Search is not available for this dataset
id
stringlengths 1
8
| text
stringlengths 72
9.81M
| addition_count
int64 0
10k
| commit_subject
stringlengths 0
3.7k
| deletion_count
int64 0
8.43k
| file_extension
stringlengths 0
32
| lang
stringlengths 1
94
| license
stringclasses 10
values | repo_name
stringlengths 9
59
|
---|---|---|---|---|---|---|---|---|
10066150 | <NME> TextArea.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using Avalonia;
using Avalonia.Controls;
using Avalonia.Controls.Presenters;
using Avalonia.Controls.Primitives;
using Avalonia.Data;
using Avalonia.Input;
using Avalonia.Input.TextInput;
using Avalonia.Interactivity;
using Avalonia.Media;
using Avalonia.Threading;
using Avalonia.VisualTree;
using AvaloniaEdit.Document;
using AvaloniaEdit.Indentation;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Search;
using AvaloniaEdit.Utils;
using System;
using System.Collections.Generic;
using System.Collections.Immutable;
using System.Collections.ObjectModel;
using System.Collections.Specialized;
using System.ComponentModel;
using System.Diagnostics;
using System.Linq;
namespace AvaloniaEdit.Editing
{
/// <summary>
/// Control that wraps a TextView and adds support for user input and the caret.
/// </summary>
public class TextArea : TemplatedControl, ITextEditorComponent, IRoutedCommandBindable, ILogicalScrollable
{
/// <summary>
/// This is the extra scrolling space that occurs after the last line.
/// </summary>
private const int AdditionalVerticalScrollAmount = 2;
private ILogicalScrollable _logicalScrollable;
private readonly TextAreaTextInputMethodClient _imClient = new TextAreaTextInputMethodClient();
#region Constructor
static TextArea()
{
KeyboardNavigation.TabNavigationProperty.OverrideDefaultValue<TextArea>(KeyboardNavigationMode.None);
FocusableProperty.OverrideDefaultValue<TextArea>(true);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
AffectsArrange<TextArea>(OffsetProperty);
AffectsRender<TextArea>(OffsetProperty);
TextInputMethodClientRequestedEvent.AddClassHandler<TextArea>((ta, e) =>
{
if (!ta.IsReadOnly)
{
e.Client = ta._imClient;
}
});
}
/// <summary>
/// Creates a new TextArea instance.
/// </summary>
public TextArea() : this(new TextView())
{
AddHandler(KeyDownEvent, OnPreviewKeyDown, RoutingStrategies.Tunnel);
AddHandler(KeyUpEvent, OnPreviewKeyUp, RoutingStrategies.Tunnel);
}
/// <summary>
/// Creates a new TextArea instance.
/// </summary>
protected TextArea(TextView textView)
{
TextView = textView ?? throw new ArgumentNullException(nameof(textView));
_logicalScrollable = textView;
Options = textView.Options;
_selection = EmptySelection = new EmptySelection(this);
textView.Services.AddService(this);
textView.LineTransformers.Add(new SelectionColorizer(this));
textView.InsertLayer(new SelectionLayer(this), KnownLayer.Selection, LayerInsertionPosition.Replace);
Caret = new Caret(this);
Caret.PositionChanged += (sender, e) => RequestSelectionValidation();
Caret.PositionChanged += CaretPositionChanged;
AttachTypingEvents();
LeftMargins.CollectionChanged += LeftMargins_CollectionChanged;
DefaultInputHandler = new TextAreaDefaultInputHandler(this);
ActiveInputHandler = DefaultInputHandler;
// TODO
//textView.GetObservable(TextBlock.FontSizeProperty).Subscribe(_ =>
//{
// TextView.SetScrollOffset(new Vector(_offset.X, _offset.Y * TextView.DefaultLineHeight));
//});
}
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
if (e.NameScope.Find("PART_CP") is ContentPresenter contentPresenter)
{
contentPresenter.Content = TextView;
}
}
internal void AddChild(IVisual visual)
{
VisualChildren.Add(visual);
InvalidateArrange();
}
internal void RemoveChild(IVisual visual)
{
VisualChildren.Remove(visual);
}
#endregion
/// <summary>
/// Defines the <see cref="IScrollable.Offset" /> property.
/// </summary>
public static readonly DirectProperty<TextArea, Vector> OffsetProperty =
AvaloniaProperty.RegisterDirect<TextArea, Vector>(
nameof(IScrollable.Offset),
o => (o as IScrollable).Offset,
(o, v) => (o as IScrollable).Offset = v);
#region InputHandler management
/// <summary>
/// Gets the default input handler.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public TextAreaDefaultInputHandler DefaultInputHandler { get; }
private ITextAreaInputHandler _activeInputHandler;
private bool _isChangingInputHandler;
/// <summary>
/// Gets/Sets the active input handler.
/// This property does not return currently active stacked input handlers. Setting this property detached all stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public ITextAreaInputHandler ActiveInputHandler
{
get => _activeInputHandler;
set
{
if (value != null && value.TextArea != this)
throw new ArgumentException("The input handler was created for a different text area than this one.");
if (_isChangingInputHandler)
throw new InvalidOperationException("Cannot set ActiveInputHandler recursively");
if (_activeInputHandler != value)
{
_isChangingInputHandler = true;
try
{
// pop the whole stack
PopStackedInputHandler(StackedInputHandlers.LastOrDefault());
Debug.Assert(StackedInputHandlers.IsEmpty);
_activeInputHandler?.Detach();
_activeInputHandler = value;
value?.Attach();
}
finally
{
_isChangingInputHandler = false;
}
ActiveInputHandlerChanged?.Invoke(this, EventArgs.Empty);
}
}
}
/// <summary>
/// Occurs when the ActiveInputHandler property changes.
/// </summary>
public event EventHandler ActiveInputHandlerChanged;
/// <summary>
/// Gets the list of currently active stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public ImmutableStack<TextAreaStackedInputHandler> StackedInputHandlers { get; private set; } = ImmutableStack<TextAreaStackedInputHandler>.Empty;
/// <summary>
/// Pushes an input handler onto the list of stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public void PushStackedInputHandler(TextAreaStackedInputHandler inputHandler)
{
if (inputHandler == null)
throw new ArgumentNullException(nameof(inputHandler));
StackedInputHandlers = StackedInputHandlers.Push(inputHandler);
inputHandler.Attach();
}
/// <summary>
/// Pops the stacked input handler (and all input handlers above it).
/// If <paramref name="inputHandler"/> is not found in the currently stacked input handlers, or is null, this method
/// does nothing.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public void PopStackedInputHandler(TextAreaStackedInputHandler inputHandler)
{
if (StackedInputHandlers.Any(i => i == inputHandler))
{
ITextAreaInputHandler oldHandler;
do
{
oldHandler = StackedInputHandlers.Peek();
StackedInputHandlers = StackedInputHandlers.Pop();
oldHandler.Detach();
} while (oldHandler != inputHandler);
}
}
#endregion
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextArea>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <inheritdoc/>
public event EventHandler DocumentChanged;
/// <summary>
/// Gets if the the document displayed by the text editor is readonly
/// </summary>
public bool IsReadOnly
{
get => ReadOnlySectionProvider == ReadOnlySectionDocument.Instance;
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextArea)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.Changing.RemoveHandler(oldValue, OnDocumentChanging);
TextDocumentWeakEventManager.Changed.RemoveHandler(oldValue, OnDocumentChanged);
TextDocumentWeakEventManager.UpdateStarted.RemoveHandler(oldValue, OnUpdateStarted);
TextDocumentWeakEventManager.UpdateFinished.RemoveHandler(oldValue, OnUpdateFinished);
}
TextView.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.Changing.AddHandler(newValue, OnDocumentChanging);
TextDocumentWeakEventManager.Changed.AddHandler(newValue, OnDocumentChanged);
TextDocumentWeakEventManager.UpdateStarted.AddHandler(newValue, OnUpdateStarted);
TextDocumentWeakEventManager.UpdateFinished.AddHandler(newValue, OnUpdateFinished);
InvalidateArrange();
}
// Reset caret location and selection: this is necessary because the caret/selection might be invalid
// in the new document (e.g. if new document is shorter than the old document).
Caret.Location = new TextLocation(1, 1);
ClearSelection();
DocumentChanged?.Invoke(this, EventArgs.Empty);
//CommandManager.InvalidateRequerySuggested();
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextArea>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
private void OnOptionChanged(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextArea)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnOptionChanged);
}
TextView.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnOptionChanged);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
#endregion
#region Caret handling on document changes
private void OnDocumentChanging(object sender, DocumentChangeEventArgs e)
{
Caret.OnDocumentChanging();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
Caret.OnDocumentChanged(e);
Selection = _selection.UpdateOnDocumentChange(e);
}
private void OnUpdateStarted(object sender, EventArgs e)
{
Document.UndoStack.PushOptional(new RestoreCaretAndSelectionUndoAction(this));
}
private void OnUpdateFinished(object sender, EventArgs e)
{
Caret.OnDocumentUpdateFinished();
}
private sealed class RestoreCaretAndSelectionUndoAction : IUndoableOperation
{
// keep textarea in weak reference because the IUndoableOperation is stored with the document
private readonly WeakReference _textAreaReference;
private readonly TextViewPosition _caretPosition;
private readonly Selection _selection;
public RestoreCaretAndSelectionUndoAction(TextArea textArea)
{
_textAreaReference = new WeakReference(textArea);
// Just save the old caret position, no need to validate here.
// If we restore it, we'll validate it anyways.
_caretPosition = textArea.Caret.NonValidatedPosition;
_selection = textArea.Selection;
}
public void Undo()
{
var textArea = (TextArea)_textAreaReference.Target;
if (textArea != null)
{
textArea.Caret.Position = _caretPosition;
textArea.Selection = _selection;
}
}
public void Redo()
{
// redo=undo: we just restore the caret/selection state
Undo();
}
}
#endregion
#region TextView property
/// <summary>
/// Gets the text view used to display text in this text area.
/// </summary>
public TextView TextView { get; }
#endregion
#region Selection property
internal readonly Selection EmptySelection;
private Selection _selection;
/// <summary>
/// Occurs when the selection has changed.
/// </summary>
public event EventHandler SelectionChanged;
/// <summary>
/// Gets/Sets the selection in this text area.
/// </summary>
public Selection Selection
{
get => _selection;
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
if (value.TextArea != this)
throw new ArgumentException("Cannot use a Selection instance that belongs to another text area.");
if (!Equals(_selection, value))
{
if (TextView != null)
{
var oldSegment = _selection.SurroundingSegment;
var newSegment = value.SurroundingSegment;
if (!Selection.EnableVirtualSpace && (_selection is SimpleSelection && value is SimpleSelection && oldSegment != null && newSegment != null))
{
// perf optimization:
// When a simple selection changes, don't redraw the whole selection, but only the changed parts.
var oldSegmentOffset = oldSegment.Offset;
var newSegmentOffset = newSegment.Offset;
if (oldSegmentOffset != newSegmentOffset)
{
TextView.Redraw(Math.Min(oldSegmentOffset, newSegmentOffset),
Math.Abs(oldSegmentOffset - newSegmentOffset));
}
var oldSegmentEndOffset = oldSegment.EndOffset;
var newSegmentEndOffset = newSegment.EndOffset;
if (oldSegmentEndOffset != newSegmentEndOffset)
{
TextView.Redraw(Math.Min(oldSegmentEndOffset, newSegmentEndOffset),
Math.Abs(oldSegmentEndOffset - newSegmentEndOffset));
}
}
else
{
TextView.Redraw(oldSegment);
TextView.Redraw(newSegment);
}
}
_selection = value;
SelectionChanged?.Invoke(this, EventArgs.Empty);
// a selection change causes commands like copy/paste/etc. to change status
//CommandManager.InvalidateRequerySuggested();
}
}
}
/// <summary>
/// Clears the current selection.
/// </summary>
public void ClearSelection()
{
Selection = EmptySelection;
}
/// <summary>
/// The <see cref="SelectionBrush"/> property.
/// </summary>
public static readonly StyledProperty<IBrush> SelectionBrushProperty =
AvaloniaProperty.Register<TextArea, IBrush>("SelectionBrush");
/// <summary>
/// Gets/Sets the background brush used for the selection.
/// </summary>
public IBrush SelectionBrush
{
get => GetValue(SelectionBrushProperty);
set => SetValue(SelectionBrushProperty, value);
}
/// <summary>
/// The <see cref="SelectionForeground"/> property.
/// </summary>
public static readonly StyledProperty<IBrush> SelectionForegroundProperty =
AvaloniaProperty.Register<TextArea, IBrush>("SelectionForeground");
/// <summary>
/// Gets/Sets the foreground brush used for selected text.
/// </summary>
public IBrush SelectionForeground
{
get => GetValue(SelectionForegroundProperty);
set => SetValue(SelectionForegroundProperty, value);
}
/// <summary>
/// The <see cref="SelectionBorder"/> property.
/// </summary>
public static readonly StyledProperty<Pen> SelectionBorderProperty =
AvaloniaProperty.Register<TextArea, Pen>("SelectionBorder");
/// <summary>
/// Gets/Sets the pen used for the border of the selection.
/// </summary>
public Pen SelectionBorder
{
get => GetValue(SelectionBorderProperty);
set => SetValue(SelectionBorderProperty, value);
}
/// <summary>
/// The <see cref="SelectionCornerRadius"/> property.
/// </summary>
public static readonly StyledProperty<double> SelectionCornerRadiusProperty =
AvaloniaProperty.Register<TextArea, double>("SelectionCornerRadius", 3.0);
/// <summary>
/// Gets/Sets the corner radius of the selection.
/// </summary>
public double SelectionCornerRadius
{
get => GetValue(SelectionCornerRadiusProperty);
set => SetValue(SelectionCornerRadiusProperty, value);
}
#endregion
#region Force caret to stay inside selection
private bool _ensureSelectionValidRequested;
private int _allowCaretOutsideSelection;
private void RequestSelectionValidation()
{
if (!_ensureSelectionValidRequested && _allowCaretOutsideSelection == 0)
{
_ensureSelectionValidRequested = true;
Dispatcher.UIThread.Post(EnsureSelectionValid);
}
}
/// <summary>
/// Code that updates only the caret but not the selection can cause confusion when
/// keys like 'Delete' delete the (possibly invisible) selected text and not the
/// text around the caret.
///
/// So we'll ensure that the caret is inside the selection.
/// (when the caret is not in the selection, we'll clear the selection)
///
/// This method is invoked using the Dispatcher so that code may temporarily violate this rule
/// (e.g. most 'extend selection' methods work by first setting the caret, then the selection),
/// it's sufficient to fix it after any event handlers have run.
/// </summary>
private void EnsureSelectionValid()
{
_ensureSelectionValidRequested = false;
if (_allowCaretOutsideSelection == 0)
{
if (!_selection.IsEmpty && !_selection.Contains(Caret.Offset))
{
ClearSelection();
}
}
}
/// <summary>
/// Temporarily allows positioning the caret outside the selection.
/// Dispose the returned IDisposable to revert the allowance.
/// </summary>
/// <remarks>
/// The text area only forces the caret to be inside the selection when other events
/// have finished running (using the dispatcher), so you don't have to use this method
/// for temporarily positioning the caret in event handlers.
/// This method is only necessary if you want to run the dispatcher, e.g. if you
/// perform a drag'n'drop operation.
/// </remarks>
public IDisposable AllowCaretOutsideSelection()
{
VerifyAccess();
_allowCaretOutsideSelection++;
return new CallbackOnDispose(
delegate
{
VerifyAccess();
_allowCaretOutsideSelection--;
RequestSelectionValidation();
});
}
#endregion
#region Properties
/// <summary>
/// Gets the Caret used for this text area.
/// </summary>
public Caret Caret { get; }
/// <summary>
/// Scrolls the text view so that the requested line is in the middle.
/// If the textview can be scrolled.
/// </summary>
/// <param name="line">The line to scroll to.</param>
public void ScrollToLine (int line)
{
var viewPortLines = (int)(this as IScrollable).Viewport.Height;
if (viewPortLines < Document.LineCount)
{
ScrollToLine(line, 2, viewPortLines / 2);
}
}
/// <summary>
/// Scrolls the textview to a position with n lines above and below it.
/// </summary>
/// <param name="line">the requested line number.</param>
/// <param name="linesEitherSide">The number of lines above and below.</param>
public void ScrollToLine(int line, int linesEitherSide)
{
ScrollToLine(line, linesEitherSide, linesEitherSide);
}
/// <summary>
/// Scrolls the textview to a position with n lines above and below it.
/// </summary>
/// <param name="line">the requested line number.</param>
/// <param name="linesAbove">The number of lines above.</param>
/// <param name="linesBelow">The number of lines below.</param>
public void ScrollToLine(int line, int linesAbove, int linesBelow)
{
var offset = line - linesAbove;
if (offset < 0)
{
offset = 0;
}
this.BringIntoView(new Rect(1, offset, 0, 1));
offset = line + linesBelow;
if (offset >= 0)
{
this.BringIntoView(new Rect(1, offset, 0, 1));
}
}
private void CaretPositionChanged(object sender, EventArgs e)
{
if (TextView == null)
return;
TextView.HighlightedLine = Caret.Line;
ScrollToLine(Caret.Line, 2);
Dispatcher.UIThread.InvokeAsync(() =>
{
(this as ILogicalScrollable).RaiseScrollInvalidated(EventArgs.Empty);
});
}
public static readonly DirectProperty<TextArea, ObservableCollection<IControl>> LeftMarginsProperty
= AvaloniaProperty.RegisterDirect<TextArea, ObservableCollection<IControl>>(nameof(LeftMargins),
c => c.LeftMargins);
/// <summary>
/// Gets the collection of margins displayed to the left of the text view.
/// </summary>
public ObservableCollection<IControl> LeftMargins { get; } = new ObservableCollection<IControl>();
private void LeftMargins_CollectionChanged(object sender, NotifyCollectionChangedEventArgs e)
{
if (e.OldItems != null)
{
foreach (var c in e.OldItems.OfType<ITextViewConnect>())
{
c.RemoveFromTextView(TextView);
}
get => _readOnlySectionProvider;
set => _readOnlySectionProvider = value ?? throw new ArgumentNullException(nameof(value));
}
#endregion
#region Focus Handling (Show/Hide Caret)
/// <summary>
/// The <see cref="RightClickMovesCaret"/> property.
/// </summary>
public static readonly StyledProperty<bool> RightClickMovesCaretProperty =
AvaloniaProperty.Register<TextArea, bool>(nameof(RightClickMovesCaret), false);
/// <summary>
/// Determines whether caret position should be changed to the mouse position when you right click or not.
/// </summary>
public bool RightClickMovesCaret
{
get => GetValue(RightClickMovesCaretProperty);
set => SetValue(RightClickMovesCaretProperty, value);
}
#endregion
#region Focus Handling (Show/Hide Caret)
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
Focus();
}
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
Caret.Show();
_imClient.SetTextArea(this);
}
protected override void OnLostFocus(RoutedEventArgs e)
{
base.OnLostFocus(e);
Caret.Hide();
_imClient.SetTextArea(null);
}
#endregion
#region OnTextInput / RemoveSelectedText / ReplaceSelectionWithText
/// <summary>
/// Occurs when the TextArea receives text input.
/// but occurs immediately before the TextArea handles the TextInput event.
/// </summary>
public event EventHandler<TextInputEventArgs> TextEntering;
/// <summary>
/// Occurs when the TextArea receives text input.
/// but occurs immediately after the TextArea handles the TextInput event.
/// </summary>
public event EventHandler<TextInputEventArgs> TextEntered;
/// <summary>
/// Raises the TextEntering event.
/// </summary>
protected virtual void OnTextEntering(TextInputEventArgs e)
{
TextEntering?.Invoke(this, e);
}
/// <summary>
/// Raises the TextEntered event.
/// </summary>
protected virtual void OnTextEntered(TextInputEventArgs e)
{
TextEntered?.Invoke(this, e);
}
protected override void OnTextInput(TextInputEventArgs e)
{
base.OnTextInput(e);
if (!e.Handled && Document != null)
{
if (string.IsNullOrEmpty(e.Text) || e.Text == "\x1b" || e.Text == "\b" || e.Text == "\u007f")
{
// TODO: check this
// ASCII 0x1b = ESC.
// produces a TextInput event with that old ASCII control char
// when Escape is pressed. We'll just ignore it.
// A deadkey followed by backspace causes a textinput event for the BS character.
// Similarly, some shortcuts like Alt+Space produce an empty TextInput event.
// We have to ignore those (not handle them) to keep the shortcut working.
return;
}
HideMouseCursor();
PerformTextInput(e);
e.Handled = true;
}
}
/// <summary>
/// Performs text input.
/// This raises the <see cref="TextEntering"/> event, replaces the selection with the text,
/// and then raises the <see cref="TextEntered"/> event.
/// </summary>
public void PerformTextInput(string text)
{
var e = new TextInputEventArgs
{
Text = text,
RoutedEvent = TextInputEvent
};
PerformTextInput(e);
}
/// <summary>
/// Performs text input.
/// This raises the <see cref="TextEntering"/> event, replaces the selection with the text,
/// and then raises the <see cref="TextEntered"/> event.
/// </summary>
public void PerformTextInput(TextInputEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
OnTextEntering(e);
if (!e.Handled)
{
if (e.Text == "\n" || e.Text == "\r" || e.Text == "\r\n")
ReplaceSelectionWithNewLine();
else
{
// TODO
//if (OverstrikeMode && Selection.IsEmpty && Document.GetLineByNumber(Caret.Line).EndOffset > Caret.Offset)
// EditingCommands.SelectRightByCharacter.Execute(null, this);
ReplaceSelectionWithText(e.Text);
}
OnTextEntered(e);
Caret.BringCaretToView();
}
}
private void ReplaceSelectionWithNewLine()
{
var newLine = TextUtilities.GetNewLineFromDocument(Document, Caret.Line);
using (Document.RunUpdate())
{
ReplaceSelectionWithText(newLine);
if (IndentationStrategy != null)
{
var line = Document.GetLineByNumber(Caret.Line);
var deletable = GetDeletableSegments(line);
if (deletable.Length == 1 && deletable[0].Offset == line.Offset && deletable[0].Length == line.Length)
{
// use indentation strategy only if the line is not read-only
IndentationStrategy.IndentLine(Document, line);
}
}
}
}
internal void RemoveSelectedText()
{
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
_selection.ReplaceSelectionWithText(string.Empty);
#if DEBUG
if (!_selection.IsEmpty)
{
foreach (var s in _selection.Segments)
{
Debug.Assert(!ReadOnlySectionProvider.GetDeletableSegments(s).Any());
}
}
#endif
}
internal void ReplaceSelectionWithText(string newText)
{
if (newText == null)
throw new ArgumentNullException(nameof(newText));
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
_selection.ReplaceSelectionWithText(newText);
}
internal ISegment[] GetDeletableSegments(ISegment segment)
{
var deletableSegments = ReadOnlySectionProvider.GetDeletableSegments(segment);
if (deletableSegments == null)
throw new InvalidOperationException("ReadOnlySectionProvider.GetDeletableSegments returned null");
var array = deletableSegments.ToArray();
var lastIndex = segment.Offset;
foreach (var t in array)
{
if (t.Offset < lastIndex)
throw new InvalidOperationException("ReadOnlySectionProvider returned incorrect segments (outside of input segment / wrong order)");
lastIndex = t.EndOffset;
}
if (lastIndex > segment.EndOffset)
throw new InvalidOperationException("ReadOnlySectionProvider returned incorrect segments (outside of input segment / wrong order)");
return array;
}
#endregion
#region IndentationStrategy property
/// <summary>
/// IndentationStrategy property.
/// </summary>
public static readonly StyledProperty<IIndentationStrategy> IndentationStrategyProperty =
AvaloniaProperty.Register<TextArea, IIndentationStrategy>("IndentationStrategy", new DefaultIndentationStrategy());
/// <summary>
/// Gets/Sets the indentation strategy used when inserting new lines.
/// </summary>
public IIndentationStrategy IndentationStrategy
{
get => GetValue(IndentationStrategyProperty);
set => SetValue(IndentationStrategyProperty, value);
}
#endregion
#region OnKeyDown/OnKeyUp
// Make life easier for text editor extensions that use a different cursor based on the pressed modifier keys.
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
TextView.InvalidateCursorIfPointerWithinTextView();
}
private void OnPreviewKeyDown(object sender, KeyEventArgs e)
{
foreach (var h in StackedInputHandlers)
{
if (e.Handled)
break;
h.OnPreviewKeyDown(e);
}
}
/// <inheritdoc/>
protected override void OnKeyUp(KeyEventArgs e)
{
base.OnKeyUp(e);
TextView.InvalidateCursorIfPointerWithinTextView();
}
private void OnPreviewKeyUp(object sender, KeyEventArgs e)
{
foreach (var h in StackedInputHandlers)
{
if (e.Handled)
break;
h.OnPreviewKeyUp(e);
}
}
#endregion
#region Hide Mouse Cursor While Typing
private bool _isMouseCursorHidden;
private void AttachTypingEvents()
{
// Use the PreviewMouseMove event in case some other editor layer consumes the MouseMove event (e.g. SD's InsertionCursorLayer)
PointerEntered += delegate { ShowMouseCursor(); };
PointerExited += delegate { ShowMouseCursor(); };
}
private void ShowMouseCursor()
{
if (_isMouseCursorHidden)
{
_isMouseCursorHidden = false;
}
}
private void HideMouseCursor()
{
if (Options.HideCursorWhileTyping && !_isMouseCursorHidden && IsPointerOver)
{
_isMouseCursorHidden = true;
}
}
#endregion
#region Overstrike mode
/// <summary>
/// The <see cref="OverstrikeMode"/> dependency property.
/// </summary>
public static readonly StyledProperty<bool> OverstrikeModeProperty =
AvaloniaProperty.Register<TextArea, bool>("OverstrikeMode");
/// <summary>
/// Gets/Sets whether overstrike mode is active.
/// </summary>
public bool OverstrikeMode
{
get => GetValue(OverstrikeModeProperty);
set => SetValue(OverstrikeModeProperty, value);
}
#endregion
/// <inheritdoc/>
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == SelectionBrushProperty
|| change.Property == SelectionBorderProperty
|| change.Property == SelectionForegroundProperty
|| change.Property == SelectionCornerRadiusProperty)
{
TextView.Redraw();
}
else if (change.Property == OverstrikeModeProperty)
{
Caret.UpdateIfVisible();
}
}
/// <summary>
/// Gets the requested service.
/// </summary>
/// <returns>Returns the requested service instance, or null if the service cannot be found.</returns>
public virtual object GetService(Type serviceType)
{
return TextView.GetService(serviceType);
}
/// <summary>
/// Occurs when text inside the TextArea was copied.
/// </summary>
public event EventHandler<TextEventArgs> TextCopied;
event EventHandler ILogicalScrollable.ScrollInvalidated
{
add { if (_logicalScrollable != null) _logicalScrollable.ScrollInvalidated += value; }
remove { if (_logicalScrollable != null) _logicalScrollable.ScrollInvalidated -= value; }
}
internal void OnTextCopied(TextEventArgs e)
{
TextCopied?.Invoke(this, e);
}
public IList<RoutedCommandBinding> CommandBindings { get; } = new List<RoutedCommandBinding>();
bool ILogicalScrollable.IsLogicalScrollEnabled => _logicalScrollable?.IsLogicalScrollEnabled ?? default(bool);
Size ILogicalScrollable.ScrollSize => _logicalScrollable?.ScrollSize ?? default(Size);
Size ILogicalScrollable.PageScrollSize => _logicalScrollable?.PageScrollSize ?? default(Size);
Size IScrollable.Extent => _logicalScrollable?.Extent ?? default(Size);
Vector IScrollable.Offset
{
get => _logicalScrollable?.Offset ?? default(Vector);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.Offset = value;
}
}
}
Size IScrollable.Viewport => _logicalScrollable?.Viewport ?? default(Size);
bool ILogicalScrollable.CanHorizontallyScroll
{
get => _logicalScrollable?.CanHorizontallyScroll ?? default(bool);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.CanHorizontallyScroll = value;
}
}
}
bool ILogicalScrollable.CanVerticallyScroll
{
get => _logicalScrollable?.CanVerticallyScroll ?? default(bool);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.CanVerticallyScroll = value;
}
}
}
public bool BringIntoView(IControl target, Rect targetRect) =>
_logicalScrollable?.BringIntoView(target, targetRect) ?? default(bool);
IControl ILogicalScrollable.GetControlInDirection(NavigationDirection direction, IControl from)
=> _logicalScrollable?.GetControlInDirection(direction, from);
public void RaiseScrollInvalidated(EventArgs e)
{
_logicalScrollable?.RaiseScrollInvalidated(e);
}
private class TextAreaTextInputMethodClient : ITextInputMethodClient
{
private TextArea _textArea;
public TextAreaTextInputMethodClient()
{
}
public event EventHandler CursorRectangleChanged;
public event EventHandler TextViewVisualChanged;
public event EventHandler SurroundingTextChanged;
public Rect CursorRectangle
{
get
{
if(_textArea == null)
{
return Rect.Empty;
}
var transform = _textArea.TextView.TransformToVisual(_textArea);
if (transform == null)
{
return default;
}
var rect = _textArea.Caret.CalculateCaretRectangle().TransformToAABB(transform.Value);
return rect;
}
}
public IVisual TextViewVisual => _textArea;
public bool SupportsPreedit => false;
public bool SupportsSurroundingText => true;
public TextInputMethodSurroundingText SurroundingText
{
get
{
if(_textArea == null)
{
return default;
}
var lineIndex = _textArea.Caret.Line;
var position = _textArea.Caret.Position;
var documentLine = _textArea.Document.GetLineByNumber(lineIndex);
var text = _textArea.Document.GetText(documentLine.Offset, documentLine.Length);
return new TextInputMethodSurroundingText
{
AnchorOffset = 0,
CursorOffset = position.Column,
Text = text
};
}
}
public void SetTextArea(TextArea textArea)
{
if(_textArea != null)
{
_textArea.Caret.PositionChanged -= Caret_PositionChanged;
_textArea.SelectionChanged -= TextArea_SelectionChanged;
}
_textArea = textArea;
if(_textArea != null)
{
_textArea.Caret.PositionChanged += Caret_PositionChanged;
_textArea.SelectionChanged += TextArea_SelectionChanged;
}
TextViewVisualChanged?.Invoke(this, EventArgs.Empty);
CursorRectangleChanged?.Invoke(this, EventArgs.Empty);
}
private void Caret_PositionChanged(object sender, EventArgs e)
{
CursorRectangleChanged?.Invoke(this, e);
}
private void TextArea_SelectionChanged(object sender, EventArgs e)
{
SurroundingTextChanged?.Invoke(this, e);
}
public void SelectInSurroundingText(int start, int end)
{
if(_textArea == null)
{
return;
}
var selection = _textArea.Selection;
_textArea.Selection = _textArea.Selection.StartSelectionOrSetEndpoint(
new TextViewPosition(selection.StartPosition.Line, start),
new TextViewPosition(selection.StartPosition.Line, end));
}
public void SetPreeditText(string text)
{
}
}
}
/// <summary>
/// EventArgs with text.
/// </summary>
public class TextEventArgs : EventArgs
{
/// <summary>
/// Gets the text.
/// </summary>
public string Text { get; }
/// <summary>
/// Creates a new TextEventArgs instance.
/// </summary>
public TextEventArgs(string text)
{
Text = text ?? throw new ArgumentNullException(nameof(text));
}
}
}
<MSG> Merge pull request #169 from Takoooooo/right-click-moves-caret-feature
You should be able to move caret by right clicking text area
<DFF> @@ -708,6 +708,21 @@ namespace AvaloniaEdit.Editing
get => _readOnlySectionProvider;
set => _readOnlySectionProvider = value ?? throw new ArgumentNullException(nameof(value));
}
+
+ /// <summary>
+ /// The <see cref="RightClickMovesCaret"/> property.
+ /// </summary>
+ public static readonly StyledProperty<bool> RightClickMovesCaretProperty =
+ AvaloniaProperty.Register<TextArea, bool>(nameof(RightClickMovesCaret), false);
+
+ /// <summary>
+ /// Determines whether caret position should be changed to the mouse position when you right click or not.
+ /// </summary>
+ public bool RightClickMovesCaret
+ {
+ get => GetValue(RightClickMovesCaretProperty);
+ set => SetValue(RightClickMovesCaretProperty, value);
+ }
#endregion
#region Focus Handling (Show/Hide Caret)
| 15 | Merge pull request #169 from Takoooooo/right-click-moves-caret-feature | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066151 | <NME> TextArea.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using Avalonia;
using Avalonia.Controls;
using Avalonia.Controls.Presenters;
using Avalonia.Controls.Primitives;
using Avalonia.Data;
using Avalonia.Input;
using Avalonia.Input.TextInput;
using Avalonia.Interactivity;
using Avalonia.Media;
using Avalonia.Threading;
using Avalonia.VisualTree;
using AvaloniaEdit.Document;
using AvaloniaEdit.Indentation;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Search;
using AvaloniaEdit.Utils;
using System;
using System.Collections.Generic;
using System.Collections.Immutable;
using System.Collections.ObjectModel;
using System.Collections.Specialized;
using System.ComponentModel;
using System.Diagnostics;
using System.Linq;
namespace AvaloniaEdit.Editing
{
/// <summary>
/// Control that wraps a TextView and adds support for user input and the caret.
/// </summary>
public class TextArea : TemplatedControl, ITextEditorComponent, IRoutedCommandBindable, ILogicalScrollable
{
/// <summary>
/// This is the extra scrolling space that occurs after the last line.
/// </summary>
private const int AdditionalVerticalScrollAmount = 2;
private ILogicalScrollable _logicalScrollable;
private readonly TextAreaTextInputMethodClient _imClient = new TextAreaTextInputMethodClient();
#region Constructor
static TextArea()
{
KeyboardNavigation.TabNavigationProperty.OverrideDefaultValue<TextArea>(KeyboardNavigationMode.None);
FocusableProperty.OverrideDefaultValue<TextArea>(true);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
AffectsArrange<TextArea>(OffsetProperty);
AffectsRender<TextArea>(OffsetProperty);
TextInputMethodClientRequestedEvent.AddClassHandler<TextArea>((ta, e) =>
{
if (!ta.IsReadOnly)
{
e.Client = ta._imClient;
}
});
}
/// <summary>
/// Creates a new TextArea instance.
/// </summary>
public TextArea() : this(new TextView())
{
AddHandler(KeyDownEvent, OnPreviewKeyDown, RoutingStrategies.Tunnel);
AddHandler(KeyUpEvent, OnPreviewKeyUp, RoutingStrategies.Tunnel);
}
/// <summary>
/// Creates a new TextArea instance.
/// </summary>
protected TextArea(TextView textView)
{
TextView = textView ?? throw new ArgumentNullException(nameof(textView));
_logicalScrollable = textView;
Options = textView.Options;
_selection = EmptySelection = new EmptySelection(this);
textView.Services.AddService(this);
textView.LineTransformers.Add(new SelectionColorizer(this));
textView.InsertLayer(new SelectionLayer(this), KnownLayer.Selection, LayerInsertionPosition.Replace);
Caret = new Caret(this);
Caret.PositionChanged += (sender, e) => RequestSelectionValidation();
Caret.PositionChanged += CaretPositionChanged;
AttachTypingEvents();
LeftMargins.CollectionChanged += LeftMargins_CollectionChanged;
DefaultInputHandler = new TextAreaDefaultInputHandler(this);
ActiveInputHandler = DefaultInputHandler;
// TODO
//textView.GetObservable(TextBlock.FontSizeProperty).Subscribe(_ =>
//{
// TextView.SetScrollOffset(new Vector(_offset.X, _offset.Y * TextView.DefaultLineHeight));
//});
}
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
if (e.NameScope.Find("PART_CP") is ContentPresenter contentPresenter)
{
contentPresenter.Content = TextView;
}
}
internal void AddChild(IVisual visual)
{
VisualChildren.Add(visual);
InvalidateArrange();
}
internal void RemoveChild(IVisual visual)
{
VisualChildren.Remove(visual);
}
#endregion
/// <summary>
/// Defines the <see cref="IScrollable.Offset" /> property.
/// </summary>
public static readonly DirectProperty<TextArea, Vector> OffsetProperty =
AvaloniaProperty.RegisterDirect<TextArea, Vector>(
nameof(IScrollable.Offset),
o => (o as IScrollable).Offset,
(o, v) => (o as IScrollable).Offset = v);
#region InputHandler management
/// <summary>
/// Gets the default input handler.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public TextAreaDefaultInputHandler DefaultInputHandler { get; }
private ITextAreaInputHandler _activeInputHandler;
private bool _isChangingInputHandler;
/// <summary>
/// Gets/Sets the active input handler.
/// This property does not return currently active stacked input handlers. Setting this property detached all stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public ITextAreaInputHandler ActiveInputHandler
{
get => _activeInputHandler;
set
{
if (value != null && value.TextArea != this)
throw new ArgumentException("The input handler was created for a different text area than this one.");
if (_isChangingInputHandler)
throw new InvalidOperationException("Cannot set ActiveInputHandler recursively");
if (_activeInputHandler != value)
{
_isChangingInputHandler = true;
try
{
// pop the whole stack
PopStackedInputHandler(StackedInputHandlers.LastOrDefault());
Debug.Assert(StackedInputHandlers.IsEmpty);
_activeInputHandler?.Detach();
_activeInputHandler = value;
value?.Attach();
}
finally
{
_isChangingInputHandler = false;
}
ActiveInputHandlerChanged?.Invoke(this, EventArgs.Empty);
}
}
}
/// <summary>
/// Occurs when the ActiveInputHandler property changes.
/// </summary>
public event EventHandler ActiveInputHandlerChanged;
/// <summary>
/// Gets the list of currently active stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public ImmutableStack<TextAreaStackedInputHandler> StackedInputHandlers { get; private set; } = ImmutableStack<TextAreaStackedInputHandler>.Empty;
/// <summary>
/// Pushes an input handler onto the list of stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public void PushStackedInputHandler(TextAreaStackedInputHandler inputHandler)
{
if (inputHandler == null)
throw new ArgumentNullException(nameof(inputHandler));
StackedInputHandlers = StackedInputHandlers.Push(inputHandler);
inputHandler.Attach();
}
/// <summary>
/// Pops the stacked input handler (and all input handlers above it).
/// If <paramref name="inputHandler"/> is not found in the currently stacked input handlers, or is null, this method
/// does nothing.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public void PopStackedInputHandler(TextAreaStackedInputHandler inputHandler)
{
if (StackedInputHandlers.Any(i => i == inputHandler))
{
ITextAreaInputHandler oldHandler;
do
{
oldHandler = StackedInputHandlers.Peek();
StackedInputHandlers = StackedInputHandlers.Pop();
oldHandler.Detach();
} while (oldHandler != inputHandler);
}
}
#endregion
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextArea>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <inheritdoc/>
public event EventHandler DocumentChanged;
/// <summary>
/// Gets if the the document displayed by the text editor is readonly
/// </summary>
public bool IsReadOnly
{
get => ReadOnlySectionProvider == ReadOnlySectionDocument.Instance;
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextArea)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.Changing.RemoveHandler(oldValue, OnDocumentChanging);
TextDocumentWeakEventManager.Changed.RemoveHandler(oldValue, OnDocumentChanged);
TextDocumentWeakEventManager.UpdateStarted.RemoveHandler(oldValue, OnUpdateStarted);
TextDocumentWeakEventManager.UpdateFinished.RemoveHandler(oldValue, OnUpdateFinished);
}
TextView.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.Changing.AddHandler(newValue, OnDocumentChanging);
TextDocumentWeakEventManager.Changed.AddHandler(newValue, OnDocumentChanged);
TextDocumentWeakEventManager.UpdateStarted.AddHandler(newValue, OnUpdateStarted);
TextDocumentWeakEventManager.UpdateFinished.AddHandler(newValue, OnUpdateFinished);
InvalidateArrange();
}
// Reset caret location and selection: this is necessary because the caret/selection might be invalid
// in the new document (e.g. if new document is shorter than the old document).
Caret.Location = new TextLocation(1, 1);
ClearSelection();
DocumentChanged?.Invoke(this, EventArgs.Empty);
//CommandManager.InvalidateRequerySuggested();
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextArea>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
private void OnOptionChanged(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextArea)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnOptionChanged);
}
TextView.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnOptionChanged);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
#endregion
#region Caret handling on document changes
private void OnDocumentChanging(object sender, DocumentChangeEventArgs e)
{
Caret.OnDocumentChanging();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
Caret.OnDocumentChanged(e);
Selection = _selection.UpdateOnDocumentChange(e);
}
private void OnUpdateStarted(object sender, EventArgs e)
{
Document.UndoStack.PushOptional(new RestoreCaretAndSelectionUndoAction(this));
}
private void OnUpdateFinished(object sender, EventArgs e)
{
Caret.OnDocumentUpdateFinished();
}
private sealed class RestoreCaretAndSelectionUndoAction : IUndoableOperation
{
// keep textarea in weak reference because the IUndoableOperation is stored with the document
private readonly WeakReference _textAreaReference;
private readonly TextViewPosition _caretPosition;
private readonly Selection _selection;
public RestoreCaretAndSelectionUndoAction(TextArea textArea)
{
_textAreaReference = new WeakReference(textArea);
// Just save the old caret position, no need to validate here.
// If we restore it, we'll validate it anyways.
_caretPosition = textArea.Caret.NonValidatedPosition;
_selection = textArea.Selection;
}
public void Undo()
{
var textArea = (TextArea)_textAreaReference.Target;
if (textArea != null)
{
textArea.Caret.Position = _caretPosition;
textArea.Selection = _selection;
}
}
public void Redo()
{
// redo=undo: we just restore the caret/selection state
Undo();
}
}
#endregion
#region TextView property
/// <summary>
/// Gets the text view used to display text in this text area.
/// </summary>
public TextView TextView { get; }
#endregion
#region Selection property
internal readonly Selection EmptySelection;
private Selection _selection;
/// <summary>
/// Occurs when the selection has changed.
/// </summary>
public event EventHandler SelectionChanged;
/// <summary>
/// Gets/Sets the selection in this text area.
/// </summary>
public Selection Selection
{
get => _selection;
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
if (value.TextArea != this)
throw new ArgumentException("Cannot use a Selection instance that belongs to another text area.");
if (!Equals(_selection, value))
{
if (TextView != null)
{
var oldSegment = _selection.SurroundingSegment;
var newSegment = value.SurroundingSegment;
if (!Selection.EnableVirtualSpace && (_selection is SimpleSelection && value is SimpleSelection && oldSegment != null && newSegment != null))
{
// perf optimization:
// When a simple selection changes, don't redraw the whole selection, but only the changed parts.
var oldSegmentOffset = oldSegment.Offset;
var newSegmentOffset = newSegment.Offset;
if (oldSegmentOffset != newSegmentOffset)
{
TextView.Redraw(Math.Min(oldSegmentOffset, newSegmentOffset),
Math.Abs(oldSegmentOffset - newSegmentOffset));
}
var oldSegmentEndOffset = oldSegment.EndOffset;
var newSegmentEndOffset = newSegment.EndOffset;
if (oldSegmentEndOffset != newSegmentEndOffset)
{
TextView.Redraw(Math.Min(oldSegmentEndOffset, newSegmentEndOffset),
Math.Abs(oldSegmentEndOffset - newSegmentEndOffset));
}
}
else
{
TextView.Redraw(oldSegment);
TextView.Redraw(newSegment);
}
}
_selection = value;
SelectionChanged?.Invoke(this, EventArgs.Empty);
// a selection change causes commands like copy/paste/etc. to change status
//CommandManager.InvalidateRequerySuggested();
}
}
}
/// <summary>
/// Clears the current selection.
/// </summary>
public void ClearSelection()
{
Selection = EmptySelection;
}
/// <summary>
/// The <see cref="SelectionBrush"/> property.
/// </summary>
public static readonly StyledProperty<IBrush> SelectionBrushProperty =
AvaloniaProperty.Register<TextArea, IBrush>("SelectionBrush");
/// <summary>
/// Gets/Sets the background brush used for the selection.
/// </summary>
public IBrush SelectionBrush
{
get => GetValue(SelectionBrushProperty);
set => SetValue(SelectionBrushProperty, value);
}
/// <summary>
/// The <see cref="SelectionForeground"/> property.
/// </summary>
public static readonly StyledProperty<IBrush> SelectionForegroundProperty =
AvaloniaProperty.Register<TextArea, IBrush>("SelectionForeground");
/// <summary>
/// Gets/Sets the foreground brush used for selected text.
/// </summary>
public IBrush SelectionForeground
{
get => GetValue(SelectionForegroundProperty);
set => SetValue(SelectionForegroundProperty, value);
}
/// <summary>
/// The <see cref="SelectionBorder"/> property.
/// </summary>
public static readonly StyledProperty<Pen> SelectionBorderProperty =
AvaloniaProperty.Register<TextArea, Pen>("SelectionBorder");
/// <summary>
/// Gets/Sets the pen used for the border of the selection.
/// </summary>
public Pen SelectionBorder
{
get => GetValue(SelectionBorderProperty);
set => SetValue(SelectionBorderProperty, value);
}
/// <summary>
/// The <see cref="SelectionCornerRadius"/> property.
/// </summary>
public static readonly StyledProperty<double> SelectionCornerRadiusProperty =
AvaloniaProperty.Register<TextArea, double>("SelectionCornerRadius", 3.0);
/// <summary>
/// Gets/Sets the corner radius of the selection.
/// </summary>
public double SelectionCornerRadius
{
get => GetValue(SelectionCornerRadiusProperty);
set => SetValue(SelectionCornerRadiusProperty, value);
}
#endregion
#region Force caret to stay inside selection
private bool _ensureSelectionValidRequested;
private int _allowCaretOutsideSelection;
private void RequestSelectionValidation()
{
if (!_ensureSelectionValidRequested && _allowCaretOutsideSelection == 0)
{
_ensureSelectionValidRequested = true;
Dispatcher.UIThread.Post(EnsureSelectionValid);
}
}
/// <summary>
/// Code that updates only the caret but not the selection can cause confusion when
/// keys like 'Delete' delete the (possibly invisible) selected text and not the
/// text around the caret.
///
/// So we'll ensure that the caret is inside the selection.
/// (when the caret is not in the selection, we'll clear the selection)
///
/// This method is invoked using the Dispatcher so that code may temporarily violate this rule
/// (e.g. most 'extend selection' methods work by first setting the caret, then the selection),
/// it's sufficient to fix it after any event handlers have run.
/// </summary>
private void EnsureSelectionValid()
{
_ensureSelectionValidRequested = false;
if (_allowCaretOutsideSelection == 0)
{
if (!_selection.IsEmpty && !_selection.Contains(Caret.Offset))
{
ClearSelection();
}
}
}
/// <summary>
/// Temporarily allows positioning the caret outside the selection.
/// Dispose the returned IDisposable to revert the allowance.
/// </summary>
/// <remarks>
/// The text area only forces the caret to be inside the selection when other events
/// have finished running (using the dispatcher), so you don't have to use this method
/// for temporarily positioning the caret in event handlers.
/// This method is only necessary if you want to run the dispatcher, e.g. if you
/// perform a drag'n'drop operation.
/// </remarks>
public IDisposable AllowCaretOutsideSelection()
{
VerifyAccess();
_allowCaretOutsideSelection++;
return new CallbackOnDispose(
delegate
{
VerifyAccess();
_allowCaretOutsideSelection--;
RequestSelectionValidation();
});
}
#endregion
#region Properties
/// <summary>
/// Gets the Caret used for this text area.
/// </summary>
public Caret Caret { get; }
/// <summary>
/// Scrolls the text view so that the requested line is in the middle.
/// If the textview can be scrolled.
/// </summary>
/// <param name="line">The line to scroll to.</param>
public void ScrollToLine (int line)
{
var viewPortLines = (int)(this as IScrollable).Viewport.Height;
if (viewPortLines < Document.LineCount)
{
ScrollToLine(line, 2, viewPortLines / 2);
}
}
/// <summary>
/// Scrolls the textview to a position with n lines above and below it.
/// </summary>
/// <param name="line">the requested line number.</param>
/// <param name="linesEitherSide">The number of lines above and below.</param>
public void ScrollToLine(int line, int linesEitherSide)
{
ScrollToLine(line, linesEitherSide, linesEitherSide);
}
/// <summary>
/// Scrolls the textview to a position with n lines above and below it.
/// </summary>
/// <param name="line">the requested line number.</param>
/// <param name="linesAbove">The number of lines above.</param>
/// <param name="linesBelow">The number of lines below.</param>
public void ScrollToLine(int line, int linesAbove, int linesBelow)
{
var offset = line - linesAbove;
if (offset < 0)
{
offset = 0;
}
this.BringIntoView(new Rect(1, offset, 0, 1));
offset = line + linesBelow;
if (offset >= 0)
{
this.BringIntoView(new Rect(1, offset, 0, 1));
}
}
private void CaretPositionChanged(object sender, EventArgs e)
{
if (TextView == null)
return;
TextView.HighlightedLine = Caret.Line;
ScrollToLine(Caret.Line, 2);
Dispatcher.UIThread.InvokeAsync(() =>
{
(this as ILogicalScrollable).RaiseScrollInvalidated(EventArgs.Empty);
});
}
public static readonly DirectProperty<TextArea, ObservableCollection<IControl>> LeftMarginsProperty
= AvaloniaProperty.RegisterDirect<TextArea, ObservableCollection<IControl>>(nameof(LeftMargins),
c => c.LeftMargins);
/// <summary>
/// Gets the collection of margins displayed to the left of the text view.
/// </summary>
public ObservableCollection<IControl> LeftMargins { get; } = new ObservableCollection<IControl>();
private void LeftMargins_CollectionChanged(object sender, NotifyCollectionChangedEventArgs e)
{
if (e.OldItems != null)
{
foreach (var c in e.OldItems.OfType<ITextViewConnect>())
{
c.RemoveFromTextView(TextView);
}
get => _readOnlySectionProvider;
set => _readOnlySectionProvider = value ?? throw new ArgumentNullException(nameof(value));
}
#endregion
#region Focus Handling (Show/Hide Caret)
/// <summary>
/// The <see cref="RightClickMovesCaret"/> property.
/// </summary>
public static readonly StyledProperty<bool> RightClickMovesCaretProperty =
AvaloniaProperty.Register<TextArea, bool>(nameof(RightClickMovesCaret), false);
/// <summary>
/// Determines whether caret position should be changed to the mouse position when you right click or not.
/// </summary>
public bool RightClickMovesCaret
{
get => GetValue(RightClickMovesCaretProperty);
set => SetValue(RightClickMovesCaretProperty, value);
}
#endregion
#region Focus Handling (Show/Hide Caret)
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
Focus();
}
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
Caret.Show();
_imClient.SetTextArea(this);
}
protected override void OnLostFocus(RoutedEventArgs e)
{
base.OnLostFocus(e);
Caret.Hide();
_imClient.SetTextArea(null);
}
#endregion
#region OnTextInput / RemoveSelectedText / ReplaceSelectionWithText
/// <summary>
/// Occurs when the TextArea receives text input.
/// but occurs immediately before the TextArea handles the TextInput event.
/// </summary>
public event EventHandler<TextInputEventArgs> TextEntering;
/// <summary>
/// Occurs when the TextArea receives text input.
/// but occurs immediately after the TextArea handles the TextInput event.
/// </summary>
public event EventHandler<TextInputEventArgs> TextEntered;
/// <summary>
/// Raises the TextEntering event.
/// </summary>
protected virtual void OnTextEntering(TextInputEventArgs e)
{
TextEntering?.Invoke(this, e);
}
/// <summary>
/// Raises the TextEntered event.
/// </summary>
protected virtual void OnTextEntered(TextInputEventArgs e)
{
TextEntered?.Invoke(this, e);
}
protected override void OnTextInput(TextInputEventArgs e)
{
base.OnTextInput(e);
if (!e.Handled && Document != null)
{
if (string.IsNullOrEmpty(e.Text) || e.Text == "\x1b" || e.Text == "\b" || e.Text == "\u007f")
{
// TODO: check this
// ASCII 0x1b = ESC.
// produces a TextInput event with that old ASCII control char
// when Escape is pressed. We'll just ignore it.
// A deadkey followed by backspace causes a textinput event for the BS character.
// Similarly, some shortcuts like Alt+Space produce an empty TextInput event.
// We have to ignore those (not handle them) to keep the shortcut working.
return;
}
HideMouseCursor();
PerformTextInput(e);
e.Handled = true;
}
}
/// <summary>
/// Performs text input.
/// This raises the <see cref="TextEntering"/> event, replaces the selection with the text,
/// and then raises the <see cref="TextEntered"/> event.
/// </summary>
public void PerformTextInput(string text)
{
var e = new TextInputEventArgs
{
Text = text,
RoutedEvent = TextInputEvent
};
PerformTextInput(e);
}
/// <summary>
/// Performs text input.
/// This raises the <see cref="TextEntering"/> event, replaces the selection with the text,
/// and then raises the <see cref="TextEntered"/> event.
/// </summary>
public void PerformTextInput(TextInputEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
OnTextEntering(e);
if (!e.Handled)
{
if (e.Text == "\n" || e.Text == "\r" || e.Text == "\r\n")
ReplaceSelectionWithNewLine();
else
{
// TODO
//if (OverstrikeMode && Selection.IsEmpty && Document.GetLineByNumber(Caret.Line).EndOffset > Caret.Offset)
// EditingCommands.SelectRightByCharacter.Execute(null, this);
ReplaceSelectionWithText(e.Text);
}
OnTextEntered(e);
Caret.BringCaretToView();
}
}
private void ReplaceSelectionWithNewLine()
{
var newLine = TextUtilities.GetNewLineFromDocument(Document, Caret.Line);
using (Document.RunUpdate())
{
ReplaceSelectionWithText(newLine);
if (IndentationStrategy != null)
{
var line = Document.GetLineByNumber(Caret.Line);
var deletable = GetDeletableSegments(line);
if (deletable.Length == 1 && deletable[0].Offset == line.Offset && deletable[0].Length == line.Length)
{
// use indentation strategy only if the line is not read-only
IndentationStrategy.IndentLine(Document, line);
}
}
}
}
internal void RemoveSelectedText()
{
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
_selection.ReplaceSelectionWithText(string.Empty);
#if DEBUG
if (!_selection.IsEmpty)
{
foreach (var s in _selection.Segments)
{
Debug.Assert(!ReadOnlySectionProvider.GetDeletableSegments(s).Any());
}
}
#endif
}
internal void ReplaceSelectionWithText(string newText)
{
if (newText == null)
throw new ArgumentNullException(nameof(newText));
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
_selection.ReplaceSelectionWithText(newText);
}
internal ISegment[] GetDeletableSegments(ISegment segment)
{
var deletableSegments = ReadOnlySectionProvider.GetDeletableSegments(segment);
if (deletableSegments == null)
throw new InvalidOperationException("ReadOnlySectionProvider.GetDeletableSegments returned null");
var array = deletableSegments.ToArray();
var lastIndex = segment.Offset;
foreach (var t in array)
{
if (t.Offset < lastIndex)
throw new InvalidOperationException("ReadOnlySectionProvider returned incorrect segments (outside of input segment / wrong order)");
lastIndex = t.EndOffset;
}
if (lastIndex > segment.EndOffset)
throw new InvalidOperationException("ReadOnlySectionProvider returned incorrect segments (outside of input segment / wrong order)");
return array;
}
#endregion
#region IndentationStrategy property
/// <summary>
/// IndentationStrategy property.
/// </summary>
public static readonly StyledProperty<IIndentationStrategy> IndentationStrategyProperty =
AvaloniaProperty.Register<TextArea, IIndentationStrategy>("IndentationStrategy", new DefaultIndentationStrategy());
/// <summary>
/// Gets/Sets the indentation strategy used when inserting new lines.
/// </summary>
public IIndentationStrategy IndentationStrategy
{
get => GetValue(IndentationStrategyProperty);
set => SetValue(IndentationStrategyProperty, value);
}
#endregion
#region OnKeyDown/OnKeyUp
// Make life easier for text editor extensions that use a different cursor based on the pressed modifier keys.
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
TextView.InvalidateCursorIfPointerWithinTextView();
}
private void OnPreviewKeyDown(object sender, KeyEventArgs e)
{
foreach (var h in StackedInputHandlers)
{
if (e.Handled)
break;
h.OnPreviewKeyDown(e);
}
}
/// <inheritdoc/>
protected override void OnKeyUp(KeyEventArgs e)
{
base.OnKeyUp(e);
TextView.InvalidateCursorIfPointerWithinTextView();
}
private void OnPreviewKeyUp(object sender, KeyEventArgs e)
{
foreach (var h in StackedInputHandlers)
{
if (e.Handled)
break;
h.OnPreviewKeyUp(e);
}
}
#endregion
#region Hide Mouse Cursor While Typing
private bool _isMouseCursorHidden;
private void AttachTypingEvents()
{
// Use the PreviewMouseMove event in case some other editor layer consumes the MouseMove event (e.g. SD's InsertionCursorLayer)
PointerEntered += delegate { ShowMouseCursor(); };
PointerExited += delegate { ShowMouseCursor(); };
}
private void ShowMouseCursor()
{
if (_isMouseCursorHidden)
{
_isMouseCursorHidden = false;
}
}
private void HideMouseCursor()
{
if (Options.HideCursorWhileTyping && !_isMouseCursorHidden && IsPointerOver)
{
_isMouseCursorHidden = true;
}
}
#endregion
#region Overstrike mode
/// <summary>
/// The <see cref="OverstrikeMode"/> dependency property.
/// </summary>
public static readonly StyledProperty<bool> OverstrikeModeProperty =
AvaloniaProperty.Register<TextArea, bool>("OverstrikeMode");
/// <summary>
/// Gets/Sets whether overstrike mode is active.
/// </summary>
public bool OverstrikeMode
{
get => GetValue(OverstrikeModeProperty);
set => SetValue(OverstrikeModeProperty, value);
}
#endregion
/// <inheritdoc/>
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == SelectionBrushProperty
|| change.Property == SelectionBorderProperty
|| change.Property == SelectionForegroundProperty
|| change.Property == SelectionCornerRadiusProperty)
{
TextView.Redraw();
}
else if (change.Property == OverstrikeModeProperty)
{
Caret.UpdateIfVisible();
}
}
/// <summary>
/// Gets the requested service.
/// </summary>
/// <returns>Returns the requested service instance, or null if the service cannot be found.</returns>
public virtual object GetService(Type serviceType)
{
return TextView.GetService(serviceType);
}
/// <summary>
/// Occurs when text inside the TextArea was copied.
/// </summary>
public event EventHandler<TextEventArgs> TextCopied;
event EventHandler ILogicalScrollable.ScrollInvalidated
{
add { if (_logicalScrollable != null) _logicalScrollable.ScrollInvalidated += value; }
remove { if (_logicalScrollable != null) _logicalScrollable.ScrollInvalidated -= value; }
}
internal void OnTextCopied(TextEventArgs e)
{
TextCopied?.Invoke(this, e);
}
public IList<RoutedCommandBinding> CommandBindings { get; } = new List<RoutedCommandBinding>();
bool ILogicalScrollable.IsLogicalScrollEnabled => _logicalScrollable?.IsLogicalScrollEnabled ?? default(bool);
Size ILogicalScrollable.ScrollSize => _logicalScrollable?.ScrollSize ?? default(Size);
Size ILogicalScrollable.PageScrollSize => _logicalScrollable?.PageScrollSize ?? default(Size);
Size IScrollable.Extent => _logicalScrollable?.Extent ?? default(Size);
Vector IScrollable.Offset
{
get => _logicalScrollable?.Offset ?? default(Vector);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.Offset = value;
}
}
}
Size IScrollable.Viewport => _logicalScrollable?.Viewport ?? default(Size);
bool ILogicalScrollable.CanHorizontallyScroll
{
get => _logicalScrollable?.CanHorizontallyScroll ?? default(bool);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.CanHorizontallyScroll = value;
}
}
}
bool ILogicalScrollable.CanVerticallyScroll
{
get => _logicalScrollable?.CanVerticallyScroll ?? default(bool);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.CanVerticallyScroll = value;
}
}
}
public bool BringIntoView(IControl target, Rect targetRect) =>
_logicalScrollable?.BringIntoView(target, targetRect) ?? default(bool);
IControl ILogicalScrollable.GetControlInDirection(NavigationDirection direction, IControl from)
=> _logicalScrollable?.GetControlInDirection(direction, from);
public void RaiseScrollInvalidated(EventArgs e)
{
_logicalScrollable?.RaiseScrollInvalidated(e);
}
private class TextAreaTextInputMethodClient : ITextInputMethodClient
{
private TextArea _textArea;
public TextAreaTextInputMethodClient()
{
}
public event EventHandler CursorRectangleChanged;
public event EventHandler TextViewVisualChanged;
public event EventHandler SurroundingTextChanged;
public Rect CursorRectangle
{
get
{
if(_textArea == null)
{
return Rect.Empty;
}
var transform = _textArea.TextView.TransformToVisual(_textArea);
if (transform == null)
{
return default;
}
var rect = _textArea.Caret.CalculateCaretRectangle().TransformToAABB(transform.Value);
return rect;
}
}
public IVisual TextViewVisual => _textArea;
public bool SupportsPreedit => false;
public bool SupportsSurroundingText => true;
public TextInputMethodSurroundingText SurroundingText
{
get
{
if(_textArea == null)
{
return default;
}
var lineIndex = _textArea.Caret.Line;
var position = _textArea.Caret.Position;
var documentLine = _textArea.Document.GetLineByNumber(lineIndex);
var text = _textArea.Document.GetText(documentLine.Offset, documentLine.Length);
return new TextInputMethodSurroundingText
{
AnchorOffset = 0,
CursorOffset = position.Column,
Text = text
};
}
}
public void SetTextArea(TextArea textArea)
{
if(_textArea != null)
{
_textArea.Caret.PositionChanged -= Caret_PositionChanged;
_textArea.SelectionChanged -= TextArea_SelectionChanged;
}
_textArea = textArea;
if(_textArea != null)
{
_textArea.Caret.PositionChanged += Caret_PositionChanged;
_textArea.SelectionChanged += TextArea_SelectionChanged;
}
TextViewVisualChanged?.Invoke(this, EventArgs.Empty);
CursorRectangleChanged?.Invoke(this, EventArgs.Empty);
}
private void Caret_PositionChanged(object sender, EventArgs e)
{
CursorRectangleChanged?.Invoke(this, e);
}
private void TextArea_SelectionChanged(object sender, EventArgs e)
{
SurroundingTextChanged?.Invoke(this, e);
}
public void SelectInSurroundingText(int start, int end)
{
if(_textArea == null)
{
return;
}
var selection = _textArea.Selection;
_textArea.Selection = _textArea.Selection.StartSelectionOrSetEndpoint(
new TextViewPosition(selection.StartPosition.Line, start),
new TextViewPosition(selection.StartPosition.Line, end));
}
public void SetPreeditText(string text)
{
}
}
}
/// <summary>
/// EventArgs with text.
/// </summary>
public class TextEventArgs : EventArgs
{
/// <summary>
/// Gets the text.
/// </summary>
public string Text { get; }
/// <summary>
/// Creates a new TextEventArgs instance.
/// </summary>
public TextEventArgs(string text)
{
Text = text ?? throw new ArgumentNullException(nameof(text));
}
}
}
<MSG> Merge pull request #169 from Takoooooo/right-click-moves-caret-feature
You should be able to move caret by right clicking text area
<DFF> @@ -708,6 +708,21 @@ namespace AvaloniaEdit.Editing
get => _readOnlySectionProvider;
set => _readOnlySectionProvider = value ?? throw new ArgumentNullException(nameof(value));
}
+
+ /// <summary>
+ /// The <see cref="RightClickMovesCaret"/> property.
+ /// </summary>
+ public static readonly StyledProperty<bool> RightClickMovesCaretProperty =
+ AvaloniaProperty.Register<TextArea, bool>(nameof(RightClickMovesCaret), false);
+
+ /// <summary>
+ /// Determines whether caret position should be changed to the mouse position when you right click or not.
+ /// </summary>
+ public bool RightClickMovesCaret
+ {
+ get => GetValue(RightClickMovesCaretProperty);
+ set => SetValue(RightClickMovesCaretProperty, value);
+ }
#endregion
#region Focus Handling (Show/Hide Caret)
| 15 | Merge pull request #169 from Takoooooo/right-click-moves-caret-feature | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066152 | <NME> TextArea.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using Avalonia;
using Avalonia.Controls;
using Avalonia.Controls.Presenters;
using Avalonia.Controls.Primitives;
using Avalonia.Data;
using Avalonia.Input;
using Avalonia.Input.TextInput;
using Avalonia.Interactivity;
using Avalonia.Media;
using Avalonia.Threading;
using Avalonia.VisualTree;
using AvaloniaEdit.Document;
using AvaloniaEdit.Indentation;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Search;
using AvaloniaEdit.Utils;
using System;
using System.Collections.Generic;
using System.Collections.Immutable;
using System.Collections.ObjectModel;
using System.Collections.Specialized;
using System.ComponentModel;
using System.Diagnostics;
using System.Linq;
namespace AvaloniaEdit.Editing
{
/// <summary>
/// Control that wraps a TextView and adds support for user input and the caret.
/// </summary>
public class TextArea : TemplatedControl, ITextEditorComponent, IRoutedCommandBindable, ILogicalScrollable
{
/// <summary>
/// This is the extra scrolling space that occurs after the last line.
/// </summary>
private const int AdditionalVerticalScrollAmount = 2;
private ILogicalScrollable _logicalScrollable;
private readonly TextAreaTextInputMethodClient _imClient = new TextAreaTextInputMethodClient();
#region Constructor
static TextArea()
{
KeyboardNavigation.TabNavigationProperty.OverrideDefaultValue<TextArea>(KeyboardNavigationMode.None);
FocusableProperty.OverrideDefaultValue<TextArea>(true);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
AffectsArrange<TextArea>(OffsetProperty);
AffectsRender<TextArea>(OffsetProperty);
TextInputMethodClientRequestedEvent.AddClassHandler<TextArea>((ta, e) =>
{
if (!ta.IsReadOnly)
{
e.Client = ta._imClient;
}
});
}
/// <summary>
/// Creates a new TextArea instance.
/// </summary>
public TextArea() : this(new TextView())
{
AddHandler(KeyDownEvent, OnPreviewKeyDown, RoutingStrategies.Tunnel);
AddHandler(KeyUpEvent, OnPreviewKeyUp, RoutingStrategies.Tunnel);
}
/// <summary>
/// Creates a new TextArea instance.
/// </summary>
protected TextArea(TextView textView)
{
TextView = textView ?? throw new ArgumentNullException(nameof(textView));
_logicalScrollable = textView;
Options = textView.Options;
_selection = EmptySelection = new EmptySelection(this);
textView.Services.AddService(this);
textView.LineTransformers.Add(new SelectionColorizer(this));
textView.InsertLayer(new SelectionLayer(this), KnownLayer.Selection, LayerInsertionPosition.Replace);
Caret = new Caret(this);
Caret.PositionChanged += (sender, e) => RequestSelectionValidation();
Caret.PositionChanged += CaretPositionChanged;
AttachTypingEvents();
LeftMargins.CollectionChanged += LeftMargins_CollectionChanged;
DefaultInputHandler = new TextAreaDefaultInputHandler(this);
ActiveInputHandler = DefaultInputHandler;
// TODO
//textView.GetObservable(TextBlock.FontSizeProperty).Subscribe(_ =>
//{
// TextView.SetScrollOffset(new Vector(_offset.X, _offset.Y * TextView.DefaultLineHeight));
//});
}
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
if (e.NameScope.Find("PART_CP") is ContentPresenter contentPresenter)
{
contentPresenter.Content = TextView;
}
}
internal void AddChild(IVisual visual)
{
VisualChildren.Add(visual);
InvalidateArrange();
}
internal void RemoveChild(IVisual visual)
{
VisualChildren.Remove(visual);
}
#endregion
/// <summary>
/// Defines the <see cref="IScrollable.Offset" /> property.
/// </summary>
public static readonly DirectProperty<TextArea, Vector> OffsetProperty =
AvaloniaProperty.RegisterDirect<TextArea, Vector>(
nameof(IScrollable.Offset),
o => (o as IScrollable).Offset,
(o, v) => (o as IScrollable).Offset = v);
#region InputHandler management
/// <summary>
/// Gets the default input handler.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public TextAreaDefaultInputHandler DefaultInputHandler { get; }
private ITextAreaInputHandler _activeInputHandler;
private bool _isChangingInputHandler;
/// <summary>
/// Gets/Sets the active input handler.
/// This property does not return currently active stacked input handlers. Setting this property detached all stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public ITextAreaInputHandler ActiveInputHandler
{
get => _activeInputHandler;
set
{
if (value != null && value.TextArea != this)
throw new ArgumentException("The input handler was created for a different text area than this one.");
if (_isChangingInputHandler)
throw new InvalidOperationException("Cannot set ActiveInputHandler recursively");
if (_activeInputHandler != value)
{
_isChangingInputHandler = true;
try
{
// pop the whole stack
PopStackedInputHandler(StackedInputHandlers.LastOrDefault());
Debug.Assert(StackedInputHandlers.IsEmpty);
_activeInputHandler?.Detach();
_activeInputHandler = value;
value?.Attach();
}
finally
{
_isChangingInputHandler = false;
}
ActiveInputHandlerChanged?.Invoke(this, EventArgs.Empty);
}
}
}
/// <summary>
/// Occurs when the ActiveInputHandler property changes.
/// </summary>
public event EventHandler ActiveInputHandlerChanged;
/// <summary>
/// Gets the list of currently active stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public ImmutableStack<TextAreaStackedInputHandler> StackedInputHandlers { get; private set; } = ImmutableStack<TextAreaStackedInputHandler>.Empty;
/// <summary>
/// Pushes an input handler onto the list of stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public void PushStackedInputHandler(TextAreaStackedInputHandler inputHandler)
{
if (inputHandler == null)
throw new ArgumentNullException(nameof(inputHandler));
StackedInputHandlers = StackedInputHandlers.Push(inputHandler);
inputHandler.Attach();
}
/// <summary>
/// Pops the stacked input handler (and all input handlers above it).
/// If <paramref name="inputHandler"/> is not found in the currently stacked input handlers, or is null, this method
/// does nothing.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public void PopStackedInputHandler(TextAreaStackedInputHandler inputHandler)
{
if (StackedInputHandlers.Any(i => i == inputHandler))
{
ITextAreaInputHandler oldHandler;
do
{
oldHandler = StackedInputHandlers.Peek();
StackedInputHandlers = StackedInputHandlers.Pop();
oldHandler.Detach();
} while (oldHandler != inputHandler);
}
}
#endregion
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextArea>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <inheritdoc/>
public event EventHandler DocumentChanged;
/// <summary>
/// Gets if the the document displayed by the text editor is readonly
/// </summary>
public bool IsReadOnly
{
get => ReadOnlySectionProvider == ReadOnlySectionDocument.Instance;
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextArea)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.Changing.RemoveHandler(oldValue, OnDocumentChanging);
TextDocumentWeakEventManager.Changed.RemoveHandler(oldValue, OnDocumentChanged);
TextDocumentWeakEventManager.UpdateStarted.RemoveHandler(oldValue, OnUpdateStarted);
TextDocumentWeakEventManager.UpdateFinished.RemoveHandler(oldValue, OnUpdateFinished);
}
TextView.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.Changing.AddHandler(newValue, OnDocumentChanging);
TextDocumentWeakEventManager.Changed.AddHandler(newValue, OnDocumentChanged);
TextDocumentWeakEventManager.UpdateStarted.AddHandler(newValue, OnUpdateStarted);
TextDocumentWeakEventManager.UpdateFinished.AddHandler(newValue, OnUpdateFinished);
InvalidateArrange();
}
// Reset caret location and selection: this is necessary because the caret/selection might be invalid
// in the new document (e.g. if new document is shorter than the old document).
Caret.Location = new TextLocation(1, 1);
ClearSelection();
DocumentChanged?.Invoke(this, EventArgs.Empty);
//CommandManager.InvalidateRequerySuggested();
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextArea>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
private void OnOptionChanged(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextArea)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnOptionChanged);
}
TextView.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnOptionChanged);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
#endregion
#region Caret handling on document changes
private void OnDocumentChanging(object sender, DocumentChangeEventArgs e)
{
Caret.OnDocumentChanging();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
Caret.OnDocumentChanged(e);
Selection = _selection.UpdateOnDocumentChange(e);
}
private void OnUpdateStarted(object sender, EventArgs e)
{
Document.UndoStack.PushOptional(new RestoreCaretAndSelectionUndoAction(this));
}
private void OnUpdateFinished(object sender, EventArgs e)
{
Caret.OnDocumentUpdateFinished();
}
private sealed class RestoreCaretAndSelectionUndoAction : IUndoableOperation
{
// keep textarea in weak reference because the IUndoableOperation is stored with the document
private readonly WeakReference _textAreaReference;
private readonly TextViewPosition _caretPosition;
private readonly Selection _selection;
public RestoreCaretAndSelectionUndoAction(TextArea textArea)
{
_textAreaReference = new WeakReference(textArea);
// Just save the old caret position, no need to validate here.
// If we restore it, we'll validate it anyways.
_caretPosition = textArea.Caret.NonValidatedPosition;
_selection = textArea.Selection;
}
public void Undo()
{
var textArea = (TextArea)_textAreaReference.Target;
if (textArea != null)
{
textArea.Caret.Position = _caretPosition;
textArea.Selection = _selection;
}
}
public void Redo()
{
// redo=undo: we just restore the caret/selection state
Undo();
}
}
#endregion
#region TextView property
/// <summary>
/// Gets the text view used to display text in this text area.
/// </summary>
public TextView TextView { get; }
#endregion
#region Selection property
internal readonly Selection EmptySelection;
private Selection _selection;
/// <summary>
/// Occurs when the selection has changed.
/// </summary>
public event EventHandler SelectionChanged;
/// <summary>
/// Gets/Sets the selection in this text area.
/// </summary>
public Selection Selection
{
get => _selection;
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
if (value.TextArea != this)
throw new ArgumentException("Cannot use a Selection instance that belongs to another text area.");
if (!Equals(_selection, value))
{
if (TextView != null)
{
var oldSegment = _selection.SurroundingSegment;
var newSegment = value.SurroundingSegment;
if (!Selection.EnableVirtualSpace && (_selection is SimpleSelection && value is SimpleSelection && oldSegment != null && newSegment != null))
{
// perf optimization:
// When a simple selection changes, don't redraw the whole selection, but only the changed parts.
var oldSegmentOffset = oldSegment.Offset;
var newSegmentOffset = newSegment.Offset;
if (oldSegmentOffset != newSegmentOffset)
{
TextView.Redraw(Math.Min(oldSegmentOffset, newSegmentOffset),
Math.Abs(oldSegmentOffset - newSegmentOffset));
}
var oldSegmentEndOffset = oldSegment.EndOffset;
var newSegmentEndOffset = newSegment.EndOffset;
if (oldSegmentEndOffset != newSegmentEndOffset)
{
TextView.Redraw(Math.Min(oldSegmentEndOffset, newSegmentEndOffset),
Math.Abs(oldSegmentEndOffset - newSegmentEndOffset));
}
}
else
{
TextView.Redraw(oldSegment);
TextView.Redraw(newSegment);
}
}
_selection = value;
SelectionChanged?.Invoke(this, EventArgs.Empty);
// a selection change causes commands like copy/paste/etc. to change status
//CommandManager.InvalidateRequerySuggested();
}
}
}
/// <summary>
/// Clears the current selection.
/// </summary>
public void ClearSelection()
{
Selection = EmptySelection;
}
/// <summary>
/// The <see cref="SelectionBrush"/> property.
/// </summary>
public static readonly StyledProperty<IBrush> SelectionBrushProperty =
AvaloniaProperty.Register<TextArea, IBrush>("SelectionBrush");
/// <summary>
/// Gets/Sets the background brush used for the selection.
/// </summary>
public IBrush SelectionBrush
{
get => GetValue(SelectionBrushProperty);
set => SetValue(SelectionBrushProperty, value);
}
/// <summary>
/// The <see cref="SelectionForeground"/> property.
/// </summary>
public static readonly StyledProperty<IBrush> SelectionForegroundProperty =
AvaloniaProperty.Register<TextArea, IBrush>("SelectionForeground");
/// <summary>
/// Gets/Sets the foreground brush used for selected text.
/// </summary>
public IBrush SelectionForeground
{
get => GetValue(SelectionForegroundProperty);
set => SetValue(SelectionForegroundProperty, value);
}
/// <summary>
/// The <see cref="SelectionBorder"/> property.
/// </summary>
public static readonly StyledProperty<Pen> SelectionBorderProperty =
AvaloniaProperty.Register<TextArea, Pen>("SelectionBorder");
/// <summary>
/// Gets/Sets the pen used for the border of the selection.
/// </summary>
public Pen SelectionBorder
{
get => GetValue(SelectionBorderProperty);
set => SetValue(SelectionBorderProperty, value);
}
/// <summary>
/// The <see cref="SelectionCornerRadius"/> property.
/// </summary>
public static readonly StyledProperty<double> SelectionCornerRadiusProperty =
AvaloniaProperty.Register<TextArea, double>("SelectionCornerRadius", 3.0);
/// <summary>
/// Gets/Sets the corner radius of the selection.
/// </summary>
public double SelectionCornerRadius
{
get => GetValue(SelectionCornerRadiusProperty);
set => SetValue(SelectionCornerRadiusProperty, value);
}
#endregion
#region Force caret to stay inside selection
private bool _ensureSelectionValidRequested;
private int _allowCaretOutsideSelection;
private void RequestSelectionValidation()
{
if (!_ensureSelectionValidRequested && _allowCaretOutsideSelection == 0)
{
_ensureSelectionValidRequested = true;
Dispatcher.UIThread.Post(EnsureSelectionValid);
}
}
/// <summary>
/// Code that updates only the caret but not the selection can cause confusion when
/// keys like 'Delete' delete the (possibly invisible) selected text and not the
/// text around the caret.
///
/// So we'll ensure that the caret is inside the selection.
/// (when the caret is not in the selection, we'll clear the selection)
///
/// This method is invoked using the Dispatcher so that code may temporarily violate this rule
/// (e.g. most 'extend selection' methods work by first setting the caret, then the selection),
/// it's sufficient to fix it after any event handlers have run.
/// </summary>
private void EnsureSelectionValid()
{
_ensureSelectionValidRequested = false;
if (_allowCaretOutsideSelection == 0)
{
if (!_selection.IsEmpty && !_selection.Contains(Caret.Offset))
{
ClearSelection();
}
}
}
/// <summary>
/// Temporarily allows positioning the caret outside the selection.
/// Dispose the returned IDisposable to revert the allowance.
/// </summary>
/// <remarks>
/// The text area only forces the caret to be inside the selection when other events
/// have finished running (using the dispatcher), so you don't have to use this method
/// for temporarily positioning the caret in event handlers.
/// This method is only necessary if you want to run the dispatcher, e.g. if you
/// perform a drag'n'drop operation.
/// </remarks>
public IDisposable AllowCaretOutsideSelection()
{
VerifyAccess();
_allowCaretOutsideSelection++;
return new CallbackOnDispose(
delegate
{
VerifyAccess();
_allowCaretOutsideSelection--;
RequestSelectionValidation();
});
}
#endregion
#region Properties
/// <summary>
/// Gets the Caret used for this text area.
/// </summary>
public Caret Caret { get; }
/// <summary>
/// Scrolls the text view so that the requested line is in the middle.
/// If the textview can be scrolled.
/// </summary>
/// <param name="line">The line to scroll to.</param>
public void ScrollToLine (int line)
{
var viewPortLines = (int)(this as IScrollable).Viewport.Height;
if (viewPortLines < Document.LineCount)
{
ScrollToLine(line, 2, viewPortLines / 2);
}
}
/// <summary>
/// Scrolls the textview to a position with n lines above and below it.
/// </summary>
/// <param name="line">the requested line number.</param>
/// <param name="linesEitherSide">The number of lines above and below.</param>
public void ScrollToLine(int line, int linesEitherSide)
{
ScrollToLine(line, linesEitherSide, linesEitherSide);
}
/// <summary>
/// Scrolls the textview to a position with n lines above and below it.
/// </summary>
/// <param name="line">the requested line number.</param>
/// <param name="linesAbove">The number of lines above.</param>
/// <param name="linesBelow">The number of lines below.</param>
public void ScrollToLine(int line, int linesAbove, int linesBelow)
{
var offset = line - linesAbove;
if (offset < 0)
{
offset = 0;
}
this.BringIntoView(new Rect(1, offset, 0, 1));
offset = line + linesBelow;
if (offset >= 0)
{
this.BringIntoView(new Rect(1, offset, 0, 1));
}
}
private void CaretPositionChanged(object sender, EventArgs e)
{
if (TextView == null)
return;
TextView.HighlightedLine = Caret.Line;
ScrollToLine(Caret.Line, 2);
Dispatcher.UIThread.InvokeAsync(() =>
{
(this as ILogicalScrollable).RaiseScrollInvalidated(EventArgs.Empty);
});
}
public static readonly DirectProperty<TextArea, ObservableCollection<IControl>> LeftMarginsProperty
= AvaloniaProperty.RegisterDirect<TextArea, ObservableCollection<IControl>>(nameof(LeftMargins),
c => c.LeftMargins);
/// <summary>
/// Gets the collection of margins displayed to the left of the text view.
/// </summary>
public ObservableCollection<IControl> LeftMargins { get; } = new ObservableCollection<IControl>();
private void LeftMargins_CollectionChanged(object sender, NotifyCollectionChangedEventArgs e)
{
if (e.OldItems != null)
{
foreach (var c in e.OldItems.OfType<ITextViewConnect>())
{
c.RemoveFromTextView(TextView);
}
get => _readOnlySectionProvider;
set => _readOnlySectionProvider = value ?? throw new ArgumentNullException(nameof(value));
}
#endregion
#region Focus Handling (Show/Hide Caret)
/// <summary>
/// The <see cref="RightClickMovesCaret"/> property.
/// </summary>
public static readonly StyledProperty<bool> RightClickMovesCaretProperty =
AvaloniaProperty.Register<TextArea, bool>(nameof(RightClickMovesCaret), false);
/// <summary>
/// Determines whether caret position should be changed to the mouse position when you right click or not.
/// </summary>
public bool RightClickMovesCaret
{
get => GetValue(RightClickMovesCaretProperty);
set => SetValue(RightClickMovesCaretProperty, value);
}
#endregion
#region Focus Handling (Show/Hide Caret)
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
Focus();
}
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
Caret.Show();
_imClient.SetTextArea(this);
}
protected override void OnLostFocus(RoutedEventArgs e)
{
base.OnLostFocus(e);
Caret.Hide();
_imClient.SetTextArea(null);
}
#endregion
#region OnTextInput / RemoveSelectedText / ReplaceSelectionWithText
/// <summary>
/// Occurs when the TextArea receives text input.
/// but occurs immediately before the TextArea handles the TextInput event.
/// </summary>
public event EventHandler<TextInputEventArgs> TextEntering;
/// <summary>
/// Occurs when the TextArea receives text input.
/// but occurs immediately after the TextArea handles the TextInput event.
/// </summary>
public event EventHandler<TextInputEventArgs> TextEntered;
/// <summary>
/// Raises the TextEntering event.
/// </summary>
protected virtual void OnTextEntering(TextInputEventArgs e)
{
TextEntering?.Invoke(this, e);
}
/// <summary>
/// Raises the TextEntered event.
/// </summary>
protected virtual void OnTextEntered(TextInputEventArgs e)
{
TextEntered?.Invoke(this, e);
}
protected override void OnTextInput(TextInputEventArgs e)
{
base.OnTextInput(e);
if (!e.Handled && Document != null)
{
if (string.IsNullOrEmpty(e.Text) || e.Text == "\x1b" || e.Text == "\b" || e.Text == "\u007f")
{
// TODO: check this
// ASCII 0x1b = ESC.
// produces a TextInput event with that old ASCII control char
// when Escape is pressed. We'll just ignore it.
// A deadkey followed by backspace causes a textinput event for the BS character.
// Similarly, some shortcuts like Alt+Space produce an empty TextInput event.
// We have to ignore those (not handle them) to keep the shortcut working.
return;
}
HideMouseCursor();
PerformTextInput(e);
e.Handled = true;
}
}
/// <summary>
/// Performs text input.
/// This raises the <see cref="TextEntering"/> event, replaces the selection with the text,
/// and then raises the <see cref="TextEntered"/> event.
/// </summary>
public void PerformTextInput(string text)
{
var e = new TextInputEventArgs
{
Text = text,
RoutedEvent = TextInputEvent
};
PerformTextInput(e);
}
/// <summary>
/// Performs text input.
/// This raises the <see cref="TextEntering"/> event, replaces the selection with the text,
/// and then raises the <see cref="TextEntered"/> event.
/// </summary>
public void PerformTextInput(TextInputEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
OnTextEntering(e);
if (!e.Handled)
{
if (e.Text == "\n" || e.Text == "\r" || e.Text == "\r\n")
ReplaceSelectionWithNewLine();
else
{
// TODO
//if (OverstrikeMode && Selection.IsEmpty && Document.GetLineByNumber(Caret.Line).EndOffset > Caret.Offset)
// EditingCommands.SelectRightByCharacter.Execute(null, this);
ReplaceSelectionWithText(e.Text);
}
OnTextEntered(e);
Caret.BringCaretToView();
}
}
private void ReplaceSelectionWithNewLine()
{
var newLine = TextUtilities.GetNewLineFromDocument(Document, Caret.Line);
using (Document.RunUpdate())
{
ReplaceSelectionWithText(newLine);
if (IndentationStrategy != null)
{
var line = Document.GetLineByNumber(Caret.Line);
var deletable = GetDeletableSegments(line);
if (deletable.Length == 1 && deletable[0].Offset == line.Offset && deletable[0].Length == line.Length)
{
// use indentation strategy only if the line is not read-only
IndentationStrategy.IndentLine(Document, line);
}
}
}
}
internal void RemoveSelectedText()
{
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
_selection.ReplaceSelectionWithText(string.Empty);
#if DEBUG
if (!_selection.IsEmpty)
{
foreach (var s in _selection.Segments)
{
Debug.Assert(!ReadOnlySectionProvider.GetDeletableSegments(s).Any());
}
}
#endif
}
internal void ReplaceSelectionWithText(string newText)
{
if (newText == null)
throw new ArgumentNullException(nameof(newText));
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
_selection.ReplaceSelectionWithText(newText);
}
internal ISegment[] GetDeletableSegments(ISegment segment)
{
var deletableSegments = ReadOnlySectionProvider.GetDeletableSegments(segment);
if (deletableSegments == null)
throw new InvalidOperationException("ReadOnlySectionProvider.GetDeletableSegments returned null");
var array = deletableSegments.ToArray();
var lastIndex = segment.Offset;
foreach (var t in array)
{
if (t.Offset < lastIndex)
throw new InvalidOperationException("ReadOnlySectionProvider returned incorrect segments (outside of input segment / wrong order)");
lastIndex = t.EndOffset;
}
if (lastIndex > segment.EndOffset)
throw new InvalidOperationException("ReadOnlySectionProvider returned incorrect segments (outside of input segment / wrong order)");
return array;
}
#endregion
#region IndentationStrategy property
/// <summary>
/// IndentationStrategy property.
/// </summary>
public static readonly StyledProperty<IIndentationStrategy> IndentationStrategyProperty =
AvaloniaProperty.Register<TextArea, IIndentationStrategy>("IndentationStrategy", new DefaultIndentationStrategy());
/// <summary>
/// Gets/Sets the indentation strategy used when inserting new lines.
/// </summary>
public IIndentationStrategy IndentationStrategy
{
get => GetValue(IndentationStrategyProperty);
set => SetValue(IndentationStrategyProperty, value);
}
#endregion
#region OnKeyDown/OnKeyUp
// Make life easier for text editor extensions that use a different cursor based on the pressed modifier keys.
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
TextView.InvalidateCursorIfPointerWithinTextView();
}
private void OnPreviewKeyDown(object sender, KeyEventArgs e)
{
foreach (var h in StackedInputHandlers)
{
if (e.Handled)
break;
h.OnPreviewKeyDown(e);
}
}
/// <inheritdoc/>
protected override void OnKeyUp(KeyEventArgs e)
{
base.OnKeyUp(e);
TextView.InvalidateCursorIfPointerWithinTextView();
}
private void OnPreviewKeyUp(object sender, KeyEventArgs e)
{
foreach (var h in StackedInputHandlers)
{
if (e.Handled)
break;
h.OnPreviewKeyUp(e);
}
}
#endregion
#region Hide Mouse Cursor While Typing
private bool _isMouseCursorHidden;
private void AttachTypingEvents()
{
// Use the PreviewMouseMove event in case some other editor layer consumes the MouseMove event (e.g. SD's InsertionCursorLayer)
PointerEntered += delegate { ShowMouseCursor(); };
PointerExited += delegate { ShowMouseCursor(); };
}
private void ShowMouseCursor()
{
if (_isMouseCursorHidden)
{
_isMouseCursorHidden = false;
}
}
private void HideMouseCursor()
{
if (Options.HideCursorWhileTyping && !_isMouseCursorHidden && IsPointerOver)
{
_isMouseCursorHidden = true;
}
}
#endregion
#region Overstrike mode
/// <summary>
/// The <see cref="OverstrikeMode"/> dependency property.
/// </summary>
public static readonly StyledProperty<bool> OverstrikeModeProperty =
AvaloniaProperty.Register<TextArea, bool>("OverstrikeMode");
/// <summary>
/// Gets/Sets whether overstrike mode is active.
/// </summary>
public bool OverstrikeMode
{
get => GetValue(OverstrikeModeProperty);
set => SetValue(OverstrikeModeProperty, value);
}
#endregion
/// <inheritdoc/>
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == SelectionBrushProperty
|| change.Property == SelectionBorderProperty
|| change.Property == SelectionForegroundProperty
|| change.Property == SelectionCornerRadiusProperty)
{
TextView.Redraw();
}
else if (change.Property == OverstrikeModeProperty)
{
Caret.UpdateIfVisible();
}
}
/// <summary>
/// Gets the requested service.
/// </summary>
/// <returns>Returns the requested service instance, or null if the service cannot be found.</returns>
public virtual object GetService(Type serviceType)
{
return TextView.GetService(serviceType);
}
/// <summary>
/// Occurs when text inside the TextArea was copied.
/// </summary>
public event EventHandler<TextEventArgs> TextCopied;
event EventHandler ILogicalScrollable.ScrollInvalidated
{
add { if (_logicalScrollable != null) _logicalScrollable.ScrollInvalidated += value; }
remove { if (_logicalScrollable != null) _logicalScrollable.ScrollInvalidated -= value; }
}
internal void OnTextCopied(TextEventArgs e)
{
TextCopied?.Invoke(this, e);
}
public IList<RoutedCommandBinding> CommandBindings { get; } = new List<RoutedCommandBinding>();
bool ILogicalScrollable.IsLogicalScrollEnabled => _logicalScrollable?.IsLogicalScrollEnabled ?? default(bool);
Size ILogicalScrollable.ScrollSize => _logicalScrollable?.ScrollSize ?? default(Size);
Size ILogicalScrollable.PageScrollSize => _logicalScrollable?.PageScrollSize ?? default(Size);
Size IScrollable.Extent => _logicalScrollable?.Extent ?? default(Size);
Vector IScrollable.Offset
{
get => _logicalScrollable?.Offset ?? default(Vector);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.Offset = value;
}
}
}
Size IScrollable.Viewport => _logicalScrollable?.Viewport ?? default(Size);
bool ILogicalScrollable.CanHorizontallyScroll
{
get => _logicalScrollable?.CanHorizontallyScroll ?? default(bool);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.CanHorizontallyScroll = value;
}
}
}
bool ILogicalScrollable.CanVerticallyScroll
{
get => _logicalScrollable?.CanVerticallyScroll ?? default(bool);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.CanVerticallyScroll = value;
}
}
}
public bool BringIntoView(IControl target, Rect targetRect) =>
_logicalScrollable?.BringIntoView(target, targetRect) ?? default(bool);
IControl ILogicalScrollable.GetControlInDirection(NavigationDirection direction, IControl from)
=> _logicalScrollable?.GetControlInDirection(direction, from);
public void RaiseScrollInvalidated(EventArgs e)
{
_logicalScrollable?.RaiseScrollInvalidated(e);
}
private class TextAreaTextInputMethodClient : ITextInputMethodClient
{
private TextArea _textArea;
public TextAreaTextInputMethodClient()
{
}
public event EventHandler CursorRectangleChanged;
public event EventHandler TextViewVisualChanged;
public event EventHandler SurroundingTextChanged;
public Rect CursorRectangle
{
get
{
if(_textArea == null)
{
return Rect.Empty;
}
var transform = _textArea.TextView.TransformToVisual(_textArea);
if (transform == null)
{
return default;
}
var rect = _textArea.Caret.CalculateCaretRectangle().TransformToAABB(transform.Value);
return rect;
}
}
public IVisual TextViewVisual => _textArea;
public bool SupportsPreedit => false;
public bool SupportsSurroundingText => true;
public TextInputMethodSurroundingText SurroundingText
{
get
{
if(_textArea == null)
{
return default;
}
var lineIndex = _textArea.Caret.Line;
var position = _textArea.Caret.Position;
var documentLine = _textArea.Document.GetLineByNumber(lineIndex);
var text = _textArea.Document.GetText(documentLine.Offset, documentLine.Length);
return new TextInputMethodSurroundingText
{
AnchorOffset = 0,
CursorOffset = position.Column,
Text = text
};
}
}
public void SetTextArea(TextArea textArea)
{
if(_textArea != null)
{
_textArea.Caret.PositionChanged -= Caret_PositionChanged;
_textArea.SelectionChanged -= TextArea_SelectionChanged;
}
_textArea = textArea;
if(_textArea != null)
{
_textArea.Caret.PositionChanged += Caret_PositionChanged;
_textArea.SelectionChanged += TextArea_SelectionChanged;
}
TextViewVisualChanged?.Invoke(this, EventArgs.Empty);
CursorRectangleChanged?.Invoke(this, EventArgs.Empty);
}
private void Caret_PositionChanged(object sender, EventArgs e)
{
CursorRectangleChanged?.Invoke(this, e);
}
private void TextArea_SelectionChanged(object sender, EventArgs e)
{
SurroundingTextChanged?.Invoke(this, e);
}
public void SelectInSurroundingText(int start, int end)
{
if(_textArea == null)
{
return;
}
var selection = _textArea.Selection;
_textArea.Selection = _textArea.Selection.StartSelectionOrSetEndpoint(
new TextViewPosition(selection.StartPosition.Line, start),
new TextViewPosition(selection.StartPosition.Line, end));
}
public void SetPreeditText(string text)
{
}
}
}
/// <summary>
/// EventArgs with text.
/// </summary>
public class TextEventArgs : EventArgs
{
/// <summary>
/// Gets the text.
/// </summary>
public string Text { get; }
/// <summary>
/// Creates a new TextEventArgs instance.
/// </summary>
public TextEventArgs(string text)
{
Text = text ?? throw new ArgumentNullException(nameof(text));
}
}
}
<MSG> Merge pull request #169 from Takoooooo/right-click-moves-caret-feature
You should be able to move caret by right clicking text area
<DFF> @@ -708,6 +708,21 @@ namespace AvaloniaEdit.Editing
get => _readOnlySectionProvider;
set => _readOnlySectionProvider = value ?? throw new ArgumentNullException(nameof(value));
}
+
+ /// <summary>
+ /// The <see cref="RightClickMovesCaret"/> property.
+ /// </summary>
+ public static readonly StyledProperty<bool> RightClickMovesCaretProperty =
+ AvaloniaProperty.Register<TextArea, bool>(nameof(RightClickMovesCaret), false);
+
+ /// <summary>
+ /// Determines whether caret position should be changed to the mouse position when you right click or not.
+ /// </summary>
+ public bool RightClickMovesCaret
+ {
+ get => GetValue(RightClickMovesCaretProperty);
+ set => SetValue(RightClickMovesCaretProperty, value);
+ }
#endregion
#region Focus Handling (Show/Hide Caret)
| 15 | Merge pull request #169 from Takoooooo/right-click-moves-caret-feature | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066153 | <NME> TextArea.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using Avalonia;
using Avalonia.Controls;
using Avalonia.Controls.Presenters;
using Avalonia.Controls.Primitives;
using Avalonia.Data;
using Avalonia.Input;
using Avalonia.Input.TextInput;
using Avalonia.Interactivity;
using Avalonia.Media;
using Avalonia.Threading;
using Avalonia.VisualTree;
using AvaloniaEdit.Document;
using AvaloniaEdit.Indentation;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Search;
using AvaloniaEdit.Utils;
using System;
using System.Collections.Generic;
using System.Collections.Immutable;
using System.Collections.ObjectModel;
using System.Collections.Specialized;
using System.ComponentModel;
using System.Diagnostics;
using System.Linq;
namespace AvaloniaEdit.Editing
{
/// <summary>
/// Control that wraps a TextView and adds support for user input and the caret.
/// </summary>
public class TextArea : TemplatedControl, ITextEditorComponent, IRoutedCommandBindable, ILogicalScrollable
{
/// <summary>
/// This is the extra scrolling space that occurs after the last line.
/// </summary>
private const int AdditionalVerticalScrollAmount = 2;
private ILogicalScrollable _logicalScrollable;
private readonly TextAreaTextInputMethodClient _imClient = new TextAreaTextInputMethodClient();
#region Constructor
static TextArea()
{
KeyboardNavigation.TabNavigationProperty.OverrideDefaultValue<TextArea>(KeyboardNavigationMode.None);
FocusableProperty.OverrideDefaultValue<TextArea>(true);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
AffectsArrange<TextArea>(OffsetProperty);
AffectsRender<TextArea>(OffsetProperty);
TextInputMethodClientRequestedEvent.AddClassHandler<TextArea>((ta, e) =>
{
if (!ta.IsReadOnly)
{
e.Client = ta._imClient;
}
});
}
/// <summary>
/// Creates a new TextArea instance.
/// </summary>
public TextArea() : this(new TextView())
{
AddHandler(KeyDownEvent, OnPreviewKeyDown, RoutingStrategies.Tunnel);
AddHandler(KeyUpEvent, OnPreviewKeyUp, RoutingStrategies.Tunnel);
}
/// <summary>
/// Creates a new TextArea instance.
/// </summary>
protected TextArea(TextView textView)
{
TextView = textView ?? throw new ArgumentNullException(nameof(textView));
_logicalScrollable = textView;
Options = textView.Options;
_selection = EmptySelection = new EmptySelection(this);
textView.Services.AddService(this);
textView.LineTransformers.Add(new SelectionColorizer(this));
textView.InsertLayer(new SelectionLayer(this), KnownLayer.Selection, LayerInsertionPosition.Replace);
Caret = new Caret(this);
Caret.PositionChanged += (sender, e) => RequestSelectionValidation();
Caret.PositionChanged += CaretPositionChanged;
AttachTypingEvents();
LeftMargins.CollectionChanged += LeftMargins_CollectionChanged;
DefaultInputHandler = new TextAreaDefaultInputHandler(this);
ActiveInputHandler = DefaultInputHandler;
// TODO
//textView.GetObservable(TextBlock.FontSizeProperty).Subscribe(_ =>
//{
// TextView.SetScrollOffset(new Vector(_offset.X, _offset.Y * TextView.DefaultLineHeight));
//});
}
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
if (e.NameScope.Find("PART_CP") is ContentPresenter contentPresenter)
{
contentPresenter.Content = TextView;
}
}
internal void AddChild(IVisual visual)
{
VisualChildren.Add(visual);
InvalidateArrange();
}
internal void RemoveChild(IVisual visual)
{
VisualChildren.Remove(visual);
}
#endregion
/// <summary>
/// Defines the <see cref="IScrollable.Offset" /> property.
