repo_name
stringlengths 4
136
| issue_id
stringlengths 5
10
| text
stringlengths 37
4.84M
|
---|---|---|
holoviz/panel | 914695488 | Title: Using Panel RangeSlider to update bounds of holoviews dynamic map
Question:
username_0: #### ALL software version info
- Windows OS
- Google Chrome
- bokeh - 2.3.2
- panel 0.11.3
- numpy 1.20.3
- pandas 1.2.3
- hvplot 0.7.1
- holoviews 1.14.3
#### Description of expected behavior and the observed behavior
We are trying to use a Panel RangeSlider to update the x-range of a hvplot dynamic map. When we have one plot, our code works fine - changing the bounds of our range slider changes the bounds of the linked plot. However, when we overlay another curve on top of our existing plot, the link between the RangeSlider and the plot bounds breaks.
The code attached below is a simpler version of our use case, which contains millions of points. Therefore, for the sake of performance, we cannot use a Bokeh RangeToolLink or use shared_axes = True. We would greatly appreciate advice on how to successfully build a JavaScript link between a Panel RangeSlider and an overlaid hvplot.
#### Complete, minimal, self-contained example code that reproduces the issue
```
import panel as pn
import numpy as np
import pandas as pd
import hvplot.pandas
from holoviews import opts
# button callback when clicked - overlays a line onto existing plot
def overlay_plot(event):
global plot1
overlay = data.hvplot.line(x = 'x', y = 'y2', width = 900, height = 360)
overlay_plot = plot1 * overlay
main_page.__setitem__(2, overlay_plot)
# range slider creation
x_slider = pn.widgets.RangeSlider(title='X-Axis Scale', start=0,
end=10, step=0.1, value=(0, 10), show_value=False)
#define data and plotting figure
x = np.linspace(0, 10, 1000)
data = pd.DataFrame({'x': x, 'y': np.sin(x), 'y2': np.cos(x)})
plot1 = data.hvplot.line(x = 'x', y = 'y', width = 900, height = 360)
# javascript code for callback
code = """
x_range.start = source.value[0]
x_range.end = source.value[1]
"""
# button to call for an overlay event
new_plot_btn = pn.widgets.Button(name='Overlay', button_type='primary', disabled=False)
new_plot_btn.on_click(overlay_plot)
# javascript link to js code to link the range slider to the existing pot to update x range bounds
x_slider.jslink(plot1, code={'value_throttled': code})
main_page = pn.Column(new_plot_btn, x_slider, plot1)
main_page.servable()
```
#### Stack traceback and/or browser JavaScript console output
No errors displayed in command line or javascript console.
#### Screenshots or screencasts of the bug in action
N/A
Answers:
username_1: So the problem here is twofold:
1) You're completely replacing the plot so the link you set up initially is still connected to the old plot.
2) However even if you were to reattach the link to the new plot I think Panel does not dynamically create a link.
For example in theory you should be able to reattach the link like this:
```python
def overlay_plot(event):
global plot1
overlay = data.hvplot.line(x = 'x', y = 'y2', width = 900, height = 360)
new_plot = plot1 * overlay
x_slider.jslink(new_plot, code={'value_throttled': code})
main_page[2] = new_plot
```
but this does not appear to work either. Will need to ensure that the JS callbacks can be created dynamically after the initial render.
username_0: @username_1 I also noticed using the same code example above if you jslink the overlayed plot before the initial render this doesn't work as well. The only thing that works before initial render is if you jslink one of the individual plots or both shown below:
**What works:**
```
plot1 = data.hvplot.line(x = 'x', y = 'y', width = 900, height = 360)
overlay = data.hvplot.line(x = 'x', y = 'y2', width = 900, height = 360)
main_plot = plot1 * overlay
x_slider.jslink(plot1, code={'value_throttled': code}) # or overlay instead of plot1
main_page = pn.Column(new_plot_btn, x_slider, main_plot)
main_page.servable()
```
**What doesn't work:**
```
plot1 = data.hvplot.line(x = 'x', y = 'y', width = 900, height = 360)
overlay = data.hvplot.line(x = 'x', y = 'y2', width = 900, height = 360)
main_plot = plot1 * overlay
x_slider.jslink(main_plot , code={'value_throttled': code}) # instead of plot1 or overlay
main_page = pn.Column(new_plot_btn, x_slider, main_plot)
main_page.servable()
```
username_0: @username_1 Any update on the issue? |
PiotrDabkowski/Js2Py | 169085033 | Title: Cannot go from .to_dict() back to Js types.
Question:
username_0: I am using the esprima translated example provided and have translated escodegen to allow me to rebuild code from the AST. I have noticed the following issue occur for certain scripts:
`js2py.base.PyJsException: TypeError: Undefiend and null dont have properties!`
This only occurs when I convert the datatype returned by esprima to a dictionary. If I then pass that converted data (without modification) back to escodegen it will throw the error above. If I don't convert to a dictionary it runs just fine. This only occurs with certain scripts, for example:
```
define(["definition"], function (foo) {
"use strict";
return foo(null, {
declaredClass: "className",
constructor: function () {
this.var = 1;
},
id: function () {
return 1;
},
type: function () {
return 1;
},
namespace: function () {
return 1;
}
});
});
```
Answers:
username_1: .to_dict() is including only enumerable properties of the object inside dict. So if some property is not enumerable it will not be included in the dict and if you convert that dict to JS it will be missing this property, hence the error.
username_0: Oh I see. However, in this scenario I cannot figure out what property is being lost. When comparing the Json generated from using esprima.js in a browser and the json representation of the dictionary generated by pyesprima no difference is present.
username_1: Is browser showing also non enumerable properties? I cant think of another reason for that error other than non enumerable properties. Do you maybe know what property failed to access?
username_0: Yes in the example provided it cannot process the null on the inheritance - `return foo(null, {`
username_1: Oh, I think I know what the reason might be. Both null and undefined are converted to None during to_dict(). Afterward when you convert that dict back, None will be converted to undefined. Maybe that is the reason. I dont have escodegen translated and I cant explore whats going on so its just a guess. I will check the details today evening when I have some time, now I am at work :D
username_0: Yes that would explain both the error message and why no difference appears when comparing the json data before and after dictionary conversion. Thanks for taking a look! Love the lib saved me many hours so far in manual translation :) |
LeetCode-Feedback/LeetCode-Feedback | 902267376 | Title: Missing Test Case - 1689. Partitioning Into Minimum Number Of Deci-Binary Numbers
Question:
username_0: <!--
Note - Any content mention below in `<!-- ->` blocks are just comments
to help you fill-up the issue. It won't be visible in the actual issue after
you click on submit.
-->
#### Your LeetCode username
<!-- Your LeetCode username -->
Niks291
#### Category of the bug
- [ ] Question
- [ ] Solution
- [ ] Language
**- [ ] Missing Test Cases**
#### Description of the bug
<!-- A clear and concise description of what the bug is. -->
When we provide large value of greater then length 10 the code becomes fails like ["`1234567888888999`"]. But solution got accepted. May be some test cases are not present.
#### Code you used for Submit/Run operation
<!--
Please make sure you wrap your code with ``` tags.
Otherwise we may reject your request.
-->
```
class Solution {
public int minPartitions(String n) {
int length = Math.min(n.length(), 10);
int max = n.charAt(0) - 48;
for (int i = 1; i < length; i++) {
if (n.charAt(i) - 48 > max) {
max = n.charAt(i) - 48;
}
}
return max;
}
}
```
#### Language used for code
<!-- C++ -->
JAVA
#### Expected behavior
<!-- A clear and concise description of what you expected to happen in
contrast with what actually happened. -->
Code should fail when we provide the value ["`1234567888888999`"] and length is greater then 10. But currently solution is getting accepted. Please refer screenshot.
#### Screenshots
<!-- If applicable, add screenshots to explain your issue. -->
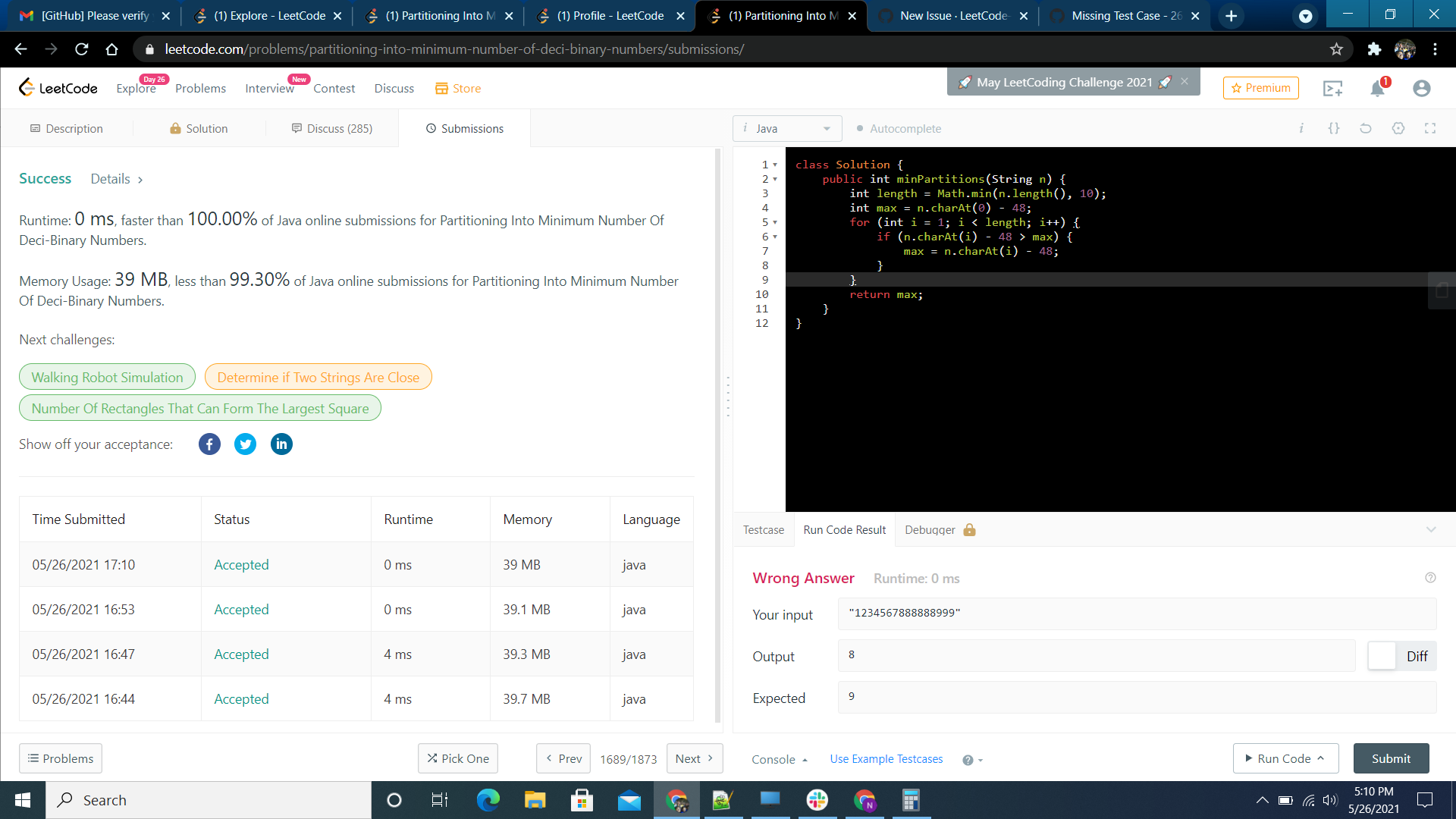
#### Additional context
<!-- Add any other additional context about the bug. -->
Status: Issue closed
Answers:
username_1: @username_0 Your solution currently fails on "111111111119". For a valid test case contribution, please provide a test case that will fail an accepted solution. Thank you for your time.
username_0: I guess someone has added this testcase after this post. Because Yesterday total test cases is 94 and all the testcases are passed as you can see in the attached snapshot. However when I tried today it shows 95 testcases. |
silexlabs/silex-templates | 37666600 | Title: Images bug/issue
Question:
username_0: I copied https://github.com/silexlabs/silex-templates/tree/gh-pages/templates/minimal-grey
folder on my dropbox
Opened the editable.html with silex
Preview in View > Preview looks perfect
But after publishing it looks like this:

The only image that gets moved is the triangle.gif
<bountysource-plugin>
---
Want to back this issue? **[Post a bounty on it!](https://www.bountysource.com/issues/3082696-images-bug-issue?utm_campaign=plugin&utm_content=tracker%2F1274069&utm_medium=issues&utm_source=github)** We accept bounties via [Bountysource](https://www.bountysource.com/?utm_campaign=plugin&utm_content=tracker%2F1274069&utm_medium=issues&utm_source=github).
</bountysource-plugin>
Answers:
username_1: should be fixed with this new release (it is online right now)
https://github.com/silexlabs/Silex/pull/366
Status: Issue closed
|
firesim/firesim | 416584054 | Title: firesim runworkload-continue command
Question:
username_0: In case you ctrl-C a running runworkload command or it crashes for some reason, support re-starting the monitoring interface (which should also let you re-copy back results).
I think this should be straightforward excluding the case where something bad happened in the middle of having a remotely mounted rootfs.
Answers:
username_0: Q: how will this handle cases where some of the instances have terminated already?
username_0: Combined with #259 this would be a user-in-the-loop way of handling faults until we have a better understanding of how these things fail at scale. |
wojtekmaj/react-pdf | 556387758 | Title: get all text for highlighting/annotating
Question:
username_0: I'm using the standard example of customTextRenderer to mark up the text of my PDF with search terms.
```javascript
<Page
key={`page_${index + 1}`}
pageNumber={index + 1}
customTextRenderer={this.makeTextRenderer(this.state.searchText)}
onMouseUp={this.selectText}
/>
```
What i'm noticing is that there seem to be arbitrary breaks in the searchText - including breaks in the middle of a word. This means the example using :
```javascript
makeTextRenderer = searchText => textItem => this.highlightPattern(textItem.str, searchText);
highlightPattern = (text,pattern) => {
console.log("text : " + text);
const splitText = text.split(pattern);
if (splitText.length <=1){
return text;
}
const matches = text.match(pattern);
return splitText.reduce((arr,element,index) => (matches[index] ? [...arr,element,<mark>{matches[index]}</mark>,
] : [...arr,element]),[]);
};
```
... doesn't work reliably as my search tokens won't necessarily match if the text is broken on a search term. Is there any way to get all the text for the document, process it for the annotations and provide it back?
thanks,
Chris
Answers:
username_1: This issue has been open for a little while. Do you have a solution to share now @username_0 ?
username_2: For this you will need to dive in into pdf.js's internals a little bit. React-PDF provides hooks to these internals in onDocumentLoad, onPageLoad etc.
Here's what I found on this topic: https://stackoverflow.com/questions/40635979/how-to-correctly-extract-text-from-a-pdf-using-pdf-js |
refinedmods/refinedstorage | 1082707599 | Title: crash when entering world
Question:
username_0: ### Describe the bug
Game crashes and you can't reenter world unless mod gets disabled.
### How can we reproduce this bug or crash?
Have a RS network running. Go several hundred meters away from controller chunk and wait for that chunk to unload.
### What Minecraft version is this happening on?
Minecraft 1.18.1
### What Forge version is this happening on?
39.0.5
### What Refined Storage version is this happening on?
latest
### Relevant log output
```shell
// I let you down. Sorry :(
Time: 12/16/21, 4:26 PM
Description: Exception in server tick loop
java.lang.IllegalStateException: No network node present at BlockPos{x=610, y=75, z=-2067}, consider removing the block at this position
at com.refinedmods.refinedstorage.blockentity.NetworkNodeBlockEntity.getNode(NetworkNodeBlockEntity.java:64) ~[refinedstorage-1.10.0-beta.2.jar%2368!:1.10.0-beta.2] {re:classloading}
at com.refinedmods.refinedstorage.blockentity.NetworkNodeBlockEntity.getNode(NetworkNodeBlockEntity.java:25) ~[refinedstorage-1.10.0-beta.2.jar%2368!:1.10.0-beta.2] {re:classloading}
at com.refinedmods.refinedstorage.util.NetworkUtils.getNodeFromBlockEntity(NetworkUtils.java:29) ~[refinedstorage-1.10.0-beta.2.jar%2368!:1.10.0-beta.2] {re:classloading}
at com.refinedmods.refinedstorage.apiimpl.network.node.NetworkNode.visit(NetworkNode.java:235) ~[refinedstorage-1.10.0-beta.2.jar%2368!:1.10.0-beta.2] {re:classloading}
at com.refinedmods.refinedstorage.apiimpl.network.NetworkNodeGraph$Visitor.visit(NetworkNodeGraph.java:116) ~[refinedstorage-1.10.0-beta.2.jar%2368!:1.10.0-beta.2] {re:classloading}
at com.refinedmods.refinedstorage.apiimpl.network.NetworkNodeGraph.invalidate(NetworkNodeGraph.java:42) ~[refinedstorage-1.10.0-beta.2.jar%2368!:1.10.0-beta.2] {re:classloading}
at com.refinedmods.refinedstorage.apiimpl.network.Network.update(Network.java:206) ~[refinedstorage-1.10.0-beta.2.jar%2368!:1.10.0-beta.2] {re:classloading}
at com.refinedmods.refinedstorage.apiimpl.network.NetworkListener.onWorldTick(NetworkListener.java:17) ~[refinedstorage-1.10.0-beta.2.jar%2368!:1.10.0-beta.2] {re:classloading}
at net.minecraftforge.eventbus.ASMEventHandler_144_NetworkListener_onWorldTick_WorldTickEvent.invoke(.dynamic) ~[?:?] {}
at net.minecraftforge.eventbus.ASMEventHandler.invoke(ASMEventHandler.java:85) ~[eventbus-5.0.3.jar%232!:?] {}
at net.minecraftforge.eventbus.EventBus.post(EventBus.java:302) ~[eventbus-5.0.3.jar%232!:?] {}
at net.minecraftforge.eventbus.EventBus.post(EventBus.java:283) ~[eventbus-5.0.3.jar%232!:?] {}
at net.minecraftforge.event.ForgeEventFactory.onPostWorldTick(ForgeEventFactory.java:866) ~[forge-1.18.1-39.0.5-universal.jar%2379!:?] {re:classloading}
at net.minecraft.server.MinecraftServer.m_5703_(MinecraftServer.java:874) ~[client-1.18.1-20211210.034407-srg.jar%2375!:?] {re:mixin,pl:accesstransformer:B,re:classloading,pl:accesstransformer:B,pl:mixin:A}
at net.minecraft.server.MinecraftServer.m_5705_(MinecraftServer.java:808) ~[client-1.18.1-20211210.034407-srg.jar%2375!:?] {re:mixin,pl:accesstransformer:B,re:classloading,pl:accesstransformer:B,pl:mixin:A}
at net.minecraft.client.server.IntegratedServer.m_5705_(IntegratedServer.java:86) ~[client-1.18.1-20211210.034407-srg.jar%2375!:?] {re:classloading,pl:runtimedistcleaner:A}
at net.minecraft.server.MinecraftServer.m_130011_(MinecraftServer.java:668) ~[client-1.18.1-20211210.034407-srg.jar%2375!:?] {re:mixin,pl:accesstransformer:B,re:classloading,pl:accesstransformer:B,pl:mixin:A}
at net.minecraft.server.MinecraftServer.m_177918_(MinecraftServer.java:258) ~[client-1.18.1-20211210.034407-srg.jar%2375!:?] {re:mixin,pl:accesstransformer:B,re:classloading,pl:accesstransformer:B,pl:mixin:A}
at java.lang.Thread.run(Thread.java:833) [?:?] {}
A detailed walkthrough of the error, its code path and all known details is as follows:
---------------------------------------------------------------------------------------
-- System Details --
Details:
Minecraft Version: 1.18.1
Minecraft Version ID: 1.18.1
Operating System: Windows 10 (amd64) version 10.0
Java Version: 17.0.1, Microsoft
Java VM Version: OpenJDK 64-Bit Server VM (mixed mode), Microsoft
[Truncated]
balm-2.3.1+0.jar |Balm |balm |2.3.1+0 |DONE |Manifest: NOSIGNATURE
silent-gear-1.18-2.8.0.jar |Silent Gear |silentgear |2.8.0 |DONE |Manifest: NOSIGNATURE
jei-1.18.1-9.1.0.46.jar |Just Enough Items |jei |9.1.0.46 |DONE |Manifest: NOSIGNATURE
JustEnoughResources-1.18.1-0.13.1.137.jar |Just Enough Resources |jeresources |0.13.1.137 |DONE |Manifest: NOSIGNATURE
forge-1.18.1-39.0.5-universal.jar |Forge |forge |39.0.5 |DONE |Manifest: 22:af:21:d8:19:82:7f:93:94:fe:2b:ac:b7:e4:41:57:68:39:87:b1:a7:5c:c6:44:f9:25:74:21:14:f5:0d:90
Bibliotheca-1.18-1.7.0.jar |Bibliotheca |bibliotheca |1.7.0 |DONE |Manifest: NOSIGNATURE
ITank-1.18-1.7.0.jar |ITank |itank |1.7.0 |DONE |Manifest: NOSIGNATURE
reap-1.18.1-1.0.0.jar |Reap Mod |reap |1.18.1-1.0.0 |DONE |Manifest: NOSIGNATURE
pipez-1.18.1-1.0.0.jar |Pipez |pipez |1.18.1-1.0.0 |DONE |Manifest: NOSIGNATURE
Xaeros_Minimap_21.22.3.1_Forge_1.18.jar |Xaero's Minimap |xaerominimap |192.168.127.12 |DONE |Manifest: NOSIGNATURE
refinedstorage-1.10.0-beta.2.jar |Refined Storage |refinedstorage |1.10.0-beta.2 |DONE |Manifest: NOSIGNATURE
ExtraStorage-1.18.1-2.0.0.jar |Extra Storage |extrastorage |2.0.0 |DONE |Manifest: NOSIGNATURE
refinedstorageaddons-0.8.0.jar |Refined Storage Addons |refinedstorageaddons |0.8.0 |DONE |Manifest: NOSIGNATURE
gravestone-1.18.1-1.0.0.jar |Gravestone Mod |gravestone |1.18.1-1.0.0 |DONE |Manifest: NOSIGNATURE
XaerosWorldMap_1.18.6.1_Forge_1.18.jar |Xaero's World Map |xaeroworldmap |1.18.6.1 |DONE |Manifest: NOSIGNATURE
NaturesCompass-1.18.1-1.9.3-forge.jar |Nature's Compass |naturescompass |1.18.1-1.9.3-forge |DONE |Manifest: NOSIGNATURE
Crash Report UUID: 377a5046-d4b1-42b0-8634-a10f3e1ff35e
FML: 39.0
Forge: net.minecraftforge:39.0.5
```
Status: Issue closed
Answers:
username_1: Sorry about that, seems like mechanics around `BlockEntity#setRemoved` changed a little bit in 1.18.x.
It is now also called when a chunk is unloaded, causing RS blocks being removed.
Thanks for the report!
username_1: I've raised an issue on Forge's side:
https://github.com/MinecraftForge/MinecraftForge/issues/8302 |
geomagpy/magpy | 263837166 | Title: extractDateFromString() will take the last date in a file name with multiple dates
Question:
username_0: Only a problem when reading files from large archives without specified filename.
Example: DSCOVR data archive files have the format _DATASTARTTIME_DATAENDTIME_DATACOMPILATIONTIME, and look like this:
oe_m1m_dscovr_**s20170911000000_e20170911235959_p20170912023324**_pub.nc
When extractDateFromString() is searching the correct file for a date, it cycles through all available number strings in the filename but only takes the last one (here, the compilation date, which is not relevant for the data in the file):
```
for i in range(len(testunder)):
try:
numberstr = re.findall(r'\d+',testunder[i])[0]
except:
numberstr = '0'
if len(numberstr) > 4:
tmpdaystring = numberstr
elif len(numberstr) == 4 and int(numberstr) > 1900: # use year at the end of string
tmpdaystring = numberstr
if len(tmpdaystring) > 8:
try: # first try whether an easy pattern can be found e.g. test12014-11-22
match = re.search(r'\d{4}-\d{2}-\d{2}', daystring)
date = datetime.strptime(match.group(), '%Y-%m-%d').date()
```
This could be remedied by testing all available `len(numberstr) > 4` strings and returning the first deciphered, but that could break the automatic reading of other formats. Will need extensive testing. Workaround for now means reading data with endtime + 1 day, so that the correct files are read, then trimming down later. |
oam-dev/oam-go-sdk | 595630599 | Title: Support OAM v1alpha2
Question:
username_0: Now oam-go-sdk is still v1alpha1 and OAM v1alpha2 is released, we must follow this up.
We could sync from [crossplane repo](https://github.com/crossplane/crossplane/tree/master/apis/oam/v1alpha2)
Answers:
username_0: Refer to https://github.com/crossplane/oam-kubernetes-runtime to see the lib for v1alpha2 lib
Status: Issue closed
|
kubernetes/kubeadm | 642601895 | Title: Kubeadm HA control plane guide - missing keepalived health check script file
Question:
username_0: <!-- Thanks for filing an issue! Before hitting the button, please answer these questions.-->
## What keywords did you search in kubeadm issues before filing this one?
- `kubeadm check_apiserver.sh missing`
- `kubeadm check_apiserver.sh`
- `kubeadm keepalived healthcheck`
## (not really a) BUG REPORT
Please see: [kubeadm docs - ha-considerations.md](https://github.com/kubernetes/kubeadm/blob/master/docs/ha-considerations.md#keepalived-configuration)
The code snipped for the keepalived configuration contains a path of the health check script `/etc/keepalived/check_apiserver.sh`.
```sh
// ...
vrrp_script check_apiserver {
script "/etc/keepalived/check_apiserver.sh"
// ...
```
Should the code of this file be in this documentation?
Thank you
Answers:
username_1: @username_2 hello, do you think this is something we should include in the guide?
username_2: Yes, I think so. So it looks like I forgot including this? I'll prepare another PR.
username_2: https://github.com/kubernetes/kubeadm/pull/2192
username_0: Thank you for the fast fix! |
HEADS-project/training | 118026526 | Title: Improve how ThingML generates it's folder structure
Question:
username_0: ThingML projects are now generated under ```thingml-gen/%PROJECT%```. Generating a project will delete the folder, and other folders in ```thingml-gen```.
I feel it would be better if the ```thingml-gen/%PROJECT%``` folder was emptied, and other folders were left alone.
Doing so will save us the trouble of going up one level and back down again if our terminal ```thingml-gen/%PROJECT%``` as current directory.<issue_closed>
Status: Issue closed |
thanethomson/httpwatcher | 837038057 | Title: please support "python -m httpwatcher"
Question:
username_0: I use "python -m http.server" a lot. your solution is exactly what I needed.
I found a no-brainer solution to support "-m" flag, just add the following file run.py:
```py
# -*- coding:utf-8 -*-
import httpwatcher
httpwatcher.watch('.')
```
and you can run "python -m httpwatcher.run"
Answers:
username_1: The easiest solution to this would be to add a `\_\_main\_\_.py` file with the exact code above. How do you feel about this? @username_2
username_2: Sure, sounds good :+1:
username_1: The latest version of the dev branch now contains a simple Python script that allows you to call:
```bash
python -m httpwatcher
``` |
MyEssentials/MyTown2 | 89691414 | Title: [Request] a way for admins to check/edit friends
Question:
username_0: One incident that happened on the server:
A resident had a disagreement with his town major and got kicked out of the town, but was still a friend with another resident in the town (he refused to tell who) so he was still able to enter the town and take stuff.
All drama could have been avoided if there was a admin command to check/edit friends of another player.
or make it so friends of a resident cant bypass the townflags.
for example you can add it as a value to a flag like the mobs flag: access[none, friends, all]
Answers:
username_1: I am thinking of removing the friends list altogether and implement a whitelist for each town that tells which player can bypass protections.
Another way to implement this is to have only the mayor granting protection bypasses when he is in a player's friends list.
Your idea is not bad but it only works if you trust ALL friends of all the residents, which is close to impossible.
Which do you think is better? Should I keep the friends list for later usage? Any other suggestions?
username_2: What about only granting friends access to plots?
So if I'm e.g. an assistant then my friends still only have access to my specific plots and they dont get my assistant rights too.
If friends need more right, they need to be invited to the town and get the right from the mayor.
But I agree with username_0 that admins should be able to see peoples friends list.
That way it gets easier to solve matters of griefing etc.
username_1: You can already add anyone as a member to the plot whether or not is a resident of the town. The issue with that right now is that any resident can make a plot in any corner of the town, so I'll have to implement the permission system for that stuff as well.
The admin manipulation of friends list will be implemented as it is a good idea.
username_2: Sounds really good.
My main concern though was that a friend get same rights (or close) which is mostly unwanted. My plot limitation was just a try to find something more suitable namely the plot assigned specifically to you and not the whole town where you could be mayor, assistant.
Well I guess you get my point. :)
For me the friends list is not important to keep, if I can just add a friend to my plot.
username_1: Okay, I may even remove the whole friends idea and just work with that. Will also modify the plot's info formatter so that you know which of the members are in the town and which aren't.
Status: Issue closed
username_1: Friends are currently not used in any kind of permission, so admin management of that would be useless. You can even look in the DB if you want to know who's friends with who.
I will close this. Reopen if there's a reason for those admin commands.
username_0: Friends did exist when i made the "Request"
All player info in the DB is stored in UUID and are kinda tedious to lookup, while a /ta command would give the actual username.
But since friends have been removed i guess there is no reason for this Request. |
TechnionYearlyProject/Roommates | 314513423 | Title: BackEnd: add route: PUT /review/city/street/userID
Question:
username_0: ok
Answers:
username_1: hi Chanan:
"PUT /review" is enough
the request body will contain all the data
username_0: ok
username_0: are you sure?
who's id is in the route?
the way it works is that each street has an entry in the database with its reviews.
therefore when i want to add a new review i look for the street using the city and street
so why would it not be reviews/:city/:street?
username_2: @username_0 , before you start with the routes please create tests for the reviews model and make sure they pass. It seems that there are bugs there.
Status: Issue closed
username_0: this issue is irelevent because of the design change
now that we save each review by its self and not for each road this route means nothing |
google/knative-gcp | 605691429 | Title: GCP Broker Component Level Benchmarks
Question:
username_0: **Problem**
Add micro benchmarks for each component
* These tests help identify bottlenecks easily
* Ideally these tests can run locally so developers can use them to find performance improvements/regression
* Nice-to-haves
* Enable a command such as /benchmark-broker-ingress in github to trigger a test against a PR. this is a convenient tool to use for any PRs that may affect performance.
* Run a continuous job and integrate with Mako so we have a history
**[Persona:](https://github.com/knative/eventing/blob/master/docs/personas.md)**
Which persona is this feature for?
**Exit Criteria**
A measurable (binary) test that would indicate that the problem has been resolved.
**Time Estimate (optional):**
How many developer-days do you think this may take to resolve?
**Additional context (optional)**
Add any other context about the feature request here.
Answers:
username_1: It would be helpful to define "performance". Historically we have latency test in Mako which is only measured by one client seeding events and one subscriber. But that doesn't seem to provide good simulation of the system running under heavy load or with various subscribers (e.g. always returning error, slow response, etc.). I would also imagine a proper simulation would incur quite some cost especially when we run it continuously. |
codeforboston/plogalong | 567272209 | Title: Spec out contact us on more screen
Question:
username_0: Page Title: Contact Us (insert below FAQ)
Topic (dropdown): Feedback / Comment / Bug / Other
Comment: (text area - 5 lines)
Name (optional): (input)
Email (optional): (input)
Answers:
username_1: @zachjohnston @username_2
How do we feel about using react-native-clean-form for this? https://github.com/esbenp/react-native-clean-form
Status: Issue closed
username_2: Closed by @k-charette in PR #184
username_0: Page Title: Contact Us (insert below FAQ)
Topic (dropdown): Feedback / Comment / Bug / Other
Comment: (text area - 5 lines)
Name (optional): (input)
Email (optional): (input)
Submit (button)
username_0: The submit button does not work.
