\n if root == None:\n return 0\n else:\n max_left_subtree_height = self.height_(root.left)\n max_right_subtree_height = self.height_(root.right)\n max_height = max(max_left_subtree_height, max_right_subtree_height) + 1\n return max_height\n\n ## height of the first node is 0 not 1\n def height(self):\n depth = self.height_(self.root)\n return depth - 1\n\n def search(self, val):\n if self.root is None:\n return False\n else:\n current = self.root\n while True:\n if val < current.data:\n if current.left:\n current = current.left\n else:\n break\n elif val > current.data:\n if current.right:\n current = current.right\n else:\n break\n if val == current.data:\n return True\n return False\n\n def get_node_path(self, val):\n if self.search(val):\n path = []\n current = self.root\n while True:\n if current is None:\n break\n else:\n if val == current.data:\n path.append(current.data)\n break\n elif val < current.data:\n if current.left:\n path.append(current.data)\n current = current.left\n else:\n break\n elif val > current.data:\n if current.right:\n path.append(current.data)\n current = current.right\n else:\n break\n return path\n return None\n\n def LCA(self, val1, val2):\n path1 = self.get_node_path(val1)\n path2 = self.get_node_path(val2)\n try:\n store = None\n if len(path1) != len(path2):\n min_list = min(path1, path2, key=len)\n max_list = max(path1, path2, key=len)\n min_list, max_list = path1, path2\n for lca1 in min_list:\n for lca2 in max_list:\n if lca1 == lca2:\n store = lca1\n return store\n except:\n return None\n\n def successor(self, root):\n current = root.right\n while True:\n if current.left:\n current = current.left\n else:\n break\n return current\n\n def Delete(self, root, val): ## driver function for delettion\n if root is None:\n return None\n else:\n if val < root.data:\n root.left = self.Delete(root.left, val)\n elif val > root.data:\n root.right = self.Delete(root.right, val)\n else:\n if not root.left:\n right = root.right\n del root\n return right\n if not root.right:\n left = root.left\n del root\n return left\n else:\n successor = self.successor(root)\n root.data = successor.data\n root.right = self.Delete(root.right, successor.data)\n return root\n\n def delete(self, val):\n if self.search(val):\n self.root = self.Delete(self.root, val)\n else:\n return \"error\"\n\n def clear(self):\n print(\"\\n\\nre-initializing\\n\")\n self.__init__()\n\n\n## creating an instance for the BST\nbst = BST()\nbst.insert(5)\nbst.insert(9)\nbst.insert(10)"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/tree/tree.rb",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n##\n# Represents a single node within a binary search tree.\nclass Node\n attr_accessor :data, :left, :right\n\n ##\n # Initializes a new node with given +data+.\n #\n # Params:\n # +data+:: Data to store within this node.\n def initialize(data)\n @data = data\n @left = nil\n @right = nil\n end\n\n def to_s\n @data\n end\nend\n\n##\n# A binary search tree made from generic nodes.\nclass BinarySearchTree\n ##\n # Initializes a new binary search tree.\n def initialize\n @root = nil\n end\n\n ##\n # Searchs this binary search tree for the provided +data+.\n #\n # Params:\n # +data+:: Data to search for\n def search(data)\n # Start at the root node\n current = @root\n puts 'Visiting all nodes...'\n\n # Iterate through all nodes\n while current.data != data\n\n puts \"Current node: #{current}\"\n\n # Determine whether to move left or right in tree\n current = if data < current.data\n current.left\n else\n current.right\n end\n\n # Occurs when the end of the tree is reached.\n if current.nil?\n puts \"Not found: #{data}\"\n return false\n end\n\n end\n\n true\n end\n\n ##\n # Inserts the given +data+ into the binary search tree.\n #\n # Params:\n # +data+:: Data to insert into BST.\n def insert(data)\n # Create new node\n node = Node.new(data)\n\n # Check if tree is empty\n if @root.nil?\n @root = node\n return\n end\n\n current = @root\n parent = nil\n\n # Traverse BST\n loop do\n parent = current\n\n # Determine whether to place left or right in the tree\n if data < parent.data\n current = current.left\n\n # Update left node\n if current.nil?\n parent.left = node\n return\n end\n else\n current = current.right\n\n # Update right node\n if current.nil?\n parent.right = node\n return\n end\n end\n end\n end\nend\n\ntree = BinarySearchTree.new\ntree.insert(15)\ntree.insert(5)\ntree.insert(30)\ntree.insert(25)\nputs tree.search(5)\nputs tree.search(10)"
}
{
"filename": "code/data_structures/src/tree/binary_tree/binary_tree/tree/tree.swift",
"content": "// Part of Cosmos by OpenGenus Foundation\npublic enum BinarySearchTree<T: Comparable> {\n case empty\n case leaf(T)\n indirect case node(BinarySearchTree, T, BinarySearchTree)\n\n public var count: Int {\n switch self {\n case .empty: return 0\n case .leaf: return 1\n case let .node(left, _, right): return left.count + 1 + right.count\n }\n }\n\n public var height: Int {\n switch self {\n case .empty: return 0\n case .leaf: return 1\n case let .node(left, _, right): return 1 + max(left.height, right.height)\n }\n }\n\n public func insert(newValue: T) -> BinarySearchTree {\n switch self {\n case .empty:\n return .leaf(newValue)\n\n case .leaf(let value):\n if newValue < value {\n return .node(.leaf(newValue), value, .empty)\n } else {\n return .node(.empty, value, .leaf(newValue))\n }\n\n case .node(let left, let value, let right):\n if newValue < value {\n return .node(left.insert(newValue), value, right)\n } else {\n return .node(left, value, right.insert(newValue))\n }\n }\n }\n\n public func search(x: T) -> BinarySearchTree? {\n switch self {\n case .empty:\n return nil\n case .leaf(let y):\n return (x == y) ? self : nil\n case let .node(left, y, right):\n if x < y {\n return left.search(x)\n } else if y < x {\n return right.search(x)\n } else {\n return self\n }\n }\n }\n\n public func contains(x: T) -> Bool {\n return search(x) != nil\n }\n \n public func minimum() -> BinarySearchTree {\n var node = self\n var prev = node\n while case let .node(next, _, _) = node {\n prev = node\n node = next\n }\n if case .leaf = node {\n return node\n }\n return prev\n }\n\n public func maximum() -> BinarySearchTree {\n var node = self\n var prev = node\n while case let .node(_, _, next) = node {\n prev = node\n node = next\n }\n if case .leaf = node {\n return node\n }\n return prev\n }\n}\n\nextension BinarySearchTree: CustomDebugStringConvertible {\n public var debugDescription: String {\n switch self {\n case .empty: return \".\"\n case .leaf(let value): return \"\\(value)\"\n case .node(let left, let value, let right):\n return \"(\\(left.debugDescription) <- \\(value) -> \\(right.debugDescription))\"\n }\n }\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/inorder_threaded_binary_search_tree/TBT_all_operations.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n#include <stdbool.h>\n\n/*\nMenu Driven Program with implementation of BST and all the fuctions\n Insert\n Traversal(all without using stack/recursion)\n preorder\n inorder\n postorder\n Delete\n Exit Program\n*/\n\nstruct node\n{\n int val;\n bool lbit;\n bool rbit;\n\n struct node *left;\n struct node *right;\n};\n\nstruct node *getNode(int val);\nstruct node *insert(struct node *head, int key);\n\nstruct node *inorderPredecessor(struct node *p);\nstruct node *inorderSuccessor(struct node *p);\n\nvoid Traversal(struct node *head);\nvoid inorder(struct node *head);\nvoid preorder(struct node *head);\n\nstruct node *findParent(struct node *p);\nstruct node *postSuccessor(struct node *p);\nvoid postorder(struct node *head);\n\n// ********************************* DELETE ********************************\nstruct node *delThreadedBST(struct node *head, int key);\nstruct node *delTwoChild(struct node *head, struct node *par, struct node *ptr);\nstruct node *delOneChild(struct node *head, struct node *par, struct node *ptr);\nstruct node *delNoChild(struct node *head, struct node *par, struct node *ptr);\n\nint \nmain()\n{\n struct node *head;\n head = (struct node *)malloc(sizeof(struct node));\n head->lbit = 0;\n head->rbit = 1; // convention for all cases\n head->right = head; // convention for all cases\n head->left = head; // head->left should point to root\n\n\n /*\n uncomment the following to create a sample tree like\n\n insert(head,10);\n insert(head,5);\n insert(head,15);\n insert(head,20);\n insert(head,13);\n insert(head,14);\n insert(head,12);\n insert(head,8);\n insert(head,3);\n insert(head, 21);\n insert(head, 22);\n insert(head, 30);\n\n will create a tree like this\n 10\n 5 15\n 3 8 13 20\n 12 14 21\n 22\n 30\n or use menu driven approach\n\n */\n\n while (1)\n {\n printf(\"\\n\\n\\n\\n****************************************************************\");\n printf(\"\\nSelect operation\");\n\n printf(\"\\n\\t1.Insert\\n\\t2.Traverse(pre in)\\n\\t3.Delete\\n\\n\\t0.Exit\\n\\nEnter your choice: \");\n int n;\n scanf(\"%d\", &n);\n int temp;\n /*system(\"cls\");*/\n\n switch (n)\n {\n case 1:\n {\n printf(\"\\nEnter number to Insert: \");\n scanf(\"%d\", &temp);\n head = insert(head, temp);\n }\n break;\n\n case 2:\n Traversal(head);\n break;\n\n case 3:\n {\n printf(\"\\nEnter number to Delete: \");\n scanf(\"%d\", &temp);\n delThreadedBST(head, temp);\n }\n break;\n\n case 0:\n exit(0);\n break;\n\n default:\n printf(\"invalid Choixe.\");\n break;\n }\n }\n\n return 0;\n}\n\n\nstruct node *\ngetNode(int val)\n{\n struct node *temp = (struct node *)malloc(sizeof(struct node));\n temp->val = val;\n temp->lbit = 0;\n temp->rbit = 0;\n temp->left = NULL;\n temp->right = NULL;\n return temp;\n}\n\nstruct node *\ninsert(struct node *head, int key)\n{\n struct node *temp = getNode(key);\n struct node *p;\n\n if (head->left == head)\n {\n head->left = temp;\n temp->left = head;\n temp->right = head;\n return head;\n }\n\n p = head->left;\n\n while (1)\n {\n if (key < p->val && p->lbit == 1)\n p = p->left;\n\n else if (key > p->val && p->rbit == 1)\n p = p->right;\n else\n break;\n }\n\n if (key < p->val)\n {\n p->lbit = 1;\n temp->left = p->left;\n temp->right = p;\n p->left = temp;\n }\n\n else if (key > p->val)\n {\n p->rbit = 1;\n // rearrange the thread after linking new node\n temp->right = p->right; // inorder successor (next)\n temp->left = p;\n p->right = temp;\n }\n\n return head;\n}\n\nstruct node *\ninorderPredecessor(struct node *p)\n{\n if (p->lbit == 0)\n return p->left;\n else if (p->lbit == 1)\n {\n p = p->left;\n while (p->rbit == 1)\n p = p->right;\n }\n return p;\n}\n\nstruct node *\ninorderSuccessor(struct node *p)\n{\n if (p->rbit == 0)\n return p->right;\n else if (p->rbit == 1)\n {\n p = p->right;\n while (p->lbit == 1)\n p = p->left;\n }\n return p;\n}\n\nvoid \ninorder(struct node *head)\n{\n struct node *p;\n p = head->left;\n while (p->lbit == 1)\n p = p->left;\n\n while (p != head)\n {\n printf(\" %d\", p->val);\n p = inorderSuccessor(p);\n }\n}\n\nvoid \npreorder(struct node *head)\n{\n struct node *p;\n p = head->left;\n\n while (p != head)\n {\n printf(\"%d \", p->val);\n\n if (p->lbit == 1)\n {\n p = p->left;\n }\n else if (p->rbit == 1)\n {\n p = p->right;\n }\n else if (p->rbit == 0)\n {\n while (p->rbit == 0)\n p = p->right;\n p = p->right;\n }\n }\n}\n\nstruct node *\nfindParent(struct node *p)\n{\n struct node *child = p;\n // ancestor of child\n while (p->rbit == 1)\n p = p->right;\n p = p->right;\n\n if (p->left == child)\n return p;\n\n p = p->left;\n while (p->right != child)\n {\n p = p->right;\n }\n return p;\n}\n\nstruct node *\npostSuccessor(struct node *p)\n{\n struct node *cur = p;\n struct node *parent = findParent(cur);\n // printf(\"suc %d\\n\", parent->val);\n\n if (parent->right == cur)\n return parent;\n\n else\n {\n while (p->rbit == 1)\n p = p->right;\n p = p->right;\n\n if (p->rbit == 1)\n {\n p = p->right;\n while (!(p->rbit == 0 && p->lbit == 0))\n {\n if (p->lbit == 1)\n p = p->left;\n else if (p->rbit == 1)\n p = p->right;\n }\n }\n // printf(\"suc %d\\n\", p->val);\n }\n return p;\n}\n\nvoid \npostorder(struct node *head)\n{\n struct node *p = head->left;\n struct node *temp = p;\n while (!(p->rbit == 0 && p->lbit == 0))\n {\n if (p->lbit == 1)\n {\n p = p->left;\n }\n else if (p->rbit == 1)\n p = p->right;\n }\n printf(\" %d\", p->val);\n\n while (p != head->left)\n {\n // printf(\" hello\\n\");\n p = postSuccessor(p);\n printf(\" %d\", p->val);\n }\n}\n\nvoid \nTraversal(struct node *head)\n{\n printf(\"\\nTraversal Type : \\n1.preorder\\n2.Inorder\\n3.PostOrder\\n\\n\\nEnter your choice: \");\n int n;\n scanf(\"%d\", &n);\n // system(\"cls\");\n switch (n)\n {\n case 1:\n printf(\"\\nPreorder:\\n\\t\");\n preorder(head);\n break;\n\n case 2:\n printf(\"\\nInorder:\\n\\t\");\n inorder(head);\n break;\n\n case 3:\n printf(\"\\nPostorder:\\n\\t\");\n postorder(head);\n break;\n\n default:\n break;\n }\n}\n\n/* ********************************* DELETE ******************************** */\n\nstruct node *\ndelThreadedBST(struct node *head, int key)\n{\n\n struct node *par = head, *ptr = head->left;\n\n bool found = 0;\n\n while (ptr != head)\n {\n if (key == ptr->val)\n {\n found = 1;\n break;\n }\n par = ptr;\n if (key < ptr->val)\n {\n if (ptr->lbit == 1)\n ptr = ptr->left;\n else\n break;\n }\n else\n {\n if (ptr->rbit == 1)\n ptr = ptr->right;\n else\n break;\n }\n }\n\n if (found == 0)\n printf(\"key not present in tree\\n\");\n\n else if (ptr->lbit == 1 && ptr->rbit == 1)\n head = delTwoChild(head, par, ptr);\n\n else if (ptr->lbit == 0 && ptr->rbit == 0)\n head = delNoChild(head, par, ptr);\n\n else\n head = delOneChild(head, par, ptr);\n\n return head;\n}\n\nstruct node *\ndelTwoChild(struct node *head, struct node *par, struct node *ptr)\n{\n\n struct node *parSuc = ptr;\n struct node *suc = ptr->right;\n\n while (suc->lbit == 1)\n {\n parSuc = suc;\n suc = suc->left;\n }\n\n ptr->val = suc->val;\n\n if (suc->lbit == 0 && suc->rbit == 0)\n head = delNoChild(head, parSuc, suc);\n\n else\n head = delOneChild(head, parSuc, suc);\n\n return head;\n}\n\nstruct node *\ndelOneChild(struct node *head, struct node *par, struct node *ptr)\n{\n\n struct node *child;\n if (ptr->lbit == 1)\n child = ptr->left;\n else\n child = ptr->right;\n\n struct node *p = inorderPredecessor(ptr);\n struct node *s = inorderSuccessor(ptr);\n\n if (ptr == par->left)\n {\n par->left = child;\n }\n else\n {\n par->right = child;\n }\n\n if (ptr->lbit == 1)\n p->right = s;\n\n else if (ptr->rbit == 1)\n s->left = p;\n\n free(ptr);\n return head;\n}\n\nstruct node *\ndelNoChild(struct node *head, struct node *par, struct node *ptr)\n{\n if (ptr == head->left)\n {\n ptr = NULL;\n }\n else if (ptr == par->left)\n {\n par->lbit = 0;\n par->left = ptr->left;\n }\n else if (ptr == par->right)\n {\n par->rbit = 0;\n par->right = ptr->right;\n }\n free(ptr);\n return head;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/rope/rope.py",
"content": "class node:\n def __init__(self, data=None):\n self.data = data\n if not data:\n self.weight = 0\n else:\n self.weight = len(data)\n self.left = None\n self.right = None\n self.parent = None\n\n def size(self):\n if self.data:\n return len(self.data)\n tmp = self.weight\n if self.right:\n return tmp + self.right.size()\n\n def addChild(self, side, node):\n node.parent = self\n nodeSize = node.size()\n\n if side == \"left\":\n self.left = node\n self.weight = nodeSize\n elif side == \"right\":\n self.right = node\n\n tmp = self\n while tmp.parent:\n if tmp.parent.left == tmp:\n tmp.parent.weight += nodeSize\n tmp = tmp.parent\n\n def removeChild(self, side):\n result = None\n if side == \"left\":\n result = self.left\n self.left = None\n self.weight = 0\n elif side == \"right\":\n result = self.right\n self.right = None\n nodeSize = result.size()\n tmp = self\n while tmp.parent:\n if tmp.parent.left == tmp:\n tmp.parent.weight -= nodeSize\n tmp = tmp.parent\n return result\n\n\nclass Rope:\n def __init__(self, text=None):\n if text:\n self.head = node(text)\n else:\n self.head = node()\n\n def Index(self, ind, node=None):\n if node is None:\n node = self.head\n if node.weight <= ind:\n return self.Index(ind - node.weight, node.right)\n elif node.left:\n return self.Index(ind, node.left)\n else:\n return node.data[ind]\n\n def concat(self, rope):\n result = Rope()\n result.head.addChild(\"left\", self.head)\n result.head.addChild(\"right\", rope.head)\n self.head = result.head\n\n def split(self, ind):\n result = Rope()\n tmp = self.head\n splitOff = list()\n # Lookup the node with the index\n while True:\n if tmp.weight <= ind and tmp.right:\n ind = ind - tmp.weight\n tmp = tmp.right\n elif tmp.left:\n tmp = tmp.left\n else:\n break\n # Split that node if the split point is in a node\n if ind != 0:\n node1 = node(tmp.data[:ind])\n node2 = node(tmp.data[ind:])\n nodeParent = node()\n nodeParent.addChildLeft(node1)\n nodeParent.addChildRight(node2)\n if tmp.parent.left == tmp:\n tmp = tmp.parent\n tmp.removeChild(\"left\")\n tmp.addChild(\"left\", nodeParent)\n tmp = nodeParent.right\n else:\n tmp = tmp.parent\n tmp.removeChild(\"right\")\n tmp.addChild(\"right\", nodeParent)\n tmp = nodeParent.right\n\n # Get everything else to the right of ind\n first = True\n while tmp.parent:\n if not tmp.parent.left:\n tmp = tmp.parent\n elif tmp.parent.left == tmp:\n if tmp.parent.right:\n splitOff.append(tmp.parent.removeChild(\"right\"))\n else:\n tmp = tmp.parent\n elif tmp.parent.right == tmp and first:\n first = False\n tmp = tmp.parent\n splitOff.append(tmp.removeChild(\"right\"))\n\n # Make a rope with the content right of ind to return\n rightRope = rope()\n while len(splitOff) > 0:\n rightRope.insert(0, splitOff.pop(0))\n return rightRope\n\n def insert(self, ind, rope):\n rightSide = self.split(ind)\n self.concat(rope)\n self.concat(rightSide)\n\n def delete(self, ind_left, ind_right):\n rightSide = self.split(ind_right)\n self.split(ind_left)\n self.concat(rightSide)\n\n\nROPE = Rope()"
}
{
"filename": "code/data_structures/src/tree/binary_tree/treap/persistent_treap.kt",
"content": "/**\n * Fully persistent Treap implementation (Allows all operations in any version)\n * Average complexity for insert, search and remove is O(log N)\n * It uses O(N * log N) memory.\n */\n\nimport java.util.*\n\n\nclass TreapNode<T : Comparable<T>> {\n val value: T\n val priority: Int\n val leftChild: TreapNode<T>?\n val rightChild: TreapNode<T>?\n\n constructor(value: T, priority: Int, leftChild: TreapNode<T>?, rightChild: TreapNode<T>?) {\n this.value = value\n this.priority = priority\n this.leftChild = leftChild\n this.rightChild = rightChild\n }\n constructor(value: T, leftChild: TreapNode<T>?, rightChild: TreapNode<T>?)\n : this(value, Random().nextInt(), leftChild, rightChild)\n\n fun cloneReplaceLeft(leftChild: TreapNode<T>?): TreapNode<T> {\n return TreapNode(this.value, this.priority, leftChild, this.rightChild)\n }\n\n fun cloneReplaceRight(rightChild: TreapNode<T>?): TreapNode<T> {\n return TreapNode(this.value, this.priority, this.leftChild, rightChild)\n }\n}\n\n\nclass PersistentTreap<T : Comparable<T>>(private val root: TreapNode<T>? = null) {\n\n fun insert(value: T): PersistentTreap<T> {\n return PersistentTreap(insert(root, value))\n }\n\n fun remove(value: T): PersistentTreap<T> {\n return PersistentTreap(remove(root, value))\n }\n\n fun search(value: T): Boolean {\n return search(root, value)\n }\n\n fun inorder(): List<T> {\n return inorder(root)\n }\n\n fun dump() {\n if (root == null)\n println(\"Empty tree\")\n else\n dump(root, 0)\n }\n\n private fun dump(currentNode: TreapNode<T>?, offset: Int) {\n if (currentNode != null) {\n dump(currentNode.rightChild, offset + 1)\n print(\" \".repeat(offset))\n print(String.format(\"%s\\t %20d\\n\", currentNode.value.toString(), currentNode.priority))\n dump(currentNode.leftChild, offset + 1)\n\n }\n }\n\n private fun inorder(currentNode: TreapNode<T>?): List<T> {\n if (currentNode == null)\n return listOf()\n val list: MutableList<T> = mutableListOf()\n list.addAll(inorder(currentNode.leftChild))\n list.add(currentNode.value)\n list.addAll(inorder(currentNode.rightChild))\n return list\n }\n\n private fun rotateLeft(currentNode: TreapNode<T>?): TreapNode<T>? {\n return currentNode?.rightChild?.cloneReplaceLeft(\n currentNode.cloneReplaceRight(currentNode.rightChild.leftChild))\n }\n\n private fun rotateRight(currentNode: TreapNode<T>?): TreapNode<T>? {\n return currentNode?.leftChild?.cloneReplaceRight(\n currentNode.cloneReplaceLeft(currentNode.leftChild.rightChild)\n )\n }\n\n private fun balance(currentNode: TreapNode<T>?): TreapNode<T>? {\n if (currentNode == null) return null\n if (currentNode.leftChild != null && currentNode.leftChild.priority > currentNode.priority)\n return rotateRight(currentNode)\n if (currentNode.rightChild != null && currentNode.rightChild.priority > currentNode.priority)\n return rotateLeft(currentNode)\n return currentNode\n }\n\n private fun insert(currentNode: TreapNode<T>?, value: T): TreapNode<T>? {\n if (currentNode == null)\n return TreapNode(value, null, null)\n return balance(\n if (value <= currentNode.value)\n currentNode.cloneReplaceLeft(insert(currentNode.leftChild, value))\n else\n currentNode.cloneReplaceRight(insert(currentNode.rightChild, value))\n )\n }\n\n private fun remove(currentNode: TreapNode<T>?, value: T): TreapNode<T>? {\n if (currentNode == null || (currentNode.leftChild == null && currentNode.rightChild == null && currentNode.value == value))\n return null\n return when {\n value < currentNode.value -> currentNode.cloneReplaceLeft(remove(currentNode.leftChild, value))\n value > currentNode.value -> currentNode.cloneReplaceRight(remove(currentNode.rightChild, value))\n else -> if (currentNode.leftChild == null ||\n (currentNode.rightChild != null && currentNode.rightChild.priority > currentNode.leftChild.priority))\n with (rotateLeft(currentNode)!!) {\n cloneReplaceLeft(remove(leftChild, value))\n }\n else\n with (rotateRight(currentNode)!!) {\n cloneReplaceRight(remove(rightChild, value))\n }\n }\n }\n\n private fun search(currentNode: TreapNode<T>?, value: T): Boolean {\n if (currentNode == null)\n return false\n return when {\n value < currentNode.value -> search(currentNode.leftChild, value)\n value > currentNode.value -> search(currentNode.rightChild, value)\n else -> true\n }\n }\n\n}\n\nfun <T : Comparable<T>> List<T>.isSorted(): Boolean {\n return (0 until this.size-1).none { this[it] > this[it +1] }\n}\n\nfun main(args: Array<String>) {\n val scan = Scanner(System.`in`)\n val treapVersions: MutableList<PersistentTreap<Int>> = mutableListOf()\n var currentTreap = PersistentTreap<Int>()\n treapVersions.add(currentTreap)\n println(\"Treap Test\\n\")\n /** Perform tree operations **/\n loop@ while (true) {\n println(\"\\nTreap Operations\\n\")\n println(\"1. insert \")\n println(\"2. delete \")\n println(\"3. search\")\n println(\"4. show tree\")\n println(\"5. exit\")\n\n print(\"Choose version: \")\n val version = scan.nextInt()\n if (version >= treapVersions.size) {\n println(\"Version $version does not exist\")\n continue\n }\n currentTreap = treapVersions[version]\n\n print(\"Choose operation: \")\n val choice = scan.nextInt()\n when (choice) {\n 1 -> {\n println(\"Enter integer element to insert\")\n currentTreap = currentTreap.insert(scan.nextInt())\n }\n 2 -> {\n println(\"Enter integer element to delete\")\n currentTreap = currentTreap.remove(scan.nextInt())\n }\n 3 -> {\n println(\"Enter integer element to search\")\n println(\"Search result : \" + currentTreap.search(scan.nextInt()))\n }\n 4 -> currentTreap.dump()\n 5 -> break@loop\n else -> println(\"Invalid operation\")\n }\n if (!currentTreap.inorder().isSorted())\n println(\"SOMETHING WENT WRONG\")\n if (choice == 1 || choice == 2) {\n println(\"Created version ${treapVersions.size}\")\n treapVersions.add(currentTreap)\n }\n }\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/treap/treap.cpp",
"content": "#include <iostream>\n#define ll long long\n#define MOD 1000000007\nusing namespace std;\n// Part of Cosmos by OpenGenus Foundation\n// structure representing a treap node\nstruct node\n{\n ll key;\n ll priority;\n node* left;\n node* right;\n node* parent;\n\n node(ll data)\n {\n key = data;\n priority = (1LL * rand()) % MOD;\n left = right = parent = NULL;\n }\n};\n\n// function to left-rotate the subtree rooted at x\nvoid left_rotate(node* &root, node* x)\n{\n node* y = x->right;\n x->right = y->left;\n\n if (y->left != NULL)\n y->left->parent = x;\n y->parent = x->parent;\n if (x->parent == NULL)\n root = y;\n else if (x->key > x->parent->key)\n x->parent->right = y;\n else\n x->parent->left = y;\n\n y->left = x;\n x->parent = y;\n}\n\n// function to right-rotate the subtree rooted at x\nvoid right_rotate(node* &root, node* x)\n{\n node* y = x->left;\n x->left = y->right;\n\n if (y->right != NULL)\n y->right->parent = x;\n y->parent = x->parent;\n if (x->parent == NULL)\n root = y;\n else if (x->key > x->parent->key)\n x->parent->right = y;\n else\n x->parent->left = y;\n\n y->right = x;\n x->parent = y;\n}\n\n// function to restore min-heap property by rotations\nvoid treap_insert_fixup(node* &root, node* z)\n{\n while (z->parent != NULL && z->parent->priority > z->priority)\n {\n // if z is a right child\n if (z->key > z->parent->key)\n left_rotate(root, z->parent);\n // if z is a left child\n else\n right_rotate(root, z->parent);\n }\n}\n\n// function to insert a node into the treap\n// performs simple BST insert and calls treap_insert_fixup\nvoid insert(node* &root, ll data)\n{\n node *x = root, *y = NULL;\n while (x != NULL)\n {\n y = x;\n if (data < x->key)\n x = x->left;\n else\n x = x->right;\n }\n\n node* z = new node(data);\n z->parent = y;\n if (y == NULL)\n root = z;\n else if (z->key > y->key)\n y->right = z;\n else\n y->left = z;\n\n treap_insert_fixup(root, z);\n}\n\nvoid preorder(node* root)\n{\n if (root)\n {\n cout << root->key << \" \";\n preorder(root->left);\n preorder(root->right);\n }\n}\n\n// free the allocated memory\nvoid delete_treap(node* root)\n{\n if (root)\n {\n delete_treap(root->left);\n delete_treap(root->right);\n delete root;\n }\n}\n\nint main()\n{\n node* root = NULL;\n int choice;\n ll key;\n while (true)\n {\n cout << \"1. Insert 2. Preorder 3. Quit\\n\";\n cin >> choice;\n if (choice == 1)\n {\n cout << \"Enter key : \";\n cin >> key;\n insert(root, key);\n }\n else if (choice == 2)\n {\n preorder(root);\n cout << endl;\n }\n else\n break;\n }\n delete_treap(root);\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/treap/treap.java",
"content": "/**\n * Part of Cosmos by OpenGenus Foundation\n **/\n \n import java.util.Scanner;\n import java.util.Random;\n \n /** Class TreapNode **/\n class TreapNode\n {\n TreapNode left, right;\n int priority, element;\n \n /** Constructor **/ \n public TreapNode()\n {\n this.element = 0;\n this.left = this;\n this.right = this;\n this.priority = Integer.MAX_VALUE;\n } \n \n /** Constructor **/ \n public TreapNode(int ele)\n {\n this(ele, null, null);\n } \n \n /** Constructor **/\n public TreapNode(int ele, TreapNode left, TreapNode right)\n {\n this.element = ele;\n this.left = left;\n this.right = right;\n this.priority = new Random().nextInt( );\n } \n }\n \n /** Class TreapTree **/\n class TreapTree\n {\n private TreapNode root;\n private static TreapNode nil = new TreapNode();\n \n /** Constructor **/\n public TreapTree()\n {\n root = nil;\n }\n \n /** Function to check if tree is empty **/\n public boolean isEmpty()\n {\n return root == nil;\n }\n \n /** Make the tree logically empty **/\n public void makeEmpty()\n {\n root = nil;\n }\n \n /** Functions to insert data **/\n public void insert(int X)\n {\n root = insert(X, root);\n }\n private TreapNode insert(int X, TreapNode T)\n {\n if (T == nil)\n return new TreapNode(X, nil, nil);\n else if (X < T.element)\n {\n T.left = insert(X, T.left);\n if (T.left.priority < T.priority)\n {\n TreapNode L = T.left;\n T.left = L.right;\n L.right = T;\n return L;\n } \n }\n else if (X > T.element)\n {\n T.right = insert(X, T.right);\n if (T.right.priority < T.priority)\n {\n TreapNode R = T.right;\n T.right = R.left;\n R.left = T;\n return R;\n }\n }\n return T;\n }\n \n /** Functions to count number of nodes **/\n public int countNodes()\n {\n return countNodes(root);\n }\n private int countNodes(TreapNode r)\n {\n if (r == nil)\n return 0;\n else\n {\n int l = 1;\n l += countNodes(r.left);\n l += countNodes(r.right);\n return l;\n }\n }\n \n /** Functions to search for an element **/\n public boolean search(int val)\n {\n return search(root, val);\n }\n private boolean search(TreapNode r, int val)\n {\n boolean found = false;\n while ((r != nil) && !found)\n {\n int rval = r.element;\n if (val < rval)\n r = r.left;\n else if (val > rval)\n r = r.right;\n else\n {\n found = true;\n break;\n }\n found = search(r, val);\n }\n return found;\n }\n \n /** Function for inorder traversal **/\n public void inorder()\n {\n inorder(root);\n }\n private void inorder(TreapNode r)\n {\n if (r != nil)\n {\n inorder(r.left);\n System.out.print(r.element +\" \");\n inorder(r.right);\n }\n }\n \n /** Function for preorder traversal **/\n public void preorder()\n {\n preorder(root);\n }\n private void preorder(TreapNode r)\n {\n if (r != nil)\n {\n System.out.print(r.element +\" \");\n preorder(r.left); \n preorder(r.right);\n }\n }\n \n /** Function for postorder traversal **/\n public void postorder()\n {\n postorder(root);\n }\n private void postorder(TreapNode r)\n {\n if (r != nil)\n {\n postorder(r.left); \n postorder(r.right);\n System.out.print(r.element +\" \");\n }\n } \n }\n \n/** Class TreapTest **/\npublic class Treap\n{\n public static void main(String[] args)\n { \n Scanner scan = new Scanner(System.in);\n /** Creating object of Treap **/\n TreapTree trpt = new TreapTree(); \n System.out.println(\"Treap Test\\n\"); \n char ch;\n /** Perform tree operations **/\n do \n {\n System.out.println(\"\\nTreap Operations\\n\");\n System.out.println(\"1. insert \");\n System.out.println(\"2. search\");\n System.out.println(\"3. count nodes\");\n System.out.println(\"4. check empty\");\n System.out.println(\"5. clear\");\n \n int choice = scan.nextInt(); \n switch (choice)\n {\n case 1 : \n System.out.println(\"Enter integer element to insert\");\n trpt.insert( scan.nextInt() ); \n break; \n case 2 : \n System.out.println(\"Enter integer element to search\");\n System.out.println(\"Search result : \"+ trpt.search( scan.nextInt() ));\n break; \n case 3 : \n System.out.println(\"Nodes = \"+ trpt.countNodes());\n break; \n case 4 : \n System.out.println(\"Empty status = \"+ trpt.isEmpty());\n break;\n case 5 : \n System.out.println(\"\\nTreap Cleared\");\n trpt.makeEmpty();\n break; \n default : \n System.out.println(\"Wrong Entry \\n \");\n break; \n }\n /** Display tree **/ \n System.out.print(\"\\nPost order : \");\n trpt.postorder();\n System.out.print(\"\\nPre order : \");\n trpt.preorder(); \n System.out.print(\"\\nIn order : \");\n trpt.inorder();\n \n System.out.println(\"\\nDo you want to continue (Type y or n) \\n\");\n ch = scan.next().charAt(0); \n } while (ch == 'Y'|| ch == 'y'); \n }\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/treap/treap.scala",
"content": "import java.util.Random\n\ncase class Treap[T](el: T, priority: Int, left: Treap[T], right: Treap[T]) { // extends Treap[T] {\n\n def contains(target: T)(implicit ord: Ordering[T]): Boolean = {\n if (target == el) {\n true\n } else if (ord.lt(target, el)) {\n if (left == null) false\n else left.contains(target)\n } else {\n if (right == null) false\n else right.contains(target)\n }\n }\n\n def insert(newEl: T)(implicit ord: Ordering[T]): Treap[T] = insert(newEl, new Random().nextInt())\n\n def insert(newEl: T, newPriority: Int)(implicit ord: Ordering[T]): Treap[T] = {\n if (newEl == el) {\n this\n } else if (ord.lt(newEl, el)) {\n val newTreap = \n if (left == null) {\n this.copy(left = Treap(newEl, newPriority, null, null))\n } else {\n this.copy(left = left.insert(newEl, newPriority))\n }\n\n if (newTreap.left.priority > priority) {\n // rotate right to raise node with higer priority\n val leftChild = newTreap.left\n leftChild.copy(right = newTreap.copy(left = leftChild.right))\n } else {\n newTreap\n }\n } else {\n val newTreap = \n if (right == null) {\n this.copy(right = Treap(newEl, newPriority, null, null))\n } else {\n this.copy(right = right.insert(newEl, newPriority))\n }\n\n if (newTreap.right.priority > priority) {\n // rotate left to raise node with higer priority\n val rightChild = newTreap.right\n rightChild.copy(left = newTreap.copy(right = rightChild.left))\n } else {\n newTreap\n }\n }\n }\n\n def inOrder(visitor: (T, Int) => Unit): Unit = {\n if (left != null) left.inOrder(visitor);\n visitor(el, priority)\n if (right != null) right.inOrder(visitor);\n }\n}\n\nobject Main {\n def main(args: Array[String]): Unit = {\n Treap(\"a\", 100, null, null).insert(\"b\").insert(\"c\").inOrder((el: String, priority: Int) => println((el, priority)))\n Treap(\"a\", 100, null, null).insert(\"c\").insert(\"c\").inOrder((el: String, priority: Int) => println((el, priority)))\n Treap(\"a\", 100, null, null).insert(\"c\").insert(\"b\").inOrder((el: String, priority: Int) => println((el, priority)))\n println(Treap(\"a\", 100, null, null).insert(\"c\").insert(\"b\").contains(\"e\"))\n println(Treap(\"a\", 100, null, null).insert(\"c\").insert(\"b\").contains(\"b\"))\n println(Treap(\"a\", 100, null, null).insert(\"c\").insert(\"b\").contains(\"a\"))\n }\n}"
}
{
"filename": "code/data_structures/src/tree/binary_tree/treap/treap.swift",
"content": "// Part of Cosmos by OpenGenus Foundation\nimport Foundation\n\npublic indirect enum Treap<Key: Comparable, Element> {\n case empty\n case node(key: Key, val: Element, p: Int, left: Treap, right: Treap)\n\n public init() {\n self = .empty\n }\n\n internal func get(_ key: Key) -> Element? {\n switch self {\n case .empty:\n return nil\n case let .node(treeKey, val, _, _, _) where treeKey == key:\n return val\n case let .node(treeKey, _, _, left, _) where key < treeKey:\n return left.get(key)\n case let .node(treeKey, _, _, _, right) where key > treeKey:\n return right.get(key)\n default:\n return nil\n }\n }\n\n public func contains(_ key: Key) -> Bool {\n switch self {\n case .empty:\n return false\n case let .node(treeKey, _, _, _, _) where treeKey == key:\n return true\n case let .node(treeKey, _, _, left, _) where key < treeKey:\n return left.contains(key)\n case let .node(treeKey, _, _, _, right) where key > treeKey:\n return right.contains(key)\n default:\n return false\n }\n }\n\n public var depth: Int {\n switch self {\n case .empty:\n return 0\n case let .node(_, _, _, left, .empty):\n return 1 + left.depth\n case let .node(_, _, _, .empty, right):\n return 1 + right.depth\n case let .node(_, _, _, left, right):\n let leftDepth = left.depth\n let rightDepth = right.depth\n return 1 + leftDepth > rightDepth ? leftDepth : rightDepth\n }\n }\n\n public var count: Int {\n return Treap.countHelper(self)\n }\n\n fileprivate static func countHelper(_ treap: Treap<Key, Element>) -> Int {\n if case let .node(_, _, _, left, right) = treap {\n return countHelper(left) + 1 + countHelper(right)\n }\n\n return 0\n }\n}\n\ninternal func leftRotate<Key: Comparable, Element>(_ tree: Treap<Key, Element>) -> Treap<Key, Element> {\n if case let .node(key, val, p, .node(leftKey, leftVal, leftP, leftLeft, leftRight), right) = tree {\n return .node(key: leftKey, val: leftVal, p: leftP, left: leftLeft,\n right: Treap.node(key: key, val: val, p: p, left: leftRight, right: right))\n } else {\n return .empty\n }\n}\n\ninternal func rightRotate<Key: Comparable, Element>(_ tree: Treap<Key, Element>) -> Treap<Key, Element> {\n if case let .node(key, val, p, left, .node(rightKey, rightVal, rightP, rightLeft, rightRight)) = tree {\n return .node(key: rightKey, val: rightVal, p: rightP,\n left: Treap.node(key: key, val: val, p: p, left: left, right: rightLeft), right: rightRight)\n } else {\n return .empty\n }\n}\n\npublic extension Treap {\n internal func set(key: Key, val: Element, p: Int = Int(arc4random())) -> Treap {\n switch self {\n case .empty:\n return .node(key: key, val: val, p: p, left: .empty, right: .empty)\n case let .node(nodeKey, nodeVal, nodeP, left, right) where key != nodeKey:\n return insertAndBalance(nodeKey, nodeVal, nodeP, left, right, key, val, p)\n case let .node(nodeKey, _, nodeP, left, right) where key == nodeKey:\n return .node(key: key, val: val, p: nodeP, left: left, right: right)\n default: \n return .empty\n }\n }\n\n fileprivate func insertAndBalance(_ nodeKey: Key, _ nodeVal: Element, _ nodeP: Int, _ left: Treap,\n _ right: Treap, _ key: Key, _ val: Element, _ p: Int) -> Treap {\n let newChild: Treap<Key, Element>\n let newNode: Treap<Key, Element>\n let rotate: (Treap) -> Treap\n if key < nodeKey {\n newChild = left.set(key: key, val: val, p: p)\n newNode = .node(key: nodeKey, val: nodeVal, p: nodeP, left: newChild, right: right)\n rotate = leftRotate\n } else if key > nodeKey {\n newChild = right.set(key: key, val: val, p: p)\n newNode = .node(key: nodeKey, val: nodeVal, p: nodeP, left: left, right: newChild)\n rotate = rightRotate\n } else {\n newChild = .empty\n newNode = .empty\n return newNode\n }\n\n if case let .node(_, _, newChildP, _, _) = newChild, newChildP < nodeP {\n return rotate(newNode)\n } else {\n return newNode\n }\n }\n\n internal func delete(key: Key) throws -> Treap {\n switch self {\n case .empty:\n throw NSError(domain: \"com.wta.treap.errorDomain\", code: -1, userInfo: nil)\n case let .node(nodeKey, val, p, left, right) where key < nodeKey:\n return try Treap.node(key: nodeKey, val: val, p: p, left: left.delete(key: key), right: right)\n case let .node(nodeKey, val, p, left, right) where key > nodeKey:\n return try Treap.node(key: nodeKey, val: val, p: p, left: left, right: right.delete(key: key))\n case let .node(_, _, _, left, right):\n return merge(left, right: right)\n }\n }\n}"
}
{
"filename": "code/data_structures/src/tree/b_tree/b_tree/b_tree_c/btree.c",
"content": "// Part of Cosmos by OpenGenus Foundation\n// Author : ABDOUS Kamel\n// Implementation of a disk-stored B-Tree\n\n#include \"btree.h\"\n#include <stdlib.h>\n\n/*\n * Binary search in a node.\n * Returns 1 in success and stores the position in i.\n * Otherwise returns 0 and i contains the position where the value is supposed to be.\n */\nint bnode_search(TVal v, int* i, BNode* n)\n{\n int bi = 0, bs = n->nb_n - 1;\n\n while(bi <= bs)\n {\n *i = (bi + bs) / 2;\n if(n->vals[*i] == v)\n return 1;\n\n else if(n->vals[*i] < v)\n bi = *i + 1;\n\n else\n bs = *i - 1;\n }\n\n *i = bi;\n return 0;\n}\n\n/*\n * Inserts v in n and keeps the values sorted.\n * rc is the right child of the value (-1 if it hasn't).\n */\nvoid bnode_ins(TVal v, int rc, BNode* n)\n{\n if(n->nb_n == MAX_N)\n return ;\n\n int i = n->nb_n - 1;\n\n while(i >= 0 && n->vals[i] > v)\n {\n n->vals[i + 1] = n->vals[i];\n n->childs[i + 2] = n->childs[i + 1];\n i--;\n }\n\n n->vals[i + 1] = v;\n n->childs[i + 2] = rc;\n\n n->nb_n++;\n}\n\n/*\n * Deletes v from the node n.\n */\nvoid bnode_del(TVal v, BNode* n)\n{\n int i = 0;\n\n if(!bnode_search(v, &i, n))\n return;\n\n n->nb_n--;\n while(i < n->nb_n)\n {\n n->vals[i] = n->vals[i + 1];\n n->childs[i + 1] = n->childs[i + 2];\n }\n}\n\nvoid inordre(BTree* f, int i)\n{\n BNode buff;\n ReadBlock(f, &buff, i);\n\n int j;\n for(j = 0; j < buff.nb_n; ++j)\n {\n if(buff.childs[j] != -1)\n inordre(f, buff.childs[j]);\n\n printf(\"%d \", buff.vals[j]);\n }\n\n if(buff.childs[j] != -1)\n inordre(f, buff.childs[j]);\n}\n\n/*\n * Returns 1 and the block that contains c in buff, and its num in i, and the position of c in j.\n * If the value doesn't exist, returns 0.\n */\nint btree_find(BTree* bt, TVal c, int* i, int* j, BNode* buff)\n{\n *i = BTreeRoot(bt);\n *j = 0;\n\n initStack(&bt->stack);\n\n while(*i != -1)\n {\n ReadBlock(bt, buff, *i);\n if(bnode_search(c, j, buff))\n return 1;\n\n pushStack(&bt->stack, *i, buff);\n *i = buff->childs[*j];\n }\n\n if(bt->stack.head != -1)\n popStack(&bt->stack, i, buff);\n\n return 0;\n}\n\nvoid btree_ins(BTree* bt, TVal c)\n{\n int i, j, k, median;\n BNode buff, brother;\n\n if(btree_find(bt, c, &i, &j, &buff))\n return;\n\n bt->fileHeader.nbVals++;\n\n if(i == -1)\n {\n bt->fileHeader.root = AllocBlock(bt);\n buff.vals[0] = c;\n buff.nb_n = 1;\n\n buff.childs[0] = buff.childs[1] = -1;\n WriteBlock(bt, &buff, BTreeRoot(bt));\n }\n\n int stop = 0, rc = -1;\n while(!stop)\n {\n if(buff.nb_n != MAX_N)\n {\n stop = 1;\n bnode_ins(c, rc, &buff);\n WriteBlock(bt, &buff, i);\n }\n\n else\n {\n for(j = MAX_N / 2 + 1, k = 0; j < MAX_N; ++j, ++k)\n {\n brother.vals[k] = buff.vals[j];\n brother.childs[k] = buff.childs[j];\n }\n\n brother.childs[k] = buff.childs[j];\n brother.nb_n = buff.nb_n = MAX_N / 2;\n\n median = buff.vals[MAX_N / 2];\n\n if(c < median)\n bnode_ins(c, rc, &buff);\n\n else\n bnode_ins(c, rc, &brother);\n\n rc = AllocBlock(bt);\n\n WriteBlock(bt, &buff, i);\n WriteBlock(bt, &brother, rc);\n\n if(bt->stack.head != -1)\n {\n c = median;\n popStack(&bt->stack, &i, &buff);\n }\n\n else\n {\n bt->fileHeader.height++;\n bt->fileHeader.root = AllocBlock(bt);\n buff.childs[0] = i;\n buff.childs[1] = rc;\n buff.vals[0] = median;\n buff.nb_n = 1;\n\n WriteBlock(bt, &buff, BTreeRoot(bt));\n stop = 1;\n }\n }\n }\n}\n\n// ------------------------------------------------------------------------ //\nvoid initStack(BStack* stack)\n{\n stack->head = -1;\n}\n\nvoid pushStack(BStack* stack, int adr, BNode* n)\n{\n if(stack->head == MAX_S - 1)\n return;\n\n stack->head++;\n stack->adrs[stack->head] = adr;\n stack->nodes[stack->head] = *n;\n}\n\nvoid popStack(BStack* stack, int* adr, BNode* n)\n{\n if(stack->head == -1)\n return;\n\n *adr = stack->adrs[stack->head];\n *n = stack->nodes[stack->head];\n\n stack->head--;\n}\n\nvoid getStackHead(BStack* stack, int* adr, BNode* n)\n{\n if(stack->head == -1)\n return;\n\n *adr = stack->adrs[stack->head];\n *n = stack->nodes[stack->head];\n}\n\n/*\n * Function to call to open a disk-stored b-tree.\n * if mode = 'N' : it creates a new btree.\n * else (you can put 'O') : it reads the btree stored in fpath.\n */\nBTree* OpenBTree(char* fpath, char mode)\n{\n BTree* bt = malloc(sizeof(BTree));\n\n if(mode == 'N')\n {\n bt->treeFile = fopen(fpath, \"wb+\");\n if(bt->treeFile == NULL)\n {\n free(bt);\n return NULL;\n }\n\n bt->fileHeader.root = bt->fileHeader.freeBlocksHead = -1;\n bt->fileHeader.nbNodes = bt->fileHeader.nbVals = bt->fileHeader.height = 0;\n\n fwrite(&(bt->fileHeader), sizeof(FileHeader), 1, bt->treeFile);\n }\n\n else\n {\n bt->treeFile = fopen(fpath, \"rb+\");\n if(bt->treeFile == NULL)\n {\n free(bt);\n return NULL;\n }\n\n fread(&(bt->fileHeader), sizeof(FileHeader), 1, bt->treeFile);\n }\n\n return bt;\n}\n\n/*\n * Close the btree.\n * Writes the tree header in the file.\n */\nvoid CloseBTree(BTree* f)\n{\n fseek(f->treeFile, 0, SEEK_SET);\n fwrite(&(f->fileHeader), sizeof(FileHeader), 1, f->treeFile);\n\n fclose(f->treeFile);\n free(f);\n}\n\nvoid ReadBlock(BTree* f, BNode* buff, int i)\n{\n fseek(f->treeFile, sizeof(FileHeader) + i * sizeof(BNode), SEEK_SET);\n fread(buff, sizeof(BNode), 1, f->treeFile);\n}\n\nvoid WriteBlock(BTree* f, BNode* buff, int i)\n{\n fseek(f->treeFile, sizeof(FileHeader) + i * sizeof(BNode), SEEK_SET);\n fwrite(buff, sizeof(BNode), 1, f->treeFile);\n}\n\n/*\n * If the freeBlocks list isn't empty, takes the head of the list as a new block.\n * Else, it allocs a new block on the disk.\n */\nint AllocBlock(BTree* f)\n{\n int i = f->fileHeader.freeBlocksHead;\n BNode buf;\n\n if(i != -1)\n {\n ReadBlock(f, &buf, i);\n f->fileHeader.freeBlocksHead = buf.childs[0];\n }\n\n else\n {\n i = f->fileHeader.nbNodes++;\n buf.nb_n = 0;\n WriteBlock(f, &buf, i);\n }\n\n return i;\n}\n\n/*\n * This function also adds the block to the freeBlocks list.\n */\nvoid FreeBlock(BTree* f, BNode* buff, int i)\n{\n buff->childs[0] = f->fileHeader.freeBlocksHead;\n f->fileHeader.freeBlocksHead = i;\n WriteBlock(f, buff, i);\n}\n\nint BTreeRoot(BTree* f)\n{\n return f->fileHeader.root;\n}"
}
{
"filename": "code/data_structures/src/tree/b_tree/b_tree/b_tree_c/btree.h",
"content": "// Part of Cosmos by OpenGenus Foundation\n// Author : ABDOUS Kamel\n// Implementation of a disk-stored B-Tree\n\n#ifndef BTREE_H_INCLUDED\n#define BTREE_H_INCLUDED\n\n#include <stdio.h>\n\n#define MIN_DEGREE 2\n#define MAX_N ((MIN_DEGREE * 2) - 1) /* Max nb of values stored in a node */\n#define MAX_C (MIN_DEGREE * 2) /* Max nb of childs of a node */\n\n#define MAX_S 20 /* Size of the stack */\n\ntypedef int TVal;\n\n/* This struct contains information about the disk-stored b-tree */\ntypedef struct\n{\n int root; /* Block num of the root */\n int nbNodes;\n int nbVals;\n int height;\n\n /* In the file that contains the b-tree, there's a list of the blocks\n that are no longer used by the tree. This field contains the block num\n of the head of this list. If this list is not empty, we use a block of the list\n when we need a new block instead of allocating a new one. */\n int freeBlocksHead;\n\n} FileHeader;\n\ntypedef struct\n{\n TVal vals[MAX_N];\n int childs[MAX_C];\n\n int nb_n; // Nb values stored in the node\n\n} BNode;\n\n/* That a static stack */\ntypedef struct\n{\n int head;\n int adrs[MAX_S];\n BNode nodes[MAX_S];\n\n} BStack;\n\n/* That's the struct to use to manipulate a b-tree */\ntypedef struct\n{\n FILE* treeFile;\n FileHeader fileHeader;\n BStack stack;\n\n} BTree;\n\n/*\n * Binary search in a node.\n * Returns 1 in success and stores the position in i.\n * Otherwise returns 0 and i contains the position where the value is supposed to be.\n */\nint bnode_search(TVal v, int* i, BNode* n);\n\n/*\n * Inserts v in n and keeps the values sorted.\n * rc is the right child of the value (-1 if it hasn't).\n */\nvoid bnode_ins(TVal v, int rc, BNode* n);\n\n/*\n * Deletes v from the node n.\n */\nvoid bnode_del(TVal v, BNode* n);\n\nvoid inordre(BTree* f, int i);\n\n/*\n * Returns 1 and the block that contains c in buff, and its num in i, and the position of c in j.\n * If the value doesn't exist, returns 0.\n */\nint btree_find(BTree* bt, TVal c, int* i, int* j, BNode* buff);\nvoid btree_ins(BTree* bt, TVal c);\n\n// ------------------------------------------------------------------- //\nvoid initStack(BStack* stack);\nvoid pushStack(BStack* stack, int adr, BNode* n);\nvoid popStack(BStack* stack, int* adr, BNode* n);\nvoid getStackHead(BStack* stack, int* adr, BNode* n);\n\n/*\n * Function to call to open a disk-stored b-tree.\n * if mode = 'N' : it creates a new btree.\n * else (you can put 'O') : it reads the btree stored in fpath.\n */\nBTree* OpenBTree(char* fpath, char mode);\n\n/*\n * Close the btree.\n * Writes the tree header in the file.\n */\nvoid CloseBTree(BTree* f);\n\nvoid ReadBlock(BTree* f, BNode* buff, int i);\nvoid WriteBlock(BTree* f, BNode* buff, int i);\n\n/*\n * If the freeBlocks list isn't empty, takes the head of the list as a new block.\n * Else, it allocs a new block on the disk.\n */\nint AllocBlock(BTree* f);\n\n/*\n * This function also adds the block to the freeBlocks list.\n */\nvoid FreeBlock(BTree* f, BNode* buff, int i);\n\nint BTreeRoot(BTree* f);\n\n#endif // BTREE_H_INCLUDED"
}
{
"filename": "code/data_structures/src/tree/b_tree/b_tree/b_tree_c/main.c",
"content": "// Part of Cosmos by OpenGenus Foundation\n// Author : ABDOUS Kamel\n// Implementation of a disk-stored B-Tree\n\n#include <stdio.h>\n#include <stdlib.h>\n#include \"btree.h\"\n\nint main()\n{\n BTree* bt = OpenBTree(\"btree.bin\", 'N');\n btree_ins(bt, 5);\n btree_ins(bt, 10);\n btree_ins(bt, 1);\n btree_ins(bt, 2);\n btree_ins(bt, 20);\n btree_ins(bt, 15);\n inordre(bt, BTreeRoot(bt));\n CloseBTree(bt);\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/b_tree/b_tree/b_tree.cpp",
"content": "// C++ implemntation of search() and traverse() methods\n#include <iostream>\nusing namespace std;\n// A BTree node\nclass BTreeNode\n{\n int *keys; // An array of keys\n int t; // Minimum degree (defines the range for number of keys)\n BTreeNode **C; // An array of child pointers\n int n; // Current number of keys\n bool leaf; // Is true when node is leaf. Otherwise false\npublic:\n BTreeNode(int _t, bool _leaf); // Constructor\n\n// A function to traverse all nodes in a subtree rooted with this node\n void traverse();\n\n// A function to search a key in subtree rooted with this node.\n BTreeNode *search(int k); // returns NULL if k is not present.\n\n// A utility function to insert a new key in this node\n// The assumption is, the node must be non-full when this\n// function is called\n void insertNonFull(int k);\n\n// A utility function to split the child y of this node\n// Note that y must be full when this function is called\n void splitChild(int i, BTreeNode *y);\n\n// Make BTree friend of this so that we can access private members of this\n// class in BTree functions\n friend class BTree;\n};\n\n// A BTree\nclass BTree\n{\n BTreeNode *root; // Pointer to root node\n int t; // Minimum degree\npublic:\n// Constructor (Initializes tree as empty)\n BTree(int _t)\n {\n root = NULL; t = _t;\n }\n\n// function to traverse the tree\n void traverse()\n {\n if (root != NULL)\n root->traverse();\n }\n\n// function to search a key in this tree\n BTreeNode* search(int k)\n {\n return (root == NULL) ? NULL : root->search(k);\n }\n\n void insert(int);\n};\n\n// Constructor for BTreeNode class\nBTreeNode::BTreeNode(int _t, bool _leaf)\n{\n // Copy the given minimum degree and leaf property\n t = _t;\n leaf = _leaf;\n\n // Allocate memory for maximum number of possible keys\n // and child pointers\n keys = new int[2 * t - 1];\n C = new BTreeNode *[2 * t];\n\n // Initialize the number of keys as 0\n n = 0;\n}\n\n// Function to traverse all nodes in a subtree rooted with this node\nvoid BTreeNode::traverse()\n{\n // There are n keys and n+1 children, travers through n keys\n // and first n children\n int i;\n for (i = 0; i < n; i++)\n {\n // If this is not leaf, then before printing key[i],\n // traverse the subtree rooted with child C[i].\n if (leaf == false)\n C[i]->traverse();\n cout << \" \" << keys[i];\n }\n\n // Print the subtree rooted with last child\n if (leaf == false)\n C[i]->traverse();\n}\n\n// Function to search key k in subtree rooted with this node\nBTreeNode *BTreeNode::search(int k)\n{\n // Find the first key greater than or equal to k\n int i = 0;\n while (i < n && k > keys[i])\n i++;\n\n // If the found key is equal to k, return this node\n if (keys[i] == k)\n return this;\n\n // If key is not found here and this is a leaf node\n if (leaf == true)\n return NULL;\n\n // Go to the appropriate child\n return C[i]->search(k);\n\n}\n\n\n\nvoid BTree::insert(int k)\n{\n // If tree is empty\n if (root == NULL)\n {\n // Allocate memory for root\n root = new BTreeNode(t, true);\n root->keys[0] = k; // Insert key\n root->n = 1; // Update number of keys in root\n }\n else // If tree is not empty\n {\n // If root is full, then tree grows in height\n if (root->n == 2 * t - 1)\n {\n // Allocate memory for new root\n BTreeNode *s = new BTreeNode(t, false);\n\n // Make old root as child of new root\n s->C[0] = root;\n\n // Split the old root and move 1 key to the new root\n s->splitChild(0, root);\n\n // New root has two children now. Decide which of the\n // two children is going to have new key\n int i = 0;\n if (s->keys[0] < k)\n i++;\n s->C[i]->insertNonFull(k);\n\n // Change root\n root = s;\n }\n else // If root is not full, call insertNonFull for root\n root->insertNonFull(k);\n }\n}\n\n// A utility function to insert a new key in this node\n// The assumption is, the node must be non-full when this\n// function is called\nvoid BTreeNode::insertNonFull(int k)\n{\n // Initialize index as index of rightmost element\n int i = n - 1;\n\n // If this is a leaf node\n if (leaf == true)\n {\n // The following loop does two things\n // a) Finds the location of new key to be inserted\n // b) Moves all greater keys to one place ahead\n while (i >= 0 && keys[i] > k)\n {\n keys[i + 1] = keys[i];\n i--;\n }\n\n // Insert the new key at found location\n keys[i + 1] = k;\n n = n + 1;\n }\n else // If this node is not leaf\n {\n // Find the child which is going to have the new key\n while (i >= 0 && keys[i] > k)\n i--;\n\n // See if the found child is full\n if (C[i + 1]->n == 2 * t - 1)\n {\n // If the child is full, then split it\n splitChild(i + 1, C[i + 1]);\n\n // After split, the middle key of C[i] goes up and\n // C[i] is splitted into two. See which of the two\n // is going to have the new key\n if (keys[i + 1] < k)\n i++;\n }\n C[i + 1]->insertNonFull(k);\n }\n}\n\n// A utility function to split the child y of this node\n// Note that y must be full when this function is called\nvoid BTreeNode::splitChild(int i, BTreeNode *y)\n{\n // Create a new node which is going to store (t-1) keys\n // of y\n BTreeNode *z = new BTreeNode(y->t, y->leaf);\n z->n = t - 1;\n\n // Copy the last (t-1) keys of y to z\n for (int j = 0; j < t - 1; j++)\n z->keys[j] = y->keys[j + t];\n\n // Copy the last t children of y to z\n if (y->leaf == false)\n for (int j = 0; j < t; j++)\n z->C[j] = y->C[j + t];\n\n // Reduce the number of keys in y\n y->n = t - 1;\n\n // Since this node is going to have a new child,\n // create space of new child\n for (int j = n; j >= i + 1; j--)\n C[j + 1] = C[j];\n\n // Link the new child to this node\n C[i + 1] = z;\n\n // A key of y will move to this node. Find location of\n // new key and move all greater keys one space ahead\n for (int j = n - 1; j >= i; j--)\n keys[j + 1] = keys[j];\n\n // Copy the middle key of y to this node\n keys[i] = y->keys[t - 1];\n\n // Increment count of keys in this node\n n = n + 1;\n}\n\n// Driver program to test above functions\nint main()\n{\n BTree t(3); // A B-Tree with minimum degree 3\n t.insert(10);\n t.insert(20);\n t.insert(5);\n t.insert(6);\n\n cout << \"Traversal of the constucted tree is \";\n t.traverse();\n\n int k = 6;\n (t.search(k) != NULL) ? cout << \"\\nPresent\" : cout << \"\\nNot Present\";\n\n k = 15;\n (t.search(k) != NULL) ? cout << \"\\nPresent\" : cout << \"\\nNot Present\";\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/b_tree/b_tree/b_tree_c/README.md",
"content": "# B tree \n\n### C implementation\n\nChange the macro MIN_DEGREE in btree.h to define the minimum degree of the btree."
}
{
"filename": "code/data_structures/src/tree/b_tree/b_tree/b_tree.py",
"content": "class BTreeNode(object):\n \"\"\"A B-Tree Node.\n \n attributes\n =====================\n leaf : boolean, determines whether this node is a leaf.\n keys : list, a list of keys internal to this node\n c : list, a list of children of this node\n \"\"\"\n\n def __init__(self, leaf=False):\n self.leaf = leaf\n self.keys = []\n self.c = []\n\n def __str__(self):\n if self.leaf:\n return \"Leaf BTreeNode with {0} keys\\n\\tK:{1}\\n\\tC:{2}\\n\".format(\n len(self.keys), self.keys, self.c\n )\n else:\n return \"Internal BTreeNode with {0} keys, {1} children\\n\\tK:{2}\\n\\n\".format(\n len(self.keys), len(self.c), self.keys, self.c\n )\n\n\nclass BTree(object):\n def __init__(self, t):\n self.root = BTreeNode(leaf=True)\n self.t = t\n\n def search(self, k, x=None):\n \"\"\"Search the B-Tree for the key k.\n \n args\n =====================\n k : Key to search for\n x : (optional) Node at which to begin search. Can be None, in which case the entire tree is searched.\n \n \"\"\"\n if isinstance(x, BTreeNode):\n i = 0\n while i < len(x.keys) and k > x.keys[i]: # look for index of k\n i += 1\n if i < len(x.keys) and k == x.keys[i]: # found exact match\n return (x, i)\n elif x.leaf: # no match in keys, and is leaf ==> no match exists\n return None\n else: # search children\n return self.search(k, x.c[i])\n else: # no node provided, search root of tree\n return self.search(k, self.root)\n\n def insert(self, k):\n r = self.root\n if len(r.keys) == (2 * self.t) - 1: # keys are full, so we must split\n s = BTreeNode()\n self.root = s\n s.c.insert(0, r) # former root is now 0th child of new root s\n self._split_child(s, 0)\n self._insert_nonfull(s, k)\n else:\n self._insert_nonfull(r, k)\n\n def _insert_nonfull(self, x, k):\n i = len(x.keys) - 1\n if x.leaf:\n # insert a key\n x.keys.append(0)\n while i >= 0 and k < x.keys[i]:\n x.keys[i + 1] = x.keys[i]\n i -= 1\n x.keys[i + 1] = k\n else:\n # insert a child\n while i >= 0 and k < x.keys[i]:\n i -= 1\n i += 1\n if len(x.c[i].keys) == (2 * self.t) - 1:\n self._split_child(x, i)\n if k > x.keys[i]:\n i += 1\n self._insert_nonfull(x.c[i], k)\n\n def _split_child(self, x, i):\n t = self.t\n y = x.c[i]\n z = BTreeNode(leaf=y.leaf)\n\n # slide all children of x to the right and insert z at i+1.\n x.c.insert(i + 1, z)\n x.keys.insert(i, y.keys[t - 1])\n\n # keys of z are t to 2t - 1,\n # y is then 0 to t-2\n z.keys = y.keys[t : (2 * t - 1)]\n y.keys = y.keys[0 : (t - 1)]\n\n # children of z are t to 2t els of y.c\n if not y.leaf:\n z.c = y.c[t : (2 * t)]\n y.c = y.c[0 : (t - 1)]\n\n def __str__(self):\n r = self.root\n return r.__str__() + \"\\n\".join([child.__str__() for child in r.c])"
}
{
"filename": "code/data_structures/src/tree/b_tree/b_tree/b_tree.swift",
"content": "// Part of Cosmos by OpenGenus Foundation\nclass BTreeNode<Key: Comparable, Value> {\n unowned var owner: BTree<Key, Value>\n\n fileprivate var keys = [Key]()\n fileprivate var values = [Value]()\n var children: [BTreeNode]?\n\n var isLeaf: Bool {\n return children == nil\n }\n\n var numberOfKeys: Int {\n return keys.count\n }\n\n init(owner: BTree<Key, Value>) {\n self.owner = owner\n }\n\n convenience init(owner: BTree<Key, Value>, keys: [Key],\n values: [Value], children: [BTreeNode]? = nil) {\n self.init(owner: owner)\n self.keys += keys\n self.values += values\n self.children = children\n }\n}\n\nextension BTreeNode {\n func value(for key: Key) -> Value? {\n var index = keys.startIndex\n\n while (index + 1) < keys.endIndex && keys[index] < key {\n index = (index + 1)\n }\n\n if key == keys[index] {\n return values[index]\n } else if key < keys[index] {\n return children?[index].value(for: key)\n } else {\n return children?[(index + 1)].value(for: key)\n }\n }\n}\n\nextension BTreeNode {\n func traverseKeysInOrder(_ process: (Key) -> Void) {\n for i in 0..<numberOfKeys {\n children?[i].traverseKeysInOrder(process)\n process(keys[i])\n }\n\n children?.last?.traverseKeysInOrder(process)\n }\n}\n\nextension BTreeNode {\n func insert(_ value: Value, for key: Key) {\n var index = keys.startIndex\n\n while index < keys.endIndex && keys[index] < key {\n index = (index + 1)\n }\n\n if index < keys.endIndex && keys[index] == key {\n values[index] = value\n return\n }\n\n if isLeaf {\n keys.insert(key, at: index)\n values.insert(value, at: index)\n owner.numberOfKeys += 1\n } else {\n children![index].insert(value, for: key)\n if children![index].numberOfKeys > owner.order * 2 {\n split(child: children![index], atIndex: index)\n }\n }\n }\n\n private func split(child: BTreeNode, atIndex index: Int) {\n let middleIndex = child.numberOfKeys / 2\n keys.insert(child.keys[middleIndex], at: index)\n values.insert(child.values[middleIndex], at: index)\n child.keys.remove(at: middleIndex)\n child.values.remove(at: middleIndex)\n\n let rightSibling = BTreeNode(\n owner: owner,\n keys: Array(child.keys[child.keys.indices.suffix(from: middleIndex)]),\n values: Array(child.values[child.values.indices.suffix(from: middleIndex)])\n )\n child.keys.removeSubrange(child.keys.indices.suffix(from: middleIndex))\n child.values.removeSubrange(child.values.indices.suffix(from: middleIndex))\n\n children!.insert(rightSibling, at: (index + 1))\n\n if child.children != nil {\n rightSibling.children = Array(\n child.children![child.children!.indices.suffix(from: (middleIndex + 1))]\n )\n child.children!.removeSubrange(child.children!.indices.suffix(from: (middleIndex + 1)))\n }\n }\n}\n\nprivate enum BTreeNodePosition {\n case left\n case right\n}\n\nextension BTreeNode {\n private var inorderPredecessor: BTreeNode {\n if isLeaf {\n return self\n } else {\n return children!.last!.inorderPredecessor\n }\n }\n\n func remove(_ key: Key) {\n var index = keys.startIndex\n\n while (index + 1) < keys.endIndex && keys[index] < key {\n index = (index + 1)\n }\n\n if keys[index] == key {\n if isLeaf {\n keys.remove(at: index)\n values.remove(at: index)\n owner.numberOfKeys -= 1\n } else {\n let predecessor = children![index].inorderPredecessor\n keys[index] = predecessor.keys.last!\n values[index] = predecessor.values.last!\n children![index].remove(keys[index])\n if children![index].numberOfKeys < owner.order {\n fix(childWithTooFewKeys: children![index], atIndex: index)\n }\n }\n } else if key < keys[index] {\n\n if let leftChild = children?[index] {\n leftChild.remove(key)\n if leftChild.numberOfKeys < owner.order {\n fix(childWithTooFewKeys: leftChild, atIndex: index)\n }\n } else {\n print(\"The key:\\(key) is not in the tree.\")\n }\n } else {\n\n if let rightChild = children?[(index + 1)] {\n rightChild.remove(key)\n if rightChild.numberOfKeys < owner.order {\n fix(childWithTooFewKeys: rightChild, atIndex: (index + 1))\n }\n } else {\n print(\"The key:\\(key) is not in the tree\")\n }\n }\n }\n\n private func fix(childWithTooFewKeys child: BTreeNode, atIndex index: Int) {\n\n if (index - 1) >= 0 && children![(index - 1)].numberOfKeys > owner.order {\n move(keyAtIndex: (index - 1), to: child, from: children![(index - 1)], at: .left)\n } else if (index + 1) < children!.count && children![(index + 1)].numberOfKeys > owner.order {\n move(keyAtIndex: index, to: child, from: children![(index + 1)], at: .right)\n } else if (index - 1) >= 0 {\n merge(child: child, atIndex: index, to: .left)\n } else {\n merge(child: child, atIndex: index, to: .right)\n }\n }\n\n private func move(keyAtIndex index: Int, to targetNode: BTreeNode,\n from node: BTreeNode, at position: BTreeNodePosition) {\n switch position {\n case .left:\n targetNode.keys.insert(keys[index], at: targetNode.keys.startIndex)\n targetNode.values.insert(values[index], at: targetNode.values.startIndex)\n keys[index] = node.keys.last!\n values[index] = node.values.last!\n node.keys.removeLast()\n node.values.removeLast()\n if !targetNode.isLeaf {\n targetNode.children!.insert(node.children!.last!,\n at: targetNode.children!.startIndex)\n node.children!.removeLast()\n }\n\n case .right:\n targetNode.keys.insert(keys[index], at: targetNode.keys.endIndex)\n targetNode.values.insert(values[index], at: targetNode.values.endIndex)\n keys[index] = node.keys.first!\n values[index] = node.values.first!\n node.keys.removeFirst()\n node.values.removeFirst()\n if !targetNode.isLeaf {\n targetNode.children!.insert(node.children!.first!,\n at: targetNode.children!.endIndex)\n node.children!.removeFirst()\n }\n }\n }\n\n private func merge(child: BTreeNode, atIndex index: Int, to position: BTreeNodePosition) {\n switch position {\n case .left:\n children![(index - 1)].keys = children![(index - 1)].keys +\n [keys[(index - 1)]] + child.keys\n\n children![(index - 1)].values = children![(index - 1)].values +\n [values[(index - 1)]] + child.values\n\n keys.remove(at: (index - 1))\n values.remove(at: (index - 1))\n\n if !child.isLeaf {\n children![(index - 1)].children =\n children![(index - 1)].children! + child.children!\n }\n\n case .right:\n children![(index + 1)].keys = child.keys + [keys[index]] +\n children![(index + 1)].keys\n\n children![(index + 1)].values = child.values + [values[index]] +\n children![(index + 1)].values\n\n keys.remove(at: index)\n values.remove(at: index)\n\n if !child.isLeaf {\n children![(index + 1)].children =\n child.children! + children![(index + 1)].children!\n }\n }\n children!.remove(at: index)\n }\n}\n\nextension BTreeNode {\n var inorderArrayFromKeys: [Key] {\n var array = [Key] ()\n\n for i in 0..<numberOfKeys {\n if let returnedArray = children?[i].inorderArrayFromKeys {\n array += returnedArray\n }\n array += [keys[i]]\n }\n\n if let returnedArray = children?.last?.inorderArrayFromKeys {\n array += returnedArray\n }\n\n return array\n }\n}\n\npublic class BTree<Key: Comparable, Value> {\n \n public let order: Int\n\n\n var rootNode: BTreeNode<Key, Value>!\n\n fileprivate(set) public var numberOfKeys = 0\n\n public init?(order: Int) {\n guard order > 0 else {\n print(\"Order has to be greater than 0.\")\n return nil\n }\n self.order = order\n rootNode = BTreeNode<Key, Value>(owner: self)\n }\n}\n\nextension BTree {\n public func traverseKeysInOrder(_ process: (Key) -> Void) {\n rootNode.traverseKeysInOrder(process)\n }\n}\n\n private func splitRoot() {\n let middleIndexOfOldRoot = rootNode.numberOfKeys / 2\n\n let newRoot = BTreeNode<Key, Value>(\n owner: self,\n keys: [rootNode.keys[middleIndexOfOldRoot]],\n values: [rootNode.values[middleIndexOfOldRoot]],\n children: [rootNode]\n )\n rootNode.keys.remove(at: middleIndexOfOldRoot)\n rootNode.values.remove(at: middleIndexOfOldRoot)\n\n let newRightChild = BTreeNode<Key, Value>(\n owner: self,\n keys: Array(rootNode.keys[rootNode.keys.indices.suffix(from: middleIndexOfOldRoot)]),\n values: Array(rootNode.values[rootNode.values.indices.suffix(from: middleIndexOfOldRoot)])\n )\n rootNode.keys.removeSubrange(rootNode.keys.indices.suffix(from: middleIndexOfOldRoot))\n rootNode.values.removeSubrange(rootNode.values.indices.suffix(from: middleIndexOfOldRoot))\n\n if rootNode.children != nil {\n newRightChild.children = Array(\n rootNode.children![rootNode.children!.indices.suffix(from: (middleIndexOfOldRoot + 1))]\n )\n rootNode.children!.removeSubrange(\n rootNode.children!.indices.suffix(from: (middleIndexOfOldRoot + 1))\n )\n }\n\n newRoot.children!.append(newRightChild)\n rootNode = newRoot\n }\n}\n\n// MARK: BTree extension: Removal\n\nextension BTree {\n /**\n * Removes `key` and the value associated with it from the tree.\n * \n * - Parameters:\n * - key: the key to remove\n */\n public func remove(_ key: Key) {\n guard rootNode.numberOfKeys > 0 else {\n return\n }\n\n rootNode.remove(key)\n\n if rootNode.numberOfKeys == 0 && !rootNode.isLeaf {\n rootNode = rootNode.children!.first!\n }\n }\n}\n\nextension BTree {\n \n public var inorderArrayFromKeys: [Key] {\n return rootNode.inorderArrayFromKeys\n }\n}\n\nextension BTree: CustomStringConvertible {\n \n public var description: String {\n return rootNode.description\n }\n}"
}
{
"filename": "code/data_structures/src/tree/b_tree/two_three_tree/twothreetree.scala",
"content": "\nsealed trait TwoThree[T] {\n\tdef insert(newEl: T): TwoThree[T]\n\tdef height(): Int\n\tdef printSideways(indent: Int): Unit\n}\n\ncase class OneLeaf[T](data: T)(implicit ord: Ordering[T]) extends TwoThree[T] {\n\tdef insert(newEl: T): TwoThree[T] = {\n\t\tif (newEl == data) this\n\t\telse if (ord.lt(newEl, data)) TwoLeaf(newEl, data)\n\t\telse \t\t\t\t\t\t TwoLeaf(data, newEl)\n\t}\t\n\tdef height(): Int = 1\n\tdef printSideways(indent: Int): Unit = {\n\t\tprintln(\"\\t\" * indent + data)\n\t}\n}\n\ncase class TwoLeaf[T](dataLeft: T, dataRight: T)(implicit ord: Ordering[T]) extends TwoThree[T] {\n\tdef insert(newEl: T): TwoThree[T] = {\n\t\tif (newEl == dataLeft || newEl == dataRight) this\n\t\telse if (ord.lt(newEl, dataLeft)) \t\t\t TwoNode(dataLeft, OneLeaf(newEl), OneLeaf(dataRight))\n\t\telse if (ord.lt(newEl, dataRight)) \t\t\t TwoNode(newEl, OneLeaf(dataLeft), OneLeaf(dataRight))\n\t\telse \t\t\t\t\t\t\t \t\t\t TwoNode(dataRight, OneLeaf(dataLeft), OneLeaf(newEl))\n\t}\t\n\tdef height(): Int = 1\n\tdef printSideways(indent: Int): Unit = {\n\t\tprintln(\"\\t\" * indent + dataRight)\n\t\tprintln(\"\\t\" * indent + dataLeft)\n\t}\n}\n\ncase class TwoNode[T](data: T, left: TwoThree[T], right: TwoThree[T])(implicit ord: Ordering[T]) extends TwoThree[T] {\n\tdef insert(newEl: T): TwoThree[T] = {\n\t\tif (newEl == data) {\n\t\t\tthis\n\t\t}\n\t\telse if (ord.lt(newEl, data)) {\n\t\t\tleft match {\n\t\t\t\tcase _: OneLeaf[T] => copy(left = left.insert(newEl))\n\t\t\t\tcase TwoLeaf(leftData, rightData) =>\n\t\t\t\t\tif (ord.lt(newEl, leftData)) {\n\t\t\t\t\t\tThreeNode(leftData, data, OneLeaf(newEl), OneLeaf(rightData), right)\n\t\t\t\t\t}\n\t\t\t\t\telse if (ord.lt(newEl, rightData)) {\n\t\t\t\t\t\tThreeNode(newEl, data, OneLeaf(leftData), OneLeaf(rightData), right)\n\t\t\t\t\t}\n\t\t\t\t\telse {\n\t\t\t\t\t\tThreeNode(rightData, data, OneLeaf(leftData), OneLeaf(newEl), right)\n\t\t\t\t\t}\n\t\t\t\tcase leftTwo: TwoNode[T] => leftTwo.insert(newEl) match {\n\t\t\t\t\t\tcase twoNodeChild: TwoNode[T] => \n\t\t\t\t\t\t\tThreeNode(twoNodeChild.data, data, twoNodeChild.left, twoNodeChild.right, right)\n\t\t\t\t\t\tcase otherChild: TwoThree[T] => this.copy(left = otherChild)\n\t\t\t\t\t}\n\t\t\t\tcase t: ThreeNode[T] => this.copy(left = t)\n\t\t\t}\n\t\t}\n\t\telse {\n\t\t\tright match {\n\t\t\t\tcase _: OneLeaf[T] => copy(right = right.insert(newEl))\n\t\t\t\tcase TwoLeaf(leftData, rightData) =>\n\t\t\t\t\tif (ord.gt(newEl, rightData)) {\n\t\t\t\t\t\tThreeNode(data, rightData, left, OneLeaf(leftData), OneLeaf(newEl))\n\t\t\t\t\t}\n\t\t\t\t\telse if (ord.gt(newEl, leftData)) {\n\t\t\t\t\t\tThreeNode(data, newEl, left, OneLeaf(leftData), OneLeaf(rightData))\n\t\t\t\t\t}\n\t\t\t\t\telse {\n\t\t\t\t\t\tThreeNode(data, leftData, left, OneLeaf(newEl), OneLeaf(rightData))\n\t\t\t\t\t}\n\t\t\t\tcase rightTwo: TwoNode[T] => rightTwo.insert(newEl) match {\n\t\t\t\t\t\tcase twoNodeChild: TwoNode[T] => \n\t\t\t\t\t\t\tThreeNode(data, twoNodeChild.data, left, twoNodeChild.left, twoNodeChild.right)\n\t\t\t\t\t\tcase otherChild: TwoThree[T] => this.copy(right = otherChild)\n\t\t\t\t\t}\n\t\t\t\tcase t: ThreeNode[T] => this.copy(right = t)\n\t\t\t}\n\t\t}\n\t}\n\tdef height(): Int = 1 + left.height()\n\tdef printSideways(indent: Int): Unit = {\n\t\tright.printSideways(indent + 1)\n\t\tprintln(\"\\t\" * indent + data)\n\t\tleft.printSideways(indent + 1)\n\t}\n}\n\ncase class ThreeNode[T](dataLeft: T, dataRight: T, left: TwoThree[T], mid: TwoThree[T], right: TwoThree[T])(implicit ord: Ordering[T]) extends TwoThree[T] {\n\tdef insert(newEl: T): TwoThree[T] = {\n\t\tif (newEl == dataLeft || newEl == dataRight) {\n\t\t\tthis\n\t\t}\n\t\telse if (ord.lt(newEl, dataLeft)) {\n\t\t\tleft.insert(newEl) match {\n\t\t\t\tcase t: TwoNode[T] =>\n\t\t\t\t\tTwoNode(dataLeft,\n\t\t\t\t\t\tt,\n\t\t\t\t\t\tTwoNode(dataRight, mid, right))\n\t\t\t\tcase t: TwoThree[T] => this.copy(left = t)\n\t\t\t}\n\t\t}\n\t\telse if (ord.lt(newEl, dataRight)) {\n\t\t\tmid.insert(newEl) match {\n\t\t\t\tcase TwoNode(d, l, r) =>\n\t\t\t\t\tTwoNode(d,\n\t\t\t\t\t\tTwoNode(dataLeft, left, l),\n\t\t\t\t\t\tTwoNode(dataRight, r, right))\n\t\t\t\tcase t: TwoThree[T] => this.copy(mid = t)\n\t\t\t}\n\t\t}\n\t\telse {\n\t\t\tright.insert(newEl) match {\n\t\t\t\tcase t: TwoNode[T] =>\n\t\t\t\t\tTwoNode(dataRight,\n\t\t\t\t\t\tTwoNode(dataLeft, left, mid),\n\t\t\t\t\t\tt)\n\t\t\t\tcase t: TwoThree[T] => this.copy(right = t)\n\t\t\t}\n\t\t}\n\t}\n\tdef height(): Int = 1 + left.height()\n\tdef printSideways(indent: Int): Unit = {\n\t\tright.printSideways(indent + 1)\n\t\tprintln(\"\\t\" * indent + dataRight)\n\t\tmid.printSideways(indent + 1)\n\t\tprintln(\"\\t\" * indent + dataLeft)\n\t\tleft.printSideways(indent + 1)\n\t}\n}\n\nobject Main {\n\tdef main(args: Array[String]): Unit = {\n\t\tTwoLeaf(-3, 5).printSideways(0)\n\t\tOneLeaf(5).printSideways(0)\n\t\tOneLeaf(5).insert(-3).printSideways(0)\n\t\tprintln(OneLeaf(5).insert(-3).insert(5).insert(6))\n\t\tprintln(OneLeaf(5).insert(-3).insert(4))\n\t\tprintln(OneLeaf(5).insert(-3).insert(4).insert(6))\n\t\tprintln(OneLeaf(5).insert(-3).insert(4).insert(6).insert(3))\n\t\tOneLeaf(5).insert(-3).insert(4).insert(6).insert(3).insert(7).printSideways(0)\n\t}\n}"
}
{
"filename": "code/data_structures/src/tree/heap/binomial_heap/binomial_heap.c",
"content": "/* C program to implement Binomial Heap tree */\n \n#include<stdio.h>\n#include<stdlib.h>\n struct node {\n int n;\n int degree;\n struct node* parent;\n struct node* child;\n struct node* sibling;\n};\n \nstruct node* MAKE_bin_HEAP();\nvoid bin_LINK(struct node*, struct node*);\nstruct node* CREATE_NODE(int);\nstruct node* bin_HEAP_UNION(struct node*, struct node*);\nstruct node* bin_HEAP_INSERT(struct node*, struct node*);\nstruct node* bin_HEAP_MERGE(struct node*, struct node*);\nstruct node* bin_HEAP_EXTRACT_MIN(struct node*);\nvoid REVERT_LIST(struct node*);\nint DISPLAY(struct node*);\nstruct node* FIND_NODE(struct node*, int);\nint bin_HEAP_DECREASE_KEY(struct node*, int, int);\nint bin_HEAP_DELETE(struct node*, int);\n \nint count = 1;\n \nstruct node* MAKE_bin_HEAP() {\n struct node* np;\n np = NULL;\n return np;\n}\n \nstruct node * H = NULL;\nstruct node *Hr = NULL;\n \nvoid bin_LINK(struct node* y, struct node* z) {\n y->parent = z;\n y->sibling = z->child;\n z->child = y;\n z->degree = z->degree + 1;\n}\n \nstruct node* CREATE_NODE(int k) {\n struct node* p;//new node;\n p = (struct node*) malloc(sizeof(struct node));\n p->n = k;\n return p;\n}\n \nstruct node* bin_HEAP_UNION(struct node* H1, struct node* H2) {\n struct node* prev_x;\n struct node* next_x;\n struct node* x;\n struct node* H = MAKE_bin_HEAP();\n H = bin_HEAP_MERGE(H1, H2);\n if (H == NULL)\n return H;\n prev_x = NULL;\n x = H;\n next_x = x->sibling;\n while (next_x != NULL) {\n if ((x->degree != next_x->degree) || ((next_x->sibling != NULL)\n && (next_x->sibling)->degree == x->degree)) {\n prev_x = x;\n x = next_x;\n } else {\n if (x->n <= next_x->n) {\n x->sibling = next_x->sibling;\n bin_LINK(next_x, x);\n } else {\n if (prev_x == NULL)\n H = next_x;\n else\n prev_x->sibling = next_x;\n bin_LINK(x, next_x);\n x = next_x;\n }\n }\n next_x = x->sibling;\n }\n return H;\n}\n \nstruct node* bin_HEAP_INSERT(struct node* H, struct node* x) {\n struct node* H1 = MAKE_bin_HEAP();\n x->parent = NULL;\n x->child = NULL;\n x->sibling = NULL;\n x->degree = 0;\n H1 = x;\n H = bin_HEAP_UNION(H, H1);\n return H;\n}\n \nstruct node* bin_HEAP_MERGE(struct node* H1, struct node* H2) {\n struct node* H = MAKE_bin_HEAP();\n struct node* y;\n struct node* z;\n struct node* a;\n struct node* b;\n y = H1;\n z = H2;\n if (y != NULL) {\n if (z != NULL && y->degree <= z->degree)\n H = y;\n else if (z != NULL && y->degree > z->degree)\n /* need some modifications here;the first and the else conditions can be merged together!!!! */\n H = z;\n else\n H = y;\n } else\n H = z;\n while (y != NULL && z != NULL) {\n if (y->degree < z->degree) {\n y = y->sibling;\n } else if (y->degree == z->degree) {\n a = y->sibling;\n y->sibling = z;\n y = a;\n } else {\n b = z->sibling;\n z->sibling = y;\n z = b;\n }\n }\n return H;\n}\n \nint DISPLAY(struct node* H) {\n struct node* p;\n if (H == NULL) {\n printf(\"\\nHEAP EMPTY\");\n return 0;\n }\n printf(\"\\nTHE ROOT NODES ARE:-\\n\");\n p = H;\n while (p != NULL) {\n printf(\"%d\", p->n);\n if (p->sibling != NULL)\n printf(\"-->\");\n p = p->sibling;\n }\n printf(\"\\n\");\n return 1;\n}\n \nstruct node* bin_HEAP_EXTRACT_MIN(struct node* H1) {\n int min;\n struct node* t = NULL;\n struct node* x = H1;\n struct node *Hr;\n struct node* p;\n Hr = NULL;\n if (x == NULL) {\n printf(\"\\nNOTHING TO EXTRACT\");\n return x;\n }\n // int min=x->n;\n p = x;\n while (p->sibling != NULL) {\n if ((p->sibling)->n < min) {\n min = (p->sibling)->n;\n t = p;\n x = p->sibling;\n }\n p = p->sibling;\n }\n if (t == NULL && x->sibling == NULL)\n H1 = NULL;\n else if (t == NULL)\n H1 = x->sibling;\n else if (t->sibling == NULL)\n t = NULL;\n else\n t->sibling = x->sibling;\n if (x->child != NULL) {\n REVERT_LIST(x->child);\n (x->child)->sibling = NULL;\n }\n H = bin_HEAP_UNION(H1, Hr);\n return x;\n}\n \nvoid REVERT_LIST(struct node* y) {\n if (y->sibling != NULL) {\n REVERT_LIST(y->sibling);\n (y->sibling)->sibling = y;\n } else {\n Hr = y;\n }\n}\n \nstruct node* FIND_NODE(struct node* H, int k) {\n struct node* x = H;\n struct node* p = NULL;\n if (x->n == k) {\n p = x;\n return p;\n }\n if (x->child != NULL && p == NULL) {\n p = FIND_NODE(x->child, k);\n }\n \n if (x->sibling != NULL && p == NULL) {\n p = FIND_NODE(x->sibling, k);\n }\n return p;\n}\n \nint bin_HEAP_DECREASE_KEY(struct node* H, int i, int k) {\n int temp;\n struct node* p;\n struct node* y;\n struct node* z;\n p = FIND_NODE(H, i);\n if (p == NULL) {\n printf(\"\\nINVALID CHOICE OF KEY TO BE REDUCED\");\n return 0;\n }\n if (k > p->n) {\n printf(\"\\nSORY!THE NEW KEY IS GREATER THAN CURRENT ONE\");\n return 0;\n }\n p->n = k;\n y = p;\n z = p->parent;\n while (z != NULL && y->n < z->n) {\n temp = y->n;\n y->n = z->n;\n z->n = temp;\n y = z;\n z = z->parent;\n }\n printf(\"\\nKEY REDUCED SUCCESSFULLY!\");\n return 1;\n}\n \nint bin_HEAP_DELETE(struct node* H, int k) {\n struct node* np;\n if (H == NULL) {\n printf(\"\\nHEAP EMPTY\");\n return 0;\n }\n \n bin_HEAP_DECREASE_KEY(H, k, -1000);\n np = bin_HEAP_EXTRACT_MIN(H);\n if (np != NULL) {\n printf(\"\\nNODE DELETED SUCCESSFULLY\");\n return 1;\n }\n return 0;\n}\n \nint main() {\n int i, n, m, l;\n struct node* p;\n struct node* np;\n char ch;\n printf(\"\\nENTER THE NUMBER OF ELEMENTS:\");\n scanf(\"%d\", &n);\n printf(\"\\nENTER THE ELEMENTS:\\n\");\n for (i = 1; i <= n; i++) {\n scanf(\"%d\", &m);\n np = CREATE_NODE(m);\n H = bin_HEAP_INSERT(H, np);\n }\n DISPLAY(H);\n do {\n printf(\"\\nMENU:-\\n\");\n printf(\n \"\\n1)INSERT AN ELEMENT\\n2)EXTRACT THE MINIMUM KEY NODE\\n3)DECREASE A NODE KEY\\n4)DELETE A NODE\\n5)QUIT\\n\");\n scanf(\"%d\", &l);\n switch (l) {\n case 1:\n do {\n printf(\"\\nENTER THE ELEMENT TO BE INSERTED:\");\n scanf(\"%d\", &m);\n p = CREATE_NODE(m);\n H = bin_HEAP_INSERT(H, p);\n printf(\"\\nNOW THE HEAP IS:\\n\");\n DISPLAY(H);\n printf(\"\\nINSERT MORE(y/Y)= \\n\");\n fflush(stdin);\n scanf(\"%c\", &ch);\n } while (ch == 'Y' || ch == 'y');\n break;\n case 2:\n do {\n printf(\"\\nEXTRACTING THE MINIMUM KEY NODE\");\n p = bin_HEAP_EXTRACT_MIN(H);\n if (p != NULL)\n printf(\"\\nTHE EXTRACTED NODE IS %d\", p->n);\n printf(\"\\nNOW THE HEAP IS:\\n\");\n DISPLAY(H);\n printf(\"\\nEXTRACT MORE(y/Y)\\n\");\n fflush(stdin);\n scanf(\"%c\", &ch);\n } while (ch == 'Y' || ch == 'y');\n break;\n case 3:\n do {\n printf(\"\\nENTER THE KEY OF THE NODE TO BE DECREASED:\");\n scanf(\"%d\", &m);\n printf(\"\\nENTER THE NEW KEY : \");\n scanf(\"%d\", &l);\n bin_HEAP_DECREASE_KEY(H, m, l);\n printf(\"\\nNOW THE HEAP IS:\\n\");\n DISPLAY(H);\n printf(\"\\nDECREASE MORE(y/Y)\\n\");\n fflush(stdin);\n scanf(\"%c\", &ch);\n } while (ch == 'Y' || ch == 'y');\n break;\n case 4:\n do {\n printf(\"\\nENTER THE KEY TO BE DELETED: \");\n scanf(\"%d\", &m);\n bin_HEAP_DELETE(H, m);\n printf(\"\\nDELETE MORE(y/Y)\\n\");\n fflush(stdin);\n scanf(\"%c\", &ch);\n } while (ch == 'y' || ch == 'Y');\n break;\n case 5:\n printf(\"\\nTHANK U SIR\\n\");\n break;\n default:\n printf(\"\\nINVALID ENTRY...TRY AGAIN....\\n\");\n }\n } while (l != 5);\n}"
}
{
"filename": "code/data_structures/src/tree/heap/binomial_heap/binomial_heap.cpp",
"content": "#include <iostream>\n#include <queue>\n#include <vector>\n\n/* Part of Cosmos by OpenGenus Foundation */\nusing namespace std;\n\nclass Node {\npublic:\n int value;\n int degree;\n Node* parent;\n Node* child;\n Node* sibling;\n Node() : value(0), degree(0), parent(0), child(0), sibling(0)\n {\n };\n ~Node()\n {\n };\n};\n\nclass BinomialHeap {\npublic:\n BinomialHeap() : head(NULL)\n {\n }\n Node* getHead()\n {\n return head;\n }\n void insert(int value)\n {\n BinomialHeap tempHeap;\n Node* tempNode = new Node();\n tempNode->value = value;\n tempHeap.setHead(&tempNode);\n bHeapUnion(tempHeap);\n }\n void deleteMin()\n {\n int min = head->value;\n Node* tmp = head;\n Node* minPre = NULL;\n Node* minCurr = head;\n\n //find the root x with the minimum key in the root list of H\n // remove x from the root list of H\n while (tmp->sibling)\n {\n if (tmp->sibling->value < min)\n {\n min = tmp->sibling->value;\n minPre = tmp;\n minCurr = tmp->sibling;\n }\n tmp = tmp->sibling;\n }\n if (!minPre && minCurr)\n head = minCurr->sibling;\n else if (minPre && minCurr)\n minPre->sibling = minCurr->sibling;\n //H' Make-BINOMIAL-HEAP()\n BinomialHeap bh;\n Node *pre, *curr;\n //reverse the order of the linked list of x's children\n pre = tmp = NULL;\n curr = minCurr->child;\n while (curr)\n {\n tmp = curr->sibling;\n curr->sibling = pre;\n curr->parent = NULL;\n pre = curr;\n curr = tmp;\n }\n //set head[H'] to point to the head of the resulting list\n bh.setHead(&pre);\n //H <- BINOMIAL-HEAP-UNION\n bHeapUnion(bh);\n }\n int getMin()\n {\n int min = 2 << 20;\n Node* tmp = head;\n while (tmp)\n {\n if (tmp->value < min)\n min = tmp->value;\n tmp = tmp->sibling;\n }\n return min;\n\n }\n void preorder()\n {\n puts(\"\");\n Node* tmp = head;\n while (tmp)\n {\n _preorder(tmp);\n tmp = tmp->sibling;\n }\n puts(\"\");\n }\n void BFS()\n {\n puts(\"\");\n queue<Node*> nodeQueue;\n Node *tmp = head;\n\n while (tmp)\n {\n nodeQueue.push(tmp);\n tmp = tmp->sibling;\n }\n\n while (!nodeQueue.empty())\n {\n Node *node = nodeQueue.front();\n nodeQueue.pop();\n\n if (node)\n printf(\"%d \", node->value);\n\n node = node->child;\n while (node)\n {\n nodeQueue.push(node);\n node = node->sibling;\n }\n }\n puts(\"\");\n\n }\n void bHeapUnion(BinomialHeap &bh)\n {\n\n _mergeHeap(bh);\n Node* prev = NULL;\n Node* x = head;\n Node* next = x->sibling;\n while (next)\n {\n if (x->degree != next->degree)\n {\n prev = x; //Case 1 and 2\n x = next; //Case 1 and 2\n }\n else if (next->sibling && next->sibling->degree == x->degree) //three BT has the same degree\n {\n if (next->value < x->value && next->value < next->sibling->value) //312, need to trans to 132\n {\n x->sibling = next->sibling;\n next->sibling = x;\n if (prev)\n prev->sibling = next;\n else\n head = next;\n\n prev = x;\n x = next;\n }\n else if (next->sibling->value < x->value && next->sibling->value < next->value) //321, need to trans to 123\n {\n x->sibling = next->sibling->sibling;\n next->sibling->sibling = next;\n if (prev)\n prev->sibling = next->sibling;\n else\n head = next->sibling;\n prev = next->sibling;\n next->sibling = x;\n x = next;\n }\n else\n {\n prev = x; //Case 1 and 2\n x = next; //Case 1 and 2\n }\n }\n else if (x->value <= next->value)\n {\n x->sibling = next->sibling; //Case 3\n _mergeTree(next, x); //Case 3\n next = x;\n }\n else\n {\n if (!prev) //Case 4\n head = next; //Case 4\n else\n prev->sibling = next; //Case 4\n _mergeTree(x, next); //Case 4\n x = next; //Case 4\n }\n next = next->sibling; //Case 4\n }\n }\n int size()\n {\n return _size(head);\n }\n void setHead(Node** node)\n {\n head = *node;\n }\n\nprivate:\n int _size(Node* node)\n {\n if (!node)\n return 0;\n return 1 + _size(node->child) + _size(node->sibling);\n }\n void _preorder(Node* node)\n {\n if (!node)\n return;\n printf(\"%d \", node->value);\n _preorder(node->child);\n if (node->parent)\n _preorder(node->sibling);\n //printf(\"%d(%d) \",node->value,node->degree);\n }\n void _mergeTree(Node* tree1, Node* tree2)\n {\n tree1->parent = tree2;\n tree1->sibling = tree2->child;\n tree2->child = tree1;\n tree2->degree++;\n }\n void _mergeHeap(BinomialHeap &bh)\n {\n Node* head2 = bh.getHead();\n Node* head1 = head;\n //for new pointer\n Node *newHead, *newCurr;\n if (!head1)\n {\n head = head2;\n return;\n }\n if (head1->degree > head2->degree)\n {\n newHead = newCurr = head2;\n head2 = head2->sibling;\n }\n else\n {\n newHead = newCurr = head1;\n head1 = head1->sibling;\n }\n //Sorted by degree into monotonically increasing order\n while (head1 && head2)\n {\n if (head1->degree < head2->degree)\n {\n newCurr->sibling = head1;\n newCurr = head1;\n head1 = head1->sibling;\n }\n else\n {\n newCurr->sibling = head2;\n newCurr = head2;\n head2 = head2->sibling;\n }\n }\n while (head1)\n {\n newCurr->sibling = head1;\n newCurr = head1;\n head1 = head1->sibling;\n }\n while (head2)\n {\n newCurr->sibling = head2;\n newCurr = head2;\n head2 = head2->sibling;\n }\n head = newHead;\n }\n\n Node* head;\n};\n\nint main()\n{\n vector<int> heap1{5, 4, 3, 2, 1};\n vector<int> heap2{4, 3, 2, 1, 8};\n BinomialHeap bh1, bh2;\n\n for (auto v: heap1)\n bh1.insert(v);\n\n for (auto v: heap2)\n bh2.insert(v);\n\n printf(\"preorder traversal of first binomialheap: \");\n bh1.preorder();\n printf(\"BFS of first binomialheap: \");\n bh1.BFS();\n\n printf(\"\\n\");\n\n printf(\"preorder traversal of second binomialheap: \");\n bh2.preorder();\n printf(\"BFS of second binomialheap: \");\n bh2.BFS();\n\n printf(\"-----------Union------------------\\n\");;\n bh1.bHeapUnion(bh2);\n printf(\"preorder traversal of union: \");\n bh1.preorder();\n printf(\"BFS of union: \");\n bh1.BFS();\n\n printf(\"-----------Delete min = 1 --------\\n\");\n bh1.deleteMin();\n printf(\"preorder traversal of delete min: \");\n bh1.preorder();\n printf(\"BFS of union: \");\n bh1.BFS();\n\n printf(\"-----------Delete min = 2 --------\\n\");\n bh1.deleteMin();\n printf(\"preorder traversal of delete min: \");\n bh1.preorder();\n printf(\"BFS of union: \");\n bh1.BFS();\n\n printf(\"binomialheap min:%d\\n\", bh1.getMin());\n printf(\"binomailheap size %d\\n\", bh1.size());\n}"
}
{
"filename": "code/data_structures/src/tree/heap/binomial_heap/binomial_heap.scala",
"content": "\nobject BinomialHeap {\n def apply[T](value: T)(implicit ord: Ordering[T], treeOrd: Ordering[BinTree[T]]) = new BinomialHeap(Map())(ord, treeOrd).add(BinTree(value))\n}\n\ncase class BinomialHeap[T](heap: Map[Int, BinTree[T]])(implicit ord: Ordering[T], treeOrd: Ordering[BinTree[T]]) {\n\n def add(tree: BinTree[T]): BinomialHeap[T] = {\n if (heap.contains(tree.order)) {\n BinomialHeap(heap - tree.order).add(tree.merge(heap(tree.order)))\n } else {\n BinomialHeap(heap + (tree.order -> tree))\n }\n }\n\n def add(value: T): BinomialHeap[T] = add(BinTree(value))\n\n def min(): T = heap.valuesIterator.min.value\n\n def deleteMin(): BinomialHeap[T] = {\n val minTree = heap.valuesIterator.min\n if (minTree.order == 0) {\n BinomialHeap(heap - minTree.order)\n } else {\n val remainingHeap = BinomialHeap(heap - minTree.order)\n minTree.kids.foldLeft(remainingHeap) { case (acc: BinomialHeap[T], tree: BinTree[T]) => acc.add(tree) }\n }\n }\n}\n\nobject BinTree {\n def apply[T](value: T)(implicit ord: Ordering[T]) = new BinTree(value, 0, IndexedSeq())\n}\n\ncase class BinTree[T](value: T, order: Int, kids: IndexedSeq[BinTree[T]])(implicit ord: Ordering[T]) {\n\n def merge(other: BinTree[T]): BinTree[T] = {\n assert(other.order == order)\n\n if (ord.lt(other.value, value)) {\n BinTree(other.value, order + 1, other.kids :+ this)\n } else {\n BinTree(value, order + 1, kids :+ other)\n }\n }\n}\n\nobject Main {\n def main(args: Array[String]): Unit = {\n implicit val ord = Ordering.by[BinTree[Int], Int](_.value)\n println(BinomialHeap(10).add(-5))\n println(BinomialHeap(10).add(-5).add(3))\n println(BinomialHeap(10).add(-5).add(3).add(13))\n println(BinomialHeap(10).add(-5).add(3).add(13).min)\n println(BinomialHeap(10).add(-5).add(3).add(13).deleteMin())\n }\n}"
}
{
"filename": "code/data_structures/src/tree/heap/max_heap/max_heap.c",
"content": "/*\n Part of Cosmos by OpenGenus Foundation\n This is a C program that forms a max heap.\n The following queries can be used:\n 0 - Print the max-heap\n 1 - Heapsort, then print the sorted array\n 2 [element] - Insert element into max-heap, then print the heap\n 3 - Remove/extract maximum element in max-heap, then print the heap\n 4 - Print maximum element of max-heap \n*/\n\n# include <stdio.h>\n int n = 0; // global variable for maintaining the sixe of the heap\n int size = 0; // global array used for heapsort\n\n/*\n The function down() is used for building the heap\n and for deleting the maximum element from the heap\n The parameters are:\n 1. pos - index of the element to be inserted\n 2. val - value to be compared with\n 3. heap - the heap array\n*/\nint down(int pos, int val, int heap[n])\n{\n int target = 0;\n while(2*pos<=n)\n {\n int left = 2*pos;\n int right = left + 1;\n if(right<=n && heap[right]>heap[left])\n {\n target = right;\n }\n else\n {\n target = left;\n }\n if(heap[target]>val)\n {\n heap[pos] = heap[target];\n pos = target;\n }\n else\n {\n break;\n }\n }\n return pos;\n}\n\n/*\n The function down2() is used for heapsort's\n delete functinality\n The parameters are:\n 1. pos - index of the element to be inserted\n 2. val - value to be compared with\n 3. heap - the heap array\n*/\nint down2(int pos, int val, int heap[size])\n{\n int target = 0;\n while(2*pos<=size)\n {\n int left = 2*pos;\n int right = left + 1;\n if(right<=size && heap[right]>heap[left])\n {\n target = right;\n }\n else\n {\n target = left;\n }\n if(heap[target]>val)\n {\n heap[pos] = heap[target];\n pos = target;\n }\n else\n {\n break;\n }\n }\n return pos;\n}\n\n/*\n The function buildheap() is used for constructing\n the max heap which uses the percolatedown method\n The parameters are:\n 1. heap - the heap array\n*/\nvoid builheap(int heap[n])\n{\n for(int i = n/2 ; i>0 ; i--)\n {\n int node = heap[i];\n int position = percolatedown(i, heap[i], heap);\n heap[position] = node;\n }\n}\n\n/*\n The function printheap() is used for printing\n the max heap\n The parameters are:\n 1. heap - the heap array\n*/\nvoid printheap(int heap[n])\n{\n for(int i = 1 ; i<=n ; i++)\n {\n printf(\"%d \", heap[i]);\n }\n}\n\n/*\n The function deletemax is used for deleting\n the maximum element from the heap\n The parameters are:\n 1. heap - the heap array\n*/\nvoid deletemax(int heap[n])\n{\n int newpos = down(1, heap[n+1], heap);\n heap[newpos] = heap[n+1];\n}\n\n/*\n The function deletemax2 is used for deleting\n the maximum element from the heap\n The parameters are:\n 1. heap - the heap array\n*/\nvoid deletemax2(int heap[size])\n{\n int newpos = down2(1, heap[size+1], heap);\n heap[newpos] = heap[size+1];\n}\n\n/*\n The function heapsort() is used for sorting\n the heap array\n The parameters are:\n 1. heap - the heap array\n 2. arr - it stores the sorted elements of the heap\n*/\nvoid heapsort(int heap[n+1], int arr[n+1])\n{\n size = n;\n int heapsortarr[100];\n for(int i = 1 ; i<=n ; i++)\n {\n heapsortarr[i] = heap[i];\n }\n for(int i = 1 ; i<=n ; i++)\n {\n arr[n-i+1] = heapsortarr[1];\n size--;\n deletemax2(heapsortarr);\n }\n}\n\nint main()\n{\n scanf(\"%d\", &n);\n int heaparr[100];\n for(int i = 0 ; i<n ; i++)\n {\n scanf(\"%d\", &heaparr[i+1]);\n }\n builheap(heaparr);\n int t = 0;\n scanf(\"%d\", &t);\n for(int j = 0 ; j<t ; j++)\n {\n int q = 0;\n scanf(\"%d\", &q);\n if(q==0) // Print the max-heap\n {\n printheap(heaparr);\n printf(\"\\n\");\n }\n else if(q==1) // Heapsort, then print the sorted array\n {\n int arr[n+1];\n heapsort(heaparr, arr);\n printheap(arr);\n printf(\"\\n\");\n }\n else if(q==2) // Insert element into max-heap, then print the heap\n {\n int a = 0;\n scanf(\"%d\", &a);\n n++;\n heaparr[n] = a;\n builheap(heaparr);\n printheap(heaparr);\n printf(\"\\n\");\n }\n else if(q==3) // Remove/extract maximum element in max-heap, then print the heap\n {\n n--;\n deletemax(heaparr);\n printheap(heaparr);\n printf(\"\\n\");\n }\n else if(q==4) // Print maximum element of max-heap\n {\n printf(\"%d\\n\", heaparr[1]);\n }\n }\n}"
}
{
"filename": "code/data_structures/src/tree/heap/max_heap/max_heap.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n/*************************************************************************************\n* ░█▀▀░█▀▀░█▀█░█▀▀░█▀▄░▀█▀░█▀▀░░░█░█░█▀▀░█▀█░█▀█░░░█▀▀░▀█▀░█▀▄░█░█░█▀▀░▀█▀░█░█░█▀▄░█▀▀\n* ░█░█░█▀▀░█░█░█▀▀░█▀▄░░█░░█░░░░░█▀█░█▀▀░█▀█░█▀▀░░░▀▀█░░█░░█▀▄░█░█░█░░░░█░░█░█░█▀▄░█▀▀\n* ░▀▀▀░▀▀▀░▀░▀░▀▀▀░▀░▀░▀▀▀░▀▀▀░░░▀░▀░▀▀▀░▀░▀░▀░░░░░▀▀▀░░▀░░▀░▀░▀▀▀░▀▀▀░░▀░░▀▀▀░▀░▀░▀▀▀\n*\n* Generic heap structure implementation using vectors instead of arrays.\n* Holds instances of HeapElement class which consist of data,key pairs.\n* Can be initialized from existing array,vector or by inserting elements one by one.\n*\n*\n* Basic operations:\n* 1.void insert(HeapElement<T> element): Insert element to heap\n* 2.HeapElement<T> deletemax(): Delete and return the maximum element from heap\n* 3.HeapElement<T> peek_max(): Return max element without deleting it from heap\n* 4.bool isEmpty(): Check if heap is Empty\n* 5.HeapElement<T> getHeapSize(): Return heap size\n* 6.void printHeap(): Print heap content\n*************************************************************************************/\n\n#include <iostream>\n#include <cstdio>\n#include <vector>\n#include <iostream>\n#include <climits>\n\nusing namespace std; //Not the best tactic, used for cleaner code and better understanding\n\ntemplate<typename P>\nclass HeapElement {\npublic:\n int key;\n P data;\n\n HeapElement()\n {\n }\n\n HeapElement(P datain, int keyin)\n {\n key = keyin;\n data = datain;\n }\n};\n\ntemplate<typename T>\nclass Heap {\nprivate:\n std::vector<HeapElement<T>> heap;\n int hpsz;\npublic:\n\n Heap()\n {\n hpsz = 0; //At first the Heap is Empty\n HeapElement<T> init;\n heap.push_back(init); //Index 0 must be initialized because we start indexing from 1\n }\n\n Heap(std::vector<HeapElement<T>>& array)\n {\n int n = array.size();\n HeapElement<T> init();\n heap.push_back(init); //The first index of heap array is 1 so we must fill 0 index with dummy content\n heap.insert(heap.end(), array.begin(), array.end());\n hpsz = n; //Heap size = Heap Array size\n for (int i = n / 2; i > 0; i--)\n combine(i);\n }\n\n void insert(HeapElement<T> elmnt)\n {\n heap.push_back(elmnt);\n hpsz++;\n int i = hpsz, parent = i / 2;\n while ((i > 1) && (heap[parent].key < heap[i].key))\n {\n iter_swap(heap.begin() + parent, heap.begin() + i);\n i = parent; parent = i / 2;\n }\n }\n\n//Get max without deleting from heap\n int peek_max()\n {\n return heap[1].data;\n }\n\n void combine (int i)\n {\n int mp = i, left = 2 * i, right = (2 * i) + 1;\n if ((left <= hpsz) && (heap[left].key > heap[mp].key))\n mp = left;\n if ((right <= hpsz) && (heap[right].key > heap[mp].key))\n mp = right;\n if (mp != i)\n {\n iter_swap(heap.begin() + i, heap.begin() + mp);\n combine(mp);\n }\n }\n\n HeapElement<T> deletemax()\n {\n\n if (isEmpty())\n {\n HeapElement<T> error; return error;\n }\n HeapElement<T> max = heap[1];\n\n heap[1] = heap[hpsz--];\n combine(1);\n return max;\n }\n\n bool isEmpty()\n {\n return hpsz == 0;\n }\n\n int getHeapSize()\n {\n return hpsz;\n }\n\n void printHeap()\n {\n for (int i = 1; i <= hpsz; i++)\n std::cout << heap[i].data;\n printf(\"\\n\");\n }\n\n};\n\nint main()\n{\n /*\n * Heap can be constructed using vector of HeapElement<T> as input\n * or array of HeapElement<T>\n */\n //HeapElement<T> a[] = {.......HeapElement<T> HERE,another HeapElement<T>.........};\n //vector<HeapElement<T>> heapin (a, a + sizeof(a) / sizeof(a[0]) ); //C++2003\n //std::vector<HeapElement<T>> heapin ({.......HeapElement<T> HERE,another HeapElement<T>.........}); //C++2011\n // std::vector<HeapElement<T>> heapin(std::begin(a), std::end(a)); //C++2003\n //Heap test(heapin);\n\n /*\n * Heap can be constructed using one by one insertion of elements\n * <-------------------------------------------------------------->\n * Heap<char> test;\n * HeapElement<char> one('A',1);\n * test.insert(one);\n *\n * HeapElement<char> two('B',3);\n * test.insert(two);\n *\n * HeapElement<char> three('C',2);\n * test.insert(three);\n *\n * HeapElement<char> four('D',5);\n * test.insert(four);\n *\n * test.printHeap(); //Heap array\n * }\n */\n\n Heap<char> test;\n\n\n int num_elements;\n std::cout << \"Enter number of elements:\";\n\n std::cin >> num_elements;\n HeapElement<char> elem; //For example we create a heap that includes celements with char values and int keys\n\n while (num_elements != 0)\n {\n num_elements--;\n std::cout << \"Enter element data:\";\n std::cin >> elem.data;\n std::cout << \"Enter element key:\";\n std::cin >> elem.key;\n test.insert(elem);\n std::cout << \"--------------\" << '\\n';\n }\n\n test.printHeap(); //Heap array\n\n\n while (!test.isEmpty())\n std::cout << \"Max after delete: \" << test.deletemax().data << '\\n';\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/heap/max_heap/max_heap.go",
"content": "/* Part of Cosmos by OpenGenus Foundation */\npackage main\n\nimport \"fmt\"\n\ntype MaxHeap struct {\n\tdata []int\n}\n\nfunc swap(x, y int) (int, int) {\n\treturn y, x\n}\n\nfunc (h *MaxHeap) Push(value int) {\n\th.data = append(h.data, value)\n\tindex := len(h.data) - 1\n\tparent := (index - 1) / 2\n\tfor index > 0 && h.data[index] > h.data[parent] {\n\t\th.data[index], h.data[parent] = swap(h.data[index], h.data[parent])\n\t\tindex = parent\n\t\tparent = (index - 1) / 2\n\t}\n}\nfunc (h *MaxHeap) Top() int {\n\treturn h.data[0]\n}\nfunc (h *MaxHeap) ExtractTop() int {\n\tindex := len(h.data) - 1\n\tmax := h.Top()\n\th.data[0], h.data[index] = swap(h.data[0], h.data[index])\n\th.data = h.data[:index]\n\th.heapify(0)\n\treturn max\n}\n\nfunc (h *MaxHeap) Pop() {\n\tindex := len(h.data) - 1\n\th.data[0], h.data[index] = swap(h.data[0], h.data[index])\n\th.data = h.data[:index]\n\th.heapify(0)\n}\n\nfunc (h *MaxHeap) heapify(index int) {\n\tleft := (index * 2) + 1\n\tright := left + 1\n\ttarget := index\n\n\tif left < len(h.data) && h.data[left] > h.data[index] {\n\t\ttarget = left\n\t}\n\tif right < len(h.data) && h.data[right] > h.data[target] {\n\t\ttarget = right\n\t}\n\tif target != index {\n\t\th.data[index], h.data[target] = swap(h.data[index], h.data[target])\n\t\th.heapify(target)\n\t}\n\n}\n\nfunc (h *MaxHeap) Empty() bool {\n\treturn len(h.data) == 0\n}\n\nfunc main() {\n\tdata := []int{45, 6, 4, 3, 2, 72, 34, 12, 456, 29, 312}\n\tvar h MaxHeap\n\n\tfor _, v := range data {\n\t\th.Push(v)\n\t}\n\n\tfmt.Println(\"Current Data is \", h.data)\n\tfor !h.Empty() {\n\t\tfmt.Println(h.Top())\n\t\th.Pop()\n\t}\n}"
}
{
"filename": "code/data_structures/src/tree/heap/max_heap/max_heap.java",
"content": "//Part of Open Genus foundation\nimport java.util.Scanner;\n\n/**\n * Created by risha on 15-10-2017.\n */\n// Priority Queue binary max_heap implementation\npublic class MaxPQ<Key extends Comparable<Key>> {\n private Key []pq;\n private int N=0;\n private int capacity;\n public MaxPQ(int capacity)\n {\n pq= (Key[]) new Comparable[capacity+1];\n this.capacity=capacity;\n }\n public boolean isEmpty()\n {\n return N==0;\n }\n public void insertKey(Key x)\n {\n if (N+1>capacity)\n resize();\n pq[++N]=x;\n swim(N);\n }\n public int size()\n {\n return N;\n }\n /* public int getCapacity()\n {\n return this.capacity;\n }*/\n private void resize()\n {\n this.capacity=2*N;\n Key[] temp= (Key[]) new Comparable[this.capacity+1];\n for (int i=1;i<=N;i++)\n temp[i]=pq[i];\n pq=temp;\n }\n public Key delMax()\n {\n if (isEmpty())\n return null;\n Key max=pq[1];\n exch(1,N--);\n sink(1);\n pq[N+1]=null;\n return max;\n }\n private void swim(int k)\n {\n while (k>1 && less(k/2,k))\n {\n exch(k,k/2);\n k=k/2;\n }\n }\n private void sink(int k)\n {\n while (2*k<=N)\n {\n int j=2*k;\n if(j<N && less(j,j+1))j++;\n if (!less(k,j))break;\n exch(k,j);\n k=j;\n }\n }\n private boolean less(int i,int j)\n {\n return pq[i].compareTo(pq[j])<0;\n }\n private void exch(int i,int j)\n {\n Key t=pq[i];\n pq[i]=pq[j];\n pq[j]=t;\n }\n public static void main(String args[])\n {\n Scanner sc=new Scanner(System.in);\n System.out.println(\"Enter the size of heap\");\n int n=sc.nextInt();\n MaxPQ<Integer> pq=new MaxPQ<Integer>(n);\n for (int i=0;i<n;i++)\n pq.insertKey(sc.nextInt());\n System.out.println(\"Size of heap: \"+pq.size());\n // System.out.println(\"Capacity: \"+pq.getCapacity());\n System.out.println(\"Maximum keys in order\");\n while (!pq.isEmpty())\n {\n System.out.println(pq.delMax());\n }\n System.out.println(pq.delMax());\n }\n}"
}
{
"filename": "code/data_structures/src/tree/heap/max_heap/max_heap.py",
"content": "# Part of Cosmos by OpenGenus Foundation\ndef left(i):\n return 2 * i + 1\n\n\ndef right(i):\n return 2 * i + 2\n\n\ndef heapify(a, i):\n l = left(i)\n r = right(i)\n length = len(a)\n if l < length and a[l] > a[i]:\n largest = l\n else:\n largest = i\n if r < length and a[r] > a[largest]:\n largest = r\n if largest != i:\n a[i], a[largest] = a[largest], a[i]\n heapify(a, largest)\n\n\ndef build_max_heap(a):\n for i in range((len(list(a)) - 1) // 2, -1, -1):\n heapify(a, i)\n\n\nif __name__ == \"__main__\":\n print(\"Enter the array of which you want to create a max heap\")\n a = [int(x) for x in input().split()]\n build_max_heap(a)\n print(\"The max heap is -\t\")\n for i in range(len(a)):\n print(a[i], end=\" \")\n print(\"\")"
}
{
"filename": "code/data_structures/src/tree/heap/min_heap/min_heap.c",
"content": "#include <stdio.h>\n\n#define asize 100\n#define min(a, b) (((a) < (b)) ? (a) : (b))\n\nstruct heap\n{\n\tint a[asize];\n\tint hsize ;\n} H;\n\nvoid \nswap(int *a, int *b)\n{\n\tint temp = *b;\n\t*b = *a;\n\t*a = temp;\n}\n\nvoid \nheapInsert(int key)\n{\n\t++H.hsize;\n\tH.a[H.hsize] = key;\n\tint i = H.hsize;\n\twhile (i > 1 && H.a[i] < H.a[i / 2]) {\n\t\tswap(&H.a[i], &H.a[i / 2]);\n\t\ti = i / 2;\n\t}\n\tprintf(\"%d is inserted in Min Heap \\n\", key);\n}\n\nvoid \ndisplayHeap()\n{\n\tint i;\n\tprintf(\"Elements in the heap:- \\n\");\n\tfor (i = 1; i <= H.hsize; i++)\n\t\tprintf(\"%d \", H.a[i]);\n\tprintf(\"\\n\");\n}\n\nvoid delMin()\n{\n\tif (H.hsize == 0) {\n\t\tprintf(\"Heap is empty,No element can be popped out \\n\");\n\t\treturn;\n\t}\n\n\tint x = H.a[1];\n\tH.a[1] = H.a[H.hsize];\n\t--H.hsize;\n\tint i = 1;\n\t\n\twhile ((2 * i) <= H.hsize) {\n\t\tif ((2 * i) + 1 <= H.hsize && H.a[i] > min(H.a[2 * i], H.a[(2 * i) + 1])) {\n\t\t\tif (H.a[(2 * i) + 1] < H.a[2 * i]) {\n\t\t\t\tswap(&H.a[i], &H.a[(2 * i) + 1]);\n\t\t\t\ti=(2 * i) + 1;\n\t\t\t}\n\t\t\telse {\n\t\t\t\tswap(&H.a[i], &H.a[(2 * i)]);\n\t\t\t\ti=2 * i;\t\n\t\t\t}\n\n\t\t}\n\t\telse if ((2 * i) + 1 > H.hsize && H.a[i] > H.a[2 * i]) {\n\t\t\tswap(&H.a[i], &H.a[(2 * i)]);\n\t\t\tbreak;\n\t\t}\n\t\telse\n\t\t\tbreak;\n\t}\n\tprintf(\"%d is popped out \\n\", x);\n\n}\n\nint \nmain()\n{\n\tint key, ch;\n\tH.hsize = 0;\n\twhile (1) {\n\t\tprintf(\"1.Insert\\n2.Display Min Heap\\n3.Pop out Minimum element\\n4.Exit\\n\");\n\t\tscanf(\"%d\", &ch);\n\t\tswitch (ch) {\n\t\t\tcase 1 :\n\t\t\t\tprintf(\"Enter integer to be inserted \\n\");\n\t\t\t\tscanf(\"%d\", &key);\n\t\t\t\theapInsert(key);\n\t\t\t\tbreak;\n\n\t\t\tcase 2 :\n\t\t\t\tdisplayHeap();\n\t\t\t\tbreak;\t\n\n\t\t\tcase 3 :\n\t\t\t\tdelMin();\n\t\t\t\tbreak;\n\n\t\t\tcase 4 :\n\t\t\t\treturn (0);\n\t\t}\n\t}\n}"
}
{
"filename": "code/data_structures/src/tree/heap/min_heap/min_heap.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n#include <iostream>\n#include <vector>\nusing namespace std;\n\nclass minHeap {\n vector<int> v;\n\n void heapify(size_t i)\n {\n size_t l = 2 * i;\n size_t r = 2 * i + 1;\n size_t min = i;\n if (l < v.size() && v[l] < v[min])\n min = l;\n if (r < v.size() && v[r] < v[min])\n min = r;\n\n if (min != i)\n {\n swap(v[i], v[min]);\n heapify(min);\n }\n }\n\npublic:\n minHeap()\n {\n v.push_back(-1);\n }\n void insert(int data)\n {\n v.push_back(data);\n int i = v.size() - 1;\n int parent = i / 2;\n\n while (v[i] < v[parent] && i > 1)\n {\n swap(v[i], v[parent]);\n i = parent;\n parent = parent / 2;\n }\n\n }\n int top()\n {\n return v[1];\n }\n\n void pop()\n {\n\n int last = v.size() - 1;\n swap(v[1], v[last]);\n v.pop_back();\n heapify(1);\n }\n\n bool isEmpty()\n {\n return v.size() == 1;\n }\n\n};\n\nint main()\n{\n\n int data;\n cout << \"\\nEnter data : \";\n cin >> data;\n minHeap h;\n while (data != -1)\n {\n h.insert(data);\n cout << \"\\nEnter data(-1 to exit) : \";\n cin >> data;\n }\n\n while (!h.isEmpty())\n {\n cout << h.top() << \" \";\n h.pop();\n }\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/heap/min_heap/min_heap.java",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n public class MinHeap\n\n {\n\n private int[] Heap;\n\n private int size;\n\n private int maxsize;\n\n \n\n private static final int FRONT = 1;\n\n \n\n public MinHeap(int maxsize)\n\n {\n\n this.maxsize = maxsize;\n\n this.size = 0;\n\n Heap = new int[this.maxsize + 1];\n\n Heap[0] = Integer.MIN_VALUE;\n\n }\n\n \n\n private int parent(int pos)\n\n {\n\n return pos / 2;\n\n }\n\n \n\n private int leftChild(int pos)\n\n {\n\n return (2 * pos);\n\n }\n\n \n\n private int rightChild(int pos)\n\n {\n\n return (2 * pos) + 1;\n\n }\n\n \n\n private boolean isLeaf(int pos)\n\n {\n\n if (pos >= (size / 2) && pos <= size)\n\n { \n\n return true;\n\n }\n\n return false;\n\n }\n\n \n\n private void swap(int fpos, int spos)\n\n {\n\n int tmp;\n\n tmp = Heap[fpos];\n\n Heap[fpos] = Heap[spos];\n\n Heap[spos] = tmp;\n\n }\n\n \n\n private void heapify(int pos)\n\n {\n\n if (!isLeaf(pos))\n\n { \n\n if ( Heap[pos] > Heap[leftChild(pos)] || Heap[pos] > Heap[rightChild(pos)])\n\n {\n\n if (Heap[leftChild(pos)] < Heap[rightChild(pos)])\n\n {\n\n swap(pos, leftChild(pos));\n\n heapify(leftChild(pos));\n\n }else\n\n {\n\n swap(pos, rightChild(pos));\n\n heapify(rightChild(pos));\n\n }\n\n }\n\n }\n\n }\n\n \n\n public void insert(int element)\n\n {\n\n Heap[++size] = element;\n\n int current = size;\n\n \n\n while (Heap[current] < Heap[parent(current)])\n\n {\n\n swap(current,parent(current));\n\n current = parent(current);\n\n }\t\n\n }\n\n \n\n public void print()\n\n {\n\n for (int i = 1; i <= size / 2; i++ )\n\n {\n\n System.out.print(\" PARENT : \" + Heap[i] + \" LEFT CHILD : \" + Heap[2*i] \n\n + \" RIGHT CHILD :\" + Heap[2 * i + 1]);\n\n System.out.println();\n\n } \n\n }\n\n \n\n public void minHeap()\n\n {\n\n for (int pos = (size / 2); pos >= 1 ; pos--)\n\n {\n\n heapify(pos);\n\n }\n\n }\n\n \n\n public int remove()\n\n {\n\n int popped = Heap[FRONT];\n\n Heap[FRONT] = Heap[size--]; \n\n heapify(FRONT);\n\n return popped;\n\n }\n\n \n\n public static void main(String...arg)\n\n {\n\n System.out.println(\"The Min Heap is \");\n\n MinHeap minHeap = new MinHeap(15);\n\n minHeap.insert(5);\n\n minHeap.insert(3);\n\n minHeap.insert(17);\n\n minHeap.insert(10);\n\n minHeap.insert(84);\n\n minHeap.insert(19);\n\n minHeap.insert(6);\n\n minHeap.insert(22);\n\n minHeap.insert(9);\n\n minHeap.minHeap();\n\n \n\n minHeap.print();\n\n System.out.println(\"The Min val is \" + minHeap.remove());\n\n }\n\n }"
}
{
"filename": "code/data_structures/src/tree/heap/min_heap/min_heap.js",
"content": "/** Source: https://github.com/brunocalou/graph-theory-js/blob/master/src/data_structures/binary_heap.js */\n\"use strict\";\n\n/**\n * The function to use when comparing variables\n * @typedef {function} comparatorFunction\n * @param {any} a - The first element to compare\n * @param {any} b - The second element to compare\n * @returns {number} A negative number, zero, or a positive number\n * as the first argument is less than, equal to, or greater than the second\n */\n\n/**\n * Comparator class\n * @constructor\n * @classdesc The Comparator class is a helper class that compares two variables\n * according to a comparator function.\n * @param {comparatorFunction} fn - The comparator function\n */\nfunction Comparator(compareFn) {\n if (compareFn) {\n /**@type {comparatorFunction} */\n this.compare = compareFn;\n }\n}\n\n/**\n * Default comparator function\n * @param {any} a - The first element to compare\n * @param {any} b - The second element to compare\n * @returns {number} A negative number, zero, or a positive number\n * as the first argument is less than, equal to, or greater than the second\n * @see comparatorFunction\n */\nComparator.prototype.compare = function(a, b) {\n if (a === b) return 0;\n return a < b ? -1 : 1;\n};\n\n/**\n * Compare if the first element is equal to the second one.\n * Override this method if you are comparing arrays, objects, ...\n * @example\n * function compare (a,b) {\n * return a[0] - b[0];\n * }\n * var a = [1,2];\n * var b = [1,2];\n *\n * var comparator = new Comparator(compare);\n *\n * //Compare just the values\n * comparator.equal(a,b);//true\n *\n * comparator.equal = function(a,b) {\n * return a == b;\n * }\n *\n * //Compare the refecence too\n * comparator.equal(a,b);//false\n *\n * var c = a;\n * comparator.equal(a,c);//true\n *\n * @param {any} a - The first element to compare\n * @param {any} b - The second element to compare\n * @returns {boolean} True if the first element is equal to\n * the second one, false otherwise\n */\nComparator.prototype.equal = function(a, b) {\n return this.compare(a, b) === 0;\n};\n\n/**\n * Compare if the first element is less than the second one\n * @param {any} a - The first element to compare\n * @param {any} b - The second element to compare\n * @returns {boolean} True if the first element is less than\n * the second one, false otherwise\n */\nComparator.prototype.lessThan = function(a, b) {\n return this.compare(a, b) < 0;\n};\n\n/**\n * Compare if the first element is less than or equal the second one\n * @param {any} a - The first element to compare\n * @param {any} b - The second element to compare\n * @returns {boolean} True if the first element is less than or equal\n * the second one, false otherwise\n */\nComparator.prototype.lessThanOrEqual = function(a, b) {\n return this.compare(a, b) < 0 || this.equal(a, b);\n};\n\n/**\n * Compare if the first element is greater than the second one\n * @param {any} a - The first element to compare\n * @param {any} b - The second element to compare\n * @returns {boolean} True if the first element is greater than\n * the second one, false otherwise\n */\nComparator.prototype.greaterThan = function(a, b) {\n return this.compare(a, b) > 0;\n};\n\n/**\n * Compare if the first element is greater than or equal the second one\n * @param {any} a - The first element to compare\n * @param {any} b - The second element to compare\n * @returns {boolean} True if the first element is greater than or equal\n * the second one, false otherwise\n */\nComparator.prototype.greaterThanOrEqual = function(a, b) {\n return this.compare(a, b) > 0 || this.equal(a, b);\n};\n\n/**\n * Change the compare function to return the opposite results\n * @example\n * // false\n * Comparator.greaterThan(1, 2);\n * @example\n * // true\n * Comparator.invert();\n * Comparator.greaterThan(1, 2);\n */\nComparator.prototype.invert = function() {\n this._originalCompare = this.compare;\n this.compare = function(a, b) {\n return this._originalCompare(b, a);\n }.bind(this);\n};\n\n/**\n * Min Binary Heap Class\n * @constructor\n * @classdesc The MinBinaryHeap class implements a minimum binary heap data structure\n * @param {function} comparator_fn - The comparator function\n */\nfunction MinBinaryHeap(comparator_fn) {\n /**@private\n * @type {array} */\n this._elements = [null]; // will not use the first index\n\n /**@public\n * @type {Comparator} */\n this.comparator = new Comparator(comparator_fn);\n\n /**@private\n * @type {number}\n */\n this._length = 0;\n\n Object.defineProperty(this, \"length\", {\n get: function() {\n return this._length;\n }\n });\n}\n\n/**\n * Changes the value of the element\n * @param {any} old_element - The element to be replaced\n * @param {any} new_element - The new element\n */\nMinBinaryHeap.prototype.changeElement = function(old_element, new_element) {\n for (var i = 1; i <= this._length; i += 1) {\n if (this.comparator.equal(old_element, this._elements[i])) {\n this._elements[i] = new_element;\n break;\n }\n }\n this._elements.shift(); //Removes the null from the first index\n this.heapify(this._elements);\n};\n\n/**\n * Removes all the elements in the heap\n */\nMinBinaryHeap.prototype.clear = function() {\n this._elements.length = 0;\n this._elements[0] = null;\n this._length = 0;\n};\n\n/**\n * The function called by the forEach method.\n * @callback MinBinaryHeap~iterateCallback\n * @function\n * @param {any} element - The current element\n * @param {number} [index] - The index of the current element\n * @param {MinBinaryHeap} [binary_heap] - The binary heap it is using\n */\n\n/**\n * Performs the fn function for all the elements of the heap, untill the callback function returns false\n * @param {iterateCallback} fn - The function the be executed on each element\n * @param {object} [this_arg] - The object to use as this when calling the fn function\n */\nMinBinaryHeap.prototype.every = function(fn, this_arg) {\n var ordered_array = this.toArray();\n var index = 0;\n\n while (ordered_array[0] !== undefined) {\n if (!fn.call(this_arg, ordered_array.shift(), index, this)) {\n break;\n }\n\n index += 1;\n }\n};\n\n/**\n * Performs the fn function for all the elements of the list\n * @param {iterateCallback} fn - The function the be executed on each element\n * @param {object} [this_arg] - The object to use as this when calling the fn function\n */\nMinBinaryHeap.prototype.forEach = function(fn, this_arg) {\n var ordered_array = this.toArray();\n var index = 0;\n\n while (ordered_array[0] !== undefined) {\n fn.call(this_arg, ordered_array.shift(), index, this);\n index += 1;\n }\n};\n\n/**\n * Transforms an array into a binary heap\n * @param {array} array - The array to transform\n */\nMinBinaryHeap.prototype.heapify = function(array) {\n this._length = array.length;\n this._elements = array;\n this._elements.unshift(null);\n for (var i = Math.floor(this._length / 2); i >= 1; i -= 1) {\n this._shiftDown(i);\n }\n};\n\n/**\n * Inserts the element to the heap\n * @param {any} element - The element to be inserted\n */\nMinBinaryHeap.prototype.insert = function(element) {\n this._elements.push(element);\n this._shiftUp();\n this._length += 1;\n};\n\n/**\n * Returns if the heap is empty\n * @returns If the heap is empty\n */\nMinBinaryHeap.prototype.isEmpty = function() {\n return !this._length;\n};\n\n/**\n * Returns the first element without removing it\n * @returns The first element without removing it\n */\nMinBinaryHeap.prototype.peek = function() {\n return this._elements[1];\n};\n\n/**\n * Inserts the element to the heap\n * This method is equivalent to insert\n * @see insert\n */\nMinBinaryHeap.prototype.push = function(element) {\n this.insert(element);\n};\n\n/**\n * Returns the first element while removing it\n * @returns {any} The first element while removing it, undefined if the heap is empty\n */\nMinBinaryHeap.prototype.pop = function() {\n var removed_element = this._elements[1];\n\n if (this._length === 1) {\n this._elements.pop();\n this._length -= 1;\n } else if (this._length > 1) {\n this._elements[1] = this._elements.pop();\n this._length -= 1;\n\n this._shiftDown();\n }\n\n return removed_element;\n};\n\n/**\n * Shifts the index to a correct position below\n * @param {number} index - The index to shift down\n */\nMinBinaryHeap.prototype._shiftDown = function(index) {\n if (index === undefined) index = 1;\n\n var parent_index = index;\n var left_child_index = parent_index * 2;\n var right_child_index = parent_index * 2 + 1;\n var smallest_child_index;\n var array_length = this._length + 1;\n while (\n parent_index < array_length &&\n (left_child_index < array_length || right_child_index < array_length)\n ) {\n if (right_child_index < array_length) {\n if (left_child_index < array_length) {\n if (\n this.comparator.lessThan(\n this._elements[left_child_index],\n this._elements[right_child_index]\n )\n ) {\n smallest_child_index = left_child_index;\n } else {\n smallest_child_index = right_child_index;\n }\n }\n } else {\n if (left_child_index < array_length) {\n smallest_child_index = left_child_index;\n }\n }\n\n if (\n this.comparator.greaterThan(\n this._elements[parent_index],\n this._elements[smallest_child_index]\n )\n ) {\n this._swap(parent_index, smallest_child_index);\n }\n\n parent_index = smallest_child_index;\n\n left_child_index = parent_index * 2;\n right_child_index = parent_index * 2 + 1;\n }\n};\n\n/**\n * Shifts the index to a correct position above\n * @param {number} index - The index to shift up\n */\nMinBinaryHeap.prototype._shiftUp = function(index) {\n if (index === undefined) index = this._elements.length - 1;\n\n var child_index = index;\n var parent_index = Math.floor(child_index / 2);\n\n while (\n child_index > 1 &&\n this.comparator.greaterThan(\n this._elements[parent_index],\n this._elements[child_index]\n )\n ) {\n this._swap(parent_index, child_index);\n child_index = parent_index;\n parent_index = Math.floor(child_index / 2);\n }\n};\n\n/**\n * Returns the size of the heap\n * @returns {number} The size of the heap\n */\nMinBinaryHeap.prototype.size = function() {\n return this._length;\n};\n\n/**\n * Swaps two elements\n * @private\n * @param {number} a - The index of the first element\n * @param {number} b - The index of the second element\n */\nMinBinaryHeap.prototype._swap = function(a, b) {\n var aux = this._elements[a];\n this._elements[a] = this._elements[b];\n this._elements[b] = aux;\n};\n\n/**\n * Converts the heap to an ordered array, without changing the heap.\n * Note that if the heap contains objects, the generated array will\n * contain references to the same objects\n * @returns {array} The converted heap in an ordered array format\n */\nMinBinaryHeap.prototype.toArray = function() {\n var array = [];\n var min_binary_heap = new MinBinaryHeap(this.comparator.compare);\n min_binary_heap._elements = this._elements.slice();\n min_binary_heap._length = this._length;\n\n while (min_binary_heap.peek() !== undefined) {\n array.push(min_binary_heap.pop());\n }\n\n return array;\n};\n\n/**\n * Max Binary Heap Class\n * @constructor\n * @extends MinBinaryHeap\n */\nfunction MaxBinaryHeap(comparator) {\n MinBinaryHeap.call(this, comparator);\n this.comparator.invert();\n}\n\nMaxBinaryHeap.prototype.toArray = function() {\n var array = [];\n var max_binary_heap = new MaxBinaryHeap();\n max_binary_heap._elements = this._elements.slice();\n max_binary_heap._length = this._length;\n max_binary_heap._comparator = this.comparator;\n\n while (max_binary_heap.peek() !== undefined) {\n array.push(max_binary_heap.pop());\n }\n\n return array;\n};\n\n/**\n * Performs an OOP like inheritance\n * @param {function} parent - The parent class\n * @param {function} child - The child class\n */\nfunction inherit(parent, child) {\n var parent_copy = Object.create(parent.prototype);\n child.prototype = parent_copy;\n child.prototype.constructor = child;\n}\n\ninherit(MinBinaryHeap, MaxBinaryHeap);\n\nmodule.exports = {\n MinBinaryHeap: MinBinaryHeap,\n MaxBinaryHeap: MaxBinaryHeap,\n Comparator: Comparator\n};"
}
{
"filename": "code/data_structures/src/tree/heap/min_heap/min_heap.py",
"content": "# Part of Cosmos by OpenGenus Foundation\ndef left(i):\n return 2 * i + 1\n\n\ndef right(i):\n return 2 * i + 2\n\n\ndef heapify(a, i):\n l = left(i)\n r = right(i)\n if l < len(a) and a[l] < a[i]:\n smallest = l\n else:\n smallest = i\n if r < len(a) and a[r] < a[smallest]:\n smallest = r\n if smallest != i:\n a[i], a[smallest] = a[smallest], a[i]\n heapify(a, smallest)\n\n\ndef build_min_heap(a):\n for i in range((len(a) - 1) // 2, -1, -1):\n heapify(a, i)\n\n\nif __name__ == \"__main__\":\n print(\"Enter the array of which you want to create a min heap\")\n a = [int(x) for x in input().split()]\n build_min_heap(a)\n print(\"The min heap is -\")\n for i in a:\n print(i, end=\" \")\n print(\"\")"
}
{
"filename": "code/data_structures/src/tree/heap/min_heap/min_heap.rb",
"content": "# Part of Cosmos by OpenGenus Foundation\n\nclass MinHeap\n attr_reader :heap\n\n def initialize\n @heap = []\n end\n\n def push(value)\n @heap.push(value)\n index = @heap.size - 1\n\n up_heap(index)\n end\n\n def pop\n min = @heap.first\n last = @heap.pop\n @heap[0] = last\n\n heapify(0)\n min\n end\n\n def empty?\n heap.empty?\n end\n\n def min\n @heap.first\n end\n\n private\n\n def up_heap(index)\n parent_index = index / 2\n\n if @heap[parent_index] > @heap[index]\n parent = @heap[parent_index]\n @heap[parent_index] = @heap[index]\n @heap[index] = parent\n\n up_heap(parent_index)\n end\n end\n\n def heapify(index)\n left = index * 2\n right = left + 1\n min = index\n\n min = left if left < @heap.size && @heap[left] < @heap[min]\n min = right if right < @heap.size && @heap[right] < @heap[min]\n\n if min != index\n min_value = @heap[min]\n @heap[min] = @heap[index]\n @heap[index] = min_value\n heapify(min)\n end\n end\nend\n\nh = MinHeap.new\nh.push(4)\nh.push(10)\nh.push(2)\np h.min\nh.push(5)\np h.pop\np h.min\nh.push(9)\nh.push(1)\np h.min"
}
{
"filename": "code/data_structures/src/tree/heap/min_heap/min_heap.swift",
"content": "\npublic func pushHeap<T>(inout xs: [T], value: T, compare: (T, T) -> Bool) {\n xs.append(value)\n siftUp(&xs, xs.startIndex, xs.endIndex, compare, xs.count)\n}\n \n\npublic func pushHeap<T: Comparable>(inout xs: [T], value: T) {\n pushHeap(&xs, value) {a, b in a < b}\n}\n \n\n\npublic func popHeap<T>(inout xs: [T], comp: (T, T) -> Bool) -> T {\n precondition(!xs.isEmpty, \"cannot pop an empty heap\")\n swap(&xs[0], &xs[xs.endIndex - 1])\n siftDown(&xs, xs.startIndex, xs.endIndex, comp, xs.count - 1, xs.startIndex)\n let result = xs.removeLast()\n return result\n}\n \n\npublic func popHeap<T: Comparable>(inout xs: [T]) -> T {\n return popHeap(&xs) {a, b in a < b}\n}\n\n\n\n// MARK: - Private\n\n\nfunc siftDown<T>(inout xs: [T],\n var first: Int,\n var last: Int,\n compare: (T, T) -> Bool,\n var len: Int,\n var start: Int)\n{\n // left-child of start is at 2 * start + 1\n // right-child of start is at 2 * start + 2\n var child = start - first\n\n if (len < 2 || (len - 2) / 2 < child) {\n return\n }\n\n child = 2 * child + 1;\n var child_i = first + child\n\n if child + 1 < len && compare(xs[child_i + 1], xs[child_i]) {\n // right-child exists and is greater than left-child\n ++child_i\n ++child\n }\n\n // check if we are in heap-order\n if compare(xs[start], xs[child_i]) {\n // we are, start is larger than it's largest child\n return\n }\n\n let top = xs[start]\n do {\n // we are not in heap-order, swap the parent with it's largest child\n xs[start] = xs[child_i]\n start = child_i;\n\n if ((len - 2) / 2 < child) {\n break\n }\n\n // recompute the child based off of the updated parent\n child = 2 * child + 1\n child_i = first + child\n\n if child + 1 < len && compare(xs[child_i + 1], xs[child_i]) {\n // right-child exists and is greater than left-child\n ++child_i\n ++child\n }\n\n // check if we are in heap-order\n } while (!compare(top, xs[child_i]))\n xs[start] = top\n}\n\n\n\n\nprivate func siftUp<T>(inout xs: [T],\n var first: Int,\n var last: Int,\n compare: (T, T) -> Bool,\n var len: Int)\n{\n if len <= 1 {\n return\n }\n len = (len - 2) / 2\n var index = first + len\n last -= 1\n if (compare(xs[last], xs[index])) {\n let t = xs[last]\n do {\n xs[last] = xs[index]\n last = index\n if len == 0 {\n break\n }\n len = (len - 1) / 2\n index = first + len\n } while (compare(t, xs[index]))\n xs[last] = t\n }\n}"
}
{
"filename": "code/data_structures/src/tree/heap/pairing_heap/pairing_heap.fs",
"content": "module PairHeap\n\ntype Heap<'a> =\n | Empty\n | Heap of 'a * (Heap<'a> list)\n\nlet findMin =\n function\n | Empty -> failwith \"empty\"\n | Heap (c, _) -> c\n\nlet merge heap1 heap2 =\n match heap1, heap2 with\n | Empty, _ -> heap2\n | _, Empty -> heap1\n | Heap (c1, sub1), Heap (c2, sub2) ->\n if c1 > c2 then\n Heap (c1, heap2::sub1)\n else\n Heap (c2, heap1::sub2)\n\nlet insert c heap =\n merge (Heap (c, [])) heap\n\nlet rec mergePairs =\n function\n | [] -> Empty\n | [sub] -> sub\n | sub1::sub2::rest -> merge (merge sub1 sub2) (mergePairs rest)\n\nlet deleteMin =\n function\n | Empty -> failwith \"empty\"\n | Heap (_, sub) -> mergePairs sub"
}
{
"filename": "code/data_structures/src/tree/heap/pairing_heap/pairing_heap.sml",
"content": "(* Pairing heap - http://en.wikipedia.org/wiki/Pairing_heap *)\n(* Part of Cosmos by OpenGenus Foundation *)\nfunctor PairingHeap(type t\n val cmp : t * t -> order) =\nstruct\n datatype 'a heap = Empty\n | Heap of 'a * 'a heap list;\n\n (* merge, O(1)\n * Merges two heaps *)\n fun merge (Empty, h) = h\n | merge (h, Empty) = h\n | merge (h1 as Heap(e1, s1), h2 as Heap(e2, s2)) =\n case cmp (e1, e2) of LESS => Heap(e1, h2 :: s1)\n | _ => Heap(e2, h1 :: s2)\n\n (* insert, O(1)\n * Inserts an element into the heap *)\n fun insert (e, h) = merge (Heap (e, []), h)\n\n (* findMin, O(1)\n * Returns the smallest element of the heap *)\n fun findMin Empty = raise Domain\n | findMin (Heap(e, _)) = e\n\n (* deleteMin, O(lg n) amortized\n * Deletes the smallest element of the heap *)\n local\n fun mergePairs [] = Empty\n | mergePairs [h] = h\n | mergePairs (h1::h2::hs) = merge (merge(h1, h2), mergePairs hs)\n in\n fun deleteMin Empty = raise Domain\n | deleteMin (Heap(_, s)) = mergePairs s\n end\n\n (* build, O(n)\n * Builds a heap from a list *)\n fun build es = foldl insert Empty es;\nend\n\nlocal\n structure IntHeap = PairingHeap(type t = int; val cmp = Int.compare);\n open IntHeap\n\n fun heapsort' Empty = []\n | heapsort' h = findMin h :: (heapsort' o deleteMin) h;\nin\n fun heapsort ls = (heapsort' o build) ls\n\n val test_0 = heapsort [] = []\n val test_1 = heapsort [1,2,3] = [1, 2, 3]\n val test_2 = heapsort [1,3,2] = [1, 2, 3]\n val test_3 = heapsort [6,2,7,5,8,1,3,4] = [1, 2, 3, 4, 5, 6, 7, 8]\nend;"
}
{
"filename": "code/data_structures/src/tree/heap/pairing_heap/README.md",
"content": "# Pairing heap\n\nA pairing heap is a heap structure, which is simple to implement in functional languages, and which provides good amortized performance.\n\n## Operations\n\n- merge - Θ(1) - Merges two heaps\n- insert - Θ(1) - Inserts an element into a heap\n- findMin - Θ(1) - Returns the minimal element from the heap\n- deleteMin - O(log n) - Deletes the minimal element from the heap\n\n## Further information\n\n- [Wikipedia](https://en.wikipedia.org/wiki/Pairing_heap)\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/data_structures/src/tree/heap/priority_queue/leftist_tree/leftist_priority_queue.cpp",
"content": "#include <iostream>\n#include <algorithm>\n\nstruct Leftist\n{\n Leftist *left, *right;\n // dis is the distance to the right-bottom side of the tree\n int dis, value, size;\n Leftist(int val = 0)\n {\n left = NULL, right = NULL;\n dis = 0, value = val, size = 1;\n }\n ~Leftist()\n {\n delete left;\n delete right;\n }\n};\n\nLeftist* merge(Leftist *x, Leftist *y)\n{\n // if x or y is NULL, return the other tree to be the answer\n if (x == NULL)\n return y;\n if (y == NULL)\n return x;\n\n if (y->value < x->value)\n std::swap(x, y);\n\n // We take x as new root, so add the size of y to x\n x->size += y->size;\n\n // merge the origin right sub-tree of x with y to construct the new right sub-tree of x\n x->right = merge(x->right, y);\n\n if (x->left == NULL && x->right != NULL)\n // if x->left is NULL pointer, swap the sub-trees to make it leftist\n std::swap(x->left, x->right);\n else if (x->right != NULL && x->left->dis < x->right->dis)\n // if the distance of left sub-tree is smaller, swap the sub-trees to make it leftist\n std::swap(x->left, x->right);\n\n // calculate the new distance\n if (x->right == NULL)\n x->dis = 0;\n else\n x->dis = x->right->dis + 1;\n return x;\n}\n\nLeftist* delete_root(Leftist *T)\n{\n //deleting root equals to make a new tree containing only left sub-tree and right sub-tree\n Leftist *my_left = T->left;\n Leftist *my_right = T->right;\n T->left = T->right = NULL;\n delete T;\n return merge(my_left, my_right);\n}\n\nint main()\n{\n\n Leftist *my_tree = new Leftist(10); // create a tree with root = 10\n // adding a node to a tree is the same as creating a new tree and merge them together\n my_tree = merge(my_tree, new Leftist(100)); // push 100\n my_tree = merge(my_tree, new Leftist(10000)); // push 10000\n my_tree = merge(my_tree, new Leftist(1)); // push 1\n my_tree = merge(my_tree, new Leftist(1266)); // push 1266\n while (my_tree != NULL)\n {\n std::cout << my_tree->value << std::endl;\n my_tree = delete_root(my_tree);\n }\n\n\n return 0;\n}\n/* the output should be\n * 1\n * 10\n * 100\n * 1266\n * 10000\n */"
}
{
"filename": "code/data_structures/src/tree/heap/priority_queue/priority_queue.js",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\nArray.prototype.swap = function(x, y) {\n let b = this[x];\n this[x] = this[y];\n this[y] = b;\n\n return this;\n};\n\n/**\n * PriorityQueue constructor\n * @param data Initial data\n * @param compareFn compare function\n * @constructor\n */\nfunction PriorityQueue(data, compareFn) {\n this.data = data || [];\n this.compareFn = compareFn || defaultCompareFn;\n\n this.push = push;\n this.pop = pop;\n this.top = top;\n this.init = init;\n this.empty = empty;\n this._up = up;\n this._down = down;\n\n this.init();\n}\n\n/**\n * Initialises the priority queue\n */\nfunction init() {\n if (this.data.length > 1) {\n let n = this.data.length;\n for (let i = Math.floor(n / 2 - 1); i >= 0; i -= 1) {\n this._down(i, n);\n }\n }\n}\n\n/**\n * Get the topmost item of the priority queue\n * @returns {*|Object}\n */\nfunction top() {\n return this.data[0];\n}\n\n/**\n * Is the queue empty?\n * @returns {boolean}\n */\nfunction empty() {\n return this.data.length === 0;\n}\n\n/**\n * Pushes the item onto the heap\n */\nfunction push(item) {\n this.data.push(item);\n this._up(this.data.length - 1);\n}\n\n/**\n * Pop removes the minimum element (according to Less) from the heap\n * and returns it.\n *\n * @returns {string|number|Object}\n */\nfunction pop() {\n if (this.data.length === 0) {\n return undefined;\n }\n\n let n = this.data.length - 1;\n this.data.swap(0, n);\n this._down(0, n);\n\n return this.data.pop();\n}\n\n/**\n * Default comparator function is for max heap\n * @param p First item to compare\n * @param q Second item to compare\n *\n * @returns {number} -1 if p < q, 1 if p > q or 0 if p == q\n */\nfunction defaultCompareFn(p, q) {\n const diff = p - q;\n\n return diff < 0 ? -1 : diff > 0 ? 1 : 0;\n}\n\n/**\n * Percolate up from index\n * @param index\n */\nfunction up(index) {\n while (index > 0) {\n let parentIndex = parent(index);\n let parentItem = this.data[parentIndex];\n let currentItem = this.data[index];\n\n if (parentIndex === index || !this.compareFn(currentItem, parentItem) < 0) {\n break;\n }\n this.data.swap(parentIndex, index);\n index = parentIndex;\n }\n}\n\n/**\n * Percolate down from index until n\n * @param index\n * @param n\n *\n * * @returns {boolean} True if a change happened. False otherwise.\n */\nfunction down(index, n) {\n let i = index;\n while (true) {\n let currentItem = this.data[i];\n let j1 = leftChild(i);\n if (j1 + 1 >= n || j1 < 0) {\n // overflows\n break;\n }\n let j = j1; // left child\n let j2 = j1 + 1; // right child\n if (j2 < n && this.compareFn(this.data[j2], this.data[j1]) < 0) {\n j = j2;\n }\n if (this.compareFn(this.data[j], currentItem) >= 0) {\n break;\n }\n this.data.swap(i, j);\n i = j;\n }\n\n return i > index;\n}\n\n/**\n * Return parent index\n * @param index current inter\n * @returns {number} parent index\n */\nfunction parent(index) {\n return Math.floor((index - 1) / 2);\n}\n\n/**\n * Return left child index\n * @param index current inter\n * @returns {number} left child index\n */\nfunction leftChild(index) {\n return index * 2 + 1;\n}\n\nfunction main() {\n const pq = new PriorityQueue([45, 6, 4, 3, 2, 72, 34, 12, 456, 29, 312]);\n pq.push(1);\n console.log(pq);\n}\n\nmain();"
}
{
"filename": "code/data_structures/src/tree/heap/priority_queue/priority_queue.py",
"content": "# Part of Cosmos by OpenGenus Foundation\nimport math\n\n\ndef default_compare_fn(p, q):\n diff = p - q\n if diff < 0:\n return -1\n elif diff > 0:\n return 1\n else:\n return 0\n\n\nclass PriorityQueue(object):\n def __init__(self, data=[], compare_fn=default_compare_fn):\n self.data = data\n self.compareFn = compare_fn\n\n self.init()\n\n def init(self):\n n = len(self.data)\n if n > 0:\n start = math.floor((n / 2) - 1)\n for i in range(int(start), 0, -1):\n self._down(i, n)\n\n def push(self, item):\n self.data.append(item)\n self._up(len(self.data) - 1)\n\n def pop(self):\n n = len(self.data)\n if n == 0:\n return None\n\n self.data[0], self.data[n - 1] = self.data[n - 1], self.data[0]\n self._down(0, n - 1)\n\n return self.data.pop()\n\n def _up(self, index):\n while index > 0:\n parent_index = int(math.floor((index - 1) / 2))\n parent_item = self.data[parent_index]\n current_item = self.data[index]\n\n if (\n parent_index == index\n or not self.compareFn(current_item, parent_item) < 0\n ):\n break\n self.data[index], self.data[parent_index] = (\n self.data[parent_index],\n self.data[index],\n )\n index = parent_index\n\n def _down(self, index, n):\n i = index\n while True:\n current_item = self.data[i]\n j1 = 2 * i + 1\n if j1 > n or j1 < 0:\n break\n j = j1\n j2 = j1 + 1\n if j2 < n and self.compareFn(self.data[j2], self.data[j1]) < 0:\n j = j2\n if self.compareFn(self.data[j], current_item) >= 0:\n break\n\n self.data[j], self.data[i] = self.data[i], self.data[j]\n i = j\n\n return i > index\n\n def top(self):\n return self.data[0]\n\n def empty(self):\n return len(self.data) == 0\n\n def __str__(self):\n return \"PriorityQueue: {}\".format(self.data)\n # return f'PriorityQueue: {self.data}'\n\n\nif __name__ == \"__main__\":\n pq = PriorityQueue([45, 6, 4, 3, 2, 72, 34, 12, 456, 29, 312])\n pq.push(1)\n pq.pop()\n print(pq)"
}
{
"filename": "code/data_structures/src/tree/heap/priority_queue/README.md",
"content": "# Priority Queue \nDescription\n---\nA priority queue is an abstract data type which is like a regular queue \nor stack data structure, but where additionally each element has a \"priority\" or a score\nassociated with it. \n\nIn a priority queue, an element with high priority \nis served before an element with low priority. \nIf two elements have the same priority, \nthey are served according to their order in the queue.\n\nThis folder contains some Priority Queue implementations using a heap\n\nPriority Queue API\n---\n- **pop()**: Removes the top element from the queue\n- **push()**: Inserts an item to the queue while maintaining the priority order\n- **top()**: Returns the top element of the queue (the item with the top priority)\n- **empty()**: Checks if the queue is empty\n\nTime Complexity\n---\npop() and push() take O(log n) and the others take O(1)."
