Search is not available for this dataset
id
stringlengths 1
8
| text
stringlengths 72
9.81M
| addition_count
int64 0
10k
| commit_subject
stringlengths 0
3.7k
| deletion_count
int64 0
8.43k
| file_extension
stringlengths 0
32
| lang
stringlengths 1
94
| license
stringclasses 10
values | repo_name
stringlengths 9
59
|
---|---|---|---|---|---|---|---|---|
1000 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, int hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
static internal extern bool SetupDiGetDeviceInterfaceDetailBuffer(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
<MSG> HWND is HANDLE which maps to C#'s IntPtr
https://docs.microsoft.com/en-us/windows/win32/winprog/windows-data-types#hwnd
<DFF> @@ -140,7 +140,7 @@ namespace HidLibrary
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
- static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, int hwndParent, int flags);
+ static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
static internal extern bool SetupDiGetDeviceInterfaceDetailBuffer(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
| 1 | HWND is HANDLE which maps to C#'s IntPtr https://docs.microsoft.com/en-us/windows/win32/winprog/windows-data-types#hwnd | 1 | .cs | cs | mit | mikeobrien/HidLibrary |
1001 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, int hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
static internal extern bool SetupDiGetDeviceInterfaceDetailBuffer(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
<MSG> HWND is HANDLE which maps to C#'s IntPtr
https://docs.microsoft.com/en-us/windows/win32/winprog/windows-data-types#hwnd
<DFF> @@ -140,7 +140,7 @@ namespace HidLibrary
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
- static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, int hwndParent, int flags);
+ static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
static internal extern bool SetupDiGetDeviceInterfaceDetailBuffer(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
| 1 | HWND is HANDLE which maps to C#'s IntPtr https://docs.microsoft.com/en-us/windows/win32/winprog/windows-data-types#hwnd | 1 | .cs | cs | mit | mikeobrien/HidLibrary |
1002 | <NME> Main.Designer.cs
<BEF> namespace TestHarness
{
partial class Main
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.Devices = new System.Windows.Forms.ListBox();
this.Refresh = new System.Windows.Forms.Button();
this.Scan = new System.Windows.Forms.Button();
this.Read = new System.Windows.Forms.Button();
this.SuccessBeep = new System.Windows.Forms.Button();
this.ErrorBeep = new System.Windows.Forms.Button();
this.Output = new System.Windows.Forms.TextBox();
this.SuspendLayout();
//
// Devices
//
this.Devices.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.Devices.FormattingEnabled = true;
this.Devices.Location = new System.Drawing.Point(0, 12);
this.Devices.Name = "Devices";
this.Devices.Size = new System.Drawing.Size(549, 147);
this.Devices.TabIndex = 0;
this.Devices.SelectedIndexChanged += new System.EventHandler(this.Devices_SelectedIndexChanged);
this.Devices.DoubleClick += new System.EventHandler(this.Devices_DoubleClick);
//
// Refresh
//
this.Refresh.Location = new System.Drawing.Point(350, 164);
this.Refresh.Name = "Refresh";
this.Refresh.Size = new System.Drawing.Size(75, 23);
this.Refresh.TabIndex = 1;
this.Refresh.Text = "Refresh";
this.Refresh.UseVisualStyleBackColor = true;
this.Refresh.Click += new System.EventHandler(this.Refresh_Click);
//
// Scan
//
this.Scan.Location = new System.Drawing.Point(93, 164);
this.Scan.Name = "Scan";
this.Scan.Size = new System.Drawing.Size(75, 23);
this.Scan.TabIndex = 2;
this.Scan.Text = "Scan";
this.Scan.UseVisualStyleBackColor = true;
this.Scan.MouseDown += new System.Windows.Forms.MouseEventHandler(this.Scan_MouseDown);
this.Scan.MouseUp += new System.Windows.Forms.MouseEventHandler(this.Scan_MouseUp);
//
// Read
//
this.Read.Location = new System.Drawing.Point(12, 164);
this.Read.Name = "Read";
this.Read.Size = new System.Drawing.Size(75, 23);
this.Read.TabIndex = 3;
this.Read.Text = "Read";
this.Read.UseVisualStyleBackColor = true;
this.Read.Click += new System.EventHandler(this.Read_Click);
//
// SuccessBeep
//
this.SuccessBeep.Location = new System.Drawing.Point(255, 164);
this.SuccessBeep.Name = "SuccessBeep";
this.SuccessBeep.Size = new System.Drawing.Size(89, 23);
this.SuccessBeep.TabIndex = 4;
this.SuccessBeep.Text = "Success Beep";
this.SuccessBeep.UseVisualStyleBackColor = true;
this.SuccessBeep.Click += new System.EventHandler(this.SuccessBeep_Click);
//
// ErrorBeep
//
this.ErrorBeep.Location = new System.Drawing.Point(174, 164);
this.ErrorBeep.Name = "ErrorBeep";
this.ErrorBeep.Size = new System.Drawing.Size(75, 23);
this.ErrorBeep.TabIndex = 5;
this.ErrorBeep.Text = "Error Beep";
this.ErrorBeep.UseVisualStyleBackColor = true;
this.ErrorBeep.Click += new System.EventHandler(this.ErrorBeep_Click);
//
// Output
//
this.Output.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
| System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.Output.Location = new System.Drawing.Point(0, 193);
this.Output.Multiline = true;
this.Output.Name = "Output";
this.Output.Size = new System.Drawing.Size(549, 274);
this.Output.TabIndex = 6;
//
// Main
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(549, 470);
this.Controls.Add(this.Output);
this.Controls.Add(this.ErrorBeep);
this.Controls.Add(this.SuccessBeep);
this.Controls.Add(this.Read);
this.Controls.Add(this.Scan);
this.Controls.Add(this.Refresh);
this.Controls.Add(this.Devices);
this.Name = "Main";
this.Text = "Hid Test Harness";
this.Load += new System.EventHandler(this.Main_Load);
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.ListBox Devices;
private System.Windows.Forms.Button Refresh;
private System.Windows.Forms.Button Scan;
private System.Windows.Forms.Button Read;
private System.Windows.Forms.Button SuccessBeep;
private System.Windows.Forms.Button ErrorBeep;
private System.Windows.Forms.TextBox Output;
}
}
<MSG> Fix merge conflicts
<DFF> @@ -29,7 +29,7 @@
private void InitializeComponent()
{
this.Devices = new System.Windows.Forms.ListBox();
- this.Refresh = new System.Windows.Forms.Button();
+ this.RefreshButton = new System.Windows.Forms.Button();
this.Scan = new System.Windows.Forms.Button();
this.Read = new System.Windows.Forms.Button();
this.SuccessBeep = new System.Windows.Forms.Button();
@@ -39,8 +39,8 @@
//
// Devices
//
- this.Devices.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
- | System.Windows.Forms.AnchorStyles.Right)));
+ this.Devices.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
+ | System.Windows.Forms.AnchorStyles.Right)));
this.Devices.FormattingEnabled = true;
this.Devices.Location = new System.Drawing.Point(0, 12);
this.Devices.Name = "Devices";
@@ -49,15 +49,15 @@
this.Devices.SelectedIndexChanged += new System.EventHandler(this.Devices_SelectedIndexChanged);
this.Devices.DoubleClick += new System.EventHandler(this.Devices_DoubleClick);
//
- // Refresh
+ // RefreshButton
//
- this.Refresh.Location = new System.Drawing.Point(350, 164);
- this.Refresh.Name = "Refresh";
- this.Refresh.Size = new System.Drawing.Size(75, 23);
- this.Refresh.TabIndex = 1;
- this.Refresh.Text = "Refresh";
- this.Refresh.UseVisualStyleBackColor = true;
- this.Refresh.Click += new System.EventHandler(this.Refresh_Click);
+ this.RefreshButton.Location = new System.Drawing.Point(350, 164);
+ this.RefreshButton.Name = "RefreshButton";
+ this.RefreshButton.Size = new System.Drawing.Size(75, 23);
+ this.RefreshButton.TabIndex = 1;
+ this.RefreshButton.Text = "Refresh";
+ this.RefreshButton.UseVisualStyleBackColor = true;
+ this.RefreshButton.Click += new System.EventHandler(this.Refresh_Click);
//
// Scan
//
@@ -102,9 +102,9 @@
//
// Output
//
- this.Output.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
- | System.Windows.Forms.AnchorStyles.Left)
- | System.Windows.Forms.AnchorStyles.Right)));
+ this.Output.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
+ | System.Windows.Forms.AnchorStyles.Left)
+ | System.Windows.Forms.AnchorStyles.Right)));
this.Output.Location = new System.Drawing.Point(0, 193);
this.Output.Multiline = true;
this.Output.Name = "Output";
@@ -121,7 +121,7 @@
this.Controls.Add(this.SuccessBeep);
this.Controls.Add(this.Read);
this.Controls.Add(this.Scan);
- this.Controls.Add(this.Refresh);
+ this.Controls.Add(this.RefreshButton);
this.Controls.Add(this.Devices);
this.Name = "Main";
this.Text = "Hid Test Harness";
@@ -134,7 +134,7 @@
#endregion
private System.Windows.Forms.ListBox Devices;
- private System.Windows.Forms.Button Refresh;
+ private System.Windows.Forms.Button RefreshButton;
private System.Windows.Forms.Button Scan;
private System.Windows.Forms.Button Read;
private System.Windows.Forms.Button SuccessBeep;
| 16 | Fix merge conflicts | 16 | .cs | Designer | mit | mikeobrien/HidLibrary |
1003 | <NME> Main.Designer.cs
<BEF> namespace TestHarness
{
partial class Main
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.Devices = new System.Windows.Forms.ListBox();
this.Refresh = new System.Windows.Forms.Button();
this.Scan = new System.Windows.Forms.Button();
this.Read = new System.Windows.Forms.Button();
this.SuccessBeep = new System.Windows.Forms.Button();
this.ErrorBeep = new System.Windows.Forms.Button();
this.Output = new System.Windows.Forms.TextBox();
this.SuspendLayout();
//
// Devices
//
this.Devices.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.Devices.FormattingEnabled = true;
this.Devices.Location = new System.Drawing.Point(0, 12);
this.Devices.Name = "Devices";
this.Devices.Size = new System.Drawing.Size(549, 147);
this.Devices.TabIndex = 0;
this.Devices.SelectedIndexChanged += new System.EventHandler(this.Devices_SelectedIndexChanged);
this.Devices.DoubleClick += new System.EventHandler(this.Devices_DoubleClick);
//
// Refresh
//
this.Refresh.Location = new System.Drawing.Point(350, 164);
this.Refresh.Name = "Refresh";
this.Refresh.Size = new System.Drawing.Size(75, 23);
this.Refresh.TabIndex = 1;
this.Refresh.Text = "Refresh";
this.Refresh.UseVisualStyleBackColor = true;
this.Refresh.Click += new System.EventHandler(this.Refresh_Click);
//
// Scan
//
this.Scan.Location = new System.Drawing.Point(93, 164);
this.Scan.Name = "Scan";
this.Scan.Size = new System.Drawing.Size(75, 23);
this.Scan.TabIndex = 2;
this.Scan.Text = "Scan";
this.Scan.UseVisualStyleBackColor = true;
this.Scan.MouseDown += new System.Windows.Forms.MouseEventHandler(this.Scan_MouseDown);
this.Scan.MouseUp += new System.Windows.Forms.MouseEventHandler(this.Scan_MouseUp);
//
// Read
//
this.Read.Location = new System.Drawing.Point(12, 164);
this.Read.Name = "Read";
this.Read.Size = new System.Drawing.Size(75, 23);
this.Read.TabIndex = 3;
this.Read.Text = "Read";
this.Read.UseVisualStyleBackColor = true;
this.Read.Click += new System.EventHandler(this.Read_Click);
//
// SuccessBeep
//
this.SuccessBeep.Location = new System.Drawing.Point(255, 164);
this.SuccessBeep.Name = "SuccessBeep";
this.SuccessBeep.Size = new System.Drawing.Size(89, 23);
this.SuccessBeep.TabIndex = 4;
this.SuccessBeep.Text = "Success Beep";
this.SuccessBeep.UseVisualStyleBackColor = true;
this.SuccessBeep.Click += new System.EventHandler(this.SuccessBeep_Click);
//
// ErrorBeep
//
this.ErrorBeep.Location = new System.Drawing.Point(174, 164);
this.ErrorBeep.Name = "ErrorBeep";
this.ErrorBeep.Size = new System.Drawing.Size(75, 23);
this.ErrorBeep.TabIndex = 5;
this.ErrorBeep.Text = "Error Beep";
this.ErrorBeep.UseVisualStyleBackColor = true;
this.ErrorBeep.Click += new System.EventHandler(this.ErrorBeep_Click);
//
// Output
//
this.Output.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
| System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right)));
this.Output.Location = new System.Drawing.Point(0, 193);
this.Output.Multiline = true;
this.Output.Name = "Output";
this.Output.Size = new System.Drawing.Size(549, 274);
this.Output.TabIndex = 6;
//
// Main
//
this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F);
this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font;
this.ClientSize = new System.Drawing.Size(549, 470);
this.Controls.Add(this.Output);
this.Controls.Add(this.ErrorBeep);
this.Controls.Add(this.SuccessBeep);
this.Controls.Add(this.Read);
this.Controls.Add(this.Scan);
this.Controls.Add(this.Refresh);
this.Controls.Add(this.Devices);
this.Name = "Main";
this.Text = "Hid Test Harness";
this.Load += new System.EventHandler(this.Main_Load);
this.ResumeLayout(false);
this.PerformLayout();
}
#endregion
private System.Windows.Forms.ListBox Devices;
private System.Windows.Forms.Button Refresh;
private System.Windows.Forms.Button Scan;
private System.Windows.Forms.Button Read;
private System.Windows.Forms.Button SuccessBeep;
private System.Windows.Forms.Button ErrorBeep;
private System.Windows.Forms.TextBox Output;
}
}
<MSG> Fix merge conflicts
<DFF> @@ -29,7 +29,7 @@
private void InitializeComponent()
{
this.Devices = new System.Windows.Forms.ListBox();
- this.Refresh = new System.Windows.Forms.Button();
+ this.RefreshButton = new System.Windows.Forms.Button();
this.Scan = new System.Windows.Forms.Button();
this.Read = new System.Windows.Forms.Button();
this.SuccessBeep = new System.Windows.Forms.Button();
@@ -39,8 +39,8 @@
//
// Devices
//
- this.Devices.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
- | System.Windows.Forms.AnchorStyles.Right)));
+ this.Devices.Anchor = ((System.Windows.Forms.AnchorStyles)(((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Left)
+ | System.Windows.Forms.AnchorStyles.Right)));
this.Devices.FormattingEnabled = true;
this.Devices.Location = new System.Drawing.Point(0, 12);
this.Devices.Name = "Devices";
@@ -49,15 +49,15 @@
this.Devices.SelectedIndexChanged += new System.EventHandler(this.Devices_SelectedIndexChanged);
this.Devices.DoubleClick += new System.EventHandler(this.Devices_DoubleClick);
//
- // Refresh
+ // RefreshButton
//
- this.Refresh.Location = new System.Drawing.Point(350, 164);
- this.Refresh.Name = "Refresh";
- this.Refresh.Size = new System.Drawing.Size(75, 23);
- this.Refresh.TabIndex = 1;
- this.Refresh.Text = "Refresh";
- this.Refresh.UseVisualStyleBackColor = true;
- this.Refresh.Click += new System.EventHandler(this.Refresh_Click);
+ this.RefreshButton.Location = new System.Drawing.Point(350, 164);
+ this.RefreshButton.Name = "RefreshButton";
+ this.RefreshButton.Size = new System.Drawing.Size(75, 23);
+ this.RefreshButton.TabIndex = 1;
+ this.RefreshButton.Text = "Refresh";
+ this.RefreshButton.UseVisualStyleBackColor = true;
+ this.RefreshButton.Click += new System.EventHandler(this.Refresh_Click);
//
// Scan
//
@@ -102,9 +102,9 @@
//
// Output
//
- this.Output.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
- | System.Windows.Forms.AnchorStyles.Left)
- | System.Windows.Forms.AnchorStyles.Right)));
+ this.Output.Anchor = ((System.Windows.Forms.AnchorStyles)((((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
+ | System.Windows.Forms.AnchorStyles.Left)
+ | System.Windows.Forms.AnchorStyles.Right)));
this.Output.Location = new System.Drawing.Point(0, 193);
this.Output.Multiline = true;
this.Output.Name = "Output";
@@ -121,7 +121,7 @@
this.Controls.Add(this.SuccessBeep);
this.Controls.Add(this.Read);
this.Controls.Add(this.Scan);
- this.Controls.Add(this.Refresh);
+ this.Controls.Add(this.RefreshButton);
this.Controls.Add(this.Devices);
this.Name = "Main";
this.Text = "Hid Test Harness";
@@ -134,7 +134,7 @@
#endregion
private System.Windows.Forms.ListBox Devices;
- private System.Windows.Forms.Button Refresh;
+ private System.Windows.Forms.Button RefreshButton;
private System.Windows.Forms.Button Scan;
private System.Windows.Forms.Button Read;
private System.Windows.Forms.Button SuccessBeep;
| 16 | Fix merge conflicts | 16 | .cs | Designer | mit | mikeobrien/HidLibrary |
1004 | <NME> IHidDevice.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace HidLibrary
{
public delegate void InsertedEventHandler();
public delegate void RemovedEventHandler();
public enum DeviceMode
{
NonOverlapped = 0,
Overlapped = 1
}
[Flags]
public enum ShareMode
{
Exclusive = 0,
ShareRead = NativeMethods.FILE_SHARE_READ,
ShareWrite = NativeMethods.FILE_SHARE_WRITE
}
public delegate void ReadCallback(HidDeviceData data);
public delegate void ReadReportCallback(HidReport report);
public delegate void WriteCallback(bool success);
public interface IHidDevice : IDisposable
{
event InsertedEventHandler Inserted;
event RemovedEventHandler Removed;
IntPtr ReadHandle { get; }
IntPtr WriteHandle { get; }
bool IsOpen { get; }
bool IsConnected { get; }
string Description { get; }
HidDeviceCapabilities Capabilities { get; }
HidDeviceAttributes Attributes { get; }
string DevicePath { get; }
bool MonitorDeviceEvents { get; set; }
void OpenDevice();
void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode);
void CloseDevice();
HidDeviceData Read();
void Read(ReadCallback callback);
void Read(ReadCallback callback, int timeout);
Task<HidDeviceData> ReadAsync(int timeout = 0);
HidDeviceData Read(int timeout);
void ReadReport(ReadReportCallback callback);
void ReadReport(ReadReportCallback callback, int timeout);
Task<HidReport> ReadReportAsync(int timeout = 0);
HidReport ReadReport(int timeout);
HidReport ReadReport();
bool ReadFeatureData(out byte[] data, byte reportId = 0);
bool ReadProduct(out byte[] data);
bool ReadManufacturer(out byte[] data);
bool ReadSerialNumber(out byte[] data);
void Write(byte[] data, WriteCallback callback);
bool Write(byte[] data);
bool Write(byte[] data, int timeout);
void Write(byte[] data, WriteCallback callback, int timeout);
Task<bool> WriteAsync(byte[] data, int timeout = 0);
void WriteReport(HidReport report, WriteCallback callback);
bool WriteReport(HidReport report);
bool WriteReport(HidReport report, int timeout);
void WriteReport(HidReport report, WriteCallback callback, int timeout);
Task<bool> WriteReportAsync(HidReport report, int timeout = 0);
HidReport CreateReport();
bool WriteFeatureData(byte[] data);
}
}
<MSG> Clean using statements
<DFF> @@ -1,7 +1,4 @@
using System;
-using System.Collections.Generic;
-using System.Linq;
-using System.Text;
using System.Threading.Tasks;
namespace HidLibrary
| 0 | Clean using statements | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
1005 | <NME> IHidDevice.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace HidLibrary
{
public delegate void InsertedEventHandler();
public delegate void RemovedEventHandler();
public enum DeviceMode
{
NonOverlapped = 0,
Overlapped = 1
}
[Flags]
public enum ShareMode
{
Exclusive = 0,
ShareRead = NativeMethods.FILE_SHARE_READ,
ShareWrite = NativeMethods.FILE_SHARE_WRITE
}
public delegate void ReadCallback(HidDeviceData data);
public delegate void ReadReportCallback(HidReport report);
public delegate void WriteCallback(bool success);
public interface IHidDevice : IDisposable
{
event InsertedEventHandler Inserted;
event RemovedEventHandler Removed;
IntPtr ReadHandle { get; }
IntPtr WriteHandle { get; }
bool IsOpen { get; }
bool IsConnected { get; }
string Description { get; }
HidDeviceCapabilities Capabilities { get; }
HidDeviceAttributes Attributes { get; }
string DevicePath { get; }
bool MonitorDeviceEvents { get; set; }
void OpenDevice();
void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode);
void CloseDevice();
HidDeviceData Read();
void Read(ReadCallback callback);
void Read(ReadCallback callback, int timeout);
Task<HidDeviceData> ReadAsync(int timeout = 0);
HidDeviceData Read(int timeout);
void ReadReport(ReadReportCallback callback);
void ReadReport(ReadReportCallback callback, int timeout);
Task<HidReport> ReadReportAsync(int timeout = 0);
HidReport ReadReport(int timeout);
HidReport ReadReport();
bool ReadFeatureData(out byte[] data, byte reportId = 0);
bool ReadProduct(out byte[] data);
bool ReadManufacturer(out byte[] data);
bool ReadSerialNumber(out byte[] data);
void Write(byte[] data, WriteCallback callback);
bool Write(byte[] data);
bool Write(byte[] data, int timeout);
void Write(byte[] data, WriteCallback callback, int timeout);
Task<bool> WriteAsync(byte[] data, int timeout = 0);
void WriteReport(HidReport report, WriteCallback callback);
bool WriteReport(HidReport report);
bool WriteReport(HidReport report, int timeout);
void WriteReport(HidReport report, WriteCallback callback, int timeout);
Task<bool> WriteReportAsync(HidReport report, int timeout = 0);
HidReport CreateReport();
bool WriteFeatureData(byte[] data);
}
}
<MSG> Clean using statements
<DFF> @@ -1,7 +1,4 @@
using System;
-using System.Collections.Generic;
-using System.Linq;
-using System.Text;
using System.Threading.Tasks;
namespace HidLibrary
| 0 | Clean using statements | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
1006 | <NME> HidLibrary.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFrameworks>net20;net35;net40;net45;netstandard2</TargetFrameworks>
<AllowUnsafeBlocks>true</AllowUnsafeBlocks>
<Version>3.2.49</Version>
<Company>Ultraviolet Catastrophe</Company>
<Copyright>Copyright © 2011-2020 Ultraviolet Catastrophe</Copyright>
<PackageId>hidlibrary</PackageId>
<Description>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</Description>
<PackageLicenseFile>LICENSE</PackageLicenseFile>
<PackageProjectUrl>https://github.com/mikeobrien/HidLibrary</PackageProjectUrl>
<PackageIconUrl>https://github.com/mikeobrien/HidLibrary/raw/master/misc/logo.png</PackageIconUrl>
<PackageTags>usb hid</PackageTags>
</PropertyGroup>
<ItemGroup>
<None Include="..\..\LICENSE" Pack="true" PackagePath=""/>
</ItemGroup>
<ItemGroup Condition=" '$(TargetFramework)' != 'net45' AND '$(TargetFramework)' != 'netstandard2' ">
<PackageReference Include="Theraot.Core" Version="1.0.3" />
</ItemGroup>
</Project>
</Project>
<MSG> Fix https://docs.microsoft.com/en-us/nuget/reference/errors-and-warnings/nu5048
<DFF> @@ -12,16 +12,17 @@
<Description>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</Description>
<PackageLicenseFile>LICENSE</PackageLicenseFile>
<PackageProjectUrl>https://github.com/mikeobrien/HidLibrary</PackageProjectUrl>
- <PackageIconUrl>https://github.com/mikeobrien/HidLibrary/raw/master/misc/logo.png</PackageIconUrl>
+ <PackageIcon>logo.png</PackageIcon>
<PackageTags>usb hid</PackageTags>
</PropertyGroup>
<ItemGroup>
- <None Include="..\..\LICENSE" Pack="true" PackagePath=""/>
+ <None Include="..\..\LICENSE" Pack="true" PackagePath="" />
+ <None Include="..\..\misc\logo.png" Pack="true" PackagePath="" />
</ItemGroup>
<ItemGroup Condition=" '$(TargetFramework)' != 'net45' AND '$(TargetFramework)' != 'netstandard2' ">
<PackageReference Include="Theraot.Core" Version="1.0.3" />
</ItemGroup>
-</Project>
+</Project>
\ No newline at end of file
| 4 | Fix https://docs.microsoft.com/en-us/nuget/reference/errors-and-warnings/nu5048 | 3 | .csproj | csproj | mit | mikeobrien/HidLibrary |
1007 | <NME> HidLibrary.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFrameworks>net20;net35;net40;net45;netstandard2</TargetFrameworks>
<AllowUnsafeBlocks>true</AllowUnsafeBlocks>
<Version>3.2.49</Version>
<Company>Ultraviolet Catastrophe</Company>
<Copyright>Copyright © 2011-2020 Ultraviolet Catastrophe</Copyright>
<PackageId>hidlibrary</PackageId>
<Description>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</Description>
<PackageLicenseFile>LICENSE</PackageLicenseFile>
<PackageProjectUrl>https://github.com/mikeobrien/HidLibrary</PackageProjectUrl>
<PackageIconUrl>https://github.com/mikeobrien/HidLibrary/raw/master/misc/logo.png</PackageIconUrl>
<PackageTags>usb hid</PackageTags>
</PropertyGroup>
<ItemGroup>
<None Include="..\..\LICENSE" Pack="true" PackagePath=""/>
</ItemGroup>
<ItemGroup Condition=" '$(TargetFramework)' != 'net45' AND '$(TargetFramework)' != 'netstandard2' ">
<PackageReference Include="Theraot.Core" Version="1.0.3" />
</ItemGroup>
</Project>
</Project>
<MSG> Fix https://docs.microsoft.com/en-us/nuget/reference/errors-and-warnings/nu5048
<DFF> @@ -12,16 +12,17 @@
<Description>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</Description>
<PackageLicenseFile>LICENSE</PackageLicenseFile>
<PackageProjectUrl>https://github.com/mikeobrien/HidLibrary</PackageProjectUrl>
- <PackageIconUrl>https://github.com/mikeobrien/HidLibrary/raw/master/misc/logo.png</PackageIconUrl>
+ <PackageIcon>logo.png</PackageIcon>
<PackageTags>usb hid</PackageTags>
</PropertyGroup>
<ItemGroup>
- <None Include="..\..\LICENSE" Pack="true" PackagePath=""/>
+ <None Include="..\..\LICENSE" Pack="true" PackagePath="" />
+ <None Include="..\..\misc\logo.png" Pack="true" PackagePath="" />
</ItemGroup>
<ItemGroup Condition=" '$(TargetFramework)' != 'net45' AND '$(TargetFramework)' != 'netstandard2' ">
<PackageReference Include="Theraot.Core" Version="1.0.3" />
</ItemGroup>
-</Project>
+</Project>
\ No newline at end of file
| 4 | Fix https://docs.microsoft.com/en-us/nuget/reference/errors-and-warnings/nu5048 | 3 | .csproj | csproj | mit | mikeobrien/HidLibrary |
1008 | <NME> TestHarness.csproj
<BEF> <?xml version="1.0" encoding="utf-8"?>
<Project ToolsVersion="4.0" DefaultTargets="Build" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
<PropertyGroup>
<Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration>
<Platform Condition=" '$(Platform)' == '' ">x86</Platform>
<ProductVersion>8.0.30703</ProductVersion>
<SchemaVersion>2.0</SchemaVersion>
<ProjectGuid>{3009AC13-6BED-44AF-92F6-8E6C802716C9}</ProjectGuid>
<OutputType>WinExe</OutputType>
<AppDesignerFolder>Properties</AppDesignerFolder>
<RootNamespace>TestHarness</RootNamespace>
<AssemblyName>TestHarness</AssemblyName>
<TargetFrameworkVersion>v4.5</TargetFrameworkVersion>
<TargetFrameworkProfile>
</TargetFrameworkProfile>
<FileAlignment>512</FileAlignment>
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|x86' ">
<PlatformTarget>AnyCPU</PlatformTarget>
<DebugSymbols>true</DebugSymbols>
<DebugType>full</DebugType>
<Optimize>false</Optimize>
<OutputPath>bin\Debug\</OutputPath>
<DefineConstants>DEBUG;TRACE</DefineConstants>
<ErrorReport>prompt</ErrorReport>
<WarningLevel>4</WarningLevel>
<Prefer32Bit>false</Prefer32Bit>
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Release|x86' ">
<PlatformTarget>x86</PlatformTarget>
<DebugType>pdbonly</DebugType>
<Optimize>true</Optimize>
<OutputPath>bin\Release\</OutputPath>
<ErrorReport>prompt</ErrorReport>
<WarningLevel>4</WarningLevel>
</PropertyGroup>
<ItemGroup>
<Reference Include="System" />
<Reference Include="System.Core" />
</PropertyGroup>
<ItemGroup>
<Reference Include="System" />
<Reference Include="System.Core" />
<Reference Include="System.Xml.Linq" />
<Reference Include="System.Data.DataSetExtensions" />
<Reference Include="Microsoft.CSharp" />
<Reference Include="System.Data" />
<Reference Include="System.Deployment" />
<Reference Include="System.Drawing" />
<Reference Include="System.Windows.Forms" />
<Reference Include="System.Xml" />
</ItemGroup>
<ItemGroup>
<Compile Include="Main.cs">
<SubType>Form</SubType>
</Compile>
<Compile Include="Main.Designer.cs">
<DependentUpon>Main.cs</DependentUpon>
</Compile>
<Compile Include="Program.cs" />
<Compile Include="Properties\AssemblyInfo.cs" />
<EmbeddedResource Include="Main.resx">
<DependentUpon>Main.cs</DependentUpon>
</EmbeddedResource>
<EmbeddedResource Include="Properties\Resources.resx">
<Generator>ResXFileCodeGenerator</Generator>
<LastGenOutput>Resources.Designer.cs</LastGenOutput>
<SubType>Designer</SubType>
</EmbeddedResource>
<Compile Include="Properties\Resources.Designer.cs">
<AutoGen>True</AutoGen>
<DependentUpon>Resources.resx</DependentUpon>
<DesignTime>True</DesignTime>
</Compile>
<None Include="app.config" />
<None Include="Properties\Settings.settings">
<Generator>SettingsSingleFileGenerator</Generator>
<LastGenOutput>Settings.Designer.cs</LastGenOutput>
</None>
<Compile Include="Properties\Settings.Designer.cs">
</ItemGroup>
<ItemGroup>
<ProjectReference Include="..\HidLibrary\HidLibrary.csproj">
<Project>{9E8F1D50-74EA-4C60-BD5C-AB2C5B53BC66}</Project>
<Name>HidLibrary</Name>
</ProjectReference>
</ItemGroup>
<Project>{9e8f1d50-74ea-4c60-bd5c-ab2c5b53bc66}</Project>
<Name>HidLibrary</Name>
</ProjectReference>
</ItemGroup>
<Import Project="$(MSBuildToolsPath)\Microsoft.CSharp.targets" />
<!-- To modify your build process, add your task inside one of the targets below and uncomment it.
Other similar extension points exist, see Microsoft.Common.targets.
<Target Name="BeforeBuild">
</Target>
<Target Name="AfterBuild">
</Target>
-->
</Project>
<MSG> Fix for OverflowException on x64
<DFF> @@ -34,6 +34,24 @@
<ErrorReport>prompt</ErrorReport>
<WarningLevel>4</WarningLevel>
</PropertyGroup>
+ <PropertyGroup Condition="'$(Configuration)|$(Platform)' == 'Debug|x64'">
+ <DebugSymbols>true</DebugSymbols>
+ <OutputPath>bin\x64\Debug\</OutputPath>
+ <DefineConstants>DEBUG;TRACE</DefineConstants>
+ <DebugType>full</DebugType>
+ <PlatformTarget>x64</PlatformTarget>
+ <ErrorReport>prompt</ErrorReport>
+ <CodeAnalysisRuleSet>MinimumRecommendedRules.ruleset</CodeAnalysisRuleSet>
+ </PropertyGroup>
+ <PropertyGroup Condition="'$(Configuration)|$(Platform)' == 'Release|x64'">
+ <OutputPath>bin\x64\Release\</OutputPath>
+ <DefineConstants>TRACE</DefineConstants>
+ <Optimize>true</Optimize>
+ <DebugType>pdbonly</DebugType>
+ <PlatformTarget>x64</PlatformTarget>
+ <ErrorReport>prompt</ErrorReport>
+ <CodeAnalysisRuleSet>MinimumRecommendedRules.ruleset</CodeAnalysisRuleSet>
+ </PropertyGroup>
<ItemGroup>
<Reference Include="System" />
<Reference Include="System.Core" />
@@ -81,7 +99,7 @@
</ItemGroup>
<ItemGroup>
<ProjectReference Include="..\HidLibrary\HidLibrary.csproj">
- <Project>{9E8F1D50-74EA-4C60-BD5C-AB2C5B53BC66}</Project>
+ <Project>{9e8f1d50-74ea-4c60-bd5c-ab2c5b53bc66}</Project>
<Name>HidLibrary</Name>
</ProjectReference>
</ItemGroup>
| 19 | Fix for OverflowException on x64 | 1 | .csproj | csproj | mit | mikeobrien/HidLibrary |
1009 | <NME> TestHarness.csproj
<BEF> <?xml version="1.0" encoding="utf-8"?>
<Project ToolsVersion="4.0" DefaultTargets="Build" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
<PropertyGroup>
<Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration>
<Platform Condition=" '$(Platform)' == '' ">x86</Platform>
<ProductVersion>8.0.30703</ProductVersion>
<SchemaVersion>2.0</SchemaVersion>
<ProjectGuid>{3009AC13-6BED-44AF-92F6-8E6C802716C9}</ProjectGuid>
<OutputType>WinExe</OutputType>
<AppDesignerFolder>Properties</AppDesignerFolder>
<RootNamespace>TestHarness</RootNamespace>
<AssemblyName>TestHarness</AssemblyName>
<TargetFrameworkVersion>v4.5</TargetFrameworkVersion>
<TargetFrameworkProfile>
</TargetFrameworkProfile>
<FileAlignment>512</FileAlignment>
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|x86' ">
<PlatformTarget>AnyCPU</PlatformTarget>
<DebugSymbols>true</DebugSymbols>
<DebugType>full</DebugType>
<Optimize>false</Optimize>
<OutputPath>bin\Debug\</OutputPath>
<DefineConstants>DEBUG;TRACE</DefineConstants>
<ErrorReport>prompt</ErrorReport>
<WarningLevel>4</WarningLevel>
<Prefer32Bit>false</Prefer32Bit>
</PropertyGroup>
<PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Release|x86' ">
<PlatformTarget>x86</PlatformTarget>
<DebugType>pdbonly</DebugType>
<Optimize>true</Optimize>
<OutputPath>bin\Release\</OutputPath>
<ErrorReport>prompt</ErrorReport>
<WarningLevel>4</WarningLevel>
</PropertyGroup>
<ItemGroup>
<Reference Include="System" />
<Reference Include="System.Core" />
</PropertyGroup>
<ItemGroup>
<Reference Include="System" />
<Reference Include="System.Core" />
<Reference Include="System.Xml.Linq" />
<Reference Include="System.Data.DataSetExtensions" />
<Reference Include="Microsoft.CSharp" />
<Reference Include="System.Data" />
<Reference Include="System.Deployment" />
<Reference Include="System.Drawing" />
<Reference Include="System.Windows.Forms" />
<Reference Include="System.Xml" />
</ItemGroup>
<ItemGroup>
<Compile Include="Main.cs">
<SubType>Form</SubType>
</Compile>
<Compile Include="Main.Designer.cs">
<DependentUpon>Main.cs</DependentUpon>
</Compile>
<Compile Include="Program.cs" />
<Compile Include="Properties\AssemblyInfo.cs" />
<EmbeddedResource Include="Main.resx">
<DependentUpon>Main.cs</DependentUpon>
</EmbeddedResource>
<EmbeddedResource Include="Properties\Resources.resx">
<Generator>ResXFileCodeGenerator</Generator>
<LastGenOutput>Resources.Designer.cs</LastGenOutput>
<SubType>Designer</SubType>
</EmbeddedResource>
<Compile Include="Properties\Resources.Designer.cs">
<AutoGen>True</AutoGen>
<DependentUpon>Resources.resx</DependentUpon>
<DesignTime>True</DesignTime>
</Compile>
<None Include="app.config" />
<None Include="Properties\Settings.settings">
<Generator>SettingsSingleFileGenerator</Generator>
<LastGenOutput>Settings.Designer.cs</LastGenOutput>
</None>
<Compile Include="Properties\Settings.Designer.cs">
</ItemGroup>
<ItemGroup>
<ProjectReference Include="..\HidLibrary\HidLibrary.csproj">
<Project>{9E8F1D50-74EA-4C60-BD5C-AB2C5B53BC66}</Project>
<Name>HidLibrary</Name>
</ProjectReference>
</ItemGroup>
<Project>{9e8f1d50-74ea-4c60-bd5c-ab2c5b53bc66}</Project>
<Name>HidLibrary</Name>
</ProjectReference>
</ItemGroup>
<Import Project="$(MSBuildToolsPath)\Microsoft.CSharp.targets" />
<!-- To modify your build process, add your task inside one of the targets below and uncomment it.
Other similar extension points exist, see Microsoft.Common.targets.
<Target Name="BeforeBuild">
</Target>
<Target Name="AfterBuild">
</Target>
-->
</Project>
<MSG> Fix for OverflowException on x64
<DFF> @@ -34,6 +34,24 @@
<ErrorReport>prompt</ErrorReport>
<WarningLevel>4</WarningLevel>
</PropertyGroup>
+ <PropertyGroup Condition="'$(Configuration)|$(Platform)' == 'Debug|x64'">
+ <DebugSymbols>true</DebugSymbols>
+ <OutputPath>bin\x64\Debug\</OutputPath>
+ <DefineConstants>DEBUG;TRACE</DefineConstants>
+ <DebugType>full</DebugType>
+ <PlatformTarget>x64</PlatformTarget>
+ <ErrorReport>prompt</ErrorReport>
+ <CodeAnalysisRuleSet>MinimumRecommendedRules.ruleset</CodeAnalysisRuleSet>
+ </PropertyGroup>
+ <PropertyGroup Condition="'$(Configuration)|$(Platform)' == 'Release|x64'">
+ <OutputPath>bin\x64\Release\</OutputPath>
+ <DefineConstants>TRACE</DefineConstants>
+ <Optimize>true</Optimize>
+ <DebugType>pdbonly</DebugType>
+ <PlatformTarget>x64</PlatformTarget>
+ <ErrorReport>prompt</ErrorReport>
+ <CodeAnalysisRuleSet>MinimumRecommendedRules.ruleset</CodeAnalysisRuleSet>
+ </PropertyGroup>
<ItemGroup>
<Reference Include="System" />
<Reference Include="System.Core" />
@@ -81,7 +99,7 @@
</ItemGroup>
<ItemGroup>
<ProjectReference Include="..\HidLibrary\HidLibrary.csproj">
- <Project>{9E8F1D50-74EA-4C60-BD5C-AB2C5B53BC66}</Project>
+ <Project>{9e8f1d50-74ea-4c60-bd5c-ab2c5b53bc66}</Project>
<Name>HidLibrary</Name>
</ProjectReference>
</ItemGroup>
| 19 | Fix for OverflowException on x64 | 1 | .csproj | csproj | mit | mikeobrien/HidLibrary |
1010 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll", CharSet = CharSet.Unicode)]
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential, CharSet=CharSet.Auto)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
static internal extern bool SetupDiGetDeviceInterfaceDetailBuffer(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll", CharSet = CharSet.Auto)]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
<MSG> CharSet.Auto is only useful on Windows 98 and ME
<DFF> @@ -27,10 +27,10 @@ namespace HidLibrary
public bool bInheritHandle;
}
- [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
+ [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Unicode)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
- [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
+ [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Unicode)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll", CharSet = CharSet.Unicode)]
@@ -106,7 +106,7 @@ namespace HidLibrary
internal IntPtr Reserved;
}
- [StructLayout(LayoutKind.Sequential, CharSet=CharSet.Auto)]
+ [StructLayout(LayoutKind.Sequential, CharSet=CharSet.Unicode)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
@@ -142,10 +142,10 @@ namespace HidLibrary
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
- [DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
+ [DllImport("setupapi.dll", CharSet = CharSet.Unicode, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
static internal extern bool SetupDiGetDeviceInterfaceDetailBuffer(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
- [DllImport("setupapi.dll", CharSet = CharSet.Auto)]
+ [DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
| 5 | CharSet.Auto is only useful on Windows 98 and ME | 5 | .cs | cs | mit | mikeobrien/HidLibrary |
1011 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll", CharSet = CharSet.Unicode)]
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential, CharSet=CharSet.Auto)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
static internal extern bool SetupDiGetDeviceInterfaceDetailBuffer(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll", CharSet = CharSet.Auto)]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
<MSG> CharSet.Auto is only useful on Windows 98 and ME
<DFF> @@ -27,10 +27,10 @@ namespace HidLibrary
public bool bInheritHandle;
}
- [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
+ [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Unicode)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
- [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Auto)]
+ [DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true, CharSet = CharSet.Unicode)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll", CharSet = CharSet.Unicode)]
@@ -106,7 +106,7 @@ namespace HidLibrary
internal IntPtr Reserved;
}
- [StructLayout(LayoutKind.Sequential, CharSet=CharSet.Auto)]
+ [StructLayout(LayoutKind.Sequential, CharSet=CharSet.Unicode)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
@@ -142,10 +142,10 @@ namespace HidLibrary
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
- [DllImport("setupapi.dll", CharSet = CharSet.Auto, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
+ [DllImport("setupapi.dll", CharSet = CharSet.Unicode, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
static internal extern bool SetupDiGetDeviceInterfaceDetailBuffer(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
- [DllImport("setupapi.dll", CharSet = CharSet.Auto)]
+ [DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
| 5 | CharSet.Auto is only useful on Windows 98 and ME | 5 | .cs | cs | mit | mikeobrien/HidLibrary |
1012 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
}
}
<MSG> Fix Native Methods to Utilize Byte Array
The methods are incorrectly passing a single byte. See the following definitions (all identical).
https://docs.microsoft.com/en-us/windows-hardware/drivers/ddi/hidsdi/nf-hidsdi-hidd_getmanufacturerstring
https://docs.microsoft.com/en-us/windows-hardware/drivers/ddi/hidsdi/nf-hidsdi-hidd_getproductstring
https://docs.microsoft.com/en-us/windows-hardware/drivers/ddi/hidsdi/nf-hidsdi-hidd_getserialnumberstring
All of these take pvoid (void pointer), so we pass the byte array, the interface will get the array pointer, and fill it accordingly. If we pass a single byte, c# won't map the array correctly in the return data.
<DFF> @@ -209,12 +209,12 @@ namespace HidLibrary
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
- internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
+ internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
- internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
+ internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
- internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
| 3 | Fix Native Methods to Utilize Byte Array | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
1013 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
}
}
<MSG> Fix Native Methods to Utilize Byte Array
The methods are incorrectly passing a single byte. See the following definitions (all identical).
https://docs.microsoft.com/en-us/windows-hardware/drivers/ddi/hidsdi/nf-hidsdi-hidd_getmanufacturerstring
https://docs.microsoft.com/en-us/windows-hardware/drivers/ddi/hidsdi/nf-hidsdi-hidd_getproductstring
https://docs.microsoft.com/en-us/windows-hardware/drivers/ddi/hidsdi/nf-hidsdi-hidd_getserialnumberstring
All of these take pvoid (void pointer), so we pass the byte array, the interface will get the array pointer, and fill it accordingly. If we pass a single byte, c# won't map the array correctly in the return data.
<DFF> @@ -209,12 +209,12 @@ namespace HidLibrary
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
- internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
+ internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
- internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int ReportBufferLength);
+ internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
- internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
| 3 | Fix Native Methods to Utilize Byte Array | 3 | .cs | cs | mit | mikeobrien/HidLibrary |
1014 | <NME> HidLibrary.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFrameworks>net20;net35;net40;net45;netstandard2</TargetFrameworks>
<GenerateAssemblyInfo>false</GenerateAssemblyInfo>
<PackageId>hidlibrary</PackageId>
<PackageVersion>3.2.49</PackageVersion>
<Title>Hid Library</Title>
<authors>Mike O'Brien</authors>
<Description>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</Description>
<PackageLicenseFile>LICENSE</PackageLicenseFile>
<PackageProjectUrl>https://github.com/mikeobrien/HidLibrary</PackageProjectUrl>
<PackageLicenseFile>LICENSE</PackageLicenseFile>
<PackageProjectUrl>https://github.com/mikeobrien/HidLibrary</PackageProjectUrl>
<PackageIcon>logo.png</PackageIcon>
<PackageTags>usb hid</PackageTags>
</PropertyGroup>
<ItemGroup>
<None Include="..\..\LICENSE" Pack="true" PackagePath="" />
<None Include="..\..\misc\logo.png" Pack="true" PackagePath="" />
</ItemGroup>
<ItemGroup Condition=" '$(TargetFramework)' != 'net45' AND '$(TargetFramework)' != 'netstandard2' ">
<PackageReference Include="Theraot.Core" Version="1.0.3" />
</ItemGroup>
</Project>
<MSG> Use project properties instead of AssemblyInfo.cs
<DFF> @@ -2,12 +2,13 @@
<PropertyGroup>
<TargetFrameworks>net20;net35;net40;net45;netstandard2</TargetFrameworks>
- <GenerateAssemblyInfo>false</GenerateAssemblyInfo>
+ <Version>3.2.49</Version>
+ <Company>Ultraviolet Catastrophe</Company>
+ <Copyright>Copyright © 2011 Ultraviolet Catastrophe</Copyright>
<PackageId>hidlibrary</PackageId>
- <PackageVersion>3.2.49</PackageVersion>
<Title>Hid Library</Title>
- <authors>Mike O'Brien</authors>
+ <Authors>Mike O'Brien</Authors>
<Description>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</Description>
<PackageLicenseFile>LICENSE</PackageLicenseFile>
<PackageProjectUrl>https://github.com/mikeobrien/HidLibrary</PackageProjectUrl>
| 4 | Use project properties instead of AssemblyInfo.cs | 3 | .csproj | csproj | mit | mikeobrien/HidLibrary |
1015 | <NME> HidLibrary.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFrameworks>net20;net35;net40;net45;netstandard2</TargetFrameworks>
<GenerateAssemblyInfo>false</GenerateAssemblyInfo>
<PackageId>hidlibrary</PackageId>
<PackageVersion>3.2.49</PackageVersion>
<Title>Hid Library</Title>
<authors>Mike O'Brien</authors>
<Description>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</Description>
<PackageLicenseFile>LICENSE</PackageLicenseFile>
<PackageProjectUrl>https://github.com/mikeobrien/HidLibrary</PackageProjectUrl>
<PackageLicenseFile>LICENSE</PackageLicenseFile>
<PackageProjectUrl>https://github.com/mikeobrien/HidLibrary</PackageProjectUrl>
<PackageIcon>logo.png</PackageIcon>
<PackageTags>usb hid</PackageTags>
</PropertyGroup>
<ItemGroup>
<None Include="..\..\LICENSE" Pack="true" PackagePath="" />
<None Include="..\..\misc\logo.png" Pack="true" PackagePath="" />
</ItemGroup>
<ItemGroup Condition=" '$(TargetFramework)' != 'net45' AND '$(TargetFramework)' != 'netstandard2' ">
<PackageReference Include="Theraot.Core" Version="1.0.3" />
</ItemGroup>
</Project>
<MSG> Use project properties instead of AssemblyInfo.cs
<DFF> @@ -2,12 +2,13 @@
<PropertyGroup>
<TargetFrameworks>net20;net35;net40;net45;netstandard2</TargetFrameworks>
- <GenerateAssemblyInfo>false</GenerateAssemblyInfo>
+ <Version>3.2.49</Version>
+ <Company>Ultraviolet Catastrophe</Company>
+ <Copyright>Copyright © 2011 Ultraviolet Catastrophe</Copyright>
<PackageId>hidlibrary</PackageId>
- <PackageVersion>3.2.49</PackageVersion>
<Title>Hid Library</Title>
- <authors>Mike O'Brien</authors>
+ <Authors>Mike O'Brien</Authors>
<Description>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</Description>
<PackageLicenseFile>LICENSE</PackageLicenseFile>
<PackageProjectUrl>https://github.com/mikeobrien/HidLibrary</PackageProjectUrl>
| 4 | Use project properties instead of AssemblyInfo.cs | 3 | .csproj | csproj | mit | mikeobrien/HidLibrary |
1016 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Unicode, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
static internal extern bool SetupDiGetDeviceInterfaceDetailBuffer(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
<MSG> Use overload instead of suffixing the p/invoke method name and overriding EntryPoint
<DFF> @@ -142,8 +142,8 @@ namespace HidLibrary
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
- [DllImport("setupapi.dll", CharSet = CharSet.Unicode, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
- static internal extern bool SetupDiGetDeviceInterfaceDetailBuffer(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
+ [DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
+ static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
| 2 | Use overload instead of suffixing the p/invoke method name and overriding EntryPoint | 2 | .cs | cs | mit | mikeobrien/HidLibrary |
1017 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll", CharSet = CharSet.Unicode, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
static internal extern bool SetupDiGetDeviceInterfaceDetailBuffer(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
<MSG> Use overload instead of suffixing the p/invoke method name and overriding EntryPoint
<DFF> @@ -142,8 +142,8 @@ namespace HidLibrary
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
- [DllImport("setupapi.dll", CharSet = CharSet.Unicode, EntryPoint = "SetupDiGetDeviceInterfaceDetail")]
- static internal extern bool SetupDiGetDeviceInterfaceDetailBuffer(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
+ [DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
+ static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll", CharSet = CharSet.Unicode)]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
| 2 | Use overload instead of suffixing the p/invoke method name and overriding EntryPoint | 2 | .cs | cs | mit | mikeobrien/HidLibrary |
1018 | <NME> README.md
<BEF> <h3>NOTE: Support is VERY limited for this library. It is almost impossible to troubleshoot issues with so many devices and configurations. The community may be able to offer some assistance <i>but you will largely be on your own</i>. If you submit an issue, please include a relevant code snippet and details about your operating system, .NET version and device. Pull requests are welcome and appreciated.</h3>
Hid Library
=============
[](http://www.nuget.org/packages/HidLibrary/) [](http://build.mikeobrien.net/viewType.html?buildTypeId=hidlibrary&guest=1)
This library enables you to enumerate and communicate with Hid compatible USB devices in .NET. It offers synchronous and asynchronous read and write functionality as well as notification of insertion and removal of a device. This library works on x86 and x64.
Installation
------------
PM> Install-Package hidlibrary
Developers
------------
| [](https://github.com/mikeobrien) | [](https://github.com/amullins83) |
|:---:|:---:|
If your interested in HID development here are a few invaluable resources:
1. [Jan Axelson's USB Hid Programming Page](http://www.lvr.com/hidpage.htm) - Excellent resource for USB Hid development. Full code samples in a number of different languages demonstrate how to use the Windows setup and Hid API.
2. [Jan Axelson's book 'USB Complete'](http://www.lvr.com/usbc.htm) - Jan Axelson's guide to USB programming. Very good covereage of Hid. Must read for anyone new to Hid programming.
| [](https://github.com/bwegman) | [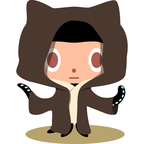](https://github.com/jwelch222) | [](https://github.com/thammer) | [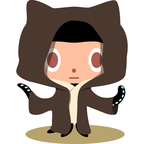](https://github.com/juliansiebert) | [](https://github.com/GeorgeHahn) |
|:---:|:---:|:---:|:---:|:---:|
| [Benjamin Wegman](https://github.com/bwegman) | [jwelch222](https://github.com/jwelch222) | [Thomas Hammer](https://github.com/thammer) | [juliansiebert](https://github.com/juliansiebert) | [George Hahn](https://github.com/GeorgeHahn) |
| [](https://github.com/rvlieshout) | [](https://github.com/ptrandem) | [](https://github.com/neilt6) | [](https://github.com/intrueder) | [](https://github.com/BrunoJuchli)
|:---:|:---:|:---:|:---:|:---:|
| [Rick van Lieshout](https://github.com/rvlieshout) | [Paul Trandem](https://github.com/ptrandem) | [Neil Thiessen](https://github.com/neilt6) | [intrueder](https://github.com/intrueder) | [Bruno Juchli](https://github.com/BrunoJuchli) |
| [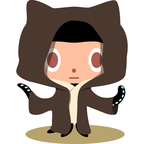](https://github.com/sblakemore) | [](https://github.com/jcyuan) | [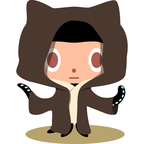](https://github.com/marekr) | [](https://github.com/bprescott) | [](https://github.com/ananth-racherla) |
|:---:|:---:|:---:|:---:|:---:|
| [sblakemore](https://github.com/sblakemore) | [J.C](https://github.com/jcyuan) | [Marek Roszko](https://github.com/marekr) | [Bill Prescott](https://github.com/bprescott) | [Ananth Racherla](https://github.com/ananth-racherla) |
Props
------------
Thanks to JetBrains for providing OSS licenses for [R#](http://www.jetbrains.com/resharper/features/code_refactoring.html) and [dotTrace](http://www.jetbrains.com/profiler/)!
Resources
------------
If your interested in HID development here are a few invaluable resources:
1. [Jan Axelson's USB Hid Programming Page](http://janaxelson.com/hidpage.htm) - Excellent resource for USB Hid development. Full code samples in a number of different languages demonstrate how to use the Windows setup and Hid API.
2. [Jan Axelson's book 'USB Complete'](http://janaxelson.com/usbc.htm) - Jan Axelson's guide to USB programming. Very good covereage of Hid. Must read for anyone new to Hid programming.
<MSG> Fixed links to Jan Axelson's page
domain moved from lvr.com to janaxelson.com
<DFF> @@ -20,5 +20,5 @@ Resources
If your interested in HID development here are a few invaluable resources:
-1. [Jan Axelson's USB Hid Programming Page](http://www.lvr.com/hidpage.htm) - Excellent resource for USB Hid development. Full code samples in a number of different languages demonstrate how to use the Windows setup and Hid API.
-2. [Jan Axelson's book 'USB Complete'](http://www.lvr.com/usbc.htm) - Jan Axelson's guide to USB programming. Very good covereage of Hid. Must read for anyone new to Hid programming.
\ No newline at end of file
+1. [Jan Axelson's USB Hid Programming Page](http://janaxelson.com/hidpage.htm) - Excellent resource for USB Hid development. Full code samples in a number of different languages demonstrate how to use the Windows setup and Hid API.
+2. [Jan Axelson's book 'USB Complete'](http://janaxelson.com/usbc.htm) - Jan Axelson's guide to USB programming. Very good covereage of Hid. Must read for anyone new to Hid programming.
| 2 | Fixed links to Jan Axelson's page | 2 | .md | md | mit | mikeobrien/HidLibrary |
1019 | <NME> README.md
<BEF> <h3>NOTE: Support is VERY limited for this library. It is almost impossible to troubleshoot issues with so many devices and configurations. The community may be able to offer some assistance <i>but you will largely be on your own</i>. If you submit an issue, please include a relevant code snippet and details about your operating system, .NET version and device. Pull requests are welcome and appreciated.</h3>
Hid Library
=============
[](http://www.nuget.org/packages/HidLibrary/) [](http://build.mikeobrien.net/viewType.html?buildTypeId=hidlibrary&guest=1)
This library enables you to enumerate and communicate with Hid compatible USB devices in .NET. It offers synchronous and asynchronous read and write functionality as well as notification of insertion and removal of a device. This library works on x86 and x64.
Installation
------------
PM> Install-Package hidlibrary
Developers
------------
| [](https://github.com/mikeobrien) | [](https://github.com/amullins83) |
|:---:|:---:|
If your interested in HID development here are a few invaluable resources:
1. [Jan Axelson's USB Hid Programming Page](http://www.lvr.com/hidpage.htm) - Excellent resource for USB Hid development. Full code samples in a number of different languages demonstrate how to use the Windows setup and Hid API.
2. [Jan Axelson's book 'USB Complete'](http://www.lvr.com/usbc.htm) - Jan Axelson's guide to USB programming. Very good covereage of Hid. Must read for anyone new to Hid programming.
| [](https://github.com/bwegman) | [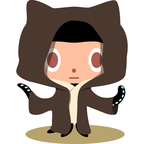](https://github.com/jwelch222) | [](https://github.com/thammer) | [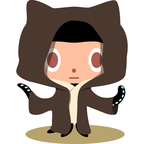](https://github.com/juliansiebert) | [](https://github.com/GeorgeHahn) |
|:---:|:---:|:---:|:---:|:---:|
| [Benjamin Wegman](https://github.com/bwegman) | [jwelch222](https://github.com/jwelch222) | [Thomas Hammer](https://github.com/thammer) | [juliansiebert](https://github.com/juliansiebert) | [George Hahn](https://github.com/GeorgeHahn) |
| [](https://github.com/rvlieshout) | [](https://github.com/ptrandem) | [](https://github.com/neilt6) | [](https://github.com/intrueder) | [](https://github.com/BrunoJuchli)
|:---:|:---:|:---:|:---:|:---:|
| [Rick van Lieshout](https://github.com/rvlieshout) | [Paul Trandem](https://github.com/ptrandem) | [Neil Thiessen](https://github.com/neilt6) | [intrueder](https://github.com/intrueder) | [Bruno Juchli](https://github.com/BrunoJuchli) |
| [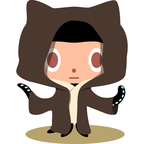](https://github.com/sblakemore) | [](https://github.com/jcyuan) | [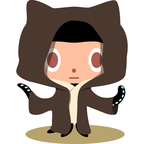](https://github.com/marekr) | [](https://github.com/bprescott) | [](https://github.com/ananth-racherla) |
|:---:|:---:|:---:|:---:|:---:|
| [sblakemore](https://github.com/sblakemore) | [J.C](https://github.com/jcyuan) | [Marek Roszko](https://github.com/marekr) | [Bill Prescott](https://github.com/bprescott) | [Ananth Racherla](https://github.com/ananth-racherla) |
Props
------------
Thanks to JetBrains for providing OSS licenses for [R#](http://www.jetbrains.com/resharper/features/code_refactoring.html) and [dotTrace](http://www.jetbrains.com/profiler/)!
Resources
------------
If your interested in HID development here are a few invaluable resources:
1. [Jan Axelson's USB Hid Programming Page](http://janaxelson.com/hidpage.htm) - Excellent resource for USB Hid development. Full code samples in a number of different languages demonstrate how to use the Windows setup and Hid API.
2. [Jan Axelson's book 'USB Complete'](http://janaxelson.com/usbc.htm) - Jan Axelson's guide to USB programming. Very good covereage of Hid. Must read for anyone new to Hid programming.
<MSG> Fixed links to Jan Axelson's page
domain moved from lvr.com to janaxelson.com
<DFF> @@ -20,5 +20,5 @@ Resources
If your interested in HID development here are a few invaluable resources:
-1. [Jan Axelson's USB Hid Programming Page](http://www.lvr.com/hidpage.htm) - Excellent resource for USB Hid development. Full code samples in a number of different languages demonstrate how to use the Windows setup and Hid API.
-2. [Jan Axelson's book 'USB Complete'](http://www.lvr.com/usbc.htm) - Jan Axelson's guide to USB programming. Very good covereage of Hid. Must read for anyone new to Hid programming.
\ No newline at end of file
+1. [Jan Axelson's USB Hid Programming Page](http://janaxelson.com/hidpage.htm) - Excellent resource for USB Hid development. Full code samples in a number of different languages demonstrate how to use the Windows setup and Hid API.
+2. [Jan Axelson's book 'USB Complete'](http://janaxelson.com/usbc.htm) - Jan Axelson's guide to USB programming. Very good covereage of Hid. Must read for anyone new to Hid programming.
| 2 | Fixed links to Jan Axelson's page | 2 | .md | md | mit | mikeobrien/HidLibrary |
1020 | <NME> TestSecurityContext.java
<BEF> package com.github.structlog4j.samples;
import com.github.structlog4j.IToLog;
import lombok.Value;
import java.security.Principal;
/**
* A Sample of an object that implements the IToLog
*/
@Value
public class TestSecurityContext implements IToLog {
private String userName;
private String tenantId;
@Override
public Object[] toLog() {
return new Object[]{"userName",getUserName(),"tenantId",getTenantId()};
}
}
<MSG> 0.3.0: added JSON formatter
<DFF> @@ -1,10 +1,8 @@
-package com.github.structlog4j.samples;
+package com.github.structlog4j.test.samples;
import com.github.structlog4j.IToLog;
import lombok.Value;
-import java.security.Principal;
-
/**
* A Sample of an object that implements the IToLog
*/
| 1 | 0.3.0: added JSON formatter | 3 | .java | java | mit | jacek99/structlog4j |
1021 | <NME> HidLibrary.nuspec
<BEF> <?xml version="1.0" encoding="UTF-8"?>
<package xmlns="http://schemas.microsoft.com/packaging/2010/07/nuspec.xsd">
<metadata>
<id>hidlibrary</id>
<version>$version$</version>
<title>Hid Library</title>
<authors>Mike O'Brien</authors>
<description>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</description>
<releaseNotes />
<copyright />
<language>en-US</language>
<licenseUrl>https://github.com/mikeobrien/HidLibrary/blob/master/LICENSE</licenseUrl>
<projectUrl>https://github.com/mikeobrien/HidLibrary</projectUrl>
<owners>Mike O'Brien</owners>
<summary>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</summary>
<iconUrl>https://github.com/mikeobrien/HidLibrary/raw/master/misc/logo.png</iconUrl>
<tags>usb hid</tags>
<dependencies>
<group targetFramework="net20">
<dependency id="Theraot.Core" version="1.0.3" />
</group>
<group targetFramework="net35">
<dependency id="Theraot.Core" version="1.0.3" />
</group>
<group targetFramework="net40">
<dependency id="Theraot.Core" version="1.0.3" />
</group>
</dependencies>
</metadata>
<files>
<file src="net20\HidLibrary.*" target="lib\net20" exclude="*.xml" />
<file src="net35\HidLibrary.*" target="lib\net35" exclude="*.xml" />
<file src="net40\HidLibrary.*" target="lib\net40" exclude="*.xml" />
<file src="net45\HidLibrary.*" target="lib\net45" exclude="*.xml" />
</files>
</package>
<MSG> Merge pull request #115 from w-michal/nuget_update
Add netstandard2 target to nuspec file.