/// </summary>
public static readonly DirectProperty<TextArea, Vector> OffsetProperty =
AvaloniaProperty.RegisterDirect<TextArea, Vector>(
nameof(IScrollable.Offset),
o => (o as IScrollable).Offset,
(o, v) => (o as IScrollable).Offset = v);
#region InputHandler management
/// <summary>
/// Gets the default input handler.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public TextAreaDefaultInputHandler DefaultInputHandler { get; }
private ITextAreaInputHandler _activeInputHandler;
private bool _isChangingInputHandler;
/// <summary>
/// Gets/Sets the active input handler.
/// This property does not return currently active stacked input handlers. Setting this property detached all stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public ITextAreaInputHandler ActiveInputHandler
{
get => _activeInputHandler;
set
{
if (value != null && value.TextArea != this)
throw new ArgumentException("The input handler was created for a different text area than this one.");
if (_isChangingInputHandler)
throw new InvalidOperationException("Cannot set ActiveInputHandler recursively");
if (_activeInputHandler != value)
{
_isChangingInputHandler = true;
try
{
// pop the whole stack
PopStackedInputHandler(StackedInputHandlers.LastOrDefault());
Debug.Assert(StackedInputHandlers.IsEmpty);
_activeInputHandler?.Detach();
_activeInputHandler = value;
value?.Attach();
}
finally
{
_isChangingInputHandler = false;
}
ActiveInputHandlerChanged?.Invoke(this, EventArgs.Empty);
}
}
}
/// <summary>
/// Occurs when the ActiveInputHandler property changes.
/// </summary>
public event EventHandler ActiveInputHandlerChanged;
/// <summary>
/// Gets the list of currently active stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public ImmutableStack<TextAreaStackedInputHandler> StackedInputHandlers { get; private set; } = ImmutableStack<TextAreaStackedInputHandler>.Empty;
/// <summary>
/// Pushes an input handler onto the list of stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public void PushStackedInputHandler(TextAreaStackedInputHandler inputHandler)
{
if (inputHandler == null)
throw new ArgumentNullException(nameof(inputHandler));
StackedInputHandlers = StackedInputHandlers.Push(inputHandler);
inputHandler.Attach();
}
/// <summary>
/// Pops the stacked input handler (and all input handlers above it).
/// If <paramref name="inputHandler"/> is not found in the currently stacked input handlers, or is null, this method
/// does nothing.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public void PopStackedInputHandler(TextAreaStackedInputHandler inputHandler)
{
if (StackedInputHandlers.Any(i => i == inputHandler))
{
ITextAreaInputHandler oldHandler;
do
{
oldHandler = StackedInputHandlers.Peek();
StackedInputHandlers = StackedInputHandlers.Pop();
oldHandler.Detach();
} while (oldHandler != inputHandler);
}
}
#endregion
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextArea>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <inheritdoc/>
public event EventHandler DocumentChanged;
/// <summary>
/// Gets if the the document displayed by the text editor is readonly
/// </summary>
public bool IsReadOnly
{
get => ReadOnlySectionProvider == ReadOnlySectionDocument.Instance;
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextArea)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.Changing.RemoveHandler(oldValue, OnDocumentChanging);
TextDocumentWeakEventManager.Changed.RemoveHandler(oldValue, OnDocumentChanged);
TextDocumentWeakEventManager.UpdateStarted.RemoveHandler(oldValue, OnUpdateStarted);
TextDocumentWeakEventManager.UpdateFinished.RemoveHandler(oldValue, OnUpdateFinished);
}
TextView.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.Changing.AddHandler(newValue, OnDocumentChanging);
TextDocumentWeakEventManager.Changed.AddHandler(newValue, OnDocumentChanged);
TextDocumentWeakEventManager.UpdateStarted.AddHandler(newValue, OnUpdateStarted);
TextDocumentWeakEventManager.UpdateFinished.AddHandler(newValue, OnUpdateFinished);
InvalidateArrange();
}
// Reset caret location and selection: this is necessary because the caret/selection might be invalid
// in the new document (e.g. if new document is shorter than the old document).
Caret.Location = new TextLocation(1, 1);
ClearSelection();
DocumentChanged?.Invoke(this, EventArgs.Empty);
//CommandManager.InvalidateRequerySuggested();
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextArea>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
private void OnOptionChanged(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextArea)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnOptionChanged);
}
TextView.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnOptionChanged);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
#endregion
#region Caret handling on document changes
private void OnDocumentChanging(object sender, DocumentChangeEventArgs e)
{
Caret.OnDocumentChanging();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
Caret.OnDocumentChanged(e);
Selection = _selection.UpdateOnDocumentChange(e);
}
private void OnUpdateStarted(object sender, EventArgs e)
{
Document.UndoStack.PushOptional(new RestoreCaretAndSelectionUndoAction(this));
}
private void OnUpdateFinished(object sender, EventArgs e)
{
Caret.OnDocumentUpdateFinished();
}
private sealed class RestoreCaretAndSelectionUndoAction : IUndoableOperation
{
// keep textarea in weak reference because the IUndoableOperation is stored with the document
private readonly WeakReference _textAreaReference;
private readonly TextViewPosition _caretPosition;
private readonly Selection _selection;
public RestoreCaretAndSelectionUndoAction(TextArea textArea)
{
_textAreaReference = new WeakReference(textArea);
// Just save the old caret position, no need to validate here.
// If we restore it, we'll validate it anyways.
_caretPosition = textArea.Caret.NonValidatedPosition;
_selection = textArea.Selection;
}
public void Undo()
{
var textArea = (TextArea)_textAreaReference.Target;
if (textArea != null)
{
textArea.Caret.Position = _caretPosition;
textArea.Selection = _selection;
}
}
public void Redo()
{
// redo=undo: we just restore the caret/selection state
Undo();
}
}
#endregion
#region TextView property
/// <summary>
/// Gets the text view used to display text in this text area.
/// </summary>
public TextView TextView { get; }
#endregion
#region Selection property
internal readonly Selection EmptySelection;
private Selection _selection;
/// <summary>
/// Occurs when the selection has changed.
/// </summary>
public event EventHandler SelectionChanged;
/// <summary>
/// Gets/Sets the selection in this text area.
/// </summary>
public Selection Selection
{
get => _selection;
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
if (value.TextArea != this)
throw new ArgumentException("Cannot use a Selection instance that belongs to another text area.");
if (!Equals(_selection, value))
{
if (TextView != null)
{
var oldSegment = _selection.SurroundingSegment;
var newSegment = value.SurroundingSegment;
if (!Selection.EnableVirtualSpace && (_selection is SimpleSelection && value is SimpleSelection && oldSegment != null && newSegment != null))
{
// perf optimization:
// When a simple selection changes, don't redraw the whole selection, but only the changed parts.
var oldSegmentOffset = oldSegment.Offset;
var newSegmentOffset = newSegment.Offset;
if (oldSegmentOffset != newSegmentOffset)
{
TextView.Redraw(Math.Min(oldSegmentOffset, newSegmentOffset),
Math.Abs(oldSegmentOffset - newSegmentOffset));
}
var oldSegmentEndOffset = oldSegment.EndOffset;
var newSegmentEndOffset = newSegment.EndOffset;
if (oldSegmentEndOffset != newSegmentEndOffset)
{
TextView.Redraw(Math.Min(oldSegmentEndOffset, newSegmentEndOffset),
Math.Abs(oldSegmentEndOffset - newSegmentEndOffset));
}
}
else
{
TextView.Redraw(oldSegment);
TextView.Redraw(newSegment);
}
}
_selection = value;
SelectionChanged?.Invoke(this, EventArgs.Empty);
// a selection change causes commands like copy/paste/etc. to change status
//CommandManager.InvalidateRequerySuggested();
}
}
}
/// <summary>
/// Clears the current selection.
/// </summary>
public void ClearSelection()
{
Selection = EmptySelection;
}
/// <summary>
/// The <see cref="SelectionBrush"/> property.
/// </summary>
public static readonly StyledProperty<IBrush> SelectionBrushProperty =
AvaloniaProperty.Register<TextArea, IBrush>("SelectionBrush");
/// <summary>
/// Gets/Sets the background brush used for the selection.
/// </summary>
public IBrush SelectionBrush
{
get => GetValue(SelectionBrushProperty);
set => SetValue(SelectionBrushProperty, value);
}
/// <summary>
/// The <see cref="SelectionForeground"/> property.
/// </summary>
public static readonly StyledProperty<IBrush> SelectionForegroundProperty =
AvaloniaProperty.Register<TextArea, IBrush>("SelectionForeground");
/// <summary>
/// Gets/Sets the foreground brush used for selected text.
/// </summary>
public IBrush SelectionForeground
{
get => GetValue(SelectionForegroundProperty);
set => SetValue(SelectionForegroundProperty, value);
}
/// <summary>
/// The <see cref="SelectionBorder"/> property.
/// </summary>
public static readonly StyledProperty<Pen> SelectionBorderProperty =
AvaloniaProperty.Register<TextArea, Pen>("SelectionBorder");
/// <summary>
/// Gets/Sets the pen used for the border of the selection.
/// </summary>
public Pen SelectionBorder
{
get => GetValue(SelectionBorderProperty);
set => SetValue(SelectionBorderProperty, value);
}
/// <summary>
/// The <see cref="SelectionCornerRadius"/> property.
/// </summary>
public static readonly StyledProperty<double> SelectionCornerRadiusProperty =
AvaloniaProperty.Register<TextArea, double>("SelectionCornerRadius", 3.0);
/// <summary>
/// Gets/Sets the corner radius of the selection.
/// </summary>
public double SelectionCornerRadius
{
get => GetValue(SelectionCornerRadiusProperty);
set => SetValue(SelectionCornerRadiusProperty, value);
}
#endregion
#region Force caret to stay inside selection
private bool _ensureSelectionValidRequested;
private int _allowCaretOutsideSelection;
private void RequestSelectionValidation()
{
if (!_ensureSelectionValidRequested && _allowCaretOutsideSelection == 0)
{
_ensureSelectionValidRequested = true;
Dispatcher.UIThread.Post(EnsureSelectionValid);
}
}
/// <summary>
/// Code that updates only the caret but not the selection can cause confusion when
/// keys like 'Delete' delete the (possibly invisible) selected text and not the
/// text around the caret.
///
/// So we'll ensure that the caret is inside the selection.
/// (when the caret is not in the selection, we'll clear the selection)
///
/// This method is invoked using the Dispatcher so that code may temporarily violate this rule
/// (e.g. most 'extend selection' methods work by first setting the caret, then the selection),
/// it's sufficient to fix it after any event handlers have run.
/// </summary>
private void EnsureSelectionValid()
{
_ensureSelectionValidRequested = false;
if (_allowCaretOutsideSelection == 0)
{
if (!_selection.IsEmpty && !_selection.Contains(Caret.Offset))
{
ClearSelection();
}
}
}
/// <summary>
/// Temporarily allows positioning the caret outside the selection.
/// Dispose the returned IDisposable to revert the allowance.
/// </summary>
/// <remarks>
/// The text area only forces the caret to be inside the selection when other events
/// have finished running (using the dispatcher), so you don't have to use this method
/// for temporarily positioning the caret in event handlers.
/// This method is only necessary if you want to run the dispatcher, e.g. if you
/// perform a drag'n'drop operation.
/// </remarks>
public IDisposable AllowCaretOutsideSelection()
{
VerifyAccess();
_allowCaretOutsideSelection++;
return new CallbackOnDispose(
delegate
{
VerifyAccess();
_allowCaretOutsideSelection--;
RequestSelectionValidation();
});
}
#endregion
#region Properties
/// <summary>
/// Gets the Caret used for this text area.
/// </summary>
public Caret Caret { get; }
/// <summary>
/// Scrolls the text view so that the requested line is in the middle.
/// If the textview can be scrolled.
/// </summary>
/// <param name="line">The line to scroll to.</param>
public void ScrollToLine (int line)
{
var viewPortLines = (int)(this as IScrollable).Viewport.Height;
if (viewPortLines < Document.LineCount)
{
ScrollToLine(line, 2, viewPortLines / 2);
}
}
/// <summary>
/// Scrolls the textview to a position with n lines above and below it.
/// </summary>
/// <param name="line">the requested line number.</param>
/// <param name="linesEitherSide">The number of lines above and below.</param>
public void ScrollToLine(int line, int linesEitherSide)
{
ScrollToLine(line, linesEitherSide, linesEitherSide);
}
/// <summary>
/// Scrolls the textview to a position with n lines above and below it.
/// </summary>
/// <param name="line">the requested line number.</param>
/// <param name="linesAbove">The number of lines above.</param>
/// <param name="linesBelow">The number of lines below.</param>
public void ScrollToLine(int line, int linesAbove, int linesBelow)
{
var offset = line - linesAbove;
if (offset < 0)
{
offset = 0;
}
this.BringIntoView(new Rect(1, offset, 0, 1));
offset = line + linesBelow;
if (offset >= 0)
{
this.BringIntoView(new Rect(1, offset, 0, 1));
}
}
private void CaretPositionChanged(object sender, EventArgs e)
{
if (TextView == null)
return;
TextView.HighlightedLine = Caret.Line;
ScrollToLine(Caret.Line, 2);
Dispatcher.UIThread.InvokeAsync(() =>
{
(this as ILogicalScrollable).RaiseScrollInvalidated(EventArgs.Empty);
});
}
public static readonly DirectProperty<TextArea, ObservableCollection<IControl>> LeftMarginsProperty
= AvaloniaProperty.RegisterDirect<TextArea, ObservableCollection<IControl>>(nameof(LeftMargins),
c => c.LeftMargins);
/// <summary>
/// Gets the collection of margins displayed to the left of the text view.
/// </summary>
public ObservableCollection<IControl> LeftMargins { get; } = new ObservableCollection<IControl>();
private void LeftMargins_CollectionChanged(object sender, NotifyCollectionChangedEventArgs e)
{
if (e.OldItems != null)
{
foreach (var c in e.OldItems.OfType<ITextViewConnect>())
{
c.RemoveFromTextView(TextView);
}
get => _readOnlySectionProvider;
set => _readOnlySectionProvider = value ?? throw new ArgumentNullException(nameof(value));
}
#endregion
#region Focus Handling (Show/Hide Caret)
/// <summary>
/// The <see cref="RightClickMovesCaret"/> property.
/// </summary>
public static readonly StyledProperty<bool> RightClickMovesCaretProperty =
AvaloniaProperty.Register<TextArea, bool>(nameof(RightClickMovesCaret), false);
/// <summary>
/// Determines whether caret position should be changed to the mouse position when you right click or not.
/// </summary>
public bool RightClickMovesCaret
{
get => GetValue(RightClickMovesCaretProperty);
set => SetValue(RightClickMovesCaretProperty, value);
}
#endregion
#region Focus Handling (Show/Hide Caret)
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
Focus();
}
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
Caret.Show();
_imClient.SetTextArea(this);
}
protected override void OnLostFocus(RoutedEventArgs e)
{
base.OnLostFocus(e);
Caret.Hide();
_imClient.SetTextArea(null);
}
#endregion
#region OnTextInput / RemoveSelectedText / ReplaceSelectionWithText
/// <summary>
/// Occurs when the TextArea receives text input.
/// but occurs immediately before the TextArea handles the TextInput event.
/// </summary>
public event EventHandler<TextInputEventArgs> TextEntering;
/// <summary>
/// Occurs when the TextArea receives text input.
/// but occurs immediately after the TextArea handles the TextInput event.
/// </summary>
public event EventHandler<TextInputEventArgs> TextEntered;
/// <summary>
/// Raises the TextEntering event.
/// </summary>
protected virtual void OnTextEntering(TextInputEventArgs e)
{
TextEntering?.Invoke(this, e);
}
/// <summary>
/// Raises the TextEntered event.
/// </summary>
protected virtual void OnTextEntered(TextInputEventArgs e)
{
TextEntered?.Invoke(this, e);
}
protected override void OnTextInput(TextInputEventArgs e)
{
base.OnTextInput(e);
if (!e.Handled && Document != null)
{
if (string.IsNullOrEmpty(e.Text) || e.Text == "\x1b" || e.Text == "\b" || e.Text == "\u007f")
{
// TODO: check this
// ASCII 0x1b = ESC.
// produces a TextInput event with that old ASCII control char
// when Escape is pressed. We'll just ignore it.
// A deadkey followed by backspace causes a textinput event for the BS character.
// Similarly, some shortcuts like Alt+Space produce an empty TextInput event.
// We have to ignore those (not handle them) to keep the shortcut working.
return;
}
HideMouseCursor();
PerformTextInput(e);
e.Handled = true;
}
}
/// <summary>
/// Performs text input.
/// This raises the <see cref="TextEntering"/> event, replaces the selection with the text,
/// and then raises the <see cref="TextEntered"/> event.
/// </summary>
public void PerformTextInput(string text)
{
var e = new TextInputEventArgs
{
Text = text,
RoutedEvent = TextInputEvent
};
PerformTextInput(e);
}
/// <summary>
/// Performs text input.
/// This raises the <see cref="TextEntering"/> event, replaces the selection with the text,
/// and then raises the <see cref="TextEntered"/> event.
/// </summary>
public void PerformTextInput(TextInputEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
OnTextEntering(e);
if (!e.Handled)
{
if (e.Text == "\n" || e.Text == "\r" || e.Text == "\r\n")
ReplaceSelectionWithNewLine();
else
{
// TODO
//if (OverstrikeMode && Selection.IsEmpty && Document.GetLineByNumber(Caret.Line).EndOffset > Caret.Offset)
// EditingCommands.SelectRightByCharacter.Execute(null, this);
ReplaceSelectionWithText(e.Text);
}
OnTextEntered(e);
Caret.BringCaretToView();
}
}
private void ReplaceSelectionWithNewLine()
{
var newLine = TextUtilities.GetNewLineFromDocument(Document, Caret.Line);
using (Document.RunUpdate())
{
ReplaceSelectionWithText(newLine);
if (IndentationStrategy != null)
{
var line = Document.GetLineByNumber(Caret.Line);
var deletable = GetDeletableSegments(line);
if (deletable.Length == 1 && deletable[0].Offset == line.Offset && deletable[0].Length == line.Length)
{
// use indentation strategy only if the line is not read-only
IndentationStrategy.IndentLine(Document, line);
}
}
}
}
internal void RemoveSelectedText()
{
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
_selection.ReplaceSelectionWithText(string.Empty);
#if DEBUG
if (!_selection.IsEmpty)
{
foreach (var s in _selection.Segments)
{
Debug.Assert(!ReadOnlySectionProvider.GetDeletableSegments(s).Any());
}
}
#endif
}
internal void ReplaceSelectionWithText(string newText)
{
if (newText == null)
throw new ArgumentNullException(nameof(newText));
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
_selection.ReplaceSelectionWithText(newText);
}
internal ISegment[] GetDeletableSegments(ISegment segment)
{
var deletableSegments = ReadOnlySectionProvider.GetDeletableSegments(segment);
if (deletableSegments == null)
throw new InvalidOperationException("ReadOnlySectionProvider.GetDeletableSegments returned null");
var array = deletableSegments.ToArray();
var lastIndex = segment.Offset;
foreach (var t in array)
{
if (t.Offset < lastIndex)
throw new InvalidOperationException("ReadOnlySectionProvider returned incorrect segments (outside of input segment / wrong order)");
lastIndex = t.EndOffset;
}
if (lastIndex > segment.EndOffset)
throw new InvalidOperationException("ReadOnlySectionProvider returned incorrect segments (outside of input segment / wrong order)");
return array;
}
#endregion
#region IndentationStrategy property
/// <summary>
/// IndentationStrategy property.
/// </summary>
public static readonly StyledProperty<IIndentationStrategy> IndentationStrategyProperty =
AvaloniaProperty.Register<TextArea, IIndentationStrategy>("IndentationStrategy", new DefaultIndentationStrategy());
/// <summary>
/// Gets/Sets the indentation strategy used when inserting new lines.
/// </summary>
public IIndentationStrategy IndentationStrategy
{
get => GetValue(IndentationStrategyProperty);
set => SetValue(IndentationStrategyProperty, value);
}
#endregion
#region OnKeyDown/OnKeyUp
// Make life easier for text editor extensions that use a different cursor based on the pressed modifier keys.
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
TextView.InvalidateCursorIfPointerWithinTextView();
}
private void OnPreviewKeyDown(object sender, KeyEventArgs e)
{
foreach (var h in StackedInputHandlers)
{
if (e.Handled)
break;
h.OnPreviewKeyDown(e);
}
}
/// <inheritdoc/>
protected override void OnKeyUp(KeyEventArgs e)
{
base.OnKeyUp(e);
TextView.InvalidateCursorIfPointerWithinTextView();
}
private void OnPreviewKeyUp(object sender, KeyEventArgs e)
{
foreach (var h in StackedInputHandlers)
{
if (e.Handled)
break;
h.OnPreviewKeyUp(e);
}
}
#endregion
#region Hide Mouse Cursor While Typing
private bool _isMouseCursorHidden;
private void AttachTypingEvents()
{
// Use the PreviewMouseMove event in case some other editor layer consumes the MouseMove event (e.g. SD's InsertionCursorLayer)
PointerEntered += delegate { ShowMouseCursor(); };
PointerExited += delegate { ShowMouseCursor(); };
}
private void ShowMouseCursor()
{
if (_isMouseCursorHidden)
{
_isMouseCursorHidden = false;
}
}
private void HideMouseCursor()
{
if (Options.HideCursorWhileTyping && !_isMouseCursorHidden && IsPointerOver)
{
_isMouseCursorHidden = true;
}
}
#endregion
#region Overstrike mode
/// <summary>
/// The <see cref="OverstrikeMode"/> dependency property.
/// </summary>
public static readonly StyledProperty<bool> OverstrikeModeProperty =
AvaloniaProperty.Register<TextArea, bool>("OverstrikeMode");
/// <summary>
/// Gets/Sets whether overstrike mode is active.
/// </summary>
public bool OverstrikeMode
{
get => GetValue(OverstrikeModeProperty);
set => SetValue(OverstrikeModeProperty, value);
}
#endregion
/// <inheritdoc/>
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == SelectionBrushProperty
|| change.Property == SelectionBorderProperty
|| change.Property == SelectionForegroundProperty
|| change.Property == SelectionCornerRadiusProperty)
{
TextView.Redraw();
}
else if (change.Property == OverstrikeModeProperty)
{
Caret.UpdateIfVisible();
}
}
/// <summary>
/// Gets the requested service.
/// </summary>
/// <returns>Returns the requested service instance, or null if the service cannot be found.</returns>
public virtual object GetService(Type serviceType)
{
return TextView.GetService(serviceType);
}
/// <summary>
/// Occurs when text inside the TextArea was copied.
/// </summary>
public event EventHandler<TextEventArgs> TextCopied;
event EventHandler ILogicalScrollable.ScrollInvalidated
{
add { if (_logicalScrollable != null) _logicalScrollable.ScrollInvalidated += value; }
remove { if (_logicalScrollable != null) _logicalScrollable.ScrollInvalidated -= value; }
}
internal void OnTextCopied(TextEventArgs e)
{
TextCopied?.Invoke(this, e);
}
public IList<RoutedCommandBinding> CommandBindings { get; } = new List<RoutedCommandBinding>();
bool ILogicalScrollable.IsLogicalScrollEnabled => _logicalScrollable?.IsLogicalScrollEnabled ?? default(bool);
Size ILogicalScrollable.ScrollSize => _logicalScrollable?.ScrollSize ?? default(Size);
Size ILogicalScrollable.PageScrollSize => _logicalScrollable?.PageScrollSize ?? default(Size);
Size IScrollable.Extent => _logicalScrollable?.Extent ?? default(Size);
Vector IScrollable.Offset
{
get => _logicalScrollable?.Offset ?? default(Vector);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.Offset = value;
}
}
}
Size IScrollable.Viewport => _logicalScrollable?.Viewport ?? default(Size);
bool ILogicalScrollable.CanHorizontallyScroll
{
get => _logicalScrollable?.CanHorizontallyScroll ?? default(bool);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.CanHorizontallyScroll = value;
}
}
}
bool ILogicalScrollable.CanVerticallyScroll
{
get => _logicalScrollable?.CanVerticallyScroll ?? default(bool);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.CanVerticallyScroll = value;
}
}
}
public bool BringIntoView(IControl target, Rect targetRect) =>
_logicalScrollable?.BringIntoView(target, targetRect) ?? default(bool);
IControl ILogicalScrollable.GetControlInDirection(NavigationDirection direction, IControl from)
=> _logicalScrollable?.GetControlInDirection(direction, from);
public void RaiseScrollInvalidated(EventArgs e)
{
_logicalScrollable?.RaiseScrollInvalidated(e);
}
private class TextAreaTextInputMethodClient : ITextInputMethodClient
{
private TextArea _textArea;
public TextAreaTextInputMethodClient()
{
}
public event EventHandler CursorRectangleChanged;
public event EventHandler TextViewVisualChanged;
public event EventHandler SurroundingTextChanged;
public Rect CursorRectangle
{
get
{
if(_textArea == null)
{
return Rect.Empty;
}
var transform = _textArea.TextView.TransformToVisual(_textArea);
if (transform == null)
{
return default;
}
var rect = _textArea.Caret.CalculateCaretRectangle().TransformToAABB(transform.Value);
return rect;
}
}
public IVisual TextViewVisual => _textArea;
public bool SupportsPreedit => false;
public bool SupportsSurroundingText => true;
public TextInputMethodSurroundingText SurroundingText
{
get
{
if(_textArea == null)
{
return default;
}
var lineIndex = _textArea.Caret.Line;
var position = _textArea.Caret.Position;
var documentLine = _textArea.Document.GetLineByNumber(lineIndex);
var text = _textArea.Document.GetText(documentLine.Offset, documentLine.Length);
return new TextInputMethodSurroundingText
{
AnchorOffset = 0,
CursorOffset = position.Column,
Text = text
};
}
}
public void SetTextArea(TextArea textArea)
{
if(_textArea != null)
{
_textArea.Caret.PositionChanged -= Caret_PositionChanged;
_textArea.SelectionChanged -= TextArea_SelectionChanged;
}
_textArea = textArea;
if(_textArea != null)
{
_textArea.Caret.PositionChanged += Caret_PositionChanged;
_textArea.SelectionChanged += TextArea_SelectionChanged;
}
TextViewVisualChanged?.Invoke(this, EventArgs.Empty);
CursorRectangleChanged?.Invoke(this, EventArgs.Empty);
}
private void Caret_PositionChanged(object sender, EventArgs e)
{
CursorRectangleChanged?.Invoke(this, e);
}
private void TextArea_SelectionChanged(object sender, EventArgs e)
{
SurroundingTextChanged?.Invoke(this, e);
}
public void SelectInSurroundingText(int start, int end)
{
if(_textArea == null)
{
return;
}
var selection = _textArea.Selection;
_textArea.Selection = _textArea.Selection.StartSelectionOrSetEndpoint(
new TextViewPosition(selection.StartPosition.Line, start),
new TextViewPosition(selection.StartPosition.Line, end));
}
public void SetPreeditText(string text)
{
}
}
}
/// <summary>
/// EventArgs with text.
/// </summary>
public class TextEventArgs : EventArgs
{
/// <summary>
/// Gets the text.
/// </summary>
public string Text { get; }
/// <summary>
/// Creates a new TextEventArgs instance.
/// </summary>
public TextEventArgs(string text)
{
Text = text ?? throw new ArgumentNullException(nameof(text));
}
}
}
<MSG> Merge pull request #169 from Takoooooo/right-click-moves-caret-feature
You should be able to move caret by right clicking text area
<DFF> @@ -708,6 +708,21 @@ namespace AvaloniaEdit.Editing
get => _readOnlySectionProvider;
set => _readOnlySectionProvider = value ?? throw new ArgumentNullException(nameof(value));
}
+
+ /// <summary>
+ /// The <see cref="RightClickMovesCaret"/> property.
+ /// </summary>
+ public static readonly StyledProperty<bool> RightClickMovesCaretProperty =
+ AvaloniaProperty.Register<TextArea, bool>(nameof(RightClickMovesCaret), false);
+
+ /// <summary>
+ /// Determines whether caret position should be changed to the mouse position when you right click or not.
+ /// </summary>
+ public bool RightClickMovesCaret
+ {
+ get => GetValue(RightClickMovesCaretProperty);
+ set => SetValue(RightClickMovesCaretProperty, value);
+ }
#endregion
#region Focus Handling (Show/Hide Caret)
| 15 | Merge pull request #169 from Takoooooo/right-click-moves-caret-feature | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066154 | <NME> TextArea.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using Avalonia;
using Avalonia.Controls;
using Avalonia.Controls.Presenters;
using Avalonia.Controls.Primitives;
using Avalonia.Data;
using Avalonia.Input;
using Avalonia.Input.TextInput;
using Avalonia.Interactivity;
using Avalonia.Media;
using Avalonia.Threading;
using Avalonia.VisualTree;
using AvaloniaEdit.Document;
using AvaloniaEdit.Indentation;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Search;
using AvaloniaEdit.Utils;
using System;
using System.Collections.Generic;
using System.Collections.Immutable;
using System.Collections.ObjectModel;
using System.Collections.Specialized;
using System.ComponentModel;
using System.Diagnostics;
using System.Linq;
namespace AvaloniaEdit.Editing
{
/// <summary>
/// Control that wraps a TextView and adds support for user input and the caret.
/// </summary>
public class TextArea : TemplatedControl, ITextEditorComponent, IRoutedCommandBindable, ILogicalScrollable
{
/// <summary>
/// This is the extra scrolling space that occurs after the last line.
/// </summary>
private const int AdditionalVerticalScrollAmount = 2;
private ILogicalScrollable _logicalScrollable;
private readonly TextAreaTextInputMethodClient _imClient = new TextAreaTextInputMethodClient();
#region Constructor
static TextArea()
{
KeyboardNavigation.TabNavigationProperty.OverrideDefaultValue<TextArea>(KeyboardNavigationMode.None);
FocusableProperty.OverrideDefaultValue<TextArea>(true);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
AffectsArrange<TextArea>(OffsetProperty);
AffectsRender<TextArea>(OffsetProperty);
TextInputMethodClientRequestedEvent.AddClassHandler<TextArea>((ta, e) =>
{
if (!ta.IsReadOnly)
{
e.Client = ta._imClient;
}
});
}
/// <summary>
/// Creates a new TextArea instance.
/// </summary>
public TextArea() : this(new TextView())
{
AddHandler(KeyDownEvent, OnPreviewKeyDown, RoutingStrategies.Tunnel);
AddHandler(KeyUpEvent, OnPreviewKeyUp, RoutingStrategies.Tunnel);
}
/// <summary>
/// Creates a new TextArea instance.
/// </summary>
protected TextArea(TextView textView)
{
TextView = textView ?? throw new ArgumentNullException(nameof(textView));
_logicalScrollable = textView;
Options = textView.Options;
_selection = EmptySelection = new EmptySelection(this);
textView.Services.AddService(this);
textView.LineTransformers.Add(new SelectionColorizer(this));
textView.InsertLayer(new SelectionLayer(this), KnownLayer.Selection, LayerInsertionPosition.Replace);
Caret = new Caret(this);
Caret.PositionChanged += (sender, e) => RequestSelectionValidation();
Caret.PositionChanged += CaretPositionChanged;
AttachTypingEvents();
LeftMargins.CollectionChanged += LeftMargins_CollectionChanged;
DefaultInputHandler = new TextAreaDefaultInputHandler(this);
ActiveInputHandler = DefaultInputHandler;
// TODO
//textView.GetObservable(TextBlock.FontSizeProperty).Subscribe(_ =>
//{
// TextView.SetScrollOffset(new Vector(_offset.X, _offset.Y * TextView.DefaultLineHeight));
//});
}
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
if (e.NameScope.Find("PART_CP") is ContentPresenter contentPresenter)
{
contentPresenter.Content = TextView;
}
}
internal void AddChild(IVisual visual)
{
VisualChildren.Add(visual);
InvalidateArrange();
}
internal void RemoveChild(IVisual visual)
{
VisualChildren.Remove(visual);
}
#endregion
/// <summary>
/// Defines the <see cref="IScrollable.Offset" /> property.
/// </summary>
public static readonly DirectProperty<TextArea, Vector> OffsetProperty =
AvaloniaProperty.RegisterDirect<TextArea, Vector>(
nameof(IScrollable.Offset),
o => (o as IScrollable).Offset,
(o, v) => (o as IScrollable).Offset = v);
#region InputHandler management
/// <summary>
/// Gets the default input handler.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public TextAreaDefaultInputHandler DefaultInputHandler { get; }
private ITextAreaInputHandler _activeInputHandler;
private bool _isChangingInputHandler;
/// <summary>
/// Gets/Sets the active input handler.
/// This property does not return currently active stacked input handlers. Setting this property detached all stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public ITextAreaInputHandler ActiveInputHandler
{
get => _activeInputHandler;
set
{
if (value != null && value.TextArea != this)
throw new ArgumentException("The input handler was created for a different text area than this one.");
if (_isChangingInputHandler)
throw new InvalidOperationException("Cannot set ActiveInputHandler recursively");
if (_activeInputHandler != value)
{
_isChangingInputHandler = true;
try
{
// pop the whole stack
PopStackedInputHandler(StackedInputHandlers.LastOrDefault());
Debug.Assert(StackedInputHandlers.IsEmpty);
_activeInputHandler?.Detach();
_activeInputHandler = value;
value?.Attach();
}
finally
{
_isChangingInputHandler = false;
}
ActiveInputHandlerChanged?.Invoke(this, EventArgs.Empty);
}
}
}
/// <summary>
/// Occurs when the ActiveInputHandler property changes.
/// </summary>
public event EventHandler ActiveInputHandlerChanged;
/// <summary>
/// Gets the list of currently active stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public ImmutableStack<TextAreaStackedInputHandler> StackedInputHandlers { get; private set; } = ImmutableStack<TextAreaStackedInputHandler>.Empty;
/// <summary>
/// Pushes an input handler onto the list of stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public void PushStackedInputHandler(TextAreaStackedInputHandler inputHandler)
{
if (inputHandler == null)
throw new ArgumentNullException(nameof(inputHandler));
StackedInputHandlers = StackedInputHandlers.Push(inputHandler);
inputHandler.Attach();
}
/// <summary>
/// Pops the stacked input handler (and all input handlers above it).
/// If <paramref name="inputHandler"/> is not found in the currently stacked input handlers, or is null, this method
/// does nothing.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public void PopStackedInputHandler(TextAreaStackedInputHandler inputHandler)
{
if (StackedInputHandlers.Any(i => i == inputHandler))
{
ITextAreaInputHandler oldHandler;
do
{
oldHandler = StackedInputHandlers.Peek();
StackedInputHandlers = StackedInputHandlers.Pop();
oldHandler.Detach();
} while (oldHandler != inputHandler);
}
}
#endregion
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextArea>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <inheritdoc/>
public event EventHandler DocumentChanged;
/// <summary>
/// Gets if the the document displayed by the text editor is readonly
/// </summary>
public bool IsReadOnly
{
get => ReadOnlySectionProvider == ReadOnlySectionDocument.Instance;
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextArea)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.Changing.RemoveHandler(oldValue, OnDocumentChanging);
TextDocumentWeakEventManager.Changed.RemoveHandler(oldValue, OnDocumentChanged);
TextDocumentWeakEventManager.UpdateStarted.RemoveHandler(oldValue, OnUpdateStarted);
TextDocumentWeakEventManager.UpdateFinished.RemoveHandler(oldValue, OnUpdateFinished);
}
TextView.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.Changing.AddHandler(newValue, OnDocumentChanging);
TextDocumentWeakEventManager.Changed.AddHandler(newValue, OnDocumentChanged);
TextDocumentWeakEventManager.UpdateStarted.AddHandler(newValue, OnUpdateStarted);
TextDocumentWeakEventManager.UpdateFinished.AddHandler(newValue, OnUpdateFinished);
InvalidateArrange();
}
// Reset caret location and selection: this is necessary because the caret/selection might be invalid
// in the new document (e.g. if new document is shorter than the old document).
Caret.Location = new TextLocation(1, 1);
ClearSelection();
DocumentChanged?.Invoke(this, EventArgs.Empty);
//CommandManager.InvalidateRequerySuggested();
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextArea>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
private void OnOptionChanged(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextArea)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnOptionChanged);
}
TextView.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnOptionChanged);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
#endregion
#region Caret handling on document changes
private void OnDocumentChanging(object sender, DocumentChangeEventArgs e)
{
Caret.OnDocumentChanging();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
Caret.OnDocumentChanged(e);
Selection = _selection.UpdateOnDocumentChange(e);
}
private void OnUpdateStarted(object sender, EventArgs e)
{
Document.UndoStack.PushOptional(new RestoreCaretAndSelectionUndoAction(this));
}
private void OnUpdateFinished(object sender, EventArgs e)
{
Caret.OnDocumentUpdateFinished();
}
private sealed class RestoreCaretAndSelectionUndoAction : IUndoableOperation
{
// keep textarea in weak reference because the IUndoableOperation is stored with the document
private readonly WeakReference _textAreaReference;
private readonly TextViewPosition _caretPosition;
private readonly Selection _selection;
public RestoreCaretAndSelectionUndoAction(TextArea textArea)
{
_textAreaReference = new WeakReference(textArea);
// Just save the old caret position, no need to validate here.
// If we restore it, we'll validate it anyways.
_caretPosition = textArea.Caret.NonValidatedPosition;
_selection = textArea.Selection;
}
public void Undo()
{
var textArea = (TextArea)_textAreaReference.Target;
if (textArea != null)
{
textArea.Caret.Position = _caretPosition;
textArea.Selection = _selection;
}
}
public void Redo()
{
// redo=undo: we just restore the caret/selection state
Undo();
}
}
#endregion
#region TextView property
/// <summary>
/// Gets the text view used to display text in this text area.
/// </summary>
public TextView TextView { get; }
#endregion
#region Selection property
internal readonly Selection EmptySelection;
private Selection _selection;
/// <summary>
/// Occurs when the selection has changed.
/// </summary>
public event EventHandler SelectionChanged;
/// <summary>
/// Gets/Sets the selection in this text area.
/// </summary>
public Selection Selection
{
get => _selection;
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
if (value.TextArea != this)
throw new ArgumentException("Cannot use a Selection instance that belongs to another text area.");
if (!Equals(_selection, value))
{
if (TextView != null)
{
var oldSegment = _selection.SurroundingSegment;
var newSegment = value.SurroundingSegment;
if (!Selection.EnableVirtualSpace && (_selection is SimpleSelection && value is SimpleSelection && oldSegment != null && newSegment != null))
{
// perf optimization:
// When a simple selection changes, don't redraw the whole selection, but only the changed parts.
var oldSegmentOffset = oldSegment.Offset;
var newSegmentOffset = newSegment.Offset;
if (oldSegmentOffset != newSegmentOffset)
{
TextView.Redraw(Math.Min(oldSegmentOffset, newSegmentOffset),
Math.Abs(oldSegmentOffset - newSegmentOffset));
}
var oldSegmentEndOffset = oldSegment.EndOffset;
var newSegmentEndOffset = newSegment.EndOffset;
if (oldSegmentEndOffset != newSegmentEndOffset)
{
TextView.Redraw(Math.Min(oldSegmentEndOffset, newSegmentEndOffset),
Math.Abs(oldSegmentEndOffset - newSegmentEndOffset));
}
}
else
{
TextView.Redraw(oldSegment);
TextView.Redraw(newSegment);
}
}
_selection = value;
SelectionChanged?.Invoke(this, EventArgs.Empty);
// a selection change causes commands like copy/paste/etc. to change status
//CommandManager.InvalidateRequerySuggested();
}
}
}
/// <summary>
/// Clears the current selection.
/// </summary>
public void ClearSelection()
{
Selection = EmptySelection;
}
/// <summary>
/// The <see cref="SelectionBrush"/> property.
/// </summary>
public static readonly StyledProperty<IBrush> SelectionBrushProperty =
AvaloniaProperty.Register<TextArea, IBrush>("SelectionBrush");
/// <summary>
/// Gets/Sets the background brush used for the selection.
/// </summary>
public IBrush SelectionBrush
{
get => GetValue(SelectionBrushProperty);
set => SetValue(SelectionBrushProperty, value);
}
/// <summary>
/// The <see cref="SelectionForeground"/> property.
/// </summary>
public static readonly StyledProperty<IBrush> SelectionForegroundProperty =
AvaloniaProperty.Register<TextArea, IBrush>("SelectionForeground");
/// <summary>
/// Gets/Sets the foreground brush used for selected text.
/// </summary>
public IBrush SelectionForeground
{
get => GetValue(SelectionForegroundProperty);
set => SetValue(SelectionForegroundProperty, value);
}
/// <summary>
/// The <see cref="SelectionBorder"/> property.
/// </summary>
public static readonly StyledProperty<Pen> SelectionBorderProperty =
AvaloniaProperty.Register<TextArea, Pen>("SelectionBorder");
/// <summary>
/// Gets/Sets the pen used for the border of the selection.
/// </summary>
public Pen SelectionBorder
{
get => GetValue(SelectionBorderProperty);
set => SetValue(SelectionBorderProperty, value);
}
/// <summary>
/// The <see cref="SelectionCornerRadius"/> property.
/// </summary>
public static readonly StyledProperty<double> SelectionCornerRadiusProperty =
AvaloniaProperty.Register<TextArea, double>("SelectionCornerRadius", 3.0);
/// <summary>
/// Gets/Sets the corner radius of the selection.
/// </summary>
public double SelectionCornerRadius
{
get => GetValue(SelectionCornerRadiusProperty);
set => SetValue(SelectionCornerRadiusProperty, value);
}
#endregion
#region Force caret to stay inside selection
private bool _ensureSelectionValidRequested;
private int _allowCaretOutsideSelection;
private void RequestSelectionValidation()
{
if (!_ensureSelectionValidRequested && _allowCaretOutsideSelection == 0)
{
_ensureSelectionValidRequested = true;
Dispatcher.UIThread.Post(EnsureSelectionValid);
}
}
/// <summary>
/// Code that updates only the caret but not the selection can cause confusion when
/// keys like 'Delete' delete the (possibly invisible) selected text and not the
/// text around the caret.
///
/// So we'll ensure that the caret is inside the selection.
/// (when the caret is not in the selection, we'll clear the selection)
///
/// This method is invoked using the Dispatcher so that code may temporarily violate this rule
/// (e.g. most 'extend selection' methods work by first setting the caret, then the selection),
/// it's sufficient to fix it after any event handlers have run.
/// </summary>
private void EnsureSelectionValid()
{
_ensureSelectionValidRequested = false;
if (_allowCaretOutsideSelection == 0)
{
if (!_selection.IsEmpty && !_selection.Contains(Caret.Offset))
{
ClearSelection();
}
}
}
/// <summary>
/// Temporarily allows positioning the caret outside the selection.
/// Dispose the returned IDisposable to revert the allowance.
/// </summary>
/// <remarks>
/// The text area only forces the caret to be inside the selection when other events
/// have finished running (using the dispatcher), so you don't have to use this method
/// for temporarily positioning the caret in event handlers.
/// This method is only necessary if you want to run the dispatcher, e.g. if you
/// perform a drag'n'drop operation.
/// </remarks>
public IDisposable AllowCaretOutsideSelection()
{
VerifyAccess();
_allowCaretOutsideSelection++;
return new CallbackOnDispose(
delegate
{
VerifyAccess();
_allowCaretOutsideSelection--;
RequestSelectionValidation();
});
}
#endregion
#region Properties
/// <summary>
/// Gets the Caret used for this text area.
/// </summary>
public Caret Caret { get; }
/// <summary>
/// Scrolls the text view so that the requested line is in the middle.
/// If the textview can be scrolled.
/// </summary>
/// <param name="line">The line to scroll to.</param>
public void ScrollToLine (int line)
{
var viewPortLines = (int)(this as IScrollable).Viewport.Height;
if (viewPortLines < Document.LineCount)
{
ScrollToLine(line, 2, viewPortLines / 2);
}
}
/// <summary>
/// Scrolls the textview to a position with n lines above and below it.
/// </summary>
/// <param name="line">the requested line number.</param>
/// <param name="linesEitherSide">The number of lines above and below.</param>
public void ScrollToLine(int line, int linesEitherSide)
{
ScrollToLine(line, linesEitherSide, linesEitherSide);
}
/// <summary>
/// Scrolls the textview to a position with n lines above and below it.
/// </summary>
/// <param name="line">the requested line number.</param>
/// <param name="linesAbove">The number of lines above.</param>
/// <param name="linesBelow">The number of lines below.</param>
public void ScrollToLine(int line, int linesAbove, int linesBelow)
{
var offset = line - linesAbove;
if (offset < 0)
{
offset = 0;
}
this.BringIntoView(new Rect(1, offset, 0, 1));
offset = line + linesBelow;
if (offset >= 0)
{
this.BringIntoView(new Rect(1, offset, 0, 1));
}
}
private void CaretPositionChanged(object sender, EventArgs e)
{
if (TextView == null)
return;
TextView.HighlightedLine = Caret.Line;
ScrollToLine(Caret.Line, 2);
Dispatcher.UIThread.InvokeAsync(() =>
{
(this as ILogicalScrollable).RaiseScrollInvalidated(EventArgs.Empty);
});
}
public static readonly DirectProperty<TextArea, ObservableCollection<IControl>> LeftMarginsProperty
= AvaloniaProperty.RegisterDirect<TextArea, ObservableCollection<IControl>>(nameof(LeftMargins),
c => c.LeftMargins);
/// <summary>
/// Gets the collection of margins displayed to the left of the text view.
/// </summary>
public ObservableCollection<IControl> LeftMargins { get; } = new ObservableCollection<IControl>();
private void LeftMargins_CollectionChanged(object sender, NotifyCollectionChangedEventArgs e)
{
if (e.OldItems != null)
{
foreach (var c in e.OldItems.OfType<ITextViewConnect>())
{
c.RemoveFromTextView(TextView);
}
get => _readOnlySectionProvider;
set => _readOnlySectionProvider = value ?? throw new ArgumentNullException(nameof(value));
}
#endregion
#region Focus Handling (Show/Hide Caret)
/// <summary>
/// The <see cref="RightClickMovesCaret"/> property.
/// </summary>
public static readonly StyledProperty<bool> RightClickMovesCaretProperty =
AvaloniaProperty.Register<TextArea, bool>(nameof(RightClickMovesCaret), false);
/// <summary>
/// Determines whether caret position should be changed to the mouse position when you right click or not.
/// </summary>
public bool RightClickMovesCaret
{
get => GetValue(RightClickMovesCaretProperty);
set => SetValue(RightClickMovesCaretProperty, value);
}
#endregion
#region Focus Handling (Show/Hide Caret)
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
Focus();
}
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
Caret.Show();
_imClient.SetTextArea(this);
}
protected override void OnLostFocus(RoutedEventArgs e)
{
base.OnLostFocus(e);
Caret.Hide();
_imClient.SetTextArea(null);
}
#endregion
#region OnTextInput / RemoveSelectedText / ReplaceSelectionWithText
/// <summary>
/// Occurs when the TextArea receives text input.
/// but occurs immediately before the TextArea handles the TextInput event.
/// </summary>
public event EventHandler<TextInputEventArgs> TextEntering;
/// <summary>
/// Occurs when the TextArea receives text input.
/// but occurs immediately after the TextArea handles the TextInput event.
/// </summary>
public event EventHandler<TextInputEventArgs> TextEntered;
/// <summary>
/// Raises the TextEntering event.
/// </summary>
protected virtual void OnTextEntering(TextInputEventArgs e)
{
TextEntering?.Invoke(this, e);
}
/// <summary>
/// Raises the TextEntered event.
/// </summary>
protected virtual void OnTextEntered(TextInputEventArgs e)
{
TextEntered?.Invoke(this, e);
}
protected override void OnTextInput(TextInputEventArgs e)
{
base.OnTextInput(e);
if (!e.Handled && Document != null)
{
if (string.IsNullOrEmpty(e.Text) || e.Text == "\x1b" || e.Text == "\b" || e.Text == "\u007f")
{
// TODO: check this
// ASCII 0x1b = ESC.
// produces a TextInput event with that old ASCII control char
// when Escape is pressed. We'll just ignore it.
// A deadkey followed by backspace causes a textinput event for the BS character.
// Similarly, some shortcuts like Alt+Space produce an empty TextInput event.
// We have to ignore those (not handle them) to keep the shortcut working.
return;
}
HideMouseCursor();
PerformTextInput(e);
e.Handled = true;
}
}
/// <summary>
/// Performs text input.
/// This raises the <see cref="TextEntering"/> event, replaces the selection with the text,
/// and then raises the <see cref="TextEntered"/> event.
/// </summary>
public void PerformTextInput(string text)
{
var e = new TextInputEventArgs
{
Text = text,
RoutedEvent = TextInputEvent
};
PerformTextInput(e);
}
/// <summary>
/// Performs text input.
/// This raises the <see cref="TextEntering"/> event, replaces the selection with the text,
/// and then raises the <see cref="TextEntered"/> event.
/// </summary>
public void PerformTextInput(TextInputEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
OnTextEntering(e);
if (!e.Handled)
{
if (e.Text == "\n" || e.Text == "\r" || e.Text == "\r\n")
ReplaceSelectionWithNewLine();
else
{
// TODO
//if (OverstrikeMode && Selection.IsEmpty && Document.GetLineByNumber(Caret.Line).EndOffset > Caret.Offset)
// EditingCommands.SelectRightByCharacter.Execute(null, this);
ReplaceSelectionWithText(e.Text);
}
OnTextEntered(e);
Caret.BringCaretToView();
}
}
private void ReplaceSelectionWithNewLine()
{
var newLine = TextUtilities.GetNewLineFromDocument(Document, Caret.Line);
using (Document.RunUpdate())
{
ReplaceSelectionWithText(newLine);
if (IndentationStrategy != null)
{
var line = Document.GetLineByNumber(Caret.Line);
var deletable = GetDeletableSegments(line);
if (deletable.Length == 1 && deletable[0].Offset == line.Offset && deletable[0].Length == line.Length)
{
// use indentation strategy only if the line is not read-only
IndentationStrategy.IndentLine(Document, line);
}
}
}
}
internal void RemoveSelectedText()
{
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
_selection.ReplaceSelectionWithText(string.Empty);
#if DEBUG
if (!_selection.IsEmpty)
{
foreach (var s in _selection.Segments)
{
Debug.Assert(!ReadOnlySectionProvider.GetDeletableSegments(s).Any());
}
}
#endif
}
internal void ReplaceSelectionWithText(string newText)
{
if (newText == null)
throw new ArgumentNullException(nameof(newText));
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
_selection.ReplaceSelectionWithText(newText);
}
internal ISegment[] GetDeletableSegments(ISegment segment)
{
var deletableSegments = ReadOnlySectionProvider.GetDeletableSegments(segment);
if (deletableSegments == null)
throw new InvalidOperationException("ReadOnlySectionProvider.GetDeletableSegments returned null");
var array = deletableSegments.ToArray();
var lastIndex = segment.Offset;
foreach (var t in array)
{
if (t.Offset < lastIndex)
throw new InvalidOperationException("ReadOnlySectionProvider returned incorrect segments (outside of input segment / wrong order)");
lastIndex = t.EndOffset;
}
if (lastIndex > segment.EndOffset)
throw new InvalidOperationException("ReadOnlySectionProvider returned incorrect segments (outside of input segment / wrong order)");
return array;
}
#endregion
#region IndentationStrategy property
/// <summary>
/// IndentationStrategy property.
/// </summary>
public static readonly StyledProperty<IIndentationStrategy> IndentationStrategyProperty =
AvaloniaProperty.Register<TextArea, IIndentationStrategy>("IndentationStrategy", new DefaultIndentationStrategy());
/// <summary>
/// Gets/Sets the indentation strategy used when inserting new lines.
/// </summary>
public IIndentationStrategy IndentationStrategy
{
get => GetValue(IndentationStrategyProperty);
set => SetValue(IndentationStrategyProperty, value);
}
#endregion
#region OnKeyDown/OnKeyUp
// Make life easier for text editor extensions that use a different cursor based on the pressed modifier keys.
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
TextView.InvalidateCursorIfPointerWithinTextView();
}
private void OnPreviewKeyDown(object sender, KeyEventArgs e)
{
foreach (var h in StackedInputHandlers)
{
if (e.Handled)
break;
h.OnPreviewKeyDown(e);
}
}
/// <inheritdoc/>
protected override void OnKeyUp(KeyEventArgs e)
{
base.OnKeyUp(e);
TextView.InvalidateCursorIfPointerWithinTextView();
}
private void OnPreviewKeyUp(object sender, KeyEventArgs e)
{
foreach (var h in StackedInputHandlers)
{
if (e.Handled)
break;
h.OnPreviewKeyUp(e);
}
}
#endregion
#region Hide Mouse Cursor While Typing
private bool _isMouseCursorHidden;
private void AttachTypingEvents()
{
// Use the PreviewMouseMove event in case some other editor layer consumes the MouseMove event (e.g. SD's InsertionCursorLayer)
PointerEntered += delegate { ShowMouseCursor(); };
PointerExited += delegate { ShowMouseCursor(); };
}
private void ShowMouseCursor()
{
if (_isMouseCursorHidden)
{
_isMouseCursorHidden = false;
}
}
private void HideMouseCursor()
{
if (Options.HideCursorWhileTyping && !_isMouseCursorHidden && IsPointerOver)
{
_isMouseCursorHidden = true;
}
}
#endregion
#region Overstrike mode
/// <summary>
/// The <see cref="OverstrikeMode"/> dependency property.
/// </summary>
public static readonly StyledProperty<bool> OverstrikeModeProperty =
AvaloniaProperty.Register<TextArea, bool>("OverstrikeMode");
/// <summary>
/// Gets/Sets whether overstrike mode is active.
/// </summary>
public bool OverstrikeMode
{
get => GetValue(OverstrikeModeProperty);
set => SetValue(OverstrikeModeProperty, value);
}
#endregion
/// <inheritdoc/>
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == SelectionBrushProperty
|| change.Property == SelectionBorderProperty
|| change.Property == SelectionForegroundProperty
|| change.Property == SelectionCornerRadiusProperty)
{
TextView.Redraw();
}
else if (change.Property == OverstrikeModeProperty)
{
Caret.UpdateIfVisible();
}
}
/// <summary>
/// Gets the requested service.
/// </summary>
/// <returns>Returns the requested service instance, or null if the service cannot be found.</returns>
public virtual object GetService(Type serviceType)
{
return TextView.GetService(serviceType);
}
/// <summary>
/// Occurs when text inside the TextArea was copied.
/// </summary>
public event EventHandler<TextEventArgs> TextCopied;
event EventHandler ILogicalScrollable.ScrollInvalidated
{
add { if (_logicalScrollable != null) _logicalScrollable.ScrollInvalidated += value; }
remove { if (_logicalScrollable != null) _logicalScrollable.ScrollInvalidated -= value; }
}
internal void OnTextCopied(TextEventArgs e)
{
TextCopied?.Invoke(this, e);
}
public IList<RoutedCommandBinding> CommandBindings { get; } = new List<RoutedCommandBinding>();
bool ILogicalScrollable.IsLogicalScrollEnabled => _logicalScrollable?.IsLogicalScrollEnabled ?? default(bool);
Size ILogicalScrollable.ScrollSize => _logicalScrollable?.ScrollSize ?? default(Size);
Size ILogicalScrollable.PageScrollSize => _logicalScrollable?.PageScrollSize ?? default(Size);
Size IScrollable.Extent => _logicalScrollable?.Extent ?? default(Size);
Vector IScrollable.Offset
{
get => _logicalScrollable?.Offset ?? default(Vector);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.Offset = value;
}
}
}
Size IScrollable.Viewport => _logicalScrollable?.Viewport ?? default(Size);
bool ILogicalScrollable.CanHorizontallyScroll
{
get => _logicalScrollable?.CanHorizontallyScroll ?? default(bool);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.CanHorizontallyScroll = value;
}
}
}
bool ILogicalScrollable.CanVerticallyScroll
{
get => _logicalScrollable?.CanVerticallyScroll ?? default(bool);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.CanVerticallyScroll = value;
}
}
}
public bool BringIntoView(IControl target, Rect targetRect) =>
_logicalScrollable?.BringIntoView(target, targetRect) ?? default(bool);
IControl ILogicalScrollable.GetControlInDirection(NavigationDirection direction, IControl from)
=> _logicalScrollable?.GetControlInDirection(direction, from);
public void RaiseScrollInvalidated(EventArgs e)
{
_logicalScrollable?.RaiseScrollInvalidated(e);
}
private class TextAreaTextInputMethodClient : ITextInputMethodClient
{
private TextArea _textArea;
public TextAreaTextInputMethodClient()
{
}
public event EventHandler CursorRectangleChanged;
public event EventHandler TextViewVisualChanged;
public event EventHandler SurroundingTextChanged;
public Rect CursorRectangle
{
get
{
if(_textArea == null)
{
return Rect.Empty;
}
var transform = _textArea.TextView.TransformToVisual(_textArea);
if (transform == null)
{
return default;
}
var rect = _textArea.Caret.CalculateCaretRectangle().TransformToAABB(transform.Value);
return rect;
}
}
public IVisual TextViewVisual => _textArea;
public bool SupportsPreedit => false;
public bool SupportsSurroundingText => true;
public TextInputMethodSurroundingText SurroundingText
{
get
{
if(_textArea == null)
{
return default;
}
var lineIndex = _textArea.Caret.Line;
var position = _textArea.Caret.Position;
var documentLine = _textArea.Document.GetLineByNumber(lineIndex);
var text = _textArea.Document.GetText(documentLine.Offset, documentLine.Length);
return new TextInputMethodSurroundingText
{
AnchorOffset = 0,
CursorOffset = position.Column,
Text = text
};
}
}
public void SetTextArea(TextArea textArea)
{
if(_textArea != null)
{
_textArea.Caret.PositionChanged -= Caret_PositionChanged;
_textArea.SelectionChanged -= TextArea_SelectionChanged;
}
_textArea = textArea;
if(_textArea != null)
{
_textArea.Caret.PositionChanged += Caret_PositionChanged;
_textArea.SelectionChanged += TextArea_SelectionChanged;
}
TextViewVisualChanged?.Invoke(this, EventArgs.Empty);
CursorRectangleChanged?.Invoke(this, EventArgs.Empty);
}
private void Caret_PositionChanged(object sender, EventArgs e)
{
CursorRectangleChanged?.Invoke(this, e);
}
private void TextArea_SelectionChanged(object sender, EventArgs e)
{
SurroundingTextChanged?.Invoke(this, e);
}
public void SelectInSurroundingText(int start, int end)
{
if(_textArea == null)
{
return;
}
var selection = _textArea.Selection;
_textArea.Selection = _textArea.Selection.StartSelectionOrSetEndpoint(
new TextViewPosition(selection.StartPosition.Line, start),
new TextViewPosition(selection.StartPosition.Line, end));
}
public void SetPreeditText(string text)
{
}
}
}
/// <summary>
/// EventArgs with text.
/// </summary>
public class TextEventArgs : EventArgs
{
/// <summary>
/// Gets the text.
/// </summary>
public string Text { get; }
/// <summary>
/// Creates a new TextEventArgs instance.
/// </summary>
public TextEventArgs(string text)
{
Text = text ?? throw new ArgumentNullException(nameof(text));
}
}
}
<MSG> Merge pull request #169 from Takoooooo/right-click-moves-caret-feature
You should be able to move caret by right clicking text area
<DFF> @@ -708,6 +708,21 @@ namespace AvaloniaEdit.Editing
get => _readOnlySectionProvider;
set => _readOnlySectionProvider = value ?? throw new ArgumentNullException(nameof(value));
}
+
+ /// <summary>
+ /// The <see cref="RightClickMovesCaret"/> property.
+ /// </summary>
+ public static readonly StyledProperty<bool> RightClickMovesCaretProperty =
+ AvaloniaProperty.Register<TextArea, bool>(nameof(RightClickMovesCaret), false);
+
+ /// <summary>
+ /// Determines whether caret position should be changed to the mouse position when you right click or not.
+ /// </summary>
+ public bool RightClickMovesCaret
+ {
+ get => GetValue(RightClickMovesCaretProperty);
+ set => SetValue(RightClickMovesCaretProperty, value);
+ }
#endregion
#region Focus Handling (Show/Hide Caret)
| 15 | Merge pull request #169 from Takoooooo/right-click-moves-caret-feature | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066155 | <NME> TextArea.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using Avalonia;
using Avalonia.Controls;
using Avalonia.Controls.Presenters;
using Avalonia.Controls.Primitives;
using Avalonia.Data;
using Avalonia.Input;
using Avalonia.Input.TextInput;
using Avalonia.Interactivity;
using Avalonia.Media;
using Avalonia.Threading;
using Avalonia.VisualTree;
using AvaloniaEdit.Document;
using AvaloniaEdit.Indentation;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Search;
using AvaloniaEdit.Utils;
using System;
using System.Collections.Generic;
using System.Collections.Immutable;
using System.Collections.ObjectModel;
using System.Collections.Specialized;
using System.ComponentModel;
using System.Diagnostics;
using System.Linq;
namespace AvaloniaEdit.Editing
{
/// <summary>
/// Control that wraps a TextView and adds support for user input and the caret.
/// </summary>
public class TextArea : TemplatedControl, ITextEditorComponent, IRoutedCommandBindable, ILogicalScrollable
{
/// <summary>
/// This is the extra scrolling space that occurs after the last line.
/// </summary>
private const int AdditionalVerticalScrollAmount = 2;
private ILogicalScrollable _logicalScrollable;
private readonly TextAreaTextInputMethodClient _imClient = new TextAreaTextInputMethodClient();
#region Constructor
static TextArea()
{
KeyboardNavigation.TabNavigationProperty.OverrideDefaultValue<TextArea>(KeyboardNavigationMode.None);
FocusableProperty.OverrideDefaultValue<TextArea>(true);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
AffectsArrange<TextArea>(OffsetProperty);
AffectsRender<TextArea>(OffsetProperty);
TextInputMethodClientRequestedEvent.AddClassHandler<TextArea>((ta, e) =>
{
if (!ta.IsReadOnly)
{
e.Client = ta._imClient;
}
});
}
/// <summary>
/// Creates a new TextArea instance.
/// </summary>
public TextArea() : this(new TextView())
{
AddHandler(KeyDownEvent, OnPreviewKeyDown, RoutingStrategies.Tunnel);
AddHandler(KeyUpEvent, OnPreviewKeyUp, RoutingStrategies.Tunnel);
}
/// <summary>
/// Creates a new TextArea instance.
/// </summary>
protected TextArea(TextView textView)
{
TextView = textView ?? throw new ArgumentNullException(nameof(textView));
_logicalScrollable = textView;
Options = textView.Options;
_selection = EmptySelection = new EmptySelection(this);
textView.Services.AddService(this);
textView.LineTransformers.Add(new SelectionColorizer(this));
textView.InsertLayer(new SelectionLayer(this), KnownLayer.Selection, LayerInsertionPosition.Replace);
Caret = new Caret(this);
Caret.PositionChanged += (sender, e) => RequestSelectionValidation();
Caret.PositionChanged += CaretPositionChanged;
AttachTypingEvents();
LeftMargins.CollectionChanged += LeftMargins_CollectionChanged;
DefaultInputHandler = new TextAreaDefaultInputHandler(this);
ActiveInputHandler = DefaultInputHandler;
// TODO
//textView.GetObservable(TextBlock.FontSizeProperty).Subscribe(_ =>
//{
// TextView.SetScrollOffset(new Vector(_offset.X, _offset.Y * TextView.DefaultLineHeight));
//});
}
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
if (e.NameScope.Find("PART_CP") is ContentPresenter contentPresenter)
{
contentPresenter.Content = TextView;
}
}
internal void AddChild(IVisual visual)
{
VisualChildren.Add(visual);
InvalidateArrange();
}
internal void RemoveChild(IVisual visual)
{
VisualChildren.Remove(visual);
}
#endregion
/// <summary>
/// Defines the <see cref="IScrollable.Offset" /> property.
/// </summary>
public static readonly DirectProperty<TextArea, Vector> OffsetProperty =
AvaloniaProperty.RegisterDirect<TextArea, Vector>(
nameof(IScrollable.Offset),
o => (o as IScrollable).Offset,
(o, v) => (o as IScrollable).Offset = v);
#region InputHandler management
/// <summary>
/// Gets the default input handler.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public TextAreaDefaultInputHandler DefaultInputHandler { get; }
private ITextAreaInputHandler _activeInputHandler;
private bool _isChangingInputHandler;
/// <summary>
/// Gets/Sets the active input handler.
/// This property does not return currently active stacked input handlers. Setting this property detached all stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public ITextAreaInputHandler ActiveInputHandler
{
get => _activeInputHandler;
set
{
if (value != null && value.TextArea != this)
throw new ArgumentException("The input handler was created for a different text area than this one.");
if (_isChangingInputHandler)
throw new InvalidOperationException("Cannot set ActiveInputHandler recursively");
if (_activeInputHandler != value)
{
_isChangingInputHandler = true;
try
{
// pop the whole stack
PopStackedInputHandler(StackedInputHandlers.LastOrDefault());
Debug.Assert(StackedInputHandlers.IsEmpty);
_activeInputHandler?.Detach();
_activeInputHandler = value;
value?.Attach();
}
finally
{
_isChangingInputHandler = false;
}
ActiveInputHandlerChanged?.Invoke(this, EventArgs.Empty);
}
}
}
/// <summary>
/// Occurs when the ActiveInputHandler property changes.
/// </summary>
public event EventHandler ActiveInputHandlerChanged;
/// <summary>
/// Gets the list of currently active stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public ImmutableStack<TextAreaStackedInputHandler> StackedInputHandlers { get; private set; } = ImmutableStack<TextAreaStackedInputHandler>.Empty;
/// <summary>
/// Pushes an input handler onto the list of stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public void PushStackedInputHandler(TextAreaStackedInputHandler inputHandler)
{
if (inputHandler == null)
throw new ArgumentNullException(nameof(inputHandler));
StackedInputHandlers = StackedInputHandlers.Push(inputHandler);
inputHandler.Attach();
}
/// <summary>
/// Pops the stacked input handler (and all input handlers above it).
/// If <paramref name="inputHandler"/> is not found in the currently stacked input handlers, or is null, this method
/// does nothing.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public void PopStackedInputHandler(TextAreaStackedInputHandler inputHandler)
{
if (StackedInputHandlers.Any(i => i == inputHandler))
{
ITextAreaInputHandler oldHandler;
do
{
oldHandler = StackedInputHandlers.Peek();
StackedInputHandlers = StackedInputHandlers.Pop();
oldHandler.Detach();
} while (oldHandler != inputHandler);
}
}
#endregion
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextArea>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <inheritdoc/>
public event EventHandler DocumentChanged;
/// <summary>
/// Gets if the the document displayed by the text editor is readonly
/// </summary>
public bool IsReadOnly
{
get => ReadOnlySectionProvider == ReadOnlySectionDocument.Instance;
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextArea)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.Changing.RemoveHandler(oldValue, OnDocumentChanging);
TextDocumentWeakEventManager.Changed.RemoveHandler(oldValue, OnDocumentChanged);
TextDocumentWeakEventManager.UpdateStarted.RemoveHandler(oldValue, OnUpdateStarted);
TextDocumentWeakEventManager.UpdateFinished.RemoveHandler(oldValue, OnUpdateFinished);
}
TextView.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.Changing.AddHandler(newValue, OnDocumentChanging);
TextDocumentWeakEventManager.Changed.AddHandler(newValue, OnDocumentChanged);
TextDocumentWeakEventManager.UpdateStarted.AddHandler(newValue, OnUpdateStarted);
TextDocumentWeakEventManager.UpdateFinished.AddHandler(newValue, OnUpdateFinished);
InvalidateArrange();
}
// Reset caret location and selection: this is necessary because the caret/selection might be invalid
// in the new document (e.g. if new document is shorter than the old document).
Caret.Location = new TextLocation(1, 1);
ClearSelection();
DocumentChanged?.Invoke(this, EventArgs.Empty);
//CommandManager.InvalidateRequerySuggested();
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextArea>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
private void OnOptionChanged(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextArea)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnOptionChanged);
}
TextView.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnOptionChanged);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
#endregion
#region Caret handling on document changes
private void OnDocumentChanging(object sender, DocumentChangeEventArgs e)
{
Caret.OnDocumentChanging();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
Caret.OnDocumentChanged(e);
Selection = _selection.UpdateOnDocumentChange(e);
}
private void OnUpdateStarted(object sender, EventArgs e)
{
Document.UndoStack.PushOptional(new RestoreCaretAndSelectionUndoAction(this));
}
private void OnUpdateFinished(object sender, EventArgs e)
{
Caret.OnDocumentUpdateFinished();
}
private sealed class RestoreCaretAndSelectionUndoAction : IUndoableOperation
{
// keep textarea in weak reference because the IUndoableOperation is stored with the document
private readonly WeakReference _textAreaReference;
private readonly TextViewPosition _caretPosition;
private readonly Selection _selection;
public RestoreCaretAndSelectionUndoAction(TextArea textArea)
{
_textAreaReference = new WeakReference(textArea);
// Just save the old caret position, no need to validate here.
// If we restore it, we'll validate it anyways.
_caretPosition = textArea.Caret.NonValidatedPosition;
_selection = textArea.Selection;
}
public void Undo()
{
var textArea = (TextArea)_textAreaReference.Target;
if (textArea != null)
{
textArea.Caret.Position = _caretPosition;
textArea.Selection = _selection;
}
}
public void Redo()
{
// redo=undo: we just restore the caret/selection state
Undo();
}
}
#endregion
#region TextView property
/// <summary>
/// Gets the text view used to display text in this text area.
/// </summary>
public TextView TextView { get; }
#endregion
#region Selection property
internal readonly Selection EmptySelection;
private Selection _selection;
/// <summary>
/// Occurs when the selection has changed.
/// </summary>
public event EventHandler SelectionChanged;
/// <summary>
/// Gets/Sets the selection in this text area.
/// </summary>
public Selection Selection
{
get => _selection;
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
if (value.TextArea != this)
throw new ArgumentException("Cannot use a Selection instance that belongs to another text area.");
if (!Equals(_selection, value))
{
if (TextView != null)
{
var oldSegment = _selection.SurroundingSegment;
var newSegment = value.SurroundingSegment;
if (!Selection.EnableVirtualSpace && (_selection is SimpleSelection && value is SimpleSelection && oldSegment != null && newSegment != null))
{
// perf optimization:
// When a simple selection changes, don't redraw the whole selection, but only the changed parts.
var oldSegmentOffset = oldSegment.Offset;
var newSegmentOffset = newSegment.Offset;
if (oldSegmentOffset != newSegmentOffset)
{
TextView.Redraw(Math.Min(oldSegmentOffset, newSegmentOffset),
Math.Abs(oldSegmentOffset - newSegmentOffset));
}
var oldSegmentEndOffset = oldSegment.EndOffset;
var newSegmentEndOffset = newSegment.EndOffset;
if (oldSegmentEndOffset != newSegmentEndOffset)
{
TextView.Redraw(Math.Min(oldSegmentEndOffset, newSegmentEndOffset),
Math.Abs(oldSegmentEndOffset - newSegmentEndOffset));
}
}
else
{
TextView.Redraw(oldSegment);
TextView.Redraw(newSegment);
}
}
_selection = value;
SelectionChanged?.Invoke(this, EventArgs.Empty);
// a selection change causes commands like copy/paste/etc. to change status
//CommandManager.InvalidateRequerySuggested();
}
}
}
/// <summary>
/// Clears the current selection.
/// </summary>
public void ClearSelection()
{
Selection = EmptySelection;
}
/// <summary>
/// The <see cref="SelectionBrush"/> property.
/// </summary>
public static readonly StyledProperty<IBrush> SelectionBrushProperty =
AvaloniaProperty.Register<TextArea, IBrush>("SelectionBrush");
/// <summary>
/// Gets/Sets the background brush used for the selection.
/// </summary>
public IBrush SelectionBrush
{
get => GetValue(SelectionBrushProperty);
set => SetValue(SelectionBrushProperty, value);
}
/// <summary>
/// The <see cref="SelectionForeground"/> property.
/// </summary>
public static readonly StyledProperty<IBrush> SelectionForegroundProperty =
AvaloniaProperty.Register<TextArea, IBrush>("SelectionForeground");
/// <summary>
/// Gets/Sets the foreground brush used for selected text.
/// </summary>
public IBrush SelectionForeground
{
get => GetValue(SelectionForegroundProperty);
set => SetValue(SelectionForegroundProperty, value);
}
/// <summary>
/// The <see cref="SelectionBorder"/> property.
/// </summary>
public static readonly StyledProperty<Pen> SelectionBorderProperty =
AvaloniaProperty.Register<TextArea, Pen>("SelectionBorder");
/// <summary>
/// Gets/Sets the pen used for the border of the selection.
/// </summary>
public Pen SelectionBorder
{
get => GetValue(SelectionBorderProperty);
set => SetValue(SelectionBorderProperty, value);
}
/// <summary>
/// The <see cref="SelectionCornerRadius"/> property.
/// </summary>
public static readonly StyledProperty<double> SelectionCornerRadiusProperty =
AvaloniaProperty.Register<TextArea, double>("SelectionCornerRadius", 3.0);
/// <summary>
/// Gets/Sets the corner radius of the selection.
/// </summary>
public double SelectionCornerRadius
{
get => GetValue(SelectionCornerRadiusProperty);
set => SetValue(SelectionCornerRadiusProperty, value);
}
#endregion
#region Force caret to stay inside selection
private bool _ensureSelectionValidRequested;
private int _allowCaretOutsideSelection;
private void RequestSelectionValidation()
{
if (!_ensureSelectionValidRequested && _allowCaretOutsideSelection == 0)
{
_ensureSelectionValidRequested = true;
Dispatcher.UIThread.Post(EnsureSelectionValid);
}
}
/// <summary>
/// Code that updates only the caret but not the selection can cause confusion when
/// keys like 'Delete' delete the (possibly invisible) selected text and not the
/// text around the caret.
///
/// So we'll ensure that the caret is inside the selection.
/// (when the caret is not in the selection, we'll clear the selection)
///
/// This method is invoked using the Dispatcher so that code may temporarily violate this rule
/// (e.g. most 'extend selection' methods work by first setting the caret, then the selection),
/// it's sufficient to fix it after any event handlers have run.
/// </summary>
private void EnsureSelectionValid()
{
_ensureSelectionValidRequested = false;
if (_allowCaretOutsideSelection == 0)
{
if (!_selection.IsEmpty && !_selection.Contains(Caret.Offset))
{
ClearSelection();
}
}
}
/// <summary>
/// Temporarily allows positioning the caret outside the selection.
/// Dispose the returned IDisposable to revert the allowance.
/// </summary>
/// <remarks>
/// The text area only forces the caret to be inside the selection when other events
/// have finished running (using the dispatcher), so you don't have to use this method
/// for temporarily positioning the caret in event handlers.
/// This method is only necessary if you want to run the dispatcher, e.g. if you
/// perform a drag'n'drop operation.
/// </remarks>
public IDisposable AllowCaretOutsideSelection()
{
VerifyAccess();
_allowCaretOutsideSelection++;
return new CallbackOnDispose(
delegate
{
VerifyAccess();
_allowCaretOutsideSelection--;
RequestSelectionValidation();
});
}
#endregion
#region Properties
/// <summary>
/// Gets the Caret used for this text area.
/// </summary>
public Caret Caret { get; }
/// <summary>
/// Scrolls the text view so that the requested line is in the middle.
/// If the textview can be scrolled.
/// </summary>
/// <param name="line">The line to scroll to.</param>
public void ScrollToLine (int line)
{
var viewPortLines = (int)(this as IScrollable).Viewport.Height;
if (viewPortLines < Document.LineCount)
{
ScrollToLine(line, 2, viewPortLines / 2);
}
}
/// <summary>
/// Scrolls the textview to a position with n lines above and below it.
/// </summary>
/// <param name="line">the requested line number.</param>
/// <param name="linesEitherSide">The number of lines above and below.</param>
public void ScrollToLine(int line, int linesEitherSide)
{
ScrollToLine(line, linesEitherSide, linesEitherSide);
}
/// <summary>
/// Scrolls the textview to a position with n lines above and below it.
/// </summary>
/// <param name="line">the requested line number.</param>
/// <param name="linesAbove">The number of lines above.</param>
/// <param name="linesBelow">The number of lines below.</param>
public void ScrollToLine(int line, int linesAbove, int linesBelow)
{
var offset = line - linesAbove;
if (offset < 0)
{
offset = 0;
}
this.BringIntoView(new Rect(1, offset, 0, 1));
offset = line + linesBelow;
if (offset >= 0)
{
this.BringIntoView(new Rect(1, offset, 0, 1));
}
}
private void CaretPositionChanged(object sender, EventArgs e)
{
if (TextView == null)
return;
TextView.HighlightedLine = Caret.Line;
ScrollToLine(Caret.Line, 2);
Dispatcher.UIThread.InvokeAsync(() =>
{
(this as ILogicalScrollable).RaiseScrollInvalidated(EventArgs.Empty);
});
}
public static readonly DirectProperty<TextArea, ObservableCollection<IControl>> LeftMarginsProperty
= AvaloniaProperty.RegisterDirect<TextArea, ObservableCollection<IControl>>(nameof(LeftMargins),
c => c.LeftMargins);
/// <summary>
/// Gets the collection of margins displayed to the left of the text view.
/// </summary>
public ObservableCollection<IControl> LeftMargins { get; } = new ObservableCollection<IControl>();
private void LeftMargins_CollectionChanged(object sender, NotifyCollectionChangedEventArgs e)
{
if (e.OldItems != null)
{
foreach (var c in e.OldItems.OfType<ITextViewConnect>())
{
c.RemoveFromTextView(TextView);
}
get => _readOnlySectionProvider;
set => _readOnlySectionProvider = value ?? throw new ArgumentNullException(nameof(value));
}
#endregion
#region Focus Handling (Show/Hide Caret)
/// <summary>
/// The <see cref="RightClickMovesCaret"/> property.
/// </summary>
public static readonly StyledProperty<bool> RightClickMovesCaretProperty =
AvaloniaProperty.Register<TextArea, bool>(nameof(RightClickMovesCaret), false);
/// <summary>
/// Determines whether caret position should be changed to the mouse position when you right click or not.
/// </summary>
public bool RightClickMovesCaret
{
get => GetValue(RightClickMovesCaretProperty);
set => SetValue(RightClickMovesCaretProperty, value);
}
#endregion
#region Focus Handling (Show/Hide Caret)
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
Focus();
}
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
Caret.Show();
_imClient.SetTextArea(this);
}
protected override void OnLostFocus(RoutedEventArgs e)
{
base.OnLostFocus(e);
Caret.Hide();
_imClient.SetTextArea(null);
}
#endregion
#region OnTextInput / RemoveSelectedText / ReplaceSelectionWithText
/// <summary>
/// Occurs when the TextArea receives text input.
/// but occurs immediately before the TextArea handles the TextInput event.
/// </summary>
public event EventHandler<TextInputEventArgs> TextEntering;
/// <summary>
/// Occurs when the TextArea receives text input.
/// but occurs immediately after the TextArea handles the TextInput event.
/// </summary>
public event EventHandler<TextInputEventArgs> TextEntered;
/// <summary>
/// Raises the TextEntering event.
/// </summary>
protected virtual void OnTextEntering(TextInputEventArgs e)
{
TextEntering?.Invoke(this, e);
}
/// <summary>
/// Raises the TextEntered event.
/// </summary>
protected virtual void OnTextEntered(TextInputEventArgs e)
{
TextEntered?.Invoke(this, e);
}
protected override void OnTextInput(TextInputEventArgs e)
{
base.OnTextInput(e);
if (!e.Handled && Document != null)
{
if (string.IsNullOrEmpty(e.Text) || e.Text == "\x1b" || e.Text == "\b" || e.Text == "\u007f")
{
// TODO: check this
// ASCII 0x1b = ESC.
// produces a TextInput event with that old ASCII control char
// when Escape is pressed. We'll just ignore it.
// A deadkey followed by backspace causes a textinput event for the BS character.
// Similarly, some shortcuts like Alt+Space produce an empty TextInput event.
// We have to ignore those (not handle them) to keep the shortcut working.
return;
}
HideMouseCursor();
PerformTextInput(e);
e.Handled = true;
}
}
/// <summary>
/// Performs text input.
/// This raises the <see cref="TextEntering"/> event, replaces the selection with the text,
/// and then raises the <see cref="TextEntered"/> event.
/// </summary>
public void PerformTextInput(string text)
{
var e = new TextInputEventArgs
{
Text = text,
RoutedEvent = TextInputEvent
};
PerformTextInput(e);
}
/// <summary>
/// Performs text input.
/// This raises the <see cref="TextEntering"/> event, replaces the selection with the text,
/// and then raises the <see cref="TextEntered"/> event.
/// </summary>
public void PerformTextInput(TextInputEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
OnTextEntering(e);
if (!e.Handled)
{
if (e.Text == "\n" || e.Text == "\r" || e.Text == "\r\n")
ReplaceSelectionWithNewLine();
else
{
// TODO
//if (OverstrikeMode && Selection.IsEmpty && Document.GetLineByNumber(Caret.Line).EndOffset > Caret.Offset)
// EditingCommands.SelectRightByCharacter.Execute(null, this);
ReplaceSelectionWithText(e.Text);
}
OnTextEntered(e);
Caret.BringCaretToView();
}
}
private void ReplaceSelectionWithNewLine()
{
var newLine = TextUtilities.GetNewLineFromDocument(Document, Caret.Line);
using (Document.RunUpdate())
{
ReplaceSelectionWithText(newLine);
if (IndentationStrategy != null)
{
var line = Document.GetLineByNumber(Caret.Line);
var deletable = GetDeletableSegments(line);
if (deletable.Length == 1 && deletable[0].Offset == line.Offset && deletable[0].Length == line.Length)
{
// use indentation strategy only if the line is not read-only
IndentationStrategy.IndentLine(Document, line);
}
}
}
}
internal void RemoveSelectedText()
{
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
_selection.ReplaceSelectionWithText(string.Empty);
#if DEBUG
if (!_selection.IsEmpty)
{
foreach (var s in _selection.Segments)
{
Debug.Assert(!ReadOnlySectionProvider.GetDeletableSegments(s).Any());
}
}
#endif
}
internal void ReplaceSelectionWithText(string newText)
{
if (newText == null)
throw new ArgumentNullException(nameof(newText));
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
_selection.ReplaceSelectionWithText(newText);
}
internal ISegment[] GetDeletableSegments(ISegment segment)
{
var deletableSegments = ReadOnlySectionProvider.GetDeletableSegments(segment);
if (deletableSegments == null)
throw new InvalidOperationException("ReadOnlySectionProvider.GetDeletableSegments returned null");
var array = deletableSegments.ToArray();
var lastIndex = segment.Offset;
foreach (var t in array)
{
if (t.Offset < lastIndex)
throw new InvalidOperationException("ReadOnlySectionProvider returned incorrect segments (outside of input segment / wrong order)");
lastIndex = t.EndOffset;
}
if (lastIndex > segment.EndOffset)
throw new InvalidOperationException("ReadOnlySectionProvider returned incorrect segments (outside of input segment / wrong order)");
return array;
}
#endregion
#region IndentationStrategy property
/// <summary>
/// IndentationStrategy property.
/// </summary>
public static readonly StyledProperty<IIndentationStrategy> IndentationStrategyProperty =
AvaloniaProperty.Register<TextArea, IIndentationStrategy>("IndentationStrategy", new DefaultIndentationStrategy());
/// <summary>
/// Gets/Sets the indentation strategy used when inserting new lines.
/// </summary>
public IIndentationStrategy IndentationStrategy
{
get => GetValue(IndentationStrategyProperty);
set => SetValue(IndentationStrategyProperty, value);
}
#endregion
#region OnKeyDown/OnKeyUp
// Make life easier for text editor extensions that use a different cursor based on the pressed modifier keys.
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
TextView.InvalidateCursorIfPointerWithinTextView();
}
private void OnPreviewKeyDown(object sender, KeyEventArgs e)
{
foreach (var h in StackedInputHandlers)
{
if (e.Handled)
break;
h.OnPreviewKeyDown(e);
}
}
/// <inheritdoc/>
protected override void OnKeyUp(KeyEventArgs e)
{
base.OnKeyUp(e);
TextView.InvalidateCursorIfPointerWithinTextView();
}
private void OnPreviewKeyUp(object sender, KeyEventArgs e)
{
foreach (var h in StackedInputHandlers)
{
if (e.Handled)
break;
h.OnPreviewKeyUp(e);
}
}
#endregion
#region Hide Mouse Cursor While Typing
private bool _isMouseCursorHidden;
private void AttachTypingEvents()
{
// Use the PreviewMouseMove event in case some other editor layer consumes the MouseMove event (e.g. SD's InsertionCursorLayer)
PointerEntered += delegate { ShowMouseCursor(); };
PointerExited += delegate { ShowMouseCursor(); };
}
private void ShowMouseCursor()
{
if (_isMouseCursorHidden)
{
_isMouseCursorHidden = false;
}
}
private void HideMouseCursor()
{
if (Options.HideCursorWhileTyping && !_isMouseCursorHidden && IsPointerOver)
{
_isMouseCursorHidden = true;
}
}
#endregion
#region Overstrike mode
/// <summary>
/// The <see cref="OverstrikeMode"/> dependency property.
/// </summary>
public static readonly StyledProperty<bool> OverstrikeModeProperty =
AvaloniaProperty.Register<TextArea, bool>("OverstrikeMode");
/// <summary>
/// Gets/Sets whether overstrike mode is active.
/// </summary>
public bool OverstrikeMode
{
get => GetValue(OverstrikeModeProperty);
set => SetValue(OverstrikeModeProperty, value);
}
#endregion
/// <inheritdoc/>
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == SelectionBrushProperty
|| change.Property == SelectionBorderProperty
|| change.Property == SelectionForegroundProperty
|| change.Property == SelectionCornerRadiusProperty)
{
TextView.Redraw();
}
else if (change.Property == OverstrikeModeProperty)
{
Caret.UpdateIfVisible();
}
}
/// <summary>
/// Gets the requested service.
/// </summary>
/// <returns>Returns the requested service instance, or null if the service cannot be found.</returns>
public virtual object GetService(Type serviceType)
{
return TextView.GetService(serviceType);
}
/// <summary>
/// Occurs when text inside the TextArea was copied.
/// </summary>
public event EventHandler<TextEventArgs> TextCopied;
event EventHandler ILogicalScrollable.ScrollInvalidated
{
add { if (_logicalScrollable != null) _logicalScrollable.ScrollInvalidated += value; }
remove { if (_logicalScrollable != null) _logicalScrollable.ScrollInvalidated -= value; }
}
internal void OnTextCopied(TextEventArgs e)
{
TextCopied?.Invoke(this, e);
}
public IList<RoutedCommandBinding> CommandBindings { get; } = new List<RoutedCommandBinding>();
bool ILogicalScrollable.IsLogicalScrollEnabled => _logicalScrollable?.IsLogicalScrollEnabled ?? default(bool);
Size ILogicalScrollable.ScrollSize => _logicalScrollable?.ScrollSize ?? default(Size);
Size ILogicalScrollable.PageScrollSize => _logicalScrollable?.PageScrollSize ?? default(Size);
Size IScrollable.Extent => _logicalScrollable?.Extent ?? default(Size);
Vector IScrollable.Offset
{
get => _logicalScrollable?.Offset ?? default(Vector);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.Offset = value;
}
}
}
Size IScrollable.Viewport => _logicalScrollable?.Viewport ?? default(Size);
bool ILogicalScrollable.CanHorizontallyScroll
{
get => _logicalScrollable?.CanHorizontallyScroll ?? default(bool);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.CanHorizontallyScroll = value;
}
}
}
bool ILogicalScrollable.CanVerticallyScroll
{
get => _logicalScrollable?.CanVerticallyScroll ?? default(bool);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.CanVerticallyScroll = value;
}
}
}
public bool BringIntoView(IControl target, Rect targetRect) =>
_logicalScrollable?.BringIntoView(target, targetRect) ?? default(bool);
IControl ILogicalScrollable.GetControlInDirection(NavigationDirection direction, IControl from)
=> _logicalScrollable?.GetControlInDirection(direction, from);
public void RaiseScrollInvalidated(EventArgs e)
{
_logicalScrollable?.RaiseScrollInvalidated(e);
}
private class TextAreaTextInputMethodClient : ITextInputMethodClient
{
private TextArea _textArea;
public TextAreaTextInputMethodClient()
{
}
public event EventHandler CursorRectangleChanged;
public event EventHandler TextViewVisualChanged;
public event EventHandler SurroundingTextChanged;
public Rect CursorRectangle
{
get
{
if(_textArea == null)
{
return Rect.Empty;
}
var transform = _textArea.TextView.TransformToVisual(_textArea);
if (transform == null)
{
return default;
}
var rect = _textArea.Caret.CalculateCaretRectangle().TransformToAABB(transform.Value);
return rect;
}
}
public IVisual TextViewVisual => _textArea;
public bool SupportsPreedit => false;
public bool SupportsSurroundingText => true;
public TextInputMethodSurroundingText SurroundingText
{
get
{
if(_textArea == null)
{
return default;
}
var lineIndex = _textArea.Caret.Line;
var position = _textArea.Caret.Position;
var documentLine = _textArea.Document.GetLineByNumber(lineIndex);
var text = _textArea.Document.GetText(documentLine.Offset, documentLine.Length);
return new TextInputMethodSurroundingText
{
AnchorOffset = 0,
CursorOffset = position.Column,
Text = text
};
}
}
public void SetTextArea(TextArea textArea)
{
if(_textArea != null)
{
_textArea.Caret.PositionChanged -= Caret_PositionChanged;
_textArea.SelectionChanged -= TextArea_SelectionChanged;
}
_textArea = textArea;
if(_textArea != null)
{
_textArea.Caret.PositionChanged += Caret_PositionChanged;
_textArea.SelectionChanged += TextArea_SelectionChanged;
}
TextViewVisualChanged?.Invoke(this, EventArgs.Empty);
CursorRectangleChanged?.Invoke(this, EventArgs.Empty);
}
private void Caret_PositionChanged(object sender, EventArgs e)
{
CursorRectangleChanged?.Invoke(this, e);
}
private void TextArea_SelectionChanged(object sender, EventArgs e)
{
SurroundingTextChanged?.Invoke(this, e);
}
public void SelectInSurroundingText(int start, int end)
{
if(_textArea == null)
{
return;
}
var selection = _textArea.Selection;
_textArea.Selection = _textArea.Selection.StartSelectionOrSetEndpoint(
new TextViewPosition(selection.StartPosition.Line, start),
new TextViewPosition(selection.StartPosition.Line, end));
}
public void SetPreeditText(string text)
{
}
}
}
/// <summary>
/// EventArgs with text.
/// </summary>
public class TextEventArgs : EventArgs
{
/// <summary>
/// Gets the text.
/// </summary>
public string Text { get; }
/// <summary>
/// Creates a new TextEventArgs instance.
/// </summary>
public TextEventArgs(string text)
{
Text = text ?? throw new ArgumentNullException(nameof(text));
}
}
}
<MSG> Merge pull request #169 from Takoooooo/right-click-moves-caret-feature
You should be able to move caret by right clicking text area
<DFF> @@ -708,6 +708,21 @@ namespace AvaloniaEdit.Editing
get => _readOnlySectionProvider;
set => _readOnlySectionProvider = value ?? throw new ArgumentNullException(nameof(value));
}
+
+ /// <summary>
+ /// The <see cref="RightClickMovesCaret"/> property.
+ /// </summary>
+ public static readonly StyledProperty<bool> RightClickMovesCaretProperty =
+ AvaloniaProperty.Register<TextArea, bool>(nameof(RightClickMovesCaret), false);
+
+ /// <summary>
+ /// Determines whether caret position should be changed to the mouse position when you right click or not.
+ /// </summary>
+ public bool RightClickMovesCaret
+ {
+ get => GetValue(RightClickMovesCaretProperty);
+ set => SetValue(RightClickMovesCaretProperty, value);
+ }
#endregion
#region Focus Handling (Show/Hide Caret)
| 15 | Merge pull request #169 from Takoooooo/right-click-moves-caret-feature | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066156 | <NME> TextArea.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using Avalonia;
using Avalonia.Controls;
using Avalonia.Controls.Presenters;
using Avalonia.Controls.Primitives;
using Avalonia.Data;
using Avalonia.Input;
using Avalonia.Input.TextInput;
using Avalonia.Interactivity;
using Avalonia.Media;
using Avalonia.Threading;
using Avalonia.VisualTree;
using AvaloniaEdit.Document;
using AvaloniaEdit.Indentation;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Search;
using AvaloniaEdit.Utils;
using System;
using System.Collections.Generic;
using System.Collections.Immutable;
using System.Collections.ObjectModel;
using System.Collections.Specialized;
using System.ComponentModel;
using System.Diagnostics;
using System.Linq;
namespace AvaloniaEdit.Editing
{
/// <summary>
/// Control that wraps a TextView and adds support for user input and the caret.
/// </summary>
public class TextArea : TemplatedControl, ITextEditorComponent, IRoutedCommandBindable, ILogicalScrollable
{
/// <summary>
/// This is the extra scrolling space that occurs after the last line.
/// </summary>
private const int AdditionalVerticalScrollAmount = 2;
private ILogicalScrollable _logicalScrollable;
private readonly TextAreaTextInputMethodClient _imClient = new TextAreaTextInputMethodClient();
#region Constructor
static TextArea()
{
KeyboardNavigation.TabNavigationProperty.OverrideDefaultValue<TextArea>(KeyboardNavigationMode.None);
FocusableProperty.OverrideDefaultValue<TextArea>(true);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
AffectsArrange<TextArea>(OffsetProperty);
AffectsRender<TextArea>(OffsetProperty);
TextInputMethodClientRequestedEvent.AddClassHandler<TextArea>((ta, e) =>
{
if (!ta.IsReadOnly)
{
e.Client = ta._imClient;
}
});
}
/// <summary>
/// Creates a new TextArea instance.
/// </summary>
public TextArea() : this(new TextView())
{
AddHandler(KeyDownEvent, OnPreviewKeyDown, RoutingStrategies.Tunnel);
AddHandler(KeyUpEvent, OnPreviewKeyUp, RoutingStrategies.Tunnel);
}
/// <summary>
/// Creates a new TextArea instance.
/// </summary>
protected TextArea(TextView textView)
{
TextView = textView ?? throw new ArgumentNullException(nameof(textView));
_logicalScrollable = textView;
Options = textView.Options;
_selection = EmptySelection = new EmptySelection(this);
textView.Services.AddService(this);
textView.LineTransformers.Add(new SelectionColorizer(this));
textView.InsertLayer(new SelectionLayer(this), KnownLayer.Selection, LayerInsertionPosition.Replace);
Caret = new Caret(this);
Caret.PositionChanged += (sender, e) => RequestSelectionValidation();
Caret.PositionChanged += CaretPositionChanged;
AttachTypingEvents();
LeftMargins.CollectionChanged += LeftMargins_CollectionChanged;
DefaultInputHandler = new TextAreaDefaultInputHandler(this);
ActiveInputHandler = DefaultInputHandler;
// TODO
//textView.GetObservable(TextBlock.FontSizeProperty).Subscribe(_ =>
//{
// TextView.SetScrollOffset(new Vector(_offset.X, _offset.Y * TextView.DefaultLineHeight));
//});
}
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
if (e.NameScope.Find("PART_CP") is ContentPresenter contentPresenter)
{
contentPresenter.Content = TextView;
}
}
internal void AddChild(IVisual visual)
{
VisualChildren.Add(visual);
InvalidateArrange();
}
internal void RemoveChild(IVisual visual)
{
VisualChildren.Remove(visual);
}
#endregion
/// <summary>
/// Defines the <see cref="IScrollable.Offset" /> property.
/// </summary>
public static readonly DirectProperty<TextArea, Vector> OffsetProperty =
AvaloniaProperty.RegisterDirect<TextArea, Vector>(
nameof(IScrollable.Offset),
o => (o as IScrollable).Offset,
(o, v) => (o as IScrollable).Offset = v);
#region InputHandler management
/// <summary>
/// Gets the default input handler.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public TextAreaDefaultInputHandler DefaultInputHandler { get; }
private ITextAreaInputHandler _activeInputHandler;
private bool _isChangingInputHandler;
/// <summary>
/// Gets/Sets the active input handler.
/// This property does not return currently active stacked input handlers. Setting this property detached all stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public ITextAreaInputHandler ActiveInputHandler
{
get => _activeInputHandler;
set
{
if (value != null && value.TextArea != this)
throw new ArgumentException("The input handler was created for a different text area than this one.");
if (_isChangingInputHandler)
throw new InvalidOperationException("Cannot set ActiveInputHandler recursively");
if (_activeInputHandler != value)
{
_isChangingInputHandler = true;
try
{
// pop the whole stack
PopStackedInputHandler(StackedInputHandlers.LastOrDefault());
Debug.Assert(StackedInputHandlers.IsEmpty);
_activeInputHandler?.Detach();
_activeInputHandler = value;
value?.Attach();
}
finally
{
_isChangingInputHandler = false;
}
ActiveInputHandlerChanged?.Invoke(this, EventArgs.Empty);
}
}
}
/// <summary>
/// Occurs when the ActiveInputHandler property changes.
/// </summary>
public event EventHandler ActiveInputHandlerChanged;
/// <summary>
/// Gets the list of currently active stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public ImmutableStack<TextAreaStackedInputHandler> StackedInputHandlers { get; private set; } = ImmutableStack<TextAreaStackedInputHandler>.Empty;
/// <summary>
/// Pushes an input handler onto the list of stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public void PushStackedInputHandler(TextAreaStackedInputHandler inputHandler)
{
if (inputHandler == null)
throw new ArgumentNullException(nameof(inputHandler));
StackedInputHandlers = StackedInputHandlers.Push(inputHandler);
inputHandler.Attach();
}
/// <summary>
/// Pops the stacked input handler (and all input handlers above it).
/// If <paramref name="inputHandler"/> is not found in the currently stacked input handlers, or is null, this method
/// does nothing.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public void PopStackedInputHandler(TextAreaStackedInputHandler inputHandler)
{
if (StackedInputHandlers.Any(i => i == inputHandler))
{
ITextAreaInputHandler oldHandler;
do
{
oldHandler = StackedInputHandlers.Peek();
StackedInputHandlers = StackedInputHandlers.Pop();
oldHandler.Detach();
} while (oldHandler != inputHandler);
}
}
#endregion
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextArea>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <inheritdoc/>
public event EventHandler DocumentChanged;
/// <summary>
/// Gets if the the document displayed by the text editor is readonly
/// </summary>
public bool IsReadOnly
{
get => ReadOnlySectionProvider == ReadOnlySectionDocument.Instance;
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextArea)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.Changing.RemoveHandler(oldValue, OnDocumentChanging);
TextDocumentWeakEventManager.Changed.RemoveHandler(oldValue, OnDocumentChanged);
TextDocumentWeakEventManager.UpdateStarted.RemoveHandler(oldValue, OnUpdateStarted);
TextDocumentWeakEventManager.UpdateFinished.RemoveHandler(oldValue, OnUpdateFinished);
}
TextView.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.Changing.AddHandler(newValue, OnDocumentChanging);
TextDocumentWeakEventManager.Changed.AddHandler(newValue, OnDocumentChanged);
TextDocumentWeakEventManager.UpdateStarted.AddHandler(newValue, OnUpdateStarted);
TextDocumentWeakEventManager.UpdateFinished.AddHandler(newValue, OnUpdateFinished);
InvalidateArrange();
}
// Reset caret location and selection: this is necessary because the caret/selection might be invalid
// in the new document (e.g. if new document is shorter than the old document).