}
{
"filename": "code/data_structures/src/tree/heap/README.md",
"content": "# Heap \nDescription\n---\nHeap is a special case of balanced binary tree data structure where the root-node key is \ncompared with its children and arranged accordingly. \n\nIf a has child node b then -\n\nkey(a) <= key(b)\n\nIf the value of parent is lesser than that of child, this property generates **Min Heap** \notherwise it generates a **Max Heap**\n\nHeap API\n---\n- **extractTop()**: Removes top element from the heap\n- **insert()**: Inserts an item to the heap\n- **top()**: Returns the top element of the heap (the minimum or maximum value)\n- **empty()**: Checks if the heap is empty\n\nTime Complexity\n---\nextractTop() and insert() take O(log n) and the others take O(1).\n\nExample folders\n---\n**max_heap**: Various implementations of a Max Heap.\n**min_heap**: Various implementations of a Min Heap\n**priority_queue**: Priority Queue implementations using a heap"
}
{
"filename": "code/data_structures/src/tree/heap/soft_heap/soft_heap.cpp",
"content": "/**\n * Soft Heap\n * Author: JonNRb\n *\n * Original paper: https://www.cs.princeton.edu/~chazelle/pubs/sheap.pdf\n * Binary heap version by Kaplan and Zwick\n */\n\n#include <functional>\n#include <iterator>\n#include <limits>\n#include <list>\n#include <memory>\n#include <stdexcept>\n\nusing namespace std;\n\n#if __cplusplus <= 201103L\nnamespace {\ntemplate <typename T, typename ... Args>\nunique_ptr<T> make_unique(Args&& ... args)\n{\n return unique_ptr<T>(new T(forward<Args>(args) ...));\n}\n} // namespace\n#endif\n\n// `T` is a comparable type and higher values of `corruption` increase the\n// orderedness of the soft heap\ntemplate <typename T>\nclass SoftHeap {\nprivate:\n// `node`s make up elements in the soft queue, which is a binary heap with\n// \"corrupted\" entries\n struct node\n {\n typename list<T>::iterator super_key;\n unsigned rank;\n\n // `target_size` is a target for the number of corrupted nodes. the number\n // increases going up the heap and should be between 1 and 2 times the\n // `target_size` of its children -- unless it is `corruption` steps from the\n // bottom, in which case it is 1.\n unsigned target_size;\n\n // `link[0]` is the left node and `link[1]` is the right node\n unique_ptr<node> link[2];\n\n // if `l` contains more than 1 entry, the node is \"corrupted\"\n list<T> l;\n };\n\n struct head\n {\n // the root of a \"soft queue\": a binary heap of nodes\n unique_ptr<node> q;\n\n // iterator to the tree following this one with the minimum `super_key` at\n // the root. may be the current `head`.\n typename list<head>::iterator suffix_min;\n };\n\n// doubly-linked list of all binary heaps (soft queues) in the soft heap\n list<head> l;\n unsigned corruption;\n\npublic:\n SoftHeap(unsigned corruption) : corruption(corruption)\n {\n }\n\n bool empty()\n {\n return l.empty();\n }\n\n void push(T item)\n {\n auto item_node = make_unique<node>();\n item_node->rank = 0;\n item_node->target_size = 1;\n item_node->link[0] = nullptr;\n item_node->link[1] = nullptr;\n item_node->l.push_front(move(item));\n item_node->super_key = item_node->l.begin();\n\n l.emplace_front();\n auto h = l.begin();\n h->q = move(item_node);\n h->suffix_min = next(h) != l.end() ? next(h)->suffix_min : h;\n\n repeated_combine(1);\n }\n\n T pop()\n {\n if (l.empty())\n throw invalid_argument(\"empty soft heap\");\n\n auto it = l.begin()->suffix_min;\n node* n = it->q.get();\n\n T ret = move(*n->l.begin());\n n->l.pop_front();\n\n if (n->l.size() < n->target_size)\n {\n if (n->link[0] || n->link[1])\n {\n sift(n);\n update_suffix_min(it);\n }\n else if (n->l.empty())\n {\n if (it == l.begin())\n l.erase(it);\n else\n {\n auto p = prev(it);\n l.erase(it);\n update_suffix_min(p);\n }\n }\n }\n\n return ret;\n }\n\nprivate:\n void update_suffix_min(typename list<head>::iterator it) const\n {\n if (it == l.end())\n return;\n while (true)\n {\n if (next(it) == l.end())\n it->suffix_min = it;\n else if (*it->q->super_key <= *next(it)->suffix_min->q->super_key)\n it->suffix_min = it;\n else\n it->suffix_min = next(it)->suffix_min;\n if (it == l.begin())\n return;\n --it;\n }\n }\n\n unique_ptr<node> combine(unique_ptr<node> a, unique_ptr<node> b) const\n {\n auto n = make_unique<node>();\n n->rank = a->rank + 1;\n n->target_size = n->rank <= corruption ? 1 : (3 * a->target_size + 1) / 2;\n n->link[0] = move(a);\n n->link[1] = move(b);\n sift(n.get());\n return n;\n }\n\n void repeated_combine(unsigned max_rank)\n {\n auto it = l.begin();\n for (auto next_it = next(l.begin()); next_it != l.end();\n it = next_it, ++next_it)\n {\n if (it->q->rank == next_it->q->rank)\n {\n if (next(next_it) == l.end() || next(next_it)->q->rank != it->q->rank)\n {\n it->q = combine(move(it->q), move(next_it->q));\n next_it = l.erase(next_it);\n if (next_it == l.end())\n break;\n }\n }\n else if (it->q->rank > max_rank)\n break;\n }\n\n update_suffix_min(it);\n }\n\n static void sift(node* n)\n {\n while (n->l.size() < n->target_size)\n {\n if (n->link[1])\n {\n if (!n->link[0] || *n->link[0]->super_key > *n->link[1]->super_key)\n swap(n->link[0], n->link[1]);\n }\n else if (!n->link[0])\n return;\n\n n->super_key = n->link[0]->super_key;\n n->l.splice(n->l.end(), n->link[0]->l);\n\n if (!n->link[0]->link[0] && !n->link[0]->link[1])\n n->link[0] = nullptr;\n else\n sift(n->link[0].get());\n }\n }\n};\n\n#include <chrono>\n#include <iostream>\n#include <random>\n#include <vector>\n\nint run_test_with_corruption(unsigned corruption, unsigned k_num_elems)\n{\n using namespace std::chrono;\n\n cout << \"making soft heap with corruption \" << corruption << \" and \"\n << k_num_elems << \" elements...\" << endl;\n SoftHeap<unsigned> sh(corruption);\n\n // create a random ordering of the numbers [0, k_num_elems)\n vector<bool> popped_nums(k_num_elems);\n vector<unsigned> nums(k_num_elems);\n random_device rd;\n mt19937 gen(rd());\n\n for (unsigned i = 0; i < k_num_elems; ++i)\n nums[i] = i;\n for (unsigned i = 0; i < k_num_elems; ++i)\n {\n uniform_int_distribution<unsigned> dis(i, k_num_elems - 1);\n swap(nums[i], nums[dis(gen)]);\n }\n\n auto start = steady_clock::now();\n\n for (unsigned i = 0; i < k_num_elems; ++i)\n sh.push(nums[i]);\n\n // this notion of `num_errs` is not rigorous in any way\n unsigned num_errs = 0;\n unsigned last = 0;\n while (!sh.empty())\n {\n unsigned i = sh.pop();\n if (popped_nums[i])\n {\n cerr << \" popped number \" << i << \" already\" << endl;\n return -1;\n }\n popped_nums[i] = true;\n if (i < last)\n ++num_errs;\n last = i;\n // cout << i << endl;\n }\n\n auto end = steady_clock::now();\n\n cout << \" error rate: \" << ((double)num_errs / k_num_elems) << endl;\n cout << \" took \" << duration_cast<milliseconds>(end - start).count() << \" ms\"\n << endl;\n\n return 0;\n}\n\nint main()\n{\n for (unsigned i = 0; i < 20; ++i)\n if (run_test_with_corruption(i, 1 << 18))\n return -1;\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/fenwick_tree/fenwick_tree.c",
"content": "#include<stdio.h>\n// Part of Cosmos by OpenGenus Foundation\nint getSum(int bit_tree[], int index)\n{\n int sum = 0; // Iniialize result\n\n // index in bit_tree[] is 1 more than the index in arr[]\n index = index + 1;\n\n // Traverse ancestors of bit_tree[index]\n while (index>0)\n {\n // Add current element of bit_tree to sum\n sum += bit_tree[index];\n\n // Move index to parent node in getSum View\n index -= index & (-index);\n }\n return sum;\n}\n\nvoid update(int bit_tree[], int n, int index, int val){\n // index in bit_tree[] is 1 more than the index in arr[]\n index = index + 1;\n\n // Traverse all ancestors and add 'val'\n while(index <=n){\n // Add 'val' to current node of BI Tree\n bit_tree[index] += val;\n\n // Update index to that of parent in update View\n index += index & (-index);\n }\n return;\n}\n\nvoid constructBITtree(int arr[], int bit_tree[], int n){\n //initialise bit_tree to 0\n for(int i=1;i<=n;i++)\n bit_tree[i] = 0;\n\n //store value in bit_tree usin update\n for(int i=0;i<n;i++)\n update(bit_tree, n, i, arr[i]);\n\n return;\n}\n\nint main(){\n int freq[] = {2, 1, 1, 3, 2, 3, 4, 5, 6, 7, 8, 9};\n int n =sizeof(freq)/sizeof(freq[0]);\n int bit_tree[n+1];\n\n constructBITtree(freq, bit_tree, n);\n\n printf(\"Sum of elements in arr[0..5] is %d\\n\",getSum(bit_tree, 5));\n\n // Let use test the update operation\n freq[3] += 6;\n update(bit_tree, n, 3, 6); //Update BIT for above change in arr[]\n\n printf(\"Sum of elements in arr[0..5] after the update is %d\\n\",getSum(bit_tree, 5));\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/fenwick_tree/fenwick_tree.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <iostream>\n#include <vector>\nusing namespace std;\n\n// Fenwick Tree (also known as Binary Indexed Tree or BIT)\nclass BIT\n{\npublic:\n int n;\n vector<int> arr;\n\n// constructor: initializes variables\n BIT(int n)\n {\n this->n = n;\n arr.resize(n + 1, 0);\n }\n\n// returns least significant bit\n int lsb(int idx)\n {\n return idx & -idx;\n }\n\n// updates the element at index idx by val\n void update(int idx, int val) // log(n) complexity\n {\n while (idx <= n)\n {\n arr[idx] += val;\n idx += lsb(idx);\n }\n }\n\n// returns prefix sum from 1 to idx\n int prefix(int idx) // log(n) complexity\n {\n int sum = 0;\n while (idx > 0)\n {\n sum += arr[idx];\n idx -= lsb(idx);\n }\n return sum;\n }\n\n// returns sum of elements between indices l and r\n int range_query(int l, int r) // log(n) complexity\n {\n return prefix(r) - prefix(l - 1);\n }\n\n};\n\nint main()\n{\n vector<int> array = {1, 2, 3, 4, 5};\n int n = array.size();\n BIT tree(n);\n\n for (int i = 0; i < n; i++)\n tree.update(i + 1, array[i]);\n\n cout << \"Range Sum from 2 to 4: \" << tree.range_query(2, 4) << endl;\n cout << \"Range Sum from 1 to 5: \" << tree.range_query(1, 5) << endl;\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/fenwick_tree/fenwick_tree.go",
"content": "package main\n\nimport \"fmt\"\n\ntype FenwickTree struct {\n\tN int\n\tarr []int\n}\n\nfunc (bit *FenwickTree) Initialize(n int) {\n\tfmt.Println(n)\n\tbit.N = n\n\tbit.arr = make([]int, n+1)\n}\n\nfunc (bit *FenwickTree) lsb(idx int) int {\n\treturn idx & -idx\n}\n\nfunc (bit *FenwickTree) Update(idx int, val int) {\n\tfor ; idx <= bit.N; idx += bit.lsb(idx) {\n\t\tbit.arr[idx] += val\n\t}\n}\n\nfunc (bit *FenwickTree) PrefixSum(idx int) int {\n\tsum := 0\n\n\tfor ; idx > 0; idx -= bit.lsb(idx) {\n\t\tsum += bit.arr[idx]\n\t}\n\n\treturn sum\n}\n\nfunc (bit *FenwickTree) RangeSum(l int, r int) int {\n\treturn bit.PrefixSum(r) - bit.PrefixSum(l-1)\n}\n\nfunc main() {\n\tarr := []int{1, 2, 3, 4, 5}\n\tn := len(arr)\n\n\tvar fenwick FenwickTree\n\tfenwick.Initialize(n)\n\n\tfor i := 0; i < n; i++ {\n\t\tfenwick.Update(i+1, arr[i])\n\t}\n\n\tfmt.Printf(\"Range sum from 2 to 4: %v\\n\", fenwick.RangeSum(2, 4))\n\tfmt.Printf(\"Range sum from 1 to 5: %v\\n\", fenwick.RangeSum(1, 5))\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/fenwick_tree/fenwick_tree_inversion_count.cpp",
"content": "#include <iostream>\n#include <cstring>\nusing namespace std;\n\nint BIT[101000], A[101000], n;\n\nint query(int i)\n{\n int ans = 0;\n for (; i > 0; i -= i & (-i))\n ans += BIT[i];\n return ans;\n}\nvoid update(int i)\n{\n for (; i <= n; i += i & (-i))\n BIT[i]++;\n}\nint main()\n{\n int ans, i;\n while (cin >> n and n)\n {\n memset(BIT, 0, (n + 1) * (sizeof(int)));\n for (int i = 0; i < n; i++)\n cin >> A[i];\n ans = 0;\n for (i = n - 1; i >= 0; i--)\n {\n ans += query(A[i] - 1);\n update(A[i]);\n }\n cout << ans << endl;\n }\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/fenwick_tree/fenwick_tree.java",
"content": "import java.util.*;\nimport java.lang.*;\nimport java.io.*;\n\nclass BinaryIndexedTree\n{\n final static int MAX = 1000; // Max tree size\n\n static int BITree[] = new int[MAX];\n\n /* n --> No. of elements present in input array. \n BITree[0..n] --> Array that represents Binary Indexed Tree.\n arr[0..n-1] --> Input array for whic prefix sum is evaluated. */\n\n // Returns sum of arr[0..index]. This function assumes\n // that the array is preprocessed and partial sums of\n // array elements are stored in BITree[].\n int getSum(int index)\n {\n int sum = 0; // Iniialize result\n \n // index in BITree[] is 1 more than the index in arr[]\n index = index + 1;\n \n // Traverse ancestors of BITree[index]\n while(index>0)\n {\n // Add current element of BITree to sum\n sum += BITree[index];\n \n // Move index to parent node in getSum View\n index -= index & (-index);\n }\n return sum;\n }\n\n // Updates a node in Binary Index Tree (BITree) at given index\n // in BITree. The given value 'val' is added to BITree[i] and \n // all of its ancestors in tree.\n public static void updateBIT(int n, int index, int val)\n {\n // index in BITree[] is 1 more than the index in arr[]\n index = index + 1;\n \n // Traverse all ancestors and add 'val'\n while(index <= n)\n {\n // Add 'val' to current node of BIT Tree\n BITree[index] += val;\n \n // Update index to that of parent in update View\n index += index & (-index);\n }\n }\n\n /* Function to construct fenwick tree from given array.*/\n void constructBITree(int arr[], int n)\n {\n // Initialize BITree[] as 0\n for(int i=1; i<=n; i++)\n BITree[i] = 0;\n \n // Store the actual values in BITree[] using update()\n for(int i=0; i<n; i++)\n updateBIT(n, i, arr[i]);\n }\n\n // The Main function\n public static void main(String args[])\n {\n int freq[] = {2, 1, 1, 3, 2, 3, 4, 5, 6, 7, 8, 9};\n int n = freq.length;\n BinaryIndexedTree tree = new BinaryIndexedTree();\n \n // Build fenwick tree from given array\n tree.constructBITree(freq, n);\n \n System.out.println(\"Sum of elements in arr[0..5] is = \" + tree.getSum(5));\n \n // Let use test the update operation\n freq[3] += 6;\n updateBIT(n, 3, 6); //Update BIT for above change in arr[]\n \n // Find sum after the value is updated\n System.out.println(\"Sum of elements in arr[0..5] after update is = \" + tree.getSum(5));\n }\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/fenwick_tree/fenwick_tree.pl",
"content": "#!/usr/bin/env perl\n\nuse utf8;\nuse 5.024;\nuse warnings;\nuse feature qw(signatures);\nno warnings qw(experimental::signatures);\n\n{\n package FenwickTree;\n\n sub new($class, %args) {\n return bless {\n arr => [ (0) x ($args{max}+1) ], # arr[k] = x[k-phi(k)+1] + ... + x[k]\n n => $args{max} + 1,\n }, 'FenwickTree';\n }\n\n sub phi($n) { return $n & -$n; }\n sub get($self, $k) { # returns x[1] + x[2] + x[3] + ... + x[k]\n return $k <= 0 ? 0 : $self->{arr}->[$k] + $self->get( $k - phi($k) );\n }\n sub add($self, $k, $c) { # x[k] += c\n return if $k > $self->{n};\n $self->{arr}->[$k] += $c;\n $self->add( $k+phi($k), $c );\n }\n}\n\nuse Test::More tests => 2;\n\nmy $tree = FenwickTree->new( max => 12 );\nmy @values = (2, 1, 1, 3, 2, 3, 4, 5, 6, 7, 8, 9);\n$tree->add($_, $values[$_-1]) for (1..12);\n\nis( $tree->get(5), 2+1+1+3+2, 'sum of first 5 elements' );\n\n$tree->add(4, 4);\n\nis( $tree->get(5), 2+1+1+(3+4)+2, 'sum after adding' );"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/fenwick_tree/fenwick_tree.py",
"content": "# Binary indexed tree or fenwick tree\n# Space Complexity: O(N) for declaring another array of N=size num_of_elements\n# Time Complexity: O(logN) for each operation(update and query as well)\n# original array for storing values for later lookup\n# Part of Cosmos by OpenGenus Foundation\narray = []\n# array to store cumulative sum\nbit = []\n\"\"\"\nindex i in the bit[] array stores the cumulative sum from the index i to i - (1<<r) + 1 (both inclusive),\n where r represents the last set bit in the index i\n\"\"\"\n\n\nclass FenwickTree:\n # To intialize list of num_of_elements+1 size\n def initialize(self, num_of_elements):\n for i in range(num_of_elements + 1):\n array.append(0)\n bit.append(0)\n\n def update(self, x, delta):\n while x <= num_of_elements:\n bit[x] = bit[x] + delta\n # x&(-x) gives the last set bit in a number x\n x = x + (x & -x)\n\n def query(self, x):\n range_sum = 0\n while x > 0:\n range_sum = range_sum + bit[x]\n # x&(-x) gives the last set bit in a number x\n x = x - (x & -x)\n return range_sum\n\n\nfenwick_tree = FenwickTree()\nnum_of_elements = int(input(\"Enter the size of list: \"))\n\nfenwick_tree.initialize(num_of_elements)\n\nfor i in range(num_of_elements):\n # storing data in orginal list\n element = int(input(\"Enter the list element: \"))\n # updating the BIT array\n fenwick_tree.update(i + 1, element)\n\nnumber_of_queries = int(input(\"Enter number of queries: \"))\n\nfor i in range(number_of_queries):\n left_index = int(input(\"Enter left index (1 indexing): \"))\n right_index = int(input(\"Enter right index (1 indexing): \"))\n if right_index < left_index:\n print(\"Invalid range \")\n continue\n print(\"Sum in range[%d,%d]: \" % (left_index, right_index))\n print(fenwick_tree.query(right_index) - fenwick_tree.query(left_index - 1))"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/fenwick_tree/README.md",
"content": "# Fenwick Tree\n\n## Explaination\nFenwick Tree / Binary indexed tree is a data structure used to process interval/range based queries. Compared to segment tree data structure, Fenwick tree uses less space and is simpler to implement.\n\nOne disadvantage of Fenwick tree is that it can be only used with an operation that is invertible. For example, addition is invertible so range sum queries can be processed, but max() is a non-invertible operation so range max queries can't be processed.\n\n## Algorithm\nLeastSignificantBit operation is critical for working of Fenwick tree and is hidden within implementation details:\n```\nfunction LSB(i):\n return i & (-i)\n```\nA Fenwick tree preforms two functions:\n* query(b) -- Queries for the range `[1, b]`. query(b) - query(a - 1) queries for range `[a, b]`.\n```\nfunction query(b):\n while b > 0:\n # process appropriately\n ...\n\n b = b - LSB(b)\n return ...\n```\n* update(pos, value) -- Updates index `pos` with `value`.\n```\nfunction update(pos, value):\n while pos <= n:\n # Update array[pos] and FenwickTree[[pos] appropriately.\n ...\n \n pos = pos + LSB(pos)\n```\n\n## Complexity\n__Space complexity__ : O(n)\n\n__Time complexity__ :\n* __Update__ : O(log<sub>2</sub>(n))\n* __Query__ : O(log<sub>2</sub>(n))\n\nWhere n is the length of array.\n\n---\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/red_black_tree/red_black_tree.c",
"content": "/**\n * author: JonNRb <[email protected]>\n * license: GPLv3\n * file: @cosmos//code/data_structures/red_black_tree/red_black.c\n * info: red black tree\n */\n\n/**\n * guided by the wisdom of\n * http://eternallyconfuzzled.com/tuts/datastructures/jsw_tut_rbtree.aspx\n */\n\n#include \"red_black_tree.h\"\n\n#include <errno.h>\n#include <stdlib.h>\n\nstatic inline int is_red(cosmos_red_black_node_t* node) {\n return node != NULL && node->red;\n}\n\nstatic int correct_rb_height(cosmos_red_black_tree_t tree) {\n if (tree == NULL) return 1; // NULL is a black node (black-height == 1)\n\n const int redness = is_red(tree);\n\n // red cannot neighbor red\n if (redness && (is_red(tree->link[0]) || is_red(tree->link[1]))) return -1;\n\n int black_height;\n\n // left and right subtrees must be correct and have same black height\n if ((black_height = correct_rb_height(tree->link[0])) < 0) return -1;\n if (black_height != correct_rb_height(tree->link[1])) return -1;\n\n return black_height + !redness;\n}\n\n/**\n * rrot = 1 (rrot = 0 is flipped)\n *\n * C B\n * / / \\\n * B => A C\n * /\n * A\n */\nstatic void rotate(cosmos_red_black_tree_t* tree, int rrot) {\n cosmos_red_black_node_t* save = (*tree)->link[!rrot];\n\n (*tree)->link[!rrot] = save->link[rrot];\n save->link[rrot] = (*tree);\n\n (*tree)->red = 1;\n save->red = 0;\n\n *tree = save;\n}\n\n/**\n * rrot = 1 (rrot = 0 is flipped)\n *\n * E E C\n * / / / \\\n * A C A E\n * \\ => / \\ => \\ /\n * C A D B D\n * / \\ \\\n * B D B\n */\nstatic void rotate_double(cosmos_red_black_tree_t* tree, int rrot) {\n if (tree == NULL) return;\n rotate(&(*tree)->link[!rrot], !rrot);\n rotate(tree, rrot);\n}\n\n/**\n * |tree| must be a black node with red children\n *\n * |path| encodes the index on the bottom layer for |tree| in the 2 level\n * subtree with root |grandparent|\n *\n * the case where |tree| has only one parent implies its parent is the root\n * which must be a black node (0, 1, 2, or 3)\n */\nstatic inline void color_flip(cosmos_red_black_tree_t* tree,\n cosmos_red_black_tree_t* parent,\n cosmos_red_black_tree_t* grandparent,\n uint8_t path) {\n if (is_red((*tree)->link[0]) && is_red((*tree)->link[1])) {\n (*tree)->red = 1;\n (*tree)->link[0]->red = 0;\n (*tree)->link[1]->red = 0;\n }\n\n if (grandparent != NULL && is_red(*parent) && is_red(*tree)) {\n if (!(path % 3)) {\n rotate(grandparent, !(path % 2));\n } else {\n rotate_double(grandparent, path % 2);\n }\n }\n}\n\nstatic void insert_impl(cosmos_red_black_tree_t* tree,\n cosmos_red_black_node_t* node) {\n cosmos_red_black_tree_t* it = tree;\n cosmos_red_black_tree_t* parent = NULL;\n cosmos_red_black_tree_t* grandparent = NULL;\n uint8_t path = 0;\n\n for (;;) {\n if (*it == NULL) {\n *it = node;\n path |= 4;\n }\n\n color_flip(it, parent, grandparent, path & 3);\n\n if (path & 4) break;\n\n int insert_right = *node->sort_key > *(*it)->sort_key;\n grandparent = parent;\n parent = it;\n it = &(*it)->link[insert_right];\n path = (path % 2) * 2 + insert_right;\n }\n}\n\nstatic cosmos_red_black_node_t* pop_impl(cosmos_red_black_tree_t* tree,\n int from_right) {\n if (*tree == NULL) return NULL;\n\n if ((*tree)->link[from_right] == NULL) {\n cosmos_red_black_node_t* ret = *tree;\n *tree = (*tree)->link[!from_right];\n return ret;\n }\n\n cosmos_red_black_tree_t* it = tree;\n cosmos_red_black_tree_t* parent = NULL;\n cosmos_red_black_node_t* sibling = NULL;\n\n for (;;) {\n // TODO(jonnrb): pop out special cases to make them easier to understand\n if (!is_red(*it) && !is_red((*it)->link[from_right])) {\n if (is_red((*it)->link[!from_right])) {\n rotate(it, from_right);\n } else if (sibling != NULL) {\n int recolor = 0;\n if (is_red(sibling->link[from_right])) {\n rotate_double(parent, from_right);\n recolor = 1;\n } else if (is_red(sibling->link[!from_right])) {\n rotate(parent, from_right);\n recolor = 1;\n } else {\n (*parent)->red = 0;\n sibling->red = 1;\n (*it)->red = 1;\n }\n\n if (recolor) {\n (*it)->red = 1;\n (*parent)->red = 1;\n (*parent)->link[0]->red = 0;\n (*parent)->link[1]->red = 0;\n }\n }\n }\n\n if ((*it)->link[from_right] == NULL) {\n cosmos_red_black_node_t* ret = *it;\n *it = (*it)->link[!from_right];\n\n (*tree)->red = 0;\n\n ret->link[0] = NULL;\n ret->link[1] = NULL;\n return ret;\n }\n\n parent = it;\n it = &(*it)->link[from_right];\n sibling = (*parent)->link[!from_right];\n }\n}\n\nvoid cosmos_red_black_construct(cosmos_red_black_node_t* node) {\n node->red = 1;\n node->link[0] = NULL;\n node->link[1] = NULL;\n}\n\nint is_rb_correct(cosmos_red_black_tree_t tree) {\n return correct_rb_height(tree) >= 0;\n}\n\nvoid cosmos_red_black_push(cosmos_red_black_tree_t* tree,\n cosmos_red_black_node_t* node) {\n if (tree == NULL || node == NULL) {\n errno = EINVAL;\n return;\n }\n\n node->link[0] = NULL;\n node->link[1] = NULL;\n\n if (*tree == NULL) {\n *tree = node;\n return;\n }\n\n insert_impl(tree, node);\n\n (*tree)->red = 0;\n}\n\ncosmos_red_black_node_t* cosmos_red_black_pop_min(\n cosmos_red_black_tree_t* tree) {\n if (tree == NULL) {\n errno = EINVAL;\n return NULL;\n }\n\n return pop_impl(tree, 0);\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/red_black_tree/red_black_tree.cpp",
"content": "#include <iostream>\n#include <memory>\n#include <cassert>\n#include <string>\n#include <stack>\nusing namespace std;\n\ntemplate<typename _Type, class _Derivative>\nclass BaseNode\n{\nprotected:\n using ValueType = _Type;\n using SPNodeType = std::shared_ptr<_Derivative>;\n\npublic:\n BaseNode(ValueType v, SPNodeType l = nullptr, SPNodeType r = nullptr)\n : value_(v), left_(l), right_(r)\n {\n }\n\n ValueType value()\n {\n return value_;\n }\n\n void value(ValueType v)\n {\n value_ = v;\n }\n\n SPNodeType &left()\n {\n return left_;\n }\n\n void left(SPNodeType l)\n {\n left_ = l;\n }\n\n SPNodeType &right()\n {\n return right_;\n }\n\n void right(SPNodeType r)\n {\n right_ = r;\n }\n\nprotected:\n ValueType value_;\n SPNodeType left_, right_;\n};\n\ntemplate<typename _Type>\nclass RBNode : public BaseNode<_Type, RBNode<_Type>>\n{\nprivate:\n using ValueType = _Type;\n using SPNodeType = std::shared_ptr<RBNode>;\n using WPNodeType = std::weak_ptr<RBNode>;\n\npublic:\n enum class Color\n {\n RED,\n BLACK\n };\n\n RBNode(ValueType v, SPNodeType l = nullptr, SPNodeType r = nullptr, SPNodeType p = nullptr)\n : BaseNode<_Type, RBNode<_Type>>(v, l, r), parent_(p), color_(Color::RED)\n {\n }\n\n SPNodeType parent()\n {\n return parent_.lock();\n }\n\n void parent(SPNodeType p)\n {\n parent_ = p;\n }\n\n Color color()\n {\n return color_;\n }\n\n void color(Color c)\n {\n color_ = c;\n }\n\nprivate:\n WPNodeType parent_;\n Color color_;\n};\n\nstruct RBTreeTest;\ntemplate<typename _Type, typename _Compare = std::less<_Type>>\nclass RBTree\n{\nprivate:\n typedef RBNode<_Type> NodeType;\n typedef std::shared_ptr<NodeType> SPNodeType;\n typedef typename RBNode<_Type>::Color Color;\n\npublic:\n RBTree() : root_(nullptr), sentinel_(std::make_shared<NodeType>(0)), compare_(_Compare())\n {\n sentinel_->left(sentinel_);\n sentinel_->right(sentinel_);\n sentinel_->parent(sentinel_);\n sentinel_->color(Color::BLACK);\n root_ = sentinel_;\n }\n\n void insert(_Type const &n);\n\n void erase(_Type const &n);\n\n SPNodeType const find(_Type const &);\n\n std::string preOrder() const;\n\n std::string inOrder() const;\n\nprivate:\n SPNodeType root_;\n SPNodeType sentinel_;\n _Compare compare_;\n\n SPNodeType &insert(SPNodeType &root, SPNodeType &pt);\n\n SPNodeType _find(_Type const &value);\n\n void rotateLeft(SPNodeType const &);\n\n void rotateRight(SPNodeType const &);\n\n void fixViolation(SPNodeType &);\n\n SPNodeType successor(SPNodeType const &);\n\n SPNodeType &sibling(SPNodeType const &);\n\n bool isLeftChild(SPNodeType const &);\n\n bool isRightChild(SPNodeType const &);\n\n void deleteOneNode(SPNodeType &);\n\n void deleteCase1(SPNodeType const &);\n\n void deleteCase2(SPNodeType const &);\n\n void deleteCase3(SPNodeType const &);\n\n void deleteCase4(SPNodeType const &);\n\n void deleteCase5(SPNodeType const &);\n\n void deleteCase6(SPNodeType const &);\n\n// for test\n friend RBTreeTest;\n};\n\ntemplate<typename _Type, typename _Compare>\nvoid\nRBTree<_Type, _Compare>::insert(_Type const &value)\n{\n SPNodeType pt = std::make_shared<NodeType>(value, sentinel_, sentinel_);\n\n root_ = insert(root_, pt);\n\n fixViolation(pt);\n}\n\ntemplate<typename _Type, typename _Compare>\nvoid\nRBTree<_Type, _Compare>::erase(_Type const &value)\n{\n SPNodeType delete_node = _find(value);\n\n if (delete_node != sentinel_)\n {\n if (delete_node->left() == sentinel_)\n deleteOneNode(delete_node);\n else\n {\n SPNodeType smallest = successor(delete_node);\n auto temp = delete_node->value();\n delete_node->value(smallest->value());\n smallest->value(temp);\n deleteOneNode(smallest);\n }\n }\n}\n\ntemplate<typename _Type, typename _Compare>\nvoid\nRBTree<_Type, _Compare>::deleteOneNode(SPNodeType &pt)\n{\n auto child = pt->left() != sentinel_ ? pt->left() : pt->right();\n if (pt->parent() == sentinel_)\n {\n root_ = child;\n root_->parent(sentinel_);\n root_->color(Color::BLACK);\n }\n else\n {\n if (isLeftChild(pt))\n pt->parent()->left(child);\n else\n pt->parent()->right(child);\n child->parent(pt->parent());\n if (pt->color() == Color::BLACK)\n {\n if (child->color() == Color::RED)\n child->color(Color::BLACK);\n else\n deleteCase1(child);\n }\n }\n}\n\ntemplate<typename _Type, typename _Compare>\nauto\nRBTree<_Type, _Compare>::find(_Type const &value)->SPNodeType const\n{\n auto pt = _find(value);\n\n return pt != sentinel_ ? pt : nullptr;\n}\n\ntemplate<typename _Type, typename _Compare>\nstd::string\nRBTree<_Type, _Compare>::preOrder() const\n{\n if (root_ == sentinel_)\n {\n return {};\n }\n std::string elem{};\n std::stack<SPNodeType> st{};\n st.push(root_);\n elem.append(std::to_string(st.top()->value()));\n while (!st.empty())\n {\n while (st.top()->left() != sentinel_)\n {\n elem.append(std::to_string(st.top()->left()->value()));\n st.push(st.top()->left());\n }\n while (!st.empty() && st.top()->right() == sentinel_)\n st.pop();\n if (!st.empty())\n {\n elem.append(std::to_string(st.top()->right()->value()));\n auto temp = st.top();\n st.pop();\n st.push(temp->right());\n }\n }\n\n return elem;\n}\n\ntemplate<typename _Type, typename _Compare>\nstd::string\nRBTree<_Type, _Compare>::inOrder() const\n{\n if (root_ == sentinel_)\n {\n return {};\n }\n std::string elem{};\n std::stack<SPNodeType> st{};\n st.push(root_);\n while (!st.empty())\n {\n while (st.top()->left() != sentinel_)\n st.push(st.top()->left());\n while (!st.empty() && st.top()->right() == sentinel_)\n {\n elem.append(std::to_string(st.top()->value()));\n st.pop();\n }\n if (!st.empty())\n {\n elem.append(std::to_string(st.top()->value()));\n auto temp = st.top();\n st.pop();\n st.push(temp->right());\n }\n }\n\n return elem;\n}\n\ntemplate<typename _Type, typename _Compare>\nauto\nRBTree<_Type, _Compare>::insert(SPNodeType &root, SPNodeType &pt)->SPNodeType &\n{\n // If the tree is empty, return a new node\n if (root == sentinel_)\n {\n pt->parent(root->parent());\n\n return pt;\n }\n\n // Otherwise, recur down the tree\n if (compare_(pt->value(), root->value()))\n {\n root->left(insert(root->left(), pt));\n root->left()->parent(root);\n }\n else if (compare_(root->value(), pt->value()))\n {\n root->right(insert(root->right(), pt));\n root->right()->parent(root);\n }\n else\n {\n pt->parent(root->parent());\n pt->left(root->left());\n pt->right(root->right());\n pt->color(root->color());\n }\n\n // return the (unchanged) node pointer\n return root;\n}\n\ntemplate<typename _Type, typename _Compare>\nauto\nRBTree<_Type, _Compare>::_find(_Type const &value)->SPNodeType\n{\n auto pt = std::make_shared<NodeType>(value);\n std::stack<SPNodeType> st{};\n st.push(root_);\n while (!st.empty())\n {\n if (compare_(st.top()->value(), pt->value()) == compare_(pt->value(), st.top()->value()))\n return st.top();\n while (st.top()->left() != sentinel_)\n {\n st.push(st.top()->left());\n if (compare_(st.top()->value(),\n pt->value()) == compare_(pt->value(), st.top()->value()))\n return st.top();\n }\n while (!st.empty() && st.top()->right() == sentinel_)\n st.pop();\n if (!st.empty())\n {\n if (compare_(st.top()->value(),\n pt->value()) == compare_(pt->value(), st.top()->value()))\n return st.top();\n else\n {\n SPNodeType &temp = st.top();\n st.pop();\n st.push(temp->right());\n }\n }\n }\n\n return sentinel_;\n}\n\ntemplate<typename _Type, typename _Compare>\nvoid\nRBTree<_Type, _Compare>::rotateLeft(SPNodeType const &pt)\n{\n auto pt_right = pt->right();\n\n pt->right() = pt_right->left();\n\n if (pt->right() != sentinel_)\n pt->right()->parent(pt);\n\n pt_right->parent(pt->parent());\n\n if (pt->parent() == sentinel_)\n root_ = pt_right;\n else if (pt == pt->parent()->left())\n pt->parent()->left(pt_right);\n else\n pt->parent()->right(pt_right);\n\n pt_right->left(pt);\n pt->parent(pt_right);\n}\n\ntemplate<typename _Type, typename _Compare>\nvoid\nRBTree<_Type, _Compare>::rotateRight(SPNodeType const &pt)\n{\n auto pt_left = pt->left();\n\n pt->left(pt_left->right());\n\n if (pt->left() != sentinel_)\n pt->left()->parent(pt);\n\n pt_left->parent(pt->parent());\n\n if (pt->parent() == sentinel_)\n root_ = pt_left;\n else if (pt == pt->parent()->left())\n pt->parent()->left(pt_left);\n else\n pt->parent()->right(pt_left);\n\n pt_left->right(pt);\n pt->parent(pt_left);\n}\n\ntemplate<typename _Type, typename _Compare>\nauto\nRBTree<_Type, _Compare>::successor(SPNodeType const &pt)->SPNodeType\n{\n auto child = sentinel_;\n if (pt->left() != sentinel_)\n {\n child = pt->left();\n while (child->right() != sentinel_)\n child = child->right();\n }\n else if (pt->right() != sentinel_)\n {\n child = pt->right();\n while (child->left() != sentinel_)\n child = child->left();\n }\n\n return child;\n}\n\ntemplate<typename _Type, typename _Compare>\nvoid\nRBTree<_Type, _Compare>::fixViolation(SPNodeType &pt)\n{\n auto parent_pt = sentinel_;\n auto grand_parent_pt = sentinel_;\n\n while (pt->color() == Color::RED && pt->parent()->color() == Color::RED)\n {\n parent_pt = pt->parent();\n grand_parent_pt = pt->parent()->parent();\n\n /*\n * Case : A\n * Parent of pt is left child of Grand-parent of pt\n */\n if (parent_pt == grand_parent_pt->left())\n {\n auto uncle_pt = grand_parent_pt->right();\n\n /*\n * Case : 1\n * The uncle of pt is also red\n * Only Recoloring required\n */\n if (uncle_pt->color() == Color::RED)\n {\n grand_parent_pt->color(Color::RED);\n parent_pt->color(Color::BLACK);\n uncle_pt->color(Color::BLACK);\n pt = grand_parent_pt;\n }\n else\n {\n /*\n * Case : 2\n * pt is right child of its parent\n * Left-rotation required\n */\n if (pt == parent_pt->right())\n {\n rotateLeft(parent_pt);\n pt = parent_pt;\n parent_pt = pt->parent();\n }\n\n /*\n * Case : 3\n * pt is left child of its parent\n * Right-rotation required\n */\n rotateRight(grand_parent_pt);\n auto temp = parent_pt->color();\n parent_pt->color(grand_parent_pt->color());\n grand_parent_pt->color(temp);\n pt = parent_pt;\n }\n }\n /*\n * Case : B\n * Parent of pt is right child of Grand-parent of pt\n */\n else\n {\n auto uncle_pt = grand_parent_pt->left();\n\n /*\n * Case : 1\n * The uncle of pt is also red\n * Only Recoloring required\n */\n if (uncle_pt->color() == Color::RED)\n {\n grand_parent_pt->color(Color::RED);\n parent_pt->color(Color::BLACK);\n uncle_pt->color(Color::BLACK);\n pt = grand_parent_pt;\n }\n else\n {\n /*\n * Case : 2\n * pt is left child of its parent\n * Right-rotation required\n */\n if (pt == parent_pt->left())\n {\n rotateRight(parent_pt);\n pt = parent_pt;\n parent_pt = pt->parent();\n }\n\n /*\n * Case : 3\n * pt is right child of its parent\n * Left-rotation required\n */\n rotateLeft(grand_parent_pt);\n auto temp = parent_pt->color();\n parent_pt->color(grand_parent_pt->color());\n grand_parent_pt->color(temp);\n pt = parent_pt;\n }\n }\n }\n\n root_->color(Color::BLACK);\n}\n\ntemplate<typename _Type, typename _Compare>\nauto\nRBTree<_Type, _Compare>::sibling(SPNodeType const &n)->SPNodeType &\n{\n return n->parent()->left() != n ? n->parent()->left() : n->parent()->right();\n}\n\ntemplate<typename _Type, typename _Compare>\nbool\nRBTree<_Type, _Compare>::isLeftChild(SPNodeType const &n)\n{\n return n == n->parent()->left();\n}\n\ntemplate<typename _Type, typename _Compare>\nbool\nRBTree<_Type, _Compare>::isRightChild(SPNodeType const &n)\n{\n return n == n->parent()->right();\n}\n\ntemplate<typename _Type, typename _Compare>\nvoid\nRBTree<_Type, _Compare>::deleteCase1(SPNodeType const &n)\n{\n if (n->parent() != sentinel_)\n deleteCase2(n);\n}\n\ntemplate<typename _Type, typename _Compare>\nvoid\nRBTree<_Type, _Compare>::deleteCase2(SPNodeType const &n)\n{\n auto s = sibling(n);\n if (s->color() == Color::RED)\n {\n n->parent()->color(Color::RED);\n s->color(Color::BLACK);\n if (isLeftChild(n))\n rotateLeft(n->parent());\n else\n rotateRight(n->parent());\n }\n deleteCase3(n);\n}\n\ntemplate<typename _Type, typename _Compare>\nvoid\nRBTree<_Type, _Compare>::deleteCase3(SPNodeType const &n)\n{\n auto s = sibling(n);\n if (n->parent()->color() == Color::BLACK\n && s->color() == Color::BLACK\n && s->left()->color() == Color::BLACK\n && s->right()->color() == Color::BLACK)\n {\n s->color(Color::RED);\n deleteCase1(n->parent());\n }\n else\n deleteCase4(n);\n}\n\ntemplate<typename _Type, typename _Compare>\nvoid\nRBTree<_Type, _Compare>::deleteCase4(SPNodeType const &n)\n{\n auto s = sibling(n);\n if (n->parent()->color() == Color::RED\n && s->color() == Color::BLACK\n && s->left()->color() == Color::BLACK\n && s->right()->color() == Color::BLACK)\n {\n s->color(Color::RED);\n n->parent()->color(Color::BLACK);\n }\n else\n deleteCase5(n);\n}\n\ntemplate<typename _Type, typename _Compare>\nvoid\nRBTree<_Type, _Compare>::deleteCase5(SPNodeType const &n)\n{\n auto s = sibling(n);\n if (s->color() == Color::BLACK)\n {\n if (isLeftChild(n)\n && s->right()->color() == Color::BLACK\n && s->left()->color() == Color::RED)\n {\n s->color(Color::RED);\n s->left()->color(Color::BLACK);\n rotateRight(s);\n }\n else if (isRightChild(n)\n && s->left()->color() == Color::BLACK\n && s->right()->color() == Color::RED)\n {\n s->color(Color::RED);\n s->right()->color(Color::BLACK);\n rotateLeft(s);\n }\n }\n deleteCase6(n);\n}\n\ntemplate<typename _Type, typename _Compare>\nvoid\nRBTree<_Type, _Compare>::deleteCase6(SPNodeType const &n)\n{\n auto s = sibling(n);\n s->color(n->parent()->color());\n n->parent()->color(Color::BLACK);\n\n if (isLeftChild(n))\n {\n s->right()->color(Color::BLACK);\n rotateLeft(n->parent());\n }\n else\n {\n s->left()->color(Color::BLACK);\n rotateRight(n->parent());\n }\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/red_black_tree/red_black_tree.h",
"content": "/**\n * author: JonNRb <[email protected]>\n * license: GPLv3\n * file: @cosmos//code/data_structures/red_black_tree/red_black.h\n * info: red black tree\n */\n\n#ifndef COSMOS_RED_BLACK_H_\n#define COSMOS_RED_BLACK_H_\n\n#include <stdint.h>\n\n/**\n * this macro subtracts off the offset of |member| within |type| from |ptr|,\n * allowing you to easily get a pointer to the containing struct of |ptr|\n *\n * ┌─────────────┬──────────┬──────┐\n * |type| -> │ XXXXXXXXXXX │ |member| │ YYYY │\n * └─────────────┴──────────┴──────┘\n * ^ ^\n * returns |ptr|\n */\n#define container_of(ptr, type, member) \\\n ({ \\\n const typeof(((type*)0)->member)* __mptr = \\\n (const typeof(((type*)0)->member)*)(__mptr); \\\n (type*)((char*)ptr - __offsetof(type, member)); \\\n })\n\n/**\n * node structure to be embedded in your data object.\n *\n * NOTE: it is expected that there will be a `uint64_t` immediately following\n * the embedded node (which will be used as a sorting key).\n */\ntypedef struct _cosmos_red_black_node cosmos_red_black_node_t;\nstruct _cosmos_red_black_node {\n cosmos_red_black_node_t* link[2];\n int red;\n uint64_t sort_key[0]; // place a `uint64_t` sort key immediately after this\n // struct in the data struct\n};\n\n/**\n * represents a whole tree. should be initialized to NULL.\n */\ntypedef cosmos_red_black_node_t* cosmos_red_black_tree_t;\n\n/**\n * initializes a node to default values\n */\nvoid cosmos_red_black_construct(cosmos_red_black_node_t* node);\n\n/**\n * takes a pointer to |tree| and |node| to insert. will update |tree| if\n * necessary.\n */\nvoid cosmos_red_black_push(cosmos_red_black_tree_t* tree,\n cosmos_red_black_node_t* node);\n\n/**\n * takes a pointer to the |tree| and returns a pointer to the minimum node in\n * the tree, which is removed from |tree|\n */\ncosmos_red_black_node_t* cosmos_red_black_pop_min(\n cosmos_red_black_tree_t* tree);\n\n#endif // COSMOS_RED_BLACK_H_"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/red_black_tree/red_black_tree.java",
"content": "public class RedBlackTree {\n// Part of Cosmos by OpenGenus Foundation\n public RedBlackTree( ) {\n header = new RedBlackNode( null );\n header.left = header.right = nullNode;\n }\n\n private final int compare( Comparable item, RedBlackNode t ) {\n if( t == header )\n return 1;\n else\n return item.compareTo( t.element );\n }\n\n public void insert( Comparable item ) {\n current = parent = grand = header;\n nullNode.element = item;\n\n while( compare( item, current ) != 0 ) {\n great = grand; grand = parent; parent = current;\n current = compare( item, current ) < 0 ?\n current.left : current.right;\n\n if( current.left.color == RED && current.right.color == RED )\n handleReorient( item );\n }\n\n if( current != nullNode )\n throw new DuplicateItemException( item.toString( ) );\n current = new RedBlackNode( item, nullNode, nullNode );\n\n if( compare( item, parent ) < 0 )\n parent.left = current;\n else\n parent.right = current;\n handleReorient( item );\n }\n\n public void remove( Comparable x ) {\n throw new UnsupportedOperationException( );\n }\n\n public Comparable findMin( ) {\n if( isEmpty( ) )\n return null;\n\n RedBlackNode itr = header.right;\n\n while( itr.left != nullNode )\n itr = itr.left;\n\n return itr.element;\n }\n\n public Comparable findMax( ) {\n if( isEmpty( ) )\n return null;\n\n RedBlackNode itr = header.right;\n\n while( itr.right != nullNode )\n itr = itr.right;\n\n return itr.element;\n }\n\n public Comparable find( Comparable x ) {\n nullNode.element = x;\n current = header.right;\n\n for( ; ; ) {\n if( x.compareTo( current.element ) < 0 )\n current = current.left;\n else if( x.compareTo( current.element ) > 0 )\n current = current.right;\n else if( current != nullNode )\n return current.element;\n else\n return null;\n }\n }\n\n public void makeEmpty( ) {\n header.right = nullNode;\n }\n\n public void printTree( ) {\n printTree( header.right );\n }\n\n private void printTree( RedBlackNode t ) {\n if( t != nullNode ) {\n printTree( t.left );\n System.out.println( t.element );\n printTree( t.right );\n }\n }\n\n public boolean isEmpty( ) {\n return header.right == nullNode;\n }\n\n private void handleReorient( Comparable item ) {\n current.color = RED;\n current.left.color = BLACK;\n current.right.color = BLACK;\n\n if( parent.color == RED )\n {\n grand.color = RED;\n if( ( compare( item, grand ) < 0 ) !=\n ( compare( item, parent ) < 0 ) )\n parent = rotate( item, grand );\n current = rotate( item, great );\n current.color = BLACK;\n }\n header.right.color = BLACK;\n }\n\n private RedBlackNode rotate( Comparable item, RedBlackNode parent ) {\n if( compare( item, parent ) < 0 )\n return parent.left = compare( item, parent.left ) < 0 ?\n rotateWithLeftChild( parent.left ) :\n rotateWithRightChild( parent.left ) ;\n else\n return parent.right = compare( item, parent.right ) < 0 ?\n rotateWithLeftChild( parent.right ) :\n rotateWithRightChild( parent.right );\n }\n\n private static RedBlackNode rotateWithLeftChild( RedBlackNode k2 ) {\n RedBlackNode k1 = k2.left;\n k2.left = k1.right;\n k1.right = k2;\n return k1;\n }\n\n private static RedBlackNode rotateWithRightChild( RedBlackNode k1 ) {\n RedBlackNode k2 = k1.right;\n k1.right = k2.left;\n k2.left = k1;\n return k2;\n }\n\n private static class RedBlackNode {\n RedBlackNode( Comparable theElement ) {\n this( theElement, null, null );\n }\n\n RedBlackNode( Comparable theElement, RedBlackNode lt, RedBlackNode rt ) {\n element = theElement;\n left = lt;\n right = rt;\n color = RedBlackTree.BLACK;\n }\n\n Comparable element;\n RedBlackNode left;\n RedBlackNode right;\n int color;\n }\n\n private RedBlackNode header;\n private static RedBlackNode nullNode;\n static\n {\n nullNode = new RedBlackNode( null );\n nullNode.left = nullNode.right = nullNode;\n }\n\n private static final int BLACK = 1;\n private static final int RED = 0;\n\n\n private static RedBlackNode current;\n private static RedBlackNode parent;\n private static RedBlackNode grand;\n private static RedBlackNode great;\n\n\n // MAIN TO TEST FOR ERRORS\n public static void main( String [ ] args ) {\n RedBlackTree t = new RedBlackTree( );\n final int NUMS = 400000;\n final int GAP = 35461;\n\n System.out.println( \"Checking... (no more output means success)\" );\n\n for( int i = GAP; i != 0; i = ( i + GAP ) % NUMS )\n t.insert( new Integer( i ) );\n\n if( ((Integer)(t.findMin( ))).intValue( ) != 1 ||\n ((Integer)(t.findMax( ))).intValue( ) != NUMS - 1 )\n System.out.println( \"FindMin or FindMax error!\" );\n\n for( int i = 1; i < NUMS; i++ )\n if( ((Integer)(t.find( new Integer( i ) ))).intValue( ) != i )\n System.out.println( \"Find error1!\" );\n }\n\n private class DuplicateItemException extends RuntimeException\n {\n public DuplicateItemException( )\n {\n super( );\n }\n\n public DuplicateItemException( String message )\n {\n super( message );\n }\n }\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/red_black_tree/red_black_tree.rb",
"content": "class Node\n attr_accessor :data, :color, :left, :right, :parent\n\n def initialize(data)\n @data = data\n @color = 'red'\n @left = nil\n @right = nil\n @parent = nil\n end\n\n def isSingle\n @left.nil? && @right.nil?\n end\n\n def addChildLeft(node)\n @left = node\n node.parent = self\n end\n\n def addChildRight(node)\n @right = node\n node.parent = self\n end\n\n def grandparent\n return nil if @parent.nil?\n @parent.parent\n end\n\n def sibling\n if @parent.nil?\n nil\n elsif @parent.left == self\n @parent.right\n else\n @parent.left\n end\n end\n\n def uncle\n if @parent.nil? || @parent.parent.nil?\n nil\n else\n @parent.sibling\n end\n end\n\n def rotateLeft\n tmp = right\n self.right = tmp.left\n tmp.left = self\n tmp.parent = parent\n if parent.left == self\n parent.left = tmp\n else\n parent.right = tmp\n end\n self.parent = tmp\n end\n\n def rotateRight\n tmp = left\n self.left = tmp.right\n tmp.right = self\n tmp.parent = parent\n if parent.left == self\n parent.left = tmp\n else\n parent.right = tmp\n end\n self.parent = tmp\n end\nend\n\nclass RedBlackTree\n attr_accessor :head\n def initialize\n @head = nil\n end\n\n def insert(value)\n tmp = Node.new(value)\n\n if @head.nil?\n @head = tmp\n else\n current = @head\n until current.nil?\n if value < current.data\n if !current.left.nil?\n current = current.left\n else\n current.left = tmp\n break\n end\n elsif value > current.data\n if !current.right.nil?\n current = current.right\n else\n current.right = tmp\n break\n end\n else\n puts \"Value #{value} already in tree\"\n return\n end\n end\n end\n insert_repair(tmp)\n end\n\n def insert_repair(node)\n uncle = node.uncle()\n grandparent = node.grandparent()\n\n if node.parent.nil?\n node.color = 'black'\n elsif node.parent.color == 'black'\n return\n elsif !uncle.nil? && (uncle.color == 'red')\n node.parent.parent.color = 'red'\n node.parent.color = 'black'\n uncle.color = 'black'\n insert_repair(node.parent.parent)\n elsif uncle.nil? || (uncle.color == 'black')\n if node == grandparent.left.right\n rotateLeft(node.parent)\n node = node.left\n elsif node == grandparent.right.left\n rotateRight(node.parent)\n node = node.right\n end\n\n if node.parent.left == node\n rotateRight(grandparent)\n else\n rotateLeft(grandparent)\n end\n node.parent.color = 'black'\n node.grandparent.color = 'red'\n end\n end\n\n def search(value)\n current = head\n until current.nil?\n if value < current.data\n current = current.left\n elsif value > current.data\n current = current.right\n else\n puts \"Element #{value} Found\"\n return\n end\n end\n puts \"Element #{value} not Found\"\n end\nend"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/red_black_tree/red_black_tree.scala",
"content": "\n// From http://scottlobdell.me/2016/02/purely-functional-red-black-trees-python/\n\ncase class RBTree[T](left: RBTree[T], value: T, right: RBTree[T], isBlack: Boolean) {\n\n val height: Int = {\n val leftHeight = if (left == null) 0 else left.height\n val rightHeight = if (right == null) 0 else right.height\n Math.max(leftHeight, rightHeight)\n }\n\n def insert(value: T)(implicit ord: Ordering[T]): RBTree[T] = update(RBTree(null, value, null, false)).blacken()\n\n def balance(): RBTree[T] = {\n if (!isBlack) {\n this\n }\n else if (left != null && !left.isBlack) {\n if (right != null && !right.isBlack) recolored()\n else if (left.left != null && !left.left.isBlack) rotateRight().recolored()\n else if (left.right != null && !left.right.isBlack) this.copy(left = left.rotateLeft()).rotateRight().recolored()\n else this\n }\n else if (right != null && !right.isBlack) {\n if (right.right != null && !right.right.isBlack) rotateLeft().recolored()\n else if (right.left != null && !right.left.isBlack) this.copy(right = right.rotateRight()).rotateLeft().recolored()\n else this\n }\n else this\n }\n\n def update(node: RBTree[T])(implicit ord: Ordering[T]): RBTree[T] = {\n if (ord.lt(node.value, value)) {\n if (left == null) this.copy(left = node.balance()).balance()\n else this.copy(left = left.update(node).balance()).balance()\n }\n else {\n if (right == null) this.copy(right = node.balance()).balance()\n else this.copy(right = right.update(node).balance()).balance()\n }\n }\n\n def blacken(): RBTree[T] = {\n if (isBlack) this\n else this.copy(isBlack = true)\n }\n\n def recolored() = RBTree(left.blacken(), value, right.blacken(), false)\n\n def rotateLeft(): RBTree[T] = right.copy(left = this.copy(right = right.left))\n def rotateRight(): RBTree[T] = left.copy(right = this.copy(left = left.right))\n\n def isMember(other: T)(implicit ord: Ordering[T]): Boolean = {\n if (ord.lt(other, value)) left.isMember(other)\n else if (ord.gt(other, value)) right.isMember(other)\n else true\n }\n}\n\nobject Main {\n def main(args: Array[String]): Unit = {\n println(RBTree(null, 7, null, false).insert(6).insert(3))\n println(RBTree(null, 1, null, false).insert(2))\n println(RBTree(null, 1, null, false).insert(2).insert(3))\n println(RBTree(null, 1, null, false).insert(2).insert(3).insert(4).insert(5))\n println(RBTree(null, 1, null, false).insert(2).insert(3).insert(4).insert(5).insert(6))\n println(RBTree(null, 1, null, false).insert(2).insert(3).insert(4).insert(5).insert(6).insert(7))\n }\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/red_black_tree/red_black_tree.test.cpp",
"content": "#include \"red_black_tree.cpp\"\n\nvoid\ntest()\n{\n std::shared_ptr<RBTree<int>> rbt;\n rbt = make_shared<RBTree<int>>();\n rbt->find(3);\n assert(rbt->preOrder() == \"\");\n assert(rbt->find(32) == nullptr);\n rbt->insert(1);\n rbt->insert(4);\n rbt->insert(7);\n rbt->insert(10);\n rbt->insert(2);\n rbt->insert(6);\n rbt->insert(8);\n rbt->insert(3);\n rbt->insert(5);\n rbt->insert(9);\n rbt->insert(100);\n assert(rbt->preOrder() == \"74213659810100\");\n rbt->insert(40);\n assert(rbt->preOrder() == \"7421365984010100\");\n rbt->insert(30);\n assert(rbt->preOrder() == \"742136598401030100\");\n rbt->insert(20);\n assert(rbt->preOrder() == \"74213659840201030100\");\n rbt->insert(15);\n assert(rbt->preOrder() == \"7421365209810154030100\");\n rbt->insert(50);\n assert(rbt->preOrder() == \"742136520981015403010050\");\n rbt->insert(60);\n assert(rbt->preOrder() == \"74213652098101540306050100\");\n rbt->insert(70);\n assert(rbt->preOrder() == \"7421365209810154030605010070\");\n rbt->insert(80);\n assert(rbt->preOrder() == \"742136520981015403060508070100\");\n rbt->insert(63);\n assert(rbt->preOrder() == \"74213652098101560403050807063100\");\n rbt->insert(67);\n assert(rbt->preOrder() == \"7421365209810156040305080676370100\");\n rbt->insert(65);\n rbt->insert(69);\n rbt->insert(37);\n rbt->insert(33);\n rbt->insert(35);\n rbt->insert(31);\n assert(rbt->inOrder() == \"1234567891015203031333537405060636567697080100\");\n assert(rbt->preOrder() == \"2074213659810156040333031373550806763657069100\");\n assert(rbt->find(31) != nullptr);\n assert(rbt->find(32) == nullptr);\n\n rbt->erase(69);\n assert(rbt->preOrder() == \"20742136598101560403330313735508067636570100\");\n rbt->erase(65);\n assert(rbt->preOrder() == \"207421365981015604033303137355080676370100\");\n rbt->erase(1);\n assert(rbt->preOrder() == \"20742365981015604033303137355080676370100\");\n rbt->erase(3);\n assert(rbt->preOrder() == \"2074265981015604033303137355080676370100\");\n rbt->erase(2);\n assert(rbt->preOrder() == \"207546981015604033303137355080676370100\");\n rbt->erase(60);\n assert(rbt->preOrder() == \"2075469810155033303137354080676370100\");\n rbt->erase(35);\n assert(rbt->preOrder() == \"20754698101550333031374080676370100\");\n rbt->erase(37);\n assert(rbt->preOrder() == \"207546981015503330314080676370100\");\n rbt->erase(40);\n assert(rbt->preOrder() == \"2075469810155031303380676370100\");\n rbt->erase(50);\n assert(rbt->preOrder() == \"20754698101567333130638070100\");\n rbt->erase(20);\n assert(rbt->preOrder() == \"157546981067333130638070100\");\n rbt->erase(15);\n assert(rbt->preOrder() == \"1075469867333130638070100\");\n rbt->erase(10);\n assert(rbt->preOrder() == \"97546867333130638070100\");\n rbt->erase(9);\n assert(rbt->preOrder() == \"8547667333130638070100\");\n rbt->erase(100);\n assert(rbt->preOrder() == \"8547667333130638070\");\n rbt->erase(70);\n assert(rbt->preOrder() == \"85476673331306380\");\n rbt->erase(80);\n assert(rbt->preOrder() == \"854763331306763\");\n}\n\n// test of RBTree methods\nstruct RBTreeTest\n{\n typedef RBTree<int> tree_type;\n using node_type = tree_type::NodeType;\n using p_node_type = tree_type::SPNodeType;\n using Color = tree_type::Color;\n\n RBTreeTest()\n {\n testSibling();\n\n // below test of delete cases need ignore invoke\n testDeleteCase2();\n testDeleteCase3();\n testDeleteCase4();\n testDeleteCase5();\n testDeleteCase6();\n }\n\n void testSibling()\n {\n tree_type t;\n p_node_type n = std::make_shared<node_type>(0), l = std::make_shared<node_type>(0),\n r = std::make_shared<node_type>(0);\n n->left(l);\n n->right(r);\n l->parent(n);\n r->parent(n);\n assert(t.sibling(l) == r);\n assert(t.sibling(r) == l);\n }\n\n void testDeleteCase2()\n {\n tree_type t;\n p_node_type n = std::make_shared<node_type>(0), p = std::make_shared<node_type>(0),\n s = std::make_shared<node_type>(0), sl = std::make_shared<node_type>(0),\n sr = std::make_shared<node_type>(0);\n\n // test n is left of parent\n p->color(Color::BLACK);\n s->color(Color::RED);\n sr->right(t.sentinel_);\n sr->left(sr->right());\n sl->right(sr->left());\n sl->left(sl->right());\n n->right(sl->left());\n n->left(n->right());\n p->parent(n->left());\n p->left(n);\n p->right(s);\n s->parent(p);\n n->parent(s->parent());\n s->left(sl);\n s->right(sr);\n sr->parent(s);\n sl->parent(sr->parent());\n t.deleteCase2(n);\n assert(p->color() == Color::RED);\n assert(s->color() == Color::BLACK);\n assert(s->parent() == t.sentinel_);\n assert(n->left() == t.sentinel_);\n assert(n->right() == t.sentinel_);\n assert(sl->left() == t.sentinel_);\n assert(sl->right() == t.sentinel_);\n assert(sr->left() == t.sentinel_);\n assert(sr->right() == t.sentinel_);\n assert(s->left() == p);\n assert(s->right() == sr);\n assert(p->parent() == s);\n assert(sr->parent() == s);\n assert(p->left() == n);\n assert(p->right() == sl);\n assert(n->parent() == p);\n assert(sl->parent() == p);\n\n // test n is right of parent\n p->color(Color::BLACK);\n s->color(Color::RED);\n\n sr->right(t.sentinel_);\n sr->left(sr->right());\n sl->right(sr->left());\n sl->left(sl->right());\n n->right(sl->left());\n n->left(n->right());\n p->parent(n->left());\n p->left(s);\n p->right(n);\n s->parent(p);\n n->parent(s->parent());\n s->left(sl);\n s->right(sr);\n sr->parent(s);\n sl->parent(sr->parent());\n t.deleteCase2(n);\n assert(p->color() == Color::RED);\n assert(s->color() == Color::BLACK);\n assert(s->parent() == t.sentinel_);\n assert(n->left() == t.sentinel_);\n assert(n->right() == t.sentinel_);\n assert(sl->left() == t.sentinel_);\n assert(sl->right() == t.sentinel_);\n assert(sr->left() == t.sentinel_);\n assert(sr->right() == t.sentinel_);\n assert(s->left() == sl);\n assert(s->right() == p);\n assert(sl->parent() == s);\n assert(p->parent() == s);\n assert(p->left() == sr);\n assert(p->right() == n);\n assert(sr->parent() == p);\n assert(n->parent() == p);\n }\n\n void testDeleteCase3()\n {\n tree_type t;\n p_node_type n = std::make_shared<node_type>(0), p = std::make_shared<node_type>(0),\n s = std::make_shared<node_type>(0), sl = std::make_shared<node_type>(0),\n sr = std::make_shared<node_type>(0);\n sr->color(Color::BLACK);\n sl->color(sr->color());\n s->color(sl->color());\n p->color(s->color());\n n->color(p->color());\n sr->right(t.sentinel_);\n sr->left(sr->right());\n sl->right(sr->left());\n sl->left(sl->right());\n n->right(sl->left());\n n->left(n->right());\n p->parent(n->left());\n p->left(n);\n p->right(s);\n s->parent(p);\n n->parent(s->parent());\n s->left(sl);\n s->right(sr);\n sr->parent(s);\n sl->parent(sr->parent());\n t.deleteCase3(n);\n assert(s->color() == Color::RED);\n assert(p->color() == Color::BLACK);\n assert(n->color() == Color::BLACK);\n assert(sl->color() == Color::BLACK);\n assert(sr->color() == Color::BLACK);\n\n sr->color(Color::BLACK);\n sl->color(sr->color());\n s->color(sl->color());\n p->color(s->color());\n n->color(p->color());\n sr->right(t.sentinel_);\n sr->left(sr->right());\n sl->right(sr->left());\n sl->left(sl->right());\n n->right(sl->left());\n n->left(n->right());\n p->parent(n->left());\n p->left(s);\n p->right(n);\n s->parent(p);\n n->parent(s->parent());\n s->left(sl);\n s->right(sr);\n sr->parent(s);\n sl->parent(sr->parent());\n t.deleteCase3(n);\n assert(s->color() == Color::RED);\n assert(p->color() == Color::BLACK);\n assert(n->color() == Color::BLACK);\n assert(sl->color() == Color::BLACK);\n assert(sr->color() == Color::BLACK);\n }\n\n void testDeleteCase4()\n {\n tree_type t;\n p_node_type n = std::make_shared<node_type>(0), p = std::make_shared<node_type>(0),\n s = std::make_shared<node_type>(0), sl = std::make_shared<node_type>(0),\n sr = std::make_shared<node_type>(0);\n sr->color(Color::BLACK);\n sl->color(sr->color());\n s->color(sl->color());\n n->color(s->color());\n p->color(Color::RED);\n sr->right(t.sentinel_);\n sr->left(sr->right());\n sl->right(sr->left());\n sl->left(sl->right());\n n->right(sl->left());\n n->left(n->right());\n p->parent(n->left());\n p->left(n);\n p->right(s);\n s->parent(p);\n n->parent(s->parent());\n s->left(sl);\n s->right(sr);\n sr->parent(s);\n sl->parent(sr->parent());\n t.deleteCase4(n);\n assert(s->color() == Color::RED);\n assert(p->color() == Color::BLACK);\n assert(n->color() == Color::BLACK);\n assert(sl->color() == Color::BLACK);\n assert(sr->color() == Color::BLACK);\n\n sr->color(Color::BLACK);\n sl->color(sr->color());\n s->color(sl->color());\n n->color(s->color());\n p->color(Color::RED);\n sr->right(t.sentinel_);\n sr->left(sr->right());\n sl->right(sr->left());\n sl->left(sl->right());\n n->right(sl->left());\n n->left(n->right());\n p->parent(n->left());\n p->left(s);\n p->right(n);\n s->parent(p);\n n->parent(s->parent());\n s->left(sl);\n s->right(sr);\n sr->parent(s);\n sl->parent(sr->parent());\n t.deleteCase4(n);\n assert(s->color() == Color::RED);\n assert(p->color() == Color::BLACK);\n assert(n->color() == Color::BLACK);\n assert(sl->color() == Color::BLACK);\n assert(sr->color() == Color::BLACK);\n }\n\n void testDeleteCase5()\n {\n tree_type t;\n p_node_type n = std::make_shared<node_type>(0), p = std::make_shared<node_type>(0),\n s = std::make_shared<node_type>(0), sl = std::make_shared<node_type>(0),\n sr = std::make_shared<node_type>(0), s_l = std::make_shared<node_type>(0),\n s_r = std::make_shared<node_type>(0);\n s_r->color(Color::BLACK);\n s_l->color(s_r->color());\n sr->color(s_l->color());\n s->color(sr->color());\n p->color(s->color());\n n->color(p->color());\n sl->color(Color::RED);\n s_r->right(t.sentinel_);\n s_r->left(s_r->right());\n s_l->right(s_r->left());\n s_l->left(s_l->right());\n sr->right(s_l->left());\n sr->left(sr->right());\n n->right(sr->left());\n n->left(n->right());\n p->parent(n->left());\n p->left(n);\n p->right(s);\n s->parent(p);\n n->parent(s->parent());\n s->left(sl);\n s->right(sr);\n sr->parent(s);\n sl->parent(sr->parent());\n sl->left(s_l);\n sl->right(s_r);\n s_r->parent(sl);\n s_l->parent(s_r->parent());\n t.deleteCase5(n);\n assert(s->color() == Color::RED);\n assert(p->color() == Color::BLACK);\n assert(n->color() == Color::BLACK);\n assert(sl->color() == Color::BLACK);\n assert(sr->color() == Color::BLACK);\n assert(s_l->color() == Color::BLACK);\n assert(s_r->color() == Color::BLACK);\n assert(p->parent() == t.sentinel_);\n assert(n->left() == t.sentinel_);\n assert(n->right() == t.sentinel_);\n assert(sr->left() == t.sentinel_);\n assert(sr->right() == t.sentinel_);\n assert(s_l->left() == t.sentinel_);\n assert(s_l->right() == t.sentinel_);\n assert(s_r->left() == t.sentinel_);\n assert(s_r->right() == t.sentinel_);\n assert(p->left() == n);\n assert(p->right() == sl);\n assert(n->parent() == p);\n assert(sl->parent() == p);\n assert(sl->left() == s_l);\n assert(sl->right() == s);\n assert(s_l->parent() == sl);\n assert(s->parent() == sl);\n assert(s->left() == s_r);\n assert(s->right() == sr);\n assert(s_r->parent() == s);\n assert(sr->parent() == s);\n\n s_r->color(Color::BLACK);\n s_l->color(s_r->color());\n sl->color(s_l->color());\n s->color(sl->color());\n p->color(s->color());\n n->color(p->color());\n sr->color(Color::RED);\n s_r->right(t.sentinel_);\n s_r->left(s_r->right());\n s_l->right(s_r->left());\n s_l->left(s_l->right());\n sl->right(s_l->left());\n sl->left(sl->right());\n n->right(sl->left());\n n->left(n->right());\n p->parent(n->left());\n p->left(s);\n p->right(n);\n s->parent(p);\n n->parent(s->parent());\n s->left(sl);\n s->right(sr);\n sr->parent(s);\n sl->parent(sr->parent());\n sr->left(s_l);\n sr->right(s_r);\n s_r->parent(sr);\n s_l->parent(s_r->parent());\n t.deleteCase5(n);\n assert(s->color() == Color::RED);\n assert(p->color() == Color::BLACK);\n assert(n->color() == Color::BLACK);\n assert(sl->color() == Color::BLACK);\n assert(sr->color() == Color::BLACK);\n assert(s_l->color() == Color::BLACK);\n assert(s_r->color() == Color::BLACK);\n assert(p->parent() == t.sentinel_);\n assert(n->left() == t.sentinel_);\n assert(n->right() == t.sentinel_);\n assert(sl->left() == t.sentinel_);\n assert(sl->right() == t.sentinel_);\n assert(s_l->left() == t.sentinel_);\n assert(s_l->right() == t.sentinel_);\n assert(s_r->left() == t.sentinel_);\n assert(s_r->right() == t.sentinel_);\n assert(p->left() == sr);\n assert(p->right() == n);\n assert(n->parent() == p);\n assert(sr->parent() == p);\n assert(sr->left() == s);\n assert(sr->right() == s_r);\n assert(s->parent() == sr);\n assert(s_r->parent() == sr);\n assert(s->left() == sl);\n assert(s->right() == s_l);\n assert(sl->parent() == s);\n assert(s_l->parent() == s);\n }\n\n void testDeleteCase6()\n {\n tree_type t;\n p_node_type n = std::make_shared<node_type>(0), p = std::make_shared<node_type>(0),\n s = std::make_shared<node_type>(0), sc = std::make_shared<node_type>(0);\n s->color(Color::BLACK);\n n->color(s->color());\n p->color(n->color());\n sc->color(Color::RED);\n sc->right(t.sentinel_);\n sc->left(sc->right());\n s->left(sc->left());\n n->right(s->left());\n n->left(n->right());\n p->parent(n->left());\n p->left(n);\n p->right(s);\n n->parent(p);\n s->parent(p);\n s->right(sc);\n sc->parent(s);\n t.deleteCase6(n);\n assert(p->color() == Color::BLACK);\n assert(n->color() == Color::BLACK);\n assert(s->color() == Color::BLACK);\n assert(sc->color() == Color::BLACK);\n assert(s->parent() == t.sentinel_);\n assert(n->left() == t.sentinel_);\n assert(n->right() == t.sentinel_);\n assert(p->right() == t.sentinel_);\n assert(sc->left() == t.sentinel_);\n assert(sc->right() == t.sentinel_);\n assert(s->left() == p);\n assert(s->right() == sc);\n assert(p->parent() == s);\n assert(sc->parent() == s);\n assert(p->left() == n);\n assert(n->parent() == p);\n\n s->color(Color::BLACK);\n n->color(s->color());\n p->color(n->color());\n sc->color(Color::RED);\n sc->right(t.sentinel_);\n sc->left(sc->right());\n s->right(sc->left());\n n->right(s->right());\n n->left(n->right());\n p->parent(n->left());\n p->left(s);\n p->right(n);\n s->parent(p);\n n->parent(p);\n s->left(sc);\n sc->parent(s);\n t.deleteCase6(n);\n assert(p->color() == Color::BLACK);\n assert(n->color() == Color::BLACK);\n assert(s->color() == Color::BLACK);\n assert(sc->color() == Color::BLACK);\n assert(s->parent() == t.sentinel_);\n assert(n->left() == t.sentinel_);\n assert(n->right() == t.sentinel_);\n assert(p->left() == t.sentinel_);\n assert(sc->left() == t.sentinel_);\n assert(sc->right() == t.sentinel_);\n assert(s->left() == sc);\n assert(s->right() == p);\n assert(sc->parent() == s);\n assert(p->parent() == s);\n assert(p->right() == n);\n assert(n->parent() == p);\n }\n};\n\n// Driver Code\nint\nmain()\n{\n RBTreeTest t;\n test();\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/splay_tree/readme.md",
"content": "# Splay Tree\n> Your personal library of every algorithm and data structure code that you will ever encounter\n\nA splay tree is a self-adjusting Binary Search Tree with the additional property that recently accessed elements are quick to access again ( O(1) time). It performs basic operations such as insertion, look-up and removal in O(log n) amortized time. For many sequences of non-random operations, splay trees perform better than other search trees, even when the specific pattern of the sequence is unknown.\n\nAll normal operations on a binary search tree are combined with one basic operation, called splaying. Splaying the tree for a certain element rearranges the tree so that the element is placed at the root of the tree.\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/splay_tree/splay_tree.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n *\n * splay tree synopsis\n *\n * template<typename _Type, class _Derivative>\n * class Node\n * {\n * protected:\n * using SPNodeType = std::shared_ptr<_Derivative>;\n * using ValueType = _Type;\n *\n * public:\n * Node(ValueType v, SPNodeType l = nullptr, SPNodeType r = nullptr);\n *\n * ValueType value();\n *\n * void value(ValueType v);\n *\n * SPNodeType left();\n *\n * void left(SPNodeType l);\n *\n * SPNodeType right();\n *\n * void right(SPNodeType r);\n *\n * protected:\n * ValueType value_;\n * std::shared_ptr<_Derivative> left_, right_;\n * };\n *\n * template<typename _Type>\n * class DerivativeNode :public Node<_Type, DerivativeNode<_Type>>\n * {\n * private:\n * using BaseNode = Node<_Type, DerivativeNode<_Type>>;\n * using SPNodeType = typename BaseNode::SPNodeType;\n * using WPNodeType = std::weak_ptr<DerivativeNode>;\n * using ValueType = typename BaseNode::ValueType;\n *\n * public:\n * DerivativeNode(ValueType v,\n * SPNodeType l = nullptr,\n * SPNodeType r = nullptr,\n * WPNodeType p = WPNodeType());\n *\n * SPNodeType parent();\n *\n * void parent(SPNodeType p);\n *\n * private:\n * WPNodeType parent_;\n * };\n *\n * template<typename _Type,\n * typename _Compare = std::less<_Type>,\n * class NodeType = DerivativeNode<_Type>>\n * class SplayTree\n * {\n * private:\n * using SPNodeType = std::shared_ptr<NodeType>;\n *\n * public:\n * using ValueType = _Type;\n * using Reference = ValueType &;\n * using ConstReference = ValueType const &;\n * using SizeType = size_t;\n * using DifferenceType = std::ptrdiff_t;\n *\n * SplayTree() :root_(nullptr), size_(0), compare_(_Compare())\n *\n * SizeType insert(ConstReference value);\n *\n * SizeType erase(ConstReference value);\n *\n * SPNodeType find(ConstReference value);\n *\n * SPNodeType minimum() const;\n *\n * SPNodeType maximum() const;\n *\n * SizeType height() const;\n *\n * SizeType size() const;\n *\n * bool empty() const;\n *\n * void inorderTravel(std::ostream &output) const;\n *\n * void preorderTravel(std::ostream &output) const;\n *\n * private:\n * SPNodeType root_;\n * SizeType size_;\n * _Compare compare_;\n *\n * SPNodeType splay(SPNodeType n);\n *\n * void leftRotate(SPNodeType n);\n *\n * void rightRotate(SPNodeType n);\n *\n * void replace(SPNodeType old, SPNodeType new_);\n *\n * SPNodeType get(ConstReference value);\n *\n * SizeType height(SPNodeType n) const;\n *\n * SPNodeType minimum(SPNodeType n) const;\n *\n * SPNodeType maximum(SPNodeType n) const;\n *\n * void inorderTravel(std::ostream &output, SPNodeType n) const;\n *\n * void preorderTravel(std::ostream &output, SPNodeType n) const;\n * };\n */\n\n#include <functional>\n#include <algorithm>\n#include <memory>\n#include <cstddef>\n\ntemplate<typename _Type, class _Derivative>\nclass Node\n{\nprotected:\n using SPNodeType = std::shared_ptr<_Derivative>;\n using ValueType = _Type;\n\npublic:\n Node(ValueType v, SPNodeType l = nullptr, SPNodeType r = nullptr)\n : value_(v), left_(l), right_(r)\n {\n };\n\n ValueType value()\n {\n return value_;\n }\n\n void value(ValueType v)\n {\n value_ = v;\n }\n\n SPNodeType left()\n {\n return left_;\n }\n\n void left(SPNodeType l)\n {\n left_ = l;\n }\n\n SPNodeType right()\n {\n return right_;\n }\n\n void right(SPNodeType r)\n {\n right_ = r;\n }\n\nprotected:\n ValueType value_;\n std::shared_ptr<_Derivative> left_, right_;\n};\n\ntemplate<typename _Type>\nclass DerivativeNode : public Node<_Type, DerivativeNode<_Type>>\n{\nprivate:\n using BaseNode = Node<_Type, DerivativeNode<_Type>>;\n using SPNodeType = typename BaseNode::SPNodeType;\n using WPNodeType = std::weak_ptr<DerivativeNode>;\n using ValueType = typename BaseNode::ValueType;\n\npublic:\n DerivativeNode(ValueType v,\n SPNodeType l = nullptr,\n SPNodeType r = nullptr,\n WPNodeType p = WPNodeType())\n : BaseNode(v, l, r), parent_(p)\n {\n };\n\n SPNodeType parent()\n {\n return parent_.lock();\n }\n\n void parent(SPNodeType p)\n {\n parent_ = p;\n }\n\nprivate:\n WPNodeType parent_;\n};\n\ntemplate<typename _Type,\n typename _Compare = std::less<_Type>,\n class NodeType = DerivativeNode<_Type>>\nclass SplayTree\n{\nprivate:\n using SPNodeType = std::shared_ptr<NodeType>;\n\npublic:\n using ValueType = _Type;\n using Reference = ValueType &;\n using ConstReference = ValueType const &;\n using SizeType = size_t;\n using DifferenceType = std::ptrdiff_t;\n\n SplayTree() : root_(nullptr), size_(0), compare_(_Compare())\n {\n ;\n }\n\n SizeType insert(ConstReference value)\n {\n SPNodeType n = root_, parent = nullptr;\n while (n)\n {\n parent = n;\n if (compare_(n->value(), value))\n n = n->right();\n else if (compare_(value, n->value()))\n n = n->left();\n else\n {\n n->value(value);\n\n return 0;\n }\n }\n n = std::make_shared<NodeType>(value);\n n->parent(parent);\n\n if (parent == nullptr)\n root_ = n;\n else if (compare_(parent->value(), n->value()))\n parent->right(n);\n else\n parent->left(n);\n splay(n);\n ++size_;\n\n return 1;\n }\n\n SizeType erase(ConstReference value)\n {\n SPNodeType n = get(value);\n if (n)\n {\n splay(n);\n if (n->left() == nullptr)\n replace(n, n->right());\n else if (n->right() == nullptr)\n replace(n, n->left());\n else\n {\n SPNodeType min = minimum(n->right());\n if (min->parent() != n)\n {\n replace(min, min->right());\n min->right(n->right());\n min->right()->parent(min);\n }\n replace(n, min);\n min->left(n->left());\n min->left()->parent(min);\n }\n --size_;\n\n return 1;\n }\n\n return 0;\n }\n\n SPNodeType find(ConstReference value)\n {\n return get(value);\n }\n\n SPNodeType minimum() const\n {\n return minimum(root_);\n }\n\n SPNodeType maximum() const\n {\n return maximum(root_);\n }\n\n SizeType height() const\n {\n return height(root_);\n }\n\n SizeType size() const\n {\n return size_;\n }\n\n bool empty() const\n {\n return size_ == 0;\n }\n\n void inorderTravel(std::ostream &output) const\n {\n inorderTravel(output, root_);\n }\n\n void preorderTravel(std::ostream &output) const\n {\n preorderTravel(output, root_);\n }\n\nprivate:\n SPNodeType root_;\n SizeType size_;\n _Compare compare_;\n\n SPNodeType splay(SPNodeType n)\n {\n while (n && n->parent())\n {\n if (!n->parent()->parent()) // zig step\n {\n if (n->parent()->left() == n)\n rightRotate(n->parent());\n else\n leftRotate(n->parent());\n }\n else if (n->parent()->left() == n && n->parent()->parent()->left() == n->parent())\n {\n rightRotate(n->parent()->parent());\n rightRotate(n->parent());\n }\n else if (n->parent()->right() == n && n->parent()->parent()->right() == n->parent())\n {\n leftRotate(n->parent()->parent());\n leftRotate(n->parent());\n }\n else if (n->parent()->right() == n && n->parent()->parent()->left() == n->parent())\n {\n leftRotate(n->parent());\n rightRotate(n->parent());\n }\n else\n {\n rightRotate(n->parent());\n leftRotate(n->parent());\n }\n }\n\n return n;\n }\n\n void leftRotate(SPNodeType n)\n {\n SPNodeType right = n->right();\n if (right)\n {\n n->right(right->left());\n if (right->left())\n right->left()->parent(n);\n right->parent(n->parent());\n }\n\n if (n->parent() == nullptr)\n root_ = right;\n else if (n == n->parent()->left())\n n->parent()->left(right);\n else\n n->parent()->right(right);\n\n if (right)\n right->left(n);\n n->parent(right);\n }\n\n void rightRotate(SPNodeType n)\n {\n SPNodeType left = n->left();\n if (left)\n {\n n->left(left->right());\n if (left->right())\n left->right()->parent(n);\n left->parent(n->parent());\n }\n\n if (n->parent() == nullptr)\n root_ = left;\n else if (n == n->parent()->left())\n n->parent()->left(left);\n else\n n->parent()->right(left);\n if (left)\n left->right(n);\n n->parent(left);\n }\n\n void replace(SPNodeType old, SPNodeType new_)\n {\n if (old->parent() == nullptr)\n root_ = new_;\n else if (old == old->parent()->left())\n old->parent()->left(new_);\n else\n old->parent()->right(new_);\n if (new_)\n new_->parent(old->parent());\n }\n\n SPNodeType get(ConstReference value)\n {\n SPNodeType n = root_;\n while (n)\n {\n if (compare_(n->value(), value))\n n = n->right();\n else if (compare_(value, n->value()))\n n = n->left();\n else\n {\n splay(n);\n\n return n;\n }\n }\n\n return nullptr;\n }\n\n SizeType height(SPNodeType n) const\n {\n if (n)\n return 1 + std::max(height(n->left()), height(n->right()));\n else\n return 0;\n }\n\n SPNodeType minimum(SPNodeType n) const\n {\n if (n)\n while (n->left())\n n = n->left();\n\n return n;\n }\n\n SPNodeType maximum(SPNodeType n) const\n {\n if (n)\n while (n->right())\n n = n->right();\n\n return n;\n }\n\n void inorderTravel(std::ostream &output, SPNodeType n) const\n {\n if (n)\n {\n inorderTravel(output, n->left());\n output << n->value() << \" \";\n inorderTravel(output, n->right());\n }\n }\n\n void preorderTravel(std::ostream &output, SPNodeType n) const\n {\n if (n)\n {\n output << n->value() << \" \";\n preorderTravel(output, n->left());\n preorderTravel(output, n->right());\n }\n }\n};\n\n/*\n * // for test\n #include <iostream>\n #include <fstream>\n * std::fstream input, ans;\n * int main() {\n * using namespace std;\n *\n * std::shared_ptr<SplayTree<int>> st = std::make_shared<SplayTree<int>>();\n *\n * input.open(\"/sample.txt\");\n * ans.open(\"/output.txt\", ios::out | ios::trunc);\n *\n * int r, ty;\n * while (input >> r)\n * {\n * input >> ty;\n *\n * // cout << r << \" \" << ty << endl;\n * if (ty == 0)\n * {\n * st->insert(r);\n * }\n * else if (ty == 1)\n * {\n * st->erase(r);\n * }\n * else\n * {\n * st->find(r);\n * }\n *\n * st->preorderTravel(ans);\n * st->inorderTravel(ans);\n * ans << endl;\n * }\n * ans << st->find(0);\n * ans << st->height();\n * ans << st->minimum();\n * ans << st->maximum();\n * ans << st->size();\n * ans << st->empty();\n *\n * return 0;\n * }\n * // */"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/splay_tree/splay_tree.go",
"content": "package splaytree\n\ntype binaryNode struct {\n\tleft, right *binaryNode\n\titem Item\n}\n\ntype splayTree struct {\n\troot *binaryNode\n\tlength int\n}\n\nfunc NewSplayTree() Tree {\n\treturn &splayTree{}\n}\n\nfunc (st *splayTree) splay(item Item) {\n\theader := &binaryNode{}\n\tl, r := header, header\n\tvar y *binaryNode\n\tt := st.root\n\n\tsplaying := true\n\tfor splaying {\n\t\tswitch {\n\t\tcase item.Less(t.item): // item might be on the left path\n\t\t\tif t.left == nil {\n\t\t\t\tsplaying = false\n\t\t\t\tbreak\n\t\t\t}\n\t\t\tif item.Less(t.left.item) { // zig-zig -> rotate right\n\t\t\t\ty = t.left\n\t\t\t\tt.left = y.right\n\t\t\t\ty.right = t\n\t\t\t\tt = y\n\t\t\t\tif t.left == nil {\n\t\t\t\t\tsplaying = false\n\t\t\t\t\tbreak\n\t\t\t\t}\n\t\t\t}\n\t\t\tr.left = t // link right\n\t\t\tr = t\n\t\t\tt = t.left\n\t\tcase t.item.Less(item): // item might be on the right path\n\t\t\tif t.right == nil {\n\t\t\t\tsplaying = false\n\t\t\t\tbreak\n\t\t\t}\n\t\t\tif t.right.item.Less(item) { // zig-zag -> rotage left\n\t\t\t\ty = t.right\n\t\t\t\tt.right = y.left\n\t\t\t\ty.left = t\n\t\t\t\tt = y\n\t\t\t\tif t.right == nil {\n\t\t\t\t\tsplaying = false\n\t\t\t\t\tbreak\n\t\t\t\t}\n\t\t\t}\n\t\t\tl.right = t\n\t\t\tl = t\n\t\t\tt = t.right\n\t\tdefault: // found the item\n\t\t\tsplaying = false\n\t\t}\n\t}\n\tl.right = t.left\n\tr.left = t.right\n\tt.left = header.right\n\tt.right = header.left\n\tst.root = t\n}\n\nfunc (st *splayTree) Get(key Item) Item {\n\tif st.root == nil {\n\t\treturn nil\n\t}\n\tst.splay(key)\n\tif st.root.item.Less(key) || key.Less(st.root.item) {\n\t\treturn nil\n\t}\n\treturn st.root.item\n}\n\nfunc (st *splayTree) Has(key Item) bool {\n\treturn st.Get(key) != nil\n}\n\nfunc (st *splayTree) ReplaceOrInsert(item Item) Item {\n\tpanic(\"not implemented\")\n}\n\nfunc (st *splayTree) insert(item Item) {\n\tn := &binaryNode{item: item}\n\tif st.root == nil {\n\t\tst.root = n\n\t\treturn\n\t}\n\tst.splay(item)\n\tswitch {\n\tcase item.Less(st.root.item):\n\t\tn.left = st.root.left\n\t\tn.right = st.root\n\t\tst.root.left = nil\n\tcase st.root.item.Less(item):\n\t\tn.right = st.root.right\n\t\tn.left = st.root\n\t\tst.root.right = nil\n\tdefault:\n\t\treturn\n\t}\n\tst.root = n\n\tst.length++\n}\n\nfunc (st *splayTree) Delete(item Item) Item {\n\tif st.length == 0 {\n\t\treturn nil\n\t}\n\n\tst.splay(item)\n\tif st.root.item.Less(item) || item.Less(st.root.item) {\n\t\treturn nil\n\t}\n\n\t// delete the root\n\tif st.root.left == nil {\n\t\tst.root = st.root.right\n\t} else {\n\t\tx := st.root.right\n\t\tst.root = st.root.left\n\t\tst.splay(item)\n\t\tst.root.right = x\n\t}\n\treturn item\n}\n\nfunc (st *splayTree) DeleteMin() Item {\n\tif st.root == nil {\n\t\treturn nil\n\t}\n\tx := st.root\n\tfor x != nil {\n\t\tx = x.left\n\t}\n\tst.splay(x.item)\n\tst.Delete(x.item)\n\treturn x.item\n}\n\nfunc (st *splayTree) DeleteMax() Item {\n\tif st.root == nil {\n\t\treturn nil\n\t}\n\tx := st.root\n\tfor x != nil {\n\t\tx = x.right\n\t}\n\tst.splay(x.item)\n\tst.Delete(x.item)\n\treturn x.item\n}\n\nfunc (st *splayTree) Len() int {\n\treturn st.length\n}\n\nfunc (st *splayTree) AscendRange(greaterOrEqual, lessThan Item, iterator ItemIterator) {\n\tpanic(\"not implemented\")\n}\n\nfunc (st *splayTree) AscendLessThan(pivot Item, iterator ItemIterator) {\n\tpanic(\"not implemented\")\n}\n\nfunc (st *splayTree) AscendGreaterOrEqual(pivot Item, iterator ItemIterator) {\n\tpanic(\"not implemented\")\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/splay_tree/splay_tree.java",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n * Public API:\n * put(): to insert a data\n * size(): to get number of nodes\n * height(): to get height of splay tree\n * remove(): to remove a data\n * get(): to find a data\n */\npublic class SplayTree<Key extends Comparable<Key>, Value> \n{\n private Node root; \n\n private class Node \n {\n private Key key; \n private Value value; \n private Node left, right; \n\n public Node(Key key, Value value) \n {\n this.key = key;\n this.value = value;\n }\n }\n\n public Value get(Key key) \n {\n root = splay(root, key);\n int cmp = key.compareTo(root.key);\n if (cmp == 0) \n \treturn root.value;\n else \n \treturn null;\n } \n\n public void put(Key key, Value value) \n {\n if (root == null) \n {\n root = new Node(key, value);\n return;\n }\n \n root = splay(root, key);\n\n int cmp = key.compareTo(root.key);\n \n if (cmp < 0) \n {\n Node n = new Node(key, value);\n n.left = root.left;\n n.right = root;\n root.left = null;\n root = n;\n }\n else if (cmp > 0) \n {\n Node n = new Node(key, value);\n n.right = root.right;\n n.left = root;\n root.right = null;\n root = n;\n }\n else \n {\n root.value = value;\n }\n\n }\n \n public void remove(Key key) \n {\n if (root == null) \n \treturn; \n \n root = splay(root, key);\n\n int cmp = key.compareTo(root.key);\n \n if (cmp == 0) \n {\n if (root.left == null) \n {\n root = root.right;\n } \n else \n {\n Node x = root.right;\n root = root.left;\n splay(root, key);\n root.right = x;\n }\n }\n }\n \n private Node splay(Node h, Key key) \n {\n if (h == null) return null;\n\n int cmp1 = key.compareTo(h.key);\n\n if (cmp1 < 0) \n {\n if (h.left == null) \n {\n return h;\n }\n int cmp2 = key.compareTo(h.left.key);\n if (cmp2 < 0) \n {\n h.left.left = splay(h.left.left, key);\n h = rotateRight(h);\n }\n else if (cmp2 > 0) \n {\n h.left.right = splay(h.left.right, key);\n if (h.left.right != null)\n h.left = rotateLeft(h.left);\n }\n \n if (h.left == null) \n \treturn h;\n else \n \treturn rotateRight(h);\n }\n\n else if (cmp1 > 0) \n { \n if (h.right == null) \n {\n return h;\n }\n\n int cmp2 = key.compareTo(h.right.key);\n if (cmp2 < 0) \n {\n h.right.left = splay(h.right.left, key);\n if (h.right.left != null)\n h.right = rotateRight(h.right);\n }\n else if (cmp2 > 0) \n {\n h.right.right = splay(h.right.right, key);\n h = rotateLeft(h);\n }\n \n if (h.right == null) \n \treturn h;\n else \n \treturn rotateLeft(h);\n }\n\n else return h;\n }\n\n public int height() \n { \n \treturn height(root); \n }\n\n private int height(Node x) \n {\n if (x == null) \n \treturn -1;\n return 1 + Math.max(height(x.left), height(x.right));\n }\n\n \n public int size() \n {\n return size(root);\n }\n \n private int size(Node x) \n {\n if (x == null) \n \treturn 0;\n else \n \treturn 1 + size(x.left) + size(x.right);\n }\n \n private Node rotateRight(Node h) \n {\n Node x = h.left;\n h.left = x.right;\n x.right = h;\n return x;\n }\n\n private Node rotateLeft(Node h) \n {\n Node x = h.right;\n h.right = x.left;\n x.left = h;\n return x;\n }\n\n public static void main(String[] args) \n { \n SplayTree<String, Integer> splay_tree = new SplayTree<String, Integer>();\n splay_tree.put(\"OpenGenus\", 1);\n splay_tree.put(\"Cosmos\", 2);\n splay_tree.put(\"Vidsum\", 3);\n splay_tree.put(\"Splay\", 4);\n System.out.println(\"Size: \" + splay_tree.size());\n splay_tree.remove(\"Splay\");\n System.out.println(\"Size: \" + splay_tree.size());\n System.out.println(splay_tree.get(\"OpenGenus\"));\n }\n\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/splay_tree/splay_tree.kt",
"content": "// Part of Cosmos by OpenGenus Foundation\n/*\n * Public API:\n * get(): to find a data\n * put(): to insert a data\n * remove(): to remove a data\n * size: to get number of nodes\n * height: to get height of splay tree\n */\n\nclass SplayTree<KeyT : Comparable<KeyT>, ValueT>() {\n private inner class Node(val key: KeyT, var value: ValueT? = null) {\n var left: Node? = null\n var right: Node? = null\n }\n private var root : Node? = null\n var size: Int = 0\n private set\n val height\n get() = height(root)\n\n private fun height(x : Node?) : Int {\n var result = -1\n if (x != null) {\n result = 1 + maxOf(height(x.left), height(x.right))\n }\n return result\n }\n private fun rotateLeft(x : Node?) : Node? {\n val y : Node? = x?.right\n x?.right = y?.left\n y?.left = x\n return y\n }\n\n private fun rotateRight(x : Node?) : Node? {\n val y : Node? = x?.left\n x?.left = y?.right\n y?.right = x\n return y\n }\n\n private fun splay(h : Node?, key : KeyT) : Node? {\n var root : Node = h ?: return null\n\n if (key < root.key) {\n val left : Node = root.left ?: return root\n\n if (key < left.key) {\n left.left = splay(left.left, key)\n root = rotateRight(root) ?: return null\n\n } else if (key > left.key) {\n left.right = splay(left.right, key)\n if (left.right != null) {\n root.left = rotateLeft(left)\n }\n }\n\n return if (root.left == null) root else rotateRight(root)\n\n } else if (key > root.key) {\n val right : Node = root.right ?: return root\n\n if (key < right.key) {\n right.left = splay(right.left, key)\n if (right.left != null) {\n root.right = rotateRight(right)\n }\n\n } else if (key > right.key) {\n right.right = splay(right.right, key)\n root = rotateLeft(root) ?: return null\n }\n\n return if (root.right == null) root else rotateLeft(root)\n\n } else {\n return root\n }\n }\n\n fun get(key: KeyT) : ValueT? {\n root = splay(root, key)\n if (root?.key == key) {\n return root?.value\n }\n return null\n }\n\n fun put(key: KeyT, value: ValueT) {\n if (root == null) {\n root = Node(key, value)\n size++\n return\n }\n\n root = splay(root, key)\n val rootKey = root?.key ?: return\n if (key < rootKey) {\n val n = Node(key, value)\n n.left = root?.left\n n.right = root\n root?.left = null\n root = n\n size++\n\n } else if (key > rootKey) {\n val n = Node(key, value)\n n.right = root?.right\n n.left = root\n root?.right = null\n root = n\n size++\n\n } else {\n root?.value = value\n }\n }\n\n fun remove(key: KeyT) {\n root = splay(root, key)\n\n val rootKey = root?.key ?: return\n if (key == rootKey) {\n size--\n if (root?.left == null) {\n root = root?.right\n } else {\n val x = root?.right\n root = root?.left\n splay(root, key)\n root?.right = x\n }\n }\n }\n}\n\nfun main(args : Array<String>) {\n val splay_tree = SplayTree<String, Int>()\n splay_tree.put(\"OpenGenus\", 1)\n splay_tree.put(\"Cosmos\", 2)\n splay_tree.put(\"Vidsum\", 3)\n splay_tree.put(\"Splay\", 4)\n\n println(\"Size Before: \" + splay_tree.size)\n println(\"Height Before: \" + splay_tree.height)\n splay_tree.remove(\"Splay\")\n println(\"Size After: \" + splay_tree.size)\n println(\"Height After: \" + splay_tree.height)\n println(splay_tree.get(\"OpenGenus\"))\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/splay_tree/splay_tree.scala",
"content": "\ncase class SplayTree[T, U](key: T, value: U, left: SplayTree[T, U], right: SplayTree[T, U]) {\n\n // Should guarantee the inserted key's node is the returned node\n def insert(newKey: T, newValue: U)(implicit ord: Ordering[T]): SplayTree[T, U] = {\n if (ord.lt(newKey, key)) {\n if (left == null) {\n SplayTree(newKey, newValue, null, this)\n } else {\n val newLeft = left.insert(newKey, newValue)\n newLeft.copy(right = this.copy(left = newLeft.right))\n }\n } else if (ord.gt(newKey, key)) {\n if (right == null) {\n SplayTree(newKey, newValue, this, null)\n } else {\n val newRight = right.insert(newKey, newValue)\n newRight.copy(left = this.copy(right = newRight.left))\n }\n } else {\n this\n }\n }\n\n // Should guarantee the get-ed key's node is the returned node\n def get(newKey: T)(implicit ord: Ordering[T]): Option[SplayTree[T, U]] = {\n if (ord.lt(newKey, key)) {\n if (left == null) {\n None\n } else {\n left.get(newKey).map { newLeft =>\n newLeft.copy(right = this.copy(left = newLeft.right))\n }\n }\n } else if (ord.gt(newKey, key)) {\n if (right == null) {\n None\n } else {\n right.get(newKey).map { newRight =>\n newRight.copy(left = this.copy(right = newRight.left))\n }\n }\n } else {\n Option(this)\n }\n }\n\n def inOrder(visitor: (T, U) => Unit): Unit = {\n if (left != null) left.inOrder(visitor);\n visitor(key, value)\n if (right != null) right.inOrder(visitor);\n }\n}\n\nobject Main {\n def main(args: Array[String]): Unit = {\n val st = SplayTree(\"a\", 100, null, null).insert(\"b\", 2).insert(\"c\", 3)\n st.inOrder((el: String, priority: Int) => println((el, priority)))\n st.get(\"b\").foreach { st2 =>\n println((st2.key, st2.value))\n }\n st.get(\"b\").get(\"c\").foreach { st2 =>\n println((st2.key, st2.value))\n }\n st.get(\"b\").get(\"c\").get(\"a\").foreach { st2 =>\n println((st2.key, st2.value))\n }\n }\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/union_find/README.md",
"content": "# Union Find\nDescription\n---\nIn computer science, a disjoint-set data structure, also called a union find data structure or merge\nfind set, is a data structure that keeps track of a set of elements partitioned into a number of disjoint\n(non-overlapping) subsets. It provides near-constant-time operations (bounded by the inverse Ackermann function)\nto add new sets, to merge existing sets, and to determine whether elements are in the same set.\n\nIn addition to many other uses (see the Applications section), disjoint-sets play a key role in\nKruskal's algorithm for finding the minimum spanning tree of a graph.\n\nUnion-Find API\n---\n- union(a, b): Add connection between a and b.\n- connected(a, b): Return true if a and b are in the same connected component.\n- find(a): Find id or root of a"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/union_find/union_find.c",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n/*\n* ░█░█░█▀█░▀█▀░█▀█░█▀█░░░░░█▀▀░▀█▀░█▀█░█▀▄\n* ░█░█░█░█░░█░░█░█░█░█░▄▄▄░█▀▀░░█░░█░█░█░█\n* ░▀▀▀░▀░▀░▀▀▀░▀▀▀░▀░▀░░░░░▀░░░▀▀▀░▀░▀░▀▀░\n*\n* Union-Find implementation using a parent array to implement set structure.\n* Basic operations:\n* 1.int find_optimal(int x): Find representative index of the set that the element with index x belongs to while compressing path for optimization\n* 2.find(int x): Find representative index of the set that the element with index x belongs to\n* 3.void union_sets(int x,int y,int parent[]): Unite sets that contain elements with id's x and y into a new set that is the union of these two\n* There should not be cycles in the graph of element nodes.\n* We are only using element indexes in parent array so elements can be of any possible type\n*/\n\n#include <stdio.h>\n#include <stdlib.h>\n\n//Function to return a parent array from a set of n elements\nint* init_parent(int a[],int length){\n int *b = (int *)malloc(length);\n for (int i = 0; i < length; i++) {\n b[i] = i;\n }\n return b;\n}\n\n//returns the representative (his input array index) of the set that includes x\nint find(int x,int parent[]) {\n while (x != parent[x]) x = parent[x];\n return(x);\n}\n\nint find_optimal(int x,int parent[]) {\n if (x != parent[x])\n parent[x] = find_optimal(parent[x],parent);\n return(parent[x]);\n}\n\n//Combines two sets in one by updating the parent array. No special policy about who will be the new representative\nvoid union_sets(int x, int y,int parent[]) {\n if (parent[x] == parent[y]) return;\n else if(find_optimal(y,parent)!= y){\n parent[find_optimal(x,parent)] = find_optimal(y,parent);\n }\n else{\n parent[find_optimal(y,parent)] = find_optimal(x,parent);\n}\n}\n\n\nint main(int argc, char const *argv[]) {\n int a[] = {1,3,6,2,7,5,0,4}; //The array can store any type. We are using int array and random integers for testing\n int a_length = sizeof(a)/sizeof(a[0]); //Num of input elements\n\n//First, all nodes are not included in any set so their parent is their self\n// int * parent_array = init_parent(a,a_length);\n\n\n/*\n* For testing, we setup our nodes to form two sets (Refering to elements with their indexes)\n 1 | 5\n / \\ | /|\\\n 0 2 | 4 6 7\n | |\n 3 |\n*/\n\nint parent_array[] = {1,1,1,2,5,5,5,5};\n printf(\"%s\\n\",\"Parent array before union: \" );\n for (int i = 0; i < a_length; i++) {\n printf(\"%d \",parent_array[i] );\n }\n printf(\"\\n\");\n\n union_sets(4,2,parent_array);\n\n printf(\"%s\\n\",\"Parent array after union: \" );\n for (int i = 0; i < a_length; i++) {\n printf(\"%d \",parent_array[i] );\n }\n printf(\"\\n\");\n\n//After the union we see that parent[5] = 1 so node 5 becomes child of node 1 and so do all of node's 5 children\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/union_find/union_find.cpp",
"content": "#include <iostream>\n#include <vector>\n\n/* Part of Cosmos by OpenGenus Foundation */\n\n// Union-find stores a graph, and allows two operations in amortized constant time:\n// * Add a new edge between two vertices.\n// * Check if two vertices belong to same component.\nclass UnionFind {\n std::vector<size_t> parent;\n std::vector<size_t> rank;\n size_t root(size_t node)\n {\n if (parent[node] == node)\n return node;\n else\n return parent[node] = getParent(parent[node]);\n }\n\n size_t getParent(size_t node)\n {\n return parent[node];\n }\n\npublic:\n// Make a graph with `size` vertices and no edges.\n UnionFind(size_t size)\n {\n parent.resize(size);\n rank.resize(size);\n for (size_t i = 0; i < size; i++)\n {\n parent[i] = i;\n rank[i] = 1;\n }\n }\n\n// Connect vertices `a` and `b`.\n void Union(size_t a, size_t b)\n {\n a = root(a);\n b = root(b);\n if (a == b)\n return;\n if (rank[a] < rank[b])\n parent[a] = b;\n else if (rank[a] > rank[b])\n parent[b] = a;\n else\n {\n parent[a] = b;\n rank[b] += 1;\n }\n }\n\n// Check if vertices `a` and `b` are in the same component.\n bool Connected(size_t a, size_t b)\n {\n return root(a) == root(b);\n }\n};\n\nint main()\n{\n UnionFind unionFind(10);\n\n unionFind.Union(3, 4);\n unionFind.Union(3, 8);\n unionFind.Union(0, 8);\n unionFind.Union(1, 3);\n unionFind.Union(7, 9);\n unionFind.Union(5, 9);\n\n // Now the components are:\n // 0 1 3 4 8\n // 5 7 9\n // 2\n // 6\n\n for (size_t i = 0; i < 10; i++)\n for (size_t j = i + 1; j < 10; j++)\n if (unionFind.Connected(i, j))\n std::cout << i << \" and \" << j << \" are in the same component\" << std::endl;\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/union_find/union_find_dynamic.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n// Union-find stores a graph, and allows two operations in amortized constant time:\n// * Add a new edge between two vertices.\n// * Check if two vertices belong to same component.\n\n#include <unordered_map>\n#include <unordered_set>\n#include <algorithm>\n\n// dynamic union find (elementary implementation)\ntemplate<typename _ValueType, typename _Hash = std::hash<_ValueType>>\nclass UnionFind\n{\npublic:\n using value_type = _ValueType;\n\n UnionFind()\n {\n }\n\n// Connect vertices `a` and `b`.\n void merge(value_type a, value_type b)\n {\n // 1. to guarantee that the set has both `a` and `b`\n auto na = nodes.find(a);\n auto nb = nodes.find(b);\n\n if (na == nodes.end())\n {\n nodes.insert(a);\n na = nodes.find(a);\n parents.insert({na, na});\n }\n\n if (nb == nodes.end())\n {\n nodes.insert(b);\n nb = nodes.find(b);\n parents.insert({nb, nb});\n }\n\n // 2. update the map\n auto pa = parents.find(na);\n\n while (pa != parents.end() && pa->first != pa->second)\n pa = parents.find(pa->second);\n\n if (pa != parents.end())\n na = pa->second;\n\n parents[na] = nb;\n }\n\n value_type find(value_type node)\n {\n auto root = nodes.find(node);\n\n // new node\n if (root == nodes.end())\n {\n // auto it = nodes.insert(node);\n nodes.insert(node);\n auto it = nodes.find(node);\n parents.insert({it, it});\n\n return node;\n }\n // existed\n else\n {\n auto pr = parents.find(root);\n while (pr != parents.end() && pr->first != pr->second)\n pr = parents.find(pr->second);\n\n return *(parents[root] = pr->second);\n }\n }\n\n bool connected(value_type a, value_type b)\n {\n return find(a) == find(b);\n }\n\nprivate:\n using Set = std::unordered_set<value_type, _Hash>;\n using SetIt = typename Set::iterator;\n\n struct SetItHash\n {\n size_t operator()(const SetIt& it) const\n {\n return std::hash<const int*>{} (&*it);\n }\n };\n\n Set nodes;\n std::unordered_map<SetIt, SetIt, SetItHash> parents;\n};"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/union_find/union_find.go",
"content": "/* Part of Cosmos by OpenGenus Foundation */\npackage main\n\nimport \"fmt\"\n\ntype UnionFind struct {\n\troot []int\n\tsize []int\n}\n\n// New returns an initialized list of Size N\nfunc New(N int) *UnionFind {\n\treturn new(UnionFind).init(N)\n}\n\n// Constructor initializes root and size arrays\nfunc (uf *UnionFind) init(N int) *UnionFind {\n\tuf = new(UnionFind)\n\tuf.root = make([]int, N)\n\tuf.size = make([]int, N)\n\n\tfor i := 0; i < N; i++ {\n\t\tuf.root[i] = i\n\t\tuf.size[i] = 1\n\t}\n\n\treturn uf\n}\n\n// Union connects p and q by finding their roots and comparing their respective\n// size arrays to keep the tree flat\nfunc (uf *UnionFind) Union(p int, q int) {\n\tqRoot := uf.Root(q)\n\tpRoot := uf.Root(p)\n\n\tif uf.size[qRoot] < uf.size[pRoot] {\n\t\tuf.root[qRoot] = uf.root[pRoot]\n\t\tuf.size[pRoot] += uf.size[qRoot]\n\t} else {\n\t\tuf.root[pRoot] = uf.root[qRoot]\n\t\tuf.size[qRoot] += uf.size[pRoot]\n\t}\n}\n\n// Root or Find traverses each parent element while compressing the\n// levels to find the root element of p\n// If we attempt to access an element outside the array it returns -1\nfunc (uf *UnionFind) Root(p int) int {\n\tif p > len(uf.root)-1 {\n\t\treturn -1\n\t}\n\n\tfor uf.root[p] != p {\n\t\tuf.root[p] = uf.root[uf.root[p]]\n\t\tp = uf.root[p]\n\t}\n\n\treturn p\n}\n\n// Root or Find\nfunc (uf *UnionFind) Find(p int) int {\n\treturn uf.Root(p)\n}\n\n// Check if items p,q are connected\nfunc (uf *UnionFind) Connected(p int, q int) bool {\n\treturn uf.Root(p) == uf.Root(q)\n}\n\nfunc main() {\n\tuf := New(10)\n\tuf.Union(3, 4)\n\tuf.Union(3, 8)\n\tuf.Union(0, 8)\n\tuf.Union(1, 3)\n\tuf.Union(7, 9)\n\tuf.Union(5, 9)\n\n\tfor i := 0; i < 10; i++ {\n\t\tfor j := i + 1; j < 10; j++ {\n\t\t\tif uf.Connected(i, j) {\n\t\t\t\tfmt.Printf(\"%d and %d are are in the same component\\n\", i, j)\n\t\t\t}\n\t\t}\n\t}\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/union_find/union_find.java",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\nclass UnionFind {\n int[] parent;\n int[] rank;\n UnionFind(int size) {\n parent = new int[size];\n rank = new int[size];\n for (int i = 0; i < size; i++) {\n parent[i] = i;\n rank[i] = 0;\n }\n }\n public int find(int v) {\n if (parent[v] == v) {\n return v;\n }\n parent[v] = find(parent[v]);\n return parent[v];\n }\n public boolean connected(int a, int b) {\n return find(a) == find(b);\n }\n public void union(int a, int b) {\n a = find(a);\n b = find(b);\n if (a != b) {\n if (rank[a] < rank[b]) {\n int tmp = a;\n a = b;\n b = tmp;\n }\n if (rank[a] == rank[b]) {\n rank[a]++;\n }\n parent[b] = a;\n }\n }\n public static void main(String[] args) {\n UnionFind unionFind = new UnionFind(10);\n\n unionFind.union(3, 4);\n unionFind.union(3, 8);\n unionFind.union(0, 8);\n unionFind.union(1, 3);\n unionFind.union(7, 9);\n unionFind.union(5, 9);\n\n // Now the components are:\n // 0 1 3 4 8\n // 5 7 9\n // 2\n // 6\n\n for (int i = 0; i < 10; i++) {\n for (int j = i + 1; j < 10; j++) {\n if (unionFind.connected(i, j)) {\n System.out.printf(\"%d and %d are in the same component\\n\", i, j);\n }\n }\n }\n }\n};"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/union_find/union_find.js",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\nclass UnionFind {\n constructor() {\n this.parents = [];\n this.ranks = [];\n }\n\n find(v) {\n if (this.parents[v] === v) {\n return v;\n }\n this.parents[v] = this.find(this.parents[v]);\n return this.parents[v];\n }\n\n union(a, b) {\n if (this.parents[a] === undefined) {\n this.parents[a] = a;\n this.ranks[a] = 0;\n }\n\n if (this.parents[b] === undefined) {\n this.parents[b] = b;\n this.ranks[b] = 0;\n }\n\n a = this.find(a);\n b = this.find(b);\n\n if (a !== b) {\n if (this.ranks[a] < this.ranks[b]) {\n [a, b] = [b, a];\n }\n\n if (this.ranks[a] === this.ranks[b]) {\n this.ranks[a]++;\n }\n\n this.parents[b] = a;\n }\n }\n\n connected(a, b) {\n return this.find(a) === this.find(b);\n }\n}\n\nconst uf = new UnionFind();\nuf.union(1, 3);\nuf.union(1, 4);\nuf.union(4, 2);\nuf.union(5, 6);\nconsole.log(uf.find(3));\nconsole.log(uf.find(4));\nconsole.log(uf.find(2));\nconsole.log(uf.find(5));"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/union_find/union_find.py",
"content": "#!/usr/bin/env python\n\n\nclass UnionFind:\n def __init__(self):\n self.parent = {}\n self.rank = {}\n\n def root(self, a):\n current_item = a\n path = []\n while self.parent[current_item] != current_item:\n path.append(current_item)\n current_item = self.parent[current_item]\n for node in path:\n self.parent[node] = current_item\n return current_item\n\n def connected(self, a, b):\n return self.root(a) == self.root(b)\n\n def find(self, a):\n return self.root(a)\n\n def create(self, a):\n if a not in self.parent:\n self.parent[a] = a\n self.rank[a] = 1\n\n def union(self, a, b):\n self.create(a)\n self.create(b)\n a_root = self.root(a)\n b_root = self.root(b)\n if self.rank[a_root] > self.rank[b_root]:\n self.parent[b_root] = a_root\n self.rank[a_root] += self.rank[b_root]\n else:\n self.parent[a_root] = b_root\n self.rank[b_root] += self.rank[a_root]\n\n def count(self, a):\n if a not in self.parent:\n return 0\n return self.rank[self.root(a)]\n\n\ndef main():\n union_find = UnionFind()\n union_find.union(1, 3)\n union_find.union(1, 4)\n union_find.union(2, 5)\n union_find.union(5, 6)\n union_find.union(7, 8)\n union_find.union(7, 9)\n union_find.union(3, 9)\n for i in range(1, 10):\n print(\n \"{} is in group {} with {} elements\".format(\n i, union_find.find(i), union_find.count(i)\n )\n )\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/union_find/union_find.scala",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\ncase class UnionFind[T](map: Map[T, T] = Map[T, T]()) {\n\n def add(element: T) = this.copy(map = map + (element -> element))\n\n def find(element: T): T = {\n val parent = map(element)\n if (parent == element) element else find(parent)\n }\n\n private def findRank(element: T, rank: Int): (T, Int) = {\n val parent = map(element)\n if (parent == element) (element, rank) else findRank(parent, rank + 1)\n }\n\n def union(x: T, y: T): UnionFind[T] = {\n val (parentX, rankX) = findRank(x, 0)\n val (parentY, rankY) = findRank(y, 0)\n val newPair = \n if (rankX > rankY) (parentY -> parentX) \n else (parentX -> parentY)\n this.copy(map = map + newPair)\n }\n}"
}
{
"filename": "code/data_structures/src/tree/multiway_tree/van_emde_boas_tree/van_emde_boas.cpp",
"content": "#include <iostream>\n#include <math.h>\n\nusing namespace std;\n\ntypedef struct VEB\n{\n int u;\n int min, max;\n int count;\n struct VEB *summary, **cluster;\n} VEB;\n\nint high(int x, int u)\n{\n return (int)(x / (int) sqrt(u));\n}\n\nint low(int x, int u)\n{\n return x % (int) sqrt(u);\n}\n\nint VEBmin(VEB*V)\n{\n return V->min;\n}\n\nint VEBmax(VEB*V)\n{\n return V->max;\n}\n\nVEB* insert(VEB*V, int x, int u)\n{\n if (!V)\n {\n V = (VEB*) malloc(sizeof(VEB));\n V->min = V->max = x;\n V->u = u;\n V->count = 1;\n if (u > 2)\n {\n V->summary = NULL;\n V->cluster = (VEB**) calloc(sqrt(u), sizeof(VEB*));\n }\n else\n {\n V->summary = NULL;\n V->cluster = NULL;\n }\n }\n else if (V->min == x || V->max == x)\n V->count = 1;\n else\n {\n if (x < V->min)\n {\n if (V->min == V->max)\n {\n V->min = x;\n V->count = 1;\n return V;\n }\n }\n else if (x > V->max)\n {\n int aux = V->max;\n V->max = x;\n x = aux;\n V->count = 1;\n if (V->min == x)\n return V;\n }\n if (V->u > 2)\n {\n if (V->cluster[high(x, V->u)] == NULL)\n V->summary = insert(V->summary, high(x, V->u), sqrt(V->u));\n V->cluster[high(x, V->u)] =\n insert(V->cluster[high(x, V->u)], low(x, V->u), sqrt(V->u));\n }\n }\n return V;\n}\n\nVEB* deleteVEB(VEB*V, int x)\n{\n if (V->min == V->max)\n {\n free(V);\n return NULL;\n }\n else if (x == V->min || x == V->max)\n {\n if (!--V->count)\n {\n if (V->summary)\n {\n int cluster = VEBmin(V->summary);\n int new_min = VEBmin(V->cluster[cluster]);\n V->min = cluster * (int) sqrt(V->u) + new_min;\n V->count = V->cluster[cluster]->count;\n (V->cluster[cluster])->count = 1;\n if ((V->cluster[cluster])->min == (V->cluster[cluster])->max)\n (V->cluster[cluster])->count = 1;\n V->cluster[cluster] = deleteVEB(V->cluster[cluster], new_min);\n if (V->cluster[cluster] == NULL)\n V->summary = deleteVEB(V->summary, cluster);\n }\n else\n {\n V->min = V->max;\n V->count = 1;\n }\n }\n }\n else\n {\n V->cluster[high(x, V->u)] = deleteVEB(V->cluster[high(x, V->u)], low(x, V->u));\n if (V->cluster[high(x, V->u)] == NULL)\n V->summary = deleteVEB(V->summary, high(x, V->u));\n }\n return V;\n}\n\nint elements(VEB*V, int x)\n{\n if (!V)\n return 0;\n else if (V->min == x || V->max == x)\n return V->count;\n else\n {\n if (V->cluster)\n return elements(V->cluster[high(x, V->u)], low(x, V->u));\n else\n return 0;\n }\n}\n\nvoid printVEB(VEB*V, int u)\n{\n for (int i = 0; i < u; ++i)\n printf(\"VEB[%d] = %d\\n\", i, elements(V, i));\n}\n\nint main()\n{\n int u = 4;\n int sqrt_u = sqrt(u);\n VEB* V = NULL;\n\n if (sqrt_u * sqrt_u != u)\n {\n printf(\"Invalid 'u' : Must be a perfect square\\n\");\n return 0;\n }\n\n V = insert(V, 0, u);\n V = insert(V, 1, u);\n V = insert(V, 2, u);\n\n printVEB(V, u);\n printf(\"\\n\\n\");\n\n V = deleteVEB(V, 0);\n V = deleteVEB(V, 1);\n\n printVEB(V, u);\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/segment_tree/generic_segment_tree.cpp",
"content": "/**\n * @file generic_segment_tree.cpp\n * @author Aryan V S (https://github.com/a-r-r-o-w)\n * @brief Implementation of a Generic Segment Tree\n */\n\nnamespace arrow {\n /**\n * @brief Support for two tree memory layouts is provided\n * \n * Binary:\n * - creates the segment tree as a full Binary tree\n * - considering the tree is built over `n` elements, the segment tree would allocate\n * `4.n` memory for internal usage\n * \n * EulerTour:\n * - more memory efficient than Binary segment tree\n * - considering the tree is build over `n` elements, the segment tree would allocate\n * `2.n` memory for internal usage\n * \n * The provided implementation defaults to Binary segment tree if no template parameter is present.\n */\n enum class TreeMemoryLayout {\n Binary,\n EulerTour\n };\n\n /**\n * @brief Generic Segment Tree\n * \n * A segment tree allows you to perform operations on an array in an efficient manner\n * \n * @tparam Type The type of values that the tree holds in its internal nodes\n * @tparam impl_type Memory layout type\n */\n template <\n class Type,\n TreeMemoryLayout impl_type = TreeMemoryLayout::Binary\n >\n class SegmentTree {\n private:\n // Internal members maintained in the segment tree\n std::vector <Type> tree_;\n \n int base_size_;\n const std::vector <Type>& base_ref_;\n\n Type (*combine_) (const Type&, const Type&);\n\n public:\n /**\n * @brief Construct a new segment tree object\n * \n * @param base_size_ Number of leaf nodes segment tree should have (`n` in the tree_memory_layout documentation)\n * @param base Base of the segment tree (leaf node values)\n * @param combine_ Function used to combine_ values of children nodes when building parent nodes\n */\n SegmentTree (int base_size, const std::vector <Type>& base, Type (*combine) (const Type&, const Type&))\n : base_size_ (base_size),\n base_ref_ (base),\n combine_ (combine) {\n if constexpr (impl_type == TreeMemoryLayout::Binary)\n tree_.resize(4 * base_size_, Type{});\n else\n tree_.resize(2 * base_size_, Type{});\n _build_impl(0, 0, base_size_ - 1);\n }\n\n /**\n * @brief Point update in segment tree. Essentially performs `array[position] = value`.\n * \n * @param position Index to be updated\n * @param value Value to be set at `position`\n */\n void pointUpdate (int position, const Type& value)\n { _point_update_impl(0, 0, base_size_ - 1, position, value); }\n\n /**\n * @brief Point query in segment tree. Essentially returns `array[position]`\n * \n * @param position Index to be queried\n * @return Type `array[position]`\n */\n Type pointQuery (int position)\n { return _point_query_impl(0, 0, base_size_ - 1, position); }\n\n /**\n * @brief Range query in segment tree. Essentially performs the `combine_` function\n * on the range `array[l, r]` (bounds are inclusive).\n * \n * @param l Left bound of query (inclusive)\n * @param r Right bound of query (inclusive)\n * @return Type Result of the query based on `combine_`\n */\n Type rangeQuery (int l, int r)\n { return _range_query_impl(0, 0, base_size_ - 1, l, r); }\n\n /**\n * @brief Custom point update in segment tree.\n * \n * The implementation for this method can be added by the user if they would like\n * to perform updates in a different sense from the norm.\n * \n * @tparam ParamTypes Types of parameters that will be passed to the update implementation\n * @param position Index to be updated\n * @param params Parameter list required by custom update implementation\n */\n template <typename... ParamTypes>\n void customPointUpdate (int position, const ParamTypes&... params)\n { _custom_point_update_impl(0, 0, base_size_ - 1, position, params...); }\n\n /**\n * @brief Custom point query in segment tree.\n * \n * The implementation for this method can be added by the user if they would like\n * to perform updates in a different sense from the norm.\n * \n * @tparam ParamTypes Types of parameters that will be passed to the query implementation\n * @param position Index to be queried\n * @param params Parameter list required by custom query implementation\n */\n template <typename... ParamTypes>\n Type customPointQuery (int position, const ParamTypes&... params)\n { _custom_point_query_impl(0, 0, base_size_ - 1, position, params...); }\n\n /**\n * @brief Custom query/update in segment tree.\n * \n * The implementation for this method can be added by the user if they would like\n * to perform queries in a different sense from the norm. It is very flexible with\n * the requirements of the user.\n * \n * This function is flexible in the sense that you can copy the body multiple times and rename\n * it to achieve different functionality as per liking.\n * \n * Operations like `lazy propagation` or `beats` or `persistence` have not been implemented, and\n * have been left to the user to do as per their liking. It can be done very easily by manipulating\n * the body of the _custom_query_impl method (and/or creating copies of this function with different\n * names for different purposes).\n * \n * @tparam ParamTypes Types of parameters that will be passed to the query/update implementation\n * @param params Parameter list required by custom query/update implementation\n */\n template <typename ReturnType, typename... ParamTypes>\n ReturnType customQuery (const ParamTypes&... params)\n { return _custom_query_impl <ReturnType> (0, 0, base_size_ - 1, params...); }\n\n private:\n /**\n * @brief Returns the index of the left child given the parent index based on\n * chosen tree_memory_layout\n * \n * @param v Index of parent\n * @param tl Left bound of parent\n * @param tr Right bound of parent\n * @return int Index of left child of parent\n */\n int _get_leftchild (int v, [[maybe_unused]] int tl, [[maybe_unused]] int tr) {\n if constexpr (impl_type == TreeMemoryLayout::Binary)\n return 2 * v + 1;\n else\n return v + 1;\n }\n\n /**\n * @brief Returns the index of the right child given the parent index based on\n * chosen tree_memory_layout\n * \n * @param v Index of parent\n * @param tl Left bound of parent\n * @param tr Right bound of parent\n * @return int Index of right child of parent\n */\n int _get_rightchild (int v, [[maybe_unused]] int tl, [[maybe_unused]] int tr) {\n if constexpr (impl_type == TreeMemoryLayout::Binary)\n return 2 * v + 2;\n else {\n int tm = (tl + tr) / 2;\n int node_count = tm - tl + 1;\n return v + 2 * node_count;\n }\n }\n\n /**\n * @brief Internal function to build the segment tree\n * \n * @param v Index of node where the segment tree starts to build\n * @param tl Left bound of node\n * @param tr Right bound of node\n */\n void _build_impl (int v, int tl, int tr) {\n if (tl == tr) {\n tree_[v] = base_ref_[tl];\n return;\n }\n\n int tm = (tl + tr) / 2;\n int lchild = _get_leftchild(v, tl, tr);\n int rchild = _get_rightchild(v, tl, tr);\n\n _build_impl(lchild, tl, tm);\n _build_impl(rchild, tm + 1, tr);\n \n tree_[v] = combine_(tree_[lchild], tree_[rchild]);\n }\n\n /**\n * @brief Internal implementation of point_update\n * \n * @param v Current node index\n * @param tl Current node left bound\n * @param tr Current node right bound\n * @param position Index to be updated\n * @param value Value to be set at `position`\n */\n void _point_update_impl (int v, int tl, int tr, int position, const Type& value) {\n if (tl == tr) {\n tree_[v] = value;\n return;\n }\n\n int tm = (tl + tr) / 2;\n int lchild = _get_leftchild(v, tl, tr);\n int rchild = _get_rightchild(v, tl, tr);\n \n // Since we need to only update a single index, we choose the correct\n // \"side\" of the segment tree to traverse to at each step\n if (position <= tm)\n _point_update_impl(lchild, tl, tm, position, value);\n else\n _point_update_impl(rchild, tm + 1, tr, position, value);\n \n tree_[v] = combine_(tree_[lchild], tree_[rchild]);\n }\n\n /**\n * @brief Internal implementation of point_query\n * \n * @param v Current node index\n * @param tl Current node left bound\n * @param tr Current node right bound\n * @param position Index to be updated\n * @return Type Value to be set at `position`\n */\n Type _point_query_impl (int v, int tl, int tr, int position) {\n if (tl == tr)\n return tree_[v];\n \n int tm = (tl + tr) / 2;\n int lchild = _get_leftchild(v, tl, tr);\n int rchild = _get_rightchild(v, tl, tr);\n \n // Since we need to only update a single index, we choose the correct\n // \"side\" of the segment tree to traverse to at each step\n if (position <= tm)\n return _point_query_impl(lchild, tl, tm, position);\n else\n return _point_query_impl(rchild, tm + 1, tr, position);\n }\n\n /**\n * @brief Internal implementation of rangeQuery\n * \n * @param v Current node index\n * @param tl Current node left bound\n * @param tr Current node right bound\n * @param l Current query left bound\n * @param r Current query right bound\n * @return Type Result of the query\n */\n Type _range_query_impl (int v, int tl, int tr, int l, int r) {\n // We are out of bounds in our search\n // Return a sentinel value which must be defaulted in the constuctor of Type\n if (l > r)\n return {};\n \n // We have found the correct range for the query\n // Return the value at current node because it's not required to process further\n if (tl == l and tr == r)\n return tree_[v];\n \n int tm = (tl + tr) / 2;\n int lchild = _get_leftchild(v, tl, tr);\n int rchild = _get_rightchild(v, tl, tr);\n\n // We have not yet found the correct range for the query\n // Get results of left child and right child and combine_\n Type lval = _range_query_impl(lchild, tl, tm, l, std::min(r, tm));\n Type rval = _range_query_impl(rchild, tm + 1, tr, std::max(l, tm + 1), r);\n \n return combine_(lval, rval);\n }\n\n /**\n * @brief Internal implementation of customPointUpdate\n * \n * Note that you need to change the definition of this function if you're passing\n * any variadic argument list in customPointUpdate\n * \n * @param v Current node index\n * @param tl Current node left bound\n * @param tr Current node right bound\n * @param position Index to be updated\n */\n void _custom_point_update_impl (int v, int tl, int tr, int position) {\n if (tl == tr) {\n throw std::runtime_error(\"undefined implementation\");\n return;\n }\n\n int tm = (tl + tr) / 2;\n int lchild = _get_leftchild(v, tl, tr);\n int rchild = _get_rightchild(v, tl, tr);\n\n // Since we need to only update a single index, we choose the correct\n // \"side\" of the segment tree to traverse to at each step\n if (position <= tm)\n _custom_point_update_impl(lchild, tl, tm, position);\n else\n _custom_point_update_impl(rchild, tm + 1, tr, position);\n \n tree_[v] = combine_(tree_[lchild], tree_[rchild]);\n }\n\n /**\n * @brief Internal implementation of customPointQuery\n * \n * Note that you need to change the definition of this function if you're passing\n * any variadic argument list in customPointQuery\n * \n * @param v Current node index\n * @param tl Current node left bound\n * @param tr Current node right bound\n * @param position Index to be queried\n * @return Type Result of the query\n */\n Type _custom_point_query_impl (int v, int tl, int tr, int position) {\n if (tl == tr) {\n throw std::runtime_error(\"undefined implementation\");\n return {};\n }\n\n int tm = (tl + tr) / 2;\n int lchild = _get_leftchild(v, tl, tr);\n int rchild = _get_rightchild(v, tl, tr);\n\n // Since we need to only update a single index, we choose the correct\n // \"side\" of the segment tree to traverse to at each step\n if (position <= tm)\n return _custom_point_query_impl(lchild, tl, tm, position);\n else\n return _custom_point_query_impl(rchild, tm + 1, tr, position);\n }\n\n /**\n * @brief Internal implementation of customQuery\n * \n * The user can create multiple copies with different names for this function and make\n * calls accordingly. Read the documentation for customQuery above.\n * \n * @tparam ReturnType Return type of the custom query/update\n * @param v Current node index\n * @param tl Current node left bound\n * @param tr Current node right bound\n * @return ReturnType Return value of the custom query/update\n */\n template <typename ReturnType>\n ReturnType _custom_query_impl (int v, int tl, int tr) {\n if (tl == tr) {\n throw std::runtime_error(\"undefined implementation\");\n return ReturnType{};\n }\n \n int tm = (tl + tr) / 2;\n int lchild = _get_leftchild(v, tl, tr);\n int rchild = _get_rightchild(v, tl, tr);\n\n // Change functionality as per liking\n if (true)\n return _custom_query_impl <ReturnType> (lchild, tl, tm);\n else\n return _custom_query_impl <ReturnType> (rchild, tm + 1, tr);\n }\n };\n};"
}
{
"filename": "code/data_structures/src/tree/segment_tree/LazySegmentTree.cpp",
"content": "// There are generally three main function for lazy propagation in segment tree\n// Lazy propagation is used when we have range update query too, without it only point \n// update is possible.\n\n// Build function\n\n// THE SEGMENT TREE WRITTEN HERE IS FOR GETTING SUM , FOR MINIMUM, MAXIMUM, XOR AND DISTINCT ELEMENT COUNT RESPECTIVE SEGMENT TREES \n// CAN BE MADE JUST BY REPLACING A FEW LINES\n\n#include <bits/stdc++.h>\nusing namespace std;\n\ntypedef long long ll;\n\nconst int N=1e5;\n\nint seg[4*N],lazy[4*N];\n\nvector<int> arr;\n\nvoid build(int node, int st, int en) {\n if (st == en) {\n // left node ,string the single array element\n seg[node] = arr[st];\n return;\n }\n\n int mid = (st + en) / 2;\n\n // recursively call for left child\n build(2 * node, st, mid);\n\n // recursively call for the right child\n build(2 * node + 1, mid + 1, en);\n\n // Updating the parent with the values of the left and right child.\n seg[node] = seg[2 * node] + seg[2 * node + 1];\n}\n\n//Above, every node represents the sum of both subtrees below it. Build function is called once per array, and the time complexity of build() is O(N).\n\n// Update Operation function\n\nvoid update(int node, int st, int en, int l, int r, int val) {\n if (lazy[node] != 0) // if node is lazy then update it\n {\n seg[node] += (en - st + 1) * lazy[node];\n\n if (st != en) // if its children exist then mark them lazy\n {\n lazy[2 * node] += lazy[node];\n lazy[2 * node + 1] += lazy[node];\n }\n lazy[node] = 0; // No longer lazy\n }\n if ((en < l) || (st > r)) // case 1\n {\n return;\n }\n if (st >= l && en <= r) // case 2\n {\n seg[node] += (en - st + 1) * val;\n if (st != en) {\n lazy[2 * node] += val; // mark its children lazy\n lazy[2 * node + 1] += val;\n }\n return;\n }\n\n // case 3\n int mid = (st + en) / 2;\n\n // recursively call for updating left child\n update(2 * node, st, mid, l, r, val);\n // recursively call for updating right child\n update(2 * node + 1, mid + 1, en, l, r, val);\n\n // Updating the parent with the values of the left and right child.\n seg[node] = (seg[2 * node] + seg[2 * node + 1]);\n}\n\n//Here above we take care of three cases for base case:\n\n// Segment lies outside the query range: in this case, we can just simply return back and terminate the call.\n// Segment lies fully inside the query range: in this case, we simply update the current node and mark the children lazy.\n// If they intersect partially, then we all update for both the child and change the values in them.\n\n// Query function\n\nint query(int node, int st, int en, int l, int r) {\n /*If the node is lazy, update it*/\n if (lazy[node] != 0) {\n\n seg[node] += (en - st + 1) * lazy[node];\n if (st != en) //Check if the child exist\n {\n // mark both the child lazy\n lazy[2 * node] += lazy[node];\n lazy[2 * node + 1] += lazy[node];\n }\n // no longer lazy\n lazy[node] = 0;\n }\n // case 1\n if (en < l || st > r) {\n return 0;\n }\n\n // case 2\n if ((l <= st) && (en <= r)) {\n return seg[node];\n }\n int mid = (st + en) / 2;\n\n //query left child \n ll q1 = query(2 * node, st, mid, l, r);\n\n // query right child\n ll q2 = query(2 * node + 1, mid + 1, en, l, r);\n\n return (q1 + q2);\n}\n\nint main(){\n int n;\n cin >> n;\n arr=vector<int>(n+1);\n\n for(int i=1;i<=n;i++)cin >> arr[i];\n\n memset(seg,0,sizeof seg);\n memset(lazy,0,sizeof lazy);\n\n build(1,1,n);\n\n\n return 0;\n}\n\n// As compared to non-lazy code only lazy code in base case is added. Also one should remember the kazy implementation only as it can\n// help u solve in non-lazy case too.\n\n// Here the insertion, query both take O(logn) time."