<DFF> @@ -25,6 +25,10 @@
<group targetFramework="net40">
<dependency id="Theraot.Core" version="1.0.3" />
</group>
+ <group targetFramework="net45">
+ </group>
+ <group targetFramework="netstandard2">
+ </group>
</dependencies>
</metadata>
<files>
@@ -32,5 +36,6 @@
<file src="net35\HidLibrary.*" target="lib\net35" exclude="*.xml" />
<file src="net40\HidLibrary.*" target="lib\net40" exclude="*.xml" />
<file src="net45\HidLibrary.*" target="lib\net45" exclude="*.xml" />
+ <file src="netstandard2\HidLibrary.*" target="lib\netstandard2" exclude="*.xml" />
</files>
</package>
\ No newline at end of file
| 5 | Merge pull request #115 from w-michal/nuget_update | 0 | .nuspec | nuspec | mit | mikeobrien/HidLibrary |
1022 | <NME> HidLibrary.nuspec
<BEF> <?xml version="1.0" encoding="UTF-8"?>
<package xmlns="http://schemas.microsoft.com/packaging/2010/07/nuspec.xsd">
<metadata>
<id>hidlibrary</id>
<version>$version$</version>
<title>Hid Library</title>
<authors>Mike O'Brien</authors>
<description>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</description>
<releaseNotes />
<copyright />
<language>en-US</language>
<licenseUrl>https://github.com/mikeobrien/HidLibrary/blob/master/LICENSE</licenseUrl>
<projectUrl>https://github.com/mikeobrien/HidLibrary</projectUrl>
<owners>Mike O'Brien</owners>
<summary>This library enables you to enumerate and communicate with Hid compatible USB devices in .NET.</summary>
<iconUrl>https://github.com/mikeobrien/HidLibrary/raw/master/misc/logo.png</iconUrl>
<tags>usb hid</tags>
<dependencies>
<group targetFramework="net20">
<dependency id="Theraot.Core" version="1.0.3" />
</group>
<group targetFramework="net35">
<dependency id="Theraot.Core" version="1.0.3" />
</group>
<group targetFramework="net40">
<dependency id="Theraot.Core" version="1.0.3" />
</group>
</dependencies>
</metadata>
<files>
<file src="net20\HidLibrary.*" target="lib\net20" exclude="*.xml" />
<file src="net35\HidLibrary.*" target="lib\net35" exclude="*.xml" />
<file src="net40\HidLibrary.*" target="lib\net40" exclude="*.xml" />
<file src="net45\HidLibrary.*" target="lib\net45" exclude="*.xml" />
</files>
</package>
<MSG> Merge pull request #115 from w-michal/nuget_update
Add netstandard2 target to nuspec file.
<DFF> @@ -25,6 +25,10 @@
<group targetFramework="net40">
<dependency id="Theraot.Core" version="1.0.3" />
</group>
+ <group targetFramework="net45">
+ </group>
+ <group targetFramework="netstandard2">
+ </group>
</dependencies>
</metadata>
<files>
@@ -32,5 +36,6 @@
<file src="net35\HidLibrary.*" target="lib\net35" exclude="*.xml" />
<file src="net40\HidLibrary.*" target="lib\net40" exclude="*.xml" />
<file src="net45\HidLibrary.*" target="lib\net45" exclude="*.xml" />
+ <file src="netstandard2\HidLibrary.*" target="lib\netstandard2" exclude="*.xml" />
</files>
</package>
\ No newline at end of file
| 5 | Merge pull request #115 from w-michal/nuget_update | 0 | .nuspec | nuspec | mit | mikeobrien/HidLibrary |
1023 | <NME> HidDevice.cs
<BEF> using System;
using System.Runtime.InteropServices;
using System.Threading;
using System.Threading.Tasks;
namespace HidLibrary
{
public class HidDevice : IHidDevice
{
public event InsertedEventHandler Inserted;
public event RemovedEventHandler Removed;
private readonly string _description;
private readonly string _devicePath;
private readonly HidDeviceAttributes _deviceAttributes;
private readonly HidDeviceCapabilities _deviceCapabilities;
private DeviceMode _deviceReadMode = DeviceMode.NonOverlapped;
private DeviceMode _deviceWriteMode = DeviceMode.NonOverlapped;
private ShareMode _deviceShareMode = ShareMode.ShareRead | ShareMode.ShareWrite;
private readonly HidDeviceEventMonitor _deviceEventMonitor;
private bool _monitorDeviceEvents;
protected delegate HidDeviceData ReadDelegate(int timeout);
protected delegate HidReport ReadReportDelegate(int timeout);
private delegate bool WriteDelegate(byte[] data, int timeout);
private delegate bool WriteReportDelegate(HidReport report, int timeout);
internal HidDevice(string devicePath, string description = null)
{
_deviceEventMonitor = new HidDeviceEventMonitor(this);
_deviceEventMonitor.Inserted += DeviceEventMonitorInserted;
_deviceEventMonitor.Removed += DeviceEventMonitorRemoved;
_devicePath = devicePath;
_description = description;
try
{
var hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
_deviceAttributes = GetDeviceAttributes(hidHandle);
_deviceCapabilities = GetDeviceCapabilities(hidHandle);
CloseDeviceIO(hidHandle);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error querying HID device '{0}'.", devicePath), exception);
}
}
public IntPtr ReadHandle { get; private set; }
public IntPtr WriteHandle { get; private set; }
public bool IsOpen { get; private set; }
public bool IsConnected { get { return HidDevices.IsConnected(_devicePath); } }
public string Description { get { return _description; } }
public HidDeviceCapabilities Capabilities { get { return _deviceCapabilities; } }
public HidDeviceAttributes Attributes { get { return _deviceAttributes; } }
public string DevicePath { get { return _devicePath; } }
public bool MonitorDeviceEvents
{
get { return _monitorDeviceEvents; }
set
{
if (value & _monitorDeviceEvents == false) _deviceEventMonitor.Init();
_monitorDeviceEvents = value;
}
}
public override string ToString()
{
return string.Format("VendorID={0}, ProductID={1}, Version={2}, DevicePath={3}",
_deviceAttributes.VendorHexId,
_deviceAttributes.ProductHexId,
_deviceAttributes.Version,
_devicePath);
}
public void OpenDevice()
{
OpenDevice(DeviceMode.NonOverlapped, DeviceMode.NonOverlapped, ShareMode.ShareRead | ShareMode.ShareWrite);
}
public void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode)
{
if (IsOpen) return;
_deviceReadMode = readMode;
_deviceWriteMode = writeMode;
_deviceShareMode = shareMode;
try
{
ReadHandle = OpenDeviceIO(_devicePath, readMode, NativeMethods.GENERIC_READ, shareMode);
WriteHandle = OpenDeviceIO(_devicePath, writeMode, NativeMethods.GENERIC_WRITE, shareMode);
}
catch (Exception exception)
{
IsOpen = false;
throw new Exception("Error opening HID device.", exception);
}
IsOpen = (ReadHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE &&
WriteHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE);
}
public void CloseDevice()
{
if (!IsOpen) return;
CloseDeviceIO(ReadHandle);
CloseDeviceIO(WriteHandle);
IsOpen = false;
}
public HidDeviceData Read()
{
return Read(0);
}
public HidDeviceData Read(int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return ReadData(timeout);
}
catch
{
return new HidDeviceData(HidDeviceData.ReadStatus.ReadError);
}
}
return new HidDeviceData(HidDeviceData.ReadStatus.NotConnected);
}
public void Read(ReadCallback callback)
{
Read(callback, 0);
}
public void Read(ReadCallback callback, int timeout)
{
var readDelegate = new ReadDelegate(Read);
var asyncState = new HidAsyncState(readDelegate, callback);
readDelegate.BeginInvoke(timeout, EndRead, asyncState);
}
public async Task<HidDeviceData> ReadAsync(int timeout = 0)
{
var readDelegate = new ReadDelegate(Read);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidDeviceData>.Factory.StartNew(() => readDelegate.Invoke(timeout));
#else
return await Task<HidDeviceData>.Factory.FromAsync(readDelegate.BeginInvoke, readDelegate.EndInvoke, timeout, null);
#endif
}
public HidReport ReadReport()
{
return ReadReport(0);
}
public HidReport ReadReport(int timeout)
{
return new HidReport(Capabilities.InputReportByteLength, Read(timeout));
}
public void ReadReport(ReadReportCallback callback)
{
ReadReport(callback, 0);
}
public void ReadReport(ReadReportCallback callback, int timeout)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
var asyncState = new HidAsyncState(readReportDelegate, callback);
readReportDelegate.BeginInvoke(timeout, EndReadReport, asyncState);
}
public async Task<HidReport> ReadReportAsync(int timeout = 0)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidReport>.Factory.StartNew(() => readReportDelegate.Invoke(timeout));
#else
return await Task<HidReport>.Factory.FromAsync(readReportDelegate.BeginInvoke, readReportDelegate.EndInvoke, timeout, null);
#endif
}
/// <summary>
/// Reads an input report from the Control channel. This method provides access to report data for devices that
/// do not use the interrupt channel to communicate for specific usages.
/// </summary>
/// <param name="reportId">The report ID to read from the device</param>
/// <returns>The HID report that is read. The report will contain the success status of the read request</returns>
///
public HidReport ReadReportSync(byte reportId)
{
byte[] cmdBuffer = new byte[Capabilities.InputReportByteLength];
cmdBuffer[0] = reportId;
bool bSuccess = NativeMethods.HidD_GetInputReport(ReadHandle, cmdBuffer, cmdBuffer.Length);
HidDeviceData deviceData = new HidDeviceData(cmdBuffer, bSuccess ? HidDeviceData.ReadStatus.Success : HidDeviceData.ReadStatus.NoDataRead);
return new HidReport(Capabilities.InputReportByteLength, deviceData);
}
public bool ReadFeatureData(out byte[] data, byte reportId = 0)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0)
{
data = new byte[0];
return false;
}
data = new byte[_deviceCapabilities.FeatureReportByteLength];
var buffer = CreateFeatureOutputBuffer();
buffer[0] = reportId;
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetFeature(hidHandle, buffer, buffer.Length);
if (success)
{
Array.Copy(buffer, 0, data, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
}
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadProduct(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetProductString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadManufacturer(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetManufacturerString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadSerialNumber(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetSerialNumberString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool Write(byte[] data)
{
return Write(data, 0);
}
public bool Write(byte[] data, int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return WriteData(data, timeout);
}
catch
{
return false;
}
}
return false;
}
public void Write(byte[] data, WriteCallback callback)
{
Write(data, callback, 0);
}
public void Write(byte[] data, WriteCallback callback, int timeout)
{
var writeDelegate = new WriteDelegate(Write);
var asyncState = new HidAsyncState(writeDelegate, callback);
writeDelegate.BeginInvoke(data, timeout, EndWrite, asyncState);
}
public async Task<bool> WriteAsync(byte[] data, int timeout = 0)
{
var writeDelegate = new WriteDelegate(Write);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeDelegate.Invoke(data, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeDelegate.BeginInvoke, writeDelegate.EndInvoke, data, timeout, null);
#endif
}
public bool WriteReport(HidReport report)
{
return WriteReport(report, 0);
}
public bool WriteReport(HidReport report, int timeout)
{
return Write(report.GetBytes(), timeout);
}
public void WriteReport(HidReport report, WriteCallback callback)
{
WriteReport(report, callback, 0);
}
public void WriteReport(HidReport report, WriteCallback callback, int timeout)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
var asyncState = new HidAsyncState(writeReportDelegate, callback);
writeReportDelegate.BeginInvoke(report, timeout, EndWriteReport, asyncState);
}
/// <summary>
/// Handle data transfers on the control channel. This method places data on the control channel for devices
/// that do not support the interupt transfers
/// </summary>
/// <param name="report">The outbound HID report</param>
/// <returns>The result of the tranfer request: true if successful otherwise false</returns>
///
public bool WriteReportSync(HidReport report)
{
if (null != report)
{
byte[] buffer = report.GetBytes();
return (NativeMethods.HidD_SetOutputReport(WriteHandle, buffer, buffer.Length));
}
else
throw new ArgumentException("The output report is null, it must be allocated before you call this method", "report");
}
public async Task<bool> WriteReportAsync(HidReport report, int timeout = 0)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeReportDelegate.Invoke(report, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeReportDelegate.BeginInvoke, writeReportDelegate.EndInvoke, report, timeout, null);
#endif
}
public HidReport CreateReport()
{
return new HidReport(Capabilities.OutputReportByteLength);
}
public bool WriteFeatureData(byte[] data)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0) return false;
}
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
}
else
{
try
{
if (IsOpen)
hidHandle = WriteHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
//var overlapped = new NativeOverlapped();
success = NativeMethods.HidD_SetFeature(hidHandle, buffer, buffer.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != WriteHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
protected static void EndRead(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadCallback)hidAsyncState.CallbackDelegate;
var data = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(data);
}
protected static void EndReadReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadReportCallback)hidAsyncState.CallbackDelegate;
var report = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(report);
}
private static void EndWrite(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private static void EndWriteReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private byte[] CreateInputBuffer()
{
return CreateBuffer(Capabilities.InputReportByteLength - 1);
}
private byte[] CreateOutputBuffer()
{
return CreateBuffer(Capabilities.OutputReportByteLength - 1);
}
private byte[] CreateFeatureOutputBuffer()
{
return CreateBuffer(Capabilities.FeatureReportByteLength - 1);
}
private static byte[] CreateBuffer(int length)
{
byte[] buffer = null;
Array.Resize(ref buffer, length + 1);
return buffer;
}
private static HidDeviceAttributes GetDeviceAttributes(IntPtr hidHandle)
{
var deviceAttributes = default(NativeMethods.HIDD_ATTRIBUTES);
deviceAttributes.Size = Marshal.SizeOf(deviceAttributes);
NativeMethods.HidD_GetAttributes(hidHandle, ref deviceAttributes);
return new HidDeviceAttributes(deviceAttributes);
}
private static HidDeviceCapabilities GetDeviceCapabilities(IntPtr hidHandle)
{
var capabilities = default(NativeMethods.HIDP_CAPS);
var preparsedDataPointer = default(IntPtr);
if (NativeMethods.HidD_GetPreparsedData(hidHandle, ref preparsedDataPointer))
{
NativeMethods.HidP_GetCaps(preparsedDataPointer, ref capabilities);
NativeMethods.HidD_FreePreparsedData(preparsedDataPointer);
}
return new HidDeviceCapabilities(capabilities);
}
private bool WriteData(byte[] data, int timeout)
{
if (_deviceCapabilities.OutputReportByteLength <= 0) return false;
var buffer = CreateOutputBuffer();
uint bytesWritten;
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.OutputReportByteLength));
if (_deviceWriteMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), "");
try
{
NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
return true;
case NativeMethods.WAIT_TIMEOUT:
return false;
case NativeMethods.WAIT_FAILED:
return false;
default:
return false;
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
return NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
}
}
protected HidDeviceData ReadData(int timeout)
{
var buffer = new byte[] { };
var status = HidDeviceData.ReadStatus.NoDataRead;
IntPtr nonManagedBuffer;
if (_deviceCapabilities.InputReportByteLength > 0)
{
uint bytesRead;
buffer = CreateInputBuffer();
nonManagedBuffer = Marshal.AllocHGlobal(buffer.Length);
if (_deviceReadMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), string.Empty);
try
{
var success = NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
if (success)
{
status = HidDeviceData.ReadStatus.Success; // No check here to see if bytesRead > 0 . Perhaps not necessary?
}
else
{
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
status = HidDeviceData.ReadStatus.Success;
NativeMethods.GetOverlappedResult(ReadHandle, ref overlapped, out bytesRead, false);
break;
case NativeMethods.WAIT_TIMEOUT:
status = HidDeviceData.ReadStatus.WaitTimedOut;
buffer = new byte[] { };
break;
case NativeMethods.WAIT_FAILED:
status = HidDeviceData.ReadStatus.WaitFail;
buffer = new byte[] { };
break;
default:
status = HidDeviceData.ReadStatus.NoDataRead;
buffer = new byte[] { };
break;
}
}
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally {
CloseDeviceIO(overlapped.EventHandle);
Marshal.FreeHGlobal(nonManagedBuffer);
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
status = HidDeviceData.ReadStatus.Success;
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally { Marshal.FreeHGlobal(nonManagedBuffer); }
}
}
return new HidDeviceData(buffer, status);
}
private static IntPtr OpenDeviceIO(string devicePath, uint deviceAccess)
{
return OpenDeviceIO(devicePath, DeviceMode.NonOverlapped, deviceAccess, ShareMode.ShareRead | ShareMode.ShareWrite);
}
private static IntPtr OpenDeviceIO(string devicePath, DeviceMode deviceMode, uint deviceAccess, ShareMode shareMode)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var flags = 0;
if (deviceMode == DeviceMode.Overlapped) flags = NativeMethods.FILE_FLAG_OVERLAPPED;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
return NativeMethods.CreateFile(devicePath, deviceAccess, (int)shareMode, ref security, NativeMethods.OPEN_EXISTING, flags, hTemplateFile: IntPtr.Zero);
}
private static void CloseDeviceIO(IntPtr handle)
{
if (Environment.OSVersion.Version.Major > 5)
{
NativeMethods.CancelIoEx(handle, IntPtr.Zero);
}
NativeMethods.CloseHandle(handle);
}
private void DeviceEventMonitorInserted()
{
if (!IsOpen) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
Inserted?.Invoke();
}
private void DeviceEventMonitorRemoved()
{
if (IsOpen) CloseDevice();
Removed?.Invoke();
}
public void Dispose()
{
if (MonitorDeviceEvents) MonitorDeviceEvents = false;
if (IsOpen) CloseDevice();
}
}
}
<MSG> Merge pull request #20 from juliansiebert/master
Fix for handle leak in HidDevice.ReadData()
<DFF> @@ -439,6 +439,7 @@ namespace HidLibrary
}
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
+ finally { CloseDeviceIO(overlapped.EventHandle); }
}
else
{
| 1 | Merge pull request #20 from juliansiebert/master | 0 | .cs | cs | mit | mikeobrien/HidLibrary |
1024 | <NME> HidDevice.cs
<BEF> using System;
using System.Runtime.InteropServices;
using System.Threading;
using System.Threading.Tasks;
namespace HidLibrary
{
public class HidDevice : IHidDevice
{
public event InsertedEventHandler Inserted;
public event RemovedEventHandler Removed;
private readonly string _description;
private readonly string _devicePath;
private readonly HidDeviceAttributes _deviceAttributes;
private readonly HidDeviceCapabilities _deviceCapabilities;
private DeviceMode _deviceReadMode = DeviceMode.NonOverlapped;
private DeviceMode _deviceWriteMode = DeviceMode.NonOverlapped;
private ShareMode _deviceShareMode = ShareMode.ShareRead | ShareMode.ShareWrite;
private readonly HidDeviceEventMonitor _deviceEventMonitor;
private bool _monitorDeviceEvents;
protected delegate HidDeviceData ReadDelegate(int timeout);
protected delegate HidReport ReadReportDelegate(int timeout);
private delegate bool WriteDelegate(byte[] data, int timeout);
private delegate bool WriteReportDelegate(HidReport report, int timeout);
internal HidDevice(string devicePath, string description = null)
{
_deviceEventMonitor = new HidDeviceEventMonitor(this);
_deviceEventMonitor.Inserted += DeviceEventMonitorInserted;
_deviceEventMonitor.Removed += DeviceEventMonitorRemoved;
_devicePath = devicePath;
_description = description;
try
{
var hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
_deviceAttributes = GetDeviceAttributes(hidHandle);
_deviceCapabilities = GetDeviceCapabilities(hidHandle);
CloseDeviceIO(hidHandle);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error querying HID device '{0}'.", devicePath), exception);
}
}
public IntPtr ReadHandle { get; private set; }
public IntPtr WriteHandle { get; private set; }
public bool IsOpen { get; private set; }
public bool IsConnected { get { return HidDevices.IsConnected(_devicePath); } }
public string Description { get { return _description; } }
public HidDeviceCapabilities Capabilities { get { return _deviceCapabilities; } }
public HidDeviceAttributes Attributes { get { return _deviceAttributes; } }
public string DevicePath { get { return _devicePath; } }
public bool MonitorDeviceEvents
{
get { return _monitorDeviceEvents; }
set
{
if (value & _monitorDeviceEvents == false) _deviceEventMonitor.Init();
_monitorDeviceEvents = value;
}
}
public override string ToString()
{
return string.Format("VendorID={0}, ProductID={1}, Version={2}, DevicePath={3}",
_deviceAttributes.VendorHexId,
_deviceAttributes.ProductHexId,
_deviceAttributes.Version,
_devicePath);
}
public void OpenDevice()
{
OpenDevice(DeviceMode.NonOverlapped, DeviceMode.NonOverlapped, ShareMode.ShareRead | ShareMode.ShareWrite);
}
public void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode)
{
if (IsOpen) return;
_deviceReadMode = readMode;
_deviceWriteMode = writeMode;
_deviceShareMode = shareMode;
try
{
ReadHandle = OpenDeviceIO(_devicePath, readMode, NativeMethods.GENERIC_READ, shareMode);
WriteHandle = OpenDeviceIO(_devicePath, writeMode, NativeMethods.GENERIC_WRITE, shareMode);
}
catch (Exception exception)
{
IsOpen = false;
throw new Exception("Error opening HID device.", exception);
}
IsOpen = (ReadHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE &&
WriteHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE);
}
public void CloseDevice()
{
if (!IsOpen) return;
CloseDeviceIO(ReadHandle);
CloseDeviceIO(WriteHandle);
IsOpen = false;
}
public HidDeviceData Read()
{
return Read(0);
}
public HidDeviceData Read(int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return ReadData(timeout);
}
catch
{
return new HidDeviceData(HidDeviceData.ReadStatus.ReadError);
}
}
return new HidDeviceData(HidDeviceData.ReadStatus.NotConnected);
}
public void Read(ReadCallback callback)
{
Read(callback, 0);
}
public void Read(ReadCallback callback, int timeout)
{
var readDelegate = new ReadDelegate(Read);
var asyncState = new HidAsyncState(readDelegate, callback);
readDelegate.BeginInvoke(timeout, EndRead, asyncState);
}
public async Task<HidDeviceData> ReadAsync(int timeout = 0)
{
var readDelegate = new ReadDelegate(Read);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidDeviceData>.Factory.StartNew(() => readDelegate.Invoke(timeout));
#else
return await Task<HidDeviceData>.Factory.FromAsync(readDelegate.BeginInvoke, readDelegate.EndInvoke, timeout, null);
#endif
}
public HidReport ReadReport()
{
return ReadReport(0);
}
public HidReport ReadReport(int timeout)
{
return new HidReport(Capabilities.InputReportByteLength, Read(timeout));
}
public void ReadReport(ReadReportCallback callback)
{
ReadReport(callback, 0);
}
public void ReadReport(ReadReportCallback callback, int timeout)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
var asyncState = new HidAsyncState(readReportDelegate, callback);
readReportDelegate.BeginInvoke(timeout, EndReadReport, asyncState);
}
public async Task<HidReport> ReadReportAsync(int timeout = 0)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidReport>.Factory.StartNew(() => readReportDelegate.Invoke(timeout));
#else
return await Task<HidReport>.Factory.FromAsync(readReportDelegate.BeginInvoke, readReportDelegate.EndInvoke, timeout, null);
#endif
}
/// <summary>
/// Reads an input report from the Control channel. This method provides access to report data for devices that
/// do not use the interrupt channel to communicate for specific usages.
/// </summary>
/// <param name="reportId">The report ID to read from the device</param>
/// <returns>The HID report that is read. The report will contain the success status of the read request</returns>
///
public HidReport ReadReportSync(byte reportId)
{
byte[] cmdBuffer = new byte[Capabilities.InputReportByteLength];
cmdBuffer[0] = reportId;
bool bSuccess = NativeMethods.HidD_GetInputReport(ReadHandle, cmdBuffer, cmdBuffer.Length);
HidDeviceData deviceData = new HidDeviceData(cmdBuffer, bSuccess ? HidDeviceData.ReadStatus.Success : HidDeviceData.ReadStatus.NoDataRead);
return new HidReport(Capabilities.InputReportByteLength, deviceData);
}
public bool ReadFeatureData(out byte[] data, byte reportId = 0)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0)
{
data = new byte[0];
return false;
}
data = new byte[_deviceCapabilities.FeatureReportByteLength];
var buffer = CreateFeatureOutputBuffer();
buffer[0] = reportId;
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetFeature(hidHandle, buffer, buffer.Length);
if (success)
{
Array.Copy(buffer, 0, data, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
}
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadProduct(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetProductString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadManufacturer(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetManufacturerString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadSerialNumber(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetSerialNumberString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool Write(byte[] data)
{
return Write(data, 0);
}
public bool Write(byte[] data, int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return WriteData(data, timeout);
}
catch
{
return false;
}
}
return false;
}
public void Write(byte[] data, WriteCallback callback)
{
Write(data, callback, 0);
}
public void Write(byte[] data, WriteCallback callback, int timeout)
{
var writeDelegate = new WriteDelegate(Write);
var asyncState = new HidAsyncState(writeDelegate, callback);
writeDelegate.BeginInvoke(data, timeout, EndWrite, asyncState);
}
public async Task<bool> WriteAsync(byte[] data, int timeout = 0)
{
var writeDelegate = new WriteDelegate(Write);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeDelegate.Invoke(data, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeDelegate.BeginInvoke, writeDelegate.EndInvoke, data, timeout, null);
#endif
}
public bool WriteReport(HidReport report)
{
return WriteReport(report, 0);
}
public bool WriteReport(HidReport report, int timeout)
{
return Write(report.GetBytes(), timeout);
}
public void WriteReport(HidReport report, WriteCallback callback)
{
WriteReport(report, callback, 0);
}
public void WriteReport(HidReport report, WriteCallback callback, int timeout)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
var asyncState = new HidAsyncState(writeReportDelegate, callback);
writeReportDelegate.BeginInvoke(report, timeout, EndWriteReport, asyncState);
}
/// <summary>
/// Handle data transfers on the control channel. This method places data on the control channel for devices
/// that do not support the interupt transfers
/// </summary>
/// <param name="report">The outbound HID report</param>
/// <returns>The result of the tranfer request: true if successful otherwise false</returns>
///
public bool WriteReportSync(HidReport report)
{
if (null != report)
{
byte[] buffer = report.GetBytes();
return (NativeMethods.HidD_SetOutputReport(WriteHandle, buffer, buffer.Length));
}
else
throw new ArgumentException("The output report is null, it must be allocated before you call this method", "report");
}
public async Task<bool> WriteReportAsync(HidReport report, int timeout = 0)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeReportDelegate.Invoke(report, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeReportDelegate.BeginInvoke, writeReportDelegate.EndInvoke, report, timeout, null);
#endif
}
public HidReport CreateReport()
{
return new HidReport(Capabilities.OutputReportByteLength);
}
public bool WriteFeatureData(byte[] data)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0) return false;
}
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
}
else
{
try
{
if (IsOpen)
hidHandle = WriteHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
//var overlapped = new NativeOverlapped();
success = NativeMethods.HidD_SetFeature(hidHandle, buffer, buffer.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != WriteHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
protected static void EndRead(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadCallback)hidAsyncState.CallbackDelegate;
var data = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(data);
}
protected static void EndReadReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadReportCallback)hidAsyncState.CallbackDelegate;
var report = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(report);
}
private static void EndWrite(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private static void EndWriteReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private byte[] CreateInputBuffer()
{
return CreateBuffer(Capabilities.InputReportByteLength - 1);
}
private byte[] CreateOutputBuffer()
{
return CreateBuffer(Capabilities.OutputReportByteLength - 1);
}
private byte[] CreateFeatureOutputBuffer()
{
return CreateBuffer(Capabilities.FeatureReportByteLength - 1);
}
private static byte[] CreateBuffer(int length)
{
byte[] buffer = null;
Array.Resize(ref buffer, length + 1);
return buffer;
}
private static HidDeviceAttributes GetDeviceAttributes(IntPtr hidHandle)
{
var deviceAttributes = default(NativeMethods.HIDD_ATTRIBUTES);
deviceAttributes.Size = Marshal.SizeOf(deviceAttributes);
NativeMethods.HidD_GetAttributes(hidHandle, ref deviceAttributes);
return new HidDeviceAttributes(deviceAttributes);
}
private static HidDeviceCapabilities GetDeviceCapabilities(IntPtr hidHandle)
{
var capabilities = default(NativeMethods.HIDP_CAPS);
var preparsedDataPointer = default(IntPtr);
if (NativeMethods.HidD_GetPreparsedData(hidHandle, ref preparsedDataPointer))
{
NativeMethods.HidP_GetCaps(preparsedDataPointer, ref capabilities);
NativeMethods.HidD_FreePreparsedData(preparsedDataPointer);
}
return new HidDeviceCapabilities(capabilities);
}
private bool WriteData(byte[] data, int timeout)
{
if (_deviceCapabilities.OutputReportByteLength <= 0) return false;
var buffer = CreateOutputBuffer();
uint bytesWritten;
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.OutputReportByteLength));
if (_deviceWriteMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), "");
try
{
NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
return true;
case NativeMethods.WAIT_TIMEOUT:
return false;
case NativeMethods.WAIT_FAILED:
return false;
default:
return false;
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
return NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
}
}
protected HidDeviceData ReadData(int timeout)
{
var buffer = new byte[] { };
var status = HidDeviceData.ReadStatus.NoDataRead;
IntPtr nonManagedBuffer;
if (_deviceCapabilities.InputReportByteLength > 0)
{
uint bytesRead;
buffer = CreateInputBuffer();
nonManagedBuffer = Marshal.AllocHGlobal(buffer.Length);
if (_deviceReadMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), string.Empty);
try
{
var success = NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
if (success)
{
status = HidDeviceData.ReadStatus.Success; // No check here to see if bytesRead > 0 . Perhaps not necessary?
}
else
{
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
status = HidDeviceData.ReadStatus.Success;
NativeMethods.GetOverlappedResult(ReadHandle, ref overlapped, out bytesRead, false);
break;
case NativeMethods.WAIT_TIMEOUT:
status = HidDeviceData.ReadStatus.WaitTimedOut;
buffer = new byte[] { };
break;
case NativeMethods.WAIT_FAILED:
status = HidDeviceData.ReadStatus.WaitFail;
buffer = new byte[] { };
break;
default:
status = HidDeviceData.ReadStatus.NoDataRead;
buffer = new byte[] { };
break;
}
}
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally {
CloseDeviceIO(overlapped.EventHandle);
Marshal.FreeHGlobal(nonManagedBuffer);
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
status = HidDeviceData.ReadStatus.Success;
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally { Marshal.FreeHGlobal(nonManagedBuffer); }
}
}
return new HidDeviceData(buffer, status);
}
private static IntPtr OpenDeviceIO(string devicePath, uint deviceAccess)
{
return OpenDeviceIO(devicePath, DeviceMode.NonOverlapped, deviceAccess, ShareMode.ShareRead | ShareMode.ShareWrite);
}
private static IntPtr OpenDeviceIO(string devicePath, DeviceMode deviceMode, uint deviceAccess, ShareMode shareMode)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var flags = 0;
if (deviceMode == DeviceMode.Overlapped) flags = NativeMethods.FILE_FLAG_OVERLAPPED;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
return NativeMethods.CreateFile(devicePath, deviceAccess, (int)shareMode, ref security, NativeMethods.OPEN_EXISTING, flags, hTemplateFile: IntPtr.Zero);
}
private static void CloseDeviceIO(IntPtr handle)
{
if (Environment.OSVersion.Version.Major > 5)
{
NativeMethods.CancelIoEx(handle, IntPtr.Zero);
}
NativeMethods.CloseHandle(handle);
}
private void DeviceEventMonitorInserted()
{
if (!IsOpen) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
Inserted?.Invoke();
}
private void DeviceEventMonitorRemoved()
{
if (IsOpen) CloseDevice();
Removed?.Invoke();
}
public void Dispose()
{
if (MonitorDeviceEvents) MonitorDeviceEvents = false;
if (IsOpen) CloseDevice();
}
}
}
<MSG> Merge pull request #20 from juliansiebert/master
Fix for handle leak in HidDevice.ReadData()
<DFF> @@ -439,6 +439,7 @@ namespace HidLibrary
}
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
+ finally { CloseDeviceIO(overlapped.EventHandle); }
}
else
{
| 1 | Merge pull request #20 from juliansiebert/master | 0 | .cs | cs | mit | mikeobrien/HidLibrary |
1025 | <NME> HidDevice.cs
<BEF> using System;
using System.Runtime.InteropServices;
using System.Threading;
using System.Threading.Tasks;
namespace HidLibrary
{
public class HidDevice : IHidDevice
{
public event InsertedEventHandler Inserted;
public event RemovedEventHandler Removed;
private readonly string _description;
private readonly string _devicePath;
private readonly HidDeviceAttributes _deviceAttributes;
private readonly HidDeviceCapabilities _deviceCapabilities;
private DeviceMode _deviceReadMode = DeviceMode.NonOverlapped;
private DeviceMode _deviceWriteMode = DeviceMode.NonOverlapped;
private ShareMode _deviceShareMode = ShareMode.ShareRead | ShareMode.ShareWrite;
private readonly HidDeviceEventMonitor _deviceEventMonitor;
private bool _monitorDeviceEvents;
protected delegate HidDeviceData ReadDelegate(int timeout);
private DeviceMode _deviceWriteMode = DeviceMode.NonOverlapped;
private readonly HidDeviceEventMonitor _deviceEventMonitor;
private bool _monitorDeviceEvents;
private delegate HidDeviceData ReadDelegate();
private delegate HidReport ReadReportDelegate();
_deviceEventMonitor.Inserted += DeviceEventMonitorInserted;
_deviceEventMonitor.Removed += DeviceEventMonitorRemoved;
_devicePath = devicePath;
_description = description;
try
{
var hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
_deviceAttributes = GetDeviceAttributes(hidHandle);
_deviceCapabilities = GetDeviceCapabilities(hidHandle);
CloseDeviceIO(hidHandle);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error querying HID device '{0}'.", devicePath), exception);
}
}
public IntPtr ReadHandle { get; private set; }
public IntPtr WriteHandle { get; private set; }
public bool IsOpen { get; private set; }
public bool IsConnected { get { return HidDevices.IsConnected(_devicePath); } }
public string Description { get { return _description; } }
public HidDeviceCapabilities Capabilities { get { return _deviceCapabilities; } }
public HidDeviceAttributes Attributes { get { return _deviceAttributes; } }
public string DevicePath { get { return _devicePath; } }
public bool MonitorDeviceEvents
{
get { return _monitorDeviceEvents; }
set
{
if (value & _monitorDeviceEvents == false) _deviceEventMonitor.Init();
_monitorDeviceEvents = value;
}
}
public override string ToString()
{
return string.Format("VendorID={0}, ProductID={1}, Version={2}, DevicePath={3}",
_deviceAttributes.VendorHexId,
_deviceAttributes.ProductHexId,
_deviceAttributes.Version,
_devicePath);
}
public void OpenDevice()
{
OpenDevice(DeviceMode.NonOverlapped, DeviceMode.NonOverlapped, ShareMode.ShareRead | ShareMode.ShareWrite);
}
public void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode)
{
if (IsOpen) return;
_deviceReadMode = readMode;
_deviceWriteMode = writeMode;
_deviceShareMode = shareMode;
try
{
ReadHandle = OpenDeviceIO(_devicePath, readMode, NativeMethods.GENERIC_READ, shareMode);
WriteHandle = OpenDeviceIO(_devicePath, writeMode, NativeMethods.GENERIC_WRITE, shareMode);
}
catch (Exception exception)
{
IsOpen = false;
throw new Exception("Error opening HID device.", exception);
}
IsOpen = (ReadHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE &&
WriteHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE);
}
public void CloseDevice()
{
if (!IsOpen) return;
CloseDeviceIO(ReadHandle);
CloseDeviceIO(WriteHandle);
IsOpen = false;
}
public HidDeviceData Read()
{
return Read(0);
}
public HidDeviceData Read(int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return ReadData(timeout);
}
catch
{
return new HidDeviceData(HidDeviceData.ReadStatus.ReadError);
}
}
return new HidDeviceData(HidDeviceData.ReadStatus.NotConnected);
}
public void Read(ReadCallback callback)
{
Read(callback, 0);
}
public void Read(ReadCallback callback, int timeout)
{
var readDelegate = new ReadDelegate(Read);
var asyncState = new HidAsyncState(readDelegate, callback);
readDelegate.BeginInvoke(timeout, EndRead, asyncState);
}
public async Task<HidDeviceData> ReadAsync(int timeout = 0)
{
var readDelegate = new ReadDelegate(Read);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidDeviceData>.Factory.StartNew(() => readDelegate.Invoke(timeout));
#else
return await Task<HidDeviceData>.Factory.FromAsync(readDelegate.BeginInvoke, readDelegate.EndInvoke, timeout, null);
#endif
}
public HidReport ReadReport()
{
return ReadReport(0);
}
public HidReport ReadReport(int timeout)
{
return new HidReport(Capabilities.InputReportByteLength, Read(timeout));
}
public void ReadReport(ReadReportCallback callback)
{
ReadReport(callback, 0);
}
public void ReadReport(ReadReportCallback callback, int timeout)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
var asyncState = new HidAsyncState(readReportDelegate, callback);
readReportDelegate.BeginInvoke(timeout, EndReadReport, asyncState);
}
public async Task<HidReport> ReadReportAsync(int timeout = 0)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidReport>.Factory.StartNew(() => readReportDelegate.Invoke(timeout));
#else
return await Task<HidReport>.Factory.FromAsync(readReportDelegate.BeginInvoke, readReportDelegate.EndInvoke, timeout, null);
#endif
}
/// <summary>
/// Reads an input report from the Control channel. This method provides access to report data for devices that
/// do not use the interrupt channel to communicate for specific usages.
/// </summary>
/// <param name="reportId">The report ID to read from the device</param>
/// <returns>The HID report that is read. The report will contain the success status of the read request</returns>
///
public HidReport ReadReportSync(byte reportId)
{
byte[] cmdBuffer = new byte[Capabilities.InputReportByteLength];
cmdBuffer[0] = reportId;
bool bSuccess = NativeMethods.HidD_GetInputReport(ReadHandle, cmdBuffer, cmdBuffer.Length);
HidDeviceData deviceData = new HidDeviceData(cmdBuffer, bSuccess ? HidDeviceData.ReadStatus.Success : HidDeviceData.ReadStatus.NoDataRead);
return new HidReport(Capabilities.InputReportByteLength, deviceData);
}
public bool ReadFeatureData(out byte[] data, byte reportId = 0)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0)
{
data = new byte[0];
return false;
}
data = new byte[_deviceCapabilities.FeatureReportByteLength];
return new HidReport(Capabilities.OutputReportByteLength);
}
private static void EndRead(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetProductString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadManufacturer(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetManufacturerString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
return CreateBuffer(Capabilities.OutputReportByteLength - 1);
}
private static byte[] CreateBuffer(int length)
{
byte[] buffer = null;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetSerialNumberString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool Write(byte[] data)
{
return Write(data, 0);
}
public bool Write(byte[] data, int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return WriteData(data, timeout);
}
catch
{
return false;
}
}
return false;
}
public void Write(byte[] data, WriteCallback callback)
{
Write(data, callback, 0);
}
public void Write(byte[] data, WriteCallback callback, int timeout)
{
var writeDelegate = new WriteDelegate(Write);
var asyncState = new HidAsyncState(writeDelegate, callback);
writeDelegate.BeginInvoke(data, timeout, EndWrite, asyncState);
}
public async Task<bool> WriteAsync(byte[] data, int timeout = 0)
{
var writeDelegate = new WriteDelegate(Write);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeDelegate.Invoke(data, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeDelegate.BeginInvoke, writeDelegate.EndInvoke, data, timeout, null);
#endif
}
public bool WriteReport(HidReport report)
{
return WriteReport(report, 0);
}
public bool WriteReport(HidReport report, int timeout)
{
return Write(report.GetBytes(), timeout);
}
public void WriteReport(HidReport report, WriteCallback callback)
{
WriteReport(report, callback, 0);
}
public void WriteReport(HidReport report, WriteCallback callback, int timeout)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
var asyncState = new HidAsyncState(writeReportDelegate, callback);
writeReportDelegate.BeginInvoke(report, timeout, EndWriteReport, asyncState);
}
/// <summary>
/// Handle data transfers on the control channel. This method places data on the control channel for devices
/// that do not support the interupt transfers
/// </summary>
/// <param name="report">The outbound HID report</param>
/// <returns>The result of the tranfer request: true if successful otherwise false</returns>
///
public bool WriteReportSync(HidReport report)
{
if (null != report)
{
byte[] buffer = report.GetBytes();
return (NativeMethods.HidD_SetOutputReport(WriteHandle, buffer, buffer.Length));
}
else
throw new ArgumentException("The output report is null, it must be allocated before you call this method", "report");
}
public async Task<bool> WriteReportAsync(HidReport report, int timeout = 0)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeReportDelegate.Invoke(report, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeReportDelegate.BeginInvoke, writeReportDelegate.EndInvoke, report, timeout, null);
#endif
}
public HidReport CreateReport()
{
return new HidReport(Capabilities.OutputReportByteLength);
}
public bool WriteFeatureData(byte[] data)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0) return false;
var buffer = CreateFeatureOutputBuffer();
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = WriteHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
//var overlapped = new NativeOverlapped();
success = NativeMethods.HidD_SetFeature(hidHandle, buffer, buffer.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != WriteHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
protected static void EndRead(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadCallback)hidAsyncState.CallbackDelegate;
var data = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(data);
}
protected static void EndReadReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadReportCallback)hidAsyncState.CallbackDelegate;
var report = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(report);
}
private static void EndWrite(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private static void EndWriteReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private byte[] CreateInputBuffer()
{
return CreateBuffer(Capabilities.InputReportByteLength - 1);
}
private byte[] CreateOutputBuffer()
{
return CreateBuffer(Capabilities.OutputReportByteLength - 1);
}
private byte[] CreateFeatureOutputBuffer()
{
return CreateBuffer(Capabilities.FeatureReportByteLength - 1);
}
private static byte[] CreateBuffer(int length)
{
byte[] buffer = null;
Array.Resize(ref buffer, length + 1);
return buffer;
}
private static HidDeviceAttributes GetDeviceAttributes(IntPtr hidHandle)
{
var deviceAttributes = default(NativeMethods.HIDD_ATTRIBUTES);
deviceAttributes.Size = Marshal.SizeOf(deviceAttributes);
NativeMethods.HidD_GetAttributes(hidHandle, ref deviceAttributes);
return new HidDeviceAttributes(deviceAttributes);
}
private static HidDeviceCapabilities GetDeviceCapabilities(IntPtr hidHandle)
{
var capabilities = default(NativeMethods.HIDP_CAPS);
var preparsedDataPointer = default(IntPtr);
if (NativeMethods.HidD_GetPreparsedData(hidHandle, ref preparsedDataPointer))
{
NativeMethods.HidP_GetCaps(preparsedDataPointer, ref capabilities);
NativeMethods.HidD_FreePreparsedData(preparsedDataPointer);
}
return new HidDeviceCapabilities(capabilities);
}
private bool WriteData(byte[] data, int timeout)
{
if (_deviceCapabilities.OutputReportByteLength <= 0) return false;
var buffer = CreateOutputBuffer();
uint bytesWritten;
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.OutputReportByteLength));
if (_deviceWriteMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), "");
try
{
NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
return true;
case NativeMethods.WAIT_TIMEOUT:
return false;
case NativeMethods.WAIT_FAILED:
return false;
default:
return false;
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
return NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
}
}
protected HidDeviceData ReadData(int timeout)
{
var buffer = new byte[] { };
var status = HidDeviceData.ReadStatus.NoDataRead;
IntPtr nonManagedBuffer;
if (_deviceCapabilities.InputReportByteLength > 0)
{
uint bytesRead;
buffer = CreateInputBuffer();
nonManagedBuffer = Marshal.AllocHGlobal(buffer.Length);
if (_deviceReadMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), string.Empty);
try
{
var success = NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
if (success)
{
status = HidDeviceData.ReadStatus.Success; // No check here to see if bytesRead > 0 . Perhaps not necessary?
}
else
{
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
status = HidDeviceData.ReadStatus.Success;
NativeMethods.GetOverlappedResult(ReadHandle, ref overlapped, out bytesRead, false);
break;
case NativeMethods.WAIT_TIMEOUT:
status = HidDeviceData.ReadStatus.WaitTimedOut;
buffer = new byte[] { };
break;
case NativeMethods.WAIT_FAILED:
status = HidDeviceData.ReadStatus.WaitFail;
buffer = new byte[] { };
break;
default:
status = HidDeviceData.ReadStatus.NoDataRead;
buffer = new byte[] { };
break;
}
}
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally {
CloseDeviceIO(overlapped.EventHandle);
Marshal.FreeHGlobal(nonManagedBuffer);
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
status = HidDeviceData.ReadStatus.Success;
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally { Marshal.FreeHGlobal(nonManagedBuffer); }
}
}
return new HidDeviceData(buffer, status);
}
private static IntPtr OpenDeviceIO(string devicePath, uint deviceAccess)
{
return OpenDeviceIO(devicePath, DeviceMode.NonOverlapped, deviceAccess, ShareMode.ShareRead | ShareMode.ShareWrite);
}
private static IntPtr OpenDeviceIO(string devicePath, DeviceMode deviceMode, uint deviceAccess, ShareMode shareMode)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var flags = 0;
if (deviceMode == DeviceMode.Overlapped) flags = NativeMethods.FILE_FLAG_OVERLAPPED;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
return NativeMethods.CreateFile(devicePath, deviceAccess, (int)shareMode, ref security, NativeMethods.OPEN_EXISTING, flags, hTemplateFile: IntPtr.Zero);
}
private static void CloseDeviceIO(IntPtr handle)
{
if (Environment.OSVersion.Version.Major > 5)
{
NativeMethods.CancelIoEx(handle, IntPtr.Zero);
}
NativeMethods.CloseHandle(handle);
}
private void DeviceEventMonitorInserted()
{
if (!IsOpen) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
Inserted?.Invoke();
}
private void DeviceEventMonitorRemoved()
{
if (IsOpen) CloseDevice();
Removed?.Invoke();
}
public void Dispose()
{
if (MonitorDeviceEvents) MonitorDeviceEvents = false;
if (IsOpen) CloseDevice();
}
}
}
<MSG> Added support for writing Hid feature data.
<DFF> @@ -26,7 +26,7 @@ namespace HidLibrary
private DeviceMode _deviceWriteMode = DeviceMode.NonOverlapped;
private readonly HidDeviceEventMonitor _deviceEventMonitor;
-
+
private bool _monitorDeviceEvents;
private delegate HidDeviceData ReadDelegate();
private delegate HidReport ReadReportDelegate();
@@ -222,6 +222,36 @@ namespace HidLibrary
return new HidReport(Capabilities.OutputReportByteLength);
}
+ public bool WriteFeatureData(byte[] data)
+ {
+ if (_deviceCapabilities.FeatureReportByteLength <= 0) return false;
+
+ var buffer = CreateFeatureOutputBuffer();
+
+ Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
+
+
+ IntPtr hidHandle = IntPtr.Zero;
+ bool success = false;
+ try
+ {
+ hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
+
+ var overlapped = new NativeOverlapped();
+ success = NativeMethods.HidD_SetFeature(hidHandle, buffer, buffer.Length);
+ }
+ catch (Exception exception)
+ {
+ throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
+ }
+ finally
+ {
+ if (hidHandle != IntPtr.Zero)
+ CloseDeviceIO(hidHandle);
+ }
+ return success;
+ }
+
private static void EndRead(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
@@ -272,6 +302,11 @@ namespace HidLibrary
return CreateBuffer(Capabilities.OutputReportByteLength - 1);
}
+ private byte[] CreateFeatureOutputBuffer()
+ {
+ return CreateBuffer(Capabilities.FeatureReportByteLength - 1);
+ }
+
private static byte[] CreateBuffer(int length)
{
byte[] buffer = null;
| 36 | Added support for writing Hid feature data. | 1 | .cs | cs | mit | mikeobrien/HidLibrary |
1026 | <NME> HidDevice.cs
<BEF> using System;
using System.Runtime.InteropServices;
using System.Threading;
using System.Threading.Tasks;
namespace HidLibrary
{
public class HidDevice : IHidDevice
{
public event InsertedEventHandler Inserted;
public event RemovedEventHandler Removed;
private readonly string _description;
private readonly string _devicePath;
private readonly HidDeviceAttributes _deviceAttributes;
private readonly HidDeviceCapabilities _deviceCapabilities;
private DeviceMode _deviceReadMode = DeviceMode.NonOverlapped;
private DeviceMode _deviceWriteMode = DeviceMode.NonOverlapped;
private ShareMode _deviceShareMode = ShareMode.ShareRead | ShareMode.ShareWrite;
private readonly HidDeviceEventMonitor _deviceEventMonitor;
private bool _monitorDeviceEvents;
protected delegate HidDeviceData ReadDelegate(int timeout);
private DeviceMode _deviceWriteMode = DeviceMode.NonOverlapped;
private readonly HidDeviceEventMonitor _deviceEventMonitor;
private bool _monitorDeviceEvents;
private delegate HidDeviceData ReadDelegate();
private delegate HidReport ReadReportDelegate();
_deviceEventMonitor.Inserted += DeviceEventMonitorInserted;
_deviceEventMonitor.Removed += DeviceEventMonitorRemoved;
_devicePath = devicePath;
_description = description;
try
{
var hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
_deviceAttributes = GetDeviceAttributes(hidHandle);
_deviceCapabilities = GetDeviceCapabilities(hidHandle);
CloseDeviceIO(hidHandle);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error querying HID device '{0}'.", devicePath), exception);
}
}
public IntPtr ReadHandle { get; private set; }
public IntPtr WriteHandle { get; private set; }
public bool IsOpen { get; private set; }
public bool IsConnected { get { return HidDevices.IsConnected(_devicePath); } }
public string Description { get { return _description; } }
public HidDeviceCapabilities Capabilities { get { return _deviceCapabilities; } }
public HidDeviceAttributes Attributes { get { return _deviceAttributes; } }
public string DevicePath { get { return _devicePath; } }
public bool MonitorDeviceEvents
{
get { return _monitorDeviceEvents; }
set
{
if (value & _monitorDeviceEvents == false) _deviceEventMonitor.Init();
_monitorDeviceEvents = value;
}
}
public override string ToString()
{
return string.Format("VendorID={0}, ProductID={1}, Version={2}, DevicePath={3}",
_deviceAttributes.VendorHexId,
_deviceAttributes.ProductHexId,
_deviceAttributes.Version,
_devicePath);
}
public void OpenDevice()
{
OpenDevice(DeviceMode.NonOverlapped, DeviceMode.NonOverlapped, ShareMode.ShareRead | ShareMode.ShareWrite);
}
public void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode)
{
if (IsOpen) return;
_deviceReadMode = readMode;
_deviceWriteMode = writeMode;
_deviceShareMode = shareMode;
try
{
ReadHandle = OpenDeviceIO(_devicePath, readMode, NativeMethods.GENERIC_READ, shareMode);
WriteHandle = OpenDeviceIO(_devicePath, writeMode, NativeMethods.GENERIC_WRITE, shareMode);
}
catch (Exception exception)
{
IsOpen = false;
throw new Exception("Error opening HID device.", exception);
}
IsOpen = (ReadHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE &&
WriteHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE);
}
public void CloseDevice()
{
if (!IsOpen) return;
CloseDeviceIO(ReadHandle);
CloseDeviceIO(WriteHandle);
IsOpen = false;
}
public HidDeviceData Read()
{
return Read(0);
}
public HidDeviceData Read(int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return ReadData(timeout);
}
catch
{
return new HidDeviceData(HidDeviceData.ReadStatus.ReadError);
}
}
return new HidDeviceData(HidDeviceData.ReadStatus.NotConnected);
}
public void Read(ReadCallback callback)
{
Read(callback, 0);
}
public void Read(ReadCallback callback, int timeout)
{
var readDelegate = new ReadDelegate(Read);
var asyncState = new HidAsyncState(readDelegate, callback);
readDelegate.BeginInvoke(timeout, EndRead, asyncState);
}
public async Task<HidDeviceData> ReadAsync(int timeout = 0)
{
var readDelegate = new ReadDelegate(Read);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidDeviceData>.Factory.StartNew(() => readDelegate.Invoke(timeout));
#else
return await Task<HidDeviceData>.Factory.FromAsync(readDelegate.BeginInvoke, readDelegate.EndInvoke, timeout, null);
#endif
}
public HidReport ReadReport()
{
return ReadReport(0);
}
public HidReport ReadReport(int timeout)
{
return new HidReport(Capabilities.InputReportByteLength, Read(timeout));
}
public void ReadReport(ReadReportCallback callback)
{
ReadReport(callback, 0);
}
public void ReadReport(ReadReportCallback callback, int timeout)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
var asyncState = new HidAsyncState(readReportDelegate, callback);
readReportDelegate.BeginInvoke(timeout, EndReadReport, asyncState);
}
public async Task<HidReport> ReadReportAsync(int timeout = 0)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidReport>.Factory.StartNew(() => readReportDelegate.Invoke(timeout));
#else
return await Task<HidReport>.Factory.FromAsync(readReportDelegate.BeginInvoke, readReportDelegate.EndInvoke, timeout, null);
#endif
}
/// <summary>
/// Reads an input report from the Control channel. This method provides access to report data for devices that
/// do not use the interrupt channel to communicate for specific usages.
/// </summary>
/// <param name="reportId">The report ID to read from the device</param>
/// <returns>The HID report that is read. The report will contain the success status of the read request</returns>
///
public HidReport ReadReportSync(byte reportId)
{
byte[] cmdBuffer = new byte[Capabilities.InputReportByteLength];
cmdBuffer[0] = reportId;
bool bSuccess = NativeMethods.HidD_GetInputReport(ReadHandle, cmdBuffer, cmdBuffer.Length);
HidDeviceData deviceData = new HidDeviceData(cmdBuffer, bSuccess ? HidDeviceData.ReadStatus.Success : HidDeviceData.ReadStatus.NoDataRead);
return new HidReport(Capabilities.InputReportByteLength, deviceData);
}
public bool ReadFeatureData(out byte[] data, byte reportId = 0)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0)
{
data = new byte[0];
return false;
}
data = new byte[_deviceCapabilities.FeatureReportByteLength];
return new HidReport(Capabilities.OutputReportByteLength);
}
private static void EndRead(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetProductString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadManufacturer(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetManufacturerString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
return CreateBuffer(Capabilities.OutputReportByteLength - 1);
}
private static byte[] CreateBuffer(int length)
{
byte[] buffer = null;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetSerialNumberString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool Write(byte[] data)
{
return Write(data, 0);
}
public bool Write(byte[] data, int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return WriteData(data, timeout);
}
catch
{
return false;
}
}
return false;
}
public void Write(byte[] data, WriteCallback callback)
{
Write(data, callback, 0);
}
public void Write(byte[] data, WriteCallback callback, int timeout)
{
var writeDelegate = new WriteDelegate(Write);
var asyncState = new HidAsyncState(writeDelegate, callback);
writeDelegate.BeginInvoke(data, timeout, EndWrite, asyncState);
}
public async Task<bool> WriteAsync(byte[] data, int timeout = 0)
{
var writeDelegate = new WriteDelegate(Write);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeDelegate.Invoke(data, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeDelegate.BeginInvoke, writeDelegate.EndInvoke, data, timeout, null);
#endif
}
public bool WriteReport(HidReport report)
{
return WriteReport(report, 0);
}
public bool WriteReport(HidReport report, int timeout)
{
return Write(report.GetBytes(), timeout);
}
public void WriteReport(HidReport report, WriteCallback callback)
{
WriteReport(report, callback, 0);
}
public void WriteReport(HidReport report, WriteCallback callback, int timeout)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
var asyncState = new HidAsyncState(writeReportDelegate, callback);
writeReportDelegate.BeginInvoke(report, timeout, EndWriteReport, asyncState);
}
/// <summary>
/// Handle data transfers on the control channel. This method places data on the control channel for devices
/// that do not support the interupt transfers
/// </summary>
/// <param name="report">The outbound HID report</param>
/// <returns>The result of the tranfer request: true if successful otherwise false</returns>
///
public bool WriteReportSync(HidReport report)
{
if (null != report)
{
byte[] buffer = report.GetBytes();
return (NativeMethods.HidD_SetOutputReport(WriteHandle, buffer, buffer.Length));
}
else
throw new ArgumentException("The output report is null, it must be allocated before you call this method", "report");
}
public async Task<bool> WriteReportAsync(HidReport report, int timeout = 0)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeReportDelegate.Invoke(report, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeReportDelegate.BeginInvoke, writeReportDelegate.EndInvoke, report, timeout, null);
#endif
}
public HidReport CreateReport()
{
return new HidReport(Capabilities.OutputReportByteLength);
}
public bool WriteFeatureData(byte[] data)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0) return false;
var buffer = CreateFeatureOutputBuffer();
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = WriteHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
//var overlapped = new NativeOverlapped();
success = NativeMethods.HidD_SetFeature(hidHandle, buffer, buffer.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != WriteHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
protected static void EndRead(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadCallback)hidAsyncState.CallbackDelegate;
var data = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(data);
}
protected static void EndReadReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadReportCallback)hidAsyncState.CallbackDelegate;
var report = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(report);
}
private static void EndWrite(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private static void EndWriteReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private byte[] CreateInputBuffer()
{
return CreateBuffer(Capabilities.InputReportByteLength - 1);
}
private byte[] CreateOutputBuffer()
{
return CreateBuffer(Capabilities.OutputReportByteLength - 1);
}
private byte[] CreateFeatureOutputBuffer()
{
return CreateBuffer(Capabilities.FeatureReportByteLength - 1);
}
private static byte[] CreateBuffer(int length)
{
byte[] buffer = null;
Array.Resize(ref buffer, length + 1);
return buffer;
}
private static HidDeviceAttributes GetDeviceAttributes(IntPtr hidHandle)
{
var deviceAttributes = default(NativeMethods.HIDD_ATTRIBUTES);
deviceAttributes.Size = Marshal.SizeOf(deviceAttributes);
NativeMethods.HidD_GetAttributes(hidHandle, ref deviceAttributes);
return new HidDeviceAttributes(deviceAttributes);
}
private static HidDeviceCapabilities GetDeviceCapabilities(IntPtr hidHandle)
{
var capabilities = default(NativeMethods.HIDP_CAPS);
var preparsedDataPointer = default(IntPtr);
if (NativeMethods.HidD_GetPreparsedData(hidHandle, ref preparsedDataPointer))
{
NativeMethods.HidP_GetCaps(preparsedDataPointer, ref capabilities);
NativeMethods.HidD_FreePreparsedData(preparsedDataPointer);
}
return new HidDeviceCapabilities(capabilities);
}
private bool WriteData(byte[] data, int timeout)
{
if (_deviceCapabilities.OutputReportByteLength <= 0) return false;
var buffer = CreateOutputBuffer();
uint bytesWritten;
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.OutputReportByteLength));
if (_deviceWriteMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), "");
try
{
NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
return true;
case NativeMethods.WAIT_TIMEOUT:
return false;
case NativeMethods.WAIT_FAILED:
return false;
default:
return false;
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
return NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
}
}
protected HidDeviceData ReadData(int timeout)
{
var buffer = new byte[] { };
var status = HidDeviceData.ReadStatus.NoDataRead;
IntPtr nonManagedBuffer;
if (_deviceCapabilities.InputReportByteLength > 0)
{
uint bytesRead;
buffer = CreateInputBuffer();
nonManagedBuffer = Marshal.AllocHGlobal(buffer.Length);
if (_deviceReadMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), string.Empty);
try
{
var success = NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
if (success)
{
status = HidDeviceData.ReadStatus.Success; // No check here to see if bytesRead > 0 . Perhaps not necessary?