Caret.Location = new TextLocation(1, 1);
ClearSelection();
DocumentChanged?.Invoke(this, EventArgs.Empty);
//CommandManager.InvalidateRequerySuggested();
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextArea>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
private void OnOptionChanged(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextArea)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnOptionChanged);
}
TextView.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnOptionChanged);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
#endregion
#region Caret handling on document changes
private void OnDocumentChanging(object sender, DocumentChangeEventArgs e)
{
Caret.OnDocumentChanging();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
Caret.OnDocumentChanged(e);
Selection = _selection.UpdateOnDocumentChange(e);
}
private void OnUpdateStarted(object sender, EventArgs e)
{
Document.UndoStack.PushOptional(new RestoreCaretAndSelectionUndoAction(this));
}
private void OnUpdateFinished(object sender, EventArgs e)
{
Caret.OnDocumentUpdateFinished();
}
private sealed class RestoreCaretAndSelectionUndoAction : IUndoableOperation
{
// keep textarea in weak reference because the IUndoableOperation is stored with the document
private readonly WeakReference _textAreaReference;
private readonly TextViewPosition _caretPosition;
private readonly Selection _selection;
public RestoreCaretAndSelectionUndoAction(TextArea textArea)
{
_textAreaReference = new WeakReference(textArea);
// Just save the old caret position, no need to validate here.
// If we restore it, we'll validate it anyways.
_caretPosition = textArea.Caret.NonValidatedPosition;
_selection = textArea.Selection;
}
public void Undo()
{
var textArea = (TextArea)_textAreaReference.Target;
if (textArea != null)
{
textArea.Caret.Position = _caretPosition;
textArea.Selection = _selection;
}
}
public void Redo()
{
// redo=undo: we just restore the caret/selection state
Undo();
}
}
#endregion
#region TextView property
/// <summary>
/// Gets the text view used to display text in this text area.
/// </summary>
public TextView TextView { get; }
#endregion
#region Selection property
internal readonly Selection EmptySelection;
private Selection _selection;
/// <summary>
/// Occurs when the selection has changed.
/// </summary>
public event EventHandler SelectionChanged;
/// <summary>
/// Gets/Sets the selection in this text area.
/// </summary>
public Selection Selection
{
get => _selection;
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
if (value.TextArea != this)
throw new ArgumentException("Cannot use a Selection instance that belongs to another text area.");
if (!Equals(_selection, value))
{
if (TextView != null)
{
var oldSegment = _selection.SurroundingSegment;
var newSegment = value.SurroundingSegment;
if (!Selection.EnableVirtualSpace && (_selection is SimpleSelection && value is SimpleSelection && oldSegment != null && newSegment != null))
{
// perf optimization:
// When a simple selection changes, don't redraw the whole selection, but only the changed parts.
var oldSegmentOffset = oldSegment.Offset;
var newSegmentOffset = newSegment.Offset;
if (oldSegmentOffset != newSegmentOffset)
{
TextView.Redraw(Math.Min(oldSegmentOffset, newSegmentOffset),
Math.Abs(oldSegmentOffset - newSegmentOffset));
}
var oldSegmentEndOffset = oldSegment.EndOffset;
var newSegmentEndOffset = newSegment.EndOffset;
if (oldSegmentEndOffset != newSegmentEndOffset)
{
TextView.Redraw(Math.Min(oldSegmentEndOffset, newSegmentEndOffset),
Math.Abs(oldSegmentEndOffset - newSegmentEndOffset));
}
}
else
{
TextView.Redraw(oldSegment);
TextView.Redraw(newSegment);
}
}
_selection = value;
SelectionChanged?.Invoke(this, EventArgs.Empty);
// a selection change causes commands like copy/paste/etc. to change status
//CommandManager.InvalidateRequerySuggested();
}
}
}
/// <summary>
/// Clears the current selection.
/// </summary>
public void ClearSelection()
{
Selection = EmptySelection;
}
/// <summary>
/// The <see cref="SelectionBrush"/> property.
/// </summary>
public static readonly StyledProperty<IBrush> SelectionBrushProperty =
AvaloniaProperty.Register<TextArea, IBrush>("SelectionBrush");
/// <summary>
/// Gets/Sets the background brush used for the selection.
/// </summary>
public IBrush SelectionBrush
{
get => GetValue(SelectionBrushProperty);
set => SetValue(SelectionBrushProperty, value);
}
/// <summary>
/// The <see cref="SelectionForeground"/> property.
/// </summary>
public static readonly StyledProperty<IBrush> SelectionForegroundProperty =
AvaloniaProperty.Register<TextArea, IBrush>("SelectionForeground");
/// <summary>
/// Gets/Sets the foreground brush used for selected text.
/// </summary>
public IBrush SelectionForeground
{
get => GetValue(SelectionForegroundProperty);
set => SetValue(SelectionForegroundProperty, value);
}
/// <summary>
/// The <see cref="SelectionBorder"/> property.
/// </summary>
public static readonly StyledProperty<Pen> SelectionBorderProperty =
AvaloniaProperty.Register<TextArea, Pen>("SelectionBorder");
/// <summary>
/// Gets/Sets the pen used for the border of the selection.
/// </summary>
public Pen SelectionBorder
{
get => GetValue(SelectionBorderProperty);
set => SetValue(SelectionBorderProperty, value);
}
/// <summary>
/// The <see cref="SelectionCornerRadius"/> property.
/// </summary>
public static readonly StyledProperty<double> SelectionCornerRadiusProperty =
AvaloniaProperty.Register<TextArea, double>("SelectionCornerRadius", 3.0);
/// <summary>
/// Gets/Sets the corner radius of the selection.
/// </summary>
public double SelectionCornerRadius
{
get => GetValue(SelectionCornerRadiusProperty);
set => SetValue(SelectionCornerRadiusProperty, value);
}
#endregion
#region Force caret to stay inside selection
private bool _ensureSelectionValidRequested;
private int _allowCaretOutsideSelection;
private void RequestSelectionValidation()
{
if (!_ensureSelectionValidRequested && _allowCaretOutsideSelection == 0)
{
_ensureSelectionValidRequested = true;
Dispatcher.UIThread.Post(EnsureSelectionValid);
}
}
/// <summary>
/// Code that updates only the caret but not the selection can cause confusion when
/// keys like 'Delete' delete the (possibly invisible) selected text and not the
/// text around the caret.
///
/// So we'll ensure that the caret is inside the selection.
/// (when the caret is not in the selection, we'll clear the selection)
///
/// This method is invoked using the Dispatcher so that code may temporarily violate this rule
/// (e.g. most 'extend selection' methods work by first setting the caret, then the selection),
/// it's sufficient to fix it after any event handlers have run.
/// </summary>
private void EnsureSelectionValid()
{
_ensureSelectionValidRequested = false;
if (_allowCaretOutsideSelection == 0)
{
if (!_selection.IsEmpty && !_selection.Contains(Caret.Offset))
{
ClearSelection();
}
}
}
/// <summary>
/// Temporarily allows positioning the caret outside the selection.
/// Dispose the returned IDisposable to revert the allowance.
/// </summary>
/// <remarks>
/// The text area only forces the caret to be inside the selection when other events
/// have finished running (using the dispatcher), so you don't have to use this method
/// for temporarily positioning the caret in event handlers.
/// This method is only necessary if you want to run the dispatcher, e.g. if you
/// perform a drag'n'drop operation.
/// </remarks>
public IDisposable AllowCaretOutsideSelection()
{
VerifyAccess();
_allowCaretOutsideSelection++;
return new CallbackOnDispose(
delegate
{
VerifyAccess();
_allowCaretOutsideSelection--;
RequestSelectionValidation();
});
}
#endregion
#region Properties
/// <summary>
/// Gets the Caret used for this text area.
/// </summary>
public Caret Caret { get; }
/// <summary>
/// Scrolls the text view so that the requested line is in the middle.
/// If the textview can be scrolled.
/// </summary>
/// <param name="line">The line to scroll to.</param>
public void ScrollToLine (int line)
{
var viewPortLines = (int)(this as IScrollable).Viewport.Height;
if (viewPortLines < Document.LineCount)
{
ScrollToLine(line, 2, viewPortLines / 2);
}
}
/// <summary>
/// Scrolls the textview to a position with n lines above and below it.
/// </summary>
/// <param name="line">the requested line number.</param>
/// <param name="linesEitherSide">The number of lines above and below.</param>
public void ScrollToLine(int line, int linesEitherSide)
{
ScrollToLine(line, linesEitherSide, linesEitherSide);
}
/// <summary>
/// Scrolls the textview to a position with n lines above and below it.
/// </summary>
/// <param name="line">the requested line number.</param>
/// <param name="linesAbove">The number of lines above.</param>
/// <param name="linesBelow">The number of lines below.</param>
public void ScrollToLine(int line, int linesAbove, int linesBelow)
{
var offset = line - linesAbove;
if (offset < 0)
{
offset = 0;
}
this.BringIntoView(new Rect(1, offset, 0, 1));
offset = line + linesBelow;
if (offset >= 0)
{
this.BringIntoView(new Rect(1, offset, 0, 1));
}
}
private void CaretPositionChanged(object sender, EventArgs e)
{
if (TextView == null)
return;
TextView.HighlightedLine = Caret.Line;
ScrollToLine(Caret.Line, 2);
Dispatcher.UIThread.InvokeAsync(() =>
{
(this as ILogicalScrollable).RaiseScrollInvalidated(EventArgs.Empty);
});
}
public static readonly DirectProperty<TextArea, ObservableCollection<IControl>> LeftMarginsProperty
= AvaloniaProperty.RegisterDirect<TextArea, ObservableCollection<IControl>>(nameof(LeftMargins),
c => c.LeftMargins);
/// <summary>
/// Gets the collection of margins displayed to the left of the text view.
/// </summary>
public ObservableCollection<IControl> LeftMargins { get; } = new ObservableCollection<IControl>();
private void LeftMargins_CollectionChanged(object sender, NotifyCollectionChangedEventArgs e)
{
if (e.OldItems != null)
{
foreach (var c in e.OldItems.OfType<ITextViewConnect>())
{
c.RemoveFromTextView(TextView);
}
get => _readOnlySectionProvider;
set => _readOnlySectionProvider = value ?? throw new ArgumentNullException(nameof(value));
}
#endregion
#region Focus Handling (Show/Hide Caret)
/// <summary>
/// The <see cref="RightClickMovesCaret"/> property.
/// </summary>
public static readonly StyledProperty<bool> RightClickMovesCaretProperty =
AvaloniaProperty.Register<TextArea, bool>(nameof(RightClickMovesCaret), false);
/// <summary>
/// Determines whether caret position should be changed to the mouse position when you right click or not.
/// </summary>
public bool RightClickMovesCaret
{
get => GetValue(RightClickMovesCaretProperty);
set => SetValue(RightClickMovesCaretProperty, value);
}
#endregion
#region Focus Handling (Show/Hide Caret)
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
Focus();
}
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
Caret.Show();
_imClient.SetTextArea(this);
}
protected override void OnLostFocus(RoutedEventArgs e)
{
base.OnLostFocus(e);
Caret.Hide();
_imClient.SetTextArea(null);
}
#endregion
#region OnTextInput / RemoveSelectedText / ReplaceSelectionWithText
/// <summary>
/// Occurs when the TextArea receives text input.
/// but occurs immediately before the TextArea handles the TextInput event.
/// </summary>
public event EventHandler<TextInputEventArgs> TextEntering;
/// <summary>
/// Occurs when the TextArea receives text input.
/// but occurs immediately after the TextArea handles the TextInput event.
/// </summary>
public event EventHandler<TextInputEventArgs> TextEntered;
/// <summary>
/// Raises the TextEntering event.
/// </summary>
protected virtual void OnTextEntering(TextInputEventArgs e)
{
TextEntering?.Invoke(this, e);
}
/// <summary>
/// Raises the TextEntered event.
/// </summary>
protected virtual void OnTextEntered(TextInputEventArgs e)
{
TextEntered?.Invoke(this, e);
}
protected override void OnTextInput(TextInputEventArgs e)
{
base.OnTextInput(e);
if (!e.Handled && Document != null)
{
if (string.IsNullOrEmpty(e.Text) || e.Text == "\x1b" || e.Text == "\b" || e.Text == "\u007f")
{
// TODO: check this
// ASCII 0x1b = ESC.
// produces a TextInput event with that old ASCII control char
// when Escape is pressed. We'll just ignore it.
// A deadkey followed by backspace causes a textinput event for the BS character.
// Similarly, some shortcuts like Alt+Space produce an empty TextInput event.
// We have to ignore those (not handle them) to keep the shortcut working.
return;
}
HideMouseCursor();
PerformTextInput(e);
e.Handled = true;
}
}
/// <summary>
/// Performs text input.
/// This raises the <see cref="TextEntering"/> event, replaces the selection with the text,
/// and then raises the <see cref="TextEntered"/> event.
/// </summary>
public void PerformTextInput(string text)
{
var e = new TextInputEventArgs
{
Text = text,
RoutedEvent = TextInputEvent
};
PerformTextInput(e);
}
/// <summary>
/// Performs text input.
/// This raises the <see cref="TextEntering"/> event, replaces the selection with the text,
/// and then raises the <see cref="TextEntered"/> event.
/// </summary>
public void PerformTextInput(TextInputEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
OnTextEntering(e);
if (!e.Handled)
{
if (e.Text == "\n" || e.Text == "\r" || e.Text == "\r\n")
ReplaceSelectionWithNewLine();
else
{
// TODO
//if (OverstrikeMode && Selection.IsEmpty && Document.GetLineByNumber(Caret.Line).EndOffset > Caret.Offset)
// EditingCommands.SelectRightByCharacter.Execute(null, this);
ReplaceSelectionWithText(e.Text);
}
OnTextEntered(e);
Caret.BringCaretToView();
}
}
private void ReplaceSelectionWithNewLine()
{
var newLine = TextUtilities.GetNewLineFromDocument(Document, Caret.Line);
using (Document.RunUpdate())
{
ReplaceSelectionWithText(newLine);
if (IndentationStrategy != null)
{
var line = Document.GetLineByNumber(Caret.Line);
var deletable = GetDeletableSegments(line);
if (deletable.Length == 1 && deletable[0].Offset == line.Offset && deletable[0].Length == line.Length)
{
// use indentation strategy only if the line is not read-only
IndentationStrategy.IndentLine(Document, line);
}
}
}
}
internal void RemoveSelectedText()
{
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
_selection.ReplaceSelectionWithText(string.Empty);
#if DEBUG
if (!_selection.IsEmpty)
{
foreach (var s in _selection.Segments)
{
Debug.Assert(!ReadOnlySectionProvider.GetDeletableSegments(s).Any());
}
}
#endif
}
internal void ReplaceSelectionWithText(string newText)
{
if (newText == null)
throw new ArgumentNullException(nameof(newText));
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
_selection.ReplaceSelectionWithText(newText);
}
internal ISegment[] GetDeletableSegments(ISegment segment)
{
var deletableSegments = ReadOnlySectionProvider.GetDeletableSegments(segment);
if (deletableSegments == null)
throw new InvalidOperationException("ReadOnlySectionProvider.GetDeletableSegments returned null");
var array = deletableSegments.ToArray();
var lastIndex = segment.Offset;
foreach (var t in array)
{
if (t.Offset < lastIndex)
throw new InvalidOperationException("ReadOnlySectionProvider returned incorrect segments (outside of input segment / wrong order)");
lastIndex = t.EndOffset;
}
if (lastIndex > segment.EndOffset)
throw new InvalidOperationException("ReadOnlySectionProvider returned incorrect segments (outside of input segment / wrong order)");
return array;
}
#endregion
#region IndentationStrategy property
/// <summary>
/// IndentationStrategy property.
/// </summary>
public static readonly StyledProperty<IIndentationStrategy> IndentationStrategyProperty =
AvaloniaProperty.Register<TextArea, IIndentationStrategy>("IndentationStrategy", new DefaultIndentationStrategy());
/// <summary>
/// Gets/Sets the indentation strategy used when inserting new lines.
/// </summary>
public IIndentationStrategy IndentationStrategy
{
get => GetValue(IndentationStrategyProperty);
set => SetValue(IndentationStrategyProperty, value);
}
#endregion
#region OnKeyDown/OnKeyUp
// Make life easier for text editor extensions that use a different cursor based on the pressed modifier keys.
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
TextView.InvalidateCursorIfPointerWithinTextView();
}
private void OnPreviewKeyDown(object sender, KeyEventArgs e)
{
foreach (var h in StackedInputHandlers)
{
if (e.Handled)
break;
h.OnPreviewKeyDown(e);
}
}
/// <inheritdoc/>
protected override void OnKeyUp(KeyEventArgs e)
{
base.OnKeyUp(e);
TextView.InvalidateCursorIfPointerWithinTextView();
}
private void OnPreviewKeyUp(object sender, KeyEventArgs e)
{
foreach (var h in StackedInputHandlers)
{
if (e.Handled)
break;
h.OnPreviewKeyUp(e);
}
}
#endregion
#region Hide Mouse Cursor While Typing
private bool _isMouseCursorHidden;
private void AttachTypingEvents()
{
// Use the PreviewMouseMove event in case some other editor layer consumes the MouseMove event (e.g. SD's InsertionCursorLayer)
PointerEntered += delegate { ShowMouseCursor(); };
PointerExited += delegate { ShowMouseCursor(); };
}
private void ShowMouseCursor()
{
if (_isMouseCursorHidden)
{
_isMouseCursorHidden = false;
}
}
private void HideMouseCursor()
{
if (Options.HideCursorWhileTyping && !_isMouseCursorHidden && IsPointerOver)
{
_isMouseCursorHidden = true;
}
}
#endregion
#region Overstrike mode
/// <summary>
/// The <see cref="OverstrikeMode"/> dependency property.
/// </summary>
public static readonly StyledProperty<bool> OverstrikeModeProperty =
AvaloniaProperty.Register<TextArea, bool>("OverstrikeMode");
/// <summary>
/// Gets/Sets whether overstrike mode is active.
/// </summary>
public bool OverstrikeMode
{
get => GetValue(OverstrikeModeProperty);
set => SetValue(OverstrikeModeProperty, value);
}
#endregion
/// <inheritdoc/>
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == SelectionBrushProperty
|| change.Property == SelectionBorderProperty
|| change.Property == SelectionForegroundProperty
|| change.Property == SelectionCornerRadiusProperty)
{
TextView.Redraw();
}
else if (change.Property == OverstrikeModeProperty)
{
Caret.UpdateIfVisible();
}
}
/// <summary>
/// Gets the requested service.
/// </summary>
/// <returns>Returns the requested service instance, or null if the service cannot be found.</returns>
public virtual object GetService(Type serviceType)
{
return TextView.GetService(serviceType);
}
/// <summary>
/// Occurs when text inside the TextArea was copied.
/// </summary>
public event EventHandler<TextEventArgs> TextCopied;
event EventHandler ILogicalScrollable.ScrollInvalidated
{
add { if (_logicalScrollable != null) _logicalScrollable.ScrollInvalidated += value; }
remove { if (_logicalScrollable != null) _logicalScrollable.ScrollInvalidated -= value; }
}
internal void OnTextCopied(TextEventArgs e)
{
TextCopied?.Invoke(this, e);
}
public IList<RoutedCommandBinding> CommandBindings { get; } = new List<RoutedCommandBinding>();
bool ILogicalScrollable.IsLogicalScrollEnabled => _logicalScrollable?.IsLogicalScrollEnabled ?? default(bool);
Size ILogicalScrollable.ScrollSize => _logicalScrollable?.ScrollSize ?? default(Size);
Size ILogicalScrollable.PageScrollSize => _logicalScrollable?.PageScrollSize ?? default(Size);
Size IScrollable.Extent => _logicalScrollable?.Extent ?? default(Size);
Vector IScrollable.Offset
{
get => _logicalScrollable?.Offset ?? default(Vector);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.Offset = value;
}
}
}
Size IScrollable.Viewport => _logicalScrollable?.Viewport ?? default(Size);
bool ILogicalScrollable.CanHorizontallyScroll
{
get => _logicalScrollable?.CanHorizontallyScroll ?? default(bool);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.CanHorizontallyScroll = value;
}
}
}
bool ILogicalScrollable.CanVerticallyScroll
{
get => _logicalScrollable?.CanVerticallyScroll ?? default(bool);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.CanVerticallyScroll = value;
}
}
}
public bool BringIntoView(IControl target, Rect targetRect) =>
_logicalScrollable?.BringIntoView(target, targetRect) ?? default(bool);
IControl ILogicalScrollable.GetControlInDirection(NavigationDirection direction, IControl from)
=> _logicalScrollable?.GetControlInDirection(direction, from);
public void RaiseScrollInvalidated(EventArgs e)
{
_logicalScrollable?.RaiseScrollInvalidated(e);
}
private class TextAreaTextInputMethodClient : ITextInputMethodClient
{
private TextArea _textArea;
public TextAreaTextInputMethodClient()
{
}
public event EventHandler CursorRectangleChanged;
public event EventHandler TextViewVisualChanged;
public event EventHandler SurroundingTextChanged;
public Rect CursorRectangle
{
get
{
if(_textArea == null)
{
return Rect.Empty;
}
var transform = _textArea.TextView.TransformToVisual(_textArea);
if (transform == null)
{
return default;
}
var rect = _textArea.Caret.CalculateCaretRectangle().TransformToAABB(transform.Value);
return rect;
}
}
public IVisual TextViewVisual => _textArea;
public bool SupportsPreedit => false;
public bool SupportsSurroundingText => true;
public TextInputMethodSurroundingText SurroundingText
{
get
{
if(_textArea == null)
{
return default;
}
var lineIndex = _textArea.Caret.Line;
var position = _textArea.Caret.Position;
var documentLine = _textArea.Document.GetLineByNumber(lineIndex);
var text = _textArea.Document.GetText(documentLine.Offset, documentLine.Length);
return new TextInputMethodSurroundingText
{
AnchorOffset = 0,
CursorOffset = position.Column,
Text = text
};
}
}
public void SetTextArea(TextArea textArea)
{
if(_textArea != null)
{
_textArea.Caret.PositionChanged -= Caret_PositionChanged;
_textArea.SelectionChanged -= TextArea_SelectionChanged;
}
_textArea = textArea;
if(_textArea != null)
{
_textArea.Caret.PositionChanged += Caret_PositionChanged;
_textArea.SelectionChanged += TextArea_SelectionChanged;
}
TextViewVisualChanged?.Invoke(this, EventArgs.Empty);
CursorRectangleChanged?.Invoke(this, EventArgs.Empty);
}
private void Caret_PositionChanged(object sender, EventArgs e)
{
CursorRectangleChanged?.Invoke(this, e);
}
private void TextArea_SelectionChanged(object sender, EventArgs e)
{
SurroundingTextChanged?.Invoke(this, e);
}
public void SelectInSurroundingText(int start, int end)
{
if(_textArea == null)
{
return;
}
var selection = _textArea.Selection;
_textArea.Selection = _textArea.Selection.StartSelectionOrSetEndpoint(
new TextViewPosition(selection.StartPosition.Line, start),
new TextViewPosition(selection.StartPosition.Line, end));
}
public void SetPreeditText(string text)
{
}
}
}
/// <summary>
/// EventArgs with text.
/// </summary>
public class TextEventArgs : EventArgs
{
/// <summary>
/// Gets the text.
/// </summary>
public string Text { get; }
/// <summary>
/// Creates a new TextEventArgs instance.
/// </summary>
public TextEventArgs(string text)
{
Text = text ?? throw new ArgumentNullException(nameof(text));
}
}
}
<MSG> Merge pull request #169 from Takoooooo/right-click-moves-caret-feature
You should be able to move caret by right clicking text area
<DFF> @@ -708,6 +708,21 @@ namespace AvaloniaEdit.Editing
get => _readOnlySectionProvider;
set => _readOnlySectionProvider = value ?? throw new ArgumentNullException(nameof(value));
}
+
+ /// <summary>
+ /// The <see cref="RightClickMovesCaret"/> property.
+ /// </summary>
+ public static readonly StyledProperty<bool> RightClickMovesCaretProperty =
+ AvaloniaProperty.Register<TextArea, bool>(nameof(RightClickMovesCaret), false);
+
+ /// <summary>
+ /// Determines whether caret position should be changed to the mouse position when you right click or not.
+ /// </summary>
+ public bool RightClickMovesCaret
+ {
+ get => GetValue(RightClickMovesCaretProperty);
+ set => SetValue(RightClickMovesCaretProperty, value);
+ }
#endregion
#region Focus Handling (Show/Hide Caret)
| 15 | Merge pull request #169 from Takoooooo/right-click-moves-caret-feature | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066157 | <NME> TextArea.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using Avalonia;
using Avalonia.Controls;
using Avalonia.Controls.Presenters;
using Avalonia.Controls.Primitives;
using Avalonia.Data;
using Avalonia.Input;
using Avalonia.Input.TextInput;
using Avalonia.Interactivity;
using Avalonia.Media;
using Avalonia.Threading;
using Avalonia.VisualTree;
using AvaloniaEdit.Document;
using AvaloniaEdit.Indentation;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Search;
using AvaloniaEdit.Utils;
using System;
using System.Collections.Generic;
using System.Collections.Immutable;
using System.Collections.ObjectModel;
using System.Collections.Specialized;
using System.ComponentModel;
using System.Diagnostics;
using System.Linq;
namespace AvaloniaEdit.Editing
{
/// <summary>
/// Control that wraps a TextView and adds support for user input and the caret.
/// </summary>
public class TextArea : TemplatedControl, ITextEditorComponent, IRoutedCommandBindable, ILogicalScrollable
{
/// <summary>
/// This is the extra scrolling space that occurs after the last line.
/// </summary>
private const int AdditionalVerticalScrollAmount = 2;
private ILogicalScrollable _logicalScrollable;
private readonly TextAreaTextInputMethodClient _imClient = new TextAreaTextInputMethodClient();
#region Constructor
static TextArea()
{
KeyboardNavigation.TabNavigationProperty.OverrideDefaultValue<TextArea>(KeyboardNavigationMode.None);
FocusableProperty.OverrideDefaultValue<TextArea>(true);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
AffectsArrange<TextArea>(OffsetProperty);
AffectsRender<TextArea>(OffsetProperty);
TextInputMethodClientRequestedEvent.AddClassHandler<TextArea>((ta, e) =>
{
if (!ta.IsReadOnly)
{
e.Client = ta._imClient;
}
});
}
/// <summary>
/// Creates a new TextArea instance.
/// </summary>
public TextArea() : this(new TextView())
{
AddHandler(KeyDownEvent, OnPreviewKeyDown, RoutingStrategies.Tunnel);
AddHandler(KeyUpEvent, OnPreviewKeyUp, RoutingStrategies.Tunnel);
}
/// <summary>
/// Creates a new TextArea instance.
/// </summary>
protected TextArea(TextView textView)
{
TextView = textView ?? throw new ArgumentNullException(nameof(textView));
_logicalScrollable = textView;
Options = textView.Options;
_selection = EmptySelection = new EmptySelection(this);
textView.Services.AddService(this);
textView.LineTransformers.Add(new SelectionColorizer(this));
textView.InsertLayer(new SelectionLayer(this), KnownLayer.Selection, LayerInsertionPosition.Replace);
Caret = new Caret(this);
Caret.PositionChanged += (sender, e) => RequestSelectionValidation();
Caret.PositionChanged += CaretPositionChanged;
AttachTypingEvents();
LeftMargins.CollectionChanged += LeftMargins_CollectionChanged;
DefaultInputHandler = new TextAreaDefaultInputHandler(this);
ActiveInputHandler = DefaultInputHandler;
// TODO
//textView.GetObservable(TextBlock.FontSizeProperty).Subscribe(_ =>
//{
// TextView.SetScrollOffset(new Vector(_offset.X, _offset.Y * TextView.DefaultLineHeight));
//});
}
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
if (e.NameScope.Find("PART_CP") is ContentPresenter contentPresenter)
{
contentPresenter.Content = TextView;
}
}
internal void AddChild(IVisual visual)
{
VisualChildren.Add(visual);
InvalidateArrange();
}
internal void RemoveChild(IVisual visual)
{
VisualChildren.Remove(visual);
}
#endregion
/// <summary>
/// Defines the <see cref="IScrollable.Offset" /> property.
/// </summary>
public static readonly DirectProperty<TextArea, Vector> OffsetProperty =
AvaloniaProperty.RegisterDirect<TextArea, Vector>(
nameof(IScrollable.Offset),
o => (o as IScrollable).Offset,
(o, v) => (o as IScrollable).Offset = v);
#region InputHandler management
/// <summary>
/// Gets the default input handler.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public TextAreaDefaultInputHandler DefaultInputHandler { get; }
private ITextAreaInputHandler _activeInputHandler;
private bool _isChangingInputHandler;
/// <summary>
/// Gets/Sets the active input handler.
/// This property does not return currently active stacked input handlers. Setting this property detached all stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public ITextAreaInputHandler ActiveInputHandler
{
get => _activeInputHandler;
set
{
if (value != null && value.TextArea != this)
throw new ArgumentException("The input handler was created for a different text area than this one.");
if (_isChangingInputHandler)
throw new InvalidOperationException("Cannot set ActiveInputHandler recursively");
if (_activeInputHandler != value)
{
_isChangingInputHandler = true;
try
{
// pop the whole stack
PopStackedInputHandler(StackedInputHandlers.LastOrDefault());
Debug.Assert(StackedInputHandlers.IsEmpty);
_activeInputHandler?.Detach();
_activeInputHandler = value;
value?.Attach();
}
finally
{
_isChangingInputHandler = false;
}
ActiveInputHandlerChanged?.Invoke(this, EventArgs.Empty);
}
}
}
/// <summary>
/// Occurs when the ActiveInputHandler property changes.
/// </summary>
public event EventHandler ActiveInputHandlerChanged;
/// <summary>
/// Gets the list of currently active stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public ImmutableStack<TextAreaStackedInputHandler> StackedInputHandlers { get; private set; } = ImmutableStack<TextAreaStackedInputHandler>.Empty;
/// <summary>
/// Pushes an input handler onto the list of stacked input handlers.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public void PushStackedInputHandler(TextAreaStackedInputHandler inputHandler)
{
if (inputHandler == null)
throw new ArgumentNullException(nameof(inputHandler));
StackedInputHandlers = StackedInputHandlers.Push(inputHandler);
inputHandler.Attach();
}
/// <summary>
/// Pops the stacked input handler (and all input handlers above it).
/// If <paramref name="inputHandler"/> is not found in the currently stacked input handlers, or is null, this method
/// does nothing.
/// </summary>
/// <remarks><inheritdoc cref="ITextAreaInputHandler"/></remarks>
public void PopStackedInputHandler(TextAreaStackedInputHandler inputHandler)
{
if (StackedInputHandlers.Any(i => i == inputHandler))
{
ITextAreaInputHandler oldHandler;
do
{
oldHandler = StackedInputHandlers.Peek();
StackedInputHandlers = StackedInputHandlers.Pop();
oldHandler.Detach();
} while (oldHandler != inputHandler);
}
}
#endregion
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextArea>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <inheritdoc/>
public event EventHandler DocumentChanged;
/// <summary>
/// Gets if the the document displayed by the text editor is readonly
/// </summary>
public bool IsReadOnly
{
get => ReadOnlySectionProvider == ReadOnlySectionDocument.Instance;
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextArea)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.Changing.RemoveHandler(oldValue, OnDocumentChanging);
TextDocumentWeakEventManager.Changed.RemoveHandler(oldValue, OnDocumentChanged);
TextDocumentWeakEventManager.UpdateStarted.RemoveHandler(oldValue, OnUpdateStarted);
TextDocumentWeakEventManager.UpdateFinished.RemoveHandler(oldValue, OnUpdateFinished);
}
TextView.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.Changing.AddHandler(newValue, OnDocumentChanging);
TextDocumentWeakEventManager.Changed.AddHandler(newValue, OnDocumentChanged);
TextDocumentWeakEventManager.UpdateStarted.AddHandler(newValue, OnUpdateStarted);
TextDocumentWeakEventManager.UpdateFinished.AddHandler(newValue, OnUpdateFinished);
InvalidateArrange();
}
// Reset caret location and selection: this is necessary because the caret/selection might be invalid
// in the new document (e.g. if new document is shorter than the old document).
Caret.Location = new TextLocation(1, 1);
ClearSelection();
DocumentChanged?.Invoke(this, EventArgs.Empty);
//CommandManager.InvalidateRequerySuggested();
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextArea>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
private void OnOptionChanged(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextArea)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnOptionChanged);
}
TextView.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnOptionChanged);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
#endregion
#region Caret handling on document changes
private void OnDocumentChanging(object sender, DocumentChangeEventArgs e)
{
Caret.OnDocumentChanging();
}
private void OnDocumentChanged(object sender, DocumentChangeEventArgs e)
{
Caret.OnDocumentChanged(e);
Selection = _selection.UpdateOnDocumentChange(e);
}
private void OnUpdateStarted(object sender, EventArgs e)
{
Document.UndoStack.PushOptional(new RestoreCaretAndSelectionUndoAction(this));
}
private void OnUpdateFinished(object sender, EventArgs e)
{
Caret.OnDocumentUpdateFinished();
}
private sealed class RestoreCaretAndSelectionUndoAction : IUndoableOperation
{
// keep textarea in weak reference because the IUndoableOperation is stored with the document
private readonly WeakReference _textAreaReference;
private readonly TextViewPosition _caretPosition;
private readonly Selection _selection;
public RestoreCaretAndSelectionUndoAction(TextArea textArea)
{
_textAreaReference = new WeakReference(textArea);
// Just save the old caret position, no need to validate here.
// If we restore it, we'll validate it anyways.
_caretPosition = textArea.Caret.NonValidatedPosition;
_selection = textArea.Selection;
}
public void Undo()
{
var textArea = (TextArea)_textAreaReference.Target;
if (textArea != null)
{
textArea.Caret.Position = _caretPosition;
textArea.Selection = _selection;
}
}
public void Redo()
{
// redo=undo: we just restore the caret/selection state
Undo();
}
}
#endregion
#region TextView property
/// <summary>
/// Gets the text view used to display text in this text area.
/// </summary>
public TextView TextView { get; }
#endregion
#region Selection property
internal readonly Selection EmptySelection;
private Selection _selection;
/// <summary>
/// Occurs when the selection has changed.
/// </summary>
public event EventHandler SelectionChanged;
/// <summary>
/// Gets/Sets the selection in this text area.
/// </summary>
public Selection Selection
{
get => _selection;
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
if (value.TextArea != this)
throw new ArgumentException("Cannot use a Selection instance that belongs to another text area.");
if (!Equals(_selection, value))
{
if (TextView != null)
{
var oldSegment = _selection.SurroundingSegment;
var newSegment = value.SurroundingSegment;
if (!Selection.EnableVirtualSpace && (_selection is SimpleSelection && value is SimpleSelection && oldSegment != null && newSegment != null))
{
// perf optimization:
// When a simple selection changes, don't redraw the whole selection, but only the changed parts.
var oldSegmentOffset = oldSegment.Offset;
var newSegmentOffset = newSegment.Offset;
if (oldSegmentOffset != newSegmentOffset)
{
TextView.Redraw(Math.Min(oldSegmentOffset, newSegmentOffset),
Math.Abs(oldSegmentOffset - newSegmentOffset));
}
var oldSegmentEndOffset = oldSegment.EndOffset;
var newSegmentEndOffset = newSegment.EndOffset;
if (oldSegmentEndOffset != newSegmentEndOffset)
{
TextView.Redraw(Math.Min(oldSegmentEndOffset, newSegmentEndOffset),
Math.Abs(oldSegmentEndOffset - newSegmentEndOffset));
}
}
else
{
TextView.Redraw(oldSegment);
TextView.Redraw(newSegment);
}
}
_selection = value;
SelectionChanged?.Invoke(this, EventArgs.Empty);
// a selection change causes commands like copy/paste/etc. to change status
//CommandManager.InvalidateRequerySuggested();
}
}
}
/// <summary>
/// Clears the current selection.
/// </summary>
public void ClearSelection()
{
Selection = EmptySelection;
}
/// <summary>
/// The <see cref="SelectionBrush"/> property.
/// </summary>
public static readonly StyledProperty<IBrush> SelectionBrushProperty =
AvaloniaProperty.Register<TextArea, IBrush>("SelectionBrush");
/// <summary>
/// Gets/Sets the background brush used for the selection.
/// </summary>
public IBrush SelectionBrush
{
get => GetValue(SelectionBrushProperty);
set => SetValue(SelectionBrushProperty, value);
}
/// <summary>
/// The <see cref="SelectionForeground"/> property.
/// </summary>
public static readonly StyledProperty<IBrush> SelectionForegroundProperty =
AvaloniaProperty.Register<TextArea, IBrush>("SelectionForeground");
/// <summary>
/// Gets/Sets the foreground brush used for selected text.
/// </summary>
public IBrush SelectionForeground
{
get => GetValue(SelectionForegroundProperty);
set => SetValue(SelectionForegroundProperty, value);
}
/// <summary>
/// The <see cref="SelectionBorder"/> property.
/// </summary>
public static readonly StyledProperty<Pen> SelectionBorderProperty =
AvaloniaProperty.Register<TextArea, Pen>("SelectionBorder");
/// <summary>
/// Gets/Sets the pen used for the border of the selection.
/// </summary>
public Pen SelectionBorder
{
get => GetValue(SelectionBorderProperty);
set => SetValue(SelectionBorderProperty, value);
}
/// <summary>
/// The <see cref="SelectionCornerRadius"/> property.
/// </summary>
public static readonly StyledProperty<double> SelectionCornerRadiusProperty =
AvaloniaProperty.Register<TextArea, double>("SelectionCornerRadius", 3.0);
/// <summary>
/// Gets/Sets the corner radius of the selection.
/// </summary>
public double SelectionCornerRadius
{
get => GetValue(SelectionCornerRadiusProperty);
set => SetValue(SelectionCornerRadiusProperty, value);
}
#endregion
#region Force caret to stay inside selection
private bool _ensureSelectionValidRequested;
private int _allowCaretOutsideSelection;
private void RequestSelectionValidation()
{
if (!_ensureSelectionValidRequested && _allowCaretOutsideSelection == 0)
{
_ensureSelectionValidRequested = true;
Dispatcher.UIThread.Post(EnsureSelectionValid);
}
}
/// <summary>
/// Code that updates only the caret but not the selection can cause confusion when
/// keys like 'Delete' delete the (possibly invisible) selected text and not the
/// text around the caret.
///
/// So we'll ensure that the caret is inside the selection.
/// (when the caret is not in the selection, we'll clear the selection)
///
/// This method is invoked using the Dispatcher so that code may temporarily violate this rule
/// (e.g. most 'extend selection' methods work by first setting the caret, then the selection),
/// it's sufficient to fix it after any event handlers have run.
/// </summary>
private void EnsureSelectionValid()
{
_ensureSelectionValidRequested = false;
if (_allowCaretOutsideSelection == 0)
{
if (!_selection.IsEmpty && !_selection.Contains(Caret.Offset))
{
ClearSelection();
}
}
}
/// <summary>
/// Temporarily allows positioning the caret outside the selection.
/// Dispose the returned IDisposable to revert the allowance.
/// </summary>
/// <remarks>
/// The text area only forces the caret to be inside the selection when other events
/// have finished running (using the dispatcher), so you don't have to use this method
/// for temporarily positioning the caret in event handlers.
/// This method is only necessary if you want to run the dispatcher, e.g. if you
/// perform a drag'n'drop operation.
/// </remarks>
public IDisposable AllowCaretOutsideSelection()
{
VerifyAccess();
_allowCaretOutsideSelection++;
return new CallbackOnDispose(
delegate
{
VerifyAccess();
_allowCaretOutsideSelection--;
RequestSelectionValidation();
});
}
#endregion
#region Properties
/// <summary>
/// Gets the Caret used for this text area.
/// </summary>
public Caret Caret { get; }
/// <summary>
/// Scrolls the text view so that the requested line is in the middle.
/// If the textview can be scrolled.
/// </summary>
/// <param name="line">The line to scroll to.</param>
public void ScrollToLine (int line)
{
var viewPortLines = (int)(this as IScrollable).Viewport.Height;
if (viewPortLines < Document.LineCount)
{
ScrollToLine(line, 2, viewPortLines / 2);
}
}
/// <summary>
/// Scrolls the textview to a position with n lines above and below it.
/// </summary>
/// <param name="line">the requested line number.</param>
/// <param name="linesEitherSide">The number of lines above and below.</param>
public void ScrollToLine(int line, int linesEitherSide)
{
ScrollToLine(line, linesEitherSide, linesEitherSide);
}
/// <summary>
/// Scrolls the textview to a position with n lines above and below it.
/// </summary>
/// <param name="line">the requested line number.</param>
/// <param name="linesAbove">The number of lines above.</param>
/// <param name="linesBelow">The number of lines below.</param>
public void ScrollToLine(int line, int linesAbove, int linesBelow)
{
var offset = line - linesAbove;
if (offset < 0)
{
offset = 0;
}
this.BringIntoView(new Rect(1, offset, 0, 1));
offset = line + linesBelow;
if (offset >= 0)
{
this.BringIntoView(new Rect(1, offset, 0, 1));
}
}
private void CaretPositionChanged(object sender, EventArgs e)
{
if (TextView == null)
return;
TextView.HighlightedLine = Caret.Line;
ScrollToLine(Caret.Line, 2);
Dispatcher.UIThread.InvokeAsync(() =>
{
(this as ILogicalScrollable).RaiseScrollInvalidated(EventArgs.Empty);
});
}
public static readonly DirectProperty<TextArea, ObservableCollection<IControl>> LeftMarginsProperty
= AvaloniaProperty.RegisterDirect<TextArea, ObservableCollection<IControl>>(nameof(LeftMargins),
c => c.LeftMargins);
/// <summary>
/// Gets the collection of margins displayed to the left of the text view.
/// </summary>
public ObservableCollection<IControl> LeftMargins { get; } = new ObservableCollection<IControl>();
private void LeftMargins_CollectionChanged(object sender, NotifyCollectionChangedEventArgs e)
{
if (e.OldItems != null)
{
foreach (var c in e.OldItems.OfType<ITextViewConnect>())
{
c.RemoveFromTextView(TextView);
}
get => _readOnlySectionProvider;
set => _readOnlySectionProvider = value ?? throw new ArgumentNullException(nameof(value));
}
#endregion
#region Focus Handling (Show/Hide Caret)
/// <summary>
/// The <see cref="RightClickMovesCaret"/> property.
/// </summary>
public static readonly StyledProperty<bool> RightClickMovesCaretProperty =
AvaloniaProperty.Register<TextArea, bool>(nameof(RightClickMovesCaret), false);
/// <summary>
/// Determines whether caret position should be changed to the mouse position when you right click or not.
/// </summary>
public bool RightClickMovesCaret
{
get => GetValue(RightClickMovesCaretProperty);
set => SetValue(RightClickMovesCaretProperty, value);
}
#endregion
#region Focus Handling (Show/Hide Caret)
protected override void OnPointerPressed(PointerPressedEventArgs e)
{
base.OnPointerPressed(e);
Focus();
}
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
Caret.Show();
_imClient.SetTextArea(this);
}
protected override void OnLostFocus(RoutedEventArgs e)
{
base.OnLostFocus(e);
Caret.Hide();
_imClient.SetTextArea(null);
}
#endregion
#region OnTextInput / RemoveSelectedText / ReplaceSelectionWithText
/// <summary>
/// Occurs when the TextArea receives text input.
/// but occurs immediately before the TextArea handles the TextInput event.
/// </summary>
public event EventHandler<TextInputEventArgs> TextEntering;
/// <summary>
/// Occurs when the TextArea receives text input.
/// but occurs immediately after the TextArea handles the TextInput event.
/// </summary>
public event EventHandler<TextInputEventArgs> TextEntered;
/// <summary>
/// Raises the TextEntering event.
/// </summary>
protected virtual void OnTextEntering(TextInputEventArgs e)
{
TextEntering?.Invoke(this, e);
}
/// <summary>
/// Raises the TextEntered event.
/// </summary>
protected virtual void OnTextEntered(TextInputEventArgs e)
{
TextEntered?.Invoke(this, e);
}
protected override void OnTextInput(TextInputEventArgs e)
{
base.OnTextInput(e);
if (!e.Handled && Document != null)
{
if (string.IsNullOrEmpty(e.Text) || e.Text == "\x1b" || e.Text == "\b" || e.Text == "\u007f")
{
// TODO: check this
// ASCII 0x1b = ESC.
// produces a TextInput event with that old ASCII control char
// when Escape is pressed. We'll just ignore it.
// A deadkey followed by backspace causes a textinput event for the BS character.
// Similarly, some shortcuts like Alt+Space produce an empty TextInput event.
// We have to ignore those (not handle them) to keep the shortcut working.
return;
}
HideMouseCursor();
PerformTextInput(e);
e.Handled = true;
}
}
/// <summary>
/// Performs text input.
/// This raises the <see cref="TextEntering"/> event, replaces the selection with the text,
/// and then raises the <see cref="TextEntered"/> event.
/// </summary>
public void PerformTextInput(string text)
{
var e = new TextInputEventArgs
{
Text = text,
RoutedEvent = TextInputEvent
};
PerformTextInput(e);
}
/// <summary>
/// Performs text input.
/// This raises the <see cref="TextEntering"/> event, replaces the selection with the text,
/// and then raises the <see cref="TextEntered"/> event.
/// </summary>
public void PerformTextInput(TextInputEventArgs e)
{
if (e == null)
throw new ArgumentNullException(nameof(e));
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
OnTextEntering(e);
if (!e.Handled)
{
if (e.Text == "\n" || e.Text == "\r" || e.Text == "\r\n")
ReplaceSelectionWithNewLine();
else
{
// TODO
//if (OverstrikeMode && Selection.IsEmpty && Document.GetLineByNumber(Caret.Line).EndOffset > Caret.Offset)
// EditingCommands.SelectRightByCharacter.Execute(null, this);
ReplaceSelectionWithText(e.Text);
}
OnTextEntered(e);
Caret.BringCaretToView();
}
}
private void ReplaceSelectionWithNewLine()
{
var newLine = TextUtilities.GetNewLineFromDocument(Document, Caret.Line);
using (Document.RunUpdate())
{
ReplaceSelectionWithText(newLine);
if (IndentationStrategy != null)
{
var line = Document.GetLineByNumber(Caret.Line);
var deletable = GetDeletableSegments(line);
if (deletable.Length == 1 && deletable[0].Offset == line.Offset && deletable[0].Length == line.Length)
{
// use indentation strategy only if the line is not read-only
IndentationStrategy.IndentLine(Document, line);
}
}
}
}
internal void RemoveSelectedText()
{
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
_selection.ReplaceSelectionWithText(string.Empty);
#if DEBUG
if (!_selection.IsEmpty)
{
foreach (var s in _selection.Segments)
{
Debug.Assert(!ReadOnlySectionProvider.GetDeletableSegments(s).Any());
}
}
#endif
}
internal void ReplaceSelectionWithText(string newText)
{
if (newText == null)
throw new ArgumentNullException(nameof(newText));
if (Document == null)
throw ThrowUtil.NoDocumentAssigned();
_selection.ReplaceSelectionWithText(newText);
}
internal ISegment[] GetDeletableSegments(ISegment segment)
{
var deletableSegments = ReadOnlySectionProvider.GetDeletableSegments(segment);
if (deletableSegments == null)
throw new InvalidOperationException("ReadOnlySectionProvider.GetDeletableSegments returned null");
var array = deletableSegments.ToArray();
var lastIndex = segment.Offset;
foreach (var t in array)
{
if (t.Offset < lastIndex)
throw new InvalidOperationException("ReadOnlySectionProvider returned incorrect segments (outside of input segment / wrong order)");
lastIndex = t.EndOffset;
}
if (lastIndex > segment.EndOffset)
throw new InvalidOperationException("ReadOnlySectionProvider returned incorrect segments (outside of input segment / wrong order)");
return array;
}
#endregion
#region IndentationStrategy property
/// <summary>
/// IndentationStrategy property.
/// </summary>
public static readonly StyledProperty<IIndentationStrategy> IndentationStrategyProperty =
AvaloniaProperty.Register<TextArea, IIndentationStrategy>("IndentationStrategy", new DefaultIndentationStrategy());
/// <summary>
/// Gets/Sets the indentation strategy used when inserting new lines.
/// </summary>
public IIndentationStrategy IndentationStrategy
{
get => GetValue(IndentationStrategyProperty);
set => SetValue(IndentationStrategyProperty, value);
}
#endregion
#region OnKeyDown/OnKeyUp
// Make life easier for text editor extensions that use a different cursor based on the pressed modifier keys.
/// <inheritdoc/>
protected override void OnKeyDown(KeyEventArgs e)
{
base.OnKeyDown(e);
TextView.InvalidateCursorIfPointerWithinTextView();
}
private void OnPreviewKeyDown(object sender, KeyEventArgs e)
{
foreach (var h in StackedInputHandlers)
{
if (e.Handled)
break;
h.OnPreviewKeyDown(e);
}
}
/// <inheritdoc/>
protected override void OnKeyUp(KeyEventArgs e)
{
base.OnKeyUp(e);
TextView.InvalidateCursorIfPointerWithinTextView();
}
private void OnPreviewKeyUp(object sender, KeyEventArgs e)
{
foreach (var h in StackedInputHandlers)
{
if (e.Handled)
break;
h.OnPreviewKeyUp(e);
}
}
#endregion
#region Hide Mouse Cursor While Typing
private bool _isMouseCursorHidden;
private void AttachTypingEvents()
{
// Use the PreviewMouseMove event in case some other editor layer consumes the MouseMove event (e.g. SD's InsertionCursorLayer)
PointerEntered += delegate { ShowMouseCursor(); };
PointerExited += delegate { ShowMouseCursor(); };
}
private void ShowMouseCursor()
{
if (_isMouseCursorHidden)
{
_isMouseCursorHidden = false;
}
}
private void HideMouseCursor()
{
if (Options.HideCursorWhileTyping && !_isMouseCursorHidden && IsPointerOver)
{
_isMouseCursorHidden = true;
}
}
#endregion
#region Overstrike mode
/// <summary>
/// The <see cref="OverstrikeMode"/> dependency property.
/// </summary>
public static readonly StyledProperty<bool> OverstrikeModeProperty =
AvaloniaProperty.Register<TextArea, bool>("OverstrikeMode");
/// <summary>
/// Gets/Sets whether overstrike mode is active.
/// </summary>
public bool OverstrikeMode
{
get => GetValue(OverstrikeModeProperty);
set => SetValue(OverstrikeModeProperty, value);
}
#endregion
/// <inheritdoc/>
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == SelectionBrushProperty
|| change.Property == SelectionBorderProperty
|| change.Property == SelectionForegroundProperty
|| change.Property == SelectionCornerRadiusProperty)
{
TextView.Redraw();
}
else if (change.Property == OverstrikeModeProperty)
{
Caret.UpdateIfVisible();
}
}
/// <summary>
/// Gets the requested service.
/// </summary>
/// <returns>Returns the requested service instance, or null if the service cannot be found.</returns>
public virtual object GetService(Type serviceType)
{
return TextView.GetService(serviceType);
}
/// <summary>
/// Occurs when text inside the TextArea was copied.
/// </summary>
public event EventHandler<TextEventArgs> TextCopied;
event EventHandler ILogicalScrollable.ScrollInvalidated
{
add { if (_logicalScrollable != null) _logicalScrollable.ScrollInvalidated += value; }
remove { if (_logicalScrollable != null) _logicalScrollable.ScrollInvalidated -= value; }
}
internal void OnTextCopied(TextEventArgs e)
{
TextCopied?.Invoke(this, e);
}
public IList<RoutedCommandBinding> CommandBindings { get; } = new List<RoutedCommandBinding>();
bool ILogicalScrollable.IsLogicalScrollEnabled => _logicalScrollable?.IsLogicalScrollEnabled ?? default(bool);
Size ILogicalScrollable.ScrollSize => _logicalScrollable?.ScrollSize ?? default(Size);
Size ILogicalScrollable.PageScrollSize => _logicalScrollable?.PageScrollSize ?? default(Size);
Size IScrollable.Extent => _logicalScrollable?.Extent ?? default(Size);
Vector IScrollable.Offset
{
get => _logicalScrollable?.Offset ?? default(Vector);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.Offset = value;
}
}
}
Size IScrollable.Viewport => _logicalScrollable?.Viewport ?? default(Size);
bool ILogicalScrollable.CanHorizontallyScroll
{
get => _logicalScrollable?.CanHorizontallyScroll ?? default(bool);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.CanHorizontallyScroll = value;
}
}
}
bool ILogicalScrollable.CanVerticallyScroll
{
get => _logicalScrollable?.CanVerticallyScroll ?? default(bool);
set
{
if (_logicalScrollable != null)
{
_logicalScrollable.CanVerticallyScroll = value;
}
}
}
public bool BringIntoView(IControl target, Rect targetRect) =>
_logicalScrollable?.BringIntoView(target, targetRect) ?? default(bool);
IControl ILogicalScrollable.GetControlInDirection(NavigationDirection direction, IControl from)
=> _logicalScrollable?.GetControlInDirection(direction, from);
public void RaiseScrollInvalidated(EventArgs e)
{
_logicalScrollable?.RaiseScrollInvalidated(e);
}
private class TextAreaTextInputMethodClient : ITextInputMethodClient
{
private TextArea _textArea;
public TextAreaTextInputMethodClient()
{
}
public event EventHandler CursorRectangleChanged;
public event EventHandler TextViewVisualChanged;
public event EventHandler SurroundingTextChanged;
public Rect CursorRectangle
{
get
{
if(_textArea == null)
{
return Rect.Empty;
}
var transform = _textArea.TextView.TransformToVisual(_textArea);
if (transform == null)
{
return default;
}
var rect = _textArea.Caret.CalculateCaretRectangle().TransformToAABB(transform.Value);
return rect;
}
}
public IVisual TextViewVisual => _textArea;
public bool SupportsPreedit => false;
public bool SupportsSurroundingText => true;
public TextInputMethodSurroundingText SurroundingText
{
get
{
if(_textArea == null)
{
return default;
}
var lineIndex = _textArea.Caret.Line;
var position = _textArea.Caret.Position;
var documentLine = _textArea.Document.GetLineByNumber(lineIndex);
var text = _textArea.Document.GetText(documentLine.Offset, documentLine.Length);
return new TextInputMethodSurroundingText
{
AnchorOffset = 0,
CursorOffset = position.Column,
Text = text
};
}
}
public void SetTextArea(TextArea textArea)
{
if(_textArea != null)
{
_textArea.Caret.PositionChanged -= Caret_PositionChanged;
_textArea.SelectionChanged -= TextArea_SelectionChanged;
}
_textArea = textArea;
if(_textArea != null)
{
_textArea.Caret.PositionChanged += Caret_PositionChanged;
_textArea.SelectionChanged += TextArea_SelectionChanged;
}
TextViewVisualChanged?.Invoke(this, EventArgs.Empty);
CursorRectangleChanged?.Invoke(this, EventArgs.Empty);
}
private void Caret_PositionChanged(object sender, EventArgs e)
{
CursorRectangleChanged?.Invoke(this, e);
}
private void TextArea_SelectionChanged(object sender, EventArgs e)
{
SurroundingTextChanged?.Invoke(this, e);
}
public void SelectInSurroundingText(int start, int end)
{
if(_textArea == null)
{
return;
}
var selection = _textArea.Selection;
_textArea.Selection = _textArea.Selection.StartSelectionOrSetEndpoint(
new TextViewPosition(selection.StartPosition.Line, start),
new TextViewPosition(selection.StartPosition.Line, end));
}
public void SetPreeditText(string text)
{
}
}
}
/// <summary>
/// EventArgs with text.
/// </summary>
public class TextEventArgs : EventArgs
{
/// <summary>
/// Gets the text.
/// </summary>
public string Text { get; }
/// <summary>
/// Creates a new TextEventArgs instance.
/// </summary>
public TextEventArgs(string text)
{
Text = text ?? throw new ArgumentNullException(nameof(text));
}
}
}
<MSG> Merge pull request #169 from Takoooooo/right-click-moves-caret-feature
You should be able to move caret by right clicking text area
<DFF> @@ -708,6 +708,21 @@ namespace AvaloniaEdit.Editing
get => _readOnlySectionProvider;
set => _readOnlySectionProvider = value ?? throw new ArgumentNullException(nameof(value));
}
+
+ /// <summary>
+ /// The <see cref="RightClickMovesCaret"/> property.
+ /// </summary>
+ public static readonly StyledProperty<bool> RightClickMovesCaretProperty =
+ AvaloniaProperty.Register<TextArea, bool>(nameof(RightClickMovesCaret), false);
+
+ /// <summary>
+ /// Determines whether caret position should be changed to the mouse position when you right click or not.
+ /// </summary>
+ public bool RightClickMovesCaret
+ {
+ get => GetValue(RightClickMovesCaretProperty);
+ set => SetValue(RightClickMovesCaretProperty, value);
+ }
#endregion
#region Focus Handling (Show/Hide Caret)
| 15 | Merge pull request #169 from Takoooooo/right-click-moves-caret-feature | 0 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066158 | <NME> view.on.js
<BEF> ADDFILE
<MSG> Tests updated to work with 'buster test' and test new event API
<DFF> @@ -0,0 +1,41 @@
+
+buster.testCase('View#on()', {
+ setUp: helpers.createView,
+
+ "Should recieve the callback when fire is called directly on a view": function() {
+ var spy = this.spy();
+
+ this.view.on('testevent', spy);
+ this.view.fire('testevent');
+ assert(spy.called);
+ },
+
+ "Should recieve the callback when event is fired on a sub view": function() {
+ var spy = this.spy();
+ var apple = this.view.module('apple');
+
+ this.view.on('testevent', spy);
+ apple.fire('testevent');
+ assert(spy.called);
+ },
+
+ "Should *not* recieve the callback when event is fired on a sub view that *doesn't* match the target": function() {
+ var spy = this.spy();
+ var apple = this.view.module('apple');
+
+ this.view.on('testevent', 'orange', spy);
+ apple.fire('testevent');
+ refute(spy.called);
+ },
+
+ "Should receive the callback when event is fired on a sub view that *does* match the target": function() {
+ var spy = this.spy();
+ var apple = this.view.module('apple');
+
+ this.view.on('testevent', 'apple', spy);
+ apple.fire('testevent');
+ assert(spy.called);
+ },
+
+ tearDown: helpers.destroyView
+});
\ No newline at end of file
| 41 | Tests updated to work with 'buster test' and test new event API | 0 | .js | on | mit | ftlabs/fruitmachine |
10066159 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
public TextEditor() : this(new TextArea())
{
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
{
}
protected TextEditor(TextArea textArea, TextDocument document)
{
this.textArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
if (Document == null)
return null;
var textView = TextArea.TextView;
return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge pull request #29 from danwalmsley/fix-text-selection
Fix text selection
<DFF> @@ -1084,7 +1084,7 @@ namespace AvaloniaEdit
if (Document == null)
return null;
var textView = TextArea.TextView;
- return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
+ return textView.GetPosition(this.TranslatePoint(point + textView.ScrollOffset, textView));
}
/// <summary>
| 1 | Merge pull request #29 from danwalmsley/fix-text-selection | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066160 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
public TextEditor() : this(new TextArea())
{
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
{
}
protected TextEditor(TextArea textArea, TextDocument document)
{
this.textArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
if (Document == null)
return null;
var textView = TextArea.TextView;
return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge pull request #29 from danwalmsley/fix-text-selection
Fix text selection
<DFF> @@ -1084,7 +1084,7 @@ namespace AvaloniaEdit
if (Document == null)
return null;
var textView = TextArea.TextView;
- return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
+ return textView.GetPosition(this.TranslatePoint(point + textView.ScrollOffset, textView));
}
/// <summary>
| 1 | Merge pull request #29 from danwalmsley/fix-text-selection | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066161 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
public TextEditor() : this(new TextArea())
{
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
{
}
protected TextEditor(TextArea textArea, TextDocument document)
{
this.textArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
if (Document == null)
return null;
var textView = TextArea.TextView;
return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge pull request #29 from danwalmsley/fix-text-selection
Fix text selection
<DFF> @@ -1084,7 +1084,7 @@ namespace AvaloniaEdit
if (Document == null)
return null;
var textView = TextArea.TextView;
- return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
+ return textView.GetPosition(this.TranslatePoint(point + textView.ScrollOffset, textView));
}
/// <summary>
| 1 | Merge pull request #29 from danwalmsley/fix-text-selection | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066162 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
public TextEditor() : this(new TextArea())
{
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
{
}
protected TextEditor(TextArea textArea, TextDocument document)
{
this.textArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
if (Document == null)
return null;
var textView = TextArea.TextView;
return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge pull request #29 from danwalmsley/fix-text-selection
Fix text selection
<DFF> @@ -1084,7 +1084,7 @@ namespace AvaloniaEdit
if (Document == null)
return null;
var textView = TextArea.TextView;
- return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
+ return textView.GetPosition(this.TranslatePoint(point + textView.ScrollOffset, textView));
}
/// <summary>
| 1 | Merge pull request #29 from danwalmsley/fix-text-selection | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066163 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
public TextEditor() : this(new TextArea())
{
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
{
}
protected TextEditor(TextArea textArea, TextDocument document)
{
this.textArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
if (Document == null)
return null;
var textView = TextArea.TextView;
return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge pull request #29 from danwalmsley/fix-text-selection
Fix text selection
<DFF> @@ -1084,7 +1084,7 @@ namespace AvaloniaEdit
if (Document == null)
return null;
var textView = TextArea.TextView;
- return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
+ return textView.GetPosition(this.TranslatePoint(point + textView.ScrollOffset, textView));
}
/// <summary>
| 1 | Merge pull request #29 from danwalmsley/fix-text-selection | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066164 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
public TextEditor() : this(new TextArea())
{
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
{
}
protected TextEditor(TextArea textArea, TextDocument document)
{
this.textArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
if (Document == null)
return null;
var textView = TextArea.TextView;
return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge pull request #29 from danwalmsley/fix-text-selection
Fix text selection
<DFF> @@ -1084,7 +1084,7 @@ namespace AvaloniaEdit
if (Document == null)
return null;
var textView = TextArea.TextView;
- return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
+ return textView.GetPosition(this.TranslatePoint(point + textView.ScrollOffset, textView));
}
/// <summary>
| 1 | Merge pull request #29 from danwalmsley/fix-text-selection | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066165 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
public TextEditor() : this(new TextArea())
{
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
{
}
protected TextEditor(TextArea textArea, TextDocument document)
{
this.textArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
if (Document == null)
return null;
var textView = TextArea.TextView;
return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge pull request #29 from danwalmsley/fix-text-selection
Fix text selection
<DFF> @@ -1084,7 +1084,7 @@ namespace AvaloniaEdit
if (Document == null)
return null;
var textView = TextArea.TextView;
- return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
+ return textView.GetPosition(this.TranslatePoint(point + textView.ScrollOffset, textView));
}
/// <summary>
| 1 | Merge pull request #29 from danwalmsley/fix-text-selection | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066166 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
public TextEditor() : this(new TextArea())
{
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
{
}
protected TextEditor(TextArea textArea, TextDocument document)
{
this.textArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
if (Document == null)
return null;
var textView = TextArea.TextView;
return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge pull request #29 from danwalmsley/fix-text-selection
Fix text selection
<DFF> @@ -1084,7 +1084,7 @@ namespace AvaloniaEdit
if (Document == null)
return null;
var textView = TextArea.TextView;
- return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
+ return textView.GetPosition(this.TranslatePoint(point + textView.ScrollOffset, textView));
}
/// <summary>
| 1 | Merge pull request #29 from danwalmsley/fix-text-selection | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066167 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
public TextEditor() : this(new TextArea())
{
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
{
}
protected TextEditor(TextArea textArea, TextDocument document)
{
this.textArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
if (Document == null)
return null;
var textView = TextArea.TextView;
return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge pull request #29 from danwalmsley/fix-text-selection
Fix text selection
<DFF> @@ -1084,7 +1084,7 @@ namespace AvaloniaEdit
if (Document == null)
return null;
var textView = TextArea.TextView;
- return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
+ return textView.GetPosition(this.TranslatePoint(point + textView.ScrollOffset, textView));
}
/// <summary>
| 1 | Merge pull request #29 from danwalmsley/fix-text-selection | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066168 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
public TextEditor() : this(new TextArea())
{
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
{
}
protected TextEditor(TextArea textArea, TextDocument document)
{
this.textArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
if (Document == null)
return null;
var textView = TextArea.TextView;
return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge pull request #29 from danwalmsley/fix-text-selection
Fix text selection
<DFF> @@ -1084,7 +1084,7 @@ namespace AvaloniaEdit
if (Document == null)
return null;
var textView = TextArea.TextView;
- return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
+ return textView.GetPosition(this.TranslatePoint(point + textView.ScrollOffset, textView));
}
/// <summary>
| 1 | Merge pull request #29 from danwalmsley/fix-text-selection | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066169 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
public TextEditor() : this(new TextArea())
{
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
{
}
protected TextEditor(TextArea textArea, TextDocument document)
{
this.textArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
if (Document == null)
return null;
var textView = TextArea.TextView;
return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge pull request #29 from danwalmsley/fix-text-selection
Fix text selection
<DFF> @@ -1084,7 +1084,7 @@ namespace AvaloniaEdit
if (Document == null)
return null;
var textView = TextArea.TextView;
- return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
+ return textView.GetPosition(this.TranslatePoint(point + textView.ScrollOffset, textView));
}
/// <summary>
| 1 | Merge pull request #29 from danwalmsley/fix-text-selection | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066170 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
public TextEditor() : this(new TextArea())
{
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
{
}
protected TextEditor(TextArea textArea, TextDocument document)
{
this.textArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
if (Document == null)
return null;
var textView = TextArea.TextView;
return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge pull request #29 from danwalmsley/fix-text-selection
Fix text selection
<DFF> @@ -1084,7 +1084,7 @@ namespace AvaloniaEdit
if (Document == null)
return null;
var textView = TextArea.TextView;
- return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
+ return textView.GetPosition(this.TranslatePoint(point + textView.ScrollOffset, textView));
}
/// <summary>
| 1 | Merge pull request #29 from danwalmsley/fix-text-selection | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066171 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
public TextEditor() : this(new TextArea())
{
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
{
}
protected TextEditor(TextArea textArea, TextDocument document)
{
this.textArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
if (Document == null)
return null;
var textView = TextArea.TextView;
return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge pull request #29 from danwalmsley/fix-text-selection
Fix text selection
<DFF> @@ -1084,7 +1084,7 @@ namespace AvaloniaEdit
if (Document == null)
return null;
var textView = TextArea.TextView;
- return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
+ return textView.GetPosition(this.TranslatePoint(point + textView.ScrollOffset, textView));
}
/// <summary>
| 1 | Merge pull request #29 from danwalmsley/fix-text-selection | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066172 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
public TextEditor() : this(new TextArea())
{
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
{
}
protected TextEditor(TextArea textArea, TextDocument document)
{
this.textArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
if (Document == null)
return null;
var textView = TextArea.TextView;
return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge pull request #29 from danwalmsley/fix-text-selection
Fix text selection
<DFF> @@ -1084,7 +1084,7 @@ namespace AvaloniaEdit
if (Document == null)
return null;
var textView = TextArea.TextView;
- return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
+ return textView.GetPosition(this.TranslatePoint(point + textView.ScrollOffset, textView));
}
/// <summary>
| 1 | Merge pull request #29 from danwalmsley/fix-text-selection | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066173 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
public TextEditor() : this(new TextArea())
{
}
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
{
}
protected TextEditor(TextArea textArea, TextDocument document)
{
this.textArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnDocumentChanged((TextDocument)e.OldValue, (TextDocument)e.NewValue);
}
private void OnDocumentChanged(TextDocument oldValue, TextDocument newValue)
{
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
if (Document == null)
return null;
var textView = TextArea.TextView;
return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge pull request #29 from danwalmsley/fix-text-selection
Fix text selection
<DFF> @@ -1084,7 +1084,7 @@ namespace AvaloniaEdit
if (Document == null)
return null;
var textView = TextArea.TextView;
- return textView.GetPosition(this.TranslatePoint(point, textView) + textView.ScrollOffset);
+ return textView.GetPosition(this.TranslatePoint(point + textView.ScrollOffset, textView));
}
/// <summary>
| 1 | Merge pull request #29 from danwalmsley/fix-text-selection | 1 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066174 | <NME> module-el.md
<BEF> ## The Module's Element
Each module has a 'root' element (`myModule.el`). This is the single element that wraps the contents of a module. It is your handle on the module once it has been [injected](view-injection.md) into the DOM. You may be familiar with the `.el` concept from [Backbone](http://backbonejs.org/). In *FruitMachine* it is similar, but due to the 'DOM free' nested rendering techniques used, the `myModule.el` is not always accessible.
In FruitMachine the `.el` property of a module is populated when `view.render()` is called.
**NOTE:** As a safety measure we do not setup modules when a module's element could not be found. This means that `myModule.el` related setup logic wont error when `myModule.el` is `undefined`.
#### Some examples
1.1. After render `module.el` will be defined
```js
var apple = new Apple();
var orange = new Orange();
apple
.add(orange)
.render();
apple.el
//=> "[object HTMLDivElement]"
orange.el
//=> "[object HTMLDivElement]"
```
1.2. Without calling `.render()` no module elements are set.
```js
var apple = new Apple();
var orange = new Orange();
apple.add(orange);
apple.el
//=> undefined
orange.el
//=> undefined
```
### FAQ
#### Why is my module.el property undefined after .render()?
The child module markup has failed to template into the parent module corrently. Check your child ids and parent markup to check they match up. See [template markup](view-template-markup.md).
#### How are module root elements found?
Internally each module has a private unique id (`myModule._fmid`). When the root element is templated, the html `id` attribute value is set to the `myModule._fmid`. When `.render()` is called on a module the HTML is templated and turned into a 'real' element in memory. We store this element and then search for descendant elements by id using `querySelector`. Server-side rendered modules being inflated on the client pick up their root element from the DOM when `.setup()` is called using `document.getElementById(module._fmid)` (super fast).
<MSG> Update module-el.md
<DFF> @@ -44,7 +44,7 @@ orange.el
#### Why is my module.el property undefined after .render()?
-The child module markup has failed to template into the parent module corrently. Check your child ids and parent markup to check they match up. See [template markup](view-template-markup.md).
+The child module markup has failed to template into the parent module correctly. Check your child ids and parent markup to check they match up. See [template markup](view-template-markup.md).
#### How are module root elements found?
| 1 | Update module-el.md | 1 | .md | md | mit | ftlabs/fruitmachine |
10066175 | <NME> defining-modules.md
<BEF> ADDFILE
<MSG> Create defining-modules.md
<DFF> @@ -0,0 +1,12 @@
+
+# Defining Modules
+
+```js
+var Apple = FruitMachine.define({
+ module: 'apple',
+ template: aTemplateFunction,
+ tag: 'section', // optional
+ classes: [ 'class-1', 'class-2'] // optional
+
+```
+
| 12 | Create defining-modules.md | 0 | .md | md | mit | ftlabs/fruitmachine |
10066176 | <NME> template.js
<BEF> ADDFILE
<MSG> Big collection example
<DFF> @@ -0,0 +1,6 @@
+
+var templateList2 = Hogan.compile(
+ "{{#collection}}" +
+ "<li class='list-2_item js-list_item' data-id='{{id}}'>{{title}}</li>" +
+ "{{/collection}}"
+);
\ No newline at end of file
| 6 | Big collection example | 0 | .js | js | mit | ftlabs/fruitmachine |
10066177 | <NME> HtmlClipboardTests.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Windows;
using AvaloniaEdit.Document;
using NUnit.Framework;
namespace AvaloniaEdit.Highlighting
{
[TestFixture]
public class HtmlClipboardTests
{
TextDocument document;
DocumentHighlighter highlighter;
public HtmlClipboardTests()
{
document = new TextDocument("using System.Text;\n\tstring text = SomeMethod();");
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
[Test, Ignore("")]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">using</span> System.Text;<br>" + Environment.NewLine +
" <span style=\"color: #ff0000; \">string</span> " +
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
[Test, Ignore("")]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">sin</span>", html);
}
}
}
<MSG> Merge branch 'master' into fix-selection-issues
<DFF> @@ -35,7 +35,7 @@ namespace AvaloniaEdit.Highlighting
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
- [Test, Ignore("")]
+ [Test]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
@@ -45,7 +45,7 @@ namespace AvaloniaEdit.Highlighting
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
- [Test, Ignore("")]
+ [Test]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
| 2 | Merge branch 'master' into fix-selection-issues | 2 | .cs | Tests/Highlighting/HtmlClipboardTests | mit | AvaloniaUI/AvaloniaEdit |
10066178 | <NME> HtmlClipboardTests.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Windows;
using AvaloniaEdit.Document;
using NUnit.Framework;
namespace AvaloniaEdit.Highlighting
{
[TestFixture]
public class HtmlClipboardTests
{
TextDocument document;
DocumentHighlighter highlighter;
public HtmlClipboardTests()
{
document = new TextDocument("using System.Text;\n\tstring text = SomeMethod();");
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
[Test, Ignore("")]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">using</span> System.Text;<br>" + Environment.NewLine +
" <span style=\"color: #ff0000; \">string</span> " +
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
[Test, Ignore("")]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">sin</span>", html);
}
}
}
<MSG> Merge branch 'master' into fix-selection-issues
<DFF> @@ -35,7 +35,7 @@ namespace AvaloniaEdit.Highlighting
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
- [Test, Ignore("")]
+ [Test]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
@@ -45,7 +45,7 @@ namespace AvaloniaEdit.Highlighting
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
- [Test, Ignore("")]
+ [Test]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
| 2 | Merge branch 'master' into fix-selection-issues | 2 | .cs | Tests/Highlighting/HtmlClipboardTests | mit | AvaloniaUI/AvaloniaEdit |
10066179 | <NME> HtmlClipboardTests.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Windows;
using AvaloniaEdit.Document;
using NUnit.Framework;
namespace AvaloniaEdit.Highlighting
{
[TestFixture]
public class HtmlClipboardTests
{
TextDocument document;
DocumentHighlighter highlighter;
public HtmlClipboardTests()
{
document = new TextDocument("using System.Text;\n\tstring text = SomeMethod();");
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
[Test, Ignore("")]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">using</span> System.Text;<br>" + Environment.NewLine +
" <span style=\"color: #ff0000; \">string</span> " +
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
[Test, Ignore("")]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">sin</span>", html);
}
}
}
<MSG> Merge branch 'master' into fix-selection-issues
<DFF> @@ -35,7 +35,7 @@ namespace AvaloniaEdit.Highlighting
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
- [Test, Ignore("")]
+ [Test]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
@@ -45,7 +45,7 @@ namespace AvaloniaEdit.Highlighting
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
- [Test, Ignore("")]
+ [Test]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
| 2 | Merge branch 'master' into fix-selection-issues | 2 | .cs | Tests/Highlighting/HtmlClipboardTests | mit | AvaloniaUI/AvaloniaEdit |
10066180 | <NME> HtmlClipboardTests.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Windows;
using AvaloniaEdit.Document;
using NUnit.Framework;
namespace AvaloniaEdit.Highlighting
{
[TestFixture]
public class HtmlClipboardTests
{
TextDocument document;
DocumentHighlighter highlighter;
public HtmlClipboardTests()
{
document = new TextDocument("using System.Text;\n\tstring text = SomeMethod();");
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
[Test, Ignore("")]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">using</span> System.Text;<br>" + Environment.NewLine +
" <span style=\"color: #ff0000; \">string</span> " +
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
[Test, Ignore("")]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">sin</span>", html);
}
}
}
<MSG> Merge branch 'master' into fix-selection-issues
<DFF> @@ -35,7 +35,7 @@ namespace AvaloniaEdit.Highlighting
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
- [Test, Ignore("")]
+ [Test]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
@@ -45,7 +45,7 @@ namespace AvaloniaEdit.Highlighting
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
- [Test, Ignore("")]
+ [Test]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
| 2 | Merge branch 'master' into fix-selection-issues | 2 | .cs | Tests/Highlighting/HtmlClipboardTests | mit | AvaloniaUI/AvaloniaEdit |
10066181 | <NME> HtmlClipboardTests.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Windows;
using AvaloniaEdit.Document;
using NUnit.Framework;
namespace AvaloniaEdit.Highlighting
{
[TestFixture]
public class HtmlClipboardTests
{
TextDocument document;
DocumentHighlighter highlighter;
public HtmlClipboardTests()
{
document = new TextDocument("using System.Text;\n\tstring text = SomeMethod();");
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
[Test, Ignore("")]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">using</span> System.Text;<br>" + Environment.NewLine +
" <span style=\"color: #ff0000; \">string</span> " +
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
[Test, Ignore("")]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">sin</span>", html);
}
}
}
<MSG> Merge branch 'master' into fix-selection-issues
<DFF> @@ -35,7 +35,7 @@ namespace AvaloniaEdit.Highlighting
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
- [Test, Ignore("")]
+ [Test]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
@@ -45,7 +45,7 @@ namespace AvaloniaEdit.Highlighting
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
- [Test, Ignore("")]
+ [Test]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
| 2 | Merge branch 'master' into fix-selection-issues | 2 | .cs | Tests/Highlighting/HtmlClipboardTests | mit | AvaloniaUI/AvaloniaEdit |
10066182 | <NME> HtmlClipboardTests.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Windows;
using AvaloniaEdit.Document;
using NUnit.Framework;
namespace AvaloniaEdit.Highlighting
{
[TestFixture]
public class HtmlClipboardTests
{
TextDocument document;
DocumentHighlighter highlighter;
public HtmlClipboardTests()
{
document = new TextDocument("using System.Text;\n\tstring text = SomeMethod();");
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
[Test, Ignore("")]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">using</span> System.Text;<br>" + Environment.NewLine +
" <span style=\"color: #ff0000; \">string</span> " +
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
[Test, Ignore("")]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">sin</span>", html);
}
}
}
<MSG> Merge branch 'master' into fix-selection-issues
<DFF> @@ -35,7 +35,7 @@ namespace AvaloniaEdit.Highlighting
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
- [Test, Ignore("")]
+ [Test]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
@@ -45,7 +45,7 @@ namespace AvaloniaEdit.Highlighting
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
- [Test, Ignore("")]
+ [Test]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
| 2 | Merge branch 'master' into fix-selection-issues | 2 | .cs | Tests/Highlighting/HtmlClipboardTests | mit | AvaloniaUI/AvaloniaEdit |
10066183 | <NME> HtmlClipboardTests.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Windows;
using AvaloniaEdit.Document;
using NUnit.Framework;
namespace AvaloniaEdit.Highlighting
{
[TestFixture]
public class HtmlClipboardTests
{
TextDocument document;
DocumentHighlighter highlighter;
public HtmlClipboardTests()
{
document = new TextDocument("using System.Text;\n\tstring text = SomeMethod();");
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
[Test, Ignore("")]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">using</span> System.Text;<br>" + Environment.NewLine +
" <span style=\"color: #ff0000; \">string</span> " +
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
[Test, Ignore("")]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">sin</span>", html);
}
}
}
<MSG> Merge branch 'master' into fix-selection-issues
<DFF> @@ -35,7 +35,7 @@ namespace AvaloniaEdit.Highlighting
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
- [Test, Ignore("")]
+ [Test]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
@@ -45,7 +45,7 @@ namespace AvaloniaEdit.Highlighting
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
- [Test, Ignore("")]
+ [Test]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
| 2 | Merge branch 'master' into fix-selection-issues | 2 | .cs | Tests/Highlighting/HtmlClipboardTests | mit | AvaloniaUI/AvaloniaEdit |
10066184 | <NME> HtmlClipboardTests.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Windows;
using AvaloniaEdit.Document;
using NUnit.Framework;
namespace AvaloniaEdit.Highlighting
{
[TestFixture]
public class HtmlClipboardTests
{
TextDocument document;
DocumentHighlighter highlighter;
public HtmlClipboardTests()
{
document = new TextDocument("using System.Text;\n\tstring text = SomeMethod();");
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
[Test, Ignore("")]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">using</span> System.Text;<br>" + Environment.NewLine +
" <span style=\"color: #ff0000; \">string</span> " +
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
[Test, Ignore("")]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">sin</span>", html);
}
}
}
<MSG> Merge branch 'master' into fix-selection-issues
<DFF> @@ -35,7 +35,7 @@ namespace AvaloniaEdit.Highlighting
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
- [Test, Ignore("")]
+ [Test]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
@@ -45,7 +45,7 @@ namespace AvaloniaEdit.Highlighting
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
- [Test, Ignore("")]
+ [Test]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
| 2 | Merge branch 'master' into fix-selection-issues | 2 | .cs | Tests/Highlighting/HtmlClipboardTests | mit | AvaloniaUI/AvaloniaEdit |
10066185 | <NME> HtmlClipboardTests.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Windows;
using AvaloniaEdit.Document;
using NUnit.Framework;
namespace AvaloniaEdit.Highlighting
{
[TestFixture]
public class HtmlClipboardTests
{
TextDocument document;
DocumentHighlighter highlighter;
public HtmlClipboardTests()
{
document = new TextDocument("using System.Text;\n\tstring text = SomeMethod();");
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
[Test, Ignore("")]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">using</span> System.Text;<br>" + Environment.NewLine +
" <span style=\"color: #ff0000; \">string</span> " +
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
[Test, Ignore("")]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">sin</span>", html);
}
}
}
<MSG> Merge branch 'master' into fix-selection-issues
<DFF> @@ -35,7 +35,7 @@ namespace AvaloniaEdit.Highlighting
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
- [Test, Ignore("")]
+ [Test]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
@@ -45,7 +45,7 @@ namespace AvaloniaEdit.Highlighting
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
- [Test, Ignore("")]
+ [Test]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
| 2 | Merge branch 'master' into fix-selection-issues | 2 | .cs | Tests/Highlighting/HtmlClipboardTests | mit | AvaloniaUI/AvaloniaEdit |
10066186 | <NME> HtmlClipboardTests.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Windows;
using AvaloniaEdit.Document;
using NUnit.Framework;
namespace AvaloniaEdit.Highlighting
{
[TestFixture]
public class HtmlClipboardTests
{
TextDocument document;
DocumentHighlighter highlighter;
public HtmlClipboardTests()
{
document = new TextDocument("using System.Text;\n\tstring text = SomeMethod();");
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
[Test, Ignore("")]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">using</span> System.Text;<br>" + Environment.NewLine +
" <span style=\"color: #ff0000; \">string</span> " +
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
[Test, Ignore("")]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">sin</span>", html);
}
}
}
<MSG> Merge branch 'master' into fix-selection-issues
<DFF> @@ -35,7 +35,7 @@ namespace AvaloniaEdit.Highlighting
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
- [Test, Ignore("")]
+ [Test]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
@@ -45,7 +45,7 @@ namespace AvaloniaEdit.Highlighting
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
- [Test, Ignore("")]
+ [Test]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
| 2 | Merge branch 'master' into fix-selection-issues | 2 | .cs | Tests/Highlighting/HtmlClipboardTests | mit | AvaloniaUI/AvaloniaEdit |
10066187 | <NME> HtmlClipboardTests.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Windows;
using AvaloniaEdit.Document;
using NUnit.Framework;
namespace AvaloniaEdit.Highlighting
{
[TestFixture]
public class HtmlClipboardTests
{
TextDocument document;
DocumentHighlighter highlighter;
public HtmlClipboardTests()
{
document = new TextDocument("using System.Text;\n\tstring text = SomeMethod();");
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
[Test, Ignore("")]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">using</span> System.Text;<br>" + Environment.NewLine +
" <span style=\"color: #ff0000; \">string</span> " +
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
[Test, Ignore("")]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">sin</span>", html);
}
}
}
<MSG> Merge branch 'master' into fix-selection-issues
<DFF> @@ -35,7 +35,7 @@ namespace AvaloniaEdit.Highlighting
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
- [Test, Ignore("")]
+ [Test]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
@@ -45,7 +45,7 @@ namespace AvaloniaEdit.Highlighting
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
- [Test, Ignore("")]
+ [Test]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
| 2 | Merge branch 'master' into fix-selection-issues | 2 | .cs | Tests/Highlighting/HtmlClipboardTests | mit | AvaloniaUI/AvaloniaEdit |
10066188 | <NME> HtmlClipboardTests.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Windows;
using AvaloniaEdit.Document;
using NUnit.Framework;
namespace AvaloniaEdit.Highlighting
{
[TestFixture]
public class HtmlClipboardTests
{
TextDocument document;
DocumentHighlighter highlighter;
public HtmlClipboardTests()
{
document = new TextDocument("using System.Text;\n\tstring text = SomeMethod();");
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
[Test, Ignore("")]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">using</span> System.Text;<br>" + Environment.NewLine +
" <span style=\"color: #ff0000; \">string</span> " +
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
[Test, Ignore("")]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">sin</span>", html);
}
}
}
<MSG> Merge branch 'master' into fix-selection-issues
<DFF> @@ -35,7 +35,7 @@ namespace AvaloniaEdit.Highlighting
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
- [Test, Ignore("")]
+ [Test]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
@@ -45,7 +45,7 @@ namespace AvaloniaEdit.Highlighting
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
- [Test, Ignore("")]
+ [Test]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
| 2 | Merge branch 'master' into fix-selection-issues | 2 | .cs | Tests/Highlighting/HtmlClipboardTests | mit | AvaloniaUI/AvaloniaEdit |
10066189 | <NME> HtmlClipboardTests.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Windows;
using AvaloniaEdit.Document;
using NUnit.Framework;
namespace AvaloniaEdit.Highlighting
{
[TestFixture]
public class HtmlClipboardTests
{
TextDocument document;
DocumentHighlighter highlighter;
public HtmlClipboardTests()
{
document = new TextDocument("using System.Text;\n\tstring text = SomeMethod();");
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
[Test, Ignore("")]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">using</span> System.Text;<br>" + Environment.NewLine +
" <span style=\"color: #ff0000; \">string</span> " +
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
[Test, Ignore("")]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">sin</span>", html);
}
}
}
<MSG> Merge branch 'master' into fix-selection-issues
<DFF> @@ -35,7 +35,7 @@ namespace AvaloniaEdit.Highlighting
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
- [Test, Ignore("")]
+ [Test]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
@@ -45,7 +45,7 @@ namespace AvaloniaEdit.Highlighting
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
- [Test, Ignore("")]
+ [Test]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
| 2 | Merge branch 'master' into fix-selection-issues | 2 | .cs | Tests/Highlighting/HtmlClipboardTests | mit | AvaloniaUI/AvaloniaEdit |
10066190 | <NME> HtmlClipboardTests.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Windows;
using AvaloniaEdit.Document;
using NUnit.Framework;
namespace AvaloniaEdit.Highlighting
{
[TestFixture]
public class HtmlClipboardTests
{
TextDocument document;
DocumentHighlighter highlighter;
public HtmlClipboardTests()
{
document = new TextDocument("using System.Text;\n\tstring text = SomeMethod();");
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
[Test, Ignore("")]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">using</span> System.Text;<br>" + Environment.NewLine +
" <span style=\"color: #ff0000; \">string</span> " +
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
[Test, Ignore("")]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">sin</span>", html);
}
}
}
<MSG> Merge branch 'master' into fix-selection-issues
<DFF> @@ -35,7 +35,7 @@ namespace AvaloniaEdit.Highlighting
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
- [Test, Ignore("")]
+ [Test]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
@@ -45,7 +45,7 @@ namespace AvaloniaEdit.Highlighting
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
- [Test, Ignore("")]
+ [Test]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
| 2 | Merge branch 'master' into fix-selection-issues | 2 | .cs | Tests/Highlighting/HtmlClipboardTests | mit | AvaloniaUI/AvaloniaEdit |
10066191 | <NME> HtmlClipboardTests.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.Windows;
using AvaloniaEdit.Document;
using NUnit.Framework;
namespace AvaloniaEdit.Highlighting
{
[TestFixture]
public class HtmlClipboardTests
{
TextDocument document;
DocumentHighlighter highlighter;
public HtmlClipboardTests()
{
document = new TextDocument("using System.Text;\n\tstring text = SomeMethod();");
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
[Test, Ignore("")]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">using</span> System.Text;<br>" + Environment.NewLine +
" <span style=\"color: #ff0000; \">string</span> " +
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
[Test, Ignore("")]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
string html = HtmlClipboard.CreateHtmlFragment(document, highlighter, segment, new HtmlOptions());
Assert.AreEqual("<span style=\"color: #008000; font-weight: bold; \">sin</span>", html);
}
}
}
<MSG> Merge branch 'master' into fix-selection-issues
<DFF> @@ -35,7 +35,7 @@ namespace AvaloniaEdit.Highlighting
highlighter = new DocumentHighlighter(document, HighlightingManager.Instance.GetDefinition("C#"));
}
- [Test, Ignore("")]
+ [Test]
public void FullDocumentTest()
{
var segment = new TextSegment { StartOffset = 0, Length = document.TextLength };
@@ -45,7 +45,7 @@ namespace AvaloniaEdit.Highlighting
"text = <span style=\"color: #191970; font-weight: bold; \">SomeMethod</span>();", html);
}
- [Test, Ignore("")]
+ [Test]
public void PartOfHighlightedWordTest()
{
var segment = new TextSegment { StartOffset = 1, Length = 3 };
| 2 | Merge branch 'master' into fix-selection-issues | 2 | .cs | Tests/Highlighting/HtmlClipboardTests | mit | AvaloniaUI/AvaloniaEdit |
10066192 | <NME> README.md
<BEF> # LoadJS
<img src="https://www.muicss.com/static/images/loadjs.svg" width="250px">
LoadJS is a tiny async loader for modern browsers (654 bytes).
[](https://david-dm.org/muicss/loadjs)
[](https://david-dm.org/muicss/loadjs#info=devDependencies)
[](https://cdnjs.com/libraries/loadjs)
## Introduction
LoadJS is a tiny async loading library for modern browsers (IE9+). It has a simple yet powerful dependency management system that lets you fetch JavaScript, CSS and image files in parallel and execute code after the dependencies have been met. The recommended way to use LoadJS is to include the minified source code of [loadjs.js](https://raw.githubusercontent.com/muicss/loadjs/master/dist/loadjs.min.js) in your <html> (possibly in the <head> tag) and then use the `loadjs` global to manage JavaScript dependencies after pageload.
LoadJS is based on the excellent [$script](https://github.com/ded/script.js) library by [Dustin Diaz](https://github.com/ded). We kept the behavior of the library the same but we re-wrote the code from scratch to add support for success/error callbacks and to optimize the library for modern browsers. LoadJS is 899 bytes (minified + gzipped).
Here's an example of what you can do with LoadJS:
```html
<script src="//unpkg.com/loadjs@latest/dist/loadjs.min.js"></script>
<script>
// define a dependency bundle and execute code when it loads
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
</script>
```
You can also use more advanced syntax for more options:
```html
<script src="//unpkg.com/loadjs@latest/dist/loadjs.min.js"></script>
<script>
// define a dependency bundle with advanced options
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
before: function(path, scriptEl) { /* execute code before fetch */ },
async: true, // load files synchronously or asynchronously (default: true)
numRetries: 3 // see caveats about using numRetries with async:false (default: 0),
returnPromise: false // return Promise object (default: false)
});
loadjs.ready('foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(depsNotFound) { /* foobar bundle load failed */ },
});
</script>
```
The latest version of LoadJS can be found in the `dist/` directory in this repository:
* [https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.js](https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.js) (for development)
* [https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.min.js](https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.min.js) (for production)
It's also available from these public CDNs:
* UNPKG
* [https://unpkg.com/[email protected]/dist/loadjs.js](https://unpkg.com/[email protected]/dist/loadjs.js) (for development)
* [https://unpkg.com/[email protected]/dist/loadjs.min.js](https://unpkg.com/[email protected]/dist/loadjs.min.js) (for production)
* CDNJS
* [https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.js](https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.js) (for development)
* [https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.min.js](https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.min.js) (for production)
You can also use it as a CJS or AMD module:
```bash
$ npm install --save loadjs
```
```javascript
var loadjs = require('loadjs');
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
```
## Browser Support
* IE9+ (`async: false` support only works in IE10+)
* Opera 12+
* Safari 5+
* Chrome
* Firefox
* iOS 6+
* Android 4.4+
LoadJS also detects script load failures from AdBlock Plus and Ghostery in:
* Safari
* Chrome
Note: LoadJS treats empty CSS files as load failures in IE9-11 and uses `rel="preload"` to load CSS files in Edge (to get around lack of support for onerror events on `<link rel="stylesheet">` tags)
## Documentation
1. Load a single file
```javascript
loadjs('/path/to/foo.js', function() {
/* foo.js loaded */
});
```
1. Fetch files in parallel and load them asynchronously
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], function() {
/* foo.js and bar.js loaded */
});
```
1. Fetch JavaScript, CSS and image files
```javascript
loadjs(['/path/to/foo.css', '/path/to/bar.png', 'path/to/thunk.js'], function() {
/* foo.css, bar.png and thunk.js loaded */
});
```
1. Force treat file as CSS stylesheet
```javascript
loadjs(['css!/path/to/cssfile.custom'], function() {
/* cssfile.custom loaded as stylesheet */
});
```
1. Force treat file as image
```javascript
loadjs(['img!/path/to/image.custom'], function() {
/* image.custom loaded */
});
```
1. Add a bundle id
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', function() {
/* foo.js & bar.js loaded */
});
```
1. Use .ready() to define bundles and callbacks separately
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
```
1. Use multiple bundles in .ready() dependency lists
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs(['/path/to/bar1.js', '/path/to/bar2.js'], 'bar');
loadjs.ready(['foo', 'bar'], function() {
/* foo.js & bar1.js & bar2.js loaded */
});
```
1. Chain .ready() together
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs('/path/to/bar.js', 'bar');
loadjs
.ready('foo', function() {
/* foo.js loaded */
})
.ready('bar', function() {
/* bar.js loaded */
});
```
1. Use Promises to register callbacks
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {returnPromise: true})
.then(function() { /* foo.js & bar.js loaded */ })
.catch(function(pathsNotFound) { /* at least one didn't load */ });
```
1. Check if bundle has already been defined
```javascript
if (!loadjs.isDefined('foobar')) {
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', function() {
/* foo.js & bar.js loaded */
});
}
```
1. Fetch files in parallel and load them in series
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {
success: function() { /* foo.js and bar.js loaded in series */ },
async: false
});
```
1. Add an error callback
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(pathsNotFound) { /* at least one path didn't load */ }
});
```
1. Retry files before calling the error callback
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(pathsNotFound) { /* at least one path didn't load */ },
numRetries: 3
});
// NOTE: Using `numRetries` with `async: false` can cause files to load out-of-sync on retries
```
1. Execute a callback before script tags are embedded
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {
success: function() {},
error: function(pathsNotFound) {},
before: function(path, scriptEl) {
/* called for each script node before being embedded */
if (path === '/path/to/foo.js') scriptEl.crossOrigin = true;
}
});
```
1. Bypass LoadJS default DOM insertion mechanism (DOM `<head>`)
```javascript
loadjs(['/path/to/foo.js'], {
success: function() {},
error: function(pathsNotFound) {},
before: function(path, scriptEl) {
document.body.appendChild(scriptEl);
/* return `false` to bypass default DOM insertion mechanism */
return false;
}
});
```
1. Use bundle ids in error callback
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs('/path/to/bar.js', 'bar');
loadjs(['/path/to/thunkor.js', '/path/to/thunky.js'], 'thunk');
// wait for multiple depdendencies
loadjs.ready(['foo', 'bar', 'thunk'], {
success: function() {
// foo.js & bar.js & thunkor.js & thunky.js loaded
},
error: function(depsNotFound) {
if (depsNotFound.indexOf('foo') > -1) {}; // foo failed
if (depsNotFound.indexOf('bar') > -1) {}; // bar failed
if (depsNotFound.indexOf('thunk') > -1) {}; // thunk failed
}
});
```
1. Use .done() for more control
```javascript
loadjs.ready(['dependency1', 'dependency2'], function() {
/* run code after dependencies have been met */
});
function fn1() {
loadjs.done('dependency1');
}
function fn2() {
loadjs.done('dependency2');
}
```
1. Reset dependency trackers
```javascript
loadjs.reset();
```
1. Implement a require-like dependency manager
```javascript
var bundles = {
'bundleA': ['/file1.js', '/file2.js'],
'bundleB': ['/file3.js', '/file4.js']
};
function require(bundleIds, callbackFn) {
bundleIds.forEach(function(bundleId) {
if (!loadjs.isDefined(bundleId)) loadjs(bundles[bundleId], bundleId);
});
loadjs.ready(bundleIds, callbackFn);
}
require(['bundleA'], function() { /* bundleA loaded */ });
require(['bundleB'], function() { /* bundleB loaded */ });
require(['bundleA', 'bundleB'], function() { /* bundleA and bundleB loaded */ });
```
## Directory structure
<pre>
loadjs/
├── dist
│ ├── loadjs.js
│ ├── loadjs.min.js
│ └── loadjs.umd.js
├── examples
├── gulpfile.js
├── LICENSE.txt
├── package.json
├── README.md
├── src
│ └── loadjs.js
├── test
└── umd-templates
</pre>
## Development Quickstart
1. Install dependencies
* [nodejs](http://nodejs.org/)
* [npm](https://www.npmjs.org/)
* http-server (via npm)
1. Clone repository
```bash
$ git clone [email protected]:muicss/loadjs.git
$ cd loadjs
```
1. Install node dependencies using npm
```bash
$ npm install
```
1. Build examples
```bash
$ npm run build-examples
```
To view the examples you can use any static file server. To use the `nodejs` http-server module:
```bash
$ npm install http-server
$ npm run http-server -- -p 3000
```
Then visit [http://localhost:3000/examples](http://localhost:3000/examples)
1. Build distribution files
```bash
$ npm run build-dist
```
The files will be located in the `dist` directory.
1. Run tests
To run the browser tests first build the `loadjs` library:
```bash
$ npm run build-tests
```
Then visit [http://localhost:3000/test](http://localhost:3000/test)
1. Build all files
```bash
$ npm run build-all
```
<MSG> Update README.md
<DFF> @@ -2,7 +2,7 @@
<img src="https://www.muicss.com/static/images/loadjs.svg" width="250px">
-LoadJS is a tiny async loader for modern browsers (654 bytes).
+LoadJS is a tiny async loader for modern browsers (692 bytes).
[](https://david-dm.org/muicss/loadjs)
[](https://david-dm.org/muicss/loadjs#info=devDependencies)
| 1 | Update README.md | 1 | .md | md | mit | muicss/loadjs |
10066193 | <NME> README.md
<BEF> # LoadJS
<img src="https://www.muicss.com/static/images/loadjs.svg" width="250px">
LoadJS is a tiny async loader for modern browsers (654 bytes).
[](https://david-dm.org/muicss/loadjs)
[](https://david-dm.org/muicss/loadjs#info=devDependencies)
[](https://cdnjs.com/libraries/loadjs)
## Introduction
LoadJS is a tiny async loading library for modern browsers (IE9+). It has a simple yet powerful dependency management system that lets you fetch JavaScript, CSS and image files in parallel and execute code after the dependencies have been met. The recommended way to use LoadJS is to include the minified source code of [loadjs.js](https://raw.githubusercontent.com/muicss/loadjs/master/dist/loadjs.min.js) in your <html> (possibly in the <head> tag) and then use the `loadjs` global to manage JavaScript dependencies after pageload.
LoadJS is based on the excellent [$script](https://github.com/ded/script.js) library by [Dustin Diaz](https://github.com/ded). We kept the behavior of the library the same but we re-wrote the code from scratch to add support for success/error callbacks and to optimize the library for modern browsers. LoadJS is 899 bytes (minified + gzipped).
