Search is not available for this dataset
id
stringlengths 1
8
| text
stringlengths 72
9.81M
| addition_count
int64 0
10k
| commit_subject
stringlengths 0
3.7k
| deletion_count
int64 0
8.43k
| file_extension
stringlengths 0
32
| lang
stringlengths 1
94
| license
stringclasses 10
values | repo_name
stringlengths 9
59
|
---|---|---|---|---|---|---|---|---|
10063750 | <NME> CompletionWindowBase.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Rendering;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Styling;
using Avalonia.Threading;
using Avalonia.VisualTree;
namespace AvaloniaEdit.CodeCompletion
{
/// <summary>
/// Base class for completion windows. Handles positioning the window at the caret.
/// </summary>
public class CompletionWindowBase : Popup, IStyleable
{
static CompletionWindowBase()
{
//BackgroundProperty.OverrideDefaultValue(typeof(CompletionWindowBase), Brushes.White);
}
Type IStyleable.StyleKey => typeof(PopupRoot);
/// <summary>
/// Gets the parent TextArea.
/// </summary>
public TextArea TextArea { get; }
private readonly Window _parentWindow;
private TextDocument _document;
/// <summary>
/// Gets/Sets the start of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int StartOffset { get; set; }
/// <summary>
/// Gets/Sets the end of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int EndOffset { get; set; }
/// <summary>
/// Gets whether the window was opened above the current line.
/// </summary>
protected bool IsUp { get; private set; }
/// <summary>
/// Creates a new CompletionWindowBase.
/// </summary>
public CompletionWindowBase(TextArea textArea) : base()
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
_parentWindow = textArea.GetVisualRoot() as Window;
AddHandler(PointerReleasedEvent, OnMouseUp, handledEventsToo: true);
StartOffset = EndOffset = TextArea.Caret.Offset;
PlacementTarget = TextArea.TextView;
StartOffset = EndOffset = TextArea.Caret.Offset;
// TODO: these events do not fire on PopupRoot
//Deactivated += OnDeactivated;
//Closed += (sender, args) => DetachEvents();
AttachEvents();
Initailize();
}
protected virtual void OnClosed()
{
DetachEvents();
}
private void Initailize()
{
if (_document != null && StartOffset != TextArea.Caret.Offset)
{
SetPosition(new TextViewPosition(_document.GetLocation(StartOffset)));
}
else
{
SetPosition(TextArea.Caret.Position);
}
}
public void Show()
{
UpdatePosition();
Open();
Height = double.NaN;
MinHeight = 0;
}
public void Hide()
{
Close();
OnClosed();
}
#region Event Handlers
private void AttachEvents()
{
((ISetLogicalParent)this).SetParent(TextArea.GetVisualRoot() as ILogical);
_document = TextArea.Document;
if (_document != null)
{
_document.Changing += TextArea_Document_Changing;
}
// LostKeyboardFocus seems to be more reliable than PreviewLostKeyboardFocus - see SD-1729
TextArea.LostFocus += TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged += TextViewScrollOffsetChanged;
TextArea.DocumentChanged += TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged += ParentWindow_LocationChanged;
_parentWindow.Deactivated += ParentWindow_Deactivated;
}
// close previous completion windows of same type
foreach (var x in TextArea.StackedInputHandlers.OfType<InputHandler>())
{
if (x.Window.GetType() == GetType())
TextArea.PopStackedInputHandler(x);
}
_myInputHandler = new InputHandler(this);
TextArea.PushStackedInputHandler(_myInputHandler);
}
/// <summary>
/// Detaches events from the text area.
/// </summary>
protected virtual void DetachEvents()
{
((ISetLogicalParent)this).SetParent(null);
if (_document != null)
{
_document.Changing -= TextArea_Document_Changing;
}
TextArea.LostFocus -= TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged -= TextViewScrollOffsetChanged;
TextArea.DocumentChanged -= TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged -= ParentWindow_LocationChanged;
_parentWindow.Deactivated -= ParentWindow_Deactivated;
}
TextArea.PopStackedInputHandler(_myInputHandler);
}
#region InputHandler
private InputHandler _myInputHandler;
/// <summary>
/// A dummy input handler (that justs invokes the default input handler).
/// This is used to ensure the completion window closes when any other input handler
/// becomes active.
/// </summary>
private sealed class InputHandler : TextAreaStackedInputHandler
{
internal readonly CompletionWindowBase Window;
public InputHandler(CompletionWindowBase window)
: base(window.TextArea)
{
Debug.Assert(window != null);
Window = window;
}
public override void Detach()
{
base.Detach();
Window.Hide();
}
public override void OnPreviewKeyDown(KeyEventArgs e)
{
// prevents crash when typing deadchar while CC window is open
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyDownEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
public override void OnPreviewKeyUp(KeyEventArgs e)
{
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyUpEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
}
#endregion
private void TextViewScrollOffsetChanged(object sender, EventArgs e)
{
ILogicalScrollable textView = TextArea;
var visibleRect = new Rect(textView.Offset.X, textView.Offset.Y, textView.Viewport.Width, textView.Viewport.Height);
//close completion window when the user scrolls so far that the anchor position is leaving the visible area
if (visibleRect.Contains(_visualLocation) || visibleRect.Contains(_visualLocationTop))
{
UpdatePosition();
}
else
{
Hide();
}
}
private void TextAreaDocumentChanged(object sender, EventArgs e)
{
Hide();
}
private void TextAreaLostFocus(object sender, RoutedEventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
private void ParentWindow_Deactivated(object sender, EventArgs e)
{
Hide();
}
private void ParentWindow_LocationChanged(object sender, EventArgs e)
{
UpdatePosition();
}
/// <inheritdoc/>
private void OnDeactivated(object sender, EventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
#endregion
/// <summary>
/// Raises a tunnel/bubble event pair for a control.
/// </summary>
/// <param name="target">The control for which the event should be raised.</param>
/// <param name="previewEvent">The tunneling event.</param>
/// <param name="event">The bubbling event.</param>
/// <param name="args">The event args to use.</param>
/// <returns>The <see cref="RoutedEventArgs.Handled"/> value of the event args.</returns>
[SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")]
protected static bool RaiseEventPair(Control target, RoutedEvent previewEvent, RoutedEvent @event, RoutedEventArgs args)
{
if (target == null)
throw new ArgumentNullException(nameof(target));
if (args == null)
throw new ArgumentNullException(nameof(args));
if (previewEvent != null)
{
args.RoutedEvent = previewEvent;
target.RaiseEvent(args);
}
args.RoutedEvent = @event ?? throw new ArgumentNullException(nameof(@event));
target.RaiseEvent(args);
return args.Handled;
}
// Special handler: handledEventsToo
private void OnMouseUp(object sender, PointerReleasedEventArgs e)
{
ActivateParentWindow();
}
/// <summary>
/// Activates the parent window.
/// </summary>
protected virtual void ActivateParentWindow()
{
_parentWindow?.Activate();
}
private void CloseIfFocusLost()
{
if (CloseOnFocusLost)
{
Debug.WriteLine("CloseIfFocusLost: this.IsFocues=" + IsFocused + " IsTextAreaFocused=" + IsTextAreaFocused);
if (!IsFocused && !IsTextAreaFocused)
{
Hide();
}
}
}
/// <summary>
/// Gets whether the completion window should automatically close when the text editor looses focus.
/// </summary>
protected virtual bool CloseOnFocusLost => true;
private bool IsTextAreaFocused
{
get
{
if (_parentWindow != null && !_parentWindow.IsActive)
return false;
return TextArea.IsFocused;
}
}
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
if (!e.Handled && e.Key == Key.Escape)
{
e.Handled = true;
Hide();
}
}
private Point _visualLocation;
private Point _visualLocationTop;
/// <summary>
/// Positions the completion window at the specified position.
/// </summary>
protected void SetPosition(TextViewPosition position)
{
var textView = TextArea.TextView;
_visualLocation = textView.GetVisualPosition(position, VisualYPosition.LineBottom);
_visualLocationTop = textView.GetVisualPosition(position, VisualYPosition.LineTop);
UpdatePosition();
}
/// <summary>
/// Updates the position of the CompletionWindow based on the parent TextView position and the screen working area.
/// It ensures that the CompletionWindow is completely visible on the screen.
/// </summary>
protected void UpdatePosition()
{
var textView = TextArea.TextView;
var position = _visualLocation - textView.ScrollOffset;
this.HorizontalOffset = position.X;
this.VerticalOffset = position.Y;
}
// TODO: check if needed
///// <inheritdoc/>
//protected override void OnRenderSizeChanged(SizeChangedInfo sizeInfo)
//{
// base.OnRenderSizeChanged(sizeInfo);
// if (sizeInfo.HeightChanged && IsUp)
// {
// this.Top += sizeInfo.PreviousSize.Height - sizeInfo.NewSize.Height;
// }
//}
/// <summary>
/// Gets/sets whether the completion window should expect text insertion at the start offset,
/// which not go into the completion region, but before it.
/// </summary>
/// <remarks>This property allows only a single insertion, it is reset to false
/// when that insertion has occurred.</remarks>
public bool ExpectInsertionBeforeStart { get; set; }
private void TextArea_Document_Changing(object sender, DocumentChangeEventArgs e)
{
if (e.Offset + e.RemovalLength == StartOffset && e.RemovalLength > 0)
{
Hide(); // removal immediately in front of completion segment: close the window
// this is necessary when pressing backspace after dot-completion
}
if (e.Offset == StartOffset && e.RemovalLength == 0 && ExpectInsertionBeforeStart)
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.AfterInsertion);
ExpectInsertionBeforeStart = false;
}
else
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.BeforeInsertion);
}
EndOffset = e.GetNewOffset(EndOffset, AnchorMovementType.AfterInsertion);
}
}
}
<MSG> detachevents
<DFF> @@ -89,9 +89,9 @@ namespace AvaloniaEdit.CodeCompletion
StartOffset = EndOffset = TextArea.Caret.Offset;
- // TODO: these events do not fire on PopupRoot
- //Deactivated += OnDeactivated;
- //Closed += (sender, args) => DetachEvents();
+ //Deactivated += OnDeactivated; //Not needed?
+
+ Closed += (sender, args) => DetachEvents();
AttachEvents();
| 3 | detachevents | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063751 | <NME> CompletionWindowBase.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Rendering;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Styling;
using Avalonia.Threading;
using Avalonia.VisualTree;
namespace AvaloniaEdit.CodeCompletion
{
/// <summary>
/// Base class for completion windows. Handles positioning the window at the caret.
/// </summary>
public class CompletionWindowBase : Popup, IStyleable
{
static CompletionWindowBase()
{
//BackgroundProperty.OverrideDefaultValue(typeof(CompletionWindowBase), Brushes.White);
}
Type IStyleable.StyleKey => typeof(PopupRoot);
/// <summary>
/// Gets the parent TextArea.
/// </summary>
public TextArea TextArea { get; }
private readonly Window _parentWindow;
private TextDocument _document;
/// <summary>
/// Gets/Sets the start of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int StartOffset { get; set; }
/// <summary>
/// Gets/Sets the end of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int EndOffset { get; set; }
/// <summary>
/// Gets whether the window was opened above the current line.
/// </summary>
protected bool IsUp { get; private set; }
/// <summary>
/// Creates a new CompletionWindowBase.
/// </summary>
public CompletionWindowBase(TextArea textArea) : base()
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
_parentWindow = textArea.GetVisualRoot() as Window;
AddHandler(PointerReleasedEvent, OnMouseUp, handledEventsToo: true);
StartOffset = EndOffset = TextArea.Caret.Offset;
PlacementTarget = TextArea.TextView;
StartOffset = EndOffset = TextArea.Caret.Offset;
// TODO: these events do not fire on PopupRoot
//Deactivated += OnDeactivated;
//Closed += (sender, args) => DetachEvents();
AttachEvents();
Initailize();
}
protected virtual void OnClosed()
{
DetachEvents();
}
private void Initailize()
{
if (_document != null && StartOffset != TextArea.Caret.Offset)
{
SetPosition(new TextViewPosition(_document.GetLocation(StartOffset)));
}
else
{
SetPosition(TextArea.Caret.Position);
}
}
public void Show()
{
UpdatePosition();
Open();
Height = double.NaN;
MinHeight = 0;
}
public void Hide()
{
Close();
OnClosed();
}
#region Event Handlers
private void AttachEvents()
{
((ISetLogicalParent)this).SetParent(TextArea.GetVisualRoot() as ILogical);
_document = TextArea.Document;
if (_document != null)
{
_document.Changing += TextArea_Document_Changing;
}
// LostKeyboardFocus seems to be more reliable than PreviewLostKeyboardFocus - see SD-1729
TextArea.LostFocus += TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged += TextViewScrollOffsetChanged;
TextArea.DocumentChanged += TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged += ParentWindow_LocationChanged;
_parentWindow.Deactivated += ParentWindow_Deactivated;
}
// close previous completion windows of same type
foreach (var x in TextArea.StackedInputHandlers.OfType<InputHandler>())
{
if (x.Window.GetType() == GetType())
TextArea.PopStackedInputHandler(x);
}
_myInputHandler = new InputHandler(this);
TextArea.PushStackedInputHandler(_myInputHandler);
}
/// <summary>
/// Detaches events from the text area.
/// </summary>
protected virtual void DetachEvents()
{
((ISetLogicalParent)this).SetParent(null);
if (_document != null)
{
_document.Changing -= TextArea_Document_Changing;
}
TextArea.LostFocus -= TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged -= TextViewScrollOffsetChanged;
TextArea.DocumentChanged -= TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged -= ParentWindow_LocationChanged;
_parentWindow.Deactivated -= ParentWindow_Deactivated;
}
TextArea.PopStackedInputHandler(_myInputHandler);
}
#region InputHandler
private InputHandler _myInputHandler;
/// <summary>
/// A dummy input handler (that justs invokes the default input handler).
/// This is used to ensure the completion window closes when any other input handler
/// becomes active.
/// </summary>
private sealed class InputHandler : TextAreaStackedInputHandler
{
internal readonly CompletionWindowBase Window;
public InputHandler(CompletionWindowBase window)
: base(window.TextArea)
{
Debug.Assert(window != null);
Window = window;
}
public override void Detach()
{
base.Detach();
Window.Hide();
}
public override void OnPreviewKeyDown(KeyEventArgs e)
{
// prevents crash when typing deadchar while CC window is open
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyDownEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
public override void OnPreviewKeyUp(KeyEventArgs e)
{
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyUpEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
}
#endregion
private void TextViewScrollOffsetChanged(object sender, EventArgs e)
{
ILogicalScrollable textView = TextArea;
var visibleRect = new Rect(textView.Offset.X, textView.Offset.Y, textView.Viewport.Width, textView.Viewport.Height);
//close completion window when the user scrolls so far that the anchor position is leaving the visible area
if (visibleRect.Contains(_visualLocation) || visibleRect.Contains(_visualLocationTop))
{
UpdatePosition();
}
else
{
Hide();
}
}
private void TextAreaDocumentChanged(object sender, EventArgs e)
{
Hide();
}
private void TextAreaLostFocus(object sender, RoutedEventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
private void ParentWindow_Deactivated(object sender, EventArgs e)
{
Hide();
}
private void ParentWindow_LocationChanged(object sender, EventArgs e)
{
UpdatePosition();
}
/// <inheritdoc/>
private void OnDeactivated(object sender, EventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
#endregion
/// <summary>
/// Raises a tunnel/bubble event pair for a control.
/// </summary>
/// <param name="target">The control for which the event should be raised.</param>
/// <param name="previewEvent">The tunneling event.</param>
/// <param name="event">The bubbling event.</param>
/// <param name="args">The event args to use.</param>
/// <returns>The <see cref="RoutedEventArgs.Handled"/> value of the event args.</returns>
[SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")]
protected static bool RaiseEventPair(Control target, RoutedEvent previewEvent, RoutedEvent @event, RoutedEventArgs args)
{
if (target == null)
throw new ArgumentNullException(nameof(target));
if (args == null)
throw new ArgumentNullException(nameof(args));
if (previewEvent != null)
{
args.RoutedEvent = previewEvent;
target.RaiseEvent(args);
}
args.RoutedEvent = @event ?? throw new ArgumentNullException(nameof(@event));
target.RaiseEvent(args);
return args.Handled;
}
// Special handler: handledEventsToo
private void OnMouseUp(object sender, PointerReleasedEventArgs e)
{
ActivateParentWindow();
}
/// <summary>
/// Activates the parent window.
/// </summary>
protected virtual void ActivateParentWindow()
{
_parentWindow?.Activate();
}
private void CloseIfFocusLost()
{
if (CloseOnFocusLost)
{
Debug.WriteLine("CloseIfFocusLost: this.IsFocues=" + IsFocused + " IsTextAreaFocused=" + IsTextAreaFocused);
if (!IsFocused && !IsTextAreaFocused)
{
Hide();
}
}
}
/// <summary>
/// Gets whether the completion window should automatically close when the text editor looses focus.
/// </summary>
protected virtual bool CloseOnFocusLost => true;
private bool IsTextAreaFocused
{
get
{
if (_parentWindow != null && !_parentWindow.IsActive)
return false;
return TextArea.IsFocused;
}
}
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
if (!e.Handled && e.Key == Key.Escape)
{
e.Handled = true;
Hide();
}
}
private Point _visualLocation;
private Point _visualLocationTop;
/// <summary>
/// Positions the completion window at the specified position.
/// </summary>
protected void SetPosition(TextViewPosition position)
{
var textView = TextArea.TextView;
_visualLocation = textView.GetVisualPosition(position, VisualYPosition.LineBottom);
_visualLocationTop = textView.GetVisualPosition(position, VisualYPosition.LineTop);
UpdatePosition();
}
/// <summary>
/// Updates the position of the CompletionWindow based on the parent TextView position and the screen working area.
/// It ensures that the CompletionWindow is completely visible on the screen.
/// </summary>
protected void UpdatePosition()
{
var textView = TextArea.TextView;
var position = _visualLocation - textView.ScrollOffset;
this.HorizontalOffset = position.X;
this.VerticalOffset = position.Y;
}
// TODO: check if needed
///// <inheritdoc/>
//protected override void OnRenderSizeChanged(SizeChangedInfo sizeInfo)
//{
// base.OnRenderSizeChanged(sizeInfo);
// if (sizeInfo.HeightChanged && IsUp)
// {
// this.Top += sizeInfo.PreviousSize.Height - sizeInfo.NewSize.Height;
// }
//}
/// <summary>
/// Gets/sets whether the completion window should expect text insertion at the start offset,
/// which not go into the completion region, but before it.
/// </summary>
/// <remarks>This property allows only a single insertion, it is reset to false
/// when that insertion has occurred.</remarks>
public bool ExpectInsertionBeforeStart { get; set; }
private void TextArea_Document_Changing(object sender, DocumentChangeEventArgs e)
{
if (e.Offset + e.RemovalLength == StartOffset && e.RemovalLength > 0)
{
Hide(); // removal immediately in front of completion segment: close the window
// this is necessary when pressing backspace after dot-completion
}
if (e.Offset == StartOffset && e.RemovalLength == 0 && ExpectInsertionBeforeStart)
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.AfterInsertion);
ExpectInsertionBeforeStart = false;
}
else
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.BeforeInsertion);
}
EndOffset = e.GetNewOffset(EndOffset, AnchorMovementType.AfterInsertion);
}
}
}
<MSG> detachevents
<DFF> @@ -89,9 +89,9 @@ namespace AvaloniaEdit.CodeCompletion
StartOffset = EndOffset = TextArea.Caret.Offset;
- // TODO: these events do not fire on PopupRoot
- //Deactivated += OnDeactivated;
- //Closed += (sender, args) => DetachEvents();
+ //Deactivated += OnDeactivated; //Not needed?
+
+ Closed += (sender, args) => DetachEvents();
AttachEvents();
| 3 | detachevents | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063752 | <NME> CompletionWindowBase.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Rendering;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Styling;
using Avalonia.Threading;
using Avalonia.VisualTree;
namespace AvaloniaEdit.CodeCompletion
{
/// <summary>
/// Base class for completion windows. Handles positioning the window at the caret.
/// </summary>
public class CompletionWindowBase : Popup, IStyleable
{
static CompletionWindowBase()
{
//BackgroundProperty.OverrideDefaultValue(typeof(CompletionWindowBase), Brushes.White);
}
Type IStyleable.StyleKey => typeof(PopupRoot);
/// <summary>
/// Gets the parent TextArea.
/// </summary>
public TextArea TextArea { get; }
private readonly Window _parentWindow;
private TextDocument _document;
/// <summary>
/// Gets/Sets the start of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int StartOffset { get; set; }
/// <summary>
/// Gets/Sets the end of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int EndOffset { get; set; }
/// <summary>
/// Gets whether the window was opened above the current line.
/// </summary>
protected bool IsUp { get; private set; }
/// <summary>
/// Creates a new CompletionWindowBase.
/// </summary>
public CompletionWindowBase(TextArea textArea) : base()
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
_parentWindow = textArea.GetVisualRoot() as Window;
AddHandler(PointerReleasedEvent, OnMouseUp, handledEventsToo: true);
StartOffset = EndOffset = TextArea.Caret.Offset;
PlacementTarget = TextArea.TextView;
StartOffset = EndOffset = TextArea.Caret.Offset;
// TODO: these events do not fire on PopupRoot
//Deactivated += OnDeactivated;
//Closed += (sender, args) => DetachEvents();
AttachEvents();
Initailize();
}
protected virtual void OnClosed()
{
DetachEvents();
}
private void Initailize()
{
if (_document != null && StartOffset != TextArea.Caret.Offset)
{
SetPosition(new TextViewPosition(_document.GetLocation(StartOffset)));
}
else
{
SetPosition(TextArea.Caret.Position);
}
}
public void Show()
{
UpdatePosition();
Open();
Height = double.NaN;
MinHeight = 0;
}
public void Hide()
{
Close();
OnClosed();
}
#region Event Handlers
private void AttachEvents()
{
((ISetLogicalParent)this).SetParent(TextArea.GetVisualRoot() as ILogical);
_document = TextArea.Document;
if (_document != null)
{
_document.Changing += TextArea_Document_Changing;
}
// LostKeyboardFocus seems to be more reliable than PreviewLostKeyboardFocus - see SD-1729
TextArea.LostFocus += TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged += TextViewScrollOffsetChanged;
TextArea.DocumentChanged += TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged += ParentWindow_LocationChanged;
_parentWindow.Deactivated += ParentWindow_Deactivated;
}
// close previous completion windows of same type
foreach (var x in TextArea.StackedInputHandlers.OfType<InputHandler>())
{
if (x.Window.GetType() == GetType())
TextArea.PopStackedInputHandler(x);
}
_myInputHandler = new InputHandler(this);
TextArea.PushStackedInputHandler(_myInputHandler);
}
/// <summary>
/// Detaches events from the text area.
/// </summary>
protected virtual void DetachEvents()
{
((ISetLogicalParent)this).SetParent(null);
if (_document != null)
{
_document.Changing -= TextArea_Document_Changing;
}
TextArea.LostFocus -= TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged -= TextViewScrollOffsetChanged;
TextArea.DocumentChanged -= TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged -= ParentWindow_LocationChanged;
_parentWindow.Deactivated -= ParentWindow_Deactivated;
}
TextArea.PopStackedInputHandler(_myInputHandler);
}
#region InputHandler
private InputHandler _myInputHandler;
/// <summary>
/// A dummy input handler (that justs invokes the default input handler).
/// This is used to ensure the completion window closes when any other input handler
/// becomes active.
/// </summary>
private sealed class InputHandler : TextAreaStackedInputHandler
{
internal readonly CompletionWindowBase Window;
public InputHandler(CompletionWindowBase window)
: base(window.TextArea)
{
Debug.Assert(window != null);
Window = window;
}
public override void Detach()
{
base.Detach();
Window.Hide();
}
public override void OnPreviewKeyDown(KeyEventArgs e)
{
// prevents crash when typing deadchar while CC window is open
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyDownEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
public override void OnPreviewKeyUp(KeyEventArgs e)
{
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyUpEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
}
#endregion
private void TextViewScrollOffsetChanged(object sender, EventArgs e)
{
ILogicalScrollable textView = TextArea;
var visibleRect = new Rect(textView.Offset.X, textView.Offset.Y, textView.Viewport.Width, textView.Viewport.Height);
//close completion window when the user scrolls so far that the anchor position is leaving the visible area
if (visibleRect.Contains(_visualLocation) || visibleRect.Contains(_visualLocationTop))
{
UpdatePosition();
}
else
{
Hide();
}
}
private void TextAreaDocumentChanged(object sender, EventArgs e)
{
Hide();
}
private void TextAreaLostFocus(object sender, RoutedEventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
private void ParentWindow_Deactivated(object sender, EventArgs e)
{
Hide();
}
private void ParentWindow_LocationChanged(object sender, EventArgs e)
{
UpdatePosition();
}
/// <inheritdoc/>
private void OnDeactivated(object sender, EventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
#endregion
/// <summary>
/// Raises a tunnel/bubble event pair for a control.
/// </summary>
/// <param name="target">The control for which the event should be raised.</param>
/// <param name="previewEvent">The tunneling event.</param>
/// <param name="event">The bubbling event.</param>
/// <param name="args">The event args to use.</param>
/// <returns>The <see cref="RoutedEventArgs.Handled"/> value of the event args.</returns>
[SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")]
protected static bool RaiseEventPair(Control target, RoutedEvent previewEvent, RoutedEvent @event, RoutedEventArgs args)
{
if (target == null)
throw new ArgumentNullException(nameof(target));
if (args == null)
throw new ArgumentNullException(nameof(args));
if (previewEvent != null)
{
args.RoutedEvent = previewEvent;
target.RaiseEvent(args);
}
args.RoutedEvent = @event ?? throw new ArgumentNullException(nameof(@event));
target.RaiseEvent(args);
return args.Handled;
}
// Special handler: handledEventsToo
private void OnMouseUp(object sender, PointerReleasedEventArgs e)
{
ActivateParentWindow();
}
/// <summary>
/// Activates the parent window.
/// </summary>
protected virtual void ActivateParentWindow()
{
_parentWindow?.Activate();
}
private void CloseIfFocusLost()
{
if (CloseOnFocusLost)
{
Debug.WriteLine("CloseIfFocusLost: this.IsFocues=" + IsFocused + " IsTextAreaFocused=" + IsTextAreaFocused);
if (!IsFocused && !IsTextAreaFocused)
{
Hide();
}
}
}
/// <summary>
/// Gets whether the completion window should automatically close when the text editor looses focus.
/// </summary>
protected virtual bool CloseOnFocusLost => true;
private bool IsTextAreaFocused
{
get
{
if (_parentWindow != null && !_parentWindow.IsActive)
return false;
return TextArea.IsFocused;
}
}
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
if (!e.Handled && e.Key == Key.Escape)
{
e.Handled = true;
Hide();
}
}
private Point _visualLocation;
private Point _visualLocationTop;
/// <summary>
/// Positions the completion window at the specified position.
/// </summary>
protected void SetPosition(TextViewPosition position)
{
var textView = TextArea.TextView;
_visualLocation = textView.GetVisualPosition(position, VisualYPosition.LineBottom);
_visualLocationTop = textView.GetVisualPosition(position, VisualYPosition.LineTop);
UpdatePosition();
}
/// <summary>
/// Updates the position of the CompletionWindow based on the parent TextView position and the screen working area.
/// It ensures that the CompletionWindow is completely visible on the screen.
/// </summary>
protected void UpdatePosition()
{
var textView = TextArea.TextView;
var position = _visualLocation - textView.ScrollOffset;
this.HorizontalOffset = position.X;
this.VerticalOffset = position.Y;
}
// TODO: check if needed
///// <inheritdoc/>
//protected override void OnRenderSizeChanged(SizeChangedInfo sizeInfo)
//{
// base.OnRenderSizeChanged(sizeInfo);
// if (sizeInfo.HeightChanged && IsUp)
// {
// this.Top += sizeInfo.PreviousSize.Height - sizeInfo.NewSize.Height;
// }
//}
/// <summary>
/// Gets/sets whether the completion window should expect text insertion at the start offset,
/// which not go into the completion region, but before it.
/// </summary>
/// <remarks>This property allows only a single insertion, it is reset to false
/// when that insertion has occurred.</remarks>
public bool ExpectInsertionBeforeStart { get; set; }
private void TextArea_Document_Changing(object sender, DocumentChangeEventArgs e)
{
if (e.Offset + e.RemovalLength == StartOffset && e.RemovalLength > 0)
{
Hide(); // removal immediately in front of completion segment: close the window
// this is necessary when pressing backspace after dot-completion
}
if (e.Offset == StartOffset && e.RemovalLength == 0 && ExpectInsertionBeforeStart)
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.AfterInsertion);
ExpectInsertionBeforeStart = false;
}
else
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.BeforeInsertion);
}
EndOffset = e.GetNewOffset(EndOffset, AnchorMovementType.AfterInsertion);
}
}
}
<MSG> detachevents
<DFF> @@ -89,9 +89,9 @@ namespace AvaloniaEdit.CodeCompletion
StartOffset = EndOffset = TextArea.Caret.Offset;
- // TODO: these events do not fire on PopupRoot
- //Deactivated += OnDeactivated;
- //Closed += (sender, args) => DetachEvents();
+ //Deactivated += OnDeactivated; //Not needed?
+
+ Closed += (sender, args) => DetachEvents();
AttachEvents();
| 3 | detachevents | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063753 | <NME> CompletionWindowBase.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Rendering;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Styling;
using Avalonia.Threading;
using Avalonia.VisualTree;
namespace AvaloniaEdit.CodeCompletion
{
/// <summary>
/// Base class for completion windows. Handles positioning the window at the caret.
/// </summary>
public class CompletionWindowBase : Popup, IStyleable
{
static CompletionWindowBase()
{
//BackgroundProperty.OverrideDefaultValue(typeof(CompletionWindowBase), Brushes.White);
}
Type IStyleable.StyleKey => typeof(PopupRoot);
/// <summary>
/// Gets the parent TextArea.
/// </summary>
public TextArea TextArea { get; }
private readonly Window _parentWindow;
private TextDocument _document;
/// <summary>
/// Gets/Sets the start of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int StartOffset { get; set; }
/// <summary>
/// Gets/Sets the end of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int EndOffset { get; set; }
/// <summary>
/// Gets whether the window was opened above the current line.
/// </summary>
protected bool IsUp { get; private set; }
/// <summary>
/// Creates a new CompletionWindowBase.
/// </summary>
public CompletionWindowBase(TextArea textArea) : base()
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
_parentWindow = textArea.GetVisualRoot() as Window;
AddHandler(PointerReleasedEvent, OnMouseUp, handledEventsToo: true);
StartOffset = EndOffset = TextArea.Caret.Offset;
PlacementTarget = TextArea.TextView;
StartOffset = EndOffset = TextArea.Caret.Offset;
// TODO: these events do not fire on PopupRoot
//Deactivated += OnDeactivated;
//Closed += (sender, args) => DetachEvents();
AttachEvents();
Initailize();
}
protected virtual void OnClosed()
{
DetachEvents();
}
private void Initailize()
{
if (_document != null && StartOffset != TextArea.Caret.Offset)
{
SetPosition(new TextViewPosition(_document.GetLocation(StartOffset)));
}
else
{
SetPosition(TextArea.Caret.Position);
}
}
public void Show()
{
UpdatePosition();
Open();
Height = double.NaN;
MinHeight = 0;
}
public void Hide()
{
Close();
OnClosed();
}
#region Event Handlers
private void AttachEvents()
{
((ISetLogicalParent)this).SetParent(TextArea.GetVisualRoot() as ILogical);
_document = TextArea.Document;
if (_document != null)
{
_document.Changing += TextArea_Document_Changing;
}
// LostKeyboardFocus seems to be more reliable than PreviewLostKeyboardFocus - see SD-1729
TextArea.LostFocus += TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged += TextViewScrollOffsetChanged;
TextArea.DocumentChanged += TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged += ParentWindow_LocationChanged;
_parentWindow.Deactivated += ParentWindow_Deactivated;
}
// close previous completion windows of same type
foreach (var x in TextArea.StackedInputHandlers.OfType<InputHandler>())
{
if (x.Window.GetType() == GetType())
TextArea.PopStackedInputHandler(x);
}
_myInputHandler = new InputHandler(this);
TextArea.PushStackedInputHandler(_myInputHandler);
}
/// <summary>
/// Detaches events from the text area.
/// </summary>
protected virtual void DetachEvents()
{
((ISetLogicalParent)this).SetParent(null);
if (_document != null)
{
_document.Changing -= TextArea_Document_Changing;
}
TextArea.LostFocus -= TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged -= TextViewScrollOffsetChanged;
TextArea.DocumentChanged -= TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged -= ParentWindow_LocationChanged;
_parentWindow.Deactivated -= ParentWindow_Deactivated;
}
TextArea.PopStackedInputHandler(_myInputHandler);
}
#region InputHandler
private InputHandler _myInputHandler;
/// <summary>
/// A dummy input handler (that justs invokes the default input handler).
/// This is used to ensure the completion window closes when any other input handler
/// becomes active.
/// </summary>
private sealed class InputHandler : TextAreaStackedInputHandler
{
internal readonly CompletionWindowBase Window;
public InputHandler(CompletionWindowBase window)
: base(window.TextArea)
{
Debug.Assert(window != null);
Window = window;
}
public override void Detach()
{
base.Detach();
Window.Hide();
}
public override void OnPreviewKeyDown(KeyEventArgs e)
{
// prevents crash when typing deadchar while CC window is open
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyDownEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
public override void OnPreviewKeyUp(KeyEventArgs e)
{
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyUpEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
}
#endregion
private void TextViewScrollOffsetChanged(object sender, EventArgs e)
{
ILogicalScrollable textView = TextArea;
var visibleRect = new Rect(textView.Offset.X, textView.Offset.Y, textView.Viewport.Width, textView.Viewport.Height);
//close completion window when the user scrolls so far that the anchor position is leaving the visible area
if (visibleRect.Contains(_visualLocation) || visibleRect.Contains(_visualLocationTop))
{
UpdatePosition();
}
else
{
Hide();
}
}
private void TextAreaDocumentChanged(object sender, EventArgs e)
{
Hide();
}
private void TextAreaLostFocus(object sender, RoutedEventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
private void ParentWindow_Deactivated(object sender, EventArgs e)
{
Hide();
}
private void ParentWindow_LocationChanged(object sender, EventArgs e)
{
UpdatePosition();
}
/// <inheritdoc/>
private void OnDeactivated(object sender, EventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
#endregion
/// <summary>
/// Raises a tunnel/bubble event pair for a control.
/// </summary>
/// <param name="target">The control for which the event should be raised.</param>
/// <param name="previewEvent">The tunneling event.</param>
/// <param name="event">The bubbling event.</param>
/// <param name="args">The event args to use.</param>
/// <returns>The <see cref="RoutedEventArgs.Handled"/> value of the event args.</returns>
[SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")]
protected static bool RaiseEventPair(Control target, RoutedEvent previewEvent, RoutedEvent @event, RoutedEventArgs args)
{
if (target == null)
throw new ArgumentNullException(nameof(target));
if (args == null)
throw new ArgumentNullException(nameof(args));
if (previewEvent != null)
{
args.RoutedEvent = previewEvent;
target.RaiseEvent(args);
}
args.RoutedEvent = @event ?? throw new ArgumentNullException(nameof(@event));
target.RaiseEvent(args);
return args.Handled;
}
// Special handler: handledEventsToo
private void OnMouseUp(object sender, PointerReleasedEventArgs e)
{
ActivateParentWindow();
}
/// <summary>
/// Activates the parent window.
/// </summary>
protected virtual void ActivateParentWindow()
{
_parentWindow?.Activate();
}
private void CloseIfFocusLost()
{
if (CloseOnFocusLost)
{
Debug.WriteLine("CloseIfFocusLost: this.IsFocues=" + IsFocused + " IsTextAreaFocused=" + IsTextAreaFocused);
if (!IsFocused && !IsTextAreaFocused)
{
Hide();
}
}
}
/// <summary>
/// Gets whether the completion window should automatically close when the text editor looses focus.
/// </summary>
protected virtual bool CloseOnFocusLost => true;
private bool IsTextAreaFocused
{
get
{
if (_parentWindow != null && !_parentWindow.IsActive)
return false;
return TextArea.IsFocused;
}
}
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
if (!e.Handled && e.Key == Key.Escape)
{
e.Handled = true;
Hide();
}
}
private Point _visualLocation;
private Point _visualLocationTop;
/// <summary>
/// Positions the completion window at the specified position.
/// </summary>
protected void SetPosition(TextViewPosition position)
{
var textView = TextArea.TextView;
_visualLocation = textView.GetVisualPosition(position, VisualYPosition.LineBottom);
_visualLocationTop = textView.GetVisualPosition(position, VisualYPosition.LineTop);
UpdatePosition();
}
/// <summary>
/// Updates the position of the CompletionWindow based on the parent TextView position and the screen working area.
/// It ensures that the CompletionWindow is completely visible on the screen.
/// </summary>
protected void UpdatePosition()
{
var textView = TextArea.TextView;
var position = _visualLocation - textView.ScrollOffset;
this.HorizontalOffset = position.X;
this.VerticalOffset = position.Y;
}
// TODO: check if needed
///// <inheritdoc/>
//protected override void OnRenderSizeChanged(SizeChangedInfo sizeInfo)
//{
// base.OnRenderSizeChanged(sizeInfo);
// if (sizeInfo.HeightChanged && IsUp)
// {
// this.Top += sizeInfo.PreviousSize.Height - sizeInfo.NewSize.Height;
// }
//}
/// <summary>
/// Gets/sets whether the completion window should expect text insertion at the start offset,
/// which not go into the completion region, but before it.
/// </summary>
/// <remarks>This property allows only a single insertion, it is reset to false
/// when that insertion has occurred.</remarks>
public bool ExpectInsertionBeforeStart { get; set; }
private void TextArea_Document_Changing(object sender, DocumentChangeEventArgs e)
{
if (e.Offset + e.RemovalLength == StartOffset && e.RemovalLength > 0)
{
Hide(); // removal immediately in front of completion segment: close the window
// this is necessary when pressing backspace after dot-completion
}
if (e.Offset == StartOffset && e.RemovalLength == 0 && ExpectInsertionBeforeStart)
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.AfterInsertion);
ExpectInsertionBeforeStart = false;
}
else
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.BeforeInsertion);
}
EndOffset = e.GetNewOffset(EndOffset, AnchorMovementType.AfterInsertion);
}
}
}
<MSG> detachevents
<DFF> @@ -89,9 +89,9 @@ namespace AvaloniaEdit.CodeCompletion
StartOffset = EndOffset = TextArea.Caret.Offset;
- // TODO: these events do not fire on PopupRoot
- //Deactivated += OnDeactivated;
- //Closed += (sender, args) => DetachEvents();
+ //Deactivated += OnDeactivated; //Not needed?
+
+ Closed += (sender, args) => DetachEvents();
AttachEvents();
| 3 | detachevents | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063754 | <NME> CompletionWindowBase.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Rendering;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Styling;
using Avalonia.Threading;
using Avalonia.VisualTree;
namespace AvaloniaEdit.CodeCompletion
{
/// <summary>
/// Base class for completion windows. Handles positioning the window at the caret.
/// </summary>
public class CompletionWindowBase : Popup, IStyleable
{
static CompletionWindowBase()
{
//BackgroundProperty.OverrideDefaultValue(typeof(CompletionWindowBase), Brushes.White);
}
Type IStyleable.StyleKey => typeof(PopupRoot);
/// <summary>
/// Gets the parent TextArea.
/// </summary>
public TextArea TextArea { get; }
private readonly Window _parentWindow;
private TextDocument _document;
/// <summary>
/// Gets/Sets the start of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int StartOffset { get; set; }
/// <summary>
/// Gets/Sets the end of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int EndOffset { get; set; }
/// <summary>
/// Gets whether the window was opened above the current line.
/// </summary>
protected bool IsUp { get; private set; }
/// <summary>
/// Creates a new CompletionWindowBase.
/// </summary>
public CompletionWindowBase(TextArea textArea) : base()
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
_parentWindow = textArea.GetVisualRoot() as Window;
AddHandler(PointerReleasedEvent, OnMouseUp, handledEventsToo: true);
StartOffset = EndOffset = TextArea.Caret.Offset;
PlacementTarget = TextArea.TextView;
StartOffset = EndOffset = TextArea.Caret.Offset;
// TODO: these events do not fire on PopupRoot
//Deactivated += OnDeactivated;
//Closed += (sender, args) => DetachEvents();
AttachEvents();
Initailize();
}
protected virtual void OnClosed()
{
DetachEvents();
}
private void Initailize()
{
if (_document != null && StartOffset != TextArea.Caret.Offset)
{
SetPosition(new TextViewPosition(_document.GetLocation(StartOffset)));
}
else
{
SetPosition(TextArea.Caret.Position);
}
}
public void Show()
{
UpdatePosition();
Open();
Height = double.NaN;
MinHeight = 0;
}
public void Hide()
{
Close();
OnClosed();
}
#region Event Handlers
private void AttachEvents()
{
((ISetLogicalParent)this).SetParent(TextArea.GetVisualRoot() as ILogical);
_document = TextArea.Document;
if (_document != null)
{
_document.Changing += TextArea_Document_Changing;
}
// LostKeyboardFocus seems to be more reliable than PreviewLostKeyboardFocus - see SD-1729
TextArea.LostFocus += TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged += TextViewScrollOffsetChanged;
TextArea.DocumentChanged += TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged += ParentWindow_LocationChanged;
_parentWindow.Deactivated += ParentWindow_Deactivated;
}
// close previous completion windows of same type
foreach (var x in TextArea.StackedInputHandlers.OfType<InputHandler>())
{
if (x.Window.GetType() == GetType())
TextArea.PopStackedInputHandler(x);
}
_myInputHandler = new InputHandler(this);
TextArea.PushStackedInputHandler(_myInputHandler);
}
/// <summary>
/// Detaches events from the text area.
/// </summary>
protected virtual void DetachEvents()
{
((ISetLogicalParent)this).SetParent(null);
if (_document != null)
{
_document.Changing -= TextArea_Document_Changing;
}
TextArea.LostFocus -= TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged -= TextViewScrollOffsetChanged;
TextArea.DocumentChanged -= TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged -= ParentWindow_LocationChanged;
_parentWindow.Deactivated -= ParentWindow_Deactivated;
}
TextArea.PopStackedInputHandler(_myInputHandler);
}
#region InputHandler
private InputHandler _myInputHandler;
/// <summary>
/// A dummy input handler (that justs invokes the default input handler).
/// This is used to ensure the completion window closes when any other input handler
/// becomes active.
/// </summary>
private sealed class InputHandler : TextAreaStackedInputHandler
{
internal readonly CompletionWindowBase Window;
public InputHandler(CompletionWindowBase window)
: base(window.TextArea)
{
Debug.Assert(window != null);
Window = window;
}
public override void Detach()
{
base.Detach();
Window.Hide();
}
public override void OnPreviewKeyDown(KeyEventArgs e)
{
// prevents crash when typing deadchar while CC window is open
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyDownEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
public override void OnPreviewKeyUp(KeyEventArgs e)
{
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyUpEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
}
#endregion
private void TextViewScrollOffsetChanged(object sender, EventArgs e)
{
ILogicalScrollable textView = TextArea;
var visibleRect = new Rect(textView.Offset.X, textView.Offset.Y, textView.Viewport.Width, textView.Viewport.Height);
//close completion window when the user scrolls so far that the anchor position is leaving the visible area
if (visibleRect.Contains(_visualLocation) || visibleRect.Contains(_visualLocationTop))
{
UpdatePosition();
}
else
{
Hide();
}
}
private void TextAreaDocumentChanged(object sender, EventArgs e)
{
Hide();
}
private void TextAreaLostFocus(object sender, RoutedEventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
private void ParentWindow_Deactivated(object sender, EventArgs e)
{
Hide();
}
private void ParentWindow_LocationChanged(object sender, EventArgs e)
{
UpdatePosition();
}
/// <inheritdoc/>
private void OnDeactivated(object sender, EventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
#endregion
/// <summary>
/// Raises a tunnel/bubble event pair for a control.
/// </summary>
/// <param name="target">The control for which the event should be raised.</param>
/// <param name="previewEvent">The tunneling event.</param>
/// <param name="event">The bubbling event.</param>
/// <param name="args">The event args to use.</param>
/// <returns>The <see cref="RoutedEventArgs.Handled"/> value of the event args.</returns>
[SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")]
protected static bool RaiseEventPair(Control target, RoutedEvent previewEvent, RoutedEvent @event, RoutedEventArgs args)
{
if (target == null)
throw new ArgumentNullException(nameof(target));
if (args == null)
throw new ArgumentNullException(nameof(args));
if (previewEvent != null)
{
args.RoutedEvent = previewEvent;
target.RaiseEvent(args);
}
args.RoutedEvent = @event ?? throw new ArgumentNullException(nameof(@event));
target.RaiseEvent(args);
return args.Handled;
}
// Special handler: handledEventsToo
private void OnMouseUp(object sender, PointerReleasedEventArgs e)
{
ActivateParentWindow();
}
/// <summary>
/// Activates the parent window.
/// </summary>
protected virtual void ActivateParentWindow()
{
_parentWindow?.Activate();
}
private void CloseIfFocusLost()
{
if (CloseOnFocusLost)
{
Debug.WriteLine("CloseIfFocusLost: this.IsFocues=" + IsFocused + " IsTextAreaFocused=" + IsTextAreaFocused);
if (!IsFocused && !IsTextAreaFocused)
{
Hide();
}
}
}
/// <summary>
/// Gets whether the completion window should automatically close when the text editor looses focus.
/// </summary>
protected virtual bool CloseOnFocusLost => true;
private bool IsTextAreaFocused
{
get
{
if (_parentWindow != null && !_parentWindow.IsActive)
return false;
return TextArea.IsFocused;
}
}
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
if (!e.Handled && e.Key == Key.Escape)
{
e.Handled = true;
Hide();
}
}
private Point _visualLocation;
private Point _visualLocationTop;
/// <summary>
/// Positions the completion window at the specified position.
/// </summary>
protected void SetPosition(TextViewPosition position)
{
var textView = TextArea.TextView;
_visualLocation = textView.GetVisualPosition(position, VisualYPosition.LineBottom);
_visualLocationTop = textView.GetVisualPosition(position, VisualYPosition.LineTop);
UpdatePosition();
}
/// <summary>
/// Updates the position of the CompletionWindow based on the parent TextView position and the screen working area.
/// It ensures that the CompletionWindow is completely visible on the screen.
/// </summary>
protected void UpdatePosition()
{
var textView = TextArea.TextView;
var position = _visualLocation - textView.ScrollOffset;
this.HorizontalOffset = position.X;
this.VerticalOffset = position.Y;
}
// TODO: check if needed
///// <inheritdoc/>
//protected override void OnRenderSizeChanged(SizeChangedInfo sizeInfo)
//{
// base.OnRenderSizeChanged(sizeInfo);
// if (sizeInfo.HeightChanged && IsUp)
// {
// this.Top += sizeInfo.PreviousSize.Height - sizeInfo.NewSize.Height;
// }
//}
/// <summary>
/// Gets/sets whether the completion window should expect text insertion at the start offset,
/// which not go into the completion region, but before it.
/// </summary>
/// <remarks>This property allows only a single insertion, it is reset to false
/// when that insertion has occurred.</remarks>
public bool ExpectInsertionBeforeStart { get; set; }
private void TextArea_Document_Changing(object sender, DocumentChangeEventArgs e)
{
if (e.Offset + e.RemovalLength == StartOffset && e.RemovalLength > 0)
{
Hide(); // removal immediately in front of completion segment: close the window
// this is necessary when pressing backspace after dot-completion
}
if (e.Offset == StartOffset && e.RemovalLength == 0 && ExpectInsertionBeforeStart)
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.AfterInsertion);
ExpectInsertionBeforeStart = false;
}
else
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.BeforeInsertion);
}
EndOffset = e.GetNewOffset(EndOffset, AnchorMovementType.AfterInsertion);
}
}
}
<MSG> detachevents
<DFF> @@ -89,9 +89,9 @@ namespace AvaloniaEdit.CodeCompletion
StartOffset = EndOffset = TextArea.Caret.Offset;
- // TODO: these events do not fire on PopupRoot
- //Deactivated += OnDeactivated;
- //Closed += (sender, args) => DetachEvents();
+ //Deactivated += OnDeactivated; //Not needed?
+
+ Closed += (sender, args) => DetachEvents();
AttachEvents();
| 3 | detachevents | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063755 | <NME> CompletionWindowBase.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Rendering;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Styling;
using Avalonia.Threading;
using Avalonia.VisualTree;
namespace AvaloniaEdit.CodeCompletion
{
/// <summary>
/// Base class for completion windows. Handles positioning the window at the caret.
/// </summary>
public class CompletionWindowBase : Popup, IStyleable
{
static CompletionWindowBase()
{
//BackgroundProperty.OverrideDefaultValue(typeof(CompletionWindowBase), Brushes.White);
}
Type IStyleable.StyleKey => typeof(PopupRoot);
/// <summary>
/// Gets the parent TextArea.
/// </summary>
public TextArea TextArea { get; }
private readonly Window _parentWindow;
private TextDocument _document;
/// <summary>
/// Gets/Sets the start of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int StartOffset { get; set; }
/// <summary>
/// Gets/Sets the end of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int EndOffset { get; set; }
/// <summary>
/// Gets whether the window was opened above the current line.
/// </summary>
protected bool IsUp { get; private set; }
/// <summary>
/// Creates a new CompletionWindowBase.
/// </summary>
public CompletionWindowBase(TextArea textArea) : base()
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
_parentWindow = textArea.GetVisualRoot() as Window;
AddHandler(PointerReleasedEvent, OnMouseUp, handledEventsToo: true);
StartOffset = EndOffset = TextArea.Caret.Offset;
PlacementTarget = TextArea.TextView;
StartOffset = EndOffset = TextArea.Caret.Offset;
// TODO: these events do not fire on PopupRoot
//Deactivated += OnDeactivated;
//Closed += (sender, args) => DetachEvents();
AttachEvents();
Initailize();
}
protected virtual void OnClosed()
{
DetachEvents();
}
private void Initailize()
{
if (_document != null && StartOffset != TextArea.Caret.Offset)
{
SetPosition(new TextViewPosition(_document.GetLocation(StartOffset)));
}
else
{
SetPosition(TextArea.Caret.Position);
}
}
public void Show()
{
UpdatePosition();
Open();
Height = double.NaN;
MinHeight = 0;
}
public void Hide()
{
Close();
OnClosed();
}
#region Event Handlers
private void AttachEvents()
{
((ISetLogicalParent)this).SetParent(TextArea.GetVisualRoot() as ILogical);
_document = TextArea.Document;
if (_document != null)
{
_document.Changing += TextArea_Document_Changing;
}
// LostKeyboardFocus seems to be more reliable than PreviewLostKeyboardFocus - see SD-1729
TextArea.LostFocus += TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged += TextViewScrollOffsetChanged;
TextArea.DocumentChanged += TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged += ParentWindow_LocationChanged;
_parentWindow.Deactivated += ParentWindow_Deactivated;
}
// close previous completion windows of same type
foreach (var x in TextArea.StackedInputHandlers.OfType<InputHandler>())
{
if (x.Window.GetType() == GetType())
TextArea.PopStackedInputHandler(x);
}
_myInputHandler = new InputHandler(this);
TextArea.PushStackedInputHandler(_myInputHandler);
}
/// <summary>
/// Detaches events from the text area.
/// </summary>
protected virtual void DetachEvents()
{
((ISetLogicalParent)this).SetParent(null);
if (_document != null)
{
_document.Changing -= TextArea_Document_Changing;
}
TextArea.LostFocus -= TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged -= TextViewScrollOffsetChanged;
TextArea.DocumentChanged -= TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged -= ParentWindow_LocationChanged;
_parentWindow.Deactivated -= ParentWindow_Deactivated;
}
TextArea.PopStackedInputHandler(_myInputHandler);
}
#region InputHandler
private InputHandler _myInputHandler;
/// <summary>
/// A dummy input handler (that justs invokes the default input handler).
/// This is used to ensure the completion window closes when any other input handler
/// becomes active.
/// </summary>
private sealed class InputHandler : TextAreaStackedInputHandler
{
internal readonly CompletionWindowBase Window;
public InputHandler(CompletionWindowBase window)
: base(window.TextArea)
{
Debug.Assert(window != null);
Window = window;
}
public override void Detach()
{
base.Detach();
Window.Hide();
}
public override void OnPreviewKeyDown(KeyEventArgs e)
{
// prevents crash when typing deadchar while CC window is open
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyDownEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
public override void OnPreviewKeyUp(KeyEventArgs e)
{
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyUpEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
}
#endregion
private void TextViewScrollOffsetChanged(object sender, EventArgs e)
{
ILogicalScrollable textView = TextArea;
var visibleRect = new Rect(textView.Offset.X, textView.Offset.Y, textView.Viewport.Width, textView.Viewport.Height);
//close completion window when the user scrolls so far that the anchor position is leaving the visible area
if (visibleRect.Contains(_visualLocation) || visibleRect.Contains(_visualLocationTop))
{
UpdatePosition();
}
else
{
Hide();
}
}
private void TextAreaDocumentChanged(object sender, EventArgs e)
{
Hide();
}
private void TextAreaLostFocus(object sender, RoutedEventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
private void ParentWindow_Deactivated(object sender, EventArgs e)
{
Hide();
}
private void ParentWindow_LocationChanged(object sender, EventArgs e)
{
UpdatePosition();
}
/// <inheritdoc/>
private void OnDeactivated(object sender, EventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
#endregion
/// <summary>
/// Raises a tunnel/bubble event pair for a control.
/// </summary>
/// <param name="target">The control for which the event should be raised.</param>
/// <param name="previewEvent">The tunneling event.</param>
/// <param name="event">The bubbling event.</param>
/// <param name="args">The event args to use.</param>
/// <returns>The <see cref="RoutedEventArgs.Handled"/> value of the event args.</returns>
[SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")]
protected static bool RaiseEventPair(Control target, RoutedEvent previewEvent, RoutedEvent @event, RoutedEventArgs args)
{
if (target == null)
throw new ArgumentNullException(nameof(target));
if (args == null)
throw new ArgumentNullException(nameof(args));
if (previewEvent != null)
{
args.RoutedEvent = previewEvent;
target.RaiseEvent(args);
}
args.RoutedEvent = @event ?? throw new ArgumentNullException(nameof(@event));
target.RaiseEvent(args);
return args.Handled;
}
// Special handler: handledEventsToo
private void OnMouseUp(object sender, PointerReleasedEventArgs e)
{
ActivateParentWindow();
}
/// <summary>
/// Activates the parent window.
/// </summary>
protected virtual void ActivateParentWindow()
{
_parentWindow?.Activate();
}
private void CloseIfFocusLost()
{
if (CloseOnFocusLost)
{
Debug.WriteLine("CloseIfFocusLost: this.IsFocues=" + IsFocused + " IsTextAreaFocused=" + IsTextAreaFocused);
if (!IsFocused && !IsTextAreaFocused)
{
Hide();
}
}
}
/// <summary>
/// Gets whether the completion window should automatically close when the text editor looses focus.
/// </summary>
protected virtual bool CloseOnFocusLost => true;
private bool IsTextAreaFocused
{
get
{
if (_parentWindow != null && !_parentWindow.IsActive)
return false;
return TextArea.IsFocused;
}
}
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
if (!e.Handled && e.Key == Key.Escape)
{
e.Handled = true;
Hide();
}
}
private Point _visualLocation;
private Point _visualLocationTop;
/// <summary>
/// Positions the completion window at the specified position.
/// </summary>
protected void SetPosition(TextViewPosition position)
{
var textView = TextArea.TextView;
_visualLocation = textView.GetVisualPosition(position, VisualYPosition.LineBottom);
_visualLocationTop = textView.GetVisualPosition(position, VisualYPosition.LineTop);
UpdatePosition();
}
/// <summary>
/// Updates the position of the CompletionWindow based on the parent TextView position and the screen working area.
/// It ensures that the CompletionWindow is completely visible on the screen.
/// </summary>
protected void UpdatePosition()
{
var textView = TextArea.TextView;
var position = _visualLocation - textView.ScrollOffset;
this.HorizontalOffset = position.X;
this.VerticalOffset = position.Y;
}
// TODO: check if needed
///// <inheritdoc/>
//protected override void OnRenderSizeChanged(SizeChangedInfo sizeInfo)
//{
// base.OnRenderSizeChanged(sizeInfo);
// if (sizeInfo.HeightChanged && IsUp)
// {
// this.Top += sizeInfo.PreviousSize.Height - sizeInfo.NewSize.Height;
// }
//}
/// <summary>
/// Gets/sets whether the completion window should expect text insertion at the start offset,
/// which not go into the completion region, but before it.
/// </summary>
/// <remarks>This property allows only a single insertion, it is reset to false
/// when that insertion has occurred.</remarks>
public bool ExpectInsertionBeforeStart { get; set; }
private void TextArea_Document_Changing(object sender, DocumentChangeEventArgs e)
{
if (e.Offset + e.RemovalLength == StartOffset && e.RemovalLength > 0)
{
Hide(); // removal immediately in front of completion segment: close the window
// this is necessary when pressing backspace after dot-completion
}
if (e.Offset == StartOffset && e.RemovalLength == 0 && ExpectInsertionBeforeStart)
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.AfterInsertion);
ExpectInsertionBeforeStart = false;
}
else
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.BeforeInsertion);
}
EndOffset = e.GetNewOffset(EndOffset, AnchorMovementType.AfterInsertion);
}
}
}
<MSG> detachevents
<DFF> @@ -89,9 +89,9 @@ namespace AvaloniaEdit.CodeCompletion
StartOffset = EndOffset = TextArea.Caret.Offset;
- // TODO: these events do not fire on PopupRoot
- //Deactivated += OnDeactivated;
- //Closed += (sender, args) => DetachEvents();
+ //Deactivated += OnDeactivated; //Not needed?
+
+ Closed += (sender, args) => DetachEvents();
AttachEvents();
| 3 | detachevents | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063756 | <NME> CompletionWindowBase.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Rendering;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Styling;
using Avalonia.Threading;
using Avalonia.VisualTree;
namespace AvaloniaEdit.CodeCompletion
{
/// <summary>
/// Base class for completion windows. Handles positioning the window at the caret.
/// </summary>
public class CompletionWindowBase : Popup, IStyleable
{
static CompletionWindowBase()
{
//BackgroundProperty.OverrideDefaultValue(typeof(CompletionWindowBase), Brushes.White);
}
Type IStyleable.StyleKey => typeof(PopupRoot);
/// <summary>
/// Gets the parent TextArea.
/// </summary>
public TextArea TextArea { get; }
private readonly Window _parentWindow;
private TextDocument _document;
/// <summary>
/// Gets/Sets the start of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int StartOffset { get; set; }
/// <summary>
/// Gets/Sets the end of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int EndOffset { get; set; }
/// <summary>
/// Gets whether the window was opened above the current line.
/// </summary>
protected bool IsUp { get; private set; }
/// <summary>
/// Creates a new CompletionWindowBase.
/// </summary>
public CompletionWindowBase(TextArea textArea) : base()
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
_parentWindow = textArea.GetVisualRoot() as Window;
AddHandler(PointerReleasedEvent, OnMouseUp, handledEventsToo: true);
StartOffset = EndOffset = TextArea.Caret.Offset;
PlacementTarget = TextArea.TextView;
StartOffset = EndOffset = TextArea.Caret.Offset;
// TODO: these events do not fire on PopupRoot
//Deactivated += OnDeactivated;
//Closed += (sender, args) => DetachEvents();
AttachEvents();
Initailize();
}
protected virtual void OnClosed()
{
DetachEvents();
}
private void Initailize()
{
if (_document != null && StartOffset != TextArea.Caret.Offset)
{
SetPosition(new TextViewPosition(_document.GetLocation(StartOffset)));
}
else
{
SetPosition(TextArea.Caret.Position);
}
}
public void Show()
{
UpdatePosition();
Open();
Height = double.NaN;
MinHeight = 0;
}
public void Hide()
{
Close();
OnClosed();
}
#region Event Handlers
private void AttachEvents()
{
((ISetLogicalParent)this).SetParent(TextArea.GetVisualRoot() as ILogical);
_document = TextArea.Document;
if (_document != null)
{
_document.Changing += TextArea_Document_Changing;
}
// LostKeyboardFocus seems to be more reliable than PreviewLostKeyboardFocus - see SD-1729
TextArea.LostFocus += TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged += TextViewScrollOffsetChanged;
TextArea.DocumentChanged += TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged += ParentWindow_LocationChanged;
_parentWindow.Deactivated += ParentWindow_Deactivated;
}
// close previous completion windows of same type
foreach (var x in TextArea.StackedInputHandlers.OfType<InputHandler>())
{
if (x.Window.GetType() == GetType())
TextArea.PopStackedInputHandler(x);
}
_myInputHandler = new InputHandler(this);
TextArea.PushStackedInputHandler(_myInputHandler);
}
/// <summary>
/// Detaches events from the text area.
/// </summary>
protected virtual void DetachEvents()
{
((ISetLogicalParent)this).SetParent(null);
if (_document != null)
{
_document.Changing -= TextArea_Document_Changing;
}
TextArea.LostFocus -= TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged -= TextViewScrollOffsetChanged;
TextArea.DocumentChanged -= TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged -= ParentWindow_LocationChanged;
_parentWindow.Deactivated -= ParentWindow_Deactivated;
}
TextArea.PopStackedInputHandler(_myInputHandler);
}
#region InputHandler
private InputHandler _myInputHandler;
/// <summary>
/// A dummy input handler (that justs invokes the default input handler).
/// This is used to ensure the completion window closes when any other input handler
/// becomes active.
/// </summary>
private sealed class InputHandler : TextAreaStackedInputHandler
{
internal readonly CompletionWindowBase Window;
public InputHandler(CompletionWindowBase window)
: base(window.TextArea)
{
Debug.Assert(window != null);
Window = window;
}
public override void Detach()
{
base.Detach();
Window.Hide();
}
public override void OnPreviewKeyDown(KeyEventArgs e)
{
// prevents crash when typing deadchar while CC window is open
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyDownEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
public override void OnPreviewKeyUp(KeyEventArgs e)
{
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyUpEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
}
#endregion
private void TextViewScrollOffsetChanged(object sender, EventArgs e)
{
ILogicalScrollable textView = TextArea;
var visibleRect = new Rect(textView.Offset.X, textView.Offset.Y, textView.Viewport.Width, textView.Viewport.Height);
//close completion window when the user scrolls so far that the anchor position is leaving the visible area
if (visibleRect.Contains(_visualLocation) || visibleRect.Contains(_visualLocationTop))
{
UpdatePosition();
}
else
{
Hide();
}
}
private void TextAreaDocumentChanged(object sender, EventArgs e)
{
Hide();
}
private void TextAreaLostFocus(object sender, RoutedEventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
private void ParentWindow_Deactivated(object sender, EventArgs e)
{
Hide();
}
private void ParentWindow_LocationChanged(object sender, EventArgs e)
{
UpdatePosition();
}
/// <inheritdoc/>
private void OnDeactivated(object sender, EventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
#endregion
/// <summary>
/// Raises a tunnel/bubble event pair for a control.
/// </summary>
/// <param name="target">The control for which the event should be raised.</param>
/// <param name="previewEvent">The tunneling event.</param>
/// <param name="event">The bubbling event.</param>
/// <param name="args">The event args to use.</param>
/// <returns>The <see cref="RoutedEventArgs.Handled"/> value of the event args.</returns>
[SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")]
protected static bool RaiseEventPair(Control target, RoutedEvent previewEvent, RoutedEvent @event, RoutedEventArgs args)
{
if (target == null)
throw new ArgumentNullException(nameof(target));
if (args == null)
throw new ArgumentNullException(nameof(args));
if (previewEvent != null)
{
args.RoutedEvent = previewEvent;
target.RaiseEvent(args);
}
args.RoutedEvent = @event ?? throw new ArgumentNullException(nameof(@event));
target.RaiseEvent(args);
return args.Handled;
}
// Special handler: handledEventsToo
private void OnMouseUp(object sender, PointerReleasedEventArgs e)
{
ActivateParentWindow();
}
/// <summary>
/// Activates the parent window.
/// </summary>
protected virtual void ActivateParentWindow()
{
_parentWindow?.Activate();
}
private void CloseIfFocusLost()
{
if (CloseOnFocusLost)
{
Debug.WriteLine("CloseIfFocusLost: this.IsFocues=" + IsFocused + " IsTextAreaFocused=" + IsTextAreaFocused);
if (!IsFocused && !IsTextAreaFocused)
{
Hide();
}
}
}
/// <summary>
/// Gets whether the completion window should automatically close when the text editor looses focus.
/// </summary>
protected virtual bool CloseOnFocusLost => true;
private bool IsTextAreaFocused
{
get
{
if (_parentWindow != null && !_parentWindow.IsActive)
return false;
return TextArea.IsFocused;
}
}
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
if (!e.Handled && e.Key == Key.Escape)
{
e.Handled = true;
Hide();
}
}
private Point _visualLocation;
private Point _visualLocationTop;
/// <summary>
/// Positions the completion window at the specified position.
/// </summary>
protected void SetPosition(TextViewPosition position)
{
var textView = TextArea.TextView;
_visualLocation = textView.GetVisualPosition(position, VisualYPosition.LineBottom);
_visualLocationTop = textView.GetVisualPosition(position, VisualYPosition.LineTop);
UpdatePosition();
}
/// <summary>
/// Updates the position of the CompletionWindow based on the parent TextView position and the screen working area.
/// It ensures that the CompletionWindow is completely visible on the screen.
/// </summary>
protected void UpdatePosition()
{
var textView = TextArea.TextView;
var position = _visualLocation - textView.ScrollOffset;
this.HorizontalOffset = position.X;
this.VerticalOffset = position.Y;
}
// TODO: check if needed
///// <inheritdoc/>
//protected override void OnRenderSizeChanged(SizeChangedInfo sizeInfo)
//{
// base.OnRenderSizeChanged(sizeInfo);
// if (sizeInfo.HeightChanged && IsUp)
// {
// this.Top += sizeInfo.PreviousSize.Height - sizeInfo.NewSize.Height;
// }
//}
/// <summary>
/// Gets/sets whether the completion window should expect text insertion at the start offset,
/// which not go into the completion region, but before it.
/// </summary>
/// <remarks>This property allows only a single insertion, it is reset to false
/// when that insertion has occurred.</remarks>
public bool ExpectInsertionBeforeStart { get; set; }
private void TextArea_Document_Changing(object sender, DocumentChangeEventArgs e)
{
if (e.Offset + e.RemovalLength == StartOffset && e.RemovalLength > 0)
{
Hide(); // removal immediately in front of completion segment: close the window
// this is necessary when pressing backspace after dot-completion
}
if (e.Offset == StartOffset && e.RemovalLength == 0 && ExpectInsertionBeforeStart)
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.AfterInsertion);
ExpectInsertionBeforeStart = false;
}
else
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.BeforeInsertion);
}
EndOffset = e.GetNewOffset(EndOffset, AnchorMovementType.AfterInsertion);
}
}
}
<MSG> detachevents
<DFF> @@ -89,9 +89,9 @@ namespace AvaloniaEdit.CodeCompletion
StartOffset = EndOffset = TextArea.Caret.Offset;
- // TODO: these events do not fire on PopupRoot
- //Deactivated += OnDeactivated;
- //Closed += (sender, args) => DetachEvents();
+ //Deactivated += OnDeactivated; //Not needed?
+
+ Closed += (sender, args) => DetachEvents();
AttachEvents();
| 3 | detachevents | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063757 | <NME> CompletionWindowBase.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Rendering;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Styling;
using Avalonia.Threading;
using Avalonia.VisualTree;
namespace AvaloniaEdit.CodeCompletion
{
/// <summary>
/// Base class for completion windows. Handles positioning the window at the caret.
/// </summary>
public class CompletionWindowBase : Popup, IStyleable
{
static CompletionWindowBase()
{
//BackgroundProperty.OverrideDefaultValue(typeof(CompletionWindowBase), Brushes.White);
}
Type IStyleable.StyleKey => typeof(PopupRoot);
/// <summary>
/// Gets the parent TextArea.
/// </summary>
public TextArea TextArea { get; }
private readonly Window _parentWindow;
private TextDocument _document;
/// <summary>
/// Gets/Sets the start of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int StartOffset { get; set; }
/// <summary>
/// Gets/Sets the end of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int EndOffset { get; set; }
/// <summary>
/// Gets whether the window was opened above the current line.
/// </summary>
protected bool IsUp { get; private set; }
/// <summary>
/// Creates a new CompletionWindowBase.
/// </summary>
public CompletionWindowBase(TextArea textArea) : base()
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
_parentWindow = textArea.GetVisualRoot() as Window;
AddHandler(PointerReleasedEvent, OnMouseUp, handledEventsToo: true);
StartOffset = EndOffset = TextArea.Caret.Offset;
PlacementTarget = TextArea.TextView;
StartOffset = EndOffset = TextArea.Caret.Offset;
// TODO: these events do not fire on PopupRoot
//Deactivated += OnDeactivated;
//Closed += (sender, args) => DetachEvents();
AttachEvents();
Initailize();
}
protected virtual void OnClosed()
{
DetachEvents();
}
private void Initailize()
{
if (_document != null && StartOffset != TextArea.Caret.Offset)
{
SetPosition(new TextViewPosition(_document.GetLocation(StartOffset)));
}
else
{
SetPosition(TextArea.Caret.Position);
}
}
public void Show()
{
UpdatePosition();
Open();
Height = double.NaN;
MinHeight = 0;
}
public void Hide()
{
Close();
OnClosed();
}
#region Event Handlers
private void AttachEvents()
{
((ISetLogicalParent)this).SetParent(TextArea.GetVisualRoot() as ILogical);
_document = TextArea.Document;
if (_document != null)
{
_document.Changing += TextArea_Document_Changing;
}
// LostKeyboardFocus seems to be more reliable than PreviewLostKeyboardFocus - see SD-1729
TextArea.LostFocus += TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged += TextViewScrollOffsetChanged;
TextArea.DocumentChanged += TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged += ParentWindow_LocationChanged;
_parentWindow.Deactivated += ParentWindow_Deactivated;
}
// close previous completion windows of same type
foreach (var x in TextArea.StackedInputHandlers.OfType<InputHandler>())
{
if (x.Window.GetType() == GetType())
TextArea.PopStackedInputHandler(x);
}
_myInputHandler = new InputHandler(this);
TextArea.PushStackedInputHandler(_myInputHandler);
}
/// <summary>
/// Detaches events from the text area.
/// </summary>
protected virtual void DetachEvents()
{
((ISetLogicalParent)this).SetParent(null);
if (_document != null)
{
_document.Changing -= TextArea_Document_Changing;
}
TextArea.LostFocus -= TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged -= TextViewScrollOffsetChanged;
TextArea.DocumentChanged -= TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged -= ParentWindow_LocationChanged;
_parentWindow.Deactivated -= ParentWindow_Deactivated;
}
TextArea.PopStackedInputHandler(_myInputHandler);
}
#region InputHandler
private InputHandler _myInputHandler;
/// <summary>
/// A dummy input handler (that justs invokes the default input handler).
/// This is used to ensure the completion window closes when any other input handler
/// becomes active.
/// </summary>
private sealed class InputHandler : TextAreaStackedInputHandler
{
internal readonly CompletionWindowBase Window;
public InputHandler(CompletionWindowBase window)
: base(window.TextArea)
{
Debug.Assert(window != null);
Window = window;
}
public override void Detach()
{
base.Detach();
Window.Hide();
}
public override void OnPreviewKeyDown(KeyEventArgs e)
{
// prevents crash when typing deadchar while CC window is open
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyDownEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
public override void OnPreviewKeyUp(KeyEventArgs e)
{
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyUpEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
}
#endregion
private void TextViewScrollOffsetChanged(object sender, EventArgs e)
{
ILogicalScrollable textView = TextArea;
var visibleRect = new Rect(textView.Offset.X, textView.Offset.Y, textView.Viewport.Width, textView.Viewport.Height);
//close completion window when the user scrolls so far that the anchor position is leaving the visible area
if (visibleRect.Contains(_visualLocation) || visibleRect.Contains(_visualLocationTop))
{
UpdatePosition();
}
else
{
Hide();
}
}
private void TextAreaDocumentChanged(object sender, EventArgs e)
{
Hide();
}
private void TextAreaLostFocus(object sender, RoutedEventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
private void ParentWindow_Deactivated(object sender, EventArgs e)
{
Hide();
}
private void ParentWindow_LocationChanged(object sender, EventArgs e)
{
UpdatePosition();
}
/// <inheritdoc/>
private void OnDeactivated(object sender, EventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
#endregion
/// <summary>
/// Raises a tunnel/bubble event pair for a control.
/// </summary>
/// <param name="target">The control for which the event should be raised.</param>
/// <param name="previewEvent">The tunneling event.</param>
/// <param name="event">The bubbling event.</param>
/// <param name="args">The event args to use.</param>
/// <returns>The <see cref="RoutedEventArgs.Handled"/> value of the event args.</returns>
[SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")]
protected static bool RaiseEventPair(Control target, RoutedEvent previewEvent, RoutedEvent @event, RoutedEventArgs args)
{
if (target == null)
throw new ArgumentNullException(nameof(target));
if (args == null)
throw new ArgumentNullException(nameof(args));
if (previewEvent != null)
{
args.RoutedEvent = previewEvent;
target.RaiseEvent(args);
}
args.RoutedEvent = @event ?? throw new ArgumentNullException(nameof(@event));
target.RaiseEvent(args);
return args.Handled;
}
// Special handler: handledEventsToo
private void OnMouseUp(object sender, PointerReleasedEventArgs e)
{
ActivateParentWindow();
}
/// <summary>
/// Activates the parent window.
/// </summary>
protected virtual void ActivateParentWindow()
{
_parentWindow?.Activate();
}
private void CloseIfFocusLost()
{
if (CloseOnFocusLost)
{
Debug.WriteLine("CloseIfFocusLost: this.IsFocues=" + IsFocused + " IsTextAreaFocused=" + IsTextAreaFocused);
if (!IsFocused && !IsTextAreaFocused)
{
Hide();
}
}
}
/// <summary>
/// Gets whether the completion window should automatically close when the text editor looses focus.
/// </summary>
protected virtual bool CloseOnFocusLost => true;
private bool IsTextAreaFocused
{
get
{
if (_parentWindow != null && !_parentWindow.IsActive)
return false;
return TextArea.IsFocused;
}
}
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
if (!e.Handled && e.Key == Key.Escape)
{
e.Handled = true;
Hide();
}
}
private Point _visualLocation;
private Point _visualLocationTop;
/// <summary>
/// Positions the completion window at the specified position.
/// </summary>
protected void SetPosition(TextViewPosition position)
{
var textView = TextArea.TextView;
_visualLocation = textView.GetVisualPosition(position, VisualYPosition.LineBottom);
_visualLocationTop = textView.GetVisualPosition(position, VisualYPosition.LineTop);
UpdatePosition();
}
/// <summary>
/// Updates the position of the CompletionWindow based on the parent TextView position and the screen working area.
/// It ensures that the CompletionWindow is completely visible on the screen.
/// </summary>
protected void UpdatePosition()
{
var textView = TextArea.TextView;
var position = _visualLocation - textView.ScrollOffset;
this.HorizontalOffset = position.X;
this.VerticalOffset = position.Y;
}
// TODO: check if needed
///// <inheritdoc/>
//protected override void OnRenderSizeChanged(SizeChangedInfo sizeInfo)
//{
// base.OnRenderSizeChanged(sizeInfo);
// if (sizeInfo.HeightChanged && IsUp)
// {
// this.Top += sizeInfo.PreviousSize.Height - sizeInfo.NewSize.Height;
// }
//}
/// <summary>
/// Gets/sets whether the completion window should expect text insertion at the start offset,
/// which not go into the completion region, but before it.
/// </summary>
/// <remarks>This property allows only a single insertion, it is reset to false
/// when that insertion has occurred.</remarks>
public bool ExpectInsertionBeforeStart { get; set; }
private void TextArea_Document_Changing(object sender, DocumentChangeEventArgs e)
{
if (e.Offset + e.RemovalLength == StartOffset && e.RemovalLength > 0)
{
Hide(); // removal immediately in front of completion segment: close the window
// this is necessary when pressing backspace after dot-completion
}
if (e.Offset == StartOffset && e.RemovalLength == 0 && ExpectInsertionBeforeStart)
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.AfterInsertion);
ExpectInsertionBeforeStart = false;
}
else
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.BeforeInsertion);
}
EndOffset = e.GetNewOffset(EndOffset, AnchorMovementType.AfterInsertion);
}
}
}
<MSG> detachevents
<DFF> @@ -89,9 +89,9 @@ namespace AvaloniaEdit.CodeCompletion
StartOffset = EndOffset = TextArea.Caret.Offset;
- // TODO: these events do not fire on PopupRoot
- //Deactivated += OnDeactivated;
- //Closed += (sender, args) => DetachEvents();
+ //Deactivated += OnDeactivated; //Not needed?
+
+ Closed += (sender, args) => DetachEvents();
AttachEvents();
| 3 | detachevents | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063758 | <NME> CompletionWindowBase.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Rendering;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Styling;
using Avalonia.Threading;
using Avalonia.VisualTree;
namespace AvaloniaEdit.CodeCompletion
{
/// <summary>
/// Base class for completion windows. Handles positioning the window at the caret.
/// </summary>
public class CompletionWindowBase : Popup, IStyleable
{
static CompletionWindowBase()
{
//BackgroundProperty.OverrideDefaultValue(typeof(CompletionWindowBase), Brushes.White);
}
Type IStyleable.StyleKey => typeof(PopupRoot);
/// <summary>
/// Gets the parent TextArea.
/// </summary>
public TextArea TextArea { get; }
private readonly Window _parentWindow;
private TextDocument _document;
/// <summary>
/// Gets/Sets the start of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int StartOffset { get; set; }
/// <summary>
/// Gets/Sets the end of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int EndOffset { get; set; }
/// <summary>
/// Gets whether the window was opened above the current line.
/// </summary>
protected bool IsUp { get; private set; }
/// <summary>
/// Creates a new CompletionWindowBase.
/// </summary>
public CompletionWindowBase(TextArea textArea) : base()
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
_parentWindow = textArea.GetVisualRoot() as Window;
AddHandler(PointerReleasedEvent, OnMouseUp, handledEventsToo: true);
StartOffset = EndOffset = TextArea.Caret.Offset;
PlacementTarget = TextArea.TextView;
StartOffset = EndOffset = TextArea.Caret.Offset;
// TODO: these events do not fire on PopupRoot
//Deactivated += OnDeactivated;
//Closed += (sender, args) => DetachEvents();
AttachEvents();
Initailize();
}
protected virtual void OnClosed()
{
DetachEvents();
}
private void Initailize()
{
if (_document != null && StartOffset != TextArea.Caret.Offset)
{
SetPosition(new TextViewPosition(_document.GetLocation(StartOffset)));
}
else
{
SetPosition(TextArea.Caret.Position);
}
}
public void Show()
{
UpdatePosition();
Open();
Height = double.NaN;
MinHeight = 0;
}
public void Hide()
{
Close();
OnClosed();
}
#region Event Handlers
private void AttachEvents()
{
((ISetLogicalParent)this).SetParent(TextArea.GetVisualRoot() as ILogical);
_document = TextArea.Document;
if (_document != null)
{
_document.Changing += TextArea_Document_Changing;
}
// LostKeyboardFocus seems to be more reliable than PreviewLostKeyboardFocus - see SD-1729
TextArea.LostFocus += TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged += TextViewScrollOffsetChanged;
TextArea.DocumentChanged += TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged += ParentWindow_LocationChanged;
_parentWindow.Deactivated += ParentWindow_Deactivated;
}
// close previous completion windows of same type
foreach (var x in TextArea.StackedInputHandlers.OfType<InputHandler>())
{
if (x.Window.GetType() == GetType())
TextArea.PopStackedInputHandler(x);
}
_myInputHandler = new InputHandler(this);
TextArea.PushStackedInputHandler(_myInputHandler);
}
/// <summary>
/// Detaches events from the text area.
/// </summary>
protected virtual void DetachEvents()
{
((ISetLogicalParent)this).SetParent(null);
if (_document != null)
{
_document.Changing -= TextArea_Document_Changing;
}
TextArea.LostFocus -= TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged -= TextViewScrollOffsetChanged;
TextArea.DocumentChanged -= TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged -= ParentWindow_LocationChanged;
_parentWindow.Deactivated -= ParentWindow_Deactivated;
}
TextArea.PopStackedInputHandler(_myInputHandler);
}
#region InputHandler
private InputHandler _myInputHandler;
/// <summary>
/// A dummy input handler (that justs invokes the default input handler).
/// This is used to ensure the completion window closes when any other input handler
/// becomes active.
/// </summary>
private sealed class InputHandler : TextAreaStackedInputHandler
{
internal readonly CompletionWindowBase Window;
public InputHandler(CompletionWindowBase window)
: base(window.TextArea)
{
Debug.Assert(window != null);
Window = window;
}
public override void Detach()
{
base.Detach();
Window.Hide();
}
public override void OnPreviewKeyDown(KeyEventArgs e)
{
// prevents crash when typing deadchar while CC window is open
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyDownEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
public override void OnPreviewKeyUp(KeyEventArgs e)
{
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyUpEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
}
#endregion
private void TextViewScrollOffsetChanged(object sender, EventArgs e)
{
ILogicalScrollable textView = TextArea;
var visibleRect = new Rect(textView.Offset.X, textView.Offset.Y, textView.Viewport.Width, textView.Viewport.Height);
//close completion window when the user scrolls so far that the anchor position is leaving the visible area
if (visibleRect.Contains(_visualLocation) || visibleRect.Contains(_visualLocationTop))
{
UpdatePosition();
}
else
{
Hide();
}
}
private void TextAreaDocumentChanged(object sender, EventArgs e)
{
Hide();
}
private void TextAreaLostFocus(object sender, RoutedEventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
private void ParentWindow_Deactivated(object sender, EventArgs e)
{
Hide();
}
private void ParentWindow_LocationChanged(object sender, EventArgs e)
{
UpdatePosition();
}
/// <inheritdoc/>
private void OnDeactivated(object sender, EventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
#endregion
/// <summary>
/// Raises a tunnel/bubble event pair for a control.
/// </summary>
/// <param name="target">The control for which the event should be raised.</param>
/// <param name="previewEvent">The tunneling event.</param>
/// <param name="event">The bubbling event.</param>
/// <param name="args">The event args to use.</param>
/// <returns>The <see cref="RoutedEventArgs.Handled"/> value of the event args.</returns>
[SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")]
protected static bool RaiseEventPair(Control target, RoutedEvent previewEvent, RoutedEvent @event, RoutedEventArgs args)
{
if (target == null)
throw new ArgumentNullException(nameof(target));
if (args == null)
throw new ArgumentNullException(nameof(args));
if (previewEvent != null)
{
args.RoutedEvent = previewEvent;
target.RaiseEvent(args);
}
args.RoutedEvent = @event ?? throw new ArgumentNullException(nameof(@event));
target.RaiseEvent(args);
return args.Handled;
}
// Special handler: handledEventsToo
private void OnMouseUp(object sender, PointerReleasedEventArgs e)
{
ActivateParentWindow();
}
/// <summary>
/// Activates the parent window.
/// </summary>
protected virtual void ActivateParentWindow()
{
_parentWindow?.Activate();
}
private void CloseIfFocusLost()
{
if (CloseOnFocusLost)
{
Debug.WriteLine("CloseIfFocusLost: this.IsFocues=" + IsFocused + " IsTextAreaFocused=" + IsTextAreaFocused);
if (!IsFocused && !IsTextAreaFocused)
{
Hide();
}
}
}
/// <summary>
/// Gets whether the completion window should automatically close when the text editor looses focus.
/// </summary>
protected virtual bool CloseOnFocusLost => true;
private bool IsTextAreaFocused
{
get
{
if (_parentWindow != null && !_parentWindow.IsActive)
return false;
return TextArea.IsFocused;
}
}
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
if (!e.Handled && e.Key == Key.Escape)
{
e.Handled = true;
Hide();
}
}
private Point _visualLocation;
private Point _visualLocationTop;
/// <summary>
/// Positions the completion window at the specified position.
/// </summary>
protected void SetPosition(TextViewPosition position)
{
var textView = TextArea.TextView;
_visualLocation = textView.GetVisualPosition(position, VisualYPosition.LineBottom);
_visualLocationTop = textView.GetVisualPosition(position, VisualYPosition.LineTop);
UpdatePosition();
}
/// <summary>
/// Updates the position of the CompletionWindow based on the parent TextView position and the screen working area.
/// It ensures that the CompletionWindow is completely visible on the screen.
/// </summary>
protected void UpdatePosition()
{
var textView = TextArea.TextView;
var position = _visualLocation - textView.ScrollOffset;
this.HorizontalOffset = position.X;
this.VerticalOffset = position.Y;
}
// TODO: check if needed
///// <inheritdoc/>
//protected override void OnRenderSizeChanged(SizeChangedInfo sizeInfo)
//{
// base.OnRenderSizeChanged(sizeInfo);
// if (sizeInfo.HeightChanged && IsUp)
// {
// this.Top += sizeInfo.PreviousSize.Height - sizeInfo.NewSize.Height;
// }
//}
/// <summary>
/// Gets/sets whether the completion window should expect text insertion at the start offset,
/// which not go into the completion region, but before it.
/// </summary>
/// <remarks>This property allows only a single insertion, it is reset to false
/// when that insertion has occurred.</remarks>
public bool ExpectInsertionBeforeStart { get; set; }
private void TextArea_Document_Changing(object sender, DocumentChangeEventArgs e)
{
if (e.Offset + e.RemovalLength == StartOffset && e.RemovalLength > 0)
{
Hide(); // removal immediately in front of completion segment: close the window
// this is necessary when pressing backspace after dot-completion
}
if (e.Offset == StartOffset && e.RemovalLength == 0 && ExpectInsertionBeforeStart)
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.AfterInsertion);
ExpectInsertionBeforeStart = false;
}
else
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.BeforeInsertion);
}
EndOffset = e.GetNewOffset(EndOffset, AnchorMovementType.AfterInsertion);
}
}
}
<MSG> detachevents
<DFF> @@ -89,9 +89,9 @@ namespace AvaloniaEdit.CodeCompletion
StartOffset = EndOffset = TextArea.Caret.Offset;
- // TODO: these events do not fire on PopupRoot
- //Deactivated += OnDeactivated;
- //Closed += (sender, args) => DetachEvents();
+ //Deactivated += OnDeactivated; //Not needed?
+
+ Closed += (sender, args) => DetachEvents();
AttachEvents();
| 3 | detachevents | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063759 | <NME> CompletionWindowBase.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Rendering;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Styling;
using Avalonia.Threading;
using Avalonia.VisualTree;
namespace AvaloniaEdit.CodeCompletion
{
/// <summary>
/// Base class for completion windows. Handles positioning the window at the caret.
/// </summary>
public class CompletionWindowBase : Popup, IStyleable
{
static CompletionWindowBase()
{
//BackgroundProperty.OverrideDefaultValue(typeof(CompletionWindowBase), Brushes.White);
}
Type IStyleable.StyleKey => typeof(PopupRoot);
/// <summary>
/// Gets the parent TextArea.
/// </summary>
public TextArea TextArea { get; }
private readonly Window _parentWindow;
private TextDocument _document;
/// <summary>
/// Gets/Sets the start of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int StartOffset { get; set; }
/// <summary>
/// Gets/Sets the end of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int EndOffset { get; set; }
/// <summary>
/// Gets whether the window was opened above the current line.
/// </summary>
protected bool IsUp { get; private set; }
/// <summary>
/// Creates a new CompletionWindowBase.
/// </summary>
public CompletionWindowBase(TextArea textArea) : base()
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
_parentWindow = textArea.GetVisualRoot() as Window;
AddHandler(PointerReleasedEvent, OnMouseUp, handledEventsToo: true);
StartOffset = EndOffset = TextArea.Caret.Offset;
PlacementTarget = TextArea.TextView;
StartOffset = EndOffset = TextArea.Caret.Offset;
// TODO: these events do not fire on PopupRoot
//Deactivated += OnDeactivated;
//Closed += (sender, args) => DetachEvents();
AttachEvents();
Initailize();
}
protected virtual void OnClosed()
{
DetachEvents();
}
private void Initailize()
{
if (_document != null && StartOffset != TextArea.Caret.Offset)
{
SetPosition(new TextViewPosition(_document.GetLocation(StartOffset)));
}
else
{
SetPosition(TextArea.Caret.Position);
}
}
public void Show()
{
UpdatePosition();
Open();
Height = double.NaN;
MinHeight = 0;
}
public void Hide()
{
Close();
OnClosed();
}
#region Event Handlers
private void AttachEvents()
{
((ISetLogicalParent)this).SetParent(TextArea.GetVisualRoot() as ILogical);
_document = TextArea.Document;
if (_document != null)
{
_document.Changing += TextArea_Document_Changing;
}
// LostKeyboardFocus seems to be more reliable than PreviewLostKeyboardFocus - see SD-1729
TextArea.LostFocus += TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged += TextViewScrollOffsetChanged;
TextArea.DocumentChanged += TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged += ParentWindow_LocationChanged;
_parentWindow.Deactivated += ParentWindow_Deactivated;
}
// close previous completion windows of same type
foreach (var x in TextArea.StackedInputHandlers.OfType<InputHandler>())
{
if (x.Window.GetType() == GetType())
TextArea.PopStackedInputHandler(x);
}
_myInputHandler = new InputHandler(this);
TextArea.PushStackedInputHandler(_myInputHandler);
}
/// <summary>
/// Detaches events from the text area.
/// </summary>
protected virtual void DetachEvents()
{
((ISetLogicalParent)this).SetParent(null);
if (_document != null)
{
_document.Changing -= TextArea_Document_Changing;
}
TextArea.LostFocus -= TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged -= TextViewScrollOffsetChanged;
TextArea.DocumentChanged -= TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged -= ParentWindow_LocationChanged;
_parentWindow.Deactivated -= ParentWindow_Deactivated;
}
TextArea.PopStackedInputHandler(_myInputHandler);
}
#region InputHandler
private InputHandler _myInputHandler;
/// <summary>
/// A dummy input handler (that justs invokes the default input handler).
/// This is used to ensure the completion window closes when any other input handler
/// becomes active.
/// </summary>
private sealed class InputHandler : TextAreaStackedInputHandler
{
internal readonly CompletionWindowBase Window;
public InputHandler(CompletionWindowBase window)
: base(window.TextArea)
{
Debug.Assert(window != null);
Window = window;
}
public override void Detach()
{
base.Detach();
Window.Hide();
}
public override void OnPreviewKeyDown(KeyEventArgs e)
{
// prevents crash when typing deadchar while CC window is open
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyDownEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
public override void OnPreviewKeyUp(KeyEventArgs e)
{
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyUpEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
}
#endregion
private void TextViewScrollOffsetChanged(object sender, EventArgs e)
{
ILogicalScrollable textView = TextArea;
var visibleRect = new Rect(textView.Offset.X, textView.Offset.Y, textView.Viewport.Width, textView.Viewport.Height);
//close completion window when the user scrolls so far that the anchor position is leaving the visible area
if (visibleRect.Contains(_visualLocation) || visibleRect.Contains(_visualLocationTop))
{
UpdatePosition();
}
else
{
Hide();
}
}
private void TextAreaDocumentChanged(object sender, EventArgs e)
{
Hide();
}
private void TextAreaLostFocus(object sender, RoutedEventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
private void ParentWindow_Deactivated(object sender, EventArgs e)
{
Hide();
}
private void ParentWindow_LocationChanged(object sender, EventArgs e)
{
UpdatePosition();
}
/// <inheritdoc/>
private void OnDeactivated(object sender, EventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
#endregion
/// <summary>
/// Raises a tunnel/bubble event pair for a control.
/// </summary>
/// <param name="target">The control for which the event should be raised.</param>
/// <param name="previewEvent">The tunneling event.</param>
/// <param name="event">The bubbling event.</param>
/// <param name="args">The event args to use.</param>
/// <returns>The <see cref="RoutedEventArgs.Handled"/> value of the event args.</returns>
[SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")]
protected static bool RaiseEventPair(Control target, RoutedEvent previewEvent, RoutedEvent @event, RoutedEventArgs args)
{
if (target == null)
throw new ArgumentNullException(nameof(target));
if (args == null)
throw new ArgumentNullException(nameof(args));
if (previewEvent != null)
{
args.RoutedEvent = previewEvent;
target.RaiseEvent(args);
}
args.RoutedEvent = @event ?? throw new ArgumentNullException(nameof(@event));
target.RaiseEvent(args);
return args.Handled;
}
// Special handler: handledEventsToo
private void OnMouseUp(object sender, PointerReleasedEventArgs e)
{
ActivateParentWindow();
}
/// <summary>
/// Activates the parent window.
/// </summary>
protected virtual void ActivateParentWindow()
{
_parentWindow?.Activate();
}
private void CloseIfFocusLost()
{
if (CloseOnFocusLost)
{
Debug.WriteLine("CloseIfFocusLost: this.IsFocues=" + IsFocused + " IsTextAreaFocused=" + IsTextAreaFocused);
if (!IsFocused && !IsTextAreaFocused)
{
Hide();
}
}
}
/// <summary>
/// Gets whether the completion window should automatically close when the text editor looses focus.
/// </summary>
protected virtual bool CloseOnFocusLost => true;
private bool IsTextAreaFocused
{
get
{
if (_parentWindow != null && !_parentWindow.IsActive)
return false;
return TextArea.IsFocused;
}
}
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
if (!e.Handled && e.Key == Key.Escape)
{
e.Handled = true;
Hide();
}
}
private Point _visualLocation;
private Point _visualLocationTop;
/// <summary>
/// Positions the completion window at the specified position.
/// </summary>
protected void SetPosition(TextViewPosition position)
{
var textView = TextArea.TextView;
_visualLocation = textView.GetVisualPosition(position, VisualYPosition.LineBottom);
_visualLocationTop = textView.GetVisualPosition(position, VisualYPosition.LineTop);
UpdatePosition();
}
/// <summary>
/// Updates the position of the CompletionWindow based on the parent TextView position and the screen working area.
/// It ensures that the CompletionWindow is completely visible on the screen.
/// </summary>
protected void UpdatePosition()
{
var textView = TextArea.TextView;
var position = _visualLocation - textView.ScrollOffset;
this.HorizontalOffset = position.X;
this.VerticalOffset = position.Y;
}
// TODO: check if needed
///// <inheritdoc/>
//protected override void OnRenderSizeChanged(SizeChangedInfo sizeInfo)
//{
// base.OnRenderSizeChanged(sizeInfo);
// if (sizeInfo.HeightChanged && IsUp)
// {
// this.Top += sizeInfo.PreviousSize.Height - sizeInfo.NewSize.Height;
// }
//}
/// <summary>
/// Gets/sets whether the completion window should expect text insertion at the start offset,
/// which not go into the completion region, but before it.
/// </summary>
/// <remarks>This property allows only a single insertion, it is reset to false
/// when that insertion has occurred.</remarks>
public bool ExpectInsertionBeforeStart { get; set; }
private void TextArea_Document_Changing(object sender, DocumentChangeEventArgs e)
{
if (e.Offset + e.RemovalLength == StartOffset && e.RemovalLength > 0)
{
Hide(); // removal immediately in front of completion segment: close the window
// this is necessary when pressing backspace after dot-completion
}
if (e.Offset == StartOffset && e.RemovalLength == 0 && ExpectInsertionBeforeStart)
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.AfterInsertion);
ExpectInsertionBeforeStart = false;
}
else
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.BeforeInsertion);
}
EndOffset = e.GetNewOffset(EndOffset, AnchorMovementType.AfterInsertion);
}
}
}
<MSG> detachevents
<DFF> @@ -89,9 +89,9 @@ namespace AvaloniaEdit.CodeCompletion
StartOffset = EndOffset = TextArea.Caret.Offset;
- // TODO: these events do not fire on PopupRoot
- //Deactivated += OnDeactivated;
- //Closed += (sender, args) => DetachEvents();
+ //Deactivated += OnDeactivated; //Not needed?
+
+ Closed += (sender, args) => DetachEvents();
AttachEvents();
| 3 | detachevents | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063760 | <NME> CompletionWindowBase.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Rendering;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Styling;
using Avalonia.Threading;
using Avalonia.VisualTree;
namespace AvaloniaEdit.CodeCompletion
{
/// <summary>
/// Base class for completion windows. Handles positioning the window at the caret.
/// </summary>
public class CompletionWindowBase : Popup, IStyleable
{
static CompletionWindowBase()
{
//BackgroundProperty.OverrideDefaultValue(typeof(CompletionWindowBase), Brushes.White);
}
Type IStyleable.StyleKey => typeof(PopupRoot);
/// <summary>
/// Gets the parent TextArea.
/// </summary>
public TextArea TextArea { get; }
private readonly Window _parentWindow;
private TextDocument _document;
/// <summary>
/// Gets/Sets the start of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int StartOffset { get; set; }
/// <summary>
/// Gets/Sets the end of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int EndOffset { get; set; }
/// <summary>
/// Gets whether the window was opened above the current line.
/// </summary>
protected bool IsUp { get; private set; }
/// <summary>
/// Creates a new CompletionWindowBase.
/// </summary>
public CompletionWindowBase(TextArea textArea) : base()
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
_parentWindow = textArea.GetVisualRoot() as Window;
AddHandler(PointerReleasedEvent, OnMouseUp, handledEventsToo: true);
StartOffset = EndOffset = TextArea.Caret.Offset;
PlacementTarget = TextArea.TextView;
StartOffset = EndOffset = TextArea.Caret.Offset;
// TODO: these events do not fire on PopupRoot
//Deactivated += OnDeactivated;
//Closed += (sender, args) => DetachEvents();
AttachEvents();
Initailize();
}
protected virtual void OnClosed()
{
DetachEvents();
}
private void Initailize()
{
if (_document != null && StartOffset != TextArea.Caret.Offset)
{
SetPosition(new TextViewPosition(_document.GetLocation(StartOffset)));
}
else
{
SetPosition(TextArea.Caret.Position);
}
}
public void Show()
{
UpdatePosition();
Open();
Height = double.NaN;
MinHeight = 0;
}
public void Hide()
{
Close();
OnClosed();
}
#region Event Handlers
private void AttachEvents()
{
((ISetLogicalParent)this).SetParent(TextArea.GetVisualRoot() as ILogical);
_document = TextArea.Document;
if (_document != null)
{
_document.Changing += TextArea_Document_Changing;
}
// LostKeyboardFocus seems to be more reliable than PreviewLostKeyboardFocus - see SD-1729
TextArea.LostFocus += TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged += TextViewScrollOffsetChanged;
TextArea.DocumentChanged += TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged += ParentWindow_LocationChanged;
_parentWindow.Deactivated += ParentWindow_Deactivated;
}
// close previous completion windows of same type
foreach (var x in TextArea.StackedInputHandlers.OfType<InputHandler>())
{
if (x.Window.GetType() == GetType())
TextArea.PopStackedInputHandler(x);
}
_myInputHandler = new InputHandler(this);
TextArea.PushStackedInputHandler(_myInputHandler);
}
/// <summary>
/// Detaches events from the text area.
/// </summary>
protected virtual void DetachEvents()
{
((ISetLogicalParent)this).SetParent(null);
if (_document != null)
{
_document.Changing -= TextArea_Document_Changing;
}
TextArea.LostFocus -= TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged -= TextViewScrollOffsetChanged;
TextArea.DocumentChanged -= TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged -= ParentWindow_LocationChanged;
_parentWindow.Deactivated -= ParentWindow_Deactivated;
}
TextArea.PopStackedInputHandler(_myInputHandler);
}
#region InputHandler
private InputHandler _myInputHandler;
/// <summary>
/// A dummy input handler (that justs invokes the default input handler).
/// This is used to ensure the completion window closes when any other input handler
/// becomes active.
/// </summary>
private sealed class InputHandler : TextAreaStackedInputHandler
{
internal readonly CompletionWindowBase Window;
public InputHandler(CompletionWindowBase window)
: base(window.TextArea)
{
Debug.Assert(window != null);
Window = window;
}
public override void Detach()
{
base.Detach();
Window.Hide();
}
public override void OnPreviewKeyDown(KeyEventArgs e)
{
// prevents crash when typing deadchar while CC window is open
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyDownEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
public override void OnPreviewKeyUp(KeyEventArgs e)
{
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyUpEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
}
#endregion
private void TextViewScrollOffsetChanged(object sender, EventArgs e)
{
ILogicalScrollable textView = TextArea;
var visibleRect = new Rect(textView.Offset.X, textView.Offset.Y, textView.Viewport.Width, textView.Viewport.Height);
//close completion window when the user scrolls so far that the anchor position is leaving the visible area
if (visibleRect.Contains(_visualLocation) || visibleRect.Contains(_visualLocationTop))
{
UpdatePosition();
}
else
{
Hide();
}
}
private void TextAreaDocumentChanged(object sender, EventArgs e)
{
Hide();
}
private void TextAreaLostFocus(object sender, RoutedEventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
private void ParentWindow_Deactivated(object sender, EventArgs e)
{
Hide();
}
private void ParentWindow_LocationChanged(object sender, EventArgs e)
{
UpdatePosition();
}
/// <inheritdoc/>
private void OnDeactivated(object sender, EventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
#endregion
/// <summary>
/// Raises a tunnel/bubble event pair for a control.
/// </summary>
/// <param name="target">The control for which the event should be raised.</param>
/// <param name="previewEvent">The tunneling event.</param>
/// <param name="event">The bubbling event.</param>
/// <param name="args">The event args to use.</param>
/// <returns>The <see cref="RoutedEventArgs.Handled"/> value of the event args.</returns>
[SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")]
protected static bool RaiseEventPair(Control target, RoutedEvent previewEvent, RoutedEvent @event, RoutedEventArgs args)
{
if (target == null)
throw new ArgumentNullException(nameof(target));
if (args == null)
throw new ArgumentNullException(nameof(args));
if (previewEvent != null)
{
args.RoutedEvent = previewEvent;
target.RaiseEvent(args);
}
args.RoutedEvent = @event ?? throw new ArgumentNullException(nameof(@event));
target.RaiseEvent(args);
return args.Handled;
}
// Special handler: handledEventsToo
private void OnMouseUp(object sender, PointerReleasedEventArgs e)
{
ActivateParentWindow();
}
/// <summary>
/// Activates the parent window.
/// </summary>
protected virtual void ActivateParentWindow()
{
_parentWindow?.Activate();
}
private void CloseIfFocusLost()
{
if (CloseOnFocusLost)
{
Debug.WriteLine("CloseIfFocusLost: this.IsFocues=" + IsFocused + " IsTextAreaFocused=" + IsTextAreaFocused);
if (!IsFocused && !IsTextAreaFocused)
{
Hide();
}
}
}
/// <summary>
/// Gets whether the completion window should automatically close when the text editor looses focus.
/// </summary>
protected virtual bool CloseOnFocusLost => true;
private bool IsTextAreaFocused
{
get
{
if (_parentWindow != null && !_parentWindow.IsActive)
return false;
return TextArea.IsFocused;
}
}
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
if (!e.Handled && e.Key == Key.Escape)
{
e.Handled = true;
Hide();
}
}
private Point _visualLocation;
private Point _visualLocationTop;
/// <summary>
/// Positions the completion window at the specified position.
/// </summary>
protected void SetPosition(TextViewPosition position)
{
var textView = TextArea.TextView;
_visualLocation = textView.GetVisualPosition(position, VisualYPosition.LineBottom);
_visualLocationTop = textView.GetVisualPosition(position, VisualYPosition.LineTop);
UpdatePosition();
}
/// <summary>
/// Updates the position of the CompletionWindow based on the parent TextView position and the screen working area.
/// It ensures that the CompletionWindow is completely visible on the screen.
/// </summary>
protected void UpdatePosition()
{
var textView = TextArea.TextView;
var position = _visualLocation - textView.ScrollOffset;
this.HorizontalOffset = position.X;
this.VerticalOffset = position.Y;
}
// TODO: check if needed
///// <inheritdoc/>
//protected override void OnRenderSizeChanged(SizeChangedInfo sizeInfo)
//{
// base.OnRenderSizeChanged(sizeInfo);
// if (sizeInfo.HeightChanged && IsUp)
// {
// this.Top += sizeInfo.PreviousSize.Height - sizeInfo.NewSize.Height;
// }
//}
/// <summary>
/// Gets/sets whether the completion window should expect text insertion at the start offset,
/// which not go into the completion region, but before it.
/// </summary>
/// <remarks>This property allows only a single insertion, it is reset to false
/// when that insertion has occurred.</remarks>
public bool ExpectInsertionBeforeStart { get; set; }
private void TextArea_Document_Changing(object sender, DocumentChangeEventArgs e)
{
if (e.Offset + e.RemovalLength == StartOffset && e.RemovalLength > 0)
{
Hide(); // removal immediately in front of completion segment: close the window
// this is necessary when pressing backspace after dot-completion
}
if (e.Offset == StartOffset && e.RemovalLength == 0 && ExpectInsertionBeforeStart)
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.AfterInsertion);
ExpectInsertionBeforeStart = false;
}
else
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.BeforeInsertion);
}
EndOffset = e.GetNewOffset(EndOffset, AnchorMovementType.AfterInsertion);
}
}
}
<MSG> detachevents
<DFF> @@ -89,9 +89,9 @@ namespace AvaloniaEdit.CodeCompletion
StartOffset = EndOffset = TextArea.Caret.Offset;
- // TODO: these events do not fire on PopupRoot
- //Deactivated += OnDeactivated;
- //Closed += (sender, args) => DetachEvents();
+ //Deactivated += OnDeactivated; //Not needed?
+
+ Closed += (sender, args) => DetachEvents();
AttachEvents();
| 3 | detachevents | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063761 | <NME> CompletionWindowBase.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Rendering;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Styling;
using Avalonia.Threading;
using Avalonia.VisualTree;
namespace AvaloniaEdit.CodeCompletion
{
/// <summary>
/// Base class for completion windows. Handles positioning the window at the caret.
/// </summary>
public class CompletionWindowBase : Popup, IStyleable
{
static CompletionWindowBase()
{
//BackgroundProperty.OverrideDefaultValue(typeof(CompletionWindowBase), Brushes.White);
}
Type IStyleable.StyleKey => typeof(PopupRoot);
/// <summary>
/// Gets the parent TextArea.
/// </summary>
public TextArea TextArea { get; }
private readonly Window _parentWindow;
private TextDocument _document;
/// <summary>
/// Gets/Sets the start of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int StartOffset { get; set; }
/// <summary>
/// Gets/Sets the end of the text range in which the completion window stays open.
/// This text portion is used to determine the text used to select an entry in the completion list by typing.
/// </summary>
public int EndOffset { get; set; }
/// <summary>
/// Gets whether the window was opened above the current line.
/// </summary>
protected bool IsUp { get; private set; }
/// <summary>
/// Creates a new CompletionWindowBase.
/// </summary>
public CompletionWindowBase(TextArea textArea) : base()
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
_parentWindow = textArea.GetVisualRoot() as Window;
AddHandler(PointerReleasedEvent, OnMouseUp, handledEventsToo: true);
StartOffset = EndOffset = TextArea.Caret.Offset;
PlacementTarget = TextArea.TextView;
StartOffset = EndOffset = TextArea.Caret.Offset;
// TODO: these events do not fire on PopupRoot
//Deactivated += OnDeactivated;
//Closed += (sender, args) => DetachEvents();
AttachEvents();
Initailize();
}
protected virtual void OnClosed()
{
DetachEvents();
}
private void Initailize()
{
if (_document != null && StartOffset != TextArea.Caret.Offset)
{
SetPosition(new TextViewPosition(_document.GetLocation(StartOffset)));
}
else
{
SetPosition(TextArea.Caret.Position);
}
}
public void Show()
{
UpdatePosition();
Open();
Height = double.NaN;
MinHeight = 0;
}
public void Hide()
{
Close();
OnClosed();
}
#region Event Handlers
private void AttachEvents()
{
((ISetLogicalParent)this).SetParent(TextArea.GetVisualRoot() as ILogical);
_document = TextArea.Document;
if (_document != null)
{
_document.Changing += TextArea_Document_Changing;
}
// LostKeyboardFocus seems to be more reliable than PreviewLostKeyboardFocus - see SD-1729
TextArea.LostFocus += TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged += TextViewScrollOffsetChanged;
TextArea.DocumentChanged += TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged += ParentWindow_LocationChanged;
_parentWindow.Deactivated += ParentWindow_Deactivated;
}
// close previous completion windows of same type
foreach (var x in TextArea.StackedInputHandlers.OfType<InputHandler>())
{
if (x.Window.GetType() == GetType())
TextArea.PopStackedInputHandler(x);
}
_myInputHandler = new InputHandler(this);
TextArea.PushStackedInputHandler(_myInputHandler);
}
/// <summary>
/// Detaches events from the text area.
/// </summary>
protected virtual void DetachEvents()
{
((ISetLogicalParent)this).SetParent(null);
if (_document != null)
{
_document.Changing -= TextArea_Document_Changing;
}
TextArea.LostFocus -= TextAreaLostFocus;
TextArea.TextView.ScrollOffsetChanged -= TextViewScrollOffsetChanged;
TextArea.DocumentChanged -= TextAreaDocumentChanged;
if (_parentWindow != null)
{
_parentWindow.PositionChanged -= ParentWindow_LocationChanged;
_parentWindow.Deactivated -= ParentWindow_Deactivated;
}
TextArea.PopStackedInputHandler(_myInputHandler);
}
#region InputHandler
private InputHandler _myInputHandler;
/// <summary>
/// A dummy input handler (that justs invokes the default input handler).
/// This is used to ensure the completion window closes when any other input handler
/// becomes active.
/// </summary>
private sealed class InputHandler : TextAreaStackedInputHandler
{
internal readonly CompletionWindowBase Window;
public InputHandler(CompletionWindowBase window)
: base(window.TextArea)
{
Debug.Assert(window != null);
Window = window;
}
public override void Detach()
{
base.Detach();
Window.Hide();
}
public override void OnPreviewKeyDown(KeyEventArgs e)
{
// prevents crash when typing deadchar while CC window is open
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyDownEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
public override void OnPreviewKeyUp(KeyEventArgs e)
{
if (e.Key == Key.DeadCharProcessed)
return;
e.Handled = RaiseEventPair(Window, null, KeyUpEvent,
new KeyEventArgs { Device = e.Device, Key = e.Key });
}
}
#endregion
private void TextViewScrollOffsetChanged(object sender, EventArgs e)
{
ILogicalScrollable textView = TextArea;
var visibleRect = new Rect(textView.Offset.X, textView.Offset.Y, textView.Viewport.Width, textView.Viewport.Height);
//close completion window when the user scrolls so far that the anchor position is leaving the visible area
if (visibleRect.Contains(_visualLocation) || visibleRect.Contains(_visualLocationTop))
{
UpdatePosition();
}
else
{
Hide();
}
}
private void TextAreaDocumentChanged(object sender, EventArgs e)
{
Hide();
}
private void TextAreaLostFocus(object sender, RoutedEventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
private void ParentWindow_Deactivated(object sender, EventArgs e)
{
Hide();
}
private void ParentWindow_LocationChanged(object sender, EventArgs e)
{
UpdatePosition();
}
/// <inheritdoc/>
private void OnDeactivated(object sender, EventArgs e)
{
Dispatcher.UIThread.Post(CloseIfFocusLost, DispatcherPriority.Background);
}
#endregion
/// <summary>
/// Raises a tunnel/bubble event pair for a control.
/// </summary>
/// <param name="target">The control for which the event should be raised.</param>
/// <param name="previewEvent">The tunneling event.</param>
/// <param name="event">The bubbling event.</param>
/// <param name="args">The event args to use.</param>
/// <returns>The <see cref="RoutedEventArgs.Handled"/> value of the event args.</returns>
[SuppressMessage("Microsoft.Design", "CA1030:UseEventsWhereAppropriate")]
protected static bool RaiseEventPair(Control target, RoutedEvent previewEvent, RoutedEvent @event, RoutedEventArgs args)
{
if (target == null)
throw new ArgumentNullException(nameof(target));
if (args == null)
throw new ArgumentNullException(nameof(args));
if (previewEvent != null)
{
args.RoutedEvent = previewEvent;
target.RaiseEvent(args);
}
args.RoutedEvent = @event ?? throw new ArgumentNullException(nameof(@event));
target.RaiseEvent(args);
return args.Handled;
}
// Special handler: handledEventsToo
private void OnMouseUp(object sender, PointerReleasedEventArgs e)
{
ActivateParentWindow();
}
/// <summary>
/// Activates the parent window.
/// </summary>
protected virtual void ActivateParentWindow()
{
_parentWindow?.Activate();
}
private void CloseIfFocusLost()
{
if (CloseOnFocusLost)
{
Debug.WriteLine("CloseIfFocusLost: this.IsFocues=" + IsFocused + " IsTextAreaFocused=" + IsTextAreaFocused);
if (!IsFocused && !IsTextAreaFocused)
{
Hide();
}
}
}
/// <summary>
/// Gets whether the completion window should automatically close when the text editor looses focus.
/// </summary>
protected virtual bool CloseOnFocusLost => true;
private bool IsTextAreaFocused
{
get
{
if (_parentWindow != null && !_parentWindow.IsActive)
return false;
return TextArea.IsFocused;
}
}
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
if (!e.Handled && e.Key == Key.Escape)
{
e.Handled = true;
Hide();
}
}
private Point _visualLocation;
private Point _visualLocationTop;
/// <summary>
/// Positions the completion window at the specified position.
/// </summary>
protected void SetPosition(TextViewPosition position)
{
var textView = TextArea.TextView;
_visualLocation = textView.GetVisualPosition(position, VisualYPosition.LineBottom);
_visualLocationTop = textView.GetVisualPosition(position, VisualYPosition.LineTop);
UpdatePosition();
}
/// <summary>
/// Updates the position of the CompletionWindow based on the parent TextView position and the screen working area.
/// It ensures that the CompletionWindow is completely visible on the screen.
/// </summary>
protected void UpdatePosition()
{
var textView = TextArea.TextView;
var position = _visualLocation - textView.ScrollOffset;
this.HorizontalOffset = position.X;
this.VerticalOffset = position.Y;
}
// TODO: check if needed
///// <inheritdoc/>
//protected override void OnRenderSizeChanged(SizeChangedInfo sizeInfo)
//{
// base.OnRenderSizeChanged(sizeInfo);
// if (sizeInfo.HeightChanged && IsUp)
// {
// this.Top += sizeInfo.PreviousSize.Height - sizeInfo.NewSize.Height;
// }
//}
/// <summary>
/// Gets/sets whether the completion window should expect text insertion at the start offset,
/// which not go into the completion region, but before it.
/// </summary>
/// <remarks>This property allows only a single insertion, it is reset to false
/// when that insertion has occurred.</remarks>
public bool ExpectInsertionBeforeStart { get; set; }
private void TextArea_Document_Changing(object sender, DocumentChangeEventArgs e)
{
if (e.Offset + e.RemovalLength == StartOffset && e.RemovalLength > 0)
{
Hide(); // removal immediately in front of completion segment: close the window
// this is necessary when pressing backspace after dot-completion
}
if (e.Offset == StartOffset && e.RemovalLength == 0 && ExpectInsertionBeforeStart)
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.AfterInsertion);
ExpectInsertionBeforeStart = false;
}
else
{
StartOffset = e.GetNewOffset(StartOffset, AnchorMovementType.BeforeInsertion);
}
EndOffset = e.GetNewOffset(EndOffset, AnchorMovementType.AfterInsertion);
}
}
}
<MSG> detachevents
<DFF> @@ -89,9 +89,9 @@ namespace AvaloniaEdit.CodeCompletion
StartOffset = EndOffset = TextArea.Caret.Offset;
- // TODO: these events do not fire on PopupRoot
- //Deactivated += OnDeactivated;
- //Closed += (sender, args) => DetachEvents();
+ //Deactivated += OnDeactivated; //Not needed?
+
+ Closed += (sender, args) => DetachEvents();
AttachEvents();
| 3 | detachevents | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063762 | <NME> finding-modules
<BEF> ADDFILE
<MSG> Create finding-modules
<DFF> @@ -0,0 +1,31 @@
+
+#Finding modules
+
+When working with a nested (DOM like) view structure, you need a way get to child view instances. FruitMachine has three APIs for this:
+
+#### View#module();
+
+Returns a single descendent View instance by module type. Similar to `Element.prototype.querySelector`.
+
+```js
+apple.module('orange');
+//=> orange
+```
+
+#### View#modules();
+
+Returns a list of descendent View instance by module type. Similar to `Element.prototype.querySelectorAll`.
+
+```js
+apple.modules('orange');
+//=> [orange, orange, ...]
+```
+
+#### View#id();
+
+Returns a single descendent View instance by id. Similar to `document.getElementById`.