}
else
{
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
status = HidDeviceData.ReadStatus.Success;
NativeMethods.GetOverlappedResult(ReadHandle, ref overlapped, out bytesRead, false);
break;
case NativeMethods.WAIT_TIMEOUT:
status = HidDeviceData.ReadStatus.WaitTimedOut;
buffer = new byte[] { };
break;
case NativeMethods.WAIT_FAILED:
status = HidDeviceData.ReadStatus.WaitFail;
buffer = new byte[] { };
break;
default:
status = HidDeviceData.ReadStatus.NoDataRead;
buffer = new byte[] { };
break;
}
}
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally {
CloseDeviceIO(overlapped.EventHandle);
Marshal.FreeHGlobal(nonManagedBuffer);
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
status = HidDeviceData.ReadStatus.Success;
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally { Marshal.FreeHGlobal(nonManagedBuffer); }
}
}
return new HidDeviceData(buffer, status);
}
private static IntPtr OpenDeviceIO(string devicePath, uint deviceAccess)
{
return OpenDeviceIO(devicePath, DeviceMode.NonOverlapped, deviceAccess, ShareMode.ShareRead | ShareMode.ShareWrite);
}
private static IntPtr OpenDeviceIO(string devicePath, DeviceMode deviceMode, uint deviceAccess, ShareMode shareMode)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var flags = 0;
if (deviceMode == DeviceMode.Overlapped) flags = NativeMethods.FILE_FLAG_OVERLAPPED;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
return NativeMethods.CreateFile(devicePath, deviceAccess, (int)shareMode, ref security, NativeMethods.OPEN_EXISTING, flags, hTemplateFile: IntPtr.Zero);
}
private static void CloseDeviceIO(IntPtr handle)
{
if (Environment.OSVersion.Version.Major > 5)
{
NativeMethods.CancelIoEx(handle, IntPtr.Zero);
}
NativeMethods.CloseHandle(handle);
}
private void DeviceEventMonitorInserted()
{
if (!IsOpen) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
Inserted?.Invoke();
}
private void DeviceEventMonitorRemoved()
{
if (IsOpen) CloseDevice();
Removed?.Invoke();
}
public void Dispose()
{
if (MonitorDeviceEvents) MonitorDeviceEvents = false;
if (IsOpen) CloseDevice();
}
}
}
<MSG> Added support for writing Hid feature data.
<DFF> @@ -26,7 +26,7 @@ namespace HidLibrary
private DeviceMode _deviceWriteMode = DeviceMode.NonOverlapped;
private readonly HidDeviceEventMonitor _deviceEventMonitor;
-
+
private bool _monitorDeviceEvents;
private delegate HidDeviceData ReadDelegate();
private delegate HidReport ReadReportDelegate();
@@ -222,6 +222,36 @@ namespace HidLibrary
return new HidReport(Capabilities.OutputReportByteLength);
}
+ public bool WriteFeatureData(byte[] data)
+ {
+ if (_deviceCapabilities.FeatureReportByteLength <= 0) return false;
+
+ var buffer = CreateFeatureOutputBuffer();
+
+ Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
+
+
+ IntPtr hidHandle = IntPtr.Zero;
+ bool success = false;
+ try
+ {
+ hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
+
+ var overlapped = new NativeOverlapped();
+ success = NativeMethods.HidD_SetFeature(hidHandle, buffer, buffer.Length);
+ }
+ catch (Exception exception)
+ {
+ throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
+ }
+ finally
+ {
+ if (hidHandle != IntPtr.Zero)
+ CloseDeviceIO(hidHandle);
+ }
+ return success;
+ }
+
private static void EndRead(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
@@ -272,6 +302,11 @@ namespace HidLibrary
return CreateBuffer(Capabilities.OutputReportByteLength - 1);
}
+ private byte[] CreateFeatureOutputBuffer()
+ {
+ return CreateBuffer(Capabilities.FeatureReportByteLength - 1);
+ }
+
private static byte[] CreateBuffer(int length)
{
byte[] buffer = null;
| 36 | Added support for writing Hid feature data. | 1 | .cs | cs | mit | mikeobrien/HidLibrary |
1027 | <NME> HidDevices.cs
<BEF> using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Linq;
using System.Runtime.InteropServices;
namespace HidLibrary
{
public class HidDevices
{
private static Guid _hidClassGuid = Guid.Empty;
public static bool IsConnected(string devicePath)
{
return EnumerateDevices().Any(x => x.Path == devicePath);
}
public static HidDevice GetDevice(string devicePath)
{
return Enumerate(devicePath).FirstOrDefault();
}
public static IEnumerable<HidDevice> Enumerate()
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description));
}
public static IEnumerable<HidDevice> Enumerate(string devicePath)
{
return EnumerateDevices().Where(x => x.Path == devicePath).Select(x => new HidDevice(x.Path, x.Description));
}
public static IEnumerable<HidDevice> Enumerate(int vendorId, params int[] productIds)
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId &&
productIds.Contains(x.Attributes.ProductId));
}
public static IEnumerable<HidDevice> Enumerate(int vendorId)
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId);
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId);
}
public static IEnumerable<HidDevice> Enumerate(ushort UsagePage)
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => (ushort)x.Capabilities.UsagePage == UsagePage);
}
internal class DeviceInfo { public string Path { get; set; } public string Description { get; set; } }
internal static IEnumerable<DeviceInfo> EnumerateDevices()
{
var devices = new List<DeviceInfo>();
var hidClass = HidClassGuid;
var deviceInfoSet = NativeMethods.SetupDiGetClassDevs(ref hidClass, null, hwndParent: IntPtr.Zero, NativeMethods.DIGCF_PRESENT | NativeMethods.DIGCF_DEVICEINTERFACE);
if (deviceInfoSet.ToInt64() != NativeMethods.INVALID_HANDLE_VALUE)
{
var deviceInfoData = CreateDeviceInfoData();
var deviceIndex = 0;
while (NativeMethods.SetupDiEnumDeviceInfo(deviceInfoSet, deviceIndex, ref deviceInfoData))
{
deviceIndex += 1;
var deviceInterfaceData = new NativeMethods.SP_DEVICE_INTERFACE_DATA();
deviceInterfaceData.cbSize = Marshal.SizeOf(deviceInterfaceData);
var deviceInterfaceIndex = 0;
while (NativeMethods.SetupDiEnumDeviceInterfaces(deviceInfoSet, ref deviceInfoData, ref hidClass, deviceInterfaceIndex, ref deviceInterfaceData))
{
deviceInterfaceIndex++;
var devicePath = GetDevicePath(deviceInfoSet, deviceInterfaceData);
var description = GetBusReportedDeviceDescription(deviceInfoSet, ref deviceInfoData) ??
GetDeviceDescription(deviceInfoSet, ref deviceInfoData);
devices.Add(new DeviceInfo { Path = devicePath, Description = description });
}
}
NativeMethods.SetupDiDestroyDeviceInfoList(deviceInfoSet);
}
return devices;
}
private static NativeMethods.SP_DEVINFO_DATA CreateDeviceInfoData()
{
var deviceInfoData = new NativeMethods.SP_DEVINFO_DATA();
deviceInfoData.cbSize = Marshal.SizeOf(deviceInfoData);
deviceInfoData.DevInst = 0;
deviceInfoData.ClassGuid = Guid.Empty;
deviceInfoData.Reserved = IntPtr.Zero;
return deviceInfoData;
}
private static string GetDevicePath(IntPtr deviceInfoSet, NativeMethods.SP_DEVICE_INTERFACE_DATA deviceInterfaceData)
{
var bufferSize = 0;
var interfaceDetail = new NativeMethods.SP_DEVICE_INTERFACE_DETAIL_DATA { Size = IntPtr.Size == 4 ? 4 + Marshal.SystemDefaultCharSize : 8 };
NativeMethods.SetupDiGetDeviceInterfaceDetail(deviceInfoSet, ref deviceInterfaceData, IntPtr.Zero, 0, ref bufferSize, IntPtr.Zero);
return NativeMethods.SetupDiGetDeviceInterfaceDetail(deviceInfoSet, ref deviceInterfaceData, ref interfaceDetail, bufferSize, ref bufferSize, IntPtr.Zero) ?
interfaceDetail.DevicePath : null;
}
private static Guid HidClassGuid
{
get
{
if (_hidClassGuid.Equals(Guid.Empty)) NativeMethods.HidD_GetHidGuid(ref _hidClassGuid);
return _hidClassGuid;
}
}
private static string GetDeviceDescription(IntPtr deviceInfoSet, ref NativeMethods.SP_DEVINFO_DATA devinfoData)
{
unsafe
{
const int charCount = 1024;
var descriptionBuffer = stackalloc char[charCount];
var requiredSize = 0;
var type = 0;
if (NativeMethods.SetupDiGetDeviceRegistryProperty(deviceInfoSet,
ref devinfoData,
NativeMethods.SPDRP_DEVICEDESC,
ref type,
descriptionBuffer,
propertyBufferSize: charCount * sizeof(char),
ref requiredSize))
{
return new string(descriptionBuffer);
}
return null;
}
}
private static string GetBusReportedDeviceDescription(IntPtr deviceInfoSet, ref NativeMethods.SP_DEVINFO_DATA devinfoData)
{
unsafe
{
const int charCount = 1024;
var descriptionBuffer = stackalloc char[charCount];
if (Environment.OSVersion.Version.Major > 5)
{
uint propertyType = 0;
var requiredSize = 0;
if (NativeMethods.SetupDiGetDeviceProperty(deviceInfoSet,
ref devinfoData,
ref NativeMethods.DEVPKEY_Device_BusReportedDeviceDesc,
ref propertyType,
descriptionBuffer,
propertyBufferSize: charCount * sizeof(char),
ref requiredSize,
0))
{
return new string(descriptionBuffer);
}
}
return null;
}
}
}
}
<MSG> Added the ability to enumerate using a specific usage page
<DFF> @@ -36,6 +36,11 @@ namespace HidLibrary
productIds.Contains(x.Attributes.ProductId));
}
+ public static IEnumerable<HidDevice> Enumerate(int vendorId, int productId, ushort UsagePage)
+ {
+ return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId &&
+ productId == (ushort)x.Attributes.ProductId && (ushort)x.Capabilities.UsagePage == UsagePage);
+ }
public static IEnumerable<HidDevice> Enumerate(int vendorId)
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId);
| 5 | Added the ability to enumerate using a specific usage page | 0 | .cs | cs | mit | mikeobrien/HidLibrary |
1028 | <NME> HidDevices.cs
<BEF> using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Linq;
using System.Runtime.InteropServices;
namespace HidLibrary
{
public class HidDevices
{
private static Guid _hidClassGuid = Guid.Empty;
public static bool IsConnected(string devicePath)
{
return EnumerateDevices().Any(x => x.Path == devicePath);
}
public static HidDevice GetDevice(string devicePath)
{
return Enumerate(devicePath).FirstOrDefault();
}
public static IEnumerable<HidDevice> Enumerate()
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description));
}
public static IEnumerable<HidDevice> Enumerate(string devicePath)
{
return EnumerateDevices().Where(x => x.Path == devicePath).Select(x => new HidDevice(x.Path, x.Description));
}
public static IEnumerable<HidDevice> Enumerate(int vendorId, params int[] productIds)
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId &&
productIds.Contains(x.Attributes.ProductId));
}
public static IEnumerable<HidDevice> Enumerate(int vendorId)
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId);
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId);
}
public static IEnumerable<HidDevice> Enumerate(ushort UsagePage)
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => (ushort)x.Capabilities.UsagePage == UsagePage);
}
internal class DeviceInfo { public string Path { get; set; } public string Description { get; set; } }
internal static IEnumerable<DeviceInfo> EnumerateDevices()
{
var devices = new List<DeviceInfo>();
var hidClass = HidClassGuid;
var deviceInfoSet = NativeMethods.SetupDiGetClassDevs(ref hidClass, null, hwndParent: IntPtr.Zero, NativeMethods.DIGCF_PRESENT | NativeMethods.DIGCF_DEVICEINTERFACE);
if (deviceInfoSet.ToInt64() != NativeMethods.INVALID_HANDLE_VALUE)
{
var deviceInfoData = CreateDeviceInfoData();
var deviceIndex = 0;
while (NativeMethods.SetupDiEnumDeviceInfo(deviceInfoSet, deviceIndex, ref deviceInfoData))
{
deviceIndex += 1;
var deviceInterfaceData = new NativeMethods.SP_DEVICE_INTERFACE_DATA();
deviceInterfaceData.cbSize = Marshal.SizeOf(deviceInterfaceData);
var deviceInterfaceIndex = 0;
while (NativeMethods.SetupDiEnumDeviceInterfaces(deviceInfoSet, ref deviceInfoData, ref hidClass, deviceInterfaceIndex, ref deviceInterfaceData))
{
deviceInterfaceIndex++;
var devicePath = GetDevicePath(deviceInfoSet, deviceInterfaceData);
var description = GetBusReportedDeviceDescription(deviceInfoSet, ref deviceInfoData) ??
GetDeviceDescription(deviceInfoSet, ref deviceInfoData);
devices.Add(new DeviceInfo { Path = devicePath, Description = description });
}
}
NativeMethods.SetupDiDestroyDeviceInfoList(deviceInfoSet);
}
return devices;
}
private static NativeMethods.SP_DEVINFO_DATA CreateDeviceInfoData()
{
var deviceInfoData = new NativeMethods.SP_DEVINFO_DATA();
deviceInfoData.cbSize = Marshal.SizeOf(deviceInfoData);
deviceInfoData.DevInst = 0;
deviceInfoData.ClassGuid = Guid.Empty;
deviceInfoData.Reserved = IntPtr.Zero;
return deviceInfoData;
}
private static string GetDevicePath(IntPtr deviceInfoSet, NativeMethods.SP_DEVICE_INTERFACE_DATA deviceInterfaceData)
{
var bufferSize = 0;
var interfaceDetail = new NativeMethods.SP_DEVICE_INTERFACE_DETAIL_DATA { Size = IntPtr.Size == 4 ? 4 + Marshal.SystemDefaultCharSize : 8 };
NativeMethods.SetupDiGetDeviceInterfaceDetail(deviceInfoSet, ref deviceInterfaceData, IntPtr.Zero, 0, ref bufferSize, IntPtr.Zero);
return NativeMethods.SetupDiGetDeviceInterfaceDetail(deviceInfoSet, ref deviceInterfaceData, ref interfaceDetail, bufferSize, ref bufferSize, IntPtr.Zero) ?
interfaceDetail.DevicePath : null;
}
private static Guid HidClassGuid
{
get
{
if (_hidClassGuid.Equals(Guid.Empty)) NativeMethods.HidD_GetHidGuid(ref _hidClassGuid);
return _hidClassGuid;
}
}
private static string GetDeviceDescription(IntPtr deviceInfoSet, ref NativeMethods.SP_DEVINFO_DATA devinfoData)
{
unsafe
{
const int charCount = 1024;
var descriptionBuffer = stackalloc char[charCount];
var requiredSize = 0;
var type = 0;
if (NativeMethods.SetupDiGetDeviceRegistryProperty(deviceInfoSet,
ref devinfoData,
NativeMethods.SPDRP_DEVICEDESC,
ref type,
descriptionBuffer,
propertyBufferSize: charCount * sizeof(char),
ref requiredSize))
{
return new string(descriptionBuffer);
}
return null;
}
}
private static string GetBusReportedDeviceDescription(IntPtr deviceInfoSet, ref NativeMethods.SP_DEVINFO_DATA devinfoData)
{
unsafe
{
const int charCount = 1024;
var descriptionBuffer = stackalloc char[charCount];
if (Environment.OSVersion.Version.Major > 5)
{
uint propertyType = 0;
var requiredSize = 0;
if (NativeMethods.SetupDiGetDeviceProperty(deviceInfoSet,
ref devinfoData,
ref NativeMethods.DEVPKEY_Device_BusReportedDeviceDesc,
ref propertyType,
descriptionBuffer,
propertyBufferSize: charCount * sizeof(char),
ref requiredSize,
0))
{
return new string(descriptionBuffer);
}
}
return null;
}
}
}
}
<MSG> Added the ability to enumerate using a specific usage page
<DFF> @@ -36,6 +36,11 @@ namespace HidLibrary
productIds.Contains(x.Attributes.ProductId));
}
+ public static IEnumerable<HidDevice> Enumerate(int vendorId, int productId, ushort UsagePage)
+ {
+ return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId &&
+ productId == (ushort)x.Attributes.ProductId && (ushort)x.Capabilities.UsagePage == UsagePage);
+ }
public static IEnumerable<HidDevice> Enumerate(int vendorId)
{
return EnumerateDevices().Select(x => new HidDevice(x.Path, x.Description)).Where(x => x.Attributes.VendorId == vendorId);
| 5 | Added the ability to enumerate using a specific usage page | 0 | .cs | cs | mit | mikeobrien/HidLibrary |
1029 | <NME> IHidDevice.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace HidLibrary
{
public enum DeviceMode
{
NonOverlapped = 0,
Overlapped = 1
}
[Flags]
public enum ShareMode
{
Exclusive = 0,
ShareRead = NativeMethods.FILE_SHARE_READ,
ShareWrite = NativeMethods.FILE_SHARE_WRITE
}
public delegate void ReadCallback(HidDeviceData data);
public delegate void ReadReportCallback(HidReport report);
public delegate void WriteCallback(bool success);
public interface IHidDevice : IDisposable
{
event InsertedEventHandler Inserted;
event RemovedEventHandler Removed;
IntPtr ReadHandle { get; }
IntPtr WriteHandle { get; }
bool IsOpen { get; }
bool IsConnected { get; }
string Description { get; }
HidDeviceCapabilities Capabilities { get; }
HidDeviceAttributes Attributes { get; }
string DevicePath { get; }
bool MonitorDeviceEvents { get; set; }
void OpenDevice();
void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode);
void CloseDevice();
HidDeviceData Read();
void Read(ReadCallback callback);
void Read(ReadCallback callback);
HidDeviceData Read(int timeout);
void ReadReport(ReadReportCallback callback);
HidReport ReadReport(int timeout);
HidReport ReadReport();
bool ReadProduct(out byte[] data);
bool ReadManufacturer(out byte[] data);
bool ReadSerialNumber(out byte[] data);
void Write(byte[] data, WriteCallback callback);
bool Write(byte[] data);
bool Write(byte[] data, int timeout);
bool Write(byte[] data, int timeout);
void WriteReport(HidReport report, WriteCallback callback);
bool WriteReport(HidReport report);
bool WriteReport(HidReport report, int timeout);
HidReport CreateReport();
bool WriteFeatureData(byte[] data);
<MSG> Add async methods to IHidDevice interface
<DFF> @@ -2,6 +2,7 @@
using System.Collections.Generic;
using System.Linq;
using System.Text;
+using System.Threading.Tasks;
namespace HidLibrary
{
@@ -51,10 +52,18 @@ namespace HidLibrary
void Read(ReadCallback callback);
+ void Read(ReadCallback callback, int timeout);
+
+ Task<HidDeviceData> ReadAsync(int timeout = 0);
+
HidDeviceData Read(int timeout);
void ReadReport(ReadReportCallback callback);
+ void ReadReport(ReadReportCallback callback, int timeout);
+
+ Task<HidReport> ReadReportAsync(int timeout = 0);
+
HidReport ReadReport(int timeout);
HidReport ReadReport();
@@ -72,12 +81,20 @@ namespace HidLibrary
bool Write(byte[] data, int timeout);
+ void Write(byte[] data, WriteCallback callback, int timeout);
+
+ Task<bool> WriteAsync(byte[] data, int timeout = 0);
+
void WriteReport(HidReport report, WriteCallback callback);
bool WriteReport(HidReport report);
bool WriteReport(HidReport report, int timeout);
+ void WriteReport(HidReport report, WriteCallback callback, int timeout);
+
+ Task<bool> WriteReportAsync(HidReport report, int timeout = 0);
+
HidReport CreateReport();
bool WriteFeatureData(byte[] data);
| 17 | Add async methods to IHidDevice interface | 0 | .cs | cs | mit | mikeobrien/HidLibrary |
1030 | <NME> IHidDevice.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace HidLibrary
{
public enum DeviceMode
{
NonOverlapped = 0,
Overlapped = 1
}
[Flags]
public enum ShareMode
{
Exclusive = 0,
ShareRead = NativeMethods.FILE_SHARE_READ,
ShareWrite = NativeMethods.FILE_SHARE_WRITE
}
public delegate void ReadCallback(HidDeviceData data);
public delegate void ReadReportCallback(HidReport report);
public delegate void WriteCallback(bool success);
public interface IHidDevice : IDisposable
{
event InsertedEventHandler Inserted;
event RemovedEventHandler Removed;
IntPtr ReadHandle { get; }
IntPtr WriteHandle { get; }
bool IsOpen { get; }
bool IsConnected { get; }
string Description { get; }
HidDeviceCapabilities Capabilities { get; }
HidDeviceAttributes Attributes { get; }
string DevicePath { get; }
bool MonitorDeviceEvents { get; set; }
void OpenDevice();
void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode);
void CloseDevice();
HidDeviceData Read();
void Read(ReadCallback callback);
void Read(ReadCallback callback);
HidDeviceData Read(int timeout);
void ReadReport(ReadReportCallback callback);
HidReport ReadReport(int timeout);
HidReport ReadReport();
bool ReadProduct(out byte[] data);
bool ReadManufacturer(out byte[] data);
bool ReadSerialNumber(out byte[] data);
void Write(byte[] data, WriteCallback callback);
bool Write(byte[] data);
bool Write(byte[] data, int timeout);
bool Write(byte[] data, int timeout);
void WriteReport(HidReport report, WriteCallback callback);
bool WriteReport(HidReport report);
bool WriteReport(HidReport report, int timeout);
HidReport CreateReport();
bool WriteFeatureData(byte[] data);
<MSG> Add async methods to IHidDevice interface
<DFF> @@ -2,6 +2,7 @@
using System.Collections.Generic;
using System.Linq;
using System.Text;
+using System.Threading.Tasks;
namespace HidLibrary
{
@@ -51,10 +52,18 @@ namespace HidLibrary
void Read(ReadCallback callback);
+ void Read(ReadCallback callback, int timeout);
+
+ Task<HidDeviceData> ReadAsync(int timeout = 0);
+
HidDeviceData Read(int timeout);
void ReadReport(ReadReportCallback callback);
+ void ReadReport(ReadReportCallback callback, int timeout);
+
+ Task<HidReport> ReadReportAsync(int timeout = 0);
+
HidReport ReadReport(int timeout);
HidReport ReadReport();
@@ -72,12 +81,20 @@ namespace HidLibrary
bool Write(byte[] data, int timeout);
+ void Write(byte[] data, WriteCallback callback, int timeout);
+
+ Task<bool> WriteAsync(byte[] data, int timeout = 0);
+
void WriteReport(HidReport report, WriteCallback callback);
bool WriteReport(HidReport report);
bool WriteReport(HidReport report, int timeout);
+ void WriteReport(HidReport report, WriteCallback callback, int timeout);
+
+ Task<bool> WriteReportAsync(HidReport report, int timeout = 0);
+
HidReport CreateReport();
bool WriteFeatureData(byte[] data);
| 17 | Add async methods to IHidDevice interface | 0 | .cs | cs | mit | mikeobrien/HidLibrary |
1031 | <NME> app.config
<BEF> ADDFILE
<MSG> Conversion to C# and added x64 support.
<DFF> @@ -0,0 +1,3 @@
+<?xml version="1.0"?>
+<configuration>
+<startup><supportedRuntime version="v4.0" sku=".NETFramework,Version=v4.0"/></startup></configuration>
| 3 | Conversion to C# and added x64 support. | 0 | .config | config | mit | mikeobrien/HidLibrary |
1032 | <NME> app.config
<BEF> ADDFILE
<MSG> Conversion to C# and added x64 support.
<DFF> @@ -0,0 +1,3 @@
+<?xml version="1.0"?>
+<configuration>
+<startup><supportedRuntime version="v4.0" sku=".NETFramework,Version=v4.0"/></startup></configuration>
| 3 | Conversion to C# and added x64 support. | 0 | .config | config | mit | mikeobrien/HidLibrary |
1033 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
namespace HidLibrary
{
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
[DllImport("hid.dll")]
static internal extern int HidP_GetValueCaps(short reportType, ref byte valueCaps, ref short valueCapsLength, IntPtr preparsedData);
}
}
<MSG> Added getters for Product, Manufacturer, and Serial Number strings
<DFF> @@ -1,5 +1,6 @@
using System;
using System.Runtime.InteropServices;
+using System.Text;
namespace HidLibrary
{
@@ -328,5 +329,14 @@ namespace HidLibrary
[DllImport("hid.dll")]
static internal extern int HidP_GetValueCaps(short reportType, ref byte valueCaps, ref short valueCapsLength, IntPtr preparsedData);
+
+ [DllImport("hid.dll", CharSet = CharSet.Unicode)]
+ internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int ReportBufferLength);
+
+ [DllImport("hid.dll", CharSet = CharSet.Unicode)]
+ internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int ReportBufferLength);
+
+ [DllImport("hid.dll", CharSet = CharSet.Unicode)]
+ internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int reportBufferLength);
}
}
| 10 | Added getters for Product, Manufacturer, and Serial Number strings | 0 | .cs | cs | mit | mikeobrien/HidLibrary |
1034 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
namespace HidLibrary
{
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
[DllImport("hid.dll")]
static internal extern int HidP_GetValueCaps(short reportType, ref byte valueCaps, ref short valueCapsLength, IntPtr preparsedData);
}
}
<MSG> Added getters for Product, Manufacturer, and Serial Number strings
<DFF> @@ -1,5 +1,6 @@
using System;
using System.Runtime.InteropServices;
+using System.Text;
namespace HidLibrary
{
@@ -328,5 +329,14 @@ namespace HidLibrary
[DllImport("hid.dll")]
static internal extern int HidP_GetValueCaps(short reportType, ref byte valueCaps, ref short valueCapsLength, IntPtr preparsedData);
+
+ [DllImport("hid.dll", CharSet = CharSet.Unicode)]
+ internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int ReportBufferLength);
+
+ [DllImport("hid.dll", CharSet = CharSet.Unicode)]
+ internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int ReportBufferLength);
+
+ [DllImport("hid.dll", CharSet = CharSet.Unicode)]
+ internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, StringBuilder lpReportBuffer, int reportBufferLength);
}
}
| 10 | Added getters for Product, Manufacturer, and Serial Number strings | 0 | .cs | cs | mit | mikeobrien/HidLibrary |
1035 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
}
[DllImport("hid.dll")]
static internal extern bool HidD_FlushQueue(int hidDeviceObject);
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetNumInputBuffers(int hidDeviceObject, ref int numberBuffers);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetNumInputBuffers(int hidDeviceObject, int numberBuffers);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
<MSG> Changed function signatures to make them easier to use from C# (without type casting)
<DFF> @@ -272,22 +272,22 @@ namespace HidLibrary
}
[DllImport("hid.dll")]
- static internal extern bool HidD_FlushQueue(int hidDeviceObject);
+ static internal extern bool HidD_FlushQueue(IntPtr hidDeviceObject);
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
- static internal extern bool HidD_GetFeature(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
- static internal extern bool HidD_GetInputReport(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
- static internal extern bool HidD_GetNumInputBuffers(int hidDeviceObject, ref int numberBuffers);
+ static internal extern bool HidD_GetNumInputBuffers(IntPtr hidDeviceObject, ref int numberBuffers);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
@@ -296,13 +296,13 @@ namespace HidLibrary
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
- static internal extern bool HidD_SetFeature(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
- static internal extern bool HidD_SetNumInputBuffers(int hidDeviceObject, int numberBuffers);
+ static internal extern bool HidD_SetNumInputBuffers(IntPtr hidDeviceObject, int numberBuffers);
[DllImport("hid.dll")]
- static internal extern bool HidD_SetOutputReport(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
| 7 | Changed function signatures to make them easier to use from C# (without type casting) | 7 | .cs | cs | mit | mikeobrien/HidLibrary |
1036 | <NME> NativeMethods.cs
<BEF> using System;
using System.Runtime.InteropServices;
[module: DefaultCharSet(CharSet.Unicode)]
namespace HidLibrary
{
internal static class NativeMethods
{
internal const int FILE_FLAG_OVERLAPPED = 0x40000000;
internal const short FILE_SHARE_READ = 0x1;
internal const short FILE_SHARE_WRITE = 0x2;
internal const uint GENERIC_READ = 0x80000000;
internal const uint GENERIC_WRITE = 0x40000000;
internal const int ACCESS_NONE = 0;
internal const int INVALID_HANDLE_VALUE = -1;
internal const short OPEN_EXISTING = 3;
internal const int WAIT_TIMEOUT = 0x102;
internal const uint WAIT_OBJECT_0 = 0;
internal const uint WAIT_FAILED = 0xffffffff;
internal const int WAIT_INFINITE = -1;
[StructLayout(LayoutKind.Sequential)]
internal struct SECURITY_ATTRIBUTES
{
public int nLength;
public IntPtr lpSecurityDescriptor;
public bool bInheritHandle;
}
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CancelIoEx(IntPtr hFile, IntPtr lpOverlapped);
[DllImport("kernel32.dll", SetLastError = true, ExactSpelling = true)]
static internal extern bool CloseHandle(IntPtr hObject);
[DllImport("kernel32.dll")]
static internal extern IntPtr CreateEvent(ref SECURITY_ATTRIBUTES securityAttributes, int bManualReset, int bInitialState, string lpName);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern IntPtr CreateFile(string lpFileName, uint dwDesiredAccess, int dwShareMode, ref SECURITY_ATTRIBUTES lpSecurityAttributes, int dwCreationDisposition, int dwFlagsAndAttributes, IntPtr hTemplateFile);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool ReadFile(IntPtr hFile, IntPtr lpBuffer, uint nNumberOfBytesToRead, out uint lpNumberOfBytesRead, [In] ref System.Threading.NativeOverlapped lpOverlapped);
[DllImport("kernel32.dll")]
static internal extern uint WaitForSingleObject(IntPtr hHandle, int dwMilliseconds);
[DllImport("kernel32.dll", SetLastError = true)]
static internal extern bool GetOverlappedResult(IntPtr hFile, [In] ref System.Threading.NativeOverlapped lpOverlapped, out uint lpNumberOfBytesTransferred, bool bWait);
[DllImport("kernel32.dll")]
static internal extern bool WriteFile(IntPtr hFile, byte[] lpBuffer, uint nNumberOfBytesToWrite, out uint lpNumberOfBytesWritten, [In] ref System.Threading.NativeOverlapped lpOverlapped);
internal const short DIGCF_PRESENT = 0x2;
internal const short DIGCF_DEVICEINTERFACE = 0x10;
internal const int DIGCF_ALLCLASSES = 0x4;
internal const int SPDRP_ADDRESS = 0x1c;
internal const int SPDRP_BUSNUMBER = 0x15;
internal const int SPDRP_BUSTYPEGUID = 0x13;
internal const int SPDRP_CAPABILITIES = 0xf;
internal const int SPDRP_CHARACTERISTICS = 0x1b;
internal const int SPDRP_CLASS = 7;
internal const int SPDRP_CLASSGUID = 8;
internal const int SPDRP_COMPATIBLEIDS = 2;
internal const int SPDRP_CONFIGFLAGS = 0xa;
internal const int SPDRP_DEVICE_POWER_DATA = 0x1e;
internal const int SPDRP_DEVICEDESC = 0;
internal const int SPDRP_DEVTYPE = 0x19;
internal const int SPDRP_DRIVER = 9;
internal const int SPDRP_ENUMERATOR_NAME = 0x16;
internal const int SPDRP_EXCLUSIVE = 0x1a;
internal const int SPDRP_FRIENDLYNAME = 0xc;
internal const int SPDRP_HARDWAREID = 1;
internal const int SPDRP_LEGACYBUSTYPE = 0x14;
internal const int SPDRP_LOCATION_INFORMATION = 0xd;
internal const int SPDRP_LOWERFILTERS = 0x12;
internal const int SPDRP_MFG = 0xb;
internal const int SPDRP_PHYSICAL_DEVICE_OBJECT_NAME = 0xe;
internal const int SPDRP_REMOVAL_POLICY = 0x1f;
internal const int SPDRP_REMOVAL_POLICY_HW_DEFAULT = 0x20;
internal const int SPDRP_REMOVAL_POLICY_OVERRIDE = 0x21;
internal const int SPDRP_SECURITY = 0x17;
internal const int SPDRP_SECURITY_SDS = 0x18;
internal const int SPDRP_SERVICE = 4;
internal const int SPDRP_UI_NUMBER = 0x10;
internal const int SPDRP_UI_NUMBER_DESC_FORMAT = 0x1d;
internal const int SPDRP_UPPERFILTERS = 0x11;
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DATA
{
internal int cbSize;
internal System.Guid InterfaceClassGuid;
internal int Flags;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVINFO_DATA
{
internal int cbSize;
internal Guid ClassGuid;
internal int DevInst;
internal IntPtr Reserved;
}
[StructLayout(LayoutKind.Sequential)]
internal struct SP_DEVICE_INTERFACE_DETAIL_DATA
{
internal int Size;
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 256)]
internal string DevicePath;
}
[StructLayout(LayoutKind.Sequential)]
internal struct DEVPROPKEY
{
public Guid fmtid;
public uint pid;
}
internal static DEVPROPKEY DEVPKEY_Device_BusReportedDeviceDesc =
new DEVPROPKEY { fmtid = new Guid(0x540b947e, 0x8b40, 0x45bc, 0xa8, 0xa2, 0x6a, 0x0b, 0x89, 0x4c, 0xbd, 0xa2), pid = 4 };
[DllImport("setupapi.dll")]
public static extern unsafe bool SetupDiGetDeviceRegistryProperty(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, int propertyVal, ref int propertyRegDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize);
[DllImport("setupapi.dll", SetLastError = true)]
public static extern unsafe bool SetupDiGetDeviceProperty(IntPtr deviceInfo, ref SP_DEVINFO_DATA deviceInfoData, ref DEVPROPKEY propkey, ref uint propertyDataType, void* propertyBuffer, int propertyBufferSize, ref int requiredSize, uint flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInfo(IntPtr deviceInfoSet, int memberIndex, ref SP_DEVINFO_DATA deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern int SetupDiDestroyDeviceInfoList(IntPtr deviceInfoSet);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiEnumDeviceInterfaces(IntPtr deviceInfoSet, ref SP_DEVINFO_DATA deviceInfoData, ref Guid interfaceClassGuid, int memberIndex, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData);
[DllImport("setupapi.dll")]
static internal extern IntPtr SetupDiGetClassDevs(ref System.Guid classGuid, string enumerator, IntPtr hwndParent, int flags);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, IntPtr deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[DllImport("setupapi.dll")]
static internal extern bool SetupDiGetDeviceInterfaceDetail(IntPtr deviceInfoSet, ref SP_DEVICE_INTERFACE_DATA deviceInterfaceData, ref SP_DEVICE_INTERFACE_DETAIL_DATA deviceInterfaceDetailData, int deviceInterfaceDetailDataSize, ref int requiredSize, IntPtr deviceInfoData);
[StructLayout(LayoutKind.Sequential)]
internal struct HIDD_ATTRIBUTES
{
internal int Size;
internal ushort VendorID;
internal ushort ProductID;
internal short VersionNumber;
}
[StructLayout(LayoutKind.Sequential)]
internal struct HIDP_CAPS
{
internal short Usage;
internal short UsagePage;
internal short InputReportByteLength;
internal short OutputReportByteLength;
internal short FeatureReportByteLength;
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 17)]
internal short[] Reserved;
internal short NumberLinkCollectionNodes;
internal short NumberInputButtonCaps;
internal short NumberInputValueCaps;
internal short NumberInputDataIndices;
internal short NumberOutputButtonCaps;
internal short NumberOutputValueCaps;
internal short NumberOutputDataIndices;
internal short NumberFeatureButtonCaps;
internal short NumberFeatureValueCaps;
internal short NumberFeatureDataIndices;
}
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
[DllImport("hid.dll")]
internal static extern bool HidD_GetProductString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetManufacturerString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int ReportBufferLength);
[DllImport("hid.dll")]
internal static extern bool HidD_GetSerialNumberString(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
}
}
}
[DllImport("hid.dll")]
static internal extern bool HidD_FlushQueue(int hidDeviceObject);
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
static internal extern bool HidD_GetFeature(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_GetInputReport(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
static internal extern bool HidD_GetNumInputBuffers(int hidDeviceObject, ref int numberBuffers);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
static internal extern bool HidD_SetFeature(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern bool HidD_SetNumInputBuffers(int hidDeviceObject, int numberBuffers);
[DllImport("hid.dll")]
static internal extern bool HidD_SetOutputReport(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
<MSG> Changed function signatures to make them easier to use from C# (without type casting)
<DFF> @@ -272,22 +272,22 @@ namespace HidLibrary
}
[DllImport("hid.dll")]
- static internal extern bool HidD_FlushQueue(int hidDeviceObject);
+ static internal extern bool HidD_FlushQueue(IntPtr hidDeviceObject);
[DllImport("hid.dll")]
static internal extern bool HidD_GetAttributes(IntPtr hidDeviceObject, ref HIDD_ATTRIBUTES attributes);
[DllImport("hid.dll")]
- static internal extern bool HidD_GetFeature(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_GetFeature(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
- static internal extern bool HidD_GetInputReport(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_GetInputReport(IntPtr hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern void HidD_GetHidGuid(ref Guid hidGuid);
[DllImport("hid.dll")]
- static internal extern bool HidD_GetNumInputBuffers(int hidDeviceObject, ref int numberBuffers);
+ static internal extern bool HidD_GetNumInputBuffers(IntPtr hidDeviceObject, ref int numberBuffers);
[DllImport("hid.dll")]
static internal extern bool HidD_GetPreparsedData(IntPtr hidDeviceObject, ref IntPtr preparsedData);
@@ -296,13 +296,13 @@ namespace HidLibrary
static internal extern bool HidD_FreePreparsedData(IntPtr preparsedData);
[DllImport("hid.dll")]
- static internal extern bool HidD_SetFeature(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_SetFeature(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
- static internal extern bool HidD_SetNumInputBuffers(int hidDeviceObject, int numberBuffers);
+ static internal extern bool HidD_SetNumInputBuffers(IntPtr hidDeviceObject, int numberBuffers);
[DllImport("hid.dll")]
- static internal extern bool HidD_SetOutputReport(int hidDeviceObject, ref byte lpReportBuffer, int reportBufferLength);
+ static internal extern bool HidD_SetOutputReport(IntPtr hidDeviceObject, byte[] lpReportBuffer, int reportBufferLength);
[DllImport("hid.dll")]
static internal extern int HidP_GetCaps(IntPtr preparsedData, ref HIDP_CAPS capabilities);
| 7 | Changed function signatures to make them easier to use from C# (without type casting) | 7 | .cs | cs | mit | mikeobrien/HidLibrary |
1037 | <NME> HidDevice.cs
<BEF> using System;
using System.Runtime.InteropServices;
using System.Threading;
using System.Threading.Tasks;
namespace HidLibrary
{
public class HidDevice : IHidDevice
{
public event InsertedEventHandler Inserted;
public event RemovedEventHandler Removed;
private readonly string _description;
private readonly string _devicePath;
private readonly HidDeviceAttributes _deviceAttributes;
private readonly HidDeviceCapabilities _deviceCapabilities;
private DeviceMode _deviceReadMode = DeviceMode.NonOverlapped;
private DeviceMode _deviceWriteMode = DeviceMode.NonOverlapped;
private ShareMode _deviceShareMode = ShareMode.ShareRead | ShareMode.ShareWrite;
private readonly HidDeviceEventMonitor _deviceEventMonitor;
private bool _monitorDeviceEvents;
protected delegate HidDeviceData ReadDelegate(int timeout);
protected delegate HidReport ReadReportDelegate(int timeout);
private delegate bool WriteDelegate(byte[] data, int timeout);
private delegate bool WriteReportDelegate(HidReport report, int timeout);
internal HidDevice(string devicePath, string description = null)
{
_deviceEventMonitor = new HidDeviceEventMonitor(this);
_deviceEventMonitor.Inserted += DeviceEventMonitorInserted;
_deviceEventMonitor.Removed += DeviceEventMonitorRemoved;
_devicePath = devicePath;
_description = description;
try
{
var hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
_deviceAttributes = GetDeviceAttributes(hidHandle);
_deviceCapabilities = GetDeviceCapabilities(hidHandle);
CloseDeviceIO(hidHandle);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error querying HID device '{0}'.", devicePath), exception);
}
}
public IntPtr ReadHandle { get; private set; }
public IntPtr WriteHandle { get; private set; }
public bool IsOpen { get; private set; }
public bool IsConnected { get { return HidDevices.IsConnected(_devicePath); } }
public string Description { get { return _description; } }
public HidDeviceCapabilities Capabilities { get { return _deviceCapabilities; } }
public HidDeviceAttributes Attributes { get { return _deviceAttributes; } }
public string DevicePath { get { return _devicePath; } }
public bool MonitorDeviceEvents
{
get { return _monitorDeviceEvents; }
set
{
if (value & _monitorDeviceEvents == false) _deviceEventMonitor.Init();
_monitorDeviceEvents = value;
}
}
public override string ToString()
{
return string.Format("VendorID={0}, ProductID={1}, Version={2}, DevicePath={3}",
_deviceAttributes.VendorHexId,
_deviceAttributes.ProductHexId,
_deviceAttributes.Version,
_devicePath);
}
public void OpenDevice()
{
OpenDevice(DeviceMode.NonOverlapped, DeviceMode.NonOverlapped, ShareMode.ShareRead | ShareMode.ShareWrite);
}
public void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode)
{
if (IsOpen) return;
_deviceReadMode = readMode;
_deviceWriteMode = writeMode;
_deviceShareMode = shareMode;
try
{
ReadHandle = OpenDeviceIO(_devicePath, readMode, NativeMethods.GENERIC_READ, shareMode);
WriteHandle = OpenDeviceIO(_devicePath, writeMode, NativeMethods.GENERIC_WRITE, shareMode);
}
catch (Exception exception)
{
IsOpen = false;
throw new Exception("Error opening HID device.", exception);
}
IsOpen = (ReadHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE &&
WriteHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE);
}
public void CloseDevice()
{
if (!IsOpen) return;
CloseDeviceIO(ReadHandle);
CloseDeviceIO(WriteHandle);
IsOpen = false;
}
public HidDeviceData Read()
{
return Read(0);
}
public HidDeviceData Read(int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return ReadData(timeout);
}
catch
{
return new HidDeviceData(HidDeviceData.ReadStatus.ReadError);
}
}
return new HidDeviceData(HidDeviceData.ReadStatus.NotConnected);
}
public void Read(ReadCallback callback)
{
Read(callback, 0);
}
public void Read(ReadCallback callback, int timeout)
{
var readDelegate = new ReadDelegate(Read);
var asyncState = new HidAsyncState(readDelegate, callback);
readDelegate.BeginInvoke(timeout, EndRead, asyncState);
}
public async Task<HidDeviceData> ReadAsync(int timeout = 0)
{
var readDelegate = new ReadDelegate(Read);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidDeviceData>.Factory.StartNew(() => readDelegate.Invoke(timeout));
#else
return await Task<HidDeviceData>.Factory.FromAsync(readDelegate.BeginInvoke, readDelegate.EndInvoke, timeout, null);
#endif
}
public HidReport ReadReport()
{
return ReadReport(0);
}
public HidReport ReadReport(int timeout)
{
return new HidReport(Capabilities.InputReportByteLength, Read(timeout));
}
public void ReadReport(ReadReportCallback callback)
{
ReadReport(callback, 0);
}
public void ReadReport(ReadReportCallback callback, int timeout)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
var asyncState = new HidAsyncState(readReportDelegate, callback);
readReportDelegate.BeginInvoke(timeout, EndReadReport, asyncState);
}
public async Task<HidReport> ReadReportAsync(int timeout = 0)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidReport>.Factory.StartNew(() => readReportDelegate.Invoke(timeout));
#else
return await Task<HidReport>.Factory.FromAsync(readReportDelegate.BeginInvoke, readReportDelegate.EndInvoke, timeout, null);
#endif
}
/// <summary>
/// Reads an input report from the Control channel. This method provides access to report data for devices that
/// do not use the interrupt channel to communicate for specific usages.
/// </summary>
/// <param name="reportId">The report ID to read from the device</param>
/// <returns>The HID report that is read. The report will contain the success status of the read request</returns>
///
public HidReport ReadReportSync(byte reportId)
{
byte[] cmdBuffer = new byte[Capabilities.InputReportByteLength];
cmdBuffer[0] = reportId;
bool bSuccess = NativeMethods.HidD_GetInputReport(ReadHandle, cmdBuffer, cmdBuffer.Length);
HidDeviceData deviceData = new HidDeviceData(cmdBuffer, bSuccess ? HidDeviceData.ReadStatus.Success : HidDeviceData.ReadStatus.NoDataRead);
return new HidReport(Capabilities.InputReportByteLength, deviceData);
}
public bool ReadFeatureData(out byte[] data, byte reportId = 0)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0)
{
data = new byte[0];
return false;
}
data = new byte[_deviceCapabilities.FeatureReportByteLength];
var buffer = CreateFeatureOutputBuffer();
buffer[0] = reportId;
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetFeature(hidHandle, buffer, buffer.Length);
if (success)
{
Array.Copy(buffer, 0, data, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
}
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadProduct(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetProductString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadManufacturer(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetManufacturerString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadSerialNumber(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetSerialNumberString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool Write(byte[] data)
{
return Write(data, 0);
}
public bool Write(byte[] data, int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return WriteData(data, timeout);
}
catch
{
return false;
}
}
return false;
}
public void Write(byte[] data, WriteCallback callback)
{
Write(data, callback, 0);
}
public void Write(byte[] data, WriteCallback callback, int timeout)
{
var writeDelegate = new WriteDelegate(Write);
var asyncState = new HidAsyncState(writeDelegate, callback);
writeDelegate.BeginInvoke(data, timeout, EndWrite, asyncState);
}
public async Task<bool> WriteAsync(byte[] data, int timeout = 0)
{
var writeDelegate = new WriteDelegate(Write);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeDelegate.Invoke(data, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeDelegate.BeginInvoke, writeDelegate.EndInvoke, data, timeout, null);
#endif
}
public bool WriteReport(HidReport report)
{
return WriteReport(report, 0);
}
public bool WriteReport(HidReport report, int timeout)
{
return Write(report.GetBytes(), timeout);
}
public void WriteReport(HidReport report, WriteCallback callback)
{
WriteReport(report, callback, 0);
}
public void WriteReport(HidReport report, WriteCallback callback, int timeout)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
var asyncState = new HidAsyncState(writeReportDelegate, callback);
writeReportDelegate.BeginInvoke(report, timeout, EndWriteReport, asyncState);
}
/// <summary>
/// Handle data transfers on the control channel. This method places data on the control channel for devices
/// that do not support the interupt transfers
/// </summary>
/// <param name="report">The outbound HID report</param>
/// <returns>The result of the tranfer request: true if successful otherwise false</returns>
///
public bool WriteReportSync(HidReport report)
{
if (null != report)
{
byte[] buffer = report.GetBytes();
return (NativeMethods.HidD_SetOutputReport(WriteHandle, buffer, buffer.Length));
}
else
throw new ArgumentException("The output report is null, it must be allocated before you call this method", "report");
}
public async Task<bool> WriteReportAsync(HidReport report, int timeout = 0)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeReportDelegate.Invoke(report, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeReportDelegate.BeginInvoke, writeReportDelegate.EndInvoke, report, timeout, null);
#endif
}
public HidReport CreateReport()
{
return new HidReport(Capabilities.OutputReportByteLength);
}
public bool WriteFeatureData(byte[] data)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0) return false;
var buffer = CreateFeatureOutputBuffer();
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = WriteHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
//var overlapped = new NativeOverlapped();
success = NativeMethods.HidD_SetFeature(hidHandle, buffer, buffer.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != WriteHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
protected static void EndRead(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadCallback)hidAsyncState.CallbackDelegate;
var data = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(data);
}
protected static void EndReadReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadReportCallback)hidAsyncState.CallbackDelegate;
var report = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(report);
}
private static void EndWrite(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private static void EndWriteReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private byte[] CreateInputBuffer()
{
return CreateBuffer(Capabilities.InputReportByteLength - 1);
}
private byte[] CreateOutputBuffer()
{
return CreateBuffer(Capabilities.OutputReportByteLength - 1);
}
private static void CloseDeviceIO(IntPtr handle)
{
NativeMethods.CancelIoEx(handle, IntPtr.Zero);
NativeMethods.CloseHandle(handle);
}
}
private static HidDeviceAttributes GetDeviceAttributes(IntPtr hidHandle)
{
var deviceAttributes = default(NativeMethods.HIDD_ATTRIBUTES);
deviceAttributes.Size = Marshal.SizeOf(deviceAttributes);
NativeMethods.HidD_GetAttributes(hidHandle, ref deviceAttributes);
return new HidDeviceAttributes(deviceAttributes);
}
private static HidDeviceCapabilities GetDeviceCapabilities(IntPtr hidHandle)
{
var capabilities = default(NativeMethods.HIDP_CAPS);
var preparsedDataPointer = default(IntPtr);
if (NativeMethods.HidD_GetPreparsedData(hidHandle, ref preparsedDataPointer))
{
NativeMethods.HidP_GetCaps(preparsedDataPointer, ref capabilities);
NativeMethods.HidD_FreePreparsedData(preparsedDataPointer);
}
return new HidDeviceCapabilities(capabilities);
}
private bool WriteData(byte[] data, int timeout)
{
if (_deviceCapabilities.OutputReportByteLength <= 0) return false;
var buffer = CreateOutputBuffer();
uint bytesWritten;
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.OutputReportByteLength));
if (_deviceWriteMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), "");
try
{
NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
return true;
case NativeMethods.WAIT_TIMEOUT:
return false;
case NativeMethods.WAIT_FAILED:
return false;
default:
return false;
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
return NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
}
}
protected HidDeviceData ReadData(int timeout)
{
var buffer = new byte[] { };
var status = HidDeviceData.ReadStatus.NoDataRead;
IntPtr nonManagedBuffer;
if (_deviceCapabilities.InputReportByteLength > 0)
{
uint bytesRead;
buffer = CreateInputBuffer();
nonManagedBuffer = Marshal.AllocHGlobal(buffer.Length);
if (_deviceReadMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), string.Empty);
try
{
var success = NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
if (success)
{
status = HidDeviceData.ReadStatus.Success; // No check here to see if bytesRead > 0 . Perhaps not necessary?
}
else
{
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
status = HidDeviceData.ReadStatus.Success;
NativeMethods.GetOverlappedResult(ReadHandle, ref overlapped, out bytesRead, false);
break;
case NativeMethods.WAIT_TIMEOUT:
status = HidDeviceData.ReadStatus.WaitTimedOut;
buffer = new byte[] { };
break;
case NativeMethods.WAIT_FAILED:
status = HidDeviceData.ReadStatus.WaitFail;
buffer = new byte[] { };
break;
default:
status = HidDeviceData.ReadStatus.NoDataRead;
buffer = new byte[] { };
break;
}
}
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally {
CloseDeviceIO(overlapped.EventHandle);
Marshal.FreeHGlobal(nonManagedBuffer);
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
status = HidDeviceData.ReadStatus.Success;
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally { Marshal.FreeHGlobal(nonManagedBuffer); }
}
}
return new HidDeviceData(buffer, status);
}
private static IntPtr OpenDeviceIO(string devicePath, uint deviceAccess)
{
return OpenDeviceIO(devicePath, DeviceMode.NonOverlapped, deviceAccess, ShareMode.ShareRead | ShareMode.ShareWrite);
}
private static IntPtr OpenDeviceIO(string devicePath, DeviceMode deviceMode, uint deviceAccess, ShareMode shareMode)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var flags = 0;
if (deviceMode == DeviceMode.Overlapped) flags = NativeMethods.FILE_FLAG_OVERLAPPED;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
return NativeMethods.CreateFile(devicePath, deviceAccess, (int)shareMode, ref security, NativeMethods.OPEN_EXISTING, flags, hTemplateFile: IntPtr.Zero);
}
private static void CloseDeviceIO(IntPtr handle)
{
if (Environment.OSVersion.Version.Major > 5)
{
NativeMethods.CancelIoEx(handle, IntPtr.Zero);
}
NativeMethods.CloseHandle(handle);
}
private void DeviceEventMonitorInserted()
{
if (!IsOpen) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
Inserted?.Invoke();
}
private void DeviceEventMonitorRemoved()
{
if (IsOpen) CloseDevice();
Removed?.Invoke();
}
public void Dispose()
{
if (MonitorDeviceEvents) MonitorDeviceEvents = false;
if (IsOpen) CloseDevice();
}
}
}
<MSG> Fixed an pre vista compatibility issue.
<DFF> @@ -518,7 +518,10 @@ namespace HidLibrary
private static void CloseDeviceIO(IntPtr handle)
{
- NativeMethods.CancelIoEx(handle, IntPtr.Zero);
+ if (Environment.OSVersion.Version.Major > 5)
+ {
+ NativeMethods.CancelIoEx(handle, IntPtr.Zero);
+ }
NativeMethods.CloseHandle(handle);
}
| 4 | Fixed an pre vista compatibility issue. | 1 | .cs | cs | mit | mikeobrien/HidLibrary |
1038 | <NME> HidDevice.cs
<BEF> using System;
using System.Runtime.InteropServices;
using System.Threading;
using System.Threading.Tasks;
namespace HidLibrary
{
public class HidDevice : IHidDevice
{
public event InsertedEventHandler Inserted;
public event RemovedEventHandler Removed;
private readonly string _description;
private readonly string _devicePath;
private readonly HidDeviceAttributes _deviceAttributes;
private readonly HidDeviceCapabilities _deviceCapabilities;
private DeviceMode _deviceReadMode = DeviceMode.NonOverlapped;
private DeviceMode _deviceWriteMode = DeviceMode.NonOverlapped;
private ShareMode _deviceShareMode = ShareMode.ShareRead | ShareMode.ShareWrite;
private readonly HidDeviceEventMonitor _deviceEventMonitor;
private bool _monitorDeviceEvents;
protected delegate HidDeviceData ReadDelegate(int timeout);
protected delegate HidReport ReadReportDelegate(int timeout);
private delegate bool WriteDelegate(byte[] data, int timeout);
private delegate bool WriteReportDelegate(HidReport report, int timeout);
internal HidDevice(string devicePath, string description = null)
{
_deviceEventMonitor = new HidDeviceEventMonitor(this);
_deviceEventMonitor.Inserted += DeviceEventMonitorInserted;
_deviceEventMonitor.Removed += DeviceEventMonitorRemoved;
_devicePath = devicePath;
_description = description;
try
{
var hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
_deviceAttributes = GetDeviceAttributes(hidHandle);
_deviceCapabilities = GetDeviceCapabilities(hidHandle);
CloseDeviceIO(hidHandle);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error querying HID device '{0}'.", devicePath), exception);
}
}
public IntPtr ReadHandle { get; private set; }
public IntPtr WriteHandle { get; private set; }
public bool IsOpen { get; private set; }
public bool IsConnected { get { return HidDevices.IsConnected(_devicePath); } }
public string Description { get { return _description; } }
public HidDeviceCapabilities Capabilities { get { return _deviceCapabilities; } }
public HidDeviceAttributes Attributes { get { return _deviceAttributes; } }
public string DevicePath { get { return _devicePath; } }
public bool MonitorDeviceEvents
{
get { return _monitorDeviceEvents; }
set
{
if (value & _monitorDeviceEvents == false) _deviceEventMonitor.Init();
_monitorDeviceEvents = value;
}
}
public override string ToString()
{
return string.Format("VendorID={0}, ProductID={1}, Version={2}, DevicePath={3}",
_deviceAttributes.VendorHexId,
_deviceAttributes.ProductHexId,
_deviceAttributes.Version,
_devicePath);
}
public void OpenDevice()
{
OpenDevice(DeviceMode.NonOverlapped, DeviceMode.NonOverlapped, ShareMode.ShareRead | ShareMode.ShareWrite);
}
public void OpenDevice(DeviceMode readMode, DeviceMode writeMode, ShareMode shareMode)
{
if (IsOpen) return;
_deviceReadMode = readMode;
_deviceWriteMode = writeMode;
_deviceShareMode = shareMode;
try
{
ReadHandle = OpenDeviceIO(_devicePath, readMode, NativeMethods.GENERIC_READ, shareMode);
WriteHandle = OpenDeviceIO(_devicePath, writeMode, NativeMethods.GENERIC_WRITE, shareMode);
}
catch (Exception exception)
{
IsOpen = false;
throw new Exception("Error opening HID device.", exception);
}
IsOpen = (ReadHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE &&
WriteHandle.ToInt32() != NativeMethods.INVALID_HANDLE_VALUE);
}
public void CloseDevice()
{
if (!IsOpen) return;
CloseDeviceIO(ReadHandle);
CloseDeviceIO(WriteHandle);
IsOpen = false;
}
public HidDeviceData Read()
{
return Read(0);
}
public HidDeviceData Read(int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return ReadData(timeout);
}
catch
{
return new HidDeviceData(HidDeviceData.ReadStatus.ReadError);
}
}
return new HidDeviceData(HidDeviceData.ReadStatus.NotConnected);
}
public void Read(ReadCallback callback)
{
Read(callback, 0);
}
public void Read(ReadCallback callback, int timeout)
{
var readDelegate = new ReadDelegate(Read);
var asyncState = new HidAsyncState(readDelegate, callback);
readDelegate.BeginInvoke(timeout, EndRead, asyncState);
}
public async Task<HidDeviceData> ReadAsync(int timeout = 0)
{
var readDelegate = new ReadDelegate(Read);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidDeviceData>.Factory.StartNew(() => readDelegate.Invoke(timeout));
#else
return await Task<HidDeviceData>.Factory.FromAsync(readDelegate.BeginInvoke, readDelegate.EndInvoke, timeout, null);
#endif
}
public HidReport ReadReport()
{
return ReadReport(0);
}
public HidReport ReadReport(int timeout)
{
return new HidReport(Capabilities.InputReportByteLength, Read(timeout));
}
public void ReadReport(ReadReportCallback callback)
{
ReadReport(callback, 0);
}
public void ReadReport(ReadReportCallback callback, int timeout)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
var asyncState = new HidAsyncState(readReportDelegate, callback);
readReportDelegate.BeginInvoke(timeout, EndReadReport, asyncState);
}
public async Task<HidReport> ReadReportAsync(int timeout = 0)
{
var readReportDelegate = new ReadReportDelegate(ReadReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<HidReport>.Factory.StartNew(() => readReportDelegate.Invoke(timeout));
#else
return await Task<HidReport>.Factory.FromAsync(readReportDelegate.BeginInvoke, readReportDelegate.EndInvoke, timeout, null);
#endif
}
/// <summary>
/// Reads an input report from the Control channel. This method provides access to report data for devices that
/// do not use the interrupt channel to communicate for specific usages.