Status: Issue closed
|
OpenConext/OpenConext-deploy | 180151097 | Title: pdp api password not properly initialized
Question:
username_0: In a clean Openconext install, I see in engineblock.ini:
```
pdp.username = pdp_admin
pdp.password = <PASSWORD>
```
and in pdp's application.ini:
```
# Internal API user (e.g. EngineBlock who call the PDP to check policies
policy.enforcement.point.user.name=pdp_admin
policy.enforcement.point.user.password=<PASSWORD>
```
which should match, but doesn't ;)
Answers:
username_0: To clarify, this is not a VM install, but a termplate-based install. So I suspect this to be a bug in the template-filling.
username_0: ```
╰─▶ grep -r engine_pdp_password * «1»
roles/engineblock/defaults/main.yml:engine_pdp_password: secret
roles/engineblock/templates/engineblock.ini.j2:pdp.password = {{ engine_pdp_password }}
╰─▶ grep policy.enforcement.point. roles/pdp/templates/application.properties.j2 policy.enforcement.point.user.name={{ pdp.username }}
policy.enforcement.point.user.password={{ pdp.password }}
```
hmm.
And it doesn't show in the vm, because the engineblock default is equal to the vm secret ("secret"). Better remove the defautl from the eb role, so we get a proper error when the variabel is not properly set, instead of falling back to a (non-functional) default.
Status: Issue closed
|
mscdex/ssh2 | 37111557 | Title: gulp exits with 'Error in plugin' when ssh2 (gulp-sftp) is used with gulp-concat and gulp.watch
Question:
username_0: ``` js
gulp.task('compile:js:remote', function(){
return gulp.src(srcJs, {base: src})
.pipe(plumber({
errorHandler: onError
}))
.pipe(removelogs())
.pipe(uglify({outSourceMap: false}))
.pipe(order([
'**/**/jquery.js',
'**/**/jquery.ui.js',
'**/**/custom.js',
'**/**/modernizr.js',
'**/**/jquery.fancybox.js',
'**/**/*.js'
]))
.pipe(concat('all.min.js'))
.pipe(gulp.dest(distScripts))
.pipe(sftp({
host: host,
auth: auth,
remotePath: remotePath + '/' + distScripts
}))
;
});
```
`compile:js:remote` is triggered after a change (`gulp.watch`) is made to source JavaScript. It should re-minify my code and upload the resulting file. However, `ssh2` is erroring out with the following. Only just introduced `gulp-concat` to this task but previously `ssh2` (`gulp-sftp`) was working without issue when I wasn't combining my JavaScript files. I've tried `{buffer: false}` but on the first run where files for this project (43 files) are uploaded `ssh2` (`gulp-sftp`) has no problem dealing with `all.min.js` (which is only 459KB). Previously created an issue for this here: https://github.com/gtg092x/gulp-sftp/issues/8#issuecomment-47936244
``` js
[14:17:33] Starting 'compile:js:remote'...
[14:17:37] Authenticating with private key.
[14:17:37] SFTP :: SFTP session closed
events.js:72
throw er; // Unhandled 'error' event
^
Error in plugin '←[36mgulp-sftp←[39m'
SFTP abrupt closure
at SFTP.<anonymous> (C:\Users\com265\AppData\Roaming\npm\node_modules\gulp-s
ftp\index.js:148:44)
at SFTP.emit (events.js:92:17)
at ChannelStream.<anonymous> (C:\Users\com265\AppData\Roaming\npm\node_modul
es\gulp-sftp\node_modules\ssh2\lib\SFTP\SFTPv3.js:58:10)
at ChannelStream.g (events.js:180:16)
at ChannelStream.emit [as _emit] (events.js:117:20)
at ChannelStream.emit (C:\Users\com265\AppData\Roaming\npm\node_modules\gulp
-sftp\node_modules\ssh2\lib\Channel.js:638:12)
at Parser.<anonymous> (C:\Users\com265\AppData\Roaming\npm\node_modules\gulp
-sftp\node_modules\ssh2\lib\Channel.js:60:20)
at Parser.g (events.js:180:16)
at Parser.emit (events.js:92:17)
at Parser.parsePacket (C:\Users\com265\AppData\Roaming\npm\node_modules\gulp
-sftp\node_modules\ssh2\lib\Parser.js:644:12)
```
Answers:
username_1: use this https://www.npmjs.com/package/gulp-sftp-up4 |
aerogear/keycloak-metrics-spi | 546230824 | Title: CLIENT_LOGIN and CLIENT_LOGIN_ERROR should be reported using keycloak_logins and keycloak_failed_login_attempts
Question:
username_0: ## Description
The events CLIENT_LOGIN and CLIENT_LOGIN_ERROR are currently exported as keycloak_user_event_CLIENT_LOGIN and keycloak_user_event_CLIENT_LOGIN_ERROR. With those metrics, only the realm is exposed, it's not possible to get the client_id. So we know only the number of CLIENT_LOGIN against a realm and not who connected through the metrics.
## Expected Behavior
I would suggest to export those metrics using the same keycloak_logins and keycloak_failed_login_attempts or create keycloak_client_logins and keycloak_failed_client_login_attempts to easily track those login including the client_id information in the metrics.
For the moment, on my side I added both events in the switch case present in MetricsEventListener. That's the only change needed BTW.
Do you want me to create a PR with this change ?
Answers:
username_1: would also like to see the ip of a causing event, would be especially interesting for failed login attemps
username_2: closing as fixed in #85
Status: Issue closed
|
ZcashFoundation/zebra | 745189708 | Title: Detect whether errors are `AlreadyVerified`
Question:
username_0: **Is your feature request related to a problem? Please describe.**
The sync logic needs to be able to programmatically detect whether an error is `AlreadyVerified` or not, to avoid restart loops.
**Describe the solution you'd like**
TBD -- @username_1 ?
**Describe alternatives you've considered**
Currently we check this (as a hack) by string comparison on the formatted error.
Answers:
username_1: Either replace the format comparisons with this
```rust
self.downcast_ref::<VerifyCheckpointError>()
.map(|e| matches!(e, VerifyCheckpointError::AlreadyVerified { .. }))
.unwrap_or(false)
```
or add a helper trait to abstract over this
```rust
use zebra_consensus::VerifyCheckpointError;
trait IsAlreadyVerified {
fn is_already_verified_error(&self) -> bool;
}
impl IsAlreadyVerified for &(dyn std::error::Error + Send + Sync + 'static) {
fn is_already_verified_error(&self) -> bool {
self.downcast_ref::<VerifyCheckpointError>()
.map(|e| matches!(e, VerifyCheckpointError::AlreadyVerified { .. }))
.unwrap_or(false)
}
}
```
username_2: Duplicate of #2339
Status: Issue closed
|
naturomics/CapsLayer | 657150649 | Title: In the provided MNIST example not all capsules are seeing activation probability
Question:
username_0: Hello naturomics! Thank you for this great code that is helping me understand capsule networks.
In the documentation i saw that all of the capsules are seeing activation, but when i ran the code 3 capsules were not activated.
Ran the code multiple times and saw that around 2-3-4 are not having any activation probability.
I've attached picture after 500 and 49500 steps and also your provided example activation chart.
Could you please help me solve this issue.
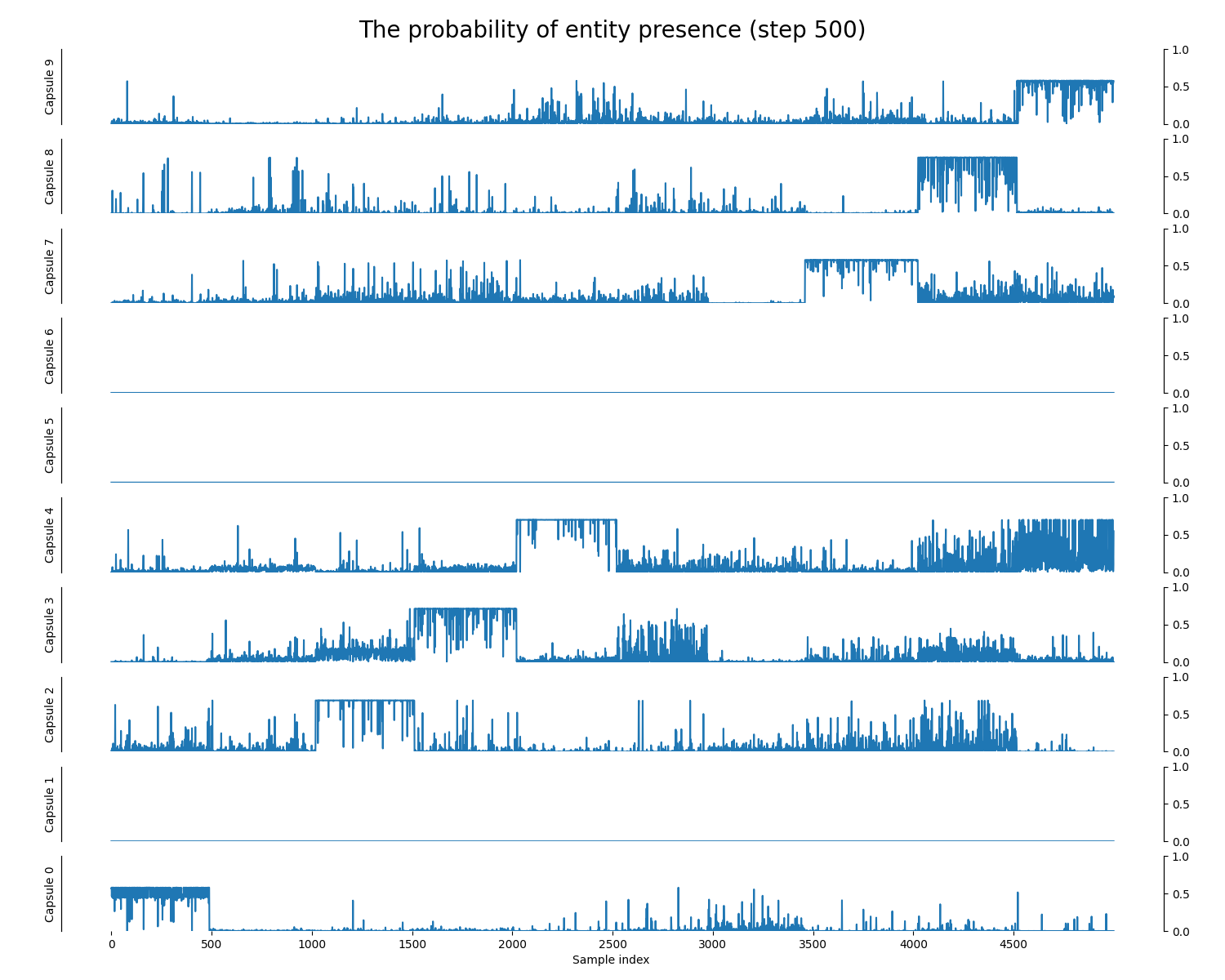
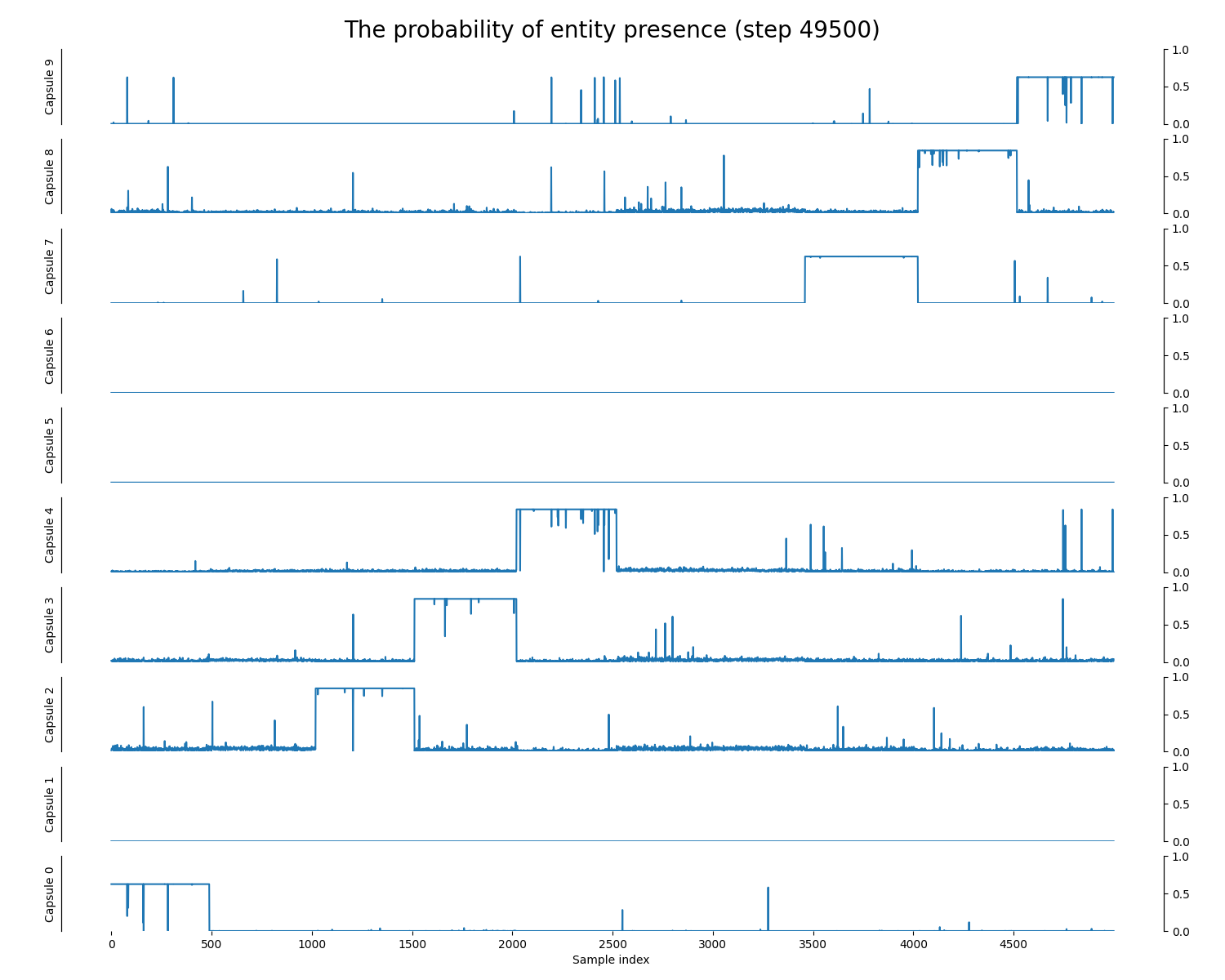
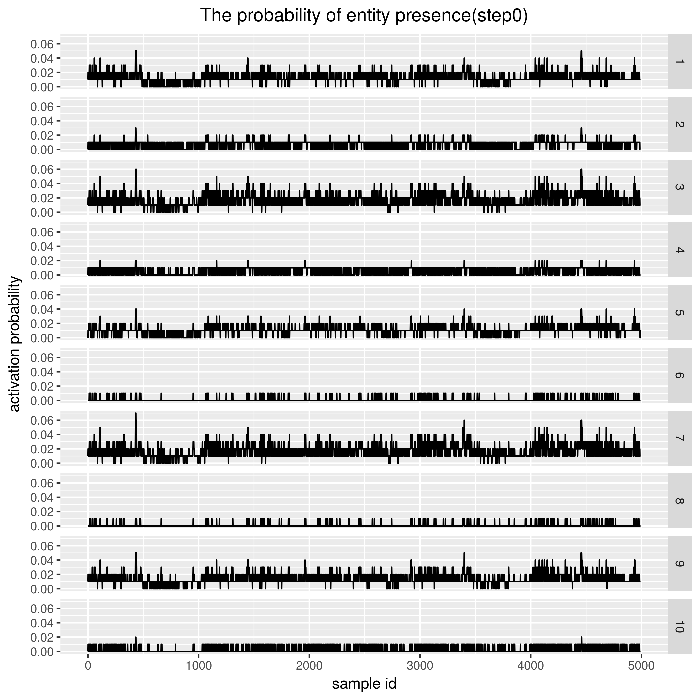 |
assemble/assemble | 157378201 | Title: assemble.data() doesn't load from a list of files
Question:
username_0: - assemble 0.12
- no assemble.js file, I use assemble inside gulpfile.js as in [this file](https://github.com/jpgcode/gulp-starterkit/blob/master/gulp/tasks/assemble.js)
- running a gulp task throws no errors
I pass an array of paths to `assemble.data()` and `assemble.cache.data` stays empty.
```javascript
gulp.task(
'load',
cb => {
app.partials( 'engine/email/partials/**/*.hbs' );
app.layouts( 'engine/email/layouts/**/*.hbs' );
app.pages( 'engine/email/*.hbs' );
app.helpers( 'engine/email/hb-helpers/**/*.js' );
app.data([
'current/**/*.yml',
'engine/email/config/**/*.yml',
'engine/email/options.yml',
'app-state.yml'
]);
cb();
}
);
```
If i pass an object instead of the strings array, it gets to `assemble.cache.data` just fine. What did I miss?
Answers:
username_1: Hmm, to narrow down the issue have you tried loading just one file from a glob, with minimal data? maybe even try a `.json`. it's possible there is a yaml error that isn't being handled correctly
username_0: It does not load a single, quite simple YAML file, but successfully loads the same file converted to JSON.
```yaml
mode: local
```
```json
{
"mode": "local"
}
```
I'm sure that all my YAML files are correct because they were loaded without any errors by `grunt-assemble` which I'm trying to upgrade at the moment.
username_2: Loading `yaml` files is not built in now.
`assemble` uses [base-data](https://github.com/node-base/base-data) now and you can add `yaml` loading by using [a custom dataLoader](https://github.com/node-base/base-data#dataloader).
```js
var yaml = require('js-yaml');
app.dataLoader('yml', function(str, fp) {
return yaml.safeLoad(str);
});
app.data('foo.yml');
//=> loads and parses `foo.yml` as yaml
```
username_0: This worked, thank you!
Status: Issue closed
username_2: Great!
username_1: @username_0 sorry I completely forgot about yaml files not being included. thanks @username_2
username_3: Awesome! This issue just saved my bottom! Can't wait for some good docs to show up for assemble especially using gulp with the project. 🍻 |
realm/realm-java | 284916090 | Title: Object Server migration required after sync failed.
Question:
username_0: Hi,
I have an issue on ROS-Android sync. it hard to reproduce but i got it few times.
i have few(1-3) schema that i need to sync, and do sync in queue (waiting for first completed than starts second)
each schema contains 3-5 tables, each table 3-7 columns and 10-500 rows
The issue appears in case user is new (he is just install the application), than app connects to the ROS and try to sync data, but in case app got time-out exception (by some reason) there is a chance that schema will be "corrupted". And in this case on next try to sync data i got "migration needed" exception.
but as i understand there is no chance to do delete-On-Migration-Needed for SyncUser.
How can i handle this?
Thank you
#### Version of Realm and tooling
Realm version(s): 4.3.1
Realm sync feature enabled: yes
Android Studio version: 3.0.1
Which Android version and device: 7.1.0, google pixel xl
Answers:
username_1: what is the full backtrace and the exception message of the "migration needed" exception?
username_0: haven't save trace :( will share it when faced issue again
Status: Issue closed
|
yellowled/yl-bp | 109883704 | Title: Migrate from grunt-autoprefixer to grunt-postcss
Question:
username_0: [How to migrate](https://github.com/nDmitry/grunt-postcss#how-to-migrate-from-grunt-autoprefixer)
`autoprefixer` can then be used as a PostCSS plugin. Plus, we might want to add other PostCSS plugins like [css-mqpacker](https://www.npmjs.com/package/css-mqpacker), [cssnano](https://www.npmjs.com/package/cssnano) or [pixrem](https://www.npmjs.com/package/pixrem).<issue_closed>
Status: Issue closed |
nkoik/vue-animated-number | 490653776 | Title: Component Name should not be single word
Question:
username_0: Component names should always be multi-word, except for root App components, and built-in components provided by Vue, such as <transition> or <component>.
[](https://vuejs.org/v2/style-guide/#Multi-word-component-names-essential)
Answers:
username_1: We could make a PR to give the possibility to change the component's name.
From this:
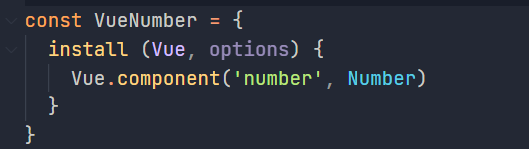
To this:
 |
petere/homebrew-postgresql | 1022854466 | Title: postgresql 14 fails to start after upgrading from postgresql 13
Question:
username_0: ```
% uname -mrsv
Darwin 20.6.0 Darwin Kernel Version 20.6.0: Mon Aug 30 06:12:21 PDT 2021; root:xnu-7195.141.6~3/RELEASE_X86_64 x86_64
```
relevant log lines from /usr/local/var/log/postgres.log
```
2021-10-11 09:49:12.206 CDT [1347] LOG: server process (PID 91405) was terminated by signal 9: Killed: 9
2021-10-11 09:49:12.206 CDT [1347] LOG: terminating any other active server processes
2021-10-11 09:49:12.206 CDT [1461] WARNING: terminating connection because of crash of another server process
2021-10-11 09:49:12.206 CDT [1461] DETAIL: The postmaster has commanded this server process to roll back the current transaction and exit, because another server process exited abnormally and possibly corrupted shared memory.
2021-10-11 09:49:12.206 CDT [1461] HINT: In a moment you should be able to reconnect to the database and repeat your command.
2021-10-11 09:49:12.218 CDT [1347] LOG: all server processes terminated; reinitializing
2021-10-11 09:49:12.230 CDT [1347] LOG: startup process (PID 91406) was terminated by signal 9: Killed: 9
2021-10-11 09:49:12.230 CDT [1347] LOG: aborting startup due to startup process failure
2021-10-11 09:49:12.232 CDT [1347] LOG: database system is shut down
2021-10-11 09:49:12.660 CDT [91407] FATAL: database files are incompatible with server
2021-10-11 09:49:12.660 CDT [91407] DETAIL: The data directory was initialized by PostgreSQL version 13, which is not compatible with this version 14.0.
```
brew postgresql-upgrade-database:
```
% brew postgresql-upgrade-database
==> Upgrading postgresql data from 13 to 14...
waiting for server to start....2021-10-11 10:28:17.679 CDT [6759] LOG: starting PostgreSQL 13.4 on x86_64-apple-darwin20.6.0, compiled by Apple clang version 13.0.0 (clang-1300.0.29.3), 64-bit
2021-10-11 10:28:17.681 CDT [6759] LOG: listening on IPv4 address "127.0.0.1", port 5432
2021-10-11 10:28:17.681 CDT [6759] LOG: listening on IPv6 address "::1", port 5432
2021-10-11 10:28:17.682 CDT [6759] LOG: listening on Unix socket "/tmp/.s.PGSQL.5432"
2021-10-11 10:28:17.695 CDT [6760] LOG: database system was shut down at 2021-10-11 10:22:01 CDT
2021-10-11 10:28:17.700 CDT [6759] LOG: database system is ready to accept connections
done
server started
waiting for server to shut down....2021-10-11 10:28:17.922 CDT [6759] LOG: received fast shutdown request
2021-10-11 10:28:17.923 CDT [6759] LOG: aborting any active transactions
2021-10-11 10:28:17.924 CDT [6759] LOG: background worker "logical replication launcher" (PID 6766) exited with exit code 1
2021-10-11 10:28:17.924 CDT [6761] LOG: shutting down
2021-10-11 10:28:17.935 CDT [6759] LOG: database system is shut down
done
server stopped
==> Moving postgresql data from /usr/local/var/postgres to /usr/local/var/postgres.old...
==> Creating database...
The files belonging to this database system will be owned by user "thomas".
This user must also own the server process.
The database cluster will be initialized with locale "C".
The default text search configuration will be set to "english".
Data page checksums are disabled.
fixing permissions on existing directory /usr/local/var/postgres ... ok
creating subdirectories ... ok
selecting dynamic shared memory implementation ... posix
selecting default max_connections ... 100
selecting default shared_buffers ... 128MB
selecting default time zone ... America/Chicago
creating configuration files ... ok
running bootstrap script ... ok
performing post-bootstrap initialization ... ok
[Truncated]
==> Moving postgresql data back from /usr/local/var/postgres.old to /usr/local/var/postgres...
Error: Failure while executing; `/usr/local/opt/postgresql/bin/pg_upgrade -r -b /usr/local/Cellar/postgresql@13/13.4/bin -B /usr/local/opt/postgresql/bin -d /usr/local/var/postgres.old -D /usr/local/var/postgres -j 12` exited with 1.
```
last lines from `/usr/local/var/log/pg_upgrade_utility.log`:
```
command: "/usr/local/opt/postgresql/bin/pg_resetwal" -o 836127 "/usr/local/var/postgres" >> "pg_upgrade_utility.log" 2>&1
pg_resetwal: error: lock file "postmaster.pid" exists
pg_resetwal: Is a server running? If not, delete the lock file and try again.
```
no postmaster.pid:
```
% ls /usr/local/var/postgres/postmaster.pid
ls: /usr/local/var/postgres/postmaster.pid: No such file or directory
```
Any guidance would be appreciated!
Answers:
username_1: Were you ever able to work around this? I am having exactly the same issue..
username_0: Unfortunately not, had to install postgresql@13 and copy the var/postgres directory to var/postgresql@13, stop my postgresql service, start postgresql@13, and I got back my database
username_1: Darn! But thanks much for the helpful workaround, I hadn't gotten to the point of figuring that out yet. :-)
username_1: It sure looks like the same issue as this one: https://github.com/Homebrew/homebrew-core/issues/73818
But the solution that worked there (setting `-j` to 1 instead of `Hardware::CPU.cores`) does not seem to help...
username_2: `brew postgresql-upgrade-database` is not something from this repository. You should report issues with it to the Homebrew core repositories.
username_1: Noted, thank you! |
delvedor/find-my-way | 1174320697 | Title: Bug: Broken routing for aliased routes ending with `<...>/::something` & `<...>/:some_var`
Question:
username_0: **Versions tested:**
- 4.5.1 (Fastify dependency: `"find-my-way": "^4.5.0"`)
- 5.2.0 (latest)
*Both contain the bug, but the symptoms are different. The bug is more severe in 5.2.0.*
**TL;DR:** There appears to be a bug for pairs of routes, one ending with `/::something` and the other with `/:some_var`. The exact behavior depends on the `find-my-way` version (*see "Observed behavior" below*), but is incorrect in either case. *See "Failure MCVE" below.*
Prefixes other than `::` seem to work correctly (tested: `~`, `@`, `$`). *See "Working MCVE" below.*
---
**Failure MCVE:** (`index.mjs`)
```javascript
import http from 'http';
// npm install --save-prod [email protected] or [email protected]
import FindMyWay from 'find-my-way';
const router = FindMyWay();
router.on('GET', '/:world_name/::articles', (req, res, params) => {
res.end('matched /:world_name/::articles\n' + JSON.stringify(params, null, 2));
});
router.on('GET', '/:world_name/:article_name', (req, res, params) => {
res.end('matched /:world_name/:article_name\n' + JSON.stringify(params, null, 2));
});
const server = http.createServer((req, res) => {
router.lookup(req, res);
});
server.listen(3000, err => {
if (err) throw err;
console.log('Server listening on: http://localhost:3000');
});
```
**Observed behavior v4.5.1**: `GET /myworld/:articles` request matches the *second* (`/:world_name/:article_name`) route (see below).
**Observed behavior v5.2.0**: Both `GET /myworld/:articles` *and* `GET /myworld/some-article` result in a 404 Not Found error. In other words, *neither* route works, as if they did not exist.
**Expected behavior**: The above request should match the *first* (`/:world_name/::articles`) route.
**Partial workaround**: No known workaround on my part for the *exact same* route, but changing `::articles` to a different prefix symbol (e.g. `~articles`) works in both 4.5.1 *and* 5.2.0.
`GET /myworld/:articles`:
```
matched /:world_name/:article_name
{
"world_name": "myworld",
"article_name": ":articles"
}
```
---
**Success MCVE:** (modified failure example, by changing `::articles` to `~articles` in the route)
```javascript
router.on('GET', '/:world_name/~articles', (req, res, params) => {
res.end('matched /:world_name/~articles\n' + JSON.stringify(params, null, 2));
});
router.on('GET', '/:world_name/:article_name', (req, res, params) => {
res.end('matched /:world_name/:article_name\n' + JSON.stringify(params, null, 2));
});
```
… (correctly) results in the following being matched for a `GET /myworld/~articles`:
```
matched /:world_name/~articles
{
"world_name": "myworld"
}
```
Answers:
username_1: You can't have ambiguous behavior. If `:` is used to detect the start of param, you can't use it as a prefix.
I'm not sure if we support it, but having `/something/\::foo` would be nice. What's the syntax of express for this case?
username_0: The only ambiguity is between `/:some_param` and `/something_not_a_param`, but this case is (to my knowledge, and definitely behavior-wise) handled by `find-my-way` anyway (by first checking the "static" routes).
Come think of it, using `/:article_name` and then checking `if(params.article_name === ':articles')` *is* a viable workaround; but it is a bit ugly, as it essentially means having another layer of routing on top of the existing router.
username_1: The invite stands: would you like to send a PR?
username_0: I can *maybe* look into it in a few days, but can't be sure I'll be able to, as my schedule is currently very busy.
So: I'll see what I can do, but no promises are made.
Status: Issue closed
|
BlueCodeSystems/smartcerv | 470960399 | Title: Make application compatible with devices that have lower specifications
Question:
username_0: Devices such as Samsung Galaxy Tab A, 7" Screen, 1.5GB RAM and 8GB ROM running on Android 5.1.1
Answers:
username_0: CIDRZ set up some devices running on Android 5 and the application is working fine they have not reported any problems. One observation is that the SmartCerv icon does not show when installed on the device instead it shows the general android icon.
For branding purposes this issue will be closed when that minor problem is fixed. |
apache/rocketmq | 697901107 | Title: vm.extra_free_kbytes 和 warmMapedFileEnable 问题
Question:
username_0: 服务异常出现busy问题,报错:cost in lock ...
Answers:
username_0: 该部分代码就是写pagecache,为啥这么慢
目前有2个方向:
1. 可用内存过小,突然的大内存申请,导致后台内存回收,慢导致延迟,调整vm.extra_free_kbytes能否解决?
2. 提前锁定内存warmMapedFileEnable 开启,是否能解决问题?
username_1: You can execute the distribution/bin/os.sh Script to optimize the operating system configuration, but the specific tuning parameters still depend on the specific machine configuration.
Issues are generally used for bug reports and feature requests, so if you have any questions about Apache RocketMQ, it is recommended to discuss it on the mailing list first, the mailing list [address](https://rocketmq.apache.org/about/contact). I will close the Issue first, but please feel free to reopen it if you have any other issues.
Status: Issue closed
|
galadril/Xam.Plugin.SimpleAppIntro | 345505305 | Title: Not show PositionIndicator
Question:
username_0: He estado probando el plugin y me parece genial lo simple que es, pero no logro mostrar la barra indicadora de slide:
`welcomePage.ShowPositionIndicator = true;`
Por cierto, ¿Puedo personalizar el color de fondo de un slide en particular?