Here's an example of what you can do with LoadJS:
```html
<script src="//unpkg.com/loadjs@latest/dist/loadjs.min.js"></script>
<script>
// define a dependency bundle and execute code when it loads
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
</script>
```
You can also use more advanced syntax for more options:
```html
<script src="//unpkg.com/loadjs@latest/dist/loadjs.min.js"></script>
<script>
// define a dependency bundle with advanced options
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
before: function(path, scriptEl) { /* execute code before fetch */ },
async: true, // load files synchronously or asynchronously (default: true)
numRetries: 3 // see caveats about using numRetries with async:false (default: 0),
returnPromise: false // return Promise object (default: false)
});
loadjs.ready('foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(depsNotFound) { /* foobar bundle load failed */ },
});
</script>
```
The latest version of LoadJS can be found in the `dist/` directory in this repository:
* [https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.js](https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.js) (for development)
* [https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.min.js](https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.min.js) (for production)
It's also available from these public CDNs:
* UNPKG
* [https://unpkg.com/[email protected]/dist/loadjs.js](https://unpkg.com/[email protected]/dist/loadjs.js) (for development)
* [https://unpkg.com/[email protected]/dist/loadjs.min.js](https://unpkg.com/[email protected]/dist/loadjs.min.js) (for production)
* CDNJS
* [https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.js](https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.js) (for development)
* [https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.min.js](https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.min.js) (for production)
You can also use it as a CJS or AMD module:
```bash
$ npm install --save loadjs
```
```javascript
var loadjs = require('loadjs');
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
```
## Browser Support
* IE9+ (`async: false` support only works in IE10+)
* Opera 12+
* Safari 5+
* Chrome
* Firefox
* iOS 6+
* Android 4.4+
LoadJS also detects script load failures from AdBlock Plus and Ghostery in:
* Safari
* Chrome
Note: LoadJS treats empty CSS files as load failures in IE9-11 and uses `rel="preload"` to load CSS files in Edge (to get around lack of support for onerror events on `<link rel="stylesheet">` tags)
## Documentation
1. Load a single file
```javascript
loadjs('/path/to/foo.js', function() {
/* foo.js loaded */
});
```
1. Fetch files in parallel and load them asynchronously
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], function() {
/* foo.js and bar.js loaded */
});
```
1. Fetch JavaScript, CSS and image files
```javascript
loadjs(['/path/to/foo.css', '/path/to/bar.png', 'path/to/thunk.js'], function() {
/* foo.css, bar.png and thunk.js loaded */
});
```
1. Force treat file as CSS stylesheet
```javascript
loadjs(['css!/path/to/cssfile.custom'], function() {
/* cssfile.custom loaded as stylesheet */
});
```
1. Force treat file as image
```javascript
loadjs(['img!/path/to/image.custom'], function() {
/* image.custom loaded */
});
```
1. Add a bundle id
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', function() {
/* foo.js & bar.js loaded */
});
```
1. Use .ready() to define bundles and callbacks separately
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
```
1. Use multiple bundles in .ready() dependency lists
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs(['/path/to/bar1.js', '/path/to/bar2.js'], 'bar');
loadjs.ready(['foo', 'bar'], function() {
/* foo.js & bar1.js & bar2.js loaded */
});
```
1. Chain .ready() together
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs('/path/to/bar.js', 'bar');
loadjs
.ready('foo', function() {
/* foo.js loaded */
})
.ready('bar', function() {
/* bar.js loaded */
});
```
1. Use Promises to register callbacks
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {returnPromise: true})
.then(function() { /* foo.js & bar.js loaded */ })
.catch(function(pathsNotFound) { /* at least one didn't load */ });
```
1. Check if bundle has already been defined
```javascript
if (!loadjs.isDefined('foobar')) {
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', function() {
/* foo.js & bar.js loaded */
});
}
```
1. Fetch files in parallel and load them in series
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {
success: function() { /* foo.js and bar.js loaded in series */ },
async: false
});
```
1. Add an error callback
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(pathsNotFound) { /* at least one path didn't load */ }
});
```
1. Retry files before calling the error callback
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(pathsNotFound) { /* at least one path didn't load */ },
numRetries: 3
});
// NOTE: Using `numRetries` with `async: false` can cause files to load out-of-sync on retries
```
1. Execute a callback before script tags are embedded
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {
success: function() {},
error: function(pathsNotFound) {},
before: function(path, scriptEl) {
/* called for each script node before being embedded */
if (path === '/path/to/foo.js') scriptEl.crossOrigin = true;
}
});
```
1. Bypass LoadJS default DOM insertion mechanism (DOM `<head>`)
```javascript
loadjs(['/path/to/foo.js'], {
success: function() {},
error: function(pathsNotFound) {},
before: function(path, scriptEl) {
document.body.appendChild(scriptEl);
/* return `false` to bypass default DOM insertion mechanism */
return false;
}
});
```
1. Use bundle ids in error callback
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs('/path/to/bar.js', 'bar');
loadjs(['/path/to/thunkor.js', '/path/to/thunky.js'], 'thunk');
// wait for multiple depdendencies
loadjs.ready(['foo', 'bar', 'thunk'], {
success: function() {
// foo.js & bar.js & thunkor.js & thunky.js loaded
},
error: function(depsNotFound) {
if (depsNotFound.indexOf('foo') > -1) {}; // foo failed
if (depsNotFound.indexOf('bar') > -1) {}; // bar failed
if (depsNotFound.indexOf('thunk') > -1) {}; // thunk failed
}
});
```
1. Use .done() for more control
```javascript
loadjs.ready(['dependency1', 'dependency2'], function() {
/* run code after dependencies have been met */
});
function fn1() {
loadjs.done('dependency1');
}
function fn2() {
loadjs.done('dependency2');
}
```
1. Reset dependency trackers
```javascript
loadjs.reset();
```
1. Implement a require-like dependency manager
```javascript
var bundles = {
'bundleA': ['/file1.js', '/file2.js'],
'bundleB': ['/file3.js', '/file4.js']
};
function require(bundleIds, callbackFn) {
bundleIds.forEach(function(bundleId) {
if (!loadjs.isDefined(bundleId)) loadjs(bundles[bundleId], bundleId);
});
loadjs.ready(bundleIds, callbackFn);
}
require(['bundleA'], function() { /* bundleA loaded */ });
require(['bundleB'], function() { /* bundleB loaded */ });
require(['bundleA', 'bundleB'], function() { /* bundleA and bundleB loaded */ });
```
## Directory structure
<pre>
loadjs/
├── dist
│ ├── loadjs.js
│ ├── loadjs.min.js
│ └── loadjs.umd.js
├── examples
├── gulpfile.js
├── LICENSE.txt
├── package.json
├── README.md
├── src
│ └── loadjs.js
├── test
└── umd-templates
</pre>
## Development Quickstart
1. Install dependencies
* [nodejs](http://nodejs.org/)
* [npm](https://www.npmjs.org/)
* http-server (via npm)
1. Clone repository
```bash
$ git clone [email protected]:muicss/loadjs.git
$ cd loadjs
```
1. Install node dependencies using npm
```bash
$ npm install
```
1. Build examples
```bash
$ npm run build-examples
```
To view the examples you can use any static file server. To use the `nodejs` http-server module:
```bash
$ npm install http-server
$ npm run http-server -- -p 3000
```
Then visit [http://localhost:3000/examples](http://localhost:3000/examples)
1. Build distribution files
```bash
$ npm run build-dist
```
The files will be located in the `dist` directory.
1. Run tests
To run the browser tests first build the `loadjs` library:
```bash
$ npm run build-tests
```
Then visit [http://localhost:3000/test](http://localhost:3000/test)
1. Build all files
```bash
$ npm run build-all
```
<MSG> Update README.md
<DFF> @@ -2,7 +2,7 @@
<img src="https://www.muicss.com/static/images/loadjs.svg" width="250px">
-LoadJS is a tiny async loader for modern browsers (654 bytes).
+LoadJS is a tiny async loader for modern browsers (692 bytes).
[](https://david-dm.org/muicss/loadjs)
[](https://david-dm.org/muicss/loadjs#info=devDependencies)
| 1 | Update README.md | 1 | .md | md | mit | muicss/loadjs |
10066194 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, new TextDocument());
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
document?.WriteTextTo(writer);
writer.Flush();
// do not close the stream
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge branch 'master' into support-multithreading
<DFF> @@ -67,14 +67,19 @@ namespace AvaloniaEdit
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
- protected TextEditor(TextArea textArea)
+ protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
+ {
+
+ }
+
+ protected TextEditor(TextArea textArea, TextDocument document)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
- SetValue(DocumentProperty, new TextDocument());
+ SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
@@ -128,7 +133,7 @@ namespace AvaloniaEdit
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
- PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
+ PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
| 8 | Merge branch 'master' into support-multithreading | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066195 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, new TextDocument());
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
document?.WriteTextTo(writer);
writer.Flush();
// do not close the stream
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge branch 'master' into support-multithreading
<DFF> @@ -67,14 +67,19 @@ namespace AvaloniaEdit
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
- protected TextEditor(TextArea textArea)
+ protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
+ {
+
+ }
+
+ protected TextEditor(TextArea textArea, TextDocument document)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
- SetValue(DocumentProperty, new TextDocument());
+ SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
@@ -128,7 +133,7 @@ namespace AvaloniaEdit
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
- PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
+ PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
| 8 | Merge branch 'master' into support-multithreading | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066196 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, new TextDocument());
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
document?.WriteTextTo(writer);
writer.Flush();
// do not close the stream
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge branch 'master' into support-multithreading
<DFF> @@ -67,14 +67,19 @@ namespace AvaloniaEdit
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
- protected TextEditor(TextArea textArea)
+ protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
+ {
+
+ }
+
+ protected TextEditor(TextArea textArea, TextDocument document)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
- SetValue(DocumentProperty, new TextDocument());
+ SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
@@ -128,7 +133,7 @@ namespace AvaloniaEdit
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
- PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
+ PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
| 8 | Merge branch 'master' into support-multithreading | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066197 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, new TextDocument());
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
document?.WriteTextTo(writer);
writer.Flush();
// do not close the stream
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge branch 'master' into support-multithreading
<DFF> @@ -67,14 +67,19 @@ namespace AvaloniaEdit
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
- protected TextEditor(TextArea textArea)
+ protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
+ {
+
+ }
+
+ protected TextEditor(TextArea textArea, TextDocument document)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
- SetValue(DocumentProperty, new TextDocument());
+ SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
@@ -128,7 +133,7 @@ namespace AvaloniaEdit
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
- PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
+ PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
| 8 | Merge branch 'master' into support-multithreading | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066198 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, new TextDocument());
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
document?.WriteTextTo(writer);
writer.Flush();
// do not close the stream
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge branch 'master' into support-multithreading
<DFF> @@ -67,14 +67,19 @@ namespace AvaloniaEdit
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
- protected TextEditor(TextArea textArea)
+ protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
+ {
+
+ }
+
+ protected TextEditor(TextArea textArea, TextDocument document)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
- SetValue(DocumentProperty, new TextDocument());
+ SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
@@ -128,7 +133,7 @@ namespace AvaloniaEdit
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
- PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
+ PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
| 8 | Merge branch 'master' into support-multithreading | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066199 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, new TextDocument());
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
document?.WriteTextTo(writer);
writer.Flush();
// do not close the stream
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge branch 'master' into support-multithreading
<DFF> @@ -67,14 +67,19 @@ namespace AvaloniaEdit
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
- protected TextEditor(TextArea textArea)
+ protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
+ {
+
+ }
+
+ protected TextEditor(TextArea textArea, TextDocument document)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
- SetValue(DocumentProperty, new TextDocument());
+ SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
@@ -128,7 +133,7 @@ namespace AvaloniaEdit
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
- PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
+ PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
| 8 | Merge branch 'master' into support-multithreading | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066200 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, new TextDocument());
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
document?.WriteTextTo(writer);
writer.Flush();
// do not close the stream
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge branch 'master' into support-multithreading
<DFF> @@ -67,14 +67,19 @@ namespace AvaloniaEdit
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
- protected TextEditor(TextArea textArea)
+ protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
+ {
+
+ }
+
+ protected TextEditor(TextArea textArea, TextDocument document)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
- SetValue(DocumentProperty, new TextDocument());
+ SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
@@ -128,7 +133,7 @@ namespace AvaloniaEdit
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
- PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
+ PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
| 8 | Merge branch 'master' into support-multithreading | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066201 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, new TextDocument());
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
document?.WriteTextTo(writer);
writer.Flush();
// do not close the stream
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge branch 'master' into support-multithreading
<DFF> @@ -67,14 +67,19 @@ namespace AvaloniaEdit
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
- protected TextEditor(TextArea textArea)
+ protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
+ {
+
+ }
+
+ protected TextEditor(TextArea textArea, TextDocument document)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
- SetValue(DocumentProperty, new TextDocument());
+ SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
@@ -128,7 +133,7 @@ namespace AvaloniaEdit
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
- PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
+ PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
| 8 | Merge branch 'master' into support-multithreading | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066202 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, new TextDocument());
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
document?.WriteTextTo(writer);
writer.Flush();
// do not close the stream
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge branch 'master' into support-multithreading
<DFF> @@ -67,14 +67,19 @@ namespace AvaloniaEdit
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
- protected TextEditor(TextArea textArea)
+ protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
+ {
+
+ }
+
+ protected TextEditor(TextArea textArea, TextDocument document)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
- SetValue(DocumentProperty, new TextDocument());
+ SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
@@ -128,7 +133,7 @@ namespace AvaloniaEdit
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
- PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
+ PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
| 8 | Merge branch 'master' into support-multithreading | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066203 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, new TextDocument());
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
document?.WriteTextTo(writer);
writer.Flush();
// do not close the stream
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge branch 'master' into support-multithreading
<DFF> @@ -67,14 +67,19 @@ namespace AvaloniaEdit
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
- protected TextEditor(TextArea textArea)
+ protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
+ {
+
+ }
+
+ protected TextEditor(TextArea textArea, TextDocument document)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
- SetValue(DocumentProperty, new TextDocument());
+ SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
@@ -128,7 +133,7 @@ namespace AvaloniaEdit
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
- PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
+ PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
| 8 | Merge branch 'master' into support-multithreading | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066204 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, new TextDocument());
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
document?.WriteTextTo(writer);
writer.Flush();
// do not close the stream
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge branch 'master' into support-multithreading
<DFF> @@ -67,14 +67,19 @@ namespace AvaloniaEdit
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
- protected TextEditor(TextArea textArea)
+ protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
+ {
+
+ }
+
+ protected TextEditor(TextArea textArea, TextDocument document)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
- SetValue(DocumentProperty, new TextDocument());
+ SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
@@ -128,7 +133,7 @@ namespace AvaloniaEdit
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
- PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
+ PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
| 8 | Merge branch 'master' into support-multithreading | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066205 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, new TextDocument());
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
document?.WriteTextTo(writer);
writer.Flush();
// do not close the stream
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge branch 'master' into support-multithreading
<DFF> @@ -67,14 +67,19 @@ namespace AvaloniaEdit
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
- protected TextEditor(TextArea textArea)
+ protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
+ {
+
+ }
+
+ protected TextEditor(TextArea textArea, TextDocument document)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
- SetValue(DocumentProperty, new TextDocument());
+ SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
@@ -128,7 +133,7 @@ namespace AvaloniaEdit
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
- PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
+ PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
| 8 | Merge branch 'master' into support-multithreading | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066206 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, new TextDocument());
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
document?.WriteTextTo(writer);
writer.Flush();
// do not close the stream
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge branch 'master' into support-multithreading
<DFF> @@ -67,14 +67,19 @@ namespace AvaloniaEdit
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
- protected TextEditor(TextArea textArea)
+ protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
+ {
+
+ }
+
+ protected TextEditor(TextArea textArea, TextDocument document)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
- SetValue(DocumentProperty, new TextDocument());
+ SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
@@ -128,7 +133,7 @@ namespace AvaloniaEdit
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
- PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
+ PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
| 8 | Merge branch 'master' into support-multithreading | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066207 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, new TextDocument());
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
document?.WriteTextTo(writer);
writer.Flush();
// do not close the stream
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge branch 'master' into support-multithreading
<DFF> @@ -67,14 +67,19 @@ namespace AvaloniaEdit
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
- protected TextEditor(TextArea textArea)
+ protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
+ {
+
+ }
+
+ protected TextEditor(TextArea textArea, TextDocument document)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
- SetValue(DocumentProperty, new TextDocument());
+ SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
@@ -128,7 +133,7 @@ namespace AvaloniaEdit
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
- PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
+ PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
| 8 | Merge branch 'master' into support-multithreading | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066208 | <NME> TextEditor.cs
<BEF> // Copyright (c) 2014 AlphaSierraPapa for the SharpDevelop Team
//
// Permission is hereby granted, free of charge, to any person obtaining a copy of this
// software and associated documentation files (the "Software"), to deal in the Software
// without restriction, including without limitation the rights to use, copy, modify, merge,
// publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons
// to whom the Software is furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all copies or
// substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR
// PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE
// FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
// DEALINGS IN THE SOFTWARE.
using System;
using System.ComponentModel;
using System.IO;
using System.Linq;
using System.Text;
using Avalonia;
using AvaloniaEdit.Document;
using AvaloniaEdit.Editing;
using AvaloniaEdit.Highlighting;
using AvaloniaEdit.Rendering;
using AvaloniaEdit.Utils;
using Avalonia.Controls;
using Avalonia.Controls.Primitives;
using Avalonia.Controls.Shapes;
using Avalonia.Input;
using Avalonia.Interactivity;
using Avalonia.LogicalTree;
using Avalonia.Media;
using Avalonia.Data;
using AvaloniaEdit.Search;
namespace AvaloniaEdit
{
/// <summary>
/// The text editor control.
/// Contains a scrollable TextArea.
/// </summary>
public class TextEditor : TemplatedControl, ITextEditorComponent
{
#region Constructors
static TextEditor()
{
FocusableProperty.OverrideDefaultValue<TextEditor>(true);
HorizontalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
VerticalScrollBarVisibilityProperty.OverrideDefaultValue<TextEditor>(ScrollBarVisibility.Auto);
OptionsProperty.Changed.Subscribe(OnOptionsChanged);
DocumentProperty.Changed.Subscribe(OnDocumentChanged);
SyntaxHighlightingProperty.Changed.Subscribe(OnSyntaxHighlightingChanged);
IsReadOnlyProperty.Changed.Subscribe(OnIsReadOnlyChanged);
IsModifiedProperty.Changed.Subscribe(OnIsModifiedChanged);
ShowLineNumbersProperty.Changed.Subscribe(OnShowLineNumbersChanged);
LineNumbersForegroundProperty.Changed.Subscribe(OnLineNumbersForegroundChanged);
FontFamilyProperty.Changed.Subscribe(OnFontFamilyPropertyChanged);
FontSizeProperty.Changed.Subscribe(OnFontSizePropertyChanged);
}
/// <summary>
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
protected TextEditor(TextArea textArea)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, new TextDocument());
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
SetValue(OptionsProperty, textArea.Options);
SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
#endregion
protected override void OnGotFocus(GotFocusEventArgs e)
{
base.OnGotFocus(e);
TextArea.Focus();
e.Handled = true;
}
#region Document property
/// <summary>
/// Document property.
/// </summary>
public static readonly StyledProperty<TextDocument> DocumentProperty
= TextView.DocumentProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the document displayed by the text editor.
/// This is a dependency property.
/// </summary>
public TextDocument Document
{
get => GetValue(DocumentProperty);
set => SetValue(DocumentProperty, value);
}
/// <summary>
/// Occurs when the document property has changed.
/// </summary>
public event EventHandler DocumentChanged;
/// <summary>
/// Raises the <see cref="DocumentChanged"/> event.
/// </summary>
protected virtual void OnDocumentChanged(EventArgs e)
{
DocumentChanged?.Invoke(this, e);
}
private static void OnDocumentChanged(AvaloniaPropertyChangedEventArgs e)
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
{
TextDocumentWeakEventManager.TextChanged.AddHandler(newValue, OnTextChanged);
PropertyChangedWeakEventManager.AddHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
OnDocumentChanged(EventArgs.Empty);
OnTextChanged(EventArgs.Empty);
}
#endregion
#region Options property
/// <summary>
/// Options property.
/// </summary>
public static readonly StyledProperty<TextEditorOptions> OptionsProperty
= TextView.OptionsProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the options currently used by the text editor.
/// </summary>
public TextEditorOptions Options
{
get => GetValue(OptionsProperty);
set => SetValue(OptionsProperty, value);
}
/// <summary>
/// Occurs when a text editor option has changed.
/// </summary>
public event PropertyChangedEventHandler OptionChanged;
/// <summary>
/// Raises the <see cref="OptionChanged"/> event.
/// </summary>
protected virtual void OnOptionChanged(PropertyChangedEventArgs e)
{
OptionChanged?.Invoke(this, e);
}
private static void OnOptionsChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnOptionsChanged((TextEditorOptions)e.OldValue, (TextEditorOptions)e.NewValue);
}
private void OnOptionsChanged(TextEditorOptions oldValue, TextEditorOptions newValue)
{
if (oldValue != null)
{
PropertyChangedWeakEventManager.RemoveHandler(oldValue, OnPropertyChangedHandler);
}
TextArea.Options = newValue;
if (newValue != null)
{
PropertyChangedWeakEventManager.AddHandler(newValue, OnPropertyChangedHandler);
}
OnOptionChanged(new PropertyChangedEventArgs(null));
}
private void OnPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
OnOptionChanged(e);
}
protected override void OnPropertyChanged(AvaloniaPropertyChangedEventArgs change)
{
base.OnPropertyChanged(change);
if (change.Property == WordWrapProperty)
{
if (WordWrap)
{
_horizontalScrollBarVisibilityBck = HorizontalScrollBarVisibility;
HorizontalScrollBarVisibility = ScrollBarVisibility.Disabled;
}
else
{
HorizontalScrollBarVisibility = _horizontalScrollBarVisibilityBck;
}
}
}
private void OnUndoStackPropertyChangedHandler(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
HandleIsOriginalChanged(e);
}
}
private void OnTextChanged(object sender, EventArgs e)
{
OnTextChanged(e);
}
#endregion
#region Text property
/// <summary>
/// Gets/Sets the text of the current document.
/// </summary>
public string Text
{
get
{
var document = Document;
return document != null ? document.Text : string.Empty;
}
set
{
var document = GetDocument();
document.Text = value ?? string.Empty;
// after replacing the full text, the caret is positioned at the end of the document
// - reset it to the beginning.
CaretOffset = 0;
document.UndoStack.ClearAll();
}
}
private TextDocument GetDocument()
{
var document = Document;
if (document == null)
throw ThrowUtil.NoDocumentAssigned();
return document;
}
/// <summary>
/// Occurs when the Text property changes.
/// </summary>
public event EventHandler TextChanged;
/// <summary>
/// Raises the <see cref="TextChanged"/> event.
/// </summary>
protected virtual void OnTextChanged(EventArgs e)
{
TextChanged?.Invoke(this, e);
}
#endregion
#region TextArea / ScrollViewer properties
private readonly TextArea textArea;
private SearchPanel searchPanel;
private bool wasSearchPanelOpened;
protected override void OnApplyTemplate(TemplateAppliedEventArgs e)
{
base.OnApplyTemplate(e);
ScrollViewer = (ScrollViewer)e.NameScope.Find("PART_ScrollViewer");
ScrollViewer.Content = TextArea;
searchPanel = SearchPanel.Install(this);
}
protected override void OnAttachedToLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnAttachedToLogicalTree(e);
if (searchPanel != null && wasSearchPanelOpened)
{
searchPanel.Open();
}
}
protected override void OnDetachedFromLogicalTree(LogicalTreeAttachmentEventArgs e)
{
base.OnDetachedFromLogicalTree(e);
if (searchPanel != null)
{
wasSearchPanelOpened = searchPanel.IsOpened;
if (searchPanel.IsOpened)
searchPanel.Close();
}
}
/// <summary>
/// Gets the text area.
/// </summary>
public TextArea TextArea => textArea;
/// <summary>
/// Gets the search panel.
/// </summary>
public SearchPanel SearchPanel => searchPanel;
/// <summary>
/// Gets the scroll viewer used by the text editor.
/// This property can return null if the template has not been applied / does not contain a scroll viewer.
/// </summary>
internal ScrollViewer ScrollViewer { get; private set; }
#endregion
#region Syntax highlighting
/// <summary>
/// The <see cref="SyntaxHighlighting"/> property.
/// </summary>
public static readonly StyledProperty<IHighlightingDefinition> SyntaxHighlightingProperty =
AvaloniaProperty.Register<TextEditor, IHighlightingDefinition>("SyntaxHighlighting");
/// <summary>
/// Gets/sets the syntax highlighting definition used to colorize the text.
/// </summary>
public IHighlightingDefinition SyntaxHighlighting
{
get => GetValue(SyntaxHighlightingProperty);
set => SetValue(SyntaxHighlightingProperty, value);
}
private IVisualLineTransformer _colorizer;
private static void OnSyntaxHighlightingChanged(AvaloniaPropertyChangedEventArgs e)
{
(e.Sender as TextEditor)?.OnSyntaxHighlightingChanged(e.NewValue as IHighlightingDefinition);
}
private void OnSyntaxHighlightingChanged(IHighlightingDefinition newValue)
{
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Remove(_colorizer);
_colorizer = null;
}
if (newValue != null)
{
_colorizer = CreateColorizer(newValue);
if (_colorizer != null)
{
textArea.TextView.LineTransformers.Insert(0, _colorizer);
}
}
}
/// <summary>
/// Creates the highlighting colorizer for the specified highlighting definition.
/// Allows derived classes to provide custom colorizer implementations for special highlighting definitions.
/// </summary>
/// <returns></returns>
protected virtual IVisualLineTransformer CreateColorizer(IHighlightingDefinition highlightingDefinition)
{
if (highlightingDefinition == null)
throw new ArgumentNullException(nameof(highlightingDefinition));
return new HighlightingColorizer(highlightingDefinition);
}
#endregion
#region WordWrap
/// <summary>
/// Word wrap dependency property.
/// </summary>
public static readonly StyledProperty<bool> WordWrapProperty =
AvaloniaProperty.Register<TextEditor, bool>("WordWrap");
/// <summary>
/// Specifies whether the text editor uses word wrapping.
/// </summary>
/// <remarks>
/// Setting WordWrap=true has the same effect as setting HorizontalScrollBarVisibility=Disabled and will override the
/// HorizontalScrollBarVisibility setting.
/// </remarks>
public bool WordWrap
{
get => GetValue(WordWrapProperty);
set => SetValue(WordWrapProperty, value);
}
#endregion
#region IsReadOnly
/// <summary>
/// IsReadOnly dependency property.
/// </summary>
public static readonly StyledProperty<bool> IsReadOnlyProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsReadOnly");
/// <summary>
/// Specifies whether the user can change the text editor content.
/// Setting this property will replace the
/// <see cref="Editing.TextArea.ReadOnlySectionProvider">TextArea.ReadOnlySectionProvider</see>.
/// </summary>
public bool IsReadOnly
{
get => GetValue(IsReadOnlyProperty);
set => SetValue(IsReadOnlyProperty, value);
}
private static void OnIsReadOnlyChanged(AvaloniaPropertyChangedEventArgs e)
{
if (e.Sender is TextEditor editor)
{
if ((bool)e.NewValue)
editor.TextArea.ReadOnlySectionProvider = ReadOnlySectionDocument.Instance;
else
editor.TextArea.ReadOnlySectionProvider = NoReadOnlySections.Instance;
}
}
#endregion
#region IsModified
/// <summary>
/// Dependency property for <see cref="IsModified"/>
/// </summary>
public static readonly StyledProperty<bool> IsModifiedProperty =
AvaloniaProperty.Register<TextEditor, bool>("IsModified");
/// <summary>
/// Gets/Sets the 'modified' flag.
/// </summary>
public bool IsModified
{
get => GetValue(IsModifiedProperty);
set => SetValue(IsModifiedProperty, value);
}
private static void OnIsModifiedChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var document = editor?.Document;
if (document != null)
{
var undoStack = document.UndoStack;
if ((bool)e.NewValue)
{
if (undoStack.IsOriginalFile)
undoStack.DiscardOriginalFileMarker();
}
else
{
undoStack.MarkAsOriginalFile();
}
}
}
private void HandleIsOriginalChanged(PropertyChangedEventArgs e)
{
if (e.PropertyName == "IsOriginalFile")
{
var document = Document;
if (document != null)
{
SetValue(IsModifiedProperty, (object)!document.UndoStack.IsOriginalFile);
}
}
}
#endregion
#region ShowLineNumbers
/// <summary>
/// ShowLineNumbers dependency property.
/// </summary>
public static readonly StyledProperty<bool> ShowLineNumbersProperty =
AvaloniaProperty.Register<TextEditor, bool>("ShowLineNumbers");
/// <summary>
/// Specifies whether line numbers are shown on the left to the text view.
/// </summary>
public bool ShowLineNumbers
{
get => GetValue(ShowLineNumbersProperty);
set => SetValue(ShowLineNumbersProperty, value);
}
private static void OnShowLineNumbersChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
if (editor == null) return;
var leftMargins = editor.TextArea.LeftMargins;
if ((bool)e.NewValue)
{
var lineNumbers = new LineNumberMargin();
var line = (Line)DottedLineMargin.Create();
leftMargins.Insert(0, lineNumbers);
leftMargins.Insert(1, line);
var lineNumbersForeground = new Binding("LineNumbersForeground") { Source = editor };
line.Bind(Shape.StrokeProperty, lineNumbersForeground);
lineNumbers.Bind(ForegroundProperty, lineNumbersForeground);
}
else
{
for (var i = 0; i < leftMargins.Count; i++)
{
if (leftMargins[i] is LineNumberMargin)
{
leftMargins.RemoveAt(i);
if (i < leftMargins.Count && DottedLineMargin.IsDottedLineMargin(leftMargins[i]))
{
leftMargins.RemoveAt(i);
}
break;
}
}
}
}
#endregion
#region LineNumbersForeground
/// <summary>
/// LineNumbersForeground dependency property.
/// </summary>
public static readonly StyledProperty<IBrush> LineNumbersForegroundProperty =
AvaloniaProperty.Register<TextEditor, IBrush>("LineNumbersForeground", Brushes.Gray);
/// <summary>
/// Gets/sets the Brush used for displaying the foreground color of line numbers.
/// </summary>
public IBrush LineNumbersForeground
{
get => GetValue(LineNumbersForegroundProperty);
set => SetValue(LineNumbersForegroundProperty, value);
}
private static void OnLineNumbersForegroundChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
var lineNumberMargin = editor?.TextArea.LeftMargins.FirstOrDefault(margin => margin is LineNumberMargin) as LineNumberMargin;
lineNumberMargin?.SetValue(ForegroundProperty, e.NewValue);
}
private static void OnFontFamilyPropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontFamilyProperty, e.NewValue);
}
private static void OnFontSizePropertyChanged(AvaloniaPropertyChangedEventArgs e)
{
var editor = e.Sender as TextEditor;
editor?.TextArea.TextView.SetValue(FontSizeProperty, e.NewValue);
}
#endregion
#region TextBoxBase-like methods
/// <summary>
/// Appends text to the end of the document.
/// </summary>
public void AppendText(string textData)
{
var document = GetDocument();
document.Insert(document.TextLength, textData);
}
/// <summary>
/// Begins a group of document changes.
/// </summary>
public void BeginChange()
{
GetDocument().BeginUpdate();
}
/// <summary>
/// Copies the current selection to the clipboard.
/// </summary>
public void Copy()
{
if (CanCopy)
{
ApplicationCommands.Copy.Execute(null, TextArea);
}
}
/// <summary>
/// Removes the current selection and copies it to the clipboard.
/// </summary>
public void Cut()
{
if (CanCut)
{
ApplicationCommands.Cut.Execute(null, TextArea);
}
}
/// <summary>
/// Begins a group of document changes and returns an object that ends the group of document
/// changes when it is disposed.
/// </summary>
public IDisposable DeclareChangeBlock()
{
return GetDocument().RunUpdate();
}
/// <summary>
/// Removes the current selection without copying it to the clipboard.
/// </summary>
public void Delete()
{
if(CanDelete)
{
ApplicationCommands.Delete.Execute(null, TextArea);
}
}
/// <summary>
/// Ends the current group of document changes.
/// </summary>
public void EndChange()
{
GetDocument().EndUpdate();
}
/// <summary>
/// Scrolls one line down.
/// </summary>
public void LineDown()
{
//if (scrollViewer != null)
// scrollViewer.LineDown();
}
/// <summary>
/// Scrolls to the left.
/// </summary>
public void LineLeft()
{
//if (scrollViewer != null)
// scrollViewer.LineLeft();
}
/// <summary>
/// Scrolls to the right.
/// </summary>
public void LineRight()
{
//if (scrollViewer != null)
// scrollViewer.LineRight();
}
/// <summary>
/// Scrolls one line up.
/// </summary>
public void LineUp()
{
//if (scrollViewer != null)
// scrollViewer.LineUp();
}
/// <summary>
/// Scrolls one page down.
/// </summary>
public void PageDown()
{
//if (scrollViewer != null)
// scrollViewer.PageDown();
}
/// <summary>
/// Scrolls one page up.
/// </summary>
public void PageUp()
{
//if (scrollViewer != null)
// scrollViewer.PageUp();
}
/// <summary>
/// Scrolls one page left.
/// </summary>
public void PageLeft()
{
//if (scrollViewer != null)
// scrollViewer.PageLeft();
}
/// <summary>
/// Scrolls one page right.
/// </summary>
public void PageRight()
{
//if (scrollViewer != null)
// scrollViewer.PageRight();
}
/// <summary>
/// Pastes the clipboard content.
/// </summary>
public void Paste()
{
if (CanPaste)
{
ApplicationCommands.Paste.Execute(null, TextArea);
}
}
/// <summary>
/// Redoes the most recent undone command.
/// </summary>
/// <returns>True is the redo operation was successful, false is the redo stack is empty.</returns>
public bool Redo()
{
if (CanRedo)
{
ApplicationCommands.Redo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Scrolls to the end of the document.
/// </summary>
public void ScrollToEnd()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToEnd();
}
/// <summary>
/// Scrolls to the start of the document.
/// </summary>
public void ScrollToHome()
{
ApplyTemplate(); // ensure scrollViewer is created
ScrollViewer?.ScrollToHome();
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToHorizontalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToHorizontalOffset(offset);
}
/// <summary>
/// Scrolls to the specified position in the document.
/// </summary>
public void ScrollToVerticalOffset(double offset)
{
ApplyTemplate(); // ensure scrollViewer is created
//if (scrollViewer != null)
// scrollViewer.ScrollToVerticalOffset(offset);
}
/// <summary>
/// Selects the entire text.
/// </summary>
public void SelectAll()
{
if (CanSelectAll)
{
ApplicationCommands.SelectAll.Execute(null, TextArea);
}
}
/// <summary>
/// Undoes the most recent command.
/// </summary>
/// <returns>True is the undo operation was successful, false is the undo stack is empty.</returns>
public bool Undo()
{
if (CanUndo)
{
ApplicationCommands.Undo.Execute(null, TextArea);
return true;
}
return false;
}
/// <summary>
/// Gets if the most recent undone command can be redone.
/// </summary>
public bool CanRedo
{
get { return ApplicationCommands.Redo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if the most recent command can be undone.
/// </summary>
public bool CanUndo
{
get { return ApplicationCommands.Undo.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be copied
/// </summary>
public bool CanCopy
{
get { return ApplicationCommands.Copy.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be cut
/// </summary>
public bool CanCut
{
get { return ApplicationCommands.Cut.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text in editor can be pasted
/// </summary>
public bool CanPaste
{
get { return ApplicationCommands.Paste.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if selected text in editor can be deleted
/// </summary>
public bool CanDelete
{
get { return ApplicationCommands.Delete.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text the editor can select all
/// </summary>
public bool CanSelectAll
{
get { return ApplicationCommands.SelectAll.CanExecute(null, TextArea); }
}
/// <summary>
/// Gets if text editor can activate the search panel
/// </summary>
public bool CanSearch
{
get { return searchPanel != null; }
}
/// <summary>
/// Gets the vertical size of the document.
/// </summary>
public double ExtentHeight => ScrollViewer?.Extent.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the current document region.
/// </summary>
public double ExtentWidth => ScrollViewer?.Extent.Width ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportHeight => ScrollViewer?.Viewport.Height ?? 0;
/// <summary>
/// Gets the horizontal size of the viewport.
/// </summary>
public double ViewportWidth => ScrollViewer?.Viewport.Width ?? 0;
/// <summary>
/// Gets the vertical scroll position.
/// </summary>
public double VerticalOffset => ScrollViewer?.Offset.Y ?? 0;
/// <summary>
/// Gets the horizontal scroll position.
/// </summary>
public double HorizontalOffset => ScrollViewer?.Offset.X ?? 0;
#endregion
#region TextBox methods
/// <summary>
/// Gets/Sets the selected text.
/// </summary>
public string SelectedText
{
get
{
// We'll get the text from the whole surrounding segment.
// This is done to ensure that SelectedText.Length == SelectionLength.
if (textArea.Document != null && !textArea.Selection.IsEmpty)
return textArea.Document.GetText(textArea.Selection.SurroundingSegment);
return string.Empty;
}
set
{
if (value == null)
throw new ArgumentNullException(nameof(value));
var textArea = TextArea;
if (textArea.Document != null)
{
var offset = SelectionStart;
var length = SelectionLength;
textArea.Document.Replace(offset, length, value);
// keep inserted text selected
textArea.Selection = Selection.Create(textArea, offset, offset + value.Length);
}
}
}
/// <summary>
/// Gets/sets the caret position.
/// </summary>
public int CaretOffset
{
get
{
return textArea.Caret.Offset;
}
set
{
textArea.Caret.Offset = value;
}
}
/// <summary>
/// Gets/sets the start position of the selection.
/// </summary>
public int SelectionStart
{
get
{
if (textArea.Selection.IsEmpty)
return textArea.Caret.Offset;
else
return textArea.Selection.SurroundingSegment.Offset;
}
set => Select(value, SelectionLength);
}
/// <summary>
/// Gets/sets the length of the selection.
/// </summary>
public int SelectionLength
{
get
{
if (!textArea.Selection.IsEmpty)
return textArea.Selection.SurroundingSegment.Length;
else
return 0;
}
set => Select(SelectionStart, value);
}
/// <summary>
/// Selects the specified text section.
/// </summary>
public void Select(int start, int length)
{
var documentLength = Document?.TextLength ?? 0;
if (start < 0 || start > documentLength)
throw new ArgumentOutOfRangeException(nameof(start), start, "Value must be between 0 and " + documentLength);
if (length < 0 || start + length > documentLength)
throw new ArgumentOutOfRangeException(nameof(length), length, "Value must be between 0 and " + (documentLength - start));
TextArea.Selection = Selection.Create(TextArea, start, start + length);
TextArea.Caret.Offset = start + length;
}
/// <summary>
/// Gets the number of lines in the document.
/// </summary>
public int LineCount
{
get
{
var document = Document;
if (document != null)
return document.LineCount;
return 1;
}
}
/// <summary>
/// Clears the text.
/// </summary>
public void Clear()
{
Text = string.Empty;
}
#endregion
#region Loading from stream
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Load(Stream stream)
{
using (var reader = FileReader.OpenStream(stream, Encoding ?? Encoding.UTF8))
{
Text = reader.ReadToEnd();
SetValue(EncodingProperty, (object)reader.CurrentEncoding);
}
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Loads the text from the stream, auto-detecting the encoding.
/// </summary>
public void Load(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO:load
//using (FileStream fs = new FileStream(fileName, FileMode.Open, FileAccess.Read, FileShare.Read))
//{
// Load(fs);
//}
}
/// <summary>
/// Encoding dependency property.
/// </summary>
public static readonly StyledProperty<Encoding> EncodingProperty =
AvaloniaProperty.Register<TextEditor, Encoding>("Encoding");
/// <summary>
/// Gets/sets the encoding used when the file is saved.
/// </summary>
/// <remarks>
/// The <see cref="Load(Stream)"/> method autodetects the encoding of the file and sets this property accordingly.
/// The <see cref="Save(Stream)"/> method uses the encoding specified in this property.
/// </remarks>
public Encoding Encoding
{
get => GetValue(EncodingProperty);
set => SetValue(EncodingProperty, value);
}
/// <summary>
/// Saves the text to the stream.
/// </summary>
/// <remarks>
/// This method sets <see cref="IsModified"/> to false.
/// </remarks>
public void Save(Stream stream)
{
if (stream == null)
throw new ArgumentNullException(nameof(stream));
var encoding = Encoding;
var document = Document;
var writer = encoding != null ? new StreamWriter(stream, encoding) : new StreamWriter(stream);
document?.WriteTextTo(writer);
writer.Flush();
// do not close the stream
SetValue(IsModifiedProperty, (object)false);
}
/// <summary>
/// Saves the text to the file.
/// </summary>
public void Save(string fileName)
{
if (fileName == null)
throw new ArgumentNullException(nameof(fileName));
// TODO: save
//using (FileStream fs = new FileStream(fileName, FileMode.Create, FileAccess.Write, FileShare.None))
//{
// Save(fs);
//}
}
#endregion
#region PointerHover events
/// <summary>
/// The PreviewPointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverEvent =
TextView.PreviewPointerHoverEvent;
/// <summary>
/// the pointerHover event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverEvent =
TextView.PointerHoverEvent;
/// <summary>
/// The PreviewPointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PreviewPointerHoverStoppedEvent =
TextView.PreviewPointerHoverStoppedEvent;
/// <summary>
/// the pointerHoverStopped event.
/// </summary>
public static readonly RoutedEvent<PointerEventArgs> PointerHoverStoppedEvent =
TextView.PointerHoverStoppedEvent;
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHover
{
add => AddHandler(PreviewPointerHoverEvent, value);
remove => RemoveHandler(PreviewPointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer has hovered over a fixed location for some time.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHover
{
add => AddHandler(PointerHoverEvent, value);
remove => RemoveHandler(PointerHoverEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PreviewPointerHoverStopped
{
add => AddHandler(PreviewPointerHoverStoppedEvent, value);
remove => RemoveHandler(PreviewPointerHoverStoppedEvent, value);
}
/// <summary>
/// Occurs when the pointer had previously hovered but now started moving again.
/// </summary>
public event EventHandler<PointerEventArgs> PointerHoverStopped
{
add => AddHandler(PointerHoverStoppedEvent, value);
remove => RemoveHandler(PointerHoverStoppedEvent, value);
}
#endregion
#region ScrollBarVisibility
/// <summary>
/// Dependency property for <see cref="HorizontalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> HorizontalScrollBarVisibilityProperty = ScrollViewer.HorizontalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the horizontal scroll bar visibility.
/// </summary>
public ScrollBarVisibility HorizontalScrollBarVisibility
{
get => GetValue(HorizontalScrollBarVisibilityProperty);
set => SetValue(HorizontalScrollBarVisibilityProperty, value);
}
private ScrollBarVisibility _horizontalScrollBarVisibilityBck = ScrollBarVisibility.Auto;
/// <summary>
/// Dependency property for <see cref="VerticalScrollBarVisibility"/>
/// </summary>
public static readonly AttachedProperty<ScrollBarVisibility> VerticalScrollBarVisibilityProperty = ScrollViewer.VerticalScrollBarVisibilityProperty.AddOwner<TextEditor>();
/// <summary>
/// Gets/Sets the vertical scroll bar visibility.
/// </summary>
public ScrollBarVisibility VerticalScrollBarVisibility
{
get => GetValue(VerticalScrollBarVisibilityProperty);
set => SetValue(VerticalScrollBarVisibilityProperty, value);
}
#endregion
object IServiceProvider.GetService(Type serviceType)
{
return TextArea.GetService(serviceType);
}
/// <summary>
/// Gets the text view position from a point inside the editor.
/// </summary>
/// <param name="point">The position, relative to top left
/// corner of TextEditor control</param>
/// <returns>The text view position, or null if the point is outside the document.</returns>
public TextViewPosition? GetPositionFromPoint(Point point)
{
if (Document == null)
return null;
var textView = TextArea.TextView;
Point tpoint = (Point)this.TranslatePoint(point + new Point(textView.ScrollOffset.X, Math.Floor(textView.ScrollOffset.Y)), textView);
return textView.GetPosition(tpoint);
}
/// <summary>
/// Scrolls to the specified line.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollToLine(int line)
{
ScrollTo(line, -1);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (layout engine must have run prior).
/// </summary>
public void ScrollTo(int line, int column)
{
const double MinimumScrollFraction = 0.3;
ScrollTo(line, column, VisualYPosition.LineMiddle,
null != ScrollViewer ? ScrollViewer.Viewport.Height / 2 : 0.0, MinimumScrollFraction);
}
/// <summary>
/// Scrolls to the specified line/column.
/// This method requires that the TextEditor was already assigned a size (WPF layout must have run prior).
/// </summary>
/// <param name="line">Line to scroll to.</param>
/// <param name="column">Column to scroll to (important if wrapping is 'on', and for the horizontal scroll position).</param>
/// <param name="yPositionMode">The mode how to reference the Y position of the line.</param>
/// <param name="referencedVerticalViewPortOffset">Offset from the top of the viewport to where the referenced line/column should be positioned.</param>
/// <param name="minimumScrollFraction">The minimum vertical and/or horizontal scroll offset, expressed as fraction of the height or width of the viewport window, respectively.</param>
public void ScrollTo(int line, int column, VisualYPosition yPositionMode,
double referencedVerticalViewPortOffset, double minimumScrollFraction)
{
TextView textView = textArea.TextView;
TextDocument document = textView.Document;
if (ScrollViewer != null && document != null)
{
if (line < 1)
line = 1;
if (line > document.LineCount)
line = document.LineCount;
ILogicalScrollable scrollInfo = textView;
if (!scrollInfo.CanHorizontallyScroll)
{
// Word wrap is enabled. Ensure that we have up-to-date info about line height so that we scroll
// to the correct position.
// This avoids that the user has to repeat the ScrollTo() call several times when there are very long lines.
VisualLine vl = textView.GetOrConstructVisualLine(document.GetLineByNumber(line));
double remainingHeight = referencedVerticalViewPortOffset;
while (remainingHeight > 0)
{
DocumentLine prevLine = vl.FirstDocumentLine.PreviousLine;
if (prevLine == null)
break;
vl = textView.GetOrConstructVisualLine(prevLine);
remainingHeight -= vl.Height;
}
}
Point p = textArea.TextView.GetVisualPosition(
new TextViewPosition(line, Math.Max(1, column)),
yPositionMode);
double targetX = ScrollViewer.Offset.X;
double targetY = ScrollViewer.Offset.Y;
double verticalPos = p.Y - referencedVerticalViewPortOffset;
if (Math.Abs(verticalPos - ScrollViewer.Offset.Y) >
minimumScrollFraction * ScrollViewer.Viewport.Height)
{
targetY = Math.Max(0, verticalPos);
}
if (column > 0)
{
if (p.X > ScrollViewer.Viewport.Width - Caret.MinimumDistanceToViewBorder * 2)
{
double horizontalPos = Math.Max(0, p.X - ScrollViewer.Viewport.Width / 2);
if (Math.Abs(horizontalPos - ScrollViewer.Offset.X) >
minimumScrollFraction * ScrollViewer.Viewport.Width)
{
targetX = 0;
}
}
else
{
targetX = 0;
}
}
if (targetX != ScrollViewer.Offset.X || targetY != ScrollViewer.Offset.Y)
ScrollViewer.Offset = new Vector(targetX, targetY);
}
}
}
}
<MSG> Merge branch 'master' into support-multithreading
<DFF> @@ -67,14 +67,19 @@ namespace AvaloniaEdit
/// <summary>
/// Creates a new TextEditor instance.
/// </summary>
- protected TextEditor(TextArea textArea)
+ protected TextEditor(TextArea textArea) : this(textArea, new TextDocument())
+ {
+
+ }
+
+ protected TextEditor(TextArea textArea, TextDocument document)
{
TextArea = textArea ?? throw new ArgumentNullException(nameof(textArea));
textArea.TextView.Services.AddService(this);
SetValue(OptionsProperty, textArea.Options);
- SetValue(DocumentProperty, new TextDocument());
+ SetValue(DocumentProperty, document);
textArea[!BackgroundProperty] = this[!BackgroundProperty];
}
@@ -128,7 +133,7 @@ namespace AvaloniaEdit
if (oldValue != null)
{
TextDocumentWeakEventManager.TextChanged.RemoveHandler(oldValue, OnTextChanged);
- PropertyChangedWeakEventManager.RemoveHandler(newValue.UndoStack, OnUndoStackPropertyChangedHandler);
+ PropertyChangedWeakEventManager.RemoveHandler(oldValue.UndoStack, OnUndoStackPropertyChangedHandler);
}
TextArea.Document = newValue;
if (newValue != null)
| 8 | Merge branch 'master' into support-multithreading | 3 | .cs | cs | mit | AvaloniaUI/AvaloniaEdit |
10066209 | <NME> README.md
<BEF> # FruitMachine [](https://travis-ci.org/ftlabs/fruitmachine) [](https://coveralls.io/r/ftlabs/fruitmachine?branch=master)
A lightweight component layout engine for client and server.
FruitMachine is designed to build rich interactive layouts from modular, reusable components. It's light and unopinionated so that it can be applied to almost any layout problem. FruitMachine is currently powering the [FT Web App](http://apps.ft.com/ftwebapp/).
```js
// Define a module
var Apple = fruitmachine.define({
name: 'apple',
template: function(){ return 'hello' }
});
// Create a module
var apple = new Apple();
// Render it
apple.render();
apple.el.outerHTML;
//=> <div class="apple">hello</div>
```
## Installation
```
$ npm install fruitmachine
```
or
```
$ bower install fruitmachine
```
or
Download the [pre-built version][built] (~2k gzipped).
[built]: http://wzrd.in/standalone/fruitmachine@latest
## Examples
- [Article viewer](http://ftlabs.github.io/fruitmachine/examples/article-viewer/)
- [TODO](http://ftlabs.github.io/fruitmachine/examples/todo/)
## Documentation
- [Introduction](docs/introduction.md)
- [Getting started](docs/getting-started.md)
- [Defining modules](docs/defining-modules.md)
- [Slots](docs/slots.md)
- [View assembly](docs/layout-assembly.md)
- [Instantiation](docs/module-instantiation.md)
- [Templates](docs/templates.md)
- [Template markup](docs/template-markup.md)
- [Rendering](docs/rendering.md)
- [DOM injection](docs/injection.md)
- [The module element](docs/module-el.md)
- [Queries](docs/queries.md)
- [Helpers](docs/module-helpers.md)
- [Removing & destroying](docs/removing-and-destroying.md)
- [Extending](docs/extending-modules.md)
- [Server-side rendering](docs/server-side-rendering.md)
- [API](docs/api.md)
- [Events](docs/events.md)
## Tests
#### With PhantomJS
```
$ npm install
$ npm test
```
#### Without PhantomJS
```
$ node_modules/.bin/buster-static
```
...then visit http://localhost:8282/ in browser
## Author
- **Wilson Page** - [@wilsonpage](http://github.com/wilsonpage)
## Contributors
- **Wilson Page** - [@wilsonpage](http://github.com/wilsonpage)
- **Matt Andrews** - [@matthew-andrews](http://github.com/matthew-andrews)
## License
Copyright (c) 2018 The Financial Times Limited
Licensed under the MIT license.
## Credits and collaboration
FruitMachine is largely unmaintained/finished. All open source code released by FT Labs is licenced under the MIT licence. We welcome comments, feedback and suggestions. Please feel free to raise an issue or pull request.
<MSG> Add dependency badge [ci skip]
<DFF> @@ -1,4 +1,4 @@
-# FruitMachine [](https://travis-ci.org/ftlabs/fruitmachine) [](https://coveralls.io/r/ftlabs/fruitmachine?branch=master)
+# FruitMachine [](https://travis-ci.org/ftlabs/fruitmachine) [](https://coveralls.io/r/ftlabs/fruitmachine?branch=master) [](https://gemnasium.com/ftlabs/fruitmachine)
A lightweight component layout engine for client and server.
| 1 | Add dependency badge [ci skip] | 1 | .md | md | mit | ftlabs/fruitmachine |
10066210 | <NME> README.md
<BEF> # LoadJS
<img src="https://www.muicss.com/static/images/loadjs.svg" width="250px">
LoadJS is a tiny async loader for modern browsers (899 bytes).
[](https://david-dm.org/muicss/loadjs)
[](https://david-dm.org/muicss/loadjs?type=dev)
## Introduction
LoadJS is a tiny async loading library for modern browsers (IE9+). It has a simple yet powerful dependency management system that lets you fetch JavaScript and CSS files in parallel and execute code after the dependencies have been met. The recommended way to use LoadJS is to include the minified source code of load.js in your <html> (possibly in the <head> tag) and then use the `loadjs` global to manage JavaScript dependencies after pageload.
LoadJS is based on the excellent [$script](https://github.com/ded/script.js) library by [Dustin Diaz](https://github.com/ded). We kept the behavior of the library the same but we re-wrote the code from scratch to add support for success/error callbacks and to optimize the library for modern browsers. LoadJS is 711 bytes (minified + gzipped).
Here's an example of what you can do with LoadJS:
```html
<script src="//unpkg.com/loadjs@latest/dist/loadjs.min.js"></script>
<script>
// define a dependency bundle and execute code when it loads
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
</script>
```
You can also use more advanced syntax for more options:
```html
<script src="//unpkg.com/loadjs@latest/dist/loadjs.min.js"></script>
<script>
// define a dependency bundle with advanced options
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
before: function(path, scriptEl) { /* execute code before fetch */ },
async: true, // load files synchronously or asynchronously (default: true)
numRetries: 3 // see caveats about using numRetries with async:false (default: 0),
returnPromise: false // return Promise object (default: false)
});
loadjs.ready('foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(depsNotFound) { /* foobar bundle load failed */ },
});
</script>
```
The latest version of LoadJS can be found in the `dist/` directory in this repository:
* [https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.js](https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.js) (for development)
* [https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.min.js](https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.min.js) (for production)
It's also available from these public CDNs:
* UNPKG
* [https://unpkg.com/[email protected]/dist/loadjs.js](https://unpkg.com/[email protected]/dist/loadjs.js) (for development)
* [https://unpkg.com/[email protected]/dist/loadjs.min.js](https://unpkg.com/[email protected]/dist/loadjs.min.js) (for production)
* CDNJS
* [https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.js](https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.js) (for development)
* [https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.min.js](https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.min.js) (for production)
You can also use it as a CJS or AMD module:
```bash
$ npm install --save loadjs
```
```javascript
var loadjs = require('loadjs');
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
```
## Browser Support
* IE9+ (`async: false` support only works in IE10+)
* Opera 12+
* Safari 5+
* Chrome
* Firefox
* iOS 6+
* Android 4.4+
LoadJS also detects script load failures from AdBlock Plus and Ghostery in:
* Safari
* Chrome
Note: LoadJS treats empty CSS files as load failures in IE9-11 and uses `rel="preload"` to load CSS files in Edge (to get around lack of support for onerror events on `<link rel="stylesheet">` tags)
## Documentation
1. Load a single file
```javascript
loadjs('/path/to/foo.js', function() {
/* foo.js loaded */
});
```
1. Fetch files in parallel and load them asynchronously
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], function() {
/* foo.js and bar.js loaded */
});
```
1. Fetch JavaScript, CSS and image files
```javascript
loadjs(['/path/to/foo.css', '/path/to/bar.png', 'path/to/thunk.js'], function() {
/* foo.css, bar.png and thunk.js loaded */
});
```
1. Force treat file as CSS stylesheet
```javascript
loadjs(['css!/path/to/cssfile.custom'], function() {
/* cssfile.custom loaded as stylesheet */
});
```
1. Force treat file as image
```javascript
loadjs(['img!/path/to/image.custom'], function() {
/* image.custom loaded */
});
```
1. Add a bundle id
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', function() {
/* foo.js & bar.js loaded */
});
```
1. Use .ready() to define bundles and callbacks separately
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
```
1. Use multiple bundles in .ready() dependency lists
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs(['/path/to/bar1.js', '/path/to/bar2.js'], 'bar');
loadjs.ready(['foo', 'bar'], function() {
/* foo.js & bar1.js & bar2.js loaded */
});
```
1. Chain .ready() together
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs('/path/to/bar.js', 'bar');
loadjs
.ready('foo', function() {
/* foo.js loaded */
})
.ready('bar', function() {
/* bar.js loaded */
});
```
1. Use Promises to register callbacks
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {returnPromise: true})
.then(function() { /* foo.js & bar.js loaded */ })
.catch(function(pathsNotFound) { /* at least one didn't load */ });
```
1. Check if bundle has already been defined
```javascript
if (!loadjs.isDefined('foobar')) {
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', function() {
/* foo.js & bar.js loaded */
});
}
```
1. Fetch files in parallel and load them in series
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {
success: function() { /* foo.js and bar.js loaded in series */ },
async: false
});
```
1. Add an error callback
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(pathsNotFound) { /* at least one path didn't load */ }
});
```
1. Retry files before calling the error callback
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(pathsNotFound) { /* at least one path didn't load */ },
numRetries: 3
});
// NOTE: Using `numRetries` with `async: false` can cause files to load out-of-sync on retries
```
1. Execute a callback before script tags are embedded
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {
success: function() {},
error: function(pathsNotFound) {},
before: function(path, scriptEl) {
/* called for each script node before being embedded */
if (path === '/path/to/foo.js') scriptEl.crossOrigin = true;
}
});
```
1. Bypass LoadJS default DOM insertion mechanism (DOM `<head>`)
```javascript
loadjs(['/path/to/foo.js'], {
success: function() {},
error: function(pathsNotFound) {},
before: function(path, scriptEl) {
document.body.appendChild(scriptEl);
/* return `false` to bypass default DOM insertion mechanism */
return false;
}
});
```
1. Use bundle ids in error callback
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs('/path/to/bar.js', 'bar');
loadjs(['/path/to/thunkor.js', '/path/to/thunky.js'], 'thunk');
// wait for multiple depdendencies
loadjs.ready(['foo', 'bar', 'thunk'], {
success: function() {
// foo.js & bar.js & thunkor.js & thunky.js loaded
},
error: function(depsNotFound) {
if (depsNotFound.indexOf('foo') > -1) {}; // foo failed
if (depsNotFound.indexOf('bar') > -1) {}; // bar failed
if (depsNotFound.indexOf('thunk') > -1) {}; // thunk failed
}
});
```
1. Use .done() for more control
```javascript
loadjs.ready(['dependency1', 'dependency2'], function() {
/* run code after dependencies have been met */
});
function fn1() {
loadjs.done('dependency1');
}
function fn2() {
loadjs.done('dependency2');
}
```
1. Reset dependency trackers
```javascript
loadjs.reset();
```
1. Implement a require-like dependency manager
```javascript
var bundles = {
'bundleA': ['/file1.js', '/file2.js'],
'bundleB': ['/file3.js', '/file4.js']
};
function require(bundleIds, callbackFn) {
bundleIds.forEach(function(bundleId) {
if (!loadjs.isDefined(bundleId)) loadjs(bundles[bundleId], bundleId);
});
loadjs.ready(bundleIds, callbackFn);
}
require(['bundleA'], function() { /* bundleA loaded */ });
require(['bundleB'], function() { /* bundleB loaded */ });
require(['bundleA', 'bundleB'], function() { /* bundleA and bundleB loaded */ });
```
## Directory structure
<pre>
loadjs/
├── dist
│ ├── loadjs.js
│ ├── loadjs.min.js
│ └── loadjs.umd.js
├── examples
├── gulpfile.js
├── LICENSE.txt
├── package.json
├── README.md
├── src
│ └── loadjs.js
├── test
└── umd-templates
</pre>
## Development Quickstart
1. Install dependencies
* [nodejs](http://nodejs.org/)
* [npm](https://www.npmjs.org/)
* http-server (via npm)
1. Clone repository
```bash
$ git clone [email protected]:muicss/loadjs.git
$ cd loadjs
```
1. Install node dependencies using npm
```bash
$ npm install
```
1. Build examples
```bash
$ npm run build-examples
```
To view the examples you can use any static file server. To use the `nodejs` http-server module:
```bash
$ npm install http-server
$ npm run http-server -- -p 3000
```
Then visit [http://localhost:3000/examples](http://localhost:3000/examples)
1. Build distribution files
```bash
$ npm run build-dist
```
The files will be located in the `dist` directory.
1. Run tests
To run the browser tests first build the `loadjs` library:
```bash
$ npm run build-tests
```
Then visit [http://localhost:3000/test](http://localhost:3000/test)
1. Build all files
```bash
$ npm run build-all
```
<MSG> Update README.md
<DFF> @@ -9,7 +9,7 @@ LoadJS is a tiny async loader for modern browsers (711 bytes).
## Introduction
-LoadJS is a tiny async loading library for modern browsers (IE9+). It has a simple yet powerful dependency management system that lets you fetch JavaScript and CSS files in parallel and execute code after the dependencies have been met. The recommended way to use LoadJS is to include the minified source code of load.js in your <html> (possibly in the <head> tag) and then use the `loadjs` global to manage JavaScript dependencies after pageload.
+LoadJS is a tiny async loading library for modern browsers (IE9+). It has a simple yet powerful dependency management system that lets you fetch JavaScript and CSS files in parallel and execute code after the dependencies have been met. The recommended way to use LoadJS is to include the minified source code of loadjs.js in your <html> (possibly in the <head> tag) and then use the `loadjs` global to manage JavaScript dependencies after pageload.
LoadJS is based on the excellent [$script](https://github.com/ded/script.js) library by [Dustin Diaz](https://github.com/ded). We kept the behavior of the library the same but we re-wrote the code from scratch to add support for success/error callbacks and to optimize the library for modern browsers. LoadJS is 711 bytes (minified + gzipped).
| 1 | Update README.md | 1 | .md | md | mit | muicss/loadjs |
10066211 | <NME> README.md
<BEF> # LoadJS
<img src="https://www.muicss.com/static/images/loadjs.svg" width="250px">
LoadJS is a tiny async loader for modern browsers (899 bytes).
[](https://david-dm.org/muicss/loadjs)
[](https://david-dm.org/muicss/loadjs?type=dev)
## Introduction
LoadJS is a tiny async loading library for modern browsers (IE9+). It has a simple yet powerful dependency management system that lets you fetch JavaScript and CSS files in parallel and execute code after the dependencies have been met. The recommended way to use LoadJS is to include the minified source code of load.js in your <html> (possibly in the <head> tag) and then use the `loadjs` global to manage JavaScript dependencies after pageload.
LoadJS is based on the excellent [$script](https://github.com/ded/script.js) library by [Dustin Diaz](https://github.com/ded). We kept the behavior of the library the same but we re-wrote the code from scratch to add support for success/error callbacks and to optimize the library for modern browsers. LoadJS is 711 bytes (minified + gzipped).
Here's an example of what you can do with LoadJS:
```html
<script src="//unpkg.com/loadjs@latest/dist/loadjs.min.js"></script>
<script>
// define a dependency bundle and execute code when it loads
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
</script>
```
You can also use more advanced syntax for more options:
```html
<script src="//unpkg.com/loadjs@latest/dist/loadjs.min.js"></script>
<script>
// define a dependency bundle with advanced options
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
before: function(path, scriptEl) { /* execute code before fetch */ },
async: true, // load files synchronously or asynchronously (default: true)
numRetries: 3 // see caveats about using numRetries with async:false (default: 0),
returnPromise: false // return Promise object (default: false)
});
loadjs.ready('foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(depsNotFound) { /* foobar bundle load failed */ },
});
</script>
```
The latest version of LoadJS can be found in the `dist/` directory in this repository:
* [https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.js](https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.js) (for development)
* [https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.min.js](https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.min.js) (for production)
It's also available from these public CDNs:
* UNPKG
* [https://unpkg.com/[email protected]/dist/loadjs.js](https://unpkg.com/[email protected]/dist/loadjs.js) (for development)
* [https://unpkg.com/[email protected]/dist/loadjs.min.js](https://unpkg.com/[email protected]/dist/loadjs.min.js) (for production)
* CDNJS
* [https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.js](https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.js) (for development)
* [https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.min.js](https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.min.js) (for production)
You can also use it as a CJS or AMD module:
```bash
$ npm install --save loadjs
```
```javascript
var loadjs = require('loadjs');
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
```
## Browser Support
* IE9+ (`async: false` support only works in IE10+)
* Opera 12+
* Safari 5+
* Chrome
* Firefox
* iOS 6+
* Android 4.4+
LoadJS also detects script load failures from AdBlock Plus and Ghostery in:
* Safari
* Chrome
Note: LoadJS treats empty CSS files as load failures in IE9-11 and uses `rel="preload"` to load CSS files in Edge (to get around lack of support for onerror events on `<link rel="stylesheet">` tags)
## Documentation
1. Load a single file
```javascript
loadjs('/path/to/foo.js', function() {
/* foo.js loaded */
});
```
1. Fetch files in parallel and load them asynchronously
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], function() {
/* foo.js and bar.js loaded */
});
```
1. Fetch JavaScript, CSS and image files
```javascript
loadjs(['/path/to/foo.css', '/path/to/bar.png', 'path/to/thunk.js'], function() {
/* foo.css, bar.png and thunk.js loaded */
});
```
1. Force treat file as CSS stylesheet
```javascript
loadjs(['css!/path/to/cssfile.custom'], function() {
/* cssfile.custom loaded as stylesheet */
});
```
1. Force treat file as image
```javascript
loadjs(['img!/path/to/image.custom'], function() {
/* image.custom loaded */
});
```
1. Add a bundle id
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', function() {
/* foo.js & bar.js loaded */
});
```
1. Use .ready() to define bundles and callbacks separately
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
```
1. Use multiple bundles in .ready() dependency lists
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs(['/path/to/bar1.js', '/path/to/bar2.js'], 'bar');
loadjs.ready(['foo', 'bar'], function() {
/* foo.js & bar1.js & bar2.js loaded */
});
```
1. Chain .ready() together
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs('/path/to/bar.js', 'bar');
loadjs
.ready('foo', function() {
/* foo.js loaded */
})
.ready('bar', function() {
/* bar.js loaded */
});
```
1. Use Promises to register callbacks
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {returnPromise: true})
.then(function() { /* foo.js & bar.js loaded */ })
.catch(function(pathsNotFound) { /* at least one didn't load */ });
```
1. Check if bundle has already been defined
```javascript
if (!loadjs.isDefined('foobar')) {
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', function() {
/* foo.js & bar.js loaded */
});
}
```
1. Fetch files in parallel and load them in series
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {
success: function() { /* foo.js and bar.js loaded in series */ },
async: false
});
```
1. Add an error callback
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(pathsNotFound) { /* at least one path didn't load */ }
});
```
1. Retry files before calling the error callback
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(pathsNotFound) { /* at least one path didn't load */ },
numRetries: 3
});
// NOTE: Using `numRetries` with `async: false` can cause files to load out-of-sync on retries
```
1. Execute a callback before script tags are embedded
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {
success: function() {},
error: function(pathsNotFound) {},
before: function(path, scriptEl) {
/* called for each script node before being embedded */
if (path === '/path/to/foo.js') scriptEl.crossOrigin = true;
}
});
```
1. Bypass LoadJS default DOM insertion mechanism (DOM `<head>`)
```javascript
loadjs(['/path/to/foo.js'], {
success: function() {},
error: function(pathsNotFound) {},
before: function(path, scriptEl) {
document.body.appendChild(scriptEl);
/* return `false` to bypass default DOM insertion mechanism */
return false;
}
});
```
1. Use bundle ids in error callback
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs('/path/to/bar.js', 'bar');
loadjs(['/path/to/thunkor.js', '/path/to/thunky.js'], 'thunk');
// wait for multiple depdendencies
loadjs.ready(['foo', 'bar', 'thunk'], {
success: function() {
// foo.js & bar.js & thunkor.js & thunky.js loaded
},
error: function(depsNotFound) {
if (depsNotFound.indexOf('foo') > -1) {}; // foo failed
if (depsNotFound.indexOf('bar') > -1) {}; // bar failed
if (depsNotFound.indexOf('thunk') > -1) {}; // thunk failed
}
});
```
1. Use .done() for more control
```javascript
loadjs.ready(['dependency1', 'dependency2'], function() {
/* run code after dependencies have been met */
});
function fn1() {
loadjs.done('dependency1');
}
function fn2() {
loadjs.done('dependency2');
}
```
1. Reset dependency trackers
```javascript
loadjs.reset();
```
1. Implement a require-like dependency manager
```javascript
var bundles = {
'bundleA': ['/file1.js', '/file2.js'],
'bundleB': ['/file3.js', '/file4.js']
};
function require(bundleIds, callbackFn) {
bundleIds.forEach(function(bundleId) {
if (!loadjs.isDefined(bundleId)) loadjs(bundles[bundleId], bundleId);
});
loadjs.ready(bundleIds, callbackFn);
}
require(['bundleA'], function() { /* bundleA loaded */ });
require(['bundleB'], function() { /* bundleB loaded */ });
require(['bundleA', 'bundleB'], function() { /* bundleA and bundleB loaded */ });
```
## Directory structure
<pre>
loadjs/
├── dist
│ ├── loadjs.js
│ ├── loadjs.min.js
│ └── loadjs.umd.js
├── examples
├── gulpfile.js
├── LICENSE.txt
├── package.json
├── README.md
├── src
│ └── loadjs.js
├── test
└── umd-templates
</pre>
## Development Quickstart
1. Install dependencies
* [nodejs](http://nodejs.org/)
* [npm](https://www.npmjs.org/)
* http-server (via npm)
1. Clone repository
```bash
$ git clone [email protected]:muicss/loadjs.git
$ cd loadjs
```
1. Install node dependencies using npm
```bash
$ npm install
```
1. Build examples
```bash
$ npm run build-examples
```
To view the examples you can use any static file server. To use the `nodejs` http-server module:
```bash
$ npm install http-server
$ npm run http-server -- -p 3000
```
Then visit [http://localhost:3000/examples](http://localhost:3000/examples)
1. Build distribution files
```bash
$ npm run build-dist
```
The files will be located in the `dist` directory.
1. Run tests
To run the browser tests first build the `loadjs` library:
```bash
$ npm run build-tests
```
Then visit [http://localhost:3000/test](http://localhost:3000/test)
1. Build all files
```bash
$ npm run build-all
```
<MSG> Update README.md
<DFF> @@ -9,7 +9,7 @@ LoadJS is a tiny async loader for modern browsers (711 bytes).
## Introduction
-LoadJS is a tiny async loading library for modern browsers (IE9+). It has a simple yet powerful dependency management system that lets you fetch JavaScript and CSS files in parallel and execute code after the dependencies have been met. The recommended way to use LoadJS is to include the minified source code of load.js in your <html> (possibly in the <head> tag) and then use the `loadjs` global to manage JavaScript dependencies after pageload.
+LoadJS is a tiny async loading library for modern browsers (IE9+). It has a simple yet powerful dependency management system that lets you fetch JavaScript and CSS files in parallel and execute code after the dependencies have been met. The recommended way to use LoadJS is to include the minified source code of loadjs.js in your <html> (possibly in the <head> tag) and then use the `loadjs` global to manage JavaScript dependencies after pageload.
LoadJS is based on the excellent [$script](https://github.com/ded/script.js) library by [Dustin Diaz](https://github.com/ded). We kept the behavior of the library the same but we re-wrote the code from scratch to add support for success/error callbacks and to optimize the library for modern browsers. LoadJS is 711 bytes (minified + gzipped).
| 1 | Update README.md | 1 | .md | md | mit | muicss/loadjs |
10066212 | <NME> README.md
<BEF> # LoadJS
<img src="https://www.muicss.com/static/images/loadjs.svg" width="250px">
LoadJS is a tiny async loader for modern browsers (899 bytes).
[](https://david-dm.org/muicss/loadjs)
[](https://david-dm.org/muicss/loadjs?type=dev)
[](https://cdnjs.com/libraries/loadjs)
## Introduction
LoadJS is a tiny async loading library for modern browsers (IE9+). It has a simple yet powerful dependency management system that lets you fetch JavaScript, CSS and image files in parallel and execute code after the dependencies have been met. The recommended way to use LoadJS is to include the minified source code of [loadjs.js](https://raw.githubusercontent.com/muicss/loadjs/master/dist/loadjs.min.js) in your <html> (possibly in the <head> tag) and then use the `loadjs` global to manage JavaScript dependencies after pageload.
LoadJS is based on the excellent [$script](https://github.com/ded/script.js) library by [Dustin Diaz](https://github.com/ded). We kept the behavior of the library the same but we re-wrote the code from scratch to add support for success/error callbacks and to optimize the library for modern browsers. LoadJS is 899 bytes (minified + gzipped).
Here's an example of what you can do with LoadJS:
```html
<script src="//unpkg.com/loadjs@latest/dist/loadjs.min.js"></script>
<script>
// define a dependency bundle and execute code when it loads
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
</script>
```
// define a dependency bundle that loads sychronously with retries
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
before: function(path, scriptEl) { /* execute code before fetch */ },
async: false, // load files synchronously or asynchronously (default: true)
numRetries: 3 // number of times to retry fetch (default: 0)
});
loadjs.ready('foobar', {
async: true, // load files synchronously or asynchronously (default: true)
numRetries: 3 // see caveats about using numRetries with async:false (default: 0),
returnPromise: false // return Promise object (default: false)
});
loadjs.ready('foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(depsNotFound) { /* foobar bundle load failed */ },
});
</script>
```
The latest version of LoadJS can be found in the `dist/` directory in this repository:
* [https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.js](https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.js) (for development)
* [https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.min.js](https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.min.js) (for production)
It's also available from these public CDNs:
* UNPKG
* [https://unpkg.com/[email protected]/dist/loadjs.js](https://unpkg.com/[email protected]/dist/loadjs.js) (for development)
* [https://unpkg.com/[email protected]/dist/loadjs.min.js](https://unpkg.com/[email protected]/dist/loadjs.min.js) (for production)
* CDNJS
* [https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.js](https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.js) (for development)
* [https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.min.js](https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.min.js) (for production)
You can also use it as a CJS or AMD module:
```bash
$ npm install --save loadjs
```
```javascript
var loadjs = require('loadjs');
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
```
## Browser Support
* IE9+ (`async: false` support only works in IE10+)
* Opera 12+
* Safari 5+
* Chrome
* Firefox
* iOS 6+
* Android 4.4+
LoadJS also detects script load failures from AdBlock Plus and Ghostery in:
* Safari
* Chrome
Note: LoadJS treats empty CSS files as load failures in IE9-11 and uses `rel="preload"` to load CSS files in Edge (to get around lack of support for onerror events on `<link rel="stylesheet">` tags)
## Documentation
1. Load a single file
```javascript
loadjs('/path/to/foo.js', function() {
/* foo.js loaded */
});
```
1. Fetch files in parallel and load them asynchronously
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], function() {
/* foo.js and bar.js loaded */
});
```
1. Fetch JavaScript, CSS and image files
```javascript
loadjs(['/path/to/foo.css', '/path/to/bar.png', 'path/to/thunk.js'], function() {
/* foo.css, bar.png and thunk.js loaded */
});
```
1. Force treat file as CSS stylesheet
```javascript
loadjs(['css!/path/to/cssfile.custom'], function() {
/* cssfile.custom loaded as stylesheet */
});
```
1. Force treat file as image
```javascript
loadjs(['img!/path/to/image.custom'], function() {
/* image.custom loaded */
});
```
1. Add a bundle id
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', function() {
/* foo.js & bar.js loaded */
});
```
1. Use .ready() to define bundles and callbacks separately
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
```
1. Use multiple bundles in .ready() dependency lists
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs(['/path/to/bar1.js', '/path/to/bar2.js'], 'bar');
loadjs.ready(['foo', 'bar'], function() {
/* foo.js & bar1.js & bar2.js loaded */
});
```
1. Chain .ready() together
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs('/path/to/bar.js', 'bar');
loadjs
.ready('foo', function() {
/* foo.js loaded */
})
.ready('bar', function() {
/* bar.js loaded */
});
```
1. Use Promises to register callbacks
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {returnPromise: true})
.then(function() { /* foo.js & bar.js loaded */ })
.catch(function(pathsNotFound) { /* at least one didn't load */ });
```
1. Check if bundle has already been defined
error: function(pathsNotFound) { /* at least one path didn't load */ },
numRetries: 3
});
```
1. Execute a callback before script tags are embedded
1. Fetch files in parallel and load them in series
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {
success: function() { /* foo.js and bar.js loaded in series */ },
async: false
});
```
1. Add an error callback
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(pathsNotFound) { /* at least one path didn't load */ }
});
```
1. Retry files before calling the error callback
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(pathsNotFound) { /* at least one path didn't load */ },
numRetries: 3
});
// NOTE: Using `numRetries` with `async: false` can cause files to load out-of-sync on retries
```
1. Execute a callback before script tags are embedded
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {
success: function() {},
error: function(pathsNotFound) {},
before: function(path, scriptEl) {
/* called for each script node before being embedded */
if (path === '/path/to/foo.js') scriptEl.crossOrigin = true;
}
});
```
1. Bypass LoadJS default DOM insertion mechanism (DOM `<head>`)
```javascript
loadjs(['/path/to/foo.js'], {
success: function() {},
error: function(pathsNotFound) {},
before: function(path, scriptEl) {
document.body.appendChild(scriptEl);
/* return `false` to bypass default DOM insertion mechanism */
return false;
}
});
```
1. Use bundle ids in error callback
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs('/path/to/bar.js', 'bar');
loadjs(['/path/to/thunkor.js', '/path/to/thunky.js'], 'thunk');
// wait for multiple depdendencies
loadjs.ready(['foo', 'bar', 'thunk'], {
success: function() {
// foo.js & bar.js & thunkor.js & thunky.js loaded
},
error: function(depsNotFound) {
if (depsNotFound.indexOf('foo') > -1) {}; // foo failed
if (depsNotFound.indexOf('bar') > -1) {}; // bar failed
if (depsNotFound.indexOf('thunk') > -1) {}; // thunk failed
}
});
```
1. Use .done() for more control
```javascript
loadjs.ready(['dependency1', 'dependency2'], function() {
/* run code after dependencies have been met */
});
function fn1() {
loadjs.done('dependency1');
}
function fn2() {
loadjs.done('dependency2');
}
```
1. Reset dependency trackers
```javascript
loadjs.reset();
```
1. Implement a require-like dependency manager
```javascript
var bundles = {
'bundleA': ['/file1.js', '/file2.js'],
'bundleB': ['/file3.js', '/file4.js']
};
function require(bundleIds, callbackFn) {
bundleIds.forEach(function(bundleId) {
if (!loadjs.isDefined(bundleId)) loadjs(bundles[bundleId], bundleId);
});
loadjs.ready(bundleIds, callbackFn);
}
require(['bundleA'], function() { /* bundleA loaded */ });
require(['bundleB'], function() { /* bundleB loaded */ });
require(['bundleA', 'bundleB'], function() { /* bundleA and bundleB loaded */ });
```
## Directory structure
<pre>
loadjs/
├── dist
│ ├── loadjs.js
│ ├── loadjs.min.js
│ └── loadjs.umd.js
├── examples
├── gulpfile.js
├── LICENSE.txt
├── package.json
├── README.md
├── src
│ └── loadjs.js
├── test
└── umd-templates
</pre>
## Development Quickstart
1. Install dependencies
* [nodejs](http://nodejs.org/)
* [npm](https://www.npmjs.org/)
* http-server (via npm)
1. Clone repository
```bash
$ git clone [email protected]:muicss/loadjs.git
$ cd loadjs
```
1. Install node dependencies using npm
```bash
$ npm install
```
1. Build examples
```bash
$ npm run build-examples
```
To view the examples you can use any static file server. To use the `nodejs` http-server module:
```bash
$ npm install http-server
$ npm run http-server -- -p 3000
```
Then visit [http://localhost:3000/examples](http://localhost:3000/examples)
1. Build distribution files
```bash
$ npm run build-dist
```
The files will be located in the `dist` directory.
1. Run tests
To run the browser tests first build the `loadjs` library:
```bash
$ npm run build-tests
```
Then visit [http://localhost:3000/test](http://localhost:3000/test)
1. Build all files
```bash
$ npm run build-all
```
<MSG> Update README.md
<DFF> @@ -30,8 +30,8 @@ You can also use more advanced syntax for more options:
// define a dependency bundle that loads sychronously with retries
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
before: function(path, scriptEl) { /* execute code before fetch */ },
- async: false, // load files synchronously or asynchronously (default: true)
- numRetries: 3 // number of times to retry fetch (default: 0)
+ async: true, // load files synchronously or asynchronously (default: true)
+ numRetries: 3 // see caveats about using numRetries with async:false (default: 0)
});
loadjs.ready('foobar', {
@@ -191,6 +191,8 @@ Note: LoadJS treats empty CSS files as load failures in IE (to get around lack o
error: function(pathsNotFound) { /* at least one path didn't load */ },
numRetries: 3
});
+
+ // NOTE: Using `numRetries` with `async: false` can cause files to load out-of-sync on retries
```
1. Execute a callback before script tags are embedded
| 4 | Update README.md | 2 | .md | md | mit | muicss/loadjs |
10066213 | <NME> README.md
<BEF> # LoadJS
<img src="https://www.muicss.com/static/images/loadjs.svg" width="250px">
LoadJS is a tiny async loader for modern browsers (899 bytes).
[](https://david-dm.org/muicss/loadjs)
[](https://david-dm.org/muicss/loadjs?type=dev)
[](https://cdnjs.com/libraries/loadjs)
## Introduction
LoadJS is a tiny async loading library for modern browsers (IE9+). It has a simple yet powerful dependency management system that lets you fetch JavaScript, CSS and image files in parallel and execute code after the dependencies have been met. The recommended way to use LoadJS is to include the minified source code of [loadjs.js](https://raw.githubusercontent.com/muicss/loadjs/master/dist/loadjs.min.js) in your <html> (possibly in the <head> tag) and then use the `loadjs` global to manage JavaScript dependencies after pageload.
LoadJS is based on the excellent [$script](https://github.com/ded/script.js) library by [Dustin Diaz](https://github.com/ded). We kept the behavior of the library the same but we re-wrote the code from scratch to add support for success/error callbacks and to optimize the library for modern browsers. LoadJS is 899 bytes (minified + gzipped).
Here's an example of what you can do with LoadJS:
```html
<script src="//unpkg.com/loadjs@latest/dist/loadjs.min.js"></script>
<script>
// define a dependency bundle and execute code when it loads
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
</script>
```
// define a dependency bundle that loads sychronously with retries
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
before: function(path, scriptEl) { /* execute code before fetch */ },
async: false, // load files synchronously or asynchronously (default: true)
numRetries: 3 // number of times to retry fetch (default: 0)
});
loadjs.ready('foobar', {
async: true, // load files synchronously or asynchronously (default: true)
numRetries: 3 // see caveats about using numRetries with async:false (default: 0),
returnPromise: false // return Promise object (default: false)
});
loadjs.ready('foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(depsNotFound) { /* foobar bundle load failed */ },
});
</script>
```
The latest version of LoadJS can be found in the `dist/` directory in this repository:
* [https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.js](https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.js) (for development)
* [https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.min.js](https://cdn.rawgit.com/muicss/loadjs/4.2.0/dist/loadjs.min.js) (for production)
It's also available from these public CDNs:
* UNPKG
* [https://unpkg.com/[email protected]/dist/loadjs.js](https://unpkg.com/[email protected]/dist/loadjs.js) (for development)
* [https://unpkg.com/[email protected]/dist/loadjs.min.js](https://unpkg.com/[email protected]/dist/loadjs.min.js) (for production)
* CDNJS
* [https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.js](https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.js) (for development)
* [https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.min.js](https://cdnjs.cloudflare.com/ajax/libs/loadjs/4.2.0/loadjs.min.js) (for production)
You can also use it as a CJS or AMD module:
```bash
$ npm install --save loadjs
```
```javascript
var loadjs = require('loadjs');
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
```
## Browser Support
* IE9+ (`async: false` support only works in IE10+)
* Opera 12+
* Safari 5+
* Chrome
* Firefox
* iOS 6+
* Android 4.4+
LoadJS also detects script load failures from AdBlock Plus and Ghostery in:
* Safari
* Chrome
Note: LoadJS treats empty CSS files as load failures in IE9-11 and uses `rel="preload"` to load CSS files in Edge (to get around lack of support for onerror events on `<link rel="stylesheet">` tags)
## Documentation
1. Load a single file
```javascript
loadjs('/path/to/foo.js', function() {
/* foo.js loaded */
});
```
1. Fetch files in parallel and load them asynchronously
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], function() {
/* foo.js and bar.js loaded */
});
```
1. Fetch JavaScript, CSS and image files
```javascript
loadjs(['/path/to/foo.css', '/path/to/bar.png', 'path/to/thunk.js'], function() {
/* foo.css, bar.png and thunk.js loaded */
});
```
1. Force treat file as CSS stylesheet
```javascript
loadjs(['css!/path/to/cssfile.custom'], function() {
/* cssfile.custom loaded as stylesheet */
});
```
1. Force treat file as image
```javascript
loadjs(['img!/path/to/image.custom'], function() {
/* image.custom loaded */
});
```
1. Add a bundle id
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', function() {
/* foo.js & bar.js loaded */
});
```
1. Use .ready() to define bundles and callbacks separately
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar');
loadjs.ready('foobar', function() {
/* foo.js & bar.js loaded */
});
```
1. Use multiple bundles in .ready() dependency lists
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs(['/path/to/bar1.js', '/path/to/bar2.js'], 'bar');
loadjs.ready(['foo', 'bar'], function() {
/* foo.js & bar1.js & bar2.js loaded */
});
```
1. Chain .ready() together
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs('/path/to/bar.js', 'bar');
loadjs
.ready('foo', function() {
/* foo.js loaded */
})
.ready('bar', function() {
/* bar.js loaded */
});
```
1. Use Promises to register callbacks
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {returnPromise: true})
.then(function() { /* foo.js & bar.js loaded */ })
.catch(function(pathsNotFound) { /* at least one didn't load */ });
```
1. Check if bundle has already been defined
error: function(pathsNotFound) { /* at least one path didn't load */ },
numRetries: 3
});
```
1. Execute a callback before script tags are embedded
1. Fetch files in parallel and load them in series
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {
success: function() { /* foo.js and bar.js loaded in series */ },
async: false
});
```
1. Add an error callback
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(pathsNotFound) { /* at least one path didn't load */ }
});
```
1. Retry files before calling the error callback
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
success: function() { /* foo.js & bar.js loaded */ },
error: function(pathsNotFound) { /* at least one path didn't load */ },
numRetries: 3
});
// NOTE: Using `numRetries` with `async: false` can cause files to load out-of-sync on retries
```
1. Execute a callback before script tags are embedded
```javascript
loadjs(['/path/to/foo.js', '/path/to/bar.js'], {
success: function() {},
error: function(pathsNotFound) {},
before: function(path, scriptEl) {
/* called for each script node before being embedded */
if (path === '/path/to/foo.js') scriptEl.crossOrigin = true;
}
});
```
1. Bypass LoadJS default DOM insertion mechanism (DOM `<head>`)
```javascript
loadjs(['/path/to/foo.js'], {
success: function() {},
error: function(pathsNotFound) {},
before: function(path, scriptEl) {
document.body.appendChild(scriptEl);
/* return `false` to bypass default DOM insertion mechanism */
return false;
}
});
```
1. Use bundle ids in error callback
```javascript
loadjs('/path/to/foo.js', 'foo');
loadjs('/path/to/bar.js', 'bar');
loadjs(['/path/to/thunkor.js', '/path/to/thunky.js'], 'thunk');
// wait for multiple depdendencies
loadjs.ready(['foo', 'bar', 'thunk'], {
success: function() {
// foo.js & bar.js & thunkor.js & thunky.js loaded
},
error: function(depsNotFound) {
if (depsNotFound.indexOf('foo') > -1) {}; // foo failed
if (depsNotFound.indexOf('bar') > -1) {}; // bar failed
if (depsNotFound.indexOf('thunk') > -1) {}; // thunk failed
}
});
```
1. Use .done() for more control
```javascript
loadjs.ready(['dependency1', 'dependency2'], function() {
/* run code after dependencies have been met */
});
function fn1() {
loadjs.done('dependency1');
}
function fn2() {
loadjs.done('dependency2');
}
```
1. Reset dependency trackers
```javascript
loadjs.reset();
```
1. Implement a require-like dependency manager
```javascript
var bundles = {
'bundleA': ['/file1.js', '/file2.js'],
'bundleB': ['/file3.js', '/file4.js']
};
function require(bundleIds, callbackFn) {
bundleIds.forEach(function(bundleId) {
if (!loadjs.isDefined(bundleId)) loadjs(bundles[bundleId], bundleId);
});
loadjs.ready(bundleIds, callbackFn);
}
require(['bundleA'], function() { /* bundleA loaded */ });
require(['bundleB'], function() { /* bundleB loaded */ });
require(['bundleA', 'bundleB'], function() { /* bundleA and bundleB loaded */ });
```
## Directory structure
<pre>
loadjs/
├── dist
│ ├── loadjs.js
│ ├── loadjs.min.js
│ └── loadjs.umd.js
├── examples
├── gulpfile.js
├── LICENSE.txt
├── package.json
├── README.md
├── src
│ └── loadjs.js
├── test
└── umd-templates
</pre>
## Development Quickstart
1. Install dependencies
* [nodejs](http://nodejs.org/)
* [npm](https://www.npmjs.org/)
* http-server (via npm)
1. Clone repository
```bash
$ git clone [email protected]:muicss/loadjs.git
$ cd loadjs
```
1. Install node dependencies using npm
```bash
$ npm install
```
1. Build examples
```bash
$ npm run build-examples
```
To view the examples you can use any static file server. To use the `nodejs` http-server module:
```bash
$ npm install http-server
$ npm run http-server -- -p 3000
```
Then visit [http://localhost:3000/examples](http://localhost:3000/examples)
1. Build distribution files
```bash
$ npm run build-dist
```
The files will be located in the `dist` directory.
1. Run tests
To run the browser tests first build the `loadjs` library:
```bash
$ npm run build-tests
```
Then visit [http://localhost:3000/test](http://localhost:3000/test)
1. Build all files
```bash
$ npm run build-all
```
<MSG> Update README.md
<DFF> @@ -30,8 +30,8 @@ You can also use more advanced syntax for more options:
// define a dependency bundle that loads sychronously with retries
loadjs(['/path/to/foo.js', '/path/to/bar.js'], 'foobar', {
before: function(path, scriptEl) { /* execute code before fetch */ },
- async: false, // load files synchronously or asynchronously (default: true)
- numRetries: 3 // number of times to retry fetch (default: 0)
+ async: true, // load files synchronously or asynchronously (default: true)
+ numRetries: 3 // see caveats about using numRetries with async:false (default: 0)
});
loadjs.ready('foobar', {
@@ -191,6 +191,8 @@ Note: LoadJS treats empty CSS files as load failures in IE (to get around lack o
error: function(pathsNotFound) { /* at least one path didn't load */ },
numRetries: 3
});
+
+ // NOTE: Using `numRetries` with `async: false` can cause files to load out-of-sync on retries
```
1. Execute a callback before script tags are embedded
| 4 | Update README.md | 2 | .md | md | mit | muicss/loadjs |
10066214 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
* Code folding.
* Code completion.
* Fully customizable and extensible.
and many,many more!
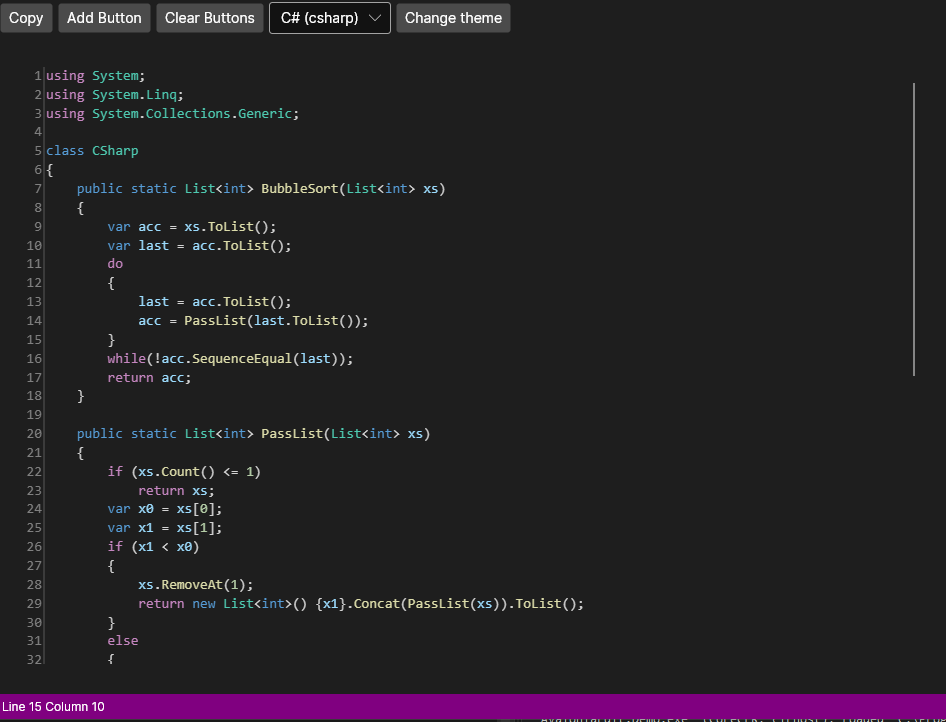
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
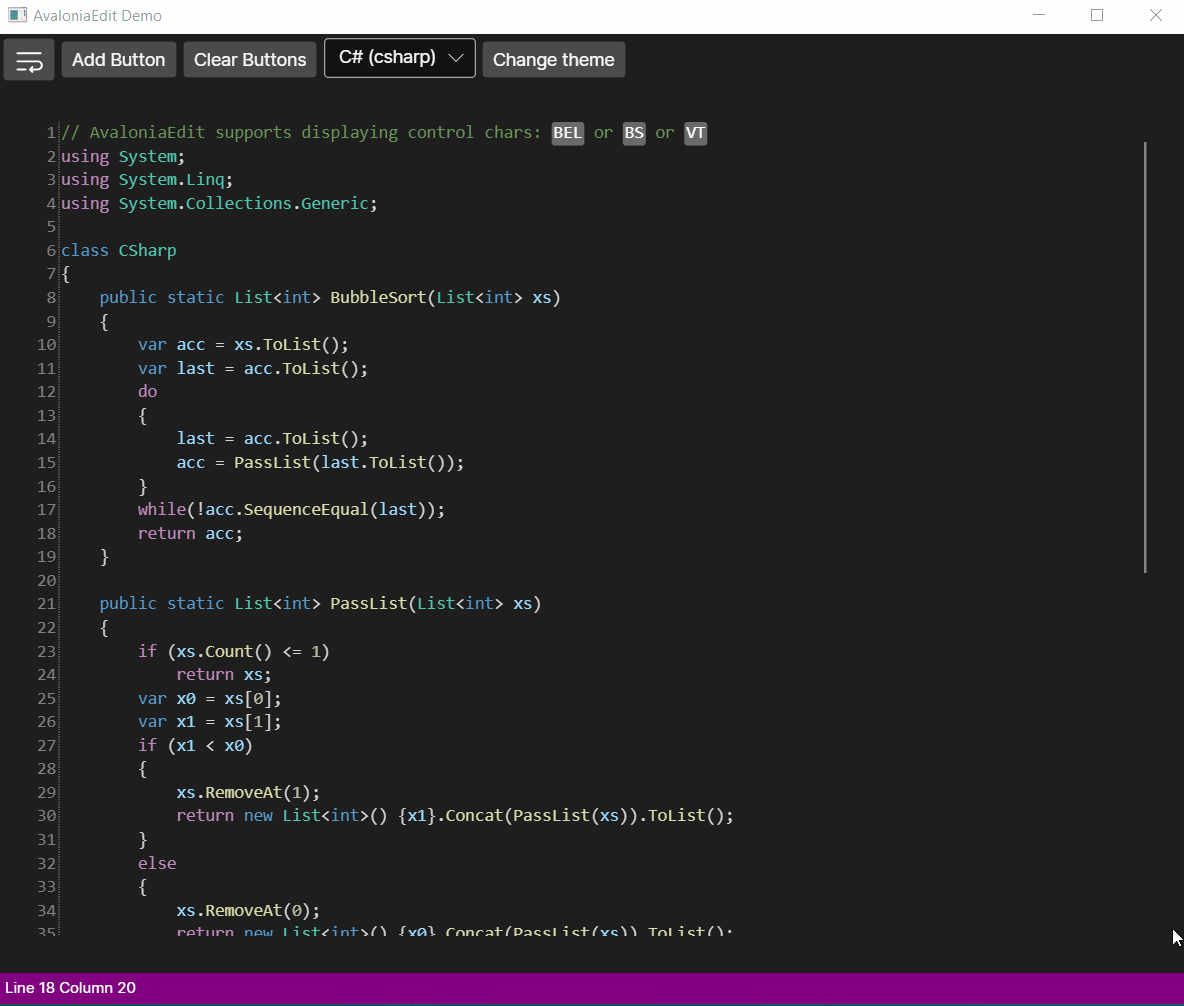
<MSG> Update readme
<DFF> @@ -13,6 +13,28 @@ AvaloniaEdit supports features like:
and many,many more!
+AvaloniaEdit currently consists of 3 packages
+ * [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
+ * [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
+ * [AvaloniaEdit.TextMate.Grammars](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) grammars for TextMate and additional infrastructure that helps you to use them.
+
+ ### How to set up theme and syntax highlighting for my project?
+First of all, if you want to use grammars that we support you should install [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) with them and [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) with TextMate integration otherwise you just install the package with TextMate integration and implement IRegistryOptions interface,that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled.
+```csharp
+//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
+var _textEditor = this.FindControl<TextEditor>("Editor");
+
+//Here we initialize RegistryOptions with the theme we want to use.
+var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
+
+//Initial setup of TextMate.
+var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
+
+//Here we are getting the lanuage by the extension and right after that we are initializing grammar with this lanuage.
+//And that all 😀, we are ready to use AvaloniaEdit with syntax highlighting!
+_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
+```
+
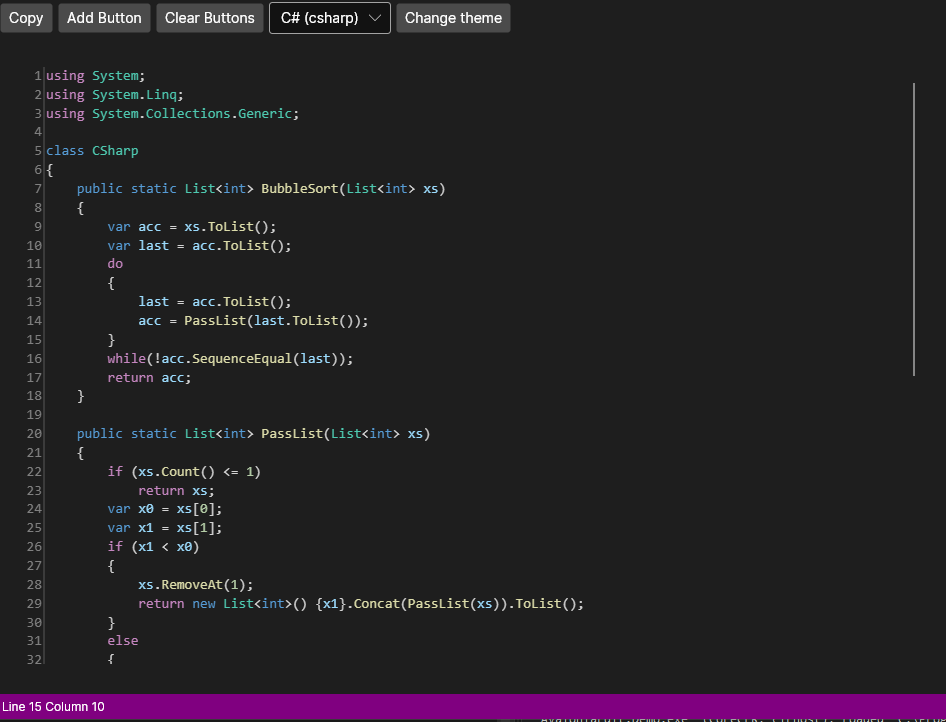
| 22 | Update readme | 0 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10066215 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
* Code folding.