/// </summary>
/// <param name="reportId">The report ID to read from the device</param>
/// <returns>The HID report that is read. The report will contain the success status of the read request</returns>
///
public HidReport ReadReportSync(byte reportId)
{
byte[] cmdBuffer = new byte[Capabilities.InputReportByteLength];
cmdBuffer[0] = reportId;
bool bSuccess = NativeMethods.HidD_GetInputReport(ReadHandle, cmdBuffer, cmdBuffer.Length);
HidDeviceData deviceData = new HidDeviceData(cmdBuffer, bSuccess ? HidDeviceData.ReadStatus.Success : HidDeviceData.ReadStatus.NoDataRead);
return new HidReport(Capabilities.InputReportByteLength, deviceData);
}
public bool ReadFeatureData(out byte[] data, byte reportId = 0)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0)
{
data = new byte[0];
return false;
}
data = new byte[_deviceCapabilities.FeatureReportByteLength];
var buffer = CreateFeatureOutputBuffer();
buffer[0] = reportId;
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetFeature(hidHandle, buffer, buffer.Length);
if (success)
{
Array.Copy(buffer, 0, data, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
}
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadProduct(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetProductString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadManufacturer(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetManufacturerString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool ReadSerialNumber(out byte[] data)
{
data = new byte[254];
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = ReadHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
success = NativeMethods.HidD_GetSerialNumberString(hidHandle, data, data.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != ReadHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
public bool Write(byte[] data)
{
return Write(data, 0);
}
public bool Write(byte[] data, int timeout)
{
if (IsConnected)
{
if (IsOpen == false) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
try
{
return WriteData(data, timeout);
}
catch
{
return false;
}
}
return false;
}
public void Write(byte[] data, WriteCallback callback)
{
Write(data, callback, 0);
}
public void Write(byte[] data, WriteCallback callback, int timeout)
{
var writeDelegate = new WriteDelegate(Write);
var asyncState = new HidAsyncState(writeDelegate, callback);
writeDelegate.BeginInvoke(data, timeout, EndWrite, asyncState);
}
public async Task<bool> WriteAsync(byte[] data, int timeout = 0)
{
var writeDelegate = new WriteDelegate(Write);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeDelegate.Invoke(data, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeDelegate.BeginInvoke, writeDelegate.EndInvoke, data, timeout, null);
#endif
}
public bool WriteReport(HidReport report)
{
return WriteReport(report, 0);
}
public bool WriteReport(HidReport report, int timeout)
{
return Write(report.GetBytes(), timeout);
}
public void WriteReport(HidReport report, WriteCallback callback)
{
WriteReport(report, callback, 0);
}
public void WriteReport(HidReport report, WriteCallback callback, int timeout)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
var asyncState = new HidAsyncState(writeReportDelegate, callback);
writeReportDelegate.BeginInvoke(report, timeout, EndWriteReport, asyncState);
}
/// <summary>
/// Handle data transfers on the control channel. This method places data on the control channel for devices
/// that do not support the interupt transfers
/// </summary>
/// <param name="report">The outbound HID report</param>
/// <returns>The result of the tranfer request: true if successful otherwise false</returns>
///
public bool WriteReportSync(HidReport report)
{
if (null != report)
{
byte[] buffer = report.GetBytes();
return (NativeMethods.HidD_SetOutputReport(WriteHandle, buffer, buffer.Length));
}
else
throw new ArgumentException("The output report is null, it must be allocated before you call this method", "report");
}
public async Task<bool> WriteReportAsync(HidReport report, int timeout = 0)
{
var writeReportDelegate = new WriteReportDelegate(WriteReport);
#if NET20 || NET35 || NET5_0_OR_GREATER
return await Task<bool>.Factory.StartNew(() => writeReportDelegate.Invoke(report, timeout));
#else
return await Task<bool>.Factory.FromAsync(writeReportDelegate.BeginInvoke, writeReportDelegate.EndInvoke, report, timeout, null);
#endif
}
public HidReport CreateReport()
{
return new HidReport(Capabilities.OutputReportByteLength);
}
public bool WriteFeatureData(byte[] data)
{
if (_deviceCapabilities.FeatureReportByteLength <= 0) return false;
var buffer = CreateFeatureOutputBuffer();
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.FeatureReportByteLength));
IntPtr hidHandle = IntPtr.Zero;
bool success = false;
try
{
if (IsOpen)
hidHandle = WriteHandle;
else
hidHandle = OpenDeviceIO(_devicePath, NativeMethods.ACCESS_NONE);
//var overlapped = new NativeOverlapped();
success = NativeMethods.HidD_SetFeature(hidHandle, buffer, buffer.Length);
}
catch (Exception exception)
{
throw new Exception(string.Format("Error accessing HID device '{0}'.", _devicePath), exception);
}
finally
{
if (hidHandle != IntPtr.Zero && hidHandle != WriteHandle)
CloseDeviceIO(hidHandle);
}
return success;
}
protected static void EndRead(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadCallback)hidAsyncState.CallbackDelegate;
var data = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(data);
}
protected static void EndReadReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (ReadReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (ReadReportCallback)hidAsyncState.CallbackDelegate;
var report = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(report);
}
private static void EndWrite(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private static void EndWriteReport(IAsyncResult ar)
{
var hidAsyncState = (HidAsyncState)ar.AsyncState;
var callerDelegate = (WriteReportDelegate)hidAsyncState.CallerDelegate;
var callbackDelegate = (WriteCallback)hidAsyncState.CallbackDelegate;
var result = callerDelegate.EndInvoke(ar);
if ((callbackDelegate != null)) callbackDelegate.Invoke(result);
}
private byte[] CreateInputBuffer()
{
return CreateBuffer(Capabilities.InputReportByteLength - 1);
}
private byte[] CreateOutputBuffer()
{
return CreateBuffer(Capabilities.OutputReportByteLength - 1);
}
private static void CloseDeviceIO(IntPtr handle)
{
NativeMethods.CancelIoEx(handle, IntPtr.Zero);
NativeMethods.CloseHandle(handle);
}
}
private static HidDeviceAttributes GetDeviceAttributes(IntPtr hidHandle)
{
var deviceAttributes = default(NativeMethods.HIDD_ATTRIBUTES);
deviceAttributes.Size = Marshal.SizeOf(deviceAttributes);
NativeMethods.HidD_GetAttributes(hidHandle, ref deviceAttributes);
return new HidDeviceAttributes(deviceAttributes);
}
private static HidDeviceCapabilities GetDeviceCapabilities(IntPtr hidHandle)
{
var capabilities = default(NativeMethods.HIDP_CAPS);
var preparsedDataPointer = default(IntPtr);
if (NativeMethods.HidD_GetPreparsedData(hidHandle, ref preparsedDataPointer))
{
NativeMethods.HidP_GetCaps(preparsedDataPointer, ref capabilities);
NativeMethods.HidD_FreePreparsedData(preparsedDataPointer);
}
return new HidDeviceCapabilities(capabilities);
}
private bool WriteData(byte[] data, int timeout)
{
if (_deviceCapabilities.OutputReportByteLength <= 0) return false;
var buffer = CreateOutputBuffer();
uint bytesWritten;
Array.Copy(data, 0, buffer, 0, Math.Min(data.Length, _deviceCapabilities.OutputReportByteLength));
if (_deviceWriteMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), "");
try
{
NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
return true;
case NativeMethods.WAIT_TIMEOUT:
return false;
case NativeMethods.WAIT_FAILED:
return false;
default:
return false;
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
return NativeMethods.WriteFile(WriteHandle, buffer, (uint)buffer.Length, out bytesWritten, ref overlapped);
}
catch { return false; }
}
}
protected HidDeviceData ReadData(int timeout)
{
var buffer = new byte[] { };
var status = HidDeviceData.ReadStatus.NoDataRead;
IntPtr nonManagedBuffer;
if (_deviceCapabilities.InputReportByteLength > 0)
{
uint bytesRead;
buffer = CreateInputBuffer();
nonManagedBuffer = Marshal.AllocHGlobal(buffer.Length);
if (_deviceReadMode == DeviceMode.Overlapped)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var overlapped = new NativeOverlapped();
var overlapTimeout = timeout <= 0 ? NativeMethods.WAIT_INFINITE : timeout;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
overlapped.OffsetLow = 0;
overlapped.OffsetHigh = 0;
overlapped.EventHandle = NativeMethods.CreateEvent(ref security, Convert.ToInt32(false), Convert.ToInt32(true), string.Empty);
try
{
var success = NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
if (success)
{
status = HidDeviceData.ReadStatus.Success; // No check here to see if bytesRead > 0 . Perhaps not necessary?
}
else
{
var result = NativeMethods.WaitForSingleObject(overlapped.EventHandle, overlapTimeout);
switch (result)
{
case NativeMethods.WAIT_OBJECT_0:
status = HidDeviceData.ReadStatus.Success;
NativeMethods.GetOverlappedResult(ReadHandle, ref overlapped, out bytesRead, false);
break;
case NativeMethods.WAIT_TIMEOUT:
status = HidDeviceData.ReadStatus.WaitTimedOut;
buffer = new byte[] { };
break;
case NativeMethods.WAIT_FAILED:
status = HidDeviceData.ReadStatus.WaitFail;
buffer = new byte[] { };
break;
default:
status = HidDeviceData.ReadStatus.NoDataRead;
buffer = new byte[] { };
break;
}
}
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally {
CloseDeviceIO(overlapped.EventHandle);
Marshal.FreeHGlobal(nonManagedBuffer);
}
}
else
{
try
{
var overlapped = new NativeOverlapped();
NativeMethods.ReadFile(ReadHandle, nonManagedBuffer, (uint)buffer.Length, out bytesRead, ref overlapped);
status = HidDeviceData.ReadStatus.Success;
Marshal.Copy(nonManagedBuffer, buffer, 0, (int)bytesRead);
}
catch { status = HidDeviceData.ReadStatus.ReadError; }
finally { Marshal.FreeHGlobal(nonManagedBuffer); }
}
}
return new HidDeviceData(buffer, status);
}
private static IntPtr OpenDeviceIO(string devicePath, uint deviceAccess)
{
return OpenDeviceIO(devicePath, DeviceMode.NonOverlapped, deviceAccess, ShareMode.ShareRead | ShareMode.ShareWrite);
}
private static IntPtr OpenDeviceIO(string devicePath, DeviceMode deviceMode, uint deviceAccess, ShareMode shareMode)
{
var security = new NativeMethods.SECURITY_ATTRIBUTES();
var flags = 0;
if (deviceMode == DeviceMode.Overlapped) flags = NativeMethods.FILE_FLAG_OVERLAPPED;
security.lpSecurityDescriptor = IntPtr.Zero;
security.bInheritHandle = true;
security.nLength = Marshal.SizeOf(security);
return NativeMethods.CreateFile(devicePath, deviceAccess, (int)shareMode, ref security, NativeMethods.OPEN_EXISTING, flags, hTemplateFile: IntPtr.Zero);
}
private static void CloseDeviceIO(IntPtr handle)
{
if (Environment.OSVersion.Version.Major > 5)
{
NativeMethods.CancelIoEx(handle, IntPtr.Zero);
}
NativeMethods.CloseHandle(handle);
}
private void DeviceEventMonitorInserted()
{
if (!IsOpen) OpenDevice(_deviceReadMode, _deviceWriteMode, _deviceShareMode);
Inserted?.Invoke();
}
private void DeviceEventMonitorRemoved()
{
if (IsOpen) CloseDevice();
Removed?.Invoke();
}
public void Dispose()
{
if (MonitorDeviceEvents) MonitorDeviceEvents = false;
if (IsOpen) CloseDevice();
}
}
}
<MSG> Fixed an pre vista compatibility issue.
<DFF> @@ -518,7 +518,10 @@ namespace HidLibrary
private static void CloseDeviceIO(IntPtr handle)
{
- NativeMethods.CancelIoEx(handle, IntPtr.Zero);
+ if (Environment.OSVersion.Version.Major > 5)
+ {
+ NativeMethods.CancelIoEx(handle, IntPtr.Zero);
+ }
NativeMethods.CloseHandle(handle);
}
| 4 | Fixed an pre vista compatibility issue. | 1 | .cs | cs | mit | mikeobrien/HidLibrary |
1039 | <NME> utils.py
<BEF> """
Utility functions for access to OS level info and URI parsing
"""
import collections
import os
import pwd
try:
from urllib import parse as _urlparse
except ImportError:
try:
from urllib import parse as _urlparse
except ImportError:
import urlparse as _urlparse
try:
from urllib.parse import unquote
except ImportError:
from urllib import unquote
LOGGER = logging.getLogger(__name__)
PARSED = collections.namedtuple('Parsed',
'scheme,netloc,path,params,query,fragment,'
'username,password,hostname,port')
PYPY = platform.python_implementation().lower() == 'pypy'
KEYWORDS = ['connect_timeout',
'client_encoding',
'options',
'application_name',
'fallback_application_name',
'keepalives',
'keepalives_idle',
'keepalives_interval',
'keepalives_count',
'sslmode',
'requiressl',
'sslcompression',
'sslcert',
'sslkey',
'sslrootcert',
'sslcrl',
'requirepeer',
'krbsrvname',
'gsslib',
:rtype: str
"""
return pwd.getpwuid(os.getuid())[0]
def parse_qs(query_string):
return getpass.getuser()
else:
try:
return pwd.getpwuid(os.getuid())[0]
except KeyError as error:
LOGGER.error('Could not get logged-in user: %s', error)
def parse_qs(query_string):
"""Return the parsed query string in a python2/3 agnostic fashion
:param str query_string: The URI query string
:rtype: dict
"""
return _urlparse.parse_qs(query_string)
def uri(host='localhost', port=5432, dbname='postgres', user='postgres',
password=None):
"""Return a PostgreSQL connection URI for the specified values.
:param str host: Host to connect to
:param int port: Port to connect on
:param str dbname: The database name
:param str user: User to connect as
:param str password: The password to use, None for no password
:return str: The PostgreSQL connection URI
"""
if port:
host = '%s:%s' % (host, port)
if password:
return 'postgresql://%s:%s@%s/%s' % (user, password, host, dbname)
return 'postgresql://%s@%s/%s' % (user, host, dbname)
def uri_to_kwargs(uri):
"""Return a URI as kwargs for connecting to PostgreSQL with psycopg2,
applying default values for non-specified areas of the URI.
:param str uri: The connection URI
:rtype: dict
"""
parsed = urlparse(uri)
default_user = get_current_user()
password = unquote(parsed.password) if parsed.password else None
kwargs = {'host': parsed.hostname,
'port': parsed.port,
'dbname': parsed.path[1:] or default_user,
'user': parsed.username or default_user,
'password': password}
values = parse_qs(parsed.query)
if 'host' in values:
kwargs['host'] = values['host'][0]
for k in [k for k in values if k in KEYWORDS]:
kwargs[k] = values[k][0] if len(values[k]) == 1 else values[k]
try:
if kwargs[k].isdigit():
kwargs[k] = int(kwargs[k])
except AttributeError:
pass
return kwargs
def urlparse(url):
"""Parse the URL in a Python2/3 independent fashion.
:param str url: The URL to parse
:rtype: Parsed
"""
value = 'http%s' % url[5:] if url[:5] == 'postgresql' else url
parsed = _urlparse.urlparse(value)
path, query = parsed.path, parsed.query
hostname = parsed.hostname if parsed.hostname else ''
return PARSED(parsed.scheme.replace('http', 'postgresql'),
parsed.netloc,
path,
parsed.params,
query,
parsed.fragment,
parsed.username,
parsed.password,
hostname.replace('%2F', '/').replace('%2f', '/'),
parsed.port)
<MSG> Merge pull request #14 from fbergr/pwd
Add alternative for pwd module
<DFF> @@ -4,7 +4,14 @@ Utility functions for access to OS level info and URI parsing
"""
import collections
import os
-import pwd
+
+# All systems do not support pwd module
+try:
+ import pwd
+except ImportError:
+ pwd = None
+ import getpass
+
try:
from urllib import parse as _urlparse
except ImportError:
@@ -47,7 +54,10 @@ def get_current_user():
:rtype: str
"""
- return pwd.getpwuid(os.getuid())[0]
+ if pwd is None:
+ return getpass.getuser()
+ else:
+ return pwd.getpwuid(os.getuid())[0]
def parse_qs(query_string):
| 12 | Merge pull request #14 from fbergr/pwd | 2 | .py | py | bsd-3-clause | gmr/queries |
1040 | <NME> utils.py
<BEF> """
Utility functions for access to OS level info and URI parsing
"""
import collections
import os
import pwd
try:
from urllib import parse as _urlparse
except ImportError:
try:
from urllib import parse as _urlparse
except ImportError:
import urlparse as _urlparse
try:
from urllib.parse import unquote
except ImportError:
from urllib import unquote
LOGGER = logging.getLogger(__name__)
PARSED = collections.namedtuple('Parsed',
'scheme,netloc,path,params,query,fragment,'
'username,password,hostname,port')
PYPY = platform.python_implementation().lower() == 'pypy'
KEYWORDS = ['connect_timeout',
'client_encoding',
'options',
'application_name',
'fallback_application_name',
'keepalives',
'keepalives_idle',
'keepalives_interval',
'keepalives_count',
'sslmode',
'requiressl',
'sslcompression',
'sslcert',
'sslkey',
'sslrootcert',
'sslcrl',
'requirepeer',
'krbsrvname',
'gsslib',
:rtype: str
"""
return pwd.getpwuid(os.getuid())[0]
def parse_qs(query_string):
return getpass.getuser()
else:
try:
return pwd.getpwuid(os.getuid())[0]
except KeyError as error:
LOGGER.error('Could not get logged-in user: %s', error)
def parse_qs(query_string):
"""Return the parsed query string in a python2/3 agnostic fashion
:param str query_string: The URI query string
:rtype: dict
"""
return _urlparse.parse_qs(query_string)
def uri(host='localhost', port=5432, dbname='postgres', user='postgres',
password=None):
"""Return a PostgreSQL connection URI for the specified values.
:param str host: Host to connect to
:param int port: Port to connect on
:param str dbname: The database name
:param str user: User to connect as
:param str password: The password to use, None for no password
:return str: The PostgreSQL connection URI
"""
if port:
host = '%s:%s' % (host, port)
if password:
return 'postgresql://%s:%s@%s/%s' % (user, password, host, dbname)
return 'postgresql://%s@%s/%s' % (user, host, dbname)
def uri_to_kwargs(uri):
"""Return a URI as kwargs for connecting to PostgreSQL with psycopg2,
applying default values for non-specified areas of the URI.
:param str uri: The connection URI
:rtype: dict
"""
parsed = urlparse(uri)
default_user = get_current_user()
password = unquote(parsed.password) if parsed.password else None
kwargs = {'host': parsed.hostname,
'port': parsed.port,
'dbname': parsed.path[1:] or default_user,
'user': parsed.username or default_user,
'password': password}
values = parse_qs(parsed.query)
if 'host' in values:
kwargs['host'] = values['host'][0]
for k in [k for k in values if k in KEYWORDS]:
kwargs[k] = values[k][0] if len(values[k]) == 1 else values[k]
try:
if kwargs[k].isdigit():
kwargs[k] = int(kwargs[k])
except AttributeError:
pass
return kwargs
def urlparse(url):
"""Parse the URL in a Python2/3 independent fashion.
:param str url: The URL to parse
:rtype: Parsed
"""
value = 'http%s' % url[5:] if url[:5] == 'postgresql' else url
parsed = _urlparse.urlparse(value)
path, query = parsed.path, parsed.query
hostname = parsed.hostname if parsed.hostname else ''
return PARSED(parsed.scheme.replace('http', 'postgresql'),
parsed.netloc,
path,
parsed.params,
query,
parsed.fragment,
parsed.username,
parsed.password,
hostname.replace('%2F', '/').replace('%2f', '/'),
parsed.port)
<MSG> Merge pull request #14 from fbergr/pwd
Add alternative for pwd module
<DFF> @@ -4,7 +4,14 @@ Utility functions for access to OS level info and URI parsing
"""
import collections
import os
-import pwd
+
+# All systems do not support pwd module
+try:
+ import pwd
+except ImportError:
+ pwd = None
+ import getpass
+
try:
from urllib import parse as _urlparse
except ImportError:
@@ -47,7 +54,10 @@ def get_current_user():
:rtype: str
"""
- return pwd.getpwuid(os.getuid())[0]
+ if pwd is None:
+ return getpass.getuser()
+ else:
+ return pwd.getpwuid(os.getuid())[0]
def parse_qs(query_string):
| 12 | Merge pull request #14 from fbergr/pwd | 2 | .py | py | bsd-3-clause | gmr/queries |
1041 | <NME> utils_tests.py
<BEF> """
Tests for functionality in the utils module
"""
import platform
import unittest
import mock
import queries
from queries import utils
class GetCurrentUserTests(unittest.TestCase):
@mock.patch('pwd.getpwuid')
def test_get_current_user(self, getpwuid):
"""get_current_user returns value from pwd.getpwuid"""
getpwuid.return_value = ['mocky']
self.assertEqual(utils.get_current_user(), 'mocky')
def test_pypy_flag(self):
"""PYPY flag is set properly"""
self.assertEqual(queries.PYPY,
platform.python_implementation() == 'PyPy')
class URICreationTests(unittest.TestCase):
def test_uri_with_password(self):
expectation = 'postgresql://foo:bar@baz:5433/qux'
self.assertEqual(queries.uri('baz', 5433, 'qux', 'foo', 'bar'),
expectation)
def test_uri_without_password(self):
expectation = 'postgresql://foo@baz:5433/qux'
self.assertEqual(queries.uri('baz', 5433, 'qux', 'foo'),
expectation)
def test_default_uri(self):
expectation = 'postgresql://postgres@localhost:5432/postgres'
self.assertEqual(queries.uri(), expectation)
class URLParseTestCase(unittest.TestCase):
URI = 'postgresql://foo:bar@baz:5444/qux'
def test_urlparse_hostname(self):
"""hostname should match expectation"""
self.assertEqual(utils.urlparse(self.URI).hostname, 'baz')
def test_urlparse_port(self):
"""port should match expectation"""
self.assertEqual(utils.urlparse(self.URI).port, 5444)
def test_urlparse_path(self):
"""path should match expectation"""
self.assertEqual(utils.urlparse(self.URI).path, '/qux')
def test_urlparse_username(self):
"""username should match expectation"""
self.assertEqual(utils.urlparse(self.URI).username, 'foo')
def test_urlparse_password(self):
"""password should match expectation"""
self.assertEqual(utils.urlparse(self.URI).password, 'bar')
class URIToKWargsTestCase(unittest.TestCase):
URI = ('postgresql://foo:c%23%5E%25%23%27%24%40%3A@baz:5444/qux?'
'options=foo&options=bar&keepalives=1&invalid=true')
def test_uri_to_kwargs_host(self):
"""hostname should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['host'], 'baz')
def test_uri_to_kwargs_port(self):
"""port should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['port'], 5444)
def test_uri_to_kwargs_dbname(self):
"""dbname should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['dbname'], 'qux')
def test_uri_to_kwargs_username(self):
"""user should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['user'], 'foo')
def test_uri_to_kwargs_password(self):
"""password should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['password'],
'c#^%#\'$@:')
def test_uri_to_kwargs_options(self):
"""options should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['options'],
['foo', 'bar'])
def test_uri_to_kwargs_keepalive(self):
"""keepalive should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['keepalives'], 1)
def test_uri_to_kwargs_invalid(self):
"""invalid query argument should not be in kwargs"""
self.assertNotIn('invaid', utils.uri_to_kwargs(self.URI))
def test_unix_socket_path_format_one(self):
socket_path = 'postgresql://%2Fvar%2Flib%2Fpostgresql/dbname'
result = utils.uri_to_kwargs(socket_path)
self.assertEqual(result['host'], '/var/lib/postgresql')
def test_unix_socket_path_format2(self):
socket_path = 'postgresql:///postgres?host=/tmp/'
result = utils.uri_to_kwargs(socket_path)
self.assertEqual(result['host'], '/tmp/')
<MSG> Constants and imports cleanup
<DFF> @@ -23,7 +23,7 @@ class PYPYDetectionTests(unittest.TestCase):
def test_pypy_flag(self):
"""PYPY flag is set properly"""
- self.assertEqual(queries.PYPY,
+ self.assertEqual(queries.utils.PYPY,
platform.python_implementation() == 'PyPy')
| 1 | Constants and imports cleanup | 1 | .py | py | bsd-3-clause | gmr/queries |
1042 | <NME> utils_tests.py
<BEF> """
Tests for functionality in the utils module
"""
import platform
import unittest
import mock
import queries
from queries import utils
class GetCurrentUserTests(unittest.TestCase):
@mock.patch('pwd.getpwuid')
def test_get_current_user(self, getpwuid):
"""get_current_user returns value from pwd.getpwuid"""
getpwuid.return_value = ['mocky']
self.assertEqual(utils.get_current_user(), 'mocky')
def test_pypy_flag(self):
"""PYPY flag is set properly"""
self.assertEqual(queries.PYPY,
platform.python_implementation() == 'PyPy')
class URICreationTests(unittest.TestCase):
def test_uri_with_password(self):
expectation = 'postgresql://foo:bar@baz:5433/qux'
self.assertEqual(queries.uri('baz', 5433, 'qux', 'foo', 'bar'),
expectation)
def test_uri_without_password(self):
expectation = 'postgresql://foo@baz:5433/qux'
self.assertEqual(queries.uri('baz', 5433, 'qux', 'foo'),
expectation)
def test_default_uri(self):
expectation = 'postgresql://postgres@localhost:5432/postgres'
self.assertEqual(queries.uri(), expectation)
class URLParseTestCase(unittest.TestCase):
URI = 'postgresql://foo:bar@baz:5444/qux'
def test_urlparse_hostname(self):
"""hostname should match expectation"""
self.assertEqual(utils.urlparse(self.URI).hostname, 'baz')
def test_urlparse_port(self):
"""port should match expectation"""
self.assertEqual(utils.urlparse(self.URI).port, 5444)
def test_urlparse_path(self):
"""path should match expectation"""
self.assertEqual(utils.urlparse(self.URI).path, '/qux')
def test_urlparse_username(self):
"""username should match expectation"""
self.assertEqual(utils.urlparse(self.URI).username, 'foo')
def test_urlparse_password(self):
"""password should match expectation"""
self.assertEqual(utils.urlparse(self.URI).password, 'bar')
class URIToKWargsTestCase(unittest.TestCase):
URI = ('postgresql://foo:c%23%5E%25%23%27%24%40%3A@baz:5444/qux?'
'options=foo&options=bar&keepalives=1&invalid=true')
def test_uri_to_kwargs_host(self):
"""hostname should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['host'], 'baz')
def test_uri_to_kwargs_port(self):
"""port should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['port'], 5444)
def test_uri_to_kwargs_dbname(self):
"""dbname should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['dbname'], 'qux')
def test_uri_to_kwargs_username(self):
"""user should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['user'], 'foo')
def test_uri_to_kwargs_password(self):
"""password should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['password'],
'c#^%#\'$@:')
def test_uri_to_kwargs_options(self):
"""options should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['options'],
['foo', 'bar'])
def test_uri_to_kwargs_keepalive(self):
"""keepalive should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['keepalives'], 1)
def test_uri_to_kwargs_invalid(self):
"""invalid query argument should not be in kwargs"""
self.assertNotIn('invaid', utils.uri_to_kwargs(self.URI))
def test_unix_socket_path_format_one(self):
socket_path = 'postgresql://%2Fvar%2Flib%2Fpostgresql/dbname'
result = utils.uri_to_kwargs(socket_path)
self.assertEqual(result['host'], '/var/lib/postgresql')
def test_unix_socket_path_format2(self):
socket_path = 'postgresql:///postgres?host=/tmp/'
result = utils.uri_to_kwargs(socket_path)
self.assertEqual(result['host'], '/tmp/')
<MSG> Constants and imports cleanup
<DFF> @@ -23,7 +23,7 @@ class PYPYDetectionTests(unittest.TestCase):
def test_pypy_flag(self):
"""PYPY flag is set properly"""
- self.assertEqual(queries.PYPY,
+ self.assertEqual(queries.utils.PYPY,
platform.python_implementation() == 'PyPy')
| 1 | Constants and imports cleanup | 1 | .py | py | bsd-3-clause | gmr/queries |
1043 | <NME> setup.py
<BEF> import os
import platform
import setuptools
# PYPY vs cpython
if platform.python_implementation() == 'PyPy':
install_requires = ['psycopg2cffi>=2.7.2,<3']
else:
install_requires = ['psycopg2>=2.5.1,<3']
# Install tornado if generating docs on readthedocs
if os.environ.get('READTHEDOCS', None) == 'True':
install_requires.append('tornado')
setuptools.setup(
name='queries',
version='2.1.0',
description='Simplified PostgreSQL client built upon Psycopg2',
long_description=open('README.rst').read(),
maintainer='Gavin M. Roy',
maintainer_email='[email protected]',
url='https://github.com/gmr/queries',
install_requires=install_requires,
extras_require={'tornado': 'tornado<6'},
license='BSD',
package_data={'': ['LICENSE', 'README.rst']},
packages=['queries'],
'Topic :: Software Development :: Libraries']
setup(name='queries',
version='1.8.2',
description="Simplified PostgreSQL client built upon Psycopg2",
maintainer="Gavin M. Roy",
maintainer_email="[email protected]",
'Programming Language :: Python :: 3',
'Programming Language :: Python :: 3.4',
'Programming Language :: Python :: 3.5',
'Programming Language :: Python :: 3.6',
'Programming Language :: Python :: 3.7',
'Programming Language :: Python :: 3.8',
'Programming Language :: Python :: Implementation :: CPython',
'Programming Language :: Python :: Implementation :: PyPy',
'Topic :: Database',
'Topic :: Software Development :: Libraries'],
zip_safe=True)
<MSG> Remove the fd from the IOLoop on OSError
<DFF> @@ -29,7 +29,7 @@ classifiers = ['Development Status :: 5 - Production/Stable',
'Topic :: Software Development :: Libraries']
setup(name='queries',
- version='1.8.2',
+ version='1.8.3',
description="Simplified PostgreSQL client built upon Psycopg2",
maintainer="Gavin M. Roy",
maintainer_email="[email protected]",
| 1 | Remove the fd from the IOLoop on OSError | 1 | .py | py | bsd-3-clause | gmr/queries |
1044 | <NME> setup.py
<BEF> import os
import platform
import setuptools
# PYPY vs cpython
if platform.python_implementation() == 'PyPy':
install_requires = ['psycopg2cffi>=2.7.2,<3']
else:
install_requires = ['psycopg2>=2.5.1,<3']
# Install tornado if generating docs on readthedocs
if os.environ.get('READTHEDOCS', None) == 'True':
install_requires.append('tornado')
setuptools.setup(
name='queries',
version='2.1.0',
description='Simplified PostgreSQL client built upon Psycopg2',
long_description=open('README.rst').read(),
maintainer='Gavin M. Roy',
maintainer_email='[email protected]',
url='https://github.com/gmr/queries',
install_requires=install_requires,
extras_require={'tornado': 'tornado<6'},
license='BSD',
package_data={'': ['LICENSE', 'README.rst']},
packages=['queries'],
'Topic :: Software Development :: Libraries']
setup(name='queries',
version='1.8.2',
description="Simplified PostgreSQL client built upon Psycopg2",
maintainer="Gavin M. Roy",
maintainer_email="[email protected]",
'Programming Language :: Python :: 3',
'Programming Language :: Python :: 3.4',
'Programming Language :: Python :: 3.5',
'Programming Language :: Python :: 3.6',
'Programming Language :: Python :: 3.7',
'Programming Language :: Python :: 3.8',
'Programming Language :: Python :: Implementation :: CPython',
'Programming Language :: Python :: Implementation :: PyPy',
'Topic :: Database',
'Topic :: Software Development :: Libraries'],
zip_safe=True)
<MSG> Remove the fd from the IOLoop on OSError
<DFF> @@ -29,7 +29,7 @@ classifiers = ['Development Status :: 5 - Production/Stable',
'Topic :: Software Development :: Libraries']
setup(name='queries',
- version='1.8.2',
+ version='1.8.3',
description="Simplified PostgreSQL client built upon Psycopg2",
maintainer="Gavin M. Roy",
maintainer_email="[email protected]",
| 1 | Remove the fd from the IOLoop on OSError | 1 | .py | py | bsd-3-clause | gmr/queries |
1045 | <NME> testing.txt
<BEF> coverage
flake8
mock
nose
codecov
tornado
<MSG> Incr counters in TornadoSession
<DFF> @@ -2,5 +2,4 @@ coverage
flake8
mock
nose
-codecov
tornado
| 0 | Incr counters in TornadoSession | 1 | .txt | txt | bsd-3-clause | gmr/queries |
1046 | <NME> testing.txt
<BEF> coverage
flake8
mock
nose
codecov
tornado
<MSG> Incr counters in TornadoSession
<DFF> @@ -2,5 +2,4 @@ coverage
flake8
mock
nose
-codecov
tornado
| 0 | Incr counters in TornadoSession | 1 | .txt | txt | bsd-3-clause | gmr/queries |
1047 | <NME> README.rst
<BEF> Queries
=======
PostgreSQL database access simplified.
Queries is an opinionated wrapper of the psycopg2_ library for interfacing with
PostgreSQL. Key features include:
- Simplified API
- Support of Python 2.6+ and 3.2+
- Asynchronous support for Tornado_
- Connection information provided by URI
- Query results delivered as a generator based iterators
- Automatically registered data-type support for UUIDs, Unicode and Unicode Arrays
- Ability to directly access psycopg2 ``connection`` and ``cursor`` objects
- Internal connection pooling
|Version| |Status| |Coverage| |License|
Documentation
-------------
Documentation is available at https://queries.readthedocs.org
Installation
------------
queries is available via pypi and can be installed with easy_install or pip:
.. code:: bash
Usage
-----
Queries provides a session based API for interacting with PostgreSQL.
Simply pass in the URI_ of the PostgreSQL server to connect to when creating
a session:
.. code:: python
session = queries.Session("postgresql://postgres@localhost:5432/postgres")
Queries built-in connection pooling will re-use connections when possible,
lowering the overhead of connecting and reconnecting.
When specifying a URI, if you omit the username and database name to connect
with, Queries will use the current OS username for both. You can also omit the
URI when connecting to connect to localhost on port 5432 as the current OS user,
connecting to a database named for the current user. For example, if your
username is ``fred`` and you omit the URI when issuing ``queries.query`` the URI
that is constructed would be ``postgresql://fred@localhost:5432/fred``.
with, Queries will use the current OS username for both. You can also omit the
URI when connecting to connect to localhost on port 5432 as the current OS user,
connecting to a database named for the current user. For example, if your
username is "fred" and you omit the URI when issuing ``queries.query`` the URI
that is constructed would be ``pgsql://fred@localhost:5432/fred``.
Here are a few examples of using the Queries simple API:
'postgresql://user:pass@server-name:5432/dbname'
Environment Variables
^^^^^^^^^^^^^^^^^^^^^
Currently Queries uses the following environment variables for tweaking various
configuration values. The supported ones are:
* ``QUERIES_MAX_POOL_SIZE`` - Modify the maximum size of the connection pool (default: 1)
Using the queries.Session class
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
To execute queries or call stored procedures, you start by creating an instance of the
``queries.Session`` class. It can act as a context manager, meaning you can
use it with the ``with`` keyword and it will take care of cleaning up after itself. For
more information on the ``with`` keyword and context managers, see PEP343_.
In addition to both the ``queries.Session.query`` and ``queries.Session.callproc``
methods that are similar to the simple API methods, the ``queries.Session`` class
provides access to the psycopg2 connection and cursor objects.
**Using queries.Session.query**
The following example shows how a ``queries.Session`` object can be used
as a context manager to query the database table:
.. code:: python
>>> import pprint
>>> import queries
>>>
>>> with queries.Session() as session:
... for row in session.query('SELECT * FROM names'):
... pprint.pprint(row)
...
{'id': 1, 'name': u'Jacob'}
{'id': 2, 'name': u'Mason'}
{'id': 3, 'name': u'Ethan'}
**Using queries.Session.callproc**
This example uses ``queries.Session.callproc`` to execute a stored
procedure and then pretty-prints the single row results as a dictionary:
.. code:: python
>>> import pprint
>>> import queries
>>> with queries.Session() as session:
... results = session.callproc('chr', [65])
... pprint.pprint(results.as_dict())
...
{'chr': u'A'}
**Asynchronous Queries with Tornado**
In addition to providing a Pythonic, synchronous client API for PostgreSQL,
Queries provides a very similar asynchronous API for use with Tornado.
The only major difference API difference between ``queries.TornadoSession`` and
``queries.Session`` is the ``TornadoSession.query`` and ``TornadoSession.callproc``
methods return the entire result set instead of acting as an iterator over
the results. The following example uses ``TornadoSession.query`` in an asynchronous
Tornado_ web application to send a JSON payload with the query result set.
.. code:: python
from tornado import gen, ioloop, web
import queries
class MainHandler(web.RequestHandler):
def initialize(self):
self.session = queries.TornadoSession()
@gen.coroutine
def get(self):
results = yield self.session.query('SELECT * FROM names')
self.finish({'data': results.items()})
results.free()
application = web.Application([
(r"/", MainHandler),
])
if __name__ == "__main__":
application.listen(8888)
ioloop.IOLoop.instance().start()
Inspiration
-----------
Queries is inspired by `Kenneth Reitz's <https://github.com/kennethreitz/>`_ awesome
work on `requests <http://docs.python-requests.org/en/latest/>`_.
Queries is a fork and enhancement of pgsql_wrapper_, which can be found in the
main GitHub repository of Queries as tags prior to version 1.2.0.
.. _psycopg2: https://pypi.python.org/pypi/psycopg2
.. _documentation: https://queries.readthedocs.org
.. _URIs: http://www.postgresql.org/docs/9.3/static/libpq-connect.html#LIBPQ-CONNSTRING
.. _psycopg2: https://pypi.python.org/pypi/psycopg2
.. _documentation: https://queries.readthedocs.org
.. _URI: http://www.postgresql.org/docs/9.3/static/libpq-connect.html#LIBPQ-CONNSTRING
.. _pgsql_wrapper: https://pypi.python.org/pypi/pgsql_wrapper
.. _Tornado: http://tornadoweb.org
.. _PEP343: http://legacy.python.org/dev/peps/pep-0343/
.. _psycopg2cffi: https://pypi.python.org/pypi/psycopg2cffi
.. |Version| image:: https://img.shields.io/pypi/v/queries.svg?
:target: https://pypi.python.org/pypi/queries
.. |Status| image:: https://img.shields.io/travis/gmr/queries.svg?
:target: https://travis-ci.org/gmr/queries
.. |Coverage| image:: https://img.shields.io/codecov/c/github/gmr/queries.svg?
:target: https://codecov.io/github/gmr/queries?branch=master
.. |License| image:: https://img.shields.io/github/license/gmr/queries.svg?
:target: https://github.com/gmr/queries
<MSG> Update intro
<DFF> @@ -1,9 +1,16 @@
-Queries
-=======
-PostgreSQL database access simplified.
+Queries: PostgreSQL Simplified
+==============================
-Queries is an opinionated wrapper of the psycopg2_ library for interfacing with
-PostgreSQL. Key features include:
+*Queries* is a BSD licensed opinionated wrapper of the psycopg2_ library for
+interacting with PostgreSQL.
+
+The popular psycopg2_ package is a full-featured python client. However there are
+multiple steps, which are often repeated, to get to the point where you can
+execute queries on the PostgreSQL server. Queries aims to reduce the complexity
+while adding additional features to make writing PostgreSQL client applications
+both fast and easy. Check out the `Usage`_ section below to see how easy it can be.
+
+Key features include:
- Simplified API
- Support of Python 2.6+ and 3.2+
@@ -23,7 +30,7 @@ Documentation is available at https://queries.readthedocs.org
Installation
------------
-queries is available via pypi and can be installed with easy_install or pip:
+Queries is available via pypi_ and can be installed with easy_install or pip:
.. code:: bash
@@ -51,7 +58,7 @@ When specifying a URI, if you omit the username and database name to connect
with, Queries will use the current OS username for both. You can also omit the
URI when connecting to connect to localhost on port 5432 as the current OS user,
connecting to a database named for the current user. For example, if your
-username is "fred" and you omit the URI when issuing ``queries.query`` the URI
+username is ``fred`` and you omit the URI when issuing ``queries.query`` the URI
that is constructed would be ``pgsql://fred@localhost:5432/fred``.
Here are a few examples of using the Queries simple API:
@@ -151,6 +158,7 @@ History
Queries is a fork and enhancement of pgsql_wrapper_, which can be found in the
main GitHub repository of Queries as tags prior to version 1.2.0.
+.. _pypi: https://pypi.python.org/pypi/queries
.. _psycopg2: https://pypi.python.org/pypi/psycopg2
.. _documentation: https://queries.readthedocs.org
.. _URIs: http://www.postgresql.org/docs/9.3/static/libpq-connect.html#LIBPQ-CONNSTRING
| 15 | Update intro | 7 | .rst | rst | bsd-3-clause | gmr/queries |
1048 | <NME> README.rst
<BEF> Queries
=======
PostgreSQL database access simplified.
Queries is an opinionated wrapper of the psycopg2_ library for interfacing with
PostgreSQL. Key features include:
- Simplified API
- Support of Python 2.6+ and 3.2+
- Asynchronous support for Tornado_
- Connection information provided by URI
- Query results delivered as a generator based iterators
- Automatically registered data-type support for UUIDs, Unicode and Unicode Arrays
- Ability to directly access psycopg2 ``connection`` and ``cursor`` objects
- Internal connection pooling
|Version| |Status| |Coverage| |License|
Documentation
-------------
Documentation is available at https://queries.readthedocs.org
Installation
------------
queries is available via pypi and can be installed with easy_install or pip:
.. code:: bash
Usage
-----
Queries provides a session based API for interacting with PostgreSQL.
Simply pass in the URI_ of the PostgreSQL server to connect to when creating
a session:
.. code:: python
session = queries.Session("postgresql://postgres@localhost:5432/postgres")
Queries built-in connection pooling will re-use connections when possible,
lowering the overhead of connecting and reconnecting.
When specifying a URI, if you omit the username and database name to connect
with, Queries will use the current OS username for both. You can also omit the
URI when connecting to connect to localhost on port 5432 as the current OS user,
connecting to a database named for the current user. For example, if your
username is ``fred`` and you omit the URI when issuing ``queries.query`` the URI
that is constructed would be ``postgresql://fred@localhost:5432/fred``.
with, Queries will use the current OS username for both. You can also omit the
URI when connecting to connect to localhost on port 5432 as the current OS user,
connecting to a database named for the current user. For example, if your
username is "fred" and you omit the URI when issuing ``queries.query`` the URI
that is constructed would be ``pgsql://fred@localhost:5432/fred``.
Here are a few examples of using the Queries simple API:
'postgresql://user:pass@server-name:5432/dbname'
Environment Variables
^^^^^^^^^^^^^^^^^^^^^
Currently Queries uses the following environment variables for tweaking various
configuration values. The supported ones are:
* ``QUERIES_MAX_POOL_SIZE`` - Modify the maximum size of the connection pool (default: 1)
Using the queries.Session class
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
To execute queries or call stored procedures, you start by creating an instance of the
``queries.Session`` class. It can act as a context manager, meaning you can
use it with the ``with`` keyword and it will take care of cleaning up after itself. For
more information on the ``with`` keyword and context managers, see PEP343_.
In addition to both the ``queries.Session.query`` and ``queries.Session.callproc``
methods that are similar to the simple API methods, the ``queries.Session`` class
provides access to the psycopg2 connection and cursor objects.
**Using queries.Session.query**
The following example shows how a ``queries.Session`` object can be used
as a context manager to query the database table:
.. code:: python
>>> import pprint
>>> import queries
>>>
>>> with queries.Session() as session:
... for row in session.query('SELECT * FROM names'):
... pprint.pprint(row)
...
{'id': 1, 'name': u'Jacob'}
{'id': 2, 'name': u'Mason'}
{'id': 3, 'name': u'Ethan'}
**Using queries.Session.callproc**
This example uses ``queries.Session.callproc`` to execute a stored
procedure and then pretty-prints the single row results as a dictionary:
.. code:: python
>>> import pprint
>>> import queries
>>> with queries.Session() as session:
... results = session.callproc('chr', [65])
... pprint.pprint(results.as_dict())
...
{'chr': u'A'}
**Asynchronous Queries with Tornado**
In addition to providing a Pythonic, synchronous client API for PostgreSQL,
Queries provides a very similar asynchronous API for use with Tornado.
The only major difference API difference between ``queries.TornadoSession`` and
``queries.Session`` is the ``TornadoSession.query`` and ``TornadoSession.callproc``
methods return the entire result set instead of acting as an iterator over
the results. The following example uses ``TornadoSession.query`` in an asynchronous
Tornado_ web application to send a JSON payload with the query result set.
.. code:: python
from tornado import gen, ioloop, web
import queries
class MainHandler(web.RequestHandler):
def initialize(self):
self.session = queries.TornadoSession()
@gen.coroutine
def get(self):
results = yield self.session.query('SELECT * FROM names')
self.finish({'data': results.items()})
results.free()
application = web.Application([
(r"/", MainHandler),
])
if __name__ == "__main__":
application.listen(8888)
ioloop.IOLoop.instance().start()
Inspiration
-----------
Queries is inspired by `Kenneth Reitz's <https://github.com/kennethreitz/>`_ awesome
work on `requests <http://docs.python-requests.org/en/latest/>`_.
Queries is a fork and enhancement of pgsql_wrapper_, which can be found in the
main GitHub repository of Queries as tags prior to version 1.2.0.
.. _psycopg2: https://pypi.python.org/pypi/psycopg2
.. _documentation: https://queries.readthedocs.org
.. _URIs: http://www.postgresql.org/docs/9.3/static/libpq-connect.html#LIBPQ-CONNSTRING
.. _psycopg2: https://pypi.python.org/pypi/psycopg2
.. _documentation: https://queries.readthedocs.org
.. _URI: http://www.postgresql.org/docs/9.3/static/libpq-connect.html#LIBPQ-CONNSTRING
.. _pgsql_wrapper: https://pypi.python.org/pypi/pgsql_wrapper
.. _Tornado: http://tornadoweb.org
.. _PEP343: http://legacy.python.org/dev/peps/pep-0343/
.. _psycopg2cffi: https://pypi.python.org/pypi/psycopg2cffi
.. |Version| image:: https://img.shields.io/pypi/v/queries.svg?
:target: https://pypi.python.org/pypi/queries
.. |Status| image:: https://img.shields.io/travis/gmr/queries.svg?
:target: https://travis-ci.org/gmr/queries
.. |Coverage| image:: https://img.shields.io/codecov/c/github/gmr/queries.svg?
:target: https://codecov.io/github/gmr/queries?branch=master
.. |License| image:: https://img.shields.io/github/license/gmr/queries.svg?
:target: https://github.com/gmr/queries
<MSG> Update intro
<DFF> @@ -1,9 +1,16 @@
-Queries
-=======
-PostgreSQL database access simplified.
+Queries: PostgreSQL Simplified
+==============================
-Queries is an opinionated wrapper of the psycopg2_ library for interfacing with
-PostgreSQL. Key features include:
+*Queries* is a BSD licensed opinionated wrapper of the psycopg2_ library for
+interacting with PostgreSQL.
+
+The popular psycopg2_ package is a full-featured python client. However there are
+multiple steps, which are often repeated, to get to the point where you can
+execute queries on the PostgreSQL server. Queries aims to reduce the complexity
+while adding additional features to make writing PostgreSQL client applications
+both fast and easy. Check out the `Usage`_ section below to see how easy it can be.
+
+Key features include:
- Simplified API
- Support of Python 2.6+ and 3.2+
@@ -23,7 +30,7 @@ Documentation is available at https://queries.readthedocs.org
Installation
------------
-queries is available via pypi and can be installed with easy_install or pip:
+Queries is available via pypi_ and can be installed with easy_install or pip:
.. code:: bash
@@ -51,7 +58,7 @@ When specifying a URI, if you omit the username and database name to connect
with, Queries will use the current OS username for both. You can also omit the
URI when connecting to connect to localhost on port 5432 as the current OS user,
connecting to a database named for the current user. For example, if your
-username is "fred" and you omit the URI when issuing ``queries.query`` the URI
+username is ``fred`` and you omit the URI when issuing ``queries.query`` the URI
that is constructed would be ``pgsql://fred@localhost:5432/fred``.
Here are a few examples of using the Queries simple API:
@@ -151,6 +158,7 @@ History
Queries is a fork and enhancement of pgsql_wrapper_, which can be found in the
main GitHub repository of Queries as tags prior to version 1.2.0.
+.. _pypi: https://pypi.python.org/pypi/queries
.. _psycopg2: https://pypi.python.org/pypi/psycopg2
.. _documentation: https://queries.readthedocs.org
.. _URIs: http://www.postgresql.org/docs/9.3/static/libpq-connect.html#LIBPQ-CONNSTRING
| 15 | Update intro | 7 | .rst | rst | bsd-3-clause | gmr/queries |
1049 | <NME> README.rst
<BEF> Queries: PostgreSQL Simplified
==============================
*Queries* is a BSD licensed opinionated wrapper of the psycopg2_ library for
interacting with PostgreSQL.
The popular psycopg2_ package is a full-featured python client. Unfortunately
as a developer, you're often repeating the same steps to get started with your
applications that use it. Queries aims to reduce the complexity of psycopg2
while adding additional features to make writing PostgreSQL client applications
both fast and easy. Check out the `Usage`_ section below to see how easy it can be.
Key features include:
- Simplified API
- Support of Python 2.6+ and 3.3+
- PyPy support via psycopg2cffi_
- Asynchronous support for Tornado_
- Connection information provided by URI
- Query results delivered as a generator based iterators
- Automatically registered data-type support for UUIDs, Unicode and Unicode Arrays
- Ability to directly access psycopg2 ``connection`` and ``cursor`` objects
- Internal connection pooling
|Version| |Status| |Coverage| |License|
Documentation
-------------
Documentation is available at https://queries.readthedocs.org
Installation
------------
Queries is available via pypi_ and can be installed with easy_install or pip:
.. code:: bash
pip install queries
Usage
-----
Queries provides a session based API for interacting with PostgreSQL.
Simply pass in the URI_ of the PostgreSQL server to connect to when creating
a session:
.. code:: python
session = queries.Session("postgresql://postgres@localhost:5432/postgres")
Queries built-in connection pooling will re-use connections when possible,
lowering the overhead of connecting and reconnecting.
When specifying a URI, if you omit the username and database name to connect
with, Queries will use the current OS username for both. You can also omit the
URI when connecting to connect to localhost on port 5432 as the current OS user,
connecting to a database named for the current user. For example, if your
username is ``fred`` and you omit the URI when issuing ``queries.query`` the URI
that is constructed would be ``postgresql://fred@localhost:5432/fred``.
If you'd rather use individual values for the connection, the queries.uri()
method provides a quick and easy way to create a URI to pass into the various
methods.
.. code:: python
>>> queries.uri("server-name", 5432, "dbname", "user", "pass")
'postgresql://user:pass@server-name:5432/dbname'
Environment Variables
^^^^^^^^^^^^^^^^^^^^^
Currently Queries uses the following environment variables for tweaking various
configuration values. The supported ones are:
* ``QUERIES_MAX_POOL_SIZE`` - Modify the maximum size of the connection pool (default: 1)
Using the queries.Session class
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
To execute queries or call stored procedures, you start by creating an instance of the
``queries.Session`` class. It can act as a context manager, meaning you can
use it with the ``with`` keyword and it will take care of cleaning up after itself. For
more information on the ``with`` keyword and context managers, see PEP343_.
In addition to both the ``queries.Session.query`` and ``queries.Session.callproc``
methods that are similar to the simple API methods, the ``queries.Session`` class
provides access to the psycopg2 connection and cursor objects.
**Using queries.Session.query**
The following example shows how a ``queries.Session`` object can be used
as a context manager to query the database table:
.. code:: python
>>> import pprint
>>> import queries
>>>
>>> with queries.Session() as session:
... for row in session.query('SELECT * FROM names'):
... pprint.pprint(row)
...
{'id': 1, 'name': u'Jacob'}
{'id': 2, 'name': u'Mason'}
{'id': 3, 'name': u'Ethan'}
**Using queries.Session.callproc**
This example uses ``queries.Session.callproc`` to execute a stored
procedure and then pretty-prints the single row results as a dictionary:
.. code:: python
>>> import pprint
>>> import queries
>>> with queries.Session() as session:
... results = session.callproc('chr', [65])
... pprint.pprint(results.as_dict())
...
{'chr': u'A'}
**Asynchronous Queries with Tornado**
In addition to providing a Pythonic, synchronous client API for PostgreSQL,
Queries provides a very similar asynchronous API for use with Tornado.
The only major difference API difference between ``queries.TornadoSession`` and
``queries.Session`` is the ``TornadoSession.query`` and ``TornadoSession.callproc``
methods return the entire result set instead of acting as an iterator over
the results. The following example uses ``TornadoSession.query`` in an asynchronous
Tornado_ web application to send a JSON payload with the query result set.
.. code:: python
from tornado import gen, ioloop, web
import queries
class MainHandler(web.RequestHandler):
def initialize(self):
self.session = queries.TornadoSession()
@gen.coroutine
def get(self):
results = yield self.session.query('SELECT * FROM names')
self.finish({'data': results.items()})
results.free()
application = web.Application([
(r"/", MainHandler),
])
if __name__ == "__main__":
application.listen(8888)
ioloop.IOLoop.instance().start()
Inspiration
-----------
Queries is inspired by `Kenneth Reitz's <https://github.com/kennethreitz/>`_ awesome
work on `requests <http://docs.python-requests.org/en/latest/>`_.
History
-------
Queries is a fork and enhancement of pgsql_wrapper_, which can be found in the
main GitHub repository of Queries as tags prior to version 1.2.0.
.. _pypi: https://pypi.python.org/pypi/queries
.. _psycopg2: https://pypi.python.org/pypi/psycopg2
.. _documentation: https://queries.readthedocs.org
.. _URI: http://www.postgresql.org/docs/9.3/static/libpq-connect.html#LIBPQ-CONNSTRING
.. _pgsql_wrapper: https://pypi.python.org/pypi/pgsql_wrapper
.. _Tornado: http://tornadoweb.org
.. _PEP343: http://legacy.python.org/dev/peps/pep-0343/
.. _psycopg2cffi: https://pypi.python.org/pypi/psycopg2cffi
.. |Version| image:: https://img.shields.io/pypi/v/queries.svg?
:target: https://pypi.python.org/pypi/queries
.. |Status| image:: https://img.shields.io/travis/gmr/queries.svg?
:target: https://travis-ci.org/gmr/queries
.. |Coverage| image:: https://img.shields.io/codecov/c/github/gmr/queries.svg?
:target: https://codecov.io/github/gmr/queries?branch=master
.. |License| image:: https://img.shields.io/github/license/gmr/queries.svg?
:target: https://github.com/gmr/queries
<MSG> Update the supported Python versions
<DFF> @@ -12,7 +12,7 @@ both fast and easy. Check out the `Usage`_ section below to see how easy it can
Key features include:
- Simplified API
-- Support of Python 2.6+ and 3.3+
+- Support of Python 2.7+ and 3.4+
- PyPy support via psycopg2cffi_
- Asynchronous support for Tornado_
- Connection information provided by URI
| 1 | Update the supported Python versions | 1 | .rst | rst | bsd-3-clause | gmr/queries |
1050 | <NME> README.rst
<BEF> Queries: PostgreSQL Simplified
==============================
*Queries* is a BSD licensed opinionated wrapper of the psycopg2_ library for
interacting with PostgreSQL.
The popular psycopg2_ package is a full-featured python client. Unfortunately
as a developer, you're often repeating the same steps to get started with your
applications that use it. Queries aims to reduce the complexity of psycopg2
while adding additional features to make writing PostgreSQL client applications
both fast and easy. Check out the `Usage`_ section below to see how easy it can be.
Key features include:
- Simplified API
- Support of Python 2.6+ and 3.3+
- PyPy support via psycopg2cffi_
- Asynchronous support for Tornado_
- Connection information provided by URI
- Query results delivered as a generator based iterators
- Automatically registered data-type support for UUIDs, Unicode and Unicode Arrays
- Ability to directly access psycopg2 ``connection`` and ``cursor`` objects
- Internal connection pooling
|Version| |Status| |Coverage| |License|
Documentation
-------------
Documentation is available at https://queries.readthedocs.org
Installation
------------
Queries is available via pypi_ and can be installed with easy_install or pip:
.. code:: bash
pip install queries
Usage
-----
Queries provides a session based API for interacting with PostgreSQL.
Simply pass in the URI_ of the PostgreSQL server to connect to when creating
a session:
.. code:: python
session = queries.Session("postgresql://postgres@localhost:5432/postgres")
Queries built-in connection pooling will re-use connections when possible,
lowering the overhead of connecting and reconnecting.
When specifying a URI, if you omit the username and database name to connect
with, Queries will use the current OS username for both. You can also omit the
URI when connecting to connect to localhost on port 5432 as the current OS user,
connecting to a database named for the current user. For example, if your
username is ``fred`` and you omit the URI when issuing ``queries.query`` the URI
that is constructed would be ``postgresql://fred@localhost:5432/fred``.
If you'd rather use individual values for the connection, the queries.uri()
method provides a quick and easy way to create a URI to pass into the various
methods.
.. code:: python
>>> queries.uri("server-name", 5432, "dbname", "user", "pass")
'postgresql://user:pass@server-name:5432/dbname'
Environment Variables
^^^^^^^^^^^^^^^^^^^^^
Currently Queries uses the following environment variables for tweaking various
configuration values. The supported ones are:
* ``QUERIES_MAX_POOL_SIZE`` - Modify the maximum size of the connection pool (default: 1)
Using the queries.Session class
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
To execute queries or call stored procedures, you start by creating an instance of the
``queries.Session`` class. It can act as a context manager, meaning you can
use it with the ``with`` keyword and it will take care of cleaning up after itself. For
more information on the ``with`` keyword and context managers, see PEP343_.
In addition to both the ``queries.Session.query`` and ``queries.Session.callproc``
methods that are similar to the simple API methods, the ``queries.Session`` class
provides access to the psycopg2 connection and cursor objects.
**Using queries.Session.query**
The following example shows how a ``queries.Session`` object can be used
as a context manager to query the database table:
.. code:: python
>>> import pprint
>>> import queries
>>>
>>> with queries.Session() as session:
... for row in session.query('SELECT * FROM names'):
... pprint.pprint(row)
...
{'id': 1, 'name': u'Jacob'}
{'id': 2, 'name': u'Mason'}
{'id': 3, 'name': u'Ethan'}
**Using queries.Session.callproc**
This example uses ``queries.Session.callproc`` to execute a stored
procedure and then pretty-prints the single row results as a dictionary:
.. code:: python
>>> import pprint
>>> import queries
>>> with queries.Session() as session:
... results = session.callproc('chr', [65])
... pprint.pprint(results.as_dict())
...
{'chr': u'A'}
**Asynchronous Queries with Tornado**
In addition to providing a Pythonic, synchronous client API for PostgreSQL,
Queries provides a very similar asynchronous API for use with Tornado.
The only major difference API difference between ``queries.TornadoSession`` and
``queries.Session`` is the ``TornadoSession.query`` and ``TornadoSession.callproc``
methods return the entire result set instead of acting as an iterator over
the results. The following example uses ``TornadoSession.query`` in an asynchronous
Tornado_ web application to send a JSON payload with the query result set.
.. code:: python
from tornado import gen, ioloop, web
import queries
class MainHandler(web.RequestHandler):
def initialize(self):
self.session = queries.TornadoSession()
@gen.coroutine
def get(self):
results = yield self.session.query('SELECT * FROM names')
self.finish({'data': results.items()})
results.free()
application = web.Application([
(r"/", MainHandler),
])
if __name__ == "__main__":
application.listen(8888)
ioloop.IOLoop.instance().start()
Inspiration
-----------
Queries is inspired by `Kenneth Reitz's <https://github.com/kennethreitz/>`_ awesome
work on `requests <http://docs.python-requests.org/en/latest/>`_.
History
-------
Queries is a fork and enhancement of pgsql_wrapper_, which can be found in the
main GitHub repository of Queries as tags prior to version 1.2.0.
.. _pypi: https://pypi.python.org/pypi/queries
.. _psycopg2: https://pypi.python.org/pypi/psycopg2
.. _documentation: https://queries.readthedocs.org
.. _URI: http://www.postgresql.org/docs/9.3/static/libpq-connect.html#LIBPQ-CONNSTRING
.. _pgsql_wrapper: https://pypi.python.org/pypi/pgsql_wrapper
.. _Tornado: http://tornadoweb.org
.. _PEP343: http://legacy.python.org/dev/peps/pep-0343/
.. _psycopg2cffi: https://pypi.python.org/pypi/psycopg2cffi
.. |Version| image:: https://img.shields.io/pypi/v/queries.svg?
:target: https://pypi.python.org/pypi/queries
.. |Status| image:: https://img.shields.io/travis/gmr/queries.svg?
:target: https://travis-ci.org/gmr/queries
.. |Coverage| image:: https://img.shields.io/codecov/c/github/gmr/queries.svg?
:target: https://codecov.io/github/gmr/queries?branch=master
.. |License| image:: https://img.shields.io/github/license/gmr/queries.svg?
:target: https://github.com/gmr/queries
<MSG> Update the supported Python versions
<DFF> @@ -12,7 +12,7 @@ both fast and easy. Check out the `Usage`_ section below to see how easy it can
Key features include:
- Simplified API
-- Support of Python 2.6+ and 3.3+
+- Support of Python 2.7+ and 3.4+
- PyPy support via psycopg2cffi_
- Asynchronous support for Tornado_
- Connection information provided by URI
| 1 | Update the supported Python versions | 1 | .rst | rst | bsd-3-clause | gmr/queries |
1051 | <NME> pool.rst
<BEF> ADDFILE
<MSG> Documentation updates
<DFF> @@ -0,0 +1,25 @@
+Connection Pooling
+==================
+The :py:class:`PoolManager <queries.pool.PoolManager>` class provides top-level
+access to the queries pooling mechanism, managing pools of connections by DSN in
+instances of the :py:class:`Pool <queries.pool.Pool>` class. The connections are
+represented by instances of the :py:class:`Connection <queries.pool.Connection>`
+class. :py:class:`Connection <queries.pool.Connection>` holds the psycopg2
+connection handle as well as lock information that lets the Pool and PoolManager
+know when connections are busy.
+
+These classes are managed automatically by the :py:class:`Session <queries.Session>`
+and should rarely be interacted with directly.
+
+If you would like to use the :py:class:`PoolManager <queries.pool.PoolManager>`
+to shutdown all connections to PostgreSQL, either reference it by class or using
+the :py:meth:`PoolManager.instance <queries.pool.PoolManager.instance>` method.
+
+.. autoclass:: queries.pool.PoolManager
+ :members:
+
+.. autoclass:: queries.pool.Pool
+ :members:
+
+.. autoclass:: queries.pool.Connection
+ :members:
| 25 | Documentation updates | 0 | .rst | rst | bsd-3-clause | gmr/queries |
1052 | <NME> pool.rst
<BEF> ADDFILE
<MSG> Documentation updates
<DFF> @@ -0,0 +1,25 @@
+Connection Pooling
+==================
+The :py:class:`PoolManager <queries.pool.PoolManager>` class provides top-level
+access to the queries pooling mechanism, managing pools of connections by DSN in
+instances of the :py:class:`Pool <queries.pool.Pool>` class. The connections are
+represented by instances of the :py:class:`Connection <queries.pool.Connection>`
+class. :py:class:`Connection <queries.pool.Connection>` holds the psycopg2
+connection handle as well as lock information that lets the Pool and PoolManager
+know when connections are busy.
+
+These classes are managed automatically by the :py:class:`Session <queries.Session>`
+and should rarely be interacted with directly.
+
+If you would like to use the :py:class:`PoolManager <queries.pool.PoolManager>`
+to shutdown all connections to PostgreSQL, either reference it by class or using
+the :py:meth:`PoolManager.instance <queries.pool.PoolManager.instance>` method.