Answers:
username_1: english please
username_0: I have been testing the plugin and it seems great how simple it is, but I can not show the slide bar (Phone model : Google pixel XL - Android version: 8.1):
`welcomePage.ShowPositionIndicator = true;`
By the way, can I customize the background color of a particular slide?
username_0: Thanks, but the bar that indicates the position of slide is not shown is a bug? or I must instantiate something in special.
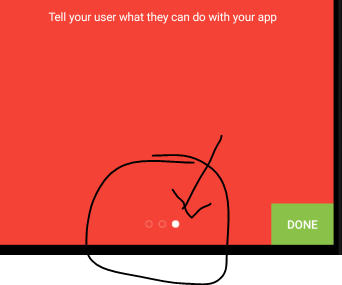
Another problem I found, is that hiding the skip button and pressing the side where it should be works the same way.
I leave you some suggestions in case you want to implement them :)
1.- To be able to change the size of the text
2.- To be able to use images for the buttons of skip and done
I would love to help with the implementation of this, but I have just started in Xamarin a couple of days ago :(
username_1: I'm using TitleStyle and BodyStyle as the styles for the text in the intro. maybe you could do something with style Overriding Global Styles in your app:
https://docs.microsoft.com/nl-nl/xamarin/xamarin-forms/user-interface/styles/xaml/application#overriding-styles
Status: Issue closed
|
nsscreencast/comments | 906464221 | Title: Parsing RSS and Atom Feeds
Question:
username_0: Written on 05/11/2019 14:37:58
URL: https://nsscreencast.com/episodes/390-podcast-app-parsing-feeds
Answers:
username_0: originally written by **<NAME>** on **01/06/2020 20:51:21**
<p>Hi, I can't get data from rss.itunes. I have the following error, </p><blockquote>`Error loading recommended podcasts: Error sending request: The certificate for this server is invalid. You might be connecting to a server that is pretending to be “<a href="http://rss.itunes.apple.com" rel="nofollow noopener" title="rss.itunes.apple.com">rss.itunes.apple.com</a>” which could put your confidential information at risk`</blockquote><p></p><p>Already tried to disable using the tips of this link, but could not.</p><p><a href="https://stackoverflow.com/questions/31254725/transport-security-has-blocked-a-cleartext-http" rel="nofollow noopener" title="https://stackoverflow.com/questions/31254725/transport-security-has-blocked-a-cleartext-http">https://stackoverflow.com/q...</a></p>
username_0: originally written by **username_0** on **01/06/2020 20:54:05**
<p>If App Transport Security was the issue, then you'd likely have a different error message. Is it possible you have a proxy in between that could be causing this? Are you able to request the URL using Paw (or cURL or similar)?</p>
username_0: originally written by **<NAME>** on **01/07/2020 14:12:27**
<p>I tried to connect on other networks but it also gave the same problem. If you access the site via browser you can. Do you have the collection to try to do via postman?</p>
username_0: originally written by **username_0** on **01/07/2020 14:41:26**
<p>I use Paw, but it does have a Postman export feature. I've exported the request and shared it here: <a href="https://nsscreencast.s3.us-east-1.amazonaws.com/shares/Top%20Podcasts%20RSS%20Feed.json" rel="nofollow noopener" title="https://nsscreencast.s3.us-east-1.amazonaws.com/shares/Top%20Podcasts%20RSS%20Feed.json">https://nsscreencast.s3.us-...</a></p>
username_0: originally written by **<NAME>** on **01/07/2020 17:07:48**
<p>Justo work in Postman, have a return. But I suspected if proxy of my work.</p>
username_0: originally written by **<NAME>** on **01/08/2020 18:21:18**
<p>Another question, I have a erro for this part of code,<code>let parser = FeedParser(data: data)<br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br>parser.parseAsync { parseResult in<br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br>let result: Swift.Result<podcast, podcastloadingerror=""><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br>do {<br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br>switch parseResult {<br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br>case .atom(let atom):<br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br>result = try .success(self.convert(atom: atom))<br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br>case .rss(let rss):<br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br>result = try .success(self.convert(rss: rss))<br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br>case .json(_): fatalError()<br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br>case .failure(let e):<br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br>result = .failure(.feedParsingError(e))<br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br>}<br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br>} </code></p><p>Have a error with pattern cannot match values of type 'Result<feed, parsererror="">'</p><p>But I parse Podcast in model, why a have Feed tag on my error?</p>
username_0: originally written by **username_0** on **01/08/2020 18:31:11**
<p>Can you post this code in gist (<a href="http://gist.github.com" rel="nofollow noopener" title="gist.github.com">gist.github.com</a>) and link it here? It's currently hard to read.</p>
username_0: originally written by **<NAME>** on **01/09/2020 14:33:31**
<p>Cannot for restriction on my network on my job, but I have a problem with pattern cannot match values of type Result<feed, parsererror=""> on case .atom line.</p>
username_0: originally written by **username_0** on **01/09/2020 15:02:15**
<p>"parsererror" means that Xcode is unable to give you the actual error because there seems to be either a typo or a type mismatch and Xcode cannot fully process the Swift code to give you a meaningful error. Often this means that the error lies elsewhere.</p><p>Without seeing _actual_ code, it's impossible for me to tell. But what was pasted above contains this line: ` let result: Swift.Result<podcast, podcastloadingerror="">`, which is invalid.</p> |
picoe/Eto | 233426551 | Title: Wpf: OnMainFormChanged
Question:
username_0: On Wpf closing a splash-screen, which is the first form of the app, causes the app to close, because changing MainForm-property to MyMainForm doesn't have an effect.
Fix:
Eto.Wpf.Forms.ApplicationHandler.OnMainFormChanged
```
public void OnMainFormChanged()
{
if (Widget.MainForm!=null)
System.Windows.Application.Current.MainWindow =Widget.MainForm.ToNative ();
}
```
Answers:
username_1: Hi, how do you manage to position your splash-screen at the center of the screen?
username_2: Fixed in #978
Status: Issue closed
|
QIICR/dcmheat | 400996336 | Title: Link to dataset broken
Question:
username_0: The script setup_datasets.sh fails because the dropbox files no longer exist.
Answers:
username_1: Thanks for reporting. This project is not feature-complete, it is the repo where I started the work, but never made time to continue... Sorry the files are gone, but there is a lot of other work that needs to be put in to make it usable. No estimate at this point if/when I will get to it. It is easier to just start using dcm2niix, which I did! |
CGATOxford/UMI-tools | 262565846 | Title: umi-tools count output lacking gene names
Question:
username_0: I'm having trouble with the umi-tools count feature, my output genes are all listed as 'R' rather than the gene name. e.g.
umi_tools count --per-gene --gene-tag=XT --per-cell -I assigned_sorted.bam -S counts.tsv
gene cell count
R AACTCCCCAATCCGAT 1
R AACTCCCTCTTGGGTA 9
R AAGACCTTCACCGGGT 4
R AAGCAGTGGTATCAAC 1
R ACAGCTAGTAATTGGA 2
Sample output of flags in my SAM file are as follows:
XT:A:R NM:i:0 AM:i:3 XS:Z:Assigned XN:i:1 XT:Z:RS1A.gene
XT:A:R NM:i:18 AM:i:2 XS:Z:Assigned XN:i:1 XT:Z:US1.gene
XT:A:R NM:i:20 AM:i:2 XS:Z:Assigned XN:i:1 XT:Z:US1.gene
NM:i:23 AM:i:7 XS:Z:Assigned XN:i:1 XT:Z:US1.gene
NM:i:1 AM:i:42 XS:Z:Assigned XN:i:1 XT:Z:US1.gene
NM:i:1 AM:i:42 XS:Z:Assigned XN:i:1 XT:Z:US1.gene
NM:i:21 AM:i:9 XS:Z:Assigned XN:i:1 XT:Z:US1.gene
NM:i:0 AM:i:44 XS:Z:Assigned XN:i:1 XT:Z:US1.gene
NM:i:2 AM:i:38 XS:Z:Assigned XN:i:1 XT:Z:US1.gene
NM:i:0 AM:i:44 XS:Z:Assigned XN:i:1 XT:Z:US1.gene
XT:A:R NM:i:15 AM:i:2 XS:Z:Unassigned_NoFeatures
XT:A:R NM:i:12 AM:i:2 XS:Z:Unassigned_NoFeatures
I thought the issue might be related to the duplicate XT entries (the first is placed there by bbmap) but stripping these has no effect on the output (moreover, several entries do not have the XT:A:R flag).
Any help would be much appreciated
Answers:
username_1: Hi,
I'm sure that the duplicated XT flags are going to cause a problem, even if its not this one! I'm not sure how pysam (the bam parsing library we use), will handle some reads having the type of the XT flag being A and some being Z (i'm guessing it takes the first instance of the flag to be the value for that read).
Will have a play and get back.
username_2: @username_0 - Would you be able to share a subset of you SAM:
`LHEAD=$(samtools view -H <BAM> |wc -l); samtools view -h <BAM> |head -$(($LHEAD + 1000))) > <OUTPUT_BAM>`
to help us work out what's going on here? You can email to me @: <EMAIL>
username_2: Hi @username_0 - I finally tracked this bug down! I believe originates from the different way featureCounts and HTSeq annotated reads which are not assigned to a gene. From what I understand, HTSeq uses just the `XF` tag and annotates each read with either the gene to which it is assigned, or a explanation as to why it was not assigned, e.g `__alignment_not_unique` ([doc here](http://htseq.readthedocs.io/en/release_0.9.1/count.html)). featureCounts uses the `XS`, `XN` and `XT` tags to describe the assignment status. number of targets, and target list.([doc here](https://rdrr.io/bioc/Rsubread/man/featureCounts.html)).
Currently, `umi_tools count` skips reads where the genes which match the `--skip-tags-regex` (default `^[__|Unassigned]`). These events are logged as "Gene skipped - matches regex". This works for HTSeq output where the gene tag and assigned tag is the same. However, for featureCounts, the assigned tag is different and for unassigned reads no XT tag is given. This should also results in the read being skipped by `umi_tools count` and the event logged as "Read skipped, no tag". However, some aligner also use the `XT` tag to describe the read alignment, e.g `XT:A:U`=unique and `XT:A:R`=repeat. Therefore, when using featureCounts, the unassigned reads retain their `XT` tag from the aligner, hence all those `R` genes. To get around this, I've introduced the `--assigned-tag` option. By defaults, this takes the same value as `--gene-tag` to support HTSeq-like output. For a featureCounts BAM, you can set this to `XS`.
Would you mind installing from the [{TS}-DebugSkipCountUnassigned branch](https://github.com/CGATOxford/UMI-tools/tree/%7BTS%7D-DebugSkipCountUnassigned) and testing this option out for me. If it works, I'll release 0.5.4 with this option immediately.
username_3: I have installed this branch. The command I use currently is.
umi_tools count --per-gene --assigned-tag=XS --gene-tag=XS --per-cell -I assigned_sorted.bam -S counts.tsv.gz
But it does not seem to work yet. Can you please explain what I am doing wrong here.
username_2: Hi @username_3. Thanks for testing out the branch. Your gene names are contained in the `XT` tag so you need to include the option `--gene-tag=XT` rather than `--gene-tag=XS` as above. I'll rename the `--assigned-tag` option to `--assigned-status-tag` to make this clearer.
Status: Issue closed
|
zeroengineteam/ZeroCore | 367000678 | Title: The SweptPlayer2D archetype from the Sample Character Controller pack has at least two problems
Question:
username_0: # Description
Repro
===
Take a look at the `SweptPlayer2D` archetype found in the **Sample Character Controller** content package available from the Market
Expected
===
The archetype has all of its settings configured properly for the SweptController to do its stuff, and also has no superfluous components
Happened
===
The SweptPlayer2D archetype's RigidBody is set to Dynamic, which doesn't affect performance, since it gets set to Kinematic in the script anyway, but it is still misleading to have it set to the wrong thing in the archetype. Additionally, it has a GravityEffect component, which does nothing.
# User Data
- **UserName**: douglasZwick
# Zero Engine Data
- **Revision**: 739
- **ChangeSet**: zeroengineteam/zerocore@671144bd3e749aa770025ef380ce0eb6f2a74a11
- **Platform**: Win32
- **Build Version Name**: 1.2.4.739 zeroengineteam/zerocore@671144bd3e749aa770025ef380ce0eb6f2a74a11 2018-02-22 Debug Win32
Status: Issue closed
Answers:
username_0: # Description
Repro
===
Take a look at the `SweptPlayer2D` archetype found in the **Sample Character Controller** content package available from the Market
Expected
===
The archetype has all of its settings configured properly for the SweptController to do its stuff, and also has no superfluous components
Happened
===
The SweptPlayer2D archetype's RigidBody is set to Dynamic, which doesn't affect performance, since it gets set to Kinematic in the script anyway, but it is still misleading to have it set to the wrong thing in the archetype. Additionally, it has a GravityEffect component, which does nothing.
# User Data
- **UserName**: douglasZwick
# Zero Engine Data
- **Revision**: 739
- **ChangeSet**: zeroengineteam/zerocore@671144bd3e749aa770025ef380ce0eb6f2a74a11
- **Platform**: Win32
- **Build Version Name**: 1.2.4.739 zeroengineteam/zerocore@671144bd3e749aa770025ef380ce0eb6f2a74a11 2018-02-22 Debug Win32 |
fedora-python/pyp2rpm | 114065349 | Title: Fedora packages naming case
Question:
username_0: It is problem to convert PyPI name to name of Fedora package in virtualenv module, because there is not strict uppercase/lowercase rule in naming of Fedora packages:
**PyPI** | **Fedora**
-----------------|----------------------
Babel | python-babel
MarkupSafe | python-markupsafe
Jinja2 | python-jinja2
Sphinx | python-sphinx
Cython | Cython
IPy | python-IPy
Answers:
username_0: This seems to be one of the most frequent reasons that generated spec file is not capable of being built. Couldn't we solve this problem or at least successfully convert more dependency names using DNF API query to check if dependency package exists in Fedora repositories?
example: we've extracted dependency MarkupSafe
```
In [68]: query = base.sack.query()
In [70]: [pkg.name for pkg in query if "MarkupSafe".lower() in pkg.name.lower()]
Out[70]: ['python-markupsafe', 'python3-markupsafe']
```
username_1: This might be the solution, but... How long does this take? If too much time, can we get the lists of Python packages from somewhere else, e.g. from portingdb? Does poritngdb have some sort of API (any sort of machine readable output)?
Back to your proposal: The logic behind this needs to be more clever than simply checking if str is in str. What if the dependency name would be simply `A`?
I see two possible better ways out (none of them happening any time soon and none of them in the direct hands of pyp2rpm project):
* Wait for the `pypi()` provides @torsava is working on
* Make the Python packaging guidelines change outlined in my previous comment happen (I've never got any feedback, maybe because I've never explicitly asked for it)
Another solution for the time being might be to get list of all Python packages in Fedora with some uppercase letters in their names (or in any other way problematic names) (there will not be so many of them I guess) and hardcode them in pyp2rpm (or put them in a YAML file, whatever). In other cases, just call `.lower()` on the Python package name, prepend it with `pythonX-` and hope for the best. (There might also be a slight issue with `python2-` prefix, because we want to encourage the use of it, but not all packages use it yet. Back to repoquery/portingdb probing I guess?)
Anyway, if you'll code some logic/magic (even based on hardcoded list), please make it happen as a separate Python module/package, reusable from outside pyp2rpm - this will make it easier to update it and also might help @torsava when filing bugs or when automating the provides generating process.
username_0: Speed doesn't seems to be problem here. Initialization of Query object takes about 0.3s. Execution of simple script including initialization of Query object, 100 iterations over it and string comparison takes still less than one second. I think it is not a disaster compared to other processes in pyp2rpm (distutils command, installation of package to virtualenv).
The example shows naive implementation to demonstrate how does DNF API query works.
The global solutions you mentioned are fine, but in my opinion name convertor would be good temporary fix of the issue. It seems not to be hard to implement it (I guess it could be ready in a week) and it might help in many cases.
I don't like idea of solution based on hardcoded list very much, so I will try to implement name convertor checking Fedora repositories directly as a separate package. The question is if DNF API or portingdb si the right choice at this moment.
username_1: If DNF repoquery is fast, go for it.
username_2: ... which results in people having all kinds of cases in requirements.txt, setup.py, pkgname arguments etc. Because RPM is case sensitive, its clear lowercase should be used.
This will only become more painful as more Python packages enter Fedora, or more people use great tools like pyp2rpm in their build strategies (containers, VMs...).
---------------------
FYI, with the current release (pyp2rpm 3.2.1) I am still seeing this case-mismatch issue.
(don't test any fixes on these PyVISA packages, there are also bugs with Sphinx document generation and module name handling which I'm looking into).
```
$ sudo dnf install python3-PyVISA-1.8-1.fc25.noarch.rpm python3-PyVISA-py-0.2-1.fc25.noarch.rpm
Error: nothing provides python3-pyvisa >= 1.8 needed by python3-PyVISA-py-0.2-1.fc25.noarch
$ rpm -qp --provides python3-PyVISA-1.8-1.fc25.noarch.rpm
python3-PyVISA = 1.8-1.fc25
python3.5dist(pyvisa) = 1.8
python3dist(pyvisa) = 1.8
$ rpm -qp --requires python3-PyVISA-py-0.2-1.fc25.noarch.rpm
python(abi) = 3.5
python3-pyvisa >= 1.8
rpmlib(CompressedFileNames) <= 3.0.4-1
rpmlib(FileDigests) <= 4.6.0-1
rpmlib(PayloadFilesHavePrefix) <= 4.0-1
rpmlib(PayloadIsXz) <= 5.2-1
```
username_2: Is this *really* needed? Given the case-insensitivity is effectively set in stone in Python-land, would this part just be baggage/confusion that people may mistakenly use or have to be maintained?
username_1: Not *really*.
username_3: Would it be possible for this to move forward based on the `pythonXYdist(name)` dependencies that are now in Fedora?
username_0: Absolutely, this issue was one of the reasons to add PyPI provides to RPM.
username_0: PR #127 was merged. There is a command line option to enable/disable the convertor based on automatic provides. It is disabled by default at this moment. I guess we can start using it by default for Fedora after F27 mass rebuild.
username_1: I see I was assigner here, sorry for not taking any action. I don't have free cycles to work on pyp2rpm :( |
apollographql/apollo-server | 554861304 | Title: RESTDataSource delete method doesn't work using context header
Question:
username_0: ```
// CODE BELOW DOESN'T WORK
const contact = await this.delete(`contacts/${contactId}`, undefined, { headers: this.context.req.headers });
// CODE BELOW WORKS
const contact = await this.delete(`contacts/${contactId}`);
```
Why I cannot set a custom header to do a delete operation? For `post` and `patch` actions works fine, but no for `delete`. Why is that so?
<!--
* ❓ Questions?
Use Stack Overflow (http://stackoverflow.com/questions/tagged/apollo)
or discuss it on our Spectrum.chat community (https://spectrum.chat/apollo).
* 💡 Feature requests?
Please search for an existing feature request before opening a new one.
If there is an existing feature request, use the 👍 to show your support
for it. If the specifics of your use case are not covered in the existing
conversation, please take the time to *add new conversation* which helps
move the feature's design forward.
* ❗️ Bug?
This is the right place! 😄
Before reporting a bug, please check for existing or closed issues
first and read the instructions for filing a bug report:
https://github.com/apollographql/apollo-server/blob/master/CONTRIBUTING.md#reporting-bugs
### This bug report should include:
- [ ] A short, but descriptive title. The title doesn't need "Apollo" in it.
- [ ] The package name and version of Apollo showing the problem.
- [ ] If applicable, the last version of Apollo where the problem did _not_ occur.
- [ ] The expected behavior.
- [ ] The actual behavior.
- [ ] A **simple**, runnable reproduction!
Please make a GitHub repository that anyone can clone and run and see the
problem. Other great ways to demonstrate a reproduction are using our
CodeSandbox template (https://codesandbox.io/s/apollo-server), or re-mixing
our Glitch template (https://glitch.com/~apollo-launchpad).
-->
Answers:
username_1: @username_0 code doesn't work means is there any error or nothing happens ? If there is an error please mention it here. |
godaddy/dmd | 637828659 | Title: Multi-line descriptions break listing tables
Question:
username_0: Command line I'm using: `jsdoc2md --plugin @godaddy/dmd -f lib/typedefs.js > API.md`
Example 1. Typedef description consists of two lines split by empty line. This syntax is recognized by every other tool I know.
```javascript
/**
* @typedef { object } Foo
* Foo line one.
*
* Foo line two.
*
* @property { boolean } [bar = false]
* Bar description.
*
* @property { boolean } [baz = false]
* Baz description.
*/
```
Example 2. Typedef description consists of two lines with linebreak escape (this syntax is recognized by VSCode).
```javascript
/**
* @typedef { object } Foo
* Foo line one. \
* Foo line two.
*
* @property { boolean } [bar = false]
* Bar description.
*
* @property { boolean } [baz = false]
* Baz description.
*/
```
While in other tables newlines are removed completely *(I wish there were a way to choose a behavior though - I want as little html as possible, but I also can tolerate `<br/>` where it makes sense)*, listings at the top of document behave differently.
`Typedefs` - description transferred to markdown output as-is in both cases, breaking the table formatting.
```
## Typedefs
Name | Description
------ | -----------
[Options] | HtmlToText options.
[Foo] | Foo line one.
Foo line two.
```
```
## Typedefs
Name | Description
------ | -----------
[Foo] | Foo line one. \
Foo line two.
```
`Functions` - with the first style description only uses the first line, with the second style description - removes newline but leaves escape symbol behind.
```
## Functions
Name | Description
------ | -----------
[foo1(bar, \[baz\])] | Foo line one.
[foo2(bar, \[baz\])] | Foo line one. \ Foo line two.
```
Answers:
username_1: @username_0 thanks for reporting! I add a fix and some docs to clarify expected behavior for the index tables over in #7
Status: Issue closed
|
ppy/osu | 597608563 | Title: Game wont open after a failed update installation
Question:
username_0: **Describe the crash:**
I opened osu and then, as usual, the latest update started downloading. After the downloading process finished I clicked to install and a couple of errors appeared. After that, I tried restarting the game to see if that fixes it but the game does not open anymore. when I click on the game to open it nothing happens at all and I cant see it running on task manager. This might be related to storage since my C drive was very low on storage when I tried to install the update (but the whole update was downloaded ). I freed up some space but that doesn't seem to help much at this point. I'm also aware that reinstalling the game would most likely fix the issue but I just wanted to let you know about this.
**Screenshots or videos showing encountered issue:**
none
**osu!lazer version:**
was running 2020.403.0
**Logs:**
<!--
*please attach logs here, which are located at:*
- `%AppData%/osu/logs` *(on Windows),*
- `~/.local/share/osu/logs` *(on Linux & macOS).*
-->
[network.log](https://github.com/ppy/osu/files/4459056/network.log)
[performance.log](https://github.com/ppy/osu/files/4459057/performance.log)
[runtime.log](https://github.com/ppy/osu/files/4459058/runtime.log)
[updater.log](https://github.com/ppy/osu/files/4459059/updater.log)
[database.log](https://github.com/ppy/osu/files/4459060/database.log)
**Computer Specifications:**
CPU: AMD Ryzen 5 2600
Motherboard: MSI Tomahawk b450
GPU: GTX 1060 6GB
RAM: 16GB
OS: Windows 10 Pro
Answers:
username_1: have you tried deleting all files in the AppData/Local/osu folder then installing again?
username_0: That fixes the problem, should I close this issue?
Status: Issue closed
username_1: Yeah for now. Squirrel is only a temporary solution for installation/updating so I would rather not track rare issues with it. |
oduwsdl/ipwb | 280582536 | Title: Sample memento doesn't work for me
Question:
username_0: A screencast:
[TODO in a few minutes]
and a copy of the initial and final ipwb stacktraces (they all seem to look the same to the naked eye):
```
C:\dnload\ipfs-etc>ipwb replay
This version of ipwb is outdated. Please run pip install --upgrade ipwb.
Executing first-run procedure on provided sample data.
Processing WARC records in salam-home.warc complete
* Running on http://localhost:5000/ (Press CTRL+C to quit)
Retrieving URI-Ms from c:\python27\lib\site-packages\ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /c:\python27\lib\site-packages\ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /c:\python27\lib\site-packages\ipwb\samples\indexes\sample-encrypted.cdxj
127.0.0.1 - - [08/Dec/2017 19:43:12] "GET / HTTP/1.1" 200 -
127.0.0.1 - - [08/Dec/2017 19:43:12] "GET /webui/webui.css HTTP/1.1" 200 -
127.0.0.1 - - [08/Dec/2017 19:43:12] "GET /webui/webui.js HTTP/1.1" 200 -
127.0.0.1 - - [08/Dec/2017 19:43:13] "GET /webui/logo.png HTTP/1.1" 200 -
127.0.0.1 - - [08/Dec/2017 19:43:13] "GET /daemon/status HTTP/1.1" 200 -
127.0.0.1 - - [08/Dec/2017 19:43:13] "GET /config/openEndedPlaceHolder HTTP/1.1" 200 -
Retrieving URI-Ms from c:\python27\lib\site-packages\ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /c:\python27\lib\site-packages\ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /c:\python27\lib\site-packages\ipwb\samples\indexes\sample-encrypted.cdxj
127.0.0.1 - - [08/Dec/2017 19:43:13] "GET / HTTP/1.1" 200 -
127.0.0.1 - - [08/Dec/2017 19:43:13] "GET /webui/favicons/apple-touch-icon.png HTTP/1.1" 200 -
127.0.0.1 - - [08/Dec/2017 19:43:13] "GET /webui/favicons/favicon-16x16.png HTTP/1.1" 200 -
127.0.0.1 - - [08/Dec/2017 19:43:13] "GET /webui/webui.css HTTP/1.1" 200 -
127.0.0.1 - - [08/Dec/2017 19:43:13] "GET /webui/webui.js HTTP/1.1" 200 -
127.0.0.1 - - [08/Dec/2017 19:43:13] "GET /webui/daemonController.js HTTP/1.1" 200 -
CDXJ Line: edu,odu,cs)/~salam 20160305192247 {"locator": "urn:ipfs/QmeVWGtnfuJ1QnpmtKKnyArVgEpq7<KEY>", "encryption_method": "xor", "encryption_key": "radon", "mime_type": "text/html", "status_code": "200"}
Unknown exception occurred while fetching from ipfs.
----------------------------------------
Exception happened during processing of request from ('127.0.0.1', 64204)
Traceback (most recent call last):
File "c:\python27\lib\SocketServer.py", line 290, in _handle_request_noblock
self.process_request(request, client_address)
File "c:\python27\lib\SocketServer.py", line 318, in process_request
self.finish_request(request, client_address)
File "c:\python27\lib\SocketServer.py", line 331, in finish_request
self.RequestHandlerClass(request, client_address, self)
File "c:\python27\lib\SocketServer.py", line 652, in __init__
self.handle()
File "c:\python27\lib\site-packages\werkzeug\serving.py", line 290, in handle
rv = BaseHTTPRequestHandler.handle(self)
File "c:\python27\lib\BaseHTTPServer.py", line 340, in handle
self.handle_one_request()
File "c:\python27\lib\site-packages\werkzeug\serving.py", line 325, in handle_one_request
return self.run_wsgi()
File "c:\python27\lib\site-packages\werkzeug\serving.py", line 267, in run_wsgi
execute(self.server.app)
File "c:\python27\lib\site-packages\werkzeug\serving.py", line 255, in execute
application_iter = app(environ, start_response)
File "c:\python27\lib\site-packages\flask\app.py", line 1997, in __call__
return self.wsgi_app(environ, start_response)
File "c:\python27\lib\site-packages\flask\app.py", line 1982, in wsgi_app
response = self.full_dispatch_request()
File "c:\python27\lib\site-packages\flask\app.py", line 1612, in full_dispatch_request
rv = self.dispatch_request()
File "c:\python27\lib\site-packages\flask\app.py", line 1598, in dispatch_request
[Truncated]
return self.run_wsgi()
File "c:\python27\lib\site-packages\werkzeug\serving.py", line 267, in run_wsgi
execute(self.server.app)
File "c:\python27\lib\site-packages\werkzeug\serving.py", line 255, in execute
application_iter = app(environ, start_response)
File "c:\python27\lib\site-packages\flask\app.py", line 1997, in __call__
return self.wsgi_app(environ, start_response)
File "c:\python27\lib\site-packages\flask\app.py", line 1982, in wsgi_app
response = self.full_dispatch_request()
File "c:\python27\lib\site-packages\flask\app.py", line 1612, in full_dispatch_request
rv = self.dispatch_request()
File "c:\python27\lib\site-packages\flask\app.py", line 1598, in dispatch_request
return self.view_functions[rule.endpoint](**req.view_args)
File "c:\python27\lib\site-packages\ipwb\replay.py", line 398, in showMementoAtDatetime
return show_uri(urir, datetime)
File "c:\python27\lib\site-packages\ipwb\replay.py", line 532, in show_uri
sys.exit()
SystemExit
----------------------------------------
```
Answers:
username_1: Oh, that's really not good. I must have broken something along the way that is not yet being tested in the Travis config.
I will look into this further, @username_0 . Are you able to replicate this with the latest build? A `git pull` will suffice as a substitute for the instructions of using pip.
username_0: ```
C:\dnload\ipfs-etc\ipwb>git log HEAD^!
fatal: ambiguous argument 'HEAD!': unknown revision or path not in the working tree.
Use '--' to separate paths from revisions, like this:
'git <command> [<revision>...] -- [<file>...]'
C:\dnload\ipfs-etc\ipwb>git log HEAD^^!
commit f17677f6a194365bd6bd7e0a20c702f1994f9ca0
Author: <NAME> <<EMAIL>>
Date: Thu Dec 7 22:00:37 2017 -0500
Fix typo
C:\dnload\ipfs-etc\ipwb>git diff
diff --git a/ipwb/__init__.py b/ipwb/__init__.py
index d41d25b..44a3f8b 100644
--- a/ipwb/__init__.py
+++ b/ipwb/__init__.py
@@ -1 +1 @@
-__version__ = '0.2017.12.07.2106'
+__version__ = '0.2017.12.07.2107'
diff --git a/ipwb/util.py b/ipwb/util.py
index 7527927..b26863c 100644
--- a/ipwb/util.py
+++ b/ipwb/util.py
@@ -40,7 +40,7 @@ def isDaemonAlive(hostAndPort="{0}:{1}".format(IPFSAPI_IP, IPFSAPI_PORT)):
try:
# OSError if ipfs not installed
- subprocess.call(['ipfs', '--version'], stdout=open(devnull, 'wb'))
+ # subprocess.call(['ipfs', '--version'], stdout=open(devnull, 'wb'))
# ConnectionError/AttributeError if IPFS daemon not running
client.id()
```
Seems still the same situation:
```
C:\dnload\ipfs-etc>\python27\python.exe ipwb/ipwb replay
This version of ipwb is outdated. Please run pip install --upgrade ipwb.
Executing first-run procedure on provided sample data.