* Code completion.
* Fully customizable and extensible.
and many,many more!
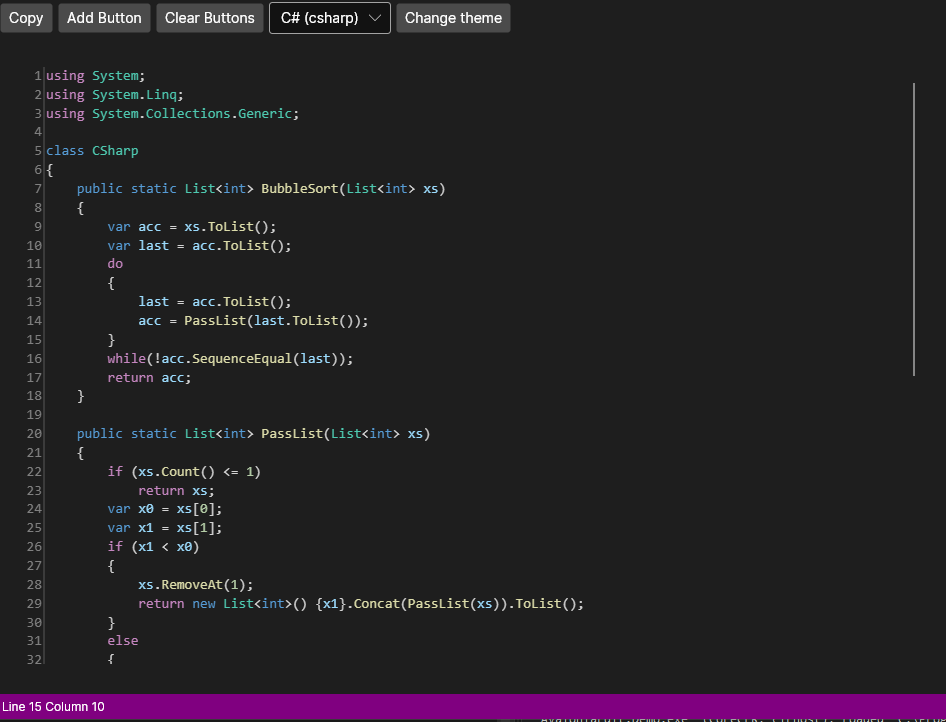
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
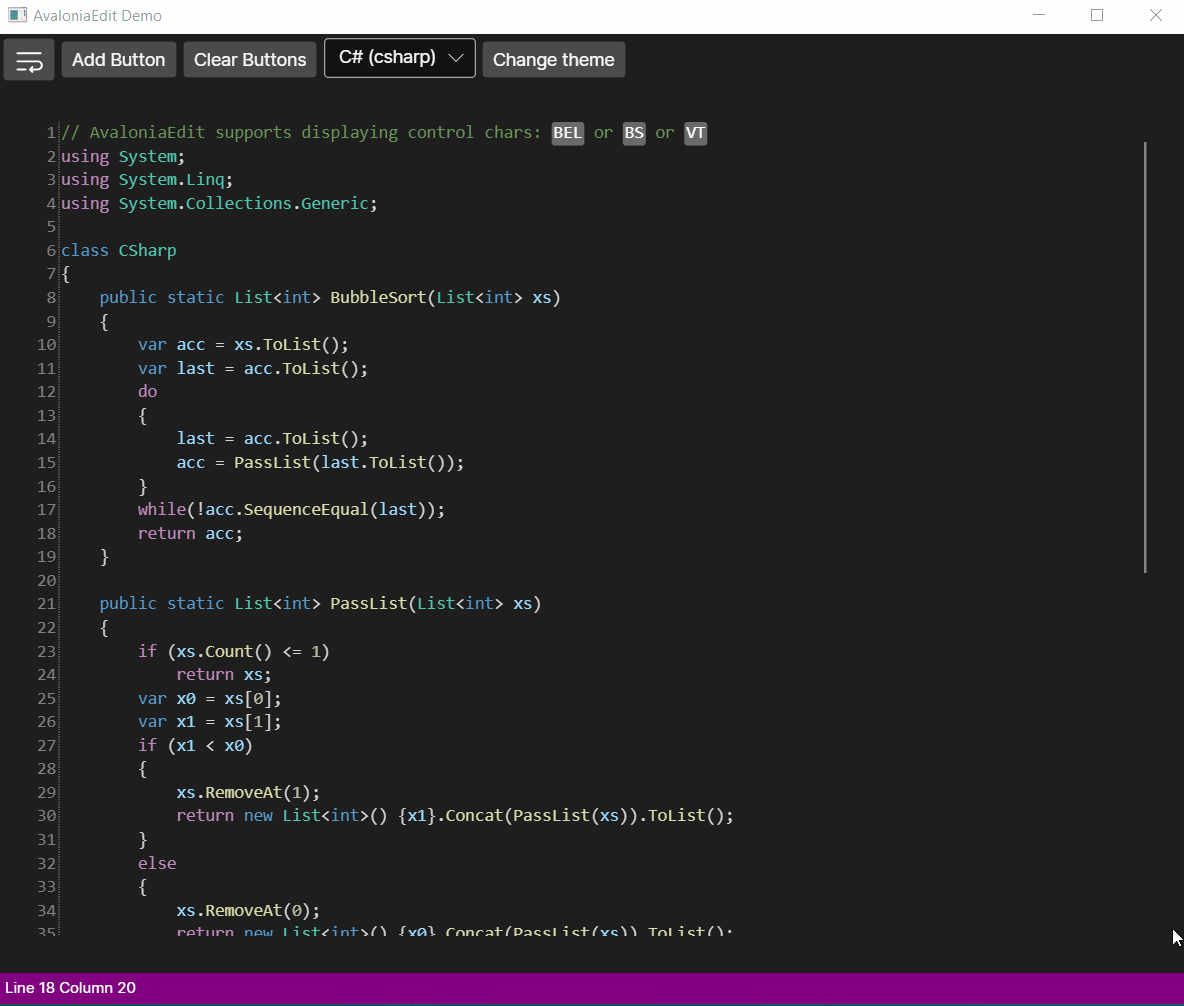
<MSG> Update readme
<DFF> @@ -13,6 +13,28 @@ AvaloniaEdit supports features like:
and many,many more!
+AvaloniaEdit currently consists of 3 packages
+ * [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
+ * [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
+ * [AvaloniaEdit.TextMate.Grammars](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) grammars for TextMate and additional infrastructure that helps you to use them.
+
+ ### How to set up theme and syntax highlighting for my project?
+First of all, if you want to use grammars that we support you should install [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) with them and [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) with TextMate integration otherwise you just install the package with TextMate integration and implement IRegistryOptions interface,that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled.
+```csharp
+//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
+var _textEditor = this.FindControl<TextEditor>("Editor");
+
+//Here we initialize RegistryOptions with the theme we want to use.
+var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
+
+//Initial setup of TextMate.
+var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
+
+//Here we are getting the lanuage by the extension and right after that we are initializing grammar with this lanuage.
+//And that all 😀, we are ready to use AvaloniaEdit with syntax highlighting!
+_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
+```
+
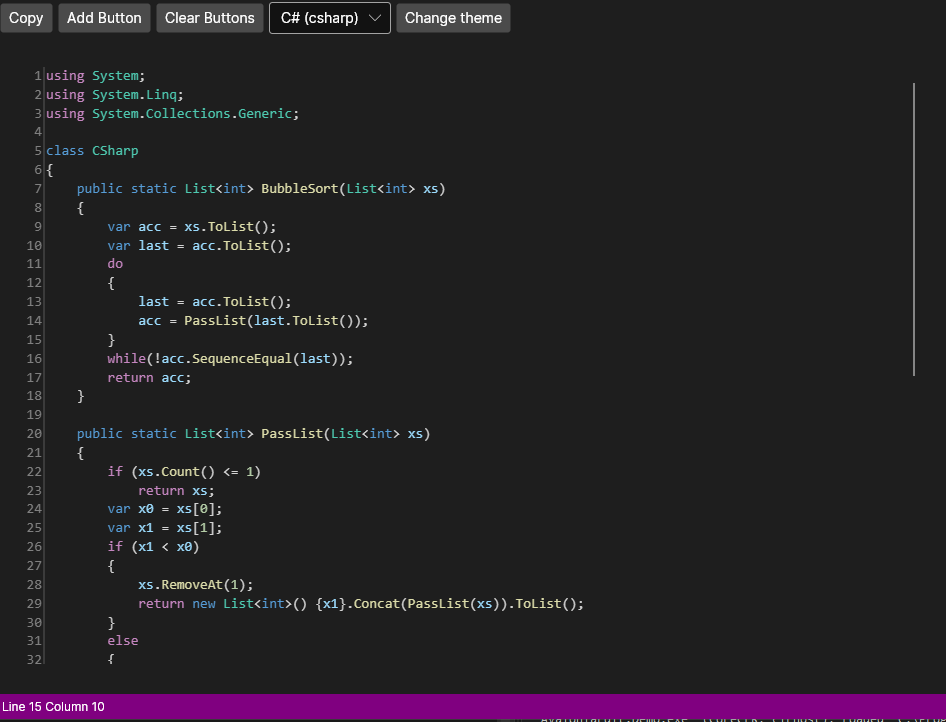
| 22 | Update readme | 0 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10066216 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
* Code folding.
* Code completion.
* Fully customizable and extensible.
and many,many more!
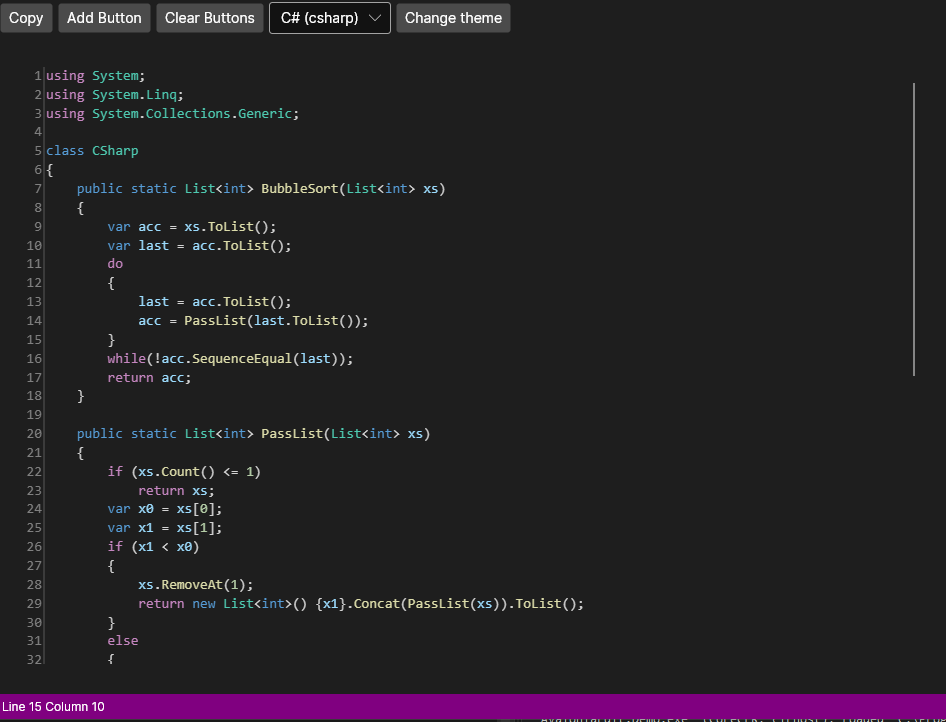
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
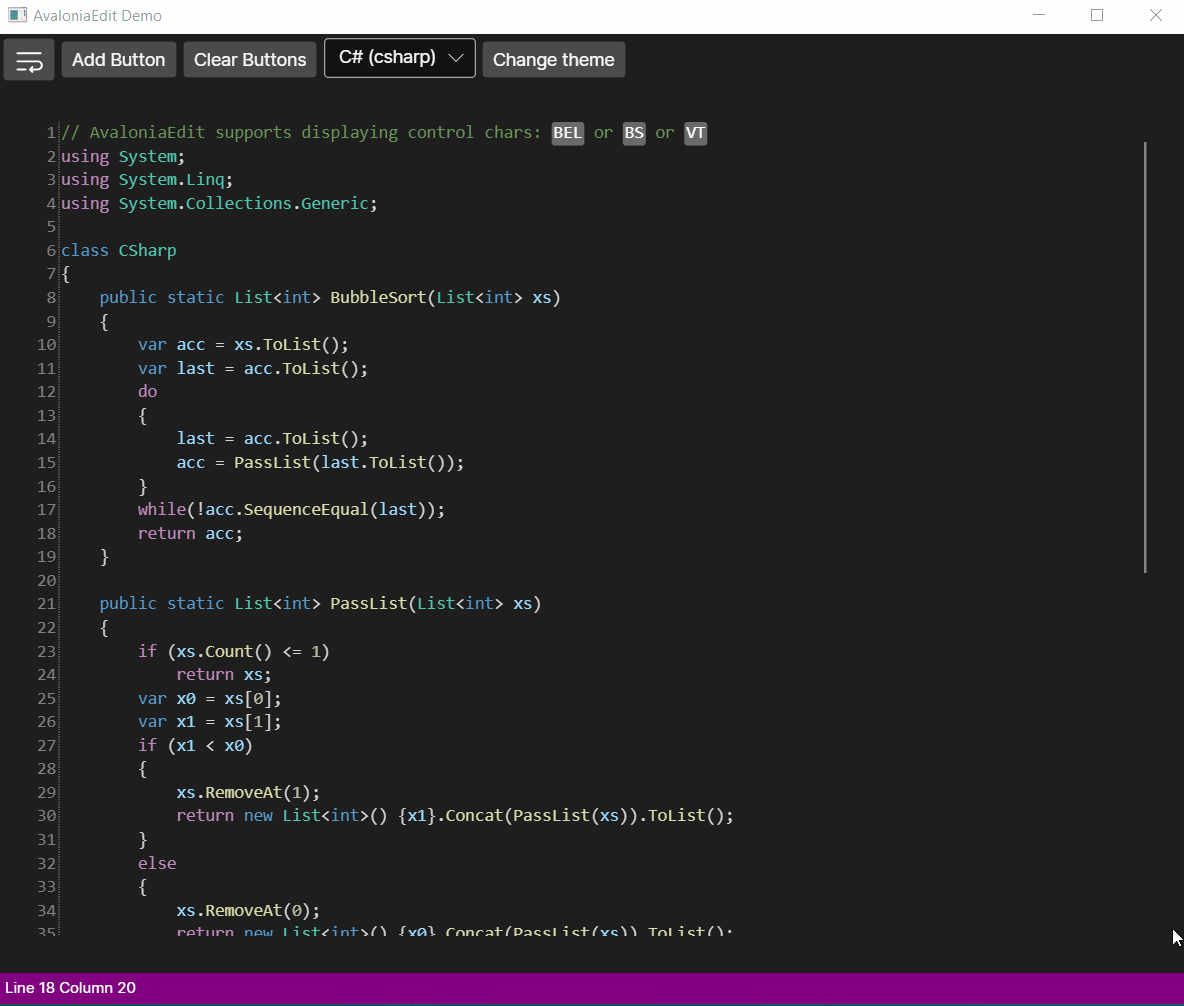
<MSG> Update readme
<DFF> @@ -13,6 +13,28 @@ AvaloniaEdit supports features like:
and many,many more!
+AvaloniaEdit currently consists of 3 packages
+ * [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
+ * [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
+ * [AvaloniaEdit.TextMate.Grammars](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) grammars for TextMate and additional infrastructure that helps you to use them.
+
+ ### How to set up theme and syntax highlighting for my project?
+First of all, if you want to use grammars that we support you should install [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) with them and [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) with TextMate integration otherwise you just install the package with TextMate integration and implement IRegistryOptions interface,that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled.
+```csharp
+//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
+var _textEditor = this.FindControl<TextEditor>("Editor");
+
+//Here we initialize RegistryOptions with the theme we want to use.
+var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
+
+//Initial setup of TextMate.
+var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
+
+//Here we are getting the lanuage by the extension and right after that we are initializing grammar with this lanuage.
+//And that all 😀, we are ready to use AvaloniaEdit with syntax highlighting!
+_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
+```
+
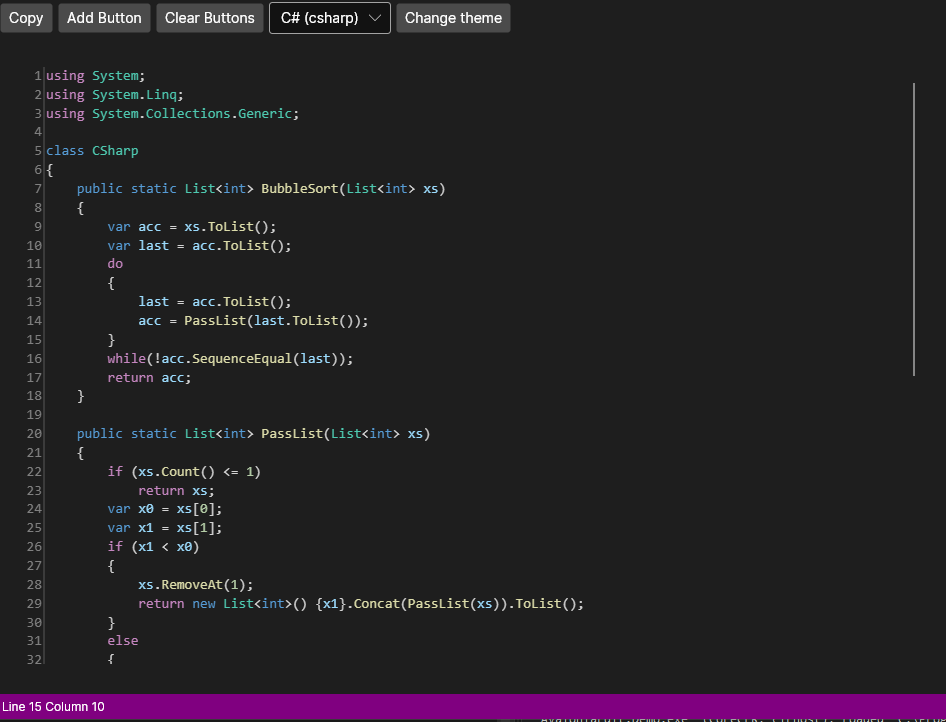
| 22 | Update readme | 0 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10066217 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
* Code folding.
* Code completion.
* Fully customizable and extensible.
and many,many more!
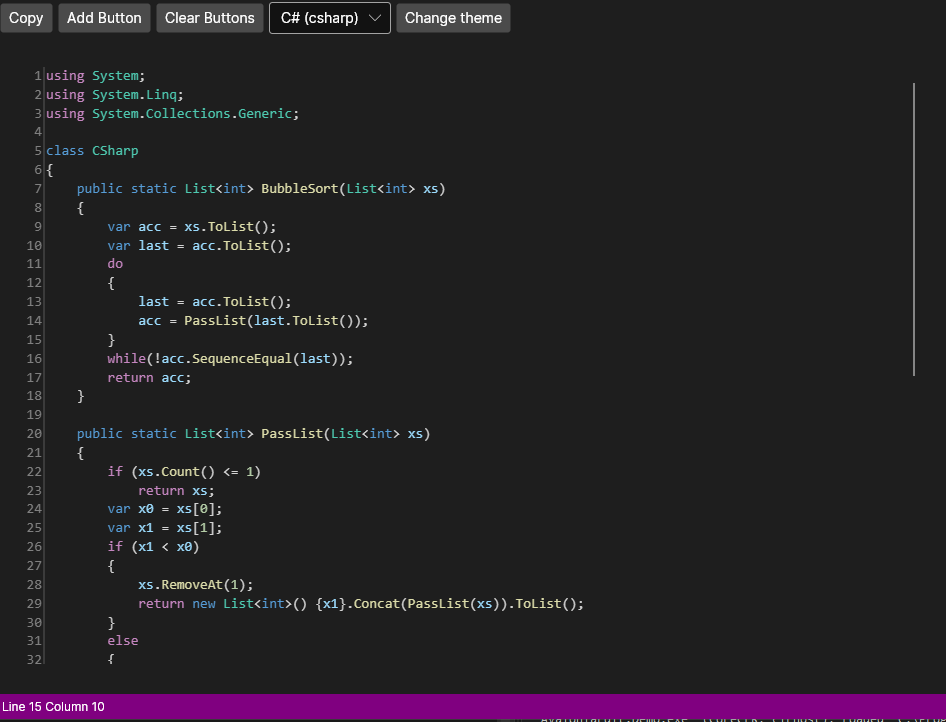
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
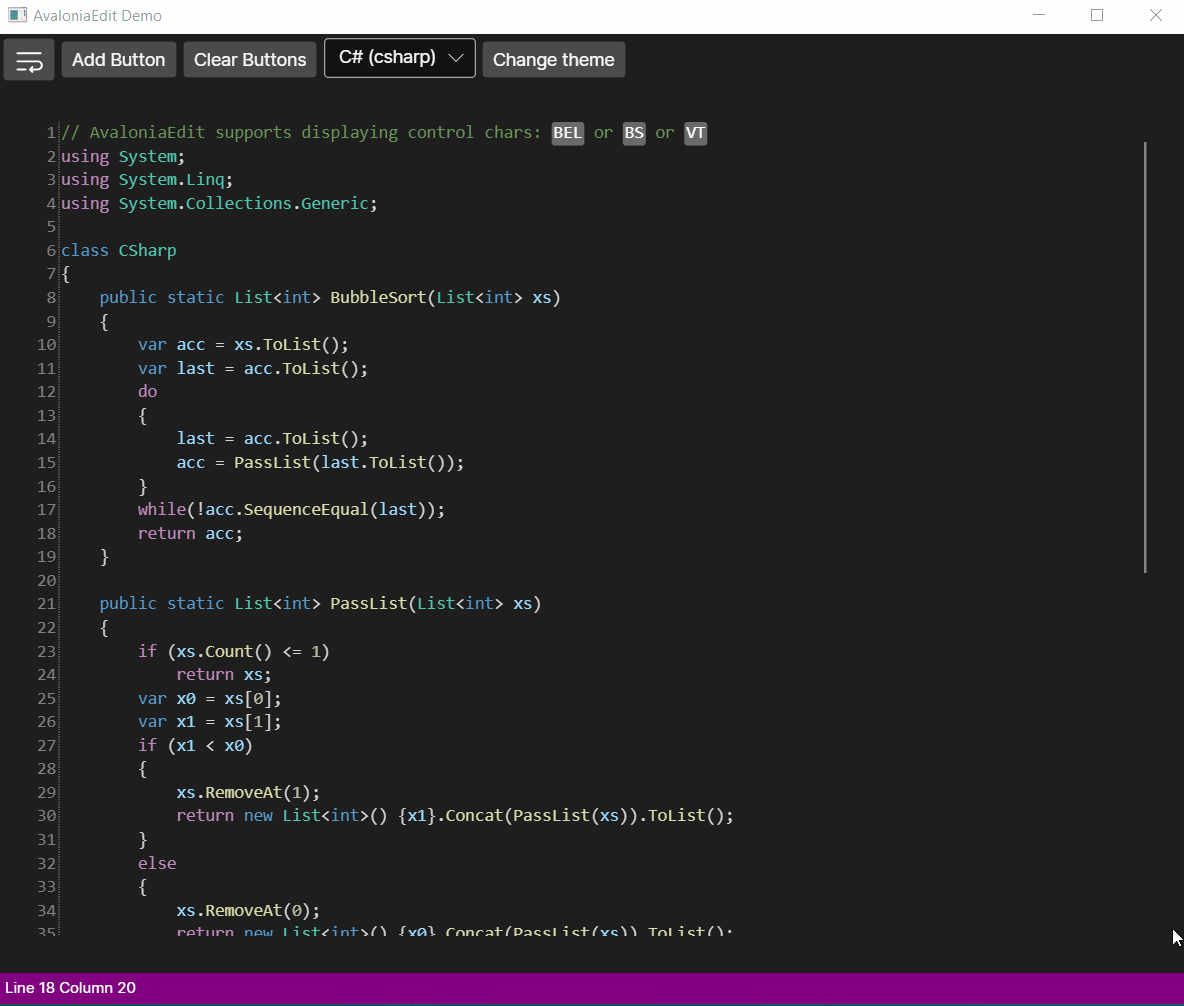
<MSG> Update readme
<DFF> @@ -13,6 +13,28 @@ AvaloniaEdit supports features like:
and many,many more!
+AvaloniaEdit currently consists of 3 packages
+ * [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
+ * [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
+ * [AvaloniaEdit.TextMate.Grammars](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) grammars for TextMate and additional infrastructure that helps you to use them.
+
+ ### How to set up theme and syntax highlighting for my project?
+First of all, if you want to use grammars that we support you should install [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) with them and [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) with TextMate integration otherwise you just install the package with TextMate integration and implement IRegistryOptions interface,that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled.
+```csharp
+//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
+var _textEditor = this.FindControl<TextEditor>("Editor");
+
+//Here we initialize RegistryOptions with the theme we want to use.
+var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
+
+//Initial setup of TextMate.
+var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
+
+//Here we are getting the lanuage by the extension and right after that we are initializing grammar with this lanuage.
+//And that all 😀, we are ready to use AvaloniaEdit with syntax highlighting!
+_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
+```
+
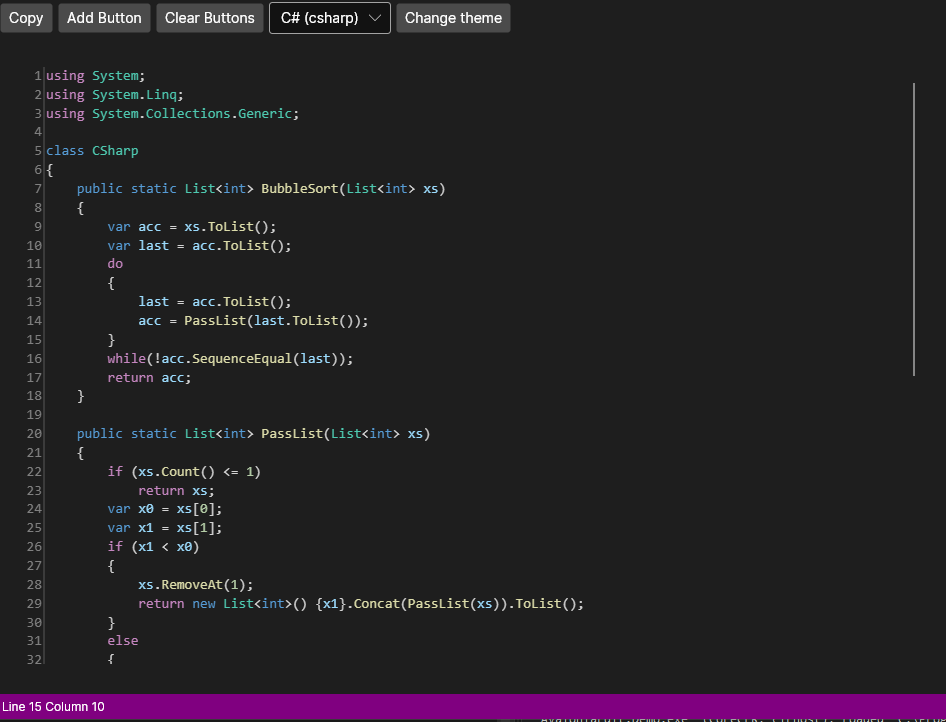
| 22 | Update readme | 0 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10066218 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
* Code folding.
* Code completion.
* Fully customizable and extensible.
and many,many more!
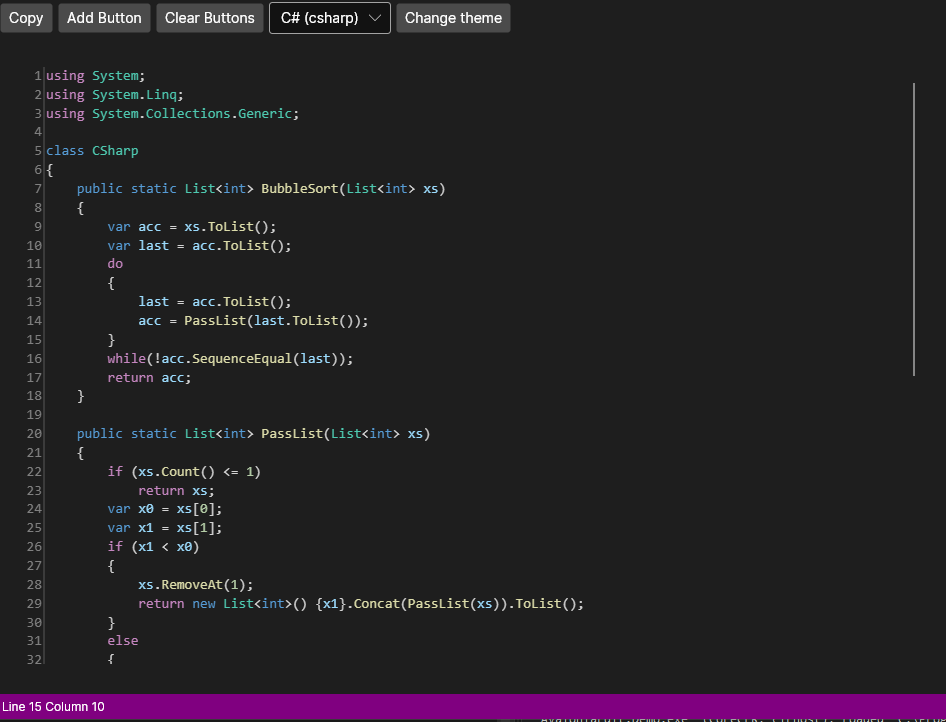
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
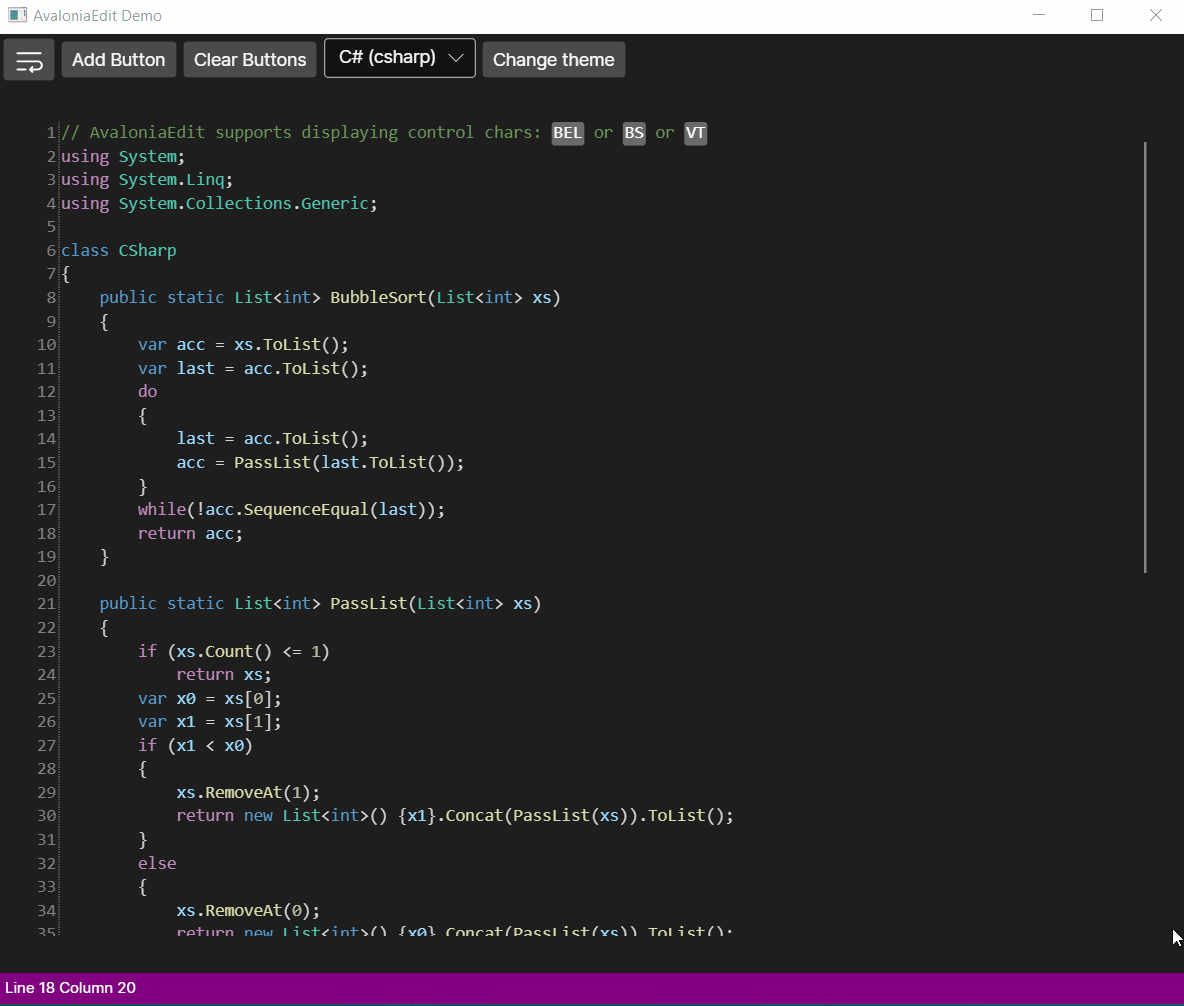
<MSG> Update readme
<DFF> @@ -13,6 +13,28 @@ AvaloniaEdit supports features like:
and many,many more!
+AvaloniaEdit currently consists of 3 packages
+ * [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
+ * [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
+ * [AvaloniaEdit.TextMate.Grammars](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) grammars for TextMate and additional infrastructure that helps you to use them.
+
+ ### How to set up theme and syntax highlighting for my project?
+First of all, if you want to use grammars that we support you should install [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) with them and [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) with TextMate integration otherwise you just install the package with TextMate integration and implement IRegistryOptions interface,that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled.
+```csharp
+//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
+var _textEditor = this.FindControl<TextEditor>("Editor");
+
+//Here we initialize RegistryOptions with the theme we want to use.
+var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
+
+//Initial setup of TextMate.
+var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
+
+//Here we are getting the lanuage by the extension and right after that we are initializing grammar with this lanuage.
+//And that all 😀, we are ready to use AvaloniaEdit with syntax highlighting!
+_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
+```
+
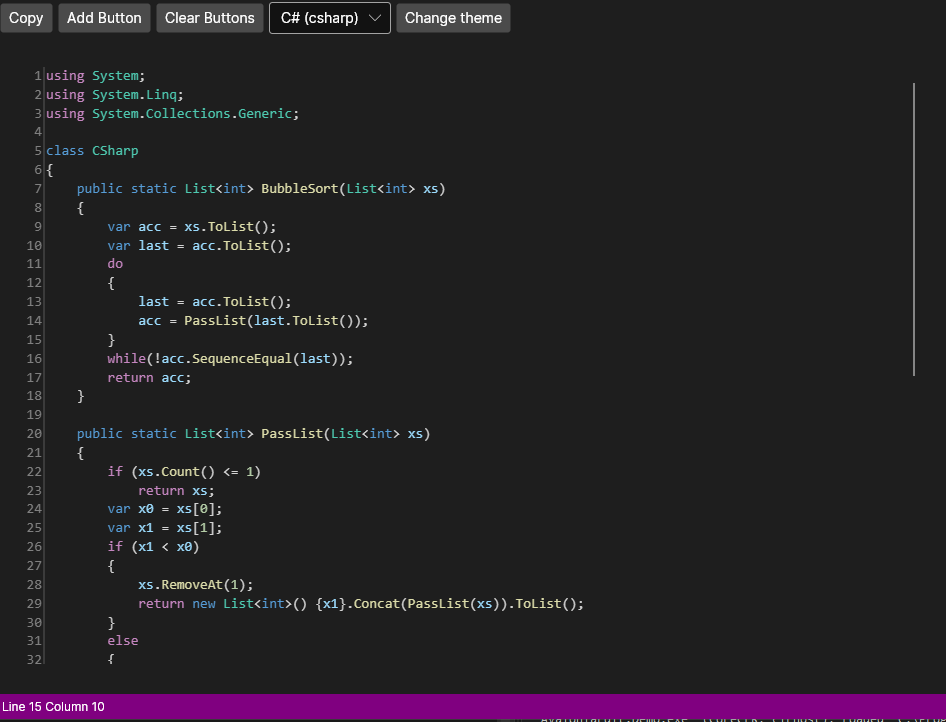
| 22 | Update readme | 0 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10066219 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
* Code folding.
* Code completion.
* Fully customizable and extensible.
and many,many more!
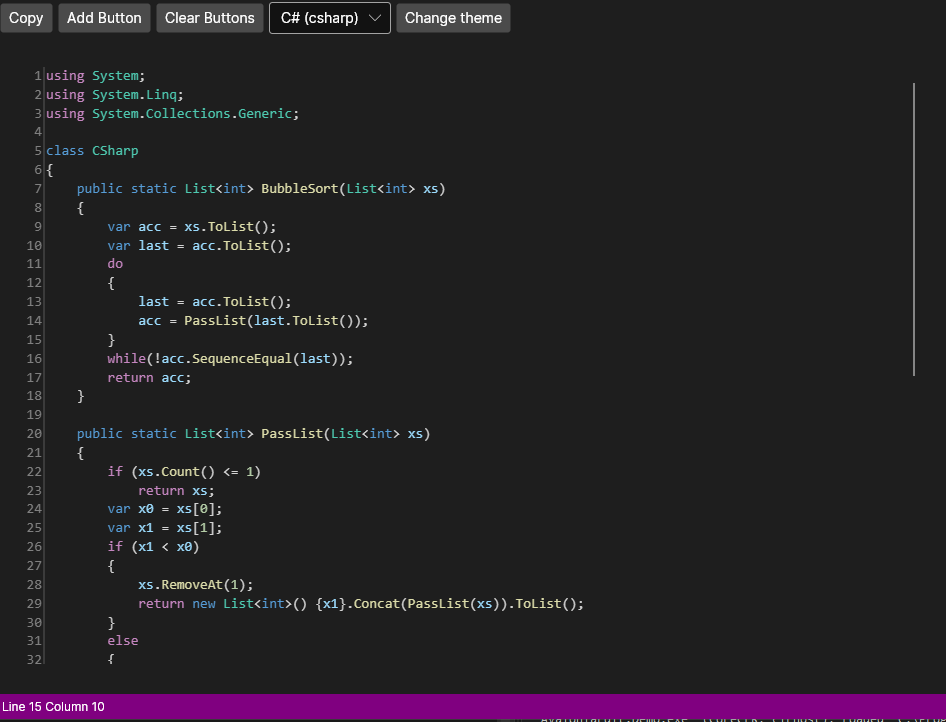
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
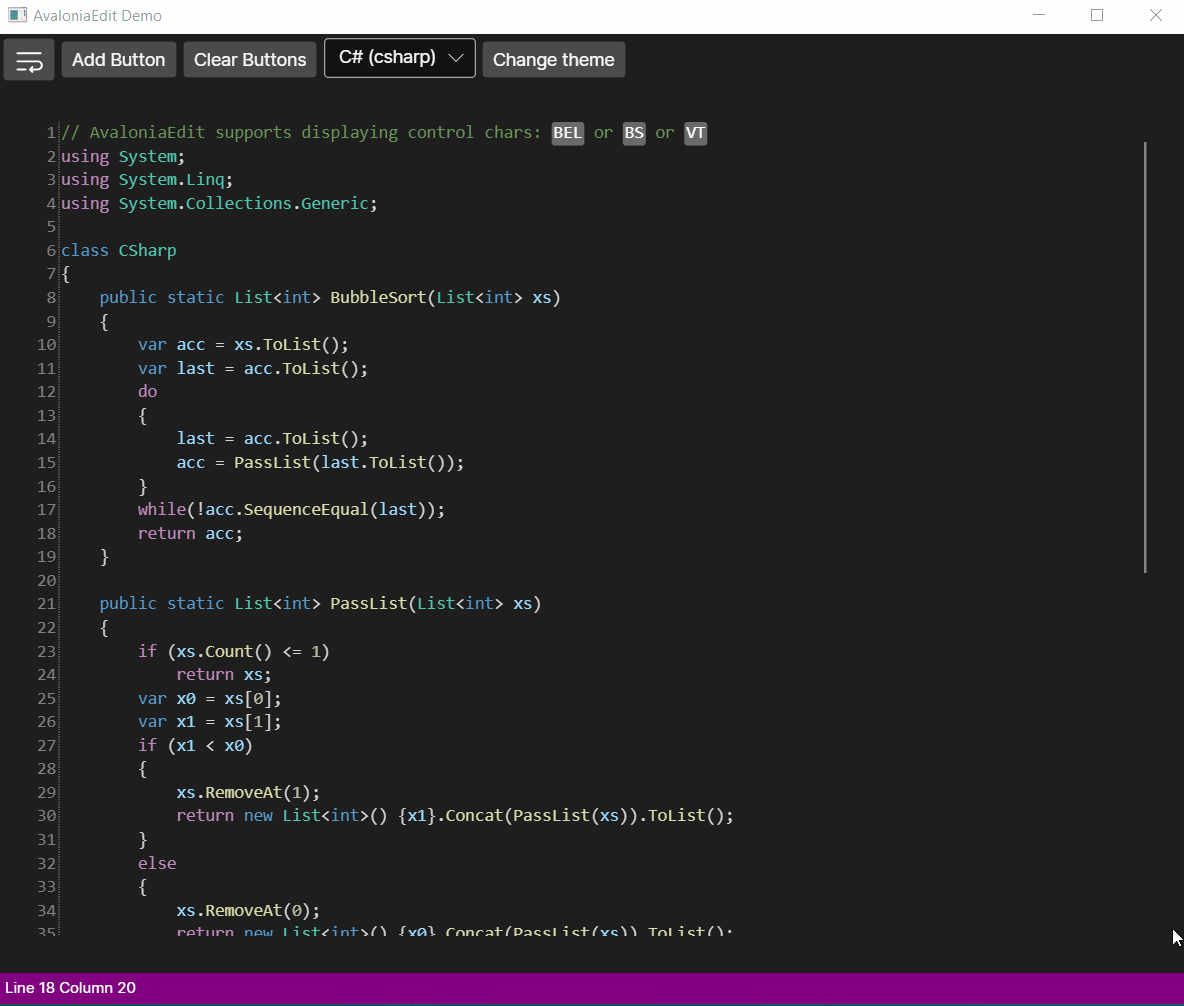
<MSG> Update readme
<DFF> @@ -13,6 +13,28 @@ AvaloniaEdit supports features like:
and many,many more!
+AvaloniaEdit currently consists of 3 packages
+ * [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
+ * [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
+ * [AvaloniaEdit.TextMate.Grammars](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) grammars for TextMate and additional infrastructure that helps you to use them.
+
+ ### How to set up theme and syntax highlighting for my project?
+First of all, if you want to use grammars that we support you should install [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) with them and [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) with TextMate integration otherwise you just install the package with TextMate integration and implement IRegistryOptions interface,that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled.
+```csharp
+//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
+var _textEditor = this.FindControl<TextEditor>("Editor");
+
+//Here we initialize RegistryOptions with the theme we want to use.
+var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
+
+//Initial setup of TextMate.
+var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
+
+//Here we are getting the lanuage by the extension and right after that we are initializing grammar with this lanuage.
+//And that all 😀, we are ready to use AvaloniaEdit with syntax highlighting!
+_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
+```
+
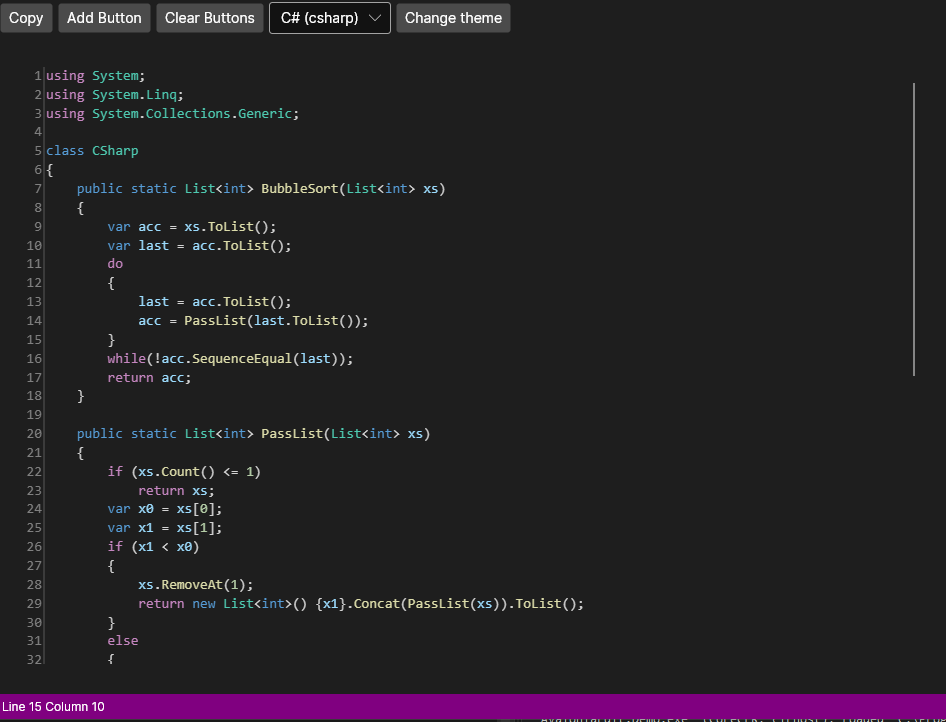
| 22 | Update readme | 0 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10066220 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
* Code folding.
* Code completion.
* Fully customizable and extensible.
and many,many more!
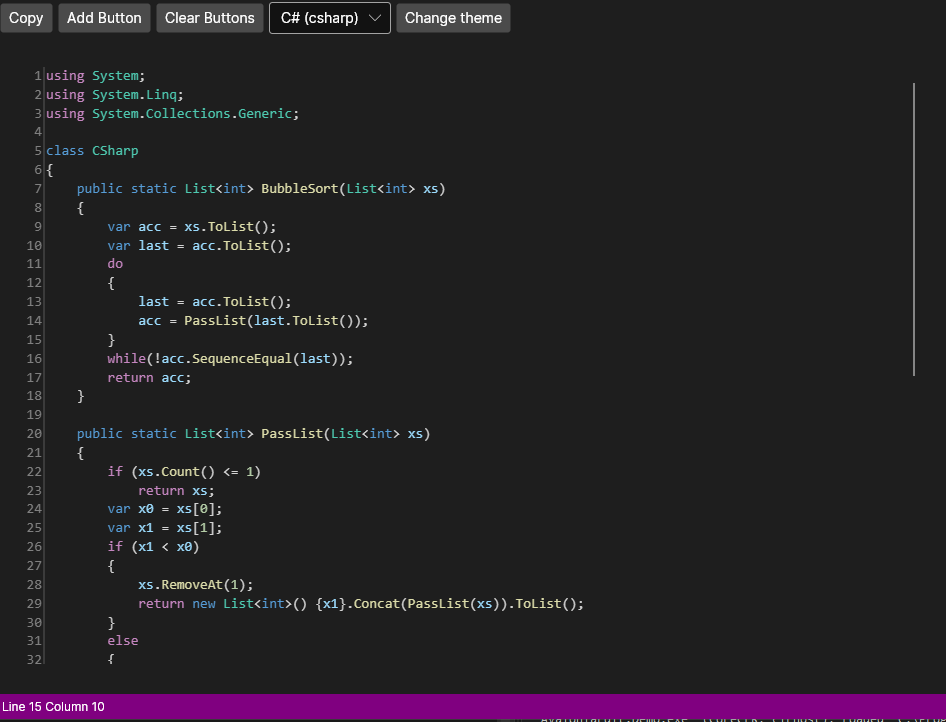
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
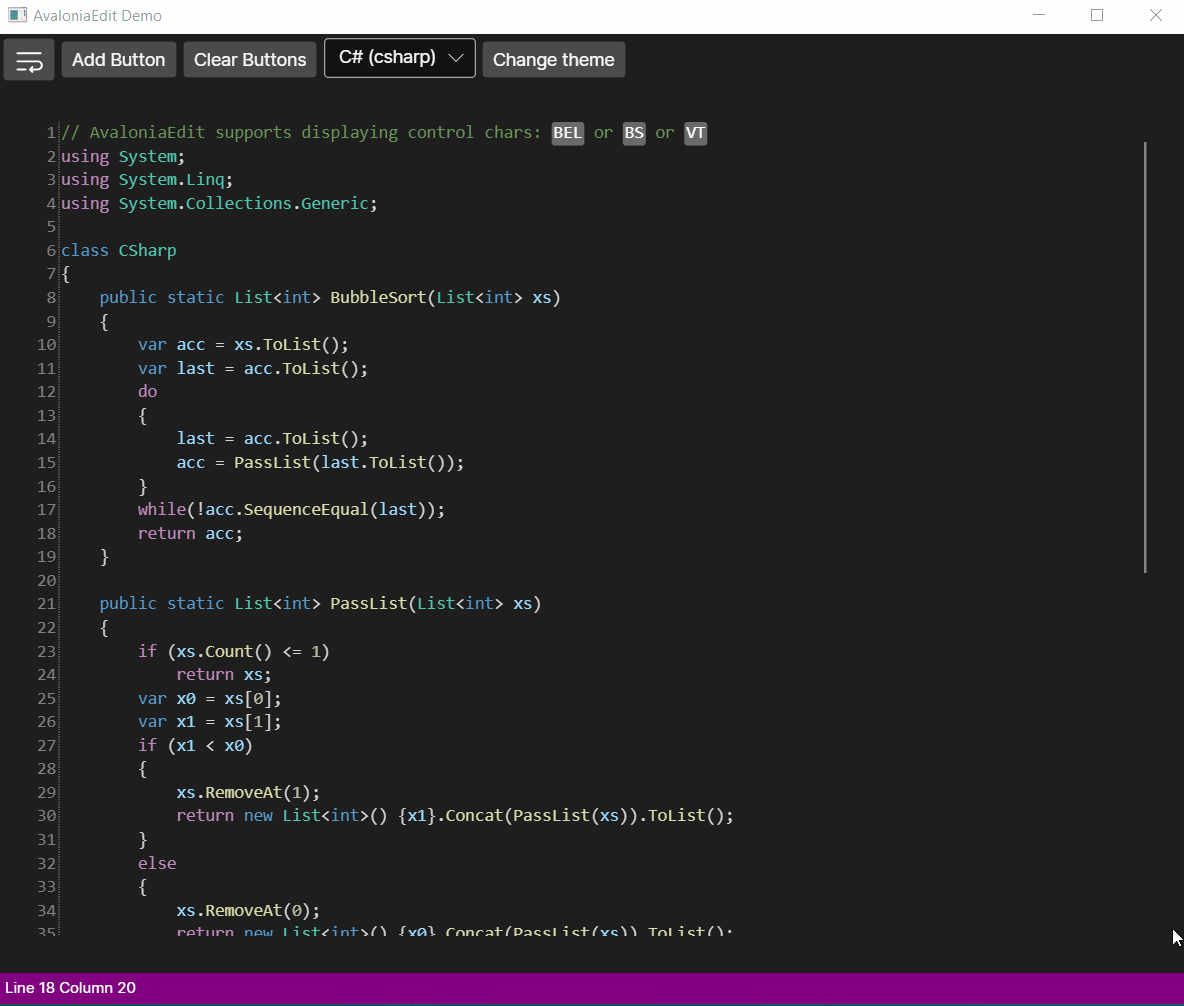
<MSG> Update readme
<DFF> @@ -13,6 +13,28 @@ AvaloniaEdit supports features like:
and many,many more!
+AvaloniaEdit currently consists of 3 packages
+ * [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
+ * [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
+ * [AvaloniaEdit.TextMate.Grammars](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) grammars for TextMate and additional infrastructure that helps you to use them.
+
+ ### How to set up theme and syntax highlighting for my project?
+First of all, if you want to use grammars that we support you should install [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) with them and [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) with TextMate integration otherwise you just install the package with TextMate integration and implement IRegistryOptions interface,that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled.
+```csharp
+//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
+var _textEditor = this.FindControl<TextEditor>("Editor");
+
+//Here we initialize RegistryOptions with the theme we want to use.
+var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
+
+//Initial setup of TextMate.
+var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
+
+//Here we are getting the lanuage by the extension and right after that we are initializing grammar with this lanuage.
+//And that all 😀, we are ready to use AvaloniaEdit with syntax highlighting!
+_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
+```
+
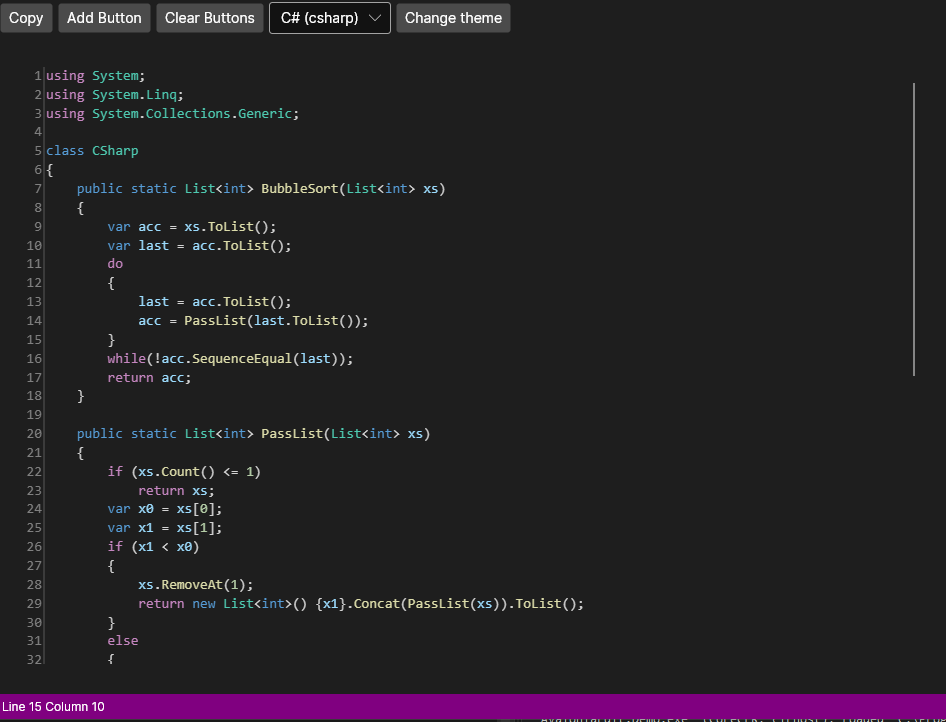
| 22 | Update readme | 0 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10066221 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
* Code folding.
* Code completion.
* Fully customizable and extensible.
and many,many more!
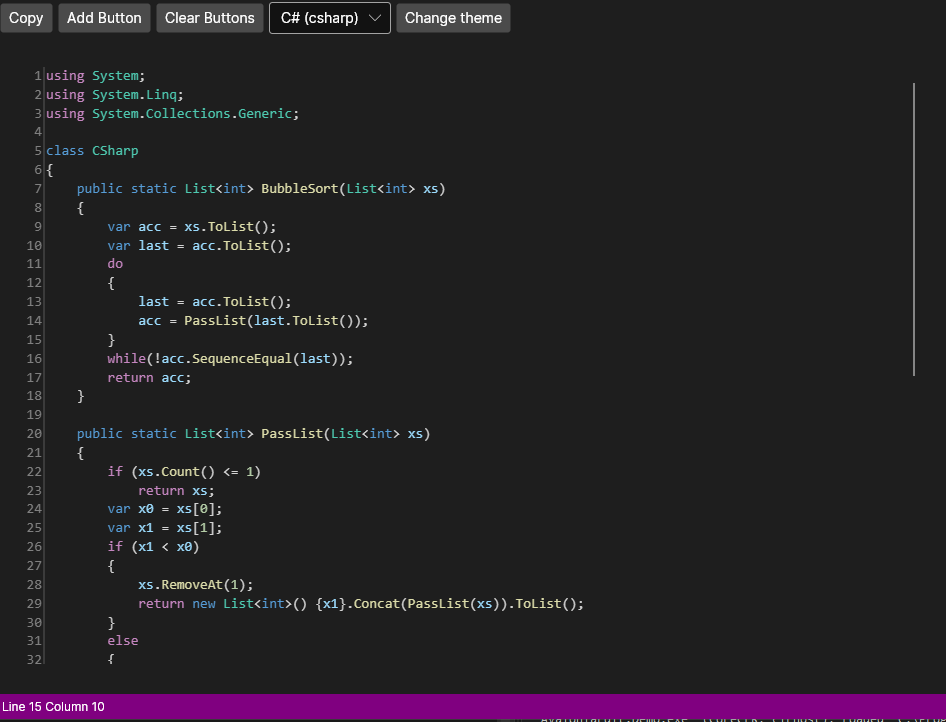
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
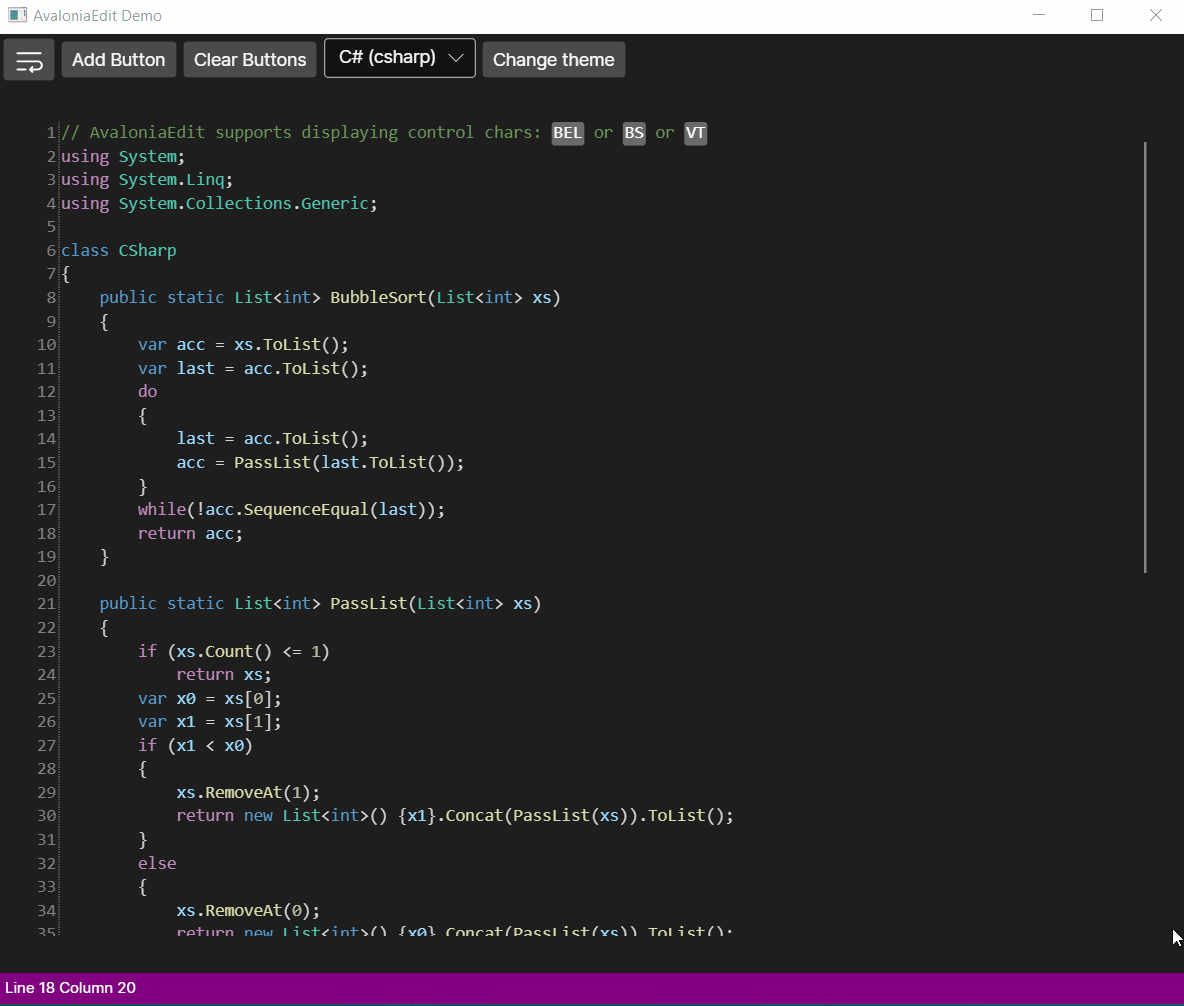
<MSG> Update readme
<DFF> @@ -13,6 +13,28 @@ AvaloniaEdit supports features like:
and many,many more!
+AvaloniaEdit currently consists of 3 packages
+ * [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
+ * [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
+ * [AvaloniaEdit.TextMate.Grammars](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) grammars for TextMate and additional infrastructure that helps you to use them.
+
+ ### How to set up theme and syntax highlighting for my project?
+First of all, if you want to use grammars that we support you should install [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) with them and [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) with TextMate integration otherwise you just install the package with TextMate integration and implement IRegistryOptions interface,that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled.
+```csharp
+//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
+var _textEditor = this.FindControl<TextEditor>("Editor");
+
+//Here we initialize RegistryOptions with the theme we want to use.
+var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
+
+//Initial setup of TextMate.
+var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
+
+//Here we are getting the lanuage by the extension and right after that we are initializing grammar with this lanuage.
+//And that all 😀, we are ready to use AvaloniaEdit with syntax highlighting!
+_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
+```
+
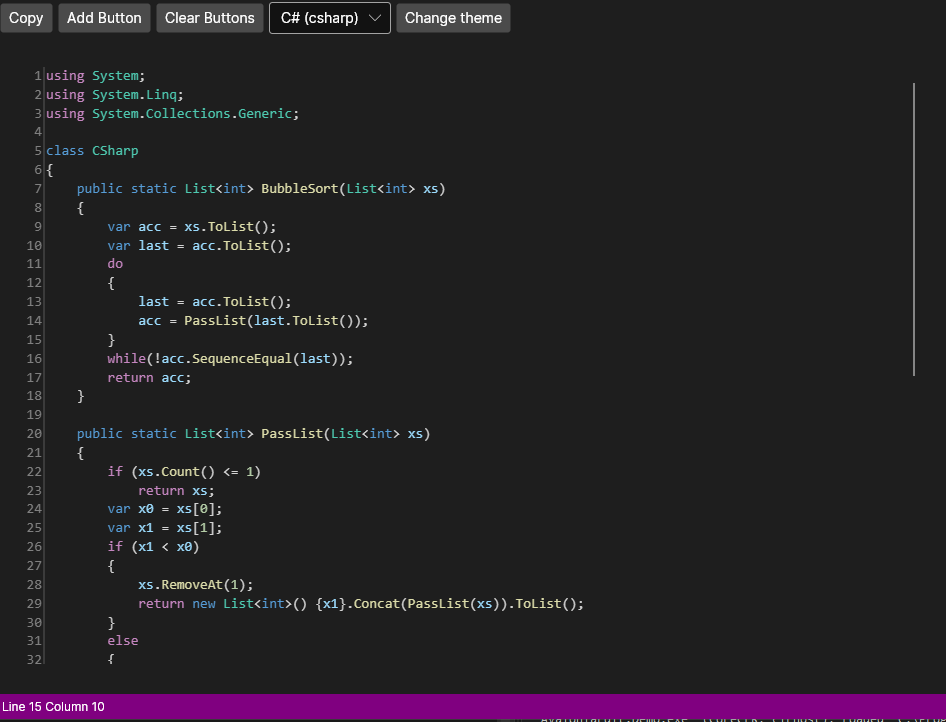
| 22 | Update readme | 0 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10066222 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
* Code folding.
* Code completion.
* Fully customizable and extensible.
and many,many more!
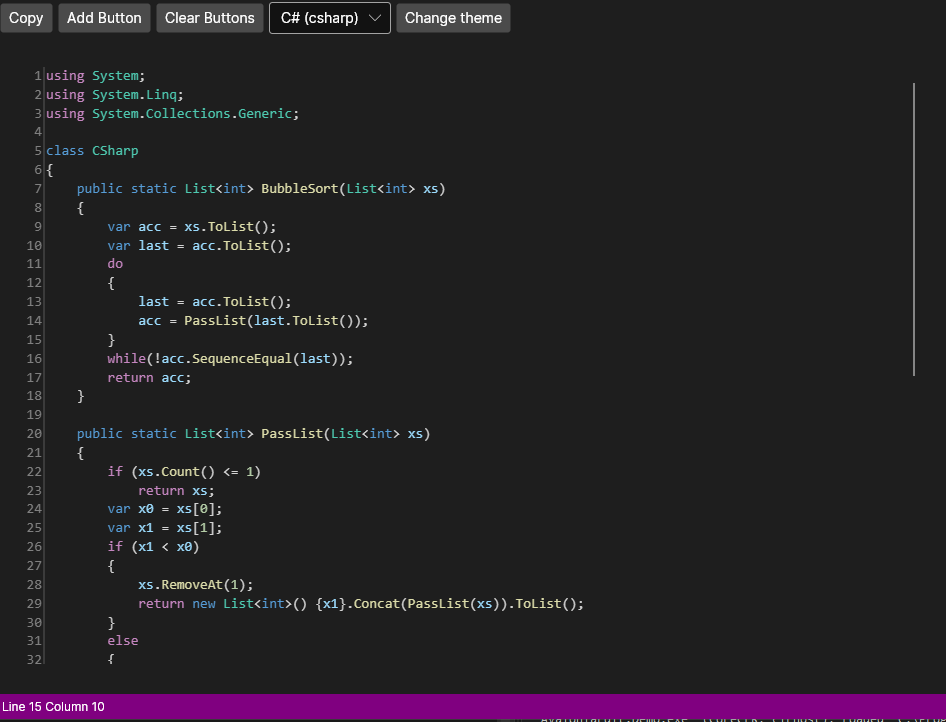
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
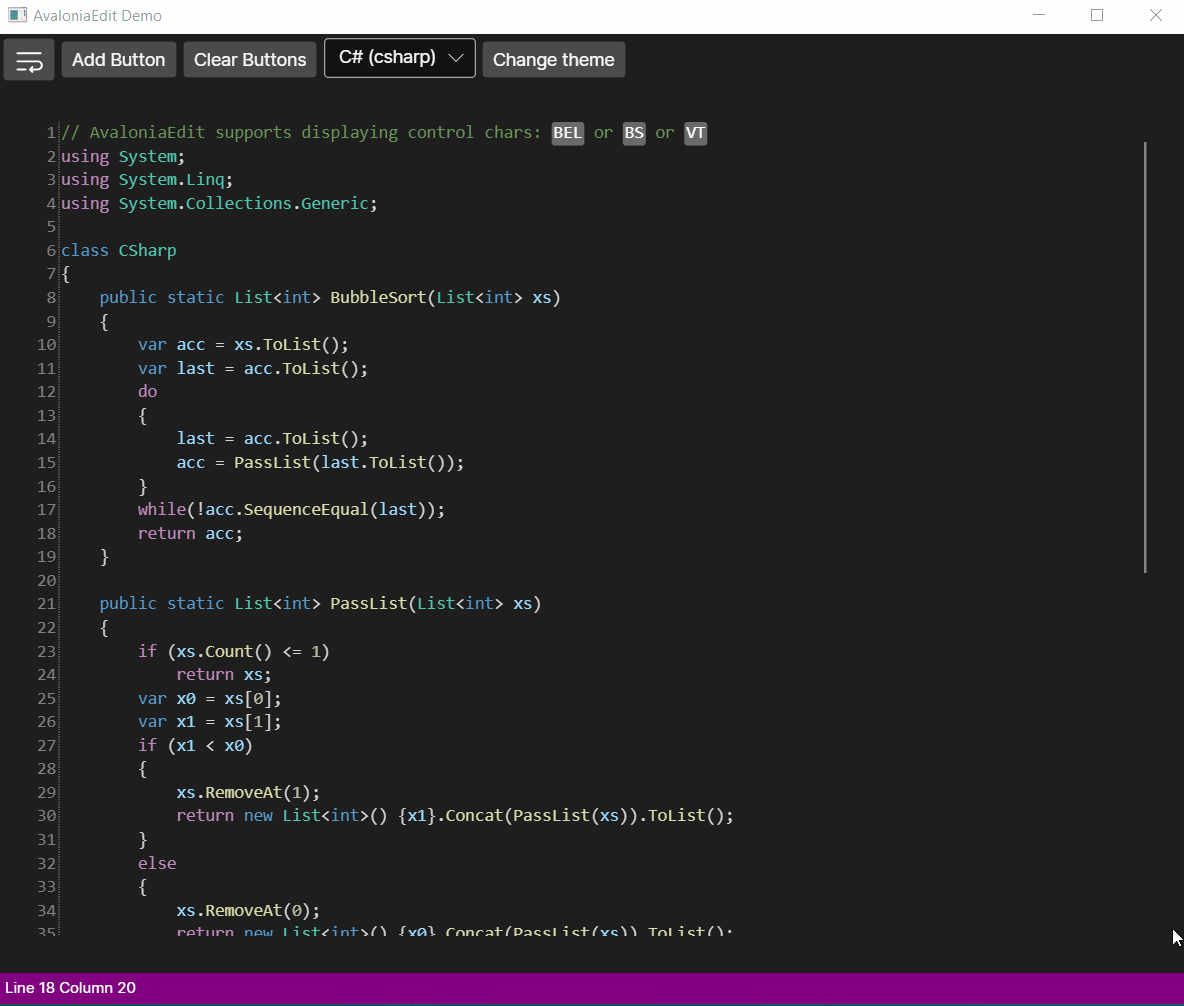
<MSG> Update readme
<DFF> @@ -13,6 +13,28 @@ AvaloniaEdit supports features like:
and many,many more!
+AvaloniaEdit currently consists of 3 packages
+ * [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
+ * [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
+ * [AvaloniaEdit.TextMate.Grammars](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) grammars for TextMate and additional infrastructure that helps you to use them.
+
+ ### How to set up theme and syntax highlighting for my project?
+First of all, if you want to use grammars that we support you should install [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) with them and [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) with TextMate integration otherwise you just install the package with TextMate integration and implement IRegistryOptions interface,that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled.
+```csharp
+//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
+var _textEditor = this.FindControl<TextEditor>("Editor");
+
+//Here we initialize RegistryOptions with the theme we want to use.
+var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
+
+//Initial setup of TextMate.
+var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
+
+//Here we are getting the lanuage by the extension and right after that we are initializing grammar with this lanuage.
+//And that all 😀, we are ready to use AvaloniaEdit with syntax highlighting!
+_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
+```
+
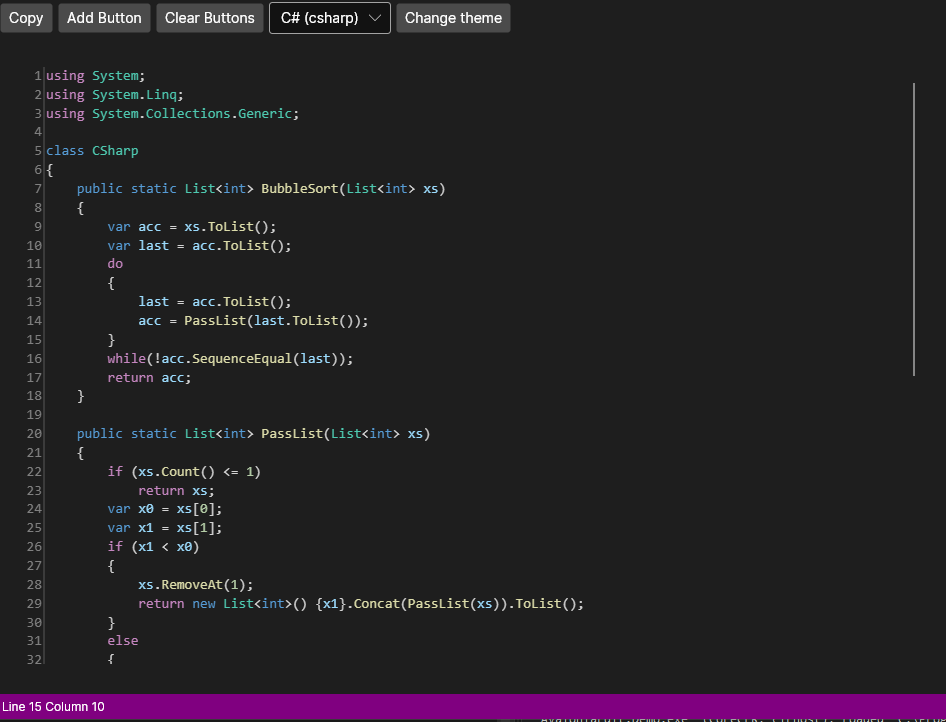
| 22 | Update readme | 0 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10066223 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
* Code folding.
* Code completion.
* Fully customizable and extensible.
and many,many more!
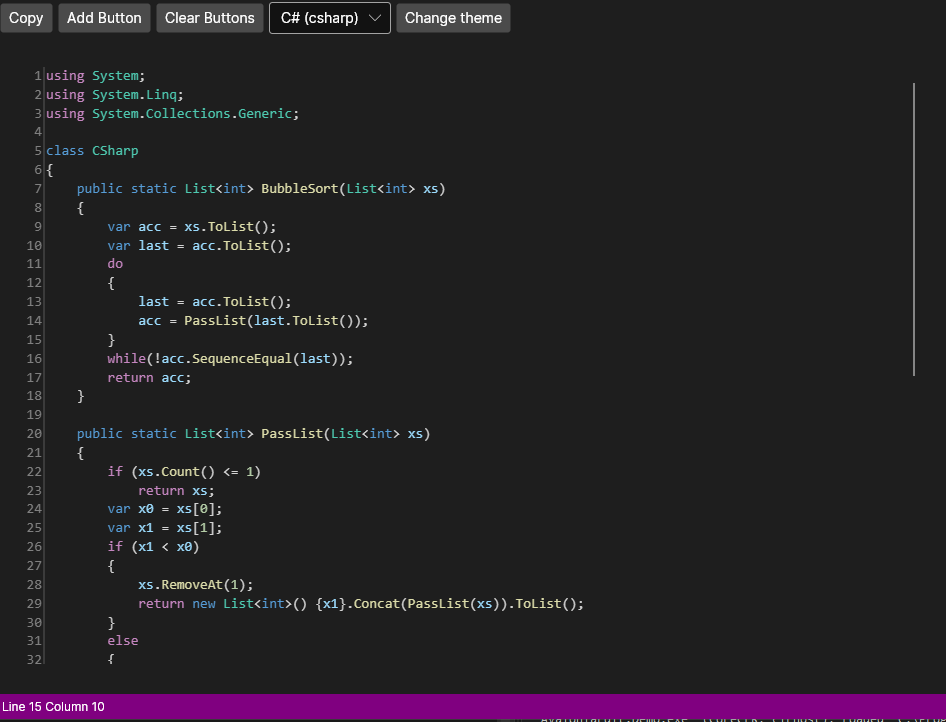
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
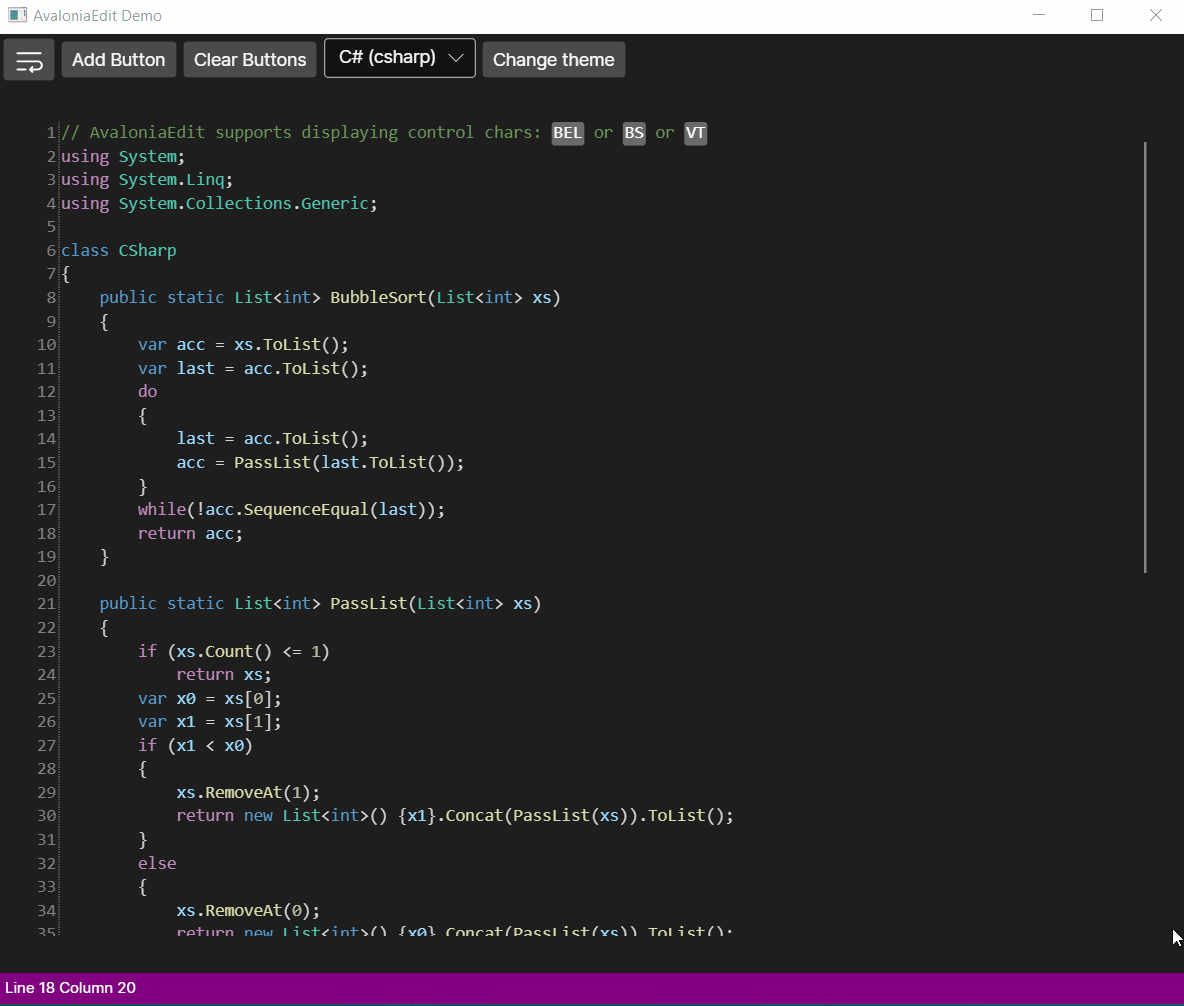
<MSG> Update readme
<DFF> @@ -13,6 +13,28 @@ AvaloniaEdit supports features like:
and many,many more!
+AvaloniaEdit currently consists of 3 packages
+ * [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
+ * [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
+ * [AvaloniaEdit.TextMate.Grammars](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) grammars for TextMate and additional infrastructure that helps you to use them.
+
+ ### How to set up theme and syntax highlighting for my project?
+First of all, if you want to use grammars that we support you should install [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) with them and [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) with TextMate integration otherwise you just install the package with TextMate integration and implement IRegistryOptions interface,that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled.
+```csharp
+//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
+var _textEditor = this.FindControl<TextEditor>("Editor");
+
+//Here we initialize RegistryOptions with the theme we want to use.
+var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
+
+//Initial setup of TextMate.
+var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
+
+//Here we are getting the lanuage by the extension and right after that we are initializing grammar with this lanuage.
+//And that all 😀, we are ready to use AvaloniaEdit with syntax highlighting!
+_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
+```
+
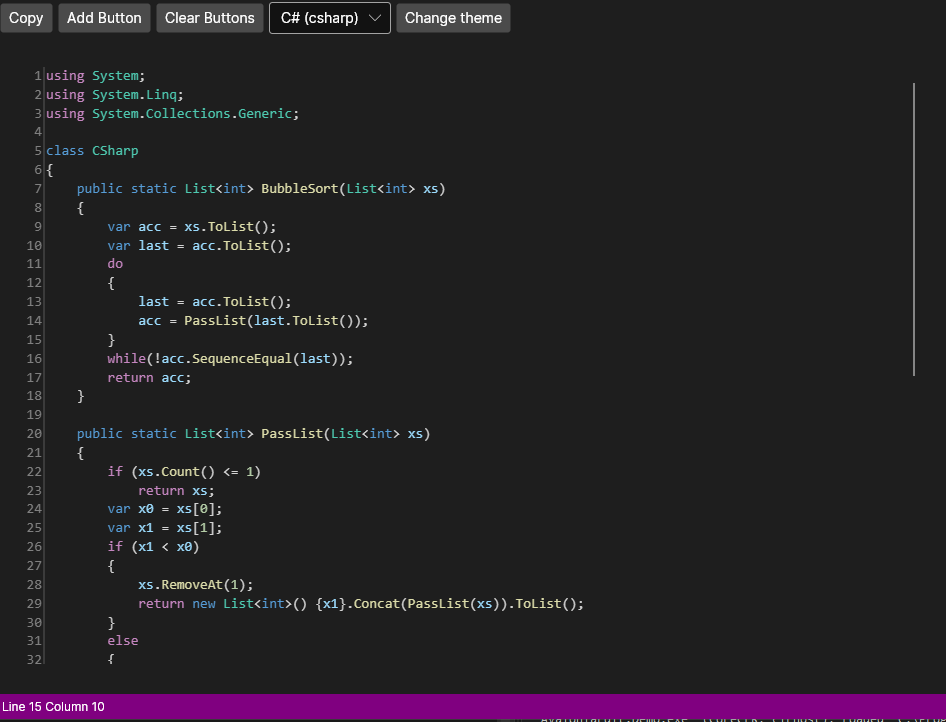
| 22 | Update readme | 0 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10066224 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
* Code folding.
* Code completion.
* Fully customizable and extensible.
and many,many more!
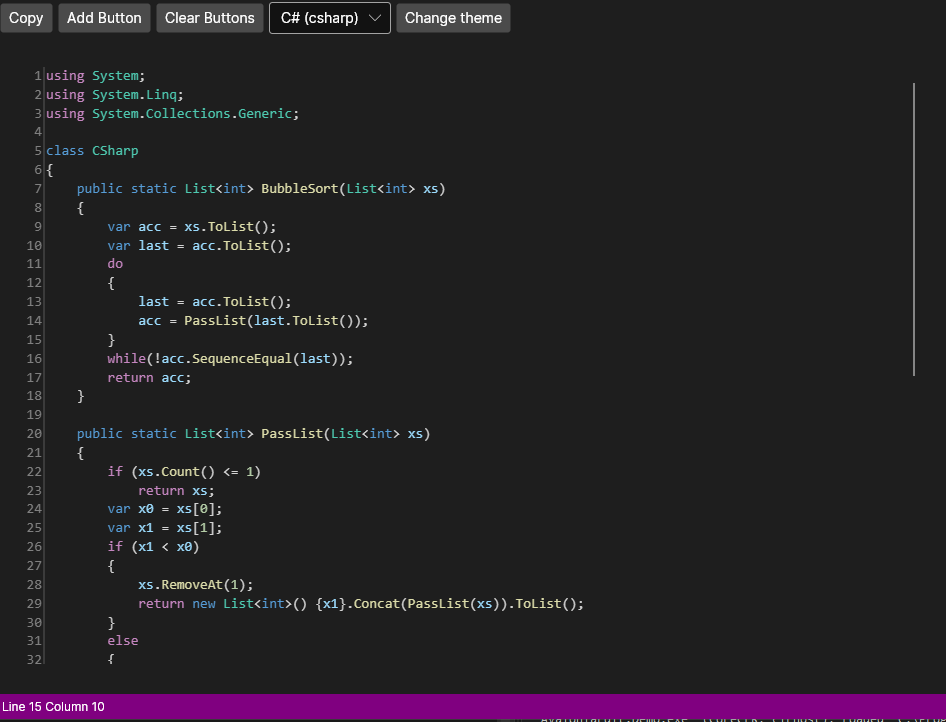
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
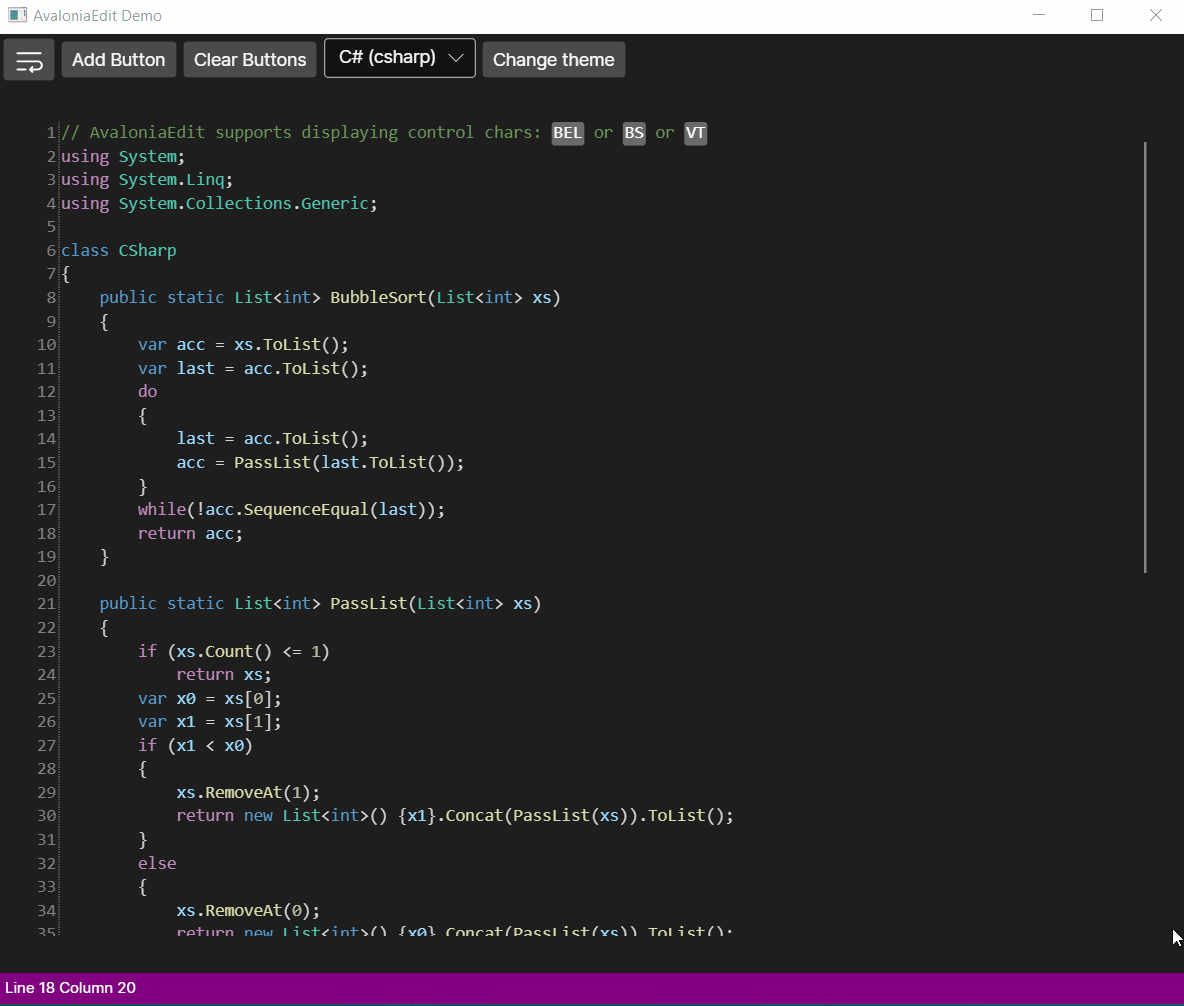
<MSG> Update readme
<DFF> @@ -13,6 +13,28 @@ AvaloniaEdit supports features like:
and many,many more!
+AvaloniaEdit currently consists of 3 packages
+ * [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
+ * [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
+ * [AvaloniaEdit.TextMate.Grammars](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) grammars for TextMate and additional infrastructure that helps you to use them.
+
+ ### How to set up theme and syntax highlighting for my project?
+First of all, if you want to use grammars that we support you should install [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) with them and [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) with TextMate integration otherwise you just install the package with TextMate integration and implement IRegistryOptions interface,that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled.
+```csharp
+//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
+var _textEditor = this.FindControl<TextEditor>("Editor");
+
+//Here we initialize RegistryOptions with the theme we want to use.
+var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
+
+//Initial setup of TextMate.
+var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
+
+//Here we are getting the lanuage by the extension and right after that we are initializing grammar with this lanuage.
+//And that all 😀, we are ready to use AvaloniaEdit with syntax highlighting!
+_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
+```
+
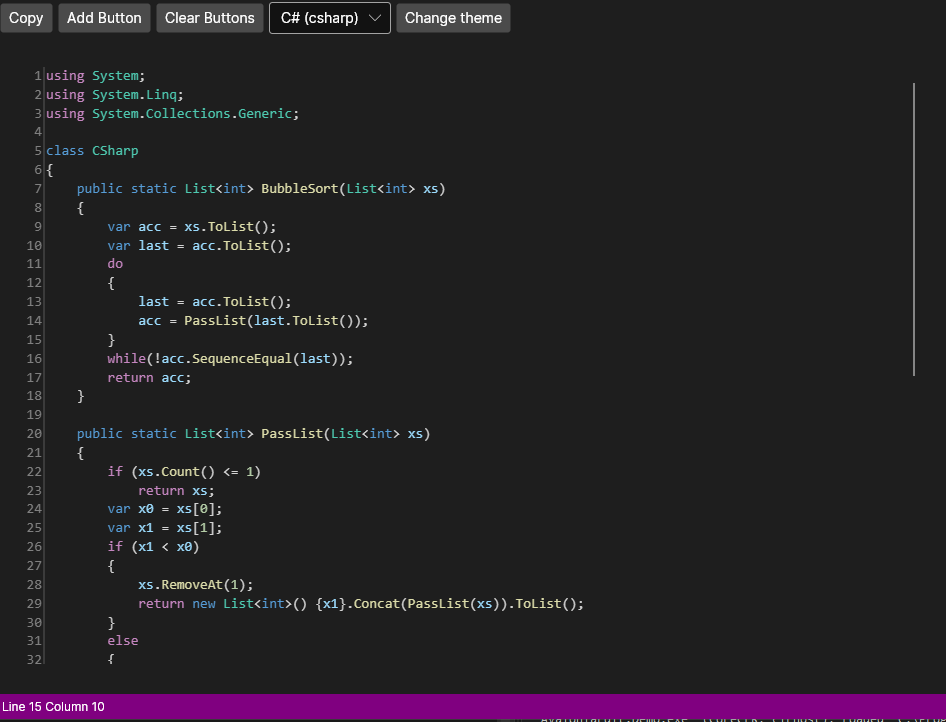
| 22 | Update readme | 0 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10066225 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
* Code folding.
* Code completion.
* Fully customizable and extensible.
and many,many more!
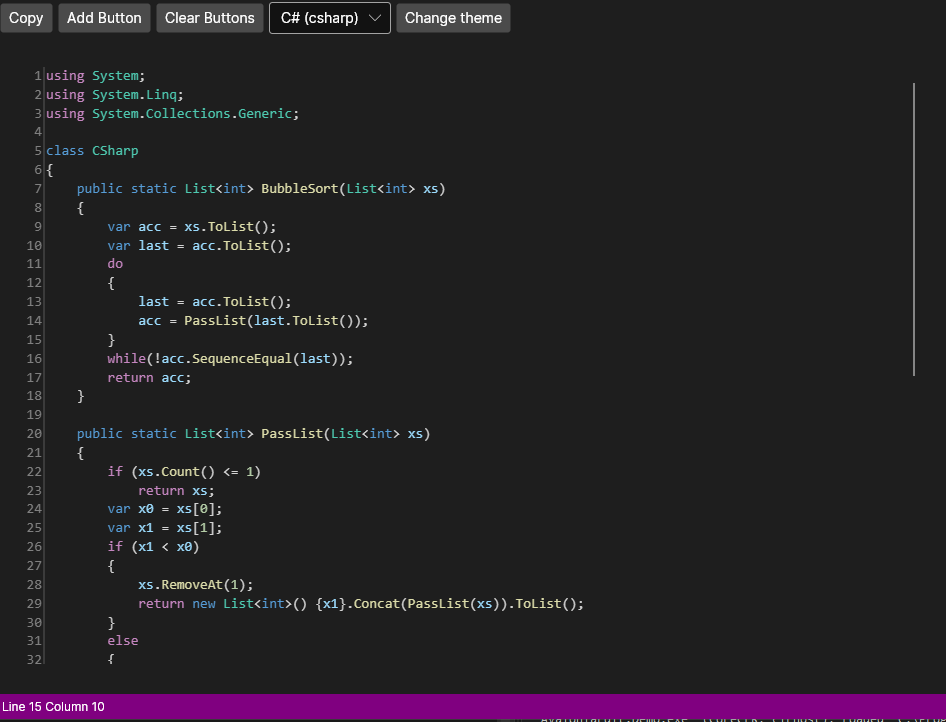
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
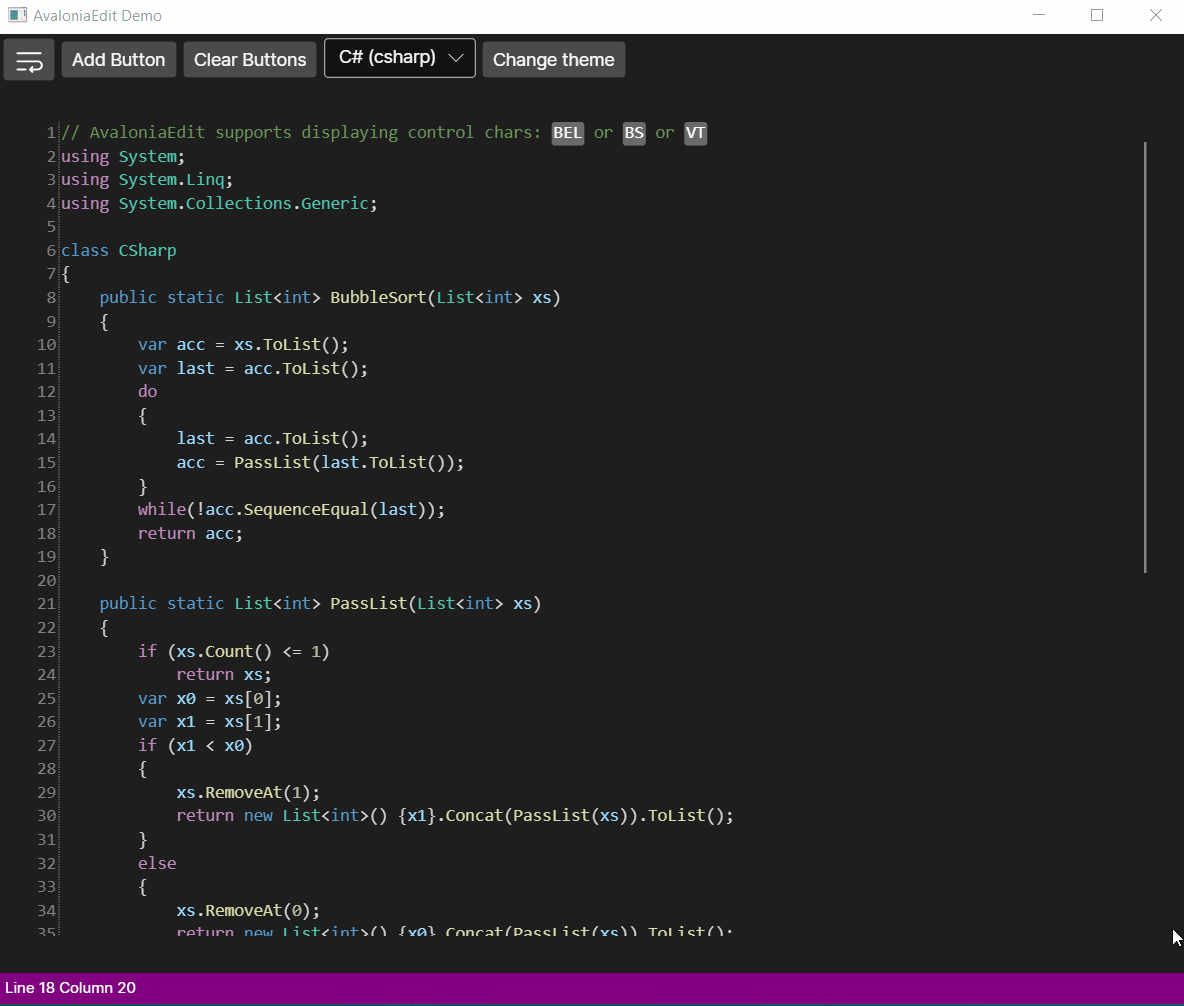
<MSG> Update readme
<DFF> @@ -13,6 +13,28 @@ AvaloniaEdit supports features like:
and many,many more!
+AvaloniaEdit currently consists of 3 packages
+ * [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
+ * [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
+ * [AvaloniaEdit.TextMate.Grammars](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) grammars for TextMate and additional infrastructure that helps you to use them.
+
+ ### How to set up theme and syntax highlighting for my project?
+First of all, if you want to use grammars that we support you should install [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) with them and [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) with TextMate integration otherwise you just install the package with TextMate integration and implement IRegistryOptions interface,that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled.
+```csharp
+//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
+var _textEditor = this.FindControl<TextEditor>("Editor");
+
+//Here we initialize RegistryOptions with the theme we want to use.
+var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
+
+//Initial setup of TextMate.
+var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
+
+//Here we are getting the lanuage by the extension and right after that we are initializing grammar with this lanuage.
+//And that all 😀, we are ready to use AvaloniaEdit with syntax highlighting!