}
{
"filename": "code/data_structures/src/tree/segment_tree/segment_tree.c",
"content": "// Program to show segment tree operations like construction, query and update\n#include <stdio.h>\n#include <math.h>\n \n// A utility function to get the middle index from corner indexes.\nint getMid(int s, int e) { return s + (e - s)/2; }\n \n/* A recursive function to get the sum of values in given range of the array.\n The following are parameters for this function.\n \n st --> Pointer to segment tree\n index --> Index of current node in the segment tree. Initially 0 is\n passed as root is always at index 0\n ss & se --> Starting and ending indexes of the segment represented by\n current node, i.e., st[index]\n qs & qe --> Starting and ending indexes of query range */\nint getSumUtil(int *st, int ss, int se, int qs, int qe, int index)\n{\n // If segment of this node is a part of given range, then return the \n // sum of the segment\n if (qs <= ss && qe >= se)\n return st[index];\n \n // If segment of this node is outside the given range\n if (se < qs || ss > qe)\n return 0;\n \n // If a part of this segment overlaps with the given range\n int mid = getMid(ss, se);\n return getSumUtil(st, ss, mid, qs, qe, 2*index+1) +\n getSumUtil(st, mid+1, se, qs, qe, 2*index+2);\n}\n \n/* A recursive function to update the nodes which have the given index in\n their range. The following are parameters\n st, index, ss and se are same as getSumUtil()\n i --> index of the element to be updated. This index is in input array.\n diff --> Value to be added to all nodes which have i in range */\nvoid updateValueUtil(int *st, int ss, int se, int i, int diff, int index)\n{\n // Base Case: If the input index lies outside the range of this segment\n if (i < ss || i > se)\n return;\n \n // If the input index is in range of this node, then update the value\n // of the node and its children\n st[index] = st[index] + diff;\n if (se != ss)\n {\n int mid = getMid(ss, se);\n updateValueUtil(st, ss, mid, i, diff, 2*index + 1);\n updateValueUtil(st, mid+1, se, i, diff, 2*index + 2);\n }\n}\n \n// The function to update a value in input array and segment tree.\n// It uses updateValueUtil() to update the value in segment tree\nvoid updateValue(int arr[], int *st, int n, int i, int new_val)\n{\n // Check for erroneous input index\n if (i < 0 || i > n-1)\n {\n printf(\"Invalid Input\");\n return;\n }\n \n // Get the difference between new value and old value\n int diff = new_val - arr[i];\n \n // Update the value in array\n arr[i] = new_val;\n \n // Update the values of nodes in segment tree\n updateValueUtil(st, 0, n-1, i, diff, 0);\n}\n \n// Return sum of elements in range from index qs (quey start) to\n// qe (query end). It mainly uses getSumUtil()\nint getSum(int *st, int n, int qs, int qe)\n{\n // Check for erroneous input values\n if (qs < 0 || qe > n-1 || qs > qe)\n {\n printf(\"Invalid Input\");\n return -1;\n }\n \n return getSumUtil(st, 0, n-1, qs, qe, 0);\n}\n \n// A recursive function that constructs Segment Tree for array[ss..se].\n// si is index of current node in segment tree st\nint constructSTUtil(int arr[], int ss, int se, int *st, int si)\n{\n // If there is one element in array, store it in current node of\n // segment tree and return\n if (ss == se)\n {\n st[si] = arr[ss];\n return arr[ss];Karan katyal\n }\n \n // If there are more than one elements, then recur for left and\n // right subtrees and store the sum of values in this node\n int mid = getMid(ss, se);\n st[si] = constructSTUtil(arr, ss, mid, st, si*2+1) +\n constructSTUtil(arr, mid+1, se, st, si*2+2);\n return st[si];\n}\n \n/* Function to construct segment tree from given array. This function\n allocates memory for segment tree and calls constructSTUtil() to\n fill the allocated memory */\nint *constructST(int arr[], int n)\n{\n // Allocate memory for segment tree\n int x = (int)(ceil(log2(n))); //Height of segment tree\n int max_size = 2*(int)pow(2, x) - 1; //Maximum size of segment tree\n int *st = new int[max_size];\n \n // Fill the allocated memory st\n constructSTUtil(arr, 0, n-1, st, 0);\n \n // Return the constructed segment tree\n return st;\n}\n \n// Driver program to test above functions\nint main()\n{\n int arr[] = {1, 3, 5, 7, 9, 11};\n int n = sizeof(arr)/sizeof(arr[0]);\n \n // Build segment tree from given array\n int *st = constructST(arr, n);\n \n // Print sum of values in array from index 1 to 3\n printf(\"Sum of values in given range = %d\\n\", getSum(st, n, 1, 3));\n \n // Update: set arr[1] = 10 and update corresponding segment\n // tree nodes\n updateValue(arr, st, n, 1, 10);\n \n // Find sum after the value is updated\n printf(\"Updated sum of values in given range = %d\\n\",\n getSum(st, n, 1, 3));\n \n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/segment_tree/segment_tree.java",
"content": "class SegmentTree {\n private int seg_t[]; // An array to store the segment tree implementation\n \n /**\n * Constructor which takes the size of the array and the array as a parameter and constructs the segment tree\n * @author Kanakalatha Vemuru (https://github.com/KanakalathaVemuru)\n * @param n is the size of the array \n * @param arr is the array on which segment tree has to be constructed\n */\n public SegmentTree(int n, int arr[]) {\n int x = (int) (Math.ceil(Math.log(n) / Math.log(2)));\n int seg_size = 2 * (int) Math.pow(2, x) - 1;\n\n this.seg_t = new int[seg_size];\n constructTree(arr, 0, n - 1, 0);\n }\n \n /** A function which will create the segment tree\n * @author Kanakalatha Vemuru (https://github.com/KanakalathaVemuru)\n * @param arr the array on which segment tree has to be constructed\n * @param start an integer representing the start of the segment represented by current node\n * @param end an integer representing the end of the segment represented by current node\n * @param index the integer representing the index of current node in segment tree\n * @return an integer representing the sum of the current segment \n */\n public int constructTree(int[] arr, int start, int end, int index) {\n if (start == end) {\n this.seg_t[index] = arr[start];\n return arr[start];\n }\n \n int mid = start + (end - start) / 2;\n this.seg_t[index] = constructTree(arr, start, mid, index*2 + 1) +\n constructTree(arr, mid + 1, end, index*2 + 2);\n return this.seg_t[index];\n }\n \n \n /** A function which will update the value at a index i. This will be called by the\n update function internally\n * @author Kanakalatha Vemuru (https://github.com/KanakalathaVemuru)\n * @param start an integer representing the start of the segment represented by current node\n * @param end an integer representing the end of the segment represented by current node\n * @param index an integer representing the index whose value has to be updated in the array\n * @param diff the difference between the value of previous value and updated value\n * @param seg_index the integer representing the index of current node in segment tree\n */\n private void updateValueUtil(int start, int end, int index, int diff, int seg_index) {\n if (index < start || index > end) {\n return;\n }\n \n this.seg_t[seg_index] += diff;\n if (start != end) {\n int mid = start + (end - start) / 2;\n updateValueUtil(start, mid, index, diff, seg_index*2 + 1);\n updateValueUtil(mid + 1, end, index, diff, seg_index*2 + 2);\n }\n }\n \n /** A function to update the value at a particular index\n * @author Kanakalatha Vemuru (https://github.com/KanakalathaVemuru)\n * @param n is the size of the array \n * @param arr is the array on which segment tree has to be constructed\n * @param index the integer representing the index whose value to be updated\n * @param value the integer representing the updated value \n */\n public void updateValue(int[] arr, int n, int index, int value) {\n if (index < 0 || index > n) {\n return;\n }\n \n int diff = value - arr[index];\n arr[index] = value;\n updateValueUtil(0, n - 1, index, diff, 0);\n }\n \n /** A function to get the sum of the elements from index q_start to index q_end. This will be called internally\n * @author Kanakalatha Vemuru (https://github.com/KanakalathaVemuru)\n * @param start an integer representing the start of the segment represented by current node\n * @param end an integer representing the end of the segment represented by current node\n * @param q_start an integer representing the start of the segment whose sum we are quering\n * @param q_end an integer representing the end of the segment whose sum we are quering\n * @param seg_index the integer representing the index of current node in segment tree\n * @return an integer representing the sum of the segment q_start to q_end\n */\n private int getSumUtil(int start, int end, int q_start, int q_end, int seg_index) {\n if (q_start <= start && q_end >= end) {\n return this.seg_t[seg_index];\n }\n \n if (q_start > end || q_end < start) {\n return 0;\n }\n \n int mid = start + (end - start)/2;\n return getSumUtil(start, mid, q_start, q_end, seg_index*2 + 1) + getSumUtil(mid + 1, end, q_start, q_end, seg_index*2 + 2);\n }\n \n /** A function to query the sum of the segment [start...end]\n * @author Kanakalatha Vemuru (https://github.com/KanakalathaVemuru)\n * @param n an integer representing the length of the array\n * @param start an integer representing the start of the segment whose sum we are quering\n * @param end an integer representing the end of the segment whose sum we are quering\n * @return an integer representing the sum of the segment start to end\n */\n public int getSum(int n, int start, int end) {\n if (start < 0 || end > n || start > end) {\n return 0;\n }\n return getSumUtil(0, n-1, start, end, 0);\n }\n\n // Driver program for testing\n public static void main(String args[])\n {\n int arr[] = {1, 3, 5, 7, 9, 11};\n int n = arr.length;\n \n SegmentTree st = new SegmentTree(n, arr);\n \n // Print sum of values in array from index 1 to 3\n System.out.println(\"Sum of values in given range = \" + st.getSum(n, 1, 3) + \".\\n\");\n \n // Update: set arr[1] = 10 and update corresponding segment\n // tree nodes\n st.updateValue(arr, n, 1, 10);\n \n // Find sum after the value is updated\n System.out.println(\"Updated sum of values in given range = \" + st.getSum(n, 1, 3) + \".\\n\");\n }\n\n}"
}
{
"filename": "code/data_structures/src/tree/segment_tree/segment_tree_optimized.cpp",
"content": "// Program to show optimised segment tree operations like construction, sum query and update\n#include <iostream>\nusing namespace std;\n\n/*Parameters for understanding code easily:\nN = size of the array for which we are making the segment tree\narr= Given array, it can be of any size\ntree=Array for the segment tree representation*/\n\nvoid createTreeOp(int *arr, int *tree, int size)\n{\n // First the array elements are fixed in the tree array from N index to 2N-1 index\n for (int i = size; i < 2 * size; i++)\n {\n tree[i] = arr[i - size];\n }\n // Now tree elements are inserted by taking sum of nodes on children index from index N-1 to 1\n for (int i = size - 1; i > 0; i--)\n {\n tree[i] = tree[2 * i] + tree[2 * i + 1];\n }\n // This approach only requires atmost 2N elements to make segment tree of N sized array\n}\n\nint sumOp(int *tree, int start, int end, int size)\n{\n start += size;\n end += size;\n int sum = 0;\n\n /*Range is set [size+start,size+end] in the beginning. At\n each step range is moved up and the value do not belonging\n to higher range are added.*/\n while (start <= end)\n {\n if (start % 2 == 1)\n {\n sum += tree[start++];\n }\n if (end % 2 == 0)\n {\n sum += tree[end--];\n }\n start = start / 2;\n end = end / 2;\n }\n return sum;\n}\n\nvoid update(int *tree, int index, int size, int newVal)\n{\n index = size + index;\n int incr = newVal - tree[index];\n // First calculate the increase in the value required in the element after the updating\n while (index >= 1)\n {\n tree[index] += incr;\n index = index / 2;\n }\n // Loop to increment the value incr in the path from root to required indexed leaf node\n}\n\nint main()\n{\n int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};\n int *tree = new int[20];\n /* In this approach we only require 2N sized tree array for storing the segment\n tree and all the operations can be achieved in iterative methods.*/\n createTreeOp(arr, tree, 10);\n cout<<\"SEGMENT TREE\"<<endl;\n for (int i = 1; i < 20; i++)\n {\n cout << tree[i] << \" \";\n }\n cout << endl<<endl;\n cout <<\"The sum of the segment from index to 3 to 5 \"<<sumOp(tree, 3, 5, 10)<<endl;\n update(tree, 0, 10, -1);\n cout <<\"Updating the value at index 0 with -1\"<<endl;\n cout<<endl<<\"SEGMENT TREE\"<<endl;\n for (int i = 1; i < 20; i++)\n {\n cout << tree[i] << \" \";\n }\n}"
}
{
"filename": "code/data_structures/src/tree/segment_tree/segment_tree_rmq.adb",
"content": "with Ada.Integer_Text_IO, Ada.Text_IO;\nuse Ada.Integer_Text_IO, Ada.Text_IO;\n-- Compile:\n-- gnatmake segment_Tree_rmq\n\n\nprocedure segment_Tree_rmq is\n\nINT_MAX: constant integer := 999999;\nMAXN : constant integer := 100005;\nv : array(0..MAXN) of integer;\ntree: array(0..4*MAXN) of integer;\nn : integer;\nq : integer;\na : integer;\nb : integer;\nc : integer;\n\nfunction min(a: integer; b: integer) return integer is\n\nbegin\n\t\n\tif a < b then return a;\n\telse return b;\n\tend if;\nend min;\n\nprocedure build(node: integer; l: integer; r: integer) is\n\n\tmid: integer;\n\nbegin\n\n\tmid := (l + r)/2;\n\t\n\tif l = r then\n\t\ttree(node) := v(l);\n\t\treturn;\n\tend if;\n\n\tbuild(2 * node, l, mid);\n\tbuild(2*node+1,mid+1,r);\n\ttree(node) := min( tree(2*node), tree(2*node + 1)); \nend build;\n\n\n--Update procedure\nprocedure update(node: integer; l: integer; r: integer; pos: integer; val: integer) is\n\n\tmid: integer := (l + r)/2;\n\nbegin\n\t\n\tif l > pos or r < pos or l > r then\n\t\treturn;\n\tend if;\n\n\tif(l = r) then\n\t\ttree(node) := val;\n\t\treturn;\n\tend if;\n\n\tif pos <= mid then\n\t\tupdate(2*node,l,mid,pos,val);\n\telse\n\t\tupdate(2*node+1,mid+1,r,pos,val);\n\tend if;\n\ttree(node) := min( tree(2*node), tree(2*node + 1)); \nend update;\n\n\n--Query function\nfunction query(node : integer; l: integer; r: integer; x: integer; y: integer) return integer is\n\t\n\tmid: integer := (l + r)/2;\n\tp1: integer; \n\tp2: integer; \nbegin\n\n\tif l > r or l > y or r < x then\n\t\treturn INT_MAX;\n\tend if;\n\tif x <= l and r <= y then\n\t\treturn tree(node);\n\tend if;\n\n\tp1 := query(2*node,l,mid,x,y);\n\tp2 := query(2*node+1,mid+1,r,x,y);\n\n\tif p1 = INT_MAX then \n\t\treturn p2;\n\tend if;\n\n\tif p2 = INT_MAX then\n\t\treturn p1;\n\tend if;\n\n\treturn min(p1, p2);\n\nend query;\n\n\nbegin\n\tPut_Line(\"Input the array range\");\n\tGet(n);\n\tPut_Line(\"Input the values\");\n\tfor i in 1..n loop\n\t\tGet(v(i));\n\tend loop;\n\n\n\tbuild(1,0,n-1);\n\n\tPut_Line(\"Input the number of operations\");\n\n\tGet(q);\n\t\n\twhile q > 0 loop\n\t\tPut_Line(\"Input 0 to query and 1 to update\");\n\t\tGet(a);\n\t\tPut_Line(\"Input the STARTING index of the query range\");\n\t\tGet(b);\n\t\tPut_Line(\"Input the ENDING index of the query range\");\n\t\tGet(c);\n\n\t\tif a = 0 then \n\t\t\tPut_Line(\"Minimum value of the given range\");\n\t\t\tPut(query(1,1,n,b,c));\n\t\t\tPut_Line(\"\");\n\t\telsif a = 1 then\n\t\t\tupdate(1,1,n,b,c);\n\t\telse\n\t\t\tPut_Line(\"Invalid Operation\");\n\t\t\tq := q + 1;\n\t\tend if;\n\t\tq := q - 1;\n\n\tend loop;\nend segment_Tree_rmq;"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/interval_tree/interval_tree.cpp",
"content": "//Part of Cosmos by OpenGenus Foundation\n\n#include <iostream>\nusing namespace std;\n\nstruct Interval\n{\n int low, high;\n};\n\n\nstruct ITNode\n{\n Interval *i;\n int max;\n ITNode *left, *right;\n};\n\n\nITNode * newNode(Interval i)\n{\n ITNode *temp = new ITNode;\n temp->i = new Interval(i);\n temp->max = i.high;\n temp->left = temp->right = NULL;\n return temp;\n};\n\n\nITNode *insert(ITNode *root, Interval i)\n{\n\n if (root == NULL)\n return newNode(i);\n\n\n int l = root->i->low;\n\n\n if (i.low < l)\n root->left = insert(root->left, i);\n\n\n else\n root->right = insert(root->right, i);\n\n\n if (root->max < i.high)\n root->max = i.high;\n\n return root;\n}\n\n\nbool doOVerlap(Interval i1, Interval i2)\n{\n if (i1.low <= i2.high && i2.low <= i1.high)\n return true;\n return false;\n}\n\nInterval *overlapSearch(ITNode *root, Interval i)\n{\n\n if (root == NULL)\n return NULL;\n\n\n if (doOVerlap(*(root->i), i))\n return root->i;\n\n\n if (root->left != NULL && root->left->max >= i.low)\n return overlapSearch(root->left, i);\n\n\n return overlapSearch(root->right, i);\n}\n\nvoid inorder(ITNode *root)\n{\n if (root == NULL)\n return;\n\n inorder(root->left);\n\n cout << \"[\" << root->i->low << \", \" << root->i->high << \"]\"\n << \" max = \" << root->max << endl;\n\n inorder(root->right);\n}\n\n\nint main()\n{\n\n Interval ints[] = {{15, 20}, {10, 30}, {17, 19},\n {5, 20}, {12, 15}, {30, 40}\n };\n int n = sizeof(ints) / sizeof(ints[0]);\n ITNode *root = NULL;\n for (int i = 0; i < n; i++)\n root = insert(root, ints[i]);\n\n cout << \"Inorder traversal of constructed Interval Tree is\\n\";\n inorder(root);\n\n Interval x = {6, 7};\n\n cout << \"\\nSearching for interval [\" << x.low << \",\" << x.high << \"]\";\n Interval *res = overlapSearch(root, x);\n if (res == NULL)\n cout << \"\\nNo Overlapping Interval\";\n else\n cout << \"\\nOverlaps with [\" << res->low << \", \" << res->high << \"]\";\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/interval_tree/interval_tree.java",
"content": "//This is a java program to implement a interval tree\nimport java.util.ArrayList;\nimport java.util.List;\nimport java.util.Map.Entry;\nimport java.util.SortedMap;\nimport java.util.SortedSet;\nimport java.util.TreeMap;\nimport java.util.TreeSet;\n \nclass Interval<Type> implements Comparable<Interval<Type>>\n{\n \n private long start;\n private long end;\n private Type data;\n \n public Interval(long start, long end, Type data)\n {\n this.start = start;\n this.end = end;\n this.data = data;\n }\n \n public long getStart()\n {\n return start;\n }\n \n public void setStart(long start)\n {\n this.start = start;\n }\n \n public long getEnd()\n {\n return end;\n }\n \n public void setEnd(long end)\n {\n this.end = end;\n }\n \n public Type getData()\n {\n return data;\n }\n \n public void setData(Type data)\n {\n this.data = data;\n }\n \n public boolean contains(long time)\n {\n return time < end && time > start;\n }\n \n public boolean intersects(Interval<?> other)\n {\n return other.getEnd() > start && other.getStart() < end;\n }\n \n public int compareTo(Interval<Type> other)\n {\n if (start < other.getStart())\n return -1;\n else if (start > other.getStart())\n return 1;\n else if (end < other.getEnd())\n return -1;\n else if (end > other.getEnd())\n return 1;\n else\n return 0;\n }\n \n}\n \nclass IntervalNode<Type>\n{\n \n private SortedMap<Interval<Type>, List<Interval<Type>>> intervals;\n private long center;\n private IntervalNode<Type> leftNode;\n private IntervalNode<Type> rightNode;\n \n public IntervalNode()\n {\n intervals = new TreeMap<Interval<Type>, List<Interval<Type>>>();\n center = 0;\n leftNode = null;\n rightNode = null;\n }\n \n public IntervalNode(List<Interval<Type>> intervalList)\n {\n \n intervals = new TreeMap<Interval<Type>, List<Interval<Type>>>();\n \n SortedSet<Long> endpoints = new TreeSet<Long>();\n \n for (Interval<Type> interval : intervalList)\n {\n endpoints.add(interval.getStart());\n endpoints.add(interval.getEnd());\n }\n \n long median = getMedian(endpoints);\n center = median;\n \n List<Interval<Type>> left = new ArrayList<Interval<Type>>();\n List<Interval<Type>> right = new ArrayList<Interval<Type>>();\n \n for (Interval<Type> interval : intervalList)\n {\n if (interval.getEnd() < median)\n left.add(interval);\n else if (interval.getStart() > median)\n right.add(interval);\n else\n {\n List<Interval<Type>> posting = intervals.get(interval);\n if (posting == null)\n {\n posting = new ArrayList<Interval<Type>>();\n intervals.put(interval, posting);\n }\n posting.add(interval);\n }\n }\n \n if (left.size() > 0)\n leftNode = new IntervalNode<Type>(left);\n if (right.size() > 0)\n rightNode = new IntervalNode<Type>(right);\n }\n \n public List<Interval<Type>> stab(long time)\n {\n List<Interval<Type>> result = new ArrayList<Interval<Type>>();\n \n for (Entry<Interval<Type>, List<Interval<Type>>> entry : intervals\n .entrySet())\n {\n if (entry.getKey().contains(time))\n for (Interval<Type> interval : entry.getValue())\n result.add(interval);\n else if (entry.getKey().getStart() > time)\n break;\n }\n \n if (time < center && leftNode != null)\n result.addAll(leftNode.stab(time));\n else if (time > center && rightNode != null)\n result.addAll(rightNode.stab(time));\n return result;\n }\n \n public List<Interval<Type>> query(Interval<?> target)\n {\n List<Interval<Type>> result = new ArrayList<Interval<Type>>();\n \n for (Entry<Interval<Type>, List<Interval<Type>>> entry : intervals\n .entrySet())\n {\n if (entry.getKey().intersects(target))\n for (Interval<Type> interval : entry.getValue())\n result.add(interval);\n else if (entry.getKey().getStart() > target.getEnd())\n break;\n }\n \n if (target.getStart() < center && leftNode != null)\n result.addAll(leftNode.query(target));\n if (target.getEnd() > center && rightNode != null)\n result.addAll(rightNode.query(target));\n return result;\n }\n \n public long getCenter()\n {\n return center;\n }\n \n public void setCenter(long center)\n {\n this.center = center;\n }\n \n public IntervalNode<Type> getLeft()\n {\n return leftNode;\n }\n \n public void setLeft(IntervalNode<Type> left)\n {\n this.leftNode = left;\n }\n \n public IntervalNode<Type> getRight()\n {\n return rightNode;\n }\n \n public void setRight(IntervalNode<Type> right)\n {\n this.rightNode = right;\n }\n \n private Long getMedian(SortedSet<Long> set)\n {\n int i = 0;\n int middle = set.size() / 2;\n for (Long point : set)\n {\n if (i == middle)\n return point;\n i++;\n }\n return null;\n }\n \n @Override\n public String toString()\n {\n StringBuffer sb = new StringBuffer();\n sb.append(center + \": \");\n for (Entry<Interval<Type>, List<Interval<Type>>> entry : intervals\n .entrySet())\n {\n sb.append(\"[\" + entry.getKey().getStart() + \",\"\n + entry.getKey().getEnd() + \"]:{\");\n for (Interval<Type> interval : entry.getValue())\n {\n sb.append(\"(\" + interval.getStart() + \",\" + interval.getEnd()\n + \",\" + interval.getData() + \")\");\n }\n sb.append(\"} \");\n }\n return sb.toString();\n }\n \n}\n \nclass IntervalTree<Type>\n{\n \n private IntervalNode<Type> head;\n private List<Interval<Type>> intervalList;\n private boolean inSync;\n private int size;\n \n public IntervalTree()\n {\n this.head = new IntervalNode<Type>();\n this.intervalList = new ArrayList<Interval<Type>>();\n this.inSync = true;\n this.size = 0;\n }\n \n public IntervalTree(List<Interval<Type>> intervalList)\n {\n this.head = new IntervalNode<Type>(intervalList);\n this.intervalList = new ArrayList<Interval<Type>>();\n this.intervalList.addAll(intervalList);\n this.inSync = true;\n this.size = intervalList.size();\n }\n \n public List<Type> get(long time)\n {\n List<Interval<Type>> intervals = getIntervals(time);\n List<Type> result = new ArrayList<Type>();\n for (Interval<Type> interval : intervals)\n result.add(interval.getData());\n return result;\n }\n \n public List<Interval<Type>> getIntervals(long time)\n {\n build();\n return head.stab(time);\n }\n \n public List<Type> get(long start, long end)\n {\n List<Interval<Type>> intervals = getIntervals(start, end);\n List<Type> result = new ArrayList<Type>();\n for (Interval<Type> interval : intervals)\n result.add(interval.getData());\n return result;\n }\n \n public List<Interval<Type>> getIntervals(long start, long end)\n {\n build();\n return head.query(new Interval<Type>(start, end, null));\n }\n \n public void addInterval(Interval<Type> interval)\n {\n intervalList.add(interval);\n inSync = false;\n }\n \n public void addInterval(long begin, long end, Type data)\n {\n intervalList.add(new Interval<Type>(begin, end, data));\n inSync = false;\n }\n \n public boolean inSync()\n {\n return inSync;\n }\n \n public void build()\n {\n if (!inSync)\n {\n head = new IntervalNode<Type>(intervalList);\n inSync = true;\n size = intervalList.size();\n }\n }\n \n public int currentSize()\n {\n return size;\n }\n \n public int listSize()\n {\n return intervalList.size();\n }\n \n @Override\n public String toString()\n {\n return nodeString(head, 0);\n }\n \n private String nodeString(IntervalNode<Type> node, int level)\n {\n if (node == null)\n return \"\";\n \n StringBuffer sb = new StringBuffer();\n for (int i = 0; i < level; i++)\n sb.append(\"\\t\");\n sb.append(node + \"\\n\");\n sb.append(nodeString(node.getLeft(), level + 1));\n sb.append(nodeString(node.getRight(), level + 1));\n return sb.toString();\n }\n}\n \npublic class IntervalTreeProblem\n{\n public static void main(String args[])\n {\n IntervalTree<Integer> it = new IntervalTree<Integer>();\n \n it.addInterval(0L, 10L, 1);\n it.addInterval(20L, 30L, 2);\n it.addInterval(15L, 17L, 3);\n it.addInterval(25L, 35L, 4);\n \n List<Integer> result1 = it.get(5L);\n List<Integer> result2 = it.get(10L);\n List<Integer> result3 = it.get(29L);\n List<Integer> result4 = it.get(5L, 15L);\n \n System.out.print(\"\\nIntervals that contain 5L:\");\n for (int r : result1)\n System.out.print(r + \" \");\n \n System.out.print(\"\\nIntervals that contain 10L:\");\n for (int r : result2)\n System.out.print(r + \" \");\n \n System.out.print(\"\\nIntervals that contain 29L:\");\n for (int r : result3)\n System.out.print(r + \" \");\n \n System.out.print(\"\\nIntervals that intersect (5L,15L):\");\n for (int r : result4)\n System.out.print(r + \" \");\n }\n}"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/interval_tree/README.md",
"content": "# Cosmos\n\nCollaborative effort by [OpenGenus](https://github.com/OpenGenus/cosmos)"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/kd_tree/kd_tree.cpp",
"content": "#include <iostream>\nusing namespace std;\n// Part of Cosmos by OpenGenus Foundation\nconst int k = 2;\n\nstruct Node\n{\n int point[k]; // To store k dimensional point\n Node *left, *right;\n};\n\nstruct Node* newNode(int arr[])\n{\n struct Node* temp = new Node;\n\n for (int i = 0; i < k; i++)\n temp->point[i] = arr[i];\n\n temp->left = temp->right = NULL;\n return temp;\n}\n\nNode *insertRec(Node *root, int point[], unsigned depth)\n{\n if (root == NULL)\n return newNode(point);\n unsigned cd = depth % k;\n if (point[cd] < (root->point[cd]))\n root->left = insertRec(root->left, point, depth + 1);\n else\n root->right = insertRec(root->right, point, depth + 1);\n return root;\n}\n\nNode* insert(Node *root, int point[])\n{\n return insertRec(root, point, 0);\n}\n\nbool arePointsSame(int point1[], int point2[])\n{\n for (int i = 0; i < k; ++i)\n if (point1[i] != point2[i])\n return false;\n\n return true;\n}\n\nbool searchRec(Node* root, int point[], unsigned depth)\n{\n if (root == NULL)\n return false;\n if (arePointsSame(root->point, point))\n return true;\n\n unsigned cd = depth % k;\n\n if (point[cd] < root->point[cd])\n return searchRec(root->left, point, depth + 1);\n\n return searchRec(root->right, point, depth + 1);\n}\n\nbool search(Node* root, int point[])\n{\n return searchRec(root, point, 0);\n}\n\nint main()\n{\n struct Node *root = NULL;\n int points[][k] = {{3, 6}, {17, 15}, {13, 15}, {6, 12},\n {9, 1}, {2, 7}, {10, 19}};\n\n int n = sizeof(points) / sizeof(points[0]);\n\n for (int i = 0; i < n; i++)\n root = insert(root, points[i]);\n\n int point1[] = {10, 19};\n (search(root, point1)) ? cout << \"Found\\n\" : cout << \"Not Found\\n\";\n\n int point2[] = {12, 19};\n (search(root, point2)) ? cout << \"Found\\n\" : cout << \"Not Found\\n\";\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/kd_tree/kd_tree.java",
"content": "import java.util.Comparator;\nimport java.util.HashSet;\n\npublic class KDTree\n{\n\tint k;\n\tKDNode root;\n\t\n\tpublic KDTree(KDPoint[] points)\n\t{\n\t\tk = 2;\n\t\troot = createNode(points,0);\n\t}\n\t\n\tpublic KDNode createNode(KDPoint[] points,int level)\n\t{\n\t\tif(points.length == 0) return null;\n\t\tint axis = level % k;\n\t\tKDPoint median = null;\n\t\tComparator<KDPoint> comparator = KDPoint.getComparator(axis);\n\t\tmedian = quickSelect(points,0,points.length-1,points.length/2+1,comparator);\n\t\tKDNode node = new KDNode(median,level);\n\t\tif(points.length>1){\n\t\t\tint tam = 0;\n\t\t\tfor(int i=0;i<points.length;i++) if(comparator.compare(points[i], median)<0) tam++;\n\t\t\tKDPoint[] p1 = new KDPoint[tam];\n\t\t\tKDPoint[] p2 = new KDPoint[points.length-tam-1];\n\t\t\tint i1 = 0;\n\t\t\tint i2 = 0;\n\t\t\tfor(int i=0;i<points.length;i++){\n\t\t\t\tif(points[i].equals(median)) continue;\n\t\t\t\telse if(comparator.compare(points[i], median)<0) p1[i1++] = points[i];\n\t\t\t\telse p2[i2++] = points[i];\n\t\t\t}\n\t\t\tKDNode l = createNode(p1, level+1);\n\t\t\tKDNode r = createNode(p2,level+1);\n\t\t\tif(l!=null) l.parent = node;\n\t\t\tif(r!=null) r.parent = node;\n\t\t\tnode.left = l;\n\t\t\tnode.right = r;\n\t\t}\n\t\treturn node;\n\t}\n\t\n\tpublic int nearestNeighbor(KDPoint p,KDNode r,HashSet<KDNode> visitados)\n\t{\t\t\n\t\tKDNode node = r;\n\t\tint res = Integer.MAX_VALUE;\n\t\tKDNode prev = null;\n\t\twhile(node!=null){\n\t\t\tint axis = node.level%k;\n\t\t\tComparator<KDPoint> comparator = KDPoint.getComparator(axis);\n\t\t\tprev = node;\n\t\t\tif(comparator.compare(p,node.point)<0 && node.left!=null) node = node.left;\n\t\t\telse if(comparator.compare(p,node.point)>0 && node.right!=null) node = node.right;\n\t\t\telse node = node.left!=null ? node.left:node.right;\n\t\t}\n\t\tnode = prev;\n\t\twhile(true){\n\t\t\tif(!node.point.equals(p)) res = Math.min(res, p.distance(node.point));\n\t\t\tint axis = node.level % k;\n\t\t\tKDNode r2 = null;\n\t\t\tif(Math.abs(p.coordinates[axis]-node.point.coordinates[axis])<res){\n\t\t\t\tr2 = prev !=null && node.left!=null && prev.equals(node.left) ? node.right:node.left; \n\t\t\t}\n\t\t\tif(r2 != null && !visitados.contains(r2)){\n\t\t\t\tres = Math.min(res, nearestNeighbor(p, r2,visitados));\n\t\t\t}\n\t\t\tvisitados.add(node);\n\t\t\tif(node.equals(r)) break;\n\t\t\tprev = node;\n\t\t\tnode = node.parent;\n\t\t}\n\t\treturn res;\n\t}\n\t\n\tpublic KDPoint quickSelect(KDPoint[] points,int left,int right,int k,Comparator<KDPoint> c)\n\t{\n\t\twhile(true){\n\t\t\tint pivotIndex = left+right/2;\n\t\t\tint pivotNewIndex = partition(points,left,right,pivotIndex,c);\n\t\t\tint pivotDist = pivotNewIndex - left + 1;\n\t\t\tif(pivotDist == k){\n\t\t\t\treturn points[pivotNewIndex];\n\t\t\t}else if(k<pivotDist){\n\t\t\t\tright= pivotNewIndex - 1;\n\t\t\t}else{\n\t\t\t\tk=k-pivotDist;\n\t\t\t\tleft=pivotNewIndex + 1;\n\t\t\t}\n\t\t}\n\t}\n\t\n\tpublic int partition(KDPoint[] points,int left,int right,int pivotIndex,Comparator<KDPoint> c)\n\t{\n\t\tKDPoint pivotValue = points[pivotIndex];\n\t\tKDPoint aux = points[pivotIndex];\n\t\tpoints[pivotIndex] = points[right];\n\t\tpoints[right] = aux;\n\t\tint storeIndex = left;\n\t\tfor(int i=left;i<right;i++){\n\t\t\tif(c.compare(points[i],pivotValue)<0){\n\t\t\t\taux = points[storeIndex];\n\t\t\t\tpoints[storeIndex] = points[i];\n\t\t\t\tpoints[i] = aux;\n\t\t\t\tstoreIndex++;\n\t\t\t}\n\t\t}\n\t\taux = points[right];\n\t\tpoints[right] = points[storeIndex];\n\t\tpoints[storeIndex] = aux;\n\t\treturn storeIndex;\n\t}\t\n\t\n\tpublic class KDNode\n\t{\n\t\tKDPoint point;\n\t\tKDNode parent;\n\t\tKDNode left;\n\t\tKDNode right;\n\t\tint level;\n\t\t\n\t\tpublic KDNode(KDPoint p,int l)\n\t\t{\n\t\t\tpoint = p;\n\t\t\tlevel = l;\n\t\t}\n\t\t\n\t\tpublic boolean equals(Object o)\n\t\t{\n\t\t\tKDNode n = (KDNode) o;\n\t\t\treturn point.equals(n.point);\n\t\t}\n\t\t\n\t\tpublic String toString()\n\t\t{\n\t\t\treturn point.toString();\n\t\t}\n\t}\n\t\n\tpublic static class KDPoint\n\t{\n\t\tint[] coordinates;\n\t\t\n\t\tpublic KDPoint(int... coordinates)\n\t\t{\n\t\t\tthis.coordinates = coordinates;\n\t\t}\n\t\t\n\t\tpublic static Comparator<KDPoint> getComparator(int axis)\n\t\t{\n\t\t\tfinal int a = axis;\n\t\t\treturn new Comparator<KDPoint>(){\n\t\t\t\tpublic int compare(KDPoint n1,KDPoint n2){\n\t\t\t\t\treturn n1.coordinates[a] - n2.coordinates[a];\n\t\t\t\t}\n\t\t\t};\n\t\t}\n\t\t\n\t\tpublic double euclideandistance(KDPoint p)\n\t\t{\n\t\t\tdouble res = 0;\n\t\t\tfor(int i=0;i<coordinates.length;i++){\n\t\t\t\tres+= Math.pow(coordinates[i] - p.coordinates[i], 2);\n\t\t\t}\n\t\t\treturn Math.sqrt(res);\n\t\t}\n\t\t\n\t\tpublic int distance(KDPoint p)\n\t\t{\n\t\t\treturn Math.abs(coordinates[0]-p.coordinates[0])+Math.abs(coordinates[1]-p.coordinates[1]);\n\t\t}\n\t\t\n\t\tpublic boolean equals(Object o)\n\t\t{\n\t\t\tKDPoint n2 = (KDPoint) o;\n\t\t\tfor(int i=0;i<coordinates.length;i++){\n\t\t\t\tif(coordinates[i]!=n2.coordinates[i]) return false;\n\t\t\t}\n\t\t\treturn true;\n\t\t}\n\t\t\n\t\tpublic String toString()\n\t\t{\n\t\t\tStringBuilder res = new StringBuilder().append(\"(\");\n\t\t\tfor(int i=0;i<coordinates.length;i++) res.append(coordinates[i]+\",\");\n\t\t\tres.setLength(res.length()-1);\n\t\t\tres.append(\")\");\n\t\t\treturn res.toString();\n\t\t}\n\t}\n}"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/oc_tree/oc_tree.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n\nclass Octant:\n \"\"\"\n UP: Y positive.\n DOWN: Y negative.\n FRONT: Z positive.\n BACK: Z negative.\n RIGHT: X positive.\n LEFT: X negative.\n \"\"\"\n\n UP_FRONT_LEFT = 0\n UP_FRONT_RIGHT = 1\n UP_BACK_LEFT = 2\n UP_BACK_RIGHT = 3\n DOWN_FRONT_LEFT = 4\n DOWN_FRONT_RIGHT = 5\n DOWN_BACK_LEFT = 6\n DOWN_BACK_RIGHT = 7\n OUT_OF_BOUNDS = -1\n\n\nclass Point3D:\n \"\"\"\n 3d point representation.\n +----+\n / /| Y\n +----+ |\n | | +\n | |/ Z\n +----+\n X\n \"\"\"\n\n def __init__(self, x, y, z):\n self.x = x\n self.y = y\n self.z = z\n\n\nclass Dimension:\n \"\"\"\n Dimension representation.\n \"\"\"\n\n def __init__(self, width, height, depth):\n self.width = width\n self.height = height\n self.depth = depth\n\n\nclass Box:\n \"\"\"\n Box Class represents boundaries.\n \"\"\"\n\n def __init__(self, x, y, z, width, height, depth):\n self.center = Point3D(x, y, z)\n self.dimension = Dimension(width, height, depth)\n self.minPos = Point3D(x - width / 2, y - height / 2, z - depth / 2)\n self.maxPos = Point3D(x + width / 2, y + height / 2, z + depth / 2)\n\n def contains(self, point3d):\n \"\"\"\n Check if box contains a point.\n 3D collision detection.\n https://developer.mozilla.org/en-US/docs/Games/Techniques/3D_collision_detection#point_vs._aabb\n \"\"\"\n return (\n point3d.x >= self.minPos.x\n and point3d.x <= self.maxPos.x\n and point3d.y >= self.minPos.y\n and point3d.y <= self.maxPos.y\n and point3d.z >= self.minPos.z\n and point3d.z <= self.maxPos.z\n )\n\n def intersect(self, box):\n \"\"\"\n Check if 2 boxes intersect.\n 3D collision detection.\n https://developer.mozilla.org/en-US/docs/Games/Techniques/3D_collision_detection#aabb_vs._aabb\n \"\"\"\n return (\n self.minPos.x <= box.maxPos.x\n and self.maxPos.x >= box.minPos.x\n and self.minPos.y <= box.maxPos.y\n and self.maxPos.y >= box.minPos.y\n and self.minPos.z <= box.maxPos.z\n and self.maxPos.z >= box.minPos.z\n )\n\n def find_octant(self, point3d):\n \"\"\"\n Find which octant contains a specific point.\n \"\"\"\n print(f\"POINT x:{point3d.x} y:{point3d.y} z:{point3d.z} IN \", end=\"\")\n\n if (\n self.center.x - self.dimension.width / 2 <= point3d.x\n and self.center.x >= point3d.x\n and self.center.y + self.dimension.height / 2 >= point3d.y\n and self.center.y <= point3d.y\n and self.center.z + self.dimension.depth / 2 >= point3d.z\n and self.center.z <= point3d.z\n ):\n print(\"UP FRONT LEFT\")\n return Octant.UP_FRONT_LEFT\n\n if (\n self.center.x + self.dimension.width / 2 >= point3d.x\n and self.center.x <= point3d.x\n and self.center.y + self.dimension.height / 2 >= point3d.y\n and self.center.y <= point3d.y\n and self.center.z + self.dimension.depth / 2 >= point3d.z\n and self.center.z <= point3d.z\n ):\n print(\"UP FRONT RIGHT\")\n return Octant.UP_FRONT_RIGHT\n\n if (\n self.center.x - self.dimension.width / 2 <= point3d.x\n and self.center.x >= point3d.x\n and self.center.y + self.dimension.height / 2 >= point3d.y\n and self.center.y <= point3d.y\n and self.center.z - self.dimension.depth / 2 <= point3d.z\n and self.center.z >= point3d.z\n ):\n print(\"UP BACK LEFT\")\n return Octant.UP_BACK_LEFT\n\n if (\n self.center.x + self.dimension.width / 2 >= point3d.x\n and self.center.x <= point3d.x\n and self.center.y + self.dimension.height / 2 >= point3d.y\n and self.center.y <= point3d.y\n and self.center.z - self.dimension.depth / 2 <= point3d.z\n and self.center.z >= point3d.z\n ):\n print(\"UP BACK RIGHT\")\n return Octant.UP_BACK_RIGHT\n\n if (\n self.center.x - self.dimension.width / 2 <= point3d.x\n and self.center.x >= point3d.x\n and self.center.y - self.dimension.height / 2 <= point3d.y\n and self.center.y >= point3d.y\n and self.center.z + self.dimension.depth / 2 >= point3d.z\n and self.center.z <= point3d.z\n ):\n print(\"DOWN FRONT LEFT\")\n return Octant.DOWN_FRONT_LEFT\n\n if (\n self.center.x + self.dimension.width / 2 >= point3d.x\n and self.center.x <= point3d.x\n and self.center.y - self.dimension.height / 2 <= point3d.y\n and self.center.y >= point3d.y\n and self.center.z + self.dimension.depth / 2 >= point3d.z\n and self.center.z <= point3d.z\n ):\n print(\"DOWN FRONT RIGHT\")\n return Octant.DOWN_FRONT_RIGHT\n\n if (\n self.center.x - self.dimension.width / 2 <= point3d.x\n and self.center.x >= point3d.x\n and self.center.y - self.dimension.height / 2 <= point3d.y\n and self.center.y >= point3d.y\n and self.center.z - self.dimension.depth / 2 <= point3d.z\n and self.center.z >= point3d.z\n ):\n print(\"DOWN BACK LEFT\")\n return Octant.DOWN_BACK_LEFT\n\n if (\n self.center.x + self.dimension.width / 2 >= point3d.x\n and self.center.x <= point3d.x\n and self.center.y - self.dimension.height / 2 <= point3d.y\n and self.center.y >= point3d.y\n and self.center.z - self.dimension.depth / 2 <= point3d.z\n and self.center.z >= point3d.z\n ):\n print(\"DOWN BACK RIGHT\")\n return Octant.DOWN_BACK_RIGHT\n\n print(\"NOT IN BOX\")\n return Octant.OUT_OF_BOUNDS\n\n\nclass OctreeNode:\n \"\"\"\n Octree node:\n - 8 octants, 8 children\n - It stores Point3D (a specific capacity)\n \"\"\"\n\n def __init__(self, box, capacity):\n self.children = [None] * 8 # each node has 8 children\n self.values = [None] * capacity\n self.boundary = box\n self.divided = False\n self.size = 0\n\n def subdivide(self):\n \"\"\"\n When the node is full, each octant (children) is initialized\n with a new center, capacity is the same.\n \"\"\"\n self.divided = True\n w = self.boundary.dimension.width / 2\n h = self.boundary.dimension.height / 2\n d = self.boundary.dimension.depth / 2\n x = self.boundary.center.x\n y = self.boundary.center.y\n z = self.boundary.center.z\n capacity = len(self.values)\n\n # UPPER PART\n self.children[Octant.UP_FRONT_LEFT] = OctreeNode(\n Box(x - w / 2, y + h / 2, z + d / 2, w, h, d), capacity\n )\n self.children[Octant.UP_FRONT_RIGHT] = OctreeNode(\n Box(x + w / 2, y + h / 2, z + d / 2, w, h, d), capacity\n )\n self.children[Octant.UP_BACK_LEFT] = OctreeNode(\n Box(x - w / 2, y + h / 2, z - d / 2, w, h, d), capacity\n )\n self.children[Octant.UP_BACK_RIGHT] = OctreeNode(\n Box(x + w / 2, y + h / 2, z - d / 2, w, h, d), capacity\n )\n\n # LOWER PART\n self.children[Octant.DOWN_FRONT_LEFT] = OctreeNode(\n Box(x - w / 2, y - h / 2, z + d / 2, w, h, d), capacity\n )\n self.children[Octant.DOWN_FRONT_RIGHT] = OctreeNode(\n Box(x + w / 2, y - h / 2, z + d / 2, w, h, d), capacity\n )\n self.children[Octant.DOWN_BACK_LEFT] = OctreeNode(\n Box(x - w / 2, y - h / 2, z - d / 2, w, h, d), capacity\n )\n self.children[Octant.DOWN_BACK_RIGHT] = OctreeNode(\n Box(x + w / 2, y - h / 2, z - d / 2, w, h, d), capacity\n )\n\n\nclass Octree:\n def __init__(self, box, capacity):\n self.root = OctreeNode(box, capacity)\n self.capacity = capacity\n\n def insert(self, point3d):\n \"\"\"\n Each node is divided in 8 octants and has a capacity.\n At first, root node octants are empty\n - If a point is not inside the boudaries, it is not inserted\n - If node is not full the we insert it\n - Otherwise we subdive the node, find which octant contains\n the point and then insert the point\n \"\"\"\n\n def insert_recursive(point3d, node):\n if node.boundary.contains(point3d) == False:\n print(f\"NOT INSERTED x:{point3d.x} y:{point3d.y} z:{point3d.z}\")\n return\n\n if node.size < self.capacity:\n node.values[node.size] = point3d\n node.size += 1\n else:\n node.subdivide()\n octant = node.boundary.find_octant(point3d)\n node = node.children[octant]\n insert_recursive(point3d, node)\n\n insert_recursive(point3d, self.root)\n\n\nif __name__ == \"__main__\":\n box = Box(x=0, y=0, z=0, width=2, height=2, depth=2)\n octree = Octree(box, capacity=4) # root\n\n # Example\n # center=(x=0,y=0,z=0)\n # +--------+\n # / /|\n # / / | height = 2\n # +--------+ | Y\n # | | |\n # | (0,0,0)| +\n # | * | / depth=2\n # | |/ Z\n # +--------+\n # width = 2\n # X\n\n # Points to be inserted\n points = [\n # 4 initial nodes, Octree does not subdivides\n Point3D(-1, 1, 1),\n Point3D(1, 1, 1),\n Point3D(-1, 1, -1),\n Point3D(1, 1, -1),\n # DOWN, Octree subdivides because node is Full\n Point3D(-1, -1, 1), # (Octant) DOWN FRONT LEFT\n Point3D(1, -1, 1), # (Octant) DOWN FRONT RIGHT\n Point3D(-1, -1, -1), # (Octant) DOWN BACK LEFT\n Point3D(1, -1, -1), # (Octant) DOWN BACK RIGHT\n Point3D(2, 2, 2), # This point is not inserted\n ]\n\n for p in points:\n octree.insert(p)\n\n # Octants\n print(\"Octants:\")\n point = Point3D(x=-1, y=1, z=1)\n assert box.find_octant(point) == Octant.UP_FRONT_LEFT, \"should be UP_FRONT_LEFT\"\n point = Point3D(x=1, y=1, z=1)\n assert box.find_octant(point) == Octant.UP_FRONT_RIGHT, \"should be UP_FRONT_RIGHT\"\n point = Point3D(x=-1, y=1, z=-1)\n assert box.find_octant(point) == Octant.UP_BACK_LEFT, \"should be UP_BACK_LEFT\"\n point = Point3D(x=1, y=1, z=-1)\n assert box.find_octant(point) == Octant.UP_BACK_RIGHT, \"should be UP_BACK_RIGHT\"\n point = Point3D(x=-1, y=-1, z=1)\n assert box.find_octant(point) == Octant.DOWN_FRONT_LEFT, \"should be DOWN_FRONT_LEFT\"\n point = Point3D(x=1, y=-1, z=1)\n assert (\n box.find_octant(point) == Octant.DOWN_FRONT_RIGHT\n ), \"should be DOWN_FRONT_RIGHT\"\n point = Point3D(x=-1, y=-1, z=-1)\n assert box.find_octant(point) == Octant.DOWN_BACK_LEFT, \"should be DOWN_BACK_LEFT\"\n point = Point3D(x=1, y=-1, z=-1)\n assert box.find_octant(point) == Octant.DOWN_BACK_RIGHT, \"should be DOWN_BACK_RIGHT\"\n\n # Intersection\n assert (\n Box(0, 0, 0, 1, 1, 1).intersect(Box(0.5, 0.5, 0.5, 1, 1, 1)) == True\n ), \"Should Intersect!\"\n assert (\n Box(1, 1, 1, 1, 1, 1).intersect(Box(1, 1, 1, 1, 1, 1)) == True\n ), \"Should Intersect!\"\n assert (\n Box(0, 0, 0, 1, 1, 1).intersect(Box(2, 2, 2, 2, 2, 2)) == False\n ), \"Should NOT Intersect!\""
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/quad_tree/Python_Implementation_Visualization/QuadTree.py",
"content": "# Part of Cosmos by OpenGenus Foundation\nfrom tkinter import *\n\n\nclass Rectangle:\n def __init__(self, x, y, h, w):\n self.x = x\n self.y = y\n self.h = h\n self.w = w\n\n\nclass Point:\n def __init__(self, x, y):\n self.x = x\n self.y = y\n\n\nclass QuadTree:\n def __init__(self, boundary, bucket):\n self.boundary = boundary\n self.bucket = bucket\n self.arr = []\n self.NorthEast = None\n self.NorthWest = None\n self.SouthEast = None\n self.SouthWest = None\n self.divided = False\n\n def contains(self, point):\n if (((point.x >= self.boundary.x - self.boundary.w) and (point.x <= self.boundary.x + self.boundary.w)) and\n ((point.y <= self.boundary.y + self.boundary.h) and (point.y >= self.boundary.y - self.boundary.h))):\n return True\n return False\n\n def subdivide(self):\n nE = Rectangle(self.boundary.x + (self.boundary.w / 2), self.boundary.y + (self.boundary.h / 2),\n self.boundary.w / 2, self.boundary.h / 2)\n nW = Rectangle(self.boundary.x - (self.boundary.w / 2), self.boundary.y + (self.boundary.h / 2),\n self.boundary.w / 2, self.boundary.h / 2)\n sE = Rectangle(self.boundary.x + (self.boundary.w / 2), self.boundary.y - (self.boundary.h / 2),\n self.boundary.w / 2, self.boundary.h / 2)\n sW = Rectangle(self.boundary.x - (self.boundary.w / 2), self.boundary.y - (self.boundary.h / 2),\n self.boundary.w / 2, self.boundary.h / 2)\n self.NorthEast = QuadTree(nE, self.bucket)\n self.NorthWest = QuadTree(nW, self.bucket)\n self.SouthEast = QuadTree(sE, self.bucket)\n self.SouthWest = QuadTree(sW, self.bucket)\n\n def insert(self, point):\n if not self.contains(point):\n return False\n\n if len(self.arr) < self.bucket:\n self.arr.append(point)\n return True\n\n if not self.divided:\n self.subdivide()\n self.divided = True\n\n if self.NorthEast.insert(point) is not None:\n return True\n if self.NorthWest.insert(point) is not None:\n return True\n if self.SouthEast.insert(point) is not None:\n return True\n if self.SouthWest.insert(point) is not None:\n return True\n\n return False\n\n def visualize(self, pointsList):\n root = Tk()\n canvas = Canvas(root, height=700, width=700, bg='#fff')\n canvas.pack()\n for point in pointsList:\n canvas.create_oval(point.x - 3, point.y - 3, point.x + 3, point.y + 3)\n self.paint(canvas)\n root.mainloop()\n\n def paint(self, canvas):\n canvas.create_rectangle(self.boundary.x - self.boundary.w, self.boundary.y + self.boundary.h,\n self.boundary.x + self.boundary.w, self.boundary.y - self.boundary.h, outline='#fb0')\n\n if self.divided:\n self.NorthEast.paint(canvas)\n self.NorthWest.paint(canvas)\n self.SouthEast.paint(canvas)\n self.SouthWest.paint(canvas)"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/quad_tree/Python_Implementation_Visualization/Visualizer.py",
"content": "# Part of Cosmos by OpenGenus Foundation\nfrom QuadTree import *\n\nif __name__ == '__main__':\n boundary = Rectangle(200, 200, 150, 150)\n QT = QuadTree(boundary, 1)\n pointsList = [Point(240, 240), Point(150, 150), Point(300, 215), Point(200, 266), Point(333, 190)]\n for point in pointsList:\n QT.insert(point)\n QT.visualize(pointsList)"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/quad_tree/quad_tree.swift",
"content": "// Part of Cosmos by OpenGenus Foundation\npublic struct Point {\n let x: Double\n let y: Double\n\n public init(_ x: Double, _ y: Double) {\n self.x = x\n self.y = y\n }\n}\n\nextension Point: CustomStringConvertible {\n public var description: String {\n return \"Point(\\(x), \\(y))\"\n }\n}\n\npublic struct Size: CustomStringConvertible {\n var xLength: Double\n var yLength: Double\n\n public init(xLength: Double, yLength: Double) {\n precondition(xLength >= 0, \"xLength can not be negative\")\n precondition(yLength >= 0, \"yLength can not be negative\")\n self.xLength = xLength\n self.yLength = yLength\n }\n\n var half: Size {\n return Size(xLength: xLength / 2, yLength: yLength / 2)\n }\n\n public var description: String {\n return \"Size(\\(xLength), \\(yLength))\"\n }\n}\n\npublic struct Rect {\n var origin: Point\n var size: Size\n\n public init(origin: Point, size: Size) {\n self.origin = origin\n self.size = size\n }\n\n var minX: Double {\n return origin.x\n }\n\n var minY: Double {\n return origin.y\n }\n\n var maxX: Double {\n return origin.x + size.xLength\n }\n\n var maxY: Double {\n return origin.y + size.yLength\n }\n\n func containts(point: Point) -> Bool {\n return (minX <= point.x && point.x <= maxX) &&\n (minY <= point.y && point.y <= maxY)\n }\n\n var leftTopRect: Rect {\n return Rect(origin: origin, size: size.half)\n }\n\n var leftBottomRect: Rect {\n return Rect(origin: Point(origin.x, origin.y + size.half.yLength), size: size.half)\n }\n\n var rightTopRect: Rect {\n return Rect(origin: Point(origin.x + size.half.xLength, origin.y), size: size.half)\n }\n\n var rightBottomRect: Rect {\n return Rect(origin: Point(origin.x + size.half.xLength, origin.y + size.half.yLength), size: size.half)\n }\n\n func intersects(rect: Rect) -> Bool {\n\n func lineSegmentsIntersect(lStart: Double, lEnd: Double, rStart: Double, rEnd: Double) -> Bool {\n return max(lStart, rStart) <= min(lEnd, rEnd)\n }\n if !lineSegmentsIntersect(lStart: minX, lEnd: maxX, rStart: rect.minX, rEnd: rect.maxX) {\n return false\n }\n\n // vertical\n return lineSegmentsIntersect(lStart: minY, lEnd: maxY, rStart: rect.minY, rEnd: rect.maxY)\n }\n}\n\nextension Rect: CustomStringConvertible {\n public var description: String {\n return \"Rect(\\(origin), \\(size))\"\n }\n}\n\nprotocol PointsContainer {\n func add(point: Point) -> Bool\n func points(inRect rect: Rect) -> [Point]\n}\n\nclass QuadTreeNode {\n\n enum NodeType {\n case leaf\n case `internal`(children: Children)\n }\n\n struct Children: Sequence {\n let leftTop: QuadTreeNode\n let leftBottom: QuadTreeNode\n let rightTop: QuadTreeNode\n let rightBottom: QuadTreeNode\n\n init(parentNode: QuadTreeNode) {\n leftTop = QuadTreeNode(rect: parentNode.rect.leftTopRect)\n leftBottom = QuadTreeNode(rect: parentNode.rect.leftBottomRect)\n rightTop = QuadTreeNode(rect: parentNode.rect.rightTopRect)\n rightBottom = QuadTreeNode(rect: parentNode.rect.rightBottomRect)\n }\n\n struct ChildrenIterator: IteratorProtocol {\n private var index = 0\n private let children: Children\n\n init(children: Children) {\n self.children = children\n }\n\n mutating func next() -> QuadTreeNode? {\n\n defer { index += 1 }\n\n switch index {\n case 0: return children.leftTop\n case 1: return children.leftBottom\n case 2: return children.rightTop\n case 3: return children.rightBottom\n default: return nil\n }\n }\n }\n\n public func makeIterator() -> ChildrenIterator {\n return ChildrenIterator(children: self)\n }\n }\n\n var points: [Point] = []\n let rect: Rect\n var type: NodeType = .leaf\n\n static let maxPointCapacity = 3\n\n init(rect: Rect) {\n self.rect = rect\n }\n\n var recursiveDescription: String {\n return recursiveDescription(withTabCount: 0)\n }\n\n private func recursiveDescription(withTabCount count: Int) -> String {\n let indent = String(repeating: \"\\t\", count: count)\n var result = \"\\(indent)\" + description + \"\\n\"\n switch type {\n case .internal(let children):\n for child in children {\n result += child.recursiveDescription(withTabCount: count + 1)\n }\n default:\n break\n }\n return result\n }\n}\n\nextension QuadTreeNode: PointsContainer {\n\n @discardableResult\n func add(point: Point) -> Bool {\n if !rect.containts(point: point) {\n return false\n }\n\n switch type {\n case .internal(let children):\n for child in children {\n if child.add(point: point) {\n return true\n }\n }\n\n fatalError(\"rect.containts evaluted to true, but none of the children added the point\")\n case .leaf:\n points.append(point)\n if points.count == QuadTreeNode.maxPointCapacity {\n subdivide()\n }\n }\n return true\n }\n\n private func subdivide() {\n switch type {\n case .leaf:\n type = .internal(children: Children(parentNode: self))\n case .internal:\n preconditionFailure(\"Calling subdivide on an internal node\")\n }\n }\n\n func points(inRect rect: Rect) -> [Point] {\n\n if !self.rect.intersects(rect: rect) {\n return []\n }\n\n var result: [Point] = []\n\n for point in points {\n if rect.containts(point: point) {\n result.append(point)\n }\n }\n\n switch type {\n case .leaf:\n break\n case .internal(let children):\n for childNode in children {\n result.append(contentsOf: childNode.points(inRect: rect))\n }\n }\n\n return result\n }\n}\n\nextension QuadTreeNode: CustomStringConvertible {\n var description: String {\n switch type {\n case .leaf:\n return \"leaf \\(rect) Points: \\(points)\"\n case .internal:\n return \"parent \\(rect) Points: \\(points)\"\n }\n }\n}\n\npublic class QuadTree: PointsContainer {\n\n let root: QuadTreeNode\n\n public init(rect: Rect) {\n self.root = QuadTreeNode(rect: rect)\n }\n\n @discardableResult\n public func add(point: Point) -> Bool {\n return root.add(point: point)\n }\n\n public func points(inRect rect: Rect) -> [Point] {\n return root.points(inRect: rect)\n }\n}\n\nextension QuadTree: CustomStringConvertible {\n public var description: String {\n return \"Quad tree\\n\" + root.recursiveDescription\n }\n}"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/segment_tree/lazy_segment_tree.java",
"content": "// Java program to demonstrate lazy propagation in segment tree\n// Part of Cosmos by OpenGenus Foundation\nclass LazySegmentTree\n{\n final int MAX = 1000; // Max tree size\n int tree[] = new int[MAX]; // To store segment tree\n int lazy[] = new int[MAX]; // To store pending updates\n \n /* index -> index of current node in segment tree\n sstart and send -> Starting and ending indexes of elements for\n which current nodes stores sum.\n ustart and uend -> starting and ending indexes of update query\n val -> which we need to add in the range ustart to uend */\n void updateRangeUtil(int index, int sstart, int send, int ustart, int uend, int val)\n {\n // If lazy value is non-zero for current node of segment\n // tree, then there are some pending updates. So we need\n // to make sure that the pending updates are done before\n // making new updates. Because this value may be used by\n // parent after recursive calls (See last line of this\n // function)\n if (lazy[index] != 0)\n {\n // Make pending updates using value stored in lazy array\n tree[index] += (send - sstart + 1) * lazy[index];\n \n // checking if it is not leaf node because if\n // it is leaf node then we cannot go further\n if (sstart != send)\n {\n // We can postpone updating children we don't\n // need their new values now.\n // Since we are not yet updating children of index,\n // we need to set lazy flags for the children\n lazy[index * 2 + 1] += lazy[index]; //left child\n lazy[index * 2 + 2] += lazy[index]; //right child\n }\n \n // Set the lazy value for current node as 0 as it\n // has been updated\n lazy[index] = 0;\n }\n \n // out of range\n if (sstart > send || sstart > uend || send < ustart)\n return;\n \n // Current segment is fully in range\n if (sstart >= ustart && send <= uend)\n {\n // Add val to current node\n tree[index] += (send - sstart + 1) * val;\n \n // same logic for checking leaf node or not\n if (sstart != send)\n {\n // This is where we store values in lazy nodes,\n // rather than updating the segment tree itelf\n // Since we don't need these updated values now\n // we postpone updates by storing values in lazy[]\n lazy[index * 2 + 1] += val;\n lazy[index * 2 + 2] += val;\n }\n return;\n }\n \n // If not completely in range, but overlaps, recur for children,\n int mid = (sstart + send) / 2;\n updateRangeUtil(index * 2 + 1, sstart, mid, ustart, uend, val);\n updateRangeUtil(index * 2 + 2, mid + 1, send, ustart, uend, val);\n \n // Use the result of children calls to update this node\n tree[index] = tree[index * 2 + 1] + tree[index * 2 + 2];\n }\n \n // Function to update a range of values in segment tree\n void updateRange(int n, int ustart, int uend, int val) {\n updateRangeUtil(0, 0, n - 1, ustart, uend, val);\n }\n \n /* A recursive function to get the sum of values in given\n range of the array. The following are parameters for\n this function.\n index --> Index of current node in the segment tree.\n Initially 0 is passed as root is always at'\n index 0\n sstart & send --> Starting and ending indexes of the\n segment represented by current node,\n i.e., tree[index]\n qstart & qend --> Starting and ending indexes of query\n range */\n int getSumUtil(int sstart, int send, int qstart, int qend, int index)\n {\n // If lazy flag is set for current node of segment tree,\n // then there are some pending updates. So we need to\n // make sure that the pending updates are done before\n // processing the sub sum query\n if (lazy[index] != 0)\n {\n // Make pending updates to this node. Note that this\n // node represents sum of elements in arr[sstart..send] and\n // all these elements must be increased by lazy[index]\n tree[index] += (send - sstart + 1) * lazy[index];\n \n // checking if it is not leaf node because if\n // it is leaf node then we cannot go further\n if (sstart != send)\n {\n // Since we are not yet updating children of index,\n // we need to set lazy values for the children\n lazy[index * 2 + 1] += lazy[index];\n lazy[index * 2 + 2] += lazy[index];\n }\n \n // update the lazy value for current node as it has\n // been updated\n lazy[index] = 0;\n }\n \n // Out of range\n if (sstart > send || sstart > qend || send < qstart)\n return 0;\n \n // At this point, pending lazy updates are done\n // for current node. So we can return value\n \n // If this segment lies in range\n if (sstart >= qstart && send <= qend)\n return tree[index];\n \n // If a part of this segment overlaps with the given range\n int mid = (sstart + send) / 2;\n return getSumUtil(sstart, mid, qstart, qend, 2 * index + 1) + getSumUtil(mid + 1, send, qstart, qend, 2 * index + 2);\n }\n \n // Return sum of elements in range from index qstart (query start)\n // to qend (query end). It mainly uses getSumUtil()\n int getSum(int n, int qstart, int qend)\n {\n // Check for erroneous input values\n if (qstart < 0 || qend > n - 1 || qstart > qend)\n {\n System.out.println(\"Invalid Input\");\n return -1;\n }\n \n return getSumUtil(0, n - 1, qstart, qend, 0);\n }\n \n /* A recursive function that constructs Segment Tree for\n array[sstart..send]. index is index of current node in segment\n tree st. */\n void constructSTUtil(int arr[], int sstart, int send, int index)\n {\n // out of range as sstart can never be greater than send\n if (sstart > send)\n return;\n \n /* If there is one element in array, store it in\n current node of segment tree and return */\n if (sstart == send)\n {\n tree[index] = arr[sstart];\n return;\n }\n \n /* If there are more than one elements, then recur\n for left and right subtrees and store the sum\n of values in this node */\n int mid = (sstart + send) / 2;\n constructSTUtil(arr, sstart, mid, index * 2 + 1);\n constructSTUtil(arr, mid + 1, send, index * 2 + 2);\n \n tree[index] = tree[index * 2 + 1] + tree[index * 2 + 2];\n }\n \n /* Function to construct segment tree from given array.\n This function allocates memory for segment tree and\n calls constructSTUtil() to fill the allocated memory */\n void constructST(int arr[], int n)\n {\n // Fill the allocated memory st\n constructSTUtil(arr, 0, n - 1, 0);\n }\n \n \n // The Main function\n public static void main(String args[])\n {\n int arr[] = {1, 3, 5, 7, 9, 11};\n int n = arr.length;\n LazySegmentTree tree = new LazySegmentTree();\n \n // Build segment tree from given array\n tree.constructST(arr, n);\n \n // Print sum of values in array from index 1 to 3\n System.out.println(\"Sum of values in given range = \" + tree.getSum(n, 1, 3));\n \n // Add 10 to all nodes at indexes from 1 to 5.\n tree.updateRange(n, 1, 5, 10);\n \n // Find sum after the value is updated\n System.out.println(\"Updated sum of values in given range = \" + tree.getSum(n, 1, 3));\n }\n}"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/segment_tree/persistent_segment_tree_sum.cpp",
"content": "// Fully persistent Segment tree with spaces. Allows to find sum in O(log size) time in any version. Uses O(n log size) memory.\n\n#include <iostream>\n#include <string>\nconst int size = 1000000000;\nconst int versionCount = 100000;\n\nstruct node\n{\n int leftBound, rightBound;\n node *leftChild, *rightChild;\n int value;\n node (int LeftBound, int RightBound)\n {\n leftBound = LeftBound;\n rightBound = RightBound;\n value = 0;\n leftChild = rightChild = 0;\n }\n node (node *vertexToClone)\n {\n leftBound = vertexToClone->leftBound;\n rightBound = vertexToClone->rightBound;\n value = vertexToClone->value;\n leftChild = vertexToClone->leftChild;\n rightChild = vertexToClone->rightChild;\n }\n} *root[versionCount];\n\nvoid add (node *vertex, int destination, int value)\n{\n if (vertex->leftBound == destination && vertex->rightBound == destination + 1)\n {\n vertex->value += value;\n return;\n }\n\n vertex->value += value;\n\n int middle = (vertex->leftBound + vertex->rightBound) / 2;\n\n if (destination < middle)\n {\n if (vertex->leftChild == 0)\n vertex->leftChild = new node (vertex->leftBound, middle);\n else\n vertex->leftChild = new node (vertex->leftChild);\n\n add (vertex->leftChild, destination, value);\n }\n else\n {\n if (vertex->rightChild == 0)\n vertex->rightChild = new node (middle, vertex->rightBound);\n else\n vertex->rightChild = new node (vertex->rightChild);\n\n add (vertex->rightChild, destination, value);\n }\n}\n\nint ask (node *vertex, int leftBound, int rightBound)\n{\n if (vertex == 0)\n return 0;\n\n if (vertex->leftBound >= leftBound && vertex->rightBound <= rightBound)\n return vertex->value;\n\n if (vertex->leftBound >= rightBound && vertex->rightBound <= leftBound)\n return 0;\n\n return ask (vertex->leftChild, leftBound, rightBound) + ask (vertex->rightChild, leftBound,\n rightBound);\n}\n\nint main ()\n{\n root[0] = new node (-size, size); // Actually allows negative numbers\n\n std::cout << \"Print number of queries!\\n\";\n\n int q;\n std::cin >> q;\n\n int currentVersion = 1;\n\n for (int _ = 0; _ < q; _++)\n {\n std::string type;\n std::cin >> type;\n\n if (type == \"add\")\n {\n int version, destination, value;\n std::cin >> version >> destination >> value;\n\n root[currentVersion] = new node (root[version]);\n add (root[currentVersion], destination, value);\n\n std::cout << \"Version \" << currentVersion << \" created succesfully!\\n\";\n ++currentVersion;\n }\n else if (type == \"ask\")\n {\n int version, leftBound, rightBound;\n std::cin >> version >> leftBound >> rightBound;\n\n std::cout << ask (root[version], leftBound, rightBound) << \"\\n\";\n }\n else\n std::cout << \"Unknown Type! Only add and ask allowed\\n\";\n }\n\n}"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/segment_tree/README.md",
"content": "# Segment Trees\n\nSegment Tree is used in cases where there are multiple range queries on array and modifications of elements of the same array. For example, finding the sum of all the elements in an array from indices **L to R**, or finding the minimum (famously known as **Range Minumum Query problem**) of all the elements in an array from indices **L to R**. These problems can be easily solved with one of the most versatile data structures, **Segment Tree**.\n\n## What is Segment Tree ?\nSegment Tree is basically a binary tree used for storing the intervals or segments. Each node in the Segment Tree represents an interval. Consider an array **A** of size **N** and a corresponding Segment Tree **T** :\n1. The root of **T** will represent the whole array `A[0:N−1]`.\n2. Each leaf in the Segment Tree **T** will represent a single element `A[i]` such that `0≤i<N`.\n3. The internal nodes in the Segment Tree **T** represents the union of elementary intervals `A[i:j]` where `0≤i<j<N`.\n\nThe root of the Segment Tree represents the whole array `A[0:N−1]`. Then it is broken down into two half intervals or segments and the two children of the root in turn represent the `A[0:(N−1)/2]` and `A[(N−1)/2+1:(N−1)]`. So in each step, the segment is divided into half and the two children represent those two halves. So the height of the segment tree will be **log N** (base 2). There are **N** leaves representing the **N** elements of the array. The number of internal nodes is **N−1**. So, the total number of nodes are **2×N−1**.\nOnce the Segment Tree is built, its structure cannot be changed. We can update the values of nodes but we cannot change its structure. Segment tree provides two operations: \n1. **Update**: To update the element of the array **A** and reflect the corresponding change in the Segment tree.\n2. **Query**: In this operation we can query on an interval or segment and return the answer to the problem (say minimum/maximum/summation in the particular segment).\n\n## Implementation :\nSince a Segment Tree is a **binary tree**, a simple linear array can be used to represent the Segment Tree. Before building the Segment Tree, one must figure **what needs to be stored in the Segment Tree's node?**\nFor example, if the question is to find the sum of all the elements in an array from indices **L to R**, then at each node (except leaf nodes) the sum of its children nodes is stored, or if the question is to find the minimum of all the elements in an array from indices **L to R**, then at each node the minimum of its children nodes is stored (**Range Minumum Query problem**).\n\nA Segment Tree can be built using **recursion (bottom-up approach)**. Start with the leaves and go up to the root and update the corresponding changes in the nodes that are in the path from leaves to root. Leaves represent a single element. In each step, the data of two children nodes are used to form an internal parent node. Each internal node will represent a union of its children’s intervals. Merging may be different for different questions. So, recursion will end up at the root node which will represent the whole array.\n\nFor **update()**, search the leaf that contains the element to update. This can be done by going to either on the left child or the right child depending on the interval which contains the element. Once the leaf is found, it is updated and again use the bottom-up approach to update the corresponding change in the path from that leaf to the root.\n\nTo make a **query()** on the Segment Tree, select a range from **L to R** (which is usually given in the question). Recurse on the tree starting from the root and check if the interval represented by the node is completely in the range from **L to R**. If the interval represented by a node is completely in the range from \n**L to R**, return that node’s value.\n\n### Example :\n\nGiven an array **A** of size **N** and some queries. There are two types of queries :\n1. **Update :** Given `idx` and `val`, update array element `A[idx]` as `A[idx]=A[idx]+val`.\n2. **Query :** Given `l` and `r` return the value of `A[l]+A[l+1]+A[l+2]+…..+A[r−1]+A[r] `such that `0≤l≤r<N`.\n\nFirst, figure what needs to be stored in the Segment Tree's node. The question asks for **summation in the interval from l to r**, so in each node, **sum of all the elements in that interval represented by the node**. Next, build the Segment Tree. The implementation with comments below explains the building process.\n```\nvoid build(int node, int start, int end)\n{\n if(start == end)\n {\n // Leaf node will have a single element\n tree[node] = A[start];\n }\n else\n {\n int mid = (start + end) / 2;\n // Recurse on the left child\n build(2*node, start, mid);\n // Recurse on the right child\n build(2*node+1, mid+1, end);\n // Internal node will have the sum of both of its children\n tree[node] = tree[2*node] + tree[2*node+1];\n }\n}\n```\nAs shown in the code above, start from the **root** and recurse on the **left** and the **right child** until a leaf node is reached. From the leaves, go back to the root and update all the nodes in the path. `node` represents the current node that is being processed. Since Segment Tree is a binary tree. `2×node` will represent the left node and `2×node+1` will represent the right node. `start` and `end` represents the interval represented by the `node`. Complexity of **build()** is **O(N).**\n\nTo update an element, look at the interval in which the element is present and recurse accordingly on the left or the right child.\n\n```\nvoid update(int node, int start, int end, int idx, int val)\n{\n if(start == end)\n {\n // Leaf node\n A[idx] += val;\n tree[node] += val;\n }\n else\n {\n int mid = (start + end) / 2;\n if(start <= idx and idx <= mid)\n {\n // If idx is in the left child, recurse on the left child\n update(2*node, start, mid, idx, val);\n }\n else\n {\n // if idx is in the right child, recurse on the right child\n update(2*node+1, mid+1, end, idx, val);\n }\n // Internal node will have the sum of both of its children\n tree[node] = tree[2*node] + tree[2*node+1];\n }\n}\n```\nComplexity of update will be **O(logN).**\n\nTo query on a given range, check 3 conditions.\n1. Range represented by a node is **completely inside** the given range.\n2. Range represented by a node is **completely outside** the given range.\n3. Range represented by a node is **partially inside and partially outside** the given range.\n\nIf the range represented by a node is completely outside the given range, simply `return 0`. If the range represented by a node is completely within the given range, return the value of the node which is the sum of all the elements in the range represented by the node. And if the range represented by a node is partially inside and partially outside the given range, return sum of the left child and the right child. Complexity of query will be **O(logN).**\n\n```\nint query(int node, int start, int end, int l, int r)\n{\n if(r < start or end < l)\n {\n // range represented by a node is completely outside the given range\n return 0;\n }\n if(l <= start and end <= r)\n {\n // range represented by a node is completely inside the given range\n return tree[node];\n }\n // range represented by a node is partially inside and partially outside the given range\n int mid = (start + end) / 2;\n int p1 = query(2*node, start, mid, l, r);\n int p2 = query(2*node+1, mid+1, end, l, r);\n return (p1 + p2);\n}\n```\n\nYou can now check out the complete code for **Segment Trees** in this same folder."
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/segment_tree/segment_tree.java",
"content": "// Part of Cosmos by OpenGenus Foundation\n\n// Java Program to show segment tree operations like construction, query and update\nclass SegmentTree {\n int st[]; // The array that stores segment tree nodes\n \n SegmentTree(int arr[], int n) {\n // Allocate memory for segment tree\n //Height of segment tree\n int x = (int) (Math.ceil(Math.log(n) / Math.log(2)));\n \n //Maximum size of segment tree\n int max_size = 2 * (int) Math.pow(2, x) - 1;\n \n st = new int[max_size]; // Memory allocation\n \n constructSTUtil(arr, 0, n - 1, 0);\n }\n \n // A utility function to get the middle index from corner indexes.\n int getMid(int s, int e) {\n return s + (e - s) / 2;\n }\n \n int getSumUtil(int ss, int se, int qs, int qe, int si) {\n // If segment of this node is a part of given range, then return\n // the sum of the segment\n if (qs <= ss && qe >= se)\n return st[si];\n \n // If segment of this node is outside the given range\n if (se < qs || ss > qe)\n return 0;\n \n // If a part of this segment overlaps with the given range\n int mid = getMid(ss, se);\n return getSumUtil(ss, mid, qs, qe, 2 * si + 1) +\n getSumUtil(mid + 1, se, qs, qe, 2 * si + 2);\n }\n \n void updateValueUtil(int ss, int se, int i, int diff, int si) {\n // Base Case: If the input index lies outside the range of \n // this segment\n if (i < ss || i > se)\n return;\n \n // If the input index is in range of this node, then update the\n // value of the node and its children\n st[si] = st[si] + diff;\n if (se != ss) {\n int mid = getMid(ss, se);\n updateValueUtil(ss, mid, i, diff, 2 * si + 1);\n updateValueUtil(mid + 1, se, i, diff, 2 * si + 2);\n }\n }\n \n void updateValue(int arr[], int n, int i, int new_val) {\n // Check for erroneous input index\n if (i < 0 || i > n - 1) {\n System.out.println(\"Invalid Input\");\n return;\n }\n \n // Get the difference between new value and old value\n int diff = new_val - arr[i];\n \n // Update the value in array\n arr[i] = new_val;\n \n // Update the values of nodes in segment tree\n updateValueUtil(0, n - 1, i, diff, 0);\n }\n \n // Return sum of elements in range from index qs (quey start) to\n // qe (query end). It mainly uses getSumUtil()\n int getSum(int n, int qs, int qe) {\n // Check for erroneous input values\n if (qs < 0 || qe > n - 1 || qs > qe) {\n System.out.println(\"Invalid Input\");\n return -1;\n }\n return getSumUtil(0, n - 1, qs, qe, 0);\n }\n \n // A recursive function that constructs Segment Tree for array[ss..se].\n // si is index of current node in segment tree st\n int constructSTUtil(int arr[], int ss, int se, int si) {\n // If there is one element in array, store it in current node of\n // segment tree and return\n if (ss == se) {\n st[si] = arr[ss];\n return arr[ss];\n }\n \n // If there are more than one elements, then recur for left and\n // right subtrees and store the sum of values in this node\n int mid = getMid(ss, se);\n st[si] = constructSTUtil(arr, ss, mid, si * 2 + 1) +\n constructSTUtil(arr, mid + 1, se, si * 2 + 2);\n return st[si];\n }\n \n // Driver program to test above functions\n public static void main(String args[]) {\n int arr[] = {1, 3, 5, 7, 9, 11};\n int n = arr.length;\n SegmentTree tree = new SegmentTree(arr, n);\n \n // Build segment tree from given array\n \n // Print sum of values in array from index 1 to 3\n System.out.println(\"Sum of values in given range = \" + tree.getSum(n, 1, 3));\n \n // Update: set arr[1] = 10 and update corresponding segment\n // tree nodes\n tree.updateValue(arr, n, 1, 10);\n \n // Find sum after the value is updated\n System.out.println(\"Updated sum of values in given range = \" +\n tree.getSum(n, 1, 3));\n }\n}"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/segment_tree/segment_tree_kth_statistics_on_segment.cpp",
"content": "#include <cassert>\n#include <iostream>\n#include <vector>\nusing namespace std;\nconst int lb = -1e9, rb = 1e9;\nstruct node\n{\n int val;\n node *l, *r;\n node()\n {\n val = 0;\n l = r = nullptr;\n }\n node(int x)\n {\n val = x;\n l = r = nullptr;\n }\n node(node* vl, node* vr)\n {\n l = vl;\n r = vr;\n if (l)\n val += l->val;\n if (r)\n val += r->val;\n }\n};\nvector<node*> tr;\nint cnt(node* v)\n{\n return v ? v->val : 0;\n}\nnode* add_impl(node* v, int tl, int tr, int x)\n{\n if (tl == tr)\n return new node(cnt(v) + 1);\n else\n {\n int tm = (tl + tr) / 2;\n if (x <= tm)\n return new node(add_impl(v ? v->l : v, tl, tm, x), v ? v->r : v);\n else\n return new node(v ? v->l : v,\n add_impl(v ? v->r : v, tm + 1, tr, x));\n }\n}\nvoid add(int x)\n{\n node* v = tr.back();\n tr.push_back(add_impl(v, lb, rb, x));\n}\nint order_of_key_impl(node* v, int tl, int tr, int l, int r)\n{\n if (!v)\n return 0;\n if (l == tl && r == tr)\n return v->val;\n int tm = (tl + tr) / 2;\n if (r <= tm)\n return order_of_key_impl(v->l, tl, tm, l, r);\n else\n return order_of_key_impl(v->l, tl, tm, l, tm) +\n order_of_key_impl(v->r, tm + 1, tr, tm + 1, r);\n}\nint order_of_key(int l, int r, int x)\n{\n int ans = order_of_key_impl(tr[r], lb, rb, lb, x - 1) -\n order_of_key_impl(tr[l - 1], lb, rb, lb, x - 1) + 1;\n return ans;\n}\nint find_by_order_impl(node* l, node* r, int tl, int tr, int x)\n{\n if (tl == tr)\n return tl;\n int tm = (tl + tr) / 2;\n if (cnt(r ? r->l : r) - cnt(l ? l->l : l) >= x)\n return find_by_order_impl(l ? l->l : l, r ? r->l : r, tl, tm, x);\n else\n return find_by_order_impl(l ? l->r : l, r ? r->r : r, tm + 1, tr,\n x - (cnt(r ? r->l : r) - cnt(l ? l->l : l)));\n}\nint find_by_order(int l, int r, int x)\n{\n assert(r - l + 1 >= x && x > 0);\n return find_by_order_impl(tr[l - 1], tr[r], lb, rb, x);\n}\nint main()\n{\n tr.push_back(nullptr);\n vector<int> a;\n int n;\n cin >> n;\n for (int i = 0; i < n; i++)\n {\n int x;\n cin >> x;\n a.push_back(x);\n add(x);\n }\n int q;\n cin >> q;\n for (int i = 0; i < q; i++)\n {\n int t; // type of question: 1 - order of key, 2 - find by order\n cin >> t;\n int l, r, x; // 1 <= l <= r <= n\n cin >> l >> r >> x;\n if (t == 1)\n cout << order_of_key(l, r, x) << endl;\n else\n cout << find_by_order(l, r, x) << endl;\n }\n}"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/segment_tree/segment_tree_lazy_propagation.cpp",
"content": "// Part of Cosmos by OpenGenus Foundation\n#include <iostream>\nusing namespace std;\n#define N 10000000\nint tree[N + 1], lazy[N + 1], A[N];\n\nvoid build(int node, int start, int end)\n{\n if (start == end)\n // Leaf node will have a single element\n tree[node] = A[start];\n else\n {\n int mid = (start + end) / 2;\n // Recurse on the left child\n build(2 * node, start, mid);\n // Recurse on the right child\n build(2 * node + 1, mid + 1, end);\n // Internal node will have the sum of both of its children\n tree[node] = tree[2 * node] + tree[2 * node + 1];\n }\n}\n\nvoid updateRange(int node, int start, int end, int l, int r, int val)\n{\n if (lazy[node] != 0)\n {\n // This node needs to be updated\n tree[node] += (end - start + 1) * lazy[node]; // Update it\n if (start != end)\n {\n lazy[node * 2] += lazy[node]; // Mark child as lazy\n lazy[node * 2 + 1] += lazy[node]; // Mark child as lazy\n }\n lazy[node] = 0; // Reset it\n }\n if (start > end or start > r or end < l) // Current segment is not within range [l, r]\n return;\n if (start >= l and end <= r)\n {\n // Segment is fully within range\n tree[node] += (end - start + 1) * val;\n if (start != end)\n {\n // Not leaf node\n lazy[node * 2] += val;\n lazy[node * 2 + 1] += val;\n }\n return;\n }\n int mid = (start + end) / 2;\n updateRange(node * 2, start, mid, l, r, val); // Updating left child\n updateRange(node * 2 + 1, mid + 1, end, l, r, val); // Updating right child\n tree[node] = tree[node * 2] + tree[node * 2 + 1]; // Updating root with max value\n}\n\nint queryRange(int node, int start, int end, int l, int r)\n{\n //cout<<endl<<node<<\" \"<<start<<\" \"<<end; For Debuging Purpose\n if (start > end or start > r or end < l)\n return 0; // Out of range\n if (lazy[node] != 0)\n {\n // This node needs to be updated\n tree[node] += (end - start + 1) * lazy[node]; // Update it\n if (start != end)\n {\n lazy[node * 2] += lazy[node]; // Mark child as lazy\n lazy[node * 2 + 1] += lazy[node]; // Mark child as lazy\n }\n lazy[node] = 0; // Reset it\n }\n if (start >= l and end <= r) // Current segment is totally within range [l, r]\n //cout<<\"tree\"<<node<<\" \"<<tree[node]<<\" \";\n return tree[node];\n int mid = (start + end) / 2;\n int p1 = queryRange(node * 2, start, mid, l, r); // Query left child\n int p2 = queryRange(node * 2 + 1, mid + 1, end, l, r); // Query right child\n return p1 + p2;\n}\n\nint main()\n{\n fill(lazy, lazy + N + 1, 0);\n fill(tree, tree + N + 1, 0);\n for (int i = 0; i < 10; i++)\n A[i] = 1;\n build(1, 0, 9);\n cout << queryRange(1, 0, 9, 3, 9);\n updateRange(1, 0, 9, 3, 7, 1);\n cout << endl\n << queryRange(1, 0, 9, 3, 9);\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/segment_tree/segment_tree_rmq.adb",
"content": "with Ada.Integer_Text_IO, Ada.Text_IO;\nuse Ada.Integer_Text_IO, Ada.Text_IO;\n-- Compile:\n-- gnatmake segment_Tree_rmq\n\n\nprocedure segment_Tree_rmq is\n\nINT_MAX: constant integer := 999999;\nMAXN : constant integer := 100005;\nv : array(0..MAXN) of integer;\ntree: array(0..4*MAXN) of integer;\nn : integer;\nq : integer;\na : integer;\nb : integer;\nc : integer;\n\nfunction min(a: integer; b: integer) return integer is\n\nbegin\n\t\n\tif a < b then return a;\n\telse return b;\n\tend if;\nend min;\n\nprocedure build(node: integer; l: integer; r: integer) is\n\n\tmid: integer;\n\nbegin\n\n\tmid := (l + r)/2;\n\t\n\tif l = r then\n\t\ttree(node) := v(l);\n\t\treturn;\n\tend if;\n\n\tbuild(2 * node, l, mid);\n\tbuild(2*node+1,mid+1,r);\n\ttree(node) := min( tree(2*node), tree(2*node + 1)); \nend build;\n\n\n--Update procedure\nprocedure update(node: integer; l: integer; r: integer; pos: integer; val: integer) is\n\n\tmid: integer := (l + r)/2;\n\nbegin\n\t\n\tif l > pos or r < pos or l > r then\n\t\treturn;\n\tend if;\n\n\tif(l = r) then\n\t\ttree(node) := val;\n\t\treturn;\n\tend if;\n\n\tif pos <= mid then\n\t\tupdate(2*node,l,mid,pos,val);\n\telse\n\t\tupdate(2*node+1,mid+1,r,pos,val);\n\tend if;\n\ttree(node) := min( tree(2*node), tree(2*node + 1)); \nend update;\n\n\n--Query function\nfunction query(node : integer; l: integer; r: integer; x: integer; y: integer) return integer is\n\t\n\tmid: integer := (l + r)/2;\n\tp1: integer; \n\tp2: integer; \nbegin\n\n\tif l > r or l > y or r < x then\n\t\treturn INT_MAX;\n\tend if;\n\tif x <= l and r <= y then\n\t\treturn tree(node);\n\tend if;\n\n\tp1 := query(2*node,l,mid,x,y);\n\tp2 := query(2*node+1,mid+1,r,x,y);\n\n\tif p1 = INT_MAX then \n\t\treturn p2;\n\tend if;\n\n\tif p2 = INT_MAX then\n\t\treturn p1;\n\tend if;\n\n\treturn min(p1, p2);\n\nend query;\n\n\nbegin\n\tPut_Line(\"Input the array range\");\n\tGet(n);\n\tPut_Line(\"Input the values\");\n\tfor i in 1..n loop\n\t\tGet(v(i));\n\tend loop;\n\n\n\tbuild(1,0,n-1);\n\n\tPut_Line(\"Input the number of operations\");\n\n\tGet(q);\n\t\n\twhile q > 0 loop\n\t\tPut_Line(\"Input 0 to query and 1 to update\");\n\t\tGet(a);\n\t\tPut_Line(\"Input the STARTING index of the query range\");\n\t\tGet(b);\n\t\tPut_Line(\"Input the ENDING index of the query range\");\n\t\tGet(c);\n\n\t\tif a = 0 then \n\t\t\tPut_Line(\"Minimum value of the given range\");\n\t\t\tPut(query(1,1,n,b,c));\n\t\t\tPut_Line(\"\");\n\t\telsif a = 1 then\n\t\t\tupdate(1,1,n,b,c);\n\t\telse\n\t\t\tPut_Line(\"Invalid Operation\");\n\t\t\tq := q + 1;\n\t\tend if;\n\t\tq := q - 1;\n\n\tend loop;\nend segment_Tree_rmq;"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/segment_tree/segment_tree_rmq.cpp",
"content": "/* Name: Mohit Khare\n * B.Tech 2nd Year\n * Computer Science and Engineering\n * MNNIT Allahabad\n */\n#include <cstdio>\n#include <climits>\n#define min(a, b) ((a) < (b) ? (a) : (b))\nusing namespace std;\n\ntypedef long long int ll;\n\n#define mod 1000000007\nconst int maxn = 1e5 + 1;\n\nvoid build(ll segtree[], ll arr[], int low, int high, int pos)\n{\n if (low == high)\n {\n segtree[pos] = arr[low];\n return;\n }\n int mid = (low + high) / 2;\n build(segtree, arr, low, mid, 2 * pos + 1);\n build(segtree, arr, mid + 1, high, 2 * pos + 2);\n segtree[pos] = min(segtree[2 * pos + 2], segtree[2 * pos + 1]);\n}\n\nll query(ll segtree[], ll arr[], int low, int high, int qs, int qe, int pos)\n{\n if (qe < low || qs > high)\n return LLONG_MAX;\n if (qs <= low && high <= qe)\n return segtree[pos];\n int mid = (low + high) / 2;\n ll left = query(segtree, arr, low, mid, qs, qe, 2 * pos + 1);\n ll right = query(segtree, arr, mid + 1, high, qs, qe, 2 * pos + 2);\n return min(right, left);\n}\nint main()\n{\n int n, m;\n printf(\"Input no of input elements and no. of queries\\n\");\n scanf(\"%d %d\", &n, &m);\n ll segtree[4 * n], arr[n];\n printf(\"Input Array elements\\n\");\n for (int i = 0; i < n; i++)\n scanf(\"%lld\", &arr[i]);\n build(segtree, arr, 0, n - 1, 0);\n for (int i = 0; i < m; i++)\n {\n int l, r;\n printf(\"Enter range - l and r\\n\");\n scanf(\"%d %d\", &l, &r);\n l--; r--;\n printf(\"Result is :%lld\\n\", query(segtree, arr, 0, n - 1, l, r, 0));\n }\n return 0;\n}\n\n/* Test Case\n *\n * 5 2\n * Input Array elements\n * 3 4 2 1 5\n * 1 2\n * Result is :3\n * 2 5\n * Result is :1\n *\n */"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/segment_tree/segment_tree_rmq.go",
"content": "/*\n * Segment Tree\n * Update: 1 point\n * Query: Range\n * Query Type: Range Minimum Query\n */\npackage main\n\nimport \"fmt\"\n\ntype SegmentTree struct {\n\tN int\n\ttree []int\n}\n\nfunc Min(x int, y int) int {\n\tif x < y {\n\t\treturn x\n\t}\n\treturn y\n}\n\nfunc (stree *SegmentTree) Initialize(n int) {\n\tstree.N = n\n\tstree.tree = make([]int, 4*n)\n}\n\nfunc (stree *SegmentTree) build(arr []int, idx int, l int, r int) {\n\tif l == r {\n\t\tstree.tree[idx] = arr[l]\n\t\treturn\n\t}\n\n\tmid := (l + r) / 2\n\n\tstree.build(arr, 2*idx+1, l, mid)\n\tstree.build(arr, 2*idx+2, mid+1, r)\n\tstree.tree[idx] = Min(stree.tree[2*idx+1], stree.tree[2*idx+2])\n}\n\nfunc (stree *SegmentTree) Build(arr []int) {\n\tstree.build(arr, 0, 0, stree.N-1)\n}\n\nfunc (stree *SegmentTree) update(idx int, l int, r int, pos int, val int) {\n\tif l == r {\n\t\tstree.tree[idx] = val\n\t\treturn\n\t}\n\n\tmid := (l + r) / 2\n\n\tif pos <= mid {\n\t\tstree.update(2*idx+1, l, mid, pos, val)\n\t} else {\n\t\tstree.update(2*idx+2, mid+1, r, pos, val)\n\t}\n\n\tstree.tree[idx] = Min(stree.tree[2*idx+1], stree.tree[2*idx+2])\n}\n\nfunc (stree *SegmentTree) Update(pos int, val int) {\n\tstree.update(0, 0, stree.N-1, pos, val)\n}\n\nfunc (stree *SegmentTree) query(idx int, l int, r int, ql int, qr int) int {\n\tif ql <= l && r <= qr {\n\t\treturn stree.tree[idx]\n\t}\n\n\tmid := (l + r) / 2\n\tminLeft := 1<<31 - 1\n\tminRight := 1<<31 - 1\n\n\tif ql <= mid {\n\t\tminLeft = stree.query(2*idx+1, l, mid, ql, qr)\n\t}\n\n\tif qr > mid {\n\t\tminRight = stree.query(2*idx+2, mid+1, r, ql, qr)\n\t}\n\n\treturn Min(minLeft, minRight)\n}\n\nfunc (stree *SegmentTree) Query(ql int, qr int) int {\n\treturn stree.query(0, 0, stree.N-1, ql, qr)\n}\n\nfunc main() {\n\tarr := []int{1, 2, 3, 4, 5}\n\tn := len(arr)\n\n\tvar stree SegmentTree\n\tstree.Initialize(n)\n\tstree.Build(arr)\n\n\tfmt.Printf(\"RMQ from 2 to 4: %v\\n\", stree.Query(1, 3))\n\tfmt.Printf(\"RMQ from 1 to 5: %v\\n\", stree.Query(0, 4))\n\n\tstree.Update(2, 7)\n\tfmt.Println(\"Change to 7\")\n\tfmt.Printf(\"RMQ from 3 to 5: %v\\n\", stree.Query(2, 4))\n\tfmt.Printf(\"RMQ from 1 to 5: %v\\n\", stree.Query(0, 4))\n}"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/segment_tree/segment_tree_rmq.py",
"content": "import math\n\n\ndef update(tree, l, r, node, index, diff):\n if l == r:\n tree[node] = diff\n return tree[l]\n mid = l + (r - l) // 2\n\n if index <= mid:\n update(tree, l, mid, (2 * node) + 1, index, diff)\n tree[node] = min(tree[node * 2 + 2], tree[node * 2 + 1])\n return diff\n else:\n update(tree, mid + 1, r, (2 * node) + 2, index, diff)\n tree[node] = min(tree[node * 2 + 2], tree[node * 2 + 1])\n\n\ndef query(tree, l, r, x, y, node):\n if l == x and r == y:\n return tree[node]\n mid = l + (r - l) // 2\n\n if x <= mid and y <= mid:\n return query(tree, l, mid, x, y, (2 * node) + 1)\n elif x > mid and y > mid:\n return query(tree, mid + 1, r, x, y, (2 * node) + 2)\n elif x <= mid and y > mid:\n left = query(tree, l, mid, x, mid, (2 * node) + 1)\n right = query(tree, mid + 1, r, mid + 1, y, (2 * node) + 2)\n return min(left, right)\n\n\ndef buildtree(tree, l, r, arr, node):\n if l == r:\n tree[node] = arr[l]\n return arr[l]\n\n mid = l + (r - l) // 2\n left = buildtree(tree, l, mid, arr, (2 * node) + 1)\n right = buildtree(tree, mid + 1, r, arr, (2 * node) + 2)\n tree[node] = min(left, right)\n return tree[node]\n\n\nn, que = map(\n int, input(\"Input total no. elements in array and No. of query :\").split(\" \")\n)\n\nprint(\"Input elements of the array : \")\narr = list(map(int, input().split(\" \")))\nx = math.ceil(math.log(n, 2))\ntotnodes = (2 * (2 ** (x + 1))) - 1\ntree = [None for i in range(totnodes)]\nbuildtree(tree, 0, n - 1, arr, 0)\nfor i in range(que):\n print(\"If you want to find query press 'q' with left and right index.\")\n print(\n \"If you want to update the array, press 'u' with index and value for the update.\"\n )\n q, x, y = input().split(\" \")\n x = int(x) - 1\n y = int(y) - 1\n if q == \"q\":\n print(\"Minimum in the given range is \" + str(query(tree, 0, n - 1, x, y, 0)))\n\n elif q == \"u\":\n y += 1\n diff = arr[x]\n arr[x] = y\n update(tree, 0, n - 1, 0, x, y)"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/segment_tree/segment_tree_rmq_with_update.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n/*\n * Processes range minimum query.\n * Query function returns index of minimum element in given interval.\n * Code assumes that length of array can be contained into integer.\n */\n\n#include <iostream>\n#include <utility>\n#include <vector>\n\nstruct Node\n{\n // store the data in variable value\n int index;\n // store the interval in a pair of integers\n std::pair<int, int> interval;\n Node *left;\n Node *right;\n};\n\n// update adds new value to array[x] rather than replace it\n\nclass SegmentTree {\nprivate:\n Node *root;\n\n int build(std::vector<int> &array, Node *node, int L, int R)\n {\n node->interval = std::make_pair(L, R);\n if (L == R)\n {\n node->index = L;\n return node->index;\n }\n\n node->left = new Node;\n node->right = new Node;\n int leftIndex = build(array, node->left, L, (L + R) / 2);\n int rightIndex = build(array, node->right, (L + R) / 2 + 1, R);\n\n node->index = (array[leftIndex] < array[rightIndex]) ? leftIndex : rightIndex;\n\n return node->index;\n }\n\n // returns the index of smallest element in the range [start, end]\n int query(Node *node, int start, int end)\n {\n if (start > end)\n return -1;\n int L = node->interval.first;\n int R = node->interval.second;\n\n if (R < start || L > end)\n return -1;\n\n if (start <= L && end >= R)\n return node->index;\n\n int leftIndex = query(node->left, start, end);\n int rightIndex = query(node->right, start, end);\n\n if (leftIndex == -1)\n return rightIndex;\n if (rightIndex == -1)\n return leftIndex;\n\n return (array[leftIndex] < array[rightIndex]) ? leftIndex : rightIndex;\n }\n\n void update(Node *node, int x, int value)\n {\n int L = node->interval.first;\n int R = node->interval.second;\n\n if (L == R)\n {\n array[L] += value;\n return;\n }\n\n if (L <= x && (L + R) / 2 >= x)\n // x is in left subtree\n update(node->left, x, value);\n else\n // x is in right subtree\n update(node->right, x, value);\n\n int leftIndex = node->left->index;\n int rightIndex = node->right->index;\n\n //update current node\n node->index = (array[leftIndex] < array[rightIndex]) ? leftIndex : rightIndex;\n }\n\n // To clear allocated memory at end of program\n void clearMem(Node *node)\n {\n int L = node->interval.first;\n int R = node->interval.second;\n\n if (L != R)\n {\n clearMem(node->left);\n clearMem(node->right);\n }\n delete node;\n }\n\npublic:\n std::vector<int> array;\n\n SegmentTree(std::vector<int> &ar)\n {\n array = ar;\n root = new Node;\n build(ar, root, 0, ar.size() - 1);\n }\n\n int query(int L, int R)\n {\n return query(root, L, R);\n }\n\n void update(int pos, int value)\n {\n return update(root, pos, value);\n }\n\n ~SegmentTree()\n {\n clearMem(root);\n }\n};\n\nint main()\n{\n // define n and array\n int n = 8;\n std::vector<int> array = {5, 4, 3, 2, 1, 0, 7, 0};\n\n SegmentTree st(array);\n\n std::cout << \"Array:\\n\";\n for (int i = 0; i < n; ++i)\n std::cout << st.array[i] << ' ';\n std::cout << '\\n';\n\n // sample query\n std::cout << \"The smallest element in the interval [1, 6] is \"\n << array[st.query(0, 5)] << '\\n'; // since array is 0 indexed.\n\n // change 0 at index 5 to 8\n st.update(5, 8);\n array[5] += 8;\n\n std::cout << \"After update, array:\\n\";\n for (int i = 0; i < n; ++i)\n std::cout << st.array[i] << ' ';\n std::cout << '\\n';\n\n std::cout << \"The smallest element in the interval [1, 6] after update is \"\n << array[st.query(0, 5)] << '\\n';\n\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/segment_tree/segment_tree.scala",
"content": "import scala.reflect.ClassTag\n\ncase class SegmentTree[T: ClassTag](input: Array[T], aggregator: (T, T) => T) {\n\n val maxArraySize = Math.pow(2.0, Math.ceil(Math.log(input.length) / Math.log(2.0))).toInt * 2 - 1\n val treeArray = populateTree((0, input.length - 1), Array.ofDim[T](maxArraySize), 0)\n\n def leftChild(index: Int) = index * 2 + 1\n def rightChild(index: Int) = index * 2 + 2\n def midIndex(left: Int, right: Int) = left + (right - left) / 2\n\n def populateTree(inputRange: (Int, Int), treeArray: Array[T], treeIndex: Int): Array[T] = {\n assert(inputRange._1 <= inputRange._2)\n\n if (inputRange._1 == inputRange._2) {\n treeArray(treeIndex) = input(inputRange._1)\n }\n else {\n val mid = midIndex(inputRange._1, inputRange._2)\n populateTree((inputRange._1, mid), treeArray, leftChild(treeIndex))\n populateTree((mid + 1, inputRange._2), treeArray, rightChild(treeIndex))\n treeArray(treeIndex) = aggregator(treeArray(leftChild(treeIndex)), treeArray(rightChild(treeIndex)))\n }\n treeArray\n }\n\n def getAggregate(start: Int, end: Int): T = {\n assert(start <= end)\n getAggregate(0, input.length - 1, start, end, 0)\n }\n\n def getAggregate(start: Int, end: Int, queryStart: Int, queryEnd: Int, treeIndex: Int): T = {\n // Query range entirely inside of range\n // println(s\"segment range: ${start}, ${end}\")\n // println(s\"query range: ${queryStart}, ${queryEnd}\")\n if (queryStart <= start && queryEnd >= end) {\n // println(\"returning single node value\")\n treeArray(treeIndex)\n }\n else {\n val mid = midIndex(start, end)\n // println(s\"${start}, ${mid}, ${end}\")\n\n if (mid < queryStart) {\n // Query range starts after mid, throw out left half\n getAggregate(mid + 1, end, queryStart, queryEnd, rightChild(treeIndex))\n }\n else if (queryEnd < mid + 1) {\n // Query range ends beefore mid, throw out right half\n getAggregate(start, mid, queryStart, queryEnd, leftChild(treeIndex))\n }\n else {\n aggregator(\n getAggregate(start, mid, queryStart, queryEnd, leftChild(treeIndex)),\n getAggregate(mid + 1, end, queryStart, queryEnd, rightChild(treeIndex)))\n }\n }\n }\n\n def update(index: Int, value: T): Unit = {\n update((0, treeArray.length - 1), index, value, 0)\n }\n\n def update(inputRange: (Int, Int), index: Int, value: T, treeIndex: Int): Unit = {\n if (inputRange._1 == inputRange._2) {\n assert(index == inputRange._1)\n treeArray(treeIndex) = value\n }\n else {\n val mid = midIndex(inputRange._1, inputRange._2)\n if (index <= mid) {\n update((inputRange._1, mid), index, value, leftChild(treeIndex))\n }\n else {\n update((mid + 1, inputRange._2), index, value, rightChild(treeIndex))\n }\n treeArray(treeIndex) = aggregator(treeArray(leftChild(treeIndex)), treeArray(rightChild(treeIndex)))\n }\n }\n}\n\nobject Main {\n def main(args: Array[String]): Unit = {\n val segTree = SegmentTree[Int](Array(1, 2, -3, 4, 5), (x: Int, y: Int) => Math.min(x, y))\n println(segTree.getAggregate(0, 13))\n val sumTree = SegmentTree[Int](Array(1, 2, -3, 4, 5), (x: Int, y: Int) => x + y)\n println(sumTree.getAggregate(1, 3))\n }\n}"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/segment_tree/segment_tree_sum.cpp",
"content": "// Segment tree with spaces. Allows to find sum in O(log size) time. Uses O(n log size) memory.\n\n#include <iostream>\n#include <string>\n\nconst int size = 1000000000;\n\nstruct node\n{\n int leftBound, rightBound;\n node *leftChild, *rightChild;\n int value;\n node (int LeftBound, int RightBound)\n {\n leftBound = LeftBound;\n rightBound = RightBound;\n value = 0;\n leftChild = rightChild = 0;\n }\n} *root;\n\nvoid add (node *vertex, int destination, int value)\n{\n if (vertex->leftBound == destination && vertex->rightBound == destination + 1)\n {\n vertex->value += value;\n return;\n }\n\n vertex->value += value;\n\n int middle = (vertex->leftBound + vertex->rightBound) / 2;\n\n if (destination < middle)\n {\n if (vertex->leftChild == 0)\n vertex->leftChild = new node (vertex->leftBound, middle);\n add (vertex->leftChild, destination, value);\n }\n else\n {\n if (vertex->rightChild == 0)\n vertex->rightChild = new node (middle, vertex->rightBound);\n add (vertex->rightChild, destination, value);\n }\n}\n\nint ask (node *vertex, int leftBound, int rightBound)\n{\n if (vertex == 0)\n return 0;\n\n if (vertex->leftBound >= leftBound && vertex->rightBound <= rightBound)\n return vertex->value;\n\n if (vertex->leftBound >= rightBound && vertex->rightBound <= leftBound)\n return 0;\n\n return ask (vertex->leftChild, leftBound, rightBound) + ask (vertex->rightChild, leftBound,\n rightBound);\n}\n\nint main ()\n{\n root = new node (-size, size); // Actually allows negative numbers\n\n std::cout << \"Print number of queries!\\n\";\n\n int q;\n std::cin >> q;\n\n for (int _ = 0; _ < q; _++)\n {\n std::string type;\n std::cin >> type;\n\n if (type == \"add\")\n {\n int destination, value;\n std::cin >> destination >> value;\n\n add (root, destination, value);\n\n std::cout << \"Added succesfully!\\n\";\n }\n else if (type == \"ask\")\n {\n int leftBound, rightBound;\n std::cin >> leftBound >> rightBound;\n\n std::cout << ask (root, leftBound, rightBound) << \"\\n\";\n }\n else\n std::cout << \"Unknown Type! Only add and ask allowed\\n\";\n }\n\n}"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/segment_tree/segment_tree_sum.go",
"content": "/*\n * Segment Tree\n * Update: 1 point\n * Query: Range\n * Query Type: Range Sum Query\n */\npackage main\n\nimport \"fmt\"\n\ntype SegmentTree struct {\n\tN int\n\ttree []int\n}\n\nfunc (stree *SegmentTree) Initialize(n int) {\n\tstree.N = n\n\tstree.tree = make([]int, 4*n)\n}\n\nfunc (stree *SegmentTree) build(arr []int, idx int, l int, r int) {\n\tif l == r {\n\t\tstree.tree[idx] = arr[l]\n\t\treturn\n\t}\n\n\tmid := (l + r) / 2\n\n\tstree.build(arr, 2*idx+1, l, mid)\n\tstree.build(arr, 2*idx+2, mid+1, r)\n\tstree.tree[idx] = stree.tree[2*idx+1] + stree.tree[2*idx+2]\n}\n\nfunc (stree *SegmentTree) Build(arr []int) {\n\tstree.build(arr, 0, 0, stree.N-1)\n}\n\nfunc (stree *SegmentTree) update(idx int, l int, r int, pos int, val int) {\n\tif l == r {\n\t\tstree.tree[idx] = val\n\t\treturn\n\t}\n\n\tmid := (l + r) / 2\n\n\tif pos <= mid {\n\t\tstree.update(2*idx+1, l, mid, pos, val)\n\t} else {\n\t\tstree.update(2*idx+2, mid+1, r, pos, val)\n\t}\n\n\tstree.tree[idx] = stree.tree[2*idx+1] + stree.tree[2*idx+2]\n}\n\nfunc (stree *SegmentTree) Update(pos int, val int) {\n\tstree.update(0, 0, stree.N-1, pos, val)\n}\n\nfunc (stree *SegmentTree) query(idx int, l int, r int, ql int, qr int) int {\n\tif ql <= l && r <= qr {\n\t\treturn stree.tree[idx]\n\t}\n\n\tmid := (l + r) / 2\n\tleft := 0\n\tright := 0\n\n\tif ql <= mid {\n\t\tleft = stree.query(2*idx+1, l, mid, ql, qr)\n\t}\n\n\tif qr > mid {\n\t\tright = stree.query(2*idx+2, mid+1, r, ql, qr)\n\t}\n\n\treturn left + right\n}\n\nfunc (stree *SegmentTree) Query(ql int, qr int) int {\n\treturn stree.query(0, 0, stree.N-1, ql, qr)\n}\n\nfunc main() {\n\tarr := []int{1, 2, 3, 4, 5}\n\tn := len(arr)\n\n\tvar stree SegmentTree\n\tstree.Initialize(n)\n\tstree.Build(arr)\n\n\tfmt.Printf(\"RSQ from 2 to 4: %v\\n\", stree.Query(1, 3))\n\tfmt.Printf(\"RSQ from 1 to 5: %v\\n\", stree.Query(0, 4))\n\n\tstree.Update(2, 7)\n\tfmt.Println(\"Change to 7\")\n\tfmt.Printf(\"RSQ from 3 to 5: %v\\n\", stree.Query(2, 4))\n\tfmt.Printf(\"RSQ from 1 to 5: %v\\n\", stree.Query(0, 4))\n}"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/segment_tree/segment_tree_sum.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n\nclass SegmentTree:\n def __init__(self, arr):\n self.n = len(arr) # number of elements in array\n self.arr = arr # stores given array\n self.segtree = [0] * (\n 4 * self.n\n ) # stores segment tree, space required is 4 * (number of elements in array)\n self.build(0, 0, self.n - 1)\n\n def build(self, node, i, j): # Time Complexity: O(n)\n if i == j:\n # Leaf node\n self.segtree[node] = self.arr[i]\n return\n # Recurse on left and right children\n mid = i + (j - i) / 2\n left = 2 * node + 1 # left child index\n right = 2 * node + 2 # right child index\n self.build(left, i, mid)\n self.build(right, mid + 1, j)\n # Internal node will be the sum of its two children\n self.segtree[node] = self.segtree[left] + self.segtree[right]\n\n def queryHelper(self, node, i, j, lo, hi):\n if j < lo or i > hi:\n # range represented by a node is completely outside the given range\n return 0\n if lo <= i and j <= hi:\n # range represented by a node is completely inside the given range\n return self.segtree[node]\n # range represented by a node is partially inside and partially outside the given range\n mid = i + (j - i) / 2\n left = 2 * node + 1 # left child index\n right = 2 * node + 2 # right child index\n q1 = self.queryHelper(left, i, mid, lo, hi)\n q2 = self.queryHelper(right, mid + 1, j, lo, hi)\n return q1 + q2\n\n # wrapper to queryHelper\n def query(self, i, j): # Time Complexity: O(log n)\n return self.queryHelper(0, 0, self.n - 1, i, j)\n\n def updateHelper(self, node, i, j, idx, val):\n if i == j:\n # Leaf Node\n self.arr[i] += val\n self.segtree[node] += val\n return\n mid = i + (j - i) / 2\n left = 2 * node + 1 # left child index\n right = 2 * node + 2 # right child index\n\n if i <= idx and idx <= mid:\n # update in left child\n self.updateHelper(left, i, mid, idx, val)\n else:\n # update in right child\n self.updateHelper(right, mid + 1, j, idx, val)\n # update internal nodes\n self.segtree[node] = self.segtree[left] + self.segtree[right]\n\n # wrapper to updateHelper\n def update(self, idx, val): # Time Complexity: O(log n)\n self.updateHelper(0, 0, self.n - 1, idx, val)\n\n\narr = [1, 2, 3, -1, 5]\ntree = SegmentTree(arr)\ntree.update(3, 5) # arr[3] = 4\nprint(tree.query(0, 3))"
}
{
"filename": "code/data_structures/src/tree/space_partitioning_tree/segment_tree/segment_tree_sum.rb",
"content": "# Part of Cosmos by OpenGenus Foundation\n\nclass SegTree\n def initialize(arr)\n @arr = arr\n @end = arr.length - 1\n @tree = Array.new(arr.length * 4, 0)\n start(0, 0, @end)\n end\n\n def start(root, left, right)\n if left == right\n @tree[root] = @arr[left]\n return 0\n end\n mid = (left + right) / 2\n node_left = 2 * root + 1\n node_right = node_left + 1\n\n start(node_left, left, mid)\n start(node_right, mid + 1, right)\n\n @tree[root] = @tree[node_right] + @tree[node_left]\n end\n\n def query_helper(root, q_left, q_right, left, right)\n if (q_left > right) || (q_right < left)\n # range represented by a node is completely outside the given range\n return 0\n end\n\n if (q_left <= left) && (q_right >= right)\n # range represented by a node is completely inside the given range\n return @tree[root]\n end\n\n mid = (left + right) / 2\n left_node = 2 * root + 1\n right_node = left_node + 1\n\n left_sum = query_helper(left_node, q_left, q_right, left, mid)\n right_sum = query_helper(right_node, q_left, q_right, mid + 1, right)\n\n left_sum + right_sum\n end\n\n # wrapper to queryHelper\n def query(query_left, query_right)\n query_helper(0, query_left, query_right, 0, @end)\n end\n\n def update_helper(root, pos, value, left, right)\n return if (pos < left) || (pos > right)\n\n if left == right\n @tree[root] = value\n return\n end\n\n mid = (left + right) / 2\n left_node = 2 * root + 1\n right_node = left_node + 1\n\n update_helper(left_node, pos, value, left, mid)\n update_helper(right_node, pos, value, mid + 1, right)\n\n # recursive update\n @tree[root] = @tree[left_node] + @tree[right_node]\n\n nil\n end\n\n # update wrapper\n def update(pos, value)\n update_helper(0, pos, value, 0, @end)\n end\n\n private :start, :query_helper, :update_helper\nend\n\narr = [1, 2, -1, 4, 5]\nseg_tree = SegTree.new arr\nseg_tree.update 2, 3 # arr[2] = 5\np seg_tree.query 0, 4"
}
{
"filename": "code/data_structures/src/tree/tree/suffix_array/suffix_array.cpp",
"content": "#include <iostream>\n#include <vector>\n#include <algorithm>\n#include <cmath>\n\n#define ll size_t\nusing namespace std;\n\nstruct ranking\n{\n ll index;\n ll first;\n ll second;\n};\n\nbool comp(ranking a, ranking b)\n{\n if (a.first == b.first)\n return a.second < b.second;\n return a.first < b.first;\n}\n\nvector<ll> build_suffix_array(string s)\n{\n const ll n = s.length();\n const ll lgn = ceil(log2(n));\n\n // vector to hold final suffix array result\n vector<ll> sa(n);\n\n // P[i][j] holds the ranking of the j-th suffix\n // after comparing the first 2^i characters\n // of that suffix\n ll P[lgn][n];\n\n // vector to store ranking tuples of suffixes\n vector<ranking> ranks(n);\n\n ll i, j, step = 1;\n for (j = 0; j < n; j++)\n P[0][j] = s[j] - 'a';\n\n for (i = 1; i <= lgn; i++, step++)\n {\n for (j = 0; j < n; j++)\n {\n ranks[j].index = j;\n ranks[j].first = P[i - 1][j];\n ranks[j].second = (j + std::pow(2, i - 1) < n) ? P[i - 1][j + (ll)(pow(2, i - 1))] : -1;\n }\n\n std::sort(ranks.begin(), ranks.end(), comp);\n\n for (j = 0; j < n; j++)\n P[i][ranks[j].index] =\n (j > 0 && ranks[j].first == ranks[j - 1].first &&\n ranks[j].second == ranks[j - 1].second) ? P[i][ranks[j - 1].index] : j;\n }\n\n step -= 1;\n\n for (i = 0; i < n; i++)\n sa[P[step][i]] = i;\n\n return sa;\n}\n\nint main()\n{\n string s;\n cin >> s;\n vector<ll> sa = build_suffix_array(s);\n for (ll i = 0; i < s.length(); i++)\n cout << i << \" : \" << sa[i] << endl;\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/tree/trie/README.md",
"content": "# Trie\nDescription\n---\nIn computer science, also called digital tree and sometimes radix tree or prefix tree \n(as they can be searched by prefixes), \nis a kind of search tree -- an ordered tree data structure that is used to store a \ndynamic set or associative array where the keys are usually strings.\n\nTrie API\n---\n- insert(text): Insert a text node into the trie.\n- contains(text) or search(text): Return true trie contains text otherwise return false\n- delete(text): Insert a text node from the trie if exists.\n\nComplexity\n---\nInsert and search costs O(key_length), \nhowever the memory requirements of Trie is \nO(ALPHABET_SIZE * key_length * N) \nwhere N is number of keys in Trie."