+
+```js
+apple.id('my_orange_1');
+//=> orange
+```
| 31 | Create finding-modules | 0 | finding-modules | mit | ftlabs/fruitmachine |
|
10063763 | <NME> jsgrid.tests.js
<BEF> $(function() {
var Grid = jsGrid.Grid,
JSGRID = "JSGrid",
JSGRID_DATA_KEY = JSGRID;
Grid.prototype.updateOnResize = false;
module("basic");
test("default creation", function() {
var gridOptions = {
simpleOption: "test",
complexOption: {
a: "subtest",
b: 1,
c: {}
}
},
grid = new Grid("#jsGrid", gridOptions);
equal(grid._container[0], $("#jsGrid")[0], "container saved");
equal(grid.simpleOption, "test", "primitive option extended");
equal(grid.complexOption, gridOptions.complexOption, "non-primitive option extended");
});
test("jquery adapter creation", function() {
var gridOptions = {
option: "test"
},
$element = $("#jsGrid"),
result = $element.jsGrid(gridOptions),
grid = $element.data(JSGRID_DATA_KEY);
equal(result, $element, "jquery fn returned source jQueryElement");
ok(grid instanceof Grid, "jsGrid saved to jquery data");
equal(grid.option, "test", "options provided");
});
test("destroy", function() {
var $element = $("#jsGrid"),
grid;
$element.jsGrid({});
grid = $element.data(JSGRID_DATA_KEY);
grid.destroy();
strictEqual($element.html(), "", "content is removed");
strictEqual($element.data(JSGRID_DATA_KEY), undefined, "jquery data is removed");
});
test("jquery adapter second call changes option value", function() {
var $element = $("#jsGrid"),
gridOptions = {
option: "test"
},
grid;
$element.jsGrid(gridOptions);
grid = $element.data(JSGRID_DATA_KEY);
gridOptions.option = "new test";
$element.jsGrid(gridOptions);
equal(grid, $element.data(JSGRID_DATA_KEY), "instance was not changed");
equal(grid.option, "new test", "option changed");
});
test("jquery adapter invokes jsGrid method", function() {
var methodResult = "",
$element = $("#jsGrid"),
gridOptions = {
method: function(str) {
methodResult = "test_" + str;
}
};
$element.jsGrid(gridOptions);
$element.jsGrid("method", "invoke");
equal(methodResult, "test_invoke", "method invoked");
});
test("onInit callback", function() {
var $element = $("#jsGrid"),
onInitArguments,
gridOptions = {
onInit: function(args) {
onInitArguments = args;
}
};
var grid = new Grid($element, gridOptions);
equal(onInitArguments.grid, grid, "grid instance is provided in onInit callback arguments");
});
test("controller methods are $.noop when not specified", function() {
var $element = $("#jsGrid"),
gridOptions = {
controller: {}
},
testOption;
$element.jsGrid(gridOptions);
deepEqual($element.data(JSGRID_DATA_KEY)._controller, {
loadData: $.noop,
insertItem: $.noop,
updateItem: $.noop,
deleteItem: $.noop
}, "controller has stub methods");
});
test("option method", function() {
var $element = $("#jsGrid"),
gridOptions = {
test: "value"
},
testOption;
$element.jsGrid(gridOptions);
testOption = $element.jsGrid("option", "test");
equal(testOption, "value", "read option value");
$element.jsGrid("option", "test", "new_value");
testOption = $element.jsGrid("option", "test");
equal(testOption, "new_value", "set option value");
});
test("fieldOption method", function() {
var dataLoadedCount = 0;
var $element = $("#jsGrid"),
gridOptions = {
loadMessage: "",
autoload: true,
controller: {
loadData: function() {
dataLoadedCount++;
return [{ prop1: "value1", prop2: "value2", prop3: "value3" }];
}
},
fields: [
{ name: "prop1", title: "_" }
]
};
$element.jsGrid(gridOptions);
var fieldOptionValue = $element.jsGrid("fieldOption", "prop1", "name");
equal(fieldOptionValue, "prop1", "read field option");
$element.jsGrid("fieldOption", "prop1", "name", "prop2");
equal($element.text(), "_value2", "set field option by field name");
equal(dataLoadedCount, 1, "data not reloaded on field option change");
$element.jsGrid("fieldOption", 0, "name", "prop3");
equal($element.text(), "_value3", "set field option by field index");
});
test("option changing event handlers", function() {
var $element = $("#jsGrid"),
optionChangingEventArgs,
optionChangedEventArgs,
gridOptions = {
test: "testValue",
another: "anotherValue",
onOptionChanging: function(e) {
optionChangingEventArgs = $.extend({}, e);
e.option = "another";
e.newValue = e.newValue + "_" + this.another;
},
onOptionChanged: function(e) {
optionChangedEventArgs = $.extend({}, e);
}
},
anotherOption;
$element.jsGrid(gridOptions);
$element.jsGrid("option", "test", "newTestValue");
equal(optionChangingEventArgs.option, "test", "option name is provided in args of optionChanging");
equal(optionChangingEventArgs.oldValue, "testValue", "old option value is provided in args of optionChanging");
equal(optionChangingEventArgs.newValue, "newTestValue", "new option value is provided in args of optionChanging");
anotherOption = $element.jsGrid("option", "another");
equal(anotherOption, "newTestValue_anotherValue", "option changing handler changed option and value");
equal(optionChangedEventArgs.option, "another", "option name is provided in args of optionChanged");
equal(optionChangedEventArgs.value, "newTestValue_anotherValue", "option value is provided in args of optionChanged");
});
test("common layout rendering", function() {
var $element = $("#jsGrid"),
grid = new Grid($element, {}),
$headerGrid,
$headerGridTable,
$bodyGrid,
$bodyGridTable;
ok($element.hasClass(grid.containerClass), "container class attached");
ok($element.children().eq(0).hasClass(grid.gridHeaderClass), "grid header");
ok($element.children().eq(1).hasClass(grid.gridBodyClass), "grid body");
ok($element.children().eq(2).hasClass(grid.pagerContainerClass), "pager container");
$headerGrid = $element.children().eq(0);
$headerGridTable = $headerGrid.children().first();
ok($headerGridTable.hasClass(grid.tableClass), "header table");
equal($headerGrid.find("." + grid.headerRowClass).length, 1, "header row");
equal($headerGrid.find("." + grid.filterRowClass).length, 1, "filter row");
equal($headerGrid.find("." + grid.insertRowClass).length, 1, "insert row");
ok(grid._headerRow.hasClass(grid.headerRowClass), "header row class");
ok(grid._filterRow.hasClass(grid.filterRowClass), "filter row class");
ok(grid._insertRow.hasClass(grid.insertRowClass), "insert row class");
$bodyGrid = $element.children().eq(1);
$bodyGridTable = $bodyGrid.children().first();
ok($bodyGridTable.hasClass(grid.tableClass), "body table");
equal(grid._content.parent()[0], $bodyGridTable[0], "content is tbody in body table");
equal($bodyGridTable.find("." + grid.noDataRowClass).length, 1, "no data row");
equal($bodyGridTable.text(), grid.noDataContent, "no data text");
});
test("set default options with setDefaults", function() {
jsGrid.setDefaults({
defaultOption: "test"
});
var $element = $("#jsGrid").jsGrid({});
equal($element.jsGrid("option", "defaultOption"), "test", "default option set");
});
module("loading");
test("loading with controller", function() {
var $element = $("#jsGrid"),
data = [
{ test: "test1" },
{ test: "test2" }
],
gridOptions = {
controller: {
loadData: function() {
return data;
}
}
},
grid = new Grid($element, gridOptions);
grid.loadData();
equal(grid.option("data"), data, "loadData loads data");
});
test("loadData throws exception when controller method not found", function() {
var $element = $("#jsGrid");
var grid = new Grid($element);
grid._controller = {};
throws(function() {
grid.loadData();
}, /loadData/, "loadData threw an exception");
});
test("onError event should be fired on controller fail", function() {
var errorArgs,
errorFired = 0,
$element = $("#jsGrid"),
gridOptions = {
controller: {
loadData: function() {
return $.Deferred().reject({ value: 1 }, "test").promise();
}
},
onError: function(args) {
errorFired++;
errorArgs = args;
}
},
grid = new Grid($element, gridOptions);
grid.loadData();
equal(errorFired, 1, "onError handler fired");
deepEqual(errorArgs, { grid: grid, args: [{ value: 1 }, "test"] }, "error has correct params");
});
asyncTest("autoload should call loadData after render", 1, function() {
new Grid($("#jsGrid"), {
autoload: true,
controller: {
loadData: function() {
ok(true, "autoload calls loadData on creation");
start();
return [];
}
}
});
});
test("loading filtered data", function() {
var filteredData,
loadingArgs,
loadedArgs,
$element = $("#jsGrid"),
data = [
{ field: "test" },
{ field: "test_another" },
{ field: "test_another" },
{ field: "test" }
],
gridOptions = {
filtering: true,
fields: [
{
name: "field",
filterValue: function(value) {
return "test";
}
}
],
onDataLoading: function(e) {
loadingArgs = $.extend(true, {}, e);
},
onDataLoaded: function(e) {
loadedArgs = $.extend(true, {}, e);
},
controller: {
loadData: function(filter) {
filteredData = $.grep(data, function(item) {
return item.field === filter.field;
});
return filteredData;
}
}
},
grid = new Grid($element, gridOptions);
grid.loadData();
equal(loadingArgs.filter.field, "test");
equal(grid.option("data").length, 2, "filtered data loaded");
deepEqual(loadedArgs.data, filteredData);
});
asyncTest("loading indication", function() {
var timeout = 10,
stage = "initial",
$element = $("#jsGrid"),
gridOptions = {
loadIndication: true,
loadIndicationDelay: timeout,
loadMessage: "loading...",
loadIndicator: function(config) {
equal(config.message, gridOptions.loadMessage, "message provided");
ok(config.container.jquery, "grid container is provided");
return {
show: function() {
stage = "started";
},
hide: function() {
stage = "finished";
}
};
},
fields: [
{ name: "field" }
],
controller: {
loadData: function() {
var deferred = $.Deferred();
equal(stage, "initial", "initial stage");
setTimeout(function() {
equal(stage, "started", "loading started");
deferred.resolve([]);
equal(stage, "finished", "loading finished");
start();
}, timeout);
return deferred.promise();
}
}
},
grid = new Grid($element, gridOptions);
grid.loadData();
});
asyncTest("loadingIndication=false should not show loading", 0, function() {
var $element = $("#jsGrid"),
timeout = 10,
gridOptions = {
loadIndication: false,
loadIndicationDelay: timeout,
loadIndicator: function() {
return {
show: function() {
ok(false, "should not call show");
},
hide: function() {
ok(false, "should not call hide");
}
};
},
fields: [
{ name: "field" }
],
controller: {
loadData: function() {
var deferred = $.Deferred();
setTimeout(function() {
deferred.resolve([]);
start();
}, timeout);
return deferred.promise();
}
}
},
grid = new Grid($element, gridOptions);
grid.loadData();
});
test("search", function() {
var $element = $("#jsGrid"),
data = [
{ field: "test" },
{ field: "test_another" },
{ field: "test_another" },
{ field: "test" }
],
gridOptions = {
pageIndex: 2,
_sortField: "field",
_sortOrder: "desc",
filtering: true,
fields: [
{
name: "field",
filterValue: function(value) {
return "test";
}
}
],
controller: {
loadData: function(filter) {
var filteredData = $.grep(data, function(item) {
return item.field === filter.field;
});
return filteredData;
}
}
},
grid = new Grid($element, gridOptions);
grid.search();
equal(grid.option("data").length, 2, "data filtered");
strictEqual(grid.option("pageIndex"), 1, "pageIndex reset");
strictEqual(grid._sortField, null, "sortField reset");
strictEqual(grid._sortOrder, "asc", "sortOrder reset");
});
test("change loadStrategy on the fly", function() {
var $element = $("#jsGrid");
var gridOptions = {
controller: {
loadData: function() {
return [];
}
}
};
var grid = new Grid($element, gridOptions);
grid.option("loadStrategy", {
firstDisplayIndex: function() {
return 0;
},
lastDisplayIndex: function() {
return 1;
},
loadParams: function() {
return [];
},
finishLoad: function() {
grid.option("data", [{}]);
}
});
grid.loadData();
equal(grid.option("data").length, 1, "new load strategy is applied");
});
module("filtering");
test("filter rendering", function() {
var $element = $("#jsGrid"),
gridOptions = {
filtering: true,
fields: [
{
name: "test",
align: "right",
filtercss: "filter-class",
filterTemplate: function() {
var result = this.filterControl = $("<input>").attr("type", "text").addClass("filter-input");
return result;
}
}
]
},
grid = new Grid($element, gridOptions);
equal(grid._filterRow.find(".filter-class").length, 1, "filtercss class is attached");
equal(grid._filterRow.find(".filter-input").length, 1, "filter control rendered");
equal(grid._filterRow.find("." + grid.cellClass).length, 1, "cell class is attached");
ok(grid._filterRow.find(".filter-class").hasClass("jsgrid-align-right"), "align class is attached");
ok(grid.fields[0].filterControl.is("input[type=text]"), "filter control saved in field");
});
test("filter get/clear", function() {
var $element = $("#jsGrid"),
gridOptions = {
filtering: true,
controller: {
loadData: function() {
return [];
}
},
fields: [
{
name: "field",
filterTemplate: function() {
return this.filterControl = $("<input>").attr("type", "text");
},
filterValue: function() {
return this.filterControl.val();
}
}
]
},
grid = new Grid($element, gridOptions);
grid.fields[0].filterControl.val("test");
deepEqual(grid.getFilter(), { field: "test" }, "get filter");
grid.clearFilter();
deepEqual(grid.getFilter(), { field: "" }, "filter cleared");
equal(grid.fields[0].filterControl.val(), "", "grid field filterControl cleared");
});
test("field without filtering", function() {
var $element = $("#jsGrid"),
jsGridFieldConfig = {
filterTemplate: function() {
var result = this.filterControl = $("<input>").attr("type", "text");
return result;
},
filterValue: function(value) {
if(!arguments.length) {
return this.filterControl.val();
}
this.filterControl.val(value);
}
},
gridOptions = {
filtering: true,
fields: [
$.extend({}, jsGridFieldConfig, {
name: "field1",
filtering: false
}),
$.extend({}, jsGridFieldConfig, {
name: "field2"
})
]
},
grid = new Grid($element, gridOptions);
grid.fields[0].filterControl.val("test1");
grid.fields[1].filterControl.val("test2");
deepEqual(grid.getFilter(), { field2: "test2" }, "field with filtering=false is not included in filter");
});
test("search with filter", function() {
var $element = $("#jsGrid"),
data = [
{ field: "test" },
{ field: "test_another" },
{ field: "test_another" },
{ field: "test" }
],
gridOptions = {
fields: [
{
name: "field"
}
],
controller: {
loadData: function(filter) {
var filteredData = $.grep(data, function(item) {
return item.field === filter.field;
});
return filteredData;
}
}
},
grid = new Grid($element, gridOptions);
grid.search({ field: "test" });
equal(grid.option("data").length, 2, "data filtered");
});
test("filtering with static data should not do actual filtering", function() {
var $element = $("#jsGrid"),
gridOptions = {
filtering: true,
fields: [
{ type: "text", name: "field" }
],
data: [
{ name: "value1" },
{ name: "value2" }
]
},
grid = new Grid($element, gridOptions);
grid._filterRow.find("input").val("1");
grid.search();
equal(grid.option("data").length, 2, "data is not filtered");
});
module("nodatarow");
test("nodatarow after bind on empty array", function() {
var $element = $("#jsGrid"),
gridOptions = {},
grid = new Grid($element, gridOptions);
grid.option("data", []);
equal(grid._content.find("." + grid.noDataRowClass).length, 1, "no data row rendered");
equal(grid._content.find("." + grid.cellClass).length, 1, "grid cell class attached");
equal(grid._content.text(), grid.noDataContent, "no data text rendered");
});
test("nodatarow customize content", function() {
var noDataMessage = "NoData Custom Content",
$element = $("#jsGrid"),
gridOptions = {
noDataContent: function() {
return noDataMessage;
}
},
grid = new Grid($element, gridOptions);
grid.option("data", []);
equal(grid._content.find("." + grid.cellClass).length, 1, "grid cell class attached");
equal(grid._content.text(), noDataMessage, "custom noDataContent");
});
module("row rendering", {
setup: function() {
this.testData = [
{ id: 1, text: "test1" },
{ id: 2, text: "test2" },
{ id: 3, text: "test3" }
];
}
});
test("rows rendered correctly", function() {
var $element = $("#jsGrid"),
gridOptions = {
data: this.testData
},
grid = new Grid($element, gridOptions);
equal(grid._content.children().length, 3, "rows rendered");
equal(grid._content.find("." + grid.oddRowClass).length, 2, "two odd rows for 3 items");
equal(grid._content.find("." + grid.evenRowClass).length, 1, "one even row for 3 items");
});
test("custom rowClass", function() {
var $element = $("#jsGrid"),
gridOptions = {
data: this.testData,
rowClass: "custom-row-cls"
},
grid = new Grid($element, gridOptions);
equal(grid._content.find("." + grid.oddRowClass).length, 2);
equal(grid._content.find("." + grid.evenRowClass).length, 1);
equal(grid._content.find(".custom-row-cls").length, 3, "custom row class");
});
test("custom rowClass callback", function() {
var $element = $("#jsGrid"),
gridOptions = {
data: this.testData,
rowClass: function(item, index) {
return item.text;
}
},
grid = new Grid($element, gridOptions);
equal(grid._content.find("." + grid.oddRowClass).length, 2);
equal(grid._content.find("." + grid.evenRowClass).length, 1);
equal(grid._content.find(".test1").length, 1, "custom row class");
equal(grid._content.find(".test2").length, 1, "custom row class");
equal(grid._content.find(".test3").length, 1, "custom row class");
});
test("rowClick standard handler", function() {
var $element = $("#jsGrid"),
$secondRow,
grid = new Grid($element, { editing: true });
grid.option("data", this.testData);
$secondRow = grid._content.find("." + grid.evenRowClass);
$secondRow.trigger("click", $.Event($secondRow));
equal(grid._editingRow.get(0), $secondRow.get(0), "clicked row is editingRow");
});
test("rowClick handler", function() {
var rowClickArgs,
$element = $("#jsGrid"),
$secondRow,
gridOptions = {
rowClick: function(args) {
rowClickArgs = args;
}
},
grid = new Grid($element, gridOptions);
grid.option("data", this.testData);
$secondRow = grid._content.find("." + grid.evenRowClass);
$secondRow.trigger("click", $.Event($secondRow));
ok(rowClickArgs.event instanceof jQuery.Event, "jquery event arg");
equal(rowClickArgs.item, this.testData[1], "item arg");
equal(rowClickArgs.itemIndex, 1, "itemIndex arg");
});
test("row selecting with selectedRowClass", function() {
var $element = $("#jsGrid"),
$secondRow,
gridOptions = {
selecting: true
},
grid = new Grid($element, gridOptions);
grid.option("data", this.testData);
$secondRow = grid._content.find("." + grid.evenRowClass);
$secondRow.trigger("mouseenter", $.Event($secondRow));
ok($secondRow.hasClass(grid.selectedRowClass), "mouseenter adds selectedRowClass");
$secondRow.trigger("mouseleave", $.Event($secondRow));
ok(!$secondRow.hasClass(grid.selectedRowClass), "mouseleave removes selectedRowClass");
});
test("no row selecting while selection is disabled", function() {
var $element = $("#jsGrid"),
$secondRow,
gridOptions = {
selecting: false
},
grid = new Grid($element, gridOptions);
grid.option("data", this.testData);
$secondRow = grid._content.find("." + grid.evenRowClass);
$secondRow.trigger("mouseenter", $.Event($secondRow));
ok(!$secondRow.hasClass(grid.selectedRowClass), "mouseenter doesn't add selectedRowClass");
});
test("refreshing and refreshed callbacks", function() {
var refreshingEventArgs,
refreshedEventArgs,
$element = $("#jsGrid"),
grid = new Grid($element, {});
grid.onRefreshing = function(e) {
refreshingEventArgs = e;
equal(grid._content.find("." + grid.oddRowClass).length, 0, "no items before refresh");
};
grid.onRefreshed = function(e) {
refreshedEventArgs = e;
equal(grid._content.find("." + grid.oddRowClass).length, 2, "items rendered after refresh");
};
grid.option("data", this.testData);
equal(refreshingEventArgs.grid, grid, "grid provided in args for refreshing event");
equal(refreshedEventArgs.grid, grid, "grid provided in args for refreshed event");
equal(grid._content.find("." + grid.oddRowClass).length, 2, "items rendered");
});
test("grid fields normalization", function() {
var CustomField = function(config) {
$.extend(true, this, config);
};
jsGrid.fields.custom = CustomField;
try {
var $element = $("#jsGrid"),
gridOptions = {
fields: [
new jsGrid.Field({
name: "text1",
title: "title1"
}),
{
name: "text2",
title: "title2"
},
{
name: "text3",
type: "custom"
}
]
},
grid = new Grid($element, gridOptions);
var field1 = grid.fields[0];
ok(field1 instanceof jsGrid.Field);
equal(field1.name, "text1", "name is set for field");
equal(field1.title, "title1", "title field");
var field2 = grid.fields[1];
ok(field2 instanceof jsGrid.Field);
equal(field2.name, "text2", "name is set for field");
equal(field2.title, "title2", "title field");
var field3 = grid.fields[2];
ok(field3 instanceof CustomField);
equal(field3.name, "text3", "name is set for field");
} finally {
delete jsGrid.fields.custom;
}
});
test("'0' itemTemplate should be rendered", function() {
var $element = $("#jsGrid"),
grid = new Grid($element, {
data: [{}],
fields: [
new jsGrid.Field({ name: "id", itemTemplate: function() { return 0; } })
]
});
equal(grid._bodyGrid.text(), "0", "item template is rendered");
});
test("grid field name used for header if title is not specified", function() {
var $element = $("#jsGrid"),
grid = new Grid($element, {
fields: [
new jsGrid.Field({ name: "id" })
]
});
equal(grid._headerRow.text(), "id", "name is rendered in header");
});
test("grid fields header and item rendering", function() {
var $element = $("#jsGrid"),
$secondRow,
gridOptions = {
fields: [
new jsGrid.Field({
name: "text",
title: "title",
css: "cell-class",
headercss: "header-class",
align: "right"
})
]
},
grid = new Grid($element, gridOptions);
grid.option("data", this.testData);
equal(grid._headerRow.text(), "title", "header rendered");
equal(grid._headerRow.find("." + grid.headerCellClass).length, 1, "header cell class is attached");
equal(grid._headerRow.find(".header-class").length, 1, "headercss class is attached");
ok(grid._headerRow.find(".header-class").hasClass("jsgrid-align-right"), "align class is attached");
$secondRow = grid._content.find("." + grid.evenRowClass);
equal($secondRow.text(), "test2", "item rendered");
equal($secondRow.find(".cell-class").length, 1, "css class added to cell");
equal($secondRow.find("." + grid.cellClass).length, 1, "cell class is attached");
ok($secondRow.find(".cell-class").hasClass("jsgrid-align-right"), "align class added to cell");
});
test("grid field cellRenderer", function() {
var testItem = { text: "test" },
args;
var $grid = $("#jsGrid");
var gridOptions = {
data: [testItem],
fields: [
{
name: "text",
cellRenderer: function(value, item) {
args = {
value: value,
item: item
};
return $("<td>").addClass("custom-class").text(value);
}
}
]
};
var grid = new Grid($grid, gridOptions);
var $customCell = $grid.find(".custom-class");
equal($customCell.length, 1, "custom cell rendered");
equal($customCell.text(), "test");
deepEqual(args, { value: "test", item: testItem }, "cellRenderer args provided");
});
test("grid field 'visible' option", function() {
var $grid = $("#jsGrid");
var gridOptions = {
editing: true,
fields: [
{ name: "id", visible: false },
{ name: "test" }
]
};
var grid = new Grid($grid, gridOptions);
equal($grid.find("." + grid.noDataRowClass).children().eq(0).prop("colspan"), 1, "no data row colspan only for visible cells");
grid.option("data", this.testData);
grid.editItem(this.testData[2]);
equal($grid.find("." + grid.headerRowClass).children().length, 1, "header single cell");
equal($grid.find("." + grid.filterRowClass).children().length, 1, "filter single cell");
equal($grid.find("." + grid.insertRowClass).children().length, 1, "insert single cell");
equal($grid.find("." + grid.editRowClass).children().length, 1, "edit single cell");
equal($grid.find("." + grid.oddRowClass).eq(0).children().length, 1, "odd data row single cell");
equal($grid.find("." + grid.evenRowClass).eq(0).children().length, 1, "even data row single cell");
});
module("inserting");
test("inserting rendering", function() {
var $element = $("#jsGrid"),
gridOptions = {
inserting: true,
fields: [
{
name: "test",
align: "right",
insertcss: "insert-class",
insertTemplate: function() {
var result = this.insertControl = $("<input>").attr("type", "text").addClass("insert-input");
return result;
}
}
]
},
grid = new Grid($element, gridOptions);
equal(grid._insertRow.find(".insert-class").length, 1, "insertcss class is attached");
equal(grid._insertRow.find(".insert-input").length, 1, "insert control rendered");
equal(grid._insertRow.find("." + grid.cellClass).length, 1, "cell class is attached");
ok(grid._insertRow.find(".insert-class").hasClass("jsgrid-align-right"), "align class is attached");
ok(grid.fields[0].insertControl.is("input[type=text]"), "insert control saved in field");
});
test("field without inserting", function() {
var $element = $("#jsGrid"),
jsGridFieldConfig = {
insertTemplate: function() {
var result = this.insertControl = $("<input>").attr("type", "text");
return result;
},
insertValue: function() {
return this.insertControl.val();
}
},
gridOptions = {
inserting: true,
fields: [
$.extend({}, jsGridFieldConfig, {
name: "field1",
inserting: false
}),
$.extend({}, jsGridFieldConfig, {
name: "field2"
})
]
},
grid = new Grid($element, gridOptions);
grid.fields[0].insertControl.val("test1");
grid.fields[1].insertControl.val("test2");
deepEqual(grid._getInsertItem(), { field2: "test2" }, "field with inserting=false is not included in inserting item");
});
test("insert data with default location", function() {
var $element = $("#jsGrid"),
inserted = false,
insertingArgs,
insertedArgs,
gridOptions = {
inserting: true,
data: [{field: "default"}],
fields: [
{
name: "field",
insertTemplate: function() {
var result = this.insertControl = $("<input>").attr("type", "text");
return result;
},
insertValue: function() {
return this.insertControl.val();
}
}
],
onItemInserting: function(e) {
insertingArgs = $.extend(true, {}, e);
},
onItemInserted: function(e) {
insertedArgs = $.extend(true, {}, e);
},
controller: {
insertItem: function() {
inserted = true;
}
}
},
grid = new Grid($element, gridOptions);
grid.fields[0].insertControl.val("test");
grid.insertItem();
equal(insertingArgs.item.field, "test", "field is provided in inserting args");
equal(grid.option("data").length, 2, "data is inserted");
ok(inserted, "controller insertItem was called");
deepEqual(grid.option("data")[1], { field: "test" }, "correct data is inserted");
equal(insertedArgs.item.field, "test", "field is provided in inserted args");
});
test("insert data with specified insert location", function() {
var $element = $("#jsGrid"),
inserted = false,
insertingArgs,
insertedArgs,
gridOptions = {
inserting: true,
insertRowLocation: "top",
data: [{field: "default"}],
fields: [
{
name: "field",
insertTemplate: function() {
var result = this.insertControl = $("<input>").attr("type", "text");
return result;
},
insertValue: function() {
return this.insertControl.val();
}
}
],
onItemInserting: function(e) {
insertingArgs = $.extend(true, {}, e);
},
onItemInserted: function(e) {
insertedArgs = $.extend(true, {}, e);
},
controller: {
insertItem: function() {
inserted = true;
}
}
},
grid = new Grid($element, gridOptions);
grid.fields[0].insertControl.val("test");
grid.insertItem();
equal(insertingArgs.item.field, "test", "field is provided in inserting args");
equal(grid.option("data").length, 2, "data is inserted");
ok(inserted, "controller insertItem was called");
deepEqual(grid.option("data")[0], { field: "test" }, "correct data is inserted at the beginning");
equal(insertedArgs.item.field, "test", "field is provided in inserted args");
});
test("insertItem accepts item to insert", function() {
var $element = $("#jsGrid"),
itemToInsert = { field: "test" },
insertedItem,
gridOptions = {
data: [],
fields: [
{
name: "field"
}
],
test("edit item", function() {
var $element = $("#jsGrid"),
updated = false,
updatingArgs,
updatingRow,
grid.insertItem(itemToInsert);
deepEqual(grid.option("data")[0], itemToInsert, "data is inserted");
deepEqual(insertedItem, itemToInsert, "controller insertItem was called with correct item");
});
module("editing");
test("editing rendering", function() {
var $element = $("#jsGrid"),
$editRow,
data = [{
test: "value"
}],
gridOptions = {
editing: true,
fields: [
{
name: "test",
align: "right",
updated = true;
}
},
onItemUpdating: function(e) {
updatingArgs = $.extend(true, {}, e);
updatingRow = grid.rowByItem(data[0])[0];
grid.option("data", data);
equal(grid._content.find("." + grid.editRowClass).length, 0, "no edit row after initial rendering");
grid.editItem(data[0]);
$editRow = grid._content.find("." + grid.editRowClass);
equal($editRow.length, 1, "edit row rendered");
grid.option("data", data);
grid.editItem(data[0]);
grid.fields[0].editControl.val("new value");
grid.updateItem();
$editRow,
data = [
{ test: "value" }
],
gridOptions = {
editing: true,
fields: [
{ name: "test" }
]
},
grid = new Grid($element, gridOptions);
grid.option("data", data);
var $row = $element.find("." + grid.oddRowClass).eq(0);
grid.editItem($row);
$editRow = grid._content.find("." + grid.editRowClass);
equal($editRow.length, 1, "edit row rendered");
grid.cancelEdit();
grid.editItem($row.get(0));
$editRow = grid._content.find("." + grid.editRowClass);
equal($editRow.length, 1, "edit row rendered");
});
test("edit item", function() {
var $element = $("#jsGrid"),
editingArgs,
editingRow,
updated = false,
updatingArgs,
updatingRow,
updatedRow,
updatedArgs,
data = [{
field: "value"
}],
gridOptions = {
editing: true,
fields: [
{
name: "field",
editTemplate: function(value) {
var result = this.editControl = $("<input>").attr("type", "text").val(value);
return result;
},
editValue: function() {
return this.editControl.val();
}
}
],
controller: {
updateItem: function(updatingItem) {
updated = true;
}
},
onItemEditing: function(e) {
editingArgs = $.extend(true, {}, e);
editingRow = grid.rowByItem(data[0])[0];
},
onItemUpdating: function(e) {
updatingArgs = $.extend(true, {}, e);
updatingRow = grid.rowByItem(data[0])[0];
},
onItemUpdated: function(e) {
updatedArgs = $.extend(true, {}, e);
updatedRow = grid.rowByItem(data[0])[0];
}
},
grid = new Grid($element, gridOptions);
grid.option("data", data);
grid.editItem(data[0]);
deepEqual(editingArgs.item, { field: "value" }, "item before editing is provided in editing event args");
equal(editingArgs.itemIndex, 0, "itemIndex is provided in editing event args");
equal(editingArgs.row[0], editingRow, "row element is provided in editing event args");
grid.fields[0].editControl.val("new value");
grid.updateItem();
deepEqual(updatingArgs.previousItem, { field: "value" }, "item before editing is provided in updating event args");
deepEqual(updatingArgs.item, { field: "new value" }, "updating item is provided in updating event args");
equal(updatingArgs.itemIndex, 0, "itemIndex is provided in updating event args");
equal(updatingArgs.row[0], updatingRow, "row element is provided in updating event args");
ok(updated, "controller updateItem called");
deepEqual(grid.option("data")[0], { field: "new value" }, "correct data updated");
equal(grid._content.find("." + grid.editRowClass).length, 0, "edit row removed");
equal(grid._content.find("." + grid.oddRowClass).length, 1, "data row rendered");
deepEqual(updatedArgs.previousItem, { field: "value" }, "item before editing is provided in updated event args");
deepEqual(updatedArgs.item, { field: "new value" }, "updated item is provided in updated event args");
equal(updatedArgs.itemIndex, 0, "itemIndex is provided in updated event args");
equal(updatedArgs.row[0], updatedRow, "row element is provided in updated event args");
});
test("failed update should not change original item", function() {
var $element = $("#jsGrid"),
data = [{
field: "value"
}],
gridOptions = {
editing: true,
fields: [
{
name: "field",
editTemplate: function(value) {
var result = this.editControl = $("<input>").attr("type", "text").val(value);
return result;
},
editValue: function() {
return this.editControl.val();
}
}
],
controller: {
updateItem: function(updatingItem) {
return $.Deferred().reject();
}
}
},
grid = new Grid($element, gridOptions);
grid.option("data", data);
grid.editItem(data[0]);
grid.fields[0].editControl.val("new value");
grid.updateItem();
deepEqual(grid.option("data")[0], { field: "value" }, "value is not updated");
});
test("cancel edit", function() {
var $element = $("#jsGrid"),
updated = false,
cancellingArgs,
cancellingRow,
data = [{
field: "value"
}],
gridOptions = {
editing: true,
fields: [
{
name: "field",
editTemplate: function(value) {
var result = this.editControl = $("<input>").attr("type", "text").val(value);
return result;
},
editValue: function() {
return this.editControl.val();
}
}
],
controller: {
updateData: function(updatingItem) {
updated = true;
}
},
onItemEditCancelling: function(e) {
cancellingArgs = $.extend(true, {}, e);
cancellingRow = grid.rowByItem(data[0])[0];
}
},
grid = new Grid($element, gridOptions);
grid.option("data", data);
grid.editItem(data[0]);
grid.fields[0].editControl.val("new value");
grid.cancelEdit();
deepEqual(cancellingArgs.item, { field: "value" }, "item before cancel is provided in cancelling event args");
equal(cancellingArgs.itemIndex, 0, "itemIndex is provided in cancelling event args");
equal(cancellingArgs.row[0], cancellingRow, "row element is provided in cancelling event args");
ok(!updated, "controller updateItem was not called");
deepEqual(grid.option("data")[0], { field: "value" }, "data were not updated");
equal(grid._content.find("." + grid.editRowClass).length, 0, "edit row removed");
equal(grid._content.find("." + grid.oddRowClass).length, 1, "data row restored");
});
test("updateItem accepts item to update and new item", function() {
var $element = $("#jsGrid"),
updatingItem,
data = [{
field: "value"
}],
gridOptions = {
fields: [
{ name: "field" }
],
controller: {
updateItem: function(item) {
return updatingItem = item;
}
}
},
grid = new Grid($element, gridOptions);
grid.option("data", data);
grid.updateItem(data[0], { field: "new value" });
deepEqual(updatingItem, { field: "new value" }, "controller updateItem called correctly");
deepEqual(grid.option("data")[0], { field: "new value" }, "correct data updated");
});
test("updateItem accepts single argument - item to update", function() {
var $element = $("#jsGrid"),
updatingItem,
data = [{
field: "value"
}],
gridOptions = {
fields: [
{ name: "field" }
],
controller: {
updateItem: function(item) {
return updatingItem = item;
}
}
},
grid = new Grid($element, gridOptions);
grid.option("data", data);
data[0].field = "new value";
grid.updateItem(data[0]);
deepEqual(updatingItem, { field: "new value" }, "controller updateItem called correctly");
deepEqual(grid.option("data")[0], { field: "new value" }, "correct data updated");
});
test("editRowRenderer", function() {
var $element = $("#jsGrid"),
data = [
{ value: "test" }
],
gridOptions = {
data: data,
editing: true,
editRowRenderer: function(item, itemIndex) {
return $("<tr>").addClass("custom-edit-row").append($("<td>").text(itemIndex + ":" + item.value));
},
fields: [
{ name: "value" }
]
},
grid = new Grid($element, gridOptions);
grid.editItem(data[0]);
var $editRow = grid._content.find(".custom-edit-row");
equal($editRow.length, 1, "edit row rendered");
equal($editRow.text(), "0:test", "custom edit row renderer rendered");
});
module("deleting");
test("delete item", function() {
var $element = $("#jsGrid"),
deleted = false,
deletingArgs,
deletedArgs,
data = [{
field: "value"
}],
gridOptions = {
confirmDeleting: false,
fields: [
{ name: "field" }
],
controller: {
deleteItem: function(deletingItem) {
deleted = true;
}
},
onItemDeleting: function(e) {
deletingArgs = $.extend(true, {}, e);
},
onItemDeleted: function(e) {
deletedArgs = $.extend(true, {}, e);
}
},
grid = new Grid($element, gridOptions);
grid.option("data", data);
grid.deleteItem(data[0]);
deepEqual(deletingArgs.item, { field: "value" }, "field and value is provided in deleting event args");
equal(deletingArgs.itemIndex, 0, "itemIndex is provided in updating event args");
equal(deletingArgs.row.length, 1, "row element is provided in updating event args");
ok(deleted, "controller deleteItem called");
equal(grid.option("data").length, 0, "data row deleted");
deepEqual(deletedArgs.item, { field: "value" }, "item is provided in updating event args");
equal(deletedArgs.itemIndex, 0, "itemIndex is provided in updating event args");
equal(deletedArgs.row.length, 1, "row element is provided in updating event args");
});
test("deleteItem accepts row", function() {
var $element = $("#jsGrid"),
deletedItem,
itemToDelete = {
field: "value"
},
data = [itemToDelete],
gridOptions = {
confirmDeleting: false,
fields: [
{ name: "field" }
],
controller: {
deleteItem: function(deletingItem) {
deletedItem = deletingItem;
}
}
},
grid = new Grid($element, gridOptions);
grid.option("data", data);
var $row = $element.find("." + grid.oddRowClass).eq(0);
grid.deleteItem($row);
deepEqual(deletedItem, itemToDelete, "controller deleteItem called correctly");
equal(grid.option("data").length, 0, "data row deleted");
});
module("paging");
test("pager is rendered if necessary", function() {
var $element = $("#jsGrid"),
grid = new Grid($element, {
data: [{}, {}, {}],
paging: false,
pageSize: 2
});
ok(grid._pagerContainer.is(":hidden"), "pager is hidden when paging=false");
equal(grid._pagerContainer.html(), "", "pager is not rendered when paging=false");
grid.option("paging", true);
ok(grid._pagerContainer.is(":visible"), "pager is visible when paging=true");
ok(grid._pagerContainer.html(), "pager is rendered when paging=true");
grid.option("data", [{}, {}]);
ok(grid._pagerContainer.is(":hidden"), "pager is hidden for single page");
ok(grid._pagerContainer.html(), "pager is rendered for single page when paging=true");
});
test("external pagerContainer", function() {
var $pagerContainer = $("<div>").appendTo("#qunit-fixture").hide(),
$element = $("#jsGrid");
new Grid($element, {
data: [{}, {}, {}],
pagerContainer: $pagerContainer,
paging: true,
pageSize: 2
});
ok($pagerContainer.is(":visible"), "external pager shown");
ok($pagerContainer.html(), "external pager rendered");
});
test("pager functionality", function() {
var $element = $("#jsGrid"),
pager,
pageChangedArgs,
grid = new Grid($element, {
data: [{}, {}, {}, {}, {}, {}, {}, {}, {}],
onPageChanged: function(args) {
pageChangedArgs = args;
},
paging: true,
pageSize: 2,
pageButtonCount: 3
});
equal(grid._pagesCount(), 5, "correct page count");
equal(grid.option("pageIndex"), 1, "pageIndex is initialized");
equal(grid._firstDisplayingPage, 1, "_firstDisplayingPage is initialized");
pager = grid._pagerContainer;
equal(pager.find("." + grid.currentPageClass).length, 1, "there is one current page");
ok(pager.find("." + grid.pageClass).eq(0).hasClass(grid.currentPageClass), "first page is current");
equal(pager.find("." + grid.pageClass).length, 3, "three pages displayed");
equal(pager.find("." + grid.pagerNavButtonClass).length, 5, "five nav buttons displayed: Fisrt Prev Next Last ...");
equal(pager.find("." + grid.pagerNavButtonInactiveClass).length, 2, "two nav buttons inactive: Fisrt Prev");
grid.openPage(2);
equal(pager.find("." + grid.currentPageClass).length, 1, "there is one current page");
ok(pager.find("." + grid.pageClass).eq(1).hasClass(grid.currentPageClass), "second page is current");
equal(pager.find("." + grid.pageClass).length, 3, "three pages displayed");
equal(pager.find("." + grid.pagerNavButtonClass).length, 5, "five nav buttons displayed: First Prev Next Last and ...");
equal(pageChangedArgs.pageIndex, 2, "onPageChanged callback provides pageIndex in arguments");
grid.showNextPages();
equal(grid._firstDisplayingPage, 3, "navigate by pages forward");
grid.showPrevPages();
equal(grid._firstDisplayingPage, 1, "navigate by pages backward");
grid.openPage(5);
equal(grid._firstDisplayingPage, 3, "opening next non-visible page moves first displaying page forward");
grid.openPage(2);
equal(grid._firstDisplayingPage, 2, "opening prev non-visible page moves first displaying page backward");
});
test("pager format", function() {
var $element = $("#jsGrid"),
grid = new Grid($element, {
data: [{}, {}, {}, {}, {}, {}],
paging: true,
pageSize: 2,
pageIndex: 2,
pageButtonCount: 1,
pagerFormat: "a {pageIndex} {first} {prev} {pages} {next} {last} {pageCount} {itemCount} z",
pagePrevText: "<",
pageNextText: ">",
pageFirstText: "<<",
pageLastText: ">>",
pageNavigatorNextText: "np",
pageNavigatorPrevText: "pp"
});
grid._firstDisplayingPage = 2;
grid._refreshPager();
equal($.trim(grid._pagerContainer.text()), "a 2 << < pp2np > >> 3 6 z", "pager text follows the format specified");
});
test("pagerRenderer", function() {
var $element = $("#jsGrid");
var pagerRendererConfig;
var pageSize = 2;
var items = [{}, {}, {}, {}, {}, {}, {}];
var pageCount = Math.ceil(items.length / pageSize);
var grid = new Grid($element, {
data: items,
paging: true,
pageSize: pageSize,
pagerRenderer: function(pagerConfig) {
pagerRendererConfig = pagerConfig;
}
});
deepEqual(pagerRendererConfig, { pageIndex: 1, pageCount: pageCount });
grid.openPage(2);
deepEqual(pagerRendererConfig, { pageIndex: 2, pageCount: pageCount });
});
test("loading by page", function() {
var $element = $("#jsGrid"),
data = [],
itemCount = 20;
for(var i = 1; i <= itemCount; i += 1) {
data.push({
value: i
});
}
var gridOptions = {
pageLoading: true,
paging: true,
pageSize: 7,
fields: [
{ name: "value" }
],
controller: {
loadData: function(filter) {
var startIndex = (filter.pageIndex - 1) * filter.pageSize,
result = data.slice(startIndex, startIndex + filter.pageSize);
return {
data: result,
itemsCount: data.length
};
}
}
};
var grid = new Grid($element, gridOptions);
grid.loadData();
var pager = grid._pagerContainer;
var gridData = grid.option("data");
equal(gridData.length, 7, "loaded one page of data");
equal(gridData[0].value, 1, "loaded right data start value");
equal(gridData[gridData.length - 1].value, 7, "loaded correct data end value");
ok(pager.find("." + grid.pageClass).eq(0).hasClass(grid.currentPageClass), "first page is current");
equal(pager.find("." + grid.pageClass).length, 3, "three pages displayed");
grid.openPage(3);
gridData = grid.option("data");
equal(gridData.length, 6, "loaded last page of data");
equal(gridData[0].value, 15, "loaded right data start value");
equal(gridData[gridData.length - 1].value, 20, "loaded right data end value");
ok(pager.find("." + grid.pageClass).eq(2).hasClass(grid.currentPageClass), "third page is current");
equal(pager.find("." + grid.pageClass).length, 3, "three pages displayed");
});
test("'openPage' method ignores indexes out of range", function() {
var $element = $("#jsGrid"),
grid = new Grid($element, {
data: [{}, {}],
paging: true,
pageSize: 1
});
grid.openPage(0);
equal(grid.option("pageIndex"), 1, "too small index is ignored");
grid.openPage(3);
equal(grid.option("pageIndex"), 1, "too big index is ignored");
});
module("sorting");
test("sorting", function() {
var $element = $("#jsGrid"),
});
module("canceling controller calls");
test("cancel controller.loadData", function() {
var $element = $("#jsGrid");
});
test("sorting with pageLoading", function() {
var $element = $("#jsGrid"),
loadFilter,
gridOptions = {
sorting: true,
pageLoading: true,
data: [
{ value: 3 },
{ value: 2 },
{ value: 1 }
],
controller: {
loadData: function(filter) {
loadFilter = filter;
return {
itemsCount: 0,
data: []
};
}
},
fields: [
{ name: "value", sorter: "number" }
]
},
grid = new Grid($element, gridOptions);
var $th = grid._headerRow.find("th").eq(0);
$th.trigger("click");
equal(grid._sortOrder, "asc", "asc sorting order for first click");
equal(grid._sortField, grid.fields[0], "sort field is set");
equal(loadFilter.sortOrder, "asc", "sort direction is provided in loadFilter");
equal(loadFilter.sortField, "value", "sort field is provided in loadFilter");
$th.trigger("click");
equal(grid._sortOrder, "desc", "desc sorting order for second click");
equal(grid._sortField, grid.fields[0], "sort field is set");
equal(loadFilter.sortOrder, "desc", "sort direction is provided in loadFilter");
equal(loadFilter.sortField, "value", "sort field is provided in loadFilter");
});
test("no sorting for column with sorting = false", function() {
var $element = $("#jsGrid");
var gridOptions = {
sorting: true,
data: [
{ value: 3 },
{ value: 2 },
{ value: 1 }
],
fields: [
{ name: "value", sorting: false }
]
};
var grid = new Grid($element, gridOptions);
var gridData = grid.option("data");
var $th = grid._headerRow.find("th").eq(0);
$th.trigger("click");
equal(grid._sortField, null, "sort field is not set for the field with sorting=false");
equal(gridData[0].value, 3);
equal(gridData[1].value, 2);
equal(gridData[2].value, 1);
equal($th.hasClass(grid.sortableClass), false, "no sorting css for field with sorting=false");
equal($th.hasClass(grid.sortAscClass), false, "no sorting css for field with sorting=false");
});
test("sort accepts sorting config", function() {
var $element = $("#jsGrid"),
gridOptions = {
sorting: true,
data: [
{ value: 3 },
{ value: 2 },
{ value: 1 }
],
fields: [
{ name: "value", sorter: "number" }
]
},
grid = new Grid($element, gridOptions);
var gridData = grid.option("data");
grid.sort({ field: "value", order: "asc" });
equal(grid._sortOrder, "asc", "asc sorting order is set");
equal(grid._sortField, grid.fields[0], "sort field is set");
equal(gridData[0].value, 1);
equal(gridData[1].value, 2);
equal(gridData[2].value, 3);
grid.sort({ field: 0 });
equal(grid._sortOrder, "desc", "desc sorting order for already set asc sorting");
equal(grid._sortField, grid.fields[0], "sort field is set");
equal(gridData[0].value, 3);
equal(gridData[1].value, 2);
equal(gridData[2].value, 1);
grid.sort("value", "asc");
equal(grid._sortOrder, "asc", "asc sorting order is set");
equal(grid._sortField, grid.fields[0], "sort field is set");
grid.sort(0);
equal(grid._sortOrder, "desc", "desc sorting order for already set asc sorting");
equal(grid._sortField, grid.fields[0], "sort field is set");
});
test("getSorting returns current sorting", function() {
var $element = $("#jsGrid"),
gridOptions = {
sorting: true,
data: [
{ value: 3 },
{ value: 2 },
{ value: 1 }
],
fields: [
{ name: "value", sorter: "number" }
]
},
grid = new Grid($element, gridOptions);
deepEqual(grid.getSorting(), { field: undefined, order: undefined }, "undefined field and order before sorting");
grid.sort("value");
deepEqual(grid.getSorting(), { field: "value", order: "asc" }, "current sorting returned");
});
test("sorting css attached correctly when a field is hidden", function() {
var $element = $("#jsGrid");
var gridOptions = {
sorting: true,
data: [],
fields: [
{ name: "field1", visible: false },
{ name: "field2" }
]
};
var grid = new Grid($element, gridOptions);
var gridData = grid.option("data");
var $th = grid._headerRow.find("th").eq(0);
$th.trigger("click");
equal($th.hasClass(grid.sortAscClass), true, "sorting css is attached to first field");
});
module("canceling events");
test("cancel item edit", function() {
var $element = $("#jsGrid");
var data = [{}];
var gridOptions = {
editing: true,
onItemEditing: function(e) {
e.cancel = true;
},
controller: {
loadData: function() {
return data;
}
},
fields: [
{ name: "test" }
]
};
var grid = new Grid($element, gridOptions);
grid.loadData();
grid.editItem(data[0]);
strictEqual(grid._editingRow, null, "no editing row");
});
test("cancel controller.loadData", function() {
var $element = $("#jsGrid");
var gridOptions = {
onDataLoading: function(e) {
e.cancel = true;
},
controller: {
loadData: function() {
return [{}];
}
},
fields: [
{ name: "test" }
]
};
var grid = new Grid($element, gridOptions);
grid.loadData();
equal(grid.option("data").length, 0, "no data loaded");
});
test("cancel controller.insertItem", function() {
var $element = $("#jsGrid");
var insertedItem = null;
var gridOptions = {
onItemInserting: function(e) {
e.cancel = true;
},
controller: {
insertItem: function(item) {
insertedItem = item;
}
},
fields: [
{ name: "test" }
]
};
var grid = new Grid($element, gridOptions);
grid.insertItem({ test: "value" });
strictEqual(insertedItem, null, "item was not inserted");
});
test("cancel controller.updateItem", function() {
var $element = $("#jsGrid");
var updatedItem = null;
var existingItem = { test: "value" };
var gridOptions = {
data: [
existingItem
],
onItemUpdating: function(e) {
e.cancel = true;
},
controller: {
updateItem: function(item) {
updatedItem = item;
}
},
fields: [
{ name: "test" }
]
};
var grid = new Grid($element, gridOptions);
grid.updateItem(existingItem, { test: "new_value" });
strictEqual(updatedItem, null, "item was not updated");
});
test("cancel controller.deleteItem", function() {
var $element = $("#jsGrid");
var deletingItem = { test: "value" };
var deletedItem = null;
var gridOptions = {
data: [
deletingItem
],
confirmDeleting: false,
onItemDeleting: function(e) {
e.cancel = true;
},
controller: {
deleteItem: function(item) {
deletedItem = item;
}
},
fields: [
{ name: "test" }
]
};
var grid = new Grid($element, gridOptions);
grid.deleteItem(deletingItem);
strictEqual(deletedItem, null, "item was not deleted");
});
module("complex properties binding");
test("rendering", function() {
var $element = $("#jsGrid");
var gridOptions = {
loadMessage: "",
data: [
{ complexProp: { prop: "test" } }
],
fields: [
{ name: "complexProp.prop", title: "" }
]
};
new Grid($element, gridOptions);
equal($element.text(), "test", "complex property value rendered");
});
test("editing", function() {
var $element = $("#jsGrid");
var gridOptions = {
editing: true,
data: [
{ complexProp: { prop: "test" } }
],
fields: [
{ type: "text", name: "complexProp.prop" }
]
};
var grid = new Grid($element, gridOptions);
grid.editItem(gridOptions.data[0]);
equal(grid.fields[0].editControl.val(), "test", "complex property value set in editor");
});
test("should not fail if property is absent", function() {
var $element = $("#jsGrid");
var gridOptions = {
loadMessage: "",
data: [
{ complexProp: { } }
],
fields: [
{ name: "complexProp.subprop.prop", title: "" }
]
};
new Grid($element, gridOptions);
equal($element.text(), "", "rendered empty value");
});
test("inserting", function() {
var $element = $("#jsGrid");
var insertingItem;
var gridOptions = {
inserting: true,
fields: [
{ type: "text", name: "complexProp.prop" }
],
onItemInserting: function(args) {
insertingItem = args.item;
}
};
var grid = new Grid($element, gridOptions);
grid.fields[0].insertControl.val("test");
grid.insertItem();
deepEqual(insertingItem, { complexProp: { prop: "test" } }, "inserting item has complex properties");
});
test("filtering", function() {
var $element = $("#jsGrid");
var loadFilter;
var gridOptions = {
filtering: true,
fields: [
{ type: "text", name: "complexProp.prop" }
],
controller: {
loadData: function(filter) {
loadFilter = filter;
}
}
};
var grid = new Grid($element, gridOptions);
grid.fields[0].filterControl.val("test");
grid.search();
deepEqual(loadFilter, { complexProp: { prop: "test" } }, "filter has complex properties");
});
test("updating", function() {
var $element = $("#jsGrid");
var updatingItem;
var gridOptions = {
editing: true,
data: [
{ complexProp: { } }
],
fields: [
{ type: "text", name: "complexProp.prop" }
],
onItemUpdating: function(args) {
updatingItem = args.item;
}
};
var grid = new Grid($element, gridOptions);
grid.editItem(gridOptions.data[0]);
grid.fields[0].editControl.val("test");
grid.updateItem();
deepEqual(updatingItem, { complexProp: { prop: "test" } }, "updating item has complex properties");
});
test("update nested prop", function() {
var $element = $("#jsGrid");
var updatingItem;
var previousItem;
var gridOptions = {
editing: true,
data: [
{ prop: { subprop1: "test1", subprop2: "test2" } }
],
fields: [
{ type: "text", name: "prop.subprop1" },
{ type: "text", name: "prop.subprop2" }
],
onItemUpdating: function(args) {
updatingItem = args.item;
}
};
var grid = new Grid($element, gridOptions);
grid.editItem(gridOptions.data[0]);
grid.fields[0].editControl.val("new_test1");
grid.updateItem();
var expectedUpdatingItem = {
prop: {
subprop1: "new_test1",
subprop2: "test2"
}
};
deepEqual(updatingItem, expectedUpdatingItem, "updating item has nested properties");
});
test("updating deeply nested prop", function() {
var $element = $("#jsGrid");
var updatingItem;
var previousItem;
var gridOptions = {
editing: true,
data: [
{ complexProp: { subprop1: { another_prop: "test" } } }
],
fields: [
{ type: "text", name: "complexProp.subprop1.prop1" },
{ type: "text", name: "complexProp.subprop1.subprop2.prop12" }
],
onItemUpdating: function(args) {
updatingItem = $.extend(true, {}, args.item);
previousItem = $.extend(true, {}, args.previousItem);
}
};
var grid = new Grid($element, gridOptions);
grid.editItem(gridOptions.data[0]);
grid.fields[0].editControl.val("test1");
grid.fields[1].editControl.val("test2");
grid.updateItem();
var expectedUpdatingItem = {
complexProp: {
subprop1: {
another_prop: "test",
prop1: "test1",
subprop2: { prop12: "test2" }
}
}
};
var expectedPreviousItem = {
complexProp: {
subprop1: {
another_prop: "test"
}
}
};
deepEqual(updatingItem, expectedUpdatingItem, "updating item has deeply nested properties");
deepEqual(previousItem, expectedPreviousItem, "previous item preserved correctly");
});
module("validation");
test("insertItem should call validation.validate", function() {
var $element = $("#jsGrid");
var fieldValidationRules = { test: "value" };
var validatingArgs;
var gridOptions = {
data: [],
inserting: true,
invalidNotify: $.noop,
validation: {
validate: function(args) {
validatingArgs = args;
return [];
}
},
fields: [
{ type: "text", name: "Name", validate: fieldValidationRules }
]
};
var grid = new Grid($element, gridOptions);
grid.fields[0].insertControl.val("test");
grid.insertItem();
deepEqual(validatingArgs, { value: "test", item: { Name: "test" }, itemIndex: -1,
row: grid._insertRow, rules: fieldValidationRules }, "validating args is provided");
});
test("insertItem rejected when data is not valid", function() {
var $element = $("#jsGrid");
var gridOptions = {
data: [],
inserting: true,
invalidNotify: $.noop,
validation: {
validate: function() {
return ["Error"];
}
},
fields: [
{ type: "text", name: "Name", validate: true }
]
};
var grid = new Grid($element, gridOptions);
grid.fields[0].insertControl.val("test");
grid.insertItem().done(function() {
ok(false, "insertItem should not be completed");
}).fail(function() {
ok(true, "insertItem should fail");
});
});
test("invalidClass is attached on invalid cell on inserting", function() {
var $element = $("#jsGrid");
var gridOptions = {
data: [],
inserting: true,
invalidNotify: $.noop,
validation: {
validate: function() {
return ["Error"];
}
},
fields: [
{ type: "text", name: "Id", visible: false },
{ type: "text", name: "Name", validate: true }
]
};
var grid = new Grid($element, gridOptions);
var $insertCell = grid._insertRow.children().eq(0);
grid.insertItem();
ok($insertCell.hasClass(grid.invalidClass), "invalid class is attached");
equal($insertCell.attr("title"), "Error", "cell tooltip contains error message");
});
test("onItemInvalid callback", function() {
var $element = $("#jsGrid");
var errors = ["Error"];
var onItemInvalidCalled = 0;
var onItemInvalidArgs;
var gridOptions = {
data: [],
inserting: true,
invalidNotify: $.noop,
onItemInvalid: function(args) {
onItemInvalidCalled++;
onItemInvalidArgs = args;
},
validation: {
validate: function(args) {
return !args.value ? errors : [];
}
},
fields: [
{ type: "text", name: "Name", validate: true }
]
};
var grid = new Grid($element, gridOptions);
grid.insertItem();
equal(onItemInvalidCalled, 1, "onItemInvalid is called, when item data is invalid");
deepEqual(onItemInvalidArgs, { grid: grid, errors: [{ field: grid.fields[0], message: "Error" }],
item: { Name: "" }, itemIndex: -1, row: grid._insertRow }, "arguments provided");
grid.fields[0].insertControl.val("test");
grid.insertItem();
equal(onItemInvalidCalled, 1, "onItemInvalid was not called, when data is valid");
});
test("invalidNotify", function() {
var $element = $("#jsGrid");
var errors = ["Error"];
var invalidNotifyCalled = 0;
var invalidNotifyArgs;
var gridOptions = {
data: [],
inserting: true,
invalidNotify: function(args) {
invalidNotifyCalled++;
invalidNotifyArgs = args;
},
validation: {
validate: function(args) {
return !args.value ? errors : [];
}
},
fields: [
{ type: "text", name: "Name", validate: true }
]
};
var grid = new Grid($element, gridOptions);
grid.insertItem();
equal(invalidNotifyCalled, 1, "invalidNotify is called, when item data is invalid");
deepEqual(invalidNotifyArgs, { grid: grid, errors: [{ field: grid.fields[0], message: "Error" }],
row: grid._insertRow, item: { Name: "" }, itemIndex: -1 }, "arguments provided");
grid.fields[0].insertControl.val("test");
grid.insertItem();
equal(invalidNotifyCalled, 1, "invalidNotify was not called, when data is valid");
});
test("invalidMessage", function() {
var $element = $("#jsGrid");
var invalidMessage;
var originalAlert = window.alert;
window.alert = function(message) {
invalidMessage = message;
};
try {
Grid.prototype.invalidMessage = "InvalidTest";
Grid.prototype.invalidNotify({ errors: [{ message: "Message1" }, { message: "Message2" }] });
var expectedInvalidMessage = ["InvalidTest", "Message1", "Message2"].join("\n");
equal(invalidMessage, expectedInvalidMessage, "message contains invalidMessage and field error messages");
} finally {
window.alert = originalAlert;
}
});
test("updateItem should call validation.validate", function() {
var $element = $("#jsGrid");
var validatingArgs;
var gridOptions = {
data: [{ Name: "" }],
editing: true,
invalidNotify: $.noop,
validation: {
validate: function(args) {
validatingArgs = args;
return ["Error"];
}
},
fields: [
{ type: "text", name: "Name", validate: "required" }
]
};
var grid = new Grid($element, gridOptions);
grid.editItem(gridOptions.data[0]);
grid.fields[0].editControl.val("test");
grid.updateItem();
deepEqual(validatingArgs, { value: "test", item: { Name: "test" }, itemIndex: 0,
row: grid._getEditRow(), rules: "required" }, "validating args is provided");
});
test("invalidClass is attached on invalid cell on updating", function() {
var $element = $("#jsGrid");
var gridOptions = {
data: [{}],
editing: true,
invalidNotify: $.noop,
validation: {
validate: function() {
return ["Error"];
}
},
fields: [
{ type: "text", name: "Name", validate: true }
]
};
var grid = new Grid($element, gridOptions);
grid.editItem(gridOptions.data[0]);
var $editCell = grid._getEditRow().children().eq(0);
grid.updateItem();
ok($editCell.hasClass(grid.invalidClass), "invalid class is attached");
equal($editCell.attr("title"), "Error", "cell tooltip contains error message");
});
test("validation should ignore not editable fields", function() {
var invalidNotifyCalled = 0;
var $element = $("#jsGrid");
var validatingArgs;
var gridOptions = {
data: [{ Name: "" }],
editing: true,
invalidNotify: function() {
invalidNotifyCalled++;
},
validation: {
validate: function() {
return ["Error"];
}
},
fields: [
{ type: "text", name: "Name", editing: false, validate: "required" }
]
};
var grid = new Grid($element, gridOptions);
grid.editItem(gridOptions.data[0]);
grid.updateItem();
equal(invalidNotifyCalled, 0, "data is valid");
});
module("api");
test("reset method should go the first page when pageLoading is truned on", function() {
var items = [{ Name: "1" }, { Name: "2" }];
var $element = $("#jsGrid");
var gridOptions = {
paging: true,
pageSize: 1,
pageLoading: true,
autoload: true,
controller: {
loadData: function(args) {
return {
data: [items[args.pageIndex - 1]],
itemsCount: items.length
};
}
},
fields: [
{ type: "text", name: "Name" }
]
};
var grid = new Grid($element, gridOptions);
grid.openPage(2);
grid.reset();
equal(grid._bodyGrid.text(), "1", "grid content reset");
});
module("i18n");
test("set locale by name", function() {
jsGrid.locales.my_lang = {
grid: {
test: "test_text"
}
};
jsGrid.locale("my_lang");
var $element = $("#jsGrid").jsGrid({});
equal($element.jsGrid("option", "test"), "test_text", "option localized");
});
test("set locale by config", function() {
jsGrid.locale( {
grid: {
test: "test_text"
}
});
var $element = $("#jsGrid").jsGrid({});
equal($element.jsGrid("option", "test"), "test_text", "option localized");
});
test("locale throws exception for unknown locale", function() {
throws(function() {
jsGrid.locale("unknown_lang");
}, /unknown_lang/, "locale threw an exception");
});
module("controller promise");
asyncTest("should support jQuery promise success callback", 1, function() {
var data = [];
var gridOptions = {
autoload: false,
controller: {
loadData: function() {
var d = $.Deferred();
setTimeout(function() {
d.resolve(data);
start();
});
return d.promise();
}
}
};
var grid = new Grid($("#jsGrid"), gridOptions);
var promise = grid._controllerCall("loadData", {}, false, $.noop);
promise.done(function(result) {
equal(result, data, "data provided to done callback");
});
});
asyncTest("should support jQuery promise fail callback", 1, function() {
var failArgs = {};
var gridOptions = {
autoload: false,
controller: {
loadData: function() {
var d = $.Deferred();
setTimeout(function() {
d.reject(failArgs);
start();
});
return d.promise();
}
}
};
var grid = new Grid($("#jsGrid"), gridOptions);
var promise = grid._controllerCall("loadData", {}, false, $.noop);
promise.fail(function(result) {
equal(result, failArgs, "fail args provided to fail callback");
});
});
asyncTest("should support JS promise success callback", 1, function() {
if(typeof Promise === "undefined") {
ok(true, "Promise not supported");
start();
return;
}
var data = [];
var gridOptions = {
autoload: false,
controller: {
loadData: function() {
return new Promise(function(resolve, reject) {
setTimeout(function() {
resolve(data);
start();
});
});
}
}
};
var grid = new Grid($("#jsGrid"), gridOptions);
var promise = grid._controllerCall("loadData", {}, false, $.noop);
promise.done(function(result) {
equal(result, data, "data provided to done callback");
});
});
asyncTest("should support JS promise fail callback", 1, function() {
if(typeof Promise === "undefined") {
ok(true, "Promise not supported");
start();
return;
}
var failArgs = {};
var gridOptions = {
autoload: false,
controller: {
loadData: function() {
return new Promise(function(resolve, reject) {
setTimeout(function() {
reject(failArgs);
start();
});
});
}
}
};
var grid = new Grid($("#jsGrid"), gridOptions);
var promise = grid._controllerCall("loadData", {}, false, $.noop);
promise.fail(function(result) {
equal(result, failArgs, "fail args provided to fail callback");
});
});
test("should support non-promise result", 1, function() {
var data = [];
var gridOptions = {
autoload: false,
controller: {
loadData: function() {
return data;
}
}
};
var grid = new Grid($("#jsGrid"), gridOptions);
var promise = grid._controllerCall("loadData", {}, false, $.noop);
promise.done(function(result) {
equal(result, data, "data provided to done callback");
});
});
module("renderTemplate");
test("should pass undefined and null arguments to the renderer", function() {
var rendererArgs;
var rendererContext;
var context = {};
var renderer = function() {
rendererArgs = arguments;
rendererContext = this;
};
Grid.prototype.renderTemplate(renderer, context, { arg1: undefined, arg2: null, arg3: "test" });
equal(rendererArgs.length, 3);
strictEqual(rendererArgs[0], undefined, "undefined passed");
strictEqual(rendererArgs[1], null, "null passed");
strictEqual(rendererArgs[2], "test", "null passed");
strictEqual(rendererContext, context, "context is preserved");
});
module("Data Export", {
setup: function() {
this.exportConfig = {};
this.exportConfig.type = "csv";
this.exportConfig.subset = "all";
this.exportConfig.delimiter = "|";
this.exportConfig.includeHeaders = true;
this.exportConfig.encapsulate = true;
this.element = $("#jsGrid");
this.gridOptions = {
width: "100%",
height: "400px",
inserting: true,
editing: true,
sorting: true,
paging: true,
pageSize: 2,
data: [
{ "Name": "Otto Clay", "Country": 1, "Married": false },
{ "Name": "Connor Johnston", "Country": 2, "Married": true },
{ "Name": "Lacey Hess", "Country": 2, "Married": false },
{ "Name": "Timothy Henson", "Country": 1, "Married": true }
],
fields: [
{ name: "Name", type: "text", width: 150, validate: "required" },
{ name: "Country", type: "select", items: [{ Name: "United States", Id: 1 },{ Name: "Canada", Id: 2 }], valueField: "Id", textField: "Name" },
{ name: "Married", type: "checkbox", title: "Is Married", sorting: false },
{ type: "control" }
]
}
}
});
/* Base Choice Criteria
type: csv
subset: all
delimiter: |
includeHeaders: true
encapsulate: true
*/
test("Should export data: Base Choice",function(){
var grid = new Grid($(this.element), this.gridOptions);
var data = grid.exportData(this.exportConfig);
var expected = "";
expected += '"Name"|"Country"|"Is Married"\r\n';
expected += '"Otto Clay"|"United States"|"false"\r\n';
expected += '"Connor Johnston"|"Canada"|"true"\r\n';
expected += '"Lacey Hess"|"Canada"|"false"\r\n';
expected += '"Timothy Henson"|"United States"|"true"\r\n';
equal(data, expected, "Output CSV configured to Base Choice Criteria -- Check Source");
});
test("Should export data: defaults = BCC",function(){
var grid = new Grid($(this.element), this.gridOptions);
var data = grid.exportData();
var expected = "";
expected += '"Name"|"Country"|"Is Married"\r\n';
expected += '"Otto Clay"|"United States"|"false"\r\n';
expected += '"Connor Johnston"|"Canada"|"true"\r\n';
expected += '"Lacey Hess"|"Canada"|"false"\r\n';
expected += '"Timothy Henson"|"United States"|"true"\r\n';
equal(data, expected, "Output CSV with all defaults -- Should be equal to Base Choice");
});
test("Should export data: subset=visible", function(){
var grid = new Grid($(this.element), this.gridOptions);
this.exportConfig.subset = "visible";
var data = grid.exportData(this.exportConfig);
var expected = "";
expected += '"Name"|"Country"|"Is Married"\r\n';
expected += '"Otto Clay"|"United States"|"false"\r\n';
expected += '"Connor Johnston"|"Canada"|"true"\r\n';
//expected += '"Lacey Hess"|"Canada"|"false"\r\n';
//expected += '"Timothy Henson"|"United States"|"true"\r\n';
equal(data, expected, "Output CSV of visible records");
});
test("Should export data: delimiter=;", function(){
var grid = new Grid($(this.element), this.gridOptions);
this.exportConfig.delimiter = ";";
var data = grid.exportData(this.exportConfig);
var expected = "";
expected += '"Name";"Country";"Is Married"\r\n';
expected += '"Otto Clay";"United States";"false"\r\n';
expected += '"Connor Johnston";"Canada";"true"\r\n';
expected += '"Lacey Hess";"Canada";"false"\r\n';
expected += '"Timothy Henson";"United States";"true"\r\n';
equal(data, expected, "Output CSV with non-default delimiter");
});
test("Should export data: headers=false", function(){
var grid = new Grid($(this.element), this.gridOptions);
this.exportConfig.includeHeaders = false;
var data = grid.exportData(this.exportConfig);
var expected = "";
//expected += '"Name"|"Country"|"Is Married"\r\n';
expected += '"Otto Clay"|"United States"|"false"\r\n';
expected += '"Connor Johnston"|"Canada"|"true"\r\n';
expected += '"Lacey Hess"|"Canada"|"false"\r\n';
expected += '"Timothy Henson"|"United States"|"true"\r\n';
equal(data, expected, "Output CSV without Headers");
});
test("Should export data: encapsulate=false", function(){
var grid = new Grid($(this.element), this.gridOptions);
this.exportConfig.encapsulate = false;
var data = grid.exportData(this.exportConfig);
var expected = "";
expected += 'Name|Country|Is Married\r\n';
expected += 'Otto Clay|United States|false\r\n';
expected += 'Connor Johnston|Canada|true\r\n';
expected += 'Lacey Hess|Canada|false\r\n';
expected += 'Timothy Henson|United States|true\r\n';
equal(data, expected, "Output CSV without encapsulation");
});
test("Should export filtered data", function(){
var grid = new Grid($(this.element), this.gridOptions);
this.exportConfig['filter'] = function(item){
if (item["Name"].indexOf("O") === 0)
return true
};
var data = grid.exportData(this.exportConfig);
var expected = "";
expected += '"Name"|"Country"|"Is Married"\r\n';
expected += '"Otto Clay"|"United States"|"false"\r\n';
//expected += '"Connor Johnston"|"Canada"|"true"\r\n';
//expected += '"Lacey Hess"|"Canada"|"false"\r\n';
//expected += '"Timothy Henson"|"United States"|"true"\r\n';
equal(data, expected, "Output CSV filtered to show names starting with O");
});
test("Should export data: transformed value", function(){
var grid = new Grid($(this.element), this.gridOptions);
this.exportConfig['transforms'] = {};
this.exportConfig.transforms['Married'] = function(value){
if (value === true) return "Yes"
if (value === false) return "No"
};
var data = grid.exportData(this.exportConfig);
var expected = "";
expected += '"Name"|"Country"|"Is Married"\r\n';
expected += '"Otto Clay"|"United States"|"No"\r\n';
expected += '"Connor Johnston"|"Canada"|"Yes"\r\n';
expected += '"Lacey Hess"|"Canada"|"No"\r\n';
expected += '"Timothy Henson"|"United States"|"Yes"\r\n';
equal(data, expected, "Output CSV column value transformed properly");
});
});
<MSG> Events: Add onItemEditing callback
Closes #147
<DFF> @@ -1176,6 +1176,8 @@ $(function() {
test("edit item", function() {
var $element = $("#jsGrid"),
+ editingArgs,
+ editingRow,
updated = false,
updatingArgs,
updatingRow,
@@ -1204,6 +1206,10 @@ $(function() {
updated = true;
}
},
+ onItemEditing: function(e) {
+ editingArgs = $.extend(true, {}, e);
+ editingRow = grid.rowByItem(data[0])[0];
+ },
onItemUpdating: function(e) {
updatingArgs = $.extend(true, {}, e);
updatingRow = grid.rowByItem(data[0])[0];
@@ -1219,6 +1225,11 @@ $(function() {
grid.option("data", data);
grid.editItem(data[0]);
+
+ deepEqual(editingArgs.item, { field: "value" }, "item before editing is provided in editing event args");
+ equal(editingArgs.itemIndex, 0, "itemIndex is provided in editing event args");
+ equal(editingArgs.row[0], editingRow, "row element is provided in editing event args");
+
grid.fields[0].editControl.val("new value");
grid.updateItem();
@@ -1786,7 +1797,36 @@ $(function() {
});
- module("canceling controller calls");
+ module("canceling events");
+
+ test("cancel item edit", function() {
+ var $element = $("#jsGrid");
+ var data = [{}];
+
+ var gridOptions = {
+ editing: true,
+
+ onItemEditing: function(e) {
+ e.cancel = true;
+ },
+
+ controller: {
+ loadData: function() {
+ return data;
+ }
+ },
+
+ fields: [
+ { name: "test" }
+ ]
+ };
+
+ var grid = new Grid($element, gridOptions);
+
+ grid.loadData();
+ grid.editItem(data[0]);
+ strictEqual(grid._editingRow, null, "no editing row");
+ });
test("cancel controller.loadData", function() {
var $element = $("#jsGrid");
| 41 | Events: Add onItemEditing callback | 1 | .js | tests | mit | tabalinas/jsgrid |
10063764 | <NME> jsgrid.tests.js
<BEF> $(function() {
var Grid = jsGrid.Grid,
JSGRID = "JSGrid",
JSGRID_DATA_KEY = JSGRID;
Grid.prototype.updateOnResize = false;
module("basic");
test("default creation", function() {
var gridOptions = {
simpleOption: "test",
complexOption: {
a: "subtest",
b: 1,
c: {}
}
},
grid = new Grid("#jsGrid", gridOptions);
equal(grid._container[0], $("#jsGrid")[0], "container saved");
equal(grid.simpleOption, "test", "primitive option extended");
equal(grid.complexOption, gridOptions.complexOption, "non-primitive option extended");
});
test("jquery adapter creation", function() {
var gridOptions = {
option: "test"
},
$element = $("#jsGrid"),
result = $element.jsGrid(gridOptions),
grid = $element.data(JSGRID_DATA_KEY);
equal(result, $element, "jquery fn returned source jQueryElement");
ok(grid instanceof Grid, "jsGrid saved to jquery data");
equal(grid.option, "test", "options provided");
});
test("destroy", function() {
var $element = $("#jsGrid"),
grid;
$element.jsGrid({});
grid = $element.data(JSGRID_DATA_KEY);
grid.destroy();
strictEqual($element.html(), "", "content is removed");
strictEqual($element.data(JSGRID_DATA_KEY), undefined, "jquery data is removed");
});
test("jquery adapter second call changes option value", function() {
var $element = $("#jsGrid"),
gridOptions = {
option: "test"
},
grid;
$element.jsGrid(gridOptions);
grid = $element.data(JSGRID_DATA_KEY);
gridOptions.option = "new test";
$element.jsGrid(gridOptions);
equal(grid, $element.data(JSGRID_DATA_KEY), "instance was not changed");
equal(grid.option, "new test", "option changed");
});
test("jquery adapter invokes jsGrid method", function() {
var methodResult = "",
$element = $("#jsGrid"),
gridOptions = {
method: function(str) {
methodResult = "test_" + str;
}
};
$element.jsGrid(gridOptions);
$element.jsGrid("method", "invoke");
equal(methodResult, "test_invoke", "method invoked");
});
test("onInit callback", function() {
var $element = $("#jsGrid"),
onInitArguments,
gridOptions = {
onInit: function(args) {
onInitArguments = args;
}
};
var grid = new Grid($element, gridOptions);
equal(onInitArguments.grid, grid, "grid instance is provided in onInit callback arguments");
});
test("controller methods are $.noop when not specified", function() {
var $element = $("#jsGrid"),
gridOptions = {
controller: {}
},
testOption;
$element.jsGrid(gridOptions);
deepEqual($element.data(JSGRID_DATA_KEY)._controller, {
loadData: $.noop,
insertItem: $.noop,
updateItem: $.noop,
deleteItem: $.noop
}, "controller has stub methods");
});
test("option method", function() {
var $element = $("#jsGrid"),
gridOptions = {
test: "value"
},
testOption;
$element.jsGrid(gridOptions);
testOption = $element.jsGrid("option", "test");
equal(testOption, "value", "read option value");
$element.jsGrid("option", "test", "new_value");
testOption = $element.jsGrid("option", "test");
equal(testOption, "new_value", "set option value");
});
test("fieldOption method", function() {
var dataLoadedCount = 0;
var $element = $("#jsGrid"),
gridOptions = {
loadMessage: "",
autoload: true,
controller: {
loadData: function() {
dataLoadedCount++;
return [{ prop1: "value1", prop2: "value2", prop3: "value3" }];
}
},
fields: [
{ name: "prop1", title: "_" }
]
};
$element.jsGrid(gridOptions);
var fieldOptionValue = $element.jsGrid("fieldOption", "prop1", "name");
equal(fieldOptionValue, "prop1", "read field option");
$element.jsGrid("fieldOption", "prop1", "name", "prop2");
equal($element.text(), "_value2", "set field option by field name");
equal(dataLoadedCount, 1, "data not reloaded on field option change");
$element.jsGrid("fieldOption", 0, "name", "prop3");
equal($element.text(), "_value3", "set field option by field index");
});
test("option changing event handlers", function() {
var $element = $("#jsGrid"),
optionChangingEventArgs,
optionChangedEventArgs,
gridOptions = {
test: "testValue",
another: "anotherValue",
onOptionChanging: function(e) {
optionChangingEventArgs = $.extend({}, e);
e.option = "another";
e.newValue = e.newValue + "_" + this.another;
},
onOptionChanged: function(e) {
optionChangedEventArgs = $.extend({}, e);
}
},
anotherOption;
$element.jsGrid(gridOptions);
$element.jsGrid("option", "test", "newTestValue");
equal(optionChangingEventArgs.option, "test", "option name is provided in args of optionChanging");
equal(optionChangingEventArgs.oldValue, "testValue", "old option value is provided in args of optionChanging");
equal(optionChangingEventArgs.newValue, "newTestValue", "new option value is provided in args of optionChanging");
anotherOption = $element.jsGrid("option", "another");
equal(anotherOption, "newTestValue_anotherValue", "option changing handler changed option and value");
equal(optionChangedEventArgs.option, "another", "option name is provided in args of optionChanged");
equal(optionChangedEventArgs.value, "newTestValue_anotherValue", "option value is provided in args of optionChanged");
});
test("common layout rendering", function() {
var $element = $("#jsGrid"),
grid = new Grid($element, {}),
$headerGrid,
$headerGridTable,
$bodyGrid,
$bodyGridTable;
ok($element.hasClass(grid.containerClass), "container class attached");
ok($element.children().eq(0).hasClass(grid.gridHeaderClass), "grid header");
ok($element.children().eq(1).hasClass(grid.gridBodyClass), "grid body");
ok($element.children().eq(2).hasClass(grid.pagerContainerClass), "pager container");
$headerGrid = $element.children().eq(0);
$headerGridTable = $headerGrid.children().first();
ok($headerGridTable.hasClass(grid.tableClass), "header table");
equal($headerGrid.find("." + grid.headerRowClass).length, 1, "header row");
equal($headerGrid.find("." + grid.filterRowClass).length, 1, "filter row");
equal($headerGrid.find("." + grid.insertRowClass).length, 1, "insert row");
ok(grid._headerRow.hasClass(grid.headerRowClass), "header row class");
ok(grid._filterRow.hasClass(grid.filterRowClass), "filter row class");
ok(grid._insertRow.hasClass(grid.insertRowClass), "insert row class");
$bodyGrid = $element.children().eq(1);
$bodyGridTable = $bodyGrid.children().first();
ok($bodyGridTable.hasClass(grid.tableClass), "body table");
equal(grid._content.parent()[0], $bodyGridTable[0], "content is tbody in body table");
equal($bodyGridTable.find("." + grid.noDataRowClass).length, 1, "no data row");
equal($bodyGridTable.text(), grid.noDataContent, "no data text");
});
test("set default options with setDefaults", function() {
jsGrid.setDefaults({
defaultOption: "test"
});
var $element = $("#jsGrid").jsGrid({});
equal($element.jsGrid("option", "defaultOption"), "test", "default option set");
});
module("loading");
test("loading with controller", function() {
var $element = $("#jsGrid"),
data = [
{ test: "test1" },
{ test: "test2" }
],
gridOptions = {
controller: {
loadData: function() {
return data;
}
}
},
grid = new Grid($element, gridOptions);
grid.loadData();
equal(grid.option("data"), data, "loadData loads data");
});
test("loadData throws exception when controller method not found", function() {
var $element = $("#jsGrid");
var grid = new Grid($element);
grid._controller = {};
throws(function() {
grid.loadData();
}, /loadData/, "loadData threw an exception");
});
test("onError event should be fired on controller fail", function() {
var errorArgs,
errorFired = 0,
$element = $("#jsGrid"),
gridOptions = {
controller: {
loadData: function() {
return $.Deferred().reject({ value: 1 }, "test").promise();
}
},
onError: function(args) {
errorFired++;
errorArgs = args;
}
},
grid = new Grid($element, gridOptions);
grid.loadData();
equal(errorFired, 1, "onError handler fired");
deepEqual(errorArgs, { grid: grid, args: [{ value: 1 }, "test"] }, "error has correct params");
});
asyncTest("autoload should call loadData after render", 1, function() {
new Grid($("#jsGrid"), {
autoload: true,
controller: {
loadData: function() {
ok(true, "autoload calls loadData on creation");
start();
return [];
}
}
});
});
test("loading filtered data", function() {
var filteredData,
loadingArgs,
loadedArgs,
$element = $("#jsGrid"),
data = [
{ field: "test" },
{ field: "test_another" },
{ field: "test_another" },
{ field: "test" }
],
gridOptions = {
filtering: true,
fields: [
{
name: "field",
filterValue: function(value) {
return "test";
}
}
],
onDataLoading: function(e) {
loadingArgs = $.extend(true, {}, e);
},
onDataLoaded: function(e) {
loadedArgs = $.extend(true, {}, e);
},
controller: {
loadData: function(filter) {
filteredData = $.grep(data, function(item) {
return item.field === filter.field;
});
return filteredData;
}
}
},
grid = new Grid($element, gridOptions);
grid.loadData();
equal(loadingArgs.filter.field, "test");
equal(grid.option("data").length, 2, "filtered data loaded");
deepEqual(loadedArgs.data, filteredData);
});
asyncTest("loading indication", function() {
var timeout = 10,
stage = "initial",
$element = $("#jsGrid"),
gridOptions = {
loadIndication: true,
loadIndicationDelay: timeout,
loadMessage: "loading...",
loadIndicator: function(config) {
equal(config.message, gridOptions.loadMessage, "message provided");
ok(config.container.jquery, "grid container is provided");
return {
show: function() {
stage = "started";
},
hide: function() {
stage = "finished";
}
};
},
fields: [
{ name: "field" }
],
controller: {
loadData: function() {
var deferred = $.Deferred();
equal(stage, "initial", "initial stage");
setTimeout(function() {
equal(stage, "started", "loading started");
deferred.resolve([]);
equal(stage, "finished", "loading finished");
start();
}, timeout);
return deferred.promise();
}
}
},
grid = new Grid($element, gridOptions);
grid.loadData();
});
asyncTest("loadingIndication=false should not show loading", 0, function() {
var $element = $("#jsGrid"),
timeout = 10,
gridOptions = {
loadIndication: false,
loadIndicationDelay: timeout,
loadIndicator: function() {
return {
show: function() {
ok(false, "should not call show");
},
hide: function() {
ok(false, "should not call hide");
}
};
},
fields: [
{ name: "field" }
],
controller: {
loadData: function() {
var deferred = $.Deferred();
setTimeout(function() {
deferred.resolve([]);
start();
}, timeout);
return deferred.promise();
}
}
},
grid = new Grid($element, gridOptions);
grid.loadData();
});
test("search", function() {
var $element = $("#jsGrid"),
data = [
{ field: "test" },
{ field: "test_another" },
{ field: "test_another" },
{ field: "test" }
],
gridOptions = {
pageIndex: 2,
_sortField: "field",
_sortOrder: "desc",
filtering: true,
fields: [
{
name: "field",
filterValue: function(value) {
return "test";
}
}
],
controller: {
loadData: function(filter) {
var filteredData = $.grep(data, function(item) {
return item.field === filter.field;
});
return filteredData;
}
}
},
grid = new Grid($element, gridOptions);
grid.search();
equal(grid.option("data").length, 2, "data filtered");
strictEqual(grid.option("pageIndex"), 1, "pageIndex reset");
strictEqual(grid._sortField, null, "sortField reset");
strictEqual(grid._sortOrder, "asc", "sortOrder reset");
});
test("change loadStrategy on the fly", function() {
var $element = $("#jsGrid");
var gridOptions = {
controller: {
loadData: function() {
return [];
}
}
};
var grid = new Grid($element, gridOptions);
grid.option("loadStrategy", {
firstDisplayIndex: function() {
return 0;
},
lastDisplayIndex: function() {
return 1;
},
loadParams: function() {
return [];
},
finishLoad: function() {
grid.option("data", [{}]);
}
});
grid.loadData();
equal(grid.option("data").length, 1, "new load strategy is applied");
});
module("filtering");
test("filter rendering", function() {
var $element = $("#jsGrid"),
gridOptions = {
filtering: true,
fields: [
{
name: "test",
align: "right",
filtercss: "filter-class",
filterTemplate: function() {
var result = this.filterControl = $("<input>").attr("type", "text").addClass("filter-input");
return result;
}
}
]
},
grid = new Grid($element, gridOptions);
equal(grid._filterRow.find(".filter-class").length, 1, "filtercss class is attached");
equal(grid._filterRow.find(".filter-input").length, 1, "filter control rendered");
equal(grid._filterRow.find("." + grid.cellClass).length, 1, "cell class is attached");
ok(grid._filterRow.find(".filter-class").hasClass("jsgrid-align-right"), "align class is attached");
ok(grid.fields[0].filterControl.is("input[type=text]"), "filter control saved in field");
});
test("filter get/clear", function() {
var $element = $("#jsGrid"),
gridOptions = {
filtering: true,
controller: {
loadData: function() {
return [];
}
},
fields: [
{
name: "field",
filterTemplate: function() {
return this.filterControl = $("<input>").attr("type", "text");
},
filterValue: function() {
return this.filterControl.val();
}
}
]
},
grid = new Grid($element, gridOptions);
grid.fields[0].filterControl.val("test");
deepEqual(grid.getFilter(), { field: "test" }, "get filter");
grid.clearFilter();
deepEqual(grid.getFilter(), { field: "" }, "filter cleared");
equal(grid.fields[0].filterControl.val(), "", "grid field filterControl cleared");
});
test("field without filtering", function() {
var $element = $("#jsGrid"),
jsGridFieldConfig = {
filterTemplate: function() {
var result = this.filterControl = $("<input>").attr("type", "text");
return result;
},
filterValue: function(value) {
if(!arguments.length) {
return this.filterControl.val();
}
this.filterControl.val(value);
}
},
gridOptions = {
filtering: true,
fields: [
$.extend({}, jsGridFieldConfig, {
name: "field1",
filtering: false
}),
$.extend({}, jsGridFieldConfig, {
name: "field2"
})
]
},
grid = new Grid($element, gridOptions);
grid.fields[0].filterControl.val("test1");
grid.fields[1].filterControl.val("test2");
deepEqual(grid.getFilter(), { field2: "test2" }, "field with filtering=false is not included in filter");
});
test("search with filter", function() {
var $element = $("#jsGrid"),
data = [
{ field: "test" },
{ field: "test_another" },
{ field: "test_another" },
{ field: "test" }
],
gridOptions = {
fields: [
{
name: "field"
}
],
controller: {
loadData: function(filter) {
var filteredData = $.grep(data, function(item) {
return item.field === filter.field;
});
return filteredData;
}
}
},
grid = new Grid($element, gridOptions);
grid.search({ field: "test" });
equal(grid.option("data").length, 2, "data filtered");
});
test("filtering with static data should not do actual filtering", function() {
var $element = $("#jsGrid"),
gridOptions = {
filtering: true,
fields: [
{ type: "text", name: "field" }
],
data: [
{ name: "value1" },
{ name: "value2" }
]
},
grid = new Grid($element, gridOptions);
grid._filterRow.find("input").val("1");
grid.search();
equal(grid.option("data").length, 2, "data is not filtered");
});
module("nodatarow");
test("nodatarow after bind on empty array", function() {
var $element = $("#jsGrid"),
gridOptions = {},
grid = new Grid($element, gridOptions);
grid.option("data", []);
equal(grid._content.find("." + grid.noDataRowClass).length, 1, "no data row rendered");
equal(grid._content.find("." + grid.cellClass).length, 1, "grid cell class attached");
equal(grid._content.text(), grid.noDataContent, "no data text rendered");
});
test("nodatarow customize content", function() {
var noDataMessage = "NoData Custom Content",
$element = $("#jsGrid"),
gridOptions = {
noDataContent: function() {
return noDataMessage;
}
},
grid = new Grid($element, gridOptions);
grid.option("data", []);
equal(grid._content.find("." + grid.cellClass).length, 1, "grid cell class attached");
equal(grid._content.text(), noDataMessage, "custom noDataContent");
});
module("row rendering", {
setup: function() {
this.testData = [
{ id: 1, text: "test1" },
{ id: 2, text: "test2" },
{ id: 3, text: "test3" }
];
}
});
test("rows rendered correctly", function() {
var $element = $("#jsGrid"),
gridOptions = {
data: this.testData
},
grid = new Grid($element, gridOptions);
equal(grid._content.children().length, 3, "rows rendered");
equal(grid._content.find("." + grid.oddRowClass).length, 2, "two odd rows for 3 items");
equal(grid._content.find("." + grid.evenRowClass).length, 1, "one even row for 3 items");
});
test("custom rowClass", function() {
var $element = $("#jsGrid"),
gridOptions = {
data: this.testData,
rowClass: "custom-row-cls"
},
grid = new Grid($element, gridOptions);
equal(grid._content.find("." + grid.oddRowClass).length, 2);
equal(grid._content.find("." + grid.evenRowClass).length, 1);
equal(grid._content.find(".custom-row-cls").length, 3, "custom row class");
});
test("custom rowClass callback", function() {
var $element = $("#jsGrid"),
gridOptions = {
data: this.testData,
rowClass: function(item, index) {
return item.text;
}
},
grid = new Grid($element, gridOptions);
equal(grid._content.find("." + grid.oddRowClass).length, 2);
equal(grid._content.find("." + grid.evenRowClass).length, 1);
equal(grid._content.find(".test1").length, 1, "custom row class");
equal(grid._content.find(".test2").length, 1, "custom row class");
equal(grid._content.find(".test3").length, 1, "custom row class");
});
test("rowClick standard handler", function() {
var $element = $("#jsGrid"),
$secondRow,
grid = new Grid($element, { editing: true });
grid.option("data", this.testData);
$secondRow = grid._content.find("." + grid.evenRowClass);
$secondRow.trigger("click", $.Event($secondRow));
equal(grid._editingRow.get(0), $secondRow.get(0), "clicked row is editingRow");
});
test("rowClick handler", function() {
var rowClickArgs,
$element = $("#jsGrid"),
$secondRow,
gridOptions = {
rowClick: function(args) {
rowClickArgs = args;
}
},
grid = new Grid($element, gridOptions);
grid.option("data", this.testData);
$secondRow = grid._content.find("." + grid.evenRowClass);
$secondRow.trigger("click", $.Event($secondRow));
ok(rowClickArgs.event instanceof jQuery.Event, "jquery event arg");
equal(rowClickArgs.item, this.testData[1], "item arg");
equal(rowClickArgs.itemIndex, 1, "itemIndex arg");
});
test("row selecting with selectedRowClass", function() {
var $element = $("#jsGrid"),
$secondRow,
gridOptions = {
selecting: true
},
grid = new Grid($element, gridOptions);
grid.option("data", this.testData);
$secondRow = grid._content.find("." + grid.evenRowClass);
$secondRow.trigger("mouseenter", $.Event($secondRow));
ok($secondRow.hasClass(grid.selectedRowClass), "mouseenter adds selectedRowClass");
$secondRow.trigger("mouseleave", $.Event($secondRow));
ok(!$secondRow.hasClass(grid.selectedRowClass), "mouseleave removes selectedRowClass");
});
test("no row selecting while selection is disabled", function() {
var $element = $("#jsGrid"),
$secondRow,
gridOptions = {
selecting: false
},
grid = new Grid($element, gridOptions);
grid.option("data", this.testData);
$secondRow = grid._content.find("." + grid.evenRowClass);
$secondRow.trigger("mouseenter", $.Event($secondRow));
ok(!$secondRow.hasClass(grid.selectedRowClass), "mouseenter doesn't add selectedRowClass");
});
test("refreshing and refreshed callbacks", function() {
var refreshingEventArgs,
refreshedEventArgs,
$element = $("#jsGrid"),
grid = new Grid($element, {});
grid.onRefreshing = function(e) {
refreshingEventArgs = e;
equal(grid._content.find("." + grid.oddRowClass).length, 0, "no items before refresh");
};
grid.onRefreshed = function(e) {
refreshedEventArgs = e;
equal(grid._content.find("." + grid.oddRowClass).length, 2, "items rendered after refresh");
};
grid.option("data", this.testData);
equal(refreshingEventArgs.grid, grid, "grid provided in args for refreshing event");
equal(refreshedEventArgs.grid, grid, "grid provided in args for refreshed event");
equal(grid._content.find("." + grid.oddRowClass).length, 2, "items rendered");
});
test("grid fields normalization", function() {
var CustomField = function(config) {
$.extend(true, this, config);
};
jsGrid.fields.custom = CustomField;
try {
var $element = $("#jsGrid"),
gridOptions = {
fields: [
new jsGrid.Field({
name: "text1",
title: "title1"
}),
{
name: "text2",
title: "title2"
},
{
name: "text3",
type: "custom"
}
]
},
grid = new Grid($element, gridOptions);
var field1 = grid.fields[0];
ok(field1 instanceof jsGrid.Field);
equal(field1.name, "text1", "name is set for field");
equal(field1.title, "title1", "title field");
var field2 = grid.fields[1];
ok(field2 instanceof jsGrid.Field);
equal(field2.name, "text2", "name is set for field");
equal(field2.title, "title2", "title field");
var field3 = grid.fields[2];
ok(field3 instanceof CustomField);
equal(field3.name, "text3", "name is set for field");
} finally {
delete jsGrid.fields.custom;
}
});
test("'0' itemTemplate should be rendered", function() {
var $element = $("#jsGrid"),
grid = new Grid($element, {
data: [{}],
fields: [
new jsGrid.Field({ name: "id", itemTemplate: function() { return 0; } })
]
});
equal(grid._bodyGrid.text(), "0", "item template is rendered");
});
test("grid field name used for header if title is not specified", function() {
var $element = $("#jsGrid"),
grid = new Grid($element, {
fields: [
new jsGrid.Field({ name: "id" })
]
});
equal(grid._headerRow.text(), "id", "name is rendered in header");
});
test("grid fields header and item rendering", function() {
var $element = $("#jsGrid"),
$secondRow,
gridOptions = {
fields: [
new jsGrid.Field({
name: "text",
title: "title",
css: "cell-class",
headercss: "header-class",
align: "right"
})
]
},
grid = new Grid($element, gridOptions);
grid.option("data", this.testData);
equal(grid._headerRow.text(), "title", "header rendered");
equal(grid._headerRow.find("." + grid.headerCellClass).length, 1, "header cell class is attached");
equal(grid._headerRow.find(".header-class").length, 1, "headercss class is attached");
ok(grid._headerRow.find(".header-class").hasClass("jsgrid-align-right"), "align class is attached");
$secondRow = grid._content.find("." + grid.evenRowClass);
equal($secondRow.text(), "test2", "item rendered");
equal($secondRow.find(".cell-class").length, 1, "css class added to cell");
equal($secondRow.find("." + grid.cellClass).length, 1, "cell class is attached");
ok($secondRow.find(".cell-class").hasClass("jsgrid-align-right"), "align class added to cell");
});
test("grid field cellRenderer", function() {
var testItem = { text: "test" },
args;
var $grid = $("#jsGrid");
var gridOptions = {
data: [testItem],
fields: [
{
name: "text",
cellRenderer: function(value, item) {
args = {
value: value,
item: item
};
return $("<td>").addClass("custom-class").text(value);
}
}
]
};
var grid = new Grid($grid, gridOptions);
var $customCell = $grid.find(".custom-class");
equal($customCell.length, 1, "custom cell rendered");
equal($customCell.text(), "test");
deepEqual(args, { value: "test", item: testItem }, "cellRenderer args provided");
});
test("grid field 'visible' option", function() {
var $grid = $("#jsGrid");
var gridOptions = {
editing: true,
fields: [
{ name: "id", visible: false },
{ name: "test" }
]
};
var grid = new Grid($grid, gridOptions);
equal($grid.find("." + grid.noDataRowClass).children().eq(0).prop("colspan"), 1, "no data row colspan only for visible cells");
grid.option("data", this.testData);
grid.editItem(this.testData[2]);
equal($grid.find("." + grid.headerRowClass).children().length, 1, "header single cell");
equal($grid.find("." + grid.filterRowClass).children().length, 1, "filter single cell");
equal($grid.find("." + grid.insertRowClass).children().length, 1, "insert single cell");
equal($grid.find("." + grid.editRowClass).children().length, 1, "edit single cell");
equal($grid.find("." + grid.oddRowClass).eq(0).children().length, 1, "odd data row single cell");
equal($grid.find("." + grid.evenRowClass).eq(0).children().length, 1, "even data row single cell");
});
module("inserting");
test("inserting rendering", function() {
var $element = $("#jsGrid"),
gridOptions = {
inserting: true,
fields: [
{
name: "test",
align: "right",
insertcss: "insert-class",
insertTemplate: function() {
var result = this.insertControl = $("<input>").attr("type", "text").addClass("insert-input");
return result;
}
}
]
},
grid = new Grid($element, gridOptions);
equal(grid._insertRow.find(".insert-class").length, 1, "insertcss class is attached");
equal(grid._insertRow.find(".insert-input").length, 1, "insert control rendered");
equal(grid._insertRow.find("." + grid.cellClass).length, 1, "cell class is attached");
ok(grid._insertRow.find(".insert-class").hasClass("jsgrid-align-right"), "align class is attached");
ok(grid.fields[0].insertControl.is("input[type=text]"), "insert control saved in field");
});
test("field without inserting", function() {
var $element = $("#jsGrid"),
jsGridFieldConfig = {
insertTemplate: function() {
var result = this.insertControl = $("<input>").attr("type", "text");
return result;
},
insertValue: function() {
return this.insertControl.val();
}
},
gridOptions = {
inserting: true,
fields: [
$.extend({}, jsGridFieldConfig, {
name: "field1",
inserting: false
}),
$.extend({}, jsGridFieldConfig, {
name: "field2"
})
]
},
grid = new Grid($element, gridOptions);
grid.fields[0].insertControl.val("test1");
grid.fields[1].insertControl.val("test2");
deepEqual(grid._getInsertItem(), { field2: "test2" }, "field with inserting=false is not included in inserting item");
});
test("insert data with default location", function() {
var $element = $("#jsGrid"),
inserted = false,
insertingArgs,
insertedArgs,
gridOptions = {
inserting: true,
data: [{field: "default"}],
fields: [
{
name: "field",
insertTemplate: function() {
var result = this.insertControl = $("<input>").attr("type", "text");
return result;
},
insertValue: function() {
return this.insertControl.val();
}
}
],
onItemInserting: function(e) {
insertingArgs = $.extend(true, {}, e);
},
onItemInserted: function(e) {
insertedArgs = $.extend(true, {}, e);
},
controller: {
insertItem: function() {
inserted = true;
}
}
},
grid = new Grid($element, gridOptions);
grid.fields[0].insertControl.val("test");
grid.insertItem();
equal(insertingArgs.item.field, "test", "field is provided in inserting args");
equal(grid.option("data").length, 2, "data is inserted");
ok(inserted, "controller insertItem was called");
deepEqual(grid.option("data")[1], { field: "test" }, "correct data is inserted");
equal(insertedArgs.item.field, "test", "field is provided in inserted args");
});
test("insert data with specified insert location", function() {
var $element = $("#jsGrid"),
inserted = false,
insertingArgs,
insertedArgs,
gridOptions = {
inserting: true,
insertRowLocation: "top",
data: [{field: "default"}],
fields: [
{
name: "field",
insertTemplate: function() {
var result = this.insertControl = $("<input>").attr("type", "text");
return result;
},
insertValue: function() {
return this.insertControl.val();
}
}
],
onItemInserting: function(e) {
insertingArgs = $.extend(true, {}, e);
},
onItemInserted: function(e) {
insertedArgs = $.extend(true, {}, e);
},
controller: {
insertItem: function() {
inserted = true;
}
}
},
grid = new Grid($element, gridOptions);
grid.fields[0].insertControl.val("test");
grid.insertItem();
equal(insertingArgs.item.field, "test", "field is provided in inserting args");
equal(grid.option("data").length, 2, "data is inserted");
ok(inserted, "controller insertItem was called");
deepEqual(grid.option("data")[0], { field: "test" }, "correct data is inserted at the beginning");
equal(insertedArgs.item.field, "test", "field is provided in inserted args");
});
test("insertItem accepts item to insert", function() {
var $element = $("#jsGrid"),
itemToInsert = { field: "test" },
insertedItem,
gridOptions = {
data: [],
fields: [
{
name: "field"
}
],
test("edit item", function() {
var $element = $("#jsGrid"),
updated = false,
updatingArgs,
updatingRow,
grid.insertItem(itemToInsert);
deepEqual(grid.option("data")[0], itemToInsert, "data is inserted");
deepEqual(insertedItem, itemToInsert, "controller insertItem was called with correct item");
});
module("editing");
test("editing rendering", function() {
var $element = $("#jsGrid"),
$editRow,
data = [{
test: "value"
}],
gridOptions = {
editing: true,
fields: [
{
name: "test",
align: "right",
updated = true;
}
},
onItemUpdating: function(e) {
updatingArgs = $.extend(true, {}, e);
updatingRow = grid.rowByItem(data[0])[0];
grid.option("data", data);
equal(grid._content.find("." + grid.editRowClass).length, 0, "no edit row after initial rendering");
grid.editItem(data[0]);
$editRow = grid._content.find("." + grid.editRowClass);
equal($editRow.length, 1, "edit row rendered");
grid.option("data", data);
grid.editItem(data[0]);
grid.fields[0].editControl.val("new value");
grid.updateItem();
$editRow,
data = [
{ test: "value" }
],
gridOptions = {
editing: true,
fields: [
{ name: "test" }
]
},
grid = new Grid($element, gridOptions);
grid.option("data", data);
var $row = $element.find("." + grid.oddRowClass).eq(0);
grid.editItem($row);
$editRow = grid._content.find("." + grid.editRowClass);
equal($editRow.length, 1, "edit row rendered");
grid.cancelEdit();
grid.editItem($row.get(0));
$editRow = grid._content.find("." + grid.editRowClass);
equal($editRow.length, 1, "edit row rendered");
});
test("edit item", function() {
var $element = $("#jsGrid"),
editingArgs,
editingRow,
updated = false,
updatingArgs,
updatingRow,
updatedRow,
updatedArgs,
data = [{
field: "value"
}],
gridOptions = {
editing: true,
fields: [
{
name: "field",
editTemplate: function(value) {
var result = this.editControl = $("<input>").attr("type", "text").val(value);
return result;
},
editValue: function() {
return this.editControl.val();
}
}
],
controller: {
updateItem: function(updatingItem) {
updated = true;
}
},
onItemEditing: function(e) {
editingArgs = $.extend(true, {}, e);
editingRow = grid.rowByItem(data[0])[0];
},
onItemUpdating: function(e) {
updatingArgs = $.extend(true, {}, e);
updatingRow = grid.rowByItem(data[0])[0];
},
onItemUpdated: function(e) {
updatedArgs = $.extend(true, {}, e);
updatedRow = grid.rowByItem(data[0])[0];
}
},
grid = new Grid($element, gridOptions);
grid.option("data", data);
grid.editItem(data[0]);
deepEqual(editingArgs.item, { field: "value" }, "item before editing is provided in editing event args");
equal(editingArgs.itemIndex, 0, "itemIndex is provided in editing event args");
equal(editingArgs.row[0], editingRow, "row element is provided in editing event args");
grid.fields[0].editControl.val("new value");
grid.updateItem();
deepEqual(updatingArgs.previousItem, { field: "value" }, "item before editing is provided in updating event args");
deepEqual(updatingArgs.item, { field: "new value" }, "updating item is provided in updating event args");
equal(updatingArgs.itemIndex, 0, "itemIndex is provided in updating event args");
equal(updatingArgs.row[0], updatingRow, "row element is provided in updating event args");
ok(updated, "controller updateItem called");
deepEqual(grid.option("data")[0], { field: "new value" }, "correct data updated");
equal(grid._content.find("." + grid.editRowClass).length, 0, "edit row removed");
equal(grid._content.find("." + grid.oddRowClass).length, 1, "data row rendered");
deepEqual(updatedArgs.previousItem, { field: "value" }, "item before editing is provided in updated event args");
deepEqual(updatedArgs.item, { field: "new value" }, "updated item is provided in updated event args");
equal(updatedArgs.itemIndex, 0, "itemIndex is provided in updated event args");
equal(updatedArgs.row[0], updatedRow, "row element is provided in updated event args");
});
test("failed update should not change original item", function() {
var $element = $("#jsGrid"),
data = [{
field: "value"
}],
gridOptions = {
editing: true,
fields: [
{
name: "field",
editTemplate: function(value) {
var result = this.editControl = $("<input>").attr("type", "text").val(value);
return result;
},
editValue: function() {
return this.editControl.val();
}
}
],
controller: {
updateItem: function(updatingItem) {
return $.Deferred().reject();
}
}
},
grid = new Grid($element, gridOptions);
grid.option("data", data);
grid.editItem(data[0]);
grid.fields[0].editControl.val("new value");
grid.updateItem();
deepEqual(grid.option("data")[0], { field: "value" }, "value is not updated");
});
test("cancel edit", function() {
var $element = $("#jsGrid"),
updated = false,
cancellingArgs,
cancellingRow,
data = [{
field: "value"
}],
gridOptions = {
editing: true,
fields: [
{
name: "field",
editTemplate: function(value) {
var result = this.editControl = $("<input>").attr("type", "text").val(value);
return result;
},
editValue: function() {
return this.editControl.val();
}
}
],
controller: {
updateData: function(updatingItem) {
updated = true;
}
},
onItemEditCancelling: function(e) {
cancellingArgs = $.extend(true, {}, e);
cancellingRow = grid.rowByItem(data[0])[0];
}
},
grid = new Grid($element, gridOptions);
grid.option("data", data);
grid.editItem(data[0]);
grid.fields[0].editControl.val("new value");
grid.cancelEdit();
deepEqual(cancellingArgs.item, { field: "value" }, "item before cancel is provided in cancelling event args");
equal(cancellingArgs.itemIndex, 0, "itemIndex is provided in cancelling event args");
equal(cancellingArgs.row[0], cancellingRow, "row element is provided in cancelling event args");
ok(!updated, "controller updateItem was not called");
deepEqual(grid.option("data")[0], { field: "value" }, "data were not updated");
equal(grid._content.find("." + grid.editRowClass).length, 0, "edit row removed");
equal(grid._content.find("." + grid.oddRowClass).length, 1, "data row restored");
});
test("updateItem accepts item to update and new item", function() {
var $element = $("#jsGrid"),
updatingItem,
data = [{
field: "value"
}],
gridOptions = {
fields: [
{ name: "field" }
],
controller: {
updateItem: function(item) {
return updatingItem = item;
}
}
},
grid = new Grid($element, gridOptions);
grid.option("data", data);
grid.updateItem(data[0], { field: "new value" });
deepEqual(updatingItem, { field: "new value" }, "controller updateItem called correctly");
deepEqual(grid.option("data")[0], { field: "new value" }, "correct data updated");
});
test("updateItem accepts single argument - item to update", function() {
var $element = $("#jsGrid"),
updatingItem,
data = [{
field: "value"
}],
gridOptions = {
fields: [
{ name: "field" }
],
controller: {
updateItem: function(item) {
return updatingItem = item;
}
}
},
grid = new Grid($element, gridOptions);
grid.option("data", data);
data[0].field = "new value";
grid.updateItem(data[0]);
deepEqual(updatingItem, { field: "new value" }, "controller updateItem called correctly");
deepEqual(grid.option("data")[0], { field: "new value" }, "correct data updated");
});
test("editRowRenderer", function() {
var $element = $("#jsGrid"),
data = [
{ value: "test" }
],
gridOptions = {
data: data,
editing: true,
editRowRenderer: function(item, itemIndex) {
return $("<tr>").addClass("custom-edit-row").append($("<td>").text(itemIndex + ":" + item.value));
},
fields: [
{ name: "value" }
]
},
grid = new Grid($element, gridOptions);
grid.editItem(data[0]);
var $editRow = grid._content.find(".custom-edit-row");
equal($editRow.length, 1, "edit row rendered");
equal($editRow.text(), "0:test", "custom edit row renderer rendered");
});
module("deleting");
test("delete item", function() {
var $element = $("#jsGrid"),
deleted = false,
deletingArgs,
deletedArgs,
data = [{
field: "value"
}],
gridOptions = {
confirmDeleting: false,
fields: [
{ name: "field" }
],
controller: {
deleteItem: function(deletingItem) {
deleted = true;
}
},
onItemDeleting: function(e) {
deletingArgs = $.extend(true, {}, e);
},
onItemDeleted: function(e) {
deletedArgs = $.extend(true, {}, e);
}
},
grid = new Grid($element, gridOptions);
grid.option("data", data);
grid.deleteItem(data[0]);
deepEqual(deletingArgs.item, { field: "value" }, "field and value is provided in deleting event args");
equal(deletingArgs.itemIndex, 0, "itemIndex is provided in updating event args");
equal(deletingArgs.row.length, 1, "row element is provided in updating event args");
ok(deleted, "controller deleteItem called");
equal(grid.option("data").length, 0, "data row deleted");
deepEqual(deletedArgs.item, { field: "value" }, "item is provided in updating event args");
equal(deletedArgs.itemIndex, 0, "itemIndex is provided in updating event args");
equal(deletedArgs.row.length, 1, "row element is provided in updating event args");
});
test("deleteItem accepts row", function() {
var $element = $("#jsGrid"),
deletedItem,
itemToDelete = {
field: "value"
},
data = [itemToDelete],
gridOptions = {
confirmDeleting: false,
fields: [
{ name: "field" }
],
controller: {
deleteItem: function(deletingItem) {
deletedItem = deletingItem;
}
}
},
grid = new Grid($element, gridOptions);
grid.option("data", data);
var $row = $element.find("." + grid.oddRowClass).eq(0);
grid.deleteItem($row);
deepEqual(deletedItem, itemToDelete, "controller deleteItem called correctly");
equal(grid.option("data").length, 0, "data row deleted");
});
module("paging");
test("pager is rendered if necessary", function() {
var $element = $("#jsGrid"),
grid = new Grid($element, {
data: [{}, {}, {}],
paging: false,
pageSize: 2
});
ok(grid._pagerContainer.is(":hidden"), "pager is hidden when paging=false");
equal(grid._pagerContainer.html(), "", "pager is not rendered when paging=false");
grid.option("paging", true);
ok(grid._pagerContainer.is(":visible"), "pager is visible when paging=true");
ok(grid._pagerContainer.html(), "pager is rendered when paging=true");
grid.option("data", [{}, {}]);
ok(grid._pagerContainer.is(":hidden"), "pager is hidden for single page");
ok(grid._pagerContainer.html(), "pager is rendered for single page when paging=true");
});
test("external pagerContainer", function() {
var $pagerContainer = $("<div>").appendTo("#qunit-fixture").hide(),
$element = $("#jsGrid");
new Grid($element, {
data: [{}, {}, {}],
pagerContainer: $pagerContainer,
paging: true,
pageSize: 2
});
ok($pagerContainer.is(":visible"), "external pager shown");
ok($pagerContainer.html(), "external pager rendered");
});
test("pager functionality", function() {
var $element = $("#jsGrid"),
pager,
pageChangedArgs,
grid = new Grid($element, {
data: [{}, {}, {}, {}, {}, {}, {}, {}, {}],
onPageChanged: function(args) {
pageChangedArgs = args;
},
paging: true,
pageSize: 2,
pageButtonCount: 3
});
equal(grid._pagesCount(), 5, "correct page count");
equal(grid.option("pageIndex"), 1, "pageIndex is initialized");
equal(grid._firstDisplayingPage, 1, "_firstDisplayingPage is initialized");
pager = grid._pagerContainer;
equal(pager.find("." + grid.currentPageClass).length, 1, "there is one current page");
ok(pager.find("." + grid.pageClass).eq(0).hasClass(grid.currentPageClass), "first page is current");
equal(pager.find("." + grid.pageClass).length, 3, "three pages displayed");
equal(pager.find("." + grid.pagerNavButtonClass).length, 5, "five nav buttons displayed: Fisrt Prev Next Last ...");
equal(pager.find("." + grid.pagerNavButtonInactiveClass).length, 2, "two nav buttons inactive: Fisrt Prev");
grid.openPage(2);
equal(pager.find("." + grid.currentPageClass).length, 1, "there is one current page");
ok(pager.find("." + grid.pageClass).eq(1).hasClass(grid.currentPageClass), "second page is current");
equal(pager.find("." + grid.pageClass).length, 3, "three pages displayed");
equal(pager.find("." + grid.pagerNavButtonClass).length, 5, "five nav buttons displayed: First Prev Next Last and ...");
equal(pageChangedArgs.pageIndex, 2, "onPageChanged callback provides pageIndex in arguments");
grid.showNextPages();
equal(grid._firstDisplayingPage, 3, "navigate by pages forward");
grid.showPrevPages();
equal(grid._firstDisplayingPage, 1, "navigate by pages backward");
grid.openPage(5);
equal(grid._firstDisplayingPage, 3, "opening next non-visible page moves first displaying page forward");
grid.openPage(2);
equal(grid._firstDisplayingPage, 2, "opening prev non-visible page moves first displaying page backward");
});
test("pager format", function() {
var $element = $("#jsGrid"),
grid = new Grid($element, {
data: [{}, {}, {}, {}, {}, {}],
paging: true,
pageSize: 2,
pageIndex: 2,
pageButtonCount: 1,
pagerFormat: "a {pageIndex} {first} {prev} {pages} {next} {last} {pageCount} {itemCount} z",
pagePrevText: "<",
pageNextText: ">",
pageFirstText: "<<",
pageLastText: ">>",
pageNavigatorNextText: "np",
pageNavigatorPrevText: "pp"
});
grid._firstDisplayingPage = 2;
grid._refreshPager();
equal($.trim(grid._pagerContainer.text()), "a 2 << < pp2np > >> 3 6 z", "pager text follows the format specified");
});
test("pagerRenderer", function() {
var $element = $("#jsGrid");
var pagerRendererConfig;
var pageSize = 2;
var items = [{}, {}, {}, {}, {}, {}, {}];
var pageCount = Math.ceil(items.length / pageSize);
var grid = new Grid($element, {
data: items,
paging: true,
pageSize: pageSize,
pagerRenderer: function(pagerConfig) {
pagerRendererConfig = pagerConfig;
}
});
deepEqual(pagerRendererConfig, { pageIndex: 1, pageCount: pageCount });
grid.openPage(2);
deepEqual(pagerRendererConfig, { pageIndex: 2, pageCount: pageCount });
});
test("loading by page", function() {
var $element = $("#jsGrid"),
data = [],
itemCount = 20;
for(var i = 1; i <= itemCount; i += 1) {
data.push({
value: i
});
}
var gridOptions = {
pageLoading: true,
paging: true,
pageSize: 7,
fields: [
{ name: "value" }
],
controller: {
loadData: function(filter) {
var startIndex = (filter.pageIndex - 1) * filter.pageSize,
result = data.slice(startIndex, startIndex + filter.pageSize);
return {
data: result,
itemsCount: data.length
};
}
}
};
var grid = new Grid($element, gridOptions);
grid.loadData();
var pager = grid._pagerContainer;
var gridData = grid.option("data");
equal(gridData.length, 7, "loaded one page of data");
equal(gridData[0].value, 1, "loaded right data start value");
equal(gridData[gridData.length - 1].value, 7, "loaded correct data end value");
ok(pager.find("." + grid.pageClass).eq(0).hasClass(grid.currentPageClass), "first page is current");
equal(pager.find("." + grid.pageClass).length, 3, "three pages displayed");
grid.openPage(3);
gridData = grid.option("data");
equal(gridData.length, 6, "loaded last page of data");
equal(gridData[0].value, 15, "loaded right data start value");
equal(gridData[gridData.length - 1].value, 20, "loaded right data end value");
ok(pager.find("." + grid.pageClass).eq(2).hasClass(grid.currentPageClass), "third page is current");
equal(pager.find("." + grid.pageClass).length, 3, "three pages displayed");
});
test("'openPage' method ignores indexes out of range", function() {
var $element = $("#jsGrid"),
grid = new Grid($element, {
data: [{}, {}],
paging: true,
pageSize: 1
});
grid.openPage(0);
equal(grid.option("pageIndex"), 1, "too small index is ignored");
grid.openPage(3);
equal(grid.option("pageIndex"), 1, "too big index is ignored");
});
module("sorting");
test("sorting", function() {
var $element = $("#jsGrid"),
});
module("canceling controller calls");
test("cancel controller.loadData", function() {
var $element = $("#jsGrid");
});
test("sorting with pageLoading", function() {
var $element = $("#jsGrid"),
loadFilter,
gridOptions = {
sorting: true,
pageLoading: true,
data: [
{ value: 3 },
{ value: 2 },
{ value: 1 }
],
controller: {
loadData: function(filter) {
loadFilter = filter;
return {
itemsCount: 0,
data: []
};
}
},
fields: [
{ name: "value", sorter: "number" }
]
},
grid = new Grid($element, gridOptions);
var $th = grid._headerRow.find("th").eq(0);
$th.trigger("click");
equal(grid._sortOrder, "asc", "asc sorting order for first click");
equal(grid._sortField, grid.fields[0], "sort field is set");
equal(loadFilter.sortOrder, "asc", "sort direction is provided in loadFilter");
equal(loadFilter.sortField, "value", "sort field is provided in loadFilter");
$th.trigger("click");
equal(grid._sortOrder, "desc", "desc sorting order for second click");
equal(grid._sortField, grid.fields[0], "sort field is set");
equal(loadFilter.sortOrder, "desc", "sort direction is provided in loadFilter");
equal(loadFilter.sortField, "value", "sort field is provided in loadFilter");
});
test("no sorting for column with sorting = false", function() {
var $element = $("#jsGrid");
var gridOptions = {
sorting: true,
data: [
{ value: 3 },
{ value: 2 },
{ value: 1 }
],
fields: [
{ name: "value", sorting: false }
]
};
var grid = new Grid($element, gridOptions);
var gridData = grid.option("data");
var $th = grid._headerRow.find("th").eq(0);
$th.trigger("click");
equal(grid._sortField, null, "sort field is not set for the field with sorting=false");
equal(gridData[0].value, 3);
equal(gridData[1].value, 2);
equal(gridData[2].value, 1);
equal($th.hasClass(grid.sortableClass), false, "no sorting css for field with sorting=false");
equal($th.hasClass(grid.sortAscClass), false, "no sorting css for field with sorting=false");
});
test("sort accepts sorting config", function() {
var $element = $("#jsGrid"),
gridOptions = {
sorting: true,
data: [
{ value: 3 },
{ value: 2 },
{ value: 1 }
],
fields: [
{ name: "value", sorter: "number" }
]
},
grid = new Grid($element, gridOptions);
var gridData = grid.option("data");
grid.sort({ field: "value", order: "asc" });
equal(grid._sortOrder, "asc", "asc sorting order is set");
equal(grid._sortField, grid.fields[0], "sort field is set");
equal(gridData[0].value, 1);
equal(gridData[1].value, 2);
equal(gridData[2].value, 3);
grid.sort({ field: 0 });
equal(grid._sortOrder, "desc", "desc sorting order for already set asc sorting");
equal(grid._sortField, grid.fields[0], "sort field is set");
equal(gridData[0].value, 3);
equal(gridData[1].value, 2);
equal(gridData[2].value, 1);
grid.sort("value", "asc");
equal(grid._sortOrder, "asc", "asc sorting order is set");
equal(grid._sortField, grid.fields[0], "sort field is set");
grid.sort(0);
equal(grid._sortOrder, "desc", "desc sorting order for already set asc sorting");
equal(grid._sortField, grid.fields[0], "sort field is set");
});
test("getSorting returns current sorting", function() {
var $element = $("#jsGrid"),
gridOptions = {
sorting: true,
data: [
{ value: 3 },
{ value: 2 },
{ value: 1 }
],
fields: [
{ name: "value", sorter: "number" }
]
},
grid = new Grid($element, gridOptions);
deepEqual(grid.getSorting(), { field: undefined, order: undefined }, "undefined field and order before sorting");
grid.sort("value");
deepEqual(grid.getSorting(), { field: "value", order: "asc" }, "current sorting returned");
});
test("sorting css attached correctly when a field is hidden", function() {
var $element = $("#jsGrid");
var gridOptions = {
sorting: true,
data: [],
fields: [
{ name: "field1", visible: false },
{ name: "field2" }
]
};
var grid = new Grid($element, gridOptions);
var gridData = grid.option("data");
var $th = grid._headerRow.find("th").eq(0);
$th.trigger("click");
equal($th.hasClass(grid.sortAscClass), true, "sorting css is attached to first field");
});
module("canceling events");
test("cancel item edit", function() {
var $element = $("#jsGrid");
var data = [{}];
var gridOptions = {
editing: true,
onItemEditing: function(e) {
e.cancel = true;
},
controller: {
loadData: function() {
return data;
}
},
fields: [
{ name: "test" }
]
};
var grid = new Grid($element, gridOptions);
grid.loadData();
grid.editItem(data[0]);
strictEqual(grid._editingRow, null, "no editing row");
});
test("cancel controller.loadData", function() {
var $element = $("#jsGrid");
var gridOptions = {
onDataLoading: function(e) {
e.cancel = true;
},
controller: {
loadData: function() {
return [{}];
}
},
fields: [
{ name: "test" }
]
};
var grid = new Grid($element, gridOptions);
grid.loadData();
equal(grid.option("data").length, 0, "no data loaded");
});
test("cancel controller.insertItem", function() {
var $element = $("#jsGrid");
var insertedItem = null;
var gridOptions = {
onItemInserting: function(e) {
e.cancel = true;
},
controller: {
insertItem: function(item) {
insertedItem = item;
}
},
fields: [
{ name: "test" }
]
};
var grid = new Grid($element, gridOptions);
grid.insertItem({ test: "value" });
strictEqual(insertedItem, null, "item was not inserted");
});
test("cancel controller.updateItem", function() {
var $element = $("#jsGrid");
var updatedItem = null;
var existingItem = { test: "value" };
var gridOptions = {
data: [
existingItem
],
onItemUpdating: function(e) {
e.cancel = true;
},
controller: {
updateItem: function(item) {
updatedItem = item;
}
},
fields: [
{ name: "test" }
]
};
var grid = new Grid($element, gridOptions);
grid.updateItem(existingItem, { test: "new_value" });
strictEqual(updatedItem, null, "item was not updated");
});
test("cancel controller.deleteItem", function() {
var $element = $("#jsGrid");
var deletingItem = { test: "value" };
var deletedItem = null;
var gridOptions = {
data: [
deletingItem
],
confirmDeleting: false,
onItemDeleting: function(e) {
e.cancel = true;
},
controller: {
deleteItem: function(item) {
deletedItem = item;
}
},
fields: [
{ name: "test" }
]
};
var grid = new Grid($element, gridOptions);
grid.deleteItem(deletingItem);
strictEqual(deletedItem, null, "item was not deleted");
});
module("complex properties binding");
test("rendering", function() {
var $element = $("#jsGrid");
var gridOptions = {
loadMessage: "",
data: [
{ complexProp: { prop: "test" } }
],
fields: [
{ name: "complexProp.prop", title: "" }
]
};
new Grid($element, gridOptions);
equal($element.text(), "test", "complex property value rendered");
});
test("editing", function() {
var $element = $("#jsGrid");
var gridOptions = {
editing: true,
data: [
{ complexProp: { prop: "test" } }
],
fields: [
{ type: "text", name: "complexProp.prop" }
]
};
var grid = new Grid($element, gridOptions);
grid.editItem(gridOptions.data[0]);
equal(grid.fields[0].editControl.val(), "test", "complex property value set in editor");
});
test("should not fail if property is absent", function() {
var $element = $("#jsGrid");
var gridOptions = {
loadMessage: "",
data: [
{ complexProp: { } }
],
fields: [
{ name: "complexProp.subprop.prop", title: "" }
]
};
new Grid($element, gridOptions);
equal($element.text(), "", "rendered empty value");
});
test("inserting", function() {
var $element = $("#jsGrid");
var insertingItem;
var gridOptions = {
inserting: true,
fields: [
{ type: "text", name: "complexProp.prop" }
],
onItemInserting: function(args) {
insertingItem = args.item;
}
};
var grid = new Grid($element, gridOptions);
grid.fields[0].insertControl.val("test");
grid.insertItem();
deepEqual(insertingItem, { complexProp: { prop: "test" } }, "inserting item has complex properties");
});
test("filtering", function() {
var $element = $("#jsGrid");
var loadFilter;
var gridOptions = {
filtering: true,
fields: [
{ type: "text", name: "complexProp.prop" }
],
controller: {
loadData: function(filter) {
loadFilter = filter;
}
}
};
var grid = new Grid($element, gridOptions);
grid.fields[0].filterControl.val("test");
grid.search();
deepEqual(loadFilter, { complexProp: { prop: "test" } }, "filter has complex properties");
});
test("updating", function() {
var $element = $("#jsGrid");
var updatingItem;
var gridOptions = {
editing: true,
data: [
{ complexProp: { } }
],
fields: [
{ type: "text", name: "complexProp.prop" }
],
onItemUpdating: function(args) {
updatingItem = args.item;
}
};
var grid = new Grid($element, gridOptions);
grid.editItem(gridOptions.data[0]);
grid.fields[0].editControl.val("test");
grid.updateItem();
deepEqual(updatingItem, { complexProp: { prop: "test" } }, "updating item has complex properties");
});
test("update nested prop", function() {
var $element = $("#jsGrid");
var updatingItem;
var previousItem;
var gridOptions = {
editing: true,
data: [
{ prop: { subprop1: "test1", subprop2: "test2" } }
],
fields: [
{ type: "text", name: "prop.subprop1" },
{ type: "text", name: "prop.subprop2" }
],
onItemUpdating: function(args) {
updatingItem = args.item;
}
};
var grid = new Grid($element, gridOptions);
grid.editItem(gridOptions.data[0]);
grid.fields[0].editControl.val("new_test1");
grid.updateItem();
var expectedUpdatingItem = {
prop: {
subprop1: "new_test1",
subprop2: "test2"
}
};
deepEqual(updatingItem, expectedUpdatingItem, "updating item has nested properties");
});
test("updating deeply nested prop", function() {
var $element = $("#jsGrid");
var updatingItem;
var previousItem;
var gridOptions = {
editing: true,
data: [
{ complexProp: { subprop1: { another_prop: "test" } } }
],
fields: [
{ type: "text", name: "complexProp.subprop1.prop1" },
{ type: "text", name: "complexProp.subprop1.subprop2.prop12" }
],
onItemUpdating: function(args) {
updatingItem = $.extend(true, {}, args.item);
previousItem = $.extend(true, {}, args.previousItem);
}
};
var grid = new Grid($element, gridOptions);
grid.editItem(gridOptions.data[0]);
grid.fields[0].editControl.val("test1");
grid.fields[1].editControl.val("test2");
grid.updateItem();
var expectedUpdatingItem = {
complexProp: {
subprop1: {
another_prop: "test",
prop1: "test1",
subprop2: { prop12: "test2" }
}
}
};
var expectedPreviousItem = {
complexProp: {
subprop1: {
another_prop: "test"
}
}
};
deepEqual(updatingItem, expectedUpdatingItem, "updating item has deeply nested properties");
deepEqual(previousItem, expectedPreviousItem, "previous item preserved correctly");
});
module("validation");
test("insertItem should call validation.validate", function() {
var $element = $("#jsGrid");
var fieldValidationRules = { test: "value" };
var validatingArgs;
var gridOptions = {
data: [],
inserting: true,
invalidNotify: $.noop,
validation: {
validate: function(args) {
validatingArgs = args;
return [];
}
},
fields: [
{ type: "text", name: "Name", validate: fieldValidationRules }
]
};
var grid = new Grid($element, gridOptions);
grid.fields[0].insertControl.val("test");
grid.insertItem();
deepEqual(validatingArgs, { value: "test", item: { Name: "test" }, itemIndex: -1,
row: grid._insertRow, rules: fieldValidationRules }, "validating args is provided");
});
test("insertItem rejected when data is not valid", function() {
var $element = $("#jsGrid");
var gridOptions = {
data: [],
inserting: true,
invalidNotify: $.noop,
validation: {
validate: function() {
return ["Error"];
}
},
fields: [
{ type: "text", name: "Name", validate: true }
]
};
var grid = new Grid($element, gridOptions);
grid.fields[0].insertControl.val("test");
grid.insertItem().done(function() {
ok(false, "insertItem should not be completed");
}).fail(function() {
ok(true, "insertItem should fail");
});
});
test("invalidClass is attached on invalid cell on inserting", function() {
var $element = $("#jsGrid");
var gridOptions = {
data: [],
inserting: true,
invalidNotify: $.noop,
validation: {
validate: function() {
return ["Error"];
}
},
fields: [
{ type: "text", name: "Id", visible: false },
{ type: "text", name: "Name", validate: true }
]
};
var grid = new Grid($element, gridOptions);
var $insertCell = grid._insertRow.children().eq(0);
grid.insertItem();
ok($insertCell.hasClass(grid.invalidClass), "invalid class is attached");
equal($insertCell.attr("title"), "Error", "cell tooltip contains error message");
});
test("onItemInvalid callback", function() {
var $element = $("#jsGrid");
var errors = ["Error"];
var onItemInvalidCalled = 0;
var onItemInvalidArgs;
var gridOptions = {
data: [],
inserting: true,
invalidNotify: $.noop,
onItemInvalid: function(args) {
onItemInvalidCalled++;
onItemInvalidArgs = args;
},
validation: {
validate: function(args) {
return !args.value ? errors : [];
}
},
fields: [
{ type: "text", name: "Name", validate: true }
]
};
var grid = new Grid($element, gridOptions);
grid.insertItem();
equal(onItemInvalidCalled, 1, "onItemInvalid is called, when item data is invalid");
deepEqual(onItemInvalidArgs, { grid: grid, errors: [{ field: grid.fields[0], message: "Error" }],
item: { Name: "" }, itemIndex: -1, row: grid._insertRow }, "arguments provided");
grid.fields[0].insertControl.val("test");
grid.insertItem();
equal(onItemInvalidCalled, 1, "onItemInvalid was not called, when data is valid");
});
test("invalidNotify", function() {
var $element = $("#jsGrid");
var errors = ["Error"];
var invalidNotifyCalled = 0;
var invalidNotifyArgs;
var gridOptions = {
data: [],
inserting: true,
invalidNotify: function(args) {
invalidNotifyCalled++;
invalidNotifyArgs = args;
},
validation: {
validate: function(args) {
return !args.value ? errors : [];
}
},
fields: [
{ type: "text", name: "Name", validate: true }
]
};
var grid = new Grid($element, gridOptions);
grid.insertItem();
equal(invalidNotifyCalled, 1, "invalidNotify is called, when item data is invalid");
deepEqual(invalidNotifyArgs, { grid: grid, errors: [{ field: grid.fields[0], message: "Error" }],
row: grid._insertRow, item: { Name: "" }, itemIndex: -1 }, "arguments provided");
grid.fields[0].insertControl.val("test");
grid.insertItem();
equal(invalidNotifyCalled, 1, "invalidNotify was not called, when data is valid");
});
test("invalidMessage", function() {
var $element = $("#jsGrid");
var invalidMessage;
var originalAlert = window.alert;
window.alert = function(message) {
invalidMessage = message;
};
try {
Grid.prototype.invalidMessage = "InvalidTest";
Grid.prototype.invalidNotify({ errors: [{ message: "Message1" }, { message: "Message2" }] });
var expectedInvalidMessage = ["InvalidTest", "Message1", "Message2"].join("\n");
equal(invalidMessage, expectedInvalidMessage, "message contains invalidMessage and field error messages");
} finally {
window.alert = originalAlert;
}
});
test("updateItem should call validation.validate", function() {
var $element = $("#jsGrid");
var validatingArgs;
var gridOptions = {
data: [{ Name: "" }],
editing: true,
invalidNotify: $.noop,
validation: {
validate: function(args) {
validatingArgs = args;
return ["Error"];
}
},
fields: [
{ type: "text", name: "Name", validate: "required" }
]
};
var grid = new Grid($element, gridOptions);
grid.editItem(gridOptions.data[0]);
grid.fields[0].editControl.val("test");
grid.updateItem();
deepEqual(validatingArgs, { value: "test", item: { Name: "test" }, itemIndex: 0,
row: grid._getEditRow(), rules: "required" }, "validating args is provided");
});
test("invalidClass is attached on invalid cell on updating", function() {
var $element = $("#jsGrid");
var gridOptions = {
data: [{}],
editing: true,
invalidNotify: $.noop,
validation: {
validate: function() {
return ["Error"];
}
},
fields: [
{ type: "text", name: "Name", validate: true }
]
};
var grid = new Grid($element, gridOptions);
grid.editItem(gridOptions.data[0]);
var $editCell = grid._getEditRow().children().eq(0);
grid.updateItem();
ok($editCell.hasClass(grid.invalidClass), "invalid class is attached");
equal($editCell.attr("title"), "Error", "cell tooltip contains error message");
});
test("validation should ignore not editable fields", function() {
var invalidNotifyCalled = 0;
var $element = $("#jsGrid");
var validatingArgs;
var gridOptions = {
data: [{ Name: "" }],
editing: true,
invalidNotify: function() {
invalidNotifyCalled++;
},
validation: {
validate: function() {
return ["Error"];
}
},
fields: [
{ type: "text", name: "Name", editing: false, validate: "required" }
]
};
var grid = new Grid($element, gridOptions);
grid.editItem(gridOptions.data[0]);
grid.updateItem();
equal(invalidNotifyCalled, 0, "data is valid");
});
module("api");
test("reset method should go the first page when pageLoading is truned on", function() {
var items = [{ Name: "1" }, { Name: "2" }];
var $element = $("#jsGrid");
var gridOptions = {
paging: true,
pageSize: 1,
pageLoading: true,
autoload: true,
controller: {
loadData: function(args) {
return {
data: [items[args.pageIndex - 1]],
itemsCount: items.length
};
}
},
fields: [
{ type: "text", name: "Name" }
]
};
var grid = new Grid($element, gridOptions);
grid.openPage(2);
grid.reset();
equal(grid._bodyGrid.text(), "1", "grid content reset");
});
module("i18n");
test("set locale by name", function() {
jsGrid.locales.my_lang = {
grid: {
test: "test_text"
}
};
jsGrid.locale("my_lang");
var $element = $("#jsGrid").jsGrid({});
equal($element.jsGrid("option", "test"), "test_text", "option localized");
});
test("set locale by config", function() {
jsGrid.locale( {
grid: {
test: "test_text"
}
});
var $element = $("#jsGrid").jsGrid({});
equal($element.jsGrid("option", "test"), "test_text", "option localized");
});
test("locale throws exception for unknown locale", function() {
throws(function() {
jsGrid.locale("unknown_lang");
}, /unknown_lang/, "locale threw an exception");
});
module("controller promise");
asyncTest("should support jQuery promise success callback", 1, function() {
var data = [];
var gridOptions = {
autoload: false,
controller: {
loadData: function() {
var d = $.Deferred();
setTimeout(function() {
d.resolve(data);
start();
});
return d.promise();
}
}
};
var grid = new Grid($("#jsGrid"), gridOptions);
var promise = grid._controllerCall("loadData", {}, false, $.noop);
promise.done(function(result) {
equal(result, data, "data provided to done callback");
});
});
asyncTest("should support jQuery promise fail callback", 1, function() {
var failArgs = {};
var gridOptions = {
autoload: false,
controller: {
loadData: function() {
var d = $.Deferred();
setTimeout(function() {
d.reject(failArgs);
start();
});
return d.promise();
}
}
};
var grid = new Grid($("#jsGrid"), gridOptions);
var promise = grid._controllerCall("loadData", {}, false, $.noop);
promise.fail(function(result) {
equal(result, failArgs, "fail args provided to fail callback");
});
});
asyncTest("should support JS promise success callback", 1, function() {
if(typeof Promise === "undefined") {
ok(true, "Promise not supported");
start();
return;
}
var data = [];
var gridOptions = {
autoload: false,
controller: {
loadData: function() {
return new Promise(function(resolve, reject) {
setTimeout(function() {
resolve(data);
start();
});
});
}
}
};
var grid = new Grid($("#jsGrid"), gridOptions);
var promise = grid._controllerCall("loadData", {}, false, $.noop);
promise.done(function(result) {
equal(result, data, "data provided to done callback");
});
});
asyncTest("should support JS promise fail callback", 1, function() {
if(typeof Promise === "undefined") {
ok(true, "Promise not supported");
start();
return;
}
var failArgs = {};
var gridOptions = {
autoload: false,
controller: {
loadData: function() {
return new Promise(function(resolve, reject) {
setTimeout(function() {
reject(failArgs);
start();
});
});
}
}
};
var grid = new Grid($("#jsGrid"), gridOptions);
var promise = grid._controllerCall("loadData", {}, false, $.noop);
promise.fail(function(result) {
equal(result, failArgs, "fail args provided to fail callback");
});
});
test("should support non-promise result", 1, function() {
var data = [];
var gridOptions = {
autoload: false,
controller: {
loadData: function() {
return data;
}
}
};
var grid = new Grid($("#jsGrid"), gridOptions);
var promise = grid._controllerCall("loadData", {}, false, $.noop);
promise.done(function(result) {
equal(result, data, "data provided to done callback");
});
});
module("renderTemplate");
test("should pass undefined and null arguments to the renderer", function() {
var rendererArgs;
var rendererContext;
var context = {};
var renderer = function() {
rendererArgs = arguments;
rendererContext = this;
};
Grid.prototype.renderTemplate(renderer, context, { arg1: undefined, arg2: null, arg3: "test" });
equal(rendererArgs.length, 3);
strictEqual(rendererArgs[0], undefined, "undefined passed");
strictEqual(rendererArgs[1], null, "null passed");
strictEqual(rendererArgs[2], "test", "null passed");
strictEqual(rendererContext, context, "context is preserved");
});
module("Data Export", {
setup: function() {
this.exportConfig = {};
this.exportConfig.type = "csv";
this.exportConfig.subset = "all";
this.exportConfig.delimiter = "|";
this.exportConfig.includeHeaders = true;
this.exportConfig.encapsulate = true;
this.element = $("#jsGrid");
this.gridOptions = {
width: "100%",
height: "400px",
inserting: true,
editing: true,
sorting: true,
paging: true,
pageSize: 2,
data: [
{ "Name": "Otto Clay", "Country": 1, "Married": false },
{ "Name": "Connor Johnston", "Country": 2, "Married": true },
{ "Name": "Lacey Hess", "Country": 2, "Married": false },
{ "Name": "Timothy Henson", "Country": 1, "Married": true }
],
fields: [
{ name: "Name", type: "text", width: 150, validate: "required" },
{ name: "Country", type: "select", items: [{ Name: "United States", Id: 1 },{ Name: "Canada", Id: 2 }], valueField: "Id", textField: "Name" },
{ name: "Married", type: "checkbox", title: "Is Married", sorting: false },
{ type: "control" }
]
}
}
});
/* Base Choice Criteria
type: csv
subset: all
delimiter: |
includeHeaders: true
encapsulate: true
*/
test("Should export data: Base Choice",function(){
var grid = new Grid($(this.element), this.gridOptions);
var data = grid.exportData(this.exportConfig);
var expected = "";
expected += '"Name"|"Country"|"Is Married"\r\n';
expected += '"Otto Clay"|"United States"|"false"\r\n';
expected += '"Connor Johnston"|"Canada"|"true"\r\n';
expected += '"Lacey Hess"|"Canada"|"false"\r\n';
expected += '"Timothy Henson"|"United States"|"true"\r\n';
equal(data, expected, "Output CSV configured to Base Choice Criteria -- Check Source");
});
test("Should export data: defaults = BCC",function(){
var grid = new Grid($(this.element), this.gridOptions);
var data = grid.exportData();
var expected = "";
expected += '"Name"|"Country"|"Is Married"\r\n';
expected += '"Otto Clay"|"United States"|"false"\r\n';
expected += '"Connor Johnston"|"Canada"|"true"\r\n';
expected += '"Lacey Hess"|"Canada"|"false"\r\n';
expected += '"Timothy Henson"|"United States"|"true"\r\n';
equal(data, expected, "Output CSV with all defaults -- Should be equal to Base Choice");
});
test("Should export data: subset=visible", function(){
var grid = new Grid($(this.element), this.gridOptions);
this.exportConfig.subset = "visible";
var data = grid.exportData(this.exportConfig);
var expected = "";
expected += '"Name"|"Country"|"Is Married"\r\n';
expected += '"Otto Clay"|"United States"|"false"\r\n';
expected += '"Connor Johnston"|"Canada"|"true"\r\n';
//expected += '"Lacey Hess"|"Canada"|"false"\r\n';
//expected += '"Timothy Henson"|"United States"|"true"\r\n';
equal(data, expected, "Output CSV of visible records");
});
test("Should export data: delimiter=;", function(){
var grid = new Grid($(this.element), this.gridOptions);
this.exportConfig.delimiter = ";";
var data = grid.exportData(this.exportConfig);
var expected = "";
expected += '"Name";"Country";"Is Married"\r\n';
expected += '"Otto Clay";"United States";"false"\r\n';
expected += '"Connor Johnston";"Canada";"true"\r\n';
expected += '"Lacey Hess";"Canada";"false"\r\n';
expected += '"Timothy Henson";"United States";"true"\r\n';
equal(data, expected, "Output CSV with non-default delimiter");
});
test("Should export data: headers=false", function(){
var grid = new Grid($(this.element), this.gridOptions);
this.exportConfig.includeHeaders = false;
var data = grid.exportData(this.exportConfig);
var expected = "";
//expected += '"Name"|"Country"|"Is Married"\r\n';
expected += '"Otto Clay"|"United States"|"false"\r\n';
expected += '"Connor Johnston"|"Canada"|"true"\r\n';
expected += '"Lacey Hess"|"Canada"|"false"\r\n';
expected += '"Timothy Henson"|"United States"|"true"\r\n';
equal(data, expected, "Output CSV without Headers");
});
test("Should export data: encapsulate=false", function(){
var grid = new Grid($(this.element), this.gridOptions);
this.exportConfig.encapsulate = false;
var data = grid.exportData(this.exportConfig);
var expected = "";
expected += 'Name|Country|Is Married\r\n';
expected += 'Otto Clay|United States|false\r\n';
expected += 'Connor Johnston|Canada|true\r\n';
expected += 'Lacey Hess|Canada|false\r\n';
expected += 'Timothy Henson|United States|true\r\n';
equal(data, expected, "Output CSV without encapsulation");
});
test("Should export filtered data", function(){
var grid = new Grid($(this.element), this.gridOptions);
this.exportConfig['filter'] = function(item){
if (item["Name"].indexOf("O") === 0)
return true
};
var data = grid.exportData(this.exportConfig);
var expected = "";
expected += '"Name"|"Country"|"Is Married"\r\n';
expected += '"Otto Clay"|"United States"|"false"\r\n';
//expected += '"Connor Johnston"|"Canada"|"true"\r\n';
//expected += '"Lacey Hess"|"Canada"|"false"\r\n';
//expected += '"Timothy Henson"|"United States"|"true"\r\n';
equal(data, expected, "Output CSV filtered to show names starting with O");
});
test("Should export data: transformed value", function(){
var grid = new Grid($(this.element), this.gridOptions);
this.exportConfig['transforms'] = {};
this.exportConfig.transforms['Married'] = function(value){
if (value === true) return "Yes"
if (value === false) return "No"
};
var data = grid.exportData(this.exportConfig);
var expected = "";
expected += '"Name"|"Country"|"Is Married"\r\n';
expected += '"Otto Clay"|"United States"|"No"\r\n';
expected += '"Connor Johnston"|"Canada"|"Yes"\r\n';
expected += '"Lacey Hess"|"Canada"|"No"\r\n';
expected += '"Timothy Henson"|"United States"|"Yes"\r\n';
equal(data, expected, "Output CSV column value transformed properly");
});
});
<MSG> Events: Add onItemEditing callback
Closes #147
<DFF> @@ -1176,6 +1176,8 @@ $(function() {
test("edit item", function() {
var $element = $("#jsGrid"),
+ editingArgs,
+ editingRow,
updated = false,
updatingArgs,
updatingRow,
@@ -1204,6 +1206,10 @@ $(function() {
updated = true;
}
},
+ onItemEditing: function(e) {
+ editingArgs = $.extend(true, {}, e);
+ editingRow = grid.rowByItem(data[0])[0];
+ },
onItemUpdating: function(e) {
updatingArgs = $.extend(true, {}, e);
updatingRow = grid.rowByItem(data[0])[0];
@@ -1219,6 +1225,11 @@ $(function() {
grid.option("data", data);
grid.editItem(data[0]);
+
+ deepEqual(editingArgs.item, { field: "value" }, "item before editing is provided in editing event args");
+ equal(editingArgs.itemIndex, 0, "itemIndex is provided in editing event args");
+ equal(editingArgs.row[0], editingRow, "row element is provided in editing event args");
+
grid.fields[0].editControl.val("new value");
grid.updateItem();
@@ -1786,7 +1797,36 @@ $(function() {
});
- module("canceling controller calls");
+ module("canceling events");
+
+ test("cancel item edit", function() {
+ var $element = $("#jsGrid");
+ var data = [{}];
+
+ var gridOptions = {
+ editing: true,
+
+ onItemEditing: function(e) {
+ e.cancel = true;
+ },
+
+ controller: {
+ loadData: function() {
+ return data;
+ }
+ },
+
+ fields: [
+ { name: "test" }
+ ]
+ };
+
+ var grid = new Grid($element, gridOptions);
+
+ grid.loadData();
+ grid.editItem(data[0]);
+ strictEqual(grid._editingRow, null, "no editing row");
+ });
test("cancel controller.loadData", function() {
var $element = $("#jsGrid");
| 41 | Events: Add onItemEditing callback | 1 | .js | tests | mit | tabalinas/jsgrid |
10063765 | <NME> jsgrid.field.tests.js
<BEF> $(function() {
var Grid = jsGrid.Grid;
module("common field config", {
setup: function() {
this.isFieldExcluded = function(FieldClass) {
return FieldClass === jsGrid.ControlField;
};
}
});
test("filtering=false prevents rendering filter template", function() {
var isFieldExcluded = this.isFieldExcluded;
$.each(jsGrid.fields, function(name, FieldClass) {
if(isFieldExcluded(FieldClass))
return;
equal(field.editTemplate("testValue"), "testValue");
strictEqual(field.filterValue(), "");
strictEqual(field.insertValue(), "");
strictEqual(field.editValue(), "");
strictEqual(field.sortingFunc, customSortingFunc);
});
var isFieldExcluded = this.isFieldExcluded;
$.each(jsGrid.fields, function(name, FieldClass) {
if(isFieldExcluded(FieldClass))
return;
var field = new FieldClass({ inserting: false });
equal(field.insertTemplate(), "", "empty insert template for field " + name);
});
});
test("editing=false renders itemTemplate", function() {
var isFieldExcluded = this.isFieldExcluded;
$.each(jsGrid.fields, function(name, FieldClass) {
if(isFieldExcluded(FieldClass))
return;
var item = {
field: "test"
};
var args;
var field = new FieldClass({
editing: false,
itemTemplate: function() {
args = arguments;
FieldClass.prototype.itemTemplate.apply(this, arguments);
}
});
var itemTemplate = field.itemTemplate("test", item);
var editTemplate = field.editTemplate("test", item);
var editTemplateContent = editTemplate instanceof jQuery ? editTemplate[0].outerHTML : editTemplate;
var itemTemplateContent = itemTemplate instanceof jQuery ? itemTemplate[0].outerHTML : itemTemplate;
equal(editTemplateContent, itemTemplateContent, "item template is rendered instead of edit template for " + name);
equal(args.length, 2, "passed both arguments for " + name);
equal(args[0], "test", "field value passed as a first argument for " + name);
equal(args[1], item, "item passed as a second argument for " + name);
});
});
module("jsGrid.field");
test("basic", function() {
var customSortingFunc = function() {
return 1;
},
field = new jsGrid.Field({
name: "testField",
title: "testTitle",
sorter: customSortingFunc
});
equal(field.headerTemplate(), "testTitle");
equal(field.itemTemplate("testValue"), "testValue");
equal(field.filterTemplate(), "");
equal(field.insertTemplate(), "");
equal(field.editTemplate("testValue"), "testValue");
strictEqual(field.filterValue(), "");
strictEqual(field.insertValue(), "");
strictEqual(field.editValue(), "testValue");
strictEqual(field.sortingFunc, customSortingFunc);
});
module("jsGrid.field.text");
test("basic", function() {
var field = new jsGrid.TextField({ name: "testField" });
equal(field.itemTemplate("testValue"), "testValue");
equal(field.filterTemplate()[0].tagName.toLowerCase(), "input");
equal(field.insertTemplate()[0].tagName.toLowerCase(), "input");
equal(field.editTemplate("testEditValue")[0].tagName.toLowerCase(), "input");
strictEqual(field.filterValue(), "");
strictEqual(field.insertValue(), "");
strictEqual(field.editValue(), "testEditValue");
});
test("set default field options with setDefaults", function() {
jsGrid.setDefaults("text", {
defaultOption: "test"
});
var $element = $("#jsGrid").jsGrid({
fields: [{ type: "text" }]
});
equal($element.jsGrid("option", "fields")[0].defaultOption, "test", "default field option set");
});
module("jsGrid.field.number");
test("basic", function() {
var field = new jsGrid.NumberField({ name: "testField" });
equal(field.itemTemplate(5), "5");
equal(field.filterTemplate()[0].tagName.toLowerCase(), "input");
equal(field.insertTemplate()[0].tagName.toLowerCase(), "input");
equal(field.editTemplate(6)[0].tagName.toLowerCase(), "input");
strictEqual(field.filterValue(), undefined);
strictEqual(field.insertValue(), undefined);
strictEqual(field.editValue(), 6);
});
module("jsGrid.field.textArea");
test("basic", function() {
var field = new jsGrid.TextAreaField({ name: "testField" });
equal(field.itemTemplate("testValue"), "testValue");
equal(field.filterTemplate()[0].tagName.toLowerCase(), "input");
equal(field.insertTemplate()[0].tagName.toLowerCase(), "textarea");
equal(field.editTemplate("testEditValue")[0].tagName.toLowerCase(), "textarea");
strictEqual(field.insertValue(), "");
strictEqual(field.editValue(), "testEditValue");
});
module("jsGrid.field.checkbox");
test("basic", function() {
var field = new jsGrid.CheckboxField({ name: "testField" }),
itemTemplate,
filterTemplate,
insertTemplate,
editTemplate;
itemTemplate = field.itemTemplate("testValue");
equal(itemTemplate[0].tagName.toLowerCase(), "input");
equal(itemTemplate.attr("type"), "checkbox");
equal(itemTemplate.attr("disabled"), "disabled");
filterTemplate = field.filterTemplate();
equal(filterTemplate[0].tagName.toLowerCase(), "input");
equal(filterTemplate.attr("type"), "checkbox");
equal(filterTemplate.prop("indeterminate"), true);
insertTemplate = field.insertTemplate();
equal(insertTemplate[0].tagName.toLowerCase(), "input");
equal(insertTemplate.attr("type"), "checkbox");
editTemplate = field.editTemplate(true);
equal(editTemplate[0].tagName.toLowerCase(), "input");
equal(editTemplate.attr("type"), "checkbox");
equal(editTemplate.is(":checked"), true);
strictEqual(field.filterValue(), undefined);
strictEqual(field.insertValue(), false);
strictEqual(field.editValue(), true);
});
module("jsGrid.field.select");
test("basic", function() {
var field,
filterTemplate,
insertTemplate,
editTemplate;
field = new jsGrid.SelectField({
name: "testField",
items: ["test1", "test2", "test3"],
selectedIndex: 1
});
equal(field.itemTemplate(1), "test2");
filterTemplate = field.filterTemplate();
equal(filterTemplate[0].tagName.toLowerCase(), "select");
equal(filterTemplate.children().length, 3);
insertTemplate = field.insertTemplate();
equal(insertTemplate[0].tagName.toLowerCase(), "select");
equal(insertTemplate.children().length, 3);
editTemplate = field.editTemplate(2);
equal(editTemplate[0].tagName.toLowerCase(), "select");
equal(editTemplate.find("option:selected").length, 1);
ok(editTemplate.children().eq(2).is(":selected"));
strictEqual(field.filterValue(), 1);
strictEqual(field.insertValue(), 1);
strictEqual(field.editValue(), 2);
});
test("items as array of integers", function() {
var field,
filterTemplate,
insertTemplate,
editTemplate;
field = new jsGrid.SelectField({
name: "testField",
items: [0, 10, 20],
selectedIndex: 0
});
strictEqual(field.itemTemplate(0), 0);
filterTemplate = field.filterTemplate();
equal(filterTemplate[0].tagName.toLowerCase(), "select");
equal(filterTemplate.children().length, 3);
insertTemplate = field.insertTemplate();
equal(insertTemplate[0].tagName.toLowerCase(), "select");
equal(insertTemplate.children().length, 3);
editTemplate = field.editTemplate(1);
equal(editTemplate[0].tagName.toLowerCase(), "select");
equal(editTemplate.find("option:selected").length, 1);
ok(editTemplate.children().eq(1).is(":selected"));
strictEqual(field.filterValue(), 0);
strictEqual(field.insertValue(), 0);
strictEqual(field.editValue(), 1);
});
test("string value type", function() {
var field = new jsGrid.SelectField({
name: "testField",
items: [
{ text: "test1", value: "1" },
{ text: "test2", value: "2" },
{ text: "test3", value: "3" }
],
textField: "text",
valueField: "value",
valueType: "string",
selectedIndex: 1
});
field.filterTemplate();
strictEqual(field.filterValue(), "2");
field.editTemplate("2");
strictEqual(field.editValue(), "2");
field.insertTemplate();
strictEqual(field.insertValue(), "2");
});
test("value type auto-defined", function() {
var field = new jsGrid.SelectField({
name: "testField",
items: [
{ text: "test1", value: "1" },
{ text: "test2", value: "2" },
{ text: "test3", value: "3" }
],
textField: "text",
valueField: "value",
selectedIndex: 1
});
strictEqual(field.sorter, "string", "sorter set according to value type");
field.filterTemplate();
strictEqual(field.filterValue(), "2");
field.editTemplate("2");
strictEqual(field.editValue(), "2");
field.insertTemplate();
strictEqual(field.insertValue(), "2");
});
test("value type defaulted to string", function() {
var field = new jsGrid.SelectField({
name: "testField",
items: [
{ text: "test1" },
{ text: "test2", value: "2" }
],
textField: "text",
valueField: "value"
});
strictEqual(field.sorter, "string", "sorter set to string if first item has no value field");
});
test("object items", function() {
var field = new jsGrid.SelectField({
name: "testField",
items: [
{ text: "test1", value: 1 },
{ text: "test2", value: 2 },
{ text: "test3", value: 3 }
]
});
strictEqual(field.itemTemplate(1), field.items[1]);
field.textField = "text";
strictEqual(field.itemTemplate(1), "test2");
field.textField = "";
field.valueField = "value";
strictEqual(field.itemTemplate(1), field.items[0]);
ok(field.editTemplate(2));
strictEqual(field.editValue(), 2);
field.textField = "text";
strictEqual(field.itemTemplate(1), "test1");
});
module("jsGrid.field.control");
test("basic", function() {
var field,
itemTemplate,
headerTemplate,
filterTemplate,
insertTemplate,
editTemplate;
field = new jsGrid.ControlField();
field._grid = {
filtering: true,
inserting: true,
option: $.noop
};
itemTemplate = field.itemTemplate("any_value");
equal(itemTemplate.filter("." + field.editButtonClass).length, 1);
equal(itemTemplate.filter("." + field.deleteButtonClass).length, 1);
headerTemplate = field.headerTemplate();
equal(headerTemplate.filter("." + field.insertModeButtonClass).length, 1);
var $modeSwitchButton = headerTemplate.filter("." + field.modeButtonClass);
$modeSwitchButton.trigger("click");
equal(headerTemplate.filter("." + field.searchModeButtonClass).length, 1);
filterTemplate = field.filterTemplate();
equal(filterTemplate.filter("." + field.searchButtonClass).length, 1);
equal(filterTemplate.filter("." + field.clearFilterButtonClass).length, 1);
insertTemplate = field.insertTemplate();
equal(insertTemplate.filter("." + field.insertButtonClass).length, 1);
editTemplate = field.editTemplate("any_value");
equal(editTemplate.filter("." + field.updateButtonClass).length, 1);
equal(editTemplate.filter("." + field.cancelEditButtonClass).length, 1);
strictEqual(field.filterValue(), "");
strictEqual(field.insertValue(), "");
strictEqual(field.editValue(), "");
});
test("switchMode button should consider filtering=false", function() {
var optionArgs = {};
var field = new jsGrid.ControlField();
field._grid = {
filtering: false,
inserting: true,
option: function(name, value) {
optionArgs = {
name: name,
value: value
};
}
};
var headerTemplate = field.headerTemplate();
equal(headerTemplate.filter("." + field.insertModeButtonClass).length, 1, "inserting switch button rendered");
var $modeSwitchButton = headerTemplate.filter("." + field.modeButtonClass);
$modeSwitchButton.trigger("click");
ok($modeSwitchButton.hasClass(field.modeOnButtonClass), "on class is attached");
equal(headerTemplate.filter("." + field.insertModeButtonClass).length, 1, "insert button rendered");
equal(headerTemplate.filter("." + field.searchModeButtonClass).length, 0, "search button not rendered");
deepEqual(optionArgs, { name: "inserting", value: true }, "turn on grid inserting mode");
$modeSwitchButton.trigger("click");
ok(!$modeSwitchButton.hasClass(field.modeOnButtonClass), "on class is detached");
deepEqual(optionArgs, { name: "inserting", value: false }, "turn off grid inserting mode");
});
test("switchMode button should consider inserting=false", function() {
var optionArgs = {};
var field = new jsGrid.ControlField();
field._grid = {
filtering: true,
inserting: false,
option: function(name, value) {
optionArgs = {
name: name,
value: value
};
}
};
var headerTemplate = field.headerTemplate();
equal(headerTemplate.filter("." + field.searchModeButtonClass).length, 1, "filtering switch button rendered");
var $modeSwitchButton = headerTemplate.filter("." + field.modeButtonClass);
$modeSwitchButton.trigger("click");
ok(!$modeSwitchButton.hasClass(field.modeOnButtonClass), "on class is detached");
equal(headerTemplate.filter("." + field.searchModeButtonClass).length, 1, "search button rendered");
equal(headerTemplate.filter("." + field.insertModeButtonClass).length, 0, "insert button not rendered");
deepEqual(optionArgs, { name: "filtering", value: false }, "turn off grid filtering mode");
$modeSwitchButton.trigger("click");
ok($modeSwitchButton.hasClass(field.modeOnButtonClass), "on class is attached");
deepEqual(optionArgs, { name: "filtering", value: true }, "turn on grid filtering mode");
});
test("switchMode is not rendered if inserting=false and filtering=false", function() {
var optionArgs = {};
var field = new jsGrid.ControlField();
field._grid = {
filtering: false,
inserting: false
};
var headerTemplate = field.headerTemplate();
strictEqual(headerTemplate, "", "empty header");
});
});
<MSG> Fields: Field 'editValue' returns value to be edited by default
<DFF> @@ -20,7 +20,7 @@ $(function() {
equal(field.editTemplate("testValue"), "testValue");
strictEqual(field.filterValue(), "");
strictEqual(field.insertValue(), "");
- strictEqual(field.editValue(), "");
+ strictEqual(field.editValue(), "testValue");
strictEqual(field.sortingFunc, customSortingFunc);
});
| 1 | Fields: Field 'editValue' returns value to be edited by default | 1 | .js | field | mit | tabalinas/jsgrid |
10063766 | <NME> jsgrid.field.tests.js
<BEF> $(function() {
var Grid = jsGrid.Grid;
module("common field config", {
setup: function() {
this.isFieldExcluded = function(FieldClass) {
return FieldClass === jsGrid.ControlField;
};
}
});
test("filtering=false prevents rendering filter template", function() {
var isFieldExcluded = this.isFieldExcluded;
$.each(jsGrid.fields, function(name, FieldClass) {
if(isFieldExcluded(FieldClass))
return;
equal(field.editTemplate("testValue"), "testValue");
strictEqual(field.filterValue(), "");
strictEqual(field.insertValue(), "");
strictEqual(field.editValue(), "");
strictEqual(field.sortingFunc, customSortingFunc);
});
var isFieldExcluded = this.isFieldExcluded;
$.each(jsGrid.fields, function(name, FieldClass) {
if(isFieldExcluded(FieldClass))
return;
var field = new FieldClass({ inserting: false });
equal(field.insertTemplate(), "", "empty insert template for field " + name);
});
});
test("editing=false renders itemTemplate", function() {
var isFieldExcluded = this.isFieldExcluded;
$.each(jsGrid.fields, function(name, FieldClass) {
if(isFieldExcluded(FieldClass))
return;
var item = {
field: "test"
};
var args;
var field = new FieldClass({
editing: false,
itemTemplate: function() {
args = arguments;
FieldClass.prototype.itemTemplate.apply(this, arguments);
}
});
var itemTemplate = field.itemTemplate("test", item);
var editTemplate = field.editTemplate("test", item);
var editTemplateContent = editTemplate instanceof jQuery ? editTemplate[0].outerHTML : editTemplate;
var itemTemplateContent = itemTemplate instanceof jQuery ? itemTemplate[0].outerHTML : itemTemplate;
equal(editTemplateContent, itemTemplateContent, "item template is rendered instead of edit template for " + name);
equal(args.length, 2, "passed both arguments for " + name);
equal(args[0], "test", "field value passed as a first argument for " + name);
equal(args[1], item, "item passed as a second argument for " + name);
});
});
module("jsGrid.field");
test("basic", function() {
var customSortingFunc = function() {
return 1;
},
field = new jsGrid.Field({
name: "testField",
title: "testTitle",
sorter: customSortingFunc
});
equal(field.headerTemplate(), "testTitle");
equal(field.itemTemplate("testValue"), "testValue");
equal(field.filterTemplate(), "");
equal(field.insertTemplate(), "");
equal(field.editTemplate("testValue"), "testValue");
strictEqual(field.filterValue(), "");
strictEqual(field.insertValue(), "");
strictEqual(field.editValue(), "testValue");
strictEqual(field.sortingFunc, customSortingFunc);
});
module("jsGrid.field.text");
test("basic", function() {
var field = new jsGrid.TextField({ name: "testField" });
equal(field.itemTemplate("testValue"), "testValue");
equal(field.filterTemplate()[0].tagName.toLowerCase(), "input");
equal(field.insertTemplate()[0].tagName.toLowerCase(), "input");
equal(field.editTemplate("testEditValue")[0].tagName.toLowerCase(), "input");
strictEqual(field.filterValue(), "");
strictEqual(field.insertValue(), "");
strictEqual(field.editValue(), "testEditValue");
});
test("set default field options with setDefaults", function() {
jsGrid.setDefaults("text", {
defaultOption: "test"
});
var $element = $("#jsGrid").jsGrid({
fields: [{ type: "text" }]
});
equal($element.jsGrid("option", "fields")[0].defaultOption, "test", "default field option set");
});
module("jsGrid.field.number");
test("basic", function() {
var field = new jsGrid.NumberField({ name: "testField" });
equal(field.itemTemplate(5), "5");
equal(field.filterTemplate()[0].tagName.toLowerCase(), "input");
equal(field.insertTemplate()[0].tagName.toLowerCase(), "input");
equal(field.editTemplate(6)[0].tagName.toLowerCase(), "input");
strictEqual(field.filterValue(), undefined);
strictEqual(field.insertValue(), undefined);
strictEqual(field.editValue(), 6);
});
module("jsGrid.field.textArea");
test("basic", function() {
var field = new jsGrid.TextAreaField({ name: "testField" });
equal(field.itemTemplate("testValue"), "testValue");
equal(field.filterTemplate()[0].tagName.toLowerCase(), "input");
equal(field.insertTemplate()[0].tagName.toLowerCase(), "textarea");
equal(field.editTemplate("testEditValue")[0].tagName.toLowerCase(), "textarea");
strictEqual(field.insertValue(), "");
strictEqual(field.editValue(), "testEditValue");
});
module("jsGrid.field.checkbox");
test("basic", function() {
var field = new jsGrid.CheckboxField({ name: "testField" }),
itemTemplate,
filterTemplate,
insertTemplate,
editTemplate;
itemTemplate = field.itemTemplate("testValue");
equal(itemTemplate[0].tagName.toLowerCase(), "input");
equal(itemTemplate.attr("type"), "checkbox");
equal(itemTemplate.attr("disabled"), "disabled");
filterTemplate = field.filterTemplate();
equal(filterTemplate[0].tagName.toLowerCase(), "input");
equal(filterTemplate.attr("type"), "checkbox");
equal(filterTemplate.prop("indeterminate"), true);
insertTemplate = field.insertTemplate();
equal(insertTemplate[0].tagName.toLowerCase(), "input");
equal(insertTemplate.attr("type"), "checkbox");
editTemplate = field.editTemplate(true);
equal(editTemplate[0].tagName.toLowerCase(), "input");
equal(editTemplate.attr("type"), "checkbox");
equal(editTemplate.is(":checked"), true);
strictEqual(field.filterValue(), undefined);
strictEqual(field.insertValue(), false);
strictEqual(field.editValue(), true);
});
module("jsGrid.field.select");
test("basic", function() {
var field,
filterTemplate,
insertTemplate,
editTemplate;
field = new jsGrid.SelectField({
name: "testField",
items: ["test1", "test2", "test3"],
selectedIndex: 1
});
equal(field.itemTemplate(1), "test2");
filterTemplate = field.filterTemplate();
equal(filterTemplate[0].tagName.toLowerCase(), "select");
equal(filterTemplate.children().length, 3);
insertTemplate = field.insertTemplate();
equal(insertTemplate[0].tagName.toLowerCase(), "select");
equal(insertTemplate.children().length, 3);
editTemplate = field.editTemplate(2);
equal(editTemplate[0].tagName.toLowerCase(), "select");
equal(editTemplate.find("option:selected").length, 1);
ok(editTemplate.children().eq(2).is(":selected"));
strictEqual(field.filterValue(), 1);
strictEqual(field.insertValue(), 1);
strictEqual(field.editValue(), 2);
});
test("items as array of integers", function() {
var field,
filterTemplate,
insertTemplate,
editTemplate;
field = new jsGrid.SelectField({
name: "testField",
items: [0, 10, 20],
selectedIndex: 0
});
strictEqual(field.itemTemplate(0), 0);
filterTemplate = field.filterTemplate();
equal(filterTemplate[0].tagName.toLowerCase(), "select");
equal(filterTemplate.children().length, 3);
insertTemplate = field.insertTemplate();
equal(insertTemplate[0].tagName.toLowerCase(), "select");
equal(insertTemplate.children().length, 3);
editTemplate = field.editTemplate(1);
equal(editTemplate[0].tagName.toLowerCase(), "select");
equal(editTemplate.find("option:selected").length, 1);
ok(editTemplate.children().eq(1).is(":selected"));
strictEqual(field.filterValue(), 0);
strictEqual(field.insertValue(), 0);
strictEqual(field.editValue(), 1);
});
test("string value type", function() {
var field = new jsGrid.SelectField({
name: "testField",
items: [
{ text: "test1", value: "1" },
{ text: "test2", value: "2" },
{ text: "test3", value: "3" }
],
textField: "text",
valueField: "value",
valueType: "string",
selectedIndex: 1
});
field.filterTemplate();
strictEqual(field.filterValue(), "2");
field.editTemplate("2");
strictEqual(field.editValue(), "2");
field.insertTemplate();
strictEqual(field.insertValue(), "2");
});
test("value type auto-defined", function() {
var field = new jsGrid.SelectField({
name: "testField",
items: [
{ text: "test1", value: "1" },
{ text: "test2", value: "2" },
{ text: "test3", value: "3" }
],
textField: "text",
valueField: "value",
selectedIndex: 1
});
strictEqual(field.sorter, "string", "sorter set according to value type");
field.filterTemplate();
strictEqual(field.filterValue(), "2");
field.editTemplate("2");
strictEqual(field.editValue(), "2");
field.insertTemplate();
strictEqual(field.insertValue(), "2");
});
test("value type defaulted to string", function() {
var field = new jsGrid.SelectField({
name: "testField",
items: [
{ text: "test1" },
{ text: "test2", value: "2" }
],
textField: "text",
valueField: "value"
});
strictEqual(field.sorter, "string", "sorter set to string if first item has no value field");
});
test("object items", function() {
var field = new jsGrid.SelectField({
name: "testField",
items: [
{ text: "test1", value: 1 },
{ text: "test2", value: 2 },
{ text: "test3", value: 3 }
]
});
strictEqual(field.itemTemplate(1), field.items[1]);
field.textField = "text";
strictEqual(field.itemTemplate(1), "test2");
field.textField = "";
field.valueField = "value";
strictEqual(field.itemTemplate(1), field.items[0]);
ok(field.editTemplate(2));
strictEqual(field.editValue(), 2);
field.textField = "text";
strictEqual(field.itemTemplate(1), "test1");
});
module("jsGrid.field.control");
test("basic", function() {
var field,
itemTemplate,
headerTemplate,
filterTemplate,
insertTemplate,
editTemplate;
field = new jsGrid.ControlField();
field._grid = {
filtering: true,
inserting: true,
option: $.noop
};
itemTemplate = field.itemTemplate("any_value");
equal(itemTemplate.filter("." + field.editButtonClass).length, 1);
equal(itemTemplate.filter("." + field.deleteButtonClass).length, 1);
headerTemplate = field.headerTemplate();
equal(headerTemplate.filter("." + field.insertModeButtonClass).length, 1);
var $modeSwitchButton = headerTemplate.filter("." + field.modeButtonClass);
$modeSwitchButton.trigger("click");
equal(headerTemplate.filter("." + field.searchModeButtonClass).length, 1);
filterTemplate = field.filterTemplate();
equal(filterTemplate.filter("." + field.searchButtonClass).length, 1);
equal(filterTemplate.filter("." + field.clearFilterButtonClass).length, 1);
insertTemplate = field.insertTemplate();
equal(insertTemplate.filter("." + field.insertButtonClass).length, 1);
editTemplate = field.editTemplate("any_value");
equal(editTemplate.filter("." + field.updateButtonClass).length, 1);
equal(editTemplate.filter("." + field.cancelEditButtonClass).length, 1);
strictEqual(field.filterValue(), "");
strictEqual(field.insertValue(), "");
strictEqual(field.editValue(), "");
});
test("switchMode button should consider filtering=false", function() {
var optionArgs = {};
var field = new jsGrid.ControlField();
field._grid = {
filtering: false,
inserting: true,
option: function(name, value) {
optionArgs = {
name: name,
value: value
};
}
};
var headerTemplate = field.headerTemplate();
equal(headerTemplate.filter("." + field.insertModeButtonClass).length, 1, "inserting switch button rendered");
var $modeSwitchButton = headerTemplate.filter("." + field.modeButtonClass);
$modeSwitchButton.trigger("click");
ok($modeSwitchButton.hasClass(field.modeOnButtonClass), "on class is attached");
equal(headerTemplate.filter("." + field.insertModeButtonClass).length, 1, "insert button rendered");
equal(headerTemplate.filter("." + field.searchModeButtonClass).length, 0, "search button not rendered");
deepEqual(optionArgs, { name: "inserting", value: true }, "turn on grid inserting mode");
$modeSwitchButton.trigger("click");
ok(!$modeSwitchButton.hasClass(field.modeOnButtonClass), "on class is detached");
deepEqual(optionArgs, { name: "inserting", value: false }, "turn off grid inserting mode");
});
test("switchMode button should consider inserting=false", function() {
var optionArgs = {};
var field = new jsGrid.ControlField();
field._grid = {
filtering: true,
inserting: false,
option: function(name, value) {
optionArgs = {
name: name,
value: value
};
}
};
var headerTemplate = field.headerTemplate();
equal(headerTemplate.filter("." + field.searchModeButtonClass).length, 1, "filtering switch button rendered");
var $modeSwitchButton = headerTemplate.filter("." + field.modeButtonClass);
$modeSwitchButton.trigger("click");
ok(!$modeSwitchButton.hasClass(field.modeOnButtonClass), "on class is detached");
equal(headerTemplate.filter("." + field.searchModeButtonClass).length, 1, "search button rendered");
equal(headerTemplate.filter("." + field.insertModeButtonClass).length, 0, "insert button not rendered");
deepEqual(optionArgs, { name: "filtering", value: false }, "turn off grid filtering mode");
$modeSwitchButton.trigger("click");
ok($modeSwitchButton.hasClass(field.modeOnButtonClass), "on class is attached");
deepEqual(optionArgs, { name: "filtering", value: true }, "turn on grid filtering mode");
});
test("switchMode is not rendered if inserting=false and filtering=false", function() {
var optionArgs = {};
var field = new jsGrid.ControlField();
field._grid = {
filtering: false,
inserting: false
};
var headerTemplate = field.headerTemplate();
strictEqual(headerTemplate, "", "empty header");
});
});
<MSG> Fields: Field 'editValue' returns value to be edited by default
<DFF> @@ -20,7 +20,7 @@ $(function() {
equal(field.editTemplate("testValue"), "testValue");
strictEqual(field.filterValue(), "");
strictEqual(field.insertValue(), "");
- strictEqual(field.editValue(), "");
+ strictEqual(field.editValue(), "testValue");
strictEqual(field.sortingFunc, customSortingFunc);
});
| 1 | Fields: Field 'editValue' returns value to be edited by default | 1 | .js | field | mit | tabalinas/jsgrid |
10063767 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
* Line numeration
* Rectangular selection
* Intra-column adornments
* Word wrapping
* Scrolling below document
* Hyperlinks
and many,many more!
AvaloniaEdit currently consists of 2 packages
* [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
* [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
### How to set up TextMate theme and syntax highlighting for my project?
First of all, if you want to use grammars supported by [TextMateSharp](https://github.com/danipen/TextMateSharp), should install the following packages:
- [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/)
- [TextMateSharp.Grammars](https://www.nuget.org/packages/TextMateSharp.Grammars/)
Alternatively, if you want to support your own grammars, you just need to install the AvaloniaEdit.TextMate package, and implement IRegistryOptions interface, that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled TextMateSharp.Grammars.
```csharp
//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
var _textEditor = this.FindControl<TextEditor>("Editor");
//Here we initialize RegistryOptions with the theme we want to use.
var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
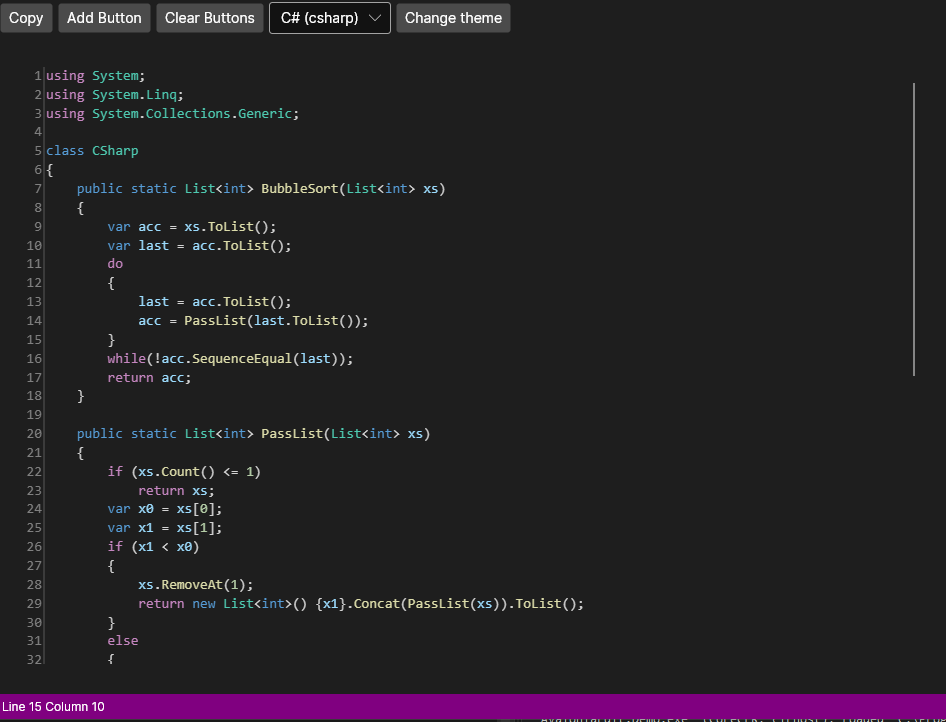
<MSG> Merge branch 'master' into feature/TextFormatterPort
<DFF> @@ -2,17 +2,22 @@
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
-This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
+This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
- * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
- * Line numeration
- * Rectangular selection
- * Intra-column adornments
- * Word wrapping
- * Scrolling below document
- * Hyperlinks
+ * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
+ * Code folding.
+ * Code completion.
+ * Fully customizable and extensible.
+ * Line numeration.
+ * Display whitespaces EOLs and tabs.
+ * Line virtualization.
+ * Multi-caret edition.
+ * Intra-column adornments.
+ * Word wrapping.
+ * Scrolling below document.
+ * Hyperlinks.
and many,many more!
@@ -41,6 +46,6 @@ var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
-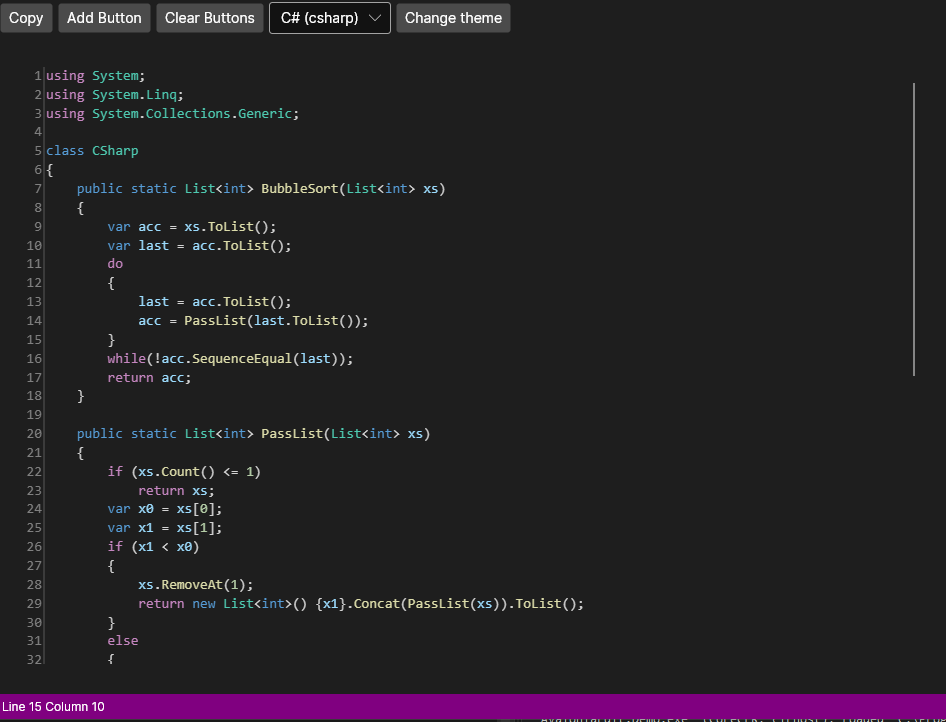
+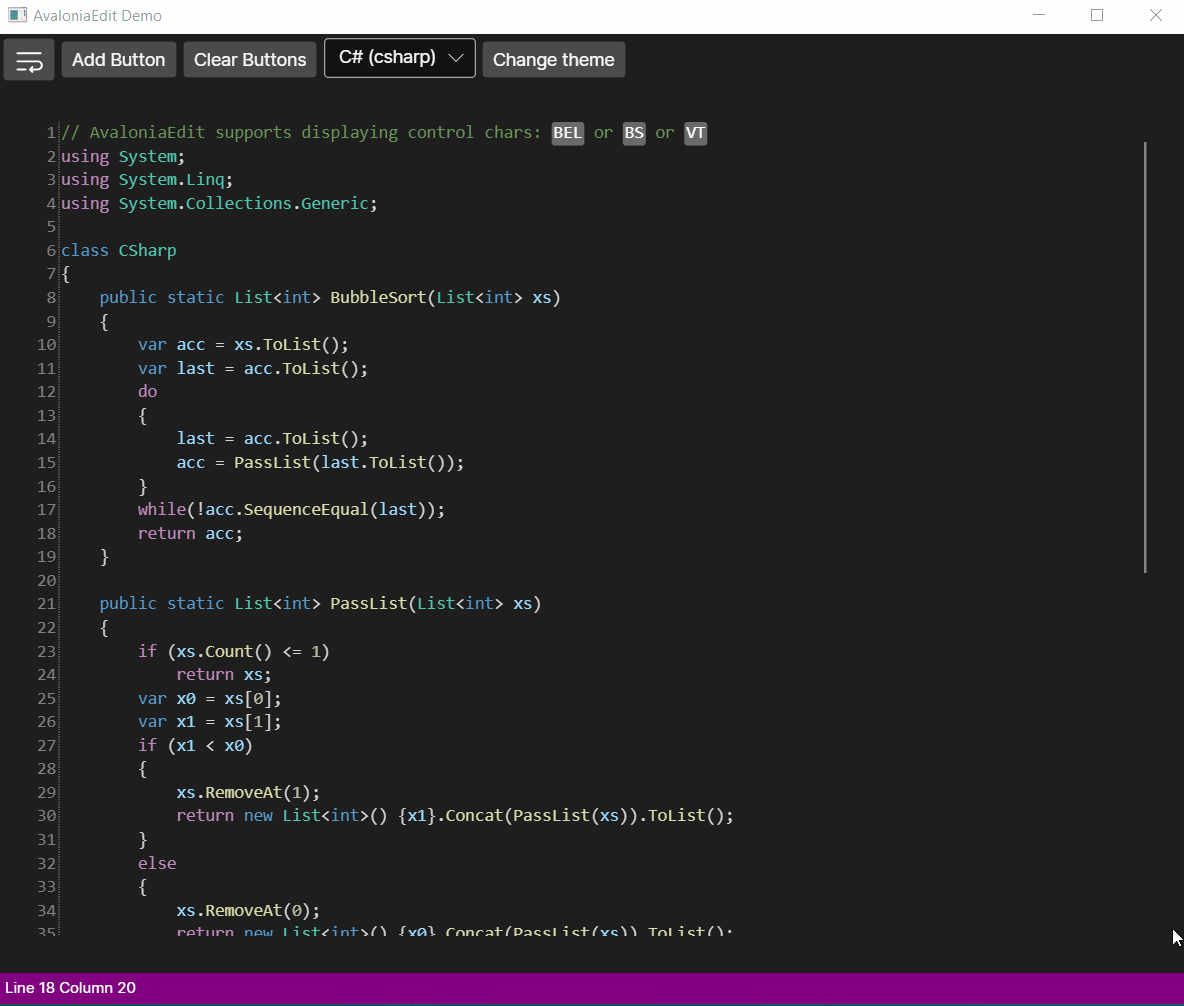
| 14 | Merge branch 'master' into feature/TextFormatterPort | 9 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10063768 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
* Line numeration
* Rectangular selection
* Intra-column adornments
* Word wrapping
* Scrolling below document
* Hyperlinks
and many,many more!
AvaloniaEdit currently consists of 2 packages
* [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
* [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
### How to set up TextMate theme and syntax highlighting for my project?
First of all, if you want to use grammars supported by [TextMateSharp](https://github.com/danipen/TextMateSharp), should install the following packages:
- [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/)
- [TextMateSharp.Grammars](https://www.nuget.org/packages/TextMateSharp.Grammars/)
Alternatively, if you want to support your own grammars, you just need to install the AvaloniaEdit.TextMate package, and implement IRegistryOptions interface, that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled TextMateSharp.Grammars.
```csharp
//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
var _textEditor = this.FindControl<TextEditor>("Editor");
//Here we initialize RegistryOptions with the theme we want to use.
var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
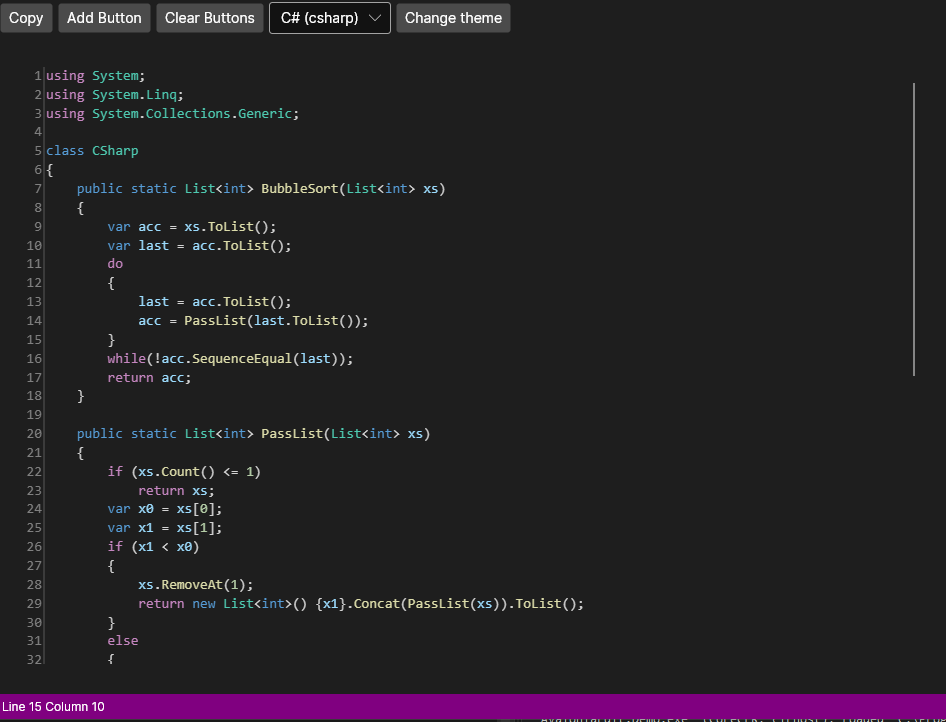
<MSG> Merge branch 'master' into feature/TextFormatterPort
<DFF> @@ -2,17 +2,22 @@
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
-This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
+This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
- * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
- * Line numeration
- * Rectangular selection
- * Intra-column adornments
- * Word wrapping
- * Scrolling below document
- * Hyperlinks
+ * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
+ * Code folding.
+ * Code completion.
+ * Fully customizable and extensible.
+ * Line numeration.
+ * Display whitespaces EOLs and tabs.
+ * Line virtualization.
+ * Multi-caret edition.
+ * Intra-column adornments.
+ * Word wrapping.
+ * Scrolling below document.
+ * Hyperlinks.
and many,many more!
@@ -41,6 +46,6 @@ var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
-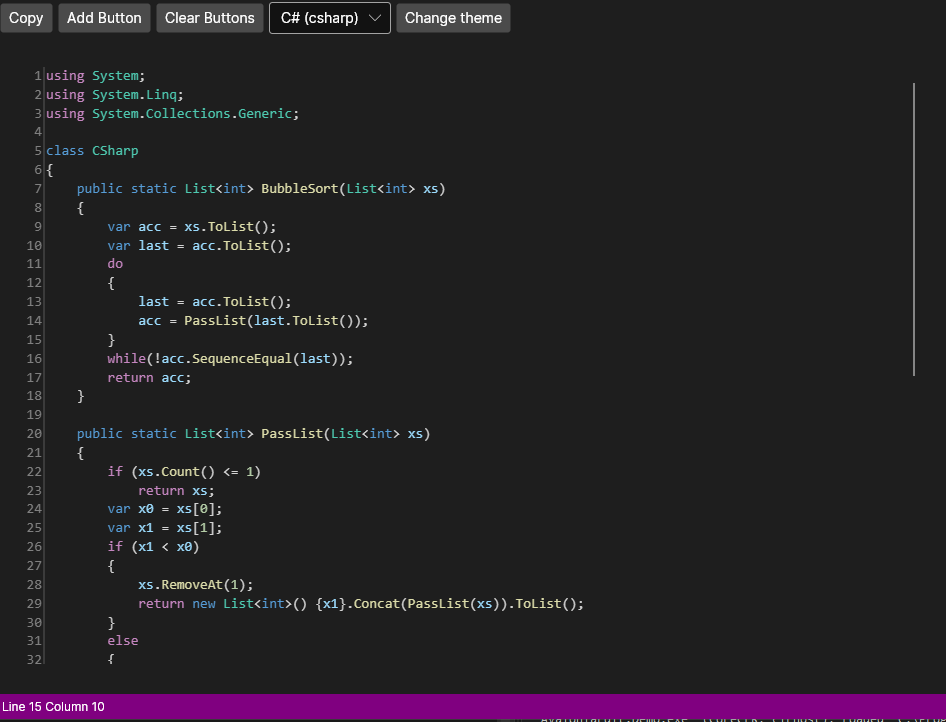
+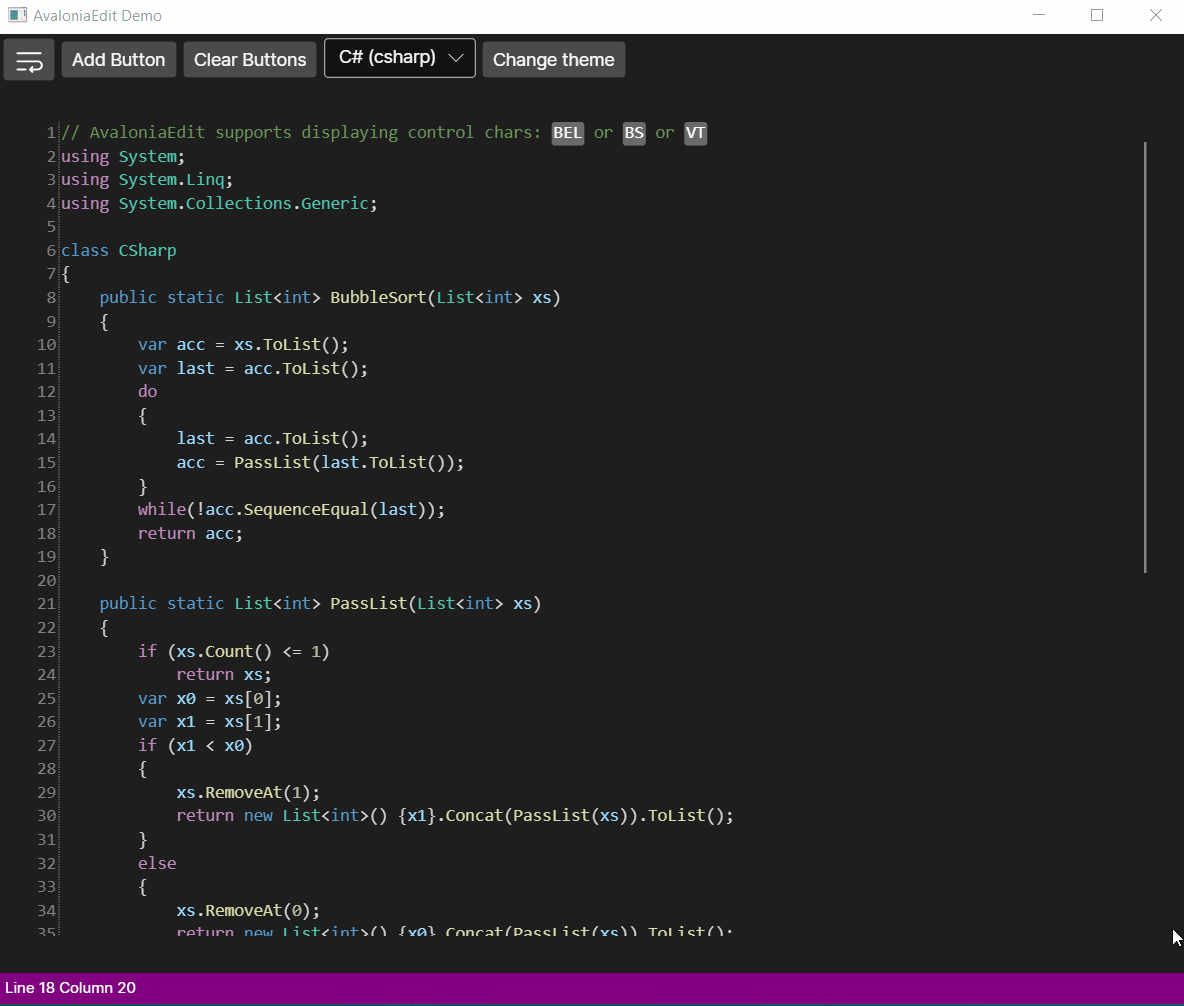
| 14 | Merge branch 'master' into feature/TextFormatterPort | 9 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10063769 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
* Line numeration
* Rectangular selection
* Intra-column adornments
* Word wrapping
* Scrolling below document
* Hyperlinks
and many,many more!
AvaloniaEdit currently consists of 2 packages
* [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
* [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
### How to set up TextMate theme and syntax highlighting for my project?
First of all, if you want to use grammars supported by [TextMateSharp](https://github.com/danipen/TextMateSharp), should install the following packages:
- [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/)
- [TextMateSharp.Grammars](https://www.nuget.org/packages/TextMateSharp.Grammars/)
Alternatively, if you want to support your own grammars, you just need to install the AvaloniaEdit.TextMate package, and implement IRegistryOptions interface, that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled TextMateSharp.Grammars.
```csharp
//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
var _textEditor = this.FindControl<TextEditor>("Editor");
//Here we initialize RegistryOptions with the theme we want to use.
var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
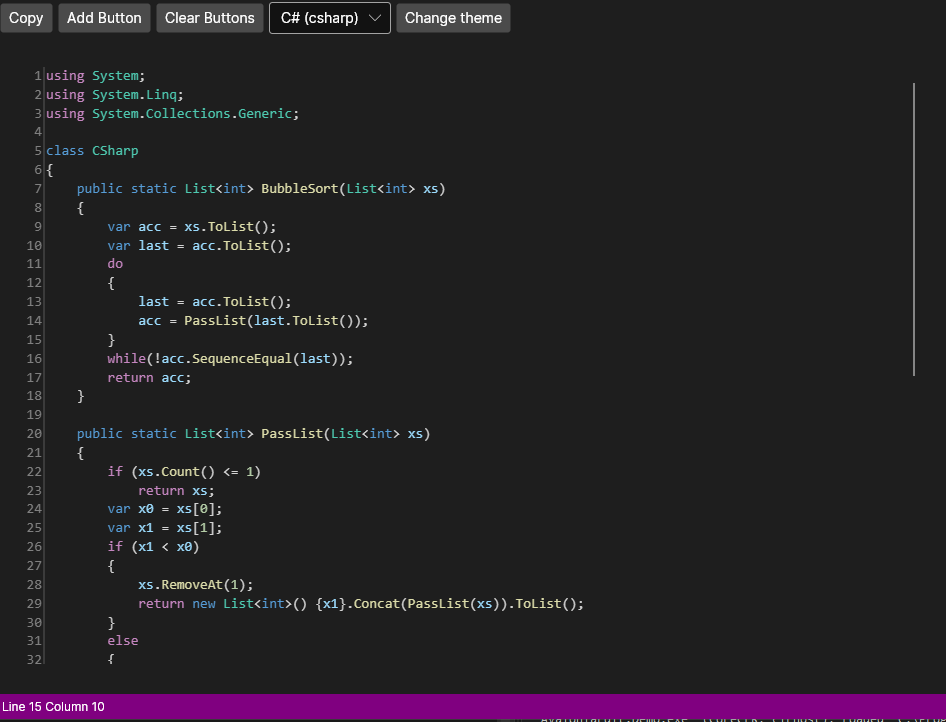
<MSG> Merge branch 'master' into feature/TextFormatterPort
<DFF> @@ -2,17 +2,22 @@
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
-This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
+This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
- * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
- * Line numeration
- * Rectangular selection
- * Intra-column adornments
- * Word wrapping
- * Scrolling below document
- * Hyperlinks
+ * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
+ * Code folding.
+ * Code completion.
+ * Fully customizable and extensible.
+ * Line numeration.
+ * Display whitespaces EOLs and tabs.
+ * Line virtualization.
+ * Multi-caret edition.
+ * Intra-column adornments.
+ * Word wrapping.
+ * Scrolling below document.
+ * Hyperlinks.
and many,many more!
@@ -41,6 +46,6 @@ var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
-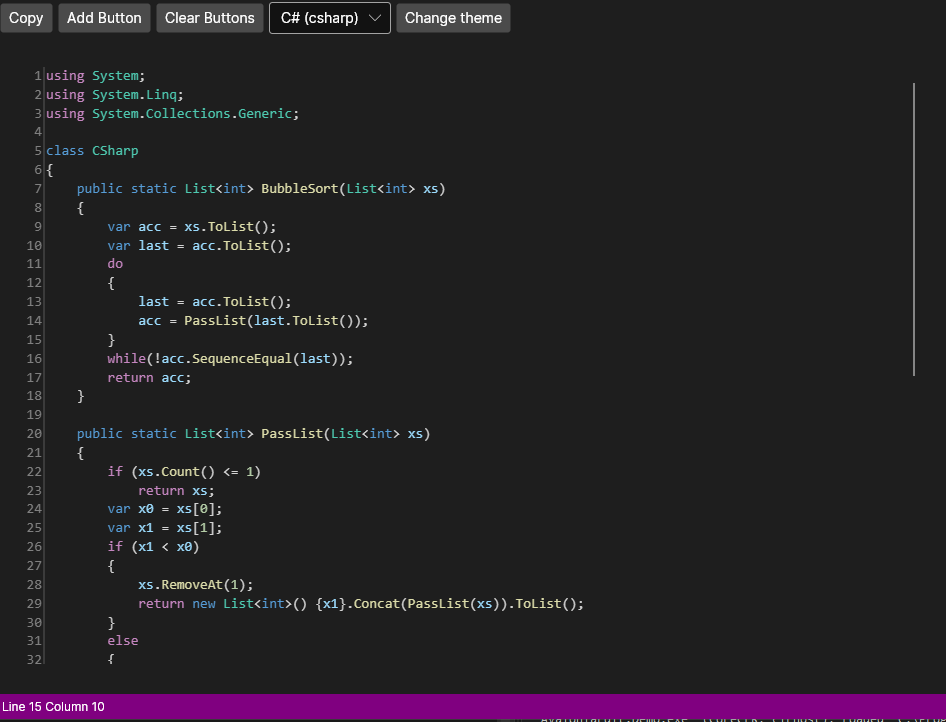
+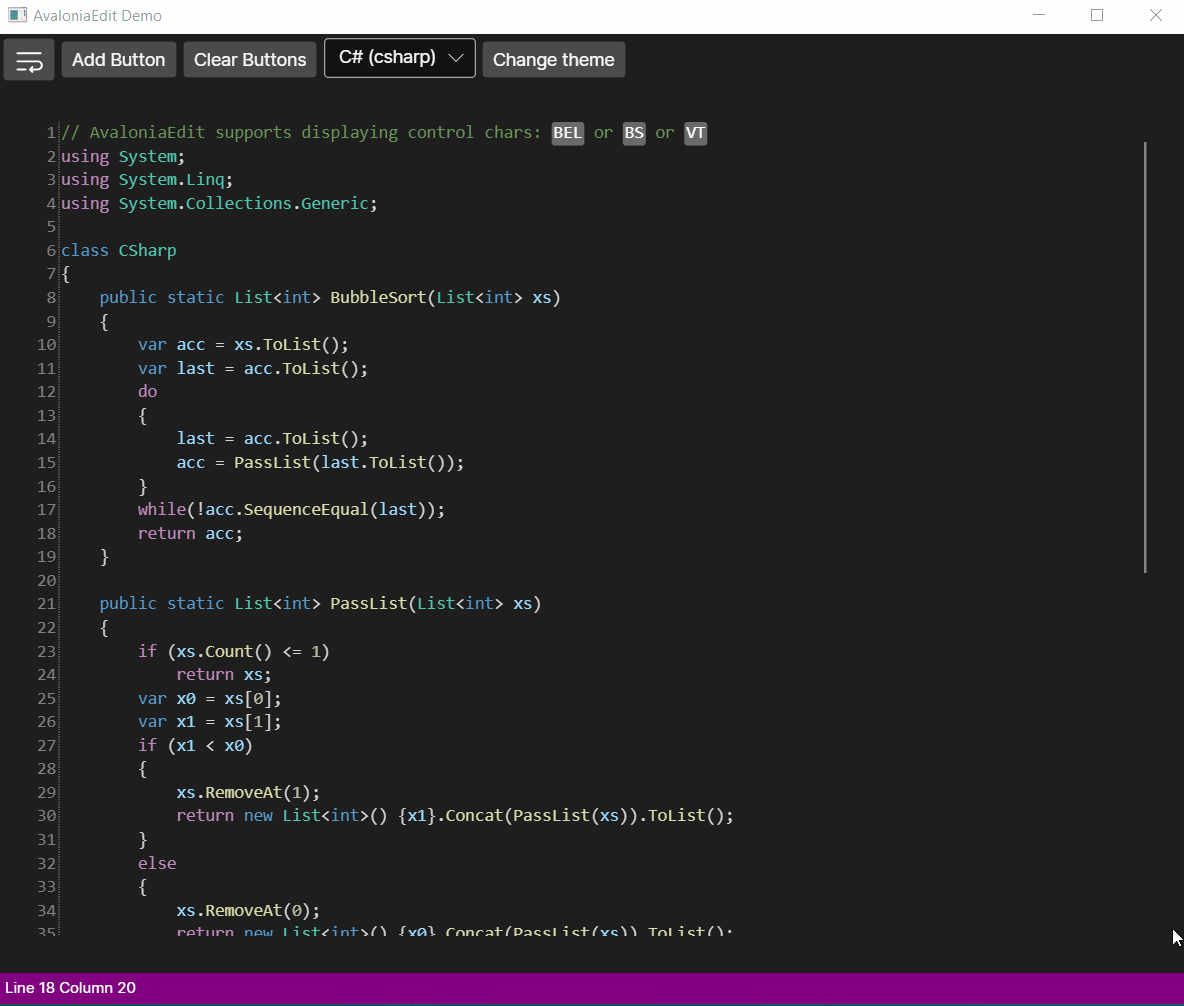
| 14 | Merge branch 'master' into feature/TextFormatterPort | 9 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10063770 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
* Line numeration
* Rectangular selection
* Intra-column adornments
* Word wrapping
* Scrolling below document
* Hyperlinks
and many,many more!
AvaloniaEdit currently consists of 2 packages
* [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
* [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
### How to set up TextMate theme and syntax highlighting for my project?
First of all, if you want to use grammars supported by [TextMateSharp](https://github.com/danipen/TextMateSharp), should install the following packages:
- [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/)
- [TextMateSharp.Grammars](https://www.nuget.org/packages/TextMateSharp.Grammars/)
Alternatively, if you want to support your own grammars, you just need to install the AvaloniaEdit.TextMate package, and implement IRegistryOptions interface, that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled TextMateSharp.Grammars.
```csharp
//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
var _textEditor = this.FindControl<TextEditor>("Editor");
//Here we initialize RegistryOptions with the theme we want to use.
var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
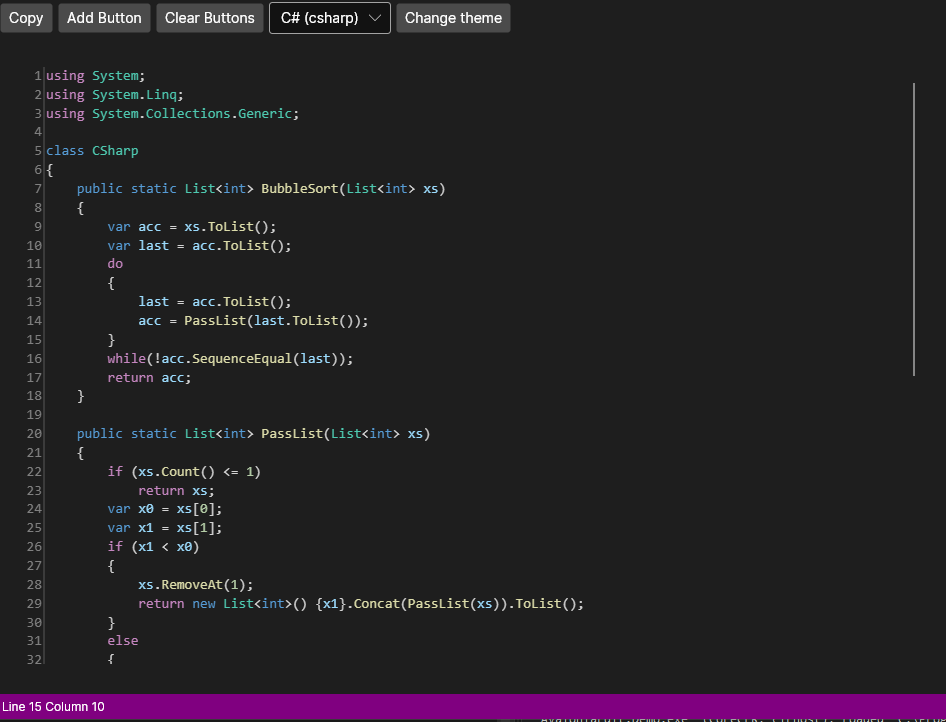
<MSG> Merge branch 'master' into feature/TextFormatterPort
<DFF> @@ -2,17 +2,22 @@
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
-This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
+This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
- * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
- * Line numeration
- * Rectangular selection
- * Intra-column adornments
- * Word wrapping
- * Scrolling below document
- * Hyperlinks
+ * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
+ * Code folding.
+ * Code completion.