+
+.. autoclass:: queries.pool.PoolManager
+ :members:
+
+.. autoclass:: queries.pool.Pool
+ :members:
+
+.. autoclass:: queries.pool.Connection
+ :members:
| 25 | Documentation updates | 0 | .rst | rst | bsd-3-clause | gmr/queries |
1053 | <NME> setup.py
<BEF> import os
import platform
import setuptools
# PYPY vs cpython
if platform.python_implementation() == 'PyPy':
install_requires = ['psycopg2cffi>=2.7.2,<2.9']
else:
install_requires = ['psycopg2>=2.5.1,<2.9']
# Install tornado if generating docs on readthedocs
if os.environ.get('READTHEDOCS', None) == 'True':
install_requires.append('tornado')
setuptools.setup(
name='queries',
version='2.1.0',
description='Simplified PostgreSQL client built upon Psycopg2',
long_description=open('README.rst').read(),
maintainer='Gavin M. Roy',
maintainer_email='[email protected]',
url='https://github.com/gmr/queries',
install_requires=install_requires,
extras_require={'tornado': 'tornado<6'},
license='BSD',
package_data={'': ['LICENSE', 'README.rst']},
packages=['queries'],
classifiers=[
'Development Status :: 5 - Production/Stable',
'Intended Audience :: Developers',
'License :: OSI Approved :: BSD License',
'Operating System :: OS Independent',
'Programming Language :: Python :: 2',
'Programming Language :: Python :: 2.7',
'Programming Language :: Python :: 3',
'Programming Language :: Python :: 3.4',
'Programming Language :: Python :: 3.5',
'Programming Language :: Python :: 3.6',
'Programming Language :: Python :: 3.7',
'Programming Language :: Python :: 3.8',
'Programming Language :: Python :: Implementation :: CPython',
'Programming Language :: Python :: Implementation :: PyPy',
'Topic :: Database',
'Topic :: Software Development :: Libraries'],
zip_safe=True)
<MSG> Merge pull request #43 from dave-shawley/bump-psycopg2
Widen psycopg2 pin to allow 2.9.x
<DFF> @@ -5,9 +5,9 @@ import setuptools
# PYPY vs cpython
if platform.python_implementation() == 'PyPy':
- install_requires = ['psycopg2cffi>=2.7.2,<2.9']
+ install_requires = ['psycopg2cffi>=2.7.2,<3']
else:
- install_requires = ['psycopg2>=2.5.1,<2.9']
+ install_requires = ['psycopg2>=2.5.1,<3']
# Install tornado if generating docs on readthedocs
if os.environ.get('READTHEDOCS', None) == 'True':
| 2 | Merge pull request #43 from dave-shawley/bump-psycopg2 | 2 | .py | py | bsd-3-clause | gmr/queries |
1054 | <NME> setup.py
<BEF> import os
import platform
import setuptools
# PYPY vs cpython
if platform.python_implementation() == 'PyPy':
install_requires = ['psycopg2cffi>=2.7.2,<2.9']
else:
install_requires = ['psycopg2>=2.5.1,<2.9']
# Install tornado if generating docs on readthedocs
if os.environ.get('READTHEDOCS', None) == 'True':
install_requires.append('tornado')
setuptools.setup(
name='queries',
version='2.1.0',
description='Simplified PostgreSQL client built upon Psycopg2',
long_description=open('README.rst').read(),
maintainer='Gavin M. Roy',
maintainer_email='[email protected]',
url='https://github.com/gmr/queries',
install_requires=install_requires,
extras_require={'tornado': 'tornado<6'},
license='BSD',
package_data={'': ['LICENSE', 'README.rst']},
packages=['queries'],
classifiers=[
'Development Status :: 5 - Production/Stable',
'Intended Audience :: Developers',
'License :: OSI Approved :: BSD License',
'Operating System :: OS Independent',
'Programming Language :: Python :: 2',
'Programming Language :: Python :: 2.7',
'Programming Language :: Python :: 3',
'Programming Language :: Python :: 3.4',
'Programming Language :: Python :: 3.5',
'Programming Language :: Python :: 3.6',
'Programming Language :: Python :: 3.7',
'Programming Language :: Python :: 3.8',
'Programming Language :: Python :: Implementation :: CPython',
'Programming Language :: Python :: Implementation :: PyPy',
'Topic :: Database',
'Topic :: Software Development :: Libraries'],
zip_safe=True)
<MSG> Merge pull request #43 from dave-shawley/bump-psycopg2
Widen psycopg2 pin to allow 2.9.x
<DFF> @@ -5,9 +5,9 @@ import setuptools
# PYPY vs cpython
if platform.python_implementation() == 'PyPy':
- install_requires = ['psycopg2cffi>=2.7.2,<2.9']
+ install_requires = ['psycopg2cffi>=2.7.2,<3']
else:
- install_requires = ['psycopg2>=2.5.1,<2.9']
+ install_requires = ['psycopg2>=2.5.1,<3']
# Install tornado if generating docs on readthedocs
if os.environ.get('READTHEDOCS', None) == 'True':
| 2 | Merge pull request #43 from dave-shawley/bump-psycopg2 | 2 | .py | py | bsd-3-clause | gmr/queries |
1055 | <NME> tornado_multiple.rst
<BEF> Concurrent Queries in Tornado
=============================
The following example issues multiple concurrent queries in a single asynchronous
request and will wait until all queries are complete before progressing:
.. code:: python
from tornado import gen, ioloop, web
import queries
class RequestHandler(web.RequestHandler):
def initialize(self):
self.session = queries.TornadoSession()
@gen.coroutine
def prepare(self):
try:
yield self.session.validate()
except queries.OperationalError as error:
logging.error('Error connecting to the database: %s', error)
raise web.HTTPError(503)
@gen.coroutine
def get(self, *args, **kwargs):
# Issue the three queries and wait for them to finish before progressing
(q1result,
q2result,
q3result) = yield [self.session.query('SELECT * FROM foo'),
self.session.query('SELECT * FROM bar'),
self.session.query('INSERT INTO requests VALUES (%s, %s, %s)',
[self.remote_ip,
self.request_uri,
self.headers.get('User-Agent', '')])]
# Close the connection
self.finish({'q1result': q1result.items(),
'q2result': q2result.items()})
# Free the results and connection locks
q1result.free()
q2result.free()
q3result.free()
if __name__ == "__main__":
application = web.Application([
(r"/", RequestHandler)
]).listen(8888)
ioloop.IOLoop.instance().start()
<MSG> Update the examples
<DFF> @@ -15,13 +15,6 @@ request and will wait until all queries are complete before progressing:
self.session = queries.TornadoSession()
@gen.coroutine
- def prepare(self):
- try:
- yield self.session.validate()
- except queries.OperationalError as error:
- logging.error('Error connecting to the database: %s', error)
- raise web.HTTPError(503)
-
@gen.coroutine
def get(self, *args, **kwargs):
| 0 | Update the examples | 7 | .rst | rst | bsd-3-clause | gmr/queries |
1056 | <NME> tornado_multiple.rst
<BEF> Concurrent Queries in Tornado
=============================
The following example issues multiple concurrent queries in a single asynchronous
request and will wait until all queries are complete before progressing:
.. code:: python
from tornado import gen, ioloop, web
import queries
class RequestHandler(web.RequestHandler):
def initialize(self):
self.session = queries.TornadoSession()
@gen.coroutine
def prepare(self):
try:
yield self.session.validate()
except queries.OperationalError as error:
logging.error('Error connecting to the database: %s', error)
raise web.HTTPError(503)
@gen.coroutine
def get(self, *args, **kwargs):
# Issue the three queries and wait for them to finish before progressing
(q1result,
q2result,
q3result) = yield [self.session.query('SELECT * FROM foo'),
self.session.query('SELECT * FROM bar'),
self.session.query('INSERT INTO requests VALUES (%s, %s, %s)',
[self.remote_ip,
self.request_uri,
self.headers.get('User-Agent', '')])]
# Close the connection
self.finish({'q1result': q1result.items(),
'q2result': q2result.items()})
# Free the results and connection locks
q1result.free()
q2result.free()
q3result.free()
if __name__ == "__main__":
application = web.Application([
(r"/", RequestHandler)
]).listen(8888)
ioloop.IOLoop.instance().start()
<MSG> Update the examples
<DFF> @@ -15,13 +15,6 @@ request and will wait until all queries are complete before progressing:
self.session = queries.TornadoSession()
@gen.coroutine
- def prepare(self):
- try:
- yield self.session.validate()
- except queries.OperationalError as error:
- logging.error('Error connecting to the database: %s', error)
- raise web.HTTPError(503)
-
@gen.coroutine
def get(self, *args, **kwargs):
| 0 | Update the examples | 7 | .rst | rst | bsd-3-clause | gmr/queries |
1057 | <NME> tornado_session_tests.py
<BEF> """
Tests for functionality in the tornado_session module
"""
import unittest
import mock
# Out of order import to ensure psycopg2cffi is registered
from queries import pool, tornado_session
from psycopg2 import extras
from tornado import concurrent, gen, ioloop, testing
class ResultsTests(unittest.TestCase):
def setUp(self):
self.cursor = mock.Mock()
self.fd = 10
self.cleanup = mock.Mock()
self.obj = tornado_session.Results(self.cursor, self.cleanup, self.fd)
def test_cursor_is_assigned(self):
self.assertEqual(self.obj.cursor, self.cursor)
def test_fd_is_assigned(self):
self.assertEqual(self.obj._fd, self.fd)
def test_cleanup_is_assigned(self):
self.assertEqual(self.obj._cleanup, self.cleanup)
@gen.coroutine
def test_free_invokes_cleanup(self):
yield self.obj.free()
self.cleanup.assert_called_once_with(self.cursor, self.fd)
class SessionInitTests(unittest.TestCase):
def setUp(self):
self.obj = tornado_session.TornadoSession()
def test_creates_empty_callback_dict(self):
self.assertDictEqual(self.obj._futures, {})
def test_creates_empty_connections_dict(self):
self.assertDictEqual(self.obj._connections, {})
def test_sets_default_cursor_factory(self):
self.assertEqual(self.obj._cursor_factory, extras.RealDictCursor)
def test_sets_tornado_ioloop_instance(self):
self.assertEqual(self.obj._ioloop, ioloop.IOLoop.instance())
def test_sets_poolmananger_instance(self):
self.assertEqual(self.obj._pool_manager, pool.PoolManager.instance())
def test_sets_uri(self):
self.assertEqual(self.obj._uri, tornado_session.session.DEFAULT_URI)
def test_creates_pool_in_manager(self):
self.assertIn(self.obj.pid, self.obj._pool_manager._pools)
def test_connection_is_none(self):
self.assertIsNone(self.obj.connection)
def test_cursor_is_none(self):
self.assertIsNone(self.obj.cursor)
class SessionConnectTests(testing.AsyncTestCase):
def setUp(self):
super(SessionConnectTests, self).setUp()
self.conn = mock.Mock()
self.conn.fileno = mock.Mock(return_value=10)
self.obj = tornado_session.TornadoSession(io_loop=self.io_loop)
def create_connection(future):
future.set_result(self.conn)
self.obj._create_connection = create_connection
@testing.gen_test
def test_connect_returns_new_connection(self):
conn = yield self.obj._connect()
self.assertEqual(conn, self.conn)
@testing.gen_test
def test_connect_returns_pooled_connection(self):
conn = yield self.obj._connect()
self.obj._pool_manager.add(self.obj.pid, conn)
second_result = yield self.obj._connect()
self.assertEqual(second_result, conn)
@testing.gen_test
def test_connect_gets_pooled_connection(self):
conn = yield self.obj._connect()
self.obj._pool_manager.add(self.obj.pid, conn)
with mock.patch.object(self.obj._pool_manager, 'get') as get:
with mock.patch.object(self.io_loop, 'add_handler'):
yield self.obj._connect()
get.assert_called_once_with(self.obj.pid, self.obj)
@testing.gen_test
def test_connect_pooled_connection_invokes_add_handler(self):
conn = yield self.obj._connect()
self.obj._pool_manager.add(self.obj.pid, conn)
with mock.patch.object(self.obj._pool_manager, 'get') as get:
get.return_value = self.conn
with mock.patch.object(self.io_loop, 'add_handler') as add_handler:
yield self.obj._connect()
add_handler.assert_called_once_with(self.conn.fileno(),
self.obj._on_io_events,
ioloop.IOLoop.WRITE)
def test_psycopg2_connect_invokes_psycopg2_connect(self):
with mock.patch('psycopg2.connect') as connect:
self.obj._psycopg2_connect({})
connect.assert_called_once_with(**{'async': True})
def test_on_io_events_returns_if_fd_not_present(self):
self.obj._on_io_events,
ioloop.IOLoop.WRITE)
@testing.gen_test
def test_psycopg2_connect_invokes_psycopg2_connect(self):
with mock.patch('psycopg2.connect') as connect:
self.obj._psycopg2_connect({})
self.obj._connections[1337] = True
self.obj._on_io_events(1337, ioloop.IOLoop.WRITE)
poll.assert_called_once_with(1337)
def test_exec_cleanup_closes_cursor(self):
with mock.patch.object(self.obj._pool_manager, 'free'):
with mock.patch.object(self.obj._ioloop, 'remove_handler'):
self.obj._connections[14] = mock.Mock()
cursor = mock.Mock()
cursor.close = mock.Mock()
self.obj._exec_cleanup(cursor, 14)
cursor.close.assert_called_once_with()
def test_exec_cleanup_frees_connection(self):
with mock.patch.object(self.obj._pool_manager, 'free') as pm_free:
with mock.patch.object(self.obj._ioloop, 'remove_handler'):
self.obj._connections[14] = conn = mock.Mock()
self.obj._exec_cleanup(mock.Mock(), 14)
pm_free.assert_called_once_with(self.obj.pid, conn)
def test_exec_cleanup_remove_handler_invoked(self):
with mock.patch.object(self.obj._pool_manager, 'free'):
with mock.patch.object(self.obj._ioloop, 'remove_handler') as rh:
self.obj._connections[14] = mock.Mock()
self.obj._exec_cleanup(mock.Mock(), 14)
rh.assert_called_once_with(14)
def test_exec_removes_connection(self):
with mock.patch.object(self.obj._pool_manager, 'free'):
with mock.patch.object(self.obj._ioloop, 'remove_handler'):
self.obj._connections[14] = mock.Mock()
self.obj._exec_cleanup(mock.Mock(), 14)
self.assertNotIn(14, self.obj._connections)
def test_exec_removes_future(self):
with mock.patch.object(self.obj._pool_manager, 'free'):
with mock.patch.object(self.obj._ioloop, 'remove_handler'):
self.obj._connections[14] = mock.Mock()
self.obj._futures[14] = mock.Mock()
self.obj._exec_cleanup(mock.Mock(), 14)
self.assertNotIn(14, self.obj._futures)
def test_pool_manager_add_failures_are_propagated(self):
futures = []
def add_future(future, callback):
futures.append((future, callback))
obj = tornado_session.TornadoSession()
obj._ioloop = mock.Mock()
obj._ioloop.add_future = add_future
future = concurrent.Future()
with mock.patch.object(obj._pool_manager, 'add') as add_method:
add_method.side_effect = pool.PoolFullError(mock.Mock())
obj._create_connection(future)
self.assertEqual(len(futures), 1)
connected_future, callback = futures.pop()
connected_future.set_result(True)
callback(connected_future)
self.assertIs(future.exception(), add_method.side_effect)
class SessionPublicMethodTests(testing.AsyncTestCase):
@testing.gen_test
def test_callproc_invokes_execute(self):
with mock.patch('queries.tornado_session.TornadoSession._execute') as \
_execute:
future = concurrent.Future()
future.set_result(True)
_execute.return_value = future
obj = tornado_session.TornadoSession(io_loop=self.io_loop)
yield obj.callproc('foo', ['bar'])
_execute.assert_called_once_with('callproc', 'foo', ['bar'])
@testing.gen_test
def test_query_invokes_execute(self):
with mock.patch('queries.tornado_session.TornadoSession._execute') as \
_execute:
future = concurrent.Future()
future.set_result(True)
_execute.return_value = future
obj = tornado_session.TornadoSession(io_loop=self.io_loop)
yield obj.query('SELECT 1')
_execute.assert_called_once_with('execute', 'SELECT 1', None)
"""
@testing.gen_test
def test_query_error_key_error(self):
obj = tornado_session.TornadoSession(io_loop=self.io_loop)
with self.assertRaises(Exception):
yield obj.query('SELECT * FROM foo WHERE bar=%(baz)s', {})
@testing.gen_test
def test_query_error_index_error(self):
obj = tornado_session.TornadoSession(io_loop=self.io_loop)
with self.assertRaises(Exception):
r = yield obj.query('SELECT * FROM foo WHERE bar=%s', [])
"""
<MSG> Test does not need to be async
<DFF> @@ -124,7 +124,6 @@ class SessionConnectTests(testing.AsyncTestCase):
self.obj._on_io_events,
ioloop.IOLoop.WRITE)
- @testing.gen_test
def test_psycopg2_connect_invokes_psycopg2_connect(self):
with mock.patch('psycopg2.connect') as connect:
self.obj._psycopg2_connect({})
| 0 | Test does not need to be async | 1 | .py | py | bsd-3-clause | gmr/queries |
1058 | <NME> tornado_session_tests.py
<BEF> """
Tests for functionality in the tornado_session module
"""
import unittest
import mock
# Out of order import to ensure psycopg2cffi is registered
from queries import pool, tornado_session
from psycopg2 import extras
from tornado import concurrent, gen, ioloop, testing
class ResultsTests(unittest.TestCase):
def setUp(self):
self.cursor = mock.Mock()
self.fd = 10
self.cleanup = mock.Mock()
self.obj = tornado_session.Results(self.cursor, self.cleanup, self.fd)
def test_cursor_is_assigned(self):
self.assertEqual(self.obj.cursor, self.cursor)
def test_fd_is_assigned(self):
self.assertEqual(self.obj._fd, self.fd)
def test_cleanup_is_assigned(self):
self.assertEqual(self.obj._cleanup, self.cleanup)
@gen.coroutine
def test_free_invokes_cleanup(self):
yield self.obj.free()
self.cleanup.assert_called_once_with(self.cursor, self.fd)
class SessionInitTests(unittest.TestCase):
def setUp(self):
self.obj = tornado_session.TornadoSession()
def test_creates_empty_callback_dict(self):
self.assertDictEqual(self.obj._futures, {})
def test_creates_empty_connections_dict(self):
self.assertDictEqual(self.obj._connections, {})
def test_sets_default_cursor_factory(self):
self.assertEqual(self.obj._cursor_factory, extras.RealDictCursor)
def test_sets_tornado_ioloop_instance(self):
self.assertEqual(self.obj._ioloop, ioloop.IOLoop.instance())
def test_sets_poolmananger_instance(self):
self.assertEqual(self.obj._pool_manager, pool.PoolManager.instance())
def test_sets_uri(self):
self.assertEqual(self.obj._uri, tornado_session.session.DEFAULT_URI)
def test_creates_pool_in_manager(self):
self.assertIn(self.obj.pid, self.obj._pool_manager._pools)
def test_connection_is_none(self):
self.assertIsNone(self.obj.connection)
def test_cursor_is_none(self):
self.assertIsNone(self.obj.cursor)
class SessionConnectTests(testing.AsyncTestCase):
def setUp(self):
super(SessionConnectTests, self).setUp()
self.conn = mock.Mock()
self.conn.fileno = mock.Mock(return_value=10)
self.obj = tornado_session.TornadoSession(io_loop=self.io_loop)
def create_connection(future):
future.set_result(self.conn)
self.obj._create_connection = create_connection
@testing.gen_test
def test_connect_returns_new_connection(self):
conn = yield self.obj._connect()
self.assertEqual(conn, self.conn)
@testing.gen_test
def test_connect_returns_pooled_connection(self):
conn = yield self.obj._connect()
self.obj._pool_manager.add(self.obj.pid, conn)
second_result = yield self.obj._connect()
self.assertEqual(second_result, conn)
@testing.gen_test
def test_connect_gets_pooled_connection(self):
conn = yield self.obj._connect()
self.obj._pool_manager.add(self.obj.pid, conn)
with mock.patch.object(self.obj._pool_manager, 'get') as get:
with mock.patch.object(self.io_loop, 'add_handler'):
yield self.obj._connect()
get.assert_called_once_with(self.obj.pid, self.obj)
@testing.gen_test
def test_connect_pooled_connection_invokes_add_handler(self):
conn = yield self.obj._connect()
self.obj._pool_manager.add(self.obj.pid, conn)
with mock.patch.object(self.obj._pool_manager, 'get') as get:
get.return_value = self.conn
with mock.patch.object(self.io_loop, 'add_handler') as add_handler:
yield self.obj._connect()
add_handler.assert_called_once_with(self.conn.fileno(),
self.obj._on_io_events,
ioloop.IOLoop.WRITE)
def test_psycopg2_connect_invokes_psycopg2_connect(self):
with mock.patch('psycopg2.connect') as connect:
self.obj._psycopg2_connect({})
connect.assert_called_once_with(**{'async': True})
def test_on_io_events_returns_if_fd_not_present(self):
self.obj._on_io_events,
ioloop.IOLoop.WRITE)
@testing.gen_test
def test_psycopg2_connect_invokes_psycopg2_connect(self):
with mock.patch('psycopg2.connect') as connect:
self.obj._psycopg2_connect({})
self.obj._connections[1337] = True
self.obj._on_io_events(1337, ioloop.IOLoop.WRITE)
poll.assert_called_once_with(1337)
def test_exec_cleanup_closes_cursor(self):
with mock.patch.object(self.obj._pool_manager, 'free'):
with mock.patch.object(self.obj._ioloop, 'remove_handler'):
self.obj._connections[14] = mock.Mock()
cursor = mock.Mock()
cursor.close = mock.Mock()
self.obj._exec_cleanup(cursor, 14)
cursor.close.assert_called_once_with()
def test_exec_cleanup_frees_connection(self):
with mock.patch.object(self.obj._pool_manager, 'free') as pm_free:
with mock.patch.object(self.obj._ioloop, 'remove_handler'):
self.obj._connections[14] = conn = mock.Mock()
self.obj._exec_cleanup(mock.Mock(), 14)
pm_free.assert_called_once_with(self.obj.pid, conn)
def test_exec_cleanup_remove_handler_invoked(self):
with mock.patch.object(self.obj._pool_manager, 'free'):
with mock.patch.object(self.obj._ioloop, 'remove_handler') as rh:
self.obj._connections[14] = mock.Mock()
self.obj._exec_cleanup(mock.Mock(), 14)
rh.assert_called_once_with(14)
def test_exec_removes_connection(self):
with mock.patch.object(self.obj._pool_manager, 'free'):
with mock.patch.object(self.obj._ioloop, 'remove_handler'):
self.obj._connections[14] = mock.Mock()
self.obj._exec_cleanup(mock.Mock(), 14)
self.assertNotIn(14, self.obj._connections)
def test_exec_removes_future(self):
with mock.patch.object(self.obj._pool_manager, 'free'):
with mock.patch.object(self.obj._ioloop, 'remove_handler'):
self.obj._connections[14] = mock.Mock()
self.obj._futures[14] = mock.Mock()
self.obj._exec_cleanup(mock.Mock(), 14)
self.assertNotIn(14, self.obj._futures)
def test_pool_manager_add_failures_are_propagated(self):
futures = []
def add_future(future, callback):
futures.append((future, callback))
obj = tornado_session.TornadoSession()
obj._ioloop = mock.Mock()
obj._ioloop.add_future = add_future
future = concurrent.Future()
with mock.patch.object(obj._pool_manager, 'add') as add_method:
add_method.side_effect = pool.PoolFullError(mock.Mock())
obj._create_connection(future)
self.assertEqual(len(futures), 1)
connected_future, callback = futures.pop()
connected_future.set_result(True)
callback(connected_future)
self.assertIs(future.exception(), add_method.side_effect)
class SessionPublicMethodTests(testing.AsyncTestCase):
@testing.gen_test
def test_callproc_invokes_execute(self):
with mock.patch('queries.tornado_session.TornadoSession._execute') as \
_execute:
future = concurrent.Future()
future.set_result(True)
_execute.return_value = future
obj = tornado_session.TornadoSession(io_loop=self.io_loop)
yield obj.callproc('foo', ['bar'])
_execute.assert_called_once_with('callproc', 'foo', ['bar'])
@testing.gen_test
def test_query_invokes_execute(self):
with mock.patch('queries.tornado_session.TornadoSession._execute') as \
_execute:
future = concurrent.Future()
future.set_result(True)
_execute.return_value = future
obj = tornado_session.TornadoSession(io_loop=self.io_loop)
yield obj.query('SELECT 1')
_execute.assert_called_once_with('execute', 'SELECT 1', None)
"""
@testing.gen_test
def test_query_error_key_error(self):
obj = tornado_session.TornadoSession(io_loop=self.io_loop)
with self.assertRaises(Exception):
yield obj.query('SELECT * FROM foo WHERE bar=%(baz)s', {})
@testing.gen_test
def test_query_error_index_error(self):
obj = tornado_session.TornadoSession(io_loop=self.io_loop)
with self.assertRaises(Exception):
r = yield obj.query('SELECT * FROM foo WHERE bar=%s', [])
"""
<MSG> Test does not need to be async
<DFF> @@ -124,7 +124,6 @@ class SessionConnectTests(testing.AsyncTestCase):
self.obj._on_io_events,
ioloop.IOLoop.WRITE)
- @testing.gen_test
def test_psycopg2_connect_invokes_psycopg2_connect(self):
with mock.patch('psycopg2.connect') as connect:
self.obj._psycopg2_connect({})
| 0 | Test does not need to be async | 1 | .py | py | bsd-3-clause | gmr/queries |
1059 | <NME> setup.py
<BEF> import os
import os
import platform
# Make the install_requires
target = platform.python_implementation()
if target == 'PyPy':
install_requires = ['psycopg2ct']
else:
install_requires = ['psycopg2>=2.5.1']
# Install tornado if generating docs on readthedocs
if os.environ.get('READTHEDOCS', None) == 'True':
if os.environ.get('READTHEDOCS', None) == 'True':
install_requires.append('tornado')
setuptools.setup(
name='queries',
version='2.1.0',
description='Simplified PostgreSQL client built upon Psycopg2',
long_description=open('README.rst').read(),
maintainer='Gavin M. Roy',
maintainer_email='[email protected]',
'Programming Language :: Python :: 3.2',
'Programming Language :: Python :: 3.3',
'Programming Language :: Python :: 3.4',
'Programming Language :: Python :: Implementation :: CPython',
'Programming Language :: Python :: Implementation :: PyPy',
'Topic :: Database',
'Topic :: Software Development :: Libraries']
setup(name='queries',
version='1.7.5',
description="Simplified PostgreSQL client built upon Psycopg2",
maintainer="Gavin M. Roy",
maintainer_email="[email protected]",
'Programming Language :: Python :: 3.4',
install_requires=install_requires,
extras_require={'tornado': 'tornado'},
license=open('LICENSE').read(),
package_data={'': ['LICENSE', 'README.md']},
packages=['queries'],
classifiers=classifiers,
zip_safe=True)
'Topic :: Software Development :: Libraries'],
zip_safe=True)
<MSG> Move to psycopg2cffi for PyPy
Move to psycopg2cffi for PyPy as psycopg2ct isn't working with PyPy 4.0.
Also add psycopg2.Warning and psycopg2.Error to the base imports.
<DFF> @@ -2,12 +2,11 @@ from setuptools import setup
import os
import platform
-# Make the install_requires
-target = platform.python_implementation()
-if target == 'PyPy':
- install_requires = ['psycopg2ct']
+# Include the proper requirements
+if platform.python_implementation() == 'PyPy':
+ install_requires = ['psycopg2cffi>=2.7.2,<3']
else:
- install_requires = ['psycopg2>=2.5.1']
+ install_requires = ['psycopg2>=2.5.1,<3']
# Install tornado if generating docs on readthedocs
if os.environ.get('READTHEDOCS', None) == 'True':
@@ -24,13 +23,14 @@ classifiers = ['Development Status :: 5 - Production/Stable',
'Programming Language :: Python :: 3.2',
'Programming Language :: Python :: 3.3',
'Programming Language :: Python :: 3.4',
+ 'Programming Language :: Python :: 3.5',
'Programming Language :: Python :: Implementation :: CPython',
'Programming Language :: Python :: Implementation :: PyPy',
'Topic :: Database',
'Topic :: Software Development :: Libraries']
setup(name='queries',
- version='1.7.5',
+ version='1.8.0',
description="Simplified PostgreSQL client built upon Psycopg2",
maintainer="Gavin M. Roy",
maintainer_email="[email protected]",
@@ -38,7 +38,7 @@ setup(name='queries',
install_requires=install_requires,
extras_require={'tornado': 'tornado'},
license=open('LICENSE').read(),
- package_data={'': ['LICENSE', 'README.md']},
+ package_data={'': ['LICENSE', 'README.rst']},
packages=['queries'],
classifiers=classifiers,
zip_safe=True)
| 7 | Move to psycopg2cffi for PyPy | 7 | .py | py | bsd-3-clause | gmr/queries |
1060 | <NME> setup.py
<BEF> import os
import os
import platform
# Make the install_requires
target = platform.python_implementation()
if target == 'PyPy':
install_requires = ['psycopg2ct']
else:
install_requires = ['psycopg2>=2.5.1']
# Install tornado if generating docs on readthedocs
if os.environ.get('READTHEDOCS', None) == 'True':
if os.environ.get('READTHEDOCS', None) == 'True':
install_requires.append('tornado')
setuptools.setup(
name='queries',
version='2.1.0',
description='Simplified PostgreSQL client built upon Psycopg2',
long_description=open('README.rst').read(),
maintainer='Gavin M. Roy',
maintainer_email='[email protected]',
'Programming Language :: Python :: 3.2',
'Programming Language :: Python :: 3.3',
'Programming Language :: Python :: 3.4',
'Programming Language :: Python :: Implementation :: CPython',
'Programming Language :: Python :: Implementation :: PyPy',
'Topic :: Database',
'Topic :: Software Development :: Libraries']
setup(name='queries',
version='1.7.5',
description="Simplified PostgreSQL client built upon Psycopg2",
maintainer="Gavin M. Roy",
maintainer_email="[email protected]",
'Programming Language :: Python :: 3.4',
install_requires=install_requires,
extras_require={'tornado': 'tornado'},
license=open('LICENSE').read(),
package_data={'': ['LICENSE', 'README.md']},
packages=['queries'],
classifiers=classifiers,
zip_safe=True)
'Topic :: Software Development :: Libraries'],
zip_safe=True)
<MSG> Move to psycopg2cffi for PyPy
Move to psycopg2cffi for PyPy as psycopg2ct isn't working with PyPy 4.0.
Also add psycopg2.Warning and psycopg2.Error to the base imports.
<DFF> @@ -2,12 +2,11 @@ from setuptools import setup
import os
import platform
-# Make the install_requires
-target = platform.python_implementation()
-if target == 'PyPy':
- install_requires = ['psycopg2ct']
+# Include the proper requirements
+if platform.python_implementation() == 'PyPy':
+ install_requires = ['psycopg2cffi>=2.7.2,<3']
else:
- install_requires = ['psycopg2>=2.5.1']
+ install_requires = ['psycopg2>=2.5.1,<3']
# Install tornado if generating docs on readthedocs
if os.environ.get('READTHEDOCS', None) == 'True':
@@ -24,13 +23,14 @@ classifiers = ['Development Status :: 5 - Production/Stable',
'Programming Language :: Python :: 3.2',
'Programming Language :: Python :: 3.3',
'Programming Language :: Python :: 3.4',
+ 'Programming Language :: Python :: 3.5',
'Programming Language :: Python :: Implementation :: CPython',
'Programming Language :: Python :: Implementation :: PyPy',
'Topic :: Database',
'Topic :: Software Development :: Libraries']
setup(name='queries',
- version='1.7.5',
+ version='1.8.0',
description="Simplified PostgreSQL client built upon Psycopg2",
maintainer="Gavin M. Roy",
maintainer_email="[email protected]",
@@ -38,7 +38,7 @@ setup(name='queries',
install_requires=install_requires,
extras_require={'tornado': 'tornado'},
license=open('LICENSE').read(),
- package_data={'': ['LICENSE', 'README.md']},
+ package_data={'': ['LICENSE', 'README.rst']},
packages=['queries'],
classifiers=classifiers,
zip_safe=True)
| 7 | Move to psycopg2cffi for PyPy | 7 | .py | py | bsd-3-clause | gmr/queries |
1061 | <NME> session.py
<BEF> """The Session class allows for a unified (and simplified) view of
interfacing with a PostgreSQL database server.
Connection details are passed in as a PostgreSQL URI and connections are pooled
by default, allowing for reuse of connections across modules in the Python
runtime without having to pass around the object handle.
While you can still access the raw `psycopg2` connection and cursor objects to
provide ultimate flexibility in how you use the queries.Session object, there
are convenience methods designed to simplify the interaction with PostgreSQL.
For `psycopg2` functionality outside of what is exposed in Session, simply
use the Session.connection or Session.cursor properties to gain access to
either object just as you would in a program using psycopg2 directly.
Example usage:
.. code:: python
import queries
with queries.Session('pgsql://postgres@localhost/postgres') as session:
for row in session.Query('SELECT * FROM table'):
print row
"""
import hashlib
import logging
import psycopg2
from psycopg2 import extensions, extras
from queries import pool, results, utils
LOGGER = logging.getLogger(__name__)
DEFAULT_ENCODING = 'UTF8'
DEFAULT_URI = 'postgresql://localhost:5432'
class Session(object):
"""The Session class allows for a unified (and simplified) view of
interfacing with a PostgreSQL database server. The Session object can
act as a context manager, providing automated cleanup and simple, Pythonic
way of interacting with the object.
:param str uri: PostgreSQL connection URI
:param psycopg2.extensions.cursor: The cursor type to use
:param int pool_idle_ttl: How long idle pools keep connections open
:param int pool_max_size: The maximum size of the pool to use
"""
_conn = None
_cursor = None
_tpc_id = None
_uri = None
# Connection status constants
INTRANS = extensions.STATUS_IN_TRANSACTION
PREPARED = extensions.STATUS_PREPARED
READY = extensions.STATUS_READY
SETUP = extensions.STATUS_SETUP
# Transaction status constants
TX_ACTIVE = extensions.TRANSACTION_STATUS_ACTIVE
TX_IDLE = extensions.TRANSACTION_STATUS_IDLE
TX_INERROR = extensions.TRANSACTION_STATUS_INERROR
TX_INTRANS = extensions.TRANSACTION_STATUS_INTRANS
TX_UNKNOWN = extensions.TRANSACTION_STATUS_UNKNOWN
def __init__(self, uri=DEFAULT_URI,
cursor_factory=extras.RealDictCursor,
pool_idle_ttl=pool.DEFAULT_IDLE_TTL,
pool_max_size=pool.DEFAULT_MAX_SIZE,
autocommit=True):
"""Connect to a PostgreSQL server using the module wide connection and
set the isolation level.
:param str uri: PostgreSQL connection URI
:param psycopg2.extensions.cursor: The cursor type to use
:param int pool_idle_ttl: How long idle pools keep connections open
:param int pool_max_size: The maximum size of the pool to use
"""
self._pool_manager = pool.PoolManager.instance()
self._uri = uri
# Ensure the pool exists in the pool manager
if self.pid not in self._pool_manager:
self._pool_manager.create(self.pid, pool_idle_ttl, pool_max_size)
self._conn = self._connect()
self._cursor_factory = cursor_factory
self._cursor = self._get_cursor(self._conn)
self._autocommit(autocommit)
@property
def backend_pid(self):
"""Return the backend process ID of the PostgreSQL server that this
session is connected to.
:rtype: int
"""
return self._conn.get_backend_pid()
def callproc(self, name, args=None):
"""Call a stored procedure on the server, returning the results in a
:py:class:`queries.Results` instance.
:param str name: The procedure name
:param list args: The list of arguments to pass in
:rtype: queries.Results
:raises: queries.DataError
:raises: queries.DatabaseError
:raises: queries.IntegrityError
:raises: queries.InternalError
:raises: queries.InterfaceError
:raises: queries.NotSupportedError
:raises: queries.ProgrammingError
"""
self._cursor.callproc(name, args)
return results.Results(self._cursor)
def close(self):
def close(self):
"""Explicitly close the connection and remove it from the connection
pool if pooling is enabled. If the connection is already closed
:raises: psycopg2.InterfaceError
"""
if not self._conn:
raise psycopg2.InterfaceError('Connection not open')
LOGGER.info('Closing connection %r in %s', self._conn, self.pid)
self._pool_manager.free(self.pid, self._conn)
self._pool_manager.remove_connection(self.pid, self._conn)
# Un-assign the connection and cursor
self._conn, self._cursor = None, None
@property
def connection(self):
"""Return the current open connection to PostgreSQL.
:rtype: psycopg2.extensions.connection
"""
return self._conn
@property
def cursor(self):
"""Return the current, active cursor for the open connection.
:rtype: psycopg2.extensions.cursor
"""
return self._cursor
@property
def encoding(self):
"""Return the current client encoding value.
:rtype: str
"""
return self._conn.encoding
@property
def notices(self):
"""Return a list of up to the last 50 server notices sent to the client.
:rtype: list
"""
return self._conn.notices
@property
def pid(self):
"""Return the pool ID used for connection pooling.
:rtype: str
"""
return hashlib.md5(':'.join([self.__class__.__name__,
self._uri]).encode('utf-8')).hexdigest()
def query(self, sql, parameters=None):
"""A generator to issue a query on the server, mogrifying the
parameters against the sql statement. Results are returned as a
:py:class:`queries.Results` object which can act as an iterator and
has multiple ways to access the result data.
:param str sql: The SQL statement
:param dict parameters: A dictionary of query parameters
:rtype: queries.Results
:raises: queries.DataError
:raises: queries.DatabaseError
:raises: queries.IntegrityError
:raises: queries.InternalError
:raises: queries.InterfaceError
:raises: queries.NotSupportedError
:raises: queries.ProgrammingError
"""
self._cursor.execute(sql, parameters)
return results.Results(self._cursor)
def set_encoding(self, value=DEFAULT_ENCODING):
def set_encoding(self, value=DEFAULT_ENCODING):
"""Set the client encoding for the session if the value specified
is different than the current client encoding.
:param str value: The encoding value to use
"""
if self._conn.encoding != value:
self._conn.set_client_encoding(value)
def __del__(self):
"""When deleting the context, ensure the instance is removed from
caches, etc.
"""
self._cleanup()
def __enter__(self):
"""For use as a context manager, return a handle to this object
instance.
:rtype: Session
"""
return self
def __exit__(self, exc_type, exc_val, exc_tb):
"""When leaving the context, ensure the instance is removed from
caches, etc.
"""
self._cleanup()
def _autocommit(self, autocommit):
"""Set the isolation level automatically to commit or not after every query
:param autocommit: Boolean (Default - True)
"""
self._conn.autocommit = autocommit
def _cleanup(self):
"""Remove the connection from the stack, closing out the cursor"""
if self._cursor:
LOGGER.debug('Closing the cursor on %s', self.pid)
self._cursor.close()
self._cursor = None
if self._conn:
LOGGER.debug('Freeing %s in the pool', self.pid)
try:
pool.PoolManager.instance().free(self.pid, self._conn)
except pool.ConnectionNotFoundError:
pass
self._conn = None
def _connect(self):
"""Connect to PostgreSQL, either by reusing a connection from the pool
if possible, or by creating the new connection.
:rtype: psycopg2.extensions.connection
:raises: pool.NoIdleConnectionsError
"""
# Attempt to get a cached connection from the connection pool
try:
connection = self._pool_manager.get(self.pid, self)
LOGGER.debug("Re-using connection for %s", self.pid)
except pool.NoIdleConnectionsError:
if self._pool_manager.is_full(self.pid):
raise
# Create a new PostgreSQL connection
kwargs = utils.uri_to_kwargs(self._uri)
LOGGER.debug("Creating a new connection for %s", self.pid)
connection = self._psycopg2_connect(kwargs)
self._pool_manager.add(self.pid, connection)
self._pool_manager.lock(self.pid, connection, self)
# Added in because psycopg2ct connects and leaves the connection in
# a weird state: consts.STATUS_DATESTYLE, returning from
# Connection._setup without setting the state as const.STATUS_OK
if utils.PYPY:
connection.reset()
# Register the custom data types
self._register_unicode(connection)
self._register_uuid(connection)
return connection
def _get_cursor(self, connection, name=None):
"""Return a cursor for the given cursor_factory. Specify a name to
use server-side cursors.
:param connection: The connection to create a cursor on
:type connection: psycopg2.extensions.connection
:param str name: A cursor name for a server side cursor
:rtype: psycopg2.extensions.cursor
"""
cursor.withhold = True
return cursor
def _psycopg2_connect(self, kwargs):
"""Return a psycopg2 connection for the specified kwargs. Extend for
use in async session adapters.
def _psycopg2_connect(self, kwargs):
"""Return a psycopg2 connection for the specified kwargs. Extend for
use in async session adapters.
:param dict kwargs: Keyword connection args
:rtype: psycopg2.extensions.connection
"""
return psycopg2.connect(**kwargs)
@staticmethod
def _register_unicode(connection):
"""Register the cursor to be able to receive Unicode string.
:type connection: psycopg2.extensions.connection
:param connection: Where to register things
"""
psycopg2.extensions.register_type(psycopg2.extensions.UNICODE,
connection)
psycopg2.extensions.register_type(psycopg2.extensions.UNICODEARRAY,
connection)
@staticmethod
def _register_uuid(connection):
"""Register the UUID extension from the psycopg2.extra module
:type connection: psycopg2.extensions.connection
:param connection: Where to register things
"""
psycopg2.extras.register_uuid(conn_or_curs=connection)
@property
def _status(self):
"""Return the current connection status as an integer value.
The status should match one of the following constants:
- queries.Session.INTRANS: Connection established, in transaction
- queries.Session.PREPARED: Prepared for second phase of transaction
- queries.Session.READY: Connected, no active transaction
:rtype: int
"""
if self._conn.status == psycopg2.extensions.STATUS_BEGIN:
return self.READY
return self._conn.status
<MSG> Metric Reporting
- Keep track of the number of query executions and exceptions per connection
- Add report function to both Pool and PoolManager
- Add methods for returning a list of busy, closed, executing, and locked connections in a Pool
- Rename Pool._connection to Pool.connection_handle
<DFF> @@ -119,7 +119,13 @@ class Session(object):
:raises: queries.ProgrammingError
"""
- self._cursor.callproc(name, args)
+ try:
+ self._cursor.callproc(name, args)
+ except psycopg2.Error as err:
+ self._incr_exceptions()
+ raise err
+ finally:
+ self._incr_executions()
return results.Results(self._cursor)
def close(self):
@@ -203,7 +209,13 @@ class Session(object):
:raises: queries.ProgrammingError
"""
- self._cursor.execute(sql, parameters)
+ try:
+ self._cursor.execute(sql, parameters)
+ except psycopg2.Error as err:
+ self._incr_exceptions()
+ raise err
+ finally:
+ self._incr_executions()
return results.Results(self._cursor)
def set_encoding(self, value=DEFAULT_ENCODING):
@@ -311,6 +323,14 @@ class Session(object):
cursor.withhold = True
return cursor
+ def _incr_exceptions(self):
+ """Increment the number of exceptions for the current connection."""
+ self._pool_manager.get_connection(self.pid, self._conn).exceptions += 1
+
+ def _incr_executions(self):
+ """Increment the number of executions for the current connection."""
+ self._pool_manager.get_connection(self.pid, self._conn).executions += 1
+
def _psycopg2_connect(self, kwargs):
"""Return a psycopg2 connection for the specified kwargs. Extend for
use in async session adapters.
| 22 | Metric Reporting | 2 | .py | py | bsd-3-clause | gmr/queries |
1062 | <NME> session.py
<BEF> """The Session class allows for a unified (and simplified) view of
interfacing with a PostgreSQL database server.
Connection details are passed in as a PostgreSQL URI and connections are pooled
by default, allowing for reuse of connections across modules in the Python
runtime without having to pass around the object handle.
While you can still access the raw `psycopg2` connection and cursor objects to
provide ultimate flexibility in how you use the queries.Session object, there
are convenience methods designed to simplify the interaction with PostgreSQL.
For `psycopg2` functionality outside of what is exposed in Session, simply
use the Session.connection or Session.cursor properties to gain access to
either object just as you would in a program using psycopg2 directly.
Example usage:
.. code:: python
import queries
with queries.Session('pgsql://postgres@localhost/postgres') as session:
for row in session.Query('SELECT * FROM table'):
print row
"""
import hashlib
import logging
import psycopg2
from psycopg2 import extensions, extras
from queries import pool, results, utils
LOGGER = logging.getLogger(__name__)
DEFAULT_ENCODING = 'UTF8'
DEFAULT_URI = 'postgresql://localhost:5432'
class Session(object):
"""The Session class allows for a unified (and simplified) view of
interfacing with a PostgreSQL database server. The Session object can
act as a context manager, providing automated cleanup and simple, Pythonic
way of interacting with the object.
:param str uri: PostgreSQL connection URI
:param psycopg2.extensions.cursor: The cursor type to use
:param int pool_idle_ttl: How long idle pools keep connections open
:param int pool_max_size: The maximum size of the pool to use
"""
_conn = None
_cursor = None
_tpc_id = None
_uri = None
# Connection status constants
INTRANS = extensions.STATUS_IN_TRANSACTION
PREPARED = extensions.STATUS_PREPARED
READY = extensions.STATUS_READY
SETUP = extensions.STATUS_SETUP
# Transaction status constants
TX_ACTIVE = extensions.TRANSACTION_STATUS_ACTIVE
TX_IDLE = extensions.TRANSACTION_STATUS_IDLE
TX_INERROR = extensions.TRANSACTION_STATUS_INERROR
TX_INTRANS = extensions.TRANSACTION_STATUS_INTRANS
TX_UNKNOWN = extensions.TRANSACTION_STATUS_UNKNOWN
def __init__(self, uri=DEFAULT_URI,
cursor_factory=extras.RealDictCursor,
pool_idle_ttl=pool.DEFAULT_IDLE_TTL,
pool_max_size=pool.DEFAULT_MAX_SIZE,
autocommit=True):
"""Connect to a PostgreSQL server using the module wide connection and
set the isolation level.
:param str uri: PostgreSQL connection URI
:param psycopg2.extensions.cursor: The cursor type to use
:param int pool_idle_ttl: How long idle pools keep connections open
:param int pool_max_size: The maximum size of the pool to use
"""
self._pool_manager = pool.PoolManager.instance()
self._uri = uri
# Ensure the pool exists in the pool manager
if self.pid not in self._pool_manager:
self._pool_manager.create(self.pid, pool_idle_ttl, pool_max_size)
self._conn = self._connect()
self._cursor_factory = cursor_factory
self._cursor = self._get_cursor(self._conn)
self._autocommit(autocommit)
@property
def backend_pid(self):
"""Return the backend process ID of the PostgreSQL server that this
session is connected to.
:rtype: int
"""
return self._conn.get_backend_pid()
def callproc(self, name, args=None):
"""Call a stored procedure on the server, returning the results in a
:py:class:`queries.Results` instance.
:param str name: The procedure name
:param list args: The list of arguments to pass in
:rtype: queries.Results
:raises: queries.DataError
:raises: queries.DatabaseError
:raises: queries.IntegrityError
:raises: queries.InternalError
:raises: queries.InterfaceError
:raises: queries.NotSupportedError
:raises: queries.ProgrammingError
"""
self._cursor.callproc(name, args)
return results.Results(self._cursor)
def close(self):
def close(self):
"""Explicitly close the connection and remove it from the connection
pool if pooling is enabled. If the connection is already closed
:raises: psycopg2.InterfaceError
"""
if not self._conn:
raise psycopg2.InterfaceError('Connection not open')
LOGGER.info('Closing connection %r in %s', self._conn, self.pid)
self._pool_manager.free(self.pid, self._conn)
self._pool_manager.remove_connection(self.pid, self._conn)
# Un-assign the connection and cursor
self._conn, self._cursor = None, None
@property
def connection(self):
"""Return the current open connection to PostgreSQL.
:rtype: psycopg2.extensions.connection
"""
return self._conn
@property
def cursor(self):
"""Return the current, active cursor for the open connection.
:rtype: psycopg2.extensions.cursor
"""
return self._cursor
@property
def encoding(self):
"""Return the current client encoding value.
:rtype: str
"""
return self._conn.encoding
@property
def notices(self):
"""Return a list of up to the last 50 server notices sent to the client.
:rtype: list
"""
return self._conn.notices
@property
def pid(self):
"""Return the pool ID used for connection pooling.
:rtype: str
"""
return hashlib.md5(':'.join([self.__class__.__name__,
self._uri]).encode('utf-8')).hexdigest()
def query(self, sql, parameters=None):
"""A generator to issue a query on the server, mogrifying the
parameters against the sql statement. Results are returned as a
:py:class:`queries.Results` object which can act as an iterator and
has multiple ways to access the result data.
:param str sql: The SQL statement
:param dict parameters: A dictionary of query parameters
:rtype: queries.Results
:raises: queries.DataError
:raises: queries.DatabaseError
:raises: queries.IntegrityError
:raises: queries.InternalError
:raises: queries.InterfaceError
:raises: queries.NotSupportedError
:raises: queries.ProgrammingError
"""
self._cursor.execute(sql, parameters)
return results.Results(self._cursor)
def set_encoding(self, value=DEFAULT_ENCODING):
def set_encoding(self, value=DEFAULT_ENCODING):
"""Set the client encoding for the session if the value specified
is different than the current client encoding.
:param str value: The encoding value to use
"""
if self._conn.encoding != value:
self._conn.set_client_encoding(value)
def __del__(self):
"""When deleting the context, ensure the instance is removed from
caches, etc.
"""
self._cleanup()
def __enter__(self):
"""For use as a context manager, return a handle to this object
instance.
:rtype: Session
"""
return self
def __exit__(self, exc_type, exc_val, exc_tb):
"""When leaving the context, ensure the instance is removed from
caches, etc.
"""
self._cleanup()
def _autocommit(self, autocommit):
"""Set the isolation level automatically to commit or not after every query
:param autocommit: Boolean (Default - True)
"""
self._conn.autocommit = autocommit
def _cleanup(self):
"""Remove the connection from the stack, closing out the cursor"""
if self._cursor:
LOGGER.debug('Closing the cursor on %s', self.pid)
self._cursor.close()
self._cursor = None
if self._conn:
LOGGER.debug('Freeing %s in the pool', self.pid)
try:
pool.PoolManager.instance().free(self.pid, self._conn)
except pool.ConnectionNotFoundError:
pass
self._conn = None
def _connect(self):
"""Connect to PostgreSQL, either by reusing a connection from the pool
if possible, or by creating the new connection.
:rtype: psycopg2.extensions.connection
:raises: pool.NoIdleConnectionsError
"""
# Attempt to get a cached connection from the connection pool
try:
connection = self._pool_manager.get(self.pid, self)
LOGGER.debug("Re-using connection for %s", self.pid)
except pool.NoIdleConnectionsError:
if self._pool_manager.is_full(self.pid):
raise
# Create a new PostgreSQL connection
kwargs = utils.uri_to_kwargs(self._uri)
LOGGER.debug("Creating a new connection for %s", self.pid)
connection = self._psycopg2_connect(kwargs)
self._pool_manager.add(self.pid, connection)
self._pool_manager.lock(self.pid, connection, self)
# Added in because psycopg2ct connects and leaves the connection in
# a weird state: consts.STATUS_DATESTYLE, returning from
# Connection._setup without setting the state as const.STATUS_OK
if utils.PYPY:
connection.reset()
# Register the custom data types
self._register_unicode(connection)
self._register_uuid(connection)
return connection
def _get_cursor(self, connection, name=None):
"""Return a cursor for the given cursor_factory. Specify a name to
use server-side cursors.
:param connection: The connection to create a cursor on
:type connection: psycopg2.extensions.connection
:param str name: A cursor name for a server side cursor
:rtype: psycopg2.extensions.cursor
"""
cursor.withhold = True
return cursor
def _psycopg2_connect(self, kwargs):
"""Return a psycopg2 connection for the specified kwargs. Extend for
use in async session adapters.
def _psycopg2_connect(self, kwargs):
"""Return a psycopg2 connection for the specified kwargs. Extend for
use in async session adapters.
:param dict kwargs: Keyword connection args
:rtype: psycopg2.extensions.connection
"""
return psycopg2.connect(**kwargs)
@staticmethod
def _register_unicode(connection):
"""Register the cursor to be able to receive Unicode string.
:type connection: psycopg2.extensions.connection
:param connection: Where to register things
"""
psycopg2.extensions.register_type(psycopg2.extensions.UNICODE,
connection)
psycopg2.extensions.register_type(psycopg2.extensions.UNICODEARRAY,
connection)
@staticmethod
def _register_uuid(connection):
"""Register the UUID extension from the psycopg2.extra module
:type connection: psycopg2.extensions.connection
:param connection: Where to register things
"""
psycopg2.extras.register_uuid(conn_or_curs=connection)
@property
def _status(self):
"""Return the current connection status as an integer value.
The status should match one of the following constants:
- queries.Session.INTRANS: Connection established, in transaction
- queries.Session.PREPARED: Prepared for second phase of transaction
- queries.Session.READY: Connected, no active transaction
:rtype: int
"""
if self._conn.status == psycopg2.extensions.STATUS_BEGIN:
return self.READY
return self._conn.status
<MSG> Metric Reporting
- Keep track of the number of query executions and exceptions per connection
- Add report function to both Pool and PoolManager
- Add methods for returning a list of busy, closed, executing, and locked connections in a Pool
- Rename Pool._connection to Pool.connection_handle
<DFF> @@ -119,7 +119,13 @@ class Session(object):
:raises: queries.ProgrammingError
"""
- self._cursor.callproc(name, args)
+ try:
+ self._cursor.callproc(name, args)
+ except psycopg2.Error as err:
+ self._incr_exceptions()
+ raise err
+ finally:
+ self._incr_executions()
return results.Results(self._cursor)
def close(self):
@@ -203,7 +209,13 @@ class Session(object):
:raises: queries.ProgrammingError
"""
- self._cursor.execute(sql, parameters)
+ try:
+ self._cursor.execute(sql, parameters)
+ except psycopg2.Error as err:
+ self._incr_exceptions()
+ raise err
+ finally:
+ self._incr_executions()
return results.Results(self._cursor)
def set_encoding(self, value=DEFAULT_ENCODING):
@@ -311,6 +323,14 @@ class Session(object):
cursor.withhold = True
return cursor
+ def _incr_exceptions(self):
+ """Increment the number of exceptions for the current connection."""
+ self._pool_manager.get_connection(self.pid, self._conn).exceptions += 1
+
+ def _incr_executions(self):
+ """Increment the number of executions for the current connection."""
+ self._pool_manager.get_connection(self.pid, self._conn).executions += 1
+
def _psycopg2_connect(self, kwargs):
"""Return a psycopg2 connection for the specified kwargs. Extend for
use in async session adapters.
| 22 | Metric Reporting | 2 | .py | py | bsd-3-clause | gmr/queries |
1063 | <NME> setup.cfg
<BEF> [flake8]
application-import-names=queries
exclude=build,env
import-order-style=google
[nosetests]
cover-html = 1
cover-html-dir = build/coverage
cover-package = queries
logging-level = DEBUG
verbosity = 2
with-coverage = 1
with-coverage = 1
detailed-errors = 1
<MSG> Update test configuration
<DFF> @@ -7,6 +7,9 @@ cover-erase = 1
cover-html = 1
cover-html-dir = build/coverage
cover-package = queries
+cover-tests = 1
logging-level = DEBUG
+stop = 1
verbosity = 2
with-coverage = 1
+detailed-errors = 1
| 3 | Update test configuration | 0 | .cfg | cfg | bsd-3-clause | gmr/queries |
1064 | <NME> setup.cfg
<BEF> [flake8]
application-import-names=queries
exclude=build,env
import-order-style=google
[nosetests]
cover-html = 1
cover-html-dir = build/coverage
cover-package = queries
logging-level = DEBUG
verbosity = 2
with-coverage = 1
with-coverage = 1
detailed-errors = 1
<MSG> Update test configuration
<DFF> @@ -7,6 +7,9 @@ cover-erase = 1
cover-html = 1
cover-html-dir = build/coverage
cover-package = queries
+cover-tests = 1
logging-level = DEBUG
+stop = 1
verbosity = 2
with-coverage = 1
+detailed-errors = 1
| 3 | Update test configuration | 0 | .cfg | cfg | bsd-3-clause | gmr/queries |
1065 | <NME> integration_tests.py
<BEF> import datetime
import os
import unittest
import unittest
import queries
from tornado import gen
from tornado import testing
@property
def pg_uri(self):
return queries.uri(os.getenv('PGHOST', 'localhost'),
int(os.getenv('PGPORT', '5432')), 'postgres')
class SessionIntegrationTests(URIMixin, unittest.TestCase):
def setUp(self):
try:
self.session = queries.Session(self.pg_uri, pool_max_size=10)
except queries.OperationalError as error:
raise unittest.SkipTest(str(error).split('\n')[0])
def tearDown(self):
self.session.close()
def test_query_returns_results_object(self):
self.assertIsInstance(self.session.query('SELECT 1 AS value'),
queries.Results)
def test_query_result_value(self):
result = self.session.query('SELECT 1 AS value')
self.assertDictEqual(result.as_dict(), {'value': 1})
def test_query_multirow_result_has_at_least_three_rows(self):
result = self.session.query('SELECT * FROM pg_stat_database')
self.assertGreaterEqual(result.count(), 3)
def test_callproc_returns_results_object(self):
timestamp = int(datetime.datetime.now().strftime('%s'))
self.assertIsInstance(self.session.callproc('to_timestamp',
[timestamp]),
queries.Results)
def test_callproc_mod_result_value(self):
result = self.session.callproc('mod', [6, 4])
self.assertEqual(6 % 4, result[0]['mod'])
class TornadoSessionIntegrationTests(URIMixin, testing.AsyncTestCase):
def setUp(self):
super(TornadoSessionIntegrationTests, self).setUp()
self.session = queries.TornadoSession(self.pg_uri,
pool_max_size=10,
io_loop=self.io_loop)
@gen.coroutine
def assertPostgresConnected(self):
try:
result = yield self.session.query('SELECT 1 AS value')
except queries.OperationalError:
raise unittest.SkipTest('PostgreSQL is not running')
self.assertIsInstance(result, queries.Results)
self.assertEqual(len(result), 1)
result.free()
@testing.gen_test
def test_successful_connection_and_query(self):
yield self.assertPostgresConnected()
@testing.gen_test
def test_query_result_value(self):
try:
result = yield self.session.query('SELECT 1 AS value')
except queries.OperationalError:
raise unittest.SkipTest('PostgreSQL is not running')
self.assertDictEqual(result.as_dict(), {'value': 1})
result.free()
@testing.gen_test
def test_query_multirow_result_has_at_least_three_rows(self):
try:
result = yield self.session.query('SELECT * FROM pg_class')
except queries.OperationalError:
raise unittest.SkipTest('PostgreSQL is not running')
self.assertGreaterEqual(result.count(), 3)
result.free()
@testing.gen_test
def test_callproc_returns_results_object(self):
timestamp = int(datetime.datetime.now().strftime('%s'))
try:
result = yield self.session.callproc('to_timestamp', [timestamp])
except queries.OperationalError:
raise unittest.SkipTest('PostgreSQL is not running')
self.assertIsInstance(result, queries.Results)
result.free()
@testing.gen_test
def test_callproc_mod_result_value(self):
try:
result = yield self.session.callproc('mod', [6, 4])
except queries.OperationalError:
raise unittest.SkipTest('PostgreSQL is not running')
self.assertEqual(6 % 4, result[0]['mod'])
result.free()
@testing.gen_test
def test_polling_stops_after_connection_error(self):
# Abort the test right away if postgres isn't running.
yield self.assertPostgresConnected()
# Use an invalid user to force an OperationalError during connection
bad_uri = queries.uri(os.getenv('PGHOST', 'localhost'),
int(os.getenv('PGPORT', '5432')), 'invalid')
session = queries.TornadoSession(bad_uri)
self.count = 0
real_poll_connection = session._poll_connection
def count_polls(*args, **kwargs):
self.count += 1
real_poll_connection(*args, **kwargs)
session._poll_connection = count_polls
with self.assertRaises(queries.OperationalError):
yield session.query('SELECT 1')
yield gen.sleep(0.05)
self.assertLess(self.count, 20)
<MSG> Remove unused import
<DFF> @@ -5,7 +5,6 @@ except ImportError:
import unittest
import queries
-from tornado import gen
from tornado import testing
| 0 | Remove unused import | 1 | .py | py | bsd-3-clause | gmr/queries |
1066 | <NME> integration_tests.py
<BEF> import datetime
import os
import unittest
import unittest
import queries
from tornado import gen
from tornado import testing
@property
def pg_uri(self):
return queries.uri(os.getenv('PGHOST', 'localhost'),
int(os.getenv('PGPORT', '5432')), 'postgres')
class SessionIntegrationTests(URIMixin, unittest.TestCase):
def setUp(self):
try:
self.session = queries.Session(self.pg_uri, pool_max_size=10)
except queries.OperationalError as error:
raise unittest.SkipTest(str(error).split('\n')[0])
def tearDown(self):
self.session.close()
def test_query_returns_results_object(self):
self.assertIsInstance(self.session.query('SELECT 1 AS value'),
queries.Results)
def test_query_result_value(self):
result = self.session.query('SELECT 1 AS value')
self.assertDictEqual(result.as_dict(), {'value': 1})
def test_query_multirow_result_has_at_least_three_rows(self):
result = self.session.query('SELECT * FROM pg_stat_database')
self.assertGreaterEqual(result.count(), 3)
def test_callproc_returns_results_object(self):
timestamp = int(datetime.datetime.now().strftime('%s'))
self.assertIsInstance(self.session.callproc('to_timestamp',
[timestamp]),
queries.Results)
def test_callproc_mod_result_value(self):
result = self.session.callproc('mod', [6, 4])
self.assertEqual(6 % 4, result[0]['mod'])
class TornadoSessionIntegrationTests(URIMixin, testing.AsyncTestCase):
def setUp(self):
super(TornadoSessionIntegrationTests, self).setUp()
self.session = queries.TornadoSession(self.pg_uri,
pool_max_size=10,
io_loop=self.io_loop)
@gen.coroutine
def assertPostgresConnected(self):
try:
result = yield self.session.query('SELECT 1 AS value')
except queries.OperationalError:
raise unittest.SkipTest('PostgreSQL is not running')
self.assertIsInstance(result, queries.Results)
self.assertEqual(len(result), 1)
result.free()
@testing.gen_test
def test_successful_connection_and_query(self):
yield self.assertPostgresConnected()
@testing.gen_test
def test_query_result_value(self):
try:
result = yield self.session.query('SELECT 1 AS value')
except queries.OperationalError:
raise unittest.SkipTest('PostgreSQL is not running')
self.assertDictEqual(result.as_dict(), {'value': 1})
result.free()
@testing.gen_test
def test_query_multirow_result_has_at_least_three_rows(self):
try:
result = yield self.session.query('SELECT * FROM pg_class')
except queries.OperationalError:
raise unittest.SkipTest('PostgreSQL is not running')
self.assertGreaterEqual(result.count(), 3)
result.free()
@testing.gen_test
def test_callproc_returns_results_object(self):
timestamp = int(datetime.datetime.now().strftime('%s'))
try:
result = yield self.session.callproc('to_timestamp', [timestamp])
except queries.OperationalError:
raise unittest.SkipTest('PostgreSQL is not running')
self.assertIsInstance(result, queries.Results)
result.free()
@testing.gen_test
def test_callproc_mod_result_value(self):
try:
result = yield self.session.callproc('mod', [6, 4])
except queries.OperationalError:
raise unittest.SkipTest('PostgreSQL is not running')
self.assertEqual(6 % 4, result[0]['mod'])
result.free()
@testing.gen_test
def test_polling_stops_after_connection_error(self):
# Abort the test right away if postgres isn't running.
yield self.assertPostgresConnected()
# Use an invalid user to force an OperationalError during connection
bad_uri = queries.uri(os.getenv('PGHOST', 'localhost'),
int(os.getenv('PGPORT', '5432')), 'invalid')
session = queries.TornadoSession(bad_uri)
self.count = 0
real_poll_connection = session._poll_connection
def count_polls(*args, **kwargs):
self.count += 1
real_poll_connection(*args, **kwargs)
session._poll_connection = count_polls
with self.assertRaises(queries.OperationalError):
yield session.query('SELECT 1')
yield gen.sleep(0.05)
self.assertLess(self.count, 20)
<MSG> Remove unused import
<DFF> @@ -5,7 +5,6 @@ except ImportError:
import unittest
import queries
-from tornado import gen
from tornado import testing
| 0 | Remove unused import | 1 | .py | py | bsd-3-clause | gmr/queries |
1067 | <NME> session.py
<BEF> """The Session class allows for a unified (and simplified) view of
interfacing with a PostgreSQL database server.