}
{
"filename": "code/data_structures/src/tree/tree/trie/trie.c",
"content": "#include <stdio.h>\n#include <stdlib.h>\n#include <string.h>\n#include <stdbool.h>\n// Part of Cosmos by OpenGenus Foundation\n#define ARRAY_SIZE(a) sizeof(a)/sizeof(a[0]) \n#define ALPHABET_SIZE (26)\n#define INDEX(c) ((int)c - (int)'a')\n#define FREE(p) \\\n free(p); \\\n p = NULL;\n \ntypedef struct trie_node trie_node_t;\n \nstruct trie_node\n{\n int value; // non zero if leaf\n trie_node_t *children[ALPHABET_SIZE];\n};\n \ntypedef struct trie trie_t;\n \nstruct trie\n{\n trie_node_t *root;\n int count;\n};\n \ntrie_node_t *getNode(void)\n{\n trie_node_t *pNode = NULL;\n pNode = (trie_node_t *)malloc(sizeof(trie_node_t));\n \n if( pNode )\n {\n int i;\n pNode->value = 0;\n \n for(i = 0; i < ALPHABET_SIZE; i++)\n {\n pNode->children[i] = NULL;\n }\n }\n return pNode;\n}\n \nvoid initialize(trie_t *pTrie)\n{\n pTrie->root = getNode();\n pTrie->count = 0;\n}\n \nvoid insert(trie_t *pTrie, char key[])\n{\n int level;\n int length = strlen(key);\n int index;\n trie_node_t *pCrawl;\n \n pTrie->count++;\n pCrawl = pTrie->root;\n \n for( level = 0; level < length; level++ )\n {\n index = INDEX(key[level]);\n \n if( pCrawl->children[index] )\n {\n // Skip current node\n pCrawl = pCrawl->children[index];\n }\n else\n {\n // Add new node\n pCrawl->children[index] = getNode();\n pCrawl = pCrawl->children[index];\n }\n }\n \n // mark last node as leaf (non zero)\n pCrawl->value = pTrie->count;\n}\n \nint search(trie_t *pTrie, char key[])\n{\n int level;\n int length = strlen(key);\n int index;\n trie_node_t *pCrawl;\n pCrawl = pTrie->root;\n \n for( level = 0; level < length; level++ )\n {\n index = INDEX(key[level]);\n if( !pCrawl->children[index] )\n {\n return 0;\n }\n pCrawl = pCrawl->children[index];\n }\n return (0 != pCrawl && pCrawl->value);\n}\n \nint leafNode(trie_node_t *pNode)\n{\n return (pNode->value != 0);\n}\n \nint isItFreeNode(trie_node_t *pNode)\n{\n int i;\n for(i = 0; i < ALPHABET_SIZE; i++)\n {\n if( pNode->children[i] )\n return 0;\n }\n return 1;\n}\n \nbool deleteHelper(trie_node_t *pNode, char key[], int level, int len)\n{\n if( pNode )\n {\n // Base case\n if( level == len )\n {\n if( pNode->value )\n {\n // Unmark leaf node\n pNode->value = 0;\n // If empty, node to be deleted\n if( isItFreeNode(pNode) )\n {\n return true;\n }\n return false;\n }\n }\n else // Recursive case\n {\n int index = INDEX(key[level]);\n if( deleteHelper(pNode->children[index], key, level+1, len) )\n {\n // last node marked, delete it\n FREE(pNode->children[index]);\n // recursively climb up, and delete eligible nodes\n return ( !leafNode(pNode) && isItFreeNode(pNode) );\n }\n }\n }\n return false;\n}\n \nvoid deleteKey(trie_t *pTrie, char key[])\n{\n int len = strlen(key);\n if( len > 0 )\n {\n deleteHelper(pTrie->root, key, 0, len);\n }\n}\n \nint main()\n{\n char keys[][8] = {\"cat\", \"case\", \"deaf\", \"dear\", \"a\", \"an\", \"the\"};\n trie_t trie;\n \n initialize(&trie);\n for(int i = 0; i < ARRAY_SIZE(keys); i++)\n {\n insert(&trie, keys[i]);\n }\n deleteKey(&trie, keys[0]);\n printf(\"%s %s\\n\", \"cat\", search(&trie, \"cat\") ? \"Present in trie\" : \"Not present in trie\");\n \n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/tree/trie/trie.cpp",
"content": "#include <cstdio>\n#include <string>\n#include <iostream>\n\n// Part of Cosmos by OpenGenus\nusing namespace std;\n#define mod 1000000007\n#define all(v) v.begin(), v.end_()\n#define rep(i, a, b) for (i = (ll)a; i < (ll)b; i++)\n#define revrep(i, a, b) for (i = (ll)a; i >= (ll)b; i--)\n#define strep(it, v) for (it = v.begin(); it != v.end_(); ++it)\n#define ii pair<ll, ll>\n#define MP make_pair\n#define pb push_back\n#define f first\n#define se second\n#define ll long long int\n#define vi vector<ll>\nll modexp(ll a, ll b)\n{\n ll res = 1; while (b > 0)\n {\n if (b & 1)\n res = (res * a);\n a = (a * a); b /= 2;\n }\n return res;\n}\n#define rs resize\nlong long readLI()\n{\n register char c; for (c = getchar(); !(c >= '0' && c <= '9'); c = getchar())\n {\n }\n register long long a = c - '0';\n for (c = getchar(); c >= '0' && c <= '9'; c = getchar())\n a = (a << 3) + (a << 1) + c - '0';\n return a;\n}\nconst ll N = 100009;\nint n, i, j, sz;\nstring a;\nll next_[27][N], end_[N];\nvoid add(string a)\n{\n int v = 0;\n for (int i = 0; i < (int)a.size(); i++)\n {\n int c = a[i] - 'a';\n if (next_[c][v] == -1)\n v = next_[c][v] = ++sz;\n else\n v = next_[c][v];\n }\n ++end_[v];\n}\nbool search(string a)\n{\n int v = 0;\n for (i = 0; i < (int)a.size(); i++)\n {\n int c = a[i] - 'a';\n if (next_[c][v] == -1)\n return false;\n v = next_[c][v];\n }\n return end_[v] > 0;\n}\nint main()\n{\n std::ios_base::sync_with_stdio(false); cin.tie(NULL);\n cin >> n;\n rep(i, 0, 27) for (int j = 0; j < N; j++)\n next_[i][j] = -1;\n rep(i, 0, n) cin >> a, add(a);\n cin >> n;\n while (n--)\n {\n cin >> a;\n cout << search(a) << endl;\n }\n return 0;\n}"
}
{
"filename": "code/data_structures/src/tree/tree/trie/trie.cs",
"content": "using System;\nusing System.Collections.Generic;\nusing Xunit;\nusing Xunit.Abstractions;\n\nnamespace Cosmos\n{\n // XUnit test to test Trie data structure.\n\tpublic class TriestTest\n\t{\n\t\tprivate readonly TrieBuilder _trieBuilder = new TrieBuilder();\n\n\t\tpublic TriestTest(ITestOutputHelper output) : base(output)\n\t\t{\n\t\t}\n\n\t\t[Fact]\n\t\tpublic void TestCreation()\n\t\t{\n\t\t\t//var words = new[] {\"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\"};\n\t\t\tvar words = new[] {\"abc\", \"abgl\"};\n\n\t\t\tTrieNode root = _trieBuilder.BuildTrie(words);\n\t\t\tConsole.WriteLine(root);\n\t\t}\n\n\t\t[Theory]\n\t\t[MemberData(nameof(GetCompleteWordData))]\n\t\tpublic void TestCompleteWordSearch(bool expected, string word, string[] source)\n\t\t{\n\t\t\tvar trieNode = _trieBuilder.BuildTrie(source);\n\t\t\tvar sut = new Trie(trieNode);\n\t\t\tvar actual = sut.SearchCompleteWord(word);\n\n\t\t\tAssert.Equal(expected, actual);\n\t\t}\n\n\t\t[Theory]\n\t\t[MemberData(nameof(GetPrefixData))]\n\t\tpublic void TestPrefixWordSearch(bool expected, string prefix, string[] source)\n\t\t{\n\t\t\tvar trieNode = _trieBuilder.BuildTrie(source);\n\t\t\tvar trie = new Trie(trieNode);\n\t\t\tvar actual = trie.SearchPrefix(prefix);\n\t\t\t\n\t\t\tAssert.Equal(expected, actual);\n\t\t}\n\n\t\tpublic static IEnumerable<object[]> GetPrefixData()\n\t\t{\n\t\t\tyield return new object[] { true, \"abc\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { true, \"ab\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { true, \"a\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { true, \"cdf\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { true, \"cd\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { true, \"c\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { false, \"\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { false, \"x\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { false, \"abd\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { false, \"abx\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { false, \"cda\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { false, \"swyx\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t}\n\n\t\tpublic static IEnumerable<object[]> GetCompleteWordData()\n\t\t{\n\t\t\tyield return new object[] { true, \"abc\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { true, \"abgl\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { true, \"cdf\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { true, \"abcd\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { true, \"lmn\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { false, \"ab\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { false, \"abg\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { false, \"cd\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { false, \"abcx\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { false, \"abglx\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { false, \"cdfx\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { false, \"abcdx\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t\tyield return new object[] { false, \"lmnx\", new[] { \"abc\", \"abgl\", \"cdf\", \"abcd\", \"lmn\" } };\n\t\t}\n\n\t}\n\n\tpublic class Trie\n\t{\n\t\tpublic TrieNode Root { get; set; }\n\n\t\tpublic Trie(TrieNode root)\n\t\t{\n\t\t\tRoot = root;\n\t\t}\n\n\t\t/// <summary>\n\t\t/// Search for a complete word, not a prefix search.\n\t\t/// </summary>\n\t\tpublic bool SearchCompleteWord(string word)\n\t\t{\n\t\t\tvar current = Root;\n\t\t\tforeach (char c in word)\n\t\t\t{\n\t\t\t\tif (current.Children.TryGetValue(c, out TrieNode node))\n\t\t\t\t\tcurrent = node;\n\t\t\t\telse\n\t\t\t\t\treturn false;\n\t\t\t}\n\n\t\t\treturn current.IsCompleteWord;\n\t\t}\n\n\t\tpublic bool SearchPrefix(string prefix)\n\t\t{\n\t\t\tif (string.IsNullOrWhiteSpace(prefix)) return false;\n\n\t\t\tvar current = Root;\n\t\t\tforeach (char c in prefix)\n\t\t\t{\n\t\t\t\tif (current.Children.TryGetValue(c, out TrieNode node))\n\t\t\t\t\tcurrent = node;\n\t\t\t\telse\n\t\t\t\t\treturn false;\n\t\t\t}\n\n\t\t\treturn true;\n\t\t}\n\t}\n\n\tpublic class TrieNode\n\t{\n\t\tpublic bool IsCompleteWord { get; set; } = false;\n\n\t\tpublic Dictionary<char, TrieNode> Children { get; } = new Dictionary<char, TrieNode>();\n\t}\n\n\t/// <summary>\n\t/// Based on https://www.youtube.com/watch?v=AXjmTQ8LEoI by Tushar Roy\n\t/// </summary>\n\tpublic class TrieBuilder\n\t{\n\t\tpublic TrieNode BuildTrie(IEnumerable<string> words)\n\t\t{\n\t\t\tTrieNode root = new TrieNode();\n\t\t\tforeach (var word in words)\n\t\t\t{\n\t\t\t\tInsert(root, word);\n\t\t\t}\n\n\t\t\treturn root;\n\t\t}\n\n\t\tprivate void Insert(TrieNode current, string word)\n\t\t{\n\t\t\tforeach (char c in word)\n\t\t\t{\n\t\t\t\tcurrent.Children.TryGetValue(c, out TrieNode node);\n\t\t\t\tif (node == null)\n\t\t\t\t{\n\t\t\t\t\tnode = new TrieNode();\n\t\t\t\t\tcurrent.Children.Add(c, node);\n\t\t\t\t}\n\t\t\t\tcurrent = node;\n\t\t\t}\n\n\t\t\tcurrent.IsCompleteWord = true;\n\t\t}\n\t}\n}"
}
{
"filename": "code/data_structures/src/tree/tree/trie/trie.go",
"content": "package main\n\nimport \"fmt\"\n\n// TrieNode has a value and a pointer to another TrieNode\ntype TrieNode struct {\n\tchildren map[rune]*TrieNode\n\tvalue interface{}\n}\n\n// New allocates and returns a new *TrieNode.\nfunc New() *TrieNode {\n\treturn &TrieNode{\n\t\tchildren: make(map[rune]*TrieNode),\n\t}\n}\n\n// Contains returns true trie contains text otherwise return false\nfunc (trie *TrieNode) Contains(key string) bool {\n\tnode := trie\n\tfor _, ch := range key {\n\t\tnode = node.children[ch]\n\t\tif node == nil {\n\t\t\treturn false\n\t\t}\n\n\t}\n\treturn node.value != nil\n}\n\nfunc (trie *TrieNode) Insert(key string, value int) bool {\n\tnode := trie\n\tfor _, ch := range key {\n\t\tchild, _ := node.children[ch]\n\n\t\tif child == nil {\n\t\t\tchild = New()\n\t\t\tnode.children[ch] = child\n\t\t}\n\n\t\tnode = child\n\t}\n\n\tisNewVal := node.value == nil\n\tnode.value = value\n\n\treturn isNewVal\n}\n\n// PathTrie node and the part string key of the child the path descends into.\ntype nodeStr struct {\n\tnode *TrieNode\n\tpart rune\n}\n\nfunc (trie *TrieNode) Delete(key string) bool {\n\tvar path []nodeStr // record ancestors to check later\n\tnode := trie\n\tfor _, ch := range key {\n\t\tpath = append(path, nodeStr{part: ch, node: node})\n\t\tnode = node.children[ch]\n\t\tif node == nil {\n\t\t\t// node does not exist\n\t\t\treturn false\n\t\t}\n\t}\n\n\t// delete the node value\n\tnode.value = nil\n\n\t// if leaf, remove it from its parent's children map. Repeat for ancestor path.\n\tif len(node.children) == 0 {\n\t\t// iterate backwards over path\n\t\tfor i := len(path) - 1; i >= 0; i-- {\n\t\t\tparent := path[i].node\n\t\t\tpart := path[i].part\n\t\t\tdelete(parent.children, part)\n\t\t\tif parent.value != nil || !(len(parent.children) == 0) {\n\t\t\t\t// parent has a value or has other children, stop\n\t\t\t\tbreak\n\t\t\t}\n\t\t}\n\t}\n\n\treturn true\n}\n\nfunc main() {\n\tkeys := []string{\"cat\", \"case\", \"deaf\", \"dear\", \"a\", \"an\", \"the\"}\n\ttr := New()\n\n\tfor i, key := range keys {\n\t\ttr.Insert(key, i)\n\t}\n\n\ttr.Delete(\"dear\")\n\tvar contains string\n\n\tif tr.Contains(\"dear\") {\n\t\tcontains = \"contains\"\n\t} else {\n\t\tcontains = \"does not contain\"\n\t}\n\tfmt.Printf(\"Trie %v %v\\n\", contains, \"dear\")\n\tif tr.Contains(\"case\") {\n\t\tcontains = \"contains\"\n\t} else {\n\t\tcontains = \"does not contain\"\n\t}\n\tfmt.Printf(\"Trie %v %v\\n\", contains, \"case\")\n}"
}
{
"filename": "code/data_structures/src/tree/tree/trie/trie.java",
"content": "// Part of Cosmos by OpenGenus Foundation\nclass TrieNode {\n char c; \n HashMap<Character, TrieNode> children = new HashMap<Character, TrieNode>();\n boolean isEnd;\n \n public TrieNode() {}\n public TrieNode(char c){ this.c = c; }\n}\n\npublic class Trie {\n private TrieNode root;\n\n public Trie() {\n root = new TrieNode();\n }\n\n // Inserts a word into the trie.\n public void insert(String word) {\n TrieNode node = root;\n\n for (int i = 0; i < word.length(); i++) {\n char c = word.charAt(i);\n if (!node.children.containsKey(c)) {\n node.children.put(c,new TrieNode(c));\n }\n node = node.children.get(c); \n }\n\n node.isEnd = true;\n }\n\n // Returns true if the word is in the trie.\n public boolean search(String word) {\n TrieNode node = searchNode(word);\n return (node == null) ? false : node.isEnd; \n }\n\n // Returns true if there is any word in the trie that starts with the given prefix.\n public boolean startsWith(String prefix) {\n TrieNode node = searchNode(prefix);\n return (node == null) ? false : true; \n }\n\n public TrieNode searchNode(String s) {\n TrieNode node = root;\n\n for (int i = 0; i < s.length(); i++) {\n char c = s.charAt(i);\n if (node.children.containsKey(c)) {\n node = node.children.get(c);\n } else {\n return null;\n }\n }\n\n if (node == root) return null;\n\n return node;\n }\n}"
}
{
"filename": "code/data_structures/src/tree/tree/trie/trie.js",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\nclass Node {\n constructor() {\n this.keys = new Map();\n this.end = false;\n }\n setEnd() {\n this.end = true;\n }\n isEnd() {\n return this.end;\n }\n}\n\nclass Trie {\n constructor() {\n this.root = new Node();\n }\n\n add(input, node = this.root) {\n if (input.length === 0) {\n node.setEnd();\n return;\n } else if (!node.keys.has(input[0])) {\n node.keys.set(input[0], new Node());\n return this.add(input.substr(1), node.keys.get(input[0]));\n } else {\n return this.add(input.substr(1), node.keys.get(input[0]));\n }\n }\n isWord(word) {\n let node = this.root;\n while (word.length > 1) {\n if (!node.keys.has(word[0])) {\n return false;\n } else {\n node = node.keys.get(word[0]);\n word = word.substr(1);\n }\n }\n return node.keys.has(word) && node.keys.get(word).isEnd() ? true : false;\n }\n print() {\n let words = [];\n const search = function(node, string) {\n if (node.keys.size !== 0) {\n for (let letter of node.keys.keys()) {\n search(node.keys.get(letter), string.concat(letter));\n }\n if (node.isEnd()) {\n words.push(string);\n }\n } else {\n string.length > 0 ? words.push(string) : undefined;\n return;\n }\n };\n search(this.root, \"\");\n return words.length > 0 ? words : mo;\n }\n}\n\n// Trie Examples\n\nmyTrie.add(\"cat\");\nmyTrie.add(\"catch\");\nmyTrie.add(\"dog\");\nmyTrie.add(\"good\");\nmyTrie.add(\"goof\");\nmyTrie.add(\"jest\");\nmyTrie.add(\"come\");\nmyTrie.add(\"comedy\");\nmyTrie.isWord(\"catc\");\nmyTrie.isWord(\"jest\");\nmyTrie.isWord(\"come\");\nmyTrie.print();"
}
{
"filename": "code/data_structures/src/tree/tree/trie/trie.py",
"content": "# An efficient information reTRIEval data structure\n\n\nclass TrieNode(object):\n def __init__(self):\n self.children = {}\n\n def __repr__(self):\n return str(self.children)\n\n\ndef insert(root_node, word):\n n = root_node\n for character in word:\n t = n.children.get(character)\n if not t:\n t = TrieNode()\n n.children[character] = t\n n = t\n\n\ndef search(root_node, search_string):\n node = root_node\n for character in search_string:\n c = node.children.get(character)\n if not c:\n return False\n node = c\n return True\n\n\nroot = TrieNode()\n\ninsert(root, \"abc\")\ninsert(root, \"pqrs\")\n\nprint(search(root, \"abc\")) # will print True\nprint(search(root, \"xyz\")) # will print False"
}
{
"filename": "code/data_structures/src/tree/tree/trie/trie.rb",
"content": "class Trie\n def insert(word)\n return if word.empty?\n\n first, rest = word.split(''.freeze, 2)\n children[first].insert(rest)\n end\n\n def search(word)\n return true if word.empty?\n\n first, rest = word.split(''.freeze, 2)\n children.key?(first) && children[first].search(rest)\n end\n\n private\n\n def children\n @children ||= Hash.new do |hash, character|\n hash[character] = Trie.new\n end\n end\nend\n\nroot = Trie.new\n\nroot.insert 'abc'\nroot.insert 'pqrs'\n\nputs root.search('abc') # => true\nputs root.search('xyz') # => false"
}
{
"filename": "code/data_structures/src/tree/tree/trie/trie.scala",
"content": "import collection.immutable.IntMap\n\nobject Trie {\n def apply(text: String): Trie = {\n if (text == \"\") {\n return new Trie(IntMap(), true)\n } else {\n return new Trie(IntMap(text.charAt(0).asInstanceOf[Int] -> apply(text.substring(1))), false)\n }\n }\n}\n\ncase class Trie(kids: IntMap[Trie], isTerminalNode: Boolean) {\n def insert(text: String): Trie = {\n if (text == \"\") {\n this.copy(isTerminalNode = true)\n } else {\n val firstLetterInt = text.charAt(0).asInstanceOf[Int]\n val restOfText = text.substring(1)\n val newKid = \n if (kids.contains(firstLetterInt))\n kids(firstLetterInt).insert(restOfText)\n else\n Trie(restOfText)\n\n this.copy(kids = kids + (firstLetterInt -> newKid))\n }\n }\n\n def hasText(text: String): Boolean = {\n if (text == \"\") {\n this.isTerminalNode\n } else {\n val firstLetterInt = text.charAt(0).asInstanceOf[Int]\n kids.contains(firstLetterInt) && kids(firstLetterInt).hasText(text.substring(1))\n }\n }\n\n def hasPrefix(prefix: String): Boolean = {\n if (prefix == \"\") {\n true\n } else {\n val firstLetterInt = prefix.charAt(0).asInstanceOf[Int]\n kids.contains(firstLetterInt) && kids(firstLetterInt).hasPrefix(prefix.substring(1))\n }\n }\n}"
}
{
"filename": "code/data_structures/src/tree/tree/trie/trie.swift",
"content": "// Part of Cosmos by OpenGenus Foundation\nimport Foundation\n\nclass TrieNode<T: Hashable> {\n var value: T?\n weak var parentNode: TrieNode?\n var children: [T: TrieNode] = [:]\n var isTerminating = false\n var isLeaf: Bool {\n return children.count == 0\n }\n\n init(value: T? = nil, parentNode: TrieNode? = nil) {\n self.value = value\n self.parentNode = parentNode\n }\n\n func add(value: T) {\n guard children[value] == nil else {\n return\n }\n children[value] = TrieNode(value: value, parentNode: self)\n }\n}\n\nclass Trie: NSObject, NSCoding {\n typealias Node = TrieNode<Character>\n public var count: Int {\n return wordCount\n }\n public var isEmpty: Bool {\n return wordCount == 0\n }\n public var words: [String] {\n return wordsInSubtrie(rootNode: root, partialWord: \"\")\n }\n fileprivate let root: Node\n fileprivate var wordCount: Int\n\n override init() {\n root = Node()\n wordCount = 0\n super.init()\n }\n\n required convenience init?(coder decoder: NSCoder) {\n self.init()\n let words = decoder.decodeObject(forKey: \"words\") as? [String]\n for word in words! {\n self.insert(word: word)\n }\n }\n\n func encode(with coder: NSCoder) {\n coder.encode(self.words, forKey: \"words\")\n }\n}\n\nextension Trie {\n\n func insert(word: String) {\n guard !word.isEmpty else {\n return\n }\n var currentNode = root\n for character in word.lowercased().characters {\n if let childNode = currentNode.children[character] {\n currentNode = childNode\n } else {\n currentNode.add(value: character)\n currentNode = currentNode.children[character]!\n }\n }\n guard !currentNode.isTerminating else {\n return\n }\n wordCount += 1\n currentNode.isTerminating = true\n }\n\n func contains(word: String) -> Bool {\n guard !word.isEmpty else {\n return false\n }\n var currentNode = root\n for character in word.lowercased().characters {\n guard let childNode = currentNode.children[character] else {\n return false\n }\n currentNode = childNode\n }\n return currentNode.isTerminating\n }\n\n private func findLastNodeOf(word: String) -> Node? {\n var currentNode = root\n for character in word.lowercased().characters {\n guard let childNode = currentNode.children[character] else {\n return nil\n }\n currentNode = childNode\n }\n return currentNode\n }\n\n private func findTerminalNodeOf(word: String) -> Node? {\n if let lastNode = findLastNodeOf(word: word) {\n return lastNode.isTerminating ? lastNode : nil\n }\n return nil\n\n }\n\n private func deleteNodesForWordEndingWith(terminalNode: Node) {\n var lastNode = terminalNode\n var character = lastNode.value\n while lastNode.isLeaf, let parentNode = lastNode.parentNode {\n lastNode = parentNode\n lastNode.children[character!] = nil\n character = lastNode.value\n if lastNode.isTerminating {\n break\n }\n }\n }\n\n func remove(word: String) {\n guard !word.isEmpty else {\n return\n }\n guard let terminalNode = findTerminalNodeOf(word: word) else {\n return\n }\n if terminalNode.isLeaf {\n deleteNodesForWordEndingWith(terminalNode: terminalNode)\n } else {\n terminalNode.isTerminating = false\n }\n wordCount -= 1\n }\n\n fileprivate func wordsInSubtrie(rootNode: Node, partialWord: String) -> [String] {\n var subtrieWords = [String]()\n var previousLetters = partialWord\n if let value = rootNode.value {\n previousLetters.append(value)\n }\n if rootNode.isTerminating {\n subtrieWords.append(previousLetters)\n }\n for childNode in rootNode.children.values {\n let childWords = wordsInSubtrie(rootNode: childNode, partialWord: previousLetters)\n subtrieWords += childWords\n }\n return subtrieWords\n }\n\n func findWordsWithPrefix(prefix: String) -> [String] {\n var words = [String]()\n let prefixLowerCased = prefix.lowercased()\n if let lastNode = findLastNodeOf(word: prefixLowerCased) {\n if lastNode.isTerminating {\n words.append(prefixLowerCased)\n }\n for childNode in lastNode.children.values {\n let childWords = wordsInSubtrie(rootNode: childNode, partialWord: prefixLowerCased)\n words += childWords\n }\n }\n return words\n }\n}"
}
{
"filename": "code/data_structures/src/tree/van_emde_boas_tree/van_emde_boas_tree.cpp",
"content": "#include <bits/stdc++.h>\nusing namespace std;\n\nclass veb\n{\n int u;\n int *min;\n int *max;\n veb *summary;\n veb **cluster;\n\npublic:\n\n veb(int u);\n void insert(int x);\n void remove(int x);\n int* pred(int x);\n int minimum();\n int maximum();\n};\n\n\nveb :: veb(int u)\n{\n this->u = u;\n this->min = NULL;\n this->max = NULL;\n\n if (u == 2)\n {\n this->summary = NULL;\n this->cluster = NULL;\n }\n else\n {\n int sub_size = sqrt(u);\n this->summary = new veb(sub_size);\n this->cluster = new veb*[sub_size];\n }\n\n}\n\n\nvoid veb::insert(int x)\n{\n if (u == 2)\n {\n if (x == 0)\n {\n if (max == NULL)\n {\n max = new int;\n min = new int;\n *max = x;\n *min = x;\n }\n else\n *min = x;\n }\n else if (x == 1)\n {\n if (min == NULL)\n {\n max = new int;\n min = new int;\n *max = x;\n *min = x;\n }\n else\n *max = x;\n }\n }\n else\n {\n if (min == NULL)\n {\n min = new int;\n max = new int;\n *max = x;\n *min = x;\n this->insert(x);\n }\n else\n {\n if ((*min) > x)\n {\n *min = x;\n this->insert(x);\n }\n else\n {\n int subsize = sqrt(u);\n int high = x / subsize, low = x % subsize;\n if (cluster[high] == NULL)\n {\n cluster[high] = new veb(subsize);\n cluster[high]->insert(low);\n summary->insert(high);\n }\n else\n cluster[high]->insert(low);\n if ((*max) < x)\n *max = x;\n }\n }\n }\n}\n\n\nvoid veb::remove(int x)\n{\n if (u == 2)\n {\n\n\n if (x == 0)\n {\n if (min != NULL)\n {\n if (*max == 0)\n {\n min = NULL;\n max = NULL;\n }\n else\n *min = 1;\n }\n }\n else if (max != NULL)\n {\n\n if (*min == 1)\n {\n min = NULL;\n max = NULL;\n }\n else\n *max = 0;\n }\n\n }\n else\n {\n\n int subsize = sqrt(u);\n int high = x / subsize, low = x % subsize;\n if (min == NULL || cluster[high] == NULL)\n return;\n if (x == *min)\n {\n\n if (x == *max)\n {\n min = NULL;\n max = NULL;\n cluster[high]->remove(low);\n return;\n }\n\n cluster[high]->remove(low);\n\n if (cluster[high]->min == NULL)\n {\n delete cluster[high];\n cluster[high] = NULL;\n summary->remove(high);\n }\n int newminhigh = summary->minimum();\n int newminlow = cluster[newminhigh]->minimum();\n *min = newminhigh * subsize + newminlow;\n }\n else\n {\n cluster[high]->remove(low);\n\n if (cluster[high]->min == NULL)\n {\n delete cluster[high];\n cluster[high] = NULL;\n summary->remove(high);\n }\n if (x == *max)\n {\n int newmaxhigh = summary->maximum();\n int newmaxlow = cluster[newmaxhigh]->maximum();\n *max = newmaxhigh * subsize + newmaxlow;\n }\n }\n\n }\n}\n\nint* veb::pred(int x)\n{\n if (u == 2)\n {\n if (x == 0)\n return NULL;\n else if (x == 1)\n {\n if (min == NULL)\n return NULL;\n else if ((*min) == 1)\n return NULL;\n else\n return min;\n }\n else\n return NULL;\n }\n else\n {\n if (min == NULL)\n return NULL;\n if ((*min) >= x)\n return NULL;\n if (x > (*max))\n return max;\n int subsize = sqrt(u);\n int high = x / subsize;\n int low = x % subsize;\n if (cluster[high] == NULL)\n {\n int *pred = summary->pred(high);\n int *ans = new int;\n *ans = (*pred) * subsize + *(cluster[*pred]->max);\n return ans;\n }\n else\n {\n int *ans_high = new int;\n int *ans_low = new int;\n\n if (low > *(cluster[high]->min))\n {\n *ans_high = high;\n ans_low = cluster[high]->pred(low);\n }\n else\n {\n ans_high = summary->pred(high);\n ans_low = cluster[(*ans_high)]->max;\n }\n\n int *ans = new int;\n *ans = (*ans_high) * subsize + (*ans_low);\n return ans;\n }\n }\n\n}\n\nint veb::minimum()\n{\n return *min;\n}\n\nint veb::maximum()\n{\n return *max;\n}\n\nvoid findpred(veb *x, int y)\n{\n int *temp = x->pred(y);\n if (temp == NULL)\n cout << \"no predecesor\" << endl;\n else\n cout << \"predecesor of \" << y << \" is \" << *temp << endl;\n\n}\n\nint main()\n{\n veb *x = new veb(16);\n x->insert(2);\n x->insert(3);\n x->insert(4);\n x->insert(5);\n x->insert(7);\n x->insert(14);\n x->insert(15);\n\n\n cout << x->minimum() << endl << x->maximum() << endl;\n\n\n\n findpred(x, 0);\n findpred(x, 1);\n findpred(x, 2);\n findpred(x, 3);\n findpred(x, 4);\n findpred(x, 5);\n findpred(x, 6);\n findpred(x, 7);\n findpred(x, 8);\n findpred(x, 9);\n findpred(x, 10);\n findpred(x, 11);\n findpred(x, 12);\n findpred(x, 13);\n findpred(x, 14);\n findpred(x, 15);\n findpred(x, 16);\n findpred(x, 7);\n\n x->remove(15);\n x->remove(5);\n\n findpred(x, 0);\n findpred(x, 1);\n findpred(x, 2);\n findpred(x, 3);\n findpred(x, 4);\n findpred(x, 5);\n findpred(x, 6);\n findpred(x, 7);\n findpred(x, 8);\n findpred(x, 9);\n findpred(x, 10);\n findpred(x, 11);\n findpred(x, 12);\n findpred(x, 13);\n findpred(x, 14);\n findpred(x, 15);\n findpred(x, 16);\n findpred(x, 7);\n\n}"
}
{
"filename": "code/data_structures/test/bag/test_bag.cpp",
"content": "//Needed for random and vector\r\n#include <vector>\r\n#include <string>\r\n#include <cstdlib>\r\n#include <iostream>\r\n\r\nusing std::cout;\r\nusing std::endl;\r\nusing std::string;\r\nusing std::vector;\r\n\r\n\r\n//Bag Class Declaration\r\nclass Bag\r\n{\r\nprivate: \r\n\tvector<string> items;\r\n\tint bagSize;\r\n\r\npublic:\r\n\tBag();\r\n\tvoid add(string);\r\n\tbool isEmpty();\r\n\tint sizeOfBag();\r\n\tstring remove();\r\n};\r\n\r\nBag::Bag()\r\n{\r\n\tbagSize = 0;\r\n}\r\n\r\nvoid Bag::add(string item)\r\n{\r\n\t//Store item in last element of vector\r\n\titems.push_back(item);\r\n\t//Increase the size\r\n\tbagSize++;\r\n}\r\n\r\nbool Bag::isEmpty(){\r\n\t//True\r\n\tif(bagSize == 0){\r\n\t\treturn true;\r\n\t}\r\n\t//False\r\n\telse{\r\n\t\treturn false;\r\n\t}\r\n}\r\n\r\nint Bag::sizeOfBag(){\r\n\treturn bagSize;\r\n}\r\n\r\nstring Bag::remove(){\r\n\t//Get random item\r\n\tint randIndx = rand() % bagSize;\r\n\tstring removed = items.at(randIndx);\r\n\t//Remove from bag\r\n\titems.erase(items.begin() + randIndx);\r\n\tbagSize--;\r\n\t//Return removed item\r\n\treturn removed;\r\n}\r\n\r\nint main()\r\n{\r\n\t//Create bag\r\n\tBag bag;\r\n\r\n\t//Get current size \r\n\tcout << \"Bag size: \" << bag.sizeOfBag() << endl;\r\n\t//Bag empty?\r\n\tcout << \"Is bag empty? \" << bag.isEmpty() << endl;\r\n\r\n\t//Add some items\r\n\tcout << \"Adding red...\" << endl;\r\n\tbag.add(\"red\");\r\n\tcout << \"Bag size: \" << bag.sizeOfBag() << endl;\r\n\tcout << \"Adding blue...\" << endl;\r\n\tbag.add(\"blue\");\r\n\tcout << \"Bag size: \" << bag.sizeOfBag() << endl;\r\n\tcout << \"Adding yellow...\" << endl;\r\n\tbag.add(\"yellow\");\r\n\tcout << \"Bag size: \" << bag.sizeOfBag() << endl;\r\n\r\n\t//Remove an item\r\n\tcout << \"Removed \" << bag.remove() << \" from bag.\" << endl;\r\n\t//Get current size \r\n\tcout << \"Bag size: \" << bag.sizeOfBag() << endl;\r\n\t//Bag empty?\r\n\tcout << \"Is bag empty? \" << bag.isEmpty() << endl;\r\n\r\n\t//Remove an item\r\n\tcout << \"Removed \" << bag.remove() << \" from bag.\" << endl;\r\n\t//Get current size \r\n\tcout << \"Bag size: \" << bag.sizeOfBag() << endl;\r\n\t//Bag empty?\r\n\tcout << \"Is bag empty? \" << bag.isEmpty() << endl;\r\n\r\n\t//Remove last item\r\n\tcout << \"Removed \" << bag.remove() << \" from bag.\" << endl;\r\n\t//Get current size \r\n\tcout << \"Bag size: \" << bag.sizeOfBag() << endl;\r\n\t//Bag empty?\r\n\tcout << \"Is bag empty? \" << bag.isEmpty() << endl;\r\n\r\n}\r"
}
{
"filename": "code/data_structures/test/list/test_list.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n *\n * test lists for std::list-like\n */\n\n#define CATCH_CONFIG_MAIN\n#ifndef XOR_LINKED_LIST_TEST_CPP\n#define XOR_LINKED_LIST_TEST_CPP\n\n#include \"../../../../test/c++/catch.hpp\"\n#include \"../../src/list/xor_linked_list/xor_linked_list.cpp\"\n#include <iostream>\n#include <list>\n#include <vector>\n\nusing vectorContainer = std::vector<int>;\nusing expectListContainer = std::list<int>;\nusing actualListContainer = XorLinkedList<int>;\nstatic size_t RandomSize;\nstatic size_t SmallRandomSize;\n\nTEST_CASE(\"init\")\n{\n srand(static_cast<unsigned int>(clock()));\n\n RandomSize = 100000 + rand() % 2;\n SmallRandomSize = RandomSize / rand() % 100 + 50;\n}\n\nvectorContainer getRandomValueContainer(size_t sz = RandomSize)\n{\n // init\n vectorContainer container(sz);\n size_t i = 0 - 1;\n while (++i < sz)\n container.at(i) = static_cast<int>(i);\n\n // randomize\n i = 0 - 1;\n while (++i < sz)\n {\n auto r = rand() % container.size();\n auto temp = container.at(i);\n container.at(i) = container.at(r);\n container.at(r) = temp;\n }\n\n return container;\n}\n\nactualListContainer copyContainerToList(const vectorContainer &container)\n{\n actualListContainer actual;\n std::for_each(container.begin(), container.end(), [&](int v)\n {\n actual.push_back(v);\n });\n\n return actual;\n}\n\nvoid copyRandomPartContainerToLists(const vectorContainer &container,\n expectListContainer &expect,\n actualListContainer &actual)\n{\n std::for_each(container.begin(), container.end(), [&](int v)\n {\n if (rand() % 2)\n {\n expect.push_back(v);\n actual.push_back(v);\n }\n });\n auto begin = container.begin();\n while (expect.size() < 10)\n {\n expect.push_back(*begin);\n actual.push_back(*begin++);\n }\n}\n\nvoid isSame(expectListContainer expect, actualListContainer actual)\n{\n CHECK(expect.size() == actual.size());\n CHECK(expect.empty() == actual.empty());\n\n auto expectIt = expect.begin();\n auto actualIt = actual.begin();\n\n while (expectIt != expect.end())\n CHECK(*expectIt++ == *actualIt++);\n}\n\nTEST_CASE(\"-ctors converts and its types\")\n{\n expectListContainer expect;\n actualListContainer actual;\n const expectListContainer cExpect;\n const actualListContainer cActual;\n\n SECTION(\"iterator\")\n {\n SECTION(\"begin\")\n {\n expectListContainer::iterator expectIt1(expect.begin());\n expectListContainer::const_iterator expectIt2(expect.begin());\n expectListContainer::reverse_iterator expectIt3(expect.begin());\n expectListContainer::const_reverse_iterator expectIt4(expect.begin());\n\n expectListContainer::const_iterator expectCIt2(expect.cbegin());\n expectListContainer::const_reverse_iterator expectCIt4(expect.cbegin());\n\n expectListContainer::reverse_iterator expectRIt3(expect.rbegin());\n expectListContainer::const_reverse_iterator expectRIt4(expect.rbegin());\n\n expectListContainer::const_reverse_iterator expectCRIt4(expect.crbegin());\n\n actualListContainer::iterator actualIt1(actual.begin());\n actualListContainer::const_iterator actualIt2(actual.begin());\n actualListContainer::reverse_iterator actualIt3(actual.begin());\n actualListContainer::const_reverse_iterator actualIt4(actual.begin());\n\n actualListContainer::const_iterator actualCIt2(actual.cbegin());\n actualListContainer::const_reverse_iterator actualCIt4(actual.cbegin());\n\n actualListContainer::reverse_iterator actualRIt3(actual.rbegin());\n actualListContainer::const_reverse_iterator actualRIt4(actual.rbegin());\n\n actualListContainer::const_reverse_iterator actualCRIt4(actual.crbegin());\n }\n\n SECTION(\"begin errors\")\n {\n // expectListContainer::iterator expectCIt1(expect.cbegin());\n // expectListContainer::reverse_iterator expectCIt3(expect.cbegin());\n //\n // expectListContainer::iterator expectRIt1(expect.rbegin());\n // expectListContainer::const_iterator expectRIt2(expect.rbegin());\n //\n // expectListContainer::iterator expectCRIt1(expect.crbegin());\n // expectListContainer::const_iterator expectCRIt2(expect.crbegin());\n // expectListContainer::reverse_iterator expectCRIt3(expect.crbegin());\n\n // actualListContainer::iterator actualCIt1(actual.cbegin());\n // actualListContainer::reverse_iterator actualCIt3(actual.cbegin());\n\n // actualListContainer::iterator actualRIt1(actual.rbegin());\n // actualListContainer::const_iterator actualRIt2(actual.rbegin());\n\n // actualListContainer::iterator actualCRIt1(actual.crbegin());\n // actualListContainer::const_iterator actualCRIt2(actual.crbegin());\n // actualListContainer::reverse_iterator actualCRIt3(actual.crbegin());\n }\n\n SECTION(\"end\")\n {\n expectListContainer::iterator expectIt1(expect.end());\n expectListContainer::const_iterator expectIt2(expect.end());\n expectListContainer::reverse_iterator expectIt3(expect.end());\n expectListContainer::const_reverse_iterator expectIt4(expect.end());\n\n expectListContainer::const_iterator expectCIt2(expect.cend());\n expectListContainer::const_reverse_iterator expectCIt4(expect.cend());\n\n expectListContainer::reverse_iterator expectRIt3(expect.rend());\n expectListContainer::const_reverse_iterator expectRIt4(expect.rend());\n\n expectListContainer::const_reverse_iterator expectCRIt4(expect.crend());\n\n actualListContainer::iterator actualIt1(actual.end());\n actualListContainer::const_iterator actualIt2(actual.end());\n actualListContainer::reverse_iterator actualIt3(actual.end());\n actualListContainer::const_reverse_iterator actualIt4(actual.end());\n\n actualListContainer::const_iterator actualCIt2(actual.cend());\n actualListContainer::const_reverse_iterator actualCIt4(actual.cend());\n\n actualListContainer::reverse_iterator actualRIt3(actual.rend());\n actualListContainer::const_reverse_iterator actualRIt4(actual.rend());\n\n actualListContainer::const_reverse_iterator actualCRIt4(actual.crend());\n }\n\n SECTION(\"end error\")\n {\n // expectListContainer::iterator expectCIt1(expect.cend());\n // expectListContainer::reverse_iterator expectCIt3(expect.cend());\n //\n // expectListContainer::iterator expectRIt1(expect.rend());\n // expectListContainer::const_iterator expectRIt2(expect.rend());\n //\n // expectListContainer::iterator expectCRIt1(expect.crend());\n // expectListContainer::const_iterator expectCRIt2(expect.crend());\n // expectListContainer::reverse_iterator expectCRIt3(expect.crend());\n\n // actualListContainer::iterator actualCIt1(actual.cend());\n // actualListContainer::reverse_iterator actualCIt3(actual.cend());\n //\n // actualListContainer::iterator actualRIt1(actual.rend());\n // actualListContainer::const_iterator actualRIt2(actual.rend());\n //\n // actualListContainer::iterator actualCRIt1(actual.crend());\n // actualListContainer::const_iterator actualCRIt2(actual.crend());\n // actualListContainer::reverse_iterator actualCRIt3(actual.crend());\n }\n\n SECTION(\"const begin\")\n {\n const expectListContainer::const_iterator expectIt2(cExpect.begin());\n const expectListContainer::const_reverse_iterator expectIt4(cExpect.begin());\n\n const expectListContainer::const_iterator expectCIt2(cExpect.cbegin());\n const expectListContainer::const_reverse_iterator expectCIt4(cExpect.cbegin());\n\n const expectListContainer::const_reverse_iterator expectRIt4(cExpect.rbegin());\n\n const expectListContainer::const_reverse_iterator expectCRIt4(cExpect.crbegin());\n\n const actualListContainer::const_iterator actualIt2(cActual.begin());\n const actualListContainer::const_reverse_iterator actualIt4(cActual.begin());\n\n const actualListContainer::const_iterator actualCIt2(cActual.cbegin());\n const actualListContainer::const_reverse_iterator actualCIt4(cActual.cbegin());\n\n const actualListContainer::const_reverse_iterator actualRIt4(cActual.rbegin());\n\n const actualListContainer::const_reverse_iterator actualCRIt4(cActual.crbegin());\n }\n\n SECTION(\"const begin errors\")\n {\n // const expectListContainer::iterator expectIt1(cExpect.begin());\n // const expectListContainer::reverse_iterator expectIt3(cExpect.begin());\n //\n // const expectListContainer::iterator expectCIt1(cExpect.cbegin());\n // const expectListContainer::reverse_iterator expectCIt3(cExpect.cbegin());\n //\n // const expectListContainer::iterator expectRIt1(cExpect.rbegin());\n // const expectListContainer::const_iterator expectRIt2(cExpect.rbegin());\n // const expectListContainer::reverse_iterator expectRIt3(cExpect.rbegin());\n //\n // const expectListContainer::iterator expectCRIt1(cExpect.crbegin());\n // const expectListContainer::const_iterator expectCRIt2(cExpect.crbegin());\n // const expectListContainer::reverse_iterator expectCRIt3(cExpect.crbegin());\n\n // const actualListContainer::iterator actualIt1(cActual.begin());\n // const actualListContainer::reverse_iterator actualIt3(cActual.begin());\n //\n // const actualListContainer::iterator actualCIt1(cActual.cbegin());\n // const actualListContainer::reverse_iterator actualCIt3(cActual.cbegin());\n //\n // const actualListContainer::iterator actualRIt1(cActual.rbegin());\n // const actualListContainer::const_iterator actualRIt2(cActual.rbegin());\n // const actualListContainer::reverse_iterator actualRIt3(cActual.rbegin());\n //\n // const actualListContainer::iterator actualCRIt1(cActual.crbegin());\n // const actualListContainer::const_iterator actualCRIt2(cActual.crbegin());\n // const actualListContainer::reverse_iterator actualCRIt3(cActual.crbegin());\n }\n\n SECTION(\"const end\")\n {\n const expectListContainer::const_iterator expectIt2(cExpect.end());\n const expectListContainer::const_reverse_iterator expectIt4(cExpect.end());\n\n const expectListContainer::const_iterator expectCIt2(cExpect.cend());\n const expectListContainer::const_reverse_iterator expectCIt4(cExpect.cend());\n\n const expectListContainer::const_reverse_iterator expectRIt4(cExpect.rend());\n\n const expectListContainer::const_reverse_iterator expectCRIt4(cExpect.crend());\n\n const actualListContainer::const_iterator actualIt2(cActual.end());\n const actualListContainer::const_reverse_iterator actualIt4(cActual.end());\n\n const actualListContainer::const_iterator actualCIt2(cActual.cend());\n const actualListContainer::const_reverse_iterator actualCIt4(cActual.cend());\n\n const actualListContainer::const_reverse_iterator actualRIt4(cActual.rend());\n\n const actualListContainer::const_reverse_iterator actualCRIt4(cActual.crend());\n }\n\n SECTION(\"const end error\")\n {\n // const expectListContainer::iterator expectIt1(cExpect.end());\n // const expectListContainer::reverse_iterator expectIt3(cExpect.end());\n //\n // const expectListContainer::iterator expectCIt1(cExpect.cend());\n // const expectListContainer::reverse_iterator expectCIt3(cExpect.cend());\n //\n // const expectListContainer::iterator expectRIt1(cExpect.rend());\n // const expectListContainer::const_iterator expectRIt2(cExpect.rend());\n // const expectListContainer::reverse_iterator expectRIt3(cExpect.rend());\n //\n // const expectListContainer::iterator expectCRIt1(cExpect.crend());\n // const expectListContainer::const_iterator expectCRIt2(cExpect.crend());\n // const expectListContainer::reverse_iterator expectCRIt3(cExpect.crend());\n\n // const actualListContainer::iterator actualIt1(cActual.end());\n // const actualListContainer::reverse_iterator actualIt3(cActual.end());\n //\n // const actualListContainer::iterator actualCIt1(cActual.cend());\n // const actualListContainer::reverse_iterator actualCIt3(cActual.cend());\n //\n // const actualListContainer::iterator actualRIt1(cActual.rend());\n // const actualListContainer::const_iterator actualRIt2(cActual.rend());\n // const actualListContainer::reverse_iterator actualRIt3(cActual.rend());\n //\n // const actualListContainer::iterator actualCRIt1(cActual.crend());\n // const actualListContainer::const_iterator actualCRIt2(cActual.crend());\n // const actualListContainer::reverse_iterator actualCRIt3(cActual.crend());\n }\n }\n\n SECTION(\"container\")\n {\n expectListContainer expect1;\n expectListContainer expect2(expect1);\n expectListContainer expect3{1, 2, 3};\n\n actualListContainer actual1;\n actualListContainer actual2(actual1);\n actualListContainer actual3{1, 2, 3};\n }\n}\n\nTEST_CASE(\"const semantic\")\n{\n expectListContainer expect;\n actualListContainer actual;\n\n const expectListContainer cExpect;\n const actualListContainer cActual;\n\n using std::is_const;\n SECTION(\"iterators\")\n {\n SECTION(\"non-const\")\n {\n CHECK(is_const<decltype(actual.begin())>() == is_const<decltype(expect.begin())>());\n CHECK(is_const<decltype(actual.begin().operator->())>()\n == is_const<decltype(expect.begin().operator->())>());\n CHECK(is_const<decltype(*actual.begin())>() == is_const<decltype(*expect.begin())>());\n CHECK(is_const<decltype(actual.end())>() == is_const<decltype(expect.end())>());\n CHECK(is_const<decltype(actual.end().operator->())>()\n == is_const<decltype(expect.end().operator->())>());\n CHECK(is_const<decltype(*actual.end())>() == is_const<decltype(*expect.end())>());\n\n CHECK(is_const<decltype(actual.cbegin())>() == is_const<decltype(expect.cbegin())>());\n CHECK(is_const<decltype(actual.cbegin().operator->())>()\n == is_const<decltype(expect.cbegin().operator->())>());\n CHECK(is_const<decltype(*actual.cbegin())>()\n == is_const<decltype(*expect.cbegin())>());\n CHECK(is_const<decltype(actual.cend())>() == is_const<decltype(expect.cend())>());\n CHECK(is_const<decltype(actual.cend().operator->())>()\n == is_const<decltype(expect.cend().operator->())>());\n CHECK(is_const<decltype(*actual.cend())>() == is_const<decltype(*expect.cend())>());\n\n CHECK(is_const<decltype(actual.rbegin())>() == is_const<decltype(expect.rbegin())>());\n CHECK(is_const<decltype(actual.rbegin().operator->())>()\n == is_const<decltype(expect.rbegin().operator->())>());\n CHECK(is_const<decltype(*actual.rbegin())>()\n == is_const<decltype(*expect.rbegin())>());\n CHECK(is_const<decltype(actual.rend())>() == is_const<decltype(expect.rend())>());\n CHECK(is_const<decltype(actual.rend().operator->())>()\n == is_const<decltype(expect.rend().operator->())>());\n CHECK(is_const<decltype(*actual.rend())>() == is_const<decltype(*expect.rend())>());\n\n CHECK(is_const<decltype(actual.crbegin())>()\n == is_const<decltype(expect.crbegin())>());\n CHECK(is_const<decltype(actual.crbegin().operator->())>()\n == is_const<decltype(expect.crbegin().operator->())>());\n CHECK(is_const<decltype(*actual.crbegin())>()\n == is_const<decltype(*expect.crbegin())>());\n CHECK(is_const<decltype(actual.crend())>() == is_const<decltype(expect.crend())>());\n CHECK(is_const<decltype(actual.crend().operator->())>()\n == is_const<decltype(expect.crend().operator->())>());\n CHECK(is_const<decltype(*actual.crend())>() == is_const<decltype(*expect.crend())>());\n\n CHECK(is_const<decltype(actual.rbegin().base())>()\n == is_const<decltype(expect.rbegin().base())>());\n CHECK(is_const<decltype(actual.rbegin().base().operator->())>()\n == is_const<decltype(expect.rbegin().base().operator->())>());\n CHECK(is_const<decltype(*actual.rbegin().base())>()\n == is_const<decltype(*expect.rbegin().base())>());\n CHECK(is_const<decltype(actual.rend().base())>()\n == is_const<decltype(expect.rend().base())>());\n CHECK(is_const<decltype(actual.rend().base().operator->())>()\n == is_const<decltype(expect.rend().base().operator->())>());\n CHECK(is_const<decltype(*actual.rend().base())>()\n == is_const<decltype(*expect.rend().base())>());\n\n CHECK(is_const<decltype(actual.crbegin().base())>()\n == is_const<decltype(expect.crbegin().base())>());\n CHECK(is_const<decltype(actual.crbegin().base().operator->())>()\n == is_const<decltype(expect.crbegin().base().operator->())>());\n CHECK(is_const<decltype(actual.crend().base())>()\n == is_const<decltype(expect.crend().base())>());\n CHECK(is_const<decltype(*actual.crbegin().base())>()\n == is_const<decltype(*expect.crbegin().base())>());\n CHECK(is_const<decltype(*actual.crbegin().base().operator->())>()\n == is_const<decltype(*expect.crbegin().base().operator->())>());\n CHECK(is_const<decltype(*actual.crend().base())>()\n == is_const<decltype(*expect.crend().base())>());\n }\n\n SECTION(\"const\")\n {\n CHECK(is_const<decltype(cActual.begin())>() == is_const<decltype(cExpect.begin())>());\n CHECK(is_const<decltype(cActual.begin().operator->())>()\n == is_const<decltype(cExpect.begin().operator->())>());\n CHECK(is_const<decltype(*cActual.begin())>() == is_const<decltype(*cExpect.begin())>());\n CHECK(is_const<decltype(cActual.end())>() == is_const<decltype(cExpect.end())>());\n CHECK(is_const<decltype(cActual.end().operator->())>()\n == is_const<decltype(cExpect.end().operator->())>());\n CHECK(is_const<decltype(*cActual.end())>() == is_const<decltype(*cExpect.end())>());\n\n CHECK(is_const<decltype(cActual.cbegin())>() == is_const<decltype(cExpect.cbegin())>());\n CHECK(is_const<decltype(cActual.cbegin().operator->())>()\n == is_const<decltype(cExpect.cbegin().operator->())>());\n CHECK(is_const<decltype(*cActual.cbegin())>()\n == is_const<decltype(*cExpect.cbegin())>());\n CHECK(is_const<decltype(cActual.cend())>() == is_const<decltype(cExpect.cend())>());\n CHECK(is_const<decltype(cActual.cend().operator->())>()\n == is_const<decltype(cExpect.cend().operator->())>());\n CHECK(is_const<decltype(*cActual.cend())>() == is_const<decltype(*cExpect.cend())>());\n\n CHECK(is_const<decltype(cActual.rbegin())>() == is_const<decltype(cExpect.rbegin())>());\n CHECK(is_const<decltype(cActual.rbegin().operator->())>()\n == is_const<decltype(cExpect.rbegin().operator->())>());\n CHECK(is_const<decltype(*cActual.rbegin())>()\n == is_const<decltype(*cExpect.rbegin())>());\n CHECK(is_const<decltype(cActual.rend())>() == is_const<decltype(cExpect.rend())>());\n CHECK(is_const<decltype(cActual.rend().operator->())>()\n == is_const<decltype(cExpect.rend().operator->())>());\n CHECK(is_const<decltype(*cActual.rend())>() == is_const<decltype(*cExpect.rend())>());\n\n CHECK(is_const<decltype(cActual.crbegin())>()\n == is_const<decltype(cExpect.crbegin())>());\n CHECK(is_const<decltype(cActual.crbegin().operator->())>()\n == is_const<decltype(cExpect.crbegin().operator->())>());\n CHECK(is_const<decltype(*cActual.crbegin())>()\n == is_const<decltype(*cExpect.crbegin())>());\n CHECK(is_const<decltype(cActual.crend())>() == is_const<decltype(cExpect.crend())>());\n CHECK(is_const<decltype(cActual.crend().operator->())>()\n == is_const<decltype(cExpect.crend().operator->())>());\n CHECK(is_const<decltype(*cActual.crend())>() == is_const<decltype(*cExpect.crend())>());\n\n CHECK(is_const<decltype(cActual.rbegin().base())>()\n == is_const<decltype(cExpect.rbegin().base())>());\n CHECK(is_const<decltype(cActual.rbegin().base().operator->())>()\n == is_const<decltype(cExpect.rbegin().base().operator->())>());\n CHECK(is_const<decltype(*cActual.rbegin().base())>()\n == is_const<decltype(*cExpect.rbegin().base())>());\n CHECK(is_const<decltype(cActual.rend().base())>()\n == is_const<decltype(cExpect.rend().base())>());\n CHECK(is_const<decltype(cActual.rend().base().operator->())>()\n == is_const<decltype(cExpect.rend().base().operator->())>());\n CHECK(is_const<decltype(*cActual.rend().base())>()\n == is_const<decltype(*cExpect.rend().base())>());\n\n CHECK(is_const<decltype(cActual.crbegin().base())>()\n == is_const<decltype(cExpect.crbegin().base())>());\n CHECK(is_const<decltype(cActual.crbegin().base().operator->())>()\n == is_const<decltype(cExpect.crbegin().base().operator->())>());\n CHECK(is_const<decltype(cActual.crend().base())>()\n == is_const<decltype(cExpect.crend().base())>());\n CHECK(is_const<decltype(*cActual.crbegin().base())>()\n == is_const<decltype(*cExpect.crbegin().base())>());\n CHECK(is_const<decltype(*cActual.crbegin().base().operator->())>()\n == is_const<decltype(*cExpect.crbegin().base().operator->())>());\n CHECK(is_const<decltype(*cActual.crend().base())>()\n == is_const<decltype(*cExpect.crend().base())>());\n }\n }\n\n SECTION(\"element access\")\n {\n SECTION(\"non-const\")\n {\n CHECK(is_const<decltype(actual.front())>() == is_const<decltype(expect.front())>());\n CHECK(is_const<decltype(actual.back())>() == is_const<decltype(expect.back())>());\n }\n\n SECTION(\"const\")\n {\n CHECK(is_const<decltype(cActual.front())>() == is_const<decltype(cExpect.front())>());\n CHECK(is_const<decltype(cActual.back())>() == is_const<decltype(cExpect.back())>());\n }\n }\n}\n\nTEST_CASE(\"element access rely on [push]\")\n{\n actualListContainer actual;\n\n SECTION(\"push one node\")\n {\n actual.push_back(1);\n CHECK(actual.front() == 1);\n CHECK(actual.back() == 1);\n }\n\n SECTION(\"push two nodes\")\n {\n actual.push_back(1);\n actual.push_back(2);\n CHECK(actual.front() == 1);\n CHECK(actual.back() == 2);\n actual.push_front(3);\n CHECK(actual.front() == 3);\n CHECK(actual.back() == 2);\n }\n}\n\nTEST_CASE(\"modifiers\")\n{\n auto randomContainer = getRandomValueContainer();\n auto randomSmallContainer = getRandomValueContainer(SmallRandomSize);\n std::initializer_list<int> randomIlist{rand(), rand(), rand(), rand(), rand()};\n expectListContainer expect;\n actualListContainer actual;\n expectListContainer::iterator expectReturnPos;\n actualListContainer::iterator actualReturnPos;\n int randomValue{};\n size_t sz{};\n\n SECTION(\"empty list\")\n {\n CHECK_THROWS_AS(actual.front(), std::out_of_range);\n CHECK_THROWS_AS(actual.back(), std::out_of_range);\n CHECK(actual.size() == 0);\n CHECK(actual.empty());\n }\n\n SECTION(\"[clear] rely on [push_back]\")\n {\n SECTION(\"while empty\")\n {\n actual.clear();\n }\n\n SECTION(\"after actions\")\n {\n actual.push_back(1);\n actual.push_back(2);\n actual.clear();\n actual.push_back(3);\n }\n\n SECTION(\"before destruct\")\n {\n actual.push_back(1);\n actual.push_back(2);\n actual.push_back(3);\n actual.clear();\n }\n\n SECTION(\"while destruct\")\n {\n actual.push_back(1);\n actual.push_back(2);\n actual.push_back(3);\n }\n }\n\n SECTION(\"[erase] rely on [push_back/begin/end/op/size]\")\n {\n SECTION(\"erase(const_iterator)\")\n {\n SECTION(\"from empty\")\n {\n /*\n * erase(end()) is undefined, refer to:\n * http://en.cppreference.com/w/cpp/container/list/erase\n */\n }\n\n SECTION(\"first one\")\n {\n SECTION(\"size is 1\")\n {\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n\n expectReturnPos = expect.erase(expect.begin());\n actualReturnPos = actual.erase(actual.begin());\n\n CHECK(expectReturnPos == expect.end());\n CHECK(actualReturnPos == actual.end());\n isSame(expect, actual);\n }\n\n SECTION(\"random size\")\n {\n copyRandomPartContainerToLists(randomContainer, expect, actual);\n\n expectReturnPos = expect.erase(expect.begin());\n actualReturnPos = actual.erase(actual.begin());\n\n CHECK(expectReturnPos == expect.begin());\n CHECK(actualReturnPos == actual.begin());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"last one\")\n {\n SECTION(\"size is 1\")\n {\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n\n expectReturnPos = expect.erase(expect.begin());\n actualReturnPos = actual.erase(actual.begin());\n\n CHECK(expectReturnPos == expect.end());\n CHECK(actualReturnPos == actual.end());\n isSame(expect, actual);\n }\n\n SECTION(\"random size\")\n {\n copyRandomPartContainerToLists(randomContainer, expect, actual);\n\n expectReturnPos = expect.erase(--expect.end());\n actualReturnPos = actual.erase(--actual.end());\n\n CHECK(expectReturnPos == expect.end());\n CHECK(actualReturnPos == actual.end());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"between of begin and end\")\n {\n copyRandomPartContainerToLists(randomContainer, expect, actual);\n\n expectReturnPos = expect.erase(---- expect.end());\n actualReturnPos = actual.erase(---- actual.end());\n\n CHECK(expectReturnPos == --expect.end());\n CHECK(actualReturnPos == --actual.end());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"erase(const_iterator, const_iterator)\")\n {\n SECTION(\"from empty\")\n {\n /*\n * erase(end(), end()) is undefined, refer to:\n * http://en.cppreference.com/w/cpp/container/list/erase\n */\n }\n\n SECTION(\"size is 1\")\n {\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n\n expectReturnPos = expect.erase(expect.begin(), expect.end());\n actualReturnPos = actual.erase(actual.begin(), actual.end());\n\n CHECK(expectReturnPos == expect.end());\n CHECK(actualReturnPos == actual.end());\n isSame(expect, actual);\n }\n\n SECTION(\"random size\")\n {\n copyRandomPartContainerToLists(randomContainer, expect, actual);\n\n SECTION(\"[begin : end)\")\n {\n expectReturnPos = expect.erase(expect.begin(), ++++++ expect.begin());\n actualReturnPos = actual.erase(actual.begin(), ++++++ actual.begin());\n\n CHECK(expectReturnPos == expect.begin());\n CHECK(actualReturnPos == actual.begin());\n isSame(expect, actual);\n }\n\n SECTION(\"(begin : end)\")\n {\n expectReturnPos = expect.erase(++++++ expect.begin(), ------ expect.end());\n actualReturnPos = actual.erase(++++++ actual.begin(), ------ actual.end());\n\n CHECK(expectReturnPos == ------ expect.end());\n CHECK(actualReturnPos == ------ actual.end());\n isSame(expect, actual);\n }\n\n SECTION(\"(begin : end]\")\n {\n expectReturnPos = expect.erase(++++++ expect.begin(), expect.end());\n actualReturnPos = actual.erase(++++++ actual.begin(), actual.end());\n\n CHECK(expectReturnPos == expect.end());\n CHECK(actualReturnPos == actual.end());\n isSame(expect, actual);\n }\n }\n }\n }\n\n SECTION(\"[insert] rely on [op/begin/end/size/push_back/clear]\")\n {\n SECTION(\"insert(const_iterator, const value_type &)\")\n {\n SECTION(\"to empty\")\n {\n randomValue = rand();\n\n expectReturnPos = expect.insert(expect.end(), randomValue);\n actualReturnPos = actual.insert(actual.end(), randomValue);\n\n CHECK(expectReturnPos == expect.begin());\n CHECK(actualReturnPos == actual.begin());\n isSame(expect, actual);\n }\n\n SECTION(\"before begin\")\n {\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n\n randomValue = rand();\n\n expectReturnPos = expect.insert(expect.begin(), randomValue);\n actualReturnPos = actual.insert(actual.begin(), randomValue);\n\n CHECK(expectReturnPos == expect.begin());\n CHECK(actualReturnPos == actual.begin());\n isSame(expect, actual);\n }\n\n SECTION(\"before end\")\n {\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n\n randomValue = rand();\n\n expectReturnPos = expect.insert(expect.end(), randomValue);\n actualReturnPos = actual.insert(actual.end(), randomValue);\n\n CHECK(expectReturnPos == --expect.end());\n CHECK(actualReturnPos == --actual.end());\n isSame(expect, actual);\n }\n\n SECTION(\"between of begin and end\")\n {\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n\n randomValue = rand();\n\n expectReturnPos = expect.insert(++expect.begin(), randomValue);\n actualReturnPos = actual.insert(++actual.begin(), randomValue);\n\n CHECK(expectReturnPos == ++expect.begin());\n CHECK(actualReturnPos == ++actual.begin());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"insert(const_iterator, value_type &&)\")\n {\n SECTION(\"to empty\")\n {\n randomValue = rand();\n\n expectReturnPos = expect.insert(expect.end(), std::move(randomValue));\n actualReturnPos = actual.insert(actual.end(), std::move(randomValue));\n\n CHECK(expectReturnPos == expect.begin());\n CHECK(actualReturnPos == actual.begin());\n isSame(expect, actual);\n }\n\n SECTION(\"before begin\")\n {\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n\n randomValue = rand();\n\n expectReturnPos = expect.insert(expect.begin(), std::move(randomValue));\n actualReturnPos = actual.insert(actual.begin(), std::move(randomValue));\n\n CHECK(expectReturnPos == expect.begin());\n CHECK(actualReturnPos == actual.begin());\n isSame(expect, actual);\n }\n\n SECTION(\"before end\")\n {\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n\n randomValue = rand();\n\n expectReturnPos = expect.insert(expect.end(), std::move(randomValue));\n actualReturnPos = actual.insert(actual.end(), std::move(randomValue));\n\n CHECK(expectReturnPos == --expect.end());\n CHECK(actualReturnPos == --actual.end());\n isSame(expect, actual);\n }\n\n SECTION(\"between of begin and end\")\n {\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n\n randomValue = rand();\n\n expectReturnPos = expect.insert(++expect.begin(), std::move(randomValue));\n actualReturnPos = actual.insert(++actual.begin(), std::move(randomValue));\n\n CHECK(expectReturnPos == ++expect.begin());\n CHECK(actualReturnPos == ++actual.begin());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"insert(const_iterator, size_type, const value_type &)\")\n {\n SECTION(\"to empty\")\n {\n SECTION(\"size is 0\")\n {\n randomValue = rand();\n sz = 0;\n\n auto expectReturnPos = expect.insert(expect.end(), sz, randomValue);\n actualReturnPos = actual.insert(actual.end(), sz, randomValue);\n\n CHECK(expectReturnPos == expect.end());\n CHECK(actualReturnPos == actual.end());\n isSame(expect, actual);\n }\n\n SECTION(\"size is non-zero\")\n {\n randomValue = rand();\n sz = rand() % 10 + SmallRandomSize;\n\n expectReturnPos = expect.insert(expect.end(), sz, randomValue);\n actualReturnPos = actual.insert(actual.end(), sz, randomValue);\n\n auto tempe = expect.end();\n auto tempa = actual.end();\n ++sz;\n while (--sz)\n {\n --tempe;\n --tempa;\n }\n CHECK(expectReturnPos == tempe);\n CHECK(actualReturnPos == tempa);\n isSame(expect, actual);\n }\n }\n\n SECTION(\"before begin\")\n {\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n\n SECTION(\"size is 0\")\n {\n randomValue = rand();\n sz = 0;\n\n expectReturnPos = expect.insert(expect.begin(), sz, randomValue);\n actualReturnPos = actual.insert(actual.begin(), sz, randomValue);\n\n CHECK(expectReturnPos == expect.begin());\n CHECK(actualReturnPos == actual.begin());\n isSame(expect, actual);\n }\n\n SECTION(\"size is non-zero\")\n {\n randomValue = rand();\n sz = rand() % 10 + SmallRandomSize;\n\n expectReturnPos = expect.insert(expect.begin(), sz, randomValue);\n actualReturnPos = actual.insert(actual.begin(), sz, randomValue);\n\n CHECK(expectReturnPos == expect.begin());\n CHECK(actualReturnPos == actual.begin());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"before end\")\n {\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n\n SECTION(\"size is 0\")\n {\n randomValue = rand();\n sz = 0;\n\n expectReturnPos = expect.insert(expect.end(), sz, randomValue);\n actualReturnPos = actual.insert(actual.end(), sz, randomValue);\n\n CHECK(expectReturnPos == expect.end());\n CHECK(actualReturnPos == actual.end());\n isSame(expect, actual);\n }\n\n SECTION(\"size is non-zero\")\n {\n randomValue = rand();\n sz = rand() % 10 + SmallRandomSize;\n\n expectReturnPos = expect.insert(expect.end(), sz, randomValue);\n actualReturnPos = actual.insert(actual.end(), sz, randomValue);\n\n auto tempe = expect.end();\n auto tempa = actual.end();\n ++sz;\n while (--sz)\n {\n --tempe;\n --tempa;\n }\n CHECK(expectReturnPos == tempe);\n CHECK(actualReturnPos == tempa);\n isSame(expect, actual);\n }\n }\n\n SECTION(\"between of begin and end\")\n {\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n\n SECTION(\"size is 0\")\n {\n randomValue = rand();\n sz = 0;\n\n expectReturnPos = expect.insert(++expect.begin(), sz, randomValue);\n actualReturnPos = actual.insert(++actual.begin(), sz, randomValue);\n\n CHECK(expectReturnPos == ++expect.begin());\n CHECK(actualReturnPos == ++actual.begin());\n isSame(expect, actual);\n }\n\n SECTION(\"size is non-zero\")\n {\n randomValue = rand();\n sz = rand() % 10 + SmallRandomSize;\n\n expectReturnPos = expect.insert(++expect.begin(), sz, randomValue);\n actualReturnPos = actual.insert(++actual.begin(), sz, randomValue);\n\n CHECK(expectReturnPos == ++expect.begin());\n CHECK(actualReturnPos == ++actual.begin());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"size is 1\")\n {\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n\n randomValue = rand();\n sz = 1;\n\n expectReturnPos = expect.insert(++expect.begin(), sz, randomValue);\n actualReturnPos = actual.insert(++actual.begin(), sz, randomValue);\n\n CHECK(expectReturnPos == ++expect.begin());\n CHECK(actualReturnPos == ++actual.begin());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"insert(const_iterator, initilizer_list)\")\n {\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n\n SECTION(\"to empty\")\n {\n expect.clear();\n actual.clear();\n SECTION(\"initializer_list is empty\")\n {\n randomIlist = {};\n\n expectReturnPos = expect.insert(expect.end(), randomIlist);\n actualReturnPos = actual.insert(actual.end(), randomIlist);\n\n CHECK(expectReturnPos == expect.end());\n CHECK(actualReturnPos == actual.end());\n isSame(expect, actual);\n }\n\n SECTION(\"initializer_list is non-empty\")\n {\n expectReturnPos = expect.insert(expect.end(), randomIlist);\n actualReturnPos = actual.insert(actual.end(), randomIlist);\n\n CHECK(expectReturnPos == expect.begin());\n CHECK(actualReturnPos == actual.begin());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"before begin\")\n {\n SECTION(\"initializer_list is empty\")\n {\n randomIlist = {};\n\n expectReturnPos = expect.insert(expect.begin(), randomIlist);\n actualReturnPos = actual.insert(actual.begin(), randomIlist);\n\n CHECK(expectReturnPos == expect.begin());\n CHECK(actualReturnPos == actual.begin());\n isSame(expect, actual);\n }\n\n SECTION(\"initializer_list is non-empty\")\n {\n expectReturnPos = expect.insert(expect.begin(), randomIlist);\n actualReturnPos = actual.insert(actual.begin(), randomIlist);\n\n CHECK(expectReturnPos == expect.begin());\n CHECK(actualReturnPos == actual.begin());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"before end\")\n {\n SECTION(\"initializer_list is empty\")\n {\n randomIlist = {};\n\n expectReturnPos = expect.insert(expect.end(), randomIlist);\n actualReturnPos = actual.insert(actual.end(), randomIlist);\n\n CHECK(expectReturnPos == expect.end());\n CHECK(actualReturnPos == actual.end());\n isSame(expect, actual);\n }\n\n SECTION(\"initializer_list is non-empty\")\n {\n expectReturnPos = expect.insert(expect.end(), randomIlist);\n actualReturnPos = actual.insert(actual.end(), randomIlist);\n\n CHECK(expectReturnPos == ++++ expect.begin());\n CHECK(actualReturnPos == ++++ actual.begin());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"between of begin and end\")\n {\n SECTION(\"initializer_list is empty\")\n {\n randomIlist = {};\n\n expectReturnPos = expect.insert(++expect.begin(), randomIlist);\n actualReturnPos = actual.insert(++actual.begin(), randomIlist);\n\n CHECK(expectReturnPos == ++expect.begin());\n CHECK(actualReturnPos == ++actual.begin());\n isSame(expect, actual);\n }\n\n SECTION(\"initializer_list is non-empty\")\n {\n expectReturnPos = expect.insert(++expect.begin(), randomIlist);\n actualReturnPos = actual.insert(++actual.begin(), randomIlist);\n\n CHECK(expectReturnPos == ++expect.begin());\n CHECK(actualReturnPos == ++actual.begin());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"size of initializer is 1\")\n {\n randomIlist = {rand()};\n\n expectReturnPos = expect.insert(++expect.begin(), randomIlist);\n actualReturnPos = actual.insert(++actual.begin(), randomIlist);\n\n CHECK(expectReturnPos == ++expect.begin());\n CHECK(actualReturnPos == ++actual.begin());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"insert(const_iterator, iterator, iterator)\")\n {\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n randomValue = rand();\n expect.push_back(randomValue);\n actual.push_back(randomValue);\n\n SECTION(\"to empty\")\n {\n expect.clear();\n actual.clear();\n\n SECTION(\"container is empty\")\n {\n randomSmallContainer = {};\n\n expectReturnPos = expect.insert(expect.end(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n actualReturnPos = actual.insert(actual.end(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n\n CHECK(expectReturnPos == expect.end());\n CHECK(actualReturnPos == actual.end());\n isSame(expect, actual);\n }\n\n SECTION(\"container is non-empty\")\n {\n expectReturnPos = expect.insert(expect.end(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n actualReturnPos = actual.insert(actual.end(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n\n CHECK(expectReturnPos == expect.begin());\n CHECK(actualReturnPos == actual.begin());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"before begin\")\n {\n SECTION(\"container is empty\")\n {\n randomSmallContainer = {};\n\n expectReturnPos = expect.insert(expect.begin(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n actualReturnPos = actual.insert(actual.begin(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n\n CHECK(expectReturnPos == expect.begin());\n CHECK(actualReturnPos == actual.begin());\n isSame(expect, actual);\n }\n\n SECTION(\"container is non-empty\")\n {\n expectReturnPos = expect.insert(expect.begin(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n actualReturnPos = actual.insert(actual.begin(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n\n CHECK(expectReturnPos == expect.begin());\n CHECK(actualReturnPos == actual.begin());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"before end\")\n {\n SECTION(\"container is empty\")\n {\n randomSmallContainer = {};\n\n expectReturnPos = expect.insert(expect.end(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n actualReturnPos = actual.insert(actual.end(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n\n CHECK(expectReturnPos == expect.end());\n CHECK(actualReturnPos == actual.end());\n isSame(expect, actual);\n }\n\n SECTION(\"container is non-empty\")\n {\n expectReturnPos = expect.insert(expect.end(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n actualReturnPos = actual.insert(actual.end(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n\n CHECK(expectReturnPos == ++++ expect.begin());\n CHECK(actualReturnPos == ++++ actual.begin());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"between of begin and end\")\n {\n SECTION(\"container is empty\")\n {\n randomSmallContainer = {};\n\n expectReturnPos = expect.insert(++expect.begin(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n actualReturnPos = actual.insert(++actual.begin(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n\n CHECK(expectReturnPos == ++expect.begin());\n CHECK(actualReturnPos == ++actual.begin());\n isSame(expect, actual);\n }\n\n SECTION(\"container is non-empty\")\n {\n expectReturnPos = expect.insert(++expect.begin(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n actualReturnPos = actual.insert(++actual.begin(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n\n CHECK(expectReturnPos == ++expect.begin());\n CHECK(actualReturnPos == ++actual.begin());\n isSame(expect, actual);\n }\n }\n\n SECTION(\"size of iterator is 1\")\n {\n randomSmallContainer = {rand()};\n\n expectReturnPos = expect.insert(++expect.begin(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n actualReturnPos = actual.insert(++actual.begin(),\n randomSmallContainer.begin(),\n randomSmallContainer.end());\n\n CHECK(expectReturnPos == ++expect.begin());\n CHECK(actualReturnPos == ++actual.begin());\n isSame(expect, actual);\n }\n }\n }\n\n SECTION(\"pop rely on [push_back/front/back]\")\n {\n // std not throws exception while invoke pop on empty list\n\n SECTION(\"pop_front\")\n {\n actual.push_back(111);\n CHECK_NOTHROW(actual.pop_front());\n CHECK_THROWS_AS(actual.front(), std::out_of_range);\n CHECK_THROWS_AS(actual.back(), std::out_of_range);\n\n actual.push_back(111);\n actual.push_back(222);\n CHECK_NOTHROW(actual.pop_front());\n CHECK_NOTHROW(actual.pop_front());\n CHECK_THROWS_AS(actual.front(), std::out_of_range);\n CHECK_THROWS_AS(actual.back(), std::out_of_range);\n\n actual.push_back(111);\n actual.push_back(222);\n actual.push_back(333);\n CHECK_NOTHROW(actual.pop_front());\n CHECK_NOTHROW(actual.pop_front());\n CHECK_NOTHROW(actual.pop_front());\n CHECK_THROWS_AS(actual.front(), std::out_of_range);\n CHECK_THROWS_AS(actual.back(), std::out_of_range);\n }\n\n SECTION(\"pop_back\")\n {\n actual.push_back(111);\n CHECK_NOTHROW(actual.pop_back());\n CHECK_THROWS_AS(actual.front(), std::out_of_range);\n CHECK_THROWS_AS(actual.back(), std::out_of_range);\n\n actual.push_back(111);\n actual.push_back(222);\n CHECK_NOTHROW(actual.pop_back());\n CHECK_NOTHROW(actual.pop_back());\n CHECK_THROWS_AS(actual.front(), std::out_of_range);\n CHECK_THROWS_AS(actual.back(), std::out_of_range);\n\n actual.push_back(111);\n actual.push_back(222);\n actual.push_back(333);\n CHECK_NOTHROW(actual.pop_back());\n CHECK_NOTHROW(actual.pop_back());\n CHECK_NOTHROW(actual.pop_back());\n CHECK_THROWS_AS(actual.front(), std::out_of_range);\n CHECK_THROWS_AS(actual.back(), std::out_of_range);\n }\n\n SECTION(\"random pop\")\n {\n copyRandomPartContainerToLists(randomContainer, expect, actual);\n\n while (!expect.empty())\n {\n CHECK(actual.front() == expect.front());\n CHECK(actual.back() == expect.back());\n if (rand() % 2)\n {\n actual.pop_back();\n expect.pop_back();\n }\n else\n {\n actual.pop_front();\n expect.pop_front();\n }\n }\n }\n }\n\n SECTION(\"[push] rely on [clear/begin/end/front/back]\")\n {\n SECTION(\"push front\")\n {\n actual.push_front(111);\n CHECK(actual.front() == 111);\n CHECK(actual.back() == 111);\n CHECK(*actual.begin() == 111);\n CHECK(*--actual.end() == 111);\n\n actual.push_front(222);\n CHECK(actual.front() == 222);\n CHECK(actual.back() == 111);\n CHECK(*actual.begin() == 222);\n CHECK(*++actual.begin() == 111);\n CHECK(*--actual.end() == 111);\n CHECK(*---- actual.end() == 222);\n\n actual.push_front(333);\n CHECK(actual.front() == 333);\n CHECK(actual.back() == 111);\n CHECK(*actual.begin() == 333);\n CHECK(*++actual.begin() == 222);\n CHECK(*++++ actual.begin() == 111);\n CHECK(*--actual.end() == 111);\n CHECK(*---- actual.end() == 222);\n CHECK(*------ actual.end() == 333);\n }\n\n SECTION(\"push back\")\n {\n actual.push_back(111);\n CHECK(actual.front() == 111);\n CHECK(actual.back() == 111);\n CHECK(*actual.begin() == 111);\n CHECK(*--actual.end() == 111);\n\n actual.push_back(222);\n CHECK(actual.front() == 111);\n CHECK(actual.back() == 222);\n CHECK(*actual.begin() == 111);\n CHECK(*++actual.begin() == 222);\n CHECK(*--actual.end() == 222);\n CHECK(*---- actual.end() == 111);\n\n actual.push_back(333);\n CHECK(actual.front() == 111);\n CHECK(actual.back() == 333);\n CHECK(*actual.begin() == 111);\n CHECK(*++actual.begin() == 222);\n CHECK(*++++ actual.begin() == 333);\n CHECK(*--actual.end() == 333);\n CHECK(*---- actual.end() == 222);\n CHECK(*------ actual.end() == 111);\n }\n\n SECTION(\"random push\")\n {\n std::for_each(randomContainer.begin(), randomContainer.end(), [&](int v)\n {\n if (rand() % 2)\n {\n actual.push_back(v);\n expect.push_back(v);\n }\n else\n {\n actual.push_front(v);\n expect.push_front(v);\n }\n\n CHECK(actual.front() == expect.front());\n CHECK(actual.back() == expect.back());\n });\n }\n }\n\n SECTION(\"random push/pop rely on [front/back]\")\n {\n SECTION(\"push is more than pop\")\n {\n std::for_each(randomContainer.begin(), randomContainer.end(), [&](int v)\n {\n if (rand() % 3)\n {\n if (rand() % 2)\n {\n actual.push_back(v);\n expect.push_back(v);\n }\n else\n {\n actual.push_front(v);\n expect.push_front(v);\n }\n }\n else\n {\n if (rand() % 2)\n {\n if (!expect.empty())\n {\n CHECK_NOTHROW(actual.pop_back());\n expect.pop_back();\n }\n }\n else if (!expect.empty())\n {\n CHECK_NOTHROW(actual.pop_front());\n expect.pop_front();\n }\n }\n if (!expect.empty())\n {\n CHECK(actual.front() == expect.front());\n CHECK(actual.back() == expect.back());\n }\n });\n }\n\n SECTION(\"pop is more than push\")\n {\n std::for_each(randomContainer.begin(), randomContainer.end(), [&](int v)\n {\n if (!(rand() % 3))\n {\n if (rand() % 2)\n {\n actual.push_back(v);\n expect.push_back(v);\n }\n else\n {\n actual.push_front(v);\n expect.push_front(v);\n }\n }\n else\n {\n if (rand() % 2)\n {\n if (!expect.empty())\n {\n CHECK_NOTHROW(actual.pop_back());\n expect.pop_back();\n }\n }\n else if (!expect.empty())\n {\n CHECK_NOTHROW(actual.pop_front());\n expect.pop_front();\n }\n }\n if (!expect.empty())\n {\n CHECK(actual.front() == expect.front());\n CHECK(actual.back() == expect.back());\n }\n });\n }\n }\n}\n\nTEST_CASE(\"capcity rely on [push/pop]\")\n{\n auto randomContainer = getRandomValueContainer();\n actualListContainer actual;\n CHECK(actual.empty());\n CHECK(actual.size() == 0);\n CHECK(actual.max_size() >= actual.size());\n\n SECTION(\"random actions\")\n {\n int expectSize = 0;\n\n std::for_each(randomContainer.begin(), randomContainer.end(), [&](int v)\n {\n if (!(rand() % 3))\n {\n if (rand() % 2)\n actual.push_back(v);\n else\n actual.push_front(v);\n ++expectSize;\n }\n else\n {\n if (rand() % 2)\n {\n if (expectSize != 0)\n {\n CHECK_NOTHROW(actual.pop_back());\n --expectSize;\n }\n }\n else if (expectSize != 0)\n {\n CHECK_NOTHROW(actual.pop_front());\n --expectSize;\n }\n }\n CHECK(actual.size() == expectSize);\n CHECK(actual.max_size() >= expectSize);\n CHECK((expectSize ^ actual.empty()));\n });\n }\n}\n\nTEST_CASE(\"iterator rely on [push_back]\")\n{\n auto expectRandomContainer = getRandomValueContainer();\n auto actual = copyContainerToList(expectRandomContainer);\n\n SECTION(\"random move (i++ more than i--) rely on [push_back]\")\n {\n SECTION(\"iterator\")\n {\n auto actualIt = actual.begin();\n auto expectIt = expectRandomContainer.begin();\n\n SECTION(\"++i/--i\")\n {\n while (expectIt < expectRandomContainer.end())\n {\n if (expectIt < expectRandomContainer.begin())\n {\n ++expectIt;\n ++actualIt;\n }\n\n if (expectIt > --expectRandomContainer.begin() && expectIt\n < expectRandomContainer.end())\n CHECK(*actualIt == *expectIt);\n\n if (rand() % 3) // times: ++ > --\n {\n auto temp = expectIt;\n if (++temp < expectRandomContainer.end()) // is not lastest\n CHECK(*(++actualIt) == *(++expectIt));\n else\n {\n ++actualIt;\n ++expectIt;\n break;\n }\n }\n else if (expectIt > expectRandomContainer.begin())\n CHECK(*(--actualIt) == *(--expectIt));\n\n if (expectIt > --expectRandomContainer.begin() && expectIt\n < expectRandomContainer.end())\n CHECK(*actualIt == *expectIt);\n }\n }\n\n SECTION(\"i++/i--\")\n {\n while (expectIt < expectRandomContainer.end())\n {\n if (expectIt < expectRandomContainer.begin())\n {\n ++expectIt;\n ++actualIt;\n }\n\n if (expectIt > --expectRandomContainer.begin() && expectIt\n < expectRandomContainer.end())\n CHECK(*actualIt == *expectIt);\n\n if (rand() % 3) // times: ++ > --\n CHECK(*(actualIt++) == *(expectIt++));\n else if (expectIt > expectRandomContainer.begin())\n CHECK(*(actualIt--) == *(expectIt--));\n\n if (expectIt > --expectRandomContainer.begin() && expectIt\n < expectRandomContainer.end())\n CHECK(*actualIt == *expectIt);\n }\n }\n }\n\n SECTION(\"reverse iterator\")\n {\n auto actualIt = actual.rbegin();\n auto expectIt = expectRandomContainer.rbegin();\n\n SECTION(\"++i/--i\")\n {\n while (expectIt < expectRandomContainer.rend())\n {\n if (expectIt < expectRandomContainer.rbegin())\n {\n ++expectIt;\n ++actualIt;\n }\n\n if (expectIt > --expectRandomContainer.rbegin() && expectIt\n < expectRandomContainer.rend())\n CHECK(*actualIt == *expectIt);\n\n if (rand() % 3) // times: ++ > --\n {\n auto temp = expectIt;\n if (++temp < expectRandomContainer.rend()) // is not lastest\n CHECK(*(++actualIt) == *(++expectIt));\n else\n {\n ++actualIt;\n ++expectIt;\n break;\n }\n }\n else if (expectIt > expectRandomContainer.rbegin())\n CHECK(*(--actualIt) == *(--expectIt));\n\n if (expectIt > --expectRandomContainer.rbegin() && expectIt\n < expectRandomContainer.rend())\n CHECK(*actualIt == *expectIt);\n }\n }\n\n SECTION(\"i++/i--\")\n {\n while (expectIt < expectRandomContainer.rend())\n {\n if (expectIt < expectRandomContainer.rbegin())\n {\n ++expectIt;\n ++actualIt;\n }\n\n if (expectIt > --expectRandomContainer.rbegin() && expectIt\n < expectRandomContainer.rend())\n CHECK(*actualIt == *expectIt);\n\n if (rand() % 3) // times: ++ > --\n CHECK(*(actualIt++) == *(expectIt++));\n else if (expectIt > expectRandomContainer.rbegin())\n CHECK(*(actualIt--) == *(expectIt--));\n\n if (expectIt > --expectRandomContainer.rbegin() && expectIt\n < expectRandomContainer.rend())\n CHECK(*actualIt == *expectIt);\n }\n }\n }\n }\n\n SECTION(\"random move (i-- more than i++) rely on [push_back]\")\n {\n SECTION(\"iterator\")\n {\n auto actualIt = --actual.end();\n auto expectIt = --expectRandomContainer.end();\n\n SECTION(\"++i/--i rely on [push_back]\")\n {\n while (expectIt > expectRandomContainer.begin())\n {\n if (expectIt >= expectRandomContainer.end())\n {\n --expectIt;\n --actualIt;\n }\n\n if (expectIt > --expectRandomContainer.begin() && expectIt\n < expectRandomContainer.end())\n CHECK(*actualIt == *expectIt);\n\n if (!(rand() % 3)) // times: ++ < --\n {\n auto temp = expectIt;\n if (++temp < expectRandomContainer.end())\n CHECK(*(++actualIt) == *(++expectIt));\n else\n {\n ++actualIt;\n ++expectIt;\n break;\n }\n }\n else if (expectIt > expectRandomContainer.begin())\n CHECK(*(--actualIt) == *(--expectIt));\n\n if (expectIt > --expectRandomContainer.begin() && expectIt\n < expectRandomContainer.end())\n CHECK(*actualIt == *expectIt);\n }\n }\n\n SECTION(\"i++/i-- rely on [push_back]\")\n {\n while (expectIt > expectRandomContainer.begin())\n {\n if (expectIt >= expectRandomContainer.end())\n {\n --expectIt;\n --actualIt;\n }\n\n if (expectIt > --expectRandomContainer.begin() && expectIt\n < expectRandomContainer.end())\n CHECK(*actualIt == *expectIt);\n\n if (!(rand() % 3)) // times: ++ < --\n CHECK(*actualIt++ == *expectIt++);\n else if (expectIt > expectRandomContainer.begin())\n CHECK(*actualIt-- == *expectIt--);\n\n if (expectIt > --expectRandomContainer.begin() && expectIt\n < expectRandomContainer.end())\n CHECK(*actualIt == *expectIt);\n }\n }\n }\n\n SECTION(\"reverse iterator\")\n {\n auto actualIt = actual.rbegin();\n auto expectIt = expectRandomContainer.rbegin();\n\n SECTION(\"++i/--i rely on [push_back]\")\n {\n while (expectIt > expectRandomContainer.rbegin())\n {\n if (expectIt >= expectRandomContainer.rend())\n {\n --expectIt;\n --actualIt;\n }\n if (expectIt > --expectRandomContainer.rbegin() && expectIt\n < expectRandomContainer.rend())\n CHECK(*actualIt == *expectIt);\n\n if (!(rand() % 3)) // times: ++ < --\n {\n auto expectItTemp = expectIt;\n if (++expectItTemp < expectRandomContainer.rend())\n CHECK(*(++actualIt) == *(++expectIt));\n else\n {\n ++actualIt;\n ++expectIt;\n break;\n }\n }\n else if (expectIt > expectRandomContainer.rbegin())\n CHECK(*(--actualIt) == *(--expectIt));\n\n if (expectIt > --expectRandomContainer.rbegin() && expectIt\n < expectRandomContainer.rend())\n CHECK(*actualIt == *expectIt);\n }\n }\n\n SECTION(\"i++/i-- rely on [push_back]\")\n {\n while (expectIt > expectRandomContainer.rbegin())\n {\n if (expectIt >= expectRandomContainer.rend())\n {\n --expectIt;\n --actualIt;\n }\n\n if (expectIt > --expectRandomContainer.rbegin() && expectIt\n < expectRandomContainer.rend())\n CHECK(*actualIt == *expectIt);\n\n if (!(rand() % 3)) // times: ++ < --\n CHECK(*(actualIt++) == *(expectIt++));\n else if (expectIt > expectRandomContainer.rbegin())\n CHECK(*(actualIt--) == *(expectIt--));\n\n if (expectIt > --expectRandomContainer.rbegin() && expectIt\n < expectRandomContainer.rend())\n CHECK(*actualIt == *expectIt);\n }\n }\n }\n }\n\n SECTION(\"[reverse iterator: base] rely on [++/--]\")\n {\n auto expectRandomContainer = getRandomValueContainer();\n actualListContainer actual = copyContainerToList(expectRandomContainer);\n vectorContainer::reverse_iterator expectReverseBegin(expectRandomContainer.begin());\n actualListContainer::const_reverse_iterator actualReverseBegin(actual.begin());\n auto expectBaseBegin = expectReverseBegin.base();\n auto actualBaseBegin = actualReverseBegin.base();\n\n while (expectBaseBegin != expectRandomContainer.end())\n {\n CHECK(*expectBaseBegin == *actualBaseBegin);\n ++expectBaseBegin;\n ++actualBaseBegin;\n }\n }\n}\n\nTEST_CASE(\"operations\")\n{\n auto randomContainer = getRandomValueContainer();\n expectListContainer expect;\n actualListContainer actual;\n\n SECTION(\"[reverse] rely on [empty/size/begin/end/front/back/push_back/push_front]\")\n {\n SECTION(\"empty\")\n {\n CHECK(actual.empty());\n CHECK(actual.size() == 0);\n CHECK(actual.begin() == actual.end());\n\n actual.reverse();\n CHECK(actual.empty());\n CHECK(actual.size() == 0);\n CHECK(actual.begin() == actual.end());\n }\n\n SECTION(\"one nodes\")\n {\n actual.push_back(1);\n CHECK(actual.front() == 1);\n CHECK(actual.back() == 1);\n CHECK(actual.size() == 1);\n CHECK_FALSE(actual.empty());\n\n actual.reverse();\n CHECK(actual.front() == 1);\n CHECK(actual.back() == 1);\n CHECK(actual.size() == 1);\n CHECK_FALSE(actual.empty());\n\n actual.pop_back();\n CHECK_THROWS_AS(actual.front(), std::out_of_range);\n CHECK_THROWS_AS(actual.back(), std::out_of_range);\n CHECK(actual.size() == 0);\n CHECK(actual.empty());\n }\n\n SECTION(\"two nodes\")\n {\n actual.push_back(1);\n actual.push_back(2);\n CHECK(actual.front() == 1);\n CHECK(actual.back() == 2);\n CHECK(actual.size() == 2);\n CHECK_FALSE(actual.empty());\n\n actual.reverse();\n CHECK(actual.front() == 2);\n CHECK(actual.back() == 1);\n CHECK(actual.size() == 2);\n CHECK_FALSE(actual.empty());\n\n actual.pop_back();\n CHECK(actual.front() == 2);\n CHECK(actual.back() == 2);\n CHECK(actual.size() == 1);\n CHECK_FALSE(actual.empty());\n\n actual.pop_back();\n CHECK_THROWS_AS(actual.front(), std::out_of_range);\n CHECK_THROWS_AS(actual.back(), std::out_of_range);\n CHECK(actual.size() == 0);\n CHECK(actual.empty());\n }\n\n SECTION(\"three nodes\")\n {\n actual.push_back(1);\n actual.push_back(2);\n actual.push_back(3);\n CHECK(actual.front() == 1);\n CHECK(actual.back() == 3);\n CHECK(actual.size() == 3);\n CHECK_FALSE(actual.empty());\n\n actual.reverse();\n CHECK(actual.front() == 3);\n CHECK(actual.back() == 1);\n CHECK(actual.size() == 3);\n CHECK_FALSE(actual.empty());\n\n actual.pop_back();\n CHECK(actual.front() == 3);\n CHECK(actual.back() == 2);\n CHECK(actual.size() == 2);\n CHECK_FALSE(actual.empty());\n\n actual.pop_back();\n CHECK(actual.front() == 3);\n CHECK(actual.back() == 3);\n CHECK(actual.size() == 1);\n CHECK_FALSE(actual.empty());\n\n actual.pop_back();\n CHECK_THROWS_AS(actual.front(), std::out_of_range);\n CHECK_THROWS_AS(actual.back(), std::out_of_range);\n CHECK(actual.size() == 0);\n CHECK(actual.empty());\n }\n\n SECTION(\"random nodes\")\n {\n while (!randomContainer.empty())\n {\n auto v = randomContainer.back();\n if (rand() % 2)\n {\n if (rand() % 2)\n {\n actual.push_back(v);\n expect.push_back(v);\n }\n else\n {\n actual.push_front(v);\n expect.push_front(v);\n }\n }\n else\n {\n if (rand() % 2)\n {\n if (!expect.empty())\n {\n actual.pop_back();\n expect.pop_back();\n }\n }\n else if (!expect.empty())\n {\n actual.pop_front();\n expect.pop_front();\n }\n }\n if (!expect.empty())\n {\n actual.reverse();\n reverse(actual.begin(), actual.end());\n actual.reverse();\n reverse(actual.begin(), actual.end());\n actual.reverse();\n reverse(expect.begin(), expect.end());\n }\n CHECK(actual.size() == expect.size());\n CHECK((expect.size() ^ actual.empty()));\n if (!expect.empty())\n {\n CHECK(expect.front() == actual.front());\n CHECK(expect.back() == actual.back());\n }\n randomContainer.pop_back();\n }\n }\n }\n}\n\nTEST_CASE(\"stl algorithm-compatible\")\n{\n vectorContainer randomContainer = getRandomValueContainer();\n expectListContainer expect;\n actualListContainer actual;\n expectListContainer::iterator expectPos;\n actualListContainer::iterator actualPos;\n int randomValue;\n size_t sz;\n auto randomSmallContainer = getRandomValueContainer(SmallRandomSize);\n copyRandomPartContainerToLists(randomContainer, expect, actual);\n\n SECTION(\"std::for_each\")\n {\n expectPos = expect.begin();\n std::for_each(actual.begin(), actual.end(), [&](int v)\n {\n CHECK(v == *expectPos++);\n });\n }\n\n SECTION(\"std::find\")\n {\n CHECK(*actual.begin() == *std::find(actual.begin(), actual.end(), *expect.begin()));\n CHECK(*++actual.begin() == *std::find(actual.begin(), actual.end(), *++expect.begin()));\n CHECK(*--actual.end() == *std::find(actual.begin(), actual.end(), *--expect.end()));\n }\n\n SECTION(\"std::equal\")\n {\n CHECK(std::equal(expect.begin(), expect.end(), actual.begin()));\n }\n}\n\nTEST_CASE(\"others\")\n{\n SECTION(\"random nodes\")\n {\n // todo: test more functions insert/find/unique/sort/erase\n\n auto randomContainer = getRandomValueContainer();\n\n actualListContainer actual;\n int expectSize = 1;\n expectListContainer expect;\n expect.push_front(randomContainer.front());\n\n actual.push_front(randomContainer.front());\n std::for_each(++randomContainer.begin(), randomContainer.end(), [&](int v)\n {\n if (rand() % 2)\n {\n if (rand() % 2)\n {\n actual.push_back(v);\n expect.push_back(v);\n }\n else\n {\n actual.push_front(v);\n expect.push_front(v);\n }\n ++expectSize;\n }\n else\n {\n if (rand() % 2)\n {\n if (expectSize != 0)\n {\n CHECK_NOTHROW(actual.pop_back());\n CHECK_NOTHROW(expect.pop_back());\n --expectSize;\n }\n }\n else if (expectSize != 0)\n {\n CHECK_NOTHROW(actual.pop_front());\n CHECK_NOTHROW(expect.pop_front());\n --expectSize;\n }\n }\n CHECK(actual.size() == expectSize);\n CHECK((expectSize ^ actual.empty()));\n if (!expect.empty())\n {\n CHECK(expect.front() == actual.front());\n CHECK(expect.back() == actual.back());\n }\n });\n }\n\n SECTION(\"efficiency\")\n {\n // todo\n }\n}\n\n#endif // XOR_LINKED_LIST_TEST_CPP"
}
{
"filename": "code/data_structures/test/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/data_structures/test/tree/binary_tree/binary_tree/diameter/test_diameter.cpp",
"content": "#define CATCH_CONFIG_MAIN\n#include \"../../../../../../../test/c++/catch.hpp\"\n#include \"../../../../../src/tree/binary_tree/binary_tree/serializer/serializer.cpp\"\n#include \"../../../../../src/tree/binary_tree/binary_tree/node/node.cpp\"\n#include \"../../../../../src/tree/binary_tree/binary_tree/diameter/diameter.cpp\"\n#include <string>\n\nTEST_CASE(\"diameter of binary tree\")\n{\n TreeSerializer ts;\n std::string str;\n\n SECTION(\"empty tree\")\n {\n str = \"#\";\n CHECK(diameter<std::shared_ptr<TreeNode<int>>>(ts.deserialize(str)) == 0);\n }\n\n SECTION(\"one-node tree\")\n {\n str = \"1 # #\";\n CHECK(diameter<std::shared_ptr<TreeNode<int>>>(ts.deserialize(str)) == 1);\n }\n\n SECTION(\"non-empty tree\")\n {\n /*\n * 1\n * / \\\n * 2 2\n * / \\\n * 3 3\n * / \\\n * 4 4\n * /\n * 5\n */\n str = \"1 2 3 4 5 # # # # 3 # 4 # # 2 # #\";\n CHECK(diameter<std::shared_ptr<TreeNode<int>>>(ts.deserialize(str)) == 6);\n\n /*\n * 1\n * / \\\n * 2 2\n * / \\\n * 3 3\n * / \\\n * 4 4\n * / \\\n * 5 5\n */\n str = \"1 2 3 4 5 # # # # 3 # 4 # 5 # # 2 # #\";\n CHECK(diameter<std::shared_ptr<TreeNode<int>>>(ts.deserialize(str)) == 7);\n\n /*\n * 1\n * / \\\n * 2 2\n * / \\ /\n * 3 3 3\n * / /\\ /\n * 4 4 4 4\n * / /\n * 5 5\n */\n str = \"1 2 3 4 5 # # # # 3 4 # # 4 # # 2 3 4 5 # # # # #\";\n CHECK(diameter<std::shared_ptr<TreeNode<int>>>(ts.deserialize(str)) == 9);\n }\n}"
}
{
"filename": "code/data_structures/test/tree/binary_tree/binary_tree/is_same/test_is_same.cpp",
"content": "#define CATCH_CONFIG_MAIN\n#ifndef TEST_TREE_COMPARER\n#define TEST_TREE_COMPARER\n\n#include <memory>\n#include <functional>\n#include <utility>\n#include \"../../../../../../../test/c++/catch.hpp\"\n#include \"../../../../../src/tree/binary_tree/binary_tree/is_same/is_same.cpp\"\n#include \"../../../../../src/tree/binary_tree/binary_tree/serializer/serializer.cpp\"\n#include \"../../../../../src/tree/binary_tree/binary_tree/node/node.cpp\"\n\nTEST_CASE(\"check two tree is same\", \"[isSameTree]\") {\n TreeSerializer serializer;\n std::shared_ptr<TreeNode<int>> root_a, root_b;\n TreeComparer<int> comparer;\n\n SECTION(\"has empty tree\") {\n root_a = serializer.deserialize(\"# \");\n root_b = serializer.deserialize(\"# \");\n REQUIRE(comparer.isSameTree(root_a, root_b));\n\n root_a = serializer.deserialize(\"1 # # \");\n root_b = serializer.deserialize(\"1 # # \");\n REQUIRE(comparer.isSameTree(root_a, root_b));\n\n root_a = serializer.deserialize(\"# \");\n root_b = serializer.deserialize(\"1 # # \");\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n std::swap(root_a, root_b);\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n }\n\n SECTION(\"has same value\") {\n root_a = serializer.deserialize(\"1 # # \");\n root_b = serializer.deserialize(\"1 # # \");\n REQUIRE(comparer.isSameTree(root_a, root_b));\n std::swap(root_a, root_b);\n REQUIRE(comparer.isSameTree(root_a, root_b));\n\n root_a = serializer.deserialize(\"1 # 2 # # \");\n root_b = serializer.deserialize(\"1 # 2 # # \");\n REQUIRE(comparer.isSameTree(root_a, root_b));\n std::swap(root_a, root_b);\n REQUIRE(comparer.isSameTree(root_a, root_b));\n\n root_a = serializer.deserialize(\"1 2 3 4 # # 5 # # # #\");\n root_b = serializer.deserialize(\"1 2 3 4 # # 5 # # # #\");\n REQUIRE(comparer.isSameTree(root_a, root_b));\n std::swap(root_a, root_b);\n REQUIRE(comparer.isSameTree(root_a, root_b));\n }\n\n SECTION(\"has not same value\") {\n root_a = serializer.deserialize(\"# \");\n root_b = serializer.deserialize(\"1 # # \");\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n std::swap(root_a, root_b);\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n\n root_a = serializer.deserialize(\"1 # # \");\n root_b = serializer.deserialize(\"2 # # \");\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n std::swap(root_a, root_b);\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n\n root_a = serializer.deserialize(\"1 # 2 # # \");\n root_b = serializer.deserialize(\"1 # 3 # # \");\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n std::swap(root_a, root_b);\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n\n root_a = serializer.deserialize(\"1 2 # # # \");\n root_b = serializer.deserialize(\"1 3 # # # \");\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n std::swap(root_a, root_b);\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n\n root_a = serializer.deserialize(\"1 2 3 4 # # 5 # # # #\");\n root_b = serializer.deserialize(\"1 2 3 4 # # 6 # # # #\");\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n std::swap(root_a, root_b);\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n }\n\n SECTION(\"has reflex node\") {\n root_a = serializer.deserialize(\"1 2 # # 3 # # \");\n root_b = serializer.deserialize(\"1 3 # # 2 # #\");\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n std::swap(root_a, root_b);\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n\n root_a = serializer.deserialize(\"1 2 # 3 # # 4 # # \");\n root_b = serializer.deserialize(\"1 4 # # 2 3 # # # \");\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n std::swap(root_a, root_b);\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n\n root_a = serializer.deserialize(\"1 2 3 # # # 4 # # \");\n root_b = serializer.deserialize(\"1 4 # # 2 # 3 # # \");\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n std::swap(root_a, root_b);\n REQUIRE_FALSE(comparer.isSameTree(root_a, root_b));\n }\n}\n\n#endif // TEST_TREE_COMPARER"
}
{
"filename": "code/data_structures/test/tree/binary_tree/binary_tree/path_sum/test_path_sum_for_sum_of_part_paths.cpp",
"content": "#define CATCH_CONFIG_MAIN\n#include \"../../../../../../../test/c++/catch.hpp\"\n#include <vector>\n#include <memory>\n#include <queue>\n#include \"../../../../../src/tree/binary_tree/binary_tree/node/node.cpp\"\n#include \"../../../../../src/tree/binary_tree/binary_tree/serializer/serializer.cpp\"\n#include \"../../../../../src/tree/binary_tree/binary_tree/path_sum/path_sum.hpp\"\n\nusing Node = TreeNode<int>;\nusing PNode = std::shared_ptr<Node>;\n\nTEST_CASE(\"sum of paths between any node\") {\n PathSum<int> sol(PathSum<int>::PathType::Part);\n size_t (PathSum<int>::* pf)(PNode, int) = &PathSum<int>::countPathsOfSum;\n\n TreeSerializer ts;\n PNode root = nullptr;\n auto res = (sol.*pf)(root, 0);\n\n SECTION(\"give empty tree\") {\n root = ts.deserialize(\"# \");\n res = (sol.*pf)(root, 0);\n CHECK(res == 0);\n }\n\n SECTION(\"give one node\") {\n root = ts.deserialize(\"1 # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 1);\n }\n\n SECTION(\"give balance tree\") {\n root = ts.deserialize(\"0 1 # # 1 # # \");\n res = (sol.*pf)(root, 0);\n CHECK(res == 1);\n res = (sol.*pf)(root, 1);\n CHECK(res == 4);\n\n root = ts.deserialize(\"1 2 # # 3 # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 1);\n res = (sol.*pf)(root, 2);\n CHECK(res == 1);\n res = (sol.*pf)(root, 3);\n CHECK(res == 2);\n res = (sol.*pf)(root, 4);\n CHECK(res == 1);\n\n root = ts.deserialize(\"0 1 1 # # # 1 1 # # # \");\n res = (sol.*pf)(root, 0);\n CHECK(res == 1);\n res = (sol.*pf)(root, 1);\n CHECK(res == 6);\n res = (sol.*pf)(root, 2);\n CHECK(res == 4);\n\n root = ts.deserialize(\"0 1 # 1 # # 1 # 1 # # \");\n res = (sol.*pf)(root, 0);\n CHECK(res == 1);\n res = (sol.*pf)(root, 1);\n CHECK(res == 6);\n res = (sol.*pf)(root, 2);\n CHECK(res == 4);\n\n root = ts.deserialize(\"1 2 4 # # # 3 5 # # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 1);\n res = (sol.*pf)(root, 2);\n CHECK(res == 1);\n res = (sol.*pf)(root, 3);\n CHECK(res == 2);\n res = (sol.*pf)(root, 4);\n CHECK(res == 2);\n res = (sol.*pf)(root, 5);\n CHECK(res == 1);\n res = (sol.*pf)(root, 6);\n CHECK(res == 1);\n res = (sol.*pf)(root, 7);\n CHECK(res == 1);\n res = (sol.*pf)(root, 8);\n CHECK(res == 1);\n res = (sol.*pf)(root, 9);\n CHECK(res == 1);\n\n root = ts.deserialize(\"1 2 4 # # # 3 # 5 # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 1);\n res = (sol.*pf)(root, 2);\n CHECK(res == 1);\n res = (sol.*pf)(root, 3);\n CHECK(res == 2);\n res = (sol.*pf)(root, 4);\n CHECK(res == 2);\n res = (sol.*pf)(root, 5);\n CHECK(res == 1);\n res = (sol.*pf)(root, 6);\n CHECK(res == 1);\n res = (sol.*pf)(root, 7);\n CHECK(res == 1);\n res = (sol.*pf)(root, 8);\n CHECK(res == 1);\n res = (sol.*pf)(root, 9);\n CHECK(res == 1);\n\n root = ts.deserialize(\"1 2 # 4 # # 3 5 # # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 1);\n res = (sol.*pf)(root, 2);\n CHECK(res == 1);\n res = (sol.*pf)(root, 3);\n CHECK(res == 2);\n res = (sol.*pf)(root, 4);\n CHECK(res == 2);\n res = (sol.*pf)(root, 5);\n CHECK(res == 1);\n res = (sol.*pf)(root, 6);\n CHECK(res == 1);\n res = (sol.*pf)(root, 7);\n CHECK(res == 1);\n res = (sol.*pf)(root, 8);\n CHECK(res == 1);\n res = (sol.*pf)(root, 9);\n CHECK(res == 1);\n\n root = ts.deserialize(\"1 2 # 4 # # 3 # 5 # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 1);\n res = (sol.*pf)(root, 2);\n CHECK(res == 1);\n res = (sol.*pf)(root, 3);\n CHECK(res == 2);\n res = (sol.*pf)(root, 4);\n CHECK(res == 2);\n res = (sol.*pf)(root, 5);\n CHECK(res == 1);\n res = (sol.*pf)(root, 6);\n CHECK(res == 1);\n res = (sol.*pf)(root, 7);\n CHECK(res == 1);\n res = (sol.*pf)(root, 8);\n CHECK(res == 1);\n res = (sol.*pf)(root, 9);\n CHECK(res == 1);\n }\n\n SECTION(\"give unbalance tree\") {\n SECTION(\"left is more deep\") {\n root = ts.deserialize(\"1 2 # # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 1);\n res = (sol.*pf)(root, 2);\n CHECK(res == 1);\n res = (sol.*pf)(root, 3);\n CHECK(res == 1);\n\n root = ts.deserialize(\"1 2 3 # # # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 1);\n res = (sol.*pf)(root, 2);\n CHECK(res == 1);\n res = (sol.*pf)(root, 3);\n CHECK(res == 2);\n res = (sol.*pf)(root, 5);\n CHECK(res == 1);\n\n root = ts.deserialize(\"1 2 # 3 # # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 1);\n res = (sol.*pf)(root, 2);\n CHECK(res == 1);\n res = (sol.*pf)(root, 3);\n CHECK(res == 2);\n res = (sol.*pf)(root, 5);\n CHECK(res == 1);\n\n root = ts.deserialize(\"1 1 1 # # # 1 # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 4);\n res = (sol.*pf)(root, 2);\n CHECK(res == 3);\n res = (sol.*pf)(root, 3);\n CHECK(res == 1);\n\n root = ts.deserialize(\"1 1 # 1 # # 1 # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 4);\n res = (sol.*pf)(root, 2);\n CHECK(res == 3);\n res = (sol.*pf)(root, 3);\n CHECK(res == 1);\n\n root = ts.deserialize(\"1 2 4 # # # 3 # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 1);\n res = (sol.*pf)(root, 2);\n CHECK(res == 1);\n res = (sol.*pf)(root, 3);\n CHECK(res == 2);\n res = (sol.*pf)(root, 4);\n CHECK(res == 2);\n res = (sol.*pf)(root, 6);\n CHECK(res == 1);\n\n root = ts.deserialize(\"1 2 # 4 # # 3 # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 1);\n res = (sol.*pf)(root, 2);\n CHECK(res == 1);\n res = (sol.*pf)(root, 3);\n CHECK(res == 2);\n res = (sol.*pf)(root, 4);\n CHECK(res == 2);\n res = (sol.*pf)(root, 6);\n CHECK(res == 1);\n }\n\n SECTION(\"right is more deep\") {\n root = ts.deserialize(\"1 # 2 # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 1);\n res = (sol.*pf)(root, 2);\n CHECK(res == 1);\n res = (sol.*pf)(root, 3);\n CHECK(res == 1);\n\n root = ts.deserialize(\"1 # 2 3 # # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 1);\n res = (sol.*pf)(root, 2);\n CHECK(res == 1);\n res = (sol.*pf)(root, 3);\n CHECK(res == 2);\n res = (sol.*pf)(root, 5);\n CHECK(res == 1);\n\n root = ts.deserialize(\"1 # 2 # 3 # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 1);\n res = (sol.*pf)(root, 2);\n CHECK(res == 1);\n res = (sol.*pf)(root, 3);\n CHECK(res == 2);\n res = (sol.*pf)(root, 5);\n CHECK(res == 1);\n\n root = ts.deserialize(\"1 1 # # 1 1 # # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 4);\n res = (sol.*pf)(root, 2);\n CHECK(res == 3);\n res = (sol.*pf)(root, 3);\n CHECK(res == 1);\n\n root = ts.deserialize(\"1 1 # # 1 # 1 # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 4);\n res = (sol.*pf)(root, 2);\n CHECK(res == 3);\n res = (sol.*pf)(root, 3);\n CHECK(res == 1);\n\n root = ts.deserialize(\"1 2 # # 3 4 # # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 1);\n res = (sol.*pf)(root, 2);\n CHECK(res == 1);\n res = (sol.*pf)(root, 3);\n CHECK(res == 2);\n res = (sol.*pf)(root, 4);\n CHECK(res == 2);\n res = (sol.*pf)(root, 7);\n CHECK(res == 1);\n\n root = ts.deserialize(\"1 2 # # 3 # 4 # # \");\n res = (sol.*pf)(root, 1);\n CHECK(res == 1);\n res = (sol.*pf)(root, 2);\n CHECK(res == 1);\n res = (sol.*pf)(root, 3);\n CHECK(res == 2);\n res = (sol.*pf)(root, 4);\n CHECK(res == 2);\n res = (sol.*pf)(root, 7);\n CHECK(res == 1);\n }\n }\n}"
}
{
"filename": "code/data_structures/test/tree/binary_tree/binary_tree/path_sum/test_path_sum_for_sum_of_whole_paths.cpp",
"content": "#define CATCH_CONFIG_MAIN\n#include \"../../../../../../../test/c++/catch.hpp\"\n#include <vector>\n#include <memory>\n#include <queue>\n#include \"../../../../../src/tree/binary_tree/binary_tree/node/node.cpp\"\n#include \"../../../../../src/tree/binary_tree/binary_tree/serializer/serializer.cpp\"\n#include \"../../../../../src/tree/binary_tree/binary_tree/path_sum/path_sum.hpp\"\n\nusing Node = TreeNode<int>;\nusing PNode = std::shared_ptr<Node>;\n\nTEST_CASE(\"sum of paths between root to bottom\") {\n PathSum<int> sol;\n size_t (PathSum<int>::* pf)(PNode, int) = &PathSum<int>::countPathsOfSum;\n\n TreeSerializer ts;\n PNode root = nullptr;\n auto res = (sol.*pf)(root, 0);\n\n SECTION(\"give empty tree\") {\n root = ts.deserialize(\"# \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n CHECK(res == 0);\n }\n }\n\n SECTION(\"give one node\") {\n root = ts.deserialize(\"1 # # \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n if (i == 1)\n CHECK(res == 1);\n else\n CHECK(res == 0);\n }\n }\n\n SECTION(\"give balance tree\") {\n root = ts.deserialize(\"1 2 # # 3 # # \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n if (i == 3 || i == 4)\n CHECK(res == 1);\n else\n CHECK(res == 0);\n }\n\n root = ts.deserialize(\"1 2 4 # # # 3 5 # # # \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n if (i == 7 || i == 9)\n CHECK(res == 1);\n else\n CHECK(res == 0);\n }\n\n root = ts.deserialize(\"1 2 4 # # # 3 # 5 # # \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n if (i == 7 || i == 9)\n CHECK(res == 1);\n else\n CHECK(res == 0);\n }\n\n root = ts.deserialize(\"1 2 # 4 # # 3 5 # # # \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n if (i == 7 || i == 9)\n CHECK(res == 1);\n else\n CHECK(res == 0);\n }\n\n root = ts.deserialize(\"1 2 # 4 # # 3 # 5 # # \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n if (i == 7 || i == 9)\n CHECK(res == 1);\n else\n CHECK(res == 0);\n }\n }\n\n SECTION(\"give unbalance tree\") {\n SECTION(\"left is more deep\") {\n root = ts.deserialize(\"1 2 # # # \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n if (i == 3)\n CHECK(res == 1);\n else\n CHECK(res == 0);\n }\n\n root = ts.deserialize(\"1 2 3 # # # # \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n if (i == 6)\n CHECK(res == 1);\n else\n CHECK(res == 0);\n }\n\n root = ts.deserialize(\"1 2 # 3 # # # \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n if (i == 6)\n CHECK(res == 1);\n else\n CHECK(res == 0);\n }\n\n root = ts.deserialize(\"1 2 4 # # # 3 # # \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n if (i == 4 || i == 7)\n CHECK(res == 1);\n else\n CHECK(res == 0);\n }\n\n root = ts.deserialize(\"1 2 # 4 # # 3 # # \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n if (i == 4 || i == 7)\n CHECK(res == 1);\n else\n CHECK(res == 0);\n }\n }\n\n SECTION(\"right is more deep\") {\n root = ts.deserialize(\"1 # 2 # # \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n if (i == 3)\n CHECK(res == 1);\n else\n CHECK(res == 0);\n }\n\n root = ts.deserialize(\"1 # 2 3 # # # \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n if (i == 6)\n CHECK(res == 1);\n else\n CHECK(res == 0);\n }\n\n root = ts.deserialize(\"1 # 2 # 3 # # \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n if (i == 6)\n CHECK(res == 1);\n else\n CHECK(res == 0);\n }\n\n root = ts.deserialize(\"1 2 # # 3 4 # # # \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n if (i == 3 || i == 8)\n CHECK(res == 1);\n else\n CHECK(res == 0);\n }\n\n root = ts.deserialize(\"1 2 # # 3 # 4 # # \");\n for (int i = -20; i < 20; ++i)\n {\n res = (sol.*pf)(root, i);\n if (i == 3 || i == 8)\n CHECK(res == 1);\n else\n CHECK(res == 0);\n }\n }\n }\n}"
}
{
"filename": "code/data_structures/test/tree/binary_tree/binary_tree/path_sum/test_path_sum_for_whole_paths.cpp",
"content": "#define CATCH_CONFIG_MAIN\n#include \"../../../../../../../test/c++/catch.hpp\"\n#include <vector>\n#include <memory>\n#include <queue>\n#include \"../../../../../src/tree/binary_tree/binary_tree/node/node.cpp\"\n#include \"../../../../../src/tree/binary_tree/binary_tree/serializer/serializer.cpp\"\n#include \"../../../../../src/tree/binary_tree/binary_tree/path_sum/path_sum.hpp\"\n\nusing Node = TreeNode<int>;\nusing PNode = std::shared_ptr<Node>;\n\nbool isSame(std::vector<std::vector<int>> &a, std::vector<std::vector<int>> &b)\n{\n std::sort(a.begin(), a.end());\n std::sort(b.begin(), b.end());\n\n if (a.size() != b.size())\n return false;\n for (size_t i = 0; i < a.size(); ++i)\n {\n if (a.at(i).size() != b.at(i).size())\n return false;\n for (size_t j = 0; j < a.at(i).size(); ++j)\n if (a.at(i).at(j) != b.at(i).at(j))\n return false;\n }\n return true;\n}\n\nTEST_CASE(\"paths between root to leaf\") {\n PathSum<int> sol;\n std::vector<std::vector<int>> (PathSum<int>::* pf)(PNode, int) = &PathSum<int>::getPathsOfSum;\n\n TreeSerializer ts;\n PNode root = nullptr;\n auto res = (sol.*pf)(root, 0);\n std::vector<std::vector<int>> expect{};\n\n SECTION(\"give empty tree\") {\n root = ts.deserialize(\"# \");\n res = (sol.*pf)(root, 0);\n expect = {};\n CHECK(isSame(res, expect));\n }\n\n SECTION(\"give one node\") {\n root = ts.deserialize(\"1 # # \");\n res = (sol.*pf)(root, 0);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 1);\n expect = {{1}};\n CHECK(isSame(res, expect));\n }\n\n SECTION(\"user define\") {\n root = ts.deserialize(\"5 4 11 7 # # 2 # # # 8 13 # # 4 5 # # 1 # # \");\n res = (sol.*pf)(root, 22);\n expect = {{5, 4, 11, 2}, {5, 8, 4, 5}};\n CHECK(isSame(res, expect));\n }\n\n SECTION(\"give balance tree\") {\n root = ts.deserialize(\"1 2 # # 3 # # \");\n res = (sol.*pf)(root, 1);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 2);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 3);\n expect = {{1, 2}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 4);\n expect = {{1, 3}};\n CHECK(isSame(res, expect));\n\n root = ts.deserialize(\"1 2 4 # # # 3 5 # # # \");\n res = (sol.*pf)(root, 7);\n expect = {{1, 2, 4}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 9);\n expect = {{1, 3, 5}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 1);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 2);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 3);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 4);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 5);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 6);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 8);\n expect = {};\n CHECK(isSame(res, expect));\n\n root = ts.deserialize(\"1 2 4 # # # 3 # 5 # # \");\n res = (sol.*pf)(root, 7);\n expect = {{1, 2, 4}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 9);\n expect = {{1, 3, 5}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 1);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 2);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 3);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 4);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 5);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 6);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 8);\n expect = {};\n CHECK(isSame(res, expect));\n\n root = ts.deserialize(\"1 2 # 4 # # 3 5 # # # \");\n res = (sol.*pf)(root, 7);\n expect = {{1, 2, 4}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 9);\n expect = {{1, 3, 5}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 1);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 2);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 3);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 4);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 5);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 6);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 8);\n expect = {};\n CHECK(isSame(res, expect));\n\n root = ts.deserialize(\"1 2 # 4 # # 3 # 5 # # \");\n res = (sol.*pf)(root, 7);\n expect = {{1, 2, 4}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 9);\n expect = {{1, 3, 5}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 1);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 2);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 3);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 4);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 5);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 6);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 8);\n expect = {};\n CHECK(isSame(res, expect));\n }\n\n SECTION(\"give unbalance tree\") {\n SECTION(\"left is more deep\") {\n root = ts.deserialize(\"1 2 # # # \");\n res = (sol.*pf)(root, 1);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 2);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 3);\n expect = {{1, 2}};\n CHECK(isSame(res, expect));\n\n root = ts.deserialize(\"1 2 3 # # # # \");\n res = (sol.*pf)(root, 1);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 2);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 3);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 5);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 6);\n expect = {{1, 2, 3}};\n CHECK(isSame(res, expect));\n\n root = ts.deserialize(\"1 2 # 3 # # # \");\n res = (sol.*pf)(root, 1);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 2);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 3);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 5);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 6);\n expect = {{1, 2, 3}};\n CHECK(isSame(res, expect));\n\n root = ts.deserialize(\"1 2 4 # # # 3 # # \");\n res = (sol.*pf)(root, 4);\n expect = {{1, 3}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 7);\n expect = {{1, 2, 4}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 1);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 2);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 3);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 5);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 6);\n expect = {};\n CHECK(isSame(res, expect));\n\n root = ts.deserialize(\"1 2 # 4 # # 3 # # \");\n res = (sol.*pf)(root, 4);\n expect = {{1, 3}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 7);\n expect = {{1, 2, 4}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 1);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 2);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 3);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 5);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 6);\n expect = {};\n CHECK(isSame(res, expect));\n }\n\n SECTION(\"right is more deep\") {\n root = ts.deserialize(\"1 # 2 # # \");\n res = (sol.*pf)(root, 1);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 2);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 3);\n expect = {{1, 2}};\n CHECK(isSame(res, expect));\n\n root = ts.deserialize(\"1 # 2 3 # # # \");\n res = (sol.*pf)(root, 1);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 2);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 3);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 5);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 6);\n expect = {{1, 2, 3}};\n CHECK(isSame(res, expect));\n\n root = ts.deserialize(\"1 # 2 # 3 # # \");\n res = (sol.*pf)(root, 1);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 2);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 3);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 5);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 6);\n expect = {{1, 2, 3}};\n CHECK(isSame(res, expect));\n\n root = ts.deserialize(\"1 2 # # 3 4 # # # \");\n res = (sol.*pf)(root, 3);\n expect = {{1, 2}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 8);\n expect = {{1, 3, 4}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 1);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 2);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 4);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 5);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 7);\n expect = {};\n CHECK(isSame(res, expect));\n\n root = ts.deserialize(\"1 2 # # 3 # 4 # # \");\n res = (sol.*pf)(root, 3);\n expect = {{1, 2}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 8);\n expect = {{1, 3, 4}};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 1);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 2);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 4);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 5);\n expect = {};\n CHECK(isSame(res, expect));\n res = (sol.*pf)(root, 7);\n expect = {};\n CHECK(isSame(res, expect));\n }\n }\n}"
}
{
"filename": "code/data_structures/test/tree/multiway_tree/red_black_tree/test_red_black.c",
"content": "/**\n * author: JonNRb <[email protected]>\n * license: GPLv3\n * file: @cosmos//code/data_structures/red_black_tree/red_black_test.c\n * info: red black tree\n */\n\n#include \"red_black_tree.h\"\n\n#include <assert.h>\n#include <inttypes.h>\n#include <math.h>\n#include <stdint.h>\n#include <stdio.h>\n#include <stdlib.h>\n#include <string.h>\n#include <time.h>\n\n#define red_printf(f, ...) printf(\"\\x1b[31m\" f \"\\x1b[0m\", __VA_ARGS__)\n\nextern int is_rb_correct(cosmos_red_black_tree_t tree);\n\ntypedef struct item {\n char label;\n\n cosmos_red_black_node_t node;\n uint64_t sort_key;\n} item_t;\n\ntypedef struct {\n char text[8];\n size_t depth;\n int red;\n} item_repr_t;\n\nvoid iot_1(cosmos_red_black_tree_t tree, size_t* size) {\n ++*size;\n if (tree != NULL) {\n iot_1(tree->link[0], size);\n iot_1(tree->link[1], size);\n }\n}\n\nvoid iot_2(cosmos_red_black_tree_t tree, item_repr_t* arr, size_t* i,\n size_t depth) {\n if (tree == NULL) {\n strncpy(arr[*i].text, \"[ NIL ]\", 8);\n arr[*i].depth = depth;\n arr[*i].red = 0;\n ++*i;\n return;\n }\n\n iot_2(tree->link[0], arr, i, depth + 1);\n\n item_t* item = container_of(tree, struct item, node);\n snprintf(arr[*i].text, 8, \"[%c,%03\" PRIu64 \"]\", item->label, item->sort_key);\n arr[*i].depth = depth;\n arr[*i].red = tree->red;\n ++*i;\n\n iot_2(tree->link[1], arr, i, depth + 1);\n}\n\nvoid print_tree(cosmos_red_black_tree_t tree) {\n size_t size = 0;\n iot_1(tree, &size);\n\n item_repr_t* arr = (item_repr_t*)malloc(size * sizeof(item_repr_t));\n size_t i = 0;\n iot_2(tree, arr, &i, 0);\n\n assert(i == size);\n\n for (size_t depth = 0, i = 0; i < size; ++depth) {\n for (size_t j = 0; j < size; ++j) {\n if (arr[j].depth == depth) {\n if (arr[j].red) {\n red_printf(\"%s\", arr[j].text);\n } else {\n printf(\"%s\", arr[j].text);\n }\n ++i;\n } else {\n printf(\" \");\n }\n }\n printf(\"\\n\");\n }\n}\n\nvoid assert_tree_invalid() {\n printf(\"checking we can check tree validity\\n\");\n\n assert(is_rb_correct(NULL));\n\n item_t items[7];\n items[0] = (item_t){'A', {{NULL, NULL}, 1}, 1};\n items[1] = (item_t){'B', {{&items[0].node, &items[2].node}, 1}, 2};\n items[2] = (item_t){'C', {{NULL, NULL}, 1}, 3};\n items[3] = (item_t){'D', {{&items[1].node, &items[5].node}, 1}, 4};\n items[4] = (item_t){'E', {{NULL, NULL}, 1}, 5};\n items[5] = (item_t){'F', {{&items[4].node, &items[6].node}, 1}, 6};\n items[6] = (item_t){'G', {{NULL, NULL}, 1}, 7};\n assert(!is_rb_correct(&items[3].node));\n}\n\nvoid print_sanity_tree() {\n item_t items[7];\n\n items[0] = (item_t){'A', {{NULL, NULL}, 0}, 1};\n items[1] = (item_t){'B', {{&items[0].node, &items[2].node}, 1}, 2};\n items[2] = (item_t){'C', {{NULL, NULL}, 0}, 3};\n items[3] = (item_t){'D', {{&items[1].node, &items[5].node}, 0}, 4};\n items[4] = (item_t){'E', {{NULL, NULL}, 0}, 5};\n items[5] = (item_t){'F', {{&items[4].node, &items[6].node}, 1}, 6};\n items[6] = (item_t){'G', {{NULL, NULL}, 0}, 7};\n\n printf(\"printing sanity tree\\n\");\n print_tree(&items[3].node);\n}\n\nvoid construct_and_insert(cosmos_red_black_tree_t* tree, item_t* item,\n char label, uint64_t sort_key) {\n item->label = label;\n item->sort_key = sort_key;\n cosmos_red_black_construct(&item->node);\n\n cosmos_red_black_push(tree, &item->node);\n\n if (!is_rb_correct(*tree)) {\n printf(\"incorrect tree inserting %\" PRIu64 \":\\n\", sort_key);\n print_tree(*tree);\n abort();\n }\n}\n\nvoid build_and_print() {\n cosmos_red_black_tree_t tree = NULL;\n item_t items[7];\n\n printf(\"no nodes\\n\");\n print_tree(tree);\n\n for (int i = 0; i < 7; ++i) {\n printf(\"%d node%s\\n\", i + 1, i != 0 ? \"s\" : \"\");\n construct_and_insert(&tree, items + i, 'A' + i, i + 1);\n print_tree(tree);\n }\n}\n\nstatic inline void swap(uint64_t* a, uint64_t* b) {\n uint64_t temp = *a;\n *a = *b;\n *b = temp;\n}\n\nvoid stress() {\n static const size_t num_nodes = 2 << 12;\n uint64_t nums[num_nodes];\n for (size_t i = 0; i < num_nodes; ++i) {\n nums[i] = i;\n }\n\n // janky shuffle\n srand(time(NULL));\n for (size_t i = 0; i < num_nodes - 1; ++i) {\n swap(nums + i, nums + i + 1 + (rand() % (num_nodes - i - 1)));\n }\n\n printf(\"stress adding %zu things in random order\\n\", num_nodes);\n cosmos_red_black_tree_t tree = NULL;\n item_t items[num_nodes];\n for (size_t i = 0; i < num_nodes; ++i) {\n construct_and_insert(&tree, items + i, 'X', nums[i]);\n }\n\n printf(\"stress popping %zu those things from front\\n\", num_nodes);\n for (size_t i = 0; i < num_nodes; ++i) {\n cosmos_red_black_node_t* popped = cosmos_red_black_pop_min(&tree);\n assert(popped != NULL);\n if (!is_rb_correct(tree)) {\n printf(\"incorrect tree popping %\" PRIu64 \"\\n\",\n container_of(popped, item_t, node)->sort_key);\n print_tree(tree);\n abort();\n }\n }\n\n assert(tree == NULL);\n}\n\nvoid time_test() {\n static const size_t max_num_nodes = 2 << 10;\n uint64_t nums[max_num_nodes];\n\n for (size_t num_nodes = 8; num_nodes < max_num_nodes; num_nodes <<= 1) {\n for (size_t i = 0; i < num_nodes; ++i) {\n nums[i] = i;\n }\n\n // janky shuffle\n srand(time(NULL));\n for (size_t i = 0; i < num_nodes - 1; ++i) {\n swap(nums + i, nums + i + 1 + (rand() % (num_nodes - i - 1)));\n }\n\n clock_t start = clock();\n cosmos_red_black_tree_t tree = NULL;\n item_t items[num_nodes];\n for (size_t i = 0; i < num_nodes; ++i) {\n construct_and_insert(&tree, items + i, 'X', nums[i]);\n }\n clock_t t = clock() - start;\n printf(\"adding %4zu things took %9\" PRId64 \" µs (log => %5.02f)\\n\",\n num_nodes, ((uint64_t)t * 1000000) / CLOCKS_PER_SEC, log((double)t));\n\n start = clock();\n for (size_t i = 0; i < num_nodes; ++i) {\n cosmos_red_black_node_t* popped = cosmos_red_black_pop_min(&tree);\n assert(popped != NULL);\n if (!is_rb_correct(tree)) {\n printf(\"incorrect tree popping %\" PRIu64 \"\\n\",\n container_of(popped, item_t, node)->sort_key);\n print_tree(tree);\n abort();\n }\n }\n t = clock() - start;\n printf(\"popping %4zu things took %9\" PRId64 \" µs (log => %5.02f)\\n\",\n num_nodes, ((uint64_t)t * 1000000) / CLOCKS_PER_SEC, log((double)t));\n\n assert(tree == NULL);\n }\n}\n\nint main() {\n print_sanity_tree();\n assert_tree_invalid();\n build_and_print();\n stress();\n time_test();\n\n return 0;\n}"
}
{
"filename": "code/data_structures/test/tree/multiway_tree/union_find/test_union_find.cpp",
"content": "#include <iostream>\n#include <cassert>\n#include \"../../../../src/tree/multiway_tree/union_find/union_find_dynamic.cpp\"\n\nint main()\n{\n UnionFind<int> unionFind;\n\n unionFind.merge(3, 4);\n unionFind.merge(3, 8);\n unionFind.merge(0, 8);\n unionFind.merge(1, 3);\n unionFind.merge(7, 9);\n unionFind.merge(5, 9);\n\n // Now the components are:\n // 0 1 3 4 8\n // 5 7 9\n assert(unionFind.connected(0, 1));\n assert(unionFind.connected(0, 3));\n assert(unionFind.connected(0, 4));\n assert(unionFind.connected(0, 8));\n assert(unionFind.connected(1, 3));\n assert(unionFind.connected(1, 4));\n assert(unionFind.connected(1, 8));\n assert(unionFind.connected(3, 4));\n assert(unionFind.connected(3, 8));\n assert(unionFind.connected(4, 8));\n assert(unionFind.connected(5, 7));\n assert(unionFind.connected(5, 9));\n assert(unionFind.connected(7, 9));\n\n assert(!unionFind.connected(0, 5));\n assert(!unionFind.connected(0, 7));\n assert(!unionFind.connected(0, 9));\n assert(!unionFind.connected(1, 5));\n assert(!unionFind.connected(1, 7));\n assert(!unionFind.connected(1, 9));\n assert(!unionFind.connected(3, 5));\n assert(!unionFind.connected(3, 7));\n assert(!unionFind.connected(3, 9));\n assert(!unionFind.connected(4, 5));\n assert(!unionFind.connected(4, 7));\n assert(!unionFind.connected(4, 9));\n assert(!unionFind.connected(8, 5));\n assert(!unionFind.connected(8, 7));\n assert(!unionFind.connected(8, 9));\n\n return 0;\n}"
}
{
"filename": "code/data_structures/test/tree/segment_tree/test_generic_segment_tree.cpp",
"content": "#include <algorithm>\n#include <iostream>\n#include <vector>\n\n// Note that the below line includes a translation unit and not header file\n\n#include \"../../../src/tree/segment_tree/generic_segment_tree.cpp\"\n\n#define ASSERT(value, expected_value, message) \\\n{ \\\n if (value != expected_value) \\\n std::cerr << \"[assertion failed]\\n\" \\\n << \" Line: \" << __LINE__ << '\\n' \\\n << \" File: \" << __FILE__ << '\\n' \\\n << \"Message: \" << message << std::endl; \\\n else \\\n std::cerr << \"[assertion passed]: \" << message << std::endl; \\\n}\n\n/**\n * This program tests the generic segment tree implementation that can be found in the include location above.\n * The implementation requires C++20 in order to compile.\n * \n * Compilation: g++ test_generic_segment_tree.cpp -o test_generic_segment_tree -Wall -Wextra -pedantic -std=c++20 -O3\n */\n\nconstexpr bool is_multitest = false;\nconstexpr int32_t inf32 = int32_t(1) << 30;\nconstexpr int64_t inf64 = int64_t(1) << 60;\n\nvoid test () {\n std::vector <int> values = { 1, 2, 3, 4, 5 };\n int n = values.size();\n\n arrow::SegmentTree <int> binaryTreeSum (n, values, [] (auto l, auto r) {\n return l + r;\n });\n\n arrow::SegmentTree <int, arrow::TreeMemoryLayout::EulerTour> eulerTourTreeSum (n, values, [] (auto l, auto r) {\n return l + r;\n });\n\n ASSERT(binaryTreeSum.rangeQuery(0, 4), 15, \"binaryTreeSum.rangeQuery(0, 4) == 15\");\n ASSERT(binaryTreeSum.rangeQuery(1, 2), 5, \"binaryTreeSum.rangeQuery(1, 2) == 5\");\n ASSERT(binaryTreeSum.rangeQuery(4, 4), 5, \"binaryTreeSum.rangeQuery(4, 4) == 5\");\n ASSERT(binaryTreeSum.rangeQuery(2, 4), 12, \"binaryTreeSum.rangeQuery(2, 4) == 12\");\n ASSERT(binaryTreeSum.rangeQuery(0, 3), 10, \"binaryTreeSum.rangeQuery(0, 3) == 10\");\n\n ASSERT(eulerTourTreeSum.rangeQuery(0, 4), 15, \"eulerTourTreeSum.rangeQuery(0, 4) == 15\");\n ASSERT(eulerTourTreeSum.rangeQuery(1, 2), 5, \"eulerTourTreeSum.rangeQuery(1, 2) == 5\");\n ASSERT(eulerTourTreeSum.rangeQuery(4, 4), 5, \"eulerTourTreeSum.rangeQuery(4, 4) == 5\");\n ASSERT(eulerTourTreeSum.rangeQuery(2, 4), 12, \"eulerTourTreeSum.rangeQuery(2, 4) == 12\");\n ASSERT(eulerTourTreeSum.rangeQuery(0, 3), 10, \"eulerTourTreeSum.rangeQuery(0, 3) == 10\");\n\n binaryTreeSum.pointUpdate(2, 10);\n eulerTourTreeSum.pointUpdate(0, 8);\n\n ASSERT(binaryTreeSum.pointQuery(2), 10, \"binaryTreeSum.pointQuery(2) == 10\");\n ASSERT(binaryTreeSum.rangeQuery(1, 3), 16, \"binaryTreeSum.rangeQuery(1, 3) == 16\");\n ASSERT(binaryTreeSum.rangeQuery(0, 4), 22, \"binaryTreeSum.rangeQuery(0, 4) == 22\");\n\n ASSERT(eulerTourTreeSum.pointQuery(0), 8, \"euler_tour_sum.pointQuery(0) == 8\");\n ASSERT(eulerTourTreeSum.rangeQuery(1, 3), 9, \"euler_tour_sum.rangeQuery(1, 3) == 9\");\n ASSERT(eulerTourTreeSum.rangeQuery(0, 4), 22, \"euler_tour_sum.rangeQuery(0, 4) == 22\");\n\n values = {\n 2, -4, 3, -1, 4, 1, -2, 5\n };\n n = values.size();\n\n struct node {\n int value;\n int maxPrefix;\n int maxSuffix;\n int maxSubsegment;\n\n node (int v = 0) {\n int m = std::max(v, 0);\n\n value = v;\n maxPrefix = m;\n maxSuffix = m;\n maxSubsegment = m;\n }\n };\n\n std::vector <node> node_values;\n\n for (auto i: values) {\n node_values.push_back(node(i));\n }\n \n arrow::SegmentTree <node> binaryTreeMaxSubsegment (n, node_values, [] (auto l, auto r) {\n node result;\n result.value = l.value + r.value;\n result.maxPrefix = std::max(l.maxPrefix, l.value + r.maxPrefix);\n result.maxSuffix = std::max(l.maxSuffix + r.value, r.maxSuffix);\n result.maxSubsegment = std::max({l.maxSubsegment, r.maxSubsegment, l.maxSuffix + r.maxPrefix});\n return result;\n });\n\n ASSERT(binaryTreeMaxSubsegment.rangeQuery(0, 7).value, 8, \"binaryTreeMaxSubsegment.rangeQuery(0, 7).value == 8\");\n ASSERT(binaryTreeMaxSubsegment.rangeQuery(3, 5).value, 4, \"binaryTreeMaxSubsegment.rangeQuery(3, 5).value == 4\");\n ASSERT(binaryTreeMaxSubsegment.rangeQuery(2, 6).value, 5, \"binaryTreeMaxSubsegment.rangeQuery(2, 6).value == 5\");\n ASSERT(binaryTreeMaxSubsegment.rangeQuery(1, 4).value, 2, \"binaryTreeMaxSubsegment.rangeQuery(1, 4).value == 2\");\n ASSERT(binaryTreeMaxSubsegment.rangeQuery(7, 7).value, 5, \"binaryTreeMaxSubsegment.rangeQuery(7, 7).value == 5\");\n ASSERT(binaryTreeMaxSubsegment.rangeQuery(0, 4).value, 4, \"binaryTreeMaxSubsegment.rangeQuery(0, 4).value == 4\");\n\n binaryTreeMaxSubsegment.pointUpdate(5, 4);\n binaryTreeMaxSubsegment.pointUpdate(3, -7);\n binaryTreeMaxSubsegment.pointUpdate(1, 3);\n \n ASSERT(binaryTreeMaxSubsegment.rangeQuery(0, 7).value, 12, \"binaryTreeMaxSubsegment.rangeQuery(0, 7).value == 12\");\n ASSERT(binaryTreeMaxSubsegment.rangeQuery(3, 5).value, 1, \"binaryTreeMaxSubsegment.rangeQuery(3, 5).value == 1\");\n ASSERT(binaryTreeMaxSubsegment.rangeQuery(2, 6).value, 2, \"binaryTreeMaxSubsegment.rangeQuery(2, 6).value == 2\");\n ASSERT(binaryTreeMaxSubsegment.rangeQuery(1, 4).value, 3, \"binaryTreeMaxSubsegment.rangeQuery(1, 4).value == 3\");\n ASSERT(binaryTreeMaxSubsegment.rangeQuery(7, 7).value, 5, \"binaryTreeMaxSubsegment.rangeQuery(7, 7).value == 5\");\n ASSERT(binaryTreeMaxSubsegment.rangeQuery(0, 4).value, 5, \"binaryTreeMaxSubsegment.rangeQuery(0, 4).value == 5\");\n}\n\nint main () {\n std::ios::sync_with_stdio(false);\n std::cin.tie(nullptr);\n\n test();\n\n return 0;\n}"
}
{
"filename": "code/design_pattern/src/builder_pattern/builder.cs",
"content": "using System;\nusing System.Collections.Generic;\n\nnamespace SandBox\n{\n\t/// <summary>\n\t/// Acts as the \"Director\" class.\n\t/// \n\t/// An intermediary between the customer (main) and the manufacturer.\n\t/// Unaware of the intracacies of building each product.\n\t/// </summary>\n\tclass Dealer\n\t{\n\t\t/// <summary>\n\t\t/// Orders a vehicle from a Manufacturer.\n\t\t/// </summary>\n\t\t/// <param name=\"manufacturer\">The manufacturer to order from</param>\n\t\tpublic Vehicle Order(Manufacturer manufacturer)\n\t\t{\n\t\t\treturn manufacturer.BuildVehicle();\n\t\t}\n\t}\n\n\t/// <summary>\n\t/// Template class for each Manufacturer to follow.\n\t/// Allows for easy extensibility, while maintaining consistency.\n\t/// </summary>\n\tabstract class Manufacturer\n\t{\n\t\tpublic Vehicle Vehicle { get; protected set; }\n\n\t\tpublic abstract Vehicle BuildVehicle();\n\t}\n\n\t/// <summary>\n\t/// 1 of 3.\n\t/// An example of one of the many possible builder classes that extend the \n\t/// abstract class Manufacturer.\n\t/// </summary>\n\tclass MotorCycleManufacturer : Manufacturer\n\t{\n\t\tpublic string ManufacturerName = \"MotorCycle\";\n\t\tpublic MotorCycleManufacturer()\n\t\t{\n\t\t}\n\n\t\tpublic override Vehicle BuildVehicle()\n\t\t{\n\t\t\tvar vehicle = new Vehicle(ManufacturerName);\n\t\t\tvehicle.Parts.Add(\"Body\", \"Bike\");\n\t\t\tvehicle.Parts.Add(\"Handlebars\", \"Stock\");\n\t\t\tvehicle.Parts.Add(\"Wheels\", \"Two-Wheeled\");\n\n\t\t\treturn vehicle;\n\t\t}\n\t}\n\n\t/// <summary>\n\t/// 2 of 3.\n\t/// An example of one of the many possible builder classes that extend the \n\t/// abstract class Manufacturer.\n\t/// </summary>\n\tclass SUVManufacturer : Manufacturer\n\t{\n\t\tprivate string ManufacturerName = \"SUV\";\n\t\tpublic SUVManufacturer()\n\t\t{\n\t\t}\n\n\t\tpublic override Vehicle BuildVehicle()\n\t\t{\n\t\t\tvar vehicle = new Vehicle(ManufacturerName);\n\t\t\tvehicle.Parts.Add(\"Body\", \"Large-Box\");\n\t\t\tvehicle.Parts.Add(\"Steering Wheel\", \"Stock\");\n\t\t\tvehicle.Parts.Add(\"Wheels\", \"Four-Wheeled\");\n\n\t\t\treturn vehicle;\n\t\t}\n\t}\n\n\t/// <summary>\n\t/// 3 of 3.\n\t/// An example of one of the many possible builder classes that extend the \n\t/// abstract class Manufacturer.\n\t/// </summary>\n\tclass ConvertibleManufacturer : Manufacturer\n\t{\n\t\tprivate string ManufacturerName = \"Convertible\";\n\t\tpublic ConvertibleManufacturer()\n\t\t{\n\t\t}\n\n\t\tpublic override Vehicle BuildVehicle()\n\t\t{\n\t\t\tvar vehicle = new Vehicle(ManufacturerName);\n\t\t\tvehicle.Parts.Add(\"Body\", \"Small-Sleek\");\n\t\t\tvehicle.Parts.Add(\"Steering Wheel\", \"Stock\");\n\t\t\tvehicle.Parts.Add(\"Wheels\", \"Four-Wheeled\");\n\t\t\tvehicle.Parts.Add(\"Roof\", \"Soft-Top\");\n\n\t\t\treturn vehicle;\n\t\t}\n\t}\n\n\t/// <summary>\n\t/// Acts as the item being built.\n\t/// \n\t/// Each item starts generic, then the manufacturer makes it unique. The\n\t/// process is abstracted from the item.\n\t/// </summary>\n\tclass Vehicle\n\t{\n\t\tpublic readonly string Make;\n\t\tpublic Dictionary<string, string> Parts = new Dictionary<string, string>();\n\n\t\tpublic Vehicle(string manufacturer)\n\t\t{\n\t\t\tthis.Make = manufacturer;\n\t\t}\n\n\t\tpublic void Show()\n\t\t{\n\t\t\tConsole.WriteLine(\"\\n---------------------------\");\n\t\t\tConsole.WriteLine(\"Vehicle Type: {0}\", Make);\n\n\t\t\tforeach (var part in Parts)\n\t\t\t\tConsole.WriteLine(\" {0} : {1}\", part.Key, part.Value);\n\t\t}\n\t}\n\n\tclass Program\n\t{\n\t\tstatic void Main(string[] args)\n\t\t{\n\t\t\tvar MotorCycleManufacturer = new MotorCycleManufacturer();\n\t\t\tvar motorCycle = MotorCycleManufacturer.BuildVehicle();\n\t\t\tmotorCycle.Show();\n\n\t\t\tvar SUVManufacturer = new SUVManufacturer();\n\t\t\tvar suv = SUVManufacturer.BuildVehicle();\n\t\t\tsuv.Show();\n\n\t\t\tvar ConvertibleManufacturer = new ConvertibleManufacturer();\n\t\t\tvar convertible = ConvertibleManufacturer.BuildVehicle();\n\t\t\tconvertible.Show();\n\t\t}\n\t}\n}"
}
{
"filename": "code/design_pattern/src/functional_patterns/functional_patterns/scala/project/build.properties",
"content": "sbt.version = 1.1.0"
}
{
"filename": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/arrows/arrow/arrow.scala",
"content": "package arrows.arrow\n\ntrait Arrow {\n\n}"
}
{
"filename": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/functors/applicative/functor/applicativefunctor.scala",
"content": "package functors.applicative.functor\n\nimport scala.language.higherKinds\n\n/**\n * Applicative is an intermediate between functor and monad.\n *\n * Its importance are dominated by:\n * - ability to apply functions of more parameters\n * For example:\n * val f = (x: Int)(y: Int) => x + y\n * If we apply the above function to a functor Future(10).map(f),\n * the result will be a Future(x => 10 + x). And you can't map that any further.\n * A way to extract the result is needed, hence applicative functors, where you can do something like:\n * val f = (x: Int)(y: Int) => x + y\n * val r: Future[Int -> Int] = Future(10).map(f)\n * Future(20).apply(r) => should return 30\n *\n * - can apply functions wrapped into a functor context\n *\n * - can combine multiple functors into one single product\n */\n\ntrait ApplicativeFunctor[F[_]] {\n def apply[A, B](fa: F[A])(f: F[A => B]): F[A] => F[B]\n}"
}
{
"filename": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/functors/bifunctor/bifunctor.scala",
"content": "package functors.bifunctor\n\nimport scala.language.higherKinds\n\n/**\n *\n * Similar to a functor, the BiFunctor must satisfy the functor laws:\n * 1. bimap f id id = id\n * first f id = id\n * second f id = id\n *\n * Applying a function over the identity, should return the identity.\n *\n * 2. bimap f g = first f . second g\n *\n * Asociativity: applying bimap f g should be equal to applying f to first and g to second.\n *\n * BiFunctors include: Either, N-Tuples\n */\ntrait BiFunctor[F[_, _]] {\n def biMap[A, B, C, D](fab: F[A, B])(f: A => C)(g: B => D): F[B, D]\n\n def first[A, C](fab: F[A, _])(f: A => C): F[C, _]\n\n def second[B, D](fab: F[_, B])(f: B => D): F[_, D]\n}"
}
{
"filename": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/functors/contravariant/contravariant.scala",
"content": "package functors.contravariant\n\ntrait Contravariant {\n\n}"
}
{
"filename": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/functors/functor/functor.scala",
"content": "package functors.functor\n\nimport scala.language.higherKinds\n\n/**\n * ADT which aspires to be a functor must preserve 2 laws:\n * 1. fmap over the identity functor of the ADT should coincide with the original ADT\n * fmap(identity, adt) === adt\n *\n * 2. The composition of 2 functions and mapping the resulting function over a functor\n * should be the same as mapping the first function over the functor and then the second one.\n * fmap(f compose g, adt) === fmap(f, fmap(g, adt))\n *\n * Some functors include: List, Set, Map, Option, etc.\n */\ntrait Functor[A, F[_]] {\n // something might be fishy with the id\n def id: A\n def fmap[B](fA: F[A])(f: A => B): F[B]\n}\n\nobject FunctorImplicits {\n implicit def listFunctor[A]: Functor[A, List] = new Functor[A, List] {\n override def id: A = ???\n\n override def fmap[B](fA: List[A])(f: A => B): List[B] = fA.map(f)\n }\n\n implicit def optionFunctor[A]: Functor[A, Option] = new Functor[A, Option] {\n override def id: A = ???\n\n override def fmap[B](fA: Option[A])(f: A => B): Option[B] = fA.map(f)\n }\n}"
}
{
"filename": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/functors/multifunctor/multifunctor.scala",
"content": "package functors.multifunctor\n\ntrait Multifunctor {\n\n}"
}
{
"filename": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/functors/profunctor/profunctor.scala",
"content": "package functors.profunctor\n\ntrait Profunctor {\n\n}"
}
{
"filename": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/main.scala",
"content": "object Main extends App{\n}"
}
{
"filename": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/monads/comonad/comonad.scala",
"content": "package monads.comonad\n\ntrait Comonad {\n\n}"
}
{
"filename": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/monads/costate/monad/costatemonad.scala",
"content": "package monads.costate.monad\n\ntrait CostateMonad {\n\n}"
}
{
"filename": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/monads/free/monad/freemonad.scala",
"content": "package monads.free.monad\n\ntrait FreeMonad {\n\n}"
}
{
"filename": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/monads/gonad/gonad.scala",
"content": "package monads.gonad\n\ntrait Gonad {\n\n}"
}
{
"filename": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/monads/io/monad/iomonad.scala",
"content": "package monads.io.monad\n\ntrait IOMonad {\n\n}"
}
{
"filename": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/monads/monad/monad.scala",
"content": "package monads.monad\n\nimport scala.language.higherKinds\n\ntrait Monad[T, M[_]] {\n def flatMap[S](fA: T => M[S])(f: M[T]): M[S]\n}\n\nobject MonadImplicits {\n implicit def listMonad[T]: Monad[T, List] = new Monad[T, List] {\n override def flatMap[S](fA: T => List[S])(f: List[T]): List[S] = f.flatMap(fA)\n }\n\n implicit def optionMonad[T]: Monad[T, Option] = new Monad[T, Option] {\n override def flatMap[S](fA: T => Option[S])(f: Option[T]): Option[S] = f.flatMap(fA)\n }\n}"
}
{
"filename": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/monads/state/monad/statemonad.scala",
"content": "package monads.state.monad\n\n/**\n * The equivalent of Memento design pattern in OOP on steroids,\n * state monads deal with maintaining previous states WHILE generating new ones.\n */\n\ntrait StateMonad[S, A] {\n def apply(f: S => (S, A)): StateMonad[S, A]\n}"
}
{
"filename": "code/design_pattern/src/functional_patterns/README.md",
"content": "# cosmos\nYour personal library of every design pattern code that you will ever encounter\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/design_pattern/src/iterator_pattern/classiterator.java",
"content": "package iterator;\n\npublic class ClassIterator implements Iterator<Student> {\n\t\n\tprivate Student[] student;\n\tprivate int i = 0;\n\t\n\tpublic ClassIterator(Student[] s){\n\t\tthis.student = s;\n\t}\n\t\n\t@Override\n\tpublic boolean hasNext() {\n\t\tif( i < student.length && student[i] != null) \n\t\t\treturn true;\n\t\telse \n\t\t\treturn false;\n\t}\n\n\t@Override\n\tpublic Student next() {\n\t\tif(this.hasNext()){\n\t\t\tStudent s = student[i];\n\t\t\ti++;\n\t\t\treturn s;\n\t\t}else return null;\t\t\n\t}\n\n\t\n}"
}
{
"filename": "code/design_pattern/src/iterator_pattern/class.java",
"content": "package iterator;\n\n\n\npublic class Class {\n\n\tprivate Student[] students = new Student[100];\n\tprivate int i = 0;\n\tprivate String class_name;\n\t\n\tpublic Class(String n) {\n\t\tthis.class_name = n;\n\t}\n\t\n\tpublic String getName() {\n\t\treturn this.class_name;\n\t}\n\t\n\tpublic void add(String name,int age) {\n\t\tStudent s = new Student(name, age);\n\t\tstudents[i] = s;\n\t\ti++;\n\t}\n\t\n\tpublic ClassIterator iterator() {\n\t\tClassIterator iterator = new ClassIterator(students);\n\t\treturn iterator;\n\t}\n\t\n}"
}
{
"filename": "code/design_pattern/src/iterator_pattern/iterator.java",
"content": "package iterator;\n\npublic interface Iterator<T> {\n\t\n\tpublic boolean hasNext();\n\tpublic Student next();\n\t\n}"
}
{
"filename": "code/design_pattern/src/iterator_pattern/main.java",
"content": "package iterator;\n\n\n\npublic class Main {\n\n\tpublic static void main(String[] args) {\n\t\tClass class1 = new Class(\"class1\");\n\t\tclass1.add(\"student1\",12);\n\t\tclass1.add(\"student2\",15);\n\t\tclass1.add(\"student3\",16);\n\t\t\t\n\t\tClassIterator it = class1.iterator();\n\t\t\n\t\twhile (it.hasNext()) {\n\t\t\tStudent student = it.next();\n\t\t\tstudent.print();\n\t\t}\n\t}\n\n}"
}
{
"filename": "code/design_pattern/src/iterator_pattern/student.java",
"content": "package iterator;\n\npublic class Student {\n\t\n\tprivate String name;\n\tprivate int age;\n\t\n\tpublic Student(String n, int a) {\n\t\tthis.name = n;\n\t\tthis.age = a;\n\t}\n\t\n\tpublic String getName() {\n\t\t\n\t\treturn this.name;\n\t\t\n\t}\n\t\n\tpublic void print() {\n\t\tSystem.out.println(\"Student: \" + this.name + \" Age: \" + this.age);\n\t}\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/adapter/adaptor.java",
"content": "package Adapter;\n\nimport Adapter.Soldiers.Adaptee;\nimport Adapter.Soldiers.General;\n\npublic class Adaptor implements Movement {\n public Adaptee adaptee;\n\n public General general;\n\n public Adaptor(Adaptee adaptee, General general) {\n this.adaptee = adaptee;\n this.general = general;\n }\n\n\n @Override\n public void walk() {\n adaptee.changeWalkStyle(general.receiveOrder());\n adaptee.walk();\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/adapter/civilian.java",
"content": "package Adapter;\n\npublic class Civilian implements Movement {\n @Override\n public void walk() {\n System.out.println(\"I'm having a beautiful stroll in my happy, little peaceful town.\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/adapter/movement.java",
"content": "package Adapter;\n\npublic interface Movement {\n void walk();\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/adapter/soldiers/adaptee.java",
"content": "package Adapter.Soldiers;\n\npublic interface Adaptee {\n void walk();\n\n void changeWalkStyle(Order newStyle);\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/adapter/soldiers/general.java",
"content": "package Adapter.Soldiers;\n\nimport Adapter.Movement;\n\nimport java.util.List;\n\npublic class General {\n private Order order;\n\n public List<Adaptee> troopsUnderCommand;\n\n public void setOrder(Order order) {\n this.order = order;\n }\n\n public Order receiveOrder() {\n if (order == null)\n return Order.AT_EASE;\n\n return order;\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/adapter/soldiers/order.java",
"content": "package Adapter.Soldiers;\n\npublic enum Order {\n WALKING, RUNNING, STROLLING, LIMPING, AT_EASE\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/adapter/soldiers/soldier.java",
"content": "package Adapter.Soldiers;\n\npublic class Soldier implements Adaptee {\n private Order order;\n\n @Override\n public void walk() {\n System.out.println(\"IM \" + order + \", SIR, YES, SIR!\");\n }\n\n @Override\n public void changeWalkStyle(Order newStyle) {\n this.order = newStyle;\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/builder/builder/nationality.java",
"content": "package builder;\n\npublic enum Nationality {\n Ro, En, Gr, Br, Ru, Aus\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/builder/builder/personbuilder.java",
"content": "package builder;\n\nimport org.omg.CORBA.DynAnyPackage.InvalidValue;\n\nimport java.text.ParseException;\nimport java.text.SimpleDateFormat;\nimport java.util.Date;\nimport java.util.function.Consumer;\n\npublic class PersonBuilder {\n private String firstname;\n private String lastname;\n\n private Date birthdate;\n private Nationality nationality = Nationality.Ro;\n private boolean isProgrammer = false;\n private Integer iq = 100;\n private boolean isPoor = false;\n private boolean isPopular = false;\n private boolean isEmployed = true;\n\n public PersonBuilder with(Consumer<PersonBuilder> builderFunction) {\n builderFunction.accept(this);\n return this;\n }\n\n public Person createPerson() {\n return new Person(firstname, lastname, birthdate, nationality, isProgrammer, iq, isPoor,\n isPopular, isEmployed);\n }\n\n public void setFirstname(String firstname) {\n this.firstname = firstname;\n }\n\n public void setLastname(String lastname) {\n this.lastname = lastname;\n }\n\n public void setBirthdate(String birthdate) throws InvalidValue, ParseException {\n SimpleDateFormat sdf = new SimpleDateFormat(\"dd-MM-yyyy\");\n Date d = sdf.parse(\"01-01-1950\");\n Date date = sdf.parse(birthdate);\n if (date.after(d))\n this.birthdate = date;\n else throw new InvalidValue(\"You're too old!!\");\n }\n\n public void setNationality(Nationality nationality) {\n this.nationality = nationality;\n }\n\n public void setProgrammer(boolean isProgrammer) {\n this.isProgrammer = isProgrammer;\n }\n\n public void setIq(Integer iq) throws InvalidValue {\n if (iq < 30)\n throw new InvalidValue(\"Don't try anything crazy.\");\n\n this.iq = iq;\n }\n\n public void setPoor(boolean isPoor) {\n this.isPoor = isPoor;\n }\n\n public void setPopular(boolean isPopular) {\n this.isPopular = isPopular;\n }\n\n public void setEmployed(boolean isEmployed) {\n this.isEmployed = isEmployed;\n }\n\n\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/builder/builder/person.java",
"content": "package builder;\n\nimport java.util.Date;\n\npublic class Person {\n private String firstname;\n private String lastname;\n\n private Date birthdate;\n private Nationality nationality;\n private boolean isProgrammer;\n private Integer iq;\n private boolean isPoor;\n private boolean isPopular;\n private boolean isEmployed;\n\n Person(String firstname,\n String lastname,\n Date birthdate,\n Nationality nationality,\n boolean isProgrammer,\n Integer iq,\n boolean isPoor, boolean isPopular, boolean isEmployed) {\n this.firstname = firstname;\n this.lastname = lastname;\n this.birthdate = birthdate;\n this.nationality = nationality;\n this.isProgrammer = isProgrammer;\n this.iq = iq;\n this.isPoor = isPoor;\n this.isPopular = isPopular;\n this.isEmployed = isEmployed;\n }\n\n @Override\n public String toString() {\n return \"Person{\" +\n \"firstname='\" + this.firstname + '\\'' +\n \", lastname='\" + this.lastname + '\\'' +\n \", birthdate=\" + this.birthdate +\n \", nationality=\" + this.nationality +\n \", isProgrammer=\" + this.isProgrammer +\n \", iq=\" + this.iq +\n \", isPoor=\" + this.isPoor +\n \", isPopular=\" + this.isPopular +\n \", isEmployed=\" + this.isEmployed +\n '}';\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/builder/main.java",
"content": "import builder.Nationality;\nimport builder.Person;\nimport builder.PersonBuilder;\nimport org.omg.CORBA.DynAnyPackage.InvalidValue;\n\nimport java.text.ParseException;\n\npublic class Main {\n\n public static void main(String[] args) {\n Person person = new PersonBuilder()\n .with(personBuilder -> {\n personBuilder.setFirstname(\"Mr.\");\n personBuilder.setLastname(\"John\");\n personBuilder.setNationality(Nationality.En);\n personBuilder.setProgrammer(true);\n try {\n personBuilder.setBirthdate(\"01-01-1900\");\n } catch (InvalidValue | ParseException invalidValue) {\n invalidValue.printStackTrace();\n }\n })\n .createPerson();\n\n System.out.println(person);\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/decorator/decorator.ts",
"content": "interface Component {\n operation(): string;\n}\n\ninterface HelloWorldDecorator {\n operation(): string;\n}\n\nclass ComponentImpl implements Component {\n public operation(): string {\n return 'This method is decorated';\n }\n}\n\n// This is the general way to implement decorators\nclass HelloWorldDecoratorImpl implements HelloWorldDecorator {\n private _component: Component;\n\n constructor(component: Component) {\n this._component = component;\n }\n\n public operation(): string {\n return `Hello world! ${this._component.operation()}`;\n }\n}\n\nlet testComponent = new ComponentImpl();\nlet decoratedTestComponent = new HelloWorldDecoratorImpl(testComponent);\nconsole.log(decoratedTestComponent.operation());\n\n// Here we use TypeScript's built in support for decorators\n// What we are using here are class decorators.\n// There are also: class property, class method, class accessor\n// and class method parameter decorators\n@helloWorldClassDecorator\nclass ComponentAltImpl implements Component {\n public operation(): string {\n return 'This message will be overriden';\n }\n}\n\nfunction helloWorldClassDecorator<T extends { new (...args: any[]): {} }>(constructor: T) {\n return class extends constructor implements HelloWorldDecorator {\n operation() {\n return `Hello world again! ${constructor.prototype.operation()}`;\n }\n };\n}\n\nlet syntacticSugarDecorator = new ComponentAltImpl();\nconsole.log(syntacticSugarDecorator.operation());"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/dailyroutinefacade.java",
"content": "package daily.tasks;\n\nimport daily.tasks.evening.routine.EveningRoutineFacade;\nimport daily.tasks.gym.GymFacade;\nimport daily.tasks.job.JobFacade;\nimport daily.tasks.morning.routine.MorningRoutineFacade;\n\npublic class DailyRoutineFacade {\n public DailyRoutineFacade() {\n new MorningRoutineFacade();\n new JobFacade();\n new GymFacade();\n new EveningRoutineFacade();\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/evening/routine/eat.java",
"content": "package daily.tasks.evening.routine;\n\nclass Eat {\n Eat() {\n System.out.println(\"Dinner - or mostly not\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/evening/routine/eveningroutinefacade.java",
"content": "package daily.tasks.evening.routine;\n\npublic class EveningRoutineFacade {\n public EveningRoutineFacade() {\n System.out.println(\"\\n\\n\\t\\tEvening Routine\\n\\n\");\n new Eat();\n new TakeAShower();\n new WriteCode();\n new WatchYoutubeVideos();\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/evening/routine/takeashower.java",
"content": "package daily.tasks.evening.routine;\n\nclass TakeAShower {\n TakeAShower() {\n System.out.println(\"Im taking a good ol' scrub\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/evening/routine/watchyoutubevideos.java",
"content": "package daily.tasks.evening.routine;\n\nclass WatchYoutubeVideos {\n WatchYoutubeVideos() {\n System.out.println(\"Im watching some youtube videos\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/evening/routine/writecode.java",
"content": "package daily.tasks.evening.routine;\n\nclass WriteCode {\n WriteCode() {\n System.out.println(\"Probably writing some Scala code\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/gym/benchpress.java",
"content": "package daily.tasks.gym;\n\nclass BenchPress {\n BenchPress() {\n System.out.println(\"Gotta bench 140 at least\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/gym/deadlift.java",
"content": "package daily.tasks.gym;\n\nclass Deadlift {\n Deadlift() {\n System.out.println(\"Deadlifting 100kg at least\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/gym/gymfacade.java",
"content": "package daily.tasks.gym;\n\npublic class GymFacade {\n public GymFacade() {\n System.out.println(\"\\n\\n\\t\\tGym Routine\\n\\n\");\n new Deadlift();\n new BenchPress();\n new Squat();\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/gym/squat.java",
"content": "package daily.tasks.gym;\n\nclass Squat {\n Squat() {\n System.out.println(\"Squatting is awesome\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/job/develop.java",
"content": "package daily.tasks.job;\n\nclass Develop {\n Develop() {\n System.out.println(\"I'm writing some basic code\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/job/eatatwork.java",
"content": "package daily.tasks.job;\n\nclass EatAtWork {\n EatAtWork() {\n System.out.println(\"This shaorma tastes great!\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/job/jobfacade.java",
"content": "package daily.tasks.job;\n\npublic class JobFacade {\n public JobFacade() {\n System.out.println(\"\\n\\n\\t\\tJob Routine\\n\\n\");\n new Develop();\n new WatchYoutubeVideos();\n new PlayFifa();\n new EatAtWork();\n new Develop();\n new Develop();\n new WatchYoutubeVideos();\n new Leave();\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/job/leave.java",
"content": "package daily.tasks.job;\n\nclass Leave {\n Leave() {\n System.out.println(\"I'm leaving home\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/job/playfifa.java",
"content": "package daily.tasks.job;\n\nclass PlayFifa {\n PlayFifa() {\n System.out.println(\"I'm playing fifa\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/job/watchyoutubevideos.java",
"content": "package daily.tasks.job;\n\nclass WatchYoutubeVideos {\n WatchYoutubeVideos() {\n System.out.println(\"I'm watching Youtube videos\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/morning/routine/dress.java",
"content": "package daily.tasks.morning.routine;\n\nclass Dress {\n Dress() {\n System.out.println(\"Dress\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/morning/routine/eat.java",
"content": "package daily.tasks.morning.routine;\n\nclass Eat {\n Eat() {\n System.out.println(\"Im eating\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/morning/routine/leave.java",
"content": "package daily.tasks.morning.routine;\n\nclass Leave {\n Leave() {\n System.out.println(\"Im leaving home\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/morning/routine/morningroutinefacade.java",
"content": "package daily.tasks.morning.routine;\n\npublic class MorningRoutineFacade {\n public MorningRoutineFacade() {\n System.out.println(\"\\n\\n\\t\\tMorning Routine\\n\\n\");\n new WakeUp();\n new Eat();\n new Dress();\n new Leave();\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/morning/routine/wakeup.java",
"content": "package daily.tasks.morning.routine;\n\nclass WakeUp {\n WakeUp() {\n System.out.println(\"Woken up\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/facade",
"content": "The whole purpose of Facade is to \"aggregate\" multiple classes and functionality into a single place, thus acting like\nwell... a Facade - beautiful on the outside, but hiding all the complexity of the \"inside building\". The example\nis taken to the extreme to showcase it properly - imagine each class that is managed has a fuckton of logic behind it\nthat is quite specific and quite hard to understand.\n\nBy using the Facade design pattern, that logic is encapsulated in the Facade and easily used."