+ * Fully customizable and extensible.
+ * Line numeration.
+ * Display whitespaces EOLs and tabs.
+ * Line virtualization.
+ * Multi-caret edition.
+ * Intra-column adornments.
+ * Word wrapping.
+ * Scrolling below document.
+ * Hyperlinks.
and many,many more!
@@ -41,6 +46,6 @@ var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
-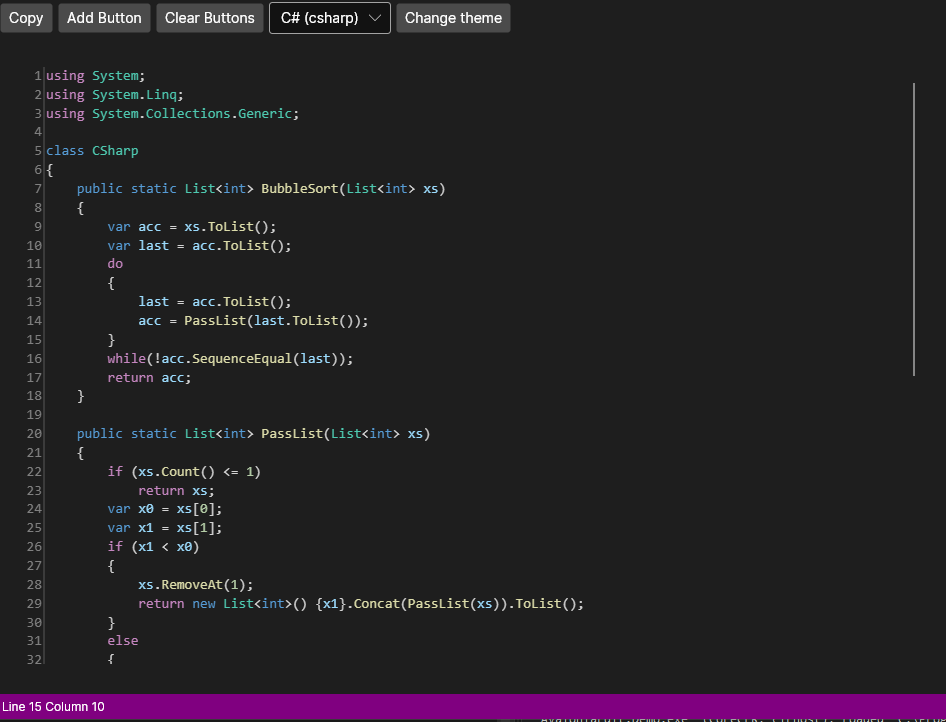
+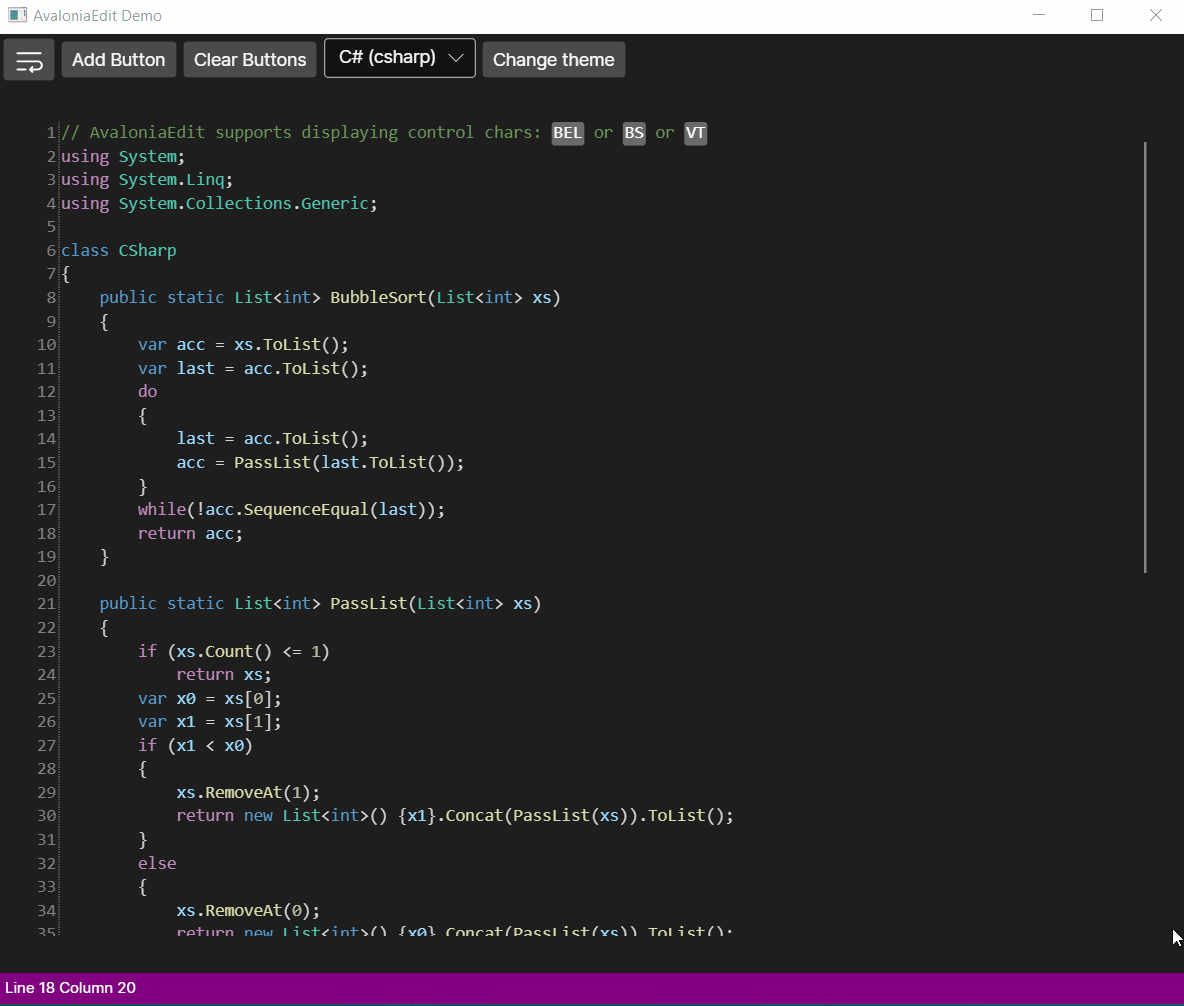
| 14 | Merge branch 'master' into feature/TextFormatterPort | 9 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10063771 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
* Line numeration
* Rectangular selection
* Intra-column adornments
* Word wrapping
* Scrolling below document
* Hyperlinks
and many,many more!
AvaloniaEdit currently consists of 2 packages
* [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
* [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
### How to set up TextMate theme and syntax highlighting for my project?
First of all, if you want to use grammars supported by [TextMateSharp](https://github.com/danipen/TextMateSharp), should install the following packages:
- [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/)
- [TextMateSharp.Grammars](https://www.nuget.org/packages/TextMateSharp.Grammars/)
Alternatively, if you want to support your own grammars, you just need to install the AvaloniaEdit.TextMate package, and implement IRegistryOptions interface, that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled TextMateSharp.Grammars.
```csharp
//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
var _textEditor = this.FindControl<TextEditor>("Editor");
//Here we initialize RegistryOptions with the theme we want to use.
var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
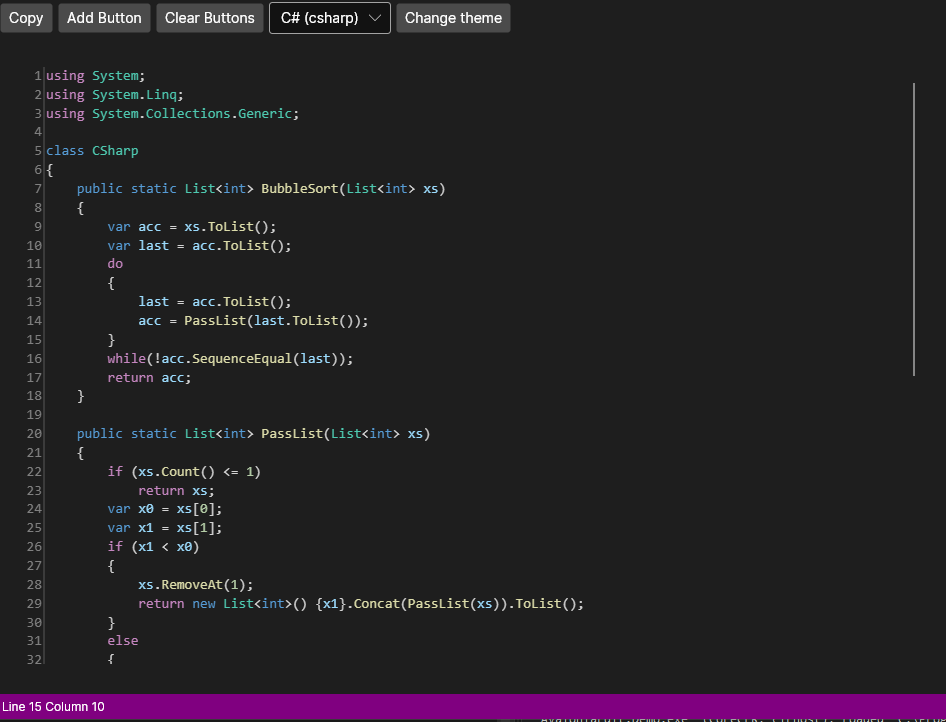
<MSG> Merge branch 'master' into feature/TextFormatterPort
<DFF> @@ -2,17 +2,22 @@
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
-This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
+This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
- * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
- * Line numeration
- * Rectangular selection
- * Intra-column adornments
- * Word wrapping
- * Scrolling below document
- * Hyperlinks
+ * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
+ * Code folding.
+ * Code completion.
+ * Fully customizable and extensible.
+ * Line numeration.
+ * Display whitespaces EOLs and tabs.
+ * Line virtualization.
+ * Multi-caret edition.
+ * Intra-column adornments.
+ * Word wrapping.
+ * Scrolling below document.
+ * Hyperlinks.
and many,many more!
@@ -41,6 +46,6 @@ var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
-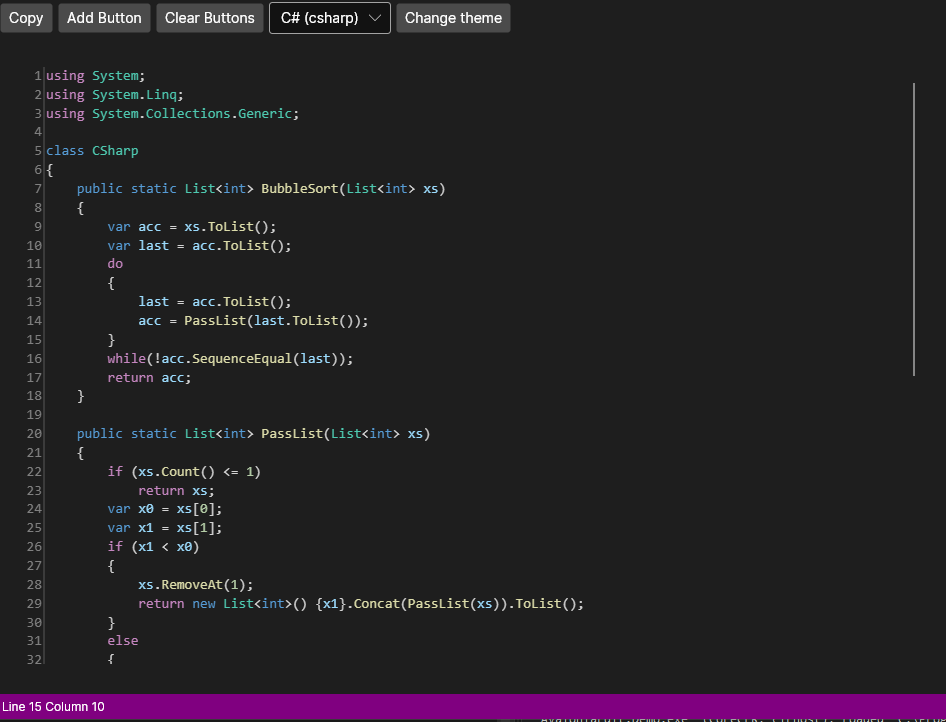
+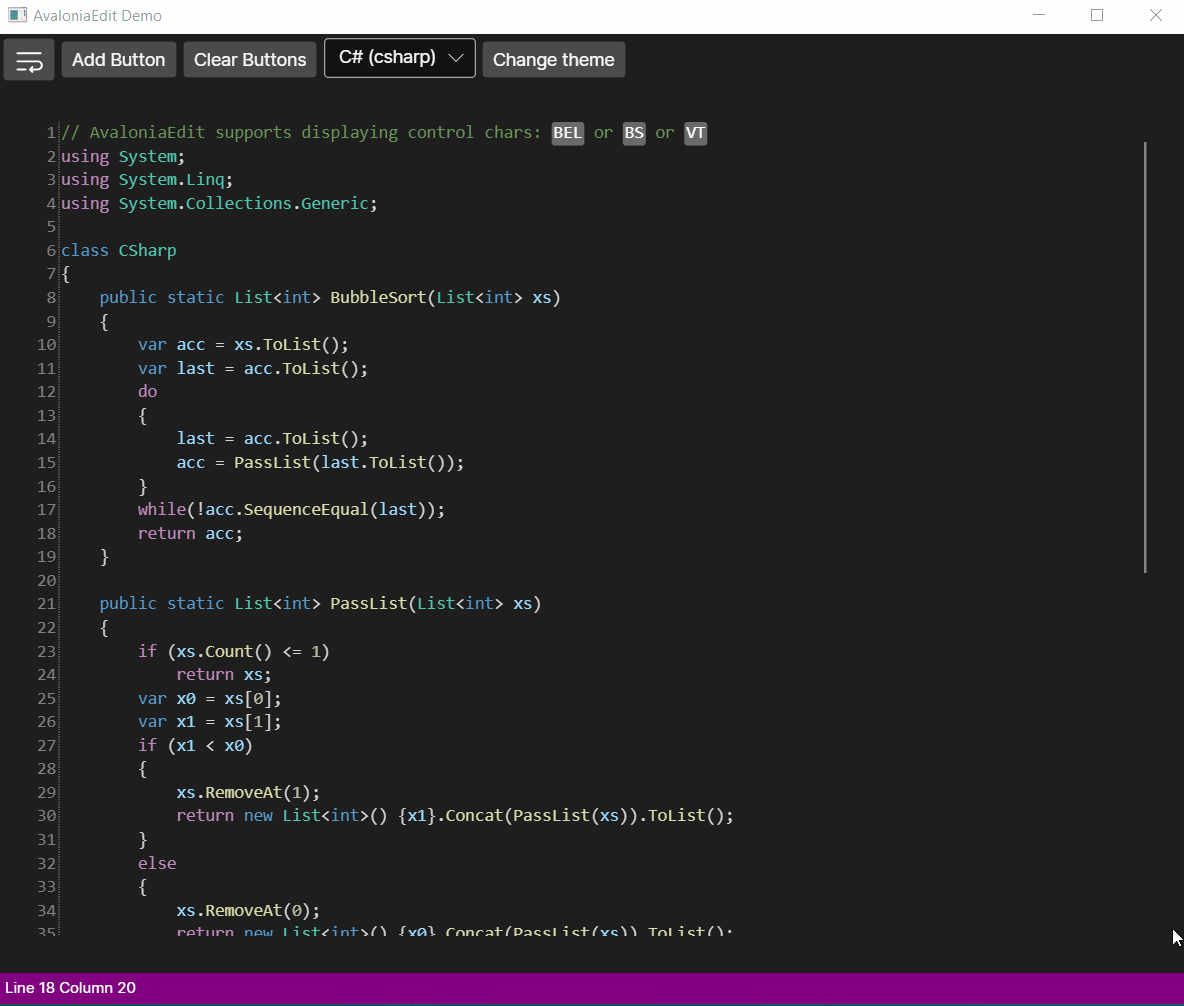
| 14 | Merge branch 'master' into feature/TextFormatterPort | 9 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10063772 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
* Line numeration
* Rectangular selection
* Intra-column adornments
* Word wrapping
* Scrolling below document
* Hyperlinks
and many,many more!
AvaloniaEdit currently consists of 2 packages
* [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
* [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
### How to set up TextMate theme and syntax highlighting for my project?
First of all, if you want to use grammars supported by [TextMateSharp](https://github.com/danipen/TextMateSharp), should install the following packages:
- [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/)
- [TextMateSharp.Grammars](https://www.nuget.org/packages/TextMateSharp.Grammars/)
Alternatively, if you want to support your own grammars, you just need to install the AvaloniaEdit.TextMate package, and implement IRegistryOptions interface, that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled TextMateSharp.Grammars.
```csharp
//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
var _textEditor = this.FindControl<TextEditor>("Editor");
//Here we initialize RegistryOptions with the theme we want to use.
var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
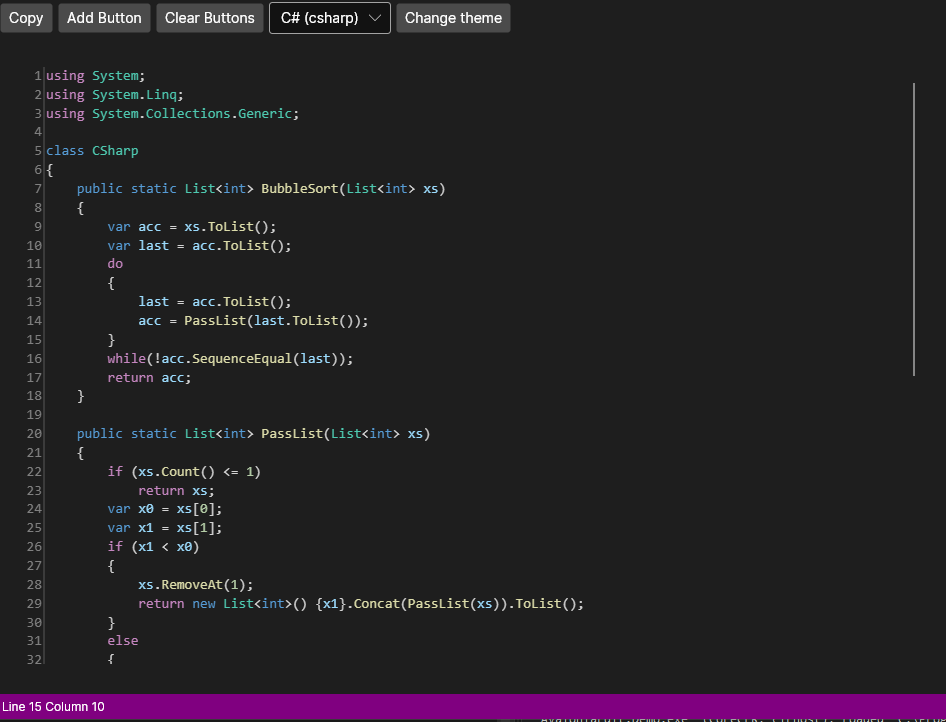
<MSG> Merge branch 'master' into feature/TextFormatterPort
<DFF> @@ -2,17 +2,22 @@
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
-This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
+This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
- * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
- * Line numeration
- * Rectangular selection
- * Intra-column adornments
- * Word wrapping
- * Scrolling below document
- * Hyperlinks
+ * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
+ * Code folding.
+ * Code completion.
+ * Fully customizable and extensible.
+ * Line numeration.
+ * Display whitespaces EOLs and tabs.
+ * Line virtualization.
+ * Multi-caret edition.
+ * Intra-column adornments.
+ * Word wrapping.
+ * Scrolling below document.
+ * Hyperlinks.
and many,many more!
@@ -41,6 +46,6 @@ var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
-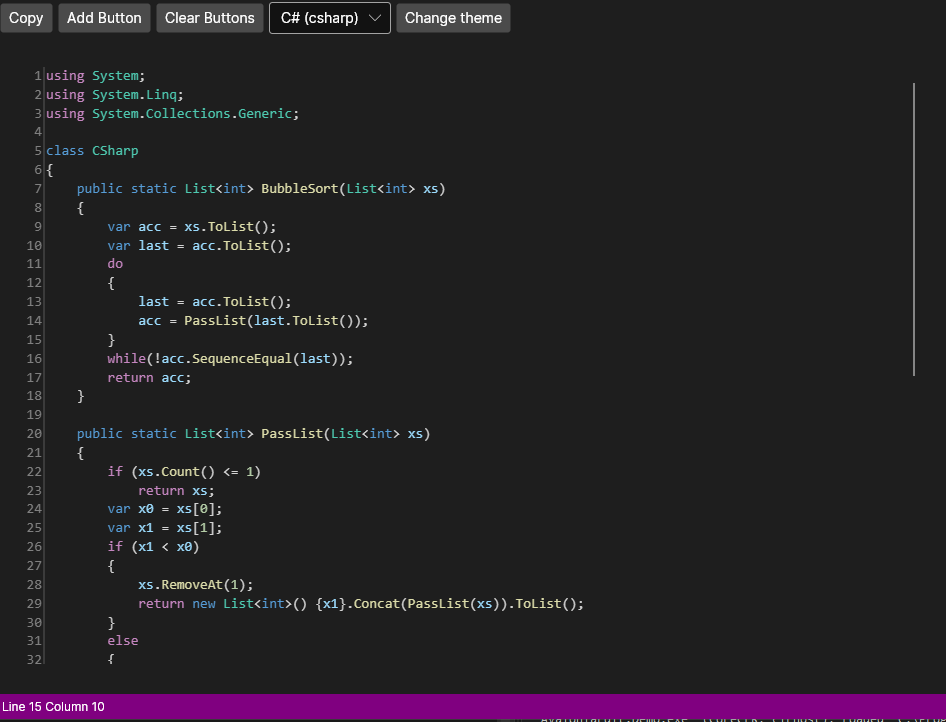
+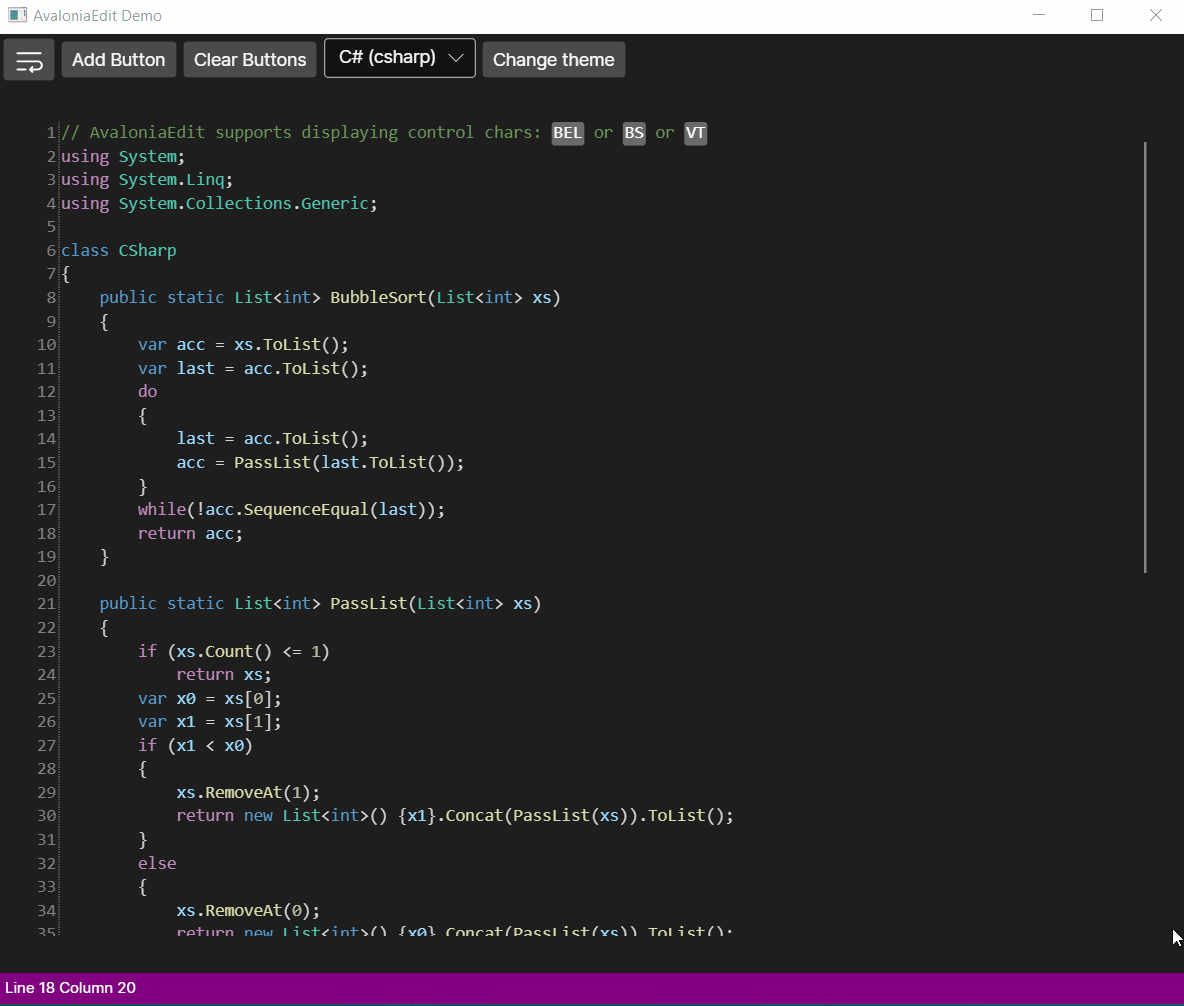
| 14 | Merge branch 'master' into feature/TextFormatterPort | 9 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10063773 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
* Line numeration
* Rectangular selection
* Intra-column adornments
* Word wrapping
* Scrolling below document
* Hyperlinks
and many,many more!
AvaloniaEdit currently consists of 2 packages
* [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
* [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
### How to set up TextMate theme and syntax highlighting for my project?
First of all, if you want to use grammars supported by [TextMateSharp](https://github.com/danipen/TextMateSharp), should install the following packages:
- [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/)
- [TextMateSharp.Grammars](https://www.nuget.org/packages/TextMateSharp.Grammars/)
Alternatively, if you want to support your own grammars, you just need to install the AvaloniaEdit.TextMate package, and implement IRegistryOptions interface, that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled TextMateSharp.Grammars.
```csharp
//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
var _textEditor = this.FindControl<TextEditor>("Editor");
//Here we initialize RegistryOptions with the theme we want to use.
var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
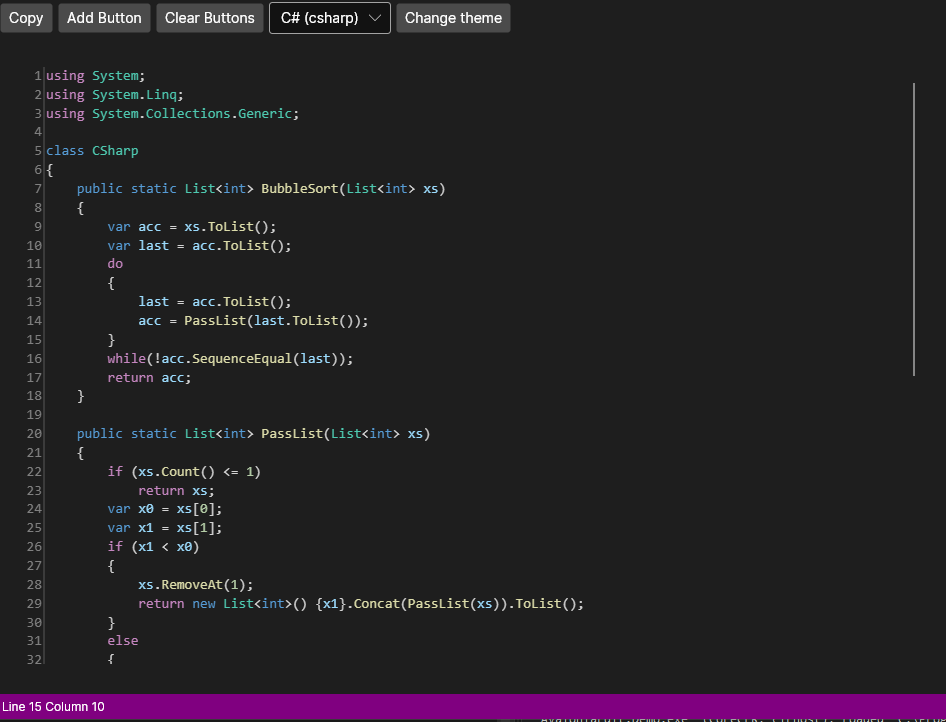
<MSG> Merge branch 'master' into feature/TextFormatterPort
<DFF> @@ -2,17 +2,22 @@
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
-This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
+This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
- * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
- * Line numeration
- * Rectangular selection
- * Intra-column adornments
- * Word wrapping
- * Scrolling below document
- * Hyperlinks
+ * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
+ * Code folding.
+ * Code completion.
+ * Fully customizable and extensible.
+ * Line numeration.
+ * Display whitespaces EOLs and tabs.
+ * Line virtualization.
+ * Multi-caret edition.
+ * Intra-column adornments.
+ * Word wrapping.
+ * Scrolling below document.
+ * Hyperlinks.
and many,many more!
@@ -41,6 +46,6 @@ var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
-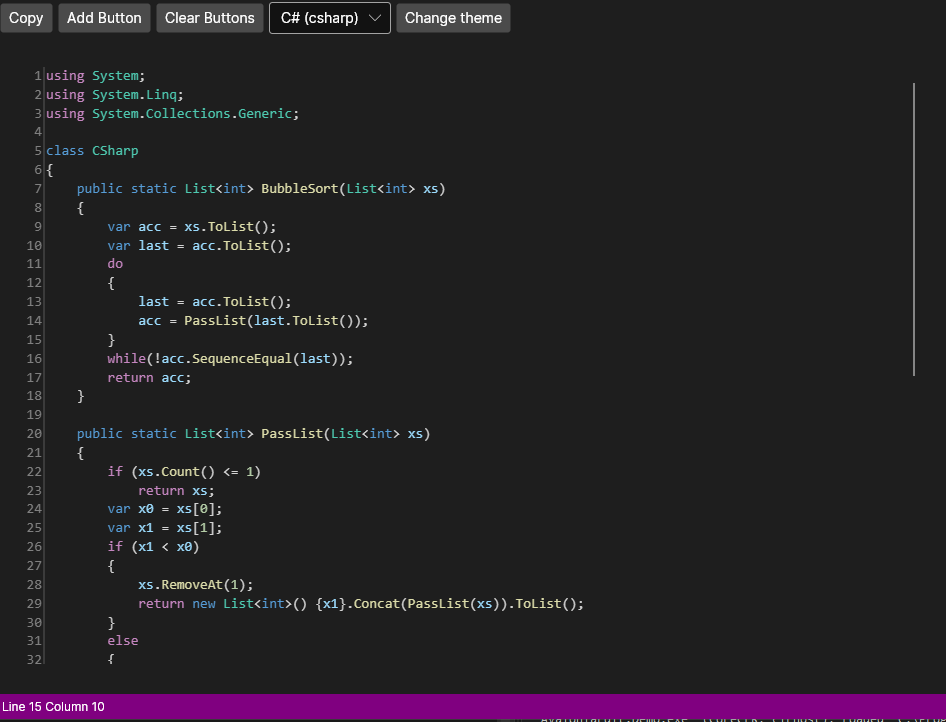
+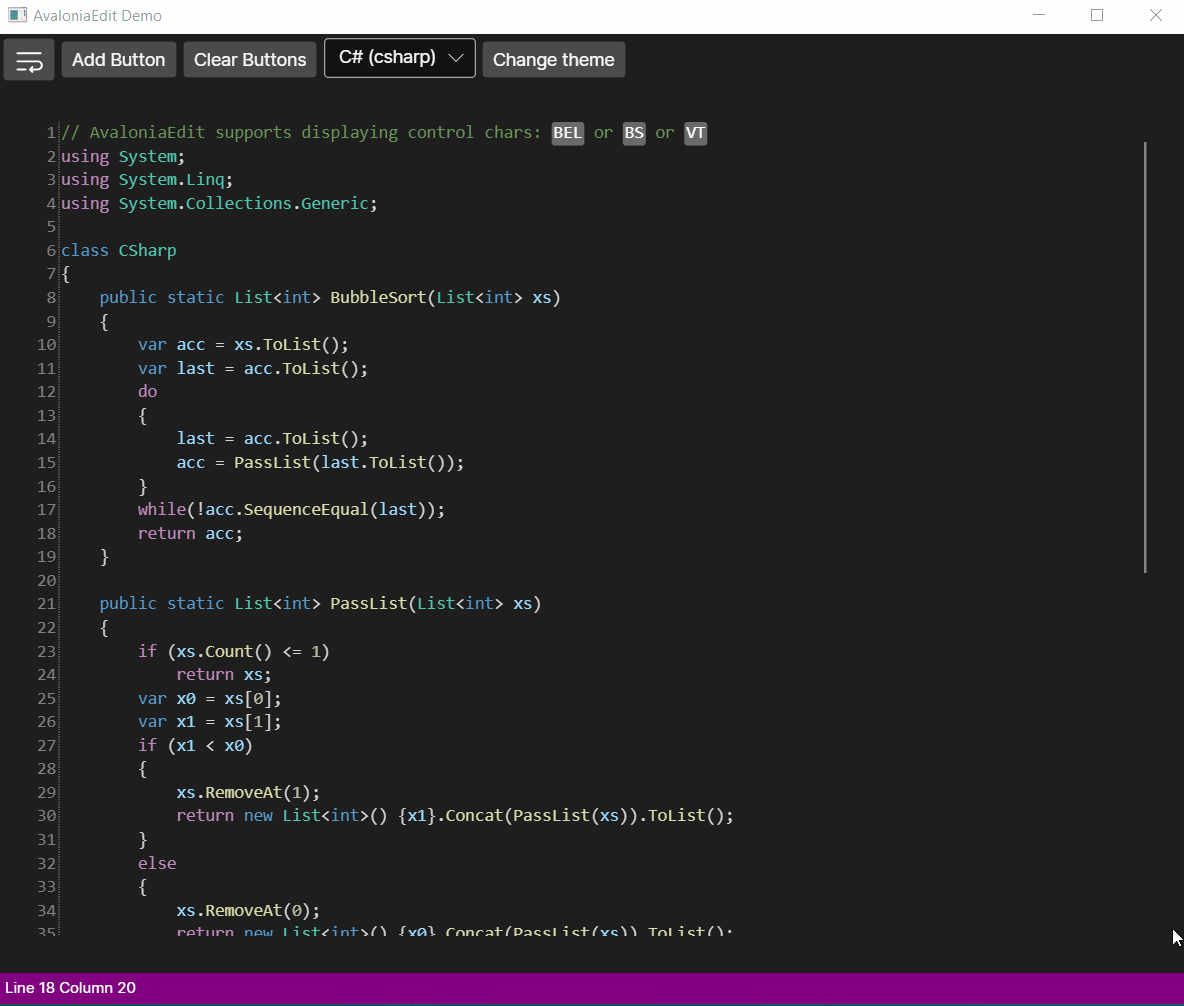
| 14 | Merge branch 'master' into feature/TextFormatterPort | 9 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10063774 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
* Line numeration
* Rectangular selection
* Intra-column adornments
* Word wrapping
* Scrolling below document
* Hyperlinks
and many,many more!
AvaloniaEdit currently consists of 2 packages
* [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
* [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
### How to set up TextMate theme and syntax highlighting for my project?
First of all, if you want to use grammars supported by [TextMateSharp](https://github.com/danipen/TextMateSharp), should install the following packages:
- [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/)
- [TextMateSharp.Grammars](https://www.nuget.org/packages/TextMateSharp.Grammars/)
Alternatively, if you want to support your own grammars, you just need to install the AvaloniaEdit.TextMate package, and implement IRegistryOptions interface, that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled TextMateSharp.Grammars.
```csharp
//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
var _textEditor = this.FindControl<TextEditor>("Editor");
//Here we initialize RegistryOptions with the theme we want to use.
var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
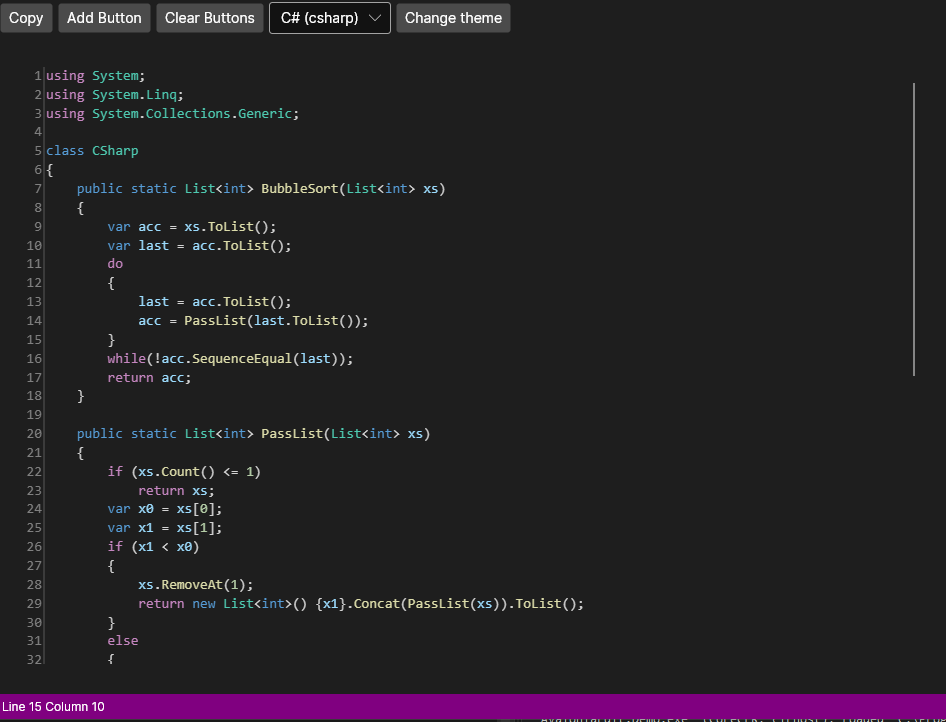
<MSG> Merge branch 'master' into feature/TextFormatterPort
<DFF> @@ -2,17 +2,22 @@
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
-This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
+This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
- * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
- * Line numeration
- * Rectangular selection
- * Intra-column adornments
- * Word wrapping
- * Scrolling below document
- * Hyperlinks
+ * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
+ * Code folding.
+ * Code completion.
+ * Fully customizable and extensible.
+ * Line numeration.
+ * Display whitespaces EOLs and tabs.
+ * Line virtualization.
+ * Multi-caret edition.
+ * Intra-column adornments.
+ * Word wrapping.
+ * Scrolling below document.
+ * Hyperlinks.
and many,many more!
@@ -41,6 +46,6 @@ var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
-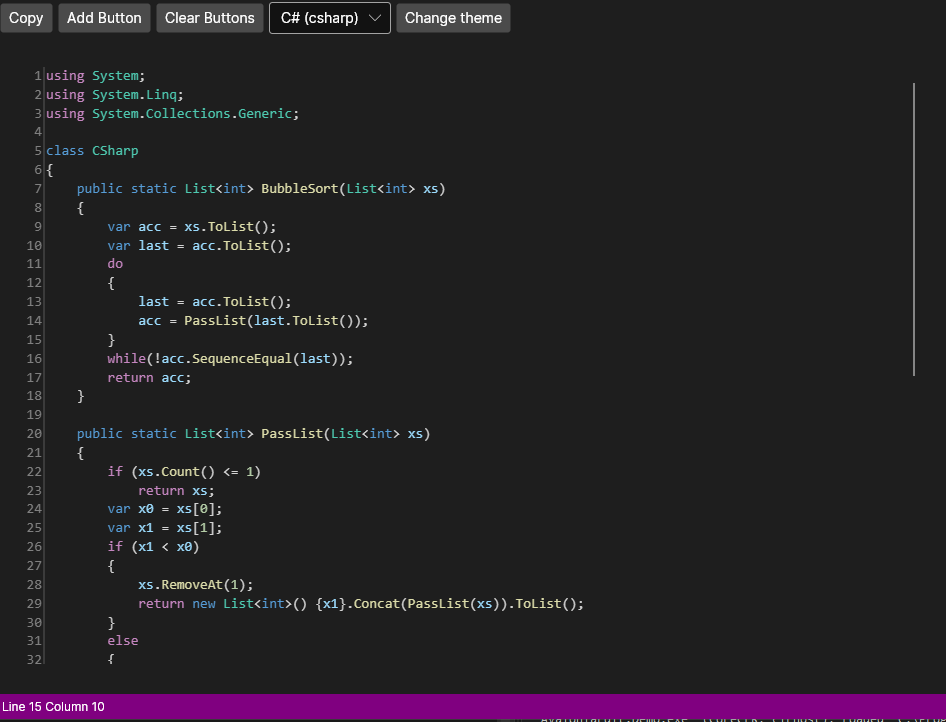
+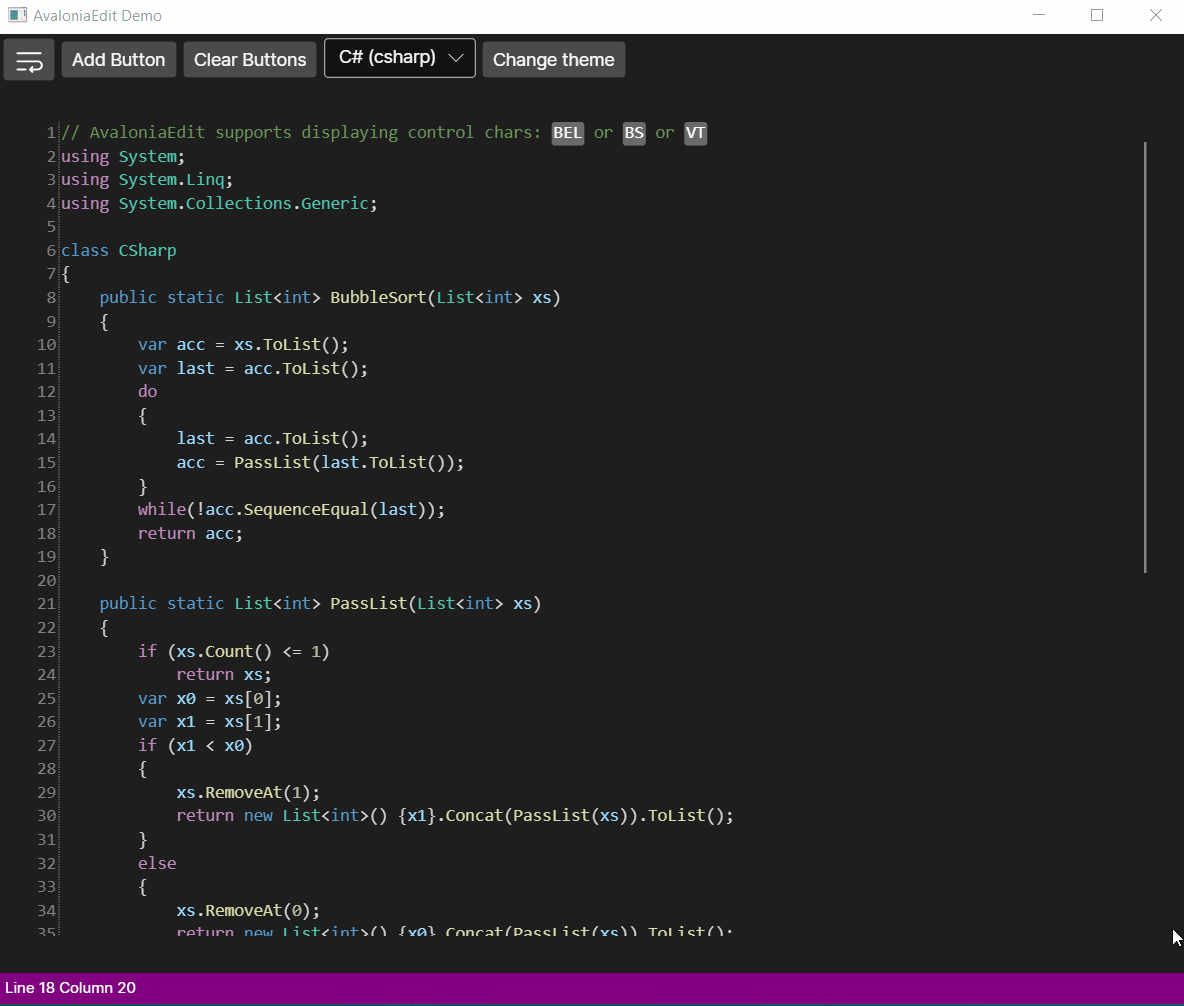
| 14 | Merge branch 'master' into feature/TextFormatterPort | 9 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10063775 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
* Line numeration
* Rectangular selection
* Intra-column adornments
* Word wrapping
* Scrolling below document
* Hyperlinks
and many,many more!
AvaloniaEdit currently consists of 2 packages
* [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
* [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
### How to set up TextMate theme and syntax highlighting for my project?
First of all, if you want to use grammars supported by [TextMateSharp](https://github.com/danipen/TextMateSharp), should install the following packages:
- [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/)
- [TextMateSharp.Grammars](https://www.nuget.org/packages/TextMateSharp.Grammars/)
Alternatively, if you want to support your own grammars, you just need to install the AvaloniaEdit.TextMate package, and implement IRegistryOptions interface, that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled TextMateSharp.Grammars.
```csharp
//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
var _textEditor = this.FindControl<TextEditor>("Editor");
//Here we initialize RegistryOptions with the theme we want to use.
var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
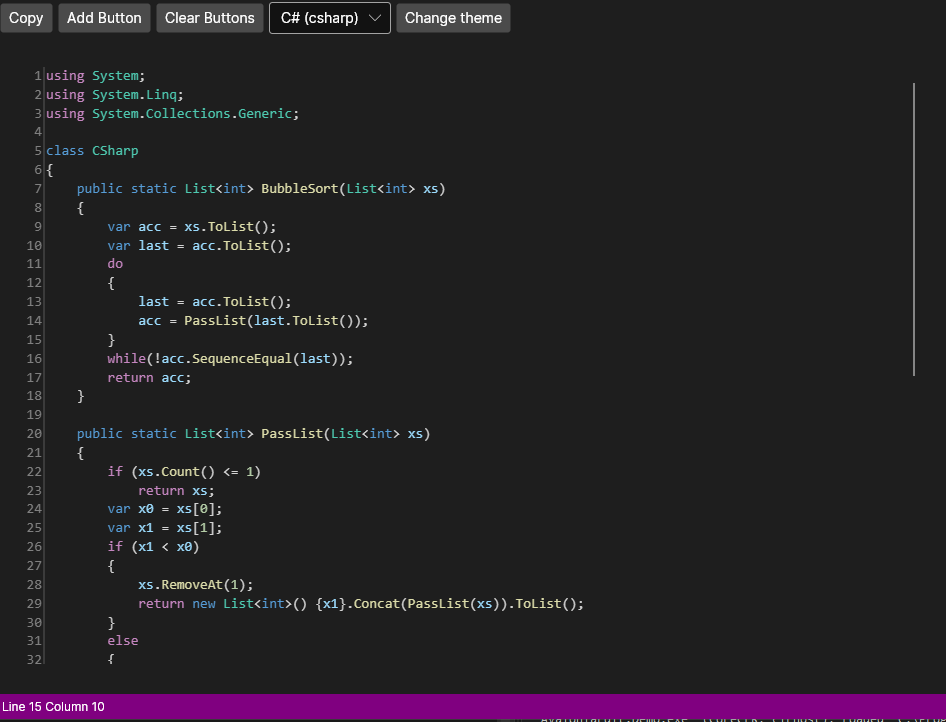
<MSG> Merge branch 'master' into feature/TextFormatterPort
<DFF> @@ -2,17 +2,22 @@
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
-This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
+This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
- * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
- * Line numeration
- * Rectangular selection
- * Intra-column adornments
- * Word wrapping
- * Scrolling below document
- * Hyperlinks
+ * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
+ * Code folding.
+ * Code completion.
+ * Fully customizable and extensible.
+ * Line numeration.
+ * Display whitespaces EOLs and tabs.
+ * Line virtualization.
+ * Multi-caret edition.
+ * Intra-column adornments.
+ * Word wrapping.
+ * Scrolling below document.
+ * Hyperlinks.
and many,many more!
@@ -41,6 +46,6 @@ var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
-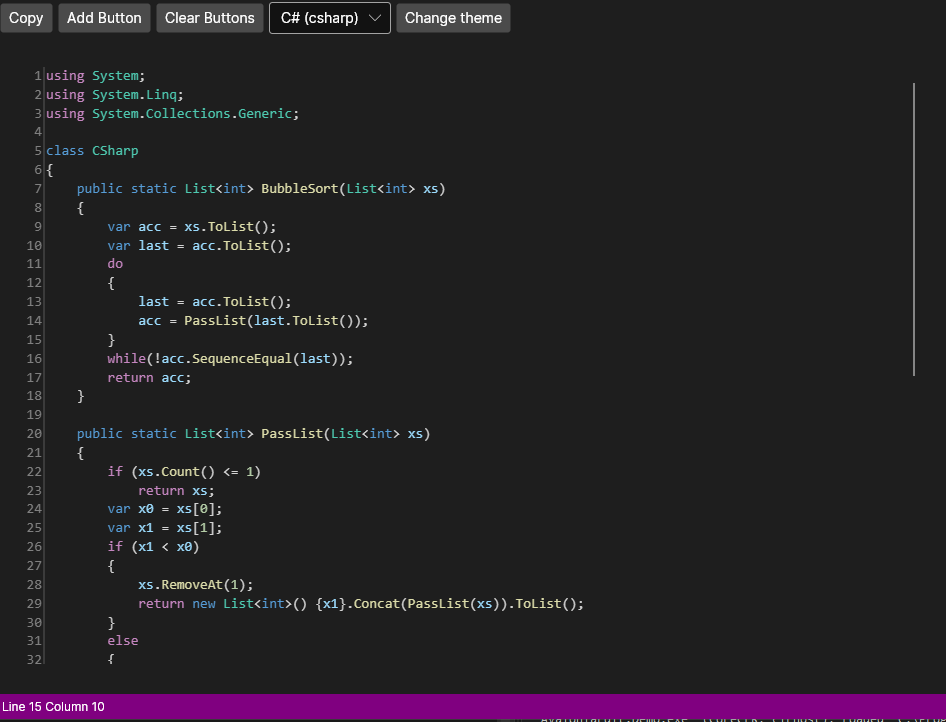
+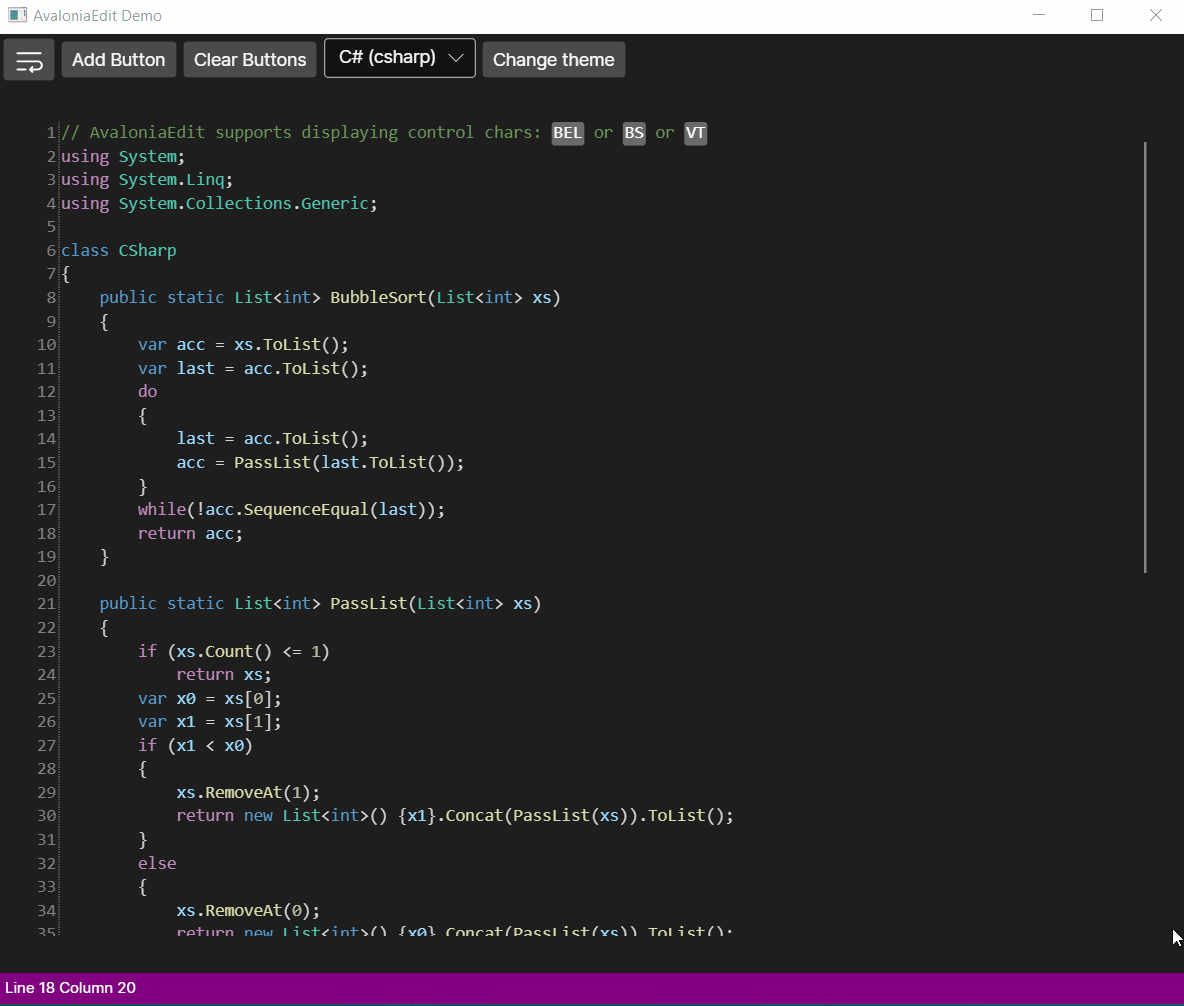
| 14 | Merge branch 'master' into feature/TextFormatterPort | 9 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10063776 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
* Line numeration
* Rectangular selection
* Intra-column adornments
* Word wrapping
* Scrolling below document
* Hyperlinks
and many,many more!
AvaloniaEdit currently consists of 2 packages
* [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
* [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
### How to set up TextMate theme and syntax highlighting for my project?
First of all, if you want to use grammars supported by [TextMateSharp](https://github.com/danipen/TextMateSharp), should install the following packages:
- [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/)
- [TextMateSharp.Grammars](https://www.nuget.org/packages/TextMateSharp.Grammars/)
Alternatively, if you want to support your own grammars, you just need to install the AvaloniaEdit.TextMate package, and implement IRegistryOptions interface, that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled TextMateSharp.Grammars.
```csharp
//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
var _textEditor = this.FindControl<TextEditor>("Editor");
//Here we initialize RegistryOptions with the theme we want to use.
var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
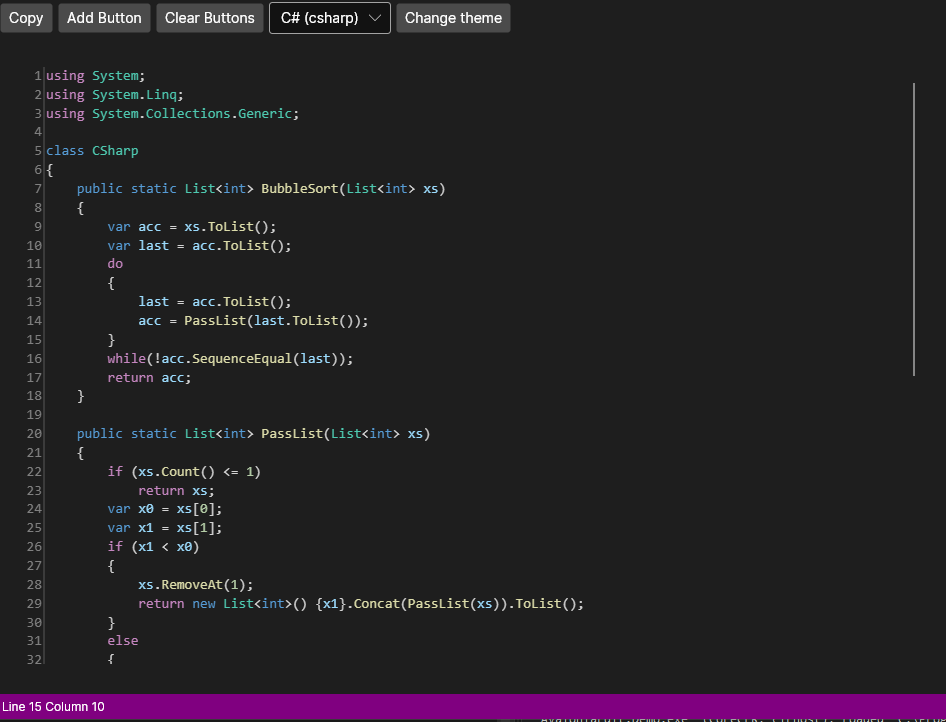
<MSG> Merge branch 'master' into feature/TextFormatterPort
<DFF> @@ -2,17 +2,22 @@
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
-This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
+This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
- * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
- * Line numeration
- * Rectangular selection
- * Intra-column adornments
- * Word wrapping
- * Scrolling below document
- * Hyperlinks
+ * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
+ * Code folding.
+ * Code completion.
+ * Fully customizable and extensible.
+ * Line numeration.
+ * Display whitespaces EOLs and tabs.
+ * Line virtualization.
+ * Multi-caret edition.
+ * Intra-column adornments.
+ * Word wrapping.
+ * Scrolling below document.
+ * Hyperlinks.
and many,many more!
@@ -41,6 +46,6 @@ var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
-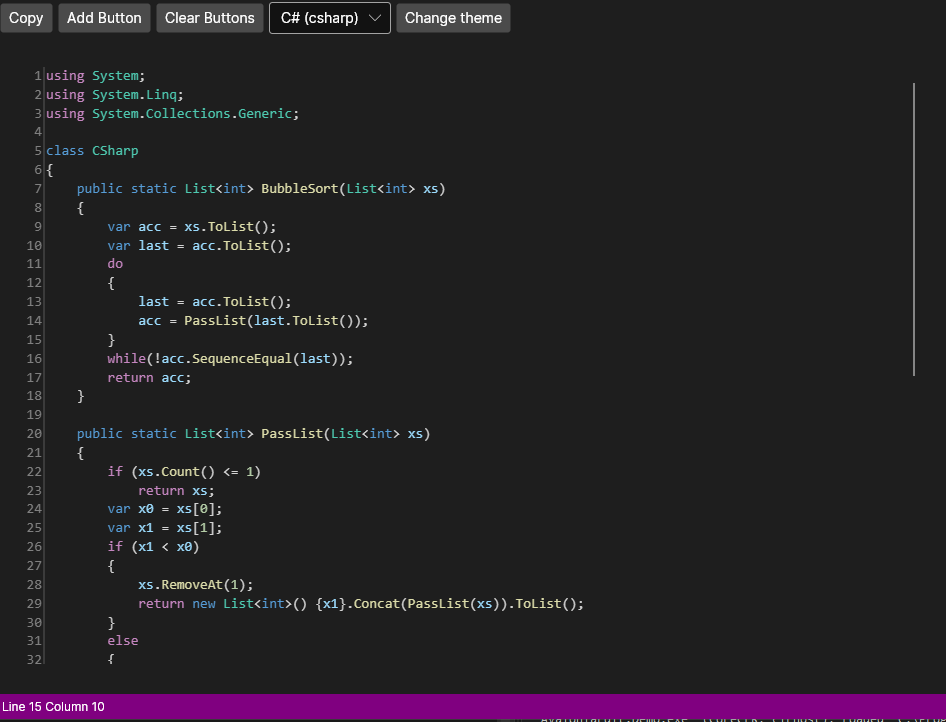
+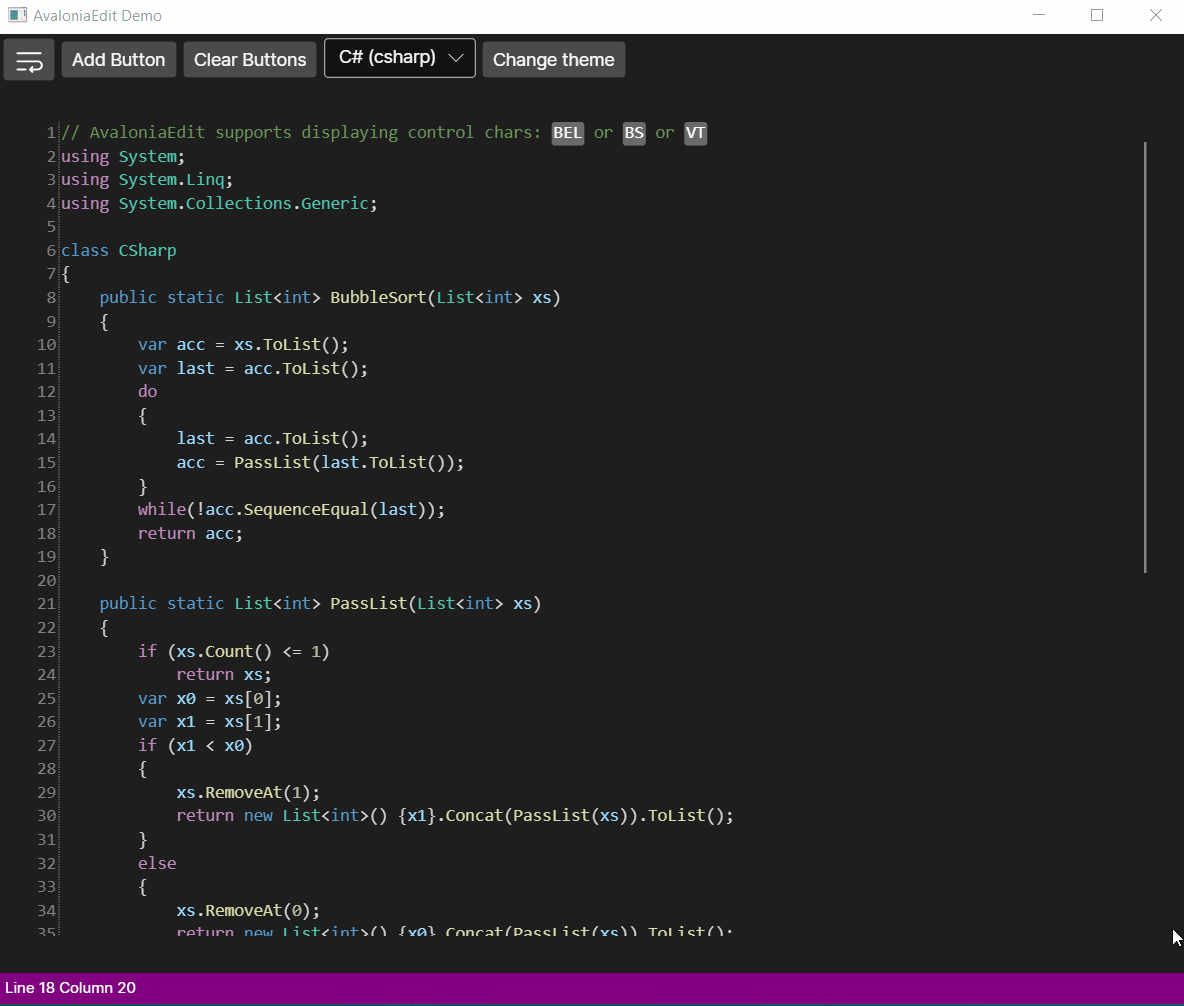
| 14 | Merge branch 'master' into feature/TextFormatterPort | 9 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10063777 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
* Line numeration
* Rectangular selection
* Intra-column adornments
* Word wrapping
* Scrolling below document
* Hyperlinks
and many,many more!
AvaloniaEdit currently consists of 2 packages
* [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
* [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
### How to set up TextMate theme and syntax highlighting for my project?
First of all, if you want to use grammars supported by [TextMateSharp](https://github.com/danipen/TextMateSharp), should install the following packages:
- [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/)
- [TextMateSharp.Grammars](https://www.nuget.org/packages/TextMateSharp.Grammars/)
Alternatively, if you want to support your own grammars, you just need to install the AvaloniaEdit.TextMate package, and implement IRegistryOptions interface, that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled TextMateSharp.Grammars.
```csharp
//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
var _textEditor = this.FindControl<TextEditor>("Editor");
//Here we initialize RegistryOptions with the theme we want to use.
var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
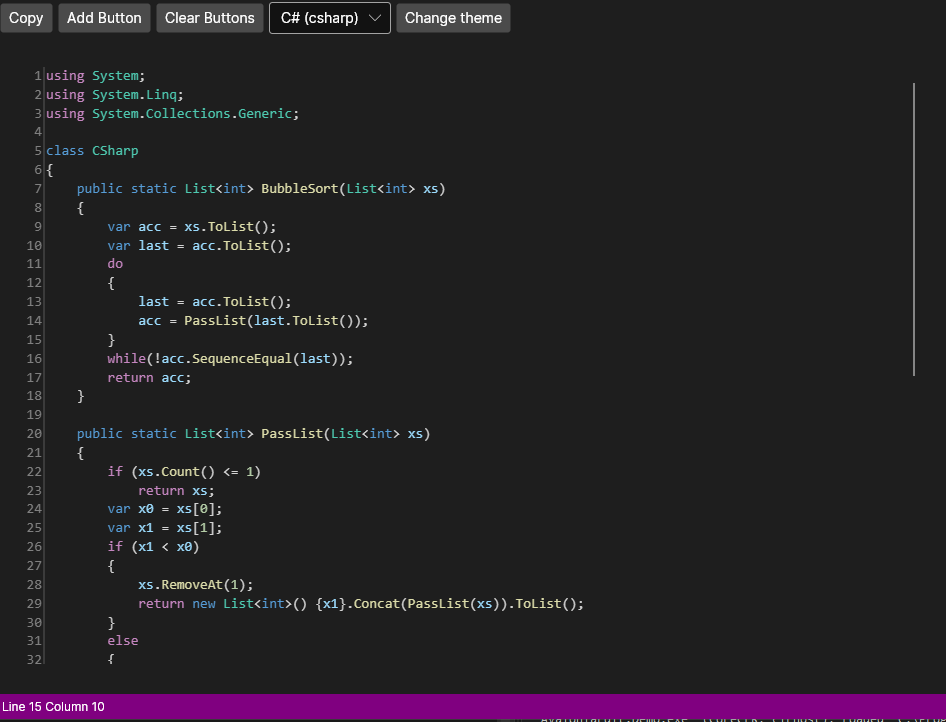
<MSG> Merge branch 'master' into feature/TextFormatterPort
<DFF> @@ -2,17 +2,22 @@
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
-This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
+This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
- * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
- * Line numeration
- * Rectangular selection
- * Intra-column adornments
- * Word wrapping
- * Scrolling below document
- * Hyperlinks
+ * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
+ * Code folding.
+ * Code completion.
+ * Fully customizable and extensible.
+ * Line numeration.
+ * Display whitespaces EOLs and tabs.
+ * Line virtualization.
+ * Multi-caret edition.
+ * Intra-column adornments.
+ * Word wrapping.
+ * Scrolling below document.
+ * Hyperlinks.
and many,many more!
@@ -41,6 +46,6 @@ var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
-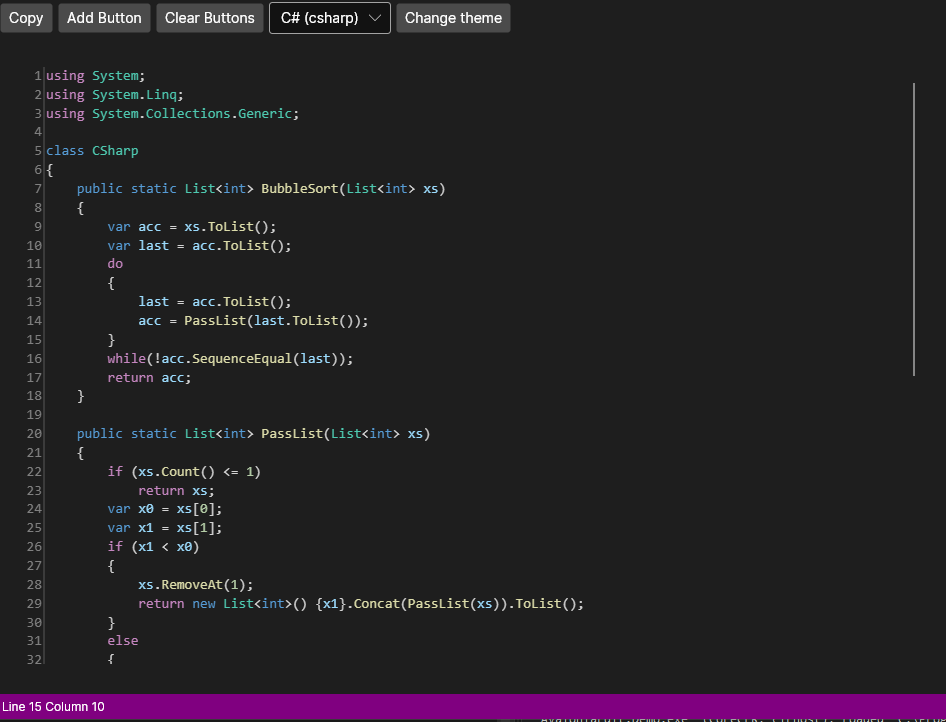
+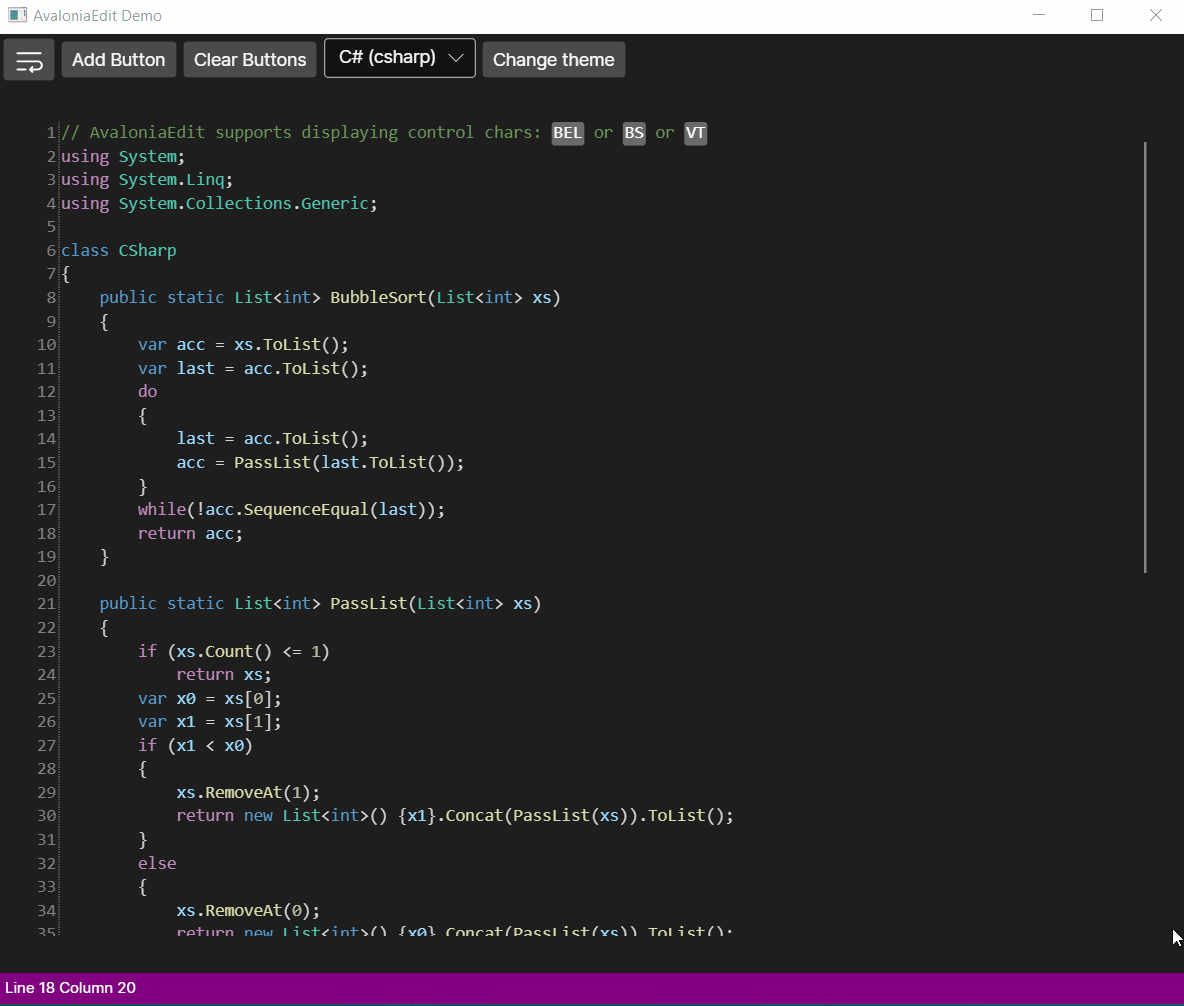
| 14 | Merge branch 'master' into feature/TextFormatterPort | 9 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10063778 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
* Line numeration
* Rectangular selection
* Intra-column adornments
* Word wrapping
* Scrolling below document
* Hyperlinks
and many,many more!
AvaloniaEdit currently consists of 2 packages
* [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
* [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
### How to set up TextMate theme and syntax highlighting for my project?
First of all, if you want to use grammars supported by [TextMateSharp](https://github.com/danipen/TextMateSharp), should install the following packages:
- [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/)
- [TextMateSharp.Grammars](https://www.nuget.org/packages/TextMateSharp.Grammars/)
Alternatively, if you want to support your own grammars, you just need to install the AvaloniaEdit.TextMate package, and implement IRegistryOptions interface, that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled TextMateSharp.Grammars.
```csharp
//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
var _textEditor = this.FindControl<TextEditor>("Editor");
//Here we initialize RegistryOptions with the theme we want to use.
var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
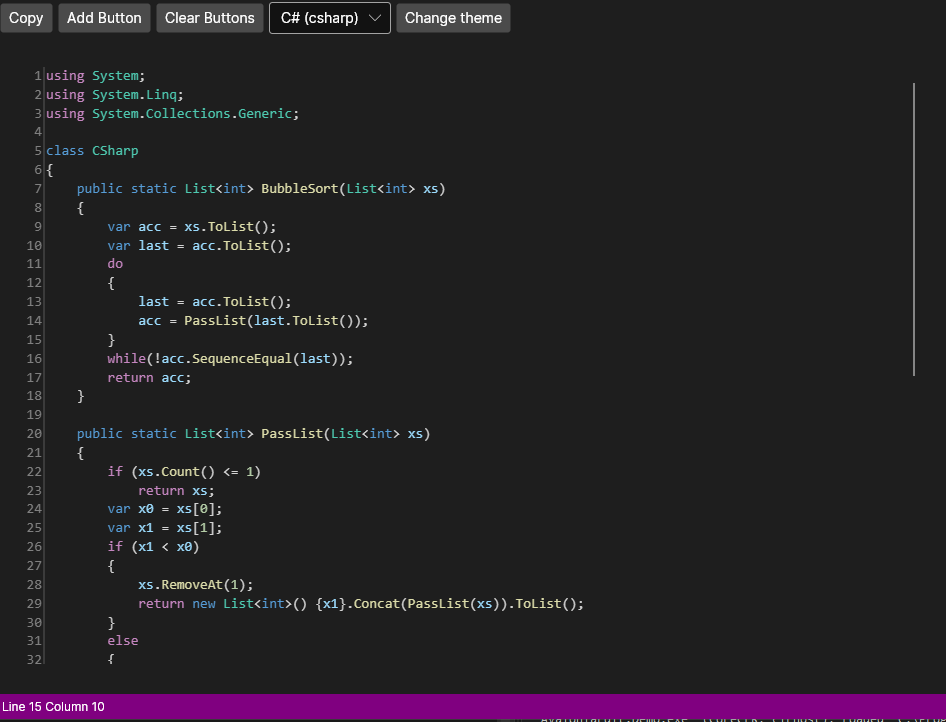
<MSG> Merge branch 'master' into feature/TextFormatterPort
<DFF> @@ -2,17 +2,22 @@
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
-This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
+This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
- * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
- * Line numeration
- * Rectangular selection
- * Intra-column adornments
- * Word wrapping
- * Scrolling below document
- * Hyperlinks
+ * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
+ * Code folding.
+ * Code completion.
+ * Fully customizable and extensible.
+ * Line numeration.
+ * Display whitespaces EOLs and tabs.
+ * Line virtualization.
+ * Multi-caret edition.
+ * Intra-column adornments.
+ * Word wrapping.
+ * Scrolling below document.
+ * Hyperlinks.
and many,many more!
@@ -41,6 +46,6 @@ var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
-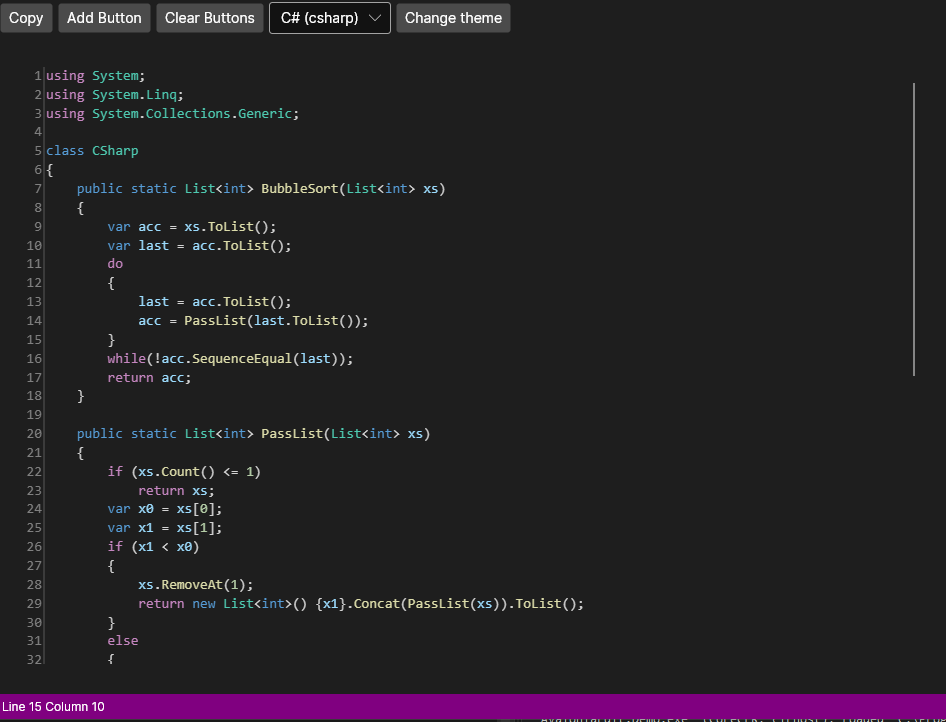
+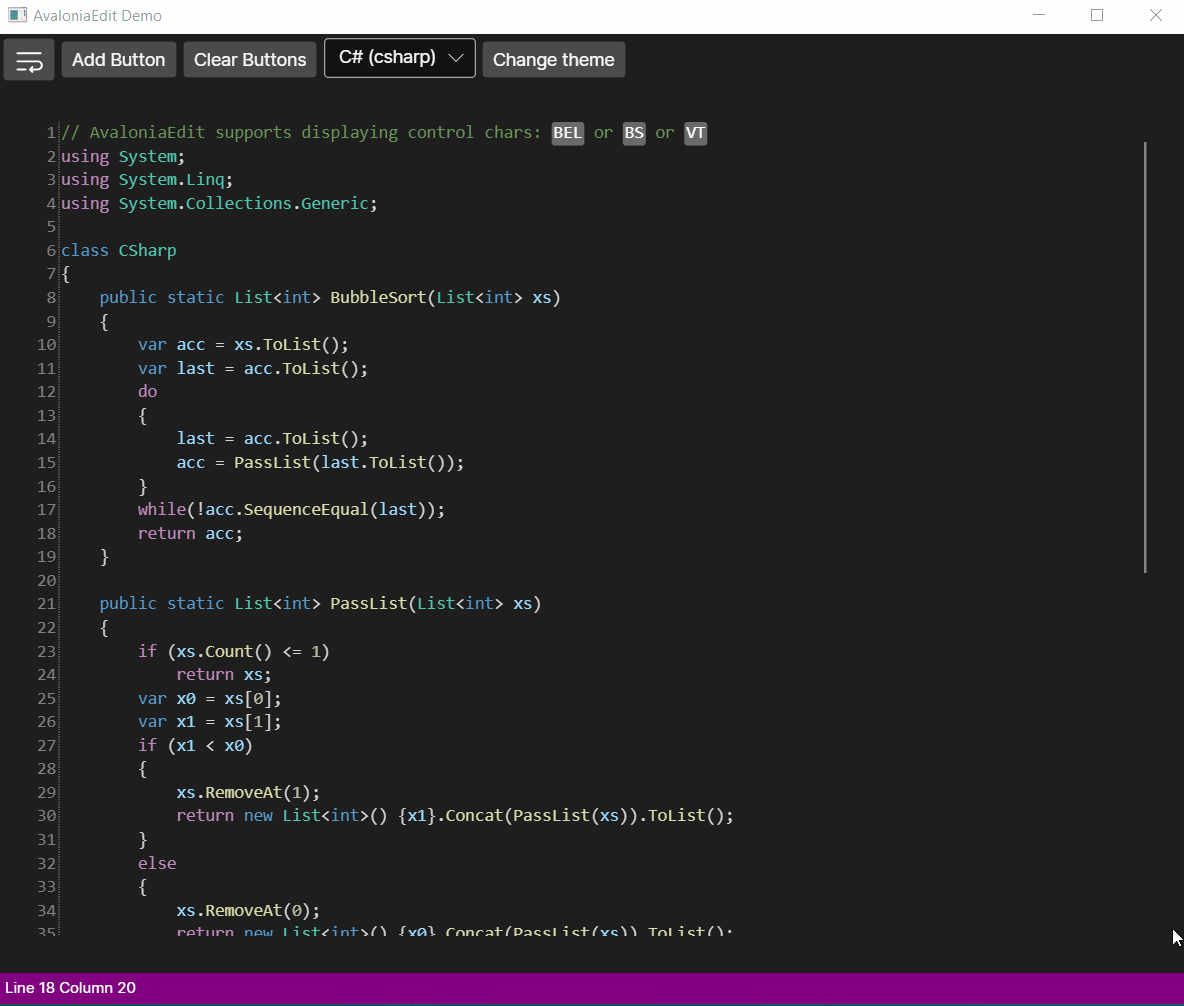
| 14 | Merge branch 'master' into feature/TextFormatterPort | 9 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10063779 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
* Line numeration
* Rectangular selection
* Intra-column adornments
* Word wrapping
* Scrolling below document
* Hyperlinks
and many,many more!
AvaloniaEdit currently consists of 2 packages
* [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
* [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
### How to set up TextMate theme and syntax highlighting for my project?
First of all, if you want to use grammars supported by [TextMateSharp](https://github.com/danipen/TextMateSharp), should install the following packages:
- [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/)
- [TextMateSharp.Grammars](https://www.nuget.org/packages/TextMateSharp.Grammars/)
Alternatively, if you want to support your own grammars, you just need to install the AvaloniaEdit.TextMate package, and implement IRegistryOptions interface, that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled TextMateSharp.Grammars.
```csharp
//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
var _textEditor = this.FindControl<TextEditor>("Editor");
//Here we initialize RegistryOptions with the theme we want to use.
var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
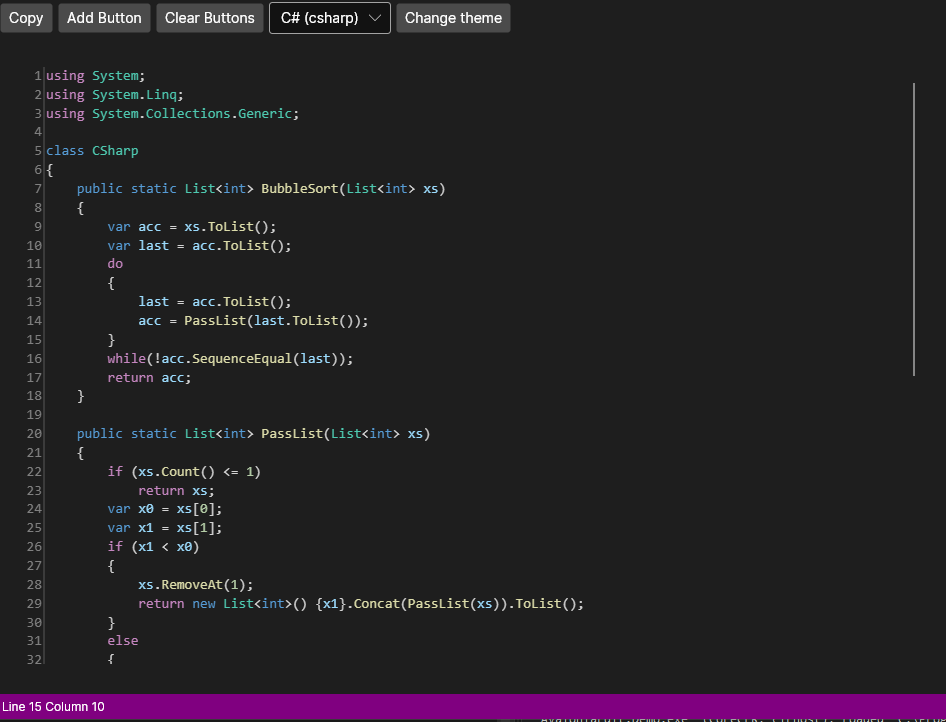
<MSG> Merge branch 'master' into feature/TextFormatterPort
<DFF> @@ -2,17 +2,22 @@
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
-This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
+This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
- * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
- * Line numeration
- * Rectangular selection
- * Intra-column adornments
- * Word wrapping
- * Scrolling below document
- * Hyperlinks
+ * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
+ * Code folding.
+ * Code completion.
+ * Fully customizable and extensible.
+ * Line numeration.
+ * Display whitespaces EOLs and tabs.
+ * Line virtualization.
+ * Multi-caret edition.
+ * Intra-column adornments.
+ * Word wrapping.
+ * Scrolling below document.
+ * Hyperlinks.
and many,many more!
@@ -41,6 +46,6 @@ var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
-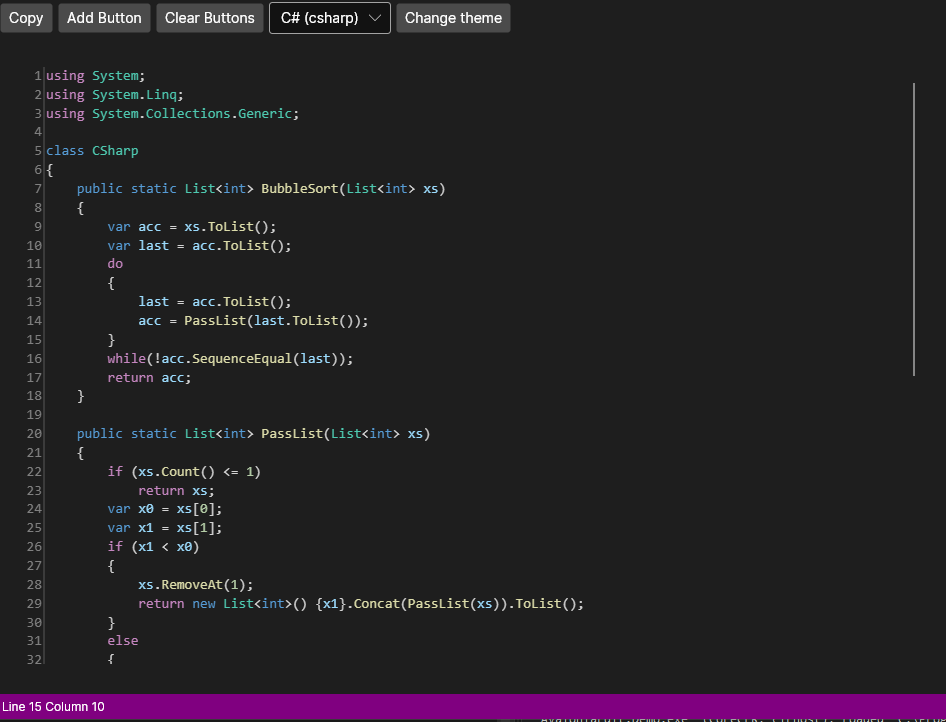
+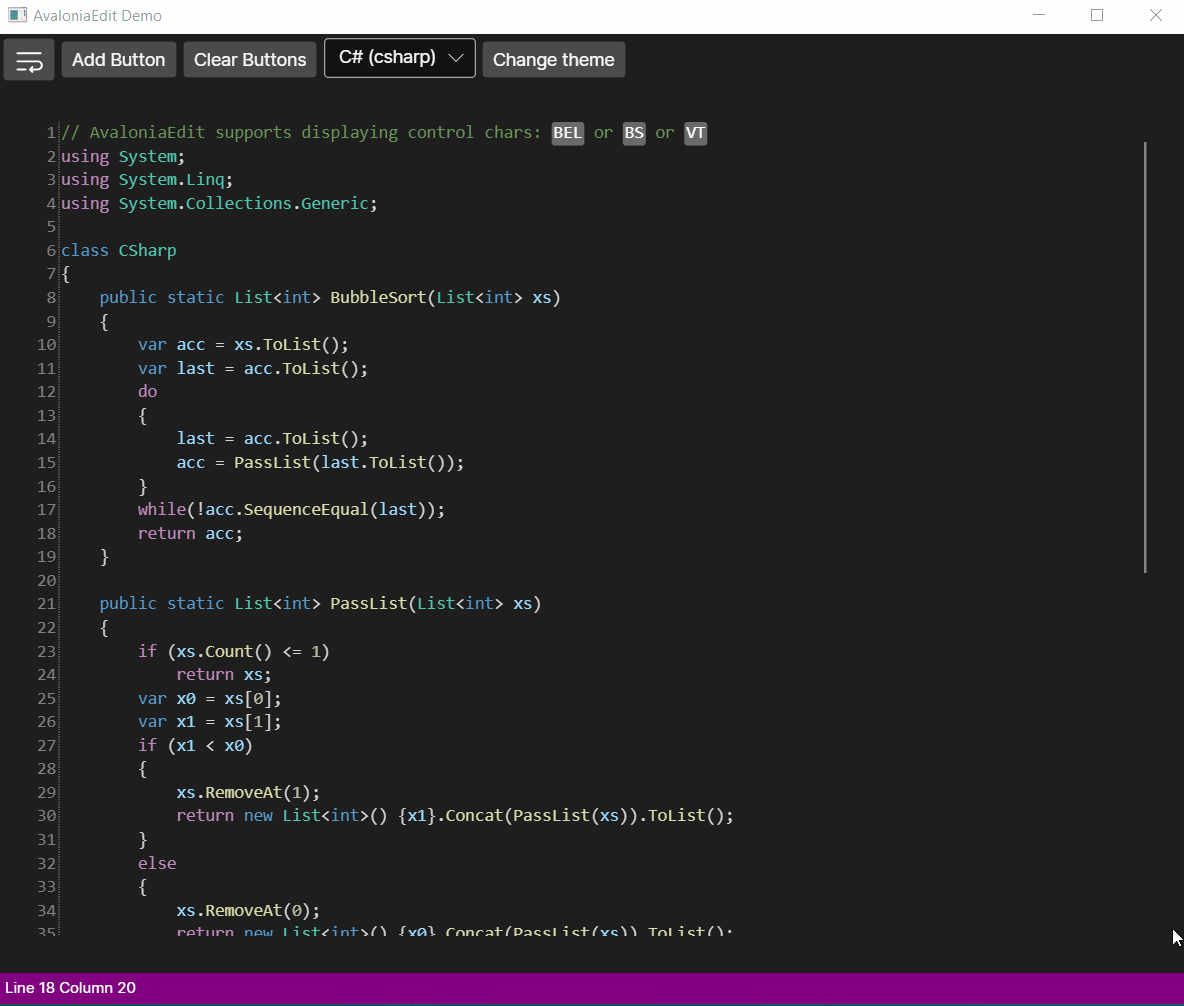
| 14 | Merge branch 'master' into feature/TextFormatterPort | 9 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10063780 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
* Line numeration
* Rectangular selection
* Intra-column adornments
* Word wrapping
* Scrolling below document
* Hyperlinks
and many,many more!
AvaloniaEdit currently consists of 2 packages
* [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
* [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
### How to set up TextMate theme and syntax highlighting for my project?
First of all, if you want to use grammars supported by [TextMateSharp](https://github.com/danipen/TextMateSharp), should install the following packages:
- [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/)
- [TextMateSharp.Grammars](https://www.nuget.org/packages/TextMateSharp.Grammars/)
Alternatively, if you want to support your own grammars, you just need to install the AvaloniaEdit.TextMate package, and implement IRegistryOptions interface, that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled TextMateSharp.Grammars.
```csharp
//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
var _textEditor = this.FindControl<TextEditor>("Editor");
//Here we initialize RegistryOptions with the theme we want to use.
var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
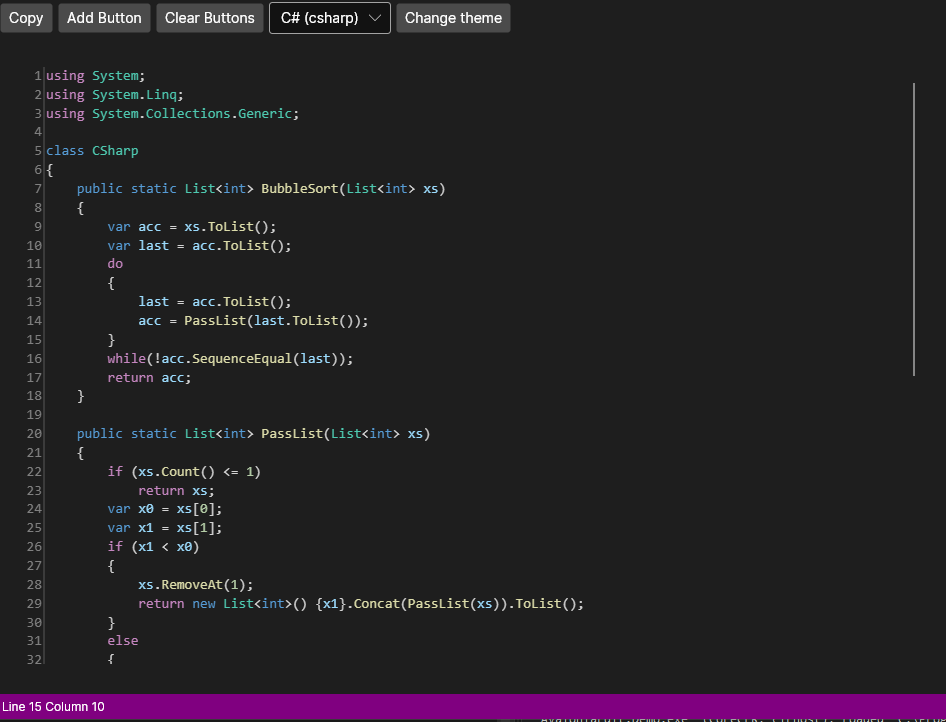
<MSG> Merge branch 'master' into feature/TextFormatterPort
<DFF> @@ -2,17 +2,22 @@
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
-This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
+This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
- * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
- * Line numeration
- * Rectangular selection
- * Intra-column adornments
- * Word wrapping
- * Scrolling below document
- * Hyperlinks
+ * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
+ * Code folding.
+ * Code completion.
+ * Fully customizable and extensible.
+ * Line numeration.
+ * Display whitespaces EOLs and tabs.
+ * Line virtualization.
+ * Multi-caret edition.
+ * Intra-column adornments.
+ * Word wrapping.
+ * Scrolling below document.
+ * Hyperlinks.
and many,many more!
@@ -41,6 +46,6 @@ var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
-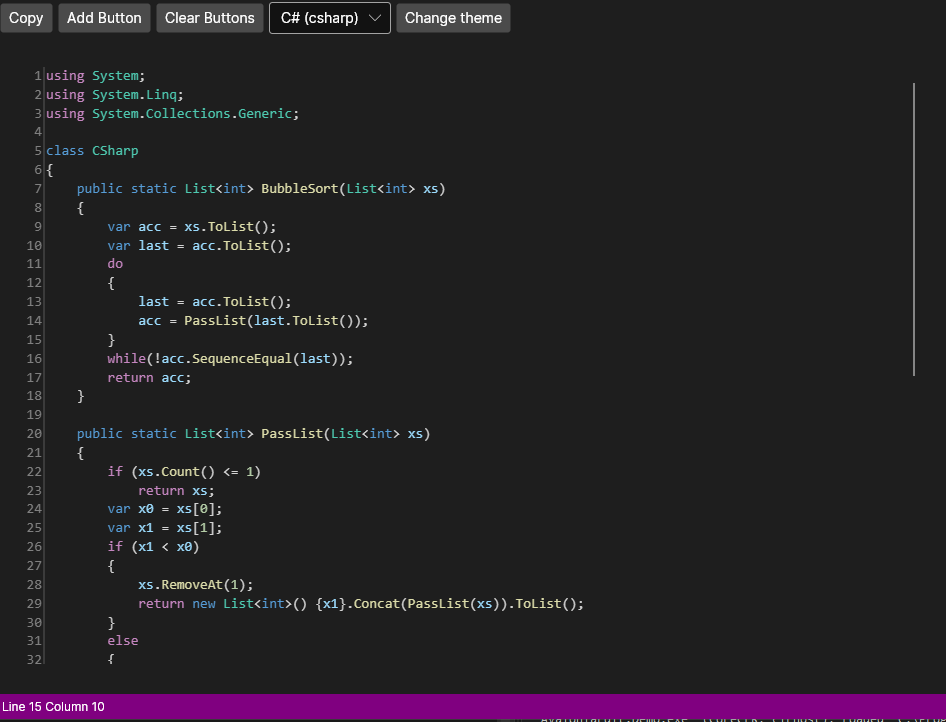
+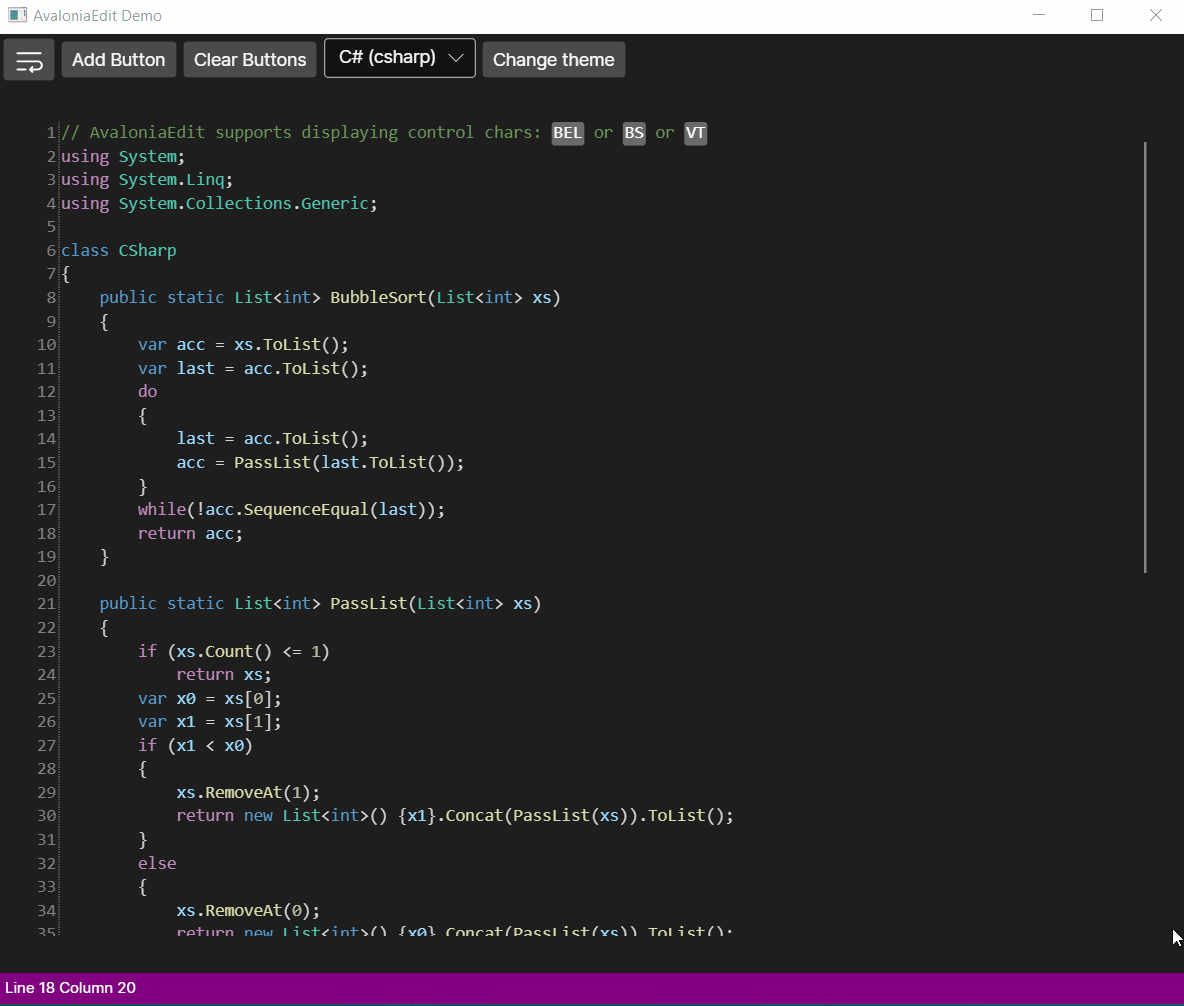
| 14 | Merge branch 'master' into feature/TextFormatterPort | 9 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10063781 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
* Line numeration
* Rectangular selection
* Intra-column adornments
* Word wrapping
* Scrolling below document
* Hyperlinks
and many,many more!
AvaloniaEdit currently consists of 2 packages
* [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
* [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
### How to set up TextMate theme and syntax highlighting for my project?
First of all, if you want to use grammars supported by [TextMateSharp](https://github.com/danipen/TextMateSharp), should install the following packages:
- [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/)
- [TextMateSharp.Grammars](https://www.nuget.org/packages/TextMateSharp.Grammars/)
Alternatively, if you want to support your own grammars, you just need to install the AvaloniaEdit.TextMate package, and implement IRegistryOptions interface, that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled TextMateSharp.Grammars.
```csharp
//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
var _textEditor = this.FindControl<TextEditor>("Editor");
//Here we initialize RegistryOptions with the theme we want to use.
var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
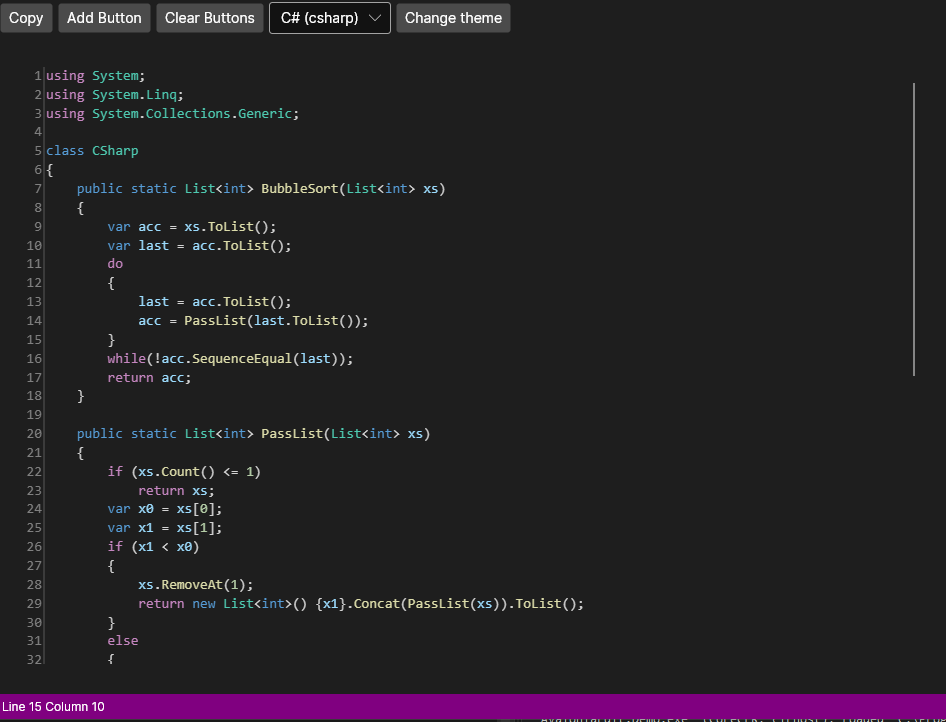
<MSG> Merge branch 'master' into feature/TextFormatterPort
<DFF> @@ -2,17 +2,22 @@
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
-This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit) WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
+This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
- * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars
- * Line numeration
- * Rectangular selection
- * Intra-column adornments
- * Word wrapping
- * Scrolling below document
- * Hyperlinks
+ * Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
+ * Code folding.
+ * Code completion.
+ * Fully customizable and extensible.
+ * Line numeration.
+ * Display whitespaces EOLs and tabs.
+ * Line virtualization.
+ * Multi-caret edition.
+ * Intra-column adornments.
+ * Word wrapping.
+ * Scrolling below document.
+ * Hyperlinks.
and many,many more!
@@ -41,6 +46,6 @@ var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
-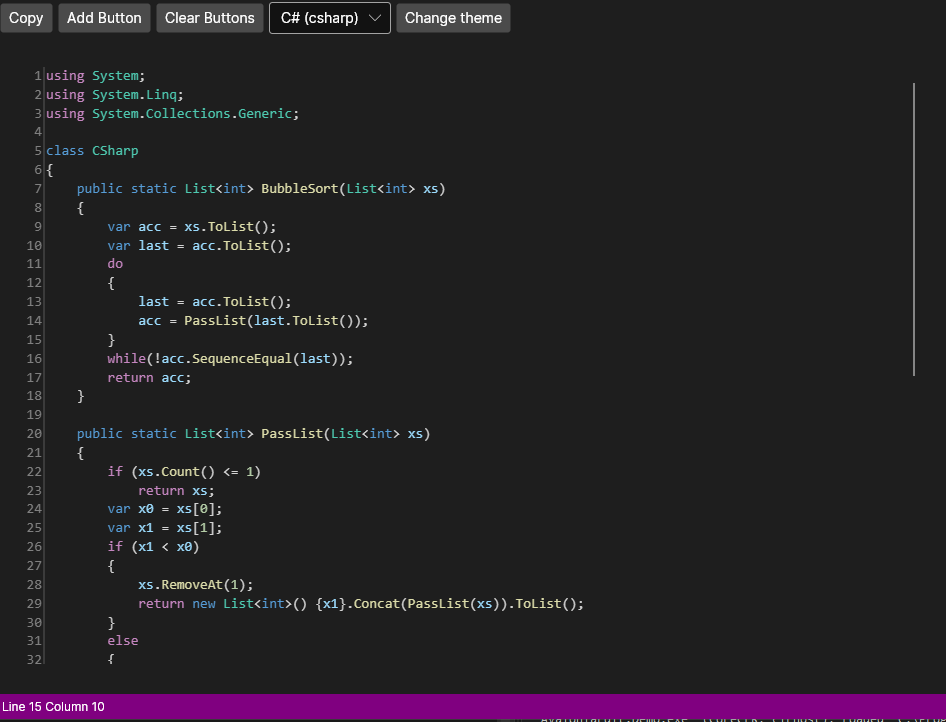
+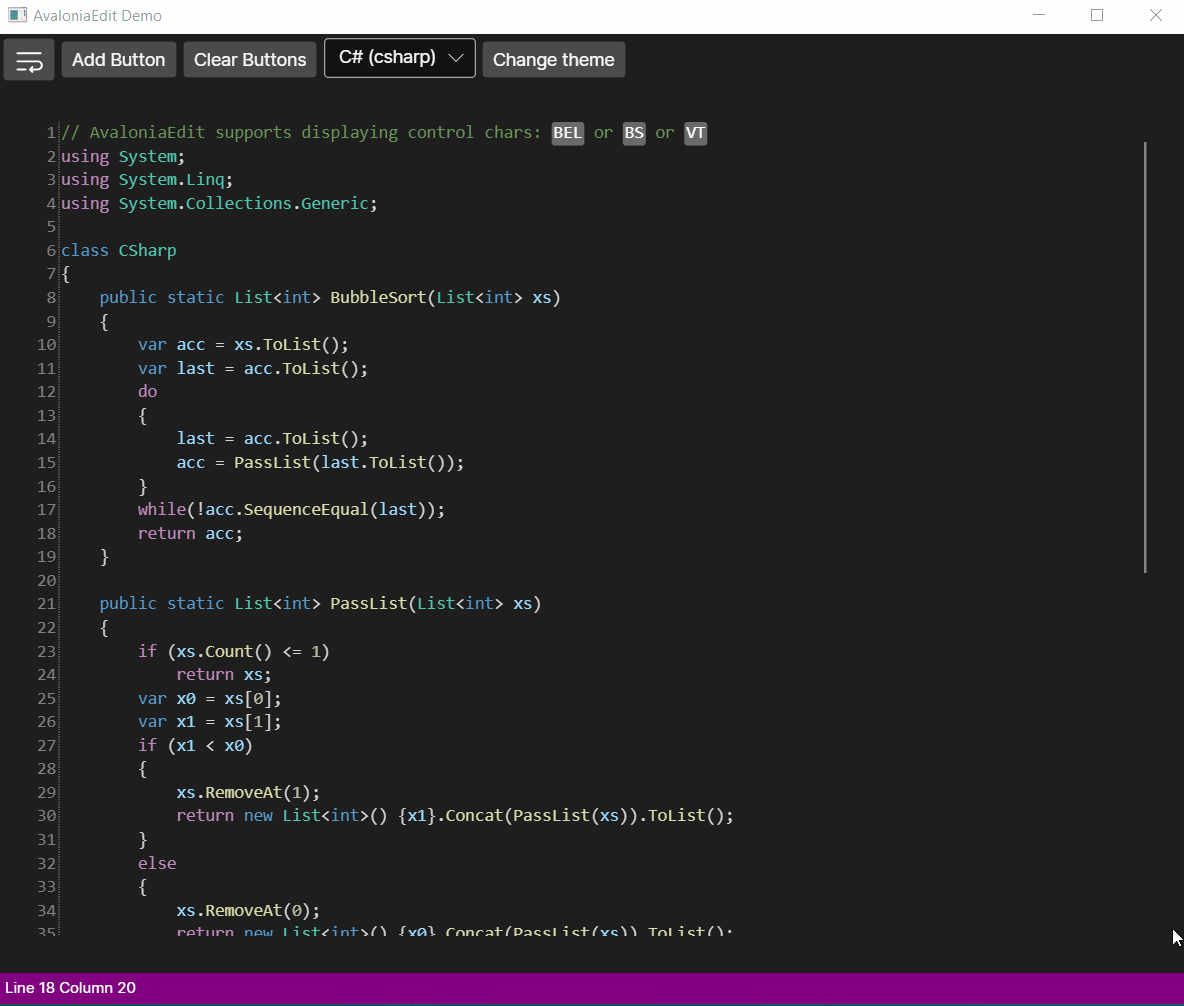
| 14 | Merge branch 'master' into feature/TextFormatterPort | 9 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10063782 | <NME> WeakEventManagerBase.cs
<BEF> using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Reactive.Disposables;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Threading;
namespace AvaloniaEdit.Utils
{
/// <summary>
/// WeakEventManager base class. Inspired by the WPF WeakEventManager class and the code in
/// https://social.msdn.microsoft.com/Forums/silverlight/en-US/34d85c3f-52ea-4adc-bb32-8297f5549042/command-binding-memory-leak?forum=silverlightbugs
/// </summary>
/// <remarks>Copied here from ReactiveUI due to bugs in its design (singleton instance for multiple events).</remarks>
/// <typeparam name="TEventManager"></typeparam>
/// <typeparam name="TEventSource">The type of the event source.</typeparam>
/// <typeparam name="TEventHandler">The type of the event handler.</typeparam>
/// <typeparam name="TEventArgs">The type of the event arguments.</typeparam>
public abstract class WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>
where TEventManager : WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>, new()
{
// ReSharper disable once StaticMemberInGenericType
private static readonly object StaticSource = new object();
/// <summary>
/// Mapping between the target of the delegate (for example a Button) and the handler (EventHandler).
/// Windows Phone needs this, otherwise the event handler gets garbage collected.
/// </summary>
private readonly ConditionalWeakTable<object, List<Delegate>> _targetToEventHandler = new ConditionalWeakTable<object, List<Delegate>>();
/// <summary>
/// Mapping from the source of the event to the list of handlers. This is a CWT to ensure it does not leak the source of the event.
/// </summary>
private readonly ConditionalWeakTable<object, WeakHandlerList> _sourceToWeakHandlers = new ConditionalWeakTable<object, WeakHandlerList>();
private static readonly Lazy<TEventManager> CurrentLazy = new Lazy<TEventManager>(() => new TEventManager());
private static TEventManager Current => CurrentLazy.Value;
/// <summary>
/// Adds a weak reference to the handler and associates it with the source.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void AddHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateAddHandler(source, handler);
}
/// <summary>
/// Removes the association between the source and the handler.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void RemoveHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateRemoveHandler(source, handler);
}
/// <summary>
/// Delivers the event to the handlers registered for the source.
/// </summary>
/// <param name="sender">The sender.</param>
/// <param name="args">The <see cref="TEventArgs"/> instance containing the event data.</param>
protected static void DeliverEvent(object sender, TEventArgs args)
{
Current.PrivateDeliverEvent(sender, args);
}
/// <summary>
/// Override this method to attach to an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StartListening(TEventSource source);
/// <summary>
/// Override this method to detach from an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StopListening(TEventSource source);
protected void PrivateAddHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("Handler must be Delegate type");
}
AddWeakHandler(source, handler);
AddTargetHandler(handler);
}
private void AddWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
weakHandlers.AddWeakHandler(source, handler);
}
else
{
weakHandlers = new WeakHandlerList();
weakHandlers.AddWeakHandler(source, handler);
_sourceToWeakHandlers.Add(source, weakHandlers);
StartListening(source);
}
Purge(source);
}
private void AddTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Add(@delegate);
}
else
{
delegates = new List<Delegate> { @delegate };
_targetToEventHandler.Add(key, delegates);
}
}
protected void PrivateRemoveHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("handler must be Delegate type");
}
RemoveWeakHandler(source, handler);
RemoveTargetHandler(handler);
}
private void RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
if (weakHandlers.RemoveWeakHandler(source, handler) && weakHandlers.Count == 0)
{
_sourceToWeakHandlers.Remove(source);
StopListening(source);
}
}
}
private void RemoveTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Remove(@delegate);
if (delegates.Count == 0)
{
_targetToEventHandler.Remove(key);
}
}
}
private void PrivateDeliverEvent(object sender, TEventArgs args)
{
var source = sender ?? StaticSource;
var hasStaleEntries = false;
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
using (weakHandlers.DeliverActive())
{
hasStaleEntries = weakHandlers.DeliverEvent(source, args);
}
}
if (hasStaleEntries)
{
Purge(source);
}
}
private void Purge(object source)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
else
{
weakHandlers.Purge();
}
}
}
internal class WeakHandler
{
private readonly WeakReference _source;
private readonly WeakReference _originalHandler;
public bool IsActive => _source != null && _source.IsAlive && _originalHandler != null && _originalHandler.IsAlive;
public TEventHandler Handler
{
get
{
if (_originalHandler == null)
{
return default(TEventHandler);
}
return (TEventHandler)_originalHandler.Target;
}
}
public WeakHandler(object source, TEventHandler originalHandler)
{
_source = new WeakReference(source);
_originalHandler = new WeakReference(originalHandler);
}
public bool Matches(object o, TEventHandler handler)
{
return _source != null &&
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
_originalHandler.Target is PropertyChangedEventHandler &&
handler is PropertyChangedEventHandler &&
Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
(handler as PropertyChangedEventHandler).Target));
}
}
internal class WeakHandlerList
{
private int _deliveries;
private readonly List<WeakHandler> _handlers;
public WeakHandlerList()
{
_handlers = new List<WeakHandler>();
}
public void AddWeakHandler(TEventSource source, TEventHandler handler)
{
var handlerSink = new WeakHandler(source, handler);
_handlers.Add(handlerSink);
}
public bool RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.Matches(source, handler))
{
return _handlers.Remove(weakHandler);
}
}
return false;
}
public WeakHandlerList Clone()
{
var newList = new WeakHandlerList();
newList._handlers.AddRange(_handlers.Where(h => h.IsActive));
return newList;
}
public int Count => _handlers.Count;
public bool IsDeliverActive => _deliveries > 0;
public IDisposable DeliverActive()
{
Interlocked.Increment(ref _deliveries);
return Disposable.Create(() => Interlocked.Decrement(ref _deliveries));
}
// ReSharper disable once MemberHidesStaticFromOuterClass
public virtual bool DeliverEvent(object sender, TEventArgs args)
{
var hasStaleEntries = false;
foreach (var handler in _handlers)
{
if (handler.IsActive)
{
var @delegate = handler.Handler as Delegate;
@delegate?.DynamicInvoke(sender, args);
}
else
{
hasStaleEntries = true;
}
}
return hasStaleEntries;
}
public void Purge()
{
for (var i = _handlers.Count - 1; i >= 0; i--)
{
if (!_handlers[i].IsActive)
{
_handlers.RemoveAt(i);
}
}
}
}
}
}
<MSG> fixes major bug in weak event manager where it would only unsubscribe
events that were of PropertyChangedHandlers meaning lots of events were being sent to the wrong instances.
<DFF> @@ -255,10 +255,9 @@ namespace AvaloniaEdit.Utils
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
- _originalHandler.Target is PropertyChangedEventHandler &&
- handler is PropertyChangedEventHandler &&
- Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
- (handler as PropertyChangedEventHandler).Target));
+ _originalHandler.Target is TEventHandler &&
+ handler is TEventHandler &&
+ handler is Delegate del && _originalHandler.Target is Delegate origDel && Equals(del.Target, origDel.Target));
}
}
| 3 | fixes major bug in weak event manager where it would only unsubscribe | 4 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063783 | <NME> WeakEventManagerBase.cs
<BEF> using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Reactive.Disposables;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Threading;
namespace AvaloniaEdit.Utils
{
/// <summary>
/// WeakEventManager base class. Inspired by the WPF WeakEventManager class and the code in
/// https://social.msdn.microsoft.com/Forums/silverlight/en-US/34d85c3f-52ea-4adc-bb32-8297f5549042/command-binding-memory-leak?forum=silverlightbugs
/// </summary>
/// <remarks>Copied here from ReactiveUI due to bugs in its design (singleton instance for multiple events).</remarks>
/// <typeparam name="TEventManager"></typeparam>
/// <typeparam name="TEventSource">The type of the event source.</typeparam>
/// <typeparam name="TEventHandler">The type of the event handler.</typeparam>
/// <typeparam name="TEventArgs">The type of the event arguments.</typeparam>
public abstract class WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>
where TEventManager : WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>, new()
{
// ReSharper disable once StaticMemberInGenericType
private static readonly object StaticSource = new object();
/// <summary>
/// Mapping between the target of the delegate (for example a Button) and the handler (EventHandler).
/// Windows Phone needs this, otherwise the event handler gets garbage collected.
/// </summary>
private readonly ConditionalWeakTable<object, List<Delegate>> _targetToEventHandler = new ConditionalWeakTable<object, List<Delegate>>();
/// <summary>
/// Mapping from the source of the event to the list of handlers. This is a CWT to ensure it does not leak the source of the event.
/// </summary>
private readonly ConditionalWeakTable<object, WeakHandlerList> _sourceToWeakHandlers = new ConditionalWeakTable<object, WeakHandlerList>();
private static readonly Lazy<TEventManager> CurrentLazy = new Lazy<TEventManager>(() => new TEventManager());
private static TEventManager Current => CurrentLazy.Value;
/// <summary>
/// Adds a weak reference to the handler and associates it with the source.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void AddHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateAddHandler(source, handler);
}
/// <summary>
/// Removes the association between the source and the handler.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void RemoveHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateRemoveHandler(source, handler);
}
/// <summary>
/// Delivers the event to the handlers registered for the source.
/// </summary>
/// <param name="sender">The sender.</param>
/// <param name="args">The <see cref="TEventArgs"/> instance containing the event data.</param>
protected static void DeliverEvent(object sender, TEventArgs args)
{
Current.PrivateDeliverEvent(sender, args);
}
/// <summary>
/// Override this method to attach to an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StartListening(TEventSource source);
/// <summary>
/// Override this method to detach from an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StopListening(TEventSource source);
protected void PrivateAddHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("Handler must be Delegate type");
}
AddWeakHandler(source, handler);
AddTargetHandler(handler);
}
private void AddWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
weakHandlers.AddWeakHandler(source, handler);
}
else
{
weakHandlers = new WeakHandlerList();
weakHandlers.AddWeakHandler(source, handler);
_sourceToWeakHandlers.Add(source, weakHandlers);
StartListening(source);
}
Purge(source);
}
private void AddTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Add(@delegate);
}
else
{
delegates = new List<Delegate> { @delegate };
_targetToEventHandler.Add(key, delegates);
}
}
protected void PrivateRemoveHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("handler must be Delegate type");
}
RemoveWeakHandler(source, handler);
RemoveTargetHandler(handler);
}
private void RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
if (weakHandlers.RemoveWeakHandler(source, handler) && weakHandlers.Count == 0)
{
_sourceToWeakHandlers.Remove(source);
StopListening(source);
}
}
}
private void RemoveTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Remove(@delegate);
if (delegates.Count == 0)
{
_targetToEventHandler.Remove(key);
}
}
}
private void PrivateDeliverEvent(object sender, TEventArgs args)
{
var source = sender ?? StaticSource;
var hasStaleEntries = false;
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
using (weakHandlers.DeliverActive())
{
hasStaleEntries = weakHandlers.DeliverEvent(source, args);
}
}
if (hasStaleEntries)
{
Purge(source);
}
}
private void Purge(object source)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
else
{
weakHandlers.Purge();
}
}
}
internal class WeakHandler
{
private readonly WeakReference _source;
private readonly WeakReference _originalHandler;
public bool IsActive => _source != null && _source.IsAlive && _originalHandler != null && _originalHandler.IsAlive;
public TEventHandler Handler
{
get
{
if (_originalHandler == null)
{
return default(TEventHandler);
}
return (TEventHandler)_originalHandler.Target;
}
}
public WeakHandler(object source, TEventHandler originalHandler)
{
_source = new WeakReference(source);
_originalHandler = new WeakReference(originalHandler);
}
public bool Matches(object o, TEventHandler handler)
{
return _source != null &&
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
_originalHandler.Target is PropertyChangedEventHandler &&
handler is PropertyChangedEventHandler &&
Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
(handler as PropertyChangedEventHandler).Target));
}
}
internal class WeakHandlerList
{
private int _deliveries;
private readonly List<WeakHandler> _handlers;
public WeakHandlerList()
{
_handlers = new List<WeakHandler>();
}
public void AddWeakHandler(TEventSource source, TEventHandler handler)
{
var handlerSink = new WeakHandler(source, handler);
_handlers.Add(handlerSink);
}
public bool RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.Matches(source, handler))
{
return _handlers.Remove(weakHandler);
}
}
return false;
}
public WeakHandlerList Clone()
{
var newList = new WeakHandlerList();
newList._handlers.AddRange(_handlers.Where(h => h.IsActive));
return newList;
}
public int Count => _handlers.Count;
public bool IsDeliverActive => _deliveries > 0;
public IDisposable DeliverActive()
{
Interlocked.Increment(ref _deliveries);
return Disposable.Create(() => Interlocked.Decrement(ref _deliveries));
}
// ReSharper disable once MemberHidesStaticFromOuterClass
public virtual bool DeliverEvent(object sender, TEventArgs args)
{
var hasStaleEntries = false;
foreach (var handler in _handlers)
{
if (handler.IsActive)
{
var @delegate = handler.Handler as Delegate;
@delegate?.DynamicInvoke(sender, args);
}
else
{
hasStaleEntries = true;
}
}
return hasStaleEntries;
}
public void Purge()
{
for (var i = _handlers.Count - 1; i >= 0; i--)
{
if (!_handlers[i].IsActive)
{
_handlers.RemoveAt(i);
}
}
}
}
}
}
<MSG> fixes major bug in weak event manager where it would only unsubscribe
events that were of PropertyChangedHandlers meaning lots of events were being sent to the wrong instances.
<DFF> @@ -255,10 +255,9 @@ namespace AvaloniaEdit.Utils
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
- _originalHandler.Target is PropertyChangedEventHandler &&
- handler is PropertyChangedEventHandler &&
- Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
- (handler as PropertyChangedEventHandler).Target));
+ _originalHandler.Target is TEventHandler &&
+ handler is TEventHandler &&
+ handler is Delegate del && _originalHandler.Target is Delegate origDel && Equals(del.Target, origDel.Target));
}
}
| 3 | fixes major bug in weak event manager where it would only unsubscribe | 4 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063784 | <NME> WeakEventManagerBase.cs
<BEF> using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Reactive.Disposables;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Threading;
namespace AvaloniaEdit.Utils
{
/// <summary>
/// WeakEventManager base class. Inspired by the WPF WeakEventManager class and the code in
/// https://social.msdn.microsoft.com/Forums/silverlight/en-US/34d85c3f-52ea-4adc-bb32-8297f5549042/command-binding-memory-leak?forum=silverlightbugs
/// </summary>
/// <remarks>Copied here from ReactiveUI due to bugs in its design (singleton instance for multiple events).</remarks>
/// <typeparam name="TEventManager"></typeparam>
/// <typeparam name="TEventSource">The type of the event source.</typeparam>
/// <typeparam name="TEventHandler">The type of the event handler.</typeparam>
/// <typeparam name="TEventArgs">The type of the event arguments.</typeparam>
public abstract class WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>
where TEventManager : WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>, new()
{
// ReSharper disable once StaticMemberInGenericType
private static readonly object StaticSource = new object();
/// <summary>
/// Mapping between the target of the delegate (for example a Button) and the handler (EventHandler).
/// Windows Phone needs this, otherwise the event handler gets garbage collected.
/// </summary>
private readonly ConditionalWeakTable<object, List<Delegate>> _targetToEventHandler = new ConditionalWeakTable<object, List<Delegate>>();
/// <summary>
/// Mapping from the source of the event to the list of handlers. This is a CWT to ensure it does not leak the source of the event.
/// </summary>
private readonly ConditionalWeakTable<object, WeakHandlerList> _sourceToWeakHandlers = new ConditionalWeakTable<object, WeakHandlerList>();
private static readonly Lazy<TEventManager> CurrentLazy = new Lazy<TEventManager>(() => new TEventManager());
private static TEventManager Current => CurrentLazy.Value;
/// <summary>
/// Adds a weak reference to the handler and associates it with the source.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void AddHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateAddHandler(source, handler);
}
/// <summary>
/// Removes the association between the source and the handler.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void RemoveHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateRemoveHandler(source, handler);
}
/// <summary>
/// Delivers the event to the handlers registered for the source.
/// </summary>
/// <param name="sender">The sender.</param>
/// <param name="args">The <see cref="TEventArgs"/> instance containing the event data.</param>
protected static void DeliverEvent(object sender, TEventArgs args)
{
Current.PrivateDeliverEvent(sender, args);
}
/// <summary>
/// Override this method to attach to an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StartListening(TEventSource source);
/// <summary>
/// Override this method to detach from an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StopListening(TEventSource source);
protected void PrivateAddHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("Handler must be Delegate type");
}
AddWeakHandler(source, handler);
AddTargetHandler(handler);
}
private void AddWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
weakHandlers.AddWeakHandler(source, handler);
}
else
{
weakHandlers = new WeakHandlerList();
weakHandlers.AddWeakHandler(source, handler);
_sourceToWeakHandlers.Add(source, weakHandlers);
StartListening(source);
}
Purge(source);
}
private void AddTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Add(@delegate);
}
else
{
delegates = new List<Delegate> { @delegate };
_targetToEventHandler.Add(key, delegates);
}
}
protected void PrivateRemoveHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("handler must be Delegate type");
}
RemoveWeakHandler(source, handler);
RemoveTargetHandler(handler);
}
private void RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
if (weakHandlers.RemoveWeakHandler(source, handler) && weakHandlers.Count == 0)
{
_sourceToWeakHandlers.Remove(source);
StopListening(source);
}
}
}
private void RemoveTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Remove(@delegate);
if (delegates.Count == 0)
{
_targetToEventHandler.Remove(key);
}
}
}
private void PrivateDeliverEvent(object sender, TEventArgs args)
{
var source = sender ?? StaticSource;
var hasStaleEntries = false;
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
using (weakHandlers.DeliverActive())
{
hasStaleEntries = weakHandlers.DeliverEvent(source, args);
}
}
if (hasStaleEntries)
{
Purge(source);
}
}
private void Purge(object source)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
else
{
weakHandlers.Purge();
}
}
}
internal class WeakHandler
{
private readonly WeakReference _source;
private readonly WeakReference _originalHandler;
public bool IsActive => _source != null && _source.IsAlive && _originalHandler != null && _originalHandler.IsAlive;
public TEventHandler Handler
{
get
{
if (_originalHandler == null)
{
return default(TEventHandler);
}
return (TEventHandler)_originalHandler.Target;
}
}
public WeakHandler(object source, TEventHandler originalHandler)
{
_source = new WeakReference(source);
_originalHandler = new WeakReference(originalHandler);
}
public bool Matches(object o, TEventHandler handler)
{
return _source != null &&
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
_originalHandler.Target is PropertyChangedEventHandler &&
handler is PropertyChangedEventHandler &&
Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
(handler as PropertyChangedEventHandler).Target));
}
}
internal class WeakHandlerList
{
private int _deliveries;
private readonly List<WeakHandler> _handlers;
public WeakHandlerList()
{
_handlers = new List<WeakHandler>();
}
public void AddWeakHandler(TEventSource source, TEventHandler handler)
{
var handlerSink = new WeakHandler(source, handler);
_handlers.Add(handlerSink);
}
public bool RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.Matches(source, handler))
{
return _handlers.Remove(weakHandler);
}
}
return false;
}
public WeakHandlerList Clone()
{
var newList = new WeakHandlerList();
newList._handlers.AddRange(_handlers.Where(h => h.IsActive));
return newList;
}
public int Count => _handlers.Count;
public bool IsDeliverActive => _deliveries > 0;
public IDisposable DeliverActive()
{
Interlocked.Increment(ref _deliveries);
return Disposable.Create(() => Interlocked.Decrement(ref _deliveries));
}
// ReSharper disable once MemberHidesStaticFromOuterClass
public virtual bool DeliverEvent(object sender, TEventArgs args)
{
var hasStaleEntries = false;
foreach (var handler in _handlers)
{
if (handler.IsActive)
{
var @delegate = handler.Handler as Delegate;
@delegate?.DynamicInvoke(sender, args);
}
else
{
hasStaleEntries = true;
}
}
return hasStaleEntries;
}
public void Purge()
{
for (var i = _handlers.Count - 1; i >= 0; i--)
{
if (!_handlers[i].IsActive)
{
_handlers.RemoveAt(i);
}
}
}
}
}
}
<MSG> fixes major bug in weak event manager where it would only unsubscribe
events that were of PropertyChangedHandlers meaning lots of events were being sent to the wrong instances.
<DFF> @@ -255,10 +255,9 @@ namespace AvaloniaEdit.Utils
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
- _originalHandler.Target is PropertyChangedEventHandler &&
- handler is PropertyChangedEventHandler &&
- Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
- (handler as PropertyChangedEventHandler).Target));
+ _originalHandler.Target is TEventHandler &&
+ handler is TEventHandler &&
+ handler is Delegate del && _originalHandler.Target is Delegate origDel && Equals(del.Target, origDel.Target));
}
}
| 3 | fixes major bug in weak event manager where it would only unsubscribe | 4 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063785 | <NME> WeakEventManagerBase.cs
<BEF> using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Reactive.Disposables;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Threading;
namespace AvaloniaEdit.Utils
{
/// <summary>
/// WeakEventManager base class. Inspired by the WPF WeakEventManager class and the code in
/// https://social.msdn.microsoft.com/Forums/silverlight/en-US/34d85c3f-52ea-4adc-bb32-8297f5549042/command-binding-memory-leak?forum=silverlightbugs
/// </summary>
/// <remarks>Copied here from ReactiveUI due to bugs in its design (singleton instance for multiple events).</remarks>
/// <typeparam name="TEventManager"></typeparam>
/// <typeparam name="TEventSource">The type of the event source.</typeparam>
/// <typeparam name="TEventHandler">The type of the event handler.</typeparam>
/// <typeparam name="TEventArgs">The type of the event arguments.</typeparam>
public abstract class WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>
where TEventManager : WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>, new()
{
// ReSharper disable once StaticMemberInGenericType
private static readonly object StaticSource = new object();
/// <summary>
/// Mapping between the target of the delegate (for example a Button) and the handler (EventHandler).
/// Windows Phone needs this, otherwise the event handler gets garbage collected.
/// </summary>
private readonly ConditionalWeakTable<object, List<Delegate>> _targetToEventHandler = new ConditionalWeakTable<object, List<Delegate>>();
/// <summary>
/// Mapping from the source of the event to the list of handlers. This is a CWT to ensure it does not leak the source of the event.
/// </summary>
private readonly ConditionalWeakTable<object, WeakHandlerList> _sourceToWeakHandlers = new ConditionalWeakTable<object, WeakHandlerList>();
private static readonly Lazy<TEventManager> CurrentLazy = new Lazy<TEventManager>(() => new TEventManager());
private static TEventManager Current => CurrentLazy.Value;
/// <summary>
/// Adds a weak reference to the handler and associates it with the source.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void AddHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateAddHandler(source, handler);
}
/// <summary>
/// Removes the association between the source and the handler.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void RemoveHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateRemoveHandler(source, handler);
}
/// <summary>
/// Delivers the event to the handlers registered for the source.
/// </summary>
/// <param name="sender">The sender.</param>
/// <param name="args">The <see cref="TEventArgs"/> instance containing the event data.</param>
protected static void DeliverEvent(object sender, TEventArgs args)
{
Current.PrivateDeliverEvent(sender, args);
}
/// <summary>
/// Override this method to attach to an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StartListening(TEventSource source);
/// <summary>
/// Override this method to detach from an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StopListening(TEventSource source);
protected void PrivateAddHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("Handler must be Delegate type");
}
AddWeakHandler(source, handler);
AddTargetHandler(handler);
}
private void AddWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
weakHandlers.AddWeakHandler(source, handler);
}
else
{
weakHandlers = new WeakHandlerList();
weakHandlers.AddWeakHandler(source, handler);
_sourceToWeakHandlers.Add(source, weakHandlers);
StartListening(source);
}
Purge(source);
}
private void AddTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Add(@delegate);
}
else
{
delegates = new List<Delegate> { @delegate };
_targetToEventHandler.Add(key, delegates);
}
}
protected void PrivateRemoveHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("handler must be Delegate type");
}
RemoveWeakHandler(source, handler);
RemoveTargetHandler(handler);
}
private void RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
if (weakHandlers.RemoveWeakHandler(source, handler) && weakHandlers.Count == 0)
{
_sourceToWeakHandlers.Remove(source);
StopListening(source);
}
}
}
private void RemoveTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Remove(@delegate);
if (delegates.Count == 0)
{
_targetToEventHandler.Remove(key);
}
}
}
private void PrivateDeliverEvent(object sender, TEventArgs args)
{
var source = sender ?? StaticSource;
var hasStaleEntries = false;
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
using (weakHandlers.DeliverActive())
{
hasStaleEntries = weakHandlers.DeliverEvent(source, args);
}
}
if (hasStaleEntries)
{
Purge(source);
}
}
private void Purge(object source)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
else
{
weakHandlers.Purge();
}
}
}
internal class WeakHandler
{
private readonly WeakReference _source;
private readonly WeakReference _originalHandler;
public bool IsActive => _source != null && _source.IsAlive && _originalHandler != null && _originalHandler.IsAlive;
public TEventHandler Handler
{
get
{
if (_originalHandler == null)
{
return default(TEventHandler);
}
return (TEventHandler)_originalHandler.Target;
}
}
public WeakHandler(object source, TEventHandler originalHandler)
{
_source = new WeakReference(source);
_originalHandler = new WeakReference(originalHandler);
}
public bool Matches(object o, TEventHandler handler)
{
return _source != null &&
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
_originalHandler.Target is PropertyChangedEventHandler &&
handler is PropertyChangedEventHandler &&
Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
(handler as PropertyChangedEventHandler).Target));
}
}
internal class WeakHandlerList
{
private int _deliveries;
private readonly List<WeakHandler> _handlers;
public WeakHandlerList()
{
_handlers = new List<WeakHandler>();
}
public void AddWeakHandler(TEventSource source, TEventHandler handler)
{
var handlerSink = new WeakHandler(source, handler);
_handlers.Add(handlerSink);
}
public bool RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.Matches(source, handler))
{
return _handlers.Remove(weakHandler);
}
}
return false;
}
public WeakHandlerList Clone()
{
var newList = new WeakHandlerList();
newList._handlers.AddRange(_handlers.Where(h => h.IsActive));
return newList;
}
public int Count => _handlers.Count;
public bool IsDeliverActive => _deliveries > 0;
public IDisposable DeliverActive()
{
Interlocked.Increment(ref _deliveries);
return Disposable.Create(() => Interlocked.Decrement(ref _deliveries));
}
// ReSharper disable once MemberHidesStaticFromOuterClass
public virtual bool DeliverEvent(object sender, TEventArgs args)
{
var hasStaleEntries = false;
foreach (var handler in _handlers)
{
if (handler.IsActive)
{
var @delegate = handler.Handler as Delegate;
@delegate?.DynamicInvoke(sender, args);
}
else
{
hasStaleEntries = true;
}
}
return hasStaleEntries;
}
public void Purge()
{
for (var i = _handlers.Count - 1; i >= 0; i--)
{
if (!_handlers[i].IsActive)
{
_handlers.RemoveAt(i);
}
}
}
}
}
}
<MSG> fixes major bug in weak event manager where it would only unsubscribe
events that were of PropertyChangedHandlers meaning lots of events were being sent to the wrong instances.
<DFF> @@ -255,10 +255,9 @@ namespace AvaloniaEdit.Utils
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
- _originalHandler.Target is PropertyChangedEventHandler &&
- handler is PropertyChangedEventHandler &&
- Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
- (handler as PropertyChangedEventHandler).Target));
+ _originalHandler.Target is TEventHandler &&
+ handler is TEventHandler &&
+ handler is Delegate del && _originalHandler.Target is Delegate origDel && Equals(del.Target, origDel.Target));
}
}
| 3 | fixes major bug in weak event manager where it would only unsubscribe | 4 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063786 | <NME> WeakEventManagerBase.cs
<BEF> using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Reactive.Disposables;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Threading;
namespace AvaloniaEdit.Utils
{
/// <summary>
/// WeakEventManager base class. Inspired by the WPF WeakEventManager class and the code in
/// https://social.msdn.microsoft.com/Forums/silverlight/en-US/34d85c3f-52ea-4adc-bb32-8297f5549042/command-binding-memory-leak?forum=silverlightbugs
/// </summary>
/// <remarks>Copied here from ReactiveUI due to bugs in its design (singleton instance for multiple events).</remarks>
/// <typeparam name="TEventManager"></typeparam>
/// <typeparam name="TEventSource">The type of the event source.</typeparam>
/// <typeparam name="TEventHandler">The type of the event handler.</typeparam>
/// <typeparam name="TEventArgs">The type of the event arguments.</typeparam>
public abstract class WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>
where TEventManager : WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>, new()
{
// ReSharper disable once StaticMemberInGenericType
private static readonly object StaticSource = new object();
/// <summary>
/// Mapping between the target of the delegate (for example a Button) and the handler (EventHandler).
/// Windows Phone needs this, otherwise the event handler gets garbage collected.
/// </summary>
private readonly ConditionalWeakTable<object, List<Delegate>> _targetToEventHandler = new ConditionalWeakTable<object, List<Delegate>>();
/// <summary>
/// Mapping from the source of the event to the list of handlers. This is a CWT to ensure it does not leak the source of the event.
/// </summary>
private readonly ConditionalWeakTable<object, WeakHandlerList> _sourceToWeakHandlers = new ConditionalWeakTable<object, WeakHandlerList>();
private static readonly Lazy<TEventManager> CurrentLazy = new Lazy<TEventManager>(() => new TEventManager());
private static TEventManager Current => CurrentLazy.Value;
/// <summary>
/// Adds a weak reference to the handler and associates it with the source.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void AddHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateAddHandler(source, handler);
}
/// <summary>
/// Removes the association between the source and the handler.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void RemoveHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateRemoveHandler(source, handler);
}
/// <summary>
/// Delivers the event to the handlers registered for the source.
/// </summary>
/// <param name="sender">The sender.</param>
/// <param name="args">The <see cref="TEventArgs"/> instance containing the event data.</param>
protected static void DeliverEvent(object sender, TEventArgs args)
{
Current.PrivateDeliverEvent(sender, args);
}
/// <summary>
/// Override this method to attach to an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StartListening(TEventSource source);
/// <summary>
/// Override this method to detach from an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StopListening(TEventSource source);
protected void PrivateAddHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("Handler must be Delegate type");
}
AddWeakHandler(source, handler);
AddTargetHandler(handler);
}
private void AddWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
weakHandlers.AddWeakHandler(source, handler);
}
else
{
weakHandlers = new WeakHandlerList();
weakHandlers.AddWeakHandler(source, handler);
_sourceToWeakHandlers.Add(source, weakHandlers);
StartListening(source);
}
Purge(source);
}
private void AddTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Add(@delegate);
}
else
{
delegates = new List<Delegate> { @delegate };
_targetToEventHandler.Add(key, delegates);
}
}
protected void PrivateRemoveHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("handler must be Delegate type");
}
RemoveWeakHandler(source, handler);
RemoveTargetHandler(handler);
}
private void RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
if (weakHandlers.RemoveWeakHandler(source, handler) && weakHandlers.Count == 0)
{
_sourceToWeakHandlers.Remove(source);
StopListening(source);
}
}
}
private void RemoveTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Remove(@delegate);
if (delegates.Count == 0)
{
_targetToEventHandler.Remove(key);
}
}
}
private void PrivateDeliverEvent(object sender, TEventArgs args)
{
var source = sender ?? StaticSource;
var hasStaleEntries = false;
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
using (weakHandlers.DeliverActive())
{
hasStaleEntries = weakHandlers.DeliverEvent(source, args);
}
}
if (hasStaleEntries)
{
Purge(source);
}
}
private void Purge(object source)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
else
{
weakHandlers.Purge();
}
}
}
internal class WeakHandler
{
private readonly WeakReference _source;
private readonly WeakReference _originalHandler;
public bool IsActive => _source != null && _source.IsAlive && _originalHandler != null && _originalHandler.IsAlive;
public TEventHandler Handler
{
get
{
if (_originalHandler == null)
{
return default(TEventHandler);
}
return (TEventHandler)_originalHandler.Target;
}
}
public WeakHandler(object source, TEventHandler originalHandler)
{
_source = new WeakReference(source);
_originalHandler = new WeakReference(originalHandler);
}
public bool Matches(object o, TEventHandler handler)
{
return _source != null &&
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
_originalHandler.Target is PropertyChangedEventHandler &&
handler is PropertyChangedEventHandler &&
Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
(handler as PropertyChangedEventHandler).Target));
}
}
internal class WeakHandlerList
{
private int _deliveries;
private readonly List<WeakHandler> _handlers;
public WeakHandlerList()
{
_handlers = new List<WeakHandler>();
}
public void AddWeakHandler(TEventSource source, TEventHandler handler)
{
var handlerSink = new WeakHandler(source, handler);
_handlers.Add(handlerSink);
}
public bool RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.Matches(source, handler))
{
return _handlers.Remove(weakHandler);
}
}
return false;
}
public WeakHandlerList Clone()
{
var newList = new WeakHandlerList();
newList._handlers.AddRange(_handlers.Where(h => h.IsActive));
return newList;
}
public int Count => _handlers.Count;
public bool IsDeliverActive => _deliveries > 0;
public IDisposable DeliverActive()
{
Interlocked.Increment(ref _deliveries);
return Disposable.Create(() => Interlocked.Decrement(ref _deliveries));
}
// ReSharper disable once MemberHidesStaticFromOuterClass
public virtual bool DeliverEvent(object sender, TEventArgs args)
{
var hasStaleEntries = false;
foreach (var handler in _handlers)
{
if (handler.IsActive)
{
var @delegate = handler.Handler as Delegate;
@delegate?.DynamicInvoke(sender, args);
}
else
{
hasStaleEntries = true;
}
}
return hasStaleEntries;
}
public void Purge()
{
for (var i = _handlers.Count - 1; i >= 0; i--)
{
if (!_handlers[i].IsActive)
{
_handlers.RemoveAt(i);
}
}
}
}
}
}
<MSG> fixes major bug in weak event manager where it would only unsubscribe
events that were of PropertyChangedHandlers meaning lots of events were being sent to the wrong instances.
<DFF> @@ -255,10 +255,9 @@ namespace AvaloniaEdit.Utils
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
- _originalHandler.Target is PropertyChangedEventHandler &&
- handler is PropertyChangedEventHandler &&
- Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
- (handler as PropertyChangedEventHandler).Target));
+ _originalHandler.Target is TEventHandler &&
+ handler is TEventHandler &&
+ handler is Delegate del && _originalHandler.Target is Delegate origDel && Equals(del.Target, origDel.Target));
}
}
| 3 | fixes major bug in weak event manager where it would only unsubscribe | 4 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063787 | <NME> WeakEventManagerBase.cs
<BEF> using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Reactive.Disposables;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Threading;
namespace AvaloniaEdit.Utils
{
/// <summary>
/// WeakEventManager base class. Inspired by the WPF WeakEventManager class and the code in
/// https://social.msdn.microsoft.com/Forums/silverlight/en-US/34d85c3f-52ea-4adc-bb32-8297f5549042/command-binding-memory-leak?forum=silverlightbugs
/// </summary>
/// <remarks>Copied here from ReactiveUI due to bugs in its design (singleton instance for multiple events).</remarks>
/// <typeparam name="TEventManager"></typeparam>
/// <typeparam name="TEventSource">The type of the event source.</typeparam>
/// <typeparam name="TEventHandler">The type of the event handler.</typeparam>
/// <typeparam name="TEventArgs">The type of the event arguments.</typeparam>
public abstract class WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>
where TEventManager : WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>, new()
{
// ReSharper disable once StaticMemberInGenericType
private static readonly object StaticSource = new object();
/// <summary>
/// Mapping between the target of the delegate (for example a Button) and the handler (EventHandler).
/// Windows Phone needs this, otherwise the event handler gets garbage collected.
/// </summary>
private readonly ConditionalWeakTable<object, List<Delegate>> _targetToEventHandler = new ConditionalWeakTable<object, List<Delegate>>();
/// <summary>
/// Mapping from the source of the event to the list of handlers. This is a CWT to ensure it does not leak the source of the event.
/// </summary>
private readonly ConditionalWeakTable<object, WeakHandlerList> _sourceToWeakHandlers = new ConditionalWeakTable<object, WeakHandlerList>();
private static readonly Lazy<TEventManager> CurrentLazy = new Lazy<TEventManager>(() => new TEventManager());
private static TEventManager Current => CurrentLazy.Value;
/// <summary>
/// Adds a weak reference to the handler and associates it with the source.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void AddHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateAddHandler(source, handler);
}
/// <summary>
/// Removes the association between the source and the handler.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void RemoveHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateRemoveHandler(source, handler);
}
/// <summary>
/// Delivers the event to the handlers registered for the source.
/// </summary>
/// <param name="sender">The sender.</param>
/// <param name="args">The <see cref="TEventArgs"/> instance containing the event data.</param>
protected static void DeliverEvent(object sender, TEventArgs args)
{
Current.PrivateDeliverEvent(sender, args);
}
/// <summary>
/// Override this method to attach to an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StartListening(TEventSource source);
/// <summary>
/// Override this method to detach from an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StopListening(TEventSource source);
protected void PrivateAddHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("Handler must be Delegate type");
}
AddWeakHandler(source, handler);
AddTargetHandler(handler);
}
private void AddWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
weakHandlers.AddWeakHandler(source, handler);
}
else
{
weakHandlers = new WeakHandlerList();
weakHandlers.AddWeakHandler(source, handler);
_sourceToWeakHandlers.Add(source, weakHandlers);
StartListening(source);
}
Purge(source);
}
private void AddTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Add(@delegate);
}
else
{
delegates = new List<Delegate> { @delegate };
_targetToEventHandler.Add(key, delegates);
}
}
protected void PrivateRemoveHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("handler must be Delegate type");
}
RemoveWeakHandler(source, handler);
RemoveTargetHandler(handler);
}
private void RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
if (weakHandlers.RemoveWeakHandler(source, handler) && weakHandlers.Count == 0)
{
_sourceToWeakHandlers.Remove(source);
StopListening(source);
}
}
}
private void RemoveTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Remove(@delegate);
if (delegates.Count == 0)
{
_targetToEventHandler.Remove(key);
}
}
}
private void PrivateDeliverEvent(object sender, TEventArgs args)
{
var source = sender ?? StaticSource;
var hasStaleEntries = false;
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
using (weakHandlers.DeliverActive())
{
hasStaleEntries = weakHandlers.DeliverEvent(source, args);
}
}
if (hasStaleEntries)
{
Purge(source);
}
}
private void Purge(object source)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
else
{
weakHandlers.Purge();
}
}
}
internal class WeakHandler
{
private readonly WeakReference _source;
private readonly WeakReference _originalHandler;
public bool IsActive => _source != null && _source.IsAlive && _originalHandler != null && _originalHandler.IsAlive;
public TEventHandler Handler
{
get
{
if (_originalHandler == null)
{
return default(TEventHandler);
}
return (TEventHandler)_originalHandler.Target;
}
}
public WeakHandler(object source, TEventHandler originalHandler)
{
_source = new WeakReference(source);
_originalHandler = new WeakReference(originalHandler);
}
public bool Matches(object o, TEventHandler handler)
{
return _source != null &&
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
_originalHandler.Target is PropertyChangedEventHandler &&
handler is PropertyChangedEventHandler &&
Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
(handler as PropertyChangedEventHandler).Target));
}
}
internal class WeakHandlerList
{
private int _deliveries;
private readonly List<WeakHandler> _handlers;
public WeakHandlerList()
{
_handlers = new List<WeakHandler>();
}
public void AddWeakHandler(TEventSource source, TEventHandler handler)
{
var handlerSink = new WeakHandler(source, handler);
_handlers.Add(handlerSink);
}
public bool RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.Matches(source, handler))
{
return _handlers.Remove(weakHandler);
}
}
return false;
}
public WeakHandlerList Clone()
{
var newList = new WeakHandlerList();
newList._handlers.AddRange(_handlers.Where(h => h.IsActive));
return newList;
}
public int Count => _handlers.Count;
public bool IsDeliverActive => _deliveries > 0;
public IDisposable DeliverActive()
{
Interlocked.Increment(ref _deliveries);
return Disposable.Create(() => Interlocked.Decrement(ref _deliveries));
}
// ReSharper disable once MemberHidesStaticFromOuterClass
public virtual bool DeliverEvent(object sender, TEventArgs args)
{
var hasStaleEntries = false;
foreach (var handler in _handlers)
{
if (handler.IsActive)
{
var @delegate = handler.Handler as Delegate;
@delegate?.DynamicInvoke(sender, args);
}
else
{
hasStaleEntries = true;
}
}
return hasStaleEntries;
}
public void Purge()
{
for (var i = _handlers.Count - 1; i >= 0; i--)
{
if (!_handlers[i].IsActive)
{
_handlers.RemoveAt(i);
}
}
}
}
}
}
<MSG> fixes major bug in weak event manager where it would only unsubscribe
events that were of PropertyChangedHandlers meaning lots of events were being sent to the wrong instances.
<DFF> @@ -255,10 +255,9 @@ namespace AvaloniaEdit.Utils
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
- _originalHandler.Target is PropertyChangedEventHandler &&
- handler is PropertyChangedEventHandler &&
- Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
- (handler as PropertyChangedEventHandler).Target));
+ _originalHandler.Target is TEventHandler &&
+ handler is TEventHandler &&
+ handler is Delegate del && _originalHandler.Target is Delegate origDel && Equals(del.Target, origDel.Target));
}
}
| 3 | fixes major bug in weak event manager where it would only unsubscribe | 4 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063788 | <NME> WeakEventManagerBase.cs
<BEF> using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Reactive.Disposables;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Threading;
namespace AvaloniaEdit.Utils
{
/// <summary>
/// WeakEventManager base class. Inspired by the WPF WeakEventManager class and the code in
/// https://social.msdn.microsoft.com/Forums/silverlight/en-US/34d85c3f-52ea-4adc-bb32-8297f5549042/command-binding-memory-leak?forum=silverlightbugs
/// </summary>
/// <remarks>Copied here from ReactiveUI due to bugs in its design (singleton instance for multiple events).</remarks>
/// <typeparam name="TEventManager"></typeparam>
/// <typeparam name="TEventSource">The type of the event source.</typeparam>
/// <typeparam name="TEventHandler">The type of the event handler.</typeparam>
/// <typeparam name="TEventArgs">The type of the event arguments.</typeparam>
public abstract class WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>
where TEventManager : WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>, new()
{
// ReSharper disable once StaticMemberInGenericType
private static readonly object StaticSource = new object();
/// <summary>
/// Mapping between the target of the delegate (for example a Button) and the handler (EventHandler).
/// Windows Phone needs this, otherwise the event handler gets garbage collected.
/// </summary>
private readonly ConditionalWeakTable<object, List<Delegate>> _targetToEventHandler = new ConditionalWeakTable<object, List<Delegate>>();
/// <summary>
/// Mapping from the source of the event to the list of handlers. This is a CWT to ensure it does not leak the source of the event.
/// </summary>
private readonly ConditionalWeakTable<object, WeakHandlerList> _sourceToWeakHandlers = new ConditionalWeakTable<object, WeakHandlerList>();
private static readonly Lazy<TEventManager> CurrentLazy = new Lazy<TEventManager>(() => new TEventManager());
private static TEventManager Current => CurrentLazy.Value;
/// <summary>
/// Adds a weak reference to the handler and associates it with the source.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void AddHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateAddHandler(source, handler);
}
/// <summary>
/// Removes the association between the source and the handler.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void RemoveHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateRemoveHandler(source, handler);
}
/// <summary>
/// Delivers the event to the handlers registered for the source.
/// </summary>
/// <param name="sender">The sender.</param>
/// <param name="args">The <see cref="TEventArgs"/> instance containing the event data.</param>
protected static void DeliverEvent(object sender, TEventArgs args)
{
Current.PrivateDeliverEvent(sender, args);
}
/// <summary>
/// Override this method to attach to an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StartListening(TEventSource source);
/// <summary>
/// Override this method to detach from an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StopListening(TEventSource source);
protected void PrivateAddHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("Handler must be Delegate type");
}
AddWeakHandler(source, handler);
AddTargetHandler(handler);
}
private void AddWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
weakHandlers.AddWeakHandler(source, handler);
}
else
{
weakHandlers = new WeakHandlerList();
weakHandlers.AddWeakHandler(source, handler);
_sourceToWeakHandlers.Add(source, weakHandlers);
StartListening(source);
}
Purge(source);
}
private void AddTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Add(@delegate);
}
else
{
delegates = new List<Delegate> { @delegate };
_targetToEventHandler.Add(key, delegates);
}
}
protected void PrivateRemoveHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("handler must be Delegate type");
}
RemoveWeakHandler(source, handler);
RemoveTargetHandler(handler);
}
private void RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
if (weakHandlers.RemoveWeakHandler(source, handler) && weakHandlers.Count == 0)
{
_sourceToWeakHandlers.Remove(source);
StopListening(source);
}
}
}
private void RemoveTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Remove(@delegate);
if (delegates.Count == 0)
{
_targetToEventHandler.Remove(key);
}
}
}
private void PrivateDeliverEvent(object sender, TEventArgs args)
{
var source = sender ?? StaticSource;
var hasStaleEntries = false;
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
using (weakHandlers.DeliverActive())
{
hasStaleEntries = weakHandlers.DeliverEvent(source, args);
}
}
if (hasStaleEntries)
{
Purge(source);
}
}
private void Purge(object source)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
else
{
weakHandlers.Purge();
}
}
}
internal class WeakHandler
{
private readonly WeakReference _source;
private readonly WeakReference _originalHandler;
public bool IsActive => _source != null && _source.IsAlive && _originalHandler != null && _originalHandler.IsAlive;
public TEventHandler Handler
{
get
{
if (_originalHandler == null)
{
return default(TEventHandler);
}
return (TEventHandler)_originalHandler.Target;
}
}
public WeakHandler(object source, TEventHandler originalHandler)
{
_source = new WeakReference(source);
_originalHandler = new WeakReference(originalHandler);
}
public bool Matches(object o, TEventHandler handler)
{
return _source != null &&
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
_originalHandler.Target is PropertyChangedEventHandler &&
handler is PropertyChangedEventHandler &&
Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
(handler as PropertyChangedEventHandler).Target));
}
}
internal class WeakHandlerList
{
private int _deliveries;
private readonly List<WeakHandler> _handlers;
public WeakHandlerList()
{
_handlers = new List<WeakHandler>();
}
public void AddWeakHandler(TEventSource source, TEventHandler handler)
{
var handlerSink = new WeakHandler(source, handler);
_handlers.Add(handlerSink);
}
public bool RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.Matches(source, handler))
{
return _handlers.Remove(weakHandler);
}
}
return false;
}
public WeakHandlerList Clone()
{
var newList = new WeakHandlerList();
newList._handlers.AddRange(_handlers.Where(h => h.IsActive));
return newList;
}
public int Count => _handlers.Count;
public bool IsDeliverActive => _deliveries > 0;
public IDisposable DeliverActive()
{
Interlocked.Increment(ref _deliveries);
return Disposable.Create(() => Interlocked.Decrement(ref _deliveries));
}
// ReSharper disable once MemberHidesStaticFromOuterClass
public virtual bool DeliverEvent(object sender, TEventArgs args)
{
var hasStaleEntries = false;
foreach (var handler in _handlers)
{
if (handler.IsActive)
{
var @delegate = handler.Handler as Delegate;
@delegate?.DynamicInvoke(sender, args);
}
else
{
hasStaleEntries = true;
}
}
return hasStaleEntries;
}
public void Purge()
{
for (var i = _handlers.Count - 1; i >= 0; i--)
{
if (!_handlers[i].IsActive)
{
_handlers.RemoveAt(i);
}
}
}
}
}
}
<MSG> fixes major bug in weak event manager where it would only unsubscribe
events that were of PropertyChangedHandlers meaning lots of events were being sent to the wrong instances.
<DFF> @@ -255,10 +255,9 @@ namespace AvaloniaEdit.Utils
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
- _originalHandler.Target is PropertyChangedEventHandler &&
- handler is PropertyChangedEventHandler &&
- Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
- (handler as PropertyChangedEventHandler).Target));
+ _originalHandler.Target is TEventHandler &&
+ handler is TEventHandler &&
+ handler is Delegate del && _originalHandler.Target is Delegate origDel && Equals(del.Target, origDel.Target));
}
}
| 3 | fixes major bug in weak event manager where it would only unsubscribe | 4 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063789 | <NME> WeakEventManagerBase.cs
<BEF> using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Reactive.Disposables;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Threading;
namespace AvaloniaEdit.Utils
{
/// <summary>
/// WeakEventManager base class. Inspired by the WPF WeakEventManager class and the code in
/// https://social.msdn.microsoft.com/Forums/silverlight/en-US/34d85c3f-52ea-4adc-bb32-8297f5549042/command-binding-memory-leak?forum=silverlightbugs
/// </summary>
/// <remarks>Copied here from ReactiveUI due to bugs in its design (singleton instance for multiple events).</remarks>
/// <typeparam name="TEventManager"></typeparam>
/// <typeparam name="TEventSource">The type of the event source.</typeparam>
/// <typeparam name="TEventHandler">The type of the event handler.</typeparam>
/// <typeparam name="TEventArgs">The type of the event arguments.</typeparam>
public abstract class WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>
where TEventManager : WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>, new()
{
// ReSharper disable once StaticMemberInGenericType
private static readonly object StaticSource = new object();
/// <summary>
/// Mapping between the target of the delegate (for example a Button) and the handler (EventHandler).
/// Windows Phone needs this, otherwise the event handler gets garbage collected.
/// </summary>
private readonly ConditionalWeakTable<object, List<Delegate>> _targetToEventHandler = new ConditionalWeakTable<object, List<Delegate>>();
/// <summary>
/// Mapping from the source of the event to the list of handlers. This is a CWT to ensure it does not leak the source of the event.
/// </summary>
private readonly ConditionalWeakTable<object, WeakHandlerList> _sourceToWeakHandlers = new ConditionalWeakTable<object, WeakHandlerList>();
private static readonly Lazy<TEventManager> CurrentLazy = new Lazy<TEventManager>(() => new TEventManager());
private static TEventManager Current => CurrentLazy.Value;
/// <summary>
/// Adds a weak reference to the handler and associates it with the source.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void AddHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateAddHandler(source, handler);
}
/// <summary>
/// Removes the association between the source and the handler.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void RemoveHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateRemoveHandler(source, handler);
}
/// <summary>
/// Delivers the event to the handlers registered for the source.