Connection details are passed in as a PostgreSQL URI and connections are pooled
by default, allowing for reuse of connections across modules in the Python
runtime without having to pass around the object handle.
While you can still access the raw `psycopg2` connection and cursor objects to
provide ultimate flexibility in how you use the queries.Session object, there
are convenience methods designed to simplify the interaction with PostgreSQL.
For `psycopg2` functionality outside of what is exposed in Session, simply
use the Session.connection or Session.cursor properties to gain access to
either object just as you would in a program using psycopg2 directly.
Example usage:
.. code:: python
import queries
with queries.Session('pgsql://postgres@localhost/postgres') as session:
for row in session.Query('SELECT * FROM table'):
print row
"""
import hashlib
import logging
import psycopg2
from psycopg2 import extensions, extras
from queries import pool, results, utils
LOGGER = logging.getLogger(__name__)
DEFAULT_ENCODING = 'UTF8'
DEFAULT_URI = 'postgresql://localhost:5432'
class Session(object):
"""The Session class allows for a unified (and simplified) view of
interfacing with a PostgreSQL database server. The Session object can
act as a context manager, providing automated cleanup and simple, Pythonic
way of interacting with the object.
class Session(object):
"""The Session class allows for a unified (and simplified) view of
interfacing with a PostgreSQL database server. The Session object can
act as a context manager, providing automated cleanup and simple, pythoic
way of interacting with the object.
:param str uri: PostgreSQL connection URI
_conn = None
_cursor = None
_tpc_id = None
_uri = None
# Connection status constants
INTRANS = extensions.STATUS_IN_TRANSACTION
PREPARED = extensions.STATUS_PREPARED
READY = extensions.STATUS_READY
SETUP = extensions.STATUS_SETUP
# Transaction status constants
TX_ACTIVE = extensions.TRANSACTION_STATUS_ACTIVE
TX_IDLE = extensions.TRANSACTION_STATUS_IDLE
TX_INERROR = extensions.TRANSACTION_STATUS_INERROR
TX_INTRANS = extensions.TRANSACTION_STATUS_INTRANS
TX_UNKNOWN = extensions.TRANSACTION_STATUS_UNKNOWN
def __init__(self, uri=DEFAULT_URI,
cursor_factory=extras.RealDictCursor,
pool_idle_ttl=pool.DEFAULT_IDLE_TTL,
pool_max_size=pool.DEFAULT_MAX_SIZE,
autocommit=True):
"""Connect to a PostgreSQL server using the module wide connection and
set the isolation level.
:param str uri: PostgreSQL connection URI
:param psycopg2.extensions.cursor: The cursor type to use
:param int pool_idle_ttl: How long idle pools keep connections open
:param int pool_max_size: The maximum size of the pool to use
"""
self._pool_manager = pool.PoolManager.instance()
self._uri = uri
# Ensure the pool exists in the pool manager
if self.pid not in self._pool_manager:
self._pool_manager.create(self.pid, pool_idle_ttl, pool_max_size)
self._conn = self._connect()
self._cursor_factory = cursor_factory
self._cursor = self._get_cursor(self._conn)
self._autocommit(autocommit)
@property
def backend_pid(self):
"""Return the backend process ID of the PostgreSQL server that this
session is connected to.
:rtype: int
"""
return self._conn.get_backend_pid()
def callproc(self, name, args=None):
"""Call a stored procedure on the server, returning the results in a
:py:class:`queries.Results` instance.
:param str name: The procedure name
:param list args: The list of arguments to pass in
:rtype: queries.Results
:raises: queries.DataError
:raises: queries.DatabaseError
:raises: queries.IntegrityError
:raises: queries.InternalError
:raises: queries.InterfaceError
:raises: queries.NotSupportedError
:raises: queries.OperationalError
:raises: queries.ProgrammingError
"""
try:
self._cursor.callproc(name, args)
except psycopg2.Error as err:
self._incr_exceptions()
raise err
finally:
self._incr_executions()
return results.Results(self._cursor)
def close(self):
"""Explicitly close the connection and remove it from the connection
pool if pooling is enabled. If the connection is already closed
:raises: psycopg2.InterfaceError
"""
if not self._conn:
raise psycopg2.InterfaceError('Connection not open')
LOGGER.info('Closing connection %r in %s', self._conn, self.pid)
self._pool_manager.free(self.pid, self._conn)
self._pool_manager.remove_connection(self.pid, self._conn)
@property
def connection(self):
"""The current open connection to PostgreSQL.
:rtype: psycopg2.extensions.connection
:rtype: psycopg2.extensions.connection
@property
def cursor(self):
"""The current, active cursor for the open connection.
:rtype: psycopg2.extensions.cursor
:rtype: psycopg2.extensions.cursor
@property
def encoding(self):
"""The current client encoding value.
:rtype: str
:rtype: str
@property
def notices(self):
"""A list of up to the last 50 server notices sent to the client.
:rtype: list
:rtype: list
@property
def pid(self):
"""Return the pool ID used for connection pooling
:rtype: str
:rtype: str
"""
return hashlib.md5(':'.join([self.__class__.__name__,
self._uri]).encode('utf-8')).hexdigest()
def query(self, sql, parameters=None):
"""A generator to issue a query on the server, mogrifying the
parameters against the sql statement. Results are returned as a
:py:class:`queries.Results` object which can act as an iterator and
has multiple ways to access the result data.
:param str sql: The SQL statement
:param dict parameters: A dictionary of query parameters
:rtype: queries.Results
:raises: queries.DataError
:raises: queries.DatabaseError
:raises: queries.IntegrityError
:raises: queries.InternalError
:raises: queries.InterfaceError
:raises: queries.NotSupportedError
:raises: queries.OperationalError
:raises: queries.ProgrammingError
"""
try:
self._cursor.execute(sql, parameters)
except psycopg2.Error as err:
self._incr_exceptions()
raise err
finally:
self._incr_executions()
return results.Results(self._cursor)
def set_encoding(self, value=DEFAULT_ENCODING):
"""Set the client encoding for the session if the value specified
is different than the current client encoding.
:param str value: The encoding value to use
"""
if self._conn.encoding != value:
self._conn.set_client_encoding(value)
def __del__(self):
"""When deleting the context, ensure the instance is removed from
caches, etc.
"""
self._cleanup()
def __enter__(self):
"""For use as a context manager, return a handle to this object
instance.
:rtype: Session
"""
return self
def __exit__(self, exc_type, exc_val, exc_tb):
"""When leaving the context, ensure the instance is removed from
caches, etc.
"""
self._cleanup()
def _autocommit(self, autocommit):
"""Set the isolation level automatically to commit or not after every query
:param autocommit: Boolean (Default - True)
"""
self._conn.autocommit = autocommit
def _cleanup(self):
"""Remove the connection from the stack, closing out the cursor"""
if self._cursor:
LOGGER.debug('Closing the cursor on %s', self.pid)
self._cursor.close()
self._cursor = None
if self._conn:
LOGGER.debug('Freeing %s in the pool', self.pid)
try:
pool.PoolManager.instance().free(self.pid, self._conn)
except pool.ConnectionNotFoundError:
pass
self._conn = None
def _connect(self):
"""Connect to PostgreSQL, either by reusing a connection from the pool
if possible, or by creating the new connection.
:rtype: psycopg2.extensions.connection
:raises: pool.NoIdleConnectionsError
"""
# Attempt to get a cached connection from the connection pool
try:
connection = self._pool_manager.get(self.pid, self)
LOGGER.debug("Re-using connection for %s", self.pid)
except pool.NoIdleConnectionsError:
if self._pool_manager.is_full(self.pid):
raise
# Create a new PostgreSQL connection
kwargs = utils.uri_to_kwargs(self._uri)
LOGGER.debug("Creating a new connection for %s", self.pid)
connection = self._psycopg2_connect(kwargs)
self._pool_manager.add(self.pid, connection)
self._pool_manager.lock(self.pid, connection, self)
# Added in because psycopg2ct connects and leaves the connection in
# a weird state: consts.STATUS_DATESTYLE, returning from
# Connection._setup without setting the state as const.STATUS_OK
if utils.PYPY:
connection.reset()
# Register the custom data types
self._register_unicode(connection)
self._register_uuid(connection)
return connection
def _get_cursor(self, connection, name=None):
"""Return a cursor for the given cursor_factory. Specify a name to
use server-side cursors.
:param connection: The connection to create a cursor on
:type connection: psycopg2.extensions.connection
:param str name: A cursor name for a server side cursor
:rtype: psycopg2.extensions.cursor
"""
cursor = connection.cursor(name=name,
cursor_factory=self._cursor_factory)
if name is not None:
cursor.scrollable = True
cursor.withhold = True
return cursor
def _incr_exceptions(self):
"""Increment the number of exceptions for the current connection."""
self._pool_manager.get_connection(self.pid, self._conn).exceptions += 1
def _incr_executions(self):
"""Increment the number of executions for the current connection."""
self._pool_manager.get_connection(self.pid, self._conn).executions += 1
def _psycopg2_connect(self, kwargs):
"""Return a psycopg2 connection for the specified kwargs. Extend for
use in async session adapters.
:param dict kwargs: Keyword connection args
:rtype: psycopg2.extensions.connection
"""
return psycopg2.connect(**kwargs)
@staticmethod
def _register_unicode(connection):
"""Register the cursor to be able to receive Unicode string.
:type connection: psycopg2.extensions.connection
:param connection: Where to register things
"""
psycopg2.extensions.register_type(psycopg2.extensions.UNICODE,
connection)
psycopg2.extensions.register_type(psycopg2.extensions.UNICODEARRAY,
connection)
@staticmethod
def _register_uuid(connection):
"""Register the UUID extension from the psycopg2.extra module
:type connection: psycopg2.extensions.connection
:param connection: Where to register things
"""
psycopg2.extras.register_uuid(conn_or_curs=connection)
@property
def _status(self):
"""Return the current connection status as an integer value.
The status should match one of the following constants:
- queries.Session.INTRANS: Connection established, in transaction
- queries.Session.PREPARED: Prepared for second phase of transaction
- queries.Session.READY: Connected, no active transaction
:rtype: int
"""
if self._conn.status == psycopg2.extensions.STATUS_BEGIN:
return self.READY
return self._conn.status
<MSG> Typos and consistency
<DFF> @@ -45,7 +45,7 @@ DEFAULT_ENCODING = 'UTF8'
class Session(object):
"""The Session class allows for a unified (and simplified) view of
interfacing with a PostgreSQL database server. The Session object can
- act as a context manager, providing automated cleanup and simple, pythoic
+ act as a context manager, providing automated cleanup and simple, Pythonic
way of interacting with the object.
:param str uri: PostgreSQL connection URI
@@ -145,7 +145,7 @@ class Session(object):
@property
def connection(self):
- """The current open connection to PostgreSQL.
+ """Return the current open connection to PostgreSQL.
:rtype: psycopg2.extensions.connection
@@ -154,7 +154,7 @@ class Session(object):
@property
def cursor(self):
- """The current, active cursor for the open connection.
+ """Return the current, active cursor for the open connection.
:rtype: psycopg2.extensions.cursor
@@ -163,7 +163,7 @@ class Session(object):
@property
def encoding(self):
- """The current client encoding value.
+ """Return the current client encoding value.
:rtype: str
@@ -172,7 +172,7 @@ class Session(object):
@property
def notices(self):
- """A list of up to the last 50 server notices sent to the client.
+ """Return a list of up to the last 50 server notices sent to the client.
:rtype: list
@@ -181,7 +181,7 @@ class Session(object):
@property
def pid(self):
- """Return the pool ID used for connection pooling
+ """Return the pool ID used for connection pooling.
:rtype: str
| 6 | Typos and consistency | 6 | .py | py | bsd-3-clause | gmr/queries |
1068 | <NME> session.py
<BEF> """The Session class allows for a unified (and simplified) view of
interfacing with a PostgreSQL database server.
Connection details are passed in as a PostgreSQL URI and connections are pooled
by default, allowing for reuse of connections across modules in the Python
runtime without having to pass around the object handle.
While you can still access the raw `psycopg2` connection and cursor objects to
provide ultimate flexibility in how you use the queries.Session object, there
are convenience methods designed to simplify the interaction with PostgreSQL.
For `psycopg2` functionality outside of what is exposed in Session, simply
use the Session.connection or Session.cursor properties to gain access to
either object just as you would in a program using psycopg2 directly.
Example usage:
.. code:: python
import queries
with queries.Session('pgsql://postgres@localhost/postgres') as session:
for row in session.Query('SELECT * FROM table'):
print row
"""
import hashlib
import logging
import psycopg2
from psycopg2 import extensions, extras
from queries import pool, results, utils
LOGGER = logging.getLogger(__name__)
DEFAULT_ENCODING = 'UTF8'
DEFAULT_URI = 'postgresql://localhost:5432'
class Session(object):
"""The Session class allows for a unified (and simplified) view of
interfacing with a PostgreSQL database server. The Session object can
act as a context manager, providing automated cleanup and simple, Pythonic
way of interacting with the object.
class Session(object):
"""The Session class allows for a unified (and simplified) view of
interfacing with a PostgreSQL database server. The Session object can
act as a context manager, providing automated cleanup and simple, pythoic
way of interacting with the object.
:param str uri: PostgreSQL connection URI
_conn = None
_cursor = None
_tpc_id = None
_uri = None
# Connection status constants
INTRANS = extensions.STATUS_IN_TRANSACTION
PREPARED = extensions.STATUS_PREPARED
READY = extensions.STATUS_READY
SETUP = extensions.STATUS_SETUP
# Transaction status constants
TX_ACTIVE = extensions.TRANSACTION_STATUS_ACTIVE
TX_IDLE = extensions.TRANSACTION_STATUS_IDLE
TX_INERROR = extensions.TRANSACTION_STATUS_INERROR
TX_INTRANS = extensions.TRANSACTION_STATUS_INTRANS
TX_UNKNOWN = extensions.TRANSACTION_STATUS_UNKNOWN
def __init__(self, uri=DEFAULT_URI,
cursor_factory=extras.RealDictCursor,
pool_idle_ttl=pool.DEFAULT_IDLE_TTL,
pool_max_size=pool.DEFAULT_MAX_SIZE,
autocommit=True):
"""Connect to a PostgreSQL server using the module wide connection and
set the isolation level.
:param str uri: PostgreSQL connection URI
:param psycopg2.extensions.cursor: The cursor type to use
:param int pool_idle_ttl: How long idle pools keep connections open
:param int pool_max_size: The maximum size of the pool to use
"""
self._pool_manager = pool.PoolManager.instance()
self._uri = uri
# Ensure the pool exists in the pool manager
if self.pid not in self._pool_manager:
self._pool_manager.create(self.pid, pool_idle_ttl, pool_max_size)
self._conn = self._connect()
self._cursor_factory = cursor_factory
self._cursor = self._get_cursor(self._conn)
self._autocommit(autocommit)
@property
def backend_pid(self):
"""Return the backend process ID of the PostgreSQL server that this
session is connected to.
:rtype: int
"""
return self._conn.get_backend_pid()
def callproc(self, name, args=None):
"""Call a stored procedure on the server, returning the results in a
:py:class:`queries.Results` instance.
:param str name: The procedure name
:param list args: The list of arguments to pass in
:rtype: queries.Results
:raises: queries.DataError
:raises: queries.DatabaseError
:raises: queries.IntegrityError
:raises: queries.InternalError
:raises: queries.InterfaceError
:raises: queries.NotSupportedError
:raises: queries.OperationalError
:raises: queries.ProgrammingError
"""
try:
self._cursor.callproc(name, args)
except psycopg2.Error as err:
self._incr_exceptions()
raise err
finally:
self._incr_executions()
return results.Results(self._cursor)
def close(self):
"""Explicitly close the connection and remove it from the connection
pool if pooling is enabled. If the connection is already closed
:raises: psycopg2.InterfaceError
"""
if not self._conn:
raise psycopg2.InterfaceError('Connection not open')
LOGGER.info('Closing connection %r in %s', self._conn, self.pid)
self._pool_manager.free(self.pid, self._conn)
self._pool_manager.remove_connection(self.pid, self._conn)
@property
def connection(self):
"""The current open connection to PostgreSQL.
:rtype: psycopg2.extensions.connection
:rtype: psycopg2.extensions.connection
@property
def cursor(self):
"""The current, active cursor for the open connection.
:rtype: psycopg2.extensions.cursor
:rtype: psycopg2.extensions.cursor
@property
def encoding(self):
"""The current client encoding value.
:rtype: str
:rtype: str
@property
def notices(self):
"""A list of up to the last 50 server notices sent to the client.
:rtype: list
:rtype: list
@property
def pid(self):
"""Return the pool ID used for connection pooling
:rtype: str
:rtype: str
"""
return hashlib.md5(':'.join([self.__class__.__name__,
self._uri]).encode('utf-8')).hexdigest()
def query(self, sql, parameters=None):
"""A generator to issue a query on the server, mogrifying the
parameters against the sql statement. Results are returned as a
:py:class:`queries.Results` object which can act as an iterator and
has multiple ways to access the result data.
:param str sql: The SQL statement
:param dict parameters: A dictionary of query parameters
:rtype: queries.Results
:raises: queries.DataError
:raises: queries.DatabaseError
:raises: queries.IntegrityError
:raises: queries.InternalError
:raises: queries.InterfaceError
:raises: queries.NotSupportedError
:raises: queries.OperationalError
:raises: queries.ProgrammingError
"""
try:
self._cursor.execute(sql, parameters)
except psycopg2.Error as err:
self._incr_exceptions()
raise err
finally:
self._incr_executions()
return results.Results(self._cursor)
def set_encoding(self, value=DEFAULT_ENCODING):
"""Set the client encoding for the session if the value specified
is different than the current client encoding.
:param str value: The encoding value to use
"""
if self._conn.encoding != value:
self._conn.set_client_encoding(value)
def __del__(self):
"""When deleting the context, ensure the instance is removed from
caches, etc.
"""
self._cleanup()
def __enter__(self):
"""For use as a context manager, return a handle to this object
instance.
:rtype: Session
"""
return self
def __exit__(self, exc_type, exc_val, exc_tb):
"""When leaving the context, ensure the instance is removed from
caches, etc.
"""
self._cleanup()
def _autocommit(self, autocommit):
"""Set the isolation level automatically to commit or not after every query
:param autocommit: Boolean (Default - True)
"""
self._conn.autocommit = autocommit
def _cleanup(self):
"""Remove the connection from the stack, closing out the cursor"""
if self._cursor:
LOGGER.debug('Closing the cursor on %s', self.pid)
self._cursor.close()
self._cursor = None
if self._conn:
LOGGER.debug('Freeing %s in the pool', self.pid)
try:
pool.PoolManager.instance().free(self.pid, self._conn)
except pool.ConnectionNotFoundError:
pass
self._conn = None
def _connect(self):
"""Connect to PostgreSQL, either by reusing a connection from the pool
if possible, or by creating the new connection.
:rtype: psycopg2.extensions.connection
:raises: pool.NoIdleConnectionsError
"""
# Attempt to get a cached connection from the connection pool
try:
connection = self._pool_manager.get(self.pid, self)
LOGGER.debug("Re-using connection for %s", self.pid)
except pool.NoIdleConnectionsError:
if self._pool_manager.is_full(self.pid):
raise
# Create a new PostgreSQL connection
kwargs = utils.uri_to_kwargs(self._uri)
LOGGER.debug("Creating a new connection for %s", self.pid)
connection = self._psycopg2_connect(kwargs)
self._pool_manager.add(self.pid, connection)
self._pool_manager.lock(self.pid, connection, self)
# Added in because psycopg2ct connects and leaves the connection in
# a weird state: consts.STATUS_DATESTYLE, returning from
# Connection._setup without setting the state as const.STATUS_OK
if utils.PYPY:
connection.reset()
# Register the custom data types
self._register_unicode(connection)
self._register_uuid(connection)
return connection
def _get_cursor(self, connection, name=None):
"""Return a cursor for the given cursor_factory. Specify a name to
use server-side cursors.
:param connection: The connection to create a cursor on
:type connection: psycopg2.extensions.connection
:param str name: A cursor name for a server side cursor
:rtype: psycopg2.extensions.cursor
"""
cursor = connection.cursor(name=name,
cursor_factory=self._cursor_factory)
if name is not None:
cursor.scrollable = True
cursor.withhold = True
return cursor
def _incr_exceptions(self):
"""Increment the number of exceptions for the current connection."""
self._pool_manager.get_connection(self.pid, self._conn).exceptions += 1
def _incr_executions(self):
"""Increment the number of executions for the current connection."""
self._pool_manager.get_connection(self.pid, self._conn).executions += 1
def _psycopg2_connect(self, kwargs):
"""Return a psycopg2 connection for the specified kwargs. Extend for
use in async session adapters.
:param dict kwargs: Keyword connection args
:rtype: psycopg2.extensions.connection
"""
return psycopg2.connect(**kwargs)
@staticmethod
def _register_unicode(connection):
"""Register the cursor to be able to receive Unicode string.
:type connection: psycopg2.extensions.connection
:param connection: Where to register things
"""
psycopg2.extensions.register_type(psycopg2.extensions.UNICODE,
connection)
psycopg2.extensions.register_type(psycopg2.extensions.UNICODEARRAY,
connection)
@staticmethod
def _register_uuid(connection):
"""Register the UUID extension from the psycopg2.extra module
:type connection: psycopg2.extensions.connection
:param connection: Where to register things
"""
psycopg2.extras.register_uuid(conn_or_curs=connection)
@property
def _status(self):
"""Return the current connection status as an integer value.
The status should match one of the following constants:
- queries.Session.INTRANS: Connection established, in transaction
- queries.Session.PREPARED: Prepared for second phase of transaction
- queries.Session.READY: Connected, no active transaction
:rtype: int
"""
if self._conn.status == psycopg2.extensions.STATUS_BEGIN:
return self.READY
return self._conn.status
<MSG> Typos and consistency
<DFF> @@ -45,7 +45,7 @@ DEFAULT_ENCODING = 'UTF8'
class Session(object):
"""The Session class allows for a unified (and simplified) view of
interfacing with a PostgreSQL database server. The Session object can
- act as a context manager, providing automated cleanup and simple, pythoic
+ act as a context manager, providing automated cleanup and simple, Pythonic
way of interacting with the object.
:param str uri: PostgreSQL connection URI
@@ -145,7 +145,7 @@ class Session(object):
@property
def connection(self):
- """The current open connection to PostgreSQL.
+ """Return the current open connection to PostgreSQL.
:rtype: psycopg2.extensions.connection
@@ -154,7 +154,7 @@ class Session(object):
@property
def cursor(self):
- """The current, active cursor for the open connection.
+ """Return the current, active cursor for the open connection.
:rtype: psycopg2.extensions.cursor
@@ -163,7 +163,7 @@ class Session(object):
@property
def encoding(self):
- """The current client encoding value.
+ """Return the current client encoding value.
:rtype: str
@@ -172,7 +172,7 @@ class Session(object):
@property
def notices(self):
- """A list of up to the last 50 server notices sent to the client.
+ """Return a list of up to the last 50 server notices sent to the client.
:rtype: list
@@ -181,7 +181,7 @@ class Session(object):
@property
def pid(self):
- """Return the pool ID used for connection pooling
+ """Return the pool ID used for connection pooling.
:rtype: str
| 6 | Typos and consistency | 6 | .py | py | bsd-3-clause | gmr/queries |
1069 | <NME> results_tests.py
<BEF> """
Tests for functionality in the results module
"""
import logging
import unittest
import mock
import psycopg2
from queries import results
LOGGER = logging.getLogger(__name__)
class ResultsTestCase(unittest.TestCase):
def setUp(self):
self.cursor = mock.MagicMock()
self.obj = results.Results(self.cursor)
def test_cursor_is_assigned(self):
self.assertEqual(self.obj.cursor, self.cursor)
def test_getitem_invokes_scroll(self):
self.cursor.scroll = mock.Mock()
self.cursor.fetchone = mock.Mock()
row = self.obj[1]
LOGGER.debug('Row: %r', row)
self.cursor.scroll.assert_called_once_with(1, 'absolute')
def test_getitem_raises_index_error(self):
self.cursor.scroll = mock.Mock(side_effect=psycopg2.ProgrammingError)
self.cursor.fetchone = mock.Mock()
def get_row():
return self.obj[1]
self.assertRaises(IndexError, get_row)
def test_getitem_invokes_fetchone(self):
self.cursor.scroll = mock.Mock()
self.cursor.fetchone = mock.Mock()
row = self.obj[1]
LOGGER.debug('Row: %r', row)
self.cursor.rowcount = 0
with mock.patch.object(self.obj, '_rewind') as rewind:
[x for x in self.obj]
assert not rewind.called, '_rewind should not be called on empty result'
def test_iter_rewinds(self):
self.cursor.__iter__ = mock.Mock(return_value=iter([1, 2, 3]))
def test_iter_rewinds(self):
self.cursor.__iter__ = mock.Mock(return_value=iter([1, 2, 3]))
with mock.patch.object(self.obj, '_rewind') as rewind:
[x for x in self.obj]
rewind.assert_called_once_with()
def test_iter_iters(self):
self.cursor.__iter__ = mock.Mock(return_value=iter([1, 2, 3]))
with mock.patch.object(self.obj, '_rewind'):
self.assertEqual([x for x in self.obj], [1, 2, 3])
def test_rowcount_value(self):
self.cursor.rowcount = 128
self.assertEqual(len(self.obj), 128)
def test_nonzero_false(self):
self.cursor.rowcount = 0
self.assertFalse(bool(self.obj))
def test_nonzero_true(self):
self.cursor.rowcount = 128
self.assertTrue(bool(self.obj))
def test_repr_str(self):
self.cursor.rowcount = 128
self.assertEqual(str(self.obj), '<queries.Results rows=128>')
def test_as_dict_no_rows(self):
self.cursor.rowcount = 0
self.assertDictEqual(self.obj.as_dict(), {})
def test_as_dict_rewinds(self):
expectation = {'foo': 'bar', 'baz': 'qux'}
self.cursor.rowcount = 1
self.cursor.fetchone = mock.Mock(return_value=expectation)
with mock.patch.object(self.obj, '_rewind') as rewind:
result = self.obj.as_dict()
LOGGER.debug('Result: %r', result)
rewind.assert_called_once_with()
def test_as_dict_value(self):
expectation = {'foo': 'bar', 'baz': 'qux'}
self.cursor.rowcount = 1
self.cursor.fetchone = mock.Mock(return_value=expectation)
with mock.patch.object(self.obj, '_rewind'):
self.assertDictEqual(self.obj.as_dict(), expectation)
def test_as_dict_with_multiple_rows_raises(self):
self.cursor.rowcount = 2
self.cursor.rowcount = 2
self.assertEqual(self.obj.count(), 2)
def test_free_raises_exception(self):
self.assertRaises(NotImplementedError, self.obj.free)
def test_items_returns_on_empty(self):
self.cursor.rowcount = 0
self.cursor.scroll = mock.Mock()
self.cursor.fetchall = mock.Mock()
self.obj.items()
assert not self.cursor.scroll.called, 'Cursor.scroll should not be called on empty result'
def test_items_invokes_scroll(self):
self.cursor.scroll = mock.Mock()
'Cursor.scroll should not be called on empty result'
def test_items_invokes_scroll(self):
self.cursor.scroll = mock.Mock()
self.cursor.fetchall = mock.Mock()
self.obj.items()
self.cursor.scroll.assert_called_once_with(0, 'absolute')
def test_items_invokes_fetchall(self):
self.cursor.scroll = mock.Mock()
self.cursor.fetchall = mock.Mock()
self.obj.items()
self.cursor.fetchall.assert_called_once_with()
def test_rownumber_value(self):
self.cursor.rownumber = 10
self.assertEqual(self.obj.rownumber, 10)
def test_query_value(self):
self.cursor.query = 'SELECT * FROM foo'
self.assertEqual(self.obj.query, 'SELECT * FROM foo')
def test_status_value(self):
self.cursor.statusmessage = 'Status message'
self.assertEqual(self.obj.status, 'Status message')
def test_rewind_invokes_scroll(self):
self.cursor.scroll = mock.Mock()
self.obj._rewind()
self.cursor.scroll.assert_called_once_with(0, 'absolute')
<MSG> Remove the NotImplemented test
<DFF> @@ -45,7 +45,8 @@ class ResultsTestCase(unittest.TestCase):
self.cursor.rowcount = 0
with mock.patch.object(self.obj, '_rewind') as rewind:
[x for x in self.obj]
- assert not rewind.called, '_rewind should not be called on empty result'
+ assert not rewind.called, \
+ '_rewind should not be called on empty result'
def test_iter_rewinds(self):
self.cursor.__iter__ = mock.Mock(return_value=iter([1, 2, 3]))
@@ -102,15 +103,13 @@ class ResultsTestCase(unittest.TestCase):
self.cursor.rowcount = 2
self.assertEqual(self.obj.count(), 2)
- def test_free_raises_exception(self):
- self.assertRaises(NotImplementedError, self.obj.free)
-
def test_items_returns_on_empty(self):
self.cursor.rowcount = 0
self.cursor.scroll = mock.Mock()
self.cursor.fetchall = mock.Mock()
self.obj.items()
- assert not self.cursor.scroll.called, 'Cursor.scroll should not be called on empty result'
+ assert not self.cursor.scroll.called, \
+ 'Cursor.scroll should not be called on empty result'
def test_items_invokes_scroll(self):
self.cursor.scroll = mock.Mock()
| 4 | Remove the NotImplemented test | 5 | .py | py | bsd-3-clause | gmr/queries |
1070 | <NME> results_tests.py
<BEF> """
Tests for functionality in the results module
"""
import logging
import unittest
import mock
import psycopg2
from queries import results
LOGGER = logging.getLogger(__name__)
class ResultsTestCase(unittest.TestCase):
def setUp(self):
self.cursor = mock.MagicMock()
self.obj = results.Results(self.cursor)
def test_cursor_is_assigned(self):
self.assertEqual(self.obj.cursor, self.cursor)
def test_getitem_invokes_scroll(self):
self.cursor.scroll = mock.Mock()
self.cursor.fetchone = mock.Mock()
row = self.obj[1]
LOGGER.debug('Row: %r', row)
self.cursor.scroll.assert_called_once_with(1, 'absolute')
def test_getitem_raises_index_error(self):
self.cursor.scroll = mock.Mock(side_effect=psycopg2.ProgrammingError)
self.cursor.fetchone = mock.Mock()
def get_row():
return self.obj[1]
self.assertRaises(IndexError, get_row)
def test_getitem_invokes_fetchone(self):
self.cursor.scroll = mock.Mock()
self.cursor.fetchone = mock.Mock()
row = self.obj[1]
LOGGER.debug('Row: %r', row)
self.cursor.rowcount = 0
with mock.patch.object(self.obj, '_rewind') as rewind:
[x for x in self.obj]
assert not rewind.called, '_rewind should not be called on empty result'
def test_iter_rewinds(self):
self.cursor.__iter__ = mock.Mock(return_value=iter([1, 2, 3]))
def test_iter_rewinds(self):
self.cursor.__iter__ = mock.Mock(return_value=iter([1, 2, 3]))
with mock.patch.object(self.obj, '_rewind') as rewind:
[x for x in self.obj]
rewind.assert_called_once_with()
def test_iter_iters(self):
self.cursor.__iter__ = mock.Mock(return_value=iter([1, 2, 3]))
with mock.patch.object(self.obj, '_rewind'):
self.assertEqual([x for x in self.obj], [1, 2, 3])
def test_rowcount_value(self):
self.cursor.rowcount = 128
self.assertEqual(len(self.obj), 128)
def test_nonzero_false(self):
self.cursor.rowcount = 0
self.assertFalse(bool(self.obj))
def test_nonzero_true(self):
self.cursor.rowcount = 128
self.assertTrue(bool(self.obj))
def test_repr_str(self):
self.cursor.rowcount = 128
self.assertEqual(str(self.obj), '<queries.Results rows=128>')
def test_as_dict_no_rows(self):
self.cursor.rowcount = 0
self.assertDictEqual(self.obj.as_dict(), {})
def test_as_dict_rewinds(self):
expectation = {'foo': 'bar', 'baz': 'qux'}
self.cursor.rowcount = 1
self.cursor.fetchone = mock.Mock(return_value=expectation)
with mock.patch.object(self.obj, '_rewind') as rewind:
result = self.obj.as_dict()
LOGGER.debug('Result: %r', result)
rewind.assert_called_once_with()
def test_as_dict_value(self):
expectation = {'foo': 'bar', 'baz': 'qux'}
self.cursor.rowcount = 1
self.cursor.fetchone = mock.Mock(return_value=expectation)
with mock.patch.object(self.obj, '_rewind'):
self.assertDictEqual(self.obj.as_dict(), expectation)
def test_as_dict_with_multiple_rows_raises(self):
self.cursor.rowcount = 2
self.cursor.rowcount = 2
self.assertEqual(self.obj.count(), 2)
def test_free_raises_exception(self):
self.assertRaises(NotImplementedError, self.obj.free)
def test_items_returns_on_empty(self):
self.cursor.rowcount = 0
self.cursor.scroll = mock.Mock()
self.cursor.fetchall = mock.Mock()
self.obj.items()
assert not self.cursor.scroll.called, 'Cursor.scroll should not be called on empty result'
def test_items_invokes_scroll(self):
self.cursor.scroll = mock.Mock()
'Cursor.scroll should not be called on empty result'
def test_items_invokes_scroll(self):
self.cursor.scroll = mock.Mock()
self.cursor.fetchall = mock.Mock()
self.obj.items()
self.cursor.scroll.assert_called_once_with(0, 'absolute')
def test_items_invokes_fetchall(self):
self.cursor.scroll = mock.Mock()
self.cursor.fetchall = mock.Mock()
self.obj.items()
self.cursor.fetchall.assert_called_once_with()
def test_rownumber_value(self):
self.cursor.rownumber = 10
self.assertEqual(self.obj.rownumber, 10)
def test_query_value(self):
self.cursor.query = 'SELECT * FROM foo'
self.assertEqual(self.obj.query, 'SELECT * FROM foo')
def test_status_value(self):
self.cursor.statusmessage = 'Status message'
self.assertEqual(self.obj.status, 'Status message')
def test_rewind_invokes_scroll(self):
self.cursor.scroll = mock.Mock()
self.obj._rewind()
self.cursor.scroll.assert_called_once_with(0, 'absolute')
<MSG> Remove the NotImplemented test
<DFF> @@ -45,7 +45,8 @@ class ResultsTestCase(unittest.TestCase):
self.cursor.rowcount = 0
with mock.patch.object(self.obj, '_rewind') as rewind:
[x for x in self.obj]
- assert not rewind.called, '_rewind should not be called on empty result'
+ assert not rewind.called, \
+ '_rewind should not be called on empty result'
def test_iter_rewinds(self):
self.cursor.__iter__ = mock.Mock(return_value=iter([1, 2, 3]))
@@ -102,15 +103,13 @@ class ResultsTestCase(unittest.TestCase):
self.cursor.rowcount = 2
self.assertEqual(self.obj.count(), 2)
- def test_free_raises_exception(self):
- self.assertRaises(NotImplementedError, self.obj.free)
-
def test_items_returns_on_empty(self):
self.cursor.rowcount = 0
self.cursor.scroll = mock.Mock()
self.cursor.fetchall = mock.Mock()
self.obj.items()
- assert not self.cursor.scroll.called, 'Cursor.scroll should not be called on empty result'
+ assert not self.cursor.scroll.called, \
+ 'Cursor.scroll should not be called on empty result'
def test_items_invokes_scroll(self):
self.cursor.scroll = mock.Mock()
| 4 | Remove the NotImplemented test | 5 | .py | py | bsd-3-clause | gmr/queries |
1071 | <NME> __init__.py
<BEF> """
Queries: PostgreSQL database access simplified
Queries is an opinionated wrapper for interfacing with PostgreSQL that offers
caching of connections and support for PyPy via psycopg2ct.
The core `queries.Queries` class will automatically register support for UUIDs,
Unicode and Unicode arrays.
"""
import logging
import sys
try:
import psycopg2cffi
import psycopg2cffi.extras
import psycopg2cffi.extensions
except ImportError:
pass
else:
sys.modules['psycopg2'] = psycopg2cffi
sys.modules['psycopg2.extras'] = psycopg2cffi.extras
sys.modules['psycopg2.extensions'] = psycopg2cffi.extensions
from queries.results import Results
from queries.session import Session
try:
from queries.tornado_session import TornadoSession
except ImportError: # pragma: nocover
TornadoSession = None
from queries.utils import uri
# For ease of access to different cursor types
from psycopg2.extras import DictCursor
from psycopg2.extras import NamedTupleCursor
from psycopg2.extras import RealDictCursor
from psycopg2.extras import LoggingCursor
from psycopg2.extras import MinTimeLoggingCursor
# Expose exceptions so clients do not need to import psycopg2 too
from psycopg2 import Warning
from psycopg2 import Error
from psycopg2 import DataError
from psycopg2 import DatabaseError
from psycopg2 import IntegrityError
from psycopg2 import InterfaceError
from psycopg2 import InternalError
from psycopg2 import NotSupportedError
from psycopg2 import OperationalError
from psycopg2 import ProgrammingError
TornadoSession = None
def uri(host='localhost', port='5432', dbname='postgres', user='postgres',
password=None):
"""Return a PostgreSQL connection URI for the specified values.
logging.getLogger('queries').addHandler(logging.NullHandler())
<MSG> Fix a bug in queries.uri() and add test coverage
<DFF> @@ -51,7 +51,7 @@ except ImportError:
TornadoSession = None
-def uri(host='localhost', port='5432', dbname='postgres', user='postgres',
+def uri(host='localhost', port=5432, dbname='postgres', user='postgres',
password=None):
"""Return a PostgreSQL connection URI for the specified values.
| 1 | Fix a bug in queries.uri() and add test coverage | 1 | .py | py | bsd-3-clause | gmr/queries |
1072 | <NME> __init__.py
<BEF> """
Queries: PostgreSQL database access simplified
Queries is an opinionated wrapper for interfacing with PostgreSQL that offers
caching of connections and support for PyPy via psycopg2ct.
The core `queries.Queries` class will automatically register support for UUIDs,
Unicode and Unicode arrays.
"""
import logging
import sys
try:
import psycopg2cffi
import psycopg2cffi.extras
import psycopg2cffi.extensions
except ImportError:
pass
else:
sys.modules['psycopg2'] = psycopg2cffi
sys.modules['psycopg2.extras'] = psycopg2cffi.extras
sys.modules['psycopg2.extensions'] = psycopg2cffi.extensions
from queries.results import Results
from queries.session import Session
try:
from queries.tornado_session import TornadoSession
except ImportError: # pragma: nocover
TornadoSession = None
from queries.utils import uri
# For ease of access to different cursor types
from psycopg2.extras import DictCursor
from psycopg2.extras import NamedTupleCursor
from psycopg2.extras import RealDictCursor
from psycopg2.extras import LoggingCursor
from psycopg2.extras import MinTimeLoggingCursor
# Expose exceptions so clients do not need to import psycopg2 too
from psycopg2 import Warning
from psycopg2 import Error
from psycopg2 import DataError
from psycopg2 import DatabaseError
from psycopg2 import IntegrityError
from psycopg2 import InterfaceError
from psycopg2 import InternalError
from psycopg2 import NotSupportedError
from psycopg2 import OperationalError
from psycopg2 import ProgrammingError
TornadoSession = None
def uri(host='localhost', port='5432', dbname='postgres', user='postgres',
password=None):
"""Return a PostgreSQL connection URI for the specified values.
logging.getLogger('queries').addHandler(logging.NullHandler())
<MSG> Fix a bug in queries.uri() and add test coverage
<DFF> @@ -51,7 +51,7 @@ except ImportError:
TornadoSession = None
-def uri(host='localhost', port='5432', dbname='postgres', user='postgres',
+def uri(host='localhost', port=5432, dbname='postgres', user='postgres',
password=None):
"""Return a PostgreSQL connection URI for the specified values.
| 1 | Fix a bug in queries.uri() and add test coverage | 1 | .py | py | bsd-3-clause | gmr/queries |
1073 | <NME> session.py
<BEF> """The Session class allows for a unified (and simplified) view of
interfacing with a PostgreSQL database server.
Connection details are passed in as a PostgreSQL URI and connections are pooled
by default, allowing for reuse of connections across modules in the Python
runtime without having to pass around the object handle.
While you can still access the raw `psycopg2` connection and cursor objects to
provide ultimate flexibility in how you use the queries.Session object, there
are convenience methods designed to simplify the interaction with PostgreSQL.
For `psycopg2` functionality outside of what is exposed in Session, simply
use the Session.connection or Session.cursor properties to gain access to
either object just as you would in a program using psycopg2 directly.
Example usage:
.. code:: python
import queries
with queries.Session('pgsql://postgres@localhost/postgres') as session:
for row in session.Query('SELECT * FROM table'):
print row
"""
import hashlib
import logging
import psycopg2
from psycopg2 import extensions, extras
from queries import pool, results, utils
LOGGER = logging.getLogger(__name__)
DEFAULT_ENCODING = 'UTF8'
DEFAULT_URI = 'postgresql://localhost:5432'
class Session(object):
"""The Session class allows for a unified (and simplified) view of
interfacing with a PostgreSQL database server. The Session object can
act as a context manager, providing automated cleanup and simple, Pythonic
way of interacting with the object.
:param str uri: PostgreSQL connection URI
:param psycopg2.extensions.cursor: The cursor type to use
:param int pool_idle_ttl: How long idle pools keep connections open
:param int pool_max_size: The maximum size of the pool to use
"""
_conn = None
_cursor = None
_tpc_id = None
_uri = None
# Connection status constants
INTRANS = extensions.STATUS_IN_TRANSACTION
PREPARED = extensions.STATUS_PREPARED
READY = extensions.STATUS_READY
SETUP = extensions.STATUS_SETUP
# Transaction status constants
TX_ACTIVE = extensions.TRANSACTION_STATUS_ACTIVE
TX_IDLE = extensions.TRANSACTION_STATUS_IDLE
TX_INERROR = extensions.TRANSACTION_STATUS_INERROR
TX_INTRANS = extensions.TRANSACTION_STATUS_INTRANS
TX_UNKNOWN = extensions.TRANSACTION_STATUS_UNKNOWN
def __init__(self, uri=DEFAULT_URI,
cursor_factory=extras.RealDictCursor,
pool_idle_ttl=pool.DEFAULT_IDLE_TTL,
pool_max_size=pool.DEFAULT_MAX_SIZE,
autocommit=True):
"""Connect to a PostgreSQL server using the module wide connection and
set the isolation level.
:param str uri: PostgreSQL connection URI
:param psycopg2.extensions.cursor: The cursor type to use
:param int pool_idle_ttl: How long idle pools keep connections open
:param int pool_max_size: The maximum size of the pool to use
"""
self._pool_manager = pool.PoolManager.instance()
:param int pool_max_size: The maximum size of the pool to use
"""
self._pool_manager = pool.PoolManager()
self._uri = uri
# Ensure the pool exists in the pool manager
self._cursor_factory = cursor_factory
self._cursor = self._get_cursor(self._conn)
self._autocommit(autocommit)
@property
def backend_pid(self):
"""Return the backend process ID of the PostgreSQL server that this
session is connected to.
:rtype: int
"""
return self._conn.get_backend_pid()
def callproc(self, name, args=None):
"""Call a stored procedure on the server, returning the results in a
:py:class:`queries.Results` instance.
:param str name: The procedure name
:param list args: The list of arguments to pass in
:rtype: queries.Results
:raises: queries.DataError
:raises: queries.DatabaseError
:raises: queries.IntegrityError
:raises: queries.InternalError
:raises: queries.InterfaceError
:raises: queries.NotSupportedError
:raises: queries.OperationalError
:raises: queries.ProgrammingError
"""
try:
self._cursor.callproc(name, args)
except psycopg2.Error as err:
self._incr_exceptions()
raise err
finally:
self._incr_executions()
return results.Results(self._cursor)
def close(self):
"""Explicitly close the connection and remove it from the connection
pool if pooling is enabled. If the connection is already closed
:raises: psycopg2.InterfaceError
"""
if not self._conn:
raise psycopg2.InterfaceError('Connection not open')
LOGGER.info('Closing connection %r in %s', self._conn, self.pid)
self._pool_manager.free(self.pid, self._conn)
self._pool_manager.remove_connection(self.pid, self._conn)
# Un-assign the connection and cursor
self._conn, self._cursor = None, None
@property
def connection(self):
"""Return the current open connection to PostgreSQL.
:rtype: psycopg2.extensions.connection
"""
return self._conn
@property
def cursor(self):
"""Return the current, active cursor for the open connection.
:rtype: psycopg2.extensions.cursor
"""
return self._cursor
@property
def encoding(self):
"""Return the current client encoding value.
:rtype: str
"""
return self._conn.encoding
@property
def notices(self):
"""Return a list of up to the last 50 server notices sent to the client.
:rtype: list
"""
return self._conn.notices
@property
def pid(self):
"""Return the pool ID used for connection pooling.
:rtype: str
"""
return hashlib.md5(':'.join([self.__class__.__name__,
self._uri]).encode('utf-8')).hexdigest()
def query(self, sql, parameters=None):
"""A generator to issue a query on the server, mogrifying the
parameters against the sql statement. Results are returned as a
:py:class:`queries.Results` object which can act as an iterator and
has multiple ways to access the result data.
:param str sql: The SQL statement
:param dict parameters: A dictionary of query parameters
:rtype: queries.Results
:raises: queries.DataError
:raises: queries.DatabaseError
:raises: queries.IntegrityError
:raises: queries.InternalError
:raises: queries.InterfaceError
:raises: queries.NotSupportedError
:raises: queries.OperationalError
:raises: queries.ProgrammingError
"""
try:
self._cursor.execute(sql, parameters)
except psycopg2.Error as err:
self._incr_exceptions()
raise err
finally:
self._incr_executions()
return results.Results(self._cursor)
def set_encoding(self, value=DEFAULT_ENCODING):
"""Set the client encoding for the session if the value specified
is different than the current client encoding.
:param str value: The encoding value to use
"""
if self._conn.encoding != value:
self._conn.set_client_encoding(value)
def __del__(self):
"""When deleting the context, ensure the instance is removed from
caches, etc.
"""
self._cleanup()
def __enter__(self):
"""For use as a context manager, return a handle to this object
instance.
:rtype: Session
"""
return self
def __exit__(self, exc_type, exc_val, exc_tb):
"""When leaving the context, ensure the instance is removed from
caches, etc.
"""
self._cleanup()
self._cursor = None
if self._conn:
self._pool_manager.free(self.pid, self._conn)
self._conn = None
self._pool_manager.clean(self.pid)
def _connect(self):
"""Connect to PostgreSQL, either by reusing a connection from the pool
LOGGER.debug('Freeing %s in the pool', self.pid)
try:
pool.PoolManager.instance().free(self.pid, self._conn)
except pool.ConnectionNotFoundError:
pass
self._conn = None
def _connect(self):
"""Connect to PostgreSQL, either by reusing a connection from the pool
if possible, or by creating the new connection.
:rtype: psycopg2.extensions.connection
:raises: pool.NoIdleConnectionsError
"""
# Attempt to get a cached connection from the connection pool
try:
connection = self._pool_manager.get(self.pid, self)
LOGGER.debug("Re-using connection for %s", self.pid)
except pool.NoIdleConnectionsError:
if self._pool_manager.is_full(self.pid):
raise
# Create a new PostgreSQL connection
kwargs = utils.uri_to_kwargs(self._uri)
LOGGER.debug("Creating a new connection for %s", self.pid)
connection = self._psycopg2_connect(kwargs)
self._pool_manager.add(self.pid, connection)
self._pool_manager.lock(self.pid, connection, self)
# Added in because psycopg2ct connects and leaves the connection in
# a weird state: consts.STATUS_DATESTYLE, returning from
# Connection._setup without setting the state as const.STATUS_OK
if utils.PYPY:
connection.reset()
# Register the custom data types
self._register_unicode(connection)
self._register_uuid(connection)
return connection
def _get_cursor(self, connection, name=None):
"""Return a cursor for the given cursor_factory. Specify a name to
use server-side cursors.
:param connection: The connection to create a cursor on
:type connection: psycopg2.extensions.connection
:param str name: A cursor name for a server side cursor
:rtype: psycopg2.extensions.cursor
"""
cursor = connection.cursor(name=name,
cursor_factory=self._cursor_factory)
if name is not None:
cursor.scrollable = True
cursor.withhold = True
return cursor
def _incr_exceptions(self):
"""Increment the number of exceptions for the current connection."""
self._pool_manager.get_connection(self.pid, self._conn).exceptions += 1
def _incr_executions(self):
"""Increment the number of executions for the current connection."""
self._pool_manager.get_connection(self.pid, self._conn).executions += 1
def _psycopg2_connect(self, kwargs):
"""Return a psycopg2 connection for the specified kwargs. Extend for
use in async session adapters.
:param dict kwargs: Keyword connection args
:rtype: psycopg2.extensions.connection
"""
return psycopg2.connect(**kwargs)
@staticmethod
def _register_unicode(connection):
"""Register the cursor to be able to receive Unicode string.
:type connection: psycopg2.extensions.connection
:param connection: Where to register things
"""
psycopg2.extensions.register_type(psycopg2.extensions.UNICODE,
connection)
psycopg2.extensions.register_type(psycopg2.extensions.UNICODEARRAY,
connection)
@staticmethod
def _register_uuid(connection):
"""Register the UUID extension from the psycopg2.extra module
:type connection: psycopg2.extensions.connection
:param connection: Where to register things
"""
psycopg2.extras.register_uuid(conn_or_curs=connection)
@property
def _status(self):
"""Return the current connection status as an integer value.
The status should match one of the following constants:
- queries.Session.INTRANS: Connection established, in transaction
- queries.Session.PREPARED: Prepared for second phase of transaction
- queries.Session.READY: Connected, no active transaction
:rtype: int
"""
if self._conn.status == psycopg2.extensions.STATUS_BEGIN:
return self.READY
return self._conn.status
<MSG> Use the singleton poolmanager instance, handle exceptions in cleanup
<DFF> @@ -85,7 +85,7 @@ class Session(object):
:param int pool_max_size: The maximum size of the pool to use
"""
- self._pool_manager = pool.PoolManager()
+ self._pool_manager = pool.PoolManager.instance()
self._uri = uri
# Ensure the pool exists in the pool manager
@@ -254,10 +254,16 @@ class Session(object):
self._cursor = None
if self._conn:
- self._pool_manager.free(self.pid, self._conn)
+ try:
+ self._pool_manager.free(self.pid, self._conn)
+ except (pool.ConnectionNotFoundError, KeyError):
+ pass
self._conn = None
- self._pool_manager.clean(self.pid)
+ try:
+ self._pool_manager.clean(self.pid)
+ except KeyError:
+ pass
def _connect(self):
"""Connect to PostgreSQL, either by reusing a connection from the pool
| 9 | Use the singleton poolmanager instance, handle exceptions in cleanup | 3 | .py | py | bsd-3-clause | gmr/queries |
1074 | <NME> session.py
<BEF> """The Session class allows for a unified (and simplified) view of
interfacing with a PostgreSQL database server.
Connection details are passed in as a PostgreSQL URI and connections are pooled
by default, allowing for reuse of connections across modules in the Python
runtime without having to pass around the object handle.
While you can still access the raw `psycopg2` connection and cursor objects to
provide ultimate flexibility in how you use the queries.Session object, there
are convenience methods designed to simplify the interaction with PostgreSQL.
For `psycopg2` functionality outside of what is exposed in Session, simply
use the Session.connection or Session.cursor properties to gain access to
either object just as you would in a program using psycopg2 directly.
Example usage:
.. code:: python
import queries
with queries.Session('pgsql://postgres@localhost/postgres') as session:
for row in session.Query('SELECT * FROM table'):
print row
"""
import hashlib
import logging
import psycopg2
from psycopg2 import extensions, extras
from queries import pool, results, utils
LOGGER = logging.getLogger(__name__)
DEFAULT_ENCODING = 'UTF8'
DEFAULT_URI = 'postgresql://localhost:5432'
class Session(object):
"""The Session class allows for a unified (and simplified) view of
interfacing with a PostgreSQL database server. The Session object can
act as a context manager, providing automated cleanup and simple, Pythonic
way of interacting with the object.
:param str uri: PostgreSQL connection URI
:param psycopg2.extensions.cursor: The cursor type to use
:param int pool_idle_ttl: How long idle pools keep connections open
:param int pool_max_size: The maximum size of the pool to use
"""
_conn = None
_cursor = None
_tpc_id = None
_uri = None
# Connection status constants
INTRANS = extensions.STATUS_IN_TRANSACTION
PREPARED = extensions.STATUS_PREPARED
READY = extensions.STATUS_READY
SETUP = extensions.STATUS_SETUP
# Transaction status constants
TX_ACTIVE = extensions.TRANSACTION_STATUS_ACTIVE
TX_IDLE = extensions.TRANSACTION_STATUS_IDLE
TX_INERROR = extensions.TRANSACTION_STATUS_INERROR
TX_INTRANS = extensions.TRANSACTION_STATUS_INTRANS
TX_UNKNOWN = extensions.TRANSACTION_STATUS_UNKNOWN
def __init__(self, uri=DEFAULT_URI,
cursor_factory=extras.RealDictCursor,
pool_idle_ttl=pool.DEFAULT_IDLE_TTL,
pool_max_size=pool.DEFAULT_MAX_SIZE,
autocommit=True):
"""Connect to a PostgreSQL server using the module wide connection and
set the isolation level.
:param str uri: PostgreSQL connection URI
:param psycopg2.extensions.cursor: The cursor type to use
:param int pool_idle_ttl: How long idle pools keep connections open
:param int pool_max_size: The maximum size of the pool to use
"""
self._pool_manager = pool.PoolManager.instance()
:param int pool_max_size: The maximum size of the pool to use
"""
self._pool_manager = pool.PoolManager()
self._uri = uri
# Ensure the pool exists in the pool manager
self._cursor_factory = cursor_factory
self._cursor = self._get_cursor(self._conn)
self._autocommit(autocommit)
@property
def backend_pid(self):
"""Return the backend process ID of the PostgreSQL server that this
session is connected to.
:rtype: int
"""
return self._conn.get_backend_pid()
def callproc(self, name, args=None):
"""Call a stored procedure on the server, returning the results in a
:py:class:`queries.Results` instance.
:param str name: The procedure name
:param list args: The list of arguments to pass in
:rtype: queries.Results
:raises: queries.DataError
:raises: queries.DatabaseError
:raises: queries.IntegrityError
:raises: queries.InternalError
:raises: queries.InterfaceError
:raises: queries.NotSupportedError
:raises: queries.OperationalError
:raises: queries.ProgrammingError
"""
try:
self._cursor.callproc(name, args)
except psycopg2.Error as err:
self._incr_exceptions()
raise err
finally:
self._incr_executions()
return results.Results(self._cursor)
def close(self):
"""Explicitly close the connection and remove it from the connection
pool if pooling is enabled. If the connection is already closed
:raises: psycopg2.InterfaceError
"""
if not self._conn:
raise psycopg2.InterfaceError('Connection not open')
LOGGER.info('Closing connection %r in %s', self._conn, self.pid)
self._pool_manager.free(self.pid, self._conn)
self._pool_manager.remove_connection(self.pid, self._conn)
# Un-assign the connection and cursor
self._conn, self._cursor = None, None
@property
def connection(self):
"""Return the current open connection to PostgreSQL.
:rtype: psycopg2.extensions.connection
"""
return self._conn
@property
def cursor(self):
"""Return the current, active cursor for the open connection.
:rtype: psycopg2.extensions.cursor
"""
return self._cursor
@property
def encoding(self):
"""Return the current client encoding value.
:rtype: str
"""
return self._conn.encoding
@property
def notices(self):
"""Return a list of up to the last 50 server notices sent to the client.
:rtype: list
"""
return self._conn.notices
@property
def pid(self):
"""Return the pool ID used for connection pooling.
:rtype: str
"""
return hashlib.md5(':'.join([self.__class__.__name__,
self._uri]).encode('utf-8')).hexdigest()
def query(self, sql, parameters=None):
"""A generator to issue a query on the server, mogrifying the
parameters against the sql statement. Results are returned as a
:py:class:`queries.Results` object which can act as an iterator and
has multiple ways to access the result data.
:param str sql: The SQL statement
:param dict parameters: A dictionary of query parameters
:rtype: queries.Results
:raises: queries.DataError
:raises: queries.DatabaseError
:raises: queries.IntegrityError
:raises: queries.InternalError
:raises: queries.InterfaceError
:raises: queries.NotSupportedError
:raises: queries.OperationalError
:raises: queries.ProgrammingError
"""
try:
self._cursor.execute(sql, parameters)
except psycopg2.Error as err:
self._incr_exceptions()
raise err
finally:
self._incr_executions()
return results.Results(self._cursor)
def set_encoding(self, value=DEFAULT_ENCODING):
"""Set the client encoding for the session if the value specified
is different than the current client encoding.
:param str value: The encoding value to use
"""
if self._conn.encoding != value:
self._conn.set_client_encoding(value)
def __del__(self):
"""When deleting the context, ensure the instance is removed from
caches, etc.
"""
self._cleanup()
def __enter__(self):
"""For use as a context manager, return a handle to this object
instance.
:rtype: Session
"""
return self
def __exit__(self, exc_type, exc_val, exc_tb):
"""When leaving the context, ensure the instance is removed from
caches, etc.
"""
self._cleanup()
self._cursor = None
if self._conn:
self._pool_manager.free(self.pid, self._conn)
self._conn = None
self._pool_manager.clean(self.pid)
def _connect(self):
"""Connect to PostgreSQL, either by reusing a connection from the pool
LOGGER.debug('Freeing %s in the pool', self.pid)
try:
pool.PoolManager.instance().free(self.pid, self._conn)
except pool.ConnectionNotFoundError:
pass
self._conn = None
def _connect(self):
"""Connect to PostgreSQL, either by reusing a connection from the pool
if possible, or by creating the new connection.
:rtype: psycopg2.extensions.connection
:raises: pool.NoIdleConnectionsError
"""
# Attempt to get a cached connection from the connection pool
try:
connection = self._pool_manager.get(self.pid, self)
LOGGER.debug("Re-using connection for %s", self.pid)
except pool.NoIdleConnectionsError:
if self._pool_manager.is_full(self.pid):
raise
# Create a new PostgreSQL connection
kwargs = utils.uri_to_kwargs(self._uri)
LOGGER.debug("Creating a new connection for %s", self.pid)
connection = self._psycopg2_connect(kwargs)
self._pool_manager.add(self.pid, connection)
self._pool_manager.lock(self.pid, connection, self)
# Added in because psycopg2ct connects and leaves the connection in
# a weird state: consts.STATUS_DATESTYLE, returning from
# Connection._setup without setting the state as const.STATUS_OK
if utils.PYPY:
connection.reset()
# Register the custom data types
self._register_unicode(connection)
self._register_uuid(connection)
return connection
def _get_cursor(self, connection, name=None):
"""Return a cursor for the given cursor_factory. Specify a name to
use server-side cursors.