}
{
"filename": "code/design_pattern/src/OOP_patterns/facade/main.java",
"content": "import daily.tasks.DailyRoutineFacade;\n\npublic class Main {\n\n public static void main(String[] args) {\n new DailyRoutineFacade();\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/factory/gifts/booze.java",
"content": "package factory.gifts;\n\npublic class Booze implements Gift {\n @Override\n public String message() {\n return \"You won booze - get drunk\";\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/factory/gifts/car.java",
"content": "package factory.gifts;\n\npublic class Car implements Gift {\n @Override\n public String message() {\n return \"Nobody wins a car, it's a prank, bro\";\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/factory/gifts/gift.java",
"content": "package factory.gifts;\n\npublic interface Gift {\n String message();\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/factory/gifts/nothing.java",
"content": "package factory.gifts;\n\npublic class Nothing implements Gift {\n @Override\n public String message() {\n return \"YOU WON NOTHING!\";\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/factory/gifts/toy.java",
"content": "package factory.gifts;\n\npublic class Toy implements Gift {\n @Override\n public String message() {\n return \"You won a toy! Be happy\";\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/factory/gifttype.java",
"content": "package factory;\n\nimport java.util.Arrays;\nimport java.util.Collections;\nimport java.util.List;\nimport java.util.Random;\n\npublic enum GiftType {\n Nothing, Car, Toy, Booze, Error;\n\n public GiftType fromString(String from) {\n if (from.equalsIgnoreCase(\"nothing\"))\n return Nothing;\n\n if (from.equalsIgnoreCase(\"car\"))\n return Car;\n\n if (from.equalsIgnoreCase(\"toy\"))\n return Toy;\n\n if (from.equalsIgnoreCase(\"booze\"))\n return Booze;\n\n return Error;\n }\n\n private static final List<GiftType> VALUES =\n Collections.unmodifiableList(Arrays.asList(values()));\n private static final int SIZE = VALUES.size();\n private static final Random RANDOM = new Random();\n\n public static GiftType randomGift() {\n return VALUES.get(RANDOM.nextInt(SIZE));\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/factory/roulette.java",
"content": "package factory;\n\nimport factory.gifts.*;\n\nimport java.util.Scanner;\n\npublic class Roulette {\n public void run() throws InterruptedException {\n while (true) {\n Scanner input = new Scanner(System.in);\n\n System.out.println(\"Let's see what the russian roulette will give you\");\n\n System.out.println(generateGift(GiftType.randomGift()).message());\n\n Thread.sleep(3000);\n }\n }\n\n public Gift generateGift(GiftType gift) {\n switch (gift) {\n case Booze:\n return new Booze();\n case Car:\n return new Car();\n case Nothing:\n return new Nothing();\n case Toy:\n return new Toy();\n case Error:\n throw new InvalidValue(\"Russian roulette is confused\");\n default:\n return new Nothing();\n }\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/observer_java/demo.java",
"content": "import observer.Observer;\nimport observer.Subject;\nimport observer.network.Artist;\nimport observer.network.Fan;\n\npublic class Demo {\n public void startDemo() {\n Observer f1 = new Fan(\"Robert\");\n Observer f2 = new Fan(\"David\");\n Observer f3 = new Fan(\"Gangplank\");\n\n Subject<String> s1 = new Artist(\"Erik Mongrain\");\n Subject<String> s2 = new Artist(\"Antoine Dufour\");\n\n s1.subscribe(f1);\n s1.subscribe(f2);\n\n s2.subscribe(f2);\n s2.subscribe(f3);\n\n s1.addNotification(\"New album coming out!!\");\n s2.addNotification(\"I'll be having a concert in Romania!\");\n\n s1.addNotification(\"Im so happy!\");\n s2.addNotification(\"How does this work?\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/observer_java/main.java",
"content": "public class Main {\n\n public static void main(String[] args) {\n new Demo().startDemo();\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/observer_java/observer/network/artist.java",
"content": "package observer.network;\n\nimport observer.Observer;\nimport observer.Subject;\n\nimport java.util.ArrayList;\nimport java.util.HashSet;\nimport java.util.List;\nimport java.util.Set;\n\npublic class Artist<T extends String> implements Subject {\n private Set<Observer> observers;\n\n private String name;\n\n private List<String> updates;\n\n public Artist(String name) {\n this.name = name;\n this.observers = new HashSet<>();\n this.updates = new ArrayList<>();\n }\n\n public Artist(Set<Observer> observers) {\n this.observers = observers;\n this.updates = new ArrayList<>();\n }\n\n @Override\n public String getLastNotification() {\n return \"[ \" + this.name + \" ] \" + this.updates.get(this.updates.size() - 1);\n }\n\n @Override\n public void addNotification(String notification) {\n System.out.println(\"[ \" + this.name + \" ] \" + notification);\n updates.add(notification);\n this.notifyObservers();\n }\n\n @Override\n public void subscribe(Observer o) {\n this.observers.add(o);\n }\n\n @Override\n public void unsubscribe(Observer o) {\n this.observers.remove(o);\n }\n\n @Override\n public void notifyObservers() {\n observers.forEach(o -> o.receiveNotification(this));\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/observer_java/observer/network/fan.java",
"content": "package observer.network;\n\nimport observer.Observer;\n\nimport java.util.*;\n\npublic class Fan implements Observer {\n private String name;\n private Set<Artist> followedSubjects;\n\n public Fan(String name) {\n this.followedSubjects = new HashSet<>();\n this.name = name;\n }\n\n public Fan(Set<Artist> subjectsToFollow) {\n this.followedSubjects = subjectsToFollow;\n }\n\n public void addSubject(Artist subject) {\n subject.subscribe(this);\n this.followedSubjects.add(subject);\n }\n\n public Set<Artist> getFollowedSubjects() {\n return new HashSet<>(followedSubjects);\n }\n\n @Override\n public void receiveNotification(observer.Subject from) {\n System.out.println(\"[\" + this.name + \"] \" + from.getLastNotification());\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/observer_java/observer/observer.java",
"content": "package observer;\n\npublic interface Observer {\n void receiveNotification(Subject from);\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/observer_java/observer/subject.java",
"content": "package observer;\n\npublic interface Subject<T extends String> {\n void subscribe(Observer o);\n\n void unsubscribe(Observer o);\n\n void notifyObservers();\n\n String getLastNotification();\n\n void addNotification(T notification);\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/observer_pattern/__init__.py",
"content": "from .observer_pattern import Notifier, Observer"
}
{
"filename": "code/design_pattern/src/OOP_patterns/observer_pattern/observer_pattern.cpp",
"content": "/*\n * observer_pattern.cpp\n *\n * Created on: 26 May 2017\n * Author: yogeshb2\n */\n\n#include <iostream>\n#include <vector>\n#include <algorithm>\n\nusing namespace std;\n\nclass IWeatherChanger\n{\npublic:\n virtual ~IWeatherChanger()\n {\n }\n virtual void onTemperatureChange(int temperature) = 0;\n};\n\nclass ITemperature\n{\npublic:\n virtual ~ITemperature()\n {\n }\n virtual void addSubscriber(IWeatherChanger* w) = 0;\n virtual void removeSubscriber(IWeatherChanger* w) = 0;\n virtual void notifyAllSubscriber() = 0;\n};\n\nclass Weather : public ITemperature\n{\npublic:\n Weather() : temperature(0)\n {\n\n }\n void addSubscriber(IWeatherChanger* w)\n {\n if (w)\n subscriberlist.push_back(w);\n }\n void removeSubscriber(IWeatherChanger* w)\n {\n //TODO\n if (w)\n {\n vector<IWeatherChanger*>::iterator it =\n find(subscriberlist.begin(), subscriberlist.end(), w);\n if (it != subscriberlist.end())\n subscriberlist.erase(it);\n else\n cout << \"Not a registered subscriber\" << endl;\n }\n }\n void notifyAllSubscriber()\n {\n if (!subscriberlist.empty())\n {\n vector<IWeatherChanger*>::iterator it;\n for (it = subscriberlist.begin(); it != subscriberlist.end(); ++it)\n (*it)->onTemperatureChange(temperature);\n }\n }\n void changeTemperature(int temp)\n {\n temperature = temp;\n notifyAllSubscriber();\n }\nprivate:\n int temperature;\n vector<IWeatherChanger*> subscriberlist;\n\n};\n\n\nclass NewsChannel : public IWeatherChanger\n{\npublic:\n NewsChannel()\n {\n\n }\n NewsChannel(string name)\n {\n this->name = name;\n }\n void onTemperatureChange(int temperature)\n {\n cout << \"Channel name : \" << name << \" Temperature : \" << temperature;\n cout << \"\\n\";\n }\nprivate:\n string name;\n\n};\n\nint main()\n{\n Weather weather;\n NewsChannel fox(\"Fox News\");\n NewsChannel times(\"Times News\");\n weather.addSubscriber(&fox);\n weather.addSubscriber(×);\n\n weather.changeTemperature(25);\n\n weather.changeTemperature(20);\n\n weather.removeSubscriber(&fox);\n\n weather.changeTemperature(10);\n\n return 0;\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/observer_pattern/observer_pattern.py",
"content": "\"\"\"\nHow to use observer:\n\n1. Decorate your observed-class with the Observer class,\n which observe some notifier.\n\n2. Implement the update member-function in your the observed-class\n which will be passed only one parameter: event and no return.\n\n3. Decorate your notified-class with the Notifier class,\n which notify each observer you registered.\n\n\nexample:\n\n class Phone():\n\n def update(self, event):\n if event == \"earthquake warning\":\n print(\"I need to run away.\")\n\n\n class App():\n\n def update(self, event):\n if event == \"it will rain\":\n print(\"I need to buy an umbrella.\")\n\n\n class EmergencyCenter():\n pass\n\n\n class WeatherCenter():\n pass\n\n\n def main():\n emergency_center = Notifier(EmergencyCenter())\n weather_center = Notifier(WeatherCenter())\n my_phone = Observer(Phone())\n my_home_weather_app = Observer(App())\n\n emergency_center.attach_observer(my_phone)\n my_home_weather_app.attach_notifier(weather_center)\n\n emergency_center.notify(\"earthquake warning\")\n weather_center.notify(\"it will rain\")\n\nnote:\n1. you can attach a notifier to an observer by itself\n or attach an observer to a notifier.\n\"\"\"\n\n\nclass Observer:\n def __init__(self, decorator=None):\n self._notifiers = {}\n self._decorator = decorator\n\n def __getattr__(self, name):\n if self._decorator is not None:\n return getattr(self._decorator, name)\n else:\n raise AttributeError\n\n def attach_notifier(self, notifier):\n \"\"\"\n notifier: Notifier-liked instance\n \"\"\"\n self._notifiers[notifier] = notifier.attach_observer(self)\n\n def detach_notifier(self, notifier):\n \"\"\"\n notifier: Notifier-liked instance\n \"\"\"\n notifier.detach_observer(self._notifiers[notifier])\n del self._notifiers[notifier]\n\n # class which be decorted needs to impelment this function\n def update(self, event):\n \"\"\"\n event: object\n \"\"\"\n return self._decorator.update(event)\n\n\nclass Notifier:\n def __init__(self, decorator=None):\n self._observers = {}\n self._decorator = decorator\n\n def __getattr__(self, name):\n if self._decorator is not None:\n return getattr(self._decorator, name)\n else:\n raise AttributeError\n\n def attach_observer(self, observer):\n \"\"\"\n observer: Observer-liked instance\n return: int\n identify the observer\n \"\"\"\n identifier = len(self._observers)\n self._observers[identifier] = observer\n return identifier\n\n def detach_observer(self, identifier):\n \"\"\"\n identifier: int\n which is returned by attach_observer member-function\n \"\"\"\n del self._observers[identifier]\n\n def notify(self, event):\n \"\"\"\n event: object\n \"\"\"\n for observer in self._observers.values():\n observer.update(event)"
}
{
"filename": "code/design_pattern/src/OOP_patterns/observer_pattern/observer_pattern.rs",
"content": "// Part of Cosmos by OpenGenus Foundation\nuse std::collections::HashMap;\n\n#[derive(Debug, Clone, Copy)]\nenum WeatherType {\n Clear,\n Rain,\n Snow,\n}\n\n#[derive(Debug, Clone, Copy)]\nenum WeatherObserverMessage {\n WeatherChanged(WeatherType),\n}\n\ntrait WeatherObserver {\n fn notify(&mut self, msg: WeatherObserverMessage);\n}\n\nstruct WeatherObserverExample {\n name: &'static str,\n}\n\nimpl WeatherObserver for WeatherObserverExample {\n fn notify(&mut self, msg: WeatherObserverMessage) {\n println!(\"{} was notified: {:?}\", self.name, msg);\n }\n}\n\nstruct Weather {\n weather: WeatherType,\n observers: HashMap<usize, Box<WeatherObserver>>,\n observer_id_counter: usize\n}\n\nimpl Weather {\n pub fn attach_observer(&mut self, observer: Box<WeatherObserver>) -> usize {\n self.observers.insert(self.observer_id_counter, observer);\n self.observer_id_counter += 1;\n self.observer_id_counter - 1\n }\n\n pub fn detach_observer(&mut self, id: usize) -> Option<Box<WeatherObserver>> {\n self.observers.remove(&id)\n }\n\n pub fn set_weather(&mut self, weather_type: WeatherType) {\n self.weather = weather_type;\n self.notify_observers(WeatherObserverMessage::WeatherChanged(weather_type));\n }\n\n pub fn notify_observers(&mut self, msg: WeatherObserverMessage) {\n for obs in self.observers.values_mut() {\n obs.notify(msg);\n }\n }\n}\n\nfn main() {\n let mut weather = Weather {\n weather: WeatherType::Clear,\n observers: HashMap::new(),\n observer_id_counter: 0\n };\n\n let first_obs = weather.attach_observer(Box::new(WeatherObserverExample {\n name: \"First Observer\",\n }));\n\n weather.set_weather(WeatherType::Rain);\n\n weather.detach_observer(first_obs);\n\n weather.attach_observer(Box::new(WeatherObserverExample {\n name: \"Second Observer\",\n }));\n\n weather.set_weather(WeatherType::Snow);\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/proxy/demo/demo.java",
"content": "package demo;\n\nimport protection.proxy.User;\nimport protection.proxy.UserProxy;\nimport virtual.proxy.UltraHDVideo;\nimport virtual.proxy.Video;\nimport virtual.proxy.VideoProxy;\n\npublic class Demo {\n public void protectionProxyRun() {\n User rob = new UserProxy(\"Robert\", \"123\");\n User david = new UserProxy(\"David\", \"pass\");\n User gangplank = new UserProxy(\"Gangplank\", \"12312312\");\n\n rob.login();\n david.login();\n gangplank.login();\n\n rob.download();\n david.download();\n gangplank.login();\n\n rob.upload();\n david.upload();\n gangplank.upload();\n }\n\n public void virtualProxyRun() throws InterruptedException {\n System.out.println(\"Trying the proxy version first - 3 videos and playing just 1\");\n long startTime = System.currentTimeMillis();\n\n Video v1 = new VideoProxy(\"Erik Mongrain - Alone in the mist\");\n Video v2 = new VideoProxy(\"Antoine Dufour - Drowning\");\n Video v3 = new VideoProxy(\"Jake McGuire - For Scale The Summit\");\n\n v3.play();\n long endTime = System.currentTimeMillis();\n\n long duration = (endTime - startTime);\n\n System.out.println(\"It took \" + (duration / 1000) + \" seconds to run\");\n System.out.println(\"Now for the not-proxy version\");\n startTime = System.currentTimeMillis();\n\n Video v4 = new UltraHDVideo(\"Erik Mongrain - Alone in the mist\");\n Video v5 = new UltraHDVideo(\"Antoine Dufour - Drowning\");\n Video v6 = new UltraHDVideo(\"Jake McGuire - For Scale The Summit\");\n\n v6.play();\n\n endTime = System.currentTimeMillis();\n\n duration = endTime - startTime;\n\n System.out.println(\"It took \" + (duration / 1000.0) + \" seconds to run\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/proxy/main.java",
"content": "import demo.Demo;\n\npublic class Main {\n\n public static void main(String[] args) throws InterruptedException {\n new Demo().protectionProxyRun();\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/proxy/protection/proxy/registeredusers.java",
"content": "package protection.proxy;\n\nimport java.util.HashMap;\nimport java.util.Map;\n\npublic class RegisteredUsers {\n static Map<String, String> registered = new HashMap<String, String>(){\n {\n put(\"Robert\", \"123\");\n put(\"David\", \"pass\");\n put(\"Gangplank\", \"Illaoi\");\n }\n };\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/proxy/protection/proxy/user.java",
"content": "package protection.proxy;\n\npublic interface User {\n void login();\n void download();\n void upload();\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/proxy/protection/proxy/userproxy.java",
"content": "package protection.proxy;\n\nimport java.util.Objects;\n\npublic class UserProxy implements User {\n private String username;\n private String password;\n Boolean isLogged;\n private User user;\n\n public UserProxy(String username, String password) {\n this.username = username;\n this.password = password;\n }\n\n\n @Override\n public void login() {\n if (Objects.equals(RegisteredUsers.registered.get(username), password)){\n this.user = new ValidUser(username, password);\n System.out.println(\"Login successful - welcome back, \" + this.username + \" !\");\n }\n else\n System.out.println(\"Invalid credentials for \" + this.username + \" !\");\n\n }\n\n @Override\n public void download() {\n if (this.user == null)\n System.out.println(\"User credentials invalid \" + this.username + \"!\");\n else\n user.download();\n }\n\n @Override\n public void upload() {\n if (this.user == null)\n System.out.println(\"User credentials invalid \" + this.username + \" !\");\n else\n user.upload();\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/proxy/protection/proxy/validuser.java",
"content": "package protection.proxy;\n\nclass ValidUser implements User {\n String username;\n String password;\n\n ValidUser(String username, String password) {\n this.username = username;\n this.password = password;\n }\n\n @Override\n public void login() {\n System.out.println(\">\" + this.username + \": Successfully logged, welcome!\");\n }\n\n @Override\n public void download() {\n System.out.println(\">\" + this.username + \": Downloading\");\n }\n\n @Override\n public void upload() {\n System.out.println(\">\" + this.username + \": Uploading\");\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/proxy/virtual/proxy/demo.java",
"content": "package virtual.proxy;\n\npublic class Demo {\n\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/proxy/virtual/proxy/ultrahdvideo.java",
"content": "package virtual.proxy;\n\npublic class UltraHDVideo implements Video {\n public String name;\n\n public UltraHDVideo(String path) throws InterruptedException {\n this.name = path;\n loadVideo();\n }\n\n @Override\n public void play() {\n System.out.println(\"4k video is being played - \" + name);\n }\n\n private void loadVideo() throws InterruptedException {\n Thread.sleep(5000);\n System.out.println(\"Video has been loaded - \" + name);\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/proxy/virtual/proxy/video.java",
"content": "package virtual.proxy;\n\npublic interface Video {\n void play();\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/proxy/virtual/proxy/videoproxy.java",
"content": "package virtual.proxy;\n\npublic class VideoProxy implements Video {\n\n public String name;\n public Video realVideo;\n\n public VideoProxy(String name) {\n this.name = name;\n }\n\n @Override\n public void play() {\n try {\n this.realVideo = new UltraHDVideo(name);\n } catch (InterruptedException e) {\n e.printStackTrace();\n }\n\n realVideo.play();\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/README.md",
"content": "# cosmos\nYour personal library of every design pattern code that you will ever encounter\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/design_pattern/src/OOP_patterns/singleton_pattern/singleton_pattern.cpp",
"content": "#include <string>\n#include <iostream>\n\ntemplate<typename T>\nclass Singleton\n{\npublic:\n static T* GetInstance();\n static void destroy();\n\nprivate:\n\n Singleton(Singleton const&)\n {\n };\n Singleton& operator=(Singleton const&)\n {\n };\n\nprotected:\n static T* m_instance;\n\n Singleton()\n {\n m_instance = static_cast <T*> (this);\n };\n ~Singleton()\n {\n };\n};\n\ntemplate<typename T>\nT * Singleton<T>::m_instance = 0;\n\ntemplate<typename T>\nT* Singleton<T>::GetInstance()\n{\n if (!m_instance)\n Singleton<T>::m_instance = new T();\n\n return m_instance;\n}\n\ntemplate<typename T>\nvoid Singleton<T>::destroy()\n{\n delete Singleton<T>::m_instance;\n Singleton<T>::m_instance = 0;\n}\n\nclass TheCow : public Singleton<TheCow>\n{\npublic:\n void SetSays(std::string &whatToSay)\n {\n whatISay = whatToSay;\n };\n void Speak(void)\n {\n std::cout << \"I say\" << whatISay << \"!\" << std::endl;\n };\nprivate:\n std::string whatISay;\n};\n\nvoid SomeFunction(void)\n{\n std::string say(\"moo\");\n TheCow::GetInstance()->SetSays(say);\n}\n\nint main ()\n{\n std::string say(\"meow\");\n TheCow::GetInstance()->SetSays(say);\n SomeFunction();\n TheCow::GetInstance()->Speak();\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/singleton_pattern/singleton_pattern.java",
"content": "\n/**\n * Singleton Design Pattern\n * \n * @author Sagar Rathod\n * @version 1.0\n * \n */\n\nclass Singleton {\n\t\n\tprivate volatile transient static Singleton singletonInstance;\n\t\n\tprivate Singleton() {}\n\t\n\tpublic static synchronized Singleton getInstance() {\n\t\t\n\t\tif ( singletonInstance == null ) {\n\t\t\tsingletonInstance = new Singleton();\n\t\t}\n\t\treturn singletonInstance;\n\t}\n}\n\npublic class SingletonPattern {\n\t\n\tpublic static void main(String[] args) {\n\t\t\n\t\tSingleton instance = Singleton.getInstance();\n\t\tSystem.out.println(instance == Singleton.getInstance());\n\t}\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/singleton_pattern/singleton_pattern.php",
"content": "<?php\n\n/**\n* Simple singleton class which could only have one instance\n* Part of Cosmos by OpenGenus Foundation\n*/\nclass Singleton\n{\n}\n\n/**\n* Class which creates instance of Singleton if it does not exists\n*/\nclass SingletonInstanter \n{\n private static $instance;\n \n public static function getInstance() {\n if (empty(self::$instance)) {\n self::$instance = new Singleton();\n }\n return self::$instance;\n }\n}"
}
{
"filename": "code/design_pattern/src/OOP_patterns/singleton_pattern/singleton_pattern.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n\nclass Singleton:\n __instance = None\n\n @property\n def x(self):\n return self.__x\n\n @x.setter\n def x(self, value):\n self.__x = value\n\n @staticmethod\n def instance():\n if not Singleton.__instance:\n Singleton.__instance = Singleton()\n return Singleton.__instance"
}
{
"filename": "code/design_pattern/src/policy_based_design/policy_design.cpp",
"content": "/*\n * policy_design.cpp\n *\n * Created: 3/15/2018 1:14:41 AM\n * Author: n-is\n * email: [email protected]\n */\n\n#include <iostream>\n\nclass Classical\n{\npublic:\n Classical(int a)\n {\n }\n\n void solve()\n {\n std::cout << \"The differential equation was solved by the classical\" <<\n \" method.\" << std::endl;\n }\n};\n\nclass Laplace\n{\npublic:\n void solve()\n {\n std::cout << \"The differential equation was solved by the Laplace\" <<\n \" Transform method.\" << std::endl;\n }\n};\n\ntemplate <class Method>\nclass DifferentialEquaton\n{\nprivate:\n Method m_;\npublic:\n DifferentialEquaton(const Method & m = Method()) :\n m_(m)\n {\n }\n\n void solve()\n {\n m_.solve();\n }\n};\n\nint main()\n{\n DifferentialEquaton<Laplace> eqn1;\n DifferentialEquaton<Classical> eqn2(Classical(1));\n\n eqn1.solve();\n eqn2.solve();\n\n return 0;\n}"
}
{
"filename": "code/design_pattern/src/policy_based_design/readme.md",
"content": "# Policy Based Design\n\nPolicy-based design, also known as policy-based class design or policy-based\nprogramming, is a computer programming paradigm based on an idiom for C++ known\nas policies.\n\n## Explanation\n\nWikipedia(<https://en.wikipedia.org/wiki/Policy-based_design>) says:\n\n>Policy-based design has been described as a compile-time variant of the strategy\npattern, and has connections with C++ template metaprogramming. It was first\npopularized by Andrei Alexandrescu with his 2001 book Modern C++ Design and his\ncolumn Generic`<Programming>` in the C/C++ Users Journal.\n\n\nThis design pattern is expecially suitable for writing library for embedded\nsystems, where a single peripheral may have various modes of operation and the\nuser may choose between the modes of operation at compile time.\n\n## Algorithm\n\nWell, a design pattern is not an algorithm, but just a solution to certain\nproblems. In the same way, policy based design a one of the best method to\nimplement strategy pattern at compile time(i.e. choose between the\nimplementations at the compile time).\n\n## Complexity\n\nImplementing Policy Based Design increases the number of classes in the program,\nso it may be hard to handle since the program will become larger. But this\ndesign pattern does not add runtime overhead while choosing the implementation.\nSo, this design pattern reduces the timing overhead. If a compiler with a very\ngood optimizer is used, the space consumed by the final program is also reduced.\n\n---\n\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/design_pattern/src/singleton_pattern/singleton_pattern.cs",
"content": "// Singleton design pattern using properties\nclass Singleton\n{\n\tprivate static readonly Singleton _instance = new Singleton();\n\tpublic static Singleton Instance { get { return _instance; } }\n\n\tprivate Singleton()\n\t{\n\t\t// Initialize object\n\t}\n}\n\nclass Program\n{\n\tstatic void Main(string[] args)\n\t{\n\t\tvar instance = Singleton.Instance;\n\t}\n}"
}
{
"filename": "code/design_pattern/src/singleton_pattern/singleton_pattern.js",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\nclass Singleton {\n constructor() {\n console.log('New Singleton');\n }\n\n static getInstance() {\n if (!Singleton.instance) {\n Singleton.instance = new Singleton();\n }\n\n return Singleton.instance;\n }\n\n foo() {\n console.log('bar');\n }\n}\n\n(function () {\n const firstInstance = Singleton.getInstance(); // New Singleton\n const secondInstance = Singleton.getInstance(); // void\n\n firstInstance.foo(); // bar\n secondInstance.foo(); // bar\n})();"
}
{
"filename": "code/design_pattern/test/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/design_pattern/test/test_observer.py",
"content": "from src.OOP_patterns.observer_pattern import Notifier, Observer\nimport unittest\nfrom enum import Enum\n\n\nclass Event(Enum):\n Earthquake = 0\n Joke = 1\n\n\nclass SomeWhere:\n def __init__(self):\n self._member = {}\n\n def add_member(self, member):\n self._member[member] = \"is here\"\n\n def remove_member(self, member):\n del self._member[member]\n\n # override update protocol\n def update(self, event):\n global res\n\n if event == Event.Earthquake:\n for member in self._member:\n res.append(\"{0} received, need to run away !!!\".format(member))\n elif event == Event.Joke:\n for member in self._member:\n res.append(\"{0} received, A false alarm ...\".format(member))\n\n\n# decorate\nclass LivingRoom(SomeWhere):\n pass\n\n\n# decorate\nclass StudyRoom(SomeWhere):\n pass\n\n\nclass School(SomeWhere):\n pass\n\n\nclass EmergencyAlart(Notifier):\n pass\n\n\nclass Kitchen(Notifier):\n pass\n\n\nres = []\n\n\nclass TestObserver(unittest.TestCase):\n def test_observer(self):\n global res\n study_room = Observer(StudyRoom())\n living_room = Observer(LivingRoom())\n school = Observer(School())\n emergency_alart = EmergencyAlart()\n kitchen = Kitchen()\n\n study_room.attach_notifier(emergency_alart)\n living_room.attach_notifier(emergency_alart)\n school.attach_notifier(emergency_alart)\n study_room.attach_notifier(kitchen)\n living_room.attach_notifier(kitchen)\n\n # My sister is studying in studyroom\n study_room.add_member(\"My sister\")\n # and I am watching TV in living room\n living_room.add_member(\"I\")\n\n # Just cooking.\n res = []\n kitchen.notify(Event.Joke)\n self.assertListEqual(\n sorted(res),\n sorted(\n [\n \"I received, A false alarm ...\",\n \"My sister received, A false alarm ...\",\n ]\n ),\n )\n\n # My sister is on her way to school.\n study_room.remove_member(\"My sister\")\n school.add_member(\"My sister\")\n res = []\n kitchen.notify(Event.Joke)\n self.assertListEqual(sorted(res), sorted([\"I received, A false alarm ...\"]))\n\n # Living room alert is no longer needed.\n living_room.detach_notifier(kitchen)\n res = []\n kitchen.notify(Event.Joke)\n self.assertListEqual(sorted(res), sorted([]))\n\n # I am studying\n living_room.remove_member(\"I\")\n study_room.add_member(\"I\")\n res = []\n kitchen.notify(Event.Joke)\n self.assertListEqual(sorted(res), sorted([\"I received, A false alarm ...\"]))\n\n # emergency alart notify you everywhere\n res = []\n emergency_alart.notify(Event.Earthquake)\n self.assertListEqual(\n sorted(res),\n sorted(\n [\n \"I received, need to run away !!!\",\n \"My sister received, need to run away !!!\",\n ]\n ),\n )"
}
{
"filename": "code/divide_conquer/src/closest_pair_of_points/closest_pair.cpp",
"content": "// divide conquer | structure to represent a point | C\n// Part of Cosmos by OpenGenus Foundation\n\n#include <vector>\n#include <cmath>\n#include <iostream>\n#include <algorithm>\n#include <cfloat>\n#define ll long long\nusing namespace std;\n\nstruct point\n{\n ll x;\n ll y;\n};\n\n// comparator function to sort points by X coordinates\nbool compX(point a, point b)\n{\n return a.x < b.x;\n}\n\n// comparator function to sort points by Y coordinates\nbool compY(point a, point b)\n{\n if (a.y < b.y)\n return true;\n if (a.y > b.y)\n return false;\n return a.x < b.x;\n}\n\npoint result1, result2;\ndouble minDist = DBL_MAX;\n\ndouble euclideanDistance(point a, point b)\n{\n return sqrt(pow(a.x - b.x, 2) + pow(a.y - b.y, 2));\n}\n\ndouble bruteMin(vector<point> &pointsByX, size_t low, size_t high)\n{\n size_t i, j;\n double dist, bestDistance = DBL_MAX;\n for (i = low; i <= high; i++)\n for (j = i + 1; j <= high; j++)\n {\n dist = euclideanDistance(pointsByX[i], pointsByX[j]);\n if (dist < bestDistance)\n {\n bestDistance = dist;\n if (bestDistance < minDist)\n {\n minDist = bestDistance;\n result1 = pointsByX[i];\n result2 = pointsByX[j];\n }\n }\n }\n return bestDistance;\n}\n\ndouble closestPair(vector<point> &pointsByX, vector<point> &pointsByY, size_t low, size_t high)\n{\n size_t i, j, n = high - low + 1;\n\n // if number of points <= 3, use brute to find min distance\n if (n <= 3)\n return bruteMin(pointsByX, low, high);\n\n size_t mid = low + (high - low) / 2;\n\n // find minimum distance among left half of points recursively\n double distLeft = closestPair(pointsByX, pointsByY, low, mid);\n\n // find minimum distance among right half of points recursively\n double distRight = closestPair(pointsByX, pointsByY, mid + 1, high);\n\n double bestDistance = min(distLeft, distRight);\n\n // store points in strip of width 2d sorted by Y coordinates\n vector<point> pointsInStrip;\n for (i = 0; i < n; i++)\n if (abs(pointsByY[i].x - pointsByX[mid].x) < bestDistance)\n pointsInStrip.push_back(pointsByY[i]);\n\n // calculate minimum distance within the strip (atmost 6 comparisons)\n for (i = 0; i < pointsInStrip.size(); i++)\n for (j = i + 1;\n j < pointsInStrip.size() &&\n abs(pointsInStrip[i].y - pointsInStrip[j].y) < bestDistance;\n j++)\n {\n double dist = euclideanDistance(pointsInStrip[i], pointsInStrip[j]);\n if (dist < bestDistance)\n {\n bestDistance = dist;\n minDist = dist;\n result1 = pointsInStrip[i];\n result2 = pointsInStrip[j];\n }\n }\n\n return bestDistance;\n}\n\nint main()\n{\n ll i, n;\n cin >> n; // number of points\n\n // pointsByX stores points sorted by X coordinates\n // pointsByY stores points sorted by Y coordinates\n vector<point> pointsByX(n), pointsByY(n);\n\n for (i = 0; i < n; i++)\n {\n cin >> pointsByX[i].x >> pointsByX[i].y;\n pointsByY[i].x = pointsByX[i].x;\n pointsByY[i].y = pointsByX[i].y;\n }\n sort(pointsByX.begin(), pointsByX.end(), compX);\n sort(pointsByY.begin(), pointsByY.end(), compY);\n\n cout << \"Shortest distance = \" << closestPair(pointsByX, pointsByY, 0, n - 1) << endl;\n cout << \"Points are (\" << result1.x << \",\" << result1.y << \") and (\" << result2.x << \",\" <<\n result2.y << \")\\n\";\n return 0;\n}"
}
{
"filename": "code/divide_conquer/src/closest_pair_of_points/closest_pair.py",
"content": "# divide conquer | structure to represent a point | Python\n# Part of Cosmos by OpenGenus Foundation\n\nimport math\n\n\ndef dist(p1, p2):\n return math.sqrt((p1[0] - p2[0]) ** 2 + (p1[1] - p2[1]) ** 2)\n\n\ndef closest_pair(ax, ay):\n # It's quicker to assign variable\n ln_ax = len(ax)\n\n if ln_ax <= 3:\n # A call to bruteforce comparison\n return brute(ax)\n\n # Division without remainder, need int\n mid = ln_ax // 2\n\n # Two-part split\n Qx = ax[:mid]\n Rx = ax[mid:]\n\n # Determine midpoint on x-axis\n midpoint = ax[mid][0]\n Qy = list()\n Ry = list()\n\n # split ay into 2 arrays using midpoint\n for x in ay:\n if x[0] <= midpoint:\n Qy.append(x)\n else:\n Ry.append(x)\n\n # Call recursively both arrays after split\n (p1, q1, mi1) = closest_pair(Qx, Qy)\n (p2, q2, mi2) = closest_pair(Rx, Ry)\n\n # Determine smaller distance between points of 2 arrays\n if mi1 <= mi2:\n d = mi1\n mn = (p1, q1)\n else:\n d = mi2\n mn = (p2, q2)\n\n # Call function to account for points on the boundary\n (p3, q3, mi3) = closest_split_pair(ax, ay, d, mn)\n\n # Determine smallest distance for the array\n if d <= mi3:\n return mn[0], mn[1], d\n else:\n return p3, q3, mi3\n\n\ndef closest_split_pair(p_x, p_y, delta, best_pair):\n # store length - quicker\n ln_x = len(p_x)\n\n # select midpoint on x-sorted array\n mx_x = p_x[ln_x // 2][0]\n\n # Create a subarray of points not further than delta from\n # midpoint on x-sorted array\n s_y = [x for x in p_y if mx_x - delta <= x[0] <= mx_x + delta]\n # assign best value to delta\n best = delta\n # store length of subarray for quickness\n ln_y = len(s_y)\n for i in range(ln_y - 1):\n for j in range(i + 1, min(i + 7, ln_y)):\n p, q = s_y[i], s_y[j]\n dst = dist(p, q)\n if dst < best:\n best_pair = p, q\n best = dst\n return best_pair[0], best_pair[1], best\n\n\ndef solution(a):\n ax = sorted(a, key=lambda x: x[0]) # Presorting x-wise\n ay = sorted(a, key=lambda x: x[1]) # Presorting y-wise\n p1, p2, mi = closest_pair(ax, ay) # Recursive D&C function\n return (p1, p2, mi)\n\n\ndef brute(ax):\n mi = dist(ax[0], ax[1])\n p1 = ax[0]\n p2 = ax[1]\n ln_ax = len(ax)\n if ln_ax == 2:\n return p1, p2, mi\n for i in range(ln_ax - 1):\n for j in range(i + 1, ln_ax):\n if i != 0 and j != 1:\n d = dist(ax[i], ax[j])\n if d < mi: # Update min_dist and points\n mi = d\n p1, p2 = ax[i], ax[j]\n return p1, p2, mi\n\n\nsolution_tuple = solution(\n [\n (0, 0),\n (7, 6),\n (2, 20),\n (12, 5),\n (16, 16),\n (5, 8),\n (19, 7),\n (14, 22),\n (8, 19),\n (7, 29),\n (10, 11),\n (1, 13),\n ]\n)\nprint(\"Point 1 =>\", solution_tuple[0])\nprint(\"Point 2 =>\", solution_tuple[1])\nprint(\"Distance =>\", solution_tuple[2])"
}
{
"filename": "code/divide_conquer/src/factorial/factorial.cpp",
"content": "#include<iostream>\n\nint factorial(int num) {\n if (num == 0 || num == 1) {\n return num;\n }\n return (num * factorial(num - 1));\n} \n\nint main() {\n int num;\n while (true) {\n std::cin >> num;\n std::cout << \"> \" << factorial(num) << '\\n';\n }\n return 0;\n}"
}
{
"filename": "code/divide_conquer/src/factorial/factorial.py",
"content": "\"\"\"\n\ndivide conquer | factorial | Python\npart of Cosmos by OpenGenus Foundation\n\n\"\"\"\n\n\ndef factorial(num):\n \"\"\"\n Returns the factorial of a given integer num.\n\n Divide and conquer is used here by organizing the function into a base and recursive case.\n\n Parameter: num is the number for which the factorial will be found\n Precondition: num is a non-negative integer\n \"\"\"\n assert (type(num)) == int\n assert num >= 0\n\n # base case\n if num == 0 or num == 1:\n return 1\n\n # recursive case\n recursive = factorial(num - 1)\n\n # output\n return num * recursive"
}
{
"filename": "code/divide_conquer/src/inversion_count/count_inversions.c",
"content": "// divide conquer | inversion count | C\n// Part of Cosmos by OpenGenus Foundation\n\n#include <stdlib.h>\n#include <stdio.h>\n\n\nint _mergeSort(int arr[], int temp[], int left, int right);\nint merge(int arr[], int temp[], int left, int mid, int right);\n\nint mergeSort(int arr[], int array_size)\n{\n int *temp = (int *)malloc(sizeof(int)*array_size);\n return _mergeSort(arr, temp, 0, array_size - 1);\n}\n\n\nint _mergeSort(int arr[], int temp[], int left, int right)\n{\n int mid, inv_count = 0;\n if (right > left)\n {\n\n mid = (right + left)/2;\n\n\n inv_count = _mergeSort(arr, temp, left, mid);\n inv_count += _mergeSort(arr, temp, mid+1, right);\n\n\n inv_count += merge(arr, temp, left, mid+1, right);\n }\n return inv_count;\n}\n\n\nint merge(int arr[], int temp[], int left, int mid, int right)\n{\n int i, j, k;\n int inv_count = 0;\n\n i = left;\n j = mid;\n k = left;\n while ((i <= mid - 1) && (j <= right))\n {\n if (arr[i] <= arr[j])\n {\n temp[k++] = arr[i++];\n }\n else\n {\n temp[k++] = arr[j++];\n\n\n inv_count = inv_count + (mid - i);\n }\n }\n\n\n while (i <= mid - 1)\n temp[k++] = arr[i++];\n\n\n while (j <= right)\n temp[k++] = arr[j++];\n\n\n for (i=left; i <= right; i++)\n arr[i] = temp[i];\n\n return inv_count;\n}\n\n\nint main(int argc, char** argv)\n{\n printf(\"Number of elements in array : \");\n int n;\n scanf(\"%d\", &n);\n int arr[n];\n printf(\"elements in array:\\n\");\n for (int x=0; x<n; x++)\n {\n scanf(\"%d\", &arr[x]);\n }\n printf(\"Number of inversions are %d \\n\", mergeSort(arr, n));\n getchar();\n return 0;\n}"
}
{
"filename": "code/divide_conquer/src/inversion_count/inversion_count.cpp",
"content": "// divide conquer | inversion count | C++\n// Part of Cosmos by OpenGenus Foundation\n\n#include <iostream>\nusing namespace std;\n\nint mergesort(int arr[], int l, int r);\nint merge(int arr[], int l, int m, int r);\n\n\n\nint main()\n{\n int n, a[100], i;\n cout << \"Enter nuber of elements : \";\n cin >> n;\n cout << \"Enter the elements : \";\n for (i = 0; i < n; i++)\n cin >> a[i];\n cout << \"Number of inversion = \" << mergesort(a, 0, n - 1);\n return 0;\n}\n\n//merge\nint merge(int arr[], int l, int m, int r)\n{\n int i, j, k, c[100], count = 0;\n i = 0;\n j = l;\n k = m;\n while (j <= m - 1 && k <= r)\n {\n if (arr[j] <= arr[k])\n c[i++] = arr[j++];\n\n else\n {\n c[i++] = arr[k++];\n count += m - j;\n\n }\n\n }\n\n while (j <= m - 1)\n c[i++] = arr[j++];\n\n while (k <= r)\n c[i++] = arr[k++];\n i = 0;\n while (l <= r)\n arr[l++] = c[i++];\n\n return count;\n}\n\n\n//mergesort\nint mergesort(int arr[], int l, int r)\n{\n int x = 0, y = 0, z = 0;\n int m = (l + r) / 2;\n if (l < r)\n {\n x += mergesort(arr, l, m);\n y += mergesort(arr, m + 1, r);\n z += merge(arr, l, m + 1, r);\n\n }\n\n return x + y + z;\n}"
}
{
"filename": "code/divide_conquer/src/inversion_count/inversion_count.java",
"content": "// divide conquer | inversion count | Java\n// Part of Cosmos by OpenGenus Foundation\n\nimport java.util.Scanner;\npublic class InversionCount {\n public static int merge(int a[], int p, int q,int r){\n\n int i = p ,j = q ,k = 0, count = 0;\n\n int temp[] = new int[r-p+1];\n\n while(i<q && j<=r){\n\n if(a[i] < a[j]){\n temp[k++] = a[i++];\n }\n else{\n temp[k++] = a[j++];\n count += (q - i);\n }\n }\n\n while(i<q){\n temp[k++] = a[i++];\n }\n\n while(j<=r){\n temp[k++] = a[j++];\n }\n\n k = 0;\n\n while(p<=r)\n a[p++] = temp[k++];\n\n return count;\n }\n public static int mergeSort(int a[],int i, int j){\n int count = 0;\n\n if(i>=j)\n return 0;\n\n int mid = (i+j)/2;\n\n count += mergeSort(a,i,mid);\n count += mergeSort(a,mid+1,j);\n count += merge(a,i,mid+1,j);\n\n return count;\n }\n public static void main(String[] args) {\n Scanner sc = new Scanner(System.in);\n\n System.out.print(\"Enter n >> \");\n int n = sc.nextInt();\n\n int a[] = new int[n];\n\n System.out.print(\"Enter elements of array >> \");\n for(int i=0;i<n;i++)\n a[i] = sc.nextInt();\n\n int count = mergeSort(a,0,a.length-1);\n\n System.out.println(\"Number of inversions : \" + count);\n\n }\n}"
}
{
"filename": "code/divide_conquer/src/inversion_count/inversion_count.js",
"content": "// divide conquer | inversion count | Javascript\n// Part of Cosmos by OpenGenus Foundation\n\nfunction mergeSort(arr, size) {\n if (array.length === 0 || array.length === 1) return 0;\n var temp = [];\n return mergeSortHelper(arr, temp, 0, size - 1);\n}\n\nfunction mergeSortHelper(arr, temp, left, right) {\n var inv_count,\n mid = 0;\n if (right > left) {\n mid = (right + left) / 2;\n\n /* sum the number of inversions from all parts */\n inv_count = mergeSortHelper(arr, temp, left, mid);\n inv_count += mergeSortHelper(arr, temp, mid + 1, right);\n\n inv_count += merge(arr, temp, left, mid + 1, right);\n }\n return inv_count;\n}\n\nfunction merge(arr, temp, left, mid, right) {\n var inv_count = 0;\n\n var i = left;\n var j = mid;\n var k = left;\n\n while (i <= mid - 1 && j <= right) {\n if (arr[i] <= arr[j]) {\n temp[k++] = arr[i++];\n } else {\n temp[k++] = arr[j++];\n\n /* remaining elements in subarray arr[i] to arr[mid] are sorted and grater than arr[j] */\n inv_count = inv_count + (mid - i);\n }\n }\n\n /* Copy remaining elements*/\n while (i <= mid - 1) temp[k++] = arr[i++];\n while (j <= right) temp[k++] = arr[j++];\n\n /*merge into original array*/\n for (i = left; i <= right; i++) arr[i] = temp[i];\n\n return inv_count;\n}\n\nconsole.log(mergeSort([5, 10, 4, 8, 2], 5));"
}
{
"filename": "code/divide_conquer/src/inversion_count/inversion_count.py",
"content": "\"\"\"\n\ndivide conquer | inversion count | Python\npart of Cosmos by OpenGenus Foundation\n\n\"\"\"\n\n\ndef merge(left, right):\n merged_arr = []\n i = 0\n j = 0\n inv_cnt = 0\n\n while i < len(left) and j < len(right):\n if left[i] <= right[j]:\n merged_arr.append(left[i])\n i += 1\n else:\n merged_arr.append(right[j])\n j += 1\n inv_cnt += len(left) - i\n\n merged_arr += left[i:]\n merged_arr += right[j:]\n\n return merged_arr, inv_cnt\n\n\ndef merge_sort(\n arr\n): # this function will return a tuple as (sorted_array, inversion_count)\n if len(arr) > 1:\n mid = len(arr) // 2\n left = arr[:mid]\n right = arr[mid:]\n\n left, left_inv_cnt = merge_sort(left)\n right, right_inv_cnt = merge_sort(right)\n merged, merged_inv_cnt = merge(left, right)\n return merged, (left_inv_cnt + right_inv_cnt + merged_inv_cnt)\n else:\n return arr, 0\n\n\narr = [1, 8, 3, 4, 9, 3]\nsorted_array, inversion_count = merge_sort(arr)\n\nprint(\"Sorted array:\", sorted_array, \" and Inversion count = %s\" % inversion_count)"
}
{
"filename": "code/divide_conquer/src/inversion_count/README.md",
"content": "# Cosmos\n\nCollaborative effort by [OpenGenus](https://github.com/OpenGenus/cosmos)"
}
{
"filename": "code/divide_conquer/src/karatsuba_multiplication/karatsuba_multiplication.py",
"content": "#!/usr/bin/env python2\n# -*- coding: utf-8 -*-\n\"\"\"\nCreated on Tue May 29 11:21:34 2018\n\n@author: Sahit\n\"\"\"\n\n\nprint(\"enter the first number\")\nn1 = input()\nprint(\"enter the second number\")\nn2 = input()\n\n\ndef Correcting(string):\n p = len(string)\n i = 1\n while i < p:\n i = i * 2\n return i\n\n\nl = max(Correcting(n1), Correcting(n2))\nnum1 = \"0\" * (l - len(n1)) + n1\nnum2 = \"0\" * (l - len(n2)) + n2\n\n\ndef Karatsuba(number_1, number_2):\n l = len(number_1)\n r1 = int(number_1)\n r2 = int(number_2)\n if len(number_1) == 1:\n return r1 * r2\n else:\n a = number_1[: l // 2]\n b = number_1[l // 2 :]\n c = number_2[: l // 2]\n d = number_2[l // 2 :]\n\n e = Karatsuba(a, c)\n f = Karatsuba(b, d)\n g = Karatsuba(b, c)\n h = Karatsuba(a, d)\n\n return ((10 ** l) * e) + (g + h) * (10 ** (l / 2)) + f\n\n\nans = Karatsuba(num1, num2)\nprint(ans)"
}
{
"filename": "code/divide_conquer/src/karatsuba_multiplication/karatsubamultiply.cpp",
"content": "// Author: Tote93\r\n#include <iostream>\r\n#include <string>\r\n#include <sstream>\r\n#include <cstdlib>\r\n\r\n\r\n/* Prototypes */\r\nint getBiggerSize(const std::string &number1, const std::string &number2);\r\nvoid checkNumbers(int size, std::string &number1, std::string &number2);\r\nstd::string KaratsubaMultiply(std::string &number1, std::string &number2, int limit);\r\nstd::string AddNumbers(std::string &number1, std::string &number2);\r\nvoid removeNonSignificantZeros(std::string &c);\r\nvoid splitString(std::string original, std::string &sub1, std::string &sub2);\r\n\r\nint main()\r\n{\r\n std::string number1, number2;\r\n\r\n std::cout << \"Insert the first number \\n\";\r\n std::cin >> number1;\r\n std::cout << \"Insert the second number \\n\";\r\n std::cin >> number2;\r\n\r\n int size = getBiggerSize(number1, number2);\r\n checkNumbers(size, number1, number2);\r\n\r\n std::string result = KaratsubaMultiply(number1, number2, number1.length());\r\n //Returns number to original without non-significant zeros\r\n removeNonSignificantZeros(number1);\r\n removeNonSignificantZeros(number2);\r\n std::cout << \"Result \" << number1 << \" * \" << number2 << \" = \" << result << \"\\n\";\r\n}\r\n\r\nint getBiggerSize(const std::string &number1, const std::string &number2)\r\n{\r\n int numb1Length = number1.length();\r\n int numb2Length = number2.length();\r\n return (numb1Length > numb2Length) ? numb1Length : numb2Length;\r\n}\r\n\r\nvoid checkNumbers(int size, std::string &number1, std::string &number2)\r\n{\r\n int newSize = 0, nZeros = 0;\r\n newSize = getBiggerSize(number1, number2);\r\n\r\n nZeros = newSize - number1.length();\r\n number1.insert(number1.begin(), nZeros, '0');\r\n nZeros = newSize - number2.length();\r\n number2.insert(number2.begin(), nZeros, '0');\r\n\r\n if (number1.length() % 2 != 0)\r\n number1.insert(number1.begin(), 1, '0');\r\n if (number2.length() % 2 != 0)\r\n number2.insert(number2.begin(), 1, '0');\r\n}\r\n\r\nstd::string KaratsubaMultiply(std::string &number1, std::string &number2, int limit)\r\n{\r\n int n = number1.length(), s;\r\n std::string w, x, y, z;\r\n std::string final1, final2, final3;\r\n std::string total;\r\n\r\n if (n == 1)\r\n {\r\n int integer1, integer2, aux;\r\n integer1 = atoi (number1.c_str());\r\n integer2 = atoi (number2.c_str());\r\n aux = integer1 * integer2;\r\n //We can replace two next lines with -> total = to_string(aux) using c++11\r\n std::ostringstream temp;\r\n temp << aux;\r\n total = temp.str();\r\n removeNonSignificantZeros (total);\r\n return total;\r\n }\r\n else\r\n {\r\n s = n / 2;\r\n splitString (number1, w, x);\r\n splitString (number2, y, z);\r\n\r\n final1 = KaratsubaMultiply (w, y, n);\r\n final1.append(2 * s, '0');\r\n\r\n std::string wz = KaratsubaMultiply (w, z, n);\r\n std::string xy = KaratsubaMultiply (x, y, n);\r\n final2 = AddNumbers (wz, xy);\r\n final2.append(s, '0');\r\n\r\n final3 = KaratsubaMultiply (x, z, n);\r\n total = AddNumbers (final1, final2);\r\n return AddNumbers (total, final3);\r\n }\r\n}\r\n\r\nvoid removeNonSignificantZeros(std::string &c)\r\n{\r\n int acum = 0;\r\n for (int i = 0; i < c.length(); ++i)\r\n {\r\n if (c[i] != '0')\r\n break;\r\n else\r\n acum++;\r\n }\r\n if (c.length() != acum)\r\n c = c.substr(acum, c.length());\r\n else\r\n c = c.substr(acum - 1, c.length());\r\n}\r\n\r\nvoid splitString(std::string original, std::string &sub1, std::string &sub2)\r\n{\r\n int n, n1, n2;\r\n sub1 = sub2 = \"\";\r\n n = original.length();\r\n\r\n if (n % 2 == 0)\r\n {\r\n n1 = n / 2;\r\n n2 = n1;\r\n }\r\n else\r\n {\r\n n1 = (n + 1) / 2;\r\n n2 = n1 - 1;\r\n }\r\n\r\n sub1 = original.substr(0, n1);\r\n sub2 = original.substr(n1, n);\r\n\r\n if (sub1.length() % 2 != 0 && sub2.length() > 2)\r\n sub1.insert(sub1.begin(), 1, '0');\r\n if (sub2.length() > 2 && sub2.length() % 2 != 0)\r\n sub2.insert(sub2.begin(), 1, '0');\r\n}\r\n\r\nstd::string AddNumbers(std::string &number1, std::string &number2)\r\n{\r\n int x, y, aux = 0, aux2 = 0;\r\n int digitos = getBiggerSize(number1, number2);\r\n std::string total, total2, cadena;\r\n int n1 = number1.length();\r\n int n2 = number2.length();\r\n\r\n if (n1 > n2)\r\n number2.insert(number2.begin(), n1 - n2, '0');\r\n else\r\n number1.insert(number1.begin(), n2 - n1, '0');\r\n\r\n for (int i = digitos - 1; i >= 0; i--)\r\n {\r\n x = number1[i] - '0';\r\n y = number2[i] - '0';\r\n aux = x + y + aux2;\r\n\r\n if (aux >= 10)\r\n {\r\n aux2 = 1;\r\n aux = aux % 10;\r\n }\r\n else\r\n aux2 = 0;\r\n\r\n total2 = '0' + aux;\r\n total.insert(0, total2);\r\n if (i == 0 && aux2 == 1)\r\n {\r\n total2 = \"1\";\r\n total.insert(0, total2);\r\n removeNonSignificantZeros(total);\r\n return total;\r\n }\r\n }\r\n removeNonSignificantZeros(total);\r\n return total;\r\n}\r"
}
{
"filename": "code/divide_conquer/src/karatsuba_multiplication/multiply.java",
"content": "// divide conquer | karatsuba multiplication | Java\n// Part of Cosmos by OpenGenus Foundation\n\nimport java.lang.*;\nimport java.util.Scanner;\n\npublic class Multiply\n{\n\tpublic static String trim(String str,int n)\n\t{\n\t\tif(str.length()>n)\n\t\t\twhile(str.charAt(0)=='0' && str.length()>n)\n\t\t\tstr=str.substring(1);\n\t\telse\n\t\t\twhile(str.length()!=n)\n\t\t\tstr=\"0\"+str;\n\t\treturn str;\n\t}\n\tpublic static String add_str(String a,String b,int n)\n\t{\n\t\ta=trim(a,n);\n\t\tb=trim(b,n);\n\t\tString val=\"\";\n\t\tint i,rem=0;\n\t\tchar []c1=a.toCharArray();\n\t\tchar []c2=b.toCharArray();\n\t\tint ans[]=new int[a.length()+1];\n\t\tfor(i=a.length();i>0;i--)\n\t\t{\n\t\tans[i]=(c1[i-1]-48+c2[i-1]-48+rem)%10;\n\t\trem=(c1[i-1]-48+c2[i-1]-48+rem)/10;\n\t\t}\n\t\tans[0]=rem;\n\t\tfor(i=0;i<ans.length;i++)\n\t\tval=val+ans[i];\n\t\tval=trim(val,a.length()+1);\n\t\treturn val;\n\t}\n\n\tpublic static String multiply(String s1,String s2,int n)\n\t{\n\t\tString a,b,c,d,ac,bd,ad_bc,ad,bc;\n\t\tint i;\n\t\tif(n==1)\n\t\t\treturn Integer.toString(Integer.parseInt(s1)*Integer.parseInt(s2));\n\t\ta=s1.substring(0,n/2);\n\t\tb=s1.substring(n/2,n);\n\t\tc=s2.substring(0,n/2);\n\t\td=s2.substring(n/2,n);\n\t\tac=multiply(a,c,n/2);\n\t\tbd=multiply(b,d,n/2);\n\t\tad=multiply(a,d,n/2);\n\t\tbc=multiply(b,c,n/2);\n\t\tad_bc=add_str(ad,bc,n);\n\n\t\tfor(i=1;i<=n;i++)\n\t\t\tac=ac+\"0\";\n\t\tfor(i=1;i<=n/2;i++)\n\t\t\tad_bc=ad_bc+\"0\";\n\t\tac=trim(ac,n*2);\n\t\tad_bc=trim(ad_bc,n*2);\n\t\tbd=trim(bd,n*2);\n\t\treturn add_str(add_str(ac,ad_bc,n*2),bd,n*2);\n\t}\n\n\tpublic static void main(String args[])\n\t{\n\t\tint n;\n\t\tScanner sc=new Scanner(System.in);\n\t\tSystem.out.print(\"Enter first number=\");\n\t\tString s1=sc.next();\n\t\tSystem.out.print(\"Enter second number=\");\n\t\tString s2=sc.next();\n\t\tn=s1.length();\n\t\tString s3=multiply(s1,s2,n);\n\t\tSystem.out.println(s3);\n\t}\n}"
}
{
"filename": "code/divide_conquer/src/maximum_contiguous_subsequence_sum/maximum_contiguous_subsequence_sum.c",
"content": "#include <stdio.h>\n\nint \nmax(int const a, int const b, const int c)\n{\n\tif (a > b)\n\t\treturn (a > c ? a : c);\n\n\treturn (b > c ? b : c);\n}\n\nint \nmaximumContiguousSubsequenceSum(const int a[], int beg, int end)\n{\n\tif (beg == end)\n\t\treturn (a[beg] > 0 ? a[beg] : 0);\n\n\tint mid = (beg + end) / 2;\n\tint leftSubProblem = maximumContiguousSubsequenceSum(a, beg, mid);\n\tint rightSubProblem = maximumContiguousSubsequenceSum(a, mid + 1, end);\n\n\tint currentSum = 0, leftSum = 0, rightSum = 0;\n\tint i;\n\n\tfor (i = mid; i >= beg; --i)\n\t{\n\t\tcurrentSum += a[i];\n\n\t\tif (leftSum < currentSum)\n\t\t\tleftSum = currentSum;\n\t\t\t\n\t}\n\tcurrentSum = 0;\n\n\tfor (i = mid + 1; i <= end; ++i)\n\t{\n\t\tcurrentSum += a[i];\n\n\t\tif (rightSum < currentSum)\n\t\t\trightSum = currentSum;\n\t}\n\n\treturn (max(leftSubProblem, rightSubProblem, leftSum + rightSum));\n}\n\nint \nmain()\n{\n\tint n;\n\tprintf(\"Enter the size of the array: \");\n\tscanf(\"%d\", &n);\n\n\tint a[n];\n\tprintf(\"Enter %d Integers \\n\", n);\n\tint i;\n\tfor (i = 0; i < n; ++i)\n\t\tscanf(\"%d\", &a[i]);\n\n\tprintf(\"Maximum Contiguous Subsequence Sum is %d \\n\", maximumContiguousSubsequenceSum(a, 0, n - 1));\n\n\treturn (0);\n}"
}
{
"filename": "code/divide_conquer/src/merge_sort_using_divide_and_conquer/inversions.c",
"content": "#include <stdio.h>\n\nconst int maxn = 1e6;\ntypedef long long unsigned llu;\n\nllu merge(int v[], int lo, int mid, int hi) {\n\tllu inv = 0;\n\tint i, j, k;\n\tint n1 = mid - lo + 1, n2 = hi - mid;\n\tint left[n1], right[n2];\n\tfor(i = 0; i < n1; i++) left[i] = v[i+lo];\n\tfor(j = 0; j < n2; j++) right[j] = v[j+mid+1];\n\n\ti = 0, j = 0, k = lo;\n\n\twhile(i < n1 && j < n2) {\n\t\tif(left[i] >= right[j]) {\n\t\t\tv[k] = left[i];\n\t\t\ti++;\n\t\t\tinv += j;\n\t\t} else {\n\t\t\tv[k] = right[j];\n\t\t\tj++;\n\t\t\tinv++;\n\t\t}\n\t\t++k;\n\t}\n\tinv -= j;\n\twhile(i < n1) {\n\t\tv[k] = left[i];\n\t\t++k; ++i;\n\t\tinv += j;\n\t}\n\twhile(j < n2) {\n\t\tv[k] = right[j];\n\t\t++k; ++j;\n\t}\n\treturn inv;\n}\n\nllu mergeSort(int v[], int lo, int hi) {\n\tllu inv = 0;\n\tif(lo < hi) {\n\t\tint mid = (lo+hi)/2;\n\t\tinv += mergeSort(v, lo, mid);\n\t\tinv += mergeSort(v, mid+1, hi);\n\t\tinv += merge(v, lo, mid, hi);\n\t}\n\treturn inv;\n}\n\nllu inversions(int v[], int lo, int hi) {\n\tint size = hi-lo+1, u[size];\n\tfor(int i = 0; i < size; i++) {\n\t\tu[i] = v[i+lo];\n\t}\n\treturn mergeSort(u, 0, size-1);\n}\n\nint main() {\n\tint n, v[maxn];\n\tscanf(\"%d\", &n);\n\tfor(int i = 0; i < n; i++) {\n\t\tscanf(\"%d\", &v[i]);\n\t}\n\tprintf(\"Inversions: %llu\\n\", inversions(v, 0, n-1));\n\treturn 0;\n}"
}
{
"filename": "code/divide_conquer/src/merge_sort_using_divide_and_conquer/merge_sort_using_divide_and_conquer.cpp",
"content": "// divide conquer | merge sort using divide and conquer | C++\n// Part of Cosmos by OpenGenus Foundation\n\n#include <cstdlib>\n#include <cstdio>\n\nint _mergeSort(int arr[], int temp[], int left, int right);\nint merge(int arr[], int temp[], int left, int mid, int right);\n\n/* This function sorts the input array and returns the\n * number of inversions in the array */\nint mergeSort(int arr[], int array_size)\n{\n int *temp = (int *)malloc(sizeof(int) * array_size);\n return _mergeSort(arr, temp, 0, array_size - 1);\n}\n\n/* An auxiliary recursive function that sorts the input array and\n * returns the number of inversions in the array. */\nint _mergeSort(int arr[], int temp[], int left, int right)\n{\n int mid, inv_count = 0;\n if (right > left)\n {\n /* Divide the array into two parts and call _mergeSortAndCountInv()\n * for each of the parts */\n mid = (right + left) / 2;\n\n /* Inversion count will be sum of inversions in left-part, right-part\n * and number of inversions in merging */\n inv_count = _mergeSort(arr, temp, left, mid);\n inv_count += _mergeSort(arr, temp, mid + 1, right);\n\n /*Merge the two parts*/\n inv_count += merge(arr, temp, left, mid + 1, right);\n }\n return inv_count;\n}\n\n/* This funt merges two sorted arrays and returns inversion count in\n * the arrays.*/\nint merge(int arr[], int temp[], int left, int mid, int right)\n{\n int i, j, k;\n int inv_count = 0;\n\n i = left; /* i is index for left subarray*/\n j = mid; /* j is index for right subarray*/\n k = left; /* k is index for resultant merged subarray*/\n while ((i <= mid - 1) && (j <= right))\n {\n if (arr[i] <= arr[j])\n temp[k++] = arr[i++];\n else\n {\n temp[k++] = arr[j++];\n\n /*this is tricky -- see above explanation/diagram for merge()*/\n inv_count = inv_count + (mid - i);\n }\n }\n\n /* Copy the remaining elements of left subarray\n * (if there are any) to temp*/\n while (i <= mid - 1)\n temp[k++] = arr[i++];\n\n /* Copy the remaining elements of right subarray\n * (if there are any) to temp*/\n while (j <= right)\n temp[k++] = arr[j++];\n\n /*Copy back the merged elements to original array*/\n for (i = left; i <= right; i++)\n arr[i] = temp[i];\n\n return inv_count;\n}\n\n/* Driver program to test above functions */\nint main()\n{\n int arr[] = {1, 20, 6, 4, 5};\n printf(\" Number of inversions are %d \\n\", mergeSort(arr, 5));\n getchar();\n return 0;\n}"
}
{
"filename": "code/divide_conquer/src/merge_sort_using_divide_and_conquer/merge_sort_using_divide_and_conquer.java",
"content": "// divide conquer | merge sort using divide and conquer | Java\n// Part of Cosmos by OpenGenus Foundation\n\npackage mergesort;\n\n/**\n *\n * @author Yatharth Shah\n */\npublic class MergeSort {\n int array[];\n int size;\n\n public MergeSort(int n) {\n size=n;\n //create array with size n\n array=new int[n];\n //asign value into the array\n for (int i=0;i<n;i++){\n array[i]=(int) Math.round(Math.random()*89+10);\n }\n }\n\n public int getSize() {\n return size;\n }\n\n\n public void merge(int left, int mid, int right) {\n int temp [] =new int[right-left+1];\n int i = left;\n int j = mid+1;\n int k = 0;\n while (i <= mid && j <= right) {\n if (array[i] <= array[j]) {\n temp[k] = array[i];\n k++;\n i++;\n } else { //array[i]>array[j]\n temp[k] = array[j];\n k++;\n j++;\n }\n }\n while(j<=right) temp[k++]=array[j++];\n while(i<=mid) temp[k++]=array[i++];\n\n for(k=0;k<temp.length;k++) array[left+k]=temp[k];\n }\n public void merge_sort(int left,int right){\n // Check if low is smaller then high, if not then the array is sorted\n if(left<right){\n // Get the index of the element which is in the middle\n int mid=(left+right)/2;\n // Sort the left side of the array\n merge_sort(left,mid);\n // Sort the right side of the array\n merge_sort(mid+1,right);\n // Combine them both\n merge(left,mid,right);\n }\n\n }\n public void print(){\n System.out.println(\"Contents of the Array\");\n for(int k=0;k<15;k++) {\n System.out.print(array[k]+\" | \");\n }\n System.out.println();\n\n }\n public static void main(String args[]){\n MergeSort m=new MergeSort(15);\n System.out.println(\"Before Sort <<<<<<<<<<<<<<<<<<<<<\");\n m.print();\n m.merge_sort(0,m.getSize()-1);\n System.out.println(\"After Sort > > > > > > > > > > > >\");\n m.print();\n System.out.println(\"=======+============+=======+============+=========\");\n MergeSort m2=new MergeSort(25);\n System.out.println(\"Before Sort <<<<<<<<<<<<<<<<<<<<<\");\n m2.print();\n m2.merge_sort(0,m2.getSize()-1);\n System.out.println(\"After Sort > > > > > > > > > > > >\");\n m2.print();\n System.out.println(\"=======+============+=======+============+=========\");\n MergeSort m3=new MergeSort(30);\n System.out.println(\"Before Sort <<<<<<<<<<<<<<<<<<<<<\");\n m3.print();\n m3.merge_sort(0,m3.getSize()-1);\n System.out.println(\"After Sort > > > > > > > > > > > >\");\n m3.print();\n System.out.println(\"=======+============+=======+============+=========\");\n\n}\n}"
}
{
"filename": "code/divide_conquer/src/merge_sort_using_divide_and_conquer/README.md",
"content": "# Cosmos\n\nCollaborative effort by [OpenGenus](https://github.com/OpenGenus/cosmos)"
}
{
"filename": "code/divide_conquer/src/power_of_a_number/power_of_a_number.cpp",
"content": "/*\nPROBLEM STATEMENT:\nGiven 2 numbers x and y, you need to find x raise to y.\n*/\n\n#include<bits/stdc++.h>\nusing namespace std;\n#define ll long long int\n#define FIO ios::sync_with_stdio(0);\nll power(ll a,ll b)\n{\n\tll res=1;\n\twhile(b!=0)\n\t{\n\t\tif(b%2!=0)\n\t\t{\n\t\t\tres=(res*a)%1000000007;\n\t\t\tb--;\n\t\t}\n\t\telse\n\t\t{\n\t\t\ta=(a*a)%1000000007;\n\t\t\tb/=2;\n\t\t}\n\t}\n\treturn res;\n}\nint main() \n{\n\tFIO;\n ll number_a,number_b,answer;\n cin >> number_a >> number_b;\n answer = power(number_a,number_b);\n cout << answer;\n\treturn 0;\n}"
}
{
"filename": "code/divide_conquer/src/power_of_a_number/power_of_a_number.py",
"content": "# Python3 program to check if\n# a given number can be expressed\n# as power\nimport math\n\n# Returns true if n can be written as x^y\ndef isPower(n) :\n\tif (n==1) :\n\t\treturn True\n\n\t# Try all numbers from 2 to sqrt(n) as base\n\tfor x in range(2,(int)(math.sqrt(n))+1) :\n\t\ty = 2\n\t\tp = (int)(math.pow(x, y))\n\n\t\t# Keep increasing y while power 'p' is smaller\n\t\t# than n.\n\t\twhile (p<=n and p>0) :\n\t\t\tif (p==n) :\n\t\t\t\treturn True\n\t\t\t\n\t\t\ty = y + 1\n\t\t\tp = math.pow(x, y)\n\t\t\n\t\t\n\treturn False\n\t\n\n# Driver Program\nfor i in range(2,100 ) :\n\tif (isPower(i)) :\n\t\tprint(i,end=\" \")\n\t\t\n\t\t"
}
{
"filename": "code/divide_conquer/src/quick_hull/quick_hull.cpp",
"content": "#include<bits/stdc++.h>\nusing namespace std;\n#define iPair pair<int, int>\nset<iPair> hull;// stores the final points to create the convex hull\n\nint findSide(iPair p1, iPair p2, iPair p)// returns the side of the hull joined points p1 and p2\n{\n int val = (p.second - p1.second) * (p2.first - p1.first) -\n (p2.second - p1.second) * (p.first - p1.first);\n \n if (val > 0)\n return 1;\n if (val < 0)\n return -1;\n return 0;\n}\n\nint line_distance(iPair p1, iPair p2, iPair p)\n{\n return abs ((p.second - p1.second) * (p2.first - p1.first) -\n (p2.second - p1.second) * (p.first - p1.first));\n}\n\nvoid quickHull(iPair arr[], int size, iPair p1, iPair p2, int side)\n{\n int ind = -1;\n int max_dist = 0;\n\n for (int i=0; i<size; i++)\n {\n int temp = line_distance(p1, p2, arr[i]);\n if (findSide(p1, p2, arr[i]) == side && temp > max_dist)\n {\n ind = i;\n max_dist = temp;\n }\n }\n\n if (ind == -1)\n {\n hull.insert(p1);\n hull.insert(p2);\n return;\n }\n\n quickHull(arr, size, arr[ind], p1, -findSide(arr[ind], p1, p2));\n quickHull(arr, size, arr[ind], p2, -findSide(arr[ind], p2, p1));\n}\n \nvoid print(iPair arr[], int size)\n{\n if (size < 3)\n {\n cout << \"Convex hull not possible\\n\";\n return;\n }\n \n int min_x = 0, max_x = 0;\n for (int i=1; i<size; i++)\n {\n if (arr[i].first < arr[min_x].first)\n min_x = i;\n if (arr[i].first > arr[max_x].first)\n max_x = i;\n }\n \n quickHull(arr, size, arr[min_x], arr[max_x], 1);\n quickHull(arr, size, arr[min_x], arr[max_x], -1);\n \n cout << \"The Convex Hull points are:\\n\";\n while (!hull.empty())\n {\n cout << \"(\" <<( *hull.begin()).first << \", \"\n << (*hull.begin()).second << \") \";\n hull.erase(hull.begin());\n }\n}\n\nint main()\n{\n iPair arr[] = {{0, 3}, {1, 1}, {2, 2}, {4, 4},\n {0, 0}, {1, 2}, {3, 1}, {3, 3}};\n int size = sizeof(arr)/sizeof(arr[0]);\n print(arr, size);\n return 0;\n}\n\n\n// OUTPUT\n// The Convex Hull points are:\n// (0, 0) (0, 3) (3, 1) (4, 4)\n\n// Code curated by Subhadeep Das(username:- Raven1233)"
}
{
"filename": "code/divide_conquer/src/quick_sort/quick_sort2.cpp",
"content": "#include <iostream>\nusing namespace std;\n\nvoid swapit(int *x, int *y)\n{\n int temp;\n temp = *x;\n *x = *y;\n *y = temp;\n}\n\nint Mpartition(int arr[], int low, int high)\n{\n int i = low - 1;\n int j = low;\n for (; j < high; j++)\n if (arr[high] < arr[j])\n {\n i++;\n swapit(&arr[j], &arr[i]);\n }\n swapit(&arr[high], &arr[i + 1]);\n return i + 1;\n\n}\n\nvoid quicksort(int arr[], int low, int high)\n{\n\n if (low < high)\n {\n int pi = Mpartition(arr, low, high);\n quicksort(arr, low, pi - 1);\n quicksort(arr, pi + 1, high);\n }\n\n}\nint main()\n{\n int arr[] = {14, 15, 13, 44, 56, 23, 8, 78, 36, 72};\n int arrsize = sizeof(arr) / sizeof(arr[0]) - 1;\n quicksort(arr, 0, arrsize);\n for (int i = 0; i < 9; i++)\n cout << arr[i] << endl;\n\n}"
}
{
"filename": "code/divide_conquer/src/quick_sort/quick_sort.c",
"content": "// divide conquer | quick sort | C\n// Part of Cosmos by OpenGenus Foundation\n\n#include<stdio.h>\n\n// A utility function to swap two elements\nvoid \nswap(int* a, int* b)\n{\n int t = *a;\n *a = *b;\n *b = t;\n}\n\n/* This function takes last element as pivot, places\n the pivot element at its correct position in sorted\n array, and places all smaller (smaller than pivot)\n to left of pivot and all greater elements to right\n of pivot */\nint \npartition (int arr[], int low, int high)\n{\n int pivot = arr[high]; // pivot\n int i = (low - 1); // Index of smaller element\n\n for (int j = low; j <= high- 1; j++)\n {\n // If current element is smaller than or\n // equal to pivot\n if (arr[j] <= pivot)\n {\n i++; // increment index of smaller element\n swap(&arr[i], &arr[j]);\n }\n }\n swap(&arr[i + 1], &arr[high]);\n return (i + 1);\n}\n\n/* The main function that implements QuickSort\n arr[] --> Array to be sorted,\n low --> Starting index,\n high --> Ending index */\nvoid \nquickSort(int arr[], int low, int high)\n{\n if (low < high)\n {\n /* pi is partitioning index, arr[p] is now\n at right place */\n int pi = partition(arr, low, high);\n\n // Separately sort elements before\n // partition and after partition\n quickSort(arr, low, pi - 1);\n quickSort(arr, pi + 1, high);\n }\n}\n\n/* Function to print an array */\nvoid \nprintArray(int arr[], int size)\n{\n int i;\n for (i = 0; i < size; i++)\n printf(\"%d \", arr[i]);\n printf(\"n\");\n}\n\n// Driver program to test above functions\nint \nmain()\n{\n int arr[] = {10, 7, 8, 9, 1, 5};\n int n = sizeof(arr)/sizeof(arr[0]);\n quickSort(arr, 0, n - 1);\n printf(\"Sorted array: n\");\n printArray(arr, n);\n return (0);\n}"
}
{
"filename": "code/divide_conquer/src/quick_sort/quick_sort.cpp",
"content": "// divide conquer | quick sort | C++\n// Part of Cosmos by OpenGenus Foundation\n\n#include <cstdio>\nusing namespace std;\n\nvoid swap(int* a, int* b)\n{\n int t = *a;\n *a = *b;\n *b = t;\n}\n\n/* This function takes last element as pivot, places\n * the pivot element at its correct position in sorted\n * array, and places all smaller (smaller than pivot)\n * to left of pivot and all greater elements to right\n * of pivot */\nint partition (int arr[], int low, int high)\n{\n int pivot = arr[high]; // pivot\n int i = (low - 1); // Index of smaller element\n\n for (int j = low; j <= high - 1; j++)\n // If current element is smaller than or\n // equal to pivot\n if (arr[j] <= pivot)\n {\n i++; // increment index of smaller element\n swap(&arr[i], &arr[j]);\n }\n swap(&arr[i + 1], &arr[high]);\n return i + 1;\n}\n\nvoid quickSort(int arr[], int low, int high)\n{\n if (low < high)\n {\n /* pi is partitioning index, arr[p] is now\n * at right place */\n int pi = partition(arr, low, high);\n\n quickSort(arr, low, pi - 1);\n quickSort(arr, pi + 1, high);\n }\n}\n\nvoid printArray(int arr[], int size)\n{\n int i;\n for (i = 0; i < size; i++)\n printf(\"%d \", arr[i]);\n printf(\"\\n\");\n}\n\nint main()\n{\n int arr[] = {10, 7, 8, 9, 1, 5};\n int n = sizeof(arr) / sizeof(arr[0]);\n quickSort(arr, 0, n - 1);\n printf(\"Sorted array: \\n\");\n printArray(arr, n);\n return 0;\n}"
}
{
"filename": "code/divide_conquer/src/quick_sort/Quick_Sort.cs",
"content": "// Part of Cosmos (OpenGenus)\nusing System;\npublic class Quick_Sort\n{\n // Conquer\n public static int Parition(int[] array, int left, int right)\n {\n int pivot = array[left];\n int index = right;\n int temp;\n\n for(int j = right; j > left; j--)\n {\n if(array[j] > pivot)\n {\n temp = array[j];\n array[j] = array[index];\n array[index] = temp;\n index--;\n }\n }\n\n array[left] = array[index];\n array[index] = pivot;\n return index;\n }\n\n // Divide array into halves\n public static void Quick(int[] array, int left, int right)\n {\n if(left < right)\n {\n int pivot = Parition(array, left, right);\n\n Quick(array, left, pivot - 1);\n Quick(array, pivot + 1, right);\n }\n }\n\n public static void QuickSort(int[] array, int size)\n {\n Quick(array, 0, size - 1);\n }\n\n // function ro print array\n public static void Print_Array(int[] array, int size)\n {\n for(int i = 0; i < size; i++)\n Console.Write(array[i] + \" \");\n\n Console.Write(\"\");\n }\n\n public static void Main()\n {\n int[] array = {2, 4, 3, 1, 6, 8, 4};\n QuickSort(array, 7);\n Print_Array(array, 7);\n }\n}\n\n// Output\n// 1 2 3 4 4 6 8"
}
{
"filename": "code/divide_conquer/src/quick_sort/quick_sort.hs",
"content": "quicksort :: (Ord a) => [a] -> [a]\nquicksort [] = []\nquicksort (x:xs) = smaller ++ [x] ++ larger\n where smaller = quicksort $ filter (<=x) xs\n larger = quicksort $ filter (>x) xs"
}
{
"filename": "code/divide_conquer/src/quick_sort/quicksort.java",
"content": "// divide conquer | quick sort | Java\n// Part of Cosmos by OpenGenus Foundation\n\npublic class QuickSort {\n\tprivate int []v;\n\tprivate int n;\n\n\tpublic String toString() {\n\t\tString result = \"\";\n\n\t\tfor(int i = 0; i < n; i++) {\n\t\t\tresult += v[i] + \" \";\n\t\t}\n\n\t\treturn result;\n\t}\n\n\tpublic void quickSort(QuickSort v, int left, int right) {\n\t\tint i = left, j = right;\n\t\tint aux;\n\t\tint pivot = (left + right) / 2;\n\n\t\twhile(i <= j) {\n\t\t\twhile(v.v[i] < v.v[pivot]) {\n\t\t\t\ti++;\n\t\t\t}\n\t\t\twhile(v.v[j] > v.v[pivot]) {\n\t\t\t\tj--;\n\t\t\t}\n\t\t\tif(i <= j) {\n\t\t\t\taux = v.v[i];\n\t\t\t\tv.v[i] = v.v[j];\n\t\t\t\tv.v[j] = aux;\n\t\t\t\ti++;\n\t\t\t\tj--;\n\t\t\t}\n\t\t}\n\n\t\tif(left < j) {\n\t\t\tquickSort(v, left, j);\n\t\t}\n\t\tif(i < right) {\n\t\t\tquickSort(v, i, right);\n\t\t}\n\n\t}\n\n\tpublic static void main(String []args) {\n\t\tQuickSort obj = new QuickSort();\n\t\tobj.n = 10;\n\t\tobj.v = new int[10];\n\n\t\tfor(int i = 0; i < 10; i++) {\n\t\t\tobj.v[i] = 10 - i;\n\t\t}\n\n\t\tSystem.out.println(obj);\n\t\tobj.quickSort(obj, 0, obj.n - 1);\n\t\tSystem.out.println(obj);\n\t}\n\n}"
}
{
"filename": "code/divide_conquer/src/quick_sort/quick_sort.py",
"content": "def partition(arr, low, high):\n i = low - 1\n pivot = arr[high]\n\n for j in range(low, high):\n\n if arr[j] <= pivot:\n\n i = i + 1\n arr[i], arr[j] = arr[j], arr[i]\n\n arr[i + 1], arr[high] = arr[high], arr[i + 1]\n return i + 1\n\n\ndef quickSort(arr, low, high):\n if low < high:\n\n pi = partition(arr, low, high)\n\n quickSort(arr, low, pi - 1)\n quickSort(arr, pi + 1, high)\n\n\narr = [10, 7, 8, 9, 1, 5]\nn = len(arr)\nquickSort(arr, 0, n - 1)\nprint(\"Sorted array is:\")\nfor i in range(n):\n print(\"%d\" % arr[i]),"
}
{
"filename": "code/divide_conquer/src/quick_sort/quick_sort.rs",
"content": "// divide conquer | quick sort | Rust\n// Part of Cosmos by OpenGenus Foundation\n\nfn quick_sort(mut arr :Vec<i32>,low :usize, high :usize) -> Vec<i32> {\n\n if low < high {\n let mid = partition(&mut arr, low, high);\n\n let arr = quick_sort(arr.clone(),low,mid);\n let arr = quick_sort(arr,mid+1,high);\n return arr\n }\n arr\n}\n\nfn partition(arr : &mut Vec<i32>, low :usize, high :usize) -> usize {\n let pivot = arr[low];\n let mut i = low ;\n let mut j = high;\n\n loop {\n while arr[i] < pivot && arr[i] != pivot {\n i += 1;\n }\n while arr[j] > pivot && arr[j] != pivot {\n j -= 1;\n }\n if i < j {\n arr.swap(i,j);\n }else{\n return j\n }\n }\n}\n\nfn main() {\n let arr = vec![10, 7, 8, 9, 1, 5];\n let len = arr.len();\n let sorted = quick_sort(arr,0,len-1);\n println!(\"Sorted array is {:?}\", sorted);\n}"
}
{
"filename": "code/divide_conquer/src/quick_sort/quick_sort.swift",
"content": "// divide conquer | quick sort | Swift\n// Part of Cosmos by OpenGenus Foundation\n\nimport Foundation;\n\nfunc partition(_ arr: inout [UInt32], _ begin: Int, _ end: Int) -> Int {\n var i = begin;\n var j = end;\n let pivot = arr[(i + j) / 2];\n\n while i <= j {\n while arr[i] < pivot {\n i += 1;\n }\n\n while arr[j] > pivot {\n j -= 1;\n }\n\n if i <= j {\n arr.swapAt(i, j);\n i += 1;\n j -= 1;\n }\n }\n arr.swapAt(i, end);\n\n return i;\n}\n\nfunc quick_sort(_ arr: inout [UInt32], begin: Int, end: Int) {\n if begin < end {\n let index = partition(&arr, begin, end);\n quick_sort(&arr, begin: begin, end: index - 1);\n quick_sort(&arr, begin: index, end: end);\n }\n}\n\nfunc test() {\n print(\"Size of array: \", terminator: \"\");\n let size = Int(readLine()!)!;\n var arr = [UInt32]();\n\n for _ in 1 ... size {\n arr.append(arc4random_uniform(100));\n }\n\n print(\"Original: \");\n print(arr);\n\n quick_sort(&arr, begin: 0, end: size - 1);\n\n print(\"Sorted: \");\n print(arr);\n}\n\ntest();"
}
{
"filename": "code/divide_conquer/src/quick_sort/README.md",
"content": "# Cosmos\n\nCollaborative effort by [OpenGenus](https://github.com/OpenGenus/cosmos)"
}
{
"filename": "code/divide_conquer/src/README.md",
"content": "# Divide and conquer\nDivide and conquer is an algorithm design paradigm based on multi-branched recursion. A divide and conquer algorithm works by recursively breaking down a problem into two or more sub-problems of the same or related type, until these become simple enough to be solved directly. The solutions to the sub-problems are then combined to give a solution to the original problem.\n\nThis divide and conquer technique is the basis of efficient algorithms for all kinds of problems, such as sorting (e.g., quicksort, merge sort), multiplying large numbers (e.g. the Karatsuba algorithm), finding the closest pair of points, syntactic analysis (e.g., top-down parsers), and computing the discrete Fourier transform (FFTs).\n# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/divide_conquer/src/search_in_a_rotated_array/search_in_a_rotated_array.cpp",
"content": "/*\r\nProblem statement:\r\nGiven a sorted and rotated array A of N distinct elements which is rotated\r\nat some point, and given an element K. The task is to find the index of\r\nthe given element K in the array A.\r\n*/\r\n\r\n#include <iostream>\r\n#include <vector>\r\n#define ll long long int\r\n\r\nll findPivot(std::vector<ll> &v, ll low, ll high) {\r\n while (low < high) {\r\n // find mid element\r\n ll mid = low + (high - low) / 2;\r\n\r\n if (v[mid] < v[0]) {\r\n high = mid;\r\n } else {\r\n low = mid + 1;\r\n }\r\n }\r\n\r\n if (v[low] < arr[0]) {\r\n return low;\r\n } else {\r\n // pivot element does not exist\r\n return -1;\r\n }\r\n}\r\n\r\nll findAns(std::vector<ll> &v, ll k, ll low, ll high) {\r\n\r\n while (low < high) {\r\n // find mid element\r\n ll mid = low + (high - low) / 2;\r\n\r\n if (v[mid] == k) {\r\n return mid;\r\n }\r\n\r\n if (v[mid] >= k) {\r\n high = mid;\r\n } else {\r\n low = mid + 1;\r\n }\r\n }\r\n\r\n if (v[low] == k) {\r\n return low;\r\n } else {\r\n // element does not exist in array\r\n return -1;\r\n }\r\n}\r\n\r\nint main() {\r\n ll test, n, k, pivot;\r\n std::cin >> test; // no. of test cases\r\n std::vector<ll> v;\r\n\r\n while (test--) {\r\n std::cin >> n; // size of array\r\n for (ll i = 0; i < n; i++) {\r\n ll num;\r\n std::cin >> num;\r\n v.push_back(num);\r\n }\r\n std::cin >> k; // element to be searched\r\n\r\n pivot = findPivot(v, 0, n - 1); // find pivot element\r\n\r\n if (pivot == -1) {\r\n // if pivot element does not exist, elements are\r\n // whole array is in ascending order\r\n std::cout << findAns(v, k, 0, n - 1) << \"\\n\";\r\n } else {\r\n if (k >= v[0]) {\r\n std::cout << findAns(v, k, 0, pivot - 1) << \"\\n\";\r\n } else {\r\n std::cout << findAns(v, k, pivot, n - 1) << \"\\n\";\r\n }\r\n }\r\n v.clear();\r\n }\r\n\r\n return 0;\r\n}\r\n\r\n/*\r\n\r\nInput:\r\n3\r\n9\r\n5 6 7 8 9 10 1 2 3\r\n10\r\n3\r\n3 1 2\r\n1\r\n4\r\n3 5 1 2\r\n6\r\n\r\nOutput:\r\n5\r\n1\r\n-1\r\n\r\n*/\r"
}
{
"filename": "code/divide_conquer/src/strassen_matrix_multiplication/main.cpp",
"content": "\n//The following program contains the strassen algorithm along with the Currently Fastest Matrix Multiplication Algorithm for sparse matrices\n// Developed by Raphael Yuster and Uri Zwick\n\n// I have also included a time calculator in case anyone wanted to check time taken by each algorithms\n\n// Although mathematcially the fast multiplication algorithm should take less time, since it is of lesser order O(n^t)\n// however it has a large preceeding constant thus the effect of the algorthm can only be seen in larger matrices\n#include <algorithm>\n#include <chrono>\n#include <iostream>\n#include <random>\n#include <vector>\n\nstd::vector<std::vector<int>> generator(int m, int n)\n{\n std::mt19937 rng;\n rng.seed(std::random_device()());\n std::uniform_int_distribution<std::mt19937::result_type> dist6(0, 1);\n std::vector<std::vector<int>> ans;\n for (int i = 0; i < m; i++)\n {\n std::vector<int> row;\n for (int j = 0; j < n; j++)\n {\n if ((i + j) % 5 != 5)\n row.push_back(dist6(rng));\n else\n row.push_back(0);\n }\n ans.push_back(row);\n }\n return ans;\n}\n\n\nint f(const std::vector<std::vector<int>>& a)\n{\n return a[0][0];\n}\nstd::vector<std::vector<int>> retriever(const std::vector<std::vector<int>>& matrix, int x, int y)\n{\n std::vector<std::vector<int>> ans;\n for (int i = 0; i < x; i++)\n {\n std::vector<int> row;\n for (int j = 0; j < y; j++)\n row.push_back(matrix[i][j]);\n ans.push_back(row);\n }\n return ans;\n}\n\n\nstd::vector<std::vector<int>> converter(const std::vector<std::vector<int>>& matrix)\n{\n auto noOfRows = matrix.size();\n auto noOfCols = matrix[0].size();\n std::vector<std::vector<int>>::size_type t = 1;\n std::vector<std::vector<int>>::size_type p;\n if (x > y)\n p = noOfRows;\n else\n p = noOfCols;\n while (t < p)\n t *= 2;\n p = t;\n int flag = 0;\n std::vector<std::vector<int>> ans;\n for (vector<vector<int>>::size_type i = 0; i < p; i++)\n {\n if (i >= noOfRows)\n flag = 1;\n std::vector<int> row;\n for (std::vector<int>::size_type j = 0; j < p; j++)\n {\n if (flag == 1)\n row.push_back(0);\n else\n {\n if (j >= noOfCols)\n row.push_back(0);\n else\n row.push_back(matrix[i][j]);\n }\n }\n ans.push_back(row);\n }\n return ans;\n}\n\nvoid print(const std::vector<std::vector<int>>& grid)\n{\n for (std::vector<std::vector<int>>::size_type i = 0; i < grid.size(); i++)\n {\n for (std::vector<int>::size_type j = 0; j < grid[i].size(); j++)\n std::cout << grid[i][j] << ' ';\n std::cout << std::endl;\n }\n}\n\nstd::vector<std::vector<int>> add(const std::vector<std::vector<int>>& a,\n const std::vector<std::vector<int>>& b)\n{\n std::vector<std::vector<int>> ans;\n for (std::vector<std::vector<int>>::size_type i = 0; i < a.size(); i++)\n {\n vector<int> row;\n for (std::vector<int>::size_type j = 0; j < a[i].size(); j++)\n {\n int temp = a[i][j] + b[i][j];\n row.push_back(temp);\n }\n ans.push_back(row);\n }\n return ans;\n}\n\nstd::vector<std::vector<int>> subtract(const std::vector<std::vector<int>>& a,\n const std::vector<std::vector<int>>& b)\n{\n std::vector<std::vector<int>> ans;\n for (std::vector<std::vector<int>>::size_type i = 0; i < a.size(); i++)\n {\n vector<int> row;\n for (std::vector<int>::size_type j = 0; j < a[i].size(); j++)\n {\n int temp = a[i][j] - b[i][j];\n row.push_back(temp);\n }\n ans.push_back(row);\n }\n return ans;\n}\n\n\n\nstd::vector<std::vector<int>> naive_multi(const std::vector<std::vector<int>>& a,\n const std::vector<std::vector<int>>& b)\n{\n int s = 0;\n std::vector<std::vector<int>> ans;\n for (std::vector<std::vector<int>>::size_type i = 0; i < a.size(); i++)\n {\n vector<int> row;\n for (std::vector<int>::size_type j = 0; j < b[i].size(); j++)\n {\n s = 0;\n for (std::vector<int>::size_type k = 0; k < a[i].size(); k++)\n s += a[i][k] * b[k][j];\n row.push_back(s);\n }\n ans.push_back(row);\n }\n return ans;\n}\n\n\nstd::vector<std::vector<int>> strassen(const std::vector<std::vector<int>>& m1,\n const std::vector<std::vector<int>>& m2)\n{\n std::vector<std::vector<int>>::size_type s1 = m1.size();\n std::vector<std::vector<int>>::size_type s2 = m2.size();\n if (s1 > 2 && s2 > 2)\n {\n std::vector<std::vector<int>> a, b, c, d, e, f, g, h, ans;\n for (std::vector<std::vector<int>>::size_type i = 0; i < m1.size() / 2; i++)\n {\n vector<int> row;\n for (std::vector<int>::size_type j = 0; j < m1[i].size() / 2; j++)\n row.push_back(m1[i][j]);\n a.push_back(row);\n }\n for (std::vector<std::vector<int>>::size_type i = 0; i < m1.size() / 2; i++)\n {\n vector<int> row;\n for (std::vector<int>::size_type j = m1[i].size() / 2; j < m1[i].size(); j++)\n row.push_back(m1[i][j]);\n b.push_back(row);\n }\n for (std::vector<std::vector<int>>::size_type i = m1.size() / 2; i < m1.size(); i++)\n {\n vector<int> row;\n for (std::vector<int>::size_type j = 0; j < m1[i].size() / 2; j++)\n row.push_back(m1[i][j]);\n c.push_back(row);\n }\n for (std::vector<std::vector<int>>::size_type i = m1.size() / 2; i < m1.size(); i++)\n {\n vector<int> row;\n for (std::vector<int>::size_type j = m1[i].size() / 2; j < m1[i].size(); j++)\n row.push_back(m1[i][j]);\n d.push_back(row);\n }\n for (std::vector<std::vector<int>>::size_type i = 0; i < m2.size() / 2; i++)\n {\n vector<int> row;\n for (std::vector<int>::size_type j = 0; j < m2[i].size() / 2; j++)\n row.push_back(m2[i][j]);\n e.push_back(row);\n }\n for (std::vector<std::vector<int>>::size_type i = 0; i < m2.size() / 2; i++)\n {\n vector<int> row;\n for (std::vector<int>::size_type j = m2[i].size() / 2; j < m2[i].size(); j++)\n row.push_back(m2[i][j]);\n f.push_back(row);\n }\n for (std::vector<std::vector<int>>::size_type i = m2.size() / 2; i < m2.size(); i++)\n {\n vector<int> row;\n for (std::vector<int>::size_type j = 0; j < m2[i].size() / 2; j++)\n row.push_back(m2[i][j]);\n g.push_back(row);\n }\n for (std::vector<std::vector<int>>::size_type i = m2.size() / 2; i < m2.size(); i++)\n {\n vector<int> row;\n for (std::vector<int>::size_type j = m2[i].size() / 2; j < m2[i].size(); j++)\n row.push_back(m2[i][j]);\n h.push_back(row);\n }\n\n\n std::vector<std::vector<int>> p1, p2, p3, p4, p5, p6, p7;\n p1 = strassen(a, subtract(f, h));\n p2 = strassen(add(a, b), h);\n p3 = strassen(add(c, d), e);\n p4 = strassen(d, subtract(g, e));\n p5 = strassen(add(a, d), add(e, h));\n p6 = strassen(subtract(b, d), add(g, h));\n p7 = strassen(subtract(a, c), add(e, f));\n std::vector<std::vector<int>> c1, c2, c3, c4;\n c1 = subtract(add(add(p5, p4), p6), p2);\n c2 = add(p1, p2);\n c3 = add(p3, p4);\n c4 = subtract(add(p1, p5), add(p3, p7));\n\n\n\n int flag1, flag2;\n for (std::vector<std::vector<int>>::size_type i = 0; i < m1.size(); i++)\n {\n std::vector<int> row;\n if (i < m1.size() / 2)\n flag1 = 0;\n else\n flag1 = 1;\n for (std::vector<int>::size_type j = 0; j < m2[i].size(); j++)\n {\n if (j < m2[i].size() / 2)\n {\n if (flag1 == 0)\n row.push_back(c1[i][j]);\n else\n row.push_back(c3[i - m1.size() / 2][j]);\n }\n else\n {\n if (flag1 == 0)\n row.push_back(c2[i][j - m2[i].size() / 2]);\n else\n row.push_back(c4[i - m1.size() / 2][j - m2[i].size() / 2]);\n }\n }\n ans.push_back(row);\n }\n return ans;\n }\n else\n {\n std::vector<std::vector<int>> v;\n v = naive_multi(m1, m2);\n return v;\n }\n}\n\nstd::vector<std::vector<int>> fast_multi(const std::vector<std::vector<int>>& m1,\n const std::vector<std::vector<int>>& m2)\n{\n std::vector<int> ranges, ranges_c, ind;\n std::vector<std::vector<int>> ans;\n for (std::vector<std::vector<int>>::size_type i = 0; i < m1.size(); i++)\n {\n int a = 0;\n int b = 0;\n for (std::vector<std::vector<int>>::size_type j = 0; j < m1[i].size(); j++)\n {\n if (m1[j][i] != 0)\n a += 1;\n if (m2[i][j] != 0)\n b += 1;\n }\n ranges.push_back(a * b);\n ranges_c.push_back(a * b);\n }\n sort(ranges.begin(), ranges.end());\n int comp, index;\n for (std::vector<std::vector<int>>::size_type i = 0; i < m1.size(); i++)\n {\n int s = 0;\n for (std::vector<std::vector<int>>::size_type j = 0; j <= i; j++)\n s += ranges[j];\n s += (m1.size() - i - 1) * m1.size() * m1.size();\n if (i == 0)\n {\n comp = s;\n index = 1;\n }\n else if (s < comp)\n {\n comp = s;\n index = i + 1;\n }\n }\n for (int g = 0; g < index; g++)\n {\n for (std::vector<std::vector<int>>::size_type i = 0; i < m1.size(); i++)\n if (ranges_c[i] == ranges[g])\n {\n ind.push_back(i);\n break;\n }\n }\n for (std::vector<vector<int>>::size_type i = 0; i < m1.size(); i++)\n {\n int flag;\n int flag_search = 0;\n for (std::vector<int>::size_type j = 0; j < ind.size(); j++)\n if (i == ind[j])\n {\n flag_search = 1;\n break;\n }\n std::vector<std::vector<int>> row, col;\n for (std::vector<int>::size_type j = 0; j < ind.size(); j++)\n {\n std::vector<int> temp1, temp2;\n temp1.push_back(m1[j][i]);\n temp2.push_back(m2[i][j]);\n col.push_back(temp1);\n row.push_back(temp2);\n }\n if (i == 0)\n {\n if (flag_search == 1)\n ans = naive_multi(col, row);\n else\n {\n std::vector<std::vector<int>> row_c, col_c;\n row_c = converter(row);\n col_c = converter(col);\n ans = retriever(strassen(col_c, row_c), m1.size(), m1.size());\n }\n }\n else\n {\n std::vector<std::vector<int>> v1, v2;\n if (flag_search == 1)\n v1 = naive_multi(col, row);\n else\n {\n std::vector<std::vector<int>> row_c, col_c;\n row_c = converter(row);\n col_c = converter(col);\n v1 = retriever(strassen(col_c, row_c), m1.size(), m1.size());\n }\n ans = add(v1, ans);\n }\n }\n return ans;\n}\n\n\nint main()\n{\n std::vector<vector<int>> matrix1, matrix2, v1, v2, v3;\n int i1, j1;\n int i2, j2;\n std::cout << \"enter the dimensions of matrix1\\n\";\n std::cin >> i1 >> j1;\n std::cout << '\\n';\n std::cout << \"enter the dimensions of matrix2\\n\";\n std::cin >> i2 >> j2;\n std::cout << '\\n';\n int x, y;\n for (x = 0; x < i1; x++)\n {\n std::vector<int> row;\n for (y = 0; y < j1; y++)\n {\n int temp;\n std::cout << \"enter the \" << x + 1 << \" , \" << y + 1 << \" element for matrix1 - \";\n std::cin >> temp;\n row.push_back(temp);\n }\n matrix1.push_back(row);\n }\n for (x = 0; x < i2; x++)\n {\n std::vector<int> row;\n for (y = 0; y < j2; y++)\n {\n int temp;\n std::cout << \"enter the \" << x + 1 << \" , \" << y + 1 << \" element for matrix2 - \";\n std::cin >> temp;\n row.push_back(temp);\n }\n matrix2.push_back(row);\n }\n //print(matrix1);\n\n std::vector<std::vector<int>> m1, m2, m3, m4;\n m1 = converter(matrix1);\n m2 = converter(matrix2);\n //print(m1);\n //cout << \"a\\n\";\n //print(m2);\n //cout <<\"a\\n\";\n /*\n * m3 = generator(1024, 1024);\n * m4 = generator(1024, 1024);\n */\n\n auto start = std::chrono::high_resolution_clock::now();\n v1 = retriever(naive_multi(m1, m2), i1, j2);\n auto stop = std::chrono::high_resolution_clock::now();\n auto duration = std::chrono::duration_cast<microseconds>(start - stop);\n\n std::cout << \"tiime taken by function\" << duration.count() << endl;\n //print(v1);\n\n\n\n auto start2 = std::chrono::high_resolution_clock::now();\n v2 = retriever(strassen(m1, m2), i1, j2);\n auto stop2 = std::chrono::high_resolution_clock::now();\n auto duration2 = std::chrono::duration_cast<microseconds>(start2 - stop2);\n\n std::cout << \"tiime taken by function\" << duration2.count() << endl;\n\n /*\n * auto start_3 = std::chrono::high_resolution_clock::now();\n * v3 = retriever(fast_multi(m1, m2), i_1, j_2);\n * auto stop_3 = std::chrono::high_resolution_clock::now();\n * auto duration_3 = std::chrono::duration_cast<microseconds>(start_3 - stop_3);\n *\n *\n * cout << \"tiime taken by function\" << duration_3.count() << endl;\n */\n\n //print(v2);\n print(v1);\n\n return 0;\n}"
}
{
"filename": "code/divide_conquer/src/strassen_matrix_multiplication/strassen.py",
"content": "#!/usr/bin/env python2\n# -*- coding: utf-8 -*-\n\"\"\"\nCreated on Tue May 29 13:36:32 2018\n\n@author: Sahit\n\"\"\"\n\nimport numpy as np\n\n\ndef Correction(matrix_1, matrix_2):\n r = max(matrix_1.shape[0], matrix_1.shape[1], matrix_2.shape[0], matrix_2.shape[1])\n i = 1\n while i < r:\n i = i * 2\n\n return i\n \"\"\"\n result = np.zeros((i,i))\n for i in xrange(matrix.shape[0]):\n for j in xrange(matrix.shape[1]):\n result[i][j] = matrix[i][j]\n return result\n \"\"\"\n\n\ndef Modification(matrix, p):\n result = np.zeros((p, p))\n for i in range(matrix.shape[0]):\n for j in range(matrix.shape[1]):\n result[i][j] = matrix[i][j]\n return result\n\n\ndef Extraction(matrix, x, y):\n result = np.zeros((x, y))\n for i in range(x):\n for j in range(y):\n result[i][j] = matrix[i][j]\n\n return result\n\n\ndef Strassen(matrix_1, matrix_2):\n r = matrix_1.shape[0] // 2\n if r == 0:\n return matrix_1.dot(matrix_2)\n else:\n\n a = matrix_1[:r, :r]\n b = matrix_1[:r, r:]\n c = matrix_1[r:, :r]\n d = matrix_1[r:, r:]\n\n e = matrix_2[:r, :r]\n f = matrix_2[:r, r:]\n g = matrix_2[r:, :r]\n h = matrix_2[r:, r:]\n\n p1 = Strassen(a, f - h)\n p2 = Strassen(a + b, h)\n p3 = Strassen(c + d, e)\n p4 = Strassen(d, g - e)\n p5 = Strassen(a + d, e + h)\n p6 = Strassen(b - d, g + h)\n p7 = Strassen(a - c, e + f)\n\n s1 = p5 + p4 + p6 - p2\n s2 = p1 + p2\n s3 = p3 + p4\n s4 = p1 + p5 - p3 - p7\n\n result = np.zeros((r * 2, r * 2))\n\n for i in range(r):\n for j in range(r):\n result[i][j] = s1[i][j]\n\n for i in range(r):\n for j in range(r):\n result[i][r + j] = s2[i][j]\n\n for i in range(r):\n for j in range(r):\n result[r + i][j] = s3[i][j]\n\n for i in range(r):\n for j in range(r):\n result[r + i][r + j] = s4[i][j]\n\n return result\n\n\nprint(\"enter the dimensions of the first matrix\")\nr1 = list(map(int, input().split()))\nx1, y1 = r1[0], r1[1]\nprint(\"enter the dimensions of the second matrix\")\nr2 = list(map(int, input().split()))\nx2, y2 = r2[0], r2[1]\n\n\nm1 = []\nfor i in range(x1):\n row = []\n for j in range(y1):\n print(\"enter the \", i + 1, j + 1, \" element of the first matrix\")\n row.append(input())\n m1.append(row)\n\nm2 = []\nfor i in range(x2):\n row = []\n for j in range(y2):\n print(\"enter the \", i + 1, j + 1, \" element of the second matrix\")\n row.append(input())\n m2.append(row)\n\n\nmatrix1 = np.array(m1)\nmatrix2 = np.array(m2)\n\nf = Correction(matrix1, matrix2)\n\nanswer = Extraction(\n Strassen(Modification(matrix1, f), Modification(matrix2, f)), x1, y2\n)\nprint(answer)"
}
{
"filename": "code/divide_conquer/src/tournament_method_to_find_min_max/tournament_method_to_find_min_max.c",
"content": "#include<stdio.h>\n\nstruct pair\n{\n int min;\n int max;\n}; \n \nstruct pair \ngetMinMax(int arr[], int low, int high)\n{\n struct pair minmax, mml, mmr; \n int mid;\n\n if (low == high) {\n minmax.max = arr[low];\n minmax.min = arr[low]; \n return (minmax);\n } \n\n if (high == low + 1) {\n if (arr[low] > arr[high]) { \n minmax.max = arr[low];\n minmax.min = arr[high];\n } \n else {\n minmax.max = arr[high];\n minmax.min = arr[low];\n } \n return (minmax);\n }\n\n mid = (low + high) / 2; \n mml = getMinMax(arr, low, mid);\n mmr = getMinMax(arr, mid + 1, high); \n\n if (mml.min < mmr.min)\n minmax.min = mml.min;\n else\n minmax.min = mmr.min; \n\n if (mml.max > mmr.max)\n minmax.max = mml.max;\n else\n minmax.max = mmr.max; \n \n return (minmax);\n}\n\nint \nmain()\n{\n int arr_size;\n printf(\"Enter size of the Array: \\n\");\n scanf(\"%d\", &arr_size);\n int arr[arr_size];\n printf(\"Enter %d Integers:- \\n\", arr_size);\n int i;\n for (i = 0; i < arr_size; ++i)\n scanf(\"%d\", &arr[i]);\n\n struct pair minmax = getMinMax(arr, 0, arr_size - 1);\n printf(\"Minimum element is %d \\n\", minmax.min);\n printf(\"Maximum element is %d \\n\", minmax.max);\n \n return (0);\n}"
}
{
"filename": "code/divide_conquer/src/warnock_algorithm/warnock_algorithm.pde",
"content": "/*\n\ndivide conquer | warnock's Algorithm | Processing\nPart of Cosmos by OpenGenus Foundation\n\nNational University from Colombia\nAuthor:Andres Vargas, Jaime\nGitub: https://github.com/jaavargasar\nwebpage: https://jaavargasar.github.io/\nLanguage: Processing\n\n\n\nDescription:\nThis is a implementation of warnock's Algorithm in 2D plane.\nThis algorithm is an implementation of divide and conquer\n*/\nimport java.util.*;\n\nvoid setup() {\n size(600, 600);\n background(250);\n createPicture1();\n createPicture2(); //another picture that you can try the warnock's algorithm\n loadPixels();\n warnockAlgorithm(0,height,width,0);\n\n}\n\n//Creating picture one\nvoid createPicture1(){\n pushStyle();\n noStroke();\n fill(#FFFF00);//yellow color\n rect(104,82,100,100);\n rect(400,422,100,100);\n fill(#FF0000); //red color\n rect(350,82,100,100);\n rect(104+60,82+45,100,100);\n popStyle();\n\n}\n\n\n//creating picture 2\nvoid createPicture2(){\n\n pushStyle();\n strokeWeight(4);\n fill(#008000); //green color\n triangle(142,82,142+200,82+60,142-20,82+100);\n noStroke();\n fill(#008080);//teal color\n rect(150+62,82+150,200,100);\n fill(#FF00FF);\n ellipse(150+62+150,82+150+50,100,100);\n fill(#FFFF00);\n rect(300,450,50,110);\n popStyle();\n\n pushStyle();\n fill(#FFFF00);\n strokeWeight(4);\n rect(300-100,450,50,110);\n popStyle();\n\n}\n\n//warnock's Algorithm\nvoid warnockAlgorithm(int x1,int y1,int y2, int x2){\n\n HashSet<Object> colors = new HashSet<Object>();\n int counter=0;\n\n int iniPixel= x2 + x1*width;\n if( iniPixel>=360000) iniPixel=359999;\n\n Object pix=pixels[iniPixel];\n colors.add(pix);\n\n for ( int y = y1-1; y >=x1; y--) { //height\n for ( int x = y2-1; x >= x2; x--) { //width\n int loc = x + y*width;\n\n //check if I've already added a color\n if( check(pixels[loc], colors) ){ colors.add(pixels[loc]); }\n\n }\n\n }\n\n counter= colors.size();\n\n if( counter>2){\n //strokeWeight(2);\n line( x2+(y2-x2)/2, x1, x2+(y2-x2)/2, y1);\n line(x2, x1+(y1-x1)/2, y2, x1+(y1-x1)/2 );\n\n //divide and conquer implementation\n warnockAlgorithm( x1+(y1-x1)/2,y1,y2,x2+(y2-x2)/2);\n warnockAlgorithm( x1,x1+(y1-x1)/2,y2,x2+(y2-x2)/2);\n warnockAlgorithm(x1,x1+(y1-x1)/2,x2+(y2-x2)/2,x2);\n warnockAlgorithm(x1+(y1-x1)/2,y1,x2+(y2-x2)/2,x2);\n\n }\n\n return;\n}\n\n\n//check if I've already have added a new color\nboolean check(Object pix,HashSet colors){\n if( colors.contains(pix) ) return false; //si ya lo contiene\n return true; //si no lo contiene\n}"
}
{
"filename": "code/divide_conquer/src/x_power_y/x_power_y.c",
"content": "#include <stdio.h>\n \nfloat \npower(float x, int y)\n{\n if (y == 0)\n return (1);\n\n float temp = power(x, y / 2);\n\n if (y % 2 == 0)\n return (temp * temp);\n\n else {\n if (y > 0)\n return (x * temp * temp);\n else\n return (temp * temp / x);\n }\n} \n \n\nint \nmain()\n{\n float x;\n printf(\"Enter x (float): \");\n scanf(\"%f\", &x);\n\n int y;\n printf(\"Enter y (int): \");\n scanf(\"%d\", &y);\n\n printf(\"%f^%d == %f\\n\", x, y, power(x, y));\n\n return (0);\n}"
}
{
"filename": "code/divide_conquer/test/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/dynamic_programming/src/array_median/array_median.c",
"content": "#include <stdio.h>\n\nvoid\nswap(int* a, int* b)\n{\n int t = *a;\n *a = *b;\n *b = t;\n}\n\nint\npartition(int arr[], int low, int high)\n{\n int pivot = arr[high]; \n int i = (low - 1); \n \n for (int j = low; j <= high- 1; j++) {\n if (arr[j] <= pivot) {\n i++; \n swap(&arr[i], &arr[j]);\n }\n }\n swap(&arr[i + 1], &arr[high]);\n return (i + 1);\n}\n\nvoid\nquickSort(int arr[], int low, int high)\n{\n if (low < high) {\n int pi = partition(arr, low, high);\n quickSort(arr, low, pi - 1);\n quickSort(arr, pi + 1, high);\n }\n}\n\nfloat\nmedian(int n, int arr[n])\n{\n\tif (n % 2 != 0)\n\t\treturn (float)arr[n / 2];\n\n\treturn ((float)(arr[(n - 1) / 2] + arr[n / 2]) / 2.0);\n}\n\nint\nmain()\n{\n\tint n;\n\tprintf(\"Enter size of the Array\\n\");\n\tscanf(\"%d\",&n);\n\tint arr[n];\n\tprintf(\"Enter %d integers\\n\",n);\n\tfor (int i = 0; i < n; i++)\n\t\tscanf(\"%d\",&arr[i]);\n\tquickSort(arr,0,n - 1);\n\tprintf(\"Median = %f\\n\",median(n,arr));\n\n\treturn (0);\n}"
}
{
"filename": "code/dynamic_programming/src/array_median/array_median.cpp",
"content": "#include <iostream>\n#include <algorithm>\n#include <vector>\nusing namespace std;\n\nfloat median(vector<int> arr)\n{\n int n = arr.size();\n if (n % 2 != 0)\n return (float)arr[n / 2];\n\n return (float)(arr[(n - 1) / 2] + arr[n / 2]) / 2;\n}\n\nint main()\n{\n int n;\n cout << \"Enter size of the Array\";\n cin >> n;\n vector<int> arr(n);\n cout << \"Enter \" << n << \" integers\";\n for (int i = 0; i < n; i++)\n cin >> arr[i];\n sort(arr.begin(), arr.end());\n cout << \"Median= \" << median(arr);\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/array_median/array_median.exs",
"content": "defmodule ArrayMedian do\n def get_median(array) when is_list(array) do\n list_nums = Enum.sort(array)\n half = length(list_nums) |> div(2)\n\n if length(list_nums) |> rem(2) != 0 do\n # odd length -> middle number\n mid = half + 1\n\n Enum.reduce_while(list_nums, 1, fn\n el, ^mid ->\n {:halt, el}\n\n _el, acc ->\n {:cont, acc + 1}\n end)\n else\n # even length -> mean of the 2 middle numbers\n {n1, n2} =\n Enum.reduce_while(list_nums, 1, fn\n el, {n1} ->\n {:halt, {n1, el}}\n\n el, ^half ->\n {:cont, {el}}\n\n _el, acc ->\n {:cont, acc + 1}\n end)\n\n (n1 + n2) / 2\n end\n end\nend\n\n# Test data from array_median.php\ntest_data = [\n [[-1], -1],\n [[0], 0],\n [[1], 1],\n [[-1, 0, 1], 0],\n [[1, 2, 3], 2],\n [[-1, -2, -3], -2],\n [[1, 2.5, 3], 2.5],\n [[-1, -2.5, -3], -2.5],\n [[1, 2, 2, 4], 2],\n [[1, 2, 3, 4], 2.5],\n [[2, 2, 1, 4], 2],\n [[3, 2, 1, 4], 2.5],\n [[-1, -2, -2, -4], -2],\n [[-1, -2, -3, -4], -2.5]\n]\n\nEnum.each(\n test_data,\n fn data = [arr, expected] ->\n result = ArrayMedian.get_median(arr)\n\n if result == expected do\n IO.puts(\"Success!!\")\n else\n IO.puts(\"Failure\")\n IO.inspect(data, label: \"Problem here\")\n IO.inspect(result, label: \"wrong\")\n end\n end\n)"
}
{
"filename": "code/dynamic_programming/src/array_median/array_median.java",
"content": "import java.util.*;\n// Part of Cosmos by OpenGenus Foundation\npublic class Median{\n public static void main(String[] args) {\n int length;\n int median;\n System.out.print(\"Enter Length of array \");\n Scanner scanner = new Scanner(System.in);\n length = scanner.nextInt();\n int array[] = new int[length];\n System.out.printf(\"Enter %d values: \\n\", length);\n for (int i=0 ; i<length ; i++){\n array[i] = scanner.nextInt();\n }\n Arrays.sort(array);\n if (array.length % 2 == 0){\n median = (((array[array.length /2]) + (array[array.length/2 - 1])) / 2);\n }\n else {\n median = array[array.length / 2];\n }\n System.out.printf(\"The median is %d\\n\", median);\n }\n}"
}
{
"filename": "code/dynamic_programming/src/array_median/array_median.php",
"content": "<?php\n\n/**\n * Part of Cosmos by OpenGenus Foundation\n */\n\n/**\n * Finds median in array of numbers\n *\n * @param array $arr array of numbers\n * @return int median\n */\nfunction median(array $arr)\n{\n sort($arr);\n $cnt = count($arr);\n if ($cnt % 2) {\n return $arr[floor(($cnt - 1) / 2)];\n }\n return ($arr[floor($cnt / 2)] + $arr[floor($cnt / 2) - 1]) / 2;\n}\n\necho \"median\\n\";\n$test_data = [\n [[-1], -1],\n [[0], 0],\n [[1], 1],\n [[-1, 0, 1], 0],\n [[1, 2, 3], 2],\n [[-1, -2, -3], -2],\n [[1, 2.5, 3], 2.5],\n [[-1, -2.5, -3], -2.5],\n [[1, 2, 2, 4], 2],\n [[1, 2, 3, 4], 2.5],\n [[2, 2, 1, 4], 2],\n [[3, 2, 1, 4], 2.5],\n [[-1, -2, -2, -4], -2],\n [[-1, -2, -3, -4], -2.5],\n];\nforeach ($test_data as $test_case) {\n $input = $test_case[0];\n $expected = $test_case[1];\n $result = median($input);\n printf(\n \" input %s, expected %s, got %s: %s\\n\",\n str_replace([\"\\n\", ' '], ['', ' '], var_export($input, true)),\n var_export($expected, true),\n var_export($result, true),\n ($expected === $result) ? 'OK' : 'FAIL'\n );\n}"
}
{
"filename": "code/dynamic_programming/src/array_median/array_median.py",
"content": "import sys\n\n# Part of Cosmos by OpenGenus Foundation\n\n\ndef median(nums):\n \"\"\"\n Calculates the median of a list of numbers.\n import median from statistics in python 3.4 and above.\n \"\"\"\n sorted_nums = sorted(nums)\n len_nums = len(nums)\n odd = len_nums % 2\n\n # If the length is odd, the median is the middle value of the sorted list.\n if odd:\n return sorted_nums[(len_nums - 1) // 2]\n\n # Otherwise it's the average of the middle two.\n return (nums[len_nums // 2] + nums[(len_nums // 2) - 1]) / 2\n\n\ndef main():\n \"\"\"Calculates median of numbers in stdin.\"\"\"\n # Read stdin until EOF\n nums_string = sys.stdin.read()\n\n # Split on whitespace\n num_strings = nums_string.split()\n\n # Make a new list of floats of all the non-whitespace values.\n nums = [float(i) for i in num_strings if i]\n\n # Print median\n if not nums:\n print(\"You didn't enter any numbers.\")\n else:\n print(median(nums))\n\n\ndef test_median():\n \"\"\"Test the median function for correctness.\"\"\"\n assert median([1, 2, 3]) == 2\n assert median([1, 2.9, 3]) == 2.9\n assert median([1, 2, 3, 4]) == 2.5\n assert median([2, 1, 4, 3]) == 2.5\n assert median([1, 3, 3, 4, 5, 6, 8, 9]) == 4.5\n\n\ndef test_main():\n \"\"\"Test the main function for correctness.\"\"\"\n # Backup stdin.read\n _stdin_read = sys.stdin.read\n\n # patch main's print\n def print_patch(arg):\n assert arg == 5.5\n\n main.__globals__[\"print\"] = print_patch\n\n # patch main's stdin\n class stdin_patch:\n def read(self):\n return \"1 2 3 4 5.5 6 7 8 9\\n\"\n\n main.__globals__[\"sys\"].stdin = stdin_patch()\n\n # invoke main with mocks\n main()\n\n # undo patches\n main.__globals__[\"sys\"].stdin.read = _stdin_read\n del main.__globals__[\"print\"]\n\n\nif __name__ == \"__main__\":\n test_median()\n # test_main()\n main()"
}
{
"filename": "code/dynamic_programming/src/array_median/array_median.rb",
"content": "# Part of Cosmos by OpenGenus Foundation\n\ndef median(*nums)\n nums.sort!\n if nums.size.odd?\n nums[(nums.size + 1) / 2 - 1]\n else\n (nums[nums.size / 2 - 1] + nums[nums.size / 2]).fdiv(2)\n end\nend"
}
{
"filename": "code/dynamic_programming/src/array_median/array_median.rs",
"content": "// Part of Cosmos by OpenGenus Foundation\n\nfn median(arr: &[i32]) -> f64 {\n let len = arr.len();\n if len % 2 == 0 {\n (arr[len / 2] + arr[len / 2 - 1]) as f64 / 2.0\n } else {\n arr[(len - 1) / 2] as f64\n }\n}\n\nfn main() {\n let arr = vec![1, 3, 3, 4, 5, 6, 8, 9];\n let median = median(&arr); //Assuming input is sorted array\n println!(\"{}\", median);\n}"
}
{
"filename": "code/dynamic_programming/src/assembly_line_scheduling/assembly_line_scheduling.cpp",
"content": "// Part of OpenGenus Cosmos\n// A C++ program to find minimum possible \n// time by the car chassis to complete \n#include <bits/stdc++.h> \nusing namespace std; \n#define NUM_LINE 2 \n#define NUM_STATION 4 \n\n// Utility function to find a minimum of two numbers \nint min(int a, int b) \n{ \n\treturn a < b ? a : b; \n} \n\nint carAssembly(int a[][NUM_STATION], \n\t\t\t\tint t[][NUM_STATION], \n\t\t\t\tint *e, int *x) \n{ \n\tint T1[NUM_STATION], T2[NUM_STATION], i; \n\n\t// time taken to leave first station in line 1 \n\tT1[0] = e[0] + a[0][0]; \n\t\n\t// time taken to leave first station in line 2 \n\tT2[0] = e[1] + a[1][0]; \n\n\t// Fill tables T1[] and T2[] using the \n\t// above given recursive relations \n\tfor (i = 1; i < NUM_STATION; ++i) \n\t{ \n\t\tT1[i] = min(T1[i - 1] + a[0][i], \n\t\t\t\t\tT2[i - 1] + t[1][i] + a[0][i]); \n\t\tT2[i] = min(T2[i - 1] + a[1][i], \n\t\t\t\t\tT1[i - 1] + t[0][i] + a[1][i]); \n\t} \n\n\t// Consider exit times and retutn minimum \n\treturn min(T1[NUM_STATION - 1] + x[0], \n\t\t\tT2[NUM_STATION - 1] + x[1]); \n} \n\n// Driver Code \nint main() \n{ \n\tint a[][NUM_STATION] = {{4, 5, 3, 2}, \n\t\t\t\t\t\t\t{2, 10, 1, 4}}; \n\tint t[][NUM_STATION] = {{0, 7, 4, 5}, \n\t\t\t\t\t\t\t{0, 9, 2, 8}}; \n\tint e[] = {10, 12}, x[] = {18, 7}; \n\n\tcout << carAssembly(a, t, e, x); \n\n\treturn 0; \n} \n\n// This is code is contributed by rathbhupendra "
}
{
"filename": "code/dynamic_programming/src/best_time_to_sell_stock_II/best_time_to_sell_stock_II.cpp",
"content": "// You are given an integer array prices where prices[i] is the price of a given stock on the ith day.\n\n// On each day, you may decide to buy and/or sell the stock. You can only hold at most one share of the stock at any time. However, you can buy it then immediately sell it on the same day.\n\n// Find and return the maximum profit you can achieve.\n\n \n\n// Example 1:\n\n// Input: prices = [7,1,5,3,6,4]\n// Output: 7\n// Explanation: Buy on day 2 (price = 1) and sell on day 3 (price = 5), profit = 5-1 = 4.\n// Then buy on day 4 (price = 3) and sell on day 5 (price = 6), profit = 6-3 = 3.\n// Total profit is 4 + 3 = 7.\n\n#include<bits/stdc++.h>\nclass Solution {\npublic:\n int maxProfit(vector<int>& arr) {\n int pr = 0;\n for(int i=1; i<arr.size(); i++){\n if(arr[i]>arr[i-1]){\n pr+= arr[i]-arr[i-1];\n }\n }\n return pr;\n }\n};"
}
{
"filename": "code/dynamic_programming/src/binomial_coefficient/binomial_coefficient.c",
"content": "/*\n * dynamic programming | binomial coefficient | C\n * Part of Cosmos by OpenGenus Foundation\n * A DP based solution that uses table C[][] to calculate the Binomial Coefficient\n */\n\n#include <stdio.h>\n\n// A utility function to return minimum of two integers\nint \nmin(int a, int b) \n{\n return (a < b ? a : b);\n}\n\n// Returns value of Binomial Coefficient C(n, k)\nint \nbinomialCoeff(int n, int k)\n{\n int C[n + 1][k + 1];\n int i, j;\n \n // Caculate value of Binomial Coefficient in bottom up manner\n for (i = 0; i <= n; i++) {\n for (j = 0; j <= min(i, k); j++) {\n // Base Cases\n if (j == 0 || j == i) {\n C[i][j] = 1;\n }\n \n // Calculate value using previosly stored values\n else {\n C[i][j] = C[i - 1][j - 1] + C[i - 1][j];\n }\n }\n }\n \n return C[n][k];\n}\n \nint main() {\n int n = 5, k = 2;\n printf (\"Value of C(%d, %d) is %d \\n\", n, k, binomialCoeff(n, k) );\n return (0);\n}"
}
{
"filename": "code/dynamic_programming/src/binomial_coefficient/binomial_coefficient.cpp",
"content": "// dynamic programming | binomial coefficient | C++\n// Part of Cosmos by OpenGenus Foundation\n\n#include <iostream>\n#include <vector>\n\nusing namespace std;\n\n/*\n * Computes C(n,k) via dynamic programming\n * C(n,k) = C(n-1, k-1) + C(n-1, k)\n * Time complexity: O(n*k)\n * Space complexity: O(k)\n */\nlong long binomialCoefficient(int n, int k)\n{\n\n long long C[k + 1];\n for (int i = 0; i < k + 1; ++i)\n C[i] = 0;\n C[0] = 1;\n\n for (int i = 1; i <= n; ++i)\n for (int j = min(i, k); j > 0; --j)\n C[j] = C[j] + C[j - 1];\n\n return C[k];\n}\n\n/*\n * More efficient algorithm to compute binomial coefficient\n * Time complexity: O(k)\n * Space complexity: O(1)\n */\nlong long binomialCoefficient_2(int n, int k)\n{\n\n long long answer = 1;\n\n k = min(k, n - k);\n\n for (int i = 1; i <= k; ++i, --n)\n {\n answer *= n;\n answer /= i;\n }\n\n return answer;\n}\n\nvoid test(vector< pair<int, int>>& testCases)\n{\n\n for (auto test : testCases)\n cout << \"C(\" << test.first << \",\" << test.second << \") = \"\n << binomialCoefficient(test.first, test.second) << \" = \"\n << binomialCoefficient_2(test.first, test.second) << '\\n';\n}\n\nint main()\n{\n\n vector< pair<int, int>> testCases = {\n {5, 2},\n {6, 6},\n {7, 5},\n {10, 6},\n {30, 15}\n };\n\n test(testCases);\n\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/binomial_coefficient/binomial_coefficient.ex",
"content": "defmodule BinomialCoefficient do\n def binn_coef(n, k) do\n cond do\n n <= 0 or k <= 0 ->\n {:error, :non_positive}\n\n n < k ->\n {:error, :k_greater}\n\n true ->\n p = n - k\n\n {rem_fact_n, div_fact} =\n if p > k do\n {\n n..(p + 1)\n |> Enum.reduce(1, &(&1 * &2)),\n 2..k\n |> Enum.reduce(1, &(&1 * &2))\n }\n else\n {\n n..(k + 1)\n |> Enum.reduce(1, &(&1 * &2)),\n 2..p\n |> Enum.reduce(1, &(&1 * &2))\n }\n end\n\n rem_fact_n / div_fact\n end\n end\nend\n\n# This code is contributed by ryguigas0"
}
{
"filename": "code/dynamic_programming/src/binomial_coefficient/binomial_coefficient.java",
"content": "// dynamic programming | binomial coefficient | Java\n// Part of Cosmos by OpenGenus Foundation\n\nimport java.io.*;\nimport java.lang.*;\nimport java.math.*;\nimport java.util.*;\n\nclass BinomialCoefficient{\n\tstatic int binomialCoeff(int n, int k) {\n\t\tif (k>n) {\n\t\t\treturn 0;\n\t\t}\n\t\t\n\t\tint c[] = new int[k+1];\n\t\tint i, j;\n\t\t\n\t\tc[0] = 1;\n\t\t\n\t\tfor (i=0; i<=n; i++) {\n\t\t\tfor (j=min(i,k); j>0; j--) {\n\t\t\t\tc[j] = c[j] + c[j-1];\n\t\t\t}\n\t\t}\n\t\t\n\t\treturn c[k];\n\t}\n\t\n\tstatic int min(int a, int b) {\n\t\treturn (a<b)? a: b;\n\t}\n\t\n\t//test case\n\tpublic static void main(String args[]) {\n\t\tint n = 5, k = 2;\n\t\tSystem.out.println(\"Value of C(\"+n+\",\"+k+\") is \"+binomialCoeff(n, k));\n\t}\n}"
}
{
"filename": "code/dynamic_programming/src/binomial_coefficient/binomial_coefficient.js",
"content": "// dynamic programming | binomial coefficient | javascript js\n// Part of Cosmos by OpenGenus Foundation\n\n/*Call the function with desired values of n and k.\n'ar' is the array name.*/\n\nfunction binomialCoefficient(n, k) {\n if (k > n) {\n console.log(\"Not possible.\");\n return;\n }\n\n const ar = [n + 1];\n for (let i = 0; i < n + 1; i++) {\n ar[i] = [n + 1];\n }\n\n for (i = 0; i < n + 1; i++) {\n for (let j = 0; j < n + 1; j++) {\n if (i == j || j == 0) {\n ar[i][j] = 1;\n } else if (j > i) {\n break;\n } else {\n ar[i][j] = ar[i - 1][j - 1] + ar[i - 1][j];\n }\n }\n }\n\n console.log(ar[n][k]);\n}"
}
{
"filename": "code/dynamic_programming/src/binomial_coefficient/binomial_coefficient.py",
"content": "# dynamic programming | binomial coefficient | Python\n# Part of Cosmos by OpenGenus Foundation\nimport numpy as np\n\n# implementation using dynamic programming\ndef binomialCoefficient(n, k, C=None):\n if k < 0 or n < 0:\n raise ValueError(\"n,k must not be less than 0\")\n\n if k > n:\n return 0\n\n k = min(k, n - k)\n\n if C is None:\n C = np.zeros((n + 1, k + 1))\n\n if k == 0:\n C[n, k] = 1\n\n if C[n, k] == 0:\n C[n, k] = binomialCoefficient(n - 1, k, C=C) + binomialCoefficient(\n n - 1, k - 1, C=C\n )\n\n return C[n, k]\n\n\n# test\n# print(binomialCoefficient(10,3))\n# print(binomialCoefficient(10,6))\n# print(binomialCoefficient(10,4))\n# print(binomialCoefficient(1,1))\n# print(binomialCoefficient(0,0))\n# print(binomialCoefficient(0,1))\n# print(binomialCoefficient(-1,1))"
}
{
"filename": "code/dynamic_programming/src/binomial_coefficient/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "",
"content": ""
}
{
"filename": "code/dynamic_programming/src/bitmask_dp/README.md",
"content": "# Bitmask DP\n\nBitmask DP is commonly used when we have smaller constraints and it's easier to try out all the possibilities to arrive at the solution.\n\nPlease feel free to add more explaination and problems for this topic!"
}
{
"filename": "code/dynamic_programming/src/boolean_parenthesization/boolean_parenthesization.c",
"content": "/*\n * dynamic programming | boolean parenthesization | C\n * Part of Cosmos by OpenGenus Foundation\n */\n\n#include <stdio.h>\n\n// Dynamic programming implementation of the boolean\n// parenthesization problem using 'T' and 'F' as characters\n// and '&', '|' and '^' as the operators\nint \nboolean_parenthesization(char c[], char o[], int n)\n{\n int i, j, k, a, t[n][n], f[n][n];\n\n for (i = 0; i < n; i++) {\n\n if (c[i] == 'T') {\n t[i][i] = 1;\n f[i][i] = 0;\n\n }\n else {\n t[i][i] = 0;\n f[i][i] = 1;\n }\n }\n\n for (i = 1; i < n; ++i) {\n\n for (j = 0, k = i; k <n; ++j,++k) {\n\n t[j][k] = 0;\n f[j][k] = 0;\n\n for (a = 0; a < i; a++) {\n int b = j + a;\n int d = t[j][b] + f[j][b];\n int e = t[b + 1][k] + f[b + 1][k];\n\n if (o[b] == '|') {\n t[j][k] += d * e - f[j][b] * f[b + 1][k];\n f[j][k] += f[j][b] * f[b + 1][k];\n }\n else if (o[b] == '&') {\n t[j][k] += t[j][b] * t[b + 1][k];\n f[j][k] += d * e - t[j][b] * t[b + 1][k];\n }\n else {\n t[j][k] += f[j][b] * t[b + 1][k] + t[j][b] * f[b + 1][k];\n f[j][k] += t[j][b] * t[b + 1][k] + f[j][b] * f[b + 1][k];\n }\n }\n }\n }\n return t[0][n - 1];\n}\n\nint main()\n{\n char c[] = \"TFTTF\";\n char s[] = \"|&|^\";\n int n = sizeof(c)-1 / sizeof(c[0]);\n printf(\"%d\\n\", boolean_parenthesization(c, s, n));\n return (0);\n}"
}
{
"filename": "code/dynamic_programming/src/boolean_parenthesization/boolean_parenthesization.cpp",
"content": "// dynamic programming | boolean parenthesization | C++\n// Part of Cosmos by OpenGenus Foundation\n\n#include <iostream>\n#include <cstring>\n\n// Dynamic programming implementation of the boolean\n// parenthesization problem using 'T' and 'F' as characters\n// and '&', '|' and '^' as the operators\nint boolean_parenthesization(char c[], char o[], int n)\n{\n int t[n][n], f[n][n];\n\n for (int i = 0; i < n; ++i)\n {\n\n if (c[i] == 'T')\n {\n t[i][i] = 1;\n f[i][i] = 0;\n\n }\n else\n {\n t[i][i] = 0;\n f[i][i] = 1;\n }\n }\n\n for (int i = 1; i < n; ++i)\n for (int j = 0, k = i; k < n; ++j, ++k)\n {\n\n t[j][k] = 0;\n f[j][k] = 0;\n\n for (int a = 0; a < i; ++a)\n {\n int b = j + a;\n int d = t[j][b] + f[j][b];\n int e = t[b + 1][k] + f[b + 1][k];\n\n if (o[b] == '|')\n {\n t[j][k] += d * e - f[j][b] * f[b + 1][k];\n f[j][k] += f[j][b] * f[b + 1][k];\n }\n else if (o[b] == '&')\n {\n t[j][k] += t[j][b] * t[b + 1][k];\n f[j][k] += d * e - t[j][b] * t[b + 1][k];\n }\n else\n {\n t[j][k] += f[j][b] * t[b + 1][k] + t[j][b] * f[b + 1][k];\n f[j][k] += t[j][b] * t[b + 1][k] + f[j][b] * f[b + 1][k];\n }\n }\n }\n return t[0][n - 1];\n}\n\nint main()\n{\n char c[] = \"TFTTF\";\n char s[] = \"|&|^\";\n int n = sizeof(c) - 1 / sizeof(c[0]);\n std::cout << boolean_parenthesization(c, s, n);\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/boolean_parenthesization/boolean_parenthesization.java",
"content": "// dynamic programming | boolean parenthesization | Java\n// Part of Cosmos by OpenGenus Foundation\n\n/*** Dynamic programming implementation of the boolean\n * parenthesization problem using 'T' and 'F' as characters\n * and '&', '|' and '^' as the operators\n ***/\n\nclass boolean_parenthesization{\n public static int boolean_parenthesization_(String symbols, String operators) {\n int noOfSymbols = symbols.length();\n int[][] trueMatrix = new int[noOfSymbols][noOfSymbols], falseMatrix = new int[noOfSymbols][noOfSymbols];\n\n for (int index=0; index < noOfSymbols; index++) {\n if (symbols.charAt(index) == 'T') {\n trueMatrix[index][index] = 1;\n falseMatrix[index][index] = 0;\n }else {\n trueMatrix[index][index] = 0;\n falseMatrix[index][index] = 1;\n }\n }\n\n for (int loopVar1=1; loopVar1 < noOfSymbols; loopVar1++) {\n for (int innerLoopVar1=0, innerLoopVar2=loopVar1; innerLoopVar2 < noOfSymbols; innerLoopVar1++, innerLoopVar2++) {\n trueMatrix[innerLoopVar1][innerLoopVar2] = 0;\n falseMatrix[innerLoopVar1][innerLoopVar2] = 0;\n int b, d, e;\n for (int a=0; a < loopVar1; a++){\n b = innerLoopVar1 + a;\n d = trueMatrix[innerLoopVar1][b] + falseMatrix[innerLoopVar1][b];\n e = trueMatrix[b+1][innerLoopVar2] + falseMatrix[b+1][innerLoopVar2];\n\n switch (operators.charAt(b)) {\n case '|':\n trueMatrix[innerLoopVar1][innerLoopVar2] += d * e - falseMatrix[innerLoopVar1][b] * falseMatrix[b+1][innerLoopVar2];\n falseMatrix[innerLoopVar1][innerLoopVar2] += falseMatrix[innerLoopVar1][b] * falseMatrix[b+1][innerLoopVar2];\n break;\n case '&':\n trueMatrix[innerLoopVar1][innerLoopVar2] += trueMatrix[innerLoopVar1][b] * trueMatrix[b+1][innerLoopVar2];\n falseMatrix[innerLoopVar1][innerLoopVar2] += d * e - trueMatrix[innerLoopVar1][b] * trueMatrix[b+1][innerLoopVar2];\n break;\n case '^':\n trueMatrix[innerLoopVar1][innerLoopVar2] += falseMatrix[innerLoopVar1][b] * trueMatrix[b+1][innerLoopVar2] + trueMatrix[innerLoopVar1][b] * falseMatrix[b+1][innerLoopVar2];\n falseMatrix[innerLoopVar1][innerLoopVar2] += trueMatrix[innerLoopVar1][b] * trueMatrix[b+1][innerLoopVar2] + falseMatrix[innerLoopVar1][b] * falseMatrix[b+1][innerLoopVar2];;\n break;\n }\n }\n }\n }\n return trueMatrix[0][noOfSymbols - 1];\n }\n public static void main(String[] args){\n String symbols = \"TFTTF\";\n String operators = \"|&|^\";\n System.out.println(boolean_parenthesization_(symbols, operators));\n }\n}"
}
{
"filename": "code/dynamic_programming/src/boolean_parenthesization/boolean_parenthesization.py",
"content": "# dynamic programming | boolean parenthesization | Python\n# Part of Cosmos by OpenGenus Foundation\n\n# Dynamic programming implementation of the boolean\n# parenthesization problem using 'T' and 'F' as characters\n# and '&', '|' and '^' as the operators\n\n\ndef boolean_parenthesization(c, o, n):\n t = [[0 for i in range(n)] for j in range(n)]\n f = [[0 for i in range(n)] for j in range(n)]\n\n for i in range(n):\n if c[i] == \"T\":\n t[i][i] = 1\n f[i][i] = 0\n else:\n t[i][i] = 0\n f[i][i] = 1\n\n for i in range(1, n):\n j, k = 0, i\n while k < n:\n\n t[j][k] = 0\n f[j][k] = 0\n\n for a in range(i):\n b = j + a\n d = t[j][b] + f[j][b]\n e = t[b + 1][k] + f[b + 1][k]\n\n if o[b] == \"|\":\n t[j][k] += d * e - f[j][b] * f[b + 1][k]\n f[j][k] += f[j][b] * f[b + 1][k]\n elif o[b] == \"&\":\n t[j][k] += t[j][b] * t[b + 1][k]\n f[j][k] += d * e - t[j][b] * t[b + 1][k]\n else:\n t[j][k] += f[j][b] * t[b + 1][k] + t[j][b] * f[b + 1][k]\n f[j][k] += t[j][b] * t[b + 1][k] + f[j][b] * f[b + 1][k]\n j += 1\n k += 1\n return t[0][n - 1]\n\n\ndef main():\n c = \"TFTTF\"\n s = \"|&|^\"\n n = len(c)\n print(boolean_parenthesization(c, s, n))\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/dynamic_programming/src/boolean_parenthesization/boolean_parenthesization.swift",
"content": "// dynamic programming | boolean parenthesization | Swift\n// Part of Cosmos by OpenGenus Foundation\n\n// Dynamic programming implementation of the boolean\n// parenthesization problem using 'T' and 'F' as characters\n// and '&', '|' and '^' as the operators\nclass BooleanParenthesization {\n \n private var characters = [Character]()\n private var operators = [Character]()\n private var memo = [[(Int, Int)]]()\n \n public func booleanParenthesization(_ expression: String) -> Int {\n \n parse(expression)\n \n memo = [[(Int, Int)]](repeating: [(Int, Int)](repeating: (-1, -1),\n count: characters.count), count: characters.count)\n \n return solve(0, characters.count - 1).0\n }\n \n private func parse(_ expression: String) {\n \n characters = []\n operators = []\n for char in expression {\n \n if char == \"T\" || char == \"F\" {\n characters.append(char)\n }\n else if char == \"&\" || char == \"|\" || char == \"^\" {\n operators.append(char)\n }\n }\n \n }\n \n private func solve(_ i: Int, _ j: Int) -> (Int, Int) {\n \n if(i == j) {\n return characters[i] == \"T\" ? (1, 0) : (0, 1)\n }\n \n if(memo[i][j] > (-1, -1)) {\n return memo[i][j]\n }\n \n var total = (0, 0)\n \n for k in i..<j {\n \n let left = solve(i, k)\n let right = solve(k+1, j)\n var answer = (0, 0)\n \n if operators[k] == \"&\" {\n answer.0 = left.0 * right.0\n answer.1 = left.0 * right.1 + left.1 * right.0 + left.1 * right.1\n }\n else if operators[k] == \"|\" {\n answer.0 = left.0 * right.0 + left.0 * right.1 + left.1 * right.0\n answer.1 = left.1 * right.1\n }\n else if operators[k] == \"^\" {\n answer.0 = left.0 * right.1 + left.1 * right.0\n answer.1 = left.0 * right.0 + left.1 * right.1\n }\n \n total.0 += answer.0\n total.1 += answer.1\n }\n \n memo[i][j] = total\n return total\n }\n \n}\n\nfunc test() {\n \n let solution = BooleanParenthesization()\n \n let testCases = [\n \"T|T&F^T\", // 4\n \"T^F|F\", // 2\n \"T&F^F|T\", // 5\n ]\n \n for test in testCases {\n print(solution.booleanParenthesization(test))\n }\n \n}\n\ntest()"
}
{
"filename": "code/dynamic_programming/src/boolean_parenthesization/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/dynamic_programming/src/box_stacking/box_stacking.cpp",
"content": "// dynamic programming | box stacking | C++\n// Part of Cosmos by OpenGenus Foundation\n\n#include <iostream>\n#include <algorithm>\n#include <vector>\n\nusing namespace std;\n\n// Struct to represent the boxes\nstruct Box\n{\n\n int height, width, length;\n\n // Initializer\n Box()\n {\n }\n Box(int h, int w, int l) : height(h), width(w), length(l)\n {\n }\n\n // Return the area of the box\n int area() const\n {\n return this->width * this->length;\n }\n\n // Return true if current box can be put\n // on the other, and false otherwise\n bool fitOn(const struct Box other)\n {\n return min(this->width, this->length) < min(other.width, other.length) &&\n max(this->width, this->length) < max(other.width, other.length);\n }\n\n // Compare the boxes based on their area\n bool operator<(const struct Box other) const\n {\n return this->area() < other.area();\n }\n};\n\n/**\n * Return the maximum height that can be\n * obtained by stacking the available boxes.\n * Time complexity: O(n^2), n = size of boxes\n */\nint maxHeight(vector<Box> boxes)\n{\n\n // Get the rotations of current boxes\n for (int i = 0, n = boxes.size(); i < n; ++i)\n {\n boxes.push_back(Box(boxes[i].width, boxes[i].length, boxes[i].height));\n boxes.push_back(Box(boxes[i].length, boxes[i].height, boxes[i].width));\n }\n\n // Sort the boxes by decreasing order of area\n sort(boxes.rbegin(), boxes.rend());\n\n int dp[boxes.size()], ans = 0;\n\n // Find the longest increasing sequence of boxes\n for (size_t i = 0; i < boxes.size(); ++i)\n {\n dp[i] = boxes[i].height;\n for (size_t j = 0; j < i; ++j)\n if (boxes[i].fitOn(boxes[j]))\n dp[i] = max(dp[i], boxes[i].height + dp[j]);\n ans = max(ans, dp[i]);\n }\n\n return ans;\n}\n\nint main()\n{\n\n // Using C++11 initializer\n vector<Box> boxes1 = {\n Box(4, 6, 7),\n Box(1, 2, 3),\n Box(4, 5, 6),\n Box(10, 12, 32)\n };\n\n vector<Box> boxes2 = {\n Box(1, 2, 3),\n Box(4, 5, 6),\n Box(3, 4, 1)\n };\n\n cout << maxHeight(boxes1) << endl; // Expected output: 60\n cout << maxHeight(boxes2) << endl; // Expected output: 15\n}"
}
{
"filename": "code/dynamic_programming/src/box_stacking/box_stacking.java",
"content": "// dynamic programming | box stacking | Java\n// Part of Cosmos by OpenGenus Foundation\n\nimport java.util.Arrays;\n\npublic class BoxStacking {\n\n public int maxHeight(Dimension[] input) {\n //get all rotations of box dimension.\n //e.g if dimension is 1,2,3 rotations will be 2,1,3 3,2,1 3,1,2 . Here length is always greater\n //or equal to width and we can do that without loss of generality.\n Dimension[] allRotationInput = new Dimension[input.length * 3];\n createAllRotation(input, allRotationInput);\n\n //sort these boxes in non increasing order by their base area.(length X width)\n Arrays.sort(allRotationInput);\n\n //apply longest increasing subsequence kind of algorithm on these sorted boxes.\n int T[] = new int[allRotationInput.length];\n int result[] = new int[allRotationInput.length];\n\n for (int i = 0; i < T.length; i++) {\n T[i] = allRotationInput[i].height;\n result[i] = i;\n }\n\n for (int i = 1; i < T.length; i++) {\n for (int j = 0; j < i; j++) {\n if (allRotationInput[i].length < allRotationInput[j].length\n && allRotationInput[i].width < allRotationInput[j].width) {\n if( T[j] + allRotationInput[i].height > T[i]){\n T[i] = T[j] + allRotationInput[i].height;\n result[i] = j;\n }\n }\n }\n }\n\n //find max in T[] and that will be our max height.\n //Result can also be found using result[] array.\n int max = Integer.MIN_VALUE;\n for(int i=0; i < T.length; i++){\n if(T[i] > max){\n max = T[i];\n }\n }\n\n return max;\n }\n\n //create all rotations of boxes, always keeping length greater or equal to width\n private void createAllRotation(Dimension[] input,\n Dimension[] allRotationInput) {\n int index = 0;\n for (int i = 0; i < input.length; i++) {\n allRotationInput[index++] = Dimension.createDimension(\n input[i].height, input[i].length, input[i].width);\n allRotationInput[index++] = Dimension.createDimension(\n input[i].length, input[i].height, input[i].width);\n allRotationInput[index++] = Dimension.createDimension(\n input[i].width, input[i].length, input[i].height);\n\n }\n }\n\n public static void main(String args[]) {\n BoxStacking bs = new BoxStacking();\n Dimension input[] = { new Dimension(3, 2, 5), new Dimension(1, 2, 4) };\n int maxHeight = bs.maxHeight(input);\n System.out.println(\"Max height is \" + maxHeight);\n assert 11 == maxHeight;\n }\n}\n\nclass Dimension implements Comparable<Dimension> {\n int height;\n int length;\n int width;\n\n Dimension(int height, int length, int width) {\n this.height = height;\n this.length = length;\n this.width = width;\n }\n\n Dimension() {\n }\n\n static Dimension createDimension(int height, int side1, int side2) {\n Dimension d = new Dimension();\n d.height = height;\n if (side1 >= side2) {\n d.length = side1;\n d.width = side2;\n } else {\n d.length = side2;\n d.width = side1;\n }\n return d;\n }\n\n /**\n * Sorts by base area(length X width)\n */\n @Override\n public int compareTo(Dimension d) {\n if (this.length * this.width >= d.length * d.width) {\n return -1;\n } else {\n return 1;\n }\n }\n\n @Override\n public String toString() {\n return \"Dimension [height=\" + height + \", length=\" + length\n + \", width=\" + width + \"]\";\n }\n}"
}
{
"filename": "code/dynamic_programming/src/box_stacking/box_stacking.py",
"content": "\"\"\"\ndynamic programming | box stacking | Python\nPart of Cosmos by OpenGenus Foundation\n\"\"\"\n\nfrom collections import namedtuple\nfrom itertools import permutations\n\ndimension = namedtuple(\"Dimension\", \"height length width\")\n\n\ndef create_rotation(given_dimensions):\n \"\"\"\n A rotation is an order wherein length is greater than or equal to width. Having this constraint avoids the\n repetition of same order, but with width and length switched.\n For e.g (height=3, width=2, length=1) is same the same box for stacking as (height=3, width=1, length=2).\n :param given_dimensions: Original box dimensions\n :return: All the possible rotations of the boxes with the condition that length >= height.\n \"\"\"\n for current_dim in given_dimensions:\n for (height, length, width) in permutations(\n (current_dim.height, current_dim.length, current_dim.width)\n ):\n if length >= width:\n yield dimension(height, length, width)\n\n\ndef sort_by_decreasing_area(rotations):\n return sorted(rotations, key=lambda dim: dim.length * dim.width, reverse=True)\n\n\ndef can_stack(box1, box2):\n return box1.length < box2.length and box1.width < box2.width\n\n\ndef box_stack_max_height(dimensions):\n boxes = sort_by_decreasing_area(\n [rotation for rotation in create_rotation(dimensions)]\n )\n num_boxes = len(boxes)\n T = [rotation.height for rotation in boxes]\n R = [idx for idx in range(num_boxes)]\n\n for i in range(1, num_boxes):\n for j in range(0, i):\n if can_stack(boxes[i], boxes[j]):\n stacked_height = T[j] + boxes[i].height\n if stacked_height > T[i]:\n T[i] = stacked_height\n R[i] = j\n\n max_height = max(T)\n start_index = T.index(max_height)\n\n # Prints the dimensions which were stored in R list.\n while True:\n print(boxes[start_index])\n next_index = R[start_index]\n if next_index == start_index:\n break\n start_index = next_index\n\n return max_height\n\n\nif __name__ == \"__main__\":\n\n d1 = dimension(3, 2, 5)\n d2 = dimension(1, 2, 4)\n\n assert 11 == box_stack_max_height([d1, d2])"
}
{
"filename": "code/dynamic_programming/src/box_stacking/README.md",
"content": "# Box Stacking\n\n## Description\n\nGiven a set of n types of 3D rectangular boxes, find the maximum height\nthat can be reached stacking instances of these boxes. Some onservations:\n\n- A box can be stacked on top of another box only if the dimensions of the\n2D base of the lower box are each strictly larger than those of the 2D base\nof the higher box.\n- The boxes can be rotated so that any side functions as its base.\n- It is allowable to use multiple instances of the same type of box.\n\n## Solution\n\nThis problem can be seen as a variation of the dynamic programming\nproblem LIS (longest increasing sequence). The steps to solve the problem are:\n\n1) Compute the rotations of the given types of boxes.\n2) Sort the boxes by decreasing order of area.\n3) Find the longest increasing sequence of boxes.\n\n---\n\n<p align=\"center\">\nA massive collaborative effort by <a href=\"https://github.com/opengenus/cosmos\">OpenGenus Foundation</a>\n</p>\n\n---"
}
{
"filename": "code/dynamic_programming/src/catalan/catalan_number.go",
"content": "package dynamic\n\nimport \"fmt\"\n\nvar errCatalan = fmt.Errorf(\"can't have a negative n-th catalan number\")\n\n// NthCatalan returns the n-th Catalan Number\n// Complexity: O(n²)\nfunc NthCatalanNumber(n int) (int64, error) {\n\tif n < 0 {\n\t\t//doesn't accept negative number\n\t\treturn 0, errCatalan\n\t}\n\n\tvar catalanNumberList []int64\n\tcatalanNumberList = append(catalanNumberList, 1) //first value is 1\n\n\tfor i := 1; i <= n; i++ {\n\t\tcatalanNumberList = append(catalanNumberList, 0) //append 0 and calculate\n\n\t\tfor j := 0; j < i; j++ {\n\t\t\tcatalanNumberList[i] += catalanNumberList[j] * catalanNumberList[i-j-1]\n\t\t}\n\t}\n\n\treturn catalanNumberList[n], nil\n}"
}
{
"filename": "",
"content": ""
}
{
"filename": "code/dynamic_programming/src/coin_change/coinchange.c",
"content": "// dynamic programming | coin change | C\n// Part of Cosmos by OpenGenus Foundation\n\n#include<stdio.h>\nint \ncount( int S[], int m, int n )\n{\n int i, j, x, y;\n \n // We need n+1 rows as the table is consturcted in bottom up manner using \n // the base case 0 value case (n = 0)\n int table[n + 1][m];\n \n // Fill the enteries for 0 value case (n = 0)\n for (i = 0; i < m; i++)\n table[0][i] = 1;\n \n // Fill rest of the table enteries in bottom up manner \n for (i = 1; i < n + 1; i++) {\n for (j = 0; j < m; j++) {\n // Count of solutions including S[j]\n x = (i - S[j] >= 0) ? table[i - S[j]][j] : 0;\n \n // Count of solutions excluding S[j]\n y = (j >= 1) ? table[i][j - 1]: 0;\n \n // total count\n table[i][j] = x + y;\n }\n }\n\n return (table[n][m - 1]);\n}\n\nint main()\n{\n\tint n;\n\tcin>>n;\n\n\tint arr[n];\n\tfor(int i=0;i<n;++i)\n\t\tcin>>arr[i];\n\n cin>>m;\n printf(\" %d \", count(arr, n, m));\n\n return (0);\n}"
}
{
"filename": "code/dynamic_programming/src/coin_change/coinchange.cpp",
"content": "// dynamic programming | coin change | C++\n// Part of Cosmos by OpenGenus Foundation\n\n#include <iostream>\n#include <vector>\nusing namespace std;\n\nint coinWays(int amt, vector<int>& coins) {\n // init the dp table\n vector<int> dp(amt+1, 0);\n int n = coins.size();\n dp[0] = 1; // base case\n for (int j = 0; j < n; ++j)\n for (int i = 1; i <= amt; ++i)\n if (i - coins[j] >= 0)\n // if coins[j] < i then add no. of ways -\n // - to form the amount by using coins[j]\n dp[i] += dp[i - coins[j]];\n\n // final result at dp[amt]\n return dp[amt];\n}\nint main() {\n vector<int> coins = {1, 2, 3}; // coin denominations\n int amount = 4; // amount\n cout << coinWays(amount, coins) << '\\n';\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/coin_change/coinchange.go",
"content": "/*\n * dynamic programming | coin change | Go\n * Part of Cosmos by OpenGenus Foundation\n */\npackage main\n\nimport \"fmt\"\n\n/*\nThere are 22 ways to combine 8 from [1 2 3 4 5 6 7 8 9 10]\n*/\n\n//DP[i] += DP[i-coint[i]]\n//j = coinset,\n//i = for each money\nfunc solveCoinChange(coins []int, target int) int {\n\n\tdp := make([]int, target+1)\n\tdp[0] = 1 //basic\n\n\tfor _, v := range coins {\n\t\tfor i := 1; i <= target; i++ {\n\t\t\tif i >= v {\n\t\t\t\tdp[i] += dp[i-v]\n\t\t\t}\n\t\t}\n\t}\n\n\treturn dp[target]\n}\nfunc main() {\n\tcoins := []int{1, 2, 3, 4, 5, 6, 7, 8, 9, 10}\n\ttarget := 8\n\n\tway := solveCoinChange(coins, target)\n\tfmt.Printf(\"There are %d ways to combine %d from %v\\n\", way, target, coins)\n}"
}
{
"filename": "code/dynamic_programming/src/coin_change/coin_change.java",
"content": "// dynamic programming | coin change | Java\n// Part of Cosmos by OpenGenus Foundation\n\npublic class WaysToCoinChange {\n\tpublic static int dynamic(int[] v, int amount) {\n\t\tint[][] solution = new int[v.length + 1][amount + 1];\n\n\t\t// if amount=0 then just return empty set to make the change\n\t\tfor (int i = 0; i <= v.length; i++) {\n\t\t\tsolution[i][0] = 1;\n\t\t}\n\n\t\t// if no coins given, 0 ways to change the amount\n\t\tfor (int i = 1; i <= amount; i++) {\n\t\t\tsolution[0][i] = 0;\n\t\t}\n\n\t\t// now fill rest of the matrix.\n\n\t\tfor (int i = 1; i <= v.length; i++) {\n\t\t\tfor (int j = 1; j <= amount; j++) {\n\t\t\t\t// check if the coin value is less than the amount needed\n\t\t\t\tif (v[i - 1] <= j) {\n\t\t\t\t\t// reduce the amount by coin value and\n\t\t\t\t\t// use the subproblem solution (amount-v[i]) and\n\t\t\t\t\t// add the solution from the top to it\n\t\t\t\t\tsolution[i][j] = solution[i - 1][j]\n\t\t\t\t\t\t\t+ solution[i][j - v[i - 1]];\n\t\t\t\t} else {\n\t\t\t\t\t// just copy the value from the top\n\t\t\t\t\tsolution[i][j] = solution[i - 1][j];\n\t\t\t\t}\n\t\t\t}\n\t\t}\n\t\treturn solution[v.length][amount];\n\t}\n\n\tpublic static void main(String[] args) {\n\t\tint amount = 5;\n\t\tint[] v = { 1, 2, 3 };\n\t\tSystem.out.println(\"By Dynamic Programming \" + dynamic(v, amount));\n\t}\n\n}"
}
{
"filename": "code/dynamic_programming/src/coin_change/coin_change.py",
"content": "#################\n## dynamic programming | coin change | Python\n## Part of Cosmos by OpenGenus Foundation\n#################\n\n\ndef coin_change(coins, amount):\n\n # init the dp table\n tab = [0 for i in range(amount + 1)]\n tab[0] = 1 # base case\n\n for j in range(len(coins)):\n for i in range(1, amount + 1):\n if coins[j] <= i:\n # if coins[j] < i then add no. of ways -\n # - to form the amount by using coins[j]\n tab[i] += tab[i - coins[j]]\n\n # final result at tab[amount]\n return tab[amount]\n\n\ndef main():\n\n coins = [1, 2, 3, 8] # coin denominations\n amount = 3 # amount of money\n\n print(\"No. of ways to change - {}\".format(coin_change(coins, amount)))\n\n return\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/dynamic_programming/src/coin_change/mincoinchange.cpp",
"content": "// dynamic programming | coin change | C++\n// Part of Cosmos by OpenGenus Foundation\n\n// A C++ program to find the minimum number of coins required from the list of given coins to make a given value\n// Given a value V, if we want to make change for V cents, and we have infinite supply of each of C = { C1, C2, .. , Cm}\n// valued coins, what is the minimum number of coins to make the change?\n#include <iostream>\nusing namespace std;\n\nint main()\n{\n int t;\n cin >> t; // no. of test cases\n while (t--)\n {\n int v, n;\n cin >> v >> n; // v is value to made and n is the number of given coins\n int c[n + 1];\n for (int i = 0; i < n; i++)\n cin >> c[i]; // c[i] holds denomination or value of different coins\n long long int dp[v + 1];\n long long int m = 100000000;\n dp[0] = 0;\n for (int i = 1; i <= v; i++)\n dp[i] = m;\n for (int i = 0; i <= n - 1; i++)\n for (int j = 1; j <= v; j++)\n if (c[i] <= j)\n if (1 + dp[j - c[i]] < dp[j])\n dp[j] = 1 + dp[j - c[i]];\n\n if (dp[v] == m)\n cout << \"-1\" << endl; // prints -1 if it is not possible to make given amount v\n else\n cout << dp[v] << endl; // dp[v] gives the min coins required to make amount v\n }\n}"
}
{
"filename": "code/dynamic_programming/src/coin_change/README.md",
"content": "# Coin Change\n\n## Description\n\nGiven a set of coins `S` with values `{ S1, S2, ..., Sm }`,\nfind the number of ways of making the change to a certain value `N`.\nThere is an infinite quantity of coins and the order of the coins doesn't matter.\n\nFor example:\n\nFor `S = {1, 2, 3}` and `N = 4`, the number of possibilities is 4, that are:\n`{1,1,1,1}`, `{1,1,2}`, `{2,2}` and `{1,3}`. Note that the sets `{1,2,1}`, `{2,1,1}`\nand `{3,1}` don't count to solution as they are permutations of the other ones.\n\n## Solution\n\nFor each set of coins, there are two possibilities for each coin:\n- The coin is not present in the set.\n- At least one coin is present.\n\nSo, being `f(S[], i, N)` the number of possibilities of making change to `N`\nwith coins from `S[0]` to `S[i]`, it can be computed by summing the number\nof possibilities of getting `N` without using `S[i]`, that is `f(S[], i-1, N)`,\nand the number of possibilities that uses `S[i]`, that is `f(S[], i, N - S[i])`.\n\nSo, we can compute `f(S[], i, N)` by doing the following:\n\n```\nf(S[], i, 0) = 1 // if N reaches 0, than we found a valid solution\n\nf(S[], i, N) = 0, if i < 0 or N < 0\n f(S[], i-1, N) + f(S[], i, N - S[i]), otherwise\n```\n\nApplying memoization to above solution, the time complexity of the algorithm\nbecomes O(N * m), where m is the number of elements in `S`.\n\n---\n\n<p align=\"center\">\nA massive collaborative effort by <a href=\"https://github.com/opengenus/cosmos\">OpenGenus Foundation</a>\n</p>\n\n---"
}
{
"filename": "code/dynamic_programming/src/Count_Subsequence_Having_Product_Less_Than_K/Solution.cpp",
"content": "#include<iostream>\n#include<cstring>\nusing namespace std;\nint dp[10005][10005];\n\nint helper(int a[], int n, int k , int product)\n{\n // base case\n if(n==0)\n {\n return 0;\n }\n if(product > k)\n {\n return 0;\n }\n if(dp[n][product] !=-1)\n {\n return dp[n][product];\n }\n\n int include = product * a[n-1];\n\n int exclude =product;\n int count =0;\n\n if( include < k)\n {\n count++;\n }\n\n count += helper(a,n-1,k,include) + helper(a,n-1,k,exclude);\n\n dp[n][product] =count;\n\n return dp[n][product];\n}\n\nint solve(int a[], int n, int k)\n{\n dp[k+1][n+1];\n memset(dp,-1,sizeof(dp));\n int product =1;\n return helper(a,n,k,product);\n \n}\nint main()\n{\n int n;\n cin>>n;\n \n int a[n];\n for(int i=0;i<n;i++)\n {\n cin>>a[i];\n }\n\n int k;\n cout<<\"Enter the value of k\"<<endl;\n cin>>k;\n\n cout<<solve(a,n,k)<<endl;\n\n return 0;\n\n}"
}
{
"filename": "code/dynamic_programming/src/die_simulation/die_simulation.cpp",
"content": "// Part of OpenGenus cosmos\n\n#define ll long long\nconst int mod = 1e9+7;\n\n// Function to add in presence of modulus. We find the modded answer \n// as the number of sequences becomes exponential fairly quickly\nll addmod(ll a, ll b)\n{ \n return (a%mod + b%mod) % mod;\n}\n\nll dieSimulator_util(int dex, vector<int>& maxroll, int noOfTimes, int prev, vector<vector<vector<ll> > >& dp, int n){\n\n // Simulations are 0 indexed ie. 0 to n-1. Hence return 1 when n is reached \n if(dex==n) \n return 1;\n\n // If for the dex'th simulation, the number of sequences has already been found, return it.\n if(dp[dex][noOfTimes][prev] != -1) \n return dp[dex][noOfTimes][prev]%mod;\n\n // Initialise answer to 0.\n int ans=0;\n \n // Put all the digits in the dex'th simulation and for each of them,\n // move forward ie. do (dex+1)th simulation. \n for(int i=0;i<6;i++)\n {\n // Check if the current digit is the same as previous one\n if(i == prev) \n { \n /*\n Check if the number of times it has occurred has become equal to the allowed number of times. If yes, then \n don't put that digit, otherwise put that digit, add one to the noOfTimes it has occurred and move on.\n */ \n if(noOfTimes < maxroll[i]) \n ans = addmod(ans, dieSimulator_util(dex + 1, maxroll, noOfTimes + 1, prev, dp, n));\n }\n\n else \n {\n ans = addmod(ans, dieSimulator_util(dex + 1, maxroll, 1, i, dp, n));\n }\n }\n\n // Record the answer\n dp[dex][noOfTimes][prev] = ans;\n\n return ans;\n} \nint dieSimulator(int n, vector<int>& maxRolls) {\n\n \n \n // Loop over maxRolls array to find the maximum number of times a digit can be rolled. \n int maxi = 0;\n for(int i=0;i<maxRolls.size();i++)\n {\n if(maxRolls[i] > maxi) \n maxi = maxRolls[i];\n }\n \n /*\n Initialise a 3d DP table with -1 where the row denotes the ith roll simulation, the \n column denotes the number of consecutive times the digit in depth has occurred and the \n depth denotes the number of distinct digits.\n */ \n vector<vector<vector<ll> > > dp(n, vector<vector<ll> >(maxi+1, vector<ll>(7, -1)));\n \n /*\n For the first call, \n current index = 0\n noOfTimes it has occurred = 0 \n no of simulation = 6\n */\n return dieSimulator_util(0, maxRolls, 0, 6, dp, n);\n}"
}
{
"filename": "code/dynamic_programming/src/die_simulation/README.md",
"content": "## Description\n\nThere is a dice simulator which generates random numbers from 0 to 5. You are given an array `constraints` where `constraints[i]` denotes the \nmaximum number of consecutive times the ith number can be rolled. You are also given `n`, the number of times the dice is to be rolled ie. the number of simulations. Find the total number of distinct sequences of length `n` which can be generated by the simulator. \nTwo sequences are considered different if at least one element differs from each other.\n\n## Thought Process\n\nThis question is tough, no doubt. So, I suggest you start with a paper and pen. Take the example `n = 3, constraints = [1,1,1,2,2,3]`. Draw three dashes for the 3 simulations (a roll of the die), \\_ \\_ \\_ and start building the sequence in an algorithmic (repetitive steps) manner.\n\n<details> \n <summary>How to fill the dashes?</summary>\n </br>\n You start filling in increasing order. <br/>\n 1=> You fill 0 _ _ . <br/>\n 2=> For the second dash, you notice that 0 can occur only 1 consecutive time. Hence you can't fill a 0 again. So you fill, 0 1 _ . <br/>\n 3=> For third blank we can choose 0. Hence 0, 1, 0. That's one sequence. <br/>\n 4=> Now, 1 can't occur more than 1 consecutive time. So you fill 2. 0 1 2. That's one sequence. <br/>\n 5=> Now you put 3 inplace of 2. So now your sequence is 0 1 3. That's another valid sequence. <br/>\n 6=> Similarly you put 4 and 5 to form 0 1 4 and 0 1 5. <br/>\n 7=> Now, you come back to 2nd place and put in 2 (recall we already considered 0). 0 2 _ . <br/>\n 8=> Can't put 2 again. Hence, we put 0, 1, 3, 4 and 5 to form 0 2 0, 0 2 1, 0 2 3, 0 2 4, 0 2 5. <br/>\n 9=> For 3, 4, 5 in 2nd place, we repeat the steps. <br/>\n 10=> Come back to 1st place. Put in 1. Now the sequence is 1 _ _ . <br/>\n 11=> In the second place, we can't put in 1. So we put 0. Do the same as above. <br/>\n 12=> After that consider 1 2 _ . But wait! Have we seen this <b>subproblem</b> somewhere before? <br/>The subproblem of 2 in the second place has occurred before.\n We found that 2 0, 2 1, 2 3, 2 4, and 2 5 (but not 2 2) were the allowed sequences after it. Since we only need the count of total sequences, it would be good \n if we record it. Hence let's assume we recorded (and we would btw) that with 2 in the 2nd place, we could form 5 distinct sequences after it. Thus while \n forming 1 _ _ , for 1 2 _ , we need not calculate further. We know that 5 sequences are on their way. <br/>\n 13=> So we try 1 3 _ . But again! We've encountered even this subsequence before in step 9! Similarly for 1 4, 1 5. <br/> \n \n</details>\n\n\n<details> \n <summary>Solution</summary>\n </br>\n We'll recurse over each dash(simulation) and do dp with memoization\n</details>\n\n<details>\n <summary>What's the time complexity?</summary>\n </br>\n Filling each cell takes O(1) time (Filling some cell require us to recurse but we can view that as filling other cells).\n There are a total of n*m*p cells where n is number of simulations, m is the length of constraint array (6 in our case) and p is the maximum constraint value,\n it takes O(6 * np) = O(np) time. \n</details>"
}
{
"filename": "code/dynamic_programming/src/digit_dp/digit_dp.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation\n * By: Sibasish Ghosh (https://github.com/sibasish14)\n */\n\n/* How many numbers x are there in the range a to b?\n * where the digit d occurs exactly k times in x?\n */\n\n#include <iostream>\n#include <vector>\n#include <string.h>\n\nusing namespace std;\n\nlong d = 3;\nlong k = 5;\n\nlong dp[25][25][2];\n/* dp[pos][count][f] = Number of valid numbers <= b from this state\n * pos = current position from left side (zero based)\n * count = number of times we have placed the digit d so far\n * f = the number we are building has already become smaller than b? [0 = no, 1 = yes]\n */\n\nlong digit_dp(string num, long pos, long count, long f)\n{\n if (count > k)\n return 0;\n\n if (pos == num.size())\n {\n if (count == k)\n return 1;\n return 0;\n }\n\n if (dp[pos][count][f] != -1)\n return dp[pos][count][f];\n\n long result = 0;\n long limit;\n\n if (f == 0)\n /* Digits we placed so far matches with the prefix of b\n * So if we place any digit > num[pos] in the current position, then\n * the number will become greater than b\n */\n limit = num[pos] - '0';\n else\n /* The number has already become smaller than b.\n * We can place any digit now.\n */\n limit = 9;\n\n // Try to place all the valid digits such that the number doesn't exceed b\n for (long dgt = 0; dgt <= limit; dgt++)\n {\n long fNext = f;\n long countNext = count;\n\n // The number is getting smaller at this position\n if (f == 0 && dgt < limit)\n fNext = 1;\n\n if (dgt == d)\n countNext++;\n\n if (countNext <= k)\n result += digit_dp(num, pos + 1, countNext, fNext);\n }\n\n return dp[pos][count][f] = result;\n}\n\nlong solve(string num)\n{\n memset(dp, -1, sizeof(dp));\n long result = digit_dp(num, 0, 0, 0);\n return result;\n}\n\nbool check(string num)\n{\n long count = 0;\n for (long i = 0; i < num.size(); i++)\n if (num[i] - '0' == d)\n count++;\n\n if (count == k)\n return true;\n else\n return false;\n}\n\nint main()\n{\n string a = \"100\", b = \"100000000\";\n long ans = solve(b) - solve(a) + check(a);\n cout << ans << \"\\n\";\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/digit_dp/DigitDP.java",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n/* How many numbers x are there in the range a to b?\n * where the digit d occurs exactly k times in x?\n */\n\nimport java.util.Arrays;\n\npublic class DigitDP {\n private static int d = 3;\n private static int k = 5;\n private static int[][][] dp = new int[25][25][2];\n /* dp[pos][count][f] = Number of valid numbers <= b from this state\n * pos = current position from left side (zero based)\n * count = number of times we have placed the digit d so far\n * f = the number we are building has already become smaller than b? [0 = no, 1 = yes]\n */\n\n public static void main(String args[]) {\n String a = \"100\";\n String b = \"100000000\";\n int ans = solve(b) - solve(a) + check(a);\n System.out.println(ans);\n }\n\n private static int check(String num) {\n int count = 0;\n for(int i = 0; i < num.length(); ++i)\n if (num.charAt(i) - '0' == d)\n count++;\n\n if (count == k)\n return 1;\n return 0;\n }\n\n private static int solve(String num) {\n for(int[][] rows: dp)\n for(int[] cols: rows)\n Arrays.fill(cols, -1);\n int ret = digit_dp(num, 0, 0, 0);\n return ret;\n }\n\n private static int digit_dp(String num, int pos, int count, int f) {\n if (count > k)\n return 0;\n if (pos == num.length()) {\n if (count == k)\n return 1;\n return 0;\n }\n if (dp[pos][count][f] != -1)\n return dp[pos][count][f];\n int res = 0;\n int limit;\n if (f == 0) {\n /* Digits we placed so far matches with the prefix of b\n * So if we place any digit > num[pos] in the current position, then\n * the number will become greater than b\n */\n limit = num.charAt(pos) - '0';\n } else {\n /* The number has already become smaller than b.\n * We can place any digit now.\n */ \n limit = 9;\n }\n\n // Try to place all the valid digits such that the number does not exceed b\n for(int dgt = 0; dgt <= limit; ++dgt) {\n int fNext = f;\n int countNext = count;\n\n // The number is getting smaller at this position\n if (f == 0 && dgt < limit)\n fNext = 1;\n if (dgt == d)\n countNext++;\n if (countNext <= k)\n res += digit_dp(num, pos + 1, countNext, fNext);\n }\n dp[pos][count][f] = res;\n return dp[pos][count][f];\n }\n}"