/// </summary>
/// <param name="sender">The sender.</param>
/// <param name="args">The <see cref="TEventArgs"/> instance containing the event data.</param>
protected static void DeliverEvent(object sender, TEventArgs args)
{
Current.PrivateDeliverEvent(sender, args);
}
/// <summary>
/// Override this method to attach to an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StartListening(TEventSource source);
/// <summary>
/// Override this method to detach from an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StopListening(TEventSource source);
protected void PrivateAddHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("Handler must be Delegate type");
}
AddWeakHandler(source, handler);
AddTargetHandler(handler);
}
private void AddWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
weakHandlers.AddWeakHandler(source, handler);
}
else
{
weakHandlers = new WeakHandlerList();
weakHandlers.AddWeakHandler(source, handler);
_sourceToWeakHandlers.Add(source, weakHandlers);
StartListening(source);
}
Purge(source);
}
private void AddTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Add(@delegate);
}
else
{
delegates = new List<Delegate> { @delegate };
_targetToEventHandler.Add(key, delegates);
}
}
protected void PrivateRemoveHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("handler must be Delegate type");
}
RemoveWeakHandler(source, handler);
RemoveTargetHandler(handler);
}
private void RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
if (weakHandlers.RemoveWeakHandler(source, handler) && weakHandlers.Count == 0)
{
_sourceToWeakHandlers.Remove(source);
StopListening(source);
}
}
}
private void RemoveTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Remove(@delegate);
if (delegates.Count == 0)
{
_targetToEventHandler.Remove(key);
}
}
}
private void PrivateDeliverEvent(object sender, TEventArgs args)
{
var source = sender ?? StaticSource;
var hasStaleEntries = false;
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
using (weakHandlers.DeliverActive())
{
hasStaleEntries = weakHandlers.DeliverEvent(source, args);
}
}
if (hasStaleEntries)
{
Purge(source);
}
}
private void Purge(object source)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
else
{
weakHandlers.Purge();
}
}
}
internal class WeakHandler
{
private readonly WeakReference _source;
private readonly WeakReference _originalHandler;
public bool IsActive => _source != null && _source.IsAlive && _originalHandler != null && _originalHandler.IsAlive;
public TEventHandler Handler
{
get
{
if (_originalHandler == null)
{
return default(TEventHandler);
}
return (TEventHandler)_originalHandler.Target;
}
}
public WeakHandler(object source, TEventHandler originalHandler)
{
_source = new WeakReference(source);
_originalHandler = new WeakReference(originalHandler);
}
public bool Matches(object o, TEventHandler handler)
{
return _source != null &&
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
_originalHandler.Target is PropertyChangedEventHandler &&
handler is PropertyChangedEventHandler &&
Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
(handler as PropertyChangedEventHandler).Target));
}
}
internal class WeakHandlerList
{
private int _deliveries;
private readonly List<WeakHandler> _handlers;
public WeakHandlerList()
{
_handlers = new List<WeakHandler>();
}
public void AddWeakHandler(TEventSource source, TEventHandler handler)
{
var handlerSink = new WeakHandler(source, handler);
_handlers.Add(handlerSink);
}
public bool RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.Matches(source, handler))
{
return _handlers.Remove(weakHandler);
}
}
return false;
}
public WeakHandlerList Clone()
{
var newList = new WeakHandlerList();
newList._handlers.AddRange(_handlers.Where(h => h.IsActive));
return newList;
}
public int Count => _handlers.Count;
public bool IsDeliverActive => _deliveries > 0;
public IDisposable DeliverActive()
{
Interlocked.Increment(ref _deliveries);
return Disposable.Create(() => Interlocked.Decrement(ref _deliveries));
}
// ReSharper disable once MemberHidesStaticFromOuterClass
public virtual bool DeliverEvent(object sender, TEventArgs args)
{
var hasStaleEntries = false;
foreach (var handler in _handlers)
{
if (handler.IsActive)
{
var @delegate = handler.Handler as Delegate;
@delegate?.DynamicInvoke(sender, args);
}
else
{
hasStaleEntries = true;
}
}
return hasStaleEntries;
}
public void Purge()
{
for (var i = _handlers.Count - 1; i >= 0; i--)
{
if (!_handlers[i].IsActive)
{
_handlers.RemoveAt(i);
}
}
}
}
}
}
<MSG> fixes major bug in weak event manager where it would only unsubscribe
events that were of PropertyChangedHandlers meaning lots of events were being sent to the wrong instances.
<DFF> @@ -255,10 +255,9 @@ namespace AvaloniaEdit.Utils
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
- _originalHandler.Target is PropertyChangedEventHandler &&
- handler is PropertyChangedEventHandler &&
- Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
- (handler as PropertyChangedEventHandler).Target));
+ _originalHandler.Target is TEventHandler &&
+ handler is TEventHandler &&
+ handler is Delegate del && _originalHandler.Target is Delegate origDel && Equals(del.Target, origDel.Target));
}
}
| 3 | fixes major bug in weak event manager where it would only unsubscribe | 4 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063790 | <NME> WeakEventManagerBase.cs
<BEF> using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Reactive.Disposables;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Threading;
namespace AvaloniaEdit.Utils
{
/// <summary>
/// WeakEventManager base class. Inspired by the WPF WeakEventManager class and the code in
/// https://social.msdn.microsoft.com/Forums/silverlight/en-US/34d85c3f-52ea-4adc-bb32-8297f5549042/command-binding-memory-leak?forum=silverlightbugs
/// </summary>
/// <remarks>Copied here from ReactiveUI due to bugs in its design (singleton instance for multiple events).</remarks>
/// <typeparam name="TEventManager"></typeparam>
/// <typeparam name="TEventSource">The type of the event source.</typeparam>
/// <typeparam name="TEventHandler">The type of the event handler.</typeparam>
/// <typeparam name="TEventArgs">The type of the event arguments.</typeparam>
public abstract class WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>
where TEventManager : WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>, new()
{
// ReSharper disable once StaticMemberInGenericType
private static readonly object StaticSource = new object();
/// <summary>
/// Mapping between the target of the delegate (for example a Button) and the handler (EventHandler).
/// Windows Phone needs this, otherwise the event handler gets garbage collected.
/// </summary>
private readonly ConditionalWeakTable<object, List<Delegate>> _targetToEventHandler = new ConditionalWeakTable<object, List<Delegate>>();
/// <summary>
/// Mapping from the source of the event to the list of handlers. This is a CWT to ensure it does not leak the source of the event.
/// </summary>
private readonly ConditionalWeakTable<object, WeakHandlerList> _sourceToWeakHandlers = new ConditionalWeakTable<object, WeakHandlerList>();
private static readonly Lazy<TEventManager> CurrentLazy = new Lazy<TEventManager>(() => new TEventManager());
private static TEventManager Current => CurrentLazy.Value;
/// <summary>
/// Adds a weak reference to the handler and associates it with the source.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void AddHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateAddHandler(source, handler);
}
/// <summary>
/// Removes the association between the source and the handler.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void RemoveHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateRemoveHandler(source, handler);
}
/// <summary>
/// Delivers the event to the handlers registered for the source.
/// </summary>
/// <param name="sender">The sender.</param>
/// <param name="args">The <see cref="TEventArgs"/> instance containing the event data.</param>
protected static void DeliverEvent(object sender, TEventArgs args)
{
Current.PrivateDeliverEvent(sender, args);
}
/// <summary>
/// Override this method to attach to an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StartListening(TEventSource source);
/// <summary>
/// Override this method to detach from an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StopListening(TEventSource source);
protected void PrivateAddHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("Handler must be Delegate type");
}
AddWeakHandler(source, handler);
AddTargetHandler(handler);
}
private void AddWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
weakHandlers.AddWeakHandler(source, handler);
}
else
{
weakHandlers = new WeakHandlerList();
weakHandlers.AddWeakHandler(source, handler);
_sourceToWeakHandlers.Add(source, weakHandlers);
StartListening(source);
}
Purge(source);
}
private void AddTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Add(@delegate);
}
else
{
delegates = new List<Delegate> { @delegate };
_targetToEventHandler.Add(key, delegates);
}
}
protected void PrivateRemoveHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("handler must be Delegate type");
}
RemoveWeakHandler(source, handler);
RemoveTargetHandler(handler);
}
private void RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
if (weakHandlers.RemoveWeakHandler(source, handler) && weakHandlers.Count == 0)
{
_sourceToWeakHandlers.Remove(source);
StopListening(source);
}
}
}
private void RemoveTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Remove(@delegate);
if (delegates.Count == 0)
{
_targetToEventHandler.Remove(key);
}
}
}
private void PrivateDeliverEvent(object sender, TEventArgs args)
{
var source = sender ?? StaticSource;
var hasStaleEntries = false;
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
using (weakHandlers.DeliverActive())
{
hasStaleEntries = weakHandlers.DeliverEvent(source, args);
}
}
if (hasStaleEntries)
{
Purge(source);
}
}
private void Purge(object source)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
else
{
weakHandlers.Purge();
}
}
}
internal class WeakHandler
{
private readonly WeakReference _source;
private readonly WeakReference _originalHandler;
public bool IsActive => _source != null && _source.IsAlive && _originalHandler != null && _originalHandler.IsAlive;
public TEventHandler Handler
{
get
{
if (_originalHandler == null)
{
return default(TEventHandler);
}
return (TEventHandler)_originalHandler.Target;
}
}
public WeakHandler(object source, TEventHandler originalHandler)
{
_source = new WeakReference(source);
_originalHandler = new WeakReference(originalHandler);
}
public bool Matches(object o, TEventHandler handler)
{
return _source != null &&
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
_originalHandler.Target is PropertyChangedEventHandler &&
handler is PropertyChangedEventHandler &&
Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
(handler as PropertyChangedEventHandler).Target));
}
}
internal class WeakHandlerList
{
private int _deliveries;
private readonly List<WeakHandler> _handlers;
public WeakHandlerList()
{
_handlers = new List<WeakHandler>();
}
public void AddWeakHandler(TEventSource source, TEventHandler handler)
{
var handlerSink = new WeakHandler(source, handler);
_handlers.Add(handlerSink);
}
public bool RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.Matches(source, handler))
{
return _handlers.Remove(weakHandler);
}
}
return false;
}
public WeakHandlerList Clone()
{
var newList = new WeakHandlerList();
newList._handlers.AddRange(_handlers.Where(h => h.IsActive));
return newList;
}
public int Count => _handlers.Count;
public bool IsDeliverActive => _deliveries > 0;
public IDisposable DeliverActive()
{
Interlocked.Increment(ref _deliveries);
return Disposable.Create(() => Interlocked.Decrement(ref _deliveries));
}
// ReSharper disable once MemberHidesStaticFromOuterClass
public virtual bool DeliverEvent(object sender, TEventArgs args)
{
var hasStaleEntries = false;
foreach (var handler in _handlers)
{
if (handler.IsActive)
{
var @delegate = handler.Handler as Delegate;
@delegate?.DynamicInvoke(sender, args);
}
else
{
hasStaleEntries = true;
}
}
return hasStaleEntries;
}
public void Purge()
{
for (var i = _handlers.Count - 1; i >= 0; i--)
{
if (!_handlers[i].IsActive)
{
_handlers.RemoveAt(i);
}
}
}
}
}
}
<MSG> fixes major bug in weak event manager where it would only unsubscribe
events that were of PropertyChangedHandlers meaning lots of events were being sent to the wrong instances.
<DFF> @@ -255,10 +255,9 @@ namespace AvaloniaEdit.Utils
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
- _originalHandler.Target is PropertyChangedEventHandler &&
- handler is PropertyChangedEventHandler &&
- Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
- (handler as PropertyChangedEventHandler).Target));
+ _originalHandler.Target is TEventHandler &&
+ handler is TEventHandler &&
+ handler is Delegate del && _originalHandler.Target is Delegate origDel && Equals(del.Target, origDel.Target));
}
}
| 3 | fixes major bug in weak event manager where it would only unsubscribe | 4 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063791 | <NME> WeakEventManagerBase.cs
<BEF> using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Reactive.Disposables;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Threading;
namespace AvaloniaEdit.Utils
{
/// <summary>
/// WeakEventManager base class. Inspired by the WPF WeakEventManager class and the code in
/// https://social.msdn.microsoft.com/Forums/silverlight/en-US/34d85c3f-52ea-4adc-bb32-8297f5549042/command-binding-memory-leak?forum=silverlightbugs
/// </summary>
/// <remarks>Copied here from ReactiveUI due to bugs in its design (singleton instance for multiple events).</remarks>
/// <typeparam name="TEventManager"></typeparam>
/// <typeparam name="TEventSource">The type of the event source.</typeparam>
/// <typeparam name="TEventHandler">The type of the event handler.</typeparam>
/// <typeparam name="TEventArgs">The type of the event arguments.</typeparam>
public abstract class WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>
where TEventManager : WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>, new()
{
// ReSharper disable once StaticMemberInGenericType
private static readonly object StaticSource = new object();
/// <summary>
/// Mapping between the target of the delegate (for example a Button) and the handler (EventHandler).
/// Windows Phone needs this, otherwise the event handler gets garbage collected.
/// </summary>
private readonly ConditionalWeakTable<object, List<Delegate>> _targetToEventHandler = new ConditionalWeakTable<object, List<Delegate>>();
/// <summary>
/// Mapping from the source of the event to the list of handlers. This is a CWT to ensure it does not leak the source of the event.
/// </summary>
private readonly ConditionalWeakTable<object, WeakHandlerList> _sourceToWeakHandlers = new ConditionalWeakTable<object, WeakHandlerList>();
private static readonly Lazy<TEventManager> CurrentLazy = new Lazy<TEventManager>(() => new TEventManager());
private static TEventManager Current => CurrentLazy.Value;
/// <summary>
/// Adds a weak reference to the handler and associates it with the source.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void AddHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateAddHandler(source, handler);
}
/// <summary>
/// Removes the association between the source and the handler.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void RemoveHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateRemoveHandler(source, handler);
}
/// <summary>
/// Delivers the event to the handlers registered for the source.
/// </summary>
/// <param name="sender">The sender.</param>
/// <param name="args">The <see cref="TEventArgs"/> instance containing the event data.</param>
protected static void DeliverEvent(object sender, TEventArgs args)
{
Current.PrivateDeliverEvent(sender, args);
}
/// <summary>
/// Override this method to attach to an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StartListening(TEventSource source);
/// <summary>
/// Override this method to detach from an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StopListening(TEventSource source);
protected void PrivateAddHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("Handler must be Delegate type");
}
AddWeakHandler(source, handler);
AddTargetHandler(handler);
}
private void AddWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
weakHandlers.AddWeakHandler(source, handler);
}
else
{
weakHandlers = new WeakHandlerList();
weakHandlers.AddWeakHandler(source, handler);
_sourceToWeakHandlers.Add(source, weakHandlers);
StartListening(source);
}
Purge(source);
}
private void AddTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Add(@delegate);
}
else
{
delegates = new List<Delegate> { @delegate };
_targetToEventHandler.Add(key, delegates);
}
}
protected void PrivateRemoveHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("handler must be Delegate type");
}
RemoveWeakHandler(source, handler);
RemoveTargetHandler(handler);
}
private void RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
if (weakHandlers.RemoveWeakHandler(source, handler) && weakHandlers.Count == 0)
{
_sourceToWeakHandlers.Remove(source);
StopListening(source);
}
}
}
private void RemoveTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Remove(@delegate);
if (delegates.Count == 0)
{
_targetToEventHandler.Remove(key);
}
}
}
private void PrivateDeliverEvent(object sender, TEventArgs args)
{
var source = sender ?? StaticSource;
var hasStaleEntries = false;
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
using (weakHandlers.DeliverActive())
{
hasStaleEntries = weakHandlers.DeliverEvent(source, args);
}
}
if (hasStaleEntries)
{
Purge(source);
}
}
private void Purge(object source)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
else
{
weakHandlers.Purge();
}
}
}
internal class WeakHandler
{
private readonly WeakReference _source;
private readonly WeakReference _originalHandler;
public bool IsActive => _source != null && _source.IsAlive && _originalHandler != null && _originalHandler.IsAlive;
public TEventHandler Handler
{
get
{
if (_originalHandler == null)
{
return default(TEventHandler);
}
return (TEventHandler)_originalHandler.Target;
}
}
public WeakHandler(object source, TEventHandler originalHandler)
{
_source = new WeakReference(source);
_originalHandler = new WeakReference(originalHandler);
}
public bool Matches(object o, TEventHandler handler)
{
return _source != null &&
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
_originalHandler.Target is PropertyChangedEventHandler &&
handler is PropertyChangedEventHandler &&
Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
(handler as PropertyChangedEventHandler).Target));
}
}
internal class WeakHandlerList
{
private int _deliveries;
private readonly List<WeakHandler> _handlers;
public WeakHandlerList()
{
_handlers = new List<WeakHandler>();
}
public void AddWeakHandler(TEventSource source, TEventHandler handler)
{
var handlerSink = new WeakHandler(source, handler);
_handlers.Add(handlerSink);
}
public bool RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.Matches(source, handler))
{
return _handlers.Remove(weakHandler);
}
}
return false;
}
public WeakHandlerList Clone()
{
var newList = new WeakHandlerList();
newList._handlers.AddRange(_handlers.Where(h => h.IsActive));
return newList;
}
public int Count => _handlers.Count;
public bool IsDeliverActive => _deliveries > 0;
public IDisposable DeliverActive()
{
Interlocked.Increment(ref _deliveries);
return Disposable.Create(() => Interlocked.Decrement(ref _deliveries));
}
// ReSharper disable once MemberHidesStaticFromOuterClass
public virtual bool DeliverEvent(object sender, TEventArgs args)
{
var hasStaleEntries = false;
foreach (var handler in _handlers)
{
if (handler.IsActive)
{
var @delegate = handler.Handler as Delegate;
@delegate?.DynamicInvoke(sender, args);
}
else
{
hasStaleEntries = true;
}
}
return hasStaleEntries;
}
public void Purge()
{
for (var i = _handlers.Count - 1; i >= 0; i--)
{
if (!_handlers[i].IsActive)
{
_handlers.RemoveAt(i);
}
}
}
}
}
}
<MSG> fixes major bug in weak event manager where it would only unsubscribe
events that were of PropertyChangedHandlers meaning lots of events were being sent to the wrong instances.
<DFF> @@ -255,10 +255,9 @@ namespace AvaloniaEdit.Utils
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
- _originalHandler.Target is PropertyChangedEventHandler &&
- handler is PropertyChangedEventHandler &&
- Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
- (handler as PropertyChangedEventHandler).Target));
+ _originalHandler.Target is TEventHandler &&
+ handler is TEventHandler &&
+ handler is Delegate del && _originalHandler.Target is Delegate origDel && Equals(del.Target, origDel.Target));
}
}
| 3 | fixes major bug in weak event manager where it would only unsubscribe | 4 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063792 | <NME> WeakEventManagerBase.cs
<BEF> using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Reactive.Disposables;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Threading;
namespace AvaloniaEdit.Utils
{
/// <summary>
/// WeakEventManager base class. Inspired by the WPF WeakEventManager class and the code in
/// https://social.msdn.microsoft.com/Forums/silverlight/en-US/34d85c3f-52ea-4adc-bb32-8297f5549042/command-binding-memory-leak?forum=silverlightbugs
/// </summary>
/// <remarks>Copied here from ReactiveUI due to bugs in its design (singleton instance for multiple events).</remarks>
/// <typeparam name="TEventManager"></typeparam>
/// <typeparam name="TEventSource">The type of the event source.</typeparam>
/// <typeparam name="TEventHandler">The type of the event handler.</typeparam>
/// <typeparam name="TEventArgs">The type of the event arguments.</typeparam>
public abstract class WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>
where TEventManager : WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>, new()
{
// ReSharper disable once StaticMemberInGenericType
private static readonly object StaticSource = new object();
/// <summary>
/// Mapping between the target of the delegate (for example a Button) and the handler (EventHandler).
/// Windows Phone needs this, otherwise the event handler gets garbage collected.
/// </summary>
private readonly ConditionalWeakTable<object, List<Delegate>> _targetToEventHandler = new ConditionalWeakTable<object, List<Delegate>>();
/// <summary>
/// Mapping from the source of the event to the list of handlers. This is a CWT to ensure it does not leak the source of the event.
/// </summary>
private readonly ConditionalWeakTable<object, WeakHandlerList> _sourceToWeakHandlers = new ConditionalWeakTable<object, WeakHandlerList>();
private static readonly Lazy<TEventManager> CurrentLazy = new Lazy<TEventManager>(() => new TEventManager());
private static TEventManager Current => CurrentLazy.Value;
/// <summary>
/// Adds a weak reference to the handler and associates it with the source.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void AddHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateAddHandler(source, handler);
}
/// <summary>
/// Removes the association between the source and the handler.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void RemoveHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateRemoveHandler(source, handler);
}
/// <summary>
/// Delivers the event to the handlers registered for the source.
/// </summary>
/// <param name="sender">The sender.</param>
/// <param name="args">The <see cref="TEventArgs"/> instance containing the event data.</param>
protected static void DeliverEvent(object sender, TEventArgs args)
{
Current.PrivateDeliverEvent(sender, args);
}
/// <summary>
/// Override this method to attach to an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StartListening(TEventSource source);
/// <summary>
/// Override this method to detach from an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StopListening(TEventSource source);
protected void PrivateAddHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("Handler must be Delegate type");
}
AddWeakHandler(source, handler);
AddTargetHandler(handler);
}
private void AddWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
weakHandlers.AddWeakHandler(source, handler);
}
else
{
weakHandlers = new WeakHandlerList();
weakHandlers.AddWeakHandler(source, handler);
_sourceToWeakHandlers.Add(source, weakHandlers);
StartListening(source);
}
Purge(source);
}
private void AddTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Add(@delegate);
}
else
{
delegates = new List<Delegate> { @delegate };
_targetToEventHandler.Add(key, delegates);
}
}
protected void PrivateRemoveHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("handler must be Delegate type");
}
RemoveWeakHandler(source, handler);
RemoveTargetHandler(handler);
}
private void RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
if (weakHandlers.RemoveWeakHandler(source, handler) && weakHandlers.Count == 0)
{
_sourceToWeakHandlers.Remove(source);
StopListening(source);
}
}
}
private void RemoveTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Remove(@delegate);
if (delegates.Count == 0)
{
_targetToEventHandler.Remove(key);
}
}
}
private void PrivateDeliverEvent(object sender, TEventArgs args)
{
var source = sender ?? StaticSource;
var hasStaleEntries = false;
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
using (weakHandlers.DeliverActive())
{
hasStaleEntries = weakHandlers.DeliverEvent(source, args);
}
}
if (hasStaleEntries)
{
Purge(source);
}
}
private void Purge(object source)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
else
{
weakHandlers.Purge();
}
}
}
internal class WeakHandler
{
private readonly WeakReference _source;
private readonly WeakReference _originalHandler;
public bool IsActive => _source != null && _source.IsAlive && _originalHandler != null && _originalHandler.IsAlive;
public TEventHandler Handler
{
get
{
if (_originalHandler == null)
{
return default(TEventHandler);
}
return (TEventHandler)_originalHandler.Target;
}
}
public WeakHandler(object source, TEventHandler originalHandler)
{
_source = new WeakReference(source);
_originalHandler = new WeakReference(originalHandler);
}
public bool Matches(object o, TEventHandler handler)
{
return _source != null &&
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
_originalHandler.Target is PropertyChangedEventHandler &&
handler is PropertyChangedEventHandler &&
Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
(handler as PropertyChangedEventHandler).Target));
}
}
internal class WeakHandlerList
{
private int _deliveries;
private readonly List<WeakHandler> _handlers;
public WeakHandlerList()
{
_handlers = new List<WeakHandler>();
}
public void AddWeakHandler(TEventSource source, TEventHandler handler)
{
var handlerSink = new WeakHandler(source, handler);
_handlers.Add(handlerSink);
}
public bool RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.Matches(source, handler))
{
return _handlers.Remove(weakHandler);
}
}
return false;
}
public WeakHandlerList Clone()
{
var newList = new WeakHandlerList();
newList._handlers.AddRange(_handlers.Where(h => h.IsActive));
return newList;
}
public int Count => _handlers.Count;
public bool IsDeliverActive => _deliveries > 0;
public IDisposable DeliverActive()
{
Interlocked.Increment(ref _deliveries);
return Disposable.Create(() => Interlocked.Decrement(ref _deliveries));
}
// ReSharper disable once MemberHidesStaticFromOuterClass
public virtual bool DeliverEvent(object sender, TEventArgs args)
{
var hasStaleEntries = false;
foreach (var handler in _handlers)
{
if (handler.IsActive)
{
var @delegate = handler.Handler as Delegate;
@delegate?.DynamicInvoke(sender, args);
}
else
{
hasStaleEntries = true;
}
}
return hasStaleEntries;
}
public void Purge()
{
for (var i = _handlers.Count - 1; i >= 0; i--)
{
if (!_handlers[i].IsActive)
{
_handlers.RemoveAt(i);
}
}
}
}
}
}
<MSG> fixes major bug in weak event manager where it would only unsubscribe
events that were of PropertyChangedHandlers meaning lots of events were being sent to the wrong instances.
<DFF> @@ -255,10 +255,9 @@ namespace AvaloniaEdit.Utils
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
- _originalHandler.Target is PropertyChangedEventHandler &&
- handler is PropertyChangedEventHandler &&
- Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
- (handler as PropertyChangedEventHandler).Target));
+ _originalHandler.Target is TEventHandler &&
+ handler is TEventHandler &&
+ handler is Delegate del && _originalHandler.Target is Delegate origDel && Equals(del.Target, origDel.Target));
}
}
| 3 | fixes major bug in weak event manager where it would only unsubscribe | 4 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063793 | <NME> WeakEventManagerBase.cs
<BEF> using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Reactive.Disposables;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Threading;
namespace AvaloniaEdit.Utils
{
/// <summary>
/// WeakEventManager base class. Inspired by the WPF WeakEventManager class and the code in
/// https://social.msdn.microsoft.com/Forums/silverlight/en-US/34d85c3f-52ea-4adc-bb32-8297f5549042/command-binding-memory-leak?forum=silverlightbugs
/// </summary>
/// <remarks>Copied here from ReactiveUI due to bugs in its design (singleton instance for multiple events).</remarks>
/// <typeparam name="TEventManager"></typeparam>
/// <typeparam name="TEventSource">The type of the event source.</typeparam>
/// <typeparam name="TEventHandler">The type of the event handler.</typeparam>
/// <typeparam name="TEventArgs">The type of the event arguments.</typeparam>
public abstract class WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>
where TEventManager : WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>, new()
{
// ReSharper disable once StaticMemberInGenericType
private static readonly object StaticSource = new object();
/// <summary>
/// Mapping between the target of the delegate (for example a Button) and the handler (EventHandler).
/// Windows Phone needs this, otherwise the event handler gets garbage collected.
/// </summary>
private readonly ConditionalWeakTable<object, List<Delegate>> _targetToEventHandler = new ConditionalWeakTable<object, List<Delegate>>();
/// <summary>
/// Mapping from the source of the event to the list of handlers. This is a CWT to ensure it does not leak the source of the event.
/// </summary>
private readonly ConditionalWeakTable<object, WeakHandlerList> _sourceToWeakHandlers = new ConditionalWeakTable<object, WeakHandlerList>();
private static readonly Lazy<TEventManager> CurrentLazy = new Lazy<TEventManager>(() => new TEventManager());
private static TEventManager Current => CurrentLazy.Value;
/// <summary>
/// Adds a weak reference to the handler and associates it with the source.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void AddHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateAddHandler(source, handler);
}
/// <summary>
/// Removes the association between the source and the handler.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void RemoveHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateRemoveHandler(source, handler);
}
/// <summary>
/// Delivers the event to the handlers registered for the source.
/// </summary>
/// <param name="sender">The sender.</param>
/// <param name="args">The <see cref="TEventArgs"/> instance containing the event data.</param>
protected static void DeliverEvent(object sender, TEventArgs args)
{
Current.PrivateDeliverEvent(sender, args);
}
/// <summary>
/// Override this method to attach to an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StartListening(TEventSource source);
/// <summary>
/// Override this method to detach from an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StopListening(TEventSource source);
protected void PrivateAddHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("Handler must be Delegate type");
}
AddWeakHandler(source, handler);
AddTargetHandler(handler);
}
private void AddWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
weakHandlers.AddWeakHandler(source, handler);
}
else
{
weakHandlers = new WeakHandlerList();
weakHandlers.AddWeakHandler(source, handler);
_sourceToWeakHandlers.Add(source, weakHandlers);
StartListening(source);
}
Purge(source);
}
private void AddTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Add(@delegate);
}
else
{
delegates = new List<Delegate> { @delegate };
_targetToEventHandler.Add(key, delegates);
}
}
protected void PrivateRemoveHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("handler must be Delegate type");
}
RemoveWeakHandler(source, handler);
RemoveTargetHandler(handler);
}
private void RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
if (weakHandlers.RemoveWeakHandler(source, handler) && weakHandlers.Count == 0)
{
_sourceToWeakHandlers.Remove(source);
StopListening(source);
}
}
}
private void RemoveTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Remove(@delegate);
if (delegates.Count == 0)
{
_targetToEventHandler.Remove(key);
}
}
}
private void PrivateDeliverEvent(object sender, TEventArgs args)
{
var source = sender ?? StaticSource;
var hasStaleEntries = false;
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
using (weakHandlers.DeliverActive())
{
hasStaleEntries = weakHandlers.DeliverEvent(source, args);
}
}
if (hasStaleEntries)
{
Purge(source);
}
}
private void Purge(object source)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
else
{
weakHandlers.Purge();
}
}
}
internal class WeakHandler
{
private readonly WeakReference _source;
private readonly WeakReference _originalHandler;
public bool IsActive => _source != null && _source.IsAlive && _originalHandler != null && _originalHandler.IsAlive;
public TEventHandler Handler
{
get
{
if (_originalHandler == null)
{
return default(TEventHandler);
}
return (TEventHandler)_originalHandler.Target;
}
}
public WeakHandler(object source, TEventHandler originalHandler)
{
_source = new WeakReference(source);
_originalHandler = new WeakReference(originalHandler);
}
public bool Matches(object o, TEventHandler handler)
{
return _source != null &&
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
_originalHandler.Target is PropertyChangedEventHandler &&
handler is PropertyChangedEventHandler &&
Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
(handler as PropertyChangedEventHandler).Target));
}
}
internal class WeakHandlerList
{
private int _deliveries;
private readonly List<WeakHandler> _handlers;
public WeakHandlerList()
{
_handlers = new List<WeakHandler>();
}
public void AddWeakHandler(TEventSource source, TEventHandler handler)
{
var handlerSink = new WeakHandler(source, handler);
_handlers.Add(handlerSink);
}
public bool RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.Matches(source, handler))
{
return _handlers.Remove(weakHandler);
}
}
return false;
}
public WeakHandlerList Clone()
{
var newList = new WeakHandlerList();
newList._handlers.AddRange(_handlers.Where(h => h.IsActive));
return newList;
}
public int Count => _handlers.Count;
public bool IsDeliverActive => _deliveries > 0;
public IDisposable DeliverActive()
{
Interlocked.Increment(ref _deliveries);
return Disposable.Create(() => Interlocked.Decrement(ref _deliveries));
}
// ReSharper disable once MemberHidesStaticFromOuterClass
public virtual bool DeliverEvent(object sender, TEventArgs args)
{
var hasStaleEntries = false;
foreach (var handler in _handlers)
{
if (handler.IsActive)
{
var @delegate = handler.Handler as Delegate;
@delegate?.DynamicInvoke(sender, args);
}
else
{
hasStaleEntries = true;
}
}
return hasStaleEntries;
}
public void Purge()
{
for (var i = _handlers.Count - 1; i >= 0; i--)
{
if (!_handlers[i].IsActive)
{
_handlers.RemoveAt(i);
}
}
}
}
}
}
<MSG> fixes major bug in weak event manager where it would only unsubscribe
events that were of PropertyChangedHandlers meaning lots of events were being sent to the wrong instances.
<DFF> @@ -255,10 +255,9 @@ namespace AvaloniaEdit.Utils
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
- _originalHandler.Target is PropertyChangedEventHandler &&
- handler is PropertyChangedEventHandler &&
- Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
- (handler as PropertyChangedEventHandler).Target));
+ _originalHandler.Target is TEventHandler &&
+ handler is TEventHandler &&
+ handler is Delegate del && _originalHandler.Target is Delegate origDel && Equals(del.Target, origDel.Target));
}
}
| 3 | fixes major bug in weak event manager where it would only unsubscribe | 4 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063794 | <NME> WeakEventManagerBase.cs
<BEF> using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Reactive.Disposables;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Threading;
namespace AvaloniaEdit.Utils
{
/// <summary>
/// WeakEventManager base class. Inspired by the WPF WeakEventManager class and the code in
/// https://social.msdn.microsoft.com/Forums/silverlight/en-US/34d85c3f-52ea-4adc-bb32-8297f5549042/command-binding-memory-leak?forum=silverlightbugs
/// </summary>
/// <remarks>Copied here from ReactiveUI due to bugs in its design (singleton instance for multiple events).</remarks>
/// <typeparam name="TEventManager"></typeparam>
/// <typeparam name="TEventSource">The type of the event source.</typeparam>
/// <typeparam name="TEventHandler">The type of the event handler.</typeparam>
/// <typeparam name="TEventArgs">The type of the event arguments.</typeparam>
public abstract class WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>
where TEventManager : WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>, new()
{
// ReSharper disable once StaticMemberInGenericType
private static readonly object StaticSource = new object();
/// <summary>
/// Mapping between the target of the delegate (for example a Button) and the handler (EventHandler).
/// Windows Phone needs this, otherwise the event handler gets garbage collected.
/// </summary>
private readonly ConditionalWeakTable<object, List<Delegate>> _targetToEventHandler = new ConditionalWeakTable<object, List<Delegate>>();
/// <summary>
/// Mapping from the source of the event to the list of handlers. This is a CWT to ensure it does not leak the source of the event.
/// </summary>
private readonly ConditionalWeakTable<object, WeakHandlerList> _sourceToWeakHandlers = new ConditionalWeakTable<object, WeakHandlerList>();
private static readonly Lazy<TEventManager> CurrentLazy = new Lazy<TEventManager>(() => new TEventManager());
private static TEventManager Current => CurrentLazy.Value;
/// <summary>
/// Adds a weak reference to the handler and associates it with the source.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void AddHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateAddHandler(source, handler);
}
/// <summary>
/// Removes the association between the source and the handler.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void RemoveHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateRemoveHandler(source, handler);
}
/// <summary>
/// Delivers the event to the handlers registered for the source.
/// </summary>
/// <param name="sender">The sender.</param>
/// <param name="args">The <see cref="TEventArgs"/> instance containing the event data.</param>
protected static void DeliverEvent(object sender, TEventArgs args)
{
Current.PrivateDeliverEvent(sender, args);
}
/// <summary>
/// Override this method to attach to an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StartListening(TEventSource source);
/// <summary>
/// Override this method to detach from an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StopListening(TEventSource source);
protected void PrivateAddHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("Handler must be Delegate type");
}
AddWeakHandler(source, handler);
AddTargetHandler(handler);
}
private void AddWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
weakHandlers.AddWeakHandler(source, handler);
}
else
{
weakHandlers = new WeakHandlerList();
weakHandlers.AddWeakHandler(source, handler);
_sourceToWeakHandlers.Add(source, weakHandlers);
StartListening(source);
}
Purge(source);
}
private void AddTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Add(@delegate);
}
else
{
delegates = new List<Delegate> { @delegate };
_targetToEventHandler.Add(key, delegates);
}
}
protected void PrivateRemoveHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("handler must be Delegate type");
}
RemoveWeakHandler(source, handler);
RemoveTargetHandler(handler);
}
private void RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
if (weakHandlers.RemoveWeakHandler(source, handler) && weakHandlers.Count == 0)
{
_sourceToWeakHandlers.Remove(source);
StopListening(source);
}
}
}
private void RemoveTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Remove(@delegate);
if (delegates.Count == 0)
{
_targetToEventHandler.Remove(key);
}
}
}
private void PrivateDeliverEvent(object sender, TEventArgs args)
{
var source = sender ?? StaticSource;
var hasStaleEntries = false;
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
using (weakHandlers.DeliverActive())
{
hasStaleEntries = weakHandlers.DeliverEvent(source, args);
}
}
if (hasStaleEntries)
{
Purge(source);
}
}
private void Purge(object source)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
else
{
weakHandlers.Purge();
}
}
}
internal class WeakHandler
{
private readonly WeakReference _source;
private readonly WeakReference _originalHandler;
public bool IsActive => _source != null && _source.IsAlive && _originalHandler != null && _originalHandler.IsAlive;
public TEventHandler Handler
{
get
{
if (_originalHandler == null)
{
return default(TEventHandler);
}
return (TEventHandler)_originalHandler.Target;
}
}
public WeakHandler(object source, TEventHandler originalHandler)
{
_source = new WeakReference(source);
_originalHandler = new WeakReference(originalHandler);
}
public bool Matches(object o, TEventHandler handler)
{
return _source != null &&
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
_originalHandler.Target is PropertyChangedEventHandler &&
handler is PropertyChangedEventHandler &&
Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
(handler as PropertyChangedEventHandler).Target));
}
}
internal class WeakHandlerList
{
private int _deliveries;
private readonly List<WeakHandler> _handlers;
public WeakHandlerList()
{
_handlers = new List<WeakHandler>();
}
public void AddWeakHandler(TEventSource source, TEventHandler handler)
{
var handlerSink = new WeakHandler(source, handler);
_handlers.Add(handlerSink);
}
public bool RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.Matches(source, handler))
{
return _handlers.Remove(weakHandler);
}
}
return false;
}
public WeakHandlerList Clone()
{
var newList = new WeakHandlerList();
newList._handlers.AddRange(_handlers.Where(h => h.IsActive));
return newList;
}
public int Count => _handlers.Count;
public bool IsDeliverActive => _deliveries > 0;
public IDisposable DeliverActive()
{
Interlocked.Increment(ref _deliveries);
return Disposable.Create(() => Interlocked.Decrement(ref _deliveries));
}
// ReSharper disable once MemberHidesStaticFromOuterClass
public virtual bool DeliverEvent(object sender, TEventArgs args)
{
var hasStaleEntries = false;
foreach (var handler in _handlers)
{
if (handler.IsActive)
{
var @delegate = handler.Handler as Delegate;
@delegate?.DynamicInvoke(sender, args);
}
else
{
hasStaleEntries = true;
}
}
return hasStaleEntries;
}
public void Purge()
{
for (var i = _handlers.Count - 1; i >= 0; i--)
{
if (!_handlers[i].IsActive)
{
_handlers.RemoveAt(i);
}
}
}
}
}
}
<MSG> fixes major bug in weak event manager where it would only unsubscribe
events that were of PropertyChangedHandlers meaning lots of events were being sent to the wrong instances.
<DFF> @@ -255,10 +255,9 @@ namespace AvaloniaEdit.Utils
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
- _originalHandler.Target is PropertyChangedEventHandler &&
- handler is PropertyChangedEventHandler &&
- Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
- (handler as PropertyChangedEventHandler).Target));
+ _originalHandler.Target is TEventHandler &&
+ handler is TEventHandler &&
+ handler is Delegate del && _originalHandler.Target is Delegate origDel && Equals(del.Target, origDel.Target));
}
}
| 3 | fixes major bug in weak event manager where it would only unsubscribe | 4 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063795 | <NME> WeakEventManagerBase.cs
<BEF> using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Reactive.Disposables;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Threading;
namespace AvaloniaEdit.Utils
{
/// <summary>
/// WeakEventManager base class. Inspired by the WPF WeakEventManager class and the code in
/// https://social.msdn.microsoft.com/Forums/silverlight/en-US/34d85c3f-52ea-4adc-bb32-8297f5549042/command-binding-memory-leak?forum=silverlightbugs
/// </summary>
/// <remarks>Copied here from ReactiveUI due to bugs in its design (singleton instance for multiple events).</remarks>
/// <typeparam name="TEventManager"></typeparam>
/// <typeparam name="TEventSource">The type of the event source.</typeparam>
/// <typeparam name="TEventHandler">The type of the event handler.</typeparam>
/// <typeparam name="TEventArgs">The type of the event arguments.</typeparam>
public abstract class WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>
where TEventManager : WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>, new()
{
// ReSharper disable once StaticMemberInGenericType
private static readonly object StaticSource = new object();
/// <summary>
/// Mapping between the target of the delegate (for example a Button) and the handler (EventHandler).
/// Windows Phone needs this, otherwise the event handler gets garbage collected.
/// </summary>
private readonly ConditionalWeakTable<object, List<Delegate>> _targetToEventHandler = new ConditionalWeakTable<object, List<Delegate>>();
/// <summary>
/// Mapping from the source of the event to the list of handlers. This is a CWT to ensure it does not leak the source of the event.
/// </summary>
private readonly ConditionalWeakTable<object, WeakHandlerList> _sourceToWeakHandlers = new ConditionalWeakTable<object, WeakHandlerList>();
private static readonly Lazy<TEventManager> CurrentLazy = new Lazy<TEventManager>(() => new TEventManager());
private static TEventManager Current => CurrentLazy.Value;
/// <summary>
/// Adds a weak reference to the handler and associates it with the source.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void AddHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateAddHandler(source, handler);
}
/// <summary>
/// Removes the association between the source and the handler.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void RemoveHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateRemoveHandler(source, handler);
}
/// <summary>
/// Delivers the event to the handlers registered for the source.
/// </summary>
/// <param name="sender">The sender.</param>
/// <param name="args">The <see cref="TEventArgs"/> instance containing the event data.</param>
protected static void DeliverEvent(object sender, TEventArgs args)
{
Current.PrivateDeliverEvent(sender, args);
}
/// <summary>
/// Override this method to attach to an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StartListening(TEventSource source);
/// <summary>
/// Override this method to detach from an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StopListening(TEventSource source);
protected void PrivateAddHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("Handler must be Delegate type");
}
AddWeakHandler(source, handler);
AddTargetHandler(handler);
}
private void AddWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
weakHandlers.AddWeakHandler(source, handler);
}
else
{
weakHandlers = new WeakHandlerList();
weakHandlers.AddWeakHandler(source, handler);
_sourceToWeakHandlers.Add(source, weakHandlers);
StartListening(source);
}
Purge(source);
}
private void AddTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Add(@delegate);
}
else
{
delegates = new List<Delegate> { @delegate };
_targetToEventHandler.Add(key, delegates);
}
}
protected void PrivateRemoveHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("handler must be Delegate type");
}
RemoveWeakHandler(source, handler);
RemoveTargetHandler(handler);
}
private void RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
if (weakHandlers.RemoveWeakHandler(source, handler) && weakHandlers.Count == 0)
{
_sourceToWeakHandlers.Remove(source);
StopListening(source);
}
}
}
private void RemoveTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Remove(@delegate);
if (delegates.Count == 0)
{
_targetToEventHandler.Remove(key);
}
}
}
private void PrivateDeliverEvent(object sender, TEventArgs args)
{
var source = sender ?? StaticSource;
var hasStaleEntries = false;
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
using (weakHandlers.DeliverActive())
{
hasStaleEntries = weakHandlers.DeliverEvent(source, args);
}
}
if (hasStaleEntries)
{
Purge(source);
}
}
private void Purge(object source)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
else
{
weakHandlers.Purge();
}
}
}
internal class WeakHandler
{
private readonly WeakReference _source;
private readonly WeakReference _originalHandler;
public bool IsActive => _source != null && _source.IsAlive && _originalHandler != null && _originalHandler.IsAlive;
public TEventHandler Handler
{
get
{
if (_originalHandler == null)
{
return default(TEventHandler);
}
return (TEventHandler)_originalHandler.Target;
}
}
public WeakHandler(object source, TEventHandler originalHandler)
{
_source = new WeakReference(source);
_originalHandler = new WeakReference(originalHandler);
}
public bool Matches(object o, TEventHandler handler)
{
return _source != null &&
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
_originalHandler.Target is PropertyChangedEventHandler &&
handler is PropertyChangedEventHandler &&
Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
(handler as PropertyChangedEventHandler).Target));
}
}
internal class WeakHandlerList
{
private int _deliveries;
private readonly List<WeakHandler> _handlers;
public WeakHandlerList()
{
_handlers = new List<WeakHandler>();
}
public void AddWeakHandler(TEventSource source, TEventHandler handler)
{
var handlerSink = new WeakHandler(source, handler);
_handlers.Add(handlerSink);
}
public bool RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.Matches(source, handler))
{
return _handlers.Remove(weakHandler);
}
}
return false;
}
public WeakHandlerList Clone()
{
var newList = new WeakHandlerList();
newList._handlers.AddRange(_handlers.Where(h => h.IsActive));
return newList;
}
public int Count => _handlers.Count;
public bool IsDeliverActive => _deliveries > 0;
public IDisposable DeliverActive()
{
Interlocked.Increment(ref _deliveries);
return Disposable.Create(() => Interlocked.Decrement(ref _deliveries));
}
// ReSharper disable once MemberHidesStaticFromOuterClass
public virtual bool DeliverEvent(object sender, TEventArgs args)
{
var hasStaleEntries = false;
foreach (var handler in _handlers)
{
if (handler.IsActive)
{
var @delegate = handler.Handler as Delegate;
@delegate?.DynamicInvoke(sender, args);
}
else
{
hasStaleEntries = true;
}
}
return hasStaleEntries;
}
public void Purge()
{
for (var i = _handlers.Count - 1; i >= 0; i--)
{
if (!_handlers[i].IsActive)
{
_handlers.RemoveAt(i);
}
}
}
}
}
}
<MSG> fixes major bug in weak event manager where it would only unsubscribe
events that were of PropertyChangedHandlers meaning lots of events were being sent to the wrong instances.
<DFF> @@ -255,10 +255,9 @@ namespace AvaloniaEdit.Utils
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
- _originalHandler.Target is PropertyChangedEventHandler &&
- handler is PropertyChangedEventHandler &&
- Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
- (handler as PropertyChangedEventHandler).Target));
+ _originalHandler.Target is TEventHandler &&
+ handler is TEventHandler &&
+ handler is Delegate del && _originalHandler.Target is Delegate origDel && Equals(del.Target, origDel.Target));
}
}
| 3 | fixes major bug in weak event manager where it would only unsubscribe | 4 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063796 | <NME> WeakEventManagerBase.cs
<BEF> using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Reactive.Disposables;
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Threading;
namespace AvaloniaEdit.Utils
{
/// <summary>
/// WeakEventManager base class. Inspired by the WPF WeakEventManager class and the code in
/// https://social.msdn.microsoft.com/Forums/silverlight/en-US/34d85c3f-52ea-4adc-bb32-8297f5549042/command-binding-memory-leak?forum=silverlightbugs
/// </summary>
/// <remarks>Copied here from ReactiveUI due to bugs in its design (singleton instance for multiple events).</remarks>
/// <typeparam name="TEventManager"></typeparam>
/// <typeparam name="TEventSource">The type of the event source.</typeparam>
/// <typeparam name="TEventHandler">The type of the event handler.</typeparam>
/// <typeparam name="TEventArgs">The type of the event arguments.</typeparam>
public abstract class WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>
where TEventManager : WeakEventManagerBase<TEventManager, TEventSource, TEventHandler, TEventArgs>, new()
{
// ReSharper disable once StaticMemberInGenericType
private static readonly object StaticSource = new object();
/// <summary>
/// Mapping between the target of the delegate (for example a Button) and the handler (EventHandler).
/// Windows Phone needs this, otherwise the event handler gets garbage collected.
/// </summary>
private readonly ConditionalWeakTable<object, List<Delegate>> _targetToEventHandler = new ConditionalWeakTable<object, List<Delegate>>();
/// <summary>
/// Mapping from the source of the event to the list of handlers. This is a CWT to ensure it does not leak the source of the event.
/// </summary>
private readonly ConditionalWeakTable<object, WeakHandlerList> _sourceToWeakHandlers = new ConditionalWeakTable<object, WeakHandlerList>();
private static readonly Lazy<TEventManager> CurrentLazy = new Lazy<TEventManager>(() => new TEventManager());
private static TEventManager Current => CurrentLazy.Value;
/// <summary>
/// Adds a weak reference to the handler and associates it with the source.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void AddHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateAddHandler(source, handler);
}
/// <summary>
/// Removes the association between the source and the handler.
/// </summary>
/// <param name="source">The source.</param>
/// <param name="handler">The handler.</param>
public static void RemoveHandler(TEventSource source, TEventHandler handler)
{
Current.PrivateRemoveHandler(source, handler);
}
/// <summary>
/// Delivers the event to the handlers registered for the source.
/// </summary>
/// <param name="sender">The sender.</param>
/// <param name="args">The <see cref="TEventArgs"/> instance containing the event data.</param>
protected static void DeliverEvent(object sender, TEventArgs args)
{
Current.PrivateDeliverEvent(sender, args);
}
/// <summary>
/// Override this method to attach to an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StartListening(TEventSource source);
/// <summary>
/// Override this method to detach from an event.
/// </summary>
/// <param name="source">The source.</param>
protected abstract void StopListening(TEventSource source);
protected void PrivateAddHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("Handler must be Delegate type");
}
AddWeakHandler(source, handler);
AddTargetHandler(handler);
}
private void AddWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
weakHandlers.AddWeakHandler(source, handler);
}
else
{
weakHandlers = new WeakHandlerList();
weakHandlers.AddWeakHandler(source, handler);
_sourceToWeakHandlers.Add(source, weakHandlers);
StartListening(source);
}
Purge(source);
}
private void AddTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Add(@delegate);
}
else
{
delegates = new List<Delegate> { @delegate };
_targetToEventHandler.Add(key, delegates);
}
}
protected void PrivateRemoveHandler(TEventSource source, TEventHandler handler)
{
if (source == null) throw new ArgumentNullException(nameof(source));
if (handler == null) throw new ArgumentNullException(nameof(handler));
if (!typeof(TEventHandler).GetTypeInfo().IsSubclassOf(typeof(Delegate)))
{
throw new ArgumentException("handler must be Delegate type");
}
RemoveWeakHandler(source, handler);
RemoveTargetHandler(handler);
}
private void RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
// clone list if we are currently delivering an event
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
if (weakHandlers.RemoveWeakHandler(source, handler) && weakHandlers.Count == 0)
{
_sourceToWeakHandlers.Remove(source);
StopListening(source);
}
}
}
private void RemoveTargetHandler(TEventHandler handler)
{
var @delegate = handler as Delegate;
var key = @delegate?.Target ?? StaticSource;
if (_targetToEventHandler.TryGetValue(key, out var delegates))
{
delegates.Remove(@delegate);
if (delegates.Count == 0)
{
_targetToEventHandler.Remove(key);
}
}
}
private void PrivateDeliverEvent(object sender, TEventArgs args)
{
var source = sender ?? StaticSource;
var hasStaleEntries = false;
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
using (weakHandlers.DeliverActive())
{
hasStaleEntries = weakHandlers.DeliverEvent(source, args);
}
}
if (hasStaleEntries)
{
Purge(source);
}
}
private void Purge(object source)
{
if (_sourceToWeakHandlers.TryGetValue(source, out var weakHandlers))
{
if (weakHandlers.IsDeliverActive)
{
weakHandlers = weakHandlers.Clone();
_sourceToWeakHandlers.Remove(source);
_sourceToWeakHandlers.Add(source, weakHandlers);
}
else
{
weakHandlers.Purge();
}
}
}
internal class WeakHandler
{
private readonly WeakReference _source;
private readonly WeakReference _originalHandler;
public bool IsActive => _source != null && _source.IsAlive && _originalHandler != null && _originalHandler.IsAlive;
public TEventHandler Handler
{
get
{
if (_originalHandler == null)
{
return default(TEventHandler);
}
return (TEventHandler)_originalHandler.Target;
}
}
public WeakHandler(object source, TEventHandler originalHandler)
{
_source = new WeakReference(source);
_originalHandler = new WeakReference(originalHandler);
}
public bool Matches(object o, TEventHandler handler)
{
return _source != null &&
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
_originalHandler.Target is PropertyChangedEventHandler &&
handler is PropertyChangedEventHandler &&
Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
(handler as PropertyChangedEventHandler).Target));
}
}
internal class WeakHandlerList
{
private int _deliveries;
private readonly List<WeakHandler> _handlers;
public WeakHandlerList()
{
_handlers = new List<WeakHandler>();
}
public void AddWeakHandler(TEventSource source, TEventHandler handler)
{
var handlerSink = new WeakHandler(source, handler);
_handlers.Add(handlerSink);
}
public bool RemoveWeakHandler(TEventSource source, TEventHandler handler)
{
foreach (var weakHandler in _handlers)
{
if (weakHandler.Matches(source, handler))
{
return _handlers.Remove(weakHandler);
}
}
return false;
}
public WeakHandlerList Clone()
{
var newList = new WeakHandlerList();
newList._handlers.AddRange(_handlers.Where(h => h.IsActive));
return newList;
}
public int Count => _handlers.Count;
public bool IsDeliverActive => _deliveries > 0;
public IDisposable DeliverActive()
{
Interlocked.Increment(ref _deliveries);
return Disposable.Create(() => Interlocked.Decrement(ref _deliveries));
}
// ReSharper disable once MemberHidesStaticFromOuterClass
public virtual bool DeliverEvent(object sender, TEventArgs args)
{
var hasStaleEntries = false;
foreach (var handler in _handlers)
{
if (handler.IsActive)
{
var @delegate = handler.Handler as Delegate;
@delegate?.DynamicInvoke(sender, args);
}
else
{
hasStaleEntries = true;
}
}
return hasStaleEntries;
}
public void Purge()
{
for (var i = _handlers.Count - 1; i >= 0; i--)
{
if (!_handlers[i].IsActive)
{
_handlers.RemoveAt(i);
}
}
}
}
}
}
<MSG> fixes major bug in weak event manager where it would only unsubscribe
events that were of PropertyChangedHandlers meaning lots of events were being sent to the wrong instances.
<DFF> @@ -255,10 +255,9 @@ namespace AvaloniaEdit.Utils
ReferenceEquals(_source.Target, o) &&
_originalHandler != null &&
(ReferenceEquals(_originalHandler.Target, handler) ||
- _originalHandler.Target is PropertyChangedEventHandler &&
- handler is PropertyChangedEventHandler &&
- Equals(((PropertyChangedEventHandler)_originalHandler.Target).Target,
- (handler as PropertyChangedEventHandler).Target));
+ _originalHandler.Target is TEventHandler &&
+ handler is TEventHandler &&
+ handler is Delegate del && _originalHandler.Target is Delegate origDel && Equals(del.Target, origDel.Target));
}
}
| 3 | fixes major bug in weak event manager where it would only unsubscribe | 4 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063797 | <NME> LumenPassport.php
<BEF> <?php
namespace Dusterio\LumenPassport;
use Illuminate\Support\Arr;
use Laravel\Passport\Passport;
use DateTimeInterface;
use Carbon\Carbon;
use Laravel\Lumen\Application;
use Laravel\Lumen\Routing\Router;
class LumenPassport
{
/**
* Allow simultaneous logins for users
*
* @var bool
*/
public static $allowMultipleTokens = false;
/**
* The date when access tokens expire (specific per password client).
*
* @var array
*/
public static $tokensExpireAt = [];
/**
* Instruct Passport to keep revoked tokens pruned.
*/
public static function allowMultipleTokens()
{
static::$allowMultipleTokens = true;
}
/**
* Get or set when access tokens expire.
*
* @param \DateTimeInterface|null $date
* @param int $clientId
* @return \DateInterval|static
*/
public static function tokensExpireIn(DateTimeInterface $date = null, $clientId = null)
{
if (! $clientId) return Passport::tokensExpireIn($date);
if (is_null($date)) {
return isset(static::$tokensExpireAt[$clientId])
? Carbon::now()->diff(static::$tokensExpireAt[$clientId])
: Passport::tokensExpireIn();
} else {
static::$tokensExpireAt[$clientId] = $date;
}
return new static;
}
/**
* Get a Passport route registrar.
*
* @param callable|Router|Application $callback
* @param array $options
* @return RouteRegistrar
*/
public static function routes($callback = null, array $options = [])
{
if ($callback instanceof Application && preg_match('/(5\.[5-8]\..*)|(6\..*)|(7\..*)|(8\..*)|(9\..*)/', $callback->version())) $callback = $callback->router;
$callback = $callback ?: function ($router) {
$router->all();
};
*/
public static function routes($callback = null, array $options = [])
{
if ($callback instanceof Application && preg_match('/5\.[5-8]\..*/', $callback->version())) $callback = $callback->router;
$callback = $callback ?: function ($router) {
$router->all();
$callback->group(Arr::except($options, ['namespace']), function ($router) use ($callback, $options) {
$routes = new RouteRegistrar($router, $options);
$routes->all();
});
}
}
<MSG> Fix regex to call router on Lumen 6
<DFF> @@ -72,7 +72,7 @@ class LumenPassport
*/
public static function routes($callback = null, array $options = [])
{
- if ($callback instanceof Application && preg_match('/5\.[5-8]\..*/', $callback->version())) $callback = $callback->router;
+ if ($callback instanceof Application && preg_match('/(5\.[5-8]\..*)|(6\..*)/', $callback->version())) $callback = $callback->router;
$callback = $callback ?: function ($router) {
$router->all();
| 1 | Fix regex to call router on Lumen 6 | 1 | .php | php | mit | dusterio/lumen-passport |
10063798 | <NME> VisualLine.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Linq;
using Avalonia;
using Avalonia.Controls;
using Avalonia.Input;
using Avalonia.Media;
using Avalonia.Media.TextFormatting;
using AvaloniaEdit.Document;
using AvaloniaEdit.Utils;
using LogicalDirection = AvaloniaEdit.Document.LogicalDirection;
namespace AvaloniaEdit.Rendering
{
/// <summary>
/// Represents a visual line in the document.
/// A visual line usually corresponds to one DocumentLine, but it can span multiple lines if
/// all but the first are collapsed.
/// </summary>
public sealed class VisualLine
{
public const int LENGTH_LIMIT = 3000;
private enum LifetimePhase : byte
{
Generating,
Transforming,
Live,
Disposed
}
private readonly TextView _textView;
private List<VisualLineElement> _elements;
internal bool HasInlineObjects;
private LifetimePhase _phase;
/// <summary>
/// Gets the document to which this VisualLine belongs.
/// </summary>
public TextDocument Document { get; }
/// <summary>
/// Gets the first document line displayed by this visual line.
/// </summary>
public DocumentLine FirstDocumentLine { get; }
/// <summary>
/// Gets the last document line displayed by this visual line.
/// </summary>
public DocumentLine LastDocumentLine { get; private set; }
/// <summary>
/// Gets a read-only collection of line elements.
/// </summary>
public ReadOnlyCollection<VisualLineElement> Elements { get; private set; }
private ReadOnlyCollection<TextLine> _textLines;
/// <summary>
/// Gets a read-only collection of text lines.
/// </summary>
public ReadOnlyCollection<TextLine> TextLines
{
get
{
if (_phase < LifetimePhase.Live)
throw new InvalidOperationException();
return _textLines;
}
}
/// <summary>
/// Gets the start offset of the VisualLine inside the document.
/// This is equivalent to <c>FirstDocumentLine.Offset</c>.
/// </summary>
public int StartOffset => FirstDocumentLine.Offset;
/// <summary>
/// Length in visual line coordinates.
/// </summary>
public int VisualLength { get; private set; }
/// <summary>
/// Length in visual line coordinates including the end of line marker, if TextEditorOptions.ShowEndOfLine is enabled.
/// </summary>
public int VisualLengthWithEndOfLineMarker
{
get
{
var length = VisualLength;
if (_textView.Options.ShowEndOfLine && LastDocumentLine.NextLine != null) length++;
return length;
}
}
/// <summary>
/// Gets the height of the visual line in device-independent pixels.
/// </summary>
public double Height { get; private set; }
/// <summary>
/// Gets the Y position of the line. This is measured in device-independent pixels relative to the start of the document.
/// </summary>
public double VisualTop { get; internal set; }
internal VisualLine(TextView textView, DocumentLine firstDocumentLine)
{
Debug.Assert(textView != null);
Debug.Assert(firstDocumentLine != null);
_textView = textView;
Document = textView.Document;
FirstDocumentLine = firstDocumentLine;
}
internal void ConstructVisualElements(ITextRunConstructionContext context, IReadOnlyList<VisualLineElementGenerator> generators)
{
Debug.Assert(_phase == LifetimePhase.Generating);
foreach (var g in generators)
{
g.StartGeneration(context);
}
_elements = new List<VisualLineElement>();
PerformVisualElementConstruction(generators);
foreach (var g in generators)
{
g.FinishGeneration();
}
var globalTextRunProperties = context.GlobalTextRunProperties;
foreach (var element in _elements)
{
element.SetTextRunProperties(new VisualLineElementTextRunProperties(globalTextRunProperties));
}
this.Elements = new ReadOnlyCollection<VisualLineElement>(_elements);
CalculateOffsets();
_phase = LifetimePhase.Transforming;
}
void PerformVisualElementConstruction(IReadOnlyList<VisualLineElementGenerator> generators)
{
var lineLength = FirstDocumentLine.Length;
var offset = FirstDocumentLine.Offset;
var currentLineEnd = offset + lineLength;
LastDocumentLine = FirstDocumentLine;
var askInterestOffset = 0; // 0 or 1
while (offset + askInterestOffset <= currentLineEnd)
{
var textPieceEndOffset = currentLineEnd;
foreach (var g in generators)
{
g.CachedInterest = g.GetFirstInterestedOffset(offset + askInterestOffset);
if (g.CachedInterest != -1)
{
if (g.CachedInterest < offset)
throw new ArgumentOutOfRangeException(g.GetType().Name + ".GetFirstInterestedOffset",
g.CachedInterest,
"GetFirstInterestedOffset must not return an offset less than startOffset. Return -1 to signal no interest.");
if (g.CachedInterest < textPieceEndOffset)
textPieceEndOffset = g.CachedInterest;
}
}
Debug.Assert(textPieceEndOffset >= offset);
if (textPieceEndOffset > offset)
{
var textPieceLength = textPieceEndOffset - offset;
_elements.Add(new VisualLineText(this, textPieceLength));
offset = textPieceEndOffset;
}
// If no elements constructed / only zero-length elements constructed:
// do not asking the generators again for the same location (would cause endless loop)
askInterestOffset = 1;
foreach (var g in generators)
{
if (g.CachedInterest == offset)
{
var element = g.ConstructElement(offset);
if (element != null)
{
_elements.Add(element);
if (element.DocumentLength > 0)
{
// a non-zero-length element was constructed
askInterestOffset = 0;
offset += element.DocumentLength;
if (offset > currentLineEnd)
{
var newEndLine = Document.GetLineByOffset(offset);
currentLineEnd = newEndLine.Offset + newEndLine.Length;
this.LastDocumentLine = newEndLine;
if (currentLineEnd < offset)
{
throw new InvalidOperationException(
"The VisualLineElementGenerator " + g.GetType().Name +
" produced an element which ends within the line delimiter");
}
}
break;
}
}
}
}
}
}
private void CalculateOffsets()
{
var visualOffset = 0;
var textOffset = 0;
foreach (var element in _elements)
{
element.VisualColumn = visualOffset;
element.RelativeTextOffset = textOffset;
visualOffset += element.VisualLength;
textOffset += element.DocumentLength;
}
VisualLength = visualOffset;
Debug.Assert(textOffset == LastDocumentLine.EndOffset - FirstDocumentLine.Offset);
}
internal void RunTransformers(ITextRunConstructionContext context, IReadOnlyList<IVisualLineTransformer> transformers)
{
Debug.Assert(_phase == LifetimePhase.Transforming);
foreach (var transformer in transformers)
{
transformer.Transform(context, _elements);
}
_phase = LifetimePhase.Live;
}
/// <summary>
/// Replaces the single element at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, params VisualLineElement[] newElements)
{
ReplaceElement(elementIndex, 1, newElements);
}
/// <summary>
/// Replaces <paramref name="count"/> elements starting at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, int count, params VisualLineElement[] newElements)
{
if (_phase != LifetimePhase.Transforming)
throw new InvalidOperationException("This method may only be called by line transformers.");
var oldDocumentLength = 0;
for (var i = elementIndex; i < elementIndex + count; i++)
{
oldDocumentLength += _elements[i].DocumentLength;
}
var newDocumentLength = 0;
foreach (var newElement in newElements)
{
newDocumentLength += newElement.DocumentLength;
}
if (oldDocumentLength != newDocumentLength)
throw new InvalidOperationException("Old elements have document length " + oldDocumentLength + ", but new elements have length " + newDocumentLength);
_elements.RemoveRange(elementIndex, count);
_elements.InsertRange(elementIndex, newElements);
CalculateOffsets();
}
internal void SetTextLines(List<TextLine> textLines)
{
_textLines = new ReadOnlyCollection<TextLine>(textLines);
Height = 0;
foreach (var line in textLines)
Height += line.Height;
}
/// <summary>
/// Gets the visual column from a document offset relative to the first line start.
/// </summary>
public int GetVisualColumn(int relativeTextOffset)
{
ThrowUtil.CheckNotNegative(relativeTextOffset, "relativeTextOffset");
foreach (var element in _elements)
{
if (element.RelativeTextOffset <= relativeTextOffset
&& element.RelativeTextOffset + element.DocumentLength >= relativeTextOffset)
{
return element.GetVisualColumn(relativeTextOffset);
}
}
return VisualLength;
}
/// <summary>
/// Gets the document offset (relative to the first line start) from a visual column.
/// </summary>
public int GetRelativeOffset(int visualColumn)
{
ThrowUtil.CheckNotNegative(visualColumn, "visualColumn");
var documentLength = 0;
foreach (var element in _elements)
{
if (element.VisualColumn <= visualColumn
&& element.VisualColumn + element.VisualLength > visualColumn)
{
return element.GetRelativeOffset(visualColumn);
}
documentLength += element.DocumentLength;
}
return documentLength;
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn)
{
return GetTextLine(visualColumn, false);
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn, bool isAtEndOfLine)
{
if (visualColumn < 0)
throw new ArgumentOutOfRangeException(nameof(visualColumn));
if (visualColumn >= VisualLengthWithEndOfLineMarker)
return TextLines[TextLines.Count - 1];
foreach (var line in TextLines)
{
if (isAtEndOfLine ? visualColumn <= line.Length : visualColumn < line.Length)
return line;
visualColumn -= line.Length;
}
throw new InvalidOperationException("Shouldn't happen (VisualLength incorrect?)");
}
/// <summary>
/// Gets the visual top from the specified text line.
/// </summary>
/// <returns>Distance in device-independent pixels
/// from the top of the document to the top of the specified text line.</returns>
public double GetTextLineVisualYPosition(TextLine textLine, VisualYPosition yPositionMode)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var pos = VisualTop;
foreach (var tl in TextLines)
{
if (tl == textLine)
{
switch (yPositionMode)
{
case VisualYPosition.LineTop:
return pos;
case VisualYPosition.LineMiddle:
return pos + tl.Height / 2;
case VisualYPosition.LineBottom:
return pos + tl.Height;
case VisualYPosition.TextTop:
return pos + tl.Baseline - _textView.DefaultBaseline;
case VisualYPosition.TextBottom:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight;
case VisualYPosition.TextMiddle:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight / 2;
case VisualYPosition.Baseline:
return pos + tl.Baseline;
default:
throw new ArgumentException("Invalid yPositionMode:" + yPositionMode);
}
}
pos += tl.Height;
}
throw new ArgumentException("textLine is not a line in this VisualLine");
}
/// <summary>
/// Gets the start visual column from the specified text line.
/// </summary>
public int GetTextLineVisualStartColumn(TextLine textLine)
{
if (!TextLines.Contains(textLine))
throw new ArgumentException("textLine is not a line in this VisualLine");
return TextLines.TakeWhile(tl => tl != textLine).Sum(tl => tl.Length);
}
/// <summary>
/// Gets a TextLine by the visual position.
/// </summary>
public TextLine GetTextLineByVisualYPosition(double visualTop)
{
const double epsilon = 0.0001;
var pos = VisualTop;
foreach (var tl in TextLines)
{
pos += tl.Height;
if (visualTop + epsilon < pos)
return tl;
}
return TextLines[TextLines.Count - 1];
}
/// <summary>
/// Gets the visual position from the specified visualColumn.
/// </summary>
/// <returns>Position in device-independent pixels
/// relative to the top left of the document.</returns>
public Point GetVisualPosition(int visualColumn, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
internal Point GetVisualPosition(int visualColumn, bool isAtEndOfLine, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn, isAtEndOfLine);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
/// <summary>
/// Gets the distance to the left border of the text area of the specified visual column.
/// The visual column must belong to the specified text line.
/// </summary>
public double GetTextLineVisualXPosition(TextLine textLine, int visualColumn)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var xPos = textLine.GetDistanceFromCharacterHit(new CharacterHit(Math.Min(visualColumn,
VisualLengthWithEndOfLineMarker)));
if (visualColumn > VisualLengthWithEndOfLineMarker)
{
xPos += (visualColumn - VisualLengthWithEndOfLineMarker) * _textView.WideSpaceWidth;
}
return xPos;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point)
{
return GetVisualColumn(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point, bool allowVirtualSpace)
{
return GetVisualColumn(GetTextLineByVisualYPosition(point.Y), point.X, allowVirtualSpace);
}
internal int GetVisualColumn(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
var vc = GetVisualColumn(textLine, point.X, allowVirtualSpace);
isAtEndOfLine = (vc >= GetTextLineVisualStartColumn(textLine) + textLine.Length);
return vc;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(TextLine textLine, double xPos, bool allowVirtualSpace)
{
if (xPos > textLine.WidthIncludingTrailingWhitespace)
{
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
var virtualX = (int)Math.Round((xPos - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth, MidpointRounding.AwayFromZero);
return VisualLengthWithEndOfLineMarker + virtualX;
}
}
var ch = textLine.GetCharacterHitFromDistance(xPos);
return ch.FirstCharacterIndex + ch.TrailingLength;
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(TextViewPosition position, bool allowVirtualSpace)
{
return ValidateVisualColumn(Document.GetOffset(position.Location), position.VisualColumn, allowVirtualSpace);
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(int offset, int visualColumn, bool allowVirtualSpace)
{
var firstDocumentLineOffset = FirstDocumentLine.Offset;
if (visualColumn < 0)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
var offsetFromVisualColumn = GetRelativeOffset(visualColumn);
offsetFromVisualColumn += firstDocumentLineOffset;
if (offsetFromVisualColumn != offset)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
if (visualColumn > VisualLength && !allowVirtualSpace)
{
return VisualLength;
}
return visualColumn;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point)
{
return GetVisualColumnFloor(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point, bool allowVirtualSpace)
{
return GetVisualColumnFloor(point, allowVirtualSpace, out _);
}
internal int GetVisualColumnFloor(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
if (point.X > textLine.WidthIncludingTrailingWhitespace)
{
isAtEndOfLine = true;
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
// clicking virtual space in the last line
var virtualX = (int)((point.X - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth);
return VisualLengthWithEndOfLineMarker + virtualX;
}
// GetCharacterHitFromDistance returns a hit with FirstCharacterIndex=last character in line
// and TrailingLength=1 when clicking behind the line, so the floor function needs to handle this case
// specially and return the line's end column instead.
return GetTextLineVisualStartColumn(textLine) + textLine.Length;
}
isAtEndOfLine = false;
var ch = textLine.GetCharacterHitFromDistance(point.X);
return ch.FirstCharacterIndex;
}
/// <summary>
/// Gets the text view position from the specified visual column.
/// </summary>
public TextViewPosition GetTextViewPosition(int visualColumn)
{
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
return new TextViewPosition(Document.GetLocation(documentOffset), visualColumn);
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is within a character, it is rounded to the next character boundary.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPosition(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumn(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is inside a character, the position in front of the character is returned.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPositionFloor(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumnFloor(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets whether the visual line was disposed.
/// </summary>
public bool IsDisposed => _phase == LifetimePhase.Disposed;
internal void Dispose()
{
if (_phase == LifetimePhase.Disposed)
{
return;
}
Debug.Assert(_phase == LifetimePhase.Live);
_phase = LifetimePhase.Disposed;
if (_visual != null)
{
((ISetLogicalParent)_visual).SetParent(null);
}
}
/// <summary>
/// Gets the next possible caret position after visualColumn, or -1 if there is no caret position.
/// </summary>
public int GetNextCaretPosition(int visualColumn, LogicalDirection direction, CaretPositioningMode mode, bool allowVirtualSpace)
{
if (!HasStopsInVirtualSpace(mode))
allowVirtualSpace = false;
if (_elements.Count == 0)
{
// special handling for empty visual lines:
if (allowVirtualSpace)
{
if (direction == LogicalDirection.Forward)
return Math.Max(0, visualColumn + 1);
if (visualColumn > 0)
return visualColumn - 1;
return -1;
}
// even though we don't have any elements,
// there's a single caret stop at visualColumn 0
if (visualColumn < 0 && direction == LogicalDirection.Forward)
return 0;
if (visualColumn > 0 && direction == LogicalDirection.Backward)
return 0;
return -1;
}
int i;
if (direction == LogicalDirection.Backward)
{
// Search Backwards:
// If the last element doesn't handle line borders, return the line end as caret stop
if (visualColumn > VisualLength && !_elements[_elements.Count - 1].HandlesLineBorders && HasImplicitStopAtLineEnd())
{
if (allowVirtualSpace)
return visualColumn - 1;
return VisualLength;
}
// skip elements that start after or at visualColumn
for (i = _elements.Count - 1; i >= 0; i--)
{
if (_elements[i].VisualColumn < visualColumn)
break;
}
// search last element that has a caret stop
for (; i >= 0; i--)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Min(visualColumn, _elements[i].VisualColumn + _elements[i].VisualLength + 1),
direction, mode);
if (pos >= 0)
return pos;
}
// If we've found nothing, and the first element doesn't handle line borders,
// return the line start as normal caret stop.
if (visualColumn > 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
}
else
{
// Search Forwards:
// If the first element doesn't handle line borders, return the line start as caret stop
if (visualColumn < 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
// skip elements that end before or at visualColumn
for (i = 0; i < _elements.Count; i++)
{
if (_elements[i].VisualColumn + _elements[i].VisualLength > visualColumn)
break;
}
// search first element that has a caret stop
for (; i < _elements.Count; i++)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Max(visualColumn, _elements[i].VisualColumn - 1),
direction, mode);
if (pos >= 0)
return pos;
}
// if we've found nothing, and the last element doesn't handle line borders,
// return the line end as caret stop
if ((allowVirtualSpace || !_elements[_elements.Count - 1].HandlesLineBorders) && HasImplicitStopAtLineEnd())
{
if (visualColumn < VisualLength)
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
return _visual ??= new VisualLineDrawingVisual(this);
}
}
{
return mode == CaretPositioningMode.Normal || mode == CaretPositioningMode.EveryCodepoint;
}
private static bool HasImplicitStopAtLineEnd() => true;
private VisualLineDrawingVisual _visual;
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
if (_visual == null)
{
_visual = new VisualLineDrawingVisual(this);
((ISetLogicalParent)_visual).SetParent(_textView);
}
return _visual;
}
}
// TODO: can inherit from Layoutable, but dev tools crash
internal sealed class VisualLineDrawingVisual : Control
{
public VisualLine VisualLine { get; }
public double LineHeight { get; }
internal bool IsAdded { get; set; }
public VisualLineDrawingVisual(VisualLine visualLine)
{
VisualLine = visualLine;
LineHeight = VisualLine.TextLines.Sum(textLine => textLine.Height);
}
public override void Render(DrawingContext context)
{
double pos = 0;
foreach (var textLine in VisualLine.TextLines)
{
textLine.Draw(context, new Point(0, pos));
pos += textLine.Height;
}
}
}
}
<MSG> Set VisualLineDrawingVisual.Parent on creation
<DFF> @@ -748,7 +748,15 @@ namespace AvaloniaEdit.Rendering
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
- return _visual ??= new VisualLineDrawingVisual(this);
+
+ if (_visual == null)
+ {
+ _visual = new VisualLineDrawingVisual(this);
+
+ ((ISetLogicalParent)_visual).SetParent(_textView);
+ }
+
+ return _visual;
}
}
| 9 | Set VisualLineDrawingVisual.Parent on creation | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063799 | <NME> VisualLine.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Linq;
using Avalonia;
using Avalonia.Controls;
using Avalonia.Input;
using Avalonia.Media;
using Avalonia.Media.TextFormatting;
using AvaloniaEdit.Document;
using AvaloniaEdit.Utils;
using LogicalDirection = AvaloniaEdit.Document.LogicalDirection;
namespace AvaloniaEdit.Rendering
{
/// <summary>
/// Represents a visual line in the document.
/// A visual line usually corresponds to one DocumentLine, but it can span multiple lines if
/// all but the first are collapsed.
/// </summary>
public sealed class VisualLine
{
public const int LENGTH_LIMIT = 3000;
private enum LifetimePhase : byte
{
Generating,
Transforming,
Live,
Disposed
}
private readonly TextView _textView;
private List<VisualLineElement> _elements;
internal bool HasInlineObjects;
private LifetimePhase _phase;
/// <summary>
/// Gets the document to which this VisualLine belongs.
/// </summary>
public TextDocument Document { get; }
/// <summary>
/// Gets the first document line displayed by this visual line.
/// </summary>
public DocumentLine FirstDocumentLine { get; }
/// <summary>
/// Gets the last document line displayed by this visual line.
/// </summary>
public DocumentLine LastDocumentLine { get; private set; }
/// <summary>
/// Gets a read-only collection of line elements.
/// </summary>
public ReadOnlyCollection<VisualLineElement> Elements { get; private set; }
private ReadOnlyCollection<TextLine> _textLines;
/// <summary>
/// Gets a read-only collection of text lines.
/// </summary>
public ReadOnlyCollection<TextLine> TextLines
{
get
{
if (_phase < LifetimePhase.Live)
throw new InvalidOperationException();
return _textLines;
}
}
/// <summary>
/// Gets the start offset of the VisualLine inside the document.
/// This is equivalent to <c>FirstDocumentLine.Offset</c>.
/// </summary>
public int StartOffset => FirstDocumentLine.Offset;
/// <summary>
/// Length in visual line coordinates.
/// </summary>
public int VisualLength { get; private set; }
/// <summary>
/// Length in visual line coordinates including the end of line marker, if TextEditorOptions.ShowEndOfLine is enabled.
/// </summary>
public int VisualLengthWithEndOfLineMarker
{
get
{
var length = VisualLength;
if (_textView.Options.ShowEndOfLine && LastDocumentLine.NextLine != null) length++;
return length;
}
}
/// <summary>
/// Gets the height of the visual line in device-independent pixels.
/// </summary>
public double Height { get; private set; }
/// <summary>
/// Gets the Y position of the line. This is measured in device-independent pixels relative to the start of the document.
/// </summary>
public double VisualTop { get; internal set; }
internal VisualLine(TextView textView, DocumentLine firstDocumentLine)
{
Debug.Assert(textView != null);
Debug.Assert(firstDocumentLine != null);
_textView = textView;
Document = textView.Document;
FirstDocumentLine = firstDocumentLine;
}
internal void ConstructVisualElements(ITextRunConstructionContext context, IReadOnlyList<VisualLineElementGenerator> generators)
{
Debug.Assert(_phase == LifetimePhase.Generating);
foreach (var g in generators)
{
g.StartGeneration(context);
}
_elements = new List<VisualLineElement>();
PerformVisualElementConstruction(generators);
foreach (var g in generators)
{
g.FinishGeneration();
}
var globalTextRunProperties = context.GlobalTextRunProperties;
foreach (var element in _elements)
{
element.SetTextRunProperties(new VisualLineElementTextRunProperties(globalTextRunProperties));
}
this.Elements = new ReadOnlyCollection<VisualLineElement>(_elements);
CalculateOffsets();
_phase = LifetimePhase.Transforming;
}
void PerformVisualElementConstruction(IReadOnlyList<VisualLineElementGenerator> generators)
{
var lineLength = FirstDocumentLine.Length;
var offset = FirstDocumentLine.Offset;
var currentLineEnd = offset + lineLength;
LastDocumentLine = FirstDocumentLine;
var askInterestOffset = 0; // 0 or 1
while (offset + askInterestOffset <= currentLineEnd)
{
var textPieceEndOffset = currentLineEnd;
foreach (var g in generators)
{
g.CachedInterest = g.GetFirstInterestedOffset(offset + askInterestOffset);
if (g.CachedInterest != -1)
{
if (g.CachedInterest < offset)
throw new ArgumentOutOfRangeException(g.GetType().Name + ".GetFirstInterestedOffset",
g.CachedInterest,
"GetFirstInterestedOffset must not return an offset less than startOffset. Return -1 to signal no interest.");
if (g.CachedInterest < textPieceEndOffset)
textPieceEndOffset = g.CachedInterest;
}
}
Debug.Assert(textPieceEndOffset >= offset);
if (textPieceEndOffset > offset)
{
var textPieceLength = textPieceEndOffset - offset;
_elements.Add(new VisualLineText(this, textPieceLength));
offset = textPieceEndOffset;
}
// If no elements constructed / only zero-length elements constructed:
// do not asking the generators again for the same location (would cause endless loop)
askInterestOffset = 1;
foreach (var g in generators)
{
if (g.CachedInterest == offset)
{
var element = g.ConstructElement(offset);
if (element != null)
{
_elements.Add(element);
if (element.DocumentLength > 0)
{
// a non-zero-length element was constructed
askInterestOffset = 0;
offset += element.DocumentLength;
if (offset > currentLineEnd)
{
var newEndLine = Document.GetLineByOffset(offset);
currentLineEnd = newEndLine.Offset + newEndLine.Length;
this.LastDocumentLine = newEndLine;
if (currentLineEnd < offset)
{
throw new InvalidOperationException(
"The VisualLineElementGenerator " + g.GetType().Name +
" produced an element which ends within the line delimiter");
}
}
break;
}
}
}
}
}
}
private void CalculateOffsets()
{
var visualOffset = 0;
var textOffset = 0;
foreach (var element in _elements)
{
element.VisualColumn = visualOffset;
element.RelativeTextOffset = textOffset;
visualOffset += element.VisualLength;
textOffset += element.DocumentLength;
}
VisualLength = visualOffset;
Debug.Assert(textOffset == LastDocumentLine.EndOffset - FirstDocumentLine.Offset);
}
internal void RunTransformers(ITextRunConstructionContext context, IReadOnlyList<IVisualLineTransformer> transformers)
{
Debug.Assert(_phase == LifetimePhase.Transforming);
foreach (var transformer in transformers)
{
transformer.Transform(context, _elements);
}
_phase = LifetimePhase.Live;
}
/// <summary>
/// Replaces the single element at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, params VisualLineElement[] newElements)
{
ReplaceElement(elementIndex, 1, newElements);
}
/// <summary>
/// Replaces <paramref name="count"/> elements starting at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, int count, params VisualLineElement[] newElements)
{
if (_phase != LifetimePhase.Transforming)
throw new InvalidOperationException("This method may only be called by line transformers.");
var oldDocumentLength = 0;
for (var i = elementIndex; i < elementIndex + count; i++)
{
oldDocumentLength += _elements[i].DocumentLength;
}
var newDocumentLength = 0;
foreach (var newElement in newElements)
{
newDocumentLength += newElement.DocumentLength;
}
if (oldDocumentLength != newDocumentLength)
throw new InvalidOperationException("Old elements have document length " + oldDocumentLength + ", but new elements have length " + newDocumentLength);
_elements.RemoveRange(elementIndex, count);
_elements.InsertRange(elementIndex, newElements);
CalculateOffsets();
}
internal void SetTextLines(List<TextLine> textLines)
{
_textLines = new ReadOnlyCollection<TextLine>(textLines);
Height = 0;
foreach (var line in textLines)
Height += line.Height;
}
/// <summary>
/// Gets the visual column from a document offset relative to the first line start.
/// </summary>
public int GetVisualColumn(int relativeTextOffset)
{
ThrowUtil.CheckNotNegative(relativeTextOffset, "relativeTextOffset");
foreach (var element in _elements)
{
if (element.RelativeTextOffset <= relativeTextOffset
&& element.RelativeTextOffset + element.DocumentLength >= relativeTextOffset)
{
return element.GetVisualColumn(relativeTextOffset);
}
}
return VisualLength;
}
/// <summary>
/// Gets the document offset (relative to the first line start) from a visual column.
/// </summary>
public int GetRelativeOffset(int visualColumn)
{
ThrowUtil.CheckNotNegative(visualColumn, "visualColumn");
var documentLength = 0;
foreach (var element in _elements)
{
if (element.VisualColumn <= visualColumn
&& element.VisualColumn + element.VisualLength > visualColumn)
{
return element.GetRelativeOffset(visualColumn);
}
documentLength += element.DocumentLength;
}
return documentLength;
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn)
{
return GetTextLine(visualColumn, false);
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn, bool isAtEndOfLine)
{
if (visualColumn < 0)
throw new ArgumentOutOfRangeException(nameof(visualColumn));
if (visualColumn >= VisualLengthWithEndOfLineMarker)
return TextLines[TextLines.Count - 1];
foreach (var line in TextLines)
{
if (isAtEndOfLine ? visualColumn <= line.Length : visualColumn < line.Length)
return line;
visualColumn -= line.Length;
}
throw new InvalidOperationException("Shouldn't happen (VisualLength incorrect?)");
}
/// <summary>
/// Gets the visual top from the specified text line.
/// </summary>
/// <returns>Distance in device-independent pixels
/// from the top of the document to the top of the specified text line.</returns>
public double GetTextLineVisualYPosition(TextLine textLine, VisualYPosition yPositionMode)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var pos = VisualTop;
foreach (var tl in TextLines)
{
if (tl == textLine)
{
switch (yPositionMode)
{
case VisualYPosition.LineTop:
return pos;
case VisualYPosition.LineMiddle:
return pos + tl.Height / 2;
case VisualYPosition.LineBottom:
return pos + tl.Height;
case VisualYPosition.TextTop:
return pos + tl.Baseline - _textView.DefaultBaseline;
case VisualYPosition.TextBottom:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight;
case VisualYPosition.TextMiddle:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight / 2;
case VisualYPosition.Baseline:
return pos + tl.Baseline;
default:
throw new ArgumentException("Invalid yPositionMode:" + yPositionMode);
}
}
pos += tl.Height;
}
throw new ArgumentException("textLine is not a line in this VisualLine");
}
/// <summary>
/// Gets the start visual column from the specified text line.
/// </summary>
public int GetTextLineVisualStartColumn(TextLine textLine)
{
if (!TextLines.Contains(textLine))
throw new ArgumentException("textLine is not a line in this VisualLine");
return TextLines.TakeWhile(tl => tl != textLine).Sum(tl => tl.Length);
}
/// <summary>
/// Gets a TextLine by the visual position.
/// </summary>
public TextLine GetTextLineByVisualYPosition(double visualTop)
{
const double epsilon = 0.0001;
var pos = VisualTop;
foreach (var tl in TextLines)
{
pos += tl.Height;
if (visualTop + epsilon < pos)
return tl;
}
return TextLines[TextLines.Count - 1];
}
/// <summary>
/// Gets the visual position from the specified visualColumn.
/// </summary>
/// <returns>Position in device-independent pixels
/// relative to the top left of the document.</returns>
public Point GetVisualPosition(int visualColumn, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
internal Point GetVisualPosition(int visualColumn, bool isAtEndOfLine, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn, isAtEndOfLine);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
/// <summary>
/// Gets the distance to the left border of the text area of the specified visual column.
/// The visual column must belong to the specified text line.
/// </summary>
public double GetTextLineVisualXPosition(TextLine textLine, int visualColumn)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var xPos = textLine.GetDistanceFromCharacterHit(new CharacterHit(Math.Min(visualColumn,
VisualLengthWithEndOfLineMarker)));
if (visualColumn > VisualLengthWithEndOfLineMarker)
{
xPos += (visualColumn - VisualLengthWithEndOfLineMarker) * _textView.WideSpaceWidth;
}
return xPos;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point)
{
return GetVisualColumn(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point, bool allowVirtualSpace)
{
return GetVisualColumn(GetTextLineByVisualYPosition(point.Y), point.X, allowVirtualSpace);
}
internal int GetVisualColumn(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
var vc = GetVisualColumn(textLine, point.X, allowVirtualSpace);
isAtEndOfLine = (vc >= GetTextLineVisualStartColumn(textLine) + textLine.Length);
return vc;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(TextLine textLine, double xPos, bool allowVirtualSpace)
{
if (xPos > textLine.WidthIncludingTrailingWhitespace)
{
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
var virtualX = (int)Math.Round((xPos - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth, MidpointRounding.AwayFromZero);
return VisualLengthWithEndOfLineMarker + virtualX;
}
}
var ch = textLine.GetCharacterHitFromDistance(xPos);
return ch.FirstCharacterIndex + ch.TrailingLength;
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(TextViewPosition position, bool allowVirtualSpace)
{
return ValidateVisualColumn(Document.GetOffset(position.Location), position.VisualColumn, allowVirtualSpace);
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(int offset, int visualColumn, bool allowVirtualSpace)
{
var firstDocumentLineOffset = FirstDocumentLine.Offset;
if (visualColumn < 0)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
var offsetFromVisualColumn = GetRelativeOffset(visualColumn);
offsetFromVisualColumn += firstDocumentLineOffset;
if (offsetFromVisualColumn != offset)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
if (visualColumn > VisualLength && !allowVirtualSpace)
{
return VisualLength;
}
return visualColumn;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point)
{
return GetVisualColumnFloor(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point, bool allowVirtualSpace)
{
return GetVisualColumnFloor(point, allowVirtualSpace, out _);
}
internal int GetVisualColumnFloor(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
if (point.X > textLine.WidthIncludingTrailingWhitespace)
{
isAtEndOfLine = true;
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
// clicking virtual space in the last line
var virtualX = (int)((point.X - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth);
return VisualLengthWithEndOfLineMarker + virtualX;
}
// GetCharacterHitFromDistance returns a hit with FirstCharacterIndex=last character in line
// and TrailingLength=1 when clicking behind the line, so the floor function needs to handle this case
// specially and return the line's end column instead.
return GetTextLineVisualStartColumn(textLine) + textLine.Length;
}
isAtEndOfLine = false;
var ch = textLine.GetCharacterHitFromDistance(point.X);
return ch.FirstCharacterIndex;
}
/// <summary>
/// Gets the text view position from the specified visual column.
/// </summary>
public TextViewPosition GetTextViewPosition(int visualColumn)
{
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
return new TextViewPosition(Document.GetLocation(documentOffset), visualColumn);
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is within a character, it is rounded to the next character boundary.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPosition(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumn(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is inside a character, the position in front of the character is returned.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPositionFloor(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumnFloor(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets whether the visual line was disposed.
/// </summary>
public bool IsDisposed => _phase == LifetimePhase.Disposed;
internal void Dispose()
{
if (_phase == LifetimePhase.Disposed)
{
return;
}
Debug.Assert(_phase == LifetimePhase.Live);
_phase = LifetimePhase.Disposed;
if (_visual != null)
{
((ISetLogicalParent)_visual).SetParent(null);
}
}
/// <summary>
/// Gets the next possible caret position after visualColumn, or -1 if there is no caret position.
/// </summary>
public int GetNextCaretPosition(int visualColumn, LogicalDirection direction, CaretPositioningMode mode, bool allowVirtualSpace)
{
if (!HasStopsInVirtualSpace(mode))
allowVirtualSpace = false;
if (_elements.Count == 0)
{
// special handling for empty visual lines:
if (allowVirtualSpace)
{
if (direction == LogicalDirection.Forward)
return Math.Max(0, visualColumn + 1);
if (visualColumn > 0)
return visualColumn - 1;
return -1;
}
// even though we don't have any elements,
// there's a single caret stop at visualColumn 0
if (visualColumn < 0 && direction == LogicalDirection.Forward)
return 0;
if (visualColumn > 0 && direction == LogicalDirection.Backward)
return 0;
return -1;
}
int i;
if (direction == LogicalDirection.Backward)
{
// Search Backwards:
// If the last element doesn't handle line borders, return the line end as caret stop
if (visualColumn > VisualLength && !_elements[_elements.Count - 1].HandlesLineBorders && HasImplicitStopAtLineEnd())
{
if (allowVirtualSpace)
return visualColumn - 1;
return VisualLength;
}
// skip elements that start after or at visualColumn
for (i = _elements.Count - 1; i >= 0; i--)
{
if (_elements[i].VisualColumn < visualColumn)
break;
}
// search last element that has a caret stop
for (; i >= 0; i--)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Min(visualColumn, _elements[i].VisualColumn + _elements[i].VisualLength + 1),
direction, mode);
if (pos >= 0)
return pos;
}
// If we've found nothing, and the first element doesn't handle line borders,
// return the line start as normal caret stop.
if (visualColumn > 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
}
else
{
// Search Forwards:
// If the first element doesn't handle line borders, return the line start as caret stop
if (visualColumn < 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
// skip elements that end before or at visualColumn
for (i = 0; i < _elements.Count; i++)
{
if (_elements[i].VisualColumn + _elements[i].VisualLength > visualColumn)
break;
}
// search first element that has a caret stop
for (; i < _elements.Count; i++)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Max(visualColumn, _elements[i].VisualColumn - 1),
direction, mode);
if (pos >= 0)
return pos;
}
// if we've found nothing, and the last element doesn't handle line borders,
// return the line end as caret stop
if ((allowVirtualSpace || !_elements[_elements.Count - 1].HandlesLineBorders) && HasImplicitStopAtLineEnd())
{
if (visualColumn < VisualLength)
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
return _visual ??= new VisualLineDrawingVisual(this);
}
}
{
return mode == CaretPositioningMode.Normal || mode == CaretPositioningMode.EveryCodepoint;
}
private static bool HasImplicitStopAtLineEnd() => true;
private VisualLineDrawingVisual _visual;
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
if (_visual == null)
{
_visual = new VisualLineDrawingVisual(this);
((ISetLogicalParent)_visual).SetParent(_textView);
}
return _visual;
}
}
// TODO: can inherit from Layoutable, but dev tools crash
internal sealed class VisualLineDrawingVisual : Control
{
public VisualLine VisualLine { get; }
public double LineHeight { get; }
internal bool IsAdded { get; set; }
public VisualLineDrawingVisual(VisualLine visualLine)
{
VisualLine = visualLine;
LineHeight = VisualLine.TextLines.Sum(textLine => textLine.Height);
}
public override void Render(DrawingContext context)
{
double pos = 0;
foreach (var textLine in VisualLine.TextLines)
{
textLine.Draw(context, new Point(0, pos));
pos += textLine.Height;
}
}
}
}
<MSG> Set VisualLineDrawingVisual.Parent on creation
<DFF> @@ -748,7 +748,15 @@ namespace AvaloniaEdit.Rendering
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
- return _visual ??= new VisualLineDrawingVisual(this);
+
+ if (_visual == null)
+ {
+ _visual = new VisualLineDrawingVisual(this);
+
+ ((ISetLogicalParent)_visual).SetParent(_textView);
+ }
+
+ return _visual;
}
}
| 9 | Set VisualLineDrawingVisual.Parent on creation | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063800 | <NME> VisualLine.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Linq;
using Avalonia;
using Avalonia.Controls;
using Avalonia.Input;
using Avalonia.Media;
using Avalonia.Media.TextFormatting;
using AvaloniaEdit.Document;
using AvaloniaEdit.Utils;
using LogicalDirection = AvaloniaEdit.Document.LogicalDirection;
namespace AvaloniaEdit.Rendering
{
/// <summary>
/// Represents a visual line in the document.
/// A visual line usually corresponds to one DocumentLine, but it can span multiple lines if
/// all but the first are collapsed.
/// </summary>
public sealed class VisualLine
{
public const int LENGTH_LIMIT = 3000;
private enum LifetimePhase : byte
{
Generating,
Transforming,
Live,
Disposed
}
private readonly TextView _textView;
private List<VisualLineElement> _elements;
internal bool HasInlineObjects;
private LifetimePhase _phase;
/// <summary>
/// Gets the document to which this VisualLine belongs.
/// </summary>
public TextDocument Document { get; }
/// <summary>
/// Gets the first document line displayed by this visual line.
/// </summary>
public DocumentLine FirstDocumentLine { get; }
/// <summary>
/// Gets the last document line displayed by this visual line.
/// </summary>
public DocumentLine LastDocumentLine { get; private set; }
/// <summary>
/// Gets a read-only collection of line elements.
/// </summary>
public ReadOnlyCollection<VisualLineElement> Elements { get; private set; }
private ReadOnlyCollection<TextLine> _textLines;
/// <summary>
/// Gets a read-only collection of text lines.
/// </summary>
public ReadOnlyCollection<TextLine> TextLines
{
get
{
if (_phase < LifetimePhase.Live)
throw new InvalidOperationException();
return _textLines;
}
}
/// <summary>
/// Gets the start offset of the VisualLine inside the document.
/// This is equivalent to <c>FirstDocumentLine.Offset</c>.
/// </summary>
public int StartOffset => FirstDocumentLine.Offset;
/// <summary>
/// Length in visual line coordinates.
/// </summary>
public int VisualLength { get; private set; }
/// <summary>
/// Length in visual line coordinates including the end of line marker, if TextEditorOptions.ShowEndOfLine is enabled.
/// </summary>
public int VisualLengthWithEndOfLineMarker
{
get
{
var length = VisualLength;
if (_textView.Options.ShowEndOfLine && LastDocumentLine.NextLine != null) length++;
return length;
}
}
/// <summary>
/// Gets the height of the visual line in device-independent pixels.
/// </summary>
public double Height { get; private set; }
/// <summary>
/// Gets the Y position of the line. This is measured in device-independent pixels relative to the start of the document.
/// </summary>
public double VisualTop { get; internal set; }
internal VisualLine(TextView textView, DocumentLine firstDocumentLine)
{
Debug.Assert(textView != null);
Debug.Assert(firstDocumentLine != null);
_textView = textView;
Document = textView.Document;
FirstDocumentLine = firstDocumentLine;
}
internal void ConstructVisualElements(ITextRunConstructionContext context, IReadOnlyList<VisualLineElementGenerator> generators)
{
Debug.Assert(_phase == LifetimePhase.Generating);
foreach (var g in generators)
{
g.StartGeneration(context);
}
_elements = new List<VisualLineElement>();
PerformVisualElementConstruction(generators);
foreach (var g in generators)
{
g.FinishGeneration();
}
var globalTextRunProperties = context.GlobalTextRunProperties;
foreach (var element in _elements)
{
element.SetTextRunProperties(new VisualLineElementTextRunProperties(globalTextRunProperties));
}
this.Elements = new ReadOnlyCollection<VisualLineElement>(_elements);
CalculateOffsets();
_phase = LifetimePhase.Transforming;
}
void PerformVisualElementConstruction(IReadOnlyList<VisualLineElementGenerator> generators)
{
var lineLength = FirstDocumentLine.Length;
var offset = FirstDocumentLine.Offset;
var currentLineEnd = offset + lineLength;
LastDocumentLine = FirstDocumentLine;
var askInterestOffset = 0; // 0 or 1
while (offset + askInterestOffset <= currentLineEnd)
{
var textPieceEndOffset = currentLineEnd;
foreach (var g in generators)
{
g.CachedInterest = g.GetFirstInterestedOffset(offset + askInterestOffset);
if (g.CachedInterest != -1)
{
if (g.CachedInterest < offset)
throw new ArgumentOutOfRangeException(g.GetType().Name + ".GetFirstInterestedOffset",
g.CachedInterest,
"GetFirstInterestedOffset must not return an offset less than startOffset. Return -1 to signal no interest.");
if (g.CachedInterest < textPieceEndOffset)
textPieceEndOffset = g.CachedInterest;
}
}
Debug.Assert(textPieceEndOffset >= offset);
if (textPieceEndOffset > offset)
{
var textPieceLength = textPieceEndOffset - offset;
_elements.Add(new VisualLineText(this, textPieceLength));
offset = textPieceEndOffset;
}
// If no elements constructed / only zero-length elements constructed:
// do not asking the generators again for the same location (would cause endless loop)
askInterestOffset = 1;
foreach (var g in generators)
{
if (g.CachedInterest == offset)
{
var element = g.ConstructElement(offset);
if (element != null)
{
_elements.Add(element);
if (element.DocumentLength > 0)
{
// a non-zero-length element was constructed
askInterestOffset = 0;
offset += element.DocumentLength;
if (offset > currentLineEnd)
{
var newEndLine = Document.GetLineByOffset(offset);
currentLineEnd = newEndLine.Offset + newEndLine.Length;
this.LastDocumentLine = newEndLine;
if (currentLineEnd < offset)
{
throw new InvalidOperationException(
"The VisualLineElementGenerator " + g.GetType().Name +
" produced an element which ends within the line delimiter");
}
}
break;
}
}
}
}
}
}
private void CalculateOffsets()
{
var visualOffset = 0;
var textOffset = 0;
foreach (var element in _elements)
{
element.VisualColumn = visualOffset;
element.RelativeTextOffset = textOffset;
visualOffset += element.VisualLength;
textOffset += element.DocumentLength;
}
VisualLength = visualOffset;
Debug.Assert(textOffset == LastDocumentLine.EndOffset - FirstDocumentLine.Offset);
}
internal void RunTransformers(ITextRunConstructionContext context, IReadOnlyList<IVisualLineTransformer> transformers)
{
Debug.Assert(_phase == LifetimePhase.Transforming);
foreach (var transformer in transformers)
{
transformer.Transform(context, _elements);
}
_phase = LifetimePhase.Live;
}
/// <summary>
/// Replaces the single element at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, params VisualLineElement[] newElements)
{
ReplaceElement(elementIndex, 1, newElements);
}
/// <summary>
/// Replaces <paramref name="count"/> elements starting at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, int count, params VisualLineElement[] newElements)
{
if (_phase != LifetimePhase.Transforming)
throw new InvalidOperationException("This method may only be called by line transformers.");
var oldDocumentLength = 0;
for (var i = elementIndex; i < elementIndex + count; i++)
{
oldDocumentLength += _elements[i].DocumentLength;
}
var newDocumentLength = 0;
foreach (var newElement in newElements)
{
newDocumentLength += newElement.DocumentLength;
}
if (oldDocumentLength != newDocumentLength)
throw new InvalidOperationException("Old elements have document length " + oldDocumentLength + ", but new elements have length " + newDocumentLength);
_elements.RemoveRange(elementIndex, count);
_elements.InsertRange(elementIndex, newElements);
CalculateOffsets();
}
internal void SetTextLines(List<TextLine> textLines)
{
_textLines = new ReadOnlyCollection<TextLine>(textLines);
Height = 0;
foreach (var line in textLines)
Height += line.Height;
}
/// <summary>
/// Gets the visual column from a document offset relative to the first line start.
/// </summary>
public int GetVisualColumn(int relativeTextOffset)
{
ThrowUtil.CheckNotNegative(relativeTextOffset, "relativeTextOffset");
foreach (var element in _elements)
{
if (element.RelativeTextOffset <= relativeTextOffset
&& element.RelativeTextOffset + element.DocumentLength >= relativeTextOffset)
{
return element.GetVisualColumn(relativeTextOffset);
}
}
return VisualLength;
}
/// <summary>
/// Gets the document offset (relative to the first line start) from a visual column.
/// </summary>
public int GetRelativeOffset(int visualColumn)
{
ThrowUtil.CheckNotNegative(visualColumn, "visualColumn");
var documentLength = 0;
foreach (var element in _elements)
{
if (element.VisualColumn <= visualColumn
&& element.VisualColumn + element.VisualLength > visualColumn)
{
return element.GetRelativeOffset(visualColumn);
}
documentLength += element.DocumentLength;
}
return documentLength;
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn)
{
return GetTextLine(visualColumn, false);
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn, bool isAtEndOfLine)
{
if (visualColumn < 0)
throw new ArgumentOutOfRangeException(nameof(visualColumn));
if (visualColumn >= VisualLengthWithEndOfLineMarker)
return TextLines[TextLines.Count - 1];
foreach (var line in TextLines)
{
if (isAtEndOfLine ? visualColumn <= line.Length : visualColumn < line.Length)
return line;
visualColumn -= line.Length;
}
throw new InvalidOperationException("Shouldn't happen (VisualLength incorrect?)");
}
/// <summary>
/// Gets the visual top from the specified text line.
/// </summary>
/// <returns>Distance in device-independent pixels
/// from the top of the document to the top of the specified text line.</returns>
public double GetTextLineVisualYPosition(TextLine textLine, VisualYPosition yPositionMode)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var pos = VisualTop;
foreach (var tl in TextLines)
{
if (tl == textLine)
{
switch (yPositionMode)
{
case VisualYPosition.LineTop:
return pos;
case VisualYPosition.LineMiddle:
return pos + tl.Height / 2;
case VisualYPosition.LineBottom:
return pos + tl.Height;
case VisualYPosition.TextTop:
return pos + tl.Baseline - _textView.DefaultBaseline;
case VisualYPosition.TextBottom:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight;
case VisualYPosition.TextMiddle:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight / 2;
case VisualYPosition.Baseline:
return pos + tl.Baseline;
default:
throw new ArgumentException("Invalid yPositionMode:" + yPositionMode);
}
}
pos += tl.Height;
}
throw new ArgumentException("textLine is not a line in this VisualLine");
}
/// <summary>
/// Gets the start visual column from the specified text line.
/// </summary>
public int GetTextLineVisualStartColumn(TextLine textLine)
{
if (!TextLines.Contains(textLine))
throw new ArgumentException("textLine is not a line in this VisualLine");
return TextLines.TakeWhile(tl => tl != textLine).Sum(tl => tl.Length);
}
/// <summary>
/// Gets a TextLine by the visual position.
/// </summary>
public TextLine GetTextLineByVisualYPosition(double visualTop)
{
const double epsilon = 0.0001;
var pos = VisualTop;
foreach (var tl in TextLines)
{
pos += tl.Height;
if (visualTop + epsilon < pos)
return tl;
}
return TextLines[TextLines.Count - 1];
}
/// <summary>
/// Gets the visual position from the specified visualColumn.
/// </summary>
/// <returns>Position in device-independent pixels
/// relative to the top left of the document.</returns>
public Point GetVisualPosition(int visualColumn, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
internal Point GetVisualPosition(int visualColumn, bool isAtEndOfLine, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn, isAtEndOfLine);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
/// <summary>
/// Gets the distance to the left border of the text area of the specified visual column.
/// The visual column must belong to the specified text line.
/// </summary>
public double GetTextLineVisualXPosition(TextLine textLine, int visualColumn)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var xPos = textLine.GetDistanceFromCharacterHit(new CharacterHit(Math.Min(visualColumn,
VisualLengthWithEndOfLineMarker)));
if (visualColumn > VisualLengthWithEndOfLineMarker)
{
xPos += (visualColumn - VisualLengthWithEndOfLineMarker) * _textView.WideSpaceWidth;
}
return xPos;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point)
{
return GetVisualColumn(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point, bool allowVirtualSpace)
{
return GetVisualColumn(GetTextLineByVisualYPosition(point.Y), point.X, allowVirtualSpace);
}
internal int GetVisualColumn(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
var vc = GetVisualColumn(textLine, point.X, allowVirtualSpace);
isAtEndOfLine = (vc >= GetTextLineVisualStartColumn(textLine) + textLine.Length);
return vc;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(TextLine textLine, double xPos, bool allowVirtualSpace)
{
if (xPos > textLine.WidthIncludingTrailingWhitespace)
{
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
var virtualX = (int)Math.Round((xPos - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth, MidpointRounding.AwayFromZero);
return VisualLengthWithEndOfLineMarker + virtualX;
}
}
var ch = textLine.GetCharacterHitFromDistance(xPos);
return ch.FirstCharacterIndex + ch.TrailingLength;
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(TextViewPosition position, bool allowVirtualSpace)
{
return ValidateVisualColumn(Document.GetOffset(position.Location), position.VisualColumn, allowVirtualSpace);
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(int offset, int visualColumn, bool allowVirtualSpace)
{
var firstDocumentLineOffset = FirstDocumentLine.Offset;
if (visualColumn < 0)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
var offsetFromVisualColumn = GetRelativeOffset(visualColumn);
offsetFromVisualColumn += firstDocumentLineOffset;
if (offsetFromVisualColumn != offset)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
if (visualColumn > VisualLength && !allowVirtualSpace)
{
return VisualLength;
}
return visualColumn;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point)
{
return GetVisualColumnFloor(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point, bool allowVirtualSpace)
{
return GetVisualColumnFloor(point, allowVirtualSpace, out _);
}
internal int GetVisualColumnFloor(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
if (point.X > textLine.WidthIncludingTrailingWhitespace)
{
isAtEndOfLine = true;
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
// clicking virtual space in the last line
var virtualX = (int)((point.X - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth);
return VisualLengthWithEndOfLineMarker + virtualX;
}
// GetCharacterHitFromDistance returns a hit with FirstCharacterIndex=last character in line
// and TrailingLength=1 when clicking behind the line, so the floor function needs to handle this case
// specially and return the line's end column instead.
return GetTextLineVisualStartColumn(textLine) + textLine.Length;
}
isAtEndOfLine = false;
var ch = textLine.GetCharacterHitFromDistance(point.X);
return ch.FirstCharacterIndex;
}
/// <summary>
/// Gets the text view position from the specified visual column.
/// </summary>
public TextViewPosition GetTextViewPosition(int visualColumn)
{
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
return new TextViewPosition(Document.GetLocation(documentOffset), visualColumn);
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is within a character, it is rounded to the next character boundary.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPosition(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumn(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is inside a character, the position in front of the character is returned.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPositionFloor(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumnFloor(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets whether the visual line was disposed.
/// </summary>
public bool IsDisposed => _phase == LifetimePhase.Disposed;
internal void Dispose()
{
if (_phase == LifetimePhase.Disposed)
{
return;
}
Debug.Assert(_phase == LifetimePhase.Live);
_phase = LifetimePhase.Disposed;
if (_visual != null)
{
((ISetLogicalParent)_visual).SetParent(null);
}
}
/// <summary>
/// Gets the next possible caret position after visualColumn, or -1 if there is no caret position.
/// </summary>
public int GetNextCaretPosition(int visualColumn, LogicalDirection direction, CaretPositioningMode mode, bool allowVirtualSpace)
{
if (!HasStopsInVirtualSpace(mode))
allowVirtualSpace = false;
if (_elements.Count == 0)
{
// special handling for empty visual lines:
if (allowVirtualSpace)
{
if (direction == LogicalDirection.Forward)
return Math.Max(0, visualColumn + 1);
if (visualColumn > 0)
return visualColumn - 1;
return -1;
}
// even though we don't have any elements,
// there's a single caret stop at visualColumn 0
if (visualColumn < 0 && direction == LogicalDirection.Forward)
return 0;
if (visualColumn > 0 && direction == LogicalDirection.Backward)
return 0;
return -1;
}
int i;
if (direction == LogicalDirection.Backward)
{
// Search Backwards:
// If the last element doesn't handle line borders, return the line end as caret stop
if (visualColumn > VisualLength && !_elements[_elements.Count - 1].HandlesLineBorders && HasImplicitStopAtLineEnd())
{
if (allowVirtualSpace)
return visualColumn - 1;
return VisualLength;
}
// skip elements that start after or at visualColumn
for (i = _elements.Count - 1; i >= 0; i--)
{
if (_elements[i].VisualColumn < visualColumn)
break;
}
// search last element that has a caret stop
for (; i >= 0; i--)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Min(visualColumn, _elements[i].VisualColumn + _elements[i].VisualLength + 1),
direction, mode);
if (pos >= 0)
return pos;
}
// If we've found nothing, and the first element doesn't handle line borders,
// return the line start as normal caret stop.
if (visualColumn > 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
}
else
{
// Search Forwards:
// If the first element doesn't handle line borders, return the line start as caret stop
if (visualColumn < 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
// skip elements that end before or at visualColumn
for (i = 0; i < _elements.Count; i++)
{
if (_elements[i].VisualColumn + _elements[i].VisualLength > visualColumn)
break;
}
// search first element that has a caret stop
for (; i < _elements.Count; i++)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Max(visualColumn, _elements[i].VisualColumn - 1),
direction, mode);
if (pos >= 0)
return pos;
}
// if we've found nothing, and the last element doesn't handle line borders,
// return the line end as caret stop
if ((allowVirtualSpace || !_elements[_elements.Count - 1].HandlesLineBorders) && HasImplicitStopAtLineEnd())
{
if (visualColumn < VisualLength)
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
return _visual ??= new VisualLineDrawingVisual(this);
}
}
{
return mode == CaretPositioningMode.Normal || mode == CaretPositioningMode.EveryCodepoint;
}
private static bool HasImplicitStopAtLineEnd() => true;
private VisualLineDrawingVisual _visual;
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
if (_visual == null)
{
_visual = new VisualLineDrawingVisual(this);
((ISetLogicalParent)_visual).SetParent(_textView);
}
return _visual;
}
}
// TODO: can inherit from Layoutable, but dev tools crash
internal sealed class VisualLineDrawingVisual : Control
{
public VisualLine VisualLine { get; }
public double LineHeight { get; }
internal bool IsAdded { get; set; }
public VisualLineDrawingVisual(VisualLine visualLine)
{
VisualLine = visualLine;
LineHeight = VisualLine.TextLines.Sum(textLine => textLine.Height);
}
public override void Render(DrawingContext context)
{
double pos = 0;
foreach (var textLine in VisualLine.TextLines)
{
textLine.Draw(context, new Point(0, pos));
pos += textLine.Height;
}
}
}
}
<MSG> Set VisualLineDrawingVisual.Parent on creation
<DFF> @@ -748,7 +748,15 @@ namespace AvaloniaEdit.Rendering
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
- return _visual ??= new VisualLineDrawingVisual(this);
+
+ if (_visual == null)
+ {
+ _visual = new VisualLineDrawingVisual(this);
+
+ ((ISetLogicalParent)_visual).SetParent(_textView);
+ }
+
+ return _visual;
}
}
| 9 | Set VisualLineDrawingVisual.Parent on creation | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063801 | <NME> VisualLine.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Linq;
using Avalonia;
using Avalonia.Controls;
using Avalonia.Input;
using Avalonia.Media;
using Avalonia.Media.TextFormatting;
using AvaloniaEdit.Document;
using AvaloniaEdit.Utils;
using LogicalDirection = AvaloniaEdit.Document.LogicalDirection;
namespace AvaloniaEdit.Rendering
{
/// <summary>
/// Represents a visual line in the document.
/// A visual line usually corresponds to one DocumentLine, but it can span multiple lines if
/// all but the first are collapsed.
/// </summary>
public sealed class VisualLine
{
public const int LENGTH_LIMIT = 3000;
private enum LifetimePhase : byte
{
Generating,
Transforming,
Live,
Disposed
}
private readonly TextView _textView;
private List<VisualLineElement> _elements;
internal bool HasInlineObjects;
private LifetimePhase _phase;
/// <summary>
/// Gets the document to which this VisualLine belongs.
/// </summary>
public TextDocument Document { get; }
/// <summary>
/// Gets the first document line displayed by this visual line.
/// </summary>
public DocumentLine FirstDocumentLine { get; }
/// <summary>
/// Gets the last document line displayed by this visual line.
/// </summary>
public DocumentLine LastDocumentLine { get; private set; }
/// <summary>
/// Gets a read-only collection of line elements.
/// </summary>
public ReadOnlyCollection<VisualLineElement> Elements { get; private set; }
private ReadOnlyCollection<TextLine> _textLines;
/// <summary>
/// Gets a read-only collection of text lines.
/// </summary>
public ReadOnlyCollection<TextLine> TextLines
{
get
{
if (_phase < LifetimePhase.Live)
throw new InvalidOperationException();
return _textLines;
}
}
/// <summary>
/// Gets the start offset of the VisualLine inside the document.
/// This is equivalent to <c>FirstDocumentLine.Offset</c>.
/// </summary>
public int StartOffset => FirstDocumentLine.Offset;
/// <summary>
/// Length in visual line coordinates.
/// </summary>
public int VisualLength { get; private set; }
/// <summary>
/// Length in visual line coordinates including the end of line marker, if TextEditorOptions.ShowEndOfLine is enabled.
/// </summary>
public int VisualLengthWithEndOfLineMarker
{
get
{
var length = VisualLength;
if (_textView.Options.ShowEndOfLine && LastDocumentLine.NextLine != null) length++;
return length;
}
}
/// <summary>
/// Gets the height of the visual line in device-independent pixels.
/// </summary>
public double Height { get; private set; }
/// <summary>
/// Gets the Y position of the line. This is measured in device-independent pixels relative to the start of the document.
/// </summary>
public double VisualTop { get; internal set; }
internal VisualLine(TextView textView, DocumentLine firstDocumentLine)
{
Debug.Assert(textView != null);
Debug.Assert(firstDocumentLine != null);
_textView = textView;
Document = textView.Document;
FirstDocumentLine = firstDocumentLine;
}
internal void ConstructVisualElements(ITextRunConstructionContext context, IReadOnlyList<VisualLineElementGenerator> generators)
{
Debug.Assert(_phase == LifetimePhase.Generating);
foreach (var g in generators)
{
g.StartGeneration(context);
}
_elements = new List<VisualLineElement>();
PerformVisualElementConstruction(generators);
foreach (var g in generators)
{
g.FinishGeneration();
}
var globalTextRunProperties = context.GlobalTextRunProperties;
foreach (var element in _elements)
{
element.SetTextRunProperties(new VisualLineElementTextRunProperties(globalTextRunProperties));
}
this.Elements = new ReadOnlyCollection<VisualLineElement>(_elements);
CalculateOffsets();
_phase = LifetimePhase.Transforming;
}
void PerformVisualElementConstruction(IReadOnlyList<VisualLineElementGenerator> generators)
{
var lineLength = FirstDocumentLine.Length;
var offset = FirstDocumentLine.Offset;
var currentLineEnd = offset + lineLength;
LastDocumentLine = FirstDocumentLine;
var askInterestOffset = 0; // 0 or 1
while (offset + askInterestOffset <= currentLineEnd)
{
var textPieceEndOffset = currentLineEnd;
foreach (var g in generators)
{
g.CachedInterest = g.GetFirstInterestedOffset(offset + askInterestOffset);
if (g.CachedInterest != -1)
{
if (g.CachedInterest < offset)
throw new ArgumentOutOfRangeException(g.GetType().Name + ".GetFirstInterestedOffset",
g.CachedInterest,
"GetFirstInterestedOffset must not return an offset less than startOffset. Return -1 to signal no interest.");
if (g.CachedInterest < textPieceEndOffset)
textPieceEndOffset = g.CachedInterest;
}
}
Debug.Assert(textPieceEndOffset >= offset);
if (textPieceEndOffset > offset)
{
var textPieceLength = textPieceEndOffset - offset;
_elements.Add(new VisualLineText(this, textPieceLength));
offset = textPieceEndOffset;
}
// If no elements constructed / only zero-length elements constructed:
// do not asking the generators again for the same location (would cause endless loop)
askInterestOffset = 1;
foreach (var g in generators)
{
if (g.CachedInterest == offset)
{
var element = g.ConstructElement(offset);
if (element != null)
{
_elements.Add(element);
if (element.DocumentLength > 0)
{
// a non-zero-length element was constructed
askInterestOffset = 0;
offset += element.DocumentLength;
if (offset > currentLineEnd)
{
var newEndLine = Document.GetLineByOffset(offset);
currentLineEnd = newEndLine.Offset + newEndLine.Length;
this.LastDocumentLine = newEndLine;
if (currentLineEnd < offset)
{
throw new InvalidOperationException(
"The VisualLineElementGenerator " + g.GetType().Name +
" produced an element which ends within the line delimiter");
}
}
break;
}
}
}
}
}
}
private void CalculateOffsets()
{
var visualOffset = 0;
var textOffset = 0;
foreach (var element in _elements)
{
element.VisualColumn = visualOffset;
element.RelativeTextOffset = textOffset;
visualOffset += element.VisualLength;
textOffset += element.DocumentLength;
}
VisualLength = visualOffset;
Debug.Assert(textOffset == LastDocumentLine.EndOffset - FirstDocumentLine.Offset);
}
internal void RunTransformers(ITextRunConstructionContext context, IReadOnlyList<IVisualLineTransformer> transformers)
{
Debug.Assert(_phase == LifetimePhase.Transforming);
foreach (var transformer in transformers)
{
transformer.Transform(context, _elements);
}
_phase = LifetimePhase.Live;
}
/// <summary>
/// Replaces the single element at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, params VisualLineElement[] newElements)
{
ReplaceElement(elementIndex, 1, newElements);
}
/// <summary>
/// Replaces <paramref name="count"/> elements starting at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, int count, params VisualLineElement[] newElements)
{
if (_phase != LifetimePhase.Transforming)
throw new InvalidOperationException("This method may only be called by line transformers.");
var oldDocumentLength = 0;
for (var i = elementIndex; i < elementIndex + count; i++)
{
oldDocumentLength += _elements[i].DocumentLength;
}
var newDocumentLength = 0;
foreach (var newElement in newElements)
{
newDocumentLength += newElement.DocumentLength;
}
if (oldDocumentLength != newDocumentLength)
throw new InvalidOperationException("Old elements have document length " + oldDocumentLength + ", but new elements have length " + newDocumentLength);
_elements.RemoveRange(elementIndex, count);
_elements.InsertRange(elementIndex, newElements);
CalculateOffsets();
}
internal void SetTextLines(List<TextLine> textLines)
{
_textLines = new ReadOnlyCollection<TextLine>(textLines);
Height = 0;
foreach (var line in textLines)
Height += line.Height;
}
/// <summary>
/// Gets the visual column from a document offset relative to the first line start.
/// </summary>
public int GetVisualColumn(int relativeTextOffset)
{
ThrowUtil.CheckNotNegative(relativeTextOffset, "relativeTextOffset");
foreach (var element in _elements)
{
if (element.RelativeTextOffset <= relativeTextOffset
&& element.RelativeTextOffset + element.DocumentLength >= relativeTextOffset)
{
return element.GetVisualColumn(relativeTextOffset);
}
}
return VisualLength;
}
/// <summary>
/// Gets the document offset (relative to the first line start) from a visual column.
/// </summary>
public int GetRelativeOffset(int visualColumn)
{
ThrowUtil.CheckNotNegative(visualColumn, "visualColumn");
var documentLength = 0;
foreach (var element in _elements)
{
if (element.VisualColumn <= visualColumn
&& element.VisualColumn + element.VisualLength > visualColumn)
{
return element.GetRelativeOffset(visualColumn);
}
documentLength += element.DocumentLength;
}
return documentLength;
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn)
{
return GetTextLine(visualColumn, false);
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn, bool isAtEndOfLine)
{
if (visualColumn < 0)
throw new ArgumentOutOfRangeException(nameof(visualColumn));
if (visualColumn >= VisualLengthWithEndOfLineMarker)
return TextLines[TextLines.Count - 1];
foreach (var line in TextLines)
{
if (isAtEndOfLine ? visualColumn <= line.Length : visualColumn < line.Length)
return line;
visualColumn -= line.Length;
}
throw new InvalidOperationException("Shouldn't happen (VisualLength incorrect?)");
}
/// <summary>
/// Gets the visual top from the specified text line.
/// </summary>
/// <returns>Distance in device-independent pixels
/// from the top of the document to the top of the specified text line.</returns>
public double GetTextLineVisualYPosition(TextLine textLine, VisualYPosition yPositionMode)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var pos = VisualTop;
foreach (var tl in TextLines)
{
if (tl == textLine)
{
switch (yPositionMode)
{
case VisualYPosition.LineTop:
return pos;
case VisualYPosition.LineMiddle:
return pos + tl.Height / 2;
case VisualYPosition.LineBottom:
return pos + tl.Height;
case VisualYPosition.TextTop:
return pos + tl.Baseline - _textView.DefaultBaseline;
case VisualYPosition.TextBottom:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight;
case VisualYPosition.TextMiddle:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight / 2;
case VisualYPosition.Baseline:
return pos + tl.Baseline;
default:
throw new ArgumentException("Invalid yPositionMode:" + yPositionMode);
}
}
pos += tl.Height;
}
throw new ArgumentException("textLine is not a line in this VisualLine");
}
/// <summary>
/// Gets the start visual column from the specified text line.
/// </summary>
public int GetTextLineVisualStartColumn(TextLine textLine)
{
if (!TextLines.Contains(textLine))
throw new ArgumentException("textLine is not a line in this VisualLine");
return TextLines.TakeWhile(tl => tl != textLine).Sum(tl => tl.Length);
}
/// <summary>
/// Gets a TextLine by the visual position.
/// </summary>
public TextLine GetTextLineByVisualYPosition(double visualTop)
{
const double epsilon = 0.0001;
var pos = VisualTop;
foreach (var tl in TextLines)
{
pos += tl.Height;
if (visualTop + epsilon < pos)
return tl;
}
return TextLines[TextLines.Count - 1];
}
/// <summary>
/// Gets the visual position from the specified visualColumn.
/// </summary>
/// <returns>Position in device-independent pixels
/// relative to the top left of the document.</returns>
public Point GetVisualPosition(int visualColumn, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
internal Point GetVisualPosition(int visualColumn, bool isAtEndOfLine, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn, isAtEndOfLine);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
/// <summary>
/// Gets the distance to the left border of the text area of the specified visual column.
/// The visual column must belong to the specified text line.
/// </summary>
public double GetTextLineVisualXPosition(TextLine textLine, int visualColumn)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var xPos = textLine.GetDistanceFromCharacterHit(new CharacterHit(Math.Min(visualColumn,
VisualLengthWithEndOfLineMarker)));
if (visualColumn > VisualLengthWithEndOfLineMarker)
{
xPos += (visualColumn - VisualLengthWithEndOfLineMarker) * _textView.WideSpaceWidth;
}
return xPos;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point)
{
return GetVisualColumn(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point, bool allowVirtualSpace)
{
return GetVisualColumn(GetTextLineByVisualYPosition(point.Y), point.X, allowVirtualSpace);
}
internal int GetVisualColumn(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
var vc = GetVisualColumn(textLine, point.X, allowVirtualSpace);
isAtEndOfLine = (vc >= GetTextLineVisualStartColumn(textLine) + textLine.Length);
return vc;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(TextLine textLine, double xPos, bool allowVirtualSpace)
{
if (xPos > textLine.WidthIncludingTrailingWhitespace)
{
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
var virtualX = (int)Math.Round((xPos - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth, MidpointRounding.AwayFromZero);
return VisualLengthWithEndOfLineMarker + virtualX;
}
}
var ch = textLine.GetCharacterHitFromDistance(xPos);
return ch.FirstCharacterIndex + ch.TrailingLength;
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(TextViewPosition position, bool allowVirtualSpace)
{
return ValidateVisualColumn(Document.GetOffset(position.Location), position.VisualColumn, allowVirtualSpace);
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(int offset, int visualColumn, bool allowVirtualSpace)
{
var firstDocumentLineOffset = FirstDocumentLine.Offset;
if (visualColumn < 0)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
var offsetFromVisualColumn = GetRelativeOffset(visualColumn);
offsetFromVisualColumn += firstDocumentLineOffset;
if (offsetFromVisualColumn != offset)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
if (visualColumn > VisualLength && !allowVirtualSpace)
{
return VisualLength;
}
return visualColumn;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point)
{
return GetVisualColumnFloor(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point, bool allowVirtualSpace)
{
return GetVisualColumnFloor(point, allowVirtualSpace, out _);
}
internal int GetVisualColumnFloor(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
if (point.X > textLine.WidthIncludingTrailingWhitespace)
{
isAtEndOfLine = true;
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
// clicking virtual space in the last line
var virtualX = (int)((point.X - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth);
return VisualLengthWithEndOfLineMarker + virtualX;
}
// GetCharacterHitFromDistance returns a hit with FirstCharacterIndex=last character in line
// and TrailingLength=1 when clicking behind the line, so the floor function needs to handle this case
// specially and return the line's end column instead.
return GetTextLineVisualStartColumn(textLine) + textLine.Length;
}
isAtEndOfLine = false;
var ch = textLine.GetCharacterHitFromDistance(point.X);
return ch.FirstCharacterIndex;
}
/// <summary>
/// Gets the text view position from the specified visual column.
/// </summary>
public TextViewPosition GetTextViewPosition(int visualColumn)
{
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
return new TextViewPosition(Document.GetLocation(documentOffset), visualColumn);
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is within a character, it is rounded to the next character boundary.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPosition(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumn(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is inside a character, the position in front of the character is returned.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPositionFloor(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumnFloor(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets whether the visual line was disposed.
/// </summary>
public bool IsDisposed => _phase == LifetimePhase.Disposed;
internal void Dispose()
{
if (_phase == LifetimePhase.Disposed)
{
return;
}
Debug.Assert(_phase == LifetimePhase.Live);
_phase = LifetimePhase.Disposed;
if (_visual != null)
{
((ISetLogicalParent)_visual).SetParent(null);
}
}
/// <summary>
/// Gets the next possible caret position after visualColumn, or -1 if there is no caret position.
/// </summary>
public int GetNextCaretPosition(int visualColumn, LogicalDirection direction, CaretPositioningMode mode, bool allowVirtualSpace)
{
if (!HasStopsInVirtualSpace(mode))
allowVirtualSpace = false;
if (_elements.Count == 0)
{
// special handling for empty visual lines:
if (allowVirtualSpace)
{
if (direction == LogicalDirection.Forward)
return Math.Max(0, visualColumn + 1);
if (visualColumn > 0)
return visualColumn - 1;
return -1;
}
// even though we don't have any elements,
// there's a single caret stop at visualColumn 0
if (visualColumn < 0 && direction == LogicalDirection.Forward)
return 0;
if (visualColumn > 0 && direction == LogicalDirection.Backward)
return 0;
return -1;
}
int i;
if (direction == LogicalDirection.Backward)
{
// Search Backwards:
// If the last element doesn't handle line borders, return the line end as caret stop
if (visualColumn > VisualLength && !_elements[_elements.Count - 1].HandlesLineBorders && HasImplicitStopAtLineEnd())
{
if (allowVirtualSpace)
return visualColumn - 1;
return VisualLength;
}
// skip elements that start after or at visualColumn
for (i = _elements.Count - 1; i >= 0; i--)
{
if (_elements[i].VisualColumn < visualColumn)
break;
}
// search last element that has a caret stop
for (; i >= 0; i--)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Min(visualColumn, _elements[i].VisualColumn + _elements[i].VisualLength + 1),
direction, mode);
if (pos >= 0)
return pos;
}
// If we've found nothing, and the first element doesn't handle line borders,
// return the line start as normal caret stop.
if (visualColumn > 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
}
else
{
// Search Forwards:
// If the first element doesn't handle line borders, return the line start as caret stop
if (visualColumn < 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
// skip elements that end before or at visualColumn
for (i = 0; i < _elements.Count; i++)
{
if (_elements[i].VisualColumn + _elements[i].VisualLength > visualColumn)
break;
}
// search first element that has a caret stop
for (; i < _elements.Count; i++)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Max(visualColumn, _elements[i].VisualColumn - 1),
direction, mode);
if (pos >= 0)
return pos;
}
// if we've found nothing, and the last element doesn't handle line borders,
// return the line end as caret stop
if ((allowVirtualSpace || !_elements[_elements.Count - 1].HandlesLineBorders) && HasImplicitStopAtLineEnd())
{
if (visualColumn < VisualLength)
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
return _visual ??= new VisualLineDrawingVisual(this);
}
}
{
return mode == CaretPositioningMode.Normal || mode == CaretPositioningMode.EveryCodepoint;
}
private static bool HasImplicitStopAtLineEnd() => true;
private VisualLineDrawingVisual _visual;
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
if (_visual == null)
{
_visual = new VisualLineDrawingVisual(this);
((ISetLogicalParent)_visual).SetParent(_textView);
}
return _visual;
}
}
// TODO: can inherit from Layoutable, but dev tools crash
internal sealed class VisualLineDrawingVisual : Control
{
public VisualLine VisualLine { get; }
public double LineHeight { get; }
internal bool IsAdded { get; set; }
public VisualLineDrawingVisual(VisualLine visualLine)
{
VisualLine = visualLine;
LineHeight = VisualLine.TextLines.Sum(textLine => textLine.Height);
}
public override void Render(DrawingContext context)
{
double pos = 0;
foreach (var textLine in VisualLine.TextLines)
{
textLine.Draw(context, new Point(0, pos));
pos += textLine.Height;
}
}
}
}
<MSG> Set VisualLineDrawingVisual.Parent on creation
<DFF> @@ -748,7 +748,15 @@ namespace AvaloniaEdit.Rendering
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
- return _visual ??= new VisualLineDrawingVisual(this);
+
+ if (_visual == null)
+ {
+ _visual = new VisualLineDrawingVisual(this);
+
+ ((ISetLogicalParent)_visual).SetParent(_textView);
+ }
+
+ return _visual;
}
}
| 9 | Set VisualLineDrawingVisual.Parent on creation | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063802 | <NME> VisualLine.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Linq;
using Avalonia;
using Avalonia.Controls;
using Avalonia.Input;
using Avalonia.Media;
using Avalonia.Media.TextFormatting;
using AvaloniaEdit.Document;
using AvaloniaEdit.Utils;
using LogicalDirection = AvaloniaEdit.Document.LogicalDirection;
namespace AvaloniaEdit.Rendering
{
/// <summary>
/// Represents a visual line in the document.
/// A visual line usually corresponds to one DocumentLine, but it can span multiple lines if
/// all but the first are collapsed.
/// </summary>
public sealed class VisualLine
{
public const int LENGTH_LIMIT = 3000;
private enum LifetimePhase : byte
{
Generating,
Transforming,
Live,
Disposed
}
private readonly TextView _textView;
private List<VisualLineElement> _elements;
internal bool HasInlineObjects;
private LifetimePhase _phase;
/// <summary>
/// Gets the document to which this VisualLine belongs.
/// </summary>
public TextDocument Document { get; }
/// <summary>
/// Gets the first document line displayed by this visual line.
/// </summary>
public DocumentLine FirstDocumentLine { get; }
/// <summary>
/// Gets the last document line displayed by this visual line.
/// </summary>
public DocumentLine LastDocumentLine { get; private set; }
/// <summary>
/// Gets a read-only collection of line elements.
/// </summary>
public ReadOnlyCollection<VisualLineElement> Elements { get; private set; }
private ReadOnlyCollection<TextLine> _textLines;
/// <summary>
/// Gets a read-only collection of text lines.
/// </summary>
public ReadOnlyCollection<TextLine> TextLines
{
get
{
if (_phase < LifetimePhase.Live)
throw new InvalidOperationException();
return _textLines;
}
}
/// <summary>
/// Gets the start offset of the VisualLine inside the document.
/// This is equivalent to <c>FirstDocumentLine.Offset</c>.
/// </summary>
public int StartOffset => FirstDocumentLine.Offset;
/// <summary>
/// Length in visual line coordinates.
/// </summary>
public int VisualLength { get; private set; }
/// <summary>
/// Length in visual line coordinates including the end of line marker, if TextEditorOptions.ShowEndOfLine is enabled.
/// </summary>
public int VisualLengthWithEndOfLineMarker
{
get
{
var length = VisualLength;
if (_textView.Options.ShowEndOfLine && LastDocumentLine.NextLine != null) length++;
return length;
}
}
/// <summary>
/// Gets the height of the visual line in device-independent pixels.
/// </summary>
public double Height { get; private set; }
/// <summary>
/// Gets the Y position of the line. This is measured in device-independent pixels relative to the start of the document.
/// </summary>
public double VisualTop { get; internal set; }
internal VisualLine(TextView textView, DocumentLine firstDocumentLine)
{
Debug.Assert(textView != null);
Debug.Assert(firstDocumentLine != null);
_textView = textView;
Document = textView.Document;
FirstDocumentLine = firstDocumentLine;
}
internal void ConstructVisualElements(ITextRunConstructionContext context, IReadOnlyList<VisualLineElementGenerator> generators)
{
Debug.Assert(_phase == LifetimePhase.Generating);
foreach (var g in generators)
{
g.StartGeneration(context);
}
_elements = new List<VisualLineElement>();
PerformVisualElementConstruction(generators);
foreach (var g in generators)
{
g.FinishGeneration();
}
var globalTextRunProperties = context.GlobalTextRunProperties;
foreach (var element in _elements)
{
element.SetTextRunProperties(new VisualLineElementTextRunProperties(globalTextRunProperties));
}
this.Elements = new ReadOnlyCollection<VisualLineElement>(_elements);
CalculateOffsets();
_phase = LifetimePhase.Transforming;
}
void PerformVisualElementConstruction(IReadOnlyList<VisualLineElementGenerator> generators)
{
var lineLength = FirstDocumentLine.Length;
var offset = FirstDocumentLine.Offset;
var currentLineEnd = offset + lineLength;
LastDocumentLine = FirstDocumentLine;
var askInterestOffset = 0; // 0 or 1
while (offset + askInterestOffset <= currentLineEnd)
{
var textPieceEndOffset = currentLineEnd;
foreach (var g in generators)
{
g.CachedInterest = g.GetFirstInterestedOffset(offset + askInterestOffset);
if (g.CachedInterest != -1)
{
if (g.CachedInterest < offset)
throw new ArgumentOutOfRangeException(g.GetType().Name + ".GetFirstInterestedOffset",
g.CachedInterest,
"GetFirstInterestedOffset must not return an offset less than startOffset. Return -1 to signal no interest.");
if (g.CachedInterest < textPieceEndOffset)
textPieceEndOffset = g.CachedInterest;
}
}
Debug.Assert(textPieceEndOffset >= offset);
if (textPieceEndOffset > offset)
{
var textPieceLength = textPieceEndOffset - offset;
_elements.Add(new VisualLineText(this, textPieceLength));
offset = textPieceEndOffset;
}
// If no elements constructed / only zero-length elements constructed:
// do not asking the generators again for the same location (would cause endless loop)
askInterestOffset = 1;
foreach (var g in generators)
{
if (g.CachedInterest == offset)
{
var element = g.ConstructElement(offset);
if (element != null)
{
_elements.Add(element);
if (element.DocumentLength > 0)
{
// a non-zero-length element was constructed
askInterestOffset = 0;
offset += element.DocumentLength;
if (offset > currentLineEnd)
{
var newEndLine = Document.GetLineByOffset(offset);
currentLineEnd = newEndLine.Offset + newEndLine.Length;
this.LastDocumentLine = newEndLine;
if (currentLineEnd < offset)
{
throw new InvalidOperationException(
"The VisualLineElementGenerator " + g.GetType().Name +
" produced an element which ends within the line delimiter");
}
}
break;
}
}
}
}
}
}
private void CalculateOffsets()
{
var visualOffset = 0;
var textOffset = 0;
foreach (var element in _elements)
{
element.VisualColumn = visualOffset;
element.RelativeTextOffset = textOffset;
visualOffset += element.VisualLength;
textOffset += element.DocumentLength;
}
VisualLength = visualOffset;
Debug.Assert(textOffset == LastDocumentLine.EndOffset - FirstDocumentLine.Offset);
}
internal void RunTransformers(ITextRunConstructionContext context, IReadOnlyList<IVisualLineTransformer> transformers)
{
Debug.Assert(_phase == LifetimePhase.Transforming);
foreach (var transformer in transformers)
{
transformer.Transform(context, _elements);
}
_phase = LifetimePhase.Live;
}
/// <summary>
/// Replaces the single element at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, params VisualLineElement[] newElements)
{
ReplaceElement(elementIndex, 1, newElements);
}
/// <summary>
/// Replaces <paramref name="count"/> elements starting at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, int count, params VisualLineElement[] newElements)
{
if (_phase != LifetimePhase.Transforming)
throw new InvalidOperationException("This method may only be called by line transformers.");
var oldDocumentLength = 0;
for (var i = elementIndex; i < elementIndex + count; i++)
{
oldDocumentLength += _elements[i].DocumentLength;
}
var newDocumentLength = 0;
foreach (var newElement in newElements)
{
newDocumentLength += newElement.DocumentLength;
}
if (oldDocumentLength != newDocumentLength)
throw new InvalidOperationException("Old elements have document length " + oldDocumentLength + ", but new elements have length " + newDocumentLength);
_elements.RemoveRange(elementIndex, count);
_elements.InsertRange(elementIndex, newElements);
CalculateOffsets();
}
internal void SetTextLines(List<TextLine> textLines)
{
_textLines = new ReadOnlyCollection<TextLine>(textLines);
Height = 0;
foreach (var line in textLines)
Height += line.Height;
}
/// <summary>
/// Gets the visual column from a document offset relative to the first line start.
/// </summary>
public int GetVisualColumn(int relativeTextOffset)
{
ThrowUtil.CheckNotNegative(relativeTextOffset, "relativeTextOffset");
foreach (var element in _elements)
{
if (element.RelativeTextOffset <= relativeTextOffset
&& element.RelativeTextOffset + element.DocumentLength >= relativeTextOffset)
{
return element.GetVisualColumn(relativeTextOffset);
}
}
return VisualLength;
}
/// <summary>
/// Gets the document offset (relative to the first line start) from a visual column.
/// </summary>
public int GetRelativeOffset(int visualColumn)
{
ThrowUtil.CheckNotNegative(visualColumn, "visualColumn");
var documentLength = 0;
foreach (var element in _elements)
{
if (element.VisualColumn <= visualColumn
&& element.VisualColumn + element.VisualLength > visualColumn)
{
return element.GetRelativeOffset(visualColumn);
}
documentLength += element.DocumentLength;
}
return documentLength;
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn)
{
return GetTextLine(visualColumn, false);
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn, bool isAtEndOfLine)
{
if (visualColumn < 0)
throw new ArgumentOutOfRangeException(nameof(visualColumn));
if (visualColumn >= VisualLengthWithEndOfLineMarker)
return TextLines[TextLines.Count - 1];
foreach (var line in TextLines)
{
if (isAtEndOfLine ? visualColumn <= line.Length : visualColumn < line.Length)
return line;
visualColumn -= line.Length;
}
throw new InvalidOperationException("Shouldn't happen (VisualLength incorrect?)");
}
/// <summary>
/// Gets the visual top from the specified text line.
/// </summary>
/// <returns>Distance in device-independent pixels
/// from the top of the document to the top of the specified text line.</returns>
public double GetTextLineVisualYPosition(TextLine textLine, VisualYPosition yPositionMode)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var pos = VisualTop;
foreach (var tl in TextLines)
{
if (tl == textLine)
{
switch (yPositionMode)
{
case VisualYPosition.LineTop:
return pos;
case VisualYPosition.LineMiddle:
return pos + tl.Height / 2;
case VisualYPosition.LineBottom:
return pos + tl.Height;
case VisualYPosition.TextTop:
return pos + tl.Baseline - _textView.DefaultBaseline;
case VisualYPosition.TextBottom:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight;
case VisualYPosition.TextMiddle:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight / 2;
case VisualYPosition.Baseline:
return pos + tl.Baseline;
default:
throw new ArgumentException("Invalid yPositionMode:" + yPositionMode);
}
}
pos += tl.Height;
}
throw new ArgumentException("textLine is not a line in this VisualLine");
}
/// <summary>
/// Gets the start visual column from the specified text line.
/// </summary>
public int GetTextLineVisualStartColumn(TextLine textLine)
{
if (!TextLines.Contains(textLine))
throw new ArgumentException("textLine is not a line in this VisualLine");
return TextLines.TakeWhile(tl => tl != textLine).Sum(tl => tl.Length);
}
/// <summary>
/// Gets a TextLine by the visual position.
/// </summary>
public TextLine GetTextLineByVisualYPosition(double visualTop)
{
const double epsilon = 0.0001;
var pos = VisualTop;
foreach (var tl in TextLines)
{
pos += tl.Height;
if (visualTop + epsilon < pos)
return tl;
}
return TextLines[TextLines.Count - 1];
}
/// <summary>
/// Gets the visual position from the specified visualColumn.
/// </summary>
/// <returns>Position in device-independent pixels
/// relative to the top left of the document.</returns>
public Point GetVisualPosition(int visualColumn, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
internal Point GetVisualPosition(int visualColumn, bool isAtEndOfLine, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn, isAtEndOfLine);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
/// <summary>
/// Gets the distance to the left border of the text area of the specified visual column.
/// The visual column must belong to the specified text line.
/// </summary>
public double GetTextLineVisualXPosition(TextLine textLine, int visualColumn)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var xPos = textLine.GetDistanceFromCharacterHit(new CharacterHit(Math.Min(visualColumn,
VisualLengthWithEndOfLineMarker)));
if (visualColumn > VisualLengthWithEndOfLineMarker)
{
xPos += (visualColumn - VisualLengthWithEndOfLineMarker) * _textView.WideSpaceWidth;
}
return xPos;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point)
{
return GetVisualColumn(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point, bool allowVirtualSpace)
{
return GetVisualColumn(GetTextLineByVisualYPosition(point.Y), point.X, allowVirtualSpace);
}
internal int GetVisualColumn(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
var vc = GetVisualColumn(textLine, point.X, allowVirtualSpace);
isAtEndOfLine = (vc >= GetTextLineVisualStartColumn(textLine) + textLine.Length);
return vc;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(TextLine textLine, double xPos, bool allowVirtualSpace)
{
if (xPos > textLine.WidthIncludingTrailingWhitespace)
{
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
var virtualX = (int)Math.Round((xPos - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth, MidpointRounding.AwayFromZero);
return VisualLengthWithEndOfLineMarker + virtualX;
}
}
var ch = textLine.GetCharacterHitFromDistance(xPos);
return ch.FirstCharacterIndex + ch.TrailingLength;
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(TextViewPosition position, bool allowVirtualSpace)
{
return ValidateVisualColumn(Document.GetOffset(position.Location), position.VisualColumn, allowVirtualSpace);
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(int offset, int visualColumn, bool allowVirtualSpace)
{
var firstDocumentLineOffset = FirstDocumentLine.Offset;
if (visualColumn < 0)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
var offsetFromVisualColumn = GetRelativeOffset(visualColumn);
offsetFromVisualColumn += firstDocumentLineOffset;
if (offsetFromVisualColumn != offset)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
if (visualColumn > VisualLength && !allowVirtualSpace)
{
return VisualLength;
}
return visualColumn;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point)
{
return GetVisualColumnFloor(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point, bool allowVirtualSpace)
{
return GetVisualColumnFloor(point, allowVirtualSpace, out _);
}
internal int GetVisualColumnFloor(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
if (point.X > textLine.WidthIncludingTrailingWhitespace)
{
isAtEndOfLine = true;
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
// clicking virtual space in the last line
var virtualX = (int)((point.X - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth);
return VisualLengthWithEndOfLineMarker + virtualX;
}
// GetCharacterHitFromDistance returns a hit with FirstCharacterIndex=last character in line
// and TrailingLength=1 when clicking behind the line, so the floor function needs to handle this case
// specially and return the line's end column instead.
return GetTextLineVisualStartColumn(textLine) + textLine.Length;
}
isAtEndOfLine = false;
var ch = textLine.GetCharacterHitFromDistance(point.X);
return ch.FirstCharacterIndex;
}
/// <summary>
/// Gets the text view position from the specified visual column.
/// </summary>
public TextViewPosition GetTextViewPosition(int visualColumn)
{
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
return new TextViewPosition(Document.GetLocation(documentOffset), visualColumn);
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is within a character, it is rounded to the next character boundary.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPosition(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumn(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is inside a character, the position in front of the character is returned.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPositionFloor(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumnFloor(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets whether the visual line was disposed.
/// </summary>
public bool IsDisposed => _phase == LifetimePhase.Disposed;
internal void Dispose()
{
if (_phase == LifetimePhase.Disposed)
{
return;
}
Debug.Assert(_phase == LifetimePhase.Live);
_phase = LifetimePhase.Disposed;
if (_visual != null)
{
((ISetLogicalParent)_visual).SetParent(null);
}
}
/// <summary>
/// Gets the next possible caret position after visualColumn, or -1 if there is no caret position.
/// </summary>
public int GetNextCaretPosition(int visualColumn, LogicalDirection direction, CaretPositioningMode mode, bool allowVirtualSpace)
{
if (!HasStopsInVirtualSpace(mode))
allowVirtualSpace = false;
if (_elements.Count == 0)
{
// special handling for empty visual lines:
if (allowVirtualSpace)
{
if (direction == LogicalDirection.Forward)
return Math.Max(0, visualColumn + 1);
if (visualColumn > 0)
return visualColumn - 1;
return -1;
}
// even though we don't have any elements,
// there's a single caret stop at visualColumn 0
if (visualColumn < 0 && direction == LogicalDirection.Forward)
return 0;
if (visualColumn > 0 && direction == LogicalDirection.Backward)
return 0;
return -1;
}
int i;
if (direction == LogicalDirection.Backward)
{
// Search Backwards:
// If the last element doesn't handle line borders, return the line end as caret stop
if (visualColumn > VisualLength && !_elements[_elements.Count - 1].HandlesLineBorders && HasImplicitStopAtLineEnd())
{
if (allowVirtualSpace)
return visualColumn - 1;
return VisualLength;
}
// skip elements that start after or at visualColumn
for (i = _elements.Count - 1; i >= 0; i--)
{
if (_elements[i].VisualColumn < visualColumn)
break;
}
// search last element that has a caret stop
for (; i >= 0; i--)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Min(visualColumn, _elements[i].VisualColumn + _elements[i].VisualLength + 1),
direction, mode);
if (pos >= 0)
return pos;
}
// If we've found nothing, and the first element doesn't handle line borders,
// return the line start as normal caret stop.
if (visualColumn > 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
}
else
{
// Search Forwards:
// If the first element doesn't handle line borders, return the line start as caret stop
if (visualColumn < 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
// skip elements that end before or at visualColumn
for (i = 0; i < _elements.Count; i++)
{
if (_elements[i].VisualColumn + _elements[i].VisualLength > visualColumn)
break;
}
// search first element that has a caret stop
for (; i < _elements.Count; i++)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Max(visualColumn, _elements[i].VisualColumn - 1),
direction, mode);
if (pos >= 0)
return pos;
}
// if we've found nothing, and the last element doesn't handle line borders,
// return the line end as caret stop
if ((allowVirtualSpace || !_elements[_elements.Count - 1].HandlesLineBorders) && HasImplicitStopAtLineEnd())
{
if (visualColumn < VisualLength)
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
return _visual ??= new VisualLineDrawingVisual(this);
}
}
{
return mode == CaretPositioningMode.Normal || mode == CaretPositioningMode.EveryCodepoint;
}
private static bool HasImplicitStopAtLineEnd() => true;
private VisualLineDrawingVisual _visual;
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
if (_visual == null)
{
_visual = new VisualLineDrawingVisual(this);
((ISetLogicalParent)_visual).SetParent(_textView);
}
return _visual;
}
}
// TODO: can inherit from Layoutable, but dev tools crash
internal sealed class VisualLineDrawingVisual : Control
{
public VisualLine VisualLine { get; }
public double LineHeight { get; }
internal bool IsAdded { get; set; }
public VisualLineDrawingVisual(VisualLine visualLine)
{
VisualLine = visualLine;
LineHeight = VisualLine.TextLines.Sum(textLine => textLine.Height);
}
public override void Render(DrawingContext context)
{
double pos = 0;
foreach (var textLine in VisualLine.TextLines)
{
textLine.Draw(context, new Point(0, pos));
pos += textLine.Height;
}
}
}
}
<MSG> Set VisualLineDrawingVisual.Parent on creation
<DFF> @@ -748,7 +748,15 @@ namespace AvaloniaEdit.Rendering
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
- return _visual ??= new VisualLineDrawingVisual(this);
+
+ if (_visual == null)
+ {
+ _visual = new VisualLineDrawingVisual(this);
+
+ ((ISetLogicalParent)_visual).SetParent(_textView);
+ }
+
+ return _visual;
}
}
| 9 | Set VisualLineDrawingVisual.Parent on creation | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063803 | <NME> VisualLine.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Linq;
using Avalonia;
using Avalonia.Controls;
using Avalonia.Input;
using Avalonia.Media;
using Avalonia.Media.TextFormatting;
using AvaloniaEdit.Document;
using AvaloniaEdit.Utils;
using LogicalDirection = AvaloniaEdit.Document.LogicalDirection;
namespace AvaloniaEdit.Rendering
{
/// <summary>
/// Represents a visual line in the document.
/// A visual line usually corresponds to one DocumentLine, but it can span multiple lines if
/// all but the first are collapsed.
/// </summary>
public sealed class VisualLine
{
public const int LENGTH_LIMIT = 3000;
private enum LifetimePhase : byte
{
Generating,
Transforming,
Live,
Disposed
}
private readonly TextView _textView;
private List<VisualLineElement> _elements;
internal bool HasInlineObjects;
private LifetimePhase _phase;
/// <summary>
/// Gets the document to which this VisualLine belongs.
/// </summary>
public TextDocument Document { get; }
/// <summary>
/// Gets the first document line displayed by this visual line.
/// </summary>
public DocumentLine FirstDocumentLine { get; }
/// <summary>
/// Gets the last document line displayed by this visual line.
/// </summary>
public DocumentLine LastDocumentLine { get; private set; }
/// <summary>
/// Gets a read-only collection of line elements.
/// </summary>
public ReadOnlyCollection<VisualLineElement> Elements { get; private set; }
private ReadOnlyCollection<TextLine> _textLines;
/// <summary>
/// Gets a read-only collection of text lines.
/// </summary>
public ReadOnlyCollection<TextLine> TextLines
{
get
{
if (_phase < LifetimePhase.Live)
throw new InvalidOperationException();
return _textLines;
}
}
/// <summary>
/// Gets the start offset of the VisualLine inside the document.
/// This is equivalent to <c>FirstDocumentLine.Offset</c>.
/// </summary>
public int StartOffset => FirstDocumentLine.Offset;
/// <summary>
/// Length in visual line coordinates.
/// </summary>
public int VisualLength { get; private set; }
/// <summary>
/// Length in visual line coordinates including the end of line marker, if TextEditorOptions.ShowEndOfLine is enabled.
/// </summary>
public int VisualLengthWithEndOfLineMarker
{
get
{
var length = VisualLength;
if (_textView.Options.ShowEndOfLine && LastDocumentLine.NextLine != null) length++;
return length;
}
}
/// <summary>
/// Gets the height of the visual line in device-independent pixels.
/// </summary>
public double Height { get; private set; }
/// <summary>
/// Gets the Y position of the line. This is measured in device-independent pixels relative to the start of the document.
/// </summary>
public double VisualTop { get; internal set; }
internal VisualLine(TextView textView, DocumentLine firstDocumentLine)
{
Debug.Assert(textView != null);
Debug.Assert(firstDocumentLine != null);
_textView = textView;
Document = textView.Document;
FirstDocumentLine = firstDocumentLine;
}
internal void ConstructVisualElements(ITextRunConstructionContext context, IReadOnlyList<VisualLineElementGenerator> generators)
{
Debug.Assert(_phase == LifetimePhase.Generating);
foreach (var g in generators)
{
g.StartGeneration(context);
}
_elements = new List<VisualLineElement>();
PerformVisualElementConstruction(generators);
foreach (var g in generators)
{
g.FinishGeneration();
}
var globalTextRunProperties = context.GlobalTextRunProperties;
foreach (var element in _elements)
{
element.SetTextRunProperties(new VisualLineElementTextRunProperties(globalTextRunProperties));
}
this.Elements = new ReadOnlyCollection<VisualLineElement>(_elements);
CalculateOffsets();
_phase = LifetimePhase.Transforming;
}
void PerformVisualElementConstruction(IReadOnlyList<VisualLineElementGenerator> generators)
{
var lineLength = FirstDocumentLine.Length;
var offset = FirstDocumentLine.Offset;
var currentLineEnd = offset + lineLength;
LastDocumentLine = FirstDocumentLine;
var askInterestOffset = 0; // 0 or 1
while (offset + askInterestOffset <= currentLineEnd)
{
var textPieceEndOffset = currentLineEnd;
foreach (var g in generators)
{
g.CachedInterest = g.GetFirstInterestedOffset(offset + askInterestOffset);
if (g.CachedInterest != -1)
{
if (g.CachedInterest < offset)
throw new ArgumentOutOfRangeException(g.GetType().Name + ".GetFirstInterestedOffset",
g.CachedInterest,
"GetFirstInterestedOffset must not return an offset less than startOffset. Return -1 to signal no interest.");
if (g.CachedInterest < textPieceEndOffset)
textPieceEndOffset = g.CachedInterest;
}
}
Debug.Assert(textPieceEndOffset >= offset);
if (textPieceEndOffset > offset)
{
var textPieceLength = textPieceEndOffset - offset;
_elements.Add(new VisualLineText(this, textPieceLength));
offset = textPieceEndOffset;
}
// If no elements constructed / only zero-length elements constructed:
// do not asking the generators again for the same location (would cause endless loop)
askInterestOffset = 1;
foreach (var g in generators)
{
if (g.CachedInterest == offset)
{
var element = g.ConstructElement(offset);
if (element != null)
{
_elements.Add(element);
if (element.DocumentLength > 0)
{
// a non-zero-length element was constructed
askInterestOffset = 0;
offset += element.DocumentLength;
if (offset > currentLineEnd)
{
var newEndLine = Document.GetLineByOffset(offset);
currentLineEnd = newEndLine.Offset + newEndLine.Length;
this.LastDocumentLine = newEndLine;
if (currentLineEnd < offset)
{
throw new InvalidOperationException(
"The VisualLineElementGenerator " + g.GetType().Name +
" produced an element which ends within the line delimiter");
}
}
break;
}
}
}
}
}
}
private void CalculateOffsets()
{
var visualOffset = 0;
var textOffset = 0;
foreach (var element in _elements)
{
element.VisualColumn = visualOffset;
element.RelativeTextOffset = textOffset;
visualOffset += element.VisualLength;
textOffset += element.DocumentLength;
}
VisualLength = visualOffset;
Debug.Assert(textOffset == LastDocumentLine.EndOffset - FirstDocumentLine.Offset);
}
internal void RunTransformers(ITextRunConstructionContext context, IReadOnlyList<IVisualLineTransformer> transformers)
{
Debug.Assert(_phase == LifetimePhase.Transforming);
foreach (var transformer in transformers)
{
transformer.Transform(context, _elements);
}
_phase = LifetimePhase.Live;
}
/// <summary>
/// Replaces the single element at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, params VisualLineElement[] newElements)
{
ReplaceElement(elementIndex, 1, newElements);
}
/// <summary>
/// Replaces <paramref name="count"/> elements starting at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, int count, params VisualLineElement[] newElements)
{
if (_phase != LifetimePhase.Transforming)
throw new InvalidOperationException("This method may only be called by line transformers.");
var oldDocumentLength = 0;
for (var i = elementIndex; i < elementIndex + count; i++)
{
oldDocumentLength += _elements[i].DocumentLength;
}
var newDocumentLength = 0;
foreach (var newElement in newElements)
{
newDocumentLength += newElement.DocumentLength;
}
if (oldDocumentLength != newDocumentLength)
throw new InvalidOperationException("Old elements have document length " + oldDocumentLength + ", but new elements have length " + newDocumentLength);
_elements.RemoveRange(elementIndex, count);
_elements.InsertRange(elementIndex, newElements);
CalculateOffsets();
}
internal void SetTextLines(List<TextLine> textLines)
{
_textLines = new ReadOnlyCollection<TextLine>(textLines);
Height = 0;
foreach (var line in textLines)
Height += line.Height;
}
/// <summary>
/// Gets the visual column from a document offset relative to the first line start.
/// </summary>
public int GetVisualColumn(int relativeTextOffset)
{
ThrowUtil.CheckNotNegative(relativeTextOffset, "relativeTextOffset");
foreach (var element in _elements)
{
if (element.RelativeTextOffset <= relativeTextOffset
&& element.RelativeTextOffset + element.DocumentLength >= relativeTextOffset)
{
return element.GetVisualColumn(relativeTextOffset);
}
}
return VisualLength;
}
/// <summary>
/// Gets the document offset (relative to the first line start) from a visual column.
/// </summary>
public int GetRelativeOffset(int visualColumn)
{
ThrowUtil.CheckNotNegative(visualColumn, "visualColumn");
var documentLength = 0;
foreach (var element in _elements)
{
if (element.VisualColumn <= visualColumn
&& element.VisualColumn + element.VisualLength > visualColumn)
{
return element.GetRelativeOffset(visualColumn);
}
documentLength += element.DocumentLength;
}
return documentLength;
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn)
{
return GetTextLine(visualColumn, false);
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn, bool isAtEndOfLine)
{
if (visualColumn < 0)
throw new ArgumentOutOfRangeException(nameof(visualColumn));
if (visualColumn >= VisualLengthWithEndOfLineMarker)
return TextLines[TextLines.Count - 1];
foreach (var line in TextLines)
{
if (isAtEndOfLine ? visualColumn <= line.Length : visualColumn < line.Length)
return line;
visualColumn -= line.Length;
}
throw new InvalidOperationException("Shouldn't happen (VisualLength incorrect?)");
}
/// <summary>
/// Gets the visual top from the specified text line.
/// </summary>
/// <returns>Distance in device-independent pixels
/// from the top of the document to the top of the specified text line.</returns>
public double GetTextLineVisualYPosition(TextLine textLine, VisualYPosition yPositionMode)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var pos = VisualTop;
foreach (var tl in TextLines)
{
if (tl == textLine)
{
switch (yPositionMode)
{
case VisualYPosition.LineTop:
return pos;
case VisualYPosition.LineMiddle:
return pos + tl.Height / 2;
case VisualYPosition.LineBottom:
return pos + tl.Height;
case VisualYPosition.TextTop:
return pos + tl.Baseline - _textView.DefaultBaseline;
case VisualYPosition.TextBottom:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight;
case VisualYPosition.TextMiddle:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight / 2;
case VisualYPosition.Baseline:
return pos + tl.Baseline;
default:
throw new ArgumentException("Invalid yPositionMode:" + yPositionMode);
}
}
pos += tl.Height;
}
throw new ArgumentException("textLine is not a line in this VisualLine");
}
/// <summary>
/// Gets the start visual column from the specified text line.
/// </summary>
public int GetTextLineVisualStartColumn(TextLine textLine)
{
if (!TextLines.Contains(textLine))
throw new ArgumentException("textLine is not a line in this VisualLine");
return TextLines.TakeWhile(tl => tl != textLine).Sum(tl => tl.Length);
}
/// <summary>
/// Gets a TextLine by the visual position.