:param connection: The connection to create a cursor on
:type connection: psycopg2.extensions.connection
:param str name: A cursor name for a server side cursor
:rtype: psycopg2.extensions.cursor
"""
cursor = connection.cursor(name=name,
cursor_factory=self._cursor_factory)
if name is not None:
cursor.scrollable = True
cursor.withhold = True
return cursor
def _incr_exceptions(self):
"""Increment the number of exceptions for the current connection."""
self._pool_manager.get_connection(self.pid, self._conn).exceptions += 1
def _incr_executions(self):
"""Increment the number of executions for the current connection."""
self._pool_manager.get_connection(self.pid, self._conn).executions += 1
def _psycopg2_connect(self, kwargs):
"""Return a psycopg2 connection for the specified kwargs. Extend for
use in async session adapters.
:param dict kwargs: Keyword connection args
:rtype: psycopg2.extensions.connection
"""
return psycopg2.connect(**kwargs)
@staticmethod
def _register_unicode(connection):
"""Register the cursor to be able to receive Unicode string.
:type connection: psycopg2.extensions.connection
:param connection: Where to register things
"""
psycopg2.extensions.register_type(psycopg2.extensions.UNICODE,
connection)
psycopg2.extensions.register_type(psycopg2.extensions.UNICODEARRAY,
connection)
@staticmethod
def _register_uuid(connection):
"""Register the UUID extension from the psycopg2.extra module
:type connection: psycopg2.extensions.connection
:param connection: Where to register things
"""
psycopg2.extras.register_uuid(conn_or_curs=connection)
@property
def _status(self):
"""Return the current connection status as an integer value.
The status should match one of the following constants:
- queries.Session.INTRANS: Connection established, in transaction
- queries.Session.PREPARED: Prepared for second phase of transaction
- queries.Session.READY: Connected, no active transaction
:rtype: int
"""
if self._conn.status == psycopg2.extensions.STATUS_BEGIN:
return self.READY
return self._conn.status
<MSG> Use the singleton poolmanager instance, handle exceptions in cleanup
<DFF> @@ -85,7 +85,7 @@ class Session(object):
:param int pool_max_size: The maximum size of the pool to use
"""
- self._pool_manager = pool.PoolManager()
+ self._pool_manager = pool.PoolManager.instance()
self._uri = uri
# Ensure the pool exists in the pool manager
@@ -254,10 +254,16 @@ class Session(object):
self._cursor = None
if self._conn:
- self._pool_manager.free(self.pid, self._conn)
+ try:
+ self._pool_manager.free(self.pid, self._conn)
+ except (pool.ConnectionNotFoundError, KeyError):
+ pass
self._conn = None
- self._pool_manager.clean(self.pid)
+ try:
+ self._pool_manager.clean(self.pid)
+ except KeyError:
+ pass
def _connect(self):
"""Connect to PostgreSQL, either by reusing a connection from the pool
| 9 | Use the singleton poolmanager instance, handle exceptions in cleanup | 3 | .py | py | bsd-3-clause | gmr/queries |
1075 | <NME> .travis.yml
<BEF> language: python
python:
- 2.6
- 2.7
- pypy
- 3.2
- 3.3
- 3.4
addons:
postgresql: '9.3'
install:
- if [[ $TRAVIS_PYTHON_VERSION == '2.6' ]]; then pip install unittest2; fi
- if [[ $TRAVIS_PYTHON_VERSION != 'pypy' ]]; then pip install -r requirements.pip;
fi
- if [[ $TRAVIS_PYTHON_VERSION == 'pypy' ]]; then pip install -r requirements.pypy;
fi
script: nosetests --with-coverage --cover-package=queries --verbose
after_success:
- coveralls
deploy:
distributions: sdist
provider: pypi
script: nosetests
after_success:
- aws s3 cp .coverage "s3://com-gavinroy-travis/queries/$TRAVIS_BUILD_NUMBER/.coverage.${TRAVIS_PYTHON_VERSION}"
jobs:
include:
- python: 2.7
- python: 3.4
- python: 3.5
- python: 3.6
- python: 3.7
- python: 3.8
- stage: coverage
if: repo = gmr/queries
services: []
python: 3.7
install:
- pip install awscli coverage codecov
script:
- mkdir coverage
- aws s3 cp --recursive s3://com-gavinroy-travis/queries/$TRAVIS_BUILD_NUMBER/
coverage
- cd coverage
- coverage combine
- cd ..
- mv coverage/.coverage .
- coverage report
after_success: codecov
- stage: deploy
if: repo = gmr/queries
python: 3.6
services: []
install: true
script: true
after_success: true
deploy:
distributions: sdist bdist_wheel
provider: pypi
user: crad
on:
tags: true
all_branches: true
password:
secure: UWQWui+QhAL1cz6oW/vqjEEp6/EPn1YOlItNJcWHNOO/WMMOlaTVYVUuXp+y+m52B+8PtYZZCTHwKCUKe97Grh291FLxgd0RJCawA40f4v1gmOFYLNKyZFBGfbC69/amxvGCcDvOPtpChHAlTIeokS5EQneVcAhXg2jXct0HTfI=
<MSG> Move to codecov and add pip caching
<DFF> @@ -1,22 +1,24 @@
+cache:
+ directories:
+ - $HOME/.pip-cache/
language: python
python:
-- 2.6
-- 2.7
-- pypy
-- 3.2
-- 3.3
-- 3.4
+ - 2.6
+ - 2.7
+ - pypy
+ - 3.2
+ - 3.3
+ - 3.4
addons:
postgresql: '9.3'
install:
-- if [[ $TRAVIS_PYTHON_VERSION == '2.6' ]]; then pip install unittest2; fi
-- if [[ $TRAVIS_PYTHON_VERSION != 'pypy' ]]; then pip install -r requirements.pip;
- fi
-- if [[ $TRAVIS_PYTHON_VERSION == 'pypy' ]]; then pip install -r requirements.pypy;
- fi
+ - if [[ $TRAVIS_PYTHON_VERSION == '2.6' ]]; then pip install unittest2 --download-cache $HOME/.pip-cache; fi
+ - if [[ $TRAVIS_PYTHON_VERSION != 'pypy' ]]; then pip install -r requirements.pip --download-cache $HOME/.pip-cache; fi
+ - if [[ $TRAVIS_PYTHON_VERSION == 'pypy' ]]; then pip install -r requirements.pypy --download-cache $HOME/.pip-cache; fi
+ - pip install codecov --download-cache $HOME/.pip-cache
script: nosetests --with-coverage --cover-package=queries --verbose
after_success:
-- coveralls
+ - codecov
deploy:
distributions: sdist
provider: pypi
| 14 | Move to codecov and add pip caching | 12 | .yml | travis | bsd-3-clause | gmr/queries |
1076 | <NME> .travis.yml
<BEF> language: python
python:
- 2.6
- 2.7
- pypy
- 3.2
- 3.3
- 3.4
addons:
postgresql: '9.3'
install:
- if [[ $TRAVIS_PYTHON_VERSION == '2.6' ]]; then pip install unittest2; fi
- if [[ $TRAVIS_PYTHON_VERSION != 'pypy' ]]; then pip install -r requirements.pip;
fi
- if [[ $TRAVIS_PYTHON_VERSION == 'pypy' ]]; then pip install -r requirements.pypy;
fi
script: nosetests --with-coverage --cover-package=queries --verbose
after_success:
- coveralls
deploy:
distributions: sdist
provider: pypi
script: nosetests
after_success:
- aws s3 cp .coverage "s3://com-gavinroy-travis/queries/$TRAVIS_BUILD_NUMBER/.coverage.${TRAVIS_PYTHON_VERSION}"
jobs:
include:
- python: 2.7
- python: 3.4
- python: 3.5
- python: 3.6
- python: 3.7
- python: 3.8
- stage: coverage
if: repo = gmr/queries
services: []
python: 3.7
install:
- pip install awscli coverage codecov
script:
- mkdir coverage
- aws s3 cp --recursive s3://com-gavinroy-travis/queries/$TRAVIS_BUILD_NUMBER/
coverage
- cd coverage
- coverage combine
- cd ..
- mv coverage/.coverage .
- coverage report
after_success: codecov
- stage: deploy
if: repo = gmr/queries
python: 3.6
services: []
install: true
script: true
after_success: true
deploy:
distributions: sdist bdist_wheel
provider: pypi
user: crad
on:
tags: true
all_branches: true
password:
secure: UWQWui+QhAL1cz6oW/vqjEEp6/EPn1YOlItNJcWHNOO/WMMOlaTVYVUuXp+y+m52B+8PtYZZCTHwKCUKe97Grh291FLxgd0RJCawA40f4v1gmOFYLNKyZFBGfbC69/amxvGCcDvOPtpChHAlTIeokS5EQneVcAhXg2jXct0HTfI=
<MSG> Move to codecov and add pip caching
<DFF> @@ -1,22 +1,24 @@
+cache:
+ directories:
+ - $HOME/.pip-cache/
language: python
python:
-- 2.6
-- 2.7
-- pypy
-- 3.2
-- 3.3
-- 3.4
+ - 2.6
+ - 2.7
+ - pypy
+ - 3.2
+ - 3.3
+ - 3.4
addons:
postgresql: '9.3'
install:
-- if [[ $TRAVIS_PYTHON_VERSION == '2.6' ]]; then pip install unittest2; fi
-- if [[ $TRAVIS_PYTHON_VERSION != 'pypy' ]]; then pip install -r requirements.pip;
- fi
-- if [[ $TRAVIS_PYTHON_VERSION == 'pypy' ]]; then pip install -r requirements.pypy;
- fi
+ - if [[ $TRAVIS_PYTHON_VERSION == '2.6' ]]; then pip install unittest2 --download-cache $HOME/.pip-cache; fi
+ - if [[ $TRAVIS_PYTHON_VERSION != 'pypy' ]]; then pip install -r requirements.pip --download-cache $HOME/.pip-cache; fi
+ - if [[ $TRAVIS_PYTHON_VERSION == 'pypy' ]]; then pip install -r requirements.pypy --download-cache $HOME/.pip-cache; fi
+ - pip install codecov --download-cache $HOME/.pip-cache
script: nosetests --with-coverage --cover-package=queries --verbose
after_success:
-- coveralls
+ - codecov
deploy:
distributions: sdist
provider: pypi
| 14 | Move to codecov and add pip caching | 12 | .yml | travis | bsd-3-clause | gmr/queries |
1077 | <NME> test-requirements.txt
<BEF> ADDFILE
<MSG> Clean up testing related things
<DFF> @@ -0,0 +1,4 @@
+coverage
+mock
+nose
+codecov
| 4 | Clean up testing related things | 0 | .txt | txt | bsd-3-clause | gmr/queries |
1078 | <NME> test-requirements.txt
<BEF> ADDFILE
<MSG> Clean up testing related things
<DFF> @@ -0,0 +1,4 @@
+coverage
+mock
+nose
+codecov
| 4 | Clean up testing related things | 0 | .txt | txt | bsd-3-clause | gmr/queries |
1079 | <NME> tornado_basic.rst
<BEF> Basic Tornado Usage
===================
The following example implements a very basic RESTful API. The following DDL will
create the table used by the API:
.. code:: sql
CREATE TABLE widgets (sku varchar(10) NOT NULL PRIMARY KEY,
name text NOT NULL,
qty integer NOT NULL);
The Tornado application provides two endpoints: /widget(/sku-value) and /widgets.
SKUs are set to be a 10 character value with the regex of ``[a-z0-9]{10}``. To
add a widget, call PUT on /widget, to update a widget call POST on /widget/[SKU].
.. code:: python
from tornado import gen, ioloop, web
import queries
class WidgetRequestHandler(web.RequestHandler):
"""Handle the CRUD methods for a widget"""
def initialize(self):
self.session = queries.TornadoSession()
def options(self, *args, **kwargs):
self.set_header('Allow', ', '.join(['DELETE', 'GET', 'POST', 'PUT']))
self.set_status(204)
self.finish()
@gen.coroutine
def delete(self, *args, **kwargs):
if 'sku' not in kwargs:
self.set_status(403)
self.finish({'error': 'missing required value: sku'})
else:
rows, data = yield self.session.query("DELETE FROM widgets WHERE sku=%(sku)s",
{'sku': kwargs['sku']})
if not results:
self.set_status(404)
self.finish({'error': 'SKU not found in system'})
else:
self.set_status(204)
self.finish()
@gen.coroutine
def get(self, *args, **kwargs):
if 'sku' not in kwargs:
self.set_status(403)
self.finish({'error': 'missing required value: sku'})
rows, data = yield self.session.query("SELECT * FROM widgets WHERE sku=%(sku)s",
{'sku': kwargs['sku']})
if not data:
self.set_status(404)
self.finish({'error': 'SKU not found in system'})
else:
self.finish(data[0])
@gen.coroutine
def post(self, *args, **kwargs):
if 'sku' not in kwargs:
self.set_status(403)
self.finish({'error': 'missing required value: sku'})
try:
rows, result = yield self.session.query("UPDATE widgets SET name=%(name)s, qty=%(qty)s WHERE sku=%(sku)s",
{'sku': kwargs['sku'],
'name': self.get_argument('name'),
'qty': self.get_argument('qty')})
except queries.DataError as error:
self.set_status(404)
self.finish({'error': {'error': error.pgerror.split('\n')[0][8:]}})
else:
if not rows:
self.set_status(404)
self.finish({'error': 'SKU not found in system'})
else:
self.set_status(204)
self.finish()
@gen.coroutine
def put(self, *args, **kwargs):
try:
yield self.session.query("INSERT INTO widgets VALUES (%s, %s, %s)",
[self.get_argument('sku'),
"""Add a widget to the database
:param list args: URI path arguments passed in by Tornado
:param list args: URI path keyword arguments passed in by Tornado
"""
try:
results = yield self.session.query("INSERT INTO widgets VALUES (%s, %s, %s)",
[self.get_argument('sku'),
class WidgetsRequestHandler(web.RequestHandler):
def initialize(self):
self.session = queries.TornadoSession()
def options(self, *args, **kwargs):
self.set_header('Allow', ', '.join(['GET']))
self.set_status(204)
self.finish()
@gen.coroutine
def get(self, *args, **kwargs):
rows, data = yield self.session.query("SELECT * FROM widgets ORDER BY sku")
self.finish({'widgets': data})
"""Get a list of all the widgets from the database
:param list args: URI path arguments passed in by Tornado
:param list args: URI path keyword arguments passed in by Tornado
"""
results = yield self.session.query('SELECT * FROM widgets ORDER BY sku')
# Tornado doesn't allow you to return a list as a JSON result by default
self.finish({'widgets': results.items()})
# Free the results and release the connection lock from session.query
results.free()
if __name__ == "__main__":
application = web.Application([
(r"/widget", WidgetRequestHandler),
(r"/widget/(?P<sku>[a-zA-Z0-9]{10})", WidgetRequestHandler),
(r"/widgets", WidgetsRequestHandler)
]).listen(8888)
ioloop.IOLoop.instance().start()
<MSG> Update the Basic Tornado example
[ci skip]
<DFF> @@ -1,5 +1,5 @@
-Basic Tornado Usage
-===================
+Basic TornadoSession Usage
+==========================
The following example implements a very basic RESTful API. The following DDL will
create the table used by the API:
@@ -23,18 +23,37 @@ add a widget, call PUT on /widget, to update a widget call POST on /widget/[SKU]
"""Handle the CRUD methods for a widget"""
def initialize(self):
+ """Setup a queries.TornadoSession object to use when the RequestHandler
+ is first initialized.
+
+ """
self.session = queries.TornadoSession()
def options(self, *args, **kwargs):
+ """Let the caller know what methods are supported
+
+ :param list args: URI path arguments passed in by Tornado
+ :param list args: URI path keyword arguments passed in by Tornado
+
+ """
self.set_header('Allow', ', '.join(['DELETE', 'GET', 'POST', 'PUT']))
- self.set_status(204)
+ self.set_status(204) # Successful request, but no data returned
self.finish()
@gen.coroutine
def delete(self, *args, **kwargs):
+ """Delete a widget from the database
+
+ :param list args: URI path arguments passed in by Tornado
+ :param list args: URI path keyword arguments passed in by Tornado
+
+ """
+ # We need a SKU, if it wasn't passed in the URL, return an error
if 'sku' not in kwargs:
self.set_status(403)
self.finish({'error': 'missing required value: sku'})
+
+ # Delete the widget from the database by SKU
else:
rows, data = yield self.session.query("DELETE FROM widgets WHERE sku=%(sku)s",
{'sku': kwargs['sku']})
@@ -42,45 +61,83 @@ add a widget, call PUT on /widget, to update a widget call POST on /widget/[SKU]
self.set_status(404)
self.finish({'error': 'SKU not found in system'})
else:
- self.set_status(204)
+ self.set_status(204) # Success, but no data returned
self.finish()
@gen.coroutine
def get(self, *args, **kwargs):
+ """Fetch a widget from the database
+
+ :param list args: URI path arguments passed in by Tornado
+ :param list args: URI path keyword arguments passed in by Tornado
+
+ """
+ # We need a SKU, if it wasn't passed in the URL, return an error
if 'sku' not in kwargs:
self.set_status(403)
self.finish({'error': 'missing required value: sku'})
- rows, data = yield self.session.query("SELECT * FROM widgets WHERE sku=%(sku)s",
- {'sku': kwargs['sku']})
- if not data:
- self.set_status(404)
- self.finish({'error': 'SKU not found in system'})
+
+ # Fetch a row from the database for the SKU
else:
- self.finish(data[0])
+ rows, data = yield self.session.query("SELECT * FROM widgets WHERE sku=%(sku)s",
+ {'sku': kwargs['sku']})
+
+ # No rows returned, send a 404 with a JSON error payload
+ if not rows:
+ self.set_status(404)
+ self.finish({'error': 'SKU not found in system'})
+
+ # Send back the row as a JSON object
+ else:
+ self.finish(data[0])
@gen.coroutine
def post(self, *args, **kwargs):
+ """Update a widget in the database
+
+ :param list args: URI path arguments passed in by Tornado
+ :param list args: URI path keyword arguments passed in by Tornado
+
+ """
+ # We need a SKU, if it wasn't passed in the URL, return an error
if 'sku' not in kwargs:
self.set_status(403)
self.finish({'error': 'missing required value: sku'})
- try:
- rows, result = yield self.session.query("UPDATE widgets SET name=%(name)s, qty=%(qty)s WHERE sku=%(sku)s",
- {'sku': kwargs['sku'],
- 'name': self.get_argument('name'),
- 'qty': self.get_argument('qty')})
- except queries.DataError as error:
- self.set_status(404)
- self.finish({'error': {'error': error.pgerror.split('\n')[0][8:]}})
+
+ # Update the widget in the database by SKU
else:
- if not rows:
- self.set_status(404)
- self.finish({'error': 'SKU not found in system'})
+
+ sql = "UPDATE widgets SET name=%(name)s, qty=%(qty)s WHERE sku=%(sku)s"
+ try:
+ rows, result = yield self.session.query(sql,
+ {'sku': kwargs['sku'],
+ 'name': self.get_argument('name'),
+ 'qty': self.get_argument('qty')})
+
+ # DataError is raised when there's a problem with the data passed in
+ except queries.DataError as error:
+ self.set_status(409)
+ self.finish({'error': {'error': error.pgerror.split('\n')[0][8:]}})
+
else:
- self.set_status(204)
- self.finish()
+ # No rows means there was no record updated
+ if not rows:
+ self.set_status(404)
+ self.finish({'error': 'SKU not found in system'})
+
+ # The record was updated
+ else:
+ self.set_status(204) # Success, but not returning data
+ self.finish()
@gen.coroutine
def put(self, *args, **kwargs):
+ """Add a widget to the database
+
+ :param list args: URI path arguments passed in by Tornado
+ :param list args: URI path keyword arguments passed in by Tornado
+
+ """
try:
yield self.session.query("INSERT INTO widgets VALUES (%s, %s, %s)",
[self.get_argument('sku'),
@@ -96,18 +153,37 @@ add a widget, call PUT on /widget, to update a widget call POST on /widget/[SKU]
class WidgetsRequestHandler(web.RequestHandler):
+ """Return a list of all of the widgets in the database"""
def initialize(self):
+ """Setup a queries.TornadoSession object to use when the RequestHandler
+ is first initialized.
+
+ """
self.session = queries.TornadoSession()
def options(self, *args, **kwargs):
+ """Let the caller know what methods are supported
+
+ :param list args: URI path arguments passed in by Tornado
+ :param list args: URI path keyword arguments passed in by Tornado
+
+ """
self.set_header('Allow', ', '.join(['GET']))
self.set_status(204)
self.finish()
@gen.coroutine
def get(self, *args, **kwargs):
+ """Get a list of all the widgets from the database
+
+ :param list args: URI path arguments passed in by Tornado
+ :param list args: URI path keyword arguments passed in by Tornado
+
+ """
rows, data = yield self.session.query("SELECT * FROM widgets ORDER BY sku")
+
+ # Tornado doesn't allow you to return a list as a JSON result by default
self.finish({'widgets': data})
| 99 | Update the Basic Tornado example | 23 | .rst | rst | bsd-3-clause | gmr/queries |
1080 | <NME> tornado_basic.rst
<BEF> Basic Tornado Usage
===================
The following example implements a very basic RESTful API. The following DDL will
create the table used by the API:
.. code:: sql
CREATE TABLE widgets (sku varchar(10) NOT NULL PRIMARY KEY,
name text NOT NULL,
qty integer NOT NULL);
The Tornado application provides two endpoints: /widget(/sku-value) and /widgets.
SKUs are set to be a 10 character value with the regex of ``[a-z0-9]{10}``. To
add a widget, call PUT on /widget, to update a widget call POST on /widget/[SKU].
.. code:: python
from tornado import gen, ioloop, web
import queries
class WidgetRequestHandler(web.RequestHandler):
"""Handle the CRUD methods for a widget"""
def initialize(self):
self.session = queries.TornadoSession()
def options(self, *args, **kwargs):
self.set_header('Allow', ', '.join(['DELETE', 'GET', 'POST', 'PUT']))
self.set_status(204)
self.finish()
@gen.coroutine
def delete(self, *args, **kwargs):
if 'sku' not in kwargs:
self.set_status(403)
self.finish({'error': 'missing required value: sku'})
else:
rows, data = yield self.session.query("DELETE FROM widgets WHERE sku=%(sku)s",
{'sku': kwargs['sku']})
if not results:
self.set_status(404)
self.finish({'error': 'SKU not found in system'})
else:
self.set_status(204)
self.finish()
@gen.coroutine
def get(self, *args, **kwargs):
if 'sku' not in kwargs:
self.set_status(403)
self.finish({'error': 'missing required value: sku'})
rows, data = yield self.session.query("SELECT * FROM widgets WHERE sku=%(sku)s",
{'sku': kwargs['sku']})
if not data:
self.set_status(404)
self.finish({'error': 'SKU not found in system'})
else:
self.finish(data[0])
@gen.coroutine
def post(self, *args, **kwargs):
if 'sku' not in kwargs:
self.set_status(403)
self.finish({'error': 'missing required value: sku'})
try:
rows, result = yield self.session.query("UPDATE widgets SET name=%(name)s, qty=%(qty)s WHERE sku=%(sku)s",
{'sku': kwargs['sku'],
'name': self.get_argument('name'),
'qty': self.get_argument('qty')})
except queries.DataError as error:
self.set_status(404)
self.finish({'error': {'error': error.pgerror.split('\n')[0][8:]}})
else:
if not rows:
self.set_status(404)
self.finish({'error': 'SKU not found in system'})
else:
self.set_status(204)
self.finish()
@gen.coroutine
def put(self, *args, **kwargs):
try:
yield self.session.query("INSERT INTO widgets VALUES (%s, %s, %s)",
[self.get_argument('sku'),
"""Add a widget to the database
:param list args: URI path arguments passed in by Tornado
:param list args: URI path keyword arguments passed in by Tornado
"""
try:
results = yield self.session.query("INSERT INTO widgets VALUES (%s, %s, %s)",
[self.get_argument('sku'),
class WidgetsRequestHandler(web.RequestHandler):
def initialize(self):
self.session = queries.TornadoSession()
def options(self, *args, **kwargs):
self.set_header('Allow', ', '.join(['GET']))
self.set_status(204)
self.finish()
@gen.coroutine
def get(self, *args, **kwargs):
rows, data = yield self.session.query("SELECT * FROM widgets ORDER BY sku")
self.finish({'widgets': data})
"""Get a list of all the widgets from the database
:param list args: URI path arguments passed in by Tornado
:param list args: URI path keyword arguments passed in by Tornado
"""
results = yield self.session.query('SELECT * FROM widgets ORDER BY sku')
# Tornado doesn't allow you to return a list as a JSON result by default
self.finish({'widgets': results.items()})
# Free the results and release the connection lock from session.query
results.free()
if __name__ == "__main__":
application = web.Application([
(r"/widget", WidgetRequestHandler),
(r"/widget/(?P<sku>[a-zA-Z0-9]{10})", WidgetRequestHandler),
(r"/widgets", WidgetsRequestHandler)
]).listen(8888)
ioloop.IOLoop.instance().start()
<MSG> Update the Basic Tornado example
[ci skip]
<DFF> @@ -1,5 +1,5 @@
-Basic Tornado Usage
-===================
+Basic TornadoSession Usage
+==========================
The following example implements a very basic RESTful API. The following DDL will
create the table used by the API:
@@ -23,18 +23,37 @@ add a widget, call PUT on /widget, to update a widget call POST on /widget/[SKU]
"""Handle the CRUD methods for a widget"""
def initialize(self):
+ """Setup a queries.TornadoSession object to use when the RequestHandler
+ is first initialized.
+
+ """
self.session = queries.TornadoSession()
def options(self, *args, **kwargs):
+ """Let the caller know what methods are supported
+
+ :param list args: URI path arguments passed in by Tornado
+ :param list args: URI path keyword arguments passed in by Tornado
+
+ """
self.set_header('Allow', ', '.join(['DELETE', 'GET', 'POST', 'PUT']))
- self.set_status(204)
+ self.set_status(204) # Successful request, but no data returned
self.finish()
@gen.coroutine
def delete(self, *args, **kwargs):
+ """Delete a widget from the database
+
+ :param list args: URI path arguments passed in by Tornado
+ :param list args: URI path keyword arguments passed in by Tornado
+
+ """
+ # We need a SKU, if it wasn't passed in the URL, return an error
if 'sku' not in kwargs:
self.set_status(403)
self.finish({'error': 'missing required value: sku'})
+
+ # Delete the widget from the database by SKU
else:
rows, data = yield self.session.query("DELETE FROM widgets WHERE sku=%(sku)s",
{'sku': kwargs['sku']})
@@ -42,45 +61,83 @@ add a widget, call PUT on /widget, to update a widget call POST on /widget/[SKU]
self.set_status(404)
self.finish({'error': 'SKU not found in system'})
else:
- self.set_status(204)
+ self.set_status(204) # Success, but no data returned
self.finish()
@gen.coroutine
def get(self, *args, **kwargs):
+ """Fetch a widget from the database
+
+ :param list args: URI path arguments passed in by Tornado
+ :param list args: URI path keyword arguments passed in by Tornado
+
+ """
+ # We need a SKU, if it wasn't passed in the URL, return an error
if 'sku' not in kwargs:
self.set_status(403)
self.finish({'error': 'missing required value: sku'})
- rows, data = yield self.session.query("SELECT * FROM widgets WHERE sku=%(sku)s",
- {'sku': kwargs['sku']})
- if not data:
- self.set_status(404)
- self.finish({'error': 'SKU not found in system'})
+
+ # Fetch a row from the database for the SKU
else:
- self.finish(data[0])
+ rows, data = yield self.session.query("SELECT * FROM widgets WHERE sku=%(sku)s",
+ {'sku': kwargs['sku']})
+
+ # No rows returned, send a 404 with a JSON error payload
+ if not rows:
+ self.set_status(404)
+ self.finish({'error': 'SKU not found in system'})
+
+ # Send back the row as a JSON object
+ else:
+ self.finish(data[0])
@gen.coroutine
def post(self, *args, **kwargs):
+ """Update a widget in the database
+
+ :param list args: URI path arguments passed in by Tornado
+ :param list args: URI path keyword arguments passed in by Tornado
+
+ """
+ # We need a SKU, if it wasn't passed in the URL, return an error
if 'sku' not in kwargs:
self.set_status(403)
self.finish({'error': 'missing required value: sku'})
- try:
- rows, result = yield self.session.query("UPDATE widgets SET name=%(name)s, qty=%(qty)s WHERE sku=%(sku)s",
- {'sku': kwargs['sku'],
- 'name': self.get_argument('name'),
- 'qty': self.get_argument('qty')})
- except queries.DataError as error:
- self.set_status(404)
- self.finish({'error': {'error': error.pgerror.split('\n')[0][8:]}})
+
+ # Update the widget in the database by SKU
else:
- if not rows:
- self.set_status(404)
- self.finish({'error': 'SKU not found in system'})
+
+ sql = "UPDATE widgets SET name=%(name)s, qty=%(qty)s WHERE sku=%(sku)s"
+ try:
+ rows, result = yield self.session.query(sql,
+ {'sku': kwargs['sku'],
+ 'name': self.get_argument('name'),
+ 'qty': self.get_argument('qty')})
+
+ # DataError is raised when there's a problem with the data passed in
+ except queries.DataError as error:
+ self.set_status(409)
+ self.finish({'error': {'error': error.pgerror.split('\n')[0][8:]}})
+
else:
- self.set_status(204)
- self.finish()
+ # No rows means there was no record updated
+ if not rows:
+ self.set_status(404)
+ self.finish({'error': 'SKU not found in system'})
+
+ # The record was updated
+ else:
+ self.set_status(204) # Success, but not returning data
+ self.finish()
@gen.coroutine
def put(self, *args, **kwargs):
+ """Add a widget to the database
+
+ :param list args: URI path arguments passed in by Tornado
+ :param list args: URI path keyword arguments passed in by Tornado
+
+ """
try:
yield self.session.query("INSERT INTO widgets VALUES (%s, %s, %s)",
[self.get_argument('sku'),
@@ -96,18 +153,37 @@ add a widget, call PUT on /widget, to update a widget call POST on /widget/[SKU]
class WidgetsRequestHandler(web.RequestHandler):
+ """Return a list of all of the widgets in the database"""
def initialize(self):
+ """Setup a queries.TornadoSession object to use when the RequestHandler
+ is first initialized.
+
+ """
self.session = queries.TornadoSession()
def options(self, *args, **kwargs):
+ """Let the caller know what methods are supported
+
+ :param list args: URI path arguments passed in by Tornado
+ :param list args: URI path keyword arguments passed in by Tornado
+
+ """
self.set_header('Allow', ', '.join(['GET']))
self.set_status(204)
self.finish()
@gen.coroutine
def get(self, *args, **kwargs):
+ """Get a list of all the widgets from the database
+
+ :param list args: URI path arguments passed in by Tornado
+ :param list args: URI path keyword arguments passed in by Tornado
+
+ """
rows, data = yield self.session.query("SELECT * FROM widgets ORDER BY sku")
+
+ # Tornado doesn't allow you to return a list as a JSON result by default
self.finish({'widgets': data})
| 99 | Update the Basic Tornado example | 23 | .rst | rst | bsd-3-clause | gmr/queries |
1081 | <NME> .travis.yml
<BEF> sudo: false
language: python
python:
- 2.7
- 3.4
- 3.5
- 3.6
- pypy
- pypy3
env:
global:
- PATH=$HOME/.local/bin:$PATH
- AWS_DEFAULT_REGION=us-east-1
- secure: "inURdx4ldkJqQXL1TyvKImC3EnL5TixC1DlNMBYi5ttygwAk+mSSSw8Yc7klB6D1m6q79xUlHRk06vbz23CsXTM4AClC5Emrk6XN2GlUKl5WI+z+A2skI59buEhLWe7e2KzhB/AVx2E3TfKa0oY7raM0UUnaOkpV1Cj+mHKPIT0="
- secure: "H32DV3713a6UUuEJujrG7SfUX4/5WrwQy/3DxeptC6L7YPlTYxHBdEsccTfN5z806EheIl4BdIoxoDtq7PU/tWQoG1Lp2ze60mpwrniHajhFnjk7zP6pHvkhGLr8flhSmAb6CQBreNFOHTLWBMGPfi7k1Q9Td9MHbRo/FsTxqsM="
install:
- pip install awscli
- pip install -r requires/testing.txt
- python setup.py develop
stages:
- test
- name: upload_coverage
if: branch = master
- name: deploy
if: tag IS present
script: nosetests
after_success:
jobs:
include:
- stage: test
services:
- postgres
script: nosetests
- stage: upload coverage
python: 3.6
install:
- pip install awscli coverage codecov
install:
- pip install awscli coverage codecov
script:
- mkdir coverage
- aws s3 cp --recursive s3://com-gavinroy-travis/queries/$TRAVIS_BUILD_NUMBER/
coverage
- cd coverage
- coverage combine
- cd ..
after_success: codecov
- stage: deploy
python: 3.6
deploy:
distributions: sdist bdist_wheel
provider: pypi
deploy:
distributions: sdist bdist_wheel
provider: pypi
user: crad
on:
tags: true
all_branches: true
password:
secure: UWQWui+QhAL1cz6oW/vqjEEp6/EPn1YOlItNJcWHNOO/WMMOlaTVYVUuXp+y+m52B+8PtYZZCTHwKCUKe97Grh291FLxgd0RJCawA40f4v1gmOFYLNKyZFBGfbC69/amxvGCcDvOPtpChHAlTIeokS5EQneVcAhXg2jXct0HTfI=
<MSG> Update to reflect findings for stages in travis
<DFF> @@ -1,31 +1,26 @@
sudo: false
language: python
-python:
- - 2.7
- - 3.4
- - 3.5
- - 3.6
- - pypy
- - pypy3
env:
global:
- - PATH=$HOME/.local/bin:$PATH
- - AWS_DEFAULT_REGION=us-east-1
- - secure: "inURdx4ldkJqQXL1TyvKImC3EnL5TixC1DlNMBYi5ttygwAk+mSSSw8Yc7klB6D1m6q79xUlHRk06vbz23CsXTM4AClC5Emrk6XN2GlUKl5WI+z+A2skI59buEhLWe7e2KzhB/AVx2E3TfKa0oY7raM0UUnaOkpV1Cj+mHKPIT0="
- - secure: "H32DV3713a6UUuEJujrG7SfUX4/5WrwQy/3DxeptC6L7YPlTYxHBdEsccTfN5z806EheIl4BdIoxoDtq7PU/tWQoG1Lp2ze60mpwrniHajhFnjk7zP6pHvkhGLr8flhSmAb6CQBreNFOHTLWBMGPfi7k1Q9Td9MHbRo/FsTxqsM="
-
-install:
- - pip install awscli
- - pip install -r requires/testing.txt
- - python setup.py develop
+ - PATH=$HOME/.local/bin:$PATH
+ - AWS_DEFAULT_REGION=us-east-1
+ - secure: "inURdx4ldkJqQXL1TyvKImC3EnL5TixC1DlNMBYi5ttygwAk+mSSSw8Yc7klB6D1m6q79xUlHRk06vbz23CsXTM4AClC5Emrk6XN2GlUKl5WI+z+A2skI59buEhLWe7e2KzhB/AVx2E3TfKa0oY7raM0UUnaOkpV1Cj+mHKPIT0="
+ - secure: "H32DV3713a6UUuEJujrG7SfUX4/5WrwQy/3DxeptC6L7YPlTYxHBdEsccTfN5z806EheIl4BdIoxoDtq7PU/tWQoG1Lp2ze60mpwrniHajhFnjk7zP6pHvkhGLr8flhSmAb6CQBreNFOHTLWBMGPfi7k1Q9Td9MHbRo/FsTxqsM="
stages:
- - test
- - name: upload_coverage
- if: branch = master
- - name: deploy
- if: tag IS present
+- test
+- name: upload coverage
+- name: deploy
+ if: tag IS present
+
+services:
+- postgres
+
+install:
+- pip install awscli
+- pip install -r requires/testing.txt
+- python setup.py develop
script: nosetests
@@ -34,11 +29,14 @@ after_success:
jobs:
include:
- - stage: test
- services:
- - postgres
- script: nosetests
+ - python: 2.7
+ - python: 3.4
+ - python: 3.5
+ - python: 3.6
+ - python: pypy
+ - python: pypy3
- stage: upload coverage
+ services: []
python: 3.6
install:
- pip install awscli coverage codecov
@@ -54,6 +52,10 @@ jobs:
after_success: codecov
- stage: deploy
python: 3.6
+ services: []
+ install: true
+ script: true
+ after_success: true
deploy:
distributions: sdist bdist_wheel
provider: pypi
| 27 | Update to reflect findings for stages in travis | 25 | .yml | travis | bsd-3-clause | gmr/queries |
1082 | <NME> .travis.yml
<BEF> sudo: false
language: python
python:
- 2.7
- 3.4
- 3.5
- 3.6
- pypy
- pypy3
env:
global:
- PATH=$HOME/.local/bin:$PATH
- AWS_DEFAULT_REGION=us-east-1
- secure: "inURdx4ldkJqQXL1TyvKImC3EnL5TixC1DlNMBYi5ttygwAk+mSSSw8Yc7klB6D1m6q79xUlHRk06vbz23CsXTM4AClC5Emrk6XN2GlUKl5WI+z+A2skI59buEhLWe7e2KzhB/AVx2E3TfKa0oY7raM0UUnaOkpV1Cj+mHKPIT0="
- secure: "H32DV3713a6UUuEJujrG7SfUX4/5WrwQy/3DxeptC6L7YPlTYxHBdEsccTfN5z806EheIl4BdIoxoDtq7PU/tWQoG1Lp2ze60mpwrniHajhFnjk7zP6pHvkhGLr8flhSmAb6CQBreNFOHTLWBMGPfi7k1Q9Td9MHbRo/FsTxqsM="
install:
- pip install awscli
- pip install -r requires/testing.txt
- python setup.py develop
stages:
- test
- name: upload_coverage
if: branch = master
- name: deploy
if: tag IS present
script: nosetests
after_success:
jobs:
include:
- stage: test
services:
- postgres
script: nosetests
- stage: upload coverage
python: 3.6
install:
- pip install awscli coverage codecov
install:
- pip install awscli coverage codecov
script:
- mkdir coverage
- aws s3 cp --recursive s3://com-gavinroy-travis/queries/$TRAVIS_BUILD_NUMBER/
coverage
- cd coverage
- coverage combine
- cd ..
after_success: codecov
- stage: deploy
python: 3.6
deploy:
distributions: sdist bdist_wheel
provider: pypi
deploy:
distributions: sdist bdist_wheel
provider: pypi
user: crad
on:
tags: true
all_branches: true
password:
secure: UWQWui+QhAL1cz6oW/vqjEEp6/EPn1YOlItNJcWHNOO/WMMOlaTVYVUuXp+y+m52B+8PtYZZCTHwKCUKe97Grh291FLxgd0RJCawA40f4v1gmOFYLNKyZFBGfbC69/amxvGCcDvOPtpChHAlTIeokS5EQneVcAhXg2jXct0HTfI=
<MSG> Update to reflect findings for stages in travis
<DFF> @@ -1,31 +1,26 @@
sudo: false
language: python
-python:
- - 2.7
- - 3.4
- - 3.5
- - 3.6
- - pypy
- - pypy3
env:
global:
- - PATH=$HOME/.local/bin:$PATH
- - AWS_DEFAULT_REGION=us-east-1
- - secure: "inURdx4ldkJqQXL1TyvKImC3EnL5TixC1DlNMBYi5ttygwAk+mSSSw8Yc7klB6D1m6q79xUlHRk06vbz23CsXTM4AClC5Emrk6XN2GlUKl5WI+z+A2skI59buEhLWe7e2KzhB/AVx2E3TfKa0oY7raM0UUnaOkpV1Cj+mHKPIT0="
- - secure: "H32DV3713a6UUuEJujrG7SfUX4/5WrwQy/3DxeptC6L7YPlTYxHBdEsccTfN5z806EheIl4BdIoxoDtq7PU/tWQoG1Lp2ze60mpwrniHajhFnjk7zP6pHvkhGLr8flhSmAb6CQBreNFOHTLWBMGPfi7k1Q9Td9MHbRo/FsTxqsM="
-
-install:
- - pip install awscli
- - pip install -r requires/testing.txt
- - python setup.py develop
+ - PATH=$HOME/.local/bin:$PATH
+ - AWS_DEFAULT_REGION=us-east-1
+ - secure: "inURdx4ldkJqQXL1TyvKImC3EnL5TixC1DlNMBYi5ttygwAk+mSSSw8Yc7klB6D1m6q79xUlHRk06vbz23CsXTM4AClC5Emrk6XN2GlUKl5WI+z+A2skI59buEhLWe7e2KzhB/AVx2E3TfKa0oY7raM0UUnaOkpV1Cj+mHKPIT0="
+ - secure: "H32DV3713a6UUuEJujrG7SfUX4/5WrwQy/3DxeptC6L7YPlTYxHBdEsccTfN5z806EheIl4BdIoxoDtq7PU/tWQoG1Lp2ze60mpwrniHajhFnjk7zP6pHvkhGLr8flhSmAb6CQBreNFOHTLWBMGPfi7k1Q9Td9MHbRo/FsTxqsM="
stages:
- - test
- - name: upload_coverage
- if: branch = master
- - name: deploy
- if: tag IS present
+- test
+- name: upload coverage
+- name: deploy
+ if: tag IS present
+
+services:
+- postgres
+
+install:
+- pip install awscli
+- pip install -r requires/testing.txt
+- python setup.py develop
script: nosetests
@@ -34,11 +29,14 @@ after_success:
jobs:
include:
- - stage: test
- services:
- - postgres
- script: nosetests
+ - python: 2.7
+ - python: 3.4
+ - python: 3.5
+ - python: 3.6
+ - python: pypy
+ - python: pypy3
- stage: upload coverage
+ services: []
python: 3.6
install:
- pip install awscli coverage codecov
@@ -54,6 +52,10 @@ jobs:
after_success: codecov
- stage: deploy
python: 3.6
+ services: []
+ install: true
+ script: true
+ after_success: true
deploy:
distributions: sdist bdist_wheel
provider: pypi
| 27 | Update to reflect findings for stages in travis | 25 | .yml | travis | bsd-3-clause | gmr/queries |
1083 | <NME> LICENSE
<BEF> Copyright (c) 2014 - 2016 Gavin M. Roy
All rights reserved.
Redistribution and use in source and binary forms, with or without modification,
are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright notice, this
list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice,
this list of conditions and the following disclaimer in the documentation
and/or other materials provided with the distribution.
* Neither the name of the queries nor the names of its
contributors may be used to endorse or promote products derived from this
software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT,
INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF
LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE
OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF
ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
<MSG> Bump license year
<DFF> @@ -1,4 +1,4 @@
-Copyright (c) 2014 - 2016 Gavin M. Roy
+Copyright (c) 2014 - 2018 Gavin M. Roy
All rights reserved.
Redistribution and use in source and binary forms, with or without modification,
| 1 | Bump license year | 1 | LICENSE | bsd-3-clause | gmr/queries |
|
1084 | <NME> LICENSE
<BEF> Copyright (c) 2014 - 2016 Gavin M. Roy
All rights reserved.
Redistribution and use in source and binary forms, with or without modification,
are permitted provided that the following conditions are met:
* Redistributions of source code must retain the above copyright notice, this
list of conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice,
this list of conditions and the following disclaimer in the documentation
and/or other materials provided with the distribution.
* Neither the name of the queries nor the names of its
contributors may be used to endorse or promote products derived from this
software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT,
INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF
LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE
OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF
ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
<MSG> Bump license year
<DFF> @@ -1,4 +1,4 @@
-Copyright (c) 2014 - 2016 Gavin M. Roy
+Copyright (c) 2014 - 2018 Gavin M. Roy
All rights reserved.
Redistribution and use in source and binary forms, with or without modification,
| 1 | Bump license year | 1 | LICENSE | bsd-3-clause | gmr/queries |
|
1085 | <NME> utils.py
<BEF> """
Utility functions for access to OS level info and URI parsing
"""
import collections
import os
import pwd
try:
from urllib import parse as _urlparse
except ImportError:
try:
from urllib import parse as _urlparse
except ImportError:
import urlparse as _urlparse
try:
from urllib.parse import unquote
except ImportError:
from urllib import unquote
LOGGER = logging.getLogger(__name__)
PARSED = collections.namedtuple('Parsed',
'scheme,netloc,path,params,query,fragment,'
'username,password,hostname,port')
PYPY = platform.python_implementation().lower() == 'pypy'
KEYWORDS = ['connect_timeout',
'client_encoding',
'options',
'application_name',
'fallback_application_name',
'keepalives',
'keepalives_idle',
'keepalives_interval',
'keepalives_count',
'sslmode',
'requiressl',
'sslcompression',
'sslcert',
'sslkey',
'sslrootcert',
'sslcrl',
'requirepeer',
'krbsrvname',
'gsslib',
:rtype: str
"""
return pwd.getpwuid(os.getuid())[0]
def parse_qs(query_string):
return getpass.getuser()
else:
try:
return pwd.getpwuid(os.getuid())[0]
except KeyError as error:
LOGGER.error('Could not get logged-in user: %s', error)
def parse_qs(query_string):
"""Return the parsed query string in a python2/3 agnostic fashion
:param str query_string: The URI query string
:rtype: dict
"""
return _urlparse.parse_qs(query_string)
def uri(host='localhost', port=5432, dbname='postgres', user='postgres',
password=None):
"""Return a PostgreSQL connection URI for the specified values.
:param str host: Host to connect to
:param int port: Port to connect on
:param str dbname: The database name
:param str user: User to connect as
:param str password: The password to use, None for no password
:return str: The PostgreSQL connection URI
"""
if port:
host = '%s:%s' % (host, port)
if password:
return 'postgresql://%s:%s@%s/%s' % (user, password, host, dbname)
return 'postgresql://%s@%s/%s' % (user, host, dbname)
def uri_to_kwargs(uri):
"""Return a URI as kwargs for connecting to PostgreSQL with psycopg2,
applying default values for non-specified areas of the URI.
:param str uri: The connection URI
:rtype: dict
"""
parsed = urlparse(uri)
default_user = get_current_user()
password = unquote(parsed.password) if parsed.password else None
kwargs = {'host': parsed.hostname,
'port': parsed.port,
'dbname': parsed.path[1:] or default_user,
'user': parsed.username or default_user,
'password': password}
values = parse_qs(parsed.query)
if 'host' in values:
kwargs['host'] = values['host'][0]
for k in [k for k in values if k in KEYWORDS]:
kwargs[k] = values[k][0] if len(values[k]) == 1 else values[k]
try:
if kwargs[k].isdigit():
kwargs[k] = int(kwargs[k])
except AttributeError:
pass
return kwargs
def urlparse(url):
"""Parse the URL in a Python2/3 independent fashion.
:param str url: The URL to parse
:rtype: Parsed
"""
value = 'http%s' % url[5:] if url[:5] == 'postgresql' else url
parsed = _urlparse.urlparse(value)
path, query = parsed.path, parsed.query
hostname = parsed.hostname if parsed.hostname else ''
return PARSED(parsed.scheme.replace('http', 'postgresql'),
parsed.netloc,
path,
parsed.params,
query,
parsed.fragment,
parsed.username,
parsed.password,
hostname.replace('%2F', '/').replace('%2f', '/'),
parsed.port)
<MSG> Add alternative for pwd module
All systems do not support pwd module. See:
https://docs.python.org/3/library/pwd.html
<DFF> @@ -4,7 +4,14 @@ Utility functions for access to OS level info and URI parsing
"""
import collections
import os
-import pwd
+
+# All systems do not support pwd module
+try:
+ import pwd
+except ImportError:
+ pwd = None
+ import getpass
+
try:
from urllib import parse as _urlparse
except ImportError:
@@ -47,7 +54,10 @@ def get_current_user():
:rtype: str
"""
- return pwd.getpwuid(os.getuid())[0]
+ if pwd is None:
+ return getpass.getuser()
+ else:
+ return pwd.getpwuid(os.getuid())[0]
def parse_qs(query_string):
| 12 | Add alternative for pwd module | 2 | .py | py | bsd-3-clause | gmr/queries |
1086 | <NME> utils.py
<BEF> """
Utility functions for access to OS level info and URI parsing
"""
import collections
import os
import pwd
try:
from urllib import parse as _urlparse
except ImportError:
try:
from urllib import parse as _urlparse
except ImportError:
import urlparse as _urlparse
try:
from urllib.parse import unquote
except ImportError:
from urllib import unquote
LOGGER = logging.getLogger(__name__)
PARSED = collections.namedtuple('Parsed',
'scheme,netloc,path,params,query,fragment,'
'username,password,hostname,port')
PYPY = platform.python_implementation().lower() == 'pypy'
KEYWORDS = ['connect_timeout',
'client_encoding',
'options',
'application_name',
'fallback_application_name',
'keepalives',
'keepalives_idle',
'keepalives_interval',
'keepalives_count',
'sslmode',
'requiressl',
'sslcompression',
'sslcert',
'sslkey',
'sslrootcert',
'sslcrl',
'requirepeer',
'krbsrvname',
'gsslib',
:rtype: str
"""
return pwd.getpwuid(os.getuid())[0]
def parse_qs(query_string):
return getpass.getuser()
else:
try:
return pwd.getpwuid(os.getuid())[0]
except KeyError as error:
LOGGER.error('Could not get logged-in user: %s', error)
def parse_qs(query_string):
"""Return the parsed query string in a python2/3 agnostic fashion
:param str query_string: The URI query string
:rtype: dict
"""
return _urlparse.parse_qs(query_string)
def uri(host='localhost', port=5432, dbname='postgres', user='postgres',
password=None):
"""Return a PostgreSQL connection URI for the specified values.
:param str host: Host to connect to
:param int port: Port to connect on
:param str dbname: The database name
:param str user: User to connect as
:param str password: The password to use, None for no password
:return str: The PostgreSQL connection URI
"""
if port:
host = '%s:%s' % (host, port)
if password:
return 'postgresql://%s:%s@%s/%s' % (user, password, host, dbname)
return 'postgresql://%s@%s/%s' % (user, host, dbname)
def uri_to_kwargs(uri):
"""Return a URI as kwargs for connecting to PostgreSQL with psycopg2,
applying default values for non-specified areas of the URI.
:param str uri: The connection URI
:rtype: dict
"""
parsed = urlparse(uri)
default_user = get_current_user()
password = unquote(parsed.password) if parsed.password else None
kwargs = {'host': parsed.hostname,
'port': parsed.port,
'dbname': parsed.path[1:] or default_user,
'user': parsed.username or default_user,
'password': password}
values = parse_qs(parsed.query)
if 'host' in values:
kwargs['host'] = values['host'][0]
for k in [k for k in values if k in KEYWORDS]:
kwargs[k] = values[k][0] if len(values[k]) == 1 else values[k]
try:
if kwargs[k].isdigit():
kwargs[k] = int(kwargs[k])
except AttributeError:
pass
return kwargs
def urlparse(url):
"""Parse the URL in a Python2/3 independent fashion.
:param str url: The URL to parse
:rtype: Parsed
"""
value = 'http%s' % url[5:] if url[:5] == 'postgresql' else url
parsed = _urlparse.urlparse(value)
path, query = parsed.path, parsed.query
hostname = parsed.hostname if parsed.hostname else ''
return PARSED(parsed.scheme.replace('http', 'postgresql'),
parsed.netloc,
path,
parsed.params,
query,
parsed.fragment,
parsed.username,
parsed.password,
hostname.replace('%2F', '/').replace('%2f', '/'),
parsed.port)
<MSG> Add alternative for pwd module
All systems do not support pwd module. See:
https://docs.python.org/3/library/pwd.html
<DFF> @@ -4,7 +4,14 @@ Utility functions for access to OS level info and URI parsing
"""
import collections
import os
-import pwd
+
+# All systems do not support pwd module
+try:
+ import pwd
+except ImportError:
+ pwd = None
+ import getpass
+
try:
from urllib import parse as _urlparse
except ImportError:
@@ -47,7 +54,10 @@ def get_current_user():
:rtype: str
"""
- return pwd.getpwuid(os.getuid())[0]
+ if pwd is None:
+ return getpass.getuser()
+ else:
+ return pwd.getpwuid(os.getuid())[0]
def parse_qs(query_string):
| 12 | Add alternative for pwd module | 2 | .py | py | bsd-3-clause | gmr/queries |
1087 | <NME> utils_tests.py
<BEF> """
Tests for functionality in the utils module
"""
import mock
import unittest
from queries import utils
class GetCurrentUserTests(unittest.TestCase):
@mock.patch('pwd.getpwuid')
def test_get_current_user(self, getpwuid):
"""get_current_user returns value from pwd.getpwuid"""
self.assertEqual(utils.get_current_user(), 'mocky')
class URLParseTestCase(unittest.TestCase):
URI = 'postgresql://foo:bar@baz:5444/qux'
URI = 'postgresql://foo:bar@baz:5444/qux'
def test_urlparse_hostname(self):
"""hostname should match expectation"""
self.assertEqual(utils.urlparse(self.URI).hostname, 'baz')
def test_urlparse_port(self):
"""port should match expectation"""
self.assertEqual(utils.urlparse(self.URI).port, 5444)
def test_urlparse_path(self):
"""path should match expectation"""
self.assertEqual(utils.urlparse(self.URI).path, '/qux')
def test_urlparse_username(self):
"""username should match expectation"""
self.assertEqual(utils.urlparse(self.URI).username, 'foo')
def test_urlparse_password(self):
"""password should match expectation"""
self.assertEqual(utils.urlparse(self.URI).password, 'bar')
class URIToKWargsTestCase(unittest.TestCase):
URI = ('postgresql://foo:c%23%5E%25%23%27%24%40%3A@baz:5444/qux?'
'options=foo&options=bar&keepalives=1&invalid=true')
def test_uri_to_kwargs_host(self):
"""hostname should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['host'], 'baz')
def test_uri_to_kwargs_port(self):
"""port should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['port'], 5444)
def test_uri_to_kwargs_dbname(self):
"""dbname should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['dbname'], 'qux')
def test_uri_to_kwargs_username(self):
"""user should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['user'], 'foo')
def test_uri_to_kwargs_password(self):
"""password should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['password'],
'c#^%#\'$@:')
def test_uri_to_kwargs_options(self):
"""options should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['options'],
['foo', 'bar'])
def test_uri_to_kwargs_keepalive(self):
"""keepalive should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['keepalives'], 1)
def test_uri_to_kwargs_invalid(self):
"""invalid query argument should not be in kwargs"""
self.assertNotIn('invaid', utils.uri_to_kwargs(self.URI))
def test_unix_socket_path_format_one(self):
socket_path = 'postgresql://%2Fvar%2Flib%2Fpostgresql/dbname'
result = utils.uri_to_kwargs(socket_path)
self.assertEqual(result['host'], '/var/lib/postgresql')
socket_path = 'postgresql:///postgres?host=/tmp/'
result = utils.uri_to_kwargs(socket_path)
self.assertEqual(result['host'], '/tmp/')
self.assertEqual(result['host'], '/tmp/')
<MSG> Change how psycopg2cffi is registered
- psycopg2cffi.compat.register doesnt muck with psycopg2.extras and psycopg2.extensions causing an issue. Change to apply the registration directly.
- Move PYPY flag to utils
- Update tests accordingly
<DFF> @@ -3,8 +3,10 @@ Tests for functionality in the utils module
"""
import mock
+import platform
import unittest
+import queries
from queries import utils
@@ -17,6 +19,31 @@ class GetCurrentUserTests(unittest.TestCase):
self.assertEqual(utils.get_current_user(), 'mocky')
+class PYPYDetectionTests(unittest.TestCase):
+
+ def test_pypy_flag(self):
+ """PYPY flag is set properly"""
+ self.assertEqual(queries.PYPY,
+ platform.python_implementation() == 'PyPy')
+
+
+class URICreationTests(unittest.TestCase):
+
+ def test_uri_with_password(self):
+ expectation = 'postgresql://foo:bar@baz:5433/qux'
+ self.assertEqual(queries.uri('baz', 5433, 'qux', 'foo', 'bar'),
+ expectation)
+
+ def test_uri_without_password(self):
+ expectation = 'postgresql://foo@baz:5433/qux'
+ self.assertEqual(queries.uri('baz', 5433, 'qux', 'foo'),
+ expectation)
+
+ def test_default_uri(self):
+ expectation = 'postgresql://postgres@localhost:5432/postgres'
+ self.assertEqual(queries.uri(), expectation)
+
+
class URLParseTestCase(unittest.TestCase):
URI = 'postgresql://foo:bar@baz:5444/qux'
@@ -90,5 +117,3 @@ class URIToKWargsTestCase(unittest.TestCase):
socket_path = 'postgresql:///postgres?host=/tmp/'
result = utils.uri_to_kwargs(socket_path)
self.assertEqual(result['host'], '/tmp/')
-
-
| 27 | Change how psycopg2cffi is registered | 2 | .py | py | bsd-3-clause | gmr/queries |
1088 | <NME> utils_tests.py
<BEF> """
Tests for functionality in the utils module
"""
import mock
import unittest
from queries import utils
class GetCurrentUserTests(unittest.TestCase):
@mock.patch('pwd.getpwuid')
def test_get_current_user(self, getpwuid):
"""get_current_user returns value from pwd.getpwuid"""
self.assertEqual(utils.get_current_user(), 'mocky')
class URLParseTestCase(unittest.TestCase):
URI = 'postgresql://foo:bar@baz:5444/qux'
URI = 'postgresql://foo:bar@baz:5444/qux'
def test_urlparse_hostname(self):
"""hostname should match expectation"""
self.assertEqual(utils.urlparse(self.URI).hostname, 'baz')
def test_urlparse_port(self):
"""port should match expectation"""
self.assertEqual(utils.urlparse(self.URI).port, 5444)
def test_urlparse_path(self):
"""path should match expectation"""
self.assertEqual(utils.urlparse(self.URI).path, '/qux')
def test_urlparse_username(self):
"""username should match expectation"""
self.assertEqual(utils.urlparse(self.URI).username, 'foo')
def test_urlparse_password(self):
"""password should match expectation"""
self.assertEqual(utils.urlparse(self.URI).password, 'bar')
class URIToKWargsTestCase(unittest.TestCase):
URI = ('postgresql://foo:c%23%5E%25%23%27%24%40%3A@baz:5444/qux?'
'options=foo&options=bar&keepalives=1&invalid=true')
def test_uri_to_kwargs_host(self):
"""hostname should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['host'], 'baz')
def test_uri_to_kwargs_port(self):
"""port should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['port'], 5444)
def test_uri_to_kwargs_dbname(self):
"""dbname should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['dbname'], 'qux')
def test_uri_to_kwargs_username(self):
"""user should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['user'], 'foo')
def test_uri_to_kwargs_password(self):
"""password should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['password'],
'c#^%#\'$@:')
def test_uri_to_kwargs_options(self):
"""options should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['options'],
['foo', 'bar'])
def test_uri_to_kwargs_keepalive(self):
"""keepalive should match expectation"""
self.assertEqual(utils.uri_to_kwargs(self.URI)['keepalives'], 1)
def test_uri_to_kwargs_invalid(self):
"""invalid query argument should not be in kwargs"""
self.assertNotIn('invaid', utils.uri_to_kwargs(self.URI))
def test_unix_socket_path_format_one(self):
socket_path = 'postgresql://%2Fvar%2Flib%2Fpostgresql/dbname'
result = utils.uri_to_kwargs(socket_path)
self.assertEqual(result['host'], '/var/lib/postgresql')
socket_path = 'postgresql:///postgres?host=/tmp/'
result = utils.uri_to_kwargs(socket_path)
self.assertEqual(result['host'], '/tmp/')
self.assertEqual(result['host'], '/tmp/')
<MSG> Change how psycopg2cffi is registered
- psycopg2cffi.compat.register doesnt muck with psycopg2.extras and psycopg2.extensions causing an issue. Change to apply the registration directly.