+_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
+```
+
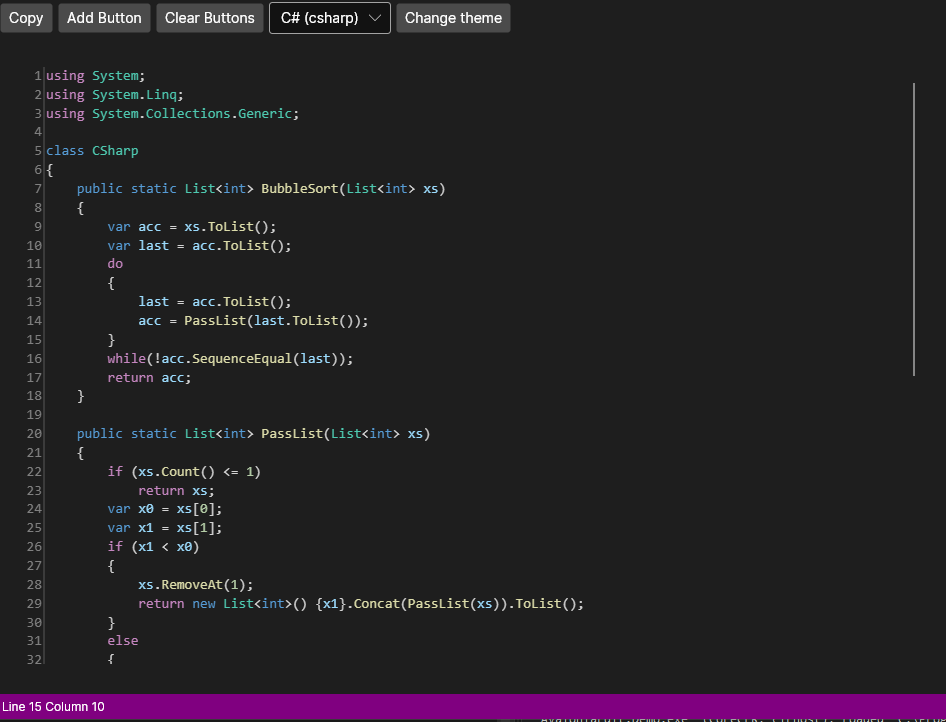
| 22 | Update readme | 0 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10066226 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
* Code folding.
* Code completion.
* Fully customizable and extensible.
and many,many more!
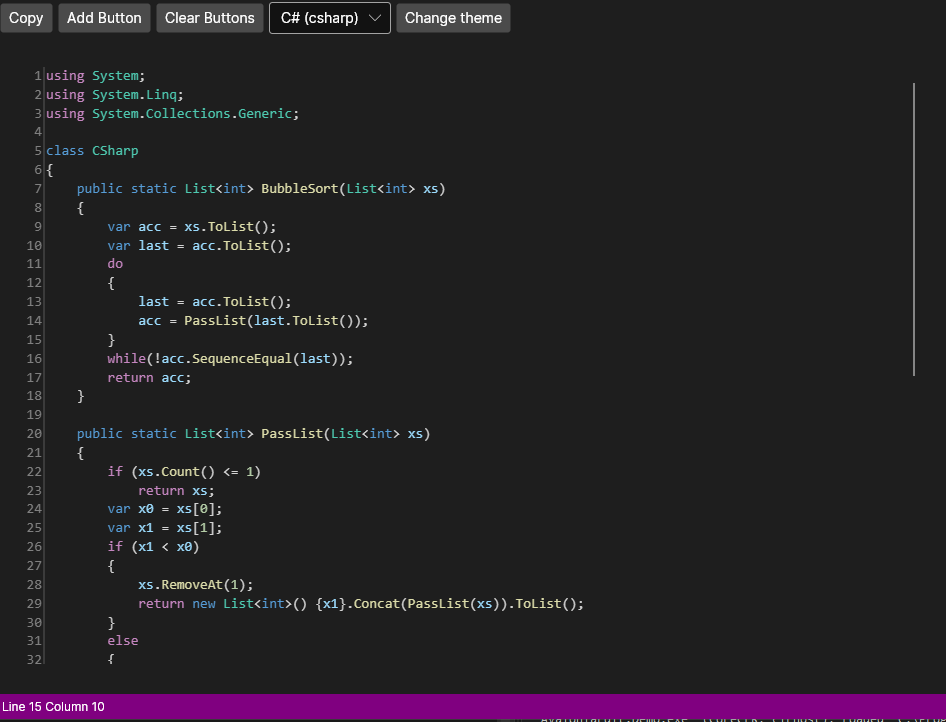
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
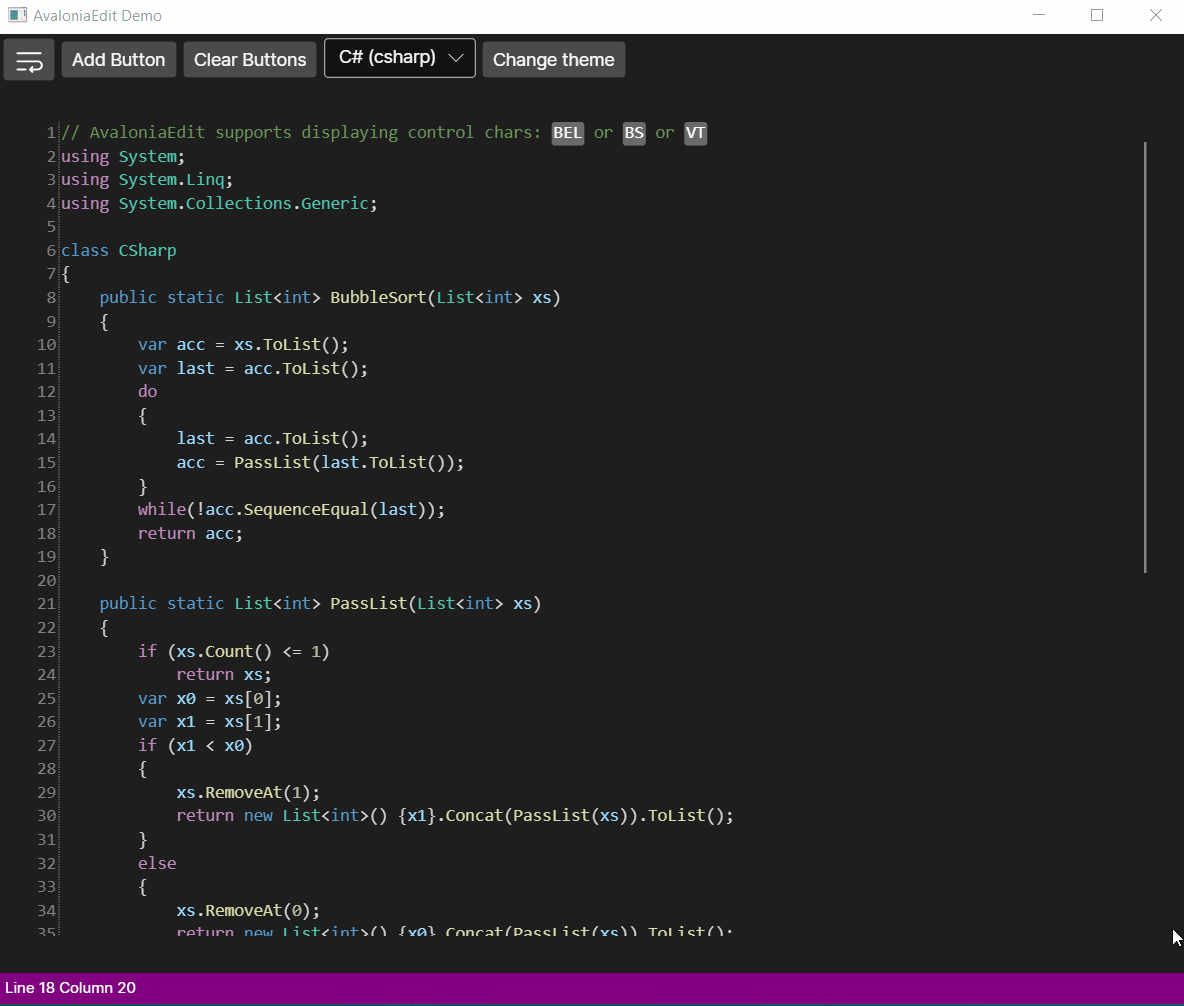
<MSG> Update readme
<DFF> @@ -13,6 +13,28 @@ AvaloniaEdit supports features like:
and many,many more!
+AvaloniaEdit currently consists of 3 packages
+ * [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
+ * [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
+ * [AvaloniaEdit.TextMate.Grammars](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) grammars for TextMate and additional infrastructure that helps you to use them.
+
+ ### How to set up theme and syntax highlighting for my project?
+First of all, if you want to use grammars that we support you should install [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) with them and [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) with TextMate integration otherwise you just install the package with TextMate integration and implement IRegistryOptions interface,that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled.
+```csharp
+//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
+var _textEditor = this.FindControl<TextEditor>("Editor");
+
+//Here we initialize RegistryOptions with the theme we want to use.
+var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
+
+//Initial setup of TextMate.
+var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
+
+//Here we are getting the lanuage by the extension and right after that we are initializing grammar with this lanuage.
+//And that all 😀, we are ready to use AvaloniaEdit with syntax highlighting!
+_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
+```
+
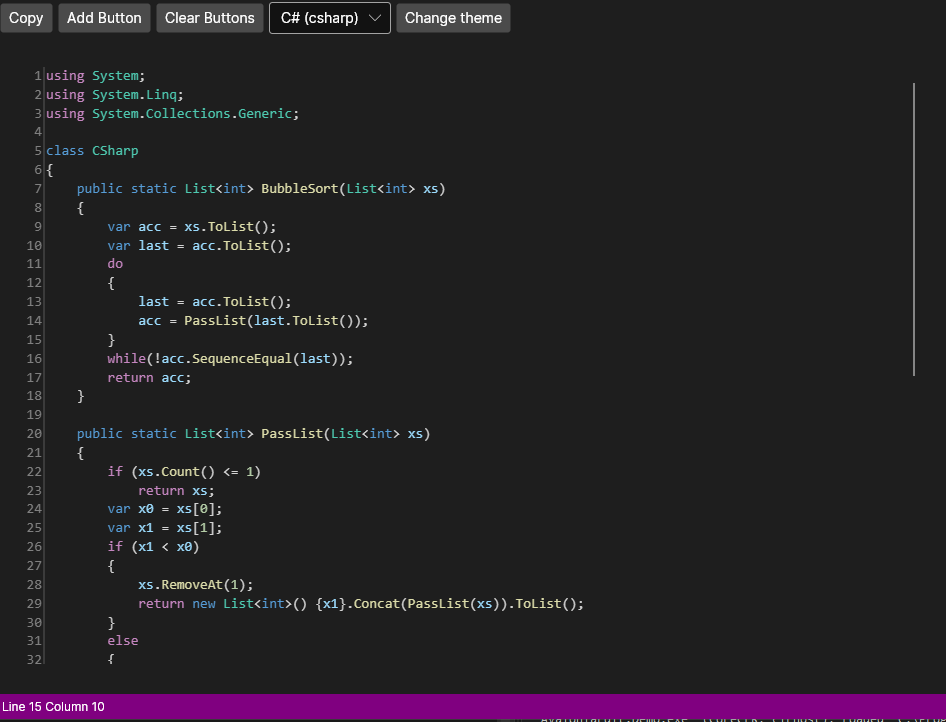
| 22 | Update readme | 0 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10066227 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
* Code folding.
* Code completion.
* Fully customizable and extensible.
and many,many more!
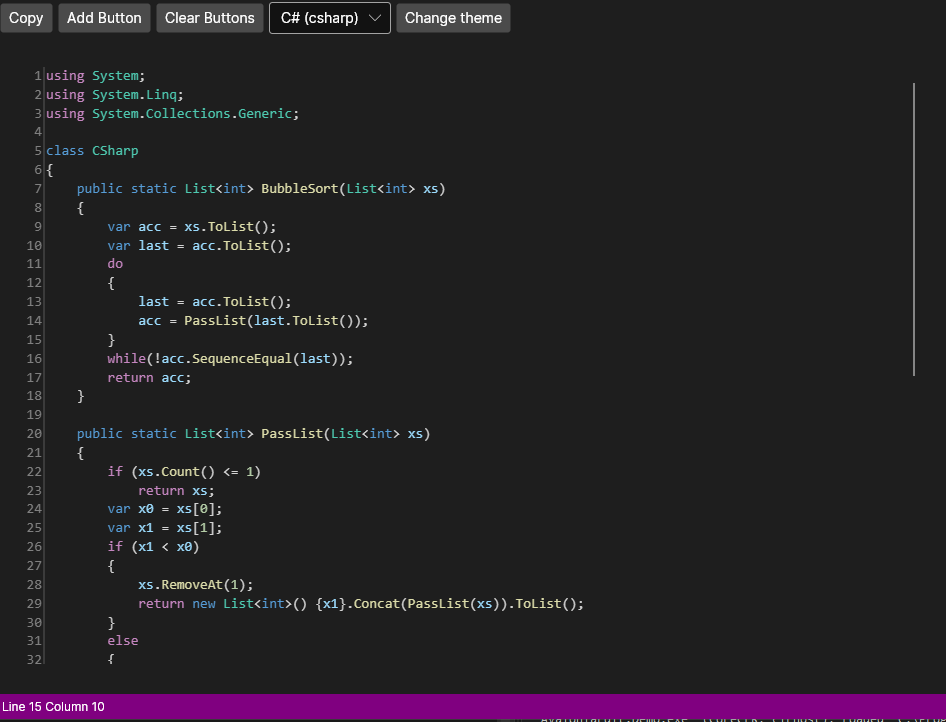
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
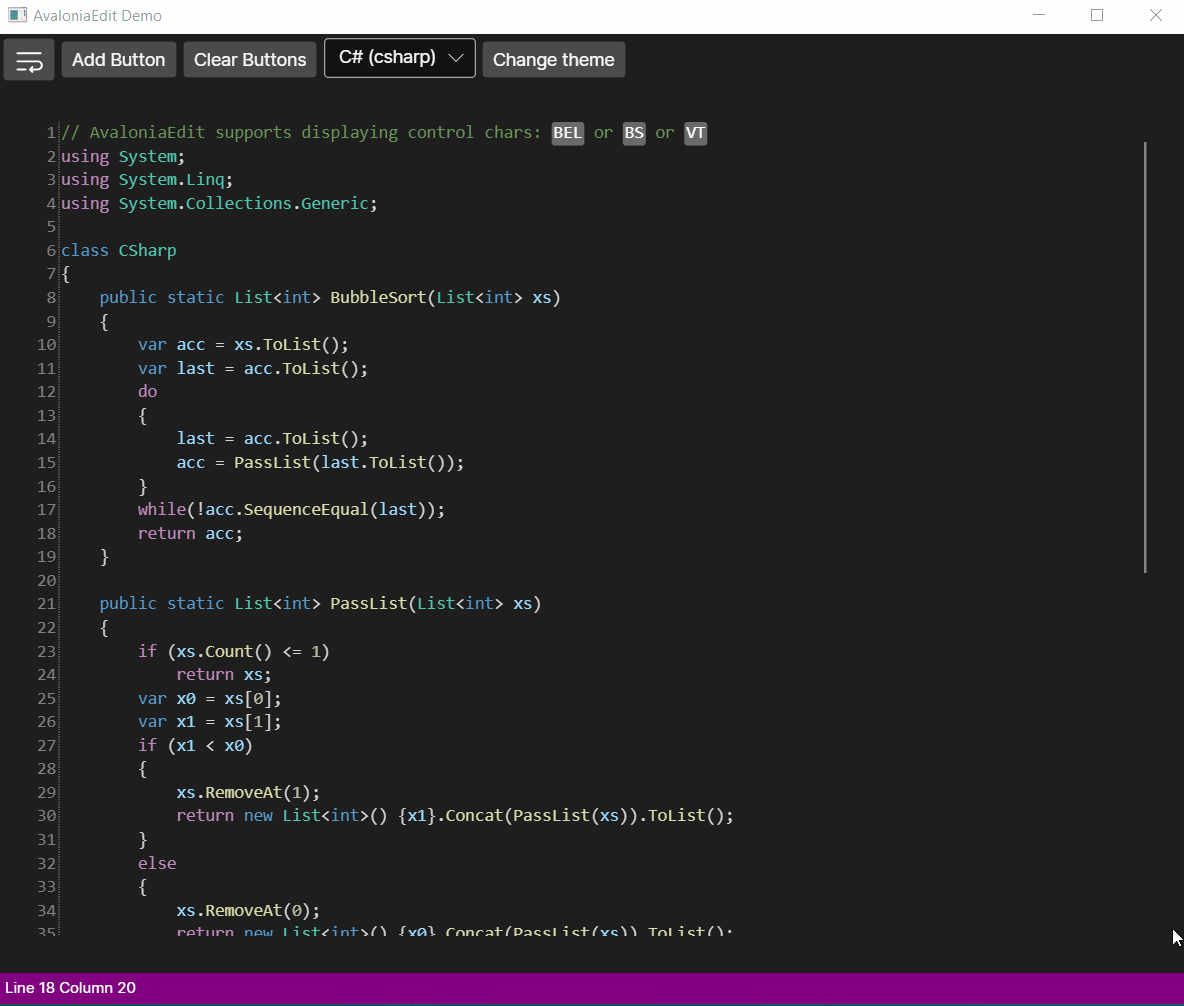
<MSG> Update readme
<DFF> @@ -13,6 +13,28 @@ AvaloniaEdit supports features like:
and many,many more!
+AvaloniaEdit currently consists of 3 packages
+ * [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
+ * [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
+ * [AvaloniaEdit.TextMate.Grammars](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) grammars for TextMate and additional infrastructure that helps you to use them.
+
+ ### How to set up theme and syntax highlighting for my project?
+First of all, if you want to use grammars that we support you should install [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) with them and [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) with TextMate integration otherwise you just install the package with TextMate integration and implement IRegistryOptions interface,that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled.
+```csharp
+//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
+var _textEditor = this.FindControl<TextEditor>("Editor");
+
+//Here we initialize RegistryOptions with the theme we want to use.
+var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
+
+//Initial setup of TextMate.
+var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
+
+//Here we are getting the lanuage by the extension and right after that we are initializing grammar with this lanuage.
+//And that all 😀, we are ready to use AvaloniaEdit with syntax highlighting!
+_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
+```
+
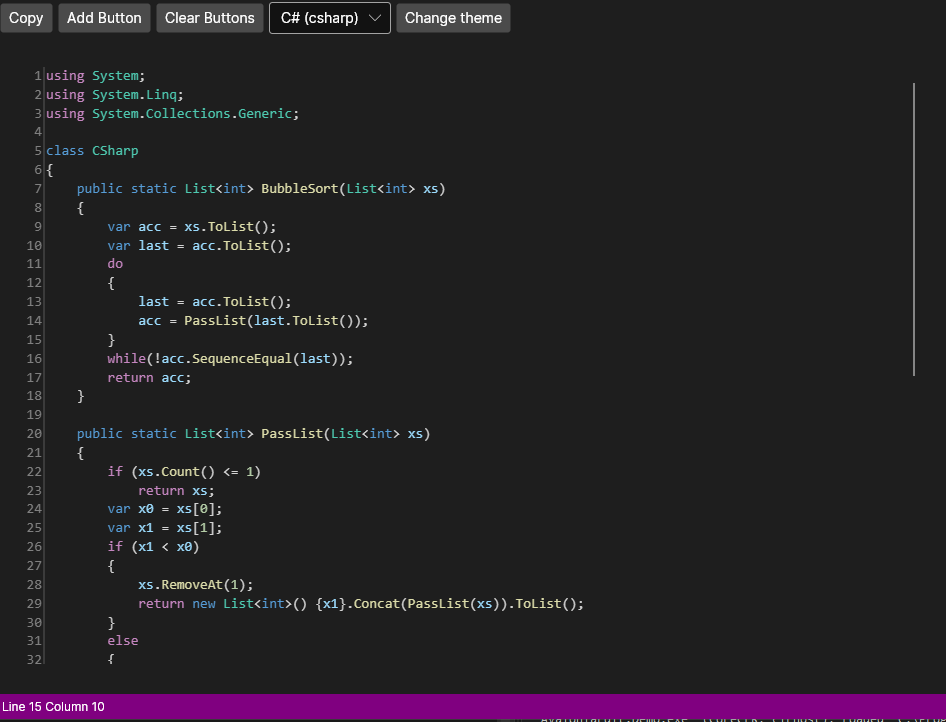
| 22 | Update readme | 0 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10066228 | <NME> README.md
<BEF> [](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
[](https://www.nuget.org/packages/Avalonia.AvaloniaEdit)
# AvaloniaEdit
This project is a port of [AvalonEdit](https://github.com/icsharpcode/AvalonEdit), a WPF-based text editor for [Avalonia](https://github.com/AvaloniaUI/Avalonia).
AvaloniaEdit supports features like:
* Syntax highlighting using [TextMate](https://github.com/danipen/TextMateSharp) grammars and themes.
* Code folding.
* Code completion.
* Fully customizable and extensible.
and many,many more!
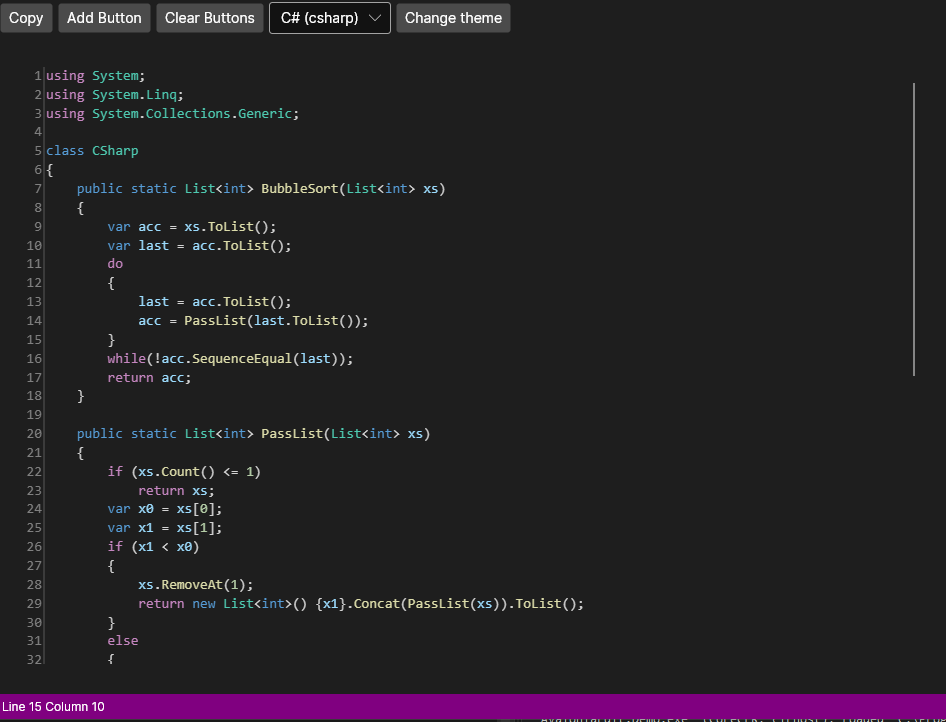
//Initial setup of TextMate.
var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
//Here we are getting the language by the extension and right after that we are initializing grammar with this language.
//And that's all 😀, you are ready to use AvaloniaEdit with syntax highlighting!
_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
```
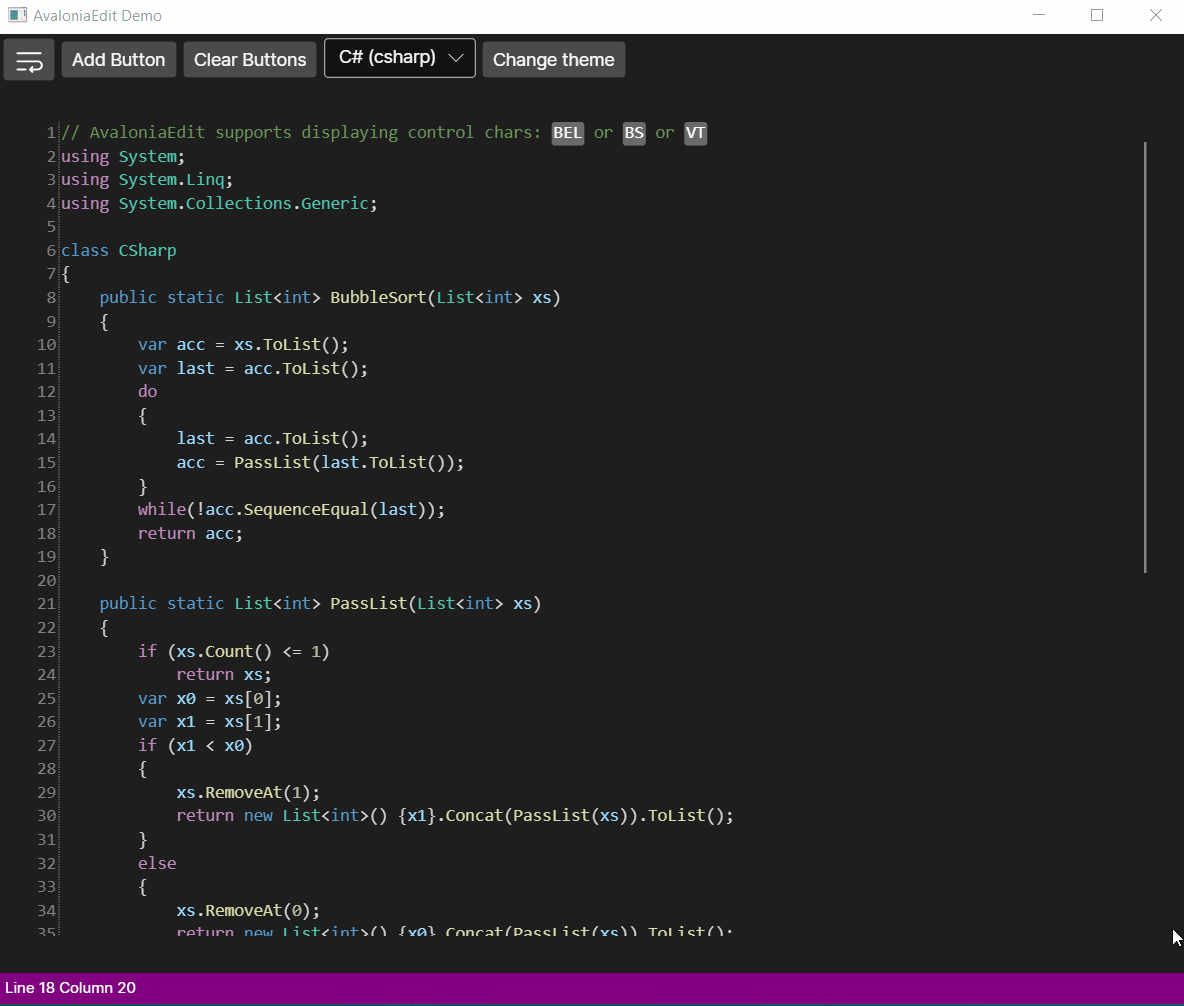
<MSG> Update readme
<DFF> @@ -13,6 +13,28 @@ AvaloniaEdit supports features like:
and many,many more!
+AvaloniaEdit currently consists of 3 packages
+ * [Avalonia.AvaloniaEdit](https://www.nuget.org/packages/Avalonia.AvaloniaEdit) well-known package that incudes text editor itself.
+ * [AvaloniaEdit.TextMate](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) package that adds TextMate integration to the AvaloniaEdit.
+ * [AvaloniaEdit.TextMate.Grammars](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) grammars for TextMate and additional infrastructure that helps you to use them.
+
+ ### How to set up theme and syntax highlighting for my project?
+First of all, if you want to use grammars that we support you should install [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate.Grammars/) with them and [package](https://www.nuget.org/packages/AvaloniaEdit.TextMate/) with TextMate integration otherwise you just install the package with TextMate integration and implement IRegistryOptions interface,that's currently the easiest way in case you want to use AvaloniaEdit with the set of grammars different from in-bundled.
+```csharp
+//First of all you need to have a reference for your TextEditor for it to be used inside AvaloniaEdit.TextMate project.
+var _textEditor = this.FindControl<TextEditor>("Editor");
+
+//Here we initialize RegistryOptions with the theme we want to use.
+var _registryOptions = new RegistryOptions(ThemeName.DarkPlus);
+
+//Initial setup of TextMate.
+var _textMateInstallation = _textEditor.InstallTextMate(_registryOptions);
+
+//Here we are getting the lanuage by the extension and right after that we are initializing grammar with this lanuage.
+//And that all 😀, we are ready to use AvaloniaEdit with syntax highlighting!
+_textMateInstallation.SetGrammar(_registryOptions.GetScopeByLanguageId(_registryOptions.GetLanguageByExtension(".cs").Id));
+```
+
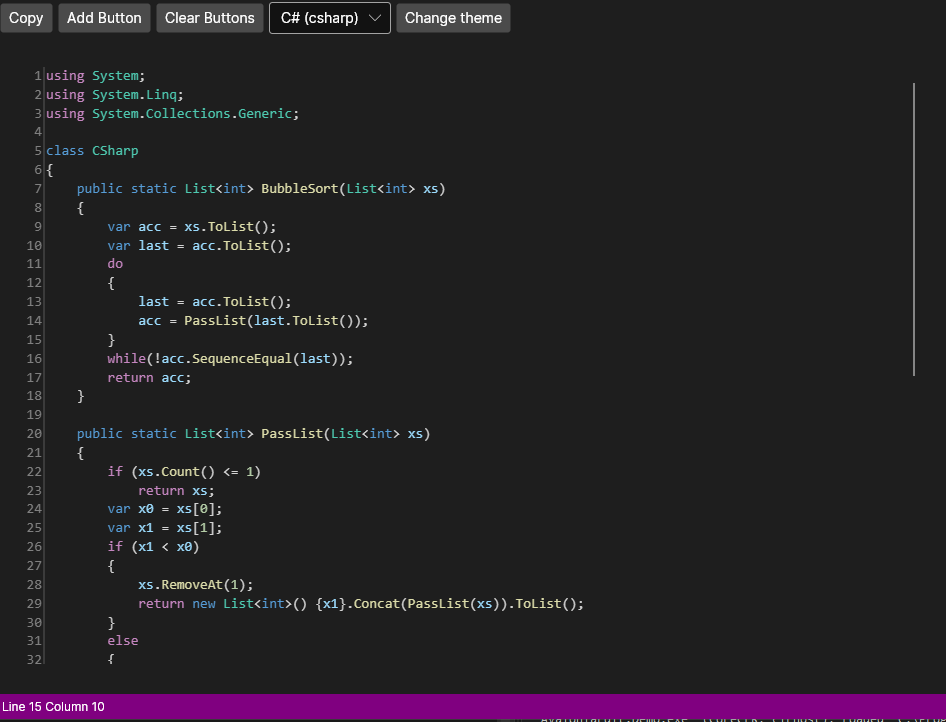
| 22 | Update readme | 0 | .md | md | mit | AvaloniaUI/AvaloniaEdit |
10066229 | <NME> define.js
<BEF>
describe('fruitmachine.define()', function() {
test("Should store the module in fruitmachine.store under module type", function() {
fruitmachine.define({ module: 'my-module-1' });
expect(fruitmachine.modules['my-module-1']).toBeDefined();
});
test("Should return an instantiable constructor", function() {
var View = fruitmachine.define({ module: 'new-module-1' });
var view = new View();
expect(view._fmid).toBeDefined();
expect(view.module()).toBe('new-module-1');
});
test("Should find module from internal module store if a `module` parameter is passed", function() {
var apple = new fruitmachine({ module: 'apple' });
expect(apple.module()).toBe('apple');
expect(apple.template).toBeDefined();
});
assert.defined(apple.template);
},
tearDown: function() {
delete FruitMachine.store['my-module'];
}
});
var View2 = fruitmachine.define(View1.extend({
module: 'new-module-2',
random: 'different',
initialize: initialize2,
setup: setup2
}));
var view1 = new View1()
.render()
.setup();
var view2 = new View2()
.render()
.setup();
expect(View1.prototype._module).toBe('new-module-1');
expect(View2.prototype._module).toBe('new-module-2');
expect(View2.prototype.random).toBe('different');
expect(initialize1.mock.calls.length).toBe(1);
expect(initialize2.mock.calls.length).toBe(1);
expect(setup1.mock.calls.length).toBe(1);
expect(setup2.mock.calls.length).toBe(1);
});
});
<MSG> Protoype now protected, child() and children() depricated soem before events added
<DFF> @@ -22,6 +22,21 @@ buster.testCase('FruitMachine.define()', {
assert.defined(apple.template);
},
+
+ "Defining reserved methods should rewrite keys with prefixed with '_'": function() {
+ var setup = this.spy();
+ var View = FruitMachine.define({
+ module: 'foobar',
+ setup: setup
+ });
+
+ var view = new View()
+ .render()
+ .setup();
+
+ assert.called(setup);
+ },
+
tearDown: function() {
delete FruitMachine.store['my-module'];
}
| 15 | Protoype now protected, child() and children() depricated soem before events added | 0 | .js | js | mit | ftlabs/fruitmachine |
10066230 | <NME> jsgrid.tests.js <BEF> $(function() { var Grid = jsGrid.Grid, JSGRID = "JSGrid", JSGRID_DATA_KEY = JSGRID; Grid.prototype.updateOnResize = false; module("basic"); test("default creation", function() { var gridOptions = { simpleOption: "test", complexOption: { a: "subtest", b: 1, c: {} } }, grid = new Grid("#jsGrid", gridOptions); equal(grid._container[0], $("#jsGrid")[0], "container saved"); equal(grid.simpleOption, "test", "primitive option extended"); equal(grid.complexOption, gridOptions.complexOption, "non-primitive option extended"); }); test("jquery adapter creation", function() { var gridOptions = { option: "test" }, $element = $("#jsGrid"), result = $element.jsGrid(gridOptions), grid = $element.data(JSGRID_DATA_KEY); equal(result, $element, "jquery fn returned source jQueryElement"); ok(grid instanceof Grid, "jsGrid saved to jquery data"); equal(grid.option, "test", "options provided"); }); test("destroy", function() { var $element = $("#jsGrid"), grid; $element.jsGrid({}); grid = $element.data(JSGRID_DATA_KEY); grid.destroy(); strictEqual($element.html(), "", "content is removed"); strictEqual($element.data(JSGRID_DATA_KEY), undefined, "jquery data is removed"); }); test("jquery adapter second call changes option value", function() { var $element = $("#jsGrid"), gridOptions = { option: "test" }, grid; $element.jsGrid(gridOptions); grid = $element.data(JSGRID_DATA_KEY); gridOptions.option = "new test"; $element.jsGrid(gridOptions); equal(grid, $element.data(JSGRID_DATA_KEY), "instance was not changed"); equal(grid.option, "new test", "option changed"); }); test("jquery adapter invokes jsGrid method", function() { var methodResult = "", $element = $("#jsGrid"), gridOptions = { method: function(str) { methodResult = "test_" + str; } }; $element.jsGrid(gridOptions); $element.jsGrid("method", "invoke"); equal(methodResult, "test_invoke", "method invoked"); }); test("onInit callback", function() { var $element = $("#jsGrid"), onInitArguments, gridOptions = { onInit: function(args) { onInitArguments = args; } }; var grid = new Grid($element, gridOptions); equal(onInitArguments.grid, grid, "grid instance is provided in onInit callback arguments"); }); test("controller methods are $.noop when not specified", function() { var $element = $("#jsGrid"), gridOptions = { controller: {} }, testOption; $element.jsGrid(gridOptions); deepEqual($element.data(JSGRID_DATA_KEY)._controller, { loadData: $.noop, insertItem: $.noop, updateItem: $.noop, deleteItem: $.noop }, "controller has stub methods"); }); test("option method", function() { var $element = $("#jsGrid"), gridOptions = { test: "value" }, testOption; $element.jsGrid(gridOptions); testOption = $element.jsGrid("option", "test"); equal(testOption, "value", "read option value"); $element.jsGrid("option", "test", "new_value"); testOption = $element.jsGrid("option", "test"); equal(testOption, "new_value", "set option value"); }); test("fieldOption method", function() { var dataLoadedCount = 0; var $element = $("#jsGrid"), gridOptions = { loadMessage: "", autoload: true, controller: { loadData: function() { dataLoadedCount++; return [{ prop1: "value1", prop2: "value2", prop3: "value3" }]; } }, fields: [ { name: "prop1", title: "_" } ] }; $element.jsGrid(gridOptions); var fieldOptionValue = $element.jsGrid("fieldOption", "prop1", "name"); equal(fieldOptionValue, "prop1", "read field option"); $element.jsGrid("fieldOption", "prop1", "name", "prop2"); equal($element.text(), "_value2", "set field option by field name"); equal(dataLoadedCount, 1, "data not reloaded on field option change"); $element.jsGrid("fieldOption", 0, "name", "prop3"); equal($element.text(), "_value3", "set field option by field index"); }); test("option changing event handlers", function() { var $element = $("#jsGrid"), optionChangingEventArgs, optionChangedEventArgs, gridOptions = { test: "testValue", another: "anotherValue", onOptionChanging: function(e) { optionChangingEventArgs = $.extend({}, e); e.option = "another"; e.newValue = e.newValue + "_" + this.another; }, onOptionChanged: function(e) { optionChangedEventArgs = $.extend({}, e); } }, anotherOption; $element.jsGrid(gridOptions); $element.jsGrid("option", "test", "newTestValue"); equal(optionChangingEventArgs.option, "test", "option name is provided in args of optionChanging"); equal(optionChangingEventArgs.oldValue, "testValue", "old option value is provided in args of optionChanging"); equal(optionChangingEventArgs.newValue, "newTestValue", "new option value is provided in args of optionChanging"); anotherOption = $element.jsGrid("option", "another"); equal(anotherOption, "newTestValue_anotherValue", "option changing handler changed option and value"); equal(optionChangedEventArgs.option, "another", "option name is provided in args of optionChanged"); equal(optionChangedEventArgs.value, "newTestValue_anotherValue", "option value is provided in args of optionChanged"); }); test("common layout rendering", function() { var $element = $("#jsGrid"), grid = new Grid($element, {}), $headerGrid, $headerGridTable, $bodyGrid, $bodyGridTable; ok($element.hasClass(grid.containerClass), "container class attached"); ok($element.children().eq(0).hasClass(grid.gridHeaderClass), "grid header"); ok($element.children().eq(1).hasClass(grid.gridBodyClass), "grid body"); ok($element.children().eq(2).hasClass(grid.pagerContainerClass), "pager container"); $headerGrid = $element.children().eq(0); $headerGridTable = $headerGrid.children().first(); ok($headerGridTable.hasClass(grid.tableClass), "header table"); equal($headerGrid.find("." + grid.headerRowClass).length, 1, "header row"); equal($headerGrid.find("." + grid.filterRowClass).length, 1, "filter row"); equal($headerGrid.find("." + grid.insertRowClass).length, 1, "insert row"); ok(grid._headerRow.hasClass(grid.headerRowClass), "header row class"); ok(grid._filterRow.hasClass(grid.filterRowClass), "filter row class"); ok(grid._insertRow.hasClass(grid.insertRowClass), "insert row class"); $bodyGrid = $element.children().eq(1); $bodyGridTable = $bodyGrid.children().first(); ok($bodyGridTable.hasClass(grid.tableClass), "body table"); equal(grid._content.parent()[0], $bodyGridTable[0], "content is tbody in body table"); equal($bodyGridTable.find("." + grid.noDataRowClass).length, 1, "no data row"); equal($bodyGridTable.text(), grid.noDataContent, "no data text"); }); test("set default options with setDefaults", function() { jsGrid.setDefaults({ defaultOption: "test" }); var $element = $("#jsGrid").jsGrid({}); equal($element.jsGrid("option", "defaultOption"), "test", "default option set"); }); module("loading"); test("loading with controller", function() { var $element = $("#jsGrid"), data = [ { test: "test1" }, { test: "test2" } ], gridOptions = { controller: { loadData: function() { return data; } } }, grid = new Grid($element, gridOptions); grid.loadData(); equal(grid.option("data"), data, "loadData loads data"); }); test("loadData throws exception when controller method not found", function() { var $element = $("#jsGrid"); var grid = new Grid($element); grid._controller = {}; throws(function() { grid.loadData(); }, /loadData/, "loadData threw an exception"); }); test("onError event should be fired on controller fail", function() { var errorArgs, errorFired = 0, $element = $("#jsGrid"), gridOptions = { controller: { loadData: function() { return $.Deferred().reject({ value: 1 }, "test").promise(); } }, onError: function(args) { errorFired++; errorArgs = args; } }, grid = new Grid($element, gridOptions); grid.loadData(); equal(errorFired, 1, "onError handler fired"); deepEqual(errorArgs, { grid: grid, args: [{ value: 1 }, "test"] }, "error has correct params"); }); asyncTest("autoload should call loadData after render", 1, function() { new Grid($("#jsGrid"), { autoload: true, controller: { loadData: function() { ok(true, "autoload calls loadData on creation"); start(); return []; } } }); }); test("loading filtered data", function() { var filteredData, loadingArgs, loadedArgs, $element = $("#jsGrid"), data = [ { field: "test" }, { field: "test_another" }, { field: "test_another" }, { field: "test" } ], gridOptions = { filtering: true, fields: [ { name: "field", filterValue: function(value) { return "test"; } } ], onDataLoading: function(e) { loadingArgs = $.extend(true, {}, e); }, onDataLoaded: function(e) { loadedArgs = $.extend(true, {}, e); }, controller: { loadData: function(filter) { filteredData = $.grep(data, function(item) { return item.field === filter.field; }); return filteredData; } } }, grid = new Grid($element, gridOptions); grid.loadData(); equal(loadingArgs.filter.field, "test"); equal(grid.option("data").length, 2, "filtered data loaded"); deepEqual(loadedArgs.data, filteredData); }); asyncTest("loading indication", function() { var timeout = 10, stage = "initial", $element = $("#jsGrid"), gridOptions = { loadIndication: true, loadIndicationDelay: timeout, loadMessage: "loading...", loadIndicator: function(config) { equal(config.message, gridOptions.loadMessage, "message provided"); ok(config.container.jquery, "grid container is provided"); return { show: function() { stage = "started"; }, hide: function() { stage = "finished"; } }; }, fields: [ { name: "field" } ], controller: { loadData: function() { var deferred = $.Deferred(); equal(stage, "initial", "initial stage"); setTimeout(function() { equal(stage, "started", "loading started"); deferred.resolve([]); equal(stage, "finished", "loading finished"); start(); }, timeout); return deferred.promise(); } } }, grid = new Grid($element, gridOptions); grid.loadData(); }); asyncTest("loadingIndication=false should not show loading", 0, function() { var $element = $("#jsGrid"), timeout = 10, gridOptions = { loadIndication: false, loadIndicationDelay: timeout, loadIndicator: function() { return { show: function() { ok(false, "should not call show"); }, hide: function() { ok(false, "should not call hide"); } }; }, fields: [ { name: "field" } ], controller: { loadData: function() { var deferred = $.Deferred(); setTimeout(function() { deferred.resolve([]); start(); }, timeout); return deferred.promise(); } } }, grid = new Grid($element, gridOptions); grid.loadData(); }); test("search", function() { var $element = $("#jsGrid"), data = [ { field: "test" }, { field: "test_another" }, { field: "test_another" }, { field: "test" } ], gridOptions = { pageIndex: 2, _sortField: "field", _sortOrder: "desc", filtering: true, fields: [ { name: "field", filterValue: function(value) { return "test"; } } ], controller: { loadData: function(filter) { var filteredData = $.grep(data, function(item) { return item.field === filter.field; }); return filteredData; } } }, grid = new Grid($element, gridOptions); grid.search(); equal(grid.option("data").length, 2, "data filtered"); strictEqual(grid.option("pageIndex"), 1, "pageIndex reset"); strictEqual(grid._sortField, null, "sortField reset"); strictEqual(grid._sortOrder, "asc", "sortOrder reset"); }); test("change loadStrategy on the fly", function() { var $element = $("#jsGrid"); var gridOptions = { controller: { loadData: function() { return []; } } }; var grid = new Grid($element, gridOptions); grid.option("loadStrategy", { firstDisplayIndex: function() { return 0; }, lastDisplayIndex: function() { return 1; }, loadParams: function() { return []; }, finishLoad: function() { grid.option("data", [{}]); } }); grid.loadData(); equal(grid.option("data").length, 1, "new load strategy is applied"); }); module("filtering"); test("filter rendering", function() { var $element = $("#jsGrid"), gridOptions = { filtering: true, fields: [ { name: "test", align: "right", filtercss: "filter-class", filterTemplate: function() { var result = this.filterControl = $("<input>").attr("type", "text").addClass("filter-input"); return result; } } ] }, grid = new Grid($element, gridOptions); equal(grid._filterRow.find(".filter-class").length, 1, "filtercss class is attached"); equal(grid._filterRow.find(".filter-input").length, 1, "filter control rendered"); equal(grid._filterRow.find("." + grid.cellClass).length, 1, "cell class is attached"); ok(grid._filterRow.find(".filter-class").hasClass("jsgrid-align-right"), "align class is attached"); ok(grid.fields[0].filterControl.is("input[type=text]"), "filter control saved in field"); }); test("filter get/clear", function() { var $element = $("#jsGrid"), gridOptions = { filtering: true, controller: { loadData: function() { return []; } }, fields: [ { name: "field", filterTemplate: function() { return this.filterControl = $("<input>").attr("type", "text"); }, filterValue: function() { return this.filterControl.val(); } } ] }, grid = new Grid($element, gridOptions); grid.fields[0].filterControl.val("test"); deepEqual(grid.getFilter(), { field: "test" }, "get filter"); }); test("grid fields normalization", function() { var $element = $("#jsGrid"), field1, field2, gridOptions = { fields: [ new jsGrid.Field("text1", { filterValue: function(value) { if(!arguments.length) { { name: "text2", title: "title2" } ] }, grid = new Grid($element, gridOptions); field1 = grid.fields[0]; ok(field1 instanceof jsGrid.Field); equal(field1.name, "text1", "name is set for field"); equal(field1.title, "title1", "title field"); field2 = grid.fields[1]; ok(field2 instanceof jsGrid.Field); equal(field2.name, "text2", "name is set for field"); equal(field2.title, "title2", "title field"); }); test("grid field name used for header if title is not specified", function() { data = [ { field: "test" }, { field: "test_another" }, { field: "test_another" }, { field: "test" } ], gridOptions = { fields: [ { name: "field" } ], controller: { loadData: function(filter) { var filteredData = $.grep(data, function(item) { return item.field === filter.field; }); return filteredData; } } }, grid = new Grid($element, gridOptions); grid.search({ field: "test" }); equal(grid.option("data").length, 2, "data filtered"); }); test("filtering with static data should not do actual filtering", function() { var $element = $("#jsGrid"), gridOptions = { filtering: true, fields: [ { type: "text", name: "field" } ], data: [ { name: "value1" }, { name: "value2" } ] }, grid = new Grid($element, gridOptions); grid._filterRow.find("input").val("1"); grid.search(); equal(grid.option("data").length, 2, "data is not filtered"); }); module("nodatarow"); test("nodatarow after bind on empty array", function() { var $element = $("#jsGrid"), gridOptions = {}, grid = new Grid($element, gridOptions); grid.option("data", []); equal(grid._content.find("." + grid.noDataRowClass).length, 1, "no data row rendered"); equal(grid._content.find("." + grid.cellClass).length, 1, "grid cell class attached"); equal(grid._content.text(), grid.noDataContent, "no data text rendered"); }); test("nodatarow customize content", function() { var noDataMessage = "NoData Custom Content", $element = $("#jsGrid"), gridOptions = { noDataContent: function() { return noDataMessage; } }, grid = new Grid($element, gridOptions); grid.option("data", []); equal(grid._content.find("." + grid.cellClass).length, 1, "grid cell class attached"); equal(grid._content.text(), noDataMessage, "custom noDataContent"); }); module("row rendering", { setup: function() { this.testData = [ { id: 1, text: "test1" }, { id: 2, text: "test2" }, { id: 3, text: "test3" } ]; } }); test("rows rendered correctly", function() { var $element = $("#jsGrid"), gridOptions = { data: this.testData }, grid = new Grid($element, gridOptions); equal(grid._content.children().length, 3, "rows rendered"); equal(grid._content.find("." + grid.oddRowClass).length, 2, "two odd rows for 3 items"); equal(grid._content.find("." + grid.evenRowClass).length, 1, "one even row for 3 items"); }); test("custom rowClass", function() { var $element = $("#jsGrid"), gridOptions = { data: this.testData, rowClass: "custom-row-cls" }, grid = new Grid($element, gridOptions); equal(grid._content.find("." + grid.oddRowClass).length, 2); equal(grid._content.find("." + grid.evenRowClass).length, 1); equal(grid._content.find(".custom-row-cls").length, 3, "custom row class"); }); test("custom rowClass callback", function() { var $element = $("#jsGrid"), gridOptions = { data: this.testData, rowClass: function(item, index) { return item.text; } }, grid = new Grid($element, gridOptions); equal(grid._content.find("." + grid.oddRowClass).length, 2); equal(grid._content.find("." + grid.evenRowClass).length, 1); equal(grid._content.find(".test1").length, 1, "custom row class"); equal(grid._content.find(".test2").length, 1, "custom row class"); equal(grid._content.find(".test3").length, 1, "custom row class"); }); test("rowClick standard handler", function() { var $element = $("#jsGrid"), $secondRow, grid = new Grid($element, { editing: true }); grid.option("data", this.testData); $secondRow = grid._content.find("." + grid.evenRowClass); $secondRow.trigger("click", $.Event($secondRow)); equal(grid._editingRow.get(0), $secondRow.get(0), "clicked row is editingRow"); }); test("rowClick handler", function() { var rowClickArgs, $element = $("#jsGrid"), $secondRow, gridOptions = { rowClick: function(args) { rowClickArgs = args; } }, grid = new Grid($element, gridOptions); grid.option("data", this.testData); $secondRow = grid._content.find("." + grid.evenRowClass); $secondRow.trigger("click", $.Event($secondRow)); ok(rowClickArgs.event instanceof jQuery.Event, "jquery event arg"); equal(rowClickArgs.item, this.testData[1], "item arg"); equal(rowClickArgs.itemIndex, 1, "itemIndex arg"); }); test("row selecting with selectedRowClass", function() { var $element = $("#jsGrid"), $secondRow, gridOptions = { selecting: true }, grid = new Grid($element, gridOptions); grid.option("data", this.testData); $secondRow = grid._content.find("." + grid.evenRowClass); $secondRow.trigger("mouseenter", $.Event($secondRow)); ok($secondRow.hasClass(grid.selectedRowClass), "mouseenter adds selectedRowClass"); $secondRow.trigger("mouseleave", $.Event($secondRow)); ok(!$secondRow.hasClass(grid.selectedRowClass), "mouseleave removes selectedRowClass"); }); test("no row selecting while selection is disabled", function() { var $element = $("#jsGrid"), $secondRow, gridOptions = { selecting: false }, grid = new Grid($element, gridOptions); grid.option("data", this.testData); $secondRow = grid._content.find("." + grid.evenRowClass); $secondRow.trigger("mouseenter", $.Event($secondRow)); ok(!$secondRow.hasClass(grid.selectedRowClass), "mouseenter doesn't add selectedRowClass"); }); test("refreshing and refreshed callbacks", function() { var refreshingEventArgs, refreshedEventArgs, $element = $("#jsGrid"), grid = new Grid($element, {}); grid.onRefreshing = function(e) { refreshingEventArgs = e; equal(grid._content.find("." + grid.oddRowClass).length, 0, "no items before refresh"); }; grid.onRefreshed = function(e) { refreshedEventArgs = e; equal(grid._content.find("." + grid.oddRowClass).length, 2, "items rendered after refresh"); }; grid.option("data", this.testData); equal(refreshingEventArgs.grid, grid, "grid provided in args for refreshing event"); equal(refreshedEventArgs.grid, grid, "grid provided in args for refreshed event"); equal(grid._content.find("." + grid.oddRowClass).length, 2, "items rendered"); }); test("grid fields normalization", function() { var CustomField = function(config) { $.extend(true, this, config); }; jsGrid.fields.custom = CustomField; try { var $element = $("#jsGrid"), gridOptions = { fields: [ new jsGrid.Field({ name: "text1", title: "title1" }), { name: "text2", title: "title2" }, { name: "text3", type: "custom" } ] }, grid = new Grid($element, gridOptions); var field1 = grid.fields[0]; ok(field1 instanceof jsGrid.Field); equal(field1.name, "text1", "name is set for field"); equal(field1.title, "title1", "title field"); var field2 = grid.fields[1]; ok(field2 instanceof jsGrid.Field); equal(field2.name, "text2", "name is set for field"); equal(field2.title, "title2", "title field"); var field3 = grid.fields[2]; ok(field3 instanceof CustomField); equal(field3.name, "text3", "name is set for field"); } finally { delete jsGrid.fields.custom; } }); test("'0' itemTemplate should be rendered", function() { var $element = $("#jsGrid"), grid = new Grid($element, { data: [{}], fields: [ new jsGrid.Field({ name: "id", itemTemplate: function() { return 0; } }) ] }); equal(grid._bodyGrid.text(), "0", "item template is rendered"); }); test("grid field name used for header if title is not specified", function() { var $element = $("#jsGrid"), grid = new Grid($element, { fields: [ new jsGrid.Field({ name: "id" }) ] }); equal(grid._headerRow.text(), "id", "name is rendered in header"); }); test("grid fields header and item rendering", function() { var $element = $("#jsGrid"), $secondRow, gridOptions = { fields: [ new jsGrid.Field({ name: "text", title: "title", css: "cell-class", headercss: "header-class", align: "right" }) ] }, grid = new Grid($element, gridOptions); grid.option("data", this.testData); equal(grid._headerRow.text(), "title", "header rendered"); equal(grid._headerRow.find("." + grid.headerCellClass).length, 1, "header cell class is attached"); equal(grid._headerRow.find(".header-class").length, 1, "headercss class is attached"); ok(grid._headerRow.find(".header-class").hasClass("jsgrid-align-right"), "align class is attached"); $secondRow = grid._content.find("." + grid.evenRowClass); equal($secondRow.text(), "test2", "item rendered"); equal($secondRow.find(".cell-class").length, 1, "css class added to cell"); equal($secondRow.find("." + grid.cellClass).length, 1, "cell class is attached"); ok($secondRow.find(".cell-class").hasClass("jsgrid-align-right"), "align class added to cell"); }); test("grid field cellRenderer", function() { var testItem = { text: "test" }, args; var $grid = $("#jsGrid"); var gridOptions = { data: [testItem], fields: [ { name: "text", cellRenderer: function(value, item) { args = { value: value, item: item }; return $("<td>").addClass("custom-class").text(value); } } ] }; var grid = new Grid($grid, gridOptions); var $customCell = $grid.find(".custom-class"); equal($customCell.length, 1, "custom cell rendered"); equal($customCell.text(), "test"); deepEqual(args, { value: "test", item: testItem }, "cellRenderer args provided"); }); test("grid field 'visible' option", function() { var $grid = $("#jsGrid"); var gridOptions = { editing: true, fields: [ { name: "id", visible: false }, { name: "test" } ] }; var grid = new Grid($grid, gridOptions); equal($grid.find("." + grid.noDataRowClass).children().eq(0).prop("colspan"), 1, "no data row colspan only for visible cells"); grid.option("data", this.testData); grid.editItem(this.testData[2]); equal($grid.find("." + grid.headerRowClass).children().length, 1, "header single cell"); equal($grid.find("." + grid.filterRowClass).children().length, 1, "filter single cell"); equal($grid.find("." + grid.insertRowClass).children().length, 1, "insert single cell"); equal($grid.find("." + grid.editRowClass).children().length, 1, "edit single cell"); equal($grid.find("." + grid.oddRowClass).eq(0).children().length, 1, "odd data row single cell"); equal($grid.find("." + grid.evenRowClass).eq(0).children().length, 1, "even data row single cell"); }); module("inserting"); test("inserting rendering", function() { var $element = $("#jsGrid"), gridOptions = { inserting: true, fields: [ { name: "test", align: "right", insertcss: "insert-class", insertTemplate: function() { var result = this.insertControl = $("<input>").attr("type", "text").addClass("insert-input"); return result; } } ] }, grid = new Grid($element, gridOptions); equal(grid._insertRow.find(".insert-class").length, 1, "insertcss class is attached"); equal(grid._insertRow.find(".insert-input").length, 1, "insert control rendered"); equal(grid._insertRow.find("." + grid.cellClass).length, 1, "cell class is attached"); ok(grid._insertRow.find(".insert-class").hasClass("jsgrid-align-right"), "align class is attached"); ok(grid.fields[0].insertControl.is("input[type=text]"), "insert control saved in field"); }); test("field without inserting", function() { var $element = $("#jsGrid"), jsGridFieldConfig = { insertTemplate: function() { var result = this.insertControl = $("<input>").attr("type", "text"); return result; }, insertValue: function() { return this.insertControl.val(); } }, gridOptions = { inserting: true, fields: [ $.extend({}, jsGridFieldConfig, { name: "field1", inserting: false }), $.extend({}, jsGridFieldConfig, { name: "field2" }) ] }, grid = new Grid($element, gridOptions); grid.fields[0].insertControl.val("test1"); grid.fields[1].insertControl.val("test2"); deepEqual(grid._getInsertItem(), { field2: "test2" }, "field with inserting=false is not included in inserting item"); }); test("insert data with default location", function() { var $element = $("#jsGrid"), inserted = false, insertingArgs, insertedArgs, gridOptions = { inserting: true, data: [{field: "default"}], fields: [ { name: "field", insertTemplate: function() { var result = this.insertControl = $("<input>").attr("type", "text"); return result; }, insertValue: function() { return this.insertControl.val(); } } ], onItemInserting: function(e) { insertingArgs = $.extend(true, {}, e); }, onItemInserted: function(e) { insertedArgs = $.extend(true, {}, e); }, controller: { insertItem: function() { inserted = true; } } }, grid = new Grid($element, gridOptions); grid.fields[0].insertControl.val("test"); grid.insertItem(); equal(insertingArgs.item.field, "test", "field is provided in inserting args"); equal(grid.option("data").length, 2, "data is inserted"); ok(inserted, "controller insertItem was called"); deepEqual(grid.option("data")[1], { field: "test" }, "correct data is inserted"); equal(insertedArgs.item.field, "test", "field is provided in inserted args"); }); test("insert data with specified insert location", function() { var $element = $("#jsGrid"), inserted = false, insertingArgs, insertedArgs, gridOptions = { inserting: true, insertRowLocation: "top", data: [{field: "default"}], fields: [ { name: "field", insertTemplate: function() { var result = this.insertControl = $("<input>").attr("type", "text"); return result; }, insertValue: function() { return this.insertControl.val(); } } ], onItemInserting: function(e) { insertingArgs = $.extend(true, {}, e); }, onItemInserted: function(e) { insertedArgs = $.extend(true, {}, e); }, controller: { insertItem: function() { inserted = true; } } }, grid = new Grid($element, gridOptions); grid.fields[0].insertControl.val("test"); grid.insertItem(); equal(insertingArgs.item.field, "test", "field is provided in inserting args"); equal(grid.option("data").length, 2, "data is inserted"); ok(inserted, "controller insertItem was called"); deepEqual(grid.option("data")[0], { field: "test" }, "correct data is inserted at the beginning"); equal(insertedArgs.item.field, "test", "field is provided in inserted args"); }); test("insertItem accepts item to insert", function() { var $element = $("#jsGrid"), itemToInsert = { field: "test" }, insertedItem, gridOptions = { data: [], fields: [ { name: "field" } ], controller: { insertItem: function(item) { insertedItem = item; } } }, grid = new Grid($element, gridOptions); grid.insertItem(itemToInsert); deepEqual(grid.option("data")[0], itemToInsert, "data is inserted"); deepEqual(insertedItem, itemToInsert, "controller insertItem was called with correct item"); }); module("editing"); test("editing rendering", function() { var $element = $("#jsGrid"), $editRow, data = [{ test: "value" }], gridOptions = { editing: true, fields: [ { name: "test", align: "right", editcss: "edit-class", editTemplate: function(value) { var result = this.editControl = $("<input>").attr("type", "text").val(value).addClass("edit-input"); return result; } } ] }, grid = new Grid($element, gridOptions); grid.option("data", data); equal(grid._content.find("." + grid.editRowClass).length, 0, "no edit row after initial rendering"); grid.editItem(data[0]); $editRow = grid._content.find("." + grid.editRowClass); equal($editRow.length, 1, "edit row rendered"); equal($editRow.find(".edit-class").length, 1, "editcss class is attached"); equal($editRow.find(".edit-input").length, 1, "edit control rendered"); equal($editRow.find("." + grid.cellClass).length, 1, "cell class is attached"); ok($editRow.find(".edit-class").hasClass("jsgrid-align-right"), "align class is attached"); ok(grid.fields[0].editControl.is("input[type=text]"), "edit control saved in field"); equal(grid.fields[0].editControl.val(), "value", "edit control value"); }); test("editItem accepts row to edit", function() { var $element = $("#jsGrid"), $editRow, data = [ { test: "value" } ], gridOptions = { editing: true, fields: [ { name: "test" } ] }, grid = new Grid($element, gridOptions); grid.option("data", data); var $row = $element.find("." + grid.oddRowClass).eq(0); grid.editItem($row); $editRow = grid._content.find("." + grid.editRowClass); equal($editRow.length, 1, "edit row rendered"); grid.cancelEdit(); grid.editItem($row.get(0)); $editRow = grid._content.find("." + grid.editRowClass); equal($editRow.length, 1, "edit row rendered"); }); test("edit item", function() { var $element = $("#jsGrid"), editingArgs, editingRow, updated = false, updatingArgs, updatingRow, updatedRow, updatedArgs, data = [{ field: "value" }], gridOptions = { editing: true, fields: [ { name: "field", editTemplate: function(value) { var result = this.editControl = $("<input>").attr("type", "text").val(value); return result; }, editValue: function() { return this.editControl.val(); } } ], controller: { updateItem: function(updatingItem) { updated = true; } }, onItemEditing: function(e) { editingArgs = $.extend(true, {}, e); editingRow = grid.rowByItem(data[0])[0]; }, onItemUpdating: function(e) { updatingArgs = $.extend(true, {}, e); updatingRow = grid.rowByItem(data[0])[0]; }, onItemUpdated: function(e) { updatedArgs = $.extend(true, {}, e); updatedRow = grid.rowByItem(data[0])[0]; } }, grid = new Grid($element, gridOptions); grid.option("data", data); grid.editItem(data[0]); deepEqual(editingArgs.item, { field: "value" }, "item before editing is provided in editing event args"); equal(editingArgs.itemIndex, 0, "itemIndex is provided in editing event args"); equal(editingArgs.row[0], editingRow, "row element is provided in editing event args"); grid.fields[0].editControl.val("new value"); grid.updateItem(); deepEqual(updatingArgs.previousItem, { field: "value" }, "item before editing is provided in updating event args"); deepEqual(updatingArgs.item, { field: "new value" }, "updating item is provided in updating event args"); equal(updatingArgs.itemIndex, 0, "itemIndex is provided in updating event args"); equal(updatingArgs.row[0], updatingRow, "row element is provided in updating event args"); ok(updated, "controller updateItem called"); deepEqual(grid.option("data")[0], { field: "new value" }, "correct data updated"); equal(grid._content.find("." + grid.editRowClass).length, 0, "edit row removed"); equal(grid._content.find("." + grid.oddRowClass).length, 1, "data row rendered"); deepEqual(updatedArgs.previousItem, { field: "value" }, "item before editing is provided in updated event args"); deepEqual(updatedArgs.item, { field: "new value" }, "updated item is provided in updated event args"); equal(updatedArgs.itemIndex, 0, "itemIndex is provided in updated event args"); equal(updatedArgs.row[0], updatedRow, "row element is provided in updated event args"); }); test("failed update should not change original item", function() { var $element = $("#jsGrid"), data = [{ field: "value" }], gridOptions = { editing: true, fields: [ { name: "field", editTemplate: function(value) { var result = this.editControl = $("<input>").attr("type", "text").val(value); return result; }, editValue: function() { return this.editControl.val(); } } ], controller: { updateItem: function(updatingItem) { return $.Deferred().reject(); } } }, grid = new Grid($element, gridOptions); grid.option("data", data); grid.editItem(data[0]); grid.fields[0].editControl.val("new value"); grid.updateItem(); deepEqual(grid.option("data")[0], { field: "value" }, "value is not updated"); }); test("cancel edit", function() { var $element = $("#jsGrid"), updated = false, cancellingArgs, cancellingRow, data = [{ field: "value" }], gridOptions = { editing: true, fields: [ { name: "field", editTemplate: function(value) { var result = this.editControl = $("<input>").attr("type", "text").val(value); return result; }, editValue: function() { return this.editControl.val(); } } ], controller: { updateData: function(updatingItem) { updated = true; } }, onItemEditCancelling: function(e) { cancellingArgs = $.extend(true, {}, e); cancellingRow = grid.rowByItem(data[0])[0]; } }, grid = new Grid($element, gridOptions); grid.option("data", data); grid.editItem(data[0]); grid.fields[0].editControl.val("new value"); grid.cancelEdit(); deepEqual(cancellingArgs.item, { field: "value" }, "item before cancel is provided in cancelling event args"); equal(cancellingArgs.itemIndex, 0, "itemIndex is provided in cancelling event args"); equal(cancellingArgs.row[0], cancellingRow, "row element is provided in cancelling event args"); ok(!updated, "controller updateItem was not called"); deepEqual(grid.option("data")[0], { field: "value" }, "data were not updated"); equal(grid._content.find("." + grid.editRowClass).length, 0, "edit row removed"); equal(grid._content.find("." + grid.oddRowClass).length, 1, "data row restored"); }); test("updateItem accepts item to update and new item", function() { var $element = $("#jsGrid"), updatingItem, data = [{ field: "value" }], gridOptions = { fields: [ { name: "field" } ], controller: { updateItem: function(item) { return updatingItem = item; } } }, grid = new Grid($element, gridOptions); grid.option("data", data); grid.updateItem(data[0], { field: "new value" }); deepEqual(updatingItem, { field: "new value" }, "controller updateItem called correctly"); deepEqual(grid.option("data")[0], { field: "new value" }, "correct data updated"); }); test("updateItem accepts single argument - item to update", function() { var $element = $("#jsGrid"), updatingItem, data = [{ field: "value" }], gridOptions = { fields: [ { name: "field" } ], controller: { updateItem: function(item) { return updatingItem = item; } } }, grid = new Grid($element, gridOptions); grid.option("data", data); data[0].field = "new value"; grid.updateItem(data[0]); deepEqual(updatingItem, { field: "new value" }, "controller updateItem called correctly"); deepEqual(grid.option("data")[0], { field: "new value" }, "correct data updated"); }); test("editRowRenderer", function() { var $element = $("#jsGrid"), data = [ { value: "test" } ], gridOptions = { data: data, editing: true, editRowRenderer: function(item, itemIndex) { return $("<tr>").addClass("custom-edit-row").append($("<td>").text(itemIndex + ":" + item.value)); }, fields: [ { name: "value" } ] }, grid = new Grid($element, gridOptions); grid.editItem(data[0]); var $editRow = grid._content.find(".custom-edit-row"); equal($editRow.length, 1, "edit row rendered"); equal($editRow.text(), "0:test", "custom edit row renderer rendered"); }); module("deleting"); test("delete item", function() { var $element = $("#jsGrid"), deleted = false, deletingArgs, deletedArgs, data = [{ field: "value" }], gridOptions = { confirmDeleting: false, fields: [ { name: "field" } ], controller: { deleteItem: function(deletingItem) { deleted = true; } }, onItemDeleting: function(e) { deletingArgs = $.extend(true, {}, e); }, onItemDeleted: function(e) { deletedArgs = $.extend(true, {}, e); } }, grid = new Grid($element, gridOptions); grid.option("data", data); grid.deleteItem(data[0]); deepEqual(deletingArgs.item, { field: "value" }, "field and value is provided in deleting event args"); equal(deletingArgs.itemIndex, 0, "itemIndex is provided in updating event args"); equal(deletingArgs.row.length, 1, "row element is provided in updating event args"); ok(deleted, "controller deleteItem called"); equal(grid.option("data").length, 0, "data row deleted"); deepEqual(deletedArgs.item, { field: "value" }, "item is provided in updating event args"); equal(deletedArgs.itemIndex, 0, "itemIndex is provided in updating event args"); equal(deletedArgs.row.length, 1, "row element is provided in updating event args"); }); test("deleteItem accepts row", function() { var $element = $("#jsGrid"), deletedItem, itemToDelete = { field: "value" }, data = [itemToDelete], gridOptions = { confirmDeleting: false, fields: [ { name: "field" } ], controller: { deleteItem: function(deletingItem) { deletedItem = deletingItem; } } }, grid = new Grid($element, gridOptions); grid.option("data", data); var $row = $element.find("." + grid.oddRowClass).eq(0); grid.deleteItem($row); deepEqual(deletedItem, itemToDelete, "controller deleteItem called correctly"); equal(grid.option("data").length, 0, "data row deleted"); }); module("paging"); test("pager is rendered if necessary", function() { var $element = $("#jsGrid"), grid = new Grid($element, { data: [{}, {}, {}], paging: false, pageSize: 2 }); ok(grid._pagerContainer.is(":hidden"), "pager is hidden when paging=false"); equal(grid._pagerContainer.html(), "", "pager is not rendered when paging=false"); grid.option("paging", true); ok(grid._pagerContainer.is(":visible"), "pager is visible when paging=true"); ok(grid._pagerContainer.html(), "pager is rendered when paging=true"); grid.option("data", [{}, {}]); ok(grid._pagerContainer.is(":hidden"), "pager is hidden for single page"); ok(grid._pagerContainer.html(), "pager is rendered for single page when paging=true"); }); test("external pagerContainer", function() { var $pagerContainer = $("<div>").appendTo("#qunit-fixture").hide(), $element = $("#jsGrid"); new Grid($element, { data: [{}, {}, {}], pagerContainer: $pagerContainer, paging: true, pageSize: 2 }); ok($pagerContainer.is(":visible"), "external pager shown"); ok($pagerContainer.html(), "external pager rendered"); }); test("pager functionality", function() { var $element = $("#jsGrid"), pager, pageChangedArgs, grid = new Grid($element, { data: [{}, {}, {}, {}, {}, {}, {}, {}, {}], onPageChanged: function(args) { pageChangedArgs = args; }, paging: true, pageSize: 2, pageButtonCount: 3 }); equal(grid._pagesCount(), 5, "correct page count"); equal(grid.option("pageIndex"), 1, "pageIndex is initialized"); equal(grid._firstDisplayingPage, 1, "_firstDisplayingPage is initialized"); pager = grid._pagerContainer; equal(pager.find("." + grid.currentPageClass).length, 1, "there is one current page"); ok(pager.find("." + grid.pageClass).eq(0).hasClass(grid.currentPageClass), "first page is current"); equal(pager.find("." + grid.pageClass).length, 3, "three pages displayed"); equal(pager.find("." + grid.pagerNavButtonClass).length, 5, "five nav buttons displayed: Fisrt Prev Next Last ..."); equal(pager.find("." + grid.pagerNavButtonInactiveClass).length, 2, "two nav buttons inactive: Fisrt Prev"); grid.openPage(2); equal(pager.find("." + grid.currentPageClass).length, 1, "there is one current page"); ok(pager.find("." + grid.pageClass).eq(1).hasClass(grid.currentPageClass), "second page is current"); equal(pager.find("." + grid.pageClass).length, 3, "three pages displayed"); equal(pager.find("." + grid.pagerNavButtonClass).length, 5, "five nav buttons displayed: First Prev Next Last and ..."); equal(pageChangedArgs.pageIndex, 2, "onPageChanged callback provides pageIndex in arguments"); grid.showNextPages(); equal(grid._firstDisplayingPage, 3, "navigate by pages forward"); grid.showPrevPages(); equal(grid._firstDisplayingPage, 1, "navigate by pages backward"); grid.openPage(5); equal(grid._firstDisplayingPage, 3, "opening next non-visible page moves first displaying page forward"); grid.openPage(2); equal(grid._firstDisplayingPage, 2, "opening prev non-visible page moves first displaying page backward"); }); test("pager format", function() { var $element = $("#jsGrid"), grid = new Grid($element, { data: [{}, {}, {}, {}, {}, {}], paging: true, pageSize: 2, pageIndex: 2, pageButtonCount: 1, pagerFormat: "a {pageIndex} {first} {prev} {pages} {next} {last} {pageCount} {itemCount} z", pagePrevText: "<", pageNextText: ">", pageFirstText: "<<", pageLastText: ">>", pageNavigatorNextText: "np", pageNavigatorPrevText: "pp" }); grid._firstDisplayingPage = 2; grid._refreshPager(); equal($.trim(grid._pagerContainer.text()), "a 2 << < pp2np > >> 3 6 z", "pager text follows the format specified"); }); test("pagerRenderer", function() { var $element = $("#jsGrid"); var pagerRendererConfig; var pageSize = 2; var items = [{}, {}, {}, {}, {}, {}, {}]; var pageCount = Math.ceil(items.length / pageSize); var grid = new Grid($element, { data: items, paging: true, pageSize: pageSize, pagerRenderer: function(pagerConfig) { pagerRendererConfig = pagerConfig; } }); deepEqual(pagerRendererConfig, { pageIndex: 1, pageCount: pageCount }); grid.openPage(2); deepEqual(pagerRendererConfig, { pageIndex: 2, pageCount: pageCount }); }); test("loading by page", function() { var $element = $("#jsGrid"), data = [], itemCount = 20; for(var i = 1; i <= itemCount; i += 1) { data.push({ value: i }); } var gridOptions = { pageLoading: true, paging: true, pageSize: 7, fields: [ { name: "value" } ], controller: { loadData: function(filter) { var startIndex = (filter.pageIndex - 1) * filter.pageSize, result = data.slice(startIndex, startIndex + filter.pageSize); return { data: result, itemsCount: data.length }; } } }; var grid = new Grid($element, gridOptions); grid.loadData(); var pager = grid._pagerContainer; var gridData = grid.option("data"); equal(gridData.length, 7, "loaded one page of data"); equal(gridData[0].value, 1, "loaded right data start value"); equal(gridData[gridData.length - 1].value, 7, "loaded correct data end value"); ok(pager.find("." + grid.pageClass).eq(0).hasClass(grid.currentPageClass), "first page is current"); equal(pager.find("." + grid.pageClass).length, 3, "three pages displayed"); grid.openPage(3); gridData = grid.option("data"); equal(gridData.length, 6, "loaded last page of data"); equal(gridData[0].value, 15, "loaded right data start value"); equal(gridData[gridData.length - 1].value, 20, "loaded right data end value"); ok(pager.find("." + grid.pageClass).eq(2).hasClass(grid.currentPageClass), "third page is current"); equal(pager.find("." + grid.pageClass).length, 3, "three pages displayed"); }); test("'openPage' method ignores indexes out of range", function() { var $element = $("#jsGrid"), grid = new Grid($element, { data: [{}, {}], paging: true, pageSize: 1 }); grid.openPage(0); equal(grid.option("pageIndex"), 1, "too small index is ignored"); grid.openPage(3); equal(grid.option("pageIndex"), 1, "too big index is ignored"); }); module("sorting"); test("sorting", function() { var $element = $("#jsGrid"), gridOptions = { sorting: true, data: [ { value: 3 }, { value: 2 }, { value: 1 } ], fields: [ { name: "value", sorter: "number" } ] }, grid = new Grid($element, gridOptions); var gridData = grid.option("data"); var $th = grid._headerRow.find("th").eq(0); $th.trigger("click"); equal(grid._sortOrder, "asc", "asc sorting order for first click"); equal(grid._sortField, grid.fields[0], "sort field is set"); equal(gridData[0].value, 1); equal(gridData[1].value, 2); equal(gridData[2].value, 3); ok($th.hasClass(grid.sortableClass)); ok($th.hasClass(grid.sortAscClass)); $th.trigger("click"); equal(grid._sortOrder, "desc", "desc sorting order for second click"); equal(grid._sortField, grid.fields[0], "sort field is set"); equal(gridData[0].value, 3); equal(gridData[1].value, 2); equal(gridData[2].value, 1); ok(!$th.hasClass(grid.sortAscClass)); ok($th.hasClass(grid.sortDescClass)); }); test("sorting with pageLoading", function() { var $element = $("#jsGrid"), loadFilter, gridOptions = { sorting: true, pageLoading: true, data: [ { value: 3 }, { value: 2 }, { value: 1 } ], controller: { loadData: function(filter) { loadFilter = filter; return { itemsCount: 0, data: [] }; } }, fields: [ { name: "value", sorter: "number" } ] }, grid = new Grid($element, gridOptions); var $th = grid._headerRow.find("th").eq(0); $th.trigger("click"); equal(grid._sortOrder, "asc", "asc sorting order for first click"); equal(grid._sortField, grid.fields[0], "sort field is set"); equal(loadFilter.sortOrder, "asc", "sort direction is provided in loadFilter"); equal(loadFilter.sortField, "value", "sort field is provided in loadFilter"); $th.trigger("click"); equal(grid._sortOrder, "desc", "desc sorting order for second click"); equal(grid._sortField, grid.fields[0], "sort field is set"); equal(loadFilter.sortOrder, "desc", "sort direction is provided in loadFilter"); equal(loadFilter.sortField, "value", "sort field is provided in loadFilter"); }); test("no sorting for column with sorting = false", function() { var $element = $("#jsGrid"); var gridOptions = { sorting: true, data: [ { value: 3 }, { value: 2 }, { value: 1 } ], fields: [ { name: "value", sorting: false } ] }; var grid = new Grid($element, gridOptions); var gridData = grid.option("data"); var $th = grid._headerRow.find("th").eq(0); $th.trigger("click"); equal(grid._sortField, null, "sort field is not set for the field with sorting=false"); equal(gridData[0].value, 3); equal(gridData[1].value, 2); equal(gridData[2].value, 1); equal($th.hasClass(grid.sortableClass), false, "no sorting css for field with sorting=false"); equal($th.hasClass(grid.sortAscClass), false, "no sorting css for field with sorting=false"); }); test("sort accepts sorting config", function() { var $element = $("#jsGrid"), gridOptions = { sorting: true, data: [ { value: 3 }, { value: 2 }, { value: 1 } ], fields: [ { name: "value", sorter: "number" } ] }, grid = new Grid($element, gridOptions); var gridData = grid.option("data"); grid.sort({ field: "value", order: "asc" }); equal(grid._sortOrder, "asc", "asc sorting order is set"); equal(grid._sortField, grid.fields[0], "sort field is set"); equal(gridData[0].value, 1); equal(gridData[1].value, 2); equal(gridData[2].value, 3); grid.sort({ field: 0 }); equal(grid._sortOrder, "desc", "desc sorting order for already set asc sorting"); equal(grid._sortField, grid.fields[0], "sort field is set"); equal(gridData[0].value, 3); equal(gridData[1].value, 2); equal(gridData[2].value, 1); grid.sort("value", "asc"); equal(grid._sortOrder, "asc", "asc sorting order is set"); equal(grid._sortField, grid.fields[0], "sort field is set"); grid.sort(0); equal(grid._sortOrder, "desc", "desc sorting order for already set asc sorting"); equal(grid._sortField, grid.fields[0], "sort field is set"); }); test("getSorting returns current sorting", function() { var $element = $("#jsGrid"), gridOptions = { sorting: true, data: [ { value: 3 }, { value: 2 }, { value: 1 } ], fields: [ { name: "value", sorter: "number" } ] }, grid = new Grid($element, gridOptions); deepEqual(grid.getSorting(), { field: undefined, order: undefined }, "undefined field and order before sorting"); grid.sort("value"); deepEqual(grid.getSorting(), { field: "value", order: "asc" }, "current sorting returned"); }); test("sorting css attached correctly when a field is hidden", function() { var $element = $("#jsGrid"); var gridOptions = { sorting: true, data: [], fields: [ { name: "field1", visible: false }, { name: "field2" } ] }; var grid = new Grid($element, gridOptions); var gridData = grid.option("data"); var $th = grid._headerRow.find("th").eq(0); $th.trigger("click"); equal($th.hasClass(grid.sortAscClass), true, "sorting css is attached to first field"); }); module("canceling events"); test("cancel item edit", function() { var $element = $("#jsGrid"); var data = [{}]; var gridOptions = { editing: true, onItemEditing: function(e) { e.cancel = true; }, controller: { loadData: function() { return data; } }, fields: [ { name: "test" } ] }; var grid = new Grid($element, gridOptions); grid.loadData(); grid.editItem(data[0]); strictEqual(grid._editingRow, null, "no editing row"); }); test("cancel controller.loadData", function() { var $element = $("#jsGrid"); var gridOptions = { onDataLoading: function(e) { e.cancel = true; }, controller: { loadData: function() { return [{}]; } }, fields: [ { name: "test" } ] }; var grid = new Grid($element, gridOptions); grid.loadData(); equal(grid.option("data").length, 0, "no data loaded"); }); test("cancel controller.insertItem", function() { var $element = $("#jsGrid"); var insertedItem = null; var gridOptions = { onItemInserting: function(e) { e.cancel = true; }, controller: { insertItem: function(item) { insertedItem = item; } }, fields: [ { name: "test" } ] }; var grid = new Grid($element, gridOptions); grid.insertItem({ test: "value" }); strictEqual(insertedItem, null, "item was not inserted"); }); test("cancel controller.updateItem", function() { var $element = $("#jsGrid"); var updatedItem = null; var existingItem = { test: "value" }; var gridOptions = { data: [ existingItem ], onItemUpdating: function(e) { e.cancel = true; }, controller: { updateItem: function(item) { updatedItem = item; } }, fields: [ { name: "test" } ] }; var grid = new Grid($element, gridOptions); grid.updateItem(existingItem, { test: "new_value" }); strictEqual(updatedItem, null, "item was not updated"); }); test("cancel controller.deleteItem", function() { var $element = $("#jsGrid"); var deletingItem = { test: "value" }; var deletedItem = null; var gridOptions = { data: [ deletingItem ], confirmDeleting: false, onItemDeleting: function(e) { e.cancel = true; }, controller: { deleteItem: function(item) { deletedItem = item; } }, fields: [ { name: "test" } ] }; var grid = new Grid($element, gridOptions); grid.deleteItem(deletingItem); strictEqual(deletedItem, null, "item was not deleted"); }); module("complex properties binding"); test("rendering", function() { var $element = $("#jsGrid"); var gridOptions = { loadMessage: "", data: [ { complexProp: { prop: "test" } } ], fields: [ { name: "complexProp.prop", title: "" } ] }; new Grid($element, gridOptions); equal($element.text(), "test", "complex property value rendered"); }); test("editing", function() { var $element = $("#jsGrid"); var gridOptions = { editing: true, data: [ { complexProp: { prop: "test" } } ], fields: [ { type: "text", name: "complexProp.prop" } ] }; var grid = new Grid($element, gridOptions); grid.editItem(gridOptions.data[0]); equal(grid.fields[0].editControl.val(), "test", "complex property value set in editor"); }); test("should not fail if property is absent", function() { var $element = $("#jsGrid"); var gridOptions = { loadMessage: "", data: [ { complexProp: { } } ], fields: [ { name: "complexProp.subprop.prop", title: "" } ] }; new Grid($element, gridOptions); equal($element.text(), "", "rendered empty value"); }); test("inserting", function() { var $element = $("#jsGrid"); var insertingItem; var gridOptions = { inserting: true, fields: [ { type: "text", name: "complexProp.prop" } ], onItemInserting: function(args) { insertingItem = args.item; } }; var grid = new Grid($element, gridOptions); grid.fields[0].insertControl.val("test"); grid.insertItem(); deepEqual(insertingItem, { complexProp: { prop: "test" } }, "inserting item has complex properties"); }); test("filtering", function() { var $element = $("#jsGrid"); var loadFilter; var gridOptions = { filtering: true, fields: [ { type: "text", name: "complexProp.prop" } ], controller: { loadData: function(filter) { loadFilter = filter; } } }; var grid = new Grid($element, gridOptions); grid.fields[0].filterControl.val("test"); grid.search(); deepEqual(loadFilter, { complexProp: { prop: "test" } }, "filter has complex properties"); }); test("updating", function() { var $element = $("#jsGrid"); var updatingItem; var gridOptions = { editing: true, data: [ { complexProp: { } } ], fields: [ { type: "text", name: "complexProp.prop" } ], onItemUpdating: function(args) { updatingItem = args.item; } }; var grid = new Grid($element, gridOptions); grid.editItem(gridOptions.data[0]); grid.fields[0].editControl.val("test"); grid.updateItem(); deepEqual(updatingItem, { complexProp: { prop: "test" } }, "updating item has complex properties"); }); test("update nested prop", function() { var $element = $("#jsGrid"); var updatingItem; var previousItem; var gridOptions = { editing: true, data: [ { prop: { subprop1: "test1", subprop2: "test2" } } ], fields: [ { type: "text", name: "prop.subprop1" }, { type: "text", name: "prop.subprop2" } ], onItemUpdating: function(args) { updatingItem = args.item; } }; var grid = new Grid($element, gridOptions); grid.editItem(gridOptions.data[0]); grid.fields[0].editControl.val("new_test1"); grid.updateItem(); var expectedUpdatingItem = { prop: { subprop1: "new_test1", subprop2: "test2" } }; deepEqual(updatingItem, expectedUpdatingItem, "updating item has nested properties"); }); test("updating deeply nested prop", function() { var $element = $("#jsGrid"); var updatingItem; var previousItem; var gridOptions = { editing: true, data: [ { complexProp: { subprop1: { another_prop: "test" } } } ], fields: [ { type: "text", name: "complexProp.subprop1.prop1" }, { type: "text", name: "complexProp.subprop1.subprop2.prop12" } ], onItemUpdating: function(args) { updatingItem = $.extend(true, {}, args.item); previousItem = $.extend(true, {}, args.previousItem); } }; var grid = new Grid($element, gridOptions); grid.editItem(gridOptions.data[0]); grid.fields[0].editControl.val("test1"); grid.fields[1].editControl.val("test2"); grid.updateItem(); var expectedUpdatingItem = { complexProp: { subprop1: { another_prop: "test", prop1: "test1", subprop2: { prop12: "test2" } } } }; var expectedPreviousItem = { complexProp: { subprop1: { another_prop: "test" } } }; deepEqual(updatingItem, expectedUpdatingItem, "updating item has deeply nested properties"); deepEqual(previousItem, expectedPreviousItem, "previous item preserved correctly"); }); module("validation"); test("insertItem should call validation.validate", function() { var $element = $("#jsGrid"); var fieldValidationRules = { test: "value" }; var validatingArgs; var gridOptions = { data: [], inserting: true, invalidNotify: $.noop, validation: { validate: function(args) { validatingArgs = args; return []; } }, fields: [ { type: "text", name: "Name", validate: fieldValidationRules } ] }; var grid = new Grid($element, gridOptions); grid.fields[0].insertControl.val("test"); grid.insertItem(); deepEqual(validatingArgs, { value: "test", item: { Name: "test" }, itemIndex: -1, row: grid._insertRow, rules: fieldValidationRules }, "validating args is provided"); }); test("insertItem rejected when data is not valid", function() { var $element = $("#jsGrid"); var gridOptions = { data: [], inserting: true, invalidNotify: $.noop, validation: { validate: function() { return ["Error"]; } }, fields: [ { type: "text", name: "Name", validate: true } ] }; var grid = new Grid($element, gridOptions); grid.fields[0].insertControl.val("test"); grid.insertItem().done(function() { ok(false, "insertItem should not be completed"); }).fail(function() { ok(true, "insertItem should fail"); }); }); test("invalidClass is attached on invalid cell on inserting", function() { var $element = $("#jsGrid"); var gridOptions = { data: [], inserting: true, invalidNotify: $.noop, validation: { validate: function() { return ["Error"]; } }, fields: [ { type: "text", name: "Id", visible: false }, { type: "text", name: "Name", validate: true } ] }; var grid = new Grid($element, gridOptions); var $insertCell = grid._insertRow.children().eq(0); grid.insertItem(); ok($insertCell.hasClass(grid.invalidClass), "invalid class is attached"); equal($insertCell.attr("title"), "Error", "cell tooltip contains error message"); }); test("onItemInvalid callback", function() { var $element = $("#jsGrid"); var errors = ["Error"]; var onItemInvalidCalled = 0; var onItemInvalidArgs; var gridOptions = { data: [], inserting: true, invalidNotify: $.noop, onItemInvalid: function(args) { onItemInvalidCalled++; onItemInvalidArgs = args; }, validation: { validate: function(args) { return !args.value ? errors : []; } }, fields: [ { type: "text", name: "Name", validate: true } ] }; var grid = new Grid($element, gridOptions); grid.insertItem(); equal(onItemInvalidCalled, 1, "onItemInvalid is called, when item data is invalid"); deepEqual(onItemInvalidArgs, { grid: grid, errors: [{ field: grid.fields[0], message: "Error" }], item: { Name: "" }, itemIndex: -1, row: grid._insertRow }, "arguments provided"); grid.fields[0].insertControl.val("test"); grid.insertItem(); equal(onItemInvalidCalled, 1, "onItemInvalid was not called, when data is valid"); }); test("invalidNotify", function() { var $element = $("#jsGrid"); var errors = ["Error"]; var invalidNotifyCalled = 0; var invalidNotifyArgs; var gridOptions = { data: [], inserting: true, invalidNotify: function(args) { invalidNotifyCalled++; invalidNotifyArgs = args; }, validation: { validate: function(args) { return !args.value ? errors : []; } }, fields: [ { type: "text", name: "Name", validate: true } ] }; var grid = new Grid($element, gridOptions); grid.insertItem(); equal(invalidNotifyCalled, 1, "invalidNotify is called, when item data is invalid"); deepEqual(invalidNotifyArgs, { grid: grid, errors: [{ field: grid.fields[0], message: "Error" }], row: grid._insertRow, item: { Name: "" }, itemIndex: -1 }, "arguments provided"); grid.fields[0].insertControl.val("test"); grid.insertItem(); equal(invalidNotifyCalled, 1, "invalidNotify was not called, when data is valid"); }); test("invalidMessage", function() { var $element = $("#jsGrid"); var invalidMessage; var originalAlert = window.alert; window.alert = function(message) { invalidMessage = message; }; try { Grid.prototype.invalidMessage = "InvalidTest"; Grid.prototype.invalidNotify({ errors: [{ message: "Message1" }, { message: "Message2" }] }); var expectedInvalidMessage = ["InvalidTest", "Message1", "Message2"].join("\n"); equal(invalidMessage, expectedInvalidMessage, "message contains invalidMessage and field error messages"); } finally { window.alert = originalAlert; } }); test("updateItem should call validation.validate", function() { var $element = $("#jsGrid"); var validatingArgs; var gridOptions = { data: [{ Name: "" }], editing: true, invalidNotify: $.noop, validation: { validate: function(args) { validatingArgs = args; return ["Error"]; } }, fields: [ { type: "text", name: "Name", validate: "required" } ] }; var grid = new Grid($element, gridOptions); grid.editItem(gridOptions.data[0]); grid.fields[0].editControl.val("test"); grid.updateItem(); deepEqual(validatingArgs, { value: "test", item: { Name: "test" }, itemIndex: 0, row: grid._getEditRow(), rules: "required" }, "validating args is provided"); }); test("invalidClass is attached on invalid cell on updating", function() { var $element = $("#jsGrid"); var gridOptions = { data: [{}], editing: true, invalidNotify: $.noop, validation: { validate: function() { return ["Error"]; } }, fields: [ { type: "text", name: "Name", validate: true } ] }; var grid = new Grid($element, gridOptions); grid.editItem(gridOptions.data[0]); var $editCell = grid._getEditRow().children().eq(0); grid.updateItem(); ok($editCell.hasClass(grid.invalidClass), "invalid class is attached"); equal($editCell.attr("title"), "Error", "cell tooltip contains error message"); }); test("validation should ignore not editable fields", function() { var invalidNotifyCalled = 0; var $element = $("#jsGrid"); var validatingArgs; var gridOptions = { data: [{ Name: "" }], editing: true, invalidNotify: function() { invalidNotifyCalled++; }, validation: { validate: function() { return ["Error"]; } }, fields: [ { type: "text", name: "Name", editing: false, validate: "required" } ] }; var grid = new Grid($element, gridOptions); grid.editItem(gridOptions.data[0]); grid.updateItem(); equal(invalidNotifyCalled, 0, "data is valid"); }); module("api"); test("reset method should go the first page when pageLoading is truned on", function() { var items = [{ Name: "1" }, { Name: "2" }]; var $element = $("#jsGrid"); var gridOptions = { paging: true, pageSize: 1, pageLoading: true, autoload: true, controller: { loadData: function(args) { return { data: [items[args.pageIndex - 1]], itemsCount: items.length }; } }, fields: [ { type: "text", name: "Name" } ] }; var grid = new Grid($element, gridOptions); grid.openPage(2); grid.reset(); equal(grid._bodyGrid.text(), "1", "grid content reset"); }); module("i18n"); test("set locale by name", function() { jsGrid.locales.my_lang = { grid: { test: "test_text" } }; jsGrid.locale("my_lang"); var $element = $("#jsGrid").jsGrid({}); equal($element.jsGrid("option", "test"), "test_text", "option localized"); }); test("set locale by config", function() { jsGrid.locale( { grid: { test: "test_text" } }); var $element = $("#jsGrid").jsGrid({}); equal($element.jsGrid("option", "test"), "test_text", "option localized"); }); test("locale throws exception for unknown locale", function() { throws(function() { jsGrid.locale("unknown_lang"); }, /unknown_lang/, "locale threw an exception"); }); module("controller promise"); asyncTest("should support jQuery promise success callback", 1, function() { var data = []; var gridOptions = { autoload: false, controller: { loadData: function() { var d = $.Deferred(); setTimeout(function() { d.resolve(data); start(); }); return d.promise(); } } }; var grid = new Grid($("#jsGrid"), gridOptions); var promise = grid._controllerCall("loadData", {}, false, $.noop); promise.done(function(result) { equal(result, data, "data provided to done callback"); }); }); asyncTest("should support jQuery promise fail callback", 1, function() { var failArgs = {}; var gridOptions = { autoload: false, controller: { loadData: function() { var d = $.Deferred(); setTimeout(function() { d.reject(failArgs); start(); }); return d.promise(); } } }; var grid = new Grid($("#jsGrid"), gridOptions); var promise = grid._controllerCall("loadData", {}, false, $.noop); promise.fail(function(result) { equal(result, failArgs, "fail args provided to fail callback"); }); }); asyncTest("should support JS promise success callback", 1, function() { if(typeof Promise === "undefined") { ok(true, "Promise not supported"); start(); return; } var data = []; var gridOptions = { autoload: false, controller: { loadData: function() { return new Promise(function(resolve, reject) { setTimeout(function() { resolve(data); start(); }); }); } } }; var grid = new Grid($("#jsGrid"), gridOptions); var promise = grid._controllerCall("loadData", {}, false, $.noop); promise.done(function(result) { equal(result, data, "data provided to done callback"); }); }); asyncTest("should support JS promise fail callback", 1, function() { if(typeof Promise === "undefined") { ok(true, "Promise not supported"); start(); return; } var failArgs = {}; var gridOptions = { autoload: false, controller: { loadData: function() { return new Promise(function(resolve, reject) { setTimeout(function() { reject(failArgs); start(); }); }); } } }; var grid = new Grid($("#jsGrid"), gridOptions); var promise = grid._controllerCall("loadData", {}, false, $.noop); promise.fail(function(result) { equal(result, failArgs, "fail args provided to fail callback"); }); }); test("should support non-promise result", 1, function() { var data = []; var gridOptions = { autoload: false, controller: { loadData: function() { return data; } } }; var grid = new Grid($("#jsGrid"), gridOptions); var promise = grid._controllerCall("loadData", {}, false, $.noop); promise.done(function(result) { equal(result, data, "data provided to done callback"); }); }); module("renderTemplate"); test("should pass undefined and null arguments to the renderer", function() { var rendererArgs; var rendererContext; var context = {}; var renderer = function() { rendererArgs = arguments; rendererContext = this; }; Grid.prototype.renderTemplate(renderer, context, { arg1: undefined, arg2: null, arg3: "test" }); equal(rendererArgs.length, 3); strictEqual(rendererArgs[0], undefined, "undefined passed"); strictEqual(rendererArgs[1], null, "null passed"); strictEqual(rendererArgs[2], "test", "null passed"); strictEqual(rendererContext, context, "context is preserved"); }); module("Data Export", { setup: function() { this.exportConfig = {}; this.exportConfig.type = "csv"; this.exportConfig.subset = "all"; this.exportConfig.delimiter = "|"; this.exportConfig.includeHeaders = true; this.exportConfig.encapsulate = true; this.element = $("#jsGrid"); this.gridOptions = { width: "100%", height: "400px", inserting: true, editing: true, sorting: true, paging: true, pageSize: 2, data: [ { "Name": "Otto Clay", "Country": 1, "Married": false }, { "Name": "Connor Johnston", "Country": 2, "Married": true }, { "Name": "Lacey Hess", "Country": 2, "Married": false }, { "Name": "Timothy Henson", "Country": 1, "Married": true } ], fields: [ { name: "Name", type: "text", width: 150, validate: "required" }, { name: "Country", type: "select", items: [{ Name: "United States", Id: 1 },{ Name: "Canada", Id: 2 }], valueField: "Id", textField: "Name" }, { name: "Married", type: "checkbox", title: "Is Married", sorting: false }, { type: "control" } ] } } }); /* Base Choice Criteria type: csv subset: all delimiter: | includeHeaders: true encapsulate: true */ test("Should export data: Base Choice",function(){ var grid = new Grid($(this.element), this.gridOptions); var data = grid.exportData(this.exportConfig); var expected = ""; expected += '"Name"|"Country"|"Is Married"\r\n'; expected += '"Otto Clay"|"United States"|"false"\r\n'; expected += '"Connor Johnston"|"Canada"|"true"\r\n'; expected += '"Lacey Hess"|"Canada"|"false"\r\n'; expected += '"Timothy Henson"|"United States"|"true"\r\n'; equal(data, expected, "Output CSV configured to Base Choice Criteria -- Check Source"); }); test("Should export data: defaults = BCC",function(){ var grid = new Grid($(this.element), this.gridOptions); var data = grid.exportData(); var expected = ""; expected += '"Name"|"Country"|"Is Married"\r\n'; expected += '"Otto Clay"|"United States"|"false"\r\n'; expected += '"Connor Johnston"|"Canada"|"true"\r\n'; expected += '"Lacey Hess"|"Canada"|"false"\r\n'; expected += '"Timothy Henson"|"United States"|"true"\r\n'; equal(data, expected, "Output CSV with all defaults -- Should be equal to Base Choice"); }); test("Should export data: subset=visible", function(){ var grid = new Grid($(this.element), this.gridOptions); this.exportConfig.subset = "visible"; var data = grid.exportData(this.exportConfig); var expected = ""; expected += '"Name"|"Country"|"Is Married"\r\n'; expected += '"Otto Clay"|"United States"|"false"\r\n'; expected += '"Connor Johnston"|"Canada"|"true"\r\n'; //expected += '"Lacey Hess"|"Canada"|"false"\r\n'; //expected += '"Timothy Henson"|"United States"|"true"\r\n'; equal(data, expected, "Output CSV of visible records"); }); test("Should export data: delimiter=;", function(){ var grid = new Grid($(this.element), this.gridOptions); this.exportConfig.delimiter = ";"; var data = grid.exportData(this.exportConfig); var expected = ""; expected += '"Name";"Country";"Is Married"\r\n'; expected += '"Otto Clay";"United States";"false"\r\n'; expected += '"Connor Johnston";"Canada";"true"\r\n'; expected += '"Lacey Hess";"Canada";"false"\r\n'; expected += '"Timothy Henson";"United States";"true"\r\n'; equal(data, expected, "Output CSV with non-default delimiter"); }); test("Should export data: headers=false", function(){ var grid = new Grid($(this.element), this.gridOptions); this.exportConfig.includeHeaders = false; var data = grid.exportData(this.exportConfig); var expected = ""; //expected += '"Name"|"Country"|"Is Married"\r\n'; expected += '"Otto Clay"|"United States"|"false"\r\n'; expected += '"Connor Johnston"|"Canada"|"true"\r\n'; expected += '"Lacey Hess"|"Canada"|"false"\r\n'; expected += '"Timothy Henson"|"United States"|"true"\r\n'; equal(data, expected, "Output CSV without Headers"); }); test("Should export data: encapsulate=false", function(){ var grid = new Grid($(this.element), this.gridOptions); this.exportConfig.encapsulate = false; var data = grid.exportData(this.exportConfig); var expected = ""; expected += 'Name|Country|Is Married\r\n'; expected += 'Otto Clay|United States|false\r\n'; expected += 'Connor Johnston|Canada|true\r\n'; expected += 'Lacey Hess|Canada|false\r\n'; expected += 'Timothy Henson|United States|true\r\n'; equal(data, expected, "Output CSV without encapsulation"); }); test("Should export filtered data", function(){ var grid = new Grid($(this.element), this.gridOptions); this.exportConfig['filter'] = function(item){ if (item["Name"].indexOf("O") === 0) return true }; var data = grid.exportData(this.exportConfig); var expected = ""; expected += '"Name"|"Country"|"Is Married"\r\n'; expected += '"Otto Clay"|"United States"|"false"\r\n'; //expected += '"Connor Johnston"|"Canada"|"true"\r\n'; //expected += '"Lacey Hess"|"Canada"|"false"\r\n'; //expected += '"Timothy Henson"|"United States"|"true"\r\n'; equal(data, expected, "Output CSV filtered to show names starting with O"); }); test("Should export data: transformed value", function(){ var grid = new Grid($(this.element), this.gridOptions); this.exportConfig['transforms'] = {}; this.exportConfig.transforms['Married'] = function(value){ if (value === true) return "Yes" if (value === false) return "No" }; var data = grid.exportData(this.exportConfig); var expected = ""; expected += '"Name"|"Country"|"Is Married"\r\n'; expected += '"Otto Clay"|"United States"|"No"\r\n'; expected += '"Connor Johnston"|"Canada"|"Yes"\r\n'; expected += '"Lacey Hess"|"Canada"|"No"\r\n'; expected += '"Timothy Henson"|"United States"|"Yes"\r\n'; equal(data, expected, "Output CSV column value transformed properly"); }); }); <MSG> Core: Field constructor shortcuts <DFF> @@ -588,9 +588,13 @@ $(function() { }); test("grid fields normalization", function() { + var CustomField = function(name, config) { + $.extend(true, this, config); + }; + + jsGrid.fields.custom = CustomField; + var $element = $("#jsGrid"), - field1, - field2, gridOptions = { fields: [ new jsGrid.Field("text1", { @@ -599,20 +603,28 @@ $(function() { { name: "text2", title: "title2" + }, + { + name: "text3", + type: "custom" } ] }, grid = new Grid($element, gridOptions); - field1 = grid.fields[0]; + var field1 = grid.fields[0]; ok(field1 instanceof jsGrid.Field); equal(field1.name, "text1", "name is set for field"); equal(field1.title, "title1", "title field"); - field2 = grid.fields[1]; + var field2 = grid.fields[1]; ok(field2 instanceof jsGrid.Field); equal(field2.name, "text2", "name is set for field"); equal(field2.title, "title2", "title field"); + + | 16 | Core: Field constructor shortcuts | 4 | .js | tests | mit | tabalinas/jsgrid |