/// </summary>
public TextLine GetTextLineByVisualYPosition(double visualTop)
{
const double epsilon = 0.0001;
var pos = VisualTop;
foreach (var tl in TextLines)
{
pos += tl.Height;
if (visualTop + epsilon < pos)
return tl;
}
return TextLines[TextLines.Count - 1];
}
/// <summary>
/// Gets the visual position from the specified visualColumn.
/// </summary>
/// <returns>Position in device-independent pixels
/// relative to the top left of the document.</returns>
public Point GetVisualPosition(int visualColumn, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
internal Point GetVisualPosition(int visualColumn, bool isAtEndOfLine, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn, isAtEndOfLine);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
/// <summary>
/// Gets the distance to the left border of the text area of the specified visual column.
/// The visual column must belong to the specified text line.
/// </summary>
public double GetTextLineVisualXPosition(TextLine textLine, int visualColumn)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var xPos = textLine.GetDistanceFromCharacterHit(new CharacterHit(Math.Min(visualColumn,
VisualLengthWithEndOfLineMarker)));
if (visualColumn > VisualLengthWithEndOfLineMarker)
{
xPos += (visualColumn - VisualLengthWithEndOfLineMarker) * _textView.WideSpaceWidth;
}
return xPos;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point)
{
return GetVisualColumn(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point, bool allowVirtualSpace)
{
return GetVisualColumn(GetTextLineByVisualYPosition(point.Y), point.X, allowVirtualSpace);
}
internal int GetVisualColumn(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
var vc = GetVisualColumn(textLine, point.X, allowVirtualSpace);
isAtEndOfLine = (vc >= GetTextLineVisualStartColumn(textLine) + textLine.Length);
return vc;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(TextLine textLine, double xPos, bool allowVirtualSpace)
{
if (xPos > textLine.WidthIncludingTrailingWhitespace)
{
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
var virtualX = (int)Math.Round((xPos - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth, MidpointRounding.AwayFromZero);
return VisualLengthWithEndOfLineMarker + virtualX;
}
}
var ch = textLine.GetCharacterHitFromDistance(xPos);
return ch.FirstCharacterIndex + ch.TrailingLength;
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(TextViewPosition position, bool allowVirtualSpace)
{
return ValidateVisualColumn(Document.GetOffset(position.Location), position.VisualColumn, allowVirtualSpace);
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(int offset, int visualColumn, bool allowVirtualSpace)
{
var firstDocumentLineOffset = FirstDocumentLine.Offset;
if (visualColumn < 0)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
var offsetFromVisualColumn = GetRelativeOffset(visualColumn);
offsetFromVisualColumn += firstDocumentLineOffset;
if (offsetFromVisualColumn != offset)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
if (visualColumn > VisualLength && !allowVirtualSpace)
{
return VisualLength;
}
return visualColumn;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point)
{
return GetVisualColumnFloor(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point, bool allowVirtualSpace)
{
return GetVisualColumnFloor(point, allowVirtualSpace, out _);
}
internal int GetVisualColumnFloor(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
if (point.X > textLine.WidthIncludingTrailingWhitespace)
{
isAtEndOfLine = true;
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
// clicking virtual space in the last line
var virtualX = (int)((point.X - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth);
return VisualLengthWithEndOfLineMarker + virtualX;
}
// GetCharacterHitFromDistance returns a hit with FirstCharacterIndex=last character in line
// and TrailingLength=1 when clicking behind the line, so the floor function needs to handle this case
// specially and return the line's end column instead.
return GetTextLineVisualStartColumn(textLine) + textLine.Length;
}
isAtEndOfLine = false;
var ch = textLine.GetCharacterHitFromDistance(point.X);
return ch.FirstCharacterIndex;
}
/// <summary>
/// Gets the text view position from the specified visual column.
/// </summary>
public TextViewPosition GetTextViewPosition(int visualColumn)
{
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
return new TextViewPosition(Document.GetLocation(documentOffset), visualColumn);
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is within a character, it is rounded to the next character boundary.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPosition(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumn(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is inside a character, the position in front of the character is returned.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPositionFloor(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumnFloor(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets whether the visual line was disposed.
/// </summary>
public bool IsDisposed => _phase == LifetimePhase.Disposed;
internal void Dispose()
{
if (_phase == LifetimePhase.Disposed)
{
return;
}
Debug.Assert(_phase == LifetimePhase.Live);
_phase = LifetimePhase.Disposed;
if (_visual != null)
{
((ISetLogicalParent)_visual).SetParent(null);
}
}
/// <summary>
/// Gets the next possible caret position after visualColumn, or -1 if there is no caret position.
/// </summary>
public int GetNextCaretPosition(int visualColumn, LogicalDirection direction, CaretPositioningMode mode, bool allowVirtualSpace)
{
if (!HasStopsInVirtualSpace(mode))
allowVirtualSpace = false;
if (_elements.Count == 0)
{
// special handling for empty visual lines:
if (allowVirtualSpace)
{
if (direction == LogicalDirection.Forward)
return Math.Max(0, visualColumn + 1);
if (visualColumn > 0)
return visualColumn - 1;
return -1;
}
// even though we don't have any elements,
// there's a single caret stop at visualColumn 0
if (visualColumn < 0 && direction == LogicalDirection.Forward)
return 0;
if (visualColumn > 0 && direction == LogicalDirection.Backward)
return 0;
return -1;
}
int i;
if (direction == LogicalDirection.Backward)
{
// Search Backwards:
// If the last element doesn't handle line borders, return the line end as caret stop
if (visualColumn > VisualLength && !_elements[_elements.Count - 1].HandlesLineBorders && HasImplicitStopAtLineEnd())
{
if (allowVirtualSpace)
return visualColumn - 1;
return VisualLength;
}
// skip elements that start after or at visualColumn
for (i = _elements.Count - 1; i >= 0; i--)
{
if (_elements[i].VisualColumn < visualColumn)
break;
}
// search last element that has a caret stop
for (; i >= 0; i--)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Min(visualColumn, _elements[i].VisualColumn + _elements[i].VisualLength + 1),
direction, mode);
if (pos >= 0)
return pos;
}
// If we've found nothing, and the first element doesn't handle line borders,
// return the line start as normal caret stop.
if (visualColumn > 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
}
else
{
// Search Forwards:
// If the first element doesn't handle line borders, return the line start as caret stop
if (visualColumn < 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
// skip elements that end before or at visualColumn
for (i = 0; i < _elements.Count; i++)
{
if (_elements[i].VisualColumn + _elements[i].VisualLength > visualColumn)
break;
}
// search first element that has a caret stop
for (; i < _elements.Count; i++)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Max(visualColumn, _elements[i].VisualColumn - 1),
direction, mode);
if (pos >= 0)
return pos;
}
// if we've found nothing, and the last element doesn't handle line borders,
// return the line end as caret stop
if ((allowVirtualSpace || !_elements[_elements.Count - 1].HandlesLineBorders) && HasImplicitStopAtLineEnd())
{
if (visualColumn < VisualLength)
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
return _visual ??= new VisualLineDrawingVisual(this);
}
}
{
return mode == CaretPositioningMode.Normal || mode == CaretPositioningMode.EveryCodepoint;
}
private static bool HasImplicitStopAtLineEnd() => true;
private VisualLineDrawingVisual _visual;
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
if (_visual == null)
{
_visual = new VisualLineDrawingVisual(this);
((ISetLogicalParent)_visual).SetParent(_textView);
}
return _visual;
}
}
// TODO: can inherit from Layoutable, but dev tools crash
internal sealed class VisualLineDrawingVisual : Control
{
public VisualLine VisualLine { get; }
public double LineHeight { get; }
internal bool IsAdded { get; set; }
public VisualLineDrawingVisual(VisualLine visualLine)
{
VisualLine = visualLine;
LineHeight = VisualLine.TextLines.Sum(textLine => textLine.Height);
}
public override void Render(DrawingContext context)
{
double pos = 0;
foreach (var textLine in VisualLine.TextLines)
{
textLine.Draw(context, new Point(0, pos));
pos += textLine.Height;
}
}
}
}
<MSG> Set VisualLineDrawingVisual.Parent on creation
<DFF> @@ -748,7 +748,15 @@ namespace AvaloniaEdit.Rendering
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
- return _visual ??= new VisualLineDrawingVisual(this);
+
+ if (_visual == null)
+ {
+ _visual = new VisualLineDrawingVisual(this);
+
+ ((ISetLogicalParent)_visual).SetParent(_textView);
+ }
+
+ return _visual;
}
}
| 9 | Set VisualLineDrawingVisual.Parent on creation | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063804 | <NME> VisualLine.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Linq;
using Avalonia;
using Avalonia.Controls;
using Avalonia.Input;
using Avalonia.Media;
using Avalonia.Media.TextFormatting;
using AvaloniaEdit.Document;
using AvaloniaEdit.Utils;
using LogicalDirection = AvaloniaEdit.Document.LogicalDirection;
namespace AvaloniaEdit.Rendering
{
/// <summary>
/// Represents a visual line in the document.
/// A visual line usually corresponds to one DocumentLine, but it can span multiple lines if
/// all but the first are collapsed.
/// </summary>
public sealed class VisualLine
{
public const int LENGTH_LIMIT = 3000;
private enum LifetimePhase : byte
{
Generating,
Transforming,
Live,
Disposed
}
private readonly TextView _textView;
private List<VisualLineElement> _elements;
internal bool HasInlineObjects;
private LifetimePhase _phase;
/// <summary>
/// Gets the document to which this VisualLine belongs.
/// </summary>
public TextDocument Document { get; }
/// <summary>
/// Gets the first document line displayed by this visual line.
/// </summary>
public DocumentLine FirstDocumentLine { get; }
/// <summary>
/// Gets the last document line displayed by this visual line.
/// </summary>
public DocumentLine LastDocumentLine { get; private set; }
/// <summary>
/// Gets a read-only collection of line elements.
/// </summary>
public ReadOnlyCollection<VisualLineElement> Elements { get; private set; }
private ReadOnlyCollection<TextLine> _textLines;
/// <summary>
/// Gets a read-only collection of text lines.
/// </summary>
public ReadOnlyCollection<TextLine> TextLines
{
get
{
if (_phase < LifetimePhase.Live)
throw new InvalidOperationException();
return _textLines;
}
}
/// <summary>
/// Gets the start offset of the VisualLine inside the document.
/// This is equivalent to <c>FirstDocumentLine.Offset</c>.
/// </summary>
public int StartOffset => FirstDocumentLine.Offset;
/// <summary>
/// Length in visual line coordinates.
/// </summary>
public int VisualLength { get; private set; }
/// <summary>
/// Length in visual line coordinates including the end of line marker, if TextEditorOptions.ShowEndOfLine is enabled.
/// </summary>
public int VisualLengthWithEndOfLineMarker
{
get
{
var length = VisualLength;
if (_textView.Options.ShowEndOfLine && LastDocumentLine.NextLine != null) length++;
return length;
}
}
/// <summary>
/// Gets the height of the visual line in device-independent pixels.
/// </summary>
public double Height { get; private set; }
/// <summary>
/// Gets the Y position of the line. This is measured in device-independent pixels relative to the start of the document.
/// </summary>
public double VisualTop { get; internal set; }
internal VisualLine(TextView textView, DocumentLine firstDocumentLine)
{
Debug.Assert(textView != null);
Debug.Assert(firstDocumentLine != null);
_textView = textView;
Document = textView.Document;
FirstDocumentLine = firstDocumentLine;
}
internal void ConstructVisualElements(ITextRunConstructionContext context, IReadOnlyList<VisualLineElementGenerator> generators)
{
Debug.Assert(_phase == LifetimePhase.Generating);
foreach (var g in generators)
{
g.StartGeneration(context);
}
_elements = new List<VisualLineElement>();
PerformVisualElementConstruction(generators);
foreach (var g in generators)
{
g.FinishGeneration();
}
var globalTextRunProperties = context.GlobalTextRunProperties;
foreach (var element in _elements)
{
element.SetTextRunProperties(new VisualLineElementTextRunProperties(globalTextRunProperties));
}
this.Elements = new ReadOnlyCollection<VisualLineElement>(_elements);
CalculateOffsets();
_phase = LifetimePhase.Transforming;
}
void PerformVisualElementConstruction(IReadOnlyList<VisualLineElementGenerator> generators)
{
var lineLength = FirstDocumentLine.Length;
var offset = FirstDocumentLine.Offset;
var currentLineEnd = offset + lineLength;
LastDocumentLine = FirstDocumentLine;
var askInterestOffset = 0; // 0 or 1
while (offset + askInterestOffset <= currentLineEnd)
{
var textPieceEndOffset = currentLineEnd;
foreach (var g in generators)
{
g.CachedInterest = g.GetFirstInterestedOffset(offset + askInterestOffset);
if (g.CachedInterest != -1)
{
if (g.CachedInterest < offset)
throw new ArgumentOutOfRangeException(g.GetType().Name + ".GetFirstInterestedOffset",
g.CachedInterest,
"GetFirstInterestedOffset must not return an offset less than startOffset. Return -1 to signal no interest.");
if (g.CachedInterest < textPieceEndOffset)
textPieceEndOffset = g.CachedInterest;
}
}
Debug.Assert(textPieceEndOffset >= offset);
if (textPieceEndOffset > offset)
{
var textPieceLength = textPieceEndOffset - offset;
_elements.Add(new VisualLineText(this, textPieceLength));
offset = textPieceEndOffset;
}
// If no elements constructed / only zero-length elements constructed:
// do not asking the generators again for the same location (would cause endless loop)
askInterestOffset = 1;
foreach (var g in generators)
{
if (g.CachedInterest == offset)
{
var element = g.ConstructElement(offset);
if (element != null)
{
_elements.Add(element);
if (element.DocumentLength > 0)
{
// a non-zero-length element was constructed
askInterestOffset = 0;
offset += element.DocumentLength;
if (offset > currentLineEnd)
{
var newEndLine = Document.GetLineByOffset(offset);
currentLineEnd = newEndLine.Offset + newEndLine.Length;
this.LastDocumentLine = newEndLine;
if (currentLineEnd < offset)
{
throw new InvalidOperationException(
"The VisualLineElementGenerator " + g.GetType().Name +
" produced an element which ends within the line delimiter");
}
}
break;
}
}
}
}
}
}
private void CalculateOffsets()
{
var visualOffset = 0;
var textOffset = 0;
foreach (var element in _elements)
{
element.VisualColumn = visualOffset;
element.RelativeTextOffset = textOffset;
visualOffset += element.VisualLength;
textOffset += element.DocumentLength;
}
VisualLength = visualOffset;
Debug.Assert(textOffset == LastDocumentLine.EndOffset - FirstDocumentLine.Offset);
}
internal void RunTransformers(ITextRunConstructionContext context, IReadOnlyList<IVisualLineTransformer> transformers)
{
Debug.Assert(_phase == LifetimePhase.Transforming);
foreach (var transformer in transformers)
{
transformer.Transform(context, _elements);
}
_phase = LifetimePhase.Live;
}
/// <summary>
/// Replaces the single element at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, params VisualLineElement[] newElements)
{
ReplaceElement(elementIndex, 1, newElements);
}
/// <summary>
/// Replaces <paramref name="count"/> elements starting at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, int count, params VisualLineElement[] newElements)
{
if (_phase != LifetimePhase.Transforming)
throw new InvalidOperationException("This method may only be called by line transformers.");
var oldDocumentLength = 0;
for (var i = elementIndex; i < elementIndex + count; i++)
{
oldDocumentLength += _elements[i].DocumentLength;
}
var newDocumentLength = 0;
foreach (var newElement in newElements)
{
newDocumentLength += newElement.DocumentLength;
}
if (oldDocumentLength != newDocumentLength)
throw new InvalidOperationException("Old elements have document length " + oldDocumentLength + ", but new elements have length " + newDocumentLength);
_elements.RemoveRange(elementIndex, count);
_elements.InsertRange(elementIndex, newElements);
CalculateOffsets();
}
internal void SetTextLines(List<TextLine> textLines)
{
_textLines = new ReadOnlyCollection<TextLine>(textLines);
Height = 0;
foreach (var line in textLines)
Height += line.Height;
}
/// <summary>
/// Gets the visual column from a document offset relative to the first line start.
/// </summary>
public int GetVisualColumn(int relativeTextOffset)
{
ThrowUtil.CheckNotNegative(relativeTextOffset, "relativeTextOffset");
foreach (var element in _elements)
{
if (element.RelativeTextOffset <= relativeTextOffset
&& element.RelativeTextOffset + element.DocumentLength >= relativeTextOffset)
{
return element.GetVisualColumn(relativeTextOffset);
}
}
return VisualLength;
}
/// <summary>
/// Gets the document offset (relative to the first line start) from a visual column.
/// </summary>
public int GetRelativeOffset(int visualColumn)
{
ThrowUtil.CheckNotNegative(visualColumn, "visualColumn");
var documentLength = 0;
foreach (var element in _elements)
{
if (element.VisualColumn <= visualColumn
&& element.VisualColumn + element.VisualLength > visualColumn)
{
return element.GetRelativeOffset(visualColumn);
}
documentLength += element.DocumentLength;
}
return documentLength;
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn)
{
return GetTextLine(visualColumn, false);
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn, bool isAtEndOfLine)
{
if (visualColumn < 0)
throw new ArgumentOutOfRangeException(nameof(visualColumn));
if (visualColumn >= VisualLengthWithEndOfLineMarker)
return TextLines[TextLines.Count - 1];
foreach (var line in TextLines)
{
if (isAtEndOfLine ? visualColumn <= line.Length : visualColumn < line.Length)
return line;
visualColumn -= line.Length;
}
throw new InvalidOperationException("Shouldn't happen (VisualLength incorrect?)");
}
/// <summary>
/// Gets the visual top from the specified text line.
/// </summary>
/// <returns>Distance in device-independent pixels
/// from the top of the document to the top of the specified text line.</returns>
public double GetTextLineVisualYPosition(TextLine textLine, VisualYPosition yPositionMode)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var pos = VisualTop;
foreach (var tl in TextLines)
{
if (tl == textLine)
{
switch (yPositionMode)
{
case VisualYPosition.LineTop:
return pos;
case VisualYPosition.LineMiddle:
return pos + tl.Height / 2;
case VisualYPosition.LineBottom:
return pos + tl.Height;
case VisualYPosition.TextTop:
return pos + tl.Baseline - _textView.DefaultBaseline;
case VisualYPosition.TextBottom:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight;
case VisualYPosition.TextMiddle:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight / 2;
case VisualYPosition.Baseline:
return pos + tl.Baseline;
default:
throw new ArgumentException("Invalid yPositionMode:" + yPositionMode);
}
}
pos += tl.Height;
}
throw new ArgumentException("textLine is not a line in this VisualLine");
}
/// <summary>
/// Gets the start visual column from the specified text line.
/// </summary>
public int GetTextLineVisualStartColumn(TextLine textLine)
{
if (!TextLines.Contains(textLine))
throw new ArgumentException("textLine is not a line in this VisualLine");
return TextLines.TakeWhile(tl => tl != textLine).Sum(tl => tl.Length);
}
/// <summary>
/// Gets a TextLine by the visual position.
/// </summary>
public TextLine GetTextLineByVisualYPosition(double visualTop)
{
const double epsilon = 0.0001;
var pos = VisualTop;
foreach (var tl in TextLines)
{
pos += tl.Height;
if (visualTop + epsilon < pos)
return tl;
}
return TextLines[TextLines.Count - 1];
}
/// <summary>
/// Gets the visual position from the specified visualColumn.
/// </summary>
/// <returns>Position in device-independent pixels
/// relative to the top left of the document.</returns>
public Point GetVisualPosition(int visualColumn, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
internal Point GetVisualPosition(int visualColumn, bool isAtEndOfLine, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn, isAtEndOfLine);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
/// <summary>
/// Gets the distance to the left border of the text area of the specified visual column.
/// The visual column must belong to the specified text line.
/// </summary>
public double GetTextLineVisualXPosition(TextLine textLine, int visualColumn)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var xPos = textLine.GetDistanceFromCharacterHit(new CharacterHit(Math.Min(visualColumn,
VisualLengthWithEndOfLineMarker)));
if (visualColumn > VisualLengthWithEndOfLineMarker)
{
xPos += (visualColumn - VisualLengthWithEndOfLineMarker) * _textView.WideSpaceWidth;
}
return xPos;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point)
{
return GetVisualColumn(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point, bool allowVirtualSpace)
{
return GetVisualColumn(GetTextLineByVisualYPosition(point.Y), point.X, allowVirtualSpace);
}
internal int GetVisualColumn(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
var vc = GetVisualColumn(textLine, point.X, allowVirtualSpace);
isAtEndOfLine = (vc >= GetTextLineVisualStartColumn(textLine) + textLine.Length);
return vc;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(TextLine textLine, double xPos, bool allowVirtualSpace)
{
if (xPos > textLine.WidthIncludingTrailingWhitespace)
{
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
var virtualX = (int)Math.Round((xPos - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth, MidpointRounding.AwayFromZero);
return VisualLengthWithEndOfLineMarker + virtualX;
}
}
var ch = textLine.GetCharacterHitFromDistance(xPos);
return ch.FirstCharacterIndex + ch.TrailingLength;
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(TextViewPosition position, bool allowVirtualSpace)
{
return ValidateVisualColumn(Document.GetOffset(position.Location), position.VisualColumn, allowVirtualSpace);
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(int offset, int visualColumn, bool allowVirtualSpace)
{
var firstDocumentLineOffset = FirstDocumentLine.Offset;
if (visualColumn < 0)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
var offsetFromVisualColumn = GetRelativeOffset(visualColumn);
offsetFromVisualColumn += firstDocumentLineOffset;
if (offsetFromVisualColumn != offset)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
if (visualColumn > VisualLength && !allowVirtualSpace)
{
return VisualLength;
}
return visualColumn;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point)
{
return GetVisualColumnFloor(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point, bool allowVirtualSpace)
{
return GetVisualColumnFloor(point, allowVirtualSpace, out _);
}
internal int GetVisualColumnFloor(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
if (point.X > textLine.WidthIncludingTrailingWhitespace)
{
isAtEndOfLine = true;
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
// clicking virtual space in the last line
var virtualX = (int)((point.X - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth);
return VisualLengthWithEndOfLineMarker + virtualX;
}
// GetCharacterHitFromDistance returns a hit with FirstCharacterIndex=last character in line
// and TrailingLength=1 when clicking behind the line, so the floor function needs to handle this case
// specially and return the line's end column instead.
return GetTextLineVisualStartColumn(textLine) + textLine.Length;
}
isAtEndOfLine = false;
var ch = textLine.GetCharacterHitFromDistance(point.X);
return ch.FirstCharacterIndex;
}
/// <summary>
/// Gets the text view position from the specified visual column.
/// </summary>
public TextViewPosition GetTextViewPosition(int visualColumn)
{
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
return new TextViewPosition(Document.GetLocation(documentOffset), visualColumn);
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is within a character, it is rounded to the next character boundary.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPosition(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumn(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is inside a character, the position in front of the character is returned.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPositionFloor(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumnFloor(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets whether the visual line was disposed.
/// </summary>
public bool IsDisposed => _phase == LifetimePhase.Disposed;
internal void Dispose()
{
if (_phase == LifetimePhase.Disposed)
{
return;
}
Debug.Assert(_phase == LifetimePhase.Live);
_phase = LifetimePhase.Disposed;
if (_visual != null)
{
((ISetLogicalParent)_visual).SetParent(null);
}
}
/// <summary>
/// Gets the next possible caret position after visualColumn, or -1 if there is no caret position.
/// </summary>
public int GetNextCaretPosition(int visualColumn, LogicalDirection direction, CaretPositioningMode mode, bool allowVirtualSpace)
{
if (!HasStopsInVirtualSpace(mode))
allowVirtualSpace = false;
if (_elements.Count == 0)
{
// special handling for empty visual lines:
if (allowVirtualSpace)
{
if (direction == LogicalDirection.Forward)
return Math.Max(0, visualColumn + 1);
if (visualColumn > 0)
return visualColumn - 1;
return -1;
}
// even though we don't have any elements,
// there's a single caret stop at visualColumn 0
if (visualColumn < 0 && direction == LogicalDirection.Forward)
return 0;
if (visualColumn > 0 && direction == LogicalDirection.Backward)
return 0;
return -1;
}
int i;
if (direction == LogicalDirection.Backward)
{
// Search Backwards:
// If the last element doesn't handle line borders, return the line end as caret stop
if (visualColumn > VisualLength && !_elements[_elements.Count - 1].HandlesLineBorders && HasImplicitStopAtLineEnd())
{
if (allowVirtualSpace)
return visualColumn - 1;
return VisualLength;
}
// skip elements that start after or at visualColumn
for (i = _elements.Count - 1; i >= 0; i--)
{
if (_elements[i].VisualColumn < visualColumn)
break;
}
// search last element that has a caret stop
for (; i >= 0; i--)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Min(visualColumn, _elements[i].VisualColumn + _elements[i].VisualLength + 1),
direction, mode);
if (pos >= 0)
return pos;
}
// If we've found nothing, and the first element doesn't handle line borders,
// return the line start as normal caret stop.
if (visualColumn > 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
}
else
{
// Search Forwards:
// If the first element doesn't handle line borders, return the line start as caret stop
if (visualColumn < 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
// skip elements that end before or at visualColumn
for (i = 0; i < _elements.Count; i++)
{
if (_elements[i].VisualColumn + _elements[i].VisualLength > visualColumn)
break;
}
// search first element that has a caret stop
for (; i < _elements.Count; i++)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Max(visualColumn, _elements[i].VisualColumn - 1),
direction, mode);
if (pos >= 0)
return pos;
}
// if we've found nothing, and the last element doesn't handle line borders,
// return the line end as caret stop
if ((allowVirtualSpace || !_elements[_elements.Count - 1].HandlesLineBorders) && HasImplicitStopAtLineEnd())
{
if (visualColumn < VisualLength)
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
return _visual ??= new VisualLineDrawingVisual(this);
}
}
{
return mode == CaretPositioningMode.Normal || mode == CaretPositioningMode.EveryCodepoint;
}
private static bool HasImplicitStopAtLineEnd() => true;
private VisualLineDrawingVisual _visual;
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
if (_visual == null)
{
_visual = new VisualLineDrawingVisual(this);
((ISetLogicalParent)_visual).SetParent(_textView);
}
return _visual;
}
}
// TODO: can inherit from Layoutable, but dev tools crash
internal sealed class VisualLineDrawingVisual : Control
{
public VisualLine VisualLine { get; }
public double LineHeight { get; }
internal bool IsAdded { get; set; }
public VisualLineDrawingVisual(VisualLine visualLine)
{
VisualLine = visualLine;
LineHeight = VisualLine.TextLines.Sum(textLine => textLine.Height);
}
public override void Render(DrawingContext context)
{
double pos = 0;
foreach (var textLine in VisualLine.TextLines)
{
textLine.Draw(context, new Point(0, pos));
pos += textLine.Height;
}
}
}
}
<MSG> Set VisualLineDrawingVisual.Parent on creation
<DFF> @@ -748,7 +748,15 @@ namespace AvaloniaEdit.Rendering
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
- return _visual ??= new VisualLineDrawingVisual(this);
+
+ if (_visual == null)
+ {
+ _visual = new VisualLineDrawingVisual(this);
+
+ ((ISetLogicalParent)_visual).SetParent(_textView);
+ }
+
+ return _visual;
}
}
| 9 | Set VisualLineDrawingVisual.Parent on creation | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063805 | <NME> VisualLine.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Linq;
using Avalonia;
using Avalonia.Controls;
using Avalonia.Input;
using Avalonia.Media;
using Avalonia.Media.TextFormatting;
using AvaloniaEdit.Document;
using AvaloniaEdit.Utils;
using LogicalDirection = AvaloniaEdit.Document.LogicalDirection;
namespace AvaloniaEdit.Rendering
{
/// <summary>
/// Represents a visual line in the document.
/// A visual line usually corresponds to one DocumentLine, but it can span multiple lines if
/// all but the first are collapsed.
/// </summary>
public sealed class VisualLine
{
public const int LENGTH_LIMIT = 3000;
private enum LifetimePhase : byte
{
Generating,
Transforming,
Live,
Disposed
}
private readonly TextView _textView;
private List<VisualLineElement> _elements;
internal bool HasInlineObjects;
private LifetimePhase _phase;
/// <summary>
/// Gets the document to which this VisualLine belongs.
/// </summary>
public TextDocument Document { get; }
/// <summary>
/// Gets the first document line displayed by this visual line.
/// </summary>
public DocumentLine FirstDocumentLine { get; }
/// <summary>
/// Gets the last document line displayed by this visual line.
/// </summary>
public DocumentLine LastDocumentLine { get; private set; }
/// <summary>
/// Gets a read-only collection of line elements.
/// </summary>
public ReadOnlyCollection<VisualLineElement> Elements { get; private set; }
private ReadOnlyCollection<TextLine> _textLines;
/// <summary>
/// Gets a read-only collection of text lines.
/// </summary>
public ReadOnlyCollection<TextLine> TextLines
{
get
{
if (_phase < LifetimePhase.Live)
throw new InvalidOperationException();
return _textLines;
}
}
/// <summary>
/// Gets the start offset of the VisualLine inside the document.
/// This is equivalent to <c>FirstDocumentLine.Offset</c>.
/// </summary>
public int StartOffset => FirstDocumentLine.Offset;
/// <summary>
/// Length in visual line coordinates.
/// </summary>
public int VisualLength { get; private set; }
/// <summary>
/// Length in visual line coordinates including the end of line marker, if TextEditorOptions.ShowEndOfLine is enabled.
/// </summary>
public int VisualLengthWithEndOfLineMarker
{
get
{
var length = VisualLength;
if (_textView.Options.ShowEndOfLine && LastDocumentLine.NextLine != null) length++;
return length;
}
}
/// <summary>
/// Gets the height of the visual line in device-independent pixels.
/// </summary>
public double Height { get; private set; }
/// <summary>
/// Gets the Y position of the line. This is measured in device-independent pixels relative to the start of the document.
/// </summary>
public double VisualTop { get; internal set; }
internal VisualLine(TextView textView, DocumentLine firstDocumentLine)
{
Debug.Assert(textView != null);
Debug.Assert(firstDocumentLine != null);
_textView = textView;
Document = textView.Document;
FirstDocumentLine = firstDocumentLine;
}
internal void ConstructVisualElements(ITextRunConstructionContext context, IReadOnlyList<VisualLineElementGenerator> generators)
{
Debug.Assert(_phase == LifetimePhase.Generating);
foreach (var g in generators)
{
g.StartGeneration(context);
}
_elements = new List<VisualLineElement>();
PerformVisualElementConstruction(generators);
foreach (var g in generators)
{
g.FinishGeneration();
}
var globalTextRunProperties = context.GlobalTextRunProperties;
foreach (var element in _elements)
{
element.SetTextRunProperties(new VisualLineElementTextRunProperties(globalTextRunProperties));
}
this.Elements = new ReadOnlyCollection<VisualLineElement>(_elements);
CalculateOffsets();
_phase = LifetimePhase.Transforming;
}
void PerformVisualElementConstruction(IReadOnlyList<VisualLineElementGenerator> generators)
{
var lineLength = FirstDocumentLine.Length;
var offset = FirstDocumentLine.Offset;
var currentLineEnd = offset + lineLength;
LastDocumentLine = FirstDocumentLine;
var askInterestOffset = 0; // 0 or 1
while (offset + askInterestOffset <= currentLineEnd)
{
var textPieceEndOffset = currentLineEnd;
foreach (var g in generators)
{
g.CachedInterest = g.GetFirstInterestedOffset(offset + askInterestOffset);
if (g.CachedInterest != -1)
{
if (g.CachedInterest < offset)
throw new ArgumentOutOfRangeException(g.GetType().Name + ".GetFirstInterestedOffset",
g.CachedInterest,
"GetFirstInterestedOffset must not return an offset less than startOffset. Return -1 to signal no interest.");
if (g.CachedInterest < textPieceEndOffset)
textPieceEndOffset = g.CachedInterest;
}
}
Debug.Assert(textPieceEndOffset >= offset);
if (textPieceEndOffset > offset)
{
var textPieceLength = textPieceEndOffset - offset;
_elements.Add(new VisualLineText(this, textPieceLength));
offset = textPieceEndOffset;
}
// If no elements constructed / only zero-length elements constructed:
// do not asking the generators again for the same location (would cause endless loop)
askInterestOffset = 1;
foreach (var g in generators)
{
if (g.CachedInterest == offset)
{
var element = g.ConstructElement(offset);
if (element != null)
{
_elements.Add(element);
if (element.DocumentLength > 0)
{
// a non-zero-length element was constructed
askInterestOffset = 0;
offset += element.DocumentLength;
if (offset > currentLineEnd)
{
var newEndLine = Document.GetLineByOffset(offset);
currentLineEnd = newEndLine.Offset + newEndLine.Length;
this.LastDocumentLine = newEndLine;
if (currentLineEnd < offset)
{
throw new InvalidOperationException(
"The VisualLineElementGenerator " + g.GetType().Name +
" produced an element which ends within the line delimiter");
}
}
break;
}
}
}
}
}
}
private void CalculateOffsets()
{
var visualOffset = 0;
var textOffset = 0;
foreach (var element in _elements)
{
element.VisualColumn = visualOffset;
element.RelativeTextOffset = textOffset;
visualOffset += element.VisualLength;
textOffset += element.DocumentLength;
}
VisualLength = visualOffset;
Debug.Assert(textOffset == LastDocumentLine.EndOffset - FirstDocumentLine.Offset);
}
internal void RunTransformers(ITextRunConstructionContext context, IReadOnlyList<IVisualLineTransformer> transformers)
{
Debug.Assert(_phase == LifetimePhase.Transforming);
foreach (var transformer in transformers)
{
transformer.Transform(context, _elements);
}
_phase = LifetimePhase.Live;
}
/// <summary>
/// Replaces the single element at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, params VisualLineElement[] newElements)
{
ReplaceElement(elementIndex, 1, newElements);
}
/// <summary>
/// Replaces <paramref name="count"/> elements starting at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, int count, params VisualLineElement[] newElements)
{
if (_phase != LifetimePhase.Transforming)
throw new InvalidOperationException("This method may only be called by line transformers.");
var oldDocumentLength = 0;
for (var i = elementIndex; i < elementIndex + count; i++)
{
oldDocumentLength += _elements[i].DocumentLength;
}
var newDocumentLength = 0;
foreach (var newElement in newElements)
{
newDocumentLength += newElement.DocumentLength;
}
if (oldDocumentLength != newDocumentLength)
throw new InvalidOperationException("Old elements have document length " + oldDocumentLength + ", but new elements have length " + newDocumentLength);
_elements.RemoveRange(elementIndex, count);
_elements.InsertRange(elementIndex, newElements);
CalculateOffsets();
}
internal void SetTextLines(List<TextLine> textLines)
{
_textLines = new ReadOnlyCollection<TextLine>(textLines);
Height = 0;
foreach (var line in textLines)
Height += line.Height;
}
/// <summary>
/// Gets the visual column from a document offset relative to the first line start.
/// </summary>
public int GetVisualColumn(int relativeTextOffset)
{
ThrowUtil.CheckNotNegative(relativeTextOffset, "relativeTextOffset");
foreach (var element in _elements)
{
if (element.RelativeTextOffset <= relativeTextOffset
&& element.RelativeTextOffset + element.DocumentLength >= relativeTextOffset)
{
return element.GetVisualColumn(relativeTextOffset);
}
}
return VisualLength;
}
/// <summary>
/// Gets the document offset (relative to the first line start) from a visual column.
/// </summary>
public int GetRelativeOffset(int visualColumn)
{
ThrowUtil.CheckNotNegative(visualColumn, "visualColumn");
var documentLength = 0;
foreach (var element in _elements)
{
if (element.VisualColumn <= visualColumn
&& element.VisualColumn + element.VisualLength > visualColumn)
{
return element.GetRelativeOffset(visualColumn);
}
documentLength += element.DocumentLength;
}
return documentLength;
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn)
{
return GetTextLine(visualColumn, false);
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn, bool isAtEndOfLine)
{
if (visualColumn < 0)
throw new ArgumentOutOfRangeException(nameof(visualColumn));
if (visualColumn >= VisualLengthWithEndOfLineMarker)
return TextLines[TextLines.Count - 1];
foreach (var line in TextLines)
{
if (isAtEndOfLine ? visualColumn <= line.Length : visualColumn < line.Length)
return line;
visualColumn -= line.Length;
}
throw new InvalidOperationException("Shouldn't happen (VisualLength incorrect?)");
}
/// <summary>
/// Gets the visual top from the specified text line.
/// </summary>
/// <returns>Distance in device-independent pixels
/// from the top of the document to the top of the specified text line.</returns>
public double GetTextLineVisualYPosition(TextLine textLine, VisualYPosition yPositionMode)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var pos = VisualTop;
foreach (var tl in TextLines)
{
if (tl == textLine)
{
switch (yPositionMode)
{
case VisualYPosition.LineTop:
return pos;
case VisualYPosition.LineMiddle:
return pos + tl.Height / 2;
case VisualYPosition.LineBottom:
return pos + tl.Height;
case VisualYPosition.TextTop:
return pos + tl.Baseline - _textView.DefaultBaseline;
case VisualYPosition.TextBottom:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight;
case VisualYPosition.TextMiddle:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight / 2;
case VisualYPosition.Baseline:
return pos + tl.Baseline;
default:
throw new ArgumentException("Invalid yPositionMode:" + yPositionMode);
}
}
pos += tl.Height;
}
throw new ArgumentException("textLine is not a line in this VisualLine");
}
/// <summary>
/// Gets the start visual column from the specified text line.
/// </summary>
public int GetTextLineVisualStartColumn(TextLine textLine)
{
if (!TextLines.Contains(textLine))
throw new ArgumentException("textLine is not a line in this VisualLine");
return TextLines.TakeWhile(tl => tl != textLine).Sum(tl => tl.Length);
}
/// <summary>
/// Gets a TextLine by the visual position.
/// </summary>
public TextLine GetTextLineByVisualYPosition(double visualTop)
{
const double epsilon = 0.0001;
var pos = VisualTop;
foreach (var tl in TextLines)
{
pos += tl.Height;
if (visualTop + epsilon < pos)
return tl;
}
return TextLines[TextLines.Count - 1];
}
/// <summary>
/// Gets the visual position from the specified visualColumn.
/// </summary>
/// <returns>Position in device-independent pixels
/// relative to the top left of the document.</returns>
public Point GetVisualPosition(int visualColumn, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
internal Point GetVisualPosition(int visualColumn, bool isAtEndOfLine, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn, isAtEndOfLine);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
/// <summary>
/// Gets the distance to the left border of the text area of the specified visual column.
/// The visual column must belong to the specified text line.
/// </summary>
public double GetTextLineVisualXPosition(TextLine textLine, int visualColumn)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var xPos = textLine.GetDistanceFromCharacterHit(new CharacterHit(Math.Min(visualColumn,
VisualLengthWithEndOfLineMarker)));
if (visualColumn > VisualLengthWithEndOfLineMarker)
{
xPos += (visualColumn - VisualLengthWithEndOfLineMarker) * _textView.WideSpaceWidth;
}
return xPos;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point)
{
return GetVisualColumn(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point, bool allowVirtualSpace)
{
return GetVisualColumn(GetTextLineByVisualYPosition(point.Y), point.X, allowVirtualSpace);
}
internal int GetVisualColumn(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
var vc = GetVisualColumn(textLine, point.X, allowVirtualSpace);
isAtEndOfLine = (vc >= GetTextLineVisualStartColumn(textLine) + textLine.Length);
return vc;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(TextLine textLine, double xPos, bool allowVirtualSpace)
{
if (xPos > textLine.WidthIncludingTrailingWhitespace)
{
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
var virtualX = (int)Math.Round((xPos - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth, MidpointRounding.AwayFromZero);
return VisualLengthWithEndOfLineMarker + virtualX;
}
}
var ch = textLine.GetCharacterHitFromDistance(xPos);
return ch.FirstCharacterIndex + ch.TrailingLength;
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(TextViewPosition position, bool allowVirtualSpace)
{
return ValidateVisualColumn(Document.GetOffset(position.Location), position.VisualColumn, allowVirtualSpace);
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(int offset, int visualColumn, bool allowVirtualSpace)
{
var firstDocumentLineOffset = FirstDocumentLine.Offset;
if (visualColumn < 0)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
var offsetFromVisualColumn = GetRelativeOffset(visualColumn);
offsetFromVisualColumn += firstDocumentLineOffset;
if (offsetFromVisualColumn != offset)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
if (visualColumn > VisualLength && !allowVirtualSpace)
{
return VisualLength;
}
return visualColumn;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point)
{
return GetVisualColumnFloor(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point, bool allowVirtualSpace)
{
return GetVisualColumnFloor(point, allowVirtualSpace, out _);
}
internal int GetVisualColumnFloor(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
if (point.X > textLine.WidthIncludingTrailingWhitespace)
{
isAtEndOfLine = true;
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
// clicking virtual space in the last line
var virtualX = (int)((point.X - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth);
return VisualLengthWithEndOfLineMarker + virtualX;
}
// GetCharacterHitFromDistance returns a hit with FirstCharacterIndex=last character in line
// and TrailingLength=1 when clicking behind the line, so the floor function needs to handle this case
// specially and return the line's end column instead.
return GetTextLineVisualStartColumn(textLine) + textLine.Length;
}
isAtEndOfLine = false;
var ch = textLine.GetCharacterHitFromDistance(point.X);
return ch.FirstCharacterIndex;
}
/// <summary>
/// Gets the text view position from the specified visual column.
/// </summary>
public TextViewPosition GetTextViewPosition(int visualColumn)
{
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
return new TextViewPosition(Document.GetLocation(documentOffset), visualColumn);
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is within a character, it is rounded to the next character boundary.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPosition(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumn(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is inside a character, the position in front of the character is returned.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPositionFloor(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumnFloor(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets whether the visual line was disposed.
/// </summary>
public bool IsDisposed => _phase == LifetimePhase.Disposed;
internal void Dispose()
{
if (_phase == LifetimePhase.Disposed)
{
return;
}
Debug.Assert(_phase == LifetimePhase.Live);
_phase = LifetimePhase.Disposed;
if (_visual != null)
{
((ISetLogicalParent)_visual).SetParent(null);
}
}
/// <summary>
/// Gets the next possible caret position after visualColumn, or -1 if there is no caret position.
/// </summary>
public int GetNextCaretPosition(int visualColumn, LogicalDirection direction, CaretPositioningMode mode, bool allowVirtualSpace)
{
if (!HasStopsInVirtualSpace(mode))
allowVirtualSpace = false;
if (_elements.Count == 0)
{
// special handling for empty visual lines:
if (allowVirtualSpace)
{
if (direction == LogicalDirection.Forward)
return Math.Max(0, visualColumn + 1);
if (visualColumn > 0)
return visualColumn - 1;
return -1;
}
// even though we don't have any elements,
// there's a single caret stop at visualColumn 0
if (visualColumn < 0 && direction == LogicalDirection.Forward)
return 0;
if (visualColumn > 0 && direction == LogicalDirection.Backward)
return 0;
return -1;
}
int i;
if (direction == LogicalDirection.Backward)
{
// Search Backwards:
// If the last element doesn't handle line borders, return the line end as caret stop
if (visualColumn > VisualLength && !_elements[_elements.Count - 1].HandlesLineBorders && HasImplicitStopAtLineEnd())
{
if (allowVirtualSpace)
return visualColumn - 1;
return VisualLength;
}
// skip elements that start after or at visualColumn
for (i = _elements.Count - 1; i >= 0; i--)
{
if (_elements[i].VisualColumn < visualColumn)
break;
}
// search last element that has a caret stop
for (; i >= 0; i--)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Min(visualColumn, _elements[i].VisualColumn + _elements[i].VisualLength + 1),
direction, mode);
if (pos >= 0)
return pos;
}
// If we've found nothing, and the first element doesn't handle line borders,
// return the line start as normal caret stop.
if (visualColumn > 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
}
else
{
// Search Forwards:
// If the first element doesn't handle line borders, return the line start as caret stop
if (visualColumn < 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
// skip elements that end before or at visualColumn
for (i = 0; i < _elements.Count; i++)
{
if (_elements[i].VisualColumn + _elements[i].VisualLength > visualColumn)
break;
}
// search first element that has a caret stop
for (; i < _elements.Count; i++)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Max(visualColumn, _elements[i].VisualColumn - 1),
direction, mode);
if (pos >= 0)
return pos;
}
// if we've found nothing, and the last element doesn't handle line borders,
// return the line end as caret stop
if ((allowVirtualSpace || !_elements[_elements.Count - 1].HandlesLineBorders) && HasImplicitStopAtLineEnd())
{
if (visualColumn < VisualLength)
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
return _visual ??= new VisualLineDrawingVisual(this);
}
}
{
return mode == CaretPositioningMode.Normal || mode == CaretPositioningMode.EveryCodepoint;
}
private static bool HasImplicitStopAtLineEnd() => true;
private VisualLineDrawingVisual _visual;
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
if (_visual == null)
{
_visual = new VisualLineDrawingVisual(this);
((ISetLogicalParent)_visual).SetParent(_textView);
}
return _visual;
}
}
// TODO: can inherit from Layoutable, but dev tools crash
internal sealed class VisualLineDrawingVisual : Control
{
public VisualLine VisualLine { get; }
public double LineHeight { get; }
internal bool IsAdded { get; set; }
public VisualLineDrawingVisual(VisualLine visualLine)
{
VisualLine = visualLine;
LineHeight = VisualLine.TextLines.Sum(textLine => textLine.Height);
}
public override void Render(DrawingContext context)
{
double pos = 0;
foreach (var textLine in VisualLine.TextLines)
{
textLine.Draw(context, new Point(0, pos));
pos += textLine.Height;
}
}
}
}
<MSG> Set VisualLineDrawingVisual.Parent on creation
<DFF> @@ -748,7 +748,15 @@ namespace AvaloniaEdit.Rendering
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
- return _visual ??= new VisualLineDrawingVisual(this);
+
+ if (_visual == null)
+ {
+ _visual = new VisualLineDrawingVisual(this);
+
+ ((ISetLogicalParent)_visual).SetParent(_textView);
+ }
+
+ return _visual;
}
}
| 9 | Set VisualLineDrawingVisual.Parent on creation | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063806 | <NME> VisualLine.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Linq;
using Avalonia;
using Avalonia.Controls;
using Avalonia.Input;
using Avalonia.Media;
using Avalonia.Media.TextFormatting;
using AvaloniaEdit.Document;
using AvaloniaEdit.Utils;
using LogicalDirection = AvaloniaEdit.Document.LogicalDirection;
namespace AvaloniaEdit.Rendering
{
/// <summary>
/// Represents a visual line in the document.
/// A visual line usually corresponds to one DocumentLine, but it can span multiple lines if
/// all but the first are collapsed.
/// </summary>
public sealed class VisualLine
{
public const int LENGTH_LIMIT = 3000;
private enum LifetimePhase : byte
{
Generating,
Transforming,
Live,
Disposed
}
private readonly TextView _textView;
private List<VisualLineElement> _elements;
internal bool HasInlineObjects;
private LifetimePhase _phase;
/// <summary>
/// Gets the document to which this VisualLine belongs.
/// </summary>
public TextDocument Document { get; }
/// <summary>
/// Gets the first document line displayed by this visual line.
/// </summary>
public DocumentLine FirstDocumentLine { get; }
/// <summary>
/// Gets the last document line displayed by this visual line.
/// </summary>
public DocumentLine LastDocumentLine { get; private set; }
/// <summary>
/// Gets a read-only collection of line elements.
/// </summary>
public ReadOnlyCollection<VisualLineElement> Elements { get; private set; }
private ReadOnlyCollection<TextLine> _textLines;
/// <summary>
/// Gets a read-only collection of text lines.
/// </summary>
public ReadOnlyCollection<TextLine> TextLines
{
get
{
if (_phase < LifetimePhase.Live)
throw new InvalidOperationException();
return _textLines;
}
}
/// <summary>
/// Gets the start offset of the VisualLine inside the document.
/// This is equivalent to <c>FirstDocumentLine.Offset</c>.
/// </summary>
public int StartOffset => FirstDocumentLine.Offset;
/// <summary>
/// Length in visual line coordinates.
/// </summary>
public int VisualLength { get; private set; }
/// <summary>
/// Length in visual line coordinates including the end of line marker, if TextEditorOptions.ShowEndOfLine is enabled.
/// </summary>
public int VisualLengthWithEndOfLineMarker
{
get
{
var length = VisualLength;
if (_textView.Options.ShowEndOfLine && LastDocumentLine.NextLine != null) length++;
return length;
}
}
/// <summary>
/// Gets the height of the visual line in device-independent pixels.
/// </summary>
public double Height { get; private set; }
/// <summary>
/// Gets the Y position of the line. This is measured in device-independent pixels relative to the start of the document.
/// </summary>
public double VisualTop { get; internal set; }
internal VisualLine(TextView textView, DocumentLine firstDocumentLine)
{
Debug.Assert(textView != null);
Debug.Assert(firstDocumentLine != null);
_textView = textView;
Document = textView.Document;
FirstDocumentLine = firstDocumentLine;
}
internal void ConstructVisualElements(ITextRunConstructionContext context, IReadOnlyList<VisualLineElementGenerator> generators)
{
Debug.Assert(_phase == LifetimePhase.Generating);
foreach (var g in generators)
{
g.StartGeneration(context);
}
_elements = new List<VisualLineElement>();
PerformVisualElementConstruction(generators);
foreach (var g in generators)
{
g.FinishGeneration();
}
var globalTextRunProperties = context.GlobalTextRunProperties;
foreach (var element in _elements)
{
element.SetTextRunProperties(new VisualLineElementTextRunProperties(globalTextRunProperties));
}
this.Elements = new ReadOnlyCollection<VisualLineElement>(_elements);
CalculateOffsets();
_phase = LifetimePhase.Transforming;
}
void PerformVisualElementConstruction(IReadOnlyList<VisualLineElementGenerator> generators)
{
var lineLength = FirstDocumentLine.Length;
var offset = FirstDocumentLine.Offset;
var currentLineEnd = offset + lineLength;
LastDocumentLine = FirstDocumentLine;
var askInterestOffset = 0; // 0 or 1
while (offset + askInterestOffset <= currentLineEnd)
{
var textPieceEndOffset = currentLineEnd;
foreach (var g in generators)
{
g.CachedInterest = g.GetFirstInterestedOffset(offset + askInterestOffset);
if (g.CachedInterest != -1)
{
if (g.CachedInterest < offset)
throw new ArgumentOutOfRangeException(g.GetType().Name + ".GetFirstInterestedOffset",
g.CachedInterest,
"GetFirstInterestedOffset must not return an offset less than startOffset. Return -1 to signal no interest.");
if (g.CachedInterest < textPieceEndOffset)
textPieceEndOffset = g.CachedInterest;
}
}
Debug.Assert(textPieceEndOffset >= offset);
if (textPieceEndOffset > offset)
{
var textPieceLength = textPieceEndOffset - offset;
_elements.Add(new VisualLineText(this, textPieceLength));
offset = textPieceEndOffset;
}
// If no elements constructed / only zero-length elements constructed:
// do not asking the generators again for the same location (would cause endless loop)
askInterestOffset = 1;
foreach (var g in generators)
{
if (g.CachedInterest == offset)
{
var element = g.ConstructElement(offset);
if (element != null)
{
_elements.Add(element);
if (element.DocumentLength > 0)
{
// a non-zero-length element was constructed
askInterestOffset = 0;
offset += element.DocumentLength;
if (offset > currentLineEnd)
{
var newEndLine = Document.GetLineByOffset(offset);
currentLineEnd = newEndLine.Offset + newEndLine.Length;
this.LastDocumentLine = newEndLine;
if (currentLineEnd < offset)
{
throw new InvalidOperationException(
"The VisualLineElementGenerator " + g.GetType().Name +
" produced an element which ends within the line delimiter");
}
}
break;
}
}
}
}
}
}
private void CalculateOffsets()
{
var visualOffset = 0;
var textOffset = 0;
foreach (var element in _elements)
{
element.VisualColumn = visualOffset;
element.RelativeTextOffset = textOffset;
visualOffset += element.VisualLength;
textOffset += element.DocumentLength;
}
VisualLength = visualOffset;
Debug.Assert(textOffset == LastDocumentLine.EndOffset - FirstDocumentLine.Offset);
}
internal void RunTransformers(ITextRunConstructionContext context, IReadOnlyList<IVisualLineTransformer> transformers)
{
Debug.Assert(_phase == LifetimePhase.Transforming);
foreach (var transformer in transformers)
{
transformer.Transform(context, _elements);
}
_phase = LifetimePhase.Live;
}
/// <summary>
/// Replaces the single element at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, params VisualLineElement[] newElements)
{
ReplaceElement(elementIndex, 1, newElements);
}
/// <summary>
/// Replaces <paramref name="count"/> elements starting at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, int count, params VisualLineElement[] newElements)
{
if (_phase != LifetimePhase.Transforming)
throw new InvalidOperationException("This method may only be called by line transformers.");
var oldDocumentLength = 0;
for (var i = elementIndex; i < elementIndex + count; i++)
{
oldDocumentLength += _elements[i].DocumentLength;
}
var newDocumentLength = 0;
foreach (var newElement in newElements)
{
newDocumentLength += newElement.DocumentLength;
}
if (oldDocumentLength != newDocumentLength)
throw new InvalidOperationException("Old elements have document length " + oldDocumentLength + ", but new elements have length " + newDocumentLength);
_elements.RemoveRange(elementIndex, count);
_elements.InsertRange(elementIndex, newElements);
CalculateOffsets();
}
internal void SetTextLines(List<TextLine> textLines)
{
_textLines = new ReadOnlyCollection<TextLine>(textLines);
Height = 0;
foreach (var line in textLines)
Height += line.Height;
}
/// <summary>
/// Gets the visual column from a document offset relative to the first line start.
/// </summary>
public int GetVisualColumn(int relativeTextOffset)
{
ThrowUtil.CheckNotNegative(relativeTextOffset, "relativeTextOffset");
foreach (var element in _elements)
{
if (element.RelativeTextOffset <= relativeTextOffset
&& element.RelativeTextOffset + element.DocumentLength >= relativeTextOffset)
{
return element.GetVisualColumn(relativeTextOffset);
}
}
return VisualLength;
}
/// <summary>
/// Gets the document offset (relative to the first line start) from a visual column.
/// </summary>
public int GetRelativeOffset(int visualColumn)
{
ThrowUtil.CheckNotNegative(visualColumn, "visualColumn");
var documentLength = 0;
foreach (var element in _elements)
{
if (element.VisualColumn <= visualColumn
&& element.VisualColumn + element.VisualLength > visualColumn)
{
return element.GetRelativeOffset(visualColumn);
}
documentLength += element.DocumentLength;
}
return documentLength;
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn)
{
return GetTextLine(visualColumn, false);
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn, bool isAtEndOfLine)
{
if (visualColumn < 0)
throw new ArgumentOutOfRangeException(nameof(visualColumn));
if (visualColumn >= VisualLengthWithEndOfLineMarker)
return TextLines[TextLines.Count - 1];
foreach (var line in TextLines)
{
if (isAtEndOfLine ? visualColumn <= line.Length : visualColumn < line.Length)
return line;
visualColumn -= line.Length;
}
throw new InvalidOperationException("Shouldn't happen (VisualLength incorrect?)");
}
/// <summary>
/// Gets the visual top from the specified text line.
/// </summary>
/// <returns>Distance in device-independent pixels
/// from the top of the document to the top of the specified text line.</returns>
public double GetTextLineVisualYPosition(TextLine textLine, VisualYPosition yPositionMode)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var pos = VisualTop;
foreach (var tl in TextLines)
{
if (tl == textLine)
{
switch (yPositionMode)
{
case VisualYPosition.LineTop:
return pos;
case VisualYPosition.LineMiddle:
return pos + tl.Height / 2;
case VisualYPosition.LineBottom:
return pos + tl.Height;
case VisualYPosition.TextTop:
return pos + tl.Baseline - _textView.DefaultBaseline;
case VisualYPosition.TextBottom:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight;
case VisualYPosition.TextMiddle:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight / 2;
case VisualYPosition.Baseline:
return pos + tl.Baseline;
default:
throw new ArgumentException("Invalid yPositionMode:" + yPositionMode);
}
}
pos += tl.Height;
}
throw new ArgumentException("textLine is not a line in this VisualLine");
}
/// <summary>
/// Gets the start visual column from the specified text line.
/// </summary>
public int GetTextLineVisualStartColumn(TextLine textLine)
{
if (!TextLines.Contains(textLine))
throw new ArgumentException("textLine is not a line in this VisualLine");
return TextLines.TakeWhile(tl => tl != textLine).Sum(tl => tl.Length);
}
/// <summary>
/// Gets a TextLine by the visual position.
/// </summary>
public TextLine GetTextLineByVisualYPosition(double visualTop)
{
const double epsilon = 0.0001;
var pos = VisualTop;
foreach (var tl in TextLines)
{
pos += tl.Height;
if (visualTop + epsilon < pos)
return tl;
}
return TextLines[TextLines.Count - 1];
}
/// <summary>
/// Gets the visual position from the specified visualColumn.
/// </summary>
/// <returns>Position in device-independent pixels
/// relative to the top left of the document.</returns>
public Point GetVisualPosition(int visualColumn, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
internal Point GetVisualPosition(int visualColumn, bool isAtEndOfLine, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn, isAtEndOfLine);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
/// <summary>
/// Gets the distance to the left border of the text area of the specified visual column.
/// The visual column must belong to the specified text line.
/// </summary>
public double GetTextLineVisualXPosition(TextLine textLine, int visualColumn)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var xPos = textLine.GetDistanceFromCharacterHit(new CharacterHit(Math.Min(visualColumn,
VisualLengthWithEndOfLineMarker)));
if (visualColumn > VisualLengthWithEndOfLineMarker)
{
xPos += (visualColumn - VisualLengthWithEndOfLineMarker) * _textView.WideSpaceWidth;
}
return xPos;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point)
{
return GetVisualColumn(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point, bool allowVirtualSpace)
{
return GetVisualColumn(GetTextLineByVisualYPosition(point.Y), point.X, allowVirtualSpace);
}
internal int GetVisualColumn(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
var vc = GetVisualColumn(textLine, point.X, allowVirtualSpace);
isAtEndOfLine = (vc >= GetTextLineVisualStartColumn(textLine) + textLine.Length);
return vc;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(TextLine textLine, double xPos, bool allowVirtualSpace)
{
if (xPos > textLine.WidthIncludingTrailingWhitespace)
{
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
var virtualX = (int)Math.Round((xPos - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth, MidpointRounding.AwayFromZero);
return VisualLengthWithEndOfLineMarker + virtualX;
}
}
var ch = textLine.GetCharacterHitFromDistance(xPos);
return ch.FirstCharacterIndex + ch.TrailingLength;
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(TextViewPosition position, bool allowVirtualSpace)
{
return ValidateVisualColumn(Document.GetOffset(position.Location), position.VisualColumn, allowVirtualSpace);
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(int offset, int visualColumn, bool allowVirtualSpace)
{
var firstDocumentLineOffset = FirstDocumentLine.Offset;
if (visualColumn < 0)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
var offsetFromVisualColumn = GetRelativeOffset(visualColumn);
offsetFromVisualColumn += firstDocumentLineOffset;
if (offsetFromVisualColumn != offset)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
if (visualColumn > VisualLength && !allowVirtualSpace)
{
return VisualLength;
}
return visualColumn;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point)
{
return GetVisualColumnFloor(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point, bool allowVirtualSpace)
{
return GetVisualColumnFloor(point, allowVirtualSpace, out _);
}
internal int GetVisualColumnFloor(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
if (point.X > textLine.WidthIncludingTrailingWhitespace)
{
isAtEndOfLine = true;
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
// clicking virtual space in the last line
var virtualX = (int)((point.X - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth);
return VisualLengthWithEndOfLineMarker + virtualX;
}
// GetCharacterHitFromDistance returns a hit with FirstCharacterIndex=last character in line
// and TrailingLength=1 when clicking behind the line, so the floor function needs to handle this case
// specially and return the line's end column instead.
return GetTextLineVisualStartColumn(textLine) + textLine.Length;
}
isAtEndOfLine = false;
var ch = textLine.GetCharacterHitFromDistance(point.X);
return ch.FirstCharacterIndex;
}
/// <summary>
/// Gets the text view position from the specified visual column.
/// </summary>
public TextViewPosition GetTextViewPosition(int visualColumn)
{
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
return new TextViewPosition(Document.GetLocation(documentOffset), visualColumn);
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is within a character, it is rounded to the next character boundary.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPosition(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumn(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is inside a character, the position in front of the character is returned.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPositionFloor(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumnFloor(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets whether the visual line was disposed.
/// </summary>
public bool IsDisposed => _phase == LifetimePhase.Disposed;
internal void Dispose()
{
if (_phase == LifetimePhase.Disposed)
{
return;
}
Debug.Assert(_phase == LifetimePhase.Live);
_phase = LifetimePhase.Disposed;
if (_visual != null)
{
((ISetLogicalParent)_visual).SetParent(null);
}
}
/// <summary>
/// Gets the next possible caret position after visualColumn, or -1 if there is no caret position.
/// </summary>
public int GetNextCaretPosition(int visualColumn, LogicalDirection direction, CaretPositioningMode mode, bool allowVirtualSpace)
{
if (!HasStopsInVirtualSpace(mode))
allowVirtualSpace = false;
if (_elements.Count == 0)
{
// special handling for empty visual lines:
if (allowVirtualSpace)
{
if (direction == LogicalDirection.Forward)
return Math.Max(0, visualColumn + 1);
if (visualColumn > 0)
return visualColumn - 1;
return -1;
}
// even though we don't have any elements,
// there's a single caret stop at visualColumn 0
if (visualColumn < 0 && direction == LogicalDirection.Forward)
return 0;
if (visualColumn > 0 && direction == LogicalDirection.Backward)
return 0;
return -1;
}
int i;
if (direction == LogicalDirection.Backward)
{
// Search Backwards:
// If the last element doesn't handle line borders, return the line end as caret stop
if (visualColumn > VisualLength && !_elements[_elements.Count - 1].HandlesLineBorders && HasImplicitStopAtLineEnd())
{
if (allowVirtualSpace)
return visualColumn - 1;
return VisualLength;
}
// skip elements that start after or at visualColumn
for (i = _elements.Count - 1; i >= 0; i--)
{
if (_elements[i].VisualColumn < visualColumn)
break;
}
// search last element that has a caret stop
for (; i >= 0; i--)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Min(visualColumn, _elements[i].VisualColumn + _elements[i].VisualLength + 1),
direction, mode);
if (pos >= 0)
return pos;
}
// If we've found nothing, and the first element doesn't handle line borders,
// return the line start as normal caret stop.
if (visualColumn > 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
}
else
{
// Search Forwards:
// If the first element doesn't handle line borders, return the line start as caret stop
if (visualColumn < 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
// skip elements that end before or at visualColumn
for (i = 0; i < _elements.Count; i++)
{
if (_elements[i].VisualColumn + _elements[i].VisualLength > visualColumn)
break;
}
// search first element that has a caret stop
for (; i < _elements.Count; i++)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Max(visualColumn, _elements[i].VisualColumn - 1),
direction, mode);
if (pos >= 0)
return pos;
}
// if we've found nothing, and the last element doesn't handle line borders,
// return the line end as caret stop
if ((allowVirtualSpace || !_elements[_elements.Count - 1].HandlesLineBorders) && HasImplicitStopAtLineEnd())
{
if (visualColumn < VisualLength)
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
return _visual ??= new VisualLineDrawingVisual(this);
}
}
{
return mode == CaretPositioningMode.Normal || mode == CaretPositioningMode.EveryCodepoint;
}
private static bool HasImplicitStopAtLineEnd() => true;
private VisualLineDrawingVisual _visual;
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
if (_visual == null)
{
_visual = new VisualLineDrawingVisual(this);
((ISetLogicalParent)_visual).SetParent(_textView);
}
return _visual;
}
}
// TODO: can inherit from Layoutable, but dev tools crash
internal sealed class VisualLineDrawingVisual : Control
{
public VisualLine VisualLine { get; }
public double LineHeight { get; }
internal bool IsAdded { get; set; }
public VisualLineDrawingVisual(VisualLine visualLine)
{
VisualLine = visualLine;
LineHeight = VisualLine.TextLines.Sum(textLine => textLine.Height);
}
public override void Render(DrawingContext context)
{
double pos = 0;
foreach (var textLine in VisualLine.TextLines)
{
textLine.Draw(context, new Point(0, pos));
pos += textLine.Height;
}
}
}
}
<MSG> Set VisualLineDrawingVisual.Parent on creation
<DFF> @@ -748,7 +748,15 @@ namespace AvaloniaEdit.Rendering
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
- return _visual ??= new VisualLineDrawingVisual(this);
+
+ if (_visual == null)
+ {
+ _visual = new VisualLineDrawingVisual(this);
+
+ ((ISetLogicalParent)_visual).SetParent(_textView);
+ }
+
+ return _visual;
}
}
| 9 | Set VisualLineDrawingVisual.Parent on creation | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063807 | <NME> VisualLine.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Linq;
using Avalonia;
using Avalonia.Controls;
using Avalonia.Input;
using Avalonia.Media;
using Avalonia.Media.TextFormatting;
using AvaloniaEdit.Document;
using AvaloniaEdit.Utils;
using LogicalDirection = AvaloniaEdit.Document.LogicalDirection;
namespace AvaloniaEdit.Rendering
{
/// <summary>
/// Represents a visual line in the document.
/// A visual line usually corresponds to one DocumentLine, but it can span multiple lines if
/// all but the first are collapsed.
/// </summary>
public sealed class VisualLine
{
public const int LENGTH_LIMIT = 3000;
private enum LifetimePhase : byte
{
Generating,
Transforming,
Live,
Disposed
}
private readonly TextView _textView;
private List<VisualLineElement> _elements;
internal bool HasInlineObjects;
private LifetimePhase _phase;
/// <summary>
/// Gets the document to which this VisualLine belongs.
/// </summary>
public TextDocument Document { get; }
/// <summary>
/// Gets the first document line displayed by this visual line.
/// </summary>
public DocumentLine FirstDocumentLine { get; }
/// <summary>
/// Gets the last document line displayed by this visual line.
/// </summary>
public DocumentLine LastDocumentLine { get; private set; }
/// <summary>
/// Gets a read-only collection of line elements.
/// </summary>
public ReadOnlyCollection<VisualLineElement> Elements { get; private set; }
private ReadOnlyCollection<TextLine> _textLines;
/// <summary>
/// Gets a read-only collection of text lines.
/// </summary>
public ReadOnlyCollection<TextLine> TextLines
{
get
{
if (_phase < LifetimePhase.Live)
throw new InvalidOperationException();
return _textLines;
}
}
/// <summary>
/// Gets the start offset of the VisualLine inside the document.
/// This is equivalent to <c>FirstDocumentLine.Offset</c>.
/// </summary>
public int StartOffset => FirstDocumentLine.Offset;
/// <summary>
/// Length in visual line coordinates.
/// </summary>
public int VisualLength { get; private set; }
/// <summary>
/// Length in visual line coordinates including the end of line marker, if TextEditorOptions.ShowEndOfLine is enabled.
/// </summary>
public int VisualLengthWithEndOfLineMarker
{
get
{
var length = VisualLength;
if (_textView.Options.ShowEndOfLine && LastDocumentLine.NextLine != null) length++;
return length;
}
}
/// <summary>
/// Gets the height of the visual line in device-independent pixels.
/// </summary>
public double Height { get; private set; }
/// <summary>
/// Gets the Y position of the line. This is measured in device-independent pixels relative to the start of the document.
/// </summary>
public double VisualTop { get; internal set; }
internal VisualLine(TextView textView, DocumentLine firstDocumentLine)
{
Debug.Assert(textView != null);
Debug.Assert(firstDocumentLine != null);
_textView = textView;
Document = textView.Document;
FirstDocumentLine = firstDocumentLine;
}
internal void ConstructVisualElements(ITextRunConstructionContext context, IReadOnlyList<VisualLineElementGenerator> generators)
{
Debug.Assert(_phase == LifetimePhase.Generating);
foreach (var g in generators)
{
g.StartGeneration(context);
}
_elements = new List<VisualLineElement>();
PerformVisualElementConstruction(generators);
foreach (var g in generators)
{
g.FinishGeneration();
}
var globalTextRunProperties = context.GlobalTextRunProperties;
foreach (var element in _elements)
{
element.SetTextRunProperties(new VisualLineElementTextRunProperties(globalTextRunProperties));
}
this.Elements = new ReadOnlyCollection<VisualLineElement>(_elements);
CalculateOffsets();
_phase = LifetimePhase.Transforming;
}
void PerformVisualElementConstruction(IReadOnlyList<VisualLineElementGenerator> generators)
{
var lineLength = FirstDocumentLine.Length;
var offset = FirstDocumentLine.Offset;
var currentLineEnd = offset + lineLength;
LastDocumentLine = FirstDocumentLine;
var askInterestOffset = 0; // 0 or 1
while (offset + askInterestOffset <= currentLineEnd)
{
var textPieceEndOffset = currentLineEnd;
foreach (var g in generators)
{
g.CachedInterest = g.GetFirstInterestedOffset(offset + askInterestOffset);
if (g.CachedInterest != -1)
{
if (g.CachedInterest < offset)
throw new ArgumentOutOfRangeException(g.GetType().Name + ".GetFirstInterestedOffset",
g.CachedInterest,
"GetFirstInterestedOffset must not return an offset less than startOffset. Return -1 to signal no interest.");
if (g.CachedInterest < textPieceEndOffset)
textPieceEndOffset = g.CachedInterest;
}
}
Debug.Assert(textPieceEndOffset >= offset);
if (textPieceEndOffset > offset)
{
var textPieceLength = textPieceEndOffset - offset;
_elements.Add(new VisualLineText(this, textPieceLength));
offset = textPieceEndOffset;
}
// If no elements constructed / only zero-length elements constructed:
// do not asking the generators again for the same location (would cause endless loop)
askInterestOffset = 1;
foreach (var g in generators)
{
if (g.CachedInterest == offset)
{
var element = g.ConstructElement(offset);
if (element != null)
{
_elements.Add(element);
if (element.DocumentLength > 0)
{
// a non-zero-length element was constructed
askInterestOffset = 0;
offset += element.DocumentLength;
if (offset > currentLineEnd)
{
var newEndLine = Document.GetLineByOffset(offset);
currentLineEnd = newEndLine.Offset + newEndLine.Length;
this.LastDocumentLine = newEndLine;
if (currentLineEnd < offset)
{
throw new InvalidOperationException(
"The VisualLineElementGenerator " + g.GetType().Name +
" produced an element which ends within the line delimiter");
}
}
break;
}
}
}
}
}
}
private void CalculateOffsets()
{
var visualOffset = 0;
var textOffset = 0;
foreach (var element in _elements)
{
element.VisualColumn = visualOffset;
element.RelativeTextOffset = textOffset;
visualOffset += element.VisualLength;
textOffset += element.DocumentLength;
}
VisualLength = visualOffset;
Debug.Assert(textOffset == LastDocumentLine.EndOffset - FirstDocumentLine.Offset);
}
internal void RunTransformers(ITextRunConstructionContext context, IReadOnlyList<IVisualLineTransformer> transformers)
{
Debug.Assert(_phase == LifetimePhase.Transforming);
foreach (var transformer in transformers)
{
transformer.Transform(context, _elements);
}
_phase = LifetimePhase.Live;
}
/// <summary>
/// Replaces the single element at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, params VisualLineElement[] newElements)
{
ReplaceElement(elementIndex, 1, newElements);
}
/// <summary>
/// Replaces <paramref name="count"/> elements starting at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, int count, params VisualLineElement[] newElements)
{
if (_phase != LifetimePhase.Transforming)
throw new InvalidOperationException("This method may only be called by line transformers.");
var oldDocumentLength = 0;
for (var i = elementIndex; i < elementIndex + count; i++)
{
oldDocumentLength += _elements[i].DocumentLength;
}
var newDocumentLength = 0;
foreach (var newElement in newElements)
{
newDocumentLength += newElement.DocumentLength;
}
if (oldDocumentLength != newDocumentLength)
throw new InvalidOperationException("Old elements have document length " + oldDocumentLength + ", but new elements have length " + newDocumentLength);
_elements.RemoveRange(elementIndex, count);
_elements.InsertRange(elementIndex, newElements);
CalculateOffsets();
}
internal void SetTextLines(List<TextLine> textLines)
{
_textLines = new ReadOnlyCollection<TextLine>(textLines);
Height = 0;
foreach (var line in textLines)
Height += line.Height;
}
/// <summary>
/// Gets the visual column from a document offset relative to the first line start.
/// </summary>
public int GetVisualColumn(int relativeTextOffset)
{
ThrowUtil.CheckNotNegative(relativeTextOffset, "relativeTextOffset");
foreach (var element in _elements)
{
if (element.RelativeTextOffset <= relativeTextOffset
&& element.RelativeTextOffset + element.DocumentLength >= relativeTextOffset)
{
return element.GetVisualColumn(relativeTextOffset);
}
}
return VisualLength;
}
/// <summary>
/// Gets the document offset (relative to the first line start) from a visual column.
/// </summary>
public int GetRelativeOffset(int visualColumn)
{
ThrowUtil.CheckNotNegative(visualColumn, "visualColumn");
var documentLength = 0;
foreach (var element in _elements)
{
if (element.VisualColumn <= visualColumn
&& element.VisualColumn + element.VisualLength > visualColumn)
{
return element.GetRelativeOffset(visualColumn);
}
documentLength += element.DocumentLength;
}
return documentLength;
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn)
{
return GetTextLine(visualColumn, false);
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn, bool isAtEndOfLine)
{
if (visualColumn < 0)
throw new ArgumentOutOfRangeException(nameof(visualColumn));
if (visualColumn >= VisualLengthWithEndOfLineMarker)
return TextLines[TextLines.Count - 1];
foreach (var line in TextLines)
{
if (isAtEndOfLine ? visualColumn <= line.Length : visualColumn < line.Length)
return line;
visualColumn -= line.Length;
}
throw new InvalidOperationException("Shouldn't happen (VisualLength incorrect?)");
}
/// <summary>
/// Gets the visual top from the specified text line.
/// </summary>
/// <returns>Distance in device-independent pixels
/// from the top of the document to the top of the specified text line.</returns>
public double GetTextLineVisualYPosition(TextLine textLine, VisualYPosition yPositionMode)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var pos = VisualTop;
foreach (var tl in TextLines)
{
if (tl == textLine)
{
switch (yPositionMode)
{
case VisualYPosition.LineTop:
return pos;
case VisualYPosition.LineMiddle:
return pos + tl.Height / 2;
case VisualYPosition.LineBottom:
return pos + tl.Height;
case VisualYPosition.TextTop:
return pos + tl.Baseline - _textView.DefaultBaseline;
case VisualYPosition.TextBottom:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight;
case VisualYPosition.TextMiddle:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight / 2;
case VisualYPosition.Baseline:
return pos + tl.Baseline;
default:
throw new ArgumentException("Invalid yPositionMode:" + yPositionMode);
}
}
pos += tl.Height;
}
throw new ArgumentException("textLine is not a line in this VisualLine");
}
/// <summary>
/// Gets the start visual column from the specified text line.
/// </summary>
public int GetTextLineVisualStartColumn(TextLine textLine)
{
if (!TextLines.Contains(textLine))
throw new ArgumentException("textLine is not a line in this VisualLine");
return TextLines.TakeWhile(tl => tl != textLine).Sum(tl => tl.Length);
}
/// <summary>
/// Gets a TextLine by the visual position.
/// </summary>
public TextLine GetTextLineByVisualYPosition(double visualTop)
{
const double epsilon = 0.0001;
var pos = VisualTop;
foreach (var tl in TextLines)
{
pos += tl.Height;
if (visualTop + epsilon < pos)
return tl;
}
return TextLines[TextLines.Count - 1];
}
/// <summary>
/// Gets the visual position from the specified visualColumn.
/// </summary>
/// <returns>Position in device-independent pixels
/// relative to the top left of the document.</returns>
public Point GetVisualPosition(int visualColumn, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
internal Point GetVisualPosition(int visualColumn, bool isAtEndOfLine, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn, isAtEndOfLine);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
/// <summary>
/// Gets the distance to the left border of the text area of the specified visual column.
/// The visual column must belong to the specified text line.
/// </summary>
public double GetTextLineVisualXPosition(TextLine textLine, int visualColumn)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var xPos = textLine.GetDistanceFromCharacterHit(new CharacterHit(Math.Min(visualColumn,
VisualLengthWithEndOfLineMarker)));
if (visualColumn > VisualLengthWithEndOfLineMarker)
{
xPos += (visualColumn - VisualLengthWithEndOfLineMarker) * _textView.WideSpaceWidth;
}
return xPos;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point)
{
return GetVisualColumn(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point, bool allowVirtualSpace)
{
return GetVisualColumn(GetTextLineByVisualYPosition(point.Y), point.X, allowVirtualSpace);
}
internal int GetVisualColumn(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
var vc = GetVisualColumn(textLine, point.X, allowVirtualSpace);
isAtEndOfLine = (vc >= GetTextLineVisualStartColumn(textLine) + textLine.Length);
return vc;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(TextLine textLine, double xPos, bool allowVirtualSpace)
{
if (xPos > textLine.WidthIncludingTrailingWhitespace)
{
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
var virtualX = (int)Math.Round((xPos - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth, MidpointRounding.AwayFromZero);
return VisualLengthWithEndOfLineMarker + virtualX;
}
}
var ch = textLine.GetCharacterHitFromDistance(xPos);
return ch.FirstCharacterIndex + ch.TrailingLength;
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(TextViewPosition position, bool allowVirtualSpace)
{
return ValidateVisualColumn(Document.GetOffset(position.Location), position.VisualColumn, allowVirtualSpace);
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(int offset, int visualColumn, bool allowVirtualSpace)
{
var firstDocumentLineOffset = FirstDocumentLine.Offset;
if (visualColumn < 0)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
var offsetFromVisualColumn = GetRelativeOffset(visualColumn);
offsetFromVisualColumn += firstDocumentLineOffset;
if (offsetFromVisualColumn != offset)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
if (visualColumn > VisualLength && !allowVirtualSpace)
{
return VisualLength;
}
return visualColumn;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point)
{
return GetVisualColumnFloor(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point, bool allowVirtualSpace)
{
return GetVisualColumnFloor(point, allowVirtualSpace, out _);
}
internal int GetVisualColumnFloor(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
if (point.X > textLine.WidthIncludingTrailingWhitespace)
{
isAtEndOfLine = true;
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
// clicking virtual space in the last line
var virtualX = (int)((point.X - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth);
return VisualLengthWithEndOfLineMarker + virtualX;
}
// GetCharacterHitFromDistance returns a hit with FirstCharacterIndex=last character in line
// and TrailingLength=1 when clicking behind the line, so the floor function needs to handle this case
// specially and return the line's end column instead.
return GetTextLineVisualStartColumn(textLine) + textLine.Length;
}
isAtEndOfLine = false;
var ch = textLine.GetCharacterHitFromDistance(point.X);
return ch.FirstCharacterIndex;
}
/// <summary>
/// Gets the text view position from the specified visual column.
/// </summary>
public TextViewPosition GetTextViewPosition(int visualColumn)
{
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
return new TextViewPosition(Document.GetLocation(documentOffset), visualColumn);
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is within a character, it is rounded to the next character boundary.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPosition(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumn(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is inside a character, the position in front of the character is returned.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPositionFloor(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumnFloor(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets whether the visual line was disposed.
/// </summary>
public bool IsDisposed => _phase == LifetimePhase.Disposed;
internal void Dispose()
{
if (_phase == LifetimePhase.Disposed)
{
return;
}
Debug.Assert(_phase == LifetimePhase.Live);
_phase = LifetimePhase.Disposed;
if (_visual != null)
{
((ISetLogicalParent)_visual).SetParent(null);
}
}
/// <summary>
/// Gets the next possible caret position after visualColumn, or -1 if there is no caret position.
/// </summary>
public int GetNextCaretPosition(int visualColumn, LogicalDirection direction, CaretPositioningMode mode, bool allowVirtualSpace)
{
if (!HasStopsInVirtualSpace(mode))
allowVirtualSpace = false;
if (_elements.Count == 0)
{
// special handling for empty visual lines:
if (allowVirtualSpace)
{
if (direction == LogicalDirection.Forward)
return Math.Max(0, visualColumn + 1);
if (visualColumn > 0)
return visualColumn - 1;
return -1;
}
// even though we don't have any elements,
// there's a single caret stop at visualColumn 0
if (visualColumn < 0 && direction == LogicalDirection.Forward)
return 0;
if (visualColumn > 0 && direction == LogicalDirection.Backward)
return 0;
return -1;
}
int i;
if (direction == LogicalDirection.Backward)
{
// Search Backwards:
// If the last element doesn't handle line borders, return the line end as caret stop
if (visualColumn > VisualLength && !_elements[_elements.Count - 1].HandlesLineBorders && HasImplicitStopAtLineEnd())
{
if (allowVirtualSpace)
return visualColumn - 1;
return VisualLength;
}
// skip elements that start after or at visualColumn
for (i = _elements.Count - 1; i >= 0; i--)
{
if (_elements[i].VisualColumn < visualColumn)
break;
}
// search last element that has a caret stop
for (; i >= 0; i--)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Min(visualColumn, _elements[i].VisualColumn + _elements[i].VisualLength + 1),
direction, mode);
if (pos >= 0)
return pos;
}
// If we've found nothing, and the first element doesn't handle line borders,
// return the line start as normal caret stop.
if (visualColumn > 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
}
else
{
// Search Forwards:
// If the first element doesn't handle line borders, return the line start as caret stop
if (visualColumn < 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
// skip elements that end before or at visualColumn
for (i = 0; i < _elements.Count; i++)
{
if (_elements[i].VisualColumn + _elements[i].VisualLength > visualColumn)
break;
}
// search first element that has a caret stop
for (; i < _elements.Count; i++)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Max(visualColumn, _elements[i].VisualColumn - 1),
direction, mode);
if (pos >= 0)
return pos;
}
// if we've found nothing, and the last element doesn't handle line borders,
// return the line end as caret stop
if ((allowVirtualSpace || !_elements[_elements.Count - 1].HandlesLineBorders) && HasImplicitStopAtLineEnd())
{
if (visualColumn < VisualLength)
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
return _visual ??= new VisualLineDrawingVisual(this);
}
}
{
return mode == CaretPositioningMode.Normal || mode == CaretPositioningMode.EveryCodepoint;
}
private static bool HasImplicitStopAtLineEnd() => true;
private VisualLineDrawingVisual _visual;
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
if (_visual == null)
{
_visual = new VisualLineDrawingVisual(this);
((ISetLogicalParent)_visual).SetParent(_textView);
}
return _visual;
}
}
// TODO: can inherit from Layoutable, but dev tools crash
internal sealed class VisualLineDrawingVisual : Control
{
public VisualLine VisualLine { get; }
public double LineHeight { get; }
internal bool IsAdded { get; set; }
public VisualLineDrawingVisual(VisualLine visualLine)
{
VisualLine = visualLine;
LineHeight = VisualLine.TextLines.Sum(textLine => textLine.Height);
}
public override void Render(DrawingContext context)
{
double pos = 0;
foreach (var textLine in VisualLine.TextLines)
{
textLine.Draw(context, new Point(0, pos));
pos += textLine.Height;
}
}
}
}
<MSG> Set VisualLineDrawingVisual.Parent on creation
<DFF> @@ -748,7 +748,15 @@ namespace AvaloniaEdit.Rendering
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
- return _visual ??= new VisualLineDrawingVisual(this);
+
+ if (_visual == null)
+ {
+ _visual = new VisualLineDrawingVisual(this);
+
+ ((ISetLogicalParent)_visual).SetParent(_textView);
+ }
+
+ return _visual;
}
}
| 9 | Set VisualLineDrawingVisual.Parent on creation | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063808 | <NME> VisualLine.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Linq;
using Avalonia;
using Avalonia.Controls;
using Avalonia.Input;
using Avalonia.Media;
using Avalonia.Media.TextFormatting;
using AvaloniaEdit.Document;
using AvaloniaEdit.Utils;
using LogicalDirection = AvaloniaEdit.Document.LogicalDirection;
namespace AvaloniaEdit.Rendering
{
/// <summary>
/// Represents a visual line in the document.
/// A visual line usually corresponds to one DocumentLine, but it can span multiple lines if
/// all but the first are collapsed.
/// </summary>
public sealed class VisualLine
{
public const int LENGTH_LIMIT = 3000;
private enum LifetimePhase : byte
{
Generating,
Transforming,
Live,
Disposed
}
private readonly TextView _textView;
private List<VisualLineElement> _elements;
internal bool HasInlineObjects;
private LifetimePhase _phase;
/// <summary>
/// Gets the document to which this VisualLine belongs.
/// </summary>
public TextDocument Document { get; }
/// <summary>
/// Gets the first document line displayed by this visual line.
/// </summary>
public DocumentLine FirstDocumentLine { get; }
/// <summary>
/// Gets the last document line displayed by this visual line.
/// </summary>
public DocumentLine LastDocumentLine { get; private set; }
/// <summary>
/// Gets a read-only collection of line elements.
/// </summary>
public ReadOnlyCollection<VisualLineElement> Elements { get; private set; }
private ReadOnlyCollection<TextLine> _textLines;
/// <summary>
/// Gets a read-only collection of text lines.
/// </summary>
public ReadOnlyCollection<TextLine> TextLines
{
get
{
if (_phase < LifetimePhase.Live)
throw new InvalidOperationException();
return _textLines;
}
}
/// <summary>
/// Gets the start offset of the VisualLine inside the document.
/// This is equivalent to <c>FirstDocumentLine.Offset</c>.
/// </summary>
public int StartOffset => FirstDocumentLine.Offset;
/// <summary>
/// Length in visual line coordinates.
/// </summary>
public int VisualLength { get; private set; }
/// <summary>
/// Length in visual line coordinates including the end of line marker, if TextEditorOptions.ShowEndOfLine is enabled.
/// </summary>
public int VisualLengthWithEndOfLineMarker
{
get
{
var length = VisualLength;
if (_textView.Options.ShowEndOfLine && LastDocumentLine.NextLine != null) length++;
return length;
}
}
/// <summary>
/// Gets the height of the visual line in device-independent pixels.
/// </summary>
public double Height { get; private set; }
/// <summary>
/// Gets the Y position of the line. This is measured in device-independent pixels relative to the start of the document.
/// </summary>
public double VisualTop { get; internal set; }
internal VisualLine(TextView textView, DocumentLine firstDocumentLine)
{
Debug.Assert(textView != null);
Debug.Assert(firstDocumentLine != null);
_textView = textView;
Document = textView.Document;
FirstDocumentLine = firstDocumentLine;
}
internal void ConstructVisualElements(ITextRunConstructionContext context, IReadOnlyList<VisualLineElementGenerator> generators)
{
Debug.Assert(_phase == LifetimePhase.Generating);
foreach (var g in generators)
{
g.StartGeneration(context);
}
_elements = new List<VisualLineElement>();
PerformVisualElementConstruction(generators);
foreach (var g in generators)
{
g.FinishGeneration();
}
var globalTextRunProperties = context.GlobalTextRunProperties;
foreach (var element in _elements)
{
element.SetTextRunProperties(new VisualLineElementTextRunProperties(globalTextRunProperties));
}
this.Elements = new ReadOnlyCollection<VisualLineElement>(_elements);
CalculateOffsets();
_phase = LifetimePhase.Transforming;
}
void PerformVisualElementConstruction(IReadOnlyList<VisualLineElementGenerator> generators)
{
var lineLength = FirstDocumentLine.Length;
var offset = FirstDocumentLine.Offset;
var currentLineEnd = offset + lineLength;
LastDocumentLine = FirstDocumentLine;
var askInterestOffset = 0; // 0 or 1
while (offset + askInterestOffset <= currentLineEnd)
{
var textPieceEndOffset = currentLineEnd;
foreach (var g in generators)
{
g.CachedInterest = g.GetFirstInterestedOffset(offset + askInterestOffset);
if (g.CachedInterest != -1)
{
if (g.CachedInterest < offset)
throw new ArgumentOutOfRangeException(g.GetType().Name + ".GetFirstInterestedOffset",
g.CachedInterest,
"GetFirstInterestedOffset must not return an offset less than startOffset. Return -1 to signal no interest.");
if (g.CachedInterest < textPieceEndOffset)
textPieceEndOffset = g.CachedInterest;
}
}
Debug.Assert(textPieceEndOffset >= offset);
if (textPieceEndOffset > offset)
{
var textPieceLength = textPieceEndOffset - offset;
_elements.Add(new VisualLineText(this, textPieceLength));
offset = textPieceEndOffset;
}
// If no elements constructed / only zero-length elements constructed:
// do not asking the generators again for the same location (would cause endless loop)
askInterestOffset = 1;
foreach (var g in generators)
{
if (g.CachedInterest == offset)
{
var element = g.ConstructElement(offset);
if (element != null)
{
_elements.Add(element);
if (element.DocumentLength > 0)
{
// a non-zero-length element was constructed
askInterestOffset = 0;
offset += element.DocumentLength;
if (offset > currentLineEnd)
{
var newEndLine = Document.GetLineByOffset(offset);
currentLineEnd = newEndLine.Offset + newEndLine.Length;
this.LastDocumentLine = newEndLine;
if (currentLineEnd < offset)
{
throw new InvalidOperationException(
"The VisualLineElementGenerator " + g.GetType().Name +
" produced an element which ends within the line delimiter");
}
}
break;
}
}
}
}
}
}
private void CalculateOffsets()
{
var visualOffset = 0;
var textOffset = 0;
foreach (var element in _elements)
{
element.VisualColumn = visualOffset;
element.RelativeTextOffset = textOffset;
visualOffset += element.VisualLength;
textOffset += element.DocumentLength;
}
VisualLength = visualOffset;
Debug.Assert(textOffset == LastDocumentLine.EndOffset - FirstDocumentLine.Offset);
}
internal void RunTransformers(ITextRunConstructionContext context, IReadOnlyList<IVisualLineTransformer> transformers)
{
Debug.Assert(_phase == LifetimePhase.Transforming);
foreach (var transformer in transformers)
{
transformer.Transform(context, _elements);
}
_phase = LifetimePhase.Live;
}
/// <summary>
/// Replaces the single element at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, params VisualLineElement[] newElements)
{
ReplaceElement(elementIndex, 1, newElements);
}
/// <summary>
/// Replaces <paramref name="count"/> elements starting at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, int count, params VisualLineElement[] newElements)
{
if (_phase != LifetimePhase.Transforming)
throw new InvalidOperationException("This method may only be called by line transformers.");
var oldDocumentLength = 0;
for (var i = elementIndex; i < elementIndex + count; i++)
{
oldDocumentLength += _elements[i].DocumentLength;
}
var newDocumentLength = 0;
foreach (var newElement in newElements)
{
newDocumentLength += newElement.DocumentLength;
}
if (oldDocumentLength != newDocumentLength)
throw new InvalidOperationException("Old elements have document length " + oldDocumentLength + ", but new elements have length " + newDocumentLength);
_elements.RemoveRange(elementIndex, count);
_elements.InsertRange(elementIndex, newElements);
CalculateOffsets();
}
internal void SetTextLines(List<TextLine> textLines)
{
_textLines = new ReadOnlyCollection<TextLine>(textLines);
Height = 0;
foreach (var line in textLines)
Height += line.Height;
}
/// <summary>
/// Gets the visual column from a document offset relative to the first line start.
/// </summary>
public int GetVisualColumn(int relativeTextOffset)
{
ThrowUtil.CheckNotNegative(relativeTextOffset, "relativeTextOffset");
foreach (var element in _elements)
{
if (element.RelativeTextOffset <= relativeTextOffset
&& element.RelativeTextOffset + element.DocumentLength >= relativeTextOffset)
{
return element.GetVisualColumn(relativeTextOffset);
}
}
return VisualLength;
}
/// <summary>
/// Gets the document offset (relative to the first line start) from a visual column.
/// </summary>
public int GetRelativeOffset(int visualColumn)
{
ThrowUtil.CheckNotNegative(visualColumn, "visualColumn");
var documentLength = 0;
foreach (var element in _elements)
{
if (element.VisualColumn <= visualColumn
&& element.VisualColumn + element.VisualLength > visualColumn)
{
return element.GetRelativeOffset(visualColumn);
}
documentLength += element.DocumentLength;
}
return documentLength;
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn)
{
return GetTextLine(visualColumn, false);
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn, bool isAtEndOfLine)
{
if (visualColumn < 0)
throw new ArgumentOutOfRangeException(nameof(visualColumn));
if (visualColumn >= VisualLengthWithEndOfLineMarker)
return TextLines[TextLines.Count - 1];
foreach (var line in TextLines)
{
if (isAtEndOfLine ? visualColumn <= line.Length : visualColumn < line.Length)
return line;
visualColumn -= line.Length;
}
throw new InvalidOperationException("Shouldn't happen (VisualLength incorrect?)");
}
/// <summary>
/// Gets the visual top from the specified text line.
/// </summary>
/// <returns>Distance in device-independent pixels
/// from the top of the document to the top of the specified text line.</returns>
public double GetTextLineVisualYPosition(TextLine textLine, VisualYPosition yPositionMode)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var pos = VisualTop;
foreach (var tl in TextLines)
{
if (tl == textLine)
{
switch (yPositionMode)
{
case VisualYPosition.LineTop:
return pos;
case VisualYPosition.LineMiddle:
return pos + tl.Height / 2;
case VisualYPosition.LineBottom:
return pos + tl.Height;
case VisualYPosition.TextTop:
return pos + tl.Baseline - _textView.DefaultBaseline;
case VisualYPosition.TextBottom:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight;
case VisualYPosition.TextMiddle:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight / 2;
case VisualYPosition.Baseline:
return pos + tl.Baseline;
default:
throw new ArgumentException("Invalid yPositionMode:" + yPositionMode);
}
}
pos += tl.Height;
}
throw new ArgumentException("textLine is not a line in this VisualLine");
}
/// <summary>
/// Gets the start visual column from the specified text line.
/// </summary>
public int GetTextLineVisualStartColumn(TextLine textLine)
{
if (!TextLines.Contains(textLine))
throw new ArgumentException("textLine is not a line in this VisualLine");
return TextLines.TakeWhile(tl => tl != textLine).Sum(tl => tl.Length);
}
/// <summary>
/// Gets a TextLine by the visual position.
/// </summary>
public TextLine GetTextLineByVisualYPosition(double visualTop)
{
const double epsilon = 0.0001;
var pos = VisualTop;
foreach (var tl in TextLines)
{
pos += tl.Height;
if (visualTop + epsilon < pos)
return tl;
}
return TextLines[TextLines.Count - 1];
}
/// <summary>
/// Gets the visual position from the specified visualColumn.
/// </summary>
/// <returns>Position in device-independent pixels
/// relative to the top left of the document.</returns>
public Point GetVisualPosition(int visualColumn, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
internal Point GetVisualPosition(int visualColumn, bool isAtEndOfLine, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn, isAtEndOfLine);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
/// <summary>
/// Gets the distance to the left border of the text area of the specified visual column.
/// The visual column must belong to the specified text line.
/// </summary>
public double GetTextLineVisualXPosition(TextLine textLine, int visualColumn)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var xPos = textLine.GetDistanceFromCharacterHit(new CharacterHit(Math.Min(visualColumn,
VisualLengthWithEndOfLineMarker)));
if (visualColumn > VisualLengthWithEndOfLineMarker)
{
xPos += (visualColumn - VisualLengthWithEndOfLineMarker) * _textView.WideSpaceWidth;
}
return xPos;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point)
{
return GetVisualColumn(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point, bool allowVirtualSpace)
{
return GetVisualColumn(GetTextLineByVisualYPosition(point.Y), point.X, allowVirtualSpace);
}
internal int GetVisualColumn(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
var vc = GetVisualColumn(textLine, point.X, allowVirtualSpace);
isAtEndOfLine = (vc >= GetTextLineVisualStartColumn(textLine) + textLine.Length);
return vc;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(TextLine textLine, double xPos, bool allowVirtualSpace)
{
if (xPos > textLine.WidthIncludingTrailingWhitespace)
{
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
var virtualX = (int)Math.Round((xPos - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth, MidpointRounding.AwayFromZero);
return VisualLengthWithEndOfLineMarker + virtualX;
}
}
var ch = textLine.GetCharacterHitFromDistance(xPos);
return ch.FirstCharacterIndex + ch.TrailingLength;
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(TextViewPosition position, bool allowVirtualSpace)
{
return ValidateVisualColumn(Document.GetOffset(position.Location), position.VisualColumn, allowVirtualSpace);
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(int offset, int visualColumn, bool allowVirtualSpace)
{
var firstDocumentLineOffset = FirstDocumentLine.Offset;
if (visualColumn < 0)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
var offsetFromVisualColumn = GetRelativeOffset(visualColumn);
offsetFromVisualColumn += firstDocumentLineOffset;
if (offsetFromVisualColumn != offset)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
if (visualColumn > VisualLength && !allowVirtualSpace)
{
return VisualLength;
}
return visualColumn;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point)
{
return GetVisualColumnFloor(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point, bool allowVirtualSpace)
{
return GetVisualColumnFloor(point, allowVirtualSpace, out _);
}
internal int GetVisualColumnFloor(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
if (point.X > textLine.WidthIncludingTrailingWhitespace)
{
isAtEndOfLine = true;
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
// clicking virtual space in the last line
var virtualX = (int)((point.X - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth);
return VisualLengthWithEndOfLineMarker + virtualX;
}
// GetCharacterHitFromDistance returns a hit with FirstCharacterIndex=last character in line
// and TrailingLength=1 when clicking behind the line, so the floor function needs to handle this case
// specially and return the line's end column instead.
return GetTextLineVisualStartColumn(textLine) + textLine.Length;
}
isAtEndOfLine = false;
var ch = textLine.GetCharacterHitFromDistance(point.X);
return ch.FirstCharacterIndex;
}
/// <summary>
/// Gets the text view position from the specified visual column.
/// </summary>
public TextViewPosition GetTextViewPosition(int visualColumn)
{
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
return new TextViewPosition(Document.GetLocation(documentOffset), visualColumn);
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is within a character, it is rounded to the next character boundary.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPosition(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumn(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is inside a character, the position in front of the character is returned.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPositionFloor(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumnFloor(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets whether the visual line was disposed.
/// </summary>
public bool IsDisposed => _phase == LifetimePhase.Disposed;
internal void Dispose()
{
if (_phase == LifetimePhase.Disposed)
{
return;
}
Debug.Assert(_phase == LifetimePhase.Live);
_phase = LifetimePhase.Disposed;
if (_visual != null)
{
((ISetLogicalParent)_visual).SetParent(null);
}
}
/// <summary>
/// Gets the next possible caret position after visualColumn, or -1 if there is no caret position.
/// </summary>
public int GetNextCaretPosition(int visualColumn, LogicalDirection direction, CaretPositioningMode mode, bool allowVirtualSpace)
{
if (!HasStopsInVirtualSpace(mode))
allowVirtualSpace = false;
if (_elements.Count == 0)
{
// special handling for empty visual lines:
if (allowVirtualSpace)
{
if (direction == LogicalDirection.Forward)
return Math.Max(0, visualColumn + 1);
if (visualColumn > 0)
return visualColumn - 1;
return -1;
}
// even though we don't have any elements,
// there's a single caret stop at visualColumn 0
if (visualColumn < 0 && direction == LogicalDirection.Forward)
return 0;
if (visualColumn > 0 && direction == LogicalDirection.Backward)
return 0;
return -1;
}
int i;
if (direction == LogicalDirection.Backward)
{
// Search Backwards:
// If the last element doesn't handle line borders, return the line end as caret stop
if (visualColumn > VisualLength && !_elements[_elements.Count - 1].HandlesLineBorders && HasImplicitStopAtLineEnd())
{
if (allowVirtualSpace)
return visualColumn - 1;
return VisualLength;
}
// skip elements that start after or at visualColumn
for (i = _elements.Count - 1; i >= 0; i--)
{
if (_elements[i].VisualColumn < visualColumn)
break;
}
// search last element that has a caret stop
for (; i >= 0; i--)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Min(visualColumn, _elements[i].VisualColumn + _elements[i].VisualLength + 1),
direction, mode);
if (pos >= 0)
return pos;
}
// If we've found nothing, and the first element doesn't handle line borders,
// return the line start as normal caret stop.
if (visualColumn > 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
}
else
{
// Search Forwards:
// If the first element doesn't handle line borders, return the line start as caret stop
if (visualColumn < 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
// skip elements that end before or at visualColumn
for (i = 0; i < _elements.Count; i++)
{
if (_elements[i].VisualColumn + _elements[i].VisualLength > visualColumn)
break;
}
// search first element that has a caret stop
for (; i < _elements.Count; i++)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Max(visualColumn, _elements[i].VisualColumn - 1),
direction, mode);
if (pos >= 0)
return pos;
}
// if we've found nothing, and the last element doesn't handle line borders,
// return the line end as caret stop
if ((allowVirtualSpace || !_elements[_elements.Count - 1].HandlesLineBorders) && HasImplicitStopAtLineEnd())
{
if (visualColumn < VisualLength)
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
return _visual ??= new VisualLineDrawingVisual(this);
}
}
{
return mode == CaretPositioningMode.Normal || mode == CaretPositioningMode.EveryCodepoint;
}
private static bool HasImplicitStopAtLineEnd() => true;
private VisualLineDrawingVisual _visual;
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
if (_visual == null)
{
_visual = new VisualLineDrawingVisual(this);
((ISetLogicalParent)_visual).SetParent(_textView);
}
return _visual;
}
}
// TODO: can inherit from Layoutable, but dev tools crash
internal sealed class VisualLineDrawingVisual : Control
{
public VisualLine VisualLine { get; }
public double LineHeight { get; }
internal bool IsAdded { get; set; }
public VisualLineDrawingVisual(VisualLine visualLine)
{
VisualLine = visualLine;
LineHeight = VisualLine.TextLines.Sum(textLine => textLine.Height);
}
public override void Render(DrawingContext context)
{
double pos = 0;
foreach (var textLine in VisualLine.TextLines)
{
textLine.Draw(context, new Point(0, pos));
pos += textLine.Height;
}
}
}
}
<MSG> Set VisualLineDrawingVisual.Parent on creation
<DFF> @@ -748,7 +748,15 @@ namespace AvaloniaEdit.Rendering
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
- return _visual ??= new VisualLineDrawingVisual(this);
+
+ if (_visual == null)
+ {
+ _visual = new VisualLineDrawingVisual(this);
+
+ ((ISetLogicalParent)_visual).SetParent(_textView);
+ }
+
+ return _visual;
}
}
| 9 | Set VisualLineDrawingVisual.Parent on creation | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063809 | <NME> VisualLine.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Linq;
using Avalonia;
using Avalonia.Controls;
using Avalonia.Input;
using Avalonia.Media;
using Avalonia.Media.TextFormatting;
using AvaloniaEdit.Document;
using AvaloniaEdit.Utils;
using LogicalDirection = AvaloniaEdit.Document.LogicalDirection;
namespace AvaloniaEdit.Rendering
{
/// <summary>
/// Represents a visual line in the document.
/// A visual line usually corresponds to one DocumentLine, but it can span multiple lines if
/// all but the first are collapsed.
/// </summary>
public sealed class VisualLine
{
public const int LENGTH_LIMIT = 3000;
private enum LifetimePhase : byte
{
Generating,
Transforming,
Live,
Disposed
}
private readonly TextView _textView;
private List<VisualLineElement> _elements;
internal bool HasInlineObjects;
private LifetimePhase _phase;
/// <summary>
/// Gets the document to which this VisualLine belongs.
/// </summary>
public TextDocument Document { get; }
/// <summary>
/// Gets the first document line displayed by this visual line.
/// </summary>
public DocumentLine FirstDocumentLine { get; }
/// <summary>
/// Gets the last document line displayed by this visual line.
/// </summary>
public DocumentLine LastDocumentLine { get; private set; }
/// <summary>
/// Gets a read-only collection of line elements.
/// </summary>
public ReadOnlyCollection<VisualLineElement> Elements { get; private set; }
private ReadOnlyCollection<TextLine> _textLines;
/// <summary>
/// Gets a read-only collection of text lines.
/// </summary>
public ReadOnlyCollection<TextLine> TextLines
{
get
{
if (_phase < LifetimePhase.Live)
throw new InvalidOperationException();
return _textLines;
}
}
/// <summary>
/// Gets the start offset of the VisualLine inside the document.
/// This is equivalent to <c>FirstDocumentLine.Offset</c>.
/// </summary>
public int StartOffset => FirstDocumentLine.Offset;
/// <summary>
/// Length in visual line coordinates.
/// </summary>
public int VisualLength { get; private set; }
/// <summary>
/// Length in visual line coordinates including the end of line marker, if TextEditorOptions.ShowEndOfLine is enabled.
/// </summary>
public int VisualLengthWithEndOfLineMarker
{
get
{
var length = VisualLength;
if (_textView.Options.ShowEndOfLine && LastDocumentLine.NextLine != null) length++;
return length;
}
}
/// <summary>
/// Gets the height of the visual line in device-independent pixels.
/// </summary>
public double Height { get; private set; }
/// <summary>
/// Gets the Y position of the line. This is measured in device-independent pixels relative to the start of the document.
/// </summary>
public double VisualTop { get; internal set; }
internal VisualLine(TextView textView, DocumentLine firstDocumentLine)
{
Debug.Assert(textView != null);
Debug.Assert(firstDocumentLine != null);
_textView = textView;
Document = textView.Document;
FirstDocumentLine = firstDocumentLine;
}
internal void ConstructVisualElements(ITextRunConstructionContext context, IReadOnlyList<VisualLineElementGenerator> generators)
{
Debug.Assert(_phase == LifetimePhase.Generating);
foreach (var g in generators)
{
g.StartGeneration(context);
}
_elements = new List<VisualLineElement>();
PerformVisualElementConstruction(generators);
foreach (var g in generators)
{
g.FinishGeneration();
}
var globalTextRunProperties = context.GlobalTextRunProperties;
foreach (var element in _elements)
{
element.SetTextRunProperties(new VisualLineElementTextRunProperties(globalTextRunProperties));
}
this.Elements = new ReadOnlyCollection<VisualLineElement>(_elements);
CalculateOffsets();
_phase = LifetimePhase.Transforming;
}
void PerformVisualElementConstruction(IReadOnlyList<VisualLineElementGenerator> generators)
{
var lineLength = FirstDocumentLine.Length;
var offset = FirstDocumentLine.Offset;
var currentLineEnd = offset + lineLength;
LastDocumentLine = FirstDocumentLine;
var askInterestOffset = 0; // 0 or 1
while (offset + askInterestOffset <= currentLineEnd)
{
var textPieceEndOffset = currentLineEnd;
foreach (var g in generators)
{
g.CachedInterest = g.GetFirstInterestedOffset(offset + askInterestOffset);
if (g.CachedInterest != -1)
{
if (g.CachedInterest < offset)
throw new ArgumentOutOfRangeException(g.GetType().Name + ".GetFirstInterestedOffset",
g.CachedInterest,
"GetFirstInterestedOffset must not return an offset less than startOffset. Return -1 to signal no interest.");
if (g.CachedInterest < textPieceEndOffset)
textPieceEndOffset = g.CachedInterest;
}
}
Debug.Assert(textPieceEndOffset >= offset);
if (textPieceEndOffset > offset)
{
var textPieceLength = textPieceEndOffset - offset;
_elements.Add(new VisualLineText(this, textPieceLength));
offset = textPieceEndOffset;
}
// If no elements constructed / only zero-length elements constructed:
// do not asking the generators again for the same location (would cause endless loop)
askInterestOffset = 1;
foreach (var g in generators)
{
if (g.CachedInterest == offset)
{
var element = g.ConstructElement(offset);
if (element != null)
{
_elements.Add(element);
if (element.DocumentLength > 0)
{
// a non-zero-length element was constructed
askInterestOffset = 0;
offset += element.DocumentLength;
if (offset > currentLineEnd)
{
var newEndLine = Document.GetLineByOffset(offset);
currentLineEnd = newEndLine.Offset + newEndLine.Length;
this.LastDocumentLine = newEndLine;
if (currentLineEnd < offset)
{
throw new InvalidOperationException(
"The VisualLineElementGenerator " + g.GetType().Name +
" produced an element which ends within the line delimiter");
}
}
break;
}
}
}
}
}
}
private void CalculateOffsets()
{
var visualOffset = 0;
var textOffset = 0;
foreach (var element in _elements)
{
element.VisualColumn = visualOffset;
element.RelativeTextOffset = textOffset;
visualOffset += element.VisualLength;
textOffset += element.DocumentLength;
}
VisualLength = visualOffset;
Debug.Assert(textOffset == LastDocumentLine.EndOffset - FirstDocumentLine.Offset);
}
internal void RunTransformers(ITextRunConstructionContext context, IReadOnlyList<IVisualLineTransformer> transformers)
{
Debug.Assert(_phase == LifetimePhase.Transforming);
foreach (var transformer in transformers)
{
transformer.Transform(context, _elements);
}
_phase = LifetimePhase.Live;
}
/// <summary>
/// Replaces the single element at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, params VisualLineElement[] newElements)
{
ReplaceElement(elementIndex, 1, newElements);
}
/// <summary>
/// Replaces <paramref name="count"/> elements starting at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, int count, params VisualLineElement[] newElements)
{
if (_phase != LifetimePhase.Transforming)
throw new InvalidOperationException("This method may only be called by line transformers.");
var oldDocumentLength = 0;
for (var i = elementIndex; i < elementIndex + count; i++)
{
oldDocumentLength += _elements[i].DocumentLength;
}
var newDocumentLength = 0;
foreach (var newElement in newElements)
{
newDocumentLength += newElement.DocumentLength;
}
if (oldDocumentLength != newDocumentLength)
throw new InvalidOperationException("Old elements have document length " + oldDocumentLength + ", but new elements have length " + newDocumentLength);
_elements.RemoveRange(elementIndex, count);
_elements.InsertRange(elementIndex, newElements);
CalculateOffsets();
}
internal void SetTextLines(List<TextLine> textLines)
{
_textLines = new ReadOnlyCollection<TextLine>(textLines);
Height = 0;
foreach (var line in textLines)
Height += line.Height;
}
/// <summary>
/// Gets the visual column from a document offset relative to the first line start.
/// </summary>
public int GetVisualColumn(int relativeTextOffset)
{
ThrowUtil.CheckNotNegative(relativeTextOffset, "relativeTextOffset");
foreach (var element in _elements)
{
if (element.RelativeTextOffset <= relativeTextOffset
&& element.RelativeTextOffset + element.DocumentLength >= relativeTextOffset)
{
return element.GetVisualColumn(relativeTextOffset);
}
}
return VisualLength;
}
/// <summary>
/// Gets the document offset (relative to the first line start) from a visual column.
/// </summary>
public int GetRelativeOffset(int visualColumn)
{
ThrowUtil.CheckNotNegative(visualColumn, "visualColumn");
var documentLength = 0;
foreach (var element in _elements)
{
if (element.VisualColumn <= visualColumn
&& element.VisualColumn + element.VisualLength > visualColumn)
{
return element.GetRelativeOffset(visualColumn);
}
documentLength += element.DocumentLength;
}
return documentLength;
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn)
{
return GetTextLine(visualColumn, false);
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn, bool isAtEndOfLine)
{
if (visualColumn < 0)
throw new ArgumentOutOfRangeException(nameof(visualColumn));
if (visualColumn >= VisualLengthWithEndOfLineMarker)
return TextLines[TextLines.Count - 1];
foreach (var line in TextLines)
{
if (isAtEndOfLine ? visualColumn <= line.Length : visualColumn < line.Length)
return line;
visualColumn -= line.Length;
}
throw new InvalidOperationException("Shouldn't happen (VisualLength incorrect?)");
}
/// <summary>
/// Gets the visual top from the specified text line.
/// </summary>
/// <returns>Distance in device-independent pixels
/// from the top of the document to the top of the specified text line.</returns>
public double GetTextLineVisualYPosition(TextLine textLine, VisualYPosition yPositionMode)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var pos = VisualTop;
foreach (var tl in TextLines)
{
if (tl == textLine)
{
switch (yPositionMode)
{
case VisualYPosition.LineTop:
return pos;
case VisualYPosition.LineMiddle:
return pos + tl.Height / 2;
case VisualYPosition.LineBottom:
return pos + tl.Height;
case VisualYPosition.TextTop:
return pos + tl.Baseline - _textView.DefaultBaseline;
case VisualYPosition.TextBottom:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight;
case VisualYPosition.TextMiddle:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight / 2;
case VisualYPosition.Baseline:
return pos + tl.Baseline;
default:
throw new ArgumentException("Invalid yPositionMode:" + yPositionMode);
}
}
pos += tl.Height;
}
throw new ArgumentException("textLine is not a line in this VisualLine");
}
/// <summary>
/// Gets the start visual column from the specified text line.
/// </summary>
public int GetTextLineVisualStartColumn(TextLine textLine)
{
if (!TextLines.Contains(textLine))
throw new ArgumentException("textLine is not a line in this VisualLine");
return TextLines.TakeWhile(tl => tl != textLine).Sum(tl => tl.Length);
}
/// <summary>
/// Gets a TextLine by the visual position.
/// </summary>
public TextLine GetTextLineByVisualYPosition(double visualTop)
{
const double epsilon = 0.0001;
var pos = VisualTop;
foreach (var tl in TextLines)
{
pos += tl.Height;
if (visualTop + epsilon < pos)
return tl;
}
return TextLines[TextLines.Count - 1];
}
/// <summary>
/// Gets the visual position from the specified visualColumn.
/// </summary>
/// <returns>Position in device-independent pixels
/// relative to the top left of the document.</returns>
public Point GetVisualPosition(int visualColumn, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
internal Point GetVisualPosition(int visualColumn, bool isAtEndOfLine, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn, isAtEndOfLine);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
/// <summary>
/// Gets the distance to the left border of the text area of the specified visual column.
/// The visual column must belong to the specified text line.
/// </summary>
public double GetTextLineVisualXPosition(TextLine textLine, int visualColumn)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var xPos = textLine.GetDistanceFromCharacterHit(new CharacterHit(Math.Min(visualColumn,
VisualLengthWithEndOfLineMarker)));
if (visualColumn > VisualLengthWithEndOfLineMarker)
{
xPos += (visualColumn - VisualLengthWithEndOfLineMarker) * _textView.WideSpaceWidth;
}
return xPos;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point)
{
return GetVisualColumn(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point, bool allowVirtualSpace)
{
return GetVisualColumn(GetTextLineByVisualYPosition(point.Y), point.X, allowVirtualSpace);
}
internal int GetVisualColumn(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
var vc = GetVisualColumn(textLine, point.X, allowVirtualSpace);
isAtEndOfLine = (vc >= GetTextLineVisualStartColumn(textLine) + textLine.Length);
return vc;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(TextLine textLine, double xPos, bool allowVirtualSpace)
{
if (xPos > textLine.WidthIncludingTrailingWhitespace)
{
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
var virtualX = (int)Math.Round((xPos - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth, MidpointRounding.AwayFromZero);
return VisualLengthWithEndOfLineMarker + virtualX;
}
}
var ch = textLine.GetCharacterHitFromDistance(xPos);
return ch.FirstCharacterIndex + ch.TrailingLength;
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(TextViewPosition position, bool allowVirtualSpace)
{
return ValidateVisualColumn(Document.GetOffset(position.Location), position.VisualColumn, allowVirtualSpace);
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(int offset, int visualColumn, bool allowVirtualSpace)
{
var firstDocumentLineOffset = FirstDocumentLine.Offset;
if (visualColumn < 0)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
var offsetFromVisualColumn = GetRelativeOffset(visualColumn);
offsetFromVisualColumn += firstDocumentLineOffset;
if (offsetFromVisualColumn != offset)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
if (visualColumn > VisualLength && !allowVirtualSpace)
{
return VisualLength;
}
return visualColumn;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point)
{
return GetVisualColumnFloor(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point, bool allowVirtualSpace)
{
return GetVisualColumnFloor(point, allowVirtualSpace, out _);
}
internal int GetVisualColumnFloor(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
if (point.X > textLine.WidthIncludingTrailingWhitespace)
{
isAtEndOfLine = true;
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
// clicking virtual space in the last line
var virtualX = (int)((point.X - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth);
return VisualLengthWithEndOfLineMarker + virtualX;
}
// GetCharacterHitFromDistance returns a hit with FirstCharacterIndex=last character in line
// and TrailingLength=1 when clicking behind the line, so the floor function needs to handle this case
// specially and return the line's end column instead.
return GetTextLineVisualStartColumn(textLine) + textLine.Length;
}
isAtEndOfLine = false;
var ch = textLine.GetCharacterHitFromDistance(point.X);
return ch.FirstCharacterIndex;
}
/// <summary>
/// Gets the text view position from the specified visual column.
/// </summary>
public TextViewPosition GetTextViewPosition(int visualColumn)
{
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
return new TextViewPosition(Document.GetLocation(documentOffset), visualColumn);
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is within a character, it is rounded to the next character boundary.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPosition(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumn(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is inside a character, the position in front of the character is returned.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPositionFloor(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumnFloor(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets whether the visual line was disposed.
/// </summary>
public bool IsDisposed => _phase == LifetimePhase.Disposed;
internal void Dispose()
{
if (_phase == LifetimePhase.Disposed)
{
return;
}
Debug.Assert(_phase == LifetimePhase.Live);
_phase = LifetimePhase.Disposed;
if (_visual != null)
{
((ISetLogicalParent)_visual).SetParent(null);
}
}
/// <summary>
/// Gets the next possible caret position after visualColumn, or -1 if there is no caret position.
/// </summary>
public int GetNextCaretPosition(int visualColumn, LogicalDirection direction, CaretPositioningMode mode, bool allowVirtualSpace)
{
if (!HasStopsInVirtualSpace(mode))
allowVirtualSpace = false;
if (_elements.Count == 0)
{
// special handling for empty visual lines:
if (allowVirtualSpace)
{
if (direction == LogicalDirection.Forward)
return Math.Max(0, visualColumn + 1);
if (visualColumn > 0)
return visualColumn - 1;
return -1;
}
// even though we don't have any elements,
// there's a single caret stop at visualColumn 0
if (visualColumn < 0 && direction == LogicalDirection.Forward)
return 0;
if (visualColumn > 0 && direction == LogicalDirection.Backward)
return 0;
return -1;
}
int i;
if (direction == LogicalDirection.Backward)
{
// Search Backwards:
// If the last element doesn't handle line borders, return the line end as caret stop
if (visualColumn > VisualLength && !_elements[_elements.Count - 1].HandlesLineBorders && HasImplicitStopAtLineEnd())
{
if (allowVirtualSpace)
return visualColumn - 1;
return VisualLength;
}
// skip elements that start after or at visualColumn
for (i = _elements.Count - 1; i >= 0; i--)
{
if (_elements[i].VisualColumn < visualColumn)
break;
}
// search last element that has a caret stop
for (; i >= 0; i--)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Min(visualColumn, _elements[i].VisualColumn + _elements[i].VisualLength + 1),
direction, mode);
if (pos >= 0)
return pos;
}
// If we've found nothing, and the first element doesn't handle line borders,
// return the line start as normal caret stop.
if (visualColumn > 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
}
else
{
// Search Forwards:
// If the first element doesn't handle line borders, return the line start as caret stop
if (visualColumn < 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
// skip elements that end before or at visualColumn
for (i = 0; i < _elements.Count; i++)
{
if (_elements[i].VisualColumn + _elements[i].VisualLength > visualColumn)
break;
}
// search first element that has a caret stop
for (; i < _elements.Count; i++)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Max(visualColumn, _elements[i].VisualColumn - 1),
direction, mode);
if (pos >= 0)
return pos;
}
// if we've found nothing, and the last element doesn't handle line borders,
// return the line end as caret stop
if ((allowVirtualSpace || !_elements[_elements.Count - 1].HandlesLineBorders) && HasImplicitStopAtLineEnd())
{
if (visualColumn < VisualLength)
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
return _visual ??= new VisualLineDrawingVisual(this);
}
}
{
return mode == CaretPositioningMode.Normal || mode == CaretPositioningMode.EveryCodepoint;
}
private static bool HasImplicitStopAtLineEnd() => true;
private VisualLineDrawingVisual _visual;
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
if (_visual == null)
{
_visual = new VisualLineDrawingVisual(this);
((ISetLogicalParent)_visual).SetParent(_textView);
}
return _visual;
}
}
// TODO: can inherit from Layoutable, but dev tools crash
internal sealed class VisualLineDrawingVisual : Control
{
public VisualLine VisualLine { get; }
public double LineHeight { get; }
internal bool IsAdded { get; set; }
public VisualLineDrawingVisual(VisualLine visualLine)
{
VisualLine = visualLine;
LineHeight = VisualLine.TextLines.Sum(textLine => textLine.Height);
}
public override void Render(DrawingContext context)
{
double pos = 0;
foreach (var textLine in VisualLine.TextLines)
{
textLine.Draw(context, new Point(0, pos));
pos += textLine.Height;
}
}
}
}
<MSG> Set VisualLineDrawingVisual.Parent on creation
<DFF> @@ -748,7 +748,15 @@ namespace AvaloniaEdit.Rendering
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
- return _visual ??= new VisualLineDrawingVisual(this);
+
+ if (_visual == null)
+ {
+ _visual = new VisualLineDrawingVisual(this);
+
+ ((ISetLogicalParent)_visual).SetParent(_textView);
+ }
+
+ return _visual;
}
}
| 9 | Set VisualLineDrawingVisual.Parent on creation | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063810 | <NME> VisualLine.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Linq;
using Avalonia;
using Avalonia.Controls;
using Avalonia.Input;
using Avalonia.Media;
using Avalonia.Media.TextFormatting;
using AvaloniaEdit.Document;
using AvaloniaEdit.Utils;
using LogicalDirection = AvaloniaEdit.Document.LogicalDirection;
namespace AvaloniaEdit.Rendering
{
/// <summary>
/// Represents a visual line in the document.
/// A visual line usually corresponds to one DocumentLine, but it can span multiple lines if
/// all but the first are collapsed.
/// </summary>
public sealed class VisualLine
{
public const int LENGTH_LIMIT = 3000;
private enum LifetimePhase : byte
{
Generating,
Transforming,
Live,
Disposed
}
private readonly TextView _textView;
private List<VisualLineElement> _elements;
internal bool HasInlineObjects;
private LifetimePhase _phase;
/// <summary>
/// Gets the document to which this VisualLine belongs.
/// </summary>
public TextDocument Document { get; }
/// <summary>
/// Gets the first document line displayed by this visual line.
/// </summary>
public DocumentLine FirstDocumentLine { get; }
/// <summary>
/// Gets the last document line displayed by this visual line.
/// </summary>
public DocumentLine LastDocumentLine { get; private set; }
/// <summary>
/// Gets a read-only collection of line elements.
/// </summary>
public ReadOnlyCollection<VisualLineElement> Elements { get; private set; }
private ReadOnlyCollection<TextLine> _textLines;
/// <summary>
/// Gets a read-only collection of text lines.
/// </summary>
public ReadOnlyCollection<TextLine> TextLines
{
get
{
if (_phase < LifetimePhase.Live)
throw new InvalidOperationException();
return _textLines;
}
}
/// <summary>
/// Gets the start offset of the VisualLine inside the document.
/// This is equivalent to <c>FirstDocumentLine.Offset</c>.
/// </summary>
public int StartOffset => FirstDocumentLine.Offset;
/// <summary>
/// Length in visual line coordinates.
/// </summary>
public int VisualLength { get; private set; }
/// <summary>
/// Length in visual line coordinates including the end of line marker, if TextEditorOptions.ShowEndOfLine is enabled.
/// </summary>
public int VisualLengthWithEndOfLineMarker
{
get
{
var length = VisualLength;
if (_textView.Options.ShowEndOfLine && LastDocumentLine.NextLine != null) length++;
return length;
}
}
/// <summary>
/// Gets the height of the visual line in device-independent pixels.
/// </summary>
public double Height { get; private set; }
/// <summary>
/// Gets the Y position of the line. This is measured in device-independent pixels relative to the start of the document.
/// </summary>
public double VisualTop { get; internal set; }
internal VisualLine(TextView textView, DocumentLine firstDocumentLine)
{
Debug.Assert(textView != null);
Debug.Assert(firstDocumentLine != null);
_textView = textView;
Document = textView.Document;
FirstDocumentLine = firstDocumentLine;
}
internal void ConstructVisualElements(ITextRunConstructionContext context, IReadOnlyList<VisualLineElementGenerator> generators)
{
Debug.Assert(_phase == LifetimePhase.Generating);
foreach (var g in generators)
{
g.StartGeneration(context);
}
_elements = new List<VisualLineElement>();
PerformVisualElementConstruction(generators);
foreach (var g in generators)
{
g.FinishGeneration();
}
var globalTextRunProperties = context.GlobalTextRunProperties;
foreach (var element in _elements)
{
element.SetTextRunProperties(new VisualLineElementTextRunProperties(globalTextRunProperties));
}
this.Elements = new ReadOnlyCollection<VisualLineElement>(_elements);
CalculateOffsets();
_phase = LifetimePhase.Transforming;
}
void PerformVisualElementConstruction(IReadOnlyList<VisualLineElementGenerator> generators)
{
var lineLength = FirstDocumentLine.Length;
var offset = FirstDocumentLine.Offset;
var currentLineEnd = offset + lineLength;
LastDocumentLine = FirstDocumentLine;
var askInterestOffset = 0; // 0 or 1
while (offset + askInterestOffset <= currentLineEnd)
{
var textPieceEndOffset = currentLineEnd;
foreach (var g in generators)
{
g.CachedInterest = g.GetFirstInterestedOffset(offset + askInterestOffset);
if (g.CachedInterest != -1)
{
if (g.CachedInterest < offset)
throw new ArgumentOutOfRangeException(g.GetType().Name + ".GetFirstInterestedOffset",
g.CachedInterest,
"GetFirstInterestedOffset must not return an offset less than startOffset. Return -1 to signal no interest.");
if (g.CachedInterest < textPieceEndOffset)
textPieceEndOffset = g.CachedInterest;
}
}
Debug.Assert(textPieceEndOffset >= offset);
if (textPieceEndOffset > offset)
{
var textPieceLength = textPieceEndOffset - offset;
_elements.Add(new VisualLineText(this, textPieceLength));
offset = textPieceEndOffset;
}
// If no elements constructed / only zero-length elements constructed:
// do not asking the generators again for the same location (would cause endless loop)
askInterestOffset = 1;
foreach (var g in generators)
{
if (g.CachedInterest == offset)
{
var element = g.ConstructElement(offset);
if (element != null)
{
_elements.Add(element);
if (element.DocumentLength > 0)
{
// a non-zero-length element was constructed
askInterestOffset = 0;
offset += element.DocumentLength;
if (offset > currentLineEnd)
{
var newEndLine = Document.GetLineByOffset(offset);
currentLineEnd = newEndLine.Offset + newEndLine.Length;
this.LastDocumentLine = newEndLine;
if (currentLineEnd < offset)
{
throw new InvalidOperationException(
"The VisualLineElementGenerator " + g.GetType().Name +
" produced an element which ends within the line delimiter");
}
}
break;
}
}
}
}
}
}
private void CalculateOffsets()
{
var visualOffset = 0;
var textOffset = 0;
foreach (var element in _elements)
{
element.VisualColumn = visualOffset;
element.RelativeTextOffset = textOffset;
visualOffset += element.VisualLength;
textOffset += element.DocumentLength;
}
VisualLength = visualOffset;
Debug.Assert(textOffset == LastDocumentLine.EndOffset - FirstDocumentLine.Offset);
}
internal void RunTransformers(ITextRunConstructionContext context, IReadOnlyList<IVisualLineTransformer> transformers)
{
Debug.Assert(_phase == LifetimePhase.Transforming);
foreach (var transformer in transformers)
{
transformer.Transform(context, _elements);
}
_phase = LifetimePhase.Live;
}
/// <summary>
/// Replaces the single element at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, params VisualLineElement[] newElements)
{
ReplaceElement(elementIndex, 1, newElements);
}
/// <summary>
/// Replaces <paramref name="count"/> elements starting at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, int count, params VisualLineElement[] newElements)
{
if (_phase != LifetimePhase.Transforming)
throw new InvalidOperationException("This method may only be called by line transformers.");
var oldDocumentLength = 0;
for (var i = elementIndex; i < elementIndex + count; i++)
{
oldDocumentLength += _elements[i].DocumentLength;
}
var newDocumentLength = 0;
foreach (var newElement in newElements)
{
newDocumentLength += newElement.DocumentLength;
}
if (oldDocumentLength != newDocumentLength)
throw new InvalidOperationException("Old elements have document length " + oldDocumentLength + ", but new elements have length " + newDocumentLength);
_elements.RemoveRange(elementIndex, count);
_elements.InsertRange(elementIndex, newElements);
CalculateOffsets();
}
internal void SetTextLines(List<TextLine> textLines)
{
_textLines = new ReadOnlyCollection<TextLine>(textLines);
Height = 0;
foreach (var line in textLines)
Height += line.Height;
}
/// <summary>
/// Gets the visual column from a document offset relative to the first line start.
/// </summary>
public int GetVisualColumn(int relativeTextOffset)
{
ThrowUtil.CheckNotNegative(relativeTextOffset, "relativeTextOffset");
foreach (var element in _elements)
{
if (element.RelativeTextOffset <= relativeTextOffset
&& element.RelativeTextOffset + element.DocumentLength >= relativeTextOffset)
{
return element.GetVisualColumn(relativeTextOffset);
}
}
return VisualLength;
}
/// <summary>
/// Gets the document offset (relative to the first line start) from a visual column.
/// </summary>
public int GetRelativeOffset(int visualColumn)
{
ThrowUtil.CheckNotNegative(visualColumn, "visualColumn");
var documentLength = 0;
foreach (var element in _elements)
{
if (element.VisualColumn <= visualColumn
&& element.VisualColumn + element.VisualLength > visualColumn)
{
return element.GetRelativeOffset(visualColumn);
}
documentLength += element.DocumentLength;
}
return documentLength;
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn)
{
return GetTextLine(visualColumn, false);
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn, bool isAtEndOfLine)
{
if (visualColumn < 0)
throw new ArgumentOutOfRangeException(nameof(visualColumn));
if (visualColumn >= VisualLengthWithEndOfLineMarker)
return TextLines[TextLines.Count - 1];
foreach (var line in TextLines)
{
if (isAtEndOfLine ? visualColumn <= line.Length : visualColumn < line.Length)
return line;
visualColumn -= line.Length;
}
throw new InvalidOperationException("Shouldn't happen (VisualLength incorrect?)");
}
/// <summary>
/// Gets the visual top from the specified text line.
/// </summary>
/// <returns>Distance in device-independent pixels
/// from the top of the document to the top of the specified text line.</returns>
public double GetTextLineVisualYPosition(TextLine textLine, VisualYPosition yPositionMode)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var pos = VisualTop;
foreach (var tl in TextLines)
{
if (tl == textLine)
{
switch (yPositionMode)
{
case VisualYPosition.LineTop:
return pos;
case VisualYPosition.LineMiddle:
return pos + tl.Height / 2;
case VisualYPosition.LineBottom:
return pos + tl.Height;
case VisualYPosition.TextTop:
return pos + tl.Baseline - _textView.DefaultBaseline;
case VisualYPosition.TextBottom:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight;
case VisualYPosition.TextMiddle:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight / 2;
case VisualYPosition.Baseline:
return pos + tl.Baseline;
default:
throw new ArgumentException("Invalid yPositionMode:" + yPositionMode);
}
}
pos += tl.Height;
}
throw new ArgumentException("textLine is not a line in this VisualLine");
}
/// <summary>
/// Gets the start visual column from the specified text line.
/// </summary>
public int GetTextLineVisualStartColumn(TextLine textLine)
{
if (!TextLines.Contains(textLine))
throw new ArgumentException("textLine is not a line in this VisualLine");
return TextLines.TakeWhile(tl => tl != textLine).Sum(tl => tl.Length);
}
/// <summary>
/// Gets a TextLine by the visual position.
/// </summary>
public TextLine GetTextLineByVisualYPosition(double visualTop)
{
const double epsilon = 0.0001;
var pos = VisualTop;
foreach (var tl in TextLines)
{
pos += tl.Height;
if (visualTop + epsilon < pos)
return tl;
}
return TextLines[TextLines.Count - 1];
}
/// <summary>
/// Gets the visual position from the specified visualColumn.
/// </summary>
/// <returns>Position in device-independent pixels
/// relative to the top left of the document.</returns>
public Point GetVisualPosition(int visualColumn, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
internal Point GetVisualPosition(int visualColumn, bool isAtEndOfLine, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn, isAtEndOfLine);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
/// <summary>
/// Gets the distance to the left border of the text area of the specified visual column.
/// The visual column must belong to the specified text line.
/// </summary>
public double GetTextLineVisualXPosition(TextLine textLine, int visualColumn)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var xPos = textLine.GetDistanceFromCharacterHit(new CharacterHit(Math.Min(visualColumn,
VisualLengthWithEndOfLineMarker)));
if (visualColumn > VisualLengthWithEndOfLineMarker)
{
xPos += (visualColumn - VisualLengthWithEndOfLineMarker) * _textView.WideSpaceWidth;
}
return xPos;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point)
{
return GetVisualColumn(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point, bool allowVirtualSpace)
{
return GetVisualColumn(GetTextLineByVisualYPosition(point.Y), point.X, allowVirtualSpace);
}
internal int GetVisualColumn(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
var vc = GetVisualColumn(textLine, point.X, allowVirtualSpace);
isAtEndOfLine = (vc >= GetTextLineVisualStartColumn(textLine) + textLine.Length);
return vc;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(TextLine textLine, double xPos, bool allowVirtualSpace)
{
if (xPos > textLine.WidthIncludingTrailingWhitespace)
{
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
var virtualX = (int)Math.Round((xPos - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth, MidpointRounding.AwayFromZero);
return VisualLengthWithEndOfLineMarker + virtualX;
}
}
var ch = textLine.GetCharacterHitFromDistance(xPos);
return ch.FirstCharacterIndex + ch.TrailingLength;
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(TextViewPosition position, bool allowVirtualSpace)
{
return ValidateVisualColumn(Document.GetOffset(position.Location), position.VisualColumn, allowVirtualSpace);
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(int offset, int visualColumn, bool allowVirtualSpace)
{
var firstDocumentLineOffset = FirstDocumentLine.Offset;
if (visualColumn < 0)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
var offsetFromVisualColumn = GetRelativeOffset(visualColumn);
offsetFromVisualColumn += firstDocumentLineOffset;
if (offsetFromVisualColumn != offset)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
if (visualColumn > VisualLength && !allowVirtualSpace)
{
return VisualLength;
}
return visualColumn;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point)
{
return GetVisualColumnFloor(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point, bool allowVirtualSpace)
{
return GetVisualColumnFloor(point, allowVirtualSpace, out _);
}
internal int GetVisualColumnFloor(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
if (point.X > textLine.WidthIncludingTrailingWhitespace)
{
isAtEndOfLine = true;
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
// clicking virtual space in the last line
var virtualX = (int)((point.X - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth);
return VisualLengthWithEndOfLineMarker + virtualX;
}
// GetCharacterHitFromDistance returns a hit with FirstCharacterIndex=last character in line
// and TrailingLength=1 when clicking behind the line, so the floor function needs to handle this case
// specially and return the line's end column instead.
return GetTextLineVisualStartColumn(textLine) + textLine.Length;
}
isAtEndOfLine = false;
var ch = textLine.GetCharacterHitFromDistance(point.X);
return ch.FirstCharacterIndex;
}
/// <summary>
/// Gets the text view position from the specified visual column.
/// </summary>
public TextViewPosition GetTextViewPosition(int visualColumn)
{
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
return new TextViewPosition(Document.GetLocation(documentOffset), visualColumn);
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is within a character, it is rounded to the next character boundary.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPosition(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumn(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is inside a character, the position in front of the character is returned.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPositionFloor(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumnFloor(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets whether the visual line was disposed.
/// </summary>
public bool IsDisposed => _phase == LifetimePhase.Disposed;
internal void Dispose()
{
if (_phase == LifetimePhase.Disposed)
{
return;
}
Debug.Assert(_phase == LifetimePhase.Live);
_phase = LifetimePhase.Disposed;
if (_visual != null)
{
((ISetLogicalParent)_visual).SetParent(null);
}
}
/// <summary>
/// Gets the next possible caret position after visualColumn, or -1 if there is no caret position.
/// </summary>
public int GetNextCaretPosition(int visualColumn, LogicalDirection direction, CaretPositioningMode mode, bool allowVirtualSpace)
{
if (!HasStopsInVirtualSpace(mode))
allowVirtualSpace = false;
if (_elements.Count == 0)
{
// special handling for empty visual lines:
if (allowVirtualSpace)
{
if (direction == LogicalDirection.Forward)
return Math.Max(0, visualColumn + 1);
if (visualColumn > 0)
return visualColumn - 1;
return -1;
}
// even though we don't have any elements,
// there's a single caret stop at visualColumn 0
if (visualColumn < 0 && direction == LogicalDirection.Forward)
return 0;
if (visualColumn > 0 && direction == LogicalDirection.Backward)
return 0;
return -1;
}
int i;
if (direction == LogicalDirection.Backward)
{
// Search Backwards:
// If the last element doesn't handle line borders, return the line end as caret stop
if (visualColumn > VisualLength && !_elements[_elements.Count - 1].HandlesLineBorders && HasImplicitStopAtLineEnd())
{
if (allowVirtualSpace)
return visualColumn - 1;
return VisualLength;
}
// skip elements that start after or at visualColumn
for (i = _elements.Count - 1; i >= 0; i--)
{
if (_elements[i].VisualColumn < visualColumn)
break;
}
// search last element that has a caret stop
for (; i >= 0; i--)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Min(visualColumn, _elements[i].VisualColumn + _elements[i].VisualLength + 1),
direction, mode);
if (pos >= 0)
return pos;
}
// If we've found nothing, and the first element doesn't handle line borders,
// return the line start as normal caret stop.
if (visualColumn > 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
}
else
{
// Search Forwards:
// If the first element doesn't handle line borders, return the line start as caret stop
if (visualColumn < 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
// skip elements that end before or at visualColumn
for (i = 0; i < _elements.Count; i++)
{
if (_elements[i].VisualColumn + _elements[i].VisualLength > visualColumn)
break;
}
// search first element that has a caret stop
for (; i < _elements.Count; i++)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Max(visualColumn, _elements[i].VisualColumn - 1),
direction, mode);
if (pos >= 0)
return pos;
}
// if we've found nothing, and the last element doesn't handle line borders,
// return the line end as caret stop
if ((allowVirtualSpace || !_elements[_elements.Count - 1].HandlesLineBorders) && HasImplicitStopAtLineEnd())
{
if (visualColumn < VisualLength)
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
return _visual ??= new VisualLineDrawingVisual(this);
}
}
{
return mode == CaretPositioningMode.Normal || mode == CaretPositioningMode.EveryCodepoint;
}
private static bool HasImplicitStopAtLineEnd() => true;
private VisualLineDrawingVisual _visual;
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
if (_visual == null)
{
_visual = new VisualLineDrawingVisual(this);
((ISetLogicalParent)_visual).SetParent(_textView);
}
return _visual;
}
}
// TODO: can inherit from Layoutable, but dev tools crash
internal sealed class VisualLineDrawingVisual : Control
{
public VisualLine VisualLine { get; }
public double LineHeight { get; }
internal bool IsAdded { get; set; }
public VisualLineDrawingVisual(VisualLine visualLine)
{
VisualLine = visualLine;
LineHeight = VisualLine.TextLines.Sum(textLine => textLine.Height);
}
public override void Render(DrawingContext context)
{
double pos = 0;
foreach (var textLine in VisualLine.TextLines)
{
textLine.Draw(context, new Point(0, pos));
pos += textLine.Height;
}
}
}
}
<MSG> Set VisualLineDrawingVisual.Parent on creation
<DFF> @@ -748,7 +748,15 @@ namespace AvaloniaEdit.Rendering
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
- return _visual ??= new VisualLineDrawingVisual(this);
+
+ if (_visual == null)
+ {
+ _visual = new VisualLineDrawingVisual(this);
+
+ ((ISetLogicalParent)_visual).SetParent(_textView);
+ }
+
+ return _visual;
}
}
| 9 | Set VisualLineDrawingVisual.Parent on creation | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063811 | <NME> VisualLine.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Linq;
using Avalonia;
using Avalonia.Controls;
using Avalonia.Input;
using Avalonia.Media;
using Avalonia.Media.TextFormatting;
using AvaloniaEdit.Document;
using AvaloniaEdit.Utils;
using LogicalDirection = AvaloniaEdit.Document.LogicalDirection;
namespace AvaloniaEdit.Rendering
{
/// <summary>
/// Represents a visual line in the document.
/// A visual line usually corresponds to one DocumentLine, but it can span multiple lines if
/// all but the first are collapsed.
/// </summary>
public sealed class VisualLine
{
public const int LENGTH_LIMIT = 3000;
private enum LifetimePhase : byte
{
Generating,
Transforming,
Live,
Disposed
}
private readonly TextView _textView;
private List<VisualLineElement> _elements;
internal bool HasInlineObjects;
private LifetimePhase _phase;
/// <summary>
/// Gets the document to which this VisualLine belongs.
/// </summary>
public TextDocument Document { get; }
/// <summary>
/// Gets the first document line displayed by this visual line.
/// </summary>
public DocumentLine FirstDocumentLine { get; }
/// <summary>
/// Gets the last document line displayed by this visual line.
/// </summary>
public DocumentLine LastDocumentLine { get; private set; }
/// <summary>
/// Gets a read-only collection of line elements.
/// </summary>
public ReadOnlyCollection<VisualLineElement> Elements { get; private set; }
private ReadOnlyCollection<TextLine> _textLines;
/// <summary>
/// Gets a read-only collection of text lines.
/// </summary>
public ReadOnlyCollection<TextLine> TextLines
{
get
{
if (_phase < LifetimePhase.Live)
throw new InvalidOperationException();
return _textLines;
}
}
/// <summary>
/// Gets the start offset of the VisualLine inside the document.
/// This is equivalent to <c>FirstDocumentLine.Offset</c>.
/// </summary>
public int StartOffset => FirstDocumentLine.Offset;
/// <summary>
/// Length in visual line coordinates.
/// </summary>
public int VisualLength { get; private set; }
/// <summary>
/// Length in visual line coordinates including the end of line marker, if TextEditorOptions.ShowEndOfLine is enabled.
/// </summary>
public int VisualLengthWithEndOfLineMarker
{
get
{
var length = VisualLength;
if (_textView.Options.ShowEndOfLine && LastDocumentLine.NextLine != null) length++;
return length;
}
}
/// <summary>
/// Gets the height of the visual line in device-independent pixels.
/// </summary>
public double Height { get; private set; }
/// <summary>
/// Gets the Y position of the line. This is measured in device-independent pixels relative to the start of the document.
/// </summary>
public double VisualTop { get; internal set; }
internal VisualLine(TextView textView, DocumentLine firstDocumentLine)
{
Debug.Assert(textView != null);
Debug.Assert(firstDocumentLine != null);
_textView = textView;
Document = textView.Document;
FirstDocumentLine = firstDocumentLine;
}
internal void ConstructVisualElements(ITextRunConstructionContext context, IReadOnlyList<VisualLineElementGenerator> generators)
{
Debug.Assert(_phase == LifetimePhase.Generating);
foreach (var g in generators)
{
g.StartGeneration(context);
}
_elements = new List<VisualLineElement>();
PerformVisualElementConstruction(generators);
foreach (var g in generators)
{
g.FinishGeneration();
}
var globalTextRunProperties = context.GlobalTextRunProperties;
foreach (var element in _elements)
{
element.SetTextRunProperties(new VisualLineElementTextRunProperties(globalTextRunProperties));
}
this.Elements = new ReadOnlyCollection<VisualLineElement>(_elements);
CalculateOffsets();
_phase = LifetimePhase.Transforming;
}
void PerformVisualElementConstruction(IReadOnlyList<VisualLineElementGenerator> generators)
{
var lineLength = FirstDocumentLine.Length;
var offset = FirstDocumentLine.Offset;
var currentLineEnd = offset + lineLength;
LastDocumentLine = FirstDocumentLine;
var askInterestOffset = 0; // 0 or 1
while (offset + askInterestOffset <= currentLineEnd)
{
var textPieceEndOffset = currentLineEnd;
foreach (var g in generators)
{
g.CachedInterest = g.GetFirstInterestedOffset(offset + askInterestOffset);
if (g.CachedInterest != -1)
{
if (g.CachedInterest < offset)
throw new ArgumentOutOfRangeException(g.GetType().Name + ".GetFirstInterestedOffset",
g.CachedInterest,
"GetFirstInterestedOffset must not return an offset less than startOffset. Return -1 to signal no interest.");
if (g.CachedInterest < textPieceEndOffset)
textPieceEndOffset = g.CachedInterest;
}
}
Debug.Assert(textPieceEndOffset >= offset);
if (textPieceEndOffset > offset)
{
var textPieceLength = textPieceEndOffset - offset;
_elements.Add(new VisualLineText(this, textPieceLength));
offset = textPieceEndOffset;
}
// If no elements constructed / only zero-length elements constructed:
// do not asking the generators again for the same location (would cause endless loop)
askInterestOffset = 1;
foreach (var g in generators)
{
if (g.CachedInterest == offset)
{
var element = g.ConstructElement(offset);
if (element != null)
{
_elements.Add(element);
if (element.DocumentLength > 0)
{
// a non-zero-length element was constructed
askInterestOffset = 0;
offset += element.DocumentLength;
if (offset > currentLineEnd)
{
var newEndLine = Document.GetLineByOffset(offset);
currentLineEnd = newEndLine.Offset + newEndLine.Length;
this.LastDocumentLine = newEndLine;
if (currentLineEnd < offset)
{
throw new InvalidOperationException(
"The VisualLineElementGenerator " + g.GetType().Name +
" produced an element which ends within the line delimiter");
}
}
break;
}
}
}
}
}
}
private void CalculateOffsets()
{
var visualOffset = 0;
var textOffset = 0;
foreach (var element in _elements)
{
element.VisualColumn = visualOffset;
element.RelativeTextOffset = textOffset;
visualOffset += element.VisualLength;
textOffset += element.DocumentLength;
}
VisualLength = visualOffset;
Debug.Assert(textOffset == LastDocumentLine.EndOffset - FirstDocumentLine.Offset);
}
internal void RunTransformers(ITextRunConstructionContext context, IReadOnlyList<IVisualLineTransformer> transformers)
{
Debug.Assert(_phase == LifetimePhase.Transforming);
foreach (var transformer in transformers)
{
transformer.Transform(context, _elements);
}
_phase = LifetimePhase.Live;
}
/// <summary>
/// Replaces the single element at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, params VisualLineElement[] newElements)
{
ReplaceElement(elementIndex, 1, newElements);
}
/// <summary>
/// Replaces <paramref name="count"/> elements starting at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, int count, params VisualLineElement[] newElements)
{
if (_phase != LifetimePhase.Transforming)
throw new InvalidOperationException("This method may only be called by line transformers.");
var oldDocumentLength = 0;
for (var i = elementIndex; i < elementIndex + count; i++)
{
oldDocumentLength += _elements[i].DocumentLength;
}
var newDocumentLength = 0;
foreach (var newElement in newElements)
{
newDocumentLength += newElement.DocumentLength;
}
if (oldDocumentLength != newDocumentLength)
throw new InvalidOperationException("Old elements have document length " + oldDocumentLength + ", but new elements have length " + newDocumentLength);
_elements.RemoveRange(elementIndex, count);
_elements.InsertRange(elementIndex, newElements);
CalculateOffsets();
}
internal void SetTextLines(List<TextLine> textLines)
{
_textLines = new ReadOnlyCollection<TextLine>(textLines);
Height = 0;
foreach (var line in textLines)
Height += line.Height;
}
/// <summary>
/// Gets the visual column from a document offset relative to the first line start.
/// </summary>
public int GetVisualColumn(int relativeTextOffset)
{
ThrowUtil.CheckNotNegative(relativeTextOffset, "relativeTextOffset");
foreach (var element in _elements)
{
if (element.RelativeTextOffset <= relativeTextOffset
&& element.RelativeTextOffset + element.DocumentLength >= relativeTextOffset)
{
return element.GetVisualColumn(relativeTextOffset);
}
}
return VisualLength;
}
/// <summary>
/// Gets the document offset (relative to the first line start) from a visual column.
/// </summary>
public int GetRelativeOffset(int visualColumn)
{
ThrowUtil.CheckNotNegative(visualColumn, "visualColumn");
var documentLength = 0;
foreach (var element in _elements)
{
if (element.VisualColumn <= visualColumn
&& element.VisualColumn + element.VisualLength > visualColumn)
{
return element.GetRelativeOffset(visualColumn);
}
documentLength += element.DocumentLength;
}
return documentLength;
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn)
{
return GetTextLine(visualColumn, false);
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn, bool isAtEndOfLine)
{
if (visualColumn < 0)
throw new ArgumentOutOfRangeException(nameof(visualColumn));
if (visualColumn >= VisualLengthWithEndOfLineMarker)
return TextLines[TextLines.Count - 1];
foreach (var line in TextLines)
{
if (isAtEndOfLine ? visualColumn <= line.Length : visualColumn < line.Length)
return line;
visualColumn -= line.Length;
}
throw new InvalidOperationException("Shouldn't happen (VisualLength incorrect?)");
}
/// <summary>
/// Gets the visual top from the specified text line.
/// </summary>
/// <returns>Distance in device-independent pixels
/// from the top of the document to the top of the specified text line.</returns>
public double GetTextLineVisualYPosition(TextLine textLine, VisualYPosition yPositionMode)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var pos = VisualTop;
foreach (var tl in TextLines)
{
if (tl == textLine)
{
switch (yPositionMode)
{
case VisualYPosition.LineTop:
return pos;
case VisualYPosition.LineMiddle:
return pos + tl.Height / 2;
case VisualYPosition.LineBottom:
return pos + tl.Height;
case VisualYPosition.TextTop:
return pos + tl.Baseline - _textView.DefaultBaseline;
case VisualYPosition.TextBottom:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight;
case VisualYPosition.TextMiddle:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight / 2;
case VisualYPosition.Baseline:
return pos + tl.Baseline;
default:
throw new ArgumentException("Invalid yPositionMode:" + yPositionMode);
}
}
pos += tl.Height;
}
throw new ArgumentException("textLine is not a line in this VisualLine");
}
/// <summary>
/// Gets the start visual column from the specified text line.
/// </summary>
public int GetTextLineVisualStartColumn(TextLine textLine)
{
if (!TextLines.Contains(textLine))
throw new ArgumentException("textLine is not a line in this VisualLine");
return TextLines.TakeWhile(tl => tl != textLine).Sum(tl => tl.Length);
}
/// <summary>
/// Gets a TextLine by the visual position.
/// </summary>
public TextLine GetTextLineByVisualYPosition(double visualTop)
{
const double epsilon = 0.0001;
var pos = VisualTop;
foreach (var tl in TextLines)
{
pos += tl.Height;
if (visualTop + epsilon < pos)
return tl;
}
return TextLines[TextLines.Count - 1];
}
/// <summary>
/// Gets the visual position from the specified visualColumn.
/// </summary>
/// <returns>Position in device-independent pixels
/// relative to the top left of the document.</returns>
public Point GetVisualPosition(int visualColumn, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
internal Point GetVisualPosition(int visualColumn, bool isAtEndOfLine, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn, isAtEndOfLine);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
/// <summary>
/// Gets the distance to the left border of the text area of the specified visual column.
/// The visual column must belong to the specified text line.
/// </summary>
public double GetTextLineVisualXPosition(TextLine textLine, int visualColumn)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var xPos = textLine.GetDistanceFromCharacterHit(new CharacterHit(Math.Min(visualColumn,
VisualLengthWithEndOfLineMarker)));
if (visualColumn > VisualLengthWithEndOfLineMarker)
{
xPos += (visualColumn - VisualLengthWithEndOfLineMarker) * _textView.WideSpaceWidth;
}
return xPos;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point)
{
return GetVisualColumn(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point, bool allowVirtualSpace)
{
return GetVisualColumn(GetTextLineByVisualYPosition(point.Y), point.X, allowVirtualSpace);
}
internal int GetVisualColumn(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
var vc = GetVisualColumn(textLine, point.X, allowVirtualSpace);
isAtEndOfLine = (vc >= GetTextLineVisualStartColumn(textLine) + textLine.Length);
return vc;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(TextLine textLine, double xPos, bool allowVirtualSpace)
{
if (xPos > textLine.WidthIncludingTrailingWhitespace)
{
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
var virtualX = (int)Math.Round((xPos - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth, MidpointRounding.AwayFromZero);
return VisualLengthWithEndOfLineMarker + virtualX;
}
}
var ch = textLine.GetCharacterHitFromDistance(xPos);
return ch.FirstCharacterIndex + ch.TrailingLength;
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(TextViewPosition position, bool allowVirtualSpace)
{
return ValidateVisualColumn(Document.GetOffset(position.Location), position.VisualColumn, allowVirtualSpace);
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(int offset, int visualColumn, bool allowVirtualSpace)
{
var firstDocumentLineOffset = FirstDocumentLine.Offset;
if (visualColumn < 0)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
var offsetFromVisualColumn = GetRelativeOffset(visualColumn);
offsetFromVisualColumn += firstDocumentLineOffset;
if (offsetFromVisualColumn != offset)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
if (visualColumn > VisualLength && !allowVirtualSpace)
{
return VisualLength;
}
return visualColumn;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point)
{
return GetVisualColumnFloor(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point, bool allowVirtualSpace)
{
return GetVisualColumnFloor(point, allowVirtualSpace, out _);
}
internal int GetVisualColumnFloor(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
if (point.X > textLine.WidthIncludingTrailingWhitespace)
{
isAtEndOfLine = true;
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
// clicking virtual space in the last line
var virtualX = (int)((point.X - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth);
return VisualLengthWithEndOfLineMarker + virtualX;
}
// GetCharacterHitFromDistance returns a hit with FirstCharacterIndex=last character in line
// and TrailingLength=1 when clicking behind the line, so the floor function needs to handle this case
// specially and return the line's end column instead.
return GetTextLineVisualStartColumn(textLine) + textLine.Length;
}
isAtEndOfLine = false;
var ch = textLine.GetCharacterHitFromDistance(point.X);
return ch.FirstCharacterIndex;
}
/// <summary>
/// Gets the text view position from the specified visual column.
/// </summary>
public TextViewPosition GetTextViewPosition(int visualColumn)
{
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
return new TextViewPosition(Document.GetLocation(documentOffset), visualColumn);
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is within a character, it is rounded to the next character boundary.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPosition(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumn(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is inside a character, the position in front of the character is returned.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPositionFloor(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumnFloor(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets whether the visual line was disposed.
/// </summary>
public bool IsDisposed => _phase == LifetimePhase.Disposed;
internal void Dispose()
{
if (_phase == LifetimePhase.Disposed)
{
return;
}
Debug.Assert(_phase == LifetimePhase.Live);
_phase = LifetimePhase.Disposed;
if (_visual != null)
{
((ISetLogicalParent)_visual).SetParent(null);
}
}
/// <summary>
/// Gets the next possible caret position after visualColumn, or -1 if there is no caret position.
/// </summary>
public int GetNextCaretPosition(int visualColumn, LogicalDirection direction, CaretPositioningMode mode, bool allowVirtualSpace)
{
if (!HasStopsInVirtualSpace(mode))
allowVirtualSpace = false;
if (_elements.Count == 0)
{
// special handling for empty visual lines:
if (allowVirtualSpace)
{
if (direction == LogicalDirection.Forward)
return Math.Max(0, visualColumn + 1);
if (visualColumn > 0)
return visualColumn - 1;
return -1;
}
// even though we don't have any elements,
// there's a single caret stop at visualColumn 0
if (visualColumn < 0 && direction == LogicalDirection.Forward)
return 0;
if (visualColumn > 0 && direction == LogicalDirection.Backward)
return 0;
return -1;
}
int i;
if (direction == LogicalDirection.Backward)
{
// Search Backwards:
// If the last element doesn't handle line borders, return the line end as caret stop
if (visualColumn > VisualLength && !_elements[_elements.Count - 1].HandlesLineBorders && HasImplicitStopAtLineEnd())
{
if (allowVirtualSpace)
return visualColumn - 1;
return VisualLength;
}
// skip elements that start after or at visualColumn
for (i = _elements.Count - 1; i >= 0; i--)
{
if (_elements[i].VisualColumn < visualColumn)
break;
}
// search last element that has a caret stop
for (; i >= 0; i--)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Min(visualColumn, _elements[i].VisualColumn + _elements[i].VisualLength + 1),
direction, mode);
if (pos >= 0)
return pos;
}
// If we've found nothing, and the first element doesn't handle line borders,
// return the line start as normal caret stop.
if (visualColumn > 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
}
else
{
// Search Forwards:
// If the first element doesn't handle line borders, return the line start as caret stop
if (visualColumn < 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
// skip elements that end before or at visualColumn
for (i = 0; i < _elements.Count; i++)
{
if (_elements[i].VisualColumn + _elements[i].VisualLength > visualColumn)
break;
}
// search first element that has a caret stop
for (; i < _elements.Count; i++)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Max(visualColumn, _elements[i].VisualColumn - 1),
direction, mode);
if (pos >= 0)
return pos;
}
// if we've found nothing, and the last element doesn't handle line borders,
// return the line end as caret stop
if ((allowVirtualSpace || !_elements[_elements.Count - 1].HandlesLineBorders) && HasImplicitStopAtLineEnd())
{
if (visualColumn < VisualLength)
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
return _visual ??= new VisualLineDrawingVisual(this);
}
}
{
return mode == CaretPositioningMode.Normal || mode == CaretPositioningMode.EveryCodepoint;
}
private static bool HasImplicitStopAtLineEnd() => true;
private VisualLineDrawingVisual _visual;
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
if (_visual == null)
{
_visual = new VisualLineDrawingVisual(this);
((ISetLogicalParent)_visual).SetParent(_textView);
}
return _visual;
}
}
// TODO: can inherit from Layoutable, but dev tools crash
internal sealed class VisualLineDrawingVisual : Control
{
public VisualLine VisualLine { get; }
public double LineHeight { get; }
internal bool IsAdded { get; set; }
public VisualLineDrawingVisual(VisualLine visualLine)
{
VisualLine = visualLine;
LineHeight = VisualLine.TextLines.Sum(textLine => textLine.Height);
}
public override void Render(DrawingContext context)
{
double pos = 0;
foreach (var textLine in VisualLine.TextLines)
{
textLine.Draw(context, new Point(0, pos));
pos += textLine.Height;
}
}
}
}
<MSG> Set VisualLineDrawingVisual.Parent on creation
<DFF> @@ -748,7 +748,15 @@ namespace AvaloniaEdit.Rendering
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
- return _visual ??= new VisualLineDrawingVisual(this);
+
+ if (_visual == null)
+ {
+ _visual = new VisualLineDrawingVisual(this);
+
+ ((ISetLogicalParent)_visual).SetParent(_textView);
+ }
+
+ return _visual;
}
}
| 9 | Set VisualLineDrawingVisual.Parent on creation | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063812 | <NME> VisualLine.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Linq;
using Avalonia;
using Avalonia.Controls;
using Avalonia.Input;
using Avalonia.Media;
using Avalonia.Media.TextFormatting;
using AvaloniaEdit.Document;
using AvaloniaEdit.Utils;
using LogicalDirection = AvaloniaEdit.Document.LogicalDirection;
namespace AvaloniaEdit.Rendering
{
/// <summary>
/// Represents a visual line in the document.
/// A visual line usually corresponds to one DocumentLine, but it can span multiple lines if
/// all but the first are collapsed.
/// </summary>
public sealed class VisualLine
{
public const int LENGTH_LIMIT = 3000;
private enum LifetimePhase : byte
{
Generating,
Transforming,
Live,
Disposed
}
private readonly TextView _textView;
private List<VisualLineElement> _elements;
internal bool HasInlineObjects;
private LifetimePhase _phase;
/// <summary>
/// Gets the document to which this VisualLine belongs.
/// </summary>
public TextDocument Document { get; }
/// <summary>
/// Gets the first document line displayed by this visual line.
/// </summary>
public DocumentLine FirstDocumentLine { get; }
/// <summary>
/// Gets the last document line displayed by this visual line.
/// </summary>
public DocumentLine LastDocumentLine { get; private set; }
/// <summary>
/// Gets a read-only collection of line elements.
/// </summary>
public ReadOnlyCollection<VisualLineElement> Elements { get; private set; }
private ReadOnlyCollection<TextLine> _textLines;
/// <summary>
/// Gets a read-only collection of text lines.
/// </summary>
public ReadOnlyCollection<TextLine> TextLines
{
get
{
if (_phase < LifetimePhase.Live)
throw new InvalidOperationException();
return _textLines;
}
}
/// <summary>
/// Gets the start offset of the VisualLine inside the document.
/// This is equivalent to <c>FirstDocumentLine.Offset</c>.
/// </summary>
public int StartOffset => FirstDocumentLine.Offset;
/// <summary>
/// Length in visual line coordinates.
/// </summary>
public int VisualLength { get; private set; }
/// <summary>
/// Length in visual line coordinates including the end of line marker, if TextEditorOptions.ShowEndOfLine is enabled.
/// </summary>
public int VisualLengthWithEndOfLineMarker
{
get
{
var length = VisualLength;
if (_textView.Options.ShowEndOfLine && LastDocumentLine.NextLine != null) length++;
return length;
}
}
/// <summary>
/// Gets the height of the visual line in device-independent pixels.
/// </summary>
public double Height { get; private set; }
/// <summary>
/// Gets the Y position of the line. This is measured in device-independent pixels relative to the start of the document.