- Move PYPY flag to utils
- Update tests accordingly
<DFF> @@ -3,8 +3,10 @@ Tests for functionality in the utils module
"""
import mock
+import platform
import unittest
+import queries
from queries import utils
@@ -17,6 +19,31 @@ class GetCurrentUserTests(unittest.TestCase):
self.assertEqual(utils.get_current_user(), 'mocky')
+class PYPYDetectionTests(unittest.TestCase):
+
+ def test_pypy_flag(self):
+ """PYPY flag is set properly"""
+ self.assertEqual(queries.PYPY,
+ platform.python_implementation() == 'PyPy')
+
+
+class URICreationTests(unittest.TestCase):
+
+ def test_uri_with_password(self):
+ expectation = 'postgresql://foo:bar@baz:5433/qux'
+ self.assertEqual(queries.uri('baz', 5433, 'qux', 'foo', 'bar'),
+ expectation)
+
+ def test_uri_without_password(self):
+ expectation = 'postgresql://foo@baz:5433/qux'
+ self.assertEqual(queries.uri('baz', 5433, 'qux', 'foo'),
+ expectation)
+
+ def test_default_uri(self):
+ expectation = 'postgresql://postgres@localhost:5432/postgres'
+ self.assertEqual(queries.uri(), expectation)
+
+
class URLParseTestCase(unittest.TestCase):
URI = 'postgresql://foo:bar@baz:5444/qux'
@@ -90,5 +117,3 @@ class URIToKWargsTestCase(unittest.TestCase):
socket_path = 'postgresql:///postgres?host=/tmp/'
result = utils.uri_to_kwargs(socket_path)
self.assertEqual(result['host'], '/tmp/')
-
-
| 27 | Change how psycopg2cffi is registered | 2 | .py | py | bsd-3-clause | gmr/queries |
1089 | <NME> setup.py
<BEF> import os
import platform
import setuptools
# PYPY vs cpython
if platform.python_implementation() == 'PyPy':
install_requires = ['psycopg2cffi>=2.7.2,<3']
else:
install_requires = ['psycopg2>=2.5.1,<3']
# Install tornado if generating docs on readthedocs
if os.environ.get('READTHEDOCS', None) == 'True':
install_requires.append('tornado')
setuptools.setup(
name='queries',
version='2.1.0',
description='Simplified PostgreSQL client built upon Psycopg2',
long_description=open('README.rst').read(),
maintainer='Gavin M. Roy',
maintainer_email='[email protected]',
url='https://github.com/gmr/queries',
install_requires=install_requires,
extras_require={'tornado': 'tornado<6'},
license='BSD',
package_data={'': ['LICENSE', 'README.rst']},
packages=['queries'],
'Topic :: Software Development :: Libraries']
setup(name='queries',
version='1.8.3',
description="Simplified PostgreSQL client built upon Psycopg2",
maintainer="Gavin M. Roy",
maintainer_email="[email protected]",
'Programming Language :: Python :: 3',
'Programming Language :: Python :: 3.4',
'Programming Language :: Python :: 3.5',
'Programming Language :: Python :: 3.6',
'Programming Language :: Python :: 3.7',
'Programming Language :: Python :: 3.8',
'Programming Language :: Python :: Implementation :: CPython',
'Programming Language :: Python :: Implementation :: PyPy',
'Topic :: Database',
'Topic :: Software Development :: Libraries'],
zip_safe=True)
<MSG> A better way to make sure the future doesnt already have an exception
<DFF> @@ -29,7 +29,7 @@ classifiers = ['Development Status :: 5 - Production/Stable',
'Topic :: Software Development :: Libraries']
setup(name='queries',
- version='1.8.3',
+ version='1.8.4',
description="Simplified PostgreSQL client built upon Psycopg2",
maintainer="Gavin M. Roy",
maintainer_email="[email protected]",
| 1 | A better way to make sure the future doesnt already have an exception | 1 | .py | py | bsd-3-clause | gmr/queries |
1090 | <NME> setup.py
<BEF> import os
import platform
import setuptools
# PYPY vs cpython
if platform.python_implementation() == 'PyPy':
install_requires = ['psycopg2cffi>=2.7.2,<3']
else:
install_requires = ['psycopg2>=2.5.1,<3']
# Install tornado if generating docs on readthedocs
if os.environ.get('READTHEDOCS', None) == 'True':
install_requires.append('tornado')
setuptools.setup(
name='queries',
version='2.1.0',
description='Simplified PostgreSQL client built upon Psycopg2',
long_description=open('README.rst').read(),
maintainer='Gavin M. Roy',
maintainer_email='[email protected]',
url='https://github.com/gmr/queries',
install_requires=install_requires,
extras_require={'tornado': 'tornado<6'},
license='BSD',
package_data={'': ['LICENSE', 'README.rst']},
packages=['queries'],
'Topic :: Software Development :: Libraries']
setup(name='queries',
version='1.8.3',
description="Simplified PostgreSQL client built upon Psycopg2",
maintainer="Gavin M. Roy",
maintainer_email="[email protected]",
'Programming Language :: Python :: 3',
'Programming Language :: Python :: 3.4',
'Programming Language :: Python :: 3.5',
'Programming Language :: Python :: 3.6',
'Programming Language :: Python :: 3.7',
'Programming Language :: Python :: 3.8',
'Programming Language :: Python :: Implementation :: CPython',
'Programming Language :: Python :: Implementation :: PyPy',
'Topic :: Database',
'Topic :: Software Development :: Libraries'],
zip_safe=True)
<MSG> A better way to make sure the future doesnt already have an exception
<DFF> @@ -29,7 +29,7 @@ classifiers = ['Development Status :: 5 - Production/Stable',
'Topic :: Software Development :: Libraries']
setup(name='queries',
- version='1.8.3',
+ version='1.8.4',
description="Simplified PostgreSQL client built upon Psycopg2",
maintainer="Gavin M. Roy",
maintainer_email="[email protected]",
| 1 | A better way to make sure the future doesnt already have an exception | 1 | .py | py | bsd-3-clause | gmr/queries |
1091 | <NME> setup.py
<BEF> import os
import platform
import setuptools
# PYPY vs cpython
if platform.python_implementation() == 'PyPy':
install_requires = ['psycopg2cffi>=2.7.2,<3']
else:
install_requires = ['psycopg2>=2.5.1,<3']
# Install tornado if generating docs on readthedocs
if os.environ.get('READTHEDOCS', None) == 'True':
install_requires.append('tornado')
setuptools.setup(
name='queries',
version='2.1.0',
description='Simplified PostgreSQL client built upon Psycopg2',
long_description=open('README.rst').read(),
maintainer='Gavin M. Roy',
maintainer_email='[email protected]',
url='https://github.com/gmr/queries',
install_requires=install_requires,
extras_require={'tornado': 'tornado<6'},
license='BSD',
package_data={'': ['LICENSE', 'README.rst']},
packages=['queries'],
'Topic :: Software Development :: Libraries']
setup(name='queries',
version='1.8.1',
description="Simplified PostgreSQL client built upon Psycopg2",
maintainer="Gavin M. Roy",
maintainer_email="[email protected]",
'Programming Language :: Python :: 3',
'Programming Language :: Python :: 3.4',
'Programming Language :: Python :: 3.5',
'Programming Language :: Python :: 3.6',
'Programming Language :: Python :: 3.7',
'Programming Language :: Python :: 3.8',
'Programming Language :: Python :: Implementation :: CPython',
'Programming Language :: Python :: Implementation :: PyPy',
'Topic :: Database',
'Topic :: Software Development :: Libraries'],
zip_safe=True)
<MSG> Dont double-raise exceptions, handle exceptions in the same place
<DFF> @@ -29,7 +29,7 @@ classifiers = ['Development Status :: 5 - Production/Stable',
'Topic :: Software Development :: Libraries']
setup(name='queries',
- version='1.8.1',
+ version='1.8.2',
description="Simplified PostgreSQL client built upon Psycopg2",
maintainer="Gavin M. Roy",
maintainer_email="[email protected]",
| 1 | Dont double-raise exceptions, handle exceptions in the same place | 1 | .py | py | bsd-3-clause | gmr/queries |
1092 | <NME> setup.py
<BEF> import os
import platform
import setuptools
# PYPY vs cpython
if platform.python_implementation() == 'PyPy':
install_requires = ['psycopg2cffi>=2.7.2,<3']
else:
install_requires = ['psycopg2>=2.5.1,<3']
# Install tornado if generating docs on readthedocs
if os.environ.get('READTHEDOCS', None) == 'True':
install_requires.append('tornado')
setuptools.setup(
name='queries',
version='2.1.0',
description='Simplified PostgreSQL client built upon Psycopg2',
long_description=open('README.rst').read(),
maintainer='Gavin M. Roy',
maintainer_email='[email protected]',
url='https://github.com/gmr/queries',
install_requires=install_requires,
extras_require={'tornado': 'tornado<6'},
license='BSD',
package_data={'': ['LICENSE', 'README.rst']},
packages=['queries'],
'Topic :: Software Development :: Libraries']
setup(name='queries',
version='1.8.1',
description="Simplified PostgreSQL client built upon Psycopg2",
maintainer="Gavin M. Roy",
maintainer_email="[email protected]",
'Programming Language :: Python :: 3',
'Programming Language :: Python :: 3.4',
'Programming Language :: Python :: 3.5',
'Programming Language :: Python :: 3.6',
'Programming Language :: Python :: 3.7',
'Programming Language :: Python :: 3.8',
'Programming Language :: Python :: Implementation :: CPython',
'Programming Language :: Python :: Implementation :: PyPy',
'Topic :: Database',
'Topic :: Software Development :: Libraries'],
zip_safe=True)
<MSG> Dont double-raise exceptions, handle exceptions in the same place
<DFF> @@ -29,7 +29,7 @@ classifiers = ['Development Status :: 5 - Production/Stable',
'Topic :: Software Development :: Libraries']
setup(name='queries',
- version='1.8.1',
+ version='1.8.2',
description="Simplified PostgreSQL client built upon Psycopg2",
maintainer="Gavin M. Roy",
maintainer_email="[email protected]",
| 1 | Dont double-raise exceptions, handle exceptions in the same place | 1 | .py | py | bsd-3-clause | gmr/queries |
1093 | <NME> README.rst
<BEF> Queries: PostgreSQL Simplified
==============================
*Queries* is a BSD licensed opinionated wrapper of the psycopg2_ library for
interacting with PostgreSQL.
The popular psycopg2_ package is a full-featured python client. Unfortunately
as a developer, you're often repeating the same steps to get started with your
applications that use it. Queries aims to reduce the complexity of psycopg2
while adding additional features to make writing PostgreSQL client applications
both fast and easy. Check out the `Usage`_ section below to see how easy it can be.
Key features include:
- Simplified API
- Support of Python 2.7+ and 3.4+
- PyPy support via psycopg2cffi_
- Asynchronous support for Tornado_
- Connection information provided by URI
- Query results delivered as a generator based iterators
- Automatically registered data-type support for UUIDs, Unicode and Unicode Arrays
- Ability to directly access psycopg2 ``connection`` and ``cursor`` objects
- Internal connection pooling
|Version| |Status| |Coverage| |License|
Documentation
-------------
Documentation is available at https://queries.readthedocs.org
Installation
------------
Queries is available via pypi_ and can be installed with easy_install or pip:
.. code:: bash
pip install queries
Usage
-----
Queries provides a session based API for interacting with PostgreSQL.
Simply pass in the URI_ of the PostgreSQL server to connect to when creating
a session:
.. code:: python
session = queries.Session("postgresql://postgres@localhost:5432/postgres")
Queries built-in connection pooling will re-use connections when possible,
lowering the overhead of connecting and reconnecting.
When specifying a URI, if you omit the username and database name to connect
with, Queries will use the current OS username for both. You can also omit the
URI when connecting to connect to localhost on port 5432 as the current OS user,
connecting to a database named for the current user. For example, if your
username is ``fred`` and you omit the URI when issuing ``queries.query`` the URI
that is constructed would be ``postgresql://fred@localhost:5432/fred``.
If you'd rather use individual values for the connection, the queries.uri()
method provides a quick and easy way to create a URI to pass into the various
methods.
.. code:: python
>>> queries.uri("server-name", 5432, "dbname", "user", "pass")
'postgresql://user:pass@server-name:5432/dbname'
Environment Variables
^^^^^^^^^^^^^^^^^^^^^
Currently Queries uses the following environment variables for tweaking various
configuration values. The supported ones are:
* ``QUERIES_MAX_POOL_SIZE`` - Modify the maximum size of the connection pool (default: 1)
Using the queries.Session class
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
To execute queries or call stored procedures, you start by creating an instance of the
``queries.Session`` class. It can act as a context manager, meaning you can
use it with the ``with`` keyword and it will take care of cleaning up after itself. For
more information on the ``with`` keyword and context managers, see PEP343_.
In addition to both the ``queries.Session.query`` and ``queries.Session.callproc``
methods that are similar to the simple API methods, the ``queries.Session`` class
provides access to the psycopg2 connection and cursor objects.
**Using queries.Session.query**
The following example shows how a ``queries.Session`` object can be used
as a context manager to query the database table:
.. code:: python
>>> import pprint
>>> import queries
>>>
>>> with queries.Session() as session:
... for row in session.query('SELECT * FROM names'):
... pprint.pprint(row)
...
{'id': 1, 'name': u'Jacob'}
{'id': 2, 'name': u'Mason'}
{'id': 3, 'name': u'Ethan'}
**Using queries.Session.callproc**
This example uses ``queries.Session.callproc`` to execute a stored
procedure and then pretty-prints the single row results as a dictionary:
.. code:: python
>>> import pprint
>>> import queries
>>> with queries.Session() as session:
... results = session.callproc('chr', [65])
... pprint.pprint(results.as_dict())
...
{'chr': u'A'}
**Asynchronous Queries with Tornado**
In addition to providing a Pythonic, synchronous client API for PostgreSQL,
Queries provides a very similar asynchronous API for use with Tornado.
The only major difference API difference between ``queries.TornadoSession`` and
``queries.Session`` is the ``TornadoSession.query`` and ``TornadoSession.callproc``
methods return the entire result set instead of acting as an iterator over
the results. The following example uses ``TornadoSession.query`` in an asynchronous
Tornado_ web application to send a JSON payload with the query result set.
.. code:: python
from tornado import gen, ioloop, web
import queries
class MainHandler(web.RequestHandler):
def initialize(self):
self.session = queries.TornadoSession()
@gen.coroutine
def get(self):
results = yield self.session.query('SELECT * FROM names')
self.finish({'data': results.items()})
results.free()
application = web.Application([
(r"/", MainHandler),
])
if __name__ == "__main__":
application.listen(8888)
ioloop.IOLoop.instance().start()
Inspiration
-----------
Queries is inspired by `Kenneth Reitz's <https://github.com/kennethreitz/>`_ awesome
work on `requests <http://docs.python-requests.org/en/latest/>`_.
History
-------
Queries is a fork and enhancement of pgsql_wrapper_, which can be found in the
main GitHub repository of Queries as tags prior to version 1.2.0.
.. _pypi: https://pypi.python.org/pypi/queries
.. _psycopg2: https://pypi.python.org/pypi/psycopg2
.. _documentation: https://queries.readthedocs.org
.. _URI: http://www.postgresql.org/docs/9.3/static/libpq-connect.html#LIBPQ-CONNSTRING
.. _pgsql_wrapper: https://pypi.python.org/pypi/pgsql_wrapper
.. _Tornado: http://tornadoweb.org
.. |Downloads| image:: https://pypip.in/d/queries/badge.svg?
:target: https://pypi.python.org/pypi/queries
.. |Coverage| image:: https://codecov.io/github/gmr/flatdict/coverage.svg?branch=master
:target: https://codecov.io/github/gmr/flatdict?branch=master
.. |Gitter| image:: https://badges.gitter.im/Join Chat.svg
:target: https://gitter.im/gmr/rabbitpy?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge
.. |Coverage| image:: https://img.shields.io/codecov/c/github/gmr/queries.svg?
:target: https://codecov.io/github/gmr/queries?branch=master
.. |License| image:: https://img.shields.io/github/license/gmr/queries.svg?
:target: https://github.com/gmr/queries
<MSG> Fix the repo for coverage info
<DFF> @@ -170,8 +170,8 @@ main GitHub repository of Queries as tags prior to version 1.2.0.
.. |Downloads| image:: https://pypip.in/d/queries/badge.svg?
:target: https://pypi.python.org/pypi/queries
-.. |Coverage| image:: https://codecov.io/github/gmr/flatdict/coverage.svg?branch=master
- :target: https://codecov.io/github/gmr/flatdict?branch=master
+.. |Coverage| image:: https://codecov.io/github/gmr/queries/coverage.svg?branch=master
+ :target: https://codecov.io/github/gmr/queries?branch=master
.. |Gitter| image:: https://badges.gitter.im/Join Chat.svg
:target: https://gitter.im/gmr/rabbitpy?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge
| 2 | Fix the repo for coverage info | 2 | .rst | rst | bsd-3-clause | gmr/queries |
1094 | <NME> README.rst
<BEF> Queries: PostgreSQL Simplified
==============================
*Queries* is a BSD licensed opinionated wrapper of the psycopg2_ library for
interacting with PostgreSQL.
The popular psycopg2_ package is a full-featured python client. Unfortunately
as a developer, you're often repeating the same steps to get started with your
applications that use it. Queries aims to reduce the complexity of psycopg2
while adding additional features to make writing PostgreSQL client applications
both fast and easy. Check out the `Usage`_ section below to see how easy it can be.
Key features include:
- Simplified API
- Support of Python 2.7+ and 3.4+
- PyPy support via psycopg2cffi_
- Asynchronous support for Tornado_
- Connection information provided by URI
- Query results delivered as a generator based iterators
- Automatically registered data-type support for UUIDs, Unicode and Unicode Arrays
- Ability to directly access psycopg2 ``connection`` and ``cursor`` objects
- Internal connection pooling
|Version| |Status| |Coverage| |License|
Documentation
-------------
Documentation is available at https://queries.readthedocs.org
Installation
------------
Queries is available via pypi_ and can be installed with easy_install or pip:
.. code:: bash
pip install queries
Usage
-----
Queries provides a session based API for interacting with PostgreSQL.
Simply pass in the URI_ of the PostgreSQL server to connect to when creating
a session:
.. code:: python
session = queries.Session("postgresql://postgres@localhost:5432/postgres")
Queries built-in connection pooling will re-use connections when possible,
lowering the overhead of connecting and reconnecting.
When specifying a URI, if you omit the username and database name to connect
with, Queries will use the current OS username for both. You can also omit the
URI when connecting to connect to localhost on port 5432 as the current OS user,
connecting to a database named for the current user. For example, if your
username is ``fred`` and you omit the URI when issuing ``queries.query`` the URI
that is constructed would be ``postgresql://fred@localhost:5432/fred``.
If you'd rather use individual values for the connection, the queries.uri()
method provides a quick and easy way to create a URI to pass into the various
methods.
.. code:: python
>>> queries.uri("server-name", 5432, "dbname", "user", "pass")
'postgresql://user:pass@server-name:5432/dbname'
Environment Variables
^^^^^^^^^^^^^^^^^^^^^
Currently Queries uses the following environment variables for tweaking various
configuration values. The supported ones are:
* ``QUERIES_MAX_POOL_SIZE`` - Modify the maximum size of the connection pool (default: 1)
Using the queries.Session class
^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
To execute queries or call stored procedures, you start by creating an instance of the
``queries.Session`` class. It can act as a context manager, meaning you can
use it with the ``with`` keyword and it will take care of cleaning up after itself. For
more information on the ``with`` keyword and context managers, see PEP343_.
In addition to both the ``queries.Session.query`` and ``queries.Session.callproc``
methods that are similar to the simple API methods, the ``queries.Session`` class
provides access to the psycopg2 connection and cursor objects.
**Using queries.Session.query**
The following example shows how a ``queries.Session`` object can be used
as a context manager to query the database table:
.. code:: python
>>> import pprint
>>> import queries
>>>
>>> with queries.Session() as session:
... for row in session.query('SELECT * FROM names'):
... pprint.pprint(row)
...
{'id': 1, 'name': u'Jacob'}
{'id': 2, 'name': u'Mason'}
{'id': 3, 'name': u'Ethan'}
**Using queries.Session.callproc**
This example uses ``queries.Session.callproc`` to execute a stored
procedure and then pretty-prints the single row results as a dictionary:
.. code:: python
>>> import pprint
>>> import queries
>>> with queries.Session() as session:
... results = session.callproc('chr', [65])
... pprint.pprint(results.as_dict())
...
{'chr': u'A'}
**Asynchronous Queries with Tornado**
In addition to providing a Pythonic, synchronous client API for PostgreSQL,
Queries provides a very similar asynchronous API for use with Tornado.
The only major difference API difference between ``queries.TornadoSession`` and
``queries.Session`` is the ``TornadoSession.query`` and ``TornadoSession.callproc``
methods return the entire result set instead of acting as an iterator over
the results. The following example uses ``TornadoSession.query`` in an asynchronous
Tornado_ web application to send a JSON payload with the query result set.
.. code:: python
from tornado import gen, ioloop, web
import queries
class MainHandler(web.RequestHandler):
def initialize(self):
self.session = queries.TornadoSession()
@gen.coroutine
def get(self):
results = yield self.session.query('SELECT * FROM names')
self.finish({'data': results.items()})
results.free()
application = web.Application([
(r"/", MainHandler),
])
if __name__ == "__main__":
application.listen(8888)
ioloop.IOLoop.instance().start()
Inspiration
-----------
Queries is inspired by `Kenneth Reitz's <https://github.com/kennethreitz/>`_ awesome
work on `requests <http://docs.python-requests.org/en/latest/>`_.
History
-------
Queries is a fork and enhancement of pgsql_wrapper_, which can be found in the
main GitHub repository of Queries as tags prior to version 1.2.0.
.. _pypi: https://pypi.python.org/pypi/queries
.. _psycopg2: https://pypi.python.org/pypi/psycopg2
.. _documentation: https://queries.readthedocs.org
.. _URI: http://www.postgresql.org/docs/9.3/static/libpq-connect.html#LIBPQ-CONNSTRING
.. _pgsql_wrapper: https://pypi.python.org/pypi/pgsql_wrapper
.. _Tornado: http://tornadoweb.org
.. |Downloads| image:: https://pypip.in/d/queries/badge.svg?
:target: https://pypi.python.org/pypi/queries
.. |Coverage| image:: https://codecov.io/github/gmr/flatdict/coverage.svg?branch=master
:target: https://codecov.io/github/gmr/flatdict?branch=master
.. |Gitter| image:: https://badges.gitter.im/Join Chat.svg
:target: https://gitter.im/gmr/rabbitpy?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge
.. |Coverage| image:: https://img.shields.io/codecov/c/github/gmr/queries.svg?
:target: https://codecov.io/github/gmr/queries?branch=master
.. |License| image:: https://img.shields.io/github/license/gmr/queries.svg?
:target: https://github.com/gmr/queries
<MSG> Fix the repo for coverage info
<DFF> @@ -170,8 +170,8 @@ main GitHub repository of Queries as tags prior to version 1.2.0.
.. |Downloads| image:: https://pypip.in/d/queries/badge.svg?
:target: https://pypi.python.org/pypi/queries
-.. |Coverage| image:: https://codecov.io/github/gmr/flatdict/coverage.svg?branch=master
- :target: https://codecov.io/github/gmr/flatdict?branch=master
+.. |Coverage| image:: https://codecov.io/github/gmr/queries/coverage.svg?branch=master
+ :target: https://codecov.io/github/gmr/queries?branch=master
.. |Gitter| image:: https://badges.gitter.im/Join Chat.svg
:target: https://gitter.im/gmr/rabbitpy?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge
| 2 | Fix the repo for coverage info | 2 | .rst | rst | bsd-3-clause | gmr/queries |
1095 | <NME> history.rst
<BEF> Version History
===============
- 1.10.4 2018-01-10
- Implement ``Results.__bool__`` to be explicit about Python 3 support.
- Catch any exception raised when using TornadoSession and invoking the execute function in psycopg2 for exceptions raised prior to sending the query to Postgres.
This could be psycopg2.Error, IndexError, KeyError, or who knows, it's not documented in psycopg2.
- 1.10.3 2017-11-01
- Remove the functionality from ``TornadoSession.validate`` and make it raise a ``DeprecationWarning``
- Catch the ``KeyError`` raised when ``PoolManager.clean()`` is invoked for a pool that doesn't exist
- 1.10.2 2017-10-26
- Ensure the pool exists when executing a query in TornadoSession, the new timeout behavior prevented that from happening.
- 1.10.1 2017-10-24
- Use an absolute time in the call to ``add_timeout``
- 1.10.0 2017-09-27
- Free when tornado_session.Result is ``__del__'d`` without ``free`` being called.
- Auto-clean the pool after Results.free TTL+1 in tornado_session.TornadoSession
- Dont raise NotImplementedError in Results.free for synchronous use, just treat as a noop
- 1.9.1 2016-10-25
- Add better exception handling around connections and getting the logged in user
- 1.9.0 2016-07-01
- Handle a potential race condition in TornadoSession when too many simultaneous new connections are made and a pool fills up
- Increase logging in various places to be more informative
- Restructure queries specific exceptions to all extend off of a base QueriesException
- Trivial code cleanup
- 1.8.10 2016-06-14
- Propagate PoolManager exceptions from TornadoSession (#20) - Fix by Dave Shawley
- 1.8.9 2015-11-11
- Move to psycopg2cffi for PyPy support
- 1.7.5 2015-09-03
- Don't let Session and TornadoSession share connections
- 1.7.1 2015-03-25
- Fix TornadoSession's use of cleanup (#8) - Fix by Oren Itamar
- 1.7.0 2015-01-13
- Implement :py:meth:`Pool.shutdown <queries.pool.Pool.shutdown>` and :py:meth:`PoolManager.shutdown <queries.pool.PoolManager.shutdown>` to
cleanly shutdown all open, non-executing connections across a Pool or all pools. Update locks in Pool operations to ensure atomicity.
- 1.6.1 2015-01-09
- Fixes an iteration error when closing a pool (#7) - Fix by Chris McGuire
- 1.6.0 2014-11-20
- Handle URI encoded password values properly
- 1.5.0 2014-10-07
- Handle empty query results in the iterator (#4) - Fix by Den Teresh
- 1.4.0 2014-09-04
- Address exception handling in tornado_session
- Address exception handling in tornado_session
<MSG> Update changelog
<DFF> @@ -1,43 +1,87 @@
Version History
===============
-- 1.10.4 2018-01-10
- - Implement ``Results.__bool__`` to be explicit about Python 3 support.
- - Catch any exception raised when using TornadoSession and invoking the execute function in psycopg2 for exceptions raised prior to sending the query to Postgres.
- This could be psycopg2.Error, IndexError, KeyError, or who knows, it's not documented in psycopg2.
-- 1.10.3 2017-11-01
- - Remove the functionality from ``TornadoSession.validate`` and make it raise a ``DeprecationWarning``
- - Catch the ``KeyError`` raised when ``PoolManager.clean()`` is invoked for a pool that doesn't exist
-- 1.10.2 2017-10-26
- - Ensure the pool exists when executing a query in TornadoSession, the new timeout behavior prevented that from happening.
-- 1.10.1 2017-10-24
- - Use an absolute time in the call to ``add_timeout``
-- 1.10.0 2017-09-27
- - Free when tornado_session.Result is ``__del__'d`` without ``free`` being called.
- - Auto-clean the pool after Results.free TTL+1 in tornado_session.TornadoSession
- - Dont raise NotImplementedError in Results.free for synchronous use, just treat as a noop
-- 1.9.1 2016-10-25
- - Add better exception handling around connections and getting the logged in user
-- 1.9.0 2016-07-01
- - Handle a potential race condition in TornadoSession when too many simultaneous new connections are made and a pool fills up
- - Increase logging in various places to be more informative
- - Restructure queries specific exceptions to all extend off of a base QueriesException
- - Trivial code cleanup
-- 1.8.10 2016-06-14
- - Propagate PoolManager exceptions from TornadoSession (#20) - Fix by Dave Shawley
-- 1.8.9 2015-11-11
- - Move to psycopg2cffi for PyPy support
-- 1.7.5 2015-09-03
- - Don't let Session and TornadoSession share connections
-- 1.7.1 2015-03-25
- - Fix TornadoSession's use of cleanup (#8) - Fix by Oren Itamar
-- 1.7.0 2015-01-13
- - Implement :py:meth:`Pool.shutdown <queries.pool.Pool.shutdown>` and :py:meth:`PoolManager.shutdown <queries.pool.PoolManager.shutdown>` to
- cleanly shutdown all open, non-executing connections across a Pool or all pools. Update locks in Pool operations to ensure atomicity.
-- 1.6.1 2015-01-09
- - Fixes an iteration error when closing a pool (#7) - Fix by Chris McGuire
-- 1.6.0 2014-11-20
- - Handle URI encoded password values properly
-- 1.5.0 2014-10-07
- - Handle empty query results in the iterator (#4) - Fix by Den Teresh
-- 1.4.0 2014-09-04
- - Address exception handling in tornado_session
+
+1.11.0 2018-01-23
+-----------------
+ - Cleanup IOLoop and internal stack in ``TornadoSession`` on connection error.
+ In the case of a connection error, the failure to do this caused CPU to peg
+ @ 100% utilization looping on a non-existent file descriptor. Thanks to
+ `cknave <https://github.com/cknave>`_ for his work on identifying the issue,
+ proposing a fix, and writing a working test case.
+ - Move the integration tests to use a local docker development environment
+ -
+
+
+
+1.10.4 2018-01-10
+-----------------
+ - Implement ``Results.__bool__`` to be explicit about Python 3 support.
+ - Catch any exception raised when using TornadoSession and invoking the execute function in psycopg2 for exceptions raised prior to sending the query to Postgres.
+ This could be psycopg2.Error, IndexError, KeyError, or who knows, it's not documented in psycopg2.
+
+1.10.3 2017-11-01
+-----------------
+ - Remove the functionality from ``TornadoSession.validate`` and make it raise a ``DeprecationWarning``
+ - Catch the ``KeyError`` raised when ``PoolManager.clean()`` is invoked for a pool that doesn't exist
+
+1.10.2 2017-10-26
+-----------------
+ - Ensure the pool exists when executing a query in TornadoSession, the new timeout behavior prevented that from happening.
+
+1.10.1 2017-10-24
+-----------------
+ - Use an absolute time in the call to ``add_timeout``
+
+1.10.0 2017-09-27
+-----------------
+ - Free when tornado_session.Result is ``__del__'d`` without ``free`` being called.
+ - Auto-clean the pool after Results.free TTL+1 in tornado_session.TornadoSession
+ - Dont raise NotImplementedError in Results.free for synchronous use, just treat as a noop
+
+1.9.1 2016-10-25
+----------------
+ - Add better exception handling around connections and getting the logged in user
+
+1.9.0 2016-07-01
+----------------
+ - Handle a potential race condition in TornadoSession when too many simultaneous new connections are made and a pool fills up
+ - Increase logging in various places to be more informative
+ - Restructure queries specific exceptions to all extend off of a base QueriesException
+ - Trivial code cleanup
+
+1.8.10 2016-06-14
+-----------------
+ - Propagate PoolManager exceptions from TornadoSession (#20) - Fix by Dave Shawley
+
+1.8.9 2015-11-11
+----------------
+ - Move to psycopg2cffi for PyPy support
+
+1.7.5 2015-09-03
+----------------
+ - Don't let Session and TornadoSession share connections
+
+1.7.1 2015-03-25
+----------------
+ - Fix TornadoSession's use of cleanup (#8) - Fix by Oren Itamar
+
+1.7.0 2015-01-13
+----------------
+ - Implement :py:meth:`Pool.shutdown <queries.pool.Pool.shutdown>` and :py:meth:`PoolManager.shutdown <queries.pool.PoolManager.shutdown>` to
+ cleanly shutdown all open, non-executing connections across a Pool or all pools. Update locks in Pool operations to ensure atomicity.
+
+1.6.1 2015-01-09
+----------------
+ - Fixes an iteration error when closing a pool (#7) - Fix by Chris McGuire
+
+1.6.0 2014-11-20
+-----------------
+ - Handle URI encoded password values properly
+
+1.5.0 2014-10-07
+----------------
+ - Handle empty query results in the iterator (#4) - Fix by Den Teresh
+
+1.4.0 2014-09-04
+----------------
+ - Address exception handling in tornado_session
| 85 | Update changelog | 41 | .rst | rst | bsd-3-clause | gmr/queries |
1096 | <NME> history.rst
<BEF> Version History
===============
- 1.10.4 2018-01-10
- Implement ``Results.__bool__`` to be explicit about Python 3 support.
- Catch any exception raised when using TornadoSession and invoking the execute function in psycopg2 for exceptions raised prior to sending the query to Postgres.
This could be psycopg2.Error, IndexError, KeyError, or who knows, it's not documented in psycopg2.
- 1.10.3 2017-11-01
- Remove the functionality from ``TornadoSession.validate`` and make it raise a ``DeprecationWarning``
- Catch the ``KeyError`` raised when ``PoolManager.clean()`` is invoked for a pool that doesn't exist
- 1.10.2 2017-10-26
- Ensure the pool exists when executing a query in TornadoSession, the new timeout behavior prevented that from happening.
- 1.10.1 2017-10-24
- Use an absolute time in the call to ``add_timeout``
- 1.10.0 2017-09-27
- Free when tornado_session.Result is ``__del__'d`` without ``free`` being called.
- Auto-clean the pool after Results.free TTL+1 in tornado_session.TornadoSession
- Dont raise NotImplementedError in Results.free for synchronous use, just treat as a noop
- 1.9.1 2016-10-25
- Add better exception handling around connections and getting the logged in user
- 1.9.0 2016-07-01
- Handle a potential race condition in TornadoSession when too many simultaneous new connections are made and a pool fills up
- Increase logging in various places to be more informative
- Restructure queries specific exceptions to all extend off of a base QueriesException
- Trivial code cleanup
- 1.8.10 2016-06-14
- Propagate PoolManager exceptions from TornadoSession (#20) - Fix by Dave Shawley
- 1.8.9 2015-11-11
- Move to psycopg2cffi for PyPy support
- 1.7.5 2015-09-03
- Don't let Session and TornadoSession share connections
- 1.7.1 2015-03-25
- Fix TornadoSession's use of cleanup (#8) - Fix by Oren Itamar
- 1.7.0 2015-01-13
- Implement :py:meth:`Pool.shutdown <queries.pool.Pool.shutdown>` and :py:meth:`PoolManager.shutdown <queries.pool.PoolManager.shutdown>` to
cleanly shutdown all open, non-executing connections across a Pool or all pools. Update locks in Pool operations to ensure atomicity.
- 1.6.1 2015-01-09
- Fixes an iteration error when closing a pool (#7) - Fix by Chris McGuire
- 1.6.0 2014-11-20
- Handle URI encoded password values properly
- 1.5.0 2014-10-07
- Handle empty query results in the iterator (#4) - Fix by Den Teresh
- 1.4.0 2014-09-04
- Address exception handling in tornado_session
- Address exception handling in tornado_session
<MSG> Update changelog
<DFF> @@ -1,43 +1,87 @@
Version History
===============
-- 1.10.4 2018-01-10
- - Implement ``Results.__bool__`` to be explicit about Python 3 support.
- - Catch any exception raised when using TornadoSession and invoking the execute function in psycopg2 for exceptions raised prior to sending the query to Postgres.
- This could be psycopg2.Error, IndexError, KeyError, or who knows, it's not documented in psycopg2.
-- 1.10.3 2017-11-01
- - Remove the functionality from ``TornadoSession.validate`` and make it raise a ``DeprecationWarning``
- - Catch the ``KeyError`` raised when ``PoolManager.clean()`` is invoked for a pool that doesn't exist
-- 1.10.2 2017-10-26
- - Ensure the pool exists when executing a query in TornadoSession, the new timeout behavior prevented that from happening.
-- 1.10.1 2017-10-24
- - Use an absolute time in the call to ``add_timeout``
-- 1.10.0 2017-09-27
- - Free when tornado_session.Result is ``__del__'d`` without ``free`` being called.
- - Auto-clean the pool after Results.free TTL+1 in tornado_session.TornadoSession
- - Dont raise NotImplementedError in Results.free for synchronous use, just treat as a noop
-- 1.9.1 2016-10-25
- - Add better exception handling around connections and getting the logged in user
-- 1.9.0 2016-07-01
- - Handle a potential race condition in TornadoSession when too many simultaneous new connections are made and a pool fills up
- - Increase logging in various places to be more informative
- - Restructure queries specific exceptions to all extend off of a base QueriesException
- - Trivial code cleanup
-- 1.8.10 2016-06-14
- - Propagate PoolManager exceptions from TornadoSession (#20) - Fix by Dave Shawley
-- 1.8.9 2015-11-11
- - Move to psycopg2cffi for PyPy support
-- 1.7.5 2015-09-03
- - Don't let Session and TornadoSession share connections
-- 1.7.1 2015-03-25
- - Fix TornadoSession's use of cleanup (#8) - Fix by Oren Itamar
-- 1.7.0 2015-01-13
- - Implement :py:meth:`Pool.shutdown <queries.pool.Pool.shutdown>` and :py:meth:`PoolManager.shutdown <queries.pool.PoolManager.shutdown>` to
- cleanly shutdown all open, non-executing connections across a Pool or all pools. Update locks in Pool operations to ensure atomicity.
-- 1.6.1 2015-01-09
- - Fixes an iteration error when closing a pool (#7) - Fix by Chris McGuire
-- 1.6.0 2014-11-20
- - Handle URI encoded password values properly
-- 1.5.0 2014-10-07
- - Handle empty query results in the iterator (#4) - Fix by Den Teresh
-- 1.4.0 2014-09-04
- - Address exception handling in tornado_session
+
+1.11.0 2018-01-23
+-----------------
+ - Cleanup IOLoop and internal stack in ``TornadoSession`` on connection error.
+ In the case of a connection error, the failure to do this caused CPU to peg
+ @ 100% utilization looping on a non-existent file descriptor. Thanks to
+ `cknave <https://github.com/cknave>`_ for his work on identifying the issue,
+ proposing a fix, and writing a working test case.
+ - Move the integration tests to use a local docker development environment
+ -
+
+
+
+1.10.4 2018-01-10
+-----------------
+ - Implement ``Results.__bool__`` to be explicit about Python 3 support.
+ - Catch any exception raised when using TornadoSession and invoking the execute function in psycopg2 for exceptions raised prior to sending the query to Postgres.
+ This could be psycopg2.Error, IndexError, KeyError, or who knows, it's not documented in psycopg2.
+
+1.10.3 2017-11-01
+-----------------
+ - Remove the functionality from ``TornadoSession.validate`` and make it raise a ``DeprecationWarning``
+ - Catch the ``KeyError`` raised when ``PoolManager.clean()`` is invoked for a pool that doesn't exist
+
+1.10.2 2017-10-26
+-----------------
+ - Ensure the pool exists when executing a query in TornadoSession, the new timeout behavior prevented that from happening.
+
+1.10.1 2017-10-24
+-----------------
+ - Use an absolute time in the call to ``add_timeout``
+
+1.10.0 2017-09-27
+-----------------
+ - Free when tornado_session.Result is ``__del__'d`` without ``free`` being called.
+ - Auto-clean the pool after Results.free TTL+1 in tornado_session.TornadoSession
+ - Dont raise NotImplementedError in Results.free for synchronous use, just treat as a noop
+
+1.9.1 2016-10-25
+----------------
+ - Add better exception handling around connections and getting the logged in user
+
+1.9.0 2016-07-01
+----------------
+ - Handle a potential race condition in TornadoSession when too many simultaneous new connections are made and a pool fills up
+ - Increase logging in various places to be more informative
+ - Restructure queries specific exceptions to all extend off of a base QueriesException
+ - Trivial code cleanup
+
+1.8.10 2016-06-14
+-----------------
+ - Propagate PoolManager exceptions from TornadoSession (#20) - Fix by Dave Shawley
+
+1.8.9 2015-11-11
+----------------
+ - Move to psycopg2cffi for PyPy support
+
+1.7.5 2015-09-03
+----------------
+ - Don't let Session and TornadoSession share connections
+
+1.7.1 2015-03-25
+----------------
+ - Fix TornadoSession's use of cleanup (#8) - Fix by Oren Itamar
+
+1.7.0 2015-01-13
+----------------
+ - Implement :py:meth:`Pool.shutdown <queries.pool.Pool.shutdown>` and :py:meth:`PoolManager.shutdown <queries.pool.PoolManager.shutdown>` to
+ cleanly shutdown all open, non-executing connections across a Pool or all pools. Update locks in Pool operations to ensure atomicity.
+
+1.6.1 2015-01-09
+----------------
+ - Fixes an iteration error when closing a pool (#7) - Fix by Chris McGuire
+
+1.6.0 2014-11-20
+-----------------
+ - Handle URI encoded password values properly
+
+1.5.0 2014-10-07
+----------------
+ - Handle empty query results in the iterator (#4) - Fix by Den Teresh
+
+1.4.0 2014-09-04
+----------------
+ - Address exception handling in tornado_session
| 85 | Update changelog | 41 | .rst | rst | bsd-3-clause | gmr/queries |
1097 | <NME> results.py
<BEF> """
query or callproc Results
"""
import logging
import psycopg2
LOGGER = logging.getLogger(__name__)
class Results(object):
"""The :py:class:`Results` class contains the results returned from
:py:meth:`Session.query <queries.Session.query>` and
:py:meth:`Session.callproc <queries.Session.callproc>`. It is able to act
as an iterator and provides many different methods for accessing the
information about and results from a query.
:param psycopg2.extensions.cursor cursor: The cursor for the results
"""
def __init__(self, cursor):
self.cursor = cursor
def __getitem__(self, item):
"""Fetch an individual row from the result set
:rtype: mixed
:raises: IndexError
"""
try:
self.cursor.scroll(item, 'absolute')
except psycopg2.ProgrammingError:
raise IndexError('No such row')
else:
return self.cursor.fetchone()
def __iter__(self):
"""Iterate through the result set
:rtype: mixed
"""
if self.cursor.rowcount:
self._rewind()
for row in self.cursor:
yield row
def __len__(self):
"""Return the number of rows that were returned from the query
:rtype: int
"""
return self.cursor.rowcount if self.cursor.rowcount >= 0 else 0
def __nonzero__(self):
return bool(self.cursor.rowcount)
def __nonzero__(self):
return bool(self.cursor.rowcount)
def __repr__(self):
return '<queries.%s rows=%s>' % (self.__class__.__name__, len(self))
multiple rows, a :py:class:`ValueError` will be raised.
:return: dict
:raises: ValueError
"""
if not self.cursor.rowcount:
return {}
self._rewind()
if self.cursor.rowcount == 1:
return dict(self.cursor.fetchone())
else:
raise ValueError('More than one row')
def count(self):
"""Return the number of rows that were returned from the query
:rtype: int
"""
return self.cursor.rowcount
def free(self):
"""Used in asynchronous sessions for freeing results and their locked
connections.
"""
LOGGER.debug('Invoking synchronous free has no effect')
def items(self):
"""Return all of the rows that are in the result set.
:rtype: list
"""
if not self.cursor.rowcount:
return []
self.cursor.scroll(0, 'absolute')
return self.cursor.fetchall()
@property
def rownumber(self):
"""Return the current offset of the result set
:rtype: int
"""
return self.cursor.rownumber
@property
def query(self):
"""Return a read-only value of the query that was submitted to
PostgreSQL.
:rtype: str
"""
return self.cursor.query
@property
def status(self):
"""Return the status message returned by PostgreSQL after the query
was executed.
:rtype: str
"""
return self.cursor.statusmessage
def _rewind(self):
"""Rewind the cursor to the first row"""
self.cursor.scroll(0, 'absolute')
<MSG> Merge pull request #27 from dave-shawley/python3-bool
Implement Results.__bool__ method.
<DFF> @@ -59,6 +59,9 @@ class Results(object):
def __nonzero__(self):
return bool(self.cursor.rowcount)
+ def __bool__(self):
+ return self.__nonzero__()
+
def __repr__(self):
return '<queries.%s rows=%s>' % (self.__class__.__name__, len(self))
| 3 | Merge pull request #27 from dave-shawley/python3-bool | 0 | .py | py | bsd-3-clause | gmr/queries |
1098 | <NME> results.py
<BEF> """
query or callproc Results
"""
import logging
import psycopg2
LOGGER = logging.getLogger(__name__)
class Results(object):
"""The :py:class:`Results` class contains the results returned from
:py:meth:`Session.query <queries.Session.query>` and
:py:meth:`Session.callproc <queries.Session.callproc>`. It is able to act
as an iterator and provides many different methods for accessing the
information about and results from a query.
:param psycopg2.extensions.cursor cursor: The cursor for the results
"""
def __init__(self, cursor):
self.cursor = cursor
def __getitem__(self, item):
"""Fetch an individual row from the result set
:rtype: mixed
:raises: IndexError
"""
try:
self.cursor.scroll(item, 'absolute')
except psycopg2.ProgrammingError:
raise IndexError('No such row')
else:
return self.cursor.fetchone()
def __iter__(self):
"""Iterate through the result set
:rtype: mixed
"""
if self.cursor.rowcount:
self._rewind()
for row in self.cursor:
yield row
def __len__(self):
"""Return the number of rows that were returned from the query
:rtype: int
"""
return self.cursor.rowcount if self.cursor.rowcount >= 0 else 0
def __nonzero__(self):
return bool(self.cursor.rowcount)
def __nonzero__(self):
return bool(self.cursor.rowcount)
def __repr__(self):
return '<queries.%s rows=%s>' % (self.__class__.__name__, len(self))
multiple rows, a :py:class:`ValueError` will be raised.
:return: dict
:raises: ValueError
"""
if not self.cursor.rowcount:
return {}
self._rewind()
if self.cursor.rowcount == 1:
return dict(self.cursor.fetchone())
else:
raise ValueError('More than one row')
def count(self):
"""Return the number of rows that were returned from the query
:rtype: int
"""
return self.cursor.rowcount
def free(self):
"""Used in asynchronous sessions for freeing results and their locked
connections.
"""
LOGGER.debug('Invoking synchronous free has no effect')
def items(self):
"""Return all of the rows that are in the result set.
:rtype: list
"""
if not self.cursor.rowcount:
return []
self.cursor.scroll(0, 'absolute')
return self.cursor.fetchall()
@property
def rownumber(self):
"""Return the current offset of the result set
:rtype: int
"""
return self.cursor.rownumber
@property
def query(self):
"""Return a read-only value of the query that was submitted to
PostgreSQL.
:rtype: str
"""
return self.cursor.query
@property
def status(self):
"""Return the status message returned by PostgreSQL after the query
was executed.
:rtype: str
"""
return self.cursor.statusmessage
def _rewind(self):
"""Rewind the cursor to the first row"""
self.cursor.scroll(0, 'absolute')
<MSG> Merge pull request #27 from dave-shawley/python3-bool
Implement Results.__bool__ method.
<DFF> @@ -59,6 +59,9 @@ class Results(object):
def __nonzero__(self):
return bool(self.cursor.rowcount)
+ def __bool__(self):
+ return self.__nonzero__()
+
def __repr__(self):
return '<queries.%s rows=%s>' % (self.__class__.__name__, len(self))
| 3 | Merge pull request #27 from dave-shawley/python3-bool | 0 | .py | py | bsd-3-clause | gmr/queries |
1099 | <NME> tornado_session.py
<BEF> """
Tornado Session Adapter
Use Queries asynchronously within the Tornado framework.
Example Use:
.. code:: python
class NameListHandler(web.RequestHandler):
def initialize(self):
self.session = queries.TornadoSession(pool_max_size=60)
@gen.coroutine
def get(self):
data = yield self.session.query('SELECT * FROM names')
if data:
self.finish({'names': data.items()})
data.free()
else:
self.set_status(500, 'Error querying the data')
"""
import logging
import socket
import warnings
from tornado import concurrent, ioloop
from psycopg2 import extras, extensions
import psycopg2
from queries import pool, results, session, utils
LOGGER = logging.getLogger(__name__)
DEFAULT_MAX_POOL_SIZE = 25
class Results(results.Results):
"""A TornadoSession specific :py:class:`queries.Results` class that adds
the :py:meth:`Results.free <queries.tornado_session.Results.free>` method.
The :py:meth:`Results.free <queries.tornado_session.Results.free>` method
**must** be called to free the connection that the results were generated
on. `Results` objects that are not freed will cause the connections to
remain locked and your application will eventually run out of connections
in the pool.
The following examples illustrate the various behaviors that the
::py:class:`queries.Results <queries.tornado_session.Requests>` class
implements:
**Using Results as an Iterator**
.. code:: python
results = yield session.query('SELECT * FROM foo')
for row in results
print row
results.free()
**Accessing an individual row by index**
.. code:: python
results = yield session.query('SELECT * FROM foo')
print results[1] # Access the second row of the results
results.free()
**Casting single row results as a dict**
.. code:: python
results = yield session.query('SELECT * FROM foo LIMIT 1')
print results.as_dict()
results.free()
**Checking to see if a query was successful**
.. code:: python
sql = "UPDATE foo SET bar='baz' WHERE qux='corgie'"
results = yield session.query(sql)
if results:
print 'Success'
results.free()
**Checking the number of rows by using len(Results)**
.. code:: python
results = yield session.query('SELECT * FROM foo')
print '%i rows' % len(results)
results.free()
"""
def __init__(self, cursor, cleanup, fd):
self.cursor = cursor
self._cleanup = cleanup
self._fd = fd
self._freed = False
def free(self):
"""Release the results and connection lock from the TornadoSession
object. This **must** be called after you finish processing the results
from :py:meth:`TornadoSession.query <queries.TornadoSession.query>` or
:py:meth:`TornadoSession.callproc <queries.TornadoSession.callproc>`
or the connection will not be able to be reused by other asynchronous
requests.
"""
self._freed = True
self._cleanup(self.cursor, self._fd)
def __del__(self):
if not self._freed:
LOGGER.warning('Auto-freeing result on deletion')
self.free()
class TornadoSession(session.Session):
"""Session class for Tornado asynchronous applications. Uses
:py:func:`tornado.gen.coroutine` to wrap API methods for use in Tornado.
Utilizes connection pooling to ensure that multiple concurrent asynchronous
queries do not block each other. Heavily trafficked services will require
a higher ``max_pool_size`` to allow for greater connection concurrency.
:py:meth:`TornadoSession.query <queries.TornadoSession.query>` and
:py:meth:`TornadoSession.callproc <queries.TornadoSession.callproc>` must
call :py:meth:`Results.free <queries.tornado_session.Results.free>`
:param str uri: PostgreSQL connection URI
:param psycopg2.extensions.cursor: The cursor type to use
:param int pool_idle_ttl: How long idle pools keep connections open
:param int pool_max_size: The maximum size of the pool to use
"""
def __init__(self, uri=session.DEFAULT_URI,
cursor_factory=extras.RealDictCursor,
pool_idle_ttl=pool.DEFAULT_IDLE_TTL,
pool_max_size=DEFAULT_MAX_POOL_SIZE,
io_loop=None):
"""Connect to a PostgreSQL server using the module wide connection and
set the isolation level.
:param str uri: PostgreSQL connection URI
:param psycopg2.extensions.cursor: The cursor type to use
:param int pool_idle_ttl: How long idle pools keep connections open
:param int pool_max_size: The maximum size of the pool to use
:param tornado.ioloop.IOLoop io_loop: IOLoop instance to use
"""
self._connections = dict()
self._cleanup_callback = None
self._cursor_factory = cursor_factory
self._futures = dict()
self._ioloop = io_loop or ioloop.IOLoop.current()
self._pool_manager = pool.PoolManager.instance()
self._pool_max_size = pool_max_size
self._pool_idle_ttl = pool_idle_ttl
self._uri = uri
self._ensure_pool_exists()
def _ensure_pool_exists(self):
"""Create the pool in the pool manager if it does not exist."""
if self.pid not in self._pool_manager:
self._pool_manager.create(self.pid, self._pool_idle_ttl,
self._pool_max_size, self._ioloop.time)
@property
def connection(self):
"""Do not use this directly with Tornado applications
:return:
"""
return None
@property
def cursor(self):
return None
def callproc(self, name, args=None):
"""Call a stored procedure asynchronously on the server, passing in the
arguments to be passed to the stored procedure, yielding the results
as a :py:class:`Results <queries.tornado_session.Results>` object.
You **must** free the results that are returned by this method to
unlock the connection used to perform the query. Failure to do so
will cause your Tornado application to run out of connections.
:param str name: The stored procedure name
:param list args: An optional list of procedure arguments
:rtype: Results
:raises: queries.DataError
:raises: queries.DatabaseError
:raises: queries.IntegrityError
:raises: queries.InternalError
:raises: queries.InterfaceError
:raises: queries.NotSupportedError
:raises: queries.OperationalError
:raises: queries.ProgrammingError
"""
return self._execute('callproc', name, args)
def query(self, sql, parameters=None):
"""Issue a query asynchronously on the server, mogrifying the
parameters against the sql statement and yielding the results
as a :py:class:`Results <queries.tornado_session.Results>` object.
You **must** free the results that are returned by this method to
unlock the connection used to perform the query. Failure to do so
will cause your Tornado application to run out of connections.
:param str sql: The SQL statement
:param dict parameters: A dictionary of query parameters
:rtype: Results
:raises: queries.DataError
:raises: queries.DatabaseError
:raises: queries.IntegrityError
:raises: queries.InternalError
:raises: queries.InterfaceError
:raises: queries.NotSupportedError
:raises: queries.OperationalError
:raises: queries.ProgrammingError
"""
return self._execute('execute', sql, parameters)
def validate(self):
"""Validate the session can connect or has open connections to
PostgreSQL. As of ``1.10.3``
.. deprecated:: 1.10.3
As of 1.10.3, this method only warns about Deprecation
:rtype: bool
"""
warnings.warn(
'All functionality removed from this method', DeprecationWarning)
def _connect(self):
"""Connect to PostgreSQL, either by reusing a connection from the pool
if possible, or by creating the new connection.
:rtype: psycopg2.extensions.connection
:raises: pool.NoIdleConnectionsError
"""
future = concurrent.Future()
# Attempt to get a cached connection from the connection pool
try:
connection = self._pool_manager.get(self.pid, self)
self._connections[connection.fileno()] = connection
future.set_result(connection)
# Add the connection to the IOLoop
self._ioloop.add_handler(connection.fileno(),
self._on_io_events,
ioloop.IOLoop.WRITE)
except pool.NoIdleConnectionsError:
self._create_connection(future)
return future
def _create_connection(self, future):
"""Create a new PostgreSQL connection
:param tornado.concurrent.Future future: future for new conn result
"""
LOGGER.debug('Creating a new connection for %s', self.pid)
# Create a new PostgreSQL connection
kwargs = utils.uri_to_kwargs(self._uri)
try:
connection = self._psycopg2_connect(kwargs)
except (psycopg2.Error, OSError, socket.error) as error:
future.set_exception(error)
return
# Add the connection for use in _poll_connection
fd = connection.fileno()
self._connections[fd] = connection
def on_connected(cf):
:param tornado.concurrent.Future future: future for new conn result
"""
# Create a new PostgreSQL connection
kwargs = utils.uri_to_kwargs(self._uri)
future.set_exception(cf.exception())
else:
try:
# Add the connection to the pool
LOGGER.debug('Connection established for %s', self.pid)
self._pool_manager.add(self.pid, connection)
except (ValueError, pool.PoolException) as err:
LOGGER.exception('Failed to add %r to the pool', self.pid)
self._cleanup_fd(fd)
future.set_exception(err)
return
self._pool_manager.lock(self.pid, connection, self)
# Added in because psycopg2cffi connects and leaves the
try:
# Add the connection to the pool
self._pool_manager.add(self.pid, connection)
except Exception as error:
LOGGER.exception('Failed to add %r to the pool', self.pid)
future.set_exception(error)
return
# Set the future result
future.set_result(connection)
# Add a future that fires once connected
self._futures[fd] = concurrent.Future()
self._ioloop.add_future(self._futures[fd], on_connected)
# Add the connection to the IOLoop
self._ioloop.add_handler(connection.fileno(),
self._on_io_events,
ioloop.IOLoop.WRITE)
def _execute(self, method, query, parameters=None):
"""Issue a query asynchronously on the server, mogrifying the
parameters against the sql statement and yielding the results
as a :py:class:`Results <queries.tornado_session.Results>` object.
This function reduces duplicate code for callproc and query by getting
the class attribute for the method passed in as the function to call.
:param str method: The method attribute to use
:param str query: The SQL statement or Stored Procedure name
:param list|dict parameters: A dictionary of query parameters
:rtype: Results
:raises: queries.DataError
:raises: queries.DatabaseError
:raises: queries.IntegrityError
:raises: queries.InternalError
:raises: queries.InterfaceError
:raises: queries.NotSupportedError
:raises: queries.OperationalError
:raises: queries.ProgrammingError
"""
future = concurrent.Future()
def on_connected(cf):
"""Invoked by the future returned by self._connect"""
if cf.exception():
future.set_exception(cf.exception())
return
# Get the psycopg2 connection object and cursor
conn = cf.result()
cursor = self._get_cursor(conn)
def completed(qf):
"""Invoked by the IOLoop when the future has completed"""
if qf.exception():
self._incr_exceptions(conn)
err = qf.exception()
LOGGER.debug('Cleaning cursor due to exception: %r', err)
self._exec_cleanup(cursor, conn.fileno())
future.set_exception(err)
else:
self._incr_executions(conn)
value = Results(cursor, self._exec_cleanup, conn.fileno())
future.set_result(value)
# Setup a callback to wait on the query result
self._futures[conn.fileno()] = concurrent.Future()
# Add the future to the IOLoop
self._ioloop.add_future(self._futures[conn.fileno()],
self._exec_cleanup(cursor, conn.fileno())
future.set_exception(error)
else:
results = Results(cursor,
self._exec_cleanup,
conn.fileno())
future.set_result(results)
# Setup a callback to wait on the query result
self._futures[conn.fileno()] = concurrent.TracebackFuture()
# Ensure the pool exists for the connection
self._ensure_pool_exists()
# Grab a connection to PostgreSQL
self._ioloop.add_future(self._connect(), on_connected)
# Return the future for the query result
return future
def _exec_cleanup(self, cursor, fd):
"""Close the cursor, remove any references to the fd in internal state
and remove the fd from the ioloop.
:param psycopg2.extensions.cursor cursor: The cursor to close
:param int fd: The connection file descriptor
"""
LOGGER.debug('Closing cursor and cleaning %s', fd)
try:
cursor.close()
except (psycopg2.Error, psycopg2.Warning) as error:
LOGGER.debug('Error closing the cursor: %s', error)
self._cleanup_fd(fd)
# If the cleanup callback exists, remove it
if self._cleanup_callback:
self._ioloop.remove_timeout(self._cleanup_callback)
# Create a new cleanup callback to clean the pool of idle connections
self._cleanup_callback = self._ioloop.add_timeout(
self._ioloop.time() + self._pool_idle_ttl + 1,
self._pool_manager.clean, self.pid)
def _cleanup_fd(self, fd, close=False):
"""Ensure the socket socket is removed from the IOLoop, the
connection stack, and futures stack.
:param int fd: The fd # to cleanup
"""
self._ioloop.remove_handler(fd)
if fd in self._connections:
try:
self._pool_manager.free(self.pid, self._connections[fd])
except pool.ConnectionNotFoundError:
pass
if close:
self._connections[fd].close()
del self._connections[fd]
if fd in self._futures:
del self._futures[fd]
def _incr_exceptions(self, conn):
"""Increment the number of exceptions for the current connection.
:param psycopg2.extensions.connection conn: the psycopg2 connection
"""
self._pool_manager.get_connection(self.pid, conn).exceptions += 1
def _incr_executions(self, conn):
"""Increment the number of executions for the current connection.
:param psycopg2.extensions.connection conn: the psycopg2 connection
"""
self._pool_manager.get_connection(self.pid, conn).executions += 1
def _on_io_events(self, fd=None, _events=None):
"""Invoked by Tornado's IOLoop when there are events for the fd
:param int fd: The file descriptor for the event
:param int _events: The events raised
"""
if fd not in self._connections:
LOGGER.warning('Received IO event for non-existing connection')
return
self._poll_connection(fd)
def _poll_connection(self, fd):
"""Check with psycopg2 to see what action to take. If the state is
POLL_OK, we should have a pending callback for that fd.
:param int fd: The socket fd for the postgresql connection
"""
try:
state = self._connections[fd].poll()
except (OSError, socket.error) as error:
self._ioloop.remove_handler(fd)
if fd in self._futures and not self._futures[fd].done():
self._futures[fd].set_exception(
psycopg2.OperationalError('Connection error (%s)' % error)
)
except (psycopg2.Error, psycopg2.Warning) as error:
if fd in self._futures and not self._futures[fd].done():
self._futures[fd].set_exception(error)
else:
if state == extensions.POLL_OK:
if fd in self._futures and not self._futures[fd].done():
self._futures[fd].set_result(True)
elif state == extensions.POLL_WRITE:
self._ioloop.update_handler(fd, ioloop.IOLoop.WRITE)
elif state == extensions.POLL_READ:
self._ioloop.update_handler(fd, ioloop.IOLoop.READ)
elif state == extensions.POLL_ERROR:
self._ioloop.remove_handler(fd)
if fd in self._futures and not self._futures[fd].done():
self._futures[fd].set_exception(
psycopg2.Error('Poll Error'))
def _psycopg2_connect(self, kwargs):
"""Return a psycopg2 connection for the specified kwargs. Extend for
use in async session adapters.
:param dict kwargs: Keyword connection args
:rtype: psycopg2.extensions.connection
"""
kwargs['async'] = True
return psycopg2.connect(**kwargs)
<MSG> Be more explicit in exception catching
- Instead of catchig a bare exception, catch the two known exception types that could be thrown when adding a connection to a pool.
- Increase logging usefulness
- Don't resue the results variable that is used as an import for queries.results
<DFF> @@ -291,6 +291,8 @@ class TornadoSession(session.Session):
:param tornado.concurrent.Future future: future for new conn result
"""
+ LOGGER.debug('Creating a new connection for %s', self.pid)
+
# Create a new PostgreSQL connection
kwargs = utils.uri_to_kwargs(self._uri)
@@ -314,8 +316,9 @@ class TornadoSession(session.Session):
try:
# Add the connection to the pool
+ LOGGER.debug('Connection established for %s', self.pid)
self._pool_manager.add(self.pid, connection)
- except Exception as error:
+ except (ValueError, pool.PoolException) as error:
LOGGER.exception('Failed to add %r to the pool', self.pid)
future.set_exception(error)
return
@@ -387,10 +390,8 @@ class TornadoSession(session.Session):
self._exec_cleanup(cursor, conn.fileno())
future.set_exception(error)
else:
- results = Results(cursor,
- self._exec_cleanup,
- conn.fileno())
- future.set_result(results)
+ value = Results(cursor, self._exec_cleanup, conn.fileno())
+ future.set_result(value)
# Setup a callback to wait on the query result
self._futures[conn.fileno()] = concurrent.TracebackFuture()
| 6 | Be more explicit in exception catching | 5 | .py | py | bsd-3-clause | gmr/queries |
Subsets and Splits