10066231 | <NME> jsgrid.tests.js <BEF> $(function() { var Grid = jsGrid.Grid, JSGRID = "JSGrid", JSGRID_DATA_KEY = JSGRID; Grid.prototype.updateOnResize = false; module("basic"); test("default creation", function() { var gridOptions = { simpleOption: "test", complexOption: { a: "subtest", b: 1, c: {} } }, grid = new Grid("#jsGrid", gridOptions); equal(grid._container[0], $("#jsGrid")[0], "container saved"); equal(grid.simpleOption, "test", "primitive option extended"); equal(grid.complexOption, gridOptions.complexOption, "non-primitive option extended"); }); test("jquery adapter creation", function() { var gridOptions = { option: "test" }, $element = $("#jsGrid"), result = $element.jsGrid(gridOptions), grid = $element.data(JSGRID_DATA_KEY); equal(result, $element, "jquery fn returned source jQueryElement"); ok(grid instanceof Grid, "jsGrid saved to jquery data"); equal(grid.option, "test", "options provided"); }); test("destroy", function() { var $element = $("#jsGrid"), grid; $element.jsGrid({}); grid = $element.data(JSGRID_DATA_KEY); grid.destroy(); strictEqual($element.html(), "", "content is removed"); strictEqual($element.data(JSGRID_DATA_KEY), undefined, "jquery data is removed"); }); test("jquery adapter second call changes option value", function() { var $element = $("#jsGrid"), gridOptions = { option: "test" }, grid; $element.jsGrid(gridOptions); grid = $element.data(JSGRID_DATA_KEY); gridOptions.option = "new test"; $element.jsGrid(gridOptions); equal(grid, $element.data(JSGRID_DATA_KEY), "instance was not changed"); equal(grid.option, "new test", "option changed"); }); test("jquery adapter invokes jsGrid method", function() { var methodResult = "", $element = $("#jsGrid"), gridOptions = { method: function(str) { methodResult = "test_" + str; } }; $element.jsGrid(gridOptions); $element.jsGrid("method", "invoke"); equal(methodResult, "test_invoke", "method invoked"); }); test("onInit callback", function() { var $element = $("#jsGrid"), onInitArguments, gridOptions = { onInit: function(args) { onInitArguments = args; } }; var grid = new Grid($element, gridOptions); equal(onInitArguments.grid, grid, "grid instance is provided in onInit callback arguments"); }); test("controller methods are $.noop when not specified", function() { var $element = $("#jsGrid"), gridOptions = { controller: {} }, testOption; $element.jsGrid(gridOptions); deepEqual($element.data(JSGRID_DATA_KEY)._controller, { loadData: $.noop, insertItem: $.noop, updateItem: $.noop, deleteItem: $.noop }, "controller has stub methods"); }); test("option method", function() { var $element = $("#jsGrid"), gridOptions = { test: "value" }, testOption; $element.jsGrid(gridOptions); testOption = $element.jsGrid("option", "test"); equal(testOption, "value", "read option value"); $element.jsGrid("option", "test", "new_value"); testOption = $element.jsGrid("option", "test"); equal(testOption, "new_value", "set option value"); }); test("fieldOption method", function() { var dataLoadedCount = 0; var $element = $("#jsGrid"), gridOptions = { loadMessage: "", autoload: true, controller: { loadData: function() { dataLoadedCount++; return [{ prop1: "value1", prop2: "value2", prop3: "value3" }]; } }, fields: [ { name: "prop1", title: "_" } ] }; $element.jsGrid(gridOptions); var fieldOptionValue = $element.jsGrid("fieldOption", "prop1", "name"); equal(fieldOptionValue, "prop1", "read field option"); $element.jsGrid("fieldOption", "prop1", "name", "prop2"); equal($element.text(), "_value2", "set field option by field name"); equal(dataLoadedCount, 1, "data not reloaded on field option change"); $element.jsGrid("fieldOption", 0, "name", "prop3"); equal($element.text(), "_value3", "set field option by field index"); }); test("option changing event handlers", function() { var $element = $("#jsGrid"), optionChangingEventArgs, optionChangedEventArgs, gridOptions = { test: "testValue", another: "anotherValue", onOptionChanging: function(e) { optionChangingEventArgs = $.extend({}, e); e.option = "another"; e.newValue = e.newValue + "_" + this.another; }, onOptionChanged: function(e) { optionChangedEventArgs = $.extend({}, e); } }, anotherOption; $element.jsGrid(gridOptions); $element.jsGrid("option", "test", "newTestValue"); equal(optionChangingEventArgs.option, "test", "option name is provided in args of optionChanging"); equal(optionChangingEventArgs.oldValue, "testValue", "old option value is provided in args of optionChanging"); equal(optionChangingEventArgs.newValue, "newTestValue", "new option value is provided in args of optionChanging"); anotherOption = $element.jsGrid("option", "another"); equal(anotherOption, "newTestValue_anotherValue", "option changing handler changed option and value"); equal(optionChangedEventArgs.option, "another", "option name is provided in args of optionChanged"); equal(optionChangedEventArgs.value, "newTestValue_anotherValue", "option value is provided in args of optionChanged"); }); test("common layout rendering", function() { var $element = $("#jsGrid"), grid = new Grid($element, {}), $headerGrid, $headerGridTable, $bodyGrid, $bodyGridTable; ok($element.hasClass(grid.containerClass), "container class attached"); ok($element.children().eq(0).hasClass(grid.gridHeaderClass), "grid header"); ok($element.children().eq(1).hasClass(grid.gridBodyClass), "grid body"); ok($element.children().eq(2).hasClass(grid.pagerContainerClass), "pager container"); $headerGrid = $element.children().eq(0); $headerGridTable = $headerGrid.children().first(); ok($headerGridTable.hasClass(grid.tableClass), "header table"); equal($headerGrid.find("." + grid.headerRowClass).length, 1, "header row"); equal($headerGrid.find("." + grid.filterRowClass).length, 1, "filter row"); equal($headerGrid.find("." + grid.insertRowClass).length, 1, "insert row"); ok(grid._headerRow.hasClass(grid.headerRowClass), "header row class"); ok(grid._filterRow.hasClass(grid.filterRowClass), "filter row class"); ok(grid._insertRow.hasClass(grid.insertRowClass), "insert row class"); $bodyGrid = $element.children().eq(1); $bodyGridTable = $bodyGrid.children().first(); ok($bodyGridTable.hasClass(grid.tableClass), "body table"); equal(grid._content.parent()[0], $bodyGridTable[0], "content is tbody in body table"); equal($bodyGridTable.find("." + grid.noDataRowClass).length, 1, "no data row"); equal($bodyGridTable.text(), grid.noDataContent, "no data text"); }); test("set default options with setDefaults", function() { jsGrid.setDefaults({ defaultOption: "test" }); var $element = $("#jsGrid").jsGrid({}); equal($element.jsGrid("option", "defaultOption"), "test", "default option set"); }); module("loading"); test("loading with controller", function() { var $element = $("#jsGrid"), data = [ { test: "test1" }, { test: "test2" } ], gridOptions = { controller: { loadData: function() { return data; } } }, grid = new Grid($element, gridOptions); grid.loadData(); equal(grid.option("data"), data, "loadData loads data"); }); test("loadData throws exception when controller method not found", function() { var $element = $("#jsGrid"); var grid = new Grid($element); grid._controller = {}; throws(function() { grid.loadData(); }, /loadData/, "loadData threw an exception"); }); test("onError event should be fired on controller fail", function() { var errorArgs, errorFired = 0, $element = $("#jsGrid"), gridOptions = { controller: { loadData: function() { return $.Deferred().reject({ value: 1 }, "test").promise(); } }, onError: function(args) { errorFired++; errorArgs = args; } }, grid = new Grid($element, gridOptions); grid.loadData(); equal(errorFired, 1, "onError handler fired"); deepEqual(errorArgs, { grid: grid, args: [{ value: 1 }, "test"] }, "error has correct params"); }); asyncTest("autoload should call loadData after render", 1, function() { new Grid($("#jsGrid"), { autoload: true, controller: { loadData: function() { ok(true, "autoload calls loadData on creation"); start(); return []; } } }); }); test("loading filtered data", function() { var filteredData, loadingArgs, loadedArgs, $element = $("#jsGrid"), data = [ { field: "test" }, { field: "test_another" }, { field: "test_another" }, { field: "test" } ], gridOptions = { filtering: true, fields: [ { name: "field", filterValue: function(value) { return "test"; } } ], onDataLoading: function(e) { loadingArgs = $.extend(true, {}, e); }, onDataLoaded: function(e) { loadedArgs = $.extend(true, {}, e); }, controller: { loadData: function(filter) { filteredData = $.grep(data, function(item) { return item.field === filter.field; }); return filteredData; } } }, grid = new Grid($element, gridOptions); grid.loadData(); equal(loadingArgs.filter.field, "test"); equal(grid.option("data").length, 2, "filtered data loaded"); deepEqual(loadedArgs.data, filteredData); }); asyncTest("loading indication", function() { var timeout = 10, stage = "initial", $element = $("#jsGrid"), gridOptions = { loadIndication: true, loadIndicationDelay: timeout, loadMessage: "loading...", loadIndicator: function(config) { equal(config.message, gridOptions.loadMessage, "message provided"); ok(config.container.jquery, "grid container is provided"); return { show: function() { stage = "started"; }, hide: function() { stage = "finished"; } }; }, fields: [ { name: "field" } ], controller: { loadData: function() { var deferred = $.Deferred(); equal(stage, "initial", "initial stage"); setTimeout(function() { equal(stage, "started", "loading started"); deferred.resolve([]); equal(stage, "finished", "loading finished"); start(); }, timeout); return deferred.promise(); } } }, grid = new Grid($element, gridOptions); grid.loadData(); }); asyncTest("loadingIndication=false should not show loading", 0, function() { var $element = $("#jsGrid"), timeout = 10, gridOptions = { loadIndication: false, loadIndicationDelay: timeout, loadIndicator: function() { return { show: function() { ok(false, "should not call show"); }, hide: function() { ok(false, "should not call hide"); } }; }, fields: [ { name: "field" } ], controller: { loadData: function() { var deferred = $.Deferred(); setTimeout(function() { deferred.resolve([]); start(); }, timeout); return deferred.promise(); } } }, grid = new Grid($element, gridOptions); grid.loadData(); }); test("search", function() { var $element = $("#jsGrid"), data = [ { field: "test" }, { field: "test_another" }, { field: "test_another" }, { field: "test" } ], gridOptions = { pageIndex: 2, _sortField: "field", _sortOrder: "desc", filtering: true, fields: [ { name: "field", filterValue: function(value) { return "test"; } } ], controller: { loadData: function(filter) { var filteredData = $.grep(data, function(item) { return item.field === filter.field; }); return filteredData; } } }, grid = new Grid($element, gridOptions); grid.search(); equal(grid.option("data").length, 2, "data filtered"); strictEqual(grid.option("pageIndex"), 1, "pageIndex reset"); strictEqual(grid._sortField, null, "sortField reset"); strictEqual(grid._sortOrder, "asc", "sortOrder reset"); }); test("change loadStrategy on the fly", function() { var $element = $("#jsGrid"); var gridOptions = { controller: { loadData: function() { return []; } } }; var grid = new Grid($element, gridOptions); grid.option("loadStrategy", { firstDisplayIndex: function() { return 0; }, lastDisplayIndex: function() { return 1; }, loadParams: function() { return []; }, finishLoad: function() { grid.option("data", [{}]); } }); grid.loadData(); equal(grid.option("data").length, 1, "new load strategy is applied"); }); module("filtering"); test("filter rendering", function() { var $element = $("#jsGrid"), gridOptions = { filtering: true, fields: [ { name: "test", align: "right", filtercss: "filter-class", filterTemplate: function() { var result = this.filterControl = $("<input>").attr("type", "text").addClass("filter-input"); return result; } } ] }, grid = new Grid($element, gridOptions); equal(grid._filterRow.find(".filter-class").length, 1, "filtercss class is attached"); equal(grid._filterRow.find(".filter-input").length, 1, "filter control rendered"); equal(grid._filterRow.find("." + grid.cellClass).length, 1, "cell class is attached"); ok(grid._filterRow.find(".filter-class").hasClass("jsgrid-align-right"), "align class is attached"); ok(grid.fields[0].filterControl.is("input[type=text]"), "filter control saved in field"); }); test("filter get/clear", function() { var $element = $("#jsGrid"), gridOptions = { filtering: true, controller: { loadData: function() { return []; } }, fields: [ { name: "field", filterTemplate: function() { return this.filterControl = $("<input>").attr("type", "text"); }, filterValue: function() { return this.filterControl.val(); } } ] }, grid = new Grid($element, gridOptions); grid.fields[0].filterControl.val("test"); deepEqual(grid.getFilter(), { field: "test" }, "get filter"); }); test("grid fields normalization", function() { var $element = $("#jsGrid"), field1, field2, gridOptions = { fields: [ new jsGrid.Field("text1", { filterValue: function(value) { if(!arguments.length) { { name: "text2", title: "title2" } ] }, grid = new Grid($element, gridOptions); field1 = grid.fields[0]; ok(field1 instanceof jsGrid.Field); equal(field1.name, "text1", "name is set for field"); equal(field1.title, "title1", "title field"); field2 = grid.fields[1]; ok(field2 instanceof jsGrid.Field); equal(field2.name, "text2", "name is set for field"); equal(field2.title, "title2", "title field"); }); test("grid field name used for header if title is not specified", function() { data = [ { field: "test" }, { field: "test_another" }, { field: "test_another" }, { field: "test" } ], gridOptions = { fields: [ { name: "field" } ], controller: { loadData: function(filter) { var filteredData = $.grep(data, function(item) { return item.field === filter.field; }); return filteredData; } } }, grid = new Grid($element, gridOptions); grid.search({ field: "test" }); equal(grid.option("data").length, 2, "data filtered"); }); test("filtering with static data should not do actual filtering", function() { var $element = $("#jsGrid"), gridOptions = { filtering: true, fields: [ { type: "text", name: "field" } ], data: [ { name: "value1" }, { name: "value2" } ] }, grid = new Grid($element, gridOptions); grid._filterRow.find("input").val("1"); grid.search(); equal(grid.option("data").length, 2, "data is not filtered"); }); module("nodatarow"); test("nodatarow after bind on empty array", function() { var $element = $("#jsGrid"), gridOptions = {}, grid = new Grid($element, gridOptions); grid.option("data", []); equal(grid._content.find("." + grid.noDataRowClass).length, 1, "no data row rendered"); equal(grid._content.find("." + grid.cellClass).length, 1, "grid cell class attached"); equal(grid._content.text(), grid.noDataContent, "no data text rendered"); }); test("nodatarow customize content", function() { var noDataMessage = "NoData Custom Content", $element = $("#jsGrid"), gridOptions = { noDataContent: function() { return noDataMessage; } }, grid = new Grid($element, gridOptions); grid.option("data", []); equal(grid._content.find("." + grid.cellClass).length, 1, "grid cell class attached"); equal(grid._content.text(), noDataMessage, "custom noDataContent"); }); module("row rendering", { setup: function() { this.testData = [ { id: 1, text: "test1" }, { id: 2, text: "test2" }, { id: 3, text: "test3" } ]; } }); test("rows rendered correctly", function() { var $element = $("#jsGrid"), gridOptions = { data: this.testData }, grid = new Grid($element, gridOptions); equal(grid._content.children().length, 3, "rows rendered"); equal(grid._content.find("." + grid.oddRowClass).length, 2, "two odd rows for 3 items"); equal(grid._content.find("." + grid.evenRowClass).length, 1, "one even row for 3 items"); }); test("custom rowClass", function() { var $element = $("#jsGrid"), gridOptions = { data: this.testData, rowClass: "custom-row-cls" }, grid = new Grid($element, gridOptions); equal(grid._content.find("." + grid.oddRowClass).length, 2); equal(grid._content.find("." + grid.evenRowClass).length, 1); equal(grid._content.find(".custom-row-cls").length, 3, "custom row class"); }); test("custom rowClass callback", function() { var $element = $("#jsGrid"), gridOptions = { data: this.testData, rowClass: function(item, index) { return item.text; } }, grid = new Grid($element, gridOptions); equal(grid._content.find("." + grid.oddRowClass).length, 2); equal(grid._content.find("." + grid.evenRowClass).length, 1); equal(grid._content.find(".test1").length, 1, "custom row class"); equal(grid._content.find(".test2").length, 1, "custom row class"); equal(grid._content.find(".test3").length, 1, "custom row class"); }); test("rowClick standard handler", function() { var $element = $("#jsGrid"), $secondRow, grid = new Grid($element, { editing: true }); grid.option("data", this.testData); $secondRow = grid._content.find("." + grid.evenRowClass); $secondRow.trigger("click", $.Event($secondRow)); equal(grid._editingRow.get(0), $secondRow.get(0), "clicked row is editingRow"); }); test("rowClick handler", function() { var rowClickArgs, $element = $("#jsGrid"), $secondRow, gridOptions = { rowClick: function(args) { rowClickArgs = args; } }, grid = new Grid($element, gridOptions); grid.option("data", this.testData); $secondRow = grid._content.find("." + grid.evenRowClass); $secondRow.trigger("click", $.Event($secondRow)); ok(rowClickArgs.event instanceof jQuery.Event, "jquery event arg"); equal(rowClickArgs.item, this.testData[1], "item arg"); equal(rowClickArgs.itemIndex, 1, "itemIndex arg"); }); test("row selecting with selectedRowClass", function() { var $element = $("#jsGrid"), $secondRow, gridOptions = { selecting: true }, grid = new Grid($element, gridOptions); grid.option("data", this.testData); $secondRow = grid._content.find("." + grid.evenRowClass); $secondRow.trigger("mouseenter", $.Event($secondRow)); ok($secondRow.hasClass(grid.selectedRowClass), "mouseenter adds selectedRowClass"); $secondRow.trigger("mouseleave", $.Event($secondRow)); ok(!$secondRow.hasClass(grid.selectedRowClass), "mouseleave removes selectedRowClass"); }); test("no row selecting while selection is disabled", function() { var $element = $("#jsGrid"), $secondRow, gridOptions = { selecting: false }, grid = new Grid($element, gridOptions); grid.option("data", this.testData); $secondRow = grid._content.find("." + grid.evenRowClass); $secondRow.trigger("mouseenter", $.Event($secondRow)); ok(!$secondRow.hasClass(grid.selectedRowClass), "mouseenter doesn't add selectedRowClass"); }); test("refreshing and refreshed callbacks", function() { var refreshingEventArgs, refreshedEventArgs, $element = $("#jsGrid"), grid = new Grid($element, {}); grid.onRefreshing = function(e) { refreshingEventArgs = e; equal(grid._content.find("." + grid.oddRowClass).length, 0, "no items before refresh"); }; grid.onRefreshed = function(e) { refreshedEventArgs = e; equal(grid._content.find("." + grid.oddRowClass).length, 2, "items rendered after refresh"); }; grid.option("data", this.testData); equal(refreshingEventArgs.grid, grid, "grid provided in args for refreshing event"); equal(refreshedEventArgs.grid, grid, "grid provided in args for refreshed event"); equal(grid._content.find("." + grid.oddRowClass).length, 2, "items rendered"); }); test("grid fields normalization", function() { var CustomField = function(config) { $.extend(true, this, config); }; jsGrid.fields.custom = CustomField; try { var $element = $("#jsGrid"), gridOptions = { fields: [ new jsGrid.Field({ name: "text1", title: "title1" }), { name: "text2", title: "title2" }, { name: "text3", type: "custom" } ] }, grid = new Grid($element, gridOptions); var field1 = grid.fields[0]; ok(field1 instanceof jsGrid.Field); equal(field1.name, "text1", "name is set for field"); equal(field1.title, "title1", "title field"); var field2 = grid.fields[1]; ok(field2 instanceof jsGrid.Field); equal(field2.name, "text2", "name is set for field"); equal(field2.title, "title2", "title field"); var field3 = grid.fields[2]; ok(field3 instanceof CustomField); equal(field3.name, "text3", "name is set for field"); } finally { delete jsGrid.fields.custom; } }); test("'0' itemTemplate should be rendered", function() { var $element = $("#jsGrid"), grid = new Grid($element, { data: [{}], fields: [ new jsGrid.Field({ name: "id", itemTemplate: function() { return 0; } }) ] }); equal(grid._bodyGrid.text(), "0", "item template is rendered"); }); test("grid field name used for header if title is not specified", function() { var $element = $("#jsGrid"), grid = new Grid($element, { fields: [ new jsGrid.Field({ name: "id" }) ] }); equal(grid._headerRow.text(), "id", "name is rendered in header"); }); test("grid fields header and item rendering", function() { var $element = $("#jsGrid"), $secondRow, gridOptions = { fields: [ new jsGrid.Field({ name: "text", title: "title", css: "cell-class", headercss: "header-class", align: "right" }) ] }, grid = new Grid($element, gridOptions); grid.option("data", this.testData); equal(grid._headerRow.text(), "title", "header rendered"); equal(grid._headerRow.find("." + grid.headerCellClass).length, 1, "header cell class is attached"); equal(grid._headerRow.find(".header-class").length, 1, "headercss class is attached"); ok(grid._headerRow.find(".header-class").hasClass("jsgrid-align-right"), "align class is attached"); $secondRow = grid._content.find("." + grid.evenRowClass); equal($secondRow.text(), "test2", "item rendered"); equal($secondRow.find(".cell-class").length, 1, "css class added to cell"); equal($secondRow.find("." + grid.cellClass).length, 1, "cell class is attached"); ok($secondRow.find(".cell-class").hasClass("jsgrid-align-right"), "align class added to cell"); }); test("grid field cellRenderer", function() { var testItem = { text: "test" }, args; var $grid = $("#jsGrid"); var gridOptions = { data: [testItem], fields: [ { name: "text", cellRenderer: function(value, item) { args = { value: value, item: item }; return $("<td>").addClass("custom-class").text(value); } } ] }; var grid = new Grid($grid, gridOptions); var $customCell = $grid.find(".custom-class"); equal($customCell.length, 1, "custom cell rendered"); equal($customCell.text(), "test"); deepEqual(args, { value: "test", item: testItem }, "cellRenderer args provided"); }); test("grid field 'visible' option", function() { var $grid = $("#jsGrid"); var gridOptions = { editing: true, fields: [ { name: "id", visible: false }, { name: "test" } ] }; var grid = new Grid($grid, gridOptions); equal($grid.find("." + grid.noDataRowClass).children().eq(0).prop("colspan"), 1, "no data row colspan only for visible cells"); grid.option("data", this.testData); grid.editItem(this.testData[2]); equal($grid.find("." + grid.headerRowClass).children().length, 1, "header single cell"); equal($grid.find("." + grid.filterRowClass).children().length, 1, "filter single cell"); equal($grid.find("." + grid.insertRowClass).children().length, 1, "insert single cell"); equal($grid.find("." + grid.editRowClass).children().length, 1, "edit single cell"); equal($grid.find("." + grid.oddRowClass).eq(0).children().length, 1, "odd data row single cell"); equal($grid.find("." + grid.evenRowClass).eq(0).children().length, 1, "even data row single cell"); }); module("inserting"); test("inserting rendering", function() { var $element = $("#jsGrid"), gridOptions = { inserting: true, fields: [ { name: "test", align: "right", insertcss: "insert-class", insertTemplate: function() { var result = this.insertControl = $("<input>").attr("type", "text").addClass("insert-input"); return result; } } ] }, grid = new Grid($element, gridOptions); equal(grid._insertRow.find(".insert-class").length, 1, "insertcss class is attached"); equal(grid._insertRow.find(".insert-input").length, 1, "insert control rendered"); equal(grid._insertRow.find("." + grid.cellClass).length, 1, "cell class is attached"); ok(grid._insertRow.find(".insert-class").hasClass("jsgrid-align-right"), "align class is attached"); ok(grid.fields[0].insertControl.is("input[type=text]"), "insert control saved in field"); }); test("field without inserting", function() { var $element = $("#jsGrid"), jsGridFieldConfig = { insertTemplate: function() { var result = this.insertControl = $("<input>").attr("type", "text"); return result; }, insertValue: function() { return this.insertControl.val(); } }, gridOptions = { inserting: true, fields: [ $.extend({}, jsGridFieldConfig, { name: "field1", inserting: false }), $.extend({}, jsGridFieldConfig, { name: "field2" }) ] }, grid = new Grid($element, gridOptions); grid.fields[0].insertControl.val("test1"); grid.fields[1].insertControl.val("test2"); deepEqual(grid._getInsertItem(), { field2: "test2" }, "field with inserting=false is not included in inserting item"); }); test("insert data with default location", function() { var $element = $("#jsGrid"), inserted = false, insertingArgs, insertedArgs, gridOptions = { inserting: true, data: [{field: "default"}], fields: [ { name: "field", insertTemplate: function() { var result = this.insertControl = $("<input>").attr("type", "text"); return result; }, insertValue: function() { return this.insertControl.val(); } } ], onItemInserting: function(e) { insertingArgs = $.extend(true, {}, e); }, onItemInserted: function(e) { insertedArgs = $.extend(true, {}, e); }, controller: { insertItem: function() { inserted = true; } } }, grid = new Grid($element, gridOptions); grid.fields[0].insertControl.val("test"); grid.insertItem(); equal(insertingArgs.item.field, "test", "field is provided in inserting args"); equal(grid.option("data").length, 2, "data is inserted"); ok(inserted, "controller insertItem was called"); deepEqual(grid.option("data")[1], { field: "test" }, "correct data is inserted"); equal(insertedArgs.item.field, "test", "field is provided in inserted args"); }); test("insert data with specified insert location", function() { var $element = $("#jsGrid"), inserted = false, insertingArgs, insertedArgs, gridOptions = { inserting: true, insertRowLocation: "top", data: [{field: "default"}], fields: [ { name: "field", insertTemplate: function() { var result = this.insertControl = $("<input>").attr("type", "text"); return result; }, insertValue: function() { return this.insertControl.val(); } } ], onItemInserting: function(e) { insertingArgs = $.extend(true, {}, e); }, onItemInserted: function(e) { insertedArgs = $.extend(true, {}, e); }, controller: { insertItem: function() { inserted = true; } } }, grid = new Grid($element, gridOptions); grid.fields[0].insertControl.val("test"); grid.insertItem(); equal(insertingArgs.item.field, "test", "field is provided in inserting args"); equal(grid.option("data").length, 2, "data is inserted"); ok(inserted, "controller insertItem was called"); deepEqual(grid.option("data")[0], { field: "test" }, "correct data is inserted at the beginning"); equal(insertedArgs.item.field, "test", "field is provided in inserted args"); }); test("insertItem accepts item to insert", function() { var $element = $("#jsGrid"), itemToInsert = { field: "test" }, insertedItem, gridOptions = { data: [], fields: [ { name: "field" } ], controller: { insertItem: function(item) { insertedItem = item; } } }, grid = new Grid($element, gridOptions); grid.insertItem(itemToInsert); deepEqual(grid.option("data")[0], itemToInsert, "data is inserted"); deepEqual(insertedItem, itemToInsert, "controller insertItem was called with correct item"); }); module("editing"); test("editing rendering", function() { var $element = $("#jsGrid"), $editRow, data = [{ test: "value" }], gridOptions = { editing: true, fields: [ { name: "test", align: "right", editcss: "edit-class", editTemplate: function(value) { var result = this.editControl = $("<input>").attr("type", "text").val(value).addClass("edit-input"); return result; } } ] }, grid = new Grid($element, gridOptions); grid.option("data", data); equal(grid._content.find("." + grid.editRowClass).length, 0, "no edit row after initial rendering"); grid.editItem(data[0]); $editRow = grid._content.find("." + grid.editRowClass); equal($editRow.length, 1, "edit row rendered"); equal($editRow.find(".edit-class").length, 1, "editcss class is attached"); equal($editRow.find(".edit-input").length, 1, "edit control rendered"); equal($editRow.find("." + grid.cellClass).length, 1, "cell class is attached"); ok($editRow.find(".edit-class").hasClass("jsgrid-align-right"), "align class is attached"); ok(grid.fields[0].editControl.is("input[type=text]"), "edit control saved in field"); equal(grid.fields[0].editControl.val(), "value", "edit control value"); }); test("editItem accepts row to edit", function() { var $element = $("#jsGrid"), $editRow, data = [ { test: "value" } ], gridOptions = { editing: true, fields: [ { name: "test" } ] }, grid = new Grid($element, gridOptions); grid.option("data", data); var $row = $element.find("." + grid.oddRowClass).eq(0); grid.editItem($row); $editRow = grid._content.find("." + grid.editRowClass); equal($editRow.length, 1, "edit row rendered"); grid.cancelEdit(); grid.editItem($row.get(0)); $editRow = grid._content.find("." + grid.editRowClass); equal($editRow.length, 1, "edit row rendered"); }); test("edit item", function() { var $element = $("#jsGrid"), editingArgs, editingRow, updated = false, updatingArgs, updatingRow, updatedRow, updatedArgs, data = [{ field: "value" }], gridOptions = { editing: true, fields: [ { name: "field", editTemplate: function(value) { var result = this.editControl = $("<input>").attr("type", "text").val(value); return result; }, editValue: function() { return this.editControl.val(); } } ], controller: { updateItem: function(updatingItem) { updated = true; } }, onItemEditing: function(e) { editingArgs = $.extend(true, {}, e); editingRow = grid.rowByItem(data[0])[0]; }, onItemUpdating: function(e) { updatingArgs = $.extend(true, {}, e); updatingRow = grid.rowByItem(data[0])[0]; }, onItemUpdated: function(e) { updatedArgs = $.extend(true, {}, e); updatedRow = grid.rowByItem(data[0])[0]; } }, grid = new Grid($element, gridOptions); grid.option("data", data); grid.editItem(data[0]); deepEqual(editingArgs.item, { field: "value" }, "item before editing is provided in editing event args"); equal(editingArgs.itemIndex, 0, "itemIndex is provided in editing event args"); equal(editingArgs.row[0], editingRow, "row element is provided in editing event args"); grid.fields[0].editControl.val("new value"); grid.updateItem(); deepEqual(updatingArgs.previousItem, { field: "value" }, "item before editing is provided in updating event args"); deepEqual(updatingArgs.item, { field: "new value" }, "updating item is provided in updating event args"); equal(updatingArgs.itemIndex, 0, "itemIndex is provided in updating event args"); equal(updatingArgs.row[0], updatingRow, "row element is provided in updating event args"); ok(updated, "controller updateItem called"); deepEqual(grid.option("data")[0], { field: "new value" }, "correct data updated"); equal(grid._content.find("." + grid.editRowClass).length, 0, "edit row removed"); equal(grid._content.find("." + grid.oddRowClass).length, 1, "data row rendered"); deepEqual(updatedArgs.previousItem, { field: "value" }, "item before editing is provided in updated event args"); deepEqual(updatedArgs.item, { field: "new value" }, "updated item is provided in updated event args"); equal(updatedArgs.itemIndex, 0, "itemIndex is provided in updated event args"); equal(updatedArgs.row[0], updatedRow, "row element is provided in updated event args"); }); test("failed update should not change original item", function() { var $element = $("#jsGrid"), data = [{ field: "value" }], gridOptions = { editing: true, fields: [ { name: "field", editTemplate: function(value) { var result = this.editControl = $("<input>").attr("type", "text").val(value); return result; }, editValue: function() { return this.editControl.val(); } } ], controller: { updateItem: function(updatingItem) { return $.Deferred().reject(); } } }, grid = new Grid($element, gridOptions); grid.option("data", data); grid.editItem(data[0]); grid.fields[0].editControl.val("new value"); grid.updateItem(); deepEqual(grid.option("data")[0], { field: "value" }, "value is not updated"); }); test("cancel edit", function() { var $element = $("#jsGrid"), updated = false, cancellingArgs, cancellingRow, data = [{ field: "value" }], gridOptions = { editing: true, fields: [ { name: "field", editTemplate: function(value) { var result = this.editControl = $("<input>").attr("type", "text").val(value); return result; }, editValue: function() { return this.editControl.val(); } } ], controller: { updateData: function(updatingItem) { updated = true; } }, onItemEditCancelling: function(e) { cancellingArgs = $.extend(true, {}, e); cancellingRow = grid.rowByItem(data[0])[0]; } }, grid = new Grid($element, gridOptions); grid.option("data", data); grid.editItem(data[0]); grid.fields[0].editControl.val("new value"); grid.cancelEdit(); deepEqual(cancellingArgs.item, { field: "value" }, "item before cancel is provided in cancelling event args"); equal(cancellingArgs.itemIndex, 0, "itemIndex is provided in cancelling event args"); equal(cancellingArgs.row[0], cancellingRow, "row element is provided in cancelling event args"); ok(!updated, "controller updateItem was not called"); deepEqual(grid.option("data")[0], { field: "value" }, "data were not updated"); equal(grid._content.find("." + grid.editRowClass).length, 0, "edit row removed"); equal(grid._content.find("." + grid.oddRowClass).length, 1, "data row restored"); }); test("updateItem accepts item to update and new item", function() { var $element = $("#jsGrid"), updatingItem, data = [{ field: "value" }], gridOptions = { fields: [ { name: "field" } ], controller: { updateItem: function(item) { return updatingItem = item; } } }, grid = new Grid($element, gridOptions); grid.option("data", data); grid.updateItem(data[0], { field: "new value" }); deepEqual(updatingItem, { field: "new value" }, "controller updateItem called correctly"); deepEqual(grid.option("data")[0], { field: "new value" }, "correct data updated"); }); test("updateItem accepts single argument - item to update", function() { var $element = $("#jsGrid"), updatingItem, data = [{ field: "value" }], gridOptions = { fields: [ { name: "field" } ], controller: { updateItem: function(item) { return updatingItem = item; } } }, grid = new Grid($element, gridOptions); grid.option("data", data); data[0].field = "new value"; grid.updateItem(data[0]); deepEqual(updatingItem, { field: "new value" }, "controller updateItem called correctly"); deepEqual(grid.option("data")[0], { field: "new value" }, "correct data updated"); }); test("editRowRenderer", function() { var $element = $("#jsGrid"), data = [ { value: "test" } ], gridOptions = { data: data, editing: true, editRowRenderer: function(item, itemIndex) { return $("<tr>").addClass("custom-edit-row").append($("<td>").text(itemIndex + ":" + item.value)); }, fields: [ { name: "value" } ] }, grid = new Grid($element, gridOptions); grid.editItem(data[0]); var $editRow = grid._content.find(".custom-edit-row"); equal($editRow.length, 1, "edit row rendered"); equal($editRow.text(), "0:test", "custom edit row renderer rendered"); }); module("deleting"); test("delete item", function() { var $element = $("#jsGrid"), deleted = false, deletingArgs, deletedArgs, data = [{ field: "value" }], gridOptions = { confirmDeleting: false, fields: [ { name: "field" } ], controller: { deleteItem: function(deletingItem) { deleted = true; } }, onItemDeleting: function(e) { deletingArgs = $.extend(true, {}, e); }, onItemDeleted: function(e) { deletedArgs = $.extend(true, {}, e); } }, grid = new Grid($element, gridOptions); grid.option("data", data); grid.deleteItem(data[0]); deepEqual(deletingArgs.item, { field: "value" }, "field and value is provided in deleting event args"); equal(deletingArgs.itemIndex, 0, "itemIndex is provided in updating event args"); equal(deletingArgs.row.length, 1, "row element is provided in updating event args"); ok(deleted, "controller deleteItem called"); equal(grid.option("data").length, 0, "data row deleted"); deepEqual(deletedArgs.item, { field: "value" }, "item is provided in updating event args"); equal(deletedArgs.itemIndex, 0, "itemIndex is provided in updating event args"); equal(deletedArgs.row.length, 1, "row element is provided in updating event args"); }); test("deleteItem accepts row", function() { var $element = $("#jsGrid"), deletedItem, itemToDelete = { field: "value" }, data = [itemToDelete], gridOptions = { confirmDeleting: false, fields: [ { name: "field" } ], controller: { deleteItem: function(deletingItem) { deletedItem = deletingItem; } } }, grid = new Grid($element, gridOptions); grid.option("data", data); var $row = $element.find("." + grid.oddRowClass).eq(0); grid.deleteItem($row); deepEqual(deletedItem, itemToDelete, "controller deleteItem called correctly"); equal(grid.option("data").length, 0, "data row deleted"); }); module("paging"); test("pager is rendered if necessary", function() { var $element = $("#jsGrid"), grid = new Grid($element, { data: [{}, {}, {}], paging: false, pageSize: 2 }); ok(grid._pagerContainer.is(":hidden"), "pager is hidden when paging=false"); equal(grid._pagerContainer.html(), "", "pager is not rendered when paging=false"); grid.option("paging", true); ok(grid._pagerContainer.is(":visible"), "pager is visible when paging=true"); ok(grid._pagerContainer.html(), "pager is rendered when paging=true"); grid.option("data", [{}, {}]); ok(grid._pagerContainer.is(":hidden"), "pager is hidden for single page"); ok(grid._pagerContainer.html(), "pager is rendered for single page when paging=true"); }); test("external pagerContainer", function() { var $pagerContainer = $("<div>").appendTo("#qunit-fixture").hide(), $element = $("#jsGrid"); new Grid($element, { data: [{}, {}, {}], pagerContainer: $pagerContainer, paging: true, pageSize: 2 }); ok($pagerContainer.is(":visible"), "external pager shown"); ok($pagerContainer.html(), "external pager rendered"); }); test("pager functionality", function() { var $element = $("#jsGrid"), pager, pageChangedArgs, grid = new Grid($element, { data: [{}, {}, {}, {}, {}, {}, {}, {}, {}], onPageChanged: function(args) { pageChangedArgs = args; }, paging: true, pageSize: 2, pageButtonCount: 3 }); equal(grid._pagesCount(), 5, "correct page count"); equal(grid.option("pageIndex"), 1, "pageIndex is initialized"); equal(grid._firstDisplayingPage, 1, "_firstDisplayingPage is initialized"); pager = grid._pagerContainer; equal(pager.find("." + grid.currentPageClass).length, 1, "there is one current page"); ok(pager.find("." + grid.pageClass).eq(0).hasClass(grid.currentPageClass), "first page is current"); equal(pager.find("." + grid.pageClass).length, 3, "three pages displayed"); equal(pager.find("." + grid.pagerNavButtonClass).length, 5, "five nav buttons displayed: Fisrt Prev Next Last ..."); equal(pager.find("." + grid.pagerNavButtonInactiveClass).length, 2, "two nav buttons inactive: Fisrt Prev"); grid.openPage(2); equal(pager.find("." + grid.currentPageClass).length, 1, "there is one current page"); ok(pager.find("." + grid.pageClass).eq(1).hasClass(grid.currentPageClass), "second page is current"); equal(pager.find("." + grid.pageClass).length, 3, "three pages displayed"); equal(pager.find("." + grid.pagerNavButtonClass).length, 5, "five nav buttons displayed: First Prev Next Last and ..."); equal(pageChangedArgs.pageIndex, 2, "onPageChanged callback provides pageIndex in arguments"); grid.showNextPages(); equal(grid._firstDisplayingPage, 3, "navigate by pages forward"); grid.showPrevPages(); equal(grid._firstDisplayingPage, 1, "navigate by pages backward"); grid.openPage(5); equal(grid._firstDisplayingPage, 3, "opening next non-visible page moves first displaying page forward"); grid.openPage(2); equal(grid._firstDisplayingPage, 2, "opening prev non-visible page moves first displaying page backward"); }); test("pager format", function() { var $element = $("#jsGrid"), grid = new Grid($element, { data: [{}, {}, {}, {}, {}, {}], paging: true, pageSize: 2, pageIndex: 2, pageButtonCount: 1, pagerFormat: "a {pageIndex} {first} {prev} {pages} {next} {last} {pageCount} {itemCount} z", pagePrevText: "<", pageNextText: ">", pageFirstText: "<<", pageLastText: ">>", pageNavigatorNextText: "np", pageNavigatorPrevText: "pp" }); grid._firstDisplayingPage = 2; grid._refreshPager(); equal($.trim(grid._pagerContainer.text()), "a 2 << < pp2np > >> 3 6 z", "pager text follows the format specified"); }); test("pagerRenderer", function() { var $element = $("#jsGrid"); var pagerRendererConfig; var pageSize = 2; var items = [{}, {}, {}, {}, {}, {}, {}]; var pageCount = Math.ceil(items.length / pageSize); var grid = new Grid($element, { data: items, paging: true, pageSize: pageSize, pagerRenderer: function(pagerConfig) { pagerRendererConfig = pagerConfig; } }); deepEqual(pagerRendererConfig, { pageIndex: 1, pageCount: pageCount }); grid.openPage(2); deepEqual(pagerRendererConfig, { pageIndex: 2, pageCount: pageCount }); }); test("loading by page", function() { var $element = $("#jsGrid"), data = [], itemCount = 20; for(var i = 1; i <= itemCount; i += 1) { data.push({ value: i }); } var gridOptions = { pageLoading: true, paging: true, pageSize: 7, fields: [ { name: "value" } ], controller: { loadData: function(filter) { var startIndex = (filter.pageIndex - 1) * filter.pageSize, result = data.slice(startIndex, startIndex + filter.pageSize); return { data: result, itemsCount: data.length }; } } }; var grid = new Grid($element, gridOptions); grid.loadData(); var pager = grid._pagerContainer; var gridData = grid.option("data"); equal(gridData.length, 7, "loaded one page of data"); equal(gridData[0].value, 1, "loaded right data start value"); equal(gridData[gridData.length - 1].value, 7, "loaded correct data end value"); ok(pager.find("." + grid.pageClass).eq(0).hasClass(grid.currentPageClass), "first page is current"); equal(pager.find("." + grid.pageClass).length, 3, "three pages displayed"); grid.openPage(3); gridData = grid.option("data"); equal(gridData.length, 6, "loaded last page of data"); equal(gridData[0].value, 15, "loaded right data start value"); equal(gridData[gridData.length - 1].value, 20, "loaded right data end value"); ok(pager.find("." + grid.pageClass).eq(2).hasClass(grid.currentPageClass), "third page is current"); equal(pager.find("." + grid.pageClass).length, 3, "three pages displayed"); }); test("'openPage' method ignores indexes out of range", function() { var $element = $("#jsGrid"), grid = new Grid($element, { data: [{}, {}], paging: true, pageSize: 1 }); grid.openPage(0); equal(grid.option("pageIndex"), 1, "too small index is ignored"); grid.openPage(3); equal(grid.option("pageIndex"), 1, "too big index is ignored"); }); module("sorting"); test("sorting", function() { var $element = $("#jsGrid"), gridOptions = { sorting: true, data: [ { value: 3 }, { value: 2 }, { value: 1 } ], fields: [ { name: "value", sorter: "number" } ] }, grid = new Grid($element, gridOptions); var gridData = grid.option("data"); var $th = grid._headerRow.find("th").eq(0); $th.trigger("click"); equal(grid._sortOrder, "asc", "asc sorting order for first click"); equal(grid._sortField, grid.fields[0], "sort field is set"); equal(gridData[0].value, 1); equal(gridData[1].value, 2); equal(gridData[2].value, 3); ok($th.hasClass(grid.sortableClass)); ok($th.hasClass(grid.sortAscClass)); $th.trigger("click"); equal(grid._sortOrder, "desc", "desc sorting order for second click"); equal(grid._sortField, grid.fields[0], "sort field is set"); equal(gridData[0].value, 3); equal(gridData[1].value, 2); equal(gridData[2].value, 1); ok(!$th.hasClass(grid.sortAscClass)); ok($th.hasClass(grid.sortDescClass)); }); test("sorting with pageLoading", function() { var $element = $("#jsGrid"), loadFilter, gridOptions = { sorting: true, pageLoading: true, data: [ { value: 3 }, { value: 2 }, { value: 1 } ], controller: { loadData: function(filter) { loadFilter = filter; return { itemsCount: 0, data: [] }; } }, fields: [ { name: "value", sorter: "number" } ] }, grid = new Grid($element, gridOptions); var $th = grid._headerRow.find("th").eq(0); $th.trigger("click"); equal(grid._sortOrder, "asc", "asc sorting order for first click"); equal(grid._sortField, grid.fields[0], "sort field is set"); equal(loadFilter.sortOrder, "asc", "sort direction is provided in loadFilter"); equal(loadFilter.sortField, "value", "sort field is provided in loadFilter"); $th.trigger("click"); equal(grid._sortOrder, "desc", "desc sorting order for second click"); equal(grid._sortField, grid.fields[0], "sort field is set"); equal(loadFilter.sortOrder, "desc", "sort direction is provided in loadFilter"); equal(loadFilter.sortField, "value", "sort field is provided in loadFilter"); }); test("no sorting for column with sorting = false", function() { var $element = $("#jsGrid"); var gridOptions = { sorting: true, data: [ { value: 3 }, { value: 2 }, { value: 1 } ], fields: [ { name: "value", sorting: false } ] }; var grid = new Grid($element, gridOptions); var gridData = grid.option("data"); var $th = grid._headerRow.find("th").eq(0); $th.trigger("click"); equal(grid._sortField, null, "sort field is not set for the field with sorting=false"); equal(gridData[0].value, 3); equal(gridData[1].value, 2); equal(gridData[2].value, 1); equal($th.hasClass(grid.sortableClass), false, "no sorting css for field with sorting=false"); equal($th.hasClass(grid.sortAscClass), false, "no sorting css for field with sorting=false"); }); test("sort accepts sorting config", function() { var $element = $("#jsGrid"), gridOptions = { sorting: true, data: [ { value: 3 }, { value: 2 }, { value: 1 } ], fields: [ { name: "value", sorter: "number" } ] }, grid = new Grid($element, gridOptions); var gridData = grid.option("data"); grid.sort({ field: "value", order: "asc" }); equal(grid._sortOrder, "asc", "asc sorting order is set"); equal(grid._sortField, grid.fields[0], "sort field is set"); equal(gridData[0].value, 1); equal(gridData[1].value, 2); equal(gridData[2].value, 3); grid.sort({ field: 0 }); equal(grid._sortOrder, "desc", "desc sorting order for already set asc sorting"); equal(grid._sortField, grid.fields[0], "sort field is set"); equal(gridData[0].value, 3); equal(gridData[1].value, 2); equal(gridData[2].value, 1); grid.sort("value", "asc"); equal(grid._sortOrder, "asc", "asc sorting order is set"); equal(grid._sortField, grid.fields[0], "sort field is set"); grid.sort(0); equal(grid._sortOrder, "desc", "desc sorting order for already set asc sorting"); equal(grid._sortField, grid.fields[0], "sort field is set"); }); test("getSorting returns current sorting", function() { var $element = $("#jsGrid"), gridOptions = { sorting: true, data: [ { value: 3 }, { value: 2 }, { value: 1 } ], fields: [ { name: "value", sorter: "number" } ] }, grid = new Grid($element, gridOptions); deepEqual(grid.getSorting(), { field: undefined, order: undefined }, "undefined field and order before sorting"); grid.sort("value"); deepEqual(grid.getSorting(), { field: "value", order: "asc" }, "current sorting returned"); }); test("sorting css attached correctly when a field is hidden", function() { var $element = $("#jsGrid"); var gridOptions = { sorting: true, data: [], fields: [ { name: "field1", visible: false }, { name: "field2" } ] }; var grid = new Grid($element, gridOptions); var gridData = grid.option("data"); var $th = grid._headerRow.find("th").eq(0); $th.trigger("click"); equal($th.hasClass(grid.sortAscClass), true, "sorting css is attached to first field"); }); module("canceling events"); test("cancel item edit", function() { var $element = $("#jsGrid"); var data = [{}]; var gridOptions = { editing: true, onItemEditing: function(e) { e.cancel = true; }, controller: { loadData: function() { return data; } }, fields: [ { name: "test" } ] }; var grid = new Grid($element, gridOptions); grid.loadData(); grid.editItem(data[0]); strictEqual(grid._editingRow, null, "no editing row"); }); test("cancel controller.loadData", function() { var $element = $("#jsGrid"); var gridOptions = { onDataLoading: function(e) { e.cancel = true; }, controller: { loadData: function() { return [{}]; } }, fields: [ { name: "test" } ] }; var grid = new Grid($element, gridOptions); grid.loadData(); equal(grid.option("data").length, 0, "no data loaded"); }); test("cancel controller.insertItem", function() { var $element = $("#jsGrid"); var insertedItem = null; var gridOptions = { onItemInserting: function(e) { e.cancel = true; }, controller: { insertItem: function(item) { insertedItem = item; } }, fields: [ { name: "test" } ] }; var grid = new Grid($element, gridOptions); grid.insertItem({ test: "value" }); strictEqual(insertedItem, null, "item was not inserted"); }); test("cancel controller.updateItem", function() { var $element = $("#jsGrid"); var updatedItem = null; var existingItem = { test: "value" }; var gridOptions = { data: [ existingItem ], onItemUpdating: function(e) { e.cancel = true; }, controller: { updateItem: function(item) { updatedItem = item; } }, fields: [ { name: "test" } ] }; var grid = new Grid($element, gridOptions); grid.updateItem(existingItem, { test: "new_value" }); strictEqual(updatedItem, null, "item was not updated"); }); test("cancel controller.deleteItem", function() { var $element = $("#jsGrid"); var deletingItem = { test: "value" }; var deletedItem = null; var gridOptions = { data: [ deletingItem ], confirmDeleting: false, onItemDeleting: function(e) { e.cancel = true; }, controller: { deleteItem: function(item) { deletedItem = item; } }, fields: [ { name: "test" } ] }; var grid = new Grid($element, gridOptions); grid.deleteItem(deletingItem); strictEqual(deletedItem, null, "item was not deleted"); }); module("complex properties binding"); test("rendering", function() { var $element = $("#jsGrid"); var gridOptions = { loadMessage: "", data: [ { complexProp: { prop: "test" } } ], fields: [ { name: "complexProp.prop", title: "" } ] }; new Grid($element, gridOptions); equal($element.text(), "test", "complex property value rendered"); }); test("editing", function() { var $element = $("#jsGrid"); var gridOptions = { editing: true, data: [ { complexProp: { prop: "test" } } ], fields: [ { type: "text", name: "complexProp.prop" } ] }; var grid = new Grid($element, gridOptions); grid.editItem(gridOptions.data[0]); equal(grid.fields[0].editControl.val(), "test", "complex property value set in editor"); }); test("should not fail if property is absent", function() { var $element = $("#jsGrid"); var gridOptions = { loadMessage: "", data: [ { complexProp: { } } ], fields: [ { name: "complexProp.subprop.prop", title: "" } ] }; new Grid($element, gridOptions); equal($element.text(), "", "rendered empty value"); }); test("inserting", function() { var $element = $("#jsGrid"); var insertingItem; var gridOptions = { inserting: true, fields: [ { type: "text", name: "complexProp.prop" } ], onItemInserting: function(args) { insertingItem = args.item; } }; var grid = new Grid($element, gridOptions); grid.fields[0].insertControl.val("test"); grid.insertItem(); deepEqual(insertingItem, { complexProp: { prop: "test" } }, "inserting item has complex properties"); }); test("filtering", function() { var $element = $("#jsGrid"); var loadFilter; var gridOptions = { filtering: true, fields: [ { type: "text", name: "complexProp.prop" } ], controller: { loadData: function(filter) { loadFilter = filter; } } }; var grid = new Grid($element, gridOptions); grid.fields[0].filterControl.val("test"); grid.search(); deepEqual(loadFilter, { complexProp: { prop: "test" } }, "filter has complex properties"); }); test("updating", function() { var $element = $("#jsGrid"); var updatingItem; var gridOptions = { editing: true, data: [ { complexProp: { } } ], fields: [ { type: "text", name: "complexProp.prop" } ], onItemUpdating: function(args) { updatingItem = args.item; } }; var grid = new Grid($element, gridOptions); grid.editItem(gridOptions.data[0]); grid.fields[0].editControl.val("test"); grid.updateItem(); deepEqual(updatingItem, { complexProp: { prop: "test" } }, "updating item has complex properties"); }); test("update nested prop", function() { var $element = $("#jsGrid"); var updatingItem; var previousItem; var gridOptions = { editing: true, data: [ { prop: { subprop1: "test1", subprop2: "test2" } } ], fields: [ { type: "text", name: "prop.subprop1" }, { type: "text", name: "prop.subprop2" } ], onItemUpdating: function(args) { updatingItem = args.item; } }; var grid = new Grid($element, gridOptions); grid.editItem(gridOptions.data[0]); grid.fields[0].editControl.val("new_test1"); grid.updateItem(); var expectedUpdatingItem = { prop: { subprop1: "new_test1", subprop2: "test2" } }; deepEqual(updatingItem, expectedUpdatingItem, "updating item has nested properties"); }); test("updating deeply nested prop", function() { var $element = $("#jsGrid"); var updatingItem; var previousItem; var gridOptions = { editing: true, data: [ { complexProp: { subprop1: { another_prop: "test" } } } ], fields: [ { type: "text", name: "complexProp.subprop1.prop1" }, { type: "text", name: "complexProp.subprop1.subprop2.prop12" } ], onItemUpdating: function(args) { updatingItem = $.extend(true, {}, args.item); previousItem = $.extend(true, {}, args.previousItem); } }; var grid = new Grid($element, gridOptions); grid.editItem(gridOptions.data[0]); grid.fields[0].editControl.val("test1"); grid.fields[1].editControl.val("test2"); grid.updateItem(); var expectedUpdatingItem = { complexProp: { subprop1: { another_prop: "test", prop1: "test1", subprop2: { prop12: "test2" } } } }; var expectedPreviousItem = { complexProp: { subprop1: { another_prop: "test" } } }; deepEqual(updatingItem, expectedUpdatingItem, "updating item has deeply nested properties"); deepEqual(previousItem, expectedPreviousItem, "previous item preserved correctly"); }); module("validation"); test("insertItem should call validation.validate", function() { var $element = $("#jsGrid"); var fieldValidationRules = { test: "value" }; var validatingArgs; var gridOptions = { data: [], inserting: true, invalidNotify: $.noop, validation: { validate: function(args) { validatingArgs = args; return []; } }, fields: [ { type: "text", name: "Name", validate: fieldValidationRules } ] }; var grid = new Grid($element, gridOptions); grid.fields[0].insertControl.val("test"); grid.insertItem(); deepEqual(validatingArgs, { value: "test", item: { Name: "test" }, itemIndex: -1, row: grid._insertRow, rules: fieldValidationRules }, "validating args is provided"); }); test("insertItem rejected when data is not valid", function() { var $element = $("#jsGrid"); var gridOptions = { data: [], inserting: true, invalidNotify: $.noop, validation: { validate: function() { return ["Error"]; } }, fields: [ { type: "text", name: "Name", validate: true } ] }; var grid = new Grid($element, gridOptions); grid.fields[0].insertControl.val("test"); grid.insertItem().done(function() { ok(false, "insertItem should not be completed"); }).fail(function() { ok(true, "insertItem should fail"); }); }); test("invalidClass is attached on invalid cell on inserting", function() { var $element = $("#jsGrid"); var gridOptions = { data: [], inserting: true, invalidNotify: $.noop, validation: { validate: function() { return ["Error"]; } }, fields: [ { type: "text", name: "Id", visible: false }, { type: "text", name: "Name", validate: true } ] }; var grid = new Grid($element, gridOptions); var $insertCell = grid._insertRow.children().eq(0); grid.insertItem(); ok($insertCell.hasClass(grid.invalidClass), "invalid class is attached"); equal($insertCell.attr("title"), "Error", "cell tooltip contains error message"); }); test("onItemInvalid callback", function() { var $element = $("#jsGrid"); var errors = ["Error"]; var onItemInvalidCalled = 0; var onItemInvalidArgs; var gridOptions = { data: [], inserting: true, invalidNotify: $.noop, onItemInvalid: function(args) { onItemInvalidCalled++; onItemInvalidArgs = args; }, validation: { validate: function(args) { return !args.value ? errors : []; } }, fields: [ { type: "text", name: "Name", validate: true } ] }; var grid = new Grid($element, gridOptions); grid.insertItem(); equal(onItemInvalidCalled, 1, "onItemInvalid is called, when item data is invalid"); deepEqual(onItemInvalidArgs, { grid: grid, errors: [{ field: grid.fields[0], message: "Error" }], item: { Name: "" }, itemIndex: -1, row: grid._insertRow }, "arguments provided"); grid.fields[0].insertControl.val("test"); grid.insertItem(); equal(onItemInvalidCalled, 1, "onItemInvalid was not called, when data is valid"); }); test("invalidNotify", function() { var $element = $("#jsGrid"); var errors = ["Error"]; var invalidNotifyCalled = 0; var invalidNotifyArgs; var gridOptions = { data: [], inserting: true, invalidNotify: function(args) { invalidNotifyCalled++; invalidNotifyArgs = args; }, validation: { validate: function(args) { return !args.value ? errors : []; } }, fields: [ { type: "text", name: "Name", validate: true } ] }; var grid = new Grid($element, gridOptions); grid.insertItem(); equal(invalidNotifyCalled, 1, "invalidNotify is called, when item data is invalid"); deepEqual(invalidNotifyArgs, { grid: grid, errors: [{ field: grid.fields[0], message: "Error" }], row: grid._insertRow, item: { Name: "" }, itemIndex: -1 }, "arguments provided"); grid.fields[0].insertControl.val("test"); grid.insertItem(); equal(invalidNotifyCalled, 1, "invalidNotify was not called, when data is valid"); }); test("invalidMessage", function() { var $element = $("#jsGrid"); var invalidMessage; var originalAlert = window.alert; window.alert = function(message) { invalidMessage = message; }; try { Grid.prototype.invalidMessage = "InvalidTest"; Grid.prototype.invalidNotify({ errors: [{ message: "Message1" }, { message: "Message2" }] }); var expectedInvalidMessage = ["InvalidTest", "Message1", "Message2"].join("\n"); equal(invalidMessage, expectedInvalidMessage, "message contains invalidMessage and field error messages"); } finally { window.alert = originalAlert; } }); test("updateItem should call validation.validate", function() { var $element = $("#jsGrid"); var validatingArgs; var gridOptions = { data: [{ Name: "" }], editing: true, invalidNotify: $.noop, validation: { validate: function(args) { validatingArgs = args; return ["Error"]; } }, fields: [ { type: "text", name: "Name", validate: "required" } ] }; var grid = new Grid($element, gridOptions); grid.editItem(gridOptions.data[0]); grid.fields[0].editControl.val("test"); grid.updateItem(); deepEqual(validatingArgs, { value: "test", item: { Name: "test" }, itemIndex: 0, row: grid._getEditRow(), rules: "required" }, "validating args is provided"); }); test("invalidClass is attached on invalid cell on updating", function() { var $element = $("#jsGrid"); var gridOptions = { data: [{}], editing: true, invalidNotify: $.noop, validation: { validate: function() { return ["Error"]; } }, fields: [ { type: "text", name: "Name", validate: true } ] }; var grid = new Grid($element, gridOptions); grid.editItem(gridOptions.data[0]); var $editCell = grid._getEditRow().children().eq(0); grid.updateItem(); ok($editCell.hasClass(grid.invalidClass), "invalid class is attached"); equal($editCell.attr("title"), "Error", "cell tooltip contains error message"); }); test("validation should ignore not editable fields", function() { var invalidNotifyCalled = 0; var $element = $("#jsGrid"); var validatingArgs; var gridOptions = { data: [{ Name: "" }], editing: true, invalidNotify: function() { invalidNotifyCalled++; }, validation: { validate: function() { return ["Error"]; } }, fields: [ { type: "text", name: "Name", editing: false, validate: "required" } ] }; var grid = new Grid($element, gridOptions); grid.editItem(gridOptions.data[0]); grid.updateItem(); equal(invalidNotifyCalled, 0, "data is valid"); }); module("api"); test("reset method should go the first page when pageLoading is truned on", function() { var items = [{ Name: "1" }, { Name: "2" }]; var $element = $("#jsGrid"); var gridOptions = { paging: true, pageSize: 1, pageLoading: true, autoload: true, controller: { loadData: function(args) { return { data: [items[args.pageIndex - 1]], itemsCount: items.length }; } }, fields: [ { type: "text", name: "Name" } ] }; var grid = new Grid($element, gridOptions); grid.openPage(2); grid.reset(); equal(grid._bodyGrid.text(), "1", "grid content reset"); }); module("i18n"); test("set locale by name", function() { jsGrid.locales.my_lang = { grid: { test: "test_text" } }; jsGrid.locale("my_lang"); var $element = $("#jsGrid").jsGrid({}); equal($element.jsGrid("option", "test"), "test_text", "option localized"); }); test("set locale by config", function() { jsGrid.locale( { grid: { test: "test_text" } }); var $element = $("#jsGrid").jsGrid({}); equal($element.jsGrid("option", "test"), "test_text", "option localized"); }); test("locale throws exception for unknown locale", function() { throws(function() { jsGrid.locale("unknown_lang"); }, /unknown_lang/, "locale threw an exception"); }); module("controller promise"); asyncTest("should support jQuery promise success callback", 1, function() { var data = []; var gridOptions = { autoload: false, controller: { loadData: function() { var d = $.Deferred(); setTimeout(function() { d.resolve(data); start(); }); return d.promise(); } } }; var grid = new Grid($("#jsGrid"), gridOptions); var promise = grid._controllerCall("loadData", {}, false, $.noop); promise.done(function(result) { equal(result, data, "data provided to done callback"); }); }); asyncTest("should support jQuery promise fail callback", 1, function() { var failArgs = {}; var gridOptions = { autoload: false, controller: { loadData: function() { var d = $.Deferred(); setTimeout(function() { d.reject(failArgs); start(); }); return d.promise(); } } }; var grid = new Grid($("#jsGrid"), gridOptions); var promise = grid._controllerCall("loadData", {}, false, $.noop); promise.fail(function(result) { equal(result, failArgs, "fail args provided to fail callback"); }); }); asyncTest("should support JS promise success callback", 1, function() { if(typeof Promise === "undefined") { ok(true, "Promise not supported"); start(); return; } var data = []; var gridOptions = { autoload: false, controller: { loadData: function() { return new Promise(function(resolve, reject) { setTimeout(function() { resolve(data); start(); }); }); } } }; var grid = new Grid($("#jsGrid"), gridOptions); var promise = grid._controllerCall("loadData", {}, false, $.noop); promise.done(function(result) { equal(result, data, "data provided to done callback"); }); }); asyncTest("should support JS promise fail callback", 1, function() { if(typeof Promise === "undefined") { ok(true, "Promise not supported"); start(); return; } var failArgs = {}; var gridOptions = { autoload: false, controller: { loadData: function() { return new Promise(function(resolve, reject) { setTimeout(function() { reject(failArgs); start(); }); }); } } }; var grid = new Grid($("#jsGrid"), gridOptions); var promise = grid._controllerCall("loadData", {}, false, $.noop); promise.fail(function(result) { equal(result, failArgs, "fail args provided to fail callback"); }); }); test("should support non-promise result", 1, function() { var data = []; var gridOptions = { autoload: false, controller: { loadData: function() { return data; } } }; var grid = new Grid($("#jsGrid"), gridOptions); var promise = grid._controllerCall("loadData", {}, false, $.noop); promise.done(function(result) { equal(result, data, "data provided to done callback"); }); }); module("renderTemplate"); test("should pass undefined and null arguments to the renderer", function() { var rendererArgs; var rendererContext; var context = {}; var renderer = function() { rendererArgs = arguments; rendererContext = this; }; Grid.prototype.renderTemplate(renderer, context, { arg1: undefined, arg2: null, arg3: "test" }); equal(rendererArgs.length, 3); strictEqual(rendererArgs[0], undefined, "undefined passed"); strictEqual(rendererArgs[1], null, "null passed"); strictEqual(rendererArgs[2], "test", "null passed"); strictEqual(rendererContext, context, "context is preserved"); }); module("Data Export", { setup: function() { this.exportConfig = {}; this.exportConfig.type = "csv"; this.exportConfig.subset = "all"; this.exportConfig.delimiter = "|"; this.exportConfig.includeHeaders = true; this.exportConfig.encapsulate = true; this.element = $("#jsGrid"); this.gridOptions = { width: "100%", height: "400px", inserting: true, editing: true, sorting: true, paging: true, pageSize: 2, data: [ { "Name": "Otto Clay", "Country": 1, "Married": false }, { "Name": "Connor Johnston", "Country": 2, "Married": true }, { "Name": "Lacey Hess", "Country": 2, "Married": false }, { "Name": "Timothy Henson", "Country": 1, "Married": true } ], fields: [ { name: "Name", type: "text", width: 150, validate: "required" }, { name: "Country", type: "select", items: [{ Name: "United States", Id: 1 },{ Name: "Canada", Id: 2 }], valueField: "Id", textField: "Name" }, { name: "Married", type: "checkbox", title: "Is Married", sorting: false }, { type: "control" } ] } } }); /* Base Choice Criteria type: csv subset: all delimiter: | includeHeaders: true encapsulate: true */ test("Should export data: Base Choice",function(){ var grid = new Grid($(this.element), this.gridOptions); var data = grid.exportData(this.exportConfig); var expected = ""; expected += '"Name"|"Country"|"Is Married"\r\n'; expected += '"Otto Clay"|"United States"|"false"\r\n'; expected += '"Connor Johnston"|"Canada"|"true"\r\n'; expected += '"Lacey Hess"|"Canada"|"false"\r\n'; expected += '"Timothy Henson"|"United States"|"true"\r\n'; equal(data, expected, "Output CSV configured to Base Choice Criteria -- Check Source"); }); test("Should export data: defaults = BCC",function(){ var grid = new Grid($(this.element), this.gridOptions); var data = grid.exportData(); var expected = ""; expected += '"Name"|"Country"|"Is Married"\r\n'; expected += '"Otto Clay"|"United States"|"false"\r\n'; expected += '"Connor Johnston"|"Canada"|"true"\r\n'; expected += '"Lacey Hess"|"Canada"|"false"\r\n'; expected += '"Timothy Henson"|"United States"|"true"\r\n'; equal(data, expected, "Output CSV with all defaults -- Should be equal to Base Choice"); }); test("Should export data: subset=visible", function(){ var grid = new Grid($(this.element), this.gridOptions); this.exportConfig.subset = "visible"; var data = grid.exportData(this.exportConfig); var expected = ""; expected += '"Name"|"Country"|"Is Married"\r\n'; expected += '"Otto Clay"|"United States"|"false"\r\n'; expected += '"Connor Johnston"|"Canada"|"true"\r\n'; //expected += '"Lacey Hess"|"Canada"|"false"\r\n'; //expected += '"Timothy Henson"|"United States"|"true"\r\n'; equal(data, expected, "Output CSV of visible records"); }); test("Should export data: delimiter=;", function(){ var grid = new Grid($(this.element), this.gridOptions); this.exportConfig.delimiter = ";"; var data = grid.exportData(this.exportConfig); var expected = ""; expected += '"Name";"Country";"Is Married"\r\n'; expected += '"Otto Clay";"United States";"false"\r\n'; expected += '"Connor Johnston";"Canada";"true"\r\n'; expected += '"Lacey Hess";"Canada";"false"\r\n'; expected += '"Timothy Henson";"United States";"true"\r\n'; equal(data, expected, "Output CSV with non-default delimiter"); }); test("Should export data: headers=false", function(){ var grid = new Grid($(this.element), this.gridOptions); this.exportConfig.includeHeaders = false; var data = grid.exportData(this.exportConfig); var expected = ""; //expected += '"Name"|"Country"|"Is Married"\r\n'; expected += '"Otto Clay"|"United States"|"false"\r\n'; expected += '"Connor Johnston"|"Canada"|"true"\r\n'; expected += '"Lacey Hess"|"Canada"|"false"\r\n'; expected += '"Timothy Henson"|"United States"|"true"\r\n'; equal(data, expected, "Output CSV without Headers"); }); test("Should export data: encapsulate=false", function(){ var grid = new Grid($(this.element), this.gridOptions); this.exportConfig.encapsulate = false; var data = grid.exportData(this.exportConfig); var expected = ""; expected += 'Name|Country|Is Married\r\n'; expected += 'Otto Clay|United States|false\r\n'; expected += 'Connor Johnston|Canada|true\r\n'; expected += 'Lacey Hess|Canada|false\r\n'; expected += 'Timothy Henson|United States|true\r\n'; equal(data, expected, "Output CSV without encapsulation"); }); test("Should export filtered data", function(){ var grid = new Grid($(this.element), this.gridOptions); this.exportConfig['filter'] = function(item){ if (item["Name"].indexOf("O") === 0) return true }; var data = grid.exportData(this.exportConfig); var expected = ""; expected += '"Name"|"Country"|"Is Married"\r\n'; expected += '"Otto Clay"|"United States"|"false"\r\n'; //expected += '"Connor Johnston"|"Canada"|"true"\r\n'; //expected += '"Lacey Hess"|"Canada"|"false"\r\n'; //expected += '"Timothy Henson"|"United States"|"true"\r\n'; equal(data, expected, "Output CSV filtered to show names starting with O"); }); test("Should export data: transformed value", function(){ var grid = new Grid($(this.element), this.gridOptions); this.exportConfig['transforms'] = {}; this.exportConfig.transforms['Married'] = function(value){ if (value === true) return "Yes" if (value === false) return "No" }; var data = grid.exportData(this.exportConfig); var expected = ""; expected += '"Name"|"Country"|"Is Married"\r\n'; expected += '"Otto Clay"|"United States"|"No"\r\n'; expected += '"Connor Johnston"|"Canada"|"Yes"\r\n'; expected += '"Lacey Hess"|"Canada"|"No"\r\n'; expected += '"Timothy Henson"|"United States"|"Yes"\r\n'; equal(data, expected, "Output CSV column value transformed properly"); }); }); <MSG> Core: Field constructor shortcuts <DFF> @@ -588,9 +588,13 @@ $(function() { }); test("grid fields normalization", function() { + var CustomField = function(name, config) { + $.extend(true, this, config); + }; + + jsGrid.fields.custom = CustomField; + var $element = $("#jsGrid"), - field1, - field2, gridOptions = { fields: [ new jsGrid.Field("text1", { @@ -599,20 +603,28 @@ $(function() { { name: "text2", title: "title2" + }, + { + name: "text3", + type: "custom" } ] }, grid = new Grid($element, gridOptions); - field1 = grid.fields[0]; + var field1 = grid.fields[0]; ok(field1 instanceof jsGrid.Field); equal(field1.name, "text1", "name is set for field"); equal(field1.title, "title1", "title field"); - field2 = grid.fields[1]; + var field2 = grid.fields[1]; ok(field2 instanceof jsGrid.Field); equal(field2.name, "text2", "name is set for field"); equal(field2.title, "title2", "title field"); + + | 16 | Core: Field constructor shortcuts | 4 | .js | tests | mit | tabalinas/jsgrid |
10066232 | <NME> jsgrid.core.js
<BEF> (function(window, $, undefined) {
var JSGRID = "JSGrid",
JSGRID_DATA_KEY = JSGRID,
JSGRID_ROW_DATA_KEY = "JSGridItem",
JSGRID_EDIT_ROW_DATA_KEY = "JSGridEditRow",
SORT_ORDER_ASC = "asc",
SORT_ORDER_DESC = "desc",
FIRST_PAGE_PLACEHOLDER = "{first}",
PAGES_PLACEHOLDER = "{pages}",
PREV_PAGE_PLACEHOLDER = "{prev}",
NEXT_PAGE_PLACEHOLDER = "{next}",
LAST_PAGE_PLACEHOLDER = "{last}",
PAGE_INDEX_PLACEHOLDER = "{pageIndex}",
PAGE_COUNT_PLACEHOLDER = "{pageCount}",
ITEM_COUNT_PLACEHOLDER = "{itemCount}",
EMPTY_HREF = "javascript:void(0);";
var getOrApply = function(value, context) {
if($.isFunction(value)) {
return value.apply(context, $.makeArray(arguments).slice(2));
}
return value;
};
var normalizePromise = function(promise) {
var d = $.Deferred();
if(promise && promise.then) {
promise.then(function() {
d.resolve.apply(d, arguments);
}, function() {
d.reject.apply(d, arguments);
});
} else {
d.resolve(promise);
}
return d.promise();
};
var defaultController = {
loadData: $.noop,
insertItem: $.noop,
updateItem: $.noop,
deleteItem: $.noop
};
function Grid(element, config) {
var $element = $(element);
$element.data(JSGRID_DATA_KEY, this);
this._container = $element;
this.data = [];
this.fields = [];
this._editingRow = null;
this._sortField = null;
this._sortOrder = SORT_ORDER_ASC;
this._firstDisplayingPage = 1;
this._init(config);
this.render();
}
Grid.prototype = {
width: "auto",
height: "auto",
updateOnResize: true,
rowClass: $.noop,
rowRenderer: null,
rowClick: function(args) {
if(this.editing) {
this.editItem($(args.event.target).closest("tr"));
}
},
rowDoubleClick: $.noop,
noDataContent: "Not found",
noDataRowClass: "jsgrid-nodata-row",
heading: true,
headerRowRenderer: null,
headerRowClass: "jsgrid-header-row",
headerCellClass: "jsgrid-header-cell",
filtering: false,
filterRowRenderer: null,
filterRowClass: "jsgrid-filter-row",
inserting: false,
insertRowLocation: "bottom",
insertRowRenderer: null,
insertRowClass: "jsgrid-insert-row",
editing: false,
editRowRenderer: null,
editRowClass: "jsgrid-edit-row",
confirmDeleting: true,
deleteConfirm: "Are you sure?",
selecting: true,
selectedRowClass: "jsgrid-selected-row",
oddRowClass: "jsgrid-row",
evenRowClass: "jsgrid-alt-row",
cellClass: "jsgrid-cell",
sorting: false,
sortableClass: "jsgrid-header-sortable",
sortAscClass: "jsgrid-header-sort jsgrid-header-sort-asc",
sortDescClass: "jsgrid-header-sort jsgrid-header-sort-desc",
paging: false,
pagerContainer: null,
pageIndex: 1,
pageSize: 20,
pageButtonCount: 15,
pagerFormat: "Pages: {first} {prev} {pages} {next} {last} {pageIndex} of {pageCount}",
pagePrevText: "Prev",
pageNextText: "Next",
pageFirstText: "First",
pageLastText: "Last",
pageNavigatorNextText: "...",
pageNavigatorPrevText: "...",
pagerContainerClass: "jsgrid-pager-container",
pagerClass: "jsgrid-pager",
pagerNavButtonClass: "jsgrid-pager-nav-button",
pagerNavButtonInactiveClass: "jsgrid-pager-nav-inactive-button",
pageClass: "jsgrid-pager-page",
currentPageClass: "jsgrid-pager-current-page",
customLoading: false,
pageLoading: false,
autoload: false,
controller: defaultController,
loadIndication: true,
loadIndicationDelay: 500,
loadMessage: "Please, wait...",
loadShading: true,
invalidMessage: "Invalid data entered!",
invalidNotify: function(args) {
var messages = $.map(args.errors, function(error) {
return error.message || null;
});
window.alert([this.invalidMessage].concat(messages).join("\n"));
},
onInit: $.noop,
onRefreshing: $.noop,
onRefreshed: $.noop,
onPageChanged: $.noop,
onItemDeleting: $.noop,
onItemDeleted: $.noop,
onItemInserting: $.noop,
onItemInserted: $.noop,
onItemEditing: $.noop,
onItemEditCancelling: $.noop,
onItemUpdating: $.noop,
onItemUpdated: $.noop,
onItemInvalid: $.noop,
onDataLoading: $.noop,
onDataLoaded: $.noop,
onDataExporting: $.noop,
onOptionChanging: $.noop,
onOptionChanged: $.noop,
onError: $.noop,
invalidClass: "jsgrid-invalid",
containerClass: "jsgrid",
tableClass: "jsgrid-table",
gridHeaderClass: "jsgrid-grid-header",
gridBodyClass: "jsgrid-grid-body",
this._controller = $.extend({}, defaultController, getOrApply(this.controller, this));
},
loadIndicator: function(config) {
return new jsGrid.LoadIndicator(config);
},
return this.pageLoading
? new jsGrid.loadStrategies.PageLoadingStrategy(this)
: new jsGrid.loadStrategies.DirectLoadingStrategy(this);
},
_initLoadStrategy: function() {
this._loadStrategy = getOrApply(this.loadStrategy, this);
},
_initController: function() {
this._controller = $.extend({}, defaultController, getOrApply(this.controller, this));
},
renderTemplate: function(source, context, config) {
var args = [];
for(var key in config) {
args.push(config[key]);
}
args.unshift(source, context);
source = getOrApply.apply(null, args);
return (source === undefined || source === null) ? "" : source;
},
loadIndicator: function(config) {
return new jsGrid.LoadIndicator(config);
},
validation: function(config) {
return jsGrid.Validation && new jsGrid.Validation(config);
},
_initFields: function() {
var self = this;
self.fields = $.map(self.fields, function(field) {
if($.isPlainObject(field)) {
var fieldConstructor = (field.type && jsGrid.fields[field.type]) || jsGrid.Field;
field = new fieldConstructor(field);
}
field._grid = self;
return field;
});
},
_attachWindowLoadResize: function() {
$(window).on("load", $.proxy(this._refreshSize, this));
},
_attachWindowResizeCallback: function() {
if(this.updateOnResize) {
$(window).on("resize", $.proxy(this._refreshSize, this));
}
},
_detachWindowResizeCallback: function() {
$(window).off("resize", this._refreshSize);
},
option: function(key, value) {
var optionChangingEventArgs,
optionChangedEventArgs;
if(arguments.length === 1)
return this[key];
optionChangingEventArgs = {
option: key,
oldValue: this[key],
newValue: value
};
this._callEventHandler(this.onOptionChanging, optionChangingEventArgs);
case "rowRenderer":
case "rowClick":
case "rowDoubleClick":
case "noDataText":
case "noDataRowClass":
case "noDataContent":
case "selecting":
this._callEventHandler(this.onOptionChanged, optionChangedEventArgs);
},
fieldOption: function(field, key, value) {
field = this._normalizeField(field);
if(arguments.length === 2)
return field[key];
field[key] = value;
this._renderGrid();
},
_handleOptionChange: function(name, value) {
this[name] = value;
switch(name) {
case "width":
case "height":
this._refreshSize();
break;
case "rowClass":
case "rowRenderer":
case "rowClick":
case "rowDoubleClick":
case "noDataRowClass":
case "noDataContent":
case "selecting":
case "selectedRowClass":
case "oddRowClass":
case "evenRowClass":
this._refreshContent();
break;
case "pageButtonCount":
case "pagerFormat":
case "pagePrevText":
case "pageNextText":
case "pageFirstText":
case "pageLastText":
case "pageNavigatorNextText":
case "pageNavigatorPrevText":
case "pagerClass":
case "pagerNavButtonClass":
case "pageClass":
case "currentPageClass":
case "pagerRenderer":
this._refreshPager();
break;
case "fields":
this._initFields();
this.render();
break;
case "data":
case "editing":
case "heading":
case "filtering":
case "inserting":
case "paging":
this.refresh();
break;
case "loadStrategy":
case "pageLoading":
this._initLoadStrategy();
this.search();
break;
case "pageIndex":
this.openPage(value);
break;
case "pageSize":
this.refresh();
this.search();
break;
case "editRowRenderer":
case "editRowClass":
this.cancelEdit();
break;
case "updateOnResize":
this._detachWindowResizeCallback();
this._attachWindowResizeCallback();
break;
case "invalidNotify":
case "invalidMessage":
break;
default:
this.render();
break;
}
},
destroy: function() {
this._detachWindowResizeCallback();
this._clear();
this._container.removeData(JSGRID_DATA_KEY);
},
render: function() {
this._renderGrid();
return this.autoload ? this.loadData() : $.Deferred().resolve().promise();
},
_renderGrid: function() {
this._clear();
this._container.addClass(this.containerClass)
.css("position", "relative")
.append(this._createHeader())
.append(this._createBody());
this._pagerContainer = this._createPagerContainer();
this._loadIndicator = this._createLoadIndicator();
this._validation = this._createValidation();
this.refresh();
},
_createLoadIndicator: function() {
return getOrApply(this.loadIndicator, this, {
message: this.loadMessage,
shading: this.loadShading,
container: this._container
});
},
_createValidation: function() {
return getOrApply(this.validation, this);
},
_clear: function() {
this.cancelEdit();
clearTimeout(this._loadingTimer);
this._pagerContainer && this._pagerContainer.empty();
this._container.empty()
.css({ position: "", width: "", height: "" });
},
_createHeader: function() {
var $headerRow = this._headerRow = this._createHeaderRow(),
$filterRow = this._filterRow = this._createFilterRow(),
$insertRow = this._insertRow = this._createInsertRow();
var $headerGrid = this._headerGrid = $("<table>").addClass(this.tableClass)
.append($headerRow)
.append($filterRow)
.append($insertRow);
var $header = this._header = $("<div>").addClass(this.gridHeaderClass)
.addClass(this._scrollBarWidth() ? "jsgrid-header-scrollbar" : "")
.append($headerGrid);
return $header;
},
_createHeaderRow: function() {
if($.isFunction(this.headerRowRenderer))
return $(this.headerRowRenderer());
var $result = $("<tr>").addClass(this.headerRowClass);
this._eachField(function(field, index) {
var $th = this._prepareCell("<th>", field, "headercss")
.append(field.headerTemplate ? field.headerTemplate() : "")
.appendTo($result);
if(this.sorting && field.sorting) {
},
_createPagerContainer: function() {
var pagerContainer = this.pagerContainer || $("<div>").appendTo(this._container);
return $(pagerContainer).addClass(this.pagerContainerClass);
},
_eachField: function(callBack) {
var self = this;
$.each(this.fields, function(index, field) {
if(field.visible) {
callBack.call(self, field, index);
}
});
},
_createFilterRow: function() {
if($.isFunction(this.filterRowRenderer))
return $(this.filterRowRenderer());
var $result = $("<tr>").addClass(this.filterRowClass);
this._eachField(function(field) {
this._prepareCell("<td>", field, "filtercss")
.append(field.filterTemplate ? field.filterTemplate() : "")
.appendTo($result);
});
$th.addClass(this.sortableClass)
.on("click", $.proxy(function() {
_createInsertRow: function() {
if($.isFunction(this.insertRowRenderer))
return $(this.insertRowRenderer());
var $result = $("<tr>").addClass(this.insertRowClass);
this._eachField(function(field) {
this._prepareCell("<td>", field, "insertcss")
.append(field.insertTemplate ? field.insertTemplate() : "")
.appendTo($result);
});
},
_createFilterRow: function() {
if($.isFunction(this.filterRowRenderer))
return $(this.renderTemplate(this.filterRowRenderer, this));
var $result = $("<tr>").addClass(this.filterRowClass);
this._eachField(function(field) {
this._prepareCell("<td>", field, "filtercss")
.append(this.renderTemplate(field.filterTemplate, field))
.appendTo($result);
});
return $result;
},
_createInsertRow: function() {
if($.isFunction(this.insertRowRenderer))
return $(this.renderTemplate(this.insertRowRenderer, this));
var $result = $("<tr>").addClass(this.insertRowClass);
this._eachField(function(field) {
this._prepareCell("<td>", field, "insertcss")
.append(this.renderTemplate(field.insertTemplate, field))
.appendTo($result);
});
return $result;
},
_callEventHandler: function(handler, eventParams) {
handler.call(this, $.extend(eventParams, {
grid: this
}));
return eventParams;
},
reset: function() {
this._resetSorting();
this._resetPager();
return this._loadStrategy.reset();
},
_resetPager: function() {
this._firstDisplayingPage = 1;
this._setPage(1);
},
_resetSorting: function() {
this._sortField = null;
this._sortOrder = SORT_ORDER_ASC;
this._clearSortingCss();
},
refresh: function() {
this._callEventHandler(this.onRefreshing);
this.cancelEdit();
this._refreshHeading();
this._refreshFiltering();
this._refreshInserting();
this._refreshContent();
this._refreshPager();
this._refreshSize();
this._callEventHandler(this.onRefreshed);
},
},
_createNoDataRow: function() {
var noDataContent = getOrApply(this.noDataContent, this);
var amountOfFields = 0;
this._eachField(function() {
amountOfFields++;
});
return $("<tr>").addClass(this.noDataRowClass)
.append($("<td>").attr("colspan", amountOfFields).append(noDataContent));
},
_createNoDataContent: function() {
return $.isFunction(this.noDataRenderer)
? this.noDataRenderer()
: this.noDataText;
},
_createRow: function(item, itemIndex) {
var $result;
if($.isFunction(this.rowRenderer)) {
$result = $(this.rowRenderer(item, itemIndex));
} else {
$result = $("<tr>");
this._renderCells($result, item);
var indexFrom = this._loadStrategy.firstDisplayIndex();
var indexTo = this._loadStrategy.lastDisplayIndex();
for(var itemIndex = indexFrom; itemIndex < indexTo; itemIndex++) {
var item = this.data[itemIndex];
$content.append(this._createRow(item, itemIndex));
}
},
_createNoDataRow: function() {
var amountOfFields = 0;
this._eachField(function() {
amountOfFields++;
});
return $("<tr>").addClass(this.noDataRowClass)
.append($("<td>").addClass(this.cellClass).attr("colspan", amountOfFields)
.append(this.renderTemplate(this.noDataContent, this)));
},
_createRow: function(item, itemIndex) {
var $result;
if($.isFunction(this.rowRenderer)) {
$result = this.renderTemplate(this.rowRenderer, this, { item: item, itemIndex: itemIndex });
} else {
$result = $("<tr>");
this._renderCells($result, item);
}
$result.addClass(this._getRowClasses(item, itemIndex))
.data(JSGRID_ROW_DATA_KEY, item)
.on("click", $.proxy(function(e) {
this.rowClick({
item: item,
itemIndex: itemIndex,
event: e
});
}, this))
.on("dblclick", $.proxy(function(e) {
this.rowDoubleClick({
item: item,
itemIndex: itemIndex,
event: e
});
}, this));
if(this.selecting) {
this._attachRowHover($result);
}
return $result;
var fieldValue = this._getItemFieldValue(item, field);
if($.isFunction(field.cellRenderer)) {
$result = $(field.cellRenderer(fieldValue, item));
} else {
$result = $("<td>").append(field.itemTemplate ? field.itemTemplate(fieldValue, item) : fieldValue);
}
return this._prepareCell($result, field);
_attachRowHover: function($row) {
var selectedRowClass = this.selectedRowClass;
$row.hover(function() {
$(this).addClass(selectedRowClass);
},
function() {
$(this).removeClass(selectedRowClass);
}
);
},
_renderCells: function($row, item) {
this._eachField(function(field) {
$row.append(this._createCell(item, field));
});
return this;
},
_createCell: function(item, field) {
var $result;
var fieldValue = this._getItemFieldValue(item, field);
var args = { value: fieldValue, item : item };
if($.isFunction(field.cellRenderer)) {
$result = this.renderTemplate(field.cellRenderer, field, args);
} else {
$result = $("<td>").append(this.renderTemplate(field.itemTemplate || fieldValue, field, args));
}
return this._prepareCell($result, field);
},
_getItemFieldValue: function(item, field) {
var props = field.name.split('.');
var result = item[props.shift()];
while(result && props.length) {
result = result[props.shift()];
}
return result;
},
_setItemFieldValue: function(item, field, value) {
var props = field.name.split('.');
var current = item;
var prop = props[0];
while(current && props.length) {
item = current;
prop = props.shift();
current = item[prop];
}
if(!current) {
while(props.length) {
item = item[prop] = {};
prop = props.shift();
}
}
item[prop] = value;
},
sort: function(field, order) {
if($.isPlainObject(field)) {
order = field.order;
field = field.field;
}
this._clearSortingCss();
this._setSortingParams(field, order);
this._setSortingCss();
return this._loadStrategy.sort();
},
_clearSortingCss: function() {
this._headerRow.find("th")
.removeClass(this.sortAscClass)
.removeClass(this.sortDescClass);
},
_setSortingParams: function(field, order) {
field = this._normalizeField(field);
order = order || ((this._sortField === field) ? this._reversedSortOrder(this._sortOrder) : SORT_ORDER_ASC);
this._sortField = field;
this._sortOrder = order;
},
_normalizeField: function(field) {
if($.isNumeric(field)) {
return this.fields[field];
}
if(typeof field === "string") {
return $.grep(this.fields, function(f) {
return f.name === field;
})[0];
}
return field;
},
_reversedSortOrder: function(order) {
return (order === SORT_ORDER_ASC ? SORT_ORDER_DESC : SORT_ORDER_ASC);
},
_setSortingCss: function() {
var fieldIndex = this._visibleFieldIndex(this._sortField);
this._headerRow.find("th").eq(fieldIndex)
.addClass(this._sortOrder === SORT_ORDER_ASC ? this.sortAscClass : this.sortDescClass);
},
_visibleFieldIndex: function(field) {
return $.inArray(field, $.grep(this.fields, function(f) { return f.visible; }));
},
_sortData: function() {
var sortFactor = this._sortFactor(),
sortField = this._sortField;
if(sortField) {
var self = this;
self.data.sort(function(item1, item2) {
var value1 = self._getItemFieldValue(item1, sortField);
var value2 = self._getItemFieldValue(item2, sortField);
return sortFactor * sortField.sortingFunc(value1, value2);
});
}
},
_sortFactor: function() {
return this._sortOrder === SORT_ORDER_ASC ? 1 : -1;
},
_itemsCount: function() {
return this._loadStrategy.itemsCount();
},
_pagesCount: function() {
var itemsCount = this._itemsCount(),
pageSize = this.pageSize;
return Math.floor(itemsCount / pageSize) + (itemsCount % pageSize ? 1 : 0);
},
_refreshPager: function() {
var $pagerContainer = this._pagerContainer;
$pagerContainer.empty();
if(this.paging) {
$pagerContainer.append(this._createPager());
}
var showPager = this.paging && this._pagesCount() > 1;
$pagerContainer.toggle(showPager);
},
_createPager: function() {
var $result;
if($.isFunction(this.pagerRenderer)) {
$result = $(this.pagerRenderer({
pageIndex: this.pageIndex,
pageCount: this._pagesCount()
}));
} else {
$result = $("<div>").append(this._createPagerByFormat());
}
$result.addClass(this.pagerClass);
return $result;
},
_createPagerByFormat: function() {
var pageIndex = this.pageIndex,
pageCount = this._pagesCount(),
itemCount = this._itemsCount(),
pagerParts = this.pagerFormat.split(" ");
return $.map(pagerParts, $.proxy(function(pagerPart) {
var result = pagerPart;
if(pagerPart === PAGES_PLACEHOLDER) {
result = this._createPages();
} else if(pagerPart === FIRST_PAGE_PLACEHOLDER) {
result = this._createPagerNavButton(this.pageFirstText, 1, pageIndex > 1);
} else if(pagerPart === PREV_PAGE_PLACEHOLDER) {
result = this._createPagerNavButton(this.pagePrevText, pageIndex - 1, pageIndex > 1);
} else if(pagerPart === NEXT_PAGE_PLACEHOLDER) {
result = this._createPagerNavButton(this.pageNextText, pageIndex + 1, pageIndex < pageCount);
} else if(pagerPart === LAST_PAGE_PLACEHOLDER) {
result = this._createPagerNavButton(this.pageLastText, pageCount, pageIndex < pageCount);
} else if(pagerPart === PAGE_INDEX_PLACEHOLDER) {
result = pageIndex;
} else if(pagerPart === PAGE_COUNT_PLACEHOLDER) {
result = pageCount;
} else if(pagerPart === ITEM_COUNT_PLACEHOLDER) {
result = itemCount;
}
return $.isArray(result) ? result.concat([" "]) : [result, " "];
}, this));
},
_createPages: function() {
var pageCount = this._pagesCount(),
pageButtonCount = this.pageButtonCount,
firstDisplayingPage = this._firstDisplayingPage,
pages = [];
if(firstDisplayingPage > 1) {
pages.push(this._createPagerPageNavButton(this.pageNavigatorPrevText, this.showPrevPages));
}
for(var i = 0, pageNumber = firstDisplayingPage; i < pageButtonCount && pageNumber <= pageCount; i++, pageNumber++) {
pages.push(pageNumber === this.pageIndex
? this._createPagerCurrentPage()
: this._createPagerPage(pageNumber));
}
if((firstDisplayingPage + pageButtonCount - 1) < pageCount) {
pages.push(this._createPagerPageNavButton(this.pageNavigatorNextText, this.showNextPages));
}
return pages;
},
_createPagerNavButton: function(text, pageIndex, isActive) {
return this._createPagerButton(text, this.pagerNavButtonClass + (isActive ? "" : " " + this.pagerNavButtonInactiveClass),
isActive ? function() { this.openPage(pageIndex); } : $.noop);
},
_createPagerPageNavButton: function(text, handler) {
return this._createPagerButton(text, this.pagerNavButtonClass, handler);
},
_createPagerPage: function(pageIndex) {
return this._createPagerButton(pageIndex, this.pageClass, function() {
this.openPage(pageIndex);
});
},
_createPagerButton: function(text, css, handler) {
var $link = $("<a>").attr("href", EMPTY_HREF)
.html(text)
.on("click", $.proxy(handler, this));
return $("<span>").addClass(css).append($link);
},
_createPagerCurrentPage: function() {
return $("<span>")
.addClass(this.pageClass)
.addClass(this.currentPageClass)
.text(this.pageIndex);
},
_refreshSize: function() {
this._refreshHeight();
this._refreshWidth();
},
_refreshWidth: function() {
var width = (this.width === "auto") ? this._getAutoWidth() : this.width;
this._container.width(width);
},
_getAutoWidth: function() {
var $headerGrid = this._headerGrid,
$header = this._header;
$headerGrid.width("auto");
var contentWidth = $headerGrid.outerWidth();
var borderWidth = $header.outerWidth() - $header.innerWidth();
$headerGrid.width("");
return contentWidth + borderWidth;
},
_scrollBarWidth: (function() {
var result;
return function() {
if(result === undefined) {
var $ghostContainer = $("<div style='width:50px;height:50px;overflow:hidden;position:absolute;top:-10000px;left:-10000px;'></div>");
var $ghostContent = $("<div style='height:100px;'></div>");
$ghostContainer.append($ghostContent).appendTo("body");
var width = $ghostContent.innerWidth();
$ghostContainer.css("overflow-y", "auto");
var widthExcludingScrollBar = $ghostContent.innerWidth();
$ghostContainer.remove();
result = width - widthExcludingScrollBar;
}
return result;
};
})(),
_refreshHeight: function() {
var container = this._container,
pagerContainer = this._pagerContainer,
height = this.height,
nonBodyHeight;
container.height(height);
if(height !== "auto") {
height = container.height();
nonBodyHeight = this._header.outerHeight(true);
if(pagerContainer.parents(container).length) {
nonBodyHeight += pagerContainer.outerHeight(true);
}
this._body.outerHeight(height - nonBodyHeight);
}
},
showPrevPages: function() {
var firstDisplayingPage = this._firstDisplayingPage,
pageButtonCount = this.pageButtonCount;
this._firstDisplayingPage = (firstDisplayingPage > pageButtonCount) ? firstDisplayingPage - pageButtonCount : 1;
this._refreshPager();
},
showNextPages: function() {
var firstDisplayingPage = this._firstDisplayingPage,
pageButtonCount = this.pageButtonCount,
pageCount = this._pagesCount();
this._firstDisplayingPage = (firstDisplayingPage + 2 * pageButtonCount > pageCount)
? pageCount - pageButtonCount + 1
: firstDisplayingPage + pageButtonCount;
this._refreshPager();
},
openPage: function(pageIndex) {
if(pageIndex < 1 || pageIndex > this._pagesCount())
return;
this._setPage(pageIndex);
this._loadStrategy.openPage(pageIndex);
},
_setPage: function(pageIndex) {
var firstDisplayingPage = this._firstDisplayingPage,
pageButtonCount = this.pageButtonCount;
this.pageIndex = pageIndex;
if(pageIndex < firstDisplayingPage) {
this._firstDisplayingPage = pageIndex;
}
if(pageIndex > firstDisplayingPage + pageButtonCount - 1) {
this._firstDisplayingPage = pageIndex - pageButtonCount + 1;
}
this._callEventHandler(this.onPageChanged, {
pageIndex: pageIndex
});
},
_controllerCall: function(method, param, isCanceled, doneCallback) {
if(isCanceled)
return $.Deferred().reject().promise();
this._showLoading();
var controller = this._controller;
if(!controller || !controller[method]) {
throw Error("controller has no method '" + method + "'");
}
return normalizePromise(controller[method](param))
.done($.proxy(doneCallback, this))
.fail($.proxy(this._errorHandler, this))
.always($.proxy(this._hideLoading, this));
},
_errorHandler: function() {
this._callEventHandler(this.onError, {
args: $.makeArray(arguments)
});
},
_showLoading: function() {
if(!this.loadIndication)
return;
clearTimeout(this._loadingTimer);
this._loadingTimer = setTimeout($.proxy(function() {
this._loadIndicator.show();
}, this), this.loadIndicationDelay);
},
_hideLoading: function() {
if(!this.loadIndication)
return;
clearTimeout(this._loadingTimer);
this._loadIndicator.hide();
},
search: function(filter) {
this._resetSorting();
this._resetPager();
return this.loadData(filter);
},
loadData: function(filter) {
filter = filter || (this.filtering ? this.getFilter() : {});
$.extend(filter, this._loadStrategy.loadParams(), this._sortingParams());
var args = this._callEventHandler(this.onDataLoading, {
filter: filter
});
return this._controllerCall("loadData", filter, args.cancel, function(loadedData) {
if(!loadedData)
return;
this._loadStrategy.finishLoad(loadedData);
this._callEventHandler(this.onDataLoaded, {
data: loadedData
});
});
},
exportData: function(exportOptions){
var options = exportOptions || {};
var type = options.type || "csv";
var result = "";
this._callEventHandler(this.onDataExporting);
switch(type){
case "csv":
result = this._dataToCsv(options);
break;
}
return result;
},
_dataToCsv: function(options){
var options = options || {};
var includeHeaders = options.hasOwnProperty("includeHeaders") ? options.includeHeaders : true;
var subset = options.subset || "all";
var filter = options.filter || undefined;
var result = [];
if (includeHeaders){
var fieldsLength = this.fields.length;
var fieldNames = {};
for(var i=0;i<fieldsLength;i++){
var field = this.fields[i];
if ("includeInDataExport" in field){
if (field.includeInDataExport === true)
fieldNames[i] = field.title || field.name;
}
}
var headerLine = this._itemToCsv(fieldNames,{},options);
result.push(headerLine);
}
var exportStartIndex = 0;
var exportEndIndex = this.data.length;
switch(subset){
case "visible":
exportEndIndex = this._firstDisplayingPage * this.pageSize;
exportStartIndex = exportEndIndex - this.pageSize;
case "all":
default:
break;
}
for (var i = exportStartIndex; i < exportEndIndex; i++){
var item = this.data[i];
var itemLine = "";
var includeItem = true;
if (filter)
if (!filter(item))
includeItem = false;
if (includeItem){
itemLine = this._itemToCsv(item, this.fields, options);