Processing WARC records in salam-home.warc complete
* Running on http://localhost:5000/ (Press CTRL+C to quit)
127.0.0.1 - - [08/Dec/2017 20:08:15] "GET /webui/favicons/apple-touch-icon.png HTTP/1.1" 200 -
127.0.0.1 - - [08/Dec/2017 20:08:15] "GET /webui/favicons/favicon-16x16.png HTTP/1.1" 200 -
Retrieving URI-Ms from ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
127.0.0.1 - - [08/Dec/2017 20:08:22] "GET / HTTP/1.1" 200 -
127.0.0.1 - - [08/Dec/2017 20:08:22] "GET /webui/webui.css HTTP/1.1" 200 -
127.0.0.1 - - [08/Dec/2017 20:08:22] "GET /webui/webui.js HTTP/1.1" 200 -
127.0.0.1 - - [08/Dec/2017 20:08:22] "GET /webui/logo.png HTTP/1.1" 200 -
127.0.0.1 - - [08/Dec/2017 20:08:22] "GET /daemon/status HTTP/1.1" 200 -
127.0.0.1 - - [08/Dec/2017 20:08:22] "GET /config/openEndedPlaceHolder HTTP/1.1" 200 -
Retrieving URI-Ms from ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
127.0.0.1 - - [08/Dec/2017 20:08:22] "GET / HTTP/1.1" 200 -
[Truncated]
File "C:\python27\lib\site-packages\werkzeug\serving.py", line 325, in handle_one_request
return self.run_wsgi()
File "C:\python27\lib\site-packages\werkzeug\serving.py", line 267, in run_wsgi
execute(self.server.app)
File "C:\python27\lib\site-packages\werkzeug\serving.py", line 255, in execute
application_iter = app(environ, start_response)
File "C:\python27\lib\site-packages\flask\app.py", line 1997, in __call__
return self.wsgi_app(environ, start_response)
File "C:\python27\lib\site-packages\flask\app.py", line 1982, in wsgi_app
response = self.full_dispatch_request()
File "C:\python27\lib\site-packages\flask\app.py", line 1612, in full_dispatch_request
rv = self.dispatch_request()
File "C:\python27\lib\site-packages\flask\app.py", line 1598, in dispatch_request
return self.view_functions[rule.endpoint](**req.view_args)
File "ipwb/ipwb\replay.py", line 398, in showMementoAtDatetime
return show_uri(urir, datetime)
File "ipwb/ipwb\replay.py", line 532, in show_uri
sys.exit()
SystemExit
```
username_1: @username_0 A bit was done with this. Please do a fresh pull and let me know if you continue to experience the above issue.
username_0: Still not OK for me, though somewhat different symptoms now: after clicking the link on welcome page, I'm taken to the below URL:
http://localhost:5000/20160305192247/cs.odu.edu/~salam
and see the following logs:
```
C:\dnload\ipfs-etc>\python27\python.exe ipwb/ipwb replay
Executing first-run procedure on provided sample data.
Processing WARC records in salam-home.warc complete
IPWB replay started on http://localhost:5000
Retrieving URI-Ms from ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
Retrieving URI-Ms from ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
Retrieving URI-Ms from ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
CDXJ Line: edu,odu,cs)/~salam 20160305192247 {"locator": "urn:ipfs/QmeVWGtnfuJ1QnpmtKKnyArVgEpq7v31kktEfh6c8mDiXE/QmZWKQRBNXNrVZ69LoGpMNJi5NU66gDhnGtQukWJepv7Kr", "encryption_method": "xor", "encryption_key": "radon", "mime_type": "text/html", "status_code": "200"}
Unknown exception occurred while fetching from ipfs.
'module' object has no attribute 'exec_info'
```
My current revision is:
```
C:\dnload\ipfs-etc\ipwb>git log -1 HEAD
commit b8f8fd4ae3ff5163ea8dd69fc0dff6b21182e88f
Merge: 1a9a518 24e3083
Author: <NAME> <<EMAIL>>
Date: Sat Dec 9 08:46:12 2017 -0500
Merge pull request #322 from oduwsdl/issue-321
Better describe replay script, fix README to align. Closes #321.
```
username_1: @username_0 Somehow `exc_info` had turned into `exec_info` at some point. This is the reason for the vague, uninformative error message you received. I fixed this in #323 thanks to your report and have pushed the changes to master. Can you re-pull and replicate the above so we can figure out what sort of exception is occurring?
username_0: Sure!
```
C:\dnload\ipfs-etc\ipwb>git log -1 HEAD
commit 866d7b484c450d43ac49fa586b6ee158ab1715a2
Merge: b8f8fd4 1947434
Author: <NAME> <<EMAIL>>
Date: Sat Dec 9 10:30:00 2017 -0500
Merge pull request #324 from oduwsdl/issue-323-new
Fixes syntax issue with reporting generic exceptions
```
Logs:
```
C:\dnload\ipfs-etc>\python27\python.exe ipwb/ipwb replay
Executing first-run procedure on provided sample data.
Processing WARC records in salam-home.warc complete
IPWB replay started on http://localhost:5000
Retrieving URI-Ms from ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
Retrieving URI-Ms from ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /ipwb/ipwb\samples\indexes\sample-encrypted.cdxj
CDXJ Line: edu,odu,cs)/~salam 20160305192247 {"locator": "urn:ipfs/QmeVWGtnfuJ1QnpmtKKnyArVgEpq7v31kktEfh6c8mDiXE/QmZWKQRBNXNrVZ69LoGpMNJi5NU66gDhnGtQukWJepv7Kr", "encryption_method": "xor", "encryption_key": "radon", "mime_type": "text/html", "status_code": "200"}
Unknown exception occurred while fetching from ipfs.
<type 'exceptions.AttributeError'>
----------------------------------------
Exception happened during processing of request from ('127.0.0.1', 56133)
Traceback (most recent call last):
File "C:\python27\lib\SocketServer.py", line 290, in _handle_request_noblock
self.process_request(request, client_address)
File "C:\python27\lib\SocketServer.py", line 318, in process_request
self.finish_request(request, client_address)
File "C:\python27\lib\SocketServer.py", line 331, in finish_request
self.RequestHandlerClass(request, client_address, self)
File "C:\python27\lib\SocketServer.py", line 652, in __init__
self.handle()
File "C:\python27\lib\site-packages\werkzeug\serving.py", line 290, in handle
rv = BaseHTTPRequestHandler.handle(self)
File "C:\python27\lib\BaseHTTPServer.py", line 340, in handle
self.handle_one_request()
File "C:\python27\lib\site-packages\werkzeug\serving.py", line 325, in handle_one_request
return self.run_wsgi()
File "C:\python27\lib\site-packages\werkzeug\serving.py", line 267, in run_wsgi
execute(self.server.app)
File "C:\python27\lib\site-packages\werkzeug\serving.py", line 255, in execute
application_iter = app(environ, start_response)
File "C:\python27\lib\site-packages\flask\app.py", line 1997, in __call__
return self.wsgi_app(environ, start_response)
File "C:\python27\lib\site-packages\flask\app.py", line 1982, in wsgi_app
response = self.full_dispatch_request()
File "C:\python27\lib\site-packages\flask\app.py", line 1612, in full_dispatch_request
rv = self.dispatch_request()
File "C:\python27\lib\site-packages\flask\app.py", line 1598, in dispatch_request
return self.view_functions[rule.endpoint](**req.view_args)
[Truncated]
execute(self.server.app)
File "C:\python27\lib\site-packages\werkzeug\serving.py", line 255, in execute
application_iter = app(environ, start_response)
File "C:\python27\lib\site-packages\flask\app.py", line 1997, in __call__
return self.wsgi_app(environ, start_response)
File "C:\python27\lib\site-packages\flask\app.py", line 1982, in wsgi_app
response = self.full_dispatch_request()
File "C:\python27\lib\site-packages\flask\app.py", line 1612, in full_dispatch_request
rv = self.dispatch_request()
File "C:\python27\lib\site-packages\flask\app.py", line 1598, in dispatch_request
return self.view_functions[rule.endpoint](**req.view_args)
File "ipwb/ipwb\replay.py", line 399, in showMementoAtDatetime
return show_uri(urir, datetime)
File "ipwb/ipwb\replay.py", line 534, in show_uri
sys.exit()
SystemExit
[...]
```
and more of similar-looking traces.
username_0: I modified `replay.py` l.533 to:
print(sys.exc_info())
and got:
(<type 'exceptions.AttributeError'>, AttributeError("'module' object has no attribute 'SIGALRM'",), <traceback object at 0x0000000004A3C548>)
username_0: Here's a diff which fixes this for me:
```diff
diff --git a/ipwb/indexer.py b/ipwb/indexer.py
index d2bfc78..da2cb61 100755
--- a/ipwb/indexer.py
+++ b/ipwb/indexer.py
@@ -33,7 +33,7 @@ from __init__ import __version__ as ipwbVersion
DEBUG = False
-IPFS_API = ipfsapi.Client(IPFSAPI_IP, IPFSAPI_PORT)
+IPFS_API = ipfsapi.Client(IPFSAPI_IP, IPFSAPI_PORT, timeout=10)
# TODO: put this method definition below indexFileAt()
diff --git a/ipwb/replay.py b/ipwb/replay.py
index cc45140..71bf34d 100755
--- a/ipwb/replay.py
+++ b/ipwb/replay.py
@@ -507,13 +507,13 @@ def show_uri(path, datetime=None):
def handler(signum, frame):
raise HashNotFoundError()
- signal.signal(signal.SIGALRM, handler)
- signal.alarm(10)
+ #signal.signal(signal.SIGALRM, handler)
+ #signal.alarm(10)
payload = IPFS_API.cat(digests[-1])
header = IPFS_API.cat(digests[-2])
- signal.alarm(0)
+ #signal.alarm(0)
except ipfsapi.exceptions.TimeoutError:
print("{0} not found at {1}".format(cdxjParts[0], digests[-1]))
@@ -530,7 +530,7 @@ def show_uri(path, datetime=None):
return '', 404
except:
print('Unknown exception occurred while fetching from ipfs.')
- print(sys.exc_info()[0])
+ print(sys.exc_info())
sys.exit()
if 'encryption_method' in jObj:
diff --git a/ipwb/util.py b/ipwb/util.py
index e92b2c9..df50b53 100644
--- a/ipwb/util.py
+++ b/ipwb/util.py
@@ -137,7 +137,7 @@ def retrieveMemCount():
def datetimeToRFC1123(digits14):
try:
- locale.setlocale(locale.LC_TIME, 'en_US')
+ locale.setlocale(locale.LC_TIME, 'english') # for Windows (see: https://stackoverflow.com/questions/19709026/how-can-i-list-all-available-windows-locales-in-python-console#comment47807449_26927374)
except locale.Error as e:
locale.setlocale(locale.LC_TIME, 'en_US.utf8')
d = datetime.datetime.strptime(digits14, '%Y%m%d%H%M%S')
```
username_1: @username_0 I originally was using the `timeout` with `ipfsapi.Client` but ran into some issues that caused the move to SIGALARM.
The locale issue should be handled more elegantly. I did not even considered Windows not supporting either of en_US or en_US.utf8. On macOS, if I swap out the line you provided, I get `locale.Error: unsupported locale setting`. The implicit case here (a result of me developing this on Mac initially) is for the try block to handle `en_US` (a macOS locale) and fall-through to `en_US.utf8` as is often found on Linux. A third condition may need to be considered in Windows' `english` locale.
username_0: Hm, actually I now see I mistakenly added the `timeout` in indexer.py; the timeout doesn't seem to work OK. Not sure how to properly inject it into *requests* via *py-ipfs-api*
username_1: @username_0 At one time we had it on the `cat()` function on the ipfsapi.client instance. That was the aforementioned problematic issue I referenced that led me to change it to use `signal` instead.
username_1: Re-tested this in a Windows 10 VM with cb4b5bc3fffa033a1b463ae71847f4acc765aa1e
```
C:\Users\Mat\Downloads\ipwb>ipwb replay
Executing first-run procedure on provided sample data.
Processing WARC records in salam-home.warc complete
IPWB replay started on http://localhost:5000
Retrieving URI-Ms from c:\python27\lib\site-packages\ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /c:\python27\lib\site-packages\ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /c:\python27\lib\site-packages\ipwb\samples\indexes\sample-encrypted.cdxj
Retrieving URI-Ms from c:\python27\lib\site-packages\ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /c:\python27\lib\site-packages\ipwb\samples\indexes\sample-encrypted.cdxj
getting index file at /c:\python27\lib\site-packages\ipwb\samples\indexes\sample-encrypted.cdxj
Getting CDXJ Lines with the URI-R cs.odu.edu/~salam from samples/indexes/sample-encrypted.cdxj
Getting CDXJ Lines with cs.odu.edu/~salam in c:\python27\lib\site-packages\ipwb\samples\indexes\sample-encrypted.cdxj
CDXJ Line: edu,odu,cs)/~salam 20160305192247 {"locator": "urn:ipfs/QmeVWGtnfuJ1QnpmtKKnyArVgEpq7v31kktEfh6c8mDiXE/QmZWKQRBNXNrVZ69LoGpMNJi5NU66gDhnGtQukWJepv7Kr", "encryption_method": "xor", "encryption_key": "radon", "mime_type": "text/html", "status_code": "200"}
Unknown exception occurred while fetching from ipfs.
<type 'exceptions.AttributeError'>
----------------------------------------
Exception happened during processing of request from ('127.0.0.1', 53156)
Traceback (most recent call last):
File "c:\python27\lib\SocketServer.py", line 290, in _handle_request_noblock
self.process_request(request, client_address)
File "c:\python27\lib\SocketServer.py", line 318, in process_request
self.finish_request(request, client_address)
File "c:\python27\lib\SocketServer.py", line 331, in finish_request
self.RequestHandlerClass(request, client_address, self)
File "c:\python27\lib\SocketServer.py", line 652, in __init__
self.handle()
File "c:\python27\lib\site-packages\werkzeug\serving.py", line 290, in handle
rv = BaseHTTPRequestHandler.handle(self)
File "c:\python27\lib\BaseHTTPServer.py", line 340, in handle
self.handle_one_request()
File "c:\python27\lib\site-packages\werkzeug\serving.py", line 325, in handle_one_request
return self.run_wsgi()
File "c:\python27\lib\site-packages\werkzeug\serving.py", line 267, in run_wsgi
execute(self.server.app)
File "c:\python27\lib\site-packages\werkzeug\serving.py", line 255, in execute
application_iter = app(environ, start_response)
File "c:\python27\lib\site-packages\flask\app.py", line 1997, in __call__
return self.wsgi_app(environ, start_response)
File "c:\python27\lib\site-packages\flask\app.py", line 1982, in wsgi_app
response = self.full_dispatch_request()
File "c:\python27\lib\site-packages\flask\app.py", line 1612, in full_dispatch_request
rv = self.dispatch_request()
File "c:\python27\lib\site-packages\flask\app.py", line 1598, in dispatch_request
return self.view_functions[rule.endpoint](**req.view_args)
File "c:\python27\lib\site-packages\ipwb\replay.py", line 218, in showMemento
return show_uri(uri, newDatetime)
File "c:\python27\lib\site-packages\ipwb\replay.py", line 593, in show_uri
sys.exit()
SystemExit
----------------------------------------
```
username_1: Again, the issue seems to stem from `signal.signal(signal.SIGALRM, handler)`
On Windows, running:
```
import signal
signal.signal(signal.SIGALRM, handler)
```
as pulled from the [signal module docs](https://docs.python.org/2/library/signal.html) produces an error:
`
AttributeError: 'module' object has no attribute 'SIGALRM'
`
username_1: An attempt was made to move to the `stopit` module and remove the `signal` dependency in https://github.com/oduwsdl/ipwb/commit/7c3076b31d90789a0a860728e866a195a5e07bdd but the timeout is never executed per the `stopit` documentation.
Perhaps a threading-based solution with a Timer would work better but the calls are currently in a god function, so some refactoring should be done to isolate these commands and any commands dependent on the `cat()` results.
username_0: Could you possibly add some kind of a "temporary workaround" for Windows users, say, an option like `--workaround-310-for-windows`, disabling the timeout completely? As of now, this makes a world of a difference for a Windows user, i.e. uncommenting the timeout makes IPWB already a *useful tool* on Windows, while keeping it makes IPWB completely unusable...
username_1: @username_0 Thanks for the continued feedback. I am working on regaining a Windows test environment but for the time being, we may just need conditional functionality within the code until this fix is properly implemented.
username_2: I think the thread approach to fetch the two pieces of data from IPFS and then synchronize those threads would eliminate the need of this alarm. However, in the interim, we can try feature-detect mechanism (potentially by exception handling) to check the presence of `SIGALARM` and silently ignore it if it is not present.
username_1: @username_0 I have added a quick-fix to prevent the SIGNAL module commands from being executed on Windows. The change has been applied to the current master branch and via the latest release. Please give it a try and report back.
username_1: As a followup on @username_2's suggestion, `signal.ALRM` on Windows produces an `AttributeError`. On Mac (and I presume Linux, untested), `signal.SIGALRM` returns an integer.
Similarly, `signal.SIGABRT` produces an integer on both Windows and Mac, so we may be able to try-catch on the call to `signal.SIGALRM` instead of explicitly conditioning on the Windows OS.
This check ought to be moot once we have the threading working correctly to re-enable the timeout functionality for Windows, but I wanted to follow-up on the previous message with the suboptimal OS-check solution. |
nklayman/vue-cli-plugin-electron-builder | 785123270 | Title: Native addon (node-addon-api) not working with webpack.
Question:
username_0: INFO Starting development server...
98% after emitting CopyPlugin
DONE Compiled successfully in 940ms 15.34.24
App running at:
- Local: http://localhost:8080/
- Network: http://192.168.0.101:8080/
Note that the development build is not optimized.
To create a production build, run npm run build.
- Bundling main process...
ERROR Failed to compile with 1 errors 15.34.25
error in ./build/Release/hhhh-native.node
Module parse failed: Unexpected character '�' (1:2)
You may need an appropriate loader to handle this file type, currently no loaders are configured to process this file. See https://webpack.js.org/concepts#loaders
(Source code omitted for this binary file)
@ ./src/cc/hhhh.js 1:14-61
@ ./src/background.js
@ multi ./src/background.js
ERROR Build failed with errors.
npm ERR! code ELIFECYCLE
npm ERR! errno 1
npm ERR! [email protected] electron:serve: `vue-cli-service electron:serve`
npm ERR! Exit status 1
npm ERR!
npm ERR! Failed at the [email protected] electron:serve script.
npm ERR! This is probably not a problem with npm. There is likely additional logging output above.
npm ERR! A complete log of this run can be found in:
npm ERR! C:\Users\Marku\AppData\Roaming\npm-cache\_logs\2021-01-13T13_34_25_092Z-debug.log
`
Here is my project structure:
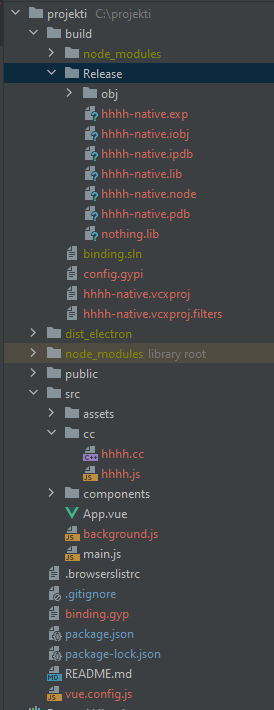
Here is my vue.config.js:
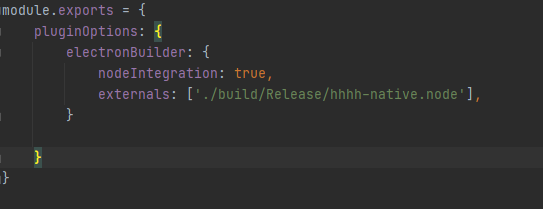
Here is my binding.gyp
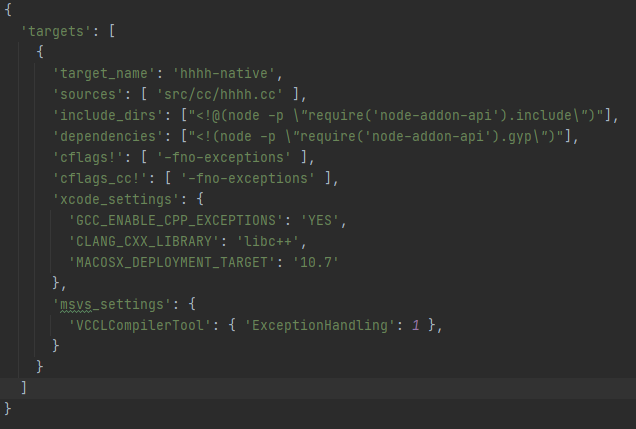<issue_closed>
Status: Issue closed |
Pokebag/pokemon-unite-data | 985365174 | Title: Verify held item changes for Patch 1.1.1.4
Question:
username_0: All held items need to be verified for Patch `1.1.1.4`. For most items, it's likely that no changes will be needed. If an item **was changed** in this patch, a data patch must be created for that item.
- [ ] Aeos Cookie
- [ ] Assault Vest
- [ ] Attack Weight
- [ ] Buddy Barrier
- [ ] Energy Amplifier
- [ ] Exp. Share
- [ ] Float Stone
- [ ] Focus Band
- [ ] Leftovers
- [ ] Muscle Band
- [ ] Rocky Helmet
- [ ] Scope Lens
- [ ] Score Shield
- [ ] Shell Bell
- [ ] Sp. Atk Specs
- [ ] Wise Glasses |
flutter/flutter | 376920458 | Title: Automatic Right-To-Left text alignment detection in Flutter
Question:
username_0: In Android `TextView` somehow detects text direction inside (RTL or LTR) by its language.
In Flutter it depends on current locale.
is there any way to make text aligment automatic per-widget?
i'm filing it as a separate issue because it's different from the one which was described in https://github.com/flutter/flutter/issues/23875
And using the same screenshots:
Flutter
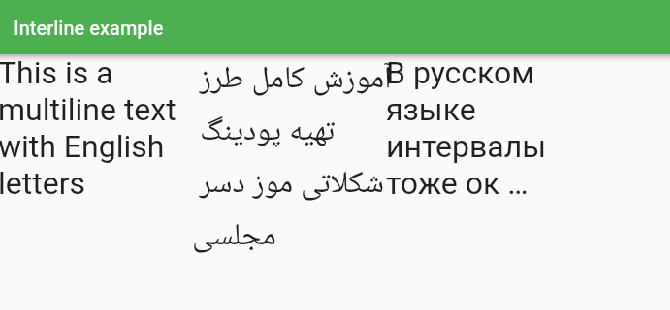
Android
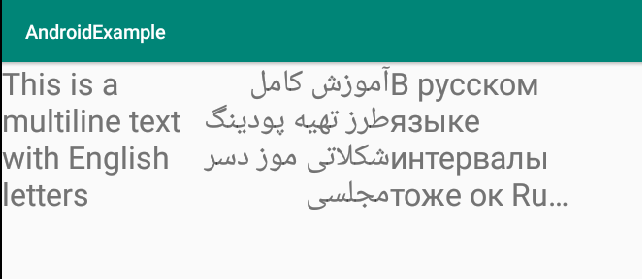
Answers:
username_1: /cc @username_3 - seems strongly related to work you've been doing.
username_2: I wanted the same effect in a textfield where when a user type in a rtl language the direction of the textfield should change, so I made a package that will automatically change the direction based on the text you give it. here is the the package [auto_direction](https://pub.dev/packages/auto_direction)
username_3: After speaking with Hixie about assuming text directions based on content, we came to the conclusion that it is extremely dangerous to do so for generic fields since basic cases like "1902شارع" will be misinterpreted as LTR in Android's way. It is much more reliable to have users or locales specify the direction.
Auto-detection may be applicable in niche circumstances where the directionality is not fixed or in multilingual situations, but we are not planning on implementing it directly within Flutter. It looks like the plugin @username_2 made is a great way to achieve this for the cases where it is necessary (thanks for making it!), though we urge you to fully consider the implications of detecting RTL.
username_3: I'm going to close this for now, but please let me know if you have any additional questions or concerns!
Status: Issue closed
username_4: What about a widget to change alignment from LTR to RTL based on locale?
username_5: I've just started using this package and it seems to do the job well. Thanks @AhmedAlYousif |
pavel-demin/red-pitaya-notes | 128193258 | Title: SDR receiver and SDR#
Question:
username_0: Hello
I'm trying to use the example of SDR receiver and SDR#.
I followed the 'getting stared' instructions and when I run SDR#, Red Pitaya doesn't appears in Source list.
I noticed that there is a plugins.xml in sdr# directory. This file seems to list some sharpPlugins.
Should I add an entry for the plugin in that file? If so, how to proceed? I didn't manage to found out.
Thanks in advance.
Cedric
Answers:
username_1: Hello Cedric,
What SDR# version are you using?
SDR# stopped supporting ExtIO plug-ins in September 2015. The latest version that supports ExtIO plug-ins is 1.0.0.1361. It can be downloaded from the [SDR receiver page](http://username_1.github.io/red-pitaya-notes/sdr-receiver) or directly from [this link](https://googledrive.com/host/0B-t5klOOymMNfmJ0bFQzTVNXQ3RtWm5SQ2NGTE1hRUlTd3V2emdSNzN6d0pYamNILW83Wmc/SDR/sdrsharp_v1.0.0.1361_with_plugins.zip).
Best regards,
Pavel
Status: Issue closed
username_0: Hi
It works with the link.
Thanks a lot |
Signbank/Global-signbank | 564041115 | Title: For ASL Signbank test_DetailViewRenders fails
Question:
username_0: Unsure why this is, because the detail view does render. Error is
```
django.urls.exceptions.NoReverseMatch: Reverse for 'public_gloss' with keyword arguments
'{'dataset_languages': <MultilingualQuerySet [<Language: American English>]>, 'selected_datasets':
<QuerySet [<Dataset: ASL>]>, 'glossid': 3289, 'SHOW_DATASET_INTERFACE_OPTIONS': False}'
not found. 1 pattern(s) tried: ['dictionary/gloss/(?P<glossid>\\d+).html$']
```
Answers:
username_1: This also fails for Global.
username_1: I caused this to break with Issue #608. It's been fixed now.
username_0: Verified that the test does not fail anymore!
Status: Issue closed
|
prometheus/alertmanager | 402308413 | Title: Unable to send email notification without SMTP authentication
Question:
username_0: Alertmanager won't send an email notification if no SMTP authentication options are configured. These messages appear repeatedly in the log file:
```
level=error ts=2019-01-23T15:49:04.898510015Z caller=notify.go:332 component=dispatcher msg="Error on notify" err="missing password for PLAIN auth mechanism; missing password for LOGIN auth mechanism"
level=error ts=2019-01-23T15:49:04.898681067Z caller=dispatch.go:177 component=dispatcher msg="Notify for alerts failed" num_alerts=1 err="missing password for PLAIN auth mechanism; missing password for LOGIN auth mechanism"
```
Config:
```yaml
global:
smtp_from: <my_email>
route:
receiver: root-route-receiver
group_by:
- alertname
group_wait: 2m
group_interval: 1m
repeat_interval: 24h
receivers:
- name: root-route-receiver
email_configs:
- to: <my_email>
smarthost: <my_smtp_host>:25
require_tls: false
```
Noticed this issue after updating to v0.16.0. My same configuration worked on 0.15.1.
Answers:
username_1: This looks related to the changes from #1608 and #1555.
Cc @glefloch @danielkucera @mxinden
username_2: I agree with @username_1's analysis. And after reading [rfc4954](https://tools.ietf.org/html/rfc4954), it isn't mandatory for the client to authenticate even if the SMTP server advertises auth mechanisms. So in case there are no credentials, AlertManager should attempt to send the mail anyway.
username_3: I just saw this, thanks. I can try to update #1713 to add better logging. It's been interesting to learn a bit of Go
Status: Issue closed
username_4: Not sure if it is related. But I have the same issue as OP after upgrading to 0.16.1 from 0.15.1
```bash
level=info ts=2019-03-25T12:40:47.545734791Z caller=silence.go:291 component=silences msg="Running maintenance failed" err="open /alertmanager/silences.395870c1a656c888: permission denied"
level=error ts=2019-03-25T12:41:11.240846092Z caller=notify.go:332 component=dispatcher msg="Error on notify" err="unknown auth mechanism: "
level=error ts=2019-03-25T12:41:11.240995145Z caller=notify.go:332 component=dispatcher msg="Error on notify" err="unknown auth mechanism: "
level=error ts=2019-03-25T12:41:11.241011693Z caller=dispatch.go:177 component=dispatcher msg="Notify for alerts failed" num_alerts=1 err="unknown auth mechanism: ; unknown auth mechanism: "
```
username_5: I have the same error..
level=info ts=2019-03-28T18:45:39.40811949Z caller=silence.go:291 component=silences msg="Running maintenance failed" err="open /alertmanager/silences.58bdc594c6f13e9b: permission denied"
level=error ts=2019-03-28T18:45:39.408236523Z caller=nflog.go:363 component=nflog msg="Running maintenance failed" err="open /alertmanager/nflog.e7b6e793c7034cc: permission denied"
level=info ts=2019-03-28T19:00:39.408124673Z caller=silence.go:291 component=silences msg="Running maintenance failed" err="open /alertmanager/silences.627a80836e089f47: permission denied" |
terascope/teraslice | 634996737 | Title: elasticsearch-state-storage metaKey is not consistently applied
Question:
username_0: A user should be able to use the set_meta_key property on elasticsearch-state-storage to use fields other than `_key` to get and set records in elasticsearch as well as the cache.
But, the metaKey property is not applied everywhere a dataEnity is created so in some instances the metaKey is missing and breaks the state-storage functions - specifically the [_updateCacheWithEs function](https://github.com/terascope/teraslice/blob/master/packages/teraslice-state-storage/src/elasticsearch-state-storage/index.ts#L172)
Perhaps metaKey is the wrong name and it should be metaField, but tests need to be implemented to test this logic as well.
Answers:
username_0: After thinking through this more the problem isn't the creating a data entity but in the getIdentifier function.
I want to retrieve a doc by something other than the _key field of the incoming doc.
For example incoming doc { _key: a, _other_field: b }, retrieved doc { _key: b }. I want to search by _other_field, but I have to save set it in the cache by its _key field.
My solution right now is that mget/ get functions can use other metadata fields for searching, but all functions that set the doc in the cache or in es use the _key field. I think this is correct.
username_1: @username_0 can we close this?
username_0: fixed by pr #1989
Status: Issue closed
|
broadinstitute/cromwell | 264698778 | Title: Custom WOM Call outputs
Question:
username_0: @username_1 commented on [Mon Sep 11 2017](https://github.com/broadinstitute/wdl4s/issues/203)
Wom currently automatically derives call outputs from TaskDefinition.
CWL separately declares call outputs and "run" outputs, which mean the former could be a subset of the latter. Make it so that WOM can support this use case.
Answers:
username_0: @username_1 is this still to-do?
username_1: yep
Status: Issue closed
|
webhippie/homebrew-webhippie | 734692932 | Title: Tap installing error with MacOS Big Sur beta
Question:
username_0: I'm attempting to use this on a MacOS Big Sur (11.0) beta, and when running `brew tap webhippie/webhippie`, I get the following error. I'm by no means a programmer, and am not very familiar with homebrew, and I have no idea how complex the fix would be, but I wanted to give you a heads up
`Error: Invalid formula: /usr/local/Homebrew/Library/Taps/webhippie/homebrew-webhippie/Formula/medialize.rb
medialize: Calling 'devel' blocks in formulae is disabled! Use 'head' blocks or @-versioned formulae instead.
Please report this issue to the webhippie/webhippie tap (not Homebrew/brew or Homebrew/core)
/usr/local/Homebrew/Library/Taps/webhippie/homebrew-webhippie/Formula/medialize.rb:21`
Status: Issue closed
Answers:
username_1: Thanks for the report, with #2 this issue should be resolved. |
kaby76/Piggy | 403866900 | Title: CSharpSerializer + Java
Question:
username_0: Just to be consistent, this is not in the csproj for CSharpSerializer.