}
{
"filename": "code/dynamic_programming/src/edit_distance/edit_distance_backtracking.cpp",
"content": "// dynamic programming | edit distance | C++\n// Part of Cosmos by OpenGenus Foundation\n\n#include <iostream>\n#include <algorithm>\n#include <vector>\n#include <string>\n#include <cmath>\n\nclass Alignment {\n std::string sx, sy, sa, sb;\n\n std::vector<std::vector<int>> A;\n int sxL, syL;\n int cost = 0;\n\n//insertion cost\n inline int insC()\n {\n return 4;\n }\n\n//deletion cost\n inline int delC()\n {\n return 4;\n }\n\n//modification cost\n inline int modC(char fr, char to)\n {\n if (fr == to)\n return 0;\n return 3;\n }\n\npublic:\n\n//constructor\n Alignment(std::string s1, std::string s2) : sx(s1), sy(s2),\n sxL(s1.length()), syL(s2.length())\n {\n //allocating size to array needed\n A.resize(s2.length() + 4, std::vector<int>(s1.length() + 4, 0));\n }\n\n//recurrence\n int rec(int i, int j)\n {\n return std::min(modC(sx[i - 1], sy[j - 1]) + A[i - 1][j - 1],\n std::min(delC() + A[i - 1][j], insC() + A[i][j - 1]));\n //i-1, j-1 in sx, sy b/c of 0-indexing in string\n }\n\n//building array of cost\n void form_array()\n {\n\n //initialising the base needed in dp\n //building up the first column, consider taking first string sx and second as null\n for (int i = 0; i <= sxL; i++)\n A[i][0] = i * (delC());\n\n //building up the first row, consider taking first string null and second as string sy\n for (int i = 0; i <= syL; i++)\n A[0][i] = i * (insC());\n\n //building up whole array from previously known values\n for (int i = 1; i <= sxL; i++)\n for (int j = 1; j <= syL; j++)\n A[i][j] = rec(i, j);\n\n cost = A[sxL][syL];\n\n }\n\n//finding the alignment\n void trace_back(int i, int j)\n {\n\n while (true)\n {\n if (i == 0 || j == 0)\n break;\n //A[i][j] will have one of the three above values from which it is derived\n //so comparing from each one\n if (i >= 0 && j >= 0 && rec(i, j) == modC(sx[i - 1], sy[j - 1]) + A[i - 1][j - 1])\n {\n sa.push_back(sx[i - 1]);\n sb.push_back(sy[j - 1]);\n i--, j--;\n }\n else if ((i - 1) >= 0 && j >= 0 && rec(i, j) == delC() + A[i - 1][j])\n {\n sa.push_back(sx[i - 1]); //0-indexing of string\n sb.push_back('-');\n i -= 1;\n }\n else if (i >= 0 && (j - 1) >= 0)\n {\n sa.push_back('-'); //0-indexing of string\n sb.push_back(sy[j - 1]);\n j -= 1;\n }\n }\n\n if (i != 0)\n while (i)\n {\n sa.push_back(sx[i - 1]);\n sb.push_back('-');\n i--;\n }\n\n else\n while (j)\n {\n sa.push_back('-');\n sb.push_back(sy[j - 1]);\n j--;\n }\n }\n\n//returning the alignment\n std::pair<std::string, std::string> alignst()\n {\n //reversing the alignments because we have formed the\n //alignments from backward(see: trace_back, i, j started from m, n respectively)\n reverse(sa.begin(), sa.end());\n reverse(sb.begin(), sb.end());\n return make_pair(sa, sb);\n }\n\n\n//returning the cost\n int kyc()\n {\n return cost;\n }\n};\n\nint main()\n{\n using namespace std;\n\n //converting sx to sy\n string sx, sy;\n sx = \"GCCCTAGCG\";\n sy = \"GCGCAATG\";\n\n //standard input stream\n //cin >> sx >> sy;\n\n pair<string, string> st;\n\n Alignment dyn(sx, sy);\n dyn.form_array();\n dyn.trace_back(sx.length(), sy.length());\n st = dyn.alignst();\n //Alignments can be different for same strings but cost will be same\n\n cout << \"Alignments of the strings\\n\";\n cout << st.first << \"\\n\";\n cout << st.second << \"\\n\";\n cout << \"Cost associated = \";\n cout << dyn.kyc() << \"\\n\";\n\n /*\tAlignments\n * M - modification, D - deletion, I - insertion for converting string1 to string2\n *\n * M M MD\n * string1\t- GCCCTAGCG\n * string2 - GCGCAAT-G\n *\n */\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/edit_distance/edit_distance.c",
"content": "/*\n * dynamic programming | edit distance | C\n * Part of Cosmos by OpenGenus Foundation\n */\n\n#include <stdio.h>\n#include <stdlib.h>\n#include <string.h>\n\nconst int MAX = 1010;\n\nint memo[MAX][MAX]; /* used for memoization */\n\nint\nmn(int x, int y)\n{\n return x < y ? x : y;\n}\n\n/* Utility function to find minimum of three numbers */\nint\nmin(int x, int y, int z)\n{\n return mn(x, mn(y, z));\n}\n\nint\neditDist(char str1[], char str2[], int m, int n)\n{\n /*\n * If first string is empty, the only option is to\n * insert all characters of second string into first\n */\n if (m == 0) return n;\n\n /*\n * If second string is empty, the only option is to\n * remove all characters of first string\n */\n if (n == 0) return m;\n\n /* If this state has already been computed, get its value */\n if (memo[m][n] > -1) return memo[m][n];\n\n /*\n * If last characters of two strings are same, nothing\n * much to do. Ignore last characters and get count for\n * remaining strings.\n */\n if (str1[m-1] == str2[n-1])\n return memo[m][n] = editDist(str1, str2, m-1, n-1);\n\n /*\n * If last characters are not same, consider all three\n * operations on last character of first string, recursively\n * compute minimum cost for all three operations and take\n * minimum of three values.\n */\n return memo[m][n] = 1 + min ( editDist(str1, str2, m, n-1), /* Insert */\n editDist(str1, str2, m-1, n), /* Remove */\n editDist(str1, str2, m-1, n-1) /* Replace */\n );\n}\n\n/* Driver program */\nint\nmain()\n{\n char str1[] = \"sunday\";\n char str2[] = \"saturday\";\n\n int str1Length = sizeof(str1) / sizeof(str1[0]);\n int str2Length = sizeof(str2) / sizeof(str2[0]);\n memset(memo, -1 ,sizeof memo);\n int minimum = editDist(str1, str2, str1Length, str2Length);\n printf(\"%d\\n\", minimum);\n\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/edit_distance/edit_distance.cpp",
"content": "// dynamic programming | edit distance | C++\n// Part of Cosmos by OpenGenus Foundation\n\n#include <iostream>\n\n#define MAX 1010\n\nusing namespace std;\n\nint memo[MAX][MAX];\n\n/*\n * A recursive approach to the edit distance problem.\n * Returns the edit distance between a[0...i] and b[0...j]\n * Time complexity: O(n*m)\n */\nint editDistance(string& a, string& b, int i, int j)\n{\n\n if (i < 0)\n return j + 1;\n if (j < 0)\n return i + 1;\n\n if (memo[i][j] > -1)\n return memo[i][j];\n\n if (a[i] == b[j])\n return memo[i][j] = editDistance(a, b, i - 1, j - 1);\n\n int c1 = editDistance(a, b, i - 1, j - 1); // replacing\n int c2 = editDistance(a, b, i - 1, j); // removing\n int c3 = editDistance(a, b, i, j - 1); // inserting\n\n return memo[i][j] = 1 + min(c1, min(c2, c3));\n}\n\nint main()\n{\n\n string a = \"opengenus\", b = \"cosmos\";\n\n for (size_t i = 0; i < MAX; ++i)\n for (size_t j = 0; j < MAX; ++j)\n memo[i][j] = -1;\n // memset(memo, -1, sizeof memo);\n cout << editDistance(a, b, a.length() - 1, b.length() - 1) << '\\n';\n}"
}
{
"filename": "code/dynamic_programming/src/edit_distance/edit_distance.go",
"content": "/*\n * dynamic programming | edit distance | Go\n * Part of Cosmos by OpenGenus Foundation\n *\n * Compile: go build edit_distance.go\n */\n\npackage main\n\nimport (\n\t\"fmt\"\n\t\"os\"\n)\n\nfunc main() {\n\n\tif len(os.Args) != 3 {\n\t\tfmt.Println(\"Wrong input, write input next to the program call\")\n\t\tfmt.Println(\"Example: ./edit_distance string1 string2\")\n\t\treturn\n\t}\n\tstr1 := os.Args[1]\n\tstr2 := os.Args[2]\n\t//str1 := \"sunday\"\n\t//str2 := \"saturday\"\n\tmin := editDistance(str1, str2, len(str1), len(str2))\n\tfmt.Println(min)\n\n}\n\nfunc min(a, b int) int {\n\tif a < b {\n\t\treturn a\n\t}\n\n\treturn b\n}\n\nfunc editDistance(str1 string, str2 string, m int, n int) int {\n\n\t// Create table to store results of DP subproblems\n\tstore := make([][]int, m+1)\n\tfor i := range store {\n\t\tstore[i] = make([]int, n+1)\n\t}\n\tfor i := 0; i <= m; i++ {\n\t\tfor j := 0; j <= n; j++ {\n\t\t\tif i == 0 {\n\t\t\t\t// When i is zero we must insert all (j) characters to get the second string\n\t\t\t\tstore[i][j] = j\n\t\t\t} else if j == 0 {\n\t\t\t\t// Remove all characters in first string\n\t\t\t\tstore[i][j] = i\n\t\t\t} else if str1[i-1] == str2[j-1] {\n\t\t\t\t// Last characters are equal, make recursion without last char\n\t\t\t\tstore[i][j] = store[i-1][j-1]\n\t\t\t} else {\n\t\t\t\t// find minimum over all possibilities\n\t\t\t\tstore[i][j] = 1 + min(min(store[i][j-1], store[i-1][j]), store[i-1][j-1])\n\t\t\t}\n\t\t}\n\t}\n\treturn store[m][n]\n}"
}
{
"filename": "code/dynamic_programming/src/edit_distance/edit_distance_hirschberg.cpp",
"content": "/*\n * dynamic programming | edit distance | C++\n * Part of Cosmos by OpenGenus Foundation\n *\n * Dynamic-Programming + Divide & conquer for getting cost and aligned strings for problem of aligning two large strings\n * Reference - Wikipedia-\"Hirschberg's algorithm\"\n */\n\n#include <string>\n#include <vector>\n#include <iostream>\n#include <algorithm>\n\nusing namespace std;\n\n//cost associated\nint cost = 0;\n\n//insertion cost\ninline int insC()\n{\n return 4;\n}\n\n//deletion cost\ninline int delC()\n{\n return 4;\n}\n\n//modification cost\ninline int modC(char fr, char to)\n{\n if (fr == to)\n return 0;\n return 3;\n}\n\n//reversing the string\nstring rever(string s)\n{\n int k = s.length();\n\n for (int i = 0; i < k / 2; i++)\n swap(s[i], s[k - i - 1]);\n return s;\n}\n\n//minimizing the sum of shortest paths (see:GainAlignment function), calculating next starting point\nint minimize(vector<int> ve1, vector<int> ve2, int le)\n{\n int sum, xmid = 0;\n\n for (int i = 0; i <= le; i++)\n {\n if (i == 0)\n sum = ve1[i] + ve2[le - i]; //reversing the array ve2 by taking its i element from last\n\n if (sum > ve1[i] + ve2[le - i])\n {\n sum = ve1[i] + ve2[le - i];\n xmid = i;\n }\n\n }\n return xmid;\n}\n\npair<string, string> stringOne(string s1, string s2)\n{\n\n //building of array for case string length one of any of the string\n string sa, sb;\n\n int m = s1.length();\n int n = s2.length();\n\n vector<vector<int>> fone(s1.length() + 5, vector<int>(2));\n\n for (int i = 0; i <= m; i++)\n fone[i][0] = i * delC();\n\n for (int j = 0; j <= n; j++)\n fone[0][j] = j * insC();\n\n for (int i = 1; i <= m; i++)\n {\n int j;\n //recurrence\n for (j = 1; j <= n; j++)\n fone[i][j] =\n min(modC(s1[i - 1], s2[j - 1]) + fone[i - 1][j - 1],\n min(delC() + fone[i - 1][j], insC() + fone[i][j - 1]));\n }\n\n int i = m, j = n;\n cost += fone[i][j];\n /*\n * This code can be shortened as beforehand we know one of the string has length one but this gives general idea of a\n * how a backtracking can be done is case of general strings length given we can use m*n space\n */\n //backtracking in case the length is one of string\n while (true)\n {\n\n if (i == 0 || j == 0)\n break;\n\n //fone[i][j] will have one of the three above values from which it is derived so comapring from each one\n\n if (i >= 0 && j >= 0 && fone[i][j] == modC(s1[i - 1], s2[j - 1]) + fone[i - 1][j - 1])\n {\n sa.push_back(s1[i - 1]);\n sb.push_back(s2[j - 1]);\n i--; j--;\n }\n else if ((i - 1) >= 0 && j >= 0 && fone[i][j] == delC() + fone[i - 1][j])\n {\n sa.push_back(s1[i - 1]);\n sb.push_back('-');\n i -= 1;\n }\n else if (i >= 0 && (j - 1) >= 0 && fone[i][j - 1] == insC() + fone[i][j - 1])\n {\n sa.push_back('-');\n sb.push_back(s2[j - 1]);\n j -= 1;\n }\n }\n\n //continue backtracking\n if (i != 0)\n while (i)\n {\n sa.push_back(s1[i - 1]);\n sb.push_back('-');\n i--;\n }\n\n else\n while (j)\n {\n sa.push_back('-');\n sb.push_back(s2[j - 1]);\n j--;\n }\n\n //strings obtained are reversed alignment because we have started from i = m, j = n\n reverse(sa.begin(), sa.end());\n reverse(sb.begin(), sb.end());\n\n return make_pair(sa, sb);\n\n}\n\n//getting the cost associated with alignment\nvector<int> SpaceEfficientAlignment(string s1, string s2)\n{\n\n //space efficient version\n int m = s1.length();\n int n = s2.length();\n\n vector<vector<int>> array2d(m + 5, vector<int>(2));\n\n //base conditions\n for (int i = 0; i <= m; i++)\n array2d[i][0] = i * (delC());\n\n for (int j = 1; j <= n; j++)\n {\n\n array2d[0][1] = j * (insC());\n\n //recurrence\n for (int i = 1; i <= m; i++)\n array2d[i][1] =\n min(modC(s1[i - 1], s2[j - 1]) + array2d[i - 1][0],\n min(delC() + array2d[i - 1][1], insC() + array2d[i][0]));\n\n for (int i = 0; i <= m; i++)\n array2d[i][0] = array2d[i][1];\n }\n\n //returning the last column to get the row element x in n/2 column: see GainAlignment function\n vector<int> vec(m + 1);\n for (int i = 0; i <= m; i++)\n vec[i] = array2d[i][1];\n\n return vec;\n}\n\npair<string, string> GainAlignment(string s1, string s2)\n{\n\n string te1, te2; //for storing alignments\n int l1 = s1.length();\n int l2 = s2.length();\n\n //trivial cases of length = 0 or length = 1\n if (l1 == 0)\n for (int i = 0; i < l2; i++)\n {\n te1.push_back('-');\n te2.push_back(s2[i]);\n cost += insC();\n }\n\n else if (l2 == 0)\n for (int i = 0; i < l1; i++)\n {\n te1.push_back(s1[i]);\n te2.push_back('-');\n cost += delC();\n }\n\n else if (l1 == 1 || l2 == 1)\n {\n pair<string, string> temp = stringOne(s1, s2);\n te1 = temp.first;\n te2 = temp.second;\n }\n //main divide and conquer\n else\n {\n int ymid = l2 / 2;\n /*\n * We know edit distance problem can be seen as shortest path from initial(0,0) to (l1,l2)\n * Now, here we are seeing it in two parts from (0,0) to (i,j) and from (i+1,j+1) to (m,n)\n * and we will see for which i it is getting minimize.\n */\n\n vector<int> ScoreL = SpaceEfficientAlignment(s1, s2.substr(0, ymid)); //for distance (0,0) to (i,j)\n vector<int> ScoreR = SpaceEfficientAlignment(rever(s1), rever(s2.substr(ymid, l2 - ymid))); //for distance (i+1,j+1) to (m,n)\n\n int xmid = minimize(ScoreL, ScoreR, l1); //minimizing the distance\n\n pair<string, string> temp = GainAlignment(s1.substr(0, xmid), s2.substr(0, ymid));\n te1 = temp.first; te2 = temp.second; //storing the alignment\n\n temp = GainAlignment(s1.substr(xmid, l1 - xmid), s2.substr(ymid, l2 - ymid));\n te1 += temp.first; te2 += temp.second; //storing the alignment\n\n }\n\n return make_pair(te1, te2);\n}\n\n\nint main()\n{\n string s1, s2;\n s1 = \"GCCCTAGCG\";\n s2 = \"GCGCAATG\";\n\n //cin >> s1 >> s2; /*standard input stream*/\n\n /*\n * If reading from strings from two files\n */\n // ifstream file1(\"file1.txt\");\n // ifstream file2(\"file2.txt\");\n // getline(file1, s1);\n // file1.close();\n\n // getline(file2, s2);\n // file2.close();\n\n pair<string, string> temp = GainAlignment(s1, s2);\n\n //Alignments can be different for same strings but cost will be same\n cout << \"Alignments of strings\\n\";\n cout << temp.first << \"\\n\";\n cout << temp.second << \"\\n\";\n cout << \"Cost associated = \" << cost << \"\\n\";\n\n /*\tAlignments\n * M - modification, D - deletion, I - insertion for converting string1 to string2\n *\n * M M MD\n * string1\t- GCCCTAGCG\n * string2 - GCGCAAT-G\n *\n */\n}"
}
{
"filename": "code/dynamic_programming/src/edit_distance/edit_distance.hs",
"content": "--- dynamic programming | edit distance | Haskell\n--- Part of Cosmos by OpenGenus\n--- Adapted from http://jelv.is/blog/Lazy-Dynamic-Programming/ \n\nmodule EditDistance where\nimport Data.Array\n\neditDistance :: String -> String -> Int\neditDistance a b = d m n\n where (m, n) = (length a, length b)\n a' = listArray (1, m) a -- 1-indexed to simplify arithmetic below\n b' = listArray (1, n) b\n d i 0 = i\n d 0 j = j\n d i j\n | a' ! i == b' ! j = table ! (i - 1, j - 1)\n | otherwise = minimum [ table ! (i - 1, j) + 1\n , table ! (i, j - 1) + 1\n , table ! (i - 1, j - 1) + 1\n ]\n table = listArray bounds\n [d i j | (i, j) <- range bounds] -- 2D array to store intermediate results\n bounds = ((0, 0), (m, n))\n\nmain = print $ editDistance \"sunday\" \"saturday\" == 3"
}
{
"filename": "code/dynamic_programming/src/edit_distance/edit_distance.java",
"content": "// dynamic programming | edit distance | Java\n// Part of Cosmos by OpenGenus Foundation\n\nimport java.util.Scanner;\n\npublic class edit_distance {\n public static int edit_DP(String s,String t){\n int l1 = s.length();\n int l2 = t.length();\n \n int dp[][] = new int[l1+1][l2+1];\n\n for(int i=0; i<=l2; i++)\n dp[0][i] = i;\n\n for(int i=0; i<=l1; i++)\n dp[i][0] = i;\n\n for(int i=1; i<=l1; i++){\n for(int j=1; j<=l2; j++){\n if(s.charAt(l1-i) == t.charAt(l2-j))\n dp[i][j] = dp[i-1][j-1];\n else\n dp[i][j] = 1 + Math.min( dp[i-1][j-1] , Math.min (dp[i-1][j] , dp[i][j-1]) );\n }\n }\n return dp[l1][l2];\n }\n\n public static void main(String[] args) {\n Scanner sc = new Scanner(System.in);\n \n System.out.print(\"Enter first string >> \");\n String a = sc.next();\n\n System.out.print(\"Enter second string >> \");\n String b = sc.next();\n \n System.out.println(\"Edit Distance : \" + edit_DP(a,b));\n }\n}"
}
{
"filename": "code/dynamic_programming/src/edit_distance/edit_distance.php",
"content": "<?php\n// dynamic programming | edit distance | PHP\n// Part of Cosmos by OpenGenus Foundation\n\nfunction editDistance($str1, $str2)\n{\n $lenStr1 = strlen($str1);\n $lenStr2 = strlen($str2);\n\n if ($lenStr1 == 0) {\n return $lenStr2;\n }\n\n if ($lenStr2 == 0) {\n return $lenStr1;\n }\n\n $distanceVectorInit = [];\n $distanceVectorFinal = [];\n\n for ($i = 0; $i < $lenStr1 + 1; $i++) {\n $distanceVectorInit[$i] = $i;\n $distanceVectorFinal[] = 0;\n }\n\n for ($i = 0; $i < $lenStr2; $i++) {\n $distanceVectorFinal[0] = $i + 1;\n // use formula to fill in the rest of the row\n for ($j = 0; $j < $lenStr1; $j++) {\n $substitutionCost = 0;\n if ($str1[$j] == $str2[$i]) {\n $substitutionCost = $distanceVectorInit[$j];\n } else {\n $substitutionCost = $distanceVectorInit[$j] + 1;\n }\n\n $distanceVectorFinal[$j+1] = min($distanceVectorInit[$j+1] + 1, min($distanceVectorFinal[$j] + 1, $substitutionCost));\n }\n\n $distanceVectorInit = $distanceVectorFinal;\n }\n\n \n return $distanceVectorFinal[$lenStr1];\n}\n\necho editDistance(\"hello\", \"hallo\");"
}
{
"filename": "code/dynamic_programming/src/edit_distance/edit_distance.py",
"content": "# dynamic programming | edit distance | Python\n# Part of Cosmos by OpenGenus Foundation\n\n# A top-down DP Python program to find minimum number\n# of operations to convert str1 to str2\ndef editDistance(str1, str2):\n def editDistance(str1, str2, m, n, memo):\n # If first string is empty, the only option is to\n # insert all characters of second string into first\n if m == 0:\n return n\n\n # If second string is empty, the only option is to\n # remove all characters of first string\n if n == 0:\n return m\n\n # If this state has already been computed, just return it\n if memo[m][n] > -1:\n return memo[m][n]\n\n # If last characters of two strings are same, nothing\n # much to do. Ignore last characters and get count for\n # remaining strings.\n if str1[m - 1] == str2[n - 1]:\n memo[m][n] = editDistance(str1, str2, m - 1, n - 1, memo)\n return memo[m][n]\n\n # If last characters are not same, consider all three\n # operations on last character of first string, recursively\n # compute minimum cost for all three operations and take\n # minimum of three values.\n memo[m][n] = 1 + min(\n editDistance(str1, str2, m, n - 1, memo), # Insert\n editDistance(str1, str2, m - 1, n, memo), # Remove\n editDistance(str1, str2, m - 1, n - 1, memo), # Replace\n )\n return memo[m][n]\n\n size = max(len(str1), len(str2)) + 1\n memo = [[-1 for _ in range(size)] for _ in range(size)]\n return editDistance(str1, str2, len(str1), len(str2), memo)\n\n\n# Driver program to test the above function\nstr1 = \"sunday\"\nstr2 = \"saturday\"\nprint(editDistance(str1, str2))"
}
{
"filename": "code/dynamic_programming/src/edit_distance/edit_distance.rs",
"content": "// dynamic programming | edit distance | Rust\n// Part of Cosmos by OpenGenus Foundation\n\nuse std::cmp;\nfn edit_distance(str1: &String, str2: &String) -> usize {\n let len_str1 = str1.len();\n let len_str2 = str2.len();\n\n if len_str1 == 0 {\n \treturn len_str2;\n }\n\n if len_str2 == 0 {\n \treturn len_str1;\n }\n\n let mut distance_vector_init = vec![0; len_str1+1];\n let mut distance_vector_final = vec![0; len_str1+1];\n\n for i in 0..len_str1+1 {\n\t\tdistance_vector_init[i] = i;\n\t}\n\t\n\n\tfor i in 0..len_str2 {\n\t\tdistance_vector_final[0] = i+1;\n\t\t// use formula to fill in the rest of the row\n for j in 0..len_str1 {\n let mut substitution_cost;\n let mut iter1 = str1.chars();\n let mut iter2 = str2.chars();\n\n let e1 = iter1.nth(j);\n let e2 = iter2.nth(i);\n\t\t\t\n if e1.is_some() && e2.is_some() && e1.unwrap() == e2.unwrap() {\n substitution_cost = distance_vector_init[j];\n } else {\n substitution_cost = distance_vector_init[j] + 1\n };\n\n distance_vector_final[j + 1] = cmp::min(distance_vector_init[j+1] + 1, cmp::min(distance_vector_final[j] + 1, substitution_cost));\n }\n\n distance_vector_init = distance_vector_final.clone();\n\t}\n\n\tdistance_vector_final[len_str1]\n}\n\nfn main() {\n println!(\"edit_distance(saturday, sunday) = {}\", edit_distance(&String::from(\"sunday\"), &String::from(\"saturday\")));\n println!(\"edit_distance(sitten, kitten) = {}\", edit_distance(&String::from(\"kitten\"), &String::from(\"sitten\")));\n println!(\"edit_distance(gumbo, gambol) = {}\", edit_distance(&String::from(\"gambol\"), &String::from(\"gumbo\")));\n}"
}
{
"filename": "code/dynamic_programming/src/edit_distance/README.md",
"content": "# Edit distance\n\n## Description\n\nEdit distance is a way of quantifying how dissimilar two strings (e.g., words) are to one another by counting the minimum number of operations required to transform one string into the other. Edit distances find applications in natural language processing, where automatic spelling correction can determine candidate corrections for a misspelled word by selecting words from a dictionary that have a low distance to the word in question. In bioinformatics, it can be used to quantify the similarity of DNA sequences, which can be viewed as strings of the letters A, C, G and T.\n\n## Example\n\nThe Levenshtein distance between \"kitten\" and \"sitting\" is 3. A minimal edit script that transforms the former into the latter is:\n\nkitten → sitten (substitution of \"s\" for \"k\")\n\nsitten → sittin (substitution of \"i\" for \"e\")\n\nsittin → sitting (insertion of \"g\" at the end).\n\nLCS distance (insertions and deletions only) gives a different distance and minimal edit script:\n\ndelete k at 0\n\ninsert s at 0\n\ndelete e at 4\n\ninsert i at 4\n\ninsert g at 6\n\nfor a total cost/distance of 5 operations.\n\n---\n\n<p align=\"center\">\n A massive collaborative effort by <a href=https://github.com/OpenGenus/cosmos>OpenGenus Foundation</a>\n</p>\n\n---"
}
{
"filename": "code/dynamic_programming/src/egg_dropping_puzzle/egg_dropping_puzzle.c",
"content": "#include <stdio.h>\n#include <string.h>\n\n#define min(a, b) (((a) < (b)) ? (a) : (b))\n#define max(a, b) (((a) > (b)) ? (a) : (b))\n\n#define MAX_EGGS 100\n#define MAX_FLOORS 100\n#define INF 1000000000\n\nint memo[MAX_EGGS][MAX_FLOORS];\n\nint \neggDrop(int n, int k)\n{ \n if (k == 0)\n return (0); \n if (k == 1)\n return (1); \n if (n == 1)\n return (k); \n\n if (memo[n][k] > -1) \n return (memo[n][k]); \n\n int ans = INF, h;\n \n for (h = 1; h <= k; ++h)\n ans = min(ans, max(eggDrop(n - 1, h - 1), eggDrop(n, k - h)));\n\n return (memo[n][k] = ans + 1); \n}\n\nint \nmain()\n{ \n memset(memo, -1, sizeof(memo));\n\n int n, k;\n printf(\"Enter number of eggs: \");\n scanf(\"%d\", &n);\n printf(\"Enter number of floors: \");\n scanf(\"%d\", &k);\n\n printf(\"Minimum Number of attempts required in Worst case = %d \\n\", eggDrop(n,k));\n\n return (0);\n}"
}
{
"filename": "code/dynamic_programming/src/egg_dropping_puzzle/egg_dropping_puzzle.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <iostream>\n\n#define MAX_EGGS 100\n#define MAX_FLOORS 100\n#define INF 1000000000\n\nusing namespace std;\n\nint memo[MAX_EGGS][MAX_FLOORS];\n\n/*\n * Returns the minimum number of attempts\n * needed in the worst case for n eggs\n * and k floors.\n * Time complexity: O(n*k^2)\n */\nint eggDrop(int n, int k)\n{\n\n // base cases\n if (k == 0)\n return 0; // if there is no floor, no attempt is necessary\n if (k == 1)\n return 1; // if there is only one floor, just one attempt is necessary\n if (n == 1)\n return k; // with only one egg, k attempts are necessary\n\n // check if it is already computed\n if (memo[n][k] > -1)\n return memo[n][k];\n\n int ans = INF;\n // attempt to drop an egg at each height from 1 to k\n for (int h = 1; h <= k; ++h)\n ans = min(ans, max( // get worst case from:\n eggDrop(n - 1, h - 1), // case in which egg breaks\n eggDrop(n, k - h) // case in which egg does not break\n ));\n\n return memo[n][k] = ans + 1; // store minimum value\n}\n\nint main()\n{\n for (int i = 0; i < MAX_EGGS; ++i)\n for (int j = 0; j < MAX_FLOORS; ++j)\n memo[i][j] = -1;\n\n cout << eggDrop(2, 100) << '\\n';\n cout << eggDrop(10, 5) << '\\n';\n cout << eggDrop(10, 100) << '\\n';\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/egg_dropping_puzzle/egg_dropping_puzzle.cs",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\nusing System;\n\nclass cosmos {\n\n\t/* Function to get minimum number of */\n\t/* trials needed in worst case with n */\n\t/* eggs and k floors */\n \n\tstatic int eggDrop(int n, int k)\n\t{\n\t\t// If there are no floors, then\n\t\t// no trials needed. OR if there\n\t\t// is one floor, one trial needed.\n \n\t\tif (k == 1 || k == 0)\n\t\t\treturn k;\n\n\t\t// We need k trials for one egg\n\t\t// and k floors\n\t\tif (n == 1)\n\t\t\treturn k;\n\n\t\tint min = int.MaxValue;\n\t\tint x, res;\n\n\t\t// Consider all droppings from\n\t\t// 1st floor to kth floor and\n\t\t// return the minimum of these\n\t\t// values plus 1.\n \n\t\tfor (x = 1; x <= k; x++) {\n\t\t\tres = Math.Max(eggDrop(n - 1, x - 1),\n\t\t\t\t\t\teggDrop(n, k - x));\n\t\t\tif (res < min)\n\t\t\t\tmin = res;\n\t\t}\n\n\t\treturn min + 1;\n\t}\n\n\t// Driver code\n \n\tstatic void Main()\n\t{\n\t\tint n = 2, k = 10;\n\t\tConsole.Write(\"Minimum number of \"\n\t\t\t\t\t+ \"trials in worst case with \"\n\t\t\t\t\t+ n + \" eggs and \" + k\n\t\t\t\t\t+ \" floors is \" + eggDrop(n, k));\n\t}\n}"
}
{
"filename": "code/dynamic_programming/src/egg_dropping_puzzle/egg_dropping_puzzle.hs",
"content": "--- Part of Cosmos by OpenGenus Foundation\neggDropping :: Int -> Int -> Int\neggDropping floors eggs = d floors eggs\n where m = floors\n n = eggs\n d floors' 1 = floors'\n d 1 _ = 1\n d 0 _ = 0\n d _ 0 = error \"No eggs left\"\n d floors' eggs' = minimum [1 + maximum [ table!(floors'-i, eggs')\n , table!(i-1, eggs'-1)\n ]\n | i <- [1..floors']\n ]\n\n table = listArray bounds\n [d i j | (i, j) <- range bounds] -- 2D array to store intermediate results\n bounds = ((0, 0), (m, n))"
}
{
"filename": "code/dynamic_programming/src/egg_dropping_puzzle/eggdroppingpuzzle.java",
"content": "\r\n//Dynamic Programming solution for the Egg Dropping Puzzle\r\n /* Returns the minimum number of attempts\r\n needed in the worst case for a eggs and b floors.*/\r\nimport java.util.*;\r\n\r\npublic class EggDroppingPuzzle { \r\n\t// min trials with a eggs and b floors \r\n\tprivate static int minTrials(int a, int b) {\r\n\t\tint eggFloor[][] = new int[a + 1][b + 1];\r\n\t \tint result, x;\r\n\t\t\r\n\t\tfor (int i = 1; i <= a; ++i) {\r\n \teggFloor[i][0] = 0; // Zero trial for zero floor.\r\n \teggFloor[i][1] = 1; // One trial for one floor \r\n\t\t}\r\n \t// j trials for only 1 egg\t\r\n \tfor (int j = 1; j <= b; ++j) {\r\n\t \teggFloor[1][j] = j;\r\n\t\t}\r\n \t\tfor (int i = 2; i <= a; ++i) {\r\n \t\tfor (int j = 2; j <= b; ++j) {\r\n\t \t\teggFloor[i][j] = Integer.MAX_VALUE;\r\n\t \t\tfor (x = 1; x <= j; ++x) { \r\n\t \t// get worst case from:case in which egg break (eggFloor[i-1][x-1]) and case in which egg does not break (eggFloor[i][j-x]).\r\n\t\t \t \t\tresult = 1 + Math.max(eggFloor[i - 1][x - 1], eggFloor[i][j - x]); \r\n\t\t\t\t\t//choose min of all values for particular x\r\n \t\t \t\tif (result < eggFloor[i][j])\r\n\t\t\t \t\teggFloor[i][j] = result;\r\n\t\t \t} \r\n\t \t\t} \r\n \t} \r\n\t\treturn eggFloor[a][b];\r\n \t}\r\n\t\t\r\n\t//testing the program\r\n \t public static void main(String args[]) { \r\n \tScanner sc = new Scanner(System.in);\r\n\t\tSystem.out.println(\"Enter no. of eggs\");\r\n\t\r\n\t\tint a = Integer.parseInt(sc.nextLine());\r\n\t\tSystem.out.println(\"Enter no. of floors\");\r\n\t\t\r\n \tint b = Integer.parseInt(sc.nextLine());\r\n \t//result outputs min no. of trials in worst case for a eggs and b floors\r\n \tint result = minTrials(a, b);\r\n \tSystem.out.println(\"Minimum number of attempts needed in Worst case with a eggs and b floor are: \" + result); \r\n \t}\r\n} \r"
}
{
"filename": "code/dynamic_programming/src/egg_dropping_puzzle/egg_dropping_puzzle.py",
"content": "# Part of Cosmos by the Open Genus Foundation\n\n\n# A Dynamic Programming based Python Program for the Egg Dropping Puzzle\nINT_MAX = 32767\n\n\ndef eggDrop(n, k):\n eggFloor = [[0 for x in range(k + 1)] for x in range(n + 1)]\n for i in range(1, n + 1):\n eggFloor[i][1] = 1\n eggFloor[i][0] = 0\n\n # We always need j trials for one egg and j floors.\n for j in range(1, k + 1):\n eggFloor[1][j] = j\n\n for i in range(2, n + 1):\n for j in range(2, k + 1):\n eggFloor[i][j] = INT_MAX\n for x in range(1, j + 1):\n res = 1 + max(eggFloor[i - 1][x - 1], eggFloor[i][j - x])\n if res < eggFloor[i][j]:\n eggFloor[i][j] = res\n\n return eggFloor[n][k]\n\n\nn = 2\nk = 36\nprint(\n \"Minimum number of trials in worst case with\"\n + str(n)\n + \"eggs and \"\n + str(k)\n + \" floors is \"\n + str(eggDrop(n, k))\n)"
}
{
"filename": "code/dynamic_programming/src/egg_dropping_puzzle/README.md",
"content": "# Egg Dropping Puzzle\n\n## Description\n\nFor a `H`-floor building, we want to know which floors are\nsafe to drop eggs from without broking them and which floors\naren't. There are a few assumptions:\n\n- An egg that survives a fall can be used again.\n- A broken egg must be discarded.\n- The effect of a fall is the same for all eggs.\n- If an egg breaks when dropped, then it would break if dropped from a higher window.\n- If an egg survives a fall, then it would survive a shorter fall.\n- It is not ruled out that the first-floor windows break eggs,\nnor is it ruled out that eggs can survive the Hth-floor windows.\n\nHaving `N` eggs available, what is the **minimum** number of egg drops needed\nto find the critical floor in the **worst case**?\n\n## Solution\n\nWe can define the state of the dynamic programming model as being a pair `(n, k)`,\nwhere `n` is the number of eggs available and `k` is the number of consecutive floors\nthat need to be tested.\n\nLet `f(n,k)` be the minimum number of attempts needed to find the critical floor in the\nworst case with `n` eggs available and with `k` consecutive floors to be tested.\n\nAttempting to drop an egg for each floor `x` leads to two possibilities:\n\n- The egg breaks from floor `x`: then we lose an egg but have to check only the floors\nlower than `x`, reducing the problem to `n-1` eggs and `x-1` floors.\n- The egg does not break: then we continue with `n` eggs at our disposal and have to\ncheck only the floors higher than `x`, reducing the problem to `n` eggs and `k-x` floors.\n\nAs we want to know the number of trials in the *worst case*, we consider the maximum of the\ntwo possibilities, that is `max( f(n-1, x-1), f(n, k-x) )`.\nAnd as we want to know the *minimum* number of trials, we choose the floor that leads to\nthe minimum number of attempts. So:\n```\nf(n, k) = 1 + min( max( f(n-1, x-1), f(n, k-x) ) , 1 <= x <= h )\n```\n\nAnd the base cases are:\n```\nf(n, 0) = 0 // if there is no floor, no attempt is necessary\nf(n, 1) = 1 // if there is only one floor, just one attempt is necessary\nf(1, k) = k // with only one egg, k attempts are necessary\n```\n\nBy using memoization or tabulation, the complexity of the algorithm becomes `O(n * k^2)`, as the number of states is `n*k` and the cost of computing a state is `O(k)`.\n\n---\n\n<p align=center>\nA massive collaborative effort by <a href=\"https://github.com/opengenus/cosmos\">OpenGenus Foundation</a>\n</p>\n\n---"
}
{
"filename": "code/dynamic_programming/src/factorial/factorial.c",
"content": "#include <stdio.h>\n\nint\nfactorial(int n)\n{\n\tif (n == 0 || n == 1)\n\t\treturn (1);\n\telse\n\t\treturn (n * factorial(n - 1));\n}\n\nint\nmain()\n{\n\tint n;\n\tprintf(\"Enter a Whole Number: \");\n\tscanf(\"%d\", &n);\n\tprintf(\"%d! = %d\\n\", n, factorial(n));\n\n\treturn (0);\n}"
}
{
"filename": "code/dynamic_programming/src/factorial/factorial.cpp",
"content": "//Part of Cosmos by OpenGenus Foundation\n#include <iostream>\nusing namespace std;\nint factorial(int n)\n{\n //base case\n if (n == 0 || n == 1)\n {\n return 1;\n }\n // recursive call\n return (n * factorial(n - 1));\n}\nint main()\n{\n int n;\n cout << \"Enter a number\";\n //Taking input\n cin >> n;\n //printing output\n cout << \"Factorial of n :\" << factorial(n) << endl;\n}"
}
{
"filename": "code/dynamic_programming/src/factorial/factorial.exs",
"content": "defmodule Factorial do\n def factorial(0), do: 1\n def factorial(1), do: 1\n def factorial(num) when num > 1 do\n num * factorial(num - 1)\n end\nend"
}
{
"filename": "code/dynamic_programming/src/factorial/factorial.go",
"content": "// Part of Cosmos by OpenGenus Foundation\npackage main\n\nimport \"fmt\"\n\nfunc factorial(num int) int {\n\tif num == 0 {\n\t\treturn 1\n\t}\n\treturn (num * factorial(num-1))\n}\n\nfunc main() {\n\tnum := 5\n\tfmt.Println(factorial(num))\n}"
}
{
"filename": "code/dynamic_programming/src/factorial/factorial.java",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n/**\n * Implements factorial using recursion\n */\npublic class Factorial {\n\n private static int factorial(int num) {\n if (num == 0) {\n return 1;\n } else {\n return (num * factorial(num - 1));\n }\n }\n\n public static void main(String[] args) {\n int number = 5;\n int result;\n result = factorial(number);\n System.out.printf(\"The factorial of %d is %d\", number, result);\n }\n \n}"
}
{
"filename": "code/dynamic_programming/src/factorial/factorial.js",
"content": "function factorialize(number) {\n if (number == 0) {\n return 1;\n } else {\n return number * factorialize(number - 1);\n }\n}\n\nfactorialize(5);\n/*replace the value of 5 with whatever value you\n *want to find the factorial of\n */"
}
{
"filename": "code/dynamic_programming/src/factorial/factorial.md",
"content": "## Fibonacci Series implemented using dynamic programming\n- If you do not know what **Fibonacci Series** is please [visit](https://iq.opengenus.org/calculate-n-fibonacci-number/) to get an idea about it.\n- If you do not know what **dynamic programming** is please [visit](https://iq.opengenus.org/introduction-to-dynamic-programming/) to get an idea about it.\n### Let's go step by step i.e code block by code block\nThe underlying concept for finding the factorial of a number *n* is :\n - ```cpp n! = n*(n-1)*(n-2)*......*2*1 ``` for all i>1\n - Which can also be written as ```cpp n! = n*((n-1)!)``` for all i>1\n - And the initial case is :\n - ```cpp 0! = 0 ``` (If you are curious about why this is the case, [checkout](https://iq.opengenus.org/factorial-of-large-numbers/))\n - ```cpp 1! = 1 ```\nThe block of code below does exactly what we discussed above but using recursion.\n```cpp \n if (n == 0 || n == 1)\n {\n return 1;\n }\n return (n * factorial(n - 1));\n```\nThe main function takes an input for the number *n* and calls the **factorial(n)** function, which has an if condition to check if it's the base condition i.e if *n* is 0 or 1, and return 1, otherwise it returns ```cpp (n * factorial(n - 1))```, which means that it call **factorial(n-1)** and waits for it to return and multiplies this with n and returns **n!**.\nThe recursion can be explained with the below diagram. \n```cpp\n main()\n | ^\n V | (return n*factorial(n-1))\n factorial(n)\n | ^\n V | (return (n-1)*factorial(n-2))\n factorial(n-1)\n | ^\n V | (return (n-2)*factorial(n-3))\n factorial(n-2)\n .\n .\n .\n .\n .\n .\n | ^\n V | (return 2*factorial(1) = 2 * 1 = 2)\n factorial(1)\n ( We know factorial(1) = 1 )\n```"
}
{
"filename": "code/dynamic_programming/src/factorial/factorial.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\nfrom functools import wraps\n\n\ndef memo(f):\n \"\"\"Memoizing decorator for dynamic programming.\"\"\"\n\n @wraps(f)\n def func(*args):\n if args not in func.cache:\n func.cache[args] = f(*args)\n return func.cache[args]\n\n func.cache = {}\n return func\n\n\n@memo\ndef factorial(num):\n \"\"\"Recursively calculate num!.\"\"\"\n if num < 0:\n raise ValueError(\"Negative numbers have no factorial.\")\n elif num == 0:\n return 1\n return num * factorial(num - 1)"
}
{
"filename": "code/dynamic_programming/src/factorial/factorial.rs",
"content": "// Part of Cosmos by OpenGenus Foundation\nfn factorial(n: u64) -> u64 {\n match n {\n 0 => 1,\n _ => n * factorial(n - 1)\n }\n}\n\nfn main() {\n for i in 1..10 {\n println!(\"factorial({}) = {}\", i, factorial(i));\n }\n}"
}
{
"filename": "code/dynamic_programming/src/factorial/factorial.scala",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\nimport scala.annotation.tailrec\n\n/**\n * Implement factorial using tail recursion\n */\nobject main {\n\n @tailrec\n private def _factorial(of: Int, current: Int): Int = {\n if (of == 0) {\n current\n } else {\n _factorial(of - 1, of * current)\n }\n }\n\n def factorial(of: Int): Int = {\n _factorial(of, 1)\n }\n\n def main(args: Array[String]) = {\n val number: Int = 5\n println(s\"The factorial of ${number} is ${factorial(number)}\")\n }\n}"
}
{
"filename": "code/dynamic_programming/src/fibonacci/fibonacci.c",
"content": "#include<stdio.h>\n \nint fib(int n)\n{\n int f[n+2]; // 1 extra to handle case, n = 0\n int i;\n \n f[0] = 0;\n f[1] = 1;\n \n for (i = 2; i <= n; i++)\n {\n f[i] = f[i-1] + f[i-2];\n }\n \n return f[n];\n}\n \nint main ()\n{\n int n = 9;\n printf(\"%d\", fib(n));\n getchar();\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/fibonacci/fibonacci.cpp",
"content": "// Part of Cosmos by OpenGenus\n#include <iostream>\nusing namespace std;\n\nint fib(int n)\n{\n int *ans = new int[n + 1]; //creating an array to store the outputs\n ans[0] = 0;\n ans[1] = 1;\n for (int i = 2; i <= n; i++)\n {\n ans[i] = ans[i - 1] + ans[i - 2]; // storing outputs for further use\n }\n return ans[n];\n}\nint main()\n{\n int n;\n cout << \"Enter the Number:\"; // taking input\n cin >> n;\n int output = fib(n);\n cout << \"Nth fibonacci no. is:\" << output << endl; //printing nth fibonacci number\n}"
}
{
"filename": "code/dynamic_programming/src/fibonacci/fibonacci.exs",
"content": "defmodule Fibonacci do\n def fib(terms) do\n if terms <= 0 do\n {:error, :non_positive}\n else\n {fib_list, _} =\n Enum.map_reduce(1..terms, {0, 1}, fn\n 1, _acc -> {1, {1, 1}}\n _t, {n1, n2} -> {n1 + n2, {n1 + n2, n1}}\n end)\n\n Enum.each(fib_list, fn f -> IO.puts(\"#{f}\") end)\n end\n end\nend"
}
{
"filename": "code/dynamic_programming/src/fibonacci/fibonacci.go",
"content": "package dynamic\n\n// NthFibonacci returns the nth Fibonacci Number\nfunc NthFibonacci(n uint) uint {\n\tif n == 0 {\n\t\treturn 0\n\t}\n\n\t// n1 and n2 are the (i-1)th and ith Fibonacci numbers, respectively\n\tvar n1, n2 uint = 0, 1\n\n\tfor i := uint(1); i < n; i++ {\n\t\tn3 := n1 + n2\n\t\tn1 = n2\n\t\tn2 = n3\n\t}\n\n\treturn n2\n}"
}
{
"filename": "code/dynamic_programming/src/fibonacci/fibonacci.java",
"content": "import java.util.Scanner;\n\npublic class Main {\n\n public static void main(String[] args) {\n Scanner input = new Scanner(System.in);\n System.out.println(\"Enter the Number:\"); // taking input\n int n = input.nextInt();\n int output = fib(n);\n System.out.println(\"Nth fibonacci no. is: \" + output);\n }\n\n public static int fib(int n) {\n int[] fibNumber = new int[n + 1]; // creating an array to store the outputs\n fibNumber[0] = 0;\n fibNumber[1] = 1;\n for (int i = 2; i <= n; i++) {\n fibNumber[i] = fibNumber[i - 1] + fibNumber[i - 2]; // storing outputs for further use\n }\n return fibNumber[n];\n }\n}"
}
{
"filename": "code/dynamic_programming/src/fibonacci/fibonacci.js",
"content": "// Problem Statement(1): Find the nth Fibonacci number \n\n// 1.a.Using Recusrion to find the nth fibonacci number\nconst FibonacciNumberRec = (num) => {\n if (num <= 1)\n return num;\n return FibonacciNumberRec(num - 1) + FibonacciNumberRec(num - 2);\n}\n\n// 1.b.Using DP to find the nth fibonacci number\n// fib[n] = fib[n - 1] + fib[n - 2]\nconst FibonacciNumberDP = (num) => {\n let fib = [0, 1] // fib[0] = 0, fib[1] = 1\n for (let i = 2; i <= num; i++) {\n fib.push(fib[i - 1] + fib[i - 2]);\n }\n\n return fib[num]; // return num'th fib number\n}\n\n// 2.Print the fibonacci series upto nth Fibonacci number\nconst FibonacciSeries = (num) => {\n let fib = [0, 1] // fib[0] = 0, fib[1] = 1\n for (let i = 2; i <= num; i++) {\n fib.push(fib[i - 1] + fib[i - 2]);\n }\n\n return fib;\n}\n\n\n// Call the functions\nlet n = 6\nconsole.log(`The ${n}th Fibonacci Number is ${FibonacciNumberRec(n)}`);\nn = 8\nconsole.log(`The ${n}th Fibonacci Number is ${FibonacciNumberDP(n)}`);\nn = 10\nconsole.log(`The Fibonacci Series upto ${n}th term is ${FibonacciSeries(n)}`);\n\n// I/O:\n// 1.a) n = 6\n// Output :\n// The 6th Fibonacci Number is 8\n// 1.b) n = 8\n// The 8th Fibonacci Number is 21\n// 2. n = 10\n// The Fibonacci Series upto 10th term is 0,1,1,2,3,5,8,13,21,34,55\n\n// Note:This is 0-based i.e, fib[0] = 0, fib[1] = 1, fib[2] = 0 + 1 = 1, fib[3] = 1 + 1 = 2"
}
{
"filename": "code/dynamic_programming/src/fibonacci/fibonacci.md",
"content": "## Fibonacci Series implemented using dynamic programming\n- If you do not know what **Fibonacci Series** is please [visit](https://iq.opengenus.org/calculate-n-fibonacci-number/) to get an idea about it.\n- If you do not know what **dynamic programming** is please [visit](https://iq.opengenus.org/n-th-fibonacci-number-using-dynamic-programming/) to get an idea about it.\n### Let's go step by step i.e code block by code block\nTo get first *n* Fibonnaci numbers, we first create an array of size n\n```cpp\n int *ans = new int[n + 1];\n``` \nSince the underlying concept for Fibonnaci Series is that :\n - ```cpp F[i] = F[i-1] + F[i-2] ``` for all i>1\n - And the initial case is :\n - ```cpp F[0] = 0 ```\n - ```cpp F[1] = 1 ```\nThe block of code below does exactly what we discussed above i.e initialise for 0,1 and then iterate from 2 to n and calculate the Fibonnaci Series using the concept of ```cpp F[i] = F[i-1] + F[i-2] ``` for all i>1.\n```cpp \n ans[0] = 0;\n ans[1] = 1;\n for (int i = 2; i <= n; i++)\n {\n ans[i] = ans[i - 1] + ans[i - 2]; \n }\n```\nThe main function takes an input for the number *n* i.e the number of first *n* Fibonacci Numbers and calls the **fib()** function to get the array of first *n* Fibonnaci Numbers."
}
{
"filename": "code/dynamic_programming/src/fibonacci/fibonacci.py",
"content": "from sys import argv\n\n\ndef fibonacci(n):\n \"\"\"\n N'th fibonacci number using Dynamic Programming\n \"\"\"\n arr = [0, 1] # 1st two numbers as 0 and 1\n\n while len(arr) < n + 1:\n arr.append(0)\n\n if n <= 1:\n return n\n else:\n if arr[n - 1] == 0:\n arr[n - 1] = fibonacci(n - 1)\n if arr[n - 2] == 0:\n arr[n - 2] = fibonacci(n - 2)\n\n arr[n] = arr[n - 2] + arr[n - 1]\n return arr[n]\n\n\nif __name__ == \"__main__\":\n if len(argv) < 2:\n print(\"Usage: python3 fibonacci.py <N>\")\n print(fibonacci(int(argv[1])))"
}
{
"filename": "code/dynamic_programming/src/friends_pairing/friends_pairing.c",
"content": "// Dynamic Programming solution to friends pairing problem in C\r\n// Contributed by Santosh Vasisht\r\n\r\n// Given n friends, each one can remain single or can be paired up with some other friend.\r\n// Each friend can be paired only once.\r\n// Find out the total number of ways in which friends can remain single or can be paired up.\r\n\r\n#include <stdio.h>\r\n#include <stdlib.h>\r\n\r\nint countFriendsPairings(int n)\r\n{\r\n int table[n+1];\r\n\r\n // Filling the table recursively in bottom-up manner\r\n for(int i = 0; i <= n; ++i)\r\n {\r\n if(i <= 2)\r\n table[i] = i;\r\n else\r\n table[i] = table[i-1] + (i-1) * table[i-2];\r\n }\r\n\r\n return table[n];\r\n}\r\n\r\n//Driver code\r\nint main()\r\n{\r\n int n = 7;\r\n printf(\"%d\\n\", countFriendsPairings(n));\r\n\r\n return 0;\r\n}"
}
{
"filename": "code/dynamic_programming/src/friends_pairing/friends_pairing.cpp",
"content": "// Dynamic Programming solution to friends pairing problem in C++\r\n// Contributed by Santosh Vasisht\r\n\r\n// Given n friends, each one can remain single or can be paired up with some other friend.\r\n// Each friend can be paired only once.\r\n// Find out the total number of ways in which friends can remain single or can be paired up.\r\n\r\n#include <bits/stdc++.h>\r\nusing namespace std;\r\n\r\nint countFriendsPairings(int n)\r\n{\r\n int table[n+1];\r\n \r\n // Filling the table recursively in bottom-up manner\r\n for(int i = 0; i <= n; ++i)\r\n {\r\n if(i <= 2)\r\n table[i] = i;\r\n else\r\n table[i] = table[i-1] + (i-1) * table[i-2];\r\n }\r\n \r\n return table[n];\r\n}\r\n\r\n// Driver code\r\nint main()\r\n{\r\n int n = 7;\r\n cout << countFriendsPairings(n) << endl;\r\n\r\n return 0;\r\n}"
}
{
"filename": "code/dynamic_programming/src/friends_pairing/friends_pairing.py",
"content": "# Dynamic Programming solution to friends pairing problem in Python3\r\n# Contributed by Santosh Vasisht\r\n\r\n# Given n friends, each one can remain single or can be paired up with some other friend.\r\n# Each friend can be paired only once.\r\n# Find out the total number of ways in which friends can remain single or can be paired up.\r\n\r\ndef countFriendsPairings(n: int) -> int:\r\n table = [0] * (n+1)\r\n \r\n # Filling the table recursively in bottom-up manner\r\n for i in range(n+1):\r\n if i <= 2:\r\n table[i] = i\r\n else:\r\n table[i] = table[i-1] + (i-1) * table[i-2]\r\n \r\n return table[n]\r\n\r\n#Driver Code\r\nif __name__ == '__main__':\r\n n = 7\r\n print(countFriendsPairings(n))"
}
{
"filename": "code/dynamic_programming/src/house_robber/HouseRobber.cpp",
"content": "// Below is the solution of the same problem using C++\nint rob(vector<int>& nums) {\n \n if(nums.size()==0)\n return 0;\n if(nums.size()==1)\n return nums[0];\n \n int res[nums.size()];\n \n res[0]=nums[0];\n res[1]=max(nums[0],nums[1]);\n \n for(int i=2;i<nums.size();i++)\n {\n res[i]=max(res[i-1],nums[i]+res[i-2]);\n }\n \n return res[nums.size()-1];\n \n }"
}
{
"filename": "code/dynamic_programming/src/house_robber/HouseRobber.java",
"content": "import java.util.Arrays;\n\nclass HouseRobber {\n public static int rob(int[] nums) {\n int len = nums.length;\n if(len == 0) return 0;\n if(len == 1) return nums[0];\n if(len == 2) return Math.max(nums[0], nums[1]);\n\n int memo[] = new int [len];\n memo[0] = nums[0];\n memo[1] = Math.max(nums[0], nums[1]);\n System.out.println(\"Memo before robbing: \" + Arrays.toString(memo) + \"\\n\");\n for(int i=2; i<len; i++){\n System.out.println(\"Visiting house no. \" + (i+1));\n int choice1 = nums[i] + memo[i-2];\n int choice2 = memo[i-1];\n System.out.println(\"choice 1 (rob this house and 2nd before) : \" + choice1);\n System.out.println(\"choice 2 (max of previous two houses) : \" + choice2);\n memo[i] = Math.max(choice1, choice2);\n System.out.println(\"Memo after robbing: \" + Arrays.toString(memo) + \"\\n\");\n }\n return memo[len-1];\n }\n public static void main(String args[]){\n int input[] = new int[]{1,2,3,4};\n System.out.println(\"Houses with given stash on a street: \" + Arrays.toString(input));\n int stolenAmount = rob(input);\n System.out.println(\"Max amount stolen by robber: \" + stolenAmount);\n }\n}"
}
{
"filename": "code/dynamic_programming/src/house_robber/HouseRobber.js",
"content": "/**\n * @param {number[]} nums\n * @return {number}\n */\nvar rob = function(nums) {\n // Tag: DP\n const dp = [];\n dp[0] = 0;\n dp[1] = 0;\n\n for (let i = 2; i < nums.length + 2; i++) {\n dp[i] = Math.max(dp[i - 2] + nums[i - 2], dp[i - 1]);\n }\n\n return dp[nums.length + 1];\n};"
}
{
"filename": "code/dynamic_programming/src/house_robber/README.md",
"content": "# House Robber\n\n## Description\n\nGiven a list of non-negative integers representing the amount of money in a house, determine the maximum amount of money a robber can rob tonight given that he cannot rob adjacent houses.\n\n## Solution\n\ne.g [1,2,3]<br>\nConsidering the constraint,<br>\nrobber has choice 1: houses with amount 1 and 3<br>\nOr choice 2: house with amount 2<br>\n\nThus, 1st choice gives the maximum amount of money.<br>\n\nConsidering n is the current house to be robbed<br>\nchoice 1: memo[n]+memo[n-2]<br>\nchoice 2: memo[n-1]<br>\n\nThe solution can be represented as the following expression:<br>\nMaximum((memo[n]+memo[n-2]), memo[n-1])<br>\n\n## Time and Space complexity\n\nTime complexity: O(n), n is number of elements in the input array<br>\nSpace complexity: O(n), space is required for the array"
}
{
"filename": "code/dynamic_programming/src/knapsack/knapsack.c",
"content": "#include <stdio.h>\n// Part of Cosmos by OpenGenus Foundation\n#define MAX 10\n#define MAXIMUM(a, b) a > b ? a : b\n\nvoid \nKnapsack(int no, int wt, int pt[MAX], int weight[MAX])\n{\n\tint knap[MAX][MAX], x[MAX] = {0};\n\n\tint i, j;\n //For item\n for (i = 0; i <=no; i++)\n knap[i][0] = 0;\n //For weight\n for (i = 0; i <=wt; i++)\n knap[0][i] = 0;\n\n\tfor (i = 1; i <= no; i++)\n\t\tfor (j = 1; j <= wt; j++) {\n\t\t\tif ((j - weight[i]) < 0)\n\t\t\t\tknap[i][j] = knap[i - 1][j];\n\t\t\telse\n\t\t\t\tknap[i][j] = MAXIMUM(knap[i - 1][j], pt[i] + knap[i - 1][j - weight[i]]);\n\t\t}\n\n\tprintf(\"Max Profit : %d \\n\", knap[no][wt]);\n\tprintf(\"Edges are :\\n\");\n\tfor (i = no; i >= 1; i--)\n\t\tif (knap[i][wt] != knap[i-1][wt]) {\n\t\t\tx[i] = 1;\n\t\t\twt -= weight[i];\n\t\t}\n\n\tfor (i = 1; i <= no; i++)\n\t\tprintf(\"%d\\t\", x[i]);\n\tprintf (\"\\n\");\n\t\n}\n\nint \nmain(int argc, char *argv[])\n{\n\tint no, wt, pt[MAX] = {0}, weight[MAX] = {0};\n\tint i;\n\n\tprintf(\"Enter the no of objects :\\n\");\n\tscanf(\"%d\", &no);\n\n\tprintf(\"Enter the total weight :\\n\");\n\tscanf(\"%d\", &wt);\n\n\tprintf(\"Enter the weights\\n\");\n\tfor (i = 1; i <= no; i ++)\n\t\tscanf(\"%d\", &weight[i]);\n\n\tprintf(\"Enter the profits\\n\");\n\tfor (i = 1; i <= no; i ++)\n\t\tscanf(\"%d\", &pt[i]);\n\n\tKnapsack(no, wt, pt, weight);\n\treturn (0);\n}"
}
{
"filename": "code/dynamic_programming/src/knapsack/knapsack.cpp",
"content": "/*\n *\n * Part of Cosmos by OpenGenus Foundation\n *\n * Description:\n * 1. We start with i = 0...N number of considered objects, where N is the\n * total number of objects we have. Similarly, for the capacity of bag we\n * go from b = 0...B where B is maximum capacity.\n * 2. Now for each pair of (i, b) we compute the subset of objects which will\n * give us the maximum value, starting from i = 1 to N and b from 0 to B.\n * 3. We compute a \"dp\" where dp[i][b] will give us the maximum value when\n * we consider the first i elements and the weight is b.\n * 4. We take a bottom-up approach, first filling dp[0][...] = 0 (we consider 0 elements)\n * and dp[...][0] = 0 (as capacity of bag is 0, value will be 0).\n * 5. Now, for each (i, b) we either include the 'i'th object or we do not.\n * dp[i][b] is the max of both these approaches. \n * Case 1: If we do not include the 'i'th object, our max value will be the same\n * as that for first (i - 1) objects to be filled in capacity b.\n * i.e. dp[i - 1][b]\n * Case 2: If we do include the 'i'th object, we need to clear space for it. For this,\n * we compute the best score when the first (i - 1) objects were made to fit in\n * a bag of capacity (b - Wi) where Wi is the weight of ith object. We add the \n * value of ith object to this score. If Vi denotes the value of ith object, the\n * best possible score after includig object i is:\n * dp[i - 1][b - Wi] + Vi\n * 6. We get the formula: dp[i][b] = max(dp[i-1][b], dp[i-1][b-Wi] + Vi)\n * As we have computed the base cases dp[0][...] and dp[...][0] we can use this formula\n * for the rest of the table and our required value will be dp[N][B].\n *\n */\n#include <utility>\n#include <iostream>\n\nusing namespace std;\n\ntypedef long long ll;\ntypedef pair<int, int> pii;\n\n#define MOD 1000000007\n#define INF 1000000000\n#define pb push_back\n#define sz size()\n#define mp make_pair\n\n//cosmos: knapsack 0-1\nint knapsack(int value[], int weight[], int n_items, int maximumWeight)\n{\n // dp[N + 1][B + 1] to accommodate base cases i = 0 and b = 0\n int dp[n_items + 1][maximumWeight + 1];\n\n // Base case, as we consider 0 items, value will be 0\n for (int w = 0; w <= maximumWeight; w++)\n dp[0][w] = 0;\n\n // We consider weight 0, therefore no items can be included\n for (int i = 0; i <= n_items; i++)\n dp[i][0] = 0;\n\n // Using formula to calculate rest of the table by base cases\n for (int i = 1; i <= n_items; i++)\n for (int w = 1; w <= maximumWeight; w++)\n {\n // Only consider object if weight of object is less than allowed weight\n if (weight[i - 1] <= w)\n dp[i][w] = max(value[i - 1] + dp[i - 1][w - weight[i - 1]], dp[i - 1][w]);\n else\n dp[i][w] = dp[i - 1][w];\n }\n\n return dp[n_items][maximumWeight];\n}\n\nint main()\n{\n ios_base::sync_with_stdio(false);\n cin.tie(NULL);\n\n int value[] = {12, 1000, 30, 10, 1000};\n int weight[] = {19, 120, 20, 1, 120};\n int n_items = 5;\n int maximumWeight = 40;\n\n cout << knapsack(value, weight, n_items, maximumWeight) << endl; //values of the items, weights, number of items and the maximum weight\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/knapsack/Knapsack_DP_all3tech.cpp",
"content": "///////////////////////////////RECURSIVE APPROACH////////////////////////////////////////////////////////\n#include <iostream>\n#include <bits/stdc++.h>\nusing namespace std;\nint knapSack(int W,int *wt,int *val,int n)\n{\n if(n==0||W==0)\n {\n return 0;\n }\n if(wt[n]<=W)\n {\n return max(val[n]+knapSack(W-wt[n],wt,val,n-1),knapSack(W,wt,val,n-1));\n //(jab le liya uss wt ko, jab nhi liya uss wt ko)\n //(liya to uska price + baki bacha hua ka price, nhi liya to baki bacha hua ka price)\n //dono ka max nikalo(...,....)\n }\n else if(wt[n]>W)\n {\n return knapSack(W,wt,val,n-1);\n //jab W bada hai to bag me aega hi nhi to baki bacha hua part pe call karenge function\n }\n}\n\n\n\nint main() {\n int val[] = { 60, 100, 120 };\n int wt[] = { 10, 20, 30 };\n int W = 50;\n int n = sizeof(val) / sizeof(val[0]);\n cout << knapSack(W, wt, val, n-1);\n\n return 0;\n}\n//////////////////////////////////////MEMOIZATION APPROACH//////////////////////////////////////////////\n\n#include <iostream>\n#include <bits/stdc++.h>\nusing namespace std;\n//memoisation = recursion + dp table ka use store karne ke liye\n\nint knapSack(int W,int *wt,int *val,int n,int **t)\n{\n if(n==0||W==0)\n {\n t[n][W]=0;\n return t[n][W];\n }\n if(t[n][W]!=-1)\n {\n return t[n][W];\n //agar -1 ki jagha ko value hai to seedha usse return karwa do\n }\n \n if(wt[n]<=W)\n {\n t[n][W]=max(val[n]+knapSack(W-wt[n],wt,val,n-1,t),knapSack(W,wt,val,n-1,t));\n return t[n][W];\n //agar -1 hai to recurssive call to karni padegi\n //aur uss jagha pe vo value store ho jaegi\n }\n else if(wt[n]>W)\n {\n t[n][W]=knapSack(W,wt,val,n-1,t);\n return t[n][W];\n //agar -1 hai to recurssive call to karni padegi\n //aur uss jagha pe vo value store ho jaegi\n \n }\n return -1;\n}\n\n\nint main() \n{\n int val[] = { 60, 100, 120 };\n int wt[] = { 10, 20, 30 };\n int W = 50;\n // int t[4][52]={-1};\n int n = sizeof(val) / sizeof(val[0]);\n ////////////////////////////////////////\n //to declare table dynamically\n int **t;\n t= new int*[n];\n \n \n for (int i = 0; i < n; i++)\n {\n t[i] = new int[W + 1];\n //we are creating cols for each row \n }\n for (int i = 0; i <n; i++)\n { \n for (int j = 0; j < W + 1; j++)\n {\n t[i][j] = -1;\n //initializing to -1\n }\n \n }\n //////////////////////////////////////\n cout <<knapSack(W, wt, val, n-1,t);\n\n return 0;\n}\n//////////////////////////////////////////TOP DOWN APPROACH/////////////////////////////////////////////\n#include <iostream>\n#include <bits/stdc++.h>\nusing namespace std;\n//top-down approach me sabse pehli baat matrix banate\n//matrix bananne se pehle recursion wala code likho\n//usme jo variable change hore honge na uski table banegi\n//table ka size n+1 w+1 karne ka rehta hai\n//recurssion me jo base condition hoti hai usko hum top down me initialize ke roop me use karte \n//that is jab T[0][0] to value 0 kar diya isme matlab (recurssion me jab n==0||w==0 then return 0 kiya tha.)\n//table me ek point like 2,3 hume kya represent karta hai\n//2 hume bataega ki bhai humne pehle 2 item liya hai\n//aur 3 hume bataega uska weight matlab 3 wt ka bag hai \n//aur item 1 item 2 diya rakha hai to ab muje max profit itna tak ka batao\n//jab hum ye store kar diye to ye stored value humko aage wale i(items n),j(W) ke liye kaam aega\n//so sabse pehle recursion fir ya to memoizaition ya top down\n//top down me stack overflow hone ka chance nhi rehta to usse best mana jata haiii!\n\nint knapSack(int W,int *wt,int *val,int n,int **t)\n{\n //yaha i denotes n (num of item) and j denotes W (weight)\n \n for(int i=0;i<n+1;i++)\n {\n for(int j=0;j<W+1;j++)\n {\n if(i==0||j==0)\n {\n t[i][j]=0;\n }\n else if(wt[i]<=W)\n {\n t[i][j]=max(val[i]+t[i-1][j-wt[i]],t[i-1][j]);\n //max(jab include kiya,include nhi kiya)\n }\n else if(wt[i]>W)\n {\n t[i][j]=t[i-1][j];\n }\n \n }\n \n }\n return t[n][W];\n}\n\n\nint main() \n{\n int val[] = { 60, 100, 120 };\n int wt[] = { 10, 20, 30 };\n int W = 50;\n // int t[4][52]={-1};\n int n = sizeof(val) / sizeof(val[0]);\n ////////////////////////////////////////\n //to declare table dynamically\n int **t;\n t= new int*[n];\n \n \n for (int i = 0; i < n; i++)\n {\n t[i] = new int[W + 1];\n //we are creating cols for each row \n }\n \n //////////////////////////////////////\n cout <<knapSack(W, wt, val, n-1,t);\n\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/knapsack/knapsack.go",
"content": "// Part of Cosmos by OpenGenus Foundation\npackage main\n\nimport \"fmt\"\n\n/*\nExptected output:\n\nThe value list is [12 34 1 23 5 8 19 10]\nThe weight list is [5 4 3 7 6 5 1 6]\nFor maxWeight 20, the max value is 89\n*/\n\nfunc max(n1, n2 int) int {\n\tif n1 > n2 {\n\t\treturn n1\n\t}\n\treturn n2\n}\n\nfunc knapsack(value, weight []int, maxWeight int) int {\n\tdp := make([][]int, len(value)+1)\n\n\tfor i := range dp {\n\t\tdp[i] = make([]int, maxWeight+1)\n\t\tfor j := range dp[i] {\n\t\t\tdp[i][j] = 0\n\t\t}\n\t}\n\n\tfor i := 1; i <= len(value); i++ {\n\t\tfor j := 1; j <= maxWeight; j++ {\n\t\t\tif weight[i-1] <= j {\n\t\t\t\tdp[i][j] = max(dp[i-1][j], value[i-1]+dp[i-1][j-weight[i-1]])\n\t\t\t} else {\n\t\t\t\tdp[i][j] = dp[i-1][j]\n\t\t\t}\n\n\t\t}\n\t}\n\n\treturn dp[len(value)][maxWeight]\n}\n\nfunc main() {\n\tvalue := []int{12, 34, 1, 23, 5, 8, 19, 10}\n\tweight := []int{5, 4, 3, 7, 6, 5, 1, 6}\n\tmaxWeight := 20\n\tfmt.Printf(\"The value list is %v\\n\", value)\n\tfmt.Printf(\"The weight list is %v\\n\", weight)\n\tfmt.Printf(\"For maxWeight %d, the max value is %d\\n\", maxWeight, knapsack(value, weight, maxWeight))\n}"
}
{
"filename": "code/dynamic_programming/src/knapsack/knapsack.java",
"content": "// Part of Cosmos by OpenGenus Foundation\n\nclass Knapsack {\n public static void main(String[] args) throws Exception {\n int val[] = {10, 40, 30, 50};\n int wt[] = {5, 4, 6, 3};\n int W = 10;\n System.out.println(knapsack(val, wt, W));\n }\n public static int knapsack(int val[], int wt[], int W) {\n int N = wt.length; // Get the total number of items. Could be wt.length or val.length. Doesn't matter\n int[][] V = new int[N + 1][W + 1]; //Create a matrix. Items are in rows and weight at in columns +1 on each side\n //What if the knapsack's capacity is 0 - Set all columns at row 0 to be 0\n for (int col = 0; col <= W; col++) {\n V[0][col] = 0;\n }\n //What if there are no items at home. Fill the first row with 0\n for (int row = 0; row <= N; row++) {\n V[row][0] = 0;\n }\n for (int item=1;item<=N;item++){\n //Let's fill the values row by row\n for (int weight=1;weight<=W;weight++){\n //Is the current items weight less than or equal to running weight\n if (wt[item-1]<=weight){\n //Given a weight, check if the value of the current item + value of the item that we could afford with the remaining weight\n //is greater than the value without the current item itself\n V[item][weight]=Math.max (val[item-1]+V[item-1][weight-wt[item-1]], V[item-1][weight]);\n }\n else {\n //If the current item's weight is more than the running weight, just carry forward the value without the current item\n V[item][weight]=V[item-1][weight];\n }\n }\n }\n //Printing the matrix\n for (int[] rows : V) {\n for (int col : rows) {\n System.out.format(\"%5d\", col);\n }\n System.out.println();\n }\n return V[N][W];\n }\n}"
}
{
"filename": "code/dynamic_programming/src/knapsack/knapsack.js",
"content": "/**\n * Part of Cosmos by OpenGenus Foundation\n */\n\n/**\n * method which returns the maximum total value in the knapsack\n * @param {array} valueArray\n * @param {array} weightArray\n * @param {integer} maximumWeight\n */\nfunction knapsack(valueArray, weightArray, maximumWeight) {\n let n = weightArray.length;\n let matrix = [];\n\n // lazy initialize the element of 2d array\n if (!matrix[0]) matrix[0] = [];\n\n // if the knapsack's capacity is 0 - Set all columns at row 0 to be 0\n for (let inc = 0; inc <= maximumWeight; inc++) {\n matrix[0][inc] = 0;\n }\n\n for (let i = 1; i < n + 1; i++) {\n // check all possible maxWeight values from 1 to W\n for (let j = 1; j < maximumWeight + 1; j++) {\n // lazy initialize the element of 2d array\n if (!matrix[j]) matrix[j] = [];\n\n // if current item weighs < j then we have two options,\n if (weightArray[i - 1] <= j) {\n // Given a weight, check if the value of the current item + value of the item that we could afford with the remaining weight\n // is greater than the value without the current item itself\n matrix[i][j] = Math.max(\n matrix[i - 1][j],\n valueArray[i - 1] + matrix[i - 1][j - weightArray[i - 1]]\n );\n } else {\n // If the current item's weight is more than the running weight, just carry forward the value without the current item\n matrix[i][j] = matrix[i - 1][j];\n }\n }\n }\n\n return matrix[n][maximumWeight];\n}\n\nconsole.log(\n \"Result - \" + knapsack([12, 1000, 30, 10, 1000], [19, 120, 20, 1, 120], 40)\n);"
}
{
"filename": "code/dynamic_programming/src/knapsack/knapsack.py",
"content": "#######################################\n# Part of Cosmos by OpenGenus Foundation\n#######################################\n\n\ndef knapsack(weights, values, W):\n\n n = len(weights)\n # create 2d DP table with zeros to store intermediate values\n tab = [[0 for i in range(W + 1)] for j in range(n + 1)]\n\n # no further init required as enitre table is already inited\n\n for i in range(1, n + 1):\n # check all possible maxWeight values from 1 to W\n for j in range(1, W + 1):\n if weights[i - 1] <= j:\n # if current item weighs < j then we have two options,\n # take max of them\n tab[i][j] = max(\n tab[i - 1][j], values[i - 1] + tab[i - 1][j - weights[i - 1]]\n )\n else:\n tab[i][j] = tab[i - 1][j]\n\n return tab[n][W]\n\n\ndef main():\n\n values = [12, 1000, 30, 10, 1000] # values of each item\n weights = [19, 120, 20, 1, 120] # weights of each item in same order\n W = 40\n\n # Call knapsack routine to compute value\n print(\"Result - {}\".format(knapsack(weights, values, W)))\n\n return\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/dynamic_programming/src/knapsack/README.md",
"content": "# 0-1 Knapsack\n\n## Description\n\nGiven `n` items, in which the `i`th item has weight `wi` and value `vi`,\nfind the maximum total value that can be put in a knapsack of capacity `W`.\nYou cannot break an item, either pick the complete item, or don't pick it.\n\n## Solution\n\nThis is a dynamic programming algorithm.\nWe first start by building a table consisting of rows of weights and columns of values.\nStarting from the first row and column, we keep on filling the table till the desired value is obtained.\n\nLet the table be represented as `M[n, w]` where `n` is the number of objects included\nand `w` is the left over space in the bag.\n\nFor every object, there can be two possibilities: \n\n- If the object's weight is greater than the leftover space in the bag, \nthen `M[n, w]` = `M[n - 1, w]`\n- Else,\nthe object might be taken or left out.\n - If it is taken, the total value of the bag becomes\n `M[n, w]` = `vn` + `M[n - 1, w - wn]`, where `vn` is value of the `n`th object and `wn` is its weight.\n - Or if the object is not included,\n `M[n, w]` = `M[n - 1, w]`\n \nThe value of `M[n,w]` is the maximum of both these cases.\n\nIn short, the optimal substructure to compute `M[n, w]` is:\n```\nM[n, w] = M[n-1, w], if wn > w\n max( M[n-1, w], vn + M[n-1, w - wn] ), otherwise\n```\n\nThe complexity of above algorithm is `O(n*W)`, as there are `n*W` states and each state is computed in `O(1)`.\n\n---\n\n<p align=\"center\">\n A massive collaborative effort by <a href=\"https://github.com/opengenus/cosmos\">OpenGenus Foundation</a>\n</p>\n\n---"
}
{
"filename": "code/dynamic_programming/src/largest_sum_contiguous_subarray/largest_sum_contiguous_subarray.c",
"content": "#include <stdio.h>\n\nint \nkadanesAlgorithm(int a[], int n)\n{\n\tint max_so_far = 0, max_ending_here = 0;\n\tint i;\n\tfor (i = 0; i < n; ++i) {\n\t max_ending_here = max_ending_here + a[i];\n\n\t if (max_so_far < max_ending_here)\n\t max_so_far = max_ending_here;\n\t\n\t if (max_ending_here < 0)\n\t max_ending_here = 0;\n\t}\n\treturn (max_so_far);\n}\n\nint \nmain()\n{\n\tint n;\n\tprintf(\"Enter size of Array: \");\n\tscanf(\"%d\", &n);\n\n\tint a[n];\n\tprintf(\"Enter %d Integers \\n\", n);\n\tint i;\n\tfor (i = 0; i < n; ++i)\n\t\tscanf(\"%d\", &a[i]);\n\n\tprintf(\"Largest Contiguous Subarray Sum: %d \\n\", kadanesAlgorithm(a, n));\n\n\treturn (0);\n}"
}
{
"filename": "code/dynamic_programming/src/largest_sum_contiguous_subarray/largest_sum_contiguous_subarray.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n *\n * BY:- https://github.com/alphaWizard\n *\n */\n\n#include <iostream>\n#include <vector>\n\nusing namespace std;\n\nint max_subarray_sum(const vector<int>& ar)\n{\n int msf = ar[0], mth = max(ar[0], 0);\n size_t p = 0;\n if (ar[0] < 0)\n ++p;\n int maxi = ar[0];\n for (size_t i = 1; i < ar.size(); i++)\n {\n maxi = max(maxi, ar[i]);\n if (ar[i] < 0)\n ++p; // for handing case of all negative array elements\n\n mth += ar[i];\n if (mth > msf)\n msf = mth;\n if (mth < 0)\n mth = 0;\n }\n return (p != ar.size()) ? msf : maxi;\n}\n\nint main()\n{\n\n cout << max_subarray_sum({-3, 2, -1, 4, -5}) << '\\n'; // Expected output: 5\n cout << max_subarray_sum({-1, -2, -3, -4, -5}) << '\\n'; // Expected output: -1\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/largest_sum_contiguous_subarray/largest_sum_contiguous_subarray.go",
"content": "// Part of Cosmos by OpenGenus Foundation\npackage main\n\nimport \"fmt\"\n\nfunc max(n1, n2 int) int {\n\tif n1 > n2 {\n\t\treturn n1\n\t}\n\treturn n2\n}\n\nfunc maxSubArraySum(data []int) int {\n\tresult := data[0]\n\tcurrent_max := max(data[0], 0)\n\n\tfor i := 1; i < len(data); i++ {\n\t\tcurrent_max += data[i]\n\t\tresult = max(result, current_max)\n\t\tcurrent_max = max(current_max, 0)\n\t}\n\n\treturn result\n}\n\nfunc main() {\n\tinput := []int{-3, 2, -1, 4, -5}\n\tinput2 := []int{-1, -2, -3, -4, -5}\n\n\tfmt.Printf(\"The max sum of %v is %d\\n\", input, maxSubArraySum(input))\n\tfmt.Printf(\"The max sum of %v is %d\\n\", input2, maxSubArraySum(input2))\n}"
}
{
"filename": "code/dynamic_programming/src/largest_sum_contiguous_subarray/largest_sum_contiguous_subarray.hs",
"content": "module LargestSum where\n\n-- Part of Cosmos by OpenGenus Foundation\n\nsublists [] = []\nsublists (a:as) = sublists as ++ [a]:(map (a:) (prefixes as))\n\nsuffixes [] = []\nsuffixes (x:xs) = (x:xs) : suffixes xs\n\nprefixes x = map reverse $ (suffixes . reverse) x\n\nlargestSum = maximum . (map sum) . sublists"
}
{
"filename": "code/dynamic_programming/src/largest_sum_contiguous_subarray/largest_sum_contiguous_subarray.java",
"content": "/* Part of Cosmos by OpenGenus Foundation */ \n\nimport java.util.Scanner;\n\npublic class Max_subarray_problem {\n\n public static void main(String[] args) {\n\n System.out.println(new int[] {-3, 2, -1, 4, -5}, 0, 4); // Expected output: 5\n System.out.println(new int[] {-1, -2, -3, -4, -5}, 0, 4); // Expected output: -1\n }\n\n private static int findmaxsum(int[] a, int l, int h) {\n int max;\n if(l==h)\n return a[l];\n else\n {\n int mid = (l + h) / 2;\n\n int leftmaxsum = findmaxsum(a, l, mid);\n int rightmaxsum = findmaxsum(a , mid + 1, h);\n int crossmaxsum = findcrosssum(a, l, mid, h);\n \n max = Math.max(Math.max(leftmaxsum, rightmaxsum), crossmaxsum);\n }\n return max;\n \n }\n\n private static int findcrosssum(int[] a, int l, int mid, int h) {\n\n int leftsum = Integer.MIN_VALUE;\n int lsum = 0;\n for(int i = mid; i >= l; i--)\n {\n lsum += a[i];\n if(lsum > leftsum)\n leftsum = lsum;\n }\n int rightsum = Integer.MIN_VALUE;\n int rsum = 0;\n for(int j = mid + 1; j <= h; j++)\n {\n rsum += a[j];\n if(rsum > rightsum)\n rightsum = rsum;\n }\n return rightsum + leftsum;\n }\n \n}"
}
{
"filename": "code/dynamic_programming/src/largest_sum_contiguous_subarray/largest_sum_contiguous_subarray.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n# Function to find the maximum contiguous subarray using Kadane's algorithm\ndef maxSubArraySum(a):\n\n max_so_far = a[0]\n max_ending_here = max(a[0], 0)\n\n for i in range(1, len(a)):\n max_ending_here += a[i]\n max_so_far = max(max_so_far, max_ending_here)\n max_ending_here = max(max_ending_here, 0)\n\n return max_so_far\n\n\ndef test():\n\n tests = [\n [-3, 2, -1, 4, -5], # Expected output: 5\n [-1, -2, -3, -4, -5], # Expected output: -1\n ]\n\n for arr in tests:\n print(\"Maximum contiguous sum of\", arr, \"is\", maxSubArraySum(arr))\n\n\ntest()"
}
{
"filename": "code/dynamic_programming/src/largest_sum_contiguous_subarray/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/dynamic_programming/src/longest_bitonic_sequence/longestbitonicseq.cpp",
"content": "#include <iostream>\n#include <vector>\nusing namespace std;\n// Part of Cosmos by OpenGenus Foundation\nint lBitconSeq(vector<int> v, int n)\n{\n vector<int> lis, lds; // lis stands for longest increasing subsequence and lds stands for longest decreasing subsequence\n if (n == 0)\n return 0; // in case tha array is empty longest length will also be empty\n // finding lis\n for (int i = 0; i < n; i++)\n lis.push_back(1);\n for (int i = 1; i < n; i++)\n for (int j = 0; j < i; j++)\n if (v[i] > v[j] && lis[j] + 1 > lis[i])\n lis[i] = lis[j] + 1;\n\n //findin lds\n for (int i = 0; i < n; i++)\n lds.push_back(1);\n for (int i = 1; i < n; i++)\n for (int j = 0; j < i; j++)\n if (v[i] < v[j] && lds[j] + 1 > lds[i])\n lds[i] = lds[j] + 1;\n\n // result wil be max(lis[i] + lds[i] -1)\n int res = lis[0] + lds[0] - 1;\n for (int i = 1; i < n; i++)\n if ((lis[i] + lds[i] - 1) > res)\n res = lis[i] + lds[i] - 1;\n return res;\n}\n\nint main()\n{\n vector<int> v;\n int n;\n cout << \"enter no. of elements in array\\n\";\n cin >> n;\n cout << \"enter elements of the array\\n\";\n for (int i = 0; i < n; i++)\n {\n int temp;\n cin >> temp;\n v.push_back(temp); // filling the array with numbers\n\n }\n cout << \"max length of the lingest bitconic sequence is \" << lBitconSeq(v, n);\n}"
}
{
"filename": "code/dynamic_programming/src/longest_bitonic_sequence/longest_bitonic_sequence.c",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <stdio.h>\n\n// helper function to get maximum of two integers\nint \nmax(int a, int b) \n{\n return (a > b ? a : b);\n}\n\n/**\n * Return the first element in v\n * that is greater than or equal\n * to x in O(log n).\n */\nint\nlower_bound(int v[], int n, int x) \n{ \n int low = 0;\n int high = n;\n while (low < high) {\n int mid = (low + high) / 2;\n if (v[mid] >= x)\n high = mid;\n else\n low = mid + 1;\n } \n return (low);\n}\n\n/**\n * Return the length of the longest\n * bitonic sequence in v.\n * Time complexity: O(n lg n).\n */\nint \nlongest_bitonic_sequence(int v[], int n) {\n \n int lis[n]; // stores the longest increasing sequence that ends at ith position\n int lds[n]; // stores the longest decreasing sequence that starts at ith position\n int tail[n], tailSize; // used to compute LIS and LDS\n int i;\n \n // Computing LIS\n tail[0] = v[0];\n tailSize = 1;\n lis[0] = 1;\n for (i = 1; i < n; ++i) {\n int idx = lower_bound(tail, tailSize, v[i]);\n if(idx == tailSize)\n tail[tailSize++] = v[i];\n else\n tail[idx] = v[i];\n lis[i] = tailSize;\n }\n\n // Computing LDS\n tailSize = 1;\n lds[n - 1] = 1;\n int i;\n for(i = n - 2; i >= 0; --i) {\n int idx = lower_bound(tail, tailSize, v[i]);\n if(idx == tailSize)\n tail[tailSize++] = v[i];\n else\n tail[idx] = v[i];\n lds[i] = tailSize;\n }\n\n // lis[i] + lds[i] - 1 is the length of the\n // longest bitonic sequence with max value\n // at position i.\n int ans = 0;\n for(i = 0; i < n; ++i)\n ans = max(ans, lis[i] + lds[i] - 1);\n\n return (ans);\n}\n\nint \nmain() \n{ \n int v1[] = {1, 2, 5, 3, 2};\n int v2[] = {1, 11, 2, 10, 4, 5, 2, 1};\n\n // Expected output: 5\n printf(\"%d\\n\", longest_bitonic_sequence(v1, sizeof(v1)/sizeof(v1[0])));\n // Expected output: 6 \n printf(\"%d\\n\", longest_bitonic_sequence(v2, sizeof(v2)/sizeof(v2[0])));\n\n return (0);\n}"
}
{
"filename": "code/dynamic_programming/src/longest_bitonic_sequence/longestbitonicsequence.java",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n/* Dynamic Programming implementation in Java for longest bitonic\n subsequence problem */\nimport java.util.*;\nimport java.lang.*;\nimport java.io.*;\n \nclass LBS\n{\n /* lbs() returns the length of the Longest Bitonic Subsequence in\n arr[] of size n. The function mainly creates two temporary arrays\n lis[] and lds[] and returns the maximum lis[i] + lds[i] - 1.\n \n lis[i] ==> Longest Increasing subsequence ending with arr[i]\n lds[i] ==> Longest decreasing subsequence starting with arr[i]\n */\n static int lbs( int arr[], int n )\n {\n int i, j;\n \n /* Allocate memory for LIS[] and initialize LIS values as 1 for\n all indexes */\n int[] lis = new int[n];\n for (i = 0; i < n; i++)\n lis[i] = 1;\n \n /* Compute LIS values from left to right */\n for (i = 1; i < n; i++)\n for (j = 0; j < i; j++)\n if (arr[i] > arr[j] && lis[i] < lis[j] + 1)\n lis[i] = lis[j] + 1;\n \n /* Allocate memory for lds and initialize LDS values for\n all indexes */\n int[] lds = new int [n];\n for (i = 0; i < n; i++)\n lds[i] = 1;\n \n /* Compute LDS values from right to left */\n for (i = n-2; i >= 0; i--)\n for (j = n-1; j > i; j--)\n if (arr[i] > arr[j] && lds[i] < lds[j] + 1)\n lds[i] = lds[j] + 1;\n \n \n /* Return the maximum value of lis[i] + lds[i] - 1*/\n int max = lis[0] + lds[0] - 1;\n for (i = 1; i < n; i++)\n if (lis[i] + lds[i] - 1 > max)\n max = lis[i] + lds[i] - 1;\n \n return max;\n }\n \n public static void main (String[] args)\n {\n int arr[] = {0, 8, 4, 12, 2, 10, 6, 14, 1, 9, 5,\n 13, 3, 11, 7, 15};\n int n = arr.length;\n System.out.println(\"Length of LBS is \"+ lbs( arr, n ));\n }\n}"
}
{
"filename": "code/dynamic_programming/src/longest_bitonic_sequence/longest_bitonic_sequence.js",
"content": "/**\n * Part of Cosmos by OpenGenus Foundation\n */\n\n/**\n * Method that returns the subsequenceLengthArray of the Longest Increasing Subsequence for the input array\n * @param {array} inputArray\n */\nfunction longestIncreasingSubsequence(inputArray) {\n // Get the length of the array\n let arrLength = inputArray.length;\n let i, j;\n\n let subsequenceLengthArray = [];\n\n for (i = 0; i < arrLength; i++) {\n subsequenceLengthArray[i] = 1;\n }\n\n for (i = 1; i < arrLength; i++) {\n for (j = 0; j < i; j++)\n if (\n inputArray[j] < inputArray[i] &&\n subsequenceLengthArray[j] + 1 > subsequenceLengthArray[i]\n ) {\n subsequenceLengthArray[i] = subsequenceLengthArray[j] + 1;\n }\n }\n\n return subsequenceLengthArray;\n}\n\n/**\n * Method that returns the maximum bitonic subsequnce length for the input array\n * @param {array} inputArray\n */\nfunction longestBitonicSubsequence(inputArray) {\n const incSubsequenceLengthArray = longestIncreasingSubsequence(inputArray);\n const decSubsequenceLengthArray = longestIncreasingSubsequence(\n inputArray.reverse()\n );\n\n /* Return the maximum value of incSubsequenceLengthArray[i] + decSubsequenceLengthArray[i] - 1*/\n let maxBitonicLength =\n incSubsequenceLengthArray[0] + decSubsequenceLengthArray[0] - 1;\n for (let i = 1; i < inputArray.length; i++) {\n if (\n incSubsequenceLengthArray[i] + decSubsequenceLengthArray[i] - 1 >\n maxBitonicLength\n ) {\n maxBitonicLength =\n incSubsequenceLengthArray[i] + decSubsequenceLengthArray[i] - 1;\n }\n }\n return maxBitonicLength;\n}\n\nconsole.log(\n `Longest Bitonic Subsequence Length - ${longestBitonicSubsequence([\n 0,\n 8,\n 4,\n 12,\n 2,\n 10,\n 6,\n 14,\n 1,\n 9,\n 5,\n 13,\n 3,\n 11,\n 7,\n 15\n ])}`\n);"
}
{
"filename": "code/dynamic_programming/src/longest_bitonic_sequence/longest_bitonic_sequence.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\nimport copy\n\n# returns the longest increasing sequence\ndef longest_increasing_seq(numbers):\n # longest increasing subsequence\n lis = []\n # base case\n lis.append([numbers[0]])\n\n # start filling using dp - forwards\n\n for i in range(1, len(numbers)):\n lis.append([])\n for j in range(i):\n if numbers[j] < numbers[i] and len(lis[j]) > len(lis[i]):\n lis[i] = copy.copy(lis[j])\n lis[i].append(numbers[i])\n return lis\n\n\n# returns longest decreasing sequence\ndef longest_decreasing_seq(numbers):\n # longest decreasing subsequence\n lds = []\n\n # to make an n sized list\n lds = [[] for _ in range(len(numbers))]\n\n # base case\n lds[-1].append(numbers[-1])\n\n # start filling using dp backwards\n for i in range(len(numbers) - 2, -1, -1):\n for j in range(len(numbers) - 1, i, -1):\n if numbers[j] < numbers[i] and len(lds[j]) > len(lds[i]):\n lds[i] = copy.copy(lds[j])\n\n lds[i].append(numbers[i])\n return lds\n\n\ndef longest_bitonic_seq(numbers):\n lis = longest_increasing_seq(numbers)\n lds = longest_decreasing_seq(numbers)\n\n # now let's find the maxmimum\n maxi = len(lis[0] + lds[0])\n output = lis[0][:-1] + lds[0][::-1]\n\n for i in range(1, len(numbers)):\n if len(lis[i] + lds[i]) > maxi:\n maxi = len(lis[i] + lds[i])\n output = lis[i][:-1] + lds[i][::-1]\n\n return output\n\n\n# you can use any input format\n# format the input such that the array of numbers looks like below\nnumbers = [1, 11, 2, 10, 4, 5, 2, 1]\n\noutput = longest_bitonic_seq(numbers)\n\nprint(output)"
}
{
"filename": "code/dynamic_programming/src/longest_bitonic_sequence/README.md",
"content": "# Longest Bitonic Sequence\n\n## Description\n\nA bitonic sequence is a sequence of numbers which is first strictly increasing\nthen after a point strictly decreasing. Find the length of the longest bitonic\nsequence in a given array `A`.\n\n## Solution\n\nThis problem is a variation of the standard longest increasing subsequence problem.\n\nWe can denote `lis(n)` as the lenght of the longest increasing subsequence that ends\nat `A[n]`, and `lds(n)` the lenght of the longest decreasing subsequence that starts\nat `A[n]`.\n\nThat way, the value of `lis(n) + lds(n) - 1` gives us the longest bitonic\nsequence in which `A[n]` is the highest value. So being `lbs(n)` the longest bitonic\nsequence from `A[0]` to `A[n]`:\n\n```\nlbs(n) = max( lis(i) + lds(i) - 1, 0 <= i <= n )\n```\n\nThe time complexity of above approach will be the same as of the algorithm\nto find the longest increasing subsequence, that can be `O(n^2)` or `O(n log n)`.\n\n---\n\n<p align=\"center\">\nA massive collaborative effort by <a href=\"https://github.com/opengenus/cosmos\">OpenGenus Foundation</a>\n</p>\n\n---"
}
{
"filename": "code/dynamic_programming/src/longest_common_increasing_subsequence/longest_common_increasing_subsequence.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <iostream>\nusing namespace std;\n\nint main()\n{\n int n, m, a[502] = {}, b[502] = {}, d[502][502] = {}, z = 0;\n cin >> n >> m;\n for (int i = 1; i <= n; i++)\n cin >> a[i];\n for (int i = 1; i <= m; i++)\n cin >> b[i];\n for (int i = 1; i <= n; i++)\n {\n int k = 0;\n for (int j = 1; j <= m; j++)\n {\n d[i][j] = d[i - 1][j];\n if (b[j] < a[i])\n k = max(k, d[i - 1][j]);\n if (b[j] == a[i])\n d[i][j] = max(d[i - 1][j], k + 1);\n z = max(z, d[i][j]);\n }\n }\n cout << z << '\\n';\n}"
}
{
"filename": "code/dynamic_programming/src/longest_common_subsequence/longest_common_subsequence.c",
"content": "/*\nProblem Statement : \nFind the length of LCS present in given two sequences.\nLCS -> Longest Common Subsequence\n*/\n\n#include <stdio.h> \n#include <string.h>\n#define MAX 1000\n\nint max(int a, int b) \n{\n return (a > b) ? a : b; \n}\n\nint lcs(char *x, char *y, int x_len, int y_len) \n{\n int dp[x_len + 1][y_len + 1]; \n\n for (int i = 0; i <= x_len; ++i) \n { \n for (int j = 0; j <= y_len; ++j) \n { \n if (i == 0 || j == 0) \n dp[i][j] = 0; \n else if (x[i - 1] == y[j - 1]) \n dp[i][j] = dp[i - 1][j - 1] + 1; \n else\n dp[i][j] = max(dp[i - 1][j], dp[i][j - 1]);\n } \n } \n\n return dp[x_len][y_len]; \n}\n\nint main() \n{\n char a[MAX], b[MAX];\n printf(\"Enter two strings : \", a, b);\n scanf(\"%s %s\", a, b);\n\n int a_len = strlen(a);\n int b_len = strlen(b);\n printf(\"\\nLength of LCS : %d\", lcs(a, b, a_len, b_len)); \n\n return 0; \n}\n\n/*\nEnter two strings : AGGTAB GXTXAYB\n\nLength of LCS : 4\n*/"
}
{
"filename": "code/dynamic_programming/src/longest_common_subsequence/longest_common_subsequence.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <iostream>\n#include <string>\n#include <cstring>\n\n#define MAX 1010\n\nusing namespace std;\n\nint memo[MAX][MAX];\n\n/* Compute the longest common subsequence\n * of strings a[0...n] and b[0...m]\n * Time complexity: O(n*m)\n */\nint lcs(const string& a, const string& b, int n, int m)\n{\n\n if (n < 0 || m < 0)\n return 0;\n\n if (memo[n][m] > -1)\n return memo[n][m];\n\n if (a[n] == b[m])\n return memo[n][m] = 1 + lcs(a, b, n - 1, m - 1);\n\n return memo[n][m] = max( lcs(a, b, n - 1, m), lcs(a, b, n, m - 1) );\n}\n\n// Helper method\nint get_lcs(const string& a, const string& b)\n{\n\n memset(memo, -1, sizeof memo);\n return lcs(a, b, a.length() - 1, b.length() - 1);\n}\n\nint main()\n{\n\n cout << get_lcs(\"cosmos\", \"opengenus\") << '\\n';\n cout << get_lcs(\"ABCDGH\", \"AEDFHR\") << '\\n';\n\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/longest_common_subsequence/longest_common_subsequence.cs",
"content": "using System; \n\nclass LongestCommonSubsequence\n{ \n static int longestCommonSubsequence(char[] x, char[] y) \n { \n int x_len = x.Length; \n int y_len = y.Length;\n int [,]dp = new int[x_len + 1, y_len + 1]; \n\n for (int i = 0; i <= x_len; i++) \n { \n for (int j = 0; j <= y_len; j++) \n { \n if (i == 0 || j == 0) \n dp[i, j] = 0; \n else if (x[i - 1] == y[j - 1]) \n dp[i, j] = dp[i - 1, j - 1] + 1; \n else\n dp[i, j] = max(dp[i - 1, j], dp[i, j - 1]); \n } \n }\n\n return dp[x_len, y_len]; \n } \n\n static int max(int a, int b) \n { \n return (a > b) ? a : b; \n } \n\n public static void Main() \n { \n String s1 = \"AGGTAB\"; \n String s2 = \"GXTXAYB\"; \n\n char[] a = s1.ToCharArray(); \n char[] b = s2.ToCharArray(); \n\n Console.Write(\"Length of LCS : \" + longestCommonSubsequence(a, b)); \n } \n} "
}
{
"filename": "code/dynamic_programming/src/longest_common_subsequence/longest_common_subsequence.go",
"content": "/* Part of Cosmos by OpenGenus Foundation */\npackage main\n\nimport \"fmt\"\n\n/*\nExpect Output\nThe length of LCS of ABCDEFGHIJKLMNOPQRABCEDG adn DEFNMJABCDEG is 9\n\n*/\n\nfunc max(n1, n2 int) int {\n\tif n1 > n2 {\n\t\treturn n1\n\t}\n\treturn n2\n}\n\nfunc LCS(str1, str2 string) int {\n\tlen1 := len(str1)\n\tlen2 := len(str2)\n\n\tdp := make([][]int, len1+1)\n\tfor v := range dp {\n\t\tdp[v] = make([]int, len2+1)\n\t}\n\n\tfor i := 1; i <= len1; i++ {\n\t\tfor j := 1; j <= len2; j++ {\n\t\t\tif str1[i-1] == str2[j-1] {\n\t\t\t\tdp[i][j] = dp[i-1][j-1] + 1\n\t\t\t} else {\n\t\t\t\tdp[i][j] = max(dp[i][j-1], dp[i-1][j])\n\t\t\t}\n\t\t}\n\t}\n\n\treturn dp[len1][len2]\n}\n\nfunc main() {\n\tstr1 := \"ABCDEFGHIJKLMNOPQRABCEDG\"\n\tstr2 := \"DEFNMJABCDEG\"\n\n\tlcs := LCS(str1, str2)\n\tfmt.Printf(\"The length of LCS of %s adn %s is %d\\n\", str1, str2, lcs)\n}"
}
{
"filename": "code/dynamic_programming/src/longest_common_subsequence/longest_common_subsequence.java",
"content": "// Part of Cosmos by OpenGenus Foundation\nclass LongestCommonSubsequence {\n /* Returns length of LCS for X[0..m-1], Y[0..n-1] */\n int lcs( char[] X, char[] Y, int m, int n ) {\n int L[][] = new int[m+1][n+1];\n \n //L[i][j] contains length of LCS of X[0..i-1] and Y[0..j-1]\n for (int i=0; i<=m; i++) {\n for (int j=0; j<=n; j++) {\n if (i == 0 || j == 0) {\n L[i][j] = 0;\n } else if (X[i-1] == Y[j-1]) {\t// if there is a match\n L[i][j] = L[i-1][j-1] + 1;\t// increate lcs value by 1\n } else {\t\t\t\t// else\n L[i][j] = Math.max(L[i-1][j], L[i][j-1]);\t// choose max value till now\n }\n }\n }\n return L[m][n];\n }\n \n public static void main(String[] args) {\n LongestCommonSubsequence lcs = new LongestCommonSubsequence();\n String s1 = \"AGGAGTCTAGCTAB\";\n String s2 = \"AGXGTTXAYBATCGAT\";\n \n char[] X=s1.toCharArray();\n char[] Y=s2.toCharArray();\n int m = X.length;\n int n = Y.length;\n \n System.out.println(\"Length of LCS is\" + \" \" + lcs.lcs( X, Y, m, n ) );\n }\n}\n "
}
{
"filename": "code/dynamic_programming/src/longest_common_subsequence/longest_common_subsequence.php",
"content": "<?php \nfunction longestCommonSubsequence($x , $y) \n{ \n $x_len = strlen($x); \n $y_len = strlen($y) ; \n\n for ($i = 0; $i <= $x_len; $i++) \n { \n for ($j = 0; $j <= $y_len; $j++) \n { \n if ($i == 0 || $j == 0) \n $dp[$i][$j] = 0; \n else if ($x[$i - 1] == $y[$j - 1]) \n $dp[$i][$j] = $dp[$i - 1][$j - 1] + 1; \n else\n $dp[$i][$j] = max($dp[$i - 1][$j], $dp[$i][$j - 1]); \n } \n } \n\n return $dp[$x_len][$y_len]; \n} \n\n$a = \"AGGTAB\"; \n$b = \"GXTXAYB\";\n\necho \"Length of LCS : \", longestCommonSubsequence($a, $b); "
}
{
"filename": "code/dynamic_programming/src/longest_common_subsequence/longest_common_subsequence.py",
"content": "# Part of Cosmos by OpenGenus Foundation\ndef lcs(X, Y):\n m = len(X)\n n = len(Y)\n dp = [[0] * (n + 1) for i in range(m + 1)]\n for i in range(m + 1):\n for j in range(n + 1):\n if i == 0 or j == 0:\n dp[i][j] = 0\n elif X[i - 1] == Y[j - 1]:\n dp[i][j] = dp[i - 1][j - 1] + 1\n else:\n dp[i][j] = max(dp[i - 1][j], dp[i][j - 1])\n return dp[m][n]\n\n\nX = \"FABCDGH\"\nY = \"AVBDC\"\n# ABC is the longest common subsequence\nprint(\"Length of LCS = \", lcs(X, Y))"
}
{
"filename": "code/dynamic_programming/src/longest_common_subsequence/longest_common_subsequence_rec.java",
"content": "// Part of Cosmos by OpenGenus Foundation\nclass LongestCommonSubsequenceRec {\n int lcs( char[] X, char[] Y, int m, int n) {\n if (m == 0 || n == 0) {\t// base case\n\t\treturn 0;\n }\n if (X[m-1] == Y[n-1]) {\t// if common element is found increase lcs length by 1\n\t\treturn 1 + lcs(X, Y, m-1, n-1);\n } else {\t// recursively move back on one string at a time\n \treturn Math.max(lcs(X, Y, m, n - 1), lcs(X, Y, m - 1, n));\n }\n }\n \n public static void main(String[] args) {\n LongestCommonSubsequenceRec lcs = new LongestCommonSubsequenceRec();\n String s1 = \"AAGTCGGTAB\";\n String s2 = \"AGXTGXAYTBC\";\n \n char[] X=s1.toCharArray();\n char[] Y=s2.toCharArray();\n int m = X.length;\n int n = Y.length;\n \n System.out.println(\"Length of LCS is\" + \" \" + lcs.lcs( X, Y, m, n ));\n }\n \n}"
}
{
"filename": "code/dynamic_programming/src/longest_common_subsequence/Printing_longest_common_subsequence.cpp",
"content": "//Printing longest common sub-sequence\r\n#include <iostream>\r\n#include<bits/stdc++.h>\r\nusing namespace std;\r\n\r\n//Helper function to print LCA\r\nstring LCA(string s1,string s2){\r\n int m=s1.length();\r\n int n=s2.length();\r\n int dp[m+1][n+1];\r\n \r\n //initialising first row\r\n for(int i=0; i<=m; i++){\r\n dp[0][i]=0;\r\n }\r\n \r\n //initialising first column\r\n for(int j=0; j<=n; j++){\r\n dp[j][0]=0;\r\n }\r\n \r\n //creating DP table\r\n for(int i=1; i<=m; i++){\r\n for(int j=1; j<=n; j++){\r\n if(s1[i-1]==s2[j-1])\r\n dp[i][j]=1+dp[i-1][j-1];\r\n else \r\n dp[i][j]=max(dp[i-1][j],dp[i][j-1]);\r\n }\r\n }\r\n \r\n int i=m,j=n;\r\n string result;\r\n \r\n while(i>0&&j>0){\r\n if(s1[i-1]==s2[j-1]){\r\n result.push_back(s1[i-1]);\r\n i--; j--;\r\n }\r\n else{\r\n if(dp[i][j-1]>dp[i-1][j])\r\n j--;\r\n else\r\n i--;\r\n }\r\n }\r\n \r\n reverse(result.begin(),result.end());\r\n return result;\r\n}\r\n\r\n\r\nint main()\r\n{\r\n string s1=\"abcdaf\";\r\n string s2=\"acbcf\";\r\n \r\n string answer=LCA(s1,s2);\r\n cout<<\"The LCA is: \"<<answer;\r\n return 0;\r\n}"
}
{
"filename": "code/dynamic_programming/src/longest_common_subsequence/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/dynamic_programming/src/longest_common_subsequence_substring/longest_common_subsequence_substring_problem.cpp",
"content": "// This is a new dynamic programming problem. I have published a research paper\n// about this problem under the guidance of Professor Rao Li (University of\n// South Carolina, Aiken) along with my friend. The paper has been accepted by\n// the Journal of Mathematics and Informatics.The problem is a variation of the\n// standard longest common subsequence problem. It says that--> \"Suppose there\n// are two strings, X and Y. Now we need to find the longest string, which is a\n// subsequence of X and a substring of Y.\"\n\n// Link of the paper--> http://www.researchmathsci.org/JMIart/JMI-v25-8.pdf\n\n\n#include <iostream>\n#include <vector>\n\nclass LCSubseqSubstr {\npublic:\n static std::string LCSS(const std::string& X, const std::string& Y, int m, int n, std::vector<std::vector<int>>& W) {\n int maxLength = 0; // keeps the max length of LCSS\n int lastIndexOnY = n; // keeps the last index of LCSS in Y\n W = std::vector<std::vector<int>>(m + 1, std::vector<int>(n + 1, 0));\n\n for (int i = 1; i <= m; i++) {\n for (int j = 1; j <= n; j++) {\n if (X[i - 1] == Y[j - 1]) {\n W[i][j] = W[i - 1][j - 1] + 1;\n } else {\n W[i][j] = W[i - 1][j];\n }\n if (W[i][j] > maxLength) {\n maxLength = W[i][j];\n lastIndexOnY = j;\n }\n }\n }\n return Y.substr(lastIndexOnY - maxLength, maxLength);\n }\n};\n\nint main() {\n std::string X, Y;\n std::cout << \"Input the first string: \";\n std::getline(std::cin, X);\n\n std::cout << \"Input the second string: \";\n std::getline(std::cin, Y);\n\n int m = X.length(), n = Y.length();\n std::vector<std::vector<int>> W1(m + 1, std::vector<int>(n + 1, 0));\n\n std::cout << \"The longest string which is a subsequence of \" << X\n << \" and a substring of \" << Y << \" is \" << LCSubseqSubstr::LCSS(X, Y, m, n, W1) << std::endl;\n\n std::cout << \"The length of the longest string which is a subsequence of \" << X\n << \" and a substring of \" << Y << \" is \" << LCSubseqSubstr::LCSS(X, Y, m, n, W1).length() << std::endl;\n\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/longest_common_substring/longest_common_substring_2.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n * finding longest common substring between two strings by dynamic programming\n */\n\n#include <string>\n#include <iostream>\n#include <cstring>\nusing namespace std;\n\nint longestCommonSubString(string s1, string s2)\n{\n int T[600][600]; //array length can be adjusted by string length of vector can be used\n memset(T, 0, sizeof(T)); //intialising T to zero\n int maximum = 0;\n\n //filling by recurrence relation\n for (int i = 1; i <= (int)s1.length(); i++)\n {\n for (int j = 1; j <= (int)s2.length(); j++)\n if (s1[i - 1] == s2[j - 1]) //matching of characters\n {\n T[i][j] = T[i - 1][j - 1] + 1;\n if (maximum < T[i][j])\n maximum = T[i][j];\n }\n }\n return maximum;\n}\n\n//longest common substring\nint main()\n{\n string s1, s2;\n\n //standard input stream\n //cin >> s1 >> s2;\n s1 = \"abcdedwwop\";\n s2 = \"abeeedcedwcdedop\";\n int maximum = longestCommonSubString(s1, s2);\n cout << \"Length of longest substring = \";\n cout << maximum << \"\\n\";\n\n //maximum length substring above -- \"cded\" -- length = 4\n}"
}
{
"filename": "code/dynamic_programming/src/longest_common_substring/Longest_Common_Substring.java",
"content": "// Space Complexity: O(n)\n// Time Complexity: O(m*n) \nimport java.util.*;\n\npublic class Longest_Common_Substring { \n \n static String LongestCommonSubstring(String str1, String str2) { \n String temp;\n // longest string is str1 and the smallest string is str2\n if (str2.length() > str1.length()){\n temp = str1;\n str1 = str2;\n str2 = temp;\n } \n int m = str1.length(); \n int n = str2.length(); \n int maxlength = 0; //length of longest common Substring \n int end = 0; //ending point of longest common Substring\n\n int consqRow[][] = new int[2][n + 1]; //store result of 2 consecutive rows\n int curr = 0; //current row in the matrix \n\n //maintaing the array for consequtive two rows\n for (int i = 1; i <= m; i++) { \n for (int j = 1; j <= n; j++) { \n if (str1.charAt(i - 1) == str2.charAt(j - 1)) { \n consqRow[curr][j] = consqRow[1 - curr][j - 1] + 1; \n if (consqRow[curr][j] > maxlength) { \n maxlength = consqRow[curr][j]; \n end = i - 1; \n } \n } \n else { \n consqRow[curr][j] = 0; \n } \n } \n curr = 1 - curr; // changing the row alternatively\n } \n\n if (maxlength == 0) { \n return \"\"; \n } \n else {\n String s = \"\";\n // string is from end-maxlength+1 to end as maxlength is the length of\n // the common substring.\n for (int i = end - maxlength + 1; i <= end; i++) {\n s += str1.charAt(i);\n }\n return s; \n }\n } \n\n public static void main(String args[]) { \n\n Scanner sc = new Scanner(System.in);\n System.out.print(\"Enter String1: \");\n String string1 = sc.nextLine(); \n System.out.print(\"Enter String2: \");\n String string2 = sc.nextLine(); \n // function call \n String temp = LongestCommonSubstring(string1, string2);\n System.out.println(\"String1: \" + string1 + \"\\nString2: \" + string2 );\n if(temp == \"\"){\n System.out.println(\"No common Substring\");\n }\n else\n System.out.println(\"Longest Common Substring: \"+temp + \" (of length: \" +temp.length()+\")\"); \n } \n} "
}
{
"filename": "code/dynamic_programming/src/longest_common_substring/Longest_Common_Substring.py",
"content": "def LongestCommonSubstring(\n string1, \n string2\n):\n # longest string is string1 and the smallest string is string2\n if len(string2) > len(string1):\n temp = string2\n string2 = string1\n string1 = temp\n\n m = len(string1)\n n = len(string2)\n consqRow = []\n\n for i in range(2):\n temp = []\n for j in range(n + 1):\n temp.append(0)\n consqRow.append(temp)\n\n curr, maxlength, end = (0, 0, 0)\n # length of longest common Substring in maxlength\n # ending point of longest common Substring\n\n # maintaing the array for consequtive two rows\n for i in range(1, m + 1):\n for j in range(1, n + 1):\n if string1[i - 1] == string2[j - 1]:\n consqRow[curr][j] = (\n consqRow[1 - curr][j - 1] + 1\n )\n if consqRow[curr][j] > maxlength:\n maxlength = consqRow[curr][j]\n end = i - 1\n else:\n consqRow[curr][j] = 0\n curr = 1 - curr # changing the row alternatively\n\n if maxlength == 0:\n return \"\"\n else:\n # string is from end-maxlength+1 to end as maxlength is the length of\n # the common substring.\n return string1[end - maxlength + 1 : end + 1]\n\n\ndef main():\n print(\"Enter String1: \")\n string1 = input()\n print(\"Enter String2: \")\n string2 = input()\n \n print(\"String1:\", string1)\n print(\"String2:\", string2)\n\n common = LongestCommonSubstring(string1, string2)\n\n if common == \"\":\n print(\"No common SubString\")\n else:\n print(\n \"Longest Common Substring:\",\n common,\n \"( of length:\",\n len(common),\n \")\",\n )\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/dynamic_programming/src/longest_common_substring/Longest_Common_Substring_rename.cpp",
"content": "// Space Complexity: O(n)\n// Time Complexity: O(m*n)\n\n#include <iostream>\n#include <vector>\n\nstd::string LongestCommonSubstring(std::string string1, std::string string2)\n{\n std::string temp;\n // longest string is string1 and the smallest string is string2\n if (string2.size() > string1.size())\n {\n temp = string1;\n string1 = string2;\n string2 = temp;\n }\n\n int m = string1.size();\n int n = string2.size();\n int maxLength = 0; // length of longest common Substring\n int end; // ending point of longest common Substring\n int curr = 0; // current row in the matrix\n\n std::vector<std::vector<int>> consecutiveRows(2, std::vector<int>(n + 1, 0)); // store result of 2 consecutive rows\n\n // maintaing the array for consequtive two rows\n for (int i = 1; i <= m; i++)\n {\n for (int j = 1; j <= n; j++)\n {\n if (string1[i - 1] == string2[j - 1])\n {\n consecutiveRows[curr][j] = consecutiveRows[1 - curr][j - 1] + 1;\n if (consecutiveRows[curr][j] > maxLength)\n {\n maxLength = consecutiveRows[curr][j];\n end = i - 1;\n }\n }\n else\n consecutiveRows[curr][j] = 0;\n }\n curr = 1 - curr; // changing the row alternatively\n }\n\n if (maxLength == 0)\n return \"\";\n else\n {\n std::string s = \"\";\n // string is from end-maxLength+1 to end as maxLength is the length of\n // the common substring.\n for (int i = end - maxLength + 1; i <= end; i++)\n s += string1[i];\n\n return s;\n }\n}\n\nint main()\n{\n std::string string1;\n std::string string2;\n std::cout << \"Enter String1: \";\n std::cin >> string1;\n std::cout << \"Enter String2: \";\n std::cin >> string2;\n std::cout << \"String1: \" << string1 << \"\\nString2: \" << string2 << \"\\n\";\n\n std::string lcsStr = LongestCommonSubstring(string1, string2);\n\n if (lcsStr == \"\")\n std::cout << \"No common substring\\n\";\n else\n std::cout << \"Longest Common Substring: \" << lcsStr << \" (of length: \" << lcsStr.size() << \")\\n\";\n\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/longest_increasing_subsequence/longest_increasing_subsequence.c",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n// LIS implementation in C\n#include<stdio.h>\n\n// Max function \nint \nmax(int a, int b)\n{\n return (a > b ? a : b);\n}\n\n// O(n^2) approach\nint \nlis(int arr[], int n)\n{\n int dp[n], ans = 0 , i = 0, j = 0;\n for (i = 0; i < n; ++i) {\n dp[i] = 1;\n for (j = 0; j < i; j++)\n if(arr[j] < arr[i])\n dp[i] = max(dp[i], 1 + dp[j]);\n ans = max(ans, dp[i]);\n }\n return (ans);\n}\n\nint \nmain() \n{\n int arr[] = {10, 22, 9, 33, 21, 50, 41, 60};\n int n = sizeof(arr) / sizeof(arr[0]);\n printf(\"LIS is : %d\\n\",lis(arr, n));\n\n return (0);\n}"
}
{
"filename": "code/dynamic_programming/src/longest_increasing_subsequence/longest_increasing_subsequence.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <iostream>\n#include <vector>\n#include <algorithm>\n\nusing namespace std;\n\n// Bottom-up O(n^2) approach\nint lis(int v[], int n)\n{\n\n int dp[n], ans = 0;\n for (int i = 0; i < n; ++i)\n {\n dp[i] = 1;\n for (int j = 0; j < i; ++j)\n if (v[j] < v[i])\n dp[i] = max(dp[i], 1 + dp[j]);\n ans = max(ans, dp[i]);\n }\n return ans;\n}\n\n// Bottom-up O(n*log(n)) approach\nint lis2(int v[], int n)\n{\n // tail[i] stores the value of the lower possible value\n // of the last element in a increasing sequence of size i\n vector<int> tail;\n for (int i = 0; i < n; ++i)\n {\n vector<int>::iterator it = lower_bound(tail.begin(), tail.end(), v[i]);\n if (it == tail.end())\n tail.push_back(v[i]);\n else\n *it = v[i];\n }\n return tail.size();\n}\n\nint main()\n{\n\n int v[9] = {10, 22, 9, 33, 21, 50, 41, 60, 80};\n cout << lis(v, 9) << \", \" << lis2(v, 9) << '\\n';\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/longest_increasing_subsequence/longest_increasing_subsequence.go",
"content": "/* Part of Cosmos by OpenGenus Foundation */\npackage main\n\nimport \"fmt\"\n\n/*\nExpteced output\n\nThe length of longest_increasing_subsequece in [10 23 5 81 36 37 12 38 51 92] is 7\n\n*/\n\nfunc max(n1, n2 int) int {\n\tif n1 > n2 {\n\t\treturn n1\n\t}\n\treturn n2\n}\n\n//O(n^2) approach\nfunc LIS(data []int) int {\n\tdp := make([]int, len(data))\n\n\tans := 0\n\tfor i := range data {\n\t\tdp[i] = 1\n\t\tfor j := 0; j < i; j++ {\n\t\t\tif data[j] < data[i] {\n\t\t\t\tdp[i] = max(dp[i], dp[j]+1)\n\t\t\t}\n\t\t}\n\t\tans = max(ans, dp[i])\n\n\t}\n\n\treturn ans\n}\n\n//O(n*logn) approach\nfunc LISV2(data []int) int {\n\ttail := make([]int, 0)\n\n\tvar find int\n\tfor i := range data {\n\n\t\tfind = -1\n\t\t//lower bound, we search from back to head\n\t\tfor v := range tail {\n\t\t\tif tail[len(tail)-v-1] > data[i] {\n\t\t\t\tfind = len(tail) - 1 - v\n\t\t\t}\n\t\t}\n\t\tif find == -1 {\n\t\t\ttail = append(tail, data[i])\n\t\t} else {\n\t\t\ttail[find] = data[i]\n\t\t}\n\t}\n\treturn len(tail)\n}\n\nfunc main() {\n\tinput := []int{10, 23, 5, 81, 36, 37, 12, 38, 51, 92}\n\tans := LIS(input)\n\tfmt.Printf(\"The length of longest_increasing_subsequece in %v is %d\\n\", input, ans)\n\tans = LISV2(input)\n\tfmt.Printf(\"The length of longest_increasing_subsequece in %v is %d\\n\", input, ans)\n}"
}
{
"filename": "code/dynamic_programming/src/longest_increasing_subsequence/longest_increasing_subsequence.java",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\nimport java.lang.Math;\n\nclass LIS\n{\n\t// returns size of the longest increasing subsequence within the given array\n\t// O(n^2) approach\n\tstatic int lis(int arr[], int n)\n\t{\n\t\tint dp[] = new int[n];\n\t\tint ans = 0;\n\t\t\n\t\tfor(int i=0; i<n; i++)\n\t\t{\n\t\t\tdp[i] = 1;\n\t\t\tfor(int j=0; j<i; j++)\n\t\t\t{\n\t\t\t\tif(arr[j] < arr[i])\n\t\t\t\t{\n\t\t\t\t\tdp[i] = Math.max(dp[i], 1+dp[j]);\n\t\t\t\t}\n\t\t\t}\n\t\t\tans = Math.max(ans, dp[i]);\n\t\t}\n\t\treturn ans;\n\t}\n\t\n\tpublic static void main (String[] args) throws java.lang.Exception\n\t{\n\t\tint arr[] = { 10, 22, 9, 33, 21, 50, 41, 60, 80 };\n int n = arr.length;\n System.out.println(\"Length of lis is \" + lis( arr, n ) + \"\\n\" );\n\t}\n}"
}
{
"filename": "code/dynamic_programming/src/longest_increasing_subsequence/longest_increasing_subsequence.js",
"content": "/**\n * Part of Cosmos by OpenGenus Foundation\n */\n\n/**\n * Method that returns the length of the Longest Increasing Subsequence for the input array\n * @param {array} inputArray\n */\nfunction longestIncreasingSubsequence(inputArray) {\n // Get the length of the array\n let arrLength = inputArray.length;\n let i, j;\n\n let subsequenceLengthArray = [];\n\n for (i = 0; i < arrLength; i++) {\n subsequenceLengthArray[i] = 1;\n }\n\n for (i = 1; i < arrLength; i++) {\n for (j = 0; j < i; j++)\n if (\n inputArray[j] < inputArray[i] &&\n subsequenceLengthArray[j] + 1 > subsequenceLengthArray[i]\n ) {\n subsequenceLengthArray[i] = subsequenceLengthArray[j] + 1;\n }\n }\n\n return Math.max(...subsequenceLengthArray);\n}\n\nconsole.log(\n `Longest Increasing Subsequence Length - ${longestIncreasingSubsequence([\n 10,\n 22,\n 9,\n 33,\n 21,\n 50,\n 41,\n 60,\n 80\n ])}`\n);"
}
{
"filename": "code/dynamic_programming/src/longest_increasing_subsequence/longest_increasing_subsequence.py",
"content": "\"\"\"LIS implementation in Python\"\"\"\n# Part of Cosmos by OpenGenus Foundation\n\n# Time Complexity: O(nlogn)\ndef length_of_lis(nums):\n \"\"\"Return the length of the Longest increasing subsequence\"\"\"\n tails = [0] * len(nums)\n size = 0\n for x in nums:\n i, j = 0, size\n while i != j:\n m = int((i + j) / 2)\n if tails[m] < x:\n i = m + 1\n else:\n j = m\n tails[i] = x\n size = max(i + 1, size)\n return size"
}
{
"filename": "code/dynamic_programming/src/longest_increasing_subsequence/longest_increasing_subsequence_using_segment_tree.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n// Finding the Longest Increasing Subsequence using Segment Tree\n\n\n#include <iostream>\n#include <algorithm>\n#include <vector>\n#include <cstring>\n#include <cmath>\n\nusing namespace std;\n\n// function to compare two pairs\nint compare(pair<int, int> p1, pair<int, int> p2)\n{\n\n if (p1.first == p2.first)\n return p1.second > p2.second;\n\n return p1.first < p2.first;\n}\n\n// Building the entire Segment tree, the root of which contains the length of the LIS\nvoid buildTree(int* tree, int pos, int low, int high, int index, int val)\n{\n\n if (index < low || index > high)\n return;\n\n if (low == high)\n {\n tree[pos] = val;\n return;\n }\n\n int mid = (high + low) / 2;\n\n buildTree(tree, 2 * pos + 1, low, mid, index, val);\n buildTree(tree, 2 * pos + 2, mid + 1, high, index, val);\n\n tree[pos] = max(tree[2 * pos + 1], tree[2 * pos + 2]);\n}\n\n// Function to query the Segment tree and return the value for a given range\nint findMax(int* tree, int pos, int low, int high, int start, int end)\n{\n\n if (low >= start && high <= end)\n return tree[pos];\n\n if (start > high || end < low)\n return 0;\n\n int mid = (high + low) / 2;\n\n return max(findMax(tree, 2 * pos + 1, low, mid, start, end),\n findMax(tree, 2 * pos + 2, mid + 1, high, start, end));\n}\n\nint findLIS(int arr[], int n)\n{\n\n pair<int, int> p[n];\n for (int i = 0; i < n; i++)\n {\n p[i].first = arr[i];\n p[i].second = i;\n }\n\n sort(p, p + n, compare);\n\n int len = pow(2, (int)(ceil(sqrt(n))) + 1) - 1;\n int tree[len];\n\n memset(tree, 0, sizeof(tree));\n\n for (int i = 0; i < n; i++)\n buildTree(tree, 0, 0, n - 1, p[i].second, findMax(tree, 0, 0, n - 1, 0, p[i].second) + 1);\n\n return tree[0];\n}\n\nint main()\n{\n int arr[] = { 10, 22, 9, 33, 21, 50, 41, 60 };\n int n = sizeof(arr) / sizeof(arr[0]);\n cout << \"Length of the LIS: \" << findLIS(arr, n);\n return 0;\n}\n\n\n// Time Complexity: O(nlogn)\n// Space Complexity: O(nlogn)"
}
{
"filename": "code/dynamic_programming/src/longest_increasing_subsequence/README.md",
"content": "# Longest Increasing Subsequence\n\n## Description\n\nGiven an array of integers `A`, find the length of the longest subsequence such\nthat all the elements in the subsequence are sorted in increasing order.\n\nExamples:\n\nThe lenght of LIS for `{3, 10, 2, 1, 20}` is 3, that is `{3, 10, 20}`.\n\nThe lenght of LIS for `{10, 22, 9, 33, 21, 50, 41, 60, 80}` is 6,\nthat is `{10, 22, 33, 50, 60, 80}`.\n\n## Solution\n\nLet's define a function `f(n)` as the longest increasing subsequence that can be\nobtained ending in `A[n]`.\n\nFor each `i` such that `i < n`, if `A[i] < A[n]`, we can add\n`A[n]` to the longest incresing subsequence that ends in `A[i]`, generating a \nsubsequence of size `1 + f(i)`. This gives us the following optimal substructure:\n\n```\nf(0) = 1 // base case\nf(n) = max(1 + f(i), i < n && A[i] < A[n])\n```\n\nUsing memoization or tabulation to store the results of the subproblems,\nthe time complexity of above approach is `O(n^2)`.\n\n---\n\n<p align=\"center\">\nA massive collaborative effort by <a href=\"https://github.com/opengenus/cosmos\">OpenGenus Foundation</a>\n</p>\n\n---"
}
{
"filename": "code/dynamic_programming/src/longest_independent_set/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/dynamic_programming/src/longest_palindromic_sequence/longest_palindromic_sequence.c",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n * @Author: Ayush Garg \n * @Date: 2017-10-13 23:11:52 \n * @Last Modified by: Ayush Garg\n * @Last Modified time: 2017-10-13 23:23:10\n */\n\n#include <stdio.h>\n#include <string.h>\n\n#define MAX 1010\n\nint dp[MAX][MAX]; // used to memoize\n\n// utility function to get max\nint max(int a, int b){\n return (a>b)?a:b;\n}\n/**\n * Return the longest palindromic subsequence\n * in s[i...j] in a top-down approach.\n * Time complexity: O(n^2), n = length of s\n */\nint lps(const char s[], int i, int j) {\n\n // if out of range\n if(i > j) return 0;\n\n // if already computed\n if(dp[i][j] > -1) return dp[i][j];\n\n // if first and last characters are equal\n if(s[i] == s[j]) {\n // number of equal characters is 2 if i != j, otherwise 1\n int equalCharacters = 2 - (i == j);\n return dp[i][j] = equalCharacters + lps(s, i+1, j-1);\n }\n // if characters are not equal, discard either s[i] or s[j]\n return dp[i][j] = max( lps(s, i+1, j), lps(s, i, j-1) );\n}\n\n// helper function\nint longest_palindrome(const char s[]) {\n\n memset(dp, -1, sizeof dp);\n return lps(s, 0, strlen(s)-1);\n}\n\nint main() {\n \n printf(\"%d\\n\",longest_palindrome(\"bbabcbcab\")); // 7: babcbab | bacbcab\n printf(\"%d\\n\",longest_palindrome(\"abbaab\")); // 4: abba | baab\n printf(\"%d\\n\",longest_palindrome(\"opengenus\")); // 3: ene | ege | ngn | nen\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/longest_palindromic_sequence/longest_palindromic_sequence.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <iostream>\n#include <cstring>\n\nusing namespace std;\n\nconst int MAX = 1010;\n\nint memo[MAX][MAX]; // used to store subproblems answers\n\n/**\n * Return the longest palindromic subsequence\n * in s[i...j] in a top-down approach.\n * Time complexity: O(n^2), n = length of s\n */\nint lps(const string& s, int i, int j)\n{\n\n // if out of range\n if (i > j)\n return 0;\n\n // if already computed\n if (memo[i][j] > -1)\n return memo[i][j];\n\n // if first and last characters are equal\n if (s[i] == s[j])\n {\n // number of equal characters is 2 if i != j, otherwise 1\n int equalCharacters = 2 - (i == j);\n return memo[i][j] = equalCharacters + lps(s, i + 1, j - 1);\n }\n // if characters are not equal, discard either s[i] or s[j]\n return memo[i][j] = max( lps(s, i + 1, j), lps(s, i, j - 1) );\n}\n\n// helper function\nint longest_palindrome(const string& s)\n{\n\n memset(memo, -1, sizeof memo);\n return lps(s, 0, s.length() - 1);\n}\n\nint main()\n{\n\n cout << longest_palindrome(\"bbabcbcab\") << '\\n'; // 7: babcbab | bacbcab\n cout << longest_palindrome(\"abbaab\") << '\\n'; // 4: abba | baab\n cout << longest_palindrome(\"opengenus\") << '\\n'; // 3: ene | ege | ngn | nen\n\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/longest_palindromic_sequence/longest_palindromic_sequence.js",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\nfunction longest_palindrome(str) {\n let longest = []; // A table to store results of subproblems\n\n // Strings of length 1 are palindrome of lentgh 1\n for (let i = 0; i < str.length; i++) {\n (longest[i] = longest[i] || [])[i] = 1;\n }\n\n for (let cl = 2; cl <= str.length; ++cl) {\n for (let i = 0; i < str.length - cl + 1; ++i) {\n let j = i + cl - 1;\n if (str[i] == str[j] && cl == 2) {\n longest[i][j] = 2;\n } else if (str[i] == str[j]) {\n longest[i][j] = longest[i + 1][j - 1] + 2;\n } else {\n longest[i][j] = Math.max(longest[i][j - 1], longest[i + 1][j]);\n }\n }\n }\n\n return longest[0][str.length - 1];\n}\n\n//test\n[\n [\"bbabcbcab\", 7], // 7: babcbab | bacbcab\n [\"abbaab\", 4], // 4: abba | baab\n [\"opengenus\", 3] // 3: ene | ege | ngn | nen\n].forEach(test => {\n console.assert(longest_palindrome(test[0]) == test[1]);\n});"
}
{
"filename": "code/dynamic_programming/src/longest_palindromic_sequence/longest_palindromic_sequence.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n\ndef longest_palindrome(text):\n \"\"\" Find the maximum length of a palindrome subsequence\n\n Dynamic Programming approach on solving the longest palindromic sequence.\n Time complexity: O(n^2), n = length of text.\n\n Args:\n text: string which will be processed\n Returns:\n Integer of maximum palindrome subsequence length\n \"\"\"\n length = len(text)\n # create palindromes length list (space O(n))\n palindromes_lengths = [1] * length\n # iterate on each substring character\n for fgap in range(1, length):\n # get pre-calculated length\n pre = palindromes_lengths[fgap]\n # reversed iteration on each substring character\n for rgap in reversed(range(0, fgap)):\n tmp = palindromes_lengths[rgap]\n if text[fgap] == text[rgap]:\n # if equal characters, update palindromes_lengths\n if rgap + 1 > fgap - 1:\n # if characters are neighbors, palindromes_lengths is 2\n palindromes_lengths[rgap] = 2\n else:\n # else they're added to the pre-calculated length\n palindromes_lengths[rgap] = 2 + pre\n else:\n # if first and last characters do not match, try the latter ones\n palindromes_lengths[rgap] = max(\n palindromes_lengths[rgap + 1], palindromes_lengths[rgap]\n )\n # update pre-calculated length\n pre = tmp\n # return the maximum palindrome length\n return palindromes_lengths[0]\n\n\ndef main():\n \"\"\" Main routine to test longest_palindrome funtion \"\"\"\n print(longest_palindrome(\"bbabcbcab\")) # 7: babcbab | bacbcab\n print(longest_palindrome(\"abbaab\")) # 4: abba | baab\n print(longest_palindrome(\"opengenus\")) # 3: ene | ege | ngn | nen\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/dynamic_programming/src/longest_palindromic_sequence/README.md",
"content": "# Longest Palindromic Sequence\n\n## Description\n\nGiven a string `S`, find the length of the longest subsequence of `S` that is also a palindrome.\n\nExamples:\n\nThe length of the longest palindromic sequence of `bbabcbcab` is 7 (`babcbab` or `bacbcab`).\n\nThe length of the longest palindromic sequence of `abbaab` is 4 (`abba` or `baab`).\n\n## Solution\n\nWe can model the state of our function as being the start and the end of the string.\nSo let `f(i,j)` be the longest palindromic sequence presented in `S[i:j]`.\n\nComparing the start and the end of `S`, we have two possibilities:\n\n- **The characters are equal:**\nSo these characters will make part of the solution and then we\nwill have to find the longest palindrome in `S[i+1:j-1]`. So,\nthe result is `f(i+1, j-1)` added by 1 or 2, depending if `i == j`.\n- **The characters are different:**\nIn this case, we will have to discard at least one of them.\nIf we discard `S[i]`, the result will be `f(i+1, j)`, and\nanalogously, if we discard `S[j]`, the result will be `f(i, j-1)`.\nAs we want the longest sequence, we take the maximum of these\npossibilities, resulting in `max( f(i+1, j), f(i, j-1) )`.\n\nFollowing is a general recursive approach:\n\n```\nif (i > j) f(i, j) = 0 // invalid range\n\nif (S[i] == S[j]) f(i, j) = f(i+1, j-1) + (1 if i == j else 2)\n\nelse f(i, j) = max( f(i+1, j), f(i, j-1) )\n```\n\nUsing memoization to store the subproblems, the time complexity of this algorithm is O(n^2), being n the length of `S`.\n\n---\n\n<p align=\"center\">\n A massive collaborative effort by <a href=https://github.com/OpenGenus/cosmos>OpenGenus Foundation</a>\n</p>\n\n---"
}
{
"filename": "code/dynamic_programming/src/longest_palindromic_substring/longest_palindromic_substring.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <iostream>\n#include <string>\n\nusing namespace std;\n\nint longestPalSubstr(string str)\n{\n int n = str.size();\n\n bool ispal[n][n];\n\n for (int i = 0; i < n; ++i)\n for (int j = 0; j < n; ++j)\n ispal[i][j] = false;\n\n // All substrings of length 1 are palindromes\n int maxLength = 1;\n for (int i = 0; i < n; ++i)\n ispal[i][i] = true;\n\n // check for sub-string of length 2.\n for (int i = 0; i < n - 1; ++i)\n if (str[i] == str[i + 1])\n {\n ispal[i][i + 1] = true;\n maxLength = 2;\n }\n\n // Check for lengths greater than 2. k is length\n // of substring\n for (int k = 3; k <= n; ++k)\n for (int i = 0; i < n - k + 1; ++i)\n {\n // Get the ending index of substring from\n // starting index i and length k\n int j = i + k - 1;\n if (ispal[i + 1][j - 1] && str[i] == str[j])\n {\n ispal[i][j] = true;\n\n if (k > maxLength)\n maxLength = k;\n }\n }\n return maxLength;\n}\n\nint main()\n{\n string str = \"hacktoberfestsefrisawesome\";\n cout << \"Length of longest palindromic substring is \" << longestPalSubstr(str);\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/longest_palindromic_substring/longest_palindromic_substring.py",
"content": "def longest_pal(test_num):\n num = str(test_num)\n # booleanArray with [start][end]\n pal_boolean_array = [[False for e in range(len(num))] for s in range(len(num))]\n # all one-letter substrings are palindromes\n for s in range(len(num)): # length one substrings are all palindromes\n pal_boolean_array[s][s] = True\n longest = 1\n\n for s in range(len(num) - 1): # check substrings of length 2\n palindrome_boolean = num[s] == num[s + 1]\n pal_boolean_array[s][s + 1] = palindrome_boolean\n if palindrome_boolean:\n longest = 2\n\n for lengths_to_check in range(3, len(num) + 1): # lengths greater than 2\n for s in range(len(num) - lengths_to_check + 1):\n other_characters_symmetry = pal_boolean_array[s + 1][\n s + lengths_to_check - 2\n ]\n palindrome_boolean = (\n num[s] == num[s + lengths_to_check - 1] and other_characters_symmetry\n )\n pal_boolean_array[s][s + lengths_to_check - 1] = palindrome_boolean\n if palindrome_boolean:\n longest = max(longest, lengths_to_check)\n return longest"
}
{
"filename": "code/dynamic_programming/src/longest_palindromic_substring/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/dynamic_programming/src/longest_repeating_subsequence/longest_repeating_subsequence.cpp",
"content": "// Part of Cosmos by OpenGenus Foundation\n\n//dynamic programming || Longest repeating subsequence\n\n#include <iostream>\n#include <string>\n#include <vector>\n\nint longestRepeatingSubsequence(std::string s)\n{\n int n = s.size(); // Obtaining the length of the string\n std::vector<std::vector<int>>dp(n + 1, std::vector<int>(n + 1, 0));\n\n // Implementation is very similar to Longest Common Subsequence problem\n for (int x = 1; x <= n; ++x)\n for (int y = 1; y <= n; ++y)\n {\n if (s[x - 1] == s[y - 1] && x != y)\n dp[x][y] = 1 + dp[x - 1][y - 1];\n else\n dp[x][y] = std::max(dp[x - 1][y], dp[x][y - 1]);\n }\n\n // Returning the value of the result\n return dp[n][n];\n}"
}
{
"filename": "code/dynamic_programming/src/matrix_chain_multiplication/matrix_chain_multiplication.c",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <stdio.h>\n#define inf_ll 2000000000000000000LL\n#define inf 1000000000\n#define eps 1e-8\n#define mod 1000000007\n#define ff first\n#define ss second\n#define N 3456789\ntypedef long long int ll;\nll dp[987][987];\nll ar[N];\n\n/*\nMatrix Chain Multiplication Problem\n\nYou are given N matrices A_1, A_2, A_3 .... A_N. You need to find product (A_1 * A_2 * A_3 .... A_N) \nAs matrix product is associative, there can be different ways of computing the same product. \nFor example (A*B*C*D) can be computed as (((A*B)*C)*D) or ((A*B)*(C*D)). \nThe cost of multiplying two matrices X and Y is given by the number of integer multiplications which \nwe need to perform while multiplying them. Among all possible ways of multiplying the given matrices \nand arriving at the same product, print the minimum total integer multiplications which\nwe need to perform.\n\nInput Format - \nThe first line contains number of matrices N\nThe second line contains N+1 integers B_0, B_1, B_2 ..... B_N.\nThe dimensions of matrix A_i is given by B_(i-1)*B_i. for every i between 1 and N\n\nOutput Format - \nPrint on a single line the answer to the above problem\n\nConstraints - \n1 <= N <= 500\n1 <= B_i <= 10000 for every i between 0 and N\n\nExample - \n\nInput - \n4\n5 5 5 5 1\n\nOutput -\n75\n\nExplanation - \nMinimum cost is achieved by multiplying matrices in the following order\n(A_1*(A_2*(A_3*A_4))). In this order, the cost of each matrix multiplication is 25.\n*/\n\nll min (ll x, ll y) {\n\treturn x < y ? x : y;\n}\n\nint main () {\n\tll n, len, i, j, k;\n\tscanf(\"%lld\", &n);\n\tn += 1;\n\tfor (i = 0; i < n; i++) scanf(\"%lld\", &ar[i]);\n\tfor (len = 2; len < n; len++) {\t\t\t// Loop on the length of the subproblem\n\t\tfor (i = 1; i < n - len + 1; i++) {\t\t// Loop on the starting index of subproblem\n\t\t\tj = i + len - 1;\n\t\t\tdp[i][j] = inf_ll;\n\t\t\tfor (k = i; k < j; k++) {\t\t// Finding the optimal solution for dp[i][j]\n\t\t\t\tdp[i][j] = min(dp[i][j], dp[i][k] + dp[k + 1][j] + ar[i-1] * ar[k] * ar[j]);\n\t\t\t}\n\t\t}\n\t}\n\tprintf(\"%lld\\n\", dp[1][n-1]);\n\treturn 0;\n}"
}
{
"filename": "code/dynamic_programming/src/matrix_chain_multiplication/matrix_chain_multiplication.cpp",
"content": "#include <climits>\n#include <cstdio>\nusing namespace std;\n// Part of Cosmos by OpenGenus Foundation\n// Matrix Ai has dimension p[i-1] x p[i] for i = 1..n\nint MatrixChainOrder(int p[], int n)\n{\n\n /* For simplicity of the program, one extra row and one\n * extra column are allocated in m[][]. 0th row and 0th\n * column of m[][] are not used */\n int m[n][n];\n\n int i, j, k, L, q;\n\n /* m[i,j] = Minimum number of scalar multiplications needed\n * to compute the matrix A[i]A[i+1]...A[j] = A[i..j] where\n * dimension of A[i] is p[i-1] x p[i] */\n\n // cost is zero when multiplying one matrix.\n for (i = 1; i < n; i++)\n m[i][i] = 0;\n\n // L is chain length.\n for (L = 2; L < n; L++)\n for (i = 1; i < n - L + 1; i++)\n {\n j = i + L - 1;\n m[i][j] = INT_MAX;\n for (k = i; k <= j - 1; k++)\n {\n // q = cost/scalar multiplications\n q = m[i][k] + m[k + 1][j] + p[i - 1] * p[k] * p[j];\n if (q < m[i][j])\n m[i][j] = q;\n }\n }\n\n return m[1][n - 1];\n}\n\nint main()\n{\n int arr[] = {1, 2, 3, 4};\n int size = sizeof(arr) / sizeof(arr[0]);\n\n printf(\"Minimum number of multiplications is %d \",\n MatrixChainOrder(arr, size));\n\n getchar();\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/matrix_chain_multiplication/matrixchainmultiplication.java",
"content": "// Dynamic Programming Python implementation of Matrix\n// Chain Multiplication.\n// Part of Cosmos by OpenGenus Foundation\nclass MatrixChainMultiplication\n{\n // Matrix Ai has dimension p[i-1] x p[i] for i = 1..n\n static int MatrixChainOrder(int p[], int n)\n {\n /* For simplicity of the program, one extra row and one\n extra column are allocated in m[][]. 0th row and 0th\n column of m[][] are not used */\n int m[][] = new int[n][n];\n \n int i, j, k, L, q;\n \n /* m[i,j] = Minimum number of scalar multiplications needed\n to compute the matrix A[i]A[i+1]...A[j] = A[i..j] where\n dimension of A[i] is p[i-1] x p[i] */\n \n // cost is zero when multiplying one matrix.\n for (i = 1; i < n; i++)\n m[i][i] = 0;\n \n // L is chain length.\n for (L=2; L<n; L++)\n {\n for (i=1; i<n-L+1; i++)\n {\n j = i+L-1;\n if(j == n) continue;\n m[i][j] = Integer.MAX_VALUE;\n for (k=i; k<=j-1; k++)\n {\n // q = cost/scalar multiplications\n q = m[i][k] + m[k+1][j] + p[i-1]*p[k]*p[j];\n if (q < m[i][j])\n m[i][j] = q;\n }\n }\n }\n \n return m[1][n-1];\n }\n \n // Driver program to test above function\n public static void main(String args[])\n {\n int arr[] = new int[] {1, 2, 3, 4};\n int size = arr.length;\n \n System.out.println(\"Minimum number of multiplications is \"+\n MatrixChainOrder(arr, size));\n }\n}"
}
{
"filename": "code/dynamic_programming/src/matrix_chain_multiplication/matrix_chain_multiplication.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n# Dynamic Programming Python implementation of Matrix Chain Multiplication.\nimport sys\nimport numpy as np\n\n# Matrix Ai has dimension p[i-1] x p[i] for i = 1..n\ndef MatrixChainOrder(p, n):\n m = [[0 for x in range(n)] for x in range(n)]\n for i in range(1, n):\n m[i][i] = 0\n\n for L in range(2, n):\n for i in range(1, n - L + 1):\n j = i + L - 1\n m[i][j] = sys.maxint\n for k in range(i, j):\n q = m[i][k] + m[k + 1][j] + p[i - 1] * p[k] * p[j]\n if q < m[i][j]:\n m[i][j] = q\n\n return m[1][n - 1]\n\n\narr = [1, 2, 3, 4]\nsize = len(arr)\n\nprint(\"Minimum number of multiplications is \" + str(MatrixChainOrder(arr, size)))\n\n\n# This Code is contributed by Debajyoti Halder in the repo"
}
{
"filename": "code/dynamic_programming/src/matrix_chain_multiplication/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/dynamic_programming/src/maximum_product_subarray/maximum_product_subarray.cpp",
"content": "/*\n\nRead Problem Description Here - https://leetcode.com/problems/maximum-product-subarray/\n\nTest Cases - \n\nInput: [2,3,-2,4]\nOutput: 6\n\nInput: [-2,0,-1]\nOutput: 0\n\n*/\n\n#include<iostream>\n#include<climits>\nusing namespace std;\n\nint maxProdSub(int* arr, int n)\n{\n\tint positiveProd = 1, negativeProd = 1;\n\tint ans = INT_MIN;\n\n\tfor(int i = 0 ; i < n ; i++)\n\t{\n\t\tint extraPositive = max(positiveProd * arr[i], max(negativeProd * arr[i], arr[i])); \n\t\t// dummy variable for getting the current max\n\t\tint extraNegative = min(positiveProd * arr[i], min(negativeProd * arr[i], arr[i])); \n\t\t// dummy variable for getting the current min \n\n\t\tpositiveProd = extraPositive; // save current max\n\t\tnegativeProd = extraNegative; // save current min\n\n\t\tif(ans < positiveProd) // if global ans is lesser than current answer, save\n\t\tans = positiveProd;\n\n\t\tif(arr[i] == 0) // if 0 is encountered we reset the values of max and min\n\t\t{\n\t\t\tpositiveProd = 1;\n\t\t\tnegativeProd = 1;\n\t\t}\n\t}\n\n\treturn ans;\n}\n\nint main()\n{\n\tint n; // size input\n\tcin>>n;\n\n\tint arr[n]; // array input\n\tfor(int i = 0 ; i < n ; i++)\n\tcin>>arr[i];\n\n\tcout<<maxProdSub(arr, n);\n}"
}
{
"filename": "code/dynamic_programming/src/maximum_subarray_sum/maximum_subarray_sum.cpp",
"content": "#include <bits/stdc++.h>\nusing namespace std;\n\nint maxSubArraySum(int a[], int size)\n{ // kedane algorithm\n int max_sum = INT_MIN, current_sum = 0;\n\n for (int i = 0; i < size; i++)\n {\n current_sum = current_sum + a[i];\n if (max_sum < current_sum)\n max_sum = current_sum;\n\n if (current_sum < 0)\n current_sum = 0;\n }\n return max_sum;\n}\n\n// Driver program to test maxSubArraySum\nint main()\n{\n int a[] = {-2, -2, 5, -1, -3, 1, 7, -3};\n int n = sizeof(a) / sizeof(a[0]);\n int max_sum = maxSubArraySum(a, n);\n cout << \"Maximum contiguous sum is \" << max_sum;\n return 0;\n}\n\n/*---------------------------------------------------------------------------------------------------------------------------------------*/\n\n// Time complexity: O(n)\n// space complexity: O(1)"
}
{
"filename": "code/dynamic_programming/src/maximum_sum_increasing_subsequence/maximum_sum_increasing_subsequence.c",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <stdio.h>\n\nint \nmaxSum(int arr[], int n)\n{\n int i, j, max = 0;\n int MSis[n];\n\n\n for (i = 0; i <= n; i++)\n MSis[i] = arr[i];\n /* Compute maximum sum values in bottom up manner */\n for (i = 1; i < n; i++)\n\t\tfor(j = 0; j < i; j++)\n\t\t\tif(arr[j] < arr[i] && MSis[i] < MSis[j] + arr[i])\n \tMSis[i] = MSis[j] + arr[i];\n\n /*Find the max value of array MSis */\n for (i = 0; i < n; i++)\n if (max < MSis[i])\n max = MSis[i];\n\n return (max);\n}\n\nint \nmain() \n{\n\tint arr[] = {4, 6, 1, 3, 8, 4, 6};\n int n = sizeof(arr) / sizeof(arr[0]);\n printf(\"Sum of maximum sum increasing subsequence is %d \\n\", maxSum(arr, n));\n\n\treturn (0);\n}"
}
{
"filename": "code/dynamic_programming/src/maximum_sum_increasing_subsequence/maximum_sum_increasing_subsequence.cpp",
"content": "#include <bits/stdc++.h>\n#include <vector>\nusing namespace std;\n\nint maxSum(int arr[], int n){\n\tint i, j, max = 0;\n\tvector<int> dp(n);\n\n\tfor (i = 0; i < n; i++){\n\t\tdp[i] = arr[i];\n\t}\n\n\tfor (i = 1; i < n; i++ ){\n\t\tfor (j = 0; j < i; j++ ){\n\t\t\tif (arr[i] > arr[j] && dp[i] < dp[j] + arr[i]){\n\t\t\t\tdp[i] = dp[j] + arr[i];\n\t\t\t}\n\t\t}\n\t}\n\n\tfor (i = 0; i < n; i++){\n\t\tif (max < dp[i]){\n\t\t\tmax = dp[i];\n\t\t}\n\t}\n\treturn max;\n}\n\n// Driver Code\nint main()\n{\n\tint arr[] = {4, 6, 1, 3, 8, 4, 6};\n\tint n = sizeof(arr) / sizeof(arr[0]);\n\tcout << \"Sum of maximum sum increasing subsequence is \" << maxSum(arr, n) << \".\\n\";\n\t\n\treturn (0);\n}"
}
{
"filename": "code/dynamic_programming/src/maximum_sum_increasing_subsequence/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/dynamic_programming/src/maximum_sum_sub_matrix/maximum_sum_sub_matrix.cpp",
"content": "// Part of Cosmos by OpenGenus Foundation\n// Author: Karan Chawla\n// 15th Oct '17\n#include <iostream>\n#include <vector>\n#include <climits>\n\nusing namespace std;\n\n//Utility function to apply kadane's algorithm\n//Returns the maximum sub in an array\nint kadane(vector<int> &nums, int &start)\n{\n //Initialize variables\n int sum = 0;\n int max_sum = INT_MAX;\n\n int finish = -1;\n int local_start = 0;\n\n for (size_t i = 0; i < nums.size(); i++)\n {\n sum += nums[i];\n if (sum < 0)\n {\n sum = 0;\n local_start = i + 1;\n }\n else if (sum > max_sum)\n {\n max_sum = sum;\n start = local_start;\n finish = i;\n }\n }\n\n if (finish == -1)\n return max_sum;\n\n //When all numbers are negative\n max_sum = nums[0];\n start = finish = 0;\n\n // Find maximum element in the array\n for (size_t i = 0; i < nums.size(); i++)\n if (nums[i] > max_sum)\n {\n max_sum = nums[i];\n start = finish = i;\n }\n\n return max_sum;\n}\n\n\n// Function to find maximum sum rectangle\nvoid findMaxSum(vector<vector<int>> &matrix)\n{\n int row = matrix.size();\n int col = matrix[0].size();\n\n int maxSum = INT_MIN;\n int final_left, final_right, final_bottom, final_top;\n\n int left, right, i, sum, start, finish = 0;\n vector<int> temp(row, 0);\n\n for (left = 0; left < col; left++)\n for (right = left; right < col; right++)\n {\n for (i = 0; i < row; i++)\n temp[i] += matrix[i][right];\n\n sum = kadane(temp, start);\n\n if (sum > maxSum)\n {\n maxSum = sum;\n final_left = left;\n final_right = right;\n final_top = start;\n final_bottom = finish;\n }\n }\n\n cout << \"(top, left)\" << final_top << \" \" << final_left;\n cout << \"(bottom, right)\" << final_bottom << \" \" << final_right;\n cout << \"Maximum sum\" << maxSum;\n\n return;\n}\n\nint main()\n{\n vector<vector<int>> nums = {{1, 2, -1, -4, -20},\n {-8, -3, 4, 2, 1},\n {3, 8, 10, 1, 3},\n {-4, -1, 1, 7, -6}};\n\n findMaxSum(nums);\n\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/maximum_sum_sub_matrix/maximum_sum_sub_matrix.java",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\nimport java.util.*;\nimport java.lang.*;\nimport java.io.*;\n \n/**\n * Given a 2D array, find the maximum sum subarray in it\n */\npublic class MaximumSubMatrixSum\n{\n public static void main (String[] args) throws java.lang.Exception\n {\n findMaxSubMatrix(new int[][] {\n {1, 2, -1, -4, -20},\n {-8, -3, 4, 2, 1},\n {3, 8, 10, 1, 3},\n {-4, -1, 1, 7, -6}\n });\n }\n \n /**\n * To find maxSum in 1d array\n * \n * return {maxSum, left, right}\n */\n public static int[] kadane(int[] a) {\n //result[0] == maxSum, result[1] == start, result[2] == end;\n int[] result = new int[]{Integer.MIN_VALUE, 0, -1};\n int currentSum = 0;\n int localStart = 0;\n \n for (int i = 0; i < a.length; i++) {\n currentSum += a[i];\n if (currentSum < 0) {\n currentSum = 0;\n localStart = i + 1;\n } else if (currentSum > result[0]) {\n result[0] = currentSum;\n result[1] = localStart;\n result[2] = i;\n }\n }\n \n //all numbers in a are negative\n if (result[2] == -1) {\n result[0] = 0;\n for (int i = 0; i < a.length; i++) {\n if (a[i] > result[0]) {\n result[0] = a[i];\n result[1] = i;\n result[2] = i;\n }\n }\n }\n \n return result;\n }\n \n /**\n * To find and print maxSum, (left, top),(right, bottom)\n */\n public static void findMaxSubMatrix(int[][] a) {\n int cols = a[0].length;\n int rows = a.length;\n int[] currentResult;\n int maxSum = Integer.MIN_VALUE;\n int left = 0;\n int top = 0;\n int right = 0;\n int bottom = 0;\n \n for (int leftCol = 0; leftCol < cols; leftCol++) {\n int[] tmp = new int[rows];\n \n for (int rightCol = leftCol; rightCol < cols; rightCol++) {\n \n for (int i = 0; i < rows; i++) {\n tmp[i] += a[i][rightCol];\n }\n currentResult = kadane(tmp);\n if (currentResult[0] > maxSum) {\n maxSum = currentResult[0];\n left = leftCol;\n top = currentResult[1];\n right = rightCol;\n bottom = currentResult[2];\n }\n }\n }\n System.out.println(\"MaxSum: \" + maxSum + \n \", range: [(\" + left + \", \" + top + \n \")(\" + right + \", \" + bottom + \")]\");\n }\n}"
}
{
"filename": "code/dynamic_programming/src/maximum_weight_independent_set_of_path_graph/maximum_weight_independent_set_of_path_graph.cpp",
"content": "#include <iostream>\nusing namespace std;\n\nint main()\n{\n int n;\n cout << \"Enter the number of vertices of the path graph : \";\n cin >> n;\n int g[n];\n cout << \"Enter the vertices of the graph : \";\n for (int i = 0; i < n; i++)\n cin >> g[i];\n int sol[n + 1];\n sol[0] = 0;\n sol[1] = g[0];\n for (int i = 2; i <= n; i++)\n sol[i] = max(sol[i - 1], sol[i - 2] + g[i - 1]);\n cout << \"The maximum weighted independent set sum is : \" << sol[n] << endl;\n int i = n;\n cout << \"The selected verices are : \";\n while (i >= 1)\n {\n if (sol[i - 1] >= sol[i - 2] + g[i - 1])\n i--;\n else\n {\n cout << g[i - 1] << \" \";\n i -= 2;\n }\n }\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/min_cost_path/min_cost_path.c",
"content": "#include <stdio.h>\n\n#define ROWS 3\n#define COLUMNS 3\n\nint \nmin(int x, int y, int z)\n{\n if (x < y)\n return ((x < z)? x : z);\n else\n return ((y < z)? y : z);\n}\n \nint \nminCost(int cost[ROWS][COLUMNS], int m, int n)\n{\n int i, j;\n int travellingCost[ROWS][COLUMNS]; \n \n travellingCost[0][0] = cost[0][0];\n \n for (i = 1; i <= m; i++)\n travellingCost[i][0] = travellingCost[i - 1][0] + cost[i][0];\n \n for (j = 1; j <= n; j++)\n travellingCost[0][j] = travellingCost[0][j - 1] + cost[0][j];\n \n for (i = 1; i <= m; i++)\n for (j = 1; j <= n; j++)\n travellingCost[i][j] = min(travellingCost[i - 1][j - 1], travellingCost[i - 1][j], travellingCost[i][j - 1]) + cost[i][j];\n \n return (travellingCost[m][n]);\n}\n\nint \nmain()\n{\n int cost[ROWS][COLUMNS] = { {1, 2, 3}, {4, 8, 2}, {1, 5, 3} };\n printf(\"%d \\n\", minCost(cost, 2, 2));\n\n return (0);\n}"
}
{
"filename": "code/dynamic_programming/src/min_cost_path/min_cost_path.cpp",
"content": "#include <cstdio>\nusing namespace std;\n#define R 3\n#define C 3\n// Part of Cosmos by OpenGenus Foundation\nint min(int x, int y, int z);\n\nint minCost(int cost[R][C], int m, int n)\n{\n int i, j;\n\n // Instead of following line, we can use int tc[m+1][n+1] or\n // dynamically allocate memoery to save space. The following line is\n // used to keep te program simple and make it working on all compilers.\n int tc[R][C];\n\n tc[0][0] = cost[0][0];\n\n /* Initialize first column of total cost(tc) array */\n for (i = 1; i <= m; i++)\n tc[i][0] = tc[i - 1][0] + cost[i][0];\n\n /* Initialize first row of tc array */\n for (j = 1; j <= n; j++)\n tc[0][j] = tc[0][j - 1] + cost[0][j];\n\n /* Construct rest of the tc array */\n for (i = 1; i <= m; i++)\n for (j = 1; j <= n; j++)\n tc[i][j] = min(tc[i - 1][j - 1],\n tc[i - 1][j],\n tc[i][j - 1]) + cost[i][j];\n\n return tc[m][n];\n}\n\nint min(int x, int y, int z)\n{\n if (x < y)\n return (x < z) ? x : z;\n else\n return (y < z) ? y : z;\n}\n\nint main()\n{\n int cost[R][C] = { {1, 2, 3},\n {4, 8, 2},\n {1, 5, 3} };\n printf(\" %d \", minCost(cost, 2, 2));\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/min_cost_path/min_cost_path.java",
"content": "// Part of Cosmos by OpenGenus Foundation\n\n// Given a cost matrix and calculate the minimum cost path to reach (m, n) \n// from (0, 0)\n\nimport java.io.*;\nimport java.lang.*;\nimport java.math.*;\nimport java.util.*;\n\nclass MinCostPath {\n\tstatic int minCost(int costMatrix[][], int m, int n) {\n\t\tint i,j;\n\t\tint tc[][] = new int[m+1][n+1];\n\t\t\n\t\ttc[0][0] = costMatrix[0][0];\n\t\t\n\t\tfor (i = 1; i <= m; i++)\n\t\t\ttc[i][0] = tc[i-1][0] + costMatrix[i][0];\n \n\t\tfor (j = 1; j <= n; j++)\n\t\t\ttc[0][j] = tc[0][j-1] + costMatrix[0][j];\n\n\t\tfor (i = 1; i <= m; i++)\n\t\t\tfor (j = 1; j <= n; j++)\n\t\t\t\ttc[i][j] = min(tc[i-1][j-1], \n\t\t\t\ttc[i-1][j], \n\t\t\t\ttc[i][j-1]) + costMatrix[i][j];\n\t\t\n\t\treturn tc[m][n];\n\t}\n\t\n\tstatic int min(int x, int y, int z) {\n\t\tif (x < y)\n\t\t\treturn (x < z)? x : z;\n\t\telse\n\t\t\treturn (y < z)? y : z;\n\t}\n\t\n\tpublic static void main(String args[]) {\n\t\tint cost[][] = \tnew int[][]{ \n\t\t\t\t\t{1, 2, 3},\n\t\t\t\t\t{4, 8, 2},\n\t\t\t\t\t{1, 5, 3} \n\t\t\t\t\t};\n\t\tSystem.out.println(minCost(cost, 2, 2));\n\t}\n}"
}
{
"filename": "code/dynamic_programming/src/min_cost_path/min_cost_path.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n\ndef minCost(costMatrix, m, n, traceback=True):\n \"\"\"Given a cost matrix and calculate the minimum cost path to reach (m, n) \n from (0, 0).\n \"\"\"\n\n tc = [[0 for x in range(m + 1)] for y in range(n + 1)]\n\n tc[0][0] = costMatrix[0][0]\n\n # Initialize first column of total cost array\n for i in range(1, m + 1):\n tc[i][0] = tc[i - 1][0] + costMatrix[i][0]\n\n # Initialize first row of total cost array\n for i in range(1, n + 1):\n tc[0][i] = tc[0][i - 1] + costMatrix[0][i]\n\n # Calculate the rest of total cost array\n for i in range(1, m + 1):\n for j in range(1, n + 1):\n tc[i][j] = (\n min(tc[i - 1][j - 1], tc[i - 1][j], tc[i][j - 1]) + costMatrix[i][j]\n )\n\n path = []\n if traceback:\n path.append((m, n))\n\n x, y = m, n\n while x != 0 and y != 0:\n prevCost = tc[x][y] - costMatrix[x][y]\n\n if x > 0 and prevCost == tc[x - 1][y]:\n x -= 1\n elif y > 0 and prevCost == tc[x][y - 1]:\n y -= 1\n else:\n x -= 1\n y -= 1\n\n path.append((x, y))\n\n path.append((0, 0))\n\n path.reverse()\n return tc[m][n], path\n\n\n# Example\n\ncostMatrix = [[1, 2, 3], [4, 8, 2], [1, 5, 3]]\n\nprint(minCost(costMatrix, 2, 2))"
}
{
"filename": "code/dynamic_programming/src/min_cost_path/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/dynamic_programming/src/minimum_cost_polygon_triangulation/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/dynamic_programming/src/minimum_insertion_palindrome/minimum_insertions_palindrome_using_lcs.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n//Number of insertion required to make a string palindrome using dynamic programming\n#include <cstring>\n#include <iostream>\n#include <algorithm>\nusing namespace std;\n\nint LCS(char *s, string r, int n, int m)\n{\n int d[n + 1][m + 1];//d[i][j] contains length of LCS of s[0..i-1] and r[0..j-1]\n\n for (int i = 0; i <= n; i++)\n for (int j = 0; j <= m; j++)\n {\n if (i == 0 || j == 0)\n d[i][j] = 0;\n else if (s[i - 1] == r[j - 1])\n d[i][j] = d[i - 1][j - 1] + 1;\n else\n d[i][j] = max(d[i - 1][j], d[i][j - 1]);\n }\n return d[n][m];\n}\nint MinInsertion(char s[], int n)\n{ //Store reverse of given string\n string rev(s, n);\n reverse(rev.begin(), rev.end());\n\n return n - LCS(s, rev, n, n);\n}\nint main()\n{\n char s[] = \"abcd\";\n cout << MinInsertion(s, strlen(s));\n return 0;\n}"
}
{
"filename": "",
"content": ""
}
{
"filename": "",
"content": ""
}
{
"filename": "",
"content": ""
}
{
"filename": "code/dynamic_programming/src/min_rests_skipped_to_reach_on_time/min_skips.cpp",
"content": "// Part of Cosmos by OpenGenus Foundation \n\n#include<bits/stdc++.h>\n\nint minSkips(vector<int>& dist, int speed, int reachTime) \n{\n /* \n dist: array containing lengths of roads\n speed: the constant speed of travel\n reachTime: the time before/at which you have to reach the office.\n You start at time=0.\n */\n\n int n = dist.size();\n double time[n];\n \n // Find times taken to cover the roads.\n for(int i=0; i<n ; i++)\n {\n time[i] = dist[i]*1.0/speed;\n }\n \n \n // Make a dp table where dp[i][j]=minimum time to cover i roads using j skips\n double dp[n][n];\n memset(dp, 0, sizeof(dp));\n \n /*\n Note that, while taking ceiling and floor of floats and doubles, we can get precision errors due to inability of \n computers to represent floating point numbers precisely in memory. This is why we subtract a very small number \n eps (epsilon) while taking ceiling of float. Eg. ceil(1.0000000001)=2 which is unintended in our case.\n */\n double eps = 0.00000001;\n \n // The dp table is going to be a lower triangular matrix as the number\n // of skips cannot exceed the number of roads.\n for(int i=0;i<n;i++)\n {\n \n for(int j=0;j<=i;j++)\n {\n \n // cover road 0 with 0 skips\n if(i == 0 && j == 0) \n dp[i][j]=time[i];\n\n // no sop is skipped\n else if (j == 0) \n dp[i][0] = ceil(dp[i-1][0]- eps)+time[i];\n\n // all the stops are skipped\n else if (i == j) \n dp[i][j] = dp[i-1][j-1]+time[i];\n\n // we've used j skips to cover i roads \n else \n dp[i][j] = min( ceil (dp[i-1][j] - eps), dp[i-1][j-1]) + time[i];\n } \n }\n \n // check the minimum number of skips required to reach on time \n for(int j=0;j<n;j++)\n {\n if( dp[n-1][j] <= reachTime ) \n return j;\n }\n \n // we cannot reach on time even if we skip all stops.\n return -1;\n}"
}
{
"filename": "code/dynamic_programming/src/min_rests_skipped_to_reach_on_time/README.md",
"content": "# Minimum Rests Skipped to Reach on Time\n\n## Description\n\nYou have to travel to your office in `reachTime` time by travelling on `n` roads given as array `dist` where `dist[i]` is the length of ith road. You have a constant speed `Speed`. After you travel road `i`, you must rest and wait for the next integer hour before you can begin traveling on the next road.\n\nFor example, if traveling a road takes 1.4 hours, you must wait until the 2 hour mark before traveling the next road. If traveling a road takes exactly 2 hours, you do not need to wait.\n\nHowever, you are allowed to skip some rests to be able to arrive on time, meaning you do not need to wait for the next integer hour. Note that this means you may finish traveling future roads at different hour marks.\n\nReturn the **minimum** number of skips required to arrive at the office on time, or -1 if it is impossible. \n\n## Thought Process\n\nLet's number the roads and stops starting from 0. So the stop after road 0 is stop 0 and so on.\nThinking in terms of subproblems and recurrence is a good start. Try answering yourself before revealing the collapsed hints. \n\n<details>\n <summary>What's the Subproblem in terms of roads and skips?</summary>\n What is the minimum time which it takes to cover the i roads using j skips.\n</details>\n\n<details>\n <summary>What's the recurrence relation?</summary>\n \n <p>There are two ways to cover i roads using j skips.\n <ol>\n\t\t <li>\tYou cover i-1 roads using j skips and don't skip at the i-1 th ie. between roads i-1 and i stop. </li>\n\t\t <li> You cover i roads using j-1 skips and skip after the ith stop. So you use j-1+1 skip. </li>\n </ol>\n </p>\n\tNow, you just need to think about the edge cases like how you would cover i roads without any skip?\n</details>\n\n## Solution\n\nCreate a 2D DP table of n rows and n columns where dp[i][j] stores the minimum time to cover till(including) road i using j skips.\n\nTo find the answer, we iterate over all columns (times taken) for road n-1. The skip count corresponding to the first time which is lesser than \nreachTime is the answer."