result.push(itemLine);
}
}
return result.join("");
},
_itemToCsv: function(item, fields, options){
var options = options || {};
var delimiter = options.delimiter || "|";
var encapsulate = options.hasOwnProperty("encapsulate") ? options.encapsulate : true;
var newline = options.newline || "\r\n";
var transforms = options.transforms || {};
var fields = fields || {};
var getItem = this._getItemFieldValue;
var result = [];
Object.keys(item).forEach(function(key,index) {
var entry = "";
//Fields.length is greater than 0 when we are matching agaisnt fields
//Field.length will be 0 when exporting header rows
if (fields.length > 0){
var field = fields[index];
//Field may be excluded from data export
if ("includeInDataExport" in field){
if (field.includeInDataExport){
//Field may be a select, which requires additional logic
if (field.type === "select"){
var selectedItem = getItem(item, field);
var resultItem = $.grep(field.items, function(item, index) {
return item[field.valueField] === selectedItem;
})[0] || "";
entry = resultItem[field.textField];
}
else{
entry = getItem(item, field);
}
}
else{
return;
}
}
else{
entry = getItem(item, field);
}
if (transforms.hasOwnProperty(field.name)){
entry = transforms[field.name](entry);
}
}
else{
entry = item[key];
}
if (encapsulate){
entry = '"'+entry+'"';
}
result.push(entry);
});
return result.join(delimiter) + newline;
},
getFilter: function() {
var result = {};
this._eachField(function(field) {
if(field.filtering) {
this._setItemFieldValue(result, field, field.filterValue());
}
});
return result;
},
_sortingParams: function() {
if(this.sorting && this._sortField) {
return {
sortField: this._sortField.name,
sortOrder: this._sortOrder
};
}
return {};
},
getSorting: function() {
var sortingParams = this._sortingParams();
return {
field: sortingParams.sortField,
order: sortingParams.sortOrder
};
},
clearFilter: function() {
var $filterRow = this._createFilterRow();
this._filterRow.replaceWith($filterRow);
this._filterRow = $filterRow;
return this.search();
},
insertItem: function(item) {
var insertingItem = item || this._getValidatedInsertItem();
if(!insertingItem)
return $.Deferred().reject().promise();
var args = this._callEventHandler(this.onItemInserting, {
item: insertingItem
});
return this._controllerCall("insertItem", insertingItem, args.cancel, function(insertedItem) {
insertedItem = insertedItem || insertingItem;
this._loadStrategy.finishInsert(insertedItem, this.insertRowLocation);
this._callEventHandler(this.onItemInserted, {
item: insertedItem
});
});
},
_getValidatedInsertItem: function() {
var item = this._getInsertItem();
return this._validateItem(item, this._insertRow) ? item : null;
},
_getInsertItem: function() {
var result = {};
this._eachField(function(field) {
if(field.inserting) {
this._setItemFieldValue(result, field, field.insertValue());
}
});
return result;
},
_validateItem: function(item, $row) {
var validationErrors = [];
var args = {
item: item,
itemIndex: this._rowIndex($row),
row: $row
};
this._eachField(function(field) {
if(!field.validate ||
($row === this._insertRow && !field.inserting) ||
($row === this._getEditRow() && !field.editing))
return;
var fieldValue = this._getItemFieldValue(item, field);
var errors = this._validation.validate($.extend({
value: fieldValue,
rules: field.validate
}, args));
this._setCellValidity($row.children().eq(this._visibleFieldIndex(field)), errors);
if(!errors.length)
return;
validationErrors.push.apply(validationErrors,
$.map(errors, function(message) {
return { field: field, message: message };
}));
});
if(!validationErrors.length)
return true;
var invalidArgs = $.extend({
errors: validationErrors
}, args);
this._callEventHandler(this.onItemInvalid, invalidArgs);
this.invalidNotify(invalidArgs);
return false;
},
_setCellValidity: function($cell, errors) {
$cell
.toggleClass(this.invalidClass, !!errors.length)
.attr("title", errors.join("\n"));
},
clearInsert: function() {
var insertRow = this._createInsertRow();
this._insertRow.replaceWith(insertRow);
this._insertRow = insertRow;
this.refresh();
},
editItem: function(item) {
var $row = this.rowByItem(item);
if($row.length) {
this._editRow($row);
}
},
rowByItem: function(item) {
if(item.jquery || item.nodeType)
return $(item);
return this._content.find("tr").filter(function() {
return $.data(this, JSGRID_ROW_DATA_KEY) === item;
});
},
_editRow: function($row) {
if(!this.editing)
return;
var item = $row.data(JSGRID_ROW_DATA_KEY);
var args = this._callEventHandler(this.onItemEditing, {
row: $row,
item: item,
itemIndex: this._itemIndex(item)
});
if(args.cancel)
return;
if(this._editingRow) {
this.cancelEdit();
}
var $editRow = this._createEditRow(item);
this._editingRow = $row;
$row.hide();
$editRow.insertBefore($row);
$row.data(JSGRID_EDIT_ROW_DATA_KEY, $editRow);
},
_createEditRow: function(item) {
if($.isFunction(this.editRowRenderer)) {
return $(this.renderTemplate(this.editRowRenderer, this, { item: item, itemIndex: this._itemIndex(item) }));
}
var $result = $("<tr>").addClass(this.editRowClass);
this._eachField(function(field) {
var fieldValue = this._getItemFieldValue(item, field);
this._prepareCell("<td>", field, "editcss")
.append(this.renderTemplate(field.editTemplate || "", field, { value: fieldValue, item: item }))
.appendTo($result);
});
return $result;
},
updateItem: function(item, editedItem) {
if(arguments.length === 1) {
editedItem = item;
}
var $row = item ? this.rowByItem(item) : this._editingRow;
editedItem = editedItem || this._getValidatedEditedItem();
if(!editedItem)
return;
return this._updateRow($row, editedItem);
},
_getValidatedEditedItem: function() {
var item = this._getEditedItem();
return this._validateItem(item, this._getEditRow()) ? item : null;
},
_updateRow: function($updatingRow, editedItem) {
var updatingItem = $updatingRow.data(JSGRID_ROW_DATA_KEY),
updatingItemIndex = this._itemIndex(updatingItem),
updatedItem = $.extend(true, {}, updatingItem, editedItem);
var args = this._callEventHandler(this.onItemUpdating, {
row: $updatingRow,
item: updatedItem,
itemIndex: updatingItemIndex,
previousItem: updatingItem
});
return this._controllerCall("updateItem", updatedItem, args.cancel, function(loadedUpdatedItem) {
var previousItem = $.extend(true, {}, updatingItem);
updatedItem = loadedUpdatedItem || $.extend(true, updatingItem, editedItem);
var $updatedRow = this._finishUpdate($updatingRow, updatedItem, updatingItemIndex);
this._callEventHandler(this.onItemUpdated, {
row: $updatedRow,
item: updatedItem,
itemIndex: updatingItemIndex,
previousItem: previousItem
});
});
},
_rowIndex: function(row) {
return this._content.children().index($(row));
},
_itemIndex: function(item) {
return $.inArray(item, this.data);
},
_finishUpdate: function($updatingRow, updatedItem, updatedItemIndex) {
this.cancelEdit();
this.data[updatedItemIndex] = updatedItem;
var $updatedRow = this._createRow(updatedItem, updatedItemIndex);
$updatingRow.replaceWith($updatedRow);
return $updatedRow;
},
_getEditedItem: function() {
var result = {};
this._eachField(function(field) {
if(field.editing) {
this._setItemFieldValue(result, field, field.editValue());
}
});
return result;
},
cancelEdit: function() {
if(!this._editingRow)
return;
var $row = this._editingRow,
editingItem = $row.data(JSGRID_ROW_DATA_KEY),
editingItemIndex = this._itemIndex(editingItem);
this._callEventHandler(this.onItemEditCancelling, {
row: $row,
item: editingItem,
itemIndex: editingItemIndex
});
this._getEditRow().remove();
this._editingRow.show();
this._editingRow = null;
},
_getEditRow: function() {
return this._editingRow && this._editingRow.data(JSGRID_EDIT_ROW_DATA_KEY);
},
deleteItem: function(item) {
var $row = this.rowByItem(item);
if(!$row.length)
return;
if(this.confirmDeleting && !window.confirm(getOrApply(this.deleteConfirm, this, $row.data(JSGRID_ROW_DATA_KEY))))
return;
return this._deleteRow($row);
},
_deleteRow: function($row) {
var deletingItem = $row.data(JSGRID_ROW_DATA_KEY),
deletingItemIndex = this._itemIndex(deletingItem);
var args = this._callEventHandler(this.onItemDeleting, {
row: $row,
item: deletingItem,
itemIndex: deletingItemIndex
});
return this._controllerCall("deleteItem", deletingItem, args.cancel, function() {
this._loadStrategy.finishDelete(deletingItem, deletingItemIndex);
this._callEventHandler(this.onItemDeleted, {
row: $row,
item: deletingItem,
itemIndex: deletingItemIndex
});
});
}
};
$.fn.jsGrid = function(config) {
var args = $.makeArray(arguments),
methodArgs = args.slice(1),
result = this;
this.each(function() {
var $element = $(this),
instance = $element.data(JSGRID_DATA_KEY),
methodResult;
if(instance) {
if(typeof config === "string") {
methodResult = instance[config].apply(instance, methodArgs);
if(methodResult !== undefined && methodResult !== instance) {
result = methodResult;
return false;
}
} else {
instance._detachWindowResizeCallback();
instance._init(config);
instance.render();
}
} else {
new Grid($element, config);
}
});
return result;
};
var fields = {};
var setDefaults = function(config) {
var componentPrototype;
if($.isPlainObject(config)) {
componentPrototype = Grid.prototype;
} else {
componentPrototype = fields[config].prototype;
config = arguments[1] || {};
}
$.extend(componentPrototype, config);
};
var locales = {};
var locale = function(lang) {
var localeConfig = $.isPlainObject(lang) ? lang : locales[lang];
if(!localeConfig)
throw Error("unknown locale " + lang);
setLocale(jsGrid, localeConfig);
};
var setLocale = function(obj, localeConfig) {
$.each(localeConfig, function(field, value) {
if($.isPlainObject(value)) {
setLocale(obj[field] || obj[field[0].toUpperCase() + field.slice(1)], value);
return;
}
if(obj.hasOwnProperty(field)) {
obj[field] = value;
} else {
obj.prototype[field] = value;
}
});
};
window.jsGrid = {
Grid: Grid,
fields: fields,
setDefaults: setDefaults,
locales: locales,
locale: locale,
version: "@VERSION"
};
}(window, jQuery));
<MSG> Code: Add renderTemplate indirection for rendering
<DFF> @@ -189,6 +189,11 @@
this._controller = $.extend({}, defaultController, getOrApply(this.controller, this));
},
+ renderTemplate: function(source, context) {
+ source = getOrApply.apply(null, arguments);
+ return source || "";
+ },
+
loadIndicator: function(config) {
return new jsGrid.LoadIndicator(config);
},
@@ -268,7 +273,6 @@
case "rowRenderer":
case "rowClick":
case "rowDoubleClick":
- case "noDataText":
case "noDataRowClass":
case "noDataContent":
case "selecting":
@@ -430,13 +434,13 @@
_createHeaderRow: function() {
if($.isFunction(this.headerRowRenderer))
- return $(this.headerRowRenderer());
+ return $(this.renderTemplate(this.headerRowRenderer, this));
var $result = $("<tr>").addClass(this.headerRowClass);
this._eachField(function(field, index) {
var $th = this._prepareCell("<th>", field, "headercss")
- .append(field.headerTemplate ? field.headerTemplate() : "")
+ .append(this.renderTemplate(field.headerTemplate, field))
.appendTo($result);
if(this.sorting && field.sorting) {
@@ -458,13 +462,13 @@
_createFilterRow: function() {
if($.isFunction(this.filterRowRenderer))
- return $(this.filterRowRenderer());
+ return $(this.renderTemplate(this.filterRowRenderer, this));
var $result = $("<tr>").addClass(this.filterRowClass);
this._eachField(function(field) {
this._prepareCell("<td>", field, "filtercss")
- .append(field.filterTemplate ? field.filterTemplate() : "")
+ .append(this.renderTemplate(field.filterTemplate, field))
.appendTo($result);
});
@@ -473,13 +477,13 @@
_createInsertRow: function() {
if($.isFunction(this.insertRowRenderer))
- return $(this.insertRowRenderer());
+ return $(this.renderTemplate(this.insertRowRenderer, this));
var $result = $("<tr>").addClass(this.insertRowClass);
this._eachField(function(field) {
this._prepareCell("<td>", field, "insertcss")
- .append(field.insertTemplate ? field.insertTemplate() : "")
+ .append(this.renderTemplate(field.insertTemplate, field))
.appendTo($result);
});
@@ -557,28 +561,21 @@
},
_createNoDataRow: function() {
- var noDataContent = getOrApply(this.noDataContent, this);
-
var amountOfFields = 0;
this._eachField(function() {
amountOfFields++;
});
return $("<tr>").addClass(this.noDataRowClass)
- .append($("<td>").attr("colspan", amountOfFields).append(noDataContent));
- },
-
- _createNoDataContent: function() {
- return $.isFunction(this.noDataRenderer)
- ? this.noDataRenderer()
- : this.noDataText;
+ .append($("<td>").attr("colspan", amountOfFields)
+ .append(this.renderTemplate(this.noDataContent, this)));
},
_createRow: function(item, itemIndex) {
var $result;
if($.isFunction(this.rowRenderer)) {
- $result = $(this.rowRenderer(item, itemIndex));
+ $result = this.renderTemplate(this.rowRenderer, this, item, itemIndex);
} else {
$result = $("<tr>");
this._renderCells($result, item);
@@ -638,9 +635,9 @@
var fieldValue = this._getItemFieldValue(item, field);
if($.isFunction(field.cellRenderer)) {
- $result = $(field.cellRenderer(fieldValue, item));
+ $result = this.renderTemplate(field.cellRenderer, field, fieldValue, item);
} else {
- $result = $("<td>").append(field.itemTemplate ? field.itemTemplate(fieldValue, item) : fieldValue);
+ $result = $("<td>").append(this.renderTemplate(field.itemTemplate || fieldValue, field, fieldValue, item));
}
return this._prepareCell($result, field);
| 16 | Code: Add renderTemplate indirection for rendering | 19 | .js | core | mit | tabalinas/jsgrid |
10066233 | <NME> jsgrid.core.js
<BEF> (function(window, $, undefined) {
var JSGRID = "JSGrid",
JSGRID_DATA_KEY = JSGRID,
JSGRID_ROW_DATA_KEY = "JSGridItem",
JSGRID_EDIT_ROW_DATA_KEY = "JSGridEditRow",
SORT_ORDER_ASC = "asc",
SORT_ORDER_DESC = "desc",
FIRST_PAGE_PLACEHOLDER = "{first}",
PAGES_PLACEHOLDER = "{pages}",
PREV_PAGE_PLACEHOLDER = "{prev}",
NEXT_PAGE_PLACEHOLDER = "{next}",
LAST_PAGE_PLACEHOLDER = "{last}",
PAGE_INDEX_PLACEHOLDER = "{pageIndex}",
PAGE_COUNT_PLACEHOLDER = "{pageCount}",
ITEM_COUNT_PLACEHOLDER = "{itemCount}",
EMPTY_HREF = "javascript:void(0);";
var getOrApply = function(value, context) {
if($.isFunction(value)) {
return value.apply(context, $.makeArray(arguments).slice(2));
}
return value;
};
var normalizePromise = function(promise) {
var d = $.Deferred();
if(promise && promise.then) {
promise.then(function() {
d.resolve.apply(d, arguments);
}, function() {
d.reject.apply(d, arguments);
});
} else {
d.resolve(promise);
}
return d.promise();
};
var defaultController = {
loadData: $.noop,
insertItem: $.noop,
updateItem: $.noop,
deleteItem: $.noop
};
function Grid(element, config) {
var $element = $(element);
$element.data(JSGRID_DATA_KEY, this);
this._container = $element;
this.data = [];
this.fields = [];
this._editingRow = null;
this._sortField = null;
this._sortOrder = SORT_ORDER_ASC;
this._firstDisplayingPage = 1;
this._init(config);
this.render();
}
Grid.prototype = {
width: "auto",
height: "auto",
updateOnResize: true,
rowClass: $.noop,
rowRenderer: null,
rowClick: function(args) {
if(this.editing) {
this.editItem($(args.event.target).closest("tr"));
}
},
rowDoubleClick: $.noop,
noDataContent: "Not found",
noDataRowClass: "jsgrid-nodata-row",
heading: true,
headerRowRenderer: null,
headerRowClass: "jsgrid-header-row",
headerCellClass: "jsgrid-header-cell",
filtering: false,
filterRowRenderer: null,
filterRowClass: "jsgrid-filter-row",
inserting: false,
insertRowLocation: "bottom",
insertRowRenderer: null,
insertRowClass: "jsgrid-insert-row",
editing: false,
editRowRenderer: null,
editRowClass: "jsgrid-edit-row",
confirmDeleting: true,
deleteConfirm: "Are you sure?",
selecting: true,
selectedRowClass: "jsgrid-selected-row",
oddRowClass: "jsgrid-row",
evenRowClass: "jsgrid-alt-row",
cellClass: "jsgrid-cell",
sorting: false,
sortableClass: "jsgrid-header-sortable",
sortAscClass: "jsgrid-header-sort jsgrid-header-sort-asc",
sortDescClass: "jsgrid-header-sort jsgrid-header-sort-desc",
paging: false,
pagerContainer: null,
pageIndex: 1,
pageSize: 20,
pageButtonCount: 15,
pagerFormat: "Pages: {first} {prev} {pages} {next} {last} {pageIndex} of {pageCount}",
pagePrevText: "Prev",
pageNextText: "Next",
pageFirstText: "First",
pageLastText: "Last",
pageNavigatorNextText: "...",
pageNavigatorPrevText: "...",
pagerContainerClass: "jsgrid-pager-container",
pagerClass: "jsgrid-pager",
pagerNavButtonClass: "jsgrid-pager-nav-button",
pagerNavButtonInactiveClass: "jsgrid-pager-nav-inactive-button",
pageClass: "jsgrid-pager-page",
currentPageClass: "jsgrid-pager-current-page",
customLoading: false,
pageLoading: false,
autoload: false,
controller: defaultController,
loadIndication: true,
loadIndicationDelay: 500,
loadMessage: "Please, wait...",
loadShading: true,
invalidMessage: "Invalid data entered!",
invalidNotify: function(args) {
var messages = $.map(args.errors, function(error) {
return error.message || null;
});
window.alert([this.invalidMessage].concat(messages).join("\n"));
},
onInit: $.noop,
onRefreshing: $.noop,
onRefreshed: $.noop,
onPageChanged: $.noop,
onItemDeleting: $.noop,
onItemDeleted: $.noop,
onItemInserting: $.noop,
onItemInserted: $.noop,
onItemEditing: $.noop,
onItemEditCancelling: $.noop,
onItemUpdating: $.noop,
onItemUpdated: $.noop,
onItemInvalid: $.noop,
onDataLoading: $.noop,
onDataLoaded: $.noop,
onDataExporting: $.noop,
onOptionChanging: $.noop,
onOptionChanged: $.noop,
onError: $.noop,
invalidClass: "jsgrid-invalid",
containerClass: "jsgrid",
tableClass: "jsgrid-table",
gridHeaderClass: "jsgrid-grid-header",
gridBodyClass: "jsgrid-grid-body",
this._controller = $.extend({}, defaultController, getOrApply(this.controller, this));
},
loadIndicator: function(config) {
return new jsGrid.LoadIndicator(config);
},
return this.pageLoading
? new jsGrid.loadStrategies.PageLoadingStrategy(this)
: new jsGrid.loadStrategies.DirectLoadingStrategy(this);
},
_initLoadStrategy: function() {
this._loadStrategy = getOrApply(this.loadStrategy, this);
},
_initController: function() {
this._controller = $.extend({}, defaultController, getOrApply(this.controller, this));
},
renderTemplate: function(source, context, config) {
var args = [];
for(var key in config) {
args.push(config[key]);
}
args.unshift(source, context);
source = getOrApply.apply(null, args);
return (source === undefined || source === null) ? "" : source;
},
loadIndicator: function(config) {
return new jsGrid.LoadIndicator(config);
},
validation: function(config) {
return jsGrid.Validation && new jsGrid.Validation(config);
},
_initFields: function() {
var self = this;
self.fields = $.map(self.fields, function(field) {
if($.isPlainObject(field)) {
var fieldConstructor = (field.type && jsGrid.fields[field.type]) || jsGrid.Field;
field = new fieldConstructor(field);
}
field._grid = self;
return field;
});
},
_attachWindowLoadResize: function() {
$(window).on("load", $.proxy(this._refreshSize, this));
},
_attachWindowResizeCallback: function() {
if(this.updateOnResize) {
$(window).on("resize", $.proxy(this._refreshSize, this));
}
},
_detachWindowResizeCallback: function() {
$(window).off("resize", this._refreshSize);
},
option: function(key, value) {
var optionChangingEventArgs,
optionChangedEventArgs;
if(arguments.length === 1)
return this[key];
optionChangingEventArgs = {
option: key,
oldValue: this[key],
newValue: value
};
this._callEventHandler(this.onOptionChanging, optionChangingEventArgs);
case "rowRenderer":
case "rowClick":
case "rowDoubleClick":
case "noDataText":
case "noDataRowClass":
case "noDataContent":
case "selecting":
this._callEventHandler(this.onOptionChanged, optionChangedEventArgs);
},
fieldOption: function(field, key, value) {
field = this._normalizeField(field);
if(arguments.length === 2)
return field[key];
field[key] = value;
this._renderGrid();
},
_handleOptionChange: function(name, value) {
this[name] = value;
switch(name) {
case "width":
case "height":
this._refreshSize();
break;
case "rowClass":
case "rowRenderer":
case "rowClick":
case "rowDoubleClick":
case "noDataRowClass":
case "noDataContent":
case "selecting":
case "selectedRowClass":
case "oddRowClass":
case "evenRowClass":
this._refreshContent();
break;
case "pageButtonCount":
case "pagerFormat":
case "pagePrevText":
case "pageNextText":
case "pageFirstText":
case "pageLastText":
case "pageNavigatorNextText":
case "pageNavigatorPrevText":
case "pagerClass":
case "pagerNavButtonClass":
case "pageClass":
case "currentPageClass":
case "pagerRenderer":
this._refreshPager();
break;
case "fields":
this._initFields();
this.render();
break;
case "data":
case "editing":
case "heading":
case "filtering":
case "inserting":
case "paging":
this.refresh();
break;
case "loadStrategy":
case "pageLoading":
this._initLoadStrategy();
this.search();
break;
case "pageIndex":
this.openPage(value);
break;
case "pageSize":
this.refresh();
this.search();
break;
case "editRowRenderer":
case "editRowClass":
this.cancelEdit();
break;
case "updateOnResize":
this._detachWindowResizeCallback();
this._attachWindowResizeCallback();
break;
case "invalidNotify":
case "invalidMessage":
break;
default:
this.render();
break;
}
},
destroy: function() {
this._detachWindowResizeCallback();
this._clear();
this._container.removeData(JSGRID_DATA_KEY);
},
render: function() {
this._renderGrid();
return this.autoload ? this.loadData() : $.Deferred().resolve().promise();
},
_renderGrid: function() {
this._clear();
this._container.addClass(this.containerClass)
.css("position", "relative")
.append(this._createHeader())
.append(this._createBody());
this._pagerContainer = this._createPagerContainer();
this._loadIndicator = this._createLoadIndicator();
this._validation = this._createValidation();
this.refresh();
},
_createLoadIndicator: function() {
return getOrApply(this.loadIndicator, this, {
message: this.loadMessage,
shading: this.loadShading,
container: this._container
});
},
_createValidation: function() {
return getOrApply(this.validation, this);
},
_clear: function() {
this.cancelEdit();
clearTimeout(this._loadingTimer);
this._pagerContainer && this._pagerContainer.empty();
this._container.empty()
.css({ position: "", width: "", height: "" });
},
_createHeader: function() {
var $headerRow = this._headerRow = this._createHeaderRow(),
$filterRow = this._filterRow = this._createFilterRow(),
$insertRow = this._insertRow = this._createInsertRow();
var $headerGrid = this._headerGrid = $("<table>").addClass(this.tableClass)
.append($headerRow)
.append($filterRow)
.append($insertRow);
var $header = this._header = $("<div>").addClass(this.gridHeaderClass)
.addClass(this._scrollBarWidth() ? "jsgrid-header-scrollbar" : "")
.append($headerGrid);
return $header;
},
_createHeaderRow: function() {
if($.isFunction(this.headerRowRenderer))
return $(this.headerRowRenderer());
var $result = $("<tr>").addClass(this.headerRowClass);
this._eachField(function(field, index) {
var $th = this._prepareCell("<th>", field, "headercss")
.append(field.headerTemplate ? field.headerTemplate() : "")
.appendTo($result);
if(this.sorting && field.sorting) {
},
_createPagerContainer: function() {
var pagerContainer = this.pagerContainer || $("<div>").appendTo(this._container);
return $(pagerContainer).addClass(this.pagerContainerClass);
},
_eachField: function(callBack) {
var self = this;
$.each(this.fields, function(index, field) {
if(field.visible) {
callBack.call(self, field, index);
}
});
},
_createFilterRow: function() {
if($.isFunction(this.filterRowRenderer))
return $(this.filterRowRenderer());
var $result = $("<tr>").addClass(this.filterRowClass);
this._eachField(function(field) {
this._prepareCell("<td>", field, "filtercss")
.append(field.filterTemplate ? field.filterTemplate() : "")
.appendTo($result);
});
$th.addClass(this.sortableClass)
.on("click", $.proxy(function() {
_createInsertRow: function() {
if($.isFunction(this.insertRowRenderer))
return $(this.insertRowRenderer());
var $result = $("<tr>").addClass(this.insertRowClass);
this._eachField(function(field) {
this._prepareCell("<td>", field, "insertcss")
.append(field.insertTemplate ? field.insertTemplate() : "")
.appendTo($result);
});
},
_createFilterRow: function() {
if($.isFunction(this.filterRowRenderer))
return $(this.renderTemplate(this.filterRowRenderer, this));
var $result = $("<tr>").addClass(this.filterRowClass);
this._eachField(function(field) {
this._prepareCell("<td>", field, "filtercss")
.append(this.renderTemplate(field.filterTemplate, field))
.appendTo($result);
});
return $result;
},
_createInsertRow: function() {
if($.isFunction(this.insertRowRenderer))
return $(this.renderTemplate(this.insertRowRenderer, this));
var $result = $("<tr>").addClass(this.insertRowClass);
this._eachField(function(field) {
this._prepareCell("<td>", field, "insertcss")
.append(this.renderTemplate(field.insertTemplate, field))
.appendTo($result);
});
return $result;
},
_callEventHandler: function(handler, eventParams) {
handler.call(this, $.extend(eventParams, {
grid: this
}));
return eventParams;
},
reset: function() {
this._resetSorting();
this._resetPager();
return this._loadStrategy.reset();
},
_resetPager: function() {
this._firstDisplayingPage = 1;
this._setPage(1);
},
_resetSorting: function() {
this._sortField = null;
this._sortOrder = SORT_ORDER_ASC;
this._clearSortingCss();
},
refresh: function() {
this._callEventHandler(this.onRefreshing);
this.cancelEdit();
this._refreshHeading();
this._refreshFiltering();
this._refreshInserting();
this._refreshContent();
this._refreshPager();
this._refreshSize();
this._callEventHandler(this.onRefreshed);
},
},
_createNoDataRow: function() {
var noDataContent = getOrApply(this.noDataContent, this);
var amountOfFields = 0;
this._eachField(function() {
amountOfFields++;
});
return $("<tr>").addClass(this.noDataRowClass)
.append($("<td>").attr("colspan", amountOfFields).append(noDataContent));
},
_createNoDataContent: function() {
return $.isFunction(this.noDataRenderer)
? this.noDataRenderer()
: this.noDataText;
},
_createRow: function(item, itemIndex) {
var $result;
if($.isFunction(this.rowRenderer)) {
$result = $(this.rowRenderer(item, itemIndex));
} else {
$result = $("<tr>");
this._renderCells($result, item);
var indexFrom = this._loadStrategy.firstDisplayIndex();
var indexTo = this._loadStrategy.lastDisplayIndex();
for(var itemIndex = indexFrom; itemIndex < indexTo; itemIndex++) {
var item = this.data[itemIndex];
$content.append(this._createRow(item, itemIndex));
}
},
_createNoDataRow: function() {
var amountOfFields = 0;
this._eachField(function() {
amountOfFields++;
});
return $("<tr>").addClass(this.noDataRowClass)
.append($("<td>").addClass(this.cellClass).attr("colspan", amountOfFields)
.append(this.renderTemplate(this.noDataContent, this)));
},
_createRow: function(item, itemIndex) {
var $result;
if($.isFunction(this.rowRenderer)) {
$result = this.renderTemplate(this.rowRenderer, this, { item: item, itemIndex: itemIndex });
} else {
$result = $("<tr>");
this._renderCells($result, item);
}
$result.addClass(this._getRowClasses(item, itemIndex))
.data(JSGRID_ROW_DATA_KEY, item)
.on("click", $.proxy(function(e) {
this.rowClick({
item: item,
itemIndex: itemIndex,
event: e
});
}, this))
.on("dblclick", $.proxy(function(e) {
this.rowDoubleClick({
item: item,
itemIndex: itemIndex,
event: e
});
}, this));
if(this.selecting) {
this._attachRowHover($result);
}
return $result;
var fieldValue = this._getItemFieldValue(item, field);
if($.isFunction(field.cellRenderer)) {
$result = $(field.cellRenderer(fieldValue, item));
} else {
$result = $("<td>").append(field.itemTemplate ? field.itemTemplate(fieldValue, item) : fieldValue);
}
return this._prepareCell($result, field);
_attachRowHover: function($row) {
var selectedRowClass = this.selectedRowClass;
$row.hover(function() {
$(this).addClass(selectedRowClass);
},
function() {
$(this).removeClass(selectedRowClass);
}
);
},
_renderCells: function($row, item) {
this._eachField(function(field) {
$row.append(this._createCell(item, field));
});
return this;
},
_createCell: function(item, field) {
var $result;
var fieldValue = this._getItemFieldValue(item, field);
var args = { value: fieldValue, item : item };
if($.isFunction(field.cellRenderer)) {
$result = this.renderTemplate(field.cellRenderer, field, args);
} else {
$result = $("<td>").append(this.renderTemplate(field.itemTemplate || fieldValue, field, args));
}
return this._prepareCell($result, field);
},
_getItemFieldValue: function(item, field) {
var props = field.name.split('.');
var result = item[props.shift()];
while(result && props.length) {
result = result[props.shift()];
}
return result;
},
_setItemFieldValue: function(item, field, value) {
var props = field.name.split('.');
var current = item;
var prop = props[0];
while(current && props.length) {
item = current;
prop = props.shift();
current = item[prop];
}
if(!current) {
while(props.length) {
item = item[prop] = {};
prop = props.shift();
}
}
item[prop] = value;
},
sort: function(field, order) {
if($.isPlainObject(field)) {
order = field.order;
field = field.field;
}
this._clearSortingCss();
this._setSortingParams(field, order);
this._setSortingCss();
return this._loadStrategy.sort();
},
_clearSortingCss: function() {
this._headerRow.find("th")
.removeClass(this.sortAscClass)
.removeClass(this.sortDescClass);
},
_setSortingParams: function(field, order) {
field = this._normalizeField(field);
order = order || ((this._sortField === field) ? this._reversedSortOrder(this._sortOrder) : SORT_ORDER_ASC);
this._sortField = field;
this._sortOrder = order;
},
_normalizeField: function(field) {
if($.isNumeric(field)) {
return this.fields[field];
}
if(typeof field === "string") {
return $.grep(this.fields, function(f) {
return f.name === field;
})[0];
}
return field;
},
_reversedSortOrder: function(order) {
return (order === SORT_ORDER_ASC ? SORT_ORDER_DESC : SORT_ORDER_ASC);
},
_setSortingCss: function() {
var fieldIndex = this._visibleFieldIndex(this._sortField);
this._headerRow.find("th").eq(fieldIndex)
.addClass(this._sortOrder === SORT_ORDER_ASC ? this.sortAscClass : this.sortDescClass);
},
_visibleFieldIndex: function(field) {
return $.inArray(field, $.grep(this.fields, function(f) { return f.visible; }));
},
_sortData: function() {
var sortFactor = this._sortFactor(),
sortField = this._sortField;
if(sortField) {
var self = this;
self.data.sort(function(item1, item2) {
var value1 = self._getItemFieldValue(item1, sortField);
var value2 = self._getItemFieldValue(item2, sortField);
return sortFactor * sortField.sortingFunc(value1, value2);
});
}
},
_sortFactor: function() {
return this._sortOrder === SORT_ORDER_ASC ? 1 : -1;
},
_itemsCount: function() {
return this._loadStrategy.itemsCount();
},
_pagesCount: function() {
var itemsCount = this._itemsCount(),
pageSize = this.pageSize;
return Math.floor(itemsCount / pageSize) + (itemsCount % pageSize ? 1 : 0);
},
_refreshPager: function() {
var $pagerContainer = this._pagerContainer;
$pagerContainer.empty();
if(this.paging) {
$pagerContainer.append(this._createPager());
}
var showPager = this.paging && this._pagesCount() > 1;
$pagerContainer.toggle(showPager);
},
_createPager: function() {
var $result;
if($.isFunction(this.pagerRenderer)) {
$result = $(this.pagerRenderer({
pageIndex: this.pageIndex,
pageCount: this._pagesCount()
}));
} else {
$result = $("<div>").append(this._createPagerByFormat());
}
$result.addClass(this.pagerClass);
return $result;
},
_createPagerByFormat: function() {
var pageIndex = this.pageIndex,
pageCount = this._pagesCount(),
itemCount = this._itemsCount(),
pagerParts = this.pagerFormat.split(" ");
return $.map(pagerParts, $.proxy(function(pagerPart) {
var result = pagerPart;
if(pagerPart === PAGES_PLACEHOLDER) {
result = this._createPages();
} else if(pagerPart === FIRST_PAGE_PLACEHOLDER) {
result = this._createPagerNavButton(this.pageFirstText, 1, pageIndex > 1);
} else if(pagerPart === PREV_PAGE_PLACEHOLDER) {
result = this._createPagerNavButton(this.pagePrevText, pageIndex - 1, pageIndex > 1);
} else if(pagerPart === NEXT_PAGE_PLACEHOLDER) {
result = this._createPagerNavButton(this.pageNextText, pageIndex + 1, pageIndex < pageCount);
} else if(pagerPart === LAST_PAGE_PLACEHOLDER) {
result = this._createPagerNavButton(this.pageLastText, pageCount, pageIndex < pageCount);
} else if(pagerPart === PAGE_INDEX_PLACEHOLDER) {
result = pageIndex;
} else if(pagerPart === PAGE_COUNT_PLACEHOLDER) {
result = pageCount;
} else if(pagerPart === ITEM_COUNT_PLACEHOLDER) {
result = itemCount;
}
return $.isArray(result) ? result.concat([" "]) : [result, " "];
}, this));
},
_createPages: function() {
var pageCount = this._pagesCount(),
pageButtonCount = this.pageButtonCount,
firstDisplayingPage = this._firstDisplayingPage,
pages = [];
if(firstDisplayingPage > 1) {
pages.push(this._createPagerPageNavButton(this.pageNavigatorPrevText, this.showPrevPages));
}
for(var i = 0, pageNumber = firstDisplayingPage; i < pageButtonCount && pageNumber <= pageCount; i++, pageNumber++) {
pages.push(pageNumber === this.pageIndex
? this._createPagerCurrentPage()
: this._createPagerPage(pageNumber));
}
if((firstDisplayingPage + pageButtonCount - 1) < pageCount) {
pages.push(this._createPagerPageNavButton(this.pageNavigatorNextText, this.showNextPages));
}
return pages;
},
_createPagerNavButton: function(text, pageIndex, isActive) {
return this._createPagerButton(text, this.pagerNavButtonClass + (isActive ? "" : " " + this.pagerNavButtonInactiveClass),
isActive ? function() { this.openPage(pageIndex); } : $.noop);
},
_createPagerPageNavButton: function(text, handler) {
return this._createPagerButton(text, this.pagerNavButtonClass, handler);
},
_createPagerPage: function(pageIndex) {
return this._createPagerButton(pageIndex, this.pageClass, function() {
this.openPage(pageIndex);
});
},
_createPagerButton: function(text, css, handler) {
var $link = $("<a>").attr("href", EMPTY_HREF)
.html(text)
.on("click", $.proxy(handler, this));
return $("<span>").addClass(css).append($link);
},
_createPagerCurrentPage: function() {
return $("<span>")
.addClass(this.pageClass)
.addClass(this.currentPageClass)
.text(this.pageIndex);
},
_refreshSize: function() {
this._refreshHeight();
this._refreshWidth();
},
_refreshWidth: function() {
var width = (this.width === "auto") ? this._getAutoWidth() : this.width;
this._container.width(width);
},
_getAutoWidth: function() {
var $headerGrid = this._headerGrid,
$header = this._header;
$headerGrid.width("auto");
var contentWidth = $headerGrid.outerWidth();
var borderWidth = $header.outerWidth() - $header.innerWidth();
$headerGrid.width("");
return contentWidth + borderWidth;
},
_scrollBarWidth: (function() {
var result;
return function() {
if(result === undefined) {
var $ghostContainer = $("<div style='width:50px;height:50px;overflow:hidden;position:absolute;top:-10000px;left:-10000px;'></div>");
var $ghostContent = $("<div style='height:100px;'></div>");
$ghostContainer.append($ghostContent).appendTo("body");
var width = $ghostContent.innerWidth();
$ghostContainer.css("overflow-y", "auto");
var widthExcludingScrollBar = $ghostContent.innerWidth();
$ghostContainer.remove();
result = width - widthExcludingScrollBar;
}
return result;
};
})(),
_refreshHeight: function() {
var container = this._container,
pagerContainer = this._pagerContainer,
height = this.height,
nonBodyHeight;
container.height(height);
if(height !== "auto") {
height = container.height();
nonBodyHeight = this._header.outerHeight(true);
if(pagerContainer.parents(container).length) {
nonBodyHeight += pagerContainer.outerHeight(true);
}
this._body.outerHeight(height - nonBodyHeight);
}
},
showPrevPages: function() {
var firstDisplayingPage = this._firstDisplayingPage,
pageButtonCount = this.pageButtonCount;
this._firstDisplayingPage = (firstDisplayingPage > pageButtonCount) ? firstDisplayingPage - pageButtonCount : 1;
this._refreshPager();
},
showNextPages: function() {
var firstDisplayingPage = this._firstDisplayingPage,
pageButtonCount = this.pageButtonCount,
pageCount = this._pagesCount();
this._firstDisplayingPage = (firstDisplayingPage + 2 * pageButtonCount > pageCount)
? pageCount - pageButtonCount + 1
: firstDisplayingPage + pageButtonCount;
this._refreshPager();
},
openPage: function(pageIndex) {
if(pageIndex < 1 || pageIndex > this._pagesCount())
return;
this._setPage(pageIndex);
this._loadStrategy.openPage(pageIndex);
},
_setPage: function(pageIndex) {
var firstDisplayingPage = this._firstDisplayingPage,
pageButtonCount = this.pageButtonCount;
this.pageIndex = pageIndex;
if(pageIndex < firstDisplayingPage) {
this._firstDisplayingPage = pageIndex;
}
if(pageIndex > firstDisplayingPage + pageButtonCount - 1) {
this._firstDisplayingPage = pageIndex - pageButtonCount + 1;
}
this._callEventHandler(this.onPageChanged, {
pageIndex: pageIndex
});
},
_controllerCall: function(method, param, isCanceled, doneCallback) {
if(isCanceled)
return $.Deferred().reject().promise();
this._showLoading();
var controller = this._controller;
if(!controller || !controller[method]) {
throw Error("controller has no method '" + method + "'");
}
return normalizePromise(controller[method](param))
.done($.proxy(doneCallback, this))
.fail($.proxy(this._errorHandler, this))
.always($.proxy(this._hideLoading, this));
},
_errorHandler: function() {
this._callEventHandler(this.onError, {
args: $.makeArray(arguments)
});
},
_showLoading: function() {
if(!this.loadIndication)
return;
clearTimeout(this._loadingTimer);
this._loadingTimer = setTimeout($.proxy(function() {
this._loadIndicator.show();
}, this), this.loadIndicationDelay);
},
_hideLoading: function() {
if(!this.loadIndication)
return;
clearTimeout(this._loadingTimer);
this._loadIndicator.hide();
},
search: function(filter) {
this._resetSorting();
this._resetPager();
return this.loadData(filter);
},
loadData: function(filter) {
filter = filter || (this.filtering ? this.getFilter() : {});
$.extend(filter, this._loadStrategy.loadParams(), this._sortingParams());
var args = this._callEventHandler(this.onDataLoading, {
filter: filter
});
return this._controllerCall("loadData", filter, args.cancel, function(loadedData) {
if(!loadedData)
return;
this._loadStrategy.finishLoad(loadedData);
this._callEventHandler(this.onDataLoaded, {
data: loadedData
});
});
},
exportData: function(exportOptions){
var options = exportOptions || {};
var type = options.type || "csv";
var result = "";
this._callEventHandler(this.onDataExporting);
switch(type){
case "csv":
result = this._dataToCsv(options);
break;
}
return result;
},
_dataToCsv: function(options){
var options = options || {};
var includeHeaders = options.hasOwnProperty("includeHeaders") ? options.includeHeaders : true;
var subset = options.subset || "all";
var filter = options.filter || undefined;
var result = [];
if (includeHeaders){
var fieldsLength = this.fields.length;
var fieldNames = {};
for(var i=0;i<fieldsLength;i++){
var field = this.fields[i];
if ("includeInDataExport" in field){
if (field.includeInDataExport === true)
fieldNames[i] = field.title || field.name;
}
}
var headerLine = this._itemToCsv(fieldNames,{},options);
result.push(headerLine);
}
var exportStartIndex = 0;
var exportEndIndex = this.data.length;
switch(subset){
case "visible":
exportEndIndex = this._firstDisplayingPage * this.pageSize;
exportStartIndex = exportEndIndex - this.pageSize;
case "all":
default:
break;
}
for (var i = exportStartIndex; i < exportEndIndex; i++){
var item = this.data[i];
var itemLine = "";
var includeItem = true;
if (filter)
if (!filter(item))
includeItem = false;
if (includeItem){
itemLine = this._itemToCsv(item, this.fields, options);
result.push(itemLine);
}
}
return result.join("");
},
_itemToCsv: function(item, fields, options){
var options = options || {};
var delimiter = options.delimiter || "|";
var encapsulate = options.hasOwnProperty("encapsulate") ? options.encapsulate : true;
var newline = options.newline || "\r\n";
var transforms = options.transforms || {};
var fields = fields || {};
var getItem = this._getItemFieldValue;
var result = [];
Object.keys(item).forEach(function(key,index) {
var entry = "";
//Fields.length is greater than 0 when we are matching agaisnt fields
//Field.length will be 0 when exporting header rows
if (fields.length > 0){
var field = fields[index];
//Field may be excluded from data export
if ("includeInDataExport" in field){
if (field.includeInDataExport){
//Field may be a select, which requires additional logic
if (field.type === "select"){
var selectedItem = getItem(item, field);
var resultItem = $.grep(field.items, function(item, index) {
return item[field.valueField] === selectedItem;
})[0] || "";
entry = resultItem[field.textField];
}
else{
entry = getItem(item, field);
}
}
else{
return;
}
}
else{
entry = getItem(item, field);
}
if (transforms.hasOwnProperty(field.name)){
entry = transforms[field.name](entry);
}
}
else{
entry = item[key];
}
if (encapsulate){
entry = '"'+entry+'"';
}
result.push(entry);
});
return result.join(delimiter) + newline;
},
getFilter: function() {
var result = {};
this._eachField(function(field) {
if(field.filtering) {
this._setItemFieldValue(result, field, field.filterValue());
}
});
return result;
},
_sortingParams: function() {
if(this.sorting && this._sortField) {
return {
sortField: this._sortField.name,
sortOrder: this._sortOrder
};
}
return {};
},
getSorting: function() {
var sortingParams = this._sortingParams();
return {
field: sortingParams.sortField,
order: sortingParams.sortOrder
};
},
clearFilter: function() {
var $filterRow = this._createFilterRow();
this._filterRow.replaceWith($filterRow);
this._filterRow = $filterRow;
return this.search();
},
insertItem: function(item) {
var insertingItem = item || this._getValidatedInsertItem();
if(!insertingItem)
return $.Deferred().reject().promise();
var args = this._callEventHandler(this.onItemInserting, {
item: insertingItem
});
return this._controllerCall("insertItem", insertingItem, args.cancel, function(insertedItem) {
insertedItem = insertedItem || insertingItem;
this._loadStrategy.finishInsert(insertedItem, this.insertRowLocation);
this._callEventHandler(this.onItemInserted, {
item: insertedItem
});
});
},
_getValidatedInsertItem: function() {
var item = this._getInsertItem();
return this._validateItem(item, this._insertRow) ? item : null;
},
_getInsertItem: function() {
var result = {};
this._eachField(function(field) {
if(field.inserting) {
this._setItemFieldValue(result, field, field.insertValue());
}
});
return result;
},
_validateItem: function(item, $row) {
var validationErrors = [];
var args = {
item: item,
itemIndex: this._rowIndex($row),
row: $row
};
this._eachField(function(field) {
if(!field.validate ||
($row === this._insertRow && !field.inserting) ||
($row === this._getEditRow() && !field.editing))
return;
var fieldValue = this._getItemFieldValue(item, field);
var errors = this._validation.validate($.extend({
value: fieldValue,
rules: field.validate
}, args));
this._setCellValidity($row.children().eq(this._visibleFieldIndex(field)), errors);
if(!errors.length)
return;
validationErrors.push.apply(validationErrors,
$.map(errors, function(message) {
return { field: field, message: message };
}));
});
if(!validationErrors.length)
return true;
var invalidArgs = $.extend({
errors: validationErrors
}, args);
this._callEventHandler(this.onItemInvalid, invalidArgs);
this.invalidNotify(invalidArgs);
return false;
},
_setCellValidity: function($cell, errors) {
$cell
.toggleClass(this.invalidClass, !!errors.length)
.attr("title", errors.join("\n"));
},
clearInsert: function() {
var insertRow = this._createInsertRow();
this._insertRow.replaceWith(insertRow);
this._insertRow = insertRow;
this.refresh();
},
editItem: function(item) {
var $row = this.rowByItem(item);
if($row.length) {
this._editRow($row);
}
},
rowByItem: function(item) {
if(item.jquery || item.nodeType)
return $(item);
return this._content.find("tr").filter(function() {
return $.data(this, JSGRID_ROW_DATA_KEY) === item;
});
},
_editRow: function($row) {
if(!this.editing)
return;
var item = $row.data(JSGRID_ROW_DATA_KEY);
var args = this._callEventHandler(this.onItemEditing, {
row: $row,
item: item,
itemIndex: this._itemIndex(item)
});
if(args.cancel)
return;
if(this._editingRow) {
this.cancelEdit();
}
var $editRow = this._createEditRow(item);
this._editingRow = $row;
$row.hide();
$editRow.insertBefore($row);
$row.data(JSGRID_EDIT_ROW_DATA_KEY, $editRow);
},
_createEditRow: function(item) {
if($.isFunction(this.editRowRenderer)) {
return $(this.renderTemplate(this.editRowRenderer, this, { item: item, itemIndex: this._itemIndex(item) }));
}
var $result = $("<tr>").addClass(this.editRowClass);
this._eachField(function(field) {
var fieldValue = this._getItemFieldValue(item, field);
this._prepareCell("<td>", field, "editcss")
.append(this.renderTemplate(field.editTemplate || "", field, { value: fieldValue, item: item }))
.appendTo($result);
});
return $result;
},
updateItem: function(item, editedItem) {
if(arguments.length === 1) {
editedItem = item;
}
var $row = item ? this.rowByItem(item) : this._editingRow;
editedItem = editedItem || this._getValidatedEditedItem();
if(!editedItem)
return;
return this._updateRow($row, editedItem);
},
_getValidatedEditedItem: function() {
var item = this._getEditedItem();
return this._validateItem(item, this._getEditRow()) ? item : null;
},
_updateRow: function($updatingRow, editedItem) {
var updatingItem = $updatingRow.data(JSGRID_ROW_DATA_KEY),
updatingItemIndex = this._itemIndex(updatingItem),
updatedItem = $.extend(true, {}, updatingItem, editedItem);
var args = this._callEventHandler(this.onItemUpdating, {
row: $updatingRow,
item: updatedItem,
itemIndex: updatingItemIndex,
previousItem: updatingItem
});
return this._controllerCall("updateItem", updatedItem, args.cancel, function(loadedUpdatedItem) {
var previousItem = $.extend(true, {}, updatingItem);
updatedItem = loadedUpdatedItem || $.extend(true, updatingItem, editedItem);
var $updatedRow = this._finishUpdate($updatingRow, updatedItem, updatingItemIndex);
this._callEventHandler(this.onItemUpdated, {
row: $updatedRow,
item: updatedItem,
itemIndex: updatingItemIndex,
previousItem: previousItem
});
});
},
_rowIndex: function(row) {
return this._content.children().index($(row));
},
_itemIndex: function(item) {
return $.inArray(item, this.data);
},
_finishUpdate: function($updatingRow, updatedItem, updatedItemIndex) {
this.cancelEdit();
this.data[updatedItemIndex] = updatedItem;
var $updatedRow = this._createRow(updatedItem, updatedItemIndex);
$updatingRow.replaceWith($updatedRow);
return $updatedRow;
},
_getEditedItem: function() {
var result = {};
this._eachField(function(field) {
if(field.editing) {
this._setItemFieldValue(result, field, field.editValue());
}
});
return result;
},
cancelEdit: function() {
if(!this._editingRow)
return;
var $row = this._editingRow,
editingItem = $row.data(JSGRID_ROW_DATA_KEY),
editingItemIndex = this._itemIndex(editingItem);
this._callEventHandler(this.onItemEditCancelling, {
row: $row,
item: editingItem,
itemIndex: editingItemIndex
});
this._getEditRow().remove();
this._editingRow.show();
this._editingRow = null;
},
_getEditRow: function() {
return this._editingRow && this._editingRow.data(JSGRID_EDIT_ROW_DATA_KEY);
},
deleteItem: function(item) {
var $row = this.rowByItem(item);
if(!$row.length)
return;
if(this.confirmDeleting && !window.confirm(getOrApply(this.deleteConfirm, this, $row.data(JSGRID_ROW_DATA_KEY))))
return;
return this._deleteRow($row);
},
_deleteRow: function($row) {
var deletingItem = $row.data(JSGRID_ROW_DATA_KEY),
deletingItemIndex = this._itemIndex(deletingItem);
var args = this._callEventHandler(this.onItemDeleting, {
row: $row,
item: deletingItem,
itemIndex: deletingItemIndex
});
return this._controllerCall("deleteItem", deletingItem, args.cancel, function() {
this._loadStrategy.finishDelete(deletingItem, deletingItemIndex);
this._callEventHandler(this.onItemDeleted, {
row: $row,
item: deletingItem,
itemIndex: deletingItemIndex
});
});
}
};
$.fn.jsGrid = function(config) {
var args = $.makeArray(arguments),
methodArgs = args.slice(1),
result = this;
this.each(function() {
var $element = $(this),
instance = $element.data(JSGRID_DATA_KEY),
methodResult;
if(instance) {
if(typeof config === "string") {
methodResult = instance[config].apply(instance, methodArgs);
if(methodResult !== undefined && methodResult !== instance) {
result = methodResult;
return false;
}
} else {
instance._detachWindowResizeCallback();
instance._init(config);
instance.render();
}
} else {
new Grid($element, config);
}
});
return result;
};
var fields = {};
var setDefaults = function(config) {
var componentPrototype;
if($.isPlainObject(config)) {
componentPrototype = Grid.prototype;
} else {
componentPrototype = fields[config].prototype;
config = arguments[1] || {};
}
$.extend(componentPrototype, config);
};
var locales = {};
var locale = function(lang) {
var localeConfig = $.isPlainObject(lang) ? lang : locales[lang];
if(!localeConfig)
throw Error("unknown locale " + lang);
setLocale(jsGrid, localeConfig);
};
var setLocale = function(obj, localeConfig) {
$.each(localeConfig, function(field, value) {
if($.isPlainObject(value)) {
setLocale(obj[field] || obj[field[0].toUpperCase() + field.slice(1)], value);
return;
}
if(obj.hasOwnProperty(field)) {
obj[field] = value;
} else {
obj.prototype[field] = value;
}
});
};
window.jsGrid = {
Grid: Grid,
fields: fields,
setDefaults: setDefaults,
locales: locales,
locale: locale,
version: "@VERSION"
};
}(window, jQuery));
<MSG> Code: Add renderTemplate indirection for rendering
<DFF> @@ -189,6 +189,11 @@
this._controller = $.extend({}, defaultController, getOrApply(this.controller, this));
},
+ renderTemplate: function(source, context) {
+ source = getOrApply.apply(null, arguments);
+ return source || "";
+ },
+
loadIndicator: function(config) {
return new jsGrid.LoadIndicator(config);
},
@@ -268,7 +273,6 @@
case "rowRenderer":
case "rowClick":
case "rowDoubleClick":
- case "noDataText":
case "noDataRowClass":
case "noDataContent":
case "selecting":
@@ -430,13 +434,13 @@
_createHeaderRow: function() {
if($.isFunction(this.headerRowRenderer))
- return $(this.headerRowRenderer());
+ return $(this.renderTemplate(this.headerRowRenderer, this));
var $result = $("<tr>").addClass(this.headerRowClass);
this._eachField(function(field, index) {
var $th = this._prepareCell("<th>", field, "headercss")
- .append(field.headerTemplate ? field.headerTemplate() : "")
+ .append(this.renderTemplate(field.headerTemplate, field))
.appendTo($result);
if(this.sorting && field.sorting) {
@@ -458,13 +462,13 @@
_createFilterRow: function() {
if($.isFunction(this.filterRowRenderer))
- return $(this.filterRowRenderer());
+ return $(this.renderTemplate(this.filterRowRenderer, this));
var $result = $("<tr>").addClass(this.filterRowClass);
this._eachField(function(field) {
this._prepareCell("<td>", field, "filtercss")
- .append(field.filterTemplate ? field.filterTemplate() : "")
+ .append(this.renderTemplate(field.filterTemplate, field))
.appendTo($result);
});
@@ -473,13 +477,13 @@
_createInsertRow: function() {
if($.isFunction(this.insertRowRenderer))
- return $(this.insertRowRenderer());
+ return $(this.renderTemplate(this.insertRowRenderer, this));
var $result = $("<tr>").addClass(this.insertRowClass);
this._eachField(function(field) {
this._prepareCell("<td>", field, "insertcss")
- .append(field.insertTemplate ? field.insertTemplate() : "")
+ .append(this.renderTemplate(field.insertTemplate, field))
.appendTo($result);
});
@@ -557,28 +561,21 @@
},
_createNoDataRow: function() {
- var noDataContent = getOrApply(this.noDataContent, this);
-
var amountOfFields = 0;
this._eachField(function() {
amountOfFields++;
});
return $("<tr>").addClass(this.noDataRowClass)
- .append($("<td>").attr("colspan", amountOfFields).append(noDataContent));
- },
-
- _createNoDataContent: function() {
- return $.isFunction(this.noDataRenderer)
- ? this.noDataRenderer()
- : this.noDataText;
+ .append($("<td>").attr("colspan", amountOfFields)
+ .append(this.renderTemplate(this.noDataContent, this)));
},
_createRow: function(item, itemIndex) {
var $result;
if($.isFunction(this.rowRenderer)) {
- $result = $(this.rowRenderer(item, itemIndex));
+ $result = this.renderTemplate(this.rowRenderer, this, item, itemIndex);
} else {
$result = $("<tr>");
this._renderCells($result, item);
@@ -638,9 +635,9 @@
var fieldValue = this._getItemFieldValue(item, field);
if($.isFunction(field.cellRenderer)) {
- $result = $(field.cellRenderer(fieldValue, item));
+ $result = this.renderTemplate(field.cellRenderer, field, fieldValue, item);
} else {
- $result = $("<td>").append(field.itemTemplate ? field.itemTemplate(fieldValue, item) : fieldValue);
+ $result = $("<td>").append(this.renderTemplate(field.itemTemplate || fieldValue, field, fieldValue, item));
}
return this._prepareCell($result, field);
| 16 | Code: Add renderTemplate indirection for rendering | 19 | .js | core | mit | tabalinas/jsgrid |
10066234 | <NME> jsgrid.load-strategies.js
<BEF> (function(jsGrid, $, undefined) {
function DirectLoadingStrategy(grid) {
this._grid = grid;
}
DirectLoadingStrategy.prototype = {
firstDisplayIndex: function() {
var grid = this._grid;
return grid.option("paging") ? (grid.option("pageIndex") - 1) * grid.option("pageSize") : 0;
},
lastDisplayIndex: function() {
var grid = this._grid;
var itemsCount = grid.option("data").length;
return grid.option("paging")
? Math.min(grid.option("pageIndex") * grid.option("pageSize"), itemsCount)
: itemsCount;
},
itemsCount: function() {
return this._grid.option("data").length;
},
openPage: function(index) {
this._grid.refresh();
},
loadParams: function() {
return {};
},
sort: function() {
this._grid._sortData();
this._grid.refresh();
},
finishLoad: function(loadedData) {
this._grid.refresh();
return $.Deferred().resolve().promise();
},
finishLoad: function(loadedData) {
this._grid.option("data", loadedData);
},
finishInsert: function(insertedItem, location) {
var grid = this._grid;
switch(location){
case "top":
grid.option("data").unshift(insertedItem);
break;
case "bottom":
default:
grid.option("data").push(insertedItem);
}
grid.refresh();
},
finishDelete: function(deletedItem, deletedItemIndex) {
var grid = this._grid;
grid.option("data").splice(deletedItemIndex, 1);
grid.reset();
}
};
function PageLoadingStrategy(grid) {
this._grid = grid;
this._itemsCount = 0;
}
PageLoadingStrategy.prototype = {
firstDisplayIndex: function() {
return 0;
},
lastDisplayIndex: function() {
return this._grid.option("data").length;
},
},
sort: function() {
this._grid.loadData();
},
finishLoad: function(loadedData) {
},
loadParams: function() {
var grid = this._grid;
return {
pageIndex: grid.option("pageIndex"),
pageSize: grid.option("pageSize")
};
},
reset: function() {
return this._grid.loadData();
},
sort: function() {
return this._grid.loadData();
},
finishLoad: function(loadedData) {
this._itemsCount = loadedData.itemsCount;
this._grid.option("data", loadedData.data);
},
finishInsert: function(insertedItem) {
this._grid.search();
},
finishDelete: function(deletedItem, deletedItemIndex) {
this._grid.search();
}
};
jsGrid.loadStrategies = {
DirectLoadingStrategy: DirectLoadingStrategy,
PageLoadingStrategy: PageLoadingStrategy
};
}(jsGrid, jQuery));
<MSG> API: Return jQuery promise from async methods
<DFF> @@ -35,6 +35,7 @@
sort: function() {
this._grid._sortData();
this._grid.refresh();
+ return $.Deferred().resolve().promise();
},
finishLoad: function(loadedData) {
@@ -86,7 +87,7 @@
},
sort: function() {
- this._grid.loadData();
+ return this._grid.loadData();
},
finishLoad: function(loadedData) {
| 2 | API: Return jQuery promise from async methods | 1 | .js | load-strategies | mit | tabalinas/jsgrid |
10066235 | <NME> jsgrid.load-strategies.js
<BEF> (function(jsGrid, $, undefined) {
function DirectLoadingStrategy(grid) {
this._grid = grid;
}
DirectLoadingStrategy.prototype = {
firstDisplayIndex: function() {
var grid = this._grid;
return grid.option("paging") ? (grid.option("pageIndex") - 1) * grid.option("pageSize") : 0;
},
lastDisplayIndex: function() {
var grid = this._grid;
var itemsCount = grid.option("data").length;
return grid.option("paging")
? Math.min(grid.option("pageIndex") * grid.option("pageSize"), itemsCount)
: itemsCount;
},
itemsCount: function() {
return this._grid.option("data").length;
},
openPage: function(index) {
this._grid.refresh();
},
loadParams: function() {
return {};
},
sort: function() {
this._grid._sortData();
this._grid.refresh();
},
finishLoad: function(loadedData) {
this._grid.refresh();
return $.Deferred().resolve().promise();
},
finishLoad: function(loadedData) {
this._grid.option("data", loadedData);
},
finishInsert: function(insertedItem, location) {
var grid = this._grid;
switch(location){
case "top":
grid.option("data").unshift(insertedItem);
break;
case "bottom":
default:
grid.option("data").push(insertedItem);
}
grid.refresh();
},
finishDelete: function(deletedItem, deletedItemIndex) {
var grid = this._grid;
grid.option("data").splice(deletedItemIndex, 1);
grid.reset();
}
};
function PageLoadingStrategy(grid) {
this._grid = grid;
this._itemsCount = 0;
}
PageLoadingStrategy.prototype = {
firstDisplayIndex: function() {
return 0;
},
lastDisplayIndex: function() {
return this._grid.option("data").length;
},
},
sort: function() {
this._grid.loadData();
},
finishLoad: function(loadedData) {
},
loadParams: function() {
var grid = this._grid;
return {
pageIndex: grid.option("pageIndex"),
pageSize: grid.option("pageSize")
};
},
reset: function() {
return this._grid.loadData();
},
sort: function() {
return this._grid.loadData();
},
finishLoad: function(loadedData) {
this._itemsCount = loadedData.itemsCount;
this._grid.option("data", loadedData.data);
},
finishInsert: function(insertedItem) {
this._grid.search();
},
finishDelete: function(deletedItem, deletedItemIndex) {
this._grid.search();
}
};
jsGrid.loadStrategies = {
DirectLoadingStrategy: DirectLoadingStrategy,
PageLoadingStrategy: PageLoadingStrategy
};
}(jsGrid, jQuery));
<MSG> API: Return jQuery promise from async methods
<DFF> @@ -35,6 +35,7 @@
sort: function() {
this._grid._sortData();
this._grid.refresh();
+ return $.Deferred().resolve().promise();
},
finishLoad: function(loadedData) {
@@ -86,7 +87,7 @@
},
sort: function() {
- this._grid.loadData();
+ return this._grid.loadData();
},
finishLoad: function(loadedData) {
| 2 | API: Return jQuery promise from async methods | 1 | .js | load-strategies | mit | tabalinas/jsgrid |
10066236 | <NME> AvaloniaEdit.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.0</TargetFramework>
<WarningsNotAsErrors>0612,0618</WarningsNotAsErrors>
<PackageId>Avalonia.AvaloniaEdit</PackageId>
</PropertyGroup>
<ItemGroup>
<EmbeddedResource Include="**\*.xshd;**\*.resx;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
<AvaloniaResource Include="**\*.xaml;Assets\*;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
</ItemGroup>
<ItemGroup>
<None Remove="Search\Assets\CaseSensitive.png" />
<None Remove="Search\Assets\CompleteWord.png" />
<None Remove="Search\Assets\FindNext.png" />
<None Remove="Search\Assets\FindPrevious.png" />
<None Remove="Search\Assets\RegularExpression.png" />
<None Remove="Search\Assets\ReplaceAll.png" />
<None Remove="Search\Assets\ReplaceNext.png" />
</ItemGroup>
<ItemGroup>
<EmbeddedResource Include="Search\Assets\CaseSensitive.png" />
<EmbeddedResource Include="Search\Assets\CompleteWord.png" />
<EmbeddedResource Include="Search\Assets\FindNext.png" />
<EmbeddedResource Include="Search\Assets\FindPrevious.png" />
<EmbeddedResource Include="Search\Assets\RegularExpression.png" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
</ItemGroup>
<ItemGroup>
<Compile Update="SR.Designer.cs">
<DesignTime>True</DesignTime>
<AutoGen>True</AutoGen>
<DependentUpon>SR.resx</DependentUpon>
</Compile>
</ItemGroup>
<ItemGroup>
<EmbeddedResource Update="SR.resx">
<Generator>PublicResXFileCodeGenerator</Generator>
<LastGenOutput>SR.Designer.cs</LastGenOutput>
</EmbeddedResource>
</ItemGroup>
<Target Name="ChangeAliasesOfSystemDrawing" BeforeTargets="FindReferenceAssembliesForReferences;ResolveReferences">
<ItemGroup>
<ReferencePath Condition="'%(FileName)' == 'Splat'">
<Aliases>SystemDrawing</Aliases>
</ReferencePath>
</ItemGroup>
</Target>
</Project>
<MSG> Upgrade to Avalonia 0.7
<DFF> @@ -31,7 +31,7 @@
</ItemGroup>
<ItemGroup>
- <PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
+ <PackageReference Include="Avalonia" Version="0.7.0" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
| 1 | Upgrade to Avalonia 0.7 | 1 | .csproj | csproj | mit | AvaloniaUI/AvaloniaEdit |
10066237 | <NME> AvaloniaEdit.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.0</TargetFramework>
<WarningsNotAsErrors>0612,0618</WarningsNotAsErrors>
<PackageId>Avalonia.AvaloniaEdit</PackageId>
</PropertyGroup>
<ItemGroup>
<EmbeddedResource Include="**\*.xshd;**\*.resx;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
<AvaloniaResource Include="**\*.xaml;Assets\*;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
</ItemGroup>
<ItemGroup>
<None Remove="Search\Assets\CaseSensitive.png" />
<None Remove="Search\Assets\CompleteWord.png" />
<None Remove="Search\Assets\FindNext.png" />
<None Remove="Search\Assets\FindPrevious.png" />
<None Remove="Search\Assets\RegularExpression.png" />
<None Remove="Search\Assets\ReplaceAll.png" />
<None Remove="Search\Assets\ReplaceNext.png" />
</ItemGroup>
<ItemGroup>
<EmbeddedResource Include="Search\Assets\CaseSensitive.png" />
<EmbeddedResource Include="Search\Assets\CompleteWord.png" />
<EmbeddedResource Include="Search\Assets\FindNext.png" />
<EmbeddedResource Include="Search\Assets\FindPrevious.png" />
<EmbeddedResource Include="Search\Assets\RegularExpression.png" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
</ItemGroup>
<ItemGroup>
<Compile Update="SR.Designer.cs">
<DesignTime>True</DesignTime>
<AutoGen>True</AutoGen>
<DependentUpon>SR.resx</DependentUpon>
</Compile>
</ItemGroup>
<ItemGroup>
<EmbeddedResource Update="SR.resx">
<Generator>PublicResXFileCodeGenerator</Generator>
<LastGenOutput>SR.Designer.cs</LastGenOutput>
</EmbeddedResource>
</ItemGroup>
<Target Name="ChangeAliasesOfSystemDrawing" BeforeTargets="FindReferenceAssembliesForReferences;ResolveReferences">
<ItemGroup>
<ReferencePath Condition="'%(FileName)' == 'Splat'">
<Aliases>SystemDrawing</Aliases>
</ReferencePath>
</ItemGroup>
</Target>
</Project>
<MSG> Upgrade to Avalonia 0.7
<DFF> @@ -31,7 +31,7 @@
</ItemGroup>
<ItemGroup>
- <PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
+ <PackageReference Include="Avalonia" Version="0.7.0" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
| 1 | Upgrade to Avalonia 0.7 | 1 | .csproj | csproj | mit | AvaloniaUI/AvaloniaEdit |
10066238 | <NME> AvaloniaEdit.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.0</TargetFramework>
<WarningsNotAsErrors>0612,0618</WarningsNotAsErrors>
<PackageId>Avalonia.AvaloniaEdit</PackageId>
</PropertyGroup>
<ItemGroup>
<EmbeddedResource Include="**\*.xshd;**\*.resx;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
<AvaloniaResource Include="**\*.xaml;Assets\*;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
</ItemGroup>
<ItemGroup>
<None Remove="Search\Assets\CaseSensitive.png" />
<None Remove="Search\Assets\CompleteWord.png" />
<None Remove="Search\Assets\FindNext.png" />
<None Remove="Search\Assets\FindPrevious.png" />
<None Remove="Search\Assets\RegularExpression.png" />
<None Remove="Search\Assets\ReplaceAll.png" />
<None Remove="Search\Assets\ReplaceNext.png" />
</ItemGroup>
<ItemGroup>
<EmbeddedResource Include="Search\Assets\CaseSensitive.png" />
<EmbeddedResource Include="Search\Assets\CompleteWord.png" />
<EmbeddedResource Include="Search\Assets\FindNext.png" />
<EmbeddedResource Include="Search\Assets\FindPrevious.png" />
<EmbeddedResource Include="Search\Assets\RegularExpression.png" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
</ItemGroup>
<ItemGroup>
<Compile Update="SR.Designer.cs">
<DesignTime>True</DesignTime>
<AutoGen>True</AutoGen>
<DependentUpon>SR.resx</DependentUpon>
</Compile>
</ItemGroup>
<ItemGroup>
<EmbeddedResource Update="SR.resx">
<Generator>PublicResXFileCodeGenerator</Generator>
<LastGenOutput>SR.Designer.cs</LastGenOutput>
</EmbeddedResource>
</ItemGroup>
<Target Name="ChangeAliasesOfSystemDrawing" BeforeTargets="FindReferenceAssembliesForReferences;ResolveReferences">
<ItemGroup>
<ReferencePath Condition="'%(FileName)' == 'Splat'">
<Aliases>SystemDrawing</Aliases>
</ReferencePath>
</ItemGroup>
</Target>
</Project>
<MSG> Upgrade to Avalonia 0.7
<DFF> @@ -31,7 +31,7 @@
</ItemGroup>
<ItemGroup>
- <PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
+ <PackageReference Include="Avalonia" Version="0.7.0" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
| 1 | Upgrade to Avalonia 0.7 | 1 | .csproj | csproj | mit | AvaloniaUI/AvaloniaEdit |
10066239 | <NME> AvaloniaEdit.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.0</TargetFramework>
<WarningsNotAsErrors>0612,0618</WarningsNotAsErrors>
<PackageId>Avalonia.AvaloniaEdit</PackageId>
</PropertyGroup>
<ItemGroup>
<EmbeddedResource Include="**\*.xshd;**\*.resx;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
<AvaloniaResource Include="**\*.xaml;Assets\*;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
</ItemGroup>
<ItemGroup>
<None Remove="Search\Assets\CaseSensitive.png" />
<None Remove="Search\Assets\CompleteWord.png" />
<None Remove="Search\Assets\FindNext.png" />
<None Remove="Search\Assets\FindPrevious.png" />
<None Remove="Search\Assets\RegularExpression.png" />
<None Remove="Search\Assets\ReplaceAll.png" />
<None Remove="Search\Assets\ReplaceNext.png" />
</ItemGroup>
<ItemGroup>
<EmbeddedResource Include="Search\Assets\CaseSensitive.png" />
<EmbeddedResource Include="Search\Assets\CompleteWord.png" />
<EmbeddedResource Include="Search\Assets\FindNext.png" />
<EmbeddedResource Include="Search\Assets\FindPrevious.png" />
<EmbeddedResource Include="Search\Assets\RegularExpression.png" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
</ItemGroup>
<ItemGroup>
<Compile Update="SR.Designer.cs">
<DesignTime>True</DesignTime>
<AutoGen>True</AutoGen>
<DependentUpon>SR.resx</DependentUpon>
</Compile>
</ItemGroup>
<ItemGroup>
<EmbeddedResource Update="SR.resx">
<Generator>PublicResXFileCodeGenerator</Generator>
<LastGenOutput>SR.Designer.cs</LastGenOutput>
</EmbeddedResource>
</ItemGroup>
<Target Name="ChangeAliasesOfSystemDrawing" BeforeTargets="FindReferenceAssembliesForReferences;ResolveReferences">
<ItemGroup>
<ReferencePath Condition="'%(FileName)' == 'Splat'">
<Aliases>SystemDrawing</Aliases>
</ReferencePath>
</ItemGroup>
</Target>
</Project>
<MSG> Upgrade to Avalonia 0.7
<DFF> @@ -31,7 +31,7 @@
</ItemGroup>
<ItemGroup>
- <PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
+ <PackageReference Include="Avalonia" Version="0.7.0" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
| 1 | Upgrade to Avalonia 0.7 | 1 | .csproj | csproj | mit | AvaloniaUI/AvaloniaEdit |
10066240 | <NME> AvaloniaEdit.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.0</TargetFramework>
<WarningsNotAsErrors>0612,0618</WarningsNotAsErrors>
<PackageId>Avalonia.AvaloniaEdit</PackageId>
</PropertyGroup>
<ItemGroup>
<EmbeddedResource Include="**\*.xshd;**\*.resx;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
<AvaloniaResource Include="**\*.xaml;Assets\*;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
</ItemGroup>
<ItemGroup>
<None Remove="Search\Assets\CaseSensitive.png" />
<None Remove="Search\Assets\CompleteWord.png" />
<None Remove="Search\Assets\FindNext.png" />
<None Remove="Search\Assets\FindPrevious.png" />
<None Remove="Search\Assets\RegularExpression.png" />
<None Remove="Search\Assets\ReplaceAll.png" />
<None Remove="Search\Assets\ReplaceNext.png" />
</ItemGroup>
<ItemGroup>
<EmbeddedResource Include="Search\Assets\CaseSensitive.png" />
<EmbeddedResource Include="Search\Assets\CompleteWord.png" />
<EmbeddedResource Include="Search\Assets\FindNext.png" />
<EmbeddedResource Include="Search\Assets\FindPrevious.png" />
<EmbeddedResource Include="Search\Assets\RegularExpression.png" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
</ItemGroup>
<ItemGroup>
<Compile Update="SR.Designer.cs">
<DesignTime>True</DesignTime>
<AutoGen>True</AutoGen>
<DependentUpon>SR.resx</DependentUpon>
</Compile>
</ItemGroup>
<ItemGroup>
<EmbeddedResource Update="SR.resx">
<Generator>PublicResXFileCodeGenerator</Generator>
<LastGenOutput>SR.Designer.cs</LastGenOutput>
</EmbeddedResource>
</ItemGroup>
<Target Name="ChangeAliasesOfSystemDrawing" BeforeTargets="FindReferenceAssembliesForReferences;ResolveReferences">
<ItemGroup>
<ReferencePath Condition="'%(FileName)' == 'Splat'">
<Aliases>SystemDrawing</Aliases>
</ReferencePath>
</ItemGroup>
</Target>
</Project>
<MSG> Upgrade to Avalonia 0.7
<DFF> @@ -31,7 +31,7 @@
</ItemGroup>
<ItemGroup>
- <PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
+ <PackageReference Include="Avalonia" Version="0.7.0" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
| 1 | Upgrade to Avalonia 0.7 | 1 | .csproj | csproj | mit | AvaloniaUI/AvaloniaEdit |
10066241 | <NME> AvaloniaEdit.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.0</TargetFramework>
<WarningsNotAsErrors>0612,0618</WarningsNotAsErrors>
<PackageId>Avalonia.AvaloniaEdit</PackageId>
</PropertyGroup>
<ItemGroup>
<EmbeddedResource Include="**\*.xshd;**\*.resx;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
<AvaloniaResource Include="**\*.xaml;Assets\*;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
</ItemGroup>
<ItemGroup>
<None Remove="Search\Assets\CaseSensitive.png" />
<None Remove="Search\Assets\CompleteWord.png" />
<None Remove="Search\Assets\FindNext.png" />
<None Remove="Search\Assets\FindPrevious.png" />
<None Remove="Search\Assets\RegularExpression.png" />
<None Remove="Search\Assets\ReplaceAll.png" />
<None Remove="Search\Assets\ReplaceNext.png" />
</ItemGroup>
<ItemGroup>
<EmbeddedResource Include="Search\Assets\CaseSensitive.png" />
<EmbeddedResource Include="Search\Assets\CompleteWord.png" />
<EmbeddedResource Include="Search\Assets\FindNext.png" />
<EmbeddedResource Include="Search\Assets\FindPrevious.png" />
<EmbeddedResource Include="Search\Assets\RegularExpression.png" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
</ItemGroup>
<ItemGroup>
<Compile Update="SR.Designer.cs">
<DesignTime>True</DesignTime>
<AutoGen>True</AutoGen>
<DependentUpon>SR.resx</DependentUpon>
</Compile>
</ItemGroup>
<ItemGroup>
<EmbeddedResource Update="SR.resx">
<Generator>PublicResXFileCodeGenerator</Generator>
<LastGenOutput>SR.Designer.cs</LastGenOutput>
</EmbeddedResource>
</ItemGroup>
<Target Name="ChangeAliasesOfSystemDrawing" BeforeTargets="FindReferenceAssembliesForReferences;ResolveReferences">
<ItemGroup>
<ReferencePath Condition="'%(FileName)' == 'Splat'">
<Aliases>SystemDrawing</Aliases>
</ReferencePath>
</ItemGroup>
</Target>
</Project>
<MSG> Upgrade to Avalonia 0.7
<DFF> @@ -31,7 +31,7 @@
</ItemGroup>
<ItemGroup>
- <PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
+ <PackageReference Include="Avalonia" Version="0.7.0" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
| 1 | Upgrade to Avalonia 0.7 | 1 | .csproj | csproj | mit | AvaloniaUI/AvaloniaEdit |
10066242 | <NME> AvaloniaEdit.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.0</TargetFramework>
<WarningsNotAsErrors>0612,0618</WarningsNotAsErrors>
<PackageId>Avalonia.AvaloniaEdit</PackageId>
</PropertyGroup>
<ItemGroup>
<EmbeddedResource Include="**\*.xshd;**\*.resx;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
<AvaloniaResource Include="**\*.xaml;Assets\*;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
</ItemGroup>
<ItemGroup>
<None Remove="Search\Assets\CaseSensitive.png" />
<None Remove="Search\Assets\CompleteWord.png" />
<None Remove="Search\Assets\FindNext.png" />
<None Remove="Search\Assets\FindPrevious.png" />
<None Remove="Search\Assets\RegularExpression.png" />
<None Remove="Search\Assets\ReplaceAll.png" />
<None Remove="Search\Assets\ReplaceNext.png" />
</ItemGroup>
<ItemGroup>
<EmbeddedResource Include="Search\Assets\CaseSensitive.png" />
<EmbeddedResource Include="Search\Assets\CompleteWord.png" />
<EmbeddedResource Include="Search\Assets\FindNext.png" />
<EmbeddedResource Include="Search\Assets\FindPrevious.png" />
<EmbeddedResource Include="Search\Assets\RegularExpression.png" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
</ItemGroup>
<ItemGroup>
<Compile Update="SR.Designer.cs">
<DesignTime>True</DesignTime>
<AutoGen>True</AutoGen>
<DependentUpon>SR.resx</DependentUpon>
</Compile>
</ItemGroup>
<ItemGroup>
<EmbeddedResource Update="SR.resx">
<Generator>PublicResXFileCodeGenerator</Generator>
<LastGenOutput>SR.Designer.cs</LastGenOutput>
</EmbeddedResource>
</ItemGroup>
<Target Name="ChangeAliasesOfSystemDrawing" BeforeTargets="FindReferenceAssembliesForReferences;ResolveReferences">
<ItemGroup>
<ReferencePath Condition="'%(FileName)' == 'Splat'">
<Aliases>SystemDrawing</Aliases>
</ReferencePath>
</ItemGroup>
</Target>
</Project>
<MSG> Upgrade to Avalonia 0.7
<DFF> @@ -31,7 +31,7 @@
</ItemGroup>
<ItemGroup>
- <PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
+ <PackageReference Include="Avalonia" Version="0.7.0" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
| 1 | Upgrade to Avalonia 0.7 | 1 | .csproj | csproj | mit | AvaloniaUI/AvaloniaEdit |
10066243 | <NME> AvaloniaEdit.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.0</TargetFramework>
<WarningsNotAsErrors>0612,0618</WarningsNotAsErrors>
<PackageId>Avalonia.AvaloniaEdit</PackageId>
</PropertyGroup>
<ItemGroup>
<EmbeddedResource Include="**\*.xshd;**\*.resx;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
<AvaloniaResource Include="**\*.xaml;Assets\*;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
</ItemGroup>
<ItemGroup>
<None Remove="Search\Assets\CaseSensitive.png" />
<None Remove="Search\Assets\CompleteWord.png" />
<None Remove="Search\Assets\FindNext.png" />
<None Remove="Search\Assets\FindPrevious.png" />
<None Remove="Search\Assets\RegularExpression.png" />
<None Remove="Search\Assets\ReplaceAll.png" />
<None Remove="Search\Assets\ReplaceNext.png" />
</ItemGroup>
<ItemGroup>
<EmbeddedResource Include="Search\Assets\CaseSensitive.png" />
<EmbeddedResource Include="Search\Assets\CompleteWord.png" />
<EmbeddedResource Include="Search\Assets\FindNext.png" />
<EmbeddedResource Include="Search\Assets\FindPrevious.png" />
<EmbeddedResource Include="Search\Assets\RegularExpression.png" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
</ItemGroup>
<ItemGroup>
<Compile Update="SR.Designer.cs">
<DesignTime>True</DesignTime>
<AutoGen>True</AutoGen>
<DependentUpon>SR.resx</DependentUpon>
</Compile>
</ItemGroup>
<ItemGroup>
<EmbeddedResource Update="SR.resx">
<Generator>PublicResXFileCodeGenerator</Generator>
<LastGenOutput>SR.Designer.cs</LastGenOutput>
</EmbeddedResource>
</ItemGroup>
<Target Name="ChangeAliasesOfSystemDrawing" BeforeTargets="FindReferenceAssembliesForReferences;ResolveReferences">
<ItemGroup>
<ReferencePath Condition="'%(FileName)' == 'Splat'">
<Aliases>SystemDrawing</Aliases>
</ReferencePath>
</ItemGroup>
</Target>
</Project>
<MSG> Upgrade to Avalonia 0.7
<DFF> @@ -31,7 +31,7 @@
</ItemGroup>
<ItemGroup>
- <PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
+ <PackageReference Include="Avalonia" Version="0.7.0" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
| 1 | Upgrade to Avalonia 0.7 | 1 | .csproj | csproj | mit | AvaloniaUI/AvaloniaEdit |
10066244 | <NME> AvaloniaEdit.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.0</TargetFramework>
<WarningsNotAsErrors>0612,0618</WarningsNotAsErrors>
<PackageId>Avalonia.AvaloniaEdit</PackageId>
</PropertyGroup>
<ItemGroup>
<EmbeddedResource Include="**\*.xshd;**\*.resx;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
<AvaloniaResource Include="**\*.xaml;Assets\*;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
</ItemGroup>
<ItemGroup>
<None Remove="Search\Assets\CaseSensitive.png" />
<None Remove="Search\Assets\CompleteWord.png" />
<None Remove="Search\Assets\FindNext.png" />
<None Remove="Search\Assets\FindPrevious.png" />
<None Remove="Search\Assets\RegularExpression.png" />
<None Remove="Search\Assets\ReplaceAll.png" />
<None Remove="Search\Assets\ReplaceNext.png" />
</ItemGroup>
<ItemGroup>
<EmbeddedResource Include="Search\Assets\CaseSensitive.png" />
<EmbeddedResource Include="Search\Assets\CompleteWord.png" />
<EmbeddedResource Include="Search\Assets\FindNext.png" />
<EmbeddedResource Include="Search\Assets\FindPrevious.png" />
<EmbeddedResource Include="Search\Assets\RegularExpression.png" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
</ItemGroup>
<ItemGroup>
<Compile Update="SR.Designer.cs">
<DesignTime>True</DesignTime>
<AutoGen>True</AutoGen>
<DependentUpon>SR.resx</DependentUpon>
</Compile>
</ItemGroup>
<ItemGroup>
<EmbeddedResource Update="SR.resx">
<Generator>PublicResXFileCodeGenerator</Generator>
<LastGenOutput>SR.Designer.cs</LastGenOutput>
</EmbeddedResource>
</ItemGroup>
<Target Name="ChangeAliasesOfSystemDrawing" BeforeTargets="FindReferenceAssembliesForReferences;ResolveReferences">
<ItemGroup>
<ReferencePath Condition="'%(FileName)' == 'Splat'">
<Aliases>SystemDrawing</Aliases>
</ReferencePath>
</ItemGroup>
</Target>
</Project>
<MSG> Upgrade to Avalonia 0.7
<DFF> @@ -31,7 +31,7 @@
</ItemGroup>
<ItemGroup>
- <PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
+ <PackageReference Include="Avalonia" Version="0.7.0" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
| 1 | Upgrade to Avalonia 0.7 | 1 | .csproj | csproj | mit | AvaloniaUI/AvaloniaEdit |
10066245 | <NME> AvaloniaEdit.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.0</TargetFramework>
<WarningsNotAsErrors>0612,0618</WarningsNotAsErrors>
<PackageId>Avalonia.AvaloniaEdit</PackageId>
</PropertyGroup>
<ItemGroup>
<EmbeddedResource Include="**\*.xshd;**\*.resx;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
<AvaloniaResource Include="**\*.xaml;Assets\*;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
</ItemGroup>
<ItemGroup>
<None Remove="Search\Assets\CaseSensitive.png" />
<None Remove="Search\Assets\CompleteWord.png" />
<None Remove="Search\Assets\FindNext.png" />
<None Remove="Search\Assets\FindPrevious.png" />
<None Remove="Search\Assets\RegularExpression.png" />
<None Remove="Search\Assets\ReplaceAll.png" />
<None Remove="Search\Assets\ReplaceNext.png" />
</ItemGroup>
<ItemGroup>
<EmbeddedResource Include="Search\Assets\CaseSensitive.png" />
<EmbeddedResource Include="Search\Assets\CompleteWord.png" />
<EmbeddedResource Include="Search\Assets\FindNext.png" />
<EmbeddedResource Include="Search\Assets\FindPrevious.png" />
<EmbeddedResource Include="Search\Assets\RegularExpression.png" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
</ItemGroup>
<ItemGroup>
<Compile Update="SR.Designer.cs">
<DesignTime>True</DesignTime>
<AutoGen>True</AutoGen>
<DependentUpon>SR.resx</DependentUpon>
</Compile>
</ItemGroup>
<ItemGroup>
<EmbeddedResource Update="SR.resx">
<Generator>PublicResXFileCodeGenerator</Generator>
<LastGenOutput>SR.Designer.cs</LastGenOutput>
</EmbeddedResource>
</ItemGroup>
<Target Name="ChangeAliasesOfSystemDrawing" BeforeTargets="FindReferenceAssembliesForReferences;ResolveReferences">
<ItemGroup>
<ReferencePath Condition="'%(FileName)' == 'Splat'">
<Aliases>SystemDrawing</Aliases>
</ReferencePath>
</ItemGroup>
</Target>
</Project>
<MSG> Upgrade to Avalonia 0.7
<DFF> @@ -31,7 +31,7 @@
</ItemGroup>
<ItemGroup>
- <PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
+ <PackageReference Include="Avalonia" Version="0.7.0" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
| 1 | Upgrade to Avalonia 0.7 | 1 | .csproj | csproj | mit | AvaloniaUI/AvaloniaEdit |
10066246 | <NME> AvaloniaEdit.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.0</TargetFramework>
<WarningsNotAsErrors>0612,0618</WarningsNotAsErrors>
<PackageId>Avalonia.AvaloniaEdit</PackageId>
</PropertyGroup>
<ItemGroup>
<EmbeddedResource Include="**\*.xshd;**\*.resx;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
<AvaloniaResource Include="**\*.xaml;Assets\*;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
</ItemGroup>
<ItemGroup>
<None Remove="Search\Assets\CaseSensitive.png" />
<None Remove="Search\Assets\CompleteWord.png" />
<None Remove="Search\Assets\FindNext.png" />
<None Remove="Search\Assets\FindPrevious.png" />
<None Remove="Search\Assets\RegularExpression.png" />
<None Remove="Search\Assets\ReplaceAll.png" />
<None Remove="Search\Assets\ReplaceNext.png" />
</ItemGroup>
<ItemGroup>
<EmbeddedResource Include="Search\Assets\CaseSensitive.png" />
<EmbeddedResource Include="Search\Assets\CompleteWord.png" />
<EmbeddedResource Include="Search\Assets\FindNext.png" />
<EmbeddedResource Include="Search\Assets\FindPrevious.png" />
<EmbeddedResource Include="Search\Assets\RegularExpression.png" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
</ItemGroup>
<ItemGroup>
<Compile Update="SR.Designer.cs">
<DesignTime>True</DesignTime>
<AutoGen>True</AutoGen>
<DependentUpon>SR.resx</DependentUpon>
</Compile>
</ItemGroup>
<ItemGroup>
<EmbeddedResource Update="SR.resx">
<Generator>PublicResXFileCodeGenerator</Generator>
<LastGenOutput>SR.Designer.cs</LastGenOutput>
</EmbeddedResource>
</ItemGroup>
<Target Name="ChangeAliasesOfSystemDrawing" BeforeTargets="FindReferenceAssembliesForReferences;ResolveReferences">
<ItemGroup>
<ReferencePath Condition="'%(FileName)' == 'Splat'">
<Aliases>SystemDrawing</Aliases>
</ReferencePath>
</ItemGroup>
</Target>
</Project>
<MSG> Upgrade to Avalonia 0.7
<DFF> @@ -31,7 +31,7 @@
</ItemGroup>
<ItemGroup>
- <PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
+ <PackageReference Include="Avalonia" Version="0.7.0" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
| 1 | Upgrade to Avalonia 0.7 | 1 | .csproj | csproj | mit | AvaloniaUI/AvaloniaEdit |
10066247 | <NME> AvaloniaEdit.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.0</TargetFramework>
<WarningsNotAsErrors>0612,0618</WarningsNotAsErrors>
<PackageId>Avalonia.AvaloniaEdit</PackageId>
</PropertyGroup>
<ItemGroup>
<EmbeddedResource Include="**\*.xshd;**\*.resx;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
<AvaloniaResource Include="**\*.xaml;Assets\*;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
</ItemGroup>
<ItemGroup>
<None Remove="Search\Assets\CaseSensitive.png" />
<None Remove="Search\Assets\CompleteWord.png" />
<None Remove="Search\Assets\FindNext.png" />
<None Remove="Search\Assets\FindPrevious.png" />
<None Remove="Search\Assets\RegularExpression.png" />
<None Remove="Search\Assets\ReplaceAll.png" />
<None Remove="Search\Assets\ReplaceNext.png" />
</ItemGroup>
<ItemGroup>
<EmbeddedResource Include="Search\Assets\CaseSensitive.png" />
<EmbeddedResource Include="Search\Assets\CompleteWord.png" />
<EmbeddedResource Include="Search\Assets\FindNext.png" />
<EmbeddedResource Include="Search\Assets\FindPrevious.png" />
<EmbeddedResource Include="Search\Assets\RegularExpression.png" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
</ItemGroup>
<ItemGroup>
<Compile Update="SR.Designer.cs">
<DesignTime>True</DesignTime>
<AutoGen>True</AutoGen>
<DependentUpon>SR.resx</DependentUpon>
</Compile>
</ItemGroup>
<ItemGroup>
<EmbeddedResource Update="SR.resx">
<Generator>PublicResXFileCodeGenerator</Generator>
<LastGenOutput>SR.Designer.cs</LastGenOutput>
</EmbeddedResource>
</ItemGroup>
<Target Name="ChangeAliasesOfSystemDrawing" BeforeTargets="FindReferenceAssembliesForReferences;ResolveReferences">
<ItemGroup>
<ReferencePath Condition="'%(FileName)' == 'Splat'">
<Aliases>SystemDrawing</Aliases>
</ReferencePath>
</ItemGroup>
</Target>
</Project>
<MSG> Upgrade to Avalonia 0.7
<DFF> @@ -31,7 +31,7 @@
</ItemGroup>
<ItemGroup>
- <PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
+ <PackageReference Include="Avalonia" Version="0.7.0" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
| 1 | Upgrade to Avalonia 0.7 | 1 | .csproj | csproj | mit | AvaloniaUI/AvaloniaEdit |
10066248 | <NME> AvaloniaEdit.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.0</TargetFramework>
<WarningsNotAsErrors>0612,0618</WarningsNotAsErrors>
<PackageId>Avalonia.AvaloniaEdit</PackageId>
</PropertyGroup>
<ItemGroup>
<EmbeddedResource Include="**\*.xshd;**\*.resx;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
<AvaloniaResource Include="**\*.xaml;Assets\*;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
</ItemGroup>
<ItemGroup>
<None Remove="Search\Assets\CaseSensitive.png" />
<None Remove="Search\Assets\CompleteWord.png" />
<None Remove="Search\Assets\FindNext.png" />
<None Remove="Search\Assets\FindPrevious.png" />
<None Remove="Search\Assets\RegularExpression.png" />
<None Remove="Search\Assets\ReplaceAll.png" />
<None Remove="Search\Assets\ReplaceNext.png" />
</ItemGroup>
<ItemGroup>
<EmbeddedResource Include="Search\Assets\CaseSensitive.png" />
<EmbeddedResource Include="Search\Assets\CompleteWord.png" />
<EmbeddedResource Include="Search\Assets\FindNext.png" />
<EmbeddedResource Include="Search\Assets\FindPrevious.png" />
<EmbeddedResource Include="Search\Assets\RegularExpression.png" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
</ItemGroup>
<ItemGroup>
<Compile Update="SR.Designer.cs">
<DesignTime>True</DesignTime>
<AutoGen>True</AutoGen>
<DependentUpon>SR.resx</DependentUpon>
</Compile>
</ItemGroup>
<ItemGroup>
<EmbeddedResource Update="SR.resx">
<Generator>PublicResXFileCodeGenerator</Generator>
<LastGenOutput>SR.Designer.cs</LastGenOutput>
</EmbeddedResource>
</ItemGroup>
<Target Name="ChangeAliasesOfSystemDrawing" BeforeTargets="FindReferenceAssembliesForReferences;ResolveReferences">
<ItemGroup>
<ReferencePath Condition="'%(FileName)' == 'Splat'">
<Aliases>SystemDrawing</Aliases>
</ReferencePath>
</ItemGroup>
</Target>
</Project>
<MSG> Upgrade to Avalonia 0.7
<DFF> @@ -31,7 +31,7 @@
</ItemGroup>
<ItemGroup>
- <PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
+ <PackageReference Include="Avalonia" Version="0.7.0" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
| 1 | Upgrade to Avalonia 0.7 | 1 | .csproj | csproj | mit | AvaloniaUI/AvaloniaEdit |
10066249 | <NME> AvaloniaEdit.csproj
<BEF> <Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.0</TargetFramework>
<WarningsNotAsErrors>0612,0618</WarningsNotAsErrors>
<PackageId>Avalonia.AvaloniaEdit</PackageId>
</PropertyGroup>
<ItemGroup>
<EmbeddedResource Include="**\*.xshd;**\*.resx;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
<AvaloniaResource Include="**\*.xaml;Assets\*;" Exclude="bin\**;obj\**;**\*.xproj;packages\**;@(EmbeddedResource)" />
</ItemGroup>
<ItemGroup>
<None Remove="Search\Assets\CaseSensitive.png" />
<None Remove="Search\Assets\CompleteWord.png" />
<None Remove="Search\Assets\FindNext.png" />
<None Remove="Search\Assets\FindPrevious.png" />
<None Remove="Search\Assets\RegularExpression.png" />
<None Remove="Search\Assets\ReplaceAll.png" />
<None Remove="Search\Assets\ReplaceNext.png" />
</ItemGroup>
<ItemGroup>
<EmbeddedResource Include="Search\Assets\CaseSensitive.png" />
<EmbeddedResource Include="Search\Assets\CompleteWord.png" />
<EmbeddedResource Include="Search\Assets\FindNext.png" />
<EmbeddedResource Include="Search\Assets\FindPrevious.png" />
<EmbeddedResource Include="Search\Assets\RegularExpression.png" />
</ItemGroup>
<ItemGroup>
<PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
</ItemGroup>
<ItemGroup>
<Compile Update="SR.Designer.cs">
<DesignTime>True</DesignTime>
<AutoGen>True</AutoGen>
<DependentUpon>SR.resx</DependentUpon>
</Compile>
</ItemGroup>
<ItemGroup>
<EmbeddedResource Update="SR.resx">
<Generator>PublicResXFileCodeGenerator</Generator>
<LastGenOutput>SR.Designer.cs</LastGenOutput>
</EmbeddedResource>
</ItemGroup>
<Target Name="ChangeAliasesOfSystemDrawing" BeforeTargets="FindReferenceAssembliesForReferences;ResolveReferences">
<ItemGroup>
<ReferencePath Condition="'%(FileName)' == 'Splat'">
<Aliases>SystemDrawing</Aliases>
</ReferencePath>
</ItemGroup>
</Target>
</Project>
<MSG> Upgrade to Avalonia 0.7
<DFF> @@ -31,7 +31,7 @@
</ItemGroup>
<ItemGroup>
- <PackageReference Include="Avalonia" Version="0.6.2-build6260-beta" />
+ <PackageReference Include="Avalonia" Version="0.7.0" />
<PackageReference Include="System.Collections.Immutable" Version="1.5.0" />
<PackageReference Include="System.Xml.ReaderWriter" Version="4.3.1" />
</ItemGroup>
| 1 | Upgrade to Avalonia 0.7 | 1 | .csproj | csproj | mit | AvaloniaUI/AvaloniaEdit |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.