/// </summary>
public double VisualTop { get; internal set; }
internal VisualLine(TextView textView, DocumentLine firstDocumentLine)
{
Debug.Assert(textView != null);
Debug.Assert(firstDocumentLine != null);
_textView = textView;
Document = textView.Document;
FirstDocumentLine = firstDocumentLine;
}
internal void ConstructVisualElements(ITextRunConstructionContext context, IReadOnlyList<VisualLineElementGenerator> generators)
{
Debug.Assert(_phase == LifetimePhase.Generating);
foreach (var g in generators)
{
g.StartGeneration(context);
}
_elements = new List<VisualLineElement>();
PerformVisualElementConstruction(generators);
foreach (var g in generators)
{
g.FinishGeneration();
}
var globalTextRunProperties = context.GlobalTextRunProperties;
foreach (var element in _elements)
{
element.SetTextRunProperties(new VisualLineElementTextRunProperties(globalTextRunProperties));
}
this.Elements = new ReadOnlyCollection<VisualLineElement>(_elements);
CalculateOffsets();
_phase = LifetimePhase.Transforming;
}
void PerformVisualElementConstruction(IReadOnlyList<VisualLineElementGenerator> generators)
{
var lineLength = FirstDocumentLine.Length;
var offset = FirstDocumentLine.Offset;
var currentLineEnd = offset + lineLength;
LastDocumentLine = FirstDocumentLine;
var askInterestOffset = 0; // 0 or 1
while (offset + askInterestOffset <= currentLineEnd)
{
var textPieceEndOffset = currentLineEnd;
foreach (var g in generators)
{
g.CachedInterest = g.GetFirstInterestedOffset(offset + askInterestOffset);
if (g.CachedInterest != -1)
{
if (g.CachedInterest < offset)
throw new ArgumentOutOfRangeException(g.GetType().Name + ".GetFirstInterestedOffset",
g.CachedInterest,
"GetFirstInterestedOffset must not return an offset less than startOffset. Return -1 to signal no interest.");
if (g.CachedInterest < textPieceEndOffset)
textPieceEndOffset = g.CachedInterest;
}
}
Debug.Assert(textPieceEndOffset >= offset);
if (textPieceEndOffset > offset)
{
var textPieceLength = textPieceEndOffset - offset;
_elements.Add(new VisualLineText(this, textPieceLength));
offset = textPieceEndOffset;
}
// If no elements constructed / only zero-length elements constructed:
// do not asking the generators again for the same location (would cause endless loop)
askInterestOffset = 1;
foreach (var g in generators)
{
if (g.CachedInterest == offset)
{
var element = g.ConstructElement(offset);
if (element != null)
{
_elements.Add(element);
if (element.DocumentLength > 0)
{
// a non-zero-length element was constructed
askInterestOffset = 0;
offset += element.DocumentLength;
if (offset > currentLineEnd)
{
var newEndLine = Document.GetLineByOffset(offset);
currentLineEnd = newEndLine.Offset + newEndLine.Length;
this.LastDocumentLine = newEndLine;
if (currentLineEnd < offset)
{
throw new InvalidOperationException(
"The VisualLineElementGenerator " + g.GetType().Name +
" produced an element which ends within the line delimiter");
}
}
break;
}
}
}
}
}
}
private void CalculateOffsets()
{
var visualOffset = 0;
var textOffset = 0;
foreach (var element in _elements)
{
element.VisualColumn = visualOffset;
element.RelativeTextOffset = textOffset;
visualOffset += element.VisualLength;
textOffset += element.DocumentLength;
}
VisualLength = visualOffset;
Debug.Assert(textOffset == LastDocumentLine.EndOffset - FirstDocumentLine.Offset);
}
internal void RunTransformers(ITextRunConstructionContext context, IReadOnlyList<IVisualLineTransformer> transformers)
{
Debug.Assert(_phase == LifetimePhase.Transforming);
foreach (var transformer in transformers)
{
transformer.Transform(context, _elements);
}
_phase = LifetimePhase.Live;
}
/// <summary>
/// Replaces the single element at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, params VisualLineElement[] newElements)
{
ReplaceElement(elementIndex, 1, newElements);
}
/// <summary>
/// Replaces <paramref name="count"/> elements starting at <paramref name="elementIndex"/> with the specified elements.
/// The replacement operation must preserve the document length, but may change the visual length.
/// </summary>
/// <remarks>
/// This method may only be called by line transformers.
/// </remarks>
public void ReplaceElement(int elementIndex, int count, params VisualLineElement[] newElements)
{
if (_phase != LifetimePhase.Transforming)
throw new InvalidOperationException("This method may only be called by line transformers.");
var oldDocumentLength = 0;
for (var i = elementIndex; i < elementIndex + count; i++)
{
oldDocumentLength += _elements[i].DocumentLength;
}
var newDocumentLength = 0;
foreach (var newElement in newElements)
{
newDocumentLength += newElement.DocumentLength;
}
if (oldDocumentLength != newDocumentLength)
throw new InvalidOperationException("Old elements have document length " + oldDocumentLength + ", but new elements have length " + newDocumentLength);
_elements.RemoveRange(elementIndex, count);
_elements.InsertRange(elementIndex, newElements);
CalculateOffsets();
}
internal void SetTextLines(List<TextLine> textLines)
{
_textLines = new ReadOnlyCollection<TextLine>(textLines);
Height = 0;
foreach (var line in textLines)
Height += line.Height;
}
/// <summary>
/// Gets the visual column from a document offset relative to the first line start.
/// </summary>
public int GetVisualColumn(int relativeTextOffset)
{
ThrowUtil.CheckNotNegative(relativeTextOffset, "relativeTextOffset");
foreach (var element in _elements)
{
if (element.RelativeTextOffset <= relativeTextOffset
&& element.RelativeTextOffset + element.DocumentLength >= relativeTextOffset)
{
return element.GetVisualColumn(relativeTextOffset);
}
}
return VisualLength;
}
/// <summary>
/// Gets the document offset (relative to the first line start) from a visual column.
/// </summary>
public int GetRelativeOffset(int visualColumn)
{
ThrowUtil.CheckNotNegative(visualColumn, "visualColumn");
var documentLength = 0;
foreach (var element in _elements)
{
if (element.VisualColumn <= visualColumn
&& element.VisualColumn + element.VisualLength > visualColumn)
{
return element.GetRelativeOffset(visualColumn);
}
documentLength += element.DocumentLength;
}
return documentLength;
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn)
{
return GetTextLine(visualColumn, false);
}
/// <summary>
/// Gets the text line containing the specified visual column.
/// </summary>
public TextLine GetTextLine(int visualColumn, bool isAtEndOfLine)
{
if (visualColumn < 0)
throw new ArgumentOutOfRangeException(nameof(visualColumn));
if (visualColumn >= VisualLengthWithEndOfLineMarker)
return TextLines[TextLines.Count - 1];
foreach (var line in TextLines)
{
if (isAtEndOfLine ? visualColumn <= line.Length : visualColumn < line.Length)
return line;
visualColumn -= line.Length;
}
throw new InvalidOperationException("Shouldn't happen (VisualLength incorrect?)");
}
/// <summary>
/// Gets the visual top from the specified text line.
/// </summary>
/// <returns>Distance in device-independent pixels
/// from the top of the document to the top of the specified text line.</returns>
public double GetTextLineVisualYPosition(TextLine textLine, VisualYPosition yPositionMode)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var pos = VisualTop;
foreach (var tl in TextLines)
{
if (tl == textLine)
{
switch (yPositionMode)
{
case VisualYPosition.LineTop:
return pos;
case VisualYPosition.LineMiddle:
return pos + tl.Height / 2;
case VisualYPosition.LineBottom:
return pos + tl.Height;
case VisualYPosition.TextTop:
return pos + tl.Baseline - _textView.DefaultBaseline;
case VisualYPosition.TextBottom:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight;
case VisualYPosition.TextMiddle:
return pos + tl.Baseline - _textView.DefaultBaseline + _textView.DefaultLineHeight / 2;
case VisualYPosition.Baseline:
return pos + tl.Baseline;
default:
throw new ArgumentException("Invalid yPositionMode:" + yPositionMode);
}
}
pos += tl.Height;
}
throw new ArgumentException("textLine is not a line in this VisualLine");
}
/// <summary>
/// Gets the start visual column from the specified text line.
/// </summary>
public int GetTextLineVisualStartColumn(TextLine textLine)
{
if (!TextLines.Contains(textLine))
throw new ArgumentException("textLine is not a line in this VisualLine");
return TextLines.TakeWhile(tl => tl != textLine).Sum(tl => tl.Length);
}
/// <summary>
/// Gets a TextLine by the visual position.
/// </summary>
public TextLine GetTextLineByVisualYPosition(double visualTop)
{
const double epsilon = 0.0001;
var pos = VisualTop;
foreach (var tl in TextLines)
{
pos += tl.Height;
if (visualTop + epsilon < pos)
return tl;
}
return TextLines[TextLines.Count - 1];
}
/// <summary>
/// Gets the visual position from the specified visualColumn.
/// </summary>
/// <returns>Position in device-independent pixels
/// relative to the top left of the document.</returns>
public Point GetVisualPosition(int visualColumn, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
internal Point GetVisualPosition(int visualColumn, bool isAtEndOfLine, VisualYPosition yPositionMode)
{
var textLine = GetTextLine(visualColumn, isAtEndOfLine);
var xPos = GetTextLineVisualXPosition(textLine, visualColumn);
var yPos = GetTextLineVisualYPosition(textLine, yPositionMode);
return new Point(xPos, yPos);
}
/// <summary>
/// Gets the distance to the left border of the text area of the specified visual column.
/// The visual column must belong to the specified text line.
/// </summary>
public double GetTextLineVisualXPosition(TextLine textLine, int visualColumn)
{
if (textLine == null)
throw new ArgumentNullException(nameof(textLine));
var xPos = textLine.GetDistanceFromCharacterHit(new CharacterHit(Math.Min(visualColumn,
VisualLengthWithEndOfLineMarker)));
if (visualColumn > VisualLengthWithEndOfLineMarker)
{
xPos += (visualColumn - VisualLengthWithEndOfLineMarker) * _textView.WideSpaceWidth;
}
return xPos;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point)
{
return GetVisualColumn(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(Point point, bool allowVirtualSpace)
{
return GetVisualColumn(GetTextLineByVisualYPosition(point.Y), point.X, allowVirtualSpace);
}
internal int GetVisualColumn(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
var vc = GetVisualColumn(textLine, point.X, allowVirtualSpace);
isAtEndOfLine = (vc >= GetTextLineVisualStartColumn(textLine) + textLine.Length);
return vc;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, rounds to the nearest column.
/// </summary>
public int GetVisualColumn(TextLine textLine, double xPos, bool allowVirtualSpace)
{
if (xPos > textLine.WidthIncludingTrailingWhitespace)
{
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
var virtualX = (int)Math.Round((xPos - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth, MidpointRounding.AwayFromZero);
return VisualLengthWithEndOfLineMarker + virtualX;
}
}
var ch = textLine.GetCharacterHitFromDistance(xPos);
return ch.FirstCharacterIndex + ch.TrailingLength;
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(TextViewPosition position, bool allowVirtualSpace)
{
return ValidateVisualColumn(Document.GetOffset(position.Location), position.VisualColumn, allowVirtualSpace);
}
/// <summary>
/// Validates the visual column and returns the correct one.
/// </summary>
public int ValidateVisualColumn(int offset, int visualColumn, bool allowVirtualSpace)
{
var firstDocumentLineOffset = FirstDocumentLine.Offset;
if (visualColumn < 0)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
var offsetFromVisualColumn = GetRelativeOffset(visualColumn);
offsetFromVisualColumn += firstDocumentLineOffset;
if (offsetFromVisualColumn != offset)
{
return GetVisualColumn(offset - firstDocumentLineOffset);
}
if (visualColumn > VisualLength && !allowVirtualSpace)
{
return VisualLength;
}
return visualColumn;
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point)
{
return GetVisualColumnFloor(point, _textView.Options.EnableVirtualSpace);
}
/// <summary>
/// Gets the visual column from a document position (relative to top left of the document).
/// If the user clicks between two visual columns, returns the first of those columns.
/// </summary>
public int GetVisualColumnFloor(Point point, bool allowVirtualSpace)
{
return GetVisualColumnFloor(point, allowVirtualSpace, out _);
}
internal int GetVisualColumnFloor(Point point, bool allowVirtualSpace, out bool isAtEndOfLine)
{
var textLine = GetTextLineByVisualYPosition(point.Y);
if (point.X > textLine.WidthIncludingTrailingWhitespace)
{
isAtEndOfLine = true;
if (allowVirtualSpace && textLine == TextLines[TextLines.Count - 1])
{
// clicking virtual space in the last line
var virtualX = (int)((point.X - textLine.WidthIncludingTrailingWhitespace) / _textView.WideSpaceWidth);
return VisualLengthWithEndOfLineMarker + virtualX;
}
// GetCharacterHitFromDistance returns a hit with FirstCharacterIndex=last character in line
// and TrailingLength=1 when clicking behind the line, so the floor function needs to handle this case
// specially and return the line's end column instead.
return GetTextLineVisualStartColumn(textLine) + textLine.Length;
}
isAtEndOfLine = false;
var ch = textLine.GetCharacterHitFromDistance(point.X);
return ch.FirstCharacterIndex;
}
/// <summary>
/// Gets the text view position from the specified visual column.
/// </summary>
public TextViewPosition GetTextViewPosition(int visualColumn)
{
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
return new TextViewPosition(Document.GetLocation(documentOffset), visualColumn);
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is within a character, it is rounded to the next character boundary.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPosition(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumn(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets the text view position from the specified visual position.
/// If the position is inside a character, the position in front of the character is returned.
/// </summary>
/// <param name="visualPosition">The position in device-independent pixels relative
/// to the top left corner of the document.</param>
/// <param name="allowVirtualSpace">Controls whether positions in virtual space may be returned.</param>
public TextViewPosition GetTextViewPositionFloor(Point visualPosition, bool allowVirtualSpace)
{
var visualColumn = GetVisualColumnFloor(visualPosition, allowVirtualSpace, out var isAtEndOfLine);
var documentOffset = GetRelativeOffset(visualColumn) + FirstDocumentLine.Offset;
var pos = new TextViewPosition(Document.GetLocation(documentOffset), visualColumn)
{
IsAtEndOfLine = isAtEndOfLine
};
return pos;
}
/// <summary>
/// Gets whether the visual line was disposed.
/// </summary>
public bool IsDisposed => _phase == LifetimePhase.Disposed;
internal void Dispose()
{
if (_phase == LifetimePhase.Disposed)
{
return;
}
Debug.Assert(_phase == LifetimePhase.Live);
_phase = LifetimePhase.Disposed;
if (_visual != null)
{
((ISetLogicalParent)_visual).SetParent(null);
}
}
/// <summary>
/// Gets the next possible caret position after visualColumn, or -1 if there is no caret position.
/// </summary>
public int GetNextCaretPosition(int visualColumn, LogicalDirection direction, CaretPositioningMode mode, bool allowVirtualSpace)
{
if (!HasStopsInVirtualSpace(mode))
allowVirtualSpace = false;
if (_elements.Count == 0)
{
// special handling for empty visual lines:
if (allowVirtualSpace)
{
if (direction == LogicalDirection.Forward)
return Math.Max(0, visualColumn + 1);
if (visualColumn > 0)
return visualColumn - 1;
return -1;
}
// even though we don't have any elements,
// there's a single caret stop at visualColumn 0
if (visualColumn < 0 && direction == LogicalDirection.Forward)
return 0;
if (visualColumn > 0 && direction == LogicalDirection.Backward)
return 0;
return -1;
}
int i;
if (direction == LogicalDirection.Backward)
{
// Search Backwards:
// If the last element doesn't handle line borders, return the line end as caret stop
if (visualColumn > VisualLength && !_elements[_elements.Count - 1].HandlesLineBorders && HasImplicitStopAtLineEnd())
{
if (allowVirtualSpace)
return visualColumn - 1;
return VisualLength;
}
// skip elements that start after or at visualColumn
for (i = _elements.Count - 1; i >= 0; i--)
{
if (_elements[i].VisualColumn < visualColumn)
break;
}
// search last element that has a caret stop
for (; i >= 0; i--)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Min(visualColumn, _elements[i].VisualColumn + _elements[i].VisualLength + 1),
direction, mode);
if (pos >= 0)
return pos;
}
// If we've found nothing, and the first element doesn't handle line borders,
// return the line start as normal caret stop.
if (visualColumn > 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
}
else
{
// Search Forwards:
// If the first element doesn't handle line borders, return the line start as caret stop
if (visualColumn < 0 && !_elements[0].HandlesLineBorders && HasImplicitStopAtLineStart(mode))
return 0;
// skip elements that end before or at visualColumn
for (i = 0; i < _elements.Count; i++)
{
if (_elements[i].VisualColumn + _elements[i].VisualLength > visualColumn)
break;
}
// search first element that has a caret stop
for (; i < _elements.Count; i++)
{
var pos = _elements[i].GetNextCaretPosition(
Math.Max(visualColumn, _elements[i].VisualColumn - 1),
direction, mode);
if (pos >= 0)
return pos;
}
// if we've found nothing, and the last element doesn't handle line borders,
// return the line end as caret stop
if ((allowVirtualSpace || !_elements[_elements.Count - 1].HandlesLineBorders) && HasImplicitStopAtLineEnd())
{
if (visualColumn < VisualLength)
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
return _visual ??= new VisualLineDrawingVisual(this);
}
}
{
return mode == CaretPositioningMode.Normal || mode == CaretPositioningMode.EveryCodepoint;
}
private static bool HasImplicitStopAtLineEnd() => true;
private VisualLineDrawingVisual _visual;
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
if (_visual == null)
{
_visual = new VisualLineDrawingVisual(this);
((ISetLogicalParent)_visual).SetParent(_textView);
}
return _visual;
}
}
// TODO: can inherit from Layoutable, but dev tools crash
internal sealed class VisualLineDrawingVisual : Control
{
public VisualLine VisualLine { get; }
public double LineHeight { get; }
internal bool IsAdded { get; set; }
public VisualLineDrawingVisual(VisualLine visualLine)
{
VisualLine = visualLine;
LineHeight = VisualLine.TextLines.Sum(textLine => textLine.Height);
}
public override void Render(DrawingContext context)
{
double pos = 0;
foreach (var textLine in VisualLine.TextLines)
{
textLine.Draw(context, new Point(0, pos));
pos += textLine.Height;
}
}
}
}
<MSG> Set VisualLineDrawingVisual.Parent on creation
<DFF> @@ -748,7 +748,15 @@ namespace AvaloniaEdit.Rendering
internal VisualLineDrawingVisual Render()
{
Debug.Assert(_phase == LifetimePhase.Live);
- return _visual ??= new VisualLineDrawingVisual(this);
+
+ if (_visual == null)
+ {
+ _visual = new VisualLineDrawingVisual(this);
+
+ ((ISetLogicalParent)_visual).SetParent(_textView);
+ }
+
+ return _visual;
}
}
| 9 | Set VisualLineDrawingVisual.Parent on creation | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063813 | <NME> fruitmachine.js
<BEF> /*jslint browser:true, node:true*/
/**
* FruitMachine
*
* Renders layouts/modules from a basic layout definition.
* If views require custom interactions devs can extend
* the basic functionality.
*
* @version 0.3.3
* @copyright The Financial Times Limited [All Rights Reserved]
* @author Wilson Page <[email protected]>
*/
'use strict';
/**
* Module Dependencies
*/
var mod = require('./module');
var define = require('./define');
var utils = require('utils');
var events = require('evt');
/**
* Creates a fruitmachine
*
* Options:
*
* - `Model` A model constructor to use (must have `.toJSON()`)
*
* @param {Object} options
*/
module.exports = function(options) {
/**
* Shortcut method for
* creating lazy views.
*
* @param {Object} options
* @return {Module}
*/
function fm(options) {
var Module = fm.modules[options.module];
if (Module) {
return new Module(options);
}
fm.create = module.exports;
fm.Model = options.Model;
fm.Module = mod(fm);
fm.define = define(fm);
fm.util = utils;
fm.define = define(fm);
fm.util = utils;
fm.modules = {};
fm.config = {
templateIterator: 'children',
templateInstance: 'child'
};
// Mixin events and return
return events(fm);
};
<MSG> Expose Events library
<DFF> @@ -49,6 +49,7 @@ module.exports = function(options) {
fm.create = module.exports;
fm.Model = options.Model;
+ fm.Events = events;
fm.Module = mod(fm);
fm.define = define(fm);
fm.util = utils;
| 1 | Expose Events library | 0 | .js | js | mit | ftlabs/fruitmachine |
10063814 | <NME> TextMate.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
using TextMateSharp.Themes;
{
public static class TextMate
{
public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
{
lock(_lock)
{
_installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
}
}
public static void DisposeTextMate(this TextEditor editor)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].Dispose();
_installations.Remove(editor);
}
}
public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetGrammar(grammar);
}
}
public static void InstallTheme(this TextEditor editor, Theme theme)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetTheme(theme);
}
}
static object _lock = new object();
static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
class TextMateInstallation
{
internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
return new Installation(editor, registryOptions, initCurrentDocument);
}
public class Installation
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
internal void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
public IRegistryOptions RegistryOptions { get { return _textMateRegistryOptions; } }
_editor.TextArea.TextView.Redraw();
}
internal void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
_editor.TextArea.TextView.Redraw();
}
internal void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
}
{
DisposeTMModel(_tmModel);
var editorModel = new TextEditorModel(_editor, _editor.Document);
_tmModel = new TMModel(editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
}
public void SetTheme(IRawTheme theme)
{
_textMateRegistry.SetTheme(theme);
GetOrCreateTransformer().SetTheme(_textMateRegistry.GetTheme());
_tmModel?.InvalidateLine(0);
_editorModel?.InvalidateViewPortLines();
}
public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
DisposeEditorModel(_editorModel);
DisposeTMModel(_tmModel, _transformer);
DisposeTransformer(_transformer);
}
}
TextEditor _editor;
IGrammar _grammar;
TMModel _tmModel;
}
_editorModel = new TextEditorModel(_editor.TextArea.TextView, _editor.Document, _exceptionHandler);
_tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
_transformer = GetOrCreateTransformer();
_transformer.SetModel(_editor.Document, _tmModel);
_tmModel.AddModelTokensChangedListener(_transformer);
}
catch (Exception ex)
{
_exceptionHandler?.Invoke(ex);
}
}
TextMateColoringTransformer GetOrCreateTransformer()
{
var transformer = _editor.TextArea.TextView.LineTransformers.OfType<TextMateColoringTransformer>().FirstOrDefault();
if (transformer is null)
{
transformer = new TextMateColoringTransformer(
_editor.TextArea.TextView, _exceptionHandler);
_editor.TextArea.TextView.LineTransformers.Add(transformer);
}
return transformer;
}
static void DisposeTransformer(TextMateColoringTransformer transformer)
{
if (transformer == null)
return;
transformer.Dispose();
}
static void DisposeTMModel(TMModel tmModel, TextMateColoringTransformer transformer)
{
if (tmModel == null)
return;
if (transformer != null)
tmModel.RemoveModelTokensChangedListener(transformer);
tmModel.Dispose();
}
static void DisposeEditorModel(TextEditorModel editorModel)
{
if (editorModel == null)
return;
editorModel.Dispose();
}
}
static Action<Exception> _exceptionHandler;
}
}
<MSG> Improve the TextMate installation API
<DFF> @@ -1,6 +1,6 @@
using System;
-using System.Collections.Generic;
using System.Linq;
+
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
@@ -9,54 +9,14 @@ namespace AvaloniaEdit.TextMate
{
public static class TextMate
{
- public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
- {
- lock(_lock)
- {
- _installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
- }
- }
-
- public static void DisposeTextMate(this TextEditor editor)
- {
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].Dispose();
- _installations.Remove(editor);
- }
- }
-
- public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
+ public static Installation InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar = null)
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetGrammar(grammar);
- }
+ return new Installation(editor, theme, grammar);
}
- public static void InstallTheme(this TextEditor editor, Theme theme)
+ public class Installation
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetTheme(theme);
- }
- }
-
- static object _lock = new object();
- static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
-
- class TextMateInstallation
- {
- internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
+ public Installation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
@@ -68,7 +28,7 @@ namespace AvaloniaEdit.TextMate
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
- internal void SetGrammar(IGrammar grammar)
+ public void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
@@ -77,14 +37,14 @@ namespace AvaloniaEdit.TextMate
_editor.TextArea.TextView.Redraw();
}
- internal void SetTheme(Theme theme)
+ public void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
- _editor.TextArea.TextView.Redraw();
+ _editorModel?.TokenizeViewPort();
}
- internal void Dispose()
+ public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
@@ -95,8 +55,8 @@ namespace AvaloniaEdit.TextMate
{
DisposeTMModel(_tmModel);
- var editorModel = new TextEditorModel(_editor, _editor.Document);
- _tmModel = new TMModel(editorModel);
+ _editorModel = new TextEditorModel(_editor, _editor.Document);
+ _tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
@@ -125,6 +85,7 @@ namespace AvaloniaEdit.TextMate
}
TextEditor _editor;
+ TextEditorModel _editorModel;
IGrammar _grammar;
TMModel _tmModel;
}
| 12 | Improve the TextMate installation API | 51 | .cs | TextMate/TextMate | mit | AvaloniaUI/AvaloniaEdit |
10063815 | <NME> TextMate.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
using TextMateSharp.Themes;
{
public static class TextMate
{
public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
{
lock(_lock)
{
_installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
}
}
public static void DisposeTextMate(this TextEditor editor)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].Dispose();
_installations.Remove(editor);
}
}
public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetGrammar(grammar);
}
}
public static void InstallTheme(this TextEditor editor, Theme theme)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetTheme(theme);
}
}
static object _lock = new object();
static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
class TextMateInstallation
{
internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
return new Installation(editor, registryOptions, initCurrentDocument);
}
public class Installation
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
internal void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
public IRegistryOptions RegistryOptions { get { return _textMateRegistryOptions; } }
_editor.TextArea.TextView.Redraw();
}
internal void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
_editor.TextArea.TextView.Redraw();
}
internal void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
}
{
DisposeTMModel(_tmModel);
var editorModel = new TextEditorModel(_editor, _editor.Document);
_tmModel = new TMModel(editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
}
public void SetTheme(IRawTheme theme)
{
_textMateRegistry.SetTheme(theme);
GetOrCreateTransformer().SetTheme(_textMateRegistry.GetTheme());
_tmModel?.InvalidateLine(0);
_editorModel?.InvalidateViewPortLines();
}
public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
DisposeEditorModel(_editorModel);
DisposeTMModel(_tmModel, _transformer);
DisposeTransformer(_transformer);
}
}
TextEditor _editor;
IGrammar _grammar;
TMModel _tmModel;
}
_editorModel = new TextEditorModel(_editor.TextArea.TextView, _editor.Document, _exceptionHandler);
_tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
_transformer = GetOrCreateTransformer();
_transformer.SetModel(_editor.Document, _tmModel);
_tmModel.AddModelTokensChangedListener(_transformer);
}
catch (Exception ex)
{
_exceptionHandler?.Invoke(ex);
}
}
TextMateColoringTransformer GetOrCreateTransformer()
{
var transformer = _editor.TextArea.TextView.LineTransformers.OfType<TextMateColoringTransformer>().FirstOrDefault();
if (transformer is null)
{
transformer = new TextMateColoringTransformer(
_editor.TextArea.TextView, _exceptionHandler);
_editor.TextArea.TextView.LineTransformers.Add(transformer);
}
return transformer;
}
static void DisposeTransformer(TextMateColoringTransformer transformer)
{
if (transformer == null)
return;
transformer.Dispose();
}
static void DisposeTMModel(TMModel tmModel, TextMateColoringTransformer transformer)
{
if (tmModel == null)
return;
if (transformer != null)
tmModel.RemoveModelTokensChangedListener(transformer);
tmModel.Dispose();
}
static void DisposeEditorModel(TextEditorModel editorModel)
{
if (editorModel == null)
return;
editorModel.Dispose();
}
}
static Action<Exception> _exceptionHandler;
}
}
<MSG> Improve the TextMate installation API
<DFF> @@ -1,6 +1,6 @@
using System;
-using System.Collections.Generic;
using System.Linq;
+
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
@@ -9,54 +9,14 @@ namespace AvaloniaEdit.TextMate
{
public static class TextMate
{
- public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
- {
- lock(_lock)
- {
- _installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
- }
- }
-
- public static void DisposeTextMate(this TextEditor editor)
- {
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].Dispose();
- _installations.Remove(editor);
- }
- }
-
- public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
+ public static Installation InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar = null)
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetGrammar(grammar);
- }
+ return new Installation(editor, theme, grammar);
}
- public static void InstallTheme(this TextEditor editor, Theme theme)
+ public class Installation
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetTheme(theme);
- }
- }
-
- static object _lock = new object();
- static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
-
- class TextMateInstallation
- {
- internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
+ public Installation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
@@ -68,7 +28,7 @@ namespace AvaloniaEdit.TextMate
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
- internal void SetGrammar(IGrammar grammar)
+ public void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
@@ -77,14 +37,14 @@ namespace AvaloniaEdit.TextMate
_editor.TextArea.TextView.Redraw();
}
- internal void SetTheme(Theme theme)
+ public void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
- _editor.TextArea.TextView.Redraw();
+ _editorModel?.TokenizeViewPort();
}
- internal void Dispose()
+ public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
@@ -95,8 +55,8 @@ namespace AvaloniaEdit.TextMate
{
DisposeTMModel(_tmModel);
- var editorModel = new TextEditorModel(_editor, _editor.Document);
- _tmModel = new TMModel(editorModel);
+ _editorModel = new TextEditorModel(_editor, _editor.Document);
+ _tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
@@ -125,6 +85,7 @@ namespace AvaloniaEdit.TextMate
}
TextEditor _editor;
+ TextEditorModel _editorModel;
IGrammar _grammar;
TMModel _tmModel;
}
| 12 | Improve the TextMate installation API | 51 | .cs | TextMate/TextMate | mit | AvaloniaUI/AvaloniaEdit |
10063816 | <NME> TextMate.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
using TextMateSharp.Themes;
{
public static class TextMate
{
public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
{
lock(_lock)
{
_installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
}
}
public static void DisposeTextMate(this TextEditor editor)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].Dispose();
_installations.Remove(editor);
}
}
public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetGrammar(grammar);
}
}
public static void InstallTheme(this TextEditor editor, Theme theme)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetTheme(theme);
}
}
static object _lock = new object();
static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
class TextMateInstallation
{
internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
return new Installation(editor, registryOptions, initCurrentDocument);
}
public class Installation
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
internal void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
public IRegistryOptions RegistryOptions { get { return _textMateRegistryOptions; } }
_editor.TextArea.TextView.Redraw();
}
internal void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
_editor.TextArea.TextView.Redraw();
}
internal void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
}
{
DisposeTMModel(_tmModel);
var editorModel = new TextEditorModel(_editor, _editor.Document);
_tmModel = new TMModel(editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
}
public void SetTheme(IRawTheme theme)
{
_textMateRegistry.SetTheme(theme);
GetOrCreateTransformer().SetTheme(_textMateRegistry.GetTheme());
_tmModel?.InvalidateLine(0);
_editorModel?.InvalidateViewPortLines();
}
public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
DisposeEditorModel(_editorModel);
DisposeTMModel(_tmModel, _transformer);
DisposeTransformer(_transformer);
}
}
TextEditor _editor;
IGrammar _grammar;
TMModel _tmModel;
}
_editorModel = new TextEditorModel(_editor.TextArea.TextView, _editor.Document, _exceptionHandler);
_tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
_transformer = GetOrCreateTransformer();
_transformer.SetModel(_editor.Document, _tmModel);
_tmModel.AddModelTokensChangedListener(_transformer);
}
catch (Exception ex)
{
_exceptionHandler?.Invoke(ex);
}
}
TextMateColoringTransformer GetOrCreateTransformer()
{
var transformer = _editor.TextArea.TextView.LineTransformers.OfType<TextMateColoringTransformer>().FirstOrDefault();
if (transformer is null)
{
transformer = new TextMateColoringTransformer(
_editor.TextArea.TextView, _exceptionHandler);
_editor.TextArea.TextView.LineTransformers.Add(transformer);
}
return transformer;
}
static void DisposeTransformer(TextMateColoringTransformer transformer)
{
if (transformer == null)
return;
transformer.Dispose();
}
static void DisposeTMModel(TMModel tmModel, TextMateColoringTransformer transformer)
{
if (tmModel == null)
return;
if (transformer != null)
tmModel.RemoveModelTokensChangedListener(transformer);
tmModel.Dispose();
}
static void DisposeEditorModel(TextEditorModel editorModel)
{
if (editorModel == null)
return;
editorModel.Dispose();
}
}
static Action<Exception> _exceptionHandler;
}
}
<MSG> Improve the TextMate installation API
<DFF> @@ -1,6 +1,6 @@
using System;
-using System.Collections.Generic;
using System.Linq;
+
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
@@ -9,54 +9,14 @@ namespace AvaloniaEdit.TextMate
{
public static class TextMate
{
- public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
- {
- lock(_lock)
- {
- _installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
- }
- }
-
- public static void DisposeTextMate(this TextEditor editor)
- {
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].Dispose();
- _installations.Remove(editor);
- }
- }
-
- public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
+ public static Installation InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar = null)
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetGrammar(grammar);
- }
+ return new Installation(editor, theme, grammar);
}
- public static void InstallTheme(this TextEditor editor, Theme theme)
+ public class Installation
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetTheme(theme);
- }
- }
-
- static object _lock = new object();
- static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
-
- class TextMateInstallation
- {
- internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
+ public Installation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
@@ -68,7 +28,7 @@ namespace AvaloniaEdit.TextMate
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
- internal void SetGrammar(IGrammar grammar)
+ public void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
@@ -77,14 +37,14 @@ namespace AvaloniaEdit.TextMate
_editor.TextArea.TextView.Redraw();
}
- internal void SetTheme(Theme theme)
+ public void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
- _editor.TextArea.TextView.Redraw();
+ _editorModel?.TokenizeViewPort();
}
- internal void Dispose()
+ public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
@@ -95,8 +55,8 @@ namespace AvaloniaEdit.TextMate
{
DisposeTMModel(_tmModel);
- var editorModel = new TextEditorModel(_editor, _editor.Document);
- _tmModel = new TMModel(editorModel);
+ _editorModel = new TextEditorModel(_editor, _editor.Document);
+ _tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
@@ -125,6 +85,7 @@ namespace AvaloniaEdit.TextMate
}
TextEditor _editor;
+ TextEditorModel _editorModel;
IGrammar _grammar;
TMModel _tmModel;
}
| 12 | Improve the TextMate installation API | 51 | .cs | TextMate/TextMate | mit | AvaloniaUI/AvaloniaEdit |
10063817 | <NME> TextMate.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
using TextMateSharp.Themes;
{
public static class TextMate
{
public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
{
lock(_lock)
{
_installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
}
}
public static void DisposeTextMate(this TextEditor editor)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].Dispose();
_installations.Remove(editor);
}
}
public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetGrammar(grammar);
}
}
public static void InstallTheme(this TextEditor editor, Theme theme)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetTheme(theme);
}
}
static object _lock = new object();
static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
class TextMateInstallation
{
internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
return new Installation(editor, registryOptions, initCurrentDocument);
}
public class Installation
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
internal void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
public IRegistryOptions RegistryOptions { get { return _textMateRegistryOptions; } }
_editor.TextArea.TextView.Redraw();
}
internal void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
_editor.TextArea.TextView.Redraw();
}
internal void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
}
{
DisposeTMModel(_tmModel);
var editorModel = new TextEditorModel(_editor, _editor.Document);
_tmModel = new TMModel(editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
}
public void SetTheme(IRawTheme theme)
{
_textMateRegistry.SetTheme(theme);
GetOrCreateTransformer().SetTheme(_textMateRegistry.GetTheme());
_tmModel?.InvalidateLine(0);
_editorModel?.InvalidateViewPortLines();
}
public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
DisposeEditorModel(_editorModel);
DisposeTMModel(_tmModel, _transformer);
DisposeTransformer(_transformer);
}
}
TextEditor _editor;
IGrammar _grammar;
TMModel _tmModel;
}
_editorModel = new TextEditorModel(_editor.TextArea.TextView, _editor.Document, _exceptionHandler);
_tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
_transformer = GetOrCreateTransformer();
_transformer.SetModel(_editor.Document, _tmModel);
_tmModel.AddModelTokensChangedListener(_transformer);
}
catch (Exception ex)
{
_exceptionHandler?.Invoke(ex);
}
}
TextMateColoringTransformer GetOrCreateTransformer()
{
var transformer = _editor.TextArea.TextView.LineTransformers.OfType<TextMateColoringTransformer>().FirstOrDefault();
if (transformer is null)
{
transformer = new TextMateColoringTransformer(
_editor.TextArea.TextView, _exceptionHandler);
_editor.TextArea.TextView.LineTransformers.Add(transformer);
}
return transformer;
}
static void DisposeTransformer(TextMateColoringTransformer transformer)
{
if (transformer == null)
return;
transformer.Dispose();
}
static void DisposeTMModel(TMModel tmModel, TextMateColoringTransformer transformer)
{
if (tmModel == null)
return;
if (transformer != null)
tmModel.RemoveModelTokensChangedListener(transformer);
tmModel.Dispose();
}
static void DisposeEditorModel(TextEditorModel editorModel)
{
if (editorModel == null)
return;
editorModel.Dispose();
}
}
static Action<Exception> _exceptionHandler;
}
}
<MSG> Improve the TextMate installation API
<DFF> @@ -1,6 +1,6 @@
using System;
-using System.Collections.Generic;
using System.Linq;
+
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
@@ -9,54 +9,14 @@ namespace AvaloniaEdit.TextMate
{
public static class TextMate
{
- public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
- {
- lock(_lock)
- {
- _installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
- }
- }
-
- public static void DisposeTextMate(this TextEditor editor)
- {
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].Dispose();
- _installations.Remove(editor);
- }
- }
-
- public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
+ public static Installation InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar = null)
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetGrammar(grammar);
- }
+ return new Installation(editor, theme, grammar);
}
- public static void InstallTheme(this TextEditor editor, Theme theme)
+ public class Installation
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetTheme(theme);
- }
- }
-
- static object _lock = new object();
- static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
-
- class TextMateInstallation
- {
- internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
+ public Installation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
@@ -68,7 +28,7 @@ namespace AvaloniaEdit.TextMate
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
- internal void SetGrammar(IGrammar grammar)
+ public void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
@@ -77,14 +37,14 @@ namespace AvaloniaEdit.TextMate
_editor.TextArea.TextView.Redraw();
}
- internal void SetTheme(Theme theme)
+ public void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
- _editor.TextArea.TextView.Redraw();
+ _editorModel?.TokenizeViewPort();
}
- internal void Dispose()
+ public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
@@ -95,8 +55,8 @@ namespace AvaloniaEdit.TextMate
{
DisposeTMModel(_tmModel);
- var editorModel = new TextEditorModel(_editor, _editor.Document);
- _tmModel = new TMModel(editorModel);
+ _editorModel = new TextEditorModel(_editor, _editor.Document);
+ _tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
@@ -125,6 +85,7 @@ namespace AvaloniaEdit.TextMate
}
TextEditor _editor;
+ TextEditorModel _editorModel;
IGrammar _grammar;
TMModel _tmModel;
}
| 12 | Improve the TextMate installation API | 51 | .cs | TextMate/TextMate | mit | AvaloniaUI/AvaloniaEdit |
10063818 | <NME> TextMate.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
using TextMateSharp.Themes;
{
public static class TextMate
{
public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
{
lock(_lock)
{
_installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
}
}
public static void DisposeTextMate(this TextEditor editor)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].Dispose();
_installations.Remove(editor);
}
}
public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetGrammar(grammar);
}
}
public static void InstallTheme(this TextEditor editor, Theme theme)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetTheme(theme);
}
}
static object _lock = new object();
static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
class TextMateInstallation
{
internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
return new Installation(editor, registryOptions, initCurrentDocument);
}
public class Installation
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
internal void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
public IRegistryOptions RegistryOptions { get { return _textMateRegistryOptions; } }
_editor.TextArea.TextView.Redraw();
}
internal void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
_editor.TextArea.TextView.Redraw();
}
internal void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
}
{
DisposeTMModel(_tmModel);
var editorModel = new TextEditorModel(_editor, _editor.Document);
_tmModel = new TMModel(editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
}
public void SetTheme(IRawTheme theme)
{
_textMateRegistry.SetTheme(theme);
GetOrCreateTransformer().SetTheme(_textMateRegistry.GetTheme());
_tmModel?.InvalidateLine(0);
_editorModel?.InvalidateViewPortLines();
}
public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
DisposeEditorModel(_editorModel);
DisposeTMModel(_tmModel, _transformer);
DisposeTransformer(_transformer);
}
}
TextEditor _editor;
IGrammar _grammar;
TMModel _tmModel;
}
_editorModel = new TextEditorModel(_editor.TextArea.TextView, _editor.Document, _exceptionHandler);
_tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
_transformer = GetOrCreateTransformer();
_transformer.SetModel(_editor.Document, _tmModel);
_tmModel.AddModelTokensChangedListener(_transformer);
}
catch (Exception ex)
{
_exceptionHandler?.Invoke(ex);
}
}
TextMateColoringTransformer GetOrCreateTransformer()
{
var transformer = _editor.TextArea.TextView.LineTransformers.OfType<TextMateColoringTransformer>().FirstOrDefault();
if (transformer is null)
{
transformer = new TextMateColoringTransformer(
_editor.TextArea.TextView, _exceptionHandler);
_editor.TextArea.TextView.LineTransformers.Add(transformer);
}
return transformer;
}
static void DisposeTransformer(TextMateColoringTransformer transformer)
{
if (transformer == null)
return;
transformer.Dispose();
}
static void DisposeTMModel(TMModel tmModel, TextMateColoringTransformer transformer)
{
if (tmModel == null)
return;
if (transformer != null)
tmModel.RemoveModelTokensChangedListener(transformer);
tmModel.Dispose();
}
static void DisposeEditorModel(TextEditorModel editorModel)
{
if (editorModel == null)
return;
editorModel.Dispose();
}
}
static Action<Exception> _exceptionHandler;
}
}
<MSG> Improve the TextMate installation API
<DFF> @@ -1,6 +1,6 @@
using System;
-using System.Collections.Generic;
using System.Linq;
+
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
@@ -9,54 +9,14 @@ namespace AvaloniaEdit.TextMate
{
public static class TextMate
{
- public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
- {
- lock(_lock)
- {
- _installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
- }
- }
-
- public static void DisposeTextMate(this TextEditor editor)
- {
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].Dispose();
- _installations.Remove(editor);
- }
- }
-
- public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
+ public static Installation InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar = null)
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetGrammar(grammar);
- }
+ return new Installation(editor, theme, grammar);
}
- public static void InstallTheme(this TextEditor editor, Theme theme)
+ public class Installation
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetTheme(theme);
- }
- }
-
- static object _lock = new object();
- static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
-
- class TextMateInstallation
- {
- internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
+ public Installation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
@@ -68,7 +28,7 @@ namespace AvaloniaEdit.TextMate
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
- internal void SetGrammar(IGrammar grammar)
+ public void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
@@ -77,14 +37,14 @@ namespace AvaloniaEdit.TextMate
_editor.TextArea.TextView.Redraw();
}
- internal void SetTheme(Theme theme)
+ public void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
- _editor.TextArea.TextView.Redraw();
+ _editorModel?.TokenizeViewPort();
}
- internal void Dispose()
+ public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
@@ -95,8 +55,8 @@ namespace AvaloniaEdit.TextMate
{
DisposeTMModel(_tmModel);
- var editorModel = new TextEditorModel(_editor, _editor.Document);
- _tmModel = new TMModel(editorModel);
+ _editorModel = new TextEditorModel(_editor, _editor.Document);
+ _tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
@@ -125,6 +85,7 @@ namespace AvaloniaEdit.TextMate
}
TextEditor _editor;
+ TextEditorModel _editorModel;
IGrammar _grammar;
TMModel _tmModel;
}
| 12 | Improve the TextMate installation API | 51 | .cs | TextMate/TextMate | mit | AvaloniaUI/AvaloniaEdit |
10063819 | <NME> TextMate.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
using TextMateSharp.Themes;
{
public static class TextMate
{
public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
{
lock(_lock)
{
_installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
}
}
public static void DisposeTextMate(this TextEditor editor)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].Dispose();
_installations.Remove(editor);
}
}
public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetGrammar(grammar);
}
}
public static void InstallTheme(this TextEditor editor, Theme theme)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetTheme(theme);
}
}
static object _lock = new object();
static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
class TextMateInstallation
{
internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
return new Installation(editor, registryOptions, initCurrentDocument);
}
public class Installation
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
internal void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
public IRegistryOptions RegistryOptions { get { return _textMateRegistryOptions; } }
_editor.TextArea.TextView.Redraw();
}
internal void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
_editor.TextArea.TextView.Redraw();
}
internal void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
}
{
DisposeTMModel(_tmModel);
var editorModel = new TextEditorModel(_editor, _editor.Document);
_tmModel = new TMModel(editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
}
public void SetTheme(IRawTheme theme)
{
_textMateRegistry.SetTheme(theme);
GetOrCreateTransformer().SetTheme(_textMateRegistry.GetTheme());
_tmModel?.InvalidateLine(0);
_editorModel?.InvalidateViewPortLines();
}
public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
DisposeEditorModel(_editorModel);
DisposeTMModel(_tmModel, _transformer);
DisposeTransformer(_transformer);
}
}
TextEditor _editor;
IGrammar _grammar;
TMModel _tmModel;
}
_editorModel = new TextEditorModel(_editor.TextArea.TextView, _editor.Document, _exceptionHandler);
_tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
_transformer = GetOrCreateTransformer();
_transformer.SetModel(_editor.Document, _tmModel);
_tmModel.AddModelTokensChangedListener(_transformer);
}
catch (Exception ex)
{
_exceptionHandler?.Invoke(ex);
}
}
TextMateColoringTransformer GetOrCreateTransformer()
{
var transformer = _editor.TextArea.TextView.LineTransformers.OfType<TextMateColoringTransformer>().FirstOrDefault();
if (transformer is null)
{
transformer = new TextMateColoringTransformer(
_editor.TextArea.TextView, _exceptionHandler);
_editor.TextArea.TextView.LineTransformers.Add(transformer);
}
return transformer;
}
static void DisposeTransformer(TextMateColoringTransformer transformer)
{
if (transformer == null)
return;
transformer.Dispose();
}
static void DisposeTMModel(TMModel tmModel, TextMateColoringTransformer transformer)
{
if (tmModel == null)
return;
if (transformer != null)
tmModel.RemoveModelTokensChangedListener(transformer);
tmModel.Dispose();
}
static void DisposeEditorModel(TextEditorModel editorModel)
{
if (editorModel == null)
return;
editorModel.Dispose();
}
}
static Action<Exception> _exceptionHandler;
}
}
<MSG> Improve the TextMate installation API
<DFF> @@ -1,6 +1,6 @@
using System;
-using System.Collections.Generic;
using System.Linq;
+
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
@@ -9,54 +9,14 @@ namespace AvaloniaEdit.TextMate
{
public static class TextMate
{
- public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
- {
- lock(_lock)
- {
- _installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
- }
- }
-
- public static void DisposeTextMate(this TextEditor editor)
- {
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].Dispose();
- _installations.Remove(editor);
- }
- }
-
- public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
+ public static Installation InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar = null)
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetGrammar(grammar);
- }
+ return new Installation(editor, theme, grammar);
}
- public static void InstallTheme(this TextEditor editor, Theme theme)
+ public class Installation
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetTheme(theme);
- }
- }
-
- static object _lock = new object();
- static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
-
- class TextMateInstallation
- {
- internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
+ public Installation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
@@ -68,7 +28,7 @@ namespace AvaloniaEdit.TextMate
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
- internal void SetGrammar(IGrammar grammar)
+ public void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
@@ -77,14 +37,14 @@ namespace AvaloniaEdit.TextMate
_editor.TextArea.TextView.Redraw();
}
- internal void SetTheme(Theme theme)
+ public void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
- _editor.TextArea.TextView.Redraw();
+ _editorModel?.TokenizeViewPort();
}
- internal void Dispose()
+ public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
@@ -95,8 +55,8 @@ namespace AvaloniaEdit.TextMate
{
DisposeTMModel(_tmModel);
- var editorModel = new TextEditorModel(_editor, _editor.Document);
- _tmModel = new TMModel(editorModel);
+ _editorModel = new TextEditorModel(_editor, _editor.Document);
+ _tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
@@ -125,6 +85,7 @@ namespace AvaloniaEdit.TextMate
}
TextEditor _editor;
+ TextEditorModel _editorModel;
IGrammar _grammar;
TMModel _tmModel;
}
| 12 | Improve the TextMate installation API | 51 | .cs | TextMate/TextMate | mit | AvaloniaUI/AvaloniaEdit |
10063820 | <NME> TextMate.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
using TextMateSharp.Themes;
{
public static class TextMate
{
public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
{
lock(_lock)
{
_installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
}
}
public static void DisposeTextMate(this TextEditor editor)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].Dispose();
_installations.Remove(editor);
}
}
public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetGrammar(grammar);
}
}
public static void InstallTheme(this TextEditor editor, Theme theme)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetTheme(theme);
}
}
static object _lock = new object();
static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
class TextMateInstallation
{
internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
return new Installation(editor, registryOptions, initCurrentDocument);
}
public class Installation
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
internal void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
public IRegistryOptions RegistryOptions { get { return _textMateRegistryOptions; } }
_editor.TextArea.TextView.Redraw();
}
internal void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
_editor.TextArea.TextView.Redraw();
}
internal void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
}
{
DisposeTMModel(_tmModel);
var editorModel = new TextEditorModel(_editor, _editor.Document);
_tmModel = new TMModel(editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
}
public void SetTheme(IRawTheme theme)
{
_textMateRegistry.SetTheme(theme);
GetOrCreateTransformer().SetTheme(_textMateRegistry.GetTheme());
_tmModel?.InvalidateLine(0);
_editorModel?.InvalidateViewPortLines();
}
public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
DisposeEditorModel(_editorModel);
DisposeTMModel(_tmModel, _transformer);
DisposeTransformer(_transformer);
}
}
TextEditor _editor;
IGrammar _grammar;
TMModel _tmModel;
}
_editorModel = new TextEditorModel(_editor.TextArea.TextView, _editor.Document, _exceptionHandler);
_tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
_transformer = GetOrCreateTransformer();
_transformer.SetModel(_editor.Document, _tmModel);
_tmModel.AddModelTokensChangedListener(_transformer);
}
catch (Exception ex)
{
_exceptionHandler?.Invoke(ex);
}
}
TextMateColoringTransformer GetOrCreateTransformer()
{
var transformer = _editor.TextArea.TextView.LineTransformers.OfType<TextMateColoringTransformer>().FirstOrDefault();
if (transformer is null)
{
transformer = new TextMateColoringTransformer(
_editor.TextArea.TextView, _exceptionHandler);
_editor.TextArea.TextView.LineTransformers.Add(transformer);
}
return transformer;
}
static void DisposeTransformer(TextMateColoringTransformer transformer)
{
if (transformer == null)
return;
transformer.Dispose();
}
static void DisposeTMModel(TMModel tmModel, TextMateColoringTransformer transformer)
{
if (tmModel == null)
return;
if (transformer != null)
tmModel.RemoveModelTokensChangedListener(transformer);
tmModel.Dispose();
}
static void DisposeEditorModel(TextEditorModel editorModel)
{
if (editorModel == null)
return;
editorModel.Dispose();
}
}
static Action<Exception> _exceptionHandler;
}
}
<MSG> Improve the TextMate installation API
<DFF> @@ -1,6 +1,6 @@
using System;
-using System.Collections.Generic;
using System.Linq;
+
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
@@ -9,54 +9,14 @@ namespace AvaloniaEdit.TextMate
{
public static class TextMate
{
- public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
- {
- lock(_lock)
- {
- _installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
- }
- }
-
- public static void DisposeTextMate(this TextEditor editor)
- {
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].Dispose();
- _installations.Remove(editor);
- }
- }
-
- public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
+ public static Installation InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar = null)
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetGrammar(grammar);
- }
+ return new Installation(editor, theme, grammar);
}
- public static void InstallTheme(this TextEditor editor, Theme theme)
+ public class Installation
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetTheme(theme);
- }
- }
-
- static object _lock = new object();
- static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
-
- class TextMateInstallation
- {
- internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
+ public Installation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
@@ -68,7 +28,7 @@ namespace AvaloniaEdit.TextMate
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
- internal void SetGrammar(IGrammar grammar)
+ public void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
@@ -77,14 +37,14 @@ namespace AvaloniaEdit.TextMate
_editor.TextArea.TextView.Redraw();
}
- internal void SetTheme(Theme theme)
+ public void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
- _editor.TextArea.TextView.Redraw();
+ _editorModel?.TokenizeViewPort();
}
- internal void Dispose()
+ public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
@@ -95,8 +55,8 @@ namespace AvaloniaEdit.TextMate
{
DisposeTMModel(_tmModel);
- var editorModel = new TextEditorModel(_editor, _editor.Document);
- _tmModel = new TMModel(editorModel);
+ _editorModel = new TextEditorModel(_editor, _editor.Document);
+ _tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
@@ -125,6 +85,7 @@ namespace AvaloniaEdit.TextMate
}
TextEditor _editor;
+ TextEditorModel _editorModel;
IGrammar _grammar;
TMModel _tmModel;
}
| 12 | Improve the TextMate installation API | 51 | .cs | TextMate/TextMate | mit | AvaloniaUI/AvaloniaEdit |
10063821 | <NME> TextMate.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
using TextMateSharp.Themes;
{
public static class TextMate
{
public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
{
lock(_lock)
{
_installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
}
}
public static void DisposeTextMate(this TextEditor editor)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].Dispose();
_installations.Remove(editor);
}
}
public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetGrammar(grammar);
}
}
public static void InstallTheme(this TextEditor editor, Theme theme)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetTheme(theme);
}
}
static object _lock = new object();
static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
class TextMateInstallation
{
internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
return new Installation(editor, registryOptions, initCurrentDocument);
}
public class Installation
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
internal void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
public IRegistryOptions RegistryOptions { get { return _textMateRegistryOptions; } }
_editor.TextArea.TextView.Redraw();
}
internal void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
_editor.TextArea.TextView.Redraw();
}
internal void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
}
{
DisposeTMModel(_tmModel);
var editorModel = new TextEditorModel(_editor, _editor.Document);
_tmModel = new TMModel(editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
}
public void SetTheme(IRawTheme theme)
{
_textMateRegistry.SetTheme(theme);
GetOrCreateTransformer().SetTheme(_textMateRegistry.GetTheme());
_tmModel?.InvalidateLine(0);
_editorModel?.InvalidateViewPortLines();
}
public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
DisposeEditorModel(_editorModel);
DisposeTMModel(_tmModel, _transformer);
DisposeTransformer(_transformer);
}
}
TextEditor _editor;
IGrammar _grammar;
TMModel _tmModel;
}
_editorModel = new TextEditorModel(_editor.TextArea.TextView, _editor.Document, _exceptionHandler);
_tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
_transformer = GetOrCreateTransformer();
_transformer.SetModel(_editor.Document, _tmModel);
_tmModel.AddModelTokensChangedListener(_transformer);
}
catch (Exception ex)
{
_exceptionHandler?.Invoke(ex);
}
}
TextMateColoringTransformer GetOrCreateTransformer()
{
var transformer = _editor.TextArea.TextView.LineTransformers.OfType<TextMateColoringTransformer>().FirstOrDefault();
if (transformer is null)
{
transformer = new TextMateColoringTransformer(
_editor.TextArea.TextView, _exceptionHandler);
_editor.TextArea.TextView.LineTransformers.Add(transformer);
}
return transformer;
}
static void DisposeTransformer(TextMateColoringTransformer transformer)
{
if (transformer == null)
return;
transformer.Dispose();
}
static void DisposeTMModel(TMModel tmModel, TextMateColoringTransformer transformer)
{
if (tmModel == null)
return;
if (transformer != null)
tmModel.RemoveModelTokensChangedListener(transformer);
tmModel.Dispose();
}
static void DisposeEditorModel(TextEditorModel editorModel)
{
if (editorModel == null)
return;
editorModel.Dispose();
}
}
static Action<Exception> _exceptionHandler;
}
}
<MSG> Improve the TextMate installation API
<DFF> @@ -1,6 +1,6 @@
using System;
-using System.Collections.Generic;
using System.Linq;
+
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
@@ -9,54 +9,14 @@ namespace AvaloniaEdit.TextMate
{
public static class TextMate
{
- public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
- {
- lock(_lock)
- {
- _installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
- }
- }
-
- public static void DisposeTextMate(this TextEditor editor)
- {
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].Dispose();
- _installations.Remove(editor);
- }
- }
-
- public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
+ public static Installation InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar = null)
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetGrammar(grammar);
- }
+ return new Installation(editor, theme, grammar);
}
- public static void InstallTheme(this TextEditor editor, Theme theme)
+ public class Installation
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetTheme(theme);
- }
- }
-
- static object _lock = new object();
- static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
-
- class TextMateInstallation
- {
- internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
+ public Installation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
@@ -68,7 +28,7 @@ namespace AvaloniaEdit.TextMate
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
- internal void SetGrammar(IGrammar grammar)
+ public void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
@@ -77,14 +37,14 @@ namespace AvaloniaEdit.TextMate
_editor.TextArea.TextView.Redraw();
}
- internal void SetTheme(Theme theme)
+ public void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
- _editor.TextArea.TextView.Redraw();
+ _editorModel?.TokenizeViewPort();
}
- internal void Dispose()
+ public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
@@ -95,8 +55,8 @@ namespace AvaloniaEdit.TextMate
{
DisposeTMModel(_tmModel);
- var editorModel = new TextEditorModel(_editor, _editor.Document);
- _tmModel = new TMModel(editorModel);
+ _editorModel = new TextEditorModel(_editor, _editor.Document);
+ _tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
@@ -125,6 +85,7 @@ namespace AvaloniaEdit.TextMate
}
TextEditor _editor;
+ TextEditorModel _editorModel;
IGrammar _grammar;
TMModel _tmModel;
}
| 12 | Improve the TextMate installation API | 51 | .cs | TextMate/TextMate | mit | AvaloniaUI/AvaloniaEdit |
10063822 | <NME> TextMate.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
using TextMateSharp.Themes;
{
public static class TextMate
{
public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
{
lock(_lock)
{
_installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
}
}
public static void DisposeTextMate(this TextEditor editor)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].Dispose();
_installations.Remove(editor);
}
}
public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetGrammar(grammar);
}
}
public static void InstallTheme(this TextEditor editor, Theme theme)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetTheme(theme);
}
}
static object _lock = new object();
static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
class TextMateInstallation
{
internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
return new Installation(editor, registryOptions, initCurrentDocument);
}
public class Installation
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
internal void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
public IRegistryOptions RegistryOptions { get { return _textMateRegistryOptions; } }
_editor.TextArea.TextView.Redraw();
}
internal void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
_editor.TextArea.TextView.Redraw();
}
internal void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
}
{
DisposeTMModel(_tmModel);
var editorModel = new TextEditorModel(_editor, _editor.Document);
_tmModel = new TMModel(editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
}
public void SetTheme(IRawTheme theme)
{
_textMateRegistry.SetTheme(theme);
GetOrCreateTransformer().SetTheme(_textMateRegistry.GetTheme());
_tmModel?.InvalidateLine(0);
_editorModel?.InvalidateViewPortLines();
}
public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
DisposeEditorModel(_editorModel);
DisposeTMModel(_tmModel, _transformer);
DisposeTransformer(_transformer);
}
}
TextEditor _editor;
IGrammar _grammar;
TMModel _tmModel;
}
_editorModel = new TextEditorModel(_editor.TextArea.TextView, _editor.Document, _exceptionHandler);
_tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
_transformer = GetOrCreateTransformer();
_transformer.SetModel(_editor.Document, _tmModel);
_tmModel.AddModelTokensChangedListener(_transformer);
}
catch (Exception ex)
{
_exceptionHandler?.Invoke(ex);
}
}
TextMateColoringTransformer GetOrCreateTransformer()
{
var transformer = _editor.TextArea.TextView.LineTransformers.OfType<TextMateColoringTransformer>().FirstOrDefault();
if (transformer is null)
{
transformer = new TextMateColoringTransformer(
_editor.TextArea.TextView, _exceptionHandler);
_editor.TextArea.TextView.LineTransformers.Add(transformer);
}
return transformer;
}
static void DisposeTransformer(TextMateColoringTransformer transformer)
{
if (transformer == null)
return;
transformer.Dispose();
}
static void DisposeTMModel(TMModel tmModel, TextMateColoringTransformer transformer)
{
if (tmModel == null)
return;
if (transformer != null)
tmModel.RemoveModelTokensChangedListener(transformer);
tmModel.Dispose();
}
static void DisposeEditorModel(TextEditorModel editorModel)
{
if (editorModel == null)
return;
editorModel.Dispose();
}
}
static Action<Exception> _exceptionHandler;
}
}
<MSG> Improve the TextMate installation API
<DFF> @@ -1,6 +1,6 @@
using System;
-using System.Collections.Generic;
using System.Linq;
+
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
@@ -9,54 +9,14 @@ namespace AvaloniaEdit.TextMate
{
public static class TextMate
{
- public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
- {
- lock(_lock)
- {
- _installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
- }
- }
-
- public static void DisposeTextMate(this TextEditor editor)
- {
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].Dispose();
- _installations.Remove(editor);
- }
- }
-
- public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
+ public static Installation InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar = null)
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetGrammar(grammar);
- }
+ return new Installation(editor, theme, grammar);
}
- public static void InstallTheme(this TextEditor editor, Theme theme)
+ public class Installation
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetTheme(theme);
- }
- }
-
- static object _lock = new object();
- static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
-
- class TextMateInstallation
- {
- internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
+ public Installation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
@@ -68,7 +28,7 @@ namespace AvaloniaEdit.TextMate
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
- internal void SetGrammar(IGrammar grammar)
+ public void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
@@ -77,14 +37,14 @@ namespace AvaloniaEdit.TextMate
_editor.TextArea.TextView.Redraw();
}
- internal void SetTheme(Theme theme)
+ public void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
- _editor.TextArea.TextView.Redraw();
+ _editorModel?.TokenizeViewPort();
}
- internal void Dispose()
+ public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
@@ -95,8 +55,8 @@ namespace AvaloniaEdit.TextMate
{
DisposeTMModel(_tmModel);
- var editorModel = new TextEditorModel(_editor, _editor.Document);
- _tmModel = new TMModel(editorModel);
+ _editorModel = new TextEditorModel(_editor, _editor.Document);
+ _tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
@@ -125,6 +85,7 @@ namespace AvaloniaEdit.TextMate
}
TextEditor _editor;
+ TextEditorModel _editorModel;
IGrammar _grammar;
TMModel _tmModel;
}
| 12 | Improve the TextMate installation API | 51 | .cs | TextMate/TextMate | mit | AvaloniaUI/AvaloniaEdit |
10063823 | <NME> TextMate.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
using TextMateSharp.Themes;
{
public static class TextMate
{
public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
{
lock(_lock)
{
_installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
}
}
public static void DisposeTextMate(this TextEditor editor)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].Dispose();
_installations.Remove(editor);
}
}
public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetGrammar(grammar);
}
}
public static void InstallTheme(this TextEditor editor, Theme theme)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetTheme(theme);
}
}
static object _lock = new object();
static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
class TextMateInstallation
{
internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
return new Installation(editor, registryOptions, initCurrentDocument);
}
public class Installation
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
internal void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
public IRegistryOptions RegistryOptions { get { return _textMateRegistryOptions; } }
_editor.TextArea.TextView.Redraw();
}
internal void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
_editor.TextArea.TextView.Redraw();
}
internal void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
}
{
DisposeTMModel(_tmModel);
var editorModel = new TextEditorModel(_editor, _editor.Document);
_tmModel = new TMModel(editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
}
public void SetTheme(IRawTheme theme)
{
_textMateRegistry.SetTheme(theme);
GetOrCreateTransformer().SetTheme(_textMateRegistry.GetTheme());
_tmModel?.InvalidateLine(0);
_editorModel?.InvalidateViewPortLines();
}
public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
DisposeEditorModel(_editorModel);
DisposeTMModel(_tmModel, _transformer);
DisposeTransformer(_transformer);
}
}
TextEditor _editor;
IGrammar _grammar;
TMModel _tmModel;
}
_editorModel = new TextEditorModel(_editor.TextArea.TextView, _editor.Document, _exceptionHandler);
_tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
_transformer = GetOrCreateTransformer();
_transformer.SetModel(_editor.Document, _tmModel);
_tmModel.AddModelTokensChangedListener(_transformer);
}
catch (Exception ex)
{
_exceptionHandler?.Invoke(ex);
}
}
TextMateColoringTransformer GetOrCreateTransformer()
{
var transformer = _editor.TextArea.TextView.LineTransformers.OfType<TextMateColoringTransformer>().FirstOrDefault();
if (transformer is null)
{
transformer = new TextMateColoringTransformer(
_editor.TextArea.TextView, _exceptionHandler);
_editor.TextArea.TextView.LineTransformers.Add(transformer);
}
return transformer;
}
static void DisposeTransformer(TextMateColoringTransformer transformer)
{
if (transformer == null)
return;
transformer.Dispose();
}
static void DisposeTMModel(TMModel tmModel, TextMateColoringTransformer transformer)
{
if (tmModel == null)
return;
if (transformer != null)
tmModel.RemoveModelTokensChangedListener(transformer);
tmModel.Dispose();
}
static void DisposeEditorModel(TextEditorModel editorModel)
{
if (editorModel == null)
return;
editorModel.Dispose();
}
}
static Action<Exception> _exceptionHandler;
}
}
<MSG> Improve the TextMate installation API
<DFF> @@ -1,6 +1,6 @@
using System;
-using System.Collections.Generic;
using System.Linq;
+
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
@@ -9,54 +9,14 @@ namespace AvaloniaEdit.TextMate
{
public static class TextMate
{
- public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
- {
- lock(_lock)
- {
- _installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
- }
- }
-
- public static void DisposeTextMate(this TextEditor editor)
- {
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].Dispose();
- _installations.Remove(editor);
- }
- }
-
- public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
+ public static Installation InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar = null)
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetGrammar(grammar);
- }
+ return new Installation(editor, theme, grammar);
}
- public static void InstallTheme(this TextEditor editor, Theme theme)
+ public class Installation
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetTheme(theme);
- }
- }
-
- static object _lock = new object();
- static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
-
- class TextMateInstallation
- {
- internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
+ public Installation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
@@ -68,7 +28,7 @@ namespace AvaloniaEdit.TextMate
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
- internal void SetGrammar(IGrammar grammar)
+ public void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
@@ -77,14 +37,14 @@ namespace AvaloniaEdit.TextMate
_editor.TextArea.TextView.Redraw();
}
- internal void SetTheme(Theme theme)
+ public void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
- _editor.TextArea.TextView.Redraw();
+ _editorModel?.TokenizeViewPort();
}
- internal void Dispose()
+ public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
@@ -95,8 +55,8 @@ namespace AvaloniaEdit.TextMate
{
DisposeTMModel(_tmModel);
- var editorModel = new TextEditorModel(_editor, _editor.Document);
- _tmModel = new TMModel(editorModel);
+ _editorModel = new TextEditorModel(_editor, _editor.Document);
+ _tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
@@ -125,6 +85,7 @@ namespace AvaloniaEdit.TextMate
}
TextEditor _editor;
+ TextEditorModel _editorModel;
IGrammar _grammar;
TMModel _tmModel;
}
| 12 | Improve the TextMate installation API | 51 | .cs | TextMate/TextMate | mit | AvaloniaUI/AvaloniaEdit |
10063824 | <NME> TextMate.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
using TextMateSharp.Themes;
{
public static class TextMate
{
public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
{
lock(_lock)
{
_installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
}
}
public static void DisposeTextMate(this TextEditor editor)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].Dispose();
_installations.Remove(editor);
}
}
public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetGrammar(grammar);
}
}
public static void InstallTheme(this TextEditor editor, Theme theme)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetTheme(theme);
}
}
static object _lock = new object();
static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
class TextMateInstallation
{
internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
return new Installation(editor, registryOptions, initCurrentDocument);
}
public class Installation
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
internal void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
public IRegistryOptions RegistryOptions { get { return _textMateRegistryOptions; } }
_editor.TextArea.TextView.Redraw();
}
internal void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
_editor.TextArea.TextView.Redraw();
}
internal void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
}
{
DisposeTMModel(_tmModel);
var editorModel = new TextEditorModel(_editor, _editor.Document);
_tmModel = new TMModel(editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
}
public void SetTheme(IRawTheme theme)
{
_textMateRegistry.SetTheme(theme);
GetOrCreateTransformer().SetTheme(_textMateRegistry.GetTheme());
_tmModel?.InvalidateLine(0);
_editorModel?.InvalidateViewPortLines();
}
public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
DisposeEditorModel(_editorModel);
DisposeTMModel(_tmModel, _transformer);
DisposeTransformer(_transformer);
}
}
TextEditor _editor;
IGrammar _grammar;
TMModel _tmModel;
}
_editorModel = new TextEditorModel(_editor.TextArea.TextView, _editor.Document, _exceptionHandler);
_tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
_transformer = GetOrCreateTransformer();
_transformer.SetModel(_editor.Document, _tmModel);
_tmModel.AddModelTokensChangedListener(_transformer);
}
catch (Exception ex)
{
_exceptionHandler?.Invoke(ex);
}
}
TextMateColoringTransformer GetOrCreateTransformer()
{
var transformer = _editor.TextArea.TextView.LineTransformers.OfType<TextMateColoringTransformer>().FirstOrDefault();
if (transformer is null)
{
transformer = new TextMateColoringTransformer(
_editor.TextArea.TextView, _exceptionHandler);
_editor.TextArea.TextView.LineTransformers.Add(transformer);
}
return transformer;
}
static void DisposeTransformer(TextMateColoringTransformer transformer)
{
if (transformer == null)
return;
transformer.Dispose();
}
static void DisposeTMModel(TMModel tmModel, TextMateColoringTransformer transformer)
{
if (tmModel == null)
return;
if (transformer != null)
tmModel.RemoveModelTokensChangedListener(transformer);
tmModel.Dispose();
}
static void DisposeEditorModel(TextEditorModel editorModel)
{
if (editorModel == null)
return;
editorModel.Dispose();
}
}
static Action<Exception> _exceptionHandler;
}
}
<MSG> Improve the TextMate installation API
<DFF> @@ -1,6 +1,6 @@
using System;
-using System.Collections.Generic;
using System.Linq;
+
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
@@ -9,54 +9,14 @@ namespace AvaloniaEdit.TextMate
{
public static class TextMate
{
- public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
- {
- lock(_lock)
- {
- _installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
- }
- }
-
- public static void DisposeTextMate(this TextEditor editor)
- {
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].Dispose();
- _installations.Remove(editor);
- }
- }
-
- public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
+ public static Installation InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar = null)
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetGrammar(grammar);
- }
+ return new Installation(editor, theme, grammar);
}
- public static void InstallTheme(this TextEditor editor, Theme theme)
+ public class Installation
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetTheme(theme);
- }
- }
-
- static object _lock = new object();
- static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
-
- class TextMateInstallation
- {
- internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
+ public Installation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
@@ -68,7 +28,7 @@ namespace AvaloniaEdit.TextMate
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
- internal void SetGrammar(IGrammar grammar)
+ public void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
@@ -77,14 +37,14 @@ namespace AvaloniaEdit.TextMate
_editor.TextArea.TextView.Redraw();
}
- internal void SetTheme(Theme theme)
+ public void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
- _editor.TextArea.TextView.Redraw();
+ _editorModel?.TokenizeViewPort();
}
- internal void Dispose()
+ public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
@@ -95,8 +55,8 @@ namespace AvaloniaEdit.TextMate
{
DisposeTMModel(_tmModel);
- var editorModel = new TextEditorModel(_editor, _editor.Document);
- _tmModel = new TMModel(editorModel);
+ _editorModel = new TextEditorModel(_editor, _editor.Document);
+ _tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
@@ -125,6 +85,7 @@ namespace AvaloniaEdit.TextMate
}
TextEditor _editor;
+ TextEditorModel _editorModel;
IGrammar _grammar;
TMModel _tmModel;
}
| 12 | Improve the TextMate installation API | 51 | .cs | TextMate/TextMate | mit | AvaloniaUI/AvaloniaEdit |
10063825 | <NME> TextMate.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
using TextMateSharp.Themes;
{
public static class TextMate
{
public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
{
lock(_lock)
{
_installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
}
}
public static void DisposeTextMate(this TextEditor editor)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].Dispose();
_installations.Remove(editor);
}
}
public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetGrammar(grammar);
}
}
public static void InstallTheme(this TextEditor editor, Theme theme)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetTheme(theme);
}
}
static object _lock = new object();
static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
class TextMateInstallation
{
internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
return new Installation(editor, registryOptions, initCurrentDocument);
}
public class Installation
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
internal void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
public IRegistryOptions RegistryOptions { get { return _textMateRegistryOptions; } }
_editor.TextArea.TextView.Redraw();
}
internal void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
_editor.TextArea.TextView.Redraw();
}
internal void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
}
{
DisposeTMModel(_tmModel);
var editorModel = new TextEditorModel(_editor, _editor.Document);
_tmModel = new TMModel(editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
}
public void SetTheme(IRawTheme theme)
{
_textMateRegistry.SetTheme(theme);
GetOrCreateTransformer().SetTheme(_textMateRegistry.GetTheme());
_tmModel?.InvalidateLine(0);
_editorModel?.InvalidateViewPortLines();
}
public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
DisposeEditorModel(_editorModel);
DisposeTMModel(_tmModel, _transformer);
DisposeTransformer(_transformer);
}
}
TextEditor _editor;
IGrammar _grammar;
TMModel _tmModel;
}
_editorModel = new TextEditorModel(_editor.TextArea.TextView, _editor.Document, _exceptionHandler);
_tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
_transformer = GetOrCreateTransformer();
_transformer.SetModel(_editor.Document, _tmModel);
_tmModel.AddModelTokensChangedListener(_transformer);
}
catch (Exception ex)
{
_exceptionHandler?.Invoke(ex);
}
}
TextMateColoringTransformer GetOrCreateTransformer()
{
var transformer = _editor.TextArea.TextView.LineTransformers.OfType<TextMateColoringTransformer>().FirstOrDefault();
if (transformer is null)
{
transformer = new TextMateColoringTransformer(
_editor.TextArea.TextView, _exceptionHandler);
_editor.TextArea.TextView.LineTransformers.Add(transformer);
}
return transformer;
}
static void DisposeTransformer(TextMateColoringTransformer transformer)
{
if (transformer == null)
return;
transformer.Dispose();
}
static void DisposeTMModel(TMModel tmModel, TextMateColoringTransformer transformer)
{
if (tmModel == null)
return;
if (transformer != null)
tmModel.RemoveModelTokensChangedListener(transformer);
tmModel.Dispose();
}
static void DisposeEditorModel(TextEditorModel editorModel)
{
if (editorModel == null)
return;
editorModel.Dispose();
}
}
static Action<Exception> _exceptionHandler;
}
}
<MSG> Improve the TextMate installation API
<DFF> @@ -1,6 +1,6 @@
using System;
-using System.Collections.Generic;
using System.Linq;
+
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
@@ -9,54 +9,14 @@ namespace AvaloniaEdit.TextMate
{
public static class TextMate
{
- public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
- {
- lock(_lock)
- {
- _installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
- }
- }
-
- public static void DisposeTextMate(this TextEditor editor)
- {
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].Dispose();
- _installations.Remove(editor);
- }
- }
-
- public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
+ public static Installation InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar = null)
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetGrammar(grammar);
- }
+ return new Installation(editor, theme, grammar);
}
- public static void InstallTheme(this TextEditor editor, Theme theme)
+ public class Installation
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetTheme(theme);
- }
- }
-
- static object _lock = new object();
- static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
-
- class TextMateInstallation
- {
- internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
+ public Installation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
@@ -68,7 +28,7 @@ namespace AvaloniaEdit.TextMate
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
- internal void SetGrammar(IGrammar grammar)
+ public void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
@@ -77,14 +37,14 @@ namespace AvaloniaEdit.TextMate
_editor.TextArea.TextView.Redraw();
}
- internal void SetTheme(Theme theme)
+ public void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
- _editor.TextArea.TextView.Redraw();
+ _editorModel?.TokenizeViewPort();
}
- internal void Dispose()
+ public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
@@ -95,8 +55,8 @@ namespace AvaloniaEdit.TextMate
{
DisposeTMModel(_tmModel);
- var editorModel = new TextEditorModel(_editor, _editor.Document);
- _tmModel = new TMModel(editorModel);
+ _editorModel = new TextEditorModel(_editor, _editor.Document);
+ _tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
@@ -125,6 +85,7 @@ namespace AvaloniaEdit.TextMate
}
TextEditor _editor;
+ TextEditorModel _editorModel;
IGrammar _grammar;
TMModel _tmModel;
}
| 12 | Improve the TextMate installation API | 51 | .cs | TextMate/TextMate | mit | AvaloniaUI/AvaloniaEdit |
10063826 | <NME> TextMate.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
using TextMateSharp.Themes;
{
public static class TextMate
{
public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
{
lock(_lock)
{
_installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
}
}
public static void DisposeTextMate(this TextEditor editor)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].Dispose();
_installations.Remove(editor);
}
}
public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetGrammar(grammar);
}
}
public static void InstallTheme(this TextEditor editor, Theme theme)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetTheme(theme);
}
}
static object _lock = new object();
static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
class TextMateInstallation
{
internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
return new Installation(editor, registryOptions, initCurrentDocument);
}
public class Installation
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
internal void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
public IRegistryOptions RegistryOptions { get { return _textMateRegistryOptions; } }
_editor.TextArea.TextView.Redraw();
}
internal void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
_editor.TextArea.TextView.Redraw();
}
internal void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
}
{
DisposeTMModel(_tmModel);
var editorModel = new TextEditorModel(_editor, _editor.Document);
_tmModel = new TMModel(editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
}
public void SetTheme(IRawTheme theme)
{
_textMateRegistry.SetTheme(theme);
GetOrCreateTransformer().SetTheme(_textMateRegistry.GetTheme());
_tmModel?.InvalidateLine(0);
_editorModel?.InvalidateViewPortLines();
}
public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
DisposeEditorModel(_editorModel);
DisposeTMModel(_tmModel, _transformer);
DisposeTransformer(_transformer);
}
}
TextEditor _editor;
IGrammar _grammar;
TMModel _tmModel;
}
_editorModel = new TextEditorModel(_editor.TextArea.TextView, _editor.Document, _exceptionHandler);
_tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
_transformer = GetOrCreateTransformer();
_transformer.SetModel(_editor.Document, _tmModel);
_tmModel.AddModelTokensChangedListener(_transformer);
}
catch (Exception ex)
{
_exceptionHandler?.Invoke(ex);
}
}
TextMateColoringTransformer GetOrCreateTransformer()
{
var transformer = _editor.TextArea.TextView.LineTransformers.OfType<TextMateColoringTransformer>().FirstOrDefault();
if (transformer is null)
{
transformer = new TextMateColoringTransformer(
_editor.TextArea.TextView, _exceptionHandler);
_editor.TextArea.TextView.LineTransformers.Add(transformer);
}
return transformer;
}
static void DisposeTransformer(TextMateColoringTransformer transformer)
{
if (transformer == null)
return;
transformer.Dispose();
}
static void DisposeTMModel(TMModel tmModel, TextMateColoringTransformer transformer)
{
if (tmModel == null)
return;
if (transformer != null)
tmModel.RemoveModelTokensChangedListener(transformer);
tmModel.Dispose();
}
static void DisposeEditorModel(TextEditorModel editorModel)
{
if (editorModel == null)
return;
editorModel.Dispose();
}
}
static Action<Exception> _exceptionHandler;
}
}
<MSG> Improve the TextMate installation API
<DFF> @@ -1,6 +1,6 @@
using System;
-using System.Collections.Generic;
using System.Linq;
+
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
@@ -9,54 +9,14 @@ namespace AvaloniaEdit.TextMate
{
public static class TextMate
{
- public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
- {
- lock(_lock)
- {
- _installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
- }
- }
-
- public static void DisposeTextMate(this TextEditor editor)
- {
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].Dispose();
- _installations.Remove(editor);
- }
- }
-
- public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
+ public static Installation InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar = null)
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetGrammar(grammar);
- }
+ return new Installation(editor, theme, grammar);
}
- public static void InstallTheme(this TextEditor editor, Theme theme)
+ public class Installation
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetTheme(theme);
- }
- }
-
- static object _lock = new object();
- static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
-
- class TextMateInstallation
- {
- internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
+ public Installation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
@@ -68,7 +28,7 @@ namespace AvaloniaEdit.TextMate
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
- internal void SetGrammar(IGrammar grammar)
+ public void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
@@ -77,14 +37,14 @@ namespace AvaloniaEdit.TextMate
_editor.TextArea.TextView.Redraw();
}
- internal void SetTheme(Theme theme)
+ public void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
- _editor.TextArea.TextView.Redraw();
+ _editorModel?.TokenizeViewPort();
}
- internal void Dispose()
+ public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
@@ -95,8 +55,8 @@ namespace AvaloniaEdit.TextMate
{
DisposeTMModel(_tmModel);
- var editorModel = new TextEditorModel(_editor, _editor.Document);
- _tmModel = new TMModel(editorModel);
+ _editorModel = new TextEditorModel(_editor, _editor.Document);
+ _tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
@@ -125,6 +85,7 @@ namespace AvaloniaEdit.TextMate
}
TextEditor _editor;
+ TextEditorModel _editorModel;
IGrammar _grammar;
TMModel _tmModel;
}
| 12 | Improve the TextMate installation API | 51 | .cs | TextMate/TextMate | mit | AvaloniaUI/AvaloniaEdit |
10063827 | <NME> TextMate.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
using TextMateSharp.Themes;
{
public static class TextMate
{
public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
{
lock(_lock)
{
_installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
}
}
public static void DisposeTextMate(this TextEditor editor)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].Dispose();
_installations.Remove(editor);
}
}
public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetGrammar(grammar);
}
}
public static void InstallTheme(this TextEditor editor, Theme theme)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetTheme(theme);
}
}
static object _lock = new object();
static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
class TextMateInstallation
{
internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
return new Installation(editor, registryOptions, initCurrentDocument);
}
public class Installation
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
internal void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
public IRegistryOptions RegistryOptions { get { return _textMateRegistryOptions; } }
_editor.TextArea.TextView.Redraw();
}
internal void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
_editor.TextArea.TextView.Redraw();
}
internal void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
}
{
DisposeTMModel(_tmModel);
var editorModel = new TextEditorModel(_editor, _editor.Document);
_tmModel = new TMModel(editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
}
public void SetTheme(IRawTheme theme)
{
_textMateRegistry.SetTheme(theme);
GetOrCreateTransformer().SetTheme(_textMateRegistry.GetTheme());
_tmModel?.InvalidateLine(0);
_editorModel?.InvalidateViewPortLines();
}
public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
DisposeEditorModel(_editorModel);
DisposeTMModel(_tmModel, _transformer);
DisposeTransformer(_transformer);
}
}
TextEditor _editor;
IGrammar _grammar;
TMModel _tmModel;
}
_editorModel = new TextEditorModel(_editor.TextArea.TextView, _editor.Document, _exceptionHandler);
_tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
_transformer = GetOrCreateTransformer();
_transformer.SetModel(_editor.Document, _tmModel);
_tmModel.AddModelTokensChangedListener(_transformer);
}
catch (Exception ex)
{
_exceptionHandler?.Invoke(ex);
}
}
TextMateColoringTransformer GetOrCreateTransformer()
{
var transformer = _editor.TextArea.TextView.LineTransformers.OfType<TextMateColoringTransformer>().FirstOrDefault();
if (transformer is null)
{
transformer = new TextMateColoringTransformer(
_editor.TextArea.TextView, _exceptionHandler);
_editor.TextArea.TextView.LineTransformers.Add(transformer);
}
return transformer;
}
static void DisposeTransformer(TextMateColoringTransformer transformer)
{
if (transformer == null)
return;
transformer.Dispose();
}
static void DisposeTMModel(TMModel tmModel, TextMateColoringTransformer transformer)
{
if (tmModel == null)
return;
if (transformer != null)
tmModel.RemoveModelTokensChangedListener(transformer);
tmModel.Dispose();
}
static void DisposeEditorModel(TextEditorModel editorModel)
{
if (editorModel == null)
return;
editorModel.Dispose();
}
}
static Action<Exception> _exceptionHandler;
}
}
<MSG> Improve the TextMate installation API
<DFF> @@ -1,6 +1,6 @@
using System;
-using System.Collections.Generic;
using System.Linq;
+
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
@@ -9,54 +9,14 @@ namespace AvaloniaEdit.TextMate
{
public static class TextMate
{
- public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
- {
- lock(_lock)
- {
- _installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
- }
- }
-
- public static void DisposeTextMate(this TextEditor editor)
- {
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].Dispose();
- _installations.Remove(editor);
- }
- }
-
- public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
+ public static Installation InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar = null)
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetGrammar(grammar);
- }
+ return new Installation(editor, theme, grammar);
}
- public static void InstallTheme(this TextEditor editor, Theme theme)
+ public class Installation
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetTheme(theme);
- }
- }
-
- static object _lock = new object();
- static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
-
- class TextMateInstallation
- {
- internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
+ public Installation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
@@ -68,7 +28,7 @@ namespace AvaloniaEdit.TextMate
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
- internal void SetGrammar(IGrammar grammar)
+ public void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
@@ -77,14 +37,14 @@ namespace AvaloniaEdit.TextMate
_editor.TextArea.TextView.Redraw();
}
- internal void SetTheme(Theme theme)
+ public void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
- _editor.TextArea.TextView.Redraw();
+ _editorModel?.TokenizeViewPort();
}
- internal void Dispose()
+ public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
@@ -95,8 +55,8 @@ namespace AvaloniaEdit.TextMate
{
DisposeTMModel(_tmModel);
- var editorModel = new TextEditorModel(_editor, _editor.Document);
- _tmModel = new TMModel(editorModel);
+ _editorModel = new TextEditorModel(_editor, _editor.Document);
+ _tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
@@ -125,6 +85,7 @@ namespace AvaloniaEdit.TextMate
}
TextEditor _editor;
+ TextEditorModel _editorModel;
IGrammar _grammar;
TMModel _tmModel;
}
| 12 | Improve the TextMate installation API | 51 | .cs | TextMate/TextMate | mit | AvaloniaUI/AvaloniaEdit |
10063828 | <NME> TextMate.cs
<BEF> using System;
using System.Collections.Generic;
using System.Linq;
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
using TextMateSharp.Themes;
{
public static class TextMate
{
public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
{
lock(_lock)
{
_installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
}
}
public static void DisposeTextMate(this TextEditor editor)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].Dispose();
_installations.Remove(editor);
}
}
public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetGrammar(grammar);
}
}
public static void InstallTheme(this TextEditor editor, Theme theme)
{
lock (_lock)
{
if (!_installations.ContainsKey(editor))
return;
_installations[editor].SetTheme(theme);
}
}
static object _lock = new object();
static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
class TextMateInstallation
{
internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
return new Installation(editor, registryOptions, initCurrentDocument);
}
public class Installation
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
internal void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
public IRegistryOptions RegistryOptions { get { return _textMateRegistryOptions; } }
_editor.TextArea.TextView.Redraw();
}
internal void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
_editor.TextArea.TextView.Redraw();
}
internal void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
{
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
}
{
DisposeTMModel(_tmModel);
var editorModel = new TextEditorModel(_editor, _editor.Document);
_tmModel = new TMModel(editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
}
public void SetTheme(IRawTheme theme)
{
_textMateRegistry.SetTheme(theme);
GetOrCreateTransformer().SetTheme(_textMateRegistry.GetTheme());
_tmModel?.InvalidateLine(0);
_editorModel?.InvalidateViewPortLines();
}
public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
DisposeEditorModel(_editorModel);
DisposeTMModel(_tmModel, _transformer);
DisposeTransformer(_transformer);
}
}
TextEditor _editor;
IGrammar _grammar;
TMModel _tmModel;
}
_editorModel = new TextEditorModel(_editor.TextArea.TextView, _editor.Document, _exceptionHandler);
_tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
_transformer = GetOrCreateTransformer();
_transformer.SetModel(_editor.Document, _tmModel);
_tmModel.AddModelTokensChangedListener(_transformer);
}
catch (Exception ex)
{
_exceptionHandler?.Invoke(ex);
}
}
TextMateColoringTransformer GetOrCreateTransformer()
{
var transformer = _editor.TextArea.TextView.LineTransformers.OfType<TextMateColoringTransformer>().FirstOrDefault();
if (transformer is null)
{
transformer = new TextMateColoringTransformer(
_editor.TextArea.TextView, _exceptionHandler);
_editor.TextArea.TextView.LineTransformers.Add(transformer);
}
return transformer;
}
static void DisposeTransformer(TextMateColoringTransformer transformer)
{
if (transformer == null)
return;
transformer.Dispose();
}
static void DisposeTMModel(TMModel tmModel, TextMateColoringTransformer transformer)
{
if (tmModel == null)
return;
if (transformer != null)
tmModel.RemoveModelTokensChangedListener(transformer);
tmModel.Dispose();
}
static void DisposeEditorModel(TextEditorModel editorModel)
{
if (editorModel == null)
return;
editorModel.Dispose();
}
}
static Action<Exception> _exceptionHandler;
}
}
<MSG> Improve the TextMate installation API
<DFF> @@ -1,6 +1,6 @@
using System;
-using System.Collections.Generic;
using System.Linq;
+
using TextMateSharp.Grammars;
using TextMateSharp.Model;
using TextMateSharp.Themes;
@@ -9,54 +9,14 @@ namespace AvaloniaEdit.TextMate
{
public static class TextMate
{
- public static void InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar)
- {
- lock(_lock)
- {
- _installations.Add(editor, new TextMateInstallation(editor, theme, grammar));
- }
- }
-
- public static void DisposeTextMate(this TextEditor editor)
- {
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].Dispose();
- _installations.Remove(editor);
- }
- }
-
- public static void InstallGrammar(this TextEditor editor, IGrammar grammar)
+ public static Installation InstallTextMate(this TextEditor editor, Theme theme, IGrammar grammar = null)
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetGrammar(grammar);
- }
+ return new Installation(editor, theme, grammar);
}
- public static void InstallTheme(this TextEditor editor, Theme theme)
+ public class Installation
{
- lock (_lock)
- {
- if (!_installations.ContainsKey(editor))
- return;
-
- _installations[editor].SetTheme(theme);
- }
- }
-
- static object _lock = new object();
- static Dictionary<TextEditor, TextMateInstallation> _installations = new Dictionary<TextEditor, TextMateInstallation>();
-
- class TextMateInstallation
- {
- internal TextMateInstallation(TextEditor editor, Theme theme, IGrammar grammar)
+ public Installation(TextEditor editor, Theme theme, IGrammar grammar)
{
_editor = editor;
@@ -68,7 +28,7 @@ namespace AvaloniaEdit.TextMate
OnEditorOnDocumentChanged(editor, EventArgs.Empty);
}
- internal void SetGrammar(IGrammar grammar)
+ public void SetGrammar(IGrammar grammar)
{
_grammar = grammar;
@@ -77,14 +37,14 @@ namespace AvaloniaEdit.TextMate
_editor.TextArea.TextView.Redraw();
}
- internal void SetTheme(Theme theme)
+ public void SetTheme(Theme theme)
{
GetOrCreateTransformer().SetTheme(theme);
- _editor.TextArea.TextView.Redraw();
+ _editorModel?.TokenizeViewPort();
}
- internal void Dispose()
+ public void Dispose()
{
_editor.DocumentChanged -= OnEditorOnDocumentChanged;
@@ -95,8 +55,8 @@ namespace AvaloniaEdit.TextMate
{
DisposeTMModel(_tmModel);
- var editorModel = new TextEditorModel(_editor, _editor.Document);
- _tmModel = new TMModel(editorModel);
+ _editorModel = new TextEditorModel(_editor, _editor.Document);
+ _tmModel = new TMModel(_editorModel);
_tmModel.SetGrammar(_grammar);
GetOrCreateTransformer().SetModel(_editor.Document, _editor.TextArea.TextView, _tmModel);
_tmModel.AddModelTokensChangedListener(GetOrCreateTransformer());
@@ -125,6 +85,7 @@ namespace AvaloniaEdit.TextMate
}
TextEditor _editor;
+ TextEditorModel _editorModel;
IGrammar _grammar;
TMModel _tmModel;
}
| 12 | Improve the TextMate installation API | 51 | .cs | TextMate/TextMate | mit | AvaloniaUI/AvaloniaEdit |
10063829 | <NME> TextSegmentCollection.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using System.Text;
using AvaloniaEdit.Utils;
using Avalonia.Threading;
namespace AvaloniaEdit.Document
{
/// <summary>
/// Interface to allow TextSegments to access the TextSegmentCollection - we cannot use a direct reference
/// because TextSegmentCollection is generic.
/// </summary>
internal interface ISegmentTree
{
void Add(TextSegment s);
void Remove(TextSegment s);
void UpdateAugmentedData(TextSegment s);
}
/// <summary>
/// <para>
/// A collection of text segments that supports efficient lookup of segments
/// intersecting with another segment.
/// </para>
/// </summary>
/// <remarks><inheritdoc cref="TextSegment"/></remarks>
/// <see cref="TextSegment"/>
public sealed class TextSegmentCollection<T> : ICollection<T>, ISegmentTree where T : TextSegment
{
// Implementation: this is basically a mixture of an augmented interval tree
// and the TextAnchorTree.
// WARNING: you need to understand interval trees (the version with the augmented 'high'/'max' field)
// and how the TextAnchorTree works before you have any chance of understanding this code.
// This means that every node holds two "segments":
// one like the segments in the text anchor tree to support efficient offset changes
// and another that is the interval as seen by the user
// So basically, the tree contains a list of contiguous node segments of the first kind,
// with interval segments starting at the end of every node segment.
// Performance:
// Add is O(lg n)
// Remove is O(lg n)
// DocumentChanged is O(m * lg n), with m the number of segments that intersect with the changed document section
// FindFirstSegmentWithStartAfter is O(m + lg n) with m being the number of segments at the same offset as the result segment
// FindIntersectingSegments is O(m + lg n) with m being the number of intersecting segments.
private TextSegment _root;
private readonly bool _isConnectedToDocument;
#region Constructor
/// <summary>
/// Creates a new TextSegmentCollection that needs manual calls to <see cref="UpdateOffsets(DocumentChangeEventArgs)"/>.
/// </summary>
public TextSegmentCollection()
{
}
/// <summary>
/// Creates a new TextSegmentCollection that updates the offsets automatically.
/// </summary>
/// <param name="textDocument">The document to which the text segments
/// that will be added to the tree belong. When the document changes, the
/// position of the text segments will be updated accordingly.</param>
public TextSegmentCollection(TextDocument textDocument)
{
if (textDocument == null)
throw new ArgumentNullException(nameof(textDocument));
Dispatcher.UIThread.VerifyAccess();
_isConnectedToDocument = true;
TextDocumentWeakEventManager.Changed.AddHandler(textDocument, OnDocumentChanged);
}
#endregion
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="e">DocumentChangeEventArgs instance describing the change to the document.</param>
public void UpdateOffsets(DocumentChangeEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
OnDocumentChanged(this, e);
CheckProperties();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
var map = e.OffsetChangeMapOrNull;
if (map != null)
{
foreach (var entry in map)
{
UpdateOffsetsInternal(entry);
}
}
else
{
UpdateOffsetsInternal(e.CreateSingleChangeMapEntry());
}
}
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="change">OffsetChangeMapEntry instance describing the change to the document.</param>
public void UpdateOffsets(OffsetChangeMapEntry change)
{
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
UpdateOffsetsInternal(change);
CheckProperties();
}
#endregion
#region UpdateOffsets (implementation)
private void UpdateOffsetsInternal(OffsetChangeMapEntry change)
{
// Special case pure insertions, because they don't always cause a text segment to increase in size when the replaced region
// is inside a segment (when offset is at start or end of a text semgent).
if (change.RemovalLength == 0)
{
InsertText(change.Offset, change.InsertionLength);
}
else
{
ReplaceText(change);
}
}
private void InsertText(int offset, int length)
{
if (length == 0)
return;
// enlarge segments that contain offset (excluding those that have offset as endpoint)
foreach (var segment in FindSegmentsContaining(offset))
{
if (segment.StartOffset < offset && offset < segment.EndOffset)
{
segment.Length += length;
}
}
// move start offsets of all segments >= offset
TextSegment node = FindFirstSegmentWithStartAfter(offset);
if (node != null)
{
node.NodeLength += length;
UpdateAugmentedData(node);
}
}
private void ReplaceText(OffsetChangeMapEntry change)
{
Debug.Assert(change.RemovalLength > 0);
var offset = change.Offset;
foreach (var segment in FindOverlappingSegments(offset, change.RemovalLength))
{
if (segment.StartOffset <= offset)
{
if (segment.EndOffset >= offset + change.RemovalLength)
{
// Replacement inside segment: adjust segment length
segment.Length += change.InsertionLength - change.RemovalLength;
}
else
{
// Replacement starting inside segment and ending after segment end: set segment end to removal position
//segment.EndOffset = offset;
segment.Length = offset - segment.StartOffset;
}
}
else
{
// Replacement starting in front of text segment and running into segment.
// Keep segment.EndOffset constant and move segment.StartOffset to the end of the replacement
var remainingLength = segment.EndOffset - (offset + change.RemovalLength);
RemoveSegment(segment);
segment.StartOffset = offset + change.RemovalLength;
segment.Length = Math.Max(0, remainingLength);
AddSegment(segment);
}
}
// move start offsets of all segments > offset
TextSegment node = FindFirstSegmentWithStartAfter(offset + 1);
if (node != null)
{
Debug.Assert(node.NodeLength >= change.RemovalLength);
node.NodeLength += change.InsertionLength - change.RemovalLength;
UpdateAugmentedData(node);
}
}
#endregion
#region Add
/// <summary>
/// Adds the specified segment to the tree. This will cause the segment to update when the
/// document changes.
/// </summary>
public void Add(T item)
{
if (item == null)
throw new ArgumentNullException(nameof(item));
if (item.OwnerTree != null)
throw new ArgumentException("The segment is already added to a SegmentCollection.");
AddSegment(item);
}
void ISegmentTree.Add(TextSegment s)
{
AddSegment(s);
}
private void AddSegment(TextSegment node)
{
var insertionOffset = node.StartOffset;
node.DistanceToMaxEnd = node.SegmentLength;
if (_root == null)
{
_root = node;
node.TotalNodeLength = node.NodeLength;
}
else if (insertionOffset >= _root.TotalNodeLength)
{
// append segment at end of tree
node.NodeLength = node.TotalNodeLength = insertionOffset - _root.TotalNodeLength;
InsertAsRight(_root.RightMost, node);
}
else
{
// insert in middle of tree
var n = FindNode(ref insertionOffset);
Debug.Assert(insertionOffset < n.NodeLength);
// split node segment 'n' at offset
node.TotalNodeLength = node.NodeLength = insertionOffset;
n.NodeLength -= insertionOffset;
InsertBefore(n, node);
}
node.OwnerTree = this;
Count++;
CheckProperties();
}
private void InsertBefore(TextSegment node, TextSegment newNode)
{
if (node.Left == null)
{
InsertAsLeft(node, newNode);
}
else
{
InsertAsRight(node.Left.RightMost, newNode);
}
}
#endregion
#region GetNextSegment / GetPreviousSegment
/// <summary>
/// Gets the next segment after the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the last segment.
/// </summary>
public T GetNextSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Successor;
}
/// <summary>
/// Gets the previous segment before the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the first segment.
/// </summary>
public T GetPreviousSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Predecessor;
}
#endregion
#region FirstSegment/LastSegment
/// <summary>
/// Returns the first segment in the collection or null, if the collection is empty.
/// </summary>
public T FirstSegment => (T)_root?.LeftMost;
/// <summary>
/// Returns the last segment in the collection or null, if the collection is empty.
/// </summary>
public T LastSegment => (T)_root?.RightMost;
#endregion
#region FindFirstSegmentWithStartAfter
/// <summary>
/// Gets the first segment with a start offset greater or equal to <paramref name="startOffset"/>.
/// Returns null if no such segment is found.
/// </summary>
public T FindFirstSegmentWithStartAfter(int startOffset)
{
if (_root == null)
return null;
if (startOffset <= 0)
return (T)_root.LeftMost;
var s = FindNode(ref startOffset);
// startOffset means that the previous segment is starting at the offset we were looking for
while (startOffset == 0)
{
var p = (s == null) ? _root.RightMost : s.Predecessor;
// There must always be a predecessor: if we were looking for the first node, we would have already
// returned it as root.LeftMost above.
Debug.Assert(p != null);
startOffset += p.NodeLength;
s = p;
}
return (T)s;
}
/// <summary>
/// Finds the node at the specified offset.
/// After the method has run, offset is relative to the beginning of the returned node.
/// </summary>
private TextSegment FindNode(ref int offset)
{
var n = _root;
while (true)
{
if (n.Left != null)
{
if (offset < n.Left.TotalNodeLength)
{
n = n.Left; // descend into left subtree
continue;
}
else
{
offset -= n.Left.TotalNodeLength; // skip left subtree
}
}
if (offset < n.NodeLength)
{
return n; // found correct node
}
else
{
offset -= n.NodeLength; // skip this node
}
if (n.Right != null)
{
n = n.Right; // descend into right subtree
}
else
{
// didn't find any node containing the offset
return null;
}
}
}
#endregion
#region FindOverlappingSegments
/// <summary>
/// Finds all segments that contain the given offset.
/// (StartOffset <= offset <= EndOffset)
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindSegmentsContaining(int offset)
{
return FindOverlappingSegments(offset, 0);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(ISegment segment)
{
if (segment == null)
throw new ArgumentNullException(nameof(segment));
return FindOverlappingSegments(segment.Offset, segment.Length);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(int offset, int length)
{
ThrowUtil.CheckNotNegative(length, "length");
var results = new List<T>();
if (_root != null)
{
FindOverlappingSegments(results, _root, offset, offset + length);
}
return new ReadOnlyCollection<T>(results);
}
private void FindOverlappingSegments(List<T> results, TextSegment node, int low, int high)
{
// low and high are relative to node.LeftMost startpos (not node.LeftMost.Offset)
if (high < 0)
{
// node is irrelevant for search because all intervals in node are after high
return;
}
// find values relative to node.Offset
var nodeLow = low - node.NodeLength;
var nodeHigh = high - node.NodeLength;
if (node.Left != null)
{
nodeLow -= node.Left.TotalNodeLength;
nodeHigh -= node.Left.TotalNodeLength;
}
if (node.DistanceToMaxEnd < nodeLow)
{
// node is irrelevant for search because all intervals in node are before low
return;
}
if (node.Left != null)
FindOverlappingSegments(results, node.Left, low, high);
if (nodeHigh < 0)
{
// node and everything in node.right is before low
return;
}
if (nodeLow <= node.SegmentLength)
{
results.Add((T)node);
}
if (node.Right != null)
FindOverlappingSegments(results, node.Right, nodeLow, nodeHigh);
}
#endregion
#region UpdateAugmentedData
private void UpdateAugmentedData(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
totalLength += node.Left.TotalNodeLength;
var leftDtme = node.Left.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
if (node.Left.Right != null)
leftDtme -= node.Left.Right.TotalNodeLength;
leftDtme -= node.NodeLength;
if (leftDtme > distanceToMaxEnd)
distanceToMaxEnd = leftDtme;
}
if (node.Right != null)
{
totalLength += node.Right.TotalNodeLength;
var rightDtme = node.Right.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
rightDtme += node.Right.NodeLength;
if (node.Right.Left != null)
rightDtme += node.Right.Left.TotalNodeLength;
if (rightDtme > distanceToMaxEnd)
distanceToMaxEnd = rightDtme;
}
if (node.TotalNodeLength != totalLength
|| node.DistanceToMaxEnd != distanceToMaxEnd)
{
node.TotalNodeLength = totalLength;
node.DistanceToMaxEnd = distanceToMaxEnd;
if (node.Parent != null)
UpdateAugmentedData(node.Parent);
}
}
void ISegmentTree.UpdateAugmentedData(TextSegment node)
{
UpdateAugmentedData(node);
}
#endregion
#region Remove
/// <summary>
/// Removes the specified segment from the tree. This will cause the segment to not update
/// anymore when the document changes.
/// </summary>
public bool Remove(T item)
{
if (!Contains(item))
return false;
RemoveSegment(item);
return true;
}
void ISegmentTree.Remove(TextSegment s)
{
RemoveSegment(s);
}
private void RemoveSegment(TextSegment s)
{
var oldOffset = s.StartOffset;
var successor = s.Successor;
if (successor != null)
successor.NodeLength += s.NodeLength;
RemoveNode(s);
if (successor != null)
UpdateAugmentedData(successor);
Disconnect(s, oldOffset);
CheckProperties();
}
private void Disconnect(TextSegment s, int offset)
{
s.Left = s.Right = s.Parent = null;
s.OwnerTree = null;
s.NodeLength = offset;
Count--;
}
/// <summary>
/// Removes all segments from the tree.
/// </summary>
public void Clear()
{
var segments = this.ToArray();
_root = null;
var offset = 0;
foreach (var s in segments)
{
offset += s.NodeLength;
Disconnect(s, offset);
}
CheckProperties();
}
#endregion
#region CheckProperties
[Conditional("DATACONSISTENCYTEST")]
internal void CheckProperties()
{
#if DEBUG
if (_root != null)
{
CheckProperties(_root);
// check red-black property:
var blackCount = -1;
CheckNodeProperties(_root, null, Red, 0, ref blackCount);
}
var expectedCount = 0;
// we cannot trust LINQ not to call ICollection.Count, so we need this loop
// to count the elements in the tree
using (var en = GetEnumerator())
{
while (en.MoveNext()) expectedCount++;
}
Debug.Assert(Count == expectedCount);
#endif
}
#if DEBUG
private void CheckProperties(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
CheckProperties(node.Left);
totalLength += node.Left.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Left.DistanceToMaxEnd + node.Left.StartOffset - node.StartOffset);
}
if (node.Right != null)
{
CheckProperties(node.Right);
totalLength += node.Right.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Right.DistanceToMaxEnd + node.Right.StartOffset - node.StartOffset);
}
Debug.Assert(node.TotalNodeLength == totalLength);
Debug.Assert(node.DistanceToMaxEnd == distanceToMaxEnd);
}
/*
1. A node is either red or black.
2. The root is black.
3. All leaves are black. (The leaves are the NIL children.)
4. Both children of every red node are black. (So every red node must have a black parent.)
5. Every simple path from a node to a descendant leaf contains the same number of black nodes. (Not counting the leaf node.)
*/
[SuppressMessage("ReSharper", "UnusedParameter.Local")]
private static void CheckNodeProperties(TextSegment node, TextSegment parentNode, bool parentColor, int blackCount, ref int expectedBlackCount)
{
if (node == null) return;
Debug.Assert(node.Parent == parentNode);
if (parentColor == Red)
{
Debug.Assert(node.Color == Black);
}
if (node.Color == Black)
{
blackCount++;
}
if (node.Left == null && node.Right == null)
{
// node is a leaf node:
if (expectedBlackCount == -1)
expectedBlackCount = blackCount;
else
Debug.Assert(expectedBlackCount == blackCount);
}
CheckNodeProperties(node.Left, node, node.Color, blackCount, ref expectedBlackCount);
CheckNodeProperties(node.Right, node, node.Color, blackCount, ref expectedBlackCount);
}
private static void AppendTreeToString(TextSegment node, StringBuilder b, int indent)
{
b.Append(node.Color == Red ? "RED " : "BLACK ");
b.AppendLine(node + node.ToDebugString());
indent += 2;
if (node.Left != null)
{
b.Append(' ', indent);
b.Append("L: ");
AppendTreeToString(node.Left, b, indent);
}
if (node.Right != null)
{
b.Append(' ', indent);
b.Append("R: ");
AppendTreeToString(node.Right, b, indent);
}
}
#endif
internal string GetTreeAsString()
{
#if DEBUG
var b = new StringBuilder();
if (_root != null)
AppendTreeToString(_root, b, 0);
return b.ToString();
#else
return "Not available in release build.";
#endif
}
#endregion
#region Red/Black Tree
internal const bool Red = true;
internal const bool Black = false;
private void InsertAsLeft(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Left == null);
parentNode.Left = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void InsertAsRight(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Right == null);
parentNode.Right = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void FixTreeOnInsert(TextSegment node)
{
Debug.Assert(node != null);
Debug.Assert(node.Color == Red);
Debug.Assert(node.Left == null || node.Left.Color == Black);
Debug.Assert(node.Right == null || node.Right.Color == Black);
var parentNode = node.Parent;
if (parentNode == null)
{
// we inserted in the root -> the node must be black
// since this is a root node, making the node black increments the number of black nodes
// on all paths by one, so it is still the same for all paths.
node.Color = Black;
return;
}
if (parentNode.Color == Black)
{
// if the parent node where we inserted was black, our red node is placed correctly.
// since we inserted a red node, the number of black nodes on each path is unchanged
// -> the tree is still balanced
return;
}
// parentNode is red, so there is a conflict here!
// because the root is black, parentNode is not the root -> there is a grandparent node
var grandparentNode = parentNode.Parent;
var uncleNode = Sibling(parentNode);
if (uncleNode != null && uncleNode.Color == Red)
{
parentNode.Color = Black;
uncleNode.Color = Black;
grandparentNode.Color = Red;
FixTreeOnInsert(grandparentNode);
return;
}
// now we know: parent is red but uncle is black
// First rotation:
if (node == parentNode.Right && parentNode == grandparentNode.Left)
{
RotateLeft(parentNode);
node = node.Left;
}
else if (node == parentNode.Left && parentNode == grandparentNode.Right)
{
RotateRight(parentNode);
node = node.Right;
}
// because node might have changed, reassign variables:
// ReSharper disable once PossibleNullReferenceException
parentNode = node.Parent;
grandparentNode = parentNode.Parent;
// Now recolor a bit:
parentNode.Color = Black;
grandparentNode.Color = Red;
// Second rotation:
if (node == parentNode.Left && parentNode == grandparentNode.Left)
{
RotateRight(grandparentNode);
}
else
{
// because of the first rotation, this is guaranteed:
Debug.Assert(node == parentNode.Right && parentNode == grandparentNode.Right);
RotateLeft(grandparentNode);
}
}
private void RemoveNode(TextSegment removedNode)
{
if (removedNode.Left != null && removedNode.Right != null)
{
// replace removedNode with it's in-order successor
var leftMost = removedNode.Right.LeftMost;
RemoveNode(leftMost); // remove leftMost from its current location
// and overwrite the removedNode with it
ReplaceNode(removedNode, leftMost);
leftMost.Left = removedNode.Left;
if (leftMost.Left != null) leftMost.Left.Parent = leftMost;
leftMost.Right = removedNode.Right;
if (leftMost.Right != null) leftMost.Right.Parent = leftMost;
leftMost.Color = removedNode.Color;
UpdateAugmentedData(leftMost);
if (leftMost.Parent != null) UpdateAugmentedData(leftMost.Parent);
return;
}
// now either removedNode.left or removedNode.right is null
// get the remaining child
var parentNode = removedNode.Parent;
var childNode = removedNode.Left ?? removedNode.Right;
ReplaceNode(removedNode, childNode);
if (parentNode != null) UpdateAugmentedData(parentNode);
if (removedNode.Color == Black)
{
if (childNode != null && childNode.Color == Red)
{
childNode.Color = Black;
}
else
{
FixTreeOnDelete(childNode, parentNode);
}
}
}
private void FixTreeOnDelete(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (parentNode == null)
return;
// warning: node may be null
var sibling = Sibling(node, parentNode);
if (sibling.Color == Red)
{
parentNode.Color = Red;
sibling.Color = Black;
if (node == parentNode.Left)
{
RotateLeft(parentNode);
}
else
{
RotateRight(parentNode);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
}
if (parentNode.Color == Black
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
FixTreeOnDelete(parentNode, parentNode.Parent);
return;
}
if (parentNode.Color == Red
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
parentNode.Color = Black;
return;
}
if (node == parentNode.Left &&
sibling.Color == Black &&
GetColor(sibling.Left) == Red &&
GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
sibling.Left.Color = Black;
RotateRight(sibling);
}
else if (node == parentNode.Right &&
sibling.Color == Black &&
GetColor(sibling.Right) == Red &&
GetColor(sibling.Left) == Black)
{
sibling.Color = Red;
sibling.Right.Color = Black;
RotateLeft(sibling);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
sibling.Color = parentNode.Color;
parentNode.Color = Black;
if (node == parentNode.Left)
{
if (sibling.Right != null)
{
Debug.Assert(sibling.Right.Color == Red);
sibling.Right.Color = Black;
}
RotateLeft(parentNode);
}
else
{
if (sibling.Left != null)
{
Debug.Assert(sibling.Left.Color == Red);
sibling.Left.Color = Black;
}
RotateRight(parentNode);
}
}
private void ReplaceNode(TextSegment replacedNode, TextSegment newNode)
{
if (replacedNode.Parent == null)
{
Debug.Assert(replacedNode == _root);
_root = newNode;
}
else
{
if (replacedNode.Parent.Left == replacedNode)
replacedNode.Parent.Left = newNode;
else
replacedNode.Parent.Right = newNode;
}
if (newNode != null)
{
newNode.Parent = replacedNode.Parent;
}
replacedNode.Parent = null;
}
private void RotateLeft(TextSegment p)
{
// let q be p's right child
var q = p.Right;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's right child to be q's left child
p.Right = q.Left;
if (p.Right != null) p.Right.Parent = p;
// set q's left child to be p
q.Left = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private void RotateRight(TextSegment p)
{
// let q be p's left child
var q = p.Left;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's left child to be q's right child
p.Left = q.Right;
if (p.Left != null) p.Left.Parent = p;
// set q's right child to be p
q.Right = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private static TextSegment Sibling(TextSegment node)
{
return node == node.Parent.Left ? node.Parent.Right : node.Parent.Left;
}
private static TextSegment Sibling(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (node == parentNode.Left)
return parentNode.Right;
else
return parentNode.Left;
}
private static bool GetColor(TextSegment node)
{
return node != null && node.Color;
}
#endregion
#region ICollection<T> implementation
/// <summary>
/// Gets the number of segments in the tree.
/// </summary>
public int Count { get; private set; }
bool ICollection<T>.IsReadOnly => false;
/// <summary>
/// Gets whether this tree contains the specified item.
/// </summary>
public bool Contains(T item)
{
return item != null && item.OwnerTree == this;
}
/// <summary>
/// Copies all segments in this SegmentTree to the specified array.
/// </summary>
public void CopyTo(T[] array, int arrayIndex)
{
if (array == null)
throw new ArgumentNullException(nameof(array));
if (array.Length < Count)
throw new ArgumentException("The array is too small", nameof(array));
if (arrayIndex < 0 || arrayIndex + Count > array.Length)
throw new ArgumentOutOfRangeException(nameof(arrayIndex), arrayIndex, "Value must be between 0 and " + (array.Length - Count));
foreach (var s in this)
{
array[arrayIndex++] = s;
}
}
/// <summary>
/// Gets an enumerator to enumerate the segments.
/// </summary>
public IEnumerator<T> GetEnumerator()
{
if (_root != null)
{
var current = _root.LeftMost;
while (current != null)
{
yield return (T)current;
// TODO: check if collection was modified during enumeration
current = current.Successor;
}
}
}
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
#endregion
}
}
<MSG> Allow a text segment collection to be disconnected from a text document.
<DFF> @@ -97,6 +97,14 @@ namespace AvaloniaEdit.Document
}
#endregion
+ public void Disconnect (TextDocument textDocument)
+ {
+ if (_isConnectedToDocument)
+ {
+ TextDocumentWeakEventManager.Changed.RemoveHandler(textDocument, OnDocumentChanged);
+ }
+ }
+
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
| 8 | Allow a text segment collection to be disconnected from a text document. | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063830 | <NME> TextSegmentCollection.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using System.Text;
using AvaloniaEdit.Utils;
using Avalonia.Threading;
namespace AvaloniaEdit.Document
{
/// <summary>
/// Interface to allow TextSegments to access the TextSegmentCollection - we cannot use a direct reference
/// because TextSegmentCollection is generic.
/// </summary>
internal interface ISegmentTree
{
void Add(TextSegment s);
void Remove(TextSegment s);
void UpdateAugmentedData(TextSegment s);
}
/// <summary>
/// <para>
/// A collection of text segments that supports efficient lookup of segments
/// intersecting with another segment.
/// </para>
/// </summary>
/// <remarks><inheritdoc cref="TextSegment"/></remarks>
/// <see cref="TextSegment"/>
public sealed class TextSegmentCollection<T> : ICollection<T>, ISegmentTree where T : TextSegment
{
// Implementation: this is basically a mixture of an augmented interval tree
// and the TextAnchorTree.
// WARNING: you need to understand interval trees (the version with the augmented 'high'/'max' field)
// and how the TextAnchorTree works before you have any chance of understanding this code.
// This means that every node holds two "segments":
// one like the segments in the text anchor tree to support efficient offset changes
// and another that is the interval as seen by the user
// So basically, the tree contains a list of contiguous node segments of the first kind,
// with interval segments starting at the end of every node segment.
// Performance:
// Add is O(lg n)
// Remove is O(lg n)
// DocumentChanged is O(m * lg n), with m the number of segments that intersect with the changed document section
// FindFirstSegmentWithStartAfter is O(m + lg n) with m being the number of segments at the same offset as the result segment
// FindIntersectingSegments is O(m + lg n) with m being the number of intersecting segments.
private TextSegment _root;
private readonly bool _isConnectedToDocument;
#region Constructor
/// <summary>
/// Creates a new TextSegmentCollection that needs manual calls to <see cref="UpdateOffsets(DocumentChangeEventArgs)"/>.
/// </summary>
public TextSegmentCollection()
{
}
/// <summary>
/// Creates a new TextSegmentCollection that updates the offsets automatically.
/// </summary>
/// <param name="textDocument">The document to which the text segments
/// that will be added to the tree belong. When the document changes, the
/// position of the text segments will be updated accordingly.</param>
public TextSegmentCollection(TextDocument textDocument)
{
if (textDocument == null)
throw new ArgumentNullException(nameof(textDocument));
Dispatcher.UIThread.VerifyAccess();
_isConnectedToDocument = true;
TextDocumentWeakEventManager.Changed.AddHandler(textDocument, OnDocumentChanged);
}
#endregion
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="e">DocumentChangeEventArgs instance describing the change to the document.</param>
public void UpdateOffsets(DocumentChangeEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
OnDocumentChanged(this, e);
CheckProperties();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
var map = e.OffsetChangeMapOrNull;
if (map != null)
{
foreach (var entry in map)
{
UpdateOffsetsInternal(entry);
}
}
else
{
UpdateOffsetsInternal(e.CreateSingleChangeMapEntry());
}
}
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="change">OffsetChangeMapEntry instance describing the change to the document.</param>
public void UpdateOffsets(OffsetChangeMapEntry change)
{
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
UpdateOffsetsInternal(change);
CheckProperties();
}
#endregion
#region UpdateOffsets (implementation)
private void UpdateOffsetsInternal(OffsetChangeMapEntry change)
{
// Special case pure insertions, because they don't always cause a text segment to increase in size when the replaced region
// is inside a segment (when offset is at start or end of a text semgent).
if (change.RemovalLength == 0)
{
InsertText(change.Offset, change.InsertionLength);
}
else
{
ReplaceText(change);
}
}
private void InsertText(int offset, int length)
{
if (length == 0)
return;
// enlarge segments that contain offset (excluding those that have offset as endpoint)
foreach (var segment in FindSegmentsContaining(offset))
{
if (segment.StartOffset < offset && offset < segment.EndOffset)
{
segment.Length += length;
}
}
// move start offsets of all segments >= offset
TextSegment node = FindFirstSegmentWithStartAfter(offset);
if (node != null)
{
node.NodeLength += length;
UpdateAugmentedData(node);
}
}
private void ReplaceText(OffsetChangeMapEntry change)
{
Debug.Assert(change.RemovalLength > 0);
var offset = change.Offset;
foreach (var segment in FindOverlappingSegments(offset, change.RemovalLength))
{
if (segment.StartOffset <= offset)
{
if (segment.EndOffset >= offset + change.RemovalLength)
{
// Replacement inside segment: adjust segment length
segment.Length += change.InsertionLength - change.RemovalLength;
}
else
{
// Replacement starting inside segment and ending after segment end: set segment end to removal position
//segment.EndOffset = offset;
segment.Length = offset - segment.StartOffset;
}
}
else
{
// Replacement starting in front of text segment and running into segment.
// Keep segment.EndOffset constant and move segment.StartOffset to the end of the replacement
var remainingLength = segment.EndOffset - (offset + change.RemovalLength);
RemoveSegment(segment);
segment.StartOffset = offset + change.RemovalLength;
segment.Length = Math.Max(0, remainingLength);
AddSegment(segment);
}
}
// move start offsets of all segments > offset
TextSegment node = FindFirstSegmentWithStartAfter(offset + 1);
if (node != null)
{
Debug.Assert(node.NodeLength >= change.RemovalLength);
node.NodeLength += change.InsertionLength - change.RemovalLength;
UpdateAugmentedData(node);
}
}
#endregion
#region Add
/// <summary>
/// Adds the specified segment to the tree. This will cause the segment to update when the
/// document changes.
/// </summary>
public void Add(T item)
{
if (item == null)
throw new ArgumentNullException(nameof(item));
if (item.OwnerTree != null)
throw new ArgumentException("The segment is already added to a SegmentCollection.");
AddSegment(item);
}
void ISegmentTree.Add(TextSegment s)
{
AddSegment(s);
}
private void AddSegment(TextSegment node)
{
var insertionOffset = node.StartOffset;
node.DistanceToMaxEnd = node.SegmentLength;
if (_root == null)
{
_root = node;
node.TotalNodeLength = node.NodeLength;
}
else if (insertionOffset >= _root.TotalNodeLength)
{
// append segment at end of tree
node.NodeLength = node.TotalNodeLength = insertionOffset - _root.TotalNodeLength;
InsertAsRight(_root.RightMost, node);
}
else
{
// insert in middle of tree
var n = FindNode(ref insertionOffset);
Debug.Assert(insertionOffset < n.NodeLength);
// split node segment 'n' at offset
node.TotalNodeLength = node.NodeLength = insertionOffset;
n.NodeLength -= insertionOffset;
InsertBefore(n, node);
}
node.OwnerTree = this;
Count++;
CheckProperties();
}
private void InsertBefore(TextSegment node, TextSegment newNode)
{
if (node.Left == null)
{
InsertAsLeft(node, newNode);
}
else
{
InsertAsRight(node.Left.RightMost, newNode);
}
}
#endregion
#region GetNextSegment / GetPreviousSegment
/// <summary>
/// Gets the next segment after the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the last segment.
/// </summary>
public T GetNextSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Successor;
}
/// <summary>
/// Gets the previous segment before the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the first segment.
/// </summary>
public T GetPreviousSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Predecessor;
}
#endregion
#region FirstSegment/LastSegment
/// <summary>
/// Returns the first segment in the collection or null, if the collection is empty.
/// </summary>
public T FirstSegment => (T)_root?.LeftMost;
/// <summary>
/// Returns the last segment in the collection or null, if the collection is empty.
/// </summary>
public T LastSegment => (T)_root?.RightMost;
#endregion
#region FindFirstSegmentWithStartAfter
/// <summary>
/// Gets the first segment with a start offset greater or equal to <paramref name="startOffset"/>.
/// Returns null if no such segment is found.
/// </summary>
public T FindFirstSegmentWithStartAfter(int startOffset)
{
if (_root == null)
return null;
if (startOffset <= 0)
return (T)_root.LeftMost;
var s = FindNode(ref startOffset);
// startOffset means that the previous segment is starting at the offset we were looking for
while (startOffset == 0)
{
var p = (s == null) ? _root.RightMost : s.Predecessor;
// There must always be a predecessor: if we were looking for the first node, we would have already
// returned it as root.LeftMost above.
Debug.Assert(p != null);
startOffset += p.NodeLength;
s = p;
}
return (T)s;
}
/// <summary>
/// Finds the node at the specified offset.
/// After the method has run, offset is relative to the beginning of the returned node.
/// </summary>
private TextSegment FindNode(ref int offset)
{
var n = _root;
while (true)
{
if (n.Left != null)
{
if (offset < n.Left.TotalNodeLength)
{
n = n.Left; // descend into left subtree
continue;
}
else
{
offset -= n.Left.TotalNodeLength; // skip left subtree
}
}
if (offset < n.NodeLength)
{
return n; // found correct node
}
else
{
offset -= n.NodeLength; // skip this node
}
if (n.Right != null)
{
n = n.Right; // descend into right subtree
}
else
{
// didn't find any node containing the offset
return null;
}
}
}
#endregion
#region FindOverlappingSegments
/// <summary>
/// Finds all segments that contain the given offset.
/// (StartOffset <= offset <= EndOffset)
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindSegmentsContaining(int offset)
{
return FindOverlappingSegments(offset, 0);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(ISegment segment)
{
if (segment == null)
throw new ArgumentNullException(nameof(segment));
return FindOverlappingSegments(segment.Offset, segment.Length);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(int offset, int length)
{
ThrowUtil.CheckNotNegative(length, "length");
var results = new List<T>();
if (_root != null)
{
FindOverlappingSegments(results, _root, offset, offset + length);
}
return new ReadOnlyCollection<T>(results);
}
private void FindOverlappingSegments(List<T> results, TextSegment node, int low, int high)
{
// low and high are relative to node.LeftMost startpos (not node.LeftMost.Offset)
if (high < 0)
{
// node is irrelevant for search because all intervals in node are after high
return;
}
// find values relative to node.Offset
var nodeLow = low - node.NodeLength;
var nodeHigh = high - node.NodeLength;
if (node.Left != null)
{
nodeLow -= node.Left.TotalNodeLength;
nodeHigh -= node.Left.TotalNodeLength;
}
if (node.DistanceToMaxEnd < nodeLow)
{
// node is irrelevant for search because all intervals in node are before low
return;
}
if (node.Left != null)
FindOverlappingSegments(results, node.Left, low, high);
if (nodeHigh < 0)
{
// node and everything in node.right is before low
return;
}
if (nodeLow <= node.SegmentLength)
{
results.Add((T)node);
}
if (node.Right != null)
FindOverlappingSegments(results, node.Right, nodeLow, nodeHigh);
}
#endregion
#region UpdateAugmentedData
private void UpdateAugmentedData(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
totalLength += node.Left.TotalNodeLength;
var leftDtme = node.Left.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
if (node.Left.Right != null)
leftDtme -= node.Left.Right.TotalNodeLength;
leftDtme -= node.NodeLength;
if (leftDtme > distanceToMaxEnd)
distanceToMaxEnd = leftDtme;
}
if (node.Right != null)
{
totalLength += node.Right.TotalNodeLength;
var rightDtme = node.Right.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
rightDtme += node.Right.NodeLength;
if (node.Right.Left != null)
rightDtme += node.Right.Left.TotalNodeLength;
if (rightDtme > distanceToMaxEnd)
distanceToMaxEnd = rightDtme;
}
if (node.TotalNodeLength != totalLength
|| node.DistanceToMaxEnd != distanceToMaxEnd)
{
node.TotalNodeLength = totalLength;
node.DistanceToMaxEnd = distanceToMaxEnd;
if (node.Parent != null)
UpdateAugmentedData(node.Parent);
}
}
void ISegmentTree.UpdateAugmentedData(TextSegment node)
{
UpdateAugmentedData(node);
}
#endregion
#region Remove
/// <summary>
/// Removes the specified segment from the tree. This will cause the segment to not update
/// anymore when the document changes.
/// </summary>
public bool Remove(T item)
{
if (!Contains(item))
return false;
RemoveSegment(item);
return true;
}
void ISegmentTree.Remove(TextSegment s)
{
RemoveSegment(s);
}
private void RemoveSegment(TextSegment s)
{
var oldOffset = s.StartOffset;
var successor = s.Successor;
if (successor != null)
successor.NodeLength += s.NodeLength;
RemoveNode(s);
if (successor != null)
UpdateAugmentedData(successor);
Disconnect(s, oldOffset);
CheckProperties();
}
private void Disconnect(TextSegment s, int offset)
{
s.Left = s.Right = s.Parent = null;
s.OwnerTree = null;
s.NodeLength = offset;
Count--;
}
/// <summary>
/// Removes all segments from the tree.
/// </summary>
public void Clear()
{
var segments = this.ToArray();
_root = null;
var offset = 0;
foreach (var s in segments)
{
offset += s.NodeLength;
Disconnect(s, offset);
}
CheckProperties();
}
#endregion
#region CheckProperties
[Conditional("DATACONSISTENCYTEST")]
internal void CheckProperties()
{
#if DEBUG
if (_root != null)
{
CheckProperties(_root);
// check red-black property:
var blackCount = -1;
CheckNodeProperties(_root, null, Red, 0, ref blackCount);
}
var expectedCount = 0;
// we cannot trust LINQ not to call ICollection.Count, so we need this loop
// to count the elements in the tree
using (var en = GetEnumerator())
{
while (en.MoveNext()) expectedCount++;
}
Debug.Assert(Count == expectedCount);
#endif
}
#if DEBUG
private void CheckProperties(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
CheckProperties(node.Left);
totalLength += node.Left.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Left.DistanceToMaxEnd + node.Left.StartOffset - node.StartOffset);
}
if (node.Right != null)
{
CheckProperties(node.Right);
totalLength += node.Right.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Right.DistanceToMaxEnd + node.Right.StartOffset - node.StartOffset);
}
Debug.Assert(node.TotalNodeLength == totalLength);
Debug.Assert(node.DistanceToMaxEnd == distanceToMaxEnd);
}
/*
1. A node is either red or black.
2. The root is black.
3. All leaves are black. (The leaves are the NIL children.)
4. Both children of every red node are black. (So every red node must have a black parent.)
5. Every simple path from a node to a descendant leaf contains the same number of black nodes. (Not counting the leaf node.)
*/
[SuppressMessage("ReSharper", "UnusedParameter.Local")]
private static void CheckNodeProperties(TextSegment node, TextSegment parentNode, bool parentColor, int blackCount, ref int expectedBlackCount)
{
if (node == null) return;
Debug.Assert(node.Parent == parentNode);
if (parentColor == Red)
{
Debug.Assert(node.Color == Black);
}
if (node.Color == Black)
{
blackCount++;
}
if (node.Left == null && node.Right == null)
{
// node is a leaf node:
if (expectedBlackCount == -1)
expectedBlackCount = blackCount;
else
Debug.Assert(expectedBlackCount == blackCount);
}
CheckNodeProperties(node.Left, node, node.Color, blackCount, ref expectedBlackCount);
CheckNodeProperties(node.Right, node, node.Color, blackCount, ref expectedBlackCount);
}
private static void AppendTreeToString(TextSegment node, StringBuilder b, int indent)
{
b.Append(node.Color == Red ? "RED " : "BLACK ");
b.AppendLine(node + node.ToDebugString());
indent += 2;
if (node.Left != null)
{
b.Append(' ', indent);
b.Append("L: ");
AppendTreeToString(node.Left, b, indent);
}
if (node.Right != null)
{
b.Append(' ', indent);
b.Append("R: ");
AppendTreeToString(node.Right, b, indent);
}
}
#endif
internal string GetTreeAsString()
{
#if DEBUG
var b = new StringBuilder();
if (_root != null)
AppendTreeToString(_root, b, 0);
return b.ToString();
#else
return "Not available in release build.";
#endif
}
#endregion
#region Red/Black Tree
internal const bool Red = true;
internal const bool Black = false;
private void InsertAsLeft(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Left == null);
parentNode.Left = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void InsertAsRight(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Right == null);
parentNode.Right = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void FixTreeOnInsert(TextSegment node)
{
Debug.Assert(node != null);
Debug.Assert(node.Color == Red);
Debug.Assert(node.Left == null || node.Left.Color == Black);
Debug.Assert(node.Right == null || node.Right.Color == Black);
var parentNode = node.Parent;
if (parentNode == null)
{
// we inserted in the root -> the node must be black
// since this is a root node, making the node black increments the number of black nodes
// on all paths by one, so it is still the same for all paths.
node.Color = Black;
return;
}
if (parentNode.Color == Black)
{
// if the parent node where we inserted was black, our red node is placed correctly.
// since we inserted a red node, the number of black nodes on each path is unchanged
// -> the tree is still balanced
return;
}
// parentNode is red, so there is a conflict here!
// because the root is black, parentNode is not the root -> there is a grandparent node
var grandparentNode = parentNode.Parent;
var uncleNode = Sibling(parentNode);
if (uncleNode != null && uncleNode.Color == Red)
{
parentNode.Color = Black;
uncleNode.Color = Black;
grandparentNode.Color = Red;
FixTreeOnInsert(grandparentNode);
return;
}
// now we know: parent is red but uncle is black
// First rotation:
if (node == parentNode.Right && parentNode == grandparentNode.Left)
{
RotateLeft(parentNode);
node = node.Left;
}
else if (node == parentNode.Left && parentNode == grandparentNode.Right)
{
RotateRight(parentNode);
node = node.Right;
}
// because node might have changed, reassign variables:
// ReSharper disable once PossibleNullReferenceException
parentNode = node.Parent;
grandparentNode = parentNode.Parent;
// Now recolor a bit:
parentNode.Color = Black;
grandparentNode.Color = Red;
// Second rotation:
if (node == parentNode.Left && parentNode == grandparentNode.Left)
{
RotateRight(grandparentNode);
}
else
{
// because of the first rotation, this is guaranteed:
Debug.Assert(node == parentNode.Right && parentNode == grandparentNode.Right);
RotateLeft(grandparentNode);
}
}
private void RemoveNode(TextSegment removedNode)
{
if (removedNode.Left != null && removedNode.Right != null)
{
// replace removedNode with it's in-order successor
var leftMost = removedNode.Right.LeftMost;
RemoveNode(leftMost); // remove leftMost from its current location
// and overwrite the removedNode with it
ReplaceNode(removedNode, leftMost);
leftMost.Left = removedNode.Left;
if (leftMost.Left != null) leftMost.Left.Parent = leftMost;
leftMost.Right = removedNode.Right;
if (leftMost.Right != null) leftMost.Right.Parent = leftMost;
leftMost.Color = removedNode.Color;
UpdateAugmentedData(leftMost);
if (leftMost.Parent != null) UpdateAugmentedData(leftMost.Parent);
return;
}
// now either removedNode.left or removedNode.right is null
// get the remaining child
var parentNode = removedNode.Parent;
var childNode = removedNode.Left ?? removedNode.Right;
ReplaceNode(removedNode, childNode);
if (parentNode != null) UpdateAugmentedData(parentNode);
if (removedNode.Color == Black)
{
if (childNode != null && childNode.Color == Red)
{
childNode.Color = Black;
}
else
{
FixTreeOnDelete(childNode, parentNode);
}
}
}
private void FixTreeOnDelete(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (parentNode == null)
return;
// warning: node may be null
var sibling = Sibling(node, parentNode);
if (sibling.Color == Red)
{
parentNode.Color = Red;
sibling.Color = Black;
if (node == parentNode.Left)
{
RotateLeft(parentNode);
}
else
{
RotateRight(parentNode);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
}
if (parentNode.Color == Black
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
FixTreeOnDelete(parentNode, parentNode.Parent);
return;
}
if (parentNode.Color == Red
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
parentNode.Color = Black;
return;
}
if (node == parentNode.Left &&
sibling.Color == Black &&
GetColor(sibling.Left) == Red &&
GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
sibling.Left.Color = Black;
RotateRight(sibling);
}
else if (node == parentNode.Right &&
sibling.Color == Black &&
GetColor(sibling.Right) == Red &&
GetColor(sibling.Left) == Black)
{
sibling.Color = Red;
sibling.Right.Color = Black;
RotateLeft(sibling);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
sibling.Color = parentNode.Color;
parentNode.Color = Black;
if (node == parentNode.Left)
{
if (sibling.Right != null)
{
Debug.Assert(sibling.Right.Color == Red);
sibling.Right.Color = Black;
}
RotateLeft(parentNode);
}
else
{
if (sibling.Left != null)
{
Debug.Assert(sibling.Left.Color == Red);
sibling.Left.Color = Black;
}
RotateRight(parentNode);
}
}
private void ReplaceNode(TextSegment replacedNode, TextSegment newNode)
{
if (replacedNode.Parent == null)
{
Debug.Assert(replacedNode == _root);
_root = newNode;
}
else
{
if (replacedNode.Parent.Left == replacedNode)
replacedNode.Parent.Left = newNode;
else
replacedNode.Parent.Right = newNode;
}
if (newNode != null)
{
newNode.Parent = replacedNode.Parent;
}
replacedNode.Parent = null;
}
private void RotateLeft(TextSegment p)
{
// let q be p's right child
var q = p.Right;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's right child to be q's left child
p.Right = q.Left;
if (p.Right != null) p.Right.Parent = p;
// set q's left child to be p
q.Left = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private void RotateRight(TextSegment p)
{
// let q be p's left child
var q = p.Left;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's left child to be q's right child
p.Left = q.Right;
if (p.Left != null) p.Left.Parent = p;
// set q's right child to be p
q.Right = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private static TextSegment Sibling(TextSegment node)
{
return node == node.Parent.Left ? node.Parent.Right : node.Parent.Left;
}
private static TextSegment Sibling(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (node == parentNode.Left)
return parentNode.Right;
else
return parentNode.Left;
}
private static bool GetColor(TextSegment node)
{
return node != null && node.Color;
}
#endregion
#region ICollection<T> implementation
/// <summary>
/// Gets the number of segments in the tree.
/// </summary>
public int Count { get; private set; }
bool ICollection<T>.IsReadOnly => false;
/// <summary>
/// Gets whether this tree contains the specified item.
/// </summary>
public bool Contains(T item)
{
return item != null && item.OwnerTree == this;
}
/// <summary>
/// Copies all segments in this SegmentTree to the specified array.
/// </summary>
public void CopyTo(T[] array, int arrayIndex)
{
if (array == null)
throw new ArgumentNullException(nameof(array));
if (array.Length < Count)
throw new ArgumentException("The array is too small", nameof(array));
if (arrayIndex < 0 || arrayIndex + Count > array.Length)
throw new ArgumentOutOfRangeException(nameof(arrayIndex), arrayIndex, "Value must be between 0 and " + (array.Length - Count));
foreach (var s in this)
{
array[arrayIndex++] = s;
}
}
/// <summary>
/// Gets an enumerator to enumerate the segments.
/// </summary>
public IEnumerator<T> GetEnumerator()
{
if (_root != null)
{
var current = _root.LeftMost;
while (current != null)
{
yield return (T)current;
// TODO: check if collection was modified during enumeration
current = current.Successor;
}
}
}
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
#endregion
}
}
<MSG> Allow a text segment collection to be disconnected from a text document.
<DFF> @@ -97,6 +97,14 @@ namespace AvaloniaEdit.Document
}
#endregion
+ public void Disconnect (TextDocument textDocument)
+ {
+ if (_isConnectedToDocument)
+ {
+ TextDocumentWeakEventManager.Changed.RemoveHandler(textDocument, OnDocumentChanged);
+ }
+ }
+
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
| 8 | Allow a text segment collection to be disconnected from a text document. | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063831 | <NME> TextSegmentCollection.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using System.Text;
using AvaloniaEdit.Utils;
using Avalonia.Threading;
namespace AvaloniaEdit.Document
{
/// <summary>
/// Interface to allow TextSegments to access the TextSegmentCollection - we cannot use a direct reference
/// because TextSegmentCollection is generic.
/// </summary>
internal interface ISegmentTree
{
void Add(TextSegment s);
void Remove(TextSegment s);
void UpdateAugmentedData(TextSegment s);
}
/// <summary>
/// <para>
/// A collection of text segments that supports efficient lookup of segments
/// intersecting with another segment.
/// </para>
/// </summary>
/// <remarks><inheritdoc cref="TextSegment"/></remarks>
/// <see cref="TextSegment"/>
public sealed class TextSegmentCollection<T> : ICollection<T>, ISegmentTree where T : TextSegment
{
// Implementation: this is basically a mixture of an augmented interval tree
// and the TextAnchorTree.
// WARNING: you need to understand interval trees (the version with the augmented 'high'/'max' field)
// and how the TextAnchorTree works before you have any chance of understanding this code.
// This means that every node holds two "segments":
// one like the segments in the text anchor tree to support efficient offset changes
// and another that is the interval as seen by the user
// So basically, the tree contains a list of contiguous node segments of the first kind,
// with interval segments starting at the end of every node segment.
// Performance:
// Add is O(lg n)
// Remove is O(lg n)
// DocumentChanged is O(m * lg n), with m the number of segments that intersect with the changed document section
// FindFirstSegmentWithStartAfter is O(m + lg n) with m being the number of segments at the same offset as the result segment
// FindIntersectingSegments is O(m + lg n) with m being the number of intersecting segments.
private TextSegment _root;
private readonly bool _isConnectedToDocument;
#region Constructor
/// <summary>
/// Creates a new TextSegmentCollection that needs manual calls to <see cref="UpdateOffsets(DocumentChangeEventArgs)"/>.
/// </summary>
public TextSegmentCollection()
{
}
/// <summary>
/// Creates a new TextSegmentCollection that updates the offsets automatically.
/// </summary>
/// <param name="textDocument">The document to which the text segments
/// that will be added to the tree belong. When the document changes, the
/// position of the text segments will be updated accordingly.</param>
public TextSegmentCollection(TextDocument textDocument)
{
if (textDocument == null)
throw new ArgumentNullException(nameof(textDocument));
Dispatcher.UIThread.VerifyAccess();
_isConnectedToDocument = true;
TextDocumentWeakEventManager.Changed.AddHandler(textDocument, OnDocumentChanged);
}
#endregion
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="e">DocumentChangeEventArgs instance describing the change to the document.</param>
public void UpdateOffsets(DocumentChangeEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
OnDocumentChanged(this, e);
CheckProperties();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
var map = e.OffsetChangeMapOrNull;
if (map != null)
{
foreach (var entry in map)
{
UpdateOffsetsInternal(entry);
}
}
else
{
UpdateOffsetsInternal(e.CreateSingleChangeMapEntry());
}
}
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="change">OffsetChangeMapEntry instance describing the change to the document.</param>
public void UpdateOffsets(OffsetChangeMapEntry change)
{
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
UpdateOffsetsInternal(change);
CheckProperties();
}
#endregion
#region UpdateOffsets (implementation)
private void UpdateOffsetsInternal(OffsetChangeMapEntry change)
{
// Special case pure insertions, because they don't always cause a text segment to increase in size when the replaced region
// is inside a segment (when offset is at start or end of a text semgent).
if (change.RemovalLength == 0)
{
InsertText(change.Offset, change.InsertionLength);
}
else
{
ReplaceText(change);
}
}
private void InsertText(int offset, int length)
{
if (length == 0)
return;
// enlarge segments that contain offset (excluding those that have offset as endpoint)
foreach (var segment in FindSegmentsContaining(offset))
{
if (segment.StartOffset < offset && offset < segment.EndOffset)
{
segment.Length += length;
}
}
// move start offsets of all segments >= offset
TextSegment node = FindFirstSegmentWithStartAfter(offset);
if (node != null)
{
node.NodeLength += length;
UpdateAugmentedData(node);
}
}
private void ReplaceText(OffsetChangeMapEntry change)
{
Debug.Assert(change.RemovalLength > 0);
var offset = change.Offset;
foreach (var segment in FindOverlappingSegments(offset, change.RemovalLength))
{
if (segment.StartOffset <= offset)
{
if (segment.EndOffset >= offset + change.RemovalLength)
{
// Replacement inside segment: adjust segment length
segment.Length += change.InsertionLength - change.RemovalLength;
}
else
{
// Replacement starting inside segment and ending after segment end: set segment end to removal position
//segment.EndOffset = offset;
segment.Length = offset - segment.StartOffset;
}
}
else
{
// Replacement starting in front of text segment and running into segment.
// Keep segment.EndOffset constant and move segment.StartOffset to the end of the replacement
var remainingLength = segment.EndOffset - (offset + change.RemovalLength);
RemoveSegment(segment);
segment.StartOffset = offset + change.RemovalLength;
segment.Length = Math.Max(0, remainingLength);
AddSegment(segment);
}
}
// move start offsets of all segments > offset
TextSegment node = FindFirstSegmentWithStartAfter(offset + 1);
if (node != null)
{
Debug.Assert(node.NodeLength >= change.RemovalLength);
node.NodeLength += change.InsertionLength - change.RemovalLength;
UpdateAugmentedData(node);
}
}
#endregion
#region Add
/// <summary>
/// Adds the specified segment to the tree. This will cause the segment to update when the
/// document changes.
/// </summary>
public void Add(T item)
{
if (item == null)
throw new ArgumentNullException(nameof(item));
if (item.OwnerTree != null)
throw new ArgumentException("The segment is already added to a SegmentCollection.");
AddSegment(item);
}
void ISegmentTree.Add(TextSegment s)
{
AddSegment(s);
}
private void AddSegment(TextSegment node)
{
var insertionOffset = node.StartOffset;
node.DistanceToMaxEnd = node.SegmentLength;
if (_root == null)
{
_root = node;
node.TotalNodeLength = node.NodeLength;
}
else if (insertionOffset >= _root.TotalNodeLength)
{
// append segment at end of tree
node.NodeLength = node.TotalNodeLength = insertionOffset - _root.TotalNodeLength;
InsertAsRight(_root.RightMost, node);
}
else
{
// insert in middle of tree
var n = FindNode(ref insertionOffset);
Debug.Assert(insertionOffset < n.NodeLength);
// split node segment 'n' at offset
node.TotalNodeLength = node.NodeLength = insertionOffset;
n.NodeLength -= insertionOffset;
InsertBefore(n, node);
}
node.OwnerTree = this;
Count++;
CheckProperties();
}
private void InsertBefore(TextSegment node, TextSegment newNode)
{
if (node.Left == null)
{
InsertAsLeft(node, newNode);
}
else
{
InsertAsRight(node.Left.RightMost, newNode);
}
}
#endregion
#region GetNextSegment / GetPreviousSegment
/// <summary>
/// Gets the next segment after the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the last segment.
/// </summary>
public T GetNextSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Successor;
}
/// <summary>
/// Gets the previous segment before the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the first segment.
/// </summary>
public T GetPreviousSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Predecessor;
}
#endregion
#region FirstSegment/LastSegment
/// <summary>
/// Returns the first segment in the collection or null, if the collection is empty.
/// </summary>
public T FirstSegment => (T)_root?.LeftMost;
/// <summary>
/// Returns the last segment in the collection or null, if the collection is empty.
/// </summary>
public T LastSegment => (T)_root?.RightMost;
#endregion
#region FindFirstSegmentWithStartAfter
/// <summary>
/// Gets the first segment with a start offset greater or equal to <paramref name="startOffset"/>.
/// Returns null if no such segment is found.
/// </summary>
public T FindFirstSegmentWithStartAfter(int startOffset)
{
if (_root == null)
return null;
if (startOffset <= 0)
return (T)_root.LeftMost;
var s = FindNode(ref startOffset);
// startOffset means that the previous segment is starting at the offset we were looking for
while (startOffset == 0)
{
var p = (s == null) ? _root.RightMost : s.Predecessor;
// There must always be a predecessor: if we were looking for the first node, we would have already
// returned it as root.LeftMost above.
Debug.Assert(p != null);
startOffset += p.NodeLength;
s = p;
}
return (T)s;
}
/// <summary>
/// Finds the node at the specified offset.
/// After the method has run, offset is relative to the beginning of the returned node.
/// </summary>
private TextSegment FindNode(ref int offset)
{
var n = _root;
while (true)
{
if (n.Left != null)
{
if (offset < n.Left.TotalNodeLength)
{
n = n.Left; // descend into left subtree
continue;
}
else
{
offset -= n.Left.TotalNodeLength; // skip left subtree
}
}
if (offset < n.NodeLength)
{
return n; // found correct node
}
else
{
offset -= n.NodeLength; // skip this node
}
if (n.Right != null)
{
n = n.Right; // descend into right subtree
}
else
{
// didn't find any node containing the offset
return null;
}
}
}
#endregion
#region FindOverlappingSegments
/// <summary>
/// Finds all segments that contain the given offset.
/// (StartOffset <= offset <= EndOffset)
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindSegmentsContaining(int offset)
{
return FindOverlappingSegments(offset, 0);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(ISegment segment)
{
if (segment == null)
throw new ArgumentNullException(nameof(segment));
return FindOverlappingSegments(segment.Offset, segment.Length);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(int offset, int length)
{
ThrowUtil.CheckNotNegative(length, "length");
var results = new List<T>();
if (_root != null)
{
FindOverlappingSegments(results, _root, offset, offset + length);
}
return new ReadOnlyCollection<T>(results);
}
private void FindOverlappingSegments(List<T> results, TextSegment node, int low, int high)
{
// low and high are relative to node.LeftMost startpos (not node.LeftMost.Offset)
if (high < 0)
{
// node is irrelevant for search because all intervals in node are after high
return;
}
// find values relative to node.Offset
var nodeLow = low - node.NodeLength;
var nodeHigh = high - node.NodeLength;
if (node.Left != null)
{
nodeLow -= node.Left.TotalNodeLength;
nodeHigh -= node.Left.TotalNodeLength;
}
if (node.DistanceToMaxEnd < nodeLow)
{
// node is irrelevant for search because all intervals in node are before low
return;
}
if (node.Left != null)
FindOverlappingSegments(results, node.Left, low, high);
if (nodeHigh < 0)
{
// node and everything in node.right is before low
return;
}
if (nodeLow <= node.SegmentLength)
{
results.Add((T)node);
}
if (node.Right != null)
FindOverlappingSegments(results, node.Right, nodeLow, nodeHigh);
}
#endregion
#region UpdateAugmentedData
private void UpdateAugmentedData(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
totalLength += node.Left.TotalNodeLength;
var leftDtme = node.Left.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
if (node.Left.Right != null)
leftDtme -= node.Left.Right.TotalNodeLength;
leftDtme -= node.NodeLength;
if (leftDtme > distanceToMaxEnd)
distanceToMaxEnd = leftDtme;
}
if (node.Right != null)
{
totalLength += node.Right.TotalNodeLength;
var rightDtme = node.Right.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
rightDtme += node.Right.NodeLength;
if (node.Right.Left != null)
rightDtme += node.Right.Left.TotalNodeLength;
if (rightDtme > distanceToMaxEnd)
distanceToMaxEnd = rightDtme;
}
if (node.TotalNodeLength != totalLength
|| node.DistanceToMaxEnd != distanceToMaxEnd)
{
node.TotalNodeLength = totalLength;
node.DistanceToMaxEnd = distanceToMaxEnd;
if (node.Parent != null)
UpdateAugmentedData(node.Parent);
}
}
void ISegmentTree.UpdateAugmentedData(TextSegment node)
{
UpdateAugmentedData(node);
}
#endregion
#region Remove
/// <summary>
/// Removes the specified segment from the tree. This will cause the segment to not update
/// anymore when the document changes.
/// </summary>
public bool Remove(T item)
{
if (!Contains(item))
return false;
RemoveSegment(item);
return true;
}
void ISegmentTree.Remove(TextSegment s)
{
RemoveSegment(s);
}
private void RemoveSegment(TextSegment s)
{
var oldOffset = s.StartOffset;
var successor = s.Successor;
if (successor != null)
successor.NodeLength += s.NodeLength;
RemoveNode(s);
if (successor != null)
UpdateAugmentedData(successor);
Disconnect(s, oldOffset);
CheckProperties();
}
private void Disconnect(TextSegment s, int offset)
{
s.Left = s.Right = s.Parent = null;
s.OwnerTree = null;
s.NodeLength = offset;
Count--;
}
/// <summary>
/// Removes all segments from the tree.
/// </summary>
public void Clear()
{
var segments = this.ToArray();
_root = null;
var offset = 0;
foreach (var s in segments)
{
offset += s.NodeLength;
Disconnect(s, offset);
}
CheckProperties();
}
#endregion
#region CheckProperties
[Conditional("DATACONSISTENCYTEST")]
internal void CheckProperties()
{
#if DEBUG
if (_root != null)
{
CheckProperties(_root);
// check red-black property:
var blackCount = -1;
CheckNodeProperties(_root, null, Red, 0, ref blackCount);
}
var expectedCount = 0;
// we cannot trust LINQ not to call ICollection.Count, so we need this loop
// to count the elements in the tree
using (var en = GetEnumerator())
{
while (en.MoveNext()) expectedCount++;
}
Debug.Assert(Count == expectedCount);
#endif
}
#if DEBUG
private void CheckProperties(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
CheckProperties(node.Left);
totalLength += node.Left.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Left.DistanceToMaxEnd + node.Left.StartOffset - node.StartOffset);
}
if (node.Right != null)
{
CheckProperties(node.Right);
totalLength += node.Right.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Right.DistanceToMaxEnd + node.Right.StartOffset - node.StartOffset);
}
Debug.Assert(node.TotalNodeLength == totalLength);
Debug.Assert(node.DistanceToMaxEnd == distanceToMaxEnd);
}
/*
1. A node is either red or black.
2. The root is black.
3. All leaves are black. (The leaves are the NIL children.)
4. Both children of every red node are black. (So every red node must have a black parent.)
5. Every simple path from a node to a descendant leaf contains the same number of black nodes. (Not counting the leaf node.)
*/
[SuppressMessage("ReSharper", "UnusedParameter.Local")]
private static void CheckNodeProperties(TextSegment node, TextSegment parentNode, bool parentColor, int blackCount, ref int expectedBlackCount)
{
if (node == null) return;
Debug.Assert(node.Parent == parentNode);
if (parentColor == Red)
{
Debug.Assert(node.Color == Black);
}
if (node.Color == Black)
{
blackCount++;
}
if (node.Left == null && node.Right == null)
{
// node is a leaf node:
if (expectedBlackCount == -1)
expectedBlackCount = blackCount;
else
Debug.Assert(expectedBlackCount == blackCount);
}
CheckNodeProperties(node.Left, node, node.Color, blackCount, ref expectedBlackCount);
CheckNodeProperties(node.Right, node, node.Color, blackCount, ref expectedBlackCount);
}
private static void AppendTreeToString(TextSegment node, StringBuilder b, int indent)
{
b.Append(node.Color == Red ? "RED " : "BLACK ");
b.AppendLine(node + node.ToDebugString());
indent += 2;
if (node.Left != null)
{
b.Append(' ', indent);
b.Append("L: ");
AppendTreeToString(node.Left, b, indent);
}
if (node.Right != null)
{
b.Append(' ', indent);
b.Append("R: ");
AppendTreeToString(node.Right, b, indent);
}
}
#endif
internal string GetTreeAsString()
{
#if DEBUG
var b = new StringBuilder();
if (_root != null)
AppendTreeToString(_root, b, 0);
return b.ToString();
#else
return "Not available in release build.";
#endif
}
#endregion
#region Red/Black Tree
internal const bool Red = true;
internal const bool Black = false;
private void InsertAsLeft(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Left == null);
parentNode.Left = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void InsertAsRight(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Right == null);
parentNode.Right = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void FixTreeOnInsert(TextSegment node)
{
Debug.Assert(node != null);
Debug.Assert(node.Color == Red);
Debug.Assert(node.Left == null || node.Left.Color == Black);
Debug.Assert(node.Right == null || node.Right.Color == Black);
var parentNode = node.Parent;
if (parentNode == null)
{
// we inserted in the root -> the node must be black
// since this is a root node, making the node black increments the number of black nodes
// on all paths by one, so it is still the same for all paths.
node.Color = Black;
return;
}
if (parentNode.Color == Black)
{
// if the parent node where we inserted was black, our red node is placed correctly.
// since we inserted a red node, the number of black nodes on each path is unchanged
// -> the tree is still balanced
return;
}
// parentNode is red, so there is a conflict here!
// because the root is black, parentNode is not the root -> there is a grandparent node
var grandparentNode = parentNode.Parent;
var uncleNode = Sibling(parentNode);
if (uncleNode != null && uncleNode.Color == Red)
{
parentNode.Color = Black;
uncleNode.Color = Black;
grandparentNode.Color = Red;
FixTreeOnInsert(grandparentNode);
return;
}
// now we know: parent is red but uncle is black
// First rotation:
if (node == parentNode.Right && parentNode == grandparentNode.Left)
{
RotateLeft(parentNode);
node = node.Left;
}
else if (node == parentNode.Left && parentNode == grandparentNode.Right)
{
RotateRight(parentNode);
node = node.Right;
}
// because node might have changed, reassign variables:
// ReSharper disable once PossibleNullReferenceException
parentNode = node.Parent;
grandparentNode = parentNode.Parent;
// Now recolor a bit:
parentNode.Color = Black;
grandparentNode.Color = Red;
// Second rotation:
if (node == parentNode.Left && parentNode == grandparentNode.Left)
{
RotateRight(grandparentNode);
}
else
{
// because of the first rotation, this is guaranteed:
Debug.Assert(node == parentNode.Right && parentNode == grandparentNode.Right);
RotateLeft(grandparentNode);
}
}
private void RemoveNode(TextSegment removedNode)
{
if (removedNode.Left != null && removedNode.Right != null)
{
// replace removedNode with it's in-order successor
var leftMost = removedNode.Right.LeftMost;
RemoveNode(leftMost); // remove leftMost from its current location
// and overwrite the removedNode with it
ReplaceNode(removedNode, leftMost);
leftMost.Left = removedNode.Left;
if (leftMost.Left != null) leftMost.Left.Parent = leftMost;
leftMost.Right = removedNode.Right;
if (leftMost.Right != null) leftMost.Right.Parent = leftMost;
leftMost.Color = removedNode.Color;
UpdateAugmentedData(leftMost);
if (leftMost.Parent != null) UpdateAugmentedData(leftMost.Parent);
return;
}
// now either removedNode.left or removedNode.right is null
// get the remaining child
var parentNode = removedNode.Parent;
var childNode = removedNode.Left ?? removedNode.Right;
ReplaceNode(removedNode, childNode);
if (parentNode != null) UpdateAugmentedData(parentNode);
if (removedNode.Color == Black)
{
if (childNode != null && childNode.Color == Red)
{
childNode.Color = Black;
}
else
{
FixTreeOnDelete(childNode, parentNode);
}
}
}
private void FixTreeOnDelete(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (parentNode == null)
return;
// warning: node may be null
var sibling = Sibling(node, parentNode);
if (sibling.Color == Red)
{
parentNode.Color = Red;
sibling.Color = Black;
if (node == parentNode.Left)
{
RotateLeft(parentNode);
}
else
{
RotateRight(parentNode);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
}
if (parentNode.Color == Black
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
FixTreeOnDelete(parentNode, parentNode.Parent);
return;
}
if (parentNode.Color == Red
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
parentNode.Color = Black;
return;
}
if (node == parentNode.Left &&
sibling.Color == Black &&
GetColor(sibling.Left) == Red &&
GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
sibling.Left.Color = Black;
RotateRight(sibling);
}
else if (node == parentNode.Right &&
sibling.Color == Black &&
GetColor(sibling.Right) == Red &&
GetColor(sibling.Left) == Black)
{
sibling.Color = Red;
sibling.Right.Color = Black;
RotateLeft(sibling);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
sibling.Color = parentNode.Color;
parentNode.Color = Black;
if (node == parentNode.Left)
{
if (sibling.Right != null)
{
Debug.Assert(sibling.Right.Color == Red);
sibling.Right.Color = Black;
}
RotateLeft(parentNode);
}
else
{
if (sibling.Left != null)
{
Debug.Assert(sibling.Left.Color == Red);
sibling.Left.Color = Black;
}
RotateRight(parentNode);
}
}
private void ReplaceNode(TextSegment replacedNode, TextSegment newNode)
{
if (replacedNode.Parent == null)
{
Debug.Assert(replacedNode == _root);
_root = newNode;
}
else
{
if (replacedNode.Parent.Left == replacedNode)
replacedNode.Parent.Left = newNode;
else
replacedNode.Parent.Right = newNode;
}
if (newNode != null)
{
newNode.Parent = replacedNode.Parent;
}
replacedNode.Parent = null;
}
private void RotateLeft(TextSegment p)
{
// let q be p's right child
var q = p.Right;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's right child to be q's left child
p.Right = q.Left;
if (p.Right != null) p.Right.Parent = p;
// set q's left child to be p
q.Left = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private void RotateRight(TextSegment p)
{
// let q be p's left child
var q = p.Left;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's left child to be q's right child
p.Left = q.Right;
if (p.Left != null) p.Left.Parent = p;
// set q's right child to be p
q.Right = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private static TextSegment Sibling(TextSegment node)
{
return node == node.Parent.Left ? node.Parent.Right : node.Parent.Left;
}
private static TextSegment Sibling(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (node == parentNode.Left)
return parentNode.Right;
else
return parentNode.Left;
}
private static bool GetColor(TextSegment node)
{
return node != null && node.Color;
}
#endregion
#region ICollection<T> implementation
/// <summary>
/// Gets the number of segments in the tree.
/// </summary>
public int Count { get; private set; }
bool ICollection<T>.IsReadOnly => false;
/// <summary>
/// Gets whether this tree contains the specified item.
/// </summary>
public bool Contains(T item)
{
return item != null && item.OwnerTree == this;
}
/// <summary>
/// Copies all segments in this SegmentTree to the specified array.
/// </summary>
public void CopyTo(T[] array, int arrayIndex)
{
if (array == null)
throw new ArgumentNullException(nameof(array));
if (array.Length < Count)
throw new ArgumentException("The array is too small", nameof(array));
if (arrayIndex < 0 || arrayIndex + Count > array.Length)
throw new ArgumentOutOfRangeException(nameof(arrayIndex), arrayIndex, "Value must be between 0 and " + (array.Length - Count));
foreach (var s in this)
{
array[arrayIndex++] = s;
}
}
/// <summary>
/// Gets an enumerator to enumerate the segments.
/// </summary>
public IEnumerator<T> GetEnumerator()
{
if (_root != null)
{
var current = _root.LeftMost;
while (current != null)
{
yield return (T)current;
// TODO: check if collection was modified during enumeration
current = current.Successor;
}
}
}
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
#endregion
}
}
<MSG> Allow a text segment collection to be disconnected from a text document.