```xml
<PropertyGroup Condition="'$(JAVA_HOME)'==''">
<JAVA_HOME>C:\Program Files\Java\jdk-11.0.1</JAVA_HOME>
</PropertyGroup>
<PropertyGroup Condition="'$(Antlr4ToolPath)'==''">
<Antlr4ToolPath>C:\Program Files\Java\javalib\antlr-4.7.2-complete.jar</Antlr4ToolPath>
</PropertyGroup>
``` |
freifunk-ffm/site-ffffm | 148351340 | Title: i18n: Anpassung in 'gluon-config-mode:reboot' wegen ungültigem SSL-Zertifikat von https://ffm.freifunk.net
Question:
username_0: Stable:Test:Dev
Nach dem Flashen der Factory-Firmware erschein eine Infoseite im Webbrowser.
Auf dieser Seite ist ein Link auf https://ffm.freifunk.net gesetzt.
Leider wird das SSL-Zertifikat als nicht vertrauenswürdig eingestuft -> doof!
Anpassen der Texte 'gluon-config-mode:reboot' in
https://github.com/freifunk-ffm/site-ffffm/blob/test/dev/de.po und https://github.com/freifunk-ffm/site-ffffm/blob/stable/i18n/en.po (auch im stable- und test-Branch).<issue_closed>
Status: Issue closed |
newamericafoundation/newamerica-cms | 735353983 | Title: Update to Wagtail 2.11
Question:
username_0: https://docs.wagtail.io/en/stable/releases/2.11.html
Answers:
username_0: This is a priority bc we're experiencing this problem and it seems silly to monkey patch when we can just upgrade https://github.com/wagtail/wagtail/issues/6656
Status: Issue closed
|
tensorflow/community | 433066735 | Title: Import Error: cannot import name 'model_utils'
Question:
username_0: import tensorflow as tf
tf.__version__( got 1.13.1)
import tensorflow_probability as tfp
got error:
File "c:\program files\python36\lib\site-packages\tensorflow_estimator\python\estimator\model_fn.py", line 33, in <module>
from tensorflow.python.saved_model import model_utils as export_utils
ImportError: cannot import name 'model_utils'
basically, there is no model_utils subfolder or file under tensorflow/python/saved_model
thanks.
Answers:
username_1: Workaround: I had a similar issue, resolved it by reinstalling tensorflow-estimator
```python
pip install -I tensorflow-estimator
```
username_2: same problem.
Comes from the tensorflowjs import. It does have a relationship with ‘estimator’, but reinstallation does not solve the problem.
username_3: yes. It shows me a similar error when I use this:
`tf.keras.estimator`
username_4: Apologies, but this issue tracker is for TF Community governance. I encourage you to address questions to the tensorflow/tensorflow issue tracker, <EMAIL> or StackOverflow.
Status: Issue closed
username_5: I had to reinstall tensorflow from 1.13 to 1.14 and that worked for me. |
flutter/flutter | 707224949 | Title: HandshakeException: Connection terminated during handshake
Question:
username_0: a follow up to this issue: https://github.com/flutter/flutter/issues/59878
Ive created a test project: https://github.com/username_0/ps_test
When running the project, this is the result:
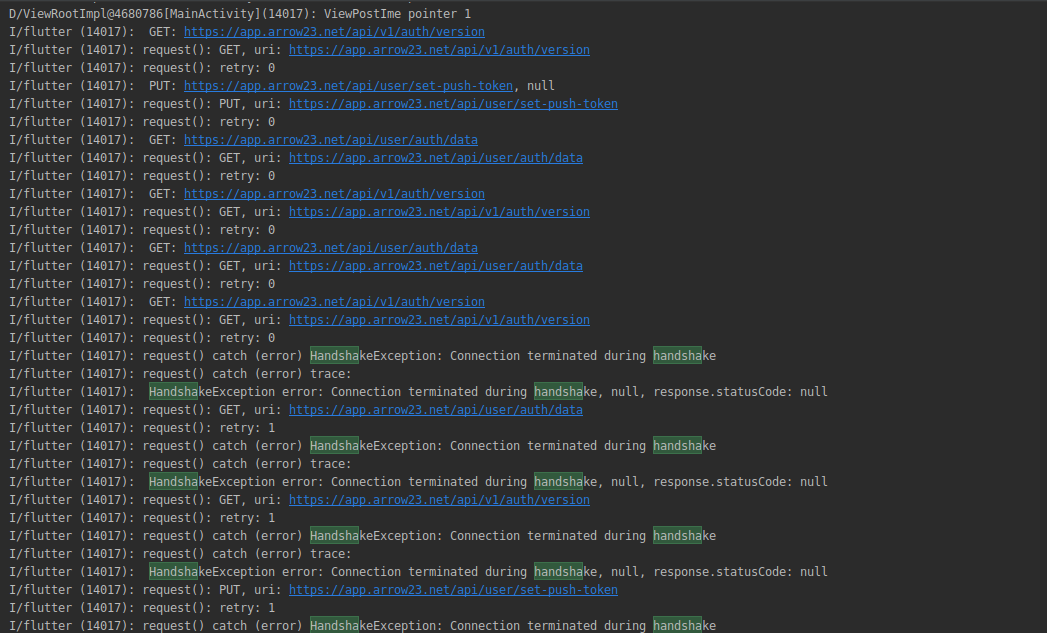
expected: no HandshakeExceptions
Answers:
username_1: Hi @username_0
From what I can see, the issue may be related to the http package rather than to Flutter itself. Please open the issue in the dedicated [repository](https://github.com/dart-lang/http).
Please see if this can help you fix the issue:
https://stackoverflow.com/questions/54285172/how-to-solve-flutter-certificate-verify-failed-error-while-performing-a-post-req
Closing, as this isn't an issue with Flutter itself.
If you disagree, please write in the comments,
providing your `flutter doctor -v`, your `flutter run -v`, your `pubspec.yaml`
a minimal reproducible code sample that does not use 3rd party plugins,
and I will reopen it.
Thank you
Status: Issue closed
username_0: This is not a certificate-verify issue, and its not reproducible within a dart environment, its only reproducible in flutter environment.
So the http team will say let the flutter guys investigate, what then? |
flutter/flutter | 459180032 | Title: Flutter run Error Throws
Question:
username_0: Flutter crash report; please file at https://github.com/flutter/flutter/issues.
## command
flutter run
## exception
ProcessException: ProcessException: Process "/Users/Mac/Dev/apps/android/gradlew" exited abnormally:
Unzipping /Users/Mac/.gradle/wrapper/dists/gradle-4.10.2-all/9fahxiiecdb76a5g3aw9oi8rv/gradle-4.10.2-all.zip to /Users/Mac/.gradle/wrapper/dists/gradle-4.10.2-all/9fahxiiecdb76a5g3aw9oi8rv
Exception in thread "main" java.util.zip.ZipException: error in opening zip file
at java.util.zip.ZipFile.open(Native Method)
at java.util.zip.ZipFile.<init>(ZipFile.java:219)
at java.util.zip.ZipFile.<init>(ZipFile.java:149)
at java.util.zip.ZipFile.<init>(ZipFile.java:163)
at org.gradle.wrapper.Install.unzip(Install.java:214)
at org.gradle.wrapper.Install.access$600(Install.java:27)
at org.gradle.wrapper.Install$1.call(Install.java:74)
at org.gradle.wrapper.Install$1.call(Install.java:48)
at org.gradle.wrapper.ExclusiveFileAccessManager.access(ExclusiveFileAccessManager.java:65)
at org.gradle.wrapper.Install.createDist(Install.java:48)
at org.gradle.wrapper.WrapperExecutor.execute(WrapperExecutor.java:128)
at org.gradle.wrapper.GradleWrapperMain.main(GradleWrapperMain.java:61)
Command: /Users/Mac/Dev/apps/android/gradlew -v
```
#0 runCheckedAsync (package:flutter_tools/src/base/process.dart:255:5)
<asynchronous suspension>
#1 _initializeGradle (package:flutter_tools/src/android/gradle.dart:225:9)
<asynchronous suspension>
#2 _ensureGradle (package:flutter_tools/src/android/gradle.dart:206:37)
<asynchronous suspension>
#3 _readGradleProject (package:flutter_tools/src/android/gradle.dart:134:31)
<asynchronous suspension>
#4 _gradleProject (package:flutter_tools/src/android/gradle.dart:111:34)
<asynchronous suspension>
#5 getGradleAppOut (package:flutter_tools/src/android/gradle.dart:105:29)
<asynchronous suspension>
#6 AndroidApk.fromAndroidProject (package:flutter_tools/src/application_package.dart:154:23)
<asynchronous suspension>
#7 ApplicationPackageFactory.getPackageForPlatform (package:flutter_tools/src/application_package.dart:46:32)
<asynchronous suspension>
#8 FlutterDevice.runHot (package:flutter_tools/src/resident_runner.dart:345:56)
<asynchronous suspension>
#9 HotRunner.run (package:flutter_tools/src/run_hot.dart:253:39)
<asynchronous suspension>
#10 RunCommand.runCommand (package:flutter_tools/src/commands/run.dart:428:37)
<asynchronous suspension>
#11 FlutterCommand.verifyThenRunCommand (package:flutter_tools/src/runner/flutter_command.dart:559:18)
#12 _asyncThenWrapperHelper.<anonymous closure> (dart:async-patch/async_patch.dart:77:64)
#13 _rootRunUnary (dart:async/zone.dart:1132:38)
#14 _CustomZone.runUnary (dart:async/zone.dart:1029:19)
#15 _FutureListener.handleValue (dart:async/future_impl.dart:126:18)
#16 Future._propagateToListeners.handleValueCallback (dart:async/future_impl.dart:639:45)
#17 Future._propagateToListeners (dart:async/future_impl.dart:668:32)
#18 Future._complete (dart:async/future_impl.dart:473:7)
#19 _SyncCompleter.complete (dart:async/future_impl.dart:51:12)
#20 _AsyncAwaitCompleter.complete (dart:async-patch/async_patch.dart:28:18)
#21 _completeOnAsyncReturn (dart:async-patch/async_patch.dart:294:13)
[Truncated]
brew install cocoapods
pod setup
[32m[✓][39m Android Studio (version 3.2)
[32m•[39m Android Studio at /Applications/Android Studio.app/Contents
[32m•[39m Flutter plugin version 29.0.2
[32m•[39m Dart plugin version 181.5540.11
[32m•[39m Java version OpenJDK Runtime Environment (build
1.8.0_152-release-1136-b06)
[32m[✓][39m VS Code (version 1.34.0)
[32m•[39m VS Code at /Applications/Visual Studio Code.app/Contents
[32m•[39m Flutter extension version 2.24.0
[32m[✓][39m Connected device (1 available)
[32m•[39m Custom Phone, 5 1 0, API 22, 768x1280 • 192.168.56.101:5555 • android-x86
• Android 5.1 (API 22)
[33m![39m Doctor found issues in 2 categories.
```
Answers:
username_0: sudo flutter run -v
Password:
Woah! You appear to be trying to run flutter as root.
We strongly recommend running the flutter tool without superuser privileges.
/
📎
[ +85 ms] executing: [/Users/Mac/development/flutter/] git log -n 1
--pretty=format:%H
[ +107 ms] Exit code 0 from: git log -n 1 --pretty=format:%H
[ +2 ms] 7a4c33425ddd78c54aba07d86f3f9a4a0051769b
[ ] executing: [/Users/Mac/development/flutter/] git describe --match
v*.*.* --first-parent --long --tags
[ +15 ms] Exit code 0 from: git describe --match v*.*.* --first-parent --long
--tags
[ +3 ms] v1.5.4-hotfix.2-0-g7a4c33425
[ +49 ms] executing: [/Users/Mac/development/flutter/] git rev-parse
--abbrev-ref --symbolic @{u}
[ +13 ms] Exit code 0 from: git rev-parse --abbrev-ref --symbolic @{u}
[ ] origin/stable
[ ] executing: [/Users/Mac/development/flutter/] git ls-remote --get-url
origin
[ +10 ms] Exit code 0 from: git ls-remote --get-url origin
[ ] https://github.com/flutter/flutter.git
[ +95 ms] executing: [/Users/Mac/development/flutter/] git rev-parse
--abbrev-ref HEAD
[ +12 ms] Exit code 0 from: git rev-parse --abbrev-ref HEAD
[ ] stable
[ +249 ms] executing: /Users/Mac/Library/Android/sdk/platform-tools/adb devices
-l
[ +14 ms] Exit code 0 from: /Users/Mac/Library/Android/sdk/platform-tools/adb
devices -l
[ +1 ms] List of devices attached
192.168.56.101:5555 device product:vbox86p
model:Custom_Phone___5_1_0___API_22___768x1280 device:vbox86p
transport_id:1
[ +16 ms] executing: idevice_id -h
[ +35 ms] /Users/Mac/Library/Android/sdk/platform-tools/adb -s
192.168.56.101:5555 shell getprop
[ +99 ms] Artifact Instance of 'FlutterWebSdk' is not required, skipping
update.
[ +6 ms] Artifact Instance of 'WindowsEngineArtifacts' is not required,
skipping update.
[ +2 ms] Artifact Instance of 'MacOSEngineArtifacts' is not required, skipping
update.
[ +2 ms] Artifact Instance of 'LinuxEngineArtifacts' is not required, skipping
update.
[ +1 ms] Artifact Instance of 'FuchsiaCacheArtifacts' is not required,
skipping update.
[ +409 ms] ro.hardware = vbox86
[ ] ro.build.characteristics = default
[ +63 ms] Launching lib/main.dart on Custom Phone, 5 1 0, API 22, 768x1280 in
debug
mode...
[ +27 ms] Initializing gradle...
[ +9 ms] Using gradle from /Users/Mac/Dev/apps/android/gradlew.
[+2398 ms] executing: /usr/bin/defaults read /Applications/Android
Studio.app/Contents/Info
[ +34 ms] Exit code 0 from: /usr/bin/defaults read /Applications/Android
Studio.app/Contents/Info
[ +1 ms] {
[Truncated]
[ +173 ms] executing: /Users/Mac/Dev/apps/android/gradlew -v
[+1530 ms]
------------------------------------------------------------
Gradle 4.10.2
------------------------------------------------------------
Build time: 2018-09-19 18:10:15 UTC
Revision: b4d8d5d170bb4ba516e88d7fe5647e2323d791dd
Kotlin DSL: 1.0-rc-6
Kotlin: 1.2.61
Groovy: 2.4.15
Ant: Apache Ant(TM) version 1.9.11 compiled on
March 23 2018
JVM: 1.8.0_152-release (JetBrains s.r.o 25.152-b06)
OS: Mac OS X 10.13.6 x86_64
[ +12 ms] Initializing gradle... (completed in 4.2s)
[ ] Resolving dependencies...
[ ] executing: [/Users/Mac/Dev/apps/android/]
/Users/Mac/Dev/apps/android/gradlew app:properties
username_1: Will track here: https://github.com/flutter/flutter/issues/36282
Status: Issue closed
|
VITA-Group/DeblurGANv2 | 963020321 | Title: MobilenetDSC
Question:
username_0: Hello! I've been trying to test out DeblurGANv2 for a personal project and have not been able to find out where the MobilenetDSC.h5 file is located? There's also no link to it in the public github. Has it not been made public yet? |
vanhoefm/krackattacks-scripts | 797151952 | Title: Which CVEs are being tested
Question:
username_0: Hi @username_0
There's 10 CVE's for krack attacks. Are this testing scripts cover all of them except for CVE-2017-13088(WNM)?
_Originally posted by @username_1 in https://github.com/username_0/krackattacks-scripts/issues/61#issuecomment-770014894_
Answers:
username_0: Several of those CVEs are more theoretical or were quite uncommon at the time and there are currently no tests for them. The README mentions which CVEs can be tested using this script.
username_1: So the tests checking only CVE-2017-13080, CVE-2017-13077, CVE-2017-13078?
username_0: Yes.
Also note the reverse situation: the tests `--replay-broadcast`, `--group --gtkinit`, and `--gtkinit` test for vulnerabilities that don't have a separate CVEs but are/were common in practice.
username_1: Understood, thanks
Status: Issue closed
|
lervag/vimtex | 186074895 | Title: Problem with delimiters
Question:
username_0: ### Explain the issue
Since one of the last commits (I am on 7b35d3782ba2284a108dc5ae3bd0b4cfe04a80c1 now) I experience problems when typing delimiters in math-mode.
1. Steps to reproduce
Open the a file with the following content:
`
\documentclass[12pt]{article}
`
\begin{document}
\begin{enumerate}
\item
\end{enumerate}
\end{document}
`
Place the cursor behind \item then type `$(`.
2. Expected behaviour
vim hangs using 100% of the CPU.
3. Observed behaviour
`(` appears.
### Minimal working example
see above
### Minimal vimrc file
Please provide a minimal vimrc file that reproduces the issue. The following
should often suffice:
```vim
set nocompatible
" Load Vimtex
let &rtp = '~/.vim/bundle/vimtex,' . &rtp
let &rtp .= ',~/.vim/bundle/vimtex/after'
" Load other plugins, if necessary
" let &rtp = '~/path/to/other/plugin,' . &rtp
filetype plugin indent on
syntax enable
" Vimtex options go here
```
Answers:
username_1: Yes, recently I have to press `^C` to interrupt the plugin and input something else, or I have to type `$$` first, and then move the cursor between two `$` symbols to type the content of math equations.
username_2: I get this as well, makes the plugin effectively unusable for me.
Status: Issue closed
username_3: Sorry about that. I think it is fixed now, please test.
username_2: Thanks, it doesn't hang for anywhere near as long now but there's still about a 0.5s delay for me every time I type any delimiter character e.g. `}`
username_3: Good to hear it's getting better!
In order for me to investigate and (perhaps) improve the efficiency further, could you please open a new issue, again with a proper minimal working example? That would be very helpful for me to investigate the mentioned delays. Note that I currently do not experience any such delays while writing myself. This could be because of faster hardware, but I think it might rather be because I hardwrap my lines (i.e. I only have short lines), or because I currently don't have that much math in my documents.
So, to summarize: Please open new issues with good MWEs to help me further investigate the delays. |
foodfight/foodfight.github.com | 312703207 | Title: STELLA Report - <NAME>'s suggested prescription for engaging with the STELLA Report
Question:
username_0: 1. Watch <NAME> - 2017 Velocity Talk: https://www.snafucatchers.com/single-post/2017/10/29/Velocity-NYC-2017-and-the-Stella-Report
2. Read Report: https://drive.google.com/file/d/0B7kFkt5WxLeDTml5cTFsWXFCb1U/view
3. <NAME> DevOps Day Detroit: https://www.youtube.com/watch?v=4jRqtciRQds |
Automattic/mongoose | 154934420 | Title: Mongoose not shutting down gracefully (on initial connection when ECONNREFUSED)
Question:
username_0: I have come into the issue of where scripts/tests won't finish/exit when the database is unavailable prior to running the script/test. Possibly related issue: https://www.bountysource.com/issues/1384221-mongoose-doesn-t-shut-down-gracefully
**Basic Example:**
```javascript
var connection = mongoose.createConnection('mongodb://localhost:27017/');
connection.on('error', function (err) {
console.log(err);
});
setTimeout(function () {
connection.close();
}, 2000);
```
If the above is run when there is a running MongoDB server, the script will exit after 2000 milliseconds, as intended.
If the above is run when there is no running MongoDB server, the script will hang for about 30 seconds (I'm assuming this is some timeout?) then the script will end.
I have tried `mongoose.disconnect()` and removing all the listeners on `connection` and `connection.db`, but they do not appear to have any effect.
How to prevent mongoose delaying the graceful termination of the script?
Answers:
username_1: Which version of mongoose? The below script executes as expected for me when no mongodb running, exits immediately:
```javascript
'use strict';
var assert = require('assert');
var mongoose = require('mongoose');
var Schema = mongoose.Schema;
var connection = mongoose.createConnection('mongodb://localhost:27017/');
connection.on('error', function (err) {
console.log(err);
});
setTimeout(function () {
connection.close();
}, 2000);
```
```
$ node gh-4151.js
{ Error: connect ECONNREFUSED 127.0.0.1:27017
at Object.exports._errnoException (util.js:896:11)
at exports._exceptionWithHostPort (util.js:919:20)
at TCPConnectWrap.afterConnect [as oncomplete] (net.js:1073:14)
name: 'MongoError',
message: 'connect ECONNREFUSED 127.0.0.1:27017' }
```
If the script hangs for about 30 seconds, I suspect you're hitting the `server.reconnectTries` and `server.reconnectInterval` limit, in which case you can try shutting off the `autoReconnect` option: http://mongoosejs.com/docs/connections.html
Status: Issue closed
|
mprokopov/docker-manager.io | 201464801 | Title: doesn't seem to work with current version of ManagerServer.exe
Question:
username_0: just now I did this
```
docker pull username_1/manager.io
docker run --rm username_1/manager.io
Manager Server [Version 16.12.31]
Copyright (c) 2016 NGSoftware. All rights reserved.
Syntax:
mono ManagerServer.exe [options]
Options:
-port [number] Set port on which HTTP server should listen on.
-path [directory] Set directory where Manager should look for data.
Examples:
mono ManagerServer.exe
mono ManagerServer.exe -port 80
mono ManagerServer.exe -port 80 -path "/root/.local/share/Manager"
[02:30:54] The type initializer for 'SQLite.SQLiteConnection' threw an exception. (TypeInitializationException)
```
This doesn't seem to be related to not specifying a volume.
I tried rebuilding the Dockerfile using 'FROM mono:latest' but that didn't work..
Answers:
username_1: That's due to issue with new sqlite library and 86/64-bit versions.
Let me check it once more.
username_1: Issue was due to wrong libe_sqlite3 library, so I've compiled manually libe_sqlite3.so and included in image.
wget -O https://raw.githubusercontent.com/ericsink/SQLitePCL.raw/master/sqlite3/sqlite3.c
gcc -shared -fPIC -O -DNDEBUG -DSQLITE_DEFAULT_FOREIGN_KEYS=1 -DSQLITE_ENABLE_FTS3_PARENTHESIS -DSQLITE_ENABLE_FTS4 -DSQLITE_ENABLE_COLUMN_METADATA -DSQLITE_ENABLE_JSON1 -DSQLITE_ENABLE_RTREE -o libe_sqlite3.so sqlite3.c
Status: Issue closed
username_0: thanks a lot of looking into this so quickly, it works now..
username_2: I'm going to temporarily remove 32-bit support from Linux version. Manager will be distributed with 64-bit Sqlite for the time being on Linux.
I've created an issue in related project to see if Sqlite binary can be distributed side-by-side for both 32-bit and 64-bit Linux.
https://github.com/ericsink/SQLitePCL.raw/issues/113 |
pulibrary/pulfalight | 693479778 | Title: Integrate the Universal Viewer into the Component Show View
Question:
username_0: This was not ported when the new design implementation for the Component Show View was merged.
Answers:
username_0: There is also behavior which should be ported from PULFA 2.0, specifically in which digital objects dynamically update the state of the UV.
username_1: Closed by #369, split out #389
Status: Issue closed
|
home-assistant/android | 684689741 | Title: No icons in widgets
Question:
username_0: <!-- READ THIS FIRST:
- Make sure you run the latest version of the Android app
- Make sure you run the latest version of Home Assistant
- Make sure to check the Companion docs for troubleshooting and configuration: https://companion.home-assistant.io/
DO NOT DELETE ANY TEXT from this template! All requested information is important.
-->
**Home Assistant Android version:**
2.2.1-274-full
**Android version:**
10
**Phone model:**
Pixel 2 XL
**Home Assistant version:**
0.114.3
**Last working Home Assistant release (if known):**
N/A
**Description of problem:**
Widgets never have icons but otherwise work perfectly
**Traceback (if applicable):**
N/A
**Screenshot of problem:**
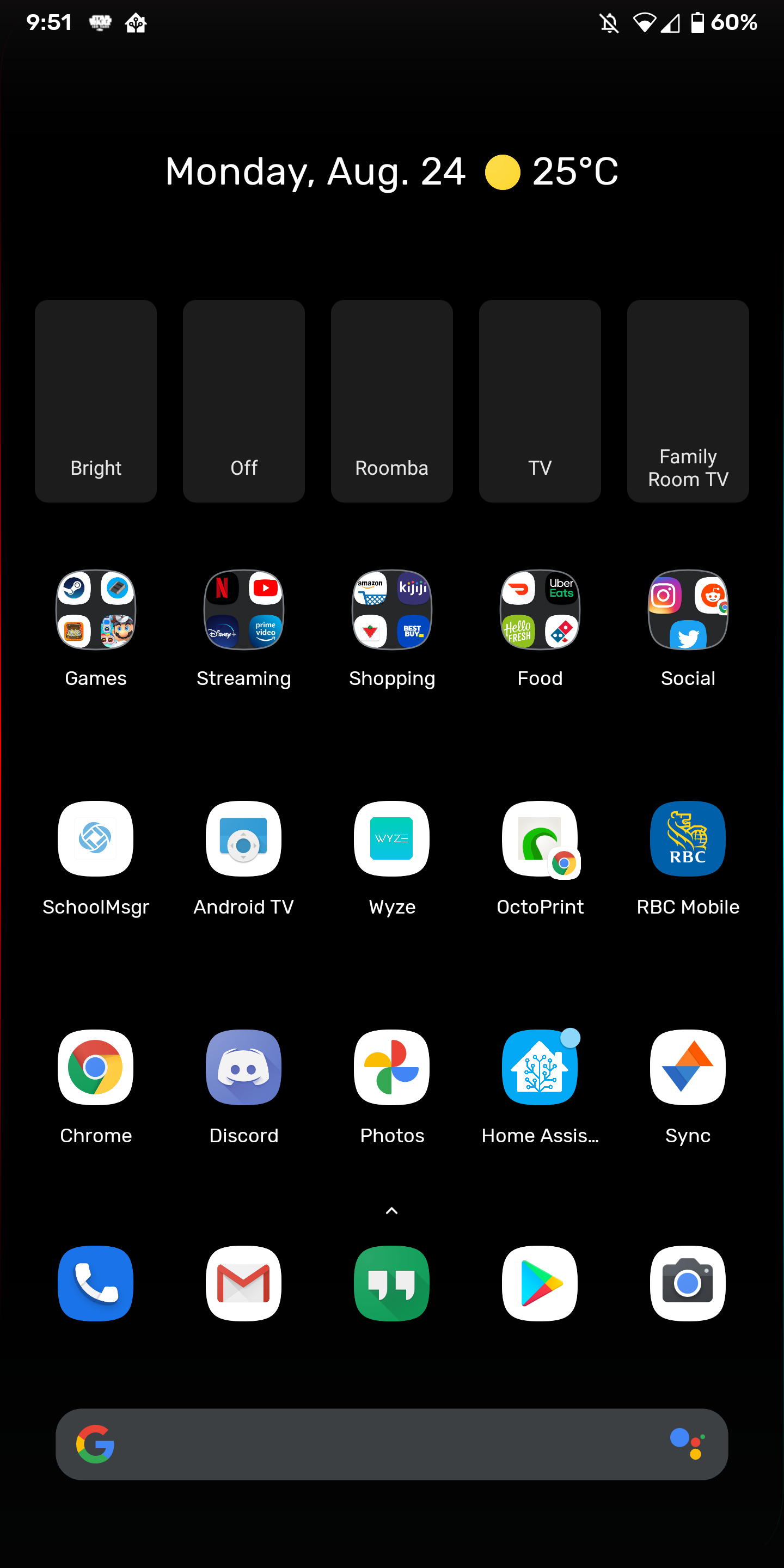
**Additional information:**
Icons were present upon initial setup of widget but dissapeared upon phone restart and have never come back!
Answers:
username_1: Were these widgets created before or after we added the material icon list? I personally have not seen the issue where they disappear upon restart. I wonder if they just need to be recreated to persist?
username_0: Yupp, looks like problem is solved with new update. Thanks guys!
Status: Issue closed
|
MicrosoftDocs/dynamics-365-customer-engagement | 371995259 | Title: Planning for transition (detailed roadmap?)
Question:
username_0: It's proving difficult to plan for the switch to UUI since there is insufficient detail on the timescale and scope of planned feature parity with Web Client. Is there a better resource than this current page to help understand which items are planned for support in UUI and some indication of planned timescale for that support? I understand not wanting to commit to deadlines, but speaking in terms of weeks, months or quarters is enough to arm system customisers with the info to plan.
For example, Xrm.Panel.loadPanel() is not supported in UUI yet. I'm mid-project and this remains the best way for me to deliver a great user experience. If it will never make it to UUI then I can plan accordingly today rather than perpetually hoping that it magically appears.
---
#### Document Details
⚠ *Do not edit this section. It is required for docs.microsoft.com ➟ GitHub issue linking.*
* ID: 9ab324e0-f5c6-64cb-d93b-85d238bf25d3
* Version Independent ID: 94fbb660-5300-51a1-9c98-4b6500800e29
* Content: [About Unified Interface Dynamics 365 Customer Engagement](https://docs.microsoft.com/en-us/dynamics365/customer-engagement/admin/about-unified-interface#capabilities-not-yet-on-unified-interface)
* Content Source: [ce/admin/about-unified-interface.md](https://github.com/MicrosoftDocs/dynamics-365-customer-engagement/blob/master/ce/admin/about-unified-interface.md)
* Service: **crm-online**
* GitHub Login: @username_2
* Microsoft Alias: **username_2**
Answers:
username_1: I agree, it becomes extremely difficult to plan for best solutions/approaches within projects not knowing what will change and when
Status: Issue closed
username_2: Thanks for the feedback. This has been passed on to the product team. One response was: UCI already supports Xrm.Panel.loadPanel().
username_2: A second response from product team: Please ask the customer to explore [Channel Integration Framework](https://docs.microsoft.com/dynamics365/customer-engagement/developer/channel-integration-framework/channel-integration-framework), to work with the panel in UCI. This provides them lot more than what they can do with the web client panel.
username_0: Thanks for the additional feedback Jim. Sadly the CIF is aligned to specific use cases (inline integrated comms) whereas I'm using the panel to load a web resource with dynamic content based on view selections and I can't see any CIF interface that would allow me to do this. Following your first comment, I need to revisit this in UCI as I believe I experienced buggy behaviour when changing URLs and docs for that function confirmed "web client only" which I may have misinterpreted as not supported in UCI".
The main point of my original comment though was to query whether this page (intends to) provide the "best" information on UCI feature parity/timing or should we be looking elsewhere for such detail? |
simple-icons/simple-icons | 551798504 | Title: WP-Rocket
Question:
username_0: Name: WP-Rocket
Website: https://wp-rocket.me/
**Official resources for icon and color:**
Color: #F56640
Main SVG Logo: https://v3b4d4f5.rocketcdn.me/wp-content/themes/wp-rocket/assets/images/logo-light.svg
Answers:
username_1: *Alexa Rank:** [~44.5k](https://www.alexa.com/siteinfo/wp-rocket.me)
username_1: 3 options here based off [this SVG](https://v3b4d4f5.rocketcdn.me/wp-content/themes/wp-rocket/assets/images/logo-dark.svg) from the website footer, each with a different depth of flame. My vote would go to number 2.