}
{
"filename": "code/dynamic_programming/src/newman_conway/newman_conway_dp.cpp",
"content": "#include <iostream>\nclass NewmanConwaySequence\n{\npublic:\n NewmanConwaySequence(unsigned int n) : n(n) {}\n\n void calculateNewmanConwaySequenceTermDP(bool flag);\n\nprivate:\n unsigned int n;\n};\nvoid NewmanConwaySequence::calculateNewmanConwaySequenceTermDP(bool flag)\n{\n if(flag == true)\n {\n unsigned int ncs[n + 1];\n ncs[0] = 0;\n ncs[1] = 1;\n ncs[2] = 1;\n\n for (int i = 3; i <= n; i++)\n ncs[i] = ncs[ncs[i - 1]] + ncs[i - ncs[i - 1]];\n std::cout << \"\\nNewman Conway Sequence with \" << n << \" elements : \";\n for(int i = 1; i <= n; i++)\n std::cout << ncs[i] << \" \";\n }\n else\n {\n unsigned int ncs[n + 1];\n ncs[0] = 0;\n ncs[1] = 1;\n ncs[2] = 1;\n\n for (int i = 3; i <= n; i++)\n ncs[i] = ncs[ncs[i - 1]] + ncs[i - ncs[i - 1]];\n\n std::cout << \"\\nNewman Conway Sequence term at \" << n << \" indexed : \";\n std::cout << ncs[n];\n }\n}\n\nint main()\n{\n unsigned int nValue, value;\n bool flag;\n std::cout << \"\\nEnter the Index of Newman Conway Sequence : \";\n std::cin >> nValue;\n NewmanConwaySequence aNewmanConwaySequenceoj(nValue);\n std::cout << \"\\nEnter the non-zero to generate the Newman Conway Sequence or zero to generate particular term in Newman Conway Sequence : \";\n std::cin >> value;\n if(value)\n flag = true;\n else\n flag = false;\n\n if(flag == true)\n aNewmanConwaySequenceoj.calculateNewmanConwaySequenceTermDP(true);\n else\n aNewmanConwaySequenceoj.calculateNewmanConwaySequenceTermDP(false);\n}"
}
{
"filename": "code/dynamic_programming/src/no_consec_ones/no_consec_1.cpp",
"content": "#include <iostream>\n#include <vector>\nusing namespace std;\n\nint countNonConsecutiveOnes(int n)\n{\n vector<int> endingZero(n), endingOne(n);\n endingZero[0] = endingOne[0] = 1;\n for (int i = 1; i < n; i++)\n {\n endingZero[i] = endingZero[i - 1] + endingOne[i - 1];\n endingOne[i] = endingZero[i - 1];\n }\n return endingZero[n - 1] + endingOne[n - 1];\n}\n\nint main()\n{\n int binaryStringLength;\n cout << \"Enter the length of binary string: \";\n cin >> binaryStringLength;\n cout << \"\\nCount of Binary representations of length \" << binaryStringLength\n << \" not having consecutive ones = \";\n cout << countNonConsecutiveOnes(binaryStringLength) << endl;\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/no_consec_ones/no_consec_ones.py",
"content": "# Part of Cosmos by Open Genus Foundation\n# Code contributed by Shamama Zehra\n\n# A dynamic programming solution for no Conseuctive Ones in Binary String problem\n\n\ndef noConsecOnes(n):\n a = [0 for x in range(n)] # number of strings ending with 0\n b = [0 for x in range(n)] # number of strings ending with 1\n\n a[0], b[0] = 1, 1\n\n for i in range(1, n):\n # number of strings ending with 0 is the previous ending with 0\n # plus the previous ending with 1 with a 0 added\n a[i] = a[i - 1] + b[i - 1]\n\n # number of strings ending with 1 is the previous ones ending with\n # 0 plus a 0\n b[i] = a[i - 1]\n\n return a[n - 1] + b[n - 1]\n\n\n# Driver program to test above function\nprint(\n \"Number of binary strings of length 5 with no consecutive ones is \"\n + str(noConsecOnes(5))\n)"
}
{
"filename": "code/dynamic_programming/src/no_consec_ones/README.md",
"content": "# Cosmos\n\n## No Consecutive Ones\n\n### Problem Statement\n\n```\nGiven a length `n`, determine the number of binary strings of length `n` that have no consecutive ones.\n\nFor example, for `n=3`, the strings of length `3` that have no consecutive ones are `000,001,010,100,101`, \nso the algorithm would return `5`.\n\nExpected time complexity: `O(2*n)`, where n is the length of the binary string with no consecutive ones.\n```\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/dynamic_programming/src/number_of_dice_rolls_with_target_sum/NumberOfDiceRollsWithTargetSum.java",
"content": "public class NumberOfDiceRollsWithTargetSum {\n\n public static void main(String[] args) {\n // Test case 1\n System.out.println(numRollsToTarget(1, 6, 3)); // it should output 1\n\n // Test case 2\n System.out.println(numRollsToTarget(30, 30, 500)); //it should output 222616187\n \n }\n\n public static int numRollsToTarget(int dices, int faces, int target) {\n /*\n * dp[i][j] = the number of possible ways modulo 10^9+7\n * i = the current i-th dice\n * j = the current number of points is j\n */\n long[][] dp = new long[35][1005];\n long MOD = (long)1e9 + 7;\n for (int i = 1; i <= faces; i++) dp[1][i] = 1; // Initialization assignment\n for (int i = 2; i <= dices; i++) {\n for (int j = i; j <= i * faces; j++) { // when j < i, it clearly does not exist.\n long count = 0;\n /*\n * Suppose the current dice i rolls the k-th face,\n * then it should be transferred from the point when the last dice was j-k\n * Note that J-K may be less than 1, so we have to limit the range of k\n */\n for (int k = 1; k <= Math.min(faces, j - 1); k++) {\n count = (count + dp[i - 1][j - k]) % MOD;\n }\n dp[i][j] = (dp[i][j] + count) % MOD;\n }\n }\n return (int)dp[dices][target];\n }\n \n}"
}
{
"filename": "code/dynamic_programming/src/number_of_substring_divisible_by_8_but_not_3/number_of_substrings.cpp",
"content": "#include <bits/stdc++.h> \nusing namespace std; \n\nint no_of_substr(string str, int len, int k) \n{ \n int count = 0; \n // iterate over the string \n for (int i = 0; i < len; i++) { \n int n = 0; \n // consider every substring starting from index 'i'\n for (int j = i; j < len; j++) { \n n = n * 10 + (str[j] - '0'); \n // if substring is divisible by k, increase the counter\n if (n % k == 0) \n count++; \n } \n } \n return count; \n} \n \nint main() \n{ \n string str;\n int len;\n cout << \"Enter the string: \";\n cin >> str;\n len = str.length(); \n cout << \"Desired Count: \" << no_of_substr(str, len, 8) - no_of_substr(str, len, 24); \n \n return 0; \n} "
}
{
"filename": "code/dynamic_programming/src/number_of_substring_divisible_by_8_but_not_3/README.md",
"content": "# Number of substrings divisible by 8 but not 3\nWe are interested to find number of substrings of a given string which are divisible by 8 but not 3.\nFor example, in \"556\", possible substrings are \"5\", \"6\", \"55\", \"56\" and \"556\". Amongst which only 56 is divisible by 8 and is not divisible by 3. Therefore, answer should be 1\n\nThis problem can be solved by first finding all the possible substrings of given string and next counting ones relevant to us but this gives us O(n^2) as time complexity. \n\nWe can use dynamic programming here to get O(n) as time complexity. \n\nFor more explanation, refer to <a href=\"https://iq.opengenus.org/number-of-substrings-divisible-by-8-but-not-3/\">number_of_substring_divisible_by_8_but_not_3</a>"
}
{
"filename": "code/dynamic_programming/src/numeric_keypad_problem/numeric_keypad_problem.cpp",
"content": "#include <bits/stdc++.h>\nusing namespace std;\n\nvector<vector<int>> nums;\n\nvoid populate()\n{\n nums.resize(10);\n nums[0].push_back(0);\n nums[0].push_back(8);\n nums[1].push_back(1);\n nums[1].push_back(2);\n nums[1].push_back(4);\n nums[2].push_back(1);\n nums[2].push_back(2);\n nums[2].push_back(3);\n nums[2].push_back(5);\n nums[3].push_back(2);\n nums[3].push_back(3);\n nums[3].push_back(6);\n nums[4].push_back(1);\n nums[4].push_back(4);\n nums[4].push_back(5);\n nums[4].push_back(7);\n nums[5].push_back(2);\n nums[5].push_back(4);\n nums[5].push_back(5);\n nums[5].push_back(6);\n nums[5].push_back(8);\n nums[6].push_back(3);\n nums[6].push_back(5);\n nums[6].push_back(6);\n nums[6].push_back(9);\n nums[7].push_back(4);\n nums[7].push_back(7);\n nums[7].push_back(8);\n nums[8].push_back(0);\n nums[8].push_back(5);\n nums[8].push_back(7);\n nums[8].push_back(8);\n nums[8].push_back(9);\n nums[9].push_back(6);\n nums[9].push_back(8);\n nums[9].push_back(9);\n}\n\nint main()\n{\n int t;\n cin >> t;\n populate();\n while (t--)\n {\n int n;\n cin >> n;\n int arr[10][n + 1];\n for (int i = 0; i < 10; i++)\n {\n arr[i][0] = 0;\n arr[i][1] = 1;\n arr[i][2] = nums[i].size();\n }\n for (int i = 3; i <= n; i++)\n for (int j = 0; j < 10; j++)\n {\n arr[j][i] = 0;\n for (std::size_t k = 0; k < nums[j].size(); k++)\n arr[j][i] += arr[nums[j][k]][i - 1];\n }\n int count = 0;\n for (int i = 0; i < 10; i++)\n count += arr[i][n];\n cout << count << endl;\n }\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/optimal_binary_search_tree/optimal_bst.py",
"content": "# Given keys and frequency, calculate the most optimal binary search tree that can be created making use of minimum cost\n\nkeys = list(map(int, input(\"Enter the list of keys\").split()))\nfreq = list(map(int, input(\"Enter the list of frequencies\").split()))\nz = []\nn = len(keys)\nfor i in range(n):\n z += [[keys[i], freq[i]]]\nz.sort()\ncost = [[10 ** 18 for i in range(n)] for j in range(n)] # initialising with infinity\nfor i in range(n):\n keys[i] = z[i][0]\n freq[i] = z[i][1]\ns = [freq[0]]\nfor i in range(n - 1):\n s += [s[i] + freq[i + 1]]\nfor i in range(n):\n cost[i][i] = freq[i]\nfor i in range(2, n + 1):\n for j in range(n - i + 1):\n l = j\n r = l + i - 1\n for k in range(l, r + 1):\n if k == l:\n cost[l][r] = min(cost[l][r], cost[l + 1][r])\n elif k == r:\n cost[l][r] = min(cost[l][r], cost[l][r - 1])\n else:\n cost[l][r] = min(cost[l][r], cost[l][k] + cost[k + 1][r])\n\n cost[l][r] += s[r] - s[l] + freq[l]\n\nprint(\"Cost of Optimal BST is : \", cost[0][n - 1])"
}
{
"filename": "code/dynamic_programming/src/palindrome_partition/palindrome_partition.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <iostream>\n#include <cstring>\n#include <string>\n\n#define MAX 1010\n\nusing namespace std;\n\nint isPal[MAX][MAX], memo[MAX];\n\n/*\n * Checks if s[i...j] is palindrome\n * in a top-down fashion. Result is\n * stored in isPal matrix.\n * Time complexity: O(n^2)\n */\nint isPalindrome(const string &s, int i, int j)\n{\n\n if (i > j)\n return isPal[i][j] = 0;\n\n if (isPal[i][j] > -1)\n return isPal[i][j];\n\n if (j - i <= 1 && s[i] == s[j])\n return isPal[i][j] = 1;\n\n if (s[i] == s[j])\n return isPal[i][j] = isPalindrome(s, i + 1, j - 1);\n\n return isPal[i][j] = 0;\n}\n\n/*\n * Compute the minimum number of cuts\n * necessary to partition the string\n * s[0...i] in a set of palindromes.\n * Time complexity: O(n^2)\n */\nint minPalPartition(const string &s, int i)\n{\n\n if (isPal[0][i])\n return memo[i] = 0;\n\n if (memo[i] > -1)\n return memo[i];\n\n int ans = i + 1;\n for (int j = 0; j < i; ++j)\n if (isPal[j + 1][i] == 1)\n ans = min(ans, 1 + minPalPartition(s, j));\n\n return memo[i] = ans;\n}\n\n// Helper function\nint solve(const string &s)\n{\n\n memset(isPal, -1, sizeof isPal);\n memset(memo, -1, sizeof memo);\n\n int n = s.length();\n for (int i = 0; i < n; ++i)\n for (int j = i; j < n; ++j)\n isPalindrome(s, i, j);\n\n return minPalPartition(s, n - 1);\n}\n\nint main()\n{\n\n cout << solve(\"aaabba\") << '\\n'; // 1: aa | abba\n cout << solve(\"ababbbabbababa\") << '\\n'; // 3: a | babbbab | b | ababa\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/palindrome_partition/palindrome_partition.js",
"content": "/**\n * Part of Cosmos by OpenGenus Foundation\n */\n\n/**\n * Method which returns the Minimum Cuts to perform Palindrome Partioning\n * @param {string} inputString\n */\nfunction palindromePartioning(inputString) {\n // Get the length of the string\n let strLength = inputString.length;\n let minCuts = 0;\n let i, L, j;\n\n /* \n Create two arrays to build the solution in bottom up manner\n cutMatrix = Minimum number of cuts needed for palindrome partitioning of substring inputString[0..i]\n palMatrix = true if substring inputString[i..j] is palindrome, else false\n */\n let palMatrix = [];\n let cutMatrix = [];\n\n // for single letter palindromes set them true (since they palindrome on there own)\n for (i = 0; i < strLength; i++) {\n // lazy initialize the element of 2d array\n if (!palMatrix[i]) palMatrix[i] = [];\n palMatrix[i][i] = true;\n }\n\n /* L is substring length. Build the solution in bottom up manner by\n considering all substrings of length starting from 2 to n. */\n for (L = 2; L <= strLength; L++) {\n // For substring of length L, set different possible starting indexes\n for (i = 0; i < strLength - L + 1; i++) {\n j = i + L - 1; // Set ending index\n\n // If L is 2, then we just need to compare two characters. Else\n // need to check two corner characters and value of palMatrix[i+1][j-1]\n if (L == 2) {\n if (!palMatrix[i]) palMatrix[i] = [];\n palMatrix[i][j] = inputString[i] == inputString[j];\n } else {\n if (!palMatrix[i]) palMatrix[i] = [];\n palMatrix[i][j] =\n inputString[i] == inputString[j] && palMatrix[i + 1][j - 1];\n }\n }\n }\n\n for (i = 0; i < strLength; i++) {\n if (palMatrix[0][i] == true) cutMatrix[i] = 0;\n else {\n cutMatrix[i] = strLength;\n for (j = 0; j < i; j++) {\n if (palMatrix[j + 1][i] == true && 1 + cutMatrix[j] < cutMatrix[i])\n cutMatrix[i] = 1 + cutMatrix[j];\n }\n }\n }\n // Return the min cut value for complete string.\n return cutMatrix[strLength - 1];\n}\n\nconsole.log(\n `Minimum Cuts to perform Palindrome Partioning - ${palindromePartioning(\n \"hallelujah\"\n )}`\n);"
}
{
"filename": "code/dynamic_programming/src/palindrome_partition/README.md",
"content": "# Palindrome Partitioning\n\n## Description\n\nGiven a string `S`, determine the minimum number of cuts necessary to partition `S` in a set of palindromes. \n\nFor example, for string `aaabbba`, minimum cuts needed is `1`, making `aa | abba`.\n\n## Solution\n\nFirst we need to know for each substring of `S`, whether it is a palindrome or not.\nLet' denote by `isPalindrome(i, j)` if `S[i:j]` is palindrome.\nThis can be computed in `O(n^2)` by the following optimal substructure:\n\n```\nisPalindrome(i, j) = false, if i > j or S[i] != S[j]\n true, if j - i <= 1 && S[i] == S[j]\n isPalindrome(i+1, j-1), otherwise\n```\n\nNow let's denote by `minPartition(n)` the minimum number of partitions needed\nto partition `S[0:n]` in a set of palindromes.\n\nFor each `i` such that `S[i+1:n]` is palindrome, we can partition at position `i`,\nand the result for `minPartition(n)` would be `1 + minPartition(i)`. Based on this:\n\n```\nminPartition(n) = min( 1 + minPartition(i), 0 <= i < n && isPalindrome(i+1, n) )\n```\n\n## Complexity\n\nThe time complexity of `isPalindrome` is `O(n^2)`, as there are `n^2` stated that are\ncomputed in `O(1)`. The time complexity of `minPartition` is also `O(n^2)`, as there\nare `n` states that are computes in `O(n)`.\n\nTherefore, the overall time complexity of such approach is `O(n^2)`.\n\n---\n<p align=\"center\">\n A massive collaborative effort by <a href=\"https://github.com/opengenus/cosmos\">OpenGenus Foundation</a>\n</p>\n\n---"
}
{
"filename": "code/dynamic_programming/src/README.md",
"content": "# Dynamic Programming \n\nDynamic programming approach is similar to divide and conquer in breaking down the problem into smaller and yet smaller possible sub-problems. But unlike, divide and conquer, these sub-problems are not solved independently. Rather, results of these smaller sub-problems are remembered and used for similar or overlapping sub-problems.\n\nDynamic programming is used where we have problems, which can be divided into similar sub-problems, so that their results can be re-used. Mostly, these algorithms are used for optimization. Before solving the in-hand sub-problem, dynamic algorithm will try to examine the results of the previously solved sub-problems. The solutions of sub-problems are combined in order to achieve the best solution.\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/dynamic_programming/src/rod_cutting/README.md",
"content": "# Rod Cutting\nGiven a rod of length n units and an array of prices p<sub>i</sub> for rods of length i = 1, 2, . . . ,n. Determine the maximum revenue r <sub>n</sub> obtainable by cutting the rod and selling the pieces.\n\n \n## Explanation\n\nLet the table of prices be - \n\nlength (i) | 1|2|3|4|5|6|7|8|9|10 \n-----------|--|-|-|-|-|--|-|--|--|------------ \nprice (p<sub>i</sub>)|1|5|8|9|10|17|17|20|24|30\n\nFor a rod of length n = 4\n\n\n\n> Image credits: ([CS Indiana](https://www.cs.indiana.edu/~achauhan/Teaching/B403/LectureNotes/images/07-rodcutting-example.jpg))\n\n\n\n> Image credits: ([Medium: Pratik Anand](https://medium.com/@pratikone/dynamic-programming-for-the-confused-rod-cutting-problem-588892796840))\n\nMaximum revenue is obtained by cutting the rod into two pieces each of length 2. Thus, maximum revenue obtained is 10.\n\nThis problem satisfies both properties of Dynamic programming and can be solved by the following recurrence relation.\n\n\n### Recurrence Relation\n\n\n## Complexity\nTime complexity : O(n^2)\n\nSpace complexity : O(n)\n\n---\n<p align=\"center\">\n\tA massive collaborative effort by <a href=\"https://github.com/OpenGenus/cosmos\">OpenGenus Foundation</a> \n</p>\n\n---"
}
{
"filename": "code/dynamic_programming/src/rod_cutting/rod_cutting.c",
"content": "#include <stdio.h>\n\n#define INT_MIN -32767 \n#define max(a, b) (((a) > (b)) ? (a) : (b))\n \nint \ncutRod(int price[], int length_of_rod)\n{\n if (length_of_rod <= 0)\n return (0);\n\n int max_val = INT_MIN, i;\n for (i = 0; i < length_of_rod; i++)\n max_val = max(max_val, price[i] + cutRod(price, length_of_rod - i - 1));\n \n return (max_val);\n}\n\nint \nmain()\n{\n int length_of_rod;\n printf(\"Enter length of rod: \");\n scanf(\"%d\", &length_of_rod);\n\n int price[length_of_rod], i;\n printf(\"Enter prices: \\n\");\n for (i = 0; i < length_of_rod; i++)\n scanf(\"%d\", &price[i]);\n\n printf(\"Maximum Obtainable Value is %d \\n\", cutRod(price, length_of_rod));\n\n return (0);\n}"
}
{
"filename": "code/dynamic_programming/src/rod_cutting/rod_cutting.cpp",
"content": "#include <iostream>\n#include <vector>\n// Part of Cosmos by OpenGenus Foundation\n// Use bottom-up DP, O(n^2) time, O(n) space.\nusing namespace std;\nint rodCutting(int rod_length, vector<int> prices)\n{\n vector<int> best_price(rod_length + 1, 0);\n best_price[0] = 0;\n for (int i = 1; i <= rod_length; ++i)\n {\n int maximum_price = INT8_MIN;\n for (int j = 1; j <= i; ++j)\n maximum_price = max(maximum_price, prices[j - 1] + best_price[i - j]);\n best_price[i] = maximum_price;\n }\n return best_price[rod_length];\n}\n\nint main()\n{\n cout << rodCutting(8, {1, 5, 8, 9, 10, 17, 17, 20, 24, 30}) << endl; // should be 22 (one length 2, another length 6)\n cout << rodCutting(10, {1, 5, 8, 9, 10, 17, 17, 20, 24, 30}) << endl; // should be 30\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/rod_cutting/rod_cutting.go",
"content": "// Part of Cosmos by OpenGenus Foundation\n// Code contributed by Marc Tibbs\n\n// A Dynamic Programming solution for Rod Cutting problem \npackage main\n\nimport (\n\t\"fmt\"\n\t\"math\"\n)\n\nconst min float64 = -1 << 63\n\nfunc rodCut(price []float64, n int) float64 {\n\tval := []float64{0}\n\tval = append(val, price...)\n\n\tfor i := 1; i < n+1; i++ {\n\t\tvar max_val = min\n\t\tfor j := 0; j < i; j++ {\n\t\t\tmax_val = math.Max(max_val, price[j] + val[i-j-1])\n\t\t}\n\t\tval[i] = max_val\n\t}\n\treturn val[n]\n}\n\n// Driver program to test rodCut function\nfunc main() {\n\tarr := []float64{1, 5, 8, 9, 10, 17, 17, 20}\n\tvar size = len(arr)\n\tfmt.Println(\"Maximum Obtainable Value is\",rodCut(arr, size))\n}"
}
{
"filename": "code/dynamic_programming/src/rod_cutting/rod_cutting.hs",
"content": "import Data.Array\nrodCutting :: Int -> [Int] -> Int\n-- Part of Cosmos by OpenGenus Foundation\n-- NOTE: great article on lazy DP http://jelv.is/blog/Lazy-Dynamic-Programming/\nrodCutting x priceList | x < 0 = 0\n | otherwise = r!x\n where priceArray = listArray (0, length priceList) priceList -- for faster indexing into prices\n r = listArray (0,x) (0: map f [1..x])\n f k = maximum [priceArray!(i-1) + rodCutting (k-i) priceList | i <- [1..k]]"
}
{
"filename": "code/dynamic_programming/src/rod_cutting/rod_cutting.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n# Code contributed by Debajyoti Halder\n\n# A Dynamic Programming solution for Rod cutting problem\nINT_MIN = -32767\n\n\ndef cutRod(price, n):\n val = [0 for x in range(n + 1)]\n val[0] = 0\n for i in range(1, n + 1):\n max_val = INT_MIN\n for j in range(i):\n max_val = max(max_val, price[j] + val[i - j - 1])\n val[i] = max_val\n\n return val[n]\n\n\n# Driver program to test above functions\narr = [1, 5, 8, 9, 10, 17, 17, 20]\nsize = len(arr)\nprint(\"Maximum Obtainable Value is \" + str(cutRod(arr, size)))"
}
{
"filename": "code/dynamic_programming/src/shortest_common_supersequence/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/dynamic_programming/src/shortest_common_supersequence/scs.java",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\nimport java.util.*;\nimport java.lang.*;\nimport java.io.*;\n\n/* A DP based program to find length\nof the shortest supersequence */\npublic class SCS {\n \n // Returns length of the shortest supersequence of X and Y\n static int superSequence(String X, String Y, int m, int n)\n {\n int dp[][] = new int[m+1][n+1];\n \n for (int i = 0; i <= m; i++)\n {\n for (int j = 0; j <= n; j++)\n {\n if (i == 0)\n dp[i][j] = j;\n else if (j == 0)\n dp[i][j] = i;\n else if (X.charAt(i-1) == Y.charAt(j-1))\n dp[i][j] = 1 + dp[i-1][j-1];\n //Since we need to minimize the length\n else\n dp[i][j] = 1 + Math.min(dp[i-1][j], dp[i][j-1]);\n }\n }\n \n return dp[m][n];\n }\n \n //Main function\n public static void main(String args[])\n {\n String X = \"ABCBDAB\";\n String Y = \"BDCABA\";\n System.out.println(\"Length of the shortest supersequence is \"+ superSequence(X, Y, X.length(),Y.length()));\n }\n}"
}
{
"filename": "code/dynamic_programming/src/shortest_common_supersequence/shortest_common_supersequence.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <iostream>\n#include <cstring>\n\nusing namespace std;\n\nconst int MAX = 1010;\n\nint memo[MAX][MAX]; // used for memoization\n\n// Function to find length of shortest common supersequence\n// between sequences X[0..m-1] and Y[0..n-1]\nint SCSLength(string& X, string& Y, int m, int n)\n{\n // if we have reached the end of either sequence, return\n // length of other sequence\n if (m == 0 || n == 0)\n return n + m;\n\n // if we have already computed this state\n if (memo[m - 1][n - 1] > -1)\n return memo[m - 1][n - 1];\n\n // if last character of X and Y matches\n if (X[m - 1] == Y[n - 1])\n return memo[m - 1][n - 1] = SCSLength(X, Y, m - 1, n - 1) + 1;\n else\n // else if last character of X and Y don't match\n return memo[m - 1][n - 1] = 1 + min(SCSLength(X, Y, m, n - 1),\n SCSLength(X, Y, m - 1, n));\n}\n\n// helper function\nint findSCSLength(string& X, string& Y)\n{\n memset(memo, -1, sizeof memo);\n return SCSLength(X, Y, X.length(), Y.length());\n}\n\n// main function\nint main()\n{\n string X = \"ABCBDAB\", Y = \"BDCABA\";\n\n cout << \"The length of shortest common supersequence is \"\n << findSCSLength(X, Y) << '\\n';\n\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/shortest_common_supersequence/shortest_common_supersequence.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n# shortest common supersequence using dp\ndef min(a, b):\n if a > b:\n return b\n else:\n return a\n\n\ndef superSeq(X, Y, m, n):\n dp = []\n for i in range(m + 1):\n l2 = []\n for j in range(n + 1):\n if not (i):\n l2.append(j)\n\n elif not (j):\n l2.append(i)\n\n elif X[i - 1] == Y[j - 1]:\n l2.append(1 + dp[i - 1][j - 1])\n\n else:\n l2.append(1 + min(dp[i - 1][j], l2[j - 1]))\n dp.append(l2)\n return dp[m][n]\n\n\nX = \"FABCDF\"\nY = \"HBABCDEF\"\n# longest supersequence can be HBFABCDEF\nprint(\"Length of the shortest supersequence = \", superSeq(X, Y, len(X), len(Y)))"
}
{
"filename": "code/dynamic_programming/src/subset_sum/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/dynamic_programming/src/subset_sum/subset_sum.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n#ifndef SUBSET_SUM\n#define SUBSET_SUM\n\n#include <iterator>\n\n/*\n * Check whether is possible to\n * get value sum from a subset\n * of the [begin:end)\n */\n\n// complexity: time: O(sum * n), space: O(sum)\ntemplate<typename _BidirectionalIter,\n typename _ValueType = typename std::iterator_traits<_BidirectionalIter>::value_type>\nbool\nisSubsetSum(_BidirectionalIter begin, _BidirectionalIter end, _ValueType sum)\n{\n auto sz = std::distance(begin, end);\n bool subset[2][sum + 1];\n\n for (int i = 0; i <= sz; i++)\n {\n auto x = begin;\n std::advance(x, i - 1);\n\n for (int j = 0; j <= sum; j++)\n {\n // A subset with sum 0 is always possible\n if (j == 0)\n subset[i % 2][j] = true;\n // If there exists no element\n // no sum is possible\n else if (i == 0)\n subset[i % 2][j] = false;\n else if (*x <= j)\n subset[i % 2][j] = subset[(i + 1) % 2][j - *x] || subset[(i + 1) % 2][j];\n else\n subset[i % 2][j] = subset[(i + 1) % 2][j];\n }\n }\n\n return subset[sz % 2][sum];\n}\n\n/*\n * // complexity: time: O(sum * n), space: O(sum * n)\n * template<typename _BidirectionalIter,\n * typename _ValueType = typename std::iterator_traits<_BidirectionalIter>::value_type>\n * bool\n * isSubsetSum(_BidirectionalIter begin, _BidirectionalIter end, _ValueType sum)\n * {\n * auto sz = std::distance(begin, end);\n * bool subset[sum + 1][sz + 1];\n *\n * for (int i = 0; i <= sz; ++i)\n * subset[0][i] = true;\n *\n * for (int i = 1; i <= sum; ++i)\n * subset[i][0] = false;\n *\n * for (int i = 1; i <= sum; ++i)\n * for (int j = 1; j <= sz; ++j)\n * {\n * auto x = begin;\n * std::advance(x, j - 1);\n *\n * subset[i][j] = subset[i][j - 1];\n * if (i >= *x)\n * subset[i][j] = subset[i][j] || subset[i - *x][j - 1];\n * }\n *\n * return subset[sum][sz];\n * }\n */\n#endif // SUBSET_SUM"
}
{
"filename": "code/dynamic_programming/src/subset_sum/subset_sum.go",
"content": "/* Part of Cosmos by OpenGenus Foundation */\npackage main\n\nimport \"fmt\"\n\nconst TargetSize int = 200\n\n/*\nExpected Output:\nCurrent subset is [1 4 8 9 34 23 17 32]\nWe can't get sum 113 from set\nWe can get sum 9 from set\nWe can't get sum 15 from set\nWe can't get sum 20 from set\nWe can get sum 31 from set\n*/\nfunc subSetSum(subset []int, dp [][]int) {\n\tfor i := range dp {\n\t\tdp[i] = make([]int, TargetSize+1)\n\t\tfor j := range dp[i] {\n\t\t\tdp[i][j] = 0\n\t\t}\n\n\t\t//If sum is 0, then it's true\n\t\tdp[i][0] = 1\n\t}\n\n\tfor i := 1; i <= len(subset); i++ {\n\t\tfor j := 0; j < TargetSize; j++ {\n\t\t\tdp[i][j] = dp[i-1][j]\n\t\t\tif j >= subset[i-1] {\n\t\t\t\tdp[i][j] += dp[i-1][j-subset[i-1]]\n\t\t\t}\n\t\t}\n\n\t}\n}\n\nfunc main() {\n\tinput := []int{1, 4, 8, 9, 34, 23, 17, 32}\n\n\t// The value of dp[i][j] will be true if there is a\n\t// dp of set[0..i-1] with sum equal to j\n\tdp := make([][]int, len(input)+1)\n\tsubSetSum(input, dp)\n\n\tsum := []int{113, 9, 15, 20, 31}\n\tfmt.Printf(\"Current subset is %v\\n\", input)\n\tfor _, v := range sum {\n\t\tif dp[len(input)][v] == 0 {\n\t\t\tfmt.Printf(\"We can't get sum %d from set\\n\", v)\n\t\t} else {\n\t\t\tfmt.Printf(\"We can get sum %d from set\\n\", v)\n\t\t}\n\t}\n}"
}
{
"filename": "code/dynamic_programming/src/subset_sum/subset_sum.java",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n// A Java programm for subset sum problem using Dynamic Programming.\nclass subset_sum\n{\n\tstatic boolean isSubsetSum(int set[], int n, int sum)\n\t{\n\t \n\t\tboolean subset[][] = new boolean[sum+1][n+1];\n\t\n\t\tfor (int i = 0; i <= n; i++)\n\t\tsubset[0][i] = true;\n\t\n\t\tfor (int i = 1; i <= sum; i++)\n\t\tsubset[i][0] = false;\n\t\n\t\tfor (int i = 1; i <= sum; i++)\n\t\t{\n\t\tfor (int j = 1; j <= n; j++)\n\t\t{\n\t\t\tsubset[i][j] = subset[i][j-1];\n\t\t\tif (i >= set[j-1])\n\t\t\tsubset[i][j] = subset[i][j]||subset[i - set[j-1]][j-1];\n\t\t}\n\t\t}\n\t\treturn subset[sum][n];\n\t}\n\t\n\tpublic static void main (String args[])\n\t{\n\t\tint set[] = {3, 34, 4, 12, 5, 7};\n\t\tint sum = 19;\n\t\tint n = set.length;\n\t\tif (isSubsetSum(set, n, sum) == true)\n\t\t\tSystem.out.println(\"Subset found\");\n\t\telse\n\t\t\tSystem.out.println(\"No subset found\");\n\t}\n}"
}
{
"filename": "code/dynamic_programming/src/subset_sum/subset_sum.py",
"content": "# Part of Cosmos by OpenGenus Foundation\n\n\ndef can_get_sum(items, target):\n dp = (target + 1) * [False]\n reachable = (target + 1) * [0]\n\n reachable[0] = 0\n dp[0] = True\n\n reachable[1] = 1\n\n i = 0\n q = 1\n\n while i < len(items) and not dp[target]:\n aux = q\n\n for j in range(0, aux):\n x = reachable[j] + items[i]\n if x <= target and not dp[x]:\n reachable[q] = x\n q += 1\n dp[x] = True\n i += 1\n\n return dp[target]\n\n\nprint(can_get_sum([1, 2, 3], 6))\nprint(can_get_sum([1, 2, 3], 7))"
}
{
"filename": "code/dynamic_programming/src/tiling_problem/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/dynamic_programming/src/tiling_problem/tiling_problem.c",
"content": "#include <stdio.h>\n\nlong long int \ntiling(int n)\n{\n\tlong long int tile[n + 1];\n\tint i;\n\t\n\ttile[0] = 1;\n\ttile[1] = 1;\n\ttile[2] = 2;\n\n\tfor (i = 3; i <= n; i++)\n\t\ttile[i] = tile[i - 1] + tile[i - 2];\n\n\treturn (tile[n]);\n}\n\nint \nmain()\n{ \n\tint n;\n\tprintf(\"Enter Number of tiles: \");\n\tscanf(\"%d\", &n);\n\tprintf(\"Number of ways to arrange tiles is %lli \\n\", tiling(n));\n\n\treturn (0);\n}"
}
{
"filename": "code/dynamic_programming/src/tiling_problem/tiling_problem.cpp",
"content": "#include <iostream>\n#include <vector>\nusing namespace std;\n// Part of Cosmos by OpenGenus Foundation\nlong long tiling(int n)\n{\n vector<long long int> tile(n + 1);\n\n tile[0] = 1;\n tile[1] = 1;\n tile[2] = 2;\n for (int i = 3; i <= n; i++)\n tile[i] = tile[i - 1] + tile[i - 2];\n return tile[n];\n}\n\nint main()\n{\n int n;\n cout << \"enter the value of n\\n\";\n cin >> n;\n cout << \"total ways to arrange the tiles is \" << tiling(n);\n}"
}
{
"filename": "code/dynamic_programming/src/tiling_problem/tiling_problem.py",
"content": "def tiling(n):\n tile = [0 for i in range(n + 1)]\n\n tile[0] = 1\n tile[1] = 1\n tile[2] = 2\n for i in range(3, n + 1):\n tile[i] = tile[i - 1] + tile[n - 2]\n\n return tile[n]\n\n\nif __name__ == \"__main__\":\n n = 10\n print(tiling(n))"
}
{
"filename": "code/dynamic_programming/src/trapping_rain_water/rainwater_trapping.cpp",
"content": "#include<iostream>\nusing namespace std;\nint main() {\n\tint n;\n\tcin>>n;\n\tint arr[n];\n\tfor(int i=0;i<n;i++){\n\t\tcin>>arr[i];\n\t}\n\tint left_highest[n];\n\tint right_highest[n];\n\tint l_high=arr[0];\n int i=0;\n\tint r_high=arr[n-1];\n\tfor(i=0;i<n;i++){\n\t\tif(arr[i]>=l_high){\n\t\tleft_highest[i]=arr[i];\n\t\tl_high=arr[i];\n\t\t}\n\t\telse\n\t\tleft_highest[i]=l_high;\n\t}\n for(i=n-1;i>=0;i--){\n if(arr[i]>=r_high){\n right_highest[i]=arr[i];\n r_high=arr[i];\n }\n else\n right_highest[i]=r_high;\n }\n int water_saved=0;\n for(i=0;i<n;i++){\n water_saved+=min(left_highest[i],right_highest[i])-arr[i];\n }\n cout<<water_saved;\n\treturn 0;\n}"
}
{
"filename": "code/dynamic_programming/src/trapping_rain_water/trapping_rain_water.cpp",
"content": "/*\n\nProblem Description - https://leetcode.com/problems/trapping-rain-water/description/\n\n\nTest Cases - \n\nInput: [0,1,0,2,1,0,1,3,2,1,2,1]\nOutput: 6\n\n\n*/\n\n#include<iostream>\n#include<vector>\nusing namespace std;\n\nint trapRainWater(int *arr, int n)\n{\n\tif(n < 3)\n return 0;\n \n vector<pair<int,int>>indexes;\n \n for(int i = 0 ; i < n ; i++)\n {\n bool check = false;\n int j = i + 1;\n \n int maxVal = 0;\n int index = i + 1;\n \n for(; j < n ; j++) // finding the corresponding value for the given start index\n {\n if(maxVal <= arr[j])\n {\n maxVal = arr[j]; \n index = j;\n \n if(maxVal >= arr[i]) // if current value exceeds or is equal to arr[i] break \n break;\n }\n }\n \n if(index != i + 1)\n {\n pair<int,int>e;\n e.first = i;\n e.second = index; // insert it as a pair to show (start, end) for our water segment\n \n indexes.push_back(e);\n i = index - 1;\n }\n }\n \n int totalVol = 0;\n for(int i = 0 ; i < indexes.size() ; i++)\n {\n pair<int,int>p = indexes[i];\n\n int minHeight = min(arr[p.first], arr[p.second]); \n // choose the minimum of the two boundaries to get max height of water enclosed\n \n // compute water stored corresponding to each cell inside a segment\n for(int j = p.first + 1 ; j < p.second ; j++)\n totalVol += abs(minHeight - arr[j]); \n }\n \n return totalVol; \n}\n\nint main()\n{\n\tint n;\n\tcin>>n;\n\n\tint arr[n];\n\tfor(int i = 0 ; i < n ; i++)\n\tcin>>arr[i];\n\n\tcout<<trapRainWater(arr, n)<<endl;\n}"
}
{
"filename": "code/dynamic_programming/src/trapping_rain_water/trapping_rain_water.java",
"content": "public class Main {\n\n public static void main(String args[]) {\n int[] heightArray = {1, 2, 1, 3, 1, 2, 1, 4, 1, 0, 0, 2, 1, 4};\n System.out.println(trap_rain_water(heightArray));\n }\n\n public static int trap_rain_water(int[] height) {\n int result = 0;\n \n if(height==null) {\n return result;\n\t\t}\n \n int left[] = new int[height.length];\n int right[] = new int[height.length];\n \n //left[i] will contain height of tallest bar on the left side of i'th bar\n left[0] = height[0];\n for(int i=1; i<height.length; i++) {\n left[i] = Math.max(left[i-1], height[i]);\n }\n \n //right[i] will contain height of tallest bar on the right side of i'th bar\n right[height.length - 1] = height[height.length - 1];\n for(int i=height.length-2; i>=0; i--) {\n right[i] = Math.max(right[i+1], height[i]);\n }\n \n //amount of water collected on i'th bar will be min(left[i], right[i]) - height[i]\n for(int i=0; i<height.length; i++) {\n result+= Math.min(left[i], right[i]) - height[i];\n }\n \n return result;\n }\n\n}"
}
{
"filename": "code/dynamic_programming/src/trapping_rain_water/trapping_rain_water.py",
"content": "# Python3 implementation of the approach\n \n# Function to return the maximum\n# water that can be stored\ndef maxWater(arr, n) :\n \n # To store the maximum water\n # that can be stored\n res = 0;\n \n # For every element of the array\n for i in range(1, n - 1) :\n \n # Find the maximum element on its left\n left = arr[i];\n for j in range(i) :\n left = max(left, arr[j]);\n \n # Find the maximum element on its right\n right = arr[i];\n \n for j in range(i + 1 , n) :\n right = max(right, arr[j]);\n \n # Update the maximum water\n res = res + (min(left, right) - arr[i]);\n \n return res;\n \n# Driver code\nif __name__ == \"__main__\" :\n \n arr = [0, 1, 0, 2, 1, 0,\n 1, 3, 2, 1, 2, 1];\n n = len(arr);\n \n print(maxWater(arr, n));"
}
{
"filename": "code/dynamic_programming/src/unique_paths/unique_paths.cpp",
"content": "// Part of Cosmos by OpenGenus Foundation\n\n#include<bits/stdc++.h>\nusing namespace std;\n\n\n //Tabulation method\n // Time complexity: O(m*n)\n int uniquePaths(int m, int n) {\n int dp[m][n];\n for(int i=0;i<m;i++){\n for(int j=0;j<n;j++){\n if(i==0 || j==0)\n dp[i][j]=1;\n else\n dp[i][j]=dp[i-1][j]+dp[i][j-1];\n }\n }\n return dp[m-1][n-1];\n }\n\n\n //nCr method\n // Time complexity: O(m+n)\n int uniquePaths1(int m, int n) {\n int N=m+n-2;\n int r=m-1;\n double res=1;\n for(int i=1;i<=r;i++){\n res=res*(N-r+i)/i;\n }\n return (int)res;\n }\n \n \n // Brute force recursive method\n // Time complexity: O(2^(m+n))\n int uniquePaths2(int m, int n) {\n if(m==1 || n==1)\n return 1;\n return uniquePaths2(m-1,n)+uniquePaths2(m,n-1);\n }\n\n //Time complexity: O(m*n)\n int solve(int i, int j, vector<vector<int>> &dp, int &m, int &n){\n if (i == m - 1 || j == n - 1) return 1;\n if (dp[i][j] != 0) return dp[i][j];\n dp[i][j] = solve(i + 1, j, dp, m, n) + solve(i, j + 1, dp, m, n);\n return dp[i][j];\n }\n int uniquePaths3(int m, int n){\n vector<vector<int>> dp(m, vector<int>(n, 0));\n return solve(0, 0, dp, m, n);\n }\n\n int main(){\n int m,n;\n cin>>m>>n;\n cout<<uniquePaths(m,n)<< endl;\n cout<<uniquePaths1(m,n) << endl;\n cout<<uniquePaths2(m,n) << endl;\n cout<<uniquePaths3(m,n) << endl;\n return 0;\n }\n "
}
{
"filename": "code/dynamic_programming/src/weighted_job_scheduling/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter\n\nCollaborative effort by [OpenGenus](https://github.com/opengenus)"
}
{
"filename": "code/dynamic_programming/src/weighted_job_scheduling/weighted_job_scheduling.cpp",
"content": "/*\n * Part of Cosmos by OpenGenus Foundation\n * C++ program for weighted job scheduling using Dynamic Programming and Binary Search\n */\n\n#include <vector>\n#include <iostream>\n#include <algorithm>\nusing namespace std;\n\n// A job has start time, finish time and profit.\n//index is used to store its given position as we will going to do sorting\nstruct Job\n{\n int start, finish, profit, index;\n};\n\n\n// A utility function that is used for sorting events\n// according to finish time\nbool jobComparator(Job s1, Job s2)\n{\n return s1.finish < s2.finish;\n}\n\n// A Binary Search based function to find the latest job\n// (before current job) that doesn't conflict with current\n// job. \"index\" is index of the current job. This function\n// returns -1 if all jobs before index conflict with it. The\n// array jobs[] is sorted in increasing order of finish time\nint latestNonConflict(const vector<Job> &jobs, int index)\n{\n // Initialize 'lo' and 'hi' for Binary Search\n int lo = 0, hi = index - 1;\n\n // Perform binary Search iteratively\n while (lo <= hi)\n {\n int mid = (lo + hi) / 2;\n if (jobs[mid].finish <= jobs[index].start)\n {\n if (jobs[mid + 1].finish <= jobs[index].start)\n lo = mid + 1;\n else\n return mid;\n }\n else\n hi = mid - 1;\n }\n\n return -11; //-11 just a flag value but kept as same to that given to index 0 see: MaxProfit func\n //so that backtracking can be done\n}\n\n// The main function that finds the subset of jobs\n// associated with maximum profit such that no two\n// jobs in the subset overlap.\nvoid MaxProfit(vector<Job> &arr)\n{\n\n int n = arr.size();\n // Sort jobs according to finish time\n sort(arr.begin(), arr.end(), jobComparator); //reason for using index\n\n // Create an array to store solutions of subproblems.\n // DP[i] stores the Jobs involved and their total profit\n // till arr[i] (including arr[i])\n vector<int> DP(n + 2);\n\n // initialize DP[0] to arr[0]\n DP[0] = arr[0].profit;\n\n //contain what is the latest non conflicting job with the current job\n vector<int> nConflict;\n\n //no job non-conflicting with one job\n nConflict.push_back(-11); //-11 is just a flag value\n\n for (int i = 1; i < n; i++)\n nConflict.push_back(latestNonConflict(arr, i));\n\n // Fill entries in DP[] using recursive property\n //recurrence\n for (int i = 1; i < n; i++)\n {\n int val = nConflict[i];\n DP[i] = max(DP[i - 1], DP[val] + arr[i].profit);\n }\n\n //finding jobs included in max profit\n int i = n - 1;\n cout << \"Optimal jobs are - \";\n while (true)\n {\n\n //terminating condition\n if (i < 0) //basically checking case of if it is equal to index of any job --(-11)\n break;\n\n //DP[i] will be one of the two values from which it is derived(shown above)\n\n if (i == 0)\n {\n cout << arr[i].index + 1;\n break;\n }\n else if (DP[i] == DP[i - 1])\n i--;\n\n else\n {\n cout << arr[i].index + 1 << \" \";\n i = nConflict[i];\n }\n }\n\n cout << \"\\nMaximum Profit = \" << DP[n - 1] << \"\\n\";\n}\n\nint main()\n{\n //example test case\n vector<Job> arr(4);\n arr[0] = {3, 5, 20, 0};\n arr[1] = {1, 2, 50, 1};\n arr[2] = {6, 19, 100, 2};\n arr[3] = {2, 100, 200, 3};\n\n MaxProfit(arr);\n\n return 0;\n}"
}
{
"filename": "code/dynamic_programming/src/weighted_job_scheduling/weighted_job_scheduling.py",
"content": "# Python3 program for weighted job scheduling using\n# Naive Recursive Method\n\n# Importing the following module to sort array\n# based on our custom comparison function\nfrom functools import cmp_to_key\n\n# A job has start time, finish time and profit\nclass Job:\n\t\n\tdef __init__(self, start, finish, profit):\n\t\t\n\t\tself.start = start\n\t\tself.finish = finish\n\t\tself.profit = profit\n\n# A utility function that is used for\n# sorting events according to finish time\ndef jobComparataor(s1, s2):\n\t\n\treturn s1.finish < s2.finish\n\n# Find the latest job (in sorted array) that\n# doesn't conflict with the job[i]. If there\n# is no compatible job, then it returns -1\ndef latestNonConflict(arr, i):\n\t\n\tfor j in range(i - 1, -1, -1):\n\t\tif arr[j].finish <= arr[i - 1].start:\n\t\t\treturn j\n\t\t\t\n\treturn -1\n\n# A recursive function that returns the\n# maximum possible profit from given\n# array of jobs. The array of jobs must\n# be sorted according to finish time\ndef findMaxProfitRec(arr, n):\n\t\n\t# Base case\n\tif n == 1:\n\t\treturn arr[n - 1].profit\n\n\t# Find profit when current job is included\n\tinclProf = arr[n - 1].profit\n\ti = latestNonConflict(arr, n)\n\t\n\tif i != -1:\n\t\tinclProf += findMaxProfitRec(arr, i + 1)\n\n\t# Find profit when current job is excluded\n\texclProf = findMaxProfitRec(arr, n - 1)\n\treturn max(inclProf, exclProf)\n\n# The main function that returns the maximum\n# possible profit from given array of jobs\ndef findMaxProfit(arr, n):\n\t\n\t# Sort jobs according to finish time\n\tarr = sorted(arr, key = cmp_to_key(jobComparataor))\n\treturn findMaxProfitRec(arr, n)\n\n# Driver code\nvalues = [ (3, 10, 20), (1, 2, 50),\n\t\t(6, 19, 100), (2, 100, 200) ]\narr = []\nfor i in values:\n\tarr.append(Job(i[0], i[1], i[2]))\n\t\nn = len(arr)\n\nprint(\"The optimal profit is\", findMaxProfit(arr, n))\n\n# This code is code contributed by Kevin Joshi"
}
{
"filename": "code/dynamic_programming/src/wildcard_matching/wildcard.cpp",
"content": "//Part of Cosmos by OpenGenus Foundation\n\nbool isMatch(string s, string p) {\n \n /*\n s is the string\n p is the pattern containing '?' and '*' apart from normal characters.\n */ \n\n int m=s.length(), n=p.length();\n\n bool dp[m+1][n+1];\n\n // Initialise each cell of the dp table with false\n for(int i=0;i<m+1;i++) \n for(int j=0;j<n+1;j++) \n dp[i][j]=false;\n\n\n for(int i=0;i<m+1;i++) \n {\n for(int j=0;j<n+1;j++) \n {\n // When both string s and pattern p is empty.\n if (i==0 && j==0) \n dp[i][j]=true;\n\n // When s is empty\n else if (i == 0)\n {\n dp[i][j]=(p[j-1]=='*' && dp[i][j-1]);\n }\n\n // When the pattern is empty\n else if (j == 0) \n dp[i][j]=false;\n\n // When the characters p[j-1] matches character s[i-1] or when \n // p[j-1] is a '?'. Both will consume 1 character of s.\n else if (p[j-1] == s[i-1] || p[j-1] == '?') \n dp[i][j]=dp[i-1][j-1];\n\n // p[j-1]='*'\n // A '*' can consume 0 or multiple characters.\n else if (p[j-1]=='*') \n dp[i][j]=dp[i-1][j] || dp[i][j-1];\n \n // If p[j-1] is any character other than those above.\n else \n dp[i][j]=false;\n }\n }\n return dp[m][n];\n }"
}
{
"filename": "code/dynamic_programming/src/wildcard_matching/wildcard.md",
"content": "# Wildcard Matching\n\nLearn more: [Wildcard Pattern Matching (Dynamic Programming)](https://iq.opengenus.org/wildcard-pattern-matching-dp/)\n\n## Description\n\nYou have a string `s` of length `m` and a pattern `p` of length `n`. With wildcard matching enabled ie. character `?` in `p` can replace 1 character of `s` and a `*` \nin `p` can replace any number of characters of `s` including 0 character, find if `s` is equivalent to `p`.\n\nReturn **true** if `s` is equivalent to `p` else **false**.\n\n## Thought Process\n\nLet's follow 1-based indexing and denote s[i,j] as the substring of s from ith to jth character including both. Same for p. So for example, if s is \"abcde\", then \ns[2,4] is the substring \"bcd\". \n\n<details> \n <summary>What's the problem in terms of s, p, m, and n?</summary>\n </br>\n Return true when s[1:m] is equivalent to p[1:n].\n \n</details>\n\n<details> \n <summary>What's the Subproblem in terms of s and p?</summary>\n </br>\n <ol>\n <li>For an index i and an index j, we want to find if s[1:i] is equivalent to p[1:j].</li>\n <li>Note that a subproblem can be formulated from the end of strings as well but then you'll need to fill the DP table from bottom to top.</li>\n </ol>\n\n</details>\n\n<details> \n <summary>Solution</summary>\n </br>\n <ol>\n <li>We iterate over all i from 1 to m. For each i, we iterate over all j from 1 to n. At each point, we check if s[1:i] is equivalent to p[1:j] and fill true\n or false in the cell dp[i][j] of the dp table. Recording this will help in finding answers to further i and j which we'll see in the recurrence relation.</li>\n <li>The final answer is dp[m][n].</li>\n </ol>\n</details>\n\n<details>\n <summary>What's the recurrence relation?</summary>\n </br>\n <ol>\n <li>If the jth character of p is the same as ith character of s, then s[1:i]=p[1:j] if s[1:i-1]=p[1:j-1] ie. dp[i][j]=dp[i-1][j-1]. Also, when jth character \n of p is ?, it will consume the ith character of s. Hence same for it.</li>\n\n <li>If the jth character of p is '*', then it can consume 0 characters ie. s[1:i]=p[1:j-1] or several characters ie. dp[i][j]=dp[i][j-1] || dp[i-1][j].</li>\n </ol>\n</details>\n\n<details>\n <summary>What's the time complexity?</summary>\n </br>\n Since we need to lookup atmost two adjacent cells to fill any cell in the dp table, filling a cell is an O(1) process. And we need to fill m*n such cells.\n Thus the complexity is O(mn).\n \n</details>\n<details>\n <summary>What's next?</summary>\n </br>\n <ol>\n <li>Think about the subproblem that each cell of the DP table handles and come up with the edge cases.</li> \n <li>What changes when you consider 0-based indexing for strings? </li>\n </ol>\n \n</details>"
}
{
"filename": "code/dynamic_programming/test/longest_repeating_subsequence/longest_repeating_subsequence_test.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#include <iostream>\n#include <string>\n#include <cassert>\n#include \"../../src/longest_repeating_subsequence/longest_repeating_subsequence.cpp\"\n\nint main()\n{\n using namespace std;\n\n std::string s1 = \"aab\";\n assert(longestRepeatingSubsequence(s1) == 1);\n}"
}
{
"filename": "code/dynamic_programming/test/README.md",
"content": "# cosmos\nYour personal library of every algorithm and data structure code that you will ever encounter"
}
{
"filename": "code/dynamic_programming/test/subset_sum/test_subset_sum.cpp",
"content": "/* Part of Cosmos by OpenGenus Foundation */\n\n#ifndef SUBSET_SUM_TEST\n#define SUBSET_SUM_TEST\n\n#include <iostream>\n#include <list>\n#include <cassert>\n#include \"../../src/subset_sum/subset_sum.cpp\"\n\nint\nmain()\n{\n using namespace std;\n\n int vz[0];\n int v[] = {1, 2, 15, 8, 5};\n list<int> l{1, 2, 15, 8, 5};\n\n assert(isSubsetSum(vz, vz, 0));\n assert(isSubsetSum(v, v + sizeof(v) / sizeof(v[0]), 13));\n assert(isSubsetSum(l.begin(), l.end(), 13));\n assert(isSubsetSum(vz, vz, 13) == false);\n assert(isSubsetSum(v, v + sizeof(v) / sizeof(v[0]), 4) == false);\n assert(isSubsetSum(l.begin(), l.end(), 4) == false);\n\n return 0;\n}\n\n#endif // SUBSET_SUM_TEST"
}
{
"filename": "code/filters/src/gaussian_filter/gaussian_filter.py",
"content": "##Author - Sagar Vakkala (@codezoned)\n\n\nfrom PIL import Image\nimport numpy\n\n\ndef main():\n ##Load the Image (Change source.jpg with your image)\n image = Image.open(\"source.jpg\")\n ##Get the width and height\n width, height = image.size\n ##Initialise a null matrix\n pix = numpy.zeros(shape=(width, height, 3), dtype=numpy.uint8)\n ##Same again\n matris = numpy.zeros(shape=(width, height, 3), dtype=numpy.uint8)\n # Neighbor matrice to be filtered pixel\n neighbour_matrice = numpy.zeros(shape=(5, 5))\n for i in range(width):\n for j in range(height):\n # Get and assign image's RGB values\n rgb_im = image.convert(\"RGB\")\n r, g, b = rgb_im.getpixel((i, j))\n pix[i][j] = r, g, b\n if i >= 2 & i < (width - 2) & j >= 2 & j < (height - 2):\n for m in range(3):\n toplam = 0.0\n for k in range(5):\n for l in range(5):\n neighbour_matrice[k][l] = matris[k + i - 2][l + j - 2][\n m\n ] # Create neighbor matrice's indexs(exclude a frame size=2)\n for k in range(5):\n for l in range(5):\n toplam = toplam + (\n neighbour_matrice[k][l] * 0.04\n ) # 0.04 is filter value.\n matris[j][i][m] = int(toplam)\n # Create an image with RGB values\n img = Image.fromarray(matris, \"RGB\")\n ##Save and show the image\n img.save(\"target.jpg\")\n img.show()\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/filters/src/median_filter/median_filter.py",
"content": "##Author - Sagar Vakkala (@codezoned)\n\nimport numpy\nfrom PIL import Image\n\n\ndef median_filter(data, filter_size):\n temp_arr = []\n index = filter_size // 2\n data_final = []\n data_final = numpy.zeros((len(data), len(data[0])))\n for i in range(len(data)):\n # Iterate over the Image Array\n for j in range(len(data[0])):\n\n for z in range(filter_size):\n if i + z - index < 0 or i + z - index > len(data) - 1:\n for c in range(filter_size):\n temp_arr.append(0)\n else:\n if j + z - index < 0 or j + index > len(data[0]) - 1:\n temp_arr.append(0)\n else:\n for k in range(filter_size):\n temp_arr.append(data[i + z - index][j + k - index])\n\n temp_arr.sort()\n data_final[i][j] = temp_arr[len(temp_arr) // 2]\n temp_arr = []\n return data_final\n\n\ndef main():\n ##Replace someImage with the noise image\n\n img = Image.open(\"someImage.png\").convert(\"L\")\n arr = numpy.array(img)\n\n ##3 defines the no of layers for the filter\n ##It also defines that the filter will be 3x3 size. Change 3 to 5 for a 5x5 filter\n\n removed_noise = median_filter(arr, 3)\n img = Image.fromarray(removed_noise)\n img.show()\n\n\nif __name__ == \"__main__\":\n main()"
}
{
"filename": "code/game_theory/src/expectimax/expectimax.py",
"content": "\"\"\"\nExpectimax game playing algorithm\nAlex Day\nPart of Cosmos by OpenGenus Foundation\n\"\"\"\n\nfrom abc import ABC, abstractmethod\nimport math\nfrom random import choice\n\n\nclass Board(ABC):\n @abstractmethod\n def terminal():\n \"\"\"\n Determine if the current state is terminal\n Returns\n -------\n bool: If the board is terminal\n \"\"\"\n return NotImplementedError()\n\n @abstractmethod\n def get_child_board(player):\n \"\"\"\n Get all possible next moves for a given player\n Parameters\n ----------\n player: int\n the player that needs to take an action (place a disc in the game)\n Returns\n -------\n List: List of all possible next board states\n \"\"\"\n return NotImplementedError()\n\n\ndef expectimax(player: int, board, depth_limit, evaluate):\n \"\"\"\n expectimax algorithm with limited search depth.\n Parameters\n ----------\n player: int\n the player that needs to take an action (place a disc in the game)\n board: the current game board instance. Should be a class that extends Board\n depth_limit: int\n the tree depth that the search algorithm needs to go further before stopping\n evaluate: fn[Board, int]\n Some function that evaluates the board at the current position\n Returns\n -------\n placement: int or None\n the column in which a disc should be placed for the specific player\n (counted from the most left as 0)\n None to give up the game\n \"\"\"\n max_player = player\n\n next_player = board.PLAYER2 if player == board.PLAYER1 else board.PLAYER1\n\n def value(board, depth_limit):\n \"\"\" Evaluate the board at the current state\n Args:\n board (Board): Current board state\n depth_limit (int): Depth limit\n Returns:\n float: Value of the board\n \"\"\"\n return evaluate(player, board)\n\n def max_value(board, depth_limit: int) -> float:\n \"\"\" Calculate the maximum value for play if players acts optimally\n Args:\n player (int): Player token to maximize value of\n board (Board): Current board state\n depth_limit (int): Depth limit\n Returns:\n float: Maximum value possible if players play optimally\n \"\"\"\n # Check if terminal or depth limit has been reached\n if depth_limit == 0 or board.terminal():\n # If leaf node return the calculated board value\n return value(board, depth_limit)\n\n # If not leaf then continue searching for maximum value\n best_value = -math.inf\n # Generate all possible moves for maximizing player and store the\n # max possible value while exploring down to terminal nodes\n for move, child_board in board.get_child_boards(player):\n best_value = max(best_value, min_value(child_board, depth_limit - 1))\n\n return best_value\n\n def min_value(board, depth_limit: int) -> float:\n \"\"\" Calculate a uniformly random move for the minplayer\n Args:\n player (int): Player token to minimize value of\n board (Board): Current board state\n depth_limit (int): Depth limit\n\n Returns:\n float: Minimum value possible if players play optimally\n \"\"\"\n # Check if terminal or depth limit has been reached\n if depth_limit == 0 or board.terminal():\n # If leaf node return the calculated board value\n return value(board, depth_limit)\n\n # If not leaf then continue searching for minimum value\n # Generate all possible moves for minimizing player and store the\n # min possible value while exploring down to terminal nodes\n move, child_board = choice(board.get_child_boards(ext_player))\n return max_value(child_board, depth_limit - 1)\n\n return best_value\n\n # Start off with the best score as low as possible. We want to maximize\n # this for our turn\n best_score = -math.inf\n placement = None\n # Generate all possible moves and boards for the current player\n for pot_move, pot_board in board.get_child_boards(player):\n # Calculate the minimum score for the player at this board\n pot_score = min_value(pot_board, depth_limit - 1)\n # If this is greater than the best_score then update\n if pot_score > best_score:\n best_score = pot_score\n placement = pot_move\n\n return placement"
}
|
makefile | #for C
CFLAGS = -Wall -Wextra -lm -lgraph -lpthread
C_SOURCES := $(shell find code -name '*.c')
c: $(C_SOURCES)
$(CC) -o $@ $^ $(CFLAGS)
|
scripts/README.md | # What's the stats script?
The stats script deals with generating a progress list of the cosmos repo, by taking into account all algorithms currently existing in the repo and categorizing them based on the languages used to implement them.
# What's the metadata generator script?
The metadata generator script deals with generating metadata(like location, files in category, last updated etc) for all categories of algorithms currently existing in the repository. The metadata is stored in the metadata directory inside the scripts directory, and it retains the directory hierarchy of the corresponding category as it's in the code directory.
# How to run?
**make sure that you're in the cosmos root directory**
### for generating the stats output in txt file :-
```bash
python3 scripts/stats.py -f txt > ./scripts/STATS.txt
```
### for generating the stats output in md file :-
```bash
python3 scripts/stats.py -f md > ./scripts/STATS.md
```
### for generating the metadata :-
```bash
python3 scripts/metadata-generator.py
```
|
scripts/STATS.md | This is the progress list of [Cosmos](https://github.com/OpenGenus/cosmos/) | Updated on: 2019-04-28
# [ARTIFICIAL INTELLIGENCE](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence)
| ARTIFICIAL INTELLIGENCE | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [a star](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/a_star/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [artificial neural network](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/artificial_neural_network/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [autoenncoder](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/autoenncoder/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [canny](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/image_processing/canny/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [convolutional neural network](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/convolutional_neural_network/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [dbscan clustering](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/dbscan_clustering/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [decision tree](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/decision_tree/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [erode dilate](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/image_processing/erode_dilate/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [factorization machines](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/factorization_machines/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [gaussian naive bayes](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/gaussian_naive_bayes/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [gbestPSO](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/particle_swarm_optimization/gbestPSO/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [hierachical clustering](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/hierachical_clustering/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [houghtransform](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/image_processing/houghtransform/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [image processing](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/image_processing/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . |
| [image stitching](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/image_processing/image_stitching/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [isodata clustering](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/isodata_clustering/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [k means](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/k_means/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [k nearest neighbours](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/k_nearest_neighbours/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [lbestPSO](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/particle_swarm_optimization/lbestPSO/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [linear regression](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/linear_regression/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [logistic regression](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/logistic_regression/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [minimax](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/minimax/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [naive bayes](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/naive_bayes/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [neural network](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/neural_network/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [neural style transfer](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/neural_style_transfer/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [nsm matlab](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/nearest_sequence_memory/nsm_matlab/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [perceptron](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/perceptron/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [prewittfilter](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/image_processing/prewittfilter/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [principal component analysis](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/principal_component_analysis/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [q learning](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/q_learning/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [restricted boltzmann machine](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/restricted_boltzmann_machine/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [sat](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/sat/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [sobelfilter](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/image_processing/sobelfilter/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [src](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [support vector machine](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/support_vector_machine/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [tsp](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/tsp/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 3 | 10 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 2 | 0 | 5 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 19 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 3 | 0 | 0 | 0 | 0 |
# [BACKTRACKING](https://github.com/OpenGenus/cosmos/tree/master/code/backtracking)
| BACKTRACKING | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [algorithm x](https://github.com/OpenGenus/cosmos/tree/master/code/backtracking/src/algorithm_x/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [crossword puzzle](https://github.com/OpenGenus/cosmos/tree/master/code/backtracking/src/crossword_puzzle/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [knight tour](https://github.com/OpenGenus/cosmos/tree/master/code/backtracking/src/knight_tour/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| [m coloring problem](https://github.com/OpenGenus/cosmos/tree/master/code/backtracking/src/m_coloring_problem/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [n queen](https://github.com/OpenGenus/cosmos/tree/master/code/backtracking/src/n_queen/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| [number of ways in maze](https://github.com/OpenGenus/cosmos/tree/master/code/backtracking/src/number_of_ways_in_maze/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| [partitions of number](https://github.com/OpenGenus/cosmos/tree/master/code/backtracking/src/partitions_of_number/) | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| [partitions of set](https://github.com/OpenGenus/cosmos/tree/master/code/backtracking/src/partitions_of_set/) | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [permutations of string](https://github.com/OpenGenus/cosmos/tree/master/code/backtracking/src/permutations_of_string/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [powerset](https://github.com/OpenGenus/cosmos/tree/master/code/backtracking/src/powerset/) | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [rat in a maze](https://github.com/OpenGenus/cosmos/tree/master/code/backtracking/src/rat_in_a_maze/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [subset sum](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/subset_sum/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [sudoku solve](https://github.com/OpenGenus/cosmos/tree/master/code/backtracking/src/sudoku_solve/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| | 0 | 0 | 7 | 10 | 0 | 0 | 0 | 0 | 0 | 0 | 8 | 0 | 1 | 6 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 6 | 0 | 0 | 0 | 5 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
# [BIT MANIPULATION](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation)
| BIT MANIPULATION | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [addition using bits](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/addition_using_bits/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . |
| [bit division](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/bit_division/) | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [byte swapper](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/byte_swapper/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [convert number binary](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/convert_number_binary/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [count set bits](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/count_set_bits/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . |
| [flip bits](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/flip_bits/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [hamming distance](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/hamming_distance/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [invert bit](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/invert_bit/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [lonely integer](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/lonely_integer/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| [magic number](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/magic_number/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [maximum xor value](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/maximum_xor_value/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [power of 2](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/power_of_2/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . |
| [subset generation](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/subset_generation/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [sum binary numbers](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/sum_binary_numbers/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [sum equals xor](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/sum_equals_xor/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [thrice unique number](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/thrice_unique_number/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [twice unique number](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/twice_unique_number/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [xor swap](https://github.com/OpenGenus/cosmos/tree/master/code/bit_manipulation/src/xor_swap/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 13 | 15 | 0 | 0 | 0 | 0 | 0 | 0 | 5 | 0 | 1 | 10 | 8 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 13 | 0 | 0 | 0 | 2 | 0 | 0 | 0 | 0 | 0 | 0 | 3 | 0 |
# [CELLULAR AUTOMATON](https://github.com/OpenGenus/cosmos/tree/master/code/cellular_automaton)
| CELLULAR AUTOMATON | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [conways game of life](https://github.com/OpenGenus/cosmos/tree/master/code/cellular_automaton/src/conways_game_of_life/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . |
| [elementary cellular automata](https://github.com/OpenGenus/cosmos/tree/master/code/cellular_automaton/src/elementary_cellular_automata/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [genetic algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/cellular_automaton/src/genetic_algorithm/) | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [langtons ant](https://github.com/OpenGenus/cosmos/tree/master/code/cellular_automaton/src/langtons_ant/) | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [von neumann cellular automata](https://github.com/OpenGenus/cosmos/tree/master/code/cellular_automaton/src/von_neumann_cellular_automata/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 2 | 3 | 0 | 0 | 0 | 0 | 0 | 0 | 2 | 1 | 1 | 4 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 3 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
# [COMPRESSION](https://github.com/OpenGenus/cosmos/tree/master/code/compression)
| COMPRESSION | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [huffman](https://github.com/OpenGenus/cosmos/tree/master/code/compression/src/lossless_compression/huffman/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [lempel ziv welch](https://github.com/OpenGenus/cosmos/tree/master/code/compression/src/lossless_compression/lempel_ziv_welch/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 0 | 2 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
# [COMPUTATIONAL GEOMETRY](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry)
| COMPUTATIONAL GEOMETRY | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [2d line intersection](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/2d_line_intersection/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . |
| [2d separating axis test](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/2d_separating_axis_test/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [area of polygon](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/area_of_polygon/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [area of triangle](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/area_of_triangle/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| [axis aligned bounding box collision](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/axis_aligned_bounding_box_collision/) | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [bresenham circle](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/bresenham_circle/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [bresenham line](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/bresenham_line/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [chans algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/chans_algorithm/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [cohen sutherland lineclip](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/cohen_sutherland_lineclip/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [dda line](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/dda_line/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [distance between points](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/distance_between_points/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| [graham scan](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/graham_scan/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [halfplane intersection](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/halfplane_intersection/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [jarvis march](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/jarvis_march/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [quickhull](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/quickhull/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [sphere tetrahedron intersection](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/sphere_tetrahedron_intersection/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [sutherland hodgeman clipping](https://github.com/OpenGenus/cosmos/tree/master/code/computational_geometry/src/sutherland_hodgeman_clipping/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 7 | 17 | 0 | 0 | 0 | 0 | 0 | 0 | 3 | 0 | 2 | 6 | 3 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 6 | 0 | 0 | 1 | 2 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 |
# [COMPUTER GRAPHICS](https://github.com/OpenGenus/cosmos/tree/master/code/computer_graphics)
| COMPUTER GRAPHICS | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [diamond square](https://github.com/OpenGenus/cosmos/tree/master/code/computer_graphics/src/diamond_square/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
# [CRYPTOGRAPHY](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography)
| CRYPTOGRAPHY | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [aes 128](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/aes_128/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [aes csharp](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/aes_128/aes_csharp/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . |
| [affine cipher](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/affine_cipher/) | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [atbash cipher](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/atbash_cipher/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [autokey cipher](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/autokey_cipher/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [baconian cipher](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/baconian_cipher/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . |
| [caesar cipher](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/caesar_cipher/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . |
| [columnar transposition cipher](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/columnar_transposition_cipher/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [example](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/aes_128/aes_csharp/example/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . |
| [huffman encoding](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/huffman_encoding/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [morse cipher](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/morse_cipher/) | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . | . | ✔️ | . | . | ✔️ | . | . | . |
| [polybius cipher](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/polybius_cipher/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [porta cipher](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/porta_cipher/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [rail fence cipher](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/rail_fence_cipher/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . |
| [rot13 cipher](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/rot13_cipher/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . |
| [rsa](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/rsa/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . |
| [rsa digital signature](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/rsa_digital_signature/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [runningkey cipher](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/runningkey_cipher/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [sha 256](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/sha/sha_256/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [vigenere cipher](https://github.com/OpenGenus/cosmos/tree/master/code/cryptography/src/vigenere_cipher/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . |
| | 0 | 1 | 7 | 10 | 0 | 0 | 0 | 0 | 0 | 0 | 2 | 1 | 2 | 8 | 5 | 1 | 1 | 1 | 1 | 0 | 0 | 0 | 4 | 0 | 0 | 0 | 15 | 0 | 0 | 6 | 0 | 0 | 2 | 0 | 0 | 1 | 0 | 4 | 0 |
# [DATA STRUCTURES](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures)
| DATA STRUCTURES | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [aa tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/aa_tree/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [abstract stack](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/stack/abstract_stack/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [arraystack](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/stack/abstract_stack/cpp/arraystack/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [avl tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/avl_tree/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [b tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/b_tree/b_tree/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [b tree c](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/b_tree/b_tree/b_tree_c/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [bag](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/bag/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [balanced expression](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/stack/balanced_expression/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [binary heap](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/binary_heap/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [binomial heap](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/heap/binomial_heap/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [bloom filter](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/hashs/bloom_filter/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . |
| [bottom view binary tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/tree/bottom_view_binary_tree/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [c](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/finite_automata/c/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [circular buffer](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/queue/circular_buffer/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [circular linked list](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/circular_linked_list/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [convert to doubly linked list](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/convert_to_doubly_linked_list/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [cpp](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/stack/abstract_stack/cpp/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [delete](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/singly_linked_list/operations/delete/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [detect cycle](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/singly_linked_list/operations/detect_cycle/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [diameter](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/diameter/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [double ended queue](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/queue/double_ended_queue/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [doubly linked list](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/doubly_linked_list/) | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [fenwick tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/multiway_tree/fenwick_tree/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [find](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/singly_linked_list/operations/find/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [from inorder and postorder](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/make_binary_tree/from_inorder_and_postorder/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [from inorder and preorder](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/make_binary_tree/from_inorder_and_preorder/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [hash table](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/hashs/hash_table/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . |
| [infix to postfix](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/stack/infix_to_postfix/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [insertion](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/singly_linked_list/operations/insertion/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [interval tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/space_partitioning_tree/interval_tree/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [is balance](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/is_balance/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [is binary tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/is_binary_tree/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [is same](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/is_same/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [kd tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/space_partitioning_tree/kd_tree/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [left view](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/traversal/preorder/left_view/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [leftist tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/heap/priority_queue/leftist_tree/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [linked list](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/linked_list/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [make mirror tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/make_mirror_tree/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [max heap](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/heap/max_heap/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [maximum height](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/maximum_height/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [menu interface](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/doubly_linked_list/menu_interface/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [merge sorted](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/singly_linked_list/operations/merge_sorted/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [min heap](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/heap/min_heap/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | . |
| [minimum height](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/minimum_height/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [n th node linked list](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/singly_linked_list/operations/n_th_node_linked_list/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [node](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/node/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [operations](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/circular_linked_list/operations/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [pairing heap](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/heap/pairing_heap/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | ✔️ |
| [path sum](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/path_sum/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [postfix evaluation](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/stack/postfix_evaluation/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | ✔️ | . | . | . | . | . | . |
| [prefix to postfix](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/stack/prefix_to_postfix/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [print reverse](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/singly_linked_list/operations/print_reverse/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [priority queue](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/heap/priority_queue/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [push](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/singly_linked_list/operations/push/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [quad tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/space_partitioning_tree/quad_tree/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [queue](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/queue/queue/) | . | . | ✔️ | ✔️ | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | ✔️ | . | . | ✔️ | . |
| [queue stream](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/queue/queue_stream/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . |
| [queue using linked list](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/queue/queue_using_linked_list/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . |
| [queue using stack](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/queue/queue_using_stack/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | ✔️ | . | . | . | . | . | . |
| [red black tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/multiway_tree/red_black_tree/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | ✔️ | . | . | . | . | . | . | . |
| [reverse](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/singly_linked_list/operations/reverse/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [reverse queue](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/queue/reverse_queue/) | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [reverse stack](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/stack/reverse_stack/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . |
| [right threaded](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/traversal/inorder/right_threaded/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [right view](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/traversal/preorder/right_view/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [rope](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/rope/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [rotate](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/singly_linked_list/operations/rotate/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [segment tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/segment_tree/) | ✔️ | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | ✔️ | . | . | . | . | . | . | . |
| [serializer](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/serializer/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [singly linked list](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/singly_linked_list/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | ✔️ | . | . | ✔️ | . |
| [skip list](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/skip_list/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | ✔️ | . | . | ✔️ | . | . | . | . |
| [soft heap](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/heap/soft_heap/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [sort](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/singly_linked_list/operations/sort/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [sort stack](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/stack/sort_stack/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [splay tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/multiway_tree/splay_tree/) | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [stack](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/stack/stack/) | . | . | ✔️ | ✔️ | . | . | ✔️ | . | ✔️ | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . |
| [suffix array](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/suffix_array/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [sum left](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/path_sum/sum_left/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [treap](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/treap/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . |
| [tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/tree/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | . |
| [trie](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/tree/trie/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | ✔️ | . | . | ✔️ | . | . | ✔️ | . |
| [two three tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/b_tree/two_three_tree/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [unclassified](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/singly_linked_list/operations/unclassified/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [union find](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/multiway_tree/union_find/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [van emde boas tree](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/van_emde_boas_tree/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [xor linked list](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/list/xor_linked_list/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [zigzag](https://github.com/OpenGenus/cosmos/tree/master/code/data_structures/src/tree/binary_tree/binary_tree/traversal/zigzag/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| | 1 | 0 | 42 | 65 | 0 | 0 | 2 | 0 | 1 | 0 | 15 | 0 | 1 | 40 | 13 | 0 | 0 | 2 | 0 | 0 | 0 | 0 | 1 | 1 | 0 | 0 | 40 | 0 | 0 | 10 | 2 | 11 | 2 | 1 | 16 | 0 | 0 | 7 | 1 |
# [DESIGN PATTERN](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern)
| DESIGN PATTERN | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [OOP patterns](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/OOP_patterns/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [adapter](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/adapter/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [arrow](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/arrows/arrow/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [bifunctor](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/functors/bifunctor/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [builder](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/builder/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [builder pattern](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/builder_pattern/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . |
| [comonad](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/monads/comonad/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [contravariant](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/functors/contravariant/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [demo](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/proxy/demo/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [facade](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/facade/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [factory](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/factory/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [functor](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/functors/functor/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [gifts](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/factory/gifts/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [gonad](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/monads/gonad/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [gym](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/facade/daily/tasks/gym/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [iterator pattern](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/iterator_pattern/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [job](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/facade/daily/tasks/job/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [monad](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/monads/costate/monad/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [multifunctor](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/functors/multifunctor/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [network](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/observer_java/observer/network/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [observer](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/observer_java/observer/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [observer java](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/observer_java/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [observer pattern](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/observer_pattern/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| [policy based design](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/policy_based_design/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [profunctor](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/functors/profunctor/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [proxy](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/proxy/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [routine](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/facade/daily/tasks/morning/routine/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [scala](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [singleton pattern](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/singleton_pattern/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . |
| [soldiers](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/adapter/soldiers/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [src](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [tasks](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/oop_patterns/facade/daily/tasks/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 0 | 3 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 17 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 4 | 0 | 0 | 0 | 1 | 10 | 0 | 0 | 0 | 0 | 0 | 2 | 0 |
# [DIVIDE CONQUER](https://github.com/OpenGenus/cosmos/tree/master/code/divide_conquer)
| DIVIDE CONQUER | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [closest pair of points](https://github.com/OpenGenus/cosmos/tree/master/code/divide_conquer/src/closest_pair_of_points/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [factorial](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/factorial/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [inversion count](https://github.com/OpenGenus/cosmos/tree/master/code/divide_conquer/src/inversion_count/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [karatsuba multiplication](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/karatsuba_multiplication/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [maximum contiguous subsequence sum](https://github.com/OpenGenus/cosmos/tree/master/code/divide_conquer/src/maximum_contiguous_subsequence_sum/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [merge sort using divide and conquer](https://github.com/OpenGenus/cosmos/tree/master/code/divide_conquer/src/merge_sort_using_divide_and_conquer/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [quick sort](https://github.com/OpenGenus/cosmos/tree/master/code/divide_conquer/src/quick_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . |
| [strassen matrix multiplication](https://github.com/OpenGenus/cosmos/tree/master/code/divide_conquer/src/strassen_matrix_multiplication/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [tournament method to find min max](https://github.com/OpenGenus/cosmos/tree/master/code/divide_conquer/src/tournament_method_to_find_min_max/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [warnock algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/divide_conquer/src/warnock_algorithm/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [x power y](https://github.com/OpenGenus/cosmos/tree/master/code/divide_conquer/src/x_power_y/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 6 | 6 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 4 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 6 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 0 |
# [DYNAMIC PROGRAMMING](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming)
| DYNAMIC PROGRAMMING | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [array median](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/array_median/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . |
| [binomial coefficient](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/binomial_coefficient/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [boolean parenthesization](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/boolean_parenthesization/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [box stacking](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/box_stacking/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [coin change](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/coin_change/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [digit dp](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/digit_dp/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [edit distance](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/edit_distance/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| [egg dropping puzzle](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/egg_dropping_puzzle/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [factorial](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/factorial/) | . | . | ✔️ | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . |
| [fibonacci](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/fibonacci/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [knapsack](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/knapsack/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [largest sum contiguous subarray](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/largest_sum_contiguous_subarray/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [longest bitonic sequence](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/longest_bitonic_sequence/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [longest common increasing subsequence](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/longest_common_increasing_subsequence/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [longest common subsequence](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/longest_common_subsequence/) | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [longest common substring](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/longest_common_substring/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [longest increasing subsequence](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/longest_increasing_subsequence/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [longest palindromic sequence](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/longest_palindromic_sequence/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [longest palindromic substring](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/longest_palindromic_substring/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [longest repeating subsequence](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/longest_repeating_subsequence/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [matrix chain multiplication](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/matrix_chain_multiplication/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [maximum sum increasing subsequence](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/maximum_sum_increasing_subsequence/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [maximum sum sub matrix](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/maximum_sum_sub_matrix/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [maximum weight independent set of path graph](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/maximum_weight_independent_set_of_path_graph/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [min cost path](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/min_cost_path/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [minimum insertion palindrome](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/minimum_insertion_palindrome/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [no consec ones](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/no_consec_ones/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [numeric keypad problem](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/numeric_keypad_problem/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [optimal binary search tree](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/optimal_binary_search_tree/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [palindrome partition](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/palindrome_partition/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [rod cutting](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/rod_cutting/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [shortest common supersequence](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/shortest_common_supersequence/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [subset sum](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/subset_sum/) | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [tiling problem](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/tiling_problem/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [weighted job scheduling](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/weighted_job_scheduling/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 17 | 31 | 0 | 0 | 1 | 0 | 0 | 0 | 9 | 0 | 4 | 19 | 7 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 2 | 0 | 0 | 0 | 24 | 0 | 0 | 1 | 3 | 1 | 0 | 0 | 1 | 0 | 0 | 0 | 0 |
# [FILTERS](https://github.com/OpenGenus/cosmos/tree/master/code/filters)
| FILTERS | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [gaussian filter](https://github.com/OpenGenus/cosmos/tree/master/code/filters/src/gaussian_filter/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [median filter](https://github.com/OpenGenus/cosmos/tree/master/code/filters/src/median_filter/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 2 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
# [GAME THEORY](https://github.com/OpenGenus/cosmos/tree/master/code/game_theory)
| GAME THEORY | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [game of nim next best move](https://github.com/OpenGenus/cosmos/tree/master/code/game_theory/src/game_of_nim_next_best_move/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [game of nim win loss prediction](https://github.com/OpenGenus/cosmos/tree/master/code/game_theory/src/game_of_nim_win_loss_prediction/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [grundy numbers kayle](https://github.com/OpenGenus/cosmos/tree/master/code/game_theory/src/grundy_numbers_kayle/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 3 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
# [GRAPH ALGORITHMS](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms)
| GRAPH ALGORITHMS | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [adjacency lists in c](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/adjacency_lists_graph_representation/adjacency_lists_in_c/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [adjacency matrix c](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/data_structures/adjacency_matrix_c/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [astar algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/astar_algorithm/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [bellman ford algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/bellman_ford_algorithm/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [biconnected components](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/biconnected_components/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [bipartite check](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/bipartite_check/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [bipartite checking](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/bipartite_checking/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [boruvka minimum spanning tree](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/boruvka_minimum_spanning_tree/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [breadth first search](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/breadth_first_search/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | . |
| [bridge tree](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/bridge_tree/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [bridges in graph](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/bridges_in_graph/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [bron kerbosch algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/bron_kerbosch_algorithm/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [centroid decomposition](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/centroid_decomposition/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [connected components](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/connected_components/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [count of ways n](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/count_of_ways_n/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [cut vertices](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/cut_vertices/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [cycle directed graph](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/cycle_directed_graph/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [cycle undirected graph](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/cycle_undirected_graph/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [data structures](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/data_structures/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [depth first search](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/depth_first_search/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . |
| [dijkstra shortest path](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/dijkstra_shortest_path/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [dinic maximum flow](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/dinic_maximum_flow/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [eulerian path](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/eulerian_path/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [floyd warshall algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/floyd_warshall_algorithm/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [ford fulkerson maximum flow](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/ford_fulkerson_maximum_flow/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [graph coloring](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/graph_coloring/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [hamiltonian cycle](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/hamiltonian_cycle/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [hamiltonian path](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/hamiltonian_path/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [johnson algorithm shortest path](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/johnson_algorithm_shortest_path/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [karger minimum cut](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/karger_minimum_cut/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [kruskal minimum spanning tree](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/kruskal_minimum_spanning_tree/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [kuhn maximum matching](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/kuhn_maximum_matching/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [kuhn munkres algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/kuhn_munkres_algorithm/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [left view binary tree](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/left_view_binary_tree/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [longest path directed acyclic graph](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/longest_path_directed_acyclic_graph/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [matrix transformation](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/matrix_transformation/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [maximum bipartite matching](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/maximum_bipartite_matching/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [postorder from inorder and preorder](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/postorder_from_inorder_and_preorder/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [prim minimum spanning tree](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/prim_minimum_spanning_tree/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [push relabel](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/push_relabel/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [steiner tree](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/steiner_tree/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [strongly connected components](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/strongly_connected_components/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [tarjan algorithm strongly connected components](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/tarjan_algorithm_strongly_connected_components/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [topological sort](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/topological_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [transitive closure graph](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/transitive_closure_graph/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [travelling salesman mst](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/travelling_salesman_mst/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 14 | 32 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 1 | 19 | 4 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 21 | 0 | 0 | 2 | 0 | 0 | 0 | 0 | 2 | 0 | 0 | 0 | 0 |
# [GREEDY ALGORITHMS](https://github.com/OpenGenus/cosmos/tree/master/code/greedy_algorithms)
| GREEDY ALGORITHMS | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [activity selection](https://github.com/OpenGenus/cosmos/tree/master/code/greedy_algorithms/src/activity_selection/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [dijkstra shortest path](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/dijkstra_shortest_path/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [egyptian fraction](https://github.com/OpenGenus/cosmos/tree/master/code/greedy_algorithms/src/egyptian_fraction/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [fractional knapsack](https://github.com/OpenGenus/cosmos/tree/master/code/greedy_algorithms/src/fractional_knapsack/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . |
| [hillclimber](https://github.com/OpenGenus/cosmos/tree/master/code/greedy_algorithms/src/hillclimber/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [huffman coding](https://github.com/OpenGenus/cosmos/tree/master/code/greedy_algorithms/src/huffman_coding/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [job sequencing](https://github.com/OpenGenus/cosmos/tree/master/code/greedy_algorithms/src/job_sequencing/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [k centers](https://github.com/OpenGenus/cosmos/tree/master/code/greedy_algorithms/src/k_centers/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [kruskal minimum spanning tree](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/kruskal_minimum_spanning_tree/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [minimum coins](https://github.com/OpenGenus/cosmos/tree/master/code/greedy_algorithms/src/minimum_coins/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [prim minimum spanning tree](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/prim_minimum_spanning_tree/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [warshall](https://github.com/OpenGenus/cosmos/tree/master/code/greedy_algorithms/src/warshall/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 9 | 9 | 0 | 0 | 0 | 0 | 0 | 0 | 2 | 0 | 2 | 5 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 10 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 |
# [MATHEMATICAL ALGORITHMS](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms)
| MATHEMATICAL ALGORITHMS | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [2sum](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/2sum/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . |
| [add polynomials](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/add_polynomials/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [amicable numbers](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/amicable_numbers/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . |
| [armstrong numbers](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/armstrong_numbers/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . |
| [automorphic numbers](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/automorphic_numbers/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | ✔️ | . | . | ✔️ | . |
| [average stream numbers](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/average_stream_numbers/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [babylonian method](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/babylonian_method/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [binomial coefficient](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/binomial_coefficient/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [catalan number](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/catalan_number/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | ✔️ | . | . | . | . | . | . | . |
| [check is square](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/check_is_square/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | ✔️ | ✔️ | . | . | ✔️ | . | . | ✔️ | . |
| [collatz conjecture sequence](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/collatz_conjecture_sequence/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [convolution](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/convolution/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [coprime numbers](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/coprime_numbers/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . |
| [count digits](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/count_digits/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | ✔️ | . | . | ✔️ | . |
| [count trailing zeroes](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/count_trailing_zeroes/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [delannoy number](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/delannoy_number/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [derangements](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/derangements/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [diophantine](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/diophantine/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [divided differences](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/divided_differences/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [elimination](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/gaussian_elimination/scala/src/main/scala/gaussian/elimination/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [euler totient](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/euler_totient/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [exponentiation by squaring](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/exponentiation_power/exponentiation_by_squaring/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [exponentiation power](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/exponentiation_power/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [factorial](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/factorial/) | . | . | ✔️ | ✔️ | ✔️ | . | ✔️ | . | ✔️ | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | ✔️ | . | . | . | . | ✔️ | ✔️ | . | . | ✔️ | . | . | ✔️ | ✔️ | ✔️ | . | . | ✔️ | . | . | ✔️ | . |
| [fast fourier transform](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/fast_fourier_transform/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [fast inverse sqrt](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/fast_inverse_sqrt/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [fermat primality test](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/primality_tests/fermat_primality_test/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [fermats little theorem](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/fermats_little_theorem/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [fibonacci number](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/fibonacci_number/) | . | . | ✔️ | ✔️ | ✔️ | . | ✔️ | . | ✔️ | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | ✔️ | ✔️ | . | . | ✔️ | . | . | ✔️ | . |
| [fractals](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/fractals/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [gaussian elimination](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/gaussian_elimination/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [gcd and lcm](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/gcd_and_lcm/) | . | . | ✔️ | ✔️ | . | . | ✔️ | . | ✔️ | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | . | . | ✔️ | . |
| [greatest digit in number](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/greatest_digit_in_number/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . |
| [hill climbing](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/hill_climbing/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [horner polynomial evaluation](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/horner_polynomial_evaluation/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [integer conversion](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/integer_conversion/) | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [integer to roman](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/integer_to_roman/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [jacobi method](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/jacobi_method/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [karatsuba multiplication](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/karatsuba_multiplication/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [largrange polynomial](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/largrange_polynomial/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [lexicographic string rank](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/lexicographic_string_rank/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [log of factorial](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/log_of_factorial/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [lucas theorem](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/lucas_theorem/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [lucky number](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/lucky_number/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [magic square](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/magic_square/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [miller rabin primality test](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/primality_tests/miller_rabin_primality_test/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [modular inverse](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/modular_inverse/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . |
| [multiply polynomial](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/multiply_polynomial/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [newman conway](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/newman_conway/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [newton polynomial](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/newton_polynomial/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [newton raphson method](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/newton_raphson_method/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [next larger number](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/next_larger_number/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [pandigital number](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/pandigital_number/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . |
| [pascal triangle](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/pascal_triangle/) | . | . | ✔️ | ✔️ | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [perfect number](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/perfect_number/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . |
| [permutation lexicographic order](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/permutation_lexicographic_order/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [poisson sample](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/poisson_sample/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [prime factors](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/prime_factors/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [prime numbers of n](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/prime_numbers_of_n/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [pythagorean triplet](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/pythagorean_triplet/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [replace 0 with 5](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/replace_0_with_5/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . |
| [reverse factorial](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/reverse_factorial/) | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | . | . |
| [reverse number](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/reverse_number/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | ✔️ | . | . | ✔️ | . |
| [russian peasant multiplication](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/russian_peasant_multiplication/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . |
| [scala](https://github.com/OpenGenus/cosmos/tree/master/code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [segmented sieve of eratosthenes](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/segmented_sieve_of_eratosthenes/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [shuffle array](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/shuffle_array/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . |
| [sieve of atkin](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/sieve_of_atkin/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [sieve of eratosthenes](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/sieve_of_eratosthenes/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . |
| [simpsons rule](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/simpsons_rule/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [smallest digit in number](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/smallest_digit_in_number/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . |
| [solovay strassen primality test](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/primality_tests/solovay_strassen_primality_test/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [square free number](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/square_free_number/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [std](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/std/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [steepest descent](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/steepest_descent/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [structures](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/gaussian_elimination/scala/src/main/scala/structures/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . |
| [sum of digits](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/sum_of_digits/) | . | . | ✔️ | ✔️ | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . |
| [taxicab numbers](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/taxicab_numbers/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [tower of hanoi](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/tower_of_hanoi/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . |
| [tribonacci numbers](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/tribonacci_numbers/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| [tridiagonal matrix](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/tridiagonal_matrix/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 48 | 61 | 2 | 0 | 5 | 0 | 3 | 0 | 27 | 0 | 10 | 46 | 29 | 0 | 0 | 1 | 0 | 1 | 0 | 0 | 15 | 1 | 0 | 0 | 48 | 0 | 0 | 19 | 11 | 10 | 0 | 0 | 7 | 0 | 0 | 16 | 0 |
# [NETWORKING](https://github.com/OpenGenus/cosmos/tree/master/code/networking)
| NETWORKING | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [determine endianess](https://github.com/OpenGenus/cosmos/tree/master/code/networking/src/determine_endianess/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [packetsniffer](https://github.com/OpenGenus/cosmos/tree/master/code/networking/src/packetsniffer/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [validate ip](https://github.com/OpenGenus/cosmos/tree/master/code/networking/src/validate_ip/) | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . |
| | 0 | 0 | 2 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 2 | 0 | 0 | 1 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 |
# [NUMERICAL ANALYSIS](https://github.com/OpenGenus/cosmos/tree/master/code/numerical_analysis)
| NUMERICAL ANALYSIS | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [src](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 1 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
# [ONLINE CHALLENGES](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges)
| ONLINE CHALLENGES | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [3D aurface area](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/hackerrank/3D_aurface_area/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [CHDIGER](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/codechef/CHDIGER/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [CHNUM](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/codechef/CHNUM/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [JAIN](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/codechef/JAIN/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [NBONACCI](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/codechef/NBONACCI/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [almost sorted](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/hackerrank/almost_sorted/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [array manipulation](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/hackerrank/array_manipulation/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [bigger is greater](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/hackerrank/bigger_is_greater/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [complement dna strand](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/rosalind/complement_dna_strand/) | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| [encryption](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/hackerrank/encryption/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 001](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_001/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| [problem 002](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_002/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 003](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_003/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 004](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_004/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 005](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_005/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 006](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_006/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 007](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_007/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 008](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_008/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 009](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_009/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 010](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_010/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 012](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_012/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 013](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_013/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 014](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_014/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 016](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_016/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 018](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_018/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 019](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_019/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 020](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_020/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 021](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_021/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 022](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_022/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 024](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_024/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 025](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_025/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 026](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_026/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 028](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_028/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 034](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_034/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 036](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_036/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 037](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_037/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 040](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_040/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 067](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_067/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [problem 102](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/project_euler/problem_102/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [the maximum subarray](https://github.com/OpenGenus/cosmos/tree/master/code/online_challenges/src/hackerrank/the_maximum_subarray/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 4 | 29 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 8 | 4 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 23 | 0 | 0 | 0 | 2 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
# [OPERATING SYSTEM](https://github.com/OpenGenus/cosmos/tree/master/code/operating_system)
| OPERATING SYSTEM | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [bankers algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/operating_system/src/deadlocks/bankers_algorithm/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [c](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/finite_automata/c/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [dining philosophers](https://github.com/OpenGenus/cosmos/tree/master/code/operating_system/src/concurrency/dining_philosophers/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [first come first serve](https://github.com/OpenGenus/cosmos/tree/master/code/operating_system/src/scheduling/first_come_first_serve/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . |
| [least recently used](https://github.com/OpenGenus/cosmos/tree/master/code/operating_system/src/memory_management/least_recently_used/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [memory mapping](https://github.com/OpenGenus/cosmos/tree/master/code/operating_system/src/memory_management/memory_mapping/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [monitors system v](https://github.com/OpenGenus/cosmos/tree/master/code/operating_system/src/concurrency/monitors/monitors_system_v/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [multi level feedback queue scheduling](https://github.com/OpenGenus/cosmos/tree/master/code/operating_system/src/scheduling/multi_level_feedback_queue_scheduling/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . |
| [peterson algorithm in c](https://github.com/OpenGenus/cosmos/tree/master/code/operating_system/src/concurrency/peterson_algorithm_for_mutual_exclusion/peterson_algorithm_in_c/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [processCreation](https://github.com/OpenGenus/cosmos/tree/master/code/operating_system/src/processCreation/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [producer consumer](https://github.com/OpenGenus/cosmos/tree/master/code/operating_system/src/concurrency/producer_consumer/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [readers writers](https://github.com/OpenGenus/cosmos/tree/master/code/operating_system/src/concurrency/readers_writers/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [round robin c](https://github.com/OpenGenus/cosmos/tree/master/code/operating_system/src/scheduling/round_robin_scheduling/round_robin_c/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [round robin scheduling](https://github.com/OpenGenus/cosmos/tree/master/code/operating_system/src/scheduling/round_robin_scheduling/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [shortest seek time first](https://github.com/OpenGenus/cosmos/tree/master/code/operating_system/src/scheduling/shortest_seek_time_first/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [smallest remaining time first](https://github.com/OpenGenus/cosmos/tree/master/code/operating_system/src/scheduling/smallest_remaining_time_first/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 10 | 6 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 2 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 1 | 0 | 1 | 0 |
# [RANDOMIZED ALGORITHMS](https://github.com/OpenGenus/cosmos/tree/master/code/randomized_algorithms)
| RANDOMIZED ALGORITHMS | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [birthday paradox](https://github.com/OpenGenus/cosmos/tree/master/code/randomized_algorithms/src/birthday_paradox/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [karger minimum cut algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/randomized_algorithms/src/karger_minimum_cut_algorithm/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [kth smallest element algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/randomized_algorithms/src/kth_smallest_element_algorithm/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [random from stream](https://github.com/OpenGenus/cosmos/tree/master/code/randomized_algorithms/src/random_from_stream/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [randomized quick sort](https://github.com/OpenGenus/cosmos/tree/master/code/randomized_algorithms/src/randomized_quick_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [reservoir sampling](https://github.com/OpenGenus/cosmos/tree/master/code/randomized_algorithms/src/reservoir_sampling/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| [shuffle an array](https://github.com/OpenGenus/cosmos/tree/master/code/randomized_algorithms/src/shuffle_an_array/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . |
| | 0 | 0 | 2 | 6 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 2 | 0 | 0 | 1 | 2 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
# [SEARCH](https://github.com/OpenGenus/cosmos/tree/master/code/search)
| SEARCH | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [binary search](https://github.com/OpenGenus/cosmos/tree/master/code/search/src/binary_search/) | . | . | ✔️ | ✔️ | . | . | ✔️ | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | ✔️ | ✔️ | . | ✔️ | ✔️ | ✔️ | ✔️ | . | ✔️ | . | . | ✔️ | . |
| [exponential search](https://github.com/OpenGenus/cosmos/tree/master/code/search/src/exponential_search/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . |
| [fibonacci search](https://github.com/OpenGenus/cosmos/tree/master/code/search/src/fibonacci_search/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [fuzzy search](https://github.com/OpenGenus/cosmos/tree/master/code/search/src/fuzzy_search/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [interpolation search](https://github.com/OpenGenus/cosmos/tree/master/code/search/src/interpolation_search/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [jump search](https://github.com/OpenGenus/cosmos/tree/master/code/search/src/jump_search/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . |
| [linear search](https://github.com/OpenGenus/cosmos/tree/master/code/search/src/linear_search/) | . | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | ✔️ | . | ✔️ | ✔️ | . | ✔️ | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | ✔️ | . | . | ✔️ | . | . | ✔️ | . |
| [ternary search](https://github.com/OpenGenus/cosmos/tree/master/code/search/src/ternary_search/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| | 0 | 0 | 7 | 7 | 1 | 0 | 1 | 0 | 0 | 0 | 6 | 0 | 2 | 6 | 8 | 0 | 0 | 3 | 0 | 1 | 1 | 0 | 7 | 0 | 0 | 0 | 7 | 1 | 1 | 3 | 5 | 2 | 1 | 0 | 4 | 0 | 0 | 2 | 0 |
# [SELECTION ALGORITHMS](https://github.com/OpenGenus/cosmos/tree/master/code/selection_algorithms)
| SELECTION ALGORITHMS | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [median of medians](https://github.com/OpenGenus/cosmos/tree/master/code/selection_algorithms/src/median_of_medians/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [src](https://github.com/OpenGenus/cosmos/tree/master/code/artificial_intelligence/src/) | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| | 0 | 0 | 1 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 1 | 1 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 2 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 0 |
# [SORTING](https://github.com/OpenGenus/cosmos/tree/master/code/sorting)
| SORTING | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [bead sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/bead_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . |
| [bogo sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/bogo_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | . | . | ✔️ | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | ✔️ |
| [bubble sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/bubble_sort/) | . | . | ✔️ | ✔️ | . | . | ✔️ | ✔️ | . | ✔️ | ✔️ | . | ✔️ | ✔️ | ✔️ | ✔️ | . | ✔️ | . | . | . | ✔️ | ✔️ | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | ✔️ | ✔️ | ✔️ | ✔️ | . | ✔️ | . |
| [bucket sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/bucket_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | ✔️ | . | . | ✔️ | . |
| [circle sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/circle_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . |
| [comb sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/comb_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [counting sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/counting_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . |
| [cycle sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/cycle_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . |
| [flash sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/flash_sort/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [gnome sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/gnome_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [heap sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/heap_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | ✔️ | . | . | ✔️ | . | . | ✔️ | . |
| [insertion sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/insertion_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | ✔️ | . | ✔️ | . | ✔️ | ✔️ | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | ✔️ | . | ✔️ | . | . | ✔️ | . |
| [intro sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/intro_sort/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [median sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/median_sort/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . |
| [merge sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/merge_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | ✔️ | . | . | . | ✔️ | ✔️ | ✔️ | . | . | ✔️ | . | . | ✔️ | ✔️ | ✔️ | ✔️ | . | ✔️ | ✔️ | . | ✔️ | ✔️ |
| [pigeonhole sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/pigeonhole_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | ✔️ | . | . | . | . | ✔️ | . | . | ✔️ | . | . | ✔️ | . |
| [quick sort](https://github.com/OpenGenus/cosmos/tree/master/code/divide_conquer/src/quick_sort/) | . | . | ✔️ | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | . | ✔️ | ✔️ | . | ✔️ | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | ✔️ | ✔️ | . | ✔️ | ✔️ | . | ✔️ | . |
| [radix sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/radix_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| [selection sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/selection_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | ✔️ | . | . | . | ✔️ | ✔️ | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | ✔️ | . | ✔️ | . | ✔️ | ✔️ | . |
| [shaker sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/shaker_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . |
| [shell sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/shell_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [sleep sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/sleep_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | ✔️ | . | . | . | . | . | ✔️ | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . | ✔️ | ✔️ | . | ✔️ | . | . | ✔️ | . |
| [stooge sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/stooge_sort/) | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [topological sort](https://github.com/OpenGenus/cosmos/tree/master/code/graph_algorithms/src/topological_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [tree sort](https://github.com/OpenGenus/cosmos/tree/master/code/sorting/src/tree_sort/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 23 | 23 | 0 | 0 | 1 | 2 | 0 | 1 | 19 | 0 | 7 | 22 | 22 | 2 | 0 | 5 | 1 | 2 | 0 | 21 | 9 | 2 | 0 | 0 | 23 | 0 | 1 | 9 | 8 | 5 | 6 | 1 | 21 | 3 | 1 | 15 | 2 |
# [SQUARE ROOT DECOMPOSITION](https://github.com/OpenGenus/cosmos/tree/master/code/square_root_decomposition)
| SQUARE ROOT DECOMPOSITION | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [mos algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/square_root_decomposition/src/mos_algorithm/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
# [STRING ALGORITHMS](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms)
| STRING ALGORITHMS | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [aho corasick algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/aho_corasick_algorithm/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [anagram search](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/anagram_search/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | ✔️ | . | . | ✔️ | . | . | ✔️ | . |
| [arithmetic on large numbers](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/arithmetic_on_large_numbers/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [boyer moore algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/boyer_moore_algorithm/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [c](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/finite_automata/c/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [finite automata](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/finite_automata/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| [kasai algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/kasai_algorithm/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [kmp algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/kmp_algorithm/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [levenshtein distance](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/levenshtein_distance/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [lipogram checker](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/lipogram_checker/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . |
| [longest palindromic substring](https://github.com/OpenGenus/cosmos/tree/master/code/dynamic_programming/src/longest_palindromic_substring/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [manachar algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/manachar_algorithm/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [morse code](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/morse_code/) | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [naive pattern search](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/naive_pattern_search/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [palindrome checker](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/palindrome_checker/) | . | . | ✔️ | ✔️ | ✔️ | ✔️ | ✔️ | . | ✔️ | . | ✔️ | . | ✔️ | ✔️ | ✔️ | . | . | ✔️ | ✔️ | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | ✔️ | ✔️ | . | ✔️ | . | ✔️ | . | . | ✔️ | . |
| [palindrome substring](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/palindrome_substring/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [pangram checker](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/pangram_checker/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | ✔️ | . | . | . | . |
| [password strength checker](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/password_strength_checker/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . |
| [rabin karp algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/rabin_karp_algorithm/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [remove dups](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/remove_dups/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | . |
| [suffix array](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/suffix_array/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [trie pattern search](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/trie_pattern_search/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [z algorithm](https://github.com/OpenGenus/cosmos/tree/master/code/string_algorithms/src/z_algorithm/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 8 | 15 | 1 | 1 | 1 | 0 | 1 | 0 | 4 | 0 | 1 | 9 | 7 | 0 | 0 | 1 | 1 | 0 | 0 | 1 | 3 | 0 | 0 | 1 | 13 | 0 | 0 | 3 | 3 | 1 | 1 | 0 | 3 | 0 | 0 | 4 | 0 |
# [THEORY OF COMPUTATION](https://github.com/OpenGenus/cosmos/tree/master/code/theory_of_computation)
| THEORY OF COMPUTATION | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [deterministic finite automaton](https://github.com/OpenGenus/cosmos/tree/master/code/theory_of_computation/src/deterministic_finite_automaton/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [nondeterministic finite atomaton](https://github.com/OpenGenus/cosmos/tree/master/code/theory_of_computation/src/nondeterministic_finite_atomaton/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 2 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 |
# [UNCLASSIFIED](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified)
| UNCLASSIFIED | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [average](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/average/) | . | . | ✔️ | ✔️ | . | . | ✔️ | . | ✔️ | . | ✔️ | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | ✔️ | ✔️ | ✔️ | ✔️ | . | ✔️ | . | . | ✔️ | . |
| [biggest of n numbers](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/biggest_of_n_numbers/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . |
| [biggest suffix](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/biggest_suffix/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [fifteen puzzle](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/fifteen_puzzle/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [jaccard similarity](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/jaccard_similarity/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [josephus problem](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/josephus_problem/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [lapindrom checker](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/lapindrom_checker/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [leap year](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/leap_year/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | ✔️ | . |
| [magic square](https://github.com/OpenGenus/cosmos/tree/master/code/mathematical_algorithms/src/magic_square/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . | . | . | . |
| [majority element](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/majority_element/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [minimum subarray size with degree](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/minimum_subarray_size_with_degree/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [no operator addition](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/no_operator_addition/) | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [optimized fibonacci](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/optimized_fibonacci/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [paint fill](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/paint_fill/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [smallest number to the left](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/smallest_number_to_the_left/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [spiral print](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/spiral_print/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [split list](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/split_list/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . |
| [tokenizer](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/tokenizer/) | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [unique number](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/unique_number/) | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . |
| [utilities](https://github.com/OpenGenus/cosmos/tree/master/code/unclassified/src/utilities/) | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | . | ✔️ | . | . | . | . | . | . |
| | 0 | 0 | 10 | 12 | 0 | 0 | 1 | 0 | 1 | 0 | 4 | 0 | 0 | 5 | 3 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | 2 | 0 | 0 | 0 | 9 | 0 | 0 | 1 | 2 | 1 | 2 | 0 | 2 | 0 | 0 | 3 | 0 |
# [UTILITY](https://github.com/OpenGenus/cosmos/tree/master/code/utility)
| UTILITY | Ada | BrainFuck | C | C++ | Clojure | Crisp | Elixir | Elm | Erlang | Fortran | Golang | HTML | Haskell | Java | JavaScript | Julia | Jupyter Notebook | Kotlin | Lua | ML | Nim | Objective-C | PHP | Perl | Processing Development Environment | PureScript | Python | Racket | Reason | Ruby | Rust | Scala | Shell Script | Standard ML | Swift | TypeScript | Visual Basic | Visual C# | Visual F# |
| ----------------------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
| [palindrome check](https://github.com/OpenGenus/cosmos/tree/master/code/utility/src/palindrome/palindrome_check/) | . | . | ✔️ | ✔️ | . | . | . | . | . | . | . | . | . | ✔️ | ✔️ | . | . | . | . | . | ✔️ | . | . | . | . | . | ✔️ | . | . | ✔️ | . | . | . | . | . | . | . | ✔️ | . |
| TOTALS | 1 | 1 | 244 | 376 | 4 | 1 | 13 | 2 | 6 | 1 | 109 | 2 | 37 | 242 | 121 | 4 | 6 | 16 | 3 | 4 | 3 | 23 | 48 | 4 | 1 | 1 | 308 | 1 | 2 | 59 | 50 | 41 | 16 | 2 | 61 | 5 | 1 | 60 | 3 |
| ------ | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- | ----------------------------------- |
|
scripts/STATS.txt | This is the progress list of Cosmos | Updated on: 2019-04-28
ARTIFICIAL INTELLIGENCE Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
a star . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
artificial neural network . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
autoenncoder . . . . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . .
canny . . x x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
convolutional neural network . . . . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . .
dbscan clustering . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
decision tree . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
erode dilate . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
factorization machines . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
gaussian naive bayes . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
gbestPSO . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
hierachical clustering . . . x . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . .
houghtransform . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
image processing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . .
image stitching . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
isodata clustering . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
k means . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . x . . . .
k nearest neighbours . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
lbestPSO . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
linear regression . . . . . . . . . . . . . x x . . . . . . . . . . . x . . . . . . . x . . . .
logistic regression . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
minimax . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
naive bayes . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . x . . . .
neural network . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
neural style transfer . . . . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . .
nsm matlab . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . .
perceptron . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
prewittfilter . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
principal component analysis . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
q learning . . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . .
restricted boltzmann machine . . . . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . .
sat . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
sobelfilter . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
src . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
support vector machine . . x . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
tsp . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 3 10 0 0 0 0 0 0 0 0 0 1 2 0 5 0 0 0 0 1 0 0 0 0 19 0 0 0 0 0 1 0 3 0 0 0 0
BACKTRACKING Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
algorithm x . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
crossword puzzle . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
knight tour . . x x . . . . . . x . . x . . . . . . . . . . . . x . . . x . . . . . . . .
m coloring problem . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
n queen . . x x . . . . . . x . x x . . . . . . . . . . . . x . . . x . . . . . . . .
number of ways in maze . . x x . . . . . . x . . x . . . . . . . . . . . . . . . . x . . . . . . . .
partitions of number . . . x . . . . . . x . . . . . . . . . . . . . . . . . . . x . . . . . . . .
partitions of set . . . x . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . .
permutations of string . . x x . . . . . . x . . . . . . x . . . . . . . . x . . . . . . . . . . . .
powerset . . x . . . . . . . x . . x . . . . . . . . . . . . . . . . . . . . . . . . .
rat in a maze . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
subset sum . . x x . . . . . . x . . x . . . . . . . . . . . . x . . . . . . . . . . . .
sudoku solve . . x x . . . . . . . . . . . . . . . . . . . . . . x . . . x . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 7 10 0 0 0 0 0 0 8 0 1 6 0 0 0 1 0 0 0 0 0 0 0 0 6 0 0 0 5 0 0 0 0 0 0 0 0
BIT MANIPULATION Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
addition using bits . . . x . . . . . . . . . . x . . . . . . . . . . . x . . . . . . . . . . x .
bit division . . x . . . . . . . x . . x x . . . . . . . . . . . x . . . . . . . . . . . .
byte swapper . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
convert number binary . . x x . . . . . . . . x x x . . . . . . . x . . . x . . . . . . . . . . . .
count set bits . . x x . . . . . . . . . x x . . . . . . . . . . . x . . . . . . . . . . x .
flip bits . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
hamming distance . . x x . . . . . . x . . x . . . . . . . . . . . . x . . . . . . . . . . . .
invert bit . . x x . . . . . . . . . . x . . . . . . . . . . . x . . . . . . . . . . . .
lonely integer . . x x . . . . . . x . . x x . . . . . . . . . . . x . . . x . . . . . . . .
magic number . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
maximum xor value . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
power of 2 . . x x . . . . . . x . . x x x . . . . . . . . . . x . . . x . . . . . . x .
subset generation . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
sum binary numbers . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
sum equals xor . . x x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
thrice unique number . . . x . . . . . . . . . x x . . . . . . . . . . . x . . . . . . . . . . . .
twice unique number . . x x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
xor swap . . x x . . . . . . x . . . . . . . . . . . . . . . x . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 13 15 0 0 0 0 0 0 5 0 1 10 8 1 0 0 0 0 0 0 1 0 0 0 13 0 0 0 2 0 0 0 0 0 0 3 0
CELLULAR AUTOMATON Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
conways game of life . . x x . . . . . . x . x x . . . . . . . . . . . . x . . x . . . . . . . . .
elementary cellular automata . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
genetic algorithm . . . x . . . . . . x . . x x . . . . . . . . . . . x . . . . . . . . . . . .
langtons ant . . . x . . . . . . . x . x . . . . . . . . . . . . x . . . . . . . . . . . .
von neumann cellular automata . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 2 3 0 0 0 0 0 0 2 1 1 4 1 0 0 0 0 0 0 0 0 0 0 0 3 0 0 1 0 0 0 0 0 0 0 0 0
COMPRESSION Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
huffman . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
lempel ziv welch . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 0 2 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0
COMPUTATIONAL GEOMETRY Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
2d line intersection . . x x . . . . . . . . x x x . . . . . . . . . . . x . . x . . . . . . . x .
2d separating axis test . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
area of polygon . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
area of triangle . . x x . . . . . . x . . x x . . . . . . . . . . . x . . . x . . . . . . . .
axis aligned bounding box collision . . . x . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . .
bresenham circle . . x x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
bresenham line . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
chans algorithm . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
cohen sutherland lineclip . . x x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
dda line . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
distance between points . . x x . . . . . . x . . x x . . . . . . . . . . . x . . . x . . . . . . . .
graham scan . . . x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
halfplane intersection . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
jarvis march . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
quickhull . . . x . . . . . . . . x x . . . . . . . . . . . . . . . . . . . . . . . . .
sphere tetrahedron intersection . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
sutherland hodgeman clipping . . x x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 7 17 0 0 0 0 0 0 3 0 2 6 3 0 0 0 0 0 0 0 0 0 0 0 6 0 0 1 2 0 0 0 0 0 0 1 0
COMPUTER GRAPHICS Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
diamond square . . . . . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0
CRYPTOGRAPHY Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
aes 128 . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
aes csharp . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x .
affine cipher . . . x . . . . . . . x . x . . . x . . . . . . . . x . . . . . . . . . . . .
atbash cipher . . . x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
autokey cipher . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
baconian cipher . . x x . . . . . . . . . x x . . . . . . . x . . . x . . x . . . . . . . . .
caesar cipher . . x x . . . . . . x . x x x . . . . . . . x . . . x . . x . . . . . . . x .
columnar transposition cipher . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
example . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x .
huffman encoding . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
morse cipher . x x x . . . . . . . . . x x . . . x . . . x . . . x . . x . . x . . x . . .
polybius cipher . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
porta cipher . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
rail fence cipher . . . x . . . . . . . . . . . . . . . . . . . . . . x . . x . . . . . . . . .
rot13 cipher . . x x . . . . . . . . . x x . . . . . . . . . . . x . . x . . x . . . . . .
rsa . . x . . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . x .
rsa digital signature . . . . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . .
runningkey cipher . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
sha 256 . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
vigenere cipher . . x x . . . . . . x . x x x x . . . . . . x . . . x . . x . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 1 7 10 0 0 0 0 0 0 2 1 2 8 5 1 1 1 1 0 0 0 4 0 0 0 15 0 0 6 0 0 2 0 0 1 0 4 0
DATA STRUCTURES Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
aa tree . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
abstract stack . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
arraystack . . x x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
avl tree . . x x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . x . . . .
b tree . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . x . . . .
b tree c . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
bag . . . . . . . . . . . . . x x . . . . . . . . . . . x . . . . . . . . . . . .
balanced expression . . x x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
binary heap . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
binomial heap . . x x . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . .
bloom filter . . x x . . . . . . x . . x x . . . . . . . . . . . x . . . . x . . x . . . .
bottom view binary tree . . . x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
c . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
circular buffer . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
circular linked list . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
convert to doubly linked list . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
cpp . . x . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
delete . . x . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
detect cycle . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
diameter . . x x . . . . . . . . x x . . . . . . . . . . . . x . . . . . . . . . . . .
double ended queue . . x x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
doubly linked list . . . x . . . . . . x . . x x . . . . . . . . . . . x . . . . . . . x . . . .
fenwick tree . . x x . . . . . . x . . x . . . . . . . . . x . . x . . . . . . . . . . . .
find . . x . . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
from inorder and postorder . . x x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
from inorder and preorder . . x x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
hash table . . x x . . . . . . x . . x x . . . . . . . . . . . x . . . x . . . x . . x .
infix to postfix . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
insertion . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
interval tree . . . x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
is balance . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
is binary tree . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
is same . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
kd tree . . . x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
left view . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
leftist tree . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
linked list . . x x . . . . . . . . . x x . . . . . . . . . . . x . . . . . . . . . . . .
make mirror tree . . x x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
max heap . . x x . . . . . . x . . x . . . . . . . . . . . . x . . . . . . . . . . . .
maximum height . . . x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
menu interface . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
merge sorted . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
min heap . . x x . . . . . . . . . x x . . . . . . . . . . . x . . x . . . . x . . . .
minimum height . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
n th node linked list . . x x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
node . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
operations . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
pairing heap . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . x
path sum . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
postfix evaluation . . x . . . . . . . . . . . . . . . . . . . . . . . x . . . . . x . . . . . .
prefix to postfix . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
print reverse . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . x . . . . . . .
priority queue . . . . . . . . . . . . . . x . . . . . . . . . . . x . . . . . . . . . . . .
push . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
quad tree . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . .
queue . . x x . . x . . . x . . x x . . . . . . . . . . . x . . x . . . . x . . x .
queue stream . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x .
queue using linked list . . x x . . . . . . . . . x . . . . . . . . . . . . x . . x . . . . . . . . .
queue using stack . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . x . . . . . .
red black tree . . x x . . . . . . . . . x . . . . . . . . . . . . . . . x . x . . . . . . .
reverse . . x x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
reverse queue . . . x . . . . . . x . . x . . . . . . . . . . . . x . . . . . . . x . . . .
reverse stack . . x x . . . . . . x . . x . . . . . . . . . . . . x . . . . . . . x . . x .
right threaded . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
right view . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
rope . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
rotate . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
segment tree x . . x . . . . . . x . . x . . . . . . . . . . . . x . . x . x . . . . . . .
serializer . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
singly linked list . . x x . . . . . . x . . x x . . . . . . . . . . . x . . x . . . . x . . x .
skip list . . x x . . . . . . . . . x . . . . . . . . . . . . . . . x . x . . x . . . .
soft heap . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
sort . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
sort stack . . x x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
splay tree . . . x . . . . . . x . . x . . . x . . . . . . . . . . . . . x . . . . . . .
stack . . x x . . x . x . x . . x x . . . . . . . x . . . x . . x x . . . x . . x .
suffix array . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
sum left . . x . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
treap . . . x . . . . . . . . . x . . . x . . . . . . . . . . . . . x . . x . . . .
tree . . x x . . . . . . x . . x x . . . . . . . . . . . x . . x . . . . x . . . .
trie . . x x . . . . . . x . . x x . . . . . . . . . . . x . . x . x . . x . . x .
two three tree . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . .
unclassified . . x x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
union find . . x x . . . . . . x . . x x . . . . . . . . . . . x . . . . x . . . . . . .
van emde boas tree . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
xor linked list . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
zigzag . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
1 0 42 65 0 0 2 0 1 0 15 0 1 40 13 0 0 2 0 0 0 0 1 1 0 0 40 0 0 10 2 11 2 1 16 0 0 7 1
DESIGN PATTERN Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
OOP patterns . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
adapter . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
arrow . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . .
bifunctor . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . .
builder . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
builder pattern . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x .
comonad . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . .
contravariant . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . .
demo . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
facade . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
factory . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
functor . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . .
gifts . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
gonad . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . .
gym . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
iterator pattern . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
job . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
monad . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . .
multifunctor . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . .
network . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
observer . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
observer java . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
observer pattern . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . x . . . . . . . .
policy based design . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
profunctor . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . .
proxy . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
routine . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
scala . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . .
singleton pattern . . . x . . . . . . . . . x . . . . . . . . x . . . x . . . . . . . . . . x .
soldiers . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
src . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
tasks . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 0 3 0 0 0 0 0 0 0 0 0 17 0 0 0 0 0 0 0 0 1 0 0 0 4 0 0 0 1 10 0 0 0 0 0 2 0
DIVIDE CONQUER Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
closest pair of points . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
factorial . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
inversion count . . x x . . . . . . . . . x x . . . . . . . . . . . x . . . . . . . . . . . .
karatsuba multiplication . . . x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
maximum contiguous subsequence sum . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
merge sort using divide and conquer . . x x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
quick sort . . x x . . . . . . . . x x . . . . . . . . . . . . x . . . x . . . x . . . .
strassen matrix multiplication . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
tournament method to find min max . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
warnock algorithm . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . . . .
x power y . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 6 6 0 0 0 0 0 0 0 0 1 4 1 0 0 0 0 0 0 0 0 0 1 0 6 0 0 0 1 0 0 0 1 0 0 0 0
DYNAMIC PROGRAMMING Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
array median . . x x . . . . . . . . . x . . . . . . . . x . . . x . . x x . . . . . . . .
binomial coefficient . . x x . . . . . . . . . x x . . . . . . . . . . . x . . . . . . . . . . . .
boolean parenthesization . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . x . . . .
box stacking . . . x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
coin change . . x x . . . . . . x . . x . . . . . . . . . . . . x . . . . . . . . . . . .
digit dp . . . x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
edit distance . . x x . . . . . . x . x x . . . . . . . . x . . . x . . . x . . . . . . . .
egg dropping puzzle . . x x . . . . . . . . x x . . . . . . . . . . . . x . . . . . . . . . . . .
factorial . . x . . . x . . . x . . x x . . . . . . . . . . . x . . . x x . . . . . . .
fibonacci . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
knapsack . . x x . . . . . . x . . x x . . . . . . . . . . . x . . . . . . . . . . . .
largest sum contiguous subarray . . x x . . . . . . x . x x . . . . . . . . . . . . x . . . . . . . . . . . .
longest bitonic sequence . . x x . . . . . . . . . x x . . . . . . . . . . . x . . . . . . . . . . . .
longest common increasing subsequence . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
longest common subsequence . . . x . . . . . . x . . x . . . . . . . . . . . . x . . . . . . . . . . . .
longest common substring . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
longest increasing subsequence . . x x . . . . . . x . . x x . . . . . . . . . . . x . . . . . . . . . . . .
longest palindromic sequence . . x x . . . . . . . . . . x . . . . . . . . . . . x . . . . . . . . . . . .
longest palindromic substring . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
longest repeating subsequence . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
matrix chain multiplication . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
maximum sum increasing subsequence . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
maximum sum sub matrix . . . x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
maximum weight independent set of path graph . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
min cost path . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
minimum insertion palindrome . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
no consec ones . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
numeric keypad problem . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
optimal binary search tree . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
palindrome partition . . . x . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . .
rod cutting . . x x . . . . . . x . x . . . . . . . . . . . . . x . . . . . . . . . . . .
shortest common supersequence . . . x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
subset sum . . . x . . . . . . x . . x . . . . . . . . . . . . x . . . . . . . . . . . .
tiling problem . . x x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
weighted job scheduling . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 17 31 0 0 1 0 0 0 9 0 4 19 7 0 0 0 0 0 0 0 2 0 0 0 24 0 0 1 3 1 0 0 1 0 0 0 0
FILTERS Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
gaussian filter . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
median filter . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 2 0 0 0 0 0 0 0 0 0 0 0 0
GAME THEORY Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
game of nim next best move . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
game of nim win loss prediction . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
grundy numbers kayle . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 3 0 0 0 0 0 0 0 0 0 0 0 0
GRAPH ALGORITHMS Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
adjacency lists in c . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
adjacency matrix c . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
astar algorithm . . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . .
bellman ford algorithm . . x x . . . . . . . . . x x . . . . . . . x . . . x . . . . . . . . . . . .
biconnected components . . . x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
bipartite check . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
bipartite checking . . . x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
boruvka minimum spanning tree . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
breadth first search . . x x . . . . . . . . . x . . . . . . . . . . . . x . . x . . . . x . . . .
bridge tree . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
bridges in graph . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
bron kerbosch algorithm . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
centroid decomposition . . . x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
connected components . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
count of ways n . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
cut vertices . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
cycle directed graph . . x x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
cycle undirected graph . . . x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
data structures . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
depth first search . . x x . . . . . . x . . x . . . x . . . . . . . . x . . x . . . . . . . . .
dijkstra shortest path . . x x . . . . . . . . . x x . . . . . . . . . . . x . . . . . . . . . . . .
dinic maximum flow . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
eulerian path . . . . . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
floyd warshall algorithm . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
ford fulkerson maximum flow . . . x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
graph coloring . . . x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
hamiltonian cycle . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
hamiltonian path . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
johnson algorithm shortest path . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
karger minimum cut . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
kruskal minimum spanning tree . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
kuhn maximum matching . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
kuhn munkres algorithm . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
left view binary tree . . . x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
longest path directed acyclic graph . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
matrix transformation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . .
maximum bipartite matching . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
postorder from inorder and preorder . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
prim minimum spanning tree . . x x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
push relabel . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
steiner tree . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
strongly connected components . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
tarjan algorithm strongly connected components . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
topological sort . . x x . . . . . . . . x x x . . . . . . . . . . . x . . . . . . . . . . . .
transitive closure graph . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
travelling salesman mst . . x x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 14 32 0 0 0 0 0 0 1 0 1 19 4 0 0 1 0 0 0 0 1 0 0 0 21 0 0 2 0 0 0 0 2 0 0 0 0
GREEDY ALGORITHMS Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
activity selection . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
dijkstra shortest path . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
egyptian fraction . . x x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
fractional knapsack . . x x . . . . . . x . . x . . . . . . . . . . . . x . . . . . . . . . . x .
hillclimber . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
huffman coding . . x x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
job sequencing . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
k centers . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
kruskal minimum spanning tree . . x x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
minimum coins . . x x . . . . . . x . x x x . . . . . . . . . . . x . . . . . . . . . . . .
prim minimum spanning tree . . x x . . . . . . . . x . . . . . . . . . . . . . x . . . . . . . . . . . .
warshall . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 9 9 0 0 0 0 0 0 2 0 2 5 1 0 0 0 0 0 0 0 0 0 0 0 10 0 0 0 0 0 0 0 0 0 0 1 0
MATHEMATICAL ALGORITHMS Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
2sum . . x x . . . . . . x . . x x . . . . . . . . . . . x . . x x . . . . . . . .
add polynomials . . x x . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . .
amicable numbers . . x x . . . . . . x . . x x . . . . . . . . . . . x . . x x . . . . . . x .
armstrong numbers . . x x . . . . . . x . . x x . . . . . . . x . . . x . . x . . . . . . . x .
automorphic numbers . . x x . . . . . . x . x x x . . . . . . . x . . . x . . x . . . . x . . x .
average stream numbers . . x x . . . . . . x . . . . . . . . . . . . . . . x . . . . . . . . . . . .
babylonian method . . x x . . . . . . x . . x x . . . . . . . . . . . x . . . . . . . . . . . .
binomial coefficient . . x x . . . . . . x . . x . . . . . . . . . . . . x . . . . . . . . . . . .
catalan number . . x x . . . . . . . . . x x . . . . . . . . . . . x . . x . x . . . . . . .
check is square . . x x . . . . . . x . . x x . . . . . . . x . . . x . . x x x . . x . . x .
collatz conjecture sequence . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
convolution . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
coprime numbers . . x x . . . . . . x . . x x . . . . . . . . . . . x . . x x . . . . . . x .
count digits . . x x . . . . . . x . x x x . . . . . . . . . . . x . . x . . . . x . . x .
count trailing zeroes . . x x . . . . . . . . . x x . . . . . . . . . . . x . . . . x . . . . . . .
delannoy number . . x x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
derangements . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
diophantine . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
divided differences . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
elimination . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . .
euler totient . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
exponentiation by squaring . . x x . . . . . . x . . . . . . . . . . . . . . . x . . . . . . . . . . . .
exponentiation power . . x x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
factorial . . x x x . x . x . x . x x x . . x . . . . x x . . x . . x x x . . x . . x .
fast fourier transform . . x . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
fast inverse sqrt . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
fermat primality test . . x . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
fermats little theorem . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
fibonacci number . . x x x . x . x . x . x x x . . . . . . . x . . . x . . x x x . . x . . x .
fractals . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
gaussian elimination . . . x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
gcd and lcm . . x x . . x . x . x . . x x . . . . . . . x . . . x . . . . x . . . . . x .
greatest digit in number . . x x . . . . . . . . x x x . . . . . . . x . . . x . . x . . . . . . . x .
hill climbing . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
horner polynomial evaluation . . . x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
integer conversion . . . x . . . . . . x . . . x . . . . . . . . . . . x . . . . . . . . . . . .
integer to roman . . . x . . . . . . . . . . x . . . . . . . . . . . x . . . . . . . . . . . .
jacobi method . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
karatsuba multiplication . . . x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
largrange polynomial . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
lexicographic string rank . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
log of factorial . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
lucas theorem . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
lucky number . . x . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
magic square . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
miller rabin primality test . . . x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
modular inverse . . . x . . . . . . . . . x . . . . . . . . . . . . x . . x . . . . . . . . .
multiply polynomial . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
newman conway . . x x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
newton polynomial . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
newton raphson method . . x x . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . . . . . .
next larger number . . . x . . . . . . . . . x . . . . . . . . x . . . x . . . . . . . . . . . .
pandigital number . . x . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . .
pascal triangle . . x x . . x . . . x . . x . . . . . . . . . . . . x . . . . . . . . . . . .
perfect number . . x x . . . . . . . . x x x . . . . . . . x . . . x . . x x . . . . . . . .
permutation lexicographic order . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
poisson sample . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
prime factors . . x x . . . . . . x . . x . . . . . . . . . . . . x . . . . . . . . . . . .
prime numbers of n . . x x . . . . . . . . . . x . . . . . . . . . . . x . . . . . . . . . . . .
pythagorean triplet . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
replace 0 with 5 . . x x . . . . . . x . . x x . . . . . . . . . . . x . . . . . . . . . . x .
reverse factorial . . x . . . . . . . x . . x x . . . . . . . . . . . x . . x . . . . . . . . .
reverse number . . x x . . . . . . x . x x x . . . . . . . x . . . x . . x . . . . x . . x .
russian peasant multiplication . . x x . . . . . . x . . . x . . . . . . . x . . . x . . . x . . . . . . x .
scala . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . .
segmented sieve of eratosthenes . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
shuffle array . . x x . . . . . . . . . . x . . . . . . . . . . . . . . x . . . . . . . . .
sieve of atkin . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
sieve of eratosthenes . . x x . . . . . . x . x x x . . . . . . . x . . . x . . . . . . . . . . x .
simpsons rule . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
smallest digit in number . . x x . . . . . . . . x x x . . . . . . . x . . . x . . x . . . . . . . x .
solovay strassen primality test . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
square free number . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
std . . x x . . . . . . x . . . x . . . . . . . . . . . x . . . . . . . . . . . .
steepest descent . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
structures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . .
sum of digits . . x x . . x . . . x . . x x . . . . . . . x . . . x . . x x . . . x . . x .
taxicab numbers . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
tower of hanoi . . x x . . . . . . x . x x x . . . . x . . . . . . x . . . x x . . . . . . .
tribonacci numbers . . x x . . . . . . x . . x x . . . . . . . . . . . x . . . x . . . . . . . .
tridiagonal matrix . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 48 61 2 0 5 0 3 0 27 0 10 46 29 0 0 1 0 1 0 0 15 1 0 0 48 0 0 19 11 10 0 0 7 0 0 16 0
NETWORKING Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
determine endianess . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
packetsniffer . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
validate ip . . x . . . . . . . x . . . x . . . . . . . x . . . x . . x . . x . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 2 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 0 0 0 1 0 0 0 2 0 0 1 0 0 1 0 0 0 0 0 0
NUMERICAL ANALYSIS Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
src . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 1 1 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0
ONLINE CHALLENGES Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
3D aurface area . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
CHDIGER . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
CHNUM . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
JAIN . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
NBONACCI . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
almost sorted . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
array manipulation . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
bigger is greater . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
complement dna strand . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . .
encryption . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
problem 001 . . x x . . . . . . . . . x x . . . . . . . . . . . x . . . x . . . . . . . .
problem 002 . . x x . . . . . . . . . x x . . . . . . . . . . . x . . . . . . . . . . . .
problem 003 . . x x . . . . . . . . . x x . . . . . . . . . . . x . . . . . . . . . . . .
problem 004 . . . x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
problem 005 . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
problem 006 . . . x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
problem 007 . . . x . . . . . . . . . . x . . . . . . . . . . . x . . . . . . . . . . . .
problem 008 . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
problem 009 . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
problem 010 . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
problem 012 . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
problem 013 . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
problem 014 . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
problem 016 . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
problem 018 . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
problem 019 . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
problem 020 . . . . . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
problem 021 . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
problem 022 . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
problem 024 . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
problem 025 . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
problem 026 . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
problem 028 . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
problem 034 . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
problem 036 . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
problem 037 . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
problem 040 . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
problem 067 . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
problem 102 . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
the maximum subarray . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 4 29 0 0 1 0 0 0 0 0 0 8 4 0 0 0 0 0 0 0 0 0 0 0 23 0 0 0 2 0 0 0 0 0 0 0 0
OPERATING SYSTEM Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
bankers algorithm . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
c . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
dining philosophers . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
first come first serve . . . x . . . . . . . . . x . . . . . . . . . . . . x . . . x . . . . . . x .
least recently used . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
memory mapping . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
monitors system v . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
multi level feedback queue scheduling . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . .
peterson algorithm in c . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
processCreation . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
producer consumer . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
readers writers . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
round robin c . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
round robin scheduling . . . x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
shortest seek time first . . x x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
smallest remaining time first . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 10 6 0 0 0 0 0 0 0 0 0 2 0 0 0 0 0 0 0 0 0 0 0 0 1 0 0 0 1 0 0 0 0 1 0 1 0
RANDOMIZED ALGORITHMS Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
birthday paradox . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
karger minimum cut algorithm . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
kth smallest element algorithm . . x x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
random from stream . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
randomized quick sort . . x x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
reservoir sampling . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . .
shuffle an array . . . x . . . . . . . . . x x . . . . . . . x . . . x . . x x . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 2 6 0 0 0 0 0 0 0 0 0 1 1 0 0 0 0 0 0 0 1 0 0 0 2 0 0 1 2 0 0 0 0 0 0 0 0
SEARCH Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
binary search . . x x . . x . . . x . x x x . . x . . . . x . . . x x . x x x x . x . . x .
exponential search . . x x . . . . . . x . . x x . . . . . . . x . . . x . . x x . . . . . . . .
fibonacci search . . x x . . . . . . . . . . x . . . . . . . . . . . x . . . . . . . x . . . .
fuzzy search . . . . . . . . . . . . . . x . . . . . . . x . . . . . . . . . . . . . . . .
interpolation search . . x x . . . . . . x . . x x . . . . . . . x . . . x . . . . . . . . . . . .
jump search . . x x . . . . . . x . . x x . . . . . . . x . . . x . . . x . . . x . . . .
linear search . . x x x . . . . . x . x x x . . x . x x . x . . . x . x x x x . . x . . x .
ternary search . . x x . . . . . . x . . x x . . x . . . . x . . . x . . . x . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 7 7 1 0 1 0 0 0 6 0 2 6 8 0 0 3 0 1 1 0 7 0 0 0 7 1 1 3 5 2 1 0 4 0 0 2 0
SELECTION ALGORITHMS Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
median of medians . . x . . . . . . . . . x . . . . . . . . . . . . . x . . . . . . . . . . . .
src . . . x . . . . . . x . . x . . . x . . . . . . . . x . . . . . . . x . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 1 1 0 0 0 0 0 0 1 0 1 1 0 0 0 1 0 0 0 0 0 0 0 0 2 0 0 0 0 0 0 0 1 0 0 0 0
SORTING Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
bead sort . . x x . . . . . . . . . x x . . . . . . x x . . . x . . . . . . . x . . x .
bogo sort . . x x . . . . . . x . . x x . . . . . . x . x . . x . . x . . . . x . . . x
bubble sort . . x x . . x x . x x . x x x x . x . . . x x . . . x . . x x . x x x x . x .
bucket sort . . x x . . . . . . x . x x x . . . . . . x x . . . x . . x . . . . x . . x .
circle sort . . x x . . . . . . . . . x x . . . . . . x . . . . x . . . . . . . x . . x .
comb sort . . x x . . . . . . x . . x x . . . . . . x . . . . x . . . . . . . x . . . .
counting sort . . x x . . . . . . x . . x x . . . . . . x . . . . x . . . . . . . x . . x .
cycle sort . . x x . . . . . . x . . x x . . . . . . x . . . . x . . . . . . . x . . x .
flash sort . . x . . . . . . . . . . . x . . . . . . x . . . . . . . . . . . . x . . . .
gnome sort . . x x . . . . . . x . . x x . . . . . . x . . . . x . . . . . . . x . . . .
heap sort . . x x . . . . . . x . . x x . . . . . . x . . . . x . . x x x . . x . . x .
insertion sort . . x x . . . . . . x . x x x . . x . x . x x . . . x . x x x . x . x . . x .
intro sort . . . x . . . . . . . . . . . . . . . . . x . . . . . . . . . . . . x . . . .
median sort . . . x . . . . . . . . . . . . . . . . . x . . . . x . . . . . . . x . . x .
merge sort . . x x . . . . . . x . x x x . . x . . . x x x . . x . . x x x x . x x . x x
pigeonhole sort . . x x . . . . . . x . . x x . . . . . . x x . . . x . . . . x . . x . . x .
quick sort . . x x . . . x . . x . x x x . . . x x . x . . . . x . . x x x x . x x . x .
radix sort . . x x . . . . . . x . x x x . . . . . . . . . . . x . . . x . . . . . . . .
selection sort . . x x . . . . . . x . x x x . . x . . . x x . . . x . . x x . x . x . x x .
shaker sort . . x x . . . . . . x . . x x . . . . . . x x . . . x . . . x . . . x . . x .
shell sort . . x x . . . . . . x . . x x . . x . . . x . . . . x . . . . . . . x . . . .
sleep sort . . x x . . . . . . x . . x x x . . . . . x x . . . x . . x . x x . x . . x .
stooge sort . . x . . . . . . . x . . x x . . . . . . . . . . . x . . . . . . . . . . . .
topological sort . . x x . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
tree sort . . x x . . . . . . x . . x x . . . . . . . . . . . x . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 23 23 0 0 1 2 0 1 19 0 7 22 22 2 0 5 1 2 0 21 9 2 0 0 23 0 1 9 8 5 6 1 21 3 1 15 2
SQUARE ROOT DECOMPOSITION Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
mos algorithm . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 0 1 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
STRING ALGORITHMS Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
aho corasick algorithm . . . x . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
anagram search . . x x . . . . . . x . . x x . . . . . . . . . . . x . . x . x . . x . . x .
arithmetic on large numbers . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
boyer moore algorithm . . x x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
c . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
finite automata . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . x . . . . . . . .
kasai algorithm . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
kmp algorithm . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
levenshtein distance . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
lipogram checker . . . x . . . . . . . . . . x . . . . . . . . . . . x . . . . . . . . . . x .
longest palindromic substring . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
manachar algorithm . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
morse code . . . x . . . . . . x . . . x . . . . . . . x . . . x . . . . . . . . . . . .
naive pattern search . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
palindrome checker . . x x x x x . x . x . x x x . . x x . . . x . . x x . . x x . x . x . . x .
palindrome substring . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
pangram checker . . x x . . . . . . x . . x x . . . . . . x x . . . x . . x . . . . x . . . .
password strength checker . . . x . . . . . . . . . x x . . . . . . . . . . . x . . . . . . . . . . x .
rabin karp algorithm . . x . . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
remove dups . . x x . . . . . . . . . . x . . . . . . . . . . . x . . . x . . . . . . . .
suffix array . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
trie pattern search . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
z algorithm . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 8 15 1 1 1 0 1 0 4 0 1 9 7 0 0 1 1 0 0 1 3 0 0 1 13 0 0 3 3 1 1 0 3 0 0 4 0
THEORY OF COMPUTATION Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
deterministic finite automaton . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
nondeterministic finite atomaton . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 2 0 0 0 0 0 0 0 0 0 0 0 0
UNCLASSIFIED Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
average . . x x . . x . x . x . . x x . . . . . . . x . . . x . . x x x x . x . . x .
biggest of n numbers . . x x . . . . . . . . . x x . . . . . . . . . . . x . . . . . . . . . . x .
biggest suffix . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
fifteen puzzle . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
jaccard similarity . . x . . . . . . . . . . x . . . . . . . . . . . . x . . . . . . . . . . . .
josephus problem . . x x . . . . . . x . . . . . . . . . . . . . . . x . . . . . . . . . . . .
lapindrom checker . . . x . . . . . . . . . . . . . . . . . . . . . . x . . . . . . . . . . . .
leap year . . x x . . . . . . x . . x . . . . . . x . . . . . x . . . x . . . . . . x .
magic square . . x . . . . . . . . . . . . . . . . . . . x . . . x . . . . . . . x . . . .
majority element . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
minimum subarray size with degree . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
no operator addition . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
optimized fibonacci . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
paint fill . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
smallest number to the left . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
spiral print . . x x . . . . . . x . . . . . . . . . . . . . . . x . . . . . . . . . . . .
split list . . . . . . . . . . . . . . x . . . . . . . . . . . x . . . . . . . . . . . .
tokenizer . . . x . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
unique number . . . . . . . . . . . . . x . . . . . . . . . . . . . . . . . . . . . . . . .
utilities . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . x . . . . . .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 10 12 0 0 1 0 1 0 4 0 0 5 3 0 0 0 0 0 1 0 2 0 0 0 9 0 0 1 2 1 2 0 2 0 0 3 0
UTILITY Ada BrainFuck C C++ Clojure Crisp Elixir Elm Erlang Fortran Golang HTML Haskell Java JavaScript Julia Jupyter Notebook Kotlin Lua ML Nim Objective-C PHP Perl Processing Development Environment PureScript Python Racket Reason Ruby Rust Scala Shell Script Standard ML Swift TypeScript Visual Basic Visual C# Visual F#
----------------------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
palindrome check . . x x . . . . . . . . . x x . . . . . x . . . . . x . . x . . . . . . . x .