<DFF> @@ -97,6 +97,14 @@ namespace AvaloniaEdit.Document
}
#endregion
+ public void Disconnect (TextDocument textDocument)
+ {
+ if (_isConnectedToDocument)
+ {
+ TextDocumentWeakEventManager.Changed.RemoveHandler(textDocument, OnDocumentChanged);
+ }
+ }
+
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
| 8 | Allow a text segment collection to be disconnected from a text document. | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063832 | <NME> TextSegmentCollection.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using System.Text;
using AvaloniaEdit.Utils;
using Avalonia.Threading;
namespace AvaloniaEdit.Document
{
/// <summary>
/// Interface to allow TextSegments to access the TextSegmentCollection - we cannot use a direct reference
/// because TextSegmentCollection is generic.
/// </summary>
internal interface ISegmentTree
{
void Add(TextSegment s);
void Remove(TextSegment s);
void UpdateAugmentedData(TextSegment s);
}
/// <summary>
/// <para>
/// A collection of text segments that supports efficient lookup of segments
/// intersecting with another segment.
/// </para>
/// </summary>
/// <remarks><inheritdoc cref="TextSegment"/></remarks>
/// <see cref="TextSegment"/>
public sealed class TextSegmentCollection<T> : ICollection<T>, ISegmentTree where T : TextSegment
{
// Implementation: this is basically a mixture of an augmented interval tree
// and the TextAnchorTree.
// WARNING: you need to understand interval trees (the version with the augmented 'high'/'max' field)
// and how the TextAnchorTree works before you have any chance of understanding this code.
// This means that every node holds two "segments":
// one like the segments in the text anchor tree to support efficient offset changes
// and another that is the interval as seen by the user
// So basically, the tree contains a list of contiguous node segments of the first kind,
// with interval segments starting at the end of every node segment.
// Performance:
// Add is O(lg n)
// Remove is O(lg n)
// DocumentChanged is O(m * lg n), with m the number of segments that intersect with the changed document section
// FindFirstSegmentWithStartAfter is O(m + lg n) with m being the number of segments at the same offset as the result segment
// FindIntersectingSegments is O(m + lg n) with m being the number of intersecting segments.
private TextSegment _root;
private readonly bool _isConnectedToDocument;
#region Constructor
/// <summary>
/// Creates a new TextSegmentCollection that needs manual calls to <see cref="UpdateOffsets(DocumentChangeEventArgs)"/>.
/// </summary>
public TextSegmentCollection()
{
}
/// <summary>
/// Creates a new TextSegmentCollection that updates the offsets automatically.
/// </summary>
/// <param name="textDocument">The document to which the text segments
/// that will be added to the tree belong. When the document changes, the
/// position of the text segments will be updated accordingly.</param>
public TextSegmentCollection(TextDocument textDocument)
{
if (textDocument == null)
throw new ArgumentNullException(nameof(textDocument));
Dispatcher.UIThread.VerifyAccess();
_isConnectedToDocument = true;
TextDocumentWeakEventManager.Changed.AddHandler(textDocument, OnDocumentChanged);
}
#endregion
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="e">DocumentChangeEventArgs instance describing the change to the document.</param>
public void UpdateOffsets(DocumentChangeEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
OnDocumentChanged(this, e);
CheckProperties();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
var map = e.OffsetChangeMapOrNull;
if (map != null)
{
foreach (var entry in map)
{
UpdateOffsetsInternal(entry);
}
}
else
{
UpdateOffsetsInternal(e.CreateSingleChangeMapEntry());
}
}
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="change">OffsetChangeMapEntry instance describing the change to the document.</param>
public void UpdateOffsets(OffsetChangeMapEntry change)
{
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
UpdateOffsetsInternal(change);
CheckProperties();
}
#endregion
#region UpdateOffsets (implementation)
private void UpdateOffsetsInternal(OffsetChangeMapEntry change)
{
// Special case pure insertions, because they don't always cause a text segment to increase in size when the replaced region
// is inside a segment (when offset is at start or end of a text semgent).
if (change.RemovalLength == 0)
{
InsertText(change.Offset, change.InsertionLength);
}
else
{
ReplaceText(change);
}
}
private void InsertText(int offset, int length)
{
if (length == 0)
return;
// enlarge segments that contain offset (excluding those that have offset as endpoint)
foreach (var segment in FindSegmentsContaining(offset))
{
if (segment.StartOffset < offset && offset < segment.EndOffset)
{
segment.Length += length;
}
}
// move start offsets of all segments >= offset
TextSegment node = FindFirstSegmentWithStartAfter(offset);
if (node != null)
{
node.NodeLength += length;
UpdateAugmentedData(node);
}
}
private void ReplaceText(OffsetChangeMapEntry change)
{
Debug.Assert(change.RemovalLength > 0);
var offset = change.Offset;
foreach (var segment in FindOverlappingSegments(offset, change.RemovalLength))
{
if (segment.StartOffset <= offset)
{
if (segment.EndOffset >= offset + change.RemovalLength)
{
// Replacement inside segment: adjust segment length
segment.Length += change.InsertionLength - change.RemovalLength;
}
else
{
// Replacement starting inside segment and ending after segment end: set segment end to removal position
//segment.EndOffset = offset;
segment.Length = offset - segment.StartOffset;
}
}
else
{
// Replacement starting in front of text segment and running into segment.
// Keep segment.EndOffset constant and move segment.StartOffset to the end of the replacement
var remainingLength = segment.EndOffset - (offset + change.RemovalLength);
RemoveSegment(segment);
segment.StartOffset = offset + change.RemovalLength;
segment.Length = Math.Max(0, remainingLength);
AddSegment(segment);
}
}
// move start offsets of all segments > offset
TextSegment node = FindFirstSegmentWithStartAfter(offset + 1);
if (node != null)
{
Debug.Assert(node.NodeLength >= change.RemovalLength);
node.NodeLength += change.InsertionLength - change.RemovalLength;
UpdateAugmentedData(node);
}
}
#endregion
#region Add
/// <summary>
/// Adds the specified segment to the tree. This will cause the segment to update when the
/// document changes.
/// </summary>
public void Add(T item)
{
if (item == null)
throw new ArgumentNullException(nameof(item));
if (item.OwnerTree != null)
throw new ArgumentException("The segment is already added to a SegmentCollection.");
AddSegment(item);
}
void ISegmentTree.Add(TextSegment s)
{
AddSegment(s);
}
private void AddSegment(TextSegment node)
{
var insertionOffset = node.StartOffset;
node.DistanceToMaxEnd = node.SegmentLength;
if (_root == null)
{
_root = node;
node.TotalNodeLength = node.NodeLength;
}
else if (insertionOffset >= _root.TotalNodeLength)
{
// append segment at end of tree
node.NodeLength = node.TotalNodeLength = insertionOffset - _root.TotalNodeLength;
InsertAsRight(_root.RightMost, node);
}
else
{
// insert in middle of tree
var n = FindNode(ref insertionOffset);
Debug.Assert(insertionOffset < n.NodeLength);
// split node segment 'n' at offset
node.TotalNodeLength = node.NodeLength = insertionOffset;
n.NodeLength -= insertionOffset;
InsertBefore(n, node);
}
node.OwnerTree = this;
Count++;
CheckProperties();
}
private void InsertBefore(TextSegment node, TextSegment newNode)
{
if (node.Left == null)
{
InsertAsLeft(node, newNode);
}
else
{
InsertAsRight(node.Left.RightMost, newNode);
}
}
#endregion
#region GetNextSegment / GetPreviousSegment
/// <summary>
/// Gets the next segment after the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the last segment.
/// </summary>
public T GetNextSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Successor;
}
/// <summary>
/// Gets the previous segment before the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the first segment.
/// </summary>
public T GetPreviousSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Predecessor;
}
#endregion
#region FirstSegment/LastSegment
/// <summary>
/// Returns the first segment in the collection or null, if the collection is empty.
/// </summary>
public T FirstSegment => (T)_root?.LeftMost;
/// <summary>
/// Returns the last segment in the collection or null, if the collection is empty.
/// </summary>
public T LastSegment => (T)_root?.RightMost;
#endregion
#region FindFirstSegmentWithStartAfter
/// <summary>
/// Gets the first segment with a start offset greater or equal to <paramref name="startOffset"/>.
/// Returns null if no such segment is found.
/// </summary>
public T FindFirstSegmentWithStartAfter(int startOffset)
{
if (_root == null)
return null;
if (startOffset <= 0)
return (T)_root.LeftMost;
var s = FindNode(ref startOffset);
// startOffset means that the previous segment is starting at the offset we were looking for
while (startOffset == 0)
{
var p = (s == null) ? _root.RightMost : s.Predecessor;
// There must always be a predecessor: if we were looking for the first node, we would have already
// returned it as root.LeftMost above.
Debug.Assert(p != null);
startOffset += p.NodeLength;
s = p;
}
return (T)s;
}
/// <summary>
/// Finds the node at the specified offset.
/// After the method has run, offset is relative to the beginning of the returned node.
/// </summary>
private TextSegment FindNode(ref int offset)
{
var n = _root;
while (true)
{
if (n.Left != null)
{
if (offset < n.Left.TotalNodeLength)
{
n = n.Left; // descend into left subtree
continue;
}
else
{
offset -= n.Left.TotalNodeLength; // skip left subtree
}
}
if (offset < n.NodeLength)
{
return n; // found correct node
}
else
{
offset -= n.NodeLength; // skip this node
}
if (n.Right != null)
{
n = n.Right; // descend into right subtree
}
else
{
// didn't find any node containing the offset
return null;
}
}
}
#endregion
#region FindOverlappingSegments
/// <summary>
/// Finds all segments that contain the given offset.
/// (StartOffset <= offset <= EndOffset)
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindSegmentsContaining(int offset)
{
return FindOverlappingSegments(offset, 0);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(ISegment segment)
{
if (segment == null)
throw new ArgumentNullException(nameof(segment));
return FindOverlappingSegments(segment.Offset, segment.Length);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(int offset, int length)
{
ThrowUtil.CheckNotNegative(length, "length");
var results = new List<T>();
if (_root != null)
{
FindOverlappingSegments(results, _root, offset, offset + length);
}
return new ReadOnlyCollection<T>(results);
}
private void FindOverlappingSegments(List<T> results, TextSegment node, int low, int high)
{
// low and high are relative to node.LeftMost startpos (not node.LeftMost.Offset)
if (high < 0)
{
// node is irrelevant for search because all intervals in node are after high
return;
}
// find values relative to node.Offset
var nodeLow = low - node.NodeLength;
var nodeHigh = high - node.NodeLength;
if (node.Left != null)
{
nodeLow -= node.Left.TotalNodeLength;
nodeHigh -= node.Left.TotalNodeLength;
}
if (node.DistanceToMaxEnd < nodeLow)
{
// node is irrelevant for search because all intervals in node are before low
return;
}
if (node.Left != null)
FindOverlappingSegments(results, node.Left, low, high);
if (nodeHigh < 0)
{
// node and everything in node.right is before low
return;
}
if (nodeLow <= node.SegmentLength)
{
results.Add((T)node);
}
if (node.Right != null)
FindOverlappingSegments(results, node.Right, nodeLow, nodeHigh);
}
#endregion
#region UpdateAugmentedData
private void UpdateAugmentedData(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
totalLength += node.Left.TotalNodeLength;
var leftDtme = node.Left.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
if (node.Left.Right != null)
leftDtme -= node.Left.Right.TotalNodeLength;
leftDtme -= node.NodeLength;
if (leftDtme > distanceToMaxEnd)
distanceToMaxEnd = leftDtme;
}
if (node.Right != null)
{
totalLength += node.Right.TotalNodeLength;
var rightDtme = node.Right.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
rightDtme += node.Right.NodeLength;
if (node.Right.Left != null)
rightDtme += node.Right.Left.TotalNodeLength;
if (rightDtme > distanceToMaxEnd)
distanceToMaxEnd = rightDtme;
}
if (node.TotalNodeLength != totalLength
|| node.DistanceToMaxEnd != distanceToMaxEnd)
{
node.TotalNodeLength = totalLength;
node.DistanceToMaxEnd = distanceToMaxEnd;
if (node.Parent != null)
UpdateAugmentedData(node.Parent);
}
}
void ISegmentTree.UpdateAugmentedData(TextSegment node)
{
UpdateAugmentedData(node);
}
#endregion
#region Remove
/// <summary>
/// Removes the specified segment from the tree. This will cause the segment to not update
/// anymore when the document changes.
/// </summary>
public bool Remove(T item)
{
if (!Contains(item))
return false;
RemoveSegment(item);
return true;
}
void ISegmentTree.Remove(TextSegment s)
{
RemoveSegment(s);
}
private void RemoveSegment(TextSegment s)
{
var oldOffset = s.StartOffset;
var successor = s.Successor;
if (successor != null)
successor.NodeLength += s.NodeLength;
RemoveNode(s);
if (successor != null)
UpdateAugmentedData(successor);
Disconnect(s, oldOffset);
CheckProperties();
}
private void Disconnect(TextSegment s, int offset)
{
s.Left = s.Right = s.Parent = null;
s.OwnerTree = null;
s.NodeLength = offset;
Count--;
}
/// <summary>
/// Removes all segments from the tree.
/// </summary>
public void Clear()
{
var segments = this.ToArray();
_root = null;
var offset = 0;
foreach (var s in segments)
{
offset += s.NodeLength;
Disconnect(s, offset);
}
CheckProperties();
}
#endregion
#region CheckProperties
[Conditional("DATACONSISTENCYTEST")]
internal void CheckProperties()
{
#if DEBUG
if (_root != null)
{
CheckProperties(_root);
// check red-black property:
var blackCount = -1;
CheckNodeProperties(_root, null, Red, 0, ref blackCount);
}
var expectedCount = 0;
// we cannot trust LINQ not to call ICollection.Count, so we need this loop
// to count the elements in the tree
using (var en = GetEnumerator())
{
while (en.MoveNext()) expectedCount++;
}
Debug.Assert(Count == expectedCount);
#endif
}
#if DEBUG
private void CheckProperties(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
CheckProperties(node.Left);
totalLength += node.Left.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Left.DistanceToMaxEnd + node.Left.StartOffset - node.StartOffset);
}
if (node.Right != null)
{
CheckProperties(node.Right);
totalLength += node.Right.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Right.DistanceToMaxEnd + node.Right.StartOffset - node.StartOffset);
}
Debug.Assert(node.TotalNodeLength == totalLength);
Debug.Assert(node.DistanceToMaxEnd == distanceToMaxEnd);
}
/*
1. A node is either red or black.
2. The root is black.
3. All leaves are black. (The leaves are the NIL children.)
4. Both children of every red node are black. (So every red node must have a black parent.)
5. Every simple path from a node to a descendant leaf contains the same number of black nodes. (Not counting the leaf node.)
*/
[SuppressMessage("ReSharper", "UnusedParameter.Local")]
private static void CheckNodeProperties(TextSegment node, TextSegment parentNode, bool parentColor, int blackCount, ref int expectedBlackCount)
{
if (node == null) return;
Debug.Assert(node.Parent == parentNode);
if (parentColor == Red)
{
Debug.Assert(node.Color == Black);
}
if (node.Color == Black)
{
blackCount++;
}
if (node.Left == null && node.Right == null)
{
// node is a leaf node:
if (expectedBlackCount == -1)
expectedBlackCount = blackCount;
else
Debug.Assert(expectedBlackCount == blackCount);
}
CheckNodeProperties(node.Left, node, node.Color, blackCount, ref expectedBlackCount);
CheckNodeProperties(node.Right, node, node.Color, blackCount, ref expectedBlackCount);
}
private static void AppendTreeToString(TextSegment node, StringBuilder b, int indent)
{
b.Append(node.Color == Red ? "RED " : "BLACK ");
b.AppendLine(node + node.ToDebugString());
indent += 2;
if (node.Left != null)
{
b.Append(' ', indent);
b.Append("L: ");
AppendTreeToString(node.Left, b, indent);
}
if (node.Right != null)
{
b.Append(' ', indent);
b.Append("R: ");
AppendTreeToString(node.Right, b, indent);
}
}
#endif
internal string GetTreeAsString()
{
#if DEBUG
var b = new StringBuilder();
if (_root != null)
AppendTreeToString(_root, b, 0);
return b.ToString();
#else
return "Not available in release build.";
#endif
}
#endregion
#region Red/Black Tree
internal const bool Red = true;
internal const bool Black = false;
private void InsertAsLeft(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Left == null);
parentNode.Left = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void InsertAsRight(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Right == null);
parentNode.Right = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void FixTreeOnInsert(TextSegment node)
{
Debug.Assert(node != null);
Debug.Assert(node.Color == Red);
Debug.Assert(node.Left == null || node.Left.Color == Black);
Debug.Assert(node.Right == null || node.Right.Color == Black);
var parentNode = node.Parent;
if (parentNode == null)
{
// we inserted in the root -> the node must be black
// since this is a root node, making the node black increments the number of black nodes
// on all paths by one, so it is still the same for all paths.
node.Color = Black;
return;
}
if (parentNode.Color == Black)
{
// if the parent node where we inserted was black, our red node is placed correctly.
// since we inserted a red node, the number of black nodes on each path is unchanged
// -> the tree is still balanced
return;
}
// parentNode is red, so there is a conflict here!
// because the root is black, parentNode is not the root -> there is a grandparent node
var grandparentNode = parentNode.Parent;
var uncleNode = Sibling(parentNode);
if (uncleNode != null && uncleNode.Color == Red)
{
parentNode.Color = Black;
uncleNode.Color = Black;
grandparentNode.Color = Red;
FixTreeOnInsert(grandparentNode);
return;
}
// now we know: parent is red but uncle is black
// First rotation:
if (node == parentNode.Right && parentNode == grandparentNode.Left)
{
RotateLeft(parentNode);
node = node.Left;
}
else if (node == parentNode.Left && parentNode == grandparentNode.Right)
{
RotateRight(parentNode);
node = node.Right;
}
// because node might have changed, reassign variables:
// ReSharper disable once PossibleNullReferenceException
parentNode = node.Parent;
grandparentNode = parentNode.Parent;
// Now recolor a bit:
parentNode.Color = Black;
grandparentNode.Color = Red;
// Second rotation:
if (node == parentNode.Left && parentNode == grandparentNode.Left)
{
RotateRight(grandparentNode);
}
else
{
// because of the first rotation, this is guaranteed:
Debug.Assert(node == parentNode.Right && parentNode == grandparentNode.Right);
RotateLeft(grandparentNode);
}
}
private void RemoveNode(TextSegment removedNode)
{
if (removedNode.Left != null && removedNode.Right != null)
{
// replace removedNode with it's in-order successor
var leftMost = removedNode.Right.LeftMost;
RemoveNode(leftMost); // remove leftMost from its current location
// and overwrite the removedNode with it
ReplaceNode(removedNode, leftMost);
leftMost.Left = removedNode.Left;
if (leftMost.Left != null) leftMost.Left.Parent = leftMost;
leftMost.Right = removedNode.Right;
if (leftMost.Right != null) leftMost.Right.Parent = leftMost;
leftMost.Color = removedNode.Color;
UpdateAugmentedData(leftMost);
if (leftMost.Parent != null) UpdateAugmentedData(leftMost.Parent);
return;
}
// now either removedNode.left or removedNode.right is null
// get the remaining child
var parentNode = removedNode.Parent;
var childNode = removedNode.Left ?? removedNode.Right;
ReplaceNode(removedNode, childNode);
if (parentNode != null) UpdateAugmentedData(parentNode);
if (removedNode.Color == Black)
{
if (childNode != null && childNode.Color == Red)
{
childNode.Color = Black;
}
else
{
FixTreeOnDelete(childNode, parentNode);
}
}
}
private void FixTreeOnDelete(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (parentNode == null)
return;
// warning: node may be null
var sibling = Sibling(node, parentNode);
if (sibling.Color == Red)
{
parentNode.Color = Red;
sibling.Color = Black;
if (node == parentNode.Left)
{
RotateLeft(parentNode);
}
else
{
RotateRight(parentNode);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
}
if (parentNode.Color == Black
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
FixTreeOnDelete(parentNode, parentNode.Parent);
return;
}
if (parentNode.Color == Red
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
parentNode.Color = Black;
return;
}
if (node == parentNode.Left &&
sibling.Color == Black &&
GetColor(sibling.Left) == Red &&
GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
sibling.Left.Color = Black;
RotateRight(sibling);
}
else if (node == parentNode.Right &&
sibling.Color == Black &&
GetColor(sibling.Right) == Red &&
GetColor(sibling.Left) == Black)
{
sibling.Color = Red;
sibling.Right.Color = Black;
RotateLeft(sibling);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
sibling.Color = parentNode.Color;
parentNode.Color = Black;
if (node == parentNode.Left)
{
if (sibling.Right != null)
{
Debug.Assert(sibling.Right.Color == Red);
sibling.Right.Color = Black;
}
RotateLeft(parentNode);
}
else
{
if (sibling.Left != null)
{
Debug.Assert(sibling.Left.Color == Red);
sibling.Left.Color = Black;
}
RotateRight(parentNode);
}
}
private void ReplaceNode(TextSegment replacedNode, TextSegment newNode)
{
if (replacedNode.Parent == null)
{
Debug.Assert(replacedNode == _root);
_root = newNode;
}
else
{
if (replacedNode.Parent.Left == replacedNode)
replacedNode.Parent.Left = newNode;
else
replacedNode.Parent.Right = newNode;
}
if (newNode != null)
{
newNode.Parent = replacedNode.Parent;
}
replacedNode.Parent = null;
}
private void RotateLeft(TextSegment p)
{
// let q be p's right child
var q = p.Right;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's right child to be q's left child
p.Right = q.Left;
if (p.Right != null) p.Right.Parent = p;
// set q's left child to be p
q.Left = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private void RotateRight(TextSegment p)
{
// let q be p's left child
var q = p.Left;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's left child to be q's right child
p.Left = q.Right;
if (p.Left != null) p.Left.Parent = p;
// set q's right child to be p
q.Right = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private static TextSegment Sibling(TextSegment node)
{
return node == node.Parent.Left ? node.Parent.Right : node.Parent.Left;
}
private static TextSegment Sibling(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (node == parentNode.Left)
return parentNode.Right;
else
return parentNode.Left;
}
private static bool GetColor(TextSegment node)
{
return node != null && node.Color;
}
#endregion
#region ICollection<T> implementation
/// <summary>
/// Gets the number of segments in the tree.
/// </summary>
public int Count { get; private set; }
bool ICollection<T>.IsReadOnly => false;
/// <summary>
/// Gets whether this tree contains the specified item.
/// </summary>
public bool Contains(T item)
{
return item != null && item.OwnerTree == this;
}
/// <summary>
/// Copies all segments in this SegmentTree to the specified array.
/// </summary>
public void CopyTo(T[] array, int arrayIndex)
{
if (array == null)
throw new ArgumentNullException(nameof(array));
if (array.Length < Count)
throw new ArgumentException("The array is too small", nameof(array));
if (arrayIndex < 0 || arrayIndex + Count > array.Length)
throw new ArgumentOutOfRangeException(nameof(arrayIndex), arrayIndex, "Value must be between 0 and " + (array.Length - Count));
foreach (var s in this)
{
array[arrayIndex++] = s;
}
}
/// <summary>
/// Gets an enumerator to enumerate the segments.
/// </summary>
public IEnumerator<T> GetEnumerator()
{
if (_root != null)
{
var current = _root.LeftMost;
while (current != null)
{
yield return (T)current;
// TODO: check if collection was modified during enumeration
current = current.Successor;
}
}
}
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
#endregion
}
}
<MSG> Allow a text segment collection to be disconnected from a text document.
<DFF> @@ -97,6 +97,14 @@ namespace AvaloniaEdit.Document
}
#endregion
+ public void Disconnect (TextDocument textDocument)
+ {
+ if (_isConnectedToDocument)
+ {
+ TextDocumentWeakEventManager.Changed.RemoveHandler(textDocument, OnDocumentChanged);
+ }
+ }
+
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
| 8 | Allow a text segment collection to be disconnected from a text document. | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063833 | <NME> TextSegmentCollection.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using System.Text;
using AvaloniaEdit.Utils;
using Avalonia.Threading;
namespace AvaloniaEdit.Document
{
/// <summary>
/// Interface to allow TextSegments to access the TextSegmentCollection - we cannot use a direct reference
/// because TextSegmentCollection is generic.
/// </summary>
internal interface ISegmentTree
{
void Add(TextSegment s);
void Remove(TextSegment s);
void UpdateAugmentedData(TextSegment s);
}
/// <summary>
/// <para>
/// A collection of text segments that supports efficient lookup of segments
/// intersecting with another segment.
/// </para>
/// </summary>
/// <remarks><inheritdoc cref="TextSegment"/></remarks>
/// <see cref="TextSegment"/>
public sealed class TextSegmentCollection<T> : ICollection<T>, ISegmentTree where T : TextSegment
{
// Implementation: this is basically a mixture of an augmented interval tree
// and the TextAnchorTree.
// WARNING: you need to understand interval trees (the version with the augmented 'high'/'max' field)
// and how the TextAnchorTree works before you have any chance of understanding this code.
// This means that every node holds two "segments":
// one like the segments in the text anchor tree to support efficient offset changes
// and another that is the interval as seen by the user
// So basically, the tree contains a list of contiguous node segments of the first kind,
// with interval segments starting at the end of every node segment.
// Performance:
// Add is O(lg n)
// Remove is O(lg n)
// DocumentChanged is O(m * lg n), with m the number of segments that intersect with the changed document section
// FindFirstSegmentWithStartAfter is O(m + lg n) with m being the number of segments at the same offset as the result segment
// FindIntersectingSegments is O(m + lg n) with m being the number of intersecting segments.
private TextSegment _root;
private readonly bool _isConnectedToDocument;
#region Constructor
/// <summary>
/// Creates a new TextSegmentCollection that needs manual calls to <see cref="UpdateOffsets(DocumentChangeEventArgs)"/>.
/// </summary>
public TextSegmentCollection()
{
}
/// <summary>
/// Creates a new TextSegmentCollection that updates the offsets automatically.
/// </summary>
/// <param name="textDocument">The document to which the text segments
/// that will be added to the tree belong. When the document changes, the
/// position of the text segments will be updated accordingly.</param>
public TextSegmentCollection(TextDocument textDocument)
{
if (textDocument == null)
throw new ArgumentNullException(nameof(textDocument));
Dispatcher.UIThread.VerifyAccess();
_isConnectedToDocument = true;
TextDocumentWeakEventManager.Changed.AddHandler(textDocument, OnDocumentChanged);
}
#endregion
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="e">DocumentChangeEventArgs instance describing the change to the document.</param>
public void UpdateOffsets(DocumentChangeEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
OnDocumentChanged(this, e);
CheckProperties();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
var map = e.OffsetChangeMapOrNull;
if (map != null)
{
foreach (var entry in map)
{
UpdateOffsetsInternal(entry);
}
}
else
{
UpdateOffsetsInternal(e.CreateSingleChangeMapEntry());
}
}
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="change">OffsetChangeMapEntry instance describing the change to the document.</param>
public void UpdateOffsets(OffsetChangeMapEntry change)
{
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
UpdateOffsetsInternal(change);
CheckProperties();
}
#endregion
#region UpdateOffsets (implementation)
private void UpdateOffsetsInternal(OffsetChangeMapEntry change)
{
// Special case pure insertions, because they don't always cause a text segment to increase in size when the replaced region
// is inside a segment (when offset is at start or end of a text semgent).
if (change.RemovalLength == 0)
{
InsertText(change.Offset, change.InsertionLength);
}
else
{
ReplaceText(change);
}
}
private void InsertText(int offset, int length)
{
if (length == 0)
return;
// enlarge segments that contain offset (excluding those that have offset as endpoint)
foreach (var segment in FindSegmentsContaining(offset))
{
if (segment.StartOffset < offset && offset < segment.EndOffset)
{
segment.Length += length;
}
}
// move start offsets of all segments >= offset
TextSegment node = FindFirstSegmentWithStartAfter(offset);
if (node != null)
{
node.NodeLength += length;
UpdateAugmentedData(node);
}
}
private void ReplaceText(OffsetChangeMapEntry change)
{
Debug.Assert(change.RemovalLength > 0);
var offset = change.Offset;
foreach (var segment in FindOverlappingSegments(offset, change.RemovalLength))
{
if (segment.StartOffset <= offset)
{
if (segment.EndOffset >= offset + change.RemovalLength)
{
// Replacement inside segment: adjust segment length
segment.Length += change.InsertionLength - change.RemovalLength;
}
else
{
// Replacement starting inside segment and ending after segment end: set segment end to removal position
//segment.EndOffset = offset;
segment.Length = offset - segment.StartOffset;
}
}
else
{
// Replacement starting in front of text segment and running into segment.
// Keep segment.EndOffset constant and move segment.StartOffset to the end of the replacement
var remainingLength = segment.EndOffset - (offset + change.RemovalLength);
RemoveSegment(segment);
segment.StartOffset = offset + change.RemovalLength;
segment.Length = Math.Max(0, remainingLength);
AddSegment(segment);
}
}
// move start offsets of all segments > offset
TextSegment node = FindFirstSegmentWithStartAfter(offset + 1);
if (node != null)
{
Debug.Assert(node.NodeLength >= change.RemovalLength);
node.NodeLength += change.InsertionLength - change.RemovalLength;
UpdateAugmentedData(node);
}
}
#endregion
#region Add
/// <summary>
/// Adds the specified segment to the tree. This will cause the segment to update when the
/// document changes.
/// </summary>
public void Add(T item)
{
if (item == null)
throw new ArgumentNullException(nameof(item));
if (item.OwnerTree != null)
throw new ArgumentException("The segment is already added to a SegmentCollection.");
AddSegment(item);
}
void ISegmentTree.Add(TextSegment s)
{
AddSegment(s);
}
private void AddSegment(TextSegment node)
{
var insertionOffset = node.StartOffset;
node.DistanceToMaxEnd = node.SegmentLength;
if (_root == null)
{
_root = node;
node.TotalNodeLength = node.NodeLength;
}
else if (insertionOffset >= _root.TotalNodeLength)
{
// append segment at end of tree
node.NodeLength = node.TotalNodeLength = insertionOffset - _root.TotalNodeLength;
InsertAsRight(_root.RightMost, node);
}
else
{
// insert in middle of tree
var n = FindNode(ref insertionOffset);
Debug.Assert(insertionOffset < n.NodeLength);
// split node segment 'n' at offset
node.TotalNodeLength = node.NodeLength = insertionOffset;
n.NodeLength -= insertionOffset;
InsertBefore(n, node);
}
node.OwnerTree = this;
Count++;
CheckProperties();
}
private void InsertBefore(TextSegment node, TextSegment newNode)
{
if (node.Left == null)
{
InsertAsLeft(node, newNode);
}
else
{
InsertAsRight(node.Left.RightMost, newNode);
}
}
#endregion
#region GetNextSegment / GetPreviousSegment
/// <summary>
/// Gets the next segment after the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the last segment.
/// </summary>
public T GetNextSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Successor;
}
/// <summary>
/// Gets the previous segment before the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the first segment.
/// </summary>
public T GetPreviousSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Predecessor;
}
#endregion
#region FirstSegment/LastSegment
/// <summary>
/// Returns the first segment in the collection or null, if the collection is empty.
/// </summary>
public T FirstSegment => (T)_root?.LeftMost;
/// <summary>
/// Returns the last segment in the collection or null, if the collection is empty.
/// </summary>
public T LastSegment => (T)_root?.RightMost;
#endregion
#region FindFirstSegmentWithStartAfter
/// <summary>
/// Gets the first segment with a start offset greater or equal to <paramref name="startOffset"/>.
/// Returns null if no such segment is found.
/// </summary>
public T FindFirstSegmentWithStartAfter(int startOffset)
{
if (_root == null)
return null;
if (startOffset <= 0)
return (T)_root.LeftMost;
var s = FindNode(ref startOffset);
// startOffset means that the previous segment is starting at the offset we were looking for
while (startOffset == 0)
{
var p = (s == null) ? _root.RightMost : s.Predecessor;
// There must always be a predecessor: if we were looking for the first node, we would have already
// returned it as root.LeftMost above.
Debug.Assert(p != null);
startOffset += p.NodeLength;
s = p;
}
return (T)s;
}
/// <summary>
/// Finds the node at the specified offset.
/// After the method has run, offset is relative to the beginning of the returned node.
/// </summary>
private TextSegment FindNode(ref int offset)
{
var n = _root;
while (true)
{
if (n.Left != null)
{
if (offset < n.Left.TotalNodeLength)
{
n = n.Left; // descend into left subtree
continue;
}
else
{
offset -= n.Left.TotalNodeLength; // skip left subtree
}
}
if (offset < n.NodeLength)
{
return n; // found correct node
}
else
{
offset -= n.NodeLength; // skip this node
}
if (n.Right != null)
{
n = n.Right; // descend into right subtree
}
else
{
// didn't find any node containing the offset
return null;
}
}
}
#endregion
#region FindOverlappingSegments
/// <summary>
/// Finds all segments that contain the given offset.
/// (StartOffset <= offset <= EndOffset)
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindSegmentsContaining(int offset)
{
return FindOverlappingSegments(offset, 0);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(ISegment segment)
{
if (segment == null)
throw new ArgumentNullException(nameof(segment));
return FindOverlappingSegments(segment.Offset, segment.Length);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(int offset, int length)
{
ThrowUtil.CheckNotNegative(length, "length");
var results = new List<T>();
if (_root != null)
{
FindOverlappingSegments(results, _root, offset, offset + length);
}
return new ReadOnlyCollection<T>(results);
}
private void FindOverlappingSegments(List<T> results, TextSegment node, int low, int high)
{
// low and high are relative to node.LeftMost startpos (not node.LeftMost.Offset)
if (high < 0)
{
// node is irrelevant for search because all intervals in node are after high
return;
}
// find values relative to node.Offset
var nodeLow = low - node.NodeLength;
var nodeHigh = high - node.NodeLength;
if (node.Left != null)
{
nodeLow -= node.Left.TotalNodeLength;
nodeHigh -= node.Left.TotalNodeLength;
}
if (node.DistanceToMaxEnd < nodeLow)
{
// node is irrelevant for search because all intervals in node are before low
return;
}
if (node.Left != null)
FindOverlappingSegments(results, node.Left, low, high);
if (nodeHigh < 0)
{
// node and everything in node.right is before low
return;
}
if (nodeLow <= node.SegmentLength)
{
results.Add((T)node);
}
if (node.Right != null)
FindOverlappingSegments(results, node.Right, nodeLow, nodeHigh);
}
#endregion
#region UpdateAugmentedData
private void UpdateAugmentedData(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
totalLength += node.Left.TotalNodeLength;
var leftDtme = node.Left.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
if (node.Left.Right != null)
leftDtme -= node.Left.Right.TotalNodeLength;
leftDtme -= node.NodeLength;
if (leftDtme > distanceToMaxEnd)
distanceToMaxEnd = leftDtme;
}
if (node.Right != null)
{
totalLength += node.Right.TotalNodeLength;
var rightDtme = node.Right.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
rightDtme += node.Right.NodeLength;
if (node.Right.Left != null)
rightDtme += node.Right.Left.TotalNodeLength;
if (rightDtme > distanceToMaxEnd)
distanceToMaxEnd = rightDtme;
}
if (node.TotalNodeLength != totalLength
|| node.DistanceToMaxEnd != distanceToMaxEnd)
{
node.TotalNodeLength = totalLength;
node.DistanceToMaxEnd = distanceToMaxEnd;
if (node.Parent != null)
UpdateAugmentedData(node.Parent);
}
}
void ISegmentTree.UpdateAugmentedData(TextSegment node)
{
UpdateAugmentedData(node);
}
#endregion
#region Remove
/// <summary>
/// Removes the specified segment from the tree. This will cause the segment to not update
/// anymore when the document changes.
/// </summary>
public bool Remove(T item)
{
if (!Contains(item))
return false;
RemoveSegment(item);
return true;
}
void ISegmentTree.Remove(TextSegment s)
{
RemoveSegment(s);
}
private void RemoveSegment(TextSegment s)
{
var oldOffset = s.StartOffset;
var successor = s.Successor;
if (successor != null)
successor.NodeLength += s.NodeLength;
RemoveNode(s);
if (successor != null)
UpdateAugmentedData(successor);
Disconnect(s, oldOffset);
CheckProperties();
}
private void Disconnect(TextSegment s, int offset)
{
s.Left = s.Right = s.Parent = null;
s.OwnerTree = null;
s.NodeLength = offset;
Count--;
}
/// <summary>
/// Removes all segments from the tree.
/// </summary>
public void Clear()
{
var segments = this.ToArray();
_root = null;
var offset = 0;
foreach (var s in segments)
{
offset += s.NodeLength;
Disconnect(s, offset);
}
CheckProperties();
}
#endregion
#region CheckProperties
[Conditional("DATACONSISTENCYTEST")]
internal void CheckProperties()
{
#if DEBUG
if (_root != null)
{
CheckProperties(_root);
// check red-black property:
var blackCount = -1;
CheckNodeProperties(_root, null, Red, 0, ref blackCount);
}
var expectedCount = 0;
// we cannot trust LINQ not to call ICollection.Count, so we need this loop
// to count the elements in the tree
using (var en = GetEnumerator())
{
while (en.MoveNext()) expectedCount++;
}
Debug.Assert(Count == expectedCount);
#endif
}
#if DEBUG
private void CheckProperties(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
CheckProperties(node.Left);
totalLength += node.Left.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Left.DistanceToMaxEnd + node.Left.StartOffset - node.StartOffset);
}
if (node.Right != null)
{
CheckProperties(node.Right);
totalLength += node.Right.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Right.DistanceToMaxEnd + node.Right.StartOffset - node.StartOffset);
}
Debug.Assert(node.TotalNodeLength == totalLength);
Debug.Assert(node.DistanceToMaxEnd == distanceToMaxEnd);
}
/*
1. A node is either red or black.
2. The root is black.
3. All leaves are black. (The leaves are the NIL children.)
4. Both children of every red node are black. (So every red node must have a black parent.)
5. Every simple path from a node to a descendant leaf contains the same number of black nodes. (Not counting the leaf node.)
*/
[SuppressMessage("ReSharper", "UnusedParameter.Local")]
private static void CheckNodeProperties(TextSegment node, TextSegment parentNode, bool parentColor, int blackCount, ref int expectedBlackCount)
{
if (node == null) return;
Debug.Assert(node.Parent == parentNode);
if (parentColor == Red)
{
Debug.Assert(node.Color == Black);
}
if (node.Color == Black)
{
blackCount++;
}
if (node.Left == null && node.Right == null)
{
// node is a leaf node:
if (expectedBlackCount == -1)
expectedBlackCount = blackCount;
else
Debug.Assert(expectedBlackCount == blackCount);
}
CheckNodeProperties(node.Left, node, node.Color, blackCount, ref expectedBlackCount);
CheckNodeProperties(node.Right, node, node.Color, blackCount, ref expectedBlackCount);
}
private static void AppendTreeToString(TextSegment node, StringBuilder b, int indent)
{
b.Append(node.Color == Red ? "RED " : "BLACK ");
b.AppendLine(node + node.ToDebugString());
indent += 2;
if (node.Left != null)
{
b.Append(' ', indent);
b.Append("L: ");
AppendTreeToString(node.Left, b, indent);
}
if (node.Right != null)
{
b.Append(' ', indent);
b.Append("R: ");
AppendTreeToString(node.Right, b, indent);
}
}
#endif
internal string GetTreeAsString()
{
#if DEBUG
var b = new StringBuilder();
if (_root != null)
AppendTreeToString(_root, b, 0);
return b.ToString();
#else
return "Not available in release build.";
#endif
}
#endregion
#region Red/Black Tree
internal const bool Red = true;
internal const bool Black = false;
private void InsertAsLeft(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Left == null);
parentNode.Left = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void InsertAsRight(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Right == null);
parentNode.Right = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void FixTreeOnInsert(TextSegment node)
{
Debug.Assert(node != null);
Debug.Assert(node.Color == Red);
Debug.Assert(node.Left == null || node.Left.Color == Black);
Debug.Assert(node.Right == null || node.Right.Color == Black);
var parentNode = node.Parent;
if (parentNode == null)
{
// we inserted in the root -> the node must be black
// since this is a root node, making the node black increments the number of black nodes
// on all paths by one, so it is still the same for all paths.
node.Color = Black;
return;
}
if (parentNode.Color == Black)
{
// if the parent node where we inserted was black, our red node is placed correctly.
// since we inserted a red node, the number of black nodes on each path is unchanged
// -> the tree is still balanced
return;
}
// parentNode is red, so there is a conflict here!
// because the root is black, parentNode is not the root -> there is a grandparent node
var grandparentNode = parentNode.Parent;
var uncleNode = Sibling(parentNode);
if (uncleNode != null && uncleNode.Color == Red)
{
parentNode.Color = Black;
uncleNode.Color = Black;
grandparentNode.Color = Red;
FixTreeOnInsert(grandparentNode);
return;
}
// now we know: parent is red but uncle is black
// First rotation:
if (node == parentNode.Right && parentNode == grandparentNode.Left)
{
RotateLeft(parentNode);
node = node.Left;
}
else if (node == parentNode.Left && parentNode == grandparentNode.Right)
{
RotateRight(parentNode);
node = node.Right;
}
// because node might have changed, reassign variables:
// ReSharper disable once PossibleNullReferenceException
parentNode = node.Parent;
grandparentNode = parentNode.Parent;
// Now recolor a bit:
parentNode.Color = Black;
grandparentNode.Color = Red;
// Second rotation:
if (node == parentNode.Left && parentNode == grandparentNode.Left)
{
RotateRight(grandparentNode);
}
else
{
// because of the first rotation, this is guaranteed:
Debug.Assert(node == parentNode.Right && parentNode == grandparentNode.Right);
RotateLeft(grandparentNode);
}
}
private void RemoveNode(TextSegment removedNode)
{
if (removedNode.Left != null && removedNode.Right != null)
{
// replace removedNode with it's in-order successor
var leftMost = removedNode.Right.LeftMost;
RemoveNode(leftMost); // remove leftMost from its current location
// and overwrite the removedNode with it
ReplaceNode(removedNode, leftMost);
leftMost.Left = removedNode.Left;
if (leftMost.Left != null) leftMost.Left.Parent = leftMost;
leftMost.Right = removedNode.Right;
if (leftMost.Right != null) leftMost.Right.Parent = leftMost;
leftMost.Color = removedNode.Color;
UpdateAugmentedData(leftMost);
if (leftMost.Parent != null) UpdateAugmentedData(leftMost.Parent);
return;
}
// now either removedNode.left or removedNode.right is null
// get the remaining child
var parentNode = removedNode.Parent;
var childNode = removedNode.Left ?? removedNode.Right;
ReplaceNode(removedNode, childNode);
if (parentNode != null) UpdateAugmentedData(parentNode);
if (removedNode.Color == Black)
{
if (childNode != null && childNode.Color == Red)
{
childNode.Color = Black;
}
else
{
FixTreeOnDelete(childNode, parentNode);
}
}
}
private void FixTreeOnDelete(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (parentNode == null)
return;
// warning: node may be null
var sibling = Sibling(node, parentNode);
if (sibling.Color == Red)
{
parentNode.Color = Red;
sibling.Color = Black;
if (node == parentNode.Left)
{
RotateLeft(parentNode);
}
else
{
RotateRight(parentNode);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
}
if (parentNode.Color == Black
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
FixTreeOnDelete(parentNode, parentNode.Parent);
return;
}
if (parentNode.Color == Red
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
parentNode.Color = Black;
return;
}
if (node == parentNode.Left &&
sibling.Color == Black &&
GetColor(sibling.Left) == Red &&
GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
sibling.Left.Color = Black;
RotateRight(sibling);
}
else if (node == parentNode.Right &&
sibling.Color == Black &&
GetColor(sibling.Right) == Red &&
GetColor(sibling.Left) == Black)
{
sibling.Color = Red;
sibling.Right.Color = Black;
RotateLeft(sibling);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
sibling.Color = parentNode.Color;
parentNode.Color = Black;
if (node == parentNode.Left)
{
if (sibling.Right != null)
{
Debug.Assert(sibling.Right.Color == Red);
sibling.Right.Color = Black;
}
RotateLeft(parentNode);
}
else
{
if (sibling.Left != null)
{
Debug.Assert(sibling.Left.Color == Red);
sibling.Left.Color = Black;
}
RotateRight(parentNode);
}
}
private void ReplaceNode(TextSegment replacedNode, TextSegment newNode)
{
if (replacedNode.Parent == null)
{
Debug.Assert(replacedNode == _root);
_root = newNode;
}
else
{
if (replacedNode.Parent.Left == replacedNode)
replacedNode.Parent.Left = newNode;
else
replacedNode.Parent.Right = newNode;
}
if (newNode != null)
{
newNode.Parent = replacedNode.Parent;
}
replacedNode.Parent = null;
}
private void RotateLeft(TextSegment p)
{
// let q be p's right child
var q = p.Right;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's right child to be q's left child
p.Right = q.Left;
if (p.Right != null) p.Right.Parent = p;
// set q's left child to be p
q.Left = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private void RotateRight(TextSegment p)
{
// let q be p's left child
var q = p.Left;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's left child to be q's right child
p.Left = q.Right;
if (p.Left != null) p.Left.Parent = p;
// set q's right child to be p
q.Right = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private static TextSegment Sibling(TextSegment node)
{
return node == node.Parent.Left ? node.Parent.Right : node.Parent.Left;
}
private static TextSegment Sibling(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (node == parentNode.Left)
return parentNode.Right;
else
return parentNode.Left;
}
private static bool GetColor(TextSegment node)
{
return node != null && node.Color;
}
#endregion
#region ICollection<T> implementation
/// <summary>
/// Gets the number of segments in the tree.
/// </summary>
public int Count { get; private set; }
bool ICollection<T>.IsReadOnly => false;
/// <summary>
/// Gets whether this tree contains the specified item.
/// </summary>
public bool Contains(T item)
{
return item != null && item.OwnerTree == this;
}
/// <summary>
/// Copies all segments in this SegmentTree to the specified array.
/// </summary>
public void CopyTo(T[] array, int arrayIndex)
{
if (array == null)
throw new ArgumentNullException(nameof(array));
if (array.Length < Count)
throw new ArgumentException("The array is too small", nameof(array));
if (arrayIndex < 0 || arrayIndex + Count > array.Length)
throw new ArgumentOutOfRangeException(nameof(arrayIndex), arrayIndex, "Value must be between 0 and " + (array.Length - Count));
foreach (var s in this)
{
array[arrayIndex++] = s;
}
}
/// <summary>
/// Gets an enumerator to enumerate the segments.
/// </summary>
public IEnumerator<T> GetEnumerator()
{
if (_root != null)
{
var current = _root.LeftMost;
while (current != null)
{
yield return (T)current;
// TODO: check if collection was modified during enumeration
current = current.Successor;
}
}
}
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
#endregion
}
}
<MSG> Allow a text segment collection to be disconnected from a text document.
<DFF> @@ -97,6 +97,14 @@ namespace AvaloniaEdit.Document
}
#endregion
+ public void Disconnect (TextDocument textDocument)
+ {
+ if (_isConnectedToDocument)
+ {
+ TextDocumentWeakEventManager.Changed.RemoveHandler(textDocument, OnDocumentChanged);
+ }
+ }
+
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
| 8 | Allow a text segment collection to be disconnected from a text document. | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063834 | <NME> TextSegmentCollection.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using System.Text;
using AvaloniaEdit.Utils;
using Avalonia.Threading;
namespace AvaloniaEdit.Document
{
/// <summary>
/// Interface to allow TextSegments to access the TextSegmentCollection - we cannot use a direct reference
/// because TextSegmentCollection is generic.
/// </summary>
internal interface ISegmentTree
{
void Add(TextSegment s);
void Remove(TextSegment s);
void UpdateAugmentedData(TextSegment s);
}
/// <summary>
/// <para>
/// A collection of text segments that supports efficient lookup of segments
/// intersecting with another segment.
/// </para>
/// </summary>
/// <remarks><inheritdoc cref="TextSegment"/></remarks>
/// <see cref="TextSegment"/>
public sealed class TextSegmentCollection<T> : ICollection<T>, ISegmentTree where T : TextSegment
{
// Implementation: this is basically a mixture of an augmented interval tree
// and the TextAnchorTree.
// WARNING: you need to understand interval trees (the version with the augmented 'high'/'max' field)
// and how the TextAnchorTree works before you have any chance of understanding this code.
// This means that every node holds two "segments":
// one like the segments in the text anchor tree to support efficient offset changes
// and another that is the interval as seen by the user
// So basically, the tree contains a list of contiguous node segments of the first kind,
// with interval segments starting at the end of every node segment.
// Performance:
// Add is O(lg n)
// Remove is O(lg n)
// DocumentChanged is O(m * lg n), with m the number of segments that intersect with the changed document section
// FindFirstSegmentWithStartAfter is O(m + lg n) with m being the number of segments at the same offset as the result segment
// FindIntersectingSegments is O(m + lg n) with m being the number of intersecting segments.
private TextSegment _root;
private readonly bool _isConnectedToDocument;
#region Constructor
/// <summary>
/// Creates a new TextSegmentCollection that needs manual calls to <see cref="UpdateOffsets(DocumentChangeEventArgs)"/>.
/// </summary>
public TextSegmentCollection()
{
}
/// <summary>
/// Creates a new TextSegmentCollection that updates the offsets automatically.
/// </summary>
/// <param name="textDocument">The document to which the text segments
/// that will be added to the tree belong. When the document changes, the
/// position of the text segments will be updated accordingly.</param>
public TextSegmentCollection(TextDocument textDocument)
{
if (textDocument == null)
throw new ArgumentNullException(nameof(textDocument));
Dispatcher.UIThread.VerifyAccess();
_isConnectedToDocument = true;
TextDocumentWeakEventManager.Changed.AddHandler(textDocument, OnDocumentChanged);
}
#endregion
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="e">DocumentChangeEventArgs instance describing the change to the document.</param>
public void UpdateOffsets(DocumentChangeEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
OnDocumentChanged(this, e);
CheckProperties();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
var map = e.OffsetChangeMapOrNull;
if (map != null)
{
foreach (var entry in map)
{
UpdateOffsetsInternal(entry);
}
}
else
{
UpdateOffsetsInternal(e.CreateSingleChangeMapEntry());
}
}
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="change">OffsetChangeMapEntry instance describing the change to the document.</param>
public void UpdateOffsets(OffsetChangeMapEntry change)
{
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
UpdateOffsetsInternal(change);
CheckProperties();
}
#endregion
#region UpdateOffsets (implementation)
private void UpdateOffsetsInternal(OffsetChangeMapEntry change)
{
// Special case pure insertions, because they don't always cause a text segment to increase in size when the replaced region
// is inside a segment (when offset is at start or end of a text semgent).
if (change.RemovalLength == 0)
{
InsertText(change.Offset, change.InsertionLength);
}
else
{
ReplaceText(change);
}
}
private void InsertText(int offset, int length)
{
if (length == 0)
return;
// enlarge segments that contain offset (excluding those that have offset as endpoint)
foreach (var segment in FindSegmentsContaining(offset))
{
if (segment.StartOffset < offset && offset < segment.EndOffset)
{
segment.Length += length;
}
}
// move start offsets of all segments >= offset
TextSegment node = FindFirstSegmentWithStartAfter(offset);
if (node != null)
{
node.NodeLength += length;
UpdateAugmentedData(node);
}
}
private void ReplaceText(OffsetChangeMapEntry change)
{
Debug.Assert(change.RemovalLength > 0);
var offset = change.Offset;
foreach (var segment in FindOverlappingSegments(offset, change.RemovalLength))
{
if (segment.StartOffset <= offset)
{
if (segment.EndOffset >= offset + change.RemovalLength)
{
// Replacement inside segment: adjust segment length
segment.Length += change.InsertionLength - change.RemovalLength;
}
else
{
// Replacement starting inside segment and ending after segment end: set segment end to removal position
//segment.EndOffset = offset;
segment.Length = offset - segment.StartOffset;
}
}
else
{
// Replacement starting in front of text segment and running into segment.
// Keep segment.EndOffset constant and move segment.StartOffset to the end of the replacement
var remainingLength = segment.EndOffset - (offset + change.RemovalLength);
RemoveSegment(segment);
segment.StartOffset = offset + change.RemovalLength;
segment.Length = Math.Max(0, remainingLength);
AddSegment(segment);
}
}
// move start offsets of all segments > offset
TextSegment node = FindFirstSegmentWithStartAfter(offset + 1);
if (node != null)
{
Debug.Assert(node.NodeLength >= change.RemovalLength);
node.NodeLength += change.InsertionLength - change.RemovalLength;
UpdateAugmentedData(node);
}
}
#endregion
#region Add
/// <summary>
/// Adds the specified segment to the tree. This will cause the segment to update when the
/// document changes.
/// </summary>
public void Add(T item)
{
if (item == null)
throw new ArgumentNullException(nameof(item));
if (item.OwnerTree != null)
throw new ArgumentException("The segment is already added to a SegmentCollection.");
AddSegment(item);
}
void ISegmentTree.Add(TextSegment s)
{
AddSegment(s);
}
private void AddSegment(TextSegment node)
{
var insertionOffset = node.StartOffset;
node.DistanceToMaxEnd = node.SegmentLength;
if (_root == null)
{
_root = node;
node.TotalNodeLength = node.NodeLength;
}
else if (insertionOffset >= _root.TotalNodeLength)
{
// append segment at end of tree
node.NodeLength = node.TotalNodeLength = insertionOffset - _root.TotalNodeLength;
InsertAsRight(_root.RightMost, node);
}
else
{
// insert in middle of tree
var n = FindNode(ref insertionOffset);
Debug.Assert(insertionOffset < n.NodeLength);
// split node segment 'n' at offset
node.TotalNodeLength = node.NodeLength = insertionOffset;
n.NodeLength -= insertionOffset;
InsertBefore(n, node);
}
node.OwnerTree = this;
Count++;
CheckProperties();
}
private void InsertBefore(TextSegment node, TextSegment newNode)
{
if (node.Left == null)
{
InsertAsLeft(node, newNode);
}
else
{
InsertAsRight(node.Left.RightMost, newNode);
}
}
#endregion
#region GetNextSegment / GetPreviousSegment
/// <summary>
/// Gets the next segment after the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the last segment.
/// </summary>
public T GetNextSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Successor;
}
/// <summary>
/// Gets the previous segment before the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the first segment.
/// </summary>
public T GetPreviousSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Predecessor;
}
#endregion
#region FirstSegment/LastSegment
/// <summary>
/// Returns the first segment in the collection or null, if the collection is empty.
/// </summary>
public T FirstSegment => (T)_root?.LeftMost;
/// <summary>
/// Returns the last segment in the collection or null, if the collection is empty.
/// </summary>
public T LastSegment => (T)_root?.RightMost;
#endregion
#region FindFirstSegmentWithStartAfter
/// <summary>
/// Gets the first segment with a start offset greater or equal to <paramref name="startOffset"/>.
/// Returns null if no such segment is found.
/// </summary>
public T FindFirstSegmentWithStartAfter(int startOffset)
{
if (_root == null)
return null;
if (startOffset <= 0)
return (T)_root.LeftMost;
var s = FindNode(ref startOffset);
// startOffset means that the previous segment is starting at the offset we were looking for
while (startOffset == 0)
{
var p = (s == null) ? _root.RightMost : s.Predecessor;
// There must always be a predecessor: if we were looking for the first node, we would have already
// returned it as root.LeftMost above.
Debug.Assert(p != null);
startOffset += p.NodeLength;
s = p;
}
return (T)s;
}
/// <summary>
/// Finds the node at the specified offset.
/// After the method has run, offset is relative to the beginning of the returned node.
/// </summary>
private TextSegment FindNode(ref int offset)
{
var n = _root;
while (true)
{
if (n.Left != null)
{
if (offset < n.Left.TotalNodeLength)
{
n = n.Left; // descend into left subtree
continue;
}
else
{
offset -= n.Left.TotalNodeLength; // skip left subtree
}
}
if (offset < n.NodeLength)
{
return n; // found correct node
}
else
{
offset -= n.NodeLength; // skip this node
}
if (n.Right != null)
{
n = n.Right; // descend into right subtree
}
else
{
// didn't find any node containing the offset
return null;
}
}
}
#endregion
#region FindOverlappingSegments
/// <summary>
/// Finds all segments that contain the given offset.
/// (StartOffset <= offset <= EndOffset)
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindSegmentsContaining(int offset)
{
return FindOverlappingSegments(offset, 0);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(ISegment segment)
{
if (segment == null)
throw new ArgumentNullException(nameof(segment));
return FindOverlappingSegments(segment.Offset, segment.Length);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(int offset, int length)
{
ThrowUtil.CheckNotNegative(length, "length");
var results = new List<T>();
if (_root != null)
{
FindOverlappingSegments(results, _root, offset, offset + length);
}
return new ReadOnlyCollection<T>(results);
}
private void FindOverlappingSegments(List<T> results, TextSegment node, int low, int high)
{
// low and high are relative to node.LeftMost startpos (not node.LeftMost.Offset)
if (high < 0)
{
// node is irrelevant for search because all intervals in node are after high
return;
}
// find values relative to node.Offset
var nodeLow = low - node.NodeLength;
var nodeHigh = high - node.NodeLength;
if (node.Left != null)
{
nodeLow -= node.Left.TotalNodeLength;
nodeHigh -= node.Left.TotalNodeLength;
}
if (node.DistanceToMaxEnd < nodeLow)
{
// node is irrelevant for search because all intervals in node are before low
return;
}
if (node.Left != null)
FindOverlappingSegments(results, node.Left, low, high);
if (nodeHigh < 0)
{
// node and everything in node.right is before low
return;
}
if (nodeLow <= node.SegmentLength)
{
results.Add((T)node);
}
if (node.Right != null)
FindOverlappingSegments(results, node.Right, nodeLow, nodeHigh);
}
#endregion
#region UpdateAugmentedData
private void UpdateAugmentedData(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
totalLength += node.Left.TotalNodeLength;
var leftDtme = node.Left.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
if (node.Left.Right != null)
leftDtme -= node.Left.Right.TotalNodeLength;
leftDtme -= node.NodeLength;
if (leftDtme > distanceToMaxEnd)
distanceToMaxEnd = leftDtme;
}
if (node.Right != null)
{
totalLength += node.Right.TotalNodeLength;
var rightDtme = node.Right.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
rightDtme += node.Right.NodeLength;
if (node.Right.Left != null)
rightDtme += node.Right.Left.TotalNodeLength;
if (rightDtme > distanceToMaxEnd)
distanceToMaxEnd = rightDtme;
}
if (node.TotalNodeLength != totalLength
|| node.DistanceToMaxEnd != distanceToMaxEnd)
{
node.TotalNodeLength = totalLength;
node.DistanceToMaxEnd = distanceToMaxEnd;
if (node.Parent != null)
UpdateAugmentedData(node.Parent);
}
}
void ISegmentTree.UpdateAugmentedData(TextSegment node)
{
UpdateAugmentedData(node);
}
#endregion
#region Remove
/// <summary>
/// Removes the specified segment from the tree. This will cause the segment to not update
/// anymore when the document changes.
/// </summary>
public bool Remove(T item)
{
if (!Contains(item))
return false;
RemoveSegment(item);
return true;
}
void ISegmentTree.Remove(TextSegment s)
{
RemoveSegment(s);
}
private void RemoveSegment(TextSegment s)
{
var oldOffset = s.StartOffset;
var successor = s.Successor;
if (successor != null)
successor.NodeLength += s.NodeLength;
RemoveNode(s);
if (successor != null)
UpdateAugmentedData(successor);
Disconnect(s, oldOffset);
CheckProperties();
}
private void Disconnect(TextSegment s, int offset)
{
s.Left = s.Right = s.Parent = null;
s.OwnerTree = null;
s.NodeLength = offset;
Count--;
}
/// <summary>
/// Removes all segments from the tree.
/// </summary>
public void Clear()
{
var segments = this.ToArray();
_root = null;
var offset = 0;
foreach (var s in segments)
{
offset += s.NodeLength;
Disconnect(s, offset);
}
CheckProperties();
}
#endregion
#region CheckProperties
[Conditional("DATACONSISTENCYTEST")]
internal void CheckProperties()
{
#if DEBUG
if (_root != null)
{
CheckProperties(_root);
// check red-black property:
var blackCount = -1;
CheckNodeProperties(_root, null, Red, 0, ref blackCount);
}
var expectedCount = 0;
// we cannot trust LINQ not to call ICollection.Count, so we need this loop
// to count the elements in the tree
using (var en = GetEnumerator())
{
while (en.MoveNext()) expectedCount++;
}
Debug.Assert(Count == expectedCount);
#endif
}
#if DEBUG
private void CheckProperties(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
CheckProperties(node.Left);
totalLength += node.Left.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Left.DistanceToMaxEnd + node.Left.StartOffset - node.StartOffset);
}
if (node.Right != null)
{
CheckProperties(node.Right);
totalLength += node.Right.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Right.DistanceToMaxEnd + node.Right.StartOffset - node.StartOffset);
}
Debug.Assert(node.TotalNodeLength == totalLength);
Debug.Assert(node.DistanceToMaxEnd == distanceToMaxEnd);
}
/*
1. A node is either red or black.
2. The root is black.
3. All leaves are black. (The leaves are the NIL children.)
4. Both children of every red node are black. (So every red node must have a black parent.)
5. Every simple path from a node to a descendant leaf contains the same number of black nodes. (Not counting the leaf node.)
*/
[SuppressMessage("ReSharper", "UnusedParameter.Local")]
private static void CheckNodeProperties(TextSegment node, TextSegment parentNode, bool parentColor, int blackCount, ref int expectedBlackCount)
{
if (node == null) return;
Debug.Assert(node.Parent == parentNode);
if (parentColor == Red)
{
Debug.Assert(node.Color == Black);
}
if (node.Color == Black)
{
blackCount++;
}
if (node.Left == null && node.Right == null)
{
// node is a leaf node:
if (expectedBlackCount == -1)
expectedBlackCount = blackCount;
else
Debug.Assert(expectedBlackCount == blackCount);
}
CheckNodeProperties(node.Left, node, node.Color, blackCount, ref expectedBlackCount);
CheckNodeProperties(node.Right, node, node.Color, blackCount, ref expectedBlackCount);
}
private static void AppendTreeToString(TextSegment node, StringBuilder b, int indent)
{
b.Append(node.Color == Red ? "RED " : "BLACK ");
b.AppendLine(node + node.ToDebugString());
indent += 2;
if (node.Left != null)
{
b.Append(' ', indent);
b.Append("L: ");
AppendTreeToString(node.Left, b, indent);
}
if (node.Right != null)
{
b.Append(' ', indent);
b.Append("R: ");
AppendTreeToString(node.Right, b, indent);
}
}
#endif
internal string GetTreeAsString()
{
#if DEBUG
var b = new StringBuilder();
if (_root != null)
AppendTreeToString(_root, b, 0);
return b.ToString();
#else
return "Not available in release build.";
#endif
}
#endregion
#region Red/Black Tree
internal const bool Red = true;
internal const bool Black = false;
private void InsertAsLeft(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Left == null);
parentNode.Left = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void InsertAsRight(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Right == null);
parentNode.Right = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void FixTreeOnInsert(TextSegment node)
{
Debug.Assert(node != null);
Debug.Assert(node.Color == Red);
Debug.Assert(node.Left == null || node.Left.Color == Black);
Debug.Assert(node.Right == null || node.Right.Color == Black);
var parentNode = node.Parent;
if (parentNode == null)
{
// we inserted in the root -> the node must be black
// since this is a root node, making the node black increments the number of black nodes
// on all paths by one, so it is still the same for all paths.
node.Color = Black;
return;
}
if (parentNode.Color == Black)
{
// if the parent node where we inserted was black, our red node is placed correctly.
// since we inserted a red node, the number of black nodes on each path is unchanged
// -> the tree is still balanced
return;
}
// parentNode is red, so there is a conflict here!
// because the root is black, parentNode is not the root -> there is a grandparent node
var grandparentNode = parentNode.Parent;
var uncleNode = Sibling(parentNode);
if (uncleNode != null && uncleNode.Color == Red)
{
parentNode.Color = Black;
uncleNode.Color = Black;
grandparentNode.Color = Red;
FixTreeOnInsert(grandparentNode);
return;
}
// now we know: parent is red but uncle is black
// First rotation:
if (node == parentNode.Right && parentNode == grandparentNode.Left)
{
RotateLeft(parentNode);
node = node.Left;
}
else if (node == parentNode.Left && parentNode == grandparentNode.Right)
{
RotateRight(parentNode);
node = node.Right;
}
// because node might have changed, reassign variables:
// ReSharper disable once PossibleNullReferenceException
parentNode = node.Parent;
grandparentNode = parentNode.Parent;
// Now recolor a bit:
parentNode.Color = Black;
grandparentNode.Color = Red;
// Second rotation:
if (node == parentNode.Left && parentNode == grandparentNode.Left)
{
RotateRight(grandparentNode);
}
else
{
// because of the first rotation, this is guaranteed:
Debug.Assert(node == parentNode.Right && parentNode == grandparentNode.Right);
RotateLeft(grandparentNode);
}
}
private void RemoveNode(TextSegment removedNode)
{
if (removedNode.Left != null && removedNode.Right != null)
{
// replace removedNode with it's in-order successor
var leftMost = removedNode.Right.LeftMost;
RemoveNode(leftMost); // remove leftMost from its current location
// and overwrite the removedNode with it
ReplaceNode(removedNode, leftMost);
leftMost.Left = removedNode.Left;
if (leftMost.Left != null) leftMost.Left.Parent = leftMost;
leftMost.Right = removedNode.Right;
if (leftMost.Right != null) leftMost.Right.Parent = leftMost;
leftMost.Color = removedNode.Color;
UpdateAugmentedData(leftMost);
if (leftMost.Parent != null) UpdateAugmentedData(leftMost.Parent);
return;
}
// now either removedNode.left or removedNode.right is null
// get the remaining child
var parentNode = removedNode.Parent;
var childNode = removedNode.Left ?? removedNode.Right;
ReplaceNode(removedNode, childNode);
if (parentNode != null) UpdateAugmentedData(parentNode);
if (removedNode.Color == Black)
{
if (childNode != null && childNode.Color == Red)
{
childNode.Color = Black;
}
else
{
FixTreeOnDelete(childNode, parentNode);
}
}
}
private void FixTreeOnDelete(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (parentNode == null)
return;
// warning: node may be null
var sibling = Sibling(node, parentNode);
if (sibling.Color == Red)
{
parentNode.Color = Red;
sibling.Color = Black;
if (node == parentNode.Left)
{
RotateLeft(parentNode);
}
else
{
RotateRight(parentNode);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
}
if (parentNode.Color == Black
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
FixTreeOnDelete(parentNode, parentNode.Parent);
return;
}
if (parentNode.Color == Red
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
parentNode.Color = Black;
return;
}
if (node == parentNode.Left &&
sibling.Color == Black &&
GetColor(sibling.Left) == Red &&
GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
sibling.Left.Color = Black;
RotateRight(sibling);
}
else if (node == parentNode.Right &&
sibling.Color == Black &&
GetColor(sibling.Right) == Red &&
GetColor(sibling.Left) == Black)
{
sibling.Color = Red;
sibling.Right.Color = Black;
RotateLeft(sibling);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
sibling.Color = parentNode.Color;
parentNode.Color = Black;
if (node == parentNode.Left)
{
if (sibling.Right != null)
{
Debug.Assert(sibling.Right.Color == Red);
sibling.Right.Color = Black;
}
RotateLeft(parentNode);
}
else
{
if (sibling.Left != null)
{
Debug.Assert(sibling.Left.Color == Red);
sibling.Left.Color = Black;
}
RotateRight(parentNode);
}
}
private void ReplaceNode(TextSegment replacedNode, TextSegment newNode)
{
if (replacedNode.Parent == null)
{
Debug.Assert(replacedNode == _root);
_root = newNode;
}
else
{
if (replacedNode.Parent.Left == replacedNode)
replacedNode.Parent.Left = newNode;
else
replacedNode.Parent.Right = newNode;
}
if (newNode != null)
{
newNode.Parent = replacedNode.Parent;
}
replacedNode.Parent = null;
}
private void RotateLeft(TextSegment p)
{
// let q be p's right child
var q = p.Right;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's right child to be q's left child
p.Right = q.Left;
if (p.Right != null) p.Right.Parent = p;
// set q's left child to be p
q.Left = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private void RotateRight(TextSegment p)
{
// let q be p's left child
var q = p.Left;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's left child to be q's right child
p.Left = q.Right;
if (p.Left != null) p.Left.Parent = p;
// set q's right child to be p
q.Right = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private static TextSegment Sibling(TextSegment node)
{
return node == node.Parent.Left ? node.Parent.Right : node.Parent.Left;
}
private static TextSegment Sibling(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (node == parentNode.Left)
return parentNode.Right;
else
return parentNode.Left;
}
private static bool GetColor(TextSegment node)
{
return node != null && node.Color;
}
#endregion
#region ICollection<T> implementation
/// <summary>
/// Gets the number of segments in the tree.
/// </summary>
public int Count { get; private set; }
bool ICollection<T>.IsReadOnly => false;
/// <summary>
/// Gets whether this tree contains the specified item.
/// </summary>
public bool Contains(T item)
{
return item != null && item.OwnerTree == this;
}
/// <summary>
/// Copies all segments in this SegmentTree to the specified array.
/// </summary>
public void CopyTo(T[] array, int arrayIndex)
{
if (array == null)
throw new ArgumentNullException(nameof(array));
if (array.Length < Count)
throw new ArgumentException("The array is too small", nameof(array));
if (arrayIndex < 0 || arrayIndex + Count > array.Length)
throw new ArgumentOutOfRangeException(nameof(arrayIndex), arrayIndex, "Value must be between 0 and " + (array.Length - Count));
foreach (var s in this)
{
array[arrayIndex++] = s;
}
}
/// <summary>
/// Gets an enumerator to enumerate the segments.
/// </summary>
public IEnumerator<T> GetEnumerator()
{
if (_root != null)
{
var current = _root.LeftMost;
while (current != null)
{
yield return (T)current;
// TODO: check if collection was modified during enumeration
current = current.Successor;
}
}
}
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
#endregion
}
}
<MSG> Allow a text segment collection to be disconnected from a text document.
<DFF> @@ -97,6 +97,14 @@ namespace AvaloniaEdit.Document
}
#endregion
+ public void Disconnect (TextDocument textDocument)
+ {
+ if (_isConnectedToDocument)
+ {
+ TextDocumentWeakEventManager.Changed.RemoveHandler(textDocument, OnDocumentChanged);
+ }
+ }
+
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
| 8 | Allow a text segment collection to be disconnected from a text document. | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063835 | <NME> TextSegmentCollection.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using System.Text;
using AvaloniaEdit.Utils;
using Avalonia.Threading;
namespace AvaloniaEdit.Document
{
/// <summary>
/// Interface to allow TextSegments to access the TextSegmentCollection - we cannot use a direct reference
/// because TextSegmentCollection is generic.
/// </summary>
internal interface ISegmentTree
{
void Add(TextSegment s);
void Remove(TextSegment s);
void UpdateAugmentedData(TextSegment s);
}
/// <summary>
/// <para>
/// A collection of text segments that supports efficient lookup of segments
/// intersecting with another segment.
/// </para>
/// </summary>
/// <remarks><inheritdoc cref="TextSegment"/></remarks>
/// <see cref="TextSegment"/>
public sealed class TextSegmentCollection<T> : ICollection<T>, ISegmentTree where T : TextSegment
{
// Implementation: this is basically a mixture of an augmented interval tree
// and the TextAnchorTree.
// WARNING: you need to understand interval trees (the version with the augmented 'high'/'max' field)
// and how the TextAnchorTree works before you have any chance of understanding this code.
// This means that every node holds two "segments":
// one like the segments in the text anchor tree to support efficient offset changes
// and another that is the interval as seen by the user
// So basically, the tree contains a list of contiguous node segments of the first kind,
// with interval segments starting at the end of every node segment.
// Performance:
// Add is O(lg n)
// Remove is O(lg n)
// DocumentChanged is O(m * lg n), with m the number of segments that intersect with the changed document section
// FindFirstSegmentWithStartAfter is O(m + lg n) with m being the number of segments at the same offset as the result segment
// FindIntersectingSegments is O(m + lg n) with m being the number of intersecting segments.
private TextSegment _root;
private readonly bool _isConnectedToDocument;
#region Constructor
/// <summary>
/// Creates a new TextSegmentCollection that needs manual calls to <see cref="UpdateOffsets(DocumentChangeEventArgs)"/>.
/// </summary>
public TextSegmentCollection()
{
}
/// <summary>
/// Creates a new TextSegmentCollection that updates the offsets automatically.
/// </summary>
/// <param name="textDocument">The document to which the text segments
/// that will be added to the tree belong. When the document changes, the
/// position of the text segments will be updated accordingly.</param>
public TextSegmentCollection(TextDocument textDocument)
{
if (textDocument == null)
throw new ArgumentNullException(nameof(textDocument));
Dispatcher.UIThread.VerifyAccess();
_isConnectedToDocument = true;
TextDocumentWeakEventManager.Changed.AddHandler(textDocument, OnDocumentChanged);
}
#endregion
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="e">DocumentChangeEventArgs instance describing the change to the document.</param>
public void UpdateOffsets(DocumentChangeEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
OnDocumentChanged(this, e);
CheckProperties();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
var map = e.OffsetChangeMapOrNull;
if (map != null)
{
foreach (var entry in map)
{
UpdateOffsetsInternal(entry);
}
}
else
{
UpdateOffsetsInternal(e.CreateSingleChangeMapEntry());
}
}
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="change">OffsetChangeMapEntry instance describing the change to the document.</param>
public void UpdateOffsets(OffsetChangeMapEntry change)
{
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
UpdateOffsetsInternal(change);
CheckProperties();
}
#endregion
#region UpdateOffsets (implementation)
private void UpdateOffsetsInternal(OffsetChangeMapEntry change)
{
// Special case pure insertions, because they don't always cause a text segment to increase in size when the replaced region
// is inside a segment (when offset is at start or end of a text semgent).
if (change.RemovalLength == 0)
{
InsertText(change.Offset, change.InsertionLength);
}
else
{
ReplaceText(change);
}
}
private void InsertText(int offset, int length)
{
if (length == 0)
return;
// enlarge segments that contain offset (excluding those that have offset as endpoint)
foreach (var segment in FindSegmentsContaining(offset))
{
if (segment.StartOffset < offset && offset < segment.EndOffset)
{
segment.Length += length;
}
}
// move start offsets of all segments >= offset
TextSegment node = FindFirstSegmentWithStartAfter(offset);
if (node != null)
{
node.NodeLength += length;
UpdateAugmentedData(node);
}
}
private void ReplaceText(OffsetChangeMapEntry change)
{
Debug.Assert(change.RemovalLength > 0);
var offset = change.Offset;
foreach (var segment in FindOverlappingSegments(offset, change.RemovalLength))
{
if (segment.StartOffset <= offset)
{
if (segment.EndOffset >= offset + change.RemovalLength)
{
// Replacement inside segment: adjust segment length
segment.Length += change.InsertionLength - change.RemovalLength;
}
else
{
// Replacement starting inside segment and ending after segment end: set segment end to removal position
//segment.EndOffset = offset;
segment.Length = offset - segment.StartOffset;
}
}
else
{
// Replacement starting in front of text segment and running into segment.
// Keep segment.EndOffset constant and move segment.StartOffset to the end of the replacement
var remainingLength = segment.EndOffset - (offset + change.RemovalLength);
RemoveSegment(segment);
segment.StartOffset = offset + change.RemovalLength;
segment.Length = Math.Max(0, remainingLength);
AddSegment(segment);
}
}
// move start offsets of all segments > offset
TextSegment node = FindFirstSegmentWithStartAfter(offset + 1);
if (node != null)
{
Debug.Assert(node.NodeLength >= change.RemovalLength);
node.NodeLength += change.InsertionLength - change.RemovalLength;
UpdateAugmentedData(node);
}
}
#endregion
#region Add
/// <summary>
/// Adds the specified segment to the tree. This will cause the segment to update when the
/// document changes.
/// </summary>
public void Add(T item)
{
if (item == null)
throw new ArgumentNullException(nameof(item));
if (item.OwnerTree != null)
throw new ArgumentException("The segment is already added to a SegmentCollection.");
AddSegment(item);
}
void ISegmentTree.Add(TextSegment s)
{
AddSegment(s);
}
private void AddSegment(TextSegment node)
{
var insertionOffset = node.StartOffset;
node.DistanceToMaxEnd = node.SegmentLength;
if (_root == null)
{
_root = node;
node.TotalNodeLength = node.NodeLength;
}
else if (insertionOffset >= _root.TotalNodeLength)
{
// append segment at end of tree
node.NodeLength = node.TotalNodeLength = insertionOffset - _root.TotalNodeLength;
InsertAsRight(_root.RightMost, node);
}
else
{
// insert in middle of tree
var n = FindNode(ref insertionOffset);
Debug.Assert(insertionOffset < n.NodeLength);
// split node segment 'n' at offset
node.TotalNodeLength = node.NodeLength = insertionOffset;
n.NodeLength -= insertionOffset;
InsertBefore(n, node);
}
node.OwnerTree = this;
Count++;
CheckProperties();
}
private void InsertBefore(TextSegment node, TextSegment newNode)
{
if (node.Left == null)
{
InsertAsLeft(node, newNode);
}
else
{
InsertAsRight(node.Left.RightMost, newNode);
}
}
#endregion
#region GetNextSegment / GetPreviousSegment
/// <summary>
/// Gets the next segment after the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the last segment.
/// </summary>
public T GetNextSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Successor;
}
/// <summary>
/// Gets the previous segment before the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the first segment.
/// </summary>
public T GetPreviousSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Predecessor;
}
#endregion
#region FirstSegment/LastSegment
/// <summary>
/// Returns the first segment in the collection or null, if the collection is empty.
/// </summary>
public T FirstSegment => (T)_root?.LeftMost;
/// <summary>
/// Returns the last segment in the collection or null, if the collection is empty.
/// </summary>
public T LastSegment => (T)_root?.RightMost;
#endregion
#region FindFirstSegmentWithStartAfter
/// <summary>
/// Gets the first segment with a start offset greater or equal to <paramref name="startOffset"/>.
/// Returns null if no such segment is found.
/// </summary>
public T FindFirstSegmentWithStartAfter(int startOffset)
{
if (_root == null)
return null;
if (startOffset <= 0)
return (T)_root.LeftMost;
var s = FindNode(ref startOffset);
// startOffset means that the previous segment is starting at the offset we were looking for
while (startOffset == 0)
{
var p = (s == null) ? _root.RightMost : s.Predecessor;
// There must always be a predecessor: if we were looking for the first node, we would have already
// returned it as root.LeftMost above.
Debug.Assert(p != null);
startOffset += p.NodeLength;
s = p;
}
return (T)s;
}
/// <summary>
/// Finds the node at the specified offset.
/// After the method has run, offset is relative to the beginning of the returned node.
/// </summary>
private TextSegment FindNode(ref int offset)
{
var n = _root;
while (true)
{
if (n.Left != null)
{
if (offset < n.Left.TotalNodeLength)
{
n = n.Left; // descend into left subtree
continue;
}
else
{
offset -= n.Left.TotalNodeLength; // skip left subtree
}
}
if (offset < n.NodeLength)
{
return n; // found correct node
}
else
{
offset -= n.NodeLength; // skip this node
}
if (n.Right != null)
{
n = n.Right; // descend into right subtree
}
else
{
// didn't find any node containing the offset
return null;
}
}
}
#endregion
#region FindOverlappingSegments
/// <summary>
/// Finds all segments that contain the given offset.
/// (StartOffset <= offset <= EndOffset)
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindSegmentsContaining(int offset)
{
return FindOverlappingSegments(offset, 0);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(ISegment segment)
{
if (segment == null)
throw new ArgumentNullException(nameof(segment));
return FindOverlappingSegments(segment.Offset, segment.Length);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(int offset, int length)
{
ThrowUtil.CheckNotNegative(length, "length");
var results = new List<T>();
if (_root != null)
{
FindOverlappingSegments(results, _root, offset, offset + length);
}
return new ReadOnlyCollection<T>(results);
}
private void FindOverlappingSegments(List<T> results, TextSegment node, int low, int high)
{
// low and high are relative to node.LeftMost startpos (not node.LeftMost.Offset)
if (high < 0)
{
// node is irrelevant for search because all intervals in node are after high
return;
}
// find values relative to node.Offset
var nodeLow = low - node.NodeLength;
var nodeHigh = high - node.NodeLength;
if (node.Left != null)
{
nodeLow -= node.Left.TotalNodeLength;
nodeHigh -= node.Left.TotalNodeLength;
}
if (node.DistanceToMaxEnd < nodeLow)
{
// node is irrelevant for search because all intervals in node are before low
return;
}
if (node.Left != null)
FindOverlappingSegments(results, node.Left, low, high);
if (nodeHigh < 0)
{
// node and everything in node.right is before low
return;
}
if (nodeLow <= node.SegmentLength)
{
results.Add((T)node);
}
if (node.Right != null)
FindOverlappingSegments(results, node.Right, nodeLow, nodeHigh);
}
#endregion
#region UpdateAugmentedData
private void UpdateAugmentedData(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
totalLength += node.Left.TotalNodeLength;
var leftDtme = node.Left.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
if (node.Left.Right != null)
leftDtme -= node.Left.Right.TotalNodeLength;
leftDtme -= node.NodeLength;
if (leftDtme > distanceToMaxEnd)
distanceToMaxEnd = leftDtme;
}
if (node.Right != null)
{
totalLength += node.Right.TotalNodeLength;
var rightDtme = node.Right.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
rightDtme += node.Right.NodeLength;
if (node.Right.Left != null)
rightDtme += node.Right.Left.TotalNodeLength;
if (rightDtme > distanceToMaxEnd)
distanceToMaxEnd = rightDtme;
}
if (node.TotalNodeLength != totalLength
|| node.DistanceToMaxEnd != distanceToMaxEnd)
{
node.TotalNodeLength = totalLength;
node.DistanceToMaxEnd = distanceToMaxEnd;
if (node.Parent != null)
UpdateAugmentedData(node.Parent);
}
}
void ISegmentTree.UpdateAugmentedData(TextSegment node)
{
UpdateAugmentedData(node);
}
#endregion
#region Remove
/// <summary>
/// Removes the specified segment from the tree. This will cause the segment to not update
/// anymore when the document changes.
/// </summary>
public bool Remove(T item)
{
if (!Contains(item))
return false;
RemoveSegment(item);
return true;
}
void ISegmentTree.Remove(TextSegment s)
{
RemoveSegment(s);
}
private void RemoveSegment(TextSegment s)
{
var oldOffset = s.StartOffset;
var successor = s.Successor;
if (successor != null)
successor.NodeLength += s.NodeLength;
RemoveNode(s);
if (successor != null)
UpdateAugmentedData(successor);
Disconnect(s, oldOffset);
CheckProperties();
}
private void Disconnect(TextSegment s, int offset)
{
s.Left = s.Right = s.Parent = null;
s.OwnerTree = null;
s.NodeLength = offset;
Count--;
}
/// <summary>
/// Removes all segments from the tree.
/// </summary>
public void Clear()
{
var segments = this.ToArray();
_root = null;
var offset = 0;
foreach (var s in segments)
{
offset += s.NodeLength;
Disconnect(s, offset);
}
CheckProperties();
}
#endregion
#region CheckProperties
[Conditional("DATACONSISTENCYTEST")]
internal void CheckProperties()
{
#if DEBUG
if (_root != null)
{
CheckProperties(_root);
// check red-black property:
var blackCount = -1;
CheckNodeProperties(_root, null, Red, 0, ref blackCount);
}
var expectedCount = 0;
// we cannot trust LINQ not to call ICollection.Count, so we need this loop
// to count the elements in the tree
using (var en = GetEnumerator())
{
while (en.MoveNext()) expectedCount++;
}
Debug.Assert(Count == expectedCount);
#endif
}
#if DEBUG
private void CheckProperties(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
CheckProperties(node.Left);
totalLength += node.Left.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Left.DistanceToMaxEnd + node.Left.StartOffset - node.StartOffset);
}
if (node.Right != null)
{
CheckProperties(node.Right);
totalLength += node.Right.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Right.DistanceToMaxEnd + node.Right.StartOffset - node.StartOffset);
}
Debug.Assert(node.TotalNodeLength == totalLength);
Debug.Assert(node.DistanceToMaxEnd == distanceToMaxEnd);
}
/*
1. A node is either red or black.
2. The root is black.
3. All leaves are black. (The leaves are the NIL children.)
4. Both children of every red node are black. (So every red node must have a black parent.)
5. Every simple path from a node to a descendant leaf contains the same number of black nodes. (Not counting the leaf node.)
*/
[SuppressMessage("ReSharper", "UnusedParameter.Local")]
private static void CheckNodeProperties(TextSegment node, TextSegment parentNode, bool parentColor, int blackCount, ref int expectedBlackCount)
{
if (node == null) return;
Debug.Assert(node.Parent == parentNode);
if (parentColor == Red)
{
Debug.Assert(node.Color == Black);
}
if (node.Color == Black)
{
blackCount++;
}
if (node.Left == null && node.Right == null)
{
// node is a leaf node:
if (expectedBlackCount == -1)
expectedBlackCount = blackCount;
else
Debug.Assert(expectedBlackCount == blackCount);
}
CheckNodeProperties(node.Left, node, node.Color, blackCount, ref expectedBlackCount);
CheckNodeProperties(node.Right, node, node.Color, blackCount, ref expectedBlackCount);
}
private static void AppendTreeToString(TextSegment node, StringBuilder b, int indent)
{
b.Append(node.Color == Red ? "RED " : "BLACK ");
b.AppendLine(node + node.ToDebugString());
indent += 2;
if (node.Left != null)
{
b.Append(' ', indent);
b.Append("L: ");
AppendTreeToString(node.Left, b, indent);
}
if (node.Right != null)
{
b.Append(' ', indent);
b.Append("R: ");
AppendTreeToString(node.Right, b, indent);
}
}
#endif
internal string GetTreeAsString()
{
#if DEBUG
var b = new StringBuilder();
if (_root != null)
AppendTreeToString(_root, b, 0);
return b.ToString();
#else
return "Not available in release build.";
#endif
}
#endregion
#region Red/Black Tree
internal const bool Red = true;
internal const bool Black = false;
private void InsertAsLeft(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Left == null);
parentNode.Left = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void InsertAsRight(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Right == null);
parentNode.Right = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void FixTreeOnInsert(TextSegment node)
{
Debug.Assert(node != null);
Debug.Assert(node.Color == Red);
Debug.Assert(node.Left == null || node.Left.Color == Black);
Debug.Assert(node.Right == null || node.Right.Color == Black);
var parentNode = node.Parent;
if (parentNode == null)
{
// we inserted in the root -> the node must be black
// since this is a root node, making the node black increments the number of black nodes
// on all paths by one, so it is still the same for all paths.
node.Color = Black;
return;
}
if (parentNode.Color == Black)
{
// if the parent node where we inserted was black, our red node is placed correctly.
// since we inserted a red node, the number of black nodes on each path is unchanged
// -> the tree is still balanced
return;
}
// parentNode is red, so there is a conflict here!
// because the root is black, parentNode is not the root -> there is a grandparent node
var grandparentNode = parentNode.Parent;
var uncleNode = Sibling(parentNode);
if (uncleNode != null && uncleNode.Color == Red)
{
parentNode.Color = Black;
uncleNode.Color = Black;
grandparentNode.Color = Red;
FixTreeOnInsert(grandparentNode);
return;
}
// now we know: parent is red but uncle is black
// First rotation:
if (node == parentNode.Right && parentNode == grandparentNode.Left)
{
RotateLeft(parentNode);
node = node.Left;
}
else if (node == parentNode.Left && parentNode == grandparentNode.Right)
{
RotateRight(parentNode);
node = node.Right;
}
// because node might have changed, reassign variables:
// ReSharper disable once PossibleNullReferenceException
parentNode = node.Parent;
grandparentNode = parentNode.Parent;
// Now recolor a bit:
parentNode.Color = Black;
grandparentNode.Color = Red;
// Second rotation:
if (node == parentNode.Left && parentNode == grandparentNode.Left)
{
RotateRight(grandparentNode);
}
else
{
// because of the first rotation, this is guaranteed:
Debug.Assert(node == parentNode.Right && parentNode == grandparentNode.Right);
RotateLeft(grandparentNode);
}
}
private void RemoveNode(TextSegment removedNode)
{
if (removedNode.Left != null && removedNode.Right != null)
{
// replace removedNode with it's in-order successor
var leftMost = removedNode.Right.LeftMost;
RemoveNode(leftMost); // remove leftMost from its current location
// and overwrite the removedNode with it
ReplaceNode(removedNode, leftMost);
leftMost.Left = removedNode.Left;
if (leftMost.Left != null) leftMost.Left.Parent = leftMost;
leftMost.Right = removedNode.Right;
if (leftMost.Right != null) leftMost.Right.Parent = leftMost;
leftMost.Color = removedNode.Color;
UpdateAugmentedData(leftMost);
if (leftMost.Parent != null) UpdateAugmentedData(leftMost.Parent);
return;
}
// now either removedNode.left or removedNode.right is null
// get the remaining child
var parentNode = removedNode.Parent;
var childNode = removedNode.Left ?? removedNode.Right;
ReplaceNode(removedNode, childNode);
if (parentNode != null) UpdateAugmentedData(parentNode);
if (removedNode.Color == Black)
{
if (childNode != null && childNode.Color == Red)
{
childNode.Color = Black;
}
else
{
FixTreeOnDelete(childNode, parentNode);
}
}
}
private void FixTreeOnDelete(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (parentNode == null)
return;
// warning: node may be null
var sibling = Sibling(node, parentNode);
if (sibling.Color == Red)
{
parentNode.Color = Red;
sibling.Color = Black;
if (node == parentNode.Left)
{
RotateLeft(parentNode);
}
else
{
RotateRight(parentNode);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
}
if (parentNode.Color == Black
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
FixTreeOnDelete(parentNode, parentNode.Parent);
return;
}
if (parentNode.Color == Red
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
parentNode.Color = Black;
return;
}
if (node == parentNode.Left &&
sibling.Color == Black &&
GetColor(sibling.Left) == Red &&
GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
sibling.Left.Color = Black;
RotateRight(sibling);
}
else if (node == parentNode.Right &&
sibling.Color == Black &&
GetColor(sibling.Right) == Red &&
GetColor(sibling.Left) == Black)
{
sibling.Color = Red;
sibling.Right.Color = Black;
RotateLeft(sibling);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
sibling.Color = parentNode.Color;
parentNode.Color = Black;
if (node == parentNode.Left)
{
if (sibling.Right != null)
{
Debug.Assert(sibling.Right.Color == Red);
sibling.Right.Color = Black;
}
RotateLeft(parentNode);
}
else
{
if (sibling.Left != null)
{
Debug.Assert(sibling.Left.Color == Red);
sibling.Left.Color = Black;
}
RotateRight(parentNode);
}
}
private void ReplaceNode(TextSegment replacedNode, TextSegment newNode)
{
if (replacedNode.Parent == null)
{
Debug.Assert(replacedNode == _root);
_root = newNode;
}
else
{
if (replacedNode.Parent.Left == replacedNode)
replacedNode.Parent.Left = newNode;
else
replacedNode.Parent.Right = newNode;
}
if (newNode != null)
{
newNode.Parent = replacedNode.Parent;
}
replacedNode.Parent = null;
}
private void RotateLeft(TextSegment p)
{
// let q be p's right child
var q = p.Right;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's right child to be q's left child
p.Right = q.Left;
if (p.Right != null) p.Right.Parent = p;
// set q's left child to be p
q.Left = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private void RotateRight(TextSegment p)
{
// let q be p's left child
var q = p.Left;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's left child to be q's right child
p.Left = q.Right;
if (p.Left != null) p.Left.Parent = p;
// set q's right child to be p
q.Right = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private static TextSegment Sibling(TextSegment node)
{
return node == node.Parent.Left ? node.Parent.Right : node.Parent.Left;
}
private static TextSegment Sibling(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (node == parentNode.Left)
return parentNode.Right;
else
return parentNode.Left;
}
private static bool GetColor(TextSegment node)
{
return node != null && node.Color;
}
#endregion
#region ICollection<T> implementation
/// <summary>
/// Gets the number of segments in the tree.
/// </summary>
public int Count { get; private set; }
bool ICollection<T>.IsReadOnly => false;
/// <summary>
/// Gets whether this tree contains the specified item.
/// </summary>
public bool Contains(T item)
{
return item != null && item.OwnerTree == this;
}
/// <summary>
/// Copies all segments in this SegmentTree to the specified array.
/// </summary>
public void CopyTo(T[] array, int arrayIndex)
{
if (array == null)
throw new ArgumentNullException(nameof(array));
if (array.Length < Count)
throw new ArgumentException("The array is too small", nameof(array));
if (arrayIndex < 0 || arrayIndex + Count > array.Length)
throw new ArgumentOutOfRangeException(nameof(arrayIndex), arrayIndex, "Value must be between 0 and " + (array.Length - Count));
foreach (var s in this)
{
array[arrayIndex++] = s;
}
}
/// <summary>
/// Gets an enumerator to enumerate the segments.
/// </summary>
public IEnumerator<T> GetEnumerator()
{
if (_root != null)
{
var current = _root.LeftMost;
while (current != null)
{
yield return (T)current;
// TODO: check if collection was modified during enumeration
current = current.Successor;
}
}
}
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
#endregion
}
}
<MSG> Allow a text segment collection to be disconnected from a text document.
<DFF> @@ -97,6 +97,14 @@ namespace AvaloniaEdit.Document
}
#endregion
+ public void Disconnect (TextDocument textDocument)
+ {
+ if (_isConnectedToDocument)
+ {
+ TextDocumentWeakEventManager.Changed.RemoveHandler(textDocument, OnDocumentChanged);
+ }
+ }
+
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
| 8 | Allow a text segment collection to be disconnected from a text document. | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063836 | <NME> TextSegmentCollection.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using System.Text;
using AvaloniaEdit.Utils;
using Avalonia.Threading;
namespace AvaloniaEdit.Document
{
/// <summary>
/// Interface to allow TextSegments to access the TextSegmentCollection - we cannot use a direct reference
/// because TextSegmentCollection is generic.
/// </summary>
internal interface ISegmentTree
{
void Add(TextSegment s);
void Remove(TextSegment s);
void UpdateAugmentedData(TextSegment s);
}
/// <summary>
/// <para>
/// A collection of text segments that supports efficient lookup of segments
/// intersecting with another segment.
/// </para>
/// </summary>
/// <remarks><inheritdoc cref="TextSegment"/></remarks>
/// <see cref="TextSegment"/>
public sealed class TextSegmentCollection<T> : ICollection<T>, ISegmentTree where T : TextSegment
{
// Implementation: this is basically a mixture of an augmented interval tree
// and the TextAnchorTree.
// WARNING: you need to understand interval trees (the version with the augmented 'high'/'max' field)
// and how the TextAnchorTree works before you have any chance of understanding this code.
// This means that every node holds two "segments":
// one like the segments in the text anchor tree to support efficient offset changes
// and another that is the interval as seen by the user
// So basically, the tree contains a list of contiguous node segments of the first kind,
// with interval segments starting at the end of every node segment.
// Performance:
// Add is O(lg n)
// Remove is O(lg n)
// DocumentChanged is O(m * lg n), with m the number of segments that intersect with the changed document section
// FindFirstSegmentWithStartAfter is O(m + lg n) with m being the number of segments at the same offset as the result segment
// FindIntersectingSegments is O(m + lg n) with m being the number of intersecting segments.
private TextSegment _root;
private readonly bool _isConnectedToDocument;
#region Constructor
/// <summary>
/// Creates a new TextSegmentCollection that needs manual calls to <see cref="UpdateOffsets(DocumentChangeEventArgs)"/>.
/// </summary>
public TextSegmentCollection()
{
}
/// <summary>
/// Creates a new TextSegmentCollection that updates the offsets automatically.
/// </summary>
/// <param name="textDocument">The document to which the text segments
/// that will be added to the tree belong. When the document changes, the
/// position of the text segments will be updated accordingly.</param>
public TextSegmentCollection(TextDocument textDocument)
{
if (textDocument == null)
throw new ArgumentNullException(nameof(textDocument));
Dispatcher.UIThread.VerifyAccess();
_isConnectedToDocument = true;
TextDocumentWeakEventManager.Changed.AddHandler(textDocument, OnDocumentChanged);
}
#endregion
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="e">DocumentChangeEventArgs instance describing the change to the document.</param>
public void UpdateOffsets(DocumentChangeEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
OnDocumentChanged(this, e);
CheckProperties();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
var map = e.OffsetChangeMapOrNull;
if (map != null)
{
foreach (var entry in map)
{
UpdateOffsetsInternal(entry);
}
}
else
{
UpdateOffsetsInternal(e.CreateSingleChangeMapEntry());
}
}
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="change">OffsetChangeMapEntry instance describing the change to the document.</param>
public void UpdateOffsets(OffsetChangeMapEntry change)
{
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
UpdateOffsetsInternal(change);
CheckProperties();
}
#endregion
#region UpdateOffsets (implementation)
private void UpdateOffsetsInternal(OffsetChangeMapEntry change)
{
// Special case pure insertions, because they don't always cause a text segment to increase in size when the replaced region
// is inside a segment (when offset is at start or end of a text semgent).
if (change.RemovalLength == 0)
{
InsertText(change.Offset, change.InsertionLength);
}
else
{
ReplaceText(change);
}
}
private void InsertText(int offset, int length)
{
if (length == 0)
return;
// enlarge segments that contain offset (excluding those that have offset as endpoint)
foreach (var segment in FindSegmentsContaining(offset))
{
if (segment.StartOffset < offset && offset < segment.EndOffset)
{
segment.Length += length;
}
}
// move start offsets of all segments >= offset
TextSegment node = FindFirstSegmentWithStartAfter(offset);
if (node != null)
{
node.NodeLength += length;
UpdateAugmentedData(node);
}
}
private void ReplaceText(OffsetChangeMapEntry change)
{
Debug.Assert(change.RemovalLength > 0);
var offset = change.Offset;
foreach (var segment in FindOverlappingSegments(offset, change.RemovalLength))
{
if (segment.StartOffset <= offset)
{
if (segment.EndOffset >= offset + change.RemovalLength)
{
// Replacement inside segment: adjust segment length
segment.Length += change.InsertionLength - change.RemovalLength;
}
else
{
// Replacement starting inside segment and ending after segment end: set segment end to removal position
//segment.EndOffset = offset;
segment.Length = offset - segment.StartOffset;
}
}
else
{
// Replacement starting in front of text segment and running into segment.
// Keep segment.EndOffset constant and move segment.StartOffset to the end of the replacement
var remainingLength = segment.EndOffset - (offset + change.RemovalLength);
RemoveSegment(segment);
segment.StartOffset = offset + change.RemovalLength;
segment.Length = Math.Max(0, remainingLength);
AddSegment(segment);
}
}
// move start offsets of all segments > offset
TextSegment node = FindFirstSegmentWithStartAfter(offset + 1);
if (node != null)
{
Debug.Assert(node.NodeLength >= change.RemovalLength);
node.NodeLength += change.InsertionLength - change.RemovalLength;
UpdateAugmentedData(node);
}
}
#endregion
#region Add
/// <summary>
/// Adds the specified segment to the tree. This will cause the segment to update when the
/// document changes.
/// </summary>
public void Add(T item)
{
if (item == null)
throw new ArgumentNullException(nameof(item));
if (item.OwnerTree != null)
throw new ArgumentException("The segment is already added to a SegmentCollection.");
AddSegment(item);
}
void ISegmentTree.Add(TextSegment s)
{
AddSegment(s);
}
private void AddSegment(TextSegment node)
{
var insertionOffset = node.StartOffset;
node.DistanceToMaxEnd = node.SegmentLength;
if (_root == null)
{
_root = node;
node.TotalNodeLength = node.NodeLength;
}
else if (insertionOffset >= _root.TotalNodeLength)
{
// append segment at end of tree
node.NodeLength = node.TotalNodeLength = insertionOffset - _root.TotalNodeLength;
InsertAsRight(_root.RightMost, node);
}
else
{
// insert in middle of tree
var n = FindNode(ref insertionOffset);
Debug.Assert(insertionOffset < n.NodeLength);
// split node segment 'n' at offset
node.TotalNodeLength = node.NodeLength = insertionOffset;
n.NodeLength -= insertionOffset;
InsertBefore(n, node);
}
node.OwnerTree = this;
Count++;
CheckProperties();
}
private void InsertBefore(TextSegment node, TextSegment newNode)
{
if (node.Left == null)
{
InsertAsLeft(node, newNode);
}
else
{
InsertAsRight(node.Left.RightMost, newNode);
}
}
#endregion
#region GetNextSegment / GetPreviousSegment
/// <summary>
/// Gets the next segment after the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the last segment.
/// </summary>
public T GetNextSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Successor;
}
/// <summary>
/// Gets the previous segment before the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the first segment.
/// </summary>
public T GetPreviousSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Predecessor;
}
#endregion
#region FirstSegment/LastSegment
/// <summary>
/// Returns the first segment in the collection or null, if the collection is empty.
/// </summary>
public T FirstSegment => (T)_root?.LeftMost;
/// <summary>
/// Returns the last segment in the collection or null, if the collection is empty.
/// </summary>
public T LastSegment => (T)_root?.RightMost;
#endregion
#region FindFirstSegmentWithStartAfter
/// <summary>
/// Gets the first segment with a start offset greater or equal to <paramref name="startOffset"/>.
/// Returns null if no such segment is found.
/// </summary>
public T FindFirstSegmentWithStartAfter(int startOffset)
{
if (_root == null)
return null;
if (startOffset <= 0)
return (T)_root.LeftMost;
var s = FindNode(ref startOffset);
// startOffset means that the previous segment is starting at the offset we were looking for
while (startOffset == 0)
{
var p = (s == null) ? _root.RightMost : s.Predecessor;
// There must always be a predecessor: if we were looking for the first node, we would have already
// returned it as root.LeftMost above.
Debug.Assert(p != null);
startOffset += p.NodeLength;
s = p;
}
return (T)s;
}
/// <summary>
/// Finds the node at the specified offset.
/// After the method has run, offset is relative to the beginning of the returned node.
/// </summary>
private TextSegment FindNode(ref int offset)
{
var n = _root;
while (true)
{
if (n.Left != null)
{
if (offset < n.Left.TotalNodeLength)
{
n = n.Left; // descend into left subtree
continue;
}
else
{
offset -= n.Left.TotalNodeLength; // skip left subtree
}
}
if (offset < n.NodeLength)
{
return n; // found correct node
}
else
{
offset -= n.NodeLength; // skip this node
}
if (n.Right != null)
{
n = n.Right; // descend into right subtree
}
else
{
// didn't find any node containing the offset
return null;
}
}
}
#endregion
#region FindOverlappingSegments
/// <summary>
/// Finds all segments that contain the given offset.
/// (StartOffset <= offset <= EndOffset)
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindSegmentsContaining(int offset)
{
return FindOverlappingSegments(offset, 0);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(ISegment segment)
{
if (segment == null)
throw new ArgumentNullException(nameof(segment));
return FindOverlappingSegments(segment.Offset, segment.Length);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(int offset, int length)
{
ThrowUtil.CheckNotNegative(length, "length");
var results = new List<T>();
if (_root != null)
{
FindOverlappingSegments(results, _root, offset, offset + length);
}
return new ReadOnlyCollection<T>(results);
}
private void FindOverlappingSegments(List<T> results, TextSegment node, int low, int high)
{
// low and high are relative to node.LeftMost startpos (not node.LeftMost.Offset)
if (high < 0)
{
// node is irrelevant for search because all intervals in node are after high
return;
}
// find values relative to node.Offset
var nodeLow = low - node.NodeLength;
var nodeHigh = high - node.NodeLength;
if (node.Left != null)
{
nodeLow -= node.Left.TotalNodeLength;
nodeHigh -= node.Left.TotalNodeLength;
}
if (node.DistanceToMaxEnd < nodeLow)
{
// node is irrelevant for search because all intervals in node are before low
return;
}
if (node.Left != null)
FindOverlappingSegments(results, node.Left, low, high);
if (nodeHigh < 0)
{
// node and everything in node.right is before low
return;
}
if (nodeLow <= node.SegmentLength)
{
results.Add((T)node);
}
if (node.Right != null)
FindOverlappingSegments(results, node.Right, nodeLow, nodeHigh);
}
#endregion
#region UpdateAugmentedData
private void UpdateAugmentedData(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
totalLength += node.Left.TotalNodeLength;
var leftDtme = node.Left.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
if (node.Left.Right != null)
leftDtme -= node.Left.Right.TotalNodeLength;
leftDtme -= node.NodeLength;
if (leftDtme > distanceToMaxEnd)
distanceToMaxEnd = leftDtme;
}
if (node.Right != null)
{
totalLength += node.Right.TotalNodeLength;
var rightDtme = node.Right.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
rightDtme += node.Right.NodeLength;
if (node.Right.Left != null)
rightDtme += node.Right.Left.TotalNodeLength;
if (rightDtme > distanceToMaxEnd)
distanceToMaxEnd = rightDtme;
}
if (node.TotalNodeLength != totalLength
|| node.DistanceToMaxEnd != distanceToMaxEnd)
{
node.TotalNodeLength = totalLength;
node.DistanceToMaxEnd = distanceToMaxEnd;
if (node.Parent != null)
UpdateAugmentedData(node.Parent);
}
}
void ISegmentTree.UpdateAugmentedData(TextSegment node)
{
UpdateAugmentedData(node);
}
#endregion
#region Remove
/// <summary>
/// Removes the specified segment from the tree. This will cause the segment to not update
/// anymore when the document changes.
/// </summary>
public bool Remove(T item)
{
if (!Contains(item))
return false;
RemoveSegment(item);
return true;
}
void ISegmentTree.Remove(TextSegment s)
{
RemoveSegment(s);
}
private void RemoveSegment(TextSegment s)
{
var oldOffset = s.StartOffset;
var successor = s.Successor;
if (successor != null)
successor.NodeLength += s.NodeLength;
RemoveNode(s);
if (successor != null)
UpdateAugmentedData(successor);
Disconnect(s, oldOffset);
CheckProperties();
}
private void Disconnect(TextSegment s, int offset)
{
s.Left = s.Right = s.Parent = null;
s.OwnerTree = null;
s.NodeLength = offset;
Count--;
}
/// <summary>
/// Removes all segments from the tree.
/// </summary>
public void Clear()
{
var segments = this.ToArray();
_root = null;
var offset = 0;
foreach (var s in segments)
{
offset += s.NodeLength;
Disconnect(s, offset);
}
CheckProperties();
}
#endregion
#region CheckProperties
[Conditional("DATACONSISTENCYTEST")]
internal void CheckProperties()
{
#if DEBUG
if (_root != null)
{
CheckProperties(_root);
// check red-black property:
var blackCount = -1;
CheckNodeProperties(_root, null, Red, 0, ref blackCount);
}
var expectedCount = 0;
// we cannot trust LINQ not to call ICollection.Count, so we need this loop
// to count the elements in the tree
using (var en = GetEnumerator())
{
while (en.MoveNext()) expectedCount++;
}
Debug.Assert(Count == expectedCount);
#endif
}
#if DEBUG
private void CheckProperties(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
CheckProperties(node.Left);
totalLength += node.Left.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Left.DistanceToMaxEnd + node.Left.StartOffset - node.StartOffset);
}
if (node.Right != null)
{
CheckProperties(node.Right);
totalLength += node.Right.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Right.DistanceToMaxEnd + node.Right.StartOffset - node.StartOffset);
}
Debug.Assert(node.TotalNodeLength == totalLength);
Debug.Assert(node.DistanceToMaxEnd == distanceToMaxEnd);
}
/*
1. A node is either red or black.
2. The root is black.
3. All leaves are black. (The leaves are the NIL children.)
4. Both children of every red node are black. (So every red node must have a black parent.)
5. Every simple path from a node to a descendant leaf contains the same number of black nodes. (Not counting the leaf node.)
*/
[SuppressMessage("ReSharper", "UnusedParameter.Local")]
private static void CheckNodeProperties(TextSegment node, TextSegment parentNode, bool parentColor, int blackCount, ref int expectedBlackCount)
{
if (node == null) return;
Debug.Assert(node.Parent == parentNode);
if (parentColor == Red)
{
Debug.Assert(node.Color == Black);
}
if (node.Color == Black)
{
blackCount++;
}
if (node.Left == null && node.Right == null)
{
// node is a leaf node:
if (expectedBlackCount == -1)
expectedBlackCount = blackCount;
else
Debug.Assert(expectedBlackCount == blackCount);
}
CheckNodeProperties(node.Left, node, node.Color, blackCount, ref expectedBlackCount);
CheckNodeProperties(node.Right, node, node.Color, blackCount, ref expectedBlackCount);
}
private static void AppendTreeToString(TextSegment node, StringBuilder b, int indent)
{
b.Append(node.Color == Red ? "RED " : "BLACK ");
b.AppendLine(node + node.ToDebugString());
indent += 2;
if (node.Left != null)
{
b.Append(' ', indent);
b.Append("L: ");
AppendTreeToString(node.Left, b, indent);
}
if (node.Right != null)
{
b.Append(' ', indent);
b.Append("R: ");
AppendTreeToString(node.Right, b, indent);
}
}
#endif
internal string GetTreeAsString()
{
#if DEBUG
var b = new StringBuilder();
if (_root != null)
AppendTreeToString(_root, b, 0);
return b.ToString();
#else
return "Not available in release build.";
#endif
}
#endregion
#region Red/Black Tree
internal const bool Red = true;
internal const bool Black = false;
private void InsertAsLeft(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Left == null);
parentNode.Left = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void InsertAsRight(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Right == null);
parentNode.Right = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void FixTreeOnInsert(TextSegment node)
{
Debug.Assert(node != null);
Debug.Assert(node.Color == Red);
Debug.Assert(node.Left == null || node.Left.Color == Black);
Debug.Assert(node.Right == null || node.Right.Color == Black);
var parentNode = node.Parent;
if (parentNode == null)
{
// we inserted in the root -> the node must be black
// since this is a root node, making the node black increments the number of black nodes
// on all paths by one, so it is still the same for all paths.
node.Color = Black;
return;
}
if (parentNode.Color == Black)
{
// if the parent node where we inserted was black, our red node is placed correctly.
// since we inserted a red node, the number of black nodes on each path is unchanged
// -> the tree is still balanced
return;
}
// parentNode is red, so there is a conflict here!
// because the root is black, parentNode is not the root -> there is a grandparent node
var grandparentNode = parentNode.Parent;
var uncleNode = Sibling(parentNode);
if (uncleNode != null && uncleNode.Color == Red)
{
parentNode.Color = Black;
uncleNode.Color = Black;
grandparentNode.Color = Red;
FixTreeOnInsert(grandparentNode);
return;
}
// now we know: parent is red but uncle is black
// First rotation:
if (node == parentNode.Right && parentNode == grandparentNode.Left)
{
RotateLeft(parentNode);
node = node.Left;
}
else if (node == parentNode.Left && parentNode == grandparentNode.Right)
{
RotateRight(parentNode);
node = node.Right;
}
// because node might have changed, reassign variables:
// ReSharper disable once PossibleNullReferenceException
parentNode = node.Parent;
grandparentNode = parentNode.Parent;
// Now recolor a bit:
parentNode.Color = Black;
grandparentNode.Color = Red;
// Second rotation:
if (node == parentNode.Left && parentNode == grandparentNode.Left)
{
RotateRight(grandparentNode);
}
else
{
// because of the first rotation, this is guaranteed:
Debug.Assert(node == parentNode.Right && parentNode == grandparentNode.Right);
RotateLeft(grandparentNode);
}
}
private void RemoveNode(TextSegment removedNode)
{
if (removedNode.Left != null && removedNode.Right != null)
{
// replace removedNode with it's in-order successor
var leftMost = removedNode.Right.LeftMost;
RemoveNode(leftMost); // remove leftMost from its current location
// and overwrite the removedNode with it
ReplaceNode(removedNode, leftMost);
leftMost.Left = removedNode.Left;
if (leftMost.Left != null) leftMost.Left.Parent = leftMost;
leftMost.Right = removedNode.Right;
if (leftMost.Right != null) leftMost.Right.Parent = leftMost;
leftMost.Color = removedNode.Color;
UpdateAugmentedData(leftMost);
if (leftMost.Parent != null) UpdateAugmentedData(leftMost.Parent);
return;
}
// now either removedNode.left or removedNode.right is null
// get the remaining child
var parentNode = removedNode.Parent;
var childNode = removedNode.Left ?? removedNode.Right;
ReplaceNode(removedNode, childNode);
if (parentNode != null) UpdateAugmentedData(parentNode);
if (removedNode.Color == Black)
{
if (childNode != null && childNode.Color == Red)
{
childNode.Color = Black;
}
else
{
FixTreeOnDelete(childNode, parentNode);
}
}
}
private void FixTreeOnDelete(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (parentNode == null)
return;
// warning: node may be null
var sibling = Sibling(node, parentNode);
if (sibling.Color == Red)
{
parentNode.Color = Red;
sibling.Color = Black;
if (node == parentNode.Left)
{
RotateLeft(parentNode);
}
else
{
RotateRight(parentNode);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
}
if (parentNode.Color == Black
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
FixTreeOnDelete(parentNode, parentNode.Parent);
return;
}
if (parentNode.Color == Red
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
parentNode.Color = Black;
return;
}
if (node == parentNode.Left &&
sibling.Color == Black &&
GetColor(sibling.Left) == Red &&
GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
sibling.Left.Color = Black;
RotateRight(sibling);
}
else if (node == parentNode.Right &&
sibling.Color == Black &&
GetColor(sibling.Right) == Red &&
GetColor(sibling.Left) == Black)
{
sibling.Color = Red;
sibling.Right.Color = Black;
RotateLeft(sibling);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
sibling.Color = parentNode.Color;
parentNode.Color = Black;
if (node == parentNode.Left)
{
if (sibling.Right != null)
{
Debug.Assert(sibling.Right.Color == Red);
sibling.Right.Color = Black;
}
RotateLeft(parentNode);
}
else
{
if (sibling.Left != null)
{
Debug.Assert(sibling.Left.Color == Red);
sibling.Left.Color = Black;
}
RotateRight(parentNode);
}
}
private void ReplaceNode(TextSegment replacedNode, TextSegment newNode)
{
if (replacedNode.Parent == null)
{
Debug.Assert(replacedNode == _root);
_root = newNode;
}
else
{
if (replacedNode.Parent.Left == replacedNode)
replacedNode.Parent.Left = newNode;
else
replacedNode.Parent.Right = newNode;
}
if (newNode != null)
{
newNode.Parent = replacedNode.Parent;
}
replacedNode.Parent = null;
}
private void RotateLeft(TextSegment p)
{
// let q be p's right child
var q = p.Right;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's right child to be q's left child
p.Right = q.Left;
if (p.Right != null) p.Right.Parent = p;
// set q's left child to be p
q.Left = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private void RotateRight(TextSegment p)
{
// let q be p's left child
var q = p.Left;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's left child to be q's right child
p.Left = q.Right;
if (p.Left != null) p.Left.Parent = p;
// set q's right child to be p
q.Right = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private static TextSegment Sibling(TextSegment node)
{
return node == node.Parent.Left ? node.Parent.Right : node.Parent.Left;
}
private static TextSegment Sibling(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (node == parentNode.Left)
return parentNode.Right;
else
return parentNode.Left;
}
private static bool GetColor(TextSegment node)
{
return node != null && node.Color;
}
#endregion
#region ICollection<T> implementation
/// <summary>
/// Gets the number of segments in the tree.
/// </summary>
public int Count { get; private set; }
bool ICollection<T>.IsReadOnly => false;
/// <summary>
/// Gets whether this tree contains the specified item.
/// </summary>
public bool Contains(T item)
{
return item != null && item.OwnerTree == this;
}
/// <summary>
/// Copies all segments in this SegmentTree to the specified array.
/// </summary>
public void CopyTo(T[] array, int arrayIndex)
{
if (array == null)
throw new ArgumentNullException(nameof(array));
if (array.Length < Count)
throw new ArgumentException("The array is too small", nameof(array));
if (arrayIndex < 0 || arrayIndex + Count > array.Length)
throw new ArgumentOutOfRangeException(nameof(arrayIndex), arrayIndex, "Value must be between 0 and " + (array.Length - Count));
foreach (var s in this)
{
array[arrayIndex++] = s;
}
}
/// <summary>
/// Gets an enumerator to enumerate the segments.
/// </summary>
public IEnumerator<T> GetEnumerator()
{
if (_root != null)
{
var current = _root.LeftMost;
while (current != null)
{
yield return (T)current;
// TODO: check if collection was modified during enumeration
current = current.Successor;
}
}
}
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
#endregion
}
}
<MSG> Allow a text segment collection to be disconnected from a text document.
<DFF> @@ -97,6 +97,14 @@ namespace AvaloniaEdit.Document
}
#endregion
+ public void Disconnect (TextDocument textDocument)
+ {
+ if (_isConnectedToDocument)
+ {
+ TextDocumentWeakEventManager.Changed.RemoveHandler(textDocument, OnDocumentChanged);
+ }
+ }
+
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
| 8 | Allow a text segment collection to be disconnected from a text document. | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063837 | <NME> TextSegmentCollection.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using System.Text;
using AvaloniaEdit.Utils;
using Avalonia.Threading;
namespace AvaloniaEdit.Document
{
/// <summary>
/// Interface to allow TextSegments to access the TextSegmentCollection - we cannot use a direct reference
/// because TextSegmentCollection is generic.
/// </summary>
internal interface ISegmentTree
{
void Add(TextSegment s);
void Remove(TextSegment s);
void UpdateAugmentedData(TextSegment s);
}
/// <summary>
/// <para>
/// A collection of text segments that supports efficient lookup of segments
/// intersecting with another segment.
/// </para>
/// </summary>
/// <remarks><inheritdoc cref="TextSegment"/></remarks>
/// <see cref="TextSegment"/>
public sealed class TextSegmentCollection<T> : ICollection<T>, ISegmentTree where T : TextSegment
{
// Implementation: this is basically a mixture of an augmented interval tree
// and the TextAnchorTree.
// WARNING: you need to understand interval trees (the version with the augmented 'high'/'max' field)
// and how the TextAnchorTree works before you have any chance of understanding this code.
// This means that every node holds two "segments":
// one like the segments in the text anchor tree to support efficient offset changes
// and another that is the interval as seen by the user
// So basically, the tree contains a list of contiguous node segments of the first kind,
// with interval segments starting at the end of every node segment.
// Performance:
// Add is O(lg n)
// Remove is O(lg n)
// DocumentChanged is O(m * lg n), with m the number of segments that intersect with the changed document section
// FindFirstSegmentWithStartAfter is O(m + lg n) with m being the number of segments at the same offset as the result segment
// FindIntersectingSegments is O(m + lg n) with m being the number of intersecting segments.
private TextSegment _root;
private readonly bool _isConnectedToDocument;
#region Constructor
/// <summary>
/// Creates a new TextSegmentCollection that needs manual calls to <see cref="UpdateOffsets(DocumentChangeEventArgs)"/>.
/// </summary>
public TextSegmentCollection()
{
}
/// <summary>
/// Creates a new TextSegmentCollection that updates the offsets automatically.
/// </summary>
/// <param name="textDocument">The document to which the text segments
/// that will be added to the tree belong. When the document changes, the
/// position of the text segments will be updated accordingly.</param>
public TextSegmentCollection(TextDocument textDocument)
{
if (textDocument == null)
throw new ArgumentNullException(nameof(textDocument));
Dispatcher.UIThread.VerifyAccess();
_isConnectedToDocument = true;
TextDocumentWeakEventManager.Changed.AddHandler(textDocument, OnDocumentChanged);
}
#endregion
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="e">DocumentChangeEventArgs instance describing the change to the document.</param>
public void UpdateOffsets(DocumentChangeEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
OnDocumentChanged(this, e);
CheckProperties();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
var map = e.OffsetChangeMapOrNull;
if (map != null)
{
foreach (var entry in map)
{
UpdateOffsetsInternal(entry);
}
}
else
{
UpdateOffsetsInternal(e.CreateSingleChangeMapEntry());
}
}
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="change">OffsetChangeMapEntry instance describing the change to the document.</param>
public void UpdateOffsets(OffsetChangeMapEntry change)
{
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
UpdateOffsetsInternal(change);
CheckProperties();
}
#endregion
#region UpdateOffsets (implementation)
private void UpdateOffsetsInternal(OffsetChangeMapEntry change)
{
// Special case pure insertions, because they don't always cause a text segment to increase in size when the replaced region
// is inside a segment (when offset is at start or end of a text semgent).
if (change.RemovalLength == 0)
{
InsertText(change.Offset, change.InsertionLength);
}
else
{
ReplaceText(change);
}
}
private void InsertText(int offset, int length)
{
if (length == 0)
return;
// enlarge segments that contain offset (excluding those that have offset as endpoint)
foreach (var segment in FindSegmentsContaining(offset))
{
if (segment.StartOffset < offset && offset < segment.EndOffset)
{
segment.Length += length;
}
}
// move start offsets of all segments >= offset
TextSegment node = FindFirstSegmentWithStartAfter(offset);
if (node != null)
{
node.NodeLength += length;
UpdateAugmentedData(node);
}
}
private void ReplaceText(OffsetChangeMapEntry change)
{
Debug.Assert(change.RemovalLength > 0);
var offset = change.Offset;
foreach (var segment in FindOverlappingSegments(offset, change.RemovalLength))
{
if (segment.StartOffset <= offset)
{
if (segment.EndOffset >= offset + change.RemovalLength)
{
// Replacement inside segment: adjust segment length
segment.Length += change.InsertionLength - change.RemovalLength;
}
else
{
// Replacement starting inside segment and ending after segment end: set segment end to removal position
//segment.EndOffset = offset;
segment.Length = offset - segment.StartOffset;
}
}
else
{
// Replacement starting in front of text segment and running into segment.
// Keep segment.EndOffset constant and move segment.StartOffset to the end of the replacement
var remainingLength = segment.EndOffset - (offset + change.RemovalLength);
RemoveSegment(segment);
segment.StartOffset = offset + change.RemovalLength;
segment.Length = Math.Max(0, remainingLength);
AddSegment(segment);
}
}
// move start offsets of all segments > offset
TextSegment node = FindFirstSegmentWithStartAfter(offset + 1);
if (node != null)
{
Debug.Assert(node.NodeLength >= change.RemovalLength);
node.NodeLength += change.InsertionLength - change.RemovalLength;
UpdateAugmentedData(node);
}
}
#endregion
#region Add
/// <summary>
/// Adds the specified segment to the tree. This will cause the segment to update when the
/// document changes.
/// </summary>
public void Add(T item)
{
if (item == null)
throw new ArgumentNullException(nameof(item));
if (item.OwnerTree != null)
throw new ArgumentException("The segment is already added to a SegmentCollection.");
AddSegment(item);
}
void ISegmentTree.Add(TextSegment s)
{
AddSegment(s);
}
private void AddSegment(TextSegment node)
{
var insertionOffset = node.StartOffset;
node.DistanceToMaxEnd = node.SegmentLength;
if (_root == null)
{
_root = node;
node.TotalNodeLength = node.NodeLength;
}
else if (insertionOffset >= _root.TotalNodeLength)
{
// append segment at end of tree
node.NodeLength = node.TotalNodeLength = insertionOffset - _root.TotalNodeLength;
InsertAsRight(_root.RightMost, node);
}
else
{
// insert in middle of tree
var n = FindNode(ref insertionOffset);
Debug.Assert(insertionOffset < n.NodeLength);
// split node segment 'n' at offset
node.TotalNodeLength = node.NodeLength = insertionOffset;
n.NodeLength -= insertionOffset;
InsertBefore(n, node);
}
node.OwnerTree = this;
Count++;
CheckProperties();
}
private void InsertBefore(TextSegment node, TextSegment newNode)
{
if (node.Left == null)
{
InsertAsLeft(node, newNode);
}
else
{
InsertAsRight(node.Left.RightMost, newNode);
}
}
#endregion
#region GetNextSegment / GetPreviousSegment
/// <summary>
/// Gets the next segment after the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the last segment.
/// </summary>
public T GetNextSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Successor;
}
/// <summary>
/// Gets the previous segment before the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the first segment.
/// </summary>
public T GetPreviousSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Predecessor;
}
#endregion
#region FirstSegment/LastSegment
/// <summary>
/// Returns the first segment in the collection or null, if the collection is empty.
/// </summary>
public T FirstSegment => (T)_root?.LeftMost;
/// <summary>
/// Returns the last segment in the collection or null, if the collection is empty.
/// </summary>
public T LastSegment => (T)_root?.RightMost;
#endregion
#region FindFirstSegmentWithStartAfter
/// <summary>
/// Gets the first segment with a start offset greater or equal to <paramref name="startOffset"/>.
/// Returns null if no such segment is found.
/// </summary>
public T FindFirstSegmentWithStartAfter(int startOffset)
{
if (_root == null)
return null;
if (startOffset <= 0)
return (T)_root.LeftMost;
var s = FindNode(ref startOffset);
// startOffset means that the previous segment is starting at the offset we were looking for
while (startOffset == 0)
{
var p = (s == null) ? _root.RightMost : s.Predecessor;
// There must always be a predecessor: if we were looking for the first node, we would have already
// returned it as root.LeftMost above.
Debug.Assert(p != null);
startOffset += p.NodeLength;
s = p;
}
return (T)s;
}
/// <summary>
/// Finds the node at the specified offset.
/// After the method has run, offset is relative to the beginning of the returned node.
/// </summary>
private TextSegment FindNode(ref int offset)
{
var n = _root;
while (true)
{
if (n.Left != null)
{
if (offset < n.Left.TotalNodeLength)
{
n = n.Left; // descend into left subtree
continue;
}
else
{
offset -= n.Left.TotalNodeLength; // skip left subtree
}
}
if (offset < n.NodeLength)
{
return n; // found correct node
}
else
{
offset -= n.NodeLength; // skip this node
}
if (n.Right != null)
{
n = n.Right; // descend into right subtree
}
else
{
// didn't find any node containing the offset
return null;
}
}
}
#endregion
#region FindOverlappingSegments
/// <summary>
/// Finds all segments that contain the given offset.
/// (StartOffset <= offset <= EndOffset)
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindSegmentsContaining(int offset)
{
return FindOverlappingSegments(offset, 0);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(ISegment segment)
{
if (segment == null)
throw new ArgumentNullException(nameof(segment));
return FindOverlappingSegments(segment.Offset, segment.Length);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(int offset, int length)
{
ThrowUtil.CheckNotNegative(length, "length");
var results = new List<T>();
if (_root != null)
{
FindOverlappingSegments(results, _root, offset, offset + length);
}
return new ReadOnlyCollection<T>(results);
}
private void FindOverlappingSegments(List<T> results, TextSegment node, int low, int high)
{
// low and high are relative to node.LeftMost startpos (not node.LeftMost.Offset)
if (high < 0)
{
// node is irrelevant for search because all intervals in node are after high
return;
}
// find values relative to node.Offset
var nodeLow = low - node.NodeLength;
var nodeHigh = high - node.NodeLength;
if (node.Left != null)
{
nodeLow -= node.Left.TotalNodeLength;
nodeHigh -= node.Left.TotalNodeLength;
}
if (node.DistanceToMaxEnd < nodeLow)
{
// node is irrelevant for search because all intervals in node are before low
return;
}
if (node.Left != null)
FindOverlappingSegments(results, node.Left, low, high);
if (nodeHigh < 0)
{
// node and everything in node.right is before low
return;
}
if (nodeLow <= node.SegmentLength)
{
results.Add((T)node);
}
if (node.Right != null)
FindOverlappingSegments(results, node.Right, nodeLow, nodeHigh);
}
#endregion
#region UpdateAugmentedData
private void UpdateAugmentedData(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
totalLength += node.Left.TotalNodeLength;
var leftDtme = node.Left.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
if (node.Left.Right != null)
leftDtme -= node.Left.Right.TotalNodeLength;
leftDtme -= node.NodeLength;
if (leftDtme > distanceToMaxEnd)
distanceToMaxEnd = leftDtme;
}
if (node.Right != null)
{
totalLength += node.Right.TotalNodeLength;
var rightDtme = node.Right.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
rightDtme += node.Right.NodeLength;
if (node.Right.Left != null)
rightDtme += node.Right.Left.TotalNodeLength;
if (rightDtme > distanceToMaxEnd)
distanceToMaxEnd = rightDtme;
}
if (node.TotalNodeLength != totalLength
|| node.DistanceToMaxEnd != distanceToMaxEnd)
{
node.TotalNodeLength = totalLength;
node.DistanceToMaxEnd = distanceToMaxEnd;
if (node.Parent != null)
UpdateAugmentedData(node.Parent);
}
}
void ISegmentTree.UpdateAugmentedData(TextSegment node)
{
UpdateAugmentedData(node);
}
#endregion
#region Remove
/// <summary>
/// Removes the specified segment from the tree. This will cause the segment to not update
/// anymore when the document changes.
/// </summary>
public bool Remove(T item)
{
if (!Contains(item))
return false;
RemoveSegment(item);
return true;
}
void ISegmentTree.Remove(TextSegment s)
{
RemoveSegment(s);
}
private void RemoveSegment(TextSegment s)
{
var oldOffset = s.StartOffset;
var successor = s.Successor;
if (successor != null)
successor.NodeLength += s.NodeLength;
RemoveNode(s);
if (successor != null)
UpdateAugmentedData(successor);
Disconnect(s, oldOffset);
CheckProperties();
}
private void Disconnect(TextSegment s, int offset)
{
s.Left = s.Right = s.Parent = null;
s.OwnerTree = null;
s.NodeLength = offset;
Count--;
}
/// <summary>
/// Removes all segments from the tree.
/// </summary>
public void Clear()
{
var segments = this.ToArray();
_root = null;
var offset = 0;
foreach (var s in segments)
{
offset += s.NodeLength;
Disconnect(s, offset);
}
CheckProperties();
}
#endregion
#region CheckProperties
[Conditional("DATACONSISTENCYTEST")]
internal void CheckProperties()
{
#if DEBUG
if (_root != null)
{
CheckProperties(_root);
// check red-black property:
var blackCount = -1;
CheckNodeProperties(_root, null, Red, 0, ref blackCount);
}
var expectedCount = 0;
// we cannot trust LINQ not to call ICollection.Count, so we need this loop
// to count the elements in the tree
using (var en = GetEnumerator())
{
while (en.MoveNext()) expectedCount++;
}
Debug.Assert(Count == expectedCount);
#endif
}
#if DEBUG
private void CheckProperties(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
CheckProperties(node.Left);
totalLength += node.Left.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Left.DistanceToMaxEnd + node.Left.StartOffset - node.StartOffset);
}
if (node.Right != null)
{
CheckProperties(node.Right);
totalLength += node.Right.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Right.DistanceToMaxEnd + node.Right.StartOffset - node.StartOffset);
}
Debug.Assert(node.TotalNodeLength == totalLength);
Debug.Assert(node.DistanceToMaxEnd == distanceToMaxEnd);
}
/*
1. A node is either red or black.
2. The root is black.
3. All leaves are black. (The leaves are the NIL children.)
4. Both children of every red node are black. (So every red node must have a black parent.)
5. Every simple path from a node to a descendant leaf contains the same number of black nodes. (Not counting the leaf node.)
*/
[SuppressMessage("ReSharper", "UnusedParameter.Local")]
private static void CheckNodeProperties(TextSegment node, TextSegment parentNode, bool parentColor, int blackCount, ref int expectedBlackCount)
{
if (node == null) return;
Debug.Assert(node.Parent == parentNode);
if (parentColor == Red)
{
Debug.Assert(node.Color == Black);
}
if (node.Color == Black)
{
blackCount++;
}
if (node.Left == null && node.Right == null)
{
// node is a leaf node:
if (expectedBlackCount == -1)
expectedBlackCount = blackCount;
else
Debug.Assert(expectedBlackCount == blackCount);
}
CheckNodeProperties(node.Left, node, node.Color, blackCount, ref expectedBlackCount);
CheckNodeProperties(node.Right, node, node.Color, blackCount, ref expectedBlackCount);
}
private static void AppendTreeToString(TextSegment node, StringBuilder b, int indent)
{
b.Append(node.Color == Red ? "RED " : "BLACK ");
b.AppendLine(node + node.ToDebugString());
indent += 2;
if (node.Left != null)
{
b.Append(' ', indent);
b.Append("L: ");
AppendTreeToString(node.Left, b, indent);
}
if (node.Right != null)
{
b.Append(' ', indent);
b.Append("R: ");
AppendTreeToString(node.Right, b, indent);
}
}
#endif
internal string GetTreeAsString()
{
#if DEBUG
var b = new StringBuilder();
if (_root != null)
AppendTreeToString(_root, b, 0);
return b.ToString();
#else
return "Not available in release build.";
#endif
}
#endregion
#region Red/Black Tree
internal const bool Red = true;
internal const bool Black = false;
private void InsertAsLeft(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Left == null);
parentNode.Left = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void InsertAsRight(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Right == null);
parentNode.Right = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void FixTreeOnInsert(TextSegment node)
{
Debug.Assert(node != null);
Debug.Assert(node.Color == Red);
Debug.Assert(node.Left == null || node.Left.Color == Black);
Debug.Assert(node.Right == null || node.Right.Color == Black);
var parentNode = node.Parent;
if (parentNode == null)
{
// we inserted in the root -> the node must be black
// since this is a root node, making the node black increments the number of black nodes
// on all paths by one, so it is still the same for all paths.
node.Color = Black;
return;
}
if (parentNode.Color == Black)
{
// if the parent node where we inserted was black, our red node is placed correctly.
// since we inserted a red node, the number of black nodes on each path is unchanged
// -> the tree is still balanced
return;
}
// parentNode is red, so there is a conflict here!
// because the root is black, parentNode is not the root -> there is a grandparent node
var grandparentNode = parentNode.Parent;
var uncleNode = Sibling(parentNode);
if (uncleNode != null && uncleNode.Color == Red)
{
parentNode.Color = Black;
uncleNode.Color = Black;
grandparentNode.Color = Red;
FixTreeOnInsert(grandparentNode);
return;
}
// now we know: parent is red but uncle is black
// First rotation:
if (node == parentNode.Right && parentNode == grandparentNode.Left)
{
RotateLeft(parentNode);
node = node.Left;
}
else if (node == parentNode.Left && parentNode == grandparentNode.Right)
{
RotateRight(parentNode);
node = node.Right;
}
// because node might have changed, reassign variables:
// ReSharper disable once PossibleNullReferenceException
parentNode = node.Parent;
grandparentNode = parentNode.Parent;
// Now recolor a bit:
parentNode.Color = Black;
grandparentNode.Color = Red;
// Second rotation:
if (node == parentNode.Left && parentNode == grandparentNode.Left)
{
RotateRight(grandparentNode);
}
else
{
// because of the first rotation, this is guaranteed:
Debug.Assert(node == parentNode.Right && parentNode == grandparentNode.Right);
RotateLeft(grandparentNode);
}
}
private void RemoveNode(TextSegment removedNode)
{
if (removedNode.Left != null && removedNode.Right != null)
{
// replace removedNode with it's in-order successor
var leftMost = removedNode.Right.LeftMost;
RemoveNode(leftMost); // remove leftMost from its current location
// and overwrite the removedNode with it
ReplaceNode(removedNode, leftMost);
leftMost.Left = removedNode.Left;
if (leftMost.Left != null) leftMost.Left.Parent = leftMost;
leftMost.Right = removedNode.Right;
if (leftMost.Right != null) leftMost.Right.Parent = leftMost;
leftMost.Color = removedNode.Color;
UpdateAugmentedData(leftMost);
if (leftMost.Parent != null) UpdateAugmentedData(leftMost.Parent);
return;
}
// now either removedNode.left or removedNode.right is null
// get the remaining child
var parentNode = removedNode.Parent;
var childNode = removedNode.Left ?? removedNode.Right;
ReplaceNode(removedNode, childNode);
if (parentNode != null) UpdateAugmentedData(parentNode);
if (removedNode.Color == Black)
{
if (childNode != null && childNode.Color == Red)
{
childNode.Color = Black;
}
else
{
FixTreeOnDelete(childNode, parentNode);
}
}
}
private void FixTreeOnDelete(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (parentNode == null)
return;
// warning: node may be null
var sibling = Sibling(node, parentNode);
if (sibling.Color == Red)
{
parentNode.Color = Red;
sibling.Color = Black;
if (node == parentNode.Left)
{
RotateLeft(parentNode);
}
else
{
RotateRight(parentNode);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
}
if (parentNode.Color == Black
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
FixTreeOnDelete(parentNode, parentNode.Parent);
return;
}
if (parentNode.Color == Red
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
parentNode.Color = Black;
return;
}
if (node == parentNode.Left &&
sibling.Color == Black &&
GetColor(sibling.Left) == Red &&
GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
sibling.Left.Color = Black;
RotateRight(sibling);
}
else if (node == parentNode.Right &&
sibling.Color == Black &&
GetColor(sibling.Right) == Red &&
GetColor(sibling.Left) == Black)
{
sibling.Color = Red;
sibling.Right.Color = Black;
RotateLeft(sibling);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
sibling.Color = parentNode.Color;
parentNode.Color = Black;
if (node == parentNode.Left)
{
if (sibling.Right != null)
{
Debug.Assert(sibling.Right.Color == Red);
sibling.Right.Color = Black;
}
RotateLeft(parentNode);
}
else
{
if (sibling.Left != null)
{
Debug.Assert(sibling.Left.Color == Red);
sibling.Left.Color = Black;
}
RotateRight(parentNode);
}
}
private void ReplaceNode(TextSegment replacedNode, TextSegment newNode)
{
if (replacedNode.Parent == null)
{
Debug.Assert(replacedNode == _root);
_root = newNode;
}
else
{
if (replacedNode.Parent.Left == replacedNode)
replacedNode.Parent.Left = newNode;
else
replacedNode.Parent.Right = newNode;
}
if (newNode != null)
{
newNode.Parent = replacedNode.Parent;
}
replacedNode.Parent = null;
}
private void RotateLeft(TextSegment p)
{
// let q be p's right child
var q = p.Right;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's right child to be q's left child
p.Right = q.Left;
if (p.Right != null) p.Right.Parent = p;
// set q's left child to be p
q.Left = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private void RotateRight(TextSegment p)
{
// let q be p's left child
var q = p.Left;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's left child to be q's right child
p.Left = q.Right;
if (p.Left != null) p.Left.Parent = p;
// set q's right child to be p
q.Right = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private static TextSegment Sibling(TextSegment node)
{
return node == node.Parent.Left ? node.Parent.Right : node.Parent.Left;
}
private static TextSegment Sibling(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (node == parentNode.Left)
return parentNode.Right;
else
return parentNode.Left;
}
private static bool GetColor(TextSegment node)
{
return node != null && node.Color;
}
#endregion
#region ICollection<T> implementation
/// <summary>
/// Gets the number of segments in the tree.
/// </summary>
public int Count { get; private set; }
bool ICollection<T>.IsReadOnly => false;
/// <summary>
/// Gets whether this tree contains the specified item.
/// </summary>
public bool Contains(T item)
{
return item != null && item.OwnerTree == this;
}
/// <summary>
/// Copies all segments in this SegmentTree to the specified array.
/// </summary>
public void CopyTo(T[] array, int arrayIndex)
{
if (array == null)
throw new ArgumentNullException(nameof(array));
if (array.Length < Count)
throw new ArgumentException("The array is too small", nameof(array));
if (arrayIndex < 0 || arrayIndex + Count > array.Length)
throw new ArgumentOutOfRangeException(nameof(arrayIndex), arrayIndex, "Value must be between 0 and " + (array.Length - Count));
foreach (var s in this)
{
array[arrayIndex++] = s;
}
}
/// <summary>
/// Gets an enumerator to enumerate the segments.
/// </summary>
public IEnumerator<T> GetEnumerator()
{
if (_root != null)
{
var current = _root.LeftMost;
while (current != null)
{
yield return (T)current;
// TODO: check if collection was modified during enumeration
current = current.Successor;
}
}
}
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
#endregion
}
}
<MSG> Allow a text segment collection to be disconnected from a text document.
<DFF> @@ -97,6 +97,14 @@ namespace AvaloniaEdit.Document
}
#endregion
+ public void Disconnect (TextDocument textDocument)
+ {
+ if (_isConnectedToDocument)
+ {
+ TextDocumentWeakEventManager.Changed.RemoveHandler(textDocument, OnDocumentChanged);
+ }
+ }
+
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
| 8 | Allow a text segment collection to be disconnected from a text document. | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063838 | <NME> TextSegmentCollection.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using System.Text;
using AvaloniaEdit.Utils;
using Avalonia.Threading;
namespace AvaloniaEdit.Document
{
/// <summary>
/// Interface to allow TextSegments to access the TextSegmentCollection - we cannot use a direct reference
/// because TextSegmentCollection is generic.
/// </summary>
internal interface ISegmentTree
{
void Add(TextSegment s);
void Remove(TextSegment s);
void UpdateAugmentedData(TextSegment s);
}
/// <summary>
/// <para>
/// A collection of text segments that supports efficient lookup of segments
/// intersecting with another segment.
/// </para>
/// </summary>
/// <remarks><inheritdoc cref="TextSegment"/></remarks>
/// <see cref="TextSegment"/>
public sealed class TextSegmentCollection<T> : ICollection<T>, ISegmentTree where T : TextSegment
{
// Implementation: this is basically a mixture of an augmented interval tree
// and the TextAnchorTree.
// WARNING: you need to understand interval trees (the version with the augmented 'high'/'max' field)
// and how the TextAnchorTree works before you have any chance of understanding this code.
// This means that every node holds two "segments":
// one like the segments in the text anchor tree to support efficient offset changes
// and another that is the interval as seen by the user
// So basically, the tree contains a list of contiguous node segments of the first kind,
// with interval segments starting at the end of every node segment.
// Performance:
// Add is O(lg n)
// Remove is O(lg n)
// DocumentChanged is O(m * lg n), with m the number of segments that intersect with the changed document section
// FindFirstSegmentWithStartAfter is O(m + lg n) with m being the number of segments at the same offset as the result segment
// FindIntersectingSegments is O(m + lg n) with m being the number of intersecting segments.
private TextSegment _root;
private readonly bool _isConnectedToDocument;
#region Constructor
/// <summary>
/// Creates a new TextSegmentCollection that needs manual calls to <see cref="UpdateOffsets(DocumentChangeEventArgs)"/>.
/// </summary>
public TextSegmentCollection()
{
}
/// <summary>
/// Creates a new TextSegmentCollection that updates the offsets automatically.
/// </summary>
/// <param name="textDocument">The document to which the text segments
/// that will be added to the tree belong. When the document changes, the
/// position of the text segments will be updated accordingly.</param>
public TextSegmentCollection(TextDocument textDocument)
{
if (textDocument == null)
throw new ArgumentNullException(nameof(textDocument));
Dispatcher.UIThread.VerifyAccess();
_isConnectedToDocument = true;
TextDocumentWeakEventManager.Changed.AddHandler(textDocument, OnDocumentChanged);
}
#endregion
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="e">DocumentChangeEventArgs instance describing the change to the document.</param>
public void UpdateOffsets(DocumentChangeEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
OnDocumentChanged(this, e);
CheckProperties();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
var map = e.OffsetChangeMapOrNull;
if (map != null)
{
foreach (var entry in map)
{
UpdateOffsetsInternal(entry);
}
}
else
{
UpdateOffsetsInternal(e.CreateSingleChangeMapEntry());
}
}
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="change">OffsetChangeMapEntry instance describing the change to the document.</param>
public void UpdateOffsets(OffsetChangeMapEntry change)
{
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
UpdateOffsetsInternal(change);
CheckProperties();
}
#endregion
#region UpdateOffsets (implementation)
private void UpdateOffsetsInternal(OffsetChangeMapEntry change)
{
// Special case pure insertions, because they don't always cause a text segment to increase in size when the replaced region
// is inside a segment (when offset is at start or end of a text semgent).
if (change.RemovalLength == 0)
{
InsertText(change.Offset, change.InsertionLength);
}
else
{
ReplaceText(change);
}
}
private void InsertText(int offset, int length)
{
if (length == 0)
return;
// enlarge segments that contain offset (excluding those that have offset as endpoint)
foreach (var segment in FindSegmentsContaining(offset))
{
if (segment.StartOffset < offset && offset < segment.EndOffset)
{
segment.Length += length;
}
}
// move start offsets of all segments >= offset
TextSegment node = FindFirstSegmentWithStartAfter(offset);
if (node != null)
{
node.NodeLength += length;
UpdateAugmentedData(node);
}
}
private void ReplaceText(OffsetChangeMapEntry change)
{
Debug.Assert(change.RemovalLength > 0);
var offset = change.Offset;
foreach (var segment in FindOverlappingSegments(offset, change.RemovalLength))
{
if (segment.StartOffset <= offset)
{
if (segment.EndOffset >= offset + change.RemovalLength)
{
// Replacement inside segment: adjust segment length
segment.Length += change.InsertionLength - change.RemovalLength;
}
else
{
// Replacement starting inside segment and ending after segment end: set segment end to removal position
//segment.EndOffset = offset;
segment.Length = offset - segment.StartOffset;
}
}
else
{
// Replacement starting in front of text segment and running into segment.
// Keep segment.EndOffset constant and move segment.StartOffset to the end of the replacement
var remainingLength = segment.EndOffset - (offset + change.RemovalLength);
RemoveSegment(segment);
segment.StartOffset = offset + change.RemovalLength;
segment.Length = Math.Max(0, remainingLength);
AddSegment(segment);
}
}
// move start offsets of all segments > offset
TextSegment node = FindFirstSegmentWithStartAfter(offset + 1);
if (node != null)
{
Debug.Assert(node.NodeLength >= change.RemovalLength);
node.NodeLength += change.InsertionLength - change.RemovalLength;
UpdateAugmentedData(node);
}
}
#endregion
#region Add
/// <summary>
/// Adds the specified segment to the tree. This will cause the segment to update when the
/// document changes.
/// </summary>
public void Add(T item)
{
if (item == null)
throw new ArgumentNullException(nameof(item));
if (item.OwnerTree != null)
throw new ArgumentException("The segment is already added to a SegmentCollection.");
AddSegment(item);
}
void ISegmentTree.Add(TextSegment s)
{
AddSegment(s);
}
private void AddSegment(TextSegment node)
{
var insertionOffset = node.StartOffset;
node.DistanceToMaxEnd = node.SegmentLength;
if (_root == null)
{
_root = node;
node.TotalNodeLength = node.NodeLength;
}
else if (insertionOffset >= _root.TotalNodeLength)
{
// append segment at end of tree
node.NodeLength = node.TotalNodeLength = insertionOffset - _root.TotalNodeLength;
InsertAsRight(_root.RightMost, node);
}
else
{
// insert in middle of tree
var n = FindNode(ref insertionOffset);
Debug.Assert(insertionOffset < n.NodeLength);
// split node segment 'n' at offset
node.TotalNodeLength = node.NodeLength = insertionOffset;
n.NodeLength -= insertionOffset;
InsertBefore(n, node);
}
node.OwnerTree = this;
Count++;
CheckProperties();
}
private void InsertBefore(TextSegment node, TextSegment newNode)
{
if (node.Left == null)
{
InsertAsLeft(node, newNode);
}
else
{
InsertAsRight(node.Left.RightMost, newNode);
}
}
#endregion
#region GetNextSegment / GetPreviousSegment
/// <summary>
/// Gets the next segment after the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the last segment.
/// </summary>
public T GetNextSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Successor;
}
/// <summary>
/// Gets the previous segment before the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the first segment.
/// </summary>
public T GetPreviousSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Predecessor;
}
#endregion
#region FirstSegment/LastSegment
/// <summary>
/// Returns the first segment in the collection or null, if the collection is empty.
/// </summary>
public T FirstSegment => (T)_root?.LeftMost;
/// <summary>
/// Returns the last segment in the collection or null, if the collection is empty.
/// </summary>
public T LastSegment => (T)_root?.RightMost;
#endregion
#region FindFirstSegmentWithStartAfter
/// <summary>
/// Gets the first segment with a start offset greater or equal to <paramref name="startOffset"/>.
/// Returns null if no such segment is found.
/// </summary>
public T FindFirstSegmentWithStartAfter(int startOffset)
{
if (_root == null)
return null;
if (startOffset <= 0)
return (T)_root.LeftMost;
var s = FindNode(ref startOffset);
// startOffset means that the previous segment is starting at the offset we were looking for
while (startOffset == 0)
{
var p = (s == null) ? _root.RightMost : s.Predecessor;
// There must always be a predecessor: if we were looking for the first node, we would have already
// returned it as root.LeftMost above.
Debug.Assert(p != null);
startOffset += p.NodeLength;
s = p;
}
return (T)s;
}
/// <summary>
/// Finds the node at the specified offset.
/// After the method has run, offset is relative to the beginning of the returned node.
/// </summary>
private TextSegment FindNode(ref int offset)
{
var n = _root;
while (true)
{
if (n.Left != null)
{
if (offset < n.Left.TotalNodeLength)
{
n = n.Left; // descend into left subtree
continue;
}
else
{
offset -= n.Left.TotalNodeLength; // skip left subtree
}
}
if (offset < n.NodeLength)
{
return n; // found correct node
}
else
{
offset -= n.NodeLength; // skip this node
}
if (n.Right != null)
{
n = n.Right; // descend into right subtree
}
else
{
// didn't find any node containing the offset
return null;
}
}
}
#endregion
#region FindOverlappingSegments
/// <summary>
/// Finds all segments that contain the given offset.
/// (StartOffset <= offset <= EndOffset)
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindSegmentsContaining(int offset)
{
return FindOverlappingSegments(offset, 0);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(ISegment segment)
{
if (segment == null)
throw new ArgumentNullException(nameof(segment));
return FindOverlappingSegments(segment.Offset, segment.Length);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(int offset, int length)
{
ThrowUtil.CheckNotNegative(length, "length");
var results = new List<T>();
if (_root != null)
{
FindOverlappingSegments(results, _root, offset, offset + length);
}
return new ReadOnlyCollection<T>(results);
}
private void FindOverlappingSegments(List<T> results, TextSegment node, int low, int high)
{
// low and high are relative to node.LeftMost startpos (not node.LeftMost.Offset)
if (high < 0)
{
// node is irrelevant for search because all intervals in node are after high
return;
}
// find values relative to node.Offset
var nodeLow = low - node.NodeLength;
var nodeHigh = high - node.NodeLength;
if (node.Left != null)
{
nodeLow -= node.Left.TotalNodeLength;
nodeHigh -= node.Left.TotalNodeLength;
}
if (node.DistanceToMaxEnd < nodeLow)
{
// node is irrelevant for search because all intervals in node are before low
return;
}
if (node.Left != null)
FindOverlappingSegments(results, node.Left, low, high);
if (nodeHigh < 0)
{
// node and everything in node.right is before low
return;
}
if (nodeLow <= node.SegmentLength)
{
results.Add((T)node);
}
if (node.Right != null)
FindOverlappingSegments(results, node.Right, nodeLow, nodeHigh);
}
#endregion
#region UpdateAugmentedData
private void UpdateAugmentedData(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
totalLength += node.Left.TotalNodeLength;
var leftDtme = node.Left.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
if (node.Left.Right != null)
leftDtme -= node.Left.Right.TotalNodeLength;
leftDtme -= node.NodeLength;
if (leftDtme > distanceToMaxEnd)
distanceToMaxEnd = leftDtme;
}
if (node.Right != null)
{
totalLength += node.Right.TotalNodeLength;
var rightDtme = node.Right.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
rightDtme += node.Right.NodeLength;
if (node.Right.Left != null)
rightDtme += node.Right.Left.TotalNodeLength;
if (rightDtme > distanceToMaxEnd)
distanceToMaxEnd = rightDtme;
}
if (node.TotalNodeLength != totalLength
|| node.DistanceToMaxEnd != distanceToMaxEnd)
{
node.TotalNodeLength = totalLength;
node.DistanceToMaxEnd = distanceToMaxEnd;
if (node.Parent != null)
UpdateAugmentedData(node.Parent);
}
}
void ISegmentTree.UpdateAugmentedData(TextSegment node)
{
UpdateAugmentedData(node);
}
#endregion
#region Remove
/// <summary>
/// Removes the specified segment from the tree. This will cause the segment to not update
/// anymore when the document changes.
/// </summary>
public bool Remove(T item)
{
if (!Contains(item))
return false;
RemoveSegment(item);
return true;
}
void ISegmentTree.Remove(TextSegment s)
{
RemoveSegment(s);
}
private void RemoveSegment(TextSegment s)
{
var oldOffset = s.StartOffset;
var successor = s.Successor;
if (successor != null)
successor.NodeLength += s.NodeLength;
RemoveNode(s);
if (successor != null)
UpdateAugmentedData(successor);
Disconnect(s, oldOffset);
CheckProperties();
}
private void Disconnect(TextSegment s, int offset)
{
s.Left = s.Right = s.Parent = null;
s.OwnerTree = null;
s.NodeLength = offset;
Count--;
}
/// <summary>
/// Removes all segments from the tree.
/// </summary>
public void Clear()
{
var segments = this.ToArray();
_root = null;
var offset = 0;
foreach (var s in segments)
{
offset += s.NodeLength;
Disconnect(s, offset);
}
CheckProperties();
}
#endregion
#region CheckProperties
[Conditional("DATACONSISTENCYTEST")]
internal void CheckProperties()
{
#if DEBUG
if (_root != null)
{
CheckProperties(_root);
// check red-black property:
var blackCount = -1;
CheckNodeProperties(_root, null, Red, 0, ref blackCount);
}
var expectedCount = 0;
// we cannot trust LINQ not to call ICollection.Count, so we need this loop
// to count the elements in the tree
using (var en = GetEnumerator())
{
while (en.MoveNext()) expectedCount++;
}
Debug.Assert(Count == expectedCount);
#endif
}
#if DEBUG
private void CheckProperties(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
CheckProperties(node.Left);
totalLength += node.Left.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Left.DistanceToMaxEnd + node.Left.StartOffset - node.StartOffset);
}
if (node.Right != null)
{
CheckProperties(node.Right);
totalLength += node.Right.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Right.DistanceToMaxEnd + node.Right.StartOffset - node.StartOffset);
}
Debug.Assert(node.TotalNodeLength == totalLength);
Debug.Assert(node.DistanceToMaxEnd == distanceToMaxEnd);
}
/*
1. A node is either red or black.
2. The root is black.
3. All leaves are black. (The leaves are the NIL children.)
4. Both children of every red node are black. (So every red node must have a black parent.)
5. Every simple path from a node to a descendant leaf contains the same number of black nodes. (Not counting the leaf node.)
*/
[SuppressMessage("ReSharper", "UnusedParameter.Local")]
private static void CheckNodeProperties(TextSegment node, TextSegment parentNode, bool parentColor, int blackCount, ref int expectedBlackCount)
{
if (node == null) return;
Debug.Assert(node.Parent == parentNode);
if (parentColor == Red)
{
Debug.Assert(node.Color == Black);
}
if (node.Color == Black)
{
blackCount++;
}
if (node.Left == null && node.Right == null)
{
// node is a leaf node:
if (expectedBlackCount == -1)
expectedBlackCount = blackCount;
else
Debug.Assert(expectedBlackCount == blackCount);
}
CheckNodeProperties(node.Left, node, node.Color, blackCount, ref expectedBlackCount);
CheckNodeProperties(node.Right, node, node.Color, blackCount, ref expectedBlackCount);
}
private static void AppendTreeToString(TextSegment node, StringBuilder b, int indent)
{
b.Append(node.Color == Red ? "RED " : "BLACK ");
b.AppendLine(node + node.ToDebugString());
indent += 2;
if (node.Left != null)
{
b.Append(' ', indent);
b.Append("L: ");
AppendTreeToString(node.Left, b, indent);
}
if (node.Right != null)
{
b.Append(' ', indent);
b.Append("R: ");
AppendTreeToString(node.Right, b, indent);
}
}
#endif
internal string GetTreeAsString()
{
#if DEBUG
var b = new StringBuilder();
if (_root != null)
AppendTreeToString(_root, b, 0);
return b.ToString();
#else
return "Not available in release build.";
#endif
}
#endregion
#region Red/Black Tree
internal const bool Red = true;
internal const bool Black = false;
private void InsertAsLeft(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Left == null);
parentNode.Left = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void InsertAsRight(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Right == null);
parentNode.Right = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void FixTreeOnInsert(TextSegment node)
{
Debug.Assert(node != null);
Debug.Assert(node.Color == Red);
Debug.Assert(node.Left == null || node.Left.Color == Black);
Debug.Assert(node.Right == null || node.Right.Color == Black);
var parentNode = node.Parent;
if (parentNode == null)
{
// we inserted in the root -> the node must be black
// since this is a root node, making the node black increments the number of black nodes
// on all paths by one, so it is still the same for all paths.
node.Color = Black;
return;
}
if (parentNode.Color == Black)
{
// if the parent node where we inserted was black, our red node is placed correctly.
// since we inserted a red node, the number of black nodes on each path is unchanged
// -> the tree is still balanced
return;
}
// parentNode is red, so there is a conflict here!
// because the root is black, parentNode is not the root -> there is a grandparent node
var grandparentNode = parentNode.Parent;
var uncleNode = Sibling(parentNode);
if (uncleNode != null && uncleNode.Color == Red)
{
parentNode.Color = Black;
uncleNode.Color = Black;
grandparentNode.Color = Red;
FixTreeOnInsert(grandparentNode);
return;
}
// now we know: parent is red but uncle is black
// First rotation:
if (node == parentNode.Right && parentNode == grandparentNode.Left)
{
RotateLeft(parentNode);
node = node.Left;
}
else if (node == parentNode.Left && parentNode == grandparentNode.Right)
{
RotateRight(parentNode);
node = node.Right;
}
// because node might have changed, reassign variables:
// ReSharper disable once PossibleNullReferenceException
parentNode = node.Parent;
grandparentNode = parentNode.Parent;
// Now recolor a bit:
parentNode.Color = Black;
grandparentNode.Color = Red;
// Second rotation:
if (node == parentNode.Left && parentNode == grandparentNode.Left)
{
RotateRight(grandparentNode);
}
else
{
// because of the first rotation, this is guaranteed:
Debug.Assert(node == parentNode.Right && parentNode == grandparentNode.Right);
RotateLeft(grandparentNode);
}
}
private void RemoveNode(TextSegment removedNode)
{
if (removedNode.Left != null && removedNode.Right != null)
{
// replace removedNode with it's in-order successor
var leftMost = removedNode.Right.LeftMost;
RemoveNode(leftMost); // remove leftMost from its current location
// and overwrite the removedNode with it
ReplaceNode(removedNode, leftMost);
leftMost.Left = removedNode.Left;
if (leftMost.Left != null) leftMost.Left.Parent = leftMost;
leftMost.Right = removedNode.Right;
if (leftMost.Right != null) leftMost.Right.Parent = leftMost;
leftMost.Color = removedNode.Color;
UpdateAugmentedData(leftMost);
if (leftMost.Parent != null) UpdateAugmentedData(leftMost.Parent);
return;
}
// now either removedNode.left or removedNode.right is null
// get the remaining child
var parentNode = removedNode.Parent;
var childNode = removedNode.Left ?? removedNode.Right;
ReplaceNode(removedNode, childNode);
if (parentNode != null) UpdateAugmentedData(parentNode);
if (removedNode.Color == Black)
{
if (childNode != null && childNode.Color == Red)
{
childNode.Color = Black;
}
else
{
FixTreeOnDelete(childNode, parentNode);
}
}
}
private void FixTreeOnDelete(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (parentNode == null)
return;
// warning: node may be null
var sibling = Sibling(node, parentNode);
if (sibling.Color == Red)
{
parentNode.Color = Red;
sibling.Color = Black;
if (node == parentNode.Left)
{
RotateLeft(parentNode);
}
else
{
RotateRight(parentNode);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
}
if (parentNode.Color == Black
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
FixTreeOnDelete(parentNode, parentNode.Parent);
return;
}
if (parentNode.Color == Red
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
parentNode.Color = Black;
return;
}
if (node == parentNode.Left &&
sibling.Color == Black &&
GetColor(sibling.Left) == Red &&
GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
sibling.Left.Color = Black;
RotateRight(sibling);
}
else if (node == parentNode.Right &&
sibling.Color == Black &&
GetColor(sibling.Right) == Red &&
GetColor(sibling.Left) == Black)
{
sibling.Color = Red;
sibling.Right.Color = Black;
RotateLeft(sibling);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
sibling.Color = parentNode.Color;
parentNode.Color = Black;
if (node == parentNode.Left)
{
if (sibling.Right != null)
{
Debug.Assert(sibling.Right.Color == Red);
sibling.Right.Color = Black;
}
RotateLeft(parentNode);
}
else
{
if (sibling.Left != null)
{
Debug.Assert(sibling.Left.Color == Red);
sibling.Left.Color = Black;
}
RotateRight(parentNode);
}
}
private void ReplaceNode(TextSegment replacedNode, TextSegment newNode)
{
if (replacedNode.Parent == null)
{
Debug.Assert(replacedNode == _root);
_root = newNode;
}
else
{
if (replacedNode.Parent.Left == replacedNode)
replacedNode.Parent.Left = newNode;
else
replacedNode.Parent.Right = newNode;
}
if (newNode != null)
{
newNode.Parent = replacedNode.Parent;
}
replacedNode.Parent = null;
}
private void RotateLeft(TextSegment p)
{
// let q be p's right child
var q = p.Right;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's right child to be q's left child
p.Right = q.Left;
if (p.Right != null) p.Right.Parent = p;
// set q's left child to be p
q.Left = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private void RotateRight(TextSegment p)
{
// let q be p's left child
var q = p.Left;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's left child to be q's right child
p.Left = q.Right;
if (p.Left != null) p.Left.Parent = p;
// set q's right child to be p
q.Right = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private static TextSegment Sibling(TextSegment node)
{
return node == node.Parent.Left ? node.Parent.Right : node.Parent.Left;
}
private static TextSegment Sibling(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (node == parentNode.Left)
return parentNode.Right;
else
return parentNode.Left;
}
private static bool GetColor(TextSegment node)
{
return node != null && node.Color;
}
#endregion
#region ICollection<T> implementation
/// <summary>
/// Gets the number of segments in the tree.