For reference the SVG they use for Safari can be found at https://v3b4d4f5.rocketcdn.me/wp-content/themes/wp-rocket/assets/favicons/safari-pinned-tab.svg
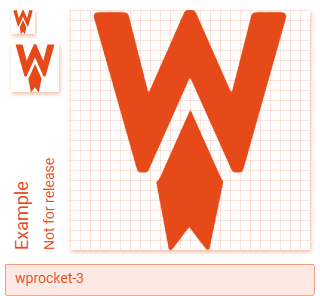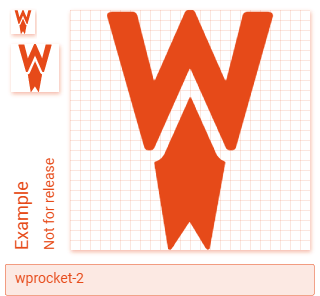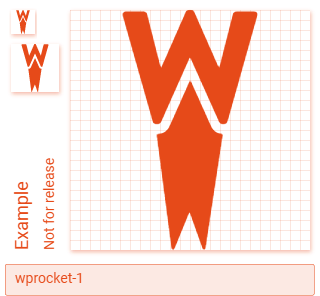
username_0: Awesome!
I also do think that #2 is best.
Thanks!
Status: Issue closed
|
cfpb/hmda-frontend | 555702566 | Title: Questions hyperlink should open an email message
Question:
username_0: The HMDA Platform homepage https://ffiec.cfpb.gov/filing/2019/ has a hyperlink at the bottom of the page entitled "Questions?". Upon clicking the hyperlink, nothing happens. Seems that the hyperlink should link to an email message to <EMAIL>.
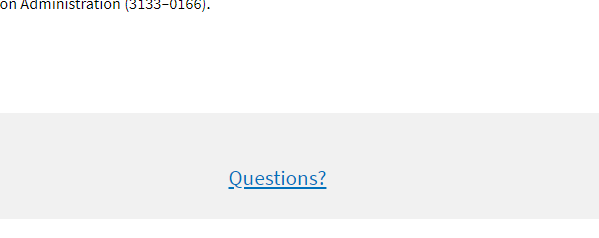
Answers:
username_1: For me this link does open a new email message in my mail app, addressed to <EMAIL>.
This could simply be a matter of end user configuration in terms of how their browser is handles email links.
username_0: Interesting @username_1, this doesn't open for me on google chrome at all (on both my machines) & upon selecting it in IE, i receive this message:
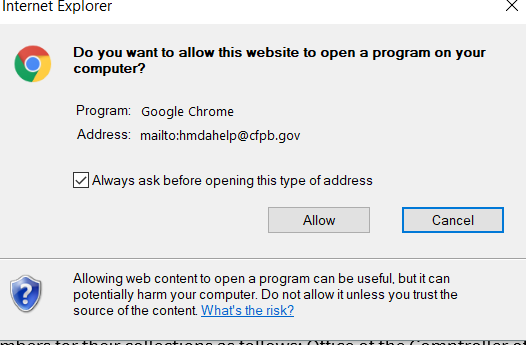
When I select Allow, it takes me to Google Chrome in an empty window.
Status: Issue closed
username_2: Yeah the `mailto:` link is set up correctly, chynna you may not have gmail configured as a mailto handler in chrome://settings/handlers and/or no default mail client configured on your computer. |
weka-2016/weka-2016 | 180535868 | Title: 2.5 Pay off some technical debt
Question:
username_0: # 2.5 Pay off some technical debt
This assignment combines:
- HTML and CSS best practices
- GitHub branching, merging and pull requests
Let's pretend that [CSS Zen Garden](http://www.csszengarden.com/) want to add some more features to their homepage.
Let's also pretend that the junior developer Sam who wrote the HTML for their page didn't have the habit of being tidy.
Before Zen Garden can add any features, they need to clean up their HTML indentation so they can see where and how to add new elements, and make sure they don't make the mess worse.
## 1. Pay off Sam's technical debt
- [ ] Start your Toggl timer.
- [ ] In Github, create a new repo called `sprint-2`.
- [ ] Clone the repo down to the EDAworkspaces directory in your VM.
- [ ] Checkout a new branch called `tidying` - this time, you'll not be pushing to the master branch. The HTML you'll be cleaning is messy, but it works. Zen Garden want to review your work to check you've not broken anything before they merge into the master branch.
- [ ] In your sprint-2 repo, add a file called `pristine-zen-garden.html`.
- [ ] Copy the [messy zen-garden html](https://github.com/dev-academy-programme/curriculum/blob/master/resources/html-with-technical-debt-CHALLENGE/zen-garden-with-debt.html) into `pristine-zen-garden.html`.
- [ ] Tidy all the indentation. There are some parts of the HTML that you'll likely not understand yet, that's ok. Your objective is just to sort out the indentation of the elements to reveal the structure of the HTML content.
- [ ] Push your `tidying` branch to GitHub.
- [ ] In Github, create a 'pull request' from your `tidying` branch to the `master` branch, with a description of the tidying you've done. Don't accept your own pull request!
- [ ] Add everyone in your cohort as collaborators to your `sprint-2` repo:
- Go to your sprint-2 repo in GitHub
- Click the settings tab up the top
- In the left nav, click 'Collaborators'
- Find all the GitHub usernames of your cohort from the students.json file in the root of your cohort repo - `www.github.com/dev-academy-programme/`yourcohort-year`/`
- Add each GitHub user as a collaborator to your `sprint-2` repo
- Your cohort now has 'write access' to your `sprint-2` repo
- [ ] Post a link to your pull request in your cohort's Slack channel with the hashtag #debtpaid.
## 2. Reflection
- [ ] Get the time it took to get to complete section '1. Pay off Sam's technical debt' from your toggl timer. At $30/hr, how much did this little piece of technical debt cost Zen Garden? Post the $ cost in the comments section below. How much would this have cost if the code was built by a person with tidy habits?
- [ ] Sam wasn't tidy with indentation, but miraculously Sam built beautifully structured 'semantic' HTML (normally poor structure flows from poor indentation - the resulting poor structure creates much more severe technical debt). Take 5 minutes to review Sam's HTML.
## 3. Roleplay
Let's pretend that you're now the technical lead on the Zen Garden 'spring tidy' project. Your job is to review 'pull requests' - to check the code is up to standard and still functioning before it is accepted onto the master branch.
- [ ] Find one other person's pull request in your cohort's Slack channel by searching for #debtpaid.
- [ ] Read through their submitted HTML code.
- [ ] If you see errors, make a comment on the pull request, asking them to resolve the issue you see and to mention you when they have pushed up a commit that resolves the issue you raised.
- [ ] Wait for a GitHub notification from them, review the pull request, and accept it if the indentation is perfect.
- [ ] Tidy their repo by deleting the `tidying` branch (they'll still have a copy locally if they need it).
Answers:
username_1: It took me 40 Minutes so if he had been tidy it would have saved zengarden $22.50
Status: Issue closed
|
QubesOS/qubes-issues | 387424554 | Title: qubes ctrl-shift-c/v collides with terminal c&p
Question:
username_0: Qubes 4.0, maybe also older.
There was already #3583 discussing this, but I'ld like to suggest 2 changes to finally resolve it and avoid having people stumble over it again and again:
@marmarek mentioned "Try Ctrl+Ins and Shift+Ins. Works for me." - it also works for me, so could go into FAQ?
About `/etc/qubes/guid.conf`:
- as people end up there, wanting to change keys, mentions ctrl-ins / shift-ins there also, so maybe they don't even need to change after reading it there.
- if there is really some need to change the keys, try and error there is rather tedious. as i did not know how to make changes in there apply without rebooting, i did reboot rather often until i found `Mod4-c` is the config value needed for "Windows-Key + c". xev names that key Super_L so it's not helpful here.
so, mention the valid names of the modifier keys there?
Answers:
username_0: key names: "Ctrl", "Shift", "Mod4" (Windows key), ... (add more verified key names)
username_0: Proposed text for the config:
```
# Before changing secure_(copy|paste)_sequence below because you need
# Ctrl-Shift-(c|v) for normal terminal copy&paste, please note that you
# can also use Ctrl-Ins and Shift-Ins for that (thus: no need to change).
# If you still want to change, here is a list of valid modifier key names:
# "Ctrl", "Shift", "Mod4" (Windows key)
```
username_1: The documentation is a community effort. Please help us improve it by submitting a pull request:
https://www.qubes-os.org/doc/doc-guidelines/
username_0: @username_1 you have that on a F-key or so? :)
I'll do, just wanted to discuss it here first to get some feedback.
username_2: I've always wondered, why is the default ctrl-shift-c? Why default to something that conflicts with terminal copy?
Wouldn't Super-v, Super-c be better? - It's memorable, it requires 2 keystrokes instead of 3, keys are close together (as opposed to Ctrl-Ins) and close to Ctrl-v/Ctrl-c (which you usually press soon after), and no conflict with keyboard shortcuts (usually Ctrl/Shift).
username_1: I have it as a "saved reply," because everyone suggests and requests documentation changes, while very few people actually submit them. Maybe I should say, "Be the change you want to see in the documentation." :slightly_smiling_face:
username_3: hi,
what terminal are you using that it conflicts with ctrl-shift-c?
--
cheers,
Holger
-------------------------------------------------------------------------------
holger@(debian|reproducible-builds|layer-acht).org
PGP fingerprint: B8BF 5413 7B09 D35C F026 FE9D 091A B856 069A AA1C
username_4: Both Konsole and gnome-terminal use Ctrl-Shift-(c|v) .
As to changing the default in Qubes, I guess any change will conflict with *someone*.
I wouldn't favour making the Qubes combo easier though, so @username_2 proposal doesn't work for me.
username_1: We've already stated that we won't be changing the default keyboard shortcuts. Users are always free to [configure them](https://www.qubes-os.org/doc/config-files/) on their own systems. The defaults are so firmly established in Qubes by this point that the costs of changing them would outweigh any potential benefits.
username_5: This pull request addresses the comment by @username_0
https://github.com/QubesOS/qubes-gui-daemon/pull/33
Additional documentation elsewhere may still be appropriate
username_6: Note that even though Ctrl+Ins and Shift+Ins works, the edit menu of gnome-terminal (the default) still shows the user "Shift+Ctrl+C" and "Shift+Ctrl+V" as being the keybinding. A new user should still be expected to believe what the menu is telling it.
Also note that I tried using edit->properties->shortcuts and changing the keys to "Ctrl+Insert" and "Shift+Insert" *does* display the correct keybinding then in the terminal edit menu, meaning this should be possible to fix by changing the default keybindings for the terminal.
username_1: Sounds like a gnome-terminal bug.
username_6: If you mean bug in the general sense that it's specific to gnome-terminal configuration then yes. In case this matters (and in case you don't already know this): the "official" keybindings are "Shift-Ctrl-C". This is what is in the default configuration on the "shortcuts" configuration screen. the Ctrl-Insert keybinding seems to be a "secret" *second* keybinding that does not show up on the shortcuts configuration screen. (i say "secret" cause it does not even appear on the gnome-terminal copy paste documentation at https://help.gnome.org/users/gnome-terminal/stable/txt-copy-paste.html )
Anyway, I was just proposing that if gnome-terminal is the default qubes terminal, and the default gnome-terminal keybinding of Shift-Ctrl-C does not work, that maybe we should set the default gnome-terminal configurable keybinding to something, giving people a chance to find it when they browse the menus.
Anyway, if someone wants to tell me where a new appVM qube gets its /home/user data to initialize the qube (I read that it does *not* copy the /home/user data from the template), I could take a shot at making a pull request for it.
username_1: /etc/skel
This is covered in the template documentation in further detail.
username_6: Thanks!
After some research i found gnome does not use files and must be run as commands. I did find the commands. They are:
```
dconf write /org/gnome/terminal/legacy/keybindings/copy "'<Primary>Insert'"
dconf write /org/gnome/terminal/legacy/keybindings/paste "'<Shift>Insert'"
```
However, if we are going to set the default, we might want to set it to something easier to use like:
```
dconf write /org/gnome/terminal/legacy/keybindings/copy "'<Primary><Super>C'"
dconf write /org/gnome/terminal/legacy/keybindings/paste "'<Primary><Super>V'"
```
So I have 2 questions:
-1- What should we set the default binding to?
-2- Since it's a command and not just a file, is putting commands in .profile to set this up on first login a ok thing to do, or is that inappropriate?
username_7: In my opinion it would make most sense for Qubes to switch to "Windows-Key/Super" for its actions, because "Ctrl+Shift+c/v" is also used in other apps. Some key combinations with "Windows-Key/Super" that might come in handy:
- copy to secure clipboard "Super+c" (`/etc/qubes/guid.conf: secure_copy_sequence = "Mod4-c";`)
- paste to secure clipboard "Super+v" (`/etc/qubes/guid.conf: secure_paste_sequence = "Mod4-v";`)
- tile window to the left "Super+Left" (Settings Manager/Window Manager/Keyboard)
- tile window to the right "Super+Right" (Settings Manager/Window Manager/Keyboard)
- maximize window "Super+Up" (Settings Manager/Window Manager/Keyboard)
- shade window "Super+Down" (Settings Manager/Window Manager/Keyboard)
An alternative solution would also be to pass consecutive key presses of "Ctrl+Shift+c/v" down to the VM. This could be implemented by comparing the secret clipboard contents with what you want to copy (if same content, keys are passed to VM) or if there is nothing to paste (if clipboard empty, keys are passed to VM).
username_1: https://github.com/QubesOS/qubes-issues/issues/4573#issuecomment-445100007
username_7: What about the idea that consecutive key presses within a short time period get passed down to the VM? The user experience would look like this:
- copy from terminal: Select text, press "Ctrl+Shift+c" twice within 500ms (first press would be an unnecessary secure copy, second press would be passed to the terminal to copy), press "Ctrl+Shift+c" again to perform secure copy
- paste into terminal: Press "Ctrl+Shift+v" twice within 500ms (first press would perform secure paste, second press would be passed to the terminal to paste)
After looking at the code (https://github.com/QubesOS/qubes-gui-daemon/blob/master/gui-daemon/xside.c#L991) this shouldn't be too difficult to implement. It would require extending `Ghandles` (adding a clipboard paste xevent timestamp and a flag to handle KeyRelease when pass-through gets triggered) and adding a configuration option for the pass-through interval into `guid.conf`.
username_6: If we assume that changing the default is not a option, then in my opinion it would make the most sense to create some kind of *standard* for a "alternate sequence". By this I mean that people who want something other then shift-control-c still have to manually change it on their own in in /etc/qubes/guid.conf, but at least we all change it to the same thing!
This basically just becomes a change in documentation.
For a initial proposal for standardized alternate keys, I would propose @username_7 's idea of:
* copy to secure clipboard "Super+c" (/etc/qubes/guid.conf: secure_copy_sequence = "Mod4-c";)
* paste to secure clipboard "Super+v" (/etc/qubes/guid.conf: secure_paste_sequence = "Mod4-v";)
If this seems reasonable to others, I can change my personal settings to try these out and let everyone know if they are actually as convenient as they sound or if there are any issues with those particular keys.
(sorry for the delay in this response)
username_6: I just tried to do a fork to create a proposed alternate in the comments of guid.conf, but found this:
# EDITING THIS FILE WILL NOT HAVE ANY EFFECT! It is not read by the GUI daemon
# anymore, and is left here for reference only. To configure the GUI daemon,
# use qvm-features (see 'man qvm-features' for details).
So It's not obvious to me where to document it anymore.
username_1: Why?
username_4: In the docs and in 'man qvm-features' for 4.1 , but I don't know what it
is you want to document.
I don't see particular value in proposing an alternative standard - as
was hashed over before, any proposed key combos will affect *someone*.
Leave it to users to choose one that works for them.
username_6: This is basically fixed in 4.1rc1
In 4.1rc1, going to "global qubes settings", has a option to select between control-shift-v and control-Meta-v .
Other then getting the message to people of where this setting is (I.E. documentation), I propose we can close this issue?
username_1: Sounds good. Closing as resolved. If anyone believes this issue is not yet resolved, or if anyone is still affected by this issue, please leave a comment, and we'll be happy to reopen it. Thank you.
Status: Issue closed
|
SignalK/signalk-java | 217083424 | Title: Chart Uploader: java.lang.NullPointerException
Question:
username_0: Attempting to upload charts fails with no user feedback via web interface. I've attempted selecting one file or multiple zip files generated by freeboard-installer.
logs follow:
```
19:42:05.909 [qtp6313372-4228 - /signalk/v1/upload] ERROR nz.co.fortytwo.signalk.processor.UploadProcessor - null
java.lang.NullPointerException
at nz.co.fortytwo.signalk.processor.UploadProcessor.install(UploadProcessor.java:167) [signalk-server-java-JIT-Deploy-30.jar:?]
at nz.co.fortytwo.signalk.processor.UploadProcessor.processUpload(UploadProcessor.java:138) [signalk-server-java-JIT-Deploy-30.jar:?]
at nz.co.fortytwo.signalk.processor.UploadProcessor.process(UploadProcessor.java:101) [signalk-server-java-JIT-Deploy-30.jar:?]
at org.apache.camel.processor.DelegateSyncProcessor.process(DelegateSyncProcessor.java:63) [camel-core-2.15.2.jar:2.15.2]
at org.apache.camel.management.InstrumentationProcessor.process(InstrumentationProcessor.java:77) [camel-core-2.15.2.jar:2.15.2]
at org.apache.camel.processor.RedeliveryErrorHandler.process(RedeliveryErrorHandler.java:448) [camel-core-2.15.2.jar:2.15.2]
at org.apache.camel.processor.CamelInternalProcessor.process(CamelInternalProcessor.java:191) [camel-core-2.15.2.jar:2.15.2]
at org.apache.camel.processor.Pipeline.process(Pipeline.java:118) [camel-core-2.15.2.jar:2.15.2]
at org.apache.camel.processor.Pipeline.process(Pipeline.java:80) [camel-core-2.15.2.jar:2.15.2]
at org.apache.camel.processor.CamelInternalProcessor.process(CamelInternalProcessor.java:191) [camel-core-2.15.2.jar:2.15.2]
at org.apache.camel.component.jetty.CamelContinuationServlet.service(CamelContinuationServlet.java:162) [camel-jetty-common-2.15.2.jar:2.15.2]
at javax.servlet.http.HttpServlet.service(HttpServlet.java:668) [geronimo-servlet_3.0_spec-1.0.jar:1.0]
at org.eclipse.jetty.servlet.ServletHolder.handle(ServletHolder.java:684) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.servlet.ServletHandler.doHandle(ServletHandler.java:503) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.server.session.SessionHandler.doHandle(SessionHandler.java:229) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.server.handler.ContextHandler.doHandle(ContextHandler.java:1086) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.servlet.ServletHandler.doScope(ServletHandler.java:429) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.server.session.SessionHandler.doScope(SessionHandler.java:193) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.server.handler.ContextHandler.doScope(ContextHandler.java:1020) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.server.handler.ScopedHandler.handle(ScopedHandler.java:135) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.security.SecurityHandler.handle(SecurityHandler.java:533) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.server.handler.HandlerWrapper.handle(HandlerWrapper.java:116) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.server.handler.ResourceHandler.handle(ResourceHandler.java:386) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.security.SecurityHandler.handle(SecurityHandler.java:533) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.server.handler.HandlerWrapper.handle(HandlerWrapper.java:116) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.server.Server.handle(Server.java:366) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.server.AbstractHttpConnection.handleRequest(AbstractHttpConnection.java:494) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.server.AbstractHttpConnection.content(AbstractHttpConnection.java:982) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.server.AbstractHttpConnection$RequestHandler.content(AbstractHttpConnection.java:1043) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.http.HttpParser.parseNext(HttpParser.java:865) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.http.HttpParser.parseAvailable(HttpParser.java:240) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.server.AsyncHttpConnection.handle(AsyncHttpConnection.java:82) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.io.nio.SelectChannelEndPoint.handle(SelectChannelEndPoint.java:696) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.io.nio.SelectChannelEndPoint$1.run(SelectChannelEndPoint.java:53) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.util.thread.QueuedThreadPool.runJob(QueuedThreadPool.java:608) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at org.eclipse.jetty.util.thread.QueuedThreadPool$3.run(QueuedThreadPool.java:543) [jetty-all-8.1.16.v20140903.jar:8.1.16.v20140903]
at java.lang.Thread.run(Thread.java:745) [?:1.8.0_65]
```
Answers:
username_1: Ive seen this before, its looking for the `Metadata.scale` entry in `tilemapresource.xml`
This is used to layer the charts so most detail goes on top.
Can you confirm you used the latest freeboard-installer pls? The early ones didnt have this.
Also some details on the KAP file you processed.
username_0: These were ZIP files I built with freboard-installer last year and used successfully with the original freeboard project. I'll attempt to re-convert them and try again.
username_0: re-converted: NOAA RNC (KAP) http://www.charts.noaa.gov/RNCs/Agreement.shtml?18652
It seems to be uploading the files now.
Status: Issue closed
|
symfony/symfony | 278485521 | Title: [DI] Local service binding conflict
Question:
username_0: | Q | A
| ---------------- | -----
| Bug report? | yes/no?
| Feature request? | no
| BC Break report? | no
| RFC? | no
| Symfony version | 3.4.0
```yaml
services:
_defaults:
bind:
$debug: '%kernel.debug%'
```
```php
use App\Debug\Debug;
class Foo
{
public function __construct(Debug $debug) {}
}
```
`
Type error: Argument 1 passed to Foo::__construct() must be an instance of App\Debug\Debug, bool given
`
Class `Debug` also exist in container. This is normal behavior or maybe it need to compare types between bind and type hinting?
Answers:
username_1: name-based bindings are winning over typehint-based autowiring
username_2: Yes, that's expected, as explained by @username_1.
Use more specific bindings.
Status: Issue closed
|
vuejs/vetur | 731026589 | Title: me too,Ctrl+K,Ctrl+F doesnot work, it has been resolved??
Question:
username_0: me too,Ctrl+K,Ctrl+F doesnot work, it has been resolved??
_Originally posted by @Gavin110 in https://github.com/vuejs/vetur/issues/622#issuecomment-357124113_
Answers:
username_1: Duplicate of #2388
At this point we can't confirm if it's a vscode upstream issue.
Status: Issue closed
|
d3fc/d3fc | 255566960 | Title: How to control annotation position
Question:
username_0: Hi,
I m using your yahoo financial chart.
By the fault, the position of annotation is set to right handle.And now, I wan to set to another position(not left handle), but I don't know how to get the particular data position. For example (cx =("d.date's position " )& cy =("d.close's position. Becuz I m trying to add a circle annotation.
Answers:
username_1: If you're referring to the charts in this blog post:
http://blog.scottlogic.com/2015/07/08/yahoo-finance-chart.html
I'm afraid the code is a little dated (using D3v3 and an older version of d3fc). I'm planning on updating that example ... when I can find the time!
Status: Issue closed
|
tr7zw/Item-NBT-API | 466416650 | Title: de.tr7zw.nbtinjector.NBTInjector.getNbtData returns null
Question:
username_0: Hey there,
I am working through an issue for a client where the method in the title seemingly returns null even if the entity has been patched, causing a NPE. No other errors were reported and the remaining class points to your plugin so I am posting it here in case you can help.
Here is the stack trace:
```
------------------------------------[ 09.07.2019 01:02:46 ]-----------------------------------
Boss 3.3.9 encountered a NullPointerException
Running CraftBukkit 1.8.8-R0.1-SNAPSHOT and Java 1.8.0_211
Plugins: WorldEdit v6.1.3;7a097ca, PlaceholderAPI v2.10.3, PermissionsEx v1.23.4, Boss v3.3.9, ViaVersion v2.1.1, NBTAPI v2.0.0-SNAPSHOT, Vault v1.7.2-b107, ProtocolLib v4.4.0, WorldGuard v6.2.1;84bc322, ASkyBlock v3.0.9.4, Essentials v172.16.58.3, DeluxeMenus v1.11.3, EssentialsChat v172.16.58.3, EssentialsGeoIP v172.16.58.3, EZPrestige v1.2.2, EssentialsSpawn v172.16.58.3, The Multiverse-Core Plugin
----------------------------------------------------------------------------------------------
More Information:
Failed to get NBT tag for SKELETON. Tag: KaBoss_KeepInside
NullPointerException (Unknown cause)
at org.mineacademy.boss.int. .COm6.native(SourceFile:185)
```
And here is the line 185:

As always, amazing library, thank you so much!
Matej
Answers:
username_1: Does the NullPointer really have nothing attatched? That sounds kinda weird.
username_0: Well we're checking if the given entity is not null on line 170, it is either null from getString or the getNbtData.
username_1: No I mean a full Stacktrace. With "you call a method and somewhere a NPE happens" I can't track down what happens 😅
username_0: Thank you, I looked into it and it appeared to by on my side, closing for now :)
Status: Issue closed
|
Azure/azure-cli | 428860105 | Title: Cortana
Question:
username_0: Has anyone thought of adding a cortana channel creation cli?
---
#### Document Details
⚠ *Do not edit this section. It is required for docs.microsoft.com ➟ GitHub issue linking.*
* ID: 55111b1f-ca0d-0eb9-7457-3cdee47a6804
* Version Independent ID: 9442f477-20be-a67b-a38a-1846dc3cfbc4
* Content: [az bot](https://docs.microsoft.com/en-us/cli/azure/bot?view=azure-cli-latest)
* Content Source: [src/command_modules/azure-cli-botservice/azure/cli/command_modules/botservice/_help.py](https://github.com/Azure/azure-cli/blob/dev/src/command_modules/azure-cli-botservice/azure/cli/command_modules/botservice/_help.py)
* Service: **bot-service**
* GitHub Login: @rloutlaw
* Microsoft Alias: **routlaw**
Answers:
username_1: Hi @username_0, this thread might be the best place to voice your support for this:
https://feedback.azure.com/forums/562027-azure-bot-service/suggestions/32039644-allow-cortana-channel-for-o365-aad-accounts
If that idea doesn't quite reflect your ask, it might make sense to post a new idea there. It seems that ideas get more traction with the service teams when they are posted to those forums.
Status: Issue closed
|
ValveSoftware/steam-for-linux | 25630777 | Title: First/Last game counters doesn't show in Profile/Games
Question:
username_0: NaN is showing instead of first/last counters in user's Games section of profile.
There is no console output related to this problem.
A problem is exists on any language settings


Status: Issue closed
Answers:
username_1: Closing as the games page of the profile was redesigned and no longer includes the affected line. |
tidyverse/reprex | 408523661 | Title: `venue = roxygen`?
Question:
username_0: I'd like to propose another option for `venue`: roxygen.
This feature would make writing `@examples` a bit easier. In particular it would avoid having to prefix the code with `#'` for the code part and `#' #>` for the output, reduce accidental commits of syntactically (or otherwise) incorrect examples, and make it easier to identify dependencies.
On the last point, it may be appropriate to assume that when `venue = 'rox'` and one is working within a package directory that the package source in the current directory is attached. (So that `library(<mypackage>)` is not necessary in the rendered output.)
I can make a PR if you'd like. I searched for roxygen in the issues list but only found references to current behaviour, not feature requests refused.
Answers:
username_1: This might be of interest to you:
https://github.com/csgillespie/roxygen2Comment
username_2: I identify with the need to format examples in roxygen comments (indent, really). But am not convinced it's best addressed in reprex.
In RStudio, you can easily walk through your examples. The only friction I feel is around whitespace. This feels more like the domain of styler (or RStudio IDE).
What do you have in mind for the roxygen venue?
Status: Issue closed
username_2: I remain unsure of the goal here.
For now, it feels like these tools provide the example-wrangling support I need:
* If I need to add `#' ` to many lines of code (i.e. convert a code snippet to roxygen syntax), I use multiple cursors in RStudio.
* I execute examples via Cmd + Enter in RStudio.
* styler now offers support applying code style to examples <https://styler.r-lib.org>
I'm sure other non-RStudio IDEs offer multiple cursors and, perhaps, example execution. So I don't see an obvious gap for reprex to fill. |
Anuken/Mindustry-Suggestions | 718655153 | Title: Seperate waves at once
Question:
username_0: **Describe the content or mechanics you are proposing.**
Seperating waves in groups you can assign to droppoints
**Describe how you think this content will improve the game. If you're proposing new content, mention how it may add more gameplay options or how it will fill a new niche.**
This will add the ability to have differant units come from differant angles, like crawler tree from the right,
dagger tree from the left, flare tree from the bottom....
Adding this wave customizability will be usefull in good map creation
Oh and another thing: --droppoints for every team--
**Before making this issue, place an `X` in the boxes below to confirm that you have acknowledged them.** *Failure to do so may result in your request being closed automatically.*
with love<3
DS3K
1. - [x] I have done a quick search in the list of suggestions to make sure this has not been suggested yet.
2. - [x] I have checked the [Trello](https://trello.com/b/aE2tcUwF/mindustry-trello) to make sure my suggestion isn't planned or implemented in a development version.
3. - [x] I am familiar with all the content already in the game or have glanced at the wiki to make sure my suggestion doesn't exist in the game yet.
Answers:
username_1: YES. PLEASE.
username_2: idc if anything else is gonna be included
**but we need this one**
username_1: Bee spam
username_0: Imagine pvp but you got wave support
Minute 3 wave for green
Minute 5 wave for purple
t-7m wave green
t-9m wave purple
and so on.. totes fun!:'D |
FACed-Off/week1-project-mango | 631233009 | Title: Form broken as it cannot find id for errorText HMTL
Question:
username_0: ### Expected outcome
Form should not send when the name has a digit in it
### Actual Outcome
The form fails as there is a bug in the code. The `js` fails here because it cannot find the id with `errorText`. This means it can't find innerHTML and the function fails. As a result the form sends. This tag needs to be added to HTML somewhere.
Note: Numbers can be allowed in names - look at Elon Musk's new kid
Answers:
username_1: Fixed within commit 890a551265a441dc352248acbcfd0ae326375e0a
In regards to the validity of the restriction, perhaps we should add a feature that asks for confirmation of form submission when adding a numerical character into the name field, as opposed to removing the ability to submit the name entirely.
Status: Issue closed
|
ragaeeb/das-js | 830770832 | Title: Update Iqamah Times for EST
Question:
username_0: Fajr, Dhuhr, and Jumu'ah iqamah times need to be updated for the time change on Sunday, March 14th, 2021 where the clock will move forward one hour into daylight saving time (EDT).
Answers:
username_0: Found an interesting bug with this one. Tried setting the Jumu'ah time to show 1:00 PM and 1:45 PM starting the 29th of march but that showed up as "1:00PM1:45PM" on the site instead of "1:00PM, 1:45PM". Do not have time to troubleshoot this so I kept it simple instead.
Status: Issue closed
|
kriasoft/react-starter-kit | 286522068 | Title: Problem async/await jest test
Question:
username_0: 7 | const result = await testAsync();
8 | expect(result).toBe('hello world');
9 | });
```
Answers:
username_0: Found a way:
In the file **jest.config.js** set:
`
setupFiles: ["<rootDir>/jest.setup.js"],
`
create new file **jest.setup.js** with this content:
`
import '@babel/polyfill';
`
Working!
Status: Issue closed
username_1: Hey, Thank you! Do you think that this should not be included?
New versions of node should support this seamplesly I believe :slightly_smiling_face:
username_0: I used node version 8.9.4 on Windows 7 and had the problem. Generally, including **polyfill** makes available using any last ES6/ES7/ES8 semantic innovations in **tests**.
username_1: you are running test with `babel-node` ?
`regeneratorRuntime` is used by babel. There is also `babel-plugin-regenerator-runtime` plugin which can inject this dependency automatically I believe..
Best will be to use simply `node` instead of `babel-node`
username_0: I'm running test with node 8.9.4.