----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- ----------------------------------- -----------------------------------
0 0 1 1 0 0 0 0 0 0 0 0 0 1 1 0 0 0 0 0 1 0 0 0 0 0 1 0 0 1 0 0 0 0 0 0 0 1 0
=============================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== =================================== ===================================
TOTALS: 1 1 244 376 4 1 13 2 6 1 109 2 37 242 121 4 6 16 3 4 3 23 48 4 1 1 308 1 2 59 50 41 16 2 61 5 1 60 3
|
scripts/build_cpp.sh | cwd=$(pwd)
cosmos_root_path="$(cd "$(dirname "${BASH_SOURCE[0]}")" ; pwd -P )/.."
cd "$cosmos_root_path"
echo ""
echo "###############################"
echo "# generating dependencies ... #"
echo "###############################"
make -f generate_dependencies.make || exit 1
echo ""
echo "#########################"
echo "# compiling sources ... #"
echo "#########################"
make -f testing.make || exit 1
cd "$cosmos_root_path/scripts"
echo ""
echo "#############################"
echo "# checking coding style ... #"
echo "#############################"
./cpp_coding_style_checker.sh || exit 1
cd $cwd
|
scripts/cpp_coding_style_checker.sh | CWD="$(pwd)"
COSMOS_ROOT="$( cd "$(dirname "${BASH_SOURCE[0]}")" ; pwd -P )/.."
COSMOS_CODE_ROOT="$COSMOS_ROOT/code"
UNCRUSTIFY_ROOT="$COSMOS_ROOT/third_party/uncrustify"
UNCRUSTIFY="$UNCRUSTIFY_ROOT/build/uncrustify"
UNCRUSTIFY_CONFIG="$UNCRUSTIFY_ROOT/../uncrustify.cfg"
total=0
error_times=0
assert()
{
error=$?
if [[ $# -eq 1 ]]; then
if [ $error -ne $1 ]; then
echo "exit ($error)"
error_code=1
exit 1
fi
else
if [ $error -ne 0 ]; then
echo "exit ($error)"
error_code=1
exit 1
fi
fi
}
echo """
###########################
# Building uncrustify ... #
###########################
"""
cd "$UNCRUSTIFY_ROOT"
tmp=`mktemp tmp.XXXXXXXXXX`
rm -rf build
mkdir build
cd build
cmake .. > $tmp
assert
cmake --build . > $tmp
assert
rm -f $tmp
assert
echo """
########################
# Formatting files ... #
########################
"""
cd "$COSMOS_CODE_ROOT"
for cpp_file in `find -name '*.cpp'`
do
# remove the output file if existed to prevent `uncrustify` is not override it
rm -f "$cpp_file.uncrustify"
if [ $? != 0 ]; then
echo "# Failed to remove file: \`$COSMOS_CODE_ROOT/$cpp_file\`."
fi;
"$UNCRUSTIFY" -q -c "$UNCRUSTIFY_CONFIG" "$COSMOS_CODE_ROOT/$cpp_file"
if [ $? != 0 ]; then
echo "# Failed to create uncrustify file: \`$COSMOS_CODE_ROOT/$cpp_file\`."
fi;
total=$(($total+1))
done
echo """
#######################
# Comparing files ... #
#######################
"""
cd "$COSMOS_CODE_ROOT"
for cpp_file in `find -name '*.cpp'`
do
d=$(diff "$cpp_file" "$cpp_file.uncrustify")
if [ $? != 0 ]; then
echo "# Failed: \`$COSMOS_CODE_ROOT/$cpp_file\`"
error_times=$(($error_times+1))
fi;
done
cd "$CWD"
echo
if [ $error_times != 0 ]; then
echo "Failed. $error_times/$total error(s) generated."
exit 1
else
echo "Passed. \`checking coding style in c++ script\` exited with 0."
fi;
|
scripts/filename_formatter.sh | # What is this?
# auto convert files' name
#
# e.g.,
# 1)
# dir="aaa/bbb/ccc/ddd.cpp"
# convert:
# ddd.cpp => DDD.cpp
# ddd.cpp => Ddd.cpp
#
# 2)
# dir="multi/path/sort/bubble_sort/BuBble-_-sort.cpp"
# convert:
# BuBble-_-sort.cpp => bubble_sort.cpp (using directory name)
# BuBble-_-sort.cpp => Bubble_Sort.cpp
# BuBble-_-sort.cpp => BubbleSort.cpp
# BuBble-_-sort.cpp => Bubble-Sort.cpp
# ,etc.
#
# How to use?
# 1. copy this script to where your directory contains files
# 2. exec
YELLOW='\033[1;33m'
NC='\033[0m'
echo "\n${YELLOW}- Warnning: if target-file-name is exist, will be override !!! ${NC}-\n"
# define
use_directory_name=0
use_type_name=1
use_default_case=0
use_lower_case=1
use_upper_case=2
use_ignore_case=3
use_default_line=0
use_under_line=1
use_dash_line=2
use_ignore_line=3
re='^[0-1]+$'
echo "Please type your choice to convert files' name ( without '.' and 'extension' )"
echo "0) Use directory name"
echo "1) Use type name"
while [ 1 ]
do
read choice
if [[ $choice =~ $re ]]; then
break
fi
echo "wrong input"
done
if [ $choice -eq $use_type_name ]; then
read name
else
name="${PWD##*/}"
fi
re='^[0-3]+$'
echo "Please type your choice to convert (alpha)"
echo "0) Use default"
echo "1) Convert upper case to lower case (default)"
echo "2) Convert lower case to upper case"
echo "3) Not convert"
while [ 1 ]
do
read alpha_choice
if [[ $alpha_choice =~ $re ]]; then
break
fi
echo "Wrong input"
done
if [ $alpha_choice -eq $use_default_case ]|| [ $alpha_choice -eq $use_lower_case ]; then
name=`echo $name | tr [:upper:] [:lower:]`
elif [ $alpha_choice -eq $use_upper_case ]; then
name=`echo $name | tr [:lower:] [:upper:]`
fi
re='^[0-3]+$'
echo "Please type your choice to convert ('-' and '_')"
echo "0) Use default"
echo "1) Convert '-' and '_' to '_' (default)"
echo "2) Convert '-' and '_' to '-'"
echo "3) Not convert"
while [ 1 ]
do
read line_choice
if [[ $line_choice =~ $re ]]; then
break
fi
echo "Wrong input"
done
if [ $line_choice -eq $use_default_line ]||[ $line_choice -eq $use_under_line ]; then
name=`echo $name | tr - _`
elif [ $line_choice -eq $use_dash_line ]; then
name=`echo $name | tr _ -`
fi
# backup
date="backup_`date`"
`mkdir "$date"`
`cp *.* "$date"`
# match
for full_file_name in *.c *.cs *.cpp *.elm *.exs *.f *.go *.hs *.java *.jl *.js *.kt *.m *.php *.py *.rb *.rs *.sh *.swift *.ts
do
temp=(${full_file_name//\./ })
# check 1: escape file if has multi-layer extension
# check 2: escape if directory not has current extension file
if [ ${#temp[@]} -ge 3 ]||[[ ${temp[0]} == '*' ]]; then
continue
fi
file_name=${temp[0]}
file_ext=${temp[1]}
# convert file name with pattern
converted_file_name=$file_name
if [ $alpha_choice -eq $use_default_case ]||[ $alpha_choice -eq $use_lower_case ]; then
converted_file_name=`echo $converted_file_name | tr [:upper:] [:lower:]`
elif [ $alpha_choice -eq $use_upper_case ]; then
converted_file_name=`echo $converted_file_name | tr [:lower:] [:upper:]`
fi
if [ $line_choice -eq $use_default_line ]||[ $line_choice -eq $use_under_line ]; then
converted_file_name=`echo $converted_file_name | tr - _`
elif [ $line_choice -eq $use_dash_line ]; then
converted_file_name=`echo $converted_file_name | tr _ -`
fi
# convert file
converted_file_name="$converted_file_name.$file_ext"
converted_target_file_name="$name.$file_ext"
# ignore line
converted_file_name=${converted_file_name//-}
converted_file_name=${converted_file_name//_}
converted_target_file_name=${converted_target_file_name//-}
converted_target_file_name=${converted_target_file_name//_}
if [[ ^$converted_file_name$ == ^$converted_target_file_name$ ]]; then
`git mv --force $full_file_name $name.$file_ext`
fi
done
|
scripts/global-metadata.py | import json
import pathlib
import collections
avoid_extensions = [
"",
# ".md",
# ".png",
# ".csv",
# ".class",
# ".data",
# ".in",
# ".jpeg",
# ".jpg",
# ".out",
# ".textclipping",
# ".properties",
# ".txt",
# ".sbt",
]
avoid_dirs = ["project", "test", "img", "image", "images"]
global_metadata = collections.defaultdict(dict)
original_paths = collections.defaultdict(str)
for path in pathlib.Path(__file__).parents[1].glob(
"scripts/metadata/code/**/**/*"):
if (path.suffix
and not any(elem in list(path.parts) for elem in avoid_dirs)
and path.suffix.lower() not in avoid_extensions):
original_paths[path.parts[-2]] = "/".join(path.parts[:-2])
for algo in original_paths:
filename = pathlib.Path("{}/{}/data.json".format(original_paths[algo],
algo))
with open(filename) as fh:
existing_data = json.load(fh)
global_metadata[original_paths[algo].split('/')[-2]][algo] = existing_data
filename = pathlib.Path("scripts/global_metadata.json")
json_dump = json.dumps(global_metadata, indent=2)
filename.write_text(json_dump)
|
scripts/global_metadata.json | {
"explainBlockchain": {
"location": "code/blockchain/explainBlockchain.txt",
"opengenus_iq:": "https://iq.opengenus.org/p/f3110420-24e9-40f8-9ee5-79b5df2f88ff/"
},
"undo in git" : {
"location" : "code/git/undochanges",
"opengenus_iq": "https://iq.opengenus.org/p/5a525c5f-e1ac-418c-abac-ff118587dd83/"
},
"view hist" : {
"location" : "code/git/viewhist",
"opengenus_iq" : "https://iq.opengenus.org/p/911697a3-030c-481a-9793-39138000febd/"
},
"bernoulli_naive_bayes": {
"location": "code/artificial_intelligence/src/Bernoulli Naive Bayes",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/bernoulli-naive-bayes/",
"files": [
"README.md",
"bernoulli.py"
],
"updated": "31-05-2020 14:30:00"
},
"largest-element-in-an-array": {
"location": "code/languages/cpp/largest-element-in-an-array",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/largest-element-in-an-array/",
"files": [
"Largest_element.cpp"
"README.md"
],
"updated": "31-05-2020 17:41:00"
}
},
"Kadane_algo": {
"location": "code/languages/Java/Kadane_algo",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/kadane-algorithm/",
"files": [
"Kadane_algo.java"
"README.md"
],
"updated": "31-05-2020 17:41:00"
}
},
"square_root_decomposition": {
"mos_algorithm": {
"location": "code/square_root_decomposition/src/mos_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"mos_algorithm.cpp"
],
"updated": "18-05-2019 08:11:05"
}
},
"filters": {
"median_filter": {
"location": "code/filters/src/median_filter",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"median_filter.py"
],
"updated": "18-05-2019 08:11:05"
},
"gaussian_filter": {
"location": "code/filters/src/gaussian_filter",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"gaussian_filter.py"
],
"updated": "18-05-2019 08:11:05"
}
},
"algorithm_applications":{
"binary_search":{
"distributing_candies":{
"location": "code/algorithm_applications/src/binary_search/distributing_candies/",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/distribute-candies/",
"files": [
"CandyDistribution.java"
],
"updated": "02-05-2020 19:40:00"
}
}
},
"dynamic_programming": {
"edit_distance": {
"location": "code/dynamic_programming/src/edit_distance",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"edit_distance.php",
"edit_distance.rs",
"edit_distance_hirschberg.cpp",
"edit_distance_backtracking.cpp",
"edit_distance.go",
"edit_distance.c",
"edit_distance.py",
"edit_distance.cpp",
"edit_distance.hs",
"edit_distance.java",
"README.md"
],
"updated": "18-05-2019 08:11:05"
},
"postmans_sort": {
"location": "code/sorting/src/postmans_sort",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/postman-sort/",
"files": [
"postmans_sort.cpp",
"readme.md"
],
"updated": "29-05-2019 20:50:07"
},
"rod_cutting": {
"location": "code/dynamic_programming/src/rod_cutting",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"rod_cutting.hs",
"rod_cutting.cpp",
"rod_cutting.py",
"README.md",
"rod_cutting.go",
"rod_cutting.c"
],
"updated": "18-05-2019 08:11:05"
},
"number_of_substring_divisible_by_8_but_not_3": {
"location": "code/dynamic_programming/src/number_of_substring_divisible_by_8_but_not_3",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/number-of-substrings-divisible-by-8-but-not-3/",
"files": [
"number_of_substrings.cpp",
"README.md"
],
"updated": "24-05-2020 08:11:05"
},
"minimum_insertion_palindrome": {
"location": "code/dynamic_programming/src/minimum_insertion_palindrome",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"minimum_insertions_palindrome_using_lcs.cpp"
],
"updated": "18-05-2019 08:11:05"
},
"weighted_job_scheduling": {
"location": "code/dynamic_programming/src/weighted_job_scheduling",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"weighted_job_scheduling.cpp"
],
"updated": "18-05-2019 08:11:05"
},
"boolean_parenthesization": {
"location": "code/dynamic_programming/src/boolean_parenthesization",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"boolean_parenthesization.py",
"boolean_parenthesization.c",
"boolean_parenthesization.swift",
"boolean_parenthesization.cpp",
"README.md",
"boolean_parenthesization.java"
],
"updated": "18-05-2019 08:11:05"
},
"shortest_common_supersequence": {
"location": "code/dynamic_programming/src/shortest_common_supersequence",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"scs.java",
"README.md",
"shortest_common_supersequence.cpp",
"shortest_common_supersequence.py"
],
"updated": "18-05-2019 08:11:05"
},
"longest_common_subsequence": {
"location": "code/dynamic_programming/src/longest_common_subsequence",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"longest_common_subsequence.py",
"longestcommonsubsequence.go",
"longestcommonsubsequencerec.java",
"README.md",
"longest_common_subsequence.cpp",
"longestcommonsubsequence.java"
],
"updated": "18-05-2019 08:11:05"
},
"fibonacci": {
"location": "code/dynamic_programming/src/fibonacci",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"fibonacci.py"
],
"updated": "30-05-2020 15:09:06"
},
"house_robber":{
"location": "code/dynamic_programming/src/house_robber",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"HouseRobber.java"
"README.md"
],
"updated": "18-05-2019 08:11:05"
},
"box_stacking": {
"location": "code/dynamic_programming/src/box_stacking",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"box_stacking.py",
"box_stacking.java",
"README.md",
"box_stacking.cpp"
],
"updated": "18-05-2019 08:11:05"
},
"coin_change": {
"location": "code/dynamic_programming/src/coin_change",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"coinchange.c",
"coin_change.py",
"mincoinchange.cpp",
"coin_change.java",
"README.md",
"coinchange.go",
"coinchange.cpp"
],
"updated": "18-05-2019 08:11:05"
},
"largest_sum_contiguous_subarray": {
"location": "code/dynamic_programming/src/largest_sum_contiguous_subarray",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"largest_sum_contiguous_subarray.go",
"largest_sum_contiguous_subarray.py",
"largest_sum_contiguous_subarray.java",
"largest_sum_contiguous_subarray.hs",
"README.md",
"largest_sum_contiguous_subarray.cpp",
"largest_sum_contiguous_subarray.c"
],
"updated": "18-05-2019 08:11:05"
},
"longest_bitonic_sequence": {
"location": "code/dynamic_programming/src/longest_bitonic_sequence",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"longest_bitonic_sequence.py",
"longest_bitonic_sequence.c",
"longest_bitonic_sequence.js",
"longestbitonicseq.cpp",
"README.md",
"longestbitonicsequence.java"
],
"updated": "18-05-2019 08:11:05"
},
"longest_common_substring": {
"location": "code/dynamic_programming/src/longest_common_substring",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"longest_common_substring.cpp"
],
"updated": "18-05-2019 08:11:05"
},
"min_cost_path": {
"location": "code/dynamic_programming/src/min_cost_path",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"min_cost_path.c",
"min_cost_path.java",
"min_cost_path.cpp",
"min_cost_path.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"knapsack": {
"location": "code/dynamic_programming/src/knapsack",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"knapsack.go",
"knapsack.js",
"knapsack.c",
"knapsack.java",
"knapsack.cpp",
"README.md",
"knapsack.py"
],
"updated": "18-05-2019 08:11:06"
},
"array_median": {
"location": "code/dynamic_programming/src/array_median",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"median.rb",
"median.cpp",
"median.java",
"median.c",
"median.php",
"median.rs",
"median.py"
],
"updated": "18-05-2019 08:11:06"
},
"minimum_cost_polygon_triangulation": {
"location": "code/dynamic_programming/src/minimum_cost_polygon_triangulation",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"optimal_binary_search_tree": {
"location": "code/dynamic_programming/src/optimal_binary_search_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"optimal_bst.py"
],
"updated": "18-05-2019 08:11:06"
},
"tiling_problem": {
"location": "code/dynamic_programming/src/tiling_problem",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"tiling.py",
"tiling.c",
"tiling.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"longest_common_increasing_subsequence": {
"location": "code/dynamic_programming/src/longest_common_increasing_subsequence",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"longest_common_increasing_subsequence.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"longest_repeating_subsequence": {
"location": "code/dynamic_programming/src/longest_repeating_subsequence",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"longest_repeating_subsequence.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"longest_independent_set": {
"location": "code/dynamic_programming/src/longest_independent_set",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"no_consec_ones": {
"location": "code/dynamic_programming/src/no_consec_ones",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"no_consec_ones.py",
"no_consec_1.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"numeric_keypad_problem": {
"location": "code/dynamic_programming/src/numeric_keypad_problem",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"numeric_keypad_problem.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"longest_palindromic_sequence": {
"location": "code/dynamic_programming/src/longest_palindromic_sequence",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"longest_palindromic_sequence.js",
"longest_palindromic_sequence.c",
"longest_palindromic_sequence.cpp",
"README.md",
"longest_palindromic_sequence.py"
],
"updated": "18-05-2019 08:11:06"
},
"maximum_weight_independent_set_of_path_graph": {
"location": "code/dynamic_programming/src/maximum_weight_independent_set_of_path_graph",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"maximum_weight_independent_set_of_path_graph.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"palindrome_partition": {
"location": "code/dynamic_programming/src/palindrome_partition",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"palindrome_partition.js",
"README.md",
"palindrome_partition.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"digit_dp": {
"location": "code/dynamic_programming/src/digit_dp",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"digit_dp.cpp",
"DigitDP.java"
],
"updated": "18-05-2019 08:11:06"
},
"maximum_sum_sub_matrix": {
"location": "code/dynamic_programming/src/maximum_sum_sub_matrix",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"maximum_sum_sub_matrix.java",
"maximum_sum_sub_matrix.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"longest_increasing_subsequence": {
"location": "code/dynamic_programming/src/longest_increasing_subsequence",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"longest_increasing_subsequence.js",
"longest_increasing_subsequence.py",
"longest_increasing_subsequence.go",
"longest_increasing_subsequence.cpp",
"longest_increasing_subsequence_using_segment_tree.cpp",
"README.md",
"longest_increasing_subsequence.java",
"longest_increasing_subsequence.c"
],
"updated": "18-05-2019 08:11:06"
},
"matrix_chain_multiplication": {
"location": "code/dynamic_programming/src/matrix_chain_multiplication",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"matrix_chain_multiplication.c",
"matrix_chain_multiplication.cpp",
"matrixchainmultiplication.java",
"README.md",
"matrix_chain_multiplication.py"
],
"updated": "18-05-2019 08:11:06"
},
"maximum_sum_increasing_subsequence": {
"location": "code/dynamic_programming/src/maximum_sum_increasing_subsequence",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"maximum_sum_increasing_subsequence.c",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"egg_dropping_puzzle": {
"location": "code/dynamic_programming/src/egg_dropping_puzzle",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"egg_dropping_puzzle.cpp",
"eggdroppingpuzzle.java",
"egg_dropping_puzzle.py",
"egg_dropping_puzzle.hs",
"README.md",
"egg_dropping_puzzle.c"
],
"updated": "18-05-2019 08:11:06"
}
},
"game_theory": {
"game_of_nim_next_best_move": {
"location": "code/game_theory/src/game_of_nim_next_best_move",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"next_best_move.py"
],
"updated": "18-05-2019 08:11:06"
},
"grundy_numbers_kayle": {
"location": "code/game_theory/src/grundy_numbers_kayle",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"grundy_numbers_kayle.py"
],
"updated": "18-05-2019 08:11:06"
},
"game_of_nim_win_loss_prediction": {
"location": "code/game_theory/src/game_of_nim_win_loss_prediction",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"win_loss_prediction.py"
],
"updated": "18-05-2019 08:11:06"
}
},
"artificial_intelligence": {
"restricted_boltzmann_machine": {
"location": "code/artificial_intelligence/src/restricted_boltzmann_machine",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"train.csv",
"test.csv",
"README.md",
"rbm.ipynb"
],
"updated": "18-05-2019 08:11:06"
},
"random_forests": {
"location": "code/artificial_intelligence/src/random_forests",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"isodata_clustering": {
"location": "code/artificial_intelligence/src/isodata_clustering",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"readme.md",
"isodata.py"
],
"updated": "18-05-2019 08:11:06"
},
"gaussian_naive_bayes": {
"location": "code/artificial_intelligence/src/gaussian_naive_bayes",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"gaussiannaivebayesmoons.py",
"graph.png"
],
"updated": "18-05-2019 08:11:06"
},
"principal_component_analysis": {
"location": "code/artificial_intelligence/src/principal_component_analysis",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"pca.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"q_learning": {
"location": "code/artificial_intelligence/src/q_learning",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"qlearning.js",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"image_processing": {
"location": "code/artificial_intelligence/src/image_processing",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"install_opencv.sh",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"gaussian_mixture_model": {
"location": "code/artificial_intelligence/src/gaussian_mixture_model",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"artificial_neural_network": {
"location": "code/artificial_intelligence/src/artificial_neural_network",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"dataset.csv",
"ann.py"
],
"updated": "18-05-2019 08:11:06"
},
"factorization_machines": {
"location": "code/artificial_intelligence/src/factorization_machines",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"matrix_factorization.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"decision_tree": {
"location": "code/artificial_intelligence/src/decision_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"decision_trees_information_gain.py",
"decision_tree.py",
"data_banknote_authentication.csv"
],
"updated": "18-05-2019 08:11:06"
},
"sat": {
"location": "code/artificial_intelligence/src/sat",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"togasat.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"a_star": {
"location": "code/artificial_intelligence/src/a_star",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"astar.py"
],
"updated": "18-05-2019 08:11:06"
},
"perceptron": {
"location": "code/artificial_intelligence/src/perceptron",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"perceptron.py"
],
"updated": "18-05-2019 08:11:06"
},
"convolutional_neural_network": {
"location": "code/artificial_intelligence/src/convolutional_neural_network",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"cnn_adversarial_examples.ipynb",
"cnn.ipynb",
"train.csv",
"test.csv"
],
"updated": "18-05-2019 08:11:06"
},
"k_means": {
"location": "code/artificial_intelligence/src/k_means",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"k_means.swift",
"k_means.cpp",
"k_means.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"neural_style_transfer": {
"location": "code/artificial_intelligence/src/neural_style_transfer",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"neural_style_transfer.ipynb"
],
"updated": "18-05-2019 08:11:06"
},
"getting_started_with_ml": {
"location": "code/artificial_intelligence/src/getting_started_with_ml",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/how-i-mastered-ml-as-fresher/",
"files": [
"README.md"
],
"updated": "29-05-2020 04:53:00"
},
"gradient_boosting_trees": {
"location": "code/artificial_intelligence/src/gradient_boosting_trees",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"k_nearest_neighbours": {
"location": "code/artificial_intelligence/src/k_nearest_neighbours",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"k_nearest_neighbours.py",
"iris.data"
],
"updated": "18-05-2019 08:11:06"
},
"autoenncoder": {
"location": "code/artificial_intelligence/src/autoenncoder",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"autoencoder.ipynb"
],
"updated": "18-05-2019 08:11:06"
},
"neural_network": {
"location": "code/artificial_intelligence/src/neural_network",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"neuralnetwork.py",
"keras_nn.py"
],
"updated": "18-05-2019 08:11:06"
},
"dbscan_clustering": {
"location": "code/artificial_intelligence/src/dbscan_clustering",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"readme.md",
"dbscan.py"
],
"updated": "18-05-2019 08:11:06"
},
"logistic_regression": {
"location": "code/artificial_intelligence/src/logistic_regression",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"logistic_regression.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"tsp": {
"location": "code/artificial_intelligence/src/tsp",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"euc_500",
"makefile",
"algo.md",
"noneuc_500",
"noneuc_100",
"salesman.cpp",
"euc_250",
"noneuc_250",
"euc_100"
],
"updated": "18-05-2019 08:11:06"
},
"naive_bayes": {
"location": "code/artificial_intelligence/src/naive_bayes",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"naive_bayes.swift",
"iris1.csv",
"gaussian_naive_bayes.py",
"README.md",
"naive_bayes.py"
],
"updated": "18-05-2019 08:11:06"
},
"support_vector_machine": {
"location": "code/artificial_intelligence/src/support_vector_machine",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"svm.py",
"svm.c",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"t_distributed_stochastic_neighbor_embedding": {
"location": "code/artificial_intelligence/src/t_distributed_stochastic_neighbor_embedding",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"minimax": {
"location": "code/artificial_intelligence/src/minimax",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"minimax.py"
],
"updated": "18-05-2019 08:11:06"
}
},
"src": {
"linear_regression": {
"location": "code/artificial_intelligence/src/linear_regression/linear_regression",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"linear_regression.js"
],
"updated": "18-05-2019 08:11:06"
},
"sobelfilter": {
"location": "code/artificial_intelligence/src/image_processing/sobelfilter",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"sobel.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"image_stitching": {
"location": "code/artificial_intelligence/src/image_processing/image_stitching",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"a.out",
"imagestitching.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"prewittfilter": {
"location": "code/artificial_intelligence/src/image_processing/prewittfilter",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"prewitt.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"canny": {
"location": "code/artificial_intelligence/src/image_processing/canny",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"canny.h",
"main.cpp",
"canny.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"erode_dilate": {
"location": "code/artificial_intelligence/src/image_processing/erode_dilate",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"main.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"houghtransform": {
"location": "code/artificial_intelligence/src/image_processing/houghtransform",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"main.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"nsm_matlab": {
"location": "code/artificial_intelligence/src/nearest_sequence_memory/nsm_matlab",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"main.m",
"simulator.m",
"nsm_agent.m"
],
"updated": "18-05-2019 08:11:06"
},
"lbestPSO": {
"location": "code/artificial_intelligence/src/particle_swarm_optimization/lbestPSO",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"Lbest2D.py",
"LBest3D.py"
],
"updated": "18-05-2019 08:11:06"
},
"gbestPSO": {
"location": "code/artificial_intelligence/src/particle_swarm_optimization/gbestPSO",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"Gbest3D.py",
"Gbest2D.py"
],
"updated": "18-05-2019 08:11:06"
},
"hierachical_clustering": {
"location": "code/artificial_intelligence/src/hierachical_clustering/hierachical_clustering",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"hierarchical_clustering.ipynb"
],
"updated": "18-05-2019 08:11:06"
},
"adjacency_matrix_c": {
"location": "code/graph_algorithms/src/data_structures/adjacency_matrix_c",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"mgraph_struct.c",
"main.c",
"mgraph_struct.h"
],
"updated": "18-05-2019 08:11:06"
},
"adjacency_lists_in_c": {
"location": "code/graph_algorithms/src/adjacency_lists_graph_representation/adjacency_lists_in_c",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"lgraph_stack.h",
"lgraph_struct.c",
"lgraph_struct.h",
"main.c",
"lgraph_stack.c",
"README.MD"
],
"updated": "18-05-2019 08:11:06"
},
"palindrome_check": {
"location": "code/utility/src/palindrome/palindrome_check",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"palindrome_check.java",
"palindrome_check.js",
"palindrome.py",
"palindrome_check.cpp",
"palindrome.nim",
"palindrome_check.c",
"palindrome_check.cs",
"palindrome_check.rb"
],
"updated": "18-05-2019 08:11:06"
},
"facade": {
"location": "code/design_pattern/src/OOP_patterns/facade",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"facade",
"main.java"
],
"updated": "18-05-2019 08:11:06"
},
"factory": {
"location": "code/design_pattern/src/OOP_patterns/factory",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"gifttype.java",
"roulette.java"
],
"updated": "18-05-2019 08:11:06"
},
"observer_java": {
"location": "code/design_pattern/src/OOP_patterns/observer_java",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"demo.java",
"main.java"
],
"updated": "18-05-2019 08:11:06"
},
"singleton_pattern": {
"location": "code/design_pattern/src/OOP_patterns/singleton_pattern",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"singleton_pattern.java",
"singleton_pattern.php",
"singleton_pattern.cpp",
"singleton_pattern.py"
],
"updated": "18-05-2019 08:11:06"
},
"observer_pattern": {
"location": "code/design_pattern/src/OOP_patterns/observer_pattern",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"observer_pattern.rs",
"observer_pattern.py",
"observer_pattern.cpp",
"__init__.py"
],
"updated": "18-05-2019 08:11:06"
},
"adapter": {
"location": "code/design_pattern/src/OOP_patterns/adapter",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"civilian.java",
"adaptor.java",
"movement.java"
],
"updated": "18-05-2019 08:11:06"
},
"complement_dna_strand": {
"location": "code/online_challenges/src/rosalind/complement_dna_strand",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"complement_dna.rs",
"complement_dna_strand.exs"
],
"updated": "18-05-2019 08:11:06"
},
"encryption": {
"location": "code/online_challenges/src/hackerrank/encryption",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"encryption.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"the_maximum_subarray": {
"location": "code/online_challenges/src/hackerrank/the_maximum_subarray",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"the_maximum_subarray.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"3D_aurface_area": {
"location": "code/online_challenges/src/hackerrank/3D_aurface_area",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"3D_surface_area.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"almost_sorted": {
"location": "code/online_challenges/src/hackerrank/almost_sorted",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"almost_sorted.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"array_manipulation": {
"location": "code/online_challenges/src/hackerrank/array_manipulation",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"array_manipulation.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"bigger_is_greater": {
"location": "code/online_challenges/src/hackerrank/bigger_is_greater",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bigger_is_greater.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_102": {
"location": "code/online_challenges/src/project_euler/problem_102",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"triangles.txt",
"README.md",
"problem_102.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"problem_022": {
"location": "code/online_challenges/src/project_euler/problem_022",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_022.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_019": {
"location": "code/online_challenges/src/project_euler/problem_019",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_019.java"
],
"updated": "18-05-2019 08:11:06"
},
"problem_007": {
"location": "code/online_challenges/src/project_euler/problem_007",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_007.js",
"problem_007.cpp",
"problem_007.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_008": {
"location": "code/online_challenges/src/project_euler/problem_008",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_008.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_037": {
"location": "code/online_challenges/src/project_euler/problem_037",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"problem_037.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"problem_004": {
"location": "code/online_challenges/src/project_euler/problem_004",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_004.cpp",
"problem_004.py",
"README.md",
"problem_004.java"
],
"updated": "18-05-2019 08:11:06"
},
"problem_014": {
"location": "code/online_challenges/src/project_euler/problem_014",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_014.py",
"problem_014.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_036": {
"location": "code/online_challenges/src/project_euler/problem_036",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_036.cpp",
"README.md",
"problem_036.py"
],
"updated": "18-05-2019 08:11:06"
},
"problem_024": {
"location": "code/online_challenges/src/project_euler/problem_024",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_024.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_005": {
"location": "code/online_challenges/src/project_euler/problem_005",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_005.java",
"problem_005.c",
"problem_005.cpp",
"README.md",
"problem_005.py"
],
"updated": "18-05-2019 08:11:06"
},
"problem_020": {
"location": "code/online_challenges/src/project_euler/problem_020",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_020.java",
"problem_020.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_028": {
"location": "code/online_challenges/src/project_euler/problem_028",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_028.cpp",
"README.md",
"problem_028.py"
],
"updated": "18-05-2019 08:11:06"
},
"problem_010": {
"location": "code/online_challenges/src/project_euler/problem_010",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_010.py",
"README.md",
"problem_010.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"problem_016": {
"location": "code/online_challenges/src/project_euler/problem_016",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_016.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_006": {
"location": "code/online_challenges/src/project_euler/problem_006",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_006.cpp",
"problem_006.java",
"README.md",
"problem_006.py"
],
"updated": "18-05-2019 08:11:06"
},
"problem_002": {
"location": "code/online_challenges/src/project_euler/problem_002",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_002.cpp",
"problem_002.c",
"problem_002.java",
"problem_002.py",
"problem_002.js",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_034": {
"location": "code/online_challenges/src/project_euler/problem_034",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_034.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_003": {
"location": "code/online_challenges/src/project_euler/problem_003",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_003.cpp",
"problem_003.py",
"problem_003.js",
"problem_003.java",
"README.md",
"problem_003.c"
],
"updated": "18-05-2019 08:11:06"
},
"problem_013": {
"location": "code/online_challenges/src/project_euler/problem_013",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_013.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_026": {
"location": "code/online_challenges/src/project_euler/problem_026",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_026.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_012": {
"location": "code/online_challenges/src/project_euler/problem_012",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_012.py",
"problem_012.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_040": {
"location": "code/online_challenges/src/project_euler/problem_040",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_040.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_018": {
"location": "code/online_challenges/src/project_euler/problem_018",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_018.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_067": {
"location": "code/online_challenges/src/project_euler/problem_067",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_067.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_021": {
"location": "code/online_challenges/src/project_euler/problem_021",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"problem_021.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"problem_009": {
"location": "code/online_challenges/src/project_euler/problem_009",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_009.py",
"problem_009.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"problem_001": {
"location": "code/online_challenges/src/project_euler/problem_001",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_001.js",
"problem_001.py",
"problem_001.java",
"problem_001.c",
"problem_001.cpp",
"README.md",
"problem_001.rs"
],
"updated": "18-05-2019 08:11:06"
},
"problem_025": {
"location": "code/online_challenges/src/project_euler/problem_025",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"problem_025.cpp",
"README.md",
"problem_025.py"
],
"updated": "18-05-2019 08:11:06"
},
"JAIN": {
"location": "code/online_challenges/src/codechef/JAIN",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"JAIN.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"NBONACCI": {
"location": "code/online_challenges/src/codechef/NBONACCI",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"NBONACCI.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"CHDIGER": {
"location": "code/online_challenges/src/codechef/CHDIGER",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"CHDIGER.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"CHNUM": {
"location": "code/online_challenges/src/codechef/CHNUM",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"CHNUM.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"fermat_primality_test": {
"location": "code/mathematical_algorithms/src/primality_tests/fermat_primality_test",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"fermat_primality_test.py",
"fermat_primality_test.c"
],
"updated": "18-05-2019 08:11:06"
},
"miller_rabin_primality_test": {
"location": "code/mathematical_algorithms/src/primality_tests/miller_rabin_primality_test",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"miller_rabin_primality_test.cpp",
"miller_rabin_primality_test.py",
"miller_rabin_primality_test.java"
],
"updated": "18-05-2019 08:11:06"
},
"solovay_strassen_primality_test": {
"location": "code/mathematical_algorithms/src/primality_tests/solovay_strassen_primality_test",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"solovay_strassen_primality_test.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"exponentiation_by_squaring": {
"location": "code/mathematical_algorithms/src/exponentiation_power/exponentiation_by_squaring",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"exponentiation_by_squaring.py",
"exponentiation_by_squaring.go",
"exponentiation_by_squaring.cpp",
"exponentiation_by_squaring.c"
],
"updated": "18-05-2019 08:11:06"
},
"scala": {
"location": "code/mathematical_algorithms/src/gaussian_elimination/scala/src/main/scala",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"main.scala"
],
"updated": "18-05-2019 08:11:06"
},
"validate_ip": {
"location": "code/networking/src/validate_ip/validate_ip",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"validate_ipv6.c",
"validate_ipv4.c"
],
"updated": "18-05-2019 08:11:06"
},
"huffman": {
"location": "code/compression/src/lossless_compression/huffman",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"huffman.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"lempel_ziv_welch": {
"location": "code/compression/src/lossless_compression/lempel_ziv_welch",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"lzw.cpp",
"README.md",
"lzw.py"
],
"updated": "18-05-2019 08:11:06"
},
"dining_philosophers": {
"location": "code/operating_system/src/concurrency/dining_philosophers",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"dining_philosophers.c",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"producer_consumer": {
"location": "code/operating_system/src/concurrency/producer_consumer",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"producer_consumer.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"readers_writers": {
"location": "code/operating_system/src/concurrency/readers_writers",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"readers_writers.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"bankers_algorithm": {
"location": "code/operating_system/src/deadlocks/bankers_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"banker_safety.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"multi_level_feedback_queue_scheduling": {
"location": "code/operating_system/src/scheduling/multi_level_feedback_queue_scheduling",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"mlfq.ts"
],
"updated": "18-05-2019 08:11:06"
},
"round_robin_scheduling": {
"location": "code/operating_system/src/scheduling/round_robin_scheduling",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"round_robin_scheduling.cpp",
"round_robin_scheduling.java"
],
"updated": "18-05-2019 08:11:06"
},
"smallest_remaining_time_first": {
"location": "code/operating_system/src/scheduling/smallest_remaining_time_first",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"srtf.c"
],
"updated": "18-05-2019 08:11:06"
},
"first_come_first_serve": {
"location": "code/operating_system/src/scheduling/first_come_first_serve",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"fcfs.cs",
"fcfs.cpp",
"fcfs.rs",
"fcfs.py",
"fcfs.java"
],
"updated": "18-05-2019 08:11:06"
},
"shortest_seek_time_first": {
"location": "code/operating_system/src/scheduling/shortest_seek_time_first",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"shortest_seek_time_first.c",
"shortest_seek_time_first.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"memory_mapping": {
"location": "code/operating_system/src/memory_management/memory_mapping",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"mapping.c"
],
"updated": "18-05-2019 08:11:06"
},
"least_recently_used": {
"location": "code/operating_system/src/memory_management/least_recently_used",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"lru.c"
],
"updated": "18-05-2019 08:11:06"
},
"bloom_filter": {
"location": "code/data_structures/src/hashs/bloom_filter",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bloom_filter.scala",
"bloom_filter.js",
"bloom_filter.java",
"bloom_filter.py",
"bloomfilter.go",
"bloom_filter.c",
"README.md",
"bloom_filter.swift",
"bloom_filter.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"hash_table": {
"location": "code/data_structures/src/hashs/hash_table",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"hash_table.rs",
"double_hashing.c",
"hash_table.swift",
"hash_table.c",
"hash_table.js",
"hash_table.py",
"hash_table.go",
"hash_table.cpp",
"README.md",
"hash_table.cs",
"hash_table.java"
],
"updated": "18-05-2019 08:11:06"
},
"infix_to_postfix": {
"location": "code/data_structures/src/stack/infix_to_postfix",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"infix_to_postfix.c",
"infix_to_postfix.java",
"infix_to_postfix.py",
"infix_to_postfix.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"postfix_evaluation": {
"location": "code/data_structures/src/stack/postfix_evaluation",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"postfix_evaluation.sh",
"README.md",
"postfix_evaluation.c",
"postfix_evaluation.py"
],
"updated": "18-05-2019 08:11:06"
},
"balanced_expression": {
"location": "code/data_structures/src/stack/balanced_expression",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"balanced_expression.cpp",
"balanced_expression.java",
"balanced_expression.c"
],
"updated": "18-05-2019 08:11:06"
},
"reverse_stack": {
"location": "code/data_structures/src/stack/reverse_stack",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"reverse_stack.swift",
"reverse_stack.cs",
"reverse_stack.c",
"reverse_stack.go",
"reverse_stack_without_extra_space.cpp",
"reverse_stack.java",
"README.md",
"reverse_stack.py"
],
"updated": "18-05-2019 08:11:06"
},
"stack": {
"location": "code/data_structures/src/stack/stack",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"stack.py",
"stack.php",
"stack.go",
"stack.js",
"stack.java",
"stack.swift",
"stack.c",
"stack.ex",
"stack.erl",
"stack.cpp",
"stack.rs",
"README.md",
"stack.rb",
"stack.cs"
],
"updated": "18-05-2019 08:11:06"
},
"prefix_to_postfix": {
"location": "code/data_structures/src/stack/prefix_to_postfix",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"prefix_to_postfix.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"abstract_stack": {
"location": "code/data_structures/src/stack/abstract_stack",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"istack.h",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"sort_stack": {
"location": "code/data_structures/src/stack/sort_stack",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"sort_stack.c",
"sort_stack.py",
"sort_stack.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"heap": {
"location": "code/data_structures/src/tree/heap",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"skip_list": {
"location": "code/data_structures/src/list/skip_list",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"skip_list.cpp",
"skip_list.swift",
"skip_list.scala",
"README.md",
"skip_list.c",
"skiplist.rb",
"skip_list.java"
],
"updated": "18-05-2019 08:11:06"
},
"doubly_linked_list": {
"location": "code/data_structures/src/list/doubly_linked_list",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"doublylinkedlist.java",
"doubly_linked_list.swift",
"doubly_linked_list.go",
"doubly_linked_list.py",
"doubly_linked_list.cpp",
"doubly_linked_list.js"
],
"updated": "18-05-2019 08:11:06"
},
"circular_linked_list": {
"location": "code/data_structures/src/list/circular_linked_list",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"circularlinkedlist.java",
"circular_linked_list.c",
"circular_linked_list.py",
"circular_linked_list.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"singly_linked_list": {
"location": "code/data_structures/src/list/singly_linked_list",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"singly_linked_list_with_classes.cpp",
"singly_linked_list.rb",
"singly_linked_list.py",
"singly_linked_list.c",
"singly_linked_list.swift",
"singly_linked_list.go",
"singly_linked_list_menu_driven.c",
"singly_linked_list.cpp",
"singly_linked_list.js",
"singly_linked_list_with_3_nodes.java",
"singly_linked_list.cs",
"singly_linked_list.java"
],
"updated": "18-05-2019 08:11:06"
},
"xor_linked_list": {
"location": "code/data_structures/src/list/xor_linked_list",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"xor_linked_list.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"queue_stream": {
"location": "code/data_structures/src/queue/queue_stream",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"queue_stream.cs"
],
"updated": "18-05-2019 08:11:06"
},
"queue_using_linked_list": {
"location": "code/data_structures/src/queue/queue_using_linked_list",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"queue_using_linked_list.rb",
"queue_using_linked_list.c",
"queue_using_linked_list.py",
"queue_using_linked_list.java",
"README.md",
"queue_using_linked_list.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"reverse_queue": {
"location": "code/data_structures/src/queue/reverse_queue",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"reverse_queue.go",
"reverse_queue.swift",
"reverse_queue.java",
"reverse_queue.cpp",
"reverse_queue.py"
],
"updated": "18-05-2019 08:11:06"
},
"circular_buffer": {
"location": "code/data_structures/src/queue/circular_buffer",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"circular_buffer.py",
"circular_buffer.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"queue_using_stack": {
"location": "code/data_structures/src/queue/queue_using_stack",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"queue_using_stack.java",
"queue_using_stack.sh",
"queue_using_stack.py",
"queue_using_stack.cpp",
"queue_using_stack.c"
],
"updated": "18-05-2019 08:11:06"
},
"double_ended_queue": {
"location": "code/data_structures/src/queue/double_ended_queue",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"deque_queue_library_function.cpp",
"deque.cpp",
"deque.c",
"deque.py"
],
"updated": "18-05-2019 08:11:06"
},
"queue": {
"location": "code/data_structures/src/queue/queue",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"queue_vector.cpp",
"queue.exs",
"queue.cs",
"queue.c",
"queue.rb",
"queue.js",
"queue.java",
"queue.swift",
"queue.py",
"README.md",
"queue.go",
"queue.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"aes_csharp": {
"location": "code/cryptography/src/aes_128/aes_csharp",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"aesdecipher.cs",
"aescipher.cs",
"helpers.cs",
"README.md",
"aeskeygen.cs",
"aesconsts.cs"
],
"updated": "18-05-2019 08:11:06"
},
"sha_256": {
"location": "code/cryptography/src/sha/sha_256",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"sha_256.py"
],
"updated": "18-05-2019 08:11:06"
}
},
"computer_graphics": {
"diamond_square": {
"location": "code/computer_graphics/src/diamond_square",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"diamond_square.py",
"diamondsquare.java"
],
"updated": "18-05-2019 08:11:06"
}
},
"languages": {
"dynamic_memory_allocation": {
"location": "code/languages/c/dynamic_memory_allocation",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"example.c"
],
"updated": "18-05-2019 08:11:06"
}
},
"2d-array-list":{
"location": "code/languages/Java/2d-array-list-java.java",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"2d-array-list-java.java"
],
"updated": "29-05-2020 05:22:42"
}
},
"Reduce_time_complexity_in_java":{
"location": "code/languages/Java/reduce_time_complexity.java",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"Reduce_Time_complexity"
],
"updated": "29-05-2020 05:22:42"
}
},
"double_to_string": {
"location": "code/languages/cpp/double_to_string",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"double_to_string.cpp"
],
"updated": "29-05-2020 14:02:06"
}
},
"2d-array":{
"location": "code/languages/Java/2d-array.java",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"2d-array.java"
],
"updated": "29-05-2020 04:26:42"
}
},
"delete_vs_free":{
"location": "code/languages/cpp/delete_vs_free",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"free_vs_delete.cpp.c"
],
"updated": "31-05-2020 16:01:43"
}
},
"delete_array":{
"location": "code/languages/c/delete_array",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"del.c"
],
"updated": "29-05-2020 02:54:16"
}
},
"begin_and_end":{
"location": "code/languages/cpp/begin_and_end",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"begin_and_end.cpp"
],
"updated": "29-05-2020 13:04:36"
}
},
"search": {
"binary_search": {
"location": "code/search/src/binary_search",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"binary_search.rs",
"binary_search.scala",
"binary_search.java",
"binary_search.php",
"binary_search.c",
"binary_search.rkt",
"binary_search.py",
"binary_search.js",
"binary_search.ex",
"binary_search.go",
"binary_search.rb",
"binary_search.hs",
"binary_search.swift",
"binary_search.cs",
"binary_search.cpp",
"binary_search.sh",
"README.md",
"binary_search_2.cpp",
"binary_search.kt"
],
"updated": "18-05-2019 08:11:06"
},
"jump_search": {
"location": "code/search/src/jump_search",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"jump_search.php",
"jump_search.js",
"jump_search.go",
"jump_search.cpp",
"jump_search.rs",
"jump_search.py",
"README.md",
"jump_search.java",
"jump_search.c",
"jump_search.swift"
],
"updated": "18-05-2019 08:11:06"
},
"linear_search": {
"location": "code/search/src/linear_search",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"linear_search.cpp",
"linear_search.rs",
"linear_search.js",
"linear_search.nim",
"linear_search.clj",
"linear_search.swift",
"linear_search.php",
"linear_search.re",
"linear_search.go",
"linear_search.ml",
"linear_search.py",
"linear_search.rb",
"linear_search.hs",
"README.md",
"linear_search.c",
"linear_search.java",
"linear_search.kt",
"linear_search.scala",
"linear_search.cs",
"sentinellinearsearch.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"ternary_search": {
"location": "code/search/src/ternary_search",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"ternary_search.cpp",
"ternary_search.php",
"ternary_search.py",
"ternary_search.js",
"ternary_search.java",
"ternary_search.rs",
"ternary_search.kt",
"README.md",
"ternary_search.c",
"ternary_search.go"
],
"updated": "18-05-2019 08:11:06"
},
"interpolation_search": {
"location": "code/search/src/interpolation_search",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"interpolation_search.go",
"interpolation_search.php",
"interpolation_search.cpp",
"interpolation_search.js",
"interpolation_search.c",
"interpolation_search.java",
"interpolation_search.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"fuzzy_search": {
"location": "code/search/src/fuzzy_search",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"fuzzy_search.js",
"fuzzy_search.php"
],
"updated": "18-05-2019 08:11:06"
},
"exponential_search": {
"location": "code/search/src/exponential_search",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"exponential_search2.cpp",
"exponential_search.py",
"exponential_search.rs",
"exponential_search.php",
"exponential_search.cpp",
"exponential_search.java",
"README.md",
"exponential_search.c",
"exponential_search.js",
"exponential_search.go",
"exponential_search.rb"
],
"updated": "18-05-2019 08:11:06"
},
"fibonacci_search": {
"location": "code/search/src/fibonacci_search",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"fibonacci_search.js",
"fibonacci_search.py",
"fibonacci_search.c",
"fibonacci_search.swift",
"fibonacci_search.cpp"
],
"updated": "18-05-2019 08:11:06"
}
},
"graph_algorithms": {
"depth_first_search": {
"location": "code/graph_algorithms/src/depth_first_search",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"dfs.cpp",
"dfs.kt",
"dfs.rb",
"dfs.java",
"dfs.go",
"depth_first_search.py",
"README.md",
"dfs.c"
],
"updated": "18-05-2019 08:11:06"
},
"strongly_connected_components": {
"location": "code/graph_algorithms/src/strongly_connected_components",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"strongly_connected_components.cpp",
"strongly_connected_components.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"connected_components": {
"location": "code/graph_algorithms/src/connected_components",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"connected_components.c"
],
"updated": "18-05-2019 08:11:06"
},
"bellman_ford_algorithm": {
"location": "code/graph_algorithms/src/bellman_ford_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bellman_ford.cpp",
"bellman_ford.c",
"bellman_ford.js",
"bellman_ford.php",
"bellman_ford.py",
"bellman_ford_adjacency_list.java",
"README.md",
"bellman_ford_edge_list.java"
],
"updated": "18-05-2019 08:11:06"
},
"left_view_binary_tree": {
"location": "code/graph_algorithms/src/left_view_binary_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"left_view_binary_tree.java",
"left_view_binary.py",
"left_view_binary_tree.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"biconnected_components": {
"location": "code/graph_algorithms/src/biconnected_components",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"biconnected_components.cpp",
"biconnected_components.java",
"biconnected_components.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"floyd_warshall_algorithm": {
"location": "code/graph_algorithms/src/floyd_warshall_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"floyd_warshall.py",
"floydwarshall.java",
"floyd_warshall.cpp",
"README.md",
"floydwarshall.c"
],
"updated": "18-05-2019 08:11:06"
},
"boruvka_minimum_spanning_tree": {
"location": "code/graph_algorithms/src/boruvka_minimum_spanning_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"boruvka_minimum_spanning_tree.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"push_relabel": {
"location": "code/graph_algorithms/src/push_relabel",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"push_relabel.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"graph_coloring": {
"location": "code/graph_algorithms/src/graph_coloring",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"graph_coloring.cpp",
"README.md",
"graph_color_greedy.py",
"graph_coloring.java"
],
"updated": "18-05-2019 08:11:06"
},
"eulerian_path": {
"location": "code/graph_algorithms/src/eulerian_path",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"eulerian.py",
"eulerian.java",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"postorder_from_inorder_and_preorder": {
"location": "code/graph_algorithms/src/postorder_from_inorder_and_preorder",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"inprepost.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"astar_algorithm": {
"location": "code/graph_algorithms/src/astar_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"astar.js"
],
"updated": "18-05-2019 08:11:06"
},
"count_of_ways_n": {
"location": "code/graph_algorithms/src/count_of_ways_n",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"count_of_ways_n.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"channel_assignment": {
"location": "code/graph_algorithms/src/channel_assignment",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"cut_vertices": {
"location": "code/graph_algorithms/src/cut_vertices",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"cut_vertices.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"steiner_tree": {
"location": "code/graph_algorithms/src/steiner_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"steiner_tree.java"
],
"updated": "18-05-2019 08:11:06"
},
"hamiltonian_cycle": {
"location": "code/graph_algorithms/src/hamiltonian_cycle",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"hamiltonian_cycle.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"tarjan_algorithm_strongly_connected_components": {
"location": "code/graph_algorithms/src/tarjan_algorithm_strongly_connected_components",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"tarjan_algorithm.c"
],
"updated": "18-05-2019 08:11:06"
},
"centroid_decomposition": {
"location": "code/graph_algorithms/src/centroid_decomposition",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"centroid_decompostition.cpp",
"centroid_decomposition.java"
],
"updated": "18-05-2019 08:11:06"
},
"fleury_algorithm_euler_path": {
"location": "code/graph_algorithms/src/fleury_algorithm_euler_path",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"johnson_algorithm_shortest_path": {
"location": "code/graph_algorithms/src/johnson_algorithm_shortest_path",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"johnsons_algo.py"
],
"updated": "18-05-2019 08:11:06"
},
"travelling_salesman_mst": {
"location": "code/graph_algorithms/src/travelling_salesman_mst",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"travelling_salesman.cpp",
"travelling_salesman.py",
"travelling_salesman_dp.c",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"vertex_cover": {
"location": "code/graph_algorithms/src/vertex_cover",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"hamiltonian_path": {
"location": "code/graph_algorithms/src/hamiltonian_path",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"hamiltonian_path.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"bridges_in_graph": {
"location": "code/graph_algorithms/src/bridges_in_graph",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"bridges.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"bipartite_check": {
"location": "code/graph_algorithms/src/bipartite_check",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bipartite_check.java"
],
"updated": "18-05-2019 08:11:06"
},
"ford_fulkerson_maximum_flow": {
"location": "code/graph_algorithms/src/ford_fulkerson_maximum_flow",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"ford_fulkerson.cpp",
"ford_fulkerson_using_bfs.cpp",
"fordfulkersonusingbfs.java",
"README.md",
"ford_fulkerson_using_bfs.py"
],
"updated": "18-05-2019 08:11:06"
},
"kuhn_maximum_matching": {
"location": "code/graph_algorithms/src/kuhn_maximum_matching",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"kuhn_maximum_matching.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"hopcroft_karp_algorithm": {
"location": "code/graph_algorithms/src/hopcroft_karp_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"cycle_directed_graph": {
"location": "code/graph_algorithms/src/cycle_directed_graph",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"cycle_directed_detection.c",
"cycle_directed_graph.c",
"cycle_directed_graph.cpp",
"cycle_directed_graph.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"bron_kerbosch_algorithm": {
"location": "code/graph_algorithms/src/bron_kerbosch_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bron_kerbosch.java"
],
"updated": "18-05-2019 08:11:06"
},
"matrix_transformation": {
"location": "code/graph_algorithms/src/matrix_transformation",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"matrix_transformation.swift"
],
"updated": "18-05-2019 08:11:06"
},
"bridge_tree": {
"location": "code/graph_algorithms/src/bridge_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bridge_tree.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"kuhn_munkres_algorithm": {
"location": "code/graph_algorithms/src/kuhn_munkres_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"kuhn_munkres_algorithm.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"shortest_path_k_edges": {
"location": "code/graph_algorithms/src/shortest_path_k_edges",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"minimum_s_t_cut": {
"location": "code/graph_algorithms/src/minimum_s_t_cut",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"maximum_bipartite_matching": {
"location": "code/graph_algorithms/src/maximum_bipartite_matching",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"max_bipartite_matching.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"breadth_first_search": {
"location": "code/graph_algorithms/src/breadth_first_search",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bfs.c",
"breadth_first_search.swift",
"bfs.cpp",
"README.md",
"breadth_first_search.py",
"bfs.java",
"bfs.rb"
],
"updated": "18-05-2019 08:11:06"
},
"cycle_undirected_graph": {
"location": "code/graph_algorithms/src/cycle_undirected_graph",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"cycle_using_union_find_datastructure.cpp",
"cyclegraph.cpp",
"cycle_undirected_graph.py",
"README.md",
"checkcycle.java"
],
"updated": "18-05-2019 08:11:06"
},
"maximum_edge_disjoint_paths": {
"location": "code/graph_algorithms/src/maximum_edge_disjoint_paths",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"karger_minimum_cut": {
"location": "code/graph_algorithms/src/karger_minimum_cut",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"karger.java"
],
"updated": "18-05-2019 08:11:06"
},
"data_structures": {
"location": "code/graph_algorithms/src/data_structures",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"adjacency_matrix.cpp",
"adjacency_list.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"bipartite_checking": {
"location": "code/graph_algorithms/src/bipartite_checking",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bipartite.cpp",
"bipartite_bfs.java",
"bipartite_checking.cpp",
"bipartite_graph_checked_adjacency_list.java",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"dinic_maximum_flow": {
"location": "code/graph_algorithms/src/dinic_maximum_flow",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"dinic_maximum_flow.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"min_operation_to_make_gcd_k": {
"location": "code/greedy_algorithms/src/min_operation_to_make_gcd_k",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/minimum-operations-to-make-gcd-k/",
"files": [
"min_operation.cpp",
"README.md"
],
"updated": "24-05-2020 08:11:06"
},
"longest_path_directed_acyclic_graph": {
"location": "code/graph_algorithms/src/longest_path_directed_acyclic_graph",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"longest_path_directed_acyclic_graph.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"transitive_closure_graph": {
"location": "code/graph_algorithms/src/transitive_closure_graph",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"transitive_closure_graph.py",
"transitive_closure_graph_floyd_warshall.cpp",
"transitive_closure.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
}
},
"min_lateness": {
"location": "code/greedy_algorithms/src/min_lateness",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/scheduling-to-minimize-lateness/",
"files": [
"README.md",
"min_lateness.cpp"
],
"updated": "18-04-2020 08:11:06"
},
"theory_of_computation": {
"nondeterministic_finite_atomaton": {
"location": "code/theory_of_computation/src/nondeterministic_finite_atomaton",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"nfa.py"
],
"updated": "18-05-2019 08:11:06"
},
"deterministic_finite_automaton": {
"location": "code/theory_of_computation/src/deterministic_finite_automaton",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"dfa.py"
],
"updated": "18-05-2019 08:11:06"
}
},
"randomized_algorithms": {
"birthday_paradox": {
"location": "code/randomized_algorithms/src/birthday_paradox",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"birthday_paradox.py"
],
"updated": "18-05-2019 08:11:06"
},
"shuffle_an_array": {
"location": "code/randomized_algorithms/src/shuffle_an_array",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"shuffle_an_array.rs",
"shuffle_library.rb",
"shuffle_an_array.rb",
"shuffle_an_array.java",
"shuffle_an_array.php",
"README.md",
"shuffle_an_array.js",
"shuffle_an_array.cpp",
"shuffle_an_array.py"
],
"updated": "18-05-2019 08:11:06"
},
"random_node_linkedlist": {
"location": "code/randomized_algorithms/src/random_node_linkedlist",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"randomized_quick_sort": {
"location": "code/randomized_algorithms/src/randomized_quick_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"randomized_quicksort.cpp",
"randomized_quicksort.c"
],
"updated": "18-05-2019 08:11:06"
},
"reservoir_sampling": {
"location": "code/randomized_algorithms/src/reservoir_sampling",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"reservoir_sampling.cpp",
"reservoir_sampling.rs",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"random_from_stream": {
"location": "code/randomized_algorithms/src/random_from_stream",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"random_number_selection_from_a_stream.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"kth_smallest_element_algorithm": {
"location": "code/randomized_algorithms/src/kth_smallest_element_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"kth_smallest_element_algorithm.cpp",
"kth_smallest_element_algorithm.c",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"karger_minimum_cut_algorithm": {
"location": "code/randomized_algorithms/src/karger_minimum_cut_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"karger_minimum_cut_algorithm.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
}
},
"design_pattern": {
"iterator_pattern": {
"location": "code/design_pattern/src/iterator_pattern",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"iterator.java",
"main.java",
"student.java",
"class.java",
"classiterator.java"
],
"updated": "18-05-2019 08:11:06"
},
"functional_patterns": {
"location": "code/design_pattern/src/functional_patterns",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"policy_based_design": {
"location": "code/design_pattern/src/policy_based_design",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"readme.md",
"policy_design.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"oop_patterns": {
"location": "code/design_pattern/src/oop_patterns",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"builder_pattern": {
"location": "code/design_pattern/src/builder_pattern",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"builder.cs"
],
"updated": "18-05-2019 08:11:06"
},
"OOP_patterns": {
"location": "code/design_pattern/src/OOP_patterns",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"__init__.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
}
},
"scala": {
"comonad": {
"location": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/monads/comonad",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"comonad.scala"
],
"updated": "18-05-2019 08:11:06"
},
"gonad": {
"location": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/monads/gonad",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"gonad.scala"
],
"updated": "18-05-2019 08:11:06"
},
"bifunctor": {
"location": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/functors/bifunctor",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bifunctor.scala"
],
"updated": "18-05-2019 08:11:06"
},
"profunctor": {
"location": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/functors/profunctor",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"profunctor.scala"
],
"updated": "18-05-2019 08:11:06"
},
"contravariant": {
"location": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/functors/contravariant",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"contravariant.scala"
],
"updated": "18-05-2019 08:11:06"
},
"multifunctor": {
"location": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/functors/multifunctor",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"multifunctor.scala"
],
"updated": "18-05-2019 08:11:06"
},
"arrow": {
"location": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/arrows/arrow",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"arrow.scala"
],
"updated": "18-05-2019 08:11:06"
},
"elimination": {
"location": "code/mathematical_algorithms/src/gaussian_elimination/scala/src/main/scala/gaussian/elimination",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"gaussianelimination.scala",
"matrixtype.scala",
"solution.scala"
],
"updated": "18-05-2019 08:11:06"
}
},
"monads": {
"monad": {
"location": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/monads/free/monad",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"freemonad.scala"
],
"updated": "18-05-2019 08:11:06"
}
},
"functors": {
"functor": {
"location": "code/design_pattern/src/functional_patterns/functional_patterns/scala/src/main/scala/functors/applicative/functor",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"applicativefunctor.scala"
],
"updated": "18-05-2019 08:11:06"
}
},
"OOP_patterns": {
"builder": {
"location": "code/design_pattern/src/OOP_patterns/builder/builder",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"personbuilder.java",
"person.java",
"nationality.java"
],
"updated": "18-05-2019 08:11:06"
},
"gifts": {
"location": "code/design_pattern/src/OOP_patterns/factory/gifts",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"car.java",
"toy.java",
"gift.java",
"booze.java",
"nothing.java"
],
"updated": "18-05-2019 08:11:06"
},
"observer": {
"location": "code/design_pattern/src/OOP_patterns/observer_java/observer",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"observer.java",
"subject.java"
],
"updated": "18-05-2019 08:11:06"
},
"demo": {
"location": "code/design_pattern/src/OOP_patterns/proxy/demo",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"demo.java"
],
"updated": "18-05-2019 08:11:06"
},
"soldiers": {
"location": "code/design_pattern/src/OOP_patterns/adapter/soldiers",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"general.java",
"soldier.java",
"order.java",
"adaptee.java"
],
"updated": "18-05-2019 08:11:06"
}
},
"facade": {
"tasks": {
"location": "code/design_pattern/src/OOP_patterns/facade/daily/tasks",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"dailyroutinefacade.java"
],
"updated": "18-05-2019 08:11:06"
}
},
"daily": {
"job": {
"location": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/job",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"develop.java",
"leave.java",
"jobfacade.java",
"playfifa.java",
"eatatwork.java",
"watchyoutubevideos.java"
],
"updated": "18-05-2019 08:11:06"
},
"gym": {
"location": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/gym",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"deadlift.java",
"squat.java",
"gymfacade.java",
"benchpress.java"
],
"updated": "18-05-2019 08:11:06"
}
},
"tasks": {
"routine": {
"location": "code/design_pattern/src/OOP_patterns/facade/daily/tasks/evening/routine",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"eveningroutinefacade.java",
"writecode.java",
"takeashower.java",
"watchyoutubevideos.java",
"eat.java"
],
"updated": "18-05-2019 08:11:06"
}
},
"observer_java": {
"network": {
"location": "code/design_pattern/src/OOP_patterns/observer_java/observer/network",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"fan.java",
"artist.java"
],
"updated": "18-05-2019 08:11:06"
}
},
"proxy": {
"proxy": {
"location": "code/design_pattern/src/OOP_patterns/proxy/protection/proxy",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"registeredusers.java",
"validuser.java",
"userproxy.java",
"user.java"
],
"updated": "18-05-2019 08:11:06"
}
},
"online_challenges": {
"rosalind": {
"location": "code/online_challenges/src/rosalind",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"project_euler": {
"location": "code/online_challenges/src/project_euler",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"documentation_guide.md",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"codechef": {
"location": "code/online_challenges/src/codechef",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
}
},
"mathematical_algorithms": {
"hill_climbing": {
"location": "code/mathematical_algorithms/src/hill_climbing",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"hill_climbing.java"
],
"updated": "18-05-2019 08:11:06"
},
"sum_of_digits": {
"location": "code/mathematical_algorithms/src/sum_of_digits",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"sum_of_digits_with_recursion.c",
"sum_of_digits.go",
"sum_of_digits.cs",
"sum_of_digits.rs",
"sum_of_digits.c",
"sum_of_digits.java",
"sum_of_digits.rb",
"sum_of_digits.php",
"sum_of_digits.py",
"sum_of_digits.ex",
"sum_of_digits.swift",
"sum_of_digits.cpp",
"sum_of_digits.js"
],
"updated": "18-05-2019 08:11:06"
},
"fibonacci_number": {
"location": "code/mathematical_algorithms/src/fibonacci_number",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"fibonacci_number.cpp",
"fibonacci_number.scala",
"fibonacci_number.hs",
"fibonacci_matrix_multiplication.py",
"fibonacci_number.go",
"fibonacci_number.js",
"fibonacci_number.swift",
"fibonacci_number.rs",
"fibonacci_number.c",
"fibonacci_number.php",
"fibonacci_number.ex",
"fibonacci_number.py",
"fibonacci_number.clj",
"fibonacci_lucas.py",
"README.md",
"fibonacci_matrix_exponentiation.cpp",
"fibonacci_for_big_numbers.cpp",
"fibonacci_number.rb",
"fibonacci_memorized.swift",
"fibonacci_number.cs",
"fibonacci_number.java",
"fast_fibonacci.c",
"fibonacci_number.erl"
],
"updated": "18-05-2019 08:11:06"
},
"multiply_polynomial": {
"location": "code/mathematical_algorithms/src/multiply_polynomial",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"multiply_polynomial.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"jacobi_method": {
"location": "code/mathematical_algorithms/src/jacobi_method",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"jacobi_method.java",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"lucky_number": {
"location": "code/mathematical_algorithms/src/lucky_number",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"lucky_number.java",
"README.md",
"lucky_number.c"
],
"updated": "18-05-2019 08:11:06"
},
"permutation_lexicographic_order": {
"location": "code/mathematical_algorithms/src/permutation_lexicographic_order",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"permutation_lexicographic_order.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"integer_conversion": {
"location": "code/mathematical_algorithms/src/integer_conversion",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"decimal_to_any_base.py",
"decimal_to_int.go",
"decimal_to_hex.cpp",
"decimal_to_bin.cpp",
"decimal_to_oct.cpp",
"decimal_to_any_base.js"
],
"updated": "18-05-2019 08:11:06"
},
"collatz_conjecture_sequence": {
"location": "code/mathematical_algorithms/src/collatz_conjecture_sequence",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"collatz_conjecture_sequence.c"
],
"updated": "18-05-2019 08:11:06"
},
"fractals": {
"location": "code/mathematical_algorithms/src/fractals",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"simple_julia.cpp",
"julia_miim.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"fermats_little_theorem": {
"location": "code/mathematical_algorithms/src/fermats_little_theorem",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"fermats_little_theorem.cpp",
"fermats_little_theorem.py"
],
"updated": "18-05-2019 08:11:06"
},
"pandigital_number": {
"location": "code/mathematical_algorithms/src/pandigital_number",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"pandigital_number.rb",
"pandigital_number.c",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"newton_raphson_method": {
"location": "code/mathematical_algorithms/src/newton_raphson_method",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"newton_raphson.php",
"newton_raphson.c",
"README.md",
"newton_raphson.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"catalan_number": {
"location": "code/mathematical_algorithms/src/catalan_number",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"catalan_number.js",
"catalan_number2.py",
"catalan_number.py",
"catalan_number_dynamic.cpp",
"catalan_number.scala",
"catalan_number.c",
"catalan_number.java",
"catalan_number.rb",
"README.md",
"catalan_number_recursive.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"pascal_triangle": {
"location": "code/mathematical_algorithms/src/pascal_triangle",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"pascal_triangle.java",
"pascal_triangle.cpp",
"pascal_triangle.go",
"pascal_triangle.exs",
"pascal_triangle.c",
"README.md",
"pascal_triangle.py"
],
"updated": "18-05-2019 08:11:06"
},
"decoding_of_string": {
"location": "code/mathematical_algorithms/src/decoding_of_string",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"taxicab_numbers": {
"location": "code/mathematical_algorithms/src/taxicab_numbers",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"taxicab_numbers.py"
],
"updated": "18-05-2019 08:11:06"
},
"average_stream_numbers": {
"location": "code/mathematical_algorithms/src/average_stream_numbers",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"average_stream_numbers.go",
"average_stream_numbers.c",
"README.md",
"average_stream_numbers.py",
"average_stream_numbers.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"automorphic_numbers": {
"location": "code/mathematical_algorithms/src/automorphic_numbers",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"automorphic_numbers.java",
"automorphic_numbers.swift",
"automorphic_numbers.php",
"automorphic_numbers.c",
"automorphic_numbers.cs",
"automorphic_numbers.py",
"automorphic_numbers.rb",
"automorphic_numbers.hs",
"automorphic_numbers.js",
"automorphic_numbers.go",
"automorphic_numbers.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"perfect_number": {
"location": "code/mathematical_algorithms/src/perfect_number",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"perfect_number.rb",
"perfect_number.hs",
"perfect_number.cpp",
"perfect_number.js",
"perfect_number.c",
"perfect_number.py",
"perfect_number.rs",
"perfect_number.php",
".gitignore",
"perfect_number.java",
"README.md",
"perfect_number_list.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"exponentiation_power": {
"location": "code/mathematical_algorithms/src/exponentiation_power",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"exponentiation_power.c",
"modulo_exponentation_power.cpp",
"exponentiation_power.cpp",
"README.md",
"exponentiation_power.java"
],
"updated": "18-05-2019 08:11:06"
},
"count_digits": {
"location": "code/mathematical_algorithms/src/count_digits",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"count_digits.cpp",
"count_digits.c",
"count_digits.hs",
"count_digits.java",
"count_digits.js",
"count_digits.swift",
"count_digits.py",
"count_digits.cs",
"count_digits.go",
"counts_digits.rb"
],
"updated": "18-05-2019 08:11:06"
},
"smallest_digit_in_number": {
"location": "code/mathematical_algorithms/src/smallest_digit_in_number",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"smallest_digit_in_number.rb",
"smallest_digit_in_number.java",
"smallest_digit_in_number.cpp",
"smallest_digit_in_number.py",
"smallest_digit_in_number.php",
"smallest_digit_in_number.cs",
"smallest_digit_in_number.hs",
"smallest_digit_in_number.js",
"smallest_digit_in_number.c"
],
"updated": "18-05-2019 08:11:06"
},
"integer_to_roman": {
"location": "code/mathematical_algorithms/src/integer_to_roman",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"integer_to_roman.py",
"integer_to_roman.js",
"integer_to_roman.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"std": {
"location": "code/mathematical_algorithms/src/std",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"std.c",
"std.js",
"std.py",
"std.go",
"std.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"euler_totient": {
"location": "code/mathematical_algorithms/src/euler_totient",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"euler_totient_sieve.py",
"euler_totient.c",
"euler_totient.java",
"euler_totient.py",
"euler_totient_sieve.cpp",
"euler_totient.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"russian_peasant_multiplication": {
"location": "code/mathematical_algorithms/src/russian_peasant_multiplication",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"russian_peasant_multiplication.py",
"russian_peasant_multiplication.cpp",
"russian_peasant_multiplication.js",
"russian_peasant_multiplication.cs",
"russian_peasant_multiplication.rs",
"russian_peasant_multiplication.php",
"README.md",
"russian_peasant_multiplication.c",
"russian_peasant_multiplication.go"
],
"updated": "18-05-2019 08:11:06"
},
"gcd_and_lcm": {
"location": "code/mathematical_algorithms/src/gcd_and_lcm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"gcd_and_lcm.cpp",
"gcd_and_lcm.erl",
"gcd_and_lcm.py",
"gcd_and_lcm.go",
"gcd_and_lcm.php",
"gcd_and_lcm.ex",
"gcd_and_lcm.scala",
"gcd_and_lcm.cs",
"gcd_and_lcm.java",
"gcd_and_lcm.c",
"gcd_and_lcm.js"
],
"updated": "18-05-2019 08:11:06"
},
"newman_conway": {
"location": "code/mathematical_algorithms/src/newman_conway",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"newman_conway_sequence.cpp",
"README.md",
"newman_conway_sequence.c"
],
"updated": "18-05-2019 08:11:06"
},
"replace_0_with_5": {
"location": "code/mathematical_algorithms/src/replace_0_with_5",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"replace_0_with_5.java",
"replace_0_with_5.py",
"replace_0_with_5.go",
"replace_0_with_5.js",
"replace_0_with_5.c",
"replace_0_with_5.cs",
"replace_0_with_5.cpp",
"0_to_5_efficent.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"diophantine": {
"location": "code/mathematical_algorithms/src/diophantine",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"diophantine.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"armstrong_numbers": {
"location": "code/mathematical_algorithms/src/armstrong_numbers",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"armstrong_numbers.cpp",
"armstrong_numbers.c",
"armstrong_numbers.py",
"armstrong_numbers.cs",
"armstrong_numbers.rb",
"README.md",
"armstrong_numbers.java",
"armstrong_number.php",
"armstrong_numbers.go",
"armstrong_numbers.js"
],
"updated": "18-05-2019 08:11:06"
},
"check_is_square": {
"location": "code/mathematical_algorithms/src/check_is_square",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"check_is_square.cs",
"check_is_square.rs",
"check_is_square.py",
"check_is_square.swift",
"check_is_square.java",
"check_is_square.c",
"check_is_square.scala",
"check_is_square.cpp",
"check_is_square.php",
"check_is_square.ruby",
"check_is_square.js",
"check_is_square.go"
],
"updated": "18-05-2019 08:11:06"
},
"fast_inverse_sqrt": {
"location": "code/mathematical_algorithms/src/fast_inverse_sqrt",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"fast_inverse_sqrt.py",
"fast_inverse_sqrt.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"greatest_digit_in_number": {
"location": "code/mathematical_algorithms/src/greatest_digit_in_number",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"greatest_digit_in_number.cpp",
"greatest_digit_in_number.php",
"greatest_digit_in_number.c",
"greatest_digit_in_number.js",
"greatest_digit_in_number.hs",
"greatest_digit_in_number.rb",
"greatest_digit_in_number.java",
"greatest_digit_in_number.py",
"greatest_digit_in_number.cs"
],
"updated": "18-05-2019 08:11:06"
},
"log_of_factorial": {
"location": "code/mathematical_algorithms/src/log_of_factorial",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"log_of_factorial.py",
"log_of_factorial.java",
"log_of_factorial.c",
"log_of_factorial.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"convolution": {
"location": "code/mathematical_algorithms/src/convolution",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"convolution.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"add_polynomials": {
"location": "code/mathematical_algorithms/src/add_polynomials",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"add_polynomials.c",
"add_polynomials.cpp",
"add_polynomials.go",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"fast_fourier_transform": {
"location": "code/mathematical_algorithms/src/fast_fourier_transform",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"fast_fourier_transform.c",
"fast_fourier_transform.java"
],
"updated": "18-05-2019 08:11:06"
},
"largrange_polynomial": {
"location": "code/mathematical_algorithms/src/largrange_polynomial",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"lagrange_polynomial.java",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"shuffle_array": {
"location": "code/mathematical_algorithms/src/shuffle_array",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"shuffle_array.rb",
"shuffle_array.c",
"shuffle_array.cpp",
"shuffle_array.js",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"newton_polynomial": {
"location": "code/mathematical_algorithms/src/newton_polynomial",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"newton_polynomial.java",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"coprime_numbers": {
"location": "code/mathematical_algorithms/src/coprime_numbers",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"coprime_numbers.js",
"coprime_numbers.cs",
"coprime_numbers.cpp",
"coprime_numbers.go",
"coprime_numbers.rb",
"coprime_numbers.py",
"coprime_numbers.java",
"coprime_numbers.c",
"coprime_numbers.rs"
],
"updated": "18-05-2019 08:11:06"
},
"lexicographic_string_rank": {
"location": "code/mathematical_algorithms/src/lexicographic_string_rank",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"lexicographic_string_rank.c",
"lexicographic_string_rank.cpp",
"lexicographic_string_rank.java",
"README.md",
"lexicographic_string_rank.py"
],
"updated": "18-05-2019 08:11:06"
},
"sieve_of_atkin": {
"location": "code/mathematical_algorithms/src/sieve_of_atkin",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"sieve_of_atkin.c",
"sieve_of_atkin.cpp",
"sieve_of_atkin.java",
"sieve_of_atkin.py"
],
"updated": "18-05-2019 08:11:06"
},
"steepest_descent": {
"location": "code/mathematical_algorithms/src/steepest_descent",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"steepest_descent.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"divided_differences": {
"location": "code/mathematical_algorithms/src/divided_differences",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"divided_differences.java",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"simpsons_rule": {
"location": "code/mathematical_algorithms/src/simpsons_rule",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"simpsons_rule.py"
],
"updated": "18-05-2019 08:11:06"
},
"binomial_coefficient": {
"location": "code/mathematical_algorithms/src/binomial_coefficient",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"binomial_coefficient.c",
"binomial_coefficient.go",
"binomial_coefficient.cpp",
"binomial_coefficient.java",
"binomial_coefficient.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"gaussian_elimination": {
"location": "code/mathematical_algorithms/src/gaussian_elimination",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"gaussian_elimination.java",
"gaussian_elimination.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"prime_numbers_of_n": {
"location": "code/mathematical_algorithms/src/prime_numbers_of_n",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"prime_numbers_of_n.cpp",
"prime_numbers_of_n.c",
"prime_numbers_of_n.js",
"prime_numbers_of_n.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"2sum": {
"location": "code/mathematical_algorithms/src/2sum",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"2sum.cpp",
"2sum.rb",
"2sum.py",
"2sum.rs",
"2sum.java",
"2sum.c",
"2sum.js",
"2sum.go"
],
"updated": "18-05-2019 08:11:06"
},
"count_trailing_zeroes": {
"location": "code/mathematical_algorithms/src/count_trailing_zeroes",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"count_trailing_zeroes_factorial.cpp",
"count_trailing_zeroes_factorial.java",
"count_trailing_zeroes.c",
"count_trailing_zeroes_factorial.py",
"count_trailing_zeroes.scala",
"count_trailing_zeroes_factorial.js"
],
"updated": "18-05-2019 08:11:06"
},
"tribonacci_numbers": {
"location": "code/mathematical_algorithms/src/tribonacci_numbers",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"tribonacci_numbers.cpp",
"tribonacci_numbers.java",
"tribonacci_numbers.js",
"tribonacci_numbers.c",
"tribonacci_numbers.rs",
"tribonacci_numbers.go",
"tribonnaci.java",
"tribonacci_numbers.py"
],
"updated": "18-05-2019 08:11:06"
},
"segmented_sieve_of_eratosthenes": {
"location": "code/mathematical_algorithms/src/segmented_sieve_of_eratosthenes",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"segmented_sieve_of_eratosthenes.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"poisson_sample": {
"location": "code/mathematical_algorithms/src/poisson_sample",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"poisson_sample.py"
],
"updated": "18-05-2019 08:11:06"
},
"horner_polynomial_evaluation": {
"location": "code/mathematical_algorithms/src/horner_polynomial_evaluation",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"horner_polynomial_evaluation.cpp",
"horner_polynomial_evaluation.java",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"amicable_numbers": {
"location": "code/mathematical_algorithms/src/amicable_numbers",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"amicable_numbers.go",
"amicable_numbers.rb",
"amicable_numbers.rs",
"amicable_numbers.js",
"amicable_numbers.java",
"amicable_numbers.cs",
"amicable_numbers.cpp",
"amicable_numbers.c",
"amicable_numbers.py"
],
"updated": "18-05-2019 08:11:06"
},
"reverse_number": {
"location": "code/mathematical_algorithms/src/reverse_number",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"reverse_number.java",
"reverse_number.rb",
"reverse_number.swift",
"reverse_number.py",
"reverse_a_number.c",
"reverse_number.c",
"reverse_number.php",
"reverse_number.hs",
"reverse_number.cpp",
"reverse_number.cs",
"reverse_number.go",
"reverse_number.js",
"reverse_number_recursion.java"
],
"updated": "18-05-2019 08:11:06"
},
"prime_factors": {
"location": "code/mathematical_algorithms/src/prime_factors",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"prime_factors.cpp",
"prime_factors.go",
"prime_factors.java",
"prime_factors.c",
"sum_of_primes.cpp",
"prime_factors.py"
],
"updated": "18-05-2019 08:11:06"
},
"delannoy_number": {
"location": "code/mathematical_algorithms/src/delannoy_number",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"delannoy_number.c",
"delannoy_number.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"dfa_division": {
"location": "code/mathematical_algorithms/src/dfa_division",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"square_free_number": {
"location": "code/mathematical_algorithms/src/square_free_number",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"square_free_number.c",
"squarefreenumber.java",
"square_free_number.cpp",
"square_free_number.py"
],
"updated": "18-05-2019 08:11:06"
},
"babylonian_method": {
"location": "code/mathematical_algorithms/src/babylonian_method",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"babylonian_method.c",
"babylonian_method.go",
"babylonian_method.py",
"babylonian_method.cpp",
"babylonian_method.js",
"README.md",
"babylonian_method.java"
],
"updated": "18-05-2019 08:11:06"
},
"derangements": {
"location": "code/mathematical_algorithms/src/derangements",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"derangements.c"
],
"updated": "18-05-2019 08:11:06"
},
"lucas_theorem": {
"location": "code/mathematical_algorithms/src/lucas_theorem",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"lucas_theorem.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"tridiagonal_matrix": {
"location": "code/mathematical_algorithms/src/tridiagonal_matrix",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"tridiagonal_matrix.java",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"next_larger_number": {
"location": "code/mathematical_algorithms/src/next_larger_number",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"next_larger_number.cpp",
"next_larger_number.php",
"next_larger_number.py",
"next_larger_number.java"
],
"updated": "18-05-2019 08:11:06"
},
"tower_of_hanoi": {
"location": "code/mathematical_algorithms/src/tower_of_hanoi",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"tower_of_hanoi_binary_solution.c",
"tower_of_hanoi.hs",
"tower_of_hanoi.ml",
"tower_of_hanoi.py",
"tower_of_hanoi.js",
"tower_of_hanoi.go",
"tower_of_hanoi.java",
"tower_of_hanoi.scala",
"tower_of_hanoi.cpp",
"tower_of_hanoi_iterative.c",
"README.md",
"tower_of_hanoi.c",
"tower_of_hanoi.rs"
],
"updated": "18-05-2019 08:11:06"
},
"modular_inverse": {
"location": "code/mathematical_algorithms/src/modular_inverse",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"modular_inverse.rb",
"modular_inverse.cpp",
"modular_inverse.py",
"modular_inverse.java"
],
"updated": "18-05-2019 08:11:06"
},
"pythagorean_triplet": {
"location": "code/mathematical_algorithms/src/pythagorean_triplet",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"pythagorean_triplet.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"reverse_factorial": {
"location": "code/mathematical_algorithms/src/reverse_factorial",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"reverse_factorial.js",
"reverse_factorial.rb",
"reverse_factorial.go",
"reverse_factorial.c",
"reverse_factorial.java",
"reverse_factorial.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"sieve_of_eratosthenes": {
"location": "code/mathematical_algorithms/src/sieve_of_eratosthenes",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"sieve_of_eratosthenes.py",
"sieve_of_eratosthenes_compact.cpp",
"sieve_of_eratosthenes.java",
"sieve_of_eratosthenes_linear.cpp",
"sieve_of_eratosthenes.php",
"sieve_of_eratosthenes.hs",
"sieve_of_eratosthenes.go",
"sieve_of_eratosthenes.cs",
"sieve_of_eratosthenes.c",
"README.md",
"sieve_of_eratosthenes.js",
"sieve_of_eratosthenes.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"minimum_operations_elements_equal": {
"location": "code/mathematical_algorithms/src/minimum_operations_elements_equal/",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/make-elements-equal/",
"files": [
"EqualizeEveryone.java"
],
"updated": "29-04-2020 19:25:00"
},
"maximum_perimeter_triangle": {
"location": "code/mathematical_algorithms/src/maximum_perimeter_triangle/",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/maximum-perimeter-of-triangle/",
"files": [
"PerimeterTriangle.java"
],
"updated": "29-04-2020 19:43:00"
}
},
"main": {
"structures": {
"location": "code/mathematical_algorithms/src/gaussian_elimination/scala/src/main/scala/structures",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"matrix.scala",
"epsilon.scala",
"regularmatrix.scala"
],
"updated": "18-05-2019 08:11:06"
}
},
"unclassified": {
"josephus_problem": {
"location": "code/unclassified/src/josephus_problem",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"josephus.go",
"josephus.c",
"josephus.py",
"README.md",
"josephus.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"minimum_subarray_size_with_degree": {
"location": "code/unclassified/src/minimum_subarray_size_with_degree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"minsubarraysizewithdegree.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"spiral_print": {
"location": "code/unclassified/src/spiral_print",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"spiral_print.c",
"spiral_print.py",
"spiral_print.go",
"README.md",
"spiral_print.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"fifteen_puzzle": {
"location": "code/unclassified/src/fifteen_puzzle",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"readme.md",
"makefile",
"log.txt",
"fifteen.c"
],
"updated": "18-05-2019 08:11:06"
},
"average": {
"location": "code/unclassified/src/average",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"readme.md",
"average.rb",
"average.go",
"average.py",
"average.js",
"average.erl",
"average.swift",
"average.rs",
"average.es6.js",
"average.ex",
"average.cpp",
"average.c",
"average.php",
"average.cs",
"average.java",
"average.sh",
"average.scala",
"average.class"
],
"updated": "18-05-2019 08:11:06"
},
"tokenizer": {
"location": "code/unclassified/src/tokenizer",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"tokenizer.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"no_operator_addition": {
"location": "code/unclassified/src/no_operator_addition",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"addition.c"
],
"updated": "18-05-2019 08:11:06"
},
"utilities": {
"location": "code/unclassified/src/utilities",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"convert2mp3.sh",
"download_link.sh"
],
"updated": "18-05-2019 08:11:06"
},
"optimized_fibonacci": {
"location": "code/unclassified/src/optimized_fibonacci",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"optimized_fibonacci.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"biggest_suffix": {
"location": "code/unclassified/src/biggest_suffix",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"readme.md",
"biggest_suffix.c"
],
"updated": "18-05-2019 08:11:06"
},
"majority_element": {
"location": "code/unclassified/src/majority_element",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"majority_element.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"smallest_number_to_the_left": {
"location": "code/unclassified/src/smallest_number_to_the_left",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"smallest.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"lapindrom_checker": {
"location": "code/unclassified/src/lapindrom_checker",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"lapindrome_checker.py",
"lapindrome_checker.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"biggest_of_n_numbers": {
"location": "code/unclassified/src/biggest_of_n_numbers",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"biggest_of_n.js",
"readme.md",
"biggest_of_n_numbers2.cpp",
"biggest_of_n_numbers.java",
"biggest_of_n_numbers.c",
"biggest_of_n_numbers.py",
"biggest_of_n_numbers.cpp",
"biggest_of_n_numbers.cs"
],
"updated": "18-05-2019 08:11:06"
},
"jaccard_similarity": {
"location": "code/unclassified/src/jaccard_similarity",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"jaccard.java",
"README.md",
"jaccard.c",
"jaccard.py"
],
"updated": "18-05-2019 08:11:06"
},
"paint_fill": {
"location": "code/unclassified/src/paint_fill",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"paint_fill.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"unique_number": {
"location": "code/unclassified/src/unique_number",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"unique_number.java"
],
"updated": "18-05-2019 08:11:06"
},
"magic_square": {
"location": "code/unclassified/src/magic_square",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"magic_square.php",
"magic_square.c",
"magic_square.swift",
"magic_square.py"
],
"updated": "18-05-2019 08:11:06"
},
"leap_year": {
"location": "code/unclassified/src/leap_year",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"leap_year.cs",
"readme.txt",
"leap_year.c",
"leap_year.nim",
"leap_year.java",
"leap_year.rs",
"leap_year.go",
"leap_year.cpp",
"leap_year.py"
],
"updated": "18-05-2019 08:11:06"
},
"split_list": {
"location": "code/unclassified/src/split_list",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"split_array.js",
"split_list.py"
],
"updated": "18-05-2019 08:11:06"
}
},
"divide_conquer": {
"inversion_count": {
"location": "code/divide_conquer/src/inversion_count",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"count_inversions.c",
"inversion_count.cpp",
"inversion_count.java",
"inversion_count.py",
"inversion_count.js",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"warnock_algorithm": {
"location": "code/divide_conquer/src/warnock_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"warnock_algorithm.pde"
],
"updated": "18-05-2019 08:11:06"
},
"factorial": {
"location": "code/divide_conquer/src/factorial",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"factorial.py"
],
"updated": "18-05-2019 08:11:06"
},
"tournament_method_to_find_min_max": {
"location": "code/divide_conquer/src/tournament_method_to_find_min_max",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"tournament_method_to_find_min_max.c"
],
"updated": "18-05-2019 08:11:06"
},
"merge_sort_using_divide_and_conquer": {
"location": "code/divide_conquer/src/merge_sort_using_divide_and_conquer",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"merge_sort_using_divide_and_conquer.cpp",
"merge_sort_using_divide_and_conquer.java",
"inversions.c",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"strassen_matrix_multiplication": {
"location": "code/divide_conquer/src/strassen_matrix_multiplication",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"main.cpp",
"strassen.py"
],
"updated": "18-05-2019 08:11:06"
},
"x_power_y": {
"location": "code/divide_conquer/src/x_power_y",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"x_power_y.c"
],
"updated": "18-05-2019 08:11:06"
},
"maximum_contiguous_subsequence_sum": {
"location": "code/divide_conquer/src/maximum_contiguous_subsequence_sum",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"maximum_contiguous_subsequence_sum.c"
],
"updated": "18-05-2019 08:11:06"
},
"closest_pair_of_points": {
"location": "code/divide_conquer/src/closest_pair_of_points",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"closest_pair.cpp",
"closest_pair.py"
],
"updated": "18-05-2019 08:11:06"
},
"karatsuba_multiplication": {
"location": "code/divide_conquer/src/karatsuba_multiplication",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"multiply.java",
"karatsuba_multiplication.py",
"karatsubamultiply.cpp"
],
"updated": "18-05-2019 08:11:06"
}
},
"selection_algorithms": {
"median_of_medians": {
"location": "code/selection_algorithms/src/median_of_medians",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"median_of_medians.py",
"median_of_medians.hs",
"median_of_medians.c"
],
"updated": "18-05-2019 08:11:06"
}
},
"cellular_automaton": {
"conways_game_of_life": {
"location": "code/cellular_automaton/src/conways_game_of_life",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"gameoflife.hs",
"life.cpp",
"life.c",
"conway.java",
"life.rb",
"life.go",
"life.py",
"README.md",
"game_of_life_c_sdl.c",
"conways_game_of_life.rb"
],
"updated": "18-05-2019 08:11:06"
},
"genetic_algorithm": {
"location": "code/cellular_automaton/src/genetic_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"genetic.cpp",
"genetic_algorithm.go",
"genetic_algorithm.java",
"genetic_algorithm.py",
"genetic_algorithm2.py",
"genetic_algorithm.js"
],
"updated": "18-05-2019 08:11:06"
},
"brians_brain": {
"location": "code/cellular_automaton/src/brians_brain",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"von_neumann_cellular_automata": {
"location": "code/cellular_automaton/src/von_neumann_cellular_automata",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"von_neumann_cellular_automata.c",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"elementary_cellular_automata": {
"location": "code/cellular_automaton/src/elementary_cellular_automata",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"elementarycellularautomaton.java",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"langtons_ant": {
"location": "code/cellular_automaton/src/langtons_ant",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"langtonant.py",
"langtonant.html",
"langtonant.cpp",
"langtonant.java",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"nobili_cellular_automata": {
"location": "code/cellular_automaton/src/nobili_cellular_automata",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
}
},
"string_algorithms": {
"z_algorithm": {
"location": "code/string_algorithms/src/z_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"z_algorithm_z_array.cpp",
"z_algorithm.py",
"z_algorithm.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"levenshtein_distance": {
"location": "code/string_algorithms/src/levenshtein_distance",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"levenshteindistance.java",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"palindrome_substring": {
"location": "code/string_algorithms/src/palindrome_substring",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"palindrome_substring.c"
],
"updated": "18-05-2019 08:11:06"
},
"anagram_search": {
"location": "code/string_algorithms/src/anagram_search",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"anagram_search.swift",
"anagram.scala",
"anagram_search.js",
"anagram_search.go",
"anagram_search.java",
"anagram_search.cs",
"anagram_search.py",
"anagram_search.rb",
"anagram_search.c",
"README.md",
"anagram_search.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"kmp_algorithm": {
"location": "code/string_algorithms/src/kmp_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"kmp.py",
"README.md",
"kmp_algorithm.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"password_strength_checker": {
"location": "code/string_algorithms/src/password_strength_checker",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"pw_checker.js",
"pw_checker.cpp",
"pw_checker.java",
"pw_checker.cs",
"README.md",
"pw_checker.py"
],
"updated": "18-05-2019 08:11:06"
},
"rabin_karp_algorithm": {
"location": "code/string_algorithms/src/rabin_karp_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"rabin_karp.c",
"rabinkarp.java",
"README.md",
"rabin_karp.py"
],
"updated": "18-05-2019 08:11:06"
},
"palindrome_checker": {
"location": "code/string_algorithms/src/palindrome_checker",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"palindrome.purs",
"palindrome.cr",
"palindrome.clj",
"palindrome.cs",
"palindrome.java",
"palindrome.hs",
"palindrome.rs",
"palindrome.py",
"palindrome.c",
"palindrome.ex",
"palindrome.erl",
"palindrome.cpp",
"palindrome.lua",
"palindrome.sh",
"palindrome.rb",
"palindrome.kt",
"palindrome.php",
"palindrome.swift",
"palindrome.go",
"palindrome.js"
],
"updated": "18-05-2019 08:11:06"
},
"manachar_algorithm": {
"location": "code/string_algorithms/src/manachar_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"manachar_longest_palindromic_subs.py",
"manachar_longest_palindromic_subs.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"morse_code": {
"location": "code/string_algorithms/src/morse_code",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"morsecode.php",
"morsecode.js",
"morsecode.py",
"morsecode.go",
"morsecode.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"trie_pattern_search": {
"location": "code/string_algorithms/src/trie_pattern_search",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"trie.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"boyer_moore_algorithm": {
"location": "code/string_algorithms/src/boyer_moore_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"boyer_moore_algorithm.c",
"boyer_moore_algorithm.cpp",
"README.md",
"boyer_moore_algorithm2.c"
],
"updated": "18-05-2019 08:11:06"
},
"remove_dups": {
"location": "code/string_algorithms/src/remove_dups",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"remove_dups.js",
"remove_dups.cpp",
"remove_dups.rs",
"remove_dumps.py",
"remove_dups.c"
],
"updated": "18-05-2019 08:11:06"
},
"kasai_algorithm": {
"location": "code/string_algorithms/src/kasai_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"kasai_algorithm.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"lipogram_checker": {
"location": "code/string_algorithms/src/lipogram_checker",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"lipogram_checker.py",
"lipogram_checker.cs",
"lipogram_checker.cpp",
"lipogram_checker.js"
],
"updated": "18-05-2019 08:11:06"
},
"aho_corasick_algorithm": {
"location": "code/string_algorithms/src/aho_corasick_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"aho_corasick_algorithm2.cpp",
"README.md",
"aho_corasick_algorithm.cpp",
"aho_corasick_algorithm.java"
],
"updated": "18-05-2019 08:11:06"
},
"longest_palindromic_substring": {
"location": "code/string_algorithms/src/longest_palindromic_substring",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"longest_palindromic_substring.py"
],
"updated": "18-05-2019 08:11:06"
},
"naive_pattern_search": {
"location": "code/string_algorithms/src/naive_pattern_search",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"naive_pattern_search.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"arithmetic_on_large_numbers": {
"location": "code/string_algorithms/src/arithmetic_on_large_numbers",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"string_factorial.cpp",
"string_multiplication.cpp",
"string_subtract.cpp",
"string_addition.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"finite_automata": {
"location": "code/string_algorithms/src/finite_automata",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"searchstringusingdfa.rs",
"searchstringusingdfa.java",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"pangram_checker": {
"location": "code/string_algorithms/src/pangram_checker",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"pangram_checker.c",
"pangram.cpp",
"pangram_checker.php",
"pangram_checker.py",
"pangram_checker.go",
"pangram_checker.m",
"pangram.java",
"pangram_checker.js",
"pangram.rb",
"README.md",
"pangram_checker.swift"
],
"updated": "18-05-2019 08:11:06"
}
},
"backtracking": {
"partitions_of_number": {
"location": "code/backtracking/src/partitions_of_number",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"partitions_of_number.rs",
"partitions_of_number.go",
"README.md",
"partitions_of_number.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"number_of_ways_in_maze": {
"location": "code/backtracking/src/number_of_ways_in_maze",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"no_of_ways_in_maze.java",
"noofwaysinmaze.c",
"no_of_ways_in_maze.rs",
"number_of_ways_in_maze.cpp",
"README.md",
"no_of_ways_in_maze.go"
],
"updated": "18-05-2019 08:11:06"
},
"m_coloring_problem": {
"location": "code/backtracking/src/m_coloring_problem",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"m_coloring.py"
],
"updated": "18-05-2019 08:11:06"
},
"algorithm_x": {
"location": "code/backtracking/src/algorithm_x",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"algo_x.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"knight_tour": {
"location": "code/backtracking/src/knight_tour",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"knight_tour.java",
"knight_tour.rs",
"knight_tour_withoutbt.c",
"knight_tour.cpp",
"knight_tour.go",
"knight_tour.c",
"knight_tour.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"rat_in_a_maze": {
"location": "code/backtracking/src/rat_in_a_maze",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"rat_in_a_maze.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"crossword_puzzle": {
"location": "code/backtracking/src/crossword_puzzle",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"crosswordpuzzle.java"
],
"updated": "18-05-2019 08:11:06"
},
"subset_sum": {
"location": "code/backtracking/src/subset_sum",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"subsetsum.java",
"subset_sum.cpp",
"subset_sum_duplicates.py",
"subsetsum.py",
"subset_sum.c",
"subset_sum.go",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"powerset": {
"location": "code/backtracking/src/powerset",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"powerset.java",
"power_set.c",
"powerset.go"
],
"updated": "18-05-2019 08:11:06"
},
"sudoku_solve": {
"location": "code/backtracking/src/sudoku_solve",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"sudoku.rs",
"README.md",
"sudoku_solve.py",
"sudokusolve.cpp",
"sudokusolve.c"
],
"updated": "18-05-2019 08:11:06"
},
"permutations_of_string": {
"location": "code/backtracking/src/permutations_of_string",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"permutations_of_string.c",
"permutations_of_string.go",
"permutations_of_string.kt",
"permutations_of_string_itertools.py",
"permutations_of_string_stl.cpp",
"permutations_of_string.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"partitions_of_set": {
"location": "code/backtracking/src/partitions_of_set",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"set_partitions.cpp",
"set_partitions.go",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"n_queen": {
"location": "code/backtracking/src/n_queen",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"nqueen.go",
"nqueen_bitimp.cpp",
"nqueen.hs",
"nqueen.py",
"nqueen_backtracking.rs",
"nqueen_backtracking.cpp",
"README.md",
"nqueen_bitset.cpp",
"nqueen.java",
"n_queen.c",
"nqueen_bit.go"
],
"updated": "18-05-2019 08:11:06"
}
},
"bit_manipulation": {
"sum_binary_numbers": {
"location": "code/bit_manipulation/src/sum_binary_numbers",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"sum_binary_numbers.c",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"maximum_xor_value": {
"location": "code/bit_manipulation/src/maximum_xor_value",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"max_xor_value.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"xor_swap": {
"location": "code/bit_manipulation/src/xor_swap",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"xor_swap.c",
"xor_swap.py",
"xor_swap.cpp",
"xorswap.go",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"count_set_bits": {
"location": "code/bit_manipulation/src/count_set_bits",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"count_set_bits.cs",
"count_set_bits.c",
"count_set_bits.cpp",
"countsetbits.java",
"count_set_bits_lookup_table.cpp",
"countsetbits.js",
"count_set_bits.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"lonely_integer": {
"location": "code/bit_manipulation/src/lonely_integer",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"lonely_integer.rs",
"lonelyinteger.cpp",
"lonelyint.java",
"lonelyinteger.py",
"lonelyinteger.go",
"README.md",
"lonelyinteger.c",
"lonelyint.js"
],
"updated": "18-05-2019 08:11:06"
},
"thrice_unique_number": {
"location": "code/bit_manipulation/src/thrice_unique_number",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"uniquenumber.py",
"thrice_unique_number.js",
"thriceuniquenumber.java",
"threeunique.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"magic_number": {
"location": "code/bit_manipulation/src/magic_number",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"magic_number.java",
"magic_number.py",
"README.md",
"magic_number.c",
"nth_magic_number.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"hamming_distance": {
"location": "code/bit_manipulation/src/hamming_distance",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"hamming_distance.java",
"hamming_distance.cpp",
"hamming_distance.go",
"hamming_distance.py",
"README.md",
"hamming_distance2.py",
"hamming_distance.c"
],
"updated": "18-05-2019 08:11:06"
},
"addition_using_bits": {
"location": "code/bit_manipulation/src/addition_using_bits",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"addition_using_bits.cpp",
"bitwise_addition.js",
"bitwise_addition.cs",
"addition_using_bits.py"
],
"updated": "18-05-2019 08:11:06"
},
"byte_swapper": {
"location": "code/bit_manipulation/src/byte_swapper",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"byteswapper.java"
],
"updated": "18-05-2019 08:11:06"
},
"invert_bit": {
"location": "code/bit_manipulation/src/invert_bit",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"invert_bit.c",
"invert_bit.cpp",
"invert_bit.js",
"invert_bit.py"
],
"updated": "18-05-2019 08:11:06"
},
"power_of_2": {
"location": "code/bit_manipulation/src/power_of_2",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"power_of_2.rs",
"power_of_2.go",
"power_of_2.jl",
"power_of_2.cpp",
"power_of_2.c",
"power_of_2.py",
"powerof2.cs",
"power_of_2.js",
"powerof2.java"
],
"updated": "18-05-2019 08:11:06"
},
"sum_equals_xor": {
"location": "code/bit_manipulation/src/sum_equals_xor",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"sum_equals_xor.py",
"sum_equals_xor.c",
"sum_equals_xor.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"subset_generation": {
"location": "code/bit_manipulation/src/subset_generation",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"subsetgeneratorusingbit.cpp",
"subset_mask_generator.cpp",
"README.md",
"subsetsum.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"convert_number_binary": {
"location": "code/bit_manipulation/src/convert_number_binary",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"convert_number_binary.cpp",
"convert_number_binary.c",
"inttobinary.py",
"binary_to_int.py",
"convert_number_binary.js",
"convert_number_binary.php",
"convertnumberbinary.java",
"convert_number_binary.hs",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"bit_division": {
"location": "code/bit_manipulation/src/bit_division",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bitdivision.c",
"bitdivison.py",
"bitdivision.go",
"bitdivision.js",
"bitdivision.java",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"flip_bits": {
"location": "code/bit_manipulation/src/flip_bits",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"flippingbits.py",
"flippingbits.c",
"README.md",
"flipbits.java",
"flippingbits.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"twice_unique_number": {
"location": "code/bit_manipulation/src/twice_unique_number",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"two_unique_numbers.c",
"README.md",
"two_unique_numbers.cpp"
],
"updated": "18-05-2019 08:11:06"
}
},
"networking": {
"packetsniffer": {
"location": "code/networking/src/packetsniffer",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"packetsniffer.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"determine_endianess": {
"location": "code/networking/src/determine_endianess",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"determine_endianess.c"
],
"updated": "18-05-2019 08:11:06"
}
},
"compression": {
"lossless_compression": {
"location": "code/compression/src/lossless_compression",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"lossy_compression": {
"location": "code/compression/src/lossy_compression",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
}
},
"computational_geometry": {
"cohen_sutherland_lineclip": {
"location": "code/computational_geometry/src/cohen_sutherland_lineclip",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"lineclip.c",
"README.md",
"cohenSutherlandAlgorithm.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"sphere_tetrahedron_intersection": {
"location": "code/computational_geometry/src/sphere_tetrahedron_intersection",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"luvector.hpp",
"sphere_tetrahedron_intersection.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"halfplane_intersection": {
"location": "code/computational_geometry/src/halfplane_intersection",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"halfplane_intersection.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"dda_line": {
"location": "code/computational_geometry/src/dda_line",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"dda_line.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"jarvis_march": {
"location": "code/computational_geometry/src/jarvis_march",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"jarvis_march.cpp",
"jarvis_march_alternative.cpp",
"jarvis_march.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"bresenham_circle": {
"location": "code/computational_geometry/src/bresenham_circle",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bresenham_circle.cpp",
"graphics.h"
],
"updated": "18-05-2019 08:11:06"
},
"graham_scan": {
"location": "code/computational_geometry/src/graham_scan",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"grahamscan.java",
"graham_scan_alternative.cpp",
"graham_scan.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"chans_algorithm": {
"location": "code/computational_geometry/src/chans_algorithm",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"chans_algorithm.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"2d_separating_axis_test": {
"location": "code/computational_geometry/src/2d_separating_axis_test",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"sat.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"bresenham_line": {
"location": "code/computational_geometry/src/bresenham_line",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bresenham_line.py",
"bresenham_line.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"area_of_polygon": {
"location": "code/computational_geometry/src/area_of_polygon",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"area_of_polygon.c",
"area_of_polygon.cpp",
"area_of_polygon.py",
"areaofpolygon.java"
],
"updated": "18-05-2019 08:11:06"
},
"sutherland_hodgeman_clipping": {
"location": "code/computational_geometry/src/sutherland_hodgeman_clipping",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"sutherland.cpp",
"README.md",
"sutherland.c"
],
"updated": "18-05-2019 08:11:06"
},
"distance_between_points": {
"location": "code/computational_geometry/src/distance_between_points",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"distance_between_points.py",
"distance_between_points.js",
"distance_between_points.cpp",
"distance_between_points.go",
"README.md",
"distancebetweenpoints.java",
"distance_between_points.rs",
"distance_between_points.c"
],
"updated": "18-05-2019 08:11:06"
},
"quickhull": {
"location": "code/computational_geometry/src/quickhull",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"test_data_soln.txt",
"quickhull_alternative.cpp",
"test_data.csv",
"quickhull.cpp",
"quickhull.java",
"test_data_soln.png",
"README.md",
"quickhull.hs"
],
"updated": "18-05-2019 08:11:06"
},
"2d_line_intersection": {
"location": "code/computational_geometry/src/2d_line_intersection",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"line.java",
"line.cpp",
"line.js",
"line.c",
"line2dintersection.hs",
"line.rb",
"line.cs",
"README.md",
"line.py"
],
"updated": "18-05-2019 08:11:06"
},
"axis_aligned_bounding_box_collision": {
"location": "code/computational_geometry/src/axis_aligned_bounding_box_collision",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"axis_aligned_bounding_box_collision.go",
"axis_aligned_bounding_box_collision.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"area_of_triangle": {
"location": "code/computational_geometry/src/area_of_triangle",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"area_of_triangle.go",
"area_of_triangle.cpp",
"areaoftriangle.java",
"area_of_triangle.rs",
"area_of_triangle.js",
"area_of_triangle.py",
"area_of_triangle.c"
],
"updated": "18-05-2019 08:11:06"
}
},
"concurrency": {
"monitors_system_v": {
"location": "code/operating_system/src/concurrency/monitors/monitors_system_v",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"monitors.c",
"monitors.h",
"main.c"
],
"updated": "18-05-2019 08:11:06"
},
"peterson_algorithm_in_c": {
"location": "code/operating_system/src/concurrency/peterson_algorithm_for_mutual_exclusion/peterson_algorithm_in_c",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"peterson_algo_mutual_exclusion_in_c.c",
"mythreads.h"
],
"updated": "18-05-2019 08:11:06"
}
},
"operating_system": {
"shell": {
"location": "code/operating_system/src/shell",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"processCreation": {
"location": "code/operating_system/src/processCreation",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"Processes.c",
"README.md"
],
"updated": "18-05-2019 08:11:06"
}
},
"scheduling": {
"round_robin_c": {
"location": "code/operating_system/src/scheduling/round_robin_scheduling/round_robin_c",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"queue.h",
"queue.c",
"README.md",
"round_robin.c"
],
"updated": "18-05-2019 08:11:06"
}
},
"data_structures": {
"binary_heap": {
"location": "code/data_structures/src/binary_heap",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"binary_heap.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"linked_list": {
"location": "code/data_structures/src/linked_list",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"linked_list.java",
"linked_list.cpp",
"linked_list.js",
"linked_list.c",
"linked_list.py"
],
"updated": "18-05-2019 08:11:06"
},
"bag": {
"location": "code/data_structures/src/bag",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bag.py",
"bag.js",
"bag.java"
],
"updated": "18-05-2019 08:11:06"
},
"other": {
"location": "code/data_structures/src/other",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"list": {
"location": "code/data_structures/src/list",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
}
},
"stack": {
"cpp": {
"location": "code/data_structures/src/stack/abstract_stack/cpp",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"istack.h",
"arraystack.java"
],
"updated": "18-05-2019 08:11:06"
}
},
"abstract_stack": {
"arraystack": {
"location": "code/data_structures/src/stack/abstract_stack/cpp/arraystack",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"arraystacktester.cpp",
"arraystack.h"
],
"updated": "18-05-2019 08:11:06"
}
},
"tree": {
"segment_tree": {
"location": "code/data_structures/src/tree/space_partitioning_tree/segment_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"lazy_segment_tree.java",
"segment_tree_lazy_propagation.cpp",
"segment_tree_rmq.adb",
"segment_tree_rmq.go",
"segment_tree_rmq.cpp",
"segment_tree_rmq_with_update.cpp",
"segment_tree_sum.py",
"segment_tree.java",
"segment_tree.scala",
"segment_tree_sum.rb",
"segment_tree_rmq.py",
"README.md",
"segment_tree_sum.cpp",
"persistent_segment_tree_sum.cpp",
"segment_tree_kth_statistics_on_segment.cpp",
"segment_tree_sum.go"
],
"updated": "18-05-2019 08:11:06"
},
"quad_tree": {
"location": "code/data_structures/src/tree/space_partitioning_tree/quad_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"quad_tree.swift"
],
"updated": "18-05-2019 08:11:06"
},
"interval_tree": {
"location": "code/data_structures/src/tree/space_partitioning_tree/interval_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"interval_tree.java",
"interval_tree.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"kd_tree": {
"location": "code/data_structures/src/tree/space_partitioning_tree/kd_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"kd_tree.cpp",
"kd_tree.java"
],
"updated": "18-05-2019 08:11:06"
},
"pairing_heap": {
"location": "code/data_structures/src/tree/heap/pairing_heap",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"pairing_heap.fs",
"pairing_heap.sml",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"max_heap": {
"location": "code/data_structures/src/tree/heap/max_heap",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"max_heap.java",
"max_heap.py",
"max_heap.cpp",
"max_heap.go",
"max_heap.c"
],
"updated": "18-05-2019 08:11:06"
},
"min_heap": {
"location": "code/data_structures/src/tree/heap/min_heap",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"min_heap.c",
"min_heap.js",
"min_heap.rb",
"min_heap.cpp",
"min_heap.py",
"min_heap.swift",
"min_heap.java"
],
"updated": "18-05-2019 08:11:06"
},
"priority_queue": {
"location": "code/data_structures/src/tree/heap/priority_queue",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"priority_queue.js",
"priority_queue.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"soft_heap": {
"location": "code/data_structures/src/tree/heap/soft_heap",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"soft_heap.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"binomial_heap": {
"location": "code/data_structures/src/tree/heap/binomial_heap",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"binomial_heap.scala",
"binomial_heap.cpp",
"binomial_heap.c"
],
"updated": "18-05-2019 08:11:06"
},
"avl_tree": {
"location": "code/data_structures/src/tree/binary_tree/avl_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"avl_tree.cpp",
"avl_tree.swift",
"avl_tree.java",
"avl_tree.c"
],
"updated": "18-05-2019 08:11:06"
},
"aa_tree": {
"location": "code/data_structures/src/tree/binary_tree/aa_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"aa_tree.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"binary_tree": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"treap": {
"location": "code/data_structures/src/tree/binary_tree/treap",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"treap.cpp",
"treap.java",
"treap.swift",
"treap.scala",
"persistent_treap.kt"
],
"updated": "18-05-2019 08:11:06"
},
"rope": {
"location": "code/data_structures/src/tree/binary_tree/rope",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"rope.py"
],
"updated": "18-05-2019 08:11:06"
},
"van_emde_boas_tree": {
"location": "code/data_structures/src/tree/multiway_tree/van_emde_boas_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"van_emde_boas.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"union_find": {
"location": "code/data_structures/src/tree/multiway_tree/union_find",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"union_find.py",
"union_find.c",
"union_find.js",
"union_find_dynamic.cpp",
"union_find.scala",
"union_find.go",
"union_find.cpp",
"README.md",
"union_find.java"
],
"updated": "18-05-2019 08:11:06"
},
"red_black_tree": {
"location": "code/data_structures/src/tree/multiway_tree/red_black_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"red_black_tree.java",
"red_black_tree.c",
"red_black_tree.scala",
"red_black_tree.test.cpp",
"red_black_tree.rb",
"red_black_tree.cpp",
"red_black_tree.h"
],
"updated": "18-05-2019 08:11:06"
},
"fenwick_tree": {
"location": "code/data_structures/src/tree/multiway_tree/fenwick_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"fenwick_tree.cpp",
"fenwick_tree.go",
"fenwick_tree.c",
"fenwick_tree_inversion_count.cpp",
"fenwick_tree.pl",
"README.md",
"fenwick_tree.py",
"fenwick_tree.java"
],
"updated": "18-05-2019 08:11:06"
},
"splay_tree": {
"location": "code/data_structures/src/tree/multiway_tree/splay_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"readme.md",
"splay_tree.go",
"splay_tree.kt",
"splay_tree.cpp",
"splay_tree.scala",
"splay_tree.java"
],
"updated": "18-05-2019 08:11:06"
},
"trie": {
"location": "code/data_structures/src/tree/tree/trie",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"trie.scala",
"trie.py",
"trie.cpp",
"trie.java",
"trie.rb",
"trie.c",
"trie.js",
"trie.cs",
"README.md",
"trie.swift",
"trie.go"
],
"updated": "18-05-2019 08:11:06"
},
"suffix_array": {
"location": "code/data_structures/src/tree/tree/suffix_array",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"suffix_array.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"two_three_tree": {
"location": "code/data_structures/src/tree/b_tree/two_three_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"twothreetree.scala"
],
"updated": "18-05-2019 08:11:06"
},
"b_tree": {
"location": "code/data_structures/src/tree/b_tree/b_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"b_tree.py",
"b_tree.swift",
"b_tree.cpp"
],
"updated": "18-05-2019 08:11:06"
}
},
"heap": {
"leftist_tree": {
"location": "code/data_structures/src/tree/heap/priority_queue/leftist_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"leftist_priority_queue.cpp"
],
"updated": "18-05-2019 08:11:06"
}
},
"binary_tree": {
"is_balance": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/is_balance",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"is_balance.java",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"maximum_height": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/maximum_height",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"maximum_height.java",
"maximum_height.py",
"maximum_height.cpp",
"maximum_height2.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"zigzag": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/traversal/zigzag",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"zigzag.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"is_same": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/is_same",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"is_same.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"path_sum": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/path_sum",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"path_sum.hpp",
"path_sum.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"sum_left": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/path_sum/sum_left",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md",
"sum_left.c",
"left_sum.py"
],
"updated": "18-05-2019 08:11:06"
},
"tree": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"tree.cpp",
"tree.py",
"tree.java",
"tree.rb",
"tree.c",
"tree.go",
"tree2.java",
"tree2.swift",
"README.md",
"tree.js",
"tree.swift"
],
"updated": "18-05-2019 08:11:06"
},
"bottom_view_binary_tree": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/tree/bottom_view_binary_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bottom_view_tree.java",
"bottom_view_tree.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"make_mirror_tree": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/make_mirror_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"make_mirror_tree.c",
"make_mirror_tree.cpp",
"README.md",
"make_mirror_tree.py"
],
"updated": "18-05-2019 08:11:06"
},
"is_binary_tree": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/is_binary_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"is_binary_tree.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"minimum_height": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/minimum_height",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"minimum_height.c",
"minimum_height.cpp",
"README.md",
"minimum_height.java",
"minimum_height.py"
],
"updated": "18-05-2019 08:11:06"
},
"diameter": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/diameter",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"diameter.java",
"diameter.py",
"diameter2.c",
"diameter.hs",
"diameter.cpp",
"README.md",
"diameter2.cpp",
"diameter.c"
],
"updated": "18-05-2019 08:11:06"
},
"convert_to_doubly_linked_list": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/convert_to_doubly_linked_list",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"convert_to_doubly_linked_list.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"serializer": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/serializer",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"serializer.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"make_binary_tree": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/make_binary_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"from_inorder_and_preorder": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/make_binary_tree/from_inorder_and_preorder",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"make_tree_from_inorder_and_preorder.cpp",
"make_tree_from_inorder_and_preorder.java",
"README.md",
"make_tree_from_inorder_and_preorder.c"
],
"updated": "18-05-2019 08:11:06"
},
"from_inorder_and_postorder": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/make_binary_tree/from_inorder_and_postorder",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"make_tree_from_inorder_and_postorder.cpp",
"make_tree_from_inorder_and_postorder.c"
],
"updated": "18-05-2019 08:11:06"
},
"node": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/node",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"node.cpp"
],
"updated": "18-05-2019 08:11:06"
}
},
"traversal": {
"right_threaded": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/traversal/inorder/right_threaded",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"right_threaded.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"right_view": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/traversal/preorder/right_view",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"right_view.py",
"right_view2.cpp",
"right_view.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"left_view": {
"location": "code/data_structures/src/tree/binary_tree/binary_tree/traversal/preorder/left_view",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"left_view.java"
],
"updated": "18-05-2019 08:11:06"
}
},
"b_tree": {
"b_tree_c": {
"location": "code/data_structures/src/tree/b_tree/b_tree/b_tree_c",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"btree.h",
"btree.c",
"main.c",
"README.md"
],
"updated": "18-05-2019 08:11:06"
}
},
"list": {
"c": {
"location": "code/data_structures/src/list/doubly_linked_list/c",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"doubly_linked_list.h",
"doubly_linked_list.c"
],
"updated": "18-05-2019 08:11:06"
},
"operations": {
"location": "code/data_structures/src/list/circular_linked_list/operations",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"is_circular.py",
"has_loop.py"
],
"updated": "18-05-2019 08:11:06"
},
"menu_interface": {
"location": "code/data_structures/src/list/singly_linked_list/menu_interface",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"link.h",
"singly_list.c"
],
"updated": "18-05-2019 08:11:06"
}
},
"singly_linked_list": {
"n_th_node_linked_list": {
"location": "code/data_structures/src/list/singly_linked_list/operations/n_th_node_linked_list",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"nth_node_from_end.cpp",
"nth_node_from_end.c"
],
"updated": "18-05-2019 08:11:06"
},
"find": {
"location": "code/data_structures/src/list/singly_linked_list/operations/find",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"search_element_python.py",
"searchelement_list.java",
"finding_if_element_is_present_in_the_list.c"
],
"updated": "18-05-2019 08:11:06"
},
"delete": {
"location": "code/data_structures/src/list/singly_linked_list/operations/delete",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"delete_node_with_key.java",
"delete_nth_node.c"
],
"updated": "18-05-2019 08:11:06"
},
"print_reverse": {
"location": "code/data_structures/src/list/singly_linked_list/operations/print_reverse",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"print_reverse.py",
"print_reverse.scala"
],
"updated": "18-05-2019 08:11:06"
},
"rotate": {
"location": "code/data_structures/src/list/singly_linked_list/operations/rotate",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"rotate.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"sort": {
"location": "code/data_structures/src/list/singly_linked_list/operations/sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bubble_sort.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"insertion": {
"location": "code/data_structures/src/list/singly_linked_list/operations/insertion",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"insertion_at_front.py",
"insertion_at_nth_node.py",
"insertion_at_ending.py"
],
"updated": "18-05-2019 08:11:06"
},
"detect_cycle": {
"location": "code/data_structures/src/list/singly_linked_list/operations/detect_cycle",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"detect_cycle.cpp",
"detect_cycle_hashmap.py"
],
"updated": "18-05-2019 08:11:06"
},
"unclassified": {
"location": "code/data_structures/src/list/singly_linked_list/operations/unclassified",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"linkedlist.java",
"linkedlisteg.java",
"union_intersection_in_list.c",
"linked_list_operations.cpp",
"union_intersection_in_list.textclipping"
],
"updated": "18-05-2019 08:11:06"
},
"push": {
"location": "code/data_structures/src/list/singly_linked_list/operations/push",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"push.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"merge_sorted": {
"location": "code/data_structures/src/list/singly_linked_list/operations/merge_sorted",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"merge_sorted.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"reverse": {
"location": "code/data_structures/src/list/singly_linked_list/operations/reverse",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"reverse.c",
"reverse.cpp",
"reverse_iteration.cpp",
"reverse_recursion2.cpp",
"reverse_recursion.cpp"
],
"updated": "18-05-2019 08:11:06"
}
},
"cryptography": {
"huffman_encoding": {
"location": "code/cryptography/src/huffman_encoding",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"huffman_encoding.c"
],
"updated": "18-05-2019 08:11:06"
},
"rsa_digital_signature": {
"location": "code/cryptography/src/rsa_digital_signature",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"rsa_digital_signature.ipynb"
],
"updated": "18-05-2019 08:11:06"
},
"baconian_cipher": {
"location": "code/cryptography/src/baconian_cipher",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"baconian.rb",
"baconian.js",
"baconian_cipher.c",
"baconian.cpp",
"README.md",
"baconian.java",
"baconian.php",
"baconian.py"
],
"updated": "18-05-2019 08:11:06"
},
"columnar_transposition_cipher": {
"location": "code/cryptography/src/columnar_transposition_cipher",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"columnar_transposition.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"aes_128": {
"location": "code/cryptography/src/aes_128",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"aes_128.py",
"aes_128.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"autokey_cipher": {
"location": "code/cryptography/src/autokey_cipher",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/auto-key-cipher/",
"files": [
"README.md",
"autokey_cipher.c",
"autokey_cipher.cpp",
"autokey_cipher.java"
],
"updated": "31-05-2020 23:14:00"
},
"vigenere_cipher": {
"location": "code/cryptography/src/vigenere_cipher",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"vigenere_cipher.rb",
"vigenere_cipher.cpp",
"vigenere_cipher.hs",
"vigenere_cipher.jl",
"vigenere_cipher.go",
"vigenere_cipher.py",
"vigenere_cipher.js",
"vigenere_cipher.c",
"README.md",
"vigenere_cipher.php",
"vigenere_cipher.java"
],
"updated": "18-05-2019 08:11:06"
},
"rot13_cipher": {
"location": "code/cryptography/src/rot13_cipher",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"rot13.sh",
"rot13.rb",
"rot13.py",
"rot13.js",
"rotn.java",
"rotn.js",
"rot13.cpp",
"README.md",
"rotn.cpp",
"rotn.c"
],
"updated": "18-05-2019 08:11:06"
},
"polybius_cipher": {
"location": "code/cryptography/src/polybius_cipher",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"polybius.py"
],
"updated": "18-05-2019 08:11:06"
},
"affine_cipher": {
"location": "code/cryptography/src/affine_cipher",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"affine.java",
"affine.py",
"affine.cpp",
"affinekotlin.kt",
"affine.htm",
"affine_cipher.py"
],
"updated": "18-05-2019 08:11:06"
},
"caesar_cipher": {
"location": "code/cryptography/src/caesar_cipher",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"decryption.cpp",
"caesar_cipher.rb",
"caesar_cipher.cpp",
"encryption.cpp",
"caesarcipher.java",
"caesar_cipher.go",
"caesar_cipher.py",
"README.md",
"caesar_cipher.cs",
"caesar_cipher.c",
"caesar_cipher.js",
"caesar_cipher.hs",
"caesar_cipher.php"
],
"updated": "18-05-2019 08:11:06"
},
"porta_cipher": {
"location": "code/cryptography/src/porta_cipher",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"porta.py"
],
"updated": "18-05-2019 08:11:06"
},
"rail_fence_cipher": {
"location": "code/cryptography/src/rail_fence_cipher",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"rail_fence.rb",
"rail_fence.py",
"rail_fence.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"atbash_cipher": {
"location": "code/cryptography/src/atbash_cipher",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"atbash_cipher.java",
"atbash_cipher.py",
"atbash_cipher.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"morse_cipher": {
"location": "code/cryptography/src/morse_cipher",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"morse_code_generator.bf",
"morse_code.sh",
"morse_code_translator.cpp",
"morse_code_translator.lua",
"morse_code_translator.php",
"morse_code_translator.ts",
"morse_code_generator.c",
"morse_code_translator.js",
"README.md",
"morse_code_translator.py",
"morse_code_generator.cpp",
"morsecode.java",
"morse_code_generator.rb"
],
"updated": "18-05-2019 08:11:06"
},
"autokey_cipher": {
"location": "code/cryptography/src/autokey_cipher",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"autokey.py"
],
"updated": "18-05-2019 08:11:06"
},
"rsa": {
"location": "code/cryptography/src/rsa",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"rsa.c",
"rsa_input.in",
"rsa.py",
"rsa.cs",
"rsa.java"
],
"updated": "18-05-2019 08:11:06"
},
"runningkey_cipher": {
"location": "code/cryptography/src/runningkey_cipher",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"runningkey.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
}
},
"aes_128": {
"example": {
"location": "code/cryptography/src/aes_128/aes_csharp/example",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"streamcipherexception.cs",
"streamcipher.cs",
"README.md"
],
"updated": "18-05-2019 08:11:06"
}
},
"greedy_algorithms": {
"prim_minimum_spanning_tree": {
"location": "code/greedy_algorithms/src/prim_minimum_spanning_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"prim_minimum_spanning_tree.cpp",
"prim_minimum_spanning_tree.c",
"README.md",
"prim_minimum_spanning_tree.py",
"prim_minimum_spanning_tree.hs"
],
"updated": "18-05-2019 08:11:06"
},
"job_sequencing": {
"location": "code/greedy_algorithms/src/job_sequencing",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"job_sequencing.cpp",
"job_sequencing.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"kruskal_minimum_spanning_tree": {
"location": "code/greedy_algorithms/src/kruskal_minimum_spanning_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"kruskal.py",
"kruskal.c",
"kruskal_using_adjacency_matrix.c",
"kruskal.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"huffman_coding": {
"location": "code/greedy_algorithms/src/huffman_coding",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"huffman_coding.cpp",
"huffman_coding.c",
"README.md",
"huffman_coding.py"
],
"updated": "18-05-2019 08:11:06"
},
"activity_selection": {
"location": "code/greedy_algorithms/src/activity_selection",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"activity_selection.java",
"activity_selection.cpp",
"activity_selection.py",
"activity_selection.c",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"minimum_coins": {
"location": "code/greedy_algorithms/src/minimum_coins",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"minimum_coins.js",
"minimum_coins.py",
"minimum_coins.c",
"minimum_coins.cpp",
"minimumcoins.java",
"minimum_coins.go",
"minimumcoins.hs",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"dijkstra_shortest_path": {
"location": "code/greedy_algorithms/src/dijkstra_shortest_path",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"dijkstra_shortest_path.java",
"dijkstra_shortest_path.c",
"dijkstra_shortest_path.py",
"README.md",
"dijkstra_shortest_path.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"warshall": {
"location": "code/greedy_algorithms/src/warshall",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"warshalls.c"
],
"updated": "18-05-2019 08:11:06"
},
"fractional_knapsack": {
"location": "code/greedy_algorithms/src/fractional_knapsack",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"fractional_knapsack.java",
"fractional_knapsack.cpp",
"fractional_knapsack.c",
"fractional_knapsack.cs",
"fractional_knapsack.py",
"README.md",
"fractional_knapsack.go"
],
"updated": "18-05-2019 08:11:06"
},
"k_centers": {
"location": "code/greedy_algorithms/src/k_centers",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"k_centers.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"hillclimber": {
"location": "code/greedy_algorithms/src/hillclimber",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"hillclimber.java"
],
"updated": "18-05-2019 08:11:06"
},
"egyptian_fraction": {
"location": "code/greedy_algorithms/src/egyptian_fraction",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"egyptian_fraction.c",
"egyptian_fraction.cpp",
"egyptian_fraction.py"
],
"updated": "18-05-2019 08:11:06"
}
},
"code": {
"src": {
"location": "code/sorting/src",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"circle_sort": {
"location": "code/sorting/circle_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:07"
}
},
"sorting": {
"topological_sort": {
"location": "code/sorting/src/topological_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"readme.md",
"topological_sort.c",
"topological_sort.java",
"topological_sort.py",
"topological_sort.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"bucket_sort": {
"location": "code/sorting/src/bucket_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bucket_sort.js",
"bucket_sort.c",
"bucket_sort.rb",
"bucket_sort.cs",
"bucket_sort.go",
"bucket_sort.php",
"bucket_sort.java",
"bucket_sort.swift",
"bucket_sort.py",
"README.md",
"bucket_sort.m",
"bucket_sort.cpp",
"bucket_sort.hs"
],
"updated": "18-05-2019 08:11:06"
},
"flash_sort": {
"location": "code/sorting/src/flash_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"flash_sort.js",
"flash_sort.c",
"flash_sort.m",
"README.md",
"flash_sort.swift"
],
"updated": "18-05-2019 08:11:06"
},
"median_sort": {
"location": "code/sorting/src/median_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"median_sort.py",
"median_sort.m",
"median_sort.swift",
"median_sort.cpp",
"median_sort_fast.cpp",
"median_sort.cs"
],
"updated": "18-05-2019 08:11:06"
},
"shell_sort": {
"location": "code/sorting/src/shell_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"shell_sort.py",
"shell_sort.c",
"shell_sort.go",
"shell_sort.js",
"shell_sort.cpp",
"shell_sort.swift",
"shellsort.go",
"shell_sort.java",
"shell_sort.kt",
"README.md",
"shell_sort.m"
],
"updated": "18-05-2019 08:11:06"
},
"quick_sort": {
"location": "code/sorting/src/quick_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"quick_sort_median_of_medians.c",
"quick_sort.py",
"quick_sort.swift",
"quick_sort.elm",
"quick_sort_extension.swift",
"quick_sort.java",
"quick_sort.m",
"quick_sort.hs",
"quick_sort.c",
"quick_sort.cs",
"quick_sort.cpp",
"quick_sort.go",
"quick_sort.ts",
"quick_sort.js",
"quick_sort.ml",
"quick_sort.scala",
"dutch_national_flag.cpp",
"quick_sort.lua",
"quick_sort.rb",
"README.md",
"quick_sort_in_place.scala",
"quick_sort_three_way.cpp",
"quick_sort.sh",
"quick_sort.rs"
],
"updated": "18-05-2019 08:11:06"
},
"selection_sort": {
"location": "code/sorting/src/selection_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"selection_sort.vb",
"selection_sort.kt",
"selection_sort.cpp",
"selection_sort.py",
"selection_sort.php",
"selection_sort.go",
"selection_sort.m",
"selection_sort_extension.swift",
"selection_sort.c",
"README.md",
"selection_sort.sh",
"selection_sort.swift",
"selection_sort.hs",
"selection_sort.js",
"selection_sort.rs",
"selection_sort.cs",
"selection_sort.rb",
"selection_sort.java"
],
"updated": "18-05-2019 08:11:06"
},
"bead_sort": {
"location": "code/sorting/src/bead_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bead_sort_numpy.py",
"bead_sort.cpp",
"bead_sort.swift",
"bead_sort.js",
"bead_sort.m",
"bead_sort.php",
"bead_sort.java",
"README.md",
"bead_sort.cs",
"bead_sort.py",
"bead_sort.c"
],
"updated": "18-05-2019 08:11:06"
},
"stooge_sort": {
"location": "code/sorting/src/stooge_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"stooge_sort.java",
"stooge_sort.js",
"stooge_sort.go",
"stooge_sort.c",
"stooge_sort.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"counting_sort": {
"location": "code/sorting/src/counting_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"counting_sort.py",
"counting_sort.go",
"counting_sort.swift",
"counting_sort.cpp",
"counting_sort.js",
"counting_sort.java",
"counting_sort.m",
"counting_sort.c",
"README.md",
"counting_sort.cs"
],
"updated": "18-05-2019 08:11:06"
},
"sleep_sort": {
"location": "code/sorting/src/sleep_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"sleep_sort.cpp",
"sleep_sort.swift",
"sleep_sort.cs",
"sleep_sort.c",
"sleep_sort.py",
"sleep_sort.sh",
"sleep_sort.js",
"sleep_sort.go",
"sleep_sort.jl",
"sleep_sort.php",
"README.md",
"sleep_sort.rb",
"sleep_sort.scala",
"sleep_sort.m",
"sleep_sort.java"
],
"updated": "18-05-2019 08:11:06"
},
"heap_sort": {
"location": "code/sorting/src/heap_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"heap_sort.py",
"heap_sort.m",
"heap_sort.swift",
"heap_sort.js",
"heap_sort.cpp",
"heapsort.go",
"heap_sort.java",
"README.md",
"heap_sort.rs",
"heap_sort.sc",
"heap_sort.rb",
"heap_sort.go",
"heap_sort.cs",
"heap_sort.c"
],
"updated": "18-05-2019 08:11:06"
},
"intro_sort": {
"location": "code/sorting/src/intro_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"intro_sort.swift",
"intro_sort.m",
"intro_sort.cpp",
"README.md"
],
"updated": "18-05-2019 08:11:06"
},
"tree_sort": {
"location": "code/sorting/src/tree_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"tree_sort.cpp",
"tree_sort.js",
"tree_sort.c",
"README.md",
"tree_sort.java",
"tree_sort.py",
"tree_sort.go"
],
"updated": "18-05-2019 08:11:06"
},
"comb_sort": {
"location": "code/sorting/src/comb_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"comb_sort.go",
"comb_sort.js",
"comb_sort.c",
"comb_sort.swift",
"comb_sort.m",
"comb_sort.java",
"README.md",
"comb_sort.cpp",
"comb_sort.py"
],
"updated": "18-05-2019 08:11:06"
},
"bogo_sort": {
"location": "code/sorting/src/bogo_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bogo_sort.java",
"bogo_sort.fs",
"bogo_sort.swift",
"bogo_sort.js",
"bogo_sort.py",
"bogo_sort.c",
"README.md",
"bogo_sort.go",
"bogo_sort.m",
"bogo_sort.pl",
"bogo_sort.rb",
"bogo_sort.cpp"
],
"updated": "18-05-2019 08:11:06"
},
"insertion_sort": {
"location": "code/sorting/src/insertion_sort",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/insertion-sort-analysis/",
"files": [
"insertion_sort_extension.swift",
"insertion_sort.hs",
"insertion_sort.py",
"insertion_sort.rb",
"insertion_sort.go",
"insertion_sort.cs",
"insertion_sort.java",
"insertion_sort.ml",
"insertion_sort.kt",
"insertion_sort.sh",
"insertion_sort.swift",
"insertion_sort.php",
"insertion_sort.c",
"insertion_sort.m",
"insertion_sort.rs",
"insertion_sort.cpp",
"README.md",
"insertion_sort.js",
"insertion_sort.re"
],
"updated": "06-05-2020 4:24:00"
},
"bubble_sort": {
"location": "code/sorting/src/bubble_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"bubble_sort.py",
"bubble_sort.sml",
"bubble_sort.go",
"bubble_sort.hs",
"bubble_sort.rb",
"bubble_sort.sh",
"bubble_sort.jl",
"bubble_sort.php",
"bubble_sort.m",
"bubble_sort.c",
"bubble_sort.swift",
"bubble_sort.cs",
"bubble_sort.cpp",
"bubble_sort.rs",
"bubble_sort.exs",
"bubble_sort_extension.swift",
"bubble_sort_efficient.cpp",
"bubble_sort.ts",
"README.md",
"bubble_sort.js",
"bubble_sort.f",
"bubble_sort.elm",
"bubble_sort.java",
"bubble_sort.kt"
],
"updated": "18-05-2019 08:11:07"
},
"merge_sort": {
"location": "code/sorting/src/merge_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"merge_sort.cs",
"merge_sort.sh",
"merge_sort.ts",
"merge_sort.kt",
"merge_sort.pl",
"merge_sort.fs",
"merge_sort.php",
"merge_sort.go",
"merge_sort_extension.swift",
"merge_sort.js",
"merge_sort.m",
"merge_sort.rs",
"merge_sort.java",
"merge_sort.py",
"merge_sort.scala",
"merge_sort.rb",
"merge_sort.c",
"merge_sort.hs",
"README.md",
"merge_sort.cpp",
"merge_sort_linked_list.c",
"merge_sort.swift"
],
"updated": "18-05-2019 08:11:07"
},
"cycle_sort": {
"location": "code/sorting/src/cycle_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"cycle_sort.py",
"cycle_sort.go",
"cycle_sort.swift",
"cycle_sort.c",
"cycle_sort.js",
"cycle_sort.java",
"README.md",
"cycle_sort.m",
"cycle_sort.cs",
"cycle_sort.cpp"
],
"updated": "18-05-2019 08:11:07"
},
"pigeonhole_sort": {
"location": "code/sorting/src/pigeonhole_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"pigeonhole_sort.cpp",
"pigeonhole_sort.php",
"pigeonhole_sort.js",
"pigeonhole_sort.go",
"pigeonhole_sort.py",
"pigeonhole_sort.m",
"pigeonhole_sort.c",
"pigeonhole_sort.swift",
"pigeonhole_sort.java",
"README.md",
"pigeonhole_sort.cs",
"pigeonholesort.scala"
],
"updated": "18-05-2019 08:11:07"
},
"radix_sort": {
"location": "code/sorting/src/radix_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"radix_sort.py",
"radix_sort.c",
"radix_sort.js",
"radix_sort.go",
"radix_sort.rs",
"radix_sort.java",
"radix_sort.cpp",
"README.md",
"radix_sort.hs"
],
"updated": "18-05-2019 08:11:07"
},
"gnome_sort": {
"location": "code/sorting/src/gnome_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"gnome_sort.java",
"gnome_sort.go",
"gnome_sort.swift",
"gnome_sort.cpp",
"README.md",
"gnome_sort.c",
"gnome_sort.js",
"gnome_sort.m",
"gnome_sort.py"
],
"updated": "18-05-2019 08:11:07"
},
"shaker_sort": {
"location": "code/sorting/src/shaker_sort",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"shaker_sort.m",
"shaker_sort.cs",
"shaker_sort.js",
"shaker_sort.cpp",
"shaker_sort.java",
"shaker_sort.c",
"shaker_sort.py",
"shaker_sort.swift",
"shaker_sort.go",
"shaker_sort.rs",
"shaker_sort.php",
"README.md"
],
"updated": "18-05-2019 08:11:07"
},
"linear_search": {
"location": "code/languages/c/linear_search",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"linear_search.c",
"linear_search_duplicates.c",
"linear_search_duplicates_linked_list.c",
"linear_search_linked_list.c "
"README.md"
],
"updated": "31-05-2020 06:50:00"
},
"initializing_multimap": {
"location": "code/languages/cpp/initializing_multimap",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"multimap.cpp"
"README.md"
],
"updated": "31-05-2020 07:18:07"
},
"languages": {
"2D_array_Numpy": {
"location": "code/languages/python/2D-array-Numpy",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"2D-array-numpy.py",
"README.md"
],
"updated": "24-04-2020 09:45:30"
},
"bootstrap-table": {
"location": "code/html/bootstrap",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/bootstrap-tables",
"files": [
"tables.html",
"Readme.MD"
],
"updated": "28-04-2020 19:15:46"
},
"Handlingexceptions": {
"location": "code/java/Handlingexceptions",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/handling-exceptions-in-java/",
"files": [
"Handlingexp.java",
"README.MD"
],
"updated": "31-05-2020 00:01:57"
},
"armstrong_num_range": {
"location": "code/mathematical_algorithms/src/armstrong_num_range",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/find-all-armstrong-numbers-in-a-range/",
"files": [
"armstrong_num_range.c",
"REAADME.md"
],
"updated": "03-05-2020 21:15:57"
},
"counter_objects": {
"location": "code/languages/python/counter_objects",
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/counter-objects-in-python/",
"files": [
"counter_obj.py",
"README.md"
],
"updated": "29-05-2020 23:50:46"
},
"VGG-11":{
"location": "code/artificial_intelligence/src/neural_network"
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/vgg-11",
"files": [
"vgg1.md"
"README.md"
],
"updated": "30-05-2020 12:03:47"
},
"Inception_Model":{
"location": "code/artificial_intelligence/src/Inception_Pre-trained_Model"
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/inception-pre-trained-cnn-model/",
"files": [
"Inception_model.md"
"README.md"
],
"updated": "30-05-2020 04:57:47"
},
"Binary_GCD_Algorithm":{
"location": "code/mathematical_algorithms/src/Binary_GCD_Algorithm"
"opengenus_discuss" : "",
"opengenus_iq": " https://iq.opengenus.org/p/27a7354e-a256-4fcd-876e-5ffdbdf9b668/",
"files": [
"Binary_GCD_Iterative.cpp"
"Binary_ GCD_Recursive.cpp"
"README.md"
],
"updated": "31-05-2020 01:08:47"
},
"this_reference":{
"location": "code/languages/Java/this_reference"
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/use-of-this-in-java/",
"files": [
"this.java"
"Readme.md"
],
"updated": "30-05-2020 11:47:47"
},
"Java_String_Class":{
"location": "code/languages/Java/String"
"opengenus_discuss": "",
"opengenus_iq": "https://iq.opengenus.org/java-lang-string-class/",
"files": [
"StringClass.java"
"Readme.md"
],
"updated": "31-05-2020 07:45:47"
}
}
|
scripts/javascript_code_style_checker.sh | #!/usr/bin/env bash
find code/ -type f -name "*.js" | xargs prettier --check
|
scripts/metadata-generator.py | import json
import pathlib
import collections
from datetime import datetime
def first_layer_files(directory):
p = pathlib.Path(directory)
files = []
for file in p.iterdir():
if file.is_file():
files.append(str(file).split("/")[-1])
return files
Path = collections.namedtuple("Entry", ("suffix", "group", "name"))
avoid_extensions = [
"",
# ".md",
# ".png",
# ".csv",
# ".class",
# ".data",
# ".in",
# ".jpeg",
# ".jpg",
# ".out",
# ".textclipping",
# ".properties",
# ".txt",
# ".sbt",
]
avoid_dirs = ["project", "test", "img", "image", "images"]
paths = []
original_paths = collections.defaultdict(str)
for path in pathlib.Path(__file__).parents[1].glob("code/**/**/*"):
if (path.suffix
and not any(elem in list(path.parts) for elem in avoid_dirs)
and path.suffix.lower() not in avoid_extensions):
original_paths[path.parts[-2]] = "/".join(path.parts[:-2])
paths.append(
Path(
suffix=path.suffix.lstrip(".").lower(),
group=path.parts[1].replace("-", " ").replace("_", " "),
name=path.parts[-2].replace("-", " ").replace("_", " "),
))
metadata = dict()
for each in paths:
x = each[-1].replace(" ", "_").rstrip()
metadata["location"] = original_paths[x] + "/" + x
metadata_path = "scripts/metadata/{}".format(metadata["location"])
pathlib.Path(metadata_path).mkdir(parents=True, exist_ok=True)
filename = pathlib.Path("{}/data.json".format(metadata_path))
# if file doesn't exist set the link values
if not filename.exists():
metadata["opengenus_discuss"] = ""
metadata["opengenus_iq"] = ""
else:
# retain previous values
with open(filename) as fh:
existing_data = json.load(fh)
metadata["opengenus_discuss"] = existing_data["opengenus_discuss"]
metadata["opengenus_iq"] = existing_data["opengenus_iq"]
filename.touch(exist_ok=True)
metadata["files"] = first_layer_files(metadata["location"])
metadata["updated"] = datetime.today().strftime("%d-%m-%Y %H:%M:%S")
json_dump = json.dumps(metadata, indent=2)
filename.write_text(json_dump)
|
scripts/metadata/code/artificial_intelligence/src/a_star/data.json | {
"location": "code/artificial_intelligence/src/a_star",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"astar.py"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/artificial_neural_network/data.json | {
"location": "code/artificial_intelligence/src/artificial_neural_network",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"dataset.csv",
"ann.py"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/autoenncoder/data.json | {
"location": "code/artificial_intelligence/src/autoenncoder",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"autoencoder.ipynb"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/convolutional_neural_network/data.json | {
"location": "code/artificial_intelligence/src/convolutional_neural_network",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"cnn_adversarial_examples.ipynb",
"cnn.ipynb",
"train.csv",
"test.csv"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/dbscan_clustering/data.json | {
"location": "code/artificial_intelligence/src/dbscan_clustering",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"readme.md",
"dbscan.py"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/decision_tree/data.json | {
"location": "code/artificial_intelligence/src/decision_tree",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"decision_trees_information_gain.py",
"decision_tree.py",
"data_banknote_authentication.csv"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/factorization_machines/data.json | {
"location": "code/artificial_intelligence/src/factorization_machines",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"matrix_factorization.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/gaussian_mixture_model/data.json | {
"location": "code/artificial_intelligence/src/gaussian_mixture_model",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/gaussian_naive_bayes/data.json | {
"location": "code/artificial_intelligence/src/gaussian_naive_bayes",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"gaussiannaivebayesmoons.py",
"graph.png"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/gradient_boosting_trees/data.json | {
"location": "code/artificial_intelligence/src/gradient_boosting_trees",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"README.md"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/hierachical_clustering/hierachical_clustering/data.json | {
"location": "code/artificial_intelligence/src/hierachical_clustering/hierachical_clustering",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"hierarchical_clustering.ipynb"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/image_processing/canny/data.json | {
"location": "code/artificial_intelligence/src/image_processing/canny",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"canny.h",
"main.cpp",
"canny.cpp"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/image_processing/data.json | {
"location": "code/artificial_intelligence/src/image_processing",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"install_opencv.sh",
"README.md"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/image_processing/erode_dilate/data.json | {
"location": "code/artificial_intelligence/src/image_processing/erode_dilate",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"main.cpp"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/image_processing/houghtransform/data.json | {
"location": "code/artificial_intelligence/src/image_processing/houghtransform",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"main.cpp"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/image_processing/image_stitching/data.json | {
"location": "code/artificial_intelligence/src/image_processing/image_stitching",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"a.out",
"imagestitching.cpp"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/image_processing/prewittfilter/data.json | {
"location": "code/artificial_intelligence/src/image_processing/prewittfilter",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"prewitt.cpp"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/image_processing/sobelfilter/data.json | {
"location": "code/artificial_intelligence/src/image_processing/sobelfilter",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"sobel.cpp"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/isodata_clustering/data.json | {
"location": "code/artificial_intelligence/src/isodata_clustering",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"readme.md",
"isodata.py"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/k_means/data.json | {
"location": "code/artificial_intelligence/src/k_means",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"k_means.swift",
"k_means.cpp",
"k_means.py",
"README.md"
],
"updated": "18-05-2019 08:11:06"
} |
scripts/metadata/code/artificial_intelligence/src/k_nearest_neighbours/data.json | {
"location": "code/artificial_intelligence/src/k_nearest_neighbours",
"opengenus_discuss": "",
"opengenus_iq": "",
"files": [
"k_nearest_neighbours.py",
"iris.data"
],
"updated": "18-05-2019 08:11:06"
} |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.