/// </summary>
public int Count { get; private set; }
bool ICollection<T>.IsReadOnly => false;
/// <summary>
/// Gets whether this tree contains the specified item.
/// </summary>
public bool Contains(T item)
{
return item != null && item.OwnerTree == this;
}
/// <summary>
/// Copies all segments in this SegmentTree to the specified array.
/// </summary>
public void CopyTo(T[] array, int arrayIndex)
{
if (array == null)
throw new ArgumentNullException(nameof(array));
if (array.Length < Count)
throw new ArgumentException("The array is too small", nameof(array));
if (arrayIndex < 0 || arrayIndex + Count > array.Length)
throw new ArgumentOutOfRangeException(nameof(arrayIndex), arrayIndex, "Value must be between 0 and " + (array.Length - Count));
foreach (var s in this)
{
array[arrayIndex++] = s;
}
}
/// <summary>
/// Gets an enumerator to enumerate the segments.
/// </summary>
public IEnumerator<T> GetEnumerator()
{
if (_root != null)
{
var current = _root.LeftMost;
while (current != null)
{
yield return (T)current;
// TODO: check if collection was modified during enumeration
current = current.Successor;
}
}
}
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
#endregion
}
}
<MSG> Allow a text segment collection to be disconnected from a text document.
<DFF> @@ -97,6 +97,14 @@ namespace AvaloniaEdit.Document
}
#endregion
+ public void Disconnect (TextDocument textDocument)
+ {
+ if (_isConnectedToDocument)
+ {
+ TextDocumentWeakEventManager.Changed.RemoveHandler(textDocument, OnDocumentChanged);
+ }
+ }
+
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
| 8 | Allow a text segment collection to be disconnected from a text document. | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063839 | <NME> TextSegmentCollection.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using System.Text;
using AvaloniaEdit.Utils;
using Avalonia.Threading;
namespace AvaloniaEdit.Document
{
/// <summary>
/// Interface to allow TextSegments to access the TextSegmentCollection - we cannot use a direct reference
/// because TextSegmentCollection is generic.
/// </summary>
internal interface ISegmentTree
{
void Add(TextSegment s);
void Remove(TextSegment s);
void UpdateAugmentedData(TextSegment s);
}
/// <summary>
/// <para>
/// A collection of text segments that supports efficient lookup of segments
/// intersecting with another segment.
/// </para>
/// </summary>
/// <remarks><inheritdoc cref="TextSegment"/></remarks>
/// <see cref="TextSegment"/>
public sealed class TextSegmentCollection<T> : ICollection<T>, ISegmentTree where T : TextSegment
{
// Implementation: this is basically a mixture of an augmented interval tree
// and the TextAnchorTree.
// WARNING: you need to understand interval trees (the version with the augmented 'high'/'max' field)
// and how the TextAnchorTree works before you have any chance of understanding this code.
// This means that every node holds two "segments":
// one like the segments in the text anchor tree to support efficient offset changes
// and another that is the interval as seen by the user
// So basically, the tree contains a list of contiguous node segments of the first kind,
// with interval segments starting at the end of every node segment.
// Performance:
// Add is O(lg n)
// Remove is O(lg n)
// DocumentChanged is O(m * lg n), with m the number of segments that intersect with the changed document section
// FindFirstSegmentWithStartAfter is O(m + lg n) with m being the number of segments at the same offset as the result segment
// FindIntersectingSegments is O(m + lg n) with m being the number of intersecting segments.
private TextSegment _root;
private readonly bool _isConnectedToDocument;
#region Constructor
/// <summary>
/// Creates a new TextSegmentCollection that needs manual calls to <see cref="UpdateOffsets(DocumentChangeEventArgs)"/>.
/// </summary>
public TextSegmentCollection()
{
}
/// <summary>
/// Creates a new TextSegmentCollection that updates the offsets automatically.
/// </summary>
/// <param name="textDocument">The document to which the text segments
/// that will be added to the tree belong. When the document changes, the
/// position of the text segments will be updated accordingly.</param>
public TextSegmentCollection(TextDocument textDocument)
{
if (textDocument == null)
throw new ArgumentNullException(nameof(textDocument));
Dispatcher.UIThread.VerifyAccess();
_isConnectedToDocument = true;
TextDocumentWeakEventManager.Changed.AddHandler(textDocument, OnDocumentChanged);
}
#endregion
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="e">DocumentChangeEventArgs instance describing the change to the document.</param>
public void UpdateOffsets(DocumentChangeEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
OnDocumentChanged(this, e);
CheckProperties();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
var map = e.OffsetChangeMapOrNull;
if (map != null)
{
foreach (var entry in map)
{
UpdateOffsetsInternal(entry);
}
}
else
{
UpdateOffsetsInternal(e.CreateSingleChangeMapEntry());
}
}
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="change">OffsetChangeMapEntry instance describing the change to the document.</param>
public void UpdateOffsets(OffsetChangeMapEntry change)
{
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
UpdateOffsetsInternal(change);
CheckProperties();
}
#endregion
#region UpdateOffsets (implementation)
private void UpdateOffsetsInternal(OffsetChangeMapEntry change)
{
// Special case pure insertions, because they don't always cause a text segment to increase in size when the replaced region
// is inside a segment (when offset is at start or end of a text semgent).
if (change.RemovalLength == 0)
{
InsertText(change.Offset, change.InsertionLength);
}
else
{
ReplaceText(change);
}
}
private void InsertText(int offset, int length)
{
if (length == 0)
return;
// enlarge segments that contain offset (excluding those that have offset as endpoint)
foreach (var segment in FindSegmentsContaining(offset))
{
if (segment.StartOffset < offset && offset < segment.EndOffset)
{
segment.Length += length;
}
}
// move start offsets of all segments >= offset
TextSegment node = FindFirstSegmentWithStartAfter(offset);
if (node != null)
{
node.NodeLength += length;
UpdateAugmentedData(node);
}
}
private void ReplaceText(OffsetChangeMapEntry change)
{
Debug.Assert(change.RemovalLength > 0);
var offset = change.Offset;
foreach (var segment in FindOverlappingSegments(offset, change.RemovalLength))
{
if (segment.StartOffset <= offset)
{
if (segment.EndOffset >= offset + change.RemovalLength)
{
// Replacement inside segment: adjust segment length
segment.Length += change.InsertionLength - change.RemovalLength;
}
else
{
// Replacement starting inside segment and ending after segment end: set segment end to removal position
//segment.EndOffset = offset;
segment.Length = offset - segment.StartOffset;
}
}
else
{
// Replacement starting in front of text segment and running into segment.
// Keep segment.EndOffset constant and move segment.StartOffset to the end of the replacement
var remainingLength = segment.EndOffset - (offset + change.RemovalLength);
RemoveSegment(segment);
segment.StartOffset = offset + change.RemovalLength;
segment.Length = Math.Max(0, remainingLength);
AddSegment(segment);
}
}
// move start offsets of all segments > offset
TextSegment node = FindFirstSegmentWithStartAfter(offset + 1);
if (node != null)
{
Debug.Assert(node.NodeLength >= change.RemovalLength);
node.NodeLength += change.InsertionLength - change.RemovalLength;
UpdateAugmentedData(node);
}
}
#endregion
#region Add
/// <summary>
/// Adds the specified segment to the tree. This will cause the segment to update when the
/// document changes.
/// </summary>
public void Add(T item)
{
if (item == null)
throw new ArgumentNullException(nameof(item));
if (item.OwnerTree != null)
throw new ArgumentException("The segment is already added to a SegmentCollection.");
AddSegment(item);
}
void ISegmentTree.Add(TextSegment s)
{
AddSegment(s);
}
private void AddSegment(TextSegment node)
{
var insertionOffset = node.StartOffset;
node.DistanceToMaxEnd = node.SegmentLength;
if (_root == null)
{
_root = node;
node.TotalNodeLength = node.NodeLength;
}
else if (insertionOffset >= _root.TotalNodeLength)
{
// append segment at end of tree
node.NodeLength = node.TotalNodeLength = insertionOffset - _root.TotalNodeLength;
InsertAsRight(_root.RightMost, node);
}
else
{
// insert in middle of tree
var n = FindNode(ref insertionOffset);
Debug.Assert(insertionOffset < n.NodeLength);
// split node segment 'n' at offset
node.TotalNodeLength = node.NodeLength = insertionOffset;
n.NodeLength -= insertionOffset;
InsertBefore(n, node);
}
node.OwnerTree = this;
Count++;
CheckProperties();
}
private void InsertBefore(TextSegment node, TextSegment newNode)
{
if (node.Left == null)
{
InsertAsLeft(node, newNode);
}
else
{
InsertAsRight(node.Left.RightMost, newNode);
}
}
#endregion
#region GetNextSegment / GetPreviousSegment
/// <summary>
/// Gets the next segment after the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the last segment.
/// </summary>
public T GetNextSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Successor;
}
/// <summary>
/// Gets the previous segment before the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the first segment.
/// </summary>
public T GetPreviousSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Predecessor;
}
#endregion
#region FirstSegment/LastSegment
/// <summary>
/// Returns the first segment in the collection or null, if the collection is empty.
/// </summary>
public T FirstSegment => (T)_root?.LeftMost;
/// <summary>
/// Returns the last segment in the collection or null, if the collection is empty.
/// </summary>
public T LastSegment => (T)_root?.RightMost;
#endregion
#region FindFirstSegmentWithStartAfter
/// <summary>
/// Gets the first segment with a start offset greater or equal to <paramref name="startOffset"/>.
/// Returns null if no such segment is found.
/// </summary>
public T FindFirstSegmentWithStartAfter(int startOffset)
{
if (_root == null)
return null;
if (startOffset <= 0)
return (T)_root.LeftMost;
var s = FindNode(ref startOffset);
// startOffset means that the previous segment is starting at the offset we were looking for
while (startOffset == 0)
{
var p = (s == null) ? _root.RightMost : s.Predecessor;
// There must always be a predecessor: if we were looking for the first node, we would have already
// returned it as root.LeftMost above.
Debug.Assert(p != null);
startOffset += p.NodeLength;
s = p;
}
return (T)s;
}
/// <summary>
/// Finds the node at the specified offset.
/// After the method has run, offset is relative to the beginning of the returned node.
/// </summary>
private TextSegment FindNode(ref int offset)
{
var n = _root;
while (true)
{
if (n.Left != null)
{
if (offset < n.Left.TotalNodeLength)
{
n = n.Left; // descend into left subtree
continue;
}
else
{
offset -= n.Left.TotalNodeLength; // skip left subtree
}
}
if (offset < n.NodeLength)
{
return n; // found correct node
}
else
{
offset -= n.NodeLength; // skip this node
}
if (n.Right != null)
{
n = n.Right; // descend into right subtree
}
else
{
// didn't find any node containing the offset
return null;
}
}
}
#endregion
#region FindOverlappingSegments
/// <summary>
/// Finds all segments that contain the given offset.
/// (StartOffset <= offset <= EndOffset)
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindSegmentsContaining(int offset)
{
return FindOverlappingSegments(offset, 0);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(ISegment segment)
{
if (segment == null)
throw new ArgumentNullException(nameof(segment));
return FindOverlappingSegments(segment.Offset, segment.Length);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(int offset, int length)
{
ThrowUtil.CheckNotNegative(length, "length");
var results = new List<T>();
if (_root != null)
{
FindOverlappingSegments(results, _root, offset, offset + length);
}
return new ReadOnlyCollection<T>(results);
}
private void FindOverlappingSegments(List<T> results, TextSegment node, int low, int high)
{
// low and high are relative to node.LeftMost startpos (not node.LeftMost.Offset)
if (high < 0)
{
// node is irrelevant for search because all intervals in node are after high
return;
}
// find values relative to node.Offset
var nodeLow = low - node.NodeLength;
var nodeHigh = high - node.NodeLength;
if (node.Left != null)
{
nodeLow -= node.Left.TotalNodeLength;
nodeHigh -= node.Left.TotalNodeLength;
}
if (node.DistanceToMaxEnd < nodeLow)
{
// node is irrelevant for search because all intervals in node are before low
return;
}
if (node.Left != null)
FindOverlappingSegments(results, node.Left, low, high);
if (nodeHigh < 0)
{
// node and everything in node.right is before low
return;
}
if (nodeLow <= node.SegmentLength)
{
results.Add((T)node);
}
if (node.Right != null)
FindOverlappingSegments(results, node.Right, nodeLow, nodeHigh);
}
#endregion
#region UpdateAugmentedData
private void UpdateAugmentedData(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
totalLength += node.Left.TotalNodeLength;
var leftDtme = node.Left.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
if (node.Left.Right != null)
leftDtme -= node.Left.Right.TotalNodeLength;
leftDtme -= node.NodeLength;
if (leftDtme > distanceToMaxEnd)
distanceToMaxEnd = leftDtme;
}
if (node.Right != null)
{
totalLength += node.Right.TotalNodeLength;
var rightDtme = node.Right.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
rightDtme += node.Right.NodeLength;
if (node.Right.Left != null)
rightDtme += node.Right.Left.TotalNodeLength;
if (rightDtme > distanceToMaxEnd)
distanceToMaxEnd = rightDtme;
}
if (node.TotalNodeLength != totalLength
|| node.DistanceToMaxEnd != distanceToMaxEnd)
{
node.TotalNodeLength = totalLength;
node.DistanceToMaxEnd = distanceToMaxEnd;
if (node.Parent != null)
UpdateAugmentedData(node.Parent);
}
}
void ISegmentTree.UpdateAugmentedData(TextSegment node)
{
UpdateAugmentedData(node);
}
#endregion
#region Remove
/// <summary>
/// Removes the specified segment from the tree. This will cause the segment to not update
/// anymore when the document changes.
/// </summary>
public bool Remove(T item)
{
if (!Contains(item))
return false;
RemoveSegment(item);
return true;
}
void ISegmentTree.Remove(TextSegment s)
{
RemoveSegment(s);
}
private void RemoveSegment(TextSegment s)
{
var oldOffset = s.StartOffset;
var successor = s.Successor;
if (successor != null)
successor.NodeLength += s.NodeLength;
RemoveNode(s);
if (successor != null)
UpdateAugmentedData(successor);
Disconnect(s, oldOffset);
CheckProperties();
}
private void Disconnect(TextSegment s, int offset)
{
s.Left = s.Right = s.Parent = null;
s.OwnerTree = null;
s.NodeLength = offset;
Count--;
}
/// <summary>
/// Removes all segments from the tree.
/// </summary>
public void Clear()
{
var segments = this.ToArray();
_root = null;
var offset = 0;
foreach (var s in segments)
{
offset += s.NodeLength;
Disconnect(s, offset);
}
CheckProperties();
}
#endregion
#region CheckProperties
[Conditional("DATACONSISTENCYTEST")]
internal void CheckProperties()
{
#if DEBUG
if (_root != null)
{
CheckProperties(_root);
// check red-black property:
var blackCount = -1;
CheckNodeProperties(_root, null, Red, 0, ref blackCount);
}
var expectedCount = 0;
// we cannot trust LINQ not to call ICollection.Count, so we need this loop
// to count the elements in the tree
using (var en = GetEnumerator())
{
while (en.MoveNext()) expectedCount++;
}
Debug.Assert(Count == expectedCount);
#endif
}
#if DEBUG
private void CheckProperties(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
CheckProperties(node.Left);
totalLength += node.Left.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Left.DistanceToMaxEnd + node.Left.StartOffset - node.StartOffset);
}
if (node.Right != null)
{
CheckProperties(node.Right);
totalLength += node.Right.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Right.DistanceToMaxEnd + node.Right.StartOffset - node.StartOffset);
}
Debug.Assert(node.TotalNodeLength == totalLength);
Debug.Assert(node.DistanceToMaxEnd == distanceToMaxEnd);
}
/*
1. A node is either red or black.
2. The root is black.
3. All leaves are black. (The leaves are the NIL children.)
4. Both children of every red node are black. (So every red node must have a black parent.)
5. Every simple path from a node to a descendant leaf contains the same number of black nodes. (Not counting the leaf node.)
*/
[SuppressMessage("ReSharper", "UnusedParameter.Local")]
private static void CheckNodeProperties(TextSegment node, TextSegment parentNode, bool parentColor, int blackCount, ref int expectedBlackCount)
{
if (node == null) return;
Debug.Assert(node.Parent == parentNode);
if (parentColor == Red)
{
Debug.Assert(node.Color == Black);
}
if (node.Color == Black)
{
blackCount++;
}
if (node.Left == null && node.Right == null)
{
// node is a leaf node:
if (expectedBlackCount == -1)
expectedBlackCount = blackCount;
else
Debug.Assert(expectedBlackCount == blackCount);
}
CheckNodeProperties(node.Left, node, node.Color, blackCount, ref expectedBlackCount);
CheckNodeProperties(node.Right, node, node.Color, blackCount, ref expectedBlackCount);
}
private static void AppendTreeToString(TextSegment node, StringBuilder b, int indent)
{
b.Append(node.Color == Red ? "RED " : "BLACK ");
b.AppendLine(node + node.ToDebugString());
indent += 2;
if (node.Left != null)
{
b.Append(' ', indent);
b.Append("L: ");
AppendTreeToString(node.Left, b, indent);
}
if (node.Right != null)
{
b.Append(' ', indent);
b.Append("R: ");
AppendTreeToString(node.Right, b, indent);
}
}
#endif
internal string GetTreeAsString()
{
#if DEBUG
var b = new StringBuilder();
if (_root != null)
AppendTreeToString(_root, b, 0);
return b.ToString();
#else
return "Not available in release build.";
#endif
}
#endregion
#region Red/Black Tree
internal const bool Red = true;
internal const bool Black = false;
private void InsertAsLeft(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Left == null);
parentNode.Left = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void InsertAsRight(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Right == null);
parentNode.Right = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void FixTreeOnInsert(TextSegment node)
{
Debug.Assert(node != null);
Debug.Assert(node.Color == Red);
Debug.Assert(node.Left == null || node.Left.Color == Black);
Debug.Assert(node.Right == null || node.Right.Color == Black);
var parentNode = node.Parent;
if (parentNode == null)
{
// we inserted in the root -> the node must be black
// since this is a root node, making the node black increments the number of black nodes
// on all paths by one, so it is still the same for all paths.
node.Color = Black;
return;
}
if (parentNode.Color == Black)
{
// if the parent node where we inserted was black, our red node is placed correctly.
// since we inserted a red node, the number of black nodes on each path is unchanged
// -> the tree is still balanced
return;
}
// parentNode is red, so there is a conflict here!
// because the root is black, parentNode is not the root -> there is a grandparent node
var grandparentNode = parentNode.Parent;
var uncleNode = Sibling(parentNode);
if (uncleNode != null && uncleNode.Color == Red)
{
parentNode.Color = Black;
uncleNode.Color = Black;
grandparentNode.Color = Red;
FixTreeOnInsert(grandparentNode);
return;
}
// now we know: parent is red but uncle is black
// First rotation:
if (node == parentNode.Right && parentNode == grandparentNode.Left)
{
RotateLeft(parentNode);
node = node.Left;
}
else if (node == parentNode.Left && parentNode == grandparentNode.Right)
{
RotateRight(parentNode);
node = node.Right;
}
// because node might have changed, reassign variables:
// ReSharper disable once PossibleNullReferenceException
parentNode = node.Parent;
grandparentNode = parentNode.Parent;
// Now recolor a bit:
parentNode.Color = Black;
grandparentNode.Color = Red;
// Second rotation:
if (node == parentNode.Left && parentNode == grandparentNode.Left)
{
RotateRight(grandparentNode);
}
else
{
// because of the first rotation, this is guaranteed:
Debug.Assert(node == parentNode.Right && parentNode == grandparentNode.Right);
RotateLeft(grandparentNode);
}
}
private void RemoveNode(TextSegment removedNode)
{
if (removedNode.Left != null && removedNode.Right != null)
{
// replace removedNode with it's in-order successor
var leftMost = removedNode.Right.LeftMost;
RemoveNode(leftMost); // remove leftMost from its current location
// and overwrite the removedNode with it
ReplaceNode(removedNode, leftMost);
leftMost.Left = removedNode.Left;
if (leftMost.Left != null) leftMost.Left.Parent = leftMost;
leftMost.Right = removedNode.Right;
if (leftMost.Right != null) leftMost.Right.Parent = leftMost;
leftMost.Color = removedNode.Color;
UpdateAugmentedData(leftMost);
if (leftMost.Parent != null) UpdateAugmentedData(leftMost.Parent);
return;
}
// now either removedNode.left or removedNode.right is null
// get the remaining child
var parentNode = removedNode.Parent;
var childNode = removedNode.Left ?? removedNode.Right;
ReplaceNode(removedNode, childNode);
if (parentNode != null) UpdateAugmentedData(parentNode);
if (removedNode.Color == Black)
{
if (childNode != null && childNode.Color == Red)
{
childNode.Color = Black;
}
else
{
FixTreeOnDelete(childNode, parentNode);
}
}
}
private void FixTreeOnDelete(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (parentNode == null)
return;
// warning: node may be null
var sibling = Sibling(node, parentNode);
if (sibling.Color == Red)
{
parentNode.Color = Red;
sibling.Color = Black;
if (node == parentNode.Left)
{
RotateLeft(parentNode);
}
else
{
RotateRight(parentNode);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
}
if (parentNode.Color == Black
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
FixTreeOnDelete(parentNode, parentNode.Parent);
return;
}
if (parentNode.Color == Red
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
parentNode.Color = Black;
return;
}
if (node == parentNode.Left &&
sibling.Color == Black &&
GetColor(sibling.Left) == Red &&
GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
sibling.Left.Color = Black;
RotateRight(sibling);
}
else if (node == parentNode.Right &&
sibling.Color == Black &&
GetColor(sibling.Right) == Red &&
GetColor(sibling.Left) == Black)
{
sibling.Color = Red;
sibling.Right.Color = Black;
RotateLeft(sibling);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
sibling.Color = parentNode.Color;
parentNode.Color = Black;
if (node == parentNode.Left)
{
if (sibling.Right != null)
{
Debug.Assert(sibling.Right.Color == Red);
sibling.Right.Color = Black;
}
RotateLeft(parentNode);
}
else
{
if (sibling.Left != null)
{
Debug.Assert(sibling.Left.Color == Red);
sibling.Left.Color = Black;
}
RotateRight(parentNode);
}
}
private void ReplaceNode(TextSegment replacedNode, TextSegment newNode)
{
if (replacedNode.Parent == null)
{
Debug.Assert(replacedNode == _root);
_root = newNode;
}
else
{
if (replacedNode.Parent.Left == replacedNode)
replacedNode.Parent.Left = newNode;
else
replacedNode.Parent.Right = newNode;
}
if (newNode != null)
{
newNode.Parent = replacedNode.Parent;
}
replacedNode.Parent = null;
}
private void RotateLeft(TextSegment p)
{
// let q be p's right child
var q = p.Right;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's right child to be q's left child
p.Right = q.Left;
if (p.Right != null) p.Right.Parent = p;
// set q's left child to be p
q.Left = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private void RotateRight(TextSegment p)
{
// let q be p's left child
var q = p.Left;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's left child to be q's right child
p.Left = q.Right;
if (p.Left != null) p.Left.Parent = p;
// set q's right child to be p
q.Right = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private static TextSegment Sibling(TextSegment node)
{
return node == node.Parent.Left ? node.Parent.Right : node.Parent.Left;
}
private static TextSegment Sibling(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (node == parentNode.Left)
return parentNode.Right;
else
return parentNode.Left;
}
private static bool GetColor(TextSegment node)
{
return node != null && node.Color;
}
#endregion
#region ICollection<T> implementation
/// <summary>
/// Gets the number of segments in the tree.
/// </summary>
public int Count { get; private set; }
bool ICollection<T>.IsReadOnly => false;
/// <summary>
/// Gets whether this tree contains the specified item.
/// </summary>
public bool Contains(T item)
{
return item != null && item.OwnerTree == this;
}
/// <summary>
/// Copies all segments in this SegmentTree to the specified array.
/// </summary>
public void CopyTo(T[] array, int arrayIndex)
{
if (array == null)
throw new ArgumentNullException(nameof(array));
if (array.Length < Count)
throw new ArgumentException("The array is too small", nameof(array));
if (arrayIndex < 0 || arrayIndex + Count > array.Length)
throw new ArgumentOutOfRangeException(nameof(arrayIndex), arrayIndex, "Value must be between 0 and " + (array.Length - Count));
foreach (var s in this)
{
array[arrayIndex++] = s;
}
}
/// <summary>
/// Gets an enumerator to enumerate the segments.
/// </summary>
public IEnumerator<T> GetEnumerator()
{
if (_root != null)
{
var current = _root.LeftMost;
while (current != null)
{
yield return (T)current;
// TODO: check if collection was modified during enumeration
current = current.Successor;
}
}
}
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
#endregion
}
}
<MSG> Allow a text segment collection to be disconnected from a text document.
<DFF> @@ -97,6 +97,14 @@ namespace AvaloniaEdit.Document
}
#endregion
+ public void Disconnect (TextDocument textDocument)
+ {
+ if (_isConnectedToDocument)
+ {
+ TextDocumentWeakEventManager.Changed.RemoveHandler(textDocument, OnDocumentChanged);
+ }
+ }
+
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
| 8 | Allow a text segment collection to be disconnected from a text document. | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063840 | <NME> TextSegmentCollection.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using System.Text;
using AvaloniaEdit.Utils;
using Avalonia.Threading;
namespace AvaloniaEdit.Document
{
/// <summary>
/// Interface to allow TextSegments to access the TextSegmentCollection - we cannot use a direct reference
/// because TextSegmentCollection is generic.
/// </summary>
internal interface ISegmentTree
{
void Add(TextSegment s);
void Remove(TextSegment s);
void UpdateAugmentedData(TextSegment s);
}
/// <summary>
/// <para>
/// A collection of text segments that supports efficient lookup of segments
/// intersecting with another segment.
/// </para>
/// </summary>
/// <remarks><inheritdoc cref="TextSegment"/></remarks>
/// <see cref="TextSegment"/>
public sealed class TextSegmentCollection<T> : ICollection<T>, ISegmentTree where T : TextSegment
{
// Implementation: this is basically a mixture of an augmented interval tree
// and the TextAnchorTree.
// WARNING: you need to understand interval trees (the version with the augmented 'high'/'max' field)
// and how the TextAnchorTree works before you have any chance of understanding this code.
// This means that every node holds two "segments":
// one like the segments in the text anchor tree to support efficient offset changes
// and another that is the interval as seen by the user
// So basically, the tree contains a list of contiguous node segments of the first kind,
// with interval segments starting at the end of every node segment.
// Performance:
// Add is O(lg n)
// Remove is O(lg n)
// DocumentChanged is O(m * lg n), with m the number of segments that intersect with the changed document section
// FindFirstSegmentWithStartAfter is O(m + lg n) with m being the number of segments at the same offset as the result segment
// FindIntersectingSegments is O(m + lg n) with m being the number of intersecting segments.
private TextSegment _root;
private readonly bool _isConnectedToDocument;
#region Constructor
/// <summary>
/// Creates a new TextSegmentCollection that needs manual calls to <see cref="UpdateOffsets(DocumentChangeEventArgs)"/>.
/// </summary>
public TextSegmentCollection()
{
}
/// <summary>
/// Creates a new TextSegmentCollection that updates the offsets automatically.
/// </summary>
/// <param name="textDocument">The document to which the text segments
/// that will be added to the tree belong. When the document changes, the
/// position of the text segments will be updated accordingly.</param>
public TextSegmentCollection(TextDocument textDocument)
{
if (textDocument == null)
throw new ArgumentNullException(nameof(textDocument));
Dispatcher.UIThread.VerifyAccess();
_isConnectedToDocument = true;
TextDocumentWeakEventManager.Changed.AddHandler(textDocument, OnDocumentChanged);
}
#endregion
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="e">DocumentChangeEventArgs instance describing the change to the document.</param>
public void UpdateOffsets(DocumentChangeEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
OnDocumentChanged(this, e);
CheckProperties();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
var map = e.OffsetChangeMapOrNull;
if (map != null)
{
foreach (var entry in map)
{
UpdateOffsetsInternal(entry);
}
}
else
{
UpdateOffsetsInternal(e.CreateSingleChangeMapEntry());
}
}
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="change">OffsetChangeMapEntry instance describing the change to the document.</param>
public void UpdateOffsets(OffsetChangeMapEntry change)
{
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
UpdateOffsetsInternal(change);
CheckProperties();
}
#endregion
#region UpdateOffsets (implementation)
private void UpdateOffsetsInternal(OffsetChangeMapEntry change)
{
// Special case pure insertions, because they don't always cause a text segment to increase in size when the replaced region
// is inside a segment (when offset is at start or end of a text semgent).
if (change.RemovalLength == 0)
{
InsertText(change.Offset, change.InsertionLength);
}
else
{
ReplaceText(change);
}
}
private void InsertText(int offset, int length)
{
if (length == 0)
return;
// enlarge segments that contain offset (excluding those that have offset as endpoint)
foreach (var segment in FindSegmentsContaining(offset))
{
if (segment.StartOffset < offset && offset < segment.EndOffset)
{
segment.Length += length;
}
}
// move start offsets of all segments >= offset
TextSegment node = FindFirstSegmentWithStartAfter(offset);
if (node != null)
{
node.NodeLength += length;
UpdateAugmentedData(node);
}
}
private void ReplaceText(OffsetChangeMapEntry change)
{
Debug.Assert(change.RemovalLength > 0);
var offset = change.Offset;
foreach (var segment in FindOverlappingSegments(offset, change.RemovalLength))
{
if (segment.StartOffset <= offset)
{
if (segment.EndOffset >= offset + change.RemovalLength)
{
// Replacement inside segment: adjust segment length
segment.Length += change.InsertionLength - change.RemovalLength;
}
else
{
// Replacement starting inside segment and ending after segment end: set segment end to removal position
//segment.EndOffset = offset;
segment.Length = offset - segment.StartOffset;
}
}
else
{
// Replacement starting in front of text segment and running into segment.
// Keep segment.EndOffset constant and move segment.StartOffset to the end of the replacement
var remainingLength = segment.EndOffset - (offset + change.RemovalLength);
RemoveSegment(segment);
segment.StartOffset = offset + change.RemovalLength;
segment.Length = Math.Max(0, remainingLength);
AddSegment(segment);
}
}
// move start offsets of all segments > offset
TextSegment node = FindFirstSegmentWithStartAfter(offset + 1);
if (node != null)
{
Debug.Assert(node.NodeLength >= change.RemovalLength);
node.NodeLength += change.InsertionLength - change.RemovalLength;
UpdateAugmentedData(node);
}
}
#endregion
#region Add
/// <summary>
/// Adds the specified segment to the tree. This will cause the segment to update when the
/// document changes.
/// </summary>
public void Add(T item)
{
if (item == null)
throw new ArgumentNullException(nameof(item));
if (item.OwnerTree != null)
throw new ArgumentException("The segment is already added to a SegmentCollection.");
AddSegment(item);
}
void ISegmentTree.Add(TextSegment s)
{
AddSegment(s);
}
private void AddSegment(TextSegment node)
{
var insertionOffset = node.StartOffset;
node.DistanceToMaxEnd = node.SegmentLength;
if (_root == null)
{
_root = node;
node.TotalNodeLength = node.NodeLength;
}
else if (insertionOffset >= _root.TotalNodeLength)
{
// append segment at end of tree
node.NodeLength = node.TotalNodeLength = insertionOffset - _root.TotalNodeLength;
InsertAsRight(_root.RightMost, node);
}
else
{
// insert in middle of tree
var n = FindNode(ref insertionOffset);
Debug.Assert(insertionOffset < n.NodeLength);
// split node segment 'n' at offset
node.TotalNodeLength = node.NodeLength = insertionOffset;
n.NodeLength -= insertionOffset;
InsertBefore(n, node);
}
node.OwnerTree = this;
Count++;
CheckProperties();
}
private void InsertBefore(TextSegment node, TextSegment newNode)
{
if (node.Left == null)
{
InsertAsLeft(node, newNode);
}
else
{
InsertAsRight(node.Left.RightMost, newNode);
}
}
#endregion
#region GetNextSegment / GetPreviousSegment
/// <summary>
/// Gets the next segment after the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the last segment.
/// </summary>
public T GetNextSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Successor;
}
/// <summary>
/// Gets the previous segment before the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the first segment.
/// </summary>
public T GetPreviousSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Predecessor;
}
#endregion
#region FirstSegment/LastSegment
/// <summary>
/// Returns the first segment in the collection or null, if the collection is empty.
/// </summary>
public T FirstSegment => (T)_root?.LeftMost;
/// <summary>
/// Returns the last segment in the collection or null, if the collection is empty.
/// </summary>
public T LastSegment => (T)_root?.RightMost;
#endregion
#region FindFirstSegmentWithStartAfter
/// <summary>
/// Gets the first segment with a start offset greater or equal to <paramref name="startOffset"/>.
/// Returns null if no such segment is found.
/// </summary>
public T FindFirstSegmentWithStartAfter(int startOffset)
{
if (_root == null)
return null;
if (startOffset <= 0)
return (T)_root.LeftMost;
var s = FindNode(ref startOffset);
// startOffset means that the previous segment is starting at the offset we were looking for
while (startOffset == 0)
{
var p = (s == null) ? _root.RightMost : s.Predecessor;
// There must always be a predecessor: if we were looking for the first node, we would have already
// returned it as root.LeftMost above.
Debug.Assert(p != null);
startOffset += p.NodeLength;
s = p;
}
return (T)s;
}
/// <summary>
/// Finds the node at the specified offset.
/// After the method has run, offset is relative to the beginning of the returned node.
/// </summary>
private TextSegment FindNode(ref int offset)
{
var n = _root;
while (true)
{
if (n.Left != null)
{
if (offset < n.Left.TotalNodeLength)
{
n = n.Left; // descend into left subtree
continue;
}
else
{
offset -= n.Left.TotalNodeLength; // skip left subtree
}
}
if (offset < n.NodeLength)
{
return n; // found correct node
}
else
{
offset -= n.NodeLength; // skip this node
}
if (n.Right != null)
{
n = n.Right; // descend into right subtree
}
else
{
// didn't find any node containing the offset
return null;
}
}
}
#endregion
#region FindOverlappingSegments
/// <summary>
/// Finds all segments that contain the given offset.
/// (StartOffset <= offset <= EndOffset)
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindSegmentsContaining(int offset)
{
return FindOverlappingSegments(offset, 0);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(ISegment segment)
{
if (segment == null)
throw new ArgumentNullException(nameof(segment));
return FindOverlappingSegments(segment.Offset, segment.Length);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(int offset, int length)
{
ThrowUtil.CheckNotNegative(length, "length");
var results = new List<T>();
if (_root != null)
{
FindOverlappingSegments(results, _root, offset, offset + length);
}
return new ReadOnlyCollection<T>(results);
}
private void FindOverlappingSegments(List<T> results, TextSegment node, int low, int high)
{
// low and high are relative to node.LeftMost startpos (not node.LeftMost.Offset)
if (high < 0)
{
// node is irrelevant for search because all intervals in node are after high
return;
}
// find values relative to node.Offset
var nodeLow = low - node.NodeLength;
var nodeHigh = high - node.NodeLength;
if (node.Left != null)
{
nodeLow -= node.Left.TotalNodeLength;
nodeHigh -= node.Left.TotalNodeLength;
}
if (node.DistanceToMaxEnd < nodeLow)
{
// node is irrelevant for search because all intervals in node are before low
return;
}
if (node.Left != null)
FindOverlappingSegments(results, node.Left, low, high);
if (nodeHigh < 0)
{
// node and everything in node.right is before low
return;
}
if (nodeLow <= node.SegmentLength)
{
results.Add((T)node);
}
if (node.Right != null)
FindOverlappingSegments(results, node.Right, nodeLow, nodeHigh);
}
#endregion
#region UpdateAugmentedData
private void UpdateAugmentedData(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
totalLength += node.Left.TotalNodeLength;
var leftDtme = node.Left.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
if (node.Left.Right != null)
leftDtme -= node.Left.Right.TotalNodeLength;
leftDtme -= node.NodeLength;
if (leftDtme > distanceToMaxEnd)
distanceToMaxEnd = leftDtme;
}
if (node.Right != null)
{
totalLength += node.Right.TotalNodeLength;
var rightDtme = node.Right.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
rightDtme += node.Right.NodeLength;
if (node.Right.Left != null)
rightDtme += node.Right.Left.TotalNodeLength;
if (rightDtme > distanceToMaxEnd)
distanceToMaxEnd = rightDtme;
}
if (node.TotalNodeLength != totalLength
|| node.DistanceToMaxEnd != distanceToMaxEnd)
{
node.TotalNodeLength = totalLength;
node.DistanceToMaxEnd = distanceToMaxEnd;
if (node.Parent != null)
UpdateAugmentedData(node.Parent);
}
}
void ISegmentTree.UpdateAugmentedData(TextSegment node)
{
UpdateAugmentedData(node);
}
#endregion
#region Remove
/// <summary>
/// Removes the specified segment from the tree. This will cause the segment to not update
/// anymore when the document changes.
/// </summary>
public bool Remove(T item)
{
if (!Contains(item))
return false;
RemoveSegment(item);
return true;
}
void ISegmentTree.Remove(TextSegment s)
{
RemoveSegment(s);
}
private void RemoveSegment(TextSegment s)
{
var oldOffset = s.StartOffset;
var successor = s.Successor;
if (successor != null)
successor.NodeLength += s.NodeLength;
RemoveNode(s);
if (successor != null)
UpdateAugmentedData(successor);
Disconnect(s, oldOffset);
CheckProperties();
}
private void Disconnect(TextSegment s, int offset)
{
s.Left = s.Right = s.Parent = null;
s.OwnerTree = null;
s.NodeLength = offset;
Count--;
}
/// <summary>
/// Removes all segments from the tree.
/// </summary>
public void Clear()
{
var segments = this.ToArray();
_root = null;
var offset = 0;
foreach (var s in segments)
{
offset += s.NodeLength;
Disconnect(s, offset);
}
CheckProperties();
}
#endregion
#region CheckProperties
[Conditional("DATACONSISTENCYTEST")]
internal void CheckProperties()
{
#if DEBUG
if (_root != null)
{
CheckProperties(_root);
// check red-black property:
var blackCount = -1;
CheckNodeProperties(_root, null, Red, 0, ref blackCount);
}
var expectedCount = 0;
// we cannot trust LINQ not to call ICollection.Count, so we need this loop
// to count the elements in the tree
using (var en = GetEnumerator())
{
while (en.MoveNext()) expectedCount++;
}
Debug.Assert(Count == expectedCount);
#endif
}
#if DEBUG
private void CheckProperties(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
CheckProperties(node.Left);
totalLength += node.Left.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Left.DistanceToMaxEnd + node.Left.StartOffset - node.StartOffset);
}
if (node.Right != null)
{
CheckProperties(node.Right);
totalLength += node.Right.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Right.DistanceToMaxEnd + node.Right.StartOffset - node.StartOffset);
}
Debug.Assert(node.TotalNodeLength == totalLength);
Debug.Assert(node.DistanceToMaxEnd == distanceToMaxEnd);
}
/*
1. A node is either red or black.
2. The root is black.
3. All leaves are black. (The leaves are the NIL children.)
4. Both children of every red node are black. (So every red node must have a black parent.)
5. Every simple path from a node to a descendant leaf contains the same number of black nodes. (Not counting the leaf node.)
*/
[SuppressMessage("ReSharper", "UnusedParameter.Local")]
private static void CheckNodeProperties(TextSegment node, TextSegment parentNode, bool parentColor, int blackCount, ref int expectedBlackCount)
{
if (node == null) return;
Debug.Assert(node.Parent == parentNode);
if (parentColor == Red)
{
Debug.Assert(node.Color == Black);
}
if (node.Color == Black)
{
blackCount++;
}
if (node.Left == null && node.Right == null)
{
// node is a leaf node:
if (expectedBlackCount == -1)
expectedBlackCount = blackCount;
else
Debug.Assert(expectedBlackCount == blackCount);
}
CheckNodeProperties(node.Left, node, node.Color, blackCount, ref expectedBlackCount);
CheckNodeProperties(node.Right, node, node.Color, blackCount, ref expectedBlackCount);
}
private static void AppendTreeToString(TextSegment node, StringBuilder b, int indent)
{
b.Append(node.Color == Red ? "RED " : "BLACK ");
b.AppendLine(node + node.ToDebugString());
indent += 2;
if (node.Left != null)
{
b.Append(' ', indent);
b.Append("L: ");
AppendTreeToString(node.Left, b, indent);
}
if (node.Right != null)
{
b.Append(' ', indent);
b.Append("R: ");
AppendTreeToString(node.Right, b, indent);
}
}
#endif
internal string GetTreeAsString()
{
#if DEBUG
var b = new StringBuilder();
if (_root != null)
AppendTreeToString(_root, b, 0);
return b.ToString();
#else
return "Not available in release build.";
#endif
}
#endregion
#region Red/Black Tree
internal const bool Red = true;
internal const bool Black = false;
private void InsertAsLeft(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Left == null);
parentNode.Left = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void InsertAsRight(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Right == null);
parentNode.Right = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void FixTreeOnInsert(TextSegment node)
{
Debug.Assert(node != null);
Debug.Assert(node.Color == Red);
Debug.Assert(node.Left == null || node.Left.Color == Black);
Debug.Assert(node.Right == null || node.Right.Color == Black);
var parentNode = node.Parent;
if (parentNode == null)
{
// we inserted in the root -> the node must be black
// since this is a root node, making the node black increments the number of black nodes
// on all paths by one, so it is still the same for all paths.
node.Color = Black;
return;
}
if (parentNode.Color == Black)
{
// if the parent node where we inserted was black, our red node is placed correctly.
// since we inserted a red node, the number of black nodes on each path is unchanged
// -> the tree is still balanced
return;
}
// parentNode is red, so there is a conflict here!
// because the root is black, parentNode is not the root -> there is a grandparent node
var grandparentNode = parentNode.Parent;
var uncleNode = Sibling(parentNode);
if (uncleNode != null && uncleNode.Color == Red)
{
parentNode.Color = Black;
uncleNode.Color = Black;
grandparentNode.Color = Red;
FixTreeOnInsert(grandparentNode);
return;
}
// now we know: parent is red but uncle is black
// First rotation:
if (node == parentNode.Right && parentNode == grandparentNode.Left)
{
RotateLeft(parentNode);
node = node.Left;
}
else if (node == parentNode.Left && parentNode == grandparentNode.Right)
{
RotateRight(parentNode);
node = node.Right;
}
// because node might have changed, reassign variables:
// ReSharper disable once PossibleNullReferenceException
parentNode = node.Parent;
grandparentNode = parentNode.Parent;
// Now recolor a bit:
parentNode.Color = Black;
grandparentNode.Color = Red;
// Second rotation:
if (node == parentNode.Left && parentNode == grandparentNode.Left)
{
RotateRight(grandparentNode);
}
else
{
// because of the first rotation, this is guaranteed:
Debug.Assert(node == parentNode.Right && parentNode == grandparentNode.Right);
RotateLeft(grandparentNode);
}
}
private void RemoveNode(TextSegment removedNode)
{
if (removedNode.Left != null && removedNode.Right != null)
{
// replace removedNode with it's in-order successor
var leftMost = removedNode.Right.LeftMost;
RemoveNode(leftMost); // remove leftMost from its current location
// and overwrite the removedNode with it
ReplaceNode(removedNode, leftMost);
leftMost.Left = removedNode.Left;
if (leftMost.Left != null) leftMost.Left.Parent = leftMost;
leftMost.Right = removedNode.Right;
if (leftMost.Right != null) leftMost.Right.Parent = leftMost;
leftMost.Color = removedNode.Color;
UpdateAugmentedData(leftMost);
if (leftMost.Parent != null) UpdateAugmentedData(leftMost.Parent);
return;
}
// now either removedNode.left or removedNode.right is null
// get the remaining child
var parentNode = removedNode.Parent;
var childNode = removedNode.Left ?? removedNode.Right;
ReplaceNode(removedNode, childNode);
if (parentNode != null) UpdateAugmentedData(parentNode);
if (removedNode.Color == Black)
{
if (childNode != null && childNode.Color == Red)
{
childNode.Color = Black;
}
else
{
FixTreeOnDelete(childNode, parentNode);
}
}
}
private void FixTreeOnDelete(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (parentNode == null)
return;
// warning: node may be null
var sibling = Sibling(node, parentNode);
if (sibling.Color == Red)
{
parentNode.Color = Red;
sibling.Color = Black;
if (node == parentNode.Left)
{
RotateLeft(parentNode);
}
else
{
RotateRight(parentNode);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
}
if (parentNode.Color == Black
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
FixTreeOnDelete(parentNode, parentNode.Parent);
return;
}
if (parentNode.Color == Red
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
parentNode.Color = Black;
return;
}
if (node == parentNode.Left &&
sibling.Color == Black &&
GetColor(sibling.Left) == Red &&
GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
sibling.Left.Color = Black;
RotateRight(sibling);
}
else if (node == parentNode.Right &&
sibling.Color == Black &&
GetColor(sibling.Right) == Red &&
GetColor(sibling.Left) == Black)
{
sibling.Color = Red;
sibling.Right.Color = Black;
RotateLeft(sibling);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
sibling.Color = parentNode.Color;
parentNode.Color = Black;
if (node == parentNode.Left)
{
if (sibling.Right != null)
{
Debug.Assert(sibling.Right.Color == Red);
sibling.Right.Color = Black;
}
RotateLeft(parentNode);
}
else
{
if (sibling.Left != null)
{
Debug.Assert(sibling.Left.Color == Red);
sibling.Left.Color = Black;
}
RotateRight(parentNode);
}
}
private void ReplaceNode(TextSegment replacedNode, TextSegment newNode)
{
if (replacedNode.Parent == null)
{
Debug.Assert(replacedNode == _root);
_root = newNode;
}
else
{
if (replacedNode.Parent.Left == replacedNode)
replacedNode.Parent.Left = newNode;
else
replacedNode.Parent.Right = newNode;
}
if (newNode != null)
{
newNode.Parent = replacedNode.Parent;
}
replacedNode.Parent = null;
}
private void RotateLeft(TextSegment p)
{
// let q be p's right child
var q = p.Right;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's right child to be q's left child
p.Right = q.Left;
if (p.Right != null) p.Right.Parent = p;
// set q's left child to be p
q.Left = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private void RotateRight(TextSegment p)
{
// let q be p's left child
var q = p.Left;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's left child to be q's right child
p.Left = q.Right;
if (p.Left != null) p.Left.Parent = p;
// set q's right child to be p
q.Right = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private static TextSegment Sibling(TextSegment node)
{
return node == node.Parent.Left ? node.Parent.Right : node.Parent.Left;
}
private static TextSegment Sibling(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (node == parentNode.Left)
return parentNode.Right;
else
return parentNode.Left;
}
private static bool GetColor(TextSegment node)
{
return node != null && node.Color;
}
#endregion
#region ICollection<T> implementation
/// <summary>
/// Gets the number of segments in the tree.
/// </summary>
public int Count { get; private set; }
bool ICollection<T>.IsReadOnly => false;
/// <summary>
/// Gets whether this tree contains the specified item.
/// </summary>
public bool Contains(T item)
{
return item != null && item.OwnerTree == this;
}
/// <summary>
/// Copies all segments in this SegmentTree to the specified array.
/// </summary>
public void CopyTo(T[] array, int arrayIndex)
{
if (array == null)
throw new ArgumentNullException(nameof(array));
if (array.Length < Count)
throw new ArgumentException("The array is too small", nameof(array));
if (arrayIndex < 0 || arrayIndex + Count > array.Length)
throw new ArgumentOutOfRangeException(nameof(arrayIndex), arrayIndex, "Value must be between 0 and " + (array.Length - Count));
foreach (var s in this)
{
array[arrayIndex++] = s;
}
}
/// <summary>
/// Gets an enumerator to enumerate the segments.
/// </summary>
public IEnumerator<T> GetEnumerator()
{
if (_root != null)
{
var current = _root.LeftMost;
while (current != null)
{
yield return (T)current;
// TODO: check if collection was modified during enumeration
current = current.Successor;
}
}
}
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
#endregion
}
}
<MSG> Allow a text segment collection to be disconnected from a text document.
<DFF> @@ -97,6 +97,14 @@ namespace AvaloniaEdit.Document
}
#endregion
+ public void Disconnect (TextDocument textDocument)
+ {
+ if (_isConnectedToDocument)
+ {
+ TextDocumentWeakEventManager.Changed.RemoveHandler(textDocument, OnDocumentChanged);
+ }
+ }
+
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
| 8 | Allow a text segment collection to be disconnected from a text document. | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063841 | <NME> TextSegmentCollection.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using System.Text;
using AvaloniaEdit.Utils;
using Avalonia.Threading;
namespace AvaloniaEdit.Document
{
/// <summary>
/// Interface to allow TextSegments to access the TextSegmentCollection - we cannot use a direct reference
/// because TextSegmentCollection is generic.
/// </summary>
internal interface ISegmentTree
{
void Add(TextSegment s);
void Remove(TextSegment s);
void UpdateAugmentedData(TextSegment s);
}
/// <summary>
/// <para>
/// A collection of text segments that supports efficient lookup of segments
/// intersecting with another segment.
/// </para>
/// </summary>
/// <remarks><inheritdoc cref="TextSegment"/></remarks>
/// <see cref="TextSegment"/>
public sealed class TextSegmentCollection<T> : ICollection<T>, ISegmentTree where T : TextSegment
{
// Implementation: this is basically a mixture of an augmented interval tree
// and the TextAnchorTree.
// WARNING: you need to understand interval trees (the version with the augmented 'high'/'max' field)
// and how the TextAnchorTree works before you have any chance of understanding this code.
// This means that every node holds two "segments":
// one like the segments in the text anchor tree to support efficient offset changes
// and another that is the interval as seen by the user
// So basically, the tree contains a list of contiguous node segments of the first kind,
// with interval segments starting at the end of every node segment.
// Performance:
// Add is O(lg n)
// Remove is O(lg n)
// DocumentChanged is O(m * lg n), with m the number of segments that intersect with the changed document section
// FindFirstSegmentWithStartAfter is O(m + lg n) with m being the number of segments at the same offset as the result segment
// FindIntersectingSegments is O(m + lg n) with m being the number of intersecting segments.
private TextSegment _root;
private readonly bool _isConnectedToDocument;
#region Constructor
/// <summary>
/// Creates a new TextSegmentCollection that needs manual calls to <see cref="UpdateOffsets(DocumentChangeEventArgs)"/>.
/// </summary>
public TextSegmentCollection()
{
}
/// <summary>
/// Creates a new TextSegmentCollection that updates the offsets automatically.
/// </summary>
/// <param name="textDocument">The document to which the text segments
/// that will be added to the tree belong. When the document changes, the
/// position of the text segments will be updated accordingly.</param>
public TextSegmentCollection(TextDocument textDocument)
{
if (textDocument == null)
throw new ArgumentNullException(nameof(textDocument));
Dispatcher.UIThread.VerifyAccess();
_isConnectedToDocument = true;
TextDocumentWeakEventManager.Changed.AddHandler(textDocument, OnDocumentChanged);
}
#endregion
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="e">DocumentChangeEventArgs instance describing the change to the document.</param>
public void UpdateOffsets(DocumentChangeEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
OnDocumentChanged(this, e);
CheckProperties();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
var map = e.OffsetChangeMapOrNull;
if (map != null)
{
foreach (var entry in map)
{
UpdateOffsetsInternal(entry);
}
}
else
{
UpdateOffsetsInternal(e.CreateSingleChangeMapEntry());
}
}
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="change">OffsetChangeMapEntry instance describing the change to the document.</param>
public void UpdateOffsets(OffsetChangeMapEntry change)
{
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
UpdateOffsetsInternal(change);
CheckProperties();
}
#endregion
#region UpdateOffsets (implementation)
private void UpdateOffsetsInternal(OffsetChangeMapEntry change)
{
// Special case pure insertions, because they don't always cause a text segment to increase in size when the replaced region
// is inside a segment (when offset is at start or end of a text semgent).
if (change.RemovalLength == 0)
{
InsertText(change.Offset, change.InsertionLength);
}
else
{
ReplaceText(change);
}
}
private void InsertText(int offset, int length)
{
if (length == 0)
return;
// enlarge segments that contain offset (excluding those that have offset as endpoint)
foreach (var segment in FindSegmentsContaining(offset))
{
if (segment.StartOffset < offset && offset < segment.EndOffset)
{
segment.Length += length;
}
}
// move start offsets of all segments >= offset
TextSegment node = FindFirstSegmentWithStartAfter(offset);
if (node != null)
{
node.NodeLength += length;
UpdateAugmentedData(node);
}
}
private void ReplaceText(OffsetChangeMapEntry change)
{
Debug.Assert(change.RemovalLength > 0);
var offset = change.Offset;
foreach (var segment in FindOverlappingSegments(offset, change.RemovalLength))
{
if (segment.StartOffset <= offset)
{
if (segment.EndOffset >= offset + change.RemovalLength)
{
// Replacement inside segment: adjust segment length
segment.Length += change.InsertionLength - change.RemovalLength;
}
else
{
// Replacement starting inside segment and ending after segment end: set segment end to removal position
//segment.EndOffset = offset;
segment.Length = offset - segment.StartOffset;
}
}
else
{
// Replacement starting in front of text segment and running into segment.
// Keep segment.EndOffset constant and move segment.StartOffset to the end of the replacement
var remainingLength = segment.EndOffset - (offset + change.RemovalLength);
RemoveSegment(segment);
segment.StartOffset = offset + change.RemovalLength;
segment.Length = Math.Max(0, remainingLength);
AddSegment(segment);
}
}
// move start offsets of all segments > offset
TextSegment node = FindFirstSegmentWithStartAfter(offset + 1);
if (node != null)
{
Debug.Assert(node.NodeLength >= change.RemovalLength);
node.NodeLength += change.InsertionLength - change.RemovalLength;
UpdateAugmentedData(node);
}
}
#endregion
#region Add
/// <summary>
/// Adds the specified segment to the tree. This will cause the segment to update when the
/// document changes.
/// </summary>
public void Add(T item)
{
if (item == null)
throw new ArgumentNullException(nameof(item));
if (item.OwnerTree != null)
throw new ArgumentException("The segment is already added to a SegmentCollection.");
AddSegment(item);
}
void ISegmentTree.Add(TextSegment s)
{
AddSegment(s);
}
private void AddSegment(TextSegment node)
{
var insertionOffset = node.StartOffset;
node.DistanceToMaxEnd = node.SegmentLength;
if (_root == null)
{
_root = node;
node.TotalNodeLength = node.NodeLength;
}
else if (insertionOffset >= _root.TotalNodeLength)
{
// append segment at end of tree
node.NodeLength = node.TotalNodeLength = insertionOffset - _root.TotalNodeLength;
InsertAsRight(_root.RightMost, node);
}
else
{
// insert in middle of tree
var n = FindNode(ref insertionOffset);
Debug.Assert(insertionOffset < n.NodeLength);
// split node segment 'n' at offset
node.TotalNodeLength = node.NodeLength = insertionOffset;
n.NodeLength -= insertionOffset;
InsertBefore(n, node);
}
node.OwnerTree = this;
Count++;
CheckProperties();
}
private void InsertBefore(TextSegment node, TextSegment newNode)
{
if (node.Left == null)
{
InsertAsLeft(node, newNode);
}
else
{
InsertAsRight(node.Left.RightMost, newNode);
}
}
#endregion
#region GetNextSegment / GetPreviousSegment
/// <summary>
/// Gets the next segment after the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the last segment.
/// </summary>
public T GetNextSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Successor;
}
/// <summary>
/// Gets the previous segment before the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the first segment.
/// </summary>
public T GetPreviousSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Predecessor;
}
#endregion
#region FirstSegment/LastSegment
/// <summary>
/// Returns the first segment in the collection or null, if the collection is empty.
/// </summary>
public T FirstSegment => (T)_root?.LeftMost;
/// <summary>
/// Returns the last segment in the collection or null, if the collection is empty.
/// </summary>
public T LastSegment => (T)_root?.RightMost;
#endregion
#region FindFirstSegmentWithStartAfter
/// <summary>
/// Gets the first segment with a start offset greater or equal to <paramref name="startOffset"/>.
/// Returns null if no such segment is found.
/// </summary>
public T FindFirstSegmentWithStartAfter(int startOffset)
{
if (_root == null)
return null;
if (startOffset <= 0)
return (T)_root.LeftMost;
var s = FindNode(ref startOffset);
// startOffset means that the previous segment is starting at the offset we were looking for
while (startOffset == 0)
{
var p = (s == null) ? _root.RightMost : s.Predecessor;
// There must always be a predecessor: if we were looking for the first node, we would have already
// returned it as root.LeftMost above.
Debug.Assert(p != null);
startOffset += p.NodeLength;
s = p;
}
return (T)s;
}
/// <summary>
/// Finds the node at the specified offset.
/// After the method has run, offset is relative to the beginning of the returned node.
/// </summary>
private TextSegment FindNode(ref int offset)
{
var n = _root;
while (true)
{
if (n.Left != null)
{
if (offset < n.Left.TotalNodeLength)
{
n = n.Left; // descend into left subtree
continue;
}
else
{
offset -= n.Left.TotalNodeLength; // skip left subtree
}
}
if (offset < n.NodeLength)
{
return n; // found correct node
}
else
{
offset -= n.NodeLength; // skip this node
}
if (n.Right != null)
{
n = n.Right; // descend into right subtree
}
else
{
// didn't find any node containing the offset
return null;
}
}
}
#endregion
#region FindOverlappingSegments
/// <summary>
/// Finds all segments that contain the given offset.
/// (StartOffset <= offset <= EndOffset)
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindSegmentsContaining(int offset)
{
return FindOverlappingSegments(offset, 0);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(ISegment segment)
{
if (segment == null)
throw new ArgumentNullException(nameof(segment));
return FindOverlappingSegments(segment.Offset, segment.Length);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(int offset, int length)
{
ThrowUtil.CheckNotNegative(length, "length");
var results = new List<T>();
if (_root != null)
{
FindOverlappingSegments(results, _root, offset, offset + length);
}
return new ReadOnlyCollection<T>(results);
}
private void FindOverlappingSegments(List<T> results, TextSegment node, int low, int high)
{
// low and high are relative to node.LeftMost startpos (not node.LeftMost.Offset)
if (high < 0)
{
// node is irrelevant for search because all intervals in node are after high
return;
}
// find values relative to node.Offset
var nodeLow = low - node.NodeLength;
var nodeHigh = high - node.NodeLength;
if (node.Left != null)
{
nodeLow -= node.Left.TotalNodeLength;
nodeHigh -= node.Left.TotalNodeLength;
}
if (node.DistanceToMaxEnd < nodeLow)
{
// node is irrelevant for search because all intervals in node are before low
return;
}
if (node.Left != null)
FindOverlappingSegments(results, node.Left, low, high);
if (nodeHigh < 0)
{
// node and everything in node.right is before low
return;
}
if (nodeLow <= node.SegmentLength)
{
results.Add((T)node);
}
if (node.Right != null)
FindOverlappingSegments(results, node.Right, nodeLow, nodeHigh);
}
#endregion
#region UpdateAugmentedData
private void UpdateAugmentedData(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
totalLength += node.Left.TotalNodeLength;
var leftDtme = node.Left.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
if (node.Left.Right != null)
leftDtme -= node.Left.Right.TotalNodeLength;
leftDtme -= node.NodeLength;
if (leftDtme > distanceToMaxEnd)
distanceToMaxEnd = leftDtme;
}
if (node.Right != null)
{
totalLength += node.Right.TotalNodeLength;
var rightDtme = node.Right.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
rightDtme += node.Right.NodeLength;
if (node.Right.Left != null)
rightDtme += node.Right.Left.TotalNodeLength;
if (rightDtme > distanceToMaxEnd)
distanceToMaxEnd = rightDtme;
}
if (node.TotalNodeLength != totalLength
|| node.DistanceToMaxEnd != distanceToMaxEnd)
{
node.TotalNodeLength = totalLength;
node.DistanceToMaxEnd = distanceToMaxEnd;
if (node.Parent != null)
UpdateAugmentedData(node.Parent);
}
}
void ISegmentTree.UpdateAugmentedData(TextSegment node)
{
UpdateAugmentedData(node);
}
#endregion
#region Remove
/// <summary>
/// Removes the specified segment from the tree. This will cause the segment to not update
/// anymore when the document changes.
/// </summary>
public bool Remove(T item)
{
if (!Contains(item))
return false;
RemoveSegment(item);
return true;
}
void ISegmentTree.Remove(TextSegment s)
{
RemoveSegment(s);
}
private void RemoveSegment(TextSegment s)
{
var oldOffset = s.StartOffset;
var successor = s.Successor;
if (successor != null)
successor.NodeLength += s.NodeLength;
RemoveNode(s);
if (successor != null)
UpdateAugmentedData(successor);
Disconnect(s, oldOffset);
CheckProperties();
}
private void Disconnect(TextSegment s, int offset)
{
s.Left = s.Right = s.Parent = null;
s.OwnerTree = null;
s.NodeLength = offset;
Count--;
}
/// <summary>
/// Removes all segments from the tree.
/// </summary>
public void Clear()
{
var segments = this.ToArray();
_root = null;
var offset = 0;
foreach (var s in segments)
{
offset += s.NodeLength;
Disconnect(s, offset);
}
CheckProperties();
}
#endregion
#region CheckProperties
[Conditional("DATACONSISTENCYTEST")]
internal void CheckProperties()
{
#if DEBUG
if (_root != null)
{
CheckProperties(_root);
// check red-black property:
var blackCount = -1;
CheckNodeProperties(_root, null, Red, 0, ref blackCount);
}
var expectedCount = 0;
// we cannot trust LINQ not to call ICollection.Count, so we need this loop
// to count the elements in the tree
using (var en = GetEnumerator())
{
while (en.MoveNext()) expectedCount++;
}
Debug.Assert(Count == expectedCount);
#endif
}
#if DEBUG
private void CheckProperties(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
CheckProperties(node.Left);
totalLength += node.Left.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Left.DistanceToMaxEnd + node.Left.StartOffset - node.StartOffset);
}
if (node.Right != null)
{
CheckProperties(node.Right);
totalLength += node.Right.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Right.DistanceToMaxEnd + node.Right.StartOffset - node.StartOffset);
}
Debug.Assert(node.TotalNodeLength == totalLength);
Debug.Assert(node.DistanceToMaxEnd == distanceToMaxEnd);
}
/*
1. A node is either red or black.
2. The root is black.
3. All leaves are black. (The leaves are the NIL children.)
4. Both children of every red node are black. (So every red node must have a black parent.)
5. Every simple path from a node to a descendant leaf contains the same number of black nodes. (Not counting the leaf node.)
*/
[SuppressMessage("ReSharper", "UnusedParameter.Local")]
private static void CheckNodeProperties(TextSegment node, TextSegment parentNode, bool parentColor, int blackCount, ref int expectedBlackCount)
{
if (node == null) return;
Debug.Assert(node.Parent == parentNode);
if (parentColor == Red)
{
Debug.Assert(node.Color == Black);
}
if (node.Color == Black)
{
blackCount++;
}
if (node.Left == null && node.Right == null)
{
// node is a leaf node:
if (expectedBlackCount == -1)
expectedBlackCount = blackCount;
else
Debug.Assert(expectedBlackCount == blackCount);
}
CheckNodeProperties(node.Left, node, node.Color, blackCount, ref expectedBlackCount);
CheckNodeProperties(node.Right, node, node.Color, blackCount, ref expectedBlackCount);
}
private static void AppendTreeToString(TextSegment node, StringBuilder b, int indent)
{
b.Append(node.Color == Red ? "RED " : "BLACK ");
b.AppendLine(node + node.ToDebugString());
indent += 2;
if (node.Left != null)
{
b.Append(' ', indent);
b.Append("L: ");
AppendTreeToString(node.Left, b, indent);
}
if (node.Right != null)
{
b.Append(' ', indent);
b.Append("R: ");
AppendTreeToString(node.Right, b, indent);
}
}
#endif
internal string GetTreeAsString()
{
#if DEBUG
var b = new StringBuilder();
if (_root != null)
AppendTreeToString(_root, b, 0);
return b.ToString();
#else
return "Not available in release build.";
#endif
}
#endregion
#region Red/Black Tree
internal const bool Red = true;
internal const bool Black = false;
private void InsertAsLeft(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Left == null);
parentNode.Left = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void InsertAsRight(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Right == null);
parentNode.Right = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void FixTreeOnInsert(TextSegment node)
{
Debug.Assert(node != null);
Debug.Assert(node.Color == Red);
Debug.Assert(node.Left == null || node.Left.Color == Black);
Debug.Assert(node.Right == null || node.Right.Color == Black);
var parentNode = node.Parent;
if (parentNode == null)
{
// we inserted in the root -> the node must be black
// since this is a root node, making the node black increments the number of black nodes
// on all paths by one, so it is still the same for all paths.
node.Color = Black;
return;
}
if (parentNode.Color == Black)
{
// if the parent node where we inserted was black, our red node is placed correctly.
// since we inserted a red node, the number of black nodes on each path is unchanged
// -> the tree is still balanced
return;
}
// parentNode is red, so there is a conflict here!
// because the root is black, parentNode is not the root -> there is a grandparent node
var grandparentNode = parentNode.Parent;
var uncleNode = Sibling(parentNode);
if (uncleNode != null && uncleNode.Color == Red)
{
parentNode.Color = Black;
uncleNode.Color = Black;
grandparentNode.Color = Red;
FixTreeOnInsert(grandparentNode);
return;
}
// now we know: parent is red but uncle is black
// First rotation:
if (node == parentNode.Right && parentNode == grandparentNode.Left)
{
RotateLeft(parentNode);
node = node.Left;
}
else if (node == parentNode.Left && parentNode == grandparentNode.Right)
{
RotateRight(parentNode);
node = node.Right;
}
// because node might have changed, reassign variables:
// ReSharper disable once PossibleNullReferenceException
parentNode = node.Parent;
grandparentNode = parentNode.Parent;
// Now recolor a bit:
parentNode.Color = Black;
grandparentNode.Color = Red;
// Second rotation:
if (node == parentNode.Left && parentNode == grandparentNode.Left)
{
RotateRight(grandparentNode);
}
else
{
// because of the first rotation, this is guaranteed:
Debug.Assert(node == parentNode.Right && parentNode == grandparentNode.Right);
RotateLeft(grandparentNode);
}
}
private void RemoveNode(TextSegment removedNode)
{
if (removedNode.Left != null && removedNode.Right != null)
{
// replace removedNode with it's in-order successor
var leftMost = removedNode.Right.LeftMost;
RemoveNode(leftMost); // remove leftMost from its current location
// and overwrite the removedNode with it
ReplaceNode(removedNode, leftMost);
leftMost.Left = removedNode.Left;
if (leftMost.Left != null) leftMost.Left.Parent = leftMost;
leftMost.Right = removedNode.Right;
if (leftMost.Right != null) leftMost.Right.Parent = leftMost;
leftMost.Color = removedNode.Color;
UpdateAugmentedData(leftMost);
if (leftMost.Parent != null) UpdateAugmentedData(leftMost.Parent);
return;
}
// now either removedNode.left or removedNode.right is null
// get the remaining child
var parentNode = removedNode.Parent;
var childNode = removedNode.Left ?? removedNode.Right;
ReplaceNode(removedNode, childNode);
if (parentNode != null) UpdateAugmentedData(parentNode);
if (removedNode.Color == Black)
{
if (childNode != null && childNode.Color == Red)
{
childNode.Color = Black;
}
else
{
FixTreeOnDelete(childNode, parentNode);
}
}
}
private void FixTreeOnDelete(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (parentNode == null)
return;
// warning: node may be null
var sibling = Sibling(node, parentNode);
if (sibling.Color == Red)
{
parentNode.Color = Red;
sibling.Color = Black;
if (node == parentNode.Left)
{
RotateLeft(parentNode);
}
else
{
RotateRight(parentNode);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
}
if (parentNode.Color == Black
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
FixTreeOnDelete(parentNode, parentNode.Parent);
return;
}
if (parentNode.Color == Red
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
parentNode.Color = Black;
return;
}
if (node == parentNode.Left &&
sibling.Color == Black &&
GetColor(sibling.Left) == Red &&
GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
sibling.Left.Color = Black;
RotateRight(sibling);
}
else if (node == parentNode.Right &&
sibling.Color == Black &&
GetColor(sibling.Right) == Red &&
GetColor(sibling.Left) == Black)
{
sibling.Color = Red;
sibling.Right.Color = Black;
RotateLeft(sibling);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
sibling.Color = parentNode.Color;
parentNode.Color = Black;
if (node == parentNode.Left)
{
if (sibling.Right != null)
{
Debug.Assert(sibling.Right.Color == Red);
sibling.Right.Color = Black;
}
RotateLeft(parentNode);
}
else
{
if (sibling.Left != null)
{
Debug.Assert(sibling.Left.Color == Red);
sibling.Left.Color = Black;
}
RotateRight(parentNode);
}
}
private void ReplaceNode(TextSegment replacedNode, TextSegment newNode)
{
if (replacedNode.Parent == null)
{
Debug.Assert(replacedNode == _root);
_root = newNode;
}
else
{
if (replacedNode.Parent.Left == replacedNode)
replacedNode.Parent.Left = newNode;
else
replacedNode.Parent.Right = newNode;
}
if (newNode != null)
{
newNode.Parent = replacedNode.Parent;
}
replacedNode.Parent = null;
}
private void RotateLeft(TextSegment p)
{
// let q be p's right child
var q = p.Right;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's right child to be q's left child
p.Right = q.Left;
if (p.Right != null) p.Right.Parent = p;
// set q's left child to be p
q.Left = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private void RotateRight(TextSegment p)
{
// let q be p's left child
var q = p.Left;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's left child to be q's right child
p.Left = q.Right;
if (p.Left != null) p.Left.Parent = p;
// set q's right child to be p
q.Right = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private static TextSegment Sibling(TextSegment node)
{
return node == node.Parent.Left ? node.Parent.Right : node.Parent.Left;
}
private static TextSegment Sibling(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (node == parentNode.Left)
return parentNode.Right;
else
return parentNode.Left;
}
private static bool GetColor(TextSegment node)
{
return node != null && node.Color;
}
#endregion
#region ICollection<T> implementation
/// <summary>
/// Gets the number of segments in the tree.
/// </summary>
public int Count { get; private set; }
bool ICollection<T>.IsReadOnly => false;
/// <summary>
/// Gets whether this tree contains the specified item.
/// </summary>
public bool Contains(T item)
{
return item != null && item.OwnerTree == this;
}
/// <summary>
/// Copies all segments in this SegmentTree to the specified array.
/// </summary>
public void CopyTo(T[] array, int arrayIndex)
{
if (array == null)
throw new ArgumentNullException(nameof(array));
if (array.Length < Count)
throw new ArgumentException("The array is too small", nameof(array));
if (arrayIndex < 0 || arrayIndex + Count > array.Length)
throw new ArgumentOutOfRangeException(nameof(arrayIndex), arrayIndex, "Value must be between 0 and " + (array.Length - Count));
foreach (var s in this)
{
array[arrayIndex++] = s;
}
}
/// <summary>
/// Gets an enumerator to enumerate the segments.
/// </summary>
public IEnumerator<T> GetEnumerator()
{
if (_root != null)
{
var current = _root.LeftMost;
while (current != null)
{
yield return (T)current;
// TODO: check if collection was modified during enumeration
current = current.Successor;
}
}
}
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
#endregion
}
}
<MSG> Allow a text segment collection to be disconnected from a text document.
<DFF> @@ -97,6 +97,14 @@ namespace AvaloniaEdit.Document
}
#endregion
+ public void Disconnect (TextDocument textDocument)
+ {
+ if (_isConnectedToDocument)
+ {
+ TextDocumentWeakEventManager.Changed.RemoveHandler(textDocument, OnDocumentChanged);
+ }
+ }
+
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
| 8 | Allow a text segment collection to be disconnected from a text document. | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063842 | <NME> TextSegmentCollection.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using System.Text;
using AvaloniaEdit.Utils;
using Avalonia.Threading;
namespace AvaloniaEdit.Document
{
/// <summary>
/// Interface to allow TextSegments to access the TextSegmentCollection - we cannot use a direct reference
/// because TextSegmentCollection is generic.
/// </summary>
internal interface ISegmentTree
{
void Add(TextSegment s);
void Remove(TextSegment s);
void UpdateAugmentedData(TextSegment s);
}
/// <summary>
/// <para>
/// A collection of text segments that supports efficient lookup of segments
/// intersecting with another segment.
/// </para>
/// </summary>
/// <remarks><inheritdoc cref="TextSegment"/></remarks>
/// <see cref="TextSegment"/>
public sealed class TextSegmentCollection<T> : ICollection<T>, ISegmentTree where T : TextSegment
{
// Implementation: this is basically a mixture of an augmented interval tree
// and the TextAnchorTree.
// WARNING: you need to understand interval trees (the version with the augmented 'high'/'max' field)
// and how the TextAnchorTree works before you have any chance of understanding this code.
// This means that every node holds two "segments":
// one like the segments in the text anchor tree to support efficient offset changes
// and another that is the interval as seen by the user
// So basically, the tree contains a list of contiguous node segments of the first kind,
// with interval segments starting at the end of every node segment.
// Performance:
// Add is O(lg n)
// Remove is O(lg n)
// DocumentChanged is O(m * lg n), with m the number of segments that intersect with the changed document section
// FindFirstSegmentWithStartAfter is O(m + lg n) with m being the number of segments at the same offset as the result segment
// FindIntersectingSegments is O(m + lg n) with m being the number of intersecting segments.
private TextSegment _root;
private readonly bool _isConnectedToDocument;
#region Constructor
/// <summary>
/// Creates a new TextSegmentCollection that needs manual calls to <see cref="UpdateOffsets(DocumentChangeEventArgs)"/>.
/// </summary>
public TextSegmentCollection()
{
}
/// <summary>
/// Creates a new TextSegmentCollection that updates the offsets automatically.
/// </summary>
/// <param name="textDocument">The document to which the text segments
/// that will be added to the tree belong. When the document changes, the
/// position of the text segments will be updated accordingly.</param>
public TextSegmentCollection(TextDocument textDocument)
{
if (textDocument == null)
throw new ArgumentNullException(nameof(textDocument));
Dispatcher.UIThread.VerifyAccess();
_isConnectedToDocument = true;
TextDocumentWeakEventManager.Changed.AddHandler(textDocument, OnDocumentChanged);
}
#endregion
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="e">DocumentChangeEventArgs instance describing the change to the document.</param>
public void UpdateOffsets(DocumentChangeEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
OnDocumentChanged(this, e);
CheckProperties();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
var map = e.OffsetChangeMapOrNull;
if (map != null)
{
foreach (var entry in map)
{
UpdateOffsetsInternal(entry);
}
}
else
{
UpdateOffsetsInternal(e.CreateSingleChangeMapEntry());
}
}
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="change">OffsetChangeMapEntry instance describing the change to the document.</param>
public void UpdateOffsets(OffsetChangeMapEntry change)
{
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
UpdateOffsetsInternal(change);
CheckProperties();
}
#endregion
#region UpdateOffsets (implementation)
private void UpdateOffsetsInternal(OffsetChangeMapEntry change)
{
// Special case pure insertions, because they don't always cause a text segment to increase in size when the replaced region
// is inside a segment (when offset is at start or end of a text semgent).
if (change.RemovalLength == 0)
{
InsertText(change.Offset, change.InsertionLength);
}
else
{
ReplaceText(change);
}
}
private void InsertText(int offset, int length)
{
if (length == 0)
return;
// enlarge segments that contain offset (excluding those that have offset as endpoint)
foreach (var segment in FindSegmentsContaining(offset))
{
if (segment.StartOffset < offset && offset < segment.EndOffset)
{
segment.Length += length;
}
}
// move start offsets of all segments >= offset
TextSegment node = FindFirstSegmentWithStartAfter(offset);
if (node != null)
{
node.NodeLength += length;
UpdateAugmentedData(node);
}
}
private void ReplaceText(OffsetChangeMapEntry change)
{
Debug.Assert(change.RemovalLength > 0);
var offset = change.Offset;
foreach (var segment in FindOverlappingSegments(offset, change.RemovalLength))
{
if (segment.StartOffset <= offset)
{
if (segment.EndOffset >= offset + change.RemovalLength)
{
// Replacement inside segment: adjust segment length
segment.Length += change.InsertionLength - change.RemovalLength;
}
else
{
// Replacement starting inside segment and ending after segment end: set segment end to removal position
//segment.EndOffset = offset;
segment.Length = offset - segment.StartOffset;
}
}
else
{
// Replacement starting in front of text segment and running into segment.
// Keep segment.EndOffset constant and move segment.StartOffset to the end of the replacement
var remainingLength = segment.EndOffset - (offset + change.RemovalLength);
RemoveSegment(segment);
segment.StartOffset = offset + change.RemovalLength;
segment.Length = Math.Max(0, remainingLength);
AddSegment(segment);
}
}
// move start offsets of all segments > offset
TextSegment node = FindFirstSegmentWithStartAfter(offset + 1);
if (node != null)
{
Debug.Assert(node.NodeLength >= change.RemovalLength);
node.NodeLength += change.InsertionLength - change.RemovalLength;
UpdateAugmentedData(node);
}
}
#endregion
#region Add
/// <summary>
/// Adds the specified segment to the tree. This will cause the segment to update when the
/// document changes.
/// </summary>
public void Add(T item)
{
if (item == null)
throw new ArgumentNullException(nameof(item));
if (item.OwnerTree != null)
throw new ArgumentException("The segment is already added to a SegmentCollection.");
AddSegment(item);
}
void ISegmentTree.Add(TextSegment s)
{
AddSegment(s);
}
private void AddSegment(TextSegment node)
{
var insertionOffset = node.StartOffset;
node.DistanceToMaxEnd = node.SegmentLength;
if (_root == null)
{
_root = node;
node.TotalNodeLength = node.NodeLength;
}
else if (insertionOffset >= _root.TotalNodeLength)
{
// append segment at end of tree
node.NodeLength = node.TotalNodeLength = insertionOffset - _root.TotalNodeLength;
InsertAsRight(_root.RightMost, node);
}
else
{
// insert in middle of tree
var n = FindNode(ref insertionOffset);
Debug.Assert(insertionOffset < n.NodeLength);
// split node segment 'n' at offset
node.TotalNodeLength = node.NodeLength = insertionOffset;
n.NodeLength -= insertionOffset;
InsertBefore(n, node);
}
node.OwnerTree = this;
Count++;
CheckProperties();
}
private void InsertBefore(TextSegment node, TextSegment newNode)
{
if (node.Left == null)
{
InsertAsLeft(node, newNode);
}
else
{
InsertAsRight(node.Left.RightMost, newNode);
}
}
#endregion
#region GetNextSegment / GetPreviousSegment
/// <summary>
/// Gets the next segment after the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the last segment.
/// </summary>
public T GetNextSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Successor;
}
/// <summary>
/// Gets the previous segment before the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the first segment.
/// </summary>
public T GetPreviousSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Predecessor;
}
#endregion
#region FirstSegment/LastSegment
/// <summary>
/// Returns the first segment in the collection or null, if the collection is empty.
/// </summary>
public T FirstSegment => (T)_root?.LeftMost;
/// <summary>
/// Returns the last segment in the collection or null, if the collection is empty.
/// </summary>
public T LastSegment => (T)_root?.RightMost;
#endregion
#region FindFirstSegmentWithStartAfter
/// <summary>
/// Gets the first segment with a start offset greater or equal to <paramref name="startOffset"/>.
/// Returns null if no such segment is found.
/// </summary>
public T FindFirstSegmentWithStartAfter(int startOffset)
{
if (_root == null)
return null;
if (startOffset <= 0)
return (T)_root.LeftMost;
var s = FindNode(ref startOffset);
// startOffset means that the previous segment is starting at the offset we were looking for
while (startOffset == 0)
{
var p = (s == null) ? _root.RightMost : s.Predecessor;
// There must always be a predecessor: if we were looking for the first node, we would have already
// returned it as root.LeftMost above.
Debug.Assert(p != null);
startOffset += p.NodeLength;
s = p;
}
return (T)s;
}
/// <summary>
/// Finds the node at the specified offset.
/// After the method has run, offset is relative to the beginning of the returned node.
/// </summary>
private TextSegment FindNode(ref int offset)
{
var n = _root;
while (true)
{
if (n.Left != null)
{
if (offset < n.Left.TotalNodeLength)
{
n = n.Left; // descend into left subtree
continue;
}
else
{
offset -= n.Left.TotalNodeLength; // skip left subtree
}
}
if (offset < n.NodeLength)
{
return n; // found correct node
}
else
{
offset -= n.NodeLength; // skip this node
}
if (n.Right != null)
{
n = n.Right; // descend into right subtree
}
else
{
// didn't find any node containing the offset
return null;
}
}
}
#endregion
#region FindOverlappingSegments
/// <summary>
/// Finds all segments that contain the given offset.
/// (StartOffset <= offset <= EndOffset)
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindSegmentsContaining(int offset)
{
return FindOverlappingSegments(offset, 0);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(ISegment segment)
{
if (segment == null)
throw new ArgumentNullException(nameof(segment));
return FindOverlappingSegments(segment.Offset, segment.Length);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(int offset, int length)
{
ThrowUtil.CheckNotNegative(length, "length");
var results = new List<T>();
if (_root != null)
{
FindOverlappingSegments(results, _root, offset, offset + length);
}
return new ReadOnlyCollection<T>(results);
}
private void FindOverlappingSegments(List<T> results, TextSegment node, int low, int high)
{
// low and high are relative to node.LeftMost startpos (not node.LeftMost.Offset)
if (high < 0)
{
// node is irrelevant for search because all intervals in node are after high
return;
}
// find values relative to node.Offset
var nodeLow = low - node.NodeLength;
var nodeHigh = high - node.NodeLength;
if (node.Left != null)
{
nodeLow -= node.Left.TotalNodeLength;
nodeHigh -= node.Left.TotalNodeLength;
}
if (node.DistanceToMaxEnd < nodeLow)
{
// node is irrelevant for search because all intervals in node are before low
return;
}
if (node.Left != null)
FindOverlappingSegments(results, node.Left, low, high);
if (nodeHigh < 0)
{
// node and everything in node.right is before low
return;
}
if (nodeLow <= node.SegmentLength)
{
results.Add((T)node);
}
if (node.Right != null)
FindOverlappingSegments(results, node.Right, nodeLow, nodeHigh);
}
#endregion
#region UpdateAugmentedData
private void UpdateAugmentedData(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
totalLength += node.Left.TotalNodeLength;
var leftDtme = node.Left.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
if (node.Left.Right != null)
leftDtme -= node.Left.Right.TotalNodeLength;
leftDtme -= node.NodeLength;
if (leftDtme > distanceToMaxEnd)
distanceToMaxEnd = leftDtme;
}
if (node.Right != null)
{
totalLength += node.Right.TotalNodeLength;
var rightDtme = node.Right.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
rightDtme += node.Right.NodeLength;
if (node.Right.Left != null)
rightDtme += node.Right.Left.TotalNodeLength;
if (rightDtme > distanceToMaxEnd)
distanceToMaxEnd = rightDtme;
}
if (node.TotalNodeLength != totalLength
|| node.DistanceToMaxEnd != distanceToMaxEnd)
{
node.TotalNodeLength = totalLength;
node.DistanceToMaxEnd = distanceToMaxEnd;
if (node.Parent != null)
UpdateAugmentedData(node.Parent);
}
}
void ISegmentTree.UpdateAugmentedData(TextSegment node)
{
UpdateAugmentedData(node);
}
#endregion
#region Remove
/// <summary>
/// Removes the specified segment from the tree. This will cause the segment to not update
/// anymore when the document changes.
/// </summary>
public bool Remove(T item)
{
if (!Contains(item))
return false;
RemoveSegment(item);
return true;
}
void ISegmentTree.Remove(TextSegment s)
{
RemoveSegment(s);
}
private void RemoveSegment(TextSegment s)
{
var oldOffset = s.StartOffset;
var successor = s.Successor;
if (successor != null)
successor.NodeLength += s.NodeLength;
RemoveNode(s);
if (successor != null)
UpdateAugmentedData(successor);
Disconnect(s, oldOffset);
CheckProperties();
}
private void Disconnect(TextSegment s, int offset)
{
s.Left = s.Right = s.Parent = null;
s.OwnerTree = null;
s.NodeLength = offset;
Count--;
}
/// <summary>
/// Removes all segments from the tree.
/// </summary>
public void Clear()
{
var segments = this.ToArray();
_root = null;
var offset = 0;
foreach (var s in segments)
{
offset += s.NodeLength;
Disconnect(s, offset);
}
CheckProperties();
}
#endregion
#region CheckProperties
[Conditional("DATACONSISTENCYTEST")]
internal void CheckProperties()
{
#if DEBUG
if (_root != null)
{
CheckProperties(_root);
// check red-black property:
var blackCount = -1;
CheckNodeProperties(_root, null, Red, 0, ref blackCount);
}
var expectedCount = 0;
// we cannot trust LINQ not to call ICollection.Count, so we need this loop
// to count the elements in the tree
using (var en = GetEnumerator())
{
while (en.MoveNext()) expectedCount++;
}
Debug.Assert(Count == expectedCount);
#endif
}
#if DEBUG
private void CheckProperties(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
CheckProperties(node.Left);
totalLength += node.Left.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Left.DistanceToMaxEnd + node.Left.StartOffset - node.StartOffset);
}
if (node.Right != null)
{
CheckProperties(node.Right);
totalLength += node.Right.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Right.DistanceToMaxEnd + node.Right.StartOffset - node.StartOffset);
}
Debug.Assert(node.TotalNodeLength == totalLength);
Debug.Assert(node.DistanceToMaxEnd == distanceToMaxEnd);
}
/*
1. A node is either red or black.
2. The root is black.
3. All leaves are black. (The leaves are the NIL children.)
4. Both children of every red node are black. (So every red node must have a black parent.)
5. Every simple path from a node to a descendant leaf contains the same number of black nodes. (Not counting the leaf node.)
*/
[SuppressMessage("ReSharper", "UnusedParameter.Local")]
private static void CheckNodeProperties(TextSegment node, TextSegment parentNode, bool parentColor, int blackCount, ref int expectedBlackCount)
{
if (node == null) return;
Debug.Assert(node.Parent == parentNode);
if (parentColor == Red)
{
Debug.Assert(node.Color == Black);
}
if (node.Color == Black)
{
blackCount++;
}
if (node.Left == null && node.Right == null)
{
// node is a leaf node:
if (expectedBlackCount == -1)
expectedBlackCount = blackCount;
else
Debug.Assert(expectedBlackCount == blackCount);
}
CheckNodeProperties(node.Left, node, node.Color, blackCount, ref expectedBlackCount);
CheckNodeProperties(node.Right, node, node.Color, blackCount, ref expectedBlackCount);
}
private static void AppendTreeToString(TextSegment node, StringBuilder b, int indent)
{
b.Append(node.Color == Red ? "RED " : "BLACK ");
b.AppendLine(node + node.ToDebugString());
indent += 2;
if (node.Left != null)
{
b.Append(' ', indent);
b.Append("L: ");
AppendTreeToString(node.Left, b, indent);
}
if (node.Right != null)
{
b.Append(' ', indent);
b.Append("R: ");
AppendTreeToString(node.Right, b, indent);
}
}
#endif
internal string GetTreeAsString()
{
#if DEBUG
var b = new StringBuilder();
if (_root != null)
AppendTreeToString(_root, b, 0);
return b.ToString();
#else
return "Not available in release build.";
#endif
}
#endregion
#region Red/Black Tree
internal const bool Red = true;
internal const bool Black = false;
private void InsertAsLeft(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Left == null);
parentNode.Left = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void InsertAsRight(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Right == null);
parentNode.Right = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void FixTreeOnInsert(TextSegment node)
{
Debug.Assert(node != null);
Debug.Assert(node.Color == Red);
Debug.Assert(node.Left == null || node.Left.Color == Black);
Debug.Assert(node.Right == null || node.Right.Color == Black);
var parentNode = node.Parent;
if (parentNode == null)
{
// we inserted in the root -> the node must be black
// since this is a root node, making the node black increments the number of black nodes
// on all paths by one, so it is still the same for all paths.
node.Color = Black;
return;
}
if (parentNode.Color == Black)
{
// if the parent node where we inserted was black, our red node is placed correctly.
// since we inserted a red node, the number of black nodes on each path is unchanged
// -> the tree is still balanced
return;
}
// parentNode is red, so there is a conflict here!
// because the root is black, parentNode is not the root -> there is a grandparent node
var grandparentNode = parentNode.Parent;
var uncleNode = Sibling(parentNode);
if (uncleNode != null && uncleNode.Color == Red)
{
parentNode.Color = Black;
uncleNode.Color = Black;
grandparentNode.Color = Red;
FixTreeOnInsert(grandparentNode);
return;
}
// now we know: parent is red but uncle is black
// First rotation:
if (node == parentNode.Right && parentNode == grandparentNode.Left)
{
RotateLeft(parentNode);
node = node.Left;
}
else if (node == parentNode.Left && parentNode == grandparentNode.Right)
{
RotateRight(parentNode);
node = node.Right;
}
// because node might have changed, reassign variables:
// ReSharper disable once PossibleNullReferenceException
parentNode = node.Parent;
grandparentNode = parentNode.Parent;
// Now recolor a bit:
parentNode.Color = Black;
grandparentNode.Color = Red;
// Second rotation:
if (node == parentNode.Left && parentNode == grandparentNode.Left)
{
RotateRight(grandparentNode);
}
else
{
// because of the first rotation, this is guaranteed:
Debug.Assert(node == parentNode.Right && parentNode == grandparentNode.Right);
RotateLeft(grandparentNode);
}
}
private void RemoveNode(TextSegment removedNode)
{
if (removedNode.Left != null && removedNode.Right != null)
{
// replace removedNode with it's in-order successor
var leftMost = removedNode.Right.LeftMost;
RemoveNode(leftMost); // remove leftMost from its current location
// and overwrite the removedNode with it
ReplaceNode(removedNode, leftMost);
leftMost.Left = removedNode.Left;
if (leftMost.Left != null) leftMost.Left.Parent = leftMost;
leftMost.Right = removedNode.Right;
if (leftMost.Right != null) leftMost.Right.Parent = leftMost;
leftMost.Color = removedNode.Color;
UpdateAugmentedData(leftMost);
if (leftMost.Parent != null) UpdateAugmentedData(leftMost.Parent);
return;
}
// now either removedNode.left or removedNode.right is null
// get the remaining child
var parentNode = removedNode.Parent;
var childNode = removedNode.Left ?? removedNode.Right;
ReplaceNode(removedNode, childNode);
if (parentNode != null) UpdateAugmentedData(parentNode);
if (removedNode.Color == Black)
{
if (childNode != null && childNode.Color == Red)
{
childNode.Color = Black;
}
else
{
FixTreeOnDelete(childNode, parentNode);
}
}
}
private void FixTreeOnDelete(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (parentNode == null)
return;
// warning: node may be null
var sibling = Sibling(node, parentNode);
if (sibling.Color == Red)
{
parentNode.Color = Red;
sibling.Color = Black;
if (node == parentNode.Left)
{
RotateLeft(parentNode);
}
else
{
RotateRight(parentNode);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
}
if (parentNode.Color == Black
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
FixTreeOnDelete(parentNode, parentNode.Parent);
return;
}
if (parentNode.Color == Red
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
parentNode.Color = Black;
return;
}
if (node == parentNode.Left &&
sibling.Color == Black &&
GetColor(sibling.Left) == Red &&
GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
sibling.Left.Color = Black;
RotateRight(sibling);
}
else if (node == parentNode.Right &&
sibling.Color == Black &&
GetColor(sibling.Right) == Red &&
GetColor(sibling.Left) == Black)
{
sibling.Color = Red;
sibling.Right.Color = Black;
RotateLeft(sibling);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
sibling.Color = parentNode.Color;
parentNode.Color = Black;
if (node == parentNode.Left)
{
if (sibling.Right != null)
{
Debug.Assert(sibling.Right.Color == Red);
sibling.Right.Color = Black;
}
RotateLeft(parentNode);
}
else
{
if (sibling.Left != null)
{
Debug.Assert(sibling.Left.Color == Red);
sibling.Left.Color = Black;
}
RotateRight(parentNode);
}
}
private void ReplaceNode(TextSegment replacedNode, TextSegment newNode)
{
if (replacedNode.Parent == null)
{
Debug.Assert(replacedNode == _root);
_root = newNode;
}
else
{
if (replacedNode.Parent.Left == replacedNode)
replacedNode.Parent.Left = newNode;
else
replacedNode.Parent.Right = newNode;
}
if (newNode != null)
{
newNode.Parent = replacedNode.Parent;
}
replacedNode.Parent = null;
}
private void RotateLeft(TextSegment p)
{
// let q be p's right child
var q = p.Right;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's right child to be q's left child
p.Right = q.Left;
if (p.Right != null) p.Right.Parent = p;
// set q's left child to be p
q.Left = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private void RotateRight(TextSegment p)
{
// let q be p's left child
var q = p.Left;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's left child to be q's right child
p.Left = q.Right;
if (p.Left != null) p.Left.Parent = p;
// set q's right child to be p
q.Right = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private static TextSegment Sibling(TextSegment node)
{
return node == node.Parent.Left ? node.Parent.Right : node.Parent.Left;
}
private static TextSegment Sibling(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (node == parentNode.Left)
return parentNode.Right;
else
return parentNode.Left;
}
private static bool GetColor(TextSegment node)
{
return node != null && node.Color;
}
#endregion
#region ICollection<T> implementation
/// <summary>
/// Gets the number of segments in the tree.
/// </summary>
public int Count { get; private set; }
bool ICollection<T>.IsReadOnly => false;
/// <summary>
/// Gets whether this tree contains the specified item.
/// </summary>
public bool Contains(T item)
{
return item != null && item.OwnerTree == this;
}
/// <summary>
/// Copies all segments in this SegmentTree to the specified array.
/// </summary>
public void CopyTo(T[] array, int arrayIndex)
{
if (array == null)
throw new ArgumentNullException(nameof(array));
if (array.Length < Count)
throw new ArgumentException("The array is too small", nameof(array));
if (arrayIndex < 0 || arrayIndex + Count > array.Length)
throw new ArgumentOutOfRangeException(nameof(arrayIndex), arrayIndex, "Value must be between 0 and " + (array.Length - Count));
foreach (var s in this)
{
array[arrayIndex++] = s;
}
}
/// <summary>
/// Gets an enumerator to enumerate the segments.
/// </summary>
public IEnumerator<T> GetEnumerator()
{
if (_root != null)
{
var current = _root.LeftMost;
while (current != null)
{
yield return (T)current;
// TODO: check if collection was modified during enumeration
current = current.Successor;
}
}
}
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
#endregion
}
}
<MSG> Allow a text segment collection to be disconnected from a text document.
<DFF> @@ -97,6 +97,14 @@ namespace AvaloniaEdit.Document
}
#endregion
+ public void Disconnect (TextDocument textDocument)
+ {
+ if (_isConnectedToDocument)
+ {
+ TextDocumentWeakEventManager.Changed.RemoveHandler(textDocument, OnDocumentChanged);
+ }
+ }
+
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
| 8 | Allow a text segment collection to be disconnected from a text document. | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063843 | <NME> TextSegmentCollection.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Diagnostics;
using System.Diagnostics.CodeAnalysis;
using System.Linq;
using System.Text;
using AvaloniaEdit.Utils;
using Avalonia.Threading;
namespace AvaloniaEdit.Document
{
/// <summary>
/// Interface to allow TextSegments to access the TextSegmentCollection - we cannot use a direct reference
/// because TextSegmentCollection is generic.
/// </summary>
internal interface ISegmentTree
{
void Add(TextSegment s);
void Remove(TextSegment s);
void UpdateAugmentedData(TextSegment s);
}
/// <summary>
/// <para>
/// A collection of text segments that supports efficient lookup of segments
/// intersecting with another segment.
/// </para>
/// </summary>
/// <remarks><inheritdoc cref="TextSegment"/></remarks>
/// <see cref="TextSegment"/>
public sealed class TextSegmentCollection<T> : ICollection<T>, ISegmentTree where T : TextSegment
{
// Implementation: this is basically a mixture of an augmented interval tree
// and the TextAnchorTree.
// WARNING: you need to understand interval trees (the version with the augmented 'high'/'max' field)
// and how the TextAnchorTree works before you have any chance of understanding this code.
// This means that every node holds two "segments":
// one like the segments in the text anchor tree to support efficient offset changes
// and another that is the interval as seen by the user
// So basically, the tree contains a list of contiguous node segments of the first kind,
// with interval segments starting at the end of every node segment.
// Performance:
// Add is O(lg n)
// Remove is O(lg n)
// DocumentChanged is O(m * lg n), with m the number of segments that intersect with the changed document section
// FindFirstSegmentWithStartAfter is O(m + lg n) with m being the number of segments at the same offset as the result segment
// FindIntersectingSegments is O(m + lg n) with m being the number of intersecting segments.
private TextSegment _root;
private readonly bool _isConnectedToDocument;
#region Constructor
/// <summary>
/// Creates a new TextSegmentCollection that needs manual calls to <see cref="UpdateOffsets(DocumentChangeEventArgs)"/>.
/// </summary>
public TextSegmentCollection()
{
}
/// <summary>
/// Creates a new TextSegmentCollection that updates the offsets automatically.
/// </summary>
/// <param name="textDocument">The document to which the text segments
/// that will be added to the tree belong. When the document changes, the
/// position of the text segments will be updated accordingly.</param>
public TextSegmentCollection(TextDocument textDocument)
{
if (textDocument == null)
throw new ArgumentNullException(nameof(textDocument));
Dispatcher.UIThread.VerifyAccess();
_isConnectedToDocument = true;
TextDocumentWeakEventManager.Changed.AddHandler(textDocument, OnDocumentChanged);
}
#endregion
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="e">DocumentChangeEventArgs instance describing the change to the document.</param>
public void UpdateOffsets(DocumentChangeEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
OnDocumentChanged(this, e);
CheckProperties();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
var map = e.OffsetChangeMapOrNull;
if (map != null)
{
foreach (var entry in map)
{
UpdateOffsetsInternal(entry);
}
}
else
{
UpdateOffsetsInternal(e.CreateSingleChangeMapEntry());
}
}
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
/// </summary>
/// <param name="change">OffsetChangeMapEntry instance describing the change to the document.</param>
public void UpdateOffsets(OffsetChangeMapEntry change)
{
if (_isConnectedToDocument)
throw new InvalidOperationException("This TextSegmentCollection will automatically update offsets; do not call UpdateOffsets manually!");
UpdateOffsetsInternal(change);
CheckProperties();
}
#endregion
#region UpdateOffsets (implementation)
private void UpdateOffsetsInternal(OffsetChangeMapEntry change)
{
// Special case pure insertions, because they don't always cause a text segment to increase in size when the replaced region
// is inside a segment (when offset is at start or end of a text semgent).
if (change.RemovalLength == 0)
{
InsertText(change.Offset, change.InsertionLength);
}
else
{
ReplaceText(change);
}
}
private void InsertText(int offset, int length)
{
if (length == 0)
return;
// enlarge segments that contain offset (excluding those that have offset as endpoint)
foreach (var segment in FindSegmentsContaining(offset))
{
if (segment.StartOffset < offset && offset < segment.EndOffset)
{
segment.Length += length;
}
}
// move start offsets of all segments >= offset
TextSegment node = FindFirstSegmentWithStartAfter(offset);
if (node != null)
{
node.NodeLength += length;
UpdateAugmentedData(node);
}
}
private void ReplaceText(OffsetChangeMapEntry change)
{
Debug.Assert(change.RemovalLength > 0);
var offset = change.Offset;
foreach (var segment in FindOverlappingSegments(offset, change.RemovalLength))
{
if (segment.StartOffset <= offset)
{
if (segment.EndOffset >= offset + change.RemovalLength)
{
// Replacement inside segment: adjust segment length
segment.Length += change.InsertionLength - change.RemovalLength;
}
else
{
// Replacement starting inside segment and ending after segment end: set segment end to removal position
//segment.EndOffset = offset;
segment.Length = offset - segment.StartOffset;
}
}
else
{
// Replacement starting in front of text segment and running into segment.
// Keep segment.EndOffset constant and move segment.StartOffset to the end of the replacement
var remainingLength = segment.EndOffset - (offset + change.RemovalLength);
RemoveSegment(segment);
segment.StartOffset = offset + change.RemovalLength;
segment.Length = Math.Max(0, remainingLength);
AddSegment(segment);
}
}
// move start offsets of all segments > offset
TextSegment node = FindFirstSegmentWithStartAfter(offset + 1);
if (node != null)
{
Debug.Assert(node.NodeLength >= change.RemovalLength);
node.NodeLength += change.InsertionLength - change.RemovalLength;
UpdateAugmentedData(node);
}
}
#endregion
#region Add
/// <summary>
/// Adds the specified segment to the tree. This will cause the segment to update when the
/// document changes.
/// </summary>
public void Add(T item)
{
if (item == null)
throw new ArgumentNullException(nameof(item));
if (item.OwnerTree != null)
throw new ArgumentException("The segment is already added to a SegmentCollection.");
AddSegment(item);
}
void ISegmentTree.Add(TextSegment s)
{
AddSegment(s);
}
private void AddSegment(TextSegment node)
{
var insertionOffset = node.StartOffset;
node.DistanceToMaxEnd = node.SegmentLength;
if (_root == null)
{
_root = node;
node.TotalNodeLength = node.NodeLength;
}
else if (insertionOffset >= _root.TotalNodeLength)
{
// append segment at end of tree
node.NodeLength = node.TotalNodeLength = insertionOffset - _root.TotalNodeLength;
InsertAsRight(_root.RightMost, node);
}
else
{
// insert in middle of tree
var n = FindNode(ref insertionOffset);
Debug.Assert(insertionOffset < n.NodeLength);
// split node segment 'n' at offset
node.TotalNodeLength = node.NodeLength = insertionOffset;
n.NodeLength -= insertionOffset;
InsertBefore(n, node);
}
node.OwnerTree = this;
Count++;
CheckProperties();
}
private void InsertBefore(TextSegment node, TextSegment newNode)
{
if (node.Left == null)
{
InsertAsLeft(node, newNode);
}
else
{
InsertAsRight(node.Left.RightMost, newNode);
}
}
#endregion
#region GetNextSegment / GetPreviousSegment
/// <summary>
/// Gets the next segment after the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the last segment.
/// </summary>
public T GetNextSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Successor;
}
/// <summary>
/// Gets the previous segment before the specified segment.
/// Segments are sorted by their start offset.
/// Returns null if segment is the first segment.
/// </summary>
public T GetPreviousSegment(T segment)
{
if (!Contains(segment))
throw new ArgumentException("segment is not inside the segment tree");
return (T)segment.Predecessor;
}
#endregion
#region FirstSegment/LastSegment
/// <summary>
/// Returns the first segment in the collection or null, if the collection is empty.
/// </summary>
public T FirstSegment => (T)_root?.LeftMost;
/// <summary>
/// Returns the last segment in the collection or null, if the collection is empty.
/// </summary>
public T LastSegment => (T)_root?.RightMost;
#endregion
#region FindFirstSegmentWithStartAfter
/// <summary>
/// Gets the first segment with a start offset greater or equal to <paramref name="startOffset"/>.
/// Returns null if no such segment is found.
/// </summary>
public T FindFirstSegmentWithStartAfter(int startOffset)
{
if (_root == null)
return null;
if (startOffset <= 0)
return (T)_root.LeftMost;
var s = FindNode(ref startOffset);
// startOffset means that the previous segment is starting at the offset we were looking for
while (startOffset == 0)
{
var p = (s == null) ? _root.RightMost : s.Predecessor;
// There must always be a predecessor: if we were looking for the first node, we would have already
// returned it as root.LeftMost above.
Debug.Assert(p != null);
startOffset += p.NodeLength;
s = p;
}
return (T)s;
}
/// <summary>
/// Finds the node at the specified offset.
/// After the method has run, offset is relative to the beginning of the returned node.
/// </summary>
private TextSegment FindNode(ref int offset)
{
var n = _root;
while (true)
{
if (n.Left != null)
{
if (offset < n.Left.TotalNodeLength)
{
n = n.Left; // descend into left subtree
continue;
}
else
{
offset -= n.Left.TotalNodeLength; // skip left subtree
}
}
if (offset < n.NodeLength)
{
return n; // found correct node
}
else
{
offset -= n.NodeLength; // skip this node
}
if (n.Right != null)
{
n = n.Right; // descend into right subtree
}
else
{
// didn't find any node containing the offset
return null;
}
}
}
#endregion
#region FindOverlappingSegments
/// <summary>
/// Finds all segments that contain the given offset.
/// (StartOffset <= offset <= EndOffset)
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindSegmentsContaining(int offset)
{
return FindOverlappingSegments(offset, 0);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(ISegment segment)
{
if (segment == null)
throw new ArgumentNullException(nameof(segment));
return FindOverlappingSegments(segment.Offset, segment.Length);
}
/// <summary>
/// Finds all segments that overlap with the given segment (including touching segments).
/// Segments are returned in the order given by GetNextSegment/GetPreviousSegment.
/// </summary>
/// <returns>Returns a new collection containing the results of the query.
/// This means it is safe to modify the TextSegmentCollection while iterating through the result collection.</returns>
public ReadOnlyCollection<T> FindOverlappingSegments(int offset, int length)
{
ThrowUtil.CheckNotNegative(length, "length");
var results = new List<T>();
if (_root != null)
{
FindOverlappingSegments(results, _root, offset, offset + length);
}
return new ReadOnlyCollection<T>(results);
}
private void FindOverlappingSegments(List<T> results, TextSegment node, int low, int high)
{
// low and high are relative to node.LeftMost startpos (not node.LeftMost.Offset)
if (high < 0)
{
// node is irrelevant for search because all intervals in node are after high
return;
}
// find values relative to node.Offset
var nodeLow = low - node.NodeLength;
var nodeHigh = high - node.NodeLength;
if (node.Left != null)
{
nodeLow -= node.Left.TotalNodeLength;
nodeHigh -= node.Left.TotalNodeLength;
}
if (node.DistanceToMaxEnd < nodeLow)
{
// node is irrelevant for search because all intervals in node are before low
return;
}
if (node.Left != null)
FindOverlappingSegments(results, node.Left, low, high);
if (nodeHigh < 0)
{
// node and everything in node.right is before low
return;
}
if (nodeLow <= node.SegmentLength)
{
results.Add((T)node);
}
if (node.Right != null)
FindOverlappingSegments(results, node.Right, nodeLow, nodeHigh);
}
#endregion
#region UpdateAugmentedData
private void UpdateAugmentedData(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
totalLength += node.Left.TotalNodeLength;
var leftDtme = node.Left.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
if (node.Left.Right != null)
leftDtme -= node.Left.Right.TotalNodeLength;
leftDtme -= node.NodeLength;
if (leftDtme > distanceToMaxEnd)
distanceToMaxEnd = leftDtme;
}
if (node.Right != null)
{
totalLength += node.Right.TotalNodeLength;
var rightDtme = node.Right.DistanceToMaxEnd;
// dtme is relative, so convert it to the coordinates of node:
rightDtme += node.Right.NodeLength;
if (node.Right.Left != null)
rightDtme += node.Right.Left.TotalNodeLength;
if (rightDtme > distanceToMaxEnd)
distanceToMaxEnd = rightDtme;
}
if (node.TotalNodeLength != totalLength
|| node.DistanceToMaxEnd != distanceToMaxEnd)
{
node.TotalNodeLength = totalLength;
node.DistanceToMaxEnd = distanceToMaxEnd;
if (node.Parent != null)
UpdateAugmentedData(node.Parent);
}
}
void ISegmentTree.UpdateAugmentedData(TextSegment node)
{
UpdateAugmentedData(node);
}
#endregion
#region Remove
/// <summary>
/// Removes the specified segment from the tree. This will cause the segment to not update
/// anymore when the document changes.
/// </summary>
public bool Remove(T item)
{
if (!Contains(item))
return false;
RemoveSegment(item);
return true;
}
void ISegmentTree.Remove(TextSegment s)
{
RemoveSegment(s);
}
private void RemoveSegment(TextSegment s)
{
var oldOffset = s.StartOffset;
var successor = s.Successor;
if (successor != null)
successor.NodeLength += s.NodeLength;
RemoveNode(s);
if (successor != null)
UpdateAugmentedData(successor);
Disconnect(s, oldOffset);
CheckProperties();
}
private void Disconnect(TextSegment s, int offset)
{
s.Left = s.Right = s.Parent = null;
s.OwnerTree = null;
s.NodeLength = offset;
Count--;
}
/// <summary>
/// Removes all segments from the tree.
/// </summary>
public void Clear()
{
var segments = this.ToArray();
_root = null;
var offset = 0;
foreach (var s in segments)
{
offset += s.NodeLength;
Disconnect(s, offset);
}
CheckProperties();
}
#endregion
#region CheckProperties
[Conditional("DATACONSISTENCYTEST")]
internal void CheckProperties()
{
#if DEBUG
if (_root != null)
{
CheckProperties(_root);
// check red-black property:
var blackCount = -1;
CheckNodeProperties(_root, null, Red, 0, ref blackCount);
}
var expectedCount = 0;
// we cannot trust LINQ not to call ICollection.Count, so we need this loop
// to count the elements in the tree
using (var en = GetEnumerator())
{
while (en.MoveNext()) expectedCount++;
}
Debug.Assert(Count == expectedCount);
#endif
}
#if DEBUG
private void CheckProperties(TextSegment node)
{
var totalLength = node.NodeLength;
var distanceToMaxEnd = node.SegmentLength;
if (node.Left != null)
{
CheckProperties(node.Left);
totalLength += node.Left.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Left.DistanceToMaxEnd + node.Left.StartOffset - node.StartOffset);
}
if (node.Right != null)
{
CheckProperties(node.Right);
totalLength += node.Right.TotalNodeLength;
distanceToMaxEnd = Math.Max(distanceToMaxEnd,
node.Right.DistanceToMaxEnd + node.Right.StartOffset - node.StartOffset);
}
Debug.Assert(node.TotalNodeLength == totalLength);
Debug.Assert(node.DistanceToMaxEnd == distanceToMaxEnd);
}
/*
1. A node is either red or black.
2. The root is black.
3. All leaves are black. (The leaves are the NIL children.)
4. Both children of every red node are black. (So every red node must have a black parent.)
5. Every simple path from a node to a descendant leaf contains the same number of black nodes. (Not counting the leaf node.)
*/
[SuppressMessage("ReSharper", "UnusedParameter.Local")]
private static void CheckNodeProperties(TextSegment node, TextSegment parentNode, bool parentColor, int blackCount, ref int expectedBlackCount)
{
if (node == null) return;
Debug.Assert(node.Parent == parentNode);
if (parentColor == Red)
{
Debug.Assert(node.Color == Black);
}
if (node.Color == Black)
{
blackCount++;
}
if (node.Left == null && node.Right == null)
{
// node is a leaf node:
if (expectedBlackCount == -1)
expectedBlackCount = blackCount;
else
Debug.Assert(expectedBlackCount == blackCount);
}
CheckNodeProperties(node.Left, node, node.Color, blackCount, ref expectedBlackCount);
CheckNodeProperties(node.Right, node, node.Color, blackCount, ref expectedBlackCount);
}
private static void AppendTreeToString(TextSegment node, StringBuilder b, int indent)
{
b.Append(node.Color == Red ? "RED " : "BLACK ");
b.AppendLine(node + node.ToDebugString());
indent += 2;
if (node.Left != null)
{
b.Append(' ', indent);
b.Append("L: ");
AppendTreeToString(node.Left, b, indent);
}
if (node.Right != null)
{
b.Append(' ', indent);
b.Append("R: ");
AppendTreeToString(node.Right, b, indent);
}
}
#endif
internal string GetTreeAsString()
{
#if DEBUG
var b = new StringBuilder();
if (_root != null)
AppendTreeToString(_root, b, 0);
return b.ToString();
#else
return "Not available in release build.";
#endif
}
#endregion
#region Red/Black Tree
internal const bool Red = true;
internal const bool Black = false;
private void InsertAsLeft(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Left == null);
parentNode.Left = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void InsertAsRight(TextSegment parentNode, TextSegment newNode)
{
Debug.Assert(parentNode.Right == null);
parentNode.Right = newNode;
newNode.Parent = parentNode;
newNode.Color = Red;
UpdateAugmentedData(parentNode);
FixTreeOnInsert(newNode);
}
private void FixTreeOnInsert(TextSegment node)
{
Debug.Assert(node != null);
Debug.Assert(node.Color == Red);
Debug.Assert(node.Left == null || node.Left.Color == Black);
Debug.Assert(node.Right == null || node.Right.Color == Black);
var parentNode = node.Parent;
if (parentNode == null)
{
// we inserted in the root -> the node must be black
// since this is a root node, making the node black increments the number of black nodes
// on all paths by one, so it is still the same for all paths.
node.Color = Black;
return;
}
if (parentNode.Color == Black)
{
// if the parent node where we inserted was black, our red node is placed correctly.
// since we inserted a red node, the number of black nodes on each path is unchanged
// -> the tree is still balanced
return;
}
// parentNode is red, so there is a conflict here!
// because the root is black, parentNode is not the root -> there is a grandparent node
var grandparentNode = parentNode.Parent;
var uncleNode = Sibling(parentNode);
if (uncleNode != null && uncleNode.Color == Red)
{
parentNode.Color = Black;
uncleNode.Color = Black;
grandparentNode.Color = Red;
FixTreeOnInsert(grandparentNode);
return;
}
// now we know: parent is red but uncle is black
// First rotation:
if (node == parentNode.Right && parentNode == grandparentNode.Left)
{
RotateLeft(parentNode);
node = node.Left;
}
else if (node == parentNode.Left && parentNode == grandparentNode.Right)
{
RotateRight(parentNode);
node = node.Right;
}
// because node might have changed, reassign variables:
// ReSharper disable once PossibleNullReferenceException
parentNode = node.Parent;
grandparentNode = parentNode.Parent;
// Now recolor a bit:
parentNode.Color = Black;
grandparentNode.Color = Red;
// Second rotation:
if (node == parentNode.Left && parentNode == grandparentNode.Left)
{
RotateRight(grandparentNode);
}
else
{
// because of the first rotation, this is guaranteed:
Debug.Assert(node == parentNode.Right && parentNode == grandparentNode.Right);
RotateLeft(grandparentNode);
}
}
private void RemoveNode(TextSegment removedNode)
{
if (removedNode.Left != null && removedNode.Right != null)
{
// replace removedNode with it's in-order successor
var leftMost = removedNode.Right.LeftMost;
RemoveNode(leftMost); // remove leftMost from its current location
// and overwrite the removedNode with it
ReplaceNode(removedNode, leftMost);
leftMost.Left = removedNode.Left;
if (leftMost.Left != null) leftMost.Left.Parent = leftMost;
leftMost.Right = removedNode.Right;
if (leftMost.Right != null) leftMost.Right.Parent = leftMost;
leftMost.Color = removedNode.Color;
UpdateAugmentedData(leftMost);
if (leftMost.Parent != null) UpdateAugmentedData(leftMost.Parent);
return;
}
// now either removedNode.left or removedNode.right is null
// get the remaining child
var parentNode = removedNode.Parent;
var childNode = removedNode.Left ?? removedNode.Right;
ReplaceNode(removedNode, childNode);
if (parentNode != null) UpdateAugmentedData(parentNode);
if (removedNode.Color == Black)
{
if (childNode != null && childNode.Color == Red)
{
childNode.Color = Black;
}
else
{
FixTreeOnDelete(childNode, parentNode);
}
}
}
private void FixTreeOnDelete(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (parentNode == null)
return;
// warning: node may be null
var sibling = Sibling(node, parentNode);
if (sibling.Color == Red)
{
parentNode.Color = Red;
sibling.Color = Black;
if (node == parentNode.Left)
{
RotateLeft(parentNode);
}
else
{
RotateRight(parentNode);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
}
if (parentNode.Color == Black
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
FixTreeOnDelete(parentNode, parentNode.Parent);
return;
}
if (parentNode.Color == Red
&& sibling.Color == Black
&& GetColor(sibling.Left) == Black
&& GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
parentNode.Color = Black;
return;
}
if (node == parentNode.Left &&
sibling.Color == Black &&
GetColor(sibling.Left) == Red &&
GetColor(sibling.Right) == Black)
{
sibling.Color = Red;
sibling.Left.Color = Black;
RotateRight(sibling);
}
else if (node == parentNode.Right &&
sibling.Color == Black &&
GetColor(sibling.Right) == Red &&
GetColor(sibling.Left) == Black)
{
sibling.Color = Red;
sibling.Right.Color = Black;
RotateLeft(sibling);
}
sibling = Sibling(node, parentNode); // update value of sibling after rotation
sibling.Color = parentNode.Color;
parentNode.Color = Black;
if (node == parentNode.Left)
{
if (sibling.Right != null)
{
Debug.Assert(sibling.Right.Color == Red);
sibling.Right.Color = Black;
}
RotateLeft(parentNode);
}
else
{
if (sibling.Left != null)
{
Debug.Assert(sibling.Left.Color == Red);
sibling.Left.Color = Black;
}
RotateRight(parentNode);
}
}
private void ReplaceNode(TextSegment replacedNode, TextSegment newNode)
{
if (replacedNode.Parent == null)
{
Debug.Assert(replacedNode == _root);
_root = newNode;
}
else
{
if (replacedNode.Parent.Left == replacedNode)
replacedNode.Parent.Left = newNode;
else
replacedNode.Parent.Right = newNode;
}
if (newNode != null)
{
newNode.Parent = replacedNode.Parent;
}
replacedNode.Parent = null;
}
private void RotateLeft(TextSegment p)
{
// let q be p's right child
var q = p.Right;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's right child to be q's left child
p.Right = q.Left;
if (p.Right != null) p.Right.Parent = p;
// set q's left child to be p
q.Left = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private void RotateRight(TextSegment p)
{
// let q be p's left child
var q = p.Left;
Debug.Assert(q != null);
Debug.Assert(q.Parent == p);
// set q to be the new root
ReplaceNode(p, q);
// set p's left child to be q's right child
p.Left = q.Right;
if (p.Left != null) p.Left.Parent = p;
// set q's right child to be p
q.Right = p;
p.Parent = q;
UpdateAugmentedData(p);
UpdateAugmentedData(q);
}
private static TextSegment Sibling(TextSegment node)
{
return node == node.Parent.Left ? node.Parent.Right : node.Parent.Left;
}
private static TextSegment Sibling(TextSegment node, TextSegment parentNode)
{
Debug.Assert(node == null || node.Parent == parentNode);
if (node == parentNode.Left)
return parentNode.Right;
else
return parentNode.Left;
}
private static bool GetColor(TextSegment node)
{
return node != null && node.Color;
}
#endregion
#region ICollection<T> implementation
/// <summary>
/// Gets the number of segments in the tree.
/// </summary>
public int Count { get; private set; }
bool ICollection<T>.IsReadOnly => false;
/// <summary>
/// Gets whether this tree contains the specified item.
/// </summary>
public bool Contains(T item)
{
return item != null && item.OwnerTree == this;
}
/// <summary>
/// Copies all segments in this SegmentTree to the specified array.
/// </summary>
public void CopyTo(T[] array, int arrayIndex)
{
if (array == null)
throw new ArgumentNullException(nameof(array));
if (array.Length < Count)
throw new ArgumentException("The array is too small", nameof(array));
if (arrayIndex < 0 || arrayIndex + Count > array.Length)
throw new ArgumentOutOfRangeException(nameof(arrayIndex), arrayIndex, "Value must be between 0 and " + (array.Length - Count));
foreach (var s in this)
{
array[arrayIndex++] = s;
}
}
/// <summary>
/// Gets an enumerator to enumerate the segments.
/// </summary>
public IEnumerator<T> GetEnumerator()
{
if (_root != null)
{
var current = _root.LeftMost;
while (current != null)
{
yield return (T)current;
// TODO: check if collection was modified during enumeration
current = current.Successor;
}
}
}
System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator()
{
return GetEnumerator();
}
#endregion
}
}
<MSG> Allow a text segment collection to be disconnected from a text document.
<DFF> @@ -97,6 +97,14 @@ namespace AvaloniaEdit.Document
}
#endregion
+ public void Disconnect (TextDocument textDocument)
+ {
+ if (_isConnectedToDocument)
+ {
+ TextDocumentWeakEventManager.Changed.RemoveHandler(textDocument, OnDocumentChanged);
+ }
+ }
+
#region OnDocumentChanged / UpdateOffsets
/// <summary>
/// Updates the start and end offsets of all segments stored in this collection.
| 8 | Allow a text segment collection to be disconnected from a text document. | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063844 | <NME> LineNumberMargin.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Globalization;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Media;
namespace AvaloniaEdit.Editing
{
/// <summary>
/// </summary>
public class LineNumberMargin : AbstractMargin
{
private TextArea _textArea;
/// <summary>
/// The typeface used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
protected FontFamily Typeface;
/// <summary>
/// The font size used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
protected double EmSize;
/// <inheritdoc/>
protected override Size MeasureOverride(Size availableSize)
protected override Size MeasureOverride(Size availableSize)
{
Typeface = this.CreateTypeface();
EmSize = GetValue(TextBlock.FontSizeProperty);
var text = TextFormatterFactory.CreateFormattedText(
this,
new string('9', MaxLineNumberLength),
Typeface,
EmSize,
GetValue(TextBlock.ForegroundProperty)
);
return new Size(text.Width, 0);
}
public override void Render(DrawingContext drawingContext)
{
var textView = TextView;
var renderSize = Bounds.Size;
if (textView is {VisualLinesValid: true}) {
var foreground = GetValue(TextBlock.ForegroundProperty);
foreach (var line in textView.VisualLines) {
var lineNumber = line.FirstDocumentLine.LineNumber;
var text = TextFormatterFactory.CreateFormattedText(
this,
lineNumber.ToString(CultureInfo.CurrentCulture),
Typeface, EmSize, foreground
);
var y = line.GetTextLineVisualYPosition(line.TextLines[0], VisualYPosition.TextTop);
drawingContext.DrawText(text, new Point(renderSize.Width - text.Width, y - textView.VerticalOffset));
}
}
}
/// <inheritdoc/>
protected override void OnTextViewChanged(TextView oldTextView, TextView newTextView)
{
if (oldTextView != null)
{
oldTextView.VisualLinesChanged -= TextViewVisualLinesChanged;
}
base.OnTextViewChanged(oldTextView, newTextView);
if (newTextView != null)
{
newTextView.VisualLinesChanged += TextViewVisualLinesChanged;
}
InvalidateVisual();
}
/// <inheritdoc/>
protected override void OnDocumentChanged(TextDocument oldDocument, TextDocument newDocument)
{
if (oldDocument != null)
{
TextDocumentWeakEventManager.LineCountChanged.RemoveHandler(oldDocument, OnDocumentLineCountChanged);
}
base.OnDocumentChanged(oldDocument, newDocument);
if (newDocument != null)
{
TextDocumentWeakEventManager.LineCountChanged.AddHandler(newDocument, OnDocumentLineCountChanged);
}
OnDocumentLineCountChanged();
}
private void OnDocumentLineCountChanged(object sender, EventArgs e)
{
OnDocumentLineCountChanged();
}
void TextViewVisualLinesChanged(object sender, EventArgs e)
{
InvalidateMeasure();
}
}
}
private AnchorSegment _selectionStart;
private bool _selecting;
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && _textArea != null)
{
e.Handled = true;
_textArea.Focus();
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
_textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
e.Device.Capture(this);
if (e.Device.Captured == this)
{
}
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
if (_textArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
_textArea.Selection = Selection.Create(_textArea, _selectionStart);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
ExtendSelection(currentSeg);
}
_textArea.Caret.BringCaretToView(5.0);
}
}
}
e.Pointer.Capture(this);
if (e.Pointer.Captured == this)
{
_selecting = true;
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.KeyModifiers.HasFlag(KeyModifiers.Shift))
{
if (TextArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
TextArea.Selection = Selection.Create(TextArea, _selectionStart);
if (e.KeyModifiers.HasFlag(KeyModifiers.Shift))
{
ExtendSelection(currentSeg);
}
TextArea.Caret.BringCaretToView(0);
}
}
}
{
if (currentSeg.Offset < _selectionStart.Offset)
{
_textArea.Caret.Offset = currentSeg.Offset;
_textArea.Selection = Selection.Create(_textArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
}
else
{
_textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
_textArea.Selection = Selection.Create(_textArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
if (_selecting && _textArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
_textArea.Caret.BringCaretToView(5.0);
}
base.OnPointerMoved(e);
}
{
TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
TextArea.Selection = Selection.Create(TextArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
if (_selecting && TextArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
TextArea.Caret.BringCaretToView(0);
}
base.OnPointerMoved(e);
}
protected override void OnPointerReleased(PointerReleasedEventArgs e)
{
if (_selecting)
{
_selecting = false;
_selectionStart = null;
e.Pointer.Capture(null);
e.Handled = true;
}
base.OnPointerReleased(e);
}
}
}
<MSG> Fix line margin interactivity
<DFF> @@ -34,19 +34,20 @@ namespace AvaloniaEdit.Editing
/// </summary>
public class LineNumberMargin : AbstractMargin
{
- private TextArea _textArea;
+ private AnchorSegment _selectionStart;
+ private bool _selecting;
/// <summary>
/// The typeface used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
- protected FontFamily Typeface;
+ protected FontFamily Typeface { get; set; }
/// <summary>
/// The font size used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
- protected double EmSize;
+ protected double EmSize { get; set; }
/// <inheritdoc/>
protected override Size MeasureOverride(Size availableSize)
@@ -129,22 +130,19 @@ namespace AvaloniaEdit.Editing
}
}
- private AnchorSegment _selectionStart;
- private bool _selecting;
-
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
- if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && _textArea != null)
+ if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && TextArea != null)
{
e.Handled = true;
- _textArea.Focus();
+ TextArea.Focus();
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
- _textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
+ TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
e.Device.Capture(this);
if (e.Device.Captured == this)
{
@@ -152,15 +150,15 @@ namespace AvaloniaEdit.Editing
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
- if (_textArea.Selection is SimpleSelection simpleSelection)
+ if (TextArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
- _textArea.Selection = Selection.Create(_textArea, _selectionStart);
+ TextArea.Selection = Selection.Create(TextArea, _selectionStart);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
ExtendSelection(currentSeg);
}
- _textArea.Caret.BringCaretToView(5.0);
+ TextArea.Caret.BringCaretToView(5.0);
}
}
}
@@ -187,26 +185,26 @@ namespace AvaloniaEdit.Editing
{
if (currentSeg.Offset < _selectionStart.Offset)
{
- _textArea.Caret.Offset = currentSeg.Offset;
- _textArea.Selection = Selection.Create(_textArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
+ TextArea.Caret.Offset = currentSeg.Offset;
+ TextArea.Selection = Selection.Create(TextArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
}
else
{
- _textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
- _textArea.Selection = Selection.Create(_textArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
+ TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
+ TextArea.Selection = Selection.Create(TextArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
- if (_selecting && _textArea != null && TextView != null)
+ if (_selecting && TextArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
- _textArea.Caret.BringCaretToView(5.0);
+ TextArea.Caret.BringCaretToView(5.0);
}
base.OnPointerMoved(e);
}
| 16 | Fix line margin interactivity | 18 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063845 | <NME> LineNumberMargin.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Globalization;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Media;
namespace AvaloniaEdit.Editing
{
/// <summary>
/// </summary>
public class LineNumberMargin : AbstractMargin
{
private TextArea _textArea;
/// <summary>
/// The typeface used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
protected FontFamily Typeface;
/// <summary>
/// The font size used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
protected double EmSize;
/// <inheritdoc/>
protected override Size MeasureOverride(Size availableSize)
protected override Size MeasureOverride(Size availableSize)
{
Typeface = this.CreateTypeface();
EmSize = GetValue(TextBlock.FontSizeProperty);
var text = TextFormatterFactory.CreateFormattedText(
this,
new string('9', MaxLineNumberLength),
Typeface,
EmSize,
GetValue(TextBlock.ForegroundProperty)
);
return new Size(text.Width, 0);
}
public override void Render(DrawingContext drawingContext)
{
var textView = TextView;
var renderSize = Bounds.Size;
if (textView is {VisualLinesValid: true}) {
var foreground = GetValue(TextBlock.ForegroundProperty);
foreach (var line in textView.VisualLines) {
var lineNumber = line.FirstDocumentLine.LineNumber;
var text = TextFormatterFactory.CreateFormattedText(
this,
lineNumber.ToString(CultureInfo.CurrentCulture),
Typeface, EmSize, foreground
);
var y = line.GetTextLineVisualYPosition(line.TextLines[0], VisualYPosition.TextTop);
drawingContext.DrawText(text, new Point(renderSize.Width - text.Width, y - textView.VerticalOffset));
}
}
}
/// <inheritdoc/>
protected override void OnTextViewChanged(TextView oldTextView, TextView newTextView)
{
if (oldTextView != null)
{
oldTextView.VisualLinesChanged -= TextViewVisualLinesChanged;
}
base.OnTextViewChanged(oldTextView, newTextView);
if (newTextView != null)
{
newTextView.VisualLinesChanged += TextViewVisualLinesChanged;
}
InvalidateVisual();
}
/// <inheritdoc/>
protected override void OnDocumentChanged(TextDocument oldDocument, TextDocument newDocument)
{
if (oldDocument != null)
{
TextDocumentWeakEventManager.LineCountChanged.RemoveHandler(oldDocument, OnDocumentLineCountChanged);
}
base.OnDocumentChanged(oldDocument, newDocument);
if (newDocument != null)
{
TextDocumentWeakEventManager.LineCountChanged.AddHandler(newDocument, OnDocumentLineCountChanged);
}
OnDocumentLineCountChanged();
}
private void OnDocumentLineCountChanged(object sender, EventArgs e)
{
OnDocumentLineCountChanged();
}
void TextViewVisualLinesChanged(object sender, EventArgs e)
{
InvalidateMeasure();
}
}
}
private AnchorSegment _selectionStart;
private bool _selecting;
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && _textArea != null)
{
e.Handled = true;
_textArea.Focus();
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
_textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
e.Device.Capture(this);
if (e.Device.Captured == this)
{
}
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
if (_textArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
_textArea.Selection = Selection.Create(_textArea, _selectionStart);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
ExtendSelection(currentSeg);
}
_textArea.Caret.BringCaretToView(5.0);
}
}
}
e.Pointer.Capture(this);
if (e.Pointer.Captured == this)
{
_selecting = true;
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.KeyModifiers.HasFlag(KeyModifiers.Shift))
{
if (TextArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
TextArea.Selection = Selection.Create(TextArea, _selectionStart);
if (e.KeyModifiers.HasFlag(KeyModifiers.Shift))
{
ExtendSelection(currentSeg);
}
TextArea.Caret.BringCaretToView(0);
}
}
}
{
if (currentSeg.Offset < _selectionStart.Offset)
{
_textArea.Caret.Offset = currentSeg.Offset;
_textArea.Selection = Selection.Create(_textArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
}
else
{
_textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
_textArea.Selection = Selection.Create(_textArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
if (_selecting && _textArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
_textArea.Caret.BringCaretToView(5.0);
}
base.OnPointerMoved(e);
}
{
TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
TextArea.Selection = Selection.Create(TextArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
if (_selecting && TextArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
TextArea.Caret.BringCaretToView(0);
}
base.OnPointerMoved(e);
}
protected override void OnPointerReleased(PointerReleasedEventArgs e)
{
if (_selecting)
{
_selecting = false;
_selectionStart = null;
e.Pointer.Capture(null);
e.Handled = true;
}
base.OnPointerReleased(e);
}
}
}
<MSG> Fix line margin interactivity
<DFF> @@ -34,19 +34,20 @@ namespace AvaloniaEdit.Editing
/// </summary>
public class LineNumberMargin : AbstractMargin
{
- private TextArea _textArea;
+ private AnchorSegment _selectionStart;
+ private bool _selecting;
/// <summary>
/// The typeface used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
- protected FontFamily Typeface;
+ protected FontFamily Typeface { get; set; }
/// <summary>
/// The font size used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
- protected double EmSize;
+ protected double EmSize { get; set; }
/// <inheritdoc/>
protected override Size MeasureOverride(Size availableSize)
@@ -129,22 +130,19 @@ namespace AvaloniaEdit.Editing
}
}
- private AnchorSegment _selectionStart;
- private bool _selecting;
-
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
- if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && _textArea != null)
+ if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && TextArea != null)
{
e.Handled = true;
- _textArea.Focus();
+ TextArea.Focus();
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
- _textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
+ TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
e.Device.Capture(this);
if (e.Device.Captured == this)
{
@@ -152,15 +150,15 @@ namespace AvaloniaEdit.Editing
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
- if (_textArea.Selection is SimpleSelection simpleSelection)
+ if (TextArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
- _textArea.Selection = Selection.Create(_textArea, _selectionStart);
+ TextArea.Selection = Selection.Create(TextArea, _selectionStart);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
ExtendSelection(currentSeg);
}
- _textArea.Caret.BringCaretToView(5.0);
+ TextArea.Caret.BringCaretToView(5.0);
}
}
}
@@ -187,26 +185,26 @@ namespace AvaloniaEdit.Editing
{
if (currentSeg.Offset < _selectionStart.Offset)
{
- _textArea.Caret.Offset = currentSeg.Offset;
- _textArea.Selection = Selection.Create(_textArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
+ TextArea.Caret.Offset = currentSeg.Offset;
+ TextArea.Selection = Selection.Create(TextArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
}
else
{
- _textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
- _textArea.Selection = Selection.Create(_textArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
+ TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
+ TextArea.Selection = Selection.Create(TextArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
- if (_selecting && _textArea != null && TextView != null)
+ if (_selecting && TextArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
- _textArea.Caret.BringCaretToView(5.0);
+ TextArea.Caret.BringCaretToView(5.0);
}
base.OnPointerMoved(e);
}
| 16 | Fix line margin interactivity | 18 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063846 | <NME> LineNumberMargin.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Globalization;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Media;
namespace AvaloniaEdit.Editing
{
/// <summary>
/// </summary>
public class LineNumberMargin : AbstractMargin
{
private TextArea _textArea;
/// <summary>
/// The typeface used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
protected FontFamily Typeface;
/// <summary>
/// The font size used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
protected double EmSize;
/// <inheritdoc/>
protected override Size MeasureOverride(Size availableSize)
protected override Size MeasureOverride(Size availableSize)
{
Typeface = this.CreateTypeface();
EmSize = GetValue(TextBlock.FontSizeProperty);
var text = TextFormatterFactory.CreateFormattedText(
this,
new string('9', MaxLineNumberLength),
Typeface,
EmSize,
GetValue(TextBlock.ForegroundProperty)
);
return new Size(text.Width, 0);
}
public override void Render(DrawingContext drawingContext)
{
var textView = TextView;
var renderSize = Bounds.Size;
if (textView is {VisualLinesValid: true}) {
var foreground = GetValue(TextBlock.ForegroundProperty);
foreach (var line in textView.VisualLines) {
var lineNumber = line.FirstDocumentLine.LineNumber;
var text = TextFormatterFactory.CreateFormattedText(
this,
lineNumber.ToString(CultureInfo.CurrentCulture),
Typeface, EmSize, foreground
);
var y = line.GetTextLineVisualYPosition(line.TextLines[0], VisualYPosition.TextTop);
drawingContext.DrawText(text, new Point(renderSize.Width - text.Width, y - textView.VerticalOffset));
}
}
}
/// <inheritdoc/>
protected override void OnTextViewChanged(TextView oldTextView, TextView newTextView)
{
if (oldTextView != null)
{
oldTextView.VisualLinesChanged -= TextViewVisualLinesChanged;
}
base.OnTextViewChanged(oldTextView, newTextView);
if (newTextView != null)
{
newTextView.VisualLinesChanged += TextViewVisualLinesChanged;
}
InvalidateVisual();
}
/// <inheritdoc/>
protected override void OnDocumentChanged(TextDocument oldDocument, TextDocument newDocument)
{
if (oldDocument != null)
{
TextDocumentWeakEventManager.LineCountChanged.RemoveHandler(oldDocument, OnDocumentLineCountChanged);
}
base.OnDocumentChanged(oldDocument, newDocument);
if (newDocument != null)
{
TextDocumentWeakEventManager.LineCountChanged.AddHandler(newDocument, OnDocumentLineCountChanged);
}
OnDocumentLineCountChanged();
}
private void OnDocumentLineCountChanged(object sender, EventArgs e)
{
OnDocumentLineCountChanged();
}
void TextViewVisualLinesChanged(object sender, EventArgs e)
{
InvalidateMeasure();
}
}
}
private AnchorSegment _selectionStart;
private bool _selecting;
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && _textArea != null)
{
e.Handled = true;
_textArea.Focus();
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
_textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
e.Device.Capture(this);
if (e.Device.Captured == this)
{
}
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
if (_textArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
_textArea.Selection = Selection.Create(_textArea, _selectionStart);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
ExtendSelection(currentSeg);
}
_textArea.Caret.BringCaretToView(5.0);
}
}
}
e.Pointer.Capture(this);
if (e.Pointer.Captured == this)
{
_selecting = true;
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.KeyModifiers.HasFlag(KeyModifiers.Shift))
{
if (TextArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
TextArea.Selection = Selection.Create(TextArea, _selectionStart);
if (e.KeyModifiers.HasFlag(KeyModifiers.Shift))
{
ExtendSelection(currentSeg);
}
TextArea.Caret.BringCaretToView(0);
}
}
}
{
if (currentSeg.Offset < _selectionStart.Offset)
{
_textArea.Caret.Offset = currentSeg.Offset;
_textArea.Selection = Selection.Create(_textArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
}
else
{
_textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
_textArea.Selection = Selection.Create(_textArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
if (_selecting && _textArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
_textArea.Caret.BringCaretToView(5.0);
}
base.OnPointerMoved(e);
}
{
TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
TextArea.Selection = Selection.Create(TextArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
if (_selecting && TextArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
TextArea.Caret.BringCaretToView(0);
}
base.OnPointerMoved(e);
}
protected override void OnPointerReleased(PointerReleasedEventArgs e)
{
if (_selecting)
{
_selecting = false;
_selectionStart = null;
e.Pointer.Capture(null);
e.Handled = true;
}
base.OnPointerReleased(e);
}
}
}
<MSG> Fix line margin interactivity
<DFF> @@ -34,19 +34,20 @@ namespace AvaloniaEdit.Editing
/// </summary>
public class LineNumberMargin : AbstractMargin
{
- private TextArea _textArea;
+ private AnchorSegment _selectionStart;
+ private bool _selecting;
/// <summary>
/// The typeface used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
- protected FontFamily Typeface;
+ protected FontFamily Typeface { get; set; }
/// <summary>
/// The font size used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
- protected double EmSize;
+ protected double EmSize { get; set; }
/// <inheritdoc/>
protected override Size MeasureOverride(Size availableSize)
@@ -129,22 +130,19 @@ namespace AvaloniaEdit.Editing
}
}
- private AnchorSegment _selectionStart;
- private bool _selecting;
-
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
- if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && _textArea != null)
+ if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && TextArea != null)
{
e.Handled = true;
- _textArea.Focus();
+ TextArea.Focus();
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
- _textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
+ TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
e.Device.Capture(this);
if (e.Device.Captured == this)
{
@@ -152,15 +150,15 @@ namespace AvaloniaEdit.Editing
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
- if (_textArea.Selection is SimpleSelection simpleSelection)
+ if (TextArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
- _textArea.Selection = Selection.Create(_textArea, _selectionStart);
+ TextArea.Selection = Selection.Create(TextArea, _selectionStart);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
ExtendSelection(currentSeg);
}
- _textArea.Caret.BringCaretToView(5.0);
+ TextArea.Caret.BringCaretToView(5.0);
}
}
}
@@ -187,26 +185,26 @@ namespace AvaloniaEdit.Editing
{
if (currentSeg.Offset < _selectionStart.Offset)
{
- _textArea.Caret.Offset = currentSeg.Offset;
- _textArea.Selection = Selection.Create(_textArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
+ TextArea.Caret.Offset = currentSeg.Offset;
+ TextArea.Selection = Selection.Create(TextArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
}
else
{
- _textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
- _textArea.Selection = Selection.Create(_textArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
+ TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
+ TextArea.Selection = Selection.Create(TextArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
- if (_selecting && _textArea != null && TextView != null)
+ if (_selecting && TextArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
- _textArea.Caret.BringCaretToView(5.0);
+ TextArea.Caret.BringCaretToView(5.0);
}
base.OnPointerMoved(e);
}
| 16 | Fix line margin interactivity | 18 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063847 | <NME> LineNumberMargin.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Globalization;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Media;
namespace AvaloniaEdit.Editing
{
/// <summary>
/// </summary>
public class LineNumberMargin : AbstractMargin
{
private TextArea _textArea;
/// <summary>
/// The typeface used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
protected FontFamily Typeface;
/// <summary>
/// The font size used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
protected double EmSize;
/// <inheritdoc/>
protected override Size MeasureOverride(Size availableSize)
protected override Size MeasureOverride(Size availableSize)
{
Typeface = this.CreateTypeface();
EmSize = GetValue(TextBlock.FontSizeProperty);
var text = TextFormatterFactory.CreateFormattedText(
this,
new string('9', MaxLineNumberLength),
Typeface,
EmSize,
GetValue(TextBlock.ForegroundProperty)
);
return new Size(text.Width, 0);
}
public override void Render(DrawingContext drawingContext)
{
var textView = TextView;
var renderSize = Bounds.Size;
if (textView is {VisualLinesValid: true}) {
var foreground = GetValue(TextBlock.ForegroundProperty);
foreach (var line in textView.VisualLines) {
var lineNumber = line.FirstDocumentLine.LineNumber;
var text = TextFormatterFactory.CreateFormattedText(
this,
lineNumber.ToString(CultureInfo.CurrentCulture),
Typeface, EmSize, foreground
);
var y = line.GetTextLineVisualYPosition(line.TextLines[0], VisualYPosition.TextTop);
drawingContext.DrawText(text, new Point(renderSize.Width - text.Width, y - textView.VerticalOffset));
}
}
}
/// <inheritdoc/>
protected override void OnTextViewChanged(TextView oldTextView, TextView newTextView)
{
if (oldTextView != null)
{
oldTextView.VisualLinesChanged -= TextViewVisualLinesChanged;
}
base.OnTextViewChanged(oldTextView, newTextView);
if (newTextView != null)
{
newTextView.VisualLinesChanged += TextViewVisualLinesChanged;
}
InvalidateVisual();
}
/// <inheritdoc/>
protected override void OnDocumentChanged(TextDocument oldDocument, TextDocument newDocument)
{
if (oldDocument != null)
{
TextDocumentWeakEventManager.LineCountChanged.RemoveHandler(oldDocument, OnDocumentLineCountChanged);
}
base.OnDocumentChanged(oldDocument, newDocument);
if (newDocument != null)
{
TextDocumentWeakEventManager.LineCountChanged.AddHandler(newDocument, OnDocumentLineCountChanged);
}
OnDocumentLineCountChanged();
}
private void OnDocumentLineCountChanged(object sender, EventArgs e)
{
OnDocumentLineCountChanged();
}
void TextViewVisualLinesChanged(object sender, EventArgs e)
{
InvalidateMeasure();
}
}
}
private AnchorSegment _selectionStart;
private bool _selecting;
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && _textArea != null)
{
e.Handled = true;
_textArea.Focus();
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
_textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
e.Device.Capture(this);
if (e.Device.Captured == this)
{
}
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
if (_textArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
_textArea.Selection = Selection.Create(_textArea, _selectionStart);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
ExtendSelection(currentSeg);
}
_textArea.Caret.BringCaretToView(5.0);
}
}
}
e.Pointer.Capture(this);
if (e.Pointer.Captured == this)
{
_selecting = true;
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.KeyModifiers.HasFlag(KeyModifiers.Shift))
{
if (TextArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
TextArea.Selection = Selection.Create(TextArea, _selectionStart);
if (e.KeyModifiers.HasFlag(KeyModifiers.Shift))
{
ExtendSelection(currentSeg);
}
TextArea.Caret.BringCaretToView(0);
}
}
}
{
if (currentSeg.Offset < _selectionStart.Offset)
{
_textArea.Caret.Offset = currentSeg.Offset;
_textArea.Selection = Selection.Create(_textArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
}
else
{
_textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
_textArea.Selection = Selection.Create(_textArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
if (_selecting && _textArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
_textArea.Caret.BringCaretToView(5.0);
}
base.OnPointerMoved(e);
}
{
TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
TextArea.Selection = Selection.Create(TextArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
if (_selecting && TextArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
TextArea.Caret.BringCaretToView(0);
}
base.OnPointerMoved(e);
}
protected override void OnPointerReleased(PointerReleasedEventArgs e)
{
if (_selecting)
{
_selecting = false;
_selectionStart = null;
e.Pointer.Capture(null);
e.Handled = true;
}
base.OnPointerReleased(e);
}
}
}
<MSG> Fix line margin interactivity
<DFF> @@ -34,19 +34,20 @@ namespace AvaloniaEdit.Editing
/// </summary>
public class LineNumberMargin : AbstractMargin
{
- private TextArea _textArea;
+ private AnchorSegment _selectionStart;
+ private bool _selecting;
/// <summary>
/// The typeface used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
- protected FontFamily Typeface;
+ protected FontFamily Typeface { get; set; }
/// <summary>
/// The font size used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
- protected double EmSize;
+ protected double EmSize { get; set; }
/// <inheritdoc/>
protected override Size MeasureOverride(Size availableSize)
@@ -129,22 +130,19 @@ namespace AvaloniaEdit.Editing
}
}
- private AnchorSegment _selectionStart;
- private bool _selecting;
-
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
- if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && _textArea != null)
+ if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && TextArea != null)
{
e.Handled = true;
- _textArea.Focus();
+ TextArea.Focus();
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
- _textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
+ TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
e.Device.Capture(this);
if (e.Device.Captured == this)
{
@@ -152,15 +150,15 @@ namespace AvaloniaEdit.Editing
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
- if (_textArea.Selection is SimpleSelection simpleSelection)
+ if (TextArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
- _textArea.Selection = Selection.Create(_textArea, _selectionStart);
+ TextArea.Selection = Selection.Create(TextArea, _selectionStart);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
ExtendSelection(currentSeg);
}
- _textArea.Caret.BringCaretToView(5.0);
+ TextArea.Caret.BringCaretToView(5.0);
}
}
}
@@ -187,26 +185,26 @@ namespace AvaloniaEdit.Editing
{
if (currentSeg.Offset < _selectionStart.Offset)
{
- _textArea.Caret.Offset = currentSeg.Offset;
- _textArea.Selection = Selection.Create(_textArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
+ TextArea.Caret.Offset = currentSeg.Offset;
+ TextArea.Selection = Selection.Create(TextArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
}
else
{
- _textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
- _textArea.Selection = Selection.Create(_textArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
+ TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
+ TextArea.Selection = Selection.Create(TextArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
- if (_selecting && _textArea != null && TextView != null)
+ if (_selecting && TextArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
- _textArea.Caret.BringCaretToView(5.0);
+ TextArea.Caret.BringCaretToView(5.0);
}
base.OnPointerMoved(e);
}
| 16 | Fix line margin interactivity | 18 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063848 | <NME> LineNumberMargin.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Globalization;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Media;
namespace AvaloniaEdit.Editing
{
/// <summary>
/// </summary>
public class LineNumberMargin : AbstractMargin
{
private TextArea _textArea;
/// <summary>
/// The typeface used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
protected FontFamily Typeface;
/// <summary>
/// The font size used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
protected double EmSize;
/// <inheritdoc/>
protected override Size MeasureOverride(Size availableSize)
protected override Size MeasureOverride(Size availableSize)
{
Typeface = this.CreateTypeface();
EmSize = GetValue(TextBlock.FontSizeProperty);
var text = TextFormatterFactory.CreateFormattedText(
this,
new string('9', MaxLineNumberLength),
Typeface,
EmSize,
GetValue(TextBlock.ForegroundProperty)
);
return new Size(text.Width, 0);
}
public override void Render(DrawingContext drawingContext)
{
var textView = TextView;
var renderSize = Bounds.Size;
if (textView is {VisualLinesValid: true}) {
var foreground = GetValue(TextBlock.ForegroundProperty);
foreach (var line in textView.VisualLines) {
var lineNumber = line.FirstDocumentLine.LineNumber;
var text = TextFormatterFactory.CreateFormattedText(
this,
lineNumber.ToString(CultureInfo.CurrentCulture),
Typeface, EmSize, foreground
);
var y = line.GetTextLineVisualYPosition(line.TextLines[0], VisualYPosition.TextTop);
drawingContext.DrawText(text, new Point(renderSize.Width - text.Width, y - textView.VerticalOffset));
}
}
}
/// <inheritdoc/>
protected override void OnTextViewChanged(TextView oldTextView, TextView newTextView)
{
if (oldTextView != null)
{
oldTextView.VisualLinesChanged -= TextViewVisualLinesChanged;
}
base.OnTextViewChanged(oldTextView, newTextView);
if (newTextView != null)
{
newTextView.VisualLinesChanged += TextViewVisualLinesChanged;
}
InvalidateVisual();
}
/// <inheritdoc/>
protected override void OnDocumentChanged(TextDocument oldDocument, TextDocument newDocument)
{
if (oldDocument != null)
{
TextDocumentWeakEventManager.LineCountChanged.RemoveHandler(oldDocument, OnDocumentLineCountChanged);
}
base.OnDocumentChanged(oldDocument, newDocument);
if (newDocument != null)
{
TextDocumentWeakEventManager.LineCountChanged.AddHandler(newDocument, OnDocumentLineCountChanged);
}
OnDocumentLineCountChanged();
}
private void OnDocumentLineCountChanged(object sender, EventArgs e)
{
OnDocumentLineCountChanged();
}
void TextViewVisualLinesChanged(object sender, EventArgs e)
{
InvalidateMeasure();
}
}
}
private AnchorSegment _selectionStart;
private bool _selecting;
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && _textArea != null)
{
e.Handled = true;
_textArea.Focus();
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
_textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
e.Device.Capture(this);
if (e.Device.Captured == this)
{
}
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
if (_textArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
_textArea.Selection = Selection.Create(_textArea, _selectionStart);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
ExtendSelection(currentSeg);
}
_textArea.Caret.BringCaretToView(5.0);
}
}
}
e.Pointer.Capture(this);
if (e.Pointer.Captured == this)
{
_selecting = true;
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.KeyModifiers.HasFlag(KeyModifiers.Shift))
{
if (TextArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
TextArea.Selection = Selection.Create(TextArea, _selectionStart);
if (e.KeyModifiers.HasFlag(KeyModifiers.Shift))
{
ExtendSelection(currentSeg);
}
TextArea.Caret.BringCaretToView(0);
}
}
}
{
if (currentSeg.Offset < _selectionStart.Offset)
{
_textArea.Caret.Offset = currentSeg.Offset;
_textArea.Selection = Selection.Create(_textArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
}
else
{
_textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
_textArea.Selection = Selection.Create(_textArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
if (_selecting && _textArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
_textArea.Caret.BringCaretToView(5.0);
}
base.OnPointerMoved(e);
}
{
TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
TextArea.Selection = Selection.Create(TextArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
if (_selecting && TextArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
TextArea.Caret.BringCaretToView(0);
}
base.OnPointerMoved(e);
}
protected override void OnPointerReleased(PointerReleasedEventArgs e)
{
if (_selecting)
{
_selecting = false;
_selectionStart = null;
e.Pointer.Capture(null);
e.Handled = true;
}
base.OnPointerReleased(e);
}
}
}
<MSG> Fix line margin interactivity
<DFF> @@ -34,19 +34,20 @@ namespace AvaloniaEdit.Editing
/// </summary>
public class LineNumberMargin : AbstractMargin
{
- private TextArea _textArea;
+ private AnchorSegment _selectionStart;
+ private bool _selecting;
/// <summary>
/// The typeface used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
- protected FontFamily Typeface;
+ protected FontFamily Typeface { get; set; }
/// <summary>
/// The font size used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
- protected double EmSize;
+ protected double EmSize { get; set; }
/// <inheritdoc/>
protected override Size MeasureOverride(Size availableSize)
@@ -129,22 +130,19 @@ namespace AvaloniaEdit.Editing
}
}
- private AnchorSegment _selectionStart;
- private bool _selecting;
-
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
- if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && _textArea != null)
+ if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && TextArea != null)
{
e.Handled = true;
- _textArea.Focus();
+ TextArea.Focus();
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
- _textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
+ TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
e.Device.Capture(this);
if (e.Device.Captured == this)
{
@@ -152,15 +150,15 @@ namespace AvaloniaEdit.Editing
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
- if (_textArea.Selection is SimpleSelection simpleSelection)
+ if (TextArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
- _textArea.Selection = Selection.Create(_textArea, _selectionStart);
+ TextArea.Selection = Selection.Create(TextArea, _selectionStart);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
ExtendSelection(currentSeg);
}
- _textArea.Caret.BringCaretToView(5.0);
+ TextArea.Caret.BringCaretToView(5.0);
}
}
}
@@ -187,26 +185,26 @@ namespace AvaloniaEdit.Editing
{
if (currentSeg.Offset < _selectionStart.Offset)
{
- _textArea.Caret.Offset = currentSeg.Offset;
- _textArea.Selection = Selection.Create(_textArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
+ TextArea.Caret.Offset = currentSeg.Offset;
+ TextArea.Selection = Selection.Create(TextArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
}
else
{
- _textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
- _textArea.Selection = Selection.Create(_textArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
+ TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
+ TextArea.Selection = Selection.Create(TextArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
- if (_selecting && _textArea != null && TextView != null)
+ if (_selecting && TextArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
- _textArea.Caret.BringCaretToView(5.0);
+ TextArea.Caret.BringCaretToView(5.0);
}
base.OnPointerMoved(e);
}
| 16 | Fix line margin interactivity | 18 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10063849 | <NME> LineNumberMargin.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Globalization;
using System.Linq;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Input;
using Avalonia.Media;
namespace AvaloniaEdit.Editing
{
/// <summary>
/// </summary>
public class LineNumberMargin : AbstractMargin
{
private TextArea _textArea;
/// <summary>
/// The typeface used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
protected FontFamily Typeface;
/// <summary>
/// The font size used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
protected double EmSize;
/// <inheritdoc/>
protected override Size MeasureOverride(Size availableSize)
protected override Size MeasureOverride(Size availableSize)
{
Typeface = this.CreateTypeface();
EmSize = GetValue(TextBlock.FontSizeProperty);
var text = TextFormatterFactory.CreateFormattedText(
this,
new string('9', MaxLineNumberLength),
Typeface,
EmSize,
GetValue(TextBlock.ForegroundProperty)
);
return new Size(text.Width, 0);
}
public override void Render(DrawingContext drawingContext)
{
var textView = TextView;
var renderSize = Bounds.Size;
if (textView is {VisualLinesValid: true}) {
var foreground = GetValue(TextBlock.ForegroundProperty);
foreach (var line in textView.VisualLines) {
var lineNumber = line.FirstDocumentLine.LineNumber;
var text = TextFormatterFactory.CreateFormattedText(
this,
lineNumber.ToString(CultureInfo.CurrentCulture),
Typeface, EmSize, foreground
);
var y = line.GetTextLineVisualYPosition(line.TextLines[0], VisualYPosition.TextTop);
drawingContext.DrawText(text, new Point(renderSize.Width - text.Width, y - textView.VerticalOffset));
}
}
}
/// <inheritdoc/>
protected override void OnTextViewChanged(TextView oldTextView, TextView newTextView)
{
if (oldTextView != null)
{
oldTextView.VisualLinesChanged -= TextViewVisualLinesChanged;
}
base.OnTextViewChanged(oldTextView, newTextView);
if (newTextView != null)
{
newTextView.VisualLinesChanged += TextViewVisualLinesChanged;
}
InvalidateVisual();
}
/// <inheritdoc/>
protected override void OnDocumentChanged(TextDocument oldDocument, TextDocument newDocument)
{
if (oldDocument != null)
{
TextDocumentWeakEventManager.LineCountChanged.RemoveHandler(oldDocument, OnDocumentLineCountChanged);
}
base.OnDocumentChanged(oldDocument, newDocument);
if (newDocument != null)
{
TextDocumentWeakEventManager.LineCountChanged.AddHandler(newDocument, OnDocumentLineCountChanged);
}
OnDocumentLineCountChanged();
}
private void OnDocumentLineCountChanged(object sender, EventArgs e)
{
OnDocumentLineCountChanged();
}
void TextViewVisualLinesChanged(object sender, EventArgs e)
{
InvalidateMeasure();
}
}
}
private AnchorSegment _selectionStart;
private bool _selecting;
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && _textArea != null)
{
e.Handled = true;
_textArea.Focus();
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
_textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
e.Device.Capture(this);
if (e.Device.Captured == this)
{
}
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
if (_textArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
_textArea.Selection = Selection.Create(_textArea, _selectionStart);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
ExtendSelection(currentSeg);
}
_textArea.Caret.BringCaretToView(5.0);
}
}
}
e.Pointer.Capture(this);
if (e.Pointer.Captured == this)
{
_selecting = true;
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.KeyModifiers.HasFlag(KeyModifiers.Shift))
{
if (TextArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
TextArea.Selection = Selection.Create(TextArea, _selectionStart);
if (e.KeyModifiers.HasFlag(KeyModifiers.Shift))
{
ExtendSelection(currentSeg);
}
TextArea.Caret.BringCaretToView(0);
}
}
}
{
if (currentSeg.Offset < _selectionStart.Offset)
{
_textArea.Caret.Offset = currentSeg.Offset;
_textArea.Selection = Selection.Create(_textArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
}
else
{
_textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
_textArea.Selection = Selection.Create(_textArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
if (_selecting && _textArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
_textArea.Caret.BringCaretToView(5.0);
}
base.OnPointerMoved(e);
}
{
TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
TextArea.Selection = Selection.Create(TextArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
if (_selecting && TextArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
TextArea.Caret.BringCaretToView(0);
}
base.OnPointerMoved(e);
}
protected override void OnPointerReleased(PointerReleasedEventArgs e)
{
if (_selecting)
{
_selecting = false;
_selectionStart = null;
e.Pointer.Capture(null);
e.Handled = true;
}
base.OnPointerReleased(e);
}
}
}
<MSG> Fix line margin interactivity
<DFF> @@ -34,19 +34,20 @@ namespace AvaloniaEdit.Editing
/// </summary>
public class LineNumberMargin : AbstractMargin
{
- private TextArea _textArea;
+ private AnchorSegment _selectionStart;
+ private bool _selecting;
/// <summary>
/// The typeface used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
- protected FontFamily Typeface;
+ protected FontFamily Typeface { get; set; }
/// <summary>
/// The font size used for rendering the line number margin.
/// This field is calculated in MeasureOverride() based on the FontFamily etc. properties.
/// </summary>
- protected double EmSize;
+ protected double EmSize { get; set; }
/// <inheritdoc/>
protected override Size MeasureOverride(Size availableSize)
@@ -129,22 +130,19 @@ namespace AvaloniaEdit.Editing
}
}
- private AnchorSegment _selectionStart;
- private bool _selecting;
-
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
- if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && _textArea != null)
+ if (!e.Handled && e.MouseButton == MouseButton.Left && TextView != null && TextArea != null)
{
e.Handled = true;
- _textArea.Focus();
+ TextArea.Focus();
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
- _textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
+ TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
e.Device.Capture(this);
if (e.Device.Captured == this)
{
@@ -152,15 +150,15 @@ namespace AvaloniaEdit.Editing
_selectionStart = new AnchorSegment(Document, currentSeg.Offset, currentSeg.Length);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
- if (_textArea.Selection is SimpleSelection simpleSelection)
+ if (TextArea.Selection is SimpleSelection simpleSelection)
_selectionStart = new AnchorSegment(Document, simpleSelection.SurroundingSegment);
}
- _textArea.Selection = Selection.Create(_textArea, _selectionStart);
+ TextArea.Selection = Selection.Create(TextArea, _selectionStart);
if (e.InputModifiers.HasFlag(InputModifiers.Shift))
{
ExtendSelection(currentSeg);
}
- _textArea.Caret.BringCaretToView(5.0);
+ TextArea.Caret.BringCaretToView(5.0);
}
}
}
@@ -187,26 +185,26 @@ namespace AvaloniaEdit.Editing
{
if (currentSeg.Offset < _selectionStart.Offset)
{
- _textArea.Caret.Offset = currentSeg.Offset;
- _textArea.Selection = Selection.Create(_textArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
+ TextArea.Caret.Offset = currentSeg.Offset;
+ TextArea.Selection = Selection.Create(TextArea, currentSeg.Offset, _selectionStart.Offset + _selectionStart.Length);
}
else
{
- _textArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
- _textArea.Selection = Selection.Create(_textArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
+ TextArea.Caret.Offset = currentSeg.Offset + currentSeg.Length;
+ TextArea.Selection = Selection.Create(TextArea, _selectionStart.Offset, currentSeg.Offset + currentSeg.Length);
}
}
protected override void OnPointerMoved(PointerEventArgs e)
{
- if (_selecting && _textArea != null && TextView != null)
+ if (_selecting && TextArea != null && TextView != null)
{
e.Handled = true;
var currentSeg = GetTextLineSegment(e);
if (currentSeg == SimpleSegment.Invalid)
return;
ExtendSelection(currentSeg);
- _textArea.Caret.BringCaretToView(5.0);
+ TextArea.Caret.BringCaretToView(5.0);
}
base.OnPointerMoved(e);
}
| 16 | Fix line margin interactivity | 18 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.