But you use **babel/polyfill** in **webpack** despite what feauteres support **node**.
username_0: Figuring out how to make the `webdriver.io + mocha` work with `RSK` found another way for solution the issue. Instead of changing **jest.config.js** and adding **jest.setup.js** file we:
`yarn add --dev @babel/[email protected] @babel/[email protected]`
and insert this row:
`plugins: [['@babel/plugin-transform-runtime', { polyfill: false }]],`
in the **.babelrc.js** file
username_1: 7 | const result = await testAsync();
8 | expect(result).toBe('hello world');
9 | });
```
username_2: yes I too would prefer to not have to compile/use polyfills for my test suite Node v 10.0.0 I still get async
``` javascript
beforeEach(async () => {
^
SyntaxError: Unexpected token (
```
Status: Issue closed
username_3: @username_0 thank you very much for crating this issue! Unfortunately, we have close it due to inactivity. Feel free to re-open it or [join](https://discord.com/invite/2nKEnKq) our [Discord](https://discord.com/invite/2nKEnKq) channel for [discussion](https://github.com/kriasoft/react-starter-kit/discussions/1950).
NOTE: The `main` branch has been updated with React Starter Kit v2, using JAM-style architecture. |
shevabam/get-rss-feed-url-extension | 1037497057 | Title: support for more sites
Question:
username_0: here's a few templates that can be used to build the feed URL...
```
BitChute: https://www.bitchute.com/feeds/rss/channel/<channel_name>
Gab: https://tv.gab.com/channel/<channel_name>/feed/rss
LBRY/Oddesy: https://lbryfeed.melroy.org/channel/<channel_name>
Steemit: http://www.hiverss.com/@<channel_name>/feed
Telegram: https://tg.i-c-a.su/rss/<channel name>
Vimeo: https://vimeo.com/<channel_name>/videos/rss
```
Answers:
username_0: regarding gab, i'm not sure if you added support already, but i just realized [tv.gab](https://tv.gab.com/channel/realstewpeters) advertises rss feeds - here's some samples from the page source...
```
<a class="uk-badge uk-badge-feed-link" href="/channel/realstewpeters/feed/rss">
<a class="uk-badge uk-badge-feed-link" href="/channel/realstewpeters/feed/atom">
<a class="uk-badge uk-badge-feed-link" href="/channel/realstewpeters/feed/json">
``` |
RobinHerbots/Inputmask | 183426841 | Title: colorMask feature does not work correctly
Question:
username_0: Generated div visually appears at the top of page, not under input — [jsfiddle](https://jsfiddle.net/username_0/x82sjn49/)
Also, generated object need class for more customization.
Answers:
username_1: any update on it
username_2: I have the same problem with this otherwise excellent plugin. I suspect it has something to do with HTML order, unfortunately, I cannot seem to find the HTML in the DOM tree of my inspector to find out what is going on :-).
username_3: @username_0 , @username_1 , @username_2 ,
In the 4.x branch the colorMask is updated. The positioning is more customizable through css and prototype fn. See readme and have a try
Status: Issue closed
username_3: @username_0 , @username_1 , @username_2 ,
Have a look at the updated jsfiddle using the 4.x branch.. You just need to add the padding in the css to make it 100%
https://jsfiddle.net/x82sjn49/34/
The colormask changes will be merged into 3.x branch also |
kubernetes/kubernetes | 195986372 | Title: 'kubectl run' supports only one '--port'?
Question:
username_0: **Is this a BUG REPORT or FEATURE REQUEST?** (choose one):
not sure
When I deploy GitLab and Jenkins using 'kubectl run ', it need more ports.
**What happened**:
I write it as --port=80 --port=9000
But only the last one is used
**What you expected to happen**:
I hope the 'kubectl run ' can provide more --port
May be the way I wrote is not right, if this is the case then how to use the ' --port' of 'kubectl run '
Answers:
username_1: @username_0, it's better to use yaml file and `kubectl create -f` to create a pod with multi-pod exposed, see this [reference](http://stackoverflow.com/questions/34502022/exposing-two-ports-in-google-container-engine)
username_0: Hi username_1,
Thank you for your help. Yeah currently I use yaml file a a workaround.
username_2: /sig cli
username_3: @username_0 hi, thanks for reporting this issue but per what I understood with run usage is that one would use it for testing or deploying something small or troubleshooting purpose. As @username_1 mentioned and you also tried, using the yaml file is the proper way to create deployment with multiple ports. I would of opinion that we can close this issue as, working as designed. /cc @thockin Thanks!! |
UFLX2MuMu/Ntupliser | 672612652 | Title: Fix 2016 crab config template to automatically change inputDBS
Question:
username_0: This feature exists already in 2017 and 2018. We just need to change the relevant line from `global` to `DBS` (https://github.com/UFLX2MuMu/Ntupliser/blob/master_2016_94X/DiMuons/crab/templates/crab_config.py#L17).
If the user doesn't specify a `DBS` name in `Samples.py` then we'll use `global`.
Answers:
username_1: PR created. (#155 )
username_0: merged closing
Status: Issue closed
|
agronholm/sqlacodegen | 708890235 | Title: columns of type citext are recognized as NullType
Question:
username_0: When using the `citext` (case-insensitive text) extension, `sqlacodegen` doesn't recognize the column. It emits a `NullType` for the column in the output.
This is easy to fix manually after running `sqlacodegen` - replacing the `NullType` instances with `Text` makes it work as expected. If I understand correctly, the `citext` extension is very primitive- it just causes all operators to use `lower()` on the values when they are of type `citext`
Would it be appropriate to add `citext` support to `sqlacodegen`? Even if all it does is emit `Text`? Hand editing it isn't a horrible task, but it would be nice if it emitted `Text` in the first place
Thanks, and thank you for the effort in making such an excellent tool!
Answers:
username_0: Adding one additional note to this- it's simple to fix the Null columns to `CIText` after running `sqlacodegen`, but it's also very easy to make a mistake and not realize that a `Null` column is a `CIText[]` type as opposed to `CIText[]`. This is the expected behavior because `CIText` is not explicitly supported (and therefore a `CIText[]` is meangingless to `sqlacodegen`) but I'm pointing it out because I've made this mistake a few times now
username_0: I added a PR for this, #125 which implements this as an extra
Status: Issue closed
|
amplab/spark-ec2 | 102058100 | Title: Feature request: Scala 2.11 support
Question:
username_0: There is work to enable multiple Scala versions support. But so far only Scala 2.10.3 is supported. Is there interest and/or a roadmap for Scala 2.11 support?
Answers:
username_1: +1
username_2: +1
username_3: +1
username_4: +1
username_5: +1
username_6: +1
username_7: +1
username_8: I think this problem will fix itself when Spark 2.0 comes out, since Scala 2.11 will become the default.
Also, GitHub tip: There is no need to add +1 comments anymore. You can now add your reaction directly to the original post by clicking on the emoji in the top right corner.
username_9: What's the status on this item? Last time I looked at the 2.0 branch it was still on 2.10..
username_10: As @username_8 said the newer 2.0.0 and 2.0.1 releases should have Scala 2.11 by default. |
jumpinjackie/mapguide-react-layout | 183949991 | Title: Error with Base Layer Group setup
Question:
username_0: Viewer does not work when Map having Base Layer Group setup with scale. In my example I have added a raster layer to Base Layer Group then I got this error message:
An error occurred during startup
scales is null
Stack Trace
deArrayifyScaleRanges@http://localhost/mapguide31/viewer2/dist/viewer.js:8840:6
deArrayifyLayers/<@http://localhost/mapguide31/viewer2/dist/viewer.js:8876:26
deArrayifyLayers@http://localhost/mapguide31/viewer2/dist/viewer.js:8862:13
deArrayifyRuntimeMap@http://localhost/mapguide31/viewer2/dist/viewer.js:8951:17
deArrayify@http://localhost/mapguide31/viewer2/dist/viewer.js:9530:17
MapGuide</MapAgentRequestBuilder</MapAgentRequestBuilder.prototype.get/</<@http://localhost/mapguide31/viewer2/dist/viewer.js:37861:26


Answers:
username_0: It seems to be fixed in #76
Status: Issue closed
|
deeplearning4j/deeplearning4j | 225295678 | Title: Cannot do forward pass: inputs not set
Question:
username_0: #### Issue Description
I'm doing regression with a computation graph and multidata set.
Upon geting to `cg.fit(dataset)` I get
```
Cannot do forward pass: inputs not set
```
Likewise, I cannot run `cg.score(dataset)` as this gives the same error.
The network and example code is given here https://gist.github.com/username_0/3df125a74c352fbc4c3ea15ea56f6360
#### Version Information
I using 8.1-SNAPSHOT
Answers:
username_1: This is most likely due to a misconfigured network, that isn't picked up correctly during validation for some reason. For example, a layer that doesn't have an input, doesn't feed into any other layer, or something similar.
I had a look at your code, but couldn't see an obvious cause - though it's a bit hard to follow the structure there.
username_2: I wonder if outputing the model config to JSON would provide insight into the issue?
username_0: @username_2 what function does this? I'm only aware of the model serializer.
username_2: ComputationGraphConfiguration.toJSON
So in your code, looks like cgconf.toJSON
Status: Issue closed
username_0: Great! Easy to find the problem. I found one merge vertex has two inputs from the same layer.
Thanks |
brantou/reading | 313981358 | Title: Linux 中的 5 个 SSH 别名例子 | Linux 中国
Question:
username_0: <b>Linux 中的 5 个 SSH 别名例子 | Linux 中国</b><br>
我们可以用 定义在你的 .bashrc 文件里的别名 或函数来大幅度缩减花在命令行界面(CLI)的时间。但这不是最佳解决之道。最佳办法是在 ssh 配置文件中使用 SSH 别名 。 -- <NAME> 有用的原文链接 请访问文末的“ 原文链接 ”获得可点击的文内链接、全尺寸原图和相关文章。 致谢 编译自…<br>
<br>
April 13, 2018 at 02:16PM<br>
via Instapaper https://mp.weixin.qq.com/s/_4EnQ2CPtNy0y7Oig9fg_w |
elixir-ecto/ecto | 257994904 | Title: Nested macros stop working when ecto updated from 2.1.5 to 2.2.3
Question:
username_0: I am filling this as a bug report as bugreport as problem appears after updating Ecto. I am far from being Elixir/Ecto expert, so please excuse my ignorance.
### Environment
* Elixir version (elixir -v): `Elixir 1.5.1`
* Database and version (PostgreSQL 9.4, MongoDB 3.2, etc.): `PostgreSQL 9.6.5`
* Ecto version (mix deps): `2.2.3`
* Database adapter and version (mix deps): `postgrex 0.13.3`
* Operating system: `Debian GNU/Linux sid`
### Current behavior
I am playing with Tilex, and https://github.com/hashrocket/tilex/blob/master/lib/tilex/stats.ex is failing to compile if Ecto is updated to `2.2.3`. It will through the following error:
```
== Compilation error in file lib/tilex/stats.ex ==
** (ArgumentError) argument error
:erlang.apply(%Macro.Env{aliases: [{SQL, Ecto.Adapters.SQL}, {Repo, Tilex.Repo}], context: nil, context_modules: [Tilex.Stats], export_vars: nil, file: "/home/username_0/temp/elixir/tilex/lib/tilex/stats.ex", function: {:hot_posts, 0}, functions: [{Ecto.Query, [exclude: 2, first: 1, first: 2, last: 1, last: 2, subquery: 1, subquery: 2]}, {Kernel, [!=: 2, !==: 2, *: 2, +: 1, +: 2, ++: 2, -: 1, -: 2, --: 2, /: 2, <: 2, <=: 2, ==: 2, ===: 2, =~: 2, >: 2, >=: 2, abs: 1, apply: 2, apply: 3, binary_part: 3, bit_size: 1, byte_size: 1, div: 2, elem: 2, exit: 1, function_exported?: 3, get_and_update_in: 3, get_in: 2, hd: 1, inspect: 1, inspect: 2, is_atom: 1, is_binary: 1, is_bitstring: 1, is_boolean: 1, is_float: 1, is_function: 1, is_function: 2, is_integer: 1, is_list: 1, ...]}], lexical_tracker: #PID<0.230.0>, line: 90, macro_aliases: [], macros: [{Ecto.Query, [distinct: 2, distinct: 3, dynamic: 1, dynamic: 2, from: 1, from: 2, group_by: 2, group_by: 3, having: 2, having: 3, join: 3, join: 4, join: 5, limit: 2, limit: 3, lock: 2, offset: 2, offset: 3, or_having: 2, or_having: 3, or_where: 2, or_where: 3, order_by: 2, order_by: 3, preload: 2, preload: 3, select: 2, select: 3, select_merge: 2, select_merge: 3, update: 2, update: 3, where: 2, where: 3]}, {Kernel, [!: 1, &&: 2, ..: 2, <>: 2, @: 1, alias!: 1, and: 2, binding: 0, binding: 1, def: 1, def: 2, defdelegate: 2, defexception: 1, defimpl: 2, defimpl: 3, defmacro: 1, defmacro: 2, defmacrop: 1, defmacrop: 2, defmodule: 2, defoverridable: 1, defp: 1, defp: 2, defprotocol: 2, defstruct: 1, destructure: 2, get_and_update_in: 2, if: 2, in: 2, is_nil: 1, match?: 2, or: 2, pop_in: 1, put_in: 2, raise: 1, raise: 2, reraise: 2, ...]}], match_vars: :warn, module: Tilex.Stats, prematch_vars: nil, requires: [Ecto.Query, Kernel, Kernel.Typespec], vars: []}, :module, [])
(elixir) lib/macro.ex:1196: Macro.do_expand_once/2
(elixir) lib/macro.ex:1252: Macro.expand_until/2
lib/ecto/query/builder.ex:490: Ecto.Query.Builder.try_expansion/5
(elixir) lib/enum.ex:1357: Enum."-map_reduce/3-lists^mapfoldl/2-0-"/3
lib/ecto/query/builder.ex:81: Ecto.Query.Builder.escape/5
lib/ecto/query/builder/select.ex:126: anonymous fn/4 in Ecto.Query.Builder.Select.escape_pairs/4
(elixir) lib/enum.ex:1357: Enum."-map_reduce/3-lists^mapfoldl/2-0-"/3
(elixir) lib/enum.ex:1357: Enum."-map_reduce/3-lists^mapfoldl/2-0-"/3
lib/ecto/query/builder/select.ex:69: Ecto.Query.Builder.Select.escape/4
lib/ecto/query/builder/select.ex:204: Ecto.Query.Builder.Select.build/5
expanding macro: Ecto.Query.select/3
lib/tilex/stats.ex:90: Tilex.Stats.hot_posts/0
expanding macro: Ecto.Query.from/2
lib/tilex/stats.ex:90: Tilex.Stats.hot_posts/0
(elixir) lib/kernel/parallel_compiler.ex:121: anonymous fn/4 in Kernel.ParallelCompiler.spawn_compilers/1
```
It compiles with Ecto version `2.1.5`. Offending line is
```
hours_age: greatest(hours_since(p.published_at), 0.1)
```
which uses 2 macros (`greatest` and `hours_since`).
```elixir
defmacro greatest(value1, value2) do
quote do
fragment("greatest(?, ?)", unquote(value1), unquote(value2))
end
end
defmacro hours_since(timestamp) do
quote do
fragment(
"extract(epoch from (current_timestamp - ?)) / 3600",
unquote(timestamp)
)
end
end
```
When rewritten to
```
hours_age: fragment("greatest(?,?)", hours_since(p.published_at), 0.1)
```
compilation is successful (and query to DB looks as expected).
### Expected behavior
Nesting of macros would work as before.
Status: Issue closed
Answers:
username_1: Thank you! Fixed on v2.2 and master branches. A new release should be out soon, just waiting for approval from @michalmuskala.
username_2: Whoops, looks like I might have introduced that when I fixed the macro's fully expanding when they shouldn't have. There was no test-case for this issue in the tests so I never saw it. ^.^;
Still no test case it seems? |
transitland/transitland-datastore | 214707407 | Title: Incorrect is_active_feed_version in api/v1/feed_versions
Question:
username_0: Hi all,
Just trying to get the latest GTFS feed from Dallas DART, and I've noticed that when using the `feed_versions` endpoint it shows all feeds that have been consumed, but an older feed still has the `is_active_feed_version` tag set to **true**, while the latest feed (and the current one) still shows as **false**.
Here's the URL I'm using: `https://transit.land/api/v1/feed_versions?feed_onestop_id=f-9vg-dallasarearapidtransit&sort_key=fetched_at&sort_order=desc&per_page=10`
And here's the data I'm getting back (edited for brevity)
```
{
"feed_versions": [
{
"sha1": "98a184a84c4c1e495d8585e0b6e95b36fad0d29e",
"earliest_calendar_date": "2017-03-13",
"latest_calendar_date": "2017-09-24",
"md5": "0eb014dedab1dd03c214a73e74f656f9",
"tags": {
"feed_lang": "en",
"feed_version": "118-119-1",
"feed_publisher_url": "http://www.dart.org",
"feed_publisher_name": "DALLAS AREA RAPID TRANSIT"
},
"fetched_at": "2017-02-28T14:00:12.068Z",
"created_at": "2017-02-28T14:00:12.131Z",
"updated_at": "2017-02-28T14:00:12.131Z",
"is_active_feed_version": false,
},
{
"sha1": "4f617b4eb34c675104717f7c882b0c14f2b8a9ea",
"earliest_calendar_date": "2017-03-13",
"latest_calendar_date": "2017-09-24",
"md5": "686369befcfca121cbb12d8b2be930ad",
"tags": {
"feed_lang": "en",
"feed_version": "118-119-1",
"feed_publisher_url": "http://www.dart.org",
"feed_publisher_name": "DALLAS AREA RAPID TRANSIT"
},
"fetched_at": "2017-02-24T15:00:11.590Z",
"created_at": "2017-02-24T15:00:11.650Z",
"updated_at": "2017-02-24T15:00:11.650Z",
"is_active_feed_version": false,
},
{
"sha1": "07f25dc0a1adc76148c99a0368451c307308bbc0",
"earliest_calendar_date": "2016-10-24",
"latest_calendar_date": "2017-03-12",
"md5": "6694fca0a0c116177292ef71249b9976",
"tags": {
"feed_lang": "en",
"feed_version": "114-112-1",
"extend_to_date": "2018-03-12",
"extend_from_date": "2017-02-12",
"feed_publisher_url": "http://www.dart.org",
"feed_publisher_name": "<NAME> TRANSIT"
},
"fetched_at": "2016-12-21T03:30:13.032Z",
"created_at": "2016-12-21T03:30:13.110Z",
"updated_at": "2017-03-05T14:13:27.210Z",
"is_active_feed_version": true,
},
...
],
"meta": {
"sort_key": "fetched_at",
"sort_order": "desc",
"offset": 0,
"per_page": 10
}
}
```
Can anyone help? I've got a script running that checks each feed for `is_active_feed_version`, and downloads the GTFS file if it exists.
Status: Issue closed
Answers:
username_1: Hi @username_0, thanks for your question.
The `is_active_feed_version` flag designates the latest version of the feed that has been imported into the Transitland Datastore. For example, when querying the `ScheduleStopPair` endpoint, you will be accessing schedule data imported from this feed version. There can be a lag between when a new feed version is fetched and when it is imported--for example, in some cases, the calendar entries are not yet active.
Using your query URL, you can just change to `per_page=1` and you will always get the latest feed version fetched (whether or not it is the latest version to be imported into the Transitland Datastore).
Alternatively, if you'd like to skip a step, give a try with an endpoint we have added recently: https://transit.land/api/v1/feeds/f-9vg-dallasarearapidtransit/download_latest_feed_version
If this does not fully answer your question, please reopen. |
microsoft/onnxruntime | 536213678 | Title: Is there a ok onnxruntime_perf_test demo there?
Question:
username_0: I use the onnxruntime_perf_test.exe to run testdata/aqueezenet/ or any other models in testdata dir, but it always can't parse the input data, Is there some sample code to demonstrate how to construct the format of input and model? Thanks~
$ ./onnxruntime_perf_test.exe -m times -e cuda -s -v ./testdata/squeezenet/model.onnx ./testdata/squeezenet/
==>
**there is no test input data for input data_0 and model .
Run failed:failed to initialize.**
I have also try onnx_run_test, only the transform\matmul_add_fusion\2Input can run successfully.
Answers:
username_1: @username_0, the model and input need to follow the same folder structure as onnx_test_runner: https://github.com/microsoft/onnxruntime/tree/master/onnxruntime/test/perftest
username_2: ```
onnxruntime_perf_test -o 99 -t 120 -x 3 C:\lotus_data\resnet_v2_50\model.onnx 1.csv
```
You may get the model and test data from onnx model zoo. For example. download https://s3.amazonaws.com/onnx-model-zoo/squeezenet/squeezenet1.1/squeezenet1.1.tar.gz and extract the whole zip.
username_0: @username_1 Thanks~
@username_2 Thanks very much, I followe your guide, download the model and execute command as you do, I'm on windows platform git bash MINGWIN64 terminal , but it still can't find the model and input data path, command:
./onnxruntime_perf_test.exe -x 3 -s -v C:/squeezenet1.1/squeezenet1.1.onnx 1.csv
I print out detailed message, find that:
in PerformanceRunner::Initialize(),
ToMBString(test_case_dir) => "."
ToMBString(model_name) => "."
in OnnxTestCase::LoadTestData:
ToMBString(test_data_dirs_[id]) => .\testdata
ToMBString(test_data_pb) => .\testdata\inputs.pb
It always find the testdata dir in the RelWithDebInfo(Release) dir, seems bug exist in path parse logic. looking forward for your further help. :)
username_2: Where does the "testdata" string come from? We didn't hardcode such a string at anywhere.
username_2: Windows uses '\\' as path separator, not '/'
```
.\onnxruntime_perf_test.exe -x 3 -s -v C:\squeezenet1.1\squeezenet1.1.onnx 1.csv
```
Status: Issue closed
|
kibaekkim/DualDecomposition.jl | 754513856 | Title: subproblem solution status
Question:
username_0: I think the current checking of solution status is too restrictive as allowing `MOI.OPTIMAL` or `MOI.LOCALLY_SOLVED` only. See https://github.com/username_0/DualDecomposition.jl/blob/master/src/LagrangeDual.jl#L106
We may want to allow more statuses.<issue_closed>
Status: Issue closed |
iberianpig/xSwipe | 125287037 | Title: Doesn't work - Dell Inspirion 5000
Question:
username_0: Hi!
I have a Dell Inspirion 5000 with Elementary OS Freya. I followed all instructions of installation, but this does not work, when I run `perl ~/xSwipe/xSwipe.pl` the command show:
```
Smartmatch is experimental at /home/username_0/xSwipe/xSwipe.pl line 121.
### $ARGV: '-m'
### $polling_interval: 30
### init_synclient
### @area_setting: [
### ' LeftEdge = 1585
',
### ' RightEdge = 5357
',
### ' TopEdge = 1446
',
### ' BottomEdge = 4408
'
### ]
### $touchpad_size_h: 2962
### $touchpad_size_w: 3772
### $x_min_thredshould: '377.2'
### $y_min_thredshould: '296.2'
### $inner_edge_left: '1710.73333333333'
### $inner_edge_right: '5231.26666666667'
### $inner_edge_top: '1742.2'
### $inner_edge_bottom: '4111.8'
### $session_name: 'pantheon'
```
When I test the touchpad it isn't work. I exec `xinput list` to see my drivers, I can see:
```
Virtual core pointer id=2 [master pointer (3)]
⎜ ↳ Virtual core XTEST pointer id=4 [slave pointer (2)]
⎜ ↳ DLL0641:00 06CB:7621 UNKNOWN id=13 [slave pointer (2)]
⎜ ↳ SynPS/2 Synaptics TouchPad id=15 [slave pointer (2)]
```
I see that I have a synaptics driver, but also have a **DLL0641:00 06CB:7621 UNKNOWN** driver, this is a Dell's driver, when I disabled this my touchpad dead, and I try enabled **SynPS/2 Synaptics TouchPad** but the touchpad keep dead.
Why xSwipe doesn't work? Did I do something bad?
PD: Sorry if my english is bad :stuck_out_tongue:
Answers:
username_1: Have you found a solution?
Having the same problem :( |
swansonk14/typed-argument-parser | 1003371851 | Title: `tap.utils.get_argument_name()` should choose a canonical argument name like `ArgumentParser.add_argument()` does
Question:
username_0: ## Motivation
`tap.tap.Tap.add_argument()` restricts the `*name_or_flags` vararg differently from `ArgumentParser.add_argument()`.
Consider this pure [argparse](https://docs.python.org/3/library/argparse.html) example:
```python
import argparse
if __name__ == "__main__":
parser = argparse.ArgumentParser()
parser.add_argument("-t", "--with", "--to",
metavar="TARGET",
dest="target_name",
help="connect to this target",
)
args = parser.parse_args()
print(args)
```
argparse will choose the first long argument name as the argument name:
```
$ python3 /tmp/scratch.py
Namespace(target_name=None)
$ python3 /tmp/scratch.py --help
usage: scratch.py [-h] [-t TARGET]
optional arguments:
-h, --help show this help message and exit
-t TARGET, --target TARGET, --to TARGET
connect to this target
```
But `Tap.add_argument()` will refuse to do the same:
```python
from tap import Tap
class ScratchParser(Tap):
target_name: str # connect to this target
def configure(self) -> None:
super().configure()
self.add_argument("-t", "--target", "--to", dest="target_name", metavar="TARGET")
if __name__ == "__main__":
args = ScratchParser().parse_args()
print(args)
```
```python
$ python3 /tmp/scratch_1.py --help
Traceback (most recent call last):
File "/tmp/scratch_1.py", line 13, in <module>
args = ScratchParser().parse_args()
File "~/lib/python3.8/site-packages/tap/tap.py", line 103, in __init__
self._configure()
[Truncated]
If `dest="target_name"` had not been specified, then I would have expected the canonical argument name to be `target` because that's what `ArgumentParser.add_argument("-t", "--target", "--to", …)` would have done.
And if it had been `ArgumentParser.add_argument("-t", "-n", …)` instead, the canonical argument name would have been `t` because that is the first name specified and there was no option that began with two hyphens to take precedence.
## Proposed Solution
`tap.utils.get_argument_name()` should be rewritten to determine the canonical argument name based on this order of preference:
1. The value of [the `dest` keyword argument](https://docs.python.org/3/library/argparse.html#dest), if it is a string / not `None`.
2. The first `*name_or_flags` that begins with `"--"`, if any.
3. The first `*name_or_flags` that begins with `"-"`.
Behaviors that should be unchanged but may be worth testing:
* If the argument is a positional argument (i.e. it does not begin with `"-"`), it must be the only item in `*name_or_flags`.
* It is an error to mix a positional argument (e.g. `"target"`) and an option (e.g. `"--target"`).
## Alternatives
I have not been able to find a way to `Tap.add_argument()` two long-named options. The alternative for this case would be to use plain argparse, but I'd lose the typing benefits offered by Typed Argument Parser.
Without `ArgumentParser.add_argument(…, dest=…)` support, the first long-named option would have stand in for `dest`. This is not ideal if I want to receive an primary option named with a reserved keyword like `ArgumentParser.add_argument("--with", "--to", dest="target_name")`.
Answers:
username_1: Great point. We'll work on this soon. Thank you so much for the thorough and extremely thoughtful write up. |
VsVim/VsVim | 4955624 | Title: Proposed mapping for C-O, C-I, C-]
Question:
username_0: I proposed that by default VsVim maps the [jump-motions](http://vimdoc.sourceforge.net/htmldoc/motion.html#jump-motions) `Ctrl-O` and `Ctrl-I` to `View.NavigateBackward` and `View.NavigateForward`.
Also, although not part of jumps motions but rather [tag-command](http://vimdoc.sourceforge.net/htmldoc/tagsrch.html#tag-commands), it seems sensible to also bind `Ctrl-]` to `Edit.GoToDefinition` given that `C-LeftMouse` is already handled by Visual Studio that way.
Meanwhile, the following nmaps would do the same:
```
nmap <C-]> :vsc Edit.GoToDefinition<CR>
nmap <C-O> :vsc View.NavigateBackward<CR>
nmap <C-I> :vsc View.NagivateForward<CR>
```
Answers:
username_1: Hi! I propose we re-open this. I agree that as a new user, Ctrl-O seems broken, specifically when jumping between files (which is my most common use case).
I expect (as is the vim use case):
with cursor over symbol, <C-]> jumps to that symbol's definition, regardless of file
<C-o> jumps back to the previous location, regardless of file. The mappings provided in @username_0's original post seem more accurate to vim behavior than the default VsVim behavior |
buff0000n/dojocad | 649638834 | Title: Problems with dry dock actual "box"
Question:
username_0: I planned my dojo to have some reactors next to the dry docks, on another floor, however, on the website it was considered valid, but on warframe it said it had no space.
So yeah, dry docks are hecking huge haha
my dojo schematics for reference
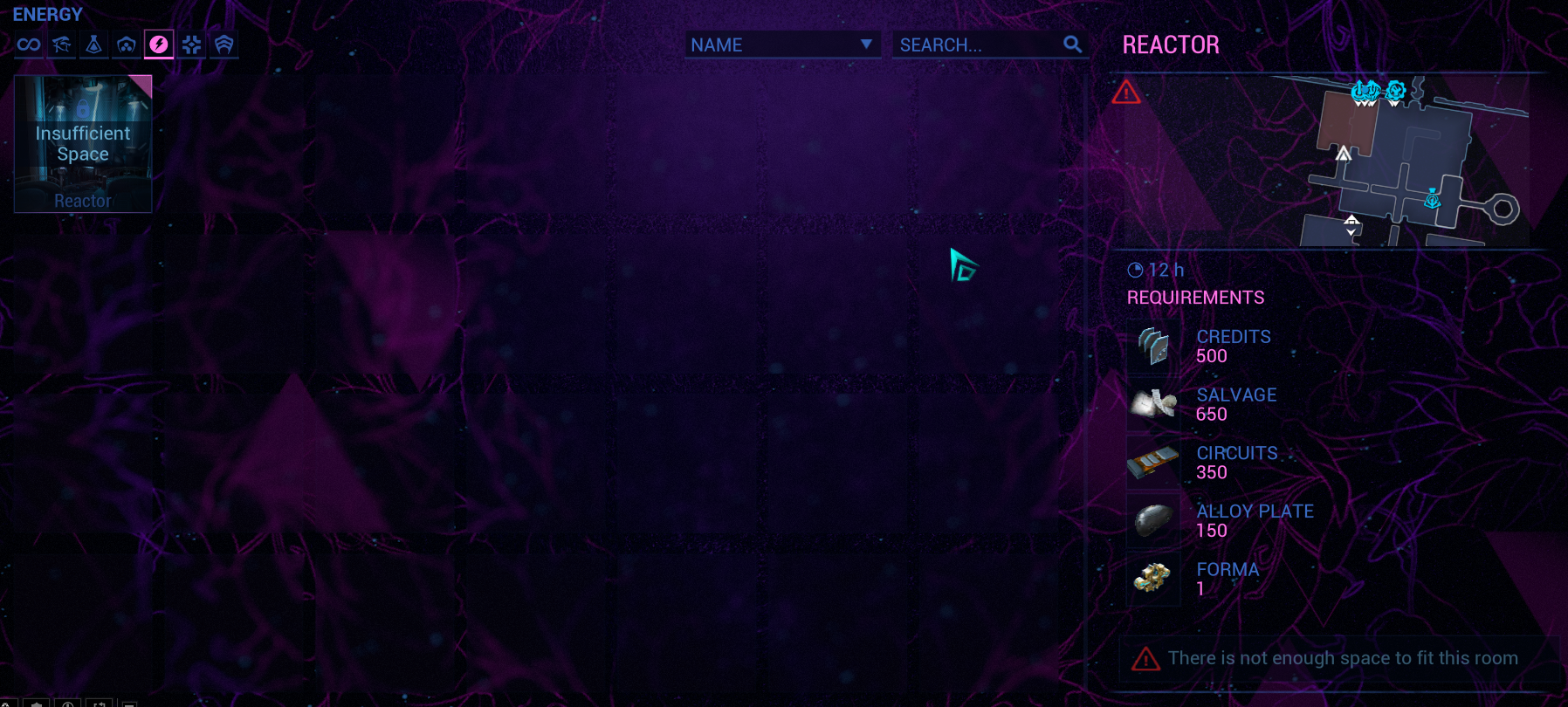
Answers:
username_0: https://username_1.github.io/dojocad/index.html?v=2,1,-222,-1&mz=BYRgNADJ0Q+gxgU2gWgCxrC8d71SAGxY6wBOyKAzAExhElhXnK0nHb0L5QOdwB7MlirhsHcMwA2ArGjpoAHI2Z5oSxnApM6fcCFjAAltEKYocKQBcw<KEY>dMn+d<KEY>bYl9oAwATcOgaGnMwGh<KEY>ZiGLi4<KEY>46C1gBAEMsGkVlKi<KEY>2<KEY>bi<KEY>zY<KEY>2V+IA
username_1: ...huh. I just built a path to that spot in my dojo and I can place a reactor there.
Are you sure your elevator goes down and not up? You can build a reactor on the floor below an Inspiration Hall, but you can't build a reactor on the floor above one.
Status: Issue closed
|
joinrpg/joinrpg-net | 387163280 | Title: Документировать API
Question:
username_0: Примерно такой гугл док нужно https://docs.google.com/document/d/1mZGwgzEM6OsxKpx_lU5sZ2bG9AQwR80nDUyv9tPbvGs/edit#
Answers:
username_0: Примерно такой гугл док нужно https://docs.google.com/document/d/1mZGwgzEM6OsxKpx_lU5sZ2bG9AQwR80nDUyv9tPbvGs/edit#
username_1: Не то что я жалуюсь, но есть некоторые сложности с тем, чтобы документировать то, что ты никогда не использовал и не знаешь, как использовать :-)
username_0: Да, поэтому я выдал тебе гуглдок, в котором ВСЕ что нужно написано :-) Просто зафигарь ее в документацию
username_0: В остальном все ок
username_1: +
Закрой, у меня прав не хватает, кажется :-)
Status: Issue closed
|
Arquisoft/radarin_en2b | 861711832 | Title: Loading tests with Gatling
Question:
username_0: I have downloaded and enable CORS as:
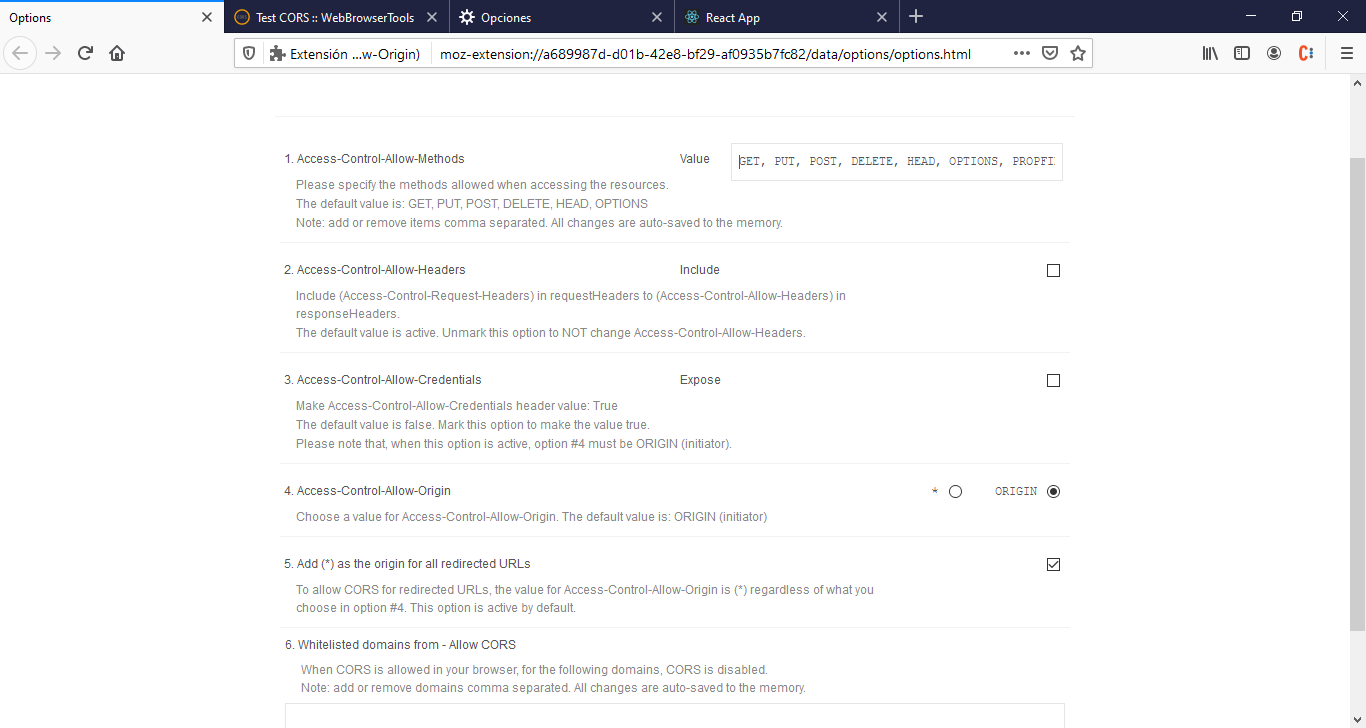
but with the proxy configured as previously shown the test CORS is:
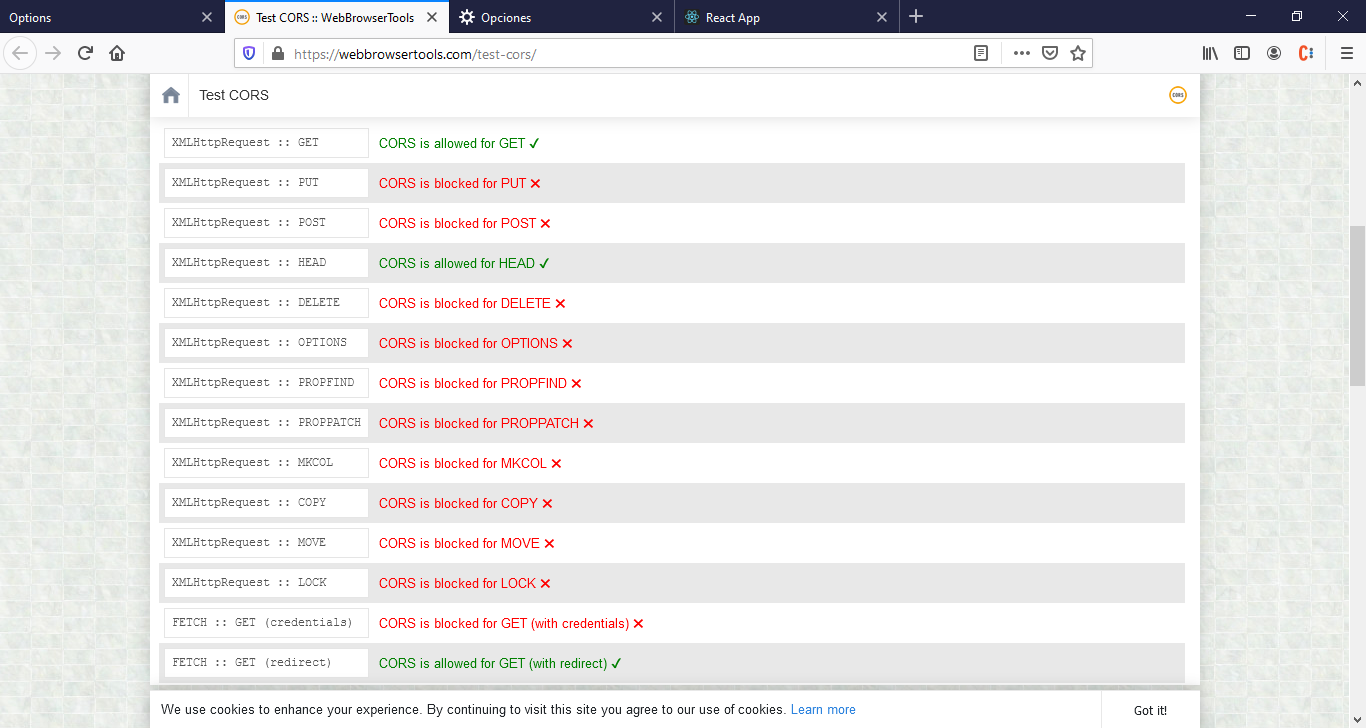
and the requests are still blocked, I do not know why @username_1
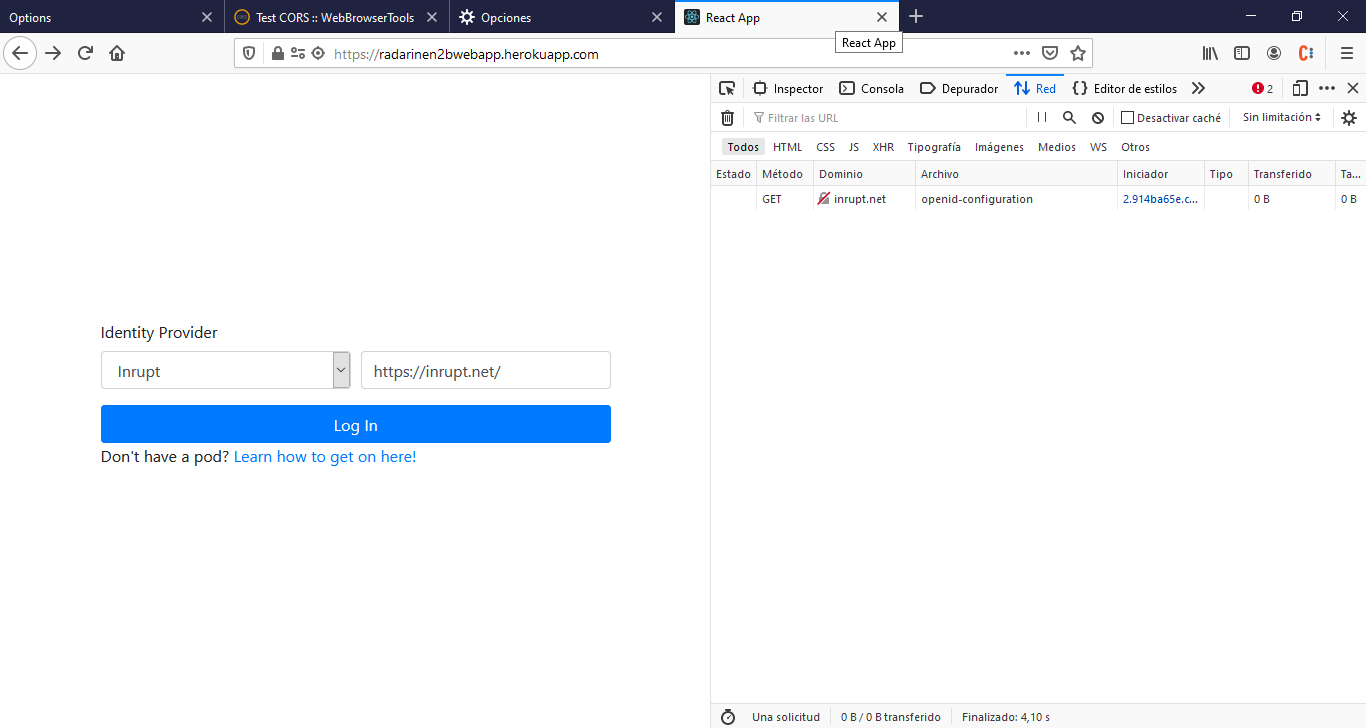
Answers:
username_1: Sorry. I forgot that I have a plugin installed in firefox that allows CORS!:
https://mybrowseraddon.com/access-control-allow-origin.html
With that, it should work.
username_0: I have downloaded and enable CORS as:
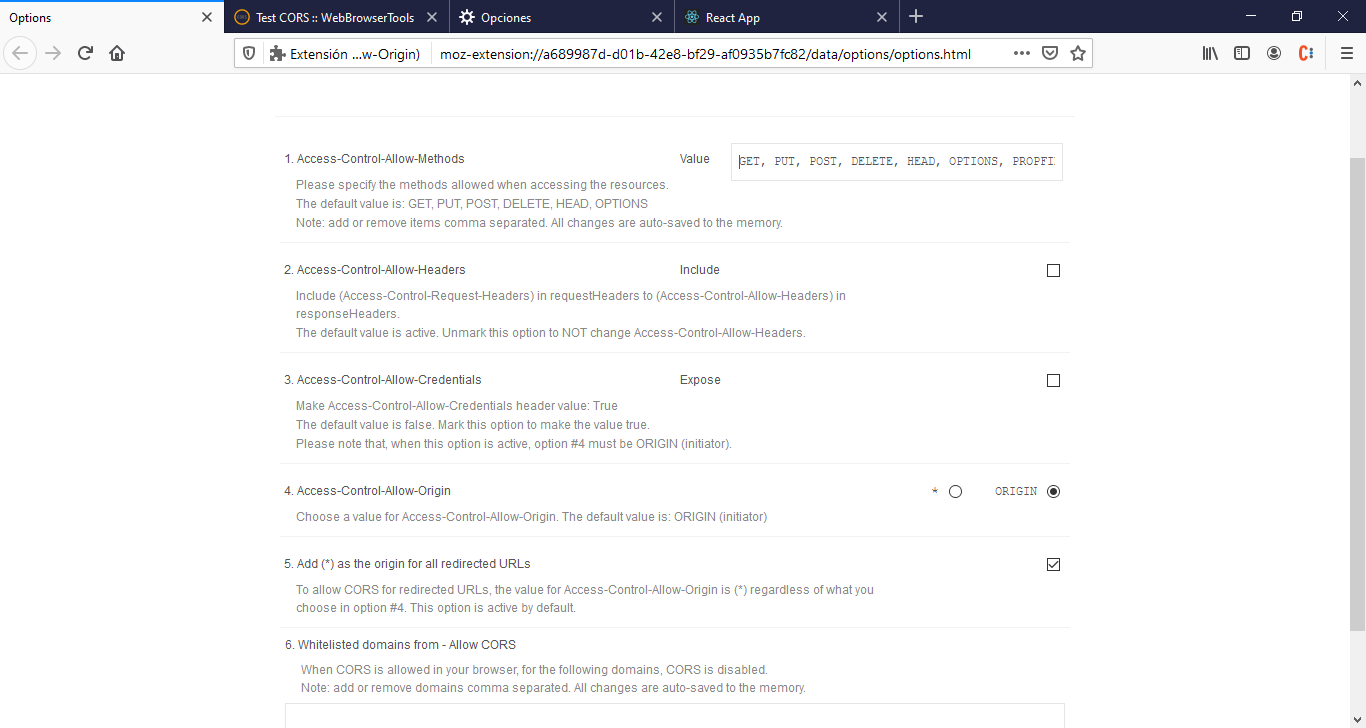
but with the proxy configured as previously shown the test CORS is:
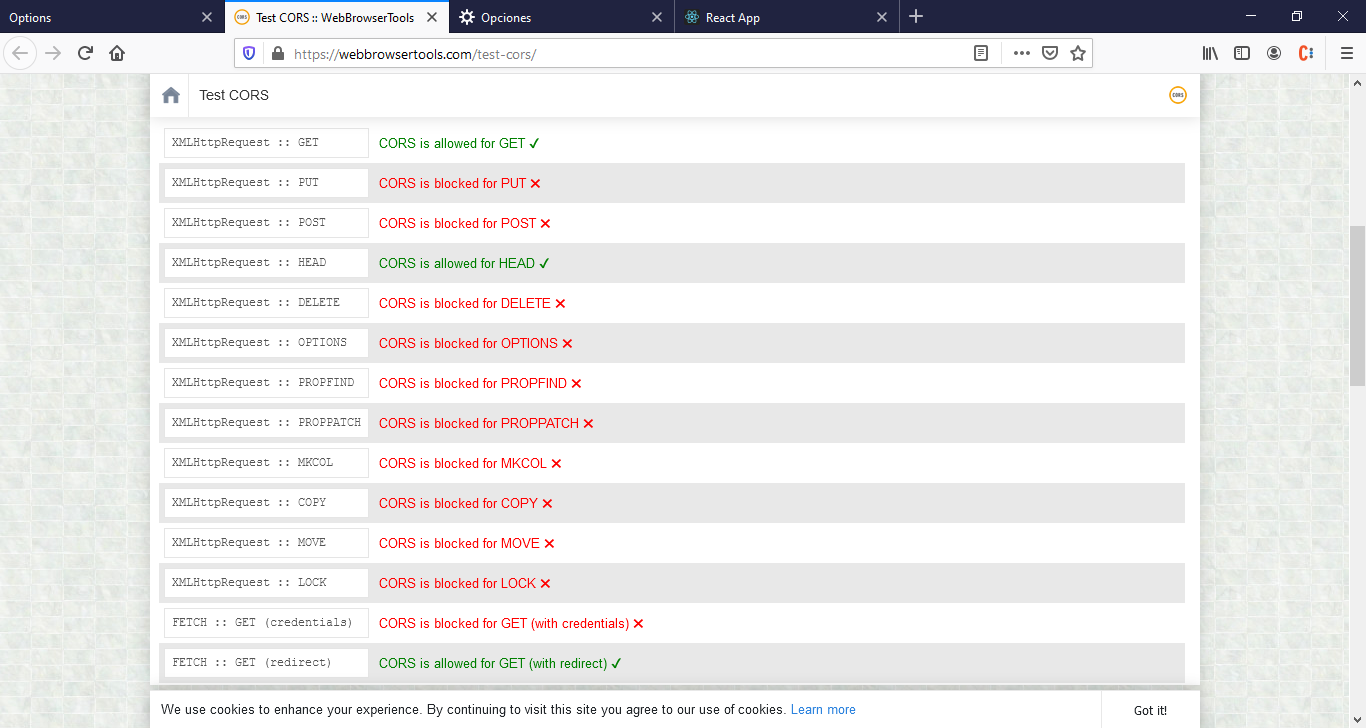
and the requests are still blocked, I do not know why @username_1
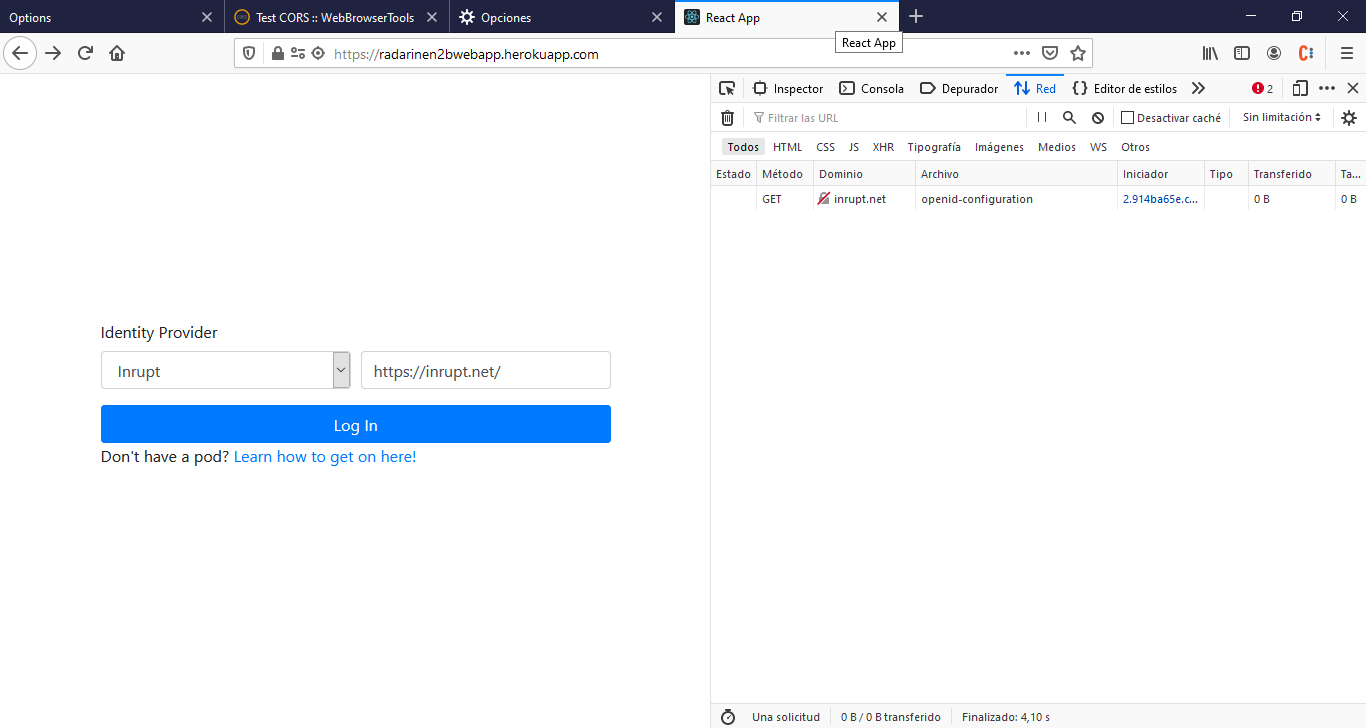
username_1: Ok. I tried uninstalling my cors plugin and everything works fine anyway. One question, does radarin0 work for you without using the gatling proxy?
username_0: Yes, without the firefox proxy configured (default) and without allow CORS extension on, both applications (Radarin 0 and RadarinEn2b) works perfectly as normal:
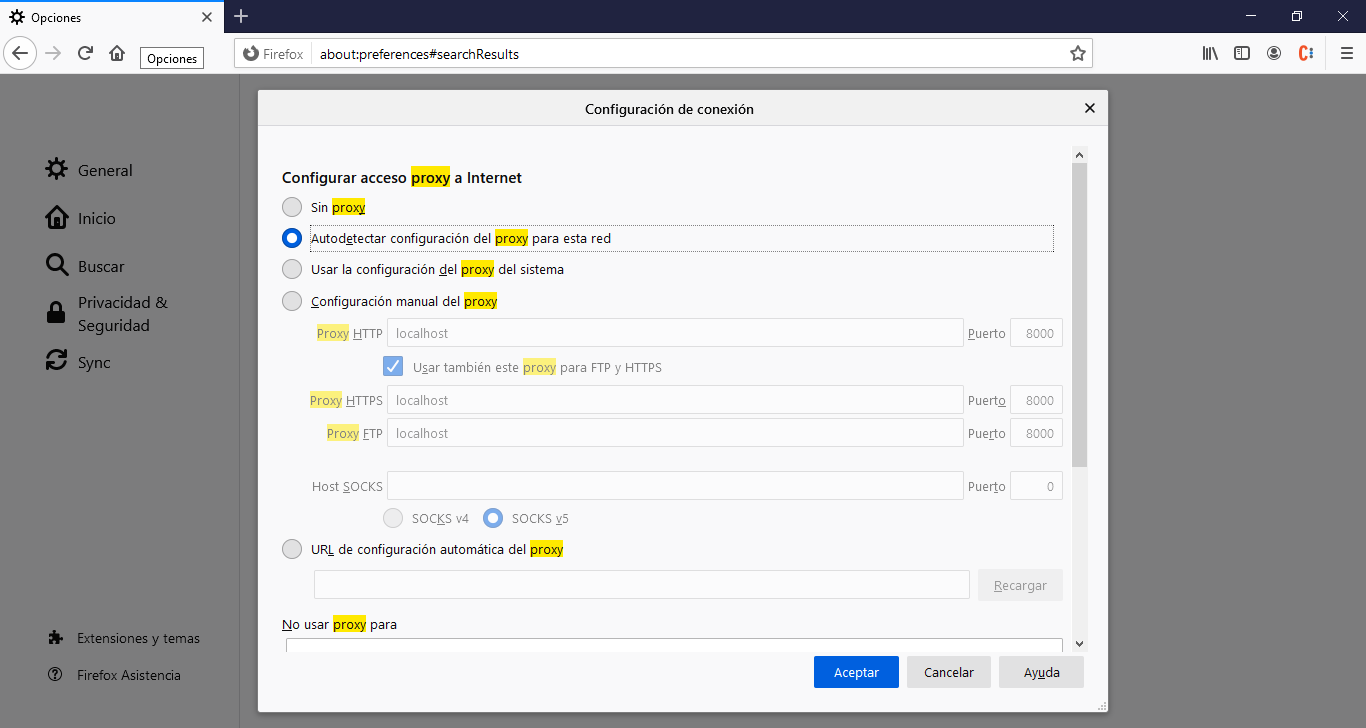
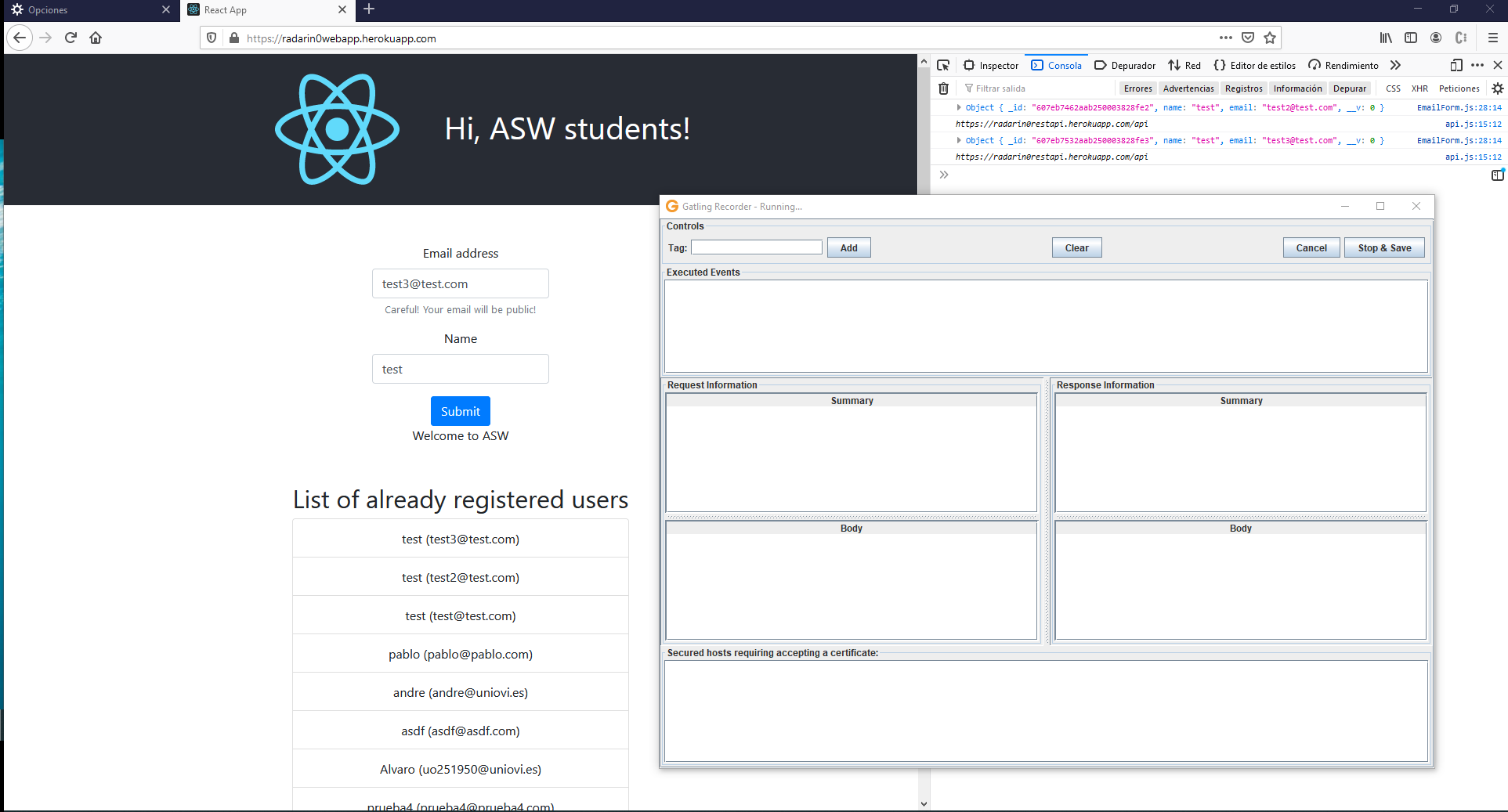
The only thing is that the Gatling recorder is not recording anything.
username_1: I think I have missed one step in my explanation on monday:
https://github.com/Arquisoft/radarin_0/tree/master/webapp#load-testing-gatling
Step 4. After generating the certificates, we must import the certificate to firefox.
Obviously as I prepared this at the beginning of the course, I already had it in my firefox... Let me know if this solves it.
username_0: It is still the same. I am sure that is my mistake and a silly one. I will ask some of my mates to try it just in case and for now I will start doing something else. Thanks.
username_2: We managed to record everything with Gatling by adding inrupt.net to the pages not used by the proxy, as it seemed that the problem was the proxy blocking some requests. |
localizely/flutter-intl-vscode | 974415899 | Title: Extension issue - Unresponsive
Question:
username_0: - Issue Type: `Bug`
- Extension Name: `flutter-intl`
- Extension Version: `1.16.0`
- OS Version: `Windows_NT x64 10.0.19043`
- VS Code version: `1.59.0`
don't know how to send more important data, extension hangs on loading project
Answers:
username_1: Hi @username_0,
I've tried to reproduce the reported issue on my machine, but everything works well.
Could you please check the Output window (`Ctrl + Shift + U`) of the VS Code? Maybe there is something useful.
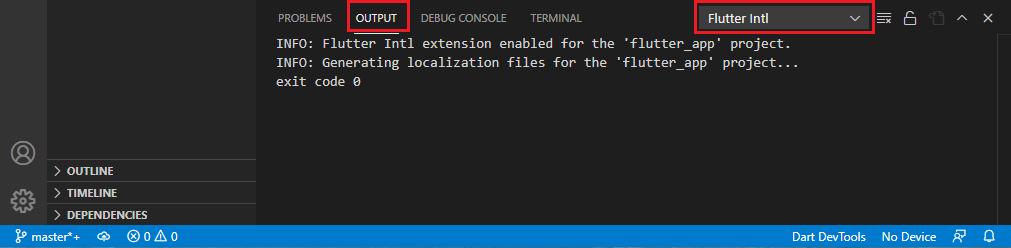
**A Large number of ARB files and string keys might affect generation performance, but it should not freeze the IDE.*
username_0: same here
don't see any unnormal
```
INFO: Flutter Intl extension enabled for the 'albero' project.
INFO: Generating localization files for the 'albero' project...
exit code 0
```
vscode wasn't freezing, only the extension...
username_1: Not sure I understand the issue. :(
Why do you mean by *"extension hangs on loading project"*?
Could you please provide us more details regarding this (e.g screen recording GIF, steps to reproduce), so we can better understand what is the problem?
username_0: that's my problem, i didn't make a screenshot of this situation in vscode. it shows a list of extension in the main view and there was an entry if this extension with the subtitle Unresponsive. I didn't see this before and find it not again... i think we should close the issue
username_1: I understand...
Thanks for reporting this and for the clarification!
I'll check if other VS Code extensions encountered similar issues, just to be sure before closing